branch_name
stringclasses 15
values | target
stringlengths 26
10.3M
| directory_id
stringlengths 40
40
| languages
listlengths 1
9
| num_files
int64 1
1.47k
| repo_language
stringclasses 34
values | repo_name
stringlengths 6
91
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
| input
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
refs/heads/master | <repo_name>akirahrkw/sinatra-jbuilder<file_sep>/lib/sinatra/jbuilder.rb
require 'sinatra/base'
require 'multi_json'
require 'jbuilder'
module Sinatra
module Jbuilder
VERSION = "1.0"
def jbuilder(object, status:200, type: :json)
content_type type
response.status = status
::Jbuilder.encode do |json|
json.set! :meta do
json.status status
end
json.set! :data do
if object.class == Array
json.array! object do |o|
o.to_json(json)
end
else
object.to_json(json)
end
end
end
end
end
Base.set :json_encoder do
::MultiJson
end
Base.helpers Jbuilder
end<file_sep>/spec/jbuilder_spec.rb
require_relative 'spec_helper'
describe Sinatra::Jbuilder do
class Human
def initialize(first_name:'akira', last_name:'hirakawa')
@last_name = last_name
@first_name = first_name
end
def to_json(json)
json.last_name @last_name
json.first_name @first_name
end
end
it 'changes http status' do
mock_app {
get('/') {
o = Human.new
jbuilder o, status:400
}
}
o = get('/')
expect(o.status).to eq 400
json = JSON.parse(o.body)['meta']
expect(json['status']).to eq 400
end
it 'changes content-type' do
mock_app {
get('/') {
o = Human.new
jbuilder o, type: :js
}
}
o = get('/')
expect(o['Content-Type']).to eq 'application/javascript;charset=utf-8'
end
it 'gets object' do
mock_app {
get('/') {
o = Human.new
jbuilder o
}
}
o = get('/')
expect(o['Content-Type']).to eq 'application/json'
json = JSON.parse(o.body)['data']
expect(json['last_name']).to eq 'hirakawa'
expect(json['first_name']).to eq 'akira'
end
it 'gets array' do
mock_app {
get('/') {
array = []
array << Human.new
array << Human.new
array << Human.new
array << Human.new
array << Human.new
jbuilder array
}
}
o = get('/')
expect(o['Content-Type']).to eq 'application/json'
json = JSON.parse(o.body)['data']
expect(json.class).to eq Array
expect(json.size).to eq 5
end
end
<file_sep>/Gemfile
source "http://rubygems.org"
gemspec
gem 'sinatra', :github => 'sinatra/sinatra'
group :development, :test do
gem 'sinatra-contrib'
gem 'jbuilder'
gem 'multi_json'
end<file_sep>/sinatra-jbuilder.gemspec
# -*- encoding: utf-8 -*-
Gem::Specification.new do |gem|
gem.authors = ["<NAME>"]
gem.email = ["<EMAIL>"]
gem.summary = %q{jbuilder for sinatra}
gem.description = %q{jbuilder for sinatra}
gem.homepage = "http://akirahrkw.com"
gem.files = `git ls-files`.split($\)
gem.test_files = gem.files.grep(%r{^(test|spec|features)/})
gem.name = "sinatra-jbuilder"
gem.require_paths = ["lib"]
gem.version = "1.0"
gem.license = "MIT"
gem.add_dependency "sinatra", '~> 1.4', '>= 1.4.0'
gem.add_dependency "multi_json"
gem.add_development_dependency "rspec", "~> 2.3"
end<file_sep>/README.md
Intro
=========
a plugin to use jbuilder in sinatra
jbuilder: https://github.com/rails/jbuilder
Concept
=========
model class takes responsibility for rendering json
Usage
=========
require 'sinatra/jbuilder'
ex:
```
class Human
...
...
# model class has to implement to_json method
def to_json(json)
json.id id
json.name name
end
end
get '/object' do
o = Human.new
jbuilder o
end
get '/array' do
array = Human.all
jbuilder array
end
```
```
{
meta: {
status: 200
},
data: {
id: 1,
name: 'akira'
}
}
```
Copyright
=========
Copyright (c) 2014 <NAME>. See LICENSE for details.
<file_sep>/spec/spec_helper.rb
require 'jbuilder'
require 'sinatra/contrib'
require 'sinatra/jbuilder'
RSpec.configure do |config|
config.expect_with :rspec
config.include Sinatra::TestHelpers
end | 55a21772aeaadf91888d94a7e047ced5d97de43b | [
"Markdown",
"Ruby"
]
| 6 | Ruby | akirahrkw/sinatra-jbuilder | 325649700782de5cb7879325603168030552ab75 | b9d90a2c8899a02e9a2f9992a89c2d455d63fc9f | |
refs/heads/master | <repo_name>nbeaudoin/Enron-Scandal-Machine-Learning<file_sep>/poi_id.py
#!/usr/bin/python
### Goal: Determine predictors of being a person of interest (POI)
### Load environment
import os
#os.chdir('/Users/nicholasbeaudoin/Desktop/OneDrive/DAND/7 Intro to Machine Learning/ud120-projects-master/final_project')
### Import packages
import sys
import pickle
sys.path.append("../tools/")
import pandas as pd
import numpy as np
from sklearn import cross_validation
import matplotlib.pyplot as plt
from sklearn.preprocessing import MinMaxScaler
from feature_format import featureFormat, targetFeatureSplit
from tester import dump_classifier_and_data
from sklearn.cross_validation import train_test_split
from sklearn.naive_bayes import GaussianNB
from sklearn.metrics import accuracy_score
from sklearn.cluster import KMeans
from sklearn.preprocessing import MinMaxScaler
from sklearn import tree
from sklearn.linear_model import LinearRegression
from sklearn.metrics import precision_score
from sklearn.metrics import recall_score
from sklearn.decomposition import PCA
from sklearn.pipeline import Pipeline
from sklearn.tree import DecisionTreeClassifier
from tester import test_classifier, dump_classifier_and_data
from sklearn.metrics import precision_score, recall_score
from sklearn.feature_selection import SelectKBest, f_classif
from sklearn.model_selection import GridSearchCV
from sklearn.model_selection import GridSearchCV, StratifiedShuffleSplit
######### Task 1: Select what features you'll use. #########
### features_list is a list of strings, each of which is a feature name.
### The first feature must be "poi".
target_label = 'poi'
email_features_list = [
'from_messages',
'from_poi_to_this_person',
'from_this_person_to_poi',
'shared_receipt_with_poi',
'to_messages',
]
financial_features_list = [
'bonus',
'deferral_payments',
'deferred_income',
'director_fees',
'exercised_stock_options',
'expenses',
'loan_advances',
'long_term_incentive',
'other',
'restricted_stock',
'restricted_stock_deferred',
'salary',
'total_payments',
'total_stock_value',
]
features_list = [target_label] + financial_features_list + email_features_list
### Load the dictionary containing the dataset
with open("final_project_dataset.pkl", "r") as data_file:
data_dict = pickle.load(data_file)
### Convert to pandas dataframe
df = pd.DataFrame(data_dict.values())
### How many features do we have?
print len(features_list)
### Exploratory Analysis
print(df.shape)
### Vizualize outliers
features_outliers = ["salary", "bonus"]
data = featureFormat(data_dict, features_outliers)
### Vizualize outliers
for point in data:
salary = point[0]
bonus = point[1]
plt.scatter( salary, bonus )
plt.xlabel("salary")
plt.ylabel("bonus")
plt.show()
### Examine outliers of salary more than $1M and bonus more than $5M
for employee in data_dict:
if (data_dict[employee]["salary"] != 'NaN') and (data_dict[employee]["bonus"] != 'NaN'):
if float(data_dict[employee]["salary"]) > 10000000 and float(data_dict[employee]["bonus"])>50000000:
print employee
data_dict.pop("TOTAL", 0)
data = featureFormat(data_dict, features_outliers)
for point in data:
salary = point[0]
bonus = point[1]
plt.scatter( salary, bonus )
plt.xlabel("salary")
plt.ylabel("bonus")
plt.show()
### Examine outliers of no salary
### Examine outliers of salary more than $1M and bonus more than $5M
for employee in data_dict:
print employee
### Remove non-employee
data_dict.pop("THE TRAVEL AGENCY IN THE PARK", 0)
### Update dataframe from dictionary
df = pd.DataFrame(data_dict.values())
feature_count = {}
for f in features_list:
feature_count[f] = df[f].size
print feature_count
# Count number of features that have NaN values
df.replace(to_replace='NaN', value=np.nan, inplace=True)
print df.isnull().sum()
# Drop anything with more than 50% missing values
# n=77 is half the observations so anything more than that we remove
#for f in features_list:
# if df.isnull().sum()[f] > 77:
# df.pop(f)
# print f
### Checking to see if we removed these features with the previous code
#for f in df.columns.values:
# print f
### Fill NA function in pandas
df = df.fillna(df.mean())
print(df.head())
### Create a new feature list with our new feautres of df
features_list_new = list(df)
# print features_list_new
features_list_new.remove('email_address')
### Create "total compensation"
df['total_comp'] = df['bonus'] + df['salary']
### Add new feature to features_list_new
features_list_new.append('total_comp')
features_list_new.remove('poi')
features_list_new[0] = 'poi'
features_list_new.append('salary')
features_list_new.append('bonus')
print features_list_new
### Take a look at the numeric data
print(df.describe())
count = 0
for key in data_dict.keys():
if (data_dict[key]["poi"]==1):
count += 1
print "Number of POIs:", count
### Select features that have less than 50% missing values
print features_list_new
### Extract features and labels from dataset for local testing
#data = featureFormat(data_dict, features_list_new, sort_keys = True)
#labels, features = targetFeatureSplit(data)
data_0 = df[features_list_new]
### Getting all cols except for POI
#features_list_new.remove('poi')
### Just want numpy array (pandas adds meta data on top of metadata and sklearn wants just matrix)
#labels = df[['poi']].values
### DF --> Dictionary
my_dataset = data_0.to_dict(orient="index")
### Extract features and labels from dataset for local testing
data = featureFormat(my_dataset, features_list_new, sort_keys = True)
labels, features = targetFeatureSplit(data)
features_train, features_test, labels_train, labels_test = \
train_test_split(data, labels, test_size=0.3, random_state=42)
### Select top 10 using K-Best
kbest = SelectKBest(f_classif, k=3)
features_selected = kbest.fit_transform(features_train, labels_train)
print(features_selected.shape)
features_list_new = [features_list_new[i+1] for i in kbest.get_support(indices=True)]
print ('Features selected by SelectKBest:')
print (features_list_new)
features_list_final = ['poi', 'deferral_payments', 'expenses', 'bonus']
data_0 = df[features_list_final]
my_dataset = data_0.to_dict(orient="index")
#data = featureFormat(my_dataset, features_list_final, sort_keys = True)
#labels, features = targetFeatureSplit(data)
features_train, features_test, labels_train, labels_test = \
train_test_split(data, labels, test_size=0.3, random_state=42)
### Naive Bayes 1
clf = GaussianNB()
clf.fit(features_train, labels_train)
gnb_pred1 = clf.predict(features_test)
gnb_score1 = clf.score(features_test, labels_test)
gnb_precision1 = precision_score(labels_test, gnb_pred1)
gnb_recall1 = recall_score(labels_test, gnb_pred1)
print ('GaussianNB 1 accuracy:', gnb_score1)
print ('GaussianNB 1 precision:', gnb_precision1)
print ('GaussianNB 1 recall:', gnb_recall1)
### Decision Tree 1
clf = DecisionTreeClassifier()
clf.fit(features_train, labels_train)
dt_pred1 = clf.predict(features_test)
dt_score1 = clf.score(features_test, labels_test)
dt_precision1 = precision_score(labels_test, dt_pred1)
dt_recall1 = recall_score(labels_test, dt_pred1)
print ('Decision Tree 1 accuracy:', dt_score1)
print ('Decision Tree 1 precision:', dt_precision1)
print ('Decision Tree 1 recall:', dt_recall1)
### Random Forest 1
from sklearn.ensemble import RandomForestClassifier
clf = RandomForestClassifier()
fit = clf.fit(features_train, labels_train)
rf_pred1 = clf.predict(features_test)
rf_score1 = clf.score(features_test, labels_test)
rf_precision1 = precision_score(labels_test, rf_pred1)
rf_recall1 = recall_score(labels_test, rf_pred1)
print ('Random Forest 1 accuracy:', rf_score1)
print ('Random Forest 1 precision:', rf_precision1)
print ('Random Forest 1 recall:', rf_recall1)
### Decision Tree 2
clf = DecisionTreeClassifier()
fit = clf.fit(features_train, labels_train)
dt_pred2 = clf.predict(features_test, labels_test)
dt_score2 = clf.score(features_test, labels_test)
dt_precision2 = precision_score(labels_test, dt_pred2)
dt_recall2 = recall_score(labels_test, dt_pred2)
print ('Decision Tree 2 accuracy:', dt_score2)
print ('Decision Tree 2 precision:', dt_precision2)
print ('Decision Tree 2 recall:', dt_recall2)
### Random Forest 2
from sklearn.ensemble import RandomForestClassifier
clf = RandomForestClassifier()
fit = clf.fit(features_train, labels_train)
rf_pred2 = clf.predict(features_test)
rf_score2 = clf.score(features_test, labels_test)
rf_precision2 = precision_score(labels_test, rf_pred2)
rf_recall2 = recall_score(labels_test, rf_pred2)
print ('Random Forest 2 accuracy:', rf_score2)
print ('Random Forest 2 precision:', rf_precision2)
print ('Random Forest 2 recall:', rf_recall2)
### GridSearch: Decision Tree 3
param_grid_dt3 = {'max_depth': np.arange(3, 10), 'min_samples_split': np.arange(3, 10),
'presort': [True, False]}
sss = StratifiedShuffleSplit(n_splits=100, test_size=0.5, random_state=0)
tree_dt3 = GridSearchCV(DecisionTreeClassifier(), param_grid_dt3)
tree_dt3.fit(features_train, labels_train)
tree_dt3.best_params_
clf = DecisionTreeClassifier(max_depth=3, min_samples_split=3, presort=True)
fit = clf.fit(features_train, labels_train)
dt_pred3 = clf.predict(features_test, labels_test)
dt_score3 = clf.score(features_test, labels_test)
dt_precision3 = precision_score(labels_test, dt_pred3)
dt_recall3 = recall_score(labels_test, dt_pred3)
print ('Decision Tree 3 accuracy:', dt_score3)
print ('Decision Tree 3 precision:', dt_precision3)
print ('Decision Tree 3 recall:', dt_recall3)
### GridSearch: Random Forest 3
param_grid_rf3 = {'max_depth': np.arange(3, 20), 'min_samples_split': np.arange(3, 10)}
sss = StratifiedShuffleSplit(n_splits=100, test_size=0.5, random_state=0)
tree_rf3 = GridSearchCV(DecisionTreeClassifier(random_state=0), param_grid_rf3, cv=sss, scoring='f1')
tree_rf3.fit(features_train, labels_train)
tree_rf3.best_params_
clf = RandomForestClassifier(max_depth=3, min_samples_split=3)
fit = clf.fit(features_train, labels_train)
rf_pred3 = clf.predict(features_test)
rf_score3 = clf.score(features_test, labels_test)
rf_precision3 = precision_score(labels_test, rf_pred3)
rf_recall3 = recall_score(labels_test, rf_pred3)
print ('Random Forest 3 accuracy:', rf_score3)
print ('Random Forest 3 precision:', rf_precision3)
print ('Random Forest 3 recall:', rf_recall3)
### Task 6: Dump your classifier, dataset, and features_list so anyone can ###
### check your results. You do not need to change anything below, but make sure
### that the version of poi_id.py that you submit can be run on its own and
### generates the necessary .pkl files for validating your results.
feature_list = features_list_final
dataset = my_dataset
test_classifier(clf, dataset, feature_list)
dump_classifier_and_data(clf, dataset, feature_list)
<file_sep>/poi_v3.py
# -*- coding: utf-8 -*-
import pickle
import pandas as pd
import numpy as np
from sklearn.cross_validation import train_test_split
from tester import test_classifier, dump_classifier_and_data
from feature_format import featureFormat, targetFeatureSplit
from sklearn.grid_search import GridSearchCV
from sklearn.svm import SVC
from sklearn.tree import DecisionTreeClassifier
import matplotlib.pyplot as plt
from sklearn.ensemble import RandomForestClassifier
from sklearn.naive_bayes import GaussianNB
### Load the dictionary containing the dataset
with open("final_project_dataset.pkl", "r") as data_file:
data_dict = pickle.load(data_file)
CLF_PICKLE_FILENAME = "my_classifier.pkl"
### Convert to pandas dataframe
df = pd.DataFrame(data_dict.values())
##############################################################################
############### EXPLORATORY ANALYSIS ##############################
##############################################################################
### First look at the data
print df.head()
# Each feature is a column
### Data shape
print df.shape
# 146 observations
# 21 features including POI
features_outliers = ["salary", "bonus"]
data = featureFormat(data_dict, features_outliers)
### Vizualize outliers
for point in data:
salary = point[0]
bonus = point[1]
plt.scatter( salary, bonus )
plt.xlabel("salary")
plt.ylabel("bonus")
plt.show()
### Examine outliers of salary more than $1M and bonus more than $5M
for employee in data_dict:
if (data_dict[employee]["salary"] != 'NaN') and (data_dict[employee]["bonus"] != 'NaN'):
if float(data_dict[employee]["salary"]) > 10000000 and float(data_dict[employee]["bonus"])>50000000:
print employee
### Looking for employees that have strange names; not empoyee?
for employee in data_dict:
print employee
### Remove non-employee and outlier "total"
data_dict.pop("TOTAL", 0)
data_dict.pop("THE TRAVEL AGENCY IN THE PARK", 0)
### Update dataframe from dictionary
df = pd.DataFrame(data_dict.values())
### Number of POI
count = 0
for key in data_dict.keys():
if (data_dict[key]["poi"]==1):
count += 1
print "Number of POIs:", count
# Replace 'NAN' strings with np.nan
df.replace(to_replace='NaN', value=np.nan, inplace=True)
### Fill NA function in pandas
df = df.fillna(df.mean())
### drop unwanted feature
del df['email_address']
##### feature list based on df, dataset as dictionary
feature_list = list(df)
feature_list[0]='poi'
feature_list[12]='bonus'
#### Scale dataframe (minmax scaling)
df_copy=df.copy()
df_copy = df_copy.drop('poi', 1)
df_copy -= df_copy.min()
df_copy /= (df_copy.max()-df_copy.min())
df_copy['poi']=df['poi']
df=df_copy
## Convert df to dictionary
dataset=df.to_dict(orient="index")
##### Clean dataset
X=featureFormat(dataset, feature_list, remove_NaN=True, remove_all_zeroes=True, remove_any_zeroes=False, sort_keys = False)
### split dataset into target and features
target,features=targetFeatureSplit(X)
### Feature selection
df_new=pd.DataFrame()
clf = DecisionTreeClassifier(min_samples_split=2,min_samples_leaf=1)
clf.fit(features, target)
tree_scores = zip(feature_list[1:], clf.feature_importances_)
for i in range(19):
if tree_scores[i][1]>0.01:
print tree_scores[i][0]
df_new[tree_scores[i][0]]=df_copy[tree_scores[i][0]]
feature_list=list(df_new)
feat=feature_list[0]
feature_list[0]='poi'
df_new['poi']=df['poi']
## Convert df to dictionary
dataset=df_new.to_dict(orient="index")
##### Clean dataset
X=featureFormat(dataset, feature_list, remove_NaN=True, remove_all_zeroes=True, remove_any_zeroes=False, sort_keys = False)
### split dataset into target and features
target,features=targetFeatureSplit(X)
###### Train Test Split
features_train, features_test, labels_train, labels_test = train_test_split(features, target, test_size=0.00, random_state=42)
################ Select Classifiers #########################
### Naive Bayes 1
clf = GaussianNB()
clf.fit(features_train, labels_train)
test_classifier(clf, dataset, feature_list)
### Decision Tree 1
clf = DecisionTreeClassifier()
clf.fit(features_train, labels_train)
test_classifier(clf, dataset, feature_list)
### Random Forest 1
clf = RandomForestClassifier()
clf.fit(features_train, labels_train)
test_classifier(clf, dataset, feature_list)
### SVC
clf_r=SVC()
clf_r.fit(features_train, labels_train)
#################### Find optimal parameters #######################
parameters = {'gamma': [1,2,3,4,5,6,7,8,9,10,10.5,11,11.5,12,12.5,13,13.5,14,14.5,15,15.5,16,16.5,17,17.5,18,18.5,19,19.5,20]}
scores = ['f1']
clf_svc=SVC(class_weight='balanced',kernel='rbf', C=10000)
A={}
for score in scores:
print("# Tuning hyper-parameters for %s" % score)
print()
clf = GridSearchCV(clf_svc,parameters,
scoring='%s_macro' % score)
clf.fit(features_train, labels_train)
print("Best parameters set found on development set:")
print()
print(clf.best_params_)
print()
print("Grid scores on development set:")
print()
A[score]=clf.best_params_
######################################
print 'FINAL RESULTS: Best Classifier'
#####################################
clf_r=SVC(class_weight='balanced', kernel='rbf', C=10000, gamma=A['f1']['gamma'])
clf_r.fit(features_train, labels_train)
print '########## Classifier tuned for f1 ################'
test_classifier(clf_r, dataset, feature_list)
dump_classifier_and_data(clf_r, dataset, feature_list)
<file_sep>/README.md
# Udacity Completed Machine Learning Capstone
Dataset Enron, courtesy of Udacity
Completed 10/1/2018
| 14aa3610b2adfda4c64bd68570baa5234c795223 | [
"Markdown",
"Python"
]
| 3 | Python | nbeaudoin/Enron-Scandal-Machine-Learning | f1079e629f13b7cc53a9b5486e4fe7e6d5f5ae4f | 51995e071021acb81f217519652f8b7e8813495d | |
refs/heads/main | <repo_name>alexchristescu/site-de-prezentare<file_sep>/src/Components/Header.js
import React,{Component} from 'react'
import "./Header.css";
import StickyHeader from 'react-sticky-header';
import Navbar from 'react-bootstrap/Navbar';
import Nav from 'react-bootstrap/Nav';
import Form from 'react-bootstrap/Form';
import FormControl from 'react-bootstrap/FormControl';
import Button from 'react-bootstrap/Button';
import NavDropdown from 'react-bootstrap/NavDropdown'
import logo from "../images/logo.svg";
import { FontAwesomeIcon } from "@fortawesome/react-fontawesome";
import {
faYoutube,
faFacebook,
faTwitter,
faInstagram,
faWhatsapp
} from "@fortawesome/free-brands-svg-icons";
export default class Header extends Component {
render(){
return(
<Navbar collapseOnSelect expand="lg" bg="dark" variant="dark" sticky="top" >
<Navbar.Brand href="#home">
<img
src= {logo}
width="50"
height="50"
className="d-inline-block align-top"
alt="React Bootstrap logo"
/>
</Navbar.Brand>
<Navbar.Toggle aria-controls="responsive-navbar-nav" />
<Navbar.Collapse id="responsive-navbar-nav" className="justify-content-center" >
<Nav >
<Nav.Link href="#features">Features</Nav.Link>
<Nav.Link href="#pricing">Pricing</Nav.Link>
<NavDropdown title="Dropdown" id="collasible-nav-dropdown">
<NavDropdown.Item href="#action/3.1">Action</NavDropdown.Item>
<NavDropdown.Item href="#action/3.2">Another action</NavDropdown.Item>
<NavDropdown.Item href="#action/3.3">Something</NavDropdown.Item>
<NavDropdown.Divider />
<NavDropdown.Item href="#action/3.4">Separated link</NavDropdown.Item>
</NavDropdown>
</Nav>
</Navbar.Collapse>
<Nav className="d-none d-md-flex" >
<Nav.Link href="#deets"><FontAwesomeIcon icon= {faFacebook} /></Nav.Link>
<Nav.Link eventKey={2} href="#memes">
<FontAwesomeIcon icon= {faInstagram} />
</Nav.Link>
<Nav.Link href="#deets"><FontAwesomeIcon icon= {faWhatsapp} /></Nav.Link>
<Nav.Link eventKey={2} href="#memes">
<FontAwesomeIcon icon= {faYoutube} />
</Nav.Link>
</Nav>
</Navbar>
)
}
}<file_sep>/src/App.js
import './App.css';
import React, {Component} from "react";
import Header from "./Components/Header";
import 'bootstrap/dist/css/bootstrap.min.css'
import Layout from "./Components/Layout"
import Home from "./Components/Home"
import Footer from "./Components/Footer"
class App extends Component {
constructor(){
super();
this.state={
}
}
render(){
return(
<Home/>
);
}
}
export default App;
| 77a1328bb4b1f05a4ed18b98acf13a12592bf1dd | [
"JavaScript"
]
| 2 | JavaScript | alexchristescu/site-de-prezentare | 256871fc91b013b9d2bf2054ef082f91e97e2dff | 020c0154fc36e1517cf97bd22dc008182bd3b5b3 | |
refs/heads/master | <file_sep>#include "agent.h"
Agent::Agent(QObject *parent, QTableWidget *tab, uint maxSteps) :
QObject(parent)
{
maxSteps_ = maxSteps;
stepsLimit = maxSteps;
stepNo = 0;
tab_ = tab;
timer.setParent(this);
timer.setInterval(5);
dtimer.setParent(this);
dtimer.setInterval(500);
connect(&timer, SIGNAL(timeout()), this, SLOT(nextStep()));
connect(&dtimer, SIGNAL(timeout()), this, SLOT(nextDemoStep()));
}
void Agent::nextStep()
{
qDebug()<< stepNo <<"step. Limit "<<maxSteps_ << "x="<<lPos.x << "y="<<lPos.y;
tab_->item(lPos.y,lPos.x)->setBackgroundColor(Qt::white);//zeruje kolor
maxSteps_--;
stepNo++;
//nastepna pozycja
position nPos = nextMove(lPos);//
updateValue(lPos, nPos);
if(tab_->item(nPos.y,nPos.x)->backgroundColor() == Qt::green)
{
qDebug()<<"znalazl wyjscie";
stop();
return;
}
tab_->item(nPos.y,nPos.x)->setBackgroundColor(Qt::yellow);
lPos = nPos;
if(maxSteps_ > 1)
{
timer.start();
}else{
stop();
qDebug()<<"limit krokow";
return;
}
}
position Agent::nextMove(position pos)
{
position nextPos = Nav::randomMove(pos);
while(1)///bedzie szukal az znajdzie nastepne dobry spot
{
if (tab_->item(nextPos.y,nextPos.x) != 0 && tab_->item(nextPos.y,nextPos.x)->backgroundColor() != Qt::gray)
return nextPos;
nextPos = Nav::randomMove(pos);
}
}
void Agent::stop()
{
timer.stop();
tab_->item(lPos.y,lPos.x)->setBackgroundColor(Qt::white);//zeruje kolor
repeats_--;
if(repeats_>0)
start(stepsLimit,repeats_);
}
void Agent::start(uint maxSteps, uint repeats)
{
reset();
stepNo = 0;
maxSteps_ = maxSteps;
repeats_ = repeats;
QList<QTableWidgetItem*> selections = tab_->selectedItems();
if (selections.count() == 0)
{
sPos = setStart();
tab_->setCurrentCell(sPos.y, sPos.x,QItemSelectionModel::Select);
}
else{
sPos.x = selections.at(0)->column();
sPos.y = selections.at(0)->row();
};
qDebug()<< "agent start at position: " <<sPos.x<<sPos.y;
lPos = sPos;
tab_->item(lPos.y,lPos.x)->setBackgroundColor(Qt::blue);
timer.start();
}
void Agent::reset()
{
itemValue v;
v.value=0;
v.numerator=0;
v.divisor=1; //wstepna wartosc to pierwsza wartosc
v.dval = 0;
v.p.clear();
int rowCount = tab_->rowCount();
for(int i = 0; i<rowCount;i++)
{
for(int j =0; j<rowCount;j++)
{
arr[i][j].divisor = 1;
arr[i][j].numerator = 0;
arr[i][j].p.clear();
arr[i][j].value = 0;
if(tab_->item(i,j)->text().toInt() > 0)
{
arr[i][j].value = tab_->item(i,j)->text().toInt();
arr[i][j].dval = tab_->item(i,j)->text().toInt();/// i tak przepisuje tylko pelne setki
};
}
}
}
void Agent::updateValue(position lastPos, position newPos)
{
itemValue it;// next item
itemValue lit;//last item
///arr podreczna tablica wartosci
///tab_ tutaj wpisujemy wynik
it = arr[newPos.y][newPos.x];// wartosc z nowego pola
lit = arr[lastPos.y][lastPos.x]; // wartosc ze starego pola
///SMA simple moving average
// int X = it.value;
// //wartosc do starego pola
// it = arr[lastPos.y][lastPos.x];
// it.numerator += X;
// it.divisor ++;
// X = it.value = it.numerator / it.divisor;
// tab_->item(lastPos.y,lastPos.x)->setText(QString::number(X));
///WMA weighted moving average
double X = arr[newPos.y][newPos.x].dval * 0.99; //bierzemy wartosc z nowego pola
double num = 0;// podreczny licznik i mianownik
double div = 0;
lit.p.append(X);
lit.n.append(stepNo);
int c = lit.p.count(); // ile elementow w kontenerach
for(int i = 0; i < c; i++)
{
num = num + lit.p.at(i)/lit.n.at(i); ///sumowanie licznika
div = div + 1/lit.n.at(i); ///sumowanie mianownika
qDebug()<< " i:" << i << "num" << num << "div" << div;
};
lit.dval = X = num / div; // nowa wartosc
qDebug()<<" X"<<X;
arr[lastPos.y][lastPos.x] = lit; // item spowrotem do starego w tablicy
tab_->item(lastPos.y,lastPos.x)->setText(QString::number(X));
}
position Agent::setStart()
{
position p;
///srodek planszy
p.x = tab_->rowCount()/2;
p.y = tab_->columnCount()/2;
for (int i= 0; i < 20; i++)
{
if(tab_->item(p.y,p.x)->backgroundColor() !=Qt::white) //sprawdza czy nie ma sciany
{
p = Nav::randomMove(p);
}else
return p;
}
return p;
}
void Agent::startDemo()
{
// sPos
tab_->item(sPos.y,sPos.x)->setBackgroundColor(Qt::blue);
lPos = sPos;
dtimer.start();
}
void Agent::nextDemoStep()
{
///tab_->item(lPos.y,lPos.x)->setBackgroundColor(Qt::white);//zeruje kolor///nie zerujemy koloru, zaznacza tam gdzie byl
if(tab_->item(lPos.y,lPos.x)->text().toInt() == 100)// jezeli koniec
{
tab_->item(lPos.y,lPos.x)->setBackgroundColor(Qt::yellow);
qDebug()<<"koniec ";
dtimer.stop();
return;
}
///wybierz najlepsze miejsce dookoła
position nextPos, temp;
double bestValue = 0;
temp.y = lPos.y - 1;
temp.x = lPos.x;
if(tab_->item(temp.y,temp.x) != 0 && tab_->item(temp.y,temp.x)->text().toDouble() > bestValue && tab_->item(temp.y,temp.x)->backgroundColor() != Qt::blue){
bestValue = tab_->item(temp.y,temp.x)->text().toDouble();
nextPos = temp;
};
temp.x = lPos.x + 1;
temp.y = lPos.y;
if(tab_->item(temp.y,temp.x) != 0 && tab_->item(temp.y,temp.x)->text().toDouble() > bestValue && tab_->item(temp.y,temp.x)->backgroundColor() != Qt::blue){
bestValue = tab_->item(temp.y,temp.x)->text().toDouble();
nextPos = temp;
};
temp.y = lPos.y + 1;
temp.x = lPos.x;
if(tab_->item(temp.y,temp.x) != 0 && tab_->item(temp.y,temp.x)->text().toDouble() > bestValue && tab_->item(temp.y,temp.x)->backgroundColor() != Qt::blue){
bestValue = tab_->item(temp.y,temp.x)->text().toDouble();
nextPos = temp;
};
temp.x = lPos.x - 1;
temp.y = lPos.y;
if(tab_->item(temp.y,temp.x) != 0 && tab_->item(temp.y,temp.x)->text().toDouble() > bestValue && tab_->item(temp.y,temp.x)->backgroundColor() != Qt::blue){
bestValue = tab_->item(temp.y,temp.x)->text().toDouble();
nextPos = temp;
};
if(bestValue == 0)
nextPos = nextMove(lPos); // zrobi losowy krok jezeli same zera dookola
if((lPos.x == nextPos.x) && (lPos.y == nextPos.y))
{
qDebug()<<"stoimy ";
tab_->item(lPos.y,lPos.x)->setBackgroundColor(Qt::red);
dtimer.stop();
}else{
qDebug()<<"best move ";
lPos = nextPos;
tab_->item(nextPos.y,nextPos.x)->setBackgroundColor(Qt::blue);
dtimer.start();
}
}
<file_sep>#ifndef AGENT_H
#define AGENT_H
#include <QObject>
#include <QTimer>
#include <QTableWidget>
#include <QDebug>
#include <QVector>
#include <array>
#include "nav.h"
#include "Helpers.h"
class Agent : public QObject
{
Q_OBJECT
public:
Agent(QObject *parent, QTableWidget *tab, uint maxSteps);
signals:
public slots:
void start(uint maxSteps, uint repeats);
void startDemo();
void reset();
void stop();
private slots:
void nextStep();
void nextDemoStep();
private:
QTimer timer;
QTimer dtimer;//demo timer
uint maxSteps_;
uint stepsLimit;
int stepNo;
QTableWidget *tab_;
uint repeats_;
position sPos;//start
position lPos;//last position
position aPos;//actual
QTableWidgetItem *prevItem;
position setStart();
position nextMove(position pos);
void updateValue(position lastPos, position newPos);
//std::array<std::array<itemValue,100>,100> arr;
itemValue arr[100][100];
};
#endif // AGENT_H
<file_sep>#ifndef NAV_H
#define NAV_H
#include "Helpers.h"
#include <QApplication>
#include <QDebug>
/// agent navigation
class Nav
{
public:
static position randomMove(position p);
};
#endif // NAV_H
<file_sep>#include "nav.h"
/// losowy wybor jednego z 4 kierunkow
position Nav::randomMove(position p)
{
int dir = qrand() % 4;
switch (dir) {
case 0:
p.x --;//lewo
qDebug()<<"left";
break;
case 1:
p.y --;//gora
qDebug()<<"up";
break;
case 2:
p.x ++;//prawo
qDebug()<<"right";
break;
case 3:
p.y ++;//dol
qDebug()<<"down";
break;
default:
break;
};
return p;
}
<file_sep>#ifndef HELPERS_H
#define HELPERS_H
#include <qvector.h>
struct position{
int x; //column
int y; //row
};
struct itemValue{
///prosta srednia wazona
int value; //wartosc pola
int numerator; //licznik
int divisor; //dzielnik,mianownik
///wazona srednia kroczaca
double dval;
QVector<double> p; //kontener wartosci
QVector<double> n;
///wlasny
};
#endif // HELPERS_H
<file_sep>#ifndef DIALOG_H
#define DIALOG_H
#include <QDialog>
#include <QDebug>
#include "agent.h"
namespace Ui {
class Dialog;
}
class Dialog : public QDialog
{
Q_OBJECT
public:
explicit Dialog(QWidget *parent = 0);
~Dialog();
private slots:
void on_spinBox_2_valueChanged(int arg1);
void on_spinBox_valueChanged(int arg1);
void on_wallGenButton_clicked();
void on_spinBox_M_valueChanged(int arg1);
void on_learnButton_clicked();
void on_runButton_clicked();
void on_refreshButton_clicked();
private:
Ui::Dialog *ui;
int worldSize;
int m;
int learningSteps;
int M;
void updateLearningSteps();
void deleteWalls();
void createItems();
void setExit(int row, int column);
void cleanWorld();
QTableWidget *tab;
Agent *agent;
};
#endif // DIALOG_H
<file_sep>#include "dialog.h"
#include "ui_dialog.h"
Dialog::Dialog(QWidget *parent) :
QDialog(parent),
ui(new Ui::Dialog)
{
ui->setupUi(this);
tab = ui->tableWidget;
tab->verticalHeader()->hide();
tab->horizontalHeader()->hide();
m = 20;
worldSize = 8;
on_spinBox_valueChanged(ui->spinBox->value());
on_spinBox_M_valueChanged(ui->spinBox_M->value());
on_spinBox_2_valueChanged(ui->spinBox_2->value());
updateLearningSteps();
agent = new Agent(this, ui->tableWidget, learningSteps);
}
Dialog::~Dialog()
{
delete ui;
}
void Dialog::updateLearningSteps()
{
learningSteps = ui->spinBox->value() * ui->spinBox_2->value();
ui->label_3->setText(QString::number(learningSteps));
}
void Dialog::on_spinBox_2_valueChanged(int arg1)
{
m = arg1;
updateLearningSteps();
}
///zmiana rozmiaru swiata
void Dialog::on_spinBox_valueChanged(int arg1)
{
worldSize = arg1;
qDebug()<< "world size changed to: " << worldSize;
ui->tableWidget->setColumnCount(worldSize);
ui->tableWidget->setRowCount(worldSize);
ui->tableWidget->setAutoFillBackground(true);
QSize tableSize, cellSize;
tableSize = ui->tableWidget->size();
cellSize.setHeight(tableSize.height() / worldSize -1);
cellSize.setWidth(tableSize.width() / worldSize -1);
qDebug()<< tableSize.width() << "tableSize " << tableSize.height();
qDebug()<< cellSize.width() << "cellSize " << cellSize.height();
for(int i=0; i < worldSize ;i++)
{
ui->tableWidget->setRowHeight(i,cellSize.height());
ui->tableWidget->setColumnWidth(i,cellSize.width());
}
createItems();
updateLearningSteps();
}
///generowanie scian na planszy
void Dialog::on_wallGenButton_clicked()
{
int prob;
int up,down,right,left;
int ws = worldSize;
prob = ui->horizontalSlider->value();
deleteWalls();
//qDebug()<< "prob value"<< prob << "qrand" << qrand() % 100;
///wall generation
for(int y =0;y < worldSize; y++){
for(int x =0;x < worldSize; x++){
y-1<0 ? up=y :up=y-1;
y+1>ws ? down=y : down=y+1;
x+1>ws ? right=x : right=x+1;
x-1<0 ? left=x :left=x-1;
//qDebug()<<"up down right left"<< up<<down<<right<<left << "cords: " << x << y;
if((qrand() % 100) < prob){
if(tab->item(up,x)->backgroundColor() != Qt::gray &&
tab->item(y,left)->backgroundColor() != Qt::gray)
tab->item(y,x)->setBackgroundColor(Qt::gray);
};
};
};
///exits generation
int egdeItems = 4*worldSize -4;
int temp;
QVector<int> exits;
//qDebug()<< "egditems: "<<egdeItems<< "exits"<< M;
for(int q=1; q <= M; q++)//////rozdanie wyjsc równomiernie wokoło klatki
{
temp = q * egdeItems/M;
//qDebug()<<"temp"<<temp;
exits.append(temp);
};
temp=0;
for(int pg=0; pg < worldSize;pg++)///gorna krawedz w prawo//////przebieg po każdej z 4 krawędzi
{
temp++;
if(temp == exits.at(0))
{
setExit(0,pg);
exits.pop_front();
}
};
for(int dp=1; dp < worldSize-1; dp++)
{
temp++;
if(temp == exits.at(0))
{
setExit(dp,worldSize-1);
exits.pop_front();
}
};
for(int ld=9; ld > -1; ld--)
{
temp++;
if(temp == exits.at(0))
{
setExit(worldSize-1,ld);
exits.pop_front();
}
};
for(int gl=8; gl > 0; gl--)
{
temp++;
if(temp == exits.at(0))
{
setExit(gl,0);
exits.pop_front();
}
};
}
/// wyczyszczenie swiata
/// 1. usuniecie sciana
/// 2. wyzerowanie textow // TODO
void Dialog::deleteWalls()
{
for(int i =0;i < worldSize; i++){
for(int j =0;j < worldSize; j++){
ui->tableWidget->item(i,j)->setBackgroundColor(Qt::white);
ui->tableWidget->item(i,j)->setText("");
};
};
agent->reset();
}
void Dialog::setExit(int row, int column)
{
tab->item(row,column)->setBackgroundColor(Qt::green);
tab->item(row,column)->setText("100");
tab->item(row,column)->setTextAlignment(Qt::AlignHCenter | Qt::AlignVCenter);
}
///alokacja itemow w tabeli
void Dialog::createItems()
{
for(int i =0;i < worldSize; i++){
for(int j =0;j < worldSize; j++){
ui->tableWidget->setItem(i, j, new QTableWidgetItem);
};
};
}
void Dialog::on_spinBox_M_valueChanged(int arg1)
{
M = arg1;
}
///proces uczenia sie
void Dialog::on_learnButton_clicked()
{
uint reps = ui->spinBox_repeats->value();
qDebug ()<<"starting "<< reps << "learning runs";
agent->start(learningSteps,reps);
}
///proces poruszania sie po "wyuczonym" swiecie
void Dialog::on_runButton_clicked()
{
agent->startDemo();
qDebug()<<"start demo";
}
/// wyczyszczenie swiata
void Dialog::on_refreshButton_clicked()
{
deleteWalls();
}
| deab348ce925e1c0aa684ef336bba204cd2b2893 | [
"C",
"C++"
]
| 7 | C++ | f8industries/ReinforcementLearning | 35eb170373cbae69454974b9729d1447fd01b625 | 6d87c53945c87dbb870c6645f6d8818930d5f5ff | |
refs/heads/main | <file_sep>import {Directive, HostBinding, HostListener} from '@angular/core';
import {style} from "@angular/animations";
@Directive({
selector: '[appRainbow]'
})
export class RainbowDirective {
@HostBinding('style.color') color ;
@HostBinding('style.borderColor') bdcolor ;
// tslint:disable-next-line:typedef
@HostListener('keyup') onKeyUp(){
this.color = '#' + (0x1000000 + (Math.random()) * 0xffffff).toString(16).substr(1, 6 ) ;
this.bdcolor = '#' + (0x1000000 + (Math.random()) * 0xffffff).toString(16).substr(1, 6 ) ;
}
constructor() { }
}
| eb2d0fdb0f27d5f3603bea750b0f41f9c66d4d79 | [
"TypeScript"
]
| 1 | TypeScript | khalilgarnaoui/TP-Angular | dd4076b9d5f04a2506ea55f565740f8d11afcbc7 | 0fa59ce7095f2ae568125661f7e7556212fadef4 | |
refs/heads/master | <repo_name>fbergmann/edit-spatial<file_sep>/LibEditSpatial/Model/DebugConfig.cs
using System.Collections.Generic;
namespace LibEditSpatial.Model
{
public class DebugConfig
{
public int Verbosity { get; set; }
public int Instationary { get; set; }
public static DebugConfig FromDict(Dictionary<string, string> dict)
{
var result = new DebugConfig
{
Verbosity = dict.Get<int>("verbosity"),
Instationary = dict.Get<int>("Instationary")
};
return result;
}
public Dictionary<string, string> ToDict()
{
var result = new Dictionary<string, string>();
result["verbosity"] = Verbosity.ToString();
result["Instationary"] = Instationary.ToString();
return result;
}
}
}<file_sep>/LibEditSpatial/Controls/CtrlPalette.cs
using System;
using System.Globalization;
using System.Windows.Forms;
using LibEditSpatial.Model;
namespace LibEditSpatial.Controls
{
public partial class CtrlPalette : UserControl
{
private DmpPalette _Palette;
public CtrlPalette()
{
InitializeComponent();
Current = new PalleteArgs();
IssuingEvents = true;
IsPainting = false;
}
public PalleteArgs Current { get; set; }
public bool IssuingEvents { get; set; }
public bool IsPainting { get; set; }
public DmpPalette Palette
{
get
{
if (_Palette == null)
_Palette = DmpPalette.Default;
return _Palette;
}
set
{
_Palette = value;
pictureBox1.Image = _Palette.ToImage(pictureBox1.Size);
}
}
public event EventHandler<PalleteArgs> PalleteValueChanged;
public event EventHandler<DmpPalette> PalleteChanged;
public void UpdateValues(double min, double current, double max)
{
if (current > max) current = max;
if (current < min) current = min;
IssuingEvents = false;
txtMin.Text = min.ToString(CultureInfo.InvariantCulture);
txtCurrent.Text = current.ToString(CultureInfo.InvariantCulture);
txtMax.Text = max.ToString(CultureInfo.InvariantCulture);
trackBar1.Minimum = (int) (min*100f);
trackBar1.Maximum = (int) (max*100f);
trackBar1.Value = (int) (current*100f);
Current.Min = min;
Current.Value = current;
Current.Max = max;
IssuingEvents = true;
}
private void OnPaletteChanged()
{
if (PalleteChanged != null && IssuingEvents)
PalleteChanged(this, Palette);
}
private void OnPaletteValueChanged()
{
if (PalleteValueChanged != null && IssuingEvents)
PalleteValueChanged(this, Current);
}
private void OnMinChanged(object sender, EventArgs e)
{
double val;
if (double.TryParse(txtMin.Text, out val))
{
Current.Min = val;
trackBar1.Minimum = (int) (val*100);
OnPaletteValueChanged();
}
}
private void SetCurrent(double val)
{
Current.Value = val;
trackBar1.Value = (int) Math.Min((Math.Max(val, trackBar1.Minimum)*100), trackBar1.Maximum);
OnPaletteValueChanged();
}
private void OnCurrentChanged(object sender, EventArgs e)
{
double val;
if (double.TryParse(txtCurrent.Text, out val))
{
SetCurrent(val);
}
}
private void OnScrollChanged(object sender, EventArgs e)
{
txtCurrent.Text = ((double) (trackBar1.Value)/100f).ToString(CultureInfo.InvariantCulture);
}
private void OnMaxChanged(object sender, EventArgs e)
{
double val;
if (double.TryParse(txtMax.Text, out val))
{
Current.Max = val;
trackBar1.Maximum = (int) (val*100);
OnPaletteValueChanged();
}
}
private void OnMouseUp(object sender, MouseEventArgs e)
{
IsPainting = false;
}
private void OnMouseDown(object sender, MouseEventArgs e)
{
IsPainting = true;
}
private void OnMouseMove(object sender, MouseEventArgs e)
{
if (Palette == null || !IsPainting) return;
var length = pictureBox1.Width/(float) Palette.Colors.Count;
float test = (int) (e.X/length);
if (test >= Palette.Colors.Count)
test = Palette.Colors.Count - 1;
var stretchX = Math.Round(Current.MapFromUnit(test / Palette.Colors.Count), 2);
SetCurrent(stretchX);
txtCurrent.Text = stretchX.ToString(CultureInfo.InvariantCulture);
//
}
private void OnMouseLeave(object sender, EventArgs e)
{
IsPainting = false;
}
private void OnMouseClick(object sender, MouseEventArgs e)
{
if (Palette == null) return;
var length = pictureBox1.Width/(float) Palette.Colors.Count;
float test = (int) (e.X/length);
if (test >= Palette.Colors.Count)
test = Palette.Colors.Count - 1;
var stretchX = Math.Round(Current.MapFromUnit(test / Palette.Colors.Count), 2);
SetCurrent(stretchX);
txtCurrent.Text = stretchX.ToString(CultureInfo.InvariantCulture);
}
public void ChangePalette(DmpPalette palette)
{
Palette = palette;
OnPaletteChanged();
}
public void ChangePalette(string filename)
{
var palette = DmpPalette.FromFile(filename);
ChangePalette(palette);
}
private void OnLoadPalette(object sender, EventArgs e)
{
using (var dialog = new OpenFileDialog
{
Title = "Open Palette",
Filter = "Palette files|*.txt|All files|*.*",
AutoUpgradeEnabled = true
})
{
if (dialog.ShowDialog() == DialogResult.OK)
{
ChangePalette(dialog.FileName);
}
}
}
}
}<file_sep>/LibEditSpatial/Util.cs
using System.Windows.Forms;
using Ookii.Dialogs;
namespace LibEditSpatial
{
public static class Util
{
public static string ToCygwin(string dir)
{
dir = dir.Replace("\\", "/");
var separator = dir.IndexOf(':');
if (separator == -1) return dir;
var drive = dir.Substring(0, separator);
var sub = dir.Substring(separator + 1);
return string.Format("/cygdrive/{0}{1}", drive, sub);
}
public static string GetDir(string baseDir)
{
using (var dlg = new VistaFolderBrowserDialog { SelectedPath = baseDir, Description = "Open Directory" })
{
if (dlg.ShowDialog() == DialogResult.OK)
return dlg.SelectedPath;
}
return baseDir;
}
}
}<file_sep>/LibEditSpatial/Model/VoidByteStringDelegate.cs
namespace LibEditSpatial.Model
{
public delegate void VoidByteStringDelegate(byte[] data, string species);
}<file_sep>/LibEditSpatial/Model/DuneConfig.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using libsbmlcs;
namespace LibEditSpatial.Model
{
public class DuneConfig
{
public bool Dirty { get; set; }
internal const string STR_GLOBAL = "Global";
internal DomainConfig _DomainConfig;
internal GlobalConfig _GlobalConfig;
internal NewtonConfig _NewtonConfig;
internal TimeLoopConfig _TimeConfig;
public Dictionary<string, string> VariableCompartmentMap { get; set; }
public List<string> CompartmentIds { get; set; }
/// <summary>
/// Initializes a new instance of the DuneConfig class.
/// </summary>
public DuneConfig()
{
Entries = new Dictionary<string, Dictionary<string, string>>();
VariableCompartmentMap = new Dictionary<string, string>();
CompartmentIds = new List<string>();
}
public string FileName { get; set; }
public Dictionary<string, Dictionary<string, string>> Entries { get; set; }
public GlobalConfig GlobalConfig
{
get
{
if (_GlobalConfig == null)
{
if (Entries.ContainsKey(STR_GLOBAL))
_GlobalConfig = GlobalConfig.FromDict(Entries[STR_GLOBAL]);
else
_GlobalConfig = GlobalConfig.Default;
}
return _GlobalConfig;
}
set
{
Dirty = true;
_GlobalConfig = value;
Entries.Remove(STR_GLOBAL);
Entries.Add(STR_GLOBAL, value.ToDict());
}
}
public NewtonConfig NewtonConfig
{
get
{
if (_NewtonConfig == null)
{
if (Entries.ContainsKey("Newton"))
_NewtonConfig = NewtonConfig.FromDict(Entries["Newton"]);
else
_NewtonConfig = NewtonConfig.Default;
}
return _NewtonConfig;
}
set
{
Dirty = true;
_NewtonConfig = value;
Entries.Remove("Newton");
Entries.Add("Newton", value.ToDict());
}
}
public DomainConfig DomainConfig
{
get
{
if (_DomainConfig == null)
{
if (Entries.ContainsKey("Domain"))
_DomainConfig = DomainConfig.FromDict(Entries["Domain"]);
else
_DomainConfig = DomainConfig.Default;
}
return _DomainConfig;
}
set
{
Dirty = true;
_DomainConfig = value;
Entries.Remove("Domain");
Entries.Add("Domain", value.ToDict());
}
}
public TimeLoopConfig TimeConfig
{
get
{
if (_TimeConfig == null)
{
if (Entries.ContainsKey("Timeloop"))
_TimeConfig = TimeLoopConfig.FromDict(Entries["Timeloop"]);
else
_TimeConfig = TimeLoopConfig.Default;
}
return _TimeConfig;
}
set
{
Dirty = true;
_TimeConfig = value;
Entries.Remove("Timeloop");
Entries.Add("Timeloop", value.ToDict());
}
}
public Dictionary<string, string> this[string key]
{
get
{
if (!Entries.ContainsKey(key))
Entries[key] = new Dictionary<string, string>();
return Entries[key];
}
}
/// <summary>
/// Returns all species in the given compartment
/// </summary>
/// <param name="compId">the compartment id</param>
/// <returns>all species in that compartment</returns>
public List<string> GetSpeciesIn(string compId)
{
return VariableCompartmentMap.Where(item => item.Value == compId).Select(item2 => item2.Key).ToList();
}
/// <summary>
/// Initialize SBML mapping of species from the given SBML file
/// </summary>
/// <param name="fileName">the sbml file</param>
public void InitFromSBML(string fileName)
{
CompartmentIds = new List<string>();
VariableCompartmentMap = new Dictionary<string, string>();
var doc = libsbml.readSBMLFromFile(fileName);
var model = doc.getModel();
if (model == null) return;
CompartmentIds = new List<string>();
VariableCompartmentMap = new Dictionary<string, string>();
for (var i = 0; i < model.getNumCompartments(); ++i)
CompartmentIds.Add(model.getCompartment(i).getId());
for (var i = 0; i < model.getNumSpecies(); ++i)
{
var species = model.getSpecies(i);
VariableCompartmentMap[species.getId()] = species.getCompartment();
}
}
/// <summary>
/// Return the name of all entries that represent variables
/// </summary>
/// <returns></returns>
public List<string> GetVariableKeys()
{
var result = new List<string>(Entries.Keys);
result.Remove(STR_GLOBAL);
result.Remove("Timeloop");
result.Remove("Verbosity");
result.Remove("Newton");
result.Remove("Data");
result.Remove("Reaction");
result.Remove("Domain");
return result;
}
private void WriteSection(StringBuilder builder, string key, Dictionary<string, string> map, string basedir = null)
{
if (!string.IsNullOrWhiteSpace(key))
builder.AppendFormat("[{0}]{1}", key, Environment.NewLine);
foreach (var item in map)
{
var val = item.Value ==null ? string.Empty : item.Value.Trim();
if (!string.IsNullOrWhiteSpace(basedir) && val.StartsWith(basedir))
val = val.Replace(basedir, "");
builder.AppendFormat("{0} = {1}{2}", item.Key, val, Environment.NewLine);
}
builder.AppendLine();
}
public void SaveAs(string fileName)
{
var baseDir = Path.GetDirectoryName(fileName);
var builder = new StringBuilder();
builder.AppendLine("# Dune Config file");
builder.AppendLine(string.Format("# written: {0} {1}", DateTime.Now.ToShortDateString(), DateTime.Now.ToShortTimeString()));
builder.AppendLine();
WriteSection(builder, "", GlobalConfig.ToDict(), baseDir);
WriteSection(builder, "Newton", NewtonConfig.ToDict(), baseDir);
if (!Entries.ContainsKey("Verbosity"))
{
var tmp = new Dictionary<string, string>();
tmp["verbosity"] = "0";
tmp["Instationary"] = "0";
Entries.Add("Verbosity", tmp);
}
WriteSection(builder, "Verbosity", Entries["Verbosity"], baseDir);
WriteSection(builder, "Timeloop", TimeConfig.ToDict(), baseDir);
WriteSection(builder, "Domain", DomainConfig.ToDict(), baseDir);
WriteSection(builder, "Reaction", Entries["Reaction"], baseDir);
foreach (var item in GetVariableKeys())
WriteSection(builder, item, Entries[item], baseDir);
if (Entries.ContainsKey("Data"))
WriteSection(builder, "Data", Entries["Data"], baseDir);
File.WriteAllText(fileName, builder.ToString());
FileName = fileName;
Dirty = false;
}
public void ApplyCompartmentMasking(string compId, string fileName)
{
var ids = GetSpeciesIn(compId);
var variables = GetVariableKeys();
var main = this["Reaction"];
foreach (var id in ids)
{
if (!variables.Contains(id)) continue;
var current = this[id];
if (current == null) continue;
current["file_compartment"] = fileName;
}
if (main == null) return;
main["in_" + compId] = fileName;
Dirty = true;
}
public static DuneConfig FromFile(string filename)
{
var lines = File.ReadAllLines(filename);
var result = new DuneConfig {
FileName = filename,
VariableCompartmentMap = new Dictionary<string, string>(),
CompartmentIds = new List<string>()
};
var current = STR_GLOBAL;
var entries = new Dictionary<string, string>();
foreach (var line in lines)
{
// skip comments
var trimmed = line.Trim();
// remove comment at the end
var commentIndex = trimmed.IndexOf("#", StringComparison.Ordinal);
if (commentIndex != -1)
{
trimmed = trimmed.Substring(0, commentIndex).Trim();
}
if (trimmed.StartsWith("#")) continue;
if (trimmed.StartsWith("[") && trimmed.EndsWith("]"))
{
result.Entries.Add(current, entries);
current = trimmed.Replace("[", "").Replace("]", "");
entries = new Dictionary<string, string>();
continue;
}
var pair = trimmed.Split(new[] {'='}, StringSplitOptions.RemoveEmptyEntries);
if (pair.Length > 0)
{
var key = pair[0].Trim();
if (!string.IsNullOrWhiteSpace(key))
if (pair.Length >= 2)
entries[key] = pair[1].Trim();
else
entries[key] = "";
}
}
result.Entries.Add(current, entries);
result.InitCompartmentMasking();
return result;
}
public void ClearCompartmentMasking()
{
var variables = GetVariableKeys();
foreach (var id in variables)
{
var current = this[id];
if (current.ContainsKey("file_compartment"))
current.Remove("file_compartment");
}
var main = this["Reaction"];
if (main == null) return;
var keys = new List<string>(main.Keys);
foreach (var key in keys)
{
if (key.StartsWith("in_"))
main.Remove(key);
}
Dirty = true;
}
public void InitCompartmentMasking()
{
var sbmlFile = GlobalConfig.SBMLFile;
if (!string.IsNullOrWhiteSpace(sbmlFile))
{
var currentDir = Path.GetDirectoryName(FileName);
if (File.Exists(sbmlFile))
{
InitFromSBML(sbmlFile);
}
else if (File.Exists(Path.Combine(currentDir, sbmlFile)))
{
InitFromSBML(Path.Combine(currentDir, sbmlFile));
}
return;
}
}
}
}<file_sep>/WFEditSpatial/Templates/main.cc
// -*- tab-width: 4; indent-tabs-mode: nil -*-
/** \file
\brief Solve two-component diffusion-reaction system
with finite volume scheme. This class tests the
multi domain grid.
*/
#ifdef HAVE_CONFIG_H
#include "config.h"
#endif
#include <math.h>
#include <fstream>
#include <iostream>
#include <vector>
#include <map>
#include <string>
#include <stdlib.h>
#include <dune/common/parallel/mpihelper.hh>
#include <dune/common/exceptions.hh>
#include <dune/common/fvector.hh>
#include <dune/common/static_assert.hh>
#include <dune/common/timer.hh>
#include <dune/common/parametertreeparser.hh>
#include <dune/grid/io/file/vtk/subsamplingvtkwriter.hh>
#include <dune/grid/io/file/gmshreader.hh>
#include <dune/grid/yaspgrid.hh>
#include <dune/grid/multidomaingrid/indexsets.hh>
#include <dune/grid/multidomaingrid/subdomainset.hh>
#include <dune/grid/multidomaingrid.hh>
#include <dune/grid/common/gridinfo.hh>
#if HAVE_ALBERTA
#include <dune/grid/albertagrid.hh>
#include <dune/grid/albertagrid/dgfparser.hh>
#endif
#if HAVE_UG
#include <dune/grid/uggrid.hh>
#endif
#if HAVE_ALUGRID
#include <dune/grid/alugrid.hh>
#include <dune/grid/io/file/dgfparser/dgfalu.hh>
#include <dune/grid/io/file/dgfparser/dgfparser.hh>
#endif
#include <dune/istl/bvector.hh>
#include <dune/istl/operators.hh>
#include <dune/istl/solvers.hh>
#include <dune/istl/preconditioners.hh>
#include <dune/istl/io.hh>
#include <dune/istl/superlu.hh>
#include <dune/copasi/utilities/newton.hh>
#include <dune/copasi/utilities/newtonutilities.hh>
#include <dune/copasi/utilities/timemanager.hh>
#include <dune/copasi/utilities/datahelper.hh>
#include <dune/copasi/utilities/sbmlhelper.hh>
#include <dune/copasi/utilities/solutioncontrol.hh>
#include <dune/pdelab/common/function.hh>
#include <dune/pdelab/common/vtkexport.hh>
#include <dune/pdelab/instationary/pvdwriter.hh>
#include <dune/pdelab/backend/istl/tags.hh>
#include <dune/pdelab/backend/istl/descriptors.hh>
#include <dune/pdelab/backend/istl/bcrsmatrixbackend.hh>
#include <dune/pdelab/backend/istlvectorbackend.hh>
#include <dune/pdelab/backend/istlmatrixbackend.hh>
#include <dune/pdelab/backend/istlsolverbackend.hh>
#include <dune/pdelab/backend/seqistlsolverbackend.hh>
#include <dune/pdelab/constraints/conforming.hh>
#include <dune/pdelab/constraints/constraints.hh>
#include <dune/pdelab/ordering/orderingbase.hh>
#include <dune/pdelab/finiteelementmap/p0fem.hh>
#include <dune/pdelab/constraints/p0.hh>
#include <dune/pdelab/constraints/constraints.hh>
#include <dune/pdelab/gridoperator/gridoperator.hh>
#include <dune/pdelab/gridoperator/onestep.hh>
#include <dune/pdelab/stationary/linearproblem.hh>
#include <dune/pdelab/instationary/onestep.hh>
#include <dune/pdelab/gridfunctionspace/subspace.hh>
#include <dune/pdelab/multidomain/multidomaingridfunctionspace.hh>
#include <dune/pdelab/multidomain/coupling.hh>
#include <dune/pdelab/multidomain/subproblemlocalfunctionspace.hh>
#include <dune/pdelab/multidomain/gridoperator.hh>
#include <dune/pdelab/multidomain/subproblem.hh>
#include <dune/pdelab/constraints/conforming.hh>
#include <dune/pdelab/multidomain/constraints.hh>
#include <dune/pdelab/multidomain/interpolate.hh>
#include <dune/pdelab/multidomain/vtk.hh>
#include "componentparameters.hh"
#include "local_operator.hh"
#include "initial_conditions.hh"
#include "reactionadapter.hh"
#include <dune/copasi/utilities/solutiontimeoutput.hh>
/**
* global data helper variable, that can be used to exchange the geometry
* via config file
*/
DataHelper* dh_geometry = NULL;
double geometry_min = 10;
double geometry_max = 10;
EVENT_SUPPORT_GLOBALS(mEvents, skipOutputUntilEvent, appliedEvent);
/** \brief Control time step after reaction.
If some concentration is negative, then it returns false.
Otherwise it returns true
\tparam V Vector backend (containing DOF)
*/
template<typename V>
bool controlReactionTimeStep (V &v)
{
for (auto it=v.begin(); it!=v.end();++it)
if (*it<0) return false;
return true;
}
template<class GV>
void run (const GV& gv, Dune::ParameterTree & param)
{
const bool verbosity = param.sub("Verbosity").get<bool>("verbosity", false);
if (verbosity)
Dune::gridinfo(gv.grid());
typedef typename GV::Grid::ctype DF;
typedef double RF;
const int dim = GV::dimension;
Dune::Timer watch;
std::string timestepping = param.get<std::string>("timestepping");
// output to vtk file (true/false)
const bool graphics = param.get<bool>("writeVTK", false);
ConvergenceAdaptiveTimeStepper<RF> timemanager(param);
// for each component parameters different classes
typedef DiffusionParameter<GV,RF> DP;
%DPCREATION%
typedef Dune::PDELab::MulticomponentDiffusion<GV,RF,DP,%NUMCOMPONENTS%> VCT;
VCT vct(%DPINITIALIZATION%);
// <<<2>>> Make grid function space
typedef Dune::PDELab::P0LocalFiniteElementMap<DF,RF,dim> FEM;
FEM fem(Dune::GeometryType(Dune::GeometryType::cube,dim));
typedef Dune::PDELab::P0ParallelConstraints CON;
typedef Dune::PDELab::ISTLVectorBackend<> VBE0;
typedef Dune::PDELab::GridFunctionSpace<GV,FEM,CON,VBE0> GFS;
typedef Dune::PDELab::ISTLVectorBackend<> VBE;
//<Dune::PDELab::ISTLParameters::static_blocking,VCT::COMPONENTS> VBE;
typedef Dune::PDELab::PowerGridFunctionSpace<GFS,VCT::COMPONENTS,VBE,
Dune::PDELab::EntityBlockedOrderingTag> TPGFS;
watch.reset();
CON con;
GFS gfs(gv,fem,con);
TPGFS tpgfs(gfs);
if (verbosity) std::cout << "=== function space setup " << watch.elapsed() << " s" << std::endl;
// some informations
tpgfs.update();
if (verbosity) std::cout << "number of DOF =" << tpgfs.globalSize() << std::endl;
watch.reset();
// <<<2b>>> make subspaces for visualization
%SUBCREATION%
if (verbosity) std::cout << "=== grid function subspaces " << watch.elapsed() << " s" << std::endl;
watch.reset();
// make constraints map and initialize it from a function (boundary conditions)
typedef typename TPGFS::template ConstraintsContainer<RF>::Type CC;
CC cg;
cg.clear();
Dune::PDELab::constraints(tpgfs,cg,false);
typedef ReactionAdapter<RF> RA;
RA ra(param);
typedef Dune::PDELab::MulticomponentCCFVSpatialDiffusionOperator<VCT,RA> LOP;
LOP lop(vct,ra); //local operator including reaction adapter
typedef Dune::PDELab::MulticomponentCCFVTemporalOperator<VCT> TLOP;
TLOP tlop(vct);
typedef Dune::PDELab::istl::BCRSMatrixBackend<> MBE;
MBE mbe(27); // Maximal number of nonzeroes per row can be cross-checked by patternStatistics().
// grid operators
typedef Dune::PDELab::GridOperator<TPGFS,TPGFS,LOP,MBE,RF,RF,RF,CC,CC> CGO0;
CGO0 cgo0(tpgfs,cg,tpgfs,cg,lop,mbe);
typedef Dune::PDELab::GridOperator<TPGFS,TPGFS,TLOP,MBE,RF,RF,RF,CC,CC> CGO1;
CGO1 cgo1(tpgfs,cg,tpgfs,cg,tlop,mbe);
// one step grid operator for instationary problems
typedef Dune::PDELab::OneStepGridOperator<CGO0, CGO1, false> EIGO;
typedef Dune::PDELab::OneStepGridOperator<CGO0, CGO1, true> DIGO;
DIGO digo(cgo0, cgo1);
EIGO eigo(cgo0, cgo1);
// <<<7>>> make vector for old time step and initialize
typedef typename Dune::PDELab::BackendVectorSelector<TPGFS, RF>::Type V;
V uold(tpgfs,0.0);
V unew(tpgfs,0.0);
// initial conditions
typedef UGeneralInitial<GV, RF> UGeneralInitialType;
%INITIALTYPECREATION%
typedef Dune::PDELab::PowerGridFunction<UGeneralInitialType, %NUMCOMPONENTS%> UInitialType;
UInitialType uinitial(%INITIALARGS%); //new
//typedef Dune::PDELab::CompositeGridFunction<%INITIALTYPES%> UInitialType; //new
//UInitialType uinitial(%INITIALARGS%); //new
Dune::PDELab::interpolate(uinitial,tpgfs,uold);
unew = uold;
if (verbosity) std::cout << "=== initial conditions " << watch.elapsed() << " s" << std::endl;
// <<<5>>> Select a linear solver backend
#if HAVE_MPI
#if HAVE_SUPERLU
typedef Dune::PDELab::ISTLBackend_BCGS_AMG_SSOR<DIGO> DLS;
DLS dls(tpgfs, param.sub("Newton").get<int>("LSMaxIterations", 100), param.sub("Newton").get<int>("LinearVerbosity", 0));
#else
typedef Dune::PDELab::ISTLBackend_OVLP_BCGS_SSORk<GFS, CC> DLS;
DLS dls(tpgfs, cc, 5000, 5, param.sub("Newton").get<int>("LinearVerbosity", 0));
#endif
#else //!parallel
#if HAVE_SUPERLU
typedef Dune::PDELab::ISTLBackend_SEQ_SuperLU DLS;
DLS dls(param.sub("Newton").get<int>("LinearVerbosity", 0));
#else
typedef Dune::PDELab::ISTLBackend_SEQ_BCGS_SSOR DLS;
DLS dls(5000, param.sub("Newton").get<int>("LinearVerbosity", 0));
#endif
#endif
typedef Dune::PDELab::ISTLBackend_OVLP_ExplicitDiagonal<TPGFS> ELS;
ELS els(tpgfs);
if (verbosity) std::cout << "=== linear solvers " << watch.elapsed() << " s" << std::endl;
// <<<6>>> Solver for non-linear problem per stage
typedef Dune::PDELab::Newton<DIGO, DLS, V> CPDESOLVER;
CPDESOLVER pdesolver(digo, dls);
pdesolver.setLineSearchStrategy(CPDESOLVER::hackbuschReuskenAcceptBest); //strategy for linesearch
typedef Dune::PDELab::NewtonParameters NewtonParameters;
NewtonParameters newtonparameters(param.sub("Newton"));
newtonparameters.set(pdesolver);
typedef Dune::PDELab::OneStepMethod<RF, DIGO, CPDESOLVER, V, V> OSMI;
Dune::PDELab::Alexander2Parameter<RF> imethod;
OSMI osmi(imethod, digo, pdesolver);
// <<<7>>> time-stepper
typedef Dune::PDELab::SimpleTimeController<RF> TC;
TC tc;
typedef Dune::PDELab::ExplicitOneStepMethod<RF, EIGO, ELS, V, V, TC> OSME;
Dune::PDELab::ExplicitEulerParameter<RF> emethod;
OSME osme(emethod, eigo, els, tc);
if (verbosity) std::cout << "=== osm " << watch.elapsed() << " s" << std::endl;
Dune::PDELab::TimeSteppingMethods<RF> timesteppingmethods;
timesteppingmethods.setTimestepMethod(osme, param.get<std::string>("explicitsolver"));
timesteppingmethods.setTimestepMethod(osmi, param.get<std::string>("implicitsolver"));
osme.setVerbosityLevel(param.sub("Verbosity").get<int>("Instationary", 0));
osmi.setVerbosityLevel(param.sub("Verbosity").get<int>("Instationary", 0));
char basename[255];
sprintf(basename,"%s-%01d",param.get<std::string>("VTKname","").c_str(),param.sub("Domain").get<int>("refine"));
typedef Dune::PVDWriter<GV> PVDWriter;
PVDWriter pvdwriter(gv,basename,Dune::VTK::conforming);
// discrete grid functions
%CREATEGRIDFUNCTIONS%
if (verbosity) std::cout << "=== output " << watch.elapsed() << " s" << std::endl;
// <<<9>>> time loop
if (graphics)
pvdwriter.write(0);
if (verbosity) std::cout << "=== output0 " << watch.elapsed() << " s" << std::endl;
EVENT_SUPPORT_INITIALIZE(gv);
double dt = timemanager.getTimeStepSize();
while (!timemanager.finalize())
{
dt = timemanager.getTimeStepSize();
if (gv.comm().rank() == 0)
{
if (verbosity)
{
std::cout << "======= solve reaction problem ======= from " << timemanager.getTime() << "\n";
std::cout << "time " << timemanager.getTime() << " dt " << timemanager.getTimeStepSize() << std::endl;
}
}
watch.reset();
try
{
// do time step
if (timestepping == "explicit")
osme.apply(timemanager.getTime(), dt, uold, unew);
else
osmi.apply(timemanager.getTime(), dt, uold, unew);
if (!controlReactionTimeStep(unew))
{
timemanager.notifyFailure();
unew = uold;
continue;
}
uold = unew;
if (gv.comm().rank() == 0 && verbosity)
std::cout << "... done\n";
}
// newton linear search error
catch (Dune::PDELab::NewtonLineSearchError) {
if (gv.comm().rank() == 0 && verbosity)
std::cout << "Newton Linesearch Error" << std::endl;
timemanager.notifyFailure();
unew = uold;
if (!verbosity)
{
std::cout << "x" << std::flush;
}
continue;
}
catch (Dune::PDELab::NewtonNotConverged) {
if (gv.comm().rank() == 0 && verbosity)
std::cout << "Newton Convergence Error" << std::endl;
timemanager.notifyFailure();
unew = uold;
if (!verbosity)
{
std::cout << "x" << std::flush;
}
continue;
}
catch (Dune::PDELab::NewtonLinearSolverError) {
if (gv.comm().rank() == 0 && verbosity )
std::cout << "Newton Linear Solver Error" << std::endl;
timemanager.notifyFailure();
unew = uold;
if (!verbosity)
{
std::cout << "x" << std::flush;
}
continue;
}
catch (Dune::ISTLError) {
if (gv.comm().rank() == 0 && verbosity )
std::cout << "ISTL Error" << std::endl;
timemanager.notifyFailure();
unew = uold;
if (!verbosity)
{
std::cout << "x" << std::flush;
}
continue;
}
if (gv.comm().rank() == 0 && verbosity)
std::cout << "... took : " << watch.elapsed() << " s" << std::endl;
// notify success in this timestep
timemanager.notifySuccess(5);
EVENT_SUPPORT_HANDLE_EVENTS(timemanager.getTime(), %NUMCOMPONENTS%, mEvents, uold, unew);
if (graphics && timemanager.isTimeForOutput())
{
if ((skipOutputUntilEvent && appliedEvent) || !skipOutputUntilEvent)
{
pvdwriter.write(timemanager.getTime());
if (!verbosity)
std::cout << "o" << std::flush;
}
else if (!verbosity)
{
std::cout << "n" << std::flush;
}
}
else if (!verbosity)
{
std::cout << "." << std::flush;
}
}
std::cout << std::endl;
}
bool isInside(const Dune::FieldVector<double, 2>& point, const Dune::FieldVector<double, 2>& dimension)
{
const auto& x = point[0];
const auto& y = point[1];
const auto& width = dimension[0];
const auto& height = dimension[1];
if (dh_geometry != NULL)
{
double val = (*dh_geometry).get((double)x, (double)y);
bool inside = val >= (geometry_min - 1e-5) && val <= (geometry_max + 1e-5);
return inside;
}
%GEOMETRY%
}
//===============================================================
// Main program with grid setup
//===============================================================
int main(int argc, char** argv)
{
try{
//Maybe initialize Mpi
Dune::MPIHelper& helper = Dune::MPIHelper::instance(argc, argv);
std::string configfile = argv[0]; configfile += ".conf";
// parse cmd line parameters
if (argc > 1 && argv[1][0] != '-')
configfile = argv[1];
for (int i = 1; i < argc; i++)
{
if (std::string(argv[i]) == "--help" || std::string(argv[i]) == "-h")
{
if(helper.rank()==0)
std::cout << "usage: ./%NAME% <configfile> [OPTIONS]" << std::endl;
return 0;
}
}
// read parameters from file
Dune::ParameterTree param;
Dune::ParameterTreeParser parser;
parser.readINITree(configfile, param);
EVENT_SUPPORT_READ_DATA(mEvents, parser, "eventinfo.ini", skipOutputUntilEvent);
int dim=param.sub("Domain").get<int>("dim", 2);
dh_geometry = DataHelper::forFile(param.sub("Domain").template get<std::string>("geometry", ""));
geometry_min = param.sub("Domain").template get<double>("geometry_min", 0);
geometry_max = param.sub("Domain").template get<double>("geometry_max", 0);
if (dim==2)
{
// make grid
Dune::FieldVector<double,2> L;
L[0] = param.get<double>("Domain.height");
L[1] = param.get<double>("Domain.width");
std::array<int,2> N;
N[0] = param.get<int>("Domain.nx");
N[1] = param.get<int>("Domain.ny");
std::bitset<2> periodic(false);
int overlap = param.get<int>("overlap", 0);
typedef Dune::YaspGrid<2> HostGrid;
HostGrid hostgrid(helper.getCommunicator(),L,N,periodic,overlap);
hostgrid.globalRefine(param.get<int>("Domain.refine",0));
typedef Dune::MultiDomainGrid<HostGrid,Dune::mdgrid::ArrayBasedTraits<2,8,8> > MDGrid;
MDGrid mdgrid(hostgrid,true);
typedef typename MDGrid::LeafGridView MDGV;
typedef typename MDGV::template Codim<0>::Iterator Iterator;
typedef typename MDGrid::SubDomainIndexType SubDomainIndexType;
MDGV mdgv = mdgrid.leafGridView();
mdgrid.startSubDomainMarking();
for(Iterator it = mdgv.template begin<0>(); it != mdgv.template end<0>(); ++it)
{
SubDomainIndexType subdomain = 0;
mdgrid.removeFromAllSubDomains(*it);
// figure out, whether it is part of the
// region, and if so add it like so:
const auto& center = it->geometry().center();
if (isInside(center, L))
{
mdgrid.addToSubDomain(subdomain,*it);
}
}
mdgrid.preUpdateSubDomains();
mdgrid.updateSubDomains();
mdgrid.postUpdateSubDomains();
typedef MDGrid::SubDomainGrid SDGrid;
const SDGrid& sdgrid = mdgrid.subDomain(0);
SDGrid::LeafGridView sdgv = sdgrid.leafGridView();
// solve problem
run(sdgv,param);
}
}
catch (Dune::Exception &e){
std::cerr << "Dune reported error: " << e << std::endl;
return 1;
}
//catch (...){
// std::cerr << "Unknown exception thrown!" << std::endl;
// return 1;
//}
}
<file_sep>/WFEditSpatial/Converter/MorpheusConverter.cs
using System;
using System.Collections.Generic;
using System.Drawing.Imaging;
using System.Globalization;
using System.IO;
using System.Linq;
using System.Text;
using System.Xml;
using EditSpatial.Model;
using libsbmlcs;
namespace EditSpatial.Converter
{
public class MorpheusConverter : IDisposable
{
private readonly Dictionary<string, string> boundaryConditions;
private readonly Dictionary<string, string> boundaryLables;
private readonly Dictionary<string, Dictionary<string, string>> boundaryValue;
private readonly Dictionary<string, string> coordinates;
private readonly Dictionary<string, string> diffusion;
private readonly Dimensions dims;
private readonly SBMLDocument document;
private readonly StringBuilder errorBuilder;
private readonly Dictionary<string, string> initial;
private readonly libsbmlcs.Model Model;
private readonly int numVariables;
public MorpheusConverter(SBMLDocument original)
{
errorBuilder = new StringBuilder();
document = original.clone();
numVariables = 0;
document.getErrorLog().setSeverityOverride(libsbml.LIBSBML_OVERRIDE_DONT_LOG);
var prop = new ConversionProperties();
prop.addOption("replaceReactions", true,
"Replace reactions with rateRules");
var status = document.convert(prop);
if (status != libsbml.LIBSBML_OPERATION_SUCCESS)
{
errorBuilder.AppendFormat("conversion of rates failed: {0}{1}", status, Environment.NewLine);
}
prop = new ConversionProperties();
prop.addOption("expandFunctionDefinitions", true);
status = document.convert(prop);
if (status != libsbml.LIBSBML_OPERATION_SUCCESS)
{
errorBuilder.AppendFormat("expanding function definitions failed: {0}{1}", status, Environment.NewLine);
}
Model = document.getModel();
boundaryLables = new Dictionary<string, string>();
coordinates = new Dictionary<string, string>();
for (var i = 0; i < Geometry.getNumCoordinateComponents(); ++i)
{
var current = Geometry.getCoordinateComponent(i);
var prefix = "x";
if (current.getType() == libsbml.SPATIAL_COORDINATEKIND_CARTESIAN_X)
prefix = "x";
else if (current.getType() == libsbml.SPATIAL_COORDINATEKIND_CARTESIAN_Y)
prefix = "y";
else if (current.getType() == libsbml.SPATIAL_COORDINATEKIND_CARTESIAN_Z)
prefix = "z";
coordinates[current.getId()] = prefix;
boundaryLables[current.getBoundaryMin().getId()] = "-" + prefix;
boundaryLables[current.getBoundaryMax().getId()] = prefix;
}
dims = new Dimensions();
dims.setWidth(GetBoundaryMaxValue(0));
dims.setHeight(GetBoundaryMaxValue(1));
dims.setDepth(GetBoundaryMaxValue(2));
boundaryConditions = new Dictionary<string, string>();
diffusion = new Dictionary<string, string>();
for (var i = 0; i < Model.getNumParameters(); ++i)
{
var current = Model.getParameter(i);
var plugin = (SpatialParameterPlugin) current.getPlugin("spatial");
if (plugin == null) continue;
if (plugin.getType() == libsbml.SBML_SPATIAL_BOUNDARYCONDITION)
{
var condition = plugin.getBoundaryCondition();
if (condition != null)
boundaryConditions[condition.getCoordinateBoundary()] = TranslateCondition(condition.getType());
}
if (plugin.getType() == libsbml.SBML_SPATIAL_DIFFUSIONCOEFFICIENT)
{
var diff = plugin.getDiffusionCoefficient();
if (diff != null)
diffusion[diff.getVariable()] = current.getValue().ToString(CultureInfo.InvariantCulture);
}
}
boundaryValue = new Dictionary<string, Dictionary<string, string>>();
initial = new Dictionary<string, string>();
for (var i = 0; i < Model.getNumSpecies(); ++i)
{
var current = Model.getSpecies(i);
var plugin = (SpatialSpeciesPlugin) current.getPlugin("spatial");
if (plugin == null || !plugin.getIsSpatial()) continue;
++numVariables;
boundaryValue[current.getId()] = new Dictionary<string, string>();
var bcValue = current.getXMinBC();
if (bcValue.HasValue && Math.Abs(bcValue.Value) > 1e-16)
boundaryValue[current.getId()]["-x"] = bcValue.Value.ToString(CultureInfo.InvariantCulture);
bcValue = current.getXMaxBC();
if (bcValue.HasValue && Math.Abs(bcValue.Value) > 1e-16)
boundaryValue[current.getId()]["x"] = bcValue.Value.ToString(CultureInfo.InvariantCulture);
bcValue = current.getYMinBC();
if (bcValue.HasValue && Math.Abs(bcValue.Value) > 1e-16)
boundaryValue[current.getId()]["-y"] = bcValue.Value.ToString(CultureInfo.InvariantCulture);
bcValue = current.getYMaxBC();
if (bcValue.HasValue && Math.Abs(bcValue.Value) > 1e-16)
boundaryValue[current.getId()]["y"] = bcValue.Value.ToString(CultureInfo.InvariantCulture);
if (current.isSetInitialConcentration())
initial[current.getId()] = current.getInitialConcentration().ToString(CultureInfo.InvariantCulture);
else if (current.isSetInitialAmount())
initial[current.getId()] = current.getInitialAmount().ToString(CultureInfo.InvariantCulture);
var assignment = Model.getInitialAssignment(current.getId());
if (assignment == null) continue;
initial[current.getId()] = TranslateExpression(assignment.getMath(), coordinates);
}
}
public Geometry Geometry
{
get
{
var plugin = (SpatialModelPlugin) Model.getPlugin("spatial");
if (plugin == null) return null;
return plugin.getGeometry();
}
}
public void Dispose()
{
if (document != null)
document.Dispose();
if (Model != null)
Model.Dispose();
if (dims != null)
dims.Dispose();
}
public static string TranslateExpression(string expression)
{
return TranslateExpression(libsbml.parseL3Formula(expression), null);
}
public static string TranslateExpression(ASTNode math, Dictionary<string, string> map)
{
if (math == null) return "";
switch (math.getType())
{
case libsbml.AST_INTEGER:
return math.getInteger().ToString();
case libsbml.AST_REAL:
case libsbml.AST_REAL_E:
return math.getReal().ToString(CultureInfo.InvariantCulture);
case libsbml.AST_DIVIDE:
{
var builder = new StringBuilder();
builder.AppendMorpheusNode("{0}", math.getChild(0), map);
builder.AppendMorpheusNode(" / {0}", math.getChild(1), map);
return builder.ToString();
}
case libsbml.AST_MINUS:
{
var builder = new StringBuilder();
if (math.getNumChildren() == 1)
{
builder.AppendMorpheusNode(" - {0}", math.getChild(0), map);
}
else
{
builder.AppendMorpheusNode("{0}", math.getChild(0), map);
builder.AppendMorpheusNode(" - {0}", math.getChild(1), map);
}
return builder.ToString();
}
case libsbml.AST_PLUS:
{
var builder = new StringBuilder();
builder.AppendMorpheusNode("{0}", math.getChild(0), map);
for (var i = 1; i < math.getNumChildren(); ++i)
{
builder.AppendMorpheusNode(" + {0}", math.getChild(i), map);
}
return builder.ToString();
}
case libsbml.AST_TIMES:
{
var builder = new StringBuilder();
builder.AppendMorpheusNode("{0}", math.getChild(0), map);
for (var i = 1; i < math.getNumChildren(); ++i)
{
builder.AppendMorpheusNode(" * {0}", math.getChild(i), map);
}
return builder.ToString();
}
case libsbml.AST_RELATIONAL_LEQ:
{
var builder = new StringBuilder();
builder.AppendMorpheusNode("{0}", math.getChild(0), map);
builder.AppendMorpheusNode(" <= {0}", math.getChild(1), map);
return builder.ToString();
}
case libsbml.AST_RELATIONAL_LT:
{
var builder = new StringBuilder();
builder.AppendMorpheusNode("{0}", math.getChild(0), map);
builder.AppendMorpheusNode(" < {0}", math.getChild(1), map);
return builder.ToString();
}
case libsbml.AST_RELATIONAL_GT:
{
var builder = new StringBuilder();
builder.AppendMorpheusNode("{0}", math.getChild(0), map);
builder.AppendMorpheusNode(" > {0}", math.getChild(1), map);
return builder.ToString();
}
case libsbml.AST_RELATIONAL_GEQ:
{
var builder = new StringBuilder();
builder.AppendMorpheusNode("{0}", math.getChild(0), map);
builder.AppendMorpheusNode(" >= {0}", math.getChild(1), map);
return builder.ToString();
}
case libsbml.AST_LOGICAL_AND:
{
var builder = new StringBuilder();
builder.AppendMorpheusNode("{0}", math.getChild(0), map);
for (var i = 1; i < math.getNumChildren(); ++i)
{
builder.AppendMorpheusNode(" and {0}", math.getChild(i), map);
}
return builder.ToString();
}
case libsbml.AST_LOGICAL_OR:
{
var builder = new StringBuilder();
builder.AppendMorpheusNode("{0}", math.getChild(0), map);
for (var i = 1; i < math.getNumChildren(); ++i)
{
builder.AppendMorpheusNode(" or {0}", math.getChild(i), map);
}
return builder.ToString();
}
case libsbml.AST_FUNCTION_PIECEWISE:
{
var builder = new StringBuilder();
builder.AppendFormat("if(");
for (var i = 0; i < math.getNumChildren() - 1; i += 2)
{
builder.AppendMorpheusNode("{0}", math.getChild(i + 1), map);
builder.AppendMorpheusNode(", {0}", math.getChild(i), map);
}
builder.AppendMorpheusNode(", {0}", math.getChild(math.getNumChildren() - 1), map);
builder.AppendFormat(")");
return builder.ToString();
}
case libsbml.AST_FUNCTION:
{
var builder = new StringBuilder();
builder.AppendFormat("{0}(", math.getName());
builder.AppendFormat("{0}", TranslateExpression(math.getChild(0), map));
for (var i = 1; i < math.getNumChildren(); ++i)
{
builder.AppendFormat(", {0}", TranslateExpression(math.getChild(i), map));
}
builder.AppendFormat(")");
return builder.ToString();
}
case libsbml.AST_FUNCTION_POWER:
case libsbml.AST_POWER:
{
var builder = new StringBuilder();
builder.AppendFormat("pow({0}", TranslateExpression(math.getChild(0), map));
builder.AppendFormat(", {0})", TranslateExpression(math.getChild(1), map));
return builder.ToString();
}
//case libsbml.AST_NAME:
default:
if (map != null && map.ContainsKey(math.getName()))
{
return "l." + map[math.getName()];
}
return math.getName();
}
}
private string TranslateCondition(string type)
{
switch (type.ToLowerInvariant())
{
default:
//case "flux":
return "constant";
case "value":
return "noflux";
}
}
private string TranslateCondition(int type)
{
switch (type)
{
default:
case libsbml.SPATIAL_BOUNDARYKIND_NEUMANN:
//case "flux":
return "constant";
case libsbml.SPATIAL_BOUNDARYKIND_DIRICHLET:
return "noflux";
}
}
public string ToMorpheus(string filename = null)
{
var settings = new XmlWriterSettings {Indent = true, Encoding = Encoding.UTF8, OmitXmlDeclaration = true};
var buffer = new StringBuilder();
var writer = XmlWriter.Create(buffer, settings);
writer.WriteStartDocument();
writer.WriteStartElement("MorpheusModel");
writer.WriteAttributeString("version", "1");
WriteDescription(writer);
WriteSpace(writer, filename);
WriteTime(writer);
WritePDE(writer);
WriteAnalysis(writer);
writer.WriteEndElement(); // MorpheusModel
writer.WriteEndDocument();
writer.Flush();
writer.Close();
return buffer.ToString();
}
private void WriteAnalysis(XmlWriter writer)
{
var multiplier = Math.Max(1, numVariables/2);
const double scale = 4;
writer.WriteStartElement("Analysis");
writer.WriteStartElement("Gnuplotter");
writer.WriteAttributeString("interval", "1");
writer.WriteAttributeString("timename", "false");
writer.WriteStartElement("Terminal");
writer.WriteAttributeString("size",
string.Format("{0} {1} {2}",
Math.Max(200*multiplier, scale*dims.getWidth()*multiplier),
Math.Max(200*multiplier, scale*dims.getHeight()*multiplier),
scale*dims.getDepth())
);
writer.WriteAttributeString("name", "png");
writer.WriteEndElement(); // Terminal
for (var i = 0; i < Model.getNumSpecies(); i++)
{
var current = Model.getSpecies(i);
var plugin = (SpatialSpeciesPlugin) current.getPlugin("spatial");
if (plugin == null) continue;
if (plugin.getIsSpatial())
{
writer.WriteStartElement("PDE");
writer.WriteAttributeString("symbol-ref", current.getId());
writer.WriteAttributeString("min", "0");
writer.WriteEndElement(); // PDE
}
}
writer.WriteEndElement(); // Gnuplotter
writer.WriteEndElement(); // Analysis
}
private double GetBoundaryMaxValue(int val, double defaultValue = 0)
{
if (val >= Geometry.getNumCoordinateComponents()) return defaultValue;
try
{
return Geometry.getCoordinateComponent(val).getBoundaryMax().getValue();
}
catch
{
return defaultValue;
}
}
private void WritePDE(XmlWriter writer)
{
writer.WriteStartElement("PDE");
for (var i = 0; i < Model.getNumSpecies(); i++)
{
var current = Model.getSpecies(i);
var plugin = (SpatialSpeciesPlugin) current.getPlugin("spatial");
if (plugin == null) continue;
if (!plugin.getIsSpatial()) continue;
writer.WriteStartElement("Layer");
writer.WriteAttributeString("symbol", current.getId());
writer.WriteAttributeString("name", current.isSetName() ? current.getName() : current.getId());
writer.WriteStartElement("Diffusion");
writer.WriteAttributeString("rate",
diffusion.ContainsKey(current.getId()) ? diffusion[current.getId()] : "0");
writer.WriteEndElement(); // Diffusion
writer.WriteStartElement("Initial");
writer.WriteStartElement("InitPDEExpression");
writer.WriteStartElement("Expression");
writer.WriteString(initial[current.getId()]);
writer.WriteEndElement(); // Expression
writer.WriteEndElement(); // InitPDEExpression
writer.WriteEndElement(); // Initial
if (boundaryValue.ContainsKey(current.getId()))
{
var bounds = boundaryValue[current.getId()];
foreach (var item in bounds)
{
writer.WriteStartElement("BoundaryValue");
writer.WriteAttributeString("boundary", item.Key);
writer.WriteAttributeString("value", item.Value);
writer.WriteEndElement(); // BoundaryValue
}
}
writer.WriteEndElement(); // Layer
}
WriteSystem(writer);
writer.WriteEndElement(); // PDE
}
private string SimplifyExpression(string expression)
{
var result = expression.Replace("+ ( - 1) *", "-");
result = result.Replace(" + - ", " - ");
result = result.Replace(" 1 * ", " ");
result = result.Replace(" * 1 ", " ");
if (result.StartsWith("1 * "))
result = result.Substring("1 * ".Length);
return result;
}
private void WriteSystem(XmlWriter writer)
{
writer.WriteStartElement("System");
writer.WriteAttributeString("solver", "runge-kutta");
writer.WriteAttributeString("time-step", "0.01");
writer.WriteAttributeString("name", Model.isSetName() ? Model.getName() : Model.getId());
for (var i = 0; i < Model.getNumCompartments(); ++i)
{
var current = Model.getCompartment(i);
writer.WriteStartElement("Constant");
writer.WriteAttributeString("symbol", current.getId());
writer.WriteAttributeString("value", current.getSize().ToString(CultureInfo.InvariantCulture));
writer.WriteEndElement(); // Constant
}
for (var i = 0; i < Model.getNumParameters(); ++i)
{
var current = Model.getParameter(i);
var plugin = (SpatialParameterPlugin) current.getPlugin("spatial");
if (plugin != null && plugin.getType() != -1) continue;
writer.WriteStartElement("Constant");
writer.WriteAttributeString("symbol", current.getId());
writer.WriteAttributeString("value", current.getValue().ToString(CultureInfo.InvariantCulture));
writer.WriteEndElement(); // Constant
}
for (var i = 0; i < Model.getNumRules(); ++i)
{
var current = Model.getRule(i);
if (current.isRate())
{
writer.WriteStartElement("DiffEqn");
writer.WriteAttributeString("symbol-ref", current.getVariable());
writer.WriteStartElement("Expression");
writer.WriteString(SimplifyExpression(TranslateExpression(current.getMath(), coordinates)));
writer.WriteEndElement(); // Expression
writer.WriteEndElement(); // DiffEqn
}
}
writer.WriteEndElement(); // System
}
private void WriteTime(XmlWriter writer)
{
writer.WriteStartElement("Time");
writer.WriteStartElement("StartTime");
writer.WriteAttributeString("unit", "sec");
writer.WriteAttributeString("value", "0");
writer.WriteEndElement(); // StartTime
writer.WriteStartElement("StopTime");
writer.WriteAttributeString("unit", "sec");
writer.WriteAttributeString("value", (60*60*24).ToString());
writer.WriteEndElement(); // StopTime
writer.WriteStartElement("SaveInterval");
writer.WriteAttributeString("value", "0");
writer.WriteEndElement(); // SaveInterval
writer.WriteStartElement("RandomSeed");
writer.WriteAttributeString("value", "2");
writer.WriteEndElement(); // RandomSeed
writer.WriteEndElement(); // Time
}
private void WriteSpace(XmlWriter writer, string filename = null)
{
writer.WriteStartElement("Space");
writer.WriteStartElement("Lattice");
writer.WriteAttributeString("class", "square");
writer.WriteStartElement("Size");
writer.WriteAttributeString("symbol", "size");
writer.WriteAttributeString("value",
string.Format("{0} {1} {2}",
dims.getWidth(),
dims.getHeight(),
dims.getDepth())
);
writer.WriteEndElement(); // Size
writer.WriteStartElement("NodeLength");
writer.WriteAttributeString("value", "1");
writer.WriteEndElement(); // NodeLength
writer.WriteStartElement("BoundaryConditions");
foreach (var entry in boundaryConditions)
{
writer.WriteStartElement("Condition");
writer.WriteAttributeString("boundary", boundaryLables[entry.Key]);
writer.WriteAttributeString("type", entry.Value);
writer.WriteEndElement(); // Condition
}
writer.WriteEndElement(); // BoundaryConditions
// write domain (if analytic)
var analyticGeometry = Geometry.GetFirstAnalyticGeometry();
if (filename != null && analyticGeometry != null && analyticGeometry.getNumAnalyticVolumes() > 0)
{
var path = Path.GetDirectoryName(filename);
var name = Path.GetFileNameWithoutExtension(filename);
if (!string.IsNullOrWhiteSpace(path) && !string.IsNullOrWhiteSpace(name))
{
var vol = analyticGeometry.getAnalyticVolume(0);
writer.WriteStartElement("Domain");
writer.WriteAttributeString("boundary-type", boundaryConditions.Values.First());
writer.WriteStartElement("Image");
var image = analyticGeometry.GenerateTiffForOrdinal(Geometry, (int) vol.getOrdinal());
image.Save(Path.Combine(path, name + ".tif"), ImageFormat.Tiff);
writer.WriteAttributeString("path", name + ".tif");
writer.WriteEndElement(); // Image
writer.WriteEndElement(); // Domain
}
}
writer.WriteEndElement(); // Lattice
writer.WriteStartElement("SpaceSymbol");
writer.WriteAttributeString("symbol", "l");
writer.WriteAttributeString("name", "location");
writer.WriteEndElement(); // SpaceSymbol
writer.WriteEndElement(); // Space
}
private void WriteDescription(XmlWriter writer)
{
writer.WriteStartElement("Description");
writer.WriteStartElement("Title");
if (Model.isSetName())
writer.WriteString(Model.getName());
else
writer.WriteString(Model.getId());
writer.WriteEndElement(); // Title
writer.WriteStartElement("Details");
var errorString = errorBuilder.ToString();
if (!string.IsNullOrWhiteSpace(errorString))
writer.WriteString("Conversion messages: " + errorString);
writer.WriteEndElement(); // Details
writer.WriteEndElement(); // Description
}
public string ToSBML()
{
return libsbml.writeSBMLToString(document);
}
}
}<file_sep>/LibEditSpatial/Model/DomainConfig.cs
using System.Collections.Generic;
namespace LibEditSpatial.Model
{
public class DomainConfig
{
public string Geometry { get; set; }
public double GeometryMin { get; set; }
public double GeometryMax { get; set; }
public int Dimensions { get; set; }
public double Width { get; set; }
public double Height { get; set; }
public double Depth { get; set; }
public int GridX { get; set; }
public int GridY { get; set; }
public int GridZ { get; set; }
public int Refinement { get; set; }
public static DomainConfig Default
{
get
{
return new DomainConfig
{
Width = 100,
Height = 100,
Depth = 1,
GridX = 64,
GridY = 64,
GridZ = 1,
Refinement = 0
};
}
}
public static DomainConfig FromDict(Dictionary<string, string> dict)
{
var result = new DomainConfig
{
Geometry = dict.Get<string>("geometry"),
GeometryMin = dict.Get<double>("geometry_min"),
GeometryMax = dict.Get<double>("geometry_max"),
Dimensions = dict.Get<int>("dim"),
Width = dict.Get<double>("width"),
Height = dict.Get<double>("height"),
Depth = dict.Get<double>("depth"),
GridX = dict.Get<int>("nx"),
GridY = dict.Get<int>("ny"),
GridZ = dict.Get<int>("nz"),
Refinement = dict.Get<int>("refine")
};
return result;
}
public Dictionary<string, string> ToDict()
{
var result = new Dictionary<string, string>();
result["geometry"] = Geometry;
result["geometry_min"] = GeometryMin.ToString();
result["geometry_max"] = GeometryMax.ToString();
result["dim"] = Dimensions.ToString();
result["width"] = Width.ToString();
result["height"] = Height.ToString();
result["depth"] = Depth.ToString();
result["nx"] = GridX.ToString();
result["ny"] = GridY.ToString();
result["nz"] = GridZ.ToString();
result["refine"] = Refinement.ToString();
return result;
}
}
}<file_sep>/WFEditSpatial/Converter/DuneConverter.cs
using System;
using System.Collections.Generic;
using System.Globalization;
using System.IO;
using System.Text;
using EditSpatial.Model;
using EditSpatial.Properties;
using libsbmlcs;
namespace EditSpatial.Converter
{
public class DuneConverter : IDisposable
{
private readonly SBMLDocument _Document;
private readonly StringBuilder _errorBuilder;
private readonly Geometry _Geometry;
private readonly libsbmlcs.Model _Model;
private readonly SBMLDocument _Original;
private readonly SpatialModelPlugin SpatialModelPlugin;
private List<string> AdditionalVars;
/// <summary>
/// This function tags all reactions in the document with a factor that indicates whether
/// it is spanning multiple compartments.
/// </summary>
/// <param name="document"></param>
private void TagReactions(SBMLDocument document)
{
AdditionalVars = new List<string>();
var model = document.getModel();
if (model == null) return;
if (model.getNumCompartments() < 2) return;
var numReactions = model.getNumReactions();
for (int i = 0; i < numReactions; ++i)
{
var compartments = new List<string>();
var reaction = model.getReaction(i);
if (!reaction.isSetKineticLaw()) continue;
var kinetics = reaction.getKineticLaw();
if (kinetics == null || !kinetics.isSetMath()) continue;
var numSubstrates = reaction.getNumReactants();
var numProducts = reaction.getNumProducts();
var numModifiers = reaction.getNumModifiers();
if (numSubstrates + numProducts < 2) continue;
for (int j = 0; j < numSubstrates; ++j)
{
var current = reaction.getReactant(j);
if (!current.isSetSpecies()) continue;
var species = model.getSpecies(current.getSpecies());
if (species == null || !species.isSetCompartment()) continue;
string compartment = species.getCompartment();
if (!compartments.Contains(compartment))
{
compartments.Add(compartment);
}
}
for (int j = 0; j < numProducts; ++j)
{
var current = reaction.getProduct(j);
if (!current.isSetSpecies()) continue;
var species = model.getSpecies(current.getSpecies());
if (species == null || !species.isSetCompartment()) continue;
string compartment = species.getCompartment();
if (!compartments.Contains(compartment))
{
compartments.Add(compartment);
}
}
for (int j = 0; j < numModifiers; ++j)
{
var current = reaction.getModifier(j);
if (!current.isSetSpecies()) continue;
var species = model.getSpecies(current.getSpecies());
if (species == null || !species.isSetCompartment()) continue;
string compartment = species.getCompartment();
if (!compartments.Contains(compartment))
{
compartments.Add(compartment);
}
}
if (compartments.Count < 2) continue;
compartments.Sort();
var formula = new StringBuilder();
foreach (var id in compartments)
{
formula.AppendFormat("isIn_{0} * ", id);
if (!AdditionalVars.Contains("isIn_" + id))
{
// create parameter temporarily to get passt validation later on
var param = model.createParameter();
param.setId("isIn_" + id);
AdditionalVars.Add("isIn_" + id);
}
}
formula.AppendFormat("({0})", libsbml.formulaToL3String(kinetics.getMath()));
kinetics.setMath(libsbml.parseL3Formula(formula.ToString()));
}
}
public DuneConverter(SBMLDocument original)
{
OdeVariables = new List<string>();
_errorBuilder = new StringBuilder();
_Document = original.clone();
_Original = original;
TagReactions(_Document);
_Document.getErrorLog().setSeverityOverride(libsbml.LIBSBML_OVERRIDE_DONT_LOG);
var prop = new ConversionProperties();
prop.addOption("promoteLocalParameters", true,
"Promotes all Local Parameters to Global ones");
var status = _Document.convert(prop);
if (status != libsbml.LIBSBML_OPERATION_SUCCESS)
{
_errorBuilder.AppendFormat("promoting local to global parameters failed: {0}{1}", status, Environment.NewLine);
}
prop = new ConversionProperties();
prop.addOption("expandFunctionDefinitions", true);
status = _Document.convert(prop);
if (status != libsbml.LIBSBML_OPERATION_SUCCESS)
{
_errorBuilder.AppendFormat("expanding function definitions failed: {0}{1}", status, Environment.NewLine);
}
prop = new ConversionProperties();
prop.addOption("replaceReactions", true,
"Replace reactions with rateRules");
status = _Document.convert(prop);
if (status != libsbml.LIBSBML_OPERATION_SUCCESS)
{
_errorBuilder.AppendFormat("conversion of rates failed: {0}{1}", status, Environment.NewLine);
}
prop = new ConversionProperties();
prop.addOption("renameSIds", true);
prop.addOption("currentIds", "default");
prop.addOption("newIds", "__default");
status = _Document.convert(prop);
if (status != libsbml.LIBSBML_OPERATION_SUCCESS)
{
_errorBuilder.AppendFormat("rename of ids failed: {0}{1}", status, Environment.NewLine);
}
_Model = _Document.getModel();
// remove parameters added only to pass validation
foreach (var id in AdditionalVars)
{
_Model.removeParameter(id);
}
for (var i = 0; i < _Model.getNumRules(); ++i)
{
var current = _Model.getRule(i);
if (current == null || current.getTypeCode() != libsbml.SBML_RATE_RULE) continue;
OdeVariables.Add(current.getVariable());
}
SpatialModelPlugin = (SpatialModelPlugin) _Model.getPlugin("spatial");
if (SpatialModelPlugin == null) return;
_Geometry = SpatialModelPlugin.getGeometry();
}
public string Errors
{
get
{
if (_errorBuilder == null) return "";
return _errorBuilder.ToString();
}
}
public double GeometryMin { get; set; }
public double GeometryMax { get; set; }
private List<string> OdeVariables { get; set; }
private List<string> ParameterIds { get; set; }
public string GeometryFile { get; set; }
public void Dispose()
{
if (_Document != null)
_Document.Dispose();
if (_Model != null)
_Model.Dispose();
if (SpatialModelPlugin != null)
SpatialModelPlugin.Dispose();
if (_Geometry != null)
_Geometry.Dispose();
}
public static string TranslateExpression(string expression, Dictionary<string, string> map = null,
libsbmlcs.Model model = null)
{
return TranslateExpression(libsbml.parseL3Formula(expression), map, model);
}
public static string TranslateExpression(ASTNode math, Dictionary<string, string> map = null,
libsbmlcs.Model model = null)
{
if (math == null) return "";
switch (math.getType())
{
case libsbml.AST_INTEGER:
return math.getInteger().ToString("E17");
case libsbml.AST_REAL:
case libsbml.AST_REAL_E:
return math.getReal().ToString("E17");
case libsbml.AST_FUNCTION_POWER:
{
{
var builder = new StringBuilder();
builder.AppendDuneNode("pow({0}", math.getChild(0), map, model);
builder.AppendDuneNode(", {0})", math.getChild(1), map, model);
return builder.ToString();
}
}
case libsbml.AST_DIVIDE:
{
var builder = new StringBuilder();
builder.AppendDuneNode("{0}", math.getChild(0), map, model);
builder.AppendDuneNode(" / {0}", math.getChild(1), map, model);
return builder.ToString();
}
case libsbml.AST_MINUS:
{
var builder = new StringBuilder();
if (math.getNumChildren() == 1)
{
builder.AppendDuneNode(" - {0}", math.getChild(0), map, model);
}
else
{
builder.AppendDuneNode("{0}", math.getChild(0), map, model);
builder.AppendDuneNode(" - {0}", math.getChild(1), map, model);
}
return builder.ToString();
}
case libsbml.AST_PLUS:
{
var builder = new StringBuilder();
builder.AppendDuneNode("{0}", math.getChild(0), map, model);
for (var i = 1; i < math.getNumChildren(); ++i)
{
builder.AppendDuneNode(" + {0}", math.getChild(i), map, model);
}
return builder.ToString();
}
case libsbml.AST_TIMES:
{
var builder = new StringBuilder();
builder.AppendDuneNode("{0}", math.getChild(0), map, model);
for (var i = 1; i < math.getNumChildren(); ++i)
{
builder.AppendDuneNode(" * {0}", math.getChild(i), map, model);
}
return builder.ToString();
}
case libsbml.AST_RELATIONAL_LEQ:
{
var builder = new StringBuilder();
builder.AppendDuneNode("{0}", math.getChild(0), map, model);
builder.AppendDuneNode(" <= {0}", math.getChild(1), map, model);
return builder.ToString();
}
case libsbml.AST_RELATIONAL_LT:
{
var builder = new StringBuilder();
builder.AppendDuneNode("{0}", math.getChild(0), map, model);
builder.AppendDuneNode(" < {0}", math.getChild(1), map, model);
return builder.ToString();
}
case libsbml.AST_RELATIONAL_GT:
{
var builder = new StringBuilder();
builder.AppendDuneNode("{0}", math.getChild(0), map, model);
builder.AppendDuneNode(" > {0}", math.getChild(1), map, model);
return builder.ToString();
}
case libsbml.AST_RELATIONAL_GEQ:
{
var builder = new StringBuilder();
builder.AppendDuneNode("{0}", math.getChild(0), map, model);
builder.AppendDuneNode(" >= {0}", math.getChild(1), map, model);
return builder.ToString();
}
case libsbml.AST_POWER:
{
var builder = new StringBuilder();
builder.AppendFormat("pow({0}", TranslateExpression(math.getChild(0), map, model));
builder.AppendFormat(", {0})", TranslateExpression(math.getChild(1), map, model));
return builder.ToString();
}
case libsbml.AST_LOGICAL_AND:
{
var builder = new StringBuilder();
builder.AppendFormat("({0}", TranslateExpression(math.getChild(0), map, model));
for (var i = 1; i < math.getNumChildren(); ++i)
{
builder.AppendFormat(" && {0}", TranslateExpression(math.getChild(i), map, model));
}
builder.AppendFormat(")");
return builder.ToString();
}
case libsbml.AST_LOGICAL_NOT:
{
var builder = new StringBuilder();
builder.AppendFormat("!({0})", TranslateExpression(math.getChild(0), map, model));
return builder.ToString();
}
case libsbml.AST_LOGICAL_OR:
{
var builder = new StringBuilder();
builder.AppendFormat("({0}", TranslateExpression(math.getChild(0), map, model));
for (var i = 1; i < math.getNumChildren(); ++i)
{
builder.AppendFormat(" || {0}", TranslateExpression(math.getChild(i), map, model));
}
builder.AppendFormat(")");
return builder.ToString();
}
case libsbml.AST_FUNCTION_PIECEWISE:
{
var builder = new StringBuilder();
builder.AppendFormat("(");
for (var i = 0; i < math.getNumChildren() - 1; i += 2)
{
builder.AppendFormat("({0}) ", TranslateExpression(math.getChild(i + 1), map, model));
builder.AppendFormat("? {0} :", TranslateExpression(math.getChild(i), map, model));
}
builder.AppendFormat(" {0}", TranslateExpression(math.getChild(math.getNumChildren() - 1), map, model));
builder.AppendFormat(")");
return builder.ToString();
}
case libsbml.AST_FUNCTION:
{
var name = math.getName();
var builder = new StringBuilder();
builder.AppendFormat("{0}(", name);
builder.AppendFormat("{0}", TranslateExpression(math.getChild(0), map, model));
for (var i = 1; i < math.getNumChildren(); ++i)
{
builder.AppendFormat(", {0}", TranslateExpression(math.getChild(i), map, model));
}
builder.AppendFormat(")");
return builder.ToString();
}
//case libsbml.AST_NAME:
default:
{
var name = math.getName();
if (map != null && map.ContainsKey(name))
{
if (map.ContainsKey("use_compartments") && model != null)
{
var species = model.getSpecies(name);
if (species == null || !species.isSetCompartment()) return map[name];
return String.Format("isIn_{0} * {1}", species.getCompartment(), map[name]);
}
return map[name];
}
return name;
}
}
}
public string ToSBML()
{
return libsbml.writeSBMLToString(_Document);
}
public void ExportTo(string filename)
{
var path = Path.GetDirectoryName(filename);
var name = Path.GetFileNameWithoutExtension(filename);
WriteCMakeLists(path, name);
WriteConfigFile(path, name);
WriteComponentParameters(path);
WriteInitialConditions(path);
WriteLocalOperator(path);
WriteMainFile(path, name);
WriteReactionAdapter(path);
if (_Original != null && path != null)
libsbml.writeSBMLToFile(_Original, Path.Combine(path, "sbml.xml"));
}
private void WriteReactionAdapter(string path)
{
var map = new Dictionary<string, string>();
var initializer = new StringBuilder();
var declarations = new StringBuilder();
var odes = new StringBuilder();
var odes2 = new StringBuilder();
foreach (var item in ParameterIds)
{
initializer.AppendFormat(
" , {0}(param.sub(\"Reaction\").template get<RF>(\"{0}\")){1}"
, item
, Environment.NewLine
);
declarations.AppendFormat(
" RF {0};{1}"
, item
, Environment.NewLine
);
}
var count = 0;
odes.AppendLine(" //// variable map: variable -> x(lsfu,...)");
var varMap = new Dictionary<string, string>();
foreach (var item in OdeVariables)
{
varMap[item] = string.Format("x(lfsu,{0})", count);
odes.AppendFormat(" // {0} -> {1}{2}", item, varMap[item], Environment.NewLine);
++count;
}
odes.AppendLine();
odes.AppendLine();
var numCompartments = _Model.getNumCompartments();
if (numCompartments > 1)
{
varMap["use_compartments"] = "1";
odes.AppendLine(" //// determine whether current location is in a compartment");
for (var i = 0; i < numCompartments; ++i)
{
var comp = _Model.getCompartment(i);
var compId = comp.getId();
initializer.AppendFormat(
" , dh_in{0}(DataHelper::forFile(param.sub(\"Reaction\").template get<std::string>(\"in_{0}\", \"\"), NearestNeighbor)){1}"
, compId
, Environment.NewLine
);
declarations.AppendFormat(
" const DataHelper* dh_in{0};{1}"
, compId
, Environment.NewLine
);
odes.AppendFormat(
" RF isIn_{0} = dh_in{0} == NULL ? 1 :(RF)dh_in{0}->get((double)pos[0], (double)pos[1]);{1}", compId,
Environment.NewLine);
}
}
odes.AppendLine();
odes.AppendLine();
odes.AppendLine(" //// calculate assignment rules");
for (var i = 0; i < _Model.getNumRules(); ++i)
{
var rule = _Model.getRule(i) as AssignmentRule;
if (rule == null || rule.getTypeCode() != libsbml.SBML_ASSIGNMENT_RULE) continue;
odes.AppendFormat(" {0} = {1};{2}",
TranslateExpression(rule.getVariable(), varMap),
TranslateExpression(rule.getMath(), varMap, _Model),
Environment.NewLine
);
}
odes.AppendLine();
odes.AppendLine();
count = 1;
odes.AppendLine(" //// calculate rate rules");
foreach (var item in OdeVariables)
{
var rule = _Model.getRateRule(item);
if (rule == null || !rule.isSetMath()) continue;
odes.AppendFormat(" // {0} {1}", item, Environment.NewLine);
var species = _Model.getSpecies(rule.getVariable());
if (species != null && numCompartments > 1 && species.isSetCompartment())
odes.AppendFormat(" RF r{0} = isIn_{1}*({2});{3}",
count,
species.getCompartment(),
TranslateExpression(rule.getMath(), varMap, _Model),
Environment.NewLine
);
else
odes.AppendFormat(" RF r{0} = {1};{2}",
count,
TranslateExpression(rule.getMath(), varMap, _Model),
Environment.NewLine
);
odes2.AppendFormat(" r.accumulate(lfsv,{0},-r{1}*eg.geometry().volume());{2}",
count - 1,
count,
Environment.NewLine
);
odes.AppendLine();
++count;
}
odes.AppendLine();
odes.AppendLine(odes2.ToString());
map["%INITIALIZER%"] = initializer.ToString();
map["%DECLARATION%"] = declarations.ToString();
map["%ODES%"] = odes.ToString();
File.WriteAllText(Path.Combine(path, "reactionadapter.hh"),
ExpandTemplate(Resources.reactionadapter, map));
}
private int GetBoundaryType(Parameter param)
{
var plug = (SpatialParameterPlugin) param.getPlugin("spatial");
if (plug == null) return -1;
var bc = plug.getBoundaryCondition();
if (bc == null) return -1;
if (bc.getType() == libsbml.SPATIAL_BOUNDARYKIND_DIRICHLET) return 1; // Dirichlet
return -1; // Neumann
}
private int GetBoundaryType(Parameter xmin, Parameter xmax, Parameter ymin, Parameter ymax)
{
if (xmin != null) return GetBoundaryType(xmin);
if (xmax != null) return GetBoundaryType(xmax);
if (ymin != null) return GetBoundaryType(ymin);
if (ymax != null) return GetBoundaryType(ymax);
return -1;
}
private void WriteConfigFile(string path, string name)
{
var builder = new StringBuilder();
var map = new Dictionary<string, string>();
map["%GEOMETRY_FILE%"] = GeometryFile;
map["%GEOMETRY_MIN%"] = GeometryMin.ToString(CultureInfo.InvariantCulture);
map["%GEOMETRY_MAX%"] = GeometryMax.ToString(CultureInfo.InvariantCulture);
map["%NAME%"] = name;
map["%TIME_TEND%"] = "500";
map["%TIME_DTMAX%"] = "1";
map["%TIME_DTPLOT%"] = "1";
map["%WORLD_WIDTH%"] = _Geometry.getCoordinateComponent(0).getBoundaryMax().getValue().ToString(CultureInfo.InvariantCulture);
map["%WORLD_HEIGHT%"] = _Geometry.getCoordinateComponent(1).getBoundaryMax().getValue().ToString(CultureInfo.InvariantCulture);
map["%GRID_X%"] = "64";
map["%GRID_Y%"] = "64";
builder.AppendLine(ExpandTemplate(Resources.config, map));
// add reaction section
builder.AppendLine("[Reaction]");
ParameterIds = new List<string>();
for (var i = 0; i < _Model.getNumParameters(); ++i)
{
var current = _Model.getParameter(i);
if (current.IsSpatial()) continue;
ParameterIds.Add(current.getId());
double currentValue = current.getValue();
if (double.IsNaN(currentValue)) currentValue = 0;
builder.AppendFormat("{0} = {1}{2}", current.getId(), currentValue, Environment.NewLine);
}
for (var i = 0; i < _Model.getNumCompartments(); ++i)
{
var current = _Model.getCompartment(i);
ParameterIds.Add(current.getId());
builder.AppendFormat("{0} = {1}{2}", current.getId(), current.getSize(), Environment.NewLine);
}
for (var i = 0; i < _Model.getNumSpecies(); ++i)
{
var current = _Model.getSpecies(i);
if (current == null || !current.getBoundaryCondition()) continue;
ParameterIds.Add(current.getId());
double currentValue = current.getInitialConcentration();
if (!current.isSetInitialConcentration()) currentValue = current.getInitialAmount();
builder.AppendFormat("{0} = {1}{2}", current.getId(), currentValue, Environment.NewLine);
}
builder.AppendLine();
// add component sections
for (var i = 0; i < OdeVariables.Count; ++i)
{
var species = _Model.getSpecies(OdeVariables[i]);
if (species == null) continue;
var plug = (SpatialSpeciesPlugin)species.getPlugin("spatial");
if (plug == null || plug.getIsSpatial() == false) continue;
var diff = species.getDiffusionX();
if (!diff.HasValue)
diff = species.getDiffusionY();
if (!diff.HasValue) diff = 0;
var Xmin = species.getSpatialParameter(libsbml.SBML_SPATIAL_BOUNDARYCONDITION, "Xmin");
var Xmax = species.getSpatialParameter(libsbml.SBML_SPATIAL_BOUNDARYCONDITION, "Xmax");
if (Xmin == null) Xmin = Xmax;
if (Xmax == null) Xmax = Xmin;
var Ymin = species.getSpatialParameter(libsbml.SBML_SPATIAL_BOUNDARYCONDITION, "Ymin");
var Ymax = species.getSpatialParameter(libsbml.SBML_SPATIAL_BOUNDARYCONDITION, "Ymax");
if (Ymin == null) Ymin = Ymax;
if (Ymax == null) Ymax = Ymin;
var type = GetBoundaryType(Xmin, Xmax, Ymin, Ymax);
builder.AppendFormat("[{0}]{1}", species.getId(), Environment.NewLine);
builder.AppendFormat("# Species '{0}' in Compartment '{1}'{2}", species.getId(), species.getCompartment(),
Environment.NewLine);
builder.AppendFormat("D = {0}{1}", diff.Value, Environment.NewLine);
builder.AppendFormat("Xmin = {0}{1}", Xmin == null ? 0 : Xmin.getValue(), Environment.NewLine);
builder.AppendFormat("Xmax = {0}{1}", Xmax == null ? 0 : Xmax.getValue(), Environment.NewLine);
builder.AppendFormat("Ymin = {0}{1}", Ymin == null ? 0 : Ymin.getValue(), Environment.NewLine);
builder.AppendFormat("Ymax = {0}{1}", Ymax == null ? 0 : Ymax.getValue(), Environment.NewLine);
builder.AppendFormat("# Dirichlet=1, Neumann=-1, Outflow=-2, None=-3{0}", Environment.NewLine);
builder.AppendFormat("BCType = {0}{1}", type, Environment.NewLine);
builder.AppendLine();
}
builder.AppendLine();
builder.AppendLine("[Data]");
builder.AppendLine("# use this section to override initial conditions of any variable");
builder.AppendLine("# by simply specifying the variable followed by a filename");
builder.AppendLine("# of the dmp file containing the initial values");
foreach (var item in OdeVariables)
builder.AppendFormat("{0} = {1}", item, Environment.NewLine);
builder.AppendLine();
File.WriteAllText(Path.Combine(path, name + ".conf"),
builder.ToString());
}
private void WriteMainFile(string path, string name)
{
var builder = new StringBuilder();
var map = new Dictionary<string, string>();
var dpCreation = new StringBuilder();
var dpInitialization = new StringBuilder();
var subCreation = new StringBuilder();
var initialTypeCreation = new StringBuilder();
var initialTypes = new StringBuilder();
var initialArgs = new StringBuilder();
var gridFunctions = new StringBuilder();
map["%NUMCOMPONENTS%"] = OdeVariables.Count.ToString();
map["%GEOMETRY%"] = GenerateGeometryExpression(path);
for (var index = 0; index < OdeVariables.Count; index++)
{
dpCreation.AppendFormat(" DP dp{0}(param, \"{1}\");{2}"
, index + 1
, OdeVariables[index]
, Environment.NewLine);
dpInitialization.AppendFormat("dp{0}", index + 1);
initialTypes.AppendFormat("U{0}InitialType", index);
initialArgs.AppendFormat("u{0}initial", index);
if (index + 1 < OdeVariables.Count)
{
dpInitialization.Append(",");
initialTypes.Append(",");
initialArgs.Append(",");
}
subCreation.AppendFormat(
" typedef Dune::PDELab::GridFunctionSubSpace<TPGFS,Dune::TypeTree::TreePath<{0}> > U{0}SUB;{1}"
, index, Environment.NewLine);
subCreation.AppendFormat(" U{0}SUB u{0}sub(tpgfs);{1}"
, index, Environment.NewLine);
initialTypeCreation.AppendFormat(" UGeneralInitialType u{0}initial(gv,param,{0});{1}"
, index, Environment.NewLine);
gridFunctions.AppendFormat(" typedef Dune::PDELab::DiscreteGridFunction<U{0}SUB,V> U{0}DGF;{1}"
, index, Environment.NewLine);
gridFunctions.AppendFormat(" U{0}DGF u{0}dgf(u{0}sub,uold);{1}"
, index, Environment.NewLine);
gridFunctions.AppendFormat(
" pvdwriter.addVertexData(new Dune::PDELab::VTKGridFunctionAdapter<U{0}DGF>(u{0}dgf,\"{1}\"));{2}"
, index
, OdeVariables[index]
, Environment.NewLine);
}
map["%DPCREATION%"] = dpCreation.ToString();
map["%SUBCREATION%"] = subCreation.ToString();
map["%DPINITIALIZATION%"] = dpInitialization.ToString();
map["%INITIALTYPECREATION%"] = initialTypeCreation.ToString();
map["%INITIALTYPES%"] = initialTypes.ToString();
map["%INITIALARGS%"] = initialArgs.ToString();
map["%CREATEGRIDFUNCTIONS%"] = gridFunctions.ToString();
map["%NAME%"] = name;
builder.AppendLine(ExpandTemplate(Resources.main, map));
File.WriteAllText(Path.Combine(path, name + ".cc"),
builder.ToString());
}
private static string GenerateGeometryExpressionForAnalyticVolume(AnalyticGeometry vol)
{
var volume = vol.getAnalyticVolume(0);
var builder = new StringBuilder();
builder.Append("\n");
builder.AppendFormat(" bool inside={0};\n",
TranslateExpression(volume.getMath()));
builder.Append(" return inside;\n");
return builder.ToString();
}
private string WriteFieldToFile(string path, SampledField data, int numSamples1, int numSamples2, int z = 0,
string basename = "geometry1.dmp")
{
var name = Path.Combine(path, basename);
var length = data.getUncompressedLength();
var array = new int[length];
data.getUncompressed(array);
using (var writer = new StreamWriter(name))
{
writer.WriteLine("{0} {1}", numSamples1, numSamples2);
for (var i = 0; i < numSamples1; ++i)
{
for (var j = 0; j < numSamples2; ++j)
{
writer.Write(array[i + numSamples1*j + numSamples1*numSamples2*z]);
writer.Write(" ");
}
writer.WriteLine();
}
}
return name;
}
private string GenerateExpressionForSampleFieldGeometry(Geometry geometry, SampledFieldGeometry sample, string path)
{
var field = geometry.getSampledField(sample.getSampledField());
var data = field;
GeometryFile = WriteFieldToFile(path,
data,
field.getNumSamples1(),
field.getNumSamples2());
var min = sample.getSampledVolume(0).getMinValue();
var max = sample.getSampledVolume(0).getMaxValue();
GeometryMin = min;
GeometryMax = max;
return "";
}
private string GenerateGeometryExpression(string path)
{
// by default return the full area
var result = "return true;";
// string result = GenerateFish(); // or the fish
if (SpatialModelPlugin == null) return result;
if (_Geometry == null || _Geometry.getNumGeometryDefinitions() == 0) return result;
var vol = _Geometry.GetFirstAnalyticGeometry();
if (vol != null && vol.getNumAnalyticVolumes() > 0)
return GenerateGeometryExpressionForAnalyticVolume(vol);
var sample = _Geometry.GetFirstSampledFieldGeometry();
if (sample != null && sample.getNumSampledVolumes() > 0)
return GenerateExpressionForSampleFieldGeometry(_Geometry, sample, path);
return result;
}
private static string GenerateFish()
{
return
" const auto& x = point[0];\n" +
" const auto& y = point[1];\n" +
"\n" +
" bool inside= (pow(0.27 * (-42. + x), 2.) + pow(0.4 * (-50. + y), 2.) < 100. ||\n" +
" (y < (-10.+ x) && y > (110. + -x) && x < 90.));\n" +
"\n" +
" return inside;\n";
}
private void WriteLocalOperator(string path)
{
File.WriteAllText(Path.Combine(path, "local_operator.hh"),
ExpandTemplate(Resources.local_operator));
}
private void WriteInitialConditions(string path)
{
var builder = new StringBuilder();
builder.AppendLine("#ifndef INITIAL_CONDITIONS_H");
builder.AppendLine("#define INITIAL_CONDITIONS_H");
builder.AppendLine();
builder.AppendLine("#include <dune/copasi/utilities/datahelper.hh>");
builder.AppendLine();
var map = new Dictionary<string, string>();
var initializer = new StringBuilder();
var declarations = new StringBuilder();
foreach (var pItem in ParameterIds)
{
initializer.AppendFormat(
" , {0}(param.sub(\"Reaction\").template get<RF>(\"{0}\")){1}"
, pItem
, Environment.NewLine
);
declarations.AppendFormat(
" RF {0};{1}"
, pItem
, Environment.NewLine
);
}
var varMap
= new Dictionary<string, string>
{
{"x", "x[0]"},
{"y", "x[1]"},
{"z", "x[2]"}
};
var additionalInitializations = new StringBuilder();
var tempBuilder = new StringBuilder();
var numCompartments = _Model.getNumCompartments();
if (numCompartments > 1)
{
additionalInitializations.AppendLine(" //// determine whether current location is in a compartment");
for (var i = 0; i < numCompartments; ++i)
{
var comp = _Model.getCompartment(i);
var compId = comp.getId();
initializer.AppendFormat(
" , dh_in{0}(DataHelper::forFile(param.sub(\"Reaction\").template get<std::string>(\"in_{0}\", \"\"), NearestNeighbor)){1}"
, compId
, Environment.NewLine
);
declarations.AppendFormat(
" const DataHelper* dh_in{0};{1}"
, compId
, Environment.NewLine
);
additionalInitializations.AppendFormat(
" RF isIn_{0} = dh_in{0} == NULL ? 1 :(RF)dh_in{0}->get((double)x[0], (double)x[1]);{1}", compId,
Environment.NewLine);
}
}
var count = 0;
foreach (var item in OdeVariables)
{
initializer.AppendFormat(
" , dh_{0}(DataHelper::forFile(param.sub(\"Data\").template get<std::string>(\"{0}\"))){1}"
, item
, Environment.NewLine
);
declarations.AppendFormat(
" const DataHelper* dh_{0};{1}"
, item
, Environment.NewLine
);
tempBuilder.AppendLine();
tempBuilder.AppendFormat(" case {0}:{1}", count, Environment.NewLine);
tempBuilder.AppendLine(" {");
tempBuilder.AppendFormat(" // initial conditions for {0}{1}", item, Environment.NewLine);
tempBuilder.AppendFormat(" if(dh_{0} != NULL){1}", item, Environment.NewLine);
tempBuilder.AppendFormat(
" __initial = (typename Traits::RangeType)(*dh_{0}).get((double)(x[0]),(double)x[1] );{1}", item,
Environment.NewLine);
tempBuilder.AppendLine(" else");
tempBuilder.Append(" __initial = ");
var species = _Model.getSpecies(item);
if (numCompartments > 1 && species != null && species.isSetCompartment())
{
tempBuilder.AppendFormat("isIn_{0} * ", species.getCompartment());
}
var initial = _Model.getInitialAssignment(item);
if (initial != null && initial.isSetMath())
{
tempBuilder.AppendFormat(
"{0};{1}",
TranslateExpression(initial.getMath(),
varMap, _Model
),
Environment.NewLine
);
tempBuilder.AppendLine();
}
else
{
if (species != null)
{
if (species.isSetInitialConcentration())
tempBuilder.AppendFormat(
"{0};{1}"
, species.getInitialConcentration().ToString("E17")
, Environment.NewLine);
else
tempBuilder.AppendFormat(
"{0};{1}"
, species.getInitialAmount().ToString("E17")
, Environment.NewLine);
}
}
tempBuilder.AppendLine(" break;");
tempBuilder.AppendLine(" }");
++count;
}
map["%INITIALIZER%"] = initializer.ToString();
map["%DECLARATION%"] = declarations.ToString();
map["%INITIALCONDITION%"] = tempBuilder.ToString();
map["%ADDITIONAL_INITIAlIZATION%"] = additionalInitializations.ToString();
builder.AppendLine(ExpandTemplate(Resources.initial_conditions, map));
builder.AppendLine();
builder.AppendLine("#endif // INITIAL_CONDITIONS_H");
File.WriteAllText(Path.Combine(path, "initial_conditions.hh"),
builder.ToString());
}
private void WriteComponentParameters(string path)
{
File.WriteAllText(Path.Combine(path, "componentparameters.hh"),
ExpandTemplate(Resources.componentparameters));
}
private void WriteCMakeLists(string path, string name)
{
File.WriteAllText(Path.Combine(path, "CMakeLists.txt"),
ExpandTemplate(Resources.CMakeLists,
new Dictionary<string, string>
{
{"%NAME%", name}
}
));
}
private string ExpandTemplate(string content,
Dictionary<string, string> map = null)
{
var final = content;
if (map != null)
foreach (var pair in map)
{
final = final.Replace(pair.Key, pair.Value);
}
return final;
}
}
}<file_sep>/WFEditSpatial/Controls/ControlDomains.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using EditSpatial.Model;
using libsbmlcs;
namespace EditSpatial.Controls
{
public partial class ControlDomains : BaseSpatialControl
{
public ControlDomains()
{
InitializeComponent();
}
private Geometry Current { get; set; }
public void InitializeFrom(Geometry geometry)
{
grid.Rows.Clear();
Current = geometry;
if (geometry == null) return;
for (long i = 0; i < geometry.getNumDomains(); ++i)
{
var domain = geometry.getDomain(i);
var domainType = domain.getDomainType();
var spatialId = domain.getId();
var builder = new StringBuilder();
for (long j = 0; j < domain.getNumInteriorPoints(); ++j)
{
var current = domain.getInteriorPoint(j);
builder.Append("(");
builder.Append(current.getCoord1());
builder.Append(";");
builder.Append(current.getCoord2());
builder.Append(";");
builder.Append(current.getCoord3());
builder.Append(")");
if (j + 1 < domain.getNumInteriorPoints())
builder.Append(", ");
}
grid.Rows.Add(spatialId, domainType, builder.ToString());
}
}
public override void SaveChanges()
{
if (Current == null) return;
for (var i = 0; i < grid.Rows.Count && i < Current.getNumDomains(); ++i)
{
var current = Current.getDomain(i);
var row = grid.Rows[i];
current.setId((string) row.Cells[0].Value);
current.setDomainType((string) row.Cells[1].Value);
var points = GetPoints((string) row.Cells[2].Value);
for (var j = 0; j < points.Count && j < current.getNumInteriorPoints(); ++j)
{
var interior = current.getInteriorPoint(j);
var point = points[j];
interior.setCoord1(point.Item1);
interior.setCoord2(point.Item2);
interior.setCoord3(point.Item3);
}
}
OnModelChanged();
}
private List<Tuple<double, double, double>> GetPoints(string value)
{
value = value.Replace("(", "");
value = value.Replace(")", "");
var points = value.Split(new[] {','}, StringSplitOptions.RemoveEmptyEntries);
return (from point in points
select point.Split(new[] {';'}, StringSplitOptions.RemoveEmptyEntries)
into coords
where coords.Length == 3
select
new Tuple<double, double, double>
(Util.SaveDouble(coords[0]),
Util.SaveDouble(coords[1]),
Util.SaveDouble(coords[2])))
.ToList();
}
public override void InvalidateSelection()
{
Current = null;
InitializeFrom(null);
}
}
}<file_sep>/LibEditSpatial/Dialogs/DlgRun.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Diagnostics;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
using LibEditSpatial.Model;
namespace LibEditSpatial.Dialogs
{
public partial class DlgRun : Form
{
private bool closing;
private Process process;
private FileSystemWatcher watcher;
public DlgRun()
{
InitializeComponent();
closing = false;
CygwinDir = @"C:\cygwin\bin";
ParaViewDir = @"E:\Program Files (x86)\ParaView 4.2.0\bin\";
}
public string CygwinDir { get; set; }
public string ParaViewDir { get; set; }
public DuneConfig Config { get; set; }
public string FileName
{
get { return txtFileName.Text; }
set { txtFileName.Text = value; }
}
private List<Tuple<string, string>> UpdateItems { get; set; }
private void OnKillClick(object sender, EventArgs e)
{
if (process == null) return;
try
{
process.Kill();
}
catch
{
}
}
private void OnAddString(string data)
{
if (closing) return;
if (string.IsNullOrEmpty(data)) return;
if (InvokeRequired)
{
Invoke(new VoidStringDelegate(OnAddString), data);
return;
}
txtResult.Text += data.Replace("\n", Environment.NewLine);
}
private void ClearWatcher()
{
if (watcher != null)
{
watcher.EnableRaisingEvents = false;
watcher.Dispose();
watcher = null;
}
}
private void ReEnableUI()
{
if (closing) return;
if (InvokeRequired)
{
Invoke(new VoidDelegate(ReEnableUI));
return;
}
progressBar1.Style = ProgressBarStyle.Continuous;
cmdRun.Enabled = true;
cmdCompile.Enabled = true;
cmdKill.Enabled = false;
ClearWatcher();
process = null;
}
private bool CheckIfEmpty(string dir)
{
var outdir = Path.Combine(dir, "vtk");
if (!Directory.Exists(outdir)) return false;
var files = Directory.GetFiles(outdir, "*.vt?", SearchOption.TopDirectoryOnly);
if (files.Length == 0) return false;
var result =
MessageBox.Show("There are already results present from a previous simulation, shall I move them away?",
"Move Existing results?", MessageBoxButtons.YesNoCancel, MessageBoxIcon.Question);
if (result == DialogResult.Yes)
{
var now = DateTime.Now;
var newDir = Path.Combine(dir, now.ToString("yyyy-MM-dd_-_HH-mm-ss") + "_old_results");
Directory.CreateDirectory(newDir);
Directory.Move(outdir, Path.Combine(newDir, "vtk"));
files = Directory.GetFiles(dir, "*.pvd");
foreach (var item in files)
{
var name = Path.GetFileName(item);
if (name == null) continue;
File.Move(item, Path.Combine(newDir, name));
}
}
if (result == DialogResult.Cancel)
return true;
return false;
}
private void OnRunClick(object sender, EventArgs e)
{
if (process != null) return;
var dir = Path.GetDirectoryName(FileName);
if (dir == null) return;
var outdir = Path.Combine(dir, "vtk");
if (CheckIfEmpty(dir)) return;
txtResult.Text = "Starting with config: temp.conf";
if (!Directory.Exists(outdir))
Directory.CreateDirectory(outdir);
var config = Path.Combine(dir, "temp.conf");
Config.SaveAs(config);
var info = new ProcessStartInfo
{
FileName = FileName,
Arguments = Util.ToCygwin(config),
WorkingDirectory = dir,
RedirectStandardError = true,
RedirectStandardOutput = true,
UseShellExecute = false,
CreateNoWindow = true
};
#pragma warning disable 4014
StartProcess(info);
#pragma warning restore 4014
}
private void QueueUpdate(string fullPath, string selectedItem)
{
UpdateItems.Add(new Tuple<string, string>(fullPath, selectedItem));
LaunchUpdate();
}
private void UpdateWorkerDoWork(object sender, DoWorkEventArgs e)
{
var arg = e.Argument as Tuple<string, string>;
if (arg == null) return;
GeneratePreview(arg.Item1, arg.Item2);
}
private void LaunchUpdate()
{
if (backgroundWorker1.IsBusy) return;
var last = UpdateItems.LastOrDefault();
UpdateItems.Clear();
if (last == null) return;
backgroundWorker1.RunWorkerAsync(last);
}
private void OnNewResult(object sender, FileSystemEventArgs e)
{
if (closing) return;
if (InvokeRequired)
{
Invoke(new FileSystemEventHandler(OnNewResult), sender, e);
return;
}
if (!chkPreview.Checked || cmbVariable.Items.Count == 0) return;
QueueUpdate(e.FullPath, cmbVariable.SelectedItem as string);
}
private void UpdatePreview(byte[] bytes, string species)
{
if (InvokeRequired)
{
Invoke(new VoidByteStringDelegate(UpdatePreview), bytes, species);
return;
}
if (!cmbVariable.SelectedItem.Equals(species))
return;
Image image;
using (var stream = new MemoryStream(bytes))
{
image = Image.FromStream(stream);
}
pictureBox1.Image = image;
}
public void GeneratePreview(string filename, string species)
{
if (string.IsNullOrWhiteSpace(species)) return;
if (!File.Exists(filename)) return;
Debug.WriteLine("Generating Preview for: {0} from {1}", species, Path.GetFileNameWithoutExtension(filename));
var tempFile = Path.Combine(Path.GetTempPath(),
Path.GetRandomFileName() + ".png");
var info = new ProcessStartInfo
{
FileName = Path.Combine(ParaViewDir, "pvpython"),
Arguments = string.Format("\"{0}\" \"{1}\" {2} \"{3}\"",
Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "pvrender.py"),
filename,
species,
tempFile
),
UseShellExecute = false,
CreateNoWindow = true,
RedirectStandardError = true,
RedirectStandardOutput = true
};
var previewProcess = new Process {StartInfo = info};
previewProcess.OutputDataReceived += (o, e2) => OnAddString(e2.Data);
previewProcess.ErrorDataReceived += (o, e3) => OnAddString(e3.Data);
previewProcess.Start();
previewProcess.EnableRaisingEvents = true;
previewProcess.BeginOutputReadLine();
previewProcess.BeginErrorReadLine();
previewProcess.WaitForExit();
previewProcess.EnableRaisingEvents = false;
if (File.Exists(tempFile))
{
var bytes = File.ReadAllBytes(tempFile);
File.Delete(tempFile);
UpdatePreview(bytes, species);
}
}
public async Task StartProcess(ProcessStartInfo info)
{
if (!string.IsNullOrEmpty(CygwinDir))
info.EnvironmentVariables["PATH"] = string.Format("{0};{1}", CygwinDir, info.EnvironmentVariables["PATH"]);
process = new Process {StartInfo = info, EnableRaisingEvents = true};
process.Exited += (o, e1) => ReEnableUI();
process.OutputDataReceived += (o, e2) => OnAddString(e2.Data);
process.ErrorDataReceived += (o, e3) => OnAddString(e3.Data);
process.Start();
Thread.Sleep(100);
process.BeginOutputReadLine();
process.BeginErrorReadLine();
cmdRun.Enabled = false;
cmdCompile.Enabled = false;
cmdKill.Enabled = true;
progressBar1.Style = ProgressBarStyle.Marquee;
ClearWatcher();
watcher = new FileSystemWatcher
{
Path = Path.Combine(info.WorkingDirectory, "vtk"),
IncludeSubdirectories = false,
Filter = "*.vt?",
NotifyFilter = NotifyFilters.FileName | NotifyFilters.LastWrite
};
watcher.Created += OnNewResult;
watcher.Changed += OnNewResult;
watcher.EnableRaisingEvents = true;
await Task.Run(() => process.WaitForExit());
}
public static void WriteBatchFiles(string dir, string cygwinDir)
{
foreach (var item in new[] { "start.bat", "compile.bat" })
{
if (File.Exists(Path.Combine(dir, "start.bat"))) continue;
var batch = File.ReadAllText(
Path.Combine(
Path.GetDirectoryName(Application.ExecutablePath), item + ".in"));
batch = batch.Replace("$$CYG_DIR$$", cygwinDir);
File.WriteAllText(Path.Combine(dir, "src\\" + item), batch);
}
}
private void OnCompileClick(object sender, EventArgs e)
{
if (process != null) return;
var dir = Path.GetDirectoryName(FileName);
if (dir == null) return;
var info = new ProcessStartInfo
{
FileName = Path.Combine(CygwinDir, "bash"),
Arguments = "-c make",
WorkingDirectory = dir,
RedirectStandardError = true,
RedirectStandardOutput = true,
UseShellExecute = false,
CreateNoWindow = true
};
#pragma warning disable 4014
StartProcess(info);
#pragma warning restore 4014
WriteBatchFiles(dir, CygwinDir);
}
private void OnBrowseClick(object sender, EventArgs e)
{
using (var dialog = new OpenFileDialog
{
FileName = FileName,
Title = "Select executable",
Filter = "All files|*.*"
})
{
if (dialog.ShowDialog() == DialogResult.OK)
FileName = dialog.FileName;
}
}
private void DlgRun_Load(object sender, EventArgs e)
{
cmbVariable.Items.Clear();
if (Config != null)
{
foreach (var v in Config.GetVariableKeys())
cmbVariable.Items.Add(v);
if (cmbVariable.Items.Count > 0)
{
cmbVariable.SelectedIndex = 0;
chkPreview.Checked = true;
}
else
chkPreview.Checked = false;
}
UpdateItems = new List<Tuple<string, string>>();
if (File.Exists(FileName))
OnRunClick(sender, e);
}
private void DlgRun_FormClosing(object sender, FormClosingEventArgs e)
{
if (process != null && !process.HasExited)
{
if (
MessageBox.Show("The simulation is still running, do you want to kill it?", "Kill Simulation?",
MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
{
if (process != null)
{
process.Kill();
}
ClearWatcher();
closing = true;
}
else e.Cancel = true;
}
}
private void OnOpenPV(object sender, EventArgs e)
{
try
{
var dir = Path.GetDirectoryName(FileName);
if (dir == null) return;
var pvd = Directory.GetFiles(dir, "*.pvd", SearchOption.TopDirectoryOnly);
if (pvd.Length > 0)
Process.Start(Path.Combine(ParaViewDir, "paraview"), pvd[0]);
}
catch
{
}
}
private void UpdateWorkerCompleted(object sender, RunWorkerCompletedEventArgs e)
{
LaunchUpdate();
}
private void OnFolderClick(object sender, EventArgs e)
{
try
{
Process.Start(Path.GetDirectoryName(FileName));
}
catch
{
}
}
}
}<file_sep>/WFEditSpatial/Program.cs
using System;
using System.IO;
using System.Windows.Forms;
using SBW;
namespace EditSpatial
{
internal static class Program
{
private static object module;
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
private static void Main(string[] args)
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
var mainForm = new MainForm();
try
{
module = mainForm;
DefaultModule.EnableServices(ref module,
"EditSpatial", // module name
"Edit Spatial", // display name
"Creates / Edits spatial models",
LowLevel.ModuleManagementType.UniqueModule,
"analysis", // service name
"Create/Edit Spatial Description", // service display name
"/Analysis", // category
"Creates / Edits spatial models", //
args
);
}
catch
{
}
if (args.Length == 1 && File.Exists(args[0]))
mainForm.OpenFile(args[0]);
Application.Run(mainForm);
}
}
}<file_sep>/WFEditSpatial/Model/ReactionInfo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using libsbmlcs;
namespace EditSpatial.Model
{
public class ReactionInfo : IDisposable
{
public ReactionInfo(Reaction reaction)
{
Reactants = new List<SpeciesReferenceInfo>();
Products = new List<SpeciesReferenceInfo>();
Reaction = reaction;
Model = reaction.getModel();
AnalyzeReaction();
}
public Reaction Reaction { get; set; }
public libsbmlcs.Model Model { get; set; }
public List<SpeciesReferenceInfo> Reactants { get; set; }
public List<SpeciesReferenceInfo> Products { get; set; }
public List<string> CompartmentIds
{
get
{
var list = Reactants.Select(sri => sri.Compartment).ToList();
list.AddRange(Products.Select(sri => sri.Compartment).ToList());
return list.Distinct().ToList();
}
}
private void AnalyzeReaction()
{
for (var i = 0; i < Reaction.getNumReactants(); ++i)
{
var reference = Reaction.getReactant(i);
if (reference != null && reference.isSetSpecies())
{
var species = Model.getSpecies(reference.getSpecies());
if (species == null || !species.isSetCompartment()) continue;
Reactants.Add(new SpeciesReferenceInfo
{
Id = species.getId(),
Compartment = species.getCompartment(),
Stoichiometry = reference.getStoichiometry()
});
}
}
for (var i = 0; i < Reaction.getNumProducts(); ++i)
{
var reference = Reaction.getProduct(i);
if (reference != null && reference.isSetSpecies())
{
var species = Model.getSpecies(reference.getSpecies());
if (species == null || !species.isSetCompartment()) continue;
Products.Add(new SpeciesReferenceInfo
{
Id = species.getId(),
Compartment = species.getCompartment(),
Stoichiometry = reference.getStoichiometry()
});
}
}
}
public void Dispose()
{
if (Model != null)
Model.Dispose();
}
}
}
<file_sep>/WFEditSpatial/Controls/ControlDisplayNode.cs
using System;
using System.Windows.Forms;
namespace EditSpatial.Controls
{
public partial class ControlDisplayNode : BaseSpatialControl
{
public ControlDisplayNode()
{
InitializeComponent();
controlText1.WordWrap = true;
controlText1.ReadOnly = true;
}
public void DisplayNode(TreeNode node)
{
var sbml = node == null ? null : (string) node.Tag;
if (string.IsNullOrWhiteSpace(sbml))
sbml = "<none>";
sbml = sbml.Replace("\n", Environment.NewLine);
controlText1.Text = sbml;
}
public override void SaveChanges()
{
// not supported ...
}
public override void InvalidateSelection()
{
DisplayNode(null);
}
}
}<file_sep>/WFEditSpatial/Model/ISBWAnalyzer.cs
using SBW;
namespace EditSpatial.Model
{
internal delegate void doAnalysisDelegate(string model);
internal interface ISBWAnalyzer
{
[Help("Loads a SBML string into EditSpatial")]
void doAnalysis(string model);
}
}<file_sep>/WFEditSpatial/Controls/ControlParameters.cs
using System.Collections.Generic;
using System.Windows.Forms;
using libsbmlcs;
namespace EditSpatial.Controls
{
public partial class ControlParameters : BaseSpatialControl
{
public ControlParameters()
{
InitializeComponent();
RowsAdded = new List<int>();
}
private SBMLDocument Current { get; set; }
public List<int> RowsAdded { get; set; }
public void InitializeFrom(SBMLDocument document)
{
grid.Rows.Clear();
Current = document;
if (document == null || document.getModel() == null) return;
try
{
IsInitializing = true;
var model = document.getModel();
CommitAddedRows(Current);
for (long i = 0; i < model.getNumParameters(); ++i)
{
var current = model.getParameter(i);
grid.Rows.Add(current.getId(),
current.getName(),
current.getValue().ToString());
}
}
finally
{
IsInitializing = false;
}
}
private void CommitAddedRows(SBMLDocument current)
{
if (RowsAdded.Count > 0 && current != null)
{
for (var i = RowsAdded.Count - 1; i >= 0; i--)
{
try
{
var index = RowsAdded[i];
if (index < 0) continue;
var row = grid.Rows[index];
var param = current.getModel().createParameter();
param.initDefaults();
param.setId(row.Cells[0].Value as string);
param.setName(row.Cells[1].Value as string);
double value;
if (double.TryParse((string) row.Cells[2].Value, out value))
param.setValue(value);
RowsAdded.RemoveAt(i);
}
catch
{
}
}
}
}
public override void SaveChanges()
{
if (Current == null) return;
CommitAddedRows(Current);
for (var i = 0; i < grid.Rows.Count; ++i)
{
var row = grid.Rows[i];
var current = Current.getModel().getParameter((string) row.Cells[0].Value);
if (current == null) continue;
current.setName((string) row.Cells[1].Value);
double value;
if (double.TryParse((string) row.Cells[2].Value, out value))
current.setValue(value);
}
OnModelChanged();
}
public override void InvalidateSelection()
{
Current = null;
InitializeFrom(null);
}
private void OnUserDeletedRow(object sender, DataGridViewRowEventArgs e)
{
if (Current == null) return;
var ids = new List<string>();
if (grid.SelectedRows.Count > 1)
{
foreach (DataGridViewRow row in grid.SelectedRows)
{
ids.Add(row.Cells[0].Value as string);
}
}
else
{
ids.Add(e.Row.Cells[0].Value as string);
}
foreach (var id in ids)
Current.getModel().removeParameter(id);
InitializeFrom(Current);
}
private void OnRowsAdded(object sender, DataGridViewRowsAddedEventArgs e)
{
if (Current == null || IsInitializing || e.RowIndex == 0) return;
RowsAdded.Add(e.RowIndex - 1);
}
}
}<file_sep>/WFEditSpatial/Model/CustomSpatialValidator.cs
using libsbmlcs;
namespace EditSpatial.Model
{
public class CustomSpatialValidator : SBMLValidator
{
public CustomSpatialValidator()
{
}
public CustomSpatialValidator(CustomSpatialValidator orig)
: base(orig)
{
}
public override SBMLValidator clone()
{
return new CustomSpatialValidator(this);
}
private bool containsSpatialMath(ASTNode node, libsbmlcs.Model model)
{
for (var i = 0; i < node.getNumChildren(); ++i)
{
if (containsSpatialMath(node.getChild(i), model))
return true;
}
if (!node.isName()) return false;
var element = model.getElementBySId(node.getName());
if (element == null) return false;
if (element.getTypeCode() == libsbml.SBML_SPECIES)
{
var plug = (SpatialSpeciesPlugin) (element.getPlugin("spatial"));
if (plug == null) return false;
return plug.getIsSpatial();
}
return false;
}
private bool dependencesAreSpatial(SBase s, libsbmlcs.Model model)
{
if (model == null || s == null || !s.isSetId()) return false;
var rule = model.getRule(s.getId());
if (rule == null || rule.getTypeCode() != libsbml.SBML_ASSIGNMENT_RULE) return false;
var ar = (AssignmentRule) (rule);
if (!ar.isSetMath()) return false;
return containsSpatialMath(ar.getMath(), model);
}
public override long validate()
{
// if we don't have a model we don't apply this validator.
if (getDocument() == null || getModel() == null)
return 0;
var numErrors = 0;
var model = getModel();
for (var i = 0; i < model.getNumSpecies(); ++i)
{
var species = model.getSpecies(i);
var plug = (SpatialSpeciesPlugin) species.getPlugin("spatial");
if (plug == null) continue;
if (plug.isSetIsSpatial() && plug.getIsSpatial()) continue;
if (dependencesAreSpatial(species, model))
{
numErrors++;
getErrorLog().add(new SBMLError(99999, 3, 1,
string.Format(
"This model has a non-spatial species, with rules involving spatial species. Please change the flag of '{0}'",
species.getId()),
0, 0,
libsbml.LIBSBML_SEV_ERROR, // or LIBSBML_SEV_ERROR if you want to stop
libsbml.LIBSBML_CAT_SBML // or whatever category you prefer
));
}
}
return numErrors;
}
}
}<file_sep>/WFEditSpatial/Templates/CMakeLists.txt
set (HEADERS
local_operator.hh
componentparameters.hh
initial_conditions.hh
reactionadapter.hh
)
set (SOURCES
%NAME%.cc
)
add_executable(%NAME% ${SOURCES})
target_link_dune_default_libraries("%NAME%")
# copy config file if we don't have it
if (NOT EXISTS "${CMAKE_CURRENT_BINARY_DIR}/%NAME%.conf")
configure_file(
${CMAKE_CURRENT_SOURCE_DIR}/%NAME%.conf
${CMAKE_CURRENT_BINARY_DIR}/%NAME%.conf COPYONLY)
endif()
# copy sbml file if we don't have it
if (NOT EXISTS "${CMAKE_CURRENT_BINARY_DIR}/sbml.xml")
configure_file(
${CMAKE_CURRENT_SOURCE_DIR}/sbml.xml
${CMAKE_CURRENT_BINARY_DIR}/sbml.xml COPYONLY)
endif()
# create vtk dir, as otherwise the executable just falls over
if (NOT EXISTS "${CMAKE_CURRENT_BINARY_DIR}/vtk")
file(MAKE_DIRECTORY "${CMAKE_CURRENT_BINARY_DIR}/vtk")
endif()
<file_sep>/LibEditSpatial/Controls/CtrlDomain.cs
using System;
using System.Globalization;
using System.IO;
using System.Windows.Forms;
using LibEditSpatial.Model;
namespace LibEditSpatial.Controls
{
public partial class CtrlDomain : UserControl
{
public CtrlDomain()
{
InitializeComponent();
}
public DomainConfig Model { get; set; }
public void LoadModel(DomainConfig model)
{
Model = model;
txtWidth.Text = Model.Width.ToString(CultureInfo.InvariantCulture);
txtHeight.Text = Model.Height.ToString(CultureInfo.InvariantCulture);
txtDepth.Text = Model.Depth.ToString(CultureInfo.InvariantCulture);
txtGridX.Text = Model.GridX.ToString();
txtGridY.Text = Model.GridY.ToString();
txtGridZ.Text = Model.GridZ.ToString();
txtRefinement.Text = Model.Refinement.ToString();
txtFile.Text = Model.Geometry;
txtMin.Text = Model.GeometryMin.ToString(CultureInfo.InvariantCulture);
txtMax.Text = Model.GeometryMax.ToString(CultureInfo.InvariantCulture);
}
private void OnWidthChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtWidth.Text, out temp))
Model.Width = temp;
}
private void OnHeightChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtHeight.Text, out temp))
Model.Height = temp;
}
private void OnDepthChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtDepth.Text, out temp))
Model.Depth = temp;
}
private void OnGridXChanged(object sender, EventArgs e)
{
if (Model == null) return;
int temp;
if (int.TryParse(txtGridX.Text, out temp))
Model.GridX = temp;
}
private void OnGridYChanged(object sender, EventArgs e)
{
if (Model == null) return;
int temp;
if (int.TryParse(txtGridY.Text, out temp))
Model.GridY = temp;
}
private void OnGridZChanged(object sender, EventArgs e)
{
if (Model == null) return;
int temp;
if (int.TryParse(txtGridZ.Text, out temp))
Model.GridZ = temp;
}
private void OnRefinementChanged(object sender, EventArgs e)
{
if (Model == null) return;
int temp;
if (int.TryParse(txtRefinement.Text, out temp))
Model.Refinement = temp;
}
private void OnFileChanged(object sender, EventArgs e)
{
if (Model == null) return;
Model.Geometry = txtFile.Text;
}
private void OnMinChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtMin.Text, out temp))
Model.GeometryMin = temp;
}
private void OnMaxChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtMax.Text, out temp))
Model.GeometryMax = temp;
}
private void OnLoadClicked(object sender, EventArgs e)
{
if (Model == null) return;
OnBrowseDmpFile();
}
public event EventHandler<string> OpenDmpFile;
public event EventHandler BrowseDmpFile;
protected virtual void OnBrowseDmpFile()
{
var handler = BrowseDmpFile;
if (handler != null) handler(this, EventArgs.Empty);
}
protected virtual void OnOpenDmpFile(string filename)
{
var handler = OpenDmpFile;
if (handler != null) handler(this, filename);
}
private void OnEditClicked(object sender, EventArgs e)
{
if (Model == null) return;
var file = Model.Geometry;
if (!File.Exists(file)) return;
try
{
OnOpenDmpFile(file);
}
catch
{
}
}
}
}<file_sep>/LibEditSpatial/Model/DmpModel.cs
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Drawing.Imaging;
using System.IO;
using System.Linq;
using System.Reflection;
using System.Text;
namespace LibEditSpatial.Model
{
public class DmpModel : ICloneable
{
public DmpModel(int cols, int rows)
{
Palette = DmpPalette.Default;
Columns = cols;
MaxX = cols;
Rows = rows;
MaxY = rows;
Data = new double[cols, rows];
Min = 0;
Max = 10;
}
/// <summary>
/// Flag indicating whether the current model needs to be saved
/// </summary>
public bool Dirty { get; set; }
/// <summary>
/// Current Palette
/// </summary>
public DmpPalette Palette { get; set; }
/// <summary>
/// Filename for the model
/// </summary>
public string FileName { get; set; }
/// <summary>
/// Number of Columns
/// </summary>
public int Columns { get; set; }
/// <summary>
/// Returns all unique values in this model
/// </summary>
public List<double> Range
{
get
{
var numbers = Data;
var unique = Enumerable.Range(0, numbers.GetUpperBound(0) + 1)
.SelectMany(x => Enumerable.Range(0, numbers.GetUpperBound(1) + 1)
.Select(y => numbers[x, y])).Distinct().ToList();
unique.Sort();
return unique;
}
}
/// <summary>
/// X range
/// </summary>
public double XRange {
get { return MaxX - MinX; }
}
/// <summary>
/// Y range
/// </summary>
public double YRange
{
get { return MaxY - MinY; }
}
/// <summary>
/// data range
/// </summary>
public double DataRange
{
get { return Max - Min; }
}
/// <summary>
/// number of rows
/// </summary>
public int Rows { get; set; }
/// <summary>
/// Saves data in form of [columns, rows]
/// </summary>
public double[,] Data { get; set; }
public double MinX { get; set; }
public double MaxX { get; set; }
public double MinY { get; set; }
public double MaxY { get; set; }
public double Min { get; set; }
public double Max { get; set; }
public bool IssueEvents { get; set; }
/// <summary>
/// Access the element at the given index position
/// </summary>
/// <param name="x">index from 0...Columns</param>
/// <param name="y">index from 0...Rows</param>
/// <returns>value at given index position</returns>
public double this[int x, int y]
{
get { return Data[x, y]; }
set
{
Data[x, y] = value;
Min = Math.Min(Min, value);
Max = Math.Max(Max, value);
OnModelChanged();
}
}
public object Clone()
{
return new DmpModel(Columns, Rows)
{
MinX = MinX,
MaxX = MaxX,
MinY = MinY,
MaxY = MaxY,
Min = Min,
Max = Max,
Data = (double[,]) Data.Clone()
};
}
//public double this[double x, double y]
//{
// get
// {
// if (x < MinX)
// x = MinX;
// if (x > MaxX)
// x = MaxX;
// if (y < MinY)
// y = MinY;
// if (y > MaxY)
// y = MaxY;
// int posX = (int)Math.Round((x - MinX)) ;
// int posY = 0;
// return Data[posX, posY];
// }
// set
// {
// Data[x, y] = value;
// Min = Math.Min(Min, value);
// Max = Math.Max(Max, value);
// OnModelChanged();
// }
//}
public event EventHandler<DmpModel> ModelChanged;
public void Invert()
{
var range = Range;
var count = range.Count/2;
for (var i = 0; i < count; i++)
{
var first = range[i];
var last = range[range.Count - 1 - i];
Transform(
x =>
{
if (Math.Abs(x - first) < 1e-10) return last;
if (Math.Abs(x - last) < 1e-10) return first;
return x;
}
);
}
}
public void OnModelChanged()
{
Dirty = true;
if (ModelChanged != null && IssueEvents)
{
ModelChanged(this, this);
}
}
public void RotateRight()
{
Data = RotateMatrixCounterClockwise(Data);
Columns = Data.GetLength(0);
Rows = Data.GetLength(1);
}
private static T[,] RotateMatrixCounterClockwise<T>(T[,] oldMatrix)
{
var newMatrix = new T[oldMatrix.GetLength(1), oldMatrix.GetLength(0)];
var newRow = 0;
for (var oldColumn = oldMatrix.GetLength(1) - 1; oldColumn >= 0; oldColumn--)
{
var newColumn = 0;
for (var oldRow = 0; oldRow < oldMatrix.GetLength(0); oldRow++)
{
newMatrix[newRow, newColumn] = oldMatrix[oldRow, oldColumn];
newColumn++;
}
newRow++;
}
return newMatrix;
}
protected T[,] ResizeArray<T>(T[,] original, int x, int y)
{
var newArray = new T[x, y];
var minX = Math.Min(original.GetLength(0), newArray.GetLength(0));
var minY = Math.Min(original.GetLength(1), newArray.GetLength(1));
for (var i = 0; i < minY; ++i)
for (var j = 0; j < minX; ++j)
newArray[j, i] = original[j, i];
return newArray;
}
public void Resize(int cols, int rows, bool scaleExisting = false)
{
if (scaleExisting)
{
ResizeByScaling(cols, rows);
return;
}
Data = ResizeArray(Data, cols, rows);
Rows = rows;
Columns = cols;
Dirty = true;
}
private static DmpPalette GetRedPaletteOrDefault()
{
try
{
string file = Path.Combine(
Path.GetDirectoryName(Assembly.GetEntryAssembly().Location),
"Palettes/black-red.txt");
if (File.Exists(file))
return DmpPalette.FromFile(
file
);
}
catch
{
}
return DmpPalette.Default;
}
private void ResizeByScalingWithPalette(int cols, int rows, DmpPalette palette)
{
var image = ToImage(palette);
var bitmap = new Bitmap(cols, rows);
using (var g = Graphics.FromImage(bitmap))
{
g.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.None;
g.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.NearestNeighbor;
g.CompositingQuality = System.Drawing.Drawing2D.CompositingQuality.HighSpeed;
g.DrawImage(image, 0, 0, cols, rows);
g.Flush(System.Drawing.Drawing2D.FlushIntention.Flush);
}
var dmp = FromImage(bitmap, (c) =>
{
var index = palette.GetIndex(c);
if (index == -1)
return 0;
return MapFromUnit((double)index / (double)palette.Colors.Count);
});
Data = dmp.Data;
Columns = dmp.Columns;
Rows = dmp.Rows;
}
/// <summary>
/// Creates a new image with required resolution,
/// and samples it again.
/// </summary>
/// <param name="cols"></param>
/// <param name="rows"></param>
public void ResizeByScaling(int cols, int rows)
{
DmpPalette palette = GetRedPaletteOrDefault();
ResizeByScalingWithPalette(cols, rows, palette);
}
public void SaveAs(string fileName)
{
var builder = new StringBuilder();
builder.AppendFormat("{0} {1}{2}", Columns, Rows, Environment.NewLine);
builder.AppendFormat("{0} {1} {2} {3} {4}", MinX, MaxX, MinY, MaxY, Environment.NewLine);
for (var y = 0; y < Rows; y++)
{
for (var x = 0; x < Columns; x++)
{
builder.Append(Data[x, y]);
if (x + 1 < Columns)
builder.Append(" ");
}
builder.AppendLine();
}
File.WriteAllText(fileName, builder.ToString());
FileName = fileName;
Dirty = false;
}
public Color GetColor(double val)
{
return Palette.GetColor(MapToUnit(val));
}
public Bitmap ToImage(DmpPalette palette)
{
if (Columns == 0 && Rows == 0)
return new Bitmap(1, 1);
var result = new Bitmap(Columns, Rows);
for (var x = 0; x < Columns; x++)
for (var y = 0; y < Rows; y++)
result.SetPixel(x, y, palette.GetColor(MapToUnit(Data[x, y])));
return result;
}
public Bitmap ToImage()
{
if (Columns == 0 && Rows == 0)
return new Bitmap(1, 1);
var result = new Bitmap(Columns, Rows);
for (var x = 0; x < Columns; x++)
for (var y = 0; y < Rows; y++)
result.SetPixel(x, y, GetColor(Data[x, y]));
return result;
}
public static bool IsImageFile(string fileName)
{
var ext = Path.GetExtension(fileName).ToLowerInvariant();
return ext.EndsWith("tif") || ext.EndsWith("tiff") || ext.EndsWith("png") || ext.EndsWith("jpg") ||
ext.EndsWith("jpeg") || ext.EndsWith("bmp");
}
public static DmpModel FromFile(string filename)
{
if (IsImageFile(filename))
return FromImage(filename);
var lines = File.ReadAllLines(filename);
var rows = 0;
var cols = 0;
if (lines.Length > 0)
{
var dims = lines[0].Split(new[] {' '}, StringSplitOptions.RemoveEmptyEntries);
if (dims.Length > 1)
{
int.TryParse(dims[0], out cols);
int.TryParse(dims[1], out rows);
}
}
var model = new DmpModel(cols, rows)
{
Min = double.MaxValue,
Max = double.MinValue,
FileName = filename
};
var start = 2;
if (lines.Length > 1)
{
var dims = lines[1].Split(new[] {' '}, StringSplitOptions.RemoveEmptyEntries);
if (dims.Length == 4)
{
double temp;
if (double.TryParse(dims[0], out temp))
model.MinX = temp;
if (double.TryParse(dims[1], out temp))
model.MaxX = temp;
if (double.TryParse(dims[2], out temp))
model.MinY = temp;
if (double.TryParse(dims[3], out temp))
model.MaxY = temp;
}
else
{
// old format
model.MinX = 0;
model.MinY = 0;
model.MaxX = model.Columns;
model.MaxY = model.Rows;
start = 1;
}
}
for (var y = start; y < Math.Min(rows + start, lines.Length); y++)
{
var entries = lines[y].Split(new[] {' '}, StringSplitOptions.RemoveEmptyEntries);
for (var x = 0; x < Math.Min(cols, entries.Length); x++)
{
double current;
if (double.TryParse(entries[x], out current))
{
model[x, y - start] = current;
}
}
}
if (Math.Abs(model.Min - model.Max) < 1e-10)
{
model.Max = model.Min + 10;
}
model.Dirty = false;
return model;
}
public static DmpModel FromImage(Bitmap bmp, Func<Color,double> mapper = null)
{
if (bmp == null) return null;
//var image = mapper == null ? ToGrayScale(bmp) : bmp;
var image = bmp;
var dmp = new DmpModel(image.Width, image.Height);
for (var y = 0; y < dmp.Rows; ++y)
for (var x = 0; x < dmp.Columns; ++x)
if (mapper == null)
dmp[x, y] = image.GetPixel(x, y).R;
else
dmp[x, y] = mapper(image.GetPixel(x, y));
dmp.Dirty = false;
return dmp;
}
public static DmpModel FromImage(string filename)
{
var bmp = Image.FromFile(filename) as Bitmap;
return FromImage(bmp);
}
public double MapToUnit(double value)
{
if (value < Min) return 0;
if (value > Max) return 1;
if (Math.Abs(DataRange) < 1e-10) return 0;
return (value-Min)/DataRange;
}
public double MapFromUnit(double value)
{
return value*DataRange + Min;
}
private Rectangle FindDimensions()
{
var minX = Columns;
var maxX = 0;
var minY = Rows;
var maxY = 0;
for (var y = 0; y < Rows; ++y)
for (var x = 0; x < Columns; ++x)
{
var current = Data[x, y];
if (Math.Abs(current) > 1e-10)
{
if (x < minX)
minX = x;
if (x > maxX)
maxX = x;
if (y < minY)
minY = y;
if (y > maxY)
maxY = y;
}
}
return new Rectangle(minX, minY, maxX, maxY);
}
public void Center()
{
// find dimensions of non-zero entries
var used = FindDimensions();
//Data[used.Y, used.X] = 3;
//Data[used.Height, used.Width] = 5;
var total = new Rectangle(0, 0, Columns, Rows);
var endDiff = new Size(total.Width - used.Width, total.Height - used.Height);
var newStart = new Size(
(int) Math.Floor((endDiff.Width + used.X)/2.0),
(int) Math.Floor((endDiff.Height + used.Y)/2.0));
//Data[newStart.Height, newStart.Width] = 7;
var difference = new Size(newStart.Width - used.X, newStart.Height - used.Y);
// Data[difference.Width, difference.Height] = 7;
var data = new double[Columns, Rows];
for (var y = used.Y; y <= used.Height; y++)
for (var x = used.X; x <= used.Width; x++)
data[x + difference.Width, y + difference.Height] = Data[x, y];
Data = data;
}
public void Transform(Func<double, double> transformation)
{
if (transformation == null) return;
for (var y = 0; y < Rows; ++y)
for (var x = 0; x < Columns; ++x)
Data[x, y] = transformation(Data[x, y]);
}
public static DmpModel operator +(DmpModel model, double value)
{
var tmp = (DmpModel) model.Clone();
tmp.Transform(x => x + value);
return tmp;
}
public static DmpModel operator +(DmpModel model, DmpModel other)
{
var tmp = (DmpModel)model.Clone();
tmp.Resize(other.Columns, other.Rows);
for (var y = 0; y < other.Rows; ++y)
for (var x = 0; x < other.Columns; ++x)
tmp[x, y] = tmp[x, y] + other[x, y];
return tmp;
}
public static DmpModel operator *(DmpModel model, double value)
{
var tmp = (DmpModel) model.Clone();
tmp.Transform(x => x*value);
return tmp;
}
public void MaskWith(DmpModel file)
{
file.Resize(Columns, Rows);
for (var y = 0; y < Rows; ++y)
for (var x = 0; x < Columns; ++x)
this[x, y] = file[x,y] * Data[x, y];
}
/// <summary>
/// Convert image to grayscale, from:
///
/// http://tech.pro/tutorial/660/csharp-tutorial-convert-a-color-image-to-grayscale
///
/// </summary>
/// <param name="original"></param>
/// <returns></returns>
public static Bitmap ToGrayScale(Bitmap original)
{
//create a blank bitmap the same size as original
Bitmap newBitmap = new Bitmap(original.Width, original.Height);
//get a graphics object from the new image
Graphics g = Graphics.FromImage(newBitmap);
//create the grayscale ColorMatrix
ColorMatrix colorMatrix = new ColorMatrix(
new float[][]
{
new float[] {.3f, .3f, .3f, 0, 0},
new float[] {.59f, .59f, .59f, 0, 0},
new float[] {.11f, .11f, .11f, 0, 0},
new float[] {0, 0, 0, 1, 0},
new float[] {0, 0, 0, 0, 1}
});
//create some image attributes
ImageAttributes attributes = new ImageAttributes();
//set the color matrix attribute
attributes.SetColorMatrix(colorMatrix);
//draw the original image on the new image
//using the grayscale color matrix
g.DrawImage(original, new Rectangle(0, 0, original.Width, original.Height),
0, 0, original.Width, original.Height, GraphicsUnit.Pixel, attributes);
//dispose the Graphics object
g.Dispose();
return newBitmap;
}
}
}
<file_sep>/WFEditSpatial/Forms/FormErrors.cs
using System;
using System.Windows.Forms;
using EditSpatial.Model;
using libsbmlcs;
namespace EditSpatial.Forms
{
public partial class FormErrors : Form
{
public FormErrors()
{
InitializeComponent();
}
private SpatialModel Model { get; set; }
public string Label
{
get { return label1.Text; }
set { label1.Text = value; }
}
private void OnValidateClick(object sender, EventArgs e)
{
ReValidate();
}
private void ReValidate()
{
if (Model == null || Model.Document == null) return;
Model.Document.clearValidators();
Model.Document.addValidator(SpatialModel.CustomSpatialValidator);
Model.Document.validateSBML();
InitializeFrom(Model);
}
public void InitializeFrom(SpatialModel model)
{
Model = model;
var haveNoDocument = model == null || model.Document == null;
var errors = haveNoDocument
? "No document loaded ..."
: model.Document.getErrorLog().toString().Replace("\n", Environment.NewLine);
controlText1.Text = errors;
if (haveNoDocument)
{
label1.Text = "";
return;
}
var numWarnings = model.Document.getNumErrors(libsbml.LIBSBML_SEV_WARNING);
var numErrors = model.Document.getNumErrors(libsbml.LIBSBML_SEV_ERROR);
var numFatals = model.Document.getNumErrors(libsbml.LIBSBML_SEV_FATAL);
if (numErrors + numFatals > 0)
{
label1.Text = "The document is invalid SBML.";
}
else if (numWarnings > 0)
{
label1.Text = "The document is valid SBML, but there were warnings.";
}
else
{
label1.Text = "The document is valid SBML.";
}
label1.Text += string.Format(" Warning(s): {0}, Error(s): {1}, Fatal Error(s): {2}", numWarnings, numErrors,
numFatals);
}
private void OnCloseClick(object sender, EventArgs e)
{
Hide();
}
private void OnFixCommonErrors(object sender, EventArgs e)
{
if (Model == null) return;
Model.FixCommonErrors();
ReValidate();
}
private void OnFormClosing(object sender, FormClosingEventArgs e)
{
e.Cancel = true;
Hide();
}
}
}<file_sep>/WFEditSpatial/Forms/FormPrepareDune.cs
using System;
using System.Diagnostics;
using System.IO;
using System.Linq;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
using EditSpatial.Model;
using LibEditSpatial.Dialogs;
using LibEditSpatial.Model;
namespace EditSpatial.Forms
{
public partial class FormPrepareDune : Form
{
private bool closing;
private Process process;
public FormPrepareDune()
{
InitializeComponent();
}
public string BuildDir
{
get { return Path.Combine(Target, "build"); }
}
public string Target
{
get
{
return Path.Combine(TargetDir, ModuleName);
}
}
public string TargetDir
{
get { return txtTargetDir.Text; }
set { txtTargetDir.Text = value; }
}
public string ModuleName
{
get { return txtName.Text; }
set { txtName.Text = value; }
}
public EditSpatialSettings Settings { get; set; }
public SpatialModel Model { get; set; }
private void OnBrowseClicke(object sender, EventArgs e)
{
txtTargetDir.Text = LibEditSpatial.Util.GetDir(txtTargetDir.Text);
}
private void DoCreateHost()
{
Util.UnzipArchive(
Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "host.zip"), Target);
txtResult.Text = "Host created in: " + Target;
}
private void OnCreateHostClick(object sender, EventArgs e)
{
if (Directory.Exists(Target))
{
var result = MessageBox.Show(
string.Format(
"The target directory '{0}' already consists if you continue, do you want to delete the directory before continuing?",
Target),
"Target dir exists", MessageBoxButtons.YesNo, MessageBoxIcon.Question);
if (result != DialogResult.Yes)
return;
try
{
Directory.Delete(Target, true);
}
catch
{
}
}
DoCreateHost();
}
private async Task RunCMake(string buildDir)
{
if (process != null) return;
var info = new ProcessStartInfo
{
FileName = Path.Combine(Settings.CygwinDir, "bash"),
Arguments = string.Format(
"-c \"cmake -DCMAKE_BUILD_TYPE=Release -DCMAKE_INSTALL_PREFIX={0} {1}\"",
LibEditSpatial.Util.ToCygwin(Settings.DuneDir),
LibEditSpatial.Util.ToCygwin(Target)
),
WorkingDirectory = buildDir,
RedirectStandardError = true,
RedirectStandardOutput = true,
UseShellExecute = false,
CreateNoWindow = true
};
#pragma warning disable 4014
await StartProcess(info);
#pragma warning restore 4014
}
private async Task RunMake(string buildDir)
{
if (process != null) return;
var info = new ProcessStartInfo
{
FileName = Path.Combine(Settings.CygwinDir, "bash"),
Arguments =
"-c make",
WorkingDirectory = buildDir,
RedirectStandardError = true,
RedirectStandardOutput = true,
UseShellExecute = false,
CreateNoWindow = true
};
#pragma warning disable 4014
await StartProcess(info);
#pragma warning restore 4014
DlgRun.WriteBatchFiles(buildDir, Settings.CygwinDir);
}
private void ReEnableUI()
{
process = null;
}
private void OnAddString(string data)
{
if (closing) return;
if (string.IsNullOrEmpty(data)) return;
if (InvokeRequired)
{
Invoke(new VoidStringDelegate(OnAddString), data);
return;
}
txtResult.AppendText(data.Replace("\n", Environment.NewLine) + Environment.NewLine);
}
public async Task StartProcess(ProcessStartInfo info)
{
if (!string.IsNullOrEmpty(Settings.CygwinDir))
info.EnvironmentVariables["PATH"] = Settings.CygwinDir + ";" + info.EnvironmentVariables["PATH"];
process = new Process {StartInfo = info, EnableRaisingEvents = true};
process.Exited += (o, e1) => ReEnableUI();
process.OutputDataReceived += (o, e2) => OnAddString(e2.Data);
process.ErrorDataReceived += (o, e3) => OnAddString(e3.Data);
process.Start();
Thread.Sleep(100);
process.BeginOutputReadLine();
process.BeginErrorReadLine();
await Task.Run(() => process.WaitForExit());
}
private void DoCompile()
{
// create build dir
if (!Directory.Exists(BuildDir))
Directory.CreateDirectory(BuildDir);
// run cmake
Task.Factory.StartNew(() => RunCMake(BuildDir)
.ContinueWith(prevTask => RunMake(BuildDir)));
}
private void OnCompileModel(object sender, EventArgs e)
{
if (!Directory.Exists(Target))
{
MessageBox.Show("Please create hosting application first", "Hosting application not present.",
MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
txtResult.Text = "Compiling Model";
DoCompile();
}
private void DoExport()
{
// export model into host/src
Model.ExportToDune(Path.Combine(Path.Combine(Target, "src"), "host.cc"));
txtResult.Text = "Dune model exported into: " + Path.Combine(Target, "src");
}
private void OnExportModel(object sender, EventArgs e)
{
if (!Directory.Exists(Target))
{
MessageBox.Show("Please create hosting application first", "Hosting application not present.",
MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
DoExport();
}
private void OnFormClosing(object sender, FormClosingEventArgs e)
{
closing = true;
}
private DuneConfig GetConfigFromDir(string directory)
{
if (!Directory.Exists(directory)) return null;
var files = Directory.GetFiles(directory, "*.conf", SearchOption.TopDirectoryOnly);
if (files.Length > 0)
{
var file = files.FirstOrDefault(f => f.Contains("temp.conf"));
if (string.IsNullOrEmpty(file))
file = files[0];
return DuneConfig.FromFile(file);
}
return null;
}
private void OnRunClick(object sender, EventArgs e)
{
if (!Directory.Exists(Target))
{
MessageBox.Show("Please create hosting application first", "Hosting application not present.",
MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
if (!Directory.Exists(BuildDir))
{
MessageBox.Show("Please compile application first", "Application not compiled.",
MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
var runDir = Path.Combine(BuildDir, "src");
var config = GetConfigFromDir(runDir);
if (config != null)
using (var dlg = new DlgRun
{
CygwinDir = Settings.CygwinDir,
ParaViewDir = Settings.ParaViewDir,
Config = config,
FileName = Directory.GetFiles(runDir, "*.exe", SearchOption.TopDirectoryOnly).FirstOrDefault()
})
{
dlg.ShowDialog(this);
}
}
private void OnEditConfig(object sender, EventArgs e)
{
if (!Directory.Exists(Target))
{
MessageBox.Show("Please create hosting application first", "Hosting application not present.",
MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
if (!Directory.Exists(Path.Combine(Target, "build")))
{
MessageBox.Show("Please compile application first", "Application not compiled.",
MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
var runDir = Path.Combine(BuildDir, "src");
var config = GetConfigFromDir(runDir);
if (config != null)
using (var dlg = new WFDuneRunner.MainForm())
{
dlg.OpenFile(config.FileName);
dlg.ShowDialog(this);
}
}
private void cmdFolder_Click(object sender, EventArgs e)
{
try
{
Process.Start(Path.Combine(txtTargetDir.Text, txtName.Text));
}
catch
{
}
}
}
}<file_sep>/WFEditSpatial/Controls/ControlRules.cs
using libsbmlcs;
namespace EditSpatial.Controls
{
public partial class ControlRules : BaseSpatialControl
{
public ControlRules()
{
InitializeComponent();
}
private SBMLDocument Current { get; set; }
private static string RuleName(int typeCode)
{
if (typeCode == libsbml.SBML_ALGEBRAIC_RULE)
return "AlgebraicRule";
if (typeCode == libsbml.SBML_RATE_RULE)
return "RateRule";
return "AssignmentRule";
}
public void InitializeFrom(SBMLDocument document)
{
grid.Rows.Clear();
Current = document;
if (document == null || document.getModel() == null) return;
var model = document.getModel();
for (long i = 0; i < model.getNumRules(); ++i)
{
var current = model.getRule(i);
grid.Rows.Add(current.getVariable(),
current.isSetMath() ? libsbml.formulaToL3String(current.getMath()) : "",
RuleName(current.getTypeCode()));
}
}
private Rule CreateRule(libsbmlcs.Model model, string type)
{
if (type == "AlgebraicRule")
return model.createAlgebraicRule();
if (type == "RateRule")
return model.createRateRule();
return model.createAssignmentRule();
}
public override void SaveChanges()
{
if (Current == null) return;
for (var i = 0; i < grid.Rows.Count; ++i)
{
var row = grid.Rows[i];
var value = (string) row.Cells[1].Value;
if (string.IsNullOrEmpty(value)) continue;
var node = libsbml.parseL3Formula(value);
if (node == null) continue;
var current = Current.getModel().getRuleByVariable((string) row.Cells[0].Value);
if (current == null)
{
current = CreateRule(Current.getModel(), (string) row.Cells[2].Value);
}
current.setVariable((string) row.Cells[0].Value);
current.setMath(node);
}
OnModelChanged();
}
public override void InvalidateSelection()
{
Current = null;
InitializeFrom(null);
}
}
}<file_sep>/LibEditSpatial/Model/NewtonConfig.cs
using System.Collections.Generic;
namespace LibEditSpatial.Model
{
public class NewtonConfig
{
public int LinearVerbosity { get; set; }
public double ReassembleThreshold { get; set; }
public int LineSearchMaxIterations { get; set; }
public int MaxIterations { get; set; }
public double AbsoluteLimit { get; set; }
public double Reduction { get; set; }
public double LinearReduction { get; set; }
public double LineSearchDampingFactor { get; set; }
public int Verbosity { get; set; }
public static NewtonConfig Default
{
get
{
return new NewtonConfig
{
LinearVerbosity = 0,
ReassembleThreshold = 0,
LineSearchMaxIterations = 5,
MaxIterations = 30,
AbsoluteLimit = 1e-18,
Reduction = 1e-8,
LinearReduction = 1e-4,
LineSearchDampingFactor = 0.5,
Verbosity = 0
};
}
}
public static NewtonConfig FromDict(Dictionary<string, string> dict)
{
var result = new NewtonConfig
{
LinearVerbosity = dict.Get<int>("LinearVerbosity"),
ReassembleThreshold = dict.Get<double>("ReassembleThreshold"),
LineSearchMaxIterations = dict.Get<int>("LineSearchMaxIterations"),
MaxIterations = dict.Get<int>("MaxIterations"),
AbsoluteLimit = dict.Get<double>("AbsoluteLimit"),
Reduction = dict.Get<double>("Reduction"),
LinearReduction = dict.Get<double>("LinearReduction"),
LineSearchDampingFactor = dict.Get<double>("LineSearchDampingFactor"),
Verbosity = dict.Get<int>("Verbosity")
};
return result;
}
public Dictionary<string, string> ToDict()
{
var result = new Dictionary<string, string>();
result["LinearVerbosity"] = LinearVerbosity.ToString();
result["ReassembleThreshold"] = ReassembleThreshold.ToString();
result["LineSearchMaxIterations"] = LineSearchMaxIterations.ToString();
result["MaxIterations"] = MaxIterations.ToString();
result["AbsoluteLimit"] = AbsoluteLimit.ToString();
result["Reduction"] = Reduction.ToString();
result["LinearReduction"] = LinearReduction.ToString();
result["LineSearchDampingFactor"] = LineSearchDampingFactor.ToString();
result["Verbosity"] = Verbosity.ToString();
return result;
}
}
}<file_sep>/WFEditSpatial/Templates/local_operator.hh
// -*- tab-width: 4; indent-tabs-mode: nil; c-basic-offset: 2 -*-
// vi: set ts=4 sw=2 et sts=2:
#ifndef DUNE_PDELAB_MULTICOMPONENTTRANSPORTOP_HH
#define DUNE_PDELAB_MULTICOMPONENTTRANSPORTOP_HH
#include<dune/common/exceptions.hh>
#include<dune/common/fvector.hh>
#include<dune/common/static_assert.hh>
#include <dune/geometry/referenceelements.hh>
#include<dune/pdelab/common/geometrywrapper.hh>
#include<dune/pdelab/common/function.hh>
#include<dune/pdelab/localoperator/defaultimp.hh>
#include<dune/pdelab/localoperator/pattern.hh>
#include<dune/pdelab/localoperator/flags.hh>
#include<dune/pdelab/localoperator/idefault.hh>
#define ANALYTICAL_JACOBIAN FALSE
namespace Dune {
namespace PDELab {
// reaction base class
// do not compute anything
class ReactionBaseAdapter
{
public:
//! constructor stores reference to the model
ReactionBaseAdapter()
{}
//! do one step
template<typename EG, typename LFSU, typename X, typename LFSV, typename R, typename DomainType>
void evaluate(const EG& eg, const LFSU& lfsu, const X& x, const LFSV& lfsv, R& r, const DomainType& x_)
{
}
template<typename RF>
void preStep(RF time, RF dt, int stages)
{
}
};
//! traits class for two phase parameter class
template<typename GV, typename RF>
struct DiffusionParameterTraits
{
//! \brief the grid view
typedef GV GridViewType;
//! \brief Enum for domain dimension
enum {
//! \brief dimension of the domain
dimDomain = GV::dimension
};
//! \brief Export type for domain field
typedef typename GV::Grid::ctype DomainFieldType;
//! \brief domain type
typedef Dune::FieldVector<DomainFieldType, dimDomain> DomainType;
//! \brief domain type
typedef Dune::FieldVector<DomainFieldType, dimDomain - 1> IntersectionDomainType;
//! \brief Export type for range field
typedef RF RangeFieldType;
//! \brief range type
typedef Dune::FieldVector<RF, GV::dimensionworld> RangeType;
//! grid types
typedef typename GV::Traits::template Codim<0>::Entity ElementType;
typedef typename GV::Intersection IntersectionType;
/** \brief Class to define the boundary condition types
*/
struct ConvectionDiffusionBoundaryConditions
{
enum Type { Dirichlet = 1, Neumann = -1, Outflow = -2, None = -3 }; // BC requiring constraints must be >0 if
// constraints assembler coming with PDELab is used
};
typedef typename ConvectionDiffusionBoundaryConditions::Type BCType;
};
//! base class for parameter class
template<class T, class Imp>
class DiffusionMulticomponentInterface
{
public:
typedef T Traits;
typedef typename Traits::BCType BCType;
//! scalar diffusion coefficient
typename Traits::RangeFieldType
D(const typename Traits::ElementType& e, const typename Traits::DomainType& x, std::size_t i) const
{
return asImp().component(i).D(e, x);
}
//! source term
typename Traits::RangeFieldType
q(const typename Traits::ElementType& e, const typename Traits::DomainType& x, std::size_t i) const
{
return asImp().component(i).q(e, x);
}
BCType
bctype(const typename Traits::IntersectionType& is, const typename Traits::IntersectionDomainType& x, std::size_t i) const
{
return asImp().component(i).bctype(is, x);
}
//! Dirichlet boundary condition on inflow
typename Traits::RangeFieldType
g(const typename Traits::IntersectionType& is, const typename Traits::IntersectionDomainType& x, std::size_t i) const
{
return asImp().component(i).g(is, x);
}
//! Neumann boundary condition
typename Traits::RangeFieldType
j(const typename Traits::IntersectionType& is, const typename Traits::IntersectionDomainType& x, std::size_t i) const
{
return asImp().component(i).j(is, x);
}
template<std::size_t i>
void setTime(typename Traits::RangeFieldType t)
{
asImp().setTime(t);
}
private:
Imp& asImp() { return static_cast<Imp &> (*this); }
const Imp& asImp() const { return static_cast<const Imp &>(*this); }
};
/** a local operator for a cell-centered finite folume scheme for
the diffusion-reaction equation
\nabla \cdot \{v u- D \nabla u \} = q in \Omega
u = g on \Gamma_D
\{v u - D \nabla u \} \cdot \nu = j on \Gamma_N
outflow on \Gamma_O
Modified version for the case
d_t (c(x,t)u(x,t)) + \nabla \cdot \{v u - D \nabla u \} = q in \Omega
where c(x,t) may become zero. We assume that the following holds:
c(x,t+dt) <= eps ==> c(x,t) <= eps
\tparam TP parameter class implementing ComponentDiffusionParameterInterface
*/
template<typename TP, typename RA = ReactionBaseAdapter>
class MulticomponentCCFVSpatialDiffusionOperator :
public NumericalJacobianVolume<MulticomponentCCFVSpatialDiffusionOperator<TP, RA> >,
public NumericalJacobianApplyVolume<MulticomponentCCFVSpatialDiffusionOperator<TP, RA> >,
public NumericalJacobianSkeleton<MulticomponentCCFVSpatialDiffusionOperator<TP, RA> >,
public NumericalJacobianBoundary<MulticomponentCCFVSpatialDiffusionOperator<TP, RA> >,
public NumericalJacobianApplySkeleton<MulticomponentCCFVSpatialDiffusionOperator<TP, RA> >,
public NumericalJacobianApplyBoundary<MulticomponentCCFVSpatialDiffusionOperator<TP, RA> >,
public FullSkeletonPattern,
public FullVolumePattern,
public LocalOperatorDefaultFlags,
public InstationaryLocalOperatorDefaultMethods<typename TP::Traits::RangeFieldType>
{
public:
// pattern assembly flags
enum { doPatternVolume = true };
enum { doPatternSkeleton = true };
// residual assembly flags
enum { doAlphaVolume = true };
enum { doAlphaSkeleton = true };
enum { doAlphaBoundary = true };
enum { dim = TP::Traits::GridViewType::dimension };
typedef typename TP::BCType BCType;
MulticomponentCCFVSpatialDiffusionOperator(TP& tp_)
: tp(tp_), ra(raDefault())
{
}
MulticomponentCCFVSpatialDiffusionOperator(TP& tp_, RA& ra_)
: tp(tp_), ra(ra_)
{
}
// volume integral depending on test and ansatz functions
template<typename EG, typename LFSU, typename X, typename LFSV, typename R>
void alpha_volume(const EG& eg, const LFSU& lfsu, const X& x, const LFSV& lfsv, R& r) const
{
typedef typename LFSV::template Child<0>::Type Space;
// domain and range field type
typedef typename Space::Traits::FiniteElementType::
Traits::LocalBasisType::Traits::DomainFieldType DF;
// dimensions
const int dim = EG::Geometry::dimension;
// cell center
const Dune::FieldVector<DF, dim>&
inside_local = Dune::ReferenceElements<DF, dim>::general(eg.entity().type()).position(0, 0);
// here could described the source (right hand side of the sytem of equations)
// for each equation (big ammount of code)
for (std::size_t k = 0; k<TP::COMPONENTS; k++)
{
// evaluate source term
typename TP::Traits::RangeFieldType q = tp.q(eg.entity(), inside_local, k);
r.accumulate(lfsv, k, -q*eg.geometry().volume());
}
ra.evaluate(eg.entity(), lfsu, x, lfsv, r, inside_local);
}
// skeleton integral depending on test and ansatz functions
// We put the Dirchlet evaluation also in the alpha term to save some geometry evaluations
template<typename IG, typename LFSU, typename X, typename LFSV, typename R>
void alpha_boundary(const IG& ig,
const LFSU& lfsu_s, const X& x_s, const LFSV& lfsv_s,
R& r_s) const
{
typedef typename LFSV::template Child<0>::Type Space;
// domain and range field type
typedef typename Space::Traits::FiniteElementType::
Traits::LocalBasisType::Traits::DomainFieldType DF;
typedef typename Space::Traits::FiniteElementType::
Traits::LocalBasisType::Traits::RangeFieldType RF;
// face geometry
const Dune::FieldVector<DF, IG::dimension - 1>&
face_local = Dune::ReferenceElements<DF, IG::dimension - 1>::general(ig.geometry().type()).position(0, 0);
RF face_volume = ig.geometry().volume();
const Dune::FieldVector<DF, IG::dimension>&
inside_local = Dune::ReferenceElements<DF, IG::dimension>::general(ig.inside()->type()).position(0, 0);
Dune::FieldVector<DF, IG::dimension>
inside_global = ig.inside()->geometry().center();
Dune::FieldVector<DF, IG::dimension>
outside_global = ig.geometry().center();
inside_global -= outside_global;
RF distance = inside_global.two_norm();
for (std::size_t k = 0; k<TP::COMPONENTS; k++)
{
typename TP::Traits::RangeFieldType D_inside = tp.D(*(ig.inside()), inside_local, k);
// evaluate boundary condition type
BCType bc = tp.bctype(ig.intersection(), face_local, k);
// do things depending on boundary condition type
if (bc == BCType::Neumann) // Neumann boundary
{
typename TP::Traits::RangeFieldType j = tp.j(ig.intersection(), face_local, k);
r_s.accumulate(lfsu_s, k, j*face_volume);
}
if (bc == BCType::Dirichlet) // Dirichlet boundary
{
typename TP::Traits::RangeFieldType g;
g = tp.g(ig.intersection(), face_local, k);
r_s.accumulate(lfsu_s, k, (-D_inside*(g - x_s(lfsu_s, k)) / distance)*face_volume);
}
}
}
#ifdef ANALYTICAL_JACOBIAN
// jacobian of boundary term
template<typename IG, typename LFSU, typename X, typename LFSV, typename M>
void jacobian_boundary(const IG& ig,
const LFSU& lfsu_s, const X& x_s, const LFSV& lfsv_s,
M& mat_ss) const
{
typedef typename LFSV::template Child<0>::Type Space;
// domain and range field type
typedef typename Space::Traits::FiniteElementType::
Traits::LocalBasisType::Traits::DomainFieldType DF;
typedef typename Space::Traits::FiniteElementType::
Traits::LocalBasisType::Traits::RangeFieldType RF;
// face geometry
const Dune::FieldVector<DF, IG::dimension - 1>&
face_local = Dune::ReferenceElements<DF, IG::dimension - 1>::general(ig.geometry().type()).position(0, 0);
RF face_volume = ig.geometry().volume();
const Dune::FieldVector<DF, IG::dimension>&
inside_local = Dune::ReferenceElements<DF, IG::dimension>::general(ig.inside()->type()).position(0, 0);
Dune::FieldVector<DF, IG::dimension>
inside_global = ig.inside()->geometry().center();
Dune::FieldVector<DF, IG::dimension>
outside_global = ig.geometry().center();
inside_global -= outside_global;
RF distance = inside_global.two_norm();
for (std::size_t k = 0; k<TP::COMPONENTS; k++)
{
// evaluate boundary condition type
int bc = tp.bctype(ig.intersection(), face_local, k);
// do things depending on boundary condition type
if (bc == BCType::Neumann) // Neumann boundary
{
return;
}
if (bc == BCType::Dirichlet) // Dirichlet boundary
{
typename TP::Traits::RangeFieldType D_inside = tp.D(*(ig.inside()), inside_local, k);
mat_ss.accumulate(lfsu_s, k, lfsu_s, k, D_inside / distance*face_volume);
return;
}
}
}
#endif //ANALYTICAL_JACOBIAN
// skeleton integral depending on test and ansatz functions
// We put the Dirchlet evaluation also in the alpha term to save some geometry evaluations
template<typename IG, typename LFSU, typename X, typename LFSV, typename R>
void alpha_skeleton(const IG& ig,
const LFSU& lfsu_s, const X& x_s, const LFSV& lfsv_s,
const LFSU& lfsu_n, const X& x_n, const LFSV& lfsv_n,
R& r_s, R& r_n) const
{
typedef typename LFSV::template Child<0>::Type Space;
// domain and range field type
typedef typename Space::Traits::FiniteElementType::
Traits::LocalBasisType::Traits::DomainFieldType DF;
typedef typename Space::Traits::FiniteElementType::
Traits::LocalBasisType::Traits::RangeFieldType RF;
// face geometry
RF face_volume = ig.geometry().volume();
const Dune::FieldVector<DF, IG::dimension>&
inside_local = Dune::ReferenceElements<DF, IG::dimension>::general(ig.inside()->type()).position(0, 0);
const Dune::FieldVector<DF, IG::dimension>&
outside_local = Dune::ReferenceElements<DF, IG::dimension>::general(ig.outside()->type()).position(0, 0);
// distance between cell centers in global coordinates
Dune::FieldVector<DF, IG::dimension>
inside_global = ig.inside()->geometry().center();
Dune::FieldVector<DF, IG::dimension>
outside_global = ig.outside()->geometry().center();
inside_global -= outside_global;
RF distance = inside_global.two_norm();
for (std::size_t k = 0; k<TP::COMPONENTS; k++)
{
// evaluate diffusion coefficients
typename TP::Traits::RangeFieldType D_inside = tp.D(*(ig.inside()), inside_local, k);
typename TP::Traits::RangeFieldType D_outside = tp.D(*(ig.outside()), outside_local, k);
typename TP::Traits::RangeFieldType D_avg = 2.0 / (1.0 / (D_inside + 1E-40) + 1.0 / (D_outside + 1E-40));
// diffusive flux
r_s.accumulate(lfsu_s, k, -(D_avg*(x_n(lfsu_n, k) - x_s(lfsu_s, k)) / distance)*face_volume);
r_n.accumulate(lfsu_n, k, (D_avg*(x_n(lfsu_n, k) - x_s(lfsu_s, k)) / distance)*face_volume);
}
}
#ifdef ANALYTICAL_JACOBIAN
// jacobian of skeleton term
template<typename IG, typename LFSU, typename X, typename LFSV, typename M>
void jacobian_skeleton(const IG& ig,
const LFSU& lfsu_s, const X& x_s, const LFSV& lfsv_s,
const LFSU& lfsu_n, const X& x_n, const LFSV& lfsv_n,
M& mat_ss, M& mat_sn,
M& mat_ns, M& mat_nn) const
{
typedef typename LFSV::template Child<0>::Type Space;
// domain and range field type
typedef typename Space::Traits::FiniteElementType::
Traits::LocalBasisType::Traits::DomainFieldType DF;
typedef typename Space::Traits::FiniteElementType::
Traits::LocalBasisType::Traits::RangeFieldType RF;
// face geometry
RF face_volume = ig.geometry().volume();
const Dune::FieldVector<DF, IG::dimension>&
inside_local = Dune::ReferenceElements<DF, IG::dimension>::general(ig.inside()->type()).position(0, 0);
const Dune::FieldVector<DF, IG::dimension>&
outside_local = Dune::ReferenceElements<DF, IG::dimension>::general(ig.outside()->type()).position(0, 0);
// distance between cell centers in global coordinates
Dune::FieldVector<DF, IG::dimension>
inside_global = ig.inside()->geometry().center();
Dune::FieldVector<DF, IG::dimension>
outside_global = ig.outside()->geometry().center();
inside_global -= outside_global;
RF distance = inside_global.two_norm();
for (std::size_t k = 0; k<TP::COMPONENTS; k++)
{
// evaluate diffusion coefficients
typename TP::Traits::RangeFieldType D_inside = tp.D(*(ig.inside()), inside_local, k);
typename TP::Traits::RangeFieldType D_outside = tp.D(*(ig.outside()), outside_local, k);
typename TP::Traits::RangeFieldType D_avg = 2.0 / (1.0 / (D_inside + 1E-40) + 1.0 / (D_outside + 1E-40));
// diffusive flux
mat_ss.accumulate(lfsu_s, k, lfsu_s, k, D_avg / distance*face_volume);
mat_sn.accumulate(lfsu_n, k, lfsu_n, k, -D_avg / distance*face_volume);
mat_ns.accumulate(lfsu_s, k, lfsu_s, k, -D_avg / distance*face_volume);
mat_nn.accumulate(lfsu_n, k, lfsu_n, k, D_avg / distance*face_volume);
}
}
#endif //ANALYTICAL_JACOBIAN
//! to be called once before each time step
void preStep(typename TP::Traits::RangeFieldType time, typename TP::Traits::RangeFieldType dt,
int stages)
{
tp.preStep(time, dt, stages);
ra.preStep(time, dt, stages);
}
//! to be called once before each stage
void preStage(typename TP::Traits::RangeFieldType time, int r)
{
}
//! to be called once at the end of each stage
void postStage()
{
}
private:
static RA & raDefault()
{
static RA ra;
return ra;
}
TP& tp;
RA& ra;
};
/** a local operator for the storage operator
*
* \f{align*}{
\int_\Omega c(x,t) uv dx
* \f}
*
* version where c(x,t) may become zero.
*/
template<class TP>
class MulticomponentCCFVTemporalOperator
: public NumericalJacobianVolume<MulticomponentCCFVTemporalOperator<TP> >,
public NumericalJacobianApplyVolume<MulticomponentCCFVTemporalOperator<TP> >,
public FullVolumePattern,
public LocalOperatorDefaultFlags,
public InstationaryLocalOperatorDefaultMethods<typename TP::Traits::RangeFieldType>
{
public:
// pattern assembly flags
enum { doPatternVolume = true };
// residual assembly flags
enum { doAlphaVolume = true };
MulticomponentCCFVTemporalOperator(TP& tp_)
: tp(tp_)
{
}
// volume integral depending on test and ansatz functions
template<typename EG, typename LFSU, typename X, typename LFSV, typename R>
void alpha_volume(const EG& eg, const LFSU& lfsu, const X& x, const LFSV& lfsv, R& r) const
{
for (std::size_t k = 0; k<TP::COMPONENTS; k++)
{
// residual contribution
r.accumulate(lfsu, k, x(lfsu, k)*eg.geometry().volume());
}
}
#ifdef ANALYTICAL_JACOBIAN
// jacobian of volume term
template<typename EG, typename LFSU, typename X, typename LFSV, typename M>
void jacobian_volume(const EG& eg, const LFSU& lfsu, const X& x, const LFSV& lfsv,
M& mat) const
{
for (std::size_t k = 0; k<TP::COMPONENTS; k++)
{
// residual contribution
mat.accumulate(lfsu, k, lfsu, k, eg.geometry().volume());
}
}
#endif //ANALYTICAL_JACOBIAN
//! to be called once before each time step
void preStep(typename TP::Traits::RangeFieldType time, typename TP::Traits::RangeFieldType dt,
int stages)
{
tp.preStep(time, dt, stages);
tp.setTimeTarget(time, dt);
}
//! to be called once before each stage
void preStage(typename TP::Traits::RangeFieldType time, int r)
{
}
//! to be called once at the end of each stage
void postStage()
{
}
//suggest time step, asked after first stage
typename TP::Traits::RangeFieldType suggestTimestep(typename TP::Traits::RangeFieldType dt) const
{
return std::numeric_limits<typename TP::Traits::RangeFieldType>::max(); //initial value should be big enough
}
private:
TP& tp;
typename TP::Traits::RangeFieldType time;
};
}
}
#endif
<file_sep>/LibEditSpatial/Dialogs/FormSettings.cs
using System;
using System.Windows.Forms;
using LibEditSpatial.Model;
namespace LibEditSpatial.Dialogs
{
public partial class FormSettings : Form
{
private EditSpatialSettings _Settings;
public FormSettings()
{
InitializeComponent();
}
public EditSpatialSettings Settings
{
get { return _Settings; }
set
{
_Settings = value;
UpdateUI();
}
}
private void UpdateUI()
{
if (_Settings == null) return;
txtCygwin.Text = _Settings.CygwinDir;
txtParaviewDir.Text = _Settings.ParaViewDir;
txtDuneDir.Text = _Settings.DuneDir;
txtDefaultDir.Text = _Settings.DefaultDir;
chkIgnoreCompartments.Checked = _Settings.IgnoreMultiCompartments;
}
private void OnBrowseCygwin(object sender, EventArgs e)
{
if (_Settings == null) return;
_Settings.CygwinDir = Util.GetDir(_Settings.CygwinDir);
txtCygwin.Text = _Settings.CygwinDir;
}
private void OnBrowsePV(object sender, EventArgs e)
{
if (_Settings == null) return;
_Settings.ParaViewDir = Util.GetDir(_Settings.ParaViewDir);
txtParaviewDir.Text = _Settings.ParaViewDir;
}
private void OnBrowseDune(object sender, EventArgs e)
{
if (_Settings == null) return;
_Settings.DuneDir = Util.GetDir(_Settings.DuneDir);
txtDuneDir.Text = _Settings.DuneDir;
}
private void txtDuneDir_TextChanged(object sender, EventArgs e)
{
if (_Settings == null) return;
_Settings.DuneDir = txtDuneDir.Text;
}
private void txtParaviewDir_TextChanged(object sender, EventArgs e)
{
if (_Settings == null) return;
_Settings.ParaViewDir = txtParaviewDir.Text;
}
private void txtCygwin_TextChanged(object sender, EventArgs e)
{
if (_Settings == null) return;
_Settings.CygwinDir = txtCygwin.Text;
}
private void chkIgnoreCompartments_CheckedChanged(object sender, EventArgs e)
{
if (_Settings == null) return;
_Settings.IgnoreMultiCompartments = chkIgnoreCompartments.Checked;
}
private void txtDefaultDir_TextChanged(object sender, EventArgs e)
{
if (_Settings == null) return;
_Settings.DefaultDir = txtDefaultDir.Text;
}
private void cmdBrowseDefaultDir_Click(object sender, EventArgs e)
{
if (_Settings == null) return;
_Settings.DefaultDir = Util.GetDir(_Settings.DefaultDir);
txtDefaultDir.Text = _Settings.DefaultDir;
}
}
}<file_sep>/LibEditSpatial/Model/DmpPalette.cs
using System;
using System.Collections.Generic;
using System.Drawing;
using System.IO;
using System.Text;
namespace LibEditSpatial.Model
{
public class DmpPalette
{
private static DmpPalette _Default;
public DmpPalette()
{
Colors = new List<Color>();
}
public static DmpPalette Default
{
get
{
if (_Default == null)
_Default = new DmpPalette
{
IsFirstTransparent = true,
Colors = new List<Color>
{
Color.Transparent
,
Color.Red
,
Color.Blue
,
Color.Green
,
Color.Yellow
,
Color.Tomato
,
Color.DarkSalmon
,
Color.YellowGreen
,
Color.DarkTurquoise
,
Color.Khaki
,
Color.MediumSeaGreen
,
Color.Purple
,
Color.Black
}
};
return _Default;
}
set { _Default = value; }
}
public List<Color> Colors { get; set; }
public bool IsFirstTransparent { get; set; }
public Color GetColor(double val)
{
var index = Math.Max(Math.Min((int) Math.Round(val*Colors.Count), Colors.Count - 1), 0);
return Colors[index];
}
public Bitmap ToImage(Size? size = null)
{
if (size == null)
size = new Size {Width = 100, Height = 30};
var image = new Bitmap(size.Value.Width, size.Value.Height);
using (var graphics = Graphics.FromImage(image))
{
var length = size.Value.Width/(float) Colors.Count;
for (var i = 0; i < Colors.Count; ++i)
{
graphics.FillRectangle(new SolidBrush(Colors[i]), length*i, 0f, length, size.Value.Height);
}
}
return image;
}
public void SaveAs(string filename)
{
var builder = new StringBuilder();
foreach (var color in Colors)
{
builder.AppendLine(ToArgbString(color));
}
File.WriteAllText(filename, builder.ToString());
}
public static string ToArgbString(Color oColor)
{
return ByteToHex(oColor.A) +
ByteToHex(oColor.R) +
ByteToHex(oColor.G) +
ByteToHex(oColor.B);
}
public static string ByteToHex(byte oByte)
{
if (oByte == 0)
return "00";
var sResult = Convert.ToString(oByte, 16);
if (sResult.Length == 1)
sResult = "0" + sResult;
return sResult;
}
public static Color ParseARGB(String argbString)
{
var oResult = Color.Empty;
if (argbString == String.Empty) return Color.Empty;
try
{
if (argbString.Length == 8)
{
int nAlpha = Convert.ToInt16(argbString.Substring(0, 2), 16);
int nRed = Convert.ToInt16(argbString.Substring(2, 2), 16);
int nGreen = Convert.ToInt16(argbString.Substring(4, 2), 16);
int nBlue = Convert.ToInt16(argbString.Substring(6, 2), 16);
oResult = Color.FromArgb(nAlpha, nRed, nGreen, nBlue);
}
else if (argbString.Length == 6)
{
int nRed = Convert.ToInt16(argbString.Substring(0, 2), 16);
int nGreen = Convert.ToInt16(argbString.Substring(2, 2), 16);
int nBlue = Convert.ToInt16(argbString.Substring(4, 2), 16);
oResult = Color.FromArgb(255, nRed, nGreen, nBlue);
}
else
{
throw new Exception("The Color string has to be in ARGB");
}
}
catch (Exception)
{
}
return oResult;
}
public static DmpPalette FromFile(string fileName)
{
var lines = File.ReadAllLines(fileName);
var result = new DmpPalette();
foreach (var line in lines)
{
try
{
var color = ParseARGB(line.Replace("#", ""));
result.Colors.Add(color);
}
catch
{
}
}
return result;
}
public int GetIndex(Color color)
{
return Colors.IndexOf(color);
}
}
}<file_sep>/WFEditSpatial/Forms/FormInitSpatial.cs
using System;
using System.Drawing;
using System.Globalization;
using System.Windows.Forms;
using EditSpatial.Model;
using libsbmlcs;
namespace EditSpatial.Forms
{
public partial class FormInitSpatial : Form
{
private AnalyticGeometry _analyticGeometry;
private Geometry _geometry;
private libsbmlcs.Model _model;
private SampledFieldGeometry _sampleGeometry;
public FormInitSpatial()
{
InitializeComponent();
_model = null;
}
public CreateModel CreateModel
{
get
{
var result = new CreateModel
{
Geometry = GeometryModel
};
foreach (var item in lstSpatialSpecies.Items)
result.Species.Add(item as SpatialSpecies);
return result;
}
}
public GeometrySettings GeometryModel
{
get
{
var geom = new GeometrySettings
{
Xmax = Util.SaveDouble(txtDimX.Text, 50),
Ymax = Util.SaveDouble(txtDimY.Text, 50),
Type = GeometryType.Default
};
if (radAnalytic.Checked)
{
geom.Type = GeometryType.Analytic;
if (_analyticGeometry != null && _analyticGeometry.getNumAnalyticVolumes() > 0)
{
_analyticGeometry.ExpandMath();
for (var i = 0; i < _analyticGeometry.getNumAnalyticVolumes(); ++i)
{
var current = _analyticGeometry.getAnalyticVolume(i);
var math = libsbml.formulaToL3String(current.getMath());
if (math.Contains(" width "))
geom.UsedSymbols.Add("width");
if (math.Contains(" height "))
geom.UsedSymbols.Add("height");
if (math.Contains(" Xmax "))
geom.UsedSymbols.Add("Xmax");
if (math.Contains(" Ymax "))
geom.UsedSymbols.Add("Ymax");
if (math.Contains(" Xmin "))
geom.UsedSymbols.Add("Xmin");
if (math.Contains(" Ymin "))
geom.UsedSymbols.Add("Ymin");
}
}
}
if (radSample.Checked)
{
geom.Type = GeometryType.Sample;
}
return
geom;
}
}
public SpatialModel SpatialModel { get; set; }
private void OnCancelClick(object sender, EventArgs e)
{
Close();
}
private void OnFinishClick(object sender, EventArgs e)
{
if (tabControl1.SelectedIndex == 1)
{
txtDimX.Text = txtWidth.Text;
txtDimY.Text = txtHeight.Text;
}
if (radAnalytic.Checked)
{
controlAnalyticGeometry1.SaveChanges();
}
if (radSample.Checked)
{
controlSampleFieldGeometry1.SaveChanges();
}
Close();
}
private void OnAllSpeciesDoubleClick(object sender, EventArgs e)
{
AddSelected();
}
private void OnSpatialSpeciesDoubleClick(object sender, EventArgs e)
{
RemoveSelected();
}
private void AddSelected()
{
var selected = lstAllSpecies.SelectedItem;
if (selected == null) return;
AddSelected(selected as string);
}
private void AddSelected(Species species)
{
if (species == null || _model == null) return;
var diffusionX = species.getDiffusionX();
var diffusionY = species.getDiffusionY();
var Xmax = species.getXMaxBC();
var Xmin = species.getXMinBC();
var Ymax = species.getYMaxBC();
var Ymin = species.getYMinBC();
var bcType = species.getBcType();
// assignments do not need to diffuse by themselves automatically
var defaultDiff =
species.getModel().
getAssignmentRuleByVariable(species.getId()) == null
? 0.001
: 0.0;
var spatialSpecies = new SpatialSpecies
{
Id = species.getId(),
DiffusionX = diffusionX.HasValue
? diffusionX.Value
: diffusionY.HasValue ? diffusionY.Value : defaultDiff,
DiffusionY = diffusionY.HasValue
? diffusionY.Value
: diffusionX.HasValue ? diffusionX.Value : defaultDiff,
MinBoundaryX = Xmin.HasValue ? Xmin.Value : 0,
MaxBoundaryX = Xmax.HasValue ? Xmax.Value : 0,
MinBoundaryY = Ymin.HasValue ? Ymin.Value : 0,
MaxBoundaryY = Ymax.HasValue ? Ymax.Value : 0,
BCType = bcType,
InitialCondition = species.getInitialExpession()
};
lstSpatialSpecies.Items.Add(spatialSpecies);
grid.Rows.Add(spatialSpecies.Id,
spatialSpecies.DiffusionX.ToString(CultureInfo.InvariantCulture),
spatialSpecies.DiffusionY.ToString(CultureInfo.InvariantCulture),
spatialSpecies.InitialCondition,
spatialSpecies.MaxBoundaryX.ToString(CultureInfo.InvariantCulture),
spatialSpecies.MaxBoundaryY.ToString(CultureInfo.InvariantCulture),
spatialSpecies.MinBoundaryX.ToString(CultureInfo.InvariantCulture),
spatialSpecies.MinBoundaryY.ToString(CultureInfo.InvariantCulture),
spatialSpecies.BCType);
}
private void AddSelected(string id)
{
if (id == null || _model == null) return;
if (lstSpatialSpecies.Items.Contains(id)) return;
AddSelected(_model.getSpecies(id));
}
private void RemoveSelected()
{
var selected = lstSpatialSpecies.SelectedItem;
if (selected == null) return;
RemoveSelected(selected as SpatialSpecies);
}
private void RemoveSelected(string id)
{
var species = GetSpecies(id);
RemoveSelected(species);
}
private SpatialSpecies GetSpecies(string id)
{
SpatialSpecies species = null;
for (var i = lstSpatialSpecies.Items.Count - 1; i >= 0; i--)
{
var current = lstSpatialSpecies.Items[i] as SpatialSpecies;
if (current != null && current.Id == id)
{
species = current;
break;
}
}
return species;
}
private void RemoveSelected(SpatialSpecies o)
{
if (o == null) return;
var current = o;
lstSpatialSpecies.Items.Remove(o);
for (var i = grid.Rows.Count - 1; i >= 0; i--)
{
if (current.Id == (string) grid.Rows[i].Cells[0].Value)
grid.Rows.RemoveAt(i);
}
}
private void OnFirstShown(object sender, EventArgs e)
{
UpdateUI();
}
private void UpdateUI()
{
if (SpatialModel == null ||
SpatialModel.Document == null ||
SpatialModel.Document.getModel() == null) return;
_model = SpatialModel.Document.getModel();
// Correct compartments & Transport
if (!MainForm.Settings.IgnoreMultiCompartments)
SpatialModel.CorrectCompartmentsAndTransport(_model);
txtDimX.Text = SpatialModel.Width.ToString();
txtDimY.Text = SpatialModel.Height.ToString();
lstSpatialSpecies.Items.Clear();
grid.Rows.Clear();
for (var i = 0; i < _model.getNumSpecies(); ++i)
{
var current = _model.getSpecies(i);
if (current == null) continue;
lstAllSpecies.Items.Add(current.getId());
if (current.getConstant() || current.getBoundaryCondition())
continue;
AddSelected(current);
}
var geom = SpatialModel.Geometry;
if (geom == null) return;
_analyticGeometry = geom.GetFirstAnalyticGeometry();
if (_analyticGeometry != null)
{
if (_analyticGeometry.getNumAnalyticVolumes() == 1 && _analyticGeometry.getAnalyticVolume(0).isSetMath()
&& (libsbml.formulaToL3String(_analyticGeometry.getAnalyticVolume(0).getMath()) == "1"))
radDefault.Checked = true;
else
radAnalytic.Checked = true;
}
_sampleGeometry = geom.GetFirstSampledFieldGeometry();
if (_sampleGeometry != null)
{
radSample.Checked = true;
}
}
private void ShowPage(int index)
{
if (index < 0) index = 0;
if (index >= tabControl1.TabCount) index = tabControl1.TabCount - 1;
var oldIndex = tabControl1.SelectedIndex;
if (oldIndex == index) return;
tabControl1.SelectedIndex = index;
}
private void OnPrevClick(object sender, EventArgs e)
{
ShowPage(tabControl1.SelectedIndex - 1);
}
private void OnNextClick(object sender, EventArgs e)
{
ShowPage(tabControl1.SelectedIndex + 1);
}
private void OnCellEndEdit(object sender, DataGridViewCellEventArgs e)
{
var row = grid.Rows[e.RowIndex];
var current = (string) row.Cells[0].Value;
var species = GetSpecies(current);
switch (e.ColumnIndex)
{
case 1:
{
species.DiffusionX = row.Cells[1].GetDouble();
break;
}
case 2:
{
species.DiffusionY = row.Cells[2].GetDouble();
break;
}
case 3:
{
var node = libsbml.parseL3Formula((string) row.Cells[3].Value);
if (node != null)
{
var formula = libsbml.formulaToL3String(node);
species.InitialCondition = formula;
row.Cells[3].Value = formula;
row.Cells[3].Style.BackColor = Color.LightGreen;
}
else
{
row.Cells[3].Style.BackColor = Color.Pink;
}
break;
}
case 4:
{
species.MaxBoundaryX = row.Cells[4].GetDouble();
break;
}
case 5:
{
species.MaxBoundaryY = row.Cells[5].GetDouble();
break;
}
case 6:
{
species.MinBoundaryX = row.Cells[6].GetDouble();
break;
}
case 7:
{
species.MinBoundaryY = row.Cells[7].GetDouble();
break;
}
case 8:
{
species.BCType = (string) row.Cells[8].Value;
break;
}
default:
break;
}
}
private void OnUserDeletingRow(object sender, DataGridViewRowCancelEventArgs e)
{
e.Cancel = true;
var id = (string) e.Row.Cells[0].Value;
RemoveSelected(id);
}
private void OnSelectedTabChanged(object sender, EventArgs e)
{
if (tabControl1.SelectedIndex == 0)
{
txtDimX.Text = txtWidth.Text;
txtDimY.Text = txtHeight.Text;
cmdNext.Enabled = true;
cmdPrev.Enabled = false;
}
else if (tabControl1.SelectedIndex == 1)
{
txtWidth.Text = txtDimX.Text;
txtHeight.Text = txtDimY.Text;
cmdNext.Enabled = false;
cmdPrev.Enabled = true;
}
}
private void radAnalytic_CheckedChanged(object sender, EventArgs e)
{
controlAnalyticGeometry1.Visible = true;
controlSampleFieldGeometry1.Visible = false;
if (!radAnalytic.Checked) return;
_geometry = SpatialModel.Geometry;
if (SpatialModel.Document == null)
return;
if (_geometry == null)
{
_geometry = new Geometry(3, 1);
}
SpatialModel.CreateCoordinateSystem(_geometry,
SpatialModel.Document.getModel(),
new GeometrySettings
{
Xmax = Util.SaveDouble(txtDimX.Text, 50),
Ymax = Util.SaveDouble(txtDimY.Text, 50)
}
);
_analyticGeometry = _geometry.GetFirstAnalyticGeometry();
if (_analyticGeometry == null)
{
_analyticGeometry = _geometry.createAnalyticGeometry();
var vol = _analyticGeometry.createAnalyticVolume();
vol.setId("vol0");
vol.setDomainType("");
vol.setFunctionType(libsbml.SPATIAL_FUNCTIONKIND_LAYERED);
vol.setOrdinal(0);
vol.setMath(libsbml.parseFormula("1"));
}
if (!_analyticGeometry.isSetId())
_analyticGeometry.setId("ana1");
controlAnalyticGeometry1.InitializeFrom(_geometry, _analyticGeometry);
}
private void radSample_CheckedChanged(object sender, EventArgs e)
{
controlAnalyticGeometry1.Visible = false;
controlSampleFieldGeometry1.Visible = true;
if (!radSample.Checked) return;
_geometry = SpatialModel.Geometry;
if (SpatialModel.Document == null) return;
if (_geometry == null)
{
_geometry = new Geometry(3, 1);
}
SpatialModel.CreateCoordinateSystem(_geometry,
SpatialModel.Document.getModel(),
new GeometrySettings
{
Xmax = Util.SaveDouble(txtDimX.Text, 50),
Ymax = Util.SaveDouble(txtDimY.Text, 50)
}
);
_sampleGeometry = _geometry.GetFirstSampledFieldGeometry();
if (_sampleGeometry == null)
{
_sampleGeometry = _geometry.createSampledFieldGeometry();
}
if (!_sampleGeometry.isSetId())
_sampleGeometry.setId("sample1");
controlSampleFieldGeometry1.InitializeFrom(_geometry, _sampleGeometry.getId());
}
private void radDefault_CheckedChanged(object sender, EventArgs e)
{
controlAnalyticGeometry1.Visible = false;
controlSampleFieldGeometry1.Visible = false;
}
private void OnApplyDiffClick(object sender, EventArgs e)
{
double defaultDiff;
if (!double.TryParse(txtDiffDefault.Text, out defaultDiff))
return;
for (var i = 0; i < grid.Rows.Count; ++i)
{
var current = grid.Rows[i];
if (current.IsNewRow) break;
current.Cells[1].Value = defaultDiff;
current.Cells[2].Value = defaultDiff;
var id = current.Cells[0].Value as string;
var species = GetSpecies(id);
if (species == null)
continue;
species.DiffusionX = defaultDiff;
species.DiffusionY = defaultDiff;
}
}
private void OnMakeIsotropic(object sender, EventArgs e)
{
for (var i = 0; i < grid.Rows.Count; ++i)
{
var current = grid.Rows[i];
if (current.IsNewRow) break;
current.Cells[2].Value = current.Cells[1].Value;
var id = current.Cells[0].Value as string;
var species = GetSpecies(id);
if (species == null)
continue;
species.DiffusionY = species.DiffusionX;
}
}
private void OnSortCompare(object sender, DataGridViewSortCompareEventArgs e)
{
if (e.CellValue1 == e.CellValue2)
{
e.SortResult = 0;
return;
}
if (e.CellValue1 == null)
{
e.SortResult = -1;
return;
}
if (e.CellValue2 == null)
{
e.SortResult = 1;
return;
}
e.SortResult = ((IComparable)e.CellValue1).CompareTo(e.CellValue2);
}
}
}<file_sep>/WFDuneRunner/MainForm.cs
using System;
using System.Collections.Generic;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Windows.Forms;
using libsbmlcs;
using LibEditSpatial.Dialogs;
using LibEditSpatial.Model;
using WFDuneRunner.Properties;
namespace WFDuneRunner
{
public partial class MainForm : Form
{
private bool _isLoading;
public MainForm()
{
InitializeComponent();
ctrlDomain1.OpenDmpFile += (o, e) => OpenDmpFile(e);
ctrlDomain1.BrowseDmpFile += (o, e) =>
{
string result;
if (BrowseDmpFile(out result))
{
ctrlDomain1.Model.Geometry = result;
ctrlDomain1.LoadModel(ctrlDomain1.Model);
}
};
NewModel();
}
public DuneConfig Config { get; set; }
public string FileName { get; set; }
public string CurrentDir { get; set; }
public string ExecutableFileName
{
get
{
var path = Path.GetDirectoryName(FileName);
if (path == null) return null;
var files = Directory.GetFiles(path, "*.exe");
if (files.Length == 0) return null;
return files[0];
}
}
public void OpenFile(string filename)
{
FileName = filename;
CurrentDir = Path.GetDirectoryName(filename);
Config = DuneConfig.FromFile(filename);
UpdateUI();
}
private string ConvertBCType(string bcString)
{
switch (bcString)
{
case "Dirichlet":
return "1";
default:
//case "Neumann":
return "-1";
case "Outflow":
return "-2";
case "None":
return "-3";
}
}
private string GetBcType(string bcCode)
{
switch (bcCode)
{
case "1":
return "Dirichlet";
default:
//case "-1":
return "Neumann";
case "-2":
return "Outflow";
case "-3":
return "None";
}
}
private void SetTitle()
{
if (string.IsNullOrWhiteSpace(FileName))
Text = "Dune Runner";
else
Text = string.Format("Dune Runner [{0}]", Path.GetFileName(FileName));
}
private void UpdateParameters()
{
gridParameters.Rows.Clear();
var parameters = Config["Reaction"];
foreach (var item in parameters)
{
gridParameters.Rows.Add(item.Key, item.Value);
}
}
private void UpdateVariables()
{
gridVariables.Rows.Clear();
var data = Config["Data"];
var varKeys = Config.GetVariableKeys();
foreach (var item in varKeys)
{
var entries = Config[item];
gridVariables.Rows.Add(item,
entries["D"],
entries.ContainsKey("BCType") ? GetBcType(entries["BCType"]) : "Neumann",
entries.ContainsKey("Xmin") ? entries["Xmin"] : "0",
entries.ContainsKey("Xmax") ? entries["Xmax"] : "0",
entries.ContainsKey("Ymin") ? entries["Ymin"] : "0",
entries.ContainsKey("Xmax") ? entries["Xmax"] : "0",
(data != null && data.ContainsKey(item)) ? data[item] : "",
null, null,
entries.ContainsKey("file_compartment") ? entries["file_compartment"] : "0"
);
}
}
private void UpdateCompartments()
{
gridCompartments.Rows.Clear();
foreach (var id in Config.CompartmentIds)
{
gridCompartments.Rows.Add(id, Config["Reaction"].Get("in_" + id, ""));
}
}
internal void UpdateUI()
{
SetTitle();
ctrlTime1.LoadModel(Config.TimeConfig);
ctrlDomain1.LoadModel(Config.DomainConfig);
ctrlNewton1.LoadModel(Config.NewtonConfig);
ctrlGlobal1.LoadModel(Config.GlobalConfig);
_isLoading = true;
UpdateParameters();
UpdateVariables();
UpdateCompartments();
cmdRun.Enabled = (!string.IsNullOrWhiteSpace(ExecutableFileName));
_isLoading = false;
}
private void NewModel()
{
FileName = null;
Config = new DuneConfig();
UpdateUI();
}
private void OnNewFile(object sender, EventArgs e)
{
NewModel();
}
private void OnOpenFile(object sender, EventArgs e)
{
using (var dialog = new OpenFileDialog
{
Title = "Open Config",
Filter = "Config files|*.conf|All files|*.*",
InitialDirectory = CurrentDir
})
{
if (dialog.ShowDialog() == DialogResult.OK)
OpenFile(dialog.FileName);
}
}
private void OnSaveFile(object sender, EventArgs e)
{
// SaveAs for now
// otherwise
// SaveFile(FileName);
OnSaveAs(sender, e);
}
private void OnAbout(object sender, EventArgs e)
{
using (var dlg = new AboutBox())
dlg.ShowDialog();
}
private void SaveFile(string fileName)
{
Config.SaveAs(fileName);
FileName = fileName;
CurrentDir = Path.GetDirectoryName(fileName);
UpdateUI();
}
private void OnSaveAs(object sender, EventArgs e)
{
using (var dialog = new SaveFileDialog
{
Title = "Save Config",
Filter = "Config files|*.conf|All files|*.*",
InitialDirectory = CurrentDir
})
{
if (dialog.ShowDialog() == DialogResult.OK)
SaveFile(dialog.FileName);
}
}
private void OnExit(object sender, EventArgs e)
{
Close();
}
private void OnRunClick(object sender, EventArgs e)
{
var fileName = ExecutableFileName;
if (fileName == null) return;
using (var dialog = new DlgRun
{
Config = Config,
FileName = fileName,
CygwinDir = Settings.CygwinDir,
ParaViewDir = Settings.ParaViewDir
})
{
dialog.ShowDialog(this);
}
}
private void OnVariableChanged(object sender, DataGridViewCellEventArgs e)
{
if (_isLoading) return;
if (e.RowIndex < 0) return;
var row = gridVariables.Rows[e.RowIndex];
if (row.IsNewRow) return;
var id = row.Cells[0].Value as string;
var entry = Config[id];
var data = Config["Data"];
switch (e.ColumnIndex)
{
case 1:
{
entry["D"] = row.Cells[1].Value as string;
break;
}
case 2:
{
entry["BCType"] = ConvertBCType(row.Cells[2].Value as string);
break;
}
case 3:
{
entry["Xmin"] = row.Cells[3].Value as string;
break;
}
case 4:
{
entry["Xmax"] = row.Cells[4].Value as string;
break;
}
case 5:
{
entry["Ymin"] = row.Cells[5].Value as string;
break;
}
case 6:
{
entry["Ymax"] = row.Cells[6].Value as string;
break;
}
case 7:
{
if (id != null)
data[id] = row.Cells[7].Value as string;
break;
}
default:
break;
}
}
private void OpenDmpFile(string file)
{
if (string.IsNullOrWhiteSpace(file)) return;
if (FileName != null && !File.Exists(file))
{
file = Path.Combine(Path.GetDirectoryName(FileName), file);
if (!File.Exists(file)) return;
}
try
{
using (var dlg = new WFEditDMP.MainForm())
{
dlg.OpenFile(file);
dlg.StartPosition = FormStartPosition.CenterParent;
dlg.ShowDialog(this);
}
}
catch
{
}
}
private bool BrowseDmpFile(out string result)
{
result = String.Empty;
var assign = false;
using (var dlg = new OpenFileDialog
{
Title = "Open file",
Filter = "DMP files|*.dmp|All files|*.*",
InitialDirectory = CurrentDir
})
{
if (dlg.ShowDialog() == DialogResult.OK)
{
var path = Path.GetDirectoryName(FileName);
var filename = dlg.FileName;
if (filename.StartsWith(path))
{
filename = filename.Replace(path, "");
while (filename.StartsWith("\\"))
filename = filename.Substring(1);
}
result = filename;
assign = true;
}
}
return assign;
}
private void OnVariableCellClick(object sender, DataGridViewCellEventArgs e)
{
if (_isLoading) return;
if (e.RowIndex < 0) return;
var row = gridVariables.Rows[e.RowIndex];
if (row.IsNewRow) return;
switch (e.ColumnIndex)
{
case 8: // open file
{
var file = row.Cells[7].Value as string;
var id = row.Cells[0].Value as string;
if (string.IsNullOrWhiteSpace(file))
{
var count = 1;
var baseName = "ic_" + id;
var name = baseName + ".dmp";
var dir = Path.GetDirectoryName(FileName);
while (File.Exists(Path.Combine(dir, name)))
{
name = string.Format("{0}_{1}.dmp", baseName, count++);
}
row.Cells[7].Value = name;
var dmpFile = new DmpModel(Config.DomainConfig.GridX, Config.DomainConfig.GridY);
dmpFile.MaxX = Config.DomainConfig.Width;
dmpFile.MaxY = Config.DomainConfig.Height;
file = Path.Combine(dir, name);
dmpFile.SaveAs(file);
}
OpenDmpFile(file);
break;
}
case 9: // browse file
{
string result;
if (BrowseDmpFile(out result))
row.Cells[7].Value = result;
break;
}
default:
break;
}
}
private void OnCompartmentAssignmentChanged(object sender, DataGridViewCellEventArgs e)
{
if (_isLoading) return;
if (e.RowIndex < 0) return;
var row = gridCompartments.Rows[e.RowIndex];
if (row.IsNewRow) return;
switch (e.ColumnIndex)
{
case 1: // edited compartmentfile
{
var file = row.Cells[1].Value as string;
var id = row.Cells[0].Value as string;
Config.ApplyCompartmentMasking(id, file);
UpdateVariables();
break;
}
default:
break;
}
}
private void OnCompartmentCellClick(object sender, DataGridViewCellEventArgs e)
{
if (_isLoading) return;
if (e.RowIndex < 0) return;
var row = gridCompartments.Rows[e.RowIndex];
if (row.IsNewRow) return;
switch (e.ColumnIndex)
{
case 2: // browse file
{
string result;
var id = row.Cells[0].Value as string;
if (BrowseDmpFile(out result))
{
row.Cells[1].Value = result;
Config.ApplyCompartmentMasking(id, result);
UpdateVariables();
}
break;
}
case 3: // view compartment
{
var file = row.Cells[1].Value as string;
var id = row.Cells[0].Value as string;
if (string.IsNullOrWhiteSpace(file))
{
var count = 1;
var baseName = "inside_" + id;
var name = baseName + ".dmp";
var dir = Path.GetDirectoryName(FileName);
while (File.Exists(Path.Combine(dir, name)))
{
name = string.Format("{0}_{1}.dmp", baseName, count++);
}
row.Cells[1].Value = name;
Config.ApplyCompartmentMasking(id, name);
UpdateVariables();
var dmpFile = new DmpModel(Config.DomainConfig.GridX, Config.DomainConfig.GridY)
{ MaxX = Config.DomainConfig.Width,
MaxY = Config.DomainConfig.Height };
file = Path.Combine(dir, name);
dmpFile.SaveAs(file);
}
OpenDmpFile(file);
break;
}
default:
break;
}
}
public void ClearExistingAssignments()
{
Config.ClearCompartmentMasking();
}
private void OnClearAllCompartmentAssignments(object sender, EventArgs e)
{
if (_isLoading) return;
ClearExistingAssignments();
}
public void ApplyCompartmentAssignments(bool clearExisting = false)
{
if (clearExisting)
ClearExistingAssignments();
foreach (DataGridViewRow row in gridCompartments.Rows)
{
var compId = row.Cells[0].Value as string;
var dmpFile = row.Cells[1].Value as string;
Config.ApplyCompartmentMasking(compId, dmpFile);
}
}
private void OnApplyCompartmentAssignments(object sender, EventArgs e)
{
if (_isLoading) return;
ApplyCompartmentAssignments();
}
private void InitFromSBML(string fileName)
{
Config.InitFromSBML(fileName);
gridCompartments.Rows.Clear();
foreach (var id in Config.CompartmentIds)
{
gridCompartments.Rows.Add(id, Config["Reaction"].Get("in_" + id, ""));
}
}
private void OnInitFromSBMLClick(object sender, EventArgs e)
{
using (var dlg = new OpenFileDialog
{
Title = "Open SBML file",
Filter = "SBML files|*.xml;*.sbml|All files|*.*",
InitialDirectory = CurrentDir
})
{
if (dlg.ShowDialog() == DialogResult.OK)
{
InitFromSBML(dlg.FileName);
}
}
}
private void OnFormClosing(object sender, FormClosingEventArgs e)
{
if (Config == null || !Config.Dirty) return;
DialogResult result =
MessageBox.Show(this,
"There are unsaved changes in the model. Would you like to save them?",
"Save changes?",
MessageBoxButtons.YesNoCancel,
MessageBoxIcon.Question,
MessageBoxDefaultButton.Button3);
if (result == DialogResult.Cancel)
{
e.Cancel = true;
}
if (result == DialogResult.Yes)
{
OnSaveFile(sender, e);
}
}
private void OnParameterValueChanged(object sender, DataGridViewCellEventArgs e)
{
if (_isLoading) return;
if (e.RowIndex < 0) return;
var row = gridParameters.Rows[e.RowIndex];
if (row.IsNewRow) return;
var id = row.Cells[0].Value as string;
if (string.IsNullOrEmpty(id)) return;
var value = row.Cells[1].Value as string;
var data = Config["Reaction"];
data[id] = value;
}
EditSpatialSettings Settings { get; set; }
public void ReadSettings()
{
Settings = EditSpatialSettings.GetDefault(Size);
if (Settings.Width < Screen.FromControl(this).WorkingArea.Width &&
Settings.Height < Screen.FromControl(this).WorkingArea.Height)
Size = new Size(Settings.Width, Settings.Height);
}
private void OnFormClosed(object sender, FormClosedEventArgs e)
{
WriteSettings();
}
private void WriteSettings()
{
if (Settings != null)
Settings.Save();
}
private void OnEditPreferencesClick(object sender, EventArgs e)
{
using (var dlg = new FormSettings { Settings = Settings })
{
if (dlg.ShowDialog(this) == DialogResult.OK)
{
dlg.Settings.Save();
ReadSettings();
}
}
}
private void OnFormLoad(object sender, EventArgs e)
{
ReadSettings();
}
#region Drag / Drop
private void MainForm_DragDrop(object sender, DragEventArgs e)
{
try
{
var sFilenames = (string[])e.Data.GetData(DataFormats.FileDrop);
var oInfo = new FileInfo(sFilenames[0]);
if (oInfo.Extension.ToLower() == ".conf")
{
OpenFile(sFilenames[0]);
}
}
catch (Exception)
{
}
}
private void MainForm_DragEnter(object sender, DragEventArgs e)
{
if (e.Data.GetDataPresent(DataFormats.FileDrop))
{
var sFilenames = (string[])e.Data.GetData(DataFormats.FileDrop);
var oInfo = new FileInfo(sFilenames[0]);
if (oInfo.Extension.ToLower() == ".conf")
{
e.Effect = DragDropEffects.Copy;
return;
}
}
e.Effect = DragDropEffects.None;
}
#endregion
}
}
<file_sep>/WFEditSpatial/Model/SpeciesReferenceInfo.cs
namespace EditSpatial.Model
{
public class SpeciesReferenceInfo
{
public string Id { get; set; }
public string Compartment { get; set; }
public double Stoichiometry { get; set; }
}
}<file_sep>/WFEditSpatial/Controls/BaseSpatialControl.cs
using System;
using System.Windows.Forms;
using EditSpatial.Model;
namespace EditSpatial.Controls
{
public partial class BaseSpatialControl : UserControl
{
public BaseSpatialControl()
{
InitializeComponent();
}
public bool IsInitializing { get; set; }
public Action UpdateAction { get; set; }
public event EventHandler ModelChanged;
public virtual void SaveChanges()
{
}
public virtual void InvalidateSelection()
{
}
protected virtual void OnModelChanged()
{
var handler = ModelChanged;
if (handler != null) handler(this, EventArgs.Empty);
}
}
}<file_sep>/WFEditSpatial/Controls/ControlSampleFieldGeometry.cs
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Drawing.Imaging;
using System.IO;
using System.Linq;
using System.Windows.Forms;
using EditSpatial.Model;
using libsbmlcs;
using Image = System.Drawing.Image;
namespace EditSpatial.Controls
{
public partial class ControlSampleFieldGeometry : BaseSpatialControl
{
private SampledField _Data;
private SampledField _Field;
public ControlSampleFieldGeometry()
{
InitializeComponent();
}
public Geometry SpatialGeometry { get; set; }
public SampledFieldGeometry Current { get; set; }
public int ThumbSize
{
get
{
int thumbSize;
if (int.TryParse(txtSize.Text, out thumbSize))
return thumbSize;
return 128;
}
}
public void InitializeFrom(Geometry geometry, string id)
{
txtId.Text = id;
grid.Rows.Clear();
thumbGeometry.Image = thumbGeometry.InitialImage;
SpatialGeometry = geometry;
if (geometry == null) return;
var sampledFieldGeometry = geometry.getGeometryDefinition(id) as SampledFieldGeometry;
Current = sampledFieldGeometry;
if (sampledFieldGeometry == null) return;
chkIsActive.Checked = Current.getIsActive();
for (long i = 0; i < sampledFieldGeometry.getNumSampledVolumes(); ++i)
{
var vol = sampledFieldGeometry.getSampledVolume(i);
var spatialId = vol.getId();
grid.Rows.Add(
spatialId,
vol.getDomainType(),
vol.getSampledValue().ToString(),
vol.getMinValue().ToString(),
vol.getMaxValue().ToString()
);
}
_Field = geometry.getSampledField( sampledFieldGeometry.getSampledField());
_Data = _Field;
var numSamples = _Field == null ? 0 : _Field.getNumSamples3() - 1;
if (SpatialGeometry.getNumCoordinateComponents() < 3 || numSamples == 0)
{
txtZ.Visible = false;
trackBar1.Visible = false;
lblZ.Visible = false;
}
else
{
txtZ.Visible = true;
trackBar1.Visible = true;
lblZ.Visible = true;
trackBar1.Minimum = 0;
trackBar1.Maximum = numSamples;
}
thumbGeometry.Image = GenerateImage(sampledFieldGeometry, geometry, ThumbSize, ThumbSize);
}
public override void SaveChanges()
{
if (Current == null || SpatialGeometry == null) return;
Current.setId(txtId.Text);
Current.setIsActive(chkIsActive.Checked);
for (var i = 0; i < grid.Rows.Count && i < Current.getNumSampledVolumes(); ++i)
{
var row = grid.Rows[i];
var current = Current.getSampledVolume(i);
current.setId((string) row.Cells[0].Value);
current.setDomainType((string) row.Cells[1].Value);
double value;
if (double.TryParse((string) row.Cells[2].Value, out value))
current.setSampledValue(value);
if (double.TryParse((string) row.Cells[3].Value, out value))
current.setMinValue(value);
if (double.TryParse((string) row.Cells[4].Value, out value))
current.setMaxValue(value);
}
OnModelChanged();
}
private List<int> GetUniqueValues(int[] data)
{
var result = new List<int>();
foreach (var t in data.Where(t => !result.Contains(t)))
{
result.Add(t);
}
return result;
}
private Image GenerateImage(SampledFieldGeometry sampledFieldGeometry, Geometry geometry, int resX = 128,
int resY = 128)
{
if (geometry == null || sampledFieldGeometry == null || geometry.getNumCoordinateComponents() < 2)
return new Bitmap(1, 1);
try
{
if (_Field == null || _Data == null)
return new Bitmap(1, 1);
var uncompressedLength = _Data.getUncompressedLength();
if (uncompressedLength == 0)
_Data.uncompress();
uncompressedLength = _Data.getUncompressedLength();
var array = new int[uncompressedLength];
_Data.getUncompressed(array);
var values = GetUniqueValues(array);
var z = Util.SaveInt(txtZ.Text, 0);
if (z >= _Field.getNumSamples3())
z = _Field.getNumSamples3() - 1;
if (z < 0) z = 0;
var result = new Bitmap(_Field.getNumSamples1(), _Field.getNumSamples2());
for (long i = 0; i < _Field.getNumSamples1(); ++i)
{
for (long j = 0; j < _Field.getNumSamples2(); ++j)
{
var index =
GetIndexFor(array[i + _Field.getNumSamples1()*j + _Field.getNumSamples1()*_Field.getNumSamples2()*z]);
result.SetPixel((int) i, (int) j, GetColorForIndex(index));
}
}
return result;
}
catch
{
}
return new Bitmap(1, 1);
}
private int GetIndexFor(int value)
{
if (Current == null) return -1;
for (long i = 0; i < Current.getNumSampledVolumes(); ++i)
{
var current = Current.getSampledVolume(i);
var currentValue = current.getSampledValue();
var currentIntValue = (uint) currentValue;
if (Math.Abs(currentValue - value) < 1e-10)
return (int) i;
if (currentIntValue == (byte) value)
return (int) i;
if (current.isSetMinValue() && current.isSetMaxValue() &&
(value >= current.getMinValue() && value <= current.getMaxValue()))
return (int) i;
}
return -1;
}
private Color GetColorForIndex(int index)
{
switch (index)
{
case -1:
return Color.Transparent;
default:
case 0:
return Color.Black;
case 1:
return Color.Red;
case 2:
return Color.Blue;
case 3:
return Color.Green;
case 4:
return Color.Yellow;
case 5:
return Color.Goldenrod;
case 6:
return Color.Lime;
case 7:
return Color.LightBlue;
case 8:
return Color.LightSalmon;
case 9:
return Color.MediumAquamarine;
}
}
private void OnReorderClick(object sender, EventArgs e)
{
}
public override void InvalidateSelection()
{
SpatialGeometry = null;
Current = null;
InitializeFrom(null, null);
}
private void OnTrackChanged(object sender, EventArgs e)
{
if (SpatialGeometry == null)
return;
if (SpatialGeometry.getNumCoordinateComponents() < 3)
return;
txtZ.Text = trackBar1.Value.ToString();
if (Current == null)
return;
InitializeFrom(SpatialGeometry, Current.getId());
}
private void OnUpdateImage(object sender, EventArgs e)
{
if (Current == null)
return;
InitializeFrom(SpatialGeometry, Current.getId());
}
private void OnReorder(object sender, EventArgs e)
{
if (Current == null)
return;
var vols = new List<SampledVolume>();
for (var i = (int) Current.getNumSampledVolumes() - 1; i >= 0; --i)
vols.Add(Current.removeSampledVolume(i));
foreach (var vol in vols)
{
Current.addSampledVolume(vol);
}
InitializeFrom(SpatialGeometry, Current.getId());
}
private void OnImageLoad(object sender, EventArgs e)
{
using (var dialog = new OpenFileDialog {Filter = "Image files|*.tif;*.png|TIFF files|*.tif|PNG files|*.png|All files|*.*" })
{
if (dialog.ShowDialog() == DialogResult.OK)
{
LoadImage(dialog.FileName);
}
}
}
private void LoadImage(string fileName)
{
var image = Image.FromFile(fileName) as Bitmap;
if (Current == null)
{
Current = SpatialGeometry.createSampledFieldGeometry();
}
Current.setId(Path.GetFileNameWithoutExtension(fileName));
_Field = SpatialGeometry.createSampledField();
var id = string.Format("sampleFiled_{0}", SpatialGeometry.getNumSampledFields());
_Field.setId(id);
Current.setSampledField(id);
_Field.setNumSamples1(image.Width);
_Field.setNumSamples2(image.Height);
_Field.setNumSamples3(1);
var data = new int[image.Width*image.Height];
var count = 0;
for (var i = 0; i < image.Width; ++i)
for (var j = 0; j < image.Height; ++j)
{
var currentColor = image.GetPixel(i, j);
var value = (int) (currentColor.GetBrightness()*10f);
//if (currentColor.B > 10 && currentColor.G > 10 && currentColor.R > 10)
//{
// value = 1;
//}
data[count++] = value;
}
var values = GetUniqueValues(data);
var col = 0;
foreach (var val in values)
{
var vol = Current.createSampledVolume();
vol.setId(string.Format("vol_{0}", col++));
vol.setSampledValue(val);
vol.setMinValue(val);
vol.setMaxValue(val);
}
_Field.setDataType(libsbml.SPATIAL_DATAKIND_UINT8);
_Field.setCompression(libsbml.SPATIAL_COMPRESSIONKIND_UNCOMPRESSED);
_Field.setSamples(data, data.Length);
_Field.setInterpolationType(libsbml.SPATIAL_INTERPOLATIONKIND_NEARESTNEIGHBOR);
_Field.uncompress();
InitializeFrom(SpatialGeometry, Current.getId());
}
private void OnImageSave(object sender, EventArgs e)
{
using (var dialog = new SaveFileDialog {Filter = "TIFF files|*.tif|All files|*.*"})
{
if (dialog.ShowDialog() == DialogResult.OK)
{
thumbGeometry.Image.Save(dialog.FileName, ImageFormat.Tiff);
}
}
}
private void OnUserDeletedRow(object sender, DataGridViewRowEventArgs e)
{
if (Current == null) return;
var id = e.Row.Cells[0].Value as string;
Current.removeSampledVolume(id);
InitializeFrom(SpatialGeometry, Current.getId());
}
}
}<file_sep>/WFEditSpatial/Model/GeometrySettings.cs
using System.Collections.Generic;
namespace EditSpatial.Model
{
public enum GeometryType
{
Default,
Analytic,
Sample
}
public class GeometrySettings
{
public GeometrySettings()
{
WrapOutside = false;
AnalyticDomains = new List<AnalyticSettings>();
UsedSymbols = new List<string>();
}
public double Xmin { get; set; }
public double Xmax { get; set; }
public double Ymin { get; set; }
public double Ymax { get; set; }
public double Zmin { get; set; }
public double Zmax { get; set; }
public List<string> UsedSymbols { get; set; }
public List<AnalyticSettings> AnalyticDomains { get; set; }
public GeometryType Type { get; set; }
public bool WrapOutside { get; set; }
}
}<file_sep>/WFEditDMP/Forms/FormAdjustDmp.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows.Forms;
using LibEditSpatial.Model;
namespace WFEditDMP.Forms
{
public partial class FormAdjustDmp : Form
{
public FormAdjustDmp()
{
InitializeComponent();
}
public DmpModel Model { get; set; }
public List<double> Numbers { get; set; }
private void UpdateUI()
{
listBox1.Items.Clear();
if (Numbers != null)
foreach (var item in Numbers)
{
listBox1.Items.Add(item);
}
if (Model != null)
{
dmpRenderControl1.LoadModel( Model);
dmpRenderControl1.DisableEditing = true;
}
}
public void InitializeFrom(List<double> selection, DmpModel model)
{
Model = model;
Numbers = selection;
UpdateUI();
}
private void cmdMerge_Click(object sender, EventArgs e)
{
if (listBox1.SelectedItems.Count < 2) return;
var target = (double) listBox1.SelectedItems[0];
for (var i = listBox1.SelectedItems.Count - 1; i >= 1; i--)
{
var current = (double) listBox1.SelectedItems[i];
Model.Transform(x => x == current ? target : x);
listBox1.Items.Remove(current);
}
Numbers = Model.Range;
Model.Min = Numbers.FirstOrDefault();
Model.Max = Numbers.LastOrDefault();
UpdateUI();
}
private void cmdReplace_Click(object sender, EventArgs e)
{
if (listBox1.SelectedItems.Count < 1) return;
double target;
if (!double.TryParse(txtReplacement.Text, out target))
return;
var list = listBox1.SelectedItems.Cast<double>().ToList();
for (var i = list.Count - 1; i >= 0; i--)
{
var current = (double)list[i];
Model.Transform(x => x == current ? target : x);
listBox1.Items.Remove(current);
Numbers.Remove(current);
}
Numbers = Model.Range;
Model.Min = Numbers.FirstOrDefault();
Model.Max = Numbers.LastOrDefault();
UpdateUI();
}
private void OnInvertClick(object sender, EventArgs e)
{
Model.Invert();
UpdateUI();
}
private void OnMultiplyClick(object sender, EventArgs e)
{
double val;
if (!double.TryParse(txtScalar.Text, out val)) return;
var list = listBox1.SelectedItems.Cast<double>().ToList();
if (!list.Any())
list.AddRange(Numbers);
Model.Transform(x => list.Contains(x) ? x * val : x);
Numbers = Model.Range;
Model.Min = Numbers.FirstOrDefault();
Model.Max = Numbers.LastOrDefault();
UpdateUI();
}
private void OnMaskClick(object sender, EventArgs e)
{
using (var dlg = new OpenFileDialog
{
Title = "Choose DMP file to mask with. ",
Filter = "All supported|*.dmp;*.tif;*.tiff;*.png;*.jpg;*.jpeg;*.bmp|DMP files|*.dmp|Image files|*.tif;*.tiff;*.png;*.jpg;*.jpeg;*.bmp|All files|*.*",
AutoUpgradeEnabled = true,
})
{
if (dlg.ShowDialog() == DialogResult.OK)
{
try
{
var file = DmpModel.FromFile(dlg.FileName);
Model.MaskWith(file);
Numbers = Model.Range;
UpdateUI();
}
catch
{
MessageBox.Show("Masking failed, ensure that the files have precisely the same dimensions", "Masking failed",
MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
}
}
}
}<file_sep>/WFEditSpatial/Controls/ControlCoordinateComponent.cs
using EditSpatial.Model;
using libsbmlcs;
namespace EditSpatial.Controls
{
public partial class ControlCoordinateComponent : BaseSpatialControl
{
public ControlCoordinateComponent()
{
InitializeComponent();
}
private CoordinateComponent Current { get; set; }
public void InitializeFrom(CoordinateComponent comp)
{
Current = comp;
if (Current == null)
return;
txtId.Text = comp.getId();
txtType.Text = libsbml.CoordinateKind_toString(comp.getType());
txtIndex.Text = comp.getType().ToString();
txtUnit.Text = comp.getUnit();
var min = comp.getBoundaryMin();
txtMinId.Text = min.getId();
txtMinValue.Text = min.getValue().ToString();
var max = comp.getBoundaryMax();
txtMaxId.Text = max.getId();
txtMaxValue.Text = max.getValue().ToString();
}
public override void SaveChanges()
{
if (Current == null) return;
Current.setId(txtId.Text);
//Current.setComponentType(txtType.Text);
Current.setType(Util.SaveInt(txtIndex.Text, Current.getType()));
if (string.IsNullOrWhiteSpace(txtUnit.Text))
Current.unsetUnit();
else
Current.setUnit(txtUnit.Text);
var min = Current.getBoundaryMin();
min.setId(txtMinId.Text);
min.setValue(Util.SaveDouble(txtMinValue.Text, min.getValue()));
var max = Current.getBoundaryMax();
max.setId(txtMaxId.Text);
max.setValue(Util.SaveDouble(txtMaxValue.Text, max.getValue()));
}
public override void InvalidateSelection()
{
Current = null;
InitializeFrom(Current);
}
}
}<file_sep>/LibEditSpatial/Model/TimeLoopConfig.cs
using System.Collections.Generic;
namespace LibEditSpatial.Model
{
public class TimeLoopConfig
{
public double Time { get; set; }
public double InitialStep { get; set; }
public double MinStep { get; set; }
public double MaxStep { get; set; }
public double PlotStep { get; set; }
public int IncreaseRate { get; set; }
public static TimeLoopConfig Default
{
get
{
return new TimeLoopConfig
{
Time = 10,
InitialStep = 0.01,
MinStep = 1e-18,
MaxStep = 0.01,
PlotStep = 0.01
};
}
}
public static TimeLoopConfig FromDict(Dictionary<string, string> dict)
{
var result = new TimeLoopConfig
{
Time = dict.Get<double>("time"),
InitialStep = dict.Get<double>("dt"),
MinStep = dict.Get<double>("dt_min"),
MaxStep = dict.Get<double>("dt_max"),
PlotStep = dict.Get<double>("dt_plot"),
IncreaseRate = dict.Get<int>("increase_rate")
};
return result;
}
public Dictionary<string, string> ToDict()
{
var result = new Dictionary<string, string>();
result["time"] = Time.ToString();
result["dt"] = InitialStep.ToString();
result["dt_min"] = MinStep.ToString();
result["dt_max"] = MaxStep.ToString();
result["dt_plot"] = PlotStep.ToString();
result["increase_rate"] = IncreaseRate.ToString();
return result;
}
}
}<file_sep>/WFEditSpatial/Model/Util.cs
using System;
using System.Collections.Generic;
using System.Drawing;
using System.IO;
using System.Text;
using System.Windows.Forms;
using EditSpatial.Converter;
using ICSharpCode.SharpZipLib.Core;
using ICSharpCode.SharpZipLib.Zip;
using ICSharpCode.SharpZipLib.Zip.Compression.Streams;
using libsbmlcs;
using Ookii.Dialogs;
using SysBio.MathKGI;
using Image = System.Drawing.Image;
namespace EditSpatial.Model
{
internal static class Util
{
public const int CHUNK_SIZE = 2048;
public static string[][] InbuiltFunctions =
{
new[]
{
"CIRCLE",
"lambda(x,y,centerX,centerY,r,piecewise(1, lt(pow(x-centerX, 2) + pow(y-centerY, 2), r*r), 0))",
"CIRCLE(x,y,25,25,10)",
"True, if inside circle with given center and radius"
},
new[]
{
"ELLIPSE",
"lambda(x,y,centerX,centerY,rx,ry,piecewise(1, lt(pow(x-centerX, 2)/pow(rx,2) + pow(y-centerY, 2)/pow(ry,2), 1), 0))",
"ELLIPSE(x,y,25,25,10, 10)",
"True, if inside ellipse with given center and radii"
},
new[]
{
"RECTANGLE",
"lambda(x,y,startX, startY, w,h,and(geq(x, startX), leq(x, startX+w), geq(y, startY), leq(y, startY+h)))",
"RECT(x,y,10,10,10, 10)",
"Generates a rectangle with given start position and width and height"
},
new[]
{
"FISH",
"lambda(x,y,w,h,piecewise(1, ((x - w * 0.42)^2 / (w * 0.37)^2 + (y - h / 2)^2 / (w * 0.25)^2 < 1 || (y < -(w * 0.1) + x && y > w * 1.1 + -x && x < w * 0.9)) && !((x - w * 0.25)^2 / (0.08 * w)^2 + (y - h * 0.45)^2 / (0.08 * h)^2 < 1), 0))",
"FISH(x,y,width,height)",
"Generates a fish in the given bounds"
}
};
public static Image GenerateTiffForOrdinal(this AnalyticGeometry analytic, Geometry geometry, int ordinal = 1,
double z = 1)
{
var range1 = geometry.getCoordinateComponent(0);
var r1Max = range1.getBoundaryMax().getValue();
var range2 = geometry.getCoordinateComponent(1);
var r2Max = range2.getBoundaryMax().getValue();
return analytic.GenerateTiff(geometry, (int) r1Max, (int) r2Max, ordinal, z);
}
public static Image GenerateTiff(this AnalyticGeometry analytic, Geometry geometry, int resX = 128, int resY = 128,
int ordinal = 1, double z = 1)
{
if (geometry == null || analytic == null || geometry.getNumCoordinateComponents() < 2)
return new Bitmap(1, 1);
try
{
var formulas = new List<Tuple<int, ASTNode>>();
for (long i = 0; i < analytic.getNumAnalyticVolumes(); ++i)
{
var current = analytic.getAnalyticVolume(i);
if (current.getOrdinal() == ordinal)
formulas.Add(new Tuple<int, ASTNode>((int) current.getOrdinal(), current.getMath()));
}
formulas.Sort((a, b) => a.Item1.CompareTo(b.Item1));
var range1 = geometry.getCoordinateComponent(0);
var r1Min = range1.getBoundaryMin().getValue();
var r1Max = range1.getBoundaryMax().getValue();
var range2 = geometry.getCoordinateComponent(1);
var r2Min = range2.getBoundaryMin().getValue();
var r2Max = range2.getBoundaryMax().getValue();
var depth = geometry.getNumCoordinateComponents() == 3
? geometry.getCoordinateComponent(2).getBoundaryMax().getValue()
: 0;
var result = new Bitmap(resX, resY);
for (var i = 0; i < resX; ++i)
{
var x = r1Min
+
(r1Max - r1Min)/
resX*i;
for (var j = 0; j < resY; ++j)
{
var y = r2Min
+
(r2Max - r2Min)/
resY*j;
for (var index = 0; index < formulas.Count; index++)
{
var item = formulas[index];
var isInside = Evaluate(item.Item2,
new List<string> {"x", "y", "z", "width", "height", "depth"},
new List<double> {x, y, z, r1Max, r2Max, depth},
new List<Tuple<string, double>>()
);
if (Math.Abs((isInside - 1.0)) < 1E-10)
{
result.SetPixel(i, j, ordinal == item.Item1 ? Color.White : Color.Black);
break;
}
}
}
}
return result;
}
catch
{
}
return new Bitmap(1, 1);
}
public static void ExpandMath(this AnalyticGeometry analytic)
{
if (analytic == null) return;
for (long i = 0; i < analytic.getNumAnalyticVolumes(); ++i)
{
var current = analytic.getAnalyticVolume(i);
if (current == null || !current.isSetMath())
continue;
var formula = libsbml.formulaToL3String(current.getMath());
for (var k = 0; k < InbuiltFunctions.Length; ++k)
{
if (formula.Contains(InbuiltFunctions[k][0]))
{
var fd = new FunctionDefinition(3, 1);
fd.setId(InbuiltFunctions[k][0]);
fd.setMath(libsbml.parseL3Formula(InbuiltFunctions[k][1]));
SBMLTransforms.replaceFD(current.getMath(), fd);
fd.Dispose();
}
}
}
}
public static List<string> GetCompartmentsFromReaction(Reaction reaction)
{
var result = new List<string>();
if (reaction == null) return result;
var model = reaction.getModel();
if (model == null) return result;
for (var i = 0; i < reaction.getNumReactants(); ++i)
{
var reference = reaction.getReactant(i);
if (reference != null && reference.isSetSpecies())
{
var species = model.getSpecies(reference.getSpecies());
if (species == null || !species.isSetCompartment()) continue;
var compartment = species.getCompartment();
if (!result.Contains(compartment))
result.Add(compartment);
}
}
for (var i = 0; i < reaction.getNumProducts(); ++i)
{
var reference = reaction.getProduct(i);
if (reference != null && reference.isSetSpecies())
{
var species = model.getSpecies(reference.getSpecies());
if (species == null || !species.isSetCompartment()) continue;
var compartment = species.getCompartment();
if (!result.Contains(compartment))
result.Add(compartment);
}
}
return result;
}
public static Dictionary<string, List<string>> GetSpeciesCompartmentMap(libsbmlcs.Model model, Reaction reaction)
{
var result = new Dictionary<string, List<string>>();
if (reaction == null) return result;
for (var i = 0; i < reaction.getNumReactants(); ++i)
{
var reference = reaction.getReactant(i);
if (reference != null && reference.isSetSpecies())
{
var species = model.getSpecies(reference.getSpecies());
if (species == null || !species.isSetCompartment()) continue;
var compartment = species.getCompartment();
if (!result.ContainsKey(compartment))
result[compartment] = new List<string>();
result[compartment].Add(species.getId());
}
}
for (var i = 0; i < reaction.getNumProducts(); ++i)
{
var reference = reaction.getProduct(i);
if (reference != null && reference.isSetSpecies())
{
var species = model.getSpecies(reference.getSpecies());
if (species == null || !species.isSetCompartment()) continue;
var compartment = species.getCompartment();
if (!result.ContainsKey(compartment))
result[compartment] = new List<string>();
result[compartment].Add(species.getId());
}
}
return result;
}
public static void MoveRuleToAssignment(this libsbmlcs.Model model, string id)
{
if (model == null) return;
var rule = model.getRuleByVariable(id) as AssignmentRule;
if (rule != null)
{
var ia = model.createInitialAssignment();
ia.setSymbol(rule.getVariable());
ia.setMath(rule.getMath());
model.removeRule(rule.getVariable());
}
}
public static AnalyticGeometry GetFirstAnalyticGeometry(this Geometry geometry)
{
if (geometry == null) return null;
for (var i = 0; i < geometry.getNumGeometryDefinitions(); ++i)
{
var analytic = geometry.getGeometryDefinition(i) as AnalyticGeometry;
if (analytic != null) return analytic;
}
return null;
}
public static SampledFieldGeometry GetFirstSampledFieldGeometry(this Geometry geometry)
{
if (geometry == null) return null;
for (var i = 0; i < geometry.getNumGeometryDefinitions(); ++i)
{
var sampledFieldGeometry = geometry.getGeometryDefinition(i) as SampledFieldGeometry;
if (sampledFieldGeometry != null) return sampledFieldGeometry;
}
return null;
}
public static void setInitialExpession(this Species species, string expression)
{
if (species == null || species.getSBMLDocument() == null || species.getSBMLDocument().getModel() == null)
return;
var model = species.getSBMLDocument().getModel();
var node = libsbml.parseL3Formula(expression);
if (node == null) return;
if (node.isNumber())
{
if (species.isSetInitialAmount())
species.setInitialAmount(GetRealValue(node));
else
species.setInitialConcentration(GetRealValue(node));
return;
}
var initial = model.getInitialAssignment(species.getId());
if (initial == null)
{
initial = model.createInitialAssignment();
initial.setSymbol(species.getId());
}
initial.setMath(node);
}
public static bool IsBasic(this ASTNode node)
{
return node.isName() || node.isFunction() ||
node.isNumber() || node.isConstant() ||
node.isBoolean() || node.getType() == libsbml.AST_TIMES ||
node.getType() == libsbml.AST_DIVIDE;
}
public static void AppendMorpheusNode(this StringBuilder builder, string format, ASTNode node,
Dictionary<string, string> map)
{
//if (!node.IsBasic())
// format = format.Replace("{0}", "({0})");
builder.AppendFormat(format, MorpheusConverter.TranslateExpression(node, map));
}
public static void AppendDuneNode(this StringBuilder builder, string format, ASTNode node,
Dictionary<string, string> map, libsbmlcs.Model model)
{
if (!node.IsBasic())
format = format.Replace("{0}", "({0})");
builder.AppendFormat(format, DuneConverter.TranslateExpression(node, map, model));
}
private static double GetRealValue(ASTNode node)
{
switch (node.getType())
{
default:
case libsbml.AST_REAL:
return node.getReal();
case libsbml.AST_REAL_E:
return Math.Pow(node.getMantissa(), node.getExponent());
case libsbml.AST_INTEGER:
return node.getInteger();
case libsbml.AST_RATIONAL:
return node.getNumerator()/(double) node.getDenominator();
}
}
public static string getInitialExpession(this Species species)
{
var result = "";
if (species == null || species.getSBMLDocument() == null || species.getSBMLDocument().getModel() == null)
return result;
var model = species.getSBMLDocument().getModel();
var initial = model.getInitialAssignment(species.getId());
if (initial != null && initial.isSetMath()) return libsbml.formulaToL3String(initial.getMath());
if (species.isSetInitialAmount())
return species.getInitialAmount().ToString();
if (species.isSetInitialConcentration())
return species.getInitialConcentration().ToString();
return result;
}
public static double? getDiffusionY(this Species species)
{
var param = species.getParameterDiffusionY();
if (param == null) return null;
return param.getValue();
}
public static double? getXMaxBC(this Species species)
{
var param = species.getBoundaryCondition("Xmax");
if (param == null) return null;
return param.getValue();
}
public static double? getYMaxBC(this Species species)
{
var param = species.getBoundaryCondition("Ymax");
if (param == null) return null;
return param.getValue();
}
public static double? getXMinBC(this Species species)
{
var param = species.getBoundaryCondition("Xmin");
if (param == null) return null;
return param.getValue();
}
public static double? getYMinBC(this Species species)
{
var param = species.getBoundaryCondition("Ymin");
if (param == null) return null;
return param.getValue();
}
public static int getBoundaryConditionType(this Parameter param, int defaultValue = libsbml.SPATIAL_BOUNDARYKIND_NEUMANN)
{
if (param == null)
return defaultValue;
var plugin = param.getPlugin("spatial") as SpatialParameterPlugin;
if (plugin == null)
return defaultValue;
var bc = plugin.getBoundaryCondition();
if (bc == null)
return defaultValue;
return bc.getType();
}
public static string getBcType(this Species species)
{
var param = species.getBoundaryCondition("Xmax");
if (param != null)
return param.getBoundaryConditionType() == libsbml.SPATIAL_BOUNDARYKIND_DIRICHLET
? "Dirichlet"
: "Neumann";
param = species.getBoundaryCondition("Xmin");
if (param != null)
return param.getBoundaryConditionType() == libsbml.SPATIAL_BOUNDARYKIND_DIRICHLET
? "Dirichlet"
: "Neumann";
param = species.getBoundaryCondition("Ymax");
if (param != null)
return param.getBoundaryConditionType() == libsbml.SPATIAL_BOUNDARYKIND_DIRICHLET
? "Dirichlet"
: "Neumann";
param = species.getBoundaryCondition("Ymin");
if (param != null)
return param.getBoundaryConditionType() == libsbml.SPATIAL_BOUNDARYKIND_DIRICHLET
? "Dirichlet"
: "Neumann";
return "Neumann";
}
public static bool IsSpatial(this Parameter parameter)
{
var plug = (SpatialParameterPlugin) parameter.getPlugin("spatial");
if (plug == null) return false;
return plug.isSpatialParameter();
}
public static Parameter setSpatialParameter(this Species species, string id, int typeCode, double value,
object box = null)
{
if (species == null || species.getSBMLDocument() == null || species.getSBMLDocument().getModel() == null)
return null;
var model = species.getSBMLDocument().getModel();
Parameter param = species.getSpatialParameter(typeCode, box);
if (param == null)
{
param = model.createParameter();
param.initDefaults();
var plugin = param.getPlugin("spatial") as SpatialParameterPlugin;
if (plugin != null && box != null)
{
switch (typeCode)
{
case libsbml.SBML_SPATIAL_DIFFUSIONCOEFFICIENT:
{
var diff = plugin.getDiffusionCoefficient();
if (diff == null)
diff = plugin.createDiffusionCoefficient();
diff.setCoordinateReference1((int) box);
diff.setVariable(species.getId());
break;
}
case libsbml.SBML_SPATIAL_BOUNDARYCONDITION:
{
var bc = plugin.getBoundaryCondition();
if (bc == null)
bc = plugin.createBoundaryCondition();
bc.setVariable(species.getId());
bc.setCoordinateBoundary((string) box);
break;
}
}
}
}
param.setId(id);
param.setValue(value);
return param;
}
public static Parameter getSpatialParameter(this Species species, int typeCode, object box = null)
{
if (species == null || species.getSBMLDocument() == null || species.getSBMLDocument().getModel() == null)
return null;
var model = species.getSBMLDocument().getModel();
for (var i = 0; i < model.getNumParameters(); ++i)
{
var parameter = model.getParameter(i);
if (parameter == null) continue;
var plugin = parameter.getPlugin("spatial") as SpatialParameterPlugin;
if (plugin == null) continue;
if (plugin.getType() != typeCode) continue;
if (typeCode == libsbml.SBML_SPATIAL_DIFFUSIONCOEFFICIENT)
{
var diff = plugin.getDiffusionCoefficient();
var index = 0;
if (box != null && box is int)
index = (int) box;
if (diff == null || diff.getCoordinateReference1() != index || diff.getVariable() != species.getId()) continue;
}
else if (typeCode == libsbml.SBML_SPATIAL_BOUNDARYCONDITION)
{
var bc = plugin.getBoundaryCondition();
if (bc == null || bc.getVariable() != species.getId()) continue;
if (box != null && box is string)
{
var bound = box as string;
if (bc.getCoordinateBoundary() != bound) continue;
}
}
return parameter;
}
return null;
}
public static Parameter getParameterDiffusionX(this Species species)
{
return species.getSpatialParameter(libsbml.SBML_SPATIAL_DIFFUSIONCOEFFICIENT, 0);
}
public static Parameter getParameterDiffusionY(this Species species)
{
return species.getSpatialParameter(libsbml.SBML_SPATIAL_DIFFUSIONCOEFFICIENT, 1);
}
public static Parameter getBoundaryCondition(this Species species, string dir)
{
return species.getSpatialParameter(libsbml.SBML_SPATIAL_BOUNDARYCONDITION, dir);
}
public static double? getDiffusionX(this Species species)
{
var param = species.getParameterDiffusionX();
if (param == null) return null;
return param.getValue();
}
internal static byte[] ToBytes(int[] array)
{
var result = new byte[array.Length];
for (var i = 0; i < array.Length; i++)
{
result[i] = (byte) (array[i]);
}
return result;
}
private static int[] ToInt(byte[] array)
{
var result = new int[array.Length];
for (var i = 0; i < array.Length; i++)
{
result[i] = array[i];
}
return result;
}
internal static int[] GetArray(SampledField data)
{
switch (data.getDataType())
{
case libsbml.SPATIAL_COMPRESSIONKIND_DEFLATED:
{
var intValue = new int[data.getSamplesLength()];
data.getSamples(intValue);
var bytes = ToBytes(intValue);
var stream = new MemoryStream(bytes);
var result = new MemoryStream();
var zipInputStream = new InflaterInputStream(stream);
var buffer = new byte[4096]; // 4K is optimum
StreamUtils.Copy(zipInputStream, result, buffer);
return ToInt(result.ToArray());
}
case libsbml.SPATIAL_COMPRESSIONKIND_UNCOMPRESSED:
default:
{
var intValue = new int[data.getSamplesLength()];
data.getSamples(intValue);
return intValue;
}
}
}
/// <summary>
/// Returns the path of the unpacked archive (temp+filename)
/// </summary>
/// <param name="archiveFilename">name of the archive file</param>
/// <param name="deleteIfExists"></param>
/// <returns>base directory with all the unzipped files</returns>
public static string UnzipArchive(string archiveFilename, string targetDir, bool deleteIfExists = true)
{
using (var inputStream = new FileStream(archiveFilename, FileMode.Open))
{
// zipped archive ...
var stream = new ZipInputStream(inputStream);
var destination = targetDir;
if (Directory.Exists(destination) && deleteIfExists)
try
{
Directory.Delete(destination, true);
}
catch
{
}
Directory.CreateDirectory(destination);
ZipEntry entry;
while ((entry = stream.GetNextEntry()) != null)
{
var sName = Path.Combine(destination, entry.Name);
var dir = Path.GetDirectoryName(sName);
if (!string.IsNullOrWhiteSpace(dir) && !Directory.Exists(dir))
Directory.CreateDirectory(dir);
if (entry.IsDirectory) continue;
var streamWriter = File.Create(sName);
var data = new byte[CHUNK_SIZE];
while (true)
{
var size = stream.Read(data, 0, data.Length);
if (size > 0)
{
streamWriter.Write(data, 0, size);
}
else
{
break;
}
}
streamWriter.Close();
}
return destination;
}
}
internal static double ComputeValueForFormula(string formula, List<string> variableIds, List<double> variableData)
{
if (formula == null && variableIds.Count > 0) formula = variableIds[0];
// if we are lucky just return a given variable column
if (variableIds.Contains(formula))
{
var index = variableIds.IndexOf(formula);
return variableData[index];
}
// at this point ... we should try and get a libSBML AST Tree and then evaluate it ...
var tree = libsbml.parseL3Formula(formula);
if (tree == null)
throw new Exception("Invalid MathML in ComputeChange::ComputeValueForFormula");
return Evaluate(tree, variableIds, variableData, new List<Tuple<string, double>>());
}
internal static double Evaluate(ASTNode tree, List<string> sVariableIds, List<double> oVariableData,
List<Tuple<string, double>> listOfParameters)
{
// no data ...
if (oVariableData == null || oVariableData.Count < 1)
{
if (tree.isReal()) return tree.getReal();
if (tree.isInteger()) return tree.getInteger();
return 0;
}
return EvaluateSingle(tree, sVariableIds, oVariableData, listOfParameters);
}
internal static double EvaluateSingle(ASTNode node, List<string> sVariableIds,
List<double> oVariableData, List<Tuple<string, double>> listOfParameters)
{
if (node == null)
return 0;
if (node.isReal()) return node.getReal();
if (node.isInteger()) return node.getInteger();
if (node.isOperator())
{
switch (node.getType())
{
case libsbml.AST_PLUS:
return
EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData, listOfParameters) +
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData, listOfParameters);
case libsbml.AST_MINUS:
if (node.getNumChildren() == 1)
return -
EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData, listOfParameters);
return
EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData, listOfParameters) -
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData, listOfParameters);
case libsbml.AST_DIVIDE:
return
EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData, listOfParameters)/
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData, listOfParameters);
case libsbml.AST_TIMES:
return
EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData, listOfParameters)*
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData, listOfParameters);
case libsbml.AST_POWER:
return
Math.Pow(
EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData, listOfParameters),
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData,
listOfParameters));
default:
break;
}
}
else if (node.isName())
{
if (sVariableIds.Contains(node.getName()))
{
var variableIndex = sVariableIds.IndexOf(node.getName());
return oVariableData[variableIndex];
}
foreach (var param in listOfParameters)
{
if (param.Item1 == node.getName())
return param.Item2;
}
}
// we are still here ... this is bad ... as last measure try a couple of inbuilts
switch (node.getType())
{
case libsbml.AST_FUNCTION_ABS:
return
Math.Abs(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCCOS:
return
Math.Acos(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCCOSH:
return
MathKGI.Acosh(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCCOT:
return
MathKGI.Acot(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCCOTH:
return
MathKGI.Acoth(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCCSC:
return
MathKGI.Acsc(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCCSCH:
return
MathKGI.Acsch(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCSEC:
return
MathKGI.Asec(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCSECH:
return
MathKGI.Asech(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCSIN:
return
Math.Asin(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCSINH:
return
MathKGI.Asinh(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCTAN:
return
Math.Atan(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ARCTANH:
return
MathKGI.Atanh(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_CEILING:
return
Math.Ceiling(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_COS:
return
Math.Cos(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_COSH:
return
Math.Cosh(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_COT:
return
MathKGI.Cot(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_COTH:
return
MathKGI.Coth(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_CSC:
return
MathKGI.Csc(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_CSCH:
return
MathKGI.Csch(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_DELAY:
return
MathKGI.Delay(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters),
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_EXP:
return
Math.Exp(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_FACTORIAL:
return
MathKGI.Factorial(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_FLOOR:
return
Math.Floor(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_LN:
return
Math.Log(
EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData, listOfParameters),
Math.E);
case libsbml.AST_FUNCTION_LOG:
return
Math.Log10(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_PIECEWISE:
{
var numChildren = (int) node.getNumChildren();
var temps = new double[numChildren];
for (var i = 0; i < numChildren; i++)
{
temps[i] = EvaluateSingle(node.getChild(i), sVariableIds, oVariableData,
listOfParameters);
}
return Piecewise(temps);
// MathKGI.Piecewise(temps);
}
case libsbml.AST_FUNCTION_POWER:
return Math.Pow(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters),
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_ROOT:
return MathKGI.Root(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters),
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_SEC:
return MathKGI.Sec(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_SECH:
return MathKGI.Sech(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_SIN:
return Math.Sin(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_SINH:
return Math.Sinh(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_TAN:
return Math.Tan(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_FUNCTION_TANH:
return Math.Tanh(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_LOGICAL_AND:
{
var numChildren = (int) node.getNumChildren();
var temps = new double[numChildren];
for (var i = 0; i < numChildren; i++)
{
temps[i] = EvaluateSingle(node.getChild(i), sVariableIds, oVariableData,
listOfParameters);
}
return
MathKGI.And(temps);
}
case libsbml.AST_LOGICAL_NOT:
{
return
MathKGI.Not(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters));
}
case libsbml.AST_LOGICAL_OR:
{
var numChildren = (int) node.getNumChildren();
var temps = new double[numChildren];
for (var i = 0; i < numChildren; i++)
{
temps[i] = EvaluateSingle(node.getChild(i), sVariableIds, oVariableData,
listOfParameters);
}
return
MathKGI.Or(temps);
}
case libsbml.AST_LOGICAL_XOR:
{
var numChildren = (int) node.getNumChildren();
var temps = new double[numChildren];
for (var i = 0; i < numChildren; i++)
{
temps[i] = EvaluateSingle(node.getChild(i), sVariableIds, oVariableData,
listOfParameters);
}
return
MathKGI.Xor(temps);
}
case libsbml.AST_RELATIONAL_EQ:
{
return
MathKGI.Eq(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters),
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData,
listOfParameters));
}
case libsbml.AST_RELATIONAL_GEQ:
return
MathKGI.Geq(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters),
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_RELATIONAL_GT:
return
MathKGI.Gt(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters),
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_RELATIONAL_LEQ:
return
MathKGI.Leq(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters),
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_RELATIONAL_LT:
return
MathKGI.Lt(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters),
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData,
listOfParameters));
case libsbml.AST_RELATIONAL_NEQ:
return
MathKGI.Neq(EvaluateSingle(node.getLeftChild(), sVariableIds, oVariableData,
listOfParameters),
EvaluateSingle(node.getRightChild(), sVariableIds, oVariableData,
listOfParameters));
default:
break;
}
return 0;
}
public static double Piecewise(double[] args)
{
double result;
for (var i = 0; i < args.Length - 1; i += 2)
{
var num = args[i + 1];
if (num == 1.0)
{
result = args[i];
return result;
}
}
result = args[args.Length - 1];
return result;
}
public static double SaveDouble(string value, double defaultValue = 0)
{
double val;
if (double.TryParse(value, out val))
return val;
return defaultValue;
}
public static long SaveInt(string value, long defaultValue)
{
long val;
if (long.TryParse(value, out val))
return val;
return defaultValue;
}
public static int SaveInt(string value, int defaultValue)
{
int val;
if (int.TryParse(value, out val))
return val;
return defaultValue;
}
public static double GetDouble(this DataGridViewCell cell, double defaultValue = 0)
{
var value = cell.Value;
if (value.GetType() == typeof (string))
{
return SaveDouble((string) value, defaultValue);
}
if (value.GetType() == typeof (double) || value.GetType() == typeof (int))
return (double) value;
return defaultValue;
}
public static CompartmentMapping getCompartmentMapping(this Compartment comp)
{
if (comp == null) return null;
var cplug = (SpatialCompartmentPlugin) comp.getPlugin("spatial");
if (cplug == null) return null;
var map = cplug.getCompartmentMapping();
if (map == null)
map = cplug.createCompartmentMapping();
return map;
}
public static int GetNumAnalyticGeometries(this Geometry geom)
{
if (geom == null) return 0;
var count = 0;
for (var i = 0; i < geom.getNumGeometryDefinitions(); ++i)
{
var current = geom.getGeometryDefinition(i);
if (current is AnalyticGeometry) ++count;
}
return count;
}
public static int GetNumSampledFieldGeometries(this Geometry geom)
{
if (geom == null) return 0;
var count = 0;
for (var i = 0; i < geom.getNumGeometryDefinitions(); ++i)
{
var current = geom.getGeometryDefinition(i);
if (current is SampledFieldGeometry) ++count;
}
return count;
}
}
}<file_sep>/WFEditSpatial/Controls/ControlAnalyticGeometry.cs
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Drawing.Imaging;
using System.Linq;
using System.Windows.Forms;
using EditSpatial.Model;
using libsbmlcs;
using LibEditSpatial.Model;
using Image = System.Drawing.Image;
namespace EditSpatial.Controls
{
public partial class ControlAnalyticGeometry : BaseSpatialControl
{
public ControlAnalyticGeometry()
{
InitializeComponent();
IsInitializing = false;
RowsAdded = new List<int>();
}
public List<int> RowsAdded { get; set; }
public Geometry SpatialGeometry { get; set; }
public AnalyticGeometry Current { get; set; }
public int ThumbSize
{
get
{
int thumbSize;
if (int.TryParse(txtSize.Text, out thumbSize))
return thumbSize;
return 128;
}
}
public double CurrentZ
{
get
{
double value;
if (double.TryParse(txtZ.Text, out value))
return value;
return 0;
}
}
private void CommitAddedRows(AnalyticGeometry analytic)
{
if (RowsAdded.Count > 0 && analytic != null)
{
for (var i = RowsAdded.Count - 1; i >= 0; i--)
{
var row = grid.Rows[RowsAdded[i]];
var vol = analytic.createAnalyticVolume();
vol.setId(row.Cells[0].Value as string);
vol.setFunctionType(libsbml.FunctionKind_fromString(row.Cells[1].Value as string));
long ordinal = 0;
vol.setOrdinal((int)ordinal);
if (long.TryParse(row.Cells[2].Value as string, out ordinal))
vol.setOrdinal((int)ordinal);
vol.setDomainType(row.Cells[3].Value as string);
vol.setMath(libsbml.parseL3Formula(row.Cells[4].Value as string));
RowsAdded.RemoveAt(i);
}
}
}
public void InitializeFrom(Geometry geometry, AnalyticGeometry analytic)
{
IsInitializing = true;
try
{
if (geometry == null)
{
txtId.Text = "";
grid.Rows.Clear();
SpatialGeometry = geometry;
thumbGeometry.Image = thumbGeometry.InitialImage;
return;
}
CommitAddedRows(analytic);
grid.Rows.Clear();
SpatialGeometry = geometry;
thumbGeometry.Image = thumbGeometry.InitialImage;
Current = analytic;
if (analytic == null) return;
chkIsActive.Checked = analytic.getIsActive();
txtId.Text = analytic.getId();
for (long i = 0; i < analytic.getNumAnalyticVolumes(); ++i)
{
var vol = analytic.getAnalyticVolume(i);
var spatialId = vol.getId();
grid.Rows.Add(spatialId, libsbml.FunctionKind_toString(vol.getFunctionType()), vol.getOrdinal().ToString(), vol.getDomainType(),
libsbml.formulaToL3String(vol.getMath()));
}
if (SpatialGeometry.getNumCoordinateComponents() < 3)
{
txtZ.Visible = false;
trackBar1.Visible = false;
lblZ.Visible = false;
}
else
{
txtZ.Visible = true;
trackBar1.Visible = true;
lblZ.Visible = true;
}
thumbGeometry.Image = GenerateImage(analytic, geometry, ThumbSize, ThumbSize);
}
finally
{
IsInitializing = false;
}
}
public void InitializeFrom(Geometry geometry, string id)
{
if (geometry == null)
{
txtId.Text = id;
grid.Rows.Clear();
SpatialGeometry = geometry;
thumbGeometry.Image = thumbGeometry.InitialImage;
return;
}
var analytic = geometry.getGeometryDefinition(id) as AnalyticGeometry;
InitializeFrom(geometry, analytic);
}
public override void SaveChanges()
{
if (Current == null || SpatialGeometry == null) return;
Current.setId(txtId.Text);
Current.setIsActive(chkIsActive.Checked);
CommitAddedRows(Current);
for (var i = 0; i < grid.Rows.Count && i < Current.getNumAnalyticVolumes(); ++i)
{
var row = grid.Rows[i];
if (row.IsNewRow) continue;
var current = Current.getAnalyticVolume(i);
current.setId((string) row.Cells[0].Value);
current.setFunctionType(libsbml.FunctionKind_fromString((string) row.Cells[1].Value));
current.setOrdinal((int)Util.SaveInt((string) row.Cells[2].Value, 0L));
current.setDomainType((string) row.Cells[3].Value);
current.setMath(libsbml.parseL3Formula((string) row.Cells[4].Value));
}
Current.ExpandMath();
OnModelChanged();
}
private DmpModel GenerateDmp(int thumbSize)
{
if (Current == null || SpatialGeometry == null) return null;
try
{
Current.ExpandMath();
var formulas = new List<Tuple<int, ASTNode>>();
for (long i = 0; i < Current.getNumAnalyticVolumes(); ++i)
{
var current = Current.getAnalyticVolume(i);
formulas.Add(new Tuple<int, ASTNode>((int) current.getOrdinal(), current.getMath()));
}
formulas.Sort((a, b) => b.Item1.CompareTo(a.Item1));
var range1 = SpatialGeometry.getCoordinateComponent(0);
var r1Min = range1.getBoundaryMin().getValue();
var r1Max = range1.getBoundaryMax().getValue();
var range2 = SpatialGeometry.getCoordinateComponent(1);
var r2Min = range2.getBoundaryMin().getValue();
var r2Max = range2.getBoundaryMax().getValue();
var r3Max = SpatialGeometry.getNumCoordinateComponents() == 3
? SpatialGeometry.getCoordinateComponent(2).getBoundaryMax().getValue()
: 0;
var r3Min = SpatialGeometry.getNumCoordinateComponents() == 3
? SpatialGeometry.getCoordinateComponent(2).getBoundaryMin().getValue()
: 0;
var resX = thumbSize;
var resY = thumbSize;
var result = new DmpModel(resX, resY)
{
MinX = r1Min,
MaxX = r1Max,
MinY = r2Min,
MaxY = r2Max
};
for (var nX = 0; nX < resX; ++nX)
{
var x = r1Min
+
(r1Max - r1Min)/
resX*nX;
for (var nY = 0; nY < resY; ++nY)
{
var y = r2Min
+
(r2Max - r2Min)/
resY*nY;
for (var index = 0; index < formulas.Count; index++)
{
var item = formulas[index];
var isInside = Util.Evaluate(item.Item2,
new List<string>
{
"x",
"y",
"z",
"width",
"height",
"depth",
"Xmin",
"Xmax",
"Ymin",
"Ymax",
"Zmin",
"Zmax"
},
new List<double> {x, y, CurrentZ, r1Max, r2Max, r3Max, r1Min, r1Max, r2Min, r2Max, r3Min, r3Max},
new List<Tuple<string, double>>()
);
if (Math.Abs((isInside - 1.0)) < 1E-10)
{
result[nX, nY] = item.Item1;
break;
}
}
}
}
return result;
}
catch
{
}
return null;
}
private void ExportDmp(string fileName, int thumbSize)
{
var dmp = GenerateDmp(thumbSize);
if (dmp != null)
dmp.SaveAs(fileName);
}
private Image GenerateImage(AnalyticGeometry analytic, Geometry geometry, int resX = 128, int resY = 128)
{
if (geometry == null || analytic == null || geometry.getNumCoordinateComponents() < 2)
return new Bitmap(1, 1);
try
{
Current.ExpandMath();
var formulas = new List<Tuple<int, ASTNode>>();
for (long i = 0; i < analytic.getNumAnalyticVolumes(); ++i)
{
var current = analytic.getAnalyticVolume(i);
formulas.Add(new Tuple<int, ASTNode>((int) current.getOrdinal(), current.getMath()));
}
formulas.Sort((a, b) => b.Item1.CompareTo(a.Item1));
var range1 = geometry.getCoordinateComponent(0);
var r1Min = range1.getBoundaryMin().getValue();
var r1Max = range1.getBoundaryMax().getValue();
var range2 = geometry.getCoordinateComponent(1);
var r2Min = range2.getBoundaryMin().getValue();
var r2Max = range2.getBoundaryMax().getValue();
var r3Max = geometry.getNumCoordinateComponents() == 3
? geometry.getCoordinateComponent(2).getBoundaryMax().getValue()
: 0;
var r3Min = geometry.getNumCoordinateComponents() == 3
? geometry.getCoordinateComponent(2).getBoundaryMin().getValue()
: 0;
var result = new Bitmap(resX, resY);
for (var i = 0; i < resX; ++i)
{
var x = r1Min
+
(r1Max - r1Min)/
resX*i;
for (var j = 0; j < resY; ++j)
{
var y = r2Min
+
(r2Max - r2Min)/
resY*j;
for (var index = 0; index < formulas.Count; index++)
{
var item = formulas[index];
var isInside = Util.Evaluate(item.Item2,
new List<string>
{
"x",
"y",
"z",
"width",
"height",
"depth",
"Xmin",
"Xmax",
"Ymin",
"Ymax",
"Zmin",
"Zmax"
},
new List<double> {x, y, CurrentZ, r1Max, r2Max, r3Max, r1Min, r1Max, r2Min, r2Max, r3Min, r3Max},
new List<Tuple<string, double>>()
);
if (Math.Abs((isInside - 1.0)) < 1E-10)
{
result.SetPixel(i, j, GetColorForIndex(item.Item1));
break;
}
}
}
}
return result;
}
catch
{
}
return new Bitmap(1, 1);
}
private Color GetColorForIndex(int index)
{
switch (index)
{
default:
case 0:
return Color.Black;
case 1:
return Color.Red;
case 2:
return Color.Blue;
case 3:
return Color.Green;
case 4:
return Color.Chocolate;
case 5:
return Color.SlateGray;
}
}
private void FlipOrder(AnalyticGeometry geometry)
{
if (geometry == null)
return;
if (geometry.getNumAnalyticVolumes() == 0)
return;
var list = new List<Tuple<int, int>>();
for (long i = 0; i < geometry.getNumAnalyticVolumes(); ++i)
{
var current = geometry.getAnalyticVolume(i);
list.Add(new Tuple<int, int>((int) i, (int) current.getOrdinal()));
}
var ordered = list.OrderByDescending(item => item.Item2);
foreach (var item in ordered)
{
var current = geometry.getAnalyticVolume(item.Item1);
current.setOrdinal(((int)geometry.getNumAnalyticVolumes() - 1) - item.Item2);
}
}
private void SortedOrder(AnalyticGeometry geometry)
{
if (geometry == null)
return;
if (geometry.getNumAnalyticVolumes() == 0)
return;
var list = new List<Tuple<int, int, string>>();
for (long i = 0; i < geometry.getNumAnalyticVolumes(); ++i)
{
var current = geometry.getAnalyticVolume(i);
list.Add(new Tuple<int, int, string>((int) i, (int) current.getOrdinal(), current.getId()));
}
var ordered = list.OrderByDescending(item => item.Item2);
var volList = geometry.getListOfAnalyticVolumes();
foreach (var item in ordered)
{
var vol = volList.remove(item.Item3);
volList.insert(item.Item2, vol);
}
}
private void OnReorderClick(object sender, EventArgs e)
{
if (Current == null)
return;
FlipOrder(Current);
InitializeFrom(SpatialGeometry, Current.getId());
}
private void OnSortClick(object sender, EventArgs e)
{
if (Current == null)
return;
SortedOrder(Current);
InitializeFrom(SpatialGeometry, Current.getId());
}
private void OnUpdateImage(object sender, EventArgs e)
{
if (Current == null)
return;
IsInitializing = true;
SaveChanges();
IsInitializing = false;
InitializeFrom(SpatialGeometry, Current.getId());
}
private void OnTrackChanged(object sender, EventArgs e)
{
if (SpatialGeometry == null)
return;
if (SpatialGeometry.getNumCoordinateComponents() < 3)
return;
var range1 = SpatialGeometry.getCoordinateComponent(2);
var r1Min = range1.getBoundaryMin().getValue();
var r1Max = range1.getBoundaryMax().getValue();
var x = r1Min
+
(r1Max - r1Min)/
trackBar1.Maximum*trackBar1.Value;
txtZ.Text = x.ToString();
if (Current == null)
return;
InitializeFrom(SpatialGeometry, Current.getId());
}
public override void InvalidateSelection()
{
Current = null;
SpatialGeometry = null;
InitializeFrom(null, string.Empty);
}
private void OnExportTiffClick(object sender, EventArgs e)
{
if (grid.SelectedRows.Count == 0) return;
long ordinal = 0;
long.TryParse(grid.SelectedRows[0].Cells[2].Value as string, out ordinal);
var range1 = SpatialGeometry.getCoordinateComponent(0);
var r1Min = range1.getBoundaryMin().getValue();
var r1Max = range1.getBoundaryMax().getValue();
var range2 = SpatialGeometry.getCoordinateComponent(1);
var r2Min = range2.getBoundaryMin().getValue();
var r2Max = range2.getBoundaryMax().getValue();
var image = Current.GenerateTiff(SpatialGeometry, (int) r1Max, (int) r2Max, (int) ordinal, CurrentZ);
using (var dialog = new SaveFileDialog {Filter = "TIFF files|*.tif|All files|*.*"})
{
if (dialog.ShowDialog() == DialogResult.OK)
{
image.Save(dialog.FileName, ImageFormat.Tiff);
}
}
}
private void OnRowsAdded(object sender, DataGridViewRowsAddedEventArgs e)
{
if (Current == null || IsInitializing || e.RowIndex - 1 < 0) return;
RowsAdded.Add(e.RowIndex - 1);
}
private void OnMakeFirstClick(object sender, EventArgs e)
{
if (Current == null || IsInitializing || grid.SelectedRows.Count != 1) return;
var selected = grid.SelectedRows[0].Cells[0].Value as string;
for (var i = 0; i < Current.getNumAnalyticVolumes(); ++i)
{
var current = Current.getAnalyticVolume(i);
if (current == null) continue;
if (current.getId() != selected) continue;
Current.removeAnalyticVolume(i);
Current.getListOfAnalyticVolumes().insertAndOwn(0, current);
InitializeFrom(SpatialGeometry, Current.getId());
break;
}
}
private void cmdExport_Click(object sender, EventArgs e)
{
using (var dlg = new SaveFileDialog {Title = "Export DMP", Filter = "DMP files|*.dmp|All files|*.*"})
{
if (dlg.ShowDialog() == DialogResult.OK)
ExportDmp(dlg.FileName, ThumbSize);
}
}
}
}<file_sep>/LibEditSpatial/Model/VoidStringDelegate.cs
namespace LibEditSpatial.Model
{
public delegate void VoidStringDelegate(string data);
}<file_sep>/WFEditSpatial/Controls/ControlText.cs
using System.Windows.Forms;
namespace EditSpatial.Controls
{
public partial class ControlText : TextBox
{
public ControlText()
{
InitializeComponent();
}
}
}<file_sep>/WFEditSpatial/Controls/ControlCoordinateComponents.cs
using System.Windows.Forms;
using libsbmlcs;
namespace EditSpatial.Controls
{
public partial class ControlCoordinateComponents : BaseSpatialControl
{
public ControlCoordinateComponents()
{
InitializeComponent();
}
public Geometry Current { get; set; }
public void InitializeFrom(Geometry geometry)
{
while (tblLayout.Controls.Count > 1)
tblLayout.Controls.RemoveAt(1);
// remove old ones
while (tblLayout.RowStyles.Count > 1)
tblLayout.RowStyles.RemoveAt(1);
Current = geometry;
if (geometry == null) return;
txtCoordSystem.Text = libsbml.GeometryKind_toString(geometry.getCoordinateSystem());
for (long i = 0; i < geometry.getNumCoordinateComponents(); ++i)
{
tblLayout.RowStyles.Add(new RowStyle
{
SizeType = SizeType.AutoSize
});
var current = geometry.getCoordinateComponent(i);
var control = new ControlCoordinateComponent {Dock = DockStyle.Fill};
control.InitializeFrom(current);
tblLayout.Controls.Add(control, 0, ((int) i) + 1);
}
}
public override void SaveChanges()
{
if (Current == null) return;
Current.setCoordinateSystem(txtCoordSystem.Text);
foreach (var control in tblLayout.Controls)
{
var current = control as BaseSpatialControl;
if (current == null) continue;
current.SaveChanges();
}
OnModelChanged();
}
public override void InvalidateSelection()
{
Current = null;
InitializeFrom(null);
}
}
}<file_sep>/LibEditSpatial/pvrender.py
# simple paraview python script for generating screenshots
from paraview.simple import *
import sys
def main(args):
if (len(args) != 4):
print("Usage: renderFile filename variable output")
return 1
filename = args[1]
variable = args[2]
result = args[3]
renderFile(filename, variable, result)
def renderFile(fileName, variable, output):
#read a file
reader = XMLUnstructuredGridReader(FileName=fileName)
# select variable
data = reader.PointData[variable]
range = data.GetRange()
reader.PointArrayStatus = [variable]
#position camera
view = GetActiveView()
if not view:
view = CreateRenderView()
#lut, pws= return_colours(variable)
dp = GetDisplayProperties()
dp.PointSize = 2
dp.Representation = "Surface"
dp.ColorArrayName = variable
dp.LookupTable = MakeBlueToRedLT(range[0], range[1])
dp.LookupTable.ColorSpace = 'Diverging'
dp.LookupTable.VectorMode = 'Magnitude'
bar = CreateScalarBar(LookupTable=dp.LookupTable, Title=variable, TitleFontSize = 10, LabelFontSize = 10)
bar.AutomaticLabelFormat = 0
bar.LabelFormat = '%6.3f'
bar.RangeLabelFormat = '%6.3f'
bar.Title = variable
bar.ComponentTitle = ''
view.Representations.append(bar)
#draw the object
Show()
# render
Render()
#save screenshot
SaveScreenshot(output)
if __name__ == '__main__':
main(sys.argv)
<file_sep>/LibEditSpatial/Model/EditSpatialSettings.cs
using System;
using System.Diagnostics;
using System.Drawing;
using System.IO;
namespace LibEditSpatial.Model
{
[Serializable]
public class EditSpatialSettings
{
public string LastDir { get; set; }
public string CygwinDir { get; set; }
public string DuneDir { get; set; }
public string ParaViewDir { get; set; }
public string DefaultDir { get; set; }
public bool IgnoreMultiCompartments { get; set; }
public int Width { get; set; }
public int Height { get; set; }
private static EditSpatialSettings _Instance;
public static EditSpatialSettings Instance
{
get
{
if (_Instance == null)
_Instance = GetDefault(new Size(1000, 600));
return _Instance;
}
set
{
_Instance = value;
_Instance.Save();
}
}
public static string ConfigFile
{
get
{
return Path.Combine(Environment.GetFolderPath(Environment.SpecialFolder.ApplicationData), "spatial_tools.xml");
}
}
public void WriteXmlToFile(string fileName)
{
var serializer = new System.Xml.Serialization.XmlSerializer(this.GetType());
using (var stream = new StringWriter())
{
serializer.Serialize(stream, this);
stream.Flush();
File.WriteAllText(fileName, stream.ToString());
}
}
public static EditSpatialSettings FromXmlFile(string fileName)
{
try
{
var serializer = new System.Xml.Serialization.XmlSerializer(typeof(EditSpatialSettings));
var text = File.ReadAllText(fileName);
using (var stringReader = new StringReader(text))
{
return (EditSpatialSettings)serializer.Deserialize(stringReader);
}
}
catch (Exception ex)
{
Debug.WriteLine("Could not load settings: " + ex.Message + "\n\n" + ex.StackTrace);
return null;
}
}
public static EditSpatialSettings GetDefault(Size current)
{
var key = FromXmlFile(ConfigFile);
if (key == null)
return new EditSpatialSettings
{
Height = current.Height,
Width = current.Width
};
//var result = new EditSpatialSettings
//{
// LastDir = (string) key.GetValue("lastDir", ""),
// CygwinDir = (string) key.GetValue("cygwinDir", ""),
// DuneDir = (string) key.GetValue("duneDir", ""),
// ParaViewDir = (string) key.GetValue("paraviewDir", ""),
// DefaultDir = (string) key.GetValue("defaultDir", ""),
// IgnoreMultiCompartments = (int) key.GetValue("ignoreComps", 0) == 1,
// Height = (int) key.GetValue("height", current.Height),
// Width = (int) key.GetValue("width", current.Width)
//};
return key;
}
public void Save()
{
WriteXmlToFile(ConfigFile);
//var key = Application.UserAppDataRegistry;
//if (key == null) return;
//
//key.SetValue("lastDir", LastDir);
//key.SetValue("cygwinDir", CygwinDir);
//key.SetValue("duneDir", DuneDir);
//key.SetValue("defaultDir", DefaultDir);
//key.SetValue("paraviewDir", ParaViewDir);
//key.SetValue("ignoreComps", IgnoreMultiCompartments ? 1 : 0);
//key.SetValue("height", Height);
//key.SetValue("width", Width);
}
}
}<file_sep>/LibEditSpatial/Controls/CtrlNewton.cs
using System;
using System.Windows.Forms;
using LibEditSpatial.Model;
namespace LibEditSpatial.Controls
{
public partial class CtrlNewton : UserControl
{
public CtrlNewton()
{
InitializeComponent();
}
public NewtonConfig Model { get; set; }
public void LoadModel(NewtonConfig model)
{
Model = model;
txtLinearVerbosity.Text = model.LinearVerbosity.ToString();
txtReassembleThreshold.Text = model.ReassembleThreshold.ToString();
txtLineSearchMaxIterations.Text = model.LineSearchMaxIterations.ToString();
txtMaxIterations.Text = model.MaxIterations.ToString();
txtAbsoluteLimit.Text = model.AbsoluteLimit.ToString();
txtReduction.Text = model.Reduction.ToString();
txtLinearReduction.Text = model.LinearReduction.ToString();
txtLineSearchDampingFactor.Text = model.LineSearchDampingFactor.ToString();
txtVerbosity.Text = model.Verbosity.ToString();
}
private void txtVerbosity_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
int temp;
if (int.TryParse(txtVerbosity.Text, out temp))
Model.Verbosity = temp;
}
private void txtLineSearchDampingFactor_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtLineSearchDampingFactor.Text, out temp))
Model.LineSearchDampingFactor = temp;
}
private void txtLinearReduction_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtLinearReduction.Text, out temp))
Model.LinearReduction = temp;
}
private void txtReduction_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtReduction.Text, out temp))
Model.Reduction = temp;
}
private void txtAbsoluteLimit_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtAbsoluteLimit.Text, out temp))
Model.AbsoluteLimit = temp;
}
private void txtMaxIterations_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
int temp;
if (int.TryParse(txtMaxIterations.Text, out temp))
Model.MaxIterations = temp;
}
private void txtLineSearchMaxIterations_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
int temp;
if (int.TryParse(txtLineSearchMaxIterations.Text, out temp))
Model.LineSearchMaxIterations = temp;
}
private void txtReassembleThreshold_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtReassembleThreshold.Text, out temp))
Model.ReassembleThreshold = temp;
}
private void txtLinearVerbosity_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
int temp;
if (int.TryParse(txtLinearVerbosity.Text, out temp))
Model.LinearVerbosity = temp;
}
}
}<file_sep>/WFEditSpatial/Controls/ControlCompartment.cs
using System.Collections.Generic;
using System.Windows.Forms;
using libsbmlcs;
namespace EditSpatial.Controls
{
public partial class ControlCompartment : BaseSpatialControl
{
public ControlCompartment()
{
InitializeComponent();
RowsAdded = new List<int>();
}
private SBMLDocument Current { get; set; }
public List<int> RowsAdded { get; set; }
public void InitializeFrom(SBMLDocument document)
{
grid.Rows.Clear();
Current = document;
if (document == null || document.getModel() == null) return;
try
{
IsInitializing = true;
var model = document.getModel();
CommitAddedRows(Current);
for (long i = 0; i < model.getNumCompartments(); ++i)
{
var current = model.getCompartment(i);
grid.Rows.Add(current.getId(),
current.getName(),
current.getSize().ToString(),
current.getSpatialDimensionsAsDouble().ToString()
);
}
}
finally
{
IsInitializing = false;
}
}
private void CommitAddedRows(SBMLDocument current)
{
if (RowsAdded.Count > 0 && current != null)
{
for (var i = RowsAdded.Count - 1; i >= 0; i--)
{
try
{
var row = grid.Rows[RowsAdded[i]];
var comp = current.getModel().createCompartment();
comp.initDefaults();
comp.setId(row.Cells[0].Value as string);
comp.setName(row.Cells[1].Value as string);
double value;
if (double.TryParse((string) row.Cells[2].Value, out value))
comp.setSize(value);
if (double.TryParse((string) row.Cells[3].Value, out value))
comp.setSpatialDimensions(value);
RowsAdded.RemoveAt(i);
}
catch
{
}
}
}
}
public override void SaveChanges()
{
if (Current == null) return;
CommitAddedRows(Current);
for (var i = 0; i < grid.Rows.Count; ++i)
{
var row = grid.Rows[i];
var current = Current.getModel().getCompartment((string) row.Cells[0].Value);
if (current == null) continue;
current.setName(row.Cells[1].Value as string);
double value;
if (double.TryParse((string) row.Cells[2].Value, out value))
current.setSize(value);
if (double.TryParse((string) row.Cells[3].Value, out value))
current.setSpatialDimensions(value);
}
OnModelChanged();
}
public override void InvalidateSelection()
{
Current = null;
InitializeFrom(null);
}
private void OnUserDeletedRow(object sender, DataGridViewRowEventArgs e)
{
if (Current == null) return;
var id = e.Row.Cells[0].Value as string;
Current.getModel().removeParameter(id);
InitializeFrom(Current);
}
private void OnRowsAdded(object sender, DataGridViewRowsAddedEventArgs e)
{
if (Current == null || IsInitializing || e.RowIndex == 0) return;
RowsAdded.Add(e.RowIndex - 1);
}
}
}<file_sep>/LibEditSpatial/Controls/DmpRenderControl.cs
using System;
using System.Drawing;
using System.Threading.Tasks;
using System.Windows.Forms;
using LibEditSpatial.Model;
namespace LibEditSpatial.Controls
{
public partial class DmpRenderControl : UserControl
{
public DmpRenderControl()
{
InitializeComponent();
Painting = false;
CurrentValue = 10;
PencilSize = 1;
}
public DmpModel Model { get; set; }
public double CurrentValue { get; set; }
private bool Painting { get; set; }
public int PencilSize { get; set; }
public bool DisableEditing { get; set; }
public bool DisableNotification { get; set; }
public event EventHandler<Point> IndexLocationChanged;
protected virtual void OnLocationChanged(Point e)
{
if (DisableNotification) return;
var handler = IndexLocationChanged;
if (handler != null) handler(this, e);
}
public event EventHandler<PointF> DataLocationChanged;
protected virtual void OnDataLocationChanged(PointF e)
{
if (DisableNotification) return;
var handler = DataLocationChanged;
if (handler != null) handler(this, e);
}
private void OnTimerTick(object sender, EventArgs e)
{
if (Model == null) return;
var bmp = Model.ToImage();
pictureBox1.Image = bmp;
timer1.Enabled = false;
}
private void UpdateUI()
{
timer1.Enabled = true;
}
public void LoadModel(DmpModel model)
{
Painting = false;
Model = model;
UpdateUI();
}
private void SetValueAround(Point point)
{
if (DisableEditing) return;
Parallel.For(0, PencilSize, i =>
{
for (var j = 0; j < PencilSize; j++)
{
var offSetX = Math.Floor(PencilSize/2f) - i;
var offSetY = Math.Floor(PencilSize/2f) - j;
var posX = (int) Math.Round(point.X - offSetX);
var posY = (int) Math.Round(point.Y - offSetY);
if (posX < 0) posX = 0;
if (posY < 0) posY = 0;
if (posX >= Model.Columns) posX = Model.Columns - 1;
if (posY >= Model.Rows) posY = Model.Rows - 1;
var distance = Math.Sqrt(Math.Pow(point.X - posX, 2) + Math.Pow(point.Y - posY, 2));
if (distance > PencilSize/2f) continue;
Model[posX, posY] = CurrentValue;
}
Application.DoEvents();
});
Model[point.X, point.Y] = CurrentValue;
UpdateUI();
}
private void OnMouseClick(object sender, MouseEventArgs e)
{
if (Model == null) return;
var point = GetPointForMouse(e.X, e.Y);
if (DisableEditing) return;
SetValueAround(point);
}
private Point GetPointForMouse(int x, int y)
{
if (Model == null) return Point.Empty;
var stretchX = (Model.Columns - 1)/(double) pictureBox1.Width;
var stretchY = (Model.Rows - 1)/(double) pictureBox1.Height;
var point = new Point(
Math.Max(Math.Min((int) Math.Round(stretchX*x + stretchX/2f), Model.Columns - 1), 0),
Math.Max(Math.Min((int) Math.Round(stretchY*y + stretchY/2f), Model.Rows - 1), 0));
OnDataLocationChanged(new PointF(
(float) ((point.X/(float) Model.Columns)*(Model.MaxX - Model.MinX) + Model.MinX),
(float) ((point.Y/(float) Model.Rows)*(Model.MaxY - Model.MinY) + Model.MinY)));
OnLocationChanged(point);
return point;
}
private void OnMouseMove(object sender, MouseEventArgs e)
{
var point = GetPointForMouse(e.X, e.Y);
if (Model == null || !Painting) return;
SetValueAround(point);
}
private void OnMouseDown(object sender, MouseEventArgs e)
{
Painting = e.Button == MouseButtons.Left;
}
private void OnMouseUp(object sender, MouseEventArgs e)
{
Painting = false;
}
private void OnMouseLeave(object sender, EventArgs e)
{
Painting = false;
}
}
}<file_sep>/LibEditSpatial/Controls/CtrlTime.cs
using System;
using System.Windows.Forms;
using LibEditSpatial.Model;
namespace LibEditSpatial.Controls
{
public partial class CtrlTime : UserControl
{
public CtrlTime()
{
InitializeComponent();
}
public TimeLoopConfig Model { get; set; }
public void LoadModel(TimeLoopConfig model)
{
Model = model;
txtTime.Text = Model.Time.ToString();
txtInitialStep.Text = Model.InitialStep.ToString();
txtMinStep.Text = Model.MinStep.ToString();
txtMaxStep.Text = Model.MaxStep.ToString();
txtPlotStep.Text = Model.PlotStep.ToString();
}
private void OnEndTimeChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtTime.Text, out temp))
Model.Time = temp;
}
private void OnInitialStepChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtInitialStep.Text, out temp))
Model.InitialStep = temp;
}
private void OnMinStepChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtMinStep.Text, out temp))
Model.MinStep = temp;
}
private void OnMaxStepChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtMaxStep.Text, out temp))
Model.MaxStep = temp;
}
private void OnPlotStepChanged(object sender, EventArgs e)
{
if (Model == null) return;
double temp;
if (double.TryParse(txtPlotStep.Text, out temp))
Model.PlotStep = temp;
}
}
}<file_sep>/LibEditSpatial/Controls/PalleteArgs.cs
using System;
namespace LibEditSpatial.Controls
{
public class PalleteArgs
{
public double Min { get; set; }
public double Max { get; set; }
public double Value { get; set; }
public double Range
{
get { return Max - Min; }
}
public double MapToUnit(double value)
{
if (value < Min) return 0;
if (value > Max) return 1;
if (Math.Abs(Range) < 1e-10) return 0;
return (value - Min) / Range;
}
public double MapFromUnit(double value)
{
return value * Range + Min;
}
}
}
<file_sep>/WFEditSpatial/Controls/ControlDomainTypes.cs
using libsbmlcs;
namespace EditSpatial.Controls
{
public partial class ControlDomainTypes : BaseSpatialControl
{
public ControlDomainTypes()
{
InitializeComponent();
}
private Geometry Current { get; set; }
public void InitializeFrom(Geometry geometry)
{
grid.Rows.Clear();
Current = geometry;
if (geometry == null) return;
for (long i = 0; i < geometry.getNumDomainTypes(); ++i)
{
var domainType = geometry.getDomainType(i);
grid.Rows.Add(domainType.getId(), domainType.getSpatialDimensions());
}
}
public override void SaveChanges()
{
if (Current == null) return;
for (var i = 0; i < grid.Rows.Count && i < Current.getNumDomainTypes(); ++i)
{
var current = Current.getDomainType(i);
var row = grid.Rows[i];
current.setId((string) row.Cells[0].Value);
current.setSpatialDimensions((int) row.Cells[1].Value);
}
OnModelChanged();
}
public override void InvalidateSelection()
{
Current = null;
InitializeFrom(null);
}
}
}<file_sep>/WFEditSpatial/Model/SpatialSpecies.cs
namespace EditSpatial.Model
{
public class SpatialSpecies
{
public string Id { get; set; }
public double MinBoundaryX { get; set; }
public double MinBoundaryY { get; set; }
public double MaxBoundaryX { get; set; }
public double MaxBoundaryY { get; set; }
public double DiffusionX { get; set; }
public double DiffusionY { get; set; }
public string BCType { get; set; }
public string InitialCondition { get; set; }
protected bool Equals(SpatialSpecies other)
{
return string.Equals(Id, other.Id);
}
public override int GetHashCode()
{
return (Id != null ? Id.GetHashCode() : 0);
}
public override string ToString()
{
return Id;
}
public override bool Equals(object obj)
{
if (ReferenceEquals(null, obj)) return false;
if (ReferenceEquals(this, obj)) return true;
if (obj is string) return Id == (string) obj;
if (obj.GetType() != GetType()) return false;
return Equals((SpatialSpecies) obj);
}
}
}<file_sep>/EditEvents.md
## Event Support
Discrete events are helpful to modify the behavior of the simulation. We added only limited support for time based events, that is events that hit after a specific time has been reached. To create events, you place a file called `eventinfo.ini`. Here an example INI file:
skipOutputUntilEvent = true
[event0]
start = 10 # start time of the event, the first accepted time point after this the event will be applied
target = 0 # index of the variable to assign to
file = event.dmp # the dmp file with the information
uniform = 0 # alternatively, the value to be assigned to it uniformly
You can add as many sections in that file as you need. Each section should contain the following entries:
* `start`: this has to be a numeric value indicating the start time, when this event ought to hit. Note that no interpolation is performed here. As soon as the start time is hit (or passed) the event will be applied.
* `target`: this is a zero based index of the model variable to be changed. To find the index for the variable to be changed you could have a look at the `host.config` file, there in the `[Data]` section all variables are listed, in the same order. (A future version will allow to use the variable names directly)
* `file`: this is a `.dmp` file containing the concentrations to be set onto the variable. In case a uniform value should be applied, this entry can be left empty.
* `uniform`: if no `file` is specified, this value will be taken when the event is applied, and the whole field is uniformly filled.
There is also a boolean entry `skipOutputUntilEvent` in the general section outside the file. If this value is set to `true`, output will be suppressed until the first event is applied.
---
<file_sep>/WFEditSpatial/Model/CreateModel.cs
using System.Collections.Generic;
namespace EditSpatial.Model
{
public class CreateModel
{
public CreateModel()
{
Species = new List<SpatialSpecies>();
Geometry = new GeometrySettings();
}
public List<SpatialSpecies> Species { get; set; }
public GeometrySettings Geometry { get; set; }
public SpatialSpecies this[string id]
{
get { return Species.Find(species => species.Id == id); }
}
}
}<file_sep>/WFEditSpatial/Model/SpatialModel.cs
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
using System.Text.RegularExpressions;
using EditSpatial.Converter;
using libsbmlcs;
using libSbl2SBML;
using SBMLSupport;
namespace EditSpatial.Model
{
public class SpatialModel
{
public bool Dirty { get; set; }
internal static CustomSpatialValidator CustomSpatialValidator = new CustomSpatialValidator();
public SpatialModel()
{
DefaultHeight = DefaultWidth = 50;
FileName = "untitled.xml";
}
public double DefaultWidth { get; set; }
public double DefaultHeight { get; set; }
public double Width
{
get
{
if (!IsSpatial || Geometry == null) return DefaultWidth;
return GetBoundaryMaxValue(0, DefaultWidth);
}
}
public double Height
{
get
{
if (!IsSpatial || Geometry == null) return DefaultHeight;
return GetBoundaryMaxValue(1, DefaultHeight);
}
}
public SBMLDocument Document { get; set; }
public Geometry Geometry
{
get
{
if (!IsSpatial) return null;
var plugin = (SpatialModelPlugin)Document.getModel().getPlugin("spatial");
if (plugin == null) return null;
return plugin.getGeometry();
}
}
public string FileName { get; set; }
public bool IsSpatial
{
get
{
if (Document == null) return false;
if (Document.getPlugin("spatial") == null) return false;
if (Document.getModel() == null) return false;
if (Document.getModel().getPlugin("spatial") == null) return false;
return true;
}
}
public bool HaveModel
{
get { return Document != null && Document.getModel() != null; }
}
public void ExportDuneSBML(string filename)
{
try
{
var converter = new DuneConverter(Document);
File.WriteAllText(filename, converter.ToSBML());
}
catch
{
}
}
private double GetBoundaryMaxValue(int val, double defaultValue = 0)
{
if (val >= Geometry.getNumCoordinateComponents()) return defaultValue;
try
{
return Geometry.getCoordinateComponent(val).getBoundaryMax().getValue();
}
catch
{
return defaultValue;
}
}
public static SpatialModel FromFile(string fileName)
{
var model = new SpatialModel
{
Document = libsbml.readSBMLFromFile(fileName),
FileName = fileName
};
if (model.Document.getNumErrors(libsbml.LIBSBML_SEV_ERROR) > 0)
{
var docString = File.ReadAllText(fileName);
if (docString.Contains("spatial:spatialId"))
{
var assembly = System.Reflection.Assembly.GetAssembly(typeof(SBMLDocument));
var converter = from type in assembly.GetTypes() where type.Name == "OldSpatialToSpatialConverter" select type;
if (converter != null && converter.Any())
model.Document = converter.First().GetMethod("convertOldSpatialSBMLFromString").Invoke(null, new object[] { docString }) as SBMLDocument;
//libsbml.Oldspat
}
}
model.Document.setConsistencyChecks(libsbml.LIBSBML_CAT_UNITS_CONSISTENCY, false);
model.Document.setConsistencyChecks(libsbml.LIBSBML_CAT_MODELING_PRACTICE, false);
model.Document.addValidator(CustomSpatialValidator);
model.Document.validateSBML();
model.Document.setConsistencyChecks(libsbml.LIBSBML_CAT_UNITS_CONSISTENCY, false);
model.Document.setConsistencyChecks(libsbml.LIBSBML_CAT_MODELING_PRACTICE, false);
return model;
}
public static SpatialModel FromJarnac(string content)
{
NOM.Namespaces = new List<string>();
var model = TModel.ReadModel(content);
model.RemoveAnnotation();
model.RemoveNotes();
return FromString(model.toSBML(false));
}
public static SpatialModel FromString(string content)
{
var model = new SpatialModel
{
Document = libsbml.readSBMLFromString(content),
FileName = "fromstring.xml"
};
model.Document.setConsistencyChecks(libsbml.LIBSBML_CAT_UNITS_CONSISTENCY, false);
model.Document.setConsistencyChecks(libsbml.LIBSBML_CAT_MODELING_PRACTICE, false);
model.Document.addValidator(CustomSpatialValidator);
model.Document.validateSBML();
model.Document.setConsistencyChecks(libsbml.LIBSBML_CAT_UNITS_CONSISTENCY, false);
model.Document.setConsistencyChecks(libsbml.LIBSBML_CAT_MODELING_PRACTICE, false);
return model;
}
public void SaveTo(string filename)
{
if (Document == null) return;
FileName = filename;
Dirty = false;
libsbml.writeSBMLToFile(Document, filename);
}
public string ToSBML()
{
if (Document == null) return "<none>";
return libsbml.writeSBMLToString(Document).Replace("\n", Environment.NewLine);
}
public string ToJarnac()
{
if (Document == null) return "";
try
{
return convertSBML.ToSBL(ToSBML());
}
catch
{
return "";
}
}
public void MoveAllRulesToAssignments()
{
if (Document == null || Document.getModel() == null)
return;
var model = Document.getModel();
for (var i = (int)model.getNumRules() - 1; i >= 0; i--)
{
var current = model.getRule(i) as AssignmentRule;
if (current == null) continue;
model.MoveRuleToAssignment(current.getVariable());
}
OnModelChanged();
}
public bool FixCommonErrors()
{
var result = false;
if (Document == null) return result;
FixUnits();
FixDiffusionCoefficients();
if (Document.getNumErrors(libsbml.LIBSBML_SEV_ERROR) == 0) return result;
for (var i = 0; i < Document.getNumErrors(); ++i)
{
var current = Document.getError(i);
var id = current.getErrorId();
var message = current.getMessage();
switch (current.getErrorId())
{
case 99109:
{
var plugin = Document.getPlugin("req");
if (plugin == null) break;
Document.setPackageRequired("req", false);
result = true;
break;
}
case 20517:
{
for (var j = 0; j < Document.getModel().getNumCompartments(); ++j)
{
var comp = Document.getModel().getCompartment(j);
if (!comp.isSetConstant())
comp.setConstant(true);
}
result = true;
break;
}
case 20623:
{
for (var j = 0; j < Document.getModel().getNumSpecies(); ++j)
{
var species = Document.getModel().getSpecies(j);
if (!species.isSetConstant())
species.setConstant(false);
}
result = true;
break;
}
case 21116:
{
for (var j = 0; j < Document.getModel().getNumReactions(); ++j)
{
var reaction = Document.getModel().getReaction(j);
for (var k = 0; k < reaction.getNumProducts(); ++k)
{
var reference = reaction.getProduct(k);
if (!reference.isSetConstant())
reference.setConstant(true);
}
for (var k = 0; k < reaction.getNumReactants(); ++k)
{
var reference = reaction.getReactant(k);
if (!reference.isSetConstant())
reference.setConstant(true);
}
}
result = true;
break;
}
case 21110:
{
for (var j = 0; j < Document.getModel().getNumReactions(); ++j)
{
var reaction = Document.getModel().getReaction(j);
if (!reaction.isSetFast())
reaction.setFast(false);
if (!reaction.isSetReversible())
reaction.setReversible(false);
}
result = true;
break;
}
case 20706:
{
for (var j = 0; j < Document.getModel().getNumParameters(); ++j)
{
var parameter = Document.getModel().getParameter(j);
if (!parameter.isSetConstant())
parameter.setConstant(false);
}
result = true;
break;
}
case 20610:
{
// have both rule and reaction on species this error is there in old versions of
// vcell code, that would export initial assignments as assignment rules
var match = Regex.Match(message.Split('\n')[2],
".*'(?<speciesId>\\w+?)'.*'(?<reactionId>\\w+?)'.*",
RegexOptions.ExplicitCapture);
if (match.Success)
{
var speciesId = match.Groups["speciesId"].Value;
Document.getModel().MoveRuleToAssignment(speciesId);
result = true;
}
break;
}
case 21121:
{
var match = Regex.Match(message, ".*'(?<speciesId>\\w+?)'.*'(?<reactionId>\\w+?)'.*",
RegexOptions.ExplicitCapture);
if (match.Success)
{
var speciesId = match.Groups["speciesId"].Value;
var reactionId = match.Groups["reactionId"].Value;
var reaction = Document.getModel().getReaction(reactionId);
if (reaction != null)
{
if (reaction.getModifier(speciesId) == null)
{
var modifier = reaction.createModifier();
modifier.setSpecies(speciesId);
result = true;
}
}
}
break;
}
case 99303:
{
// remove the unitdefinition referenced by an element
var match = Regex.Match(message, ".*<(?<type>\\w+?)>.*'(?<id>\\w+?)'.*",
RegexOptions.ExplicitCapture);
if (match.Success)
{
var type = match.Groups["type"].Value;
var elementId = match.Groups["id"].Value;
switch (type)
{
case "parameter":
{
var param = Document.getModel().getParameter(elementId);
if (param != null)
{
param.unsetUnits();
result = true;
}
break;
}
default:
{
Debug.WriteLine("Need to fix type '{0}' und id '{1}'", type, elementId);
break;
}
}
}
break;
}
default:
Debug.WriteLine("Don't know what to do with: {0}: {1}", id, message);
break;
}
}
Document.getErrorLog().clearLog();
if (result)
OnModelChanged();
return result;
}
public void FixUnits()
{
if (Document == null) return;
var model = Document.getModel();
if (model == null) return;
var needUpdate = false;
if (!model.isSetLengthUnits())
{
model.setLengthUnits("length");
needUpdate = true;
}
if (!model.isSetVolumeUnits())
{
model.setVolumeUnits("volume");
needUpdate = true;
}
if (!model.isSetSubstanceUnits())
{
model.setSubstanceUnits("substance");
needUpdate = true;
}
if (!model.isSetTimeUnits())
{
model.setTimeUnits("time");
needUpdate = true;
}
var unit = model.getUnitDefinition("length");
if (unit == null)
{
unit = model.createUnitDefinition();
unit.setId("length");
var kind = unit.createUnit();
kind.setKind(libsbml.UNIT_KIND_METRE);
kind.setExponent(1);
kind.setScale(0);
kind.setMultiplier(1e-06);
needUpdate = true;
}
unit = model.getUnitDefinition("volume");
if (unit == null)
{
unit = model.createUnitDefinition();
unit.setId("volume");
var kind = unit.createUnit();
kind.setKind(libsbml.UNIT_KIND_LITRE);
kind.setExponent(1);
kind.setScale(-3);
kind.setMultiplier(1);
model.setVolumeUnits("volume");
needUpdate = true;
}
unit = model.getUnitDefinition("substance");
if (unit == null)
{
unit = model.createUnitDefinition();
unit.setId("substance");
var kind = unit.createUnit();
kind.setKind(libsbml.UNIT_KIND_MOLE);
kind.setExponent(1);
kind.setScale(-6);
kind.setMultiplier(1);
model.setSubstanceUnits("substance");
needUpdate = true;
}
unit = model.getUnitDefinition("time");
if (unit == null)
{
unit = model.createUnitDefinition();
unit.setId("time");
var kind = unit.createUnit();
kind.setKind(libsbml.UNIT_KIND_SECOND);
kind.setExponent(1);
kind.setScale(0);
kind.setMultiplier(60);
model.setTimeUnits("time");
needUpdate = true;
}
if (needUpdate)
OnModelChanged();
}
public void FixDiffusionCoefficients()
{
if (Document == null || !IsSpatial || Geometry == null) return;
var numCooords = Geometry.getNumCoordinateComponents();
var dict = new Dictionary<string, List<Tuple<int, Parameter>>>();
for (var i = 0; i < Document.getModel().getNumParameters(); ++i)
{
var current = Document.getModel().getParameter(i);
if (current == null) continue;
var plug = current.getPlugin("spatial") as SpatialParameterPlugin;
if (plug == null) continue;
if (plug.getType() != libsbml.SBML_SPATIAL_DIFFUSIONCOEFFICIENT) continue;
var diff = plug.getDiffusionCoefficient();
if (diff == null || !diff.isSetCoordinateReference1()) continue;
if (!dict.ContainsKey(diff.getVariable()))
dict[diff.getVariable()] = new List<Tuple<int, Parameter>>();
dict[diff.getVariable()].Add(new Tuple<int, Parameter>((int)diff.getCoordinateReference1(), current));
}
foreach (var key in dict.Keys)
{
var current = dict[key];
if (current == null || current.Count == 0 || current.Count == numCooords) continue;
foreach (var entry in current)
{
var param = entry.Item2;
if (param == null) continue;
var plug = param.getPlugin("spatial") as SpatialParameterPlugin;
if (plug == null) continue;
if (plug.getType() != libsbml.SBML_SPATIAL_DIFFUSIONCOEFFICIENT) continue;
var diff = plug.getDiffusionCoefficient();
if (diff == null || !diff.isSetCoordinateReference1()) continue;
diff.unsetCoordinateReference1();
}
//for (int i = 0; i < 3; ++i)
//{
// var param = current.FirstOrDefault(e => e.Item1 == i);
// if (param != null) continue;
//}
}
}
public bool ConvertToL3()
{
if (Document.getLevel() < 3)
{
var prop = new ConversionProperties(new SBMLNamespaces(3, 1));
prop.addOption("strict", false);
prop.addOption("setLevelAndVersion", true);
prop.addOption("ignorePackages", true);
if (Document.convert(prop) != libsbml.LIBSBML_OPERATION_SUCCESS)
{
return false;
}
}
Document.enablePackage(SpatialExtension.getXmlnsL3V1V1(), "spatial", true);
Document.setPackageRequired("spatial", true);
//Document.enablePackage(RequiredElementsExtension.getXmlnsL3V1V1(), "req", true);
//Document.setPackageRequired("req", false);
return true;
}
public bool ConvertToSpatial(CreateModel createModel)
{
if (Document == null) return true;
if (!ConvertToL3()) return false;
var model = Document.getModel();
var plugin = (SpatialModelPlugin)model.getPlugin("spatial");
if (plugin == null)
return false;
var geometry = plugin.getGeometry();
if (geometry == null)
geometry = plugin.createGeometry();
if (geometry == null)
return false;
// create coordinate components
CreateCoordinateSystem(geometry, model, createModel.Geometry);
//if (geometry.getNumGeometryDefinitions()==0)
if (!SetupGeometry(model, geometry, createModel)) return false;
SetupSpecies(createModel, model);
SetIsLocalOnReactions(model);
return true;
}
private void SplitReaction(libsbmlcs.Model model, Reaction reaction)
{
var info = new ReactionInfo(reaction);
if (info.CompartmentIds.Count < 2) return;
if (info.CompartmentIds.Count == 2)
{
// straight transport
var comp1Id = info.CompartmentIds[0];
var comp1 = model.getCompartment(comp1Id);
if (comp1 == null || comp1.getSpatialDimensions() == 2) return;
var comp2Id = info.CompartmentIds[1];
var comp2 = model.getCompartment(comp2Id);
if (comp2 == null || comp2.getSpatialDimensions() == 2) return;
var memId = String.Format("mem_{0}_{1}", comp2Id, comp1Id);
var memComp = model.getCompartment(memId);
if (memComp == null)
{
memId = String.Format("mem_{0}_{1}", comp1Id, comp2Id);
memComp = model.getCompartment(memId);
}
if (memComp == null)
{
memComp = model.createCompartment();
memComp.initDefaults();
memComp.setId(memId);
memComp.setSize(1);
memComp.setUnits("area");
memComp.setSpatialDimensions(2);
}
var math = new ASTNode(reaction.getKineticLaw().getMath());
var react1 = model.createReaction();
react1.initDefaults();
react1.setReversible(reaction.getReversible());
react1.setId(String.Format("mem_{0}_{1}", comp1Id, reaction.getId()));
var react2builder = new StringBuilder("1");
var react2 = model.createReaction();
react2.initDefaults();
react2.setReversible(reaction.getReversible());
react2.setId(String.Format("mem_{0}_{1}", comp2Id, reaction.getId()));
for (var i = 0; i < reaction.getNumReactants(); ++i)
{
var reference = reaction.getReactant(i);
var species = model.getSpecies(reference.getSpecies());
if (species.getCompartment() == comp1Id)
{
var newRef = react1.createReactant();
newRef.setSpecies(reference.getSpecies());
newRef.setStoichiometry(reference.getStoichiometry());
}
else
{
var newId = "mem_" + species.getId();
var newSpecies = model.getSpecies(newId);
if (newSpecies == null)
{
newSpecies = model.createSpecies();
newSpecies.initDefaults();
newSpecies.setId(newId);
newSpecies.setName(species.getId());
newSpecies.setCompartment(memId);
newSpecies.setInitialConcentration(0);
}
var newRef = react2.createProduct();
newRef.setSpecies(newSpecies.getId());
newRef.setStoichiometry(reference.getStoichiometry());
newRef = react1.createReactant();
newRef.setSpecies(newSpecies.getId());
newRef.setStoichiometry(reference.getStoichiometry());
react2builder.Append(" * " + newSpecies.getId());
math.renameSIdRefs(species.getId(), newSpecies.getId());
}
}
for (var i = 0; i < reaction.getNumProducts(); ++i)
{
var reference = reaction.getProduct(i);
var species = model.getSpecies(reference.getSpecies());
if (species.getCompartment() == comp1Id)
{
var newRef = react1.createProduct();
newRef.setSpecies(species.getId());
newRef.setStoichiometry(reference.getStoichiometry());
}
else
{
var newId = "mem_" + species.getId();
var newSpecies = model.getSpecies(newId);
if (newSpecies == null)
{
newSpecies = model.createSpecies();
newSpecies.initDefaults();
newSpecies.setId(newId);
newSpecies.setName(species.getId());
newSpecies.setCompartment(memId);
newSpecies.setInitialConcentration(0);
}
var newRef = react1.createProduct();
newRef.setSpecies(newSpecies.getId());
newRef.setStoichiometry(reference.getStoichiometry());
newRef = react2.createReactant();
newRef.setSpecies(newSpecies.getId());
newRef.setStoichiometry(reference.getStoichiometry());
react2builder.Append(" * " + newSpecies.getId());
newRef = react2.createProduct();
newRef.setSpecies(species.getId());
newRef.setStoichiometry(reference.getStoichiometry());
math.renameSIdRefs(species.getId(), newSpecies.getId());
}
}
var law = react2.createKineticLaw();
law.setFormula(react2builder.ToString());
law = react1.createKineticLaw();
for (var l = 0; l < reaction.getKineticLaw().getNumLocalParameters(); ++l)
{
var param = reaction.getKineticLaw().getParameter(l);
var newParam = law.createParameter();
newParam.initDefaults();
newParam.setId(param.getId());
newParam.setValue(param.getValue());
}
law.setMath(math);
model.removeReaction(reaction.getId());
reaction.Dispose();
}
}
/// <summary>
/// This method first goes ahead, and looks through reactions,
/// whether a transport reaction can be found. If so, it tests
/// whether the compartments differ in spatialDimensions (i.e
/// checks that the transport goes via a membrane), if it does
/// not, it will create a membrane compartment, and split the
/// reaction such that it will go from the compartment -> to
/// the membrane -> to the other compartment.
/// </summary>
/// <param name="model">the model to check</param>
internal void CorrectCompartmentsAndTransport(libsbmlcs.Model model)
{
for (var i = (int)model.getNumReactions() - 1; i >= 0; --i)
{
var reaction = model.getReaction(i);
var comps = Util.GetCompartmentsFromReaction(reaction);
if (comps.Count < 2)
continue;
SplitReaction(model, reaction);
}
}
private bool SetupGeometry(libsbmlcs.Model model, Geometry geometry,
CreateModel createModel)
{
var numCompartments = model.getNumCompartments();
if (numCompartments == 1 || MainForm.Settings.IgnoreMultiCompartments)
return SetupUnicompartmentalGeometry(model, geometry, createModel);
var num3DComps = 0;
for (var index = 0; index < numCompartments; ++index)
{
var current = model.getCompartment(index);
if (current == null || !current.isSetSpatialDimensions()) continue;
if (current.getSpatialDimensions() == 3) num3DComps++;
}
var length = createModel.Geometry.Xmax / num3DComps;
var def = geometry.createAnalyticGeometry();
def.setId("geometry");
var order = OrderCompartments(model);
var i = 0;
AdjacentDomains lastAdjacent = null;
Domain memDomain = null;
for (var j = 0; j < order.Count; j++)
{
var comp = model.getCompartment(order[j]);
var cplug = (SpatialCompartmentPlugin)comp.getPlugin("spatial");
if (cplug == null)
return false;
var domainType = geometry.createDomainType();
domainType.setId("domainType_" + order[j]);
domainType.setSpatialDimensions((int)comp.getSpatialDimensionsAsDouble());
var domain = geometry.createDomain();
domain.setId("domain_" + order[j]);
domain.setDomainType("domainType_" + order[j]);
var point = domain.createInteriorPoint();
point.setCoord1(i * length + length / 2.0);
point.setCoord2(createModel.Geometry.Ymax / 2.0);
point.setCoord3(0);
if (lastAdjacent != null)
{
var adj = geometry.createAdjacentDomains();
adj.setId(String.Format("adj_{0}_{1}", memDomain.getId(), domain.getId()));
adj.setDomain1(memDomain.getId());
adj.setDomain2(domain.getId());
}
var vol = def.createAnalyticVolume();
vol.setId("vol_" + order[j]);
vol.setDomainType("domainType_" + order[j]);
vol.setFunctionType(libsbml.SPATIAL_FUNCTIONKIND_LAYERED);
vol.setOrdinal(i);
vol.setMath(libsbml.parseFormula(i == 0
? "1" // first compartment gets all
: j == order.Count - 1
? string.Format("geq(x, {0})", (i * length)) // last compartment gets rest
: string.Format("and(geq(x, {0}), lt(x, {1}))", (i * length), ((i + 1) * length))));
var map = cplug.getCompartmentMapping();
if (map == null)
map = cplug.createCompartmentMapping();
map.setId("mapping_" + order[j]);
map.setDomainType(domainType.getId());
map.setUnitSize(1);
if (i + 1 < numCompartments)
{
if (j + 1 < order.Count && model.getCompartment(order[j + 1]).getSpatialDimensions() == 2)
{
// next compartment is a 2D compartment!
domainType = geometry.createDomainType();
domainType.setId("domainType_" + order[j + 1]);
domainType.setSpatialDimensions(2);
comp = model.getCompartment(order[j + 1]);
cplug = (SpatialCompartmentPlugin)comp.getPlugin("spatial");
if (cplug == null)
return false;
map = cplug.getCompartmentMapping();
if (map == null)
map = cplug.createCompartmentMapping();
map.setId("mapping_" + comp.getId());
map.setDomainType(domainType.getId());
map.setUnitSize(1);
memDomain = geometry.createDomain();
memDomain.setId("domain_mem_" + order[j]);
memDomain.setDomainType(domainType.getId());
lastAdjacent = geometry.createAdjacentDomains();
lastAdjacent.setId(String.Format("adj_{0}_{1}", memDomain.getId(), domain.getId()));
lastAdjacent.setDomain1(memDomain.getId());
lastAdjacent.setDomain2(domain.getId());
++j; //++i;
//vol = def.createAnalyticVolume();
//vol.setId("vol_" + order[j]);
//vol.setDomainType("domainType_" + order[j]);
//vol.setFunctionType(libsbml.SPATIAL_FUNCTIONKIND_LAYERED);
//vol.setOrdinal(i);
//vol.setMath(libsbml.parseFormula(i == 0 ? "1" : // string.Format(
// //"and(geq(x, {0}), lt(x, {1}))", (i * length), ((i + 1) * length))
//string.Format(//"and(and(geq(x, {0}), lt(x, {1})), and(geq(y, {0}), lt(y, {1})))", (i * length), ((i + 1) * length))
//"and(geq(x, {0}), lt(x, {1}))", (i * length), ((i + 1) * length))));
}
else
{
domainType = geometry.createDomainType();
domainType.setId("mem_" + order[j]);
domainType.setSpatialDimensions(2);
comp = model.createCompartment();
comp.initDefaults();
comp.setId("c_mem_" + order[j]);
comp.setSize(1);
cplug = (SpatialCompartmentPlugin)comp.getPlugin("spatial");
if (cplug == null)
return false;
map = cplug.getCompartmentMapping();
if (map == null)
map = cplug.createCompartmentMapping();
map.setId("mapping_" + comp.getId());
map.setDomainType(domainType.getId());
map.setUnitSize(1);
memDomain = geometry.createDomain();
memDomain.setId("domain_mem_" + order[j]);
memDomain.setDomainType("mem_" + order[j]);
lastAdjacent = geometry.createAdjacentDomains();
lastAdjacent.setId(String.Format("adj_{0}_{1}", memDomain.getId(), domain.getId()));
lastAdjacent.setDomain1(memDomain.getId());
lastAdjacent.setDomain2(domain.getId());
}
}
++i;
}
return true;
}
private static bool SetupUnicompartmentalGeometry(libsbmlcs.Model model, Geometry geometry, CreateModel createModel)
{
var domainType = geometry.createDomainType();
domainType.setId("domainType0");
domainType.setSpatialDimensions(3);
var domain = geometry.createDomain();
domain.setId("domain0");
domain.setDomainType("domainType0");
var point = domain.createInteriorPoint();
point.setCoord1(createModel.Geometry.Xmax / 2.0);
point.setCoord2(createModel.Geometry.Ymax / 2.0);
point.setCoord3(0);
AnalyticVolume vol = null;
AnalyticGeometry def = null;
def = geometry.GetFirstAnalyticGeometry();
if (def == null)
{
def = geometry.createAnalyticGeometry();
def.setId("geometry0");
}
vol = def.getAnalyticVolume(0);
if (vol == null)
{
vol = def.createAnalyticVolume();
vol.setId("vol0");
vol.setOrdinal(0);
}
if (!vol.isSetMath() || string.IsNullOrWhiteSpace(libsbml.formulaToL3String(vol.getMath())))
vol.setMath(libsbml.parseL3Formula("1"));
vol.setDomainType("domainType0");
vol.setFunctionType(libsbml.SPATIAL_FUNCTIONKIND_LAYERED);
var comp = model.getCompartment(0);
var cplug = (SpatialCompartmentPlugin)comp.getPlugin("spatial");
if (cplug == null)
return false;
var map = cplug.getCompartmentMapping();
if (map == null)
map = cplug.createCompartmentMapping();
map.setId("mapping0");
map.setDomainType(domainType.getId());
map.setUnitSize(1);
if (createModel.Geometry.WrapOutside)
{
comp = model.createCompartment();
comp.initDefaults();
comp.setId("__outside");
cplug = (SpatialCompartmentPlugin)comp.getPlugin("spatial");
domainType = geometry.createDomainType();
domainType.setId("domainType1");
domainType.setSpatialDimensions(3);
domain = geometry.createDomain();
domain.setId("domain1");
domain.setDomainType("domainType1");
point = domain.createInteriorPoint();
point.setCoord1(0);
point.setCoord2(0);
point.setCoord3(0);
map = cplug.getCompartmentMapping();
if (map == null)
map = cplug.createCompartmentMapping();
map.setId("mapping1");
map.setDomainType(domainType.getId());
map.setUnitSize(1);
vol.setOrdinal(1);
vol.setMath(
libsbml.parseFormula(
string.Format("piecewise(1, and(geq(x, {0}), leq(x, {1}), geq(y, {2}), leq(y, {3})), 0)",
createModel.Geometry.Xmin + 1, createModel.Geometry.Xmax - 1,
createModel.Geometry.Ymin + 1, createModel.Geometry.Ymax - 1
)
));
vol = def.createAnalyticVolume();
vol.setId("vol1");
vol.setDomainType("domainType1");
vol.setFunctionType(libsbml.SPATIAL_FUNCTIONKIND_LAYERED);
vol.setOrdinal(0);
vol.setMath(libsbml.parseFormula("1"));
// membrane
comp = model.createCompartment();
comp.initDefaults();
comp.setId("__membrane");
cplug = (SpatialCompartmentPlugin)comp.getPlugin("spatial");
domainType = geometry.createDomainType();
domainType.setId("domainType2");
domainType.setSpatialDimensions(2);
domain = geometry.createDomain();
domain.setId("domain2");
domain.setDomainType("domainType2");
map = cplug.getCompartmentMapping();
if (map == null)
map = cplug.createCompartmentMapping();
map.setId("mapping2");
map.setDomainType(domainType.getId());
map.setUnitSize(1);
var adjacent = geometry.createAdjacentDomains();
adjacent.setId("adj_1");
adjacent.setDomain1("domain2");
adjacent.setDomain2("domain1");
adjacent = geometry.createAdjacentDomains();
adjacent.setId("adj_2");
adjacent.setDomain1("domain2");
adjacent.setDomain2("domain0");
}
return true;
}
private static void SetupSpecies(CreateModel createModel, libsbmlcs.Model model)
{
var spatialElements = (from s in createModel.Species select s.Id).ToList();
for (var i = 0; i < model.getNumSpecies(); i++)
{
var species = model.getSpecies(i);
var splug = (SpatialSpeciesPlugin)species.getPlugin("spatial");
if (splug == null) continue;
splug.setIsSpatial(false);
var id = species.getId();
if (!spatialElements.Contains(id)) continue;
var currentSpecies = createModel[id];
species.setInitialExpession(currentSpecies.InitialCondition);
splug.setIsSpatial(true);
SetRequiredElements(species);
var temp = species.getParameterDiffusionX();
if (temp != null && temp.isSetId())
model.removeParameter(temp.getId());
var param = model.createParameter();
param.initDefaults();
param.setId(id + "_diff_X");
param.setValue(currentSpecies.DiffusionX);
var pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
var diff = pplug.getDiffusionCoefficient();
if (diff == null)
diff = pplug.createDiffusionCoefficient();
diff.setVariable(id);
diff.setType(libsbml.SPATIAL_DIFFUSIONKIND_ANISOTROPIC);
diff.setCoordinateReference1(0);
SetRequiredElements(param);
temp = species.getParameterDiffusionY();
if (temp != null && temp.isSetId())
model.removeParameter(temp.getId());
model.removeParameter(id + "_diff_Y");
param = model.createParameter();
param.initDefaults();
param.setId(id + "_diff_Y");
param.setValue(currentSpecies.DiffusionY);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
diff = pplug.getDiffusionCoefficient();
if (diff == null)
diff = pplug.createDiffusionCoefficient();
diff.setVariable(id);
diff.setType(libsbml.SPATIAL_DIFFUSIONKIND_ANISOTROPIC);
diff.setCoordinateReference1(1);
SetRequiredElements(param);
temp = species.getSpatialParameter(libsbml.SBML_SPATIAL_BOUNDARYCONDITION, "Xmin");
if (temp != null && temp.isSetId())
model.removeParameter(temp.getId());
param = model.createParameter();
param.initDefaults();
param.setId(id + "_BC_Xmin");
param.setValue(currentSpecies.MinBoundaryX);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
var bc = pplug.getBoundaryCondition();
if (bc == null)
bc = pplug.createBoundaryCondition();
bc.setVariable(id);
bc.setCoordinateBoundary("Xmin");
bc.setType(currentSpecies.BCType == "Dirichlet" ? libsbml.SPATIAL_BOUNDARYKIND_DIRICHLET : libsbml.SPATIAL_BOUNDARYKIND_NEUMANN);
SetRequiredElements(param);
temp = species.getSpatialParameter(libsbml.SBML_SPATIAL_BOUNDARYCONDITION, "Xmax");
if (temp != null && temp.isSetId())
model.removeParameter(temp.getId());
param = model.createParameter();
param.initDefaults();
param.setId(id + "_BC_Xmax");
param.setValue(currentSpecies.MaxBoundaryX);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
bc = pplug.getBoundaryCondition();
if (bc == null)
bc = pplug.createBoundaryCondition();
bc.setVariable(id);
bc.setCoordinateBoundary("Xmax");
bc.setType(currentSpecies.BCType == "Dirichlet" ? libsbml.SPATIAL_BOUNDARYKIND_DIRICHLET : libsbml.SPATIAL_BOUNDARYKIND_NEUMANN);
SetRequiredElements(param);
temp = species.getSpatialParameter(libsbml.SBML_SPATIAL_BOUNDARYCONDITION, "Ymin");
if (temp != null && temp.isSetId())
model.removeParameter(temp.getId());
param = model.createParameter();
param.initDefaults();
param.setId(id + "_BC_Ymin");
param.setValue(currentSpecies.MinBoundaryY);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
bc = pplug.getBoundaryCondition();
if (bc == null)
bc = pplug.createBoundaryCondition();
bc.setVariable(id);
bc.setCoordinateBoundary("Ymin");
bc.setType(currentSpecies.BCType == "Dirichlet" ? libsbml.SPATIAL_BOUNDARYKIND_DIRICHLET : libsbml.SPATIAL_BOUNDARYKIND_NEUMANN);
SetRequiredElements(param);
temp = species.getSpatialParameter(libsbml.SBML_SPATIAL_BOUNDARYCONDITION, "Ymax");
if (temp != null && temp.isSetId())
model.removeParameter(temp.getId());
param = model.createParameter();
param.initDefaults();
param.setId(id + "_BC_Ymax");
param.setValue(currentSpecies.MaxBoundaryY);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
bc = pplug.getBoundaryCondition();
if (bc == null)
bc = pplug.createBoundaryCondition();
bc.setVariable(id);
bc.setCoordinateBoundary("Ymax");
bc.setType(currentSpecies.BCType == "Dirichlet" ? libsbml.SPATIAL_BOUNDARYKIND_DIRICHLET : libsbml.SPATIAL_BOUNDARYKIND_NEUMANN);
SetRequiredElements(param);
}
}
private void SetIsLocalOnReactions(libsbmlcs.Model model)
{
for (var i = 0; i < model.getNumReactions(); i++)
{
var reaction = model.getReaction(i);
var rplug = (SpatialReactionPlugin)reaction.getPlugin("spatial");
if (rplug == null) continue;
SetRequiredElements(reaction);
var idsContainedIn = GetSpeciesReferenceIdsContainedIn(reaction);
var isLocal = idsContainedIn.Count > 0;
rplug.setIsLocal(isLocal);
}
}
public void CreateCoordinateSystem(Geometry geometry, libsbmlcs.Model model,
GeometrySettings settings)
{
geometry.setCoordinateSystem(libsbml.SPATIAL_GEOMETRYKIND_CARTESIAN);
var coord = geometry.getCoordinateComponent("x");
if (coord == null)
{
coord = geometry.createCoordinateComponent();
}
var unitDef = model.getUnitDefinition("um");
if (unitDef == null)
{
unitDef = model.createUnitDefinition();
unitDef.setId("um");
var kind = unitDef.createUnit();
kind.setExponent(1);
kind.setMultiplier(1e-6);
kind.setScale(0);
kind.setKind(libsbml.UNIT_KIND_METRE);
}
coord.setId("x");
coord.setUnit("um");
coord.setType(libsbml.SPATIAL_COORDINATEKIND_CARTESIAN_X);
var min = coord.getBoundaryMin();
if (min == null)
min = coord.createBoundaryMin();
min.setId("Xmin");
min.setValue(settings.Xmin);
var max = coord.getBoundaryMax();
if (max == null) max = coord.createBoundaryMax();
max.setId("Xmax");
max.setValue(settings.Xmax);
coord = geometry.getCoordinateComponent("y");
if (coord == null)
coord = geometry.createCoordinateComponent();
coord.setId("y");
coord.setUnit("um");
coord.setType(libsbml.SPATIAL_COORDINATEKIND_CARTESIAN_Y);
min = coord.getBoundaryMin();
if (min == null)
min = coord.createBoundaryMin();
min.setId("Ymin");
min.setValue(settings.Ymin);
max = coord.getBoundaryMax();
if (max == null)
max = coord.createBoundaryMax();
max.setId("Ymax");
max.setValue(settings.Ymax);
model.removeParameter("x");
var param = model.createParameter();
param.initDefaults();
param.setId("x");
param.setValue(0);
var pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
var symbol = pplug.createSpatialSymbolReference();
symbol.setSpatialRef("x");
SetRequiredElements(param, false);
model.removeParameter("y");
param = model.createParameter();
param.initDefaults();
param.setId("y");
param.setValue(0);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
symbol = pplug.createSpatialSymbolReference();
symbol.setSpatialRef("y");
SetRequiredElements(param, false);
if (settings.UsedSymbols.Contains("width"))
{
model.removeParameter("width");
param = model.createParameter();
param.initDefaults();
param.setId("width");
param.setValue(settings.Xmax);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
symbol = pplug.createSpatialSymbolReference();
symbol.setSpatialRef("Xmax");
SetRequiredElements(param, false);
}
if (settings.UsedSymbols.Contains("height"))
{
model.removeParameter("height");
param = model.createParameter();
param.initDefaults();
param.setId("height");
param.setValue(settings.Ymax);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
symbol = pplug.createSpatialSymbolReference();
symbol.setSpatialRef("Ymax");
SetRequiredElements(param, false);
}
if (settings.UsedSymbols.Contains("Xmax"))
{
model.removeParameter("Xmax");
param = model.createParameter();
param.initDefaults();
param.setId("Xmax");
param.setValue(settings.Xmax);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
symbol = pplug.createSpatialSymbolReference();
symbol.setSpatialRef("Xmax");
SetRequiredElements(param, false);
}
if (settings.UsedSymbols.Contains("Ymax"))
{
model.removeParameter("Ymax");
param = model.createParameter();
param.initDefaults();
param.setId("Ymax");
param.setValue(settings.Ymax);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
symbol = pplug.createSpatialSymbolReference();
symbol.setSpatialRef("Ymax");
SetRequiredElements(param, false);
}
if (settings.UsedSymbols.Contains("Xmin"))
{
model.removeParameter("Xmin");
param = model.createParameter();
param.initDefaults();
param.setId("Xmin");
param.setValue(settings.Xmin);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
symbol = pplug.createSpatialSymbolReference();
symbol.setSpatialRef("Xmin");
SetRequiredElements(param, false);
}
if (settings.UsedSymbols.Contains("Ymin"))
{
model.removeParameter("Ymin");
param = model.createParameter();
param.initDefaults();
param.setId("Ymin");
param.setValue(settings.Ymin);
pplug = (SpatialParameterPlugin)param.getPlugin("spatial");
symbol = pplug.createSpatialSymbolReference();
symbol.setSpatialRef("Ymin");
SetRequiredElements(param, false);
}
}
private List<string> OrderCompartments(libsbmlcs.Model model)
{
var list = new List<string>();
var orders = new Dictionary<string, List<string>>();
var matrix = new int[model.getNumReactions(), model.getNumCompartments()];
var reactions = new List<string>();
for (var i = 0; i < model.getNumReactions(); i++)
reactions.Add(model.getReaction(i).getId());
var compartments = new List<string>();
for (var i = 0; i < model.getNumCompartments(); i++)
compartments.Add(model.getCompartment(i).getId());
for (var i = 0; i < model.getNumReactions(); i++)
{
var reaction = model.getReaction(i);
var id = reaction.getId();
for (var j = 0; j < reaction.getNumReactants(); j++)
{
var reference = reaction.getReactant(j);
if (!reference.isSetSpecies()) continue;
var species = model.getSpecies(reference.getSpecies());
if (species == null) continue;
if (!orders.ContainsKey(id))
orders[id] = new List<string>();
if (!orders[id].Contains(species.getCompartment()))
orders[id].Add(species.getCompartment());
matrix[reactions.IndexOf(id), compartments.IndexOf(species.getCompartment())] = 1;
}
for (var j = 0; j < reaction.getNumProducts(); j++)
{
var reference = reaction.getProduct(j);
if (!reference.isSetSpecies()) continue;
var species = model.getSpecies(reference.getSpecies());
if (species == null) continue;
if (!orders.ContainsKey(id))
orders[id] = new List<string>();
if (!orders[id].Contains(species.getCompartment()))
orders[id].Add(species.getCompartment());
matrix[reactions.IndexOf(id), compartments.IndexOf(species.getCompartment())] = 1;
}
for (var j = 0; j < reaction.getNumModifiers(); j++)
{
var reference = reaction.getModifier(j);
if (!reference.isSetSpecies()) continue;
var species = model.getSpecies(reference.getSpecies());
if (species == null) continue;
if (!orders.ContainsKey(id))
orders[id] = new List<string>();
if (!orders[id].Contains(species.getCompartment()))
orders[id].Add(species.getCompartment());
matrix[reactions.IndexOf(id), compartments.IndexOf(species.getCompartment())] = 1;
}
}
foreach (var order in orders.Values)
order.Sort();
var uniqueOrders = new List<List<string>>();
foreach (var order in orders.Values)
{
if (!Contains(uniqueOrders, order))
uniqueOrders.Add(order);
}
for (var i = uniqueOrders.Count - 1; i >= 0; i--)
if (uniqueOrders[i].Count < 2)
uniqueOrders.RemoveAt(i);
var counts = CountOccurances(uniqueOrders);
var max = counts.Any() ? counts.Values.Max() : 0;
while (max > 1)
{
// get first one that occurs only once
var current = counts.FirstOrDefault(e => e.Value == 1);
var id = current.Key;
if (string.IsNullOrEmpty(id)) break;
var order = RemoveIdFrom(uniqueOrders, id);
if (list.Contains(id))
{
if (counts.ContainsKey(id))
counts.Remove(id);
continue;
}
list.Add(id);
list.AddRange(order.Where(item => !item.Equals(id)));
counts = CountOccurances(uniqueOrders);
if (counts.ContainsKey(id))
counts.Remove(id);
max = counts.Any() ? counts.Values.Max() : 0;
}
// add remaining
for (var i = 0; i < model.getNumCompartments(); ++i)
{
var current = model.getCompartment(i);
if (!list.Contains(current.getId())) list.Add(current.getId());
}
return list;
}
private List<string> RemoveIdFrom(List<List<string>> uniqueOrders, string id)
{
var item = uniqueOrders.FirstOrDefault(e => e.Contains(id));
if (item == null) return null;
uniqueOrders.Remove(item);
return item;
}
private static Dictionary<string, int> CountOccurances(List<List<string>> orders)
{
var counts = new Dictionary<string, int>();
foreach (var entries in orders)
{
foreach (var key in entries)
{
if (!counts.ContainsKey(key))
counts[key] = 0;
counts[key]++;
}
}
return counts;
}
private bool Contains(List<List<string>> uniqueOrders, List<string> order)
{
var stringOrder = Combine(order);
foreach (var item in uniqueOrders)
{
if (Combine(item) == stringOrder)
return true;
}
return false;
}
private string Combine(List<string> order)
{
var builder = new StringBuilder();
for (var i = 0; i < order.Count; i++)
{
builder.Append(order[i]);
if (i + 1 < order.Count)
builder.Append(", ");
}
return builder.ToString();
}
/// <summary>
/// Verifies whether the reaction uses any spatial elements
/// </summary>
/// <param name="reaction"></param>
/// <returns></returns>
private List<string> GetSpeciesReferenceIdsContainedIn(Reaction reaction)
{
var result = new List<string>();
if (reaction == null || reaction.getSBMLDocument() == null) return result;
var model = reaction.getSBMLDocument().getModel();
if (model == null) return result;
for (var i = 0; i < reaction.getNumReactants(); ++i)
{
var currentRef = reaction.getReactant(i);
var id = currentRef.getSpecies();
var current = model.getSpecies(id);
if (current == null) continue;
var plug = (SpatialSpeciesPlugin)current.getPlugin("spatial");
var isSpatial = plug != null && plug.getIsSpatial();
if (isSpatial && !result.Contains(id))
result.Add(id);
}
for (var i = 0; i < reaction.getNumProducts(); ++i)
{
var currentRef = reaction.getProduct(i);
var id = currentRef.getSpecies();
var current = model.getSpecies(id);
if (current == null) continue;
var plug = (SpatialSpeciesPlugin)current.getPlugin("spatial");
var isSpatial = plug != null && plug.getIsSpatial();
if (isSpatial && !result.Contains(id))
result.Add(id);
}
for (var i = 0; i < reaction.getNumModifiers(); ++i)
{
var currentRef = reaction.getModifier(i);
var id = currentRef.getSpecies();
var current = model.getSpecies(id);
if (current == null) continue;
var plug = (SpatialSpeciesPlugin)current.getPlugin("spatial");
var isSpatial = plug != null && plug.getIsSpatial();
if (isSpatial && !result.Contains(id))
result.Add(id);
}
return result;
}
public event EventHandler ModelChanged;
protected virtual void OnModelChanged()
{
var handler = ModelChanged;
if (handler != null) handler(this, EventArgs.Empty);
}
private static void SetRequiredElements(SBase sbase, bool hasAlternativeMath = true)
{
//var req = (RequiredElementsSBasePlugin) sbase.getPlugin("req");
//if (req == null) return;
//req.setCoreHasAlternateMath(hasAlternativeMath);
//req.setMathOverridden("spatial");
}
public string ToMorpheus(string filename = null)
{
var converter = new MorpheusConverter(Document);
return converter.ToMorpheus(filename);
}
public void ExportToDune(string filename)
{
var converter = new DuneConverter(Document);
converter.ExportTo(filename);
}
}
}<file_sep>/WFDuneRunner/Program.cs
using System;
using System.IO;
using System.Windows.Forms;
namespace WFDuneRunner
{
internal static class Program
{
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
private static void Main(string[] args)
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
var form = new MainForm();
foreach (var file in args)
{
if (File.Exists(file))
{
form.OpenFile(file);
break;
}
}
Application.Run(form);
}
}
}<file_sep>/LibEditSpatial/Controls/CtrlGlobal.cs
using System;
using System.Windows.Forms;
using LibEditSpatial.Model;
namespace LibEditSpatial.Controls
{
public partial class CtrlGlobal : UserControl
{
public CtrlGlobal()
{
InitializeComponent();
}
public GlobalConfig Model { get; set; }
public void LoadModel(GlobalConfig model)
{
Model = model;
chkWriteVTK.Checked = Model.WriteVTK;
txtVTKname.Text = Model.VTKname;
txtOverlap.Text = Model.Overlap.ToString();
txtIntegrationorder.Text = Model.IntegrationOrder.ToString();
txtSubsampling.Text = Model.SubSampling.ToString();
if (Model.TimeStepping == "explicit")
radExplicit.Checked = true;
else
radImplicit.Checked = true;
txtExplicitsolver.Text = Model.ExplicitSolver;
txtImplicitSovler.Text = Model.ImplicitSolver;
}
private void chkWriteVTK_CheckedChanged(object sender, EventArgs e)
{
if (Model == null) return;
Model.WriteVTK = chkWriteVTK.Checked;
}
private void txtVTKname_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
Model.VTKname = txtVTKname.Text;
}
private void txtOverlap_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
int temp;
if (int.TryParse(txtOverlap.Text, out temp))
Model.Overlap = temp;
}
private void txtIntegrationorder_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
int temp;
if (int.TryParse(txtIntegrationorder.Text, out temp))
Model.IntegrationOrder = temp;
}
private void txtSubsampling_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
int temp;
if (int.TryParse(txtSubsampling.Text, out temp))
Model.SubSampling = temp;
}
private void radImplicit_CheckedChanged(object sender, EventArgs e)
{
if (Model == null) return;
if (radImplicit.Checked)
Model.TimeStepping = "implicit";
else
Model.TimeStepping = "explicit";
}
private void radExplicit_CheckedChanged(object sender, EventArgs e)
{
if (Model == null) return;
if (radExplicit.Checked)
Model.TimeStepping = "explicit";
else
Model.TimeStepping = "implicit";
}
private void txtExplicitsolver_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
Model.ExplicitSolver = txtExplicitsolver.Text;
}
private void txtImplicitSovler_TextChanged(object sender, EventArgs e)
{
if (Model == null) return;
Model.ImplicitSolver = txtImplicitSovler.Text;
}
}
}<file_sep>/WFEditSpatial/Controls/ControlAjacentDomains.cs
using libsbmlcs;
namespace EditSpatial.Controls
{
public partial class ControlAdjacentDomains : BaseSpatialControl
{
public ControlAdjacentDomains()
{
InitializeComponent();
}
public Geometry Current { get; set; }
public void InitializeFrom(Geometry geometry)
{
grid.Rows.Clear();
Current = geometry;
if (geometry == null) return;
for (long i = 0; i < geometry.getNumAdjacentDomains(); ++i)
{
var domain = geometry.getAdjacentDomains(i);
var spatialId = domain.getId();
grid.Rows.Add(spatialId, domain.getDomain1(), domain.getDomain2());
}
}
public override void SaveChanges()
{
if (Current == null) return;
for (var i = 0; i < grid.Rows.Count && i < Current.getNumAdjacentDomains(); ++i)
{
var domain = Current.getAdjacentDomains(i);
var row = grid.Rows[i];
if (domain == null) continue;
domain.setId((string) row.Cells[0].Value);
domain.setDomain1((string) row.Cells[1].Value);
domain.setDomain2((string) row.Cells[2].Value);
}
OnModelChanged();
}
public override void InvalidateSelection()
{
Current = null;
InitializeFrom(null);
}
}
}<file_sep>/WFEditSpatial/MainForm.cs
using System;
using System.Drawing;
using System.IO;
using System.Windows.Forms;
using EditSpatial.Controls;
using EditSpatial.Forms;
using EditSpatial.Model;
using libsbmlcs;
using LibEditSpatial.Dialogs;
using LibEditSpatial.Model;
using SBW;
using SBW.Utils;
namespace EditSpatial
{
public partial class MainForm : Form, ISBWAnalyzer
{
private const string NODE_COORDINATES = "nodeCoordinateComponents";
private const string NODE_DOMAINTYPES = "nodeOfDomainTypes";
private const string NODE_DOMAINS = "nodeOfDomains";
private const string NODE_ADJACENTDOMAINS = "nodeOfAdjacentDomains";
private const string NODE_GEOMS = "nodeOfGeometryDefinitions";
private const string NODE_COMPARTMENTS = "nodeCompartments";
private const string NODE_SPECIES = "nodeSpecies";
private const string NODE_PARAMETERS = "nodeParameters";
private const string NODE_REACTIONS = "nodeReactions";
private const string NODE_RULES = "nodeRules";
private const string NODE_INITIAL_ASSIGNMENTS = "nodeInitialAssignments";
private const string NODE_COMPARTMENT_MAPPINGS = "nodeCompartmentMappings";
private readonly SBWFavorites favs;
private readonly SBWMenu menu;
private bool haveAkira;
public MainForm()
{
InitializeComponent();
ErrorForm = new FormErrors();
Annotation = new FormSpatialAnnotation();
menu = new SBWMenu(mnuSBW, "Edit Spatial", () =>
{
if (Model == null) return "";
if (Model.Document == null) return "";
return libsbml.writeSBMLToString(Model.Document);
});
favs = new SBWFavorites(() =>
{
if (Model == null) return "";
if (Model.Document == null) return "";
return libsbml.writeSBMLToString(Model.Document);
}, toolStrip1);
controlInitialAssignments1.UpdateAction = () =>
{
if (Model.Document != null && Model.Document.getModel() != null)
UpdateTreeWithInitialAssignments(Model.Document.getModel());
};
controlAdjacentDomains1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
controlAnalyticGeometry1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
controlCompartment1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
controlCoordinateComponents1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
controlDomains1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
controlDomainTypes1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
controlInitialAssignments1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
controlMapCompartments1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
controlParameters1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
controlRules1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
controlSampleFieldGeometry1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
controlSpecies1.ModelChanged += (e, o) =>
{ if (Model != null) Model.Dirty = true; };
NewModel();
}
public static EditSpatialSettings Settings { get; set; }
public SpatialModel Model { get; set; }
public FormErrors ErrorForm { get; set; }
public FormSpatialAnnotation Annotation { get; set; }
public void doAnalysis(string model)
{
if (InvokeRequired)
{
Invoke(new doAnalysisDelegate(doAnalysis), model);
}
else
{
LoadFromString(model);
Model.Dirty = true;
}
}
public void InvalidateCoreAll()
{
foreach (var control in splitInitial.Panel2.Controls)
{
var current = control as BaseSpatialControl;
if (current == null) continue;
current.InvalidateSelection();
}
}
public void SaveCoreAll()
{
foreach (var control in splitInitial.Panel2.Controls)
{
var current = control as BaseSpatialControl;
if (current == null) continue;
current.SaveChanges();
if (current.UpdateAction != null)
current.UpdateAction();
}
}
public void InvalidateGeometryAll()
{
foreach (var control in splitGeometry.Panel2.Controls)
{
var current = control as BaseSpatialControl;
if (current == null) continue;
current.InvalidateSelection();
}
}
public void SaveGeormetryAll()
{
foreach (var control in splitGeometry.Panel2.Controls)
{
var current = control as BaseSpatialControl;
if (current == null) continue;
current.SaveChanges();
if (current.UpdateAction != null)
current.UpdateAction();
}
}
private void OnGeometrySelect(object sender, TreeViewEventArgs e)
{
var node = e.Node;
SaveGeormetryAll();
InvalidateGeometryAll();
SelectGeometryNode(node);
}
private void SelectGeometryNode(TreeNode node)
{
if (node.Name == NODE_COORDINATES)
{
controlAnalyticGeometry1.Visible = false;
controlAdjacentDomains1.Visible = false;
controlDomainTypes1.Visible = false;
controlDomains1.Visible = false;
controlDisplayNode1.Visible = false;
controlSampleFieldGeometry1.Visible = false;
controlCoordinateComponents1.Visible = true;
controlMapCompartments1.Visible = false;
controlCoordinateComponents1.InitializeFrom(Model.Geometry);
}
else if (node.Name == NODE_DOMAINTYPES)
{
controlAnalyticGeometry1.Visible = false;
controlAdjacentDomains1.Visible = false;
controlDomainTypes1.Visible = true;
controlDomains1.Visible = false;
controlDisplayNode1.Visible = false;
controlSampleFieldGeometry1.Visible = false;
controlCoordinateComponents1.Visible = false;
controlMapCompartments1.Visible = false;
controlDomainTypes1.InitializeFrom(Model.Geometry);
}
else if (node.Name == NODE_DOMAINS)
{
controlAnalyticGeometry1.Visible = false;
controlAdjacentDomains1.Visible = false;
controlDomainTypes1.Visible = false;
controlDomains1.Visible = true;
controlDisplayNode1.Visible = false;
controlSampleFieldGeometry1.Visible = false;
controlCoordinateComponents1.Visible = false;
controlMapCompartments1.Visible = false;
controlDomains1.InitializeFrom(Model.Geometry);
}
else if (node.Name == NODE_ADJACENTDOMAINS)
{
controlAnalyticGeometry1.Visible = false;
controlAdjacentDomains1.Visible = true;
controlDomainTypes1.Visible = false;
controlDomains1.Visible = false;
controlDisplayNode1.Visible = false;
controlSampleFieldGeometry1.Visible = false;
controlCoordinateComponents1.Visible = false;
controlMapCompartments1.Visible = false;
controlAdjacentDomains1.InitializeFrom(Model.Geometry);
}
else if (node.Name == NODE_COMPARTMENT_MAPPINGS)
{
controlAnalyticGeometry1.Visible = false;
controlAdjacentDomains1.Visible = false;
controlDomainTypes1.Visible = false;
controlDomains1.Visible = false;
controlDisplayNode1.Visible = false;
controlSampleFieldGeometry1.Visible = false;
controlCoordinateComponents1.Visible = false;
controlMapCompartments1.Visible = true;
controlMapCompartments1.InitializeFrom(Model.Document.getModel());
}
else if (node.Parent != null && node.Parent.Name == NODE_GEOMS &&
Model.Geometry != null &&
Model.Geometry.getGeometryDefinition(node.Text) is AnalyticGeometry)
{
controlAnalyticGeometry1.Visible = true;
controlAdjacentDomains1.Visible = false;
controlDomainTypes1.Visible = false;
controlDomains1.Visible = false;
controlDisplayNode1.Visible = false;
controlSampleFieldGeometry1.Visible = false;
controlCoordinateComponents1.Visible = false;
controlMapCompartments1.Visible = false;
controlAnalyticGeometry1.InitializeFrom(Model.Geometry, node.Text);
}
else if (node.Parent != null && node.Parent.Name == NODE_GEOMS &&
Model.Geometry != null &&
Model.Geometry.getGeometryDefinition(node.Text) is SampledFieldGeometry)
{
controlAnalyticGeometry1.Visible = false;
controlAdjacentDomains1.Visible = false;
controlDomainTypes1.Visible = false;
controlDomains1.Visible = false;
controlDisplayNode1.Visible = false;
controlSampleFieldGeometry1.Visible = true;
controlCoordinateComponents1.Visible = false;
controlMapCompartments1.Visible = false;
controlSampleFieldGeometry1.InitializeFrom(Model.Geometry, node.Text);
}
else
{
controlAnalyticGeometry1.Visible = false;
controlAdjacentDomains1.Visible = false;
controlDomainTypes1.Visible = false;
controlDomains1.Visible = false;
controlDisplayNode1.Visible = true;
controlSampleFieldGeometry1.Visible = false;
controlCoordinateComponents1.Visible = false;
controlMapCompartments1.Visible = false;
controlDisplayNode1.DisplayNode(node);
}
}
private void SetFilename(string filename)
{
Text = string.Format("Edit Spatial: [ {0} ]", Path.GetFileName(filename));
}
public void UpdateUI()
{
if (Model == null) return;
InvalidateGeometryAll();
splitGeometry.Enabled = Model.IsSpatial;
cmdAkira.Enabled = Model.IsSpatial;
cmdPrepareDune.Enabled = Model.IsSpatial;
FillGeometryFromModel(Model);
FillCoreTreeFromModel(Model);
controlDisplayNode1.ResetText();
controlDisplayNode2.ResetText();
txtSBML.Text = Model.ToSBML();
txtJarnac.Text = Model.HaveModel ? Model.ToJarnac() : "";
SetFilename(Model.FileName);
treeCore.SelectedNode =
treeCore.Nodes[NODE_INITIAL_ASSIGNMENTS];
treeSpatial.SelectedNode =
treeSpatial.Nodes[NODE_GEOMS].Nodes.Count > 0
? treeSpatial.Nodes[NODE_GEOMS].FirstNode
: treeSpatial.Nodes[NODE_GEOMS];
}
private void ClearCoreTree()
{
treeCore.Nodes[NODE_COMPARTMENTS].Nodes.Clear();
treeCore.Nodes[NODE_SPECIES].Nodes.Clear();
treeCore.Nodes[NODE_PARAMETERS].Nodes.Clear();
treeCore.Nodes[NODE_REACTIONS].Nodes.Clear();
treeCore.Nodes[NODE_RULES].Nodes.Clear();
treeCore.Nodes[NODE_INITIAL_ASSIGNMENTS].Nodes.Clear();
}
private void FillCoreTreeFromModel(SpatialModel spatialModel)
{
if (spatialModel.Document == null)
{
ClearCoreTree();
return;
}
var model = spatialModel.Document.getModel();
if (model == null)
{
ClearCoreTree();
return;
}
FillTreeWithCompartments(model);
FillTreeWithSpecies(model);
FillTreeWithParameters(model);
FillTreeWithReactions(model);
FillTreeWithRules(model);
FillTreeWithInitialAssignments(model);
}
private void UpdateTreeWithInitialAssignments(libsbmlcs.Model model)
{
var root = treeCore.Nodes[NODE_INITIAL_ASSIGNMENTS];
foreach (TreeNode item in root.Nodes)
{
var current = model.getInitialAssignment(item.Text);
if (current == null) continue;
item.Tag = current.toSBML();
}
}
private void FillTreeWithInitialAssignments(libsbmlcs.Model model)
{
var root = treeCore.Nodes[NODE_INITIAL_ASSIGNMENTS];
root.Nodes.Clear();
for (long i = 0; i < model.getNumInitialAssignments(); ++i)
{
var current = model.getInitialAssignment(i);
var node = new TreeNode(current.getSymbol()) {Tag = current.toSBML()};
root.Nodes.Add(node);
}
}
private void FillTreeWithRules(libsbmlcs.Model model)
{
var root = treeCore.Nodes[NODE_RULES];
root.Nodes.Clear();
for (long i = 0; i < model.getNumRules(); ++i)
{
var current = model.getRule(i);
var node = new TreeNode(current.getId()) {Tag = current.toSBML()};
root.Nodes.Add(node);
}
}
private void FillTreeWithReactions(libsbmlcs.Model model)
{
var root = treeCore.Nodes[NODE_REACTIONS];
root.Nodes.Clear();
for (long i = 0; i < model.getNumReactions(); ++i)
{
var current = model.getReaction(i);
var node = new TreeNode(current.getId()) {Tag = current.toSBML()};
root.Nodes.Add(node);
}
}
private void FillTreeWithParameters(libsbmlcs.Model model)
{
var root = treeCore.Nodes[NODE_PARAMETERS];
root.Nodes.Clear();
for (long i = 0; i < model.getNumParameters(); ++i)
{
var current = model.getParameter(i);
var node = new TreeNode(current.getId()) {Tag = current.toSBML()};
root.Nodes.Add(node);
}
}
private void FillTreeWithSpecies(libsbmlcs.Model model)
{
var root = treeCore.Nodes[NODE_SPECIES];
root.Nodes.Clear();
for (long i = 0; i < model.getNumSpecies(); ++i)
{
var current = model.getSpecies(i);
var node = new TreeNode(current.getId()) {Tag = current.toSBML()};
root.Nodes.Add(node);
}
}
private void FillTreeWithCompartments(libsbmlcs.Model model)
{
var root = treeCore.Nodes[NODE_COMPARTMENTS];
root.Nodes.Clear();
for (long i = 0; i < model.getNumCompartments(); ++i)
{
var current = model.getCompartment(i);
var node = new TreeNode(current.getId()) {Tag = current.toSBML()};
root.Nodes.Add(node);
}
}
private void FillGeometryFromModel(SpatialModel model)
{
var geom = model.Geometry;
if (geom == null)
{
treeSpatial.Nodes[NODE_COORDINATES].Nodes.Clear();
treeSpatial.Nodes[NODE_DOMAINTYPES].Nodes.Clear();
treeSpatial.Nodes[NODE_DOMAINS].Nodes.Clear();
treeSpatial.Nodes[NODE_ADJACENTDOMAINS].Nodes.Clear();
treeSpatial.Nodes[NODE_GEOMS].Nodes.Clear();
return;
}
var root = treeSpatial.Nodes[NODE_COORDINATES];
root.Nodes.Clear();
for (long i = 0; i < geom.getNumCoordinateComponents(); ++i)
{
var current = geom.getCoordinateComponent(i);
var node = new TreeNode(current.getId()) {Tag = current.toSBML()};
root.Nodes.Add(node);
}
root = treeSpatial.Nodes[NODE_DOMAINTYPES];
root.Nodes.Clear();
for (long i = 0; i < geom.getNumDomainTypes(); ++i)
{
var current = geom.getDomainType(i);
var node = new TreeNode(current.getId()) {Tag = current.toSBML()};
root.Nodes.Add(node);
}
root = treeSpatial.Nodes[NODE_DOMAINS];
root.Nodes.Clear();
for (long i = 0; i < geom.getNumDomains(); ++i)
{
var current = geom.getDomain(i);
var node = new TreeNode(current.getId()) {Tag = current.toSBML()};
root.Nodes.Add(node);
}
root = treeSpatial.Nodes[NODE_ADJACENTDOMAINS];
root.Nodes.Clear();
for (long i = 0; i < geom.getNumAdjacentDomains(); ++i)
{
var current = geom.getAdjacentDomains(i);
var node = new TreeNode(current.getId()) {Tag = current.toSBML()};
root.Nodes.Add(node);
}
root = treeSpatial.Nodes[NODE_GEOMS];
root.Nodes.Clear();
for (long i = 0; i < geom.getNumGeometryDefinitions(); ++i)
{
var current = geom.getGeometryDefinition(i);
var node = new TreeNode(current.getId()) {Tag = current.toSBML()};
var analytic = current as AnalyticGeometry;
if (analytic != null)
{
for (long j = 0; j < analytic.getNumAnalyticVolumes(); ++j)
{
var vol = analytic.getAnalyticVolume(j);
var volNode = new TreeNode(vol.getId()) {Tag = vol.toSBML()};
node.Nodes.Add(volNode);
}
}
var sample = current as SampledFieldGeometry;
if (sample != null)
{
var field = geom.getSampledField(sample.getSampledField());
if (field != null)
{
var fieldNode = new TreeNode(field.getId()) {Tag = field.toSBML()};
node.Nodes.Add(fieldNode);
for (long j = 0; j < sample.getNumSampledVolumes(); ++j)
{
var vol = sample.getSampledVolume(j);
var volNode = new TreeNode(vol.getId()) {Tag = vol.toSBML()};
node.Nodes.Add(volNode);
}
}
}
root.Nodes.Add(node);
}
}
private void OnNewModel(object sender, EventArgs e)
{
if (SaveModelIfDirtyOrCancel()) return;
NewModel();
}
private void NewModel()
{
Model = new SpatialModel();
Model.ModelChanged += (o, args) => UpdateUI();
UpdateUI();
}
private void OnOpenFile(object sender, EventArgs e)
{
if (SaveModelIfDirtyOrCancel()) return;
var dialog = new OpenFileDialog
{
Title = "Open Spatial SBML",
Filter = "SBML files|*.xml;*.sbml|All files|*.*",
AutoUpgradeEnabled = true
};
if (dialog.ShowDialog() != DialogResult.OK)
return;
OpenFile(dialog.FileName);
}
public void LoadFromString(string content)
{
OpenModel(SpatialModel.FromString(content));
}
public void LoadFromJarnac(string content)
{
OpenModel(SpatialModel.FromJarnac(content));
}
public void OpenFile(string fileName)
{
OpenModel(SpatialModel.FromFile(fileName));
}
private void OpenModel(SpatialModel model)
{
try
{
Model = model;
Model.ModelChanged += (o, args) => UpdateUI();
UpdateUI();
if (Model != null && Model.Document.getNumErrors(libsbml.LIBSBML_SEV_ERROR) > 0)
{
ShowErrors();
}
else
{
ErrorForm.Hide();
}
if (Model != null && !Model.IsSpatial)
{
Model.ConvertToL3();
ConvertToSpatial();
}
}
catch (Exception ex)
{
MessageBox.Show(
string.Format("Could not load the given model.{0}{1}", Environment.NewLine, ex.Message),
"Model could not be loaded",
MessageBoxButtons.OK,
MessageBoxIcon.Error
);
}
}
private void ShowErrors()
{
ErrorForm.InitializeFrom(Model);
ErrorForm.Show();
}
private void OnSaveFile(object sender, EventArgs e)
{
var dialog = new SaveFileDialog
{
Title = "Save Spatial SBML",
Filter = "SBML files|*.xml;*.sbml|All files|*.*",
AutoUpgradeEnabled = true
};
if (dialog.ShowDialog() != DialogResult.OK)
return;
SaveGeormetryAll();
Model.SaveTo(dialog.FileName);
UpdateUI();
}
private void OnPrint(object sender, EventArgs e)
{
}
private void OnCut(object sender, EventArgs e)
{
}
private void OnCopy(object sender, EventArgs e)
{
}
private void OnPaste(object sender, EventArgs e)
{
}
private void OnAbout(object sender, EventArgs e)
{
new FormAbout().ShowDialog(this);
}
private void OnExit(object sender, EventArgs e)
{
ErrorForm.Dispose();
ErrorForm = null;
Application.Exit();
}
private void OnCoreSelect(object sender, TreeViewEventArgs e)
{
var node = e.Node;
SaveCoreAll();
InvalidateCoreAll();
SelectCoreNode(node);
controlDisplayNode2.DisplayNode(e.Node);
}
private void SelectCoreNode(TreeNode node)
{
if (node.Name == NODE_INITIAL_ASSIGNMENTS)
{
controlDisplayNode2.Visible = false;
controlInitialAssignments1.Visible = true;
controlInitialAssignments1.InitializeFrom(Model.Document);
controlParameters1.Visible = false;
controlSpecies1.Visible = false;
controlCompartment1.Visible = false;
controlRules1.Visible = false;
}
else if (node.Name == NODE_PARAMETERS)
{
controlDisplayNode2.Visible = false;
controlInitialAssignments1.Visible = false;
controlParameters1.Visible = true;
controlSpecies1.Visible = false;
controlCompartment1.Visible = false;
controlRules1.Visible = false;
controlParameters1.InitializeFrom(Model.Document);
}
else if (node.Name == NODE_SPECIES)
{
controlDisplayNode2.Visible = false;
controlInitialAssignments1.Visible = false;
controlParameters1.Visible = false;
controlSpecies1.Visible = true;
controlCompartment1.Visible = false;
controlSpecies1.InitializeFrom(Model.Document);
controlRules1.Visible = false;
}
else if (node.Name == NODE_COMPARTMENTS)
{
controlDisplayNode2.Visible = false;
controlInitialAssignments1.Visible = false;
controlParameters1.Visible = false;
controlSpecies1.Visible = false;
controlCompartment1.Visible = true;
controlCompartment1.InitializeFrom(Model.Document);
controlRules1.Visible = false;
}
else if (node.Name == NODE_RULES)
{
controlDisplayNode2.Visible = false;
controlInitialAssignments1.Visible = false;
controlParameters1.Visible = false;
controlSpecies1.Visible = false;
controlCompartment1.Visible = false;
controlRules1.Visible = true;
controlRules1.InitializeFrom(Model.Document);
}
else
{
controlDisplayNode2.Visible = true;
controlInitialAssignments1.Visible = false;
controlParameters1.Visible = false;
controlSpecies1.Visible = false;
controlCompartment1.Visible = false;
controlRules1.Visible = false;
controlDisplayNode2.DisplayNode(node);
}
}
private void OnLoad(object sender, EventArgs e)
{
ReadSettings();
var remove = false;
haveAkira = false;
try
{
favs.Update();
menu.UpdateSBWMenu();
SBWExporter.SetupImport(
mnuImport, doAnalysis, s => SetFilename(s));
foreach (ToolStripItem item in mnuSBW.DropDownItems)
{
haveAkira |= haveAkira || item.Text == "Spatial SBML";
}
}
catch
{
remove = true;
}
if (remove || mnuSBW.DropDownItems.Count == 0)
{
favs.RemoveFromToolStrip();
mnuSBW.Visible = false;
mnuExportDune.Visible = false;
}
cmdAkira.Visible = haveAkira;
}
public void ReadSettings()
{
Settings = EditSpatialSettings.GetDefault(Size);
if (Settings.Width < Screen.FromControl(this).WorkingArea.Width &&
Settings.Height < Screen.FromControl(this).WorkingArea.Height)
Size = new Size(Settings.Width, Settings.Height);
chkMultipleCompartments.Checked = Settings.IgnoreMultiCompartments;
}
private void OnFormClosed(object sender, FormClosedEventArgs e)
{
WriteSettings();
}
private void WriteSettings()
{
Settings.Save();
}
private void OnShowWarnings(object sender, EventArgs e)
{
ShowErrors();
}
private void OnApplyJarnacClick(object sender, EventArgs e)
{
LoadFromJarnac(txtJarnac.Text);
}
private void OnExportMorpheusClick(object sender, EventArgs e)
{
if (Model == null || Model.Document == null)
return;
Model.Document.clearValidators();
Model.Document.addValidator(SpatialModel.CustomSpatialValidator);
Model.Document.checkConsistency();
if (Model.Document.getNumErrors(libsbml.LIBSBML_SEV_ERROR) > 0)
{
MessageBox.Show(
"Unfortunately, the SBML model contains a number of errors. These need to be corrected, before the model can be exported to Morpheus.",
"Invalid Model", MessageBoxButtons.OK, MessageBoxIcon.Error);
ShowErrors();
return;
}
var dialog = new SaveFileDialog
{
Title = "Save Morpheus Configuration File",
Filter = "Configuration file|*.xml|All files|*.*",
AutoUpgradeEnabled = true
};
if (dialog.ShowDialog() != DialogResult.OK)
return;
ExportMorpheusFile(dialog.FileName);
}
public void ExportMorpheusFile(string fileName)
{
File.WriteAllText(fileName, Model.ToMorpheus(fileName));
}
private void ConvertToSpatial()
{
var dialog = new FormInitSpatial { SpatialModel = Model };
if (dialog.ShowDialog(this) == DialogResult.OK)
{
var selection = dialog.CreateModel;
if (!Model.ConvertToSpatial(selection))
{
ShowErrors();
}
else
{
UpdateUI();
}
}
}
private void OnShowSpatialWizard(object sender, EventArgs e)
{
ConvertToSpatial();
}
private void OnExportDuneSBMLClick(object sender, EventArgs e)
{
if (Model == null || Model.Document == null)
return;
Model.Document.clearValidators();
Model.Document.addValidator(SpatialModel.CustomSpatialValidator);
Model.Document.checkConsistency();
if (Model.Document.getNumErrors(libsbml.LIBSBML_SEV_ERROR) > 0)
{
MessageBox.Show(
"Unfortunately, the SBML model contains a number of errors. These need to be corrected, before the model can be exported to Dune.",
"Invalid Model", MessageBoxButtons.OK, MessageBoxIcon.Error);
ShowErrors();
return;
}
var dialog = new SaveFileDialog
{
Title = "Export DUNE SBML Model",
Filter = "SBML files|*.xml;*.sbml|All files|*.*",
AutoUpgradeEnabled = true
};
if (dialog.ShowDialog() != DialogResult.OK)
return;
Model.ExportDuneSBML(dialog.FileName);
}
private void OnExportDuneClick(object sender, EventArgs e)
{
if (Model == null || Model.Document == null)
return;
Model.Document.clearValidators();
Model.Document.addValidator(SpatialModel.CustomSpatialValidator);
Model.Document.checkConsistency();
if (Model.Document.getNumErrors(libsbml.LIBSBML_SEV_ERROR) > 0)
{
MessageBox.Show(
"Unfortunately, the SBML model contains a number of errors. These need to be corrected, before the model can be exported to Dune.",
"Invalid Model", MessageBoxButtons.OK, MessageBoxIcon.Error);
ShowErrors();
return;
}
var dialog = new SaveFileDialog
{
Title = "Export Model to DUNE",
Filter = "Main Implementation file|*.cc|All files|*.*",
AutoUpgradeEnabled = true
};
if (dialog.ShowDialog() != DialogResult.OK)
return;
Model.ExportToDune(dialog.FileName);
}
private void OnAddSampledFieldGeometry(object sender, EventArgs e)
{
if (Model.Geometry == null) return;
var geom = Model.Geometry.createSampledFieldGeometry();
geom.setId(String.Format("sampledFieldGeometry{0}", Model.Geometry.GetNumSampledFieldGeometries()));
Model.Dirty = true;
UpdateUI();
}
private void OnAddAnalyticGeometry(object sender, EventArgs e)
{
if (Model.Geometry == null) return;
var geom = Model.Geometry.createAnalyticGeometry();
geom.setId(String.Format("analyticGeometry{0}", Model.Geometry.GetNumAnalyticGeometries()));
Model.Dirty = true;
UpdateUI();
}
private void OnSpatialItemDeleteClick(object sender, EventArgs e)
{
var selected = treeSpatial.SelectedNode;
if (selected == null || selected.Level == 0) return;
var selectedId = selected.Text;
var parent = selected.Parent;
if (Model.Geometry == null) return;
Model.Dirty = true;
if (selected.Level == 1)
switch (parent.Name)
{
case NODE_DOMAINS:
{
Model.Geometry.removeDomain(selectedId);
UpdateUI();
break;
}
case NODE_DOMAINTYPES:
{
Model.Geometry.removeDomainType(selectedId);
UpdateUI();
break;
}
case NODE_GEOMS:
{
Model.Geometry.removeGeometryDefinition(selectedId);
UpdateUI();
break;
}
case NODE_ADJACENTDOMAINS:
{
Model.Geometry.removeAdjacentDomains(selectedId);
UpdateUI();
break;
}
case NODE_COORDINATES:
{
Model.Geometry.removeCoordinateComponent(selectedId);
UpdateUI();
break;
}
}
if (selected.Level == 2)
{
switch (parent.Parent.Name)
{
case NODE_GEOMS:
{
var geom = Model.Geometry.getGeometryDefinition(parent.Text);
if (geom is AnalyticGeometry)
{
var ageom = geom as AnalyticGeometry;
ageom.removeAnalyticVolume(selectedId);
UpdateUI();
}
else if (geom is SampledFieldGeometry)
{
var sgeom = geom as SampledFieldGeometry;
sgeom.removeSampledVolume(selectedId);
UpdateUI();
}
break;
}
}
}
treeSpatial.SelectedNode = parent;
}
private void OnCoreItemDeleteClick(object sender, EventArgs e)
{
var selected = treeCore.SelectedNode;
if (selected == null || selected.Level == 0) return;
var selectedId = selected.Text;
var parent = selected.Parent;
switch (parent.Name)
{
case NODE_PARAMETERS:
{
Model.Document.getModel().removeParameter(selectedId);
UpdateUI();
break;
}
case NODE_COMPARTMENTS:
{
Model.Document.getModel().removeCompartment(selectedId);
UpdateUI();
break;
}
case NODE_SPECIES:
{
Model.Document.getModel().removeSpecies(selectedId);
UpdateUI();
break;
}
case NODE_REACTIONS:
{
Model.Document.getModel().removeSpecies(selectedId);
UpdateUI();
break;
}
case NODE_RULES:
{
Model.Document.getModel().removeRule(selectedId);
UpdateUI();
break;
}
case NODE_INITIAL_ASSIGNMENTS:
{
Model.Document.getModel().removeInitialAssignment(selectedId);
UpdateUI();
break;
}
}
treeCore.SelectedNode = parent;
}
private void OnMoveARtoIAClick(object sender, EventArgs e)
{
if (Model == null) return;
Model.MoveAllRulesToAssignments();
Model.Dirty = true;
UpdateUI();
}
private void OnEditSpatialAnnotationClick(object sender, EventArgs e)
{
if (Model == null || Model.Document == null) return;
Annotation.InitFrom(Model);
Annotation.ShowDialog(this);
if (Annotation.Status == DialogResult.OK)
{
Annotation.SaveToModel(Model.Document.getModel());
}
}
private void OnSimulateWithAkiraClick(object sender, EventArgs e)
{
if (!haveAkira) return;
if (Model == null || Model.Document == null) return;
if (!Model.IsSpatial) return;
try
{
HighLevel.Send("Spatial SBML", "Spatial SBML", "void doAnalysis(string)", Model.ToSBML());
}
catch
{
}
}
private void OnCheckMultipleCompartmentsClick(object sender, EventArgs e)
{
Settings.IgnoreMultiCompartments = chkMultipleCompartments.Checked;
Settings.Save();
}
private void OnEditPreferencesClick(object sender, EventArgs e)
{
using (var dlg = new FormSettings {Settings = Settings})
{
if (dlg.ShowDialog(this) == DialogResult.OK)
{
dlg.Settings.Save();
ReadSettings();
}
}
}
private void OnPrepareDuneClick(object sender, EventArgs e)
{
if (Model == null || Model.Document == null) return;
if (!Model.IsSpatial) return;
using (var dlg = new FormPrepareDune
{
Settings = Settings,
Model = Model,
TargetDir = Settings.DefaultDir,
ModuleName = Path.GetFileNameWithoutExtension(Model.FileName)
})
{
dlg.ShowDialog(this);
}
}
#region Drag / Drop
private void MainForm_DragDrop(object sender, DragEventArgs e)
{
try
{
var sFilenames = (string[]) e.Data.GetData(DataFormats.FileDrop);
var oInfo = new FileInfo(sFilenames[0]);
if (oInfo.Extension.ToLower() == ".xml" || oInfo.Extension.ToLower() == ".sbml")
{
OpenFile(sFilenames[0]);
}
}
catch (Exception)
{
}
}
private void MainForm_DragEnter(object sender, DragEventArgs e)
{
if (e.Data.GetDataPresent(DataFormats.FileDrop))
{
var sFilenames = (string[]) e.Data.GetData(DataFormats.FileDrop);
var oInfo = new FileInfo(sFilenames[0]);
if (oInfo.Extension.ToLower() == ".xml" || oInfo.Extension.ToLower() == ".sbml")
{
e.Effect = DragDropEffects.Copy;
return;
}
}
e.Effect = DragDropEffects.None;
}
#endregion
/// <summary>
/// This function asks a user whether the model should be saved
/// </summary>
/// <returns>true, if model is dirty and the user pressed cancel, false otherwise</returns>
private bool SaveModelIfDirtyOrCancel()
{
if (Model == null || !Model.Dirty) return false;
DialogResult result =
MessageBox.Show(this,
"There are unsaved changes in the model. Would you like to save them?",
"Save changes?",
MessageBoxButtons.YesNoCancel,
MessageBoxIcon.Question,
MessageBoxDefaultButton.Button3);
if (result == DialogResult.Cancel)
{
return true;
}
if (result == DialogResult.Yes)
{
OnSaveFile(this, EventArgs.Empty);
}
return false;
}
private void OnFormClosing(object sender, FormClosingEventArgs e)
{
e.Cancel = SaveModelIfDirtyOrCancel();
}
}
}
<file_sep>/WFEditSpatial/Forms/FormSpatialAnnotation.cs
using System;
using System.Collections.Generic;
using System.Globalization;
using System.Windows.Forms;
using EditSpatial.Model;
using libsbmlcs;
namespace EditSpatial.Forms
{
public partial class FormSpatialAnnotation : Form
{
public const string SPATIAL_ANNOTATION_URL = "http://fbergmann.github.io/spatial-sbml/settings";
private readonly Random rand;
public FormSpatialAnnotation()
{
InitializeComponent();
rand = new Random();
}
public libsbmlcs.Model Model { get; set; }
public DialogResult Status { get; set; }
private void InitializeIdCombo(libsbmlcs.Model model)
{
colId.Items.Clear();
for (var i = 0; i < model.getNumSpecies(); i++)
{
colId.Items.Add(model.getSpecies(i).getId());
}
}
private XMLNode getAnnotationNode(libsbmlcs.Model model, string ns)
{
if (model == null || !model.isSetAnnotation()) return null;
var parent = model.getAnnotation();
var again = true;
while (again)
{
again = false;
for (var i = 0; i < parent.getNumChildren(); ++i)
{
var current = parent.getChild(i);
if (current.getName() == "annotation")
{
again = true;
parent = current;
break;
}
if (current.hasNamespaceURI(ns))
return current;
}
}
return null;
}
private string Clean(string original)
{
var result = original.Replace("<notes>", "");
result = result.Replace("</notes>", "");
result = result.Replace("</body>", "");
result = result.Replace("<pre>", "");
result = result.Replace("</pre>", "");
result = result.Replace("<body xmlns=\"http://www.w3.org/1999/xhtml\">", "");
result = result.Replace("\n", "");
result = result.Trim();
return result;
}
private void AddRowForNote(string text, Species species)
{
text = Clean(text);
if (string.IsNullOrWhiteSpace(text)) return;
if (!text.Contains("spatial")) return;
var palette = "black-blue";
if (text.Contains("GFP"))
palette = "black-green";
if (text.Contains("RFP"))
palette = "black-red";
double max = 6;
double scale = 10;
if (species.isSetInitialConcentration() && species.getInitialConcentration() > 0)
max = scale*species.getInitialConcentration();
else if (species.isSetInitialAmount() && species.getInitialAmount() > 0)
max = scale*species.getInitialAmount();
grid.Rows.Add(species.getId(), palette, max.ToString(CultureInfo.InvariantCulture));
}
private void InitializeFromNotes(libsbmlcs.Model model)
{
grid.Rows.Clear();
if (model == null) return;
Model = model;
for (var i = 0; i < model.getNumSpecies(); ++i)
{
var current = model.getSpecies(i);
if (current == null || !current.isSetNotes()) continue;
AddRowForNote(current.getNotesString(), current);
}
}
private void InitializeFromAnnotation(libsbmlcs.Model model)
{
var node = getAnnotationNode(model, SPATIAL_ANNOTATION_URL);
if (node == null) return;
var update = node.getChild("update");
if (update.getName() == "update")
{
txtStep.Text = update.getAttrValue("step");
txtUpdate.Text = update.getAttrValue("freq");
}
grid.Rows.Clear();
var items = node.getChild("items");
if (items.getName() == "items")
{
for (var i = 0; i < items.getNumChildren(); ++i)
{
var item = items.getChild(i);
var id = item.getAttrValue("sbmlId");
var palette = item.getAttrValue("palette");
var max = item.getAttrValue("max");
grid.Rows.Add(id, palette, max);
}
}
}
public void SaveToModel(libsbmlcs.Model model)
{
if (model == null) return;
var node = new XMLNode(new XMLTriple("spatialInfo", SPATIAL_ANNOTATION_URL, ""), new XMLAttributes());
node.addAttr("xmlns", SPATIAL_ANNOTATION_URL);
// save stepsize
var update = new XMLNode(new XMLTriple("update", SPATIAL_ANNOTATION_URL, ""), new XMLAttributes());
update.addAttr("step", txtStep.Text);
update.addAttr("freq", txtUpdate.Text);
node.addChild(update);
// save assignments
if (grid.Rows.Count > 0)
{
var items = new XMLNode(new XMLTriple("items", SPATIAL_ANNOTATION_URL, ""), new XMLAttributes());
for (var i = 0; i < grid.Rows.Count; ++i)
{
var current = grid.Rows[i];
var item = new XMLNode(new XMLTriple("item", SPATIAL_ANNOTATION_URL, ""), new XMLAttributes());
var id = current.Cells[0].Value as string;
if (string.IsNullOrWhiteSpace(id)) continue;
item.addAttr("sbmlId", id);
item.addAttr("palette", current.Cells[1].Value as string);
item.addAttr("max", current.Cells[2].Value as string);
items.addChild(item);
}
node.addChild(items);
}
if (model.isSetAnnotation())
{
var annot = model.getAnnotation();
var num = (int) annot.getNumChildren();
for (var i = num - 1; i >= 0; i--)
{
var child = annot.getChild(i);
if (child.getName() == "spatialInfo" &&
(child.getNamespaceURI() == SPATIAL_ANNOTATION_URL || child.getNamespaceURI() == ""))
annot.removeChild(i);
}
}
model.removeTopLevelAnnotationElement("spatialInfo", SPATIAL_ANNOTATION_URL, false);
model.appendAnnotation(node);
}
public void InitFrom(SpatialModel spatialModel)
{
Status = DialogResult.Cancel;
colId.Items.Clear();
grid.Rows.Clear();
if (spatialModel == null || spatialModel.Document == null || spatialModel.Document.getModel() == null)
return;
Model = spatialModel.Document.getModel();
if (Model == null)
return;
InitializeIdCombo(Model);
InitializeFromAnnotation(Model);
if (grid.Rows.Count < 2)
InitializeFromNotes(Model);
}
private void FormSpatialAnnotation_FormClosing(object sender, FormClosingEventArgs e)
{
e.Cancel = true;
Hide();
}
private void OnInitFromNotesClick(object sender, EventArgs e)
{
InitializeFromNotes(Model);
}
private void OnClearClick(object sender, EventArgs e)
{
grid.Rows.Clear();
}
private void cmdOK_Click(object sender, EventArgs e)
{
Status = DialogResult.OK;
}
private void cmdCombine_Click(object sender, EventArgs e)
{
var selected = grid.SelectedRows;
if (selected == null || selected.Count < 2) return;
// get common properties
var palette = selected[0].Cells[1].Value as string;
var max = selected[0].Cells[2].Value as string;
// remove rows
var ids = new List<string>();
for (var i = selected.Count - 1; i >= 0; i--)
{
ids.Insert(0, selected[i].Cells[0].Value as string);
grid.Rows.Remove(selected[i]);
}
// add species with assignment rule
var species = Model.createSpecies();
species.initDefaults();
var name = "combined_" + ids[0];
var formula = ids[0];
colId.Items.Remove(ids[0]);
for (var i = 1; i < ids.Count; i++)
{
name = name + "_" + ids[i];
formula = formula + " + " + ids[i];
colId.Items.Remove(ids[i]);
}
species.setId(name);
species.setCompartment(Model.getSpecies(ids[0]).getCompartment());
var plug = species.getPlugin("spatial") as SpatialSpeciesPlugin;
if (plug != null)
plug.setIsSpatial(true);
var assignment = Model.createAssignmentRule();
assignment.setVariable(species.getId());
assignment.setFormula(formula);
colId.Items.Add(species.getId());
// add new row
var index = grid.Rows.Add(species.getId(), palette, max);
// select row
grid.Rows[index].Selected = true;
}
private string getRandomPalette()
{
switch (rand.Next(3))
{
default:
return "black-green";
case 2:
return "black-red";
case 1:
return "black-blue";
}
}
private void OnAddAllClick(object sender, EventArgs e)
{
OnClearClick(sender, e);
for (var i = 0; i < Model.getNumSpecies(); i++)
{
var species = Model.getSpecies(i);
double max = 6;
double scale = 10;
if (species.isSetInitialConcentration() && species.getInitialConcentration() > 0)
max = scale*species.getInitialConcentration();
else if (species.isSetInitialAmount() && species.getInitialAmount() > 0)
max = scale*species.getInitialAmount();
grid.Rows.Add(species.getId(), getRandomPalette(), max);
}
}
}
}<file_sep>/WFEditDMP/MainForm.cs
using System;
using System.Drawing;
using System.Drawing.Imaging;
using System.Globalization;
using System.IO;
using System.Windows.Forms;
using LibEditSpatial.Dialogs;
using LibEditSpatial.Model;
using WFEditDMP.Forms;
namespace WFEditDMP
{
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
NewModel();
ctrlPalette1.PalleteValueChanged += (o, args) => { dmpRenderControl1.CurrentValue = args.Value; };
ctrlPalette1.PalleteChanged += (o, args) =>
{
if (Model != null)
{
Model.Palette = args;
UpdateUI();
}
DmpPalette.Default = args;
};
dmpRenderControl1.IndexLocationChanged +=
(o, args) => { lblPosition.Text = string.Format("nx={0}, ny={1}", args.X, args.Y); };
dmpRenderControl1.DataLocationChanged +=
(o, args) => { lblData.Text = string.Format("x={0}, y={1}", args.X, args.Y); };
}
public DmpModel Model { get; set; }
public string LastOpenDir { get; set; }
public String[] PaletteFiles { get; set; }
public string CurrentPalette { get; set; }
private void SetTitle(string fileName)
{
if (!string.IsNullOrEmpty(fileName))
Text = String.Format("Edit DMP - [{0}]", Path.GetFileName(fileName));
else
Text = "Edit DMP";
}
private void UpdateUI()
{
if (Model == null)
{
SetTitle(null);
lblMessage.Text = "no model";
return;
}
lblSize.Text = string.Format("{0} x {1}", Model.Columns, Model.Rows);
txtCols.Text = Model.Columns.ToString();
txtRows.Text = Model.Rows.ToString();
txtMinX.Text = Model.MinX.ToString(CultureInfo.InvariantCulture);
txtMaxX.Text = Model.MaxX.ToString(CultureInfo.InvariantCulture);
txtMinY.Text = Model.MinY.ToString(CultureInfo.InvariantCulture);
txtMaxY.Text = Model.MaxY.ToString(CultureInfo.InvariantCulture);
ctrlPalette1.UpdateValues(Model.Min, ctrlPalette1.Current.Value, Model.Max);
dmpRenderControl1.LoadModel(Model);
SetTitle(Model.FileName);
}
public void OpenFile(string filename)
{
LastOpenDir = Path.GetDirectoryName(filename);
Model = DmpModel.FromFile(filename);
ctrlPalette1.UpdateValues(Model.Min, ctrlPalette1.Current.Value, Model.Max);
dmpRenderControl1.CurrentValue = Model.Max;
Model.Palette = ctrlPalette1.Palette;
UpdateUI();
}
private void NewModel()
{
NewModel(new Size(50, 50), new RectangleF(0, 50, 0, 50));
}
private void NewModel(Size size, RectangleF rect)
{
Model = new DmpModel(50, 50);
ctrlPalette1.UpdateValues(Model.Min, ctrlPalette1.Current.Value, Model.Max);
dmpRenderControl1.CurrentValue = Model.Max;
Model.Palette = ctrlPalette1.Palette;
UpdateUI();
}
private void OnNewClick(object sender, EventArgs e)
{
if (SaveModelIfDirtyOrCancel())
return;
using (var dlg = new FormResize {
Dimensions = new Size(Model.Columns, Model.Rows),
CanvasBounds = new RectangleF((float)Model.MinX, (float)Model.MinY, (float)Model.MaxX, (float)Model.MaxY)
} )
{
if (dlg.ShowDialog(this) == DialogResult.OK)
{
NewModel(dlg.Dimensions, dlg.CanvasBounds);
}
}
}
private void OnOpenClick(object sender, EventArgs e)
{
if (SaveModelIfDirtyOrCancel())
return;
using (var dialog = new OpenFileDialog
{
Title = "Open file",
Filter = "DMP files|*.dmp|Image files|*.tif;*.tiff;*.png;*.jpg;*.jpeg;*.bmp|All files|*.*",
AutoUpgradeEnabled = true,
InitialDirectory = LastOpenDir
})
{
if (dialog.ShowDialog() == DialogResult.OK)
{
OpenFile(dialog.FileName);
}
}
}
private void OnSaveClick(object sender, EventArgs e)
{
if (string.IsNullOrWhiteSpace(Model.FileName))
{
OnSaveAsClick(sender, e);
return;
}
SaveAs(Model.FileName);
}
private void OnExitClick(object sender, EventArgs e)
{
Close();
}
private void OnAboutClick(object sender, EventArgs e)
{
using (var dlg = new AboutBox())
{
dlg.ShowDialog();
}
}
private void SaveAs(string fileName)
{
if (Model == null) return;
string lower = fileName.ToLowerInvariant();
if (lower.EndsWith(".tif") || lower.EndsWith(".tiff"))
Model.ToImage().Save(fileName);
else
Model.SaveAs(fileName);
UpdateUI();
}
private void OnSaveAsClick(object sender, EventArgs e)
{
if (Model == null) return;
using (var dialog = new SaveFileDialog
{
Title = "Save file",
Filter = "DMP files|*.dmp|TIFF files|*.tif;*.tiff|All files|*.*",
AutoUpgradeEnabled = true,
InitialDirectory = LastOpenDir
})
{
if (dialog.ShowDialog() == DialogResult.OK)
SaveAs(dialog.FileName);
}
}
private void LoadPalettes(string baseDirectory)
{
PaletteFiles = Directory.GetFiles(baseDirectory, "*.txt", SearchOption.TopDirectoryOnly);
cmbPalettes.Items.Clear();
cmbPalettes.Items.Add("Default");
foreach (var file in PaletteFiles)
{
cmbPalettes.Items.Add(Path.GetFileNameWithoutExtension(file));
}
}
private void OnLoad(object sender, EventArgs e)
{
ctrlPalette1.Palette = DmpPalette.Default;
LoadPalettes(Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "Palettes"));
}
private void OnPaletteChanged(object sender, EventArgs e)
{
var index = cmbPalettes.SelectedIndex;
if (index < 0) return;
if ((string) cmbPalettes.Items[index] == CurrentPalette)
return;
if (index == 0)
{
ctrlPalette1.ChangePalette(DmpPalette.Default);
}
else
try
{
ctrlPalette1.ChangePalette(PaletteFiles[index - 1]);
}
catch
{
}
CurrentPalette = (string) cmbPalettes.Items[index];
}
private void OnSizeChanged(object sender, EventArgs e)
{
int val;
if (int.TryParse(txtSize.Text, out val))
{
dmpRenderControl1.PencilSize = val;
}
}
private void OnResizeClicked(object sender, EventArgs e)
{
int rows, cols;
if (int.TryParse(txtCols.Text, out cols) && int.TryParse(txtRows.Text, out rows))
{
Model.Resize(cols, rows);
UpdateUI();
}
}
private void OnRotateLeft(object sender, EventArgs e)
{
Model.RotateRight();
Model.RotateRight();
Model.RotateRight();
UpdateUI();
}
private void OnRotateRight(object sender, EventArgs e)
{
Model.RotateRight();
UpdateUI();
}
private void OnCenterClick(object sender, EventArgs e)
{
Model.Center();
UpdateUI();
}
private void OnMinXChanged(object sender, EventArgs e)
{
double temp;
if (Model != null && double.TryParse(txtMinX.Text, out temp))
Model.MinX = temp;
}
private void OnMaxXChanged(object sender, EventArgs e)
{
double temp;
if (Model != null && double.TryParse(txtMaxX.Text, out temp))
Model.MaxX = temp;
}
private void OnMinYChanged(object sender, EventArgs e)
{
double temp;
if (Model != null && double.TryParse(txtMinY.Text, out temp))
Model.MinY = temp;
}
private void OnMaxYChanged(object sender, EventArgs e)
{
double temp;
if (Model != null && double.TryParse(txtMaxY.Text, out temp))
Model.MaxY = temp;
}
private void OnAdjustClick(object sender, EventArgs e)
{
if (Model == null) return;
var range = Model.Range;
using (var dlg = new FormAdjustDmp())
{
dlg.InitializeFrom(range, (DmpModel) Model.Clone());
if (dlg.ShowDialog(this) == DialogResult.OK)
{
Model = dlg.Model;
UpdateUI();
}
}
}
private int FindHCF(int m, int n)
{
int temp, reminder;
if (m < n)
{
temp = m;
m = n;
n = temp;
}
while (true)
{
reminder = m % n;
if (reminder == 0)
return n;
else
m = n;
n = reminder;
}
}
private void OnAspectClick(object sender, EventArgs e)
{
// resizes the window to match the aspect ratio
var screen = Screen.FromControl(this);
StartPosition = FormStartPosition.Manual;
var bounds = screen.Bounds;
Location = bounds.Location;
int hcf = FindHCF((int)Math.Round(Model.MaxX), (int)Math.Round(Model.MaxY));
double width = Math.Round(Model.MaxX);
double height = Math.Round(Model.MaxY);
double factorX = width / (double)hcf;
double factorY = height / (double)hcf;
double maxWidth = bounds.Width - 50;
double maxHeight = bounds.Height - 50;
double min = Math.Min(maxWidth, maxHeight);
if (factorX > factorY)
{
double newHeight = height / width * min;
Size = new System.Drawing.Size((int)min, (int)newHeight);
}
else
{
double newWidth = width / height * min;
Size = new System.Drawing.Size((int)newWidth, (int)min);
}
}
/// <summary>
/// This function asks a user whether the model should be saved
/// </summary>
/// <returns>true, if model is dirty and the user pressed cancel, false otherwise</returns>
private bool SaveModelIfDirtyOrCancel()
{
if (Model == null || !Model.Dirty) return false;
DialogResult result =
MessageBox.Show(this,
"There are unsaved changes in the model. Would you like to save them?",
"Save changes?",
MessageBoxButtons.YesNoCancel,
MessageBoxIcon.Question,
MessageBoxDefaultButton.Button3);
if (result == DialogResult.Cancel)
{
return true;
}
if (result == DialogResult.Yes)
{
OnSaveClick(this, EventArgs.Empty);
}
return false;
}
private void OnFormClosing(object sender, FormClosingEventArgs e)
{
e.Cancel = SaveModelIfDirtyOrCancel();
}
private void OnResizeClick(object sender, EventArgs e)
{
using (var dlg = new FormResize
{
Dimensions = new Size(Model.Columns, Model.Rows),
CanvasBounds = new RectangleF((float) Model.MinX, (float) Model.MinY, (float) Model.MaxX, (float) Model.MaxY)
})
{
if (dlg.ShowDialog(this) == DialogResult.OK)
{
Model.Resize(dlg.Dimensions.Width, dlg.Dimensions.Height, dlg.ScaleContents);
Model.MinX = dlg.CanvasBounds.X;
Model.MinY = dlg.CanvasBounds.Y;
Model.MaxX = dlg.CanvasBounds.Width;
Model.MaxY = dlg.CanvasBounds.Height;
UpdateUI();
}
}
}
private void OnMaskWithCompartmentClick(object sender, EventArgs e)
{
using (var dlg = new OpenFileDialog {
Title = "Choose DMP file to mask with. ",
Filter = "All supported|*.dmp;*.tif;*.tiff;*.png;*.jpg;*.jpeg;*.bmp|DMP files|*.dmp|Image files|*.tif;*.tiff;*.png;*.jpg;*.jpeg;*.bmp|All files|*.*",
AutoUpgradeEnabled = true,
InitialDirectory = this.LastOpenDir})
{
if (dlg.ShowDialog() == DialogResult.OK)
{
try
{
var file = DmpModel.FromFile(dlg.FileName);
Model.MaskWith(file);
UpdateUI();
}
catch
{
MessageBox.Show("Masking failed, ensure that the files have precisely the same dimensions", "Masking failed",
MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
}
}
#region Drag / Drop
private void MainForm_DragDrop(object sender, DragEventArgs e)
{
try
{
var sFilenames = (string[])e.Data.GetData(DataFormats.FileDrop);
var oInfo = new FileInfo(sFilenames[0]);
if (oInfo.Extension.ToLower() == ".dmp" || DmpModel.IsImageFile(sFilenames[0]))
{
OpenFile(sFilenames[0]);
}
}
catch (Exception)
{
}
}
private void MainForm_DragEnter(object sender, DragEventArgs e)
{
if (e.Data.GetDataPresent(DataFormats.FileDrop))
{
var sFilenames = (string[])e.Data.GetData(DataFormats.FileDrop);
var oInfo = new FileInfo(sFilenames[0]);
if (oInfo.Extension.ToLower() == ".dmp" || DmpModel.IsImageFile(sFilenames[0]))
{
e.Effect = DragDropEffects.Copy;
return;
}
}
e.Effect = DragDropEffects.None;
}
#endregion
}
}<file_sep>/LibEditSpatial/Model/StaticHelper.cs
using System.Collections.Generic;
namespace LibEditSpatial.Model
{
public static class StaticHelper
{
public static bool GetBool(this Dictionary<string, string> dict, string option)
{
if (!dict.ContainsKey(option)) return false;
switch (dict[option].ToLowerInvariant())
{
case "yes":
case "on":
return true;
default:
return false;
}
}
public static string GetString(this Dictionary<string, string> dict, string option)
{
if (!dict.ContainsKey(option)) return "";
return dict[option];
}
public static T Get<T>(this Dictionary<string, string> dict, string option, T defaultValue = default(T))
{
if (!dict.ContainsKey(option)) return defaultValue;
switch (typeof (T).ToString())
{
case "System.Boolean":
return (T) (object) GetBool(dict, option);
case "System.Int16":
case "System.Int32":
case "System.Int64":
{
int temp;
if (int.TryParse(dict[option], out temp))
return (T) (object) temp;
break;
}
case "System.Single":
{
float temp;
if (float.TryParse(dict[option], out temp))
return (T) (object) temp;
break;
}
case "System.Double":
{
double temp;
if (double.TryParse(dict[option], out temp))
return (T) (object) temp;
break;
}
case "System.String":
{
return (T) (object) dict[option];
}
default:
return defaultValue;
}
return defaultValue;
}
}
}<file_sep>/WFEditSpatial/Templates/componentparameters.hh
// -*- tab-width: 4; indent-tabs-mode: nil; c-basic-offset: 2 -*-
// vi: set ts=4 sw=2 et sts=2:
#ifndef DUNE_COPASI_DIFFUSIONPARAMETERS_HH
#define DUNE_COPASI_DIFFUSIONPARAMETERS_HH
#include <dune/copasi/utilities/componentparameters.hh>
#include <dune/copasi/utilities/datahelper.hh>
#include "local_operator.hh"
/**
* Generic DiffusionParameter for all variables.
*
* It is to be initialized from a config file, with the following parameters:
*
* - D -> double, the diffusion coefficient
* - Xmin -> double, boundary condition at left boundary
* - Xmax -> double, boundary condition at right boundary
* - Ymin -> double, boundary condition at top boundary
* - Ymax -> double, boundary condition at bottom boundary
*
* - BCType: int, the type of boundary condition
* Dirichlet=1, Neumann=-1, Outflow=-2, None=-3
*
* Additionally the values returned can be overwritten by files. For that set the
* parameters below to dmp files.
*
* - file_bcType, a file containing bctypes for all coordinates
* - file_dc, a file containing the diffusion coefficients for the coordinates
* - file_neumann, a file containing Neumann boundary values for the coordinates
* - file_dirichlet, a file containing Dirichlet boundary values for the coordinates
* - file_compartment, a file 1 wherever inside compartment and 0 outside for the coordinates
*/
template<typename GV, typename RF>
class DiffusionParameter :
public Dune::PDELab::DiffusionMulticomponentInterface<Dune::PDELab::DiffusionParameterTraits<GV, RF>,
DiffusionParameter<GV, RF> >
{
enum { dim = GV::Grid::dimension };
public:
typedef Dune::PDELab::DiffusionParameterTraits<GV, RF> Traits;
typedef typename Traits::BCType BCType;
DiffusionParameter(const Dune::ParameterTree & param, const std::string cname)
: time(0.)
, Dt(param.sub(cname).template get<RF>("D"))
, Xmin(param.sub(cname).template get<RF>("Xmin", 0))
, Xmax(param.sub(cname).template get<RF>("Xmax", 0))
, Ymin(param.sub(cname).template get<RF>("Ymin", 0))
, Ymax(param.sub(cname).template get<RF>("Ymax", 0))
, width(param.sub("Domain").template get<int>("width", 0))
, height(param.sub("Domain").template get<int>("height", 0))
, boundarytype(BCType::Neumann)
, dh_bcType(DataHelper::forFile(param.sub(cname).template get<std::string>("file_bcType", "")))
, dh_neumann(DataHelper::forFile(param.sub(cname).template get<std::string>("file_neumann", "")))
, dh_dirichlet(DataHelper::forFile(param.sub(cname).template get<std::string>("file_dirichlet", ""), NearestNeighbor))
, dh_dc(DataHelper::forFile(param.sub(cname).template get<std::string>("file_dc", "")))
, dh_compartment(DataHelper::forFile(param.sub(cname).template get<std::string>("file_compartment", ""), NearestNeighbor))
{
int bc = param.sub(cname).template get<int>("BCType");
switch (bc) {
case 1:
boundarytype = BCType::Dirichlet;
break;
case -1:
boundarytype = BCType::Neumann;
break;
case -2:
boundarytype = BCType::Outflow;
break;
case -3:
boundarytype = BCType::None;
break;
}
}
//! tensor permeability
typename Traits::RangeFieldType
D(const typename Traits::ElementType& e, const typename Traits::DomainType& x_) const
{
typename Traits::DomainType x = e.geometry().global(x_);
if (dh_dc == NULL)
{
if (dh_compartment == NULL) return Dt;
return Dt * dh_compartment->get(double(x[0]), double(x[1]));
}
return (typename Traits::RangeFieldType)dh_dc->get(double(x[0]), double(x[1]));
}
//! source/reaction term
typename Traits::RangeFieldType q(const typename Traits::ElementType& e, const typename Traits::DomainType&) const
{
return 0.0;
}
//! boundary condition type function
BCType
bctype(const typename Traits::IntersectionType& is, const typename Traits::IntersectionDomainType& x_) const
{
if (dh_bcType == NULL) return boundarytype;
typename Traits::DomainType x = is.geometry().global(x_);
return (BCType)dh_bcType->get(double(x[0]), double(x[1]));
}
bool
isDirichlet(const typename Traits::IntersectionType& is, const typename Traits::IntersectionDomainType& x) const
{
return bctype(is, x) == BCType::Dirichlet;
}
//! Dirichlet boundary condition value
typename Traits::RangeFieldType
g(const typename Traits::IntersectionType& is, const typename Traits::IntersectionDomainType& x_) const
{
if (!is.boundary() || bctype(is, x_) != BCType::Dirichlet)
return 0; // Dirichlet is zero
typename Traits::DomainType x = is.geometry().global(x_);
if (dh_dirichlet != NULL)
{
return (typename Traits::RangeFieldType)dh_dirichlet->get(double(x[0]), double(x[1]));
}
if (x[0] < 1e-6)
return Xmin;
if (x[0] > width - 1e-6)
return Xmax;
if (x[1] < 1e-6)
return Ymin;
if (x[1] > height - 1e-6)
return Ymax;
return 0.0;
}
//! Neumann boundary condition
typename Traits::RangeFieldType
j(const typename Traits::IntersectionType& is, const typename Traits::IntersectionDomainType& x_) const
{
if (!is.boundary() || bctype(is, x_) != BCType::Neumann)
return 0.0;
typename Traits::DomainType x = is.geometry().global(x_);
if (dh_neumann != NULL)
{
return (typename Traits::RangeFieldType)dh_neumann->get(double(x[0]), double(x[1]));
}
if (x[0] < 1e-6)
return Xmin;
if (x[0] > width - 1e-6)
return Xmax;
if (x[1] < 1e-6)
return Ymin;
if (x[1] > height - 1e-6)
return Ymax;
return 0.0;
}
//! set time for subsequent evaluation
void setTime(RF t)
{
time = t;
}
void setTimeTarget(RF time_, RF dt_)
{
tend = time_;
}
//! to be called once before each time step
void preStep(RF time_, RF dt_, int stages)
{
}
private:
RF time, tend, dt;
const RF Dt;
const RF Xmin;
const RF Xmax;
const RF Ymin;
const RF Ymax;
const RF width;
const RF height;
BCType boundarytype;
const DataHelper* dh_bcType;
const DataHelper* dh_neumann;
const DataHelper* dh_dirichlet;
const DataHelper* dh_dc;
const DataHelper* dh_compartment;
};
#endif
<file_sep>/LibEditSpatial/Model/GlobalConfig.cs
using System.Collections.Generic;
namespace LibEditSpatial.Model
{
public class GlobalConfig
{
public bool WriteVTK { get; set; }
public string VTKname { get; set; }
public int Overlap { get; set; }
public int IntegrationOrder { get; set; }
public int SubSampling { get; set; }
public string TimeStepping { get; set; }
public string ExplicitSolver { get; set; }
public string ImplicitSolver { get; set; }
public string SBMLFile { get; set; }
public static GlobalConfig Default
{
get
{
return new GlobalConfig
{
WriteVTK = true,
ExplicitSolver = "RK4",
ImplicitSolver = "Alexander2",
IntegrationOrder = 2,
SubSampling = 2,
Overlap = 1,
TimeStepping = "implicit"
};
}
}
public static GlobalConfig FromDict(Dictionary<string, string> dict)
{
var result = new GlobalConfig
{
WriteVTK = dict.GetBool("writeVTK"),
VTKname = dict.GetString("VTKname"),
Overlap = dict.Get<int>("overlap"),
IntegrationOrder = dict.Get<int>("integrationorder"),
SubSampling = dict.Get<int>("subsampling"),
TimeStepping = dict.GetString("timestepping"),
ExplicitSolver = dict.GetString("explicitsolver"),
ImplicitSolver = dict.GetString("implicitsolver"),
SBMLFile = dict.GetString("sbmlfile")
};
return result;
}
public Dictionary<string, string> ToDict()
{
var result = new Dictionary<string, string>();
result["writeVTK"] = WriteVTK ? "yes" : "no";
result["VTKname"] = VTKname;
result["overlap"] = Overlap.ToString();
result["integrationorder"] = IntegrationOrder.ToString();
result["subsampling"] = SubSampling.ToString();
result["timestepping"] = TimeStepping;
result["explicitsolver"] = ExplicitSolver;
result["implicitsolver"] = ImplicitSolver;
result["sbmlfile"] = SBMLFile;
return result;
}
}
}<file_sep>/WFEditSpatial/Templates/reactionadapter.h
#ifndef REACTIONADAPTER_H
#define REACTIONADAPTER_H
#include <dune/copasi/utilities/sbmlhelper.hh>
//! Adapter for system of equations
/*!
\tparam M model type
This class is used for adapting system of ODE's (right side)
to system of PDE's (as source term)
What need to be implemented is only the evaluate function
*/
template<typename RF>
class ReactionAdapter
{
public:
//! constructor stores reference to the model
ReactionAdapter(const Dune::ParameterTree & param_)
: param(param_)
%INITIALIZER%
{
}
void preStep(RF time,RF dt,int stages)
{
}
//! evaluate model in entity eg with local values x
template<typename EG, typename LFSU, typename X, typename LFSV, typename R, typename DomainType>
void evaluate(const EG& eg, const LFSU& lfsu, const X& x, const LFSV& lfsv, R& r, const DomainType& x_)
{
const auto& pos = eg.geometry().global(x_);
%ODES%
}
private:
const Dune::ParameterTree & param;
%DECLARATION%
};
#endif // REACTIONADAPTER_H
<file_sep>/README.md
# Edit Spatial
This project contains a prototype editor for manipulating models using SBML Models using [SBML Level 3](http://sbml.org/Documents/Specifications) with the [Spatial package](http://sbml.org/Community/Wiki/SBML_Level_3_Proposals/Spatial_Geometries_and_Spatial_Processes "Spatial package").
## Using the Editor
There is not much there just yet, basically just open the SBML model in it and inspect / modify the geometry. Currently supported geometries are images and analytic geometries. To make it easier to compose analytic geometries. The following predefined functions can be used:
* CIRCLE: use like: `CIRCLE(x,y,25,25,10)`
* ELLIPSE: use like: `ELLIPSE(x,y,25,25,10,10)`
* RECTANGLE: use like: `RECT(x,y,10,10,10,10)`
* FISH: use like: `FISH(x,y,width,height)`
## How to build
The project is a .NET project simply open and compile `EditSpatial.sln` with either Visual Studio or MonoDevelop.
## Libraries
This project requires the following libraries:
- [libSBML](http://sbml.org/Software/libSBML) along with a supported xml library (xml2, expat, xerces-c)
- [Ookii.Dialogs](http://www.ookii.org/) used for browsing folders, easily
## License
This project is licensed under the BSD license:
```
Copyright (c) 2015-2018, <NAME>
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are
met:
Redistributions of source code must retain the above copyright notice,
this list of conditions and the following disclaimer. Redistributions in
binary form must reproduce the above copyright notice, this list of
conditions and the following disclaimer in the documentation and/or
other materials provided with the distribution. THIS SOFTWARE IS
PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY
EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR
CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
```
<file_sep>/WFEditSpatial/Templates/initial_conditions.h
/** \brief A function for initial values of the model
*/
template<typename GV, typename RF>
class UGeneralInitial
: public Dune::PDELab::GridFunctionBase<Dune::PDELab::
GridFunctionTraits<GV, RF, 1, Dune::FieldVector<RF, 1> >, UGeneralInitial<GV, RF> >
{
const GV& gv;
public:
typedef Dune::PDELab::GridFunctionTraits<GV,RF,1,Dune::FieldVector<RF,1> > Traits;
UGeneralInitial(const GV& gv_)
: gv(gv_)
, param()
, mComponentIndex(0)
{}
//! construct from grid view
UGeneralInitial(const GV& gv_, const Dune::ParameterTree & param_, int componentIndex)
: gv(gv_)
, param(param_)
, mComponentIndex(componentIndex)
%INITIALIZER%
{}
//! evaluate extended function on element
inline void evaluate (const typename Traits::ElementType& e,
const typename Traits::DomainType& xlocal,
typename Traits::RangeType& __initial) const
{
const auto& x = e.geometry().global(xlocal);
%ADDITIONAL_INITIAlIZATION%
switch(mComponentIndex)
{
%INITIALCONDITION%
default:
{
// this cannot happen
Dune::PDELab::Exception ex;
ex.message("Unexpected Index.");
throw ex;
}
}
}
//! get a reference to the grid view
inline const GV& getGridView () {return gv;}
private:
const Dune::ParameterTree & param;
int mComponentIndex;
%DECLARATION%
};
<file_sep>/WFEditSpatial/Model/AnalyticSettings.cs
namespace EditSpatial.Model
{
public class AnalyticSettings
{
public string Type { get; set; }
public string Math { get; set; }
}
}<file_sep>/WFEditSpatial/Controls/ControlMapCompartments.cs
using EditSpatial.Model;
using libsbmlcs;
namespace EditSpatial.Controls
{
public partial class ControlMapCompartments : BaseSpatialControl
{
public ControlMapCompartments()
{
InitializeComponent();
}
private libsbmlcs.Model Current { get; set; }
public void InitializeFrom(libsbmlcs.Model model)
{
grid.Rows.Clear();
Current = model;
colDomainType.Items.Clear();
if (model == null) return;
var mplug = (SpatialModelPlugin) model.getPlugin("spatial");
if (mplug != null)
{
var dtypes = mplug.getGeometry().getListOfDomainTypes();
for (var i = 0; i < dtypes.size(); ++i)
{
var dtype = dtypes.get(i);
if (dtype == null) continue;
colDomainType.Items.Add(dtype.getId());
}
}
for (long i = 0; i < model.getNumCompartments(); ++i)
{
var comp = model.getCompartment(i);
var map = comp.getCompartmentMapping();
grid.Rows.Add(comp.getId(), (map == null) ? "" : map.getDomainType());
}
}
public override void SaveChanges()
{
if (Current == null) return;
for (var i = 0; i < grid.Rows.Count && i < Current.getNumCompartments(); ++i)
{
var row = grid.Rows[i];
var current = Current.getCompartment((string) row.Cells[0].Value);
var map = current.getCompartmentMapping();
if (map == null) continue;
map.setDomainType((string) row.Cells[1].Value);
}
OnModelChanged();
}
public override void InvalidateSelection()
{
Current = null;
InitializeFrom(null);
}
}
}<file_sep>/LibEditSpatial/Dialogs/FormResize.cs
using System.Drawing;
using System.Globalization;
using System.Windows.Forms;
namespace LibEditSpatial.Dialogs
{
public partial class FormResize : Form
{
public RectangleF CanvasBounds
{
get {
float minX; float.TryParse(txtMinX.Text, out minX);
float maxX; float.TryParse(txtMaxX.Text, out maxX);
float minY; float.TryParse(txtMinY.Text, out minY);
float maxY; float.TryParse(txtMaxY.Text, out maxY);
return new RectangleF(minX, minY, maxX, maxY);
}
set
{
txtMinX.Text = value.X.ToString(CultureInfo.InvariantCulture);
txtMinY.Text = value.Y.ToString(CultureInfo.InvariantCulture);
txtMaxX.Text = value.Width.ToString(CultureInfo.InvariantCulture);
txtMaxY.Text = value.Height.ToString(CultureInfo.InvariantCulture);
}
}
public Size Dimensions
{
get {
int width; int.TryParse(txtColumns.Text, out width);
int height; int.TryParse(txtRows.Text, out height);
return new Size(width, height);
}
set {
txtColumns.Text = value.Width.ToString();
txtRows.Text = value.Height.ToString();
}
}
public bool ScaleContents
{
get { return chkScaleContents.Checked; }
set { chkScaleContents.Checked = value; }
}
public FormResize()
{
InitializeComponent();
}
}
}
<file_sep>/LibEditSpatial/Model/VoidDelegate.cs
namespace LibEditSpatial.Model
{
public delegate void VoidDelegate();
} | 31bc48816959528d5218b0e1e440b55244ed0619 | [
"CMake",
"Markdown",
"C#",
"Python",
"C++"
]
| 69 | C# | fbergmann/edit-spatial | 7a8e56c1a89320514d78b360f49b517fd9de37f1 | 7cb5a2dbd541bab283a9de8fc1b6e87ad9807689 | |
refs/heads/master | <repo_name>marc001-ai/checkpwnedemails<file_sep>/checkpwnedemails.py
__author__ = "<NAME>"
__version__ = "1.2.3"
from argparse import ArgumentParser
from time import sleep
import json
import requests
import sys
PWNED_API_URL = "https://haveibeenpwned.com/api/v3/%s/%s?truncateResponse=%s"
HEADERS = {
"User-Agent": "checkpwnedemails",
"hibp-api-key": "",
}
EMAILINDEX = 0
PWNEDINDEX = 1
DATAINDEX = 2
BREACHED = "breachedaccount"
PASTEBIN = "pasteaccount"
RATE_LIMIT = 1.6 # in seconds
class PwnedArgParser(ArgumentParser):
def error(self, message):
sys.stderr.write('error: %s\n' %message)
self.print_help()
sys.exit(2)
def get_args():
parser = PwnedArgParser()
parser.add_argument('-a', dest='apikey_path', help='Path to text file that contains your HIBP API key.')
parser.add_argument('-b', action="store_true", dest='only_breaches', help='Return results for breaches only.')
parser.add_argument('-i', dest='input_path', help='Path to text file that lists email addresses.')
parser.add_argument('-n', action="store_true", dest='names_only', help='Return the name of the breach(es) only.')
parser.add_argument('-o', dest='output_path', help='Path to output (tab deliminated) text file.')
parser.add_argument('-p', action="store_true", dest='only_pwned', help='Print only the pwned email addresses.')
parser.add_argument('-s', dest="single_email", help='Send query for just one email address.')
parser.add_argument('-t', action="store_true", dest='only_pastebins', help='Return results for pastebins only.')
if len(sys.argv) == 1: # If no arguments were provided, then print help and exit.
parser.print_help()
sys.exit(1)
return parser.parse_args()
# Used for removing the trailing '\n' character on each email.
def clean_list(list_of_strings):
return [str(x).strip() for x in list_of_strings]
# This function will print the appropriate output string based on the
# HTTP error code what was passed in. If an invalid HIBP API key was used
# (error code 401), then checkpwnedemails.py should stop running.
def printHTTPErrorOutput(http_error_code, hibp_api_key, email=None):
ERROR_CODE_OUTPUT = {
400: "HTTP Error 400. %s does not appear to be a valid email address." % (email),
401: "HTTP Error 401. Unauthorised - the API key provided (%s) was not valid." % (hibp_api_key),
403: "HTTP Error 403. Forbidden - no user agent has been specified in the request.",
429: "HTTP Error 429. Too many requests; the rate limit has been exceeded.",
503: "HTTP Error 503. Service unavailable."
}
try:
print(ERROR_CODE_OUTPUT[http_error_code])
except KeyError:
print("HTTP Error %s" % (http_error_code))
if http_error_code == 401:
sys.exit(1)
def get_results(email_list, service, opts, hibp_api_key):
results = [] # list of tuples (email adress, been pwned?, json data)
for email in email_list:
email = email.strip()
data = []
names_only = "true" if opts.names_only else "false"
try:
response = requests.get(url=PWNED_API_URL % (service, email, names_only), headers=HEADERS)
is_pwned = True
# Before parsing the response (for JSON), check if any content was returned.
# Otherwise, a json.decoder.JSONDecodeError will be thrown because we were trying
# to parse JSON from an empty response.
if response.content:
data = response.json()
else:
data = None # No results came back for this email. According to HIBP, this email was not pwned.
is_pwned = False
results.append( (email, is_pwned, data) )
except requests.exceptions.HTTPError as e:
if e.code == 404 and not opts.only_pwned:
results.append( (email, False, data) ) # No results came back for this email. According to HIBP, this email was not pwned.
elif e.code != 404:
printHTTPErrorOutput(e.code, hibp_api_key, email)
sleep(RATE_LIMIT) # This delay is for rate limiting.
if not opts.output_path:
try:
last_result = results[-1]
if not last_result[PWNEDINDEX]:
if service == BREACHED:
print("Email address %s not pwned. Yay!" % (email))
else:
print("Email address %s was not found in any pastes. Yay!" %(email))
else:
print("\n%s pwned!\n==========" % (email))
print(json.dumps(data, indent=4))
print('\n')
except IndexError:
pass
return results
# This function will convert every item, in dlist, into a string and
# encode any unicode strings into an 8-bit string.
def clean_and_encode(dlist):
cleaned_list = []
for d in dlist:
try:
cleaned_list.append(str(d))
except UnicodeEncodeError:
cleaned_list.append(str(d.encode('utf-8'))) # Clean the data.
return cleaned_list
def tab_delimited_string(data):
DATACLASSES = 'DataClasses'
begining_sub_str = data[EMAILINDEX] + '\t' + str(data[PWNEDINDEX])
output_list = []
if data[DATAINDEX]:
for bp in data[DATAINDEX]: # bp stands for breaches/pastbins
d = bp
try:
flat_data_classes = [str(x) for x in d[DATACLASSES]]
d[DATACLASSES] = flat_data_classes
except KeyError:
pass # Not processing a string for a breach.
flat_d = clean_and_encode(d.values())
output_list.append(begining_sub_str + '\t' + "\t".join(flat_d))
else:
output_list.append(begining_sub_str)
return '\n'.join(output_list)
def write_results_to_file(filename, results, opts):
BREACHESTXT = "_breaches.txt"
PASTESTXT = "_pastes.txt"
BREACH_HEADER = ("Email Address", "Is Pwned", "Name", "Title", "Domain", "Breach Date", "Added Date", "Modified Date", "Pwn Count", "Description", "Logo Path", "Data Classes", "Is Verified", "Is Fabricated", "Is Sensitive", "Is Retired", "Is SpamList")
PASTES_HEADER = ("Email Address", "Is Pwned", "ID", "Source", "Title", "Date", "Email Count")
files = []
file_headers = {
BREACHESTXT: "\t".join(BREACH_HEADER),
PASTESTXT: "\t".join(PASTES_HEADER)
}
if opts.only_breaches:
files.append(BREACHESTXT)
elif opts.only_pastebins:
files.append(PASTESTXT)
else:
files.append(BREACHESTXT)
files.append(PASTESTXT)
if filename.rfind('.') > -1:
filename = filename[:filename.rfind('.')]
for res, f in zip(results, files):
outfile = open(filename + f, 'w', encoding='utf-8')
outfile.write(file_headers[f] + '\n')
for r in res:
outfile.write(tab_delimited_string(r) + '\n')
outfile.close()
def main():
hibp_api_key = ""
email_list = []
opts = get_args()
if not opts.apikey_path:
print("\nThe path to the file containing the HaveIBeenPwned API key was not found.")
print("Please provide the file path with the -a switch and try again.\n")
sys.exit(1)
else:
try:
with open(opts.apikey_path) as apikey_file:
hibp_api_key = apikey_file.readline().strip()
HEADERS["hibp-api-key"] = hibp_api_key
except IOError:
print("\nCould not read file:", opts.apikey_path)
print("Check if the file path is valid, and try again.\n")
sys.exit(1)
if opts.single_email:
email_list = [opts.single_email]
elif opts.input_path:
email_list_file = open(opts.input_path, 'r')
email_list = clean_list(email_list_file.readlines())
email_list_file.close()
else:
print("\nNo email addresses were provided.")
print("Please provide a single email address (using -s) or a list of email addresses (using -i).\n")
sys.exit(1)
results = []
if opts.only_breaches:
results.append(get_results(email_list, BREACHED, opts, hibp_api_key))
elif opts.only_pastebins:
results.append(get_results(email_list, PASTEBIN, opts, hibp_api_key))
else:
results.append(get_results(email_list, BREACHED, opts, hibp_api_key))
results.append(get_results(email_list, PASTEBIN, opts, hibp_api_key))
if opts.output_path:
write_results_to_file(opts.output_path, results, opts)
if __name__ == '__main__':
main()
| 033a7449308a49fb96daede58a911413eb74455c | [
"Python"
]
| 1 | Python | marc001-ai/checkpwnedemails | f254fba5c8994c86ac797053e25825b678793092 | 97ca202b0d2cf12a81df706ef67b7123fba64ded | |
refs/heads/master | <file_sep>package four.elite.tournament;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
import com.nostra13.universalimageloader.core.ImageLoader;
import java.util.ArrayList;
import java.util.List;
public class TournamentResults extends AppCompatActivity {
TextView playerName;
TextView teamName;
ImageView playerImage;
Gson gson;
Player player;
ArrayList<Game> listOfPlayerMatches;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_tournament_results);
Intent intent = getIntent();
Bundle bundle = intent.getExtras();
gson = new Gson();
playerImage = (ImageView)findViewById(R.id.playerLogo);
playerName = (TextView)findViewById(R.id.playerName);
teamName = (TextView)findViewById(R.id.teamName);
listOfPlayerMatches = gson.fromJson(bundle.getString("matches"), new TypeToken<ArrayList<Game>>(){}.getType());
player = gson.fromJson(bundle.getString("winningPlayer"), Player.class);
playerName.setText(player.getName());
teamName.setText(player.getTeamName());
ImageLoader imageLoader = ImageLoader.getInstance();
imageLoader.displayImage(player.getImageUrl(), playerImage);
}
public void viewMatchHistory(View v){
Intent intent = new Intent(TournamentResults.this, MatchHistory.class);
intent.putExtra("matches",gson.toJson(listOfPlayerMatches));
startActivity(intent);
}
public void leave(View v){
finish();
}
}
<file_sep>package four.elite.tournament;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
import com.google.gson.Gson;
import java.util.ArrayList;
import java.util.List;
public class MainCreate extends AppCompatActivity implements AdapterView.OnItemClickListener {
TextView tournamentName;
ListView playersListView;
Tournament newTournament;
List<Player> players = new ArrayList<Player>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_create);
tournamentName = (TextView)findViewById(R.id.tournamentNameLabel);
playersListView = (ListView)findViewById(R.id.playerListView);
populatePlayers();
}
public void addingNewPlayer(View v){
Intent intent=new Intent(MainCreate.this,PlayerCreation.class);
startActivityForResult(intent, 200);
}
public void populatePlayers(){
String[] names = new String[players.size()];
String[] teams = new String[players.size()];
String[] imageUrls = new String[players.size()];
int[] rankings = new int[players.size()];
for(int i=0; i< players.size(); i++){
names[i] = players.get(i).getName();
teams[i] = players.get(i).getTeamName();
imageUrls[i] = players.get(i).getImageUrl();
}
MySimpleArrayAdapter arrayAdapter = new MySimpleArrayAdapter(getApplicationContext(), names, teams, rankings, imageUrls);
playersListView.setAdapter(arrayAdapter);
playersListView.setOnItemClickListener(this);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if(requestCode == 200 && resultCode == 200){
String playerName = data.getStringExtra("Player Name");
String teamName = data.getStringExtra("Team Name");
String imageUrl = data.getStringExtra("Image URL");
Player playerToAdd = new Player(teamName, playerName, imageUrl);
players.add(playerToAdd);
populatePlayers();
}
}
@Override
public void onItemClick(AdapterView<?> parent, View view, final int position, long id) {
new AlertDialog.Builder(this)
.setMessage("Are you sure you want to remove this user?")
.setCancelable(false)
.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
players.remove(position);
populatePlayers();
}
})
.setNegativeButton("No", null)
.show();
}
public void finishCreation(View v){
if(players.size() < 2){
Toast toast = Toast.makeText(getApplicationContext(), "Need at least 2 players", Toast.LENGTH_LONG);
toast.show();
}else if (tournamentName.getText().toString().equals("")){
Toast toast = Toast.makeText(getApplicationContext(), "Not a valid Tournament name", Toast.LENGTH_LONG);
toast.show();
}else{
AlertDialog levelDialog;
final CharSequence[] options = {"Round Robin","Knockout","Round Robin & Knockout"};
AlertDialog.Builder dialog = new AlertDialog.Builder(this);
dialog.setTitle("Select type of tournament!");
dialog.setSingleChoiceItems(options, -1, new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int item) {
//Setup intent
final Intent intent = new Intent();
Tournament newTournament;
Gson gson = new Gson();
switch (item) {
case 0:
newTournament = new Tournament(tournamentName.getText().toString(),players,options[0].toString());
intent.putExtra("tournament-object", gson.toJson(newTournament));
setResult(200, intent);
finish();
break;
case 1:
if((players.size() & players.size()-1) != 0){
Toast toast = Toast.makeText(getApplicationContext(), "Number of Players Must be a Power of 2", Toast.LENGTH_LONG);
toast.show();
}
else {
newTournament = new Tournament(tournamentName.getText().toString(), players, options[1].toString());
intent.putExtra("tournament-object", gson.toJson(newTournament));
setResult(200, intent);
finish();
}
break;
case 2:
newTournament = new Tournament(tournamentName.getText().toString(),players,options[2].toString());
intent.putExtra("tournament-object", gson.toJson(newTournament));
setResult(200, intent);
finish();
break;
}
}
});
dialog.setNegativeButton("Cancel", null);
levelDialog = dialog.create();
levelDialog.show();
}
}
}
<file_sep>package four.elite.tournament;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import android.widget.NumberPicker;
import android.widget.TextView;
import com.google.gson.Gson;
import com.nostra13.universalimageloader.core.ImageLoader;
public class MatchActivity extends AppCompatActivity {
NumberPicker homeTeamScore;
NumberPicker awayTeamScore;
TextView homeTeamLabel;
TextView awayTeamLabel;
TextView homePlayerName;
TextView awayPlayerName;
ImageView homeImage;
ImageView awayImage;
Game game;
Gson gson;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_match);
Intent intent = getIntent();
Bundle bundle = intent.getExtras();
gson = new Gson();
String json = bundle.getString("game");
game = gson.fromJson(json, Game.class);
//init all textviews and images
homeTeamLabel = (TextView)findViewById(R.id.homeTeamLabel);
awayTeamLabel = (TextView)findViewById(R.id.awayTeamLabel);
homePlayerName = (TextView)findViewById(R.id.homePlayerLabel);
awayPlayerName = (TextView)findViewById(R.id.awayPlayerLabel);
homeTeamLabel.setText(game.getHomeTeam().getTeamName());
awayTeamLabel.setText(game.getAwayTeam().getTeamName());
homePlayerName.setText(game.getHomeTeam().getName());
awayPlayerName.setText(game.getAwayTeam().getName());
//init number pickers
homeTeamScore = (NumberPicker)findViewById(R.id.homeTeamScore);
homeTeamScore.setMaxValue(50);
homeTeamScore.setMinValue(0);
awayTeamScore = (NumberPicker)findViewById(R.id.awayTeamScore);
awayTeamScore.setMaxValue(50);
awayTeamScore.setMinValue(0);
//init image loader
ImageLoader imageLoader = ImageLoader.getInstance();
homeImage = (ImageView)findViewById(R.id.homeTeamImage);
awayImage = (ImageView)findViewById(R.id.awayTeamImage);
imageLoader.displayImage(game.getHomeTeam().getImageUrl(),homeImage);
imageLoader.displayImage(game.getAwayTeam().getImageUrl(),awayImage);
}
public void cancel(View v){
finish();
}
public void confirmScore(View v){
Intent intent = new Intent();
intent.putExtra("homeScore", homeTeamScore.getValue());
intent.putExtra("awayScore", awayTeamScore.getValue());
setResult(200, intent);
finish();
}
}
<file_sep>package four.elite.tournament;
import android.content.Context;
import java.util.ArrayList;
import java.util.List;
/**
* Created by Brandon on 2015-12-03.
*/
public class Testing {
public static void setupFakeTournaments(Context context){
List<Tournament> tournaments = new ArrayList<Tournament>();
List<Player> laLigaPlayers = new ArrayList<Player>();
Player messi = new Player("Barcelona","<NAME>", null);
Player ronaldo = new Player("<NAME>","<NAME>", null);
laLigaPlayers.add(messi);
laLigaPlayers.add(ronaldo);
Tournament laLiga = new Tournament("La Liga", laLigaPlayers, "Round Robin");
DataManager.saveTournaments(context, tournaments);
}
}
<file_sep>package four.elite.tournament;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
public class Player implements Comparable<Player>{
private String teamName,playerName,imageUrl;
private int ranking,gamesPlayed, gamesWon, totalGoalsFor, totalGoalsAgainst;
public Player(){}
public Player(String teamName, String playerName, String url){
this.teamName = teamName;
this.playerName = playerName;
this.imageUrl = url;
this.gamesWon = 0;
this.gamesPlayed = 0;
if(url == null){
this.imageUrl = "http://dogr.io/doge.png";
}
}
public String getName(){return this.playerName;}
public String getImageUrl(){
return this.imageUrl;
}
public void setImageUrl(String url){
this.imageUrl = url;
}
public String getTeamName(){
return this.teamName;
}
public int getGamesPlayed(){
return this.gamesPlayed;
}
public int getGamesWon(){
return this.gamesWon;
}
public int getRanking(){
return this.ranking;
}
public void setName(String name){
this.playerName = name;
}
public void setTeamName(String teamName){
this.teamName = teamName;
}
public void setRanking(int rank){
this.ranking = rank;
}
public void incrementGamesWon(){
this.gamesWon++;
}
public void incrementGamesPlayed(){
this.gamesPlayed++;
}
public void addGoalsFor(int goals)
{
totalGoalsFor += goals;
}
public void addGoalsAgainst(int goalsAgainst)
{
totalGoalsAgainst += goalsAgainst;
}
public int getTotalGoalsFor()
{
return totalGoalsFor;
}
public int getTotalGoalsAgainst()
{
return totalGoalsAgainst;
}
public boolean equals(Object obj){
Player temp = (Player) obj;
if(this.teamName.equals(temp.getTeamName()) && this.playerName.equals(temp.getName()) && this.imageUrl.equals(temp.getImageUrl())){
return true;
}
return false;
}
@Override
public int compareTo(Player another) {
if (this.gamesWon > another.getGamesWon()) {
return -1;
} else if (this.gamesWon < another.getGamesWon()) {
return 1;
} else{
if (this.totalGoalsFor > another.getTotalGoalsFor()) {
return -1;
} else if (this.totalGoalsFor < another.getTotalGoalsAgainst()) {
return 1;
}
else{
if (this.totalGoalsAgainst < another.getTotalGoalsAgainst()) {
return -1;
}
else if (this.totalGoalsAgainst < another.getTotalGoalsAgainst()) {
return 1;
}
else{
return 0;
}
}
}
}
}
<file_sep>package four.elite.tournament;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.AdapterView;
import android.widget.Button;
import android.widget.ListView;
import android.widget.NumberPicker;
import android.widget.TextView;
import android.widget.Toast;
import com.google.gson.Gson;
import java.util.List;
public class TournamentMain extends AppCompatActivity implements AdapterView.OnItemClickListener
{
public Tournament tournament;
TextView tourneyNameLabel;
ListView listView;
Button nextMatch;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_tournament_main);
Intent intent = getIntent();
Bundle bundle = intent.getExtras();
tourneyNameLabel = (TextView)findViewById(R.id.tourneyName);
listView = (ListView)findViewById(R.id.playerList);
tournament = DataManager.getTournamentByName(getApplicationContext(),bundle.getString("Tournament Name"));
tourneyNameLabel.setText(tournament.getName());
if(tournament.isCompleted()){
nextMatch = (Button)findViewById(R.id.nextMatch);
nextMatch.setText("Results");
}
populatePlayers();
}
private void populatePlayers(){
String[] names = new String[tournament.getPlayers().size()];
String[] teams = new String[tournament.getPlayers().size()];
String[] urls = new String[tournament.getPlayers().size()];
int[] rankings = new int[tournament.getPlayers().size()];
for(int i=0; i< tournament.getPlayers().size(); i++){
names[i] = tournament.getPlayers().get(i).getName();
teams[i] = tournament.getPlayers().get(i).getTeamName();
rankings[i] = tournament.getPlayers().get(i).getRanking();
urls[i] = tournament.getPlayers().get(i).getImageUrl();
}
MySimpleArrayAdapter arrayAdapter = new MySimpleArrayAdapter(getApplicationContext(), names, teams, rankings, urls);
listView.setAdapter(arrayAdapter);
listView.setOnItemClickListener(this);
}
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intent = new Intent(TournamentMain.this,PlayerInfo.class);
intent.putExtra("Tournament Name", tournament.getName());
intent.putExtra("Player Name", tournament.getPlayers().get(position).getName());
intent.putExtra("Player Team", tournament.getPlayers().get(position).getTeamName());
intent.putExtra("Player Ranking", tournament.getPlayers().get(position).getRanking());
intent.putExtra("Player Games", tournament.getPlayers().get(position).getGamesPlayed());
intent.putExtra("Player Wins", tournament.getPlayers().get(position).getGamesWon());
intent.putExtra("Image URL", tournament.getPlayers().get(position).getImageUrl());
this.startActivity(intent);
}
public void viewingNextMatch(View v){
Intent intent = new Intent(TournamentMain.this,MatchActivity.class);
Gson gson = new Gson();
//Store anything in bundle here
Game nextGame = tournament.getNextGame();
if(nextGame != null){
intent.putExtra("game", gson.toJson(nextGame));
this.startActivityForResult(intent, 200);
}else{
if(tournament.getType().equals("Round Robin") || tournament.getType().equals("Knockout")){
tournament.complete(true);
Intent tourneyResultIntent = new Intent(TournamentMain.this, TournamentResults.class);
tourneyResultIntent.putExtra("winningPlayer", gson.toJson(tournament.getPlayer(0)));
tourneyResultIntent.putExtra("matches", gson.toJson(tournament.getPlayedGames()));
startActivity(tourneyResultIntent);
}
else{
final AlertDialog numDlg;
final NumberPicker numPkr;
AlertDialog.Builder dialogBuilder = new AlertDialog.Builder(this);
numPkr = new NumberPicker(dialogBuilder.getContext());
numPkr.setMinValue(0);
numPkr.setMaxValue(tournament.getPlayers().size());
dialogBuilder.setTitle("Player in Knockout Stage");
dialogBuilder.setView(numPkr);
dialogBuilder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
});
dialogBuilder.setPositiveButton("Set", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
int numProceeding = numPkr.getValue();
tournament.sortRankings();
while(tournament.getPlayers().size() > numProceeding){
tournament.getPlayers().remove(tournament.getPlayers().size() - 1);
}
tournament = new Tournament(tournament.getName(), tournament.getPlayers(), "Knockout");
MainActivity.updateTournaments(getApplicationContext(), tournament);
populatePlayers();
}
});
numDlg = dialogBuilder.create();
numDlg.show();
}
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
//matchActivity result
if(requestCode == 200 && resultCode == 200){
//handle return values
tournament.getNextGame().setHomeTeamScore(data.getIntExtra("homeScore", -1));
tournament.getNextGame().setAwayTeamScore(data.getIntExtra("awayScore", -1));
tournament.getNextGame().setPlayed(true);
tournament.updatePlayer();
tournament.sortRankings();
tournament.setRank();
populatePlayers();
System.out.println(tournament.getNextGame().getHomeTeam().getGamesPlayed());
MainActivity.updateTournaments(getApplicationContext(), tournament);
System.out.println(tournament.getNextGame().getHomeTeam().getGamesPlayed());
tournament.incrementGameCounter();
MainActivity.updateTournaments(getApplicationContext(), tournament);
}
}
public void viewMatches(View v){
Intent intent = new Intent(TournamentMain.this,MatchHistory.class);
Gson gson = new Gson();
String matchesSTR = gson.toJson(tournament.getPlayedGames());
intent.putExtra("matches",matchesSTR);
this.startActivity(intent);
}
}
| 615a9988453394c5bb955045146db13b59e794fa | [
"Java"
]
| 6 | Java | BrandonDanis/tournament-maker | 2d8aeb89927590b1ea8a79c4a1c78353339d155e | 6ed077e2c4ab604e2e134c291a69a4e3f1ad0c4b | |
refs/heads/master | <file_sep>public class Node{
Node next;
int data;
int index;
public Node(int data, int index){
this.data = data;
this.index = index;
}
public int getIndex(){
return this.index;
}
} | aeac8843adb5799ee0ba61d11a3b4e3948af45bf | [
"Java"
]
| 1 | Java | Sleeepybear1/WillisJavaPractice | 13465cc2e1d1a7273bad1258bd4095f44d511c08 | 4ba8a44d0b2c7d2e9a464702b522e9abd16e3d00 | |
refs/heads/master | <repo_name>malikxomer/static<file_sep>/6.Project one-Circle of Squares/1.Circle of Squares(My Logic).py
import turtle
my_turtle = turtle.Turtle()
my_turtle.speed(0)
def fun(length,angle,angle2):
my_turtle.forward(length)
my_turtle.right(angle)
my_turtle.forward(length)
my_turtle.right(angle)
my_turtle.forward(length)
my_turtle.right(angle)
my_turtle.forward(length)
my_turtle.right(angle2)
for square in range(100): #360/11 = ... # we choose a prime number so the circle is dense
fun(100,90, 101)
<file_sep>/10.Excersices/10.Rock paper scissor solution by Qazi.py
import random
COMPUTER_SCORE = 0
HUMAN_SCORE = 0
human_choice = ""
computer_choice = ""
def choice_to_number(choice):
"""Convert Choice to number."""
my_dict = {'rock':0 , 'paper':1 , 'scissors':2}
return my_dict[choice]
def number_to_choice(number):
my_dict = {0:'rock' , 1:'paper' , 2:'scissors'}
return my_dict[number]
def random_computer_choice():
"""Choose randomly for computer"""
random.choice(['rock' , 'paper' , 'scissors'])
def choice_result(human_choice,computer_choice):
"""Return the result of who wins"""
global COMPUTER_SCORE
global HUMAN_SCORE
human_number = choice_to_number(human_choice)
computer_choice = choice_to_number(computer_choice)
# Anything % 3 == a number between 0-2
# [0,1,2] == [rock, paper, scissors]
# rocks vs scissors
# (0-2) % 3 == 1
# (-2) % 3 == 1
# 1 == 1
# rock wins
#
#paper vs scissors
# (paper - scissors) % 3 == 1
# (1-2) % 3 == 1
# 2 == 1
# scissors wins
#
# paper vs rocks
# (paper - rocks) % 3 == 1
# (1-0) % 3 == 1
# 1 %3 == 1
# 1 == 1
# paper wins
if (human_number - computer_number) % 3 == 1:
COMPUTER_SCORE +=1
elif human_number == computer_number:
print('Tie')
else:
HUMAN_SCORE +=1
###############################
def test_number_to_choice():
assert number_to_choice(0) == 'rock'
assert number_to_choice(1) == 'paper'
assert number_to_choice(2) == 'scissors'
def test_choice_to_number():
assert choice_to_number('rock') == 0
assert choice_to_number('paper') == 1
assert choice_to_number('scissors') == 2
def test_all():
test_choice_to_number()
test_number_to_choice()
#Uncomment the line below to test your code
test_all()
<file_sep>/10.Excersices/2.Square of number.py
def square_number(number):
# return number*number
return number ** 2 # **2 is used to get square of a number
print(square_number(3))
assert square_number(0) == 0 #To check the answer is true or false
assert square_number(1) == 1 #To check the answer is true or false
assert square_number(2) == 4 #To check the answer is true or false
assert square_number(3) == 9 #To check the answer is true or false
assert square_number(4) == 16 #To check the answer is true or false
assert square_number(5) == 25 #To check the answer is true or false
assert square_number(6) == 36 #To check the answer is true or false
assert square_number(7) == 49 #To check the answer is true or false
assert square_number(8) == 64 #To check the answer is true or false
assert square_number(9) == 81 #To check the answer is true or false
assert square_number(10) == 100 #To check the answer is true or false
<file_sep>/9.Loops/1.For Loops.py
#Do something 5 times
for i in range(5):
print(i)
print(list(range(5))) #show what is the range of 5 --> [0,1,2,3,4]
#Range takes starting and ending point, excluding the ending point
#range(Start,Stop)
list(range(0,2)) #gives back [0,1]
for number in range(20,41):
print(number)
#Add numbers in a range
#Range(1,4) contains [1,2,3]
#We have to add 1+2+3 and return a 6
count = 0
for number in range(1,4):
count = count+number
print(count)
#Write a function that sums all elements of a list and return them
def sum_list(my_list):
count=0
for number in my_list:
count = count+number
return count
#assert gives us an error if statement is false, and nothing if it is true
assert sum_list([1,2,3]) == 6
assert sum_list([1,2,3,4])==10
<file_sep>/8.Boolean Algebra/1.Boolean Algebra.py
# If condition
# Generates true or False as a result i-e
if True:
print('Hello')
if False:
print('hi')
# == equals
# < Smaller than
# > Greater than
# <= Smaller than or equals
# >= Greater than or equals
# Not equals !=
johnny_hours_worked = 40
if johnny_hours_worked >40:
print('pay him overtime')
johnny_hours_worked = 41
if johnny_hours_worked >40:
print('pay him overtime now')
<file_sep>/7.Dictionaries/1.Dictionary Basics.py
#dictionay is made up of to things i-e key and value
# In java we call them maps of graphs
#Dictionay[key] --> value
# dictionart = {'name':'Omer' , 'Age':'24'}
#1 : Simple Dictionary ==
phone_book = {
'omer' : '000-123-456-789',
'Basil' : '111-123-456-789',
'Iftikhar' : '222-123-456-789'
}
print(phone_book['omer'])
#2 : Dictionary keys containing Lists ==
#Each key contains multiple values
phone_book = {
'omer' : ['000-111-222-333','<EMAIL>','Rawalpindi'],
'basil' : ['666-777-888-999','<EMAIL>','Gujrat'],
'iftikhar' : ['555-555-666-555','<EMAIL>','Peshawar']
}
print(phone_book['omer'][1])
<file_sep>/2.loops/for loop.py
import turtle
my_turtle=turtle.Turtle()
def square(length,angle):
my_turtle.forward(length)
my_turtle.left(angle)
my_turtle.forward(length)
my_turtle.left(angle)
my_turtle.forward(length)
my_turtle.left(angle)
my_turtle.forward(length)
for i in range(4):
square(50,90)
<file_sep>/10.Excersices/3.Check is Even.py
def check_even(number):
if number%2 == 0:
return print('number is even')
elif number%2 != 0:
return print('number is odd')
check_even(13)
<file_sep>/10.Excersices/4.Length of a string.py
#My way:
def string_length(Str):
return len(Str)
print(string_length('<NAME>'))
#Qazi's way:
def string_len(my_string):
count=0
for letter in my_string:
#print(letter)
count+=1
return count
print(string_len('hello'))
<file_sep>/10.Excersices/9.Rock Paper Scissors(my way).py
#Rules for the game
# rock vs rock == draw
# rock vs paper == paper wins
# rock vs scissor == rock wins
# paper vs paper == draw
# paper vs rock == paper wins
# paper vs scissors == scissors wins
# scissors vs scissors == draw
# scissors vs paper == scissors wins
# scissors vs rock == rock wins
import random
#Global variable thal all function know about.
#DO NOT EDIT THESE GLOBAL VARIABLES
#OR YOUR GAME WILL BREAK
COMPUTER_SCORE = 0
HUMAN_SCORE = 0
human_choice = ""
computer_choice = ""
def choice_to_number(choice):
"""Convert Choice to number."""
#If choice is rock, give back 0
#If choice is paper, give back 1
#If choice is scissors, give back 2
return {'rock':0 , 'paper':1 , 'scissors':2}[choice]
def number_to_choice(number):
"""Convert Number to choice."""
#If choice is 0, give back rock
#If choice is 1, give back paper
#If choice is 2, give back scissors
return {0:'rock' , 1:'paper' , 2:'scissors'}[number]
def random_computer_choice():
"""Choose randomly for computer"""
#lookup random.choice()
choice = random.randint(0,2)
return number_to_choice(choice)
# or we can use return random.choice(['rock' , 'paper' , 'scissors'])
def choice_result(human_choice,computer_choice):
"""Return the result of who wins"""
#DO NOT REMOVE THESE GLOBAL VARIABLE LINES.
#we specified them to say that we want the variables in the main program to change their values
global COMPUTER_SCORE
global HUMAN_SCORE
# based on the given human_choice and computer_choice
# determine who won and increment their score by 1.
# if tie, then don't increment anyone's score
#example code
# if human_choice == 'rock' and computer_choice == 'paper':
# COMPUTER_SCORE = COMPUTER_SCORE +1
if human_choice == 'rock' and computer_choice == 'paper':
COMPUTER_SCORE = COMPUTER_SCORE +1
return COMPUTER_SCORE
elif human_choice == 'rock' and computer_choice == 'scissors':
HUMAN_SCORE = HUMAN_SCORE+1
return HUMAN_SCORE
elif human_choice == 'rock' and computer_choice == 'rock':
return 'same'
elif human_choice == 'paper' and computer_choice == 'rock':
HUMAN_SCORE = HUMAN_SCORE+1
return HUMAN_SCORE
elif human_choice == 'paper' and computer_choice == 'scissors':
COMPUTER_SCORE = COMPUTER_SCORE +1
return COMPUTER_SCORE
elif human_choice == 'paper' and computer_choice == 'paper':
return 'same'
elif human_choice == 'scissors' and computer_choice == 'paper':
HUMAN_SCORE = HUMAN_SCORE+1
return HUMAN_SCORE
elif human_choice == 'scissors' and computer_choice == 'rock':
COMPUTER_SCORE = COMPUTER_SCORE +1
return COMPUTER_SCORE
elif human_choice == 'scissors' and computer_choice == 'scissors':
return 'same'
else:
print('Hello')
###############################
#DO NOT REMOVE THESE TEST FUNCTIONS.
#THEY WILL TEST YOUR CODE
def test_number_to_choice():
assert number_to_choice(0) == 'rock'
assert number_to_choice(1) == 'paper'
assert number_to_choice(2) == 'scissors'
def test_choice_to_number():
assert choice_to_number('rock') == 0
assert choice_to_number('paper') == 1
assert choice_to_number('scissors') == 2
def test_all():
test_choice_to_number()
test_number_to_choice()
#Uncomment the line below to test your code
test_all()
###############################
human_choice = number_to_choice(1)
computer_choice = random_computer_choice()
choice_result(human_choice,computer_choice)
print("computer score == ",COMPUTER_SCORE)
print("human score == ",HUMAN_SCORE)
print('human choice == ',human_choice)
print("random computer choice == ",computer_choice)
<file_sep>/8.Boolean Algebra/2.Logical operators.py
#AND operator
5 and 5 #True
True and True #True
True and False #False
johnny_homework=True
throw_out_garbage=True
if johnny_homework and throw_out_garbage: #Returns true
print('hello')
#OR operator
poison = False
pizza = True
pizza or poison #True
#NOT operator
not False #True
not True #False
not(True or False) #False
<file_sep>/5.Lists/2.Slicing in List.py
# Slicing in Lists
# We are going to slice elements of a String
# They will be stored in a List of Strings
'what-is-going-on'.split()
greetings = 'what-is-going-on'.split('-')
greetings
greetings[0]
greetings[1]
greetings[2]
greetings[3]
greetings[-1]
greetings[-2]
greetings[-3]
# We can also do slicing like in Strings using [Start: Stop: Step]
race = ['john','bob','timothy']
race[0:]
race[:]
race[0:2]
race[0:-1]
race[0:-1]
<file_sep>/4.String_Slicing/1.stringSlicing.py
#Gets the first letter of hello, which is 'h'
'hello'[0]
#[Start:Stop]
'hello'[0:4]
#we can skip the start, if it is zero
'hello'[:3]
#if we skip the stop,it will take the last index
'hello'[0:]
#if we skip start and stop it will go from 0 to last index i-e this [:] will be [0:last index]
'hello'[:]
# [-1] will show the last index i-e 'o'
'hello'[-1]
#All of the below do the same task and delete the last 'o' from hello
'hello'[0:-1]
'hello'[0:4]
'hello'[:4]
'hello'[:-1]
<file_sep>/4.String_Slicing/4.Reverse a string.py
# By using string slicing
data = "atoyot"
stringlength = len(data) # calculates length of string
slicedstring = data[stringlength::-1] # Reverse the String
print (slicedstring) # Print the string
# By Using a loop, Returns the string in the form of a list
data = "ikuzus"
reversedString = []
index = len(data) # Calculate length of string and save in index
while index> 0:
reversedString += data[index-1] #save the value of data[index-1] in reverseString
index = index - 1
print(reversedString)
# By using join condition
data = 'adnoH' #initial string
reversed=''.join(reversed(data)) # .join() method merges all of the characters resulting from the reversed iteration into a new string
print(reversed) #print the reversed string
<file_sep>/4.String_Slicing/3.startstopstep.py
#start:stop:step
#step is used to jump the object
greeting = 'Hi how are you doing, it is very nice to meet you.'
greeting
greeting[0:-1:1]
#step=1 is by default so it won't affect the text
greeting[0:-1:2]
greeting[0:-1:3]
<file_sep>/10.Excersices/11.Square a list.py
numbers = [1,2,3,4,5,6,7,8,9]
squared_numbers = []
for number in numbers:
squared_numbers.append(number ** 2)
print(squared_numbers)
<file_sep>/9.Loops/2.while loops.py
# We use while loops when we don't know when it is going to stop
# We use for loops when we know when it is going to stop
# While ( CONDITION ) --> True
# THEN THIS
# Condtion example:
# 5<6 --> True, so the loop will run
# 5>6 --> False, so the loop won't run
count = 0
while count < 100:
print(count)
count=count+1
# if count == 70:
# break
# ============= Practice =============
# Print from 100 to 1 and instead of zero print 'blast off'
count = 100
while count>0:
print(count)
count=count-1
if count==0:
print('blast off')
<file_sep>/5.Lists/5.Using Append method to add.py
# You can use Append on List
# Append makes the Lists more powerful and awesome
numbers = [1,2,3,4,5]
# Append is used to add something at the end of list
numbers.append(6)
numbers.append(7)
# we can add by using a for loop , numbers from 8 to 20
list(range(8,21)) #checking the numbers in this range
for number in range(8,21):
numbers.append(number)
#Reverse
number[::-1]
<file_sep>/5.Lists/4.Xbox example using split.py
data = 'XBOX 360 | 150 | NEW'
details = data.split('|')
print(details)
product_name=details[0]
product_price=details[1]
product_condition=details[2]
print(product_name)
print(product_price)
print(product_condition)
#Easiest way of doing the same
product,price,condition = data.split('|')
print(product)
print(price)
print(condition)
<file_sep>/10.Excersices/1.Sum two numbers.py
def sum_numbers(number1,number2):
return number1+number2
print(sum_numbers(2,3))
<file_sep>/5.Lists/3.Reversing a List Using Split.py
#We will use slicing to reverse a list here
race = ['john','bob','timothy']
race[0:] # will give us the whole list
race[::-1] #It will REVERSE the string
data = [1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20]
data[::-1]
<file_sep>/10.Excersices/7.Find the bigger guy from three.py
# My solution
def find_max(num1,num2,num3):
if num1>num2:
if num1>3:
return num1
elif num1<num3:
return num3
else:
return 'num1 and num3 are equal'
elif num2>num1:
if num2>num3:
return num2
elif num2<num3:
return num3
else:
return 'num2 and num3 are equal'
else:
return 'All numbers are equal'
print(find_max(8,8,8))
#######################
# Qazi's solution
def find_bigger(guy1,guy2,guy3):
if guy1>guy2:
bigger_guy = guy1
else:
bigger_guy = guy2
if bigger_guy > guy3:
biggest_guy=bigger_guy
else:
biggest_guy=guy3
return biggest_guy
print(find_bigger(16,234,111))
##########################
# One line solution
def bigger_guy(num1,num2):
if num1>num2:
return num1
else:
return num2
def biggest_guy(num1,num2,num3):
return bigger_guy(bigger_guy(num1,num2),num3)
print(biggest_guy(50,40,30))
#########################
#Python built in function
def big_num(number1,number2,number3,number4):
return max(number1,number2,number3,number4)
print(max(30,10,40,20))
<file_sep>/10.Excersices/5.last letter of a string.py
def last_letter(my_string):
return my_string[-1]
print(last_letter('omer!'))
<file_sep>/3.primitive data types/Primitive_datatypes.py
#string data types
'hello'
"hello"
#Integers
3
4
5
#float
40.22234
2.46345
1234.12341
<file_sep>/10.Excersices/6.Find the bigger guy from two.py
def find_max(num1,num2):
if num1>num2:
return num1
elif num2>num1:
return num2
else:
return 'both numbers are equal'
print(find_max(3,6))
<file_sep>/1.functions/function.py
import turtle
my_turtle=turtle.Turtle()
def square():
my_turtle.forward(100)
my_turtle.left(90)
my_turtle.forward(100)
my_turtle.left(90)
my_turtle.forward(100)
my_turtle.left(90)
my_turtle.forward(100)
square()
<file_sep>/11.Tuples/Tuples.py
#Lists
groceries = ['banana' , 'apple', 'grapes']
#Tuples
#We cant change things in a tuple, no adding, no deleting
social_security_number = ('12345','12345','12346234')
groceries.append('cheese')
print(groceries)
#We can't do the above operations to a Tuple
# It is used in cases where you need data to never be changed
<file_sep>/10.Excersices/8.Usain Bolt races me and a guy.py
# give choice to get back a name
def number_to_choice(choice):
if choice==1:
return 'Usain'
elif choice==2:
return 'Me'
elif choice==3:
return 'Qazi'
else:
return 'Not a member of race'
# give name to get back a position
def choice_to_number(choice):
if choice=='Usain':
return 1
elif choice=='Me':
return 2
elif choice=='Qazi':
return 3
else:
return 'Not a member of race'
print(number_to_choice(2))
print(choice_to_number('Qazi'))
##########################
#Using dictionary to solve the same problem
def num_to_choice(number):
return {1:'Usain',2:'Me',3:'Qazi'}[number]
def choice_to_num(name):
return {'Usain':1,'Me':2,'Qazi':3}[name]
print(num_to_choice(3))
print(choice_to_num('Usain'))
<file_sep>/8.Boolean Algebra/3.if-else-elseif.py
#if
#else
#else if or elif in python
#Rock paper Scissors--> Rock wins in this game
human = 'rock'
computer = 'scissors'
if human == 'rock' and computer == 'scissors':
human_score = 1
# Now checking with else if
computer = 'banana'
if human == 'rock' and computer == 'scissors':
human_score = 1
elif human == 'rock' and computer == 'banana':
computer_score=0
human_score=0
print('you cant pick anything other than rock and scissors')
# Checking if anyone picked wrong
if human == 'banana' or computer == 'banana':
print('wrong input')
#Checking by other way
if computer != 'rock' or computer !='scissors' or computer !='paper':
print("WRONG CHOICE,PICK AGAIN")
<file_sep>/5.Lists/1.Creating Lists and accessing index.py
# Creating a list of different data types
things = ['apple','banana',4,5,'oranges']
things
things[0]
things[1]
things[2]
things[3]
things[4]
things[-1]
things[-2]
things[-3]
things[-4]
<file_sep>/4.String_Slicing/2.stringSlicingOnRealData.py
#index('|') is used to get the index number of '|'
# First Way: This is manual and not good for too much data i-e spread sheet
data = 'XBOX 360 | 150 | New'
product_name = data[:data.index('|')]
product_price = data[11:15]
product_condition = data[16:]
print("product name = "+product_name)
print("product price = "+product_price)
print("product condition ="+product_condition)
# Second way: But this is too length so we have a third way
data = 'XBOX 360 | 150 | New'
product_name = data[:data.index('|')]
print("product name = "+product_name)
new_data = data[data.index('|')+1:]
product_price = new_data[:new_data.index('|')]
print("product price = "+product_price)
product_condition = new_data[new_data.index('|')+1:]
print("product condition ="+product_condition)
#Third way: By using find(), it is short and fast
data = 'XBOX 360 | 150 | New'
product_name_index = data.find('|')
product_name = data[:product_name_index]
print("product name = "+product_name)
#start finding for '|' and starts from index 10 i-e product_name_index+1
product_price_index = data.find('|',product_name_index+1)
product_price = data[product_name_index+1:product_price_index]
print("product price = "+product_price)
#start finding for '|' and starts from index 16 i-e product_price_index+1
product_condition = data[product_price_index+1:]
print("product condition ="+product_condition)
# WE CAN DIRECTLY FIND FROM PIPE TO PIPE I-E ('|') TO ('|')
product_price = data[data.find('|')+1 : data.find('|',10)]
print(product_price)
| 5b8170bdb5e0f330bebf7213bec753bc3f607588 | [
"Python"
]
| 31 | Python | malikxomer/static | dc169c91227641e0f741502f6f6ebfb66f798ab4 | 62605dcdce12daa9b6d2bac6a097237440cc2b35 | |
refs/heads/master | <repo_name>jeffbaars/rcm_python<file_sep>/plot_wrfout_grid.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re, math
import numpy as np
#import matplotlib.pyplot as plt
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
from utils_cmap import *
import pickle
from collections import defaultdict
from make_cmap import *
import matplotlib as mpl
mpl.use('Agg')
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
run_dirs = rcm_dir + '/rundirs'
geo_em = rcm_dir + '/data' + '/geo_em.d02.nc'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#vars = ['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
vars = ['PREC', 'T2MAX', 'T2MIN']
sdt = '197001'
edt = '209912'
models = ['access1.0', 'access1.3', 'bcc-csm1.1', 'canesm2', \
'ccsm4', 'csiro-mk3.6.0', 'fgoals-g2', 'gfdl-cm3', \
'giss-e2-h', 'miroc5', 'mri-cgcm3', 'noresm1-m']
#---------------------------------------------------------------------------
# Make run directory, load geo_em file.
#---------------------------------------------------------------------------
rundir = run_dirs + '/plot_wrfout_grid.' + str(os.getpid())
print 'making ', rundir
os.makedirs(rundir)
print 'Loading ', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
#---------------------------------------------------------------------------
# For each model, make a maps of every season...
#---------------------------------------------------------------------------
for m in range(len(models)):
model = models[m]
#--- Get list of extracted files for this model.
files = get_extract_files(data_dirs, model, sdt, edt, 0)
#--- Get files broken out by year and season.
(spr_files, sum_files, fal_files, win_files, ann_files, years) = \
get_seasonal_files(files)
#--- Loop over year, making maps of each
for y in range(len(years)):
yyyy = years[y]
#--- Winter plots.
if (win_files[yyyy] == win_files[yyyy]):
iret = run_mapper('T2MAX', model, rundir, 'winter', win_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('T2MIN', model, rundir, 'winter', win_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('PREC', model, rundir, 'winter', win_files,\
yyyy, lat, lon, levs_pcp, cmap_pcp)
#--- Summer plots.
if (sum_files[yyyy] == sum_files[yyyy]):
iret = run_mapper('T2MAX', model, rundir, 'summer', sum_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('T2MIN', model, rundir, 'summer', sum_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('PREC', model, rundir, 'summer', sum_files,\
yyyy, lat, lon, levs_pcp, cmap_pcp)
#--- Spring plots.
if (spr_files[yyyy] == spr_files[yyyy]):
iret = run_mapper('T2MAX', model, rundir, 'spring', spr_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('T2MIN', model, rundir, 'spring', spr_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('PREC', model, rundir, 'spring', spr_files,\
yyyy, lat, lon, levs_pcp, cmap_pcp)
#--- Fall plots.
if (fal_files[yyyy] == fal_files[yyyy]):
iret = run_mapper('T2MAX', model, rundir, 'fall', fal_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('T2MIN', model, rundir, 'fall', fal_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('PREC', model, rundir, 'fall', fal_files,\
yyyy, lat, lon, levs_pcp, cmap_pcp)
#--- Annual plots.
if (ann_files[yyyy] == ann_files[yyyy]):
iret = run_mapper('T2MAX', model, rundir, 'annual', ann_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('T2MIN', model, rundir, 'annual', ann_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('PREC', model, rundir, 'annual', ann_files,\
yyyy, lat, lon, levs_pcp, cmap_pcp)
sys.exit()
<file_sep>/utils_snotel_obs.py
#!/usr/bin/python
import os, sys, glob, re
import numpy as np
import csv
in2mm = 25.4
#---------------------------------------------------------------------------
# Read in a swe stations file.
#---------------------------------------------------------------------------
def read_swe_stations_file(station_file):
stns = []
latpts = []
lonpts = []
elevs = []
stn_names = []
with open(station_file) as csv_file:
csv_reader = csv.reader(csv_file, delimiter=',')
line_count = 0
for row in csv_reader:
stns.append(row[0])
latpts.append(float(row[1]))
lonpts.append(float(row[2]))
elevs.append(float(row[3]))
s = row[4]
s = s.strip(' "\'\t\r\n')
stn_names.append(s)
return stns, latpts, lonpts, elevs, stn_names
#---------------------------------------------------------------------------
# Read in a snotel data file. Columns are Water Year,Day,Oct,Nov,Dec,Jan,
# Feb,Mar,Apr,May,Jun,Jul,Aug,Sep.
#---------------------------------------------------------------------------
def read_snotel(swe_file):
yyyy = []
swe_obs = []
with open(swe_file) as csv_file:
csv_reader = csv.reader(csv_file, delimiter=',')
line_count = 0
for row in csv_reader:
#--- Skip comments.
if row[0].startswith('#'):
continue
#--- Skip non-1st-of-the-month data.
if row[1] != '01':
continue
#--- Skip if there's no actual value for this year.
if not row[8]:
continue
#--- Grab April (1st).
yyyy.append(row[0])
swe_obs.append(float(row[8]) * in2mm)
return yyyy, swe_obs
<file_sep>/old/utils_wrfout_20190523.py
#!/usr/bin/python
import os, sys, glob, re, math
import numpy as np
from netCDF4 import Dataset as NetCDFFile
import matplotlib as mpl
mpl.use('Agg')
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
from collections import defaultdict
from utils_cmap import *
import pickle
from utils_date import *
from utils_ghcnd_obs import *
dtfmt = "%Y%m%d%H"
#seasons = ['winter']
#seasons = ['annual', 'spring', 'summer', 'fall', 'winter']
seasons = ['spring', 'summer', 'fall', 'winter', 'annual']
seasons_mo = ['ALL', 'MAM', 'JJA', 'SON', 'DJF']
seasons_lab = {
'annual': 'Jan-Dec',
'spring': 'Mar-Apr-May',
'summer': 'Jun-Jul-Aug',
'fall': 'Sep-Oct-Nov',
'winter': 'Dec-Jan-Feb'
}
var_lab = {
'T2MAX': 'Average Max Temperature ($^\circ$C)',
'T2MIN': 'Average Min Temperature ($^\circ$C)',
'PREC': 'Total Precipitation (in.)',
'SPDUV10MEAN': 'Average Wind Speed (m/s)',
'SPDUV10MAX': 'Maximum Wind Speed (m/s)',
'SNOW': 'Snow Water Equivalent (mm)',
'SWDOWN': 'Shortwave Down Radiation (W/m^2)'
}
ylims = {
'PRECmin': [0, 1.0],
'PRECmax': [0, 5.0],
'PRECavg': [0, 45.0],
'PRECtot': [0, 40.0],
'T2MAXavg': [-5, 40.0],
'T2MAXmax': [0, 50.0],
'T2MINmin': [-30.0, 25.0],
'T2MINmax': [-30.0, 25.0],
'T2MINavg': [-20, 25.0],
'SPDUV10MEANavg': [0, 5.0],
'SPDUV10MAXmax': [0, 20.0]
}
labels = {
'PRECtot': 'Total Precipitation (in.)',
'PRECmax': 'Maximum Precipitation (in.)',
'PRECmin': 'Minimum Precipitation (in.)',
'PRECavg': 'Average Precipitation (in.)',
'T2MAXavg': 'Average Maximum Temperature ($^\circ$C)',
'T2MAXmax': 'Max Maximum Temperature ($^\circ$C)',
'T2MAXmin': 'Min Maximum Temperature ($^\circ$C)',
'T2MINavg': 'Average Minimum Temperature ($^\circ$C)',
'T2MINmax': 'Max Minimum Temperature ($^\circ$C)',
'T2MINmin': 'Min Minimum Temperature ($^\circ$C)',
'SPDUV10MEANavg': 'Average Wind Speed (m/s)',
'SPDUV10MAXmax': 'Maximum Wind Speed (m/s)'
}
colorbar_labs = {
'T2MAX': 'Temperature ($^\circ$C)',
'T2MIN': 'Temperature ($^\circ$C)',
'PREC': 'Precipitation (in.)',
'SPDUV10MEAN': 'Wind Speed (m/s)',
'SPDUV10MAX': 'Wind Speed (m/s)',
'SNOW': 'Snow Water Equivalent (mm)',
'SWDOWN': 'Shortwave Down Radiation (W/m^2)'
}
zooms = ['Z1', 'Z2']
target_lat = 47.44472
target_lon = -122.31361
fudge_lat = 1.7
fudge_lon = 2.1
minlatZ2 = target_lat - fudge_lat
maxlatZ2 = target_lat + fudge_lat
minlonZ2 = target_lon - fudge_lon
maxlonZ2 = target_lon + fudge_lon
minlat = {
'Z1': 39.0,
'Z2': minlatZ2 }
maxlat = {
'Z1': 51.0,
'Z2': maxlatZ2 }
minlon = {
'Z1': -131.0,
'Z2': minlonZ2 }
maxlon = {
'Z1': -108.0,
'Z2': maxlonZ2 }
mm2in = 0.0393700787
min_season_days = 85
std_lapse = 0.0065 #-- std. atmos. lapse rate
smooth_fact = 5 #-- odd number, used for smoothing time series plots.
fs = 9
titlefs = 9
width = 10
height = 8
maplw = 1.0
mvc = -9999.0
#---------------------------------------------------------------------------
# Load geo_em file.
#---------------------------------------------------------------------------
def load_geo_em(geo_em):
nc = NetCDFFile(geo_em, 'r')
lat = nc.variables['XLAT_M'][0,:,:]
lon = nc.variables['XLONG_M'][0,:,:]
hgt = nc.variables['HGT_M'][0,:,:]
lat = np.transpose(lat)
lon = np.transpose(lon)
hgt = np.transpose(hgt)
nc.close()
return lat, lon, hgt
#---------------------------------------------------------------------------
# Get netcdf dimension
#---------------------------------------------------------------------------
def get_nc_dim(ncin, dimname):
nc = NetCDFFile(ncin, 'r')
dim = len(nc.dimensions[dimname])
nc.close()
return dim
#---------------------------------------------------------------------------
# Load wrfout file.
#---------------------------------------------------------------------------
def load_wrfout(wrfout, vars):
nc = NetCDFFile(wrfout, 'r')
ntimes = len(nc.dimensions['Time'])
dataout = {}
for v in range(len(vars)):
var = vars[v]
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
dataout[var] = dat_tmp
#--- Get date from global attributes and clean it up and trim it down.
dt_all = nc.START_DATE
dt = dt_all.replace('-', '')
dt = dt.replace(':', '')
dt = dt.replace('_', '')
dt = dt[0:10]
nc.close()
return dataout, ntimes, dt
#---------------------------------------------------------------------------
# Load SWE wrfout file.
#---------------------------------------------------------------------------
def load_wrfout_swe(wrfout, vars):
nc = NetCDFFile(wrfout, 'r')
ntimes = len(nc.dimensions['Time'])
dataout = {}
for v in range(len(vars)):
var = vars[v]
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
dataout[var] = dat_tmp
nc.close()
return dataout, ntimes
#---------------------------------------------------------------------------
# Load wrfout file.
#---------------------------------------------------------------------------
def sum_wrfout_slice(files, vars, maski, maskj):
sums = {}
cnts = {}
for f in range(len(files)):
print 'reading ', files[f]
nc = NetCDFFile(files[f], 'r')
ntimes = len(nc.dimensions['Time'])
for v in range(len(vars)):
var = vars[v]
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
sum_c = 0
cnt_c = 0
for n in range(len(maski)):
sum_c = sum_c + sum(dat_tmp[maski[n], maskj[n],:])
cnt_c = cnt_c + len(dat_tmp[maski[n], maskj[n],:])
if (var in sums):
sums[var] = sums[var] + sum_c
cnts[var] = cnts[var] + cnt_c
else:
sums[var] = sum_c
cnts[var] = cnt_c
return sums, cnts
#---------------------------------------------------------------------------
# For ensemble standard deviation plots....
#---------------------------------------------------------------------------
def get_ensmean_std(var, models, years, season, \
sum_files, spr_files, win_files, fal_files, ann_files, \
pickle_file):
nyears = 0
nmodels = len(models)
files = []
dts_all = []
for y in range(len(years)):
yyyy = years[y]
for m in range(nmodels):
model = models[m]
sum_files_c = sum_files[model]
spr_files_c = spr_files[model]
win_files_c = win_files[model]
fal_files_c = fal_files[model]
ann_files_c = ann_files[model]
if season == 'summer':
if (sum_files_c[yyyy] == sum_files_c[yyyy]):
nyears += 1
for f in range(len(sum_files_c[yyyy])):
files.append(sum_files_c[yyyy][f])
else:
continue
elif season == 'spring':
if (spr_files_c[yyyy] == spr_files_c[yyyy]):
nyears += 1
for f in range(len(spr_files_c[yyyy])):
files.append(spr_files_c[yyyy][f])
else:
continue
elif season == 'winter':
if (win_files_c[yyyy] == win_files_c[yyyy]):
nyears += 1
for f in range(len(win_files_c[yyyy])):
files.append(win_files_c[yyyy][f])
else:
continue
elif season == 'fall':
if (fal_files_c[yyyy] == fal_files_c[yyyy]):
nyears += 1
for f in range(len(fal_files_c[yyyy])):
files.append(fal_files_c[yyyy][f])
else:
continue
elif season == 'annual':
if (ann_files_c[yyyy] == ann_files_c[yyyy]):
nyears += 1
for f in range(len(ann_files_c[yyyy])):
files.append(ann_files_c[yyyy][f])
else:
continue
print 'files = ', files
#--- Get sorted, unique set of dates from files found above.
nfiles = len(files)
dts_all = []
for f in range(nfiles):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
dts_all.append(dt_c)
dts_all = list(sorted(set(dts_all)))
#--- For each unique date, read in files for each model.
for d in range(len(dts_all)):
#--- First make sure all members have a file.
files_c = []
print dts_all[d]
for f in range(nfiles):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
if dt_c == dts_all[d]:
files_c.append(files[f])
if len(files_c) != nmodels:
print 'missing member(s) for date = ', dts_all[d]
sys.exit()
#--- Now read in files for each member.
for n in range(len(files_c)):
print 'loading ', files_c[n]
(vardat, ntimes, dt) = load_wrfout(files_c[n], [var])
plotdat = vardat[var][:,:,:]
nx,ny,nt = plotdat.shape
if n == 0:
dat_all = np.ones((nx, ny, nt, nmodels)) * np.nan
stdevs = np.ones((nx, ny, nt)) * np.nan
dat_all[:,:,:,n] = plotdat
#--- For each ntimes (these should be monthly files, so ntimes are
#--- days and will be ~30 days), calculate a standard deviation.
for nt in range(ntimes):
dat_c = np.squeeze(dat_all[:,:,nt,:])
stdevs[:,:,nt] = np.std(dat_c, axis=2)
print stdevs
print stdevs.shape
sys.exit()
# #--- Calculate the mean of the standard deviations.
# if d == 0:
# stdevmean = np.ones((nx, ny, len(dts_all))) * np.nan
# stdevmean[:,:,d] = np.mean(stdevs, axis=2)
#--- Create pickle file.
pickle.dump((varmean), open(pickle_file,'wb'), -1)
return varmean, nyears
#---------------------------------------------------------------------------
# For ensemble mean plots, get system nco command.
#---------------------------------------------------------------------------
def get_ensmean_syscom(file_plot, var, models, years, season, \
sum_files, spr_files, \
win_files, fal_files, ann_files, ncocom):
syscom = ncocom
nyears = 0
for m in range(len(models)):
model = models[m]
sum_files_c = sum_files[model]
spr_files_c = spr_files[model]
win_files_c = win_files[model]
fal_files_c = fal_files[model]
ann_files_c = ann_files[model]
for y in range(len(years)):
yyyy = years[y]
if season == 'summer':
if (sum_files_c[yyyy] == sum_files_c[yyyy]):
nyears += 1
for f in range(len(sum_files_c[yyyy])):
syscom = syscom + ' ' + sum_files_c[yyyy][f]
else:
continue
elif season == 'spring':
if (spr_files_c[yyyy] == spr_files_c[yyyy]):
nyears += 1
for f in range(len(spr_files_c[yyyy])):
syscom = syscom + ' ' + spr_files_c[yyyy][f]
else:
continue
elif season == 'winter':
if (win_files_c[yyyy] == win_files_c[yyyy]):
nyears += 1
for f in range(len(win_files_c[yyyy])):
syscom = syscom + ' ' + win_files_c[yyyy][f]
else:
continue
elif season == 'fall':
if (fal_files_c[yyyy] == fal_files_c[yyyy]):
nyears += 1
for f in range(len(fal_files_c[yyyy])):
syscom = syscom + ' ' + fal_files_c[yyyy][f]
else:
continue
elif season == 'annual':
if (ann_files_c[yyyy] == ann_files_c[yyyy]):
nyears += 1
for f in range(len(ann_files_c[yyyy])):
syscom = syscom + ' ' + ann_files_c[yyyy][f]
else:
continue
return syscom, nyears
#---------------------------------------------------------------------------
# For ensemble mean plots, run system nco command.
#---------------------------------------------------------------------------
def run_ensmean_syscom(file_plot, var, ncocom, syscom, yyyy_s, yyyy_e, \
season, rundir, ):
#--- If system command is too big (too many files to send
#--- to nco), break it up into 1000 piece chunks.
files_c = syscom.replace(ncocom, '')
scsplit = files_c.split()
nfiles = len(scsplit)
niter = 1000
if nfiles > niter:
nt = int(math.ceil(float(nfiles) / float(niter)))
files_all = []
for n in range(nt):
file_c = rundir + '/' + var + '_' + season + '_' + \
yyyy_s + '_' + yyyy_e + '_subset' + str(n) + '.nc'
if os.path.isfile(file_c):
print 'this file already exists: ', file_c
files_all.append(file_c)
continue
nb = n * niter
ne = (n+1) * niter
syscom = ncocom + ' ' + ' '.join(scsplit[nb:ne]) + ' ' + file_c
print syscom
iret = os.system(syscom)
if iret != 0:
print 'problem running nco! iret = ', iret
return mvc
files_all.append(file_c)
syscom = ncocom + ' ' + ' '.join(files_all) + ' ' + file_plot
print syscom
iret = os.system(syscom)
if iret != 0:
print 'problem running nco! iret = ', iret
return mvc
else:
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
iret = os.system(syscom)
if iret != 0:
print 'problem running nco! iret = ', iret
return mvc
return 1
#---------------------------------------------------------------------------
# Get plot file name.
#---------------------------------------------------------------------------
def get_plotfname(plot_dir, stn, sdt, edt, season, var, stat):
plotfname = plot_dir + '/' + stn + '_' + sdt + '_' + edt + '_' + \
season + '_' + var + '_' + stat + '.png'
return plotfname
#---------------------------------------------------------------------------
# Get plot title.
#---------------------------------------------------------------------------
def get_title(season, stn, var, stat, years):
titleout = station_name_dict[stn] + ' (' + stn.upper() + '), ' + \
seasons_lab[season] + ' ' + labels[var+stat] + ', ' + \
str(years[0]) + ' - ' + str(years[len(years)-1])
return titleout
#---------------------------------------------------------------------------
# Get list of extracted wrfout files between sdt_yyyymm and edt_yyyymm.
#---------------------------------------------------------------------------
def get_extract_files(data_dirs, model, sdt_yyyymm, edt_yyyymm, snowrad):
files_out = []
for d in range(len(data_dirs)):
path_c = data_dirs[d] + '/' + model + '/extract/*.nc'
files = glob.glob(path_c)
for f in range(len(files)):
if snowrad == 0:
if 'snowrad' in files[f]:
continue
else:
if 'snowrad' not in files[f]:
continue
dt_c = re.findall(r'\.(\d{10})\.', files[f])
if (dt_c):
dt_c = dt_c[0]
yyyymm_c = dt_c[0:6]
if (yyyymm_c >= sdt_yyyymm and yyyymm_c <= edt_yyyymm):
files_out.append(files[f])
if (len(files_out) == 0):
sys.exit('get_extract_files: no files found!')
return list(sorted(set(files_out)))
#---------------------------------------------------------------------------
# Get seasonal stats.
#---------------------------------------------------------------------------
def get_seasonal_files(files):
springs = defaultdict(list)
summers = defaultdict(list)
falls = defaultdict(list)
winters = defaultdict(list)
annuals = defaultdict(list)
spring_ndays = {}
summer_ndays = {}
fall_ndays = {}
winter_ndays = {}
annual_ndays = {}
years_all = []
#--- Loop over all files, binning them into seasons / years.
for f in range(len(files)):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
if (dt_c):
yyyy = dt_c[0:4]
mm = dt_c[4:6]
years_all.append(yyyy)
if (mm == '03' or mm == '04' or mm == '05'):
if ((yyyy) in spring_ndays):
spring_ndays[yyyy] += 1
else:
spring_ndays[yyyy] = 1
springs[yyyy].append(files[f])
elif (mm == '06' or mm == '07' or mm == '08'):
if ((yyyy) in summer_ndays):
summer_ndays[yyyy] += 1
else:
summer_ndays[yyyy] = 1
summers[yyyy].append(files[f])
elif (mm == '09' or mm == '10' or mm == '11'):
if ((yyyy) in fall_ndays):
fall_ndays[yyyy] += 1
else:
fall_ndays[yyyy] = 1
falls[yyyy].append(files[f])
elif (mm == '12'):
yyyyw = str(int(yyyy) + 1)
if ((yyyyw) in winter_ndays):
winter_ndays[yyyyw] += 1
else:
winter_ndays[yyyyw] = 1
winters[yyyyw].append(files[f])
elif (mm == '01' or mm == '02'):
if ((yyyy) in winter_ndays):
winter_ndays[yyyy] += 1
else:
winter_ndays[yyyy] = 1
winters[yyyy].append(files[f])
if ((yyyy) in annual_ndays):
annual_ndays[yyyy] += 1
else:
annual_ndays[yyyy] = 1
annuals[yyyy].append(files[f])
#--- Get list of unique, sorted years found above.
years = list(sorted(set(years_all)))
#--- Check number of data points per unique years and nan out
#--- ones that do not have enough (< min_season_days).
for y in range(len(years)):
yyyy = years[y]
if (yyyy in spring_ndays):
if (spring_ndays[yyyy] < 3):
springs[yyyy] = np.nan
else:
springs[yyyy] = np.nan
if (yyyy in summer_ndays):
if (summer_ndays[yyyy] < 3):
summers[yyyy] = np.nan
else:
summers[yyyy] = np.nan
if (yyyy in fall_ndays):
if (fall_ndays[yyyy] < 3):
falls[yyyy] = np.nan
else:
falls[yyyy] = np.nan
if (yyyy in winter_ndays):
if (winter_ndays[yyyy] < 3):
winters[yyyy] = np.nan
else:
winters[yyyy] = np.nan
if (yyyy in annual_ndays):
if (annual_ndays[yyyy] < 3):
annuals[yyyy] = np.nan
else:
annuals[yyyy] = np.nan
return springs, summers, falls, winters, annuals, years
#---------------------------------------------------------------------------
# Get seasonal files (new)
#---------------------------------------------------------------------------
def get_seasonal_files_new(files):
seafiles = defaultdict(list)
ndays = {}
# springs = defaultdict(list)
# summers = defaultdict(list)
# falls = defaultdict(list)
# winters = defaultdict(list)
# spring_ndays = {}
# summer_ndays = {}
# fall_ndays = {}
# winter_ndays = {}
years_all = []
#--- Loop over all files, binning them into seasons / years.
for f in range(len(files)):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
if (dt_c):
yyyy = dt_c[0:4]
mm = dt_c[4:6]
if mm == '12':
yyyy = str(int(yyyy) + 1)
else:
yyyy = yyyy
years_all.append(yyyy)
if (mm == '03' or mm == '04' or mm == '05'):
season = 'spring'
if (mm == '06' or mm == '07' or mm == '08'):
season = 'summer'
if (mm == '09' or mm == '10' or mm == '11'):
season = 'fall'
if (mm == '12' or mm == '01' or mm == '02'):
season = 'winter'
if ((yyyy) in ndays):
ndays[season+yyyy] += 1
else:
ndays[season+yyyy] = 1
seafiles[season,yyyy].append(files[f])
#--- Get list of unique, sorted years found above.
years = list(sorted(set(years_all)))
return seafiles, years
'''
#--- Check number of data points per unique years and nan out
#--- ones that do not have enough (< min_season_days).
for y in range(len(years)):
yyyy = years[y]
if (yyyy in spring_ndays):
if (spring_ndays[yyyy] < 3):
springs[yyyy] = np.nan
else:
springs[yyyy] = np.nan
if (yyyy in summer_ndays):
if (summer_ndays[yyyy] < 3):
summers[yyyy] = np.nan
else:
summers[yyyy] = np.nan
if (yyyy in fall_ndays):
if (fall_ndays[yyyy] < 3):
falls[yyyy] = np.nan
else:
falls[yyyy] = np.nan
if (yyyy in winter_ndays):
if (winter_ndays[yyyy] < 3):
winters[yyyy] = np.nan
else:
winters[yyyy] = np.nan
'''
#---------------------------------------------------------------------------
# Get seasonal stats (totals or averages currently).
#---------------------------------------------------------------------------
def get_seasonal_stats(data_all, dts_all, models, stns, var, stat):
out_sum = {}
out_avg = {}
out_max = {}
out_min = {}
years_all = []
stn_pr = 'KSEA'
for m in range(len(models)):
mod = models[m]
data_all_c = data_all[mod]
for s in range(len(stns)):
stn = stns[s]
ndays = {}
years_mod_stn = []
for d in range(len(dts_all)):
yyyy = dts_all[d][0:4]
mm = dts_all[d][4:6]
years_mod_stn.append(yyyy)
if ((var,stn,dts_all[d]) in data_all_c):
dat_c = data_all_c[var,stn,dts_all[d]]
else:
ndays[season+yyyy] = np.nan
out_sum[season,mod,stn,yyyy] = np.nan
out_max[season,mod,stn,yyyy] = np.nan
out_min[season,mod,stn,yyyy] = np.nan
continue
#--- Spring.
if (mm == '03' or mm == '04' or mm == '05'):
season = 'spring'
#--- Count number of days for this year's spring.
if (season+yyyy in ndays):
ndays[season+yyyy] += 1
else:
ndays[season+yyyy] = 1
if ((season,mod,stn,yyyy) in out_sum):
out_sum[season,mod,stn,yyyy] = out_sum[\
season,mod,stn,yyyy] + dat_c
else:
out_sum[season,mod,stn,yyyy] = dat_c
#--- Get maximum.
if ((season,mod,stn,yyyy) in out_max):
if (dat_c > out_max[season,mod,stn,yyyy]):
out_max[season,mod,stn,yyyy] = dat_c
# if (stn == stn_pr):
# print '-----'
# print stn, mod, yyyy, dts_all[d], dat_c
# print 'out_max[season,mod,stn,yyyy] = ', \
# out_max[season,mod,stn,yyyy]
else:
out_max[season,mod,stn,yyyy] = dat_c
#--- Get minimum.
if ((season,mod,stn,yyyy) in out_min):
if (dat_c < out_min[season,mod,stn,yyyy]):
out_min[season,mod,stn,yyyy] = dat_c
else:
out_min[season,mod,stn,yyyy] = dat_c
#--- Summer.
if (mm == '06' or mm == '07' or mm == '08'):
season = 'summer'
#--- Count number of days for this year's summer.
if (season+yyyy in ndays):
ndays[season+yyyy] += 1
else:
ndays[season+yyyy] = 1
if ((season,mod,stn,yyyy) in out_sum):
out_sum[season,mod,stn,yyyy] = out_sum[\
season,mod,stn,yyyy] + dat_c
else:
out_sum[season,mod,stn,yyyy] = dat_c
#--- Get maximum.
if ((season,mod,stn,yyyy) in out_max):
if (dat_c > out_max[season,mod,stn,yyyy]):
out_max[season,mod,stn,yyyy] = dat_c
else:
out_max[season,mod,stn,yyyy] = dat_c
#--- Get minimum.
if ((season,mod,stn,yyyy) in out_min):
if (dat_c < out_min[season,mod,stn,yyyy]):
out_min[season,mod,stn,yyyy] = dat_c
else:
out_min[season,mod,stn,yyyy] = dat_c
#--- Fall.
if (mm == '09' or mm == '10' or mm == '11'):
season = 'fall'
#--- Count number of days for this year's fall.
if (season+yyyy in ndays):
ndays[season+yyyy] += 1
else:
ndays[season+yyyy] = 1
if ((season,mod,stn,yyyy) in out_sum):
out_sum[season,mod,stn,yyyy] = out_sum[\
season,mod,stn,yyyy] + dat_c
else:
out_sum[season,mod,stn,yyyy] = dat_c
#--- Get maximum.
if ((season,mod,stn,yyyy) in out_max):
if (dat_c > out_max[season,mod,stn,yyyy]):
out_max[season,mod,stn,yyyy] = dat_c
else:
out_max[season,mod,stn,yyyy] = dat_c
#--- Get minimum.
if ((season,mod,stn,yyyy) in out_min):
if (dat_c < out_min[season,mod,stn,yyyy]):
out_min[season,mod,stn,yyyy] = dat_c
else:
out_min[season,mod,stn,yyyy] = dat_c
#--- Winter, December only. Put December winter data into
#--- next year's winter-- naming winters by their Jan/Feb
#--- year rather than their Dec year.
if (mm == '12'):
season = 'winter'
yyyyw = str(int(yyyy) + 1)
#--- Count number of days for this year's winter.
if (season+yyyyw in ndays):
ndays[season+yyyyw] += 1
else:
ndays[season+yyyyw] = 1
if ((season,mod,stn,yyyyw) in out_sum):
out_sum[season,mod,stn,yyyyw] = out_sum[\
season,mod,stn,yyyyw] + dat_c
else:
out_sum[season,mod,stn,yyyyw] = dat_c
#--- Get maximum.
if ((season,mod,stn,yyyyw) in out_max):
if (dat_c > out_max[season,mod,stn,yyyyw]):
out_max[season,mod,stn,yyyyw] = dat_c
else:
out_max[season,mod,stn,yyyyw] = dat_c
#--- Get minimum.
if ((season,mod,stn,yyyyw) in out_min):
if (dat_c < out_min[season,mod,stn,yyyyw]):
out_min[season,mod,stn,yyyyw] = dat_c
else:
out_min[season,mod,stn,yyyyw] = dat_c
#--- Winter, Jan & Feb.
if (mm == '01' or mm == '02'):
season = 'winter'
#--- Count number of days for this year's winter.
if (season+yyyy in ndays):
ndays[season+yyyy] += 1
else:
ndays[season+yyyy] = 1
if ((season,mod,stn,yyyy) in out_sum):
out_sum[season,mod,stn,yyyy] = out_sum[\
season,mod,stn,yyyy] + dat_c
else:
out_sum[season,mod,stn,yyyy] = dat_c
#--- Get maximum.
if ((season,mod,stn,yyyy) in out_max):
if (dat_c > out_max[season,mod,stn,yyyy]):
out_max[season,mod,stn,yyyy] = dat_c
else:
out_max[season,mod,stn,yyyy] = dat_c
#--- Get minimum.
if ((season,mod,stn,yyyy) in out_min):
if (dat_c < out_min[season,mod,stn,yyyy]):
out_min[season,mod,stn,yyyy] = dat_c
else:
out_min[season,mod,stn,yyyy] = dat_c
#--- Get list of unique, sorted years found above.
years = list(sorted(set(years_mod_stn)))
# if (mod == 'bcc-csm1.1' and stn == 'KSEA'):
# for yyyy in years:
# if ((season+yyyy) in ndays):
# print season,yyyy, ndays[season+yyyy]
# else:
# print season,yyyy, 'missing'
#--- Check number of data points per unique years and nan out
#--- ones that do not have enough (< min_season_days).
for y in range(len(years)):
yyyy = years[y]
for s in range(len(seasons)):
season = seasons[s]
if ((season+yyyy) in ndays):
if (ndays[season+yyyy] < min_season_days):
out_sum[season,mod,stn,yyyy] = np.nan
out_max[season,mod,stn,yyyy] = np.nan
out_min[season,mod,stn,yyyy] = np.nan
else:
ndays[season+yyyy] = np.nan
out_sum[season,mod,stn,yyyy] = np.nan
out_max[season,mod,stn,yyyy] = np.nan
out_min[season,mod,stn,yyyy] = np.nan
#--- if stat requested was 'avg', do averaging.
if (stat == 'avg' or stat == 'max' or stat == 'min'):
for y in range(len(years)):
yyyy = years[y]
for s in range(len(seasons)):
season = seasons[s]
out_avg[season,mod,stn,yyyy] = out_sum[\
season,mod,stn,yyyy] / ndays[season+yyyy]
#--- Keep around all years found for this model and station.
years_all.append(years_mod_stn)
#--- Final, unique, sorted list of years found.
years = list(sorted(set(years_all[0])))
if (stat == 'avg'):
return out_avg, years
elif (stat == 'max'):
return out_max, years
elif (stat == 'min'):
return out_min, years
else:
return out_sum, years
#---------------------------------------------------------------------------
# Make time series of sent-in data for a set of stations for one model.
#---------------------------------------------------------------------------
def ts_stns(statplot, years, stns, mod, titlein, plotfname, var, stat, \
cols, seasonsplot):
fig, ax = plt.subplots( figsize=(10,6) )
xlabs = []
for s in range(len(stns)):
ptot = []
stn = stns[s]
for y in range(len(years)):
yyyy = years[y]
for ss in range(len(seasons)):
season = seasons[ss]
if (season in seasonsplot):
if (var == 'PREC'):
ptot.append(statplot[season,mod,stns[s],yyyy] * mm2in)
elif (var == 'T2MAX' or var == 'T2MIN'):
ptot.append(statplot[season,mod,stns[s],yyyy] - 273.15)
elif (var.find('SPD') >= 0):
ptot.append(statplot[season,mod,stns[s],yyyy])
if (s == 0):
xlabs.append(season.title() + ' ' + years[y])
plt.plot(ptot, alpha = 0.3, label = stn, color = cols[s])
lab_c = stn + ', ' + str(smooth_fact) + '-pt smoothing'
plt.plot(smooth(ptot, smooth_fact), label = lab_c, color = cols[s])
#--- y-axis labeling.
plt.ylim(ylims[var+stat])
plt.ylabel('Seasonal ' + labels[var+stat], fontsize=fs+1)
plt.tick_params(axis='y', which='major', labelsize=fs+1)
#--- x-axis labels.
xticks_c = range(0,len(xlabs), 4)
xlabs_c = [ xlabs[i] for i in xticks_c ]
plt.xticks(xticks_c)
plt.tick_params(axis='x', which='major', labelsize=fs-1)
ax.set_xticklabels(xlabs_c, rotation=90)
plt.xlabel('Season', fontsize=fs+1)
plt.title(titlein, fontsize=titlefs, fontweight='bold')
plt.tight_layout()
plt.grid()
plt.legend(fontsize = fs-1, loc = 'best')
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
return 1
#---------------------------------------------------------------------------
# Make time series of sent-in data for a set of models for one station.
#---------------------------------------------------------------------------
def ts_mods(statplot, years, obsplot, years_obs, stn, models, titlein, \
plotfname, var, stat, cols, seasonsplot):
fig, ax = plt.subplots( figsize=(10,6) )
lw_sm = 1.8
#--- Plot models.
xlabs = []
mod_all = np.ones((len(models),len(years))) * np.nan
for m in range(len(models)):
ptot = []
mod = models[m]
for y in range(len(years)):
yyyy = years[y]
for ss in range(len(seasons)):
season = seasons[ss]
key = (season,mod,stn,yyyy)
if (season in seasonsplot):
if (var == 'PREC'):
ptot_c = statplot[key] * mm2in
elif (var == 'T2MAX' or var == 'T2MIN'):
ptot_c = statplot[key] - 273.15
elif (var.find('SPD') >= 0):
ptot_c = statplot[key]
if (m == 0):
xlabs.append(years[y])
ptot.append(ptot_c)
mod_all[m,y] = ptot_c
# plt.plot(ptot, alpha = 0.20, label = mod.upper(), color = cols[m], \
# linewidth=lw_sm)
lab_c = mod.upper() + ', ' + str(smooth_fact) + '-pt smoothing'
plt.plot(smooth(ptot, smooth_fact), label = lab_c, color = cols[m], \
linewidth=lw_sm, alpha = 0.23)
#--- Plot ensemble mean.
plt.plot(np.mean(mod_all, axis=0), label = 'Ensemble Mean', \
color = 'darkgreen', linewidth=2.2)
#--- Plot observations.
otot = []
for y in range(len(years_obs)):
# yyyy = years[y]
yyyy = years_obs[y]
for ss in range(len(seasons)):
season = seasons[ss]
key = (season,stn,yyyy)
if (season in seasonsplot):
otot.append(obsplot[key])
plt.plot(otot, alpha=0.5, label='Observed', color='black', \
linestyle='None', marker='o', markersize=3)
lab_c = 'Observed ' + str(smooth_fact) + '-pt smoothing'
plt.plot(smooth(otot,smooth_fact), label=lab_c, color='black', \
linewidth=lw_sm)
#--- y-axis labeling.
plt.ylim(ylims[var+stat])
plt.ylabel('Seasonal ' + labels[var+stat], fontsize=fs+1)
plt.tick_params(axis='y', which='major', labelsize=fs+1)
#--- x-axis labels.
xticks_c = range(0,len(xlabs), 4)
xlabs_c = [ xlabs[i] for i in xticks_c ]
plt.xticks(xticks_c)
plt.tick_params(axis='x', which='major', labelsize=fs-1)
ax.set_xticklabels(xlabs_c, rotation=90)
plt.xlabel('Year', fontsize=fs+1)
plt.title(titlein, fontsize=titlefs, fontweight='bold')
plt.tight_layout()
plt.grid()
plt.legend(fontsize = fs-1, loc = 'best')
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
return 1
#---------------------------------------------------------------------------
# Loop over each models, grabbing data from extract wrfout files.
#---------------------------------------------------------------------------
def load_extract_data(geo_em, stns, latpts, lonpts, elevs, models, \
data_dirs, sdt, edt, vars, pickle_dir, load_pickle):
#--- Load geo em file to get lat/lon/elev grids. Also interpolate
#--- model elevation to stns lat/lon point locations.
print 'Loading:\n', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
elevs_mod = []
elevs_diff = []
for ns in range(len(stns)):
dat_interp = bilinear_interpolate(lat, lon, hgt, \
latpts[ns], lonpts[ns], 0)
elevs_mod.append(dat_interp)
elevs_diff.append(elevs[ns] - dat_interp)
for m in range(len(models)):
data_all = {}
dts_all = []
model = models[m]
pickle_file = pickle_dir + '/extract_' + model + '_' + \
sdt + '_' + edt + '.pickle'
#--- Get list of extracted files for this model.
files = get_extract_files(data_dirs, model, sdt, edt, 0)
files = list(sorted(set(files)))
print 'Loading: '
#--- Loop over all extract files, reading them in.
for f in range(len(files)):
(vardat, ntimes, dt) = load_wrfout(files[f], vars)
print files[f], ntimes
#--- Loop over each vars, interpolating data to stns for
#--- each ntimes.
for v in range(len(vars)):
var = vars[v]
vardat_c = vardat[var]
#--- Loop over each ntime, grabbing interpolated data for
#--- variable var for each stns.
for n in range(ntimes):
dt_c = time_increment(dt, n, dtfmt)
for ns in range(len(stns)):
dat_interp = bilinear_interpolate(lat, lon, \
vardat_c[:,:,n],\
latpts[ns], \
lonpts[ns], 0)
#--- Do lapse rate correction for temperature.
if (var == 'T2MAX' or var == 'T2MIN'):
dat_c = dat_interp - std_lapse * elevs_diff[ns]
else:
dat_c = dat_interp
data_all[var, stns[ns], dt_c] = dat_c
dts_all.append(dt_c)
#--- Get sorted unique list of all dates seen.
dts_unique = list(sorted(set(dts_all)))
#--- Create pickle file.
pickle.dump((model, data_all, dts_unique, vars, stns), \
open(pickle_file,'wb'), -1)
return data_all, dts_unique, models, vars, stns
#---------------------------------------------------------------------------
# Interpolate from a sent-in grid to a sent in lat/lon point.
#---------------------------------------------------------------------------
def bilinear_interpolate(latgrid, longrid, datagrid, latpt, lonpt, deb):
totdiff = abs(latgrid - latpt) + abs(longrid - lonpt)
(i,j) = np.unravel_index(totdiff.argmin(), totdiff.shape)
#--- Get lat/lon box in which latpt,lonpt resides.
if (latpt >= latgrid[i,j] and lonpt >= longrid[i,j]):
iif = i
jjf = j
elif (latpt < latgrid[i,j] and lonpt < longrid[i,j]):
iif = i-1
jjf = j-1
elif (latpt >= latgrid[i,j] and lonpt < longrid[i,j]):
iif = i-1
jjf = j
elif (latpt < latgrid[i,j] and lonpt >= longrid[i,j]):
iif = i
jjf = j-1
(nx, ny) = np.shape(latgrid)
if (deb == 1):
print 'nx, ny = ', nx, ny
print 'iif,jjf = ', iif,jjf
print 'latgrid[iif+1,jjf] = ', latgrid[iif+1,jjf]
print 'latgrid[iif,jjf] = ', latgrid[iif,jjf]
if (iif >= (nx-1) or jjf >= (ny-1) or iif < 0 or jjf < 0):
return mvc
#--- Do bilinear interpolation to latpt,lonpt.
dlat = latgrid[iif,jjf+1] - latgrid[iif,jjf]
dlon = longrid[iif+1,jjf] - longrid[iif,jjf]
dslat = latgrid[iif,jjf+1] - latpt
dslon = longrid[iif+1,jjf] - lonpt
wrgt = 1 - (dslon/dlon)
wup = 1 - (dslat/dlat)
vll = datagrid[iif,jjf]
vlr = datagrid[iif,jjf+1]
vul = datagrid[iif+1,jjf]
vur = datagrid[iif+1,jjf+1]
if (deb > 1):
print 'll lat, lon, val = ', latgrid[iif,jjf], longrid[iif,jjf], vll
print 'lr lat, lon, val = ', latgrid[iif+1,jjf], longrid[iif+1,jjf], vlr
print 'ur lat, lon, val = ', latgrid[iif+1,jjf+1], longrid[iif+1,jjf+1],vur
print 'ul lat, lon, val = ', latgrid[iif,jjf+1], longrid[iif,jjf+1], vul
print 'latpt, lonpt = ', latpt, lonpt
print 'vll = ', vll
print 'vlr = ', vlr
print 'vul = ', vul
print 'vur = ', vur
datout = (1-wrgt) * ((1-wup) * vll + wup * vul) + \
(wrgt) * ((1-wup) * vlr + wup * vur)
# if (deb == 1):
# print 'datout = ', datout
# sys.exit()
# if (datout == 0.0):
# print 'nx, ny = ', nx, ny
# print 'iif,jjf = ', iif,jjf
# print 'latgrid[iif+1,jjf] = ', latgrid[iif+1,jjf]
# print 'latgrid[iif,jjf] = ', latgrid[iif,jjf]
#
# print 'll lat, lon, val = ', latgrid[iif,jjf], longrid[iif,jjf], vll
# print 'lr lat, lon, val = ', latgrid[iif+1,jjf], longrid[iif+1,jjf], vl#r
# print 'ur lat, lon, val = ', latgrid[iif+1,jjf+1], longrid[iif+1,jjf+1],vur
# print 'ul lat, lon, val = ', latgrid[iif,jjf+1], longrid[iif,jjf+1], vu#l
# print 'latpt, lonpt = ', latpt, lonpt
#
# print 'vll = ', vll
# print 'vlr = ', vlr
# print 'vul = ', vul
# print 'vur = ', vur
#
# sys.exit()
return datout
#---------------------------------------------------------------------------
# Run mapper.
#---------------------------------------------------------------------------
def run_mapper(var, model, rundir, season, files, yyyy, \
lat, lon, levs_c, my_cmap ):
file_plot = rundir + '/' + model + '_' + season + '_' + yyyy + '_' + \
var + '.nc'
if (var == 'T2MAX' or var == 'T2MIN'):
if (os.path.exists(file_plot)):
print 'nc file ', file_plot, ' already exists-- using it'
else:
syscom = 'ncra'
for f in range(len(files[yyyy])):
print yyyy, files[yyyy][f]
syscom = syscom + ' ' + files[yyyy][f]
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
os.system(syscom)
elif (var == 'PREC'):
if (os.path.exists(file_plot)):
print 'nc file ', file_plot, ' already exists-- using it'
else:
syscom = 'ncra -y ttl'
for f in range(len(files[yyyy])):
print yyyy, files[yyyy][f]
syscom = syscom + ' ' + files[yyyy][f]
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
os.system(syscom)
print 'Loading ', var, ' from ', file_plot
(vardat, ntimes, dt) = load_wrfout(file_plot, [var])
syscom = 'rm -f ' + file_plot
os.system(syscom)
titlein = model.upper() + ', ' + var_lab[var] + ', ' + \
seasons_lab[season] + ' ' + yyyy
plotdat = vardat[var][:,:,0]
if (var == 'PREC'):
plotdat = plotdat * mm2in
else:
plotdat = plotdat - 273.15
for z in range(len(zooms)):
plotfname = rundir + '/' + model + '_' + yyyy + '_' + season + '_' + \
var + '_' + zooms[z] + '.png'
print 'colorbar_labs[var] = ', colorbar_labs[var]
mapper(var, lat, lon, plotdat, levs_c, my_cmap, maplw, \
colorbar_labs[var], titlein, plotfname, zooms[z])
return 1
#---------------------------------------------------------------------------
# Mapper.
#---------------------------------------------------------------------------
def mapper(var, lat, lon, grid, levs, cmap_in, maplw, colorbar_lab, titlein, \
plotfname, zoom):
ur_lat = maxlat[zoom]
ur_lon = maxlon[zoom]
ll_lat = minlat[zoom]
ll_lon = minlon[zoom]
lat_ctr = ll_lat + ((ur_lat - ll_lat) * 0.5)
lon_ctr = ll_lon + ((ur_lon - ll_lon) * 0.5)
if (zoom == 'Z1'):
res = 'i'
elif (zoom == 'Z2'):
res = 'h'
fig = plt.figure(figsize=(width,height))
# left, bottom, width, height:
ax = fig.add_axes([0.00,0.05,0.99,0.91])
map = Basemap(resolution = res,projection='lcc',\
llcrnrlon= ll_lon, llcrnrlat=ll_lat,\
urcrnrlon= ur_lon, urcrnrlat= ur_lat,\
lat_0=lat_ctr,lon_0=lon_ctr,lat_1=(ur_lat - ll_lat))
#--- Get lat and lon data in map's x/y coordinates.
x,y = map(lon, lat)
#--- Draw coastlines, country boundaries, fill continents.
map.drawcoastlines(linewidth = maplw)
map.drawstates(linewidth = maplw)
map.drawcountries(linewidth = maplw)
#--- Draw the edge of the map projection region (the projection limb)
map.drawmapboundary(linewidth = maplw)
#--- Draw lat/lon grid lines every 30 degrees.
map.drawmeridians(np.arange(0, 360, 30), linewidth = maplw)
map.drawparallels(np.arange(-90, 90, 30), linewidth = maplw)
cs = plt.contourf(x, y, grid, levs, cmap=cmap_in)
cbar = map.colorbar(cs, location='bottom', pad="3%", size=0.1, ticks=levs)
cbar.set_label(colorbar_lab, fontsize=fs, size=fs-1)
cbar.ax.tick_params(labelsize=fs-2)
if var != 'SNOW':
csl = plt.contour(x, y, grid, levs, colors = 'black', linewidths=0.8)
plt.clabel(csl, inline=1, fontsize=10, fmt='%2.1f', fontweights='bold')
plt.title(titlein, fontsize=titlefs, fontweight='bold')
#--- Save plot.
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
#---------------------------------------------------------------------------
# Gaussian smoother, taken from
# https://www.swharden.com/wp/2008-11-17-linear-data-smoothing-in-python/
#---------------------------------------------------------------------------
def smoothListGaussian(list,strippedXs=False,degree=5):
window=degree*2-1
weight=np.array([1.0]*window)
weightGauss=[]
for i in range(window):
i=i-degree+1
frac=i/float(window)
gauss=1/(np.exp((4*(frac))**2))
weightGauss.append(gauss)
weight=np.array(weightGauss)*weight
smoothed=[0.0]*(len(list)-window)
for i in range(len(smoothed)):
smoothed[i]=sum(np.array(list[i:i+window])*weight)/sum(weight)
return smoothed
#def running_mean(x, N):
# cumsum = np.cumsum(np.insert(x, 0, 0))
# return (cumsum[N:] - cumsum[:-N]) / float(N)
#---------------------------------------------------------------------------
# Smoother like Matlab's running average smoother 'smooth', taken from
# https://stackoverflow.com/questions/40443020/matlabs-smooth-implementation-n-point-moving-average-in-numpy-python
#---------------------------------------------------------------------------
def smooth(a,WSZ):
# a: NumPy 1-D array containing the data to be smoothed
# WSZ: smoothing window size needs, which must be odd number,
# as in the original MATLAB implementation
out0 = np.convolve(a,np.ones(WSZ,dtype=int),'valid')/WSZ
r = np.arange(1,WSZ-1,2)
start = np.cumsum(a[:WSZ-1])[::2]/r
stop = (np.cumsum(a[:-WSZ:-1])[::2]/r)[::-1]
return np.concatenate(( start , out0, stop ))
<file_sep>/old/old_old/test_read_ghcnd_obs_20180412.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
#from make_cmap import *
#from netCDF4 import Dataset as NetCDFFile
#from utils_wrfout import *
#from utils_date import *
#import pickle
#from ghcndextractor import *
#from ghcndextractor import ghcndextractor
import codecs
from utils_ghcnd_obs import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
#ghcnFolder = rcm_dir + '/obs'
ghcnd_dir = rcm_dir + '/obs/ghcnd_all'
data_dir = '/home/disk/r2d2/steed/cmip5/rcp8.5'
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
vars = ['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
#sdt = '202001'
#edt = '202912'
sdt = '197001'
edt = '209912'
dtfmt = "%Y%m%d%H"
stns = ['KSEA', 'KSMP', 'KYKM', 'KGEG', 'KPDX', 'KMFR', 'KHQM']
models = ['gfdl-cm3', 'miroc5']
stn_cols = ['b', 'r', 'g', 'c', 'm', 'k', 'grey']
mod_cols = ['b', 'r']
latpts = [47.44472, 47.27667, 46.56417, 47.62139, 45.59083, \
42.38111, 46.97278]
lonpts = [-122.31361, -121.33722, -120.53361, -117.52778, -122.60028, \
-122.87222, -123.93028]
elevs = [130.0, 1207.0, 333.0, 735.0, 8.0, 405.0, 4.0]
ghcnd_dict = {
'KSEA': 'USW00024233',
'KSMP': 'USW00024237',
'KYKM': 'USW00024243',
'KGEG': 'USW00024157',
'KPDX': 'USW00024229',
'KMFR': 'USW00024225',
'KHQM': 'USW00094225'}
#---------------------------------------------------------------------------
#
#---------------------------------------------------------------------------
elements = ['TMAX', 'TMIN', 'PRCP']
for s in range(len(stns)):
stn_ghcnd = ghcnd_dict[stns[s]]
file_c = ghcnd_dir + '/' + stn_ghcnd + '.dly'
if os.path.isfile(file_c):
print 'reading ', file_c
(datag, dts_all) = read_ghcnd(file_c, elements)
test = get_seasonal_stats_ghcnd(datag, dts_all)
# for dt in dts_all:
# print dt
#
# for key in datag.keys():
# print(key)
#
# sys.exit()
#
# el = 'TMAX'
# dt = '19701205'
# key = (el,dt)
## if (el,yr,mo,dy) in datag:
# if key in datag:
# print 'datag[key] = ', datag[key]
# else:
# print 'cannot see key in datag! key = ', key
# sys.exit()
sys.exit()
<file_sep>/old/test_read_ghcnd_obs.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
#from make_cmap import *
#from netCDF4 import Dataset as NetCDFFile
#from utils_wrfout import *
#from utils_date import *
import pickle
#from ghcndextractor import *
#from ghcndextractor import ghcndextractor
import codecs
from utils_ghcnd_obs import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
ghcnd_dir = rcm_dir + '/obs/ghcnd_all'
data_dir = '/home/disk/r2d2/steed/cmip5/rcp8.5'
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
vars = ['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
#sdt = '202001'
#edt = '202912'
sdt = '197001'
edt = '201712'
#dtfmt = "%Y%m%d%H"
#stns = ['KSEA', 'KSMP', 'KYKM', 'KGEG', 'KPDX', 'KMFR', 'KHQM']
stns = ['KSEA']
models = ['gfdl-cm3', 'miroc5']
stn_cols = ['b', 'r', 'g', 'c', 'm', 'k', 'grey']
mod_cols = ['b', 'r']
#latpts = [47.44472, 47.27667, 46.56417, 47.62139, 45.59083, \
# 42.38111, 46.97278]
#lonpts = [-122.31361, -121.33722, -120.53361, -117.52778, -122.60028, \
# -122.87222, -123.93028]
#elevs = [130.0, 1207.0, 333.0, 735.0, 8.0, 405.0, 4.0]
latpts = [47.44472]
lonpts = [-122.31361]
elevs = [130.0]
elements = ['TMAX']
#---------------------------------------------------------------------------
#
#---------------------------------------------------------------------------
(data_all, dts_all) = read_ghcnd(stns, elements, ghcnd_dir, sdt, edt)
tc = data_all['TMAX','KSEA','20170101']
print (tc * (9./5.)) + 32
tc = data_all['TMAX','KSEA','20170701']
print (tc * (9./5.)) + 32
tc = data_all['TMAX','KSEA','20170702']
print (tc * (9./5.)) + 32
tc = data_all['TMAX','KSEA','20170703']
print (tc * (9./5.)) + 32
##---------------------------------------------------------------------------
##
##---------------------------------------------------------------------------
#elements = ['TMAX', 'TMIN', 'PRCP']
#
#load_pickle = 1
#pickle_file = pickle_dir + '/ghcnd_test.pickle'
#if load_pickle and os.path.isfile(pickle_file):
# print 'reading pickle file ', pickle_file
# (data_all, dts_all, elements, stns) = \
# pickle.load(open(pickle_file, 'rb'))
# print 'done!'
#else:
# (data_all, dts_all) = read_ghcnd(stns, elements, ghcnd_dir, sdt, edt)
# pickle.dump((data_all, dts_all, elements, stns), \
# open(pickle_file,'wb'), -1)
#
#var = 'TMAX'
#(tmx_mx, years) = get_seasonal_stats_ghcnd(data_all, dts_all, stns, var, 'max')
#(tmx_avg, years) = get_seasonal_stats_ghcnd(data_all, dts_all, stns, var, 'avg')
#var = 'TMIN'
#(tmn_mn, years) = get_seasonal_stats_ghcnd(data_all, dts_all, stns, var, 'min')
#(tmn_avg, years) = get_seasonal_stats_ghcnd(data_all, dts_all, stns, var, 'avg')
#var = 'PRCP'
#(pcp_mx, years) = get_seasonal_stats_ghcnd(data_all, dts_all, stns, var, 'max')
#(pcp_tot, years) = get_seasonal_stats_ghcnd(data_all, dts_all, stns, var, 'tot')
#
#for year in years:
# key = ('fall','KSEA',year)
# if (key in pcp_tot):
# print year, pcp_tot[key]
# else:
# print key, ' not in there'
sys.exit()
<file_sep>/igtg_check_miroc5.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
from igtg_check_utils_miroc5 import *
import matplotlib.pyplot as plt
#import pickle
from make_cmap import *
from Scientific.IO.NetCDF import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
data_dir = '/home/disk/mass/jbaars/cmip5/rcp8.5/miroc5/test_unstaggertos'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
varname = 'tos'
file_old = data_dir + '/' + 'inlatlon'
#file_new = data_dir + '/' + 'indata'
file_new = data_dir + '/' + 'testdata'
itime = 1
ilev = 1
title = 'both'
plotfname = './both8.png'
#title = 'inlatlon'
#plotfname = './inlatlon.png'
#title = 'indata'
#plotfname = './indata.png'
#i1 = 4
#j1 = 107
#i2 = 174
#j2 = 183
i1 = 147
j1 = 92
i2 = 1
j2 = 1
#minlat = 50.0
#maxlat = 70.0
#minlon = -10.0
#maxlon = 20.0
#minlat = 55.0
#maxlat = 65.0
#minlon = 0.0
#maxlon = 10.0
#--- account for python counters starting at 0.
i1 = i1 - 1
j1 = j1 - 1
#---------------------------------------------------------------------------
# Load old and new files.
#---------------------------------------------------------------------------
(lat_old, lon_old, tos_old) = load_nc(file_old, 0)
(lat_new, lon_new, tos_new) = load_nc(file_new, 1)
print 'lat_old = ', lat_old
print 'lat_new = ', lat_new
print ''
print 'tos_old = ', tos_old
print 'tos_new = ', tos_new
print ''
print 'lon_old = ', lon_old
print 'lon_new = ', lon_new
#sys.exit()
#nx,ny = np.shape(lon_old)
#print 'nx, ny = ', nx, ny
#for ii in range(nx):
# for jj in range(ny):
#
# if (lat_old[ii,jj] > minlat and lat_old[ii,jj] < maxlat):
#
# lon_c = lon_old[ii,jj]
# if (lon_c > 180.0):
# lon_c = lon_c - 360
#
# print 'is ', minlon, ' < ', lon_c, ' < ', maxlon
# if (lon_c > minlon and lon_c < maxlon):
# print 'yes'
# else:
# print 'no'
#sys.exit()
lat_thresh = 4.0
lon_thresh = 5.0
print 'lat_old[i1,j1], lon_old[i1,j1] = ', lat_old[i1,j1], lon_old[i1,j1]
maxlat = lat_old[i1,j1] + lat_thresh
minlat = lat_old[i1,j1] - lat_thresh
maxlon = lon_old[i1,j1] + lon_thresh - 360
minlon = lon_old[i1,j1] - lon_thresh - 360
print 'minlat, maxlat = ', minlat, maxlat
print 'minlon, maxlon = ', minlon, maxlon
#sys.exit()
#---------------------------------------------------------------------------
# Plot old data.
#---------------------------------------------------------------------------
mapper(i1, j1, i2, j2, lat_old, lon_old, lat_new, lon_new, tos_new, \
minlat, maxlat, minlon, maxlon, title, plotfname)
sys.exit()
<file_sep>/old/old_old/utils_wrfout_20180116.py
#!/usr/bin/python
import os, sys, glob, re
import numpy as np
from netCDF4 import Dataset as NetCDFFile
#from datetime import date, timedelta, datetime
#import time, stat
#from collections import defaultdict
#from utils_date import *
#from mpl_toolkits.basemap import Basemap, cm
#import matplotlib.pyplot as plt
mm2in = 0.0393700787
#---------------------------------------------------------------------------
# Load geo_em file.
#---------------------------------------------------------------------------
def load_geo_em(geo_em):
nc = NetCDFFile(geo_em, 'r')
lat = nc.variables['XLAT_M'][0,:,:]
lon = nc.variables['XLONG_M'][0,:,:]
lat = np.transpose(lat)
lon = np.transpose(lon)
nc.close()
return lat, lon
#---------------------------------------------------------------------------
# Load wrfout file.
#---------------------------------------------------------------------------
def load_wrfout(wrfout, var):
nc = NetCDFFile(wrfout, 'r')
ntimes = len(nc.dimensions['Time'])
dataout = nc.variables[var][:,:,:]
dataout = np.transpose(dataout)
#--- Get date from global attributes and clean it up and trim it down.
dt_all = nc.START_DATE
dt = dt_all.replace('-', '')
dt = dt.replace(':', '')
dt = dt.replace('_', '')
dt = dt[0:10]
nc.close()
return dataout, ntimes, dt
#---------------------------------------------------------------------------
# Get list of extracted wrfout files between sdt_yyyymm and edt_yyyymm.
#---------------------------------------------------------------------------
def get_extract_files(data_dir, model, sdt_yyyymm, edt_yyyymm):
files_out = []
files = glob.glob(data_dir + '/' + model + '*' + '.nc')
for f in range(len(files)):
dt_c = re.findall(r'\.(\d{10})\.', files[f])
if (dt_c):
dt_c = dt_c[0]
yyyymm_c = dt_c[0:6]
if (yyyymm_c >= sdt_yyyymm and yyyymm_c <= edt_yyyymm):
files_out.append(files[f])
return files_out
#---------------------------------------------------------------------------
# Get seasonal totals. NEED TO SORT OUT HOW TO HANDLE WINTERS AT THE
# EDGES (FIRST YEAR AND LAST) WHICH WON"T HAVE A FULL 3 MONTHS.
#---------------------------------------------------------------------------
def get_seasonal_totals(data_all, dts_all, models, stns):
springs = {}
summers = {}
falls = {}
winters = {}
for s in range(len(stns)):
stn = stns[s]
for m in range(len(models)):
mod = models[m]
for d in range(len(dts_all)):
yyyy = dts_all[d][0:4]
mm = dts_all[d][4:6]
if (mm == '03' or mm == '04' or mm == '05'):
# if (stn == stn_pr and yyyy == yyyy_pr):
# print dts_all[d], mm, data_all[mod,stn,dts_all[d]]
if ((mod,stn,yyyy) in springs):
springs[mod,stn,yyyy] = springs[mod,stn,yyyy] + \
data_all[mod,stn,dts_all[d]]
else:
springs[mod,stn,yyyy] = data_all[mod,stn,dts_all[d]]
# if (stn == stn_pr and yyyy == yyyy_pr):
# print 'springs tot = ', springs[mod,stn,yyyy]
if (mm == '06' or mm == '07' or mm == '08'):
if ((mod,stn,yyyy) in summers):
summers[mod,stn,yyyy] = summers[mod,stn,yyyy] + \
data_all[mod,stn,dts_all[d]]
else:
summers[mod,stn,yyyy] = data_all[mod,stn,dts_all[d]]
if (mm == '09' or mm == '10' or mm == '11'):
if ((mod,stn,yyyy) in falls):
falls[mod,stn,yyyy] = falls[mod,stn,yyyy] + \
data_all[mod,stn,dts_all[d]]
else:
falls[mod,stn,yyyy] = data_all[mod,stn,dts_all[d]]
if (mm == '12' or mm == '01' or mm == '02'):
if ((mod,stn,yyyy) in winters):
winters[mod,stn,yyyy] = winters[mod,stn,yyyy] + \
data_all[mod,stn,dts_all[d]]
else:
winters[mod,stn,yyyy] = data_all[mod,stn,dts_all[d]]
return springs, summers, falls, winters
#---------------------------------------------------------------------------
# Interpolate from a sent-in grid to a sent in lat/lon point.
#---------------------------------------------------------------------------
def bilinear_interpolate(latgrid, longrid, datagrid, latpt, lonpt, deb):
totdiff = abs(latgrid - latpt) + abs(longrid - lonpt)
(i,j) = np.unravel_index(totdiff.argmin(), totdiff.shape)
#--- Get lat/lon box in which latpt,lonpt resides.
if (latpt >= latgrid[i,j] and lonpt >= longrid[i,j]):
iif = i
jjf = j
elif (latpt < latgrid[i,j] and lonpt < longrid[i,j]):
iif = i-1
jjf = j-1
elif (latpt >= latgrid[i,j] and lonpt < longrid[i,j]):
iif = i-1
jjf = j
elif (latpt < latgrid[i,j] and lonpt >= longrid[i,j]):
iif = i
jjf = j-1
(nx, ny) = np.shape(latgrid)
if (deb == 1):
print 'nx, ny = ', nx, ny
print 'iif,jjf = ', iif,jjf
print 'latgrid[iif+1,jjf] = ', latgrid[iif+1,jjf]
print 'latgrid[iif,jjf] = ', latgrid[iif,jjf]
if (iif >= (nx-1) or jjf >= (ny-1) or iif < 0 or jjf < 0):
return mvc
#--- Do bilinear interpolation to latpt,lonpt.
dlat = latgrid[iif,jjf+1] - latgrid[iif,jjf]
dlon = longrid[iif+1,jjf] - longrid[iif,jjf]
dslat = latgrid[iif,jjf+1] - latpt
dslon = longrid[iif+1,jjf] - lonpt
wrgt = 1 - (dslon/dlon)
wup = 1 - (dslat/dlat)
vll = datagrid[iif,jjf]
vlr = datagrid[iif,jjf+1]
vul = datagrid[iif+1,jjf]
vur = datagrid[iif+1,jjf+1]
if (deb > 1):
print 'll lat, lon, val = ', latgrid[iif,jjf], longrid[iif,jjf], vll
print 'lr lat, lon, val = ', latgrid[iif+1,jjf], longrid[iif+1,jjf], vlr
print 'ur lat, lon, val = ', latgrid[iif+1,jjf+1], longrid[iif+1,jjf+1],vur
print 'ul lat, lon, val = ', latgrid[iif,jjf+1], longrid[iif,jjf+1], vul
print 'latpt, lonpt = ', latpt, lonpt
print 'vll = ', vll
print 'vlr = ', vlr
print 'vul = ', vul
print 'vur = ', vur
datout = (1-wrgt) * ((1-wup) * vll + wup * vul) + \
(wrgt) * ((1-wup) * vlr + wup * vur)
# if (deb == 1):
# print 'datout = ', datout
# sys.exit()
# if (datout == 0.0):
# print 'nx, ny = ', nx, ny
# print 'iif,jjf = ', iif,jjf
# print 'latgrid[iif+1,jjf] = ', latgrid[iif+1,jjf]
# print 'latgrid[iif,jjf] = ', latgrid[iif,jjf]
#
# print 'll lat, lon, val = ', latgrid[iif,jjf], longrid[iif,jjf], vll
# print 'lr lat, lon, val = ', latgrid[iif+1,jjf], longrid[iif+1,jjf], vl#r
# print 'ur lat, lon, val = ', latgrid[iif+1,jjf+1], longrid[iif+1,jjf+1],vur
# print 'ul lat, lon, val = ', latgrid[iif,jjf+1], longrid[iif,jjf+1], vu#l
# print 'latpt, lonpt = ', latpt, lonpt
#
# print 'vll = ', vll
# print 'vlr = ', vlr
# print 'vul = ', vul
# print 'vur = ', vur
#
# sys.exit()
return datout
<file_sep>/create_wrf_state_mask.py
#!/usr/bin/python
import os, sys, glob, re
import numpy as np
from netCDF4 import Dataset as NetCDFFile
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
import pickle
from utils_date import *
import csv
ll_lat = 45.3
ur_lat = 49.3
ll_lon = -125.0
ur_lon = -116.5
fs = 9
titlefs = 9
width = 10
height = 8
#width = 30
#height = 24
maplw = 1.0
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
mask_file = '/home/disk/spock/jbaars/rcm/data/mask_pts.dat'
#---------------------------------------------------------------------------
# Read mask lat/lon points file.
#---------------------------------------------------------------------------
mask = open(mask_file)
csvReader = csv.reader(mask)
maski = []
maskj = []
for row in csvReader:
maski.append(int(row[0]))
maskj.append(int(row[1]))
#---------------------------------------------------------------------------
# Load geo_em file.
#---------------------------------------------------------------------------
print 'Loading ', geo_em
nc = NetCDFFile(geo_em, 'r')
lat = nc.variables['XLAT_M'][0,:,:]
lon = nc.variables['XLONG_M'][0,:,:]
hgt = nc.variables['HGT_M'][0,:,:]
lat = np.transpose(lat)
lon = np.transpose(lon)
hgt = np.transpose(hgt)
nc.close()
#---------------------------------------------------------------------------
#
#---------------------------------------------------------------------------
lat_ctr = ll_lat + ((ur_lat - ll_lat) * 0.5)
lon_ctr = ll_lon + ((ur_lon - ll_lon) * 0.5)
res = 'i'
#res = 'h'
fig = plt.figure(figsize=(width,height))
# left, bottom, width, height:
ax = fig.add_axes([0.00,0.05,0.99,0.91])
map = Basemap(resolution = res,projection='lcc',\
llcrnrlon= ll_lon, llcrnrlat=ll_lat,\
urcrnrlon= ur_lon, urcrnrlat= ur_lat,\
lat_0=lat_ctr,lon_0=lon_ctr,lat_1=(ur_lat - ll_lat))
#--- Get lat and lon data in map's x/y coordinates.
x,y = map(lon, lat)
#--- Draw coastlines, country boundaries, fill continents.
map.drawcoastlines(linewidth = maplw)
map.drawstates(linewidth = maplw)
map.drawcountries(linewidth = maplw)
#--- Draw the edge of the map projection region (the projection limb)
map.drawmapboundary(linewidth = maplw)
#-- Add dots grid points.
(nx,ny) = lon.shape
#for x in range(nx):
# for y in range(ny):
# lat_c = lat[x,y]
# lon_c = lon[x,y]
# if (lat_c >= ll_lat and lat_c <= ur_lat and \
# lon_c >= ll_lon and lon_c <= ur_lon):
# xs, ys = map(lon_c, lat_c)
# plt.plot(xs, ys, 'ro', markersize = 3)
# plt.text(xs, ys, str(x) + ',' + str(y), fontsize=8)
plt.title('WA state mask grid points', fontsize=titlefs, fontweight='bold')
for m in range(len(maski)):
lat_c = lat[maski[m],maskj[m]]
lon_c = lon[maski[m],maskj[m]]
xs, ys = map(lon_c, lat_c)
plt.plot(xs, ys, 'ro', markersize = 4)
#--- Save plot.
plotfname = './WA_mask.png'
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
sys.exit()
<file_sep>/old/old_old/plot_wrfout_grid_20180703.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re, math
import numpy as np
#import matplotlib.pyplot as plt
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
import pickle
from collections import defaultdict
from make_cmap import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
run_dirs = rcm_dir + '/rundirs'
data_dir = '/home/disk/r2d2/steed/cmip5/rcp8.5'
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#vars = ['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
vars = ['PREC', 'T2MAX', 'T2MIN']
vars = ['T2MIN']
#sdt = '202001'
#edt = '202912'
#sdt = '197001'
sdt = '204501'
edt = '209912'
#models = ['gfdl-cm3']
models = ['miroc5']
dtfmt = "%Y%m%d%H"
#var_c = 'T2MIN'
##print 'levs[var_c] = ', levs[var_c]
#print 'cmaps[var_c] = ', cmaps[var_c]
#test = cmaps[var_c].extend(cmaps[var_c])
#print 'test = ', test
#sys.exit()
#---------------------------------------------------------------------------
# Make a color map using make_cmap.
#---------------------------------------------------------------------------
levs_norm_c = []
for i in range(len(levs_temp)):
x = float(levs_temp[i])
norm_c = (x - min(levs_temp)) / (max(levs_temp) - min(levs_temp))
levs_norm_c.append(norm_c)
cmap_temp = make_cmap(cmap_temp, bit = True, position = levs_norm_c)
levs_norm_c = []
for i in range(len(levs_pcp)):
x = float(levs_pcp[i])
norm_c = (x - min(levs_pcp)) / (max(levs_pcp) - min(levs_pcp))
levs_norm_c.append(norm_c)
cmap_pcp = make_cmap(cmap_pcp, bit = True, position = levs_norm_c)
#---------------------------------------------------------------------------
# Make run directory, load geo_em file.
#---------------------------------------------------------------------------
rundir = run_dirs + '/plot_wrfout_grid.' + str(os.getpid())
print 'making ', rundir
os.makedirs(rundir)
print 'Loading ', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
#---------------------------------------------------------------------------
# For each model, make a maps of every season...
#---------------------------------------------------------------------------
for m in range(len(models)):
model = models[m]
data_dir_c = data_dir + '/' + model + '/extract'
#--- Get list of extracted files for this model.
files = get_extract_files(data_dir_c, model, sdt, edt)
#--- Get files broken out by year and season.
(spr_files, sum_files, fal_files, win_files, years) = \
get_seasonal_files(files)
#--- Loop over year, making maps of each
for y in range(len(years)):
yyyy = years[y]
#--- Winter plots.
if (win_files[yyyy] == win_files[yyyy]):
iret = run_mapper('T2MAX', model, rundir, 'winter', win_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('T2MIN', model, rundir, 'winter', win_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('PREC', model, rundir, 'winter', win_files,\
yyyy, lat, lon, levs_pcp, cmap_pcp)
#--- Summer plots.
if (sum_files[yyyy] == sum_files[yyyy]):
iret = run_mapper('T2MAX', model, rundir, 'summer', sum_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('T2MIN', model, rundir, 'summer', sum_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('PREC', model, rundir, 'summer', sum_files,\
yyyy, lat, lon, levs_pcp, cmap_pcp)
#--- Spring plots.
if (spr_files[yyyy] == spr_files[yyyy]):
iret = run_mapper('T2MAX', model, rundir, 'spring', spr_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('T2MIN', model, rundir, 'spring', spr_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('PREC', model, rundir, 'spring', spr_files,\
yyyy, lat, lon, levs_pcp, cmap_pcp)
#--- Fall plots.
if (fal_files[yyyy] == fal_files[yyyy]):
iret = run_mapper('T2MAX', model, rundir, 'fall', fal_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('T2MIN', model, rundir, 'fall', fal_files,\
yyyy, lat, lon, levs_temp, cmap_temp)
iret = run_mapper('PREC', model, rundir, 'fall', fal_files,\
yyyy, lat, lon, levs_pcp, cmap_pcp)
# #--- Winter plots.
# if (win_files[yyyy] == win_files[yyyy]):
# for v in range(len(vars)):
# var_c = vars[v]
# iret = run_mapper(vars[v], model, rundir, 'winter', win_files,\
# yyyy, lat, lon, levs[var_c], cmaps[var_c])
#
# #--- Summer plots.
# if (sum_files[yyyy] == sum_files[yyyy]):
# for v in range(len(vars)):
# var_c = vars[v]
# iret = run_mapper(var_c, model, rundir, 'summer', sum_files,\
# yyyy, lat, lon, \
# levs[var_c], cmaps[str(var_c)])
#
# #--- Spring plots.
# if (spr_files[yyyy] == spr_files[yyyy]):
# for v in range(len(vars)):
# var_c = vars[v]
# iret = run_mapper(vars[v], model, rundir, 'spring', spr_files,\
# yyyy, lat, lon, levs[var_c], cmaps[var_c])
#
# #--- Fall plots.
# if (fal_files[yyyy] == fal_files[yyyy]):
# for v in range(len(vars)):
# var_c = vars[v]
# iret = run_mapper(vars[v], model, rundir, 'fall', fal_files,\
# yyyy, lat, lon, levs[var_c], cmaps[var_c])
#
# sys.exit()
sys.exit()
<file_sep>/old/old_old/plot_wrfout_grid_20180322.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re, math
import numpy as np
#import matplotlib.pyplot as plt
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
import pickle
from collections import defaultdict
from make_cmap import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
run_dirs = rcm_dir + '/rundirs'
data_dir = '/home/disk/r2d2/steed/cmip5/rcp8.5'
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
vars = ['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
#sdt = '202001'
#edt = '202912'
sdt = '197001'
edt = '209912'
models = ['gfdl-cm3']
dtfmt = "%Y%m%d%H"
#---------------------------------------------------------------------------
# Make a color map using make_cmap.
#---------------------------------------------------------------------------
#if (varname == 'tos'):
# levs_c = levs_temp
# cmap_c = cmap_temp
#elif(varname == 'ua'):
# levs_c = levs_prec
# cmap_c = cmap_prec
levs_c = levs_temp
cmap_c = cmap_temp
levs_norm_c = []
for i in range(len(levs_c)):
x = float(levs_c[i])
norm_c = (x - min(levs_c)) / (max(levs_c) - min(levs_c))
levs_norm_c.append(norm_c)
my_cmap = make_cmap(cmap_c, bit = True, position = levs_norm_c)
#---------------------------------------------------------------------------
# Make run directory, load geo_em file.
#---------------------------------------------------------------------------
rundir = run_dirs + '/plot_wrfout_grid.' + str(os.getpid())
print 'making ', rundir
os.makedirs(rundir)
print 'Loading ', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
#---------------------------------------------------------------------------
# For each model, make a maps of every season...
#---------------------------------------------------------------------------
for m in range(len(models)):
model = models[m]
data_dir_c = data_dir + '/' + model + '/extract'
#--- Get list of extracted files for this model.
files = get_extract_files(data_dir_c, model, sdt, edt)
#--- Get files broken out by year and season.
(spr_files, sum_files, fal_files, win_files, years) = \
get_seasonal_files(files)
#--- Loop over year, making maps of each
for y in range(len(years)):
yyyy = years[y]
#--- Winter plots.
if (win_files[yyyy] == win_files[yyyy]):
test = run_mapper('T2MAX', model, rundir, 'winter', win_files, \
yyyy, 'Temperature (K)', lat, lon, levs_c, \
my_cmap)
if (sum_files[yyyy] == sum_files[yyyy]):
test = run_mapper('T2MAX', model, rundir, 'summer', sum_files, \
yyyy, 'Temperature (K)', lat, lon, levs_c, \
my_cmap)
test = run_mapper('PREC', model, rundir, 'summer', sum_files, \
yyyy, 'Precipitation (in.)', lat, lon, levs_c, \
my_cmap)
sys.exit()
sys.exit()
# file_plot = rundir + '/sum_' + yyyy + '.nc'
# syscom = 'ncra'
# for f in range(len(sum_files[yyyy])):
# print yyyy, sum_files[yyyy][f]
# syscom = syscom + ' ' + sum_files[yyyy][f]
# syscom = syscom + ' ' + file_plot
# print 'syscom = ', syscom
# os.system(syscom)
#
# var = 'T2MAX'
# print 'Loading ', var, ' from ', file_plot
# (vardat, ntimes, dt) = load_wrfout(file_plot, [var])
#
# colorbar_lab = 'Temperature (K)'
# titlein = model.upper() + \
# ', Average Max Temperature (K), Summer (JJA) ' + yyyy
#
# plotdat = vardat[var][:,:,0]
# for z in range(len(zooms)):
# plotfname = rundir + '/sum_' + var + '_' + yyyy + '_' + \
# zooms[z] + '.png'
# mapper(lat, lon, plotdat, levs_c, my_cmap, maplw, \
# colorbar_lab, titlein, plotfname, zooms[z])
sys.exit()
sys.exit()
<file_sep>/plot_lat_tos.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
from igtg_check_utils import *
import matplotlib.pyplot as plt
#import pickle
from make_cmap import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
x = range(140,161)
dat = [ 285.5343, 285.7745, 286.0928, 286.3722, 286.6160, 286.6160, \
286.8224, 286.3380, 286.5203, 286.7137, 286.8461, 286.9745, \
287.1689, 287.3819, 287.6205, 287.6205, 287.4508, 287.9211, \
288.3215, 288.6165, 288.8190 ]
#x = range(0,len(dat))
plt.plot(x, dat, 'b')
plt.plot(x, dat, 'bo')
plt.grid()
plt.title('j = 92, i = 140 - 160')
plt.savefig('lat_tos_test.png')
plt.close()
sys.exit()
<file_sep>/utils_load_data.py
#!/usr/bin/python
import os, sys, glob, re, math
import numpy as np
from collections import defaultdict
from utils_cmap import *
import pickle
from utils_date import *
from utils_stats import *
from utils_ghcnd_obs import *
#---------------------------------------------------------------------------
# Get model pickle file name
#---------------------------------------------------------------------------
def get_mod_pkl_name (pdir, model, sdt, edt):
pf = pdir + '/extract_' + model + '_' + sdt + '_' + edt + '.pkl'
return pf
#---------------------------------------------------------------------------
# Load geo_em file.
#---------------------------------------------------------------------------
def load_geo_em(geo_em):
nc = NetCDFFile(geo_em, 'r')
lat = nc.variables['XLAT_M'][0,:,:]
lon = nc.variables['XLONG_M'][0,:,:]
hgt = nc.variables['HGT_M'][0,:,:]
lat = np.transpose(lat)
lon = np.transpose(lon)
hgt = np.transpose(hgt)
nc.close()
return lat, lon, hgt
#---------------------------------------------------------------------------
# Get netcdf dimension
#---------------------------------------------------------------------------
def get_nc_dim(ncin, dimname):
nc = NetCDFFile(ncin, 'r')
dim = len(nc.dimensions[dimname])
nc.close()
return dim
#---------------------------------------------------------------------------
# Load wrfout file.
#---------------------------------------------------------------------------
def load_wrfout(wrfout, vars):
nc = NetCDFFile(wrfout, 'r')
ntimes = len(nc.dimensions['Time'])
dataout = {}
for v in range(len(vars)):
var = vars[v]
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
dataout[var] = dat_tmp
#--- Get date from global attributes and clean it up and trim it down.
dt_all = nc.START_DATE
dt = dt_all.replace('-', '')
dt = dt.replace(':', '')
dt = dt.replace('_', '')
dt = dt[0:10]
nc.close()
return dataout, ntimes, dt
#---------------------------------------------------------------------------
# Load SWE wrfout file.
#---------------------------------------------------------------------------
def load_wrfout_swe(wrfout, vars):
nc = NetCDFFile(wrfout, 'r')
ntimes = len(nc.dimensions['Time'])
dataout = {}
for v in range(len(vars)):
var = vars[v]
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
dataout[var] = dat_tmp
nc.close()
return dataout, ntimes
#---------------------------------------------------------------------------
# Get list of extracted wrfout files between sdt_yyyymm and edt_yyyymm.
#---------------------------------------------------------------------------
def get_extract_files(data_dirs, model, sdt_yyyymm, edt_yyyymm, snowrad):
files_out = []
for d in range(len(data_dirs)):
path_c = data_dirs[d] + '/' + model + '/extract/*.nc'
files = glob.glob(path_c)
for f in range(len(files)):
if snowrad == 0:
if 'snowrad' in files[f]:
continue
else:
if 'snowrad' not in files[f]:
continue
dt_c = re.findall(r'\.(\d{10})\.', files[f])
if (dt_c):
dt_c = dt_c[0]
yyyymm_c = dt_c[0:6]
if (yyyymm_c >= sdt_yyyymm and yyyymm_c <= edt_yyyymm):
files_out.append(files[f])
if (len(files_out) == 0):
sys.exit('get_extract_files: no files found!')
return list(sorted(set(files_out)))
#---------------------------------------------------------------------------
# Get seasonal stats.
#---------------------------------------------------------------------------
def get_seasonal_files(files):
springs = defaultdict(list)
summers = defaultdict(list)
falls = defaultdict(list)
winters = defaultdict(list)
annuals = defaultdict(list)
spring_ndays = {}
summer_ndays = {}
fall_ndays = {}
winter_ndays = {}
annual_ndays = {}
years_all = []
#--- Loop over all files, binning them into seasons / years.
for f in range(len(files)):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
if (dt_c):
yyyy = dt_c[0:4]
mm = dt_c[4:6]
years_all.append(yyyy)
if (mm == '03' or mm == '04' or mm == '05'):
if ((yyyy) in spring_ndays):
spring_ndays[yyyy] += 1
else:
spring_ndays[yyyy] = 1
springs[yyyy].append(files[f])
elif (mm == '06' or mm == '07' or mm == '08'):
if ((yyyy) in summer_ndays):
summer_ndays[yyyy] += 1
else:
summer_ndays[yyyy] = 1
summers[yyyy].append(files[f])
elif (mm == '09' or mm == '10' or mm == '11'):
if ((yyyy) in fall_ndays):
fall_ndays[yyyy] += 1
else:
fall_ndays[yyyy] = 1
falls[yyyy].append(files[f])
elif (mm == '12'):
yyyyw = str(int(yyyy) + 1)
if ((yyyyw) in winter_ndays):
winter_ndays[yyyyw] += 1
else:
winter_ndays[yyyyw] = 1
winters[yyyyw].append(files[f])
elif (mm == '01' or mm == '02'):
if ((yyyy) in winter_ndays):
winter_ndays[yyyy] += 1
else:
winter_ndays[yyyy] = 1
winters[yyyy].append(files[f])
if ((yyyy) in annual_ndays):
annual_ndays[yyyy] += 1
else:
annual_ndays[yyyy] = 1
annuals[yyyy].append(files[f])
#--- Get list of unique, sorted years found above.
years = list(sorted(set(years_all)))
#--- Check number of data points per unique years and nan out
#--- ones that do not have enough (< min_season_days).
for y in range(len(years)):
yyyy = years[y]
if (yyyy in spring_ndays):
if (spring_ndays[yyyy] < 3):
springs[yyyy] = np.nan
else:
springs[yyyy] = np.nan
if (yyyy in summer_ndays):
if (summer_ndays[yyyy] < 3):
summers[yyyy] = np.nan
else:
summers[yyyy] = np.nan
if (yyyy in fall_ndays):
if (fall_ndays[yyyy] < 3):
falls[yyyy] = np.nan
else:
falls[yyyy] = np.nan
if (yyyy in winter_ndays):
if (winter_ndays[yyyy] < 3):
winters[yyyy] = np.nan
else:
winters[yyyy] = np.nan
if (yyyy in annual_ndays):
if (annual_ndays[yyyy] < 3):
annuals[yyyy] = np.nan
else:
annuals[yyyy] = np.nan
return springs, summers, falls, winters, annuals, years
#---------------------------------------------------------------------------
# Get seasonal files (new)
#---------------------------------------------------------------------------
def get_seasonal_files_new(files):
seafiles = defaultdict(list)
ndays = {}
years_all = []
#--- Loop over all files, binning them into seasons / years.
for f in range(len(files)):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
if (dt_c):
yyyy = dt_c[0:4]
mm = dt_c[4:6]
if mm == '12':
yyyy = str(int(yyyy) + 1)
else:
yyyy = yyyy
years_all.append(yyyy)
if (mm == '03' or mm == '04' or mm == '05'):
season = 'spring'
if (mm == '06' or mm == '07' or mm == '08'):
season = 'summer'
if (mm == '09' or mm == '10' or mm == '11'):
season = 'fall'
if (mm == '12' or mm == '01' or mm == '02'):
season = 'winter'
if ((yyyy) in ndays):
ndays[season+yyyy] += 1
else:
ndays[season+yyyy] = 1
seafiles[season,yyyy].append(files[f])
#--- Get list of unique, sorted years found above.
years = list(sorted(set(years_all)))
return seafiles, years
#---------------------------------------------------------------------------
# Loop over each models, grabbing data from extract wrfout files.
#---------------------------------------------------------------------------
def load_extract_data(geo_em, stns, latpts, lonpts, elevs, models, \
data_dirs, sdt, edt, varsin, pickle_dir, load_pickle):
#--- Create temporary list of variables, removing 'T2MEAN' if in original
#--- list (we'll calculate T2MEAN if necessary after loading data).
vars_tmp = list(varsin)
if 'T2MEAN' in vars_tmp:
i = vars_tmp.index('T2MEAN')
del vars_tmp[i]
#--- Load geo em file to get lat/lon/elev grids. Also interpolate
#--- model elevation to stns lat/lon point locations.
print 'Loading:\n', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
elevs_mod = []
elevs_diff = []
for ns in range(len(stns)):
dat_interp = bilinear_interpolate(lat, lon, hgt, \
latpts[ns], lonpts[ns], 0)
elevs_mod.append(dat_interp)
elevs_diff.append(elevs[ns] - dat_interp)
for m in range(len(models)):
data_all = {}
dts_all = []
model = models[m]
pickle_file = get_mod_pkl_name(pickle_dir, model, sdt, edt)
#--- Get list of extracted files for this model.
print 'model = ', model
files = get_extract_files(data_dirs, model, sdt, edt, 0)
files = list(sorted(set(files)))
print 'Loading: '
#--- Loop over all extract files, reading them in.
for f in range(len(files)):
(vardat, ntimes, dt) = load_wrfout(files[f], vars_tmp)
print files[f], ntimes
#--- Loop over each vars_tmp, interpolating data to stns for
#--- each ntimes.
for v in range(len(vars_tmp)):
var = vars_tmp[v]
vardat_c = vardat[var]
#--- Loop over each ntime, grabbing interpolated data for
#--- variable var for each stns.
for n in range(ntimes):
dt_c = time_increment(dt, n, dtfmt)
for ns in range(len(stns)):
dat_interp = bilinear_interpolate(lat, lon, \
vardat_c[:,:,n],\
latpts[ns], \
lonpts[ns], 0)
#--- Do lapse rate correction for temperature.
if (var == 'T2MAX' or var == 'T2MIN'):
dat_c = dat_interp - std_lapse * elevs_diff[ns]
else:
dat_c = dat_interp
data_all[var, stns[ns], dt_c] = dat_c
dts_all.append(dt_c)
#--- Get sorted unique list of all dates seen.
dts_unique = list(sorted(set(dts_all)))
#--- If T2MEAN was requested, calculate it from T2MAX and T2MIN for
#--- every station and date.
if 'T2MEAN' in varsin:
for ns in range(len(stns)):
for d in range(len(dts_unique)):
keymax = ('T2MAX', stns[ns], dts_unique[d])
keymin = ('T2MIN', stns[ns], dts_unique[d])
if keymax in data_all and keymin in data_all:
keymean = ('T2MEAN', stns[ns], dts_unique[d])
# if data_all[keymax] < (273-60) or \
# data_all[keymax] > (273+60) or \
# data_all[keymin] < (273-60) or \
# data_all[keymin] > (273+60):
# print 'stns[ns] = ', stns[ns]
# print 'dts_unique[d] = ', dts_unique[d]
# print 'max = ', data_all[keymax]
# print 'min = ', data_all[keymin]
## sys.exit()
data_all[keymean] = np.mean([data_all[keymax], \
data_all[keymin]])
#--- Create pickle file.
pickle.dump((model, data_all, dts_unique, varsin, stns), \
open(pickle_file,'wb'), -1)
return data_all, dts_unique, models, varsin, stns
<file_sep>/plot_sst_map.py
#!/usr/bin/python
import os, sys, glob, re
import numpy as np
from netCDF4 import Dataset as NetCDFFile
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
from collections import defaultdict
from utils_cmap import *
import pickle
from utils_date import *
from utils_wrfout import *
from matplotlib import colors as c
import numpy.ma as ma
#----------------------------------------------------------------------------
#----------------------------------------------------------------------------
model = 'ccsm4'
dt = '1999072900'
#geo_em = '/home/disk/spock/jbaars/rcm/data/geo_em.d02.nc'
geo_em = '/home/disk/riker/steed/geo_em.d02.nc.urban.water.nc'
wrfout = './wrf2d.d02.1hr.' + dt + '.nc'
gcm = '/home/disk/r2d2/steed/cmip5/rcp8.5/ccsm4/data/ncungrib/' + \
'ncFILE:1999-07-01_00'
##----------------------------------------------------------------------------
## Read gcm.
##----------------------------------------------------------------------------
#nc = NetCDFFile(gcm, 'r')
#lat_gcm = nc.variables['lat'][:]
#lon_gcm = nc.variables['lon'][:]
#lm_gcm = nc.variables['LANDSEA'][:,:]
#nc.close()
#
#xs, ys = np.meshgrid(lon_gcm, lat_gcm)
#
##----------------------------------------------------------------------------
## Plot it.
##----------------------------------------------------------------------------
#titlein = 'GCM Land Mask, ' + model + ', ' + dt
#plotfname = './gcm_landmask.png'
#zoom = 'Z1'
#
#ur_lat = maxlat[zoom]
#ur_lon = maxlon[zoom]
#ll_lat = minlat[zoom]
#ll_lon = minlon[zoom]
#lat_ctr = ll_lat + ((ur_lat - ll_lat) * 0.5)
#lon_ctr = ll_lon + ((ur_lon - ll_lon) * 0.5)
#
#if (zoom == 'Z1'):
# res = 'i'
#elif (zoom == 'Z2'):
# res = 'h'
#
#fig = plt.figure(figsize=(width,height))
## left, bottom, width, height:
#ax = fig.add_axes([0.00,0.05,0.99,0.91])
#map = Basemap(resolution = res,projection='lcc',\
# llcrnrlon= ll_lon, llcrnrlat=ll_lat,\
# urcrnrlon= ur_lon, urcrnrlat= ur_lat,\
# lat_0=lat_ctr,lon_0=lon_ctr,lat_1=(ur_lat - ll_lat))
#
##--- Get lat and lon data in map's x/y coordinates.
##x,y = map(lon_gcm, lat_gcm)
#x,y = map(xs, ys)
#
##--- Draw coastlines, country boundaries, fill continents.
#map.drawcoastlines(linewidth = maplw)
#map.drawstates(linewidth = maplw)
#map.drawcountries(linewidth = maplw)
#
##--- Draw the edge of the map projection region (the projection limb)
#map.drawmapboundary(linewidth = maplw)
##--- Draw lat/lon grid lines every 30 degrees.
#map.drawmeridians(np.arange(0, 360, 30), linewidth = maplw)
#map.drawparallels(np.arange(-90, 90, 30), linewidth = maplw)
#
#cMap = c.ListedColormap(['cornflowerblue','darkkhaki'])
#plt.pcolormesh(x, y, lm_gcm, cmap=cMap)
#
#plt.title(titlein, fontsize=titlefs, fontweight='bold')
#
##--- Save plot.
#print 'xli ', plotfname, ' &'
#plt.savefig(plotfname)
#
#plt.close()
#
#sys.exit()
#----------------------------------------------------------------------------
# Read geo_em.
#----------------------------------------------------------------------------
nc = NetCDFFile(geo_em, 'r')
lat = nc.variables['XLAT_M'][0,:,:]
lon = nc.variables['XLONG_M'][0,:,:]
hgt = nc.variables['HGT_M'][0,:,:]
lm = nc.variables['LANDMASK'][0,:,:]
luse = nc.variables['LU_INDEX'][0,:,:]
lat = np.transpose(lat)
lon = np.transpose(lon)
hgt = np.transpose(hgt)
lm = np.transpose(lm)
luse = np.transpose(luse)
nc.close()
urban = 13
water = 17
luse[luse == urban] = 100
luse[luse == water] = 101
luse[luse < 100] = 102
#----------------------------------------------------------------------------
# Read wrfout.
#----------------------------------------------------------------------------
nc = NetCDFFile(wrfout, 'r')
sst = nc.variables['SST'][0,:,:]
sst = np.transpose(sst)
nc.close()
sst = ((sst - 273.15) * 9.0/5.0) + 32
#----------------------------------------------------------------------------
# Plot it.
#----------------------------------------------------------------------------
plotfname = './test.png'
titlein = 'Surface Temperature, Land Mask, Urban Land Use, ' + model + \
', ' + dt
zoom = 'Z2'
minlatZ2 = 46.5
maxlatZ2 = 49.0
minlonZ2 = -124.0
maxlonZ2 = -121.0
#minlatZ2 = 44.0
#maxlatZ2 = 47.0
#minlonZ2 = -125.0
#maxlonZ2 = -120.0
#ur_lat = maxlat[zoom]
#ur_lon = maxlon[zoom]
#ll_lat = minlat[zoom]
#ll_lon = minlon[zoom]
ur_lat = maxlatZ2
ur_lon = maxlonZ2
ll_lat = minlatZ2
ll_lon = minlonZ2
lat_ctr = ll_lat + ((ur_lat - ll_lat) * 0.5)
lon_ctr = ll_lon + ((ur_lon - ll_lon) * 0.5)
if (zoom == 'Z1'):
res = 'i'
elif (zoom == 'Z2'):
res = 'h'
fig = plt.figure(figsize=(width,height))
# left, bottom, width, height:
ax = fig.add_axes([0.00,0.05,0.99,0.91])
map = Basemap(resolution = res,projection='lcc',\
llcrnrlon= ll_lon, llcrnrlat=ll_lat,\
urcrnrlon= ur_lon, urcrnrlat= ur_lat,\
lat_0=lat_ctr,lon_0=lon_ctr,lat_1=(ur_lat - ll_lat))
#--- Get lat and lon data in map's x/y coordinates.
x,y = map(lon, lat)
#--- Draw coastlines, country boundaries, fill continents.
map.drawcoastlines(linewidth = maplw)
map.drawstates(linewidth = maplw)
map.drawcountries(linewidth = maplw)
#--- Draw the edge of the map projection region (the projection limb)
map.drawmapboundary(linewidth = maplw)
#--- Draw lat/lon grid lines every 30 degrees.
map.drawmeridians(np.arange(0, 360, 30), linewidth = maplw)
map.drawparallels(np.arange(-90, 90, 30), linewidth = maplw)
cMap = c.ListedColormap(['darkgray', 'royalblue','darkkhaki'])
CS = plt.contour(x, y, sst, [52,56,60,64,68,72,76,80], color = 'white')
plt.clabel(CS, inline=1, fontsize=10, fmt='%2.0f')
plt.pcolormesh(x, y, luse, cmap=cMap)
#--- Plot station locations.
stn_plot = ['KPDX', 'KSEA']
stn_lats = [45.59083, 47.44472]
stn_lons = [-122.60028, -122.31361]
for sp in range(len(stn_plot)):
xs, ys = map(stn_lons[sp], stn_lats[sp])
plt.plot(xs, ys, 'rs', markersize = 5, markeredgewidth = 1.1)
plt.text(xs, ys, stn_plot[sp])
plt.title(titlein, fontsize=titlefs, fontweight='bold')
#--- Save plot.
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
#plt.show()
plt.close()
<file_sep>/plot_rcm_stns_cei.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
from utils_wrfout import *
from utils_load_data import *
from utils_plot import *
from utils_date import *
from utils_ghcnd_obs import *
from utils_cei import *
import cPickle as pickle #-- cPickle is faster, but deprecated in python 3.0
import csv
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
#plot_dir = rcm_dir + '/plots'
plot_dir = rcm_dir + '/plots/new'
pickle_dir = rcm_dir + '/pickle'
data_dir = rcm_dir + '/data'
ghcnd_dir = data_dir + '/ghcnd/ghcnd_all'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
geo_em = data_dir + '/geo_em.d02.nc'
station_file = data_dir + '/station_file.txt'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#vars_mod =['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
vars_mod = ['PREC', 'T2MAX', 'T2MIN', 'T2MEAN']
sdt = '197001'
#edt = '209912'
edt = '197912'
#--- GHCND data downloaded through June 2019, so 2019 is a partial year, so
#--- only include obs through 2018.
#edt_obs = '201812'
edt_obs = '197912'
var_obs_dict = {
'PREC': 'PRCP',
'T2MAX': 'TMAX',
'T2MIN': 'TMIN',
'T2MEAN': 'T2MEAN'
}
stats_dict = {
'PREC': ['max', 'tot'],
'T2MAX': ['max', 'avg'],
'T2MIN': ['min', 'max', 'avg'],
'T2MEAN': ['min', 'max', 'avg'],
}
#models = ['access1.0', 'access1.3', 'bcc-csm1.1', 'canesm2', \
# 'ccsm4', 'csiro-mk3.6.0', 'fgoals-g2', 'gfdl-cm3', \
# 'giss-e2-h', 'miroc5', 'mri-cgcm3', 'noresm1-m']
models = ['access1.0', 'access1.3']
mod_cols = ['indigo', 'blue', 'deepskyblue', \
'darkgreen', 'lime', 'yellow', \
'magenta', 'red', 'salmon', 'gray', 'darkgray', 'lightblue']
#---------------------------------------------------------------------------
# Load stations file.
#---------------------------------------------------------------------------
stns = []
latpts = []
lonpts = []
elevs = []
with open(station_file) as csv_file:
csv_reader = csv.reader(csv_file, delimiter=',')
line_count = 0
for row in csv_reader:
stns.append(row[0])
latpts.append(float(row[1]))
lonpts.append(float(row[2]))
elevs.append(float(row[3]))
##---------------------------------------------------------------------------
## Load GHCND obs.
##---------------------------------------------------------------------------
#vars_obs = []
#for var in vars_mod:
# vars_obs.append(var_obs_dict[var])
#
#pf = pickle_dir + '/obs_daily_test.pkl'
#if os.path.isfile(pf):
# print 'Loading ', pf
# (obs_all, obs_dts_all, stns, latpts, lonpts, elevs) = \
# pickle.load(open(pf, 'rb'))
#else:
# (obs_all, obs_dts_all) = read_ghcnd(stns, vars_obs, ghcnd_dir, sdt, edt_obs)
# print 'Creating ', pf
# pickle.dump((obs_all, obs_dts_all, stns, latpts, lonpts, elevs), \
# open(pf,'wb'), -1)
#
#---------------------------------------------------------------------------
# Load model data.
#---------------------------------------------------------------------------
#pf_all = pickle_dir + '/mod_daily.pkl'
pf_all = 'junk'
if os.path.isfile(pf_all):
print 'Loading ', pf_all
(mod_all, mod_dts_all) = pickle.load(open(pf_all, 'rb'))
else:
mod_all = {}
mod_dts_all = []
for m in range(len(models)):
model = models[m]
pf = get_mod_pkl_name(pickle_dir, model, sdt, edt)
print 'Loading ', pf
(model, data_c, dts_unique, vars, stns) = pickle.load(open(pf, 'rb'))
mod_all[model] = data_c
mod_dts_all = mod_dts_all + dts_unique
# print 'Creating ', pf_all
# pickle.dump((mod_all, mod_dts_all), open(pf_all,'wb'), -1)
#---------------------------------------------------------------------------
#
#---------------------------------------------------------------------------
pf = pickle_dir + '/cei_daily_perc_mxmn.pkl'
if os.path.isfile(pf):
(daily_perc_mxmn) = pickle.load(open(pf, 'rb'))
else:
daily_perc_mxmn = get_daily_perc_mxmn(models, stns, mod_all, sdt)
print 'Creating ', pf
pickle.dump((daily_perc_mxmn), open(pf,'wb'), -1)
test = get_monthly_mxmn(daily_perc_mxmn, mod_all, models, stns, sdt)
sys.exit()
<file_sep>/igtg_check_utils.py
#!/usr/bin/python
import os, os.path, time, glob, re, math
import sys, string, readline, h5py
from Scientific.IO.NetCDF import *
import numpy as np
#from datetime import datetime, timedelta, time
from datetime import datetime
import matplotlib as mpl
mpl.use('Agg')
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
#from rw_plotsettings import *
import pickle
colorbar_lab = 'm/s';
maplw = 0.8
#levs_spd = [ 0.0, 2.5, 5.0, 7.5, 10.0, 12.5, 15.0, 17.5, 20.0, 22.5,
# 25.0, 27.5, 30.0, 32.5, 35.0, 37.5 ]
levs_spd = [ -24, -21, -18, -15, -12, -9, -6, -3, 3, 6, 9, 12, 15, 18, \
21, 24]
cmap_spd = [(255,255,255), \
(192,192,192), \
(128,128,128 ), \
(0,255,255), \
(32,178,170), \
(0,255,0), \
(0,128,0), \
(255,0,204), \
(199,21,133), \
(0,0,255), \
(0,0,128), \
(255,255,0), \
(255,204,17), \
(255,69,0), \
(0,0,0),\
(255,255,255)]
levs_tos = [ 264, 266, 268, 270, 272, 274, 276, 278, 280, 282, 284, 286, \
288, 290, 292, 294]
cmap_tos = [(255,255,255), \
(192,192,192), \
(128,128,128 ), \
(0,255,255), \
(32,178,170), \
(0,255,0), \
(0,128,0), \
(255,0,204), \
(199,21,133), \
(0,0,255), \
(0,0,128), \
(255,255,0), \
(255,204,17), \
(255,69,0), \
(0,0,0),\
(255,255,255)]
#---------------------------------------------------------------------------
# Load 2-D lat/lon netcdf data file.
#---------------------------------------------------------------------------
def load_nc(filein, varname, itime, ilev):
print 'opening ', filein
f = NetCDFFile(filein, 'r')
ll_shape = f.variables['lat'].shape
data_all = f.variables[varname]
fillval = data_all._FillValue[0]
ndims = len(f.dimensions)
dims = f.dimensions
dimnames = f.dimensions.keys()
for d in range(ndims):
print d, dimnames[d], dims[dimnames[d]]
#--- Find ny dimension.
if (dimnames[d] == 'lat'):
ny = dims[dimnames[d]]
elif (dimnames[d] == 'j'):
ny = dims[dimnames[d]]
#--- Find nx dimension.
if (dimnames[d] == 'lon'):
nx = dims[dimnames[d]]
elif (dimnames[d] == 'i'):
nx = dims[dimnames[d]]
#--- Fill in 2-D lat / lon terr arrays, if needed (if read-in
#--- lat/lon are 1-D).
if (len(ll_shape) == 1):
lon_tmp = f.variables['lon'][:]
lat_tmp = f.variables['lat'][:]
lonout = np.empty([nx,ny])
latout = np.empty([nx,ny])
for y in range(ny):
latout[:,y] = lat_tmp[y]
for x in range(nx):
lonout[x,:] = lon_tmp[x]
elif (len(ll_shape) == 2):
lonout = f.variables['lon'][:,:]
latout = f.variables['lat'][:,:]
lonout = np.transpose(lonout)
latout = np.transpose(latout)
#--- Convert from 0-to-360 longitudes to -180-to-180.
rows,cols = np.where(lonout >= 180.0)
lonout[rows,cols] = lonout[rows,cols] - 360.0
#--- If data has 3 dimensions, assume it's (nx,ny,time).
if (len(data_all.shape) == 3):
data_tmp = f.variables[varname][itime,:,:]
#--- If data has 4 dimensions, assume it's (nx,ny,time,levs).
elif (len(data_all.shape) == 4):
data_tmp = f.variables[varname][itime,ilev,:,:]
f.close()
#--- transpose data array to be x/y (lon/lat).
dataout = np.transpose(data_tmp)
dataout[dataout == fillval] = np.NAN
# print 'latout.shape = ', latout.shape
# print 'lonout.shape = ', lonout.shape
# print 'dataout.shape = ', dataout.shape
#
# sys.exit()
return latout, lonout, dataout
##---------------------------------------------------------------------------
## Load netcdf data file.
##---------------------------------------------------------------------------
#def load_nc(filein, varname, itime, ilev):
# file = NetCDFFile(filein, 'r')
# lat_tmp = file.variables['lat'][:]
# lon_tmp = file.variables['lon'][:]
# data_all = file.variables[varname]
# fillval = data_all._FillValue[0]
# data_tmp = file.variables[varname][itime,ilev,:,:]
# file.close()
#
# #--- Convert from 0-to-360 longitudes to -180-to-180.
# ipos = np.where(lon_tmp >= 180.0)
# iwest = ipos[0]
# lon_tmp[iwest] = lon_tmp[iwest] - 360.0
#
# #--- transpose data array to be x/y (lon/lat).
# dataout = np.transpose(data_tmp)
# dataout[dataout == fillval] = np.NAN
#
# #--- Fill in 2-D lat / lon terr arrays.
# nx = len(lon_tmp)
# ny = len(lat_tmp)
#
# lonout = np.empty([nx,ny])
# latout = np.empty([nx,ny])
# for y in range(ny):
# latout[:,y] = lat_tmp[y]
# for x in range(nx):
# lonout[x,:] = lon_tmp[x]
#
# return latout, lonout, dataout
#---------------------------------------------------------------------------
# Mapper.
#---------------------------------------------------------------------------
def mapper(lat, lon, grid, minlat, maxlat, minlon, maxlon, \
levs, cmap, maplw, colorbar_lab, title, plotfname):
ur_lat = maxlat
ur_lon = maxlon
ll_lat = minlat
ll_lon = minlon
lat_ctr = ll_lat + ((ur_lat - ll_lat) * 0.5)
lon_ctr = ll_lon + ((ur_lon - ll_lon) * 0.5)
width = 6.5
height = 6.5
fs = 9
titlefs = 9
fig = plt.figure(figsize=(width,height))
# left, bottom, width, height:
ax = fig.add_axes([0.00,0.05,0.99,0.91])
#---
# map = Basemap(projection='ortho',lat_0=45,lon_0=-100,resolution='l')
map = Basemap(resolution='i',projection='lcc',\
llcrnrlon= ll_lon, llcrnrlat=ll_lat,\
urcrnrlon= ur_lon, urcrnrlat= ur_lat,\
lat_0=lat_ctr,lon_0=lon_ctr,lat_1=(ur_lat - ll_lat))
#--- Get lat and lon data in map's x/y coordinates.
x,y = map(lon, lat)
#--- Draw coastlines, country boundaries, fill continents.
map.drawcoastlines(linewidth = maplw)
map.drawstates(linewidth = maplw)
map.drawcountries(linewidth = maplw)
#--- Draw the edge of the map projection region (the projection limb)
map.drawmapboundary(linewidth = maplw)
#--- Draw lat/lon grid lines every 30 degrees.
map.drawmeridians(np.arange(0, 360, 30), linewidth = maplw)
map.drawparallels(np.arange(-90, 90, 30), linewidth = maplw)
cs = plt.contourf(x, y, grid, levs, cmap=cmap)
cbar = map.colorbar(cs, location='bottom', pad="3%", size=0.1, ticks=levs)
cbar.set_label(colorbar_lab, fontsize=fs, size=fs-1)
cbar.ax.tick_params(labelsize=fs-1)
plt.title(title, fontsize=titlefs, fontweight='bold')
#--- Save plot.
print 'creating ', plotfname
plt.savefig(plotfname)
plt.close()
<file_sep>/old/old_old/utils_wrfout_20180117.py
#!/usr/bin/python
import os, sys, glob, re
import numpy as np
from netCDF4 import Dataset as NetCDFFile
import matplotlib.pyplot as plt
#from datetime import date, timedelta, datetime
#import time, stat
#from collections import defaultdict
#from utils_date import *
#from mpl_toolkits.basemap import Basemap, cm
mm2in = 0.0393700787
min_season_days = 85
fs = 9
titlefs = 9
width = 10
height = 6
#---------------------------------------------------------------------------
# Load geo_em file.
#---------------------------------------------------------------------------
def load_geo_em(geo_em):
nc = NetCDFFile(geo_em, 'r')
lat = nc.variables['XLAT_M'][0,:,:]
lon = nc.variables['XLONG_M'][0,:,:]
lat = np.transpose(lat)
lon = np.transpose(lon)
nc.close()
return lat, lon
#---------------------------------------------------------------------------
# Load wrfout file.
#---------------------------------------------------------------------------
def load_wrfout(wrfout, var):
nc = NetCDFFile(wrfout, 'r')
ntimes = len(nc.dimensions['Time'])
dataout = nc.variables[var][:,:,:]
dataout = np.transpose(dataout)
#--- Get date from global attributes and clean it up and trim it down.
dt_all = nc.START_DATE
dt = dt_all.replace('-', '')
dt = dt.replace(':', '')
dt = dt.replace('_', '')
dt = dt[0:10]
nc.close()
return dataout, ntimes, dt
#---------------------------------------------------------------------------
# Get list of extracted wrfout files between sdt_yyyymm and edt_yyyymm.
#---------------------------------------------------------------------------
def get_extract_files(data_dir, model, sdt_yyyymm, edt_yyyymm):
files_out = []
files = glob.glob(data_dir + '/' + model + '*' + '.nc')
for f in range(len(files)):
dt_c = re.findall(r'\.(\d{10})\.', files[f])
if (dt_c):
dt_c = dt_c[0]
yyyymm_c = dt_c[0:6]
if (yyyymm_c >= sdt_yyyymm and yyyymm_c <= edt_yyyymm):
files_out.append(files[f])
return files_out
#---------------------------------------------------------------------------
# Get seasonal totals.
#---------------------------------------------------------------------------
def get_seasonal_totals(data_all, dts_all, models, stns):
springs = {}
summers = {}
falls = {}
winters = {}
years_all = []
for s in range(len(stns)):
stn = stns[s]
for m in range(len(models)):
mod = models[m]
spring_ndays = {}
summer_ndays = {}
fall_ndays = {}
winter_ndays = {}
years_mod_stn = []
for d in range(len(dts_all)):
yyyy = dts_all[d][0:4]
mm = dts_all[d][4:6]
years_mod_stn.append(yyyy)
#--- Spring.
if (mm == '03' or mm == '04' or mm == '05'):
#--- Count number of days for this year's spring.
if ((yyyy) in spring_ndays):
spring_ndays[yyyy] += 1
else:
spring_ndays[yyyy] = 1
if ((mod,stn,yyyy) in springs):
springs[mod,stn,yyyy] = springs[mod,stn,yyyy] + \
data_all[mod,stn,dts_all[d]]
else:
springs[mod,stn,yyyy] = data_all[mod,stn,dts_all[d]]
#--- Summer.
if (mm == '06' or mm == '07' or mm == '08'):
#--- Count number of days for this year's summer.
if ((yyyy) in summer_ndays):
summer_ndays[yyyy] += 1
else:
summer_ndays[yyyy] = 1
if ((mod,stn,yyyy) in summers):
summers[mod,stn,yyyy] = summers[mod,stn,yyyy] + \
data_all[mod,stn,dts_all[d]]
else:
summers[mod,stn,yyyy] = data_all[mod,stn,dts_all[d]]
#--- Fall.
if (mm == '09' or mm == '10' or mm == '11'):
#--- Count number of days for this year's fall.
if ((yyyy) in fall_ndays):
fall_ndays[yyyy] += 1
else:
fall_ndays[yyyy] = 1
if ((mod,stn,yyyy) in falls):
falls[mod,stn,yyyy] = falls[mod,stn,yyyy] + \
data_all[mod,stn,dts_all[d]]
else:
falls[mod,stn,yyyy] = data_all[mod,stn,dts_all[d]]
#--- Winter, December only. Put December winter data into
#--- next year's winter-- naming winters by their Jan/Feb
#--- year rather than their Dec year.
if (mm == '12'):
yyyyw = str(int(yyyy) + 1)
#--- Count number of days for this year's winter.
if ((yyyyw) in winter_ndays):
winter_ndays[yyyyw] += 1
else:
winter_ndays[yyyyw] = 1
if ((mod,stn,yyyyw) in winters):
winters[mod,stn,yyyyw] = winters[mod,stn,yyyyw] + \
data_all[mod,stn,dts_all[d]]
else:
winters[mod,stn,yyyyw] = data_all[mod,stn,dts_all[d]]
#--- Winter, Jan & Feb.
if (mm == '01' or mm == '02'):
#--- Count number of days for this year's winter.
if ((yyyy) in winter_ndays):
winter_ndays[yyyy] += 1
else:
winter_ndays[yyyy] = 1
if ((mod,stn,yyyy) in winters):
winters[mod,stn,yyyy] = winters[mod,stn,yyyy] + \
data_all[mod,stn,dts_all[d]]
else:
winters[mod,stn,yyyy] = data_all[mod,stn,dts_all[d]]
years = list(sorted(set(years_mod_stn)))
for y in range(len(years)):
yyyy = years[y]
if (spring_ndays[yyyy] < min_season_days):
springs[mod,stn,yyyy] = np.nan
if (summer_ndays[yyyy] < min_season_days):
summers[mod,stn,yyyy] = np.nan
if (fall_ndays[yyyy] < min_season_days):
falls[mod,stn,yyyy] = np.nan
if (winter_ndays[yyyy] < min_season_days):
winters[mod,stn,yyyy] = np.nan
years_all.append(years_mod_stn)
years = list(sorted(set(years_all[0])))
return springs, summers, falls, winters, years
#---------------------------------------------------------------------------
# Make time series for seasonsal total precip for a set of stations.
#---------------------------------------------------------------------------
def ts(winters, springs, summers, falls, years, stns, mod, titlein, plotfname):
fig, ax = plt.subplots( figsize=(10,6) )
title1 = mod + ', ' + str(years[0]) + ' - ' + str(years[len(years)-1])
xlabs = []
for s in range(len(stns)):
ptot = []
stn = stns[s]
print stn
for y in range(len(years)):
yyyy = years[y]
ptot.append(winters[mod,stns[s],yyyy] * mm2in)
ptot.append(springs[mod,stns[s],yyyy] * mm2in)
ptot.append(summers[mod,stns[s],yyyy] * mm2in)
ptot.append(falls[mod,stns[s],yyyy] * mm2in)
if (s == 0):
xlabs.append('Winter ' + years[y])
xlabs.append('Spring ' + years[y])
xlabs.append('Summer ' + years[y])
xlabs.append('Fall ' + years[y])
print stn, ptot
plt.plot(ptot, label = stn)
plt.ylim([0, 40.0])
plt.xlabel('Season', fontsize=fs+1)
plt.ylabel('Forecast Seasonal Total Precipitation (in.)', fontsize=fs+1)
plt.xticks(range(0,len(xlabs)))
plt.tick_params(axis='x', which='major', labelsize=fs-1)
plt.tick_params(axis='y', which='major', labelsize=fs+1)
plt.title(title1, fontsize=titlefs, fontweight='bold')
ax.set_xticklabels( xlabs, rotation=90)
plt.tight_layout()
plt.grid()
plt.legend(fontsize = fs, loc = 'best')
print 'creating ', plotfname
plt.savefig(plotfname)
return 1
#---------------------------------------------------------------------------
# Interpolate from a sent-in grid to a sent in lat/lon point.
#---------------------------------------------------------------------------
def bilinear_interpolate(latgrid, longrid, datagrid, latpt, lonpt, deb):
totdiff = abs(latgrid - latpt) + abs(longrid - lonpt)
(i,j) = np.unravel_index(totdiff.argmin(), totdiff.shape)
#--- Get lat/lon box in which latpt,lonpt resides.
if (latpt >= latgrid[i,j] and lonpt >= longrid[i,j]):
iif = i
jjf = j
elif (latpt < latgrid[i,j] and lonpt < longrid[i,j]):
iif = i-1
jjf = j-1
elif (latpt >= latgrid[i,j] and lonpt < longrid[i,j]):
iif = i-1
jjf = j
elif (latpt < latgrid[i,j] and lonpt >= longrid[i,j]):
iif = i
jjf = j-1
(nx, ny) = np.shape(latgrid)
if (deb == 1):
print 'nx, ny = ', nx, ny
print 'iif,jjf = ', iif,jjf
print 'latgrid[iif+1,jjf] = ', latgrid[iif+1,jjf]
print 'latgrid[iif,jjf] = ', latgrid[iif,jjf]
if (iif >= (nx-1) or jjf >= (ny-1) or iif < 0 or jjf < 0):
return mvc
#--- Do bilinear interpolation to latpt,lonpt.
dlat = latgrid[iif,jjf+1] - latgrid[iif,jjf]
dlon = longrid[iif+1,jjf] - longrid[iif,jjf]
dslat = latgrid[iif,jjf+1] - latpt
dslon = longrid[iif+1,jjf] - lonpt
wrgt = 1 - (dslon/dlon)
wup = 1 - (dslat/dlat)
vll = datagrid[iif,jjf]
vlr = datagrid[iif,jjf+1]
vul = datagrid[iif+1,jjf]
vur = datagrid[iif+1,jjf+1]
if (deb > 1):
print 'll lat, lon, val = ', latgrid[iif,jjf], longrid[iif,jjf], vll
print 'lr lat, lon, val = ', latgrid[iif+1,jjf], longrid[iif+1,jjf], vlr
print 'ur lat, lon, val = ', latgrid[iif+1,jjf+1], longrid[iif+1,jjf+1],vur
print 'ul lat, lon, val = ', latgrid[iif,jjf+1], longrid[iif,jjf+1], vul
print 'latpt, lonpt = ', latpt, lonpt
print 'vll = ', vll
print 'vlr = ', vlr
print 'vul = ', vul
print 'vur = ', vur
datout = (1-wrgt) * ((1-wup) * vll + wup * vul) + \
(wrgt) * ((1-wup) * vlr + wup * vur)
# if (deb == 1):
# print 'datout = ', datout
# sys.exit()
# if (datout == 0.0):
# print 'nx, ny = ', nx, ny
# print 'iif,jjf = ', iif,jjf
# print 'latgrid[iif+1,jjf] = ', latgrid[iif+1,jjf]
# print 'latgrid[iif,jjf] = ', latgrid[iif,jjf]
#
# print 'll lat, lon, val = ', latgrid[iif,jjf], longrid[iif,jjf], vll
# print 'lr lat, lon, val = ', latgrid[iif+1,jjf], longrid[iif+1,jjf], vl#r
# print 'ur lat, lon, val = ', latgrid[iif+1,jjf+1], longrid[iif+1,jjf+1],vur
# print 'ul lat, lon, val = ', latgrid[iif,jjf+1], longrid[iif,jjf+1], vu#l
# print 'latpt, lonpt = ', latpt, lonpt
#
# print 'vll = ', vll
# print 'vlr = ', vlr
# print 'vul = ', vul
# print 'vur = ', vur
#
# sys.exit()
return datout
<file_sep>/plot_wrfout_stns.py~
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
from utils_wrfout import *
from utils_date import *
from utils_ghcnd_obs import *
import cPickle as pickle #-- cPickle is faster, but deprecated in python 3.0
import csv
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
#plot_dir = rcm_dir + '/plots'
plot_dir = rcm_dir + '/plots/new'
pickle_dir = rcm_dir + '/pickle'
data_dir = rcm_dir + '/data'
obs_dir = rcm_dir + '/obs'
ghcnd_dir = obs_dir + '/ghcnd_all'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
geo_em = data_dir + '/geo_em.d02.nc'
station_file = obs_dir + '/station_file.txt'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#vars_mod =['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
vars_mod = ['PREC', 'T2MAX', 'T2MIN', 'T2MEAN']
sdt = '197001'
edt = '209912'
#--- GHCND data downloaded through June 2019, so 2019 is a partial year, so
#--- only include obs through 2018.
edt_obs = '201812'
var_obs_dict = {
'PREC': 'PRCP',
'T2MAX': 'TMAX',
'T2MIN': 'TMIN',
'T2MEAN': 'T2MEAN'
}
stats_dict = {
'PREC': ['max', 'tot'],
'T2MAX': ['max', 'avg'],
'T2MIN': ['min', 'max', 'avg'],
'T2MEAN': ['min', 'max', 'avg'],
}
models = ['access1.0', 'access1.3', 'bcc-csm1.1', 'canesm2', \
'ccsm4', 'csiro-mk3.6.0', 'fgoals-g2', 'gfdl-cm3', \
'giss-e2-h', 'miroc5', 'mri-cgcm3', 'noresm1-m']
mod_cols = ['indigo', 'blue', 'deepskyblue', \
'darkgreen', 'lime', 'yellow', \
'magenta', 'red', 'salmon', 'gray', 'darkgray', 'lightblue']
#---------------------------------------------------------------------------
# Load stations file.
#---------------------------------------------------------------------------
stns = []
latpts = []
lonpts = []
elevs = []
with open(station_file) as csv_file:
csv_reader = csv.reader(csv_file, delimiter=',')
line_count = 0
for row in csv_reader:
stns.append(row[0])
latpts.append(float(row[1]))
lonpts.append(float(row[2]))
elevs.append(float(row[3]))
#---------------------------------------------------------------------------
# Load GHCND obs.
#---------------------------------------------------------------------------
load_obs_all = 0
pickle_file = pickle_dir + '/obs_stats.pkl'
if load_obs_all == 0:
#--- If not loading all obs, just load stats pickle file.
if os.path.isfile(pickle_file):
print 'reading pickle file ', pickle_file
(obs,yy_obs) = pickle.load(open(pickle_file, 'rb'))
else:
sys.exit('cannot see ' + pickle_file)
else:
vars_obs = []
for var in vars_mod:
vars_obs.append(var_obs_dict[var])
#--- Load GHCND obs; get seasonal stats on them.
(data_all, dts_all) = read_ghcnd(stns, vars_obs, ghcnd_dir, sdt, edt_obs)
obs = {}
yy_obs = {}
for var in vars_mod:
varo = var_obs_dict[var]
stats = stats_dict[var]
for stat in stats:
(obs[varo,stat], yy_obs[varo,stat]) = get_seasonal_stats_ghcnd(\
data_all,dts_all,stns,varo,stat)
pickle.dump((obs,yy_obs), open(pickle_file,'wb'), -1)
#---------------------------------------------------------------------------
# Load model data.
#---------------------------------------------------------------------------
load_mod_all = 0
pf_mod_stats = pickle_dir + '/mod_stats.pkl'
if load_mod_all == 0:
#--- If not loading all model data, just load stats pickle file.
if os.path.isfile(pf_mod_stats):
print 'reading pickle file ', pf_mod_stats
(mod,yy_mod) = pickle.load(open(pf_mod_stats, 'rb'))
else:
sys.exit('cannot see ' + pf_mod_stats)
else:
# (data_all, dts_unique, models, vars_all, stns) = \
# load_extract_data(geo_em, stns, latpts, lonpts, elevs, models, \
# data_dirs, sdt, edt, vars_mod, pickle_dir, 0)
data_all = {}
dts_all = []
for m in range(len(models)):
model = models[m]
pf = get_mod_pkl_name(pickle_dir, model, sdt, edt)
print 'loading ', pf
(model, data_c, dts_unique, vars, stns) = pickle.load(open(pf, 'rb'))
data_all[model] = data_c
dts_all = dts_all + dts_unique
mod = {}
yy_mod = {}
for var in vars_mod:
stats = stats_dict[var]
for stat in stats:
(mod[var,stat], yy_mod[var,stat]) = get_seasonal_stats(\
data_all, dts_unique, models, stns, var, stat)
pickle.dump((mod,yy_mod), open(pf_mod_stats,'wb'), -1)
#---------------------------------------------------------------------------
#
#---------------------------------------------------------------------------
'''
var = 'T2MEAN'
stat = 'max'
statplot = mod[(var,stat)]
stn = 'KSEA'
season = 'annual'
for m in range(len(models)):
mod = models[m]
print '-----'
yyyy = '2097'
key = (season,mod,stn,yyyy)
print mod, var, stat, stn, yyyy, season, statplot[key]
yyyy = '2098'
key = (season,mod,stn,yyyy)
print mod, var, stat, stn, yyyy, season, statplot[key]
yyyy = '2099'
key = (season,mod,stn,yyyy)
print mod, var, stat, stn, yyyy, season, statplot[key]
#sys.exit()
'''
#---------------------------------------------------------------------------
# Make a time series plots of each station, variable, stat and season.
#---------------------------------------------------------------------------
#--- Do multiple models on a plot for one station.
for ss in range(len(stns)):
stn = stns[ss]
for v in range(len(vars_mod)):
var = vars_mod[v]
varo = var_obs_dict[var]
stats = stats_dict[var]
for st in range(len(stats)):
stat = stats[st]
for s in range(len(seasons)):
season = seasons[s]
plotfname = get_plotfname(plot_dir,stn,sdt,edt,season,var,stat)
titlein = get_title(season,stn,var,stat,yy_mod[var,stat])
iret = ts_mods(mod[var,stat],yy_mod[var,stat], \
obs[varo,stat],yy_obs[varo,stat], stn, \
models, titlein, plotfname, var, stat, \
mod_cols, [season])
# sys.exit()
# sys.exit()
sys.exit()
<file_sep>/old/old_old/plot_wrfout_stns_20181108.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
from utils_ghcnd_obs import *
import pickle
import csv
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
data_dir = rcm_dir + '/data'
obs_dir = rcm_dir + '/obs'
ghcnd_dir = obs_dir + '/ghcnd_all'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
geo_em = data_dir + '/geo_em.d02.nc'
station_file = obs_dir + '/station_file.txt'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#vars_mod =['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
vars_mod = ['PREC', 'T2MAX', 'T2MIN']
sdt = '197001'
edt = '209912'
var_obs_dict = {
'PREC': 'PRCP',
'T2MAX': 'TMAX',
'T2MIN': 'TMIN'
}
stats_dict = {
'PREC': ['max', 'tot'],
'T2MAX': ['max', 'avg'],
'T2MIN': ['min', 'max', 'avg']
}
models = ['gfdl-cm3', 'miroc5', 'bcc-csm1.1', \
'access1.3', 'canesm2', 'noresm1-m', \
'ccsm4', 'csiro-mk3.6.0', 'fgoals-g2' ]
mod_cols = ['indigo', 'blue', 'deepskyblue', \
'darkgreen', 'lime', 'yellow', \
'magenta', 'red', 'salmon']
stns = []
latpts = []
lonpts = []
elevs = []
with open(station_file) as csv_file:
csv_reader = csv.reader(csv_file, delimiter=',')
line_count = 0
for row in csv_reader:
stns.append(row[0])
latpts.append(float(row[1]))
lonpts.append(float(row[2]))
elevs.append(float(row[3]))
stn_cols = ['b', 'r', 'g', 'c', 'm', 'k', 'grey']
#---------------------------------------------------------------------------
# Load obs.
#---------------------------------------------------------------------------
load_obs_all = 0
pickle_file = pickle_dir + '/obs_stats.pickle'
if load_obs_all == 0:
#--- If not loading all obs, just load stats pickle file.
if os.path.isfile(pickle_file):
print 'reading pickle file ', pickle_file
(obs,yy_obs) = pickle.load(open(pickle_file, 'rb'))
else:
sys.exit('cannot see ' + pickle_file)
else:
vars_obs = []
for var in vars_mod:
vars_obs.append(var_obs_dict[var])
#--- Load GHCND obs; get seasonal stats on them.
(data_all, dts_all) = read_ghcnd(stns, vars_obs, ghcnd_dir, sdt, edt)
obs = {}
yy_obs = {}
for var in vars_mod:
varo = var_obs_dict[var]
stats = stats_dict[var]
for stat in stats:
(obs[varo,stat], yy_obs[varo,stat]) = get_seasonal_stats_ghcnd(\
data_all,dts_all,stns,varo,stat)
pickle.dump((obs,yy_obs), open(pickle_file,'wb'), -1)
#---------------------------------------------------------------------------
# Load model data.
#---------------------------------------------------------------------------
load_mod_all = 1
pickle_file = pickle_dir + '/mod_stats.pickle'
if load_mod_all == 0:
#--- If not loading all model data, just load stats pickle file.
if os.path.isfile(pickle_file):
print 'reading pickle file ', pickle_file
(mod,yy_mod) = pickle.load(open(pickle_file, 'rb'))
else:
sys.exit('cannot see ' + pickle_file)
else:
pickle_file_all = pickle_dir + '/extract_' + sdt + '_' + edt + '.pickle'
pickle_file_all = 'testjunk'
print 'reading pickle file ', pickle_file_all
if os.path.isfile(pickle_file_all):
(data_all, dts_unique, models, vars, stns) = \
pickle.load(open(pickle_file_all, 'rb'))
print 'done!'
else:
(data_all, dts_unique, models, vars_all, stns) = \
load_extract_data(geo_em, stns, latpts, lonpts, elevs, \
models, data_dirs, sdt, edt, vars_mod, \
pickle_dir, 0)
mod = {}
yy_mod = {}
for var in vars_mod:
stats = stats_dict[var]
for stat in stats:
(mod[var,stat], yy_mod[var,stat]) = get_seasonal_stats(\
data_all,dts_unique,models,stns,var,stat)
pickle.dump((mod,yy_mod), open(pickle_file,'wb'), -1)
#var = 'T2MAX'
#stat = 'avg'
#key = (var,stat)
#test = mod[key]
#years = yy_mod[key]
#season = 'winter'
##model = 'bcc-csm1.1'
#model = 'gfdl-cm3'
#stn = 'KSEA'
#
#for yyyy in years:
# key = (season,model,stn,yyyy)
# dat_c = test[key]
# print yyyy, dat_c
#for key in mod:
# print key, mod[key]
sys.exit()
#---------------------------------------------------------------------------
# Get seasonal precipitation totals and make a time series plot of them.
#---------------------------------------------------------------------------
##--- Do multiple stations on a plot for one model.
#mod = 'miroc5'
#for s in range(len(seasons)):
# season = seasons[s]
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_tot.png'
# titlein = 'Total ' + seasons_lab[season] + ' Precipitation, ' + \
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_stns(pcp_tot, years, stns, mod, titlein, plotfname, 'PREC', \
# 'tot', stn_cols, [season])
#
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_max.png'
# titlein = 'Maximum 24-h ' + seasons_lab[season] + ' Precipitation, ' + \
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_stns(pcp_max, years, stns, mod, titlein, plotfname, 'PREC', \
# 'max', stn_cols, [season])
#--- Do multiple models on a plot for one station.
for ss in range(len(stns)):
stn = stns[ss]
for v in range(len(vars_mod)):
var = vars_mod[v]
varo = var_obs_dict[var]
stats = stats_dict[var]
for st in range(len(stats)):
stat = stats[st]
for s in range(len(seasons)):
season = seasons[s]
plotfname = get_plotfname(plot_dir,stn,sdt,edt,season,var,stat)
titlein = get_title(season,stn,var,stat,yy_mod[var,stat])
iret = ts_mods(mod[var,stat],yy_mod[var,stat], \
obs[varo,stat],yy_obs[varo,stat], stn, \
models, titlein, plotfname, var, stat, \
mod_cols, [season])
# sys.exit()
sys.exit()
#---------------------------------------------------------------------------
# Get seasonal temperature maxes and mins.
#---------------------------------------------------------------------------
#--- Do multiple stations on a plot for one model.
#mod = 'miroc5'
#for s in range(len(seasons)):
# season = seasons[s]
#
# #--- Max temperature.
# var = 'T2MAX'
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_max.png'
# titlein = 'Maximum Seasonal Temperature, ' + seasons_lab[season] + ', ' +\
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_stns(tmx_max, years, stns, mod, titlein, plotfname, var, 'max', \
# stn_cols, [season])
#
# #--- Min temperature.
# var = 'T2MIN'
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_min.png'
# titlein = 'Minimum Seasonal Temperature, ' + seasons_lab[season] + ', ' +\
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_stns(tmn_min, years, stns, mod, titlein, plotfname, var, 'min', \
# stn_cols, [season])
#
#sys.exit()
#
##--- Do multiple models on a plot for one station.
#for ss in range(len(stns)):
# stn = stns[ss]
# for s in range(len(seasons)):
# season = seasons[s]
#
# #--- Max temperature.
# var = 'T2MAX'
# plotfname = plot_dir + '/' + stn + '_' + sdt + '_' + edt + '_' + \
# season + '_' + var + '_max.png'
# titlein = 'Maximum Seasonal Temperature, ' + seasons_lab[season] + \
# ', ' + stn.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_mods(tmx_max, years, stn, models, titlein, plotfname, \
# var, 'max', stn_cols, [season])
#
# #--- Min temperature.
# var = 'T2MIN'
# plotfname = plot_dir + '/' + stn + '_' + sdt + '_' + edt + '_' + \
# season + '_' + var + '_min.png'
# titlein = 'Minimum Seasonal Temperature, ' + seasons_lab[season] + \
# ', ' + stn.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_mods(tmn_min, years, stn, models, titlein, plotfname, \
# var, 'min', stn_cols, [season])
#
##---------------------------------------------------------------------------
## Get seasonal temperature averages.
##---------------------------------------------------------------------------
#(tmx_avg, years) = get_seasonal_stats(data_all, dts_unique, models, stns, \
# 'T2MAX', 'avg')
#(tmn_avg, years) = get_seasonal_stats(data_all, dts_unique, models, stns, \
# 'T2MIN', 'avg')
#
##--- Do multiple stations on a plot for one model.
#mod = 'gfdl-cm3'
#for s in range(len(seasons)):
# season = seasons[s]
#
# #--- Max temperature.
# var = 'T2MAX'
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_avg.png'
# titlein = 'Average Maximum Temperature, ' + seasons_lab[season] + ', ' +\
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_stns(tmx_avg, years, stns, mod, titlein, plotfname, var, 'avg', \
# stn_cols, [season])
#
# #--- Min temperature.
# var = 'T2MIN'
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_avg.png'
# titlein = 'Average Minimum Temperature, ' + seasons_lab[season] + ', ' +\
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_stns(tmn_avg, years, stns, mod, titlein, plotfname, var, 'avg', \
# stn_cols, [season])
#
#
##--- Do multiple models on a plot for one station.
#for ss in range(len(stns)):
# stn = stns[ss]
# for s in range(len(seasons)):
# season = seasons[s]
#
# #--- Max temperature.
# var = 'T2MAX'
# plotfname = plot_dir + '/' + stn + '_' + sdt + '_' + edt + '_' + \
# season + '_' + var + '_avg.png'
# titlein = 'Average Maximum Temperature, ' + seasons_lab[season] + \
# ', ' + stn.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_mods(tmx_avg, years, stn, models, titlein, plotfname, \
# var, 'avg', stn_cols, [season])
#
# #--- Min temperature.
# var = 'T2MIN'
# plotfname = plot_dir + '/' + stn + '_' + sdt + '_' + edt + '_' + \
# season + '_' + var + '_avg.png'
# titlein = 'Average Minimum Temperature, ' + seasons_lab[season] + \
# ', ' + stn.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_mods(tmn_avg, years, stn, models, titlein, plotfname, \
# var, 'avg', stn_cols, [season])
#
#sys.exit()
#
##---------------------------------------------------------------------------
## Wind Speed.
##---------------------------------------------------------------------------
#mod = 'gfdl-cm3'
#var = 'SPDUV10MEAN'
#(spdavg_avg, years) = get_seasonal_stats(data_all, dts_unique, models, stns, \
# var, 'avg')
#for s in range(len(seasons)):
# season = seasons[s]
#
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_avg.png'
# titlein = 'Average Wind Speed, ' + seasons_lab[season] + ', ' +\
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_stns(spdavg_avg, years, stns, mod, titlein, plotfname, var, \
# 'avg', stn_cols, [season])
#
#var = 'SPDUV10MAX'
#(spdmax_max, years) = get_seasonal_stats(data_all, dts_unique, models, stns, \
# var, 'max')
#for s in range(len(seasons)):
# season = seasons[s]
#
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_max.png'
# titlein = 'Maximum Wind Speed, ' + seasons_lab[season] + ', ' +\
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts_stns(spdmax_max, years, stns, mod, titlein, plotfname, var, \
# 'max', stn_cols, [season])
#
#
#sys.exit()
<file_sep>/igtg_check_miroc5_v2.py
#!/usr/bin/python
import os, os.path, time, glob, re, math
import sys, string, readline, h5py
from Scientific.IO.NetCDF import *
import numpy as np
#from datetime import datetime, timedelta, time
from datetime import datetime
import matplotlib as mpl
mpl.use('Agg')
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
#from rw_plotsettings import *
import pickle
import csv
from numpy import genfromtxt
maplw = 0.8
plotfname = './test_pts2.png'
title = 'pts'
#ptsfile = '/home/disk/mass/jbaars/cmip5/rcp8.5/miroc5/test_unstaggertos/pts.txt'
ptsfile = '/home/disk/mass/jbaars/cmip5/rcp8.5/miroc5/test_unstaggertos/pts3.txt'
indices = []
lats = []
lons = []
f = open(ptsfile, 'rt')
try:
reader = csv.reader(f)
for index, lat, lon in reader:
indices.append(float(index))
lats.append(float(lat))
lons.append(float(lon))
finally:
f.close()
maxlat = max(lats) + 0.5
minlat = min(lats) - 0.5
maxlon = max(lons) + 0.5
minlon = min(lons) - 0.5
ur_lat = maxlat
ur_lon = maxlon
ll_lat = minlat
ll_lon = minlon
lat_ctr = ll_lat + ((ur_lat - ll_lat) * 0.5)
lon_ctr = ll_lon + ((ur_lon - ll_lon) * 0.5)
width = 12
height = 4
fs = 9
titlefs = 9
fig = plt.figure(figsize=(width,height))
# left, bottom, width, height:
ax = fig.add_axes([0.00,0.05,0.99,0.91])
#---
map = Basemap(resolution='i',projection='lcc',\
llcrnrlon= ll_lon, llcrnrlat=ll_lat,\
urcrnrlon= ur_lon, urcrnrlat= ur_lat,\
lat_0=lat_ctr,lon_0=lon_ctr,lat_1=(ur_lat - ll_lat))
#--- Draw coastlines, country boundaries, fill continents.
map.drawcoastlines(linewidth = maplw)
map.drawstates(linewidth = maplw)
map.drawcountries(linewidth = maplw)
#--- Draw the edge of the map projection region (the projection limb)
map.drawmapboundary(linewidth = maplw)
#--- Draw lat/lon grid lines every 30 degrees.
map.drawmeridians(np.arange(0, 360, 30), linewidth = maplw)
map.drawparallels(np.arange(-90, 90, 30), linewidth = maplw)
#-- Add dots for grid points for 2nd grid.
for i in range(len(lats)):
xs, ys = map(lons[i], lats[i])
if(indices[i] == 2):
mark = 'gx'
ms = 5.0
plt.plot(xs, ys, mark, markersize = 3, mew=ms)
xs2 = xs
ys2 = ys
if (indices[i] == 1):
mark = 'ro'
ms = 1.0
plt.plot([xs,xs2], [ys,ys2], linewidth=2, color='g')
else:
mark = 'bo'
ms = 1.0
plt.plot(xs, ys, mark, markersize = 6, mew=ms)
# strp = "%d,%d,%.3f" % (ii+1, jj+1, tos_new[ii+1,jj+1])
# plt.text(xs, ys, strp, fontsize=6)
plt.title(title, fontsize=titlefs, fontweight='bold')
#--- Save plot.
print 'creating ', plotfname
plt.savefig(plotfname)
plt.close()
<file_sep>/old/old_old/igtg_check_20161103.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
from igtg_check_utils import *
import matplotlib.pyplot as plt
#import pickle
from make_cmap import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
data_dir = rcm_dir + '/igtg.nml.works.3d.4d'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
varname = 'ua'
file_old = data_dir + '/' + varname + '.1990010106.1991010100.nc'
file_new = data_dir + '/' + varname + '_new.nc'
itime = 1
ilev = 1
title_old = varname + ' OLD, itime = ' + str(itime) + ', ilev = ' + \
str(ilev)
plotfname_old = './' + varname + '_old_itime' + str(itime) + '_ilev' + \
str(ilev) + '.png'
title_new = varname + ' NEW, itime = ' + str(itime) + ', ilev = ' + \
str(ilev)
plotfname_new = './' + varname + '_new_itime' + str(itime) + '_ilev' + \
str(ilev) + '.png'
minlat = 20.0
maxlat = 50.0
minlon = -120.0
maxlon = -50.0
#minlat = 20.0
#maxlat = 90.0
#minlon = -175.0
#maxlon = -85.0
#---------------------------------------------------------------------------
# Make a color map using make_cmap.
#---------------------------------------------------------------------------
levs_norm_spd = []
for i in range(len(levs_spd)):
x = float(levs_spd[i])
norm_c = (x - min(levs_spd)) / (max(levs_spd) - min(levs_spd))
levs_norm_spd.append(norm_c)
my_cmap = make_cmap(cmap_spd, bit = True, position = levs_norm_spd)
#---------------------------------------------------------------------------
# Load old and new files.
#---------------------------------------------------------------------------
(lat_old, lon_old, data_old) = load_nc(file_old, varname, itime, ilev)
(lat_new, lon_new, data_new) = load_nc(file_new, varname, itime, ilev)
#---------------------------------------------------------------------------
# Plot old data.
#---------------------------------------------------------------------------
#mapper(lat_old, lon_old, data_old, minlat, maxlat, minlon, maxlon, \
# levs_spd, my_cmap, maplw, colorbar_lab, title_old, plotfname_old)
#
#sys.exit()
mapper(lat_new, lon_new, data_new, minlat, maxlat, minlon, maxlon, \
levs_spd, my_cmap, maplw, colorbar_lab, title_new, plotfname_new)
sys.exit()
<file_sep>/utils_plot.py
#!/usr/bin/python
import os, sys, glob, re, math
import numpy as np
import matplotlib as mpl
mpl.use('Agg')
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
from utils_cmap import *
from utils_date import *
zooms = ['Z1', 'Z2']
seasons = ['spring', 'summer', 'fall', 'winter', 'annual']
seasons_mo = ['ALL', 'MAM', 'JJA', 'SON', 'DJF']
seasons_lab = {
'annual': 'Jan-Dec',
'spring': 'Mar-Apr-May',
'summer': 'Jun-Jul-Aug',
'fall': 'Sep-Oct-Nov',
'winter': 'Dec-Jan-Feb'
}
var_lab = {
'T2MAX': 'Average Max Temperature ($^\circ$C)',
'T2MIN': 'Average Min Temperature ($^\circ$C)',
'T2MEAN': 'Average Mean Temperature ($^\circ$C)',
'PREC': 'Total Precipitation (in.)',
'SPDUV10MEAN': 'Average Wind Speed (m/s)',
'SPDUV10MAX': 'Maximum Wind Speed (m/s)',
'SNOW': 'Snow Water Equivalent (mm)',
'SWDOWN': 'Shortwave Down Radiation (W/m^2)'
}
ylims = {
'PRECmin': [0, 1.0],
'PRECmax': [0, 5.0],
'PRECavg': [0, 45.0],
'PRECtot': [0, 40.0],
'T2MAXavg': [-5, 40.0],
'T2MAXmax': [0, 50.0],
'T2MINmin': [-30.0, 25.0],
'T2MINmax': [-30.0, 25.0],
'T2MINavg': [-20, 25.0],
'T2MEANmin': [-30.0, 25.0],
'T2MEANmax': [-10.0, 50.0],
'T2MEANavg': [-10.0, 50.0],
'SPDUV10MEANavg': [0, 5.0],
'SPDUV10MAXmax': [0, 20.0],
'SNOWtot': [0, 2000.0]
}
labels = {
'PRECtot': 'Total Precipitation (in.)',
'PRECmax': 'Maximum Precipitation (in.)',
'PRECmin': 'Minimum Precipitation (in.)',
'PRECavg': 'Average Precipitation (in.)',
'T2MAXavg': 'Average Maximum Temperature ($^\circ$C)',
'T2MAXmax': 'Max Maximum Temperature ($^\circ$C)',
'T2MAXmin': 'Min Maximum Temperature ($^\circ$C)',
'T2MINavg': 'Average Minimum Temperature ($^\circ$C)',
'T2MINmax': 'Max Minimum Temperature ($^\circ$C)',
'T2MINmin': 'Min Minimum Temperature ($^\circ$C)',
'T2MEANavg': 'Average Mean Temperature ($^\circ$C)',
'T2MEANmax': 'Max Mean Temperature ($^\circ$C)',
'T2MEANmin': 'Min Mean Temperature ($^\circ$C)',
'SPDUV10MEANavg': 'Average Wind Speed (m/s)',
'SPDUV10MAXmax': 'Maximum Wind Speed (m/s)',
'SNOWtot': 'Snow Water Equivalent (mm)'
}
colorbar_labs = {
'T2MAX': 'Temperature ($^\circ$C)',
'T2MIN': 'Temperature ($^\circ$C)',
'T2MEAN': 'Temperature ($^\circ$C)',
'PREC': 'Precipitation (in.)',
'SPDUV10MEAN': 'Wind Speed (m/s)',
'SPDUV10MAX': 'Wind Speed (m/s)',
'SNOW': 'Snow Water Equivalent (mm)',
'SWDOWN': 'Shortwave Down Radiation (W/m^2)'
}
target_lat = 47.44472
target_lon = -122.31361
fudge_lat = 1.7
fudge_lon = 2.1
minlatZ2 = target_lat - fudge_lat
maxlatZ2 = target_lat + fudge_lat
minlonZ2 = target_lon - fudge_lon
maxlonZ2 = target_lon + fudge_lon
minlat = {
'Z1': 39.0,
'Z2': minlatZ2 }
maxlat = {
'Z1': 51.0,
'Z2': maxlatZ2 }
minlon = {
'Z1': -131.0,
'Z2': minlonZ2 }
maxlon = {
'Z1': -108.0,
'Z2': maxlonZ2 }
fs = 9
titlefs = 9
width = 10
height = 8
maplw = 1.0
#---------------------------------------------------------------------------
# Get plot file name.
#---------------------------------------------------------------------------
def get_plotfname(plot_dir, stn, sdt, edt, season, var, stat):
plotfname = plot_dir + '/' + stn + '_' + sdt + '_' + edt + '_' + \
season + '_' + var + '_' + stat + '.png'
return plotfname
#---------------------------------------------------------------------------
# Get plot title.
#---------------------------------------------------------------------------
def get_title(season, stn, var, stat, years):
titleout = station_name_dict[stn] + ' (' + stn.upper() + '), ' + \
seasons_lab[season] + ' ' + labels[var+stat] + ', ' + \
str(years[0]) + ' - ' + str(years[len(years)-1])
return titleout
#---------------------------------------------------------------------------
# Make time series of sent-in data for a set of stations for one model.
#---------------------------------------------------------------------------
def ts_stns(statplot, years, stns, mod, titlein, plotfname, var, stat, \
cols, seasonsplot):
fig, ax = plt.subplots( figsize=(10,6) )
xlabs = []
for s in range(len(stns)):
ptot = []
stn = stns[s]
for y in range(len(years)):
yyyy = years[y]
for ss in range(len(seasons)):
season = seasons[ss]
if (season in seasonsplot):
if (var == 'PREC'):
ptot.append(statplot[season,mod,stns[s],yyyy] * mm2in)
elif (var == 'T2MAX' or var == 'T2MIN' or var == 'T2MEAN'):
ptot.append(statplot[season,mod,stns[s],yyyy] - 273.15)
elif (var.find('SPD') >= 0):
ptot.append(statplot[season,mod,stns[s],yyyy])
if (s == 0):
xlabs.append(season.title() + ' ' + years[y])
plt.plot(ptot, alpha = 0.3, label = stn, color = cols[s])
plt.plot(ptot, label = stn, color = cols[s])
#--- y-axis labeling.
plt.ylim(ylims[var+stat])
plt.ylabel('Seasonal ' + labels[var+stat], fontsize=fs+1)
plt.tick_params(axis='y', which='major', labelsize=fs+1)
#--- x-axis labels.
xticks_c = range(0,len(xlabs), 4)
xlabs_c = [ xlabs[i] for i in xticks_c ]
plt.xticks(xticks_c)
plt.tick_params(axis='x', which='major', labelsize=fs-1)
ax.set_xticklabels(xlabs_c, rotation=90)
plt.xlabel('Season', fontsize=fs+1)
plt.title(titlein, fontsize=titlefs, fontweight='bold')
plt.tight_layout()
plt.grid()
plt.legend(fontsize = fs-1, loc = 'best')
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
return 1
#---------------------------------------------------------------------------
# Make time series of sent-in data for a set of models for one station.
#---------------------------------------------------------------------------
def ts_mods(statplot, years, obsplot, years_obs, stn, models, titlein, \
plotfname, var, stat, cols, seasonsplot):
fig, ax = plt.subplots( figsize=(10,6) )
lw_sm = 1.8
#--- Plot models.
xlabs = []
mod_all = np.ones((len(models),len(years))) * np.nan
for m in range(len(models)):
ptot = []
mod = models[m]
for y in range(len(years)):
yyyy = years[y]
for ss in range(len(seasons)):
season = seasons[ss]
key = (season,mod,stn,yyyy)
if (season in seasonsplot):
if (var == 'PREC'):
ptot_c = statplot[key] * mm2in
elif (var == 'T2MAX' or var == 'T2MIN' or var == 'T2MEAN'):
ptot_c = statplot[key] - 273.15
elif (var.find('SPD') >= 0):
ptot_c = statplot[key]
if (m == 0):
xlabs.append(years[y])
ptot.append(ptot_c)
mod_all[m,y] = ptot_c
plt.plot(ptot, label = mod.upper(), \
color = cols[m], linewidth=lw_sm, alpha = 0.23)
#--- Plot ensemble mean.
plt.plot(np.nanmean(mod_all, axis=0), label = 'Ensemble Mean', \
color = 'darkgreen', linewidth=2.2)
#--- Plot observations.
otot = []
for y in range(len(years_obs)):
yyyy = years_obs[y]
for ss in range(len(seasons)):
season = seasons[ss]
key = (season,stn,yyyy)
if (season in seasonsplot):
otot.append(obsplot[key])
plt.plot(otot, label='Observed', color='black', \
linestyle='None', marker='o', markersize=3)
#--- y-axis labeling.
plt.ylim(ylims[var+stat])
plt.ylabel('Seasonal ' + labels[var+stat], fontsize=fs+1)
plt.tick_params(axis='y', which='major', labelsize=fs+1)
#--- x-axis labels.
xticks_c = range(0,len(xlabs), 4)
xlabs_c = [ xlabs[i] for i in xticks_c ]
plt.xticks(xticks_c)
plt.tick_params(axis='x', which='major', labelsize=fs-1)
ax.set_xticklabels(xlabs_c, rotation=90)
plt.xlabel('Year', fontsize=fs+1)
plt.title(titlein, fontsize=titlefs, fontweight='bold')
plt.tight_layout()
plt.grid()
plt.legend(fontsize = fs-1, loc = 'best')
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
return 1
#---------------------------------------------------------------------------
# Make time series of sent-in data for a set of models for one station.
#---------------------------------------------------------------------------
def ts_swe(swe_all, years, swe_obs, years_obs, stn, s, models, titlein, \
plotfname, var, stat, cols):
(nm,ns,nf) = swe_all.shape
fig, ax = plt.subplots( figsize=(10,6) )
lw_sm = 1.8
#--- Plot models.
xlabs = []
mod_all = np.ones((len(models),len(years))) * np.nan
for m in range(len(models)):
mod = models[m]
swe_plot = []
for f in range(nf):
swe_plot.append(swe_all[m,s,f])
plt.plot(swe_plot, label = mod.upper(), color = cols[m], \
linewidth=lw_sm, alpha = 0.23)
#--- Plot ensemble mean.
plt.plot(np.nanmean(swe_all[:,s,:], axis=0), label = 'Ensemble Mean', \
color = 'darkgreen', linewidth=2.2)
#--- Plot observations.
obs_plot = []
obs_yyyy_plot = []
for y in range(len(years)):
if years[y] in years_obs:
i = years_obs.index(years[y])
obs_plot.append(swe_obs[i])
else:
obs_plot.append(np.nan)
plt.plot(obs_plot, label='Observed', color='black', \
linestyle='None', marker='o', markersize=3)
#--- y-axis labeling.
plt.ylim(ylims[var+stat])
plt.ylabel(labels[var+stat], fontsize=fs+1)
plt.tick_params(axis='y', which='major', labelsize=fs+1)
#--- x-axis labels.
xticks_c = range(0,len(years), 4)
xlabs_c = [ years[i] for i in xticks_c ]
plt.xticks(xticks_c)
plt.tick_params(axis='x', which='major', labelsize=fs-1)
ax.set_xticklabels(xlabs_c, rotation=90)
plt.xlabel('Year', fontsize=fs+1)
plt.title(titlein, fontsize=titlefs, fontweight='bold')
plt.tight_layout()
plt.grid()
plt.legend(fontsize = fs-1, loc = 'best')
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
return 1
#---------------------------------------------------------------------------
# Run mapper.
#---------------------------------------------------------------------------
def run_mapper(var, model, rundir, season, files, yyyy, \
lat, lon, levs_c, my_cmap ):
file_plot = rundir + '/' + model + '_' + season + '_' + yyyy + '_' + \
var + '.nc'
if (var == 'T2MAX' or var == 'T2MIN'):
if (os.path.exists(file_plot)):
print 'nc file ', file_plot, ' already exists-- using it'
else:
syscom = 'ncra'
for f in range(len(files[yyyy])):
print yyyy, files[yyyy][f]
syscom = syscom + ' ' + files[yyyy][f]
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
os.system(syscom)
elif (var == 'PREC'):
if (os.path.exists(file_plot)):
print 'nc file ', file_plot, ' already exists-- using it'
else:
syscom = 'ncra -y ttl'
for f in range(len(files[yyyy])):
print yyyy, files[yyyy][f]
syscom = syscom + ' ' + files[yyyy][f]
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
os.system(syscom)
print 'Loading ', var, ' from ', file_plot
(vardat, ntimes, dt) = load_wrfout(file_plot, [var])
syscom = 'rm -f ' + file_plot
os.system(syscom)
titlein = model.upper() + ', ' + var_lab[var] + ', ' + \
seasons_lab[season] + ' ' + yyyy
plotdat = vardat[var][:,:,0]
if (var == 'PREC'):
plotdat = plotdat * mm2in
else:
plotdat = plotdat - 273.15
for z in range(len(zooms)):
plotfname = rundir + '/' + model + '_' + yyyy + '_' + season + '_' + \
var + '_' + zooms[z] + '.png'
print 'colorbar_labs[var] = ', colorbar_labs[var]
mapper(var, lat, lon, plotdat, levs_c, my_cmap, maplw, \
colorbar_labs[var], titlein, plotfname, zooms[z])
return 1
#---------------------------------------------------------------------------
# Mapper.
#---------------------------------------------------------------------------
def mapper(var, lat, lon, grid, levs, cmap_in, maplw, colorbar_lab, titlein, \
plotfname, zoom):
ur_lat = maxlat[zoom]
ur_lon = maxlon[zoom]
ll_lat = minlat[zoom]
ll_lon = minlon[zoom]
lat_ctr = ll_lat + ((ur_lat - ll_lat) * 0.5)
lon_ctr = ll_lon + ((ur_lon - ll_lon) * 0.5)
if (zoom == 'Z1'):
res = 'i'
elif (zoom == 'Z2'):
res = 'h'
fig = plt.figure(figsize=(width,height))
# left, bottom, width, height:
ax = fig.add_axes([0.00,0.05,0.99,0.91])
map = Basemap(resolution = res,projection='lcc',\
llcrnrlon= ll_lon, llcrnrlat=ll_lat,\
urcrnrlon= ur_lon, urcrnrlat= ur_lat,\
lat_0=lat_ctr,lon_0=lon_ctr,lat_1=(ur_lat - ll_lat))
#--- Get lat and lon data in map's x/y coordinates.
x,y = map(lon, lat)
#--- Draw coastlines, country boundaries, fill continents.
map.drawcoastlines(linewidth = maplw)
map.drawstates(linewidth = maplw)
map.drawcountries(linewidth = maplw)
#--- Draw the edge of the map projection region (the projection limb)
map.drawmapboundary(linewidth = maplw)
#--- Draw lat/lon grid lines every 30 degrees.
map.drawmeridians(np.arange(0, 360, 30), linewidth = maplw)
map.drawparallels(np.arange(-90, 90, 30), linewidth = maplw)
cs = plt.contourf(x, y, grid, levs, cmap=cmap_in)
cbar = map.colorbar(cs, location='bottom', pad="3%", size=0.1, ticks=levs)
cbar.set_label(colorbar_lab, fontsize=fs, size=fs-1)
cbar.ax.tick_params(labelsize=fs-2)
if var != 'SNOW':
csl = plt.contour(x, y, grid, levs, colors = 'black', linewidths=0.8)
plt.clabel(csl, inline=1, fontsize=10, fmt='%2.1f', fontweights='bold')
plt.title(titlein, fontsize=titlefs, fontweight='bold')
#--- Save plot.
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
#---------------------------------------------------------------------------
# Smoother like Matlab's running average smoother 'smooth', taken from
# https://stackoverflow.com/questions/40443020/matlabs-smooth-implementation-n-point-moving-average-in-numpy-python
#---------------------------------------------------------------------------
def smooth(a,WSZ):
# a: NumPy 1-D array containing the data to be smoothed
# WSZ: smoothing window size needs, which must be odd number,
# as in the original MATLAB implementation
out0 = np.convolve(a,np.ones(WSZ,dtype=int),'valid')/WSZ
r = np.arange(1,WSZ-1,2)
start = np.cumsum(a[:WSZ-1])[::2]/r
stop = (np.cumsum(a[:-WSZ:-1])[::2]/r)[::-1]
return np.concatenate(( start , out0, stop ))
<file_sep>/plot_wrfout_grid_avg_snowrad.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re, math
import numpy as np
#import matplotlib.pyplot as plt
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
from utils_cmap import *
import pickle
from collections import defaultdict
from make_cmap import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
run_dirs = rcm_dir + '/rundirs'
geo_em = rcm_dir + '/data' + '/geo_em.d02.nc'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#vars = ['SNOW', 'SWDOWN']
vars = ['SNOW']
sdts = ['197001', '203001', '207001']
edts = ['200012', '206012', '209912']
models = ['access1.0', 'access1.3', 'bcc-csm1.1', 'canesm2', \
'ccsm4', 'csiro-mk3.6.0', 'fgoals-g2', 'gfdl-cm3', \
'giss-e2-h', 'miroc5', 'mri-cgcm3', 'noresm1-m']
#models = ['ccsm4']
#---------------------------------------------------------------------------
# Make run directory, load geo_em file.
#---------------------------------------------------------------------------
rundir = run_dirs + '/plot_wrfout_grid_avg_snowrad.' + str(os.getpid())
print 'making ', rundir
os.makedirs(rundir)
#rundir = '/home/disk/spock/jbaars/rcm/rundirs/plot_wrfout_grid_avg.4149'
print 'Loading ', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
#---------------------------------------------------------------------------
# For each model, make a maps short-wave down radiation (SWDOWN).
#---------------------------------------------------------------------------
#var = 'SWDOWN'
#for m in range(len(models)):
# model = models[m]
# for n in range(len(sdts)):
# sdt = sdts[n]
# edt = edts[n]
# yyyy_s = sdt[0:4]
# yyyy_e = edt[0:4]
#
# #--- Get list of extracted files for this model.
# files = get_extract_files(data_dirs, model, sdt, edt, 1)
#
# #--- Get files broken out by year and season.
# (spr_files, sum_files, fal_files, win_files, years) = \
# get_seasonal_files(files)
#
# for season in seasons:
# file_plot = rundir + '/' + model + '_' + var + '_' + season + \
# '_' + yyyy_s + '_' + yyyy_e + '.nc'
# syscom = 'ncra'
# nyears = 0
# for y in range(len(years)):
# yyyy = years[y]
#
# if season == 'summer':
# if (sum_files[yyyy] == sum_files[yyyy]):
# nyears += 1
# for f in range(len(sum_files[yyyy])):
# syscom = syscom + ' ' + sum_files[yyyy][f]
# else:
# continue
# elif season == 'spring':
# if (spr_files[yyyy] == spr_files[yyyy]):
# nyears += 1
# for f in range(len(spr_files[yyyy])):
# syscom = syscom + ' ' + spr_files[yyyy][f]
# else:
# continue
# elif season == 'winter':
# if (win_files[yyyy] == win_files[yyyy]):
# nyears += 1
# for f in range(len(win_files[yyyy])):
# syscom = syscom + ' ' + win_files[yyyy][f]
# else:
# continue
# elif season == 'fall':
# if (fal_files[yyyy] == fal_files[yyyy]):
# nyears += 1
# for f in range(len(fal_files[yyyy])):
# syscom = syscom + ' ' + fal_files[yyyy][f]
# else:
# continue
#
# syscom = syscom + ' ' + file_plot
# print 'syscom = ', syscom
# os.system(syscom)
#
# print 'Loading ', var, ' from ', file_plot
# nc = NetCDFFile(file_plot, 'r')
# ntimes = len(nc.dimensions['Time'])
# vardat = {}
# dat_tmp = nc.variables[var][:,:,:]
# dat_tmp = np.transpose(dat_tmp)
# vardat[var] = dat_tmp
# nc.close()
#
# titlein = model.upper() + ', ' + var_lab[var] + ', ' + \
# seasons_lab[season] + ', ' + yyyy_s + ' - ' + yyyy_e
#
# plotdat = vardat[var][:,:,0]
# if (var == 'PREC'):
# plotdat = plotdat * mm2in
#
# for z in range(len(zooms)):
# plotfname = rundir + '/' + model + '_' + sdt + '_' + \
# edt + '_' + season + '_' + var + '_' + \
# zooms[z] + '.png'
# mapper(var, lat, lon, plotdat, levs_swdown, cmap_swdown, \
# maplw, colorbar_labs[var], titlein, plotfname, \
# zooms[z])
## sys.exit()
'''
#---------------------------------------------------------------------------
# For each model, make a maps Snow Water Equivalent.
#---------------------------------------------------------------------------
var = 'SNOW'
for m in range(len(models)):
model = models[m]
for n in range(len(sdts)):
sdt = sdts[n]
edt = edts[n]
yyyy_s = sdt[0:4]
yyyy_e = edt[0:4]
#--- Get list of extracted files for this model.
files = []
for d in range(len(data_dirs)):
# path_c = data_dirs[d] + '/' + model + '/extract/*0401*.nc'
path_c = data_dirs[d] + '/' + model + '/swe/*0401*.nc'
files_c = glob.glob(path_c)
for f in range(len(files_c)):
if 'snowrad' not in files_c[f]:
continue
dt_c = re.findall(r'\.(\d{10})\.', files_c[f])
if (dt_c):
dt_c = dt_c[0]
yyyymm_c = dt_c[0:6]
if (yyyymm_c >= sdt and yyyymm_c <= edt):
files.append(files_c[f])
#--- Make plot for each zooms.
file_plot = rundir + '/' + model + '_' + var + '_apr1_' + \
yyyy_s + '_' + yyyy_e + '.nc'
syscom = 'ncra'
for f in range(len(files)):
syscom = syscom + ' ' + files[f]
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
os.system(syscom)
print 'Loading ', var, ' from ', file_plot
nc = NetCDFFile(file_plot, 'r')
ntimes = len(nc.dimensions['Time'])
vardat = {}
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
vardat[var] = dat_tmp
nc.close()
titlein = model.upper() + ', ' + var_lab[var] + ', ' + \
'Apr 1st, ' + yyyy_s + ' - ' + yyyy_e
plotdat = vardat[var][:,:,0]
for z in range(len(zooms)):
plotfname = rundir + '/' + model + '_' + sdt + '_' + \
edt + '_apr1_' + var + '_' + \
zooms[z] + '.png'
mapper(var, lat, lon, plotdat, levs_swe, cmap_swe, \
maplw, colorbar_labs[var], titlein, plotfname, \
zooms[z])
'''
#---------------------------------------------------------------------------
# Make map of Snow Water Equivalent for ensemble mean.
#---------------------------------------------------------------------------
#(nx,ny) = lat.shape
#snowrad_mean = np.ones((nx, ny, len(sdts))) * np.nan
#var = 'SNOW'
#for n in range(len(sdts)):
# sdt = sdts[n]
# edt = edts[n]
# yyyy_s = sdt[0:4]
# yyyy_e = edt[0:4]
#
# files = []
# dts_all = []
# for m in range(len(models)):
# model = models[m]
# for d in range(len(data_dirs)):
# path_c = data_dirs[d] + '/' + model + '/swe/*0401*.nc'
# files_c = glob.glob(path_c)
#
# for f in range(len(files_c)):
# if 'snowrad' not in files_c[f]:
# continue
#
# dt_c = re.findall(r'\.(\d{10})\.', files_c[f])
#
# if (dt_c):
# dt_c = dt_c[0]
# yyyymm_c = dt_c[0:6]
# if (yyyymm_c >= sdt and yyyymm_c <= edt):
# files.append(files_c[f])
# dts_all.append(dt_c)
#
# #--- Make plot for each zooms.
## file_plot = rundir + '/ensmean_' + var + '_apr1_' + \
## yyyy_s + '_' + yyyy_e + '.nc'
#
# for f in range(len(files)):
# print files[f], dts_all[f]
# (vardat, ntimes) = load_wrfout_swe(files[f], [var])
# plotdat = vardat[var][:,:,0]
# nx,ny = plotdat.shape
# if f == 0:
# dat_all = np.ones((nx, ny, len(files))) * np.nan
# dat_all[:,:,f] = plotdat
# print 'np.amax(plotdat) = ', np.amax(plotdat)
#
# snowrad_mean[:,:,n] = np.mean(dat_all, axis=2)
# snowrad_std = np.std(dat_all, axis=2)
#
# print 'np.amax(snowrad_std) = ', np.amax(snowrad_std)
# print 'np.amax(snowrad_mean) = ', np.amax(snowrad_mean[:,:,n])
# print 'np.amax(dat_all[:,:,0]) = ', np.amax(dat_all[:,:,0])
# print 'np.amax(dat_all[:,:,1]) = ', np.amax(dat_all[:,:,1])
#
# for z in range(len(zooms)):
# titlein = 'Ensemble Mean, ' + var_lab[var] + ', ' + \
# 'Apr 1st, ' + yyyy_s + ' - ' + yyyy_e
# plotfname = rundir + '/ensmean_' + sdt + '_' + \
# edt + '_apr1_' + var + '_' + zooms[z] + '.png'
# mapper(var, lat, lon, snowrad_mean[:,:,n], levs_swe, cmap_swe, \
# maplw, colorbar_labs[var], titlein, plotfname, \
# zooms[z])
#
# titlein = 'Ensemble Standard Deviation, ' + var_lab[var] + ', ' + \
# 'Apr 1st, ' + yyyy_s + ' - ' + yyyy_e
# plotfname = rundir + '/ensstd_' + sdt + '_' + \
# edt + '_apr1_' + var + '_' + zooms[z] + '.png'
# mapper(var, lat, lon, snowrad_std, levs_swe, cmap_swe, \
# maplw, colorbar_labs[var], titlein, plotfname, \
# zooms[z])
#
pf = pickle_dir + '/swe_mean.pkl'
#pickle.dump((snowrad_mean), open(pf,'wb'), -1)
(snowrad_mean) = pickle.load(open(pf, 'rb'))
var = 'SNOW'
for n in range(1,len(sdts)):
diff_c = snowrad_mean[:,:,n] - snowrad_mean[:,:,0]
dttit = '(' + sdts[n][0:4] + '-' + edts[n][0:4] + ') - ' + \
'(' + sdts[0][0:4] + '-' + edts[0][0:4] + ')'
print diff_c
print np.amin(diff_c)
print np.amax(diff_c)
print diff_c.shape
for z in range(len(zooms)):
titlein = 'Apr 1st ' + var_lab[var] + \
', Ensemble Mean Difference, ' + ', ' + dttit
plotfname = rundir + '/ensmean_diff_' + sdts[n] + '_' + \
edts[n] + '_apr1_' + var + '_' + zooms[z] + '.png'
mapper(var, lat, lon, diff_c, levs_swe_diff, cmap_swe_diff, \
maplw, colorbar_labs[var], titlein, plotfname, \
zooms[z])
##---------------------------------------------------------------------------
## Make map of difference.
##---------------------------------------------------------------------------
#for m in range(len(models)):
# model = models[m]
#
# syscom = 'ncdiff'
# file_diff = rundir + '/' + model + '_' + var + '_apr1_' + 'diff.nc'
#
# for n in range(len(sdts)):
# sdt = sdts[n]
# edt = edts[n]
# yyyy_s = sdt[0:4]
# yyyy_e = edt[0:4]
# file_plot = rundir + '/' + model + '_' + var + '_apr1_' + \
# yyyy_s + '_' + yyyy_e + '.nc'
# if os.path.isfile(file_plot):
# syscom = syscom + ' ' + file_plot
#
# syscom = syscom + ' ' + file_diff
#
# print 'syscom = ', syscom
# os.system(syscom)
#
# print 'Loading ', var, ' from ', file_diff
# nc = NetCDFFile(file_diff, 'r')
# ntimes = len(nc.dimensions['Time'])
# vardat = {}
# dat_tmp = nc.variables[var][:,:,:]
# dat_tmp = np.transpose(dat_tmp)
# vardat[var] = dat_tmp
# nc.close()
#
# titlein = model.upper() + ', ' + var_lab[var] + ', ' + \
# 'Apr 1st, Difference, ' + yyyy_s + ' - ' + yyyy_e
#
# plotdat = vardat[var][:,:,0]
#
# for z in range(len(zooms)):
# plotfname = rundir + '/' + model + '_' + sdt + '_' + \
# edt + '_apr1_diff_' + var + '_' + \
# zooms[z] + '.png'
# mapper(var, lat, lon, plotdat, levs_swe, cmap_swe, \
# maplw, colorbar_labs[var], titlein, plotfname, \
# zooms[z])
sys.exit()
<file_sep>/utils_cei.py
#!/usr/bin/python
#----------------------------------------------------------------------------
# Climate extreme indices based on Rick's code which is based on:
# http://etccdi.pacificclimate.org/list_27_indices.shtml
#----------------------------------------------------------------------------
import os, sys, glob, re, math
import numpy as np
from utils_date import *
from utils_ghcnd_obs import *
#basefirst = 1971
#baselast = 2000
#first = 1970
#last = 2099
basefirst = 1971
baselast = 1974
first = 1970
last = 1979
#--- percentages used for...
percs = [0.1, 0.9]
dpm = [ 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 ]
#----------------------------------------------------------------------------
# calculate daily percentages of the historical period.
#----------------------------------------------------------------------------
def get_daily_perc_mxmn(models, stns, mod_all_in, sdt):
bdt = sdt + '0100'
daily_perc = {}
#--- Loop over models.
for m in range(len(models)):
model = models[m]
mod_all = mod_all_in[model]
#--- Loop over stations.
for s in range(len(stns)):
stn = stns[s]
#--- Loop over each day of the year.
for d in range(0,364):
fdate = time_increment(bdt, d, dtfmt)
basemmddhh = fdate[4:10]
txunsorted = []
tnunsorted = []
#--- Loop over every year within the "base" period.
for y in range(basefirst, baselast+1):
basedate = str(y) + basemmddhh
#--- Per CEI definition of daily percentage calc, Looks
#--- +/- 2 days from basedate, presumably to increase
#--- sample size(?).
for n in range(-2, 3):
dt = time_increment(basedate, n, dtfmt)
key = ('T2MAX', stn, dt)
if key in mod_all:
txunsorted.append(mod_all['T2MAX', stn, dt])
key = ('T2MIN', stn, dt)
if key in mod_all:
tnunsorted.append(mod_all['T2MIN', stn, dt])
#--- Sort lists of maxes and mins.
txsorted = list(sorted(txunsorted))
tnsorted = list(sorted(tnunsorted))
#--- Get percentage (percentages defined in percs) for
#--- max and min.
for p in range(len(percs)):
perc = percs[p]
np = int(round(float(len(txsorted)+1) * perc))
key = (model, 'T2MAX', stn, fdate, str(perc))
daily_perc[key] = txsorted[np]
np = int(round(float(len(tnsorted)+1) * perc))
key = (model, 'T2MIN', stn, fdate, str(perc))
daily_perc[key] = tnsorted[np]
return daily_perc
#----------------------------------------------------------------------------
# Calculate monthly max and min climate index.
#----------------------------------------------------------------------------
def get_monthly_mxmn(daily_perc, mod_all, models, stns, sdt):
model = models[0]
stn = stns[0]
for m in range(0,12):
mstr = str(m+1).zfill(2)
for y in range(first, last+1):
bdt = str(y) + mstr + '0100'
yyyymm = str(y) + mstr
for d in range(0, dpm[m]):
fdate = time_increment(bdt, d, dtfmt)
basedate = '1970' + fdate[4:10]
key = ('T2MAX', stn, fdate)
keydp = (model, 'T2MAX', stn, fdate)
if mod_all[key] > daily_perc[keydp]
print fdate
sys.exit()
# #--- Loop over models.
# for m in range(len(models)):
# model = models[m]
# mod_all = mod_all_in[model]
# #--- Loop over stations.
# for s in range(len(stns)):
# stn = stns[s]
#
# for m in range(0,12):
# print m
# for y in range(first, last+1):
# print y
sys.exit()
<file_sep>/old/old_old/plot_wrfout_stns_20180117.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
#import matplotlib.pyplot as plt
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
import pickle
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
data_dir = '/home/disk/r2d2/steed/cmip5/rcp8.5'
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
var = 'PREC'
sdt = '202001'
edt = '202912'
models = ['gfdl-cm3']
dtfmt = "%Y%m%d%H"
stns = ['KSEA', 'KSMP', 'KYKM']
latpts = [47.44472, 47.27667, 46.56417]
lonpts = [-122.31361, -121.33722, -120.53361]
#stns = ['KSEA']
#latpts = [47.44472]
#lonpts = [-122.31361]
#stns = ['KSMP']
#latpts = [47.27667]
#lonpts = [-121.33722]
#---------------------------------------------------------------------------
# Loop over each models, grabbing data from extract wrfout files.
#---------------------------------------------------------------------------
pickle_file = pickle_dir + '/' + var + '_' + sdt + '_' + edt + '.pickle'
if os.path.isfile(pickle_file):
(data_all, dts_unique, models, stns) = pickle.load(open(pickle_file, 'rb'))
else:
print 'Loading ', geo_em
(lat, lon) = load_geo_em(geo_em)
data_all = {}
dts_all = []
for m in range(len(models)):
model = models[m]
data_dir_c = data_dir + '/' + model + '/extract'
#--- Get list of extracted files for this model.
files = get_extract_files(data_dir_c, model, sdt, edt)
#--- Loop over all extract files, reading them in.
for f in range(len(files)):
print 'Loading ', files[f]
(vardat, ntimes, dt) = load_wrfout(files[f], var)
#--- Loop over each ntime, grabbing interpolated data for
#--- variable var for each stns.
for n in range(ntimes):
dt_c = time_increment(dt, n, dtfmt)
for ns in range(len(stns)):
prec_interp = bilinear_interpolate(lat, lon, vardat[:,:,n],\
latpts[ns], lonpts[ns],\
0)
data_all[model, stns[ns], dt_c] = prec_interp
dts_all.append(dt_c)
#--- Get sorted unique list of all dates seen.
dts_unique = list(sorted(set(dts_all)))
#--- Create pickle file.
pickle.dump((data_all, dts_unique, models, stns),open(pickle_file,'wb'), -1)
#---------------------------------------------------------------------------
# Get seasonal totals.
#---------------------------------------------------------------------------
(springs, summers, falls, winters, years) = \
get_seasonal_totals(data_all, dts_unique, models, stns)
#---------------------------------------------------------------------------
# Plot times series of total precip for each station.
#---------------------------------------------------------------------------
mod = 'gfdl-cm3'
plotfname = 'test.png'
titlein = mod + ', ' + str(years[0]) + ' - ' + str(years[len(years)-1])
iret = ts(winters, springs, summers, falls, years, stns, mod, \
titlein, plotfname)
#---------------------------------------------------------------------------
#
#---------------------------------------------------------------------------
sys.exit()
<file_sep>/plot_rcm_stns_swe.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re, math
import numpy as np
#import matplotlib.pyplot as plt
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_load_data import *
from utils_plot import *
from utils_date import *
from utils_cmap import *
from utils_snotel_obs import *
import pickle
from collections import defaultdict
from make_cmap import *
import csv
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
data_dir = rcm_dir + '/data'
snotel_dir = data_dir + '/snotel'
run_dirs = rcm_dir + '/rundirs'
geo_em = rcm_dir + '/data' + '/geo_em.d02.nc'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
station_file = data_dir + '/station_file_swe.txt'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
varswe = 'SNOW'
stat = 'tot'
sdt = '197001'
edt = '209912'
models = ['access1.0', 'access1.3', 'bcc-csm1.1', 'canesm2', \
'ccsm4', 'csiro-mk3.6.0', 'fgoals-g2', 'gfdl-cm3', \
'giss-e2-h', 'miroc5', 'mri-cgcm3', 'noresm1-m']
mod_cols = ['indigo', 'blue', 'deepskyblue', \
'darkgreen', 'lime', 'yellow', \
'magenta', 'red', 'salmon', 'gray', 'darkgray', 'lightblue']
yyyy_s = sdt[0:4]
yyyy_e = edt[0:4]
#---------------------------------------------------------------------------
# Load stations file.
#---------------------------------------------------------------------------
print 'Loading ', station_file
(stns, latpts, lonpts, elevs, stn_names) = read_swe_stations_file(station_file)
#---------------------------------------------------------------------------
# Load SNOTEL data for each stns, if data exists.
#---------------------------------------------------------------------------
swe_yyyy = {}
swe_obs = {}
for s in range(len(stns)):
stn = stns[s]
swe_file = snotel_dir + '/' + stns[s] + '.csv'
if os.path.isfile(swe_file):
print 'Loading ', swe_file
(swe_yyyy[stn], swe_obs[stn]) = read_snotel(swe_file)
#---------------------------------------------------------------------------
# Load geo_em file.
#---------------------------------------------------------------------------
print 'Loading ', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
#---------------------------------------------------------------------------
# Get model station elevations.
#---------------------------------------------------------------------------
stn_mod_elevs = []
print 'stn, actual elev, mod elev'
for s in range(len(stns)):
elev_c = bilinear_interpolate(lat, lon, hgt, latpts[s], lonpts[s], 0)
stn_mod_elevs.append(elev_c)
print stns[s], elevs[s], stn_mod_elevs[s]
#---------------------------------------------------------------------------
# For each model, read in Snow Water Equivalent data for all stns.
#---------------------------------------------------------------------------
pfswe = pickle_dir + '/swe_stns.pkl'
if os.path.isfile(pfswe):
print 'Loading ', pfswe
(stns, dts_all, swe_all) = pickle.load(open(pfswe, 'rb'))
else:
for m in range(len(models)):
model = models[m]
#--- Get list of extracted files for this model.
files = []
for d in range(len(data_dirs)):
path_c = data_dirs[d] + '/' + model + '/swe/*0401*.nc'
files_c = glob.glob(path_c)
for f in range(len(files_c)):
if 'snowrad' not in files_c[f]:
continue
dt_c = re.findall(r'\.(\d{10})\.', files_c[f])
if (dt_c):
dt_c = dt_c[0]
yyyymm_c = dt_c[0:6]
if (yyyymm_c >= sdt and yyyymm_c <= edt):
files.append(files_c[f])
#--- Initialize our big SWE array.
if 'swe_all' not in locals():
swe_all = np.ones((len(models), len(stns), len(files))) * np.nan
#--- Loop over files found above reading in Apr 1 SWE for every station.
dts_c = []
for f in range(len(files)):
print files[f]
(vardat, ntimes) = load_wrfout_swe(files[f], [varswe])
dt_c = re.findall(r'\.(\d{10})\.', files[f])
dts_c.append(dt_c)
for s in range(len(stns)):
dat_interp = bilinear_interpolate(lat, lon, \
vardat[varswe][:,:,0], \
latpts[s], lonpts[s], 0)
swe_all[m,s,f] = dat_interp
#--- Initialize our dates array if this is the first model. Otherwise
#--- do a date check to make sure # of dates are identical to first
#--- model's. Bomb otherwise, as that means a model is missing a file.
if 'dts_all' not in locals():
dts_all = dts_c
else:
if sorted(dts_c) != sorted(dts_all):
print 'dts_c = ', dts_c
print 'dts_all = ', dts_all
sys.exit('dts problem!')
pickle.dump((stns, dts_all, swe_all), open(pfswe,'wb'), -1)
#---------------------------------------------------------------------------
# nan out 1970, because that's a partial winter given models started 01/01/70.
#---------------------------------------------------------------------------
swe_all[:,:,0] = np.nan
#---------------------------------------------------------------------------
# Get years from dts_all.
#---------------------------------------------------------------------------
years = []
for d in range(len(dts_all)):
years.append(dts_all[d][0][0:4])
#---------------------------------------------------------------------------
# Make plots using ts_swe.
#---------------------------------------------------------------------------
(nm,ns,nf) = swe_all.shape
for s in range(len(stns)):
stn = stns[s]
plotfname = plot_dir + '/' + stn + '_' + sdt + '_' + edt + '_swe.png'
titlein = stn_names[s] + ' (' + stn.upper() + '), ' + \
'(model elev: ' + str(int(stn_mod_elevs[s])) + '-m, ' + \
'actual elev: ' + str(int(elevs[s])) + '-m), ' + \
labels[varswe+stat] + ', ' + \
str(years[0]) + ' - ' + str(years[len(years)-1])
iret = ts_swe(swe_all, years, swe_obs[stn], swe_yyyy[stn], stn, s, \
models, titlein, plotfname, varswe, stat, mod_cols)
sys.exit()
<file_sep>/old/old_old/plot_wrfout_stns_20180123.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
#import matplotlib.pyplot as plt
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
import pickle
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
data_dir = '/home/disk/r2d2/steed/cmip5/rcp8.5'
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
vars = ['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
sdt = '202001'
edt = '202912'
models = ['gfdl-cm3']
dtfmt = "%Y%m%d%H"
stns = ['KSEA', 'KSMP', 'KYKM']
latpts = [47.44472, 47.27667, 46.56417]
lonpts = [-122.31361, -121.33722, -120.53361]
#---------------------------------------------------------------------------
# Loop over each models, grabbing data from extract wrfout files.
#---------------------------------------------------------------------------
pickle_file = pickle_dir + '/extract_' + sdt + '_' + edt + '.pickle'
if os.path.isfile(pickle_file):
(data_all, dts_unique, models, vars, stns) = \
pickle.load(open(pickle_file, 'rb'))
else:
print 'Loading ', geo_em
(lat, lon) = load_geo_em(geo_em)
data_all = {}
dts_all = []
for m in range(len(models)):
model = models[m]
data_dir_c = data_dir + '/' + model + '/extract'
#--- Get list of extracted files for this model.
files = get_extract_files(data_dir_c, model, sdt, edt)
#--- Loop over all extract files, reading them in.
for f in range(len(files)):
print 'Loading ', files[f]
(vardat, ntimes, dt) = load_wrfout(files[f], vars)
#--- Loop over each vars, interpolating data to stns for
#--- each ntimes.
for v in range(len(vars)):
var = vars[v]
vardat_c = vardat[var]
#--- Loop over each ntime, grabbing interpolated data for
#--- variable var for each stns.
for n in range(ntimes):
dt_c = time_increment(dt, n, dtfmt)
for ns in range(len(stns)):
dat_interp = bilinear_interpolate(lat, lon, \
vardat_c[:,:,n],\
latpts[ns], \
lonpts[ns], 0)
data_all[model, var, stns[ns], dt_c] = dat_interp
dts_all.append(dt_c)
#--- Get sorted unique list of all dates seen.
dts_unique = list(sorted(set(dts_all)))
#--- Create pickle file.
pickle.dump((data_all, dts_unique, models, vars, stns), \
open(pickle_file,'wb'), -1)
#---------------------------------------------------------------------------
# Get seasonal precipitation totals and make a time series plot of them.
#---------------------------------------------------------------------------
(spr_pcp_tot, sum_pcp_tot, fal_pcp_tot, win_pcp_tot, years) = \
get_seasonal_stats(data_all, dts_unique, models, stns, 'PREC', 'tot')
mod = 'gfdl-cm3'
plotfname = plot_dir + '/' + mod + '_season_precip.png'
titlein = mod + ', ' + str(years[0]) + ' - ' + str(years[len(years)-1])
iret = ts(win_pcp_tot, spr_pcp_tot, sum_pcp_tot, fal_pcp_tot, years, \
stns, mod, titlein, plotfname, 'PREC')
#---------------------------------------------------------------------------
# Get seasonal temperature averages.
#---------------------------------------------------------------------------
(spr_tmx_avg, sum_tmx_avg, fal_tmx_avg, win_tmx_avg, years) = \
get_seasonal_stats(data_all, dts_unique, models, stns, 'T2MAX', 'avg')
(spr_tmn_avg, sum_tmn_avg, fal_tmn_avg, win_tmn_avg, years) = \
get_seasonal_stats(data_all, dts_unique, models, stns, 'T2MIN', 'avg')
(spr_spdavg_avg, sum_spdavg_avg, fal_spdavg_avg, win_spdavg_avg, years) = \
get_seasonal_stats(data_all, dts_unique, models, stns, \
'SPDUV10MEAN', 'avg')
mod = 'gfdl-cm3'
plotfname = plot_dir + '/' + mod + '_season_tmx_avg.png'
titlein = mod + ', TMAX Average, ' + str(years[0]) + ' - ' + \
str(years[len(years)-1])
iret = ts(win_tmx_avg, spr_tmx_avg, sum_tmx_avg, fal_tmx_avg, years, \
stns, mod, titlein, plotfname, 'T2MAX')
mod = 'gfdl-cm3'
plotfname = plot_dir + '/' + mod + '_season_tmn_avg.png'
titlein = mod + ', TMIN Average, ' + str(years[0]) + ' - ' + \
str(years[len(years)-1])
iret = ts(win_tmn_avg, spr_tmn_avg, sum_tmn_avg, fal_tmn_avg, years, \
stns, mod, titlein, plotfname, 'T2MIN')
#---------------------------------------------------------------------------
#
#---------------------------------------------------------------------------
sys.exit()
<file_sep>/old/plot_wrfout_grid_avg_20190516.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re, math
import numpy as np
#import matplotlib.pyplot as plt
#from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
import pickle
from collections import defaultdict
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
run_dirs = rcm_dir + '/rundirs'
geo_em = rcm_dir + '/data' + '/geo_em.d02.nc'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#vars = ['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
#vars = ['T2MAX', 'T2MIN', 'PREC']
vars = ['T2MAX', 'T2MIN']
#vars = ['PREC']
#vars = ['SPDUV10MAX', 'SPDUV10MEAN']
sdts = ['197001', '203001', '207001']
edts = ['200012', '206012', '209912']
models = ['access1.0', 'access1.3', 'bcc-csm1.1', 'canesm2', \
'ccsm4', 'csiro-mk3.6.0', 'fgoals-g2', 'gfdl-cm3', \
'giss-e2-h', 'miroc5', 'mri-cgcm3', 'noresm1-m']
dtfmt = "%Y%m%d%H"
#---------------------------------------------------------------------------
# Make run directory, load geo_em file.
#---------------------------------------------------------------------------
rundir = run_dirs + '/plot_wrfout_grid_avg.' + str(os.getpid())
print 'making ', rundir
os.makedirs(rundir)
#rundir = run_dirs + '/plot_wrfout_grid_avg.23227' #
#rundir = run_dirs + '/plot_wrfout_grid_avg.16978' # PREC
#rundir = run_dirs + '/plot_wrfout_grid_avg.27465'
print 'Loading ', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
'''
#---------------------------------------------------------------------------
# For each model, make a map of every season...
#---------------------------------------------------------------------------
for m in range(len(models)):
model = models[m]
for n in range(len(sdts)):
sdt = sdts[n]
edt = edts[n]
yyyy_s = sdt[0:4]
yyyy_e = edt[0:4]
#--- Get list of extracted files for this model.
files = get_extract_files(data_dirs, model, sdt, edt, 0)
#--- Get files broken out by year and season.
(spr_files, sum_files, fal_files, win_files, ann_files, years) = \
get_seasonal_files(files)
for var in vars:
nyears_ann = 0
for season in seasons:
file_plot = rundir + '/' + model + '_' + var + '_' + season + \
'_' + yyyy_s + '_' + yyyy_e + '.nc'
if (var == 'T2MAX' or var == 'T2MIN'):
syscom = 'ncra'
elif (var == 'PREC'):
syscom = 'ncra -y ttl'
nyears = 0
for y in range(len(years)):
yyyy = years[y]
if season == 'summer':
if (sum_files[yyyy] == sum_files[yyyy]):
nyears += 1
for f in range(len(sum_files[yyyy])):
syscom = syscom + ' ' + sum_files[yyyy][f]
else:
continue
elif season == 'spring':
if (spr_files[yyyy] == spr_files[yyyy]):
nyears += 1
for f in range(len(spr_files[yyyy])):
syscom = syscom + ' ' + spr_files[yyyy][f]
else:
continue
elif season == 'winter':
if (win_files[yyyy] == win_files[yyyy]):
nyears += 1
for f in range(len(win_files[yyyy])):
syscom = syscom + ' ' + win_files[yyyy][f]
else:
continue
elif season == 'fall':
if (fal_files[yyyy] == fal_files[yyyy]):
nyears += 1
for f in range(len(fal_files[yyyy])):
syscom = syscom + ' ' + fal_files[yyyy][f]
else:
continue
elif season == 'annual':
if (ann_files[yyyy] == ann_files[yyyy]):
nyears += 1
for f in range(len(ann_files[yyyy])):
syscom = syscom + ' ' + ann_files[yyyy][f]
else:
continue
nyears_ann = nyears_ann + nyears
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
os.system(syscom)
print 'Loading ', var, ' from ', file_plot
(vardat, ntimes, dt) = load_wrfout(file_plot, [var])
titlein = model.upper() + ', Average ' + var_lab[var] + ', ' + \
seasons_lab[season] + ', ' + yyyy_s + ' - ' + yyyy_e
plotdat = vardat[var][:,:,0]
if (var == 'PREC'):
if 'prec_tot' in locals():
prec_tot = np.add(prec_tot, plotdat)
else:
prec_tot = plotdat
plotdat = (plotdat / nyears) * mm2in
levs_c = levs_pcp
cmap_c = cmap_pcp
else:
plotdat = plotdat - 273.15
levs_c = levs_temp
cmap_c = cmap_temp
for z in range(len(zooms)):
plotfname = rundir + '/' + model + '_' + sdt + '_' + \
edt + '_' + season + '_' + var + '_' + \
zooms[z] + '.png'
mapper(var, lat, lon, plotdat, levs_c, cmap_c, \
maplw, colorbar_labs[var], titlein, plotfname, \
zooms[z])
# sys.exit()
if (var == 'PREC'):
file_plot = rundir + '/' + model + '_' + var + '_annual_' + \
yyyy_s + '_' + yyyy_e + '.nc'
f = NetCDFFile(file_plot, 'w')
(nx,ny) = prec_tot.shape
f.createDimension('nx', nx)
f.createDimension('ny', ny)
pcpnc = f.createVariable('pcp', 'f', ('nx','ny'))
pcpnc[:,:] = prec_tot
pcpnc.description = 'total annual precip'
pcpnc.units = 'mm'
f.close()
sys.exit()
'''
#---------------------------------------------------------------------------
# Ensemble spread plots for every season...
#---------------------------------------------------------------------------
for n in range(len(sdts)):
spr_files = {}
sum_files = {}
fal_files = {}
win_files = {}
ann_files = {}
sdt = sdts[n]
edt = edts[n]
yyyy_s = sdt[0:4]
yyyy_e = edt[0:4]
#--- Fill seasonal file dictionaries for each model.
for m in range(len(models)):
model = models[m]
#--- Get list of extracted files for this model.
files = get_extract_files(data_dirs, model, sdt, edt, 0)
#--- Get files broken out by year and season.
(spr_files[model], sum_files[model], fal_files[model], \
win_files[model], ann_files[model], years) = get_seasonal_files(files)
#--- For each variable and season, make a plot.
for var in vars:
if (var == 'T2MAX' or var == 'T2MIN' or var == 'SPDUV10MAX' or \
var == 'SPDUV10MEAN'):
ncocom = 'ncra'
elif (var == 'PREC'):
ncocom = 'ncra -y ttl'
for season in seasons:
file_plot = rundir + '/' + var + '_' + season + \
'_' + yyyy_s + '_' + yyyy_e + '.nc'
# (ensstd, nyears) = get_ensmean_std(file_plot, var, models, \
# years, season, \
# sum_files, spr_files, \
# win_files, fal_files, \
# ann_files, ncocom)
(ensstd, nyears) = get_ensmean_std(file_plot, var, models, \
years, season, files)
print ensstd
# if os.path.isfile(file_plot):
# print 'file_plot already exists. file_plot = ', file_plot
# else:
# iret = run_ensmean_syscom(file_plot, var, ncocom, syscom, \
# yyyy_s, yyyy_e, season, rundir)
#--- Add number of years (nmodels * years) as dimension to nc file.
syscom = 'ncap2 -s \'defdim(\"nmodyears\",' + str(nyears) + \
')\'' + ' ' + file_plot
print syscom
os.system(syscom)
print 'Loading ', var, ' from ', file_plot
(vardat, ntimes, dt) = load_wrfout(file_plot, [var])
titlein = 'Ensemble Mean, ' + var_lab[var] + ', ' + \
seasons_lab[season] + ', ' + yyyy_s + ' - ' + yyyy_e
plotdat = vardat[var][:,:,0]
if (var == 'PREC'):
plotdat = (plotdat / nyears) * mm2in
levs_c = levs_pcp
cmap_c = cmap_pcp
elif var == 'T2MAX' or var == 'T2MIN':
plotdat = plotdat - 273.15
levs_c = levs_temp
cmap_c = cmap_temp
elif var == 'SPDUV10MAX':
levs_c = levs_spd
cmap_c = cmap_spd
for z in range(len(zooms)):
plotfname = rundir + '/map_all_' + sdt + '_' + edt + '_' + \
season + '_' + var + '_' + zooms[z] + '.png'
print var, lat, lon, plotdat, levs_c, cmap_c, \
maplw, colorbar_labs[var], titlein, plotfname, \
zooms[z]
mapper(var, lat, lon, plotdat, levs_c, cmap_c, \
maplw, colorbar_labs[var], titlein, plotfname, \
zooms[z])
#sys.exit()
sys.exit()
'''
#---------------------------------------------------------------------------
# Ensemble mean plots for every season...
#---------------------------------------------------------------------------
for n in range(len(sdts)):
spr_files = {}
sum_files = {}
fal_files = {}
win_files = {}
ann_files = {}
sdt = sdts[n]
edt = edts[n]
yyyy_s = sdt[0:4]
yyyy_e = edt[0:4]
#--- Fill seasonal file dictionaries for each model.
for m in range(len(models)):
model = models[m]
#--- Get list of extracted files for this model.
files = get_extract_files(data_dirs, model, sdt, edt, 0)
#--- Get files broken out by year and season.
(spr_files[model], sum_files[model], fal_files[model], \
win_files[model], ann_files[model], years) = get_seasonal_files(files)
#--- For each variable and season, make a plot.
for var in vars:
if (var == 'T2MAX' or var == 'T2MIN' or var == 'SPDUV10MAX' or \
var == 'SPDUV10MEAN'):
ncocom = 'ncra'
elif (var == 'PREC'):
ncocom = 'ncra -y ttl'
for season in seasons:
file_plot = rundir + '/' + var + '_' + season + \
'_' + yyyy_s + '_' + yyyy_e + '.nc'
(syscom, nyears) = get_ensmean_syscom(file_plot, var, models, \
years, season, \
sum_files, spr_files, \
win_files, fal_files, \
ann_files, ncocom)
if os.path.isfile(file_plot):
print 'file_plot already exists. file_plot = ', file_plot
else:
iret = run_ensmean_syscom(file_plot, var, ncocom, syscom, \
yyyy_s, yyyy_e, season, rundir)
#--- Add number of years (nmodels * years) as dimension to nc file.
syscom = 'ncap2 -s \'defdim(\"nmodyears\",' + str(nyears) + \
')\'' + ' ' + file_plot
print syscom
os.system(syscom)
print 'Loading ', var, ' from ', file_plot
(vardat, ntimes, dt) = load_wrfout(file_plot, [var])
titlein = 'Ensemble Mean, ' + var_lab[var] + ', ' + \
seasons_lab[season] + ', ' + yyyy_s + ' - ' + yyyy_e
plotdat = vardat[var][:,:,0]
if (var == 'PREC'):
plotdat = (plotdat / nyears) * mm2in
levs_c = levs_pcp
cmap_c = cmap_pcp
elif var == 'T2MAX' or var == 'T2MIN':
plotdat = plotdat - 273.15
levs_c = levs_temp
cmap_c = cmap_temp
elif var == 'SPDUV10MAX':
levs_c = levs_spd
cmap_c = cmap_spd
for z in range(len(zooms)):
plotfname = rundir + '/map_all_' + sdt + '_' + edt + '_' + \
season + '_' + var + '_' + zooms[z] + '.png'
print var, lat, lon, plotdat, levs_c, cmap_c, \
maplw, colorbar_labs[var], titlein, plotfname, \
zooms[z]
mapper(var, lat, lon, plotdat, levs_c, cmap_c, \
maplw, colorbar_labs[var], titlein, plotfname, \
zooms[z])
#sys.exit()
#sys.exit()
'''
#---------------------------------------------------------------------------
# Get difference plots for the two sdts / edts periods.
#---------------------------------------------------------------------------
for nd in range(1,len(sdts)):
print nd, sdts[nd]
sdt_hist = sdts[0]
edt_hist = edts[0]
yyyy_s_hist = sdt_hist[0:4]
yyyy_e_hist = edt_hist[0:4]
sdt_fore = sdts[nd]
edt_fore = edts[nd]
yyyy_s_fore = sdt_fore[0:4]
yyyy_e_fore = edt_fore[0:4]
for var in vars:
for season in seasons:
#--- Get diff between forecast and historical for var and season.
file_hist = rundir + '/' + var + '_' + season + \
'_' + yyyy_s_hist + '_' + yyyy_e_hist + '.nc'
forename = yyyy_s_fore + '_' + yyyy_e_fore
file_fore = rundir + '/' + var + '_' + season + \
'_' + forename + '.nc'
file_diff = rundir + '/' + var + '_' + season + '_' + \
forename + '_diff.nc'
syscom = 'ncdiff -O ' + file_fore + ' ' + file_hist + ' ' +file_diff
print 'syscom = ', syscom
os.system(syscom)
#--- Load diff file.
print 'Loading ', var, ' from ', file_diff
(vardat, ntimes, dt) = load_wrfout(file_diff, [var])
plotdat = vardat[var][:,:,0]
nameadd = ''
if (var == 'PREC'):
(vardat, ntimes, dt) = load_wrfout(file_hist, [var])
vardat_hist = vardat[var][:,:,0]
nmodyears = get_nc_dim(file_hist, 'nmodyears')
vardat_hist = (vardat_hist / nmodyears) * mm2in
(vardat, ntimes, dt) = load_wrfout(file_fore, [var])
vardat_fore = vardat[var][:,:,0]
nmodyears = get_nc_dim(file_fore, 'nmodyears')
vardat_fore = (vardat_fore / nmodyears) * mm2in
#--- Do plot of percentage difference:
plotdat = ((vardat_fore - vardat_hist) / \
((vardat_fore + vardat_hist) / 2)) * 100
levs_c = levs_pcp_percdiff
cmap_c = cmap_pcp_percdiff
var_long = 'Total Precipitation'
cb_lab = 'Total Precip Percentage Difference (%)'
stat_c = 'Percentage Difference'
units_c = '%'
nameadd = '_PERCDIFF'
# #--- Do plot of actual difference:
# plotdat = vardat_fore - vardat_hist
# cb_lab = colorbar_labs[var]
# if season == 'annual' or season == 'fall' or season == 'winter':
# levs_c = levs_pcp_diff_annual
# cmap_c = cmap_pcp_diff_annual
# else:
# levs_c = levs_pcp_diff
# cmap_c = cmap_pcp_diff
# var_long = 'Total Precipitation'
# stat_c = 'Difference'
# units_c = 'in.'
elif var == 'T2MAX':
cb_lab = colorbar_labs[var]
levs_c = levs_temp_diff
cmap_c = cmap_temp_diff
var_long = 'Average Maximum Temperature'
stat_c = 'Difference'
units_c = '$^\circ$C'
elif var == 'T2MIN':
cb_lab = colorbar_labs[var]
levs_c = levs_temp_diff
cmap_c = cmap_temp_diff
var_long = 'Average Minimum Temperature'
stat_c = 'Difference'
units_c = '$^\circ$C'
elif var == 'SPDUV10MAX':
diff_c = plotdat
(vardat, ntimes, dt) = load_wrfout(file_hist, [var])
vardat_hist = vardat[var][:,:,0]
(vardat, ntimes, dt) = load_wrfout(file_fore, [var])
vardat_fore = vardat[var][:,:,0]
plotdat = ((vardat_fore - vardat_hist) / \
((vardat_fore + vardat_hist) / 2)) * 100
levs_c = levs_spd_diff
cmap_c = cmap_spd_diff
var_long = 'Average Maximum Wind Speed'
cb_lab = colorbar_labs[var]
stat_c = 'Difference'
units_c = 'm/s'
# titlein = 'Ensemble Mean, ' + var_lab[var] + ', ' + \
# seasons_lab[season] + ', ' + yyyy_s + ' - ' + yyyy_e
titlein = seasons_lab[season] + ' ' + var_long + ' ' + stat_c + \
' (' + units_c + ')' + \
', (' + yyyy_s_fore + ' - ' + yyyy_e_fore + ') ' + \
' - ' + \
'(' + yyyy_s_hist + ' - ' + yyyy_e_hist + ')'
for z in range(len(zooms)):
plotfname = rundir + '/map_diff_' + sdt_fore + '_' + \
edt_fore + '_' + season + '_' + var + nameadd + \
'_' + zooms[z] + '.png'
mapper(var, lat, lon, plotdat, levs_c, cmap_c, \
maplw, cb_lab, titlein, plotfname, zooms[z])
#sys.exit()
sys.exit()
<file_sep>/old/old_old/test_read_ghcnd_obs_20180411.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
#from make_cmap import *
#from netCDF4 import Dataset as NetCDFFile
#from utils_wrfout import *
#from utils_date import *
#import pickle
#from ghcndextractor import *
#from ghcndextractor import ghcndextractor
import codecs
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
#ghcnFolder = rcm_dir + '/obs'
ghcnd_dir = rcm_dir + '/obs/ghcnd_all'
data_dir = '/home/disk/r2d2/steed/cmip5/rcp8.5'
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
vars = ['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
#sdt = '202001'
#edt = '202912'
sdt = '197001'
edt = '209912'
dtfmt = "%Y%m%d%H"
stns = ['KSEA', 'KSMP', 'KYKM', 'KGEG', 'KPDX', 'KMFR', 'KHQM']
models = ['gfdl-cm3', 'miroc5']
stn_cols = ['b', 'r', 'g', 'c', 'm', 'k', 'grey']
mod_cols = ['b', 'r']
latpts = [47.44472, 47.27667, 46.56417, 47.62139, 45.59083, \
42.38111, 46.97278]
lonpts = [-122.31361, -121.33722, -120.53361, -117.52778, -122.60028, \
-122.87222, -123.93028]
elevs = [130.0, 1207.0, 333.0, 735.0, 8.0, 405.0, 4.0]
#---------------------------------------------------------------------------
#
#---------------------------------------------------------------------------
stn_ghcnd = 'USW00024233'
file_c = ghcnd_dir + '/' + stn_ghcnd + '.dly'
if not os.path.isfile(file_c):
print 'i do not see ', file_c
sys.exit()
print 'i see ', file_c
readLoc = codecs.open(file_c, "r", "utf-8")
allLines = readLoc.readlines()
readLoc.close
for lineOfData in allLines:
print lineOfData
countryCode = lineOfData[0:2]
stationID = lineOfData[0:11]
stationMonthCode = lineOfData[0:17]
year = lineOfData[11:15]
month = lineOfData[15:17]
element = lineOfData[17:21]
# print 'year, month, element = ', year, month, element
for x in range(0, 30):
dayOM = x + 1
offsetStart = (x*8)+21
offsetEnd = offsetStart + 8
ld = lineOfData[offsetStart:offsetEnd]
val = ld[0:5]
mflag = ld[5:6]
qflag = ld[6:7]
sflag = ld[7:8]
# print '-----'
# print 'ld = ', element, ld
# print 'val = ', val
# print 'mflag = ', mflag
# print 'qflag = ', qflag
# print 'sflag = ', sflag
if val == -9999.0:
val = np.nan
if element == 'TMAX' or element == 'TMIN':
#--- Temperatures are in tenths of degrees C.
valout = float(val) / 10.0
elif element == 'PRCP':
#--- Precip is in tenths of a mm. Converting to inches.
valout = (float(val) / 10.0) * 0.0393701
if qflag and qflag.strip():
print 'qflag is not empty, mflag, qflag, sflag = ', \
mflag, ',', qflag, ',', sflag
valout = np.nan
print element, year, month, valout
# sys.exit()
# sys.exit()
#readLoc = codecs.open(file_c, "r", "utf-8")
#allLines = readLoc.readlines()
#readLoc.close
#
#for eachReadLine in allLines:
# readRow(eachReadLine)
#ghcndextractor.countries = ["US"]
#ghcndextractor.states = ["NJ"]
#ghcndextractor.ghcnFolder = "/Users/d035331/Documents/Demo/DemoData_Weather/ghcnd_all"
#
#ghcndextractor.getStationsFromFiles()
#stations = ghcndextractor.getCSVStationMetaData()
#ghcndextractor.readDailyFiles()
#dayCSV = ghcndextractor.getDailyDataCSV(["12"], ["25"], ["USW00014780"])
#print(dayCSV)
#
#
#
#
#
#stn_ghcnd = 'USW00024233'
#
##file_c = ghcnd_dir + '/' + stn_ghcnd + '.dly'
#
#stationIDCodes = ['USW00024233']
#iret = readDailyFiles()
sys.exit()
<file_sep>/old/old_old/utils_test.py
#!/usr/bin/python
import os, sys, glob, re
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
#from netCDF4 import Dataset as NetCDFFile
#from collections import defaultdict
#from utils_cmap import *
#import pickle
#from utils_date import *
def plot_it(data_all, dts_all, models, stns, var, stat, cols, \
data_obs, dts_obs):
mod = 'gfdl-cm3'
var = 'T2MAX'
stn = 'KSEA'
stat = 'avg'
plotfname = 'test.png'
fig, ax = plt.subplots( figsize=(10,6) )
lw_sm = 1.8
for m in range(len(models)):
ptot = []
mod = models[m]
for d in range(len(dts_all)):
dt_c = dts_all[d]
yyyy = dt_c[0:4]
mm = dt_c[4:6]
if (yyyy == '1999' and (mm >= '06' and mm <= '08')):
key = (mod,var,stn,dt_c)
if (key in data_all):
mod_c = data_all[key]
ptot.append(mod_c - 273.15)
lab_c = mod.upper()
plt.plot(ptot, label = lab_c, color = cols[m], linewidth=lw_sm)
ptot = []
for d in range(len(dts_obs)):
dat_c = data_obs['TMAX','KSEA',dts_obs[d]]
ptot.append(dat_c)
lab_c = 'Obs'
plt.plot(ptot, label = lab_c, color = 'black', linewidth=2.1)
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
return -9999
<file_sep>/igtg_check.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
from igtg_check_utils import *
import matplotlib.pyplot as plt
#import pickle
from make_cmap import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
#data_dir = rcm_dir + '/igtg.nml.works.3d.4d'
data_dir = '/home/disk/mass/steed/cmip5/rcp4.5/access1.0/common/' \
'igtg.nml.works.3d.tos'
#data_dir = '/home/disk/mass/steed/cmip5/rcp4.5/access1.0/common/testdata'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#varname = 'ua'
varname = 'tos'
#file_old = data_dir + '/' + varname + '.1990010106.1991010100.nc'
#file_new = data_dir + '/' + varname + '_new.nc'
file_old = data_dir + '/' + 'indata'
file_new = data_dir + '/' + 'outdata'
itime = 1
ilev = 1
title_old = varname + ' OLD, itime = ' + str(itime) + ', ilev = ' + \
str(ilev)
plotfname_old = './' + varname + '_old_itime' + str(itime) + '_ilev' + \
str(ilev) + '.png'
title_new = varname + ' NEW, itime = ' + str(itime) + ', ilev = ' + \
str(ilev)
plotfname_new = './' + varname + '_new_itime' + str(itime) + '_ilev' + \
str(ilev) + '.png'
#minlat = 20.0
#maxlat = 50.0
#minlon = -120.0
#maxlon = -50.0
minlat = 20.0
maxlat = 70.0
minlon = -175.0
maxlon = -85.0
#---------------------------------------------------------------------------
# Make a color map using make_cmap.
#---------------------------------------------------------------------------
if (varname == 'tos'):
levs_c = levs_tos
cmap_c = cmap_tos
elif(varname == 'ua'):
levs_c = levs_spd
cmap_c = cmap_spd
levs_norm_c = []
for i in range(len(levs_c)):
x = float(levs_c[i])
norm_c = (x - min(levs_c)) / (max(levs_c) - min(levs_c))
levs_norm_c.append(norm_c)
my_cmap = make_cmap(cmap_c, bit = True, position = levs_norm_c)
#---------------------------------------------------------------------------
# Load old and new files.
#---------------------------------------------------------------------------
(lat_old, lon_old, data_old) = load_nc(file_old, varname, itime, ilev)
print 'lat_old, lon_old, data_old = ', lat_old, lon_old, data_old
(lat_new, lon_new, data_new) = load_nc(file_new, varname, itime, ilev)
print 'lat_new, lon_new, data_new = ', lat_new, lon_new, data_new
#sys.exit()
#---------------------------------------------------------------------------
# Plot old data.
#---------------------------------------------------------------------------
mapper(lat_old, lon_old, data_old, minlat, maxlat, minlon, maxlon, \
levs_c, my_cmap, maplw, colorbar_lab, title_old, plotfname_old)
mapper(lat_new, lon_new, data_new, minlat, maxlat, minlon, maxlon, \
levs_c, my_cmap, maplw, colorbar_lab, title_new, plotfname_new)
sys.exit()
<file_sep>/old/old_old/areal_cdd_compare_20180524.py
#!/usr/bin/python
import os, sys, glob, re
import numpy as np
from netCDF4 import Dataset as NetCDFFile
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
import pickle
from utils_date import *
from utils_wrfout import *
import csv
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
data_dir = rcm_dir + '/data'
cdd_data = data_dir + '/mask_pts.dat'
mask_file = data_dir + '/mask_pts.dat'
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
pickle_file = pickle_dir + '/T2MAX_slice.pickle'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
sdt = '197001'
edt = '201712'
#edt = '198012'
#vars_mod = ['PREC', 'T2MAX', 'T2MIN']
vars_mod = ['T2MAX']
models = ['gfdl-cm3', 'miroc5', 'bcc-csm1.1', 'access1.3']
mod_cols = ['b', 'r', 'g', 'y']
#---------------------------------------------------------------------------
# Read mask lat/lon points file.
#---------------------------------------------------------------------------
mask = open(mask_file)
csvReader = csv.reader(mask)
maski = []
maskj = []
for row in csvReader:
maski.append(int(row[0]))
maskj.append(int(row[1]))
mask.close()
#---------------------------------------------------------------------------
# Read Climate Division Data file.
#---------------------------------------------------------------------------
cdd_yyyy = {}
cdd_tmx_avg = {}
for s in range(len(seasons)):
sm = seasons_mo[s]
season = seasons[s]
cdd_file = data_dir + '/cdd_' + sm + '_WA.dat'
print 'reading ', cdd_file
cdd = open(cdd_file)
csvReader = csv.reader(cdd)
yyyy = []
tmx_avg = []
for row in csvReader:
(yyyy_c,val) = row[0].split()
yyyy.append(int(yyyy_c))
if float(val) < -100:
tmx_avg.append(np.nan)
else:
tmx_avg.append(float(val))
cdd.close()
cdd_yyyy[season] = yyyy
cdd_tmx_avg[season] = tmx_avg
#---------------------------------------------------------------------------
# For each model, read in grids and keep "slice" of it as defined by
# mask_file read in above.
#---------------------------------------------------------------------------
#sums_all = {}
#cnts_all = {}
#years_all = []
#for m in range(len(models)):
# mod = models[m]
# files = get_extract_files(data_dirs, mod, sdt, edt)
# files = list(sorted(set(files)))
# (seafiles, years) = get_seasonal_files_new(files)
#
# years_all.append(years)
#
# for y in range(len(years)):
# yyyy = years[y]
# print 'loading ', mod, yyyy
# for s in range(len(seasons)):
# season = seasons[s]
# if (seafiles[season,yyyy] == seafiles[season,yyyy]):
# (sums, cnts) = sum_wrfout_slice(seafiles[season,yyyy], \
# vars_mod, maski, maskj)
# for var in vars_mod:
# key = (mod,season,var,yyyy)
# #--- Winter of first year will only have Jan-Feb. So
# #--- nan it out.
# if (y == 0 and season == 'winter'):
# sums_all[key] = np.nan
# cnts_all[key] = np.nan
# else:
# if (var in sums):
# sums_all[key] = sums[var]
# cnts_all[key] = cnts[var]
#
#years_all = list(sorted(set(years_all[0])))
#pickle.dump((sums_all,cnts_all,years_all), open(pickle_file,'wb'), -1)
#
#sys.exit()
if os.path.isfile(pickle_file):
print 'reading pickle file ', pickle_file
(sums_all,cnts_all,years_all) = pickle.load(open(pickle_file, 'rb'))
#---------------------------------------------------------------------------
# Make plot for each seasons.
#---------------------------------------------------------------------------
var = 'T2MAX'
stat = 'avg'
lw_sm = 1.8
smooth_fact = 5 #-- odd number, used for smoothing time series plots.
for s in range(len(seasons)):
fig, ax = plt.subplots( figsize=(10,6) )
season = seasons[s]
cdd_yyyy_c = cdd_yyyy[season]
test = np.asarray(cdd_tmx_avg[season])
cdd_tmx_avg_c = (test - 32.0) * (5./9.)
# plt.plot(cdd_tmx_avg_c, alpha=0.4, label='Observed', color='black', \
# linestyle='None', marker='o', markersize=3)
lab_c = 'Observed ' + str(smooth_fact) + '-pt smoothing'
plt.plot(smooth(cdd_tmx_avg_c, smooth_fact), label=lab_c, color='black', \
linewidth=lw_sm)
for m in range(len(models)):
mod = models[m]
modp = []
xlabs = []
for y in range(len(cdd_yyyy_c)):
yyyy = cdd_yyyy_c[y]
xlabs.append(yyyy)
key = (mod,season,var,str(yyyy))
if key in sums_all:
tc = (sums_all[key] / cnts_all[key]) - 273.15
tf = (tc * 9./5.) + 32.0
modp.append(tc)
else:
modp.append(np.nan)
# plt.plot(modp, alpha = 0.17, label = mod.upper(), color = mod_cols[m], \
# linewidth=lw_sm)
lab_c = mod.upper() + ', ' + str(smooth_fact) + '-pt smoothing'
plt.plot(smooth(modp, smooth_fact), label = lab_c, color = mod_cols[m],\
linewidth=lw_sm)
#--- y-axis labeling.
# plt.ylim(ylims[var+stat])
plt.ylabel('Seasonal ' + labels[var+stat], fontsize=fs+1)
plt.tick_params(axis='y', which='major', labelsize=fs+1)
#--- x-axis labels.
xticks_c = range(0,len(xlabs), 1)
xlabs_c = [ xlabs[i] for i in xticks_c ]
plt.xticks(xticks_c)
plt.tick_params(axis='x', which='major', labelsize=fs-1)
ax.set_xticklabels(xlabs_c, rotation=90)
plt.xlabel('Year', fontsize=fs+1)
titlein = 'Climate Division Data Areal Verification, WA state, ' + \
seasons_lab[season] + ', ' + labels[var+stat] + ', ' + \
sdt + ' - ' + edt
plt.title(titlein, fontsize=titlefs, fontweight='bold')
plt.tight_layout()
plt.grid()
plt.legend(fontsize = fs-1, loc = 'best')
plotfname = plot_dir + '/areal_' + season + '.png'
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
sys.exit()
<file_sep>/utils_stats.py
#!/usr/bin/python
import os, sys, glob, re, math
import numpy as np
from collections import defaultdict
import pickle
from utils_date import *
from utils_plot import *
from utils_ghcnd_obs import *
dtfmt = "%Y%m%d%H"
mm2in = 0.0393700787
min_season_days = 85
std_lapse = 0.0065 #-- std. atmos. lapse rate
mvc = -9999.0
#---------------------------------------------------------------------------
# Load wrfout file.
#---------------------------------------------------------------------------
def sum_wrfout_slice(files, vars, maski, maskj):
sums = {}
cnts = {}
for f in range(len(files)):
print 'reading ', files[f]
nc = NetCDFFile(files[f], 'r')
ntimes = len(nc.dimensions['Time'])
for v in range(len(vars)):
var = vars[v]
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
sum_c = 0
cnt_c = 0
for n in range(len(maski)):
sum_c = sum_c + sum(dat_tmp[maski[n], maskj[n],:])
cnt_c = cnt_c + len(dat_tmp[maski[n], maskj[n],:])
if (var in sums):
sums[var] = sums[var] + sum_c
cnts[var] = cnts[var] + cnt_c
else:
sums[var] = sum_c
cnts[var] = cnt_c
return sums, cnts
#---------------------------------------------------------------------------
# For ensemble standard deviation plots....
#---------------------------------------------------------------------------
def get_ensmean_std(var, models, years, season, \
sum_files, spr_files, win_files, fal_files, ann_files, \
pickle_file):
nyears = 0
nmodels = len(models)
files = []
dts_all = []
for y in range(len(years)):
yyyy = years[y]
for m in range(nmodels):
model = models[m]
sum_files_c = sum_files[model]
spr_files_c = spr_files[model]
win_files_c = win_files[model]
fal_files_c = fal_files[model]
ann_files_c = ann_files[model]
if season == 'summer':
if (sum_files_c[yyyy] == sum_files_c[yyyy]):
nyears += 1
for f in range(len(sum_files_c[yyyy])):
files.append(sum_files_c[yyyy][f])
else:
continue
elif season == 'spring':
if (spr_files_c[yyyy] == spr_files_c[yyyy]):
nyears += 1
for f in range(len(spr_files_c[yyyy])):
files.append(spr_files_c[yyyy][f])
else:
continue
elif season == 'winter':
if (win_files_c[yyyy] == win_files_c[yyyy]):
nyears += 1
for f in range(len(win_files_c[yyyy])):
files.append(win_files_c[yyyy][f])
else:
continue
elif season == 'fall':
if (fal_files_c[yyyy] == fal_files_c[yyyy]):
nyears += 1
for f in range(len(fal_files_c[yyyy])):
files.append(fal_files_c[yyyy][f])
else:
continue
elif season == 'annual':
if (ann_files_c[yyyy] == ann_files_c[yyyy]):
nyears += 1
for f in range(len(ann_files_c[yyyy])):
files.append(ann_files_c[yyyy][f])
else:
continue
print 'files = ', files
#--- Get sorted, unique set of dates from files found above.
nfiles = len(files)
dts_all = []
for f in range(nfiles):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
dts_all.append(dt_c)
dts_all = list(sorted(set(dts_all)))
#--- For each unique date, read in files for each model.
for d in range(len(dts_all)):
#--- First make sure all members have a file.
files_c = []
print dts_all[d]
for f in range(nfiles):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
if dt_c == dts_all[d]:
files_c.append(files[f])
if len(files_c) != nmodels:
print 'missing member(s) for date = ', dts_all[d]
sys.exit()
#--- Now read in files for each member.
for n in range(len(files_c)):
print 'loading ', files_c[n]
(vardat, ntimes, dt) = load_wrfout(files_c[n], [var])
plotdat = vardat[var][:,:,:]
nx,ny,nt = plotdat.shape
if n == 0:
dat_all = np.ones((nx, ny, nt, nmodels)) * np.nan
stdevs_tmp = np.ones((nx, ny, nt)) * np.nan
if n == 0 and d == 0:
stdevs = np.ones((nx, ny, nt)) * np.nan
dat_all[:,:,:,n] = plotdat
#--- For each ntimes (these should be monthly files, so ntimes are
#--- days and will be ~30 days), calculate a standard deviation.
for nt in range(ntimes):
dat_c = np.squeeze(dat_all[:,:,nt,:])
stdevs_tmp[:,:,nt] = np.std(dat_c, axis=2)
#--- Add this month's standard deviation grids to our final array,
#--- stdevs.
if d == 0:
stdevs = stdevs_tmp
else:
stdevs = np.concatenate((stdevs, stdevs_tmp), axis = 2)
#--- Calculate the mean of the daily standard deviations.
stdevmean = np.mean(stdevs, axis=2)
#--- Create pickle file.
pickle.dump((stdevmean), open(pickle_file,'wb'), -1)
return stdevmean
#---------------------------------------------------------------------------
# For ensemble mean plots, get system nco command.
#---------------------------------------------------------------------------
def get_ensmean_syscom(file_plot, var, models, years, season, \
sum_files, spr_files, \
win_files, fal_files, ann_files, ncocom):
syscom = ncocom
nyears = 0
for m in range(len(models)):
model = models[m]
sum_files_c = sum_files[model]
spr_files_c = spr_files[model]
win_files_c = win_files[model]
fal_files_c = fal_files[model]
ann_files_c = ann_files[model]
for y in range(len(years)):
yyyy = years[y]
if season == 'summer':
if (sum_files_c[yyyy] == sum_files_c[yyyy]):
nyears += 1
for f in range(len(sum_files_c[yyyy])):
syscom = syscom + ' ' + sum_files_c[yyyy][f]
else:
continue
elif season == 'spring':
if (spr_files_c[yyyy] == spr_files_c[yyyy]):
nyears += 1
for f in range(len(spr_files_c[yyyy])):
syscom = syscom + ' ' + spr_files_c[yyyy][f]
else:
continue
elif season == 'winter':
if (win_files_c[yyyy] == win_files_c[yyyy]):
nyears += 1
for f in range(len(win_files_c[yyyy])):
syscom = syscom + ' ' + win_files_c[yyyy][f]
else:
continue
elif season == 'fall':
if (fal_files_c[yyyy] == fal_files_c[yyyy]):
nyears += 1
for f in range(len(fal_files_c[yyyy])):
syscom = syscom + ' ' + fal_files_c[yyyy][f]
else:
continue
elif season == 'annual':
if (ann_files_c[yyyy] == ann_files_c[yyyy]):
nyears += 1
for f in range(len(ann_files_c[yyyy])):
syscom = syscom + ' ' + ann_files_c[yyyy][f]
else:
continue
return syscom, nyears
#---------------------------------------------------------------------------
# For ensemble mean plots, run system nco command.
#---------------------------------------------------------------------------
def run_ensmean_syscom(file_plot, var, ncocom, syscom, yyyy_s, yyyy_e, \
season, rundir, ):
#--- If system command is too big (too many files to send
#--- to nco), break it up into 1000 piece chunks.
files_c = syscom.replace(ncocom, '')
scsplit = files_c.split()
nfiles = len(scsplit)
niter = 1000
if nfiles > niter:
nt = int(math.ceil(float(nfiles) / float(niter)))
files_all = []
for n in range(nt):
file_c = rundir + '/' + var + '_' + season + '_' + \
yyyy_s + '_' + yyyy_e + '_subset' + str(n) + '.nc'
if os.path.isfile(file_c):
print 'this file already exists: ', file_c
files_all.append(file_c)
continue
nb = n * niter
ne = (n+1) * niter
syscom = ncocom + ' ' + ' '.join(scsplit[nb:ne]) + ' ' + file_c
print syscom
iret = os.system(syscom)
if iret != 0:
print 'problem running nco! iret = ', iret
return mvc
files_all.append(file_c)
syscom = ncocom + ' ' + ' '.join(files_all) + ' ' + file_plot
print syscom
iret = os.system(syscom)
if iret != 0:
print 'problem running nco! iret = ', iret
return mvc
else:
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
iret = os.system(syscom)
if iret != 0:
print 'problem running nco! iret = ', iret
return mvc
return 1
#---------------------------------------------------------------------------
# Get seasonal stats (totals or averages currently).
#---------------------------------------------------------------------------
def get_seasonal_stats(data_all, dts_all, models, stns, var, stat):
out_sum = {}
out_avg = {}
out_max = {}
out_min = {}
years_all = []
for m in range(len(models)):
mod = models[m]
data_all_c = data_all[mod]
for s in range(len(stns)):
stn = stns[s]
ndays = {}
years_mod_stn = []
for d in range(len(dts_all)):
yyyy = dts_all[d][0:4]
mm = dts_all[d][4:6]
years_mod_stn.append(yyyy)
#--- Very first date of all model runs will have unusable
#--- (all 0's) in the fields. So don't try and use it.
if dts_all[d] == '1970010100':
continue
if ((var,stn,dts_all[d]) in data_all_c):
dat_c = data_all_c[var,stn,dts_all[d]]
else:
ndays[season+yyyy] = np.nan
out_sum[season,mod,stn,yyyy] = np.nan
out_max[season,mod,stn,yyyy] = np.nan
out_min[season,mod,stn,yyyy] = np.nan
continue
#--- annual is all days so get sum/max/min for every dts_all.
(ndays, out_sum, out_max, out_min) = \
get_season_summaxmin(dat_c, mod, stn, 'annual',yyyy,mm,\
ndays, out_sum, out_max, out_min)
if (mm == '03' or mm == '04' or mm == '05'):
season = 'spring'
if (mm == '06' or mm == '07' or mm == '08'):
season = 'summer'
if (mm == '09' or mm == '10' or mm == '11'):
season = 'fall'
if (mm == '12' or mm == '01' or mm == '02'):
season = 'winter'
(ndays, out_sum, out_max, out_min) = \
get_season_summaxmin(dat_c, mod, stn, season, yyyy, mm,\
ndays, out_sum, out_max, out_min)
#--- Get list of unique, sorted years found above.
years = list(sorted(set(years_mod_stn)))
#--- Check number of data points per unique years and nan out
#--- ones that do not have enough (< min_season_days).
for y in range(len(years)):
yyyy = years[y]
for s in range(len(seasons)):
season = seasons[s]
if ((season+yyyy) in ndays):
if (ndays[season+yyyy] < min_season_days):
out_sum[season,mod,stn,yyyy] = np.nan
out_max[season,mod,stn,yyyy] = np.nan
out_min[season,mod,stn,yyyy] = np.nan
else:
ndays[season+yyyy] = np.nan
out_sum[season,mod,stn,yyyy] = np.nan
out_max[season,mod,stn,yyyy] = np.nan
out_min[season,mod,stn,yyyy] = np.nan
#--- if stat requested was 'avg', do averaging.
if (stat == 'avg' or stat == 'max' or stat == 'min'):
for y in range(len(years)):
yyyy = years[y]
for s in range(len(seasons)):
season = seasons[s]
out_avg[season,mod,stn,yyyy] = out_sum[\
season,mod,stn,yyyy] / ndays[season+yyyy]
#--- Keep around all years found for this model and station.
years_all.append(years_mod_stn)
#--- Final, unique, sorted list of years found.
years = list(sorted(set(years_all[0])))
if (stat == 'avg'):
return out_avg, years
elif (stat == 'max'):
return out_max, years
elif (stat == 'min'):
return out_min, years
else:
return out_sum, years
#---------------------------------------------------------------------------
# Interpolate from a sent-in grid to a sent in lat/lon point.
#---------------------------------------------------------------------------
def bilinear_interpolate(latgrid, longrid, datagrid, latpt, lonpt, deb):
totdiff = abs(latgrid - latpt) + abs(longrid - lonpt)
(i,j) = np.unravel_index(totdiff.argmin(), totdiff.shape)
#--- Get lat/lon box in which latpt,lonpt resides.
if (latpt >= latgrid[i,j] and lonpt >= longrid[i,j]):
iif = i
jjf = j
elif (latpt < latgrid[i,j] and lonpt < longrid[i,j]):
iif = i-1
jjf = j-1
elif (latpt >= latgrid[i,j] and lonpt < longrid[i,j]):
iif = i-1
jjf = j
elif (latpt < latgrid[i,j] and lonpt >= longrid[i,j]):
iif = i
jjf = j-1
(nx, ny) = np.shape(latgrid)
if (deb == 1):
print 'nx, ny = ', nx, ny
print 'iif,jjf = ', iif,jjf
print 'latgrid[iif+1,jjf] = ', latgrid[iif+1,jjf]
print 'latgrid[iif,jjf] = ', latgrid[iif,jjf]
if (iif >= (nx-1) or jjf >= (ny-1) or iif < 0 or jjf < 0):
return mvc
#--- Do bilinear interpolation to latpt,lonpt.
dlat = latgrid[iif,jjf+1] - latgrid[iif,jjf]
dlon = longrid[iif+1,jjf] - longrid[iif,jjf]
dslat = latgrid[iif,jjf+1] - latpt
dslon = longrid[iif+1,jjf] - lonpt
wrgt = 1 - (dslon/dlon)
wup = 1 - (dslat/dlat)
vll = datagrid[iif,jjf]
vlr = datagrid[iif,jjf+1]
vul = datagrid[iif+1,jjf]
vur = datagrid[iif+1,jjf+1]
if (deb > 1):
print 'll lat, lon, val = ', latgrid[iif,jjf], longrid[iif,jjf], vll
print 'lr lat, lon, val = ', latgrid[iif+1,jjf], longrid[iif+1,jjf], vlr
print 'ur lat, lon, val = ', latgrid[iif+1,jjf+1], longrid[iif+1,jjf+1],vur
print 'ul lat, lon, val = ', latgrid[iif,jjf+1], longrid[iif,jjf+1], vul
print 'latpt, lonpt = ', latpt, lonpt
print 'vll = ', vll
print 'vlr = ', vlr
print 'vul = ', vul
print 'vur = ', vur
datout = (1-wrgt) * ((1-wup) * vll + wup * vul) + \
(wrgt) * ((1-wup) * vlr + wup * vur)
# if (deb == 1):
# print 'datout = ', datout
# sys.exit()
# if (datout == 0.0):
# print 'nx, ny = ', nx, ny
# print 'iif,jjf = ', iif,jjf
# print 'latgrid[iif+1,jjf] = ', latgrid[iif+1,jjf]
# print 'latgrid[iif,jjf] = ', latgrid[iif,jjf]
#
# print 'll lat, lon, val = ', latgrid[iif,jjf], longrid[iif,jjf], vll
# print 'lr lat, lon, val = ', latgrid[iif+1,jjf], longrid[iif+1,jjf], vl#r
# print 'ur lat, lon, val = ', latgrid[iif+1,jjf+1], longrid[iif+1,jjf+1],vur
# print 'ul lat, lon, val = ', latgrid[iif,jjf+1], longrid[iif,jjf+1], vu#l
# print 'latpt, lonpt = ', latpt, lonpt
#
# print 'vll = ', vll
# print 'vlr = ', vlr
# print 'vul = ', vul
# print 'vur = ', vur
#
# sys.exit()
return datout
#----------------------------------------------------------------------------
# Add data to sums, max's and min's arrays.
#----------------------------------------------------------------------------
def get_season_summaxmin(dat_c, mod, stn, season, yyyyin, month, ndays, \
out_sum, out_max, out_min):
#--- Look out for cases where data is NaN (e.g. the 31st of June) and
#--- do nothing (return) in those cases.
if np.isnan(dat_c):
return ndays, out_sum, out_max, out_min
#--- Put December winter data into next year's winter-- naming
#--- winters by their Jan/Feb year rather than their Dec year.
if month == '12':
yyyy = str(int(yyyyin) + 1)
else:
yyyy = yyyyin
#--- Count number of days for this season and year.
if (season+yyyy) in ndays:
ndays[season+yyyy] += 1
else:
ndays[season+yyyy] = 1
key = (season,mod,stn,yyyy)
#--- Get sum of sent in data.
if key in out_sum:
out_sum[key] = out_sum[key] + dat_c
else:
out_sum[key] = dat_c
#--- Get maximum.
if key in out_max:
if dat_c > out_max[key]:
out_max[key] = dat_c
else:
out_max[key] = dat_c
#--- Get minimum.
if key in out_min:
if dat_c < out_min[key]:
out_min[key] = dat_c
else:
out_min[key] = dat_c
return ndays, out_sum, out_max, out_min
<file_sep>/old/old_old/igtg_check_utils_20161103.py
#!/usr/bin/python
import os, os.path, time, glob, re, math
import sys, string, readline, h5py
from Scientific.IO.NetCDF import *
import numpy as np
#from datetime import datetime, timedelta, time
from datetime import datetime
import matplotlib as mpl
mpl.use('Agg')
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
#from rw_plotsettings import *
import pickle
colorbar_lab = 'm/s';
maplw = 0.8
#levs_spd = [ 0.0, 2.5, 5.0, 7.5, 10.0, 12.5, 15.0, 17.5, 20.0, 22.5,
# 25.0, 27.5, 30.0, 32.5, 35.0, 37.5 ]
levs_spd = [ -24, -21, -18, -15, -12, -9, -6, -3, 3, 6, 9, 12, 15, 18, \
21, 24]
cmap_spd = [(255,255,255), \
(192,192,192), \
(128,128,128 ), \
(0,255,255), \
(32,178,170), \
(0,255,0), \
(0,128,0), \
(255,0,204), \
(199,21,133), \
(0,0,255), \
(0,0,128), \
(255,255,0), \
(255,204,17), \
(255,69,0), \
(0,0,0),\
(255,255,255)]
#---------------------------------------------------------------------------
# Load geog file.
#---------------------------------------------------------------------------
def load_nc(filein, varname, itime, ilev):
file = NetCDFFile(filein, 'r')
lat_tmp = file.variables['lat'][:]
lon_tmp = file.variables['lon'][:]
data_all = file.variables[varname]
fillval = data_all._FillValue[0]
data_tmp = file.variables[varname][itime,ilev,:,:]
file.close()
#--- Convert from 0-to-360 longitudes to -180-to-180.
ipos = np.where(lon_tmp >= 180.0)
iwest = ipos[0]
lon_tmp[iwest] = lon_tmp[iwest] - 360.0
#--- transpose data array to be x/y (lon/lat).
dataout = np.transpose(data_tmp)
dataout[dataout == fillval] = np.NAN
#--- Fill in 2-D lat / lon terr arrays.
nx = len(lon_tmp)
ny = len(lat_tmp)
lonout = np.empty([nx,ny])
latout = np.empty([nx,ny])
for y in range(ny):
latout[:,y] = lat_tmp[y]
for x in range(nx):
lonout[x,:] = lon_tmp[x]
return latout, lonout, dataout
#---------------------------------------------------------------------------
# Mapper.
#---------------------------------------------------------------------------
def mapper(lat, lon, grid, minlat, maxlat, minlon, maxlon, \
levs, cmap, maplw, colorbar_lab, title, plotfname):
ur_lat = maxlat
ur_lon = maxlon
ll_lat = minlat
ll_lon = minlon
lat_ctr = ll_lat + ((ur_lat - ll_lat) * 0.5)
lon_ctr = ll_lon + ((ur_lon - ll_lon) * 0.5)
width = 6.5
height = 6.5
fs = 9
titlefs = 9
fig = plt.figure(figsize=(width,height))
# left, bottom, width, height:
ax = fig.add_axes([0.00,0.05,0.99,0.91])
#---
map = Basemap(projection='ortho',lat_0=45,lon_0=-100,resolution='l')
# map = Basemap(resolution='i',projection='lcc',\
# llcrnrlon= ll_lon, llcrnrlat=ll_lat,\
# urcrnrlon= ur_lon, urcrnrlat= ur_lat,\
# lat_0=lat_ctr,lon_0=lon_ctr,lat_1=(ur_lat - ll_lat))
#--- Get lat and lon data in map's x/y coordinates.
x,y = map(lon, lat)
#--- Draw coastlines, country boundaries, fill continents.
map.drawcoastlines(linewidth = maplw)
map.drawstates(linewidth = maplw)
map.drawcountries(linewidth = maplw)
#--- Draw the edge of the map projection region (the projection limb)
map.drawmapboundary(linewidth = maplw)
#--- Draw lat/lon grid lines every 30 degrees.
map.drawmeridians(np.arange(0, 360, 30), linewidth = maplw)
map.drawparallels(np.arange(-90, 90, 30), linewidth = maplw)
cs = plt.contourf(x, y, grid, levs, cmap=cmap)
cbar = map.colorbar(cs, location='bottom', pad="3%", size=0.1, ticks=levs)
cbar.set_label(colorbar_lab, fontsize=fs, size=fs-1)
cbar.ax.tick_params(labelsize=fs-1)
plt.title(title, fontsize=titlefs, fontweight='bold')
#--- Save plot.
print 'creating ', plotfname
plt.savefig(plotfname)
plt.close()
<file_sep>/old/old_old/areal_cdd_compare_20180523.py
#!/usr/bin/python
import os, sys, glob, re
import numpy as np
from netCDF4 import Dataset as NetCDFFile
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
import pickle
from utils_date import *
from utils_wrfout import *
import csv
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
data_dir = rcm_dir + '/data'
cdd_data = data_dir + '/mask_pts.dat'
mask_file = data_dir + '/mask_pts.dat'
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
pickle_file = pickle_dir + '/T2MAX_slice.pickle'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
sdt = '197001'
#edt = '201712'
edt = '197512'
#vars_mod = ['PREC', 'T2MAX', 'T2MIN']
vars_mod = ['T2MAX']
models = ['gfdl-cm3', 'miroc5', 'bcc-csm1.1', 'access1.3']
mod_cols = ['b', 'r', 'g', 'y']
#---------------------------------------------------------------------------
# Read mask lat/lon points file.
#---------------------------------------------------------------------------
mask = open(mask_file)
csvReader = csv.reader(mask)
maski = []
maskj = []
for row in csvReader:
maski.append(int(row[0]))
maskj.append(int(row[1]))
mask.close()
#---------------------------------------------------------------------------
# Read Climate Division Data file.
#---------------------------------------------------------------------------
cdd_yyyy = {}
cdd_tmx_avg = {}
for s in range(len(seasons)):
sm = seasons_mo[s]
season = seasons[s]
cdd_file = data_dir + '/cdd_' + sm + '_WA.dat'
cdd = open(cdd_file)
csvReader = csv.reader(cdd)
yyyy = []
tmx_avg = []
for row in csvReader:
(yyyy_c,val) = row[0].split()
yyyy.append(int(yyyy_c))
tmx_avg.append(float(val))
cdd.close()
cdd_yyyy[season] = yyyy
cdd_tmx_avg[season] = tmx_avg
##---------------------------------------------------------------------------
## Load geo_em file.
##---------------------------------------------------------------------------
#print 'Loading ', geo_em
#nc = NetCDFFile(geo_em, 'r')
#lat = nc.variables['XLAT_M'][0,:,:]
#lon = nc.variables['XLONG_M'][0,:,:]
#hgt = nc.variables['HGT_M'][0,:,:]
#lat = np.transpose(lat)
#lon = np.transpose(lon)
#hgt = np.transpose(hgt)
#nc.close()
#---------------------------------------------------------------------------
#
#---------------------------------------------------------------------------
sums_all = {}
cnts_all = {}
years_all = []
for m in range(len(models)):
mod = models[m]
print 'Loading slice for model ', mod
files = get_extract_files(data_dirs, mod, sdt, edt)
files = list(sorted(set(files)))
(seafiles, years) = get_seasonal_files_new(files)
years_all.append(years)
for y in range(len(years)):
yyyy = years[y]
print 'loading year ', yyyy
for s in range(len(seasons)):
season = seasons[s]
if (seafiles[season,yyyy] == seafiles[season,yyyy]):
(sums, cnts) = sum_wrfout_slice(seafiles[season,yyyy], \
vars_mod, maski, maskj)
for var in vars_mod:
key = (mod,season,var,yyyy)
if (var in sums):
sums_all[key] = sums[var]
cnts_all[key] = cnts[var]
years_all = list(sorted(set(years_all[0])))
pickle.dump((sums_all,cnts_all,years_all), open(pickle_file,'wb'), -1)
sys.exit()
#---------------------------------------------------------------------------
#
#---------------------------------------------------------------------------
var = 'T2MAX'
stat = 'avg'
fig, ax = plt.subplots( figsize=(10,6) )
lw_sm = 1.8
smooth_fact = 5 #-- odd number, used for smoothing time series plots.
for s in range(len(seasons)):
season = seasons[s]
cdd_yyyy_c = cdd_yyyy[season]
cdd_tmx_avg_c = (cdd_tmx_avg[season] - 32.0) * (5./9.)
plt.plot(cdd_tmx_avg_c, alpha=0.6, label='Observed', color='black', \
linestyle='None', marker='o', markersize=3)
lab_c = 'Observed ' + str(smooth_fact) + '-pt smoothing'
plt.plot(smooth(cdd_tmx_avg_c, smooth_fact), label=lab_c, color='black', \
linewidth=lw_sm)
for m in range(len(models)):
mod = models[m]
modp = []
xlabs = []
for y in range(len(cdd_yyyy_c)):
yyyy = cdd_yyyy_c[y]
xlabs.append(yyyy)
key = (mod,season,var,yyyy)
if key in sums_all:
tc = sums_all[key] / cnts_all[key]
tf = (tc * 9./5.) + 32.0
modp.append(tf)
else:
modp.append(np.nan)
plt.plot(modp, alpha = 0.25, label = mod.upper(), color = mod_cols[m], \
linewidth=lw_sm)
lab_c = mod.upper() + ', ' + str(smooth_fact) + '-pt smoothing'
plt.plot(smooth(modp, smooth_fact), label = lab_c, color = mod_cols[m],\
linewidth=lw_sm)
#--- y-axis labeling.
plt.ylim(ylims[15,90])
plt.ylabel('Seasonal ' + labels[var+stat], fontsize=fs+1)
plt.tick_params(axis='y', which='major', labelsize=fs+1)
#--- x-axis labels.
xticks_c = range(0,len(xlabs), 4)
xlabs_c = [ xlabs[i] for i in xticks_c ]
plt.xticks(xticks_c)
plt.tick_params(axis='x', which='major', labelsize=fs-1)
ax.set_xticklabels(xlabs_c, rotation=90)
plt.xlabel('Year', fontsize=fs+1)
titlein = 'test'
plt.title(titlein, fontsize=titlefs, fontweight='bold')
plt.tight_layout()
plt.grid()
plt.legend(fontsize = fs-1, loc = 'best')
plotfname = './test.png'
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
sys.exit()
'''
#--- Loop over year...
for y in range(len(years)):
# sums = {}
# cnts = {}
yyyy = years[y]
print 'loading year ', yyyy
if (win_files[yyyy] == win_files[yyyy]):
season = 'winter'
# (sums, cnts) = sum_wrfout_slice(win_files[yyyy], vars_mod, \
# maski, maskj, sums, cnts)
(sums, cnts) = sum_wrfout_slice(win_files[yyyy], vars_mod, \
maski, maskj)
for var in vars_mod:
key = (mod,season,var,yyyy)
sums_all[key] = sums[var]
cnts_all[key] = cnts[var]
if (spr_files[yyyy] == spr_files[yyyy]):
season = 'spring'
# (sums, cnts) = sum_wrfout_slice(spr_files[yyyy], vars_mod, \
# maski, maskj, sums, cnts)
(sums, cnts) = sum_wrfout_slice(spr_files[yyyy], vars_mod, \
maski, maskj)
for var in vars_mod:
key = (mod,season,var,yyyy)
sums_all[key] = sums[var]
cnts_all[key] = cnts[var]
if (sum_files[yyyy] == sum_files[yyyy]):
season = 'summer'
# (sums, cnts) = sum_wrfout_slice(sum_files[yyyy], vars_mod, \
# maski, maskj, sums, cnts)
(sums, cnts) = sum_wrfout_slice(sum_files[yyyy], vars_mod, \
maski, maskj)
for var in vars_mod:
key = (mod,season,var,yyyy)
sums_all[key] = sums[var]
cnts_all[key] = cnts[var]
if (fal_files[yyyy] == fal_files[yyyy]):
season = 'fall'
# (sums, cnts) = sum_wrfout_slice(fal_files[yyyy], vars_mod, \
# maski, maskj, sums, cnts)
(sums, cnts) = sum_wrfout_slice(fal_files[yyyy], vars_mod, \
maski, maskj)
for var in vars_mod:
key = (mod,season,var,yyyy)
sums_all[key] = sums[var]
cnts_all[key] = cnts[var]
years_all = list(sorted(set(years_all[0])))
pickle.dump((sums_all,cnts_all,years_all), open(pickle_file,'wb'), -1)
sys.exit()
'''
#---------------------------------------------------------------------------
#
#---------------------------------------------------------------------------
(sums_all,cnts_all,years_all) = pickle.load(open(pickle_file, 'rb'))
fig, ax = plt.subplots( figsize=(10,6) )
plotfname = './test.png'
season = 'summer'
var = 'T2MAX'
for m in range(len(models)):
mod = models[m]
vals = []
for yyyy in years_all:
key = (mod,season,var,yyyy)
if key in sums_all:
avg_c = sums_all[key] / float(cnts_all[key])
if (var == 'PREC'):
avg_pr = avg_c * mm2in
elif (var == 'T2MAX' or var == 'T2MIN'):
avg_pr = ((avg_c - 273.15) * (9./5.)) + 32.0
print mod, yyyy, season, var, avg_pr
else:
avg_pr = np.nan
vals.append(avg_pr)
plt.plot(vals, label = mod.upper(), color = mod_cols[m], linewidth=2)
# sys.exit()
plt.tight_layout()
plt.grid()
plt.legend(fontsize = fs-1, loc = 'best')
print 'xli ', plotfname, ' &'
plt.savefig(plotfname)
plt.close()
sys.exit()
<file_sep>/old/old_old/plot_wrfout_grid_avg_20181030.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re, math
import numpy as np
#import matplotlib.pyplot as plt
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
import pickle
from collections import defaultdict
from make_cmap import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
run_dirs = rcm_dir + '/rundirs'
geo_em = rcm_dir + '/data' + '/geo_em.d02.nc'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#vars = ['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
vars = ['PREC', 'T2MAX', 'T2MIN']
#vars = ['T2MAX', 'T2MIN']
#vars = ['SWDOWN']
#vars = ['PREC']
sdts = ['197001', '207001']
edts = ['200012', '209912']
#sdts = ['207001']
#edts = ['209912']
#models = ['gfdl-cm3', 'miroc5', 'bcc-csm1.1', 'access1.3', 'canesm2',
# 'noresm1-m', 'ccsm4']
#models = ['ccsm4']
models = ['csiro-mk3.6.0', 'fgoals-g2']
dtfmt = "%Y%m%d%H"
#---------------------------------------------------------------------------
# Make a color map using make_cmap.
#---------------------------------------------------------------------------
levs_norm_c = []
for i in range(len(levs_temp)):
x = float(levs_temp[i])
norm_c = (x - min(levs_temp)) / (max(levs_temp) - min(levs_temp))
levs_norm_c.append(norm_c)
cmap_temp = make_cmap(cmap_temp, bit = True, position = levs_norm_c)
levs_norm_c = []
for i in range(len(levs_pcp)):
x = float(levs_pcp[i])
norm_c = (x - min(levs_pcp)) / (max(levs_pcp) - min(levs_pcp))
levs_norm_c.append(norm_c)
cmap_pcp = make_cmap(cmap_pcp, bit = True, position = levs_norm_c)
#---------------------------------------------------------------------------
# Make run directory, load geo_em file.
#---------------------------------------------------------------------------
rundir = run_dirs + '/plot_wrfout_grid_avg.' + str(os.getpid())
print 'making ', rundir
os.makedirs(rundir)
print 'Loading ', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
#---------------------------------------------------------------------------
# For each model, make a maps of every season...
#---------------------------------------------------------------------------
for m in range(len(models)):
model = models[m]
for n in range(len(sdts)):
sdt = sdts[n]
edt = edts[n]
yyyy_s = sdt[0:4]
yyyy_e = edt[0:4]
#--- Get list of extracted files for this model.
files = get_extract_files(data_dirs, model, sdt, edt, 0)
#--- Get files broken out by year and season.
(spr_files, sum_files, fal_files, win_files, years) = \
get_seasonal_files(files)
for var in vars:
for season in seasons:
file_plot = rundir + '/' + model + '_' + var + '_' + season + \
'_' + yyyy_s + '_' + yyyy_e + '.nc'
if (var == 'T2MAX' or var == 'T2MIN'):
syscom = 'ncra'
elif (var == 'PREC'):
syscom = 'ncra -y ttl'
nyears = 0
for y in range(len(years)):
yyyy = years[y]
if season == 'summer':
if (sum_files[yyyy] == sum_files[yyyy]):
nyears += 1
for f in range(len(sum_files[yyyy])):
syscom = syscom + ' ' + sum_files[yyyy][f]
else:
continue
elif season == 'spring':
if (spr_files[yyyy] == spr_files[yyyy]):
nyears += 1
for f in range(len(spr_files[yyyy])):
syscom = syscom + ' ' + spr_files[yyyy][f]
else:
continue
elif season == 'winter':
if (win_files[yyyy] == win_files[yyyy]):
nyears += 1
for f in range(len(win_files[yyyy])):
syscom = syscom + ' ' + win_files[yyyy][f]
else:
continue
elif season == 'fall':
if (fal_files[yyyy] == fal_files[yyyy]):
nyears += 1
for f in range(len(fal_files[yyyy])):
syscom = syscom + ' ' + fal_files[yyyy][f]
else:
continue
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
os.system(syscom)
print 'Loading ', var, ' from ', file_plot
(vardat, ntimes, dt) = load_wrfout(file_plot, [var])
titlein = model.upper() + ', ' + var_lab[var] + ', ' + \
seasons_lab[season] + ', ' + yyyy_s + ' - ' + yyyy_e
plotdat = vardat[var][:,:,0]
if (var == 'PREC'):
plotdat = (plotdat / nyears) * mm2in
levs_c = levs_pcp
cmap_c = cmap_pcp
else:
levs_c = levs_temp
cmap_c = cmap_temp
for z in range(len(zooms)):
plotfname = rundir + '/' + model + '_' + sdt + '_' + \
edt + '_' + season + '_' + var + '_' + \
zooms[z] + '.png'
mapper(var, lat, lon, plotdat, levs_c, cmap_c, \
maplw, colorbar_labs[var], titlein, plotfname, \
zooms[z])
# sys.exit()
# sys.exit()
# if (var == 'T2MAX' or var == 'T2MIN'):
# if (os.path.exists(file_plot)):
# print 'nc file ', file_plot, ' already exists-- using it'
# else:
# syscom = 'ncra'
# for f in range(len(files[yyyy])):
# print yyyy, files[yyyy][f]
# syscom = syscom + ' ' + files[yyyy][f]
# syscom = syscom + ' ' + file_plot
# print 'syscom = ', syscom
# os.system(syscom)
# elif (var == 'PREC'):
# if (os.path.exists(file_plot)):
# print 'nc file ', file_plot, ' already exists-- using it'
# else:
# syscom = 'ncra -y ttl'
# for f in range(len(files[yyyy])):
# print yyyy, files[yyyy][f]
# syscom = syscom + ' ' + files[yyyy][f]
# syscom = syscom + ' ' + file_plot
# print 'syscom = ', syscom
# os.system(syscom)
# #--- Loop over year, making maps of each
# for y in range(len(years)):
# yyyy = years[y]
#
# #--- Winter plots.
# if (win_files[yyyy] == win_files[yyyy]):
# iret = run_mapper('T2MAX', model, rundir, 'winter', win_files,\
# yyyy, lat, lon, levs_temp, cmap_temp)
# iret = run_mapper('T2MIN', model, rundir, 'winter', win_files,\
# yyyy, lat, lon, levs_temp, cmap_temp)
# iret = run_mapper('PREC', model, rundir, 'winter', win_files,\
# yyyy, lat, lon, levs_pcp, cmap_pcp)
#
# #--- Summer plots.
# if (sum_files[yyyy] == sum_files[yyyy]):
# iret = run_mapper('T2MAX', model, rundir, 'summer', sum_files,\
# yyyy, lat, lon, levs_temp, cmap_temp)
# iret = run_mapper('T2MIN', model, rundir, 'summer', sum_files,\
# yyyy, lat, lon, levs_temp, cmap_temp)
# iret = run_mapper('PREC', model, rundir, 'summer', sum_files,\
# yyyy, lat, lon, levs_pcp, cmap_pcp)
#
# #--- Spring plots.
# if (spr_files[yyyy] == spr_files[yyyy]):
# iret = run_mapper('T2MAX', model, rundir, 'spring', spr_files,\
# yyyy, lat, lon, levs_temp, cmap_temp)
# iret = run_mapper('T2MIN', model, rundir, 'spring', spr_files,\
# yyyy, lat, lon, levs_temp, cmap_temp)
# iret = run_mapper('PREC', model, rundir, 'spring', spr_files,\
# yyyy, lat, lon, levs_pcp, cmap_pcp)
#
# #--- Fall plots.
# if (fal_files[yyyy] == fal_files[yyyy]):
# iret = run_mapper('T2MAX', model, rundir, 'fall', fal_files,\
# yyyy, lat, lon, levs_temp, cmap_temp)
# iret = run_mapper('T2MIN', model, rundir, 'fall', fal_files,\
# yyyy, lat, lon, levs_temp, cmap_temp)
# iret = run_mapper('PREC', model, rundir, 'fall', fal_files,\
# yyyy, lat, lon, levs_pcp, cmap_pcp)
sys.exit()
<file_sep>/utils_wrfout.py
#!/usr/bin/python
import os, sys, glob, re, math
import numpy as np
from netCDF4 import Dataset as NetCDFFile
import matplotlib as mpl
mpl.use('Agg')
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
from collections import defaultdict
from utils_cmap import *
import pickle
from utils_date import *
from utils_ghcnd_obs import *
dtfmt = "%Y%m%d%H"
#seasons = ['winter']
#seasons = ['annual', 'spring', 'summer', 'fall', 'winter']
seasons = ['spring', 'summer', 'fall', 'winter', 'annual']
seasons_mo = ['ALL', 'MAM', 'JJA', 'SON', 'DJF']
seasons_lab = {
'annual': 'Jan-Dec',
'spring': 'Mar-Apr-May',
'summer': 'Jun-Jul-Aug',
'fall': 'Sep-Oct-Nov',
'winter': 'Dec-Jan-Feb'
}
zooms = ['Z1', 'Z2']
mm2in = 0.0393700787
min_season_days = 85
std_lapse = 0.0065 #-- std. atmos. lapse rate
#smooth_fact = 5 #-- odd number, used for smoothing time series plots.
smooth_fact = 1 #-- odd number, used for smoothing time series plots.
fs = 9
titlefs = 9
width = 10
height = 8
maplw = 1.0
mvc = -9999.0
#---------------------------------------------------------------------------
# Get model pickle file name
#---------------------------------------------------------------------------
def get_mod_pkl_name (pdir, model, sdt, edt):
pf = pdir + '/extract_' + model + '_' + sdt + '_' + edt + '.pkl'
return pf
#---------------------------------------------------------------------------
# Load geo_em file.
#---------------------------------------------------------------------------
def load_geo_em(geo_em):
nc = NetCDFFile(geo_em, 'r')
lat = nc.variables['XLAT_M'][0,:,:]
lon = nc.variables['XLONG_M'][0,:,:]
hgt = nc.variables['HGT_M'][0,:,:]
lat = np.transpose(lat)
lon = np.transpose(lon)
hgt = np.transpose(hgt)
nc.close()
return lat, lon, hgt
#---------------------------------------------------------------------------
# Get netcdf dimension
#---------------------------------------------------------------------------
def get_nc_dim(ncin, dimname):
nc = NetCDFFile(ncin, 'r')
dim = len(nc.dimensions[dimname])
nc.close()
return dim
#---------------------------------------------------------------------------
# Load wrfout file.
#---------------------------------------------------------------------------
def load_wrfout(wrfout, vars):
nc = NetCDFFile(wrfout, 'r')
ntimes = len(nc.dimensions['Time'])
dataout = {}
for v in range(len(vars)):
var = vars[v]
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
dataout[var] = dat_tmp
#--- Get date from global attributes and clean it up and trim it down.
dt_all = nc.START_DATE
dt = dt_all.replace('-', '')
dt = dt.replace(':', '')
dt = dt.replace('_', '')
dt = dt[0:10]
nc.close()
return dataout, ntimes, dt
#---------------------------------------------------------------------------
# Load SWE wrfout file.
#---------------------------------------------------------------------------
def load_wrfout_swe(wrfout, vars):
nc = NetCDFFile(wrfout, 'r')
ntimes = len(nc.dimensions['Time'])
dataout = {}
for v in range(len(vars)):
var = vars[v]
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
dataout[var] = dat_tmp
nc.close()
return dataout, ntimes
#---------------------------------------------------------------------------
# Load wrfout file.
#---------------------------------------------------------------------------
def sum_wrfout_slice(files, vars, maski, maskj):
sums = {}
cnts = {}
for f in range(len(files)):
print 'reading ', files[f]
nc = NetCDFFile(files[f], 'r')
ntimes = len(nc.dimensions['Time'])
for v in range(len(vars)):
var = vars[v]
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
sum_c = 0
cnt_c = 0
for n in range(len(maski)):
sum_c = sum_c + sum(dat_tmp[maski[n], maskj[n],:])
cnt_c = cnt_c + len(dat_tmp[maski[n], maskj[n],:])
if (var in sums):
sums[var] = sums[var] + sum_c
cnts[var] = cnts[var] + cnt_c
else:
sums[var] = sum_c
cnts[var] = cnt_c
return sums, cnts
#---------------------------------------------------------------------------
# For ensemble standard deviation plots....
#---------------------------------------------------------------------------
def get_ensmean_std(var, models, years, season, \
sum_files, spr_files, win_files, fal_files, ann_files, \
pickle_file):
nyears = 0
nmodels = len(models)
files = []
dts_all = []
for y in range(len(years)):
yyyy = years[y]
for m in range(nmodels):
model = models[m]
sum_files_c = sum_files[model]
spr_files_c = spr_files[model]
win_files_c = win_files[model]
fal_files_c = fal_files[model]
ann_files_c = ann_files[model]
if season == 'summer':
if (sum_files_c[yyyy] == sum_files_c[yyyy]):
nyears += 1
for f in range(len(sum_files_c[yyyy])):
files.append(sum_files_c[yyyy][f])
else:
continue
elif season == 'spring':
if (spr_files_c[yyyy] == spr_files_c[yyyy]):
nyears += 1
for f in range(len(spr_files_c[yyyy])):
files.append(spr_files_c[yyyy][f])
else:
continue
elif season == 'winter':
if (win_files_c[yyyy] == win_files_c[yyyy]):
nyears += 1
for f in range(len(win_files_c[yyyy])):
files.append(win_files_c[yyyy][f])
else:
continue
elif season == 'fall':
if (fal_files_c[yyyy] == fal_files_c[yyyy]):
nyears += 1
for f in range(len(fal_files_c[yyyy])):
files.append(fal_files_c[yyyy][f])
else:
continue
elif season == 'annual':
if (ann_files_c[yyyy] == ann_files_c[yyyy]):
nyears += 1
for f in range(len(ann_files_c[yyyy])):
files.append(ann_files_c[yyyy][f])
else:
continue
print 'files = ', files
#--- Get sorted, unique set of dates from files found above.
nfiles = len(files)
dts_all = []
for f in range(nfiles):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
dts_all.append(dt_c)
dts_all = list(sorted(set(dts_all)))
#--- For each unique date, read in files for each model.
for d in range(len(dts_all)):
#--- First make sure all members have a file.
files_c = []
print dts_all[d]
for f in range(nfiles):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
if dt_c == dts_all[d]:
files_c.append(files[f])
if len(files_c) != nmodels:
print 'missing member(s) for date = ', dts_all[d]
sys.exit()
#--- Now read in files for each member.
for n in range(len(files_c)):
print 'loading ', files_c[n]
(vardat, ntimes, dt) = load_wrfout(files_c[n], [var])
plotdat = vardat[var][:,:,:]
nx,ny,nt = plotdat.shape
if n == 0:
dat_all = np.ones((nx, ny, nt, nmodels)) * np.nan
stdevs_tmp = np.ones((nx, ny, nt)) * np.nan
if n == 0 and d == 0:
stdevs = np.ones((nx, ny, nt)) * np.nan
dat_all[:,:,:,n] = plotdat
#--- For each ntimes (these should be monthly files, so ntimes are
#--- days and will be ~30 days), calculate a standard deviation.
for nt in range(ntimes):
dat_c = np.squeeze(dat_all[:,:,nt,:])
stdevs_tmp[:,:,nt] = np.std(dat_c, axis=2)
#--- Add this month's standard deviation grids to our final array,
#--- stdevs.
if d == 0:
stdevs = stdevs_tmp
else:
stdevs = np.concatenate((stdevs, stdevs_tmp), axis = 2)
#--- Calculate the mean of the daily standard deviations.
stdevmean = np.mean(stdevs, axis=2)
#--- Create pickle file.
pickle.dump((stdevmean), open(pickle_file,'wb'), -1)
return stdevmean
#---------------------------------------------------------------------------
# For ensemble mean plots, get system nco command.
#---------------------------------------------------------------------------
def get_ensmean_syscom(file_plot, var, models, years, season, \
sum_files, spr_files, \
win_files, fal_files, ann_files, ncocom):
syscom = ncocom
nyears = 0
for m in range(len(models)):
model = models[m]
sum_files_c = sum_files[model]
spr_files_c = spr_files[model]
win_files_c = win_files[model]
fal_files_c = fal_files[model]
ann_files_c = ann_files[model]
for y in range(len(years)):
yyyy = years[y]
if season == 'summer':
if (sum_files_c[yyyy] == sum_files_c[yyyy]):
nyears += 1
for f in range(len(sum_files_c[yyyy])):
syscom = syscom + ' ' + sum_files_c[yyyy][f]
else:
continue
elif season == 'spring':
if (spr_files_c[yyyy] == spr_files_c[yyyy]):
nyears += 1
for f in range(len(spr_files_c[yyyy])):
syscom = syscom + ' ' + spr_files_c[yyyy][f]
else:
continue
elif season == 'winter':
if (win_files_c[yyyy] == win_files_c[yyyy]):
nyears += 1
for f in range(len(win_files_c[yyyy])):
syscom = syscom + ' ' + win_files_c[yyyy][f]
else:
continue
elif season == 'fall':
if (fal_files_c[yyyy] == fal_files_c[yyyy]):
nyears += 1
for f in range(len(fal_files_c[yyyy])):
syscom = syscom + ' ' + fal_files_c[yyyy][f]
else:
continue
elif season == 'annual':
if (ann_files_c[yyyy] == ann_files_c[yyyy]):
nyears += 1
for f in range(len(ann_files_c[yyyy])):
syscom = syscom + ' ' + ann_files_c[yyyy][f]
else:
continue
return syscom, nyears
#---------------------------------------------------------------------------
# For ensemble mean plots, run system nco command.
#---------------------------------------------------------------------------
def run_ensmean_syscom(file_plot, var, ncocom, syscom, yyyy_s, yyyy_e, \
season, rundir, ):
#--- If system command is too big (too many files to send
#--- to nco), break it up into 1000 piece chunks.
files_c = syscom.replace(ncocom, '')
scsplit = files_c.split()
nfiles = len(scsplit)
niter = 1000
if nfiles > niter:
nt = int(math.ceil(float(nfiles) / float(niter)))
files_all = []
for n in range(nt):
file_c = rundir + '/' + var + '_' + season + '_' + \
yyyy_s + '_' + yyyy_e + '_subset' + str(n) + '.nc'
if os.path.isfile(file_c):
print 'this file already exists: ', file_c
files_all.append(file_c)
continue
nb = n * niter
ne = (n+1) * niter
syscom = ncocom + ' ' + ' '.join(scsplit[nb:ne]) + ' ' + file_c
print syscom
iret = os.system(syscom)
if iret != 0:
print 'problem running nco! iret = ', iret
return mvc
files_all.append(file_c)
syscom = ncocom + ' ' + ' '.join(files_all) + ' ' + file_plot
print syscom
iret = os.system(syscom)
if iret != 0:
print 'problem running nco! iret = ', iret
return mvc
else:
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
iret = os.system(syscom)
if iret != 0:
print 'problem running nco! iret = ', iret
return mvc
return 1
#---------------------------------------------------------------------------
# Get plot file name.
#---------------------------------------------------------------------------
def get_plotfname(plot_dir, stn, sdt, edt, season, var, stat):
plotfname = plot_dir + '/' + stn + '_' + sdt + '_' + edt + '_' + \
season + '_' + var + '_' + stat + '.png'
return plotfname
#---------------------------------------------------------------------------
# Get plot title.
#---------------------------------------------------------------------------
def get_title(season, stn, var, stat, years):
titleout = station_name_dict[stn] + ' (' + stn.upper() + '), ' + \
seasons_lab[season] + ' ' + labels[var+stat] + ', ' + \
str(years[0]) + ' - ' + str(years[len(years)-1])
return titleout
#---------------------------------------------------------------------------
# Get list of extracted wrfout files between sdt_yyyymm and edt_yyyymm.
#---------------------------------------------------------------------------
def get_extract_files(data_dirs, model, sdt_yyyymm, edt_yyyymm, snowrad):
files_out = []
for d in range(len(data_dirs)):
path_c = data_dirs[d] + '/' + model + '/extract/*.nc'
files = glob.glob(path_c)
for f in range(len(files)):
if snowrad == 0:
if 'snowrad' in files[f]:
continue
else:
if 'snowrad' not in files[f]:
continue
dt_c = re.findall(r'\.(\d{10})\.', files[f])
if (dt_c):
dt_c = dt_c[0]
yyyymm_c = dt_c[0:6]
if (yyyymm_c >= sdt_yyyymm and yyyymm_c <= edt_yyyymm):
files_out.append(files[f])
if (len(files_out) == 0):
sys.exit('get_extract_files: no files found!')
return list(sorted(set(files_out)))
#---------------------------------------------------------------------------
# Get seasonal stats.
#---------------------------------------------------------------------------
def get_seasonal_files(files):
springs = defaultdict(list)
summers = defaultdict(list)
falls = defaultdict(list)
winters = defaultdict(list)
annuals = defaultdict(list)
spring_ndays = {}
summer_ndays = {}
fall_ndays = {}
winter_ndays = {}
annual_ndays = {}
years_all = []
#--- Loop over all files, binning them into seasons / years.
for f in range(len(files)):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
if (dt_c):
yyyy = dt_c[0:4]
mm = dt_c[4:6]
years_all.append(yyyy)
if (mm == '03' or mm == '04' or mm == '05'):
if ((yyyy) in spring_ndays):
spring_ndays[yyyy] += 1
else:
spring_ndays[yyyy] = 1
springs[yyyy].append(files[f])
elif (mm == '06' or mm == '07' or mm == '08'):
if ((yyyy) in summer_ndays):
summer_ndays[yyyy] += 1
else:
summer_ndays[yyyy] = 1
summers[yyyy].append(files[f])
elif (mm == '09' or mm == '10' or mm == '11'):
if ((yyyy) in fall_ndays):
fall_ndays[yyyy] += 1
else:
fall_ndays[yyyy] = 1
falls[yyyy].append(files[f])
elif (mm == '12'):
yyyyw = str(int(yyyy) + 1)
if ((yyyyw) in winter_ndays):
winter_ndays[yyyyw] += 1
else:
winter_ndays[yyyyw] = 1
winters[yyyyw].append(files[f])
elif (mm == '01' or mm == '02'):
if ((yyyy) in winter_ndays):
winter_ndays[yyyy] += 1
else:
winter_ndays[yyyy] = 1
winters[yyyy].append(files[f])
if ((yyyy) in annual_ndays):
annual_ndays[yyyy] += 1
else:
annual_ndays[yyyy] = 1
annuals[yyyy].append(files[f])
#--- Get list of unique, sorted years found above.
years = list(sorted(set(years_all)))
#--- Check number of data points per unique years and nan out
#--- ones that do not have enough (< min_season_days).
for y in range(len(years)):
yyyy = years[y]
if (yyyy in spring_ndays):
if (spring_ndays[yyyy] < 3):
springs[yyyy] = np.nan
else:
springs[yyyy] = np.nan
if (yyyy in summer_ndays):
if (summer_ndays[yyyy] < 3):
summers[yyyy] = np.nan
else:
summers[yyyy] = np.nan
if (yyyy in fall_ndays):
if (fall_ndays[yyyy] < 3):
falls[yyyy] = np.nan
else:
falls[yyyy] = np.nan
if (yyyy in winter_ndays):
if (winter_ndays[yyyy] < 3):
winters[yyyy] = np.nan
else:
winters[yyyy] = np.nan
if (yyyy in annual_ndays):
if (annual_ndays[yyyy] < 3):
annuals[yyyy] = np.nan
else:
annuals[yyyy] = np.nan
return springs, summers, falls, winters, annuals, years
#---------------------------------------------------------------------------
# Get seasonal files (new)
#---------------------------------------------------------------------------
def get_seasonal_files_new(files):
seafiles = defaultdict(list)
ndays = {}
# springs = defaultdict(list)
# summers = defaultdict(list)
# falls = defaultdict(list)
# winters = defaultdict(list)
# spring_ndays = {}
# summer_ndays = {}
# fall_ndays = {}
# winter_ndays = {}
years_all = []
#--- Loop over all files, binning them into seasons / years.
for f in range(len(files)):
dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
if (dt_c):
yyyy = dt_c[0:4]
mm = dt_c[4:6]
if mm == '12':
yyyy = str(int(yyyy) + 1)
else:
yyyy = yyyy
years_all.append(yyyy)
if (mm == '03' or mm == '04' or mm == '05'):
season = 'spring'
if (mm == '06' or mm == '07' or mm == '08'):
season = 'summer'
if (mm == '09' or mm == '10' or mm == '11'):
season = 'fall'
if (mm == '12' or mm == '01' or mm == '02'):
season = 'winter'
if ((yyyy) in ndays):
ndays[season+yyyy] += 1
else:
ndays[season+yyyy] = 1
seafiles[season,yyyy].append(files[f])
#--- Get list of unique, sorted years found above.
years = list(sorted(set(years_all)))
return seafiles, years
'''
#--- Check number of data points per unique years and nan out
#--- ones that do not have enough (< min_season_days).
for y in range(len(years)):
yyyy = years[y]
if (yyyy in spring_ndays):
if (spring_ndays[yyyy] < 3):
springs[yyyy] = np.nan
else:
springs[yyyy] = np.nan
if (yyyy in summer_ndays):
if (summer_ndays[yyyy] < 3):
summers[yyyy] = np.nan
else:
summers[yyyy] = np.nan
if (yyyy in fall_ndays):
if (fall_ndays[yyyy] < 3):
falls[yyyy] = np.nan
else:
falls[yyyy] = np.nan
if (yyyy in winter_ndays):
if (winter_ndays[yyyy] < 3):
winters[yyyy] = np.nan
else:
winters[yyyy] = np.nan
'''
#---------------------------------------------------------------------------
# Get seasonal stats (totals or averages currently).
#---------------------------------------------------------------------------
def get_seasonal_stats(data_all, dts_all, models, stns, var, stat):
out_sum = {}
out_avg = {}
out_max = {}
out_min = {}
years_all = []
for m in range(len(models)):
mod = models[m]
data_all_c = data_all[mod]
for s in range(len(stns)):
stn = stns[s]
ndays = {}
years_mod_stn = []
for d in range(len(dts_all)):
yyyy = dts_all[d][0:4]
mm = dts_all[d][4:6]
years_mod_stn.append(yyyy)
#--- Very first date of all model runs will have unusable
#--- (all 0's) in the fields. So don't try and use it.
if dts_all[d] == '1970010100':
continue
if ((var,stn,dts_all[d]) in data_all_c):
dat_c = data_all_c[var,stn,dts_all[d]]
else:
ndays[season+yyyy] = np.nan
out_sum[season,mod,stn,yyyy] = np.nan
out_max[season,mod,stn,yyyy] = np.nan
out_min[season,mod,stn,yyyy] = np.nan
continue
#--- annual is all days so get sum/max/min for every dts_all.
(ndays, out_sum, out_max, out_min) = \
get_season_summaxmin(dat_c, mod, stn, 'annual',yyyy,mm,\
ndays, out_sum, out_max, out_min)
if (mm == '03' or mm == '04' or mm == '05'):
season = 'spring'
if (mm == '06' or mm == '07' or mm == '08'):
season = 'summer'
if (mm == '09' or mm == '10' or mm == '11'):
season = 'fall'
if (mm == '12' or mm == '01' or mm == '02'):
season = 'winter'
(ndays, out_sum, out_max, out_min) = \
get_season_summaxmin(dat_c, mod, stn, season, yyyy, mm,\
ndays, out_sum, out_max, out_min)
#--- Get list of unique, sorted years found above.
years = list(sorted(set(years_mod_stn)))
#--- Check number of data points per unique years and nan out
#--- ones that do not have enough (< min_season_days).
for y in range(len(years)):
yyyy = years[y]
for s in range(len(seasons)):
season = seasons[s]
if ((season+yyyy) in ndays):
if (ndays[season+yyyy] < min_season_days):
out_sum[season,mod,stn,yyyy] = np.nan
out_max[season,mod,stn,yyyy] = np.nan
out_min[season,mod,stn,yyyy] = np.nan
else:
ndays[season+yyyy] = np.nan
out_sum[season,mod,stn,yyyy] = np.nan
out_max[season,mod,stn,yyyy] = np.nan
out_min[season,mod,stn,yyyy] = np.nan
#--- if stat requested was 'avg', do averaging.
if (stat == 'avg' or stat == 'max' or stat == 'min'):
for y in range(len(years)):
yyyy = years[y]
for s in range(len(seasons)):
season = seasons[s]
out_avg[season,mod,stn,yyyy] = out_sum[\
season,mod,stn,yyyy] / ndays[season+yyyy]
#--- Keep around all years found for this model and station.
years_all.append(years_mod_stn)
#--- Final, unique, sorted list of years found.
years = list(sorted(set(years_all[0])))
if (stat == 'avg'):
return out_avg, years
elif (stat == 'max'):
return out_max, years
elif (stat == 'min'):
return out_min, years
else:
return out_sum, years
#---------------------------------------------------------------------------
# Loop over each models, grabbing data from extract wrfout files.
#---------------------------------------------------------------------------
def load_extract_data(geo_em, stns, latpts, lonpts, elevs, models, \
data_dirs, sdt, edt, varsin, pickle_dir, load_pickle):
#--- Create temporary list of variables, removing 'T2MEAN' if in original
#--- list (we'll calculate T2MEAN if necessary after loading data).
vars_tmp = list(varsin)
if 'T2MEAN' in vars_tmp:
i = vars_tmp.index('T2MEAN')
del vars_tmp[i]
#--- Load geo em file to get lat/lon/elev grids. Also interpolate
#--- model elevation to stns lat/lon point locations.
print 'Loading:\n', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
elevs_mod = []
elevs_diff = []
for ns in range(len(stns)):
dat_interp = bilinear_interpolate(lat, lon, hgt, \
latpts[ns], lonpts[ns], 0)
elevs_mod.append(dat_interp)
elevs_diff.append(elevs[ns] - dat_interp)
for m in range(len(models)):
data_all = {}
dts_all = []
model = models[m]
pickle_file = get_mod_pkl_name(pickle_dir, model, sdt, edt)
#--- Get list of extracted files for this model.
print 'model = ', model
files = get_extract_files(data_dirs, model, sdt, edt, 0)
files = list(sorted(set(files)))
print 'Loading: '
#--- Loop over all extract files, reading them in.
for f in range(len(files)):
(vardat, ntimes, dt) = load_wrfout(files[f], vars_tmp)
print files[f], ntimes
#--- Loop over each vars_tmp, interpolating data to stns for
#--- each ntimes.
for v in range(len(vars_tmp)):
var = vars_tmp[v]
vardat_c = vardat[var]
#--- Loop over each ntime, grabbing interpolated data for
#--- variable var for each stns.
for n in range(ntimes):
dt_c = time_increment(dt, n, dtfmt)
for ns in range(len(stns)):
dat_interp = bilinear_interpolate(lat, lon, \
vardat_c[:,:,n],\
latpts[ns], \
lonpts[ns], 0)
#--- Do lapse rate correction for temperature.
if (var == 'T2MAX' or var == 'T2MIN'):
dat_c = dat_interp - std_lapse * elevs_diff[ns]
else:
dat_c = dat_interp
data_all[var, stns[ns], dt_c] = dat_c
dts_all.append(dt_c)
#--- Get sorted unique list of all dates seen.
dts_unique = list(sorted(set(dts_all)))
#--- If T2MEAN was requested, calculate it from T2MAX and T2MIN for
#--- every station and date.
if 'T2MEAN' in varsin:
for ns in range(len(stns)):
for d in range(len(dts_unique)):
keymax = ('T2MAX', stns[ns], dts_unique[d])
keymin = ('T2MIN', stns[ns], dts_unique[d])
if keymax in data_all and keymin in data_all:
keymean = ('T2MEAN', stns[ns], dts_unique[d])
# if data_all[keymax] < (273-60) or \
# data_all[keymax] > (273+60) or \
# data_all[keymin] < (273-60) or \
# data_all[keymin] > (273+60):
# print 'stns[ns] = ', stns[ns]
# print 'dts_unique[d] = ', dts_unique[d]
# print 'max = ', data_all[keymax]
# print 'min = ', data_all[keymin]
## sys.exit()
data_all[keymean] = np.mean([data_all[keymax], \
data_all[keymin]])
#--- Create pickle file.
pickle.dump((model, data_all, dts_unique, varsin, stns), \
open(pickle_file,'wb'), -1)
return data_all, dts_unique, models, varsin, stns
#---------------------------------------------------------------------------
# Interpolate from a sent-in grid to a sent in lat/lon point.
#---------------------------------------------------------------------------
def bilinear_interpolate(latgrid, longrid, datagrid, latpt, lonpt, deb):
totdiff = abs(latgrid - latpt) + abs(longrid - lonpt)
(i,j) = np.unravel_index(totdiff.argmin(), totdiff.shape)
#--- Get lat/lon box in which latpt,lonpt resides.
if (latpt >= latgrid[i,j] and lonpt >= longrid[i,j]):
iif = i
jjf = j
elif (latpt < latgrid[i,j] and lonpt < longrid[i,j]):
iif = i-1
jjf = j-1
elif (latpt >= latgrid[i,j] and lonpt < longrid[i,j]):
iif = i-1
jjf = j
elif (latpt < latgrid[i,j] and lonpt >= longrid[i,j]):
iif = i
jjf = j-1
(nx, ny) = np.shape(latgrid)
if (deb == 1):
print 'nx, ny = ', nx, ny
print 'iif,jjf = ', iif,jjf
print 'latgrid[iif+1,jjf] = ', latgrid[iif+1,jjf]
print 'latgrid[iif,jjf] = ', latgrid[iif,jjf]
if (iif >= (nx-1) or jjf >= (ny-1) or iif < 0 or jjf < 0):
return mvc
#--- Do bilinear interpolation to latpt,lonpt.
dlat = latgrid[iif,jjf+1] - latgrid[iif,jjf]
dlon = longrid[iif+1,jjf] - longrid[iif,jjf]
dslat = latgrid[iif,jjf+1] - latpt
dslon = longrid[iif+1,jjf] - lonpt
wrgt = 1 - (dslon/dlon)
wup = 1 - (dslat/dlat)
vll = datagrid[iif,jjf]
vlr = datagrid[iif,jjf+1]
vul = datagrid[iif+1,jjf]
vur = datagrid[iif+1,jjf+1]
if (deb > 1):
print 'll lat, lon, val = ', latgrid[iif,jjf], longrid[iif,jjf], vll
print 'lr lat, lon, val = ', latgrid[iif+1,jjf], longrid[iif+1,jjf], vlr
print 'ur lat, lon, val = ', latgrid[iif+1,jjf+1], longrid[iif+1,jjf+1],vur
print 'ul lat, lon, val = ', latgrid[iif,jjf+1], longrid[iif,jjf+1], vul
print 'latpt, lonpt = ', latpt, lonpt
print 'vll = ', vll
print 'vlr = ', vlr
print 'vul = ', vul
print 'vur = ', vur
datout = (1-wrgt) * ((1-wup) * vll + wup * vul) + \
(wrgt) * ((1-wup) * vlr + wup * vur)
# if (deb == 1):
# print 'datout = ', datout
# sys.exit()
# if (datout == 0.0):
# print 'nx, ny = ', nx, ny
# print 'iif,jjf = ', iif,jjf
# print 'latgrid[iif+1,jjf] = ', latgrid[iif+1,jjf]
# print 'latgrid[iif,jjf] = ', latgrid[iif,jjf]
#
# print 'll lat, lon, val = ', latgrid[iif,jjf], longrid[iif,jjf], vll
# print 'lr lat, lon, val = ', latgrid[iif+1,jjf], longrid[iif+1,jjf], vl#r
# print 'ur lat, lon, val = ', latgrid[iif+1,jjf+1], longrid[iif+1,jjf+1],vur
# print 'ul lat, lon, val = ', latgrid[iif,jjf+1], longrid[iif,jjf+1], vu#l
# print 'latpt, lonpt = ', latpt, lonpt
#
# print 'vll = ', vll
# print 'vlr = ', vlr
# print 'vul = ', vul
# print 'vur = ', vur
#
# sys.exit()
return datout
#----------------------------------------------------------------------------
# Add data to sums, max's and min's arrays.
#----------------------------------------------------------------------------
def get_season_summaxmin(dat_c, mod, stn, season, yyyyin, month, ndays, \
out_sum, out_max, out_min):
#--- Look out for cases where data is NaN (e.g. the 31st of June) and
#--- do nothing (return) in those cases.
if np.isnan(dat_c):
return ndays, out_sum, out_max, out_min
#--- Put December winter data into next year's winter-- naming
#--- winters by their Jan/Feb year rather than their Dec year.
if month == '12':
yyyy = str(int(yyyyin) + 1)
else:
yyyy = yyyyin
#--- Count number of days for this season and year.
if (season+yyyy) in ndays:
ndays[season+yyyy] += 1
else:
ndays[season+yyyy] = 1
key = (season,mod,stn,yyyy)
#--- Get sum of sent in data.
if key in out_sum:
out_sum[key] = out_sum[key] + dat_c
else:
out_sum[key] = dat_c
#--- Get maximum.
if key in out_max:
if dat_c > out_max[key]:
out_max[key] = dat_c
else:
out_max[key] = dat_c
#--- Get minimum.
if key in out_min:
if dat_c < out_min[key]:
out_min[key] = dat_c
else:
out_min[key] = dat_c
return ndays, out_sum, out_max, out_min
<file_sep>/old/old_old/plot_wrfout_grid_20180130.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re, math
import numpy as np
#import matplotlib.pyplot as plt
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
import pickle
from collections import defaultdict
from make_cmap import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
run_dirs = rcm_dir + '/rundirs'
data_dir = '/home/disk/r2d2/steed/cmip5/rcp8.5'
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
vars = ['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
#sdt = '202001'
#edt = '202912'
sdt = '197001'
edt = '209912'
models = ['gfdl-cm3']
dtfmt = "%Y%m%d%H"
#---------------------------------------------------------------------------
# Make a color map using make_cmap.
#---------------------------------------------------------------------------
#if (varname == 'tos'):
# levs_c = levs_temp
# cmap_c = cmap_temp
#elif(varname == 'ua'):
# levs_c = levs_prec
# cmap_c = cmap_prec
levs_c = levs_temp
cmap_c = cmap_temp
levs_norm_c = []
for i in range(len(levs_c)):
x = float(levs_c[i])
norm_c = (x - min(levs_c)) / (max(levs_c) - min(levs_c))
levs_norm_c.append(norm_c)
my_cmap = make_cmap(cmap_c, bit = True, position = levs_norm_c)
#---------------------------------------------------------------------------
# Make run directory, load geo_em file.
#---------------------------------------------------------------------------
rundir = run_dirs + '/plot_wrfout_grid.' + str(os.getpid())
print 'making ', rundir
os.makedirs(rundir)
print 'Loading ', geo_em
(lat, lon) = load_geo_em(geo_em)
#---------------------------------------------------------------------------
# For each model, make a maps of every season...
#---------------------------------------------------------------------------
for m in range(len(models)):
model = models[m]
data_dir_c = data_dir + '/' + model + '/extract'
#--- Get list of extracted files for this model.
files = get_extract_files(data_dir_c, model, sdt, edt)
#--- Get files broken out by year and season.
(spr_files, sum_files, fal_files, win_files, years) = \
get_seasonal_files(files)
#--- Loop over year, making maps of each
for y in range(len(years)):
yyyy = years[y]
#--- Winter plots.
if (win_files[yyyy] == win_files[yyyy]):
file_plot = rundir + '/win_' + yyyy + '.nc'
syscom = 'ncra'
for f in range(len(win_files[yyyy])):
print yyyy, win_files[yyyy][f]
syscom = syscom + ' ' + win_files[yyyy][f]
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
os.system(syscom)
var = 'T2MAX'
print 'Loading ', var, ' from ', file_plot
(vardat, ntimes, dt) = load_wrfout(file_plot, [var])
colorbar_lab = 'Temperature (K)'
titlein = model + ', Average Max Temperature (K), DJF ' + yyyy
plotfname = rundir + '/win_' + var + '_' + yyyy + '.png'
plotdat = vardat[var][:,:,0]
mapper(lat, lon, plotdat, minlat, maxlat, minlon, maxlon, \
levs_c, my_cmap, maplw, colorbar_lab, titlein, plotfname)
if (sum_files[yyyy] == sum_files[yyyy]):
file_plot = rundir + '/sum_' + yyyy + '.nc'
syscom = 'ncra'
for f in range(len(sum_files[yyyy])):
print yyyy, sum_files[yyyy][f]
syscom = syscom + ' ' + sum_files[yyyy][f]
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
os.system(syscom)
var = 'T2MAX'
print 'Loading ', var, ' from ', file_plot
(vardat, ntimes, dt) = load_wrfout(file_plot, [var])
colorbar_lab = 'Temperature (K)'
titlein = model + ', Average Max Temperature (K), JJA ' + yyyy
plotfname = rundir + '/sum_' + var + '_' + yyyy + '.png'
plotdat = vardat[var][:,:,0]
mapper(lat, lon, plotdat, minlat, maxlat, minlon, maxlon, \
levs_c, my_cmap, maplw, colorbar_lab, titlein, plotfname)
sys.exit()
# #--- Loop over all extract files, reading them in.
# springs = defaultdict(list)
# summers = defaultdict(list)
# falls = defaultdict(list)
# winters = defaultdict(list)
# spring_ndays = {}
# summer_ndays = {}
# fall_ndays = {}
# winter_ndays = {}
#
# for f in range(len(files)):
## print files[f]
# dt_c = re.findall(r'\.(\d{10})\.', files[f])[0]
# if (dt_c):
# yyyy = dt_c[0:4]
# mm = dt_c[4:6]
# years_mod_stn.append(yyyy)
#
# print dt_c, yyyy, mm
# if (mm == '03' or mm == '04' or mm == '05'):
# if ((yyyy) in spring_ndays):
# spring_ndays[yyyy] += 1
# else:
# spring_ndays[yyyy] = 1
# springs[yyyy].append(files[f])
# elif (mm == '06' or mm == '07' or mm == '08'):
# if ((yyyy) in summer_ndays):
# summer_ndays[yyyy] += 1
# else:
# summer_ndays[yyyy] = 1
# summers[yyyy].append(files[f])
# elif (mm == '09' or mm == '10' or mm == '11'):
# if ((yyyy) in fall_ndays):
# fall_ndays[yyyy] += 1
# else:
# fall_ndays[yyyy] = 1
# falls[yyyy].append(files[f])
# elif (mm == '12'):
# if ((yyyyw) in winter_ndays):
# winter_ndays[yyyyw] += 1
# else:
# winter_ndays[yyyyw] = 1
# yyyyw = str(int(yyyy) + 1)
# winters[yyyyw].append(files[f])
# elif (mm == '01' or mm == '02'):
# if ((yyyy) in winter_ndays):
# winter_ndays[yyyy] += 1
# else:
# winter_ndays[yyyy] = 1
# winters[yyyy].append(files[f])
#
# print springs
# print 'springs[2020] = ', springs['2020']
# print 'summers[2020] = ', summers['2020']
# print 'winters[2020] = ', winters['2020']
#
# sys.exit()
##---------------------------------------------------------------------------
## Loop over each models, grabbing data from extract wrfout files.
##---------------------------------------------------------------------------
#pickle_file = pickle_dir + '/extract_' + sdt + '_' + edt + '.pickle'
#if os.path.isfile(pickle_file):
# (data_all, dts_unique, models, vars, stns) = \
# pickle.load(open(pickle_file, 'rb'))
#else:
# print 'Loading ', geo_em
# (lat, lon) = load_geo_em(geo_em)
#
# data_all = {}
# dts_all = []
# for m in range(len(models)):
#
# model = models[m]
# data_dir_c = data_dir + '/' + model + '/extract'
#
# #--- Get list of extracted files for this model.
# files = get_extract_files(data_dir_c, model, sdt, edt)
#
# #--- Loop over all extract files, reading them in.
# for f in range(len(files)):
#
# print 'Loading ', files[f]
# (vardat, ntimes, dt) = load_wrfout(files[f], vars)
#
# #--- Loop over each vars, interpolating data to stns for
# #--- each ntimes.
# for v in range(len(vars)):
#
# var = vars[v]
# vardat_c = vardat[var]
#
# #--- Loop over each ntime, grabbing interpolated data for
# #--- variable var for each stns.
# for n in range(ntimes):
# dt_c = time_increment(dt, n, dtfmt)
# for ns in range(len(stns)):
# dat_interp = bilinear_interpolate(lat, lon, \
# vardat_c[:,:,n],\
# latpts[ns], \
# lonpts[ns], 0)
# data_all[model, var, stns[ns], dt_c] = dat_interp
# dts_all.append(dt_c)
#
# #--- Get sorted unique list of all dates seen.
# dts_unique = list(sorted(set(dts_all)))
#
# #--- Create pickle file.
# pickle.dump((data_all, dts_unique, models, vars, stns), \
# open(pickle_file,'wb'), -1)
#
##---------------------------------------------------------------------------
## Get seasonal precipitation totals and make a time series plot of them.
##---------------------------------------------------------------------------
#(spr_pcp_tot, sum_pcp_tot, fal_pcp_tot, win_pcp_tot, years) = \
# get_seasonal_stats(data_all, dts_unique, models, stns, 'PREC', 'tot')
#
#mod = 'gfdl-cm3'
#plotfname = plot_dir + '/' + mod + '_season_precip.png'
#titlein = mod + ', ' + str(years[0]) + ' - ' + str(years[len(years)-1])
#iret = ts(win_pcp_tot, spr_pcp_tot, sum_pcp_tot, fal_pcp_tot, years, \
# stns, mod, titlein, plotfname, 'PREC')
#
##---------------------------------------------------------------------------
## Get seasonal temperature averages.
##---------------------------------------------------------------------------
#(spr_tmx_avg, sum_tmx_avg, fal_tmx_avg, win_tmx_avg, years) = \
# get_seasonal_stats(data_all, dts_unique, models, stns, 'T2MAX', 'avg')
#(spr_tmn_avg, sum_tmn_avg, fal_tmn_avg, win_tmn_avg, years) = \
# get_seasonal_stats(data_all, dts_unique, models, stns, 'T2MIN', 'avg')
#
#(spr_spdavg_avg, sum_spdavg_avg, fal_spdavg_avg, win_spdavg_avg, years) = \
# get_seasonal_stats(data_all, dts_unique, models, stns, \
# 'SPDUV10MEAN', 'avg')
#
#mod = 'gfdl-cm3'
#plotfname = plot_dir + '/' + mod + '_season_tmx_avg.png'
#titlein = mod + ', TMAX Average, ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
#iret = ts(win_tmx_avg, spr_tmx_avg, sum_tmx_avg, fal_tmx_avg, years, \
# stns, mod, titlein, plotfname, 'T2MAX')
#
#mod = 'gfdl-cm3'
#plotfname = plot_dir + '/' + mod + '_season_tmn_avg.png'
#titlein = mod + ', TMIN Average, ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
#iret = ts(win_tmn_avg, spr_tmn_avg, sum_tmn_avg, fal_tmn_avg, years, \
# stns, mod, titlein, plotfname, 'T2MIN')
sys.exit()
<file_sep>/old/old_old/plot_wrfout_grid_avg_snowrad_20180926.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re, math
import numpy as np
#import matplotlib.pyplot as plt
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
import pickle
from collections import defaultdict
from make_cmap import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
run_dirs = rcm_dir + '/rundirs'
geo_em = rcm_dir + '/data' + '/geo_em.d02.nc'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#vars = ['SNOW', 'SWDOWN']
#vars = ['SNOW']
vars = ['SWDOWN']
sdts = ['197001', '207001']
edts = ['200012', '209912']
#sdts = ['207001']
#edts = ['209912']
#models = ['gfdl-cm3', 'miroc5', 'bcc-csm1.1', 'access1.3']
#models = ['gfdl-cm3', 'miroc5', 'access1.3']
#models = ['gfdl-cm3']
models = ['access1.3']
dtfmt = "%Y%m%d%H"
#---------------------------------------------------------------------------
# Make a color map using make_cmap.
#---------------------------------------------------------------------------
levs_norm_c = []
for i in range(len(levs_temp)):
x = float(levs_temp[i])
norm_c = (x - min(levs_temp)) / (max(levs_temp) - min(levs_temp))
levs_norm_c.append(norm_c)
cmap_temp = make_cmap(cmap_temp, bit = True, position = levs_norm_c)
levs_norm_c = []
for i in range(len(levs_pcp)):
x = float(levs_pcp[i])
norm_c = (x - min(levs_pcp)) / (max(levs_pcp) - min(levs_pcp))
levs_norm_c.append(norm_c)
cmap_pcp = make_cmap(cmap_pcp, bit = True, position = levs_norm_c)
levs_norm_c = []
for i in range(len(levs_swe)):
x = float(levs_swe[i])
norm_c = (x - min(levs_swe)) / (max(levs_swe) - min(levs_swe))
levs_norm_c.append(norm_c)
cmap_swe = make_cmap(cmap_swe, bit = True, position = levs_norm_c)
levs_norm_c = []
for i in range(len(levs_swdown)):
x = float(levs_swdown[i])
norm_c = (x - min(levs_swdown)) / (max(levs_swdown) - min(levs_swdown))
levs_norm_c.append(norm_c)
cmap_swdown = make_cmap(cmap_swdown, bit = True, position = levs_norm_c)
#---------------------------------------------------------------------------
# Make run directory, load geo_em file.
#---------------------------------------------------------------------------
rundir = run_dirs + '/plot_wrfout_grid_avg.' + str(os.getpid())
print 'making ', rundir
os.makedirs(rundir)
#rundir = '/home/disk/spock/jbaars/rcm/rundirs/plot_wrfout_grid_avg.4149'
print 'Loading ', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
#---------------------------------------------------------------------------
# For each model, make a maps short-wave down radiation (SWDOWN).
#---------------------------------------------------------------------------
var = 'SWDOWN'
for m in range(len(models)):
model = models[m]
for n in range(len(sdts)):
sdt = sdts[n]
edt = edts[n]
yyyy_s = sdt[0:4]
yyyy_e = edt[0:4]
#--- Get list of extracted files for this model.
files = get_extract_files(data_dirs, model, sdt, edt, 1)
#--- Get files broken out by year and season.
(spr_files, sum_files, fal_files, win_files, years) = \
get_seasonal_files(files)
for season in seasons:
file_plot = rundir + '/' + model + '_' + var + '_' + season + \
'_' + yyyy_s + '_' + yyyy_e + '.nc'
syscom = 'ncra'
nyears = 0
for y in range(len(years)):
yyyy = years[y]
if season == 'summer':
if (sum_files[yyyy] == sum_files[yyyy]):
nyears += 1
for f in range(len(sum_files[yyyy])):
syscom = syscom + ' ' + sum_files[yyyy][f]
else:
continue
elif season == 'spring':
if (spr_files[yyyy] == spr_files[yyyy]):
nyears += 1
for f in range(len(spr_files[yyyy])):
syscom = syscom + ' ' + spr_files[yyyy][f]
else:
continue
elif season == 'winter':
if (win_files[yyyy] == win_files[yyyy]):
nyears += 1
for f in range(len(win_files[yyyy])):
syscom = syscom + ' ' + win_files[yyyy][f]
else:
continue
elif season == 'fall':
if (fal_files[yyyy] == fal_files[yyyy]):
nyears += 1
for f in range(len(fal_files[yyyy])):
syscom = syscom + ' ' + fal_files[yyyy][f]
else:
continue
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
os.system(syscom)
print 'Loading ', var, ' from ', file_plot
nc = NetCDFFile(file_plot, 'r')
ntimes = len(nc.dimensions['Time'])
vardat = {}
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
vardat[var] = dat_tmp
nc.close()
titlein = model.upper() + ', ' + var_lab[var] + ', ' + \
seasons_lab[season] + ', ' + yyyy_s + ' - ' + yyyy_e
plotdat = vardat[var][:,:,0]
if (var == 'PREC'):
plotdat = plotdat * mm2in
for z in range(len(zooms)):
plotfname = rundir + '/' + model + '_' + sdt + '_' + \
edt + '_' + season + '_' + var + '_' + \
zooms[z] + '.png'
mapper(var, lat, lon, plotdat, levs_swdown, cmap_swdown, \
maplw, colorbar_labs[var], titlein, plotfname, \
zooms[z])
# sys.exit()
##---------------------------------------------------------------------------
## For each model, make a maps Snow Water Equivalent.
##---------------------------------------------------------------------------
#var = 'SNOW'
#for m in range(len(models)):
#
# model = models[m]
#
# for n in range(len(sdts)):
# sdt = sdts[n]
# edt = edts[n]
# yyyy_s = sdt[0:4]
# yyyy_e = edt[0:4]
#
# #--- Get list of extracted files for this model.
# files = []
# for d in range(len(data_dirs)):
# path_c = data_dirs[d] + '/' + model + '/extract/*0401*.nc'
# files_c = glob.glob(path_c)
#
# for f in range(len(files_c)):
# if 'snowrad' not in files_c[f]:
# continue
#
# dt_c = re.findall(r'\.(\d{10})\.', files_c[f])
# if (dt_c):
# dt_c = dt_c[0]
# yyyymm_c = dt_c[0:6]
# if (yyyymm_c >= sdt and yyyymm_c <= edt):
# files.append(files_c[f])
#
# #--- Make plot for each zooms.
# file_plot = rundir + '/' + model + '_' + var + '_apr1_' + \
# yyyy_s + '_' + yyyy_e + '.nc'
# syscom = 'ncra'
# for f in range(len(files)):
# syscom = syscom + ' ' + files[f]
#
# syscom = syscom + ' ' + file_plot
# print 'syscom = ', syscom
# os.system(syscom)
#
# print 'Loading ', var, ' from ', file_plot
# nc = NetCDFFile(file_plot, 'r')
# ntimes = len(nc.dimensions['Time'])
# vardat = {}
# dat_tmp = nc.variables[var][:,:,:]
# dat_tmp = np.transpose(dat_tmp)
# vardat[var] = dat_tmp
# nc.close()
# titlein = model.upper() + ', ' + var_lab[var] + ', ' + \
# 'Apr 1st, ' + yyyy_s + ' - ' + yyyy_e
#
# plotdat = vardat[var][:,:,0]
#
# for z in range(len(zooms)):
# plotfname = rundir + '/' + model + '_' + sdt + '_' + \
# edt + '_apr1_' + var + '_' + \
# zooms[z] + '.png'
# mapper(var, lat, lon, plotdat, levs_swe, cmap_swe, \
# maplw, colorbar_labs[var], titlein, plotfname, \
# zooms[z])
#
##---------------------------------------------------------------------------
## Make map of difference.
##---------------------------------------------------------------------------
#for m in range(len(models)):
# model = models[m]
#
# syscom = 'ncdiff'
# file_diff = rundir + '/' + model + '_' + var + '_apr1_' + 'diff.nc'
#
# for n in range(len(sdts)):
# sdt = sdts[n]
# edt = edts[n]
# yyyy_s = sdt[0:4]
# yyyy_e = edt[0:4]
# file_plot = rundir + '/' + model + '_' + var + '_apr1_' + \
# yyyy_s + '_' + yyyy_e + '.nc'
# if os.path.isfile(file_plot):
# syscom = syscom + ' ' + file_plot
#
# syscom = syscom + ' ' + file_diff
#
# print 'syscom = ', syscom
# os.system(syscom)
#
# print 'Loading ', var, ' from ', file_diff
# nc = NetCDFFile(file_diff, 'r')
# ntimes = len(nc.dimensions['Time'])
# vardat = {}
# dat_tmp = nc.variables[var][:,:,:]
# dat_tmp = np.transpose(dat_tmp)
# vardat[var] = dat_tmp
# nc.close()
#
# titlein = model.upper() + ', ' + var_lab[var] + ', ' + \
# 'Apr 1st, Difference, ' + yyyy_s + ' - ' + yyyy_e
#
# plotdat = vardat[var][:,:,0]
#
# for z in range(len(zooms)):
# plotfname = rundir + '/' + model + '_' + sdt + '_' + \
# edt + '_apr1_diff_' + var + '_' + \
# zooms[z] + '.png'
# mapper(var, lat, lon, plotdat, levs_swe, cmap_swe, \
# maplw, colorbar_labs[var], titlein, plotfname, \
# zooms[z])
sys.exit()
<file_sep>/old/old_old/plot_wrfout_stns_20180329.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
import pickle
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
data_dir = '/home/disk/r2d2/steed/cmip5/rcp8.5'
geo_em = '/home/disk/a125/steed/run/geo_em.d02.nc'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
vars = ['PREC', 'T2MAX', 'T2MIN', 'SPDUV10MAX', 'SPDUV10MEAN', 'SPDUV10STD']
#sdt = '202001'
#edt = '202912'
#sdt = '197001'
#edt = '209912'
sdt = '199809'
edt = '200804'
models = ['gfdl-cm3', 'miroc5']
dtfmt = "%Y%m%d%H"
stns = ['KSEA', 'KSMP', 'KYKM']
stn_cols = ['b', 'r', 'g']
latpts = [47.44472, 47.27667, 46.56417]
lonpts = [-122.31361, -121.33722, -120.53361]
elevs = [130.0, 1207.0, 333.0]
##---------------------------------------------------------------------------
## Test.
##---------------------------------------------------------------------------
#x = range(0,len(vars),2)
#print 'x = ', x
#
##test = vars[x]
##print 'test = ', test
#
#test = [ vars[i] for i in x ]
#print 'test = ', test
#
#sys.exit()
#ndays = {}
#season = 'winter'
#yyyy = '1970'
#ndays[season+yyyy] = 26.0
#if (season+yyyy in ndays):
# print 'hi'
#else:
# print 'bye'
#sys.exit()
#season = 'winter'
#seasonsin = ['winter', 'spring']
#if (season in seasonsin):
# print 'hi'
#else:
# print 'bye'
#
#sys.exit()
#---------------------------------------------------------------------------
# Loop over each models, grabbing data from extract wrfout files.
#---------------------------------------------------------------------------
load_pickle = 1
pickle_file = pickle_dir + '/extract_' + sdt + '_' + edt + '.pickle'
if load_pickle and os.path.isfile(pickle_file):
(data_all, dts_unique, models, vars, stns) = \
pickle.load(open(pickle_file, 'rb'))
else:
#--- Load geo em file to get lat/lon/elev grids. Also interpolate model
#--- elevation to stns lat/lon point locations.
print 'Loading:\n', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
elevs_mod = []
elevs_diff = []
for ns in range(len(stns)):
dat_interp = bilinear_interpolate(lat, lon, hgt, \
latpts[ns], lonpts[ns], 0)
elevs_mod.append(dat_interp)
elevs_diff.append(elevs[ns] - dat_interp)
data_all = {}
dts_all = []
for m in range(len(models)):
model = models[m]
data_dir_c = data_dir + '/' + model + '/extract'
#--- Get list of extracted files for this model.
files = get_extract_files(data_dir_c, model, sdt, edt)
print 'Loading: '
#--- Loop over all extract files, reading them in.
for f in range(len(files)):
print files[f]
(vardat, ntimes, dt) = load_wrfout(files[f], vars)
#--- Loop over each vars, interpolating data to stns for
#--- each ntimes.
for v in range(len(vars)):
var = vars[v]
vardat_c = vardat[var]
#--- Loop over each ntime, grabbing interpolated data for
#--- variable var for each stns.
for n in range(ntimes):
dt_c = time_increment(dt, n, dtfmt)
for ns in range(len(stns)):
dat_interp = bilinear_interpolate(lat, lon, \
vardat_c[:,:,n],\
latpts[ns], \
lonpts[ns], 0)
#--- Do lapse rate correction for temperature.
if (var == 'T2MAX' or var == 'T2MIN'):
dat_c = dat_interp - std_lapse * elevs_diff[ns]
else:
dat_c = dat_interp
data_all[model, var, stns[ns], dt_c] = dat_c
dts_all.append(dt_c)
#--- Get sorted unique list of all dates seen.
dts_unique = list(sorted(set(dts_all)))
#--- Create pickle file.
pickle.dump((data_all, dts_unique, models, vars, stns), \
open(pickle_file,'wb'), -1)
##---------------------------------------------------------------------------
## Get seasonal precipitation totals and make a time series plot of them.
##---------------------------------------------------------------------------
#var = 'PREC'
#(pcp_tot, years) = get_seasonal_stats(data_all, dts_unique, models, \
# stns, var, 'tot')
#(pcp_max, years) = get_seasonal_stats(data_all, dts_unique, models, \
# stns, var, 'max')
#mod = 'gfdl-cm3'
#for s in range(len(seasons)):
# season = seasons[s]
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_tot.png'
# titlein = 'Total ' + seasons_lab[season] + ' Precipitation, ' + \
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts(pcp_tot, years, stns, mod, titlein, plotfname, 'PREC', 'tot', \
# stn_cols, [season])
#
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_max.png'
# titlein = 'Maximum 24-h ' + seasons_lab[season] + ' Precipitation, ' + \
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts(pcp_max, years, stns, mod, titlein, plotfname, 'PREC', 'max', \
# stn_cols, [season])
#
#---------------------------------------------------------------------------
# Get seasonal temperature maxes and mins.
#---------------------------------------------------------------------------
(tmx_max, years) = get_seasonal_stats(data_all, dts_unique, models, stns, \
'T2MAX', 'max')
(tmn_min, years) = get_seasonal_stats(data_all, dts_unique, models, stns, \
'T2MIN', 'min')
#mod = 'gfdl-cm3'
#for s in range(len(seasons)):
# season = seasons[s]
#
# #--- Max temperature.
# var = 'T2MAX'
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_max.png'
# titlein = 'Maximum Seasonal Temperature, ' + seasons_lab[season] + ', ' +\
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts(tmx_max, years, stns, mod, titlein, plotfname, var, 'max', \
# stn_cols, [season])
#
# #--- Min temperature.
# var = 'T2MIN'
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_min.png'
# titlein = 'Minimum Seasonal Temperature, ' + seasons_lab[season] + ', ' +\
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts(tmn_min, years, stns, mod, titlein, plotfname, var, 'min', \
# stn_cols, [season])
#
##---------------------------------------------------------------------------
## Get seasonal temperature averages.
##---------------------------------------------------------------------------
#(tmx_avg, years) = get_seasonal_stats(data_all, dts_unique, models, stns, \
# 'T2MAX', 'avg')
#(tmn_avg, years) = get_seasonal_stats(data_all, dts_unique, models, stns, \
# 'T2MIN', 'avg')
#
#mod = 'gfdl-cm3'
#for s in range(len(seasons)):
# season = seasons[s]
#
# #--- Max temperature.
# var = 'T2MAX'
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_avg.png'
# titlein = 'Average Maximum Temperature, ' + seasons_lab[season] + ', ' +\
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts(tmx_avg, years, stns, mod, titlein, plotfname, var, 'avg', \
# stn_cols, [season])
#
# #--- Min temperature.
# var = 'T2MIN'
# plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
# '_' + var + '_avg.png'
# titlein = 'Average Minimum Temperature, ' + seasons_lab[season] + ', ' +\
# mod.upper() + ', ' + str(years[0]) + ' - ' + \
# str(years[len(years)-1])
# iret = ts(tmn_avg, years, stns, mod, titlein, plotfname, var, 'avg', \
# stn_cols, [season])
#
#sys.exit()
#---------------------------------------------------------------------------
# Wind Speed.
#---------------------------------------------------------------------------
mod = 'gfdl-cm3'
var = 'SPDUV10MEAN'
(spdavg_avg, years) = get_seasonal_stats(data_all, dts_unique, models, stns, \
var, 'avg')
for s in range(len(seasons)):
season = seasons[s]
plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
'_' + var + '_avg.png'
titlein = 'Average Wind Speed, ' + seasons_lab[season] + ', ' +\
mod.upper() + ', ' + str(years[0]) + ' - ' + \
str(years[len(years)-1])
iret = ts(spdavg_avg, years, stns, mod, titlein, plotfname, var, 'avg', \
stn_cols, [season])
var = 'SPDUV10MAX'
(spdmax_max, years) = get_seasonal_stats(data_all, dts_unique, models, stns, \
var, 'max')
for s in range(len(seasons)):
season = seasons[s]
plotfname = plot_dir + '/' + mod + '_' + sdt + '_' + edt + '_' + season + \
'_' + var + '_max.png'
titlein = 'Maximum Wind Speed, ' + seasons_lab[season] + ', ' +\
mod.upper() + ', ' + str(years[0]) + ' - ' + \
str(years[len(years)-1])
iret = ts(spdmax_max, years, stns, mod, titlein, plotfname, var, 'max', \
stn_cols, [season])
sys.exit()
<file_sep>/old/old_old/plot_wrfout_grid_avg_snowrad_20180801.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re, math
import numpy as np
#import matplotlib.pyplot as plt
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
import pickle
from collections import defaultdict
from make_cmap import *
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
plot_dir = rcm_dir + '/plots'
pickle_dir = rcm_dir + '/pickle'
run_dirs = rcm_dir + '/rundirs'
geo_em = rcm_dir + '/data' + '/geo_em.d02.nc'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#vars = ['SNOW', 'SWDOWN']
vars = ['SNOW']
sdts = ['197001', '207001']
edts = ['200012', '209912']
#sdts = ['207001']
#edts = ['209912']
#models = ['gfdl-cm3', 'miroc5', 'bcc-csm1.1', 'access1.3']
#models = ['gfdl-cm3', 'miroc5', 'access1.3']
models = ['gfdl-cm3']
#models = ['access1.3']
dtfmt = "%Y%m%d%H"
#---------------------------------------------------------------------------
# Make a color map using make_cmap.
#---------------------------------------------------------------------------
levs_norm_c = []
for i in range(len(levs_temp)):
x = float(levs_temp[i])
norm_c = (x - min(levs_temp)) / (max(levs_temp) - min(levs_temp))
levs_norm_c.append(norm_c)
cmap_temp = make_cmap(cmap_temp, bit = True, position = levs_norm_c)
levs_norm_c = []
for i in range(len(levs_pcp)):
x = float(levs_pcp[i])
norm_c = (x - min(levs_pcp)) / (max(levs_pcp) - min(levs_pcp))
levs_norm_c.append(norm_c)
cmap_pcp = make_cmap(cmap_pcp, bit = True, position = levs_norm_c)
levs_norm_c = []
for i in range(len(levs_swe)):
x = float(levs_swe[i])
norm_c = (x - min(levs_swe)) / (max(levs_swe) - min(levs_swe))
levs_norm_c.append(norm_c)
cmap_swe = make_cmap(cmap_swe, bit = True, position = levs_norm_c)
#---------------------------------------------------------------------------
# Make run directory, load geo_em file.
#---------------------------------------------------------------------------
rundir = run_dirs + '/plot_wrfout_grid_avg.' + str(os.getpid())
print 'making ', rundir
os.makedirs(rundir)
#rundir = '/home/disk/spock/jbaars/rcm/rundirs/plot_wrfout_grid_avg.4149'
print 'Loading ', geo_em
(lat, lon, hgt) = load_geo_em(geo_em)
#---------------------------------------------------------------------------
# For each model, make a maps of every season...
#---------------------------------------------------------------------------
for m in range(len(models)):
model = models[m]
for n in range(len(sdts)):
sdt = sdts[n]
edt = edts[n]
yyyy_s = sdt[0:4]
yyyy_e = edt[0:4]
#--- Get list of extracted files for this model.
files = []
for d in range(len(data_dirs)):
path_c = data_dirs[d] + '/' + model + '/extract/*0401*.nc'
files_c = glob.glob(path_c)
for f in range(len(files_c)):
if 'snowrad' not in files_c[f]:
continue
dt_c = re.findall(r'\.(\d{10})\.', files_c[f])
if (dt_c):
dt_c = dt_c[0]
yyyymm_c = dt_c[0:6]
if (yyyymm_c >= sdt and yyyymm_c <= edt):
files.append(files_c[f])
#--- Make plot for each vars and zooms.
for var in vars:
file_plot = rundir + '/' + model + '_' + var + '_apr1_' + \
yyyy_s + '_' + yyyy_e + '.nc'
syscom = 'ncra'
for f in range(len(files)):
syscom = syscom + ' ' + files[f]
syscom = syscom + ' ' + file_plot
print 'syscom = ', syscom
os.system(syscom)
print 'Loading ', var, ' from ', file_plot
nc = NetCDFFile(file_plot, 'r')
ntimes = len(nc.dimensions['Time'])
vardat = {}
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
vardat[var] = dat_tmp
nc.close()
titlein = model.upper() + ', ' + var_lab[var] + ', ' + \
'Apr 1st, ' + yyyy_s + ' - ' + yyyy_e
plotdat = vardat[var][:,:,0]
for z in range(len(zooms)):
plotfname = rundir + '/' + model + '_' + sdt + '_' + \
edt + '_apr1_' + var + '_' + \
zooms[z] + '.png'
mapper(var, lat, lon, plotdat, levs_swe, cmap_swe, \
maplw, colorbar_labs[var], titlein, plotfname, \
zooms[z])
#---------------------------------------------------------------------------
# Make map of difference.
#---------------------------------------------------------------------------
for m in range(len(models)):
model = models[m]
for var in vars:
syscom = 'ncdiff'
file_diff = rundir + '/' + model + '_' + var + '_apr1_' + 'diff.nc'
for n in range(len(sdts)):
sdt = sdts[n]
edt = edts[n]
yyyy_s = sdt[0:4]
yyyy_e = edt[0:4]
file_plot = rundir + '/' + model + '_' + var + '_apr1_' + \
yyyy_s + '_' + yyyy_e + '.nc'
if os.path.isfile(file_plot):
syscom = syscom + ' ' + file_plot
syscom = syscom + ' ' + file_diff
print 'syscom = ', syscom
os.system(syscom)
print 'Loading ', var, ' from ', file_diff
nc = NetCDFFile(file_diff, 'r')
ntimes = len(nc.dimensions['Time'])
vardat = {}
dat_tmp = nc.variables[var][:,:,:]
dat_tmp = np.transpose(dat_tmp)
vardat[var] = dat_tmp
nc.close()
titlein = model.upper() + ', ' + var_lab[var] + ', ' + \
'Apr 1st, Difference, ' + yyyy_s + ' - ' + yyyy_e
plotdat = vardat[var][:,:,0]
for z in range(len(zooms)):
plotfname = rundir + '/' + model + '_' + sdt + '_' + \
edt + '_apr1_diff_' + var + '_' + \
zooms[z] + '.png'
mapper(var, lat, lon, plotdat, levs_swe, cmap_swe, \
maplw, colorbar_labs[var], titlein, plotfname, \
zooms[z])
sys.exit()
<file_sep>/igtg_check_utils_miroc5.py
#!/usr/bin/python
import os, os.path, time, glob, re, math
import sys, string, readline, h5py
from Scientific.IO.NetCDF import *
import numpy as np
#from datetime import datetime, timedelta, time
from datetime import datetime
import matplotlib as mpl
mpl.use('Agg')
import matplotlib.pyplot as plt
from mpl_toolkits.basemap import Basemap, cm, shiftgrid
#from rw_plotsettings import *
import pickle
colorbar_lab = 'm/s';
maplw = 0.8
#---------------------------------------------------------------------------
# Load 2-D lat/lon netcdf data file.
#---------------------------------------------------------------------------
def load_nc(filein, latlon2d):
print 'reading ', filein
f = NetCDFFile(filein, 'r')
ndims = len(f.dimensions)
dims = f.dimensions
dimnames = f.dimensions.keys()
for d in range(ndims):
print d, dimnames[d], dims[dimnames[d]]
#--- Find ny dimension.
if (dimnames[d] == 'lat' or dimnames[d] == 'rlat'):
ny = dims[dimnames[d]]
#--- Find nx dimension.
if (dimnames[d] == 'lon' or dimnames[d] == 'rlon'):
nx = dims[dimnames[d]]
if (latlon2d == 1):
lon_tmp = f.variables['lon'][:,:]
lat_tmp = f.variables['lat'][:,:]
# tos_tmp = f.variables['tos'][0,:,:]
tos_tmp = f.variables['tos'][:,:]
lonout = np.transpose(lon_tmp)
latout = np.transpose(lat_tmp)
tosout = np.transpose(tos_tmp)
else:
lon_tmp = f.variables['lon'][:]
lat_tmp = f.variables['lat'][:]
lonout = np.empty([nx,ny])
latout = np.empty([nx,ny])
for y in range(ny):
latout[:,y] = lat_tmp[y]
for x in range(nx):
lonout[x,:] = lon_tmp[x]
tosout = -9999
f.close()
return latout, lonout, tosout
#---------------------------------------------------------------------------
# Mapper.
#---------------------------------------------------------------------------
def mapper(i1, j1, i2, j2, lat1, lon1, lat2, lon2, tos_new,
minlat, maxlat, minlon, maxlon, title, plotfname):
ur_lat = maxlat
ur_lon = maxlon
ll_lat = minlat
ll_lon = minlon
lat_ctr = ll_lat + ((ur_lat - ll_lat) * 0.5)
lon_ctr = ll_lon + ((ur_lon - ll_lon) * 0.5)
width = 6.5
height = 6.5
fs = 9
titlefs = 9
fig = plt.figure(figsize=(width,height))
# left, bottom, width, height:
ax = fig.add_axes([0.00,0.05,0.99,0.91])
#---
map = Basemap(resolution='i',projection='lcc',\
llcrnrlon= ll_lon, llcrnrlat=ll_lat,\
urcrnrlon= ur_lon, urcrnrlat= ur_lat,\
lat_0=lat_ctr,lon_0=lon_ctr,lat_1=(ur_lat - ll_lat))
#--- Draw coastlines, country boundaries, fill continents.
map.drawcoastlines(linewidth = maplw)
map.drawstates(linewidth = maplw)
map.drawcountries(linewidth = maplw)
#--- Draw the edge of the map projection region (the projection limb)
map.drawmapboundary(linewidth = maplw)
#--- Draw lat/lon grid lines every 30 degrees.
map.drawmeridians(np.arange(0, 360, 30), linewidth = maplw)
map.drawparallels(np.arange(-90, 90, 30), linewidth = maplw)
# #--- Get lat and lon data in map's x/y coordinates.
# x1,y1 = map(lon1, lat1)
# x2,y2 = map(lon2, lat2)
#-- Add dots for grid points for 1st grid.
nx,ny = np.shape(lon1)
print 'nx, ny = ', nx, ny
for ii in range(nx):
for jj in range(ny):
if (lat1[ii,jj] > minlat and lat1[ii,jj] < maxlat):
lon_c = lon1[ii,jj]
if (lon_c > 180.0):
lon_c = lon_c - 360
if (lon_c > minlon and lon_c < maxlon):
if (ii == i1 and jj == j1):
mark = 'ro'
else:
mark = 'bo'
xs, ys = map(lon1[ii,jj], lat1[ii,jj])
plt.plot(xs, ys, mark, markersize = 5)
# plt.text(xs, ys, str(ii) + ',' + str(jj), fontsize=7)
plt.text(xs, ys, str(ii+1) + ',' + str(jj+1), fontsize=7)
#-- Add dots for grid points for 2nd grid.
nx,ny = np.shape(lon2)
print 'nx, ny = ', nx, ny
for ii in range(nx):
for jj in range(ny):
if (lat2[ii,jj] > minlat and lat2[ii,jj] < maxlat):
lon_c = lon2[ii,jj]
if (lon_c > 180.0):
lon_c = lon_c - 360
if (lon_c > minlon and lon_c < maxlon):
if (ii == i2 and jj == j2):
mark = 'rx'
ms = 2.0
else:
mark = 'kx'
ms = 1.2
# iip = ii - 1
# jjp = jj - 1
# xs, ys = map(lon2[iip,jjp], lat2[iip,jjp])
xs, ys = map(lon2[ii,jj], lat2[ii,jj])
plt.plot(xs, ys, mark, markersize = 6, mew=ms)
# plt.text(xs, ys, str(ii) + ',' + str(jj), fontsize=6)
strp = "%d,%d,%.3f" % (ii+1, jj+1, tos_new[ii+1,jj+1])
# strp = "%d,%d,%.3f" % (ii-1, jj-1, tos_new[ii-1,jj-1])
# strp = "%d,%d,%.3f" % (iip, jjp, tos_new[iip,jjp])
# strp = "%d,%d,%.3f" % (ii+2, jj+2, tos_new[ii+2,jj+2])
# strp = "%d,%d,%.3f" % (ii+6, jj+6, tos_new[ii+6,jj+6])
# strp = "%d,%d,%.3f" % (ii+3, jj+3, tos_new[ii+3,jj+3])
# strp = "%d,%d,%.3f" % (ii-2, jj-2, tos_new[ii-2,jj-2])
# strp = "%d,%d,%.3f" % (ii-3, jj-3, tos_new[ii-3,jj-3])
plt.text(xs, ys, strp, fontsize=6)
plt.title(title, fontsize=titlefs, fontweight='bold')
#--- Save plot.
print 'creating ', plotfname
plt.savefig(plotfname)
plt.close()
<file_sep>/check_extract_files.py
#!/usr/bin/python
import sys, os, os.path, time, glob, re
import numpy as np
from make_cmap import *
from netCDF4 import Dataset as NetCDFFile
from utils_wrfout import *
from utils_date import *
from utils_ghcnd_obs import *
import pickle
import csv
import json
#---------------------------------------------------------------------------
# Paths.
#---------------------------------------------------------------------------
rcm_dir = '/home/disk/spock/jbaars/rcm'
py_dir = rcm_dir + '/python'
#plot_dir = rcm_dir + '/plots'
plot_dir = rcm_dir + '/plots/new'
pickle_dir = rcm_dir + '/pickle'
data_dir = rcm_dir + '/data'
ghcnd_dir = data_dir + '/ghcnd/ghcnd_all'
data_dirs = ['/home/disk/r2d2/steed/cmip5/rcp8.5', \
'/home/disk/vader/steed/cmip5/rcp8.5', \
'/home/disk/jabba/steed/cmip5/rcp8.5']
geo_em = data_dir + '/geo_em.d02.nc'
station_file = data_dir + '/station_file.txt'
#---------------------------------------------------------------------------
# Settings.
#---------------------------------------------------------------------------
#vars_mod = ['PREC', 'T2MAX', 'T2MIN']
vars_mod = ['T2MAX']
sdt = '197001'
edt = '209912'
#--- GHCND data downloaded through June 2019, so 2019 is a partial year, so
#--- only include obs through 2018.
edt_obs = '201812'
var_obs_dict = {
'PREC': 'PRCP',
'T2MAX': 'TMAX',
'T2MIN': 'TMIN',
'T2MEAN': 'T2MEAN'
}
stats_dict = {
'PREC': ['max', 'tot'],
'T2MAX': ['max', 'avg'],
'T2MIN': ['min', 'max', 'avg'],
'T2MEAN': ['min', 'max', 'avg'],
}
models = ['access1.0', 'access1.3', 'bcc-csm1.1', 'canesm2', \
'ccsm4', 'csiro-mk3.6.0', 'fgoals-g2', 'gfdl-cm3', \
'giss-e2-h', 'miroc5', 'mri-cgcm3', 'noresm1-m']
models = ['access1.0']
temp_max = 273 + 60
temp_min = 273 - 60
for m in range(len(models)):
data_all = {}
dts_all = []
model = models[m]
#--- Get list of extracted files for this model.
print 'model = ', model
files = get_extract_files(data_dirs, model, sdt, edt, 0)
files = list(sorted(set(files)))
#--- Loop over all extract files, reading them in.
for f in range(len(files)):
(vardat, ntimes, dt) = load_wrfout(files[f], vars_mod)
for v in range(len(vars_mod)):
var = vars_mod[v]
dat_c = vardat[var]
x,y,z = dat_c.shape
for k in range(z):
max_c = np.amax(dat_c[:,:,k])
if max_c < temp_min or max_c > temp_max:
print model, ', ', files[f], ', ', k
'''
#---------------------------------------------------------------------------
# Load model data.
#---------------------------------------------------------------------------
load_mod_all = 1
pf_mod_stats = pickle_dir + '/mod_stats.pkl'
if load_mod_all == 0:
#--- If not loading all model data, just load stats pickle file.
if os.path.isfile(pf_mod_stats):
print 'reading pickle file ', pf_mod_stats
(mod,yy_mod) = pickle.load(open(pf_mod_stats, 'rb'))
else:
sys.exit('cannot see ' + pf_mod_stats)
else:
(data_all, dts_unique, models, vars_all, stns) = \
load_extract_data(geo_em, stns, latpts, lonpts, elevs, models, \
data_dirs, sdt, edt, vars_mod, pickle_dir, 0)
sys.exit()
data_all = {}
dts_all = []
for m in range(len(models)):
model = models[m]
pf = get_mod_pkl_name(pickle_dir, model, sdt, edt)
print 'loading ', pf
(model, data_c, dts_unique, vars, stns) = pickle.load(open(pf, 'rb'))
data_all[model] = data_c
dts_all = dts_all + dts_unique
mod = {}
yy_mod = {}
for var in vars_mod:
stats = stats_dict[var]
for stat in stats:
(mod[var,stat], yy_mod[var,stat]) = get_seasonal_stats(\
data_all, dts_unique, models, stns, var, stat)
pickle.dump((mod,yy_mod), open(pf_mod_stats,'wb'), -1)
sys.exit()
#---------------------------------------------------------------------------
# Make a time series plots of each station, variable, stat and season.
#---------------------------------------------------------------------------
#--- Do multiple models on a plot for one station.
for ss in range(len(stns)):
stn = stns[ss]
for v in range(len(vars_mod)):
var = vars_mod[v]
varo = var_obs_dict[var]
stats = stats_dict[var]
for st in range(len(stats)):
stat = stats[st]
for s in range(len(seasons)):
season = seasons[s]
plotfname = get_plotfname(plot_dir,stn,sdt,edt,season,var,stat)
titlein = get_title(season,stn,var,stat,yy_mod[var,stat])
iret = ts_mods(mod[var,stat],yy_mod[var,stat], \
obs[varo,stat],yy_obs[varo,stat], stn, \
models, titlein, plotfname, var, stat, \
mod_cols, [season])
# sys.exit()
sys.exit()
sys.exit()
'''
| 4edc5c4ff3301fb9c73cac48e6799ea6c1837618 | [
"Python"
]
| 42 | Python | jeffbaars/rcm_python | d0352233be9f3364b1e05f3728cfadc7fbee44a9 | 1892dda746054e5f0621bd5fecee770c7ba49279 | |
refs/heads/master | <repo_name>inyaJohnson/php-api<file_sep>/app/repo/Animal.php
<?php
/**
* Created by PhpStorm.
* User: tujailer
* Date: 10/18/19
* Time: 8:35 PM
*/
namespace App\Repo;
interface Animal{
public function eat();
}<file_sep>/app/repo/ProductBind.php
<?php
/**
* Created by PhpStorm.
* User: tujailer
* Date: 10/18/19
* Time: 7:30 PM
*/
namespace App\Repo;
use App\Model\Product;
use App\Model\Review;
class ProductBind extends Product
{
function reviews(){
return $this->hasMany(Review::class);
}
}<file_sep>/routes/web.php
<?php
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
use App\Model\Product;
//Route::get('/', function () {
// return view('welcome');
//});
Route::get('/', function () {
$products = Product::find(4)->reviews;
// dd($products);
return view('output', ['data' => $products]);
});
Auth::routes();
Route::get('/home', 'HomeController@index')->name('home');
| c4d5de7ad1eba5d85ae920af8751a3b724449715 | [
"PHP"
]
| 3 | PHP | inyaJohnson/php-api | da9e0693c96858b5584bafe35a88f3256ba72125 | c4103a8142df21c2620413d35358762c99c94c65 | |
refs/heads/master | <repo_name>Noushid/nice-web<file_sep>/application/views/templates/footer.php
<!--footer 1 start -->
<footer class="footer footer-one">
<div class="primary-footer brand-bg">
<div class="container">
<a href="#top" class="page-scroll btn-floating btn-large pink back-top waves-effect waves-light tt-animate btt" data-section="#top">
<i class="material-icons"></i>
</a>
<div class="row">
<div class="col-md-4 widget clearfix">
<h2 class="white-text">About Classic International</h2>
<p>Classic International is Leading Typing and Translation Service Provider in India and Kuwait. Also We offer Health Insurances And Educational Insurances, etc..</p>
<ul class="social-link tt-animate ltr">
<li><a href="https://www.facebook.com/ClassicInternational" target="_blank"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div><!-- /.col-md-3 -->
<div class="col-md-3 widget" style="padding-left: 80px;">
<h2 class="white-text">First Look</h2>
<ul class="footer-list">
<li><a href="about"><i class="fa fa-chevron-right mr-10"></i>About Us</a></li>
<li><a href="home#WhatWeDo"><i class="fa fa-chevron-right mr-10"></i>What We Do</a></li>
<li><a href="home#appointment"><i class="fa fa-chevron-right mr-10"></i>Make An Appointment</a></li>
<li><a href="contact#getintouch"><i class="fa fa-chevron-right mr-10"></i>Contact Us</a></li>
</ul>
</div><!-- /.col-md-3 -->
<div class="col-md-2 widget">
<h2 class="white-text">Quick links</h2>
<ul class="footer-list">
<li><a href="services"><i class="fa fa-chevron-right mr-10"></i>Our Services</a></li>
<li><a href="home#latestnews"><i class="fa fa-chevron-right mr-10"></i>Latest News</a></li>
<li><a href="blog"><i class="fa fa-chevron-right mr-10"></i>Our Blogs</a></li>
<li><a href="contact#getintouch"><i class="fa fa-chevron-right mr-10"></i>Get In Touch</a></li>
</ul>
</div><!-- /.col-md-3 -->
<div class="col-md-3 widget">
<h2 class="white-text">Subscribe Me</h2>
<form id="subscribeForm" method="post" action="">
<div class="form-group clearfix">
<label class="sr-only" for="subscribe">Email address</label>
<input type="email" class="form-control" id="mail" name="email" placeholder="Email address">
<button type="submit" class="tt-animate ltr" id="subscribe"><i class="fa fa-long-arrow-right"></i></button>
</div>
</form>
<div class="col-md-10 hide" id="sub_msg">
<div class="alert alert-success">
Subscribed
</div>
</div>
<div class="col-md-10 hide" id="sub_error">
<div class="alert alert-success" id="error_content">
Subscription failed!try again later
</div>
</div>
<div class="widget-tags">
<a href="moments">Our Moments</a>
<a href="login">Admin Login</a>
</div><!-- /.widget-tags -->
</div><!-- /.col-md-3 -->
</div><!-- /.row -->
</div><!-- /.container -->
</div><!-- /.primary-footer -->
<div class="secondary-footer brand-bg darken-2">
<div class="container">
<span class="copy-text">Copyright © <span id="year"></span> <a href="home">Classic International info</a> | All Rights Reserved | Designed By <a href="http://psybotechnologies.com/">Psybo Technologies</a></span>
</div><!-- /.container -->
</div><!-- /.secondary-footer -->
</footer>
<!--footer 1 end-->
<!-- Preloader -->
<div id="preloader">
<div class="preloader-position">
<img src="<?php echo public_url()?>assets/img/logo-colored.png" alt="logo" >
<div class="progress">
<div class="indeterminate"></div>
</div>
</div>
</div>
<!-- End Preloader -->
<!-- Applay Modal Dialog Box
===================================== -->
<div id="applayServices" class="modal fade" role="dialog">
<div class="modal-dialog">
<!-- Modal content-->
<div class="modal-content">
<div class="modal-header bg-gray">
<button type="button" class="close" data-dismiss="modal">×</button>
<h5 class="modal-title text-center"><i class="fa fa-list fa-fw"></i>Get A Quote Now</h5>
</div>
<div class="modal-body">
<form class="form-horizontal" name="quoteForm" id="quoteForm" method="POST" action="request-quote">
<div class="">
<div class="col-sm-offset-1 col-md-10">
<div class="input-field mt-20">
<input type="text" name="name" class="validate" id="name" required="">
<label for="name">Full Name*</label>
</div>
</div>
<div class="col-sm-offset-1 col-md-10">
<div class="input-field mt-20">
<input id="email" type="email" name="email" class="validate" required="">
<label for="email" data-error="wrong" data-success="right">Email Address*</label>
</div>
</div>
<div class="col-sm-offset-1 col-md-10">
<div class="input-field mt-20">
<input type="text" name="people" class="validate" id="phone">
<label for="people">Contact Number*</label>
</div>
</div>
<div class="col-sm-offset-1 col-md-10">
<div class="input-field mt-20">
<select class="text-capitalize selectpicker form-control required selectbox" name="service" data-style="g-select" data-width="100%">
<option value="0" selected="">Select Service</option>
<option value="Typing Services">Typing Services</option>
<option value="Services for Ministry of Health">Services for Ministry of Health</option>
<option value="Services for Higher & Lower Education">Services for Higher & Lower Education</option>
<option value="Medical Fingerprint Assists Services">Medical Fingerprint Assists Services</option>
<option value="Insurance Services">Insurance Services</option>
<option value="Health Insurance Services">Health Insurance Services</option>
<option value="Education Services">Education Services</option>
<option value="Transportation services">Transportation services </option>
<option value="Pan Card Services">Pan Card Services</option>
<option value="Air Tickets and Related Services">Air Tickets and Related Services</option>
<option value="Courier Services">Courier Services</option>
<option value="Miscellaneous Services">Miscellaneous Services</option>
</select>
</div>
</div>
<div class="col-sm-offset-1 col-md-10">
<div class="input-field mt-20">
<textarea name="message" id="message" class="materialize-textarea" ></textarea>
<label for="message">Additional note</label>
</div>
</div>
</div><!-- /.row -->
<div class="form-group mt40 text-right">
<div class="col-sm-10">
<div class="col-sm-8 col-sm-offset-2" id="statusMsg">
</div>
<div class="col-sm-2 pull-right">
<button type="submit" class="waves-effect waves-light btn">Submit</button>
</div>
</div>
</div>
</form>
</div>
<div class="modal-footer bg-gray">
<span class="pull-left color-pink"><span><i class="fa fa-phone color-cyan fa-fw ml-10"></i></span>9953 01 77</span>
<span class="pull-right color-pink"><span><i class="fa fa-envelope color-cyan fa-fw mr-10"></i></span><EMAIL></span>
</div>
</div>
</div>
</div>
<!-- jQuery -->
<script src="<?php echo public_url()?>assets/js/jquery-2.1.3.min.js"></script>
<script src="<?php echo public_url()?>assets/bootstrap/js/bootstrap.min.js"></script>
<script src="<?php echo public_url()?>assets/materialize/js/materialize.min.js"></script>
<script src="<?php echo public_url()?>assets/js/jquery.easing.min.js"></script>
<script src="<?php echo public_url()?>assets/js/jquery.sticky.min.js"></script>
<script src="<?php echo public_url()?>assets/js/smoothscroll.min.js"></script>
<script src="<?php echo public_url()?>assets/js/imagesloaded.js"></script>
<script src="<?php echo public_url()?>assets/js/jquery.stellar.min.js"></script>
<script src="<?php echo public_url()?>assets/js/jquery.inview.min.js"></script>
<script src="<?php echo public_url()?>assets/js/jquery.shuffle.min.js"></script>
<script src="<?php echo public_url()?>assets/js/menuzord.js"></script>
<script src="<?php echo public_url()?>assets/js/bootstrap-tabcollapse.min.js"></script>
<script src="<?php echo public_url()?>assets/owl.carousel/owl.carousel.js"></script>
<script src="<?php echo public_url()?>assets/flexSlider/jquery.flexslider-min.js"></script>
<script src="<?php echo public_url()?>assets/magnific-popup/jquery.magnific-popup.min.js"></script>
<script src="<?php echo public_url()?>assets/js/scripts.js"></script>
<script src="<?php echo public_url()?>assets/js/masonry.pkgd.min.js"></script>
<script src="https://maps.googleapis.com/maps/api/js"></script>
<!-- RS5.0 Core JS Files -->
<script src="<?php echo public_url()?>assets/revolution/js/jquery.themepunch.tools.min.js"></script>
<script src="<?php echo public_url()?>assets/revolution/js/jquery.themepunch.revolution.min.js"></script>
<!-- RS5.0 Init -->
<script type="text/javascript">
jQuery(document).ready(function () {
jQuery(".materialize-slider").revolution({
sliderType: "standard",
sliderLayout: "fullscreen",
delay: 9000,
navigation: {
keyboardNavigation: "on",
keyboard_direction: "horizontal",
mouseScrollNavigation: "off",
onHoverStop: "off",
touch: {
touchenabled: "on",
swipe_threshold: 75,
swipe_min_touches: 1,
swipe_direction: "horizontal",
drag_block_vertical: false
},
arrows: {
style: "gyges",
enable: true,
hide_onmobile: false,
hide_onleave: true,
tmp: '',
left: {
h_align: "left",
v_align: "center",
h_offset: 10,
v_offset: 0
},
right: {
h_align: "right",
v_align: "center",
h_offset: 10,
v_offset: 0
}
}
},
responsiveLevels: [1240, 1024, 778, 480],
gridwidth: [1240, 1024, 778, 480],
gridheight: [700, 600, 500, 500],
disableProgressBar: "on",
parallax: {
type: "mouse",
origo: "slidercenter",
speed: 2000,
levels: [2, 3, 4, 5, 6, 7, 12, 16, 10, 50]
}
});
});
</script>
<!-- SLIDER REVOLUTION 5.0 EXTENSIONS (Load Extensions only on Local File Systems! The following part can be removed on Server for On Demand Loading) -->
<script type="text/javascript" src="<?php echo public_url()?>assets/revolution/js/extensions/revolution.extension.video.min.js"></script>
<script type="text/javascript" src="<?php echo public_url()?>assets/revolution/js/extensions/revolution.extension.slideanims.min.js"></script>
<script type="text/javascript" src="<?php echo public_url()?>assets/revolution/js/extensions/revolution.extension.actions.min.js"></script>
<script type="text/javascript" src="<?php echo public_url()?>assets/revolution/js/extensions/revolution.extension.layeranimation.min.js"></script>
<script type="text/javascript" src="<?php echo public_url()?>assets/revolution/js/extensions/revolution.extension.kenburn.min.js"></script>
<script type="text/javascript" src="<?php echo public_url()?>assets/revolution/js/extensions/revolution.extension.navigation.min.js"></script>
<script type="text/javascript" src="<?php echo public_url()?>assets/revolution/js/extensions/revolution.extension.migration.min.js"></script>
<script type="text/javascript" src="<?php echo public_url()?>assets/revolution/js/extensions/revolution.extension.parallax.min.js"></script>
<!-- year scrpt -->
<script type="text/javascript">
n = new Date();
y = n.getFullYear();
document.getElementById("year").innerHTML = y;
</script>
<!-- Google Map Customization -->
<script type="text/javascript">
jQuery(document).ready(function() {
//set your google maps parameters
var $latitude = 29.26708, //Visit http://www.latlong.net/convert-address-to-lat-long.html for generate your Lat. Long.
$longitude = 47.941092,
$map_zoom = 14 /* ZOOM SETTING */
//google map custom marker icon
var $marker_url = 'assets/img/pin.png';
//we define here the style of the map
var style = [{
styles: [
{
"elementType": "geometry",
"stylers": [
{
"color": "#212121"
}
]
},
{
"elementType": "labels.icon",
"stylers": [
{
"visibility": "off"
}
]
},
{
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#757575"
}
]
},
{
"elementType": "labels.text.stroke",
"stylers": [
{
"color": "#212121"
}
]
},
{
"featureType": "administrative",
"elementType": "geometry",
"stylers": [
{
"color": "#757575"
}
]
},
{
"featureType": "administrative.country",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#9e9e9e"
}
]
},
{
"featureType": "administrative.land_parcel",
"stylers": [
{
"visibility": "off"
}
]
},
{
"featureType": "administrative.locality",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#bdbdbd"
}
]
},
{
"featureType": "poi",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#757575"
}
]
},
{
"featureType": "poi.park",
"elementType": "geometry",
"stylers": [
{
"color": "#181818"
}
]
},
{
"featureType": "poi.park",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#616161"
}
]
},
{
"featureType": "poi.park",
"elementType": "labels.text.stroke",
"stylers": [
{
"color": "#1b1b1b"
}
]
},
{
"featureType": "road",
"elementType": "geometry.fill",
"stylers": [
{
"color": "#2c2c2c"
}
]
},
{
"featureType": "road",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#8a8a8a"
}
]
},
{
"featureType": "road.arterial",
"elementType": "geometry",
"stylers": [
{
"color": "#373737"
}
]
},
{
"featureType": "road.highway",
"elementType": "geometry",
"stylers": [
{
"color": "#3c3c3c"
}
]
},
{
"featureType": "road.highway.controlled_access",
"elementType": "geometry",
"stylers": [
{
"color": "#4e4e4e"
}
]
},
{
"featureType": "road.local",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#616161"
}
]
},
{
"featureType": "transit",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#757575"
}
]
},
{
"featureType": "water",
"elementType": "geometry",
"stylers": [
{
"color": "#000000"
}
]
},
{
"featureType": "water",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#3d3d3d"
}
]
}
]
}];
//set google map options
var map_options = {
center: new google.maps.LatLng($latitude, $longitude),
zoom: $map_zoom,
panControl: true,
zoomControl: true,
mapTypeControl: true,
streetViewControl: true,
mapTypeId: google.maps.MapTypeId.ROADMAP,
scrollwheel: false,
styles: style
}
//inizialize the map
var map = new google.maps.Map(document.getElementById('myMap'), map_options);
//add a custom marker to the map
var marker = new google.maps.Marker({
position: new google.maps.LatLng($latitude, $longitude),
map: map,
visible: true,
icon: $marker_url
});
var contentString = '<div id="mapcontent">' + '<p><strong>Classic Typing Centre</strong> <br> Jleeb Al shuyoukh, Abbassiya, Kuwait <br> Mob: 99 530 177</p></div>';
var infowindow = new google.maps.InfoWindow({
maxWidth: 320,
content: contentString
});
google.maps.event.addListener(marker, 'click', function() {
infowindow.open(map, marker);
});
});
</script>
<!--Subscribe-->
</body>
</html><file_sep>/application/views/errors/html/error_404.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="description" content="materialize is a material design based mutipurpose responsive template">
<meta name="keywords" content="material design, card style, material template, portfolio, corporate, business, creative, agency">
<meta name="author" content="trendytheme.net">
<title>404</title>
<!-- favicon -->
<link rel="shortcut icon" href="assets/img/ico/favicon.png">
<!-- apple-touch-icon -->
<link rel="apple-touch-icon-precomposed" sizes="144x144" href="assets/img/ico/apple-touch-icon-144-precomposed.png">
<link rel="apple-touch-icon-precomposed" sizes="114x114" href="assets/img/ico/apple-touch-icon-114-precomposed.png">
<link rel="apple-touch-icon-precomposed" sizes="72x72" href="assets/img/ico/apple-touch-icon-72-precomposed.png">
<link rel="apple-touch-icon-precomposed" href="assets/img/ico/apple-touch-icon-57-precomposed.png">
<link href='https://fonts.googleapis.com/css?family=Raleway:400,300,500,700,900' rel='stylesheet' type='text/css'>
<!-- FontAwesome CSS -->
<link href="assets/fonts/font-awesome/css/font-awesome.min.css" rel="stylesheet">
<!-- materialize -->
<link href="assets/materialize/css/materialize.min.css" rel="stylesheet">
<!-- Bootstrap -->
<link href="assets/bootstrap/css/bootstrap.min.css" rel="stylesheet">
<!-- shortcodes -->
<link href="assets/css/shortcodes/shortcodes.css" rel="stylesheet">
<!-- Style CSS -->
<link href="assets/css/style.css" rel="stylesheet">
<!-- HTML5 shim and Respond.js for IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<section class="fullscreen-banner valign-wrapper error-wrapper-alt">
<div class="valign-cell">
<div class="container">
<div class="text-center">
<h1 class="mb-30">404</h1>
<span class="error-sub">OOPS! <?php echo $heading; ?></span>
<p>Sorry, <?php echo $message; ?></p>
<a class="btn btn-lg waves-effect waves-light" href="home">Take Me Home</a>
</div>
</div><!-- /.container -->
</div><!-- /.valign-cell -->
</section>
<!-- Preloader -->
<div id="preloader">
<div class="preloader-position">
<img src="assets/img/logo-colored.png" alt="logo" >
<div class="progress">
<div class="indeterminate"></div>
</div>
</div>
</div>
<!-- End Preloader -->
<!-- jQuery -->
<script src="assets/js/jquery-2.1.3.min.js"></script>
<script src="assets/bootstrap/js/bootstrap.min.js"></script>
<script src="assets/materialize/js/materialize.min.js"></script>
<script src="assets/js/menuzord.js"></script>
<script src="assets/js/jquery.easing.min.js"></script>
<script src="assets/js/smoothscroll.min.js"></script>
<script src="assets/js/twitterFetcher.min.js"></script>
<script src="assets/js/scripts.js"></script>
</body>
</html><file_sep>/application/views/admin/brochure.php
<div class="col-md-12">
<div class="row">
<div class="box" style="margin-left: 14px;">
<button class="btn btn-primary" ng-click="newBrochure()"><i class="fa fa-plus"></i> Add</button>
<form class="form-horizontal" method="POST" ng-show="showform" ng-submit="addBrochure()">
<h3>New Brochure</h3>
<div class="form-group">
<label for="" class="control-label col-md-1">Name</label>
<div class="col-md-4">
<input type="text" class="form-control" name="name" ng-model="newbrochure.name" required=""/>
</div>
<label for="" class="control-label col-md-1">subject</label>
<div class="col-md-4">
<input class="form-control" type="text" ng-model="newbrochure.subject" name="subject" />
</div>
</div>
<div class="form-group">
<label class="control-label col-md-1" for="date">Date</label>
<div class="col-md-4">
<p class="input-group">
<input type="text" class="form-control" name="date" uib-datepicker-popup="dd-MMMM-yyyy" ng-model="date" is-open="popup2.opened" datepicker-options="dateOptions" ng-required="true" close-text="Close" readonly show-button-bar="false"/>
<span class="input-group-btn">
<button type="button" class="btn btn-default" ng-click="open2()"><i class="glyphicon glyphicon-calendar"></i></button>
</span>
</p>
</div>
</div>
<div class="form-group">
<label for="" class="control-label col-md-1">brochure</label>
<div class="col-md-4">
<button ngf-select="uploadFiles($files, $invalidFiles)"
accept="application/pdf,.csv, application/vnd.openxmlformats-officedocument.spreadsheetml.sheet, application/vnd.ms-excel,text/plain,application/msword, application/vnd.ms-excel, application/vnd.ms-powerpoint,application/vnd.oasis.opendocument.text"
ngf-max-height="1000" ngf-max-size="10MB">
Select Files
</button>
<span class="alert alert-danger"ng-show="fileValidation.status == true">{{fileValidation.msg}}</span>
</div>
<div class="col-md-12">
<div class="row">
<ul class="list-group">
<li ng-repeat="f in files" style="font:smaller" class="list-group-item">
<div class="row">
<div class="col-sm-12">
<div class="col-sm-2">
<img ngf-src="f.$ngfBlobUrl" class="thumbnail" width="100px" ngf-no-object-url="true" >
<span>{{f.name}} {{f.$errorParam}}</span>
</div>
<div class="col-sm-6">
<div class="row">
<div class="col-sm-8">
<div class="progress" ng-show="f.progress >= 0" ng-class="{cancel: uploadstatus == 1}">
<div ng-show="uploadstatus == 1">{{f.progressmsg}}</div>
<div class="progress-bar progress-bar-success" role="progressbar" aria-valuenow="40"
aria-valuemin="0" aria-valuemax="100" style="width:{{f.progress}}%" ng-show="uploadstatus != 1">
{{f.progress}}% Complete
</div>
</div>
</div>
<div class="col-sm-4">
<button class="btn btn-danger" type="button" ng-click="abort()">cancel</button>
</div>
</div>
</div>
</div>
</div>
</li>
<li ng-repeat="f in errFiles" style="font:smaller" class="list-group-item">
{{f.name}} {{f.$error}} {{f.$errorParam}}
</li>
</ul>
</div>
</div>
{{errorMsg}}
<!----- ***for existing Brochure **---->
<div class="clearfix"></div>
<div class="row" ng-show="newbrochure.file_name">
<a href="{{newbrochure.fileUrl}}">{{newbrochure.file_name}}</a>
</div>
</div>
<div class="form-group text-center">
<button class="btn btn-primary" type="submit">Save</button>
<button class="btn btn-danger" type="button" ng-click="hideForm()">Cancel</button>
</div>
</form>
<div class="row">
<form class="form-inline" ng-show="showtable">
<div class="form-group">
<label for="" class="control-label col-md-2">Show</label>
<div class="col-md-3">
<select name="numPerPage" ng-model="numPerPage" class="form-control"
ng-options="num for num in paginations">{{num}}
</select>
</div>
<label class="control-label col-md-2">Search</label>
<div class="col-md-3">
<input type="text" ng-model="search" class="form-control" placeholder="Search">
</div>
</div>
</form>
</div>
</div>
</div>
<div class="help-block" ng-show="!showtable">{{message}}</div>
<table class="table table-bordered" ng-show="showtable">
<thead>
<tr>
<th>#</th>
<th>Name</th>
<th>Subject</th>
<th>Date</th>
<th>File</th>
<th>action</th>
</tr>
</thead>
<tbody>
<tr dir-paginate="brochure in brochures | filter:search | limitTo:pageSize | itemsPerPage:numPerPage">
<td>{{$index+1}}</td>
<td>{{brochure.name}}</td>
<td>{{brochure.subject}}</td>
<td>{{brochure.date | date:'dd-MMM-yyyy'}}</td>
<td>{{brochure.file_name}}</td>
<td>
<div class="btn-group btn-group-xs" role="group">
<button type="button" class="btn btn-info" ng-click="showForm(brochure)">
<i class="fa fa-pencil"></i>
</button>
<button type="button" class="btn btn-danger" confirmed-click="deleteBrochure(brochure)" ng-confirm-click="Would you like to delete this item?!">
<i class="fa fa-trash-o"></i>
</button>
</div>
</td>
</tr>
</tbody>
</table>
<dir-pagination-controls
max-size="10"
direction-links="true"
boundary-links="true" >
</dir-pagination-controls>
</div>
<div id="loading" ng-show="loading">
<div id="loading-image">
<img src="<?php echo public_url() . 'assets/admin/img/loading.gif' ?>" alt=""/>
<h4>Please wait...</h4>
</div>
</div><file_sep>/application/views/index.php
<!--header start-->
<header id="header" class="tt-nav nav-border-bottom">
<div class="header-sticky light-header ">
<div class="container">
<div class="search-wrapper">
<div class="search-trigger pull-right">
<div class='search-btn'></div>
<i class="material-icons"></i>
</div>
<!-- Modal Search Form -->
<i class="search-close material-icons"></i>
<div class="search-form-wrapper">
<form action="#" class="white-form">
<div class="input-field">
<input type="text" name="search" id="search">
<label for="search" class="">Search Here...</label>
</div>
<button class="btn pink search-button waves-effect waves-light" type="submit"><i class="material-icons"></i></button>
</form>
</div>
</div><!-- /.search-wrapper -->
<div id="materialize-menu" class="menuzord">
<!--logo start-->
<a href="home" class="logo-brand">
<img src="<?php echo public_url()?>assets/img/logo.png" alt="" >
</a>
<!--logo end-->
<!--mega menu start-->
<ul class="menuzord-menu pull-right">
<!-- <li class="active"><a href="index.html">Home</a></li>-->
<!-- <li><a href="about">About Us</a>-->
<!-- <ul class="dropdown">-->
<!-- <li><a href="about#aboutus">About Company</a></li>-->
<!-- <li><a href="team">Our Teams</a></li>-->
<!-- </ul>-->
<!-- </li>-->
<!-- <li><a href="services">Our Services</a>-->
<!-- <ul class="dropdown">-->
<!-- <li><a href="services#services1">Typing Services</a></li>-->
<!-- <li><a href="services#services2">Services for Ministry of Health</a></li>-->
<!-- <li><a href="services#services3">Services for Higher & Lower Education</a></li>-->
<!-- <li><a href="services#services4">Medical Fingerprint Assists Services</a></li>-->
<!-- <li><a href="services#services5">Insurance Services</a></li>-->
<!-- <li><a href="services#services6">Health Insurance Services</a></li>-->
<!-- <li><a href="services#services7">Education Services</a></li>-->
<!-- <li><a href="services#services8">Transportation services </a></li>-->
<!-- <li><a href="services#services9">Pan Card Services</a></li>-->
<!-- <li><a href="services#services10">Air Tickets and Related Services</a></li>-->
<!-- <li><a href="services#services11">Courier Services</a></li>-->
<!-- <li><a href="services#services12">Miscellaneous Services</a></li>-->
<!-- </ul>-->
<!-- </li>-->
<!-- <li><a href="gallery">Moments</a></li>-->
<!-- <li><a href="blog">Blog</a></li>-->
<!-- <li><a href="contact">Contact Us</a>-->
<!-- </li>-->
<?php echo menu('Home') ?>
</ul>
<!--mega menu end-->
</div>
</div>
</div>
</header>
<!--header end-->
<!-- start revolution slider 5.0 -->
<section class="rev_slider_wrapper">
<div class="rev_slider materialize-slider">
<ul>
<!-- slide 1 start -->
<li data-transition="fade" data-slotamount="default" data-easein="Power4.easeInOut" data-easeout="Power4.easeInOut" data-masterspeed="2000" data-thumb="<?php echo public_url()?>assets/img/banner/1.jpg" data-rotate="0" data-fstransition="fade" data-fsmasterspeed="1500" data-fsslotamount="7" data-saveperformance="off" data-title="materialize Material" data-description="">
<!-- MAIN IMAGE -->
<img src="<?php echo public_url()?>assets/img/banner/1.jpg" alt="" data-bgposition="center center" data-bgfit="cover" data-bgrepeat="no-repeat" data-bgparallax="10" class="rev-slidebg" data-no-retina>
<!-- LAYER NR. 1 -->
<div class="tp-caption rev-heading text-extrabold dark-text tp-resizeme"
data-x="right"
data-y="center" data-voffset="-50"
data-fontsize="['60','60','60','45']"
data-lineheight="['60','60','60','50']"
data-width="none"
data-height="none"
data-whitespace="nowrap"
data-transform_idle="o:1;"
data-transform_in="y:[100%];z:0;rX:0deg;rY:0;rZ:0;sX:1;sY:1;skX:0;skY:0;opacity:0;s:600;e:Power4.easeInOut;"
data-transform_out="y:[100%];s:1000;e:Power2.easeInOut;s:1000;e:Power2.easeInOut;"
data-mask_in="x:0px;y:[100%];s:inherit;e:inherit;"
data-mask_out="x:inherit;y:inherit;s:inherit;e:inherit;"
data-start="800"
data-splitin="none"
data-splitout="none"
data-responsive_offset="on"
style="z-index: 5; white-space: nowrap;">We Serve Your Needs
</div>
<!-- LAYER NR. 3 -->
<div class="tp-caption tp-resizeme rev-btn"
data-x="['right','right','right','right']" data-hoffset="['0','0','0','0']"
data-y="['middle','middle','middle','middle']" data-voffset="['130','130','130','130']"
data-width="none"
data-height="none"
data-whitespace="nowrap"
data-transform_idle="o:1;"
data-style_hover="cursor:default;"
data-transform_in="y:[100%];z:0;rX:0deg;rY:0;rZ:0;sX:1;sY:1;skX:0;skY:0;opacity:0;s:600;e:Power4.easeInOut;"
data-transform_out="y:[100%];s:1000;e:Power2.easeInOut;s:1000;e:Power2.easeInOut;"
data-mask_in="x:0px;y:[100%];s:inherit;e:inherit;"
data-mask_out="x:inherit;y:inherit;s:inherit;e:inherit;"
data-start="1200"
data-splitin="none"
data-splitout="none"
data-responsive_offset="on"
style="z-index: 7; white-space: nowrap;">
<a href="contact" class="btn btn-lg waves-effect waves-light">Contact Now</a>
</div>
</li>
<!-- slide 1 end -->
<!-- slide 2 start -->
<li data-transition="fade" data-slotamount="default" data-easein="Power4.easeInOut" data-easeout="Power4.easeInOut" data-masterspeed="2000" data-thumb="<?php echo public_url()?>assets/img/banner/3.jpg" data-rotate="0" data-fstransition="fade" data-fsmasterspeed="1500" data-fsslotamount="7" data-saveperformance="off" data-title="Unique Design" data-description="">
<!-- MAIN IMAGE -->
<img src="<?php echo public_url()?>assets/img/banner/2.jpg" alt="" data-bgposition="center center" data-bgfit="cover" data-bgrepeat="no-repeat" data-bgparallax="10" class="rev-slidebg" data-no-retina>
<!-- LAYER NR. 1 -->
<div class="tp-caption rev-heading text-extrabold dark-text tp-resizeme"
data-x="center"
data-y="center" data-voffset="-50"
data-fontsize="['60','60','60','45']"
data-lineheight="['60','60','60','50']"
data-width="none"
data-height="none"
data-whitespace="nowrap"
data-transform_idle="o:1;"
data-transform_in="y:[100%];z:0;rX:0deg;rY:0;rZ:0;sX:1;sY:1;skX:0;skY:0;opacity:0;s:600;e:Power4.easeInOut;"
data-transform_out="y:[100%];s:1000;e:Power2.easeInOut;s:1000;e:Power2.easeInOut;"
data-mask_in="x:0px;y:[100%];s:inherit;e:inherit;"
data-mask_out="x:inherit;y:inherit;s:inherit;e:inherit;"
data-start="800"
data-splitin="none"
data-splitout="none"
data-responsive_offset="on"
style="z-index: 5; white-space: nowrap;">We Solve Your Problem Easy
</div>
<!-- LAYER NR. 3 -->
<div class="tp-caption tp-resizeme rev-btn"
data-x="['center','center','center','center']" data-hoffset="['0','0','0','0']"
data-y="['middle','middle','middle','middle']" data-voffset="['130','130','130','130']"
data-width="none"
data-height="none"
data-whitespace="nowrap"
data-transform_idle="o:1;"
data-style_hover="cursor:default;"
data-transform_in="y:[100%];z:0;rX:0deg;rY:0;rZ:0;sX:1;sY:1;skX:0;skY:0;opacity:0;s:600;e:Power4.easeInOut;"
data-transform_out="y:[100%];s:1000;e:Power2.easeInOut;s:1000;e:Power2.easeInOut;"
data-mask_in="x:0px;y:[100%];s:inherit;e:inherit;"
data-mask_out="x:inherit;y:inherit;s:inherit;e:inherit;"
data-start="1200"
data-splitin="none"
data-splitout="none"
data-responsive_offset="on"
style="z-index: 7; white-space: nowrap;">
<a href="home#appointment" class="btn btn-lg waves-effect waves-light">Request Quotes now</a>
</div>
</li>
<!-- slide 2 end -->
<!-- slide 3 start -->
<li data-transition="fade" data-slotamount="default" data-easein="Power4.easeInOut" data-easeout="Power4.easeInOut" data-masterspeed="2000" data-thumb="<?php echo public_url()?>assets/img/banner/3.jpg" data-rotate="0" data-fstransition="fade" data-fsmasterspeed="1500" data-fsslotamount="7" data-saveperformance="off" data-title="Unique Design" data-description="">
<!-- MAIN IMAGE -->
<img src="<?php echo public_url()?>assets/img/banner/3.jpg" alt="" data-bgposition="center center" data-bgfit="cover" data-bgrepeat="no-repeat" data-bgparallax="10" class="rev-slidebg" data-no-retina>
<!-- LAYER NR. 1 -->
<div class="tp-caption rev-heading text-extrabold dark-text tp-resizeme"
data-x="left"
data-y="center" data-voffset="-50"
data-fontsize="['60','60','60','45']"
data-lineheight="['60','60','60','50']"
data-width="none"
data-height="none"
data-whitespace="nowrap"
data-transform_idle="o:1;"
data-transform_in="y:[100%];z:0;rX:0deg;rY:0;rZ:0;sX:1;sY:1;skX:0;skY:0;opacity:0;s:600;e:Power4.easeInOut;"
data-transform_out="y:[100%];s:1000;e:Power2.easeInOut;s:1000;e:Power2.easeInOut;"
data-mask_in="x:0px;y:[100%];s:inherit;e:inherit;"
data-mask_out="x:inherit;y:inherit;s:inherit;e:inherit;"
data-start="800"
data-splitin="none"
data-splitout="none"
data-responsive_offset="on"
style="z-index: 5; white-space: nowrap;">Call Now : 99 530 177
</div>
<!-- LAYER NR. 3 -->
<div class="tp-caption tp-resizeme rev-btn"
data-x="['left','left','left','left']" data-hoffset="['0','0','0','0']"
data-y="['middle','middle','middle','middle']" data-voffset="['130','130','130','130']"
data-width="none"
data-height="none"
data-whitespace="nowrap"
data-transform_idle="o:1;"
data-style_hover="cursor:default;"
data-transform_in="y:[100%];z:0;rX:0deg;rY:0;rZ:0;sX:1;sY:1;skX:0;skY:0;opacity:0;s:600;e:Power4.easeInOut;"
data-transform_out="y:[100%];s:1000;e:Power2.easeInOut;s:1000;e:Power2.easeInOut;"
data-mask_in="x:0px;y:[100%];s:inherit;e:inherit;"
data-mask_out="x:inherit;y:inherit;s:inherit;e:inherit;"
data-start="1200"
data-splitin="none"
data-splitout="none"
data-responsive_offset="on"
style="z-index: 7; white-space: nowrap;">
<a href="contact" class="btn btn-lg waves-effect waves-light">Connect with us</a>
</div>
</li>
<!-- slide 3 end -->
<!-- slide 4 start -->
<li data-transition="fade" data-slotamount="default" data-easein="Power4.easeInOut" data-easeout="Power4.easeInOut" data-masterspeed="2000" data-thumb="<?php echo public_url()?>assets/img/banner/4.jpg" data-rotate="0" data-fstransition="fade" data-fsmasterspeed="1500" data-fsslotamount="7" data-saveperformance="off" data-title="Unique Design" data-description="">
<!-- MAIN IMAGE -->
<img src="<?php echo public_url()?>assets/img/banner/4.jpg" alt="" data-bgposition="center center" data-bgfit="cover" data-bgrepeat="no-repeat" data-bgparallax="10" class="rev-slidebg" data-no-retina>
<!-- LAYER NR. 1 -->
<div class="tp-caption rev-heading text-extrabold dark-text tp-resizeme"
data-x="left"
data-y="center" data-voffset="-50"
data-fontsize="['60','60','60','45']"
data-lineheight="['60','60','60','50']"
data-width="none"
data-height="none"
data-whitespace="nowrap"
data-transform_idle="o:1;"
data-transform_in="y:[100%];z:0;rX:0deg;rY:0;rZ:0;sX:1;sY:1;skX:0;skY:0;opacity:0;s:600;e:Power4.easeInOut;"
data-transform_out="y:[100%];s:1000;e:Power2.easeInOut;s:1000;e:Power2.easeInOut;"
data-mask_in="x:0px;y:[100%];s:inherit;e:inherit;"
data-mask_out="x:inherit;y:inherit;s:inherit;e:inherit;"
data-start="800"
data-splitin="none"
data-splitout="none"
data-responsive_offset="on"
style="z-index: 5; white-space: nowrap;">Request A Quote Now
</div>
<!-- LAYER NR. 3 -->
<div class="tp-caption tp-resizeme rev-btn"
data-x="['left','left','left','left']" data-hoffset="['0','0','0','0']"
data-y="['middle','middle','middle','middle']" data-voffset="['130','130','130','130']"
data-width="none"
data-height="none"
data-whitespace="nowrap"
data-transform_idle="o:1;"
data-style_hover="cursor:default;"
data-transform_in="y:[100%];z:0;rX:0deg;rY:0;rZ:0;sX:1;sY:1;skX:0;skY:0;opacity:0;s:600;e:Power4.easeInOut;"
data-transform_out="y:[100%];s:1000;e:Power2.easeInOut;s:1000;e:Power2.easeInOut;"
data-mask_in="x:0px;y:[100%];s:inherit;e:inherit;"
data-mask_out="x:inherit;y:inherit;s:inherit;e:inherit;"
data-start="1200"
data-splitin="none"
data-splitout="none"
data-responsive_offset="on"
style="z-index: 7; white-space: nowrap;">
<a href="home#appointment" class="btn btn-lg waves-effect waves-light">Make A Quote Now</a>
</div>
</li>
<!-- slide 4 end -->
</ul>
</div><!-- end revolution slider -->
</section><!-- end of slider wrapper -->
<!-- Welcome head start -->
<section>
<div class="container">
<div class="row padding-top-60">
<div class="col-md-7 wow fadeInUp">
<h2 class="font-30 text-bold mb-30">Welcome to Classic International Info</h2>
<p>
The reputed leading typing and service center in state of Kuwait, located in Jleeb Al Shuyoukh, Abbassiya- for all your document production requirements, directed by our leadereminent Mr. Musthafa , competent withvast experience in this field thorough the updatedknowledge and changes of legal procedures and formalities in different Ministries.Classic typing Center has grown as an ideal figure in the area of services like typing, proof reading, editing etc.We believe that quality is born by combining experience to knowledge.
</p>
</div>
<div class="col-sm-offset-2 col-md-3">
<img src="<?php echo public_url()?>assets/img/bg/Classic.png" class="img-responsive " alt="Image">
</div>
</div>
</div><!-- /.container -->
</section>
<!-- Welcome head End -->
<section class="full-width promo-box brand-bg ptb-50">
<div class="container">
<div class="col-md-12">
<div class="promo-info">
<span class="white-text text-uppercase">Do you ever feel stuck in your business? </span>
<h2 class="white-text text-bold text-uppercase no-margin">Get solution for your all Problems</h2>
</div>
<?php
if (isset($brochure) and $brochure != false) { ?>
<div class="promo-btn">
<a href="<?php echo (isset($brochure->fileUrl) ? $brochure->fileUrl : '')?>" download class="btn white waves-effect waves-grey">Download Our Brochure</a>
</div>
<?php }
?>
</div>
</div>
</section>
<!-- Featured SEO Carousel -->
<section id="WhatWeDo" class="section-padding">
<div class="container">
<div class="text-center mb-80">
<h2 class="section-title">What We Do</h2>
<p class="section-sub">We aim to later all needs of our beloved clients, assuring the managent system will meet the expectations of our customers in regards with the service undergo in our hands</p>
</div>
<div class="seo-featured-carousel brand-dot">
<div class="featured-item seo-service">
<div class="icon">
<img class="img-responsive" src="<?php echo public_url()?>assets/img/bg/TypingService.jpg" alt="">
</div>
<div class="desc">
<h2>Typing services</h2>
</div>
</div><!-- /.featured-item -->
<div class="featured-item seo-service">
<div class="icon">
<img class="img-responsive" src="<?php echo public_url()?>assets/img/bg/health.jpg" alt="">
</div>
<div class="desc">
<h2>Services for Ministry of health</h2>
</div>
</div><!-- /.featured-item -->
<div class="featured-item seo-service">
<div class="icon">
<img class="img-responsive" src="<?php echo public_url()?>assets/img/bg/education.jpg" alt="">
</div>
<div class="desc">
<h2>Services for higher & lower education</h2>
</div>
</div><!-- /.featured-item -->
<div class="featured-item seo-service">
<div class="icon">
<img class="img-responsive" src="<?php echo public_url()?>assets/img/bg/Medical-finger.jpg" alt="">
</div>
<div class="desc">
<h2>Medical finger print assists services</h2>
</div>
</div><!-- /.featured-item -->
<div class="featured-item seo-service">
<div class="icon">
<img class="img-responsive" src="<?php echo public_url()?>assets/img/bg/Hinsurance.jpg" alt="">
</div>
<div class="desc">
<h2>Health insurance services</h2>
</div>
</div><!-- /.featured-item -->
<div class="featured-item seo-service">
<div class="icon">
<img class="img-responsive" src="<?php echo public_url()?>assets/img/bg/education2.jpg" alt="">
</div>
<div class="desc">
<h2>Education services</h2>
</div>
</div><!-- /.featured-item -->
<div class="featured-item seo-service">
<div class="icon">
<img class="img-responsive" src="<?php echo public_url()?>assets/img/bg/Transportation.png" alt="">
</div>
<div class="desc">
<h2>Transportation services</h2>
</div>
</div><!-- /.featured-item -->
<div class="featured-item seo-service">
<div class="icon">
<img class="img-responsive" src="<?php echo public_url()?>assets/img/bg/PAN_card.jpg" alt="">
</div>
<div class="desc">
<h2>Pan card services</h2>
</div>
</div><!-- /.featured-item -->
<div class="featured-item seo-service">
<div class="icon">
<img class="img-responsive" src="<?php echo public_url()?>assets/img/bg/air.jpg" alt="">
</div>
<div class="desc">
<h2>Air tickets and related services</h2>
</div>
</div><!-- /.featured-item -->
<div class="featured-item seo-service">
<div class="icon">
<img class="img-responsive" src="<?php echo public_url()?>assets/img/bg/Courier.jpg" alt="">
</div>
<div class="desc">
<h2>Courier services</h2>
</div>
</div><!-- /.featured-item -->
<div class="featured-item seo-service">
<div class="icon">
<img class="img-responsive" src="<?php echo public_url()?>assets/img/bg/miscellaneous.jpg" alt="">
</div>
<div class="desc">
<h2>Miscellaneous Services</h2>
</div>
</div><!-- /.featured-item -->
</div><!-- /.seo-featured -->
</div><!-- /.container -->
</section>
<!-- Featured Carousel End -->
<section class="section-padding bg-fixed parallax-bg pre-banner-2 overlay dark-5" data-stellar-background-ratio="0.5">
<div id="appointment" class="container">
<div class="text-center">
<h2 class="section-title text-capitalize white-text">Make an Appointment</h2>
</div>
<div class="row">
<div class="col-md-4 contact-info">
<address>
<i class="material-icons brand-color"></i>
<div class="address">
Jleeb Al shuyoukh, Abbassiya, <br>
Kuwait
<p><br></p>
</div>
<i class="material-icons brand-color"></i>
<div class="phone">
<p>Mob: 995 301 77 , 555 301 77 , <br> 979 746 31 ,
607 581 13<br>
Fax: 24344318 <br>
Office: 24 310 783 </p>
</div>
<i class="material-icons brand-color"></i>
<div class="mail">
<p><a href="mailto:<EMAIL>"><EMAIL></a>
<br><a href="mailto:<EMAIL>"><EMAIL></a></p>
</div>
<i class="material-icons brand-color"></i>
<div class="mail">
<p><a href="#">Ministry of Commercial Registration No: 4180/2004</a></p>
</div>
</address>
</div><!-- /.col-md-4 -->
<div class="col-md-8">
<form class="white-form" name="contact-form" id="appForm" action="appointment-request" method="POST">
<div class="row">
<div class="col-md-6">
<div class="input-field">
<input type="text" name="name" class="validate" id="name" required="">
<label for="name">Full Name*</label>
</div>
</div><!-- /.col-md-4 -->
<div class="col-md-6">
<div class="input-field">
<input id="email" type="email" name="email" class="validate" required="">
<label for="email" data-error="wrong" data-success="right">Email Address*</label>
</div>
</div><!-- /.col-md-4 -->
</div><!-- /.row -->
<div class="row">
<div class="col-md-6">
<div class="input-field">
<input type="text" name="phone" class="validate" id="people">
<label for="people">Contact Number*</label>
</div>
</div><!-- /.col-md-4 -->
<div class="col-md-6">
<div class="input-field">
<select class="text-capitalize selectpicker form-control required selectbox" name="service" data-style="g-select" data-width="100%" style="background-color: transparent;">
<option value="0" selected="">Select Service</option>
<option value="Typing Services">Typing Services</option>
<option value="Services for Ministry of Health">Services for Ministry of Health</option>
<option value="Services for Higher & Lower Education">Services for Higher & Lower Education</option>
<option value="Medical Fingerprint Assists Services">Medical Fingerprint Assists Services</option>
<option value="Insurance Services">Insurance Services</option>
<option value="Health Insurance Services">Health Insurance Services</option>
<option value="Education Services">Education Services</option>
<option value="Transportation services">Transportation services </option>
<option value="Pan Card Services">Pan Card Services</option>
<option value="Air Tickets and Related Services">Air Tickets and Related Services</option>
<option value="Courier Services">Courier Services</option>
<option value="Miscellaneous Services">Miscellaneous Services</option>
</select>
</div>
</div><!-- /.col-md-4 -->
</div><!-- /.row -->
<div class="input-field">
<textarea name="message" id="message" class="materialize-textarea" ></textarea>
<label for="message">Aditional note</label>
</div>
<div class="text-center mb-80">
<button type="submit" name="submit" class="waves-effect waves-light btn btn-lg text-medium mt-30 text-capitalize">Book Now</button>
</div>
</form>
</div>
</div>
</div><!-- /.container -->
</section>
<!-- Feature Accordion -->
<section class="section-padding">
<div class="container">
<div class="text-center mb-80">
<h2 class="section-title text-uppercase">Our Features</h2>
<p class="section-sub">Classic International Info, The reputed leading service centre in state of Kuwait, located in Jleeb Al Shuyoukh, Abbassiya- for all your document production requirements, directed by our leader Mr. Musthafa Who is the eminent, competent with vast experience in this field, Thorough knowledge on updated changes of legal procdures and formalities in different Ministries.</p>
</div>
<div class="row">
<div class="col-md-6">
<div class="panel-group feature-accordion brand-accordion icon angle-icon" id="accordion-one">
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title">
<a data-toggle="collapse" data-parent="#accordion-one" href="#collapse-one">
We have
</a>
</h3>
</div>
<div id="collapse-one" class="panel-collapse collapse">
<div class="panel-body">
20 years’ of service experience in same field.
</div>
</div>
</div>
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title">
<a class="collapsed" data-toggle="collapse" data-parent="#accordion-one" href="#collapse-two">
Our Hard Working Team
</a>
</h3>
</div>
<div id="collapse-two" class="panel-collapse collapse">
<div class="panel-body">
All staffs are well grounded in their areas. Our administrative staffs are working with a customer driven focus.
</div>
</div>
</div>
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title">
<a class="collapsed" data-toggle="collapse" data-parent="#accordion-one" href="#collapse-three">
Our Motto
</a>
</h3>
</div>
<div id="collapse-three" class="panel-collapse collapse">
<div class="panel-body">
Trust is our motto.
</div>
</div>
</div>
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title">
<a class="collapsed" data-toggle="collapse" data-parent="#accordion-one" href="#collapse-four">
Our Goodwill
</a>
</h3>
</div>
<div id="collapse-four" class="panel-collapse collapse">
<div class="panel-body">
Candidates satisfaction is our goodwill.
</div>
</div>
</div>
</div>
</div><!-- /.col-md-6 -->
<div class="col-md-6">
<div class="panel-group feature-accordion brand-accordion icon angle-icon" id="accordion-one">
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title">
<a data-toggle="collapse" data-parent="#accordion-two" href="#1collapse-one">
We Made
</a>
</h3>
</div>
<div id="1collapse-one" class="panel-collapse collapse">
<div class="panel-body">
Transportation facilities are arranged from all location in Kuwait.
</div>
</div>
</div>
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title">
<a class="collapsed" data-toggle="collapse" data-parent="#accordion-two" href="#1collapse-two">
Creative Assistence
</a>
</h3>
</div>
<div id="1collapse-two" class="panel-collapse collapse">
<div class="panel-body">
Certificate verification from ministry of lower education and higher education for various ministry department.
</div>
</div>
</div>
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title">
<a class="collapsed" data-toggle="collapse" data-parent="#accordion-two" href="#1collapse-three">
Awesome Customer Servicers
</a>
</h3>
</div>
<div id="1collapse-three" class="panel-collapse collapse">
<div class="panel-body">
We arrange certificate attestation facility in India(HRD, Ministry of External affairs in Delhi and Kuwait Embassy in Delhi) & good conduct certificate.
</div>
</div>
</div>
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title">
<a class="collapsed" data-toggle="collapse" data-parent="#accordion-two" href="#1collapse-four">
Also we are
</a>
</h3>
</div>
<div id="1collapse-four" class="panel-collapse collapse">
<div class="panel-body">
Also we are doing all kind of typing works.
</div>
</div>
</div>
</div>
</div><!-- /.col-md-6 -->
</div><!-- /.row -->
</div><!-- /.container -->
</section>
<section id="latestnews" class="section-padding latest-news-card gray-bg">
<div class="container">
<div class="text-center mb-80">
<h2 class="section-title text-uppercase">Latest News</h2>
<!-- <p class="section-sub">Quisque non erat mi. Etiam congue et augue sed tempus. Aenean sed ipsum luctus, scelerisque ipsum nec, iaculis justo. Sed at vestibulum purus, sit amet viverra diam. Nulla ac nisi rhoncus,</p> -->
</div>
<div class="blog-carousel">
<?php
if (isset($news) and $news != false) {
foreach ($news as $value) {
?>
<article class="card">
<div class="card-image waves-effect waves-block waves-light">
<img class="activator" src="<?php echo(isset($value->thumbImgUrl) ? $value->thumbImgUrl : ''); ?>" alt="image">
</div>
<div class="card-content">
<h2 class="entry-title activator"><?php echo $value->heading ?></h2>
</div>
<div class="card-reveal overlay-blue">
<span class="card-title close-button"><i class="fa fa-times"></i></span>
<p><?php echo $value->content ?></p>
</div>
</article><!-- /.card -->
<?php
}
}
?>
</div>
</div><!-- /.container -->
</section>
<file_sep>/application/views/about.php
<!--header start-->
<header id="header" class="tt-nav nav-border-bottom">
<div class="header-sticky light-header ">
<div class="container">
<div class="search-wrapper">
<div class="search-trigger pull-right">
<div class='search-btn'></div>
<i class="material-icons"></i>
</div>
<!-- Modal Search Form -->
<i class="search-close material-icons"></i>
<div class="search-form-wrapper">
<form action="#" class="white-form">
<div class="input-field">
<input type="text" name="search" id="search">
<label for="search" class="">Search Here...</label>
</div>
<button class="btn pink search-button waves-effect waves-light" type="submit"><i class="material-icons"></i></button>
</form>
</div>
</div><!-- /.search-wrapper -->
<div id="materialize-menu" class="menuzord">
<!--logo start-->
<a href="home" class="logo-brand">
<img src="<?php echo public_url()?>assets/img/logo.png" alt="" >
</a>
<!--logo end-->
<!--mega menu start-->
<ul class="menuzord-menu pull-right">
<!-- <li class="active"><a href="index.html">Home</a></li>
<li><a href="about.html">About Us</a></li>
<li><a href="service.html">Our Services</a></li>
<li><a href="gallery.html">Moments</a></li>
<li><a href="blog.html">Blog</a></li>
<li><a href="contact.html">Contact Us</a></li> -->
<?php echo menu('About Us') ?>
</ul>
<!--mega menu end-->
</div>
</div>
</div>
</header>
<!--header end-->
<!--page title start-->
<section id="aboutus" class="page-title pattern-bg ptb-50">
<div class="container">
<div class="row">
<div class="col-md-12">
<h2>Classic International</h2>
<span>About Us</span>
<ol class="breadcrumb">
<li><a href="#">Home</a></li>
<li class="active">About Us</li>
</ol>
</div>
</div>
</div>
</section>
<!--page title end-->
<section class="section-padding">
<div class="container">
<h2 class="font-30 text-medium mb-30">Welcome to Classic International Group</h2>
<div class="row">
<div class="col-md-7">
<p>
Welcome to <strong>Classic InternationalGroup</strong>, the reputed leading typing and service center in state of Kuwait, located in Jleeb Al Shuyoukh, Abbassiya- for all your document production requirements, directed by our leader eminent <strong>Mr. Musthafa</strong> , competent with vast experience in this field thorough the updated knowledge and changes of legal procedures and formalities in different Ministries. <strong>Classic typing Center</strong> has grown as an ideal figure in the area of services like typing, proof reading, editing etc. We believe that quality is born by combining experience to knowledge.
</p>
<p>
So we provide the best high quality services that an organization may need. Let us help you achieve your goals through our highly-experienced staff assuring your expenses in limited budget. We offer professional typing services, including proof reading at competitive rates. We have been providing quality based services for more than twenty years. We are thrilled and honored to have the support of our well-respected customer’s group to help us to provide them quality service.
</p>
</div><!-- /.col-md-7 -->
<div class="col-md-5">
<div class="gallery-thumb">
<ul class="slides">
<li data-thumb="<?php echo public_url()?>assets/img/blog/1.jpg">
<img src="<?php echo public_url()?>assets/img/blog/1.jpg" alt="image">
</li>
<li data-thumb="<?php echo public_url()?>assets/img/blog/1.jpg">
<img src="<?php echo public_url()?>assets/img/blog/1.jpg" alt="image">
</li>
<li data-thumb="<?php echo public_url()?>assets/img/blog/1.jpg">
<img src="<?php echo public_url()?>assets/img/blog/1.jpg" alt="image">
</li>
<li data-thumb="<?php echo public_url()?>assets/img/blog/1.jpg">
<img src="<?php echo public_url()?>assets/img/blog/1.jpg" alt="image">
</li>
</ul>
</div><!-- /.gallery-thumb -->
</div><!-- /.col-md-5 -->
</div><!-- /.row -->
<div class="row">
<div class="col-md-12">
<p>
We have a long list of satisfied customers, who still keeps coming back to us for further work. They are pleased with the value we give to their time and money. Classic Typingguarantees the best typing service you would find in Kuwait
</p>
</div>
</div>
</div><!-- /.container -->
</section>
<section id="whatwedo" class="padding-top-100">
<div class="container">
<div class="text-center mb-80">
<h2 class="section-title text-uppercase">More About Us</h2>
</div>
<div class="vertical-tab">
<div class="row">
<div class="col-sm-3">
<!-- Nav tabs -->
<ul class="nav nav-tabs nav-stacked" role="tablist">
<li role="presentation" class="active"><a href="#tab-6" class="waves-effect waves-light" role="tab" data-toggle="tab">What We Do</a></li>
<li role="presentation"><a href="#tab-7" class="waves-effect waves-light" role="tab" data-toggle="tab">Our Mission</a></li>
<li role="presentation"><a href="#tab-8" class="waves-effect waves-light" role="tab" data-toggle="tab">Our Team</a></li>
<li role="presentation"><a href="#tab-9" class="waves-effect waves-light" role="tab" data-toggle="tab">Our Services</a></li>
</ul>
</div><!-- /.col-md-3 -->
<div class="col-sm-9">
<!-- Tab panes -->
<div class="panel-body">
<div class="tab-content">
<div role="tabpanel" class="tab-pane fade in active" id="tab-6">
<h2>What We Do</h2>
<img class="alignright" src="<?php echo public_url()?>assets/img/busy_man.png" alt="">
<p>We servce English, Arabic, Malayalam and Urdu Typing, We will arrange translation from authorized translators in Kuwait, Special Services for Nurses and Technical Staffs, We can assist you to process your family's medical, finger and reports, We provide educational services for students, professionals and businesses across the globe, All kind of Visa typing, Passport, shuoonand <a href="https://www.paci.gov.kw/Default.aspx">civil id</a> forms typing, Insurance Services, <a href="http://www.mohe.edu.kw/equivalencynew/home.aspx">Education</a> Services and also <a href="https://www.paci.gov.kw/Default.aspx">Civil id related Kuwait</a>, <a href="http://www.csc.net.kw:8888/csc/ar/home.jsp">Civil commission Kuwait</a>, <a href="http://cr.lsgkerala.gov.in/">Birth death marriage related in India</a> and <a href="http://www.noorkaroots.net">nookra</a> related services available here.</p>
</div>
<div role="tabpanel" class="tab-pane fade" id="tab-7">
<h2>Our Mission</h2>
<img class="alignright" src="<?php echo public_url()?>assets/img/mission.png" alt="">
<p>We aim to later all needs of our beloved clients, assuring the managent system will meet the expectations of our customers in regards with the service undergo in our hands.</p>
<p>We serve your needs, So we provide the best high quality services that an organization may need. Let us help you achieve your goals through our highly-experienced staff assuring your expenses in limited budget.</p>
</div>
<div role="tabpanel" class="tab-pane fade" id="tab-8">
<h2>Our Team</h2>
<img class="alignright" src="<?php echo public_url()?>assets/img/business.png" alt="">
<p>We have a long list of <a href="team">Professional Staffs</a>, They are pleased with the value we give to their time and money. Classic Typingguarantees the best typing service you would find in Kuwait.</p>
<p>We have been providing quality based services for more than twenty years. We are thrilled and honored to have the support of our well-respected customer’s group to help us to provide them quality service.</p>
</div>
<div role="tabpanel" class="tab-pane fade" id="tab-9">
<h2>Our Services</h2>
<img class="alignright mt-20" src="<?php echo public_url()?>assets/img/data.png" alt="">
<p>
<a href="services#services1">Typing Services</a>
<br><a href="services#services2">Services for Ministry of Health</a> , <a href="services#services4">Medical Fingerprint Assists Services</a>
<br><a href="services#services3">Services for Higher & Lower Education</a> , <a href="services#services7">Education Services</a>
<br><a href="services#services5">Insurance Services</a>, <a href="services#services6">Health Insurance Services</a>
<br><a href="services#services8">Transportation services</a> , <a href="services#services10">Air Tickets and Related Services</a>
<br><a href="services#services9">Pan Card Services</a>
<br><a href="services#services11">Courier Services</a> , <a href="services#services12">Miscellaneous Services</a>
</p>
</div>
</div>
</div>
</div>
</div><!-- /.row -->
</div><!-- /.vertical-tab -->
</div><!-- /.container -->
</section>
<section class="section-padding">
<div class="container">
<div class="team-tab" role="tabpanel">
<!-- Tab panes -->
<div class="panel-body">
<div class="tab-content">
<div role="tabpanel" class="tab-pane fade in active">
<div class="row">
<div class="col-md-4 col-sm-3">
<figure class="team-img text-center">
<img src="<?php echo public_url()?>assets/img/bg/musthafa.png" class="img-responsive" alt="Image">
<ul class="team-social-links list-inline">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-google-plus"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</figure>
</div><!-- /.col-md-4 -->
<div class="col-md-8 col-sm-9">
<div class="team-intro">
<h3>Mr. Musthafa<small>Co-Founder</small></h3>
<p>A id a torquent tortor at lacus et donec platea eu scelerisque maecenas ac eros a adipiscing id lobortis cum lacus erat. Parturient eleifend adipiscing ultrices a cursus est feugiat porta a at condimentum fames adipiscing odio in nisi venenatis suspendisse suspendisse parturient. Leo congue sociosqu maecenas ligula eu penatibus at suscipit mus scelerisque.</p>
</div>
</div> <!-- col-md-8 -->
</div> <!-- row -->
</div> <!--team-1 end-->
</div> <!--tab-content end -->
</div>
</div> <!--tab-pan end -->
</div><!-- /.container -->
</section>
<file_sep>/application/views/contact.php
<!--header start-->
<header id="header" class="tt-nav nav-border-bottom">
<div class="header-sticky light-header ">
<div class="container">
<div class="search-wrapper">
<div class="search-trigger pull-right">
<div class='search-btn'></div>
<i class="material-icons"></i>
</div>
<!-- Modal Search Form -->
<i class="search-close material-icons"></i>
<div class="search-form-wrapper">
<form action="#" class="white-form">
<div class="input-field">
<input type="text" name="search" id="search">
<label for="search" class="">Search Here...</label>
</div>
<button class="btn pink search-button waves-effect waves-light" type="submit"><i class="material-icons"></i></button>
</form>
</div>
</div><!-- /.search-wrapper -->
<div id="materialize-menu" class="menuzord">
<!--logo start-->
<a href="home" class="logo-brand">
<img src="<?php echo public_url()?>assets/img/logo.png" alt="" >
</a>
<!--logo end-->
<!--mega menu start-->
<ul class="menuzord-menu pull-right">
<!-- <li class="active"><a href="index.html">Home</a></li>
<li><a href="about.html">About Us</a></li>
<li><a href="service.html">Our Services</a></li>
<li><a href="gallery.html">Moments</a></li>
<li><a href="blog.html">Blog</a></li>
<li><a href="contact.html">Contact Us</a></li> -->
<?php echo menu('Contact') ?>
</ul>
<!--mega menu end-->
</div>
</div>
</div>
</header>
<!--header end-->
<!--page title start-->
<section class="page-title pattern-bg ptb-50">
<div class="container">
<div class="row">
<div class="col-md-12">
<h2>Contact Us</h2>
<span>24*7 Hours Services</span>
<ol class="breadcrumb">
<li><a href="#">Home</a></li>
<li class="active">Contact Us</li>
</ol>
</div>
</div>
</div>
</section>
<!--page title end-->
<!-- contact-form-section -->
<section id="getintouch" class="section-padding">
<div class="container">
<div class="text-center mb-80">
<h2 class="section-title text-uppercase">Get in touch</h2>
<p class="section-sub">24-7 Technical Support from Kuwait. An online support to help your problems go away.A 24 hour, reliable service to help solve problems your face with any of our services.We are just a phone call or message us away.</p>
</div>
<div class="row mb-80">
<div class="col-md-8">
<form name="contact-form" id="contactForm" action="contact-request" method="POST">
<div class="row">
<div class="col-md-6">
<div class="input-field">
<input type="text" name="name" class="validate" id="name" required="">
<label for="name">Name</label>
</div>
</div><!-- /.col-md-6 -->
<div class="col-md-6">
<div class="input-field">
<label class="sr-only" for="email">Email</label>
<input id="email" type="email" name="email" class="validate" required="">
<label for="email" data-error="wrong" data-success="right">Email</label>
</div>
</div><!-- /.col-md-6 -->
</div><!-- /.row -->
<div class="row">
<div class="col-md-6">
<div class="input-field">
<input id="phone" type="tel" name="phone" class="validate" >
<label for="phone">Phone Number</label>
</div>
</div><!-- /.col-md-6 -->
<div class="col-md-6">
<div class="input-field">
<input id="website" type="text" name="website" class="validate" >
<label for="website">Your Website</label>
</div>
</div><!-- /.col-md-6 -->
</div><!-- /.row -->
<div class="input-field">
<textarea name="message" id="message" class="materialize-textarea" ></textarea>
<label for="message">Message</label>
</div>
<button type="submit" name="submit" class="waves-effect waves-light btn submit-button pink mt-30">Send Message</button>
</form>
</div><!-- /.col-md-8 -->
<div class="col-md-4 contact-info">
<address>
<i class="material-icons brand-color"></i>
<div class="address">
Block 4, Street 5 Jleeb Al-Shuyoukh,<br>
Abbassiya, Kuwait Police Station Road, <br>
Behind BEC & Lulu Exchange,<br> Near Orma Jewellery
<hr>
</div>
<i class="material-icons brand-color"></i>
<div class="phone">
<p>Fax: 24 34 43 18<br>
Telephone: 24 31 07 83 <br> Mobile: 99 53 01 77 <br> Whatsapp: 99 53 01 77 <strong>,</strong> <span class="ml-10">99 25 38 41</span></p>
</div>
<i class="material-icons brand-color"></i>
<div class="mail">
<p><a href="mailto:<EMAIL>"><EMAIL></a><br>
<a href="mailto:<EMAIL>"><EMAIL></a> <br>
<a href="mailto:<EMAIL>"><EMAIL></a></p>
</div>
</address>
</div><!-- /.col-md-4 -->
</div><!-- /.row -->
</div>
</section>
<!-- contact-form-section End -->
<!-- map-section -->
<div id="myMap" class="height-450"></div>
<!-- /.map-section -->
<file_sep>/application/controllers/Home.php
<?php
/**
* Created by PhpStorm.
* User: psybo-03
* Date: 25/4/17
* Time: 5:57 PM
*/
defined('BASEPATH') or exit('No Direct Script Access Allowed');
class Home extends CI_Controller
{
protected $header = 'templates/header';
protected $footer = 'templates/footer';
function __construct()
{
parent::__construct();
$this->load->library('session');
$this->load->model('Brochures_Model', 'brochure');
$this->load->model('News_Model', 'news');
$this->load->model('Subscription_Model', 'subscription');
$this->load->model('Slide_Image_Model', 'slide_image');
$this->load->model('Gallery_Files_Model', 'gallery_files');
$this->load->model('Blog_Model', 'blog');
}
public function login()
{
if ($this->session->userdata('logged_in') == TRUE) {
redirect(base_url('admin'));
return FALSE;
}
$this->load->view('login');
}
public function test($page = 'test')
{
$this->load->view($this->header);
$this->load->view($page);
$this->load->view($this->footer);
}
public function index($page = 'index')
{
$data['slide_image'] = $this->_get_slide_images();
$data['brochure'] = $this->_get_latest_brochure()[0];
$data['news'] = $this->_get_news();
$this->load->view($this->header);
$this->load->view($page,$data);
$this->load->view($this->footer);
}
public function about($page = 'about')
{
$this->load->view($this->header);
$this->load->view($page);
$this->load->view($this->footer);
}
public function team($page = 'team')
{
$this->load->view($this->header);
$this->load->view($page);
$this->load->view($this->footer);
}
public function service($page = 'service')
{
$this->load->view($this->header);
$this->load->view($page);
$this->load->view($this->footer);
}
public function moments($page = 'gallery')
{
$data['gallery'] = $this->_get_gallery();
$this->load->view($this->header);
$this->load->view($page,$data);
$this->load->view($this->footer);
}
public function blog($page = 'blog')
{
$data['blog'] = $this->_get_blog();
$this->load->view($this->header);
$this->load->view($page,$data);
$this->load->view($this->footer);
}
public function blogView($id)
{
$data['blog'] = $this->_get_blog($id);
$data['blog_latest'] = $this->_get_latest_blog();
$this->load->view($this->header);
$this->load->view('blogView', $data);
$this->load->view($this->footer);
}
public function contact($page = 'contact')
{
$this->load->view($this->header);
$this->load->view($page);
$this->load->view($this->footer);
}
public function _get_latest_brochure()
{
$data = $this->brochure->select($limit = 1, $order = 'DESC');
return $data;
}
public function _get_news()
{
$data = $this->news->select($limit = 6, $order = 'DESC');
return $data;
}
public function subscribe()
{
$this->form_validation->set_rules('email', 'Email', 'required|valid_email|callback_check_mail');
if ($this->form_validation->run() == FALSE) {
$this->output->set_status_header(400, 'Validation Error');
$this->output->set_output(validation_errors());
} else {
$data['email'] = $this->input->post('email');
$data['ip'] = $this->get_client_ip();
$data['date'] = date('Y-m-d');
if ($this->subscription->add($data)) {
$this->output->set_output('subscription success');
} else {
$this->output->set_status_header(500, 'Server Error');
$this->output->set_output('subscription success');
}
}
}
public function check_mail($mail)
{
if ($this->subscription->select_where(['email' => $mail]) == FALSE) {
return TRUE;
} else {
$this->form_validation->set_error_delimiters('<div style="color: #ff0000">', '</div>');
$this->form_validation->set_message('check_mail', 'You Have Already Subscribed');
return FALSE;
}
}
// Function to get the client IP address
function get_client_ip() {
$ipaddress = '';
if (isset($_SERVER['HTTP_CLIENT_IP']))
$ipaddress = $_SERVER['HTTP_CLIENT_IP'];
else if(isset($_SERVER['HTTP_X_FORWARDED_FOR']))
$ipaddress = $_SERVER['HTTP_X_FORWARDED_FOR'];
else if(isset($_SERVER['HTTP_X_FORWARDED']))
$ipaddress = $_SERVER['HTTP_X_FORWARDED'];
else if(isset($_SERVER['HTTP_FORWARDED_FOR']))
$ipaddress = $_SERVER['HTTP_FORWARDED_FOR'];
else if(isset($_SERVER['HTTP_FORWARDED']))
$ipaddress = $_SERVER['HTTP_FORWARDED'];
else if(isset($_SERVER['REMOTE_ADDR']))
$ipaddress = $_SERVER['REMOTE_ADDR'];
else
$ipaddress = 'UNKNOWN';
return $ipaddress;
}
public function unsubscribe($param1)
{
if ($this->subscription->unsub(['active' => 0],['email' => $param1])) {
redirect(base_url(), 'refresh');
}
}
public function _get_slide_images()
{
$data = $this->slide_image->select();
return $data;
}
public function _get_gallery()
{
$data = $this->gallery_files->select();
return $data;
}
public function _get_blog($id="")
{
if ($id != "") {
$data = $this->blog->select_where(['id' => $id]);
return $data;
} else {
$data = $this->blog->select();
return $data;
}
}
public function _get_latest_blog()
{
$data = $this->blog->select($limit = 8, $order = 'DESC');
return $data;
}
public function request_quote()
{
$this->form_validation->set_rules('name', 'Name', 'required');
$this->form_validation->set_rules('email', 'Email', 'required|valid_email');
if ($this->form_validation->run() === FALSE) {
$this->output->set_status_header(400, 'Validation error');
} else {
$name = $this->input->post('name');
$email = $this->input->post('email');
$phone = $this->input->post('phone');
$service = $this->input->post('service');
$message = $this->input->post('message');
$message = wordwrap($message, 70, "\n");
$subject = 'Quote From : ' . $name;
$content = 'name : ' . $name . PHP_EOL . PHP_EOL;
$content .= 'Quote from : ' . $email . PHP_EOL . PHP_EOL;
$content .= 'Phone : ' . $phone . PHP_EOL . PHP_EOL;
$content .= 'Requested Service : ' . $service . PHP_EOL . PHP_EOL;
$content .= 'Message : ' . $message . PHP_EOL . PHP_EOL;
$content = str_replace("\n.", "\n.", $content);
$to = '<EMAIL>';
$headers = 'From:<EMAIL>';
if (mail($to, $subject, $content, $headers)) {
$this->output->set_output("We will contact you soon!\n Thank you.");
} else {
$this->output->set_status_header(400, 'Unable to send mail');
$this->output->set_output("Please try again later.");
}
}
}
public function appointment_request()
{
$this->form_validation->set_rules('name', 'Name', 'required');
$this->form_validation->set_rules('email', 'Email', 'required|valid_email');
if ($this->form_validation->run() === FALSE) {
$this->output->set_status_header(400, 'Validation error');
} else {
$name = $this->input->post('name');
$email = $this->input->post('email');
$phone = $this->input->post('phone');
$service = $this->input->post('service');
$message = $this->input->post('message');
$message = wordwrap($message, 70, "\n");
$subject = 'Appointment From : ' . $name;
$content = 'name : ' . $name . PHP_EOL . PHP_EOL;
$content .= 'appointment from : ' . $email . PHP_EOL . PHP_EOL;
$content .= 'Phone : ' . $phone . PHP_EOL . PHP_EOL;
$content .= 'Requested Service : ' . $service . PHP_EOL . PHP_EOL;
$content .= 'Message : ' . $message . PHP_EOL . PHP_EOL;
$content = str_replace("\n.", "\n.", $content);
$to = '<EMAIL>';
$headers = 'From:<EMAIL>';
if (mail($to, $subject, $content, $headers)) {
$this->output->set_output("We will contact you soon!\n Thank you.");
} else {
$this->output->set_status_header(400, 'Unable to send mail');
$this->output->set_output("Please try again later.");
}
}
}
public function contact_request()
{
$this->form_validation->set_rules('name', 'Name', 'required');
$this->form_validation->set_rules('email', 'Email', 'required|valid_email');
if ($this->form_validation->run() === FALSE) {
$this->output->set_status_header(400, 'Validation error');
} else {
$name = $this->input->post('name');
$email = $this->input->post('email');
$phone = $this->input->post('phone');
$website = $this->input->post('website');
$message = $this->input->post('message');
$message = wordwrap($message, 70, "\n");
$subject = 'Contact Request From : ' . $name;
$content = 'name : ' . $name . PHP_EOL . PHP_EOL;
$content .= 'contact request : ' . $email . PHP_EOL . PHP_EOL;
$content .= 'Phone : ' . $phone . PHP_EOL . PHP_EOL;
$content .= 'Website : ' . $website . PHP_EOL . PHP_EOL;
$content .= 'Message : ' . $message . PHP_EOL . PHP_EOL;
$content = str_replace("\n.", "\n.", $content);
$to = '<EMAIL>';
$headers = 'From:<EMAIL>';
if (mail($to, $subject, $content, $headers)) {
$this->output->set_output("We will contact you soon!\n Thank you.");
} else {
$this->output->set_status_header(400, 'Unable to send mail');
$this->output->set_output("Please try again later.");
}
}
}
}<file_sep>/application/controllers/App.php
<?php
/**
* Created by PhpStorm.
* User: psybo-03
* Date: 25/4/17
* Time: 3:36 PM
*/
defined('BASEPATH') OR exit('No direct script access allowed');
/**
* Class App
*
*/
class App extends CI_Controller
{
protected $limit = 10;
function __construct()
{
parent::__construct();
require_once '../vendor/fzaninotto/faker/src/autoload.php';
// can only be called from the command line!
if (!$this->input->is_cli_request()) {
exit('Direct Access Not Allowed');
}
// can only run in the development environment
if (ENVIRONMENT !== 'development') {
exit('Wowesers! You don\'t want to do that');
}
// initiate faker
$this->faker = Faker\Factory::create();
// Required Models
$this->load->model('Blog_Model', 'blog');
$this->load->model('Brochures_Model', 'brochure');
$this->load->model('Files_Model', 'file');
$this->load->model('Galleries_Model', 'gallery');
$this->load->model('Gallery_Files_Model', 'gallery_file');
$this->load->model('Helpful_Links_Model', 'helpful_link');
$this->load->model('News_Model', 'news');
$this->load->model('Slide_Image_Model', 'slide_image');
$this->load->model('Users_Model', 'user');
}
function truncate()
{
$this->_truncate_db();
}
/**
* Seed local database
*/
function seed($tabel = "")
{
$this->truncate();
$this->_seed_users(1);
$this->_seed_blog($this->limit);
$this->_seed_brochure($this->limit);
$this->_seed_files(5);
$this->_seed_gallery($this->limit);
$this->_seed_gallery_files($this->limit);
$this->_seed_helpful_link($this->limit);
$this->_seed_news($this->limit);
$this->_seed_slide_image($this->limit);
}
function _seed_users($limit)
{
echo "seeding $limit users";
// create a bunch of base buyer accounts
for ($i = 0; $i < $limit; $i++) {
echo ".";
$data = array(
'username' => 'admin', // get a unique nickname
'password' => hash('<PASSWORD>', '<PASSWORD>'), // run this via your password hashing function
);
$this->user->add($data);
}
echo PHP_EOL;
}
function _seed_news($limit)
{
echo "seeding $limit news";
for ($i = 0; $i < $limit; $i++) {
echo ".";
$data = [
'heading' => $this->faker->sentence(4),
'content' => $this->faker->paragraph(3),
'date' => $this->faker->date(),
'file_id' => $this->faker->randomElement([1, 2, 3, 4, 5]),
];
$this->news->add($data);
}
echo PHP_EOL;
}
function _seed_slide_image($limit)
{
echo "seeding $limit slide_images";
for ($i = 0; $i < $limit; $i++) {
echo ".";
$data = [
'file_id' => $this->faker->randomElement([1, 2, 3, 4, 5]),
];
$this->slide_image->add($data);
}
echo PHP_EOL;
}
function _seed_brochure($limit)
{
echo "seeding $limit brochures";
for ($i = 0; $i < $limit; $i++) {
echo ".";
$data = [
'name' => $this->faker->name,
'subject' => $this->faker->sentence(3),
'date' => $this->faker->date(),
'file_id' => $this->faker->randomElement([1, 2, 3, 4, 5]),
];
$this->brochure->add($data);
}
echo PHP_EOL;
}
function _seed_gallery($limit)
{
echo "seeding $limit gallery";
for ($i = 0; $i < $limit; $i++) {
echo ".";
$data = [
'gallery_name' => $this->faker->name,
'description' => $this->faker->sentence(5),
];
$this->gallery->add($data);
}
echo PHP_EOL;
}
function _seed_gallery_files($limit)
{
echo "seeding $limit gallery_files";
for ($i = 0; $i < $limit; $i++) {
echo ".";
$data = [
'gallery_id' => $this->faker->randomElement([1, 2, 3, 4, 5, 6, 7, 8, 9, 10]),
'file_id' => $this->faker->randomElement([1, 2, 3, 4, 5]),
];
$this->gallery_file->add($data);
}
echo PHP_EOL;
}
function _seed_helpful_link($limit)
{
echo "seeding $limit helpful links";
for ($i = 0; $i < $limit; $i++) {
echo ".";
$data = [
'name' => $this->faker->name,
'link' => $this->faker->url,
];
$this->helpful_link->add($data);
}
echo PHP_EOL;
}
function _seed_blog($limit)
{
echo "seeding $limit blogs";
for ($i = 0; $i < $limit; $i++) {
echo ".";
$data = [
'name' => $this->faker->name,
'heading' => $this->faker->sentence(3),
'content' => $this->faker->paragraph(4),
'date' => $this->faker->date(),
'file_id' => $this->faker->randomElement([1, 2, 3, 4, 5]),
];
$this->blog->add($data);
}
echo PHP_EOL;
}
function _seed_files($limit)
{
echo "seeding $limit files";
for ($i = 0; $i < $limit; $i++) {
echo ".";
$data = [
'file_name' => $this->faker->image($dir = FCPATH . 'uploads', $width = 640, $height = 480, 'cats', false),
'file_type' => $this->faker->fileExtension,
'size' => $this->faker->randomElement([1200, 500, 6000, 5654]),
'date' => $this->faker->date()
];
$this->file->add($data);
}
echo PHP_EOL;
}
function _truncate_db()
{
$this->user->trunc();
$this->blog->trunc();
$this->brochure->trunc();
$this->file->trunc();
$this->gallery->trunc();
$this->gallery_file->trunc();
$this->helpful_link->trunc();
$this->news->trunc();
$this->slide_image->trunc();
}
}<file_sep>/application/helpers/menu_helper.php
<?php
/**
* Created by PhpStorm.
* User: psybo-03
* Date: 9/2/17
* Time: 5:04 PM
*/
function dashboard_menu()
{
$menu = [
'dashboard' => [
'title' => 'dashboard',
'icon' => 'fa-tachometer',
'link' => '/'
],
'slide image' => [
'title' => 'slide image',
'icon' => 'fa-picture-o',
'link' => 'slide-images'
],
'news' => [
'title' => 'news',
'icon' => 'fa-newspaper-o',
'link' => 'news'
],
'brochure' => [
'title' => 'brochure',
'icon' => 'fa-file-pdf-o',
'link' => 'brochures'
],
'gallery' => [
'title' => 'gallery',
'icon' => 'fa-picture-o',
'link' => 'gallery'
],
'helpful link' => [
'title' => 'helpful link',
'icon' => 'fa-external-link',
'link' => 'helpful-links'
],
'blog' => [
'title' => 'blog',
'icon' => 'fa-comment',
'link' => 'blog'
]
];
$html = '<nav class="sidebar-left">
<div class="">
<ul class="menu-left">
<li>
<div class="user-img">
<img class="img-responsive img-circle center-block" src="' . public_url() . 'assets/img/logo/logo.png" alt="User">
</div>
<div class="user-id text-center">
<span class=""><NAME></span>
</div>
</li>';
foreach ($menu as $key => $value) {
$html .= '<li><a href="' . base_url('admin/#'.$value['link']) . '" ng-class="{active : url == \'' . $value['link'] . '\'}">'.ucfirst($value['title']).' <i class="menu-icon fa '.$value['icon'].' pull-right"></i></a></li>';
}
$html .='</ul>
</div>
</nav>';
return $html;
}
function menu($current)
{
$menu = [
'Home' => [
'title' => 'Home',
'icon' => '',
'link' => 'home'
],
'About Us' => [
'title' => 'About Us',
'icon' => '',
'link' => 'about',
'sub' => [
'about us' => [
'title' => 'about company',
'icon' => '',
'link' => 'about#aboutus',
],
'team' => [
'title' => 'our team',
'icon' => '',
'link' => 'team',
]
]
],
'Our Services' => [
'title' => 'Our Services',
'icon' => '',
'link' => 'services',
'sub' => [
'typing services' => [
'title' => 'typing service',
'icon' => '',
'link' => 'services#services1',
],
'services for ministry health' => [
'title' => 'services for ministry health',
'icon' => '',
'link' => 'services#services2',
],
'services for higher & lower education' => [
'title' => 'services for higher & lower education',
'icon' => '',
'link' => 'services#services3',
],
'medical fingerprint assists services' => [
'title' => 'medical fingerprint assists services',
'icon' => '',
'link' => 'services#services4',
],
'insurance services' => [
'title' => 'insurance services',
'icon' => '',
'link' => 'services#services5',
],
'health insurance services' => [
'title' => 'health insurance services',
'icon' => '',
'link' => 'services#services6',
],
'education services' => [
'title' => 'education services',
'icon' => '',
'link' => 'services#services7',
],
'transportation services' => [
'title' => 'transportation services',
'icon' => '',
'link' => 'services#services8',
],
'pan card services' => [
'title' => 'pan card services',
'icon' => '',
'link' => 'services#services9',
],
'air tickets and related services' => [
'title' => 'air tickets and related services',
'icon' => '',
'link' => 'services#services10',
],
'courier services' => [
'title' => 'courier services',
'icon' => '',
'link' => 'services#services11',
],
'miscellaneous services' => [
'title' => 'miscellaneous services',
'icon' => '',
'link' => 'services#services12',
]
]
],
'Moments' => [
'title' => 'Moments',
'icon' => '',
'link' => 'moments'
],
'Blog' => [
'title' => 'Blog',
'icon' => '',
'link' => 'blog'
],
'Contact' => [
'title' => 'Contact',
'link' => 'contact'
]
];
$html = '';
foreach ($menu as $key=>$value) {
if (isset($value['sub'])) {
if ($key == $current) {
$html .= '<li class="active">' .PHP_EOL.
'<a href="' . $value['link'] . '">' . ucwords($value['title']) . '</a>' . PHP_EOL .
'<ul class="dropdown">' . PHP_EOL;
foreach ($value['sub'] as $sub) {
$html .= '<li><a href="' . $sub['link'] . '">' . ucwords($sub['title']) . '</a></li>'. PHP_EOL;
}
} else {
$html .= '<li>' .PHP_EOL.
'<a href="' . $value['link'] . '">' . ucwords($value['title']) . '</a>' . PHP_EOL .
'<ul class="dropdown">' . PHP_EOL;
foreach ($value['sub'] as $sub) {
$html .= '<li><a href="' . $sub['link'] . '">' . ucwords($sub['title']) . '</a></li>'. PHP_EOL;
}
}
$html .= '</ul>' . PHP_EOL;
$html .= '</li>' . PHP_EOL;
}else{
if ($key == $current) {
$html .= '<li class="active"><a href="' . base_url($value['link']) . '">' . $key . '</a></li>' . PHP_EOL;
}else{
$html .= '<li><a href="' . base_url($value['link']) . '">' . $key . '</a></li>' . PHP_EOL;
}
}
}
return $html;
}
<file_sep>/application/models/Gallery_Files_Model.php
<?php
/**
* Created by PhpStorm.
* User: psybo-03
* Date: 25/4/17
* Time: 4:48 PM
*/
defined('BASEPATH') or exit ('No direct script access allowed');
require_once APPPATH . 'core/My_Model.php';
class Gallery_Files_Model extends My_Model
{
protected $table = 'gallery_files';
function __construct()
{
parent::__construct();
}
/**
* Return ALL galleries with their files ie,album model
*
*/
public function select($limit = null, $order = null)
{
$this->db->from('galleries');
if ($limit != null) {
$this->db->limit($limit);
}
if ($order != null) {
$this->db->order_by('id', 'DESC');
}
$query = $this->db->get();
if ($query->num_rows() > 0) {
$galleries = $query->result();
foreach ($galleries as $value) {
$this->db->from('gallery_files');
$this->db->where('gallery_id', $value->id);
$glr_fls_query = $this->db->get();
$gallery_files = $glr_fls_query->result();
foreach ($gallery_files as $val) {
$this->db->from('files');
$this->db->where('id', $val->file_id);
$files_query = $this->db->get();
$files = $files_query->result();
foreach ($files as $file) {
$val->file_name = $file->file_name;
$val->file_type = $file->file_type;
$val->gallery_file_id = $val->id;
$val->thumbImgUrl = public_url() . 'uploads/thumb/thumb_' . $file->file_name;
$val->imgUrl = public_url() . 'uploads/' . $file->file_name;
}
}
$value->files = $gallery_files;
}
return $galleries;
}else
return FALSE;
}
/**
* Return ONE gallery with their files ie,album model
*
*/
public function select_where($galery_id, $limit = null, $order = null)
{
$this->db->from('galleries');
$this->db->where('id', $galery_id);
if ($limit != null) {
$this->db->limit($limit);
}
if ($order != null) {
$this->db->order_by('id', 'DESC');
}
$query = $this->db->get();
if ($query->num_rows() > 0) {
$galleries = $query->result();
foreach ($galleries as $value) {
$this->db->from('gallery_files');
$this->db->where('gallery_id', $value->id);
$glr_fls_query = $this->db->get();
$gallery_files = $glr_fls_query->result();
foreach ($gallery_files as $val) {
$this->db->from('files');
$this->db->where('id', $val->file_id);
$files_query = $this->db->get();
$files = $files_query->result();
foreach ($files as $file) {
$val->file_name = $file->file_name;
$val->file_type = $file->file_type;
$val->gallery_file_id = $val->id;
}
}
$value->files = $gallery_files;
}
return $galleries;
}else
return FALSE;
}
/**
*return all gallery files one by one
*
*/
public function select_all($limit = null, $order = null)
{
$this->db->from('galleries');
if ($limit != null) {
$this->db->limit($limit);
}
if ($order != null) {
$this->db->order_by($order, 'DESC');
}
$all_files = [];
$query = $this->db->get();
if ($query->num_rows() > 0) {
$galleries = $query->result();
foreach ($galleries as $value) {
$this->db->from('gallery_files');
$this->db->where('gallery_id', $value->id);
$glr_fls_query = $this->db->get();
$gallery_files = $glr_fls_query->result();
foreach ($gallery_files as $val) {
$this->db->from('files');
$this->db->where('id', $val->file_id);
$files_query = $this->db->get();
$files = $files_query->result();
foreach ($files as $file) {
$val->thumbUrl = public_url() . 'uploads/thumb/thumb_' . $file->file_name;
$val->url = public_url() . 'uploads/' . $file->file_name;
$val->alt = 'gallery';
$val->category = $value->name;
$val->description = $value->description;
array_push($all_files, $val);
}
}
}
return $all_files;
}else
return FALSE;
}
public function select_gl_fl_where(array $where)
{
$this->db->select(['gallery_files.*', 'files.id as filId', 'files.file_name', 'files.file_type']);
$this->db->from($this->table);
$this->db->join('files', 'files.id = gallery_files.file_id', 'full');
$this->db->where($where);
$query = $this->db->get();
if ($query->num_rows() > 0) {
return $query->result();
}else
return FALSE;
}
public function add($data)
{
return $this->insert($data);
}
public function edit($data, $id)
{
return $this->update($data, $id);
}
public function remove($id)
{
return $this->drop($id);
}
public function trunc()
{
return $this->truncate();
}
}
<file_sep>/resources/js/angularscript.js
/**
* Created by psybo-03 on 25/4/17.
*/
var app = angular.module('myApp', ['ngRoute', 'ui.bootstrap', 'angularUtils.directives.dirPagination', 'ngFileUpload']);
app.config(['$routeProvider', '$locationProvider','$qProvider', function ($routeProvider, $locationProvider, $qProvider) {
$qProvider.errorOnUnhandledRejections(false);
$locationProvider.hashPrefix('');
$routeProvider
.when('/#/', {
templateUrl: '',
controller:'dashboardController'
})
.when('/news', {
templateUrl: 'news',
controller:'newsController'
})
.when('/brochures', {
templateUrl: 'brochures',
controller:'brochureController'
})
.when('/helpful-links', {
templateUrl: 'helpful-links',
controller:'helpfulLinkController'
})
.when('/gallery', {
templateUrl: 'gallery',
controller:'galleryController'
})
.when('/slide-images', {
templateUrl: 'slide-images',
controller:'slideImageController'
})
.when('/blog', {
templateUrl: 'blog',
controller:'blogController'
})
.when('/edit-profile', {
templateUrl: 'change'
})
}]);
//Pagination filter
app.filter('startFrom', function() {
return function(input, start) {
start = +start; //parse to int
return input.slice(start);
}
});
app.directive('fileModel', ['$parse', function ($parse) {
return {
restrict: 'A',
link: function(scope, element, attrs) {
var model = $parse(attrs.fileModel);
var modelSetter = model.assign;
scope.filespre = [];
element.bind('change', function(){
var values = [];
angular.forEach(element[0].files, function (item) {
//url
item.url = URL.createObjectURL(item);
item.model = attrs.fileModel;
scope.filespre.push(item);
});
scope.$apply(function(){
modelSetter(scope, element[0].files);
//old
//modelSetter(scope, element[0].files[0]);
});
});
}
};
}]);
app.directive('ngConfirmClick', [
function () {
return {
link: function (scope, element, attr) {
var msg = attr.ngConfirmClick || "Are you sure?";
var clickAction = attr.confirmedClick;
element.bind('click', function (event) {
if (window.confirm(msg)) {
scope.$eval(clickAction)
}
});
}
};
}
]);
<file_sep>/application/views/blog.php
<!--header start-->
<header id="header" class="tt-nav nav-border-bottom">
<div class="header-sticky light-header ">
<div class="container">
<div class="search-wrapper">
<div class="search-trigger pull-right">
<div class='search-btn'></div>
<i class="material-icons"></i>
</div>
<!-- Modal Search Form -->
<i class="search-close material-icons"></i>
<div class="search-form-wrapper">
<form action="#" class="white-form">
<div class="input-field">
<input type="text" name="search" id="search">
<label for="search" class="">Search Here...</label>
</div>
<button class="btn pink search-button waves-effect waves-light" type="submit"><i class="material-icons"></i></button>
</form>
</div>
</div><!-- /.search-wrapper -->
<div id="materialize-menu" class="menuzord">
<!--logo start-->
<a href="home" class="logo-brand">
<img src="<?php echo public_url()?>assets/img/logo.png" alt="" >
</a>
<!--logo end-->
<!--mega menu start-->
<ul class="menuzord-menu pull-right">
<!-- <li class="active"><a href="index.html">Home</a></li>
<li><a href="about.html">About Us</a></li>
<li><a href="service.html">Our Services</a></li>
<li><a href="gallery.html">Moments</a></li>
<li><a href="blog.html">Blog</a></li>
<li><a href="contact.html">Contact Us</a></li> -->
<?php echo menu('Blog') ?>
</ul>
<!--mega menu end-->
</div>
</div>
</div>
</header>
<!--header end-->
<!--page title start-->
<section class="page-title pattern-bg ptb-50">
<div class="container">
<div class="row">
<div class="col-md-12">
<h2>Classic International</h2>
<span>Our Blog Page</span>
<ol class="breadcrumb">
<li><a href="home">Home</a></li>
<li class="active">Blog</li>
</ol>
</div>
</div>
</div>
</section>
<!--page title end-->
<!-- Grid News -->
<section class="section-padding grid-news-hover grid-blog">
<div class="container">
<div class="row">
<div id="blogGrid">
<!-- blog one start -->
<?php
if (isset($blog) and $blog != FALSE) {
foreach ($blog as $value) {
?>
<div class="col-xs-12 col-sm-6 col-md-4 blog-grid-item">
<article class="post-wrapper">
<div class="thumb-wrapper waves-effect waves-block waves-light">
<a href="<?php echo 'blogView/' . $value->id ?>"><img src="<?php echo $value->imgUrl ?>" class="img-responsive"
alt=""></a>
<div class="post-date">
<?php echo $value->day?><span><?php echo $value->month?></span>
</div>
</div>
<!-- .post-thumb -->
<div class="blog-content">
<div class="hover-overlay light-blue"></div>
<header class="entry-header-wrapper">
<div class="entry-header">
<h2 class="entry-title pcut"><a href="<?php echo 'blogView/' . $value->id ?>"><?php echo $value->heading ?></a></h2>
</div>
<!-- /.entry-header -->
</header>
<!-- /.entry-header-wrapper -->
<div class="entry-content">
<p class="pcut"> <?php echo $value->content ?></p>
</div>
<!-- .entry-content -->
</div>
<!-- /.blog-content -->
</article>
<!-- /.post-wrapper -->
</div><!-- /.col-md-4 -->
<?php
}
}?>
<!-- blog one end -->
</div><!-- /#blogGrid -->
</div><!-- /.row -->
<!-- <ul class="pagination post-pagination text-center mt-50">-->
<!-- <li><a href="#." class="waves-effect waves-light"><i class="fa fa-angle-left"></i></a></li>-->
<!-- <li><span class="current waves-effect waves-light">1</span></li>-->
<!-- <li><a href="#." class="waves-effect waves-light">2</a></li>-->
<!-- <li><a href="#." class="waves-effect waves-light"><i class="fa fa-angle-right"></i></a></li>-->
<!-- </ul>-->
</div><!-- /.container -->
</section>
<!-- Grid News End -->
<file_sep>/application/controllers/Slide_Image_Controller.php
<?php
/**
* Created by PhpStorm.
* User: psybo-03
* Date: 4/5/17
* Time: 1:02 PM
*/
defined('BASEPATH') or exit('No Direct Script Access Allowed');
require_once(APPPATH . 'core/Check_Logged.php');
class Slide_Image_Controller extends Check_Logged
{
function __construct()
{
parent::__construct();
$this->load->model('Slide_Image_Model', 'slide_image');
$this->load->model('Files_Model', 'file');
$this->load->library(['upload', 'image_lib']);
if (!$this->logged) {
redirect(base_url('login'));
}
}
function index()
{
}
function get_all()
{
$data = $this->slide_image->select();
$this->output->set_content_type('application/json')->set_output(json_encode($data));
}
function store()
{
if ($this->input->server('REQUEST_METHOD') == 'POST') {
$uploaded = json_decode($this->input->post('uploaded'));
if (!empty($uploaded)) {
foreach ($uploaded as $value) {
$data = [];
$data['file_name'] = $value->file_name;
$data['file_type'] = $value->file_type;
$data['size'] = $value->file_size;
$data['date'] = date('Y-m-d');
$file_id = $this->file->add($data);
if ($file_id) {
$slide_data['file_id'] = $file_id;
if ($this->slide_image->add($slide_data)) {
/************resize and create thumbnail image*******/
if ($value->image_width > 1920 OR $value->image_width < 1920) {
$img_cfg['image_library'] = 'gd2';
$img_cfg['source_image'] = getwdir() . 'uploads/' . $value->file_name;
$img_cfg['maintain_ratio'] = TRUE;
$img_cfg['new_image'] = getwdir() . 'uploads/' . $value->file_name;
$img_cfg['width'] = 1920;
$img_cfg['quality'] = 80;
$img_cfg['master_dim'] = 'width';
$this->image_lib->initialize($img_cfg);
if (!$this->image_lib->resize()) {
$resize_error[] = $this->image_lib->display_errors();
}
$this->image_lib->clear();
/********End resize*********/
$this->output->set_content_type('application/json')->set_output(json_encode('Upload success'));
}
}else{
$this->output->set_status_header(400, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode('Add error.try again later'));
}
}
}
} else {
$this->output->set_status_header(400, 'Validation Error');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Select an image']));
}
} else {
show_404();
}
}
function update($id)
{
}
function upload()
{
$config['upload_path'] = getwdir() . 'uploads';
$config['allowed_types'] = 'jpg|png|jpeg|JPG|JPEG';
$config['max_size'] = 4096;
$config['file_name'] = 'S_' . rand();
$config['multi'] = 'ignore';
$this->upload->initialize($config);
if ($this->upload->do_upload('file')) {
$this->output->set_content_type('application/json')->set_output(json_encode($this->upload->data()));
}else{
$this->output->set_status_header(401, 'File Upload Error');
$this->output->set_content_type('application/json')->set_output(json_encode($this->upload->display_errors()));
}
}
public function delete($id)
{
$slide_image = $this->slide_image->select_where(['slide_images.id' => $id]);
if ($slide_image) {
if ($this->slide_image->remove($id)) {
if ($this->file->remove($slide_image[0]->file_id) && file_exists(getwdir() . 'uploads/' . $slide_image[0]->file_name)) {
unlink(getwdir() . 'uploads/' . $slide_image[0]->file_name);
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Slide Image Deleted']));
} else {
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Slide Image Deleted and file delete error']));
}
} else {
$this->output->set_status_header(500, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Delete Error']));
}
} else {
$this->output->set_status_header(400, '404');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Record Not found']));
}
}
}<file_sep>/application/views/admin/slide_image.php
<div class="col-md-12" ng-class="{disable : loading} ">
<div class="row" style="padding-left: 14px">
<div class="box">
<button class="btn btn-primary" ng-click="newSlideImage()"><i class="fa fa-plus"></i> Add</button>
<form class="form-horizontal" method="POST" ng-submit="addSlideImage()" ng-show="showform" name="addform" enctype="multipart/form-data">
<h3>New Slide Image</h3>
<div class="form-group">
<label for="" class="control-label col-md-1">Photo</label>
<div class="col-md-4">
<button ngf-select="uploadFiles($files, $invalidFiles)"
accept="image/*"
ngf-max-height="5000"
ngf-max-size="5MB"
ngf-multiple="true">
Select Files
</button>
<span class="alert alert-danger"ng-show="fileValidation.status == true">{{fileValidation.msg}}</span>
<br><br>
Files:
<ul>
<li ng-repeat="f in files" style="font:smaller">
<img ngf-src="f.$ngfBlobUrl" class="thumbnail" width="100px" ngf-no-object-url="true" >
{{f.name}} {{f.$errorParam}}
<div class="progress" ng-show="f.progress >= 0">
<div class="progress-bar progress-bar-success" role="progressbar" aria-valuenow="40"
aria-valuemin="0" aria-valuemax="100" style="width:{{f.progress}}%">
{{f.progress}}% Complete
</div>
</div>
</li>
<li ng-repeat="f in errFiles" style="font:smaller">{{f.name}} {{f.$error}} {{f.$errorParam}}
</li>
</ul>
{{errorMsg}}
</div>
<span class="alert alert-warning">Image should be 100*100</span>
<!----for existing image----->
<div class="clearfix"></div>
<div class="clearfix"></div>
</div>
<div class="container" ng-show="show_error">
<div class="row">
<div class="col-md-6">
<div class="alert alert-danger alert-dismissable fade in ">
<a href="" class="close" data-dismiss="alert" arial-label="close">×</a>
<h4>following files are not uploaded</h4>
<p ng-repeat-start="err in error">{{err}}</p>
<hr ng-repeat-end />
</div>
</div>
</div>
</div>
<div class="form-group text-center">
<button class="btn btn-primary" type="submit">Save</button>
<button class="btn btn-danger" type="button" ng-click="hideForm()">Cancel</button>
</div>
</form>
</div>
<div class="clearfix"></div>
<hr/>
<div class="row">
<div class="container-fluid">
<div class="row">
<div class="row" style="margin-left: 14px">
<div class="col-md-2" dir-paginate="(key,preview) in slideimages | filter:search | limitTo:pageSize | itemsPerPage:numPerPage">
<div class="thumbnail cus-thumb" ng-mouseover="showcaption=true" ng-mouseleave="showcaption=false" style="max-height: 142px;min-height: 142px">
<div class="caption" ng-show="showcaption">
<div id="content">
<a href="{{preview.imgUrl}}" class="label label-warning" rel="tooltip" title="Show">Show</a>
<a href="" class="label label-danger" rel="tooltip" title="Delete" confirmed-click="deleteImage(preview)" ng-confirm-click="Would you like to delete this item?!">Delete</a>
</div>
</div>
<img src="{{preview.imgUrl}}" alt="thumbnails">
</div>
</div>
</div>
</div>
<div class="pull-right">
<dir-pagination-controls
max-size="10"
direction-links="true"
boundary-links="true">
</dir-pagination-controls>
</div>
</div>
</div>
</div>
</div>
<div id="loading" ng-show="loading">
<div id="loading-image">
<img src="<?php echo public_url() . 'assets/admin/img/loading.gif' ?>" alt=""/>
<h4>Please wait...</h4>
</div>
</div><file_sep>/application/controllers/News_Controller.php
<?php
/**
* Created by PhpStorm.
* User: psybo-03
* Date: 26/4/17
* Time: 10:27 AM
*/
defined('BASEPATH') or exit('No Direct Script Access Allowed');
require_once(APPPATH . 'core/Check_Logged.php');
class News_Controller extends Check_Logged
{
function __construct()
{
parent::__construct();
$this->load->model('News_Model', 'news');
$this->load->model('Files_Model', 'file');
$this->load->library(['upload', 'image_lib']);
if (!$this->logged) {
redirect(base_url('login'));
}
}
function index()
{
}
function get_all()
{
$data = $this->news->select();
$this->output->set_content_type('application/json')->set_output(json_encode($data));
}
function store()
{
$this->form_validation->set_rules('heading', 'Heading', 'required');
$this->form_validation->set_rules('content', 'Content', 'required');
$this->form_validation->set_rules('date', 'Date', 'required');
if ($this->form_validation->run() === FALSE) {
$this->output->set_status_header(400, 'Validation Error');
$this->output->set_content_type('application/json')->set_output(json_encode(validation_errors()));
} else {
$post_data = $this->input->post();
$uploaded = json_decode($post_data['uploaded']);
unset($post_data['uploaded']);
if (!empty($uploaded)) {
/*INSERT FILE DATA TO DB*/
$file_data['file_name'] = $uploaded->file_name;
$file_data['file_type'] = $uploaded->file_type;
$file_data['size'] = $uploaded->file_size;
$file_data['date'] = $this->input->post('date');
$file_id = $this->file->add($file_data);
$post_data['file_id'] = $file_id;
$resize_error = [];
if ($file_id and $this->news->add($post_data)) {
/*****Create Thumb Image****/
$img_cfg['source_image'] = getwdir() . 'uploads/' . $uploaded->file_name;
$img_cfg['maintain_ratio'] = TRUE;
$img_cfg['new_image'] = getwdir() . 'uploads/thumb/thumb_' . $uploaded->file_name;
$img_cfg['quality'] = 99;
$img_cfg['master_dim'] = 'height';
$this->image_lib->initialize($img_cfg);
if (!$this->image_lib->resize()) {
$resize_error[] = $this->image_lib->display_errors();
}
$this->image_lib->clear();
/********End Thumb*********/
/*resize and create thumbnail image*/
if ($uploaded->file_size > 1024) {
$img_cfg['image_library'] = 'gd2';
$img_cfg['source_image'] = getwdir() . 'uploads/' . $uploaded->file_name;
$img_cfg['maintain_ratio'] = TRUE;
$img_cfg['new_image'] = getwdir() . 'uploads/' . $uploaded->file_name;
$img_cfg['height'] = 500;
$img_cfg['quality'] = 100;
$img_cfg['master_dim'] = 'height';
$this->image_lib->initialize($img_cfg);
if (!$this->image_lib->resize()) {
$resize_error[] = $this->image_lib->display_errors();
}
$this->image_lib->clear();
/********End resize*********/
if (empty($resize_error)) {
$this->output->set_content_type('application/json')->set_output(json_encode($post_data));
} else {
$this->output->set_status_header(402, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode($resize_error));
}
} else {
$this->output->set_content_type('application/json')->set_output(json_encode($post_data));
}
}
} else {
if ($this->news->add($post_data)) {
$this->output->set_content_type('application/json')->set_output(json_encode($post_data));
} else {
$this->output->set_status_header(402, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Add Error']));
}
}
}
}
function update($id)
{
$this->form_validation->set_rules('heading', 'Heading', 'required');
$this->form_validation->set_rules('content', 'Content', 'required');
if ($this->form_validation->run() === FALSE) {
$this->output->set_status_header(400, 'Validation Error');
$this->output->set_content_type('application/json')->set_output(json_encode(validation_errors()));
} else {
if ($this->input->post('date') == '0000-00-00') {
$date = null;
}else{
$date = $this->input->post('date');
}
$uploaded = json_decode($this->input->post('uploaded'));
if (empty($uploaded)) {
$data['heading'] = $this->input->post('heading');
$data['content'] = $this->input->post('content');
$data['date'] = $date;
if ($this->news->edit($data,$id)) {
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Updated']));
} else {
$this->output->set_status_header(500, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'server Down']));
}
} else {
$file_data['file_name'] = $uploaded->file_name;
$file_data['file_type'] = $uploaded->file_type;
$file_data['size'] = $uploaded->file_size;
$file_data['date'] = $date;
$file_id = $this->file->add($file_data);
if ($file_id) {
$data = [];
$data['heading'] = $this->input->post('heading');
$data['content'] = $this->input->post('content');
$data['date'] = $date;
$data['file_id'] = $file_id;
if ($this->news->edit($data, $id)) {
if ($this->input->post('file_id') != 'null') {
if ($this->file->remove($this->input->post('file_id'))) {
if (file_exists(getwdir() . 'uploads/' . $this->input->post('file_name'))) {
unlink(getwdir() . 'uploads/' . $this->input->post('file_name'));
}
}
}
/*****Create Thumb Image****/
$img_cfg['source_image'] = getwdir() . 'uploads/' . $uploaded->file_name;
$img_cfg['maintain_ratio'] = TRUE;
$img_cfg['new_image'] = getwdir() . 'uploads/thumb/thumb_' . $uploaded->file_name;
$img_cfg['quality'] = 99;
$img_cfg['master_dim'] = 'height';
$this->image_lib->initialize($img_cfg);
if (!$this->image_lib->resize()) {
$resize_error[] = $this->image_lib->display_errors();
}
$this->image_lib->clear();
/********End Thumb*********/
/*resize and create thumbnail image*/
if ($uploaded->file_size > 1024) {
$img_cfg['image_library'] = 'gd2';
$img_cfg['source_image'] = getwdir() . 'uploads/' . $uploaded->file_name;
$img_cfg['maintain_ratio'] = TRUE;
$img_cfg['new_image'] = getwdir() . 'uploads/' . $uploaded->file_name;
$img_cfg['height'] = 500;
$img_cfg['quality'] = 100;
$img_cfg['master_dim'] = 'height';
$this->image_lib->initialize($img_cfg);
if (!$this->image_lib->resize()) {
$resize_error[] = $this->image_lib->display_errors();
}
$this->image_lib->clear();
/********End resize*********/
if (empty($resize_error)) {
$this->output->set_content_type('application/json')->set_output(json_encode($date));
} else {
$this->output->set_status_header(402, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode($resize_error));
}
} else {
$this->output->set_content_type('application/json')->set_output(json_encode($data));
}
}else{
$this->output->set_status_header(402, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Server Error']));
}
}
}
}
}
function delete_image($id)
{
$news = $this->news->select_where(['id' => $id]);
if ($this->file->remove($news[0]->file_id)) {
$data['file_id'] = null;
if ($this->news->edit($data,$id)) {
if (file_exists(getwdir() . 'uploads/' . $news[0]->file_name)) {
unlink(getwdir() . 'uploads/' . $news[0]->file_name);
}
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Image Delete']));
}
}else{
$this->output->set_status_header(400, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Try again later']));
}
}
function upload()
{
$config['upload_path'] = getwdir() . 'uploads';
$config['allowed_types'] = 'jpg|png|jpeg|JPG|JPEG';
$config['max_size'] = 4096;
$config['file_name'] = 'N_' . rand();
$config['multi'] = 'ignore';
$this->upload->initialize($config);
if ($this->upload->do_upload('file')) {
$this->output->set_content_type('application/json')->set_output(json_encode($this->upload->data()));
}else{
$this->output->set_status_header(401, 'File Upload Error');
$this->output->set_content_type('application/json')->set_output(json_encode($this->upload->display_errors()));
}
}
public function delete($id)
{
$news = $this->news->select_where(['id' => $id]);
if ($this->news->remove($id)) {
if ($this->file->remove($news[0]->file_id) && file_exists(getwdir() . 'uploads/' . $news[0]->file_name)) {
unlink(getwdir() . 'uploads/' . $news[0]->file_name);
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'News Deleted']));
} else {
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'News Deleted and file delete error']));
}
} else {
$this->output->set_status_header(500, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Delete Error']));
}
}
}<file_sep>/application/views/admin/news.php
<div class="col-md-12">
<div class="row">
<button class="btn btn-primary" ng-click="newNews()"><i class="fa fa-plus"></i> Add</button>
<form class="form-horizontal" method="POST" ng-show="showform" ng-submit="addNews()">
<h3>New News</h3><br/>
<div class="form-group">
<label for="" class="control-label col-md-1">Heading</label>
<div class="col-md-8">
<textarea class="form-control" name="heading" ng-model="newnews.heading" required=""></textarea>
</div>
</div>
<div class="form-group">
<label for="" class="control-label col-md-1">Content</label>
<div class="col-md-8">
<textarea class="form-control" ng-model="newnews.content" name="content" required=""></textarea>
</div>
</div>
<div class="form-group">
<label class="control-label col-md-1" for="date">Date</label>
<div class="col-md-4">
<p class="input-group">
<input type="text" class="form-control" name="date" uib-datepicker-popup="dd-MMMM-yyyy" ng-model="date" is-open="popup2.opened" datepicker-options="dateOptions" ng-required="true" close-text="Close" readonly show-button-bar="false"/>
<span class="input-group-btn">
<button type="button" class="btn btn-default" ng-click="open2()"><i class="glyphicon glyphicon-calendar"></i></button>
</span>
</p>
</div>
</div>
<div class="form-group">
<label for="" class="control-label col-md-1">Photo</label>
<div class="col-md-4">
<button ngf-select="uploadFiles($files, $invalidFiles)" accept="image/*" ngf-max-height="5000" ngf-max-size="5MB">Select Files</button>
</div>
<div class="col-md-12">
Files:
<div class="row">
<ul class="list-group">
<li ng-repeat="f in files" style="font:smaller" class="list-group-item">
<div class="row">
<div class="col-sm-12">
<div class="col-sm-2">
<img ngf-src="f.$ngfBlobUrl" class="thumbnail" width="100px" ngf-no-object-url="true">
<span>{{f.name}} {{f.$errorParam}}</span>
</div>
<div class="col-sm-6">
<div class="row">
<div class="col-sm-8">
<div class="progress" ng-show="f.progress >= 0" ng-class="{cancel: uploadstatus == 1}">
<div ng-show="uploadstatus == 1">{{f.progressmsg}}</div>
<div class="progress-bar progress-bar-success" role="progressbar" aria-valuenow="40"
aria-valuemin="0" aria-valuemax="100" style="width:{{f.progress}}%" ng-show="uploadstatus != 1">
{{f.progress}}% Complete
</div>
</div>
</div>
<div class="col-sm-4">
<button class="btn btn-danger" type="button" ng-click="abort()">cancel</button>
</div>
</div>
</div>
</div>
</div>
</li>
<li ng-repeat="f in errFiles" style="font:smaller" class="list-group-item">{{f.name}} {{f.$error}} {{f.$errorParam}}
</li>
</ul>
</div>
{{errorMsg}}
</div>
<!-- for existing image-->
<div class="clearfix"></div>
<div class="row" ng-show="newnews.file_name" style="margin-left: 14px">
<div class="col-md-2">
<div class="thumbnail cus-thumb" ng-mouseover="showcaption=true" ng-mouseleave="showcaption=false">
<div class="caption" ng-show="showcaption">
<div id="content">
<a href="" class="label label-warning" rel="tooltip" title="Show">Show</a>
<a href="" class="label label-danger" rel="tooltip" title="delete" confirmed-click="deleteImage(newnews)" ng-confirm-click="Would you like to delete this item?!">delete</a>
</div>
</div>
<img src="{{newnews.thumbImgUrl}}" alt="thumbnails">
</div>
</div>
</div>
<div class="clearfix"></div>
</div>
<div class="form-group text-center">
<button class="btn btn-primary" type="submit">Save</button>
<button class="btn btn-danger" type="button" ng-click="hideForm()">Cancel</button>
</div>
</form>
<div class="row">
<form class="form-inline" ng-show="showtable">
<div class="form-group">
<label for="" class="control-label col-md-2">Show</label>
<div class="col-md-3">
<select name="numPerPage" ng-model="numPerPage" class="form-control"
ng-options="num for num in paginations">{{num}}
</select>
</div>
<label class="control-label col-md-2">Search</label>
<div class="col-md-3">
<input type="text" ng-model="search" class="form-control" placeholder="Search">
</div>
</div>
</form>
</div>
</div>
<div class="help-block" ng-show="!showtable">{{message}}</div>
<table class="table table-bordered" ng-show="showtable">
<thead>
<tr>
<th>#</th>
<th>Heading</th>
<th>Content</th>
<th>Date</th>
<th>image</th>
<th>action</th>
</tr>
</thead>
<tbody>
<tr dir-paginate="news in newses | filter:search | limitTo:pageSize | itemsPerPage:numPerPage">
<td>{{$index+1}}</td>
<td>{{news.heading}}</td>
<td><p class="description" popover-placement="top" uib-popover="{{news.content}}" popover-trigger="'mouseenter'">{{news.content}}</p></td></td>
<td><span ng-if="news.date!='0000-00-00'">{{news.date |date:'dd-MMM-yyyy'}}</span></td>
<td><a href="{{news.imgUrl}}"><img class="img img-thumbnail" src="{{news.thumbImgUrl}}" alt="thumbnail" width="25px" height="25px"/></a></td>
<td>
<div class="btn-group btn-group-xs" role="group">
<button type="button" class="btn btn-info" ng-click="showForm(news)">
<i class="fa fa-pencil"></i>
</button>
<button type="button" class="btn btn-danger" confirmed-click="deleteNews(news)" ng-confirm-click="Would you like to delete this item?!">
<i class="fa fa-trash-o"></i>
</button>
</div>
</td>
</tr>
</tbody>
</table>
<dir-pagination-controls
max-size="10"
direction-links="true"
boundary-links="true" >
</dir-pagination-controls>
</div>
<div id="loading" ng-show="loading">
<div id="loading-image">
<img src="<?php echo public_url() . 'assets/admin/img/loading.gif' ?>" alt=""/>
<h4>Please wait...</h4>
</div>
</div><file_sep>/application/views/team.php
<!--header start-->
<header id="header" class="tt-nav nav-border-bottom">
<div class="header-sticky light-header ">
<div class="container">
<div class="search-wrapper">
<div class="search-trigger pull-right">
<div class='search-btn'></div>
<i class="material-icons"></i>
</div>
<!-- Modal Search Form -->
<i class="search-close material-icons"></i>
<div class="search-form-wrapper">
<form action="#" class="white-form">
<div class="input-field">
<input type="text" name="search" id="search">
<label for="search" class="">Search Here...</label>
</div>
<button class="btn pink search-button waves-effect waves-light" type="submit"><i class="material-icons"></i></button>
</form>
</div>
</div><!-- /.search-wrapper -->
<div id="materialize-menu" class="menuzord">
<!--logo start-->
<a href="home" class="logo-brand">
<img src="<?php echo public_url()?>assets/img/logo.png" alt="" >
</a>
<!--logo end-->
<!--mega menu start-->
<ul class="menuzord-menu pull-right">
<!-- <li><a href="index.html">Home</a></li>
<li><a href="about.html">About Us</a></li>
<li><a href="service.html">Our Services</a></li>
<li><a href="gallery.html">Moments</a></li>
<li class="active"><a href="blog.html">Blog</a></li>
<li><a href="contact.html">Contact Us</a></li> -->
<?php echo menu('Blog') ?>
</ul>
<!--mega menu end-->
</div>
</div>
</div>
</header>
<!--header end-->
<!--page title start-->
<section class="page-title pattern-bg ptb-50">
<div class="container">
<div class="row">
<div class="col-md-12">
<h2>Classic International</h2>
<span>Our Professional Teams</span>
<ol class="breadcrumb">
<li><a href="home">Home</a></li>
<li><a href="about">About Us</a></li>
<li class="active">Our Teams</li>
</ol>
</div>
</div>
</div>
</section>
<!--page title end-->
<section class="section-padding">
<div class="container">
<div class="text-center mb-80">
<h2 class="section-title text-uppercase">Our Team</h2>
<p class="section-sub">"Individual commitment to a group effort... That is what makes a Team work, a Company work, a Society work, a Civilization work."</p>
</div>
<div class="row">
<div class="col-md-3" style="margin-left: 38%;">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/a1.jpg" class="img-responsive" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
</div><!-- /.row -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/inner.png" alt="image">
</div>
<div class="row">
<div class="col-md-3" style="margin-left: 25%;">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/b1.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
<div class="col-md-3">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/b2.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
</div><!-- /.row -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/inner.png" alt="image">
</div>
<div class="row">
<div class="col-md-3" style="margin-left: 13%;">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/c2.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
<div class="col-md-3">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/c3.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
<div class="col-md-3">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/c4.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
</div><!-- /.row -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/inner.png" alt="image">
</div>
<div class="row">
<div class="col-md-3" style="margin-left: 25%;">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/d1.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
<div class="col-md-3">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/d2.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
</div><!-- /.row -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/inner.png" alt="image">
</div>
<div class="text-center mb-80">
<h2>Our Data Entry Operators</h2>
</div>
<div class="row">
<div class="col-md-3">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/e1.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
<div class="col-md-3">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/e2.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
<div class="col-md-3">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/e3.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
<div class="col-md-3">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/e4.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
<div class="col-md-3">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/e5.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
<div class="col-md-3">
<div class="team-wrapper">
<div class="team-img">
<a href="#"><img src="<?php echo public_url()?>assets/img/teams/e6.jpg" class="img-responsive mw80" alt="Image"></a>
</div><!-- /.team-img -->
<ul class="team-social-links list-inline text-center">
<li><a href="#"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
</ul>
</div>
</div>
</div><!-- /.row -->
</div><!-- /.container -->
</section><file_sep>/application/views/admin/gallery.php
<div class="col-md-12" ng-class="{disable : loading} ">
<div class="row" style="padding-left: 14px">
<div class="box">
<button class="btn btn-primary" ng-click="newGallery()"><i class="fa fa-plus"></i> Add</button>
<form class="form-horizontal" method="POST" ng-submit="addGallery()" ng-show="showform" name="addform" enctype="multipart/form-data">
<h3>New Gallery</h3>
<div class="form-group">
<label for="" class="control-label col-md-1">Name</label>
<div class="col-md-4">
<input type="text" class="form-control" name="name" ng-model="newgallery.gallery_name" placeholder="special character Not allowed ( !@#$%^&*() )"/>
</div>
<label for="" class="control-label col-md-1">Description</label>
<div class="col-md-4">
<textarea class="form-control" name="description" ng-model="newgallery.description"></textarea>
</div>
</div>
<div class="form-group">
<label for="" class="control-label col-md-1">Photo</label>
<div class="col-md-4">
<button ngf-select="uploadFiles($files, $invalidFiles)"
accept="image/*"
ngf-max-height="5000"
ngf-max-size="5MB"
ngf-multiple="true">
Select Files
</button>
<span class="alert alert-danger" ng-show="fileValidation.status == true">{{fileValidation.msg}}</span>
</div>
<div class="col-md-12">
Files:
<div class="row">
<ul class="list-group">
<li ng-repeat="f in files" style="font:smaller" class="list-group-item">
<div class="row">
<div class="col-sm-12">
<div class="col-sm-2">
<img ngf-src="f.$ngfBlobUrl" class="thumbnail" width="100px" ngf-no-object-url="true">
<span>{{f.name}} {{f.$errorParam}}</span>
</div>
<div class="col-sm-6">
<div class="row">
<div class="col-sm-8">
<div class="progress" ng-show="f.progress >= 0" ng-class="{cancel: uploadstatus == 1}">
<div ng-show="uploadstatus == 1">{{f.progressmsg}}</div>
<div class="progress-bar progress-bar-success" role="progressbar" aria-valuenow="40"
aria-valuemin="0" aria-valuemax="100" style="width:{{f.progress}}%" ng-show="uploadstatus != 1">
{{f.progress}}% Complete
</div>
</div>
</div>
<div class="col-sm-4">
<!-- <button class="btn btn-danger" type="button" ng-click="abort()">cancel</button>-->
</div>
</div>
</div>
</div>
</div>
</li>
<li class="bg-danger" ng-repeat="f in errFiles" style="font:smaller" class="list-group-item">{{f.name}} {{f.$error}} {{f.$errorParam}}
</li>
</ul>
</div>
<div class="row" ng-show="errorMsg">
<div class="alert alert-danger">
{{errorMsg}}
</div>
</div>
</div>
<span class="alert alert-warning">Image should be 100*100</span>
<!----for existing image----->
<div class="clearfix"></div>
<div class="row">
<div class="col-md-2" ng-repeat="(key,preview) in item_files">
<div class="thumbnail cus-thumb" ng-mouseover="showcaption=true" ng-mouseleave="showcaption=false" style="max-height: 142px;min-height: 142px">
<div class="caption" ng-show="showcaption">
<div id="content">
<a href="" class="label label-warning" rel="tooltip" title="Show">Show</a>
<a href="" class="label label-danger" rel="tooltip" title="Delete" confirmed-click="deleteImage(preview)" ng-confirm-click="Would you like to delete this item?!">Delete</a>
</div>
</div>
<img src="{{preview.thumbImgUrl}}" alt="thumbnails">
</div>
</div>
</div>
<div class="clearfix"></div>
</div>
<div class="container" ng-show="show_error">
<div class="row">
<div class="col-md-6">
<div class="alert alert-danger alert-dismissable fade in ">
<a href="" class="close" data-dismiss="alert" arial-label="close">×</a>
<h4>following files are not uploaded</h4>
<p ng-repeat-start="err in error">{{err}}</p>
<hr ng-repeat-end />
</div>
</div>
</div>
</div>
<div class="form-group text-center">
<button class="btn btn-primary" type="submit">Save</button>
<button class="btn btn-danger" type="button" ng-click="hideForm()">Cancel</button>
</div>
</form>
</div>
<div class="clearfix"></div>
<hr/>
<div class="row">
<div class="container-fluid">
<div class="row">
<div class="col-lg-3" dir-paginate="gallery in galleries | filter:search | limitTo:pageSize | itemsPerPage:numPerPage">
<div class="cuadro_intro_hover thumbnail" style="background-color:#cccccc;">
<p style="text-align:center;">
<img class="img-responsive" style="cursor:pointer;" src="{{gallery.files[0].thumbImgUrl}}" alt="{{gallery.name}}" />
</p>
<div class="caption">
<div class="blur"></div>
<div class="caption-text">
<h3 style="border-top:2px solid white; border-bottom:2px solid white; padding:10px;">{{gallery.gallery_name}}</h3>
<p>{{gallery.description}}</p>
<button type="button" style="color: initial;" class="btn btn-default" data-toggle="modal" data-target="#gallery" ng-click="showGalleryFiles(gallery)">Open</button>
<button type="button" style="color: initial;" class="btn btn-info" ng-click="ShowForm(gallery)">edit</button>
<button type="button" style="color: initial;" class="btn btn-danger" confirmed-click="deleteGallery(gallery)" ng-confirm-click="Would you like to delete this item?!">delete</button>
</div>
</div>
</div>
</div>
</div>
<div class="pull-right">
<dir-pagination-controls
max-size="10"
direction-links="true"
boundary-links="true">
</dir-pagination-controls>
</div>
<div class="modal" id="gallery" role="dialog">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4>{{galleryfiles.gallery_name}}</h4>
</div>
<div class="modal-body">
<div class="row">
<div class="col-md-2" ng-repeat="file in galleryfiles.files">
<div class="thumbnail img-responsive">
<a href="{{file.imgUrl}}">
<img src="{{file.thumbImgUrl}}" alt="{{file.file_name}}"/>
</a>
</div>
</div>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">close</button>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<div id="loading" ng-show="loading">
<div id="loading-image">
<img src="<?php echo public_url() . 'assets/admin/img/loading.gif' ?>" alt=""/>
<h4>Please wait...</h4>
</div>
</div><file_sep>/application/controllers/Brochure_Controller.php
<?php
/**
* Created by PhpStorm.
* User: psybo-03
* Date: 4/5/17
* Time: 1:02 PM
*/
defined('BASEPATH') or exit('No Direct Script Access Allowed');
require_once(APPPATH . 'core/Check_Logged.php');
class Brochure_Controller extends Check_Logged
{
function __construct()
{
parent::__construct();
$this->load->model('Brochures_Model', 'brochure');
$this->load->model('Files_Model', 'file');
$this->load->library(['upload', 'image_lib']);
if (!$this->logged) {
redirect(base_url('login'));
}
}
function index()
{
}
function get_all()
{
$data = $this->brochure->select();
$this->output->set_content_type('application/json')->set_output(json_encode($data));
}
function store()
{
$this->form_validation->set_rules('name', 'Name', 'required');
$this->form_validation->set_rules('date', 'Date', 'required');
if ($this->form_validation->run() === FALSE) {
$this->output->set_status_header(400, 'Validation Error');
$this->output->set_content_type('application/json')->set_output(json_encode(validation_errors()));
} else {
$post_data = $this->input->post();
$uploaded = json_decode($post_data['uploaded']);
unset($post_data['uploaded']);
if (!empty($uploaded)) {
/*INSERT FILE DATA TO DB*/
$file_data['file_name'] = $uploaded->file_name;
$file_data['file_type'] = $uploaded->file_type;
$file_data['size'] = $uploaded->file_size;
$file_data['date'] = $this->input->post('date');
$file_id = $this->file->add($file_data);
$post_data['file_id'] = $file_id;
if ($file_id and $this->brochure->add($post_data)) {
$this->output->set_content_type('application/json')->set_output(json_encode($post_data));
}
} else {
$this->output->set_status_header(403, 'Validation Error');
$this->output->set_content_type('application/json')->set_output(json_encode(['validation_error' => 'select any file']));
}
}
}
function update($id)
{
$this->form_validation->set_rules('name', 'Name', 'required');
$this->form_validation->set_rules('date', 'Date', 'required');
if ($this->form_validation->run() === FALSE) {
$this->output->set_status_header(400, 'Validation Error');
$this->output->set_content_type('application/json')->set_output(json_encode(validation_errors()));
} else {
if ($this->input->post('date') == '0000-00-00') {
$date = null;
}else{
$date = $this->input->post('date');
}
$uploaded = json_decode($this->input->post('uploaded'));
if (empty($uploaded)) {
$data['name'] = $this->input->post('name');
$data['subject'] = $this->input->post('subject');
$data['date'] = $date;
if ($this->brochure->edit($data,$id)) {
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Updated']));
} else {
$this->output->set_status_header(500, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'server Down']));
}
} else {
$file_data['file_name'] = $uploaded->file_name;
$file_data['file_type'] = $uploaded->file_type;
$file_data['size'] = $uploaded->file_size;
$file_data['date'] = $date;
$file_id = $this->file->add($file_data);
if ($file_id) {
$data = [];
$data['name'] = $this->input->post('name');
$data['subject'] = $this->input->post('subject');
$data['date'] = $date;
$data['file_id'] = $file_id;
if ($this->brochure->edit($data, $id)) {
if ($this->input->post('file_id') != 'null') {
if ($this->file->remove($this->input->post('file_id'))) {
if (file_exists(getwdir() . 'uploads/' . $this->input->post('file_name'))) {
unlink(getwdir() . 'uploads/' . $this->input->post('file_name'));
}
}
$this->output->set_content_type('application/json')->set_output(json_encode($data));
} else {
$this->output->set_content_type('application/json')->set_output(json_encode($data));
}
}else{
$this->output->set_status_header(402, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Server Error']));
}
}
}
}
}
function upload()
{
$config['upload_path'] = getwdir() . 'uploads';
$config['allowed_types'] = 'pdf|docx|doc|xlsx|word|csv|odt|odp|ods';
$config['max_size'] = 4096;
$config['file_name'] = 'B_' . rand();
$config['multi'] = 'ignore';
$this->upload->initialize($config);
if ($this->upload->do_upload('file')) {
$this->output->set_content_type('application/json')->set_output(json_encode($this->upload->data()));
}else{
$this->output->set_status_header(401, 'File Upload Error');
$this->output->set_content_type('application/json')->set_output(json_encode($this->upload->display_errors()));
}
}
public function delete($id)
{
$brochure = $this->brochure->select_where(['id' => $id]);
if ($this->brochure->remove($id)) {
if ($this->file->remove($brochure[0]->file_id) && file_exists(getwdir() . 'uploads/' . $brochure[0]->file_name)) {
unlink(getwdir() . 'uploads/' . $brochure[0]->file_name);
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Brochure Deleted']));
} else {
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Brochure Deleted and file delete error']));
}
} else {
$this->output->set_status_header(500, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Delete Error']));
}
}
}<file_sep>/application/controllers/Helpful_Link_Controller.php
<?php
/**
* Created by PhpStorm.
* User: psybo-03
* Date: 4/5/17
* Time: 1:02 PM
*/
defined('BASEPATH') or exit('No Direct Script Access Allowed');
require_once(APPPATH . 'core/Check_Logged.php');
class Helpful_Link_Controller extends Check_Logged
{
function __construct()
{
parent::__construct();
$this->load->model('Helpful_Links_Model', 'helpful_link');
if (!$this->logged) {
redirect(base_url('login'));
}
}
function index()
{
}
function get_all()
{
$data = $this->helpful_link->select();
$this->output->set_content_type('application/json')->set_output(json_encode($data));
}
function store()
{
$this->form_validation->set_rules('name', 'Name', 'required');
$this->form_validation->set_rules('link', 'Link', 'required');
if ($this->form_validation->run() === FALSE) {
$this->output->set_status_header(400, 'Validation Error');
$this->output->set_content_type('application/json')->set_output(json_encode(validation_errors()));
} else {
$post_data = $this->input->post();
if ($this->helpful_link->add($post_data)) {
$this->output->set_content_type('application/json')->set_output(json_encode($post_data));
}
else {
$this->output->set_status_header(500, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Add error.Try again later']));
}
}
}
function update($id)
{
$this->form_validation->set_rules('name', 'Name', 'required');
$this->form_validation->set_rules('link', 'Link', 'required');
if ($this->form_validation->run() === FALSE) {
$this->output->set_status_header(400, 'Validation Error');
$this->output->set_content_type('application/json')->set_output(json_encode(validation_errors()));
} else {
$data['name'] = $this->input->post('name');
$data['link'] = $this->input->post('link');
if ($this->helpful_link->edit($data, $id)) {
$this->output->set_content_type('application/json')->set_output(json_encode($data));
}else{
$this->output->set_status_header(402, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Server Error']));
}
}
}
public function delete($id)
{
$helpful_link = $this->helpful_link->select_where(['id' => $id]);
if ($helpful_link != FALSE) {
if ($this->helpful_link->remove($id)) {
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Brochure Deleted.']));
} else {
$this->output->set_status_header(500, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Delete Error']));
}
}else{
$this->output->set_status_header(404, 'Not found');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'The record Not found']));
}
}
}<file_sep>/application/views/service.php
<!--header start-->
<header id="header" class="tt-nav nav-border-bottom">
<div class="header-sticky light-header ">
<div class="container">
<div class="search-wrapper">
<div class="search-trigger pull-right">
<div class='search-btn'></div>
<i class="material-icons"></i>
</div>
<!-- Modal Search Form -->
<i class="search-close material-icons"></i>
<div class="search-form-wrapper">
<form action="#" class="white-form">
<div class="input-field">
<input type="text" name="search" id="search">
<label for="search" class="">Search Here...</label>
</div>
<button class="btn pink search-button waves-effect waves-light" type="submit"><i class="material-icons"></i></button>
</form>
</div>
</div><!-- /.search-wrapper -->
<div id="materialize-menu" class="menuzord">
<!--logo start-->
<a href="home" class="logo-brand">
<img src="<?php echo public_url()?>assets/img/logo.png" alt="" >
</a>
<!--logo end-->
<!--mega menu start-->
<ul class="menuzord-menu pull-right">
<!-- <li class="active"><a href="index.html">Home</a></li>
<li><a href="about.html">About Us</a></li>
<li><a href="service.html">Our Services</a></li>
<li><a href="gallery.html">Moments</a></li>
<li><a href="blog.html">Blog</a></li>
<li><a href="contact.html">Contact Us</a></li> -->
<?php echo menu('Our Services') ?>
</ul>
<!--mega menu end-->
</div>
</div>
</div>
</header>
<!--header end-->
<!--page title start-->
<section class="page-title pattern-bg ptb-50">
<div class="container">
<div class="row">
<div class="col-md-12">
<h2>Classic International</h2>
<span>We Serve Your Needs</span>
<ol class="breadcrumb">
<li><a href="#">Home</a></li>
<li class="active">Our Services</li>
</ol>
</div>
</div>
</div>
</section>
<section id="count3" class="facts-two">
<div class="container">
<div class="row text-center">
<div class="col-sm-3 counter-wrap">
<i class="material-icons brand-color"></i>
<span class="timer">1545</span>
<span class="count-description">Client Work With Us</span>
</div> <!-- /.col-sm-3 -->
<div class="col-sm-3 counter-wrap">
<i class="material-icons brand-color"></i>
<span class="timer">535</span>
<span class="count-description">Years of Experience</span>
</div><!-- /.col-sm-3 -->
<div class="col-sm-3 counter-wrap">
<i class="material-icons brand-color"></i>
<span class="timer">1544</span>
<span class="count-description">Cups Of Coffee</span>
</div><!-- /.col-sm-3 -->
<div class="col-sm-3 counter-wrap">
<i class="material-icons brand-color"></i>
<span class="timer">111</span>
<span class="count-description">Awards Won</span>
</div><!-- /.col-sm-3 -->
</div>
</div><!-- /.container -->
</section>
<!--page title end-->
<section class="section-padding banner-6 parallax-bg bg-fixed overlay light-9" data-stellar-background-ratio="0.5">
<div class="container">
<div class="text-center">
<h2 class="section-title text-uppercase">Our Services</h2>
<p class="section-sub">Classic International is Leading Typing and Translation Service Provider in India and Kuwait. Also We offer Health Insurances And Educational Insurances. </p>
</div>
</div><!-- /.container -->
</section>
<section id="services1" class="padding-top-70">
<div class="container">
<div class="row">
<div class="col-md-8">
<h2 class="font-30 mb-30">Typing Services</h2>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua.
</p>
<div class="row">
<div class="col-md-6">
<ul class="check-circle-list">
<li><i class="fa fa-check-square mr-10"></i> English Arabic and Malayalam typing </li>
<li><i class="fa fa-check-square mr-10"></i> All kind of Visa typing </li>
<li><i class="fa fa-check-square mr-10"></i> Passport, shuoonand civil id forms typing </li>
<li><i class="fa fa-check-square mr-10"></i> Medical and finger print office forms typing </li>
<li><i class="fa fa-check-square mr-10"></i> Indian/Pakistan embassy forms typing </li>
<li><i class="fa fa-check-square mr-10"></i> Different types of CV, bio data and all kind of documents typing </li>
</ul>
</div>
<div class="col-md-6">
<ul class="check-circle-list">
<li><i class="fa fa-check-square mr-10"></i> School admission and related forms typing </li>
<li><i class="fa fa-check-square mr-10"></i> All kind of job applications typing </li>
<li><i class="fa fa-check-square mr-10"></i> Driving license application and related forms typing </li>
<li><i class="fa fa-check-square mr-10"></i> Marriage Affidavits, Relation/Non Relation Affidavits </li>
<li><i class="fa fa-check-square mr-10"></i> Employment Contracts for Servants/Maids </li>
</ul>
</div>
</div>
</div>
<div class="col-md-4">
<img src="<?php echo public_url()?>assets/img/bg/typingServices.png" class="img-responsive animated slideInLeft" alt="Image">
</div>
</div>
</div><!-- /.container -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<section id="services2" class="padding-top-70 animated fadeInLeft">
<div class="container">
<div class="row">
<div class="col-md-4">
<img src="<?php echo public_url()?>assets/img/bg/ministryHealth.png" class="img-responsive animated slideInRight mt-50" alt="Image">
</div>
<div class="col-md-8">
<h2 class="font-30 mb-30">Services for Ministry of Health</h2>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua.
</p>
<div class="row">
<div class="col-md-8">
<ul class="check-circle-list">
<li><i class="fa fa-check-square mr-10"></i> Arrange Bio data in order as per ministry requirements </li>
<li><i class="fa fa-check-square mr-10"></i> Certificate attestation from all department in India </li>
<li><i class="fa fa-check-square mr-10"></i> Good conduct certificate from Nursing council in India and assisting you throughout the selection process </li>
<li><i class="fa fa-check-square mr-10"></i> Arabic Class (it will be conducted during processing time) </li>
<li><i class="fa fa-check-square mr-10"></i> Certificate verification from Ministry of Lower education and Ministry of Higher education of Kuwait. </li>
<li><i class="fa fa-check-square mr-10"></i> Submission for Civil service commission, Medical + Finger + Medical license's related processing Report submission </li>
</ul>
</div>
<div class="col-md-4">
<ul class="check-circle-list">
<li><i class="fa fa-check-square mr-10"></i> Passport submission </li>
<li><i class="fa fa-check-square mr-10"></i> Getting appointment Order </li>
<li><i class="fa fa-check-square mr-10"></i> File closing </li>
<li><i class="fa fa-check-square mr-10"></i> File opening </li>
<li><i class="fa fa-check-square mr-10"></i> Bank account opening process </li>
<li><i class="fa fa-check-square mr-10"></i> Salary Fixation(Budgeting) </li>
</ul>
</div>
</div>
</div>
</div>
</div><!-- /.container -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<section id="services3" class="padding-top-70">
<div class="container">
<div class="row">
<div class="col-md-7">
<h2 class="font-30 mb-30 mt-60">Services for Higher & Lower Education</h2>
<p>
Certificate verification from Ministry of Lower education and Ministry of Higher education of Kuwait. We prepare online application, scanning and uploading your certificates, related to your qualification.
</p>
</div>
<div class="col-md-5">
<img src="<?php echo public_url()?>assets/img/bg/edu.png" class="img-responsive animated1 fadeInDownBig" alt="Image">
</div>
</div>
</div><!-- /.container -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<section id="services4" class="padding-top-70">
<div class="container">
<div class="row">
<div class="col-md-5">
<img src="<?php echo public_url()?>assets/img/bg/fingerprint.png" class="img-responsive animated slideInLeft" alt="Image">
</div>
<div class="col-md-7">
<h2 class="font-30 mb-30 mt-60">Medical Fingerprint Assists Services</h2>
<p>
We can assist you to process your family's medical, finger prints and related reports.
</p>
</div>
</div>
</div><!-- /.container -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<section id="services5" class="padding-top-70">
<div class="container">
<div class="row">
<div class="col-md-7">
<h2 class="font-30 mb-30 mt-60">Insurance Services</h2>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua.
</p>
<div class="row">
<div class="col-md-12">
<ul class="check-circle-list">
<li><i class="fa fa-check-square mr-10"></i> For all your car insurance </li>
<li><i class="fa fa-check-square mr-10"></i> Industrial commercial and individual insurance needs…. </li>
<li><i class="fa fa-check-square mr-10"></i> c/o KIBB sulthan group </li>
</ul>
</div>
</div>
</div>
<div class="col-md-5">
<img src="<?php echo public_url()?>assets/img/bg/insurance.png" class="img-responsive animated fadeInDownBig" alt="Image">
</div>
</div>
</div><!-- /.container --><!-- -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<section id="services6" class="padding-top-70">
<div class="container">
<div class="row">
<div class="col-md-5">
<img src="<?php echo public_url()?>assets/img/bg/Health-Insurance.png" class="img-responsive animated fadeInDownBig" alt="Image">
</div>
<div class="col-md-7">
<h2 class="font-30 mb-30 mt-60">Health Insurance Services</h2>
<p>
We provide you primary details from form filling and also help for money transactions until the final stage.
</p>
</div>
</div>
</div><!-- /.container -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<section id="services7" class="padding-top-70">
<div class="container">
<div class="row">
<div class="col-md-7">
<h2 class="font-30 mb-30 mt-60">Education Services</h2>
<p>
We are sure to support your education plan by giving essential guidance for admission procedures .
</p>
</div>
<div class="col-md-5">
<img src="<?php echo public_url()?>assets/img/bg/Education.png" class="img-responsive animated fadeInDownBig" alt="Image">
</div>
</div>
</div><!-- /.container -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<section id="services8" class="padding-top-70">
<div class="container">
<div class="row">
<div class="col-md-5">
<img src="<?php echo public_url()?>assets/img/bg/transportation.png" class="img-responsive animated fadeInDownBig" alt="Image">
</div>
<div class="col-md-7">
<h2 class="font-30 mb-30 mt-60">Transportation services </h2>
<p>
Wherever you plan to go, we will serve you by reserving and booking taxi services in Kuwait.
</p>
</div>
</div>
</div><!-- /.container -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<section id="services9" class="padding-top-70">
<div class="container">
<div class="row">
<div class="col-md-7">
<h2 class="font-30 mb-30 mt-80">Pan Card Services</h2>
<p>
We will support you in your economic progress by issuing pan card.
</p>
</div>
<div class="col-md-5">
<img src="<?php echo public_url()?>assets/img/bg/pan.png" class="img-responsive animated fadeInDownBig" alt="Image" style="max-width: 80%;margin-bottom: 19px;">
</div>
</div>
</div><!-- /.container -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<section id="services10" class="padding-top-70">
<div class="container">
<div class="row">
<div class="col-md-5">
<img src="<?php echo public_url()?>assets/img/bg/air-tickets.png" class="img-responsive animated slideInLeft" alt="Image">
</div>
<div class="col-md-7">
<h2 class="font-30 mb-30">Air Tickets and Related Services</h2>
<p>
Whatever may be destination in your dream world, you can fly with us bybooking cheap flight tickets to there with our price beat guarantee. Find exclusive student/youth fares and exciting Round The World tickets. From simple weekend getaways to complex travel that includes multiple destinations. Classic Travel handles every detail with efficiency, courtesy, and credibility while always keeping your needs, desires, and comfort in mind. Where in the world do you want to go? Depend on classic Travel to get you there while also satisfying your expectations.
</p>
</div>
</div>
</div><!-- /.container -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<section id="services11" class="padding-top-70">
<div class="container">
<div class="row">
<div class="col-md-7">
<h2 class="font-30 mb-30 mt-40">Courier Services</h2>
<p>
We send mail by PROFESSIONAL Courier Service, hand to hand delivery all over the world within 72 working hours. We also send International Fax, Email, Attachment of Big document and any other electronic file; if you are looking for any of those services you can contact us immediately. We are ready to serve you.
</p>
</div>
<div class="col-md-5">
<img src="<?php echo public_url()?>assets/img/bg/courier.png" class="img-responsive animated fadeInDownBig" alt="Image">
</div>
</div>
</div><!-- /.container -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<section id="services12" class="padding-top-70">
<div class="container">
<div class="row">
<div class="col-md-4">
<img src="<?php echo public_url()?>assets/img/bg/mis.png" class="img-responsive animated fadeInLeftBig" alt="Image">
</div>
<div class="col-md-8">
<h2 class="font-30 mb-30">Miscellaneous Services</h2>
<p>
Ministry type & arrange by order bio data, certificate attestation from all department in India, Good conduct certificate from Nursing council in India and assisting you during and after selection.
</p>
<div class="row">
<div class="col-md-6">
<ul class="check-circle-list">
<li><i class="fa fa-check-square mr-10"></i> Photostat </li>
<li><i class="fa fa-check-square mr-10"></i> Color and black and white printing </li>
<li><i class="fa fa-check-square mr-10"></i> Scanning and mailing </li>
<li><i class="fa fa-check-square mr-10"></i> Health insurance </li>
<li><i class="fa fa-check-square mr-10"></i> Medical and finger print Service(Family Visa, Company Visa, Domestic Visa) </li>
<li><i class="fa fa-check-square mr-10"></i> English Arabic and Malayalam typing </li>
<li><i class="fa fa-check-square mr-10"></i> All kind of job applications typing </li>
</ul>
</div>
<div class="col-md-6">
<ul class="check-circle-list">
<li><i class="fa fa-check-square mr-10"></i> Passport, shuoon and civil id forms typing </li>
<li><i class="fa fa-check-square mr-10"></i> Medical and finger print office forms typing </li>
<li><i class="fa fa-check-square mr-10"></i> Indian Pakistan embassy forms typing </li>
<li><i class="fa fa-check-square mr-10"></i> School admission and related forms typing </li>
<li><i class="fa fa-check-square mr-10"></i> Driving license application and related forms typing </li>
<li><i class="fa fa-check-square mr-10"></i> Different types of CV and bio data typing </li>
</ul>
</div>
</div>
</div>
</div>
</div><!-- /.container -->
<div class="mocup-wrapper text-center">
<img src="<?php echo public_url()?>assets/img/bg/bottom.png" alt="image">
</div>
</section>
<div class="text-center mt-80 mb-80">
<h2 class="mb-30 text-uppercase">For any other queries. contact us here.</h2>
<a href="#" class="btn btn-lg waves-effect waves-light" data-toggle="modal" data-target="#applayServices">Contact Support</a>
</div>
<file_sep>/application/views/admin/helpful_links.php
<div class="col-md-12">
<div class="row">
<div class="box" style="margin-left: 14px;">
<button class="btn btn-primary" ng-click="newLink()"><i class="fa fa-plus"></i> Add</button>
<form class="form-horizontal" method="POST" ng-show="showform" ng-submit="addLink()">
<h3>New Link</h3>
<div class="form-group">
<label for="" class="control-label col-md-1">Name</label>
<div class="col-md-4">
<input type="text" class="form-control" name="name" ng-model="newlink.name" required=""/>
</div>
<label for="" class="control-label col-md-1">Link</label>
<div class="col-md-4">
<input class="form-control" type="text" ng-model="newlink.link" name="link" pattern="[-a-zA-Z0-9@:%_\+.~#?&//=]{2,256}\.[a-z]{2,4}\b(\/[-a-zA-Z0-9@:%_\+.~#?&//=]*)?" onblur="checkURL(this);" required=""/>
</div>
</div>
<div class="form-group text-center">
<button class="btn btn-primary" type="submit">Save</button>
<button class="btn btn-danger" type="button" ng-click="hideForm()">Cancel</button>
</div>
</form>
<form class="form-inline" ng-show="showtable">
<div class="form-group">
<label for="" class="control-label col-md-2">Show</label>
<div class="col-md-3">
<select name="numPerPage" ng-model="numPerPage" class="form-control"
ng-options="num for num in paginations">{{num}}
</select>
</div>
<label class="control-label col-md-2">Search</label>
<div class="col-md-3">
<input type="text" ng-model="search" class="form-control" placeholder="Search">
</div>
</div>
</form>
</div>
</div>
<div class="help-block" ng-show="!showtable">{{message}}</div>
<table class="table table-bordered" ng-show="showtable">
<thead>
<tr>
<th>#</th>
<th>Name</th>
<th>Link</th>
<th>action</th>
</tr>
</thead>
<tbody>
<tr dir-paginate="link in links | filter:search | limitTo:pageSize | itemsPerPage:numPerPage">
<td>{{$index+1}}</td>
<td>{{link.name}}</td>
<td>{{link.link}}</td>
<td>
<div class="btn-group btn-group-xs" role="group">
<button type="button" class="btn btn-info" ng-click="showForm(link)">
<i class="fa fa-pencil"></i>
</button>
<button type="button" class="btn btn-danger" confirmed-click="deleteLink(link)" ng-confirm-click="Would you like to delete this item?!">
<i class="fa fa-trash-o"></i>
</button>
</div>
</td>
</tr>
</tbody>
</table>
<dir-pagination-controls
max-size="10"
direction-links="true"
boundary-links="true" >
</dir-pagination-controls>
</div>
<div id="loading" ng-show="loading">
<div id="loading-image">
<img src="<?php echo public_url() . 'assets/admin/img/loading.gif' ?>" alt=""/>
<h4>Please wait...</h4>
</div>
</div><file_sep>/application/controllers/Gallery_Controller.php
<?php
/**
* Created by PhpStorm.
* User: psybo-03
* Date: 4/5/17
* Time: 4:38 PM
*/
defined('BASEPATH') or exit('No Direct Script Access Allowed');
require_once(APPPATH . 'core/Check_Logged.php');
class Gallery_Controller extends Check_Logged
{
function __construct()
{
parent::__construct();
$this->load->model('Galleries_Model', 'gallery');
$this->load->model('Gallery_Files_Model', 'gallery_files');
$this->load->model('Files_Model', 'file');
$this->load->library(['upload', 'image_lib']);
if (!$this->logged) {
redirect(base_url('login'));
}
}
function index()
{
}
function get_all()
{
$data = $this->gallery_files->select();
$this->output->set_content_type('application/json')->set_output(json_encode($data));
}
function store()
{
$this->form_validation->set_rules('gallery_name', 'Name', 'required');
if ($this->form_validation->run() === FALSE) {
$this->output->set_status_header(400, 'Validation Error');
$this->output->set_content_type('application/json')->set_output(json_encode(validation_errors()));
} else {
$post_data = $this->input->post();
$uploaded = json_decode($post_data['uploaded']);
unset($post_data['uploaded']);
if (!empty($uploaded) ) {
$gallery_id = $this->gallery->add($post_data);
if ($gallery_id) {
/*INSERT FILE DATA TO DB*/
foreach ($uploaded as $value) {
$file_data['file_name'] = $value->file_name;
$file_data['file_type'] = $value->file_type;
$file_data['size'] = $value->file_size;
$file_data['date'] = date('Y-m-d');
$file_id = $this->file->add($file_data);
$gallery_files_data['gallery_id'] = $gallery_id;
$gallery_files_data['file_id'] = $file_id;
if ($this->gallery_files->add($gallery_files_data)) {
/*****Create Thumb Image****/
$img_cfg['source_image'] = getwdir() . 'uploads/' . $value->file_name;
$img_cfg['maintain_ratio'] = TRUE;
$img_cfg['new_image'] = getwdir() . 'uploads/thumb/thumb_' . $value->file_name;
$img_cfg['quality'] = 99;
$img_cfg['master_dim'] = 'height';
$this->image_lib->initialize($img_cfg);
if (!$this->image_lib->resize()) {
$resize_error[] = $this->image_lib->display_errors();
}
$this->image_lib->clear();
/********End Thumb*********/
/*resize and create thumbnail image*/
if ($value->file_size > 1024) {
$img_cfg['image_library'] = 'gd2';
$img_cfg['source_image'] = getwdir() . 'uploads/' . $value->file_name;
$img_cfg['maintain_ratio'] = TRUE;
$img_cfg['new_image'] = getwdir() . 'uploads/' . $value->file_name;
$img_cfg['height'] = 500;
$img_cfg['quality'] = 100;
$img_cfg['master_dim'] = 'height';
$this->image_lib->initialize($img_cfg);
if (!$this->image_lib->resize()) {
$resize_error[] = $this->image_lib->display_errors();
}
$this->image_lib->clear();
/********End resize*********/
}
}
$resize_error = [];
if (empty($resize_error)) {
$this->output->set_content_type('application/json')->set_output(json_encode($post_data));
} else {
// $this->output->set_status_header(402, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode($resize_error));
}
}
}
} else {
$this->output->set_status_header(400, 'Validation Error');
$this->output->set_content_type('application/json')->set_output(json_encode(['validation_error' => 'Please select images.']));
}
}
}
function update($id){
$this->form_validation->set_rules('gallery_name', 'Name', 'required');
if ($this->form_validation->run() === FALSE) {
$this->output->set_status_header(400, 'Validation Error');
$this->output->set_content_type('application/json')->set_output(json_encode(validation_errors()));
} else {
$post_data = $this->input->post();
$uploaded = json_decode($post_data['uploaded']);
unset($post_data['uploaded']);
unset($post_data['files']);
if ($this->gallery->edit($post_data,$id)) {
if (!empty($uploaded)) {
/*INSERT FILE DATA TO DB*/
foreach ($uploaded as $value) {
$file_data['file_name'] = $value->file_name;
$file_data['file_type'] = $value->file_type;
$file_data['size'] = $value->file_size;
$file_data['date'] = $this->input->post('date');
$file_id = $this->file->add($file_data);
$gallery_files_data['gallery_id'] = $id;
$gallery_files_data['file_id'] = $file_id;
if ($this->gallery_files->add($gallery_files_data)) {
/*****Create Thumb Image****/
$img_cfg['source_image'] = getwdir() . 'uploads/' . $value->file_name;
$img_cfg['maintain_ratio'] = TRUE;
$img_cfg['new_image'] = getwdir() . 'uploads/thumb/thumb_' . $value->file_name;
$img_cfg['quality'] = 99;
$img_cfg['master_dim'] = 'height';
$this->image_lib->initialize($img_cfg);
if (!$this->image_lib->resize()) {
$resize_error[] = $this->image_lib->display_errors();
}
$this->image_lib->clear();
/********End Thumb*********/
/*resize and create thumbnail image*/
if ($value->file_size > 1024) {
$img_cfg['image_library'] = 'gd2';
$img_cfg['source_image'] = getwdir() . 'uploads/' . $value->file_name;
$img_cfg['maintain_ratio'] = TRUE;
$img_cfg['new_image'] = getwdir() . 'uploads/' . $value->file_name;
$img_cfg['height'] = 500;
$img_cfg['quality'] = 100;
$img_cfg['master_dim'] = 'height';
$this->image_lib->initialize($img_cfg);
if (!$this->image_lib->resize()) {
$resize_error[] = $this->image_lib->display_errors();
}
$this->image_lib->clear();
/********End resize*********/
}
}
$resize_error = [];
if (empty($resize_error)) {
$this->output->set_content_type('application/json')->set_output(json_encode($post_data));
} else {
$this->output->set_content_type('application/json')->set_output(json_encode($resize_error));
}
}
} else {
$this->output->set_content_type('application/json')->set_output(json_encode($post_data));
}
} else {
$this->output->set_status_header(500, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['validation_error' => 'Please select images.']));
}
}
}
function delete_image($id)
{
$gallery_files = $this->gallery_files->select_gl_fl_where(['gallery_files.id' => $id]);
if ($this->file->remove($gallery_files[0]->file_id) AND $this->gallery_files->remove($gallery_files[0]->id)) {
if (file_exists(getwdir() . 'uploads/' . $gallery_files[0]->file_name)) {
unlink(getwdir() . 'uploads/' . $gallery_files[0]->file_name);
}
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Image Delete']));
}else{
$this->output->set_status_header(400, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Try again later']));
}
}
function upload()
{
$config['upload_path'] = getwdir() . 'uploads';
$config['allowed_types'] = 'jpg|png|jpeg|JPG|JPEG';
$config['max_size'] = 4096;
$config['file_name'] = 'G_' . rand();
$config['multi'] = 'ignore';
$this->upload->initialize($config);
if ($this->upload->do_upload('file')) {
$this->output->set_content_type('application/json')->set_output(json_encode($this->upload->data()));
}else{
$this->output->set_status_header(401, 'File Upload Error');
$this->output->set_content_type('application/json')->set_output(json_encode($this->upload->display_errors()));
}
}
public function delete($id)
{
$gallery_files = $this->gallery_files->select_where($id);
if ($gallery_files) {
if (!empty($gallery_files[0]->files)) {
foreach ($gallery_files[0]->files as $file) {
if ($this->gallery_files->remove($file->id)) {
if ($this->file->remove($file->file_id) && file_exists(getwdir() . 'uploads/' . $file->file_name)) {
unlink(getwdir() . 'uploads/' . $file->file_name);
$status = 1;
} else {
$status = 0;
}
}
}
if ($status == 1) {
if ($this->gallery->remove($id)) {
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Gallery Deleted']));
}
} elseif ($status == 0) {
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Gallery not deleted but some files are deleted']));
}
} else {
if ($this->gallery->remove($id)) {
$this->output->set_content_type('application/json')->set_output(json_encode(['msg' => 'Gallery Deleted']));
} else {
$this->output->set_status_header(500, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'Delete Error']));
}
}
} else {
$this->output->set_status_header(500, 'Server Down');
$this->output->set_content_type('application/json')->set_output(json_encode(['error' => 'The Record Not found']));
}
}
}<file_sep>/application/views/templates/header.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="description" content="Classic International is Leading Typing and Translation Service Provider in India and Kuwait. Also We offer Health Insurances and Educational Insurances">
<meta name="keywords" content="Typing services, Services for Ministry of health, Services for higher & lower education, Medical finger print assists services, Health insurance services, Education services, Transportation services, Pan card services, Air tickets and related services, Courier services, Services in Kuwait">
<meta name="author" content="Psybo Technologies">
<title>Home | Classic International</title>
<!-- favicon -->
<link rel="shortcut icon" href="<?php echo public_url()?>assets/img/favicon.png">
<!-- apple-touch-icon -->
<link rel="apple-touch-icon-precomposed" sizes="144x144" href="<?php echo public_url()?>assets/img/ico/apple-touch-icon-144-precomposed.png">
<link rel="apple-touch-icon-precomposed" sizes="114x114" href="<?php echo public_url()?>assets/img/ico/apple-touch-icon-114-precomposed.png">
<link rel="apple-touch-icon-precomposed" sizes="72x72" href="<?php echo public_url()?>assets/img/ico/apple-touch-icon-72-precomposed.png">
<link rel="apple-touch-icon-precomposed" href="<?php echo public_url()?>assets/img/ico/apple-touch-icon-57-precomposed.png">
<link href='https://fonts.googleapis.com/css?family=Raleway:400,300,500,700,900' rel='stylesheet' type='text/css'>
<!-- FontAwesome CSS -->
<link href="<?php echo public_url()?>assets/fonts/font-awesome/css/font-awesome.min.css" rel="stylesheet">
<!-- Material Icons CSS -->
<link href="<?php echo public_url()?>assets/fonts/iconfont/material-icons.css" rel="stylesheet">
<!-- magnific-popup -->
<link href="<?php echo public_url()?>assets/magnific-popup/magnific-popup.css" rel="stylesheet">
<!-- owl.carousel -->
<link href="<?php echo public_url()?>assets/owl.carousel/assets/owl.carousel.css" rel="stylesheet">
<link href="<?php echo public_url()?>assets/owl.carousel/assets/owl.theme.default.min.css" rel="stylesheet">
<!-- flexslider -->
<link href="<?php echo public_url()?>assets/flexSlider/flexslider.css" rel="stylesheet">
<!-- materialize -->
<link href="<?php echo public_url()?>assets/materialize/css/materialize.min.css" rel="stylesheet">
<!-- Bootstrap -->
<link href="assets/bootstrap/css/bootstrap.css" rel="stylesheet">
<!-- shortcodes -->
<link href="<?php echo public_url()?>assets/css/shortcodes/shortcodes.css" rel="stylesheet">
<!-- Style CSS -->
<link href="<?php echo public_url()?>assets/css/style.css" rel="stylesheet">
<!-- RS5.0 Main Stylesheet -->
<link rel="stylesheet" type="text/css" href="<?php echo public_url()?>assets/revolution/css/settings.css">
<!-- RS5.0 Layers and Navigation Styles -->
<link rel="stylesheet" type="text/css" href="<?php echo public_url()?>assets/revolution/css/layers.css">
<link rel="stylesheet" type="text/css" href="<?php echo public_url()?>assets/revolution/css/navigation.css">
<!-- HTML5 shim and Respond.js for IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body id="top" class="has-header-search">
<!-- Top bar -->
<div class="top-bar dark-bg lighten-2 visible-md visible-lg">
<div class="container">
<div class="row">
<div class="col-md-6 text-left">
<ul class="topbar-cta no-margin">
<li class="mr-20">
<a><i class="material-icons mr-10"></i><EMAIL></a>
</li>
<li>
<a><i class="material-icons mr-10"></i> 99 530 177</a>
</li>
</ul>
</div>
<!-- Social Icon -->
<div class="col-md-6 text-right">
<!-- Social Icon -->
<ul class="list-inline social-top tt-animate btt">
<li><a href="https://www.facebook.com/ClassicInternational" target="_blank"><i class="fa fa-facebook"></i></a></li>
<li><a href="#"><i class="fa fa-twitter"></i></a></li>
<li><a href="#"><i class="fa fa-linkedin"></i></a></li>
<li class="link"><a class="thm-btn" href="#" data-toggle="modal" data-target="#applayServices">get a quote</a></li>
<!-- <li class="link hide" id="status"><a class="thm-btn" href="#">Sending...</a></li>-->
<!-- <li class="link hide"><a class="thm-btn" href="#">Sent</a></li>-->
</ul>
<!-- <div class="link">
<a href="#" class="thm-btn">get a quote</a>
</div> -->
</div>
</div><!-- /.row -->
</div><!-- /.container -->
</div><!-- /.top-bar -->
<file_sep>/application/views/gallery.php
<!--header start-->
<header id="header" class="tt-nav nav-border-bottom">
<div class="header-sticky light-header ">
<div class="container">
<div class="search-wrapper">
<div class="search-trigger pull-right">
<div class='search-btn'></div>
<i class="material-icons"></i>
</div>
<!-- Modal Search Form -->
<i class="search-close material-icons"></i>
<div class="search-form-wrapper">
<form action="#" class="white-form">
<div class="input-field">
<input type="text" name="search" id="search">
<label for="search" class="">Search Here...</label>
</div>
<button class="btn pink search-button waves-effect waves-light" type="submit"><i class="material-icons"></i></button>
</form>
</div>
</div><!-- /.search-wrapper -->
<div id="materialize-menu" class="menuzord">
<!--logo start-->
<a href="home" class="logo-brand">
<img src="<?php echo public_url()?>assets/img/logo.png" alt="" >
</a>
<!--logo end-->
<!--mega menu start-->
<ul class="menuzord-menu pull-right">
<!-- <li class="active"><a href="index.html">Home</a></li>
<li><a href="about.html">About Us</a></li>
<li><a href="service.html">Our Services</a></li>
<li><a href="gallery.html">Moments</a></li>
<li><a href="blog.html">Blog</a></li>
<li><a href="contact.html">Contact Us</a></li> -->
<?php echo menu('Moments') ?>
</ul>
<!--mega menu end-->
</div>
</div>
</div>
</header>
<!--header end-->
<!--page title start-->
<section class="page-title pattern-bg ptb-50">
<div class="container">
<div class="row">
<div class="col-md-12">
<h2>Classic International</h2>
<span>Life is not made up of minutes, hours, days, weeks, months, or years, but of moments.</span>
<ol class="breadcrumb">
<li><a href="#">Home</a></li>
<li class="active">Our Moments</li>
</ol>
</div>
</div>
</div>
</section>
<!--page title end-->
<section class="section-padding">
<div class="text-center mb-50">
<h2 class="section-title text-uppercase">Our Moments</h2>
<!-- <p class="section-sub">Quisque non erat mi. Etiam congue et augue sed tempus. Aenean sed ipsum luctus, scelerisque ipsum nec, iaculis justo. Sed at vestibulum purus, sit amet vived at vestibulum purus erra at vestibulum purus diam. Nulla ac nisi rhoncus,</p> -->
</div>
<div class="portfolio-container text-center">
<div class="portfolio col-4 mtb-50">
<!-- add "gutter" class for add spacing -->
<?php
if (isset($gallery) and $gallery != false) {
foreach ($gallery as $value) {
?>
<div class="portfolio-item">
<div class="portfolio-wrapper">
<div class="thumb">
<div class="bg-overlay"></div>
<div class="portfolio-slider" data-direction="vertical">
<ul class="slides">
<?php
foreach ($value->files as $file) { ?>
<li>
<a href="<?php echo $file->imgUrl ?>" title="materialize Unique Design">
<img src="<?php echo $file->imgUrl ?>" alt="">
</a>
</li>
<?php } ?>
</ul>
</div>
<div class="portfolio-intro">
<div class="action-btn">
<a href="#"> <i class="fa fa-search"></i> </a>
</div>
</div>
</div>
<!-- thumb -->
</div>
<!-- /.portfolio-wrapper -->
</div><!-- /.portfolio-item -->
<?php
}
}?>
</div><!-- /.portfolio -->
</div><!-- portfolio-container -->
</section>
<file_sep>/application/config/routes.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
/*
| -------------------------------------------------------------------------
| URI ROUTING
| -------------------------------------------------------------------------
| This file lets you re-map URI requests to specific controller functions.
|
| Typically there is a one-to-one relationship between a URL string
| and its corresponding controller class/method. The segments in a
| URL normally follow this pattern:
|
| example.com/class/method/id/
|
| In some instances, however, you may want to remap this relationship
| so that a different class/function is called than the one
| corresponding to the URL.
|
| Please see the user guide for complete details:
|
| https://codeigniter.com/user_guide/general/routing.html
|
| -------------------------------------------------------------------------
| RESERVED ROUTES
| -------------------------------------------------------------------------
|
| There are three reserved routes:
|
| $route['default_controller'] = 'welcome';
|
| This route indicates which controller class should be loaded if the
| URI contains no data. In the above example, the "welcome" class
| would be loaded.
|
| $route['404_override'] = 'errors/page_missing';
|
| This route will tell the Router which controller/method to use if those
| provided in the URL cannot be matched to a valid route.
|
| $route['translate_uri_dashes'] = FALSE;
|
| This is not exactly a route, but allows you to automatically route
| controller and method names that contain dashes. '-' isn't a valid
| class or method name character, so it requires translation.
| When you set this option to TRUE, it will replace ALL dashes in the
| controller and method URI segments.
|
| Examples: my-controller/index -> my_controller/index
| my-controller/my-method -> my_controller/my_method
*/
$route['default_controller'] = 'Home';
$route['login'] = 'Home/login';
/****HOME ROUTES START******/
$route['home'] = 'Home/index';
$route['about'] = 'Home/about';
$route['team'] = 'Home/team';
$route['services'] = 'Home/service';
$route['moments'] = 'Home/moments';
$route['blog'] = 'Home/blog';
$route['blogView/(:num)'] = 'Home/blogView/$1';
$route['contact'] = 'Home/contact';
$route['subscribe'] = 'Home/subscribe';
$route['request-quote'] = 'Home/request_quote';
$route['appointment-request'] = 'Home/appointment_request';
$route['contact-request'] = 'Home/contact_request';
$route['test'] = 'Home/test';
/****HOME ROUTES END******/
/****ADMIN******/
$route['admin'] = 'Dashboard';
$route['login/verify'] = 'Dashboard/verify';
$route['logout'] = 'Dashboard/logout';
$route['admin/check-thumb'] = 'Dashboard/thumbnail_check';
$route['admin/user'] = 'Dashboard/get_user';
$route['admin/change'] = 'Dashboard/change_profile';
$route['admin/change/submit'] = 'Dashboard/edit_profile';
/*NEWS*/
$route['admin/news'] = 'Dashboard/news';
$route['admin/news/get-all'] = 'News_Controller/get_all';
$route['admin/news/add'] = 'News_Controller/store';
$route['admin/news/edit/(:num)'] = 'News_Controller/update/$1';
$route['admin/news/upload'] = 'News_Controller/upload';
$route['admin/news/delete-image/(:num)'] = 'News_Controller/delete_image/$1';
$route['admin/news/delete/(:num)'] = 'News_Controller/delete/$1';
/*BROCHURE*/
$route['admin/brochures'] = 'Dashboard/brochure';
$route['admin/brochure/get-all'] = 'Brochure_Controller/get_all';
$route['admin/brochure/add'] = 'Brochure_Controller/store';
$route['admin/brochure/edit/(:num)'] = 'Brochure_Controller/update/$1';
$route['admin/brochure/upload'] = 'Brochure_Controller/upload';
$route['admin/brochure/delete-image/(:num)'] = 'Brochure_Controller/delete_image/$1';
$route['admin/brochure/delete/(:num)'] = 'Brochure_Controller/delete/$1';
/*HELPFUL-LINKS*/
$route['admin/helpful-links'] = 'Dashboard/helpful_link';
$route['admin/helpful-link/get-all'] = 'Helpful_Link_Controller/get_all';
$route['admin/helpful-link/add'] = 'Helpful_Link_Controller/store';
$route['admin/helpful-link/edit/(:num)'] = 'Helpful_Link_Controller/update/$1';
$route['admin/helpful-link/delete/(:num)'] = 'Helpful_Link_Controller/delete/$1';
/*GALLERY*/
$route['admin/gallery'] = 'Dashboard/gallery';
$route['admin/gallery/get-all'] = 'Gallery_Controller/get_all';
$route['admin/gallery/add'] = 'Gallery_Controller/store';
$route['admin/gallery/edit/(:num)'] = 'Gallery_Controller/update/$1';
$route['admin/gallery/upload'] = 'Gallery_Controller/upload';
$route['admin/gallery/delete-image/(:num)'] = 'Gallery_Controller/delete_image/$1';
$route['admin/gallery/delete/(:num)'] = 'Gallery_Controller/delete/$1';
/*SLIDE IMAGE*/
$route['admin/slide-images'] = 'Dashboard/slide_image';
$route['admin/slide-images/get-all'] = 'Slide_Image_Controller/get_all';
$route['admin/slide-images/upload'] = 'Slide_Image_Controller/upload';
$route['admin/slide-images/add'] = 'Slide_Image_Controller/store';
$route['admin/slide-images/delete/(:num)'] = 'Slide_Image_Controller/delete/$1';
/*BLOG*/
$route['admin/blog'] = 'Dashboard/blog';
$route['admin/blog/get-all'] = 'Blog_Controller/get_all';
$route['admin/blog/add'] = 'Blog_Controller/store';
$route['admin/blog/edit/(:num)'] = 'Blog_Controller/update/$1';
$route['admin/blog/upload'] = 'Blog_Controller/upload';
$route['admin/blog/delete-image/(:num)'] = 'Blog_Controller/delete_image/$1';
$route['admin/blog/delete/(:num)'] = 'Blog_Controller/delete/$1';
$route['404_override'] = '';
$route['translate_uri_dashes'] = FALSE;
<file_sep>/application/views/blogView.php
<!--header start-->
<header id="header" class="tt-nav nav-border-bottom">
<div class="header-sticky light-header ">
<div class="container">
<div class="search-wrapper">
<div class="search-trigger pull-right">
<div class='search-btn'></div>
<i class="material-icons"></i>
</div>
<!-- Modal Search Form -->
<i class="search-close material-icons"></i>
<div class="search-form-wrapper">
<form action="#" class="white-form">
<div class="input-field">
<input type="text" name="search" id="search">
<label for="search" class="">Search Here...</label>
</div>
<button class="btn pink search-button waves-effect waves-light" type="submit"><i class="material-icons"></i></button>
</form>
</div>
</div><!-- /.search-wrapper -->
<div id="materialize-menu" class="menuzord">
<!--logo start-->
<a href="home" class="logo-brand">
<img src="<?php echo public_url()?>assets/img/logo.png" alt="" >
</a>
<!--logo end-->
<!--mega menu start-->
<ul class="menuzord-menu pull-right">
<!-- <li><a href="index.html">Home</a></li>
<li><a href="about.html">About Us</a></li>
<li><a href="service.html">Our Services</a></li>
<li><a href="gallery.html">Moments</a></li>
<li class="active"><a href="blog.html">Blog</a></li>
<li><a href="contact.html">Contact Us</a></li> -->
<?php echo menu('Blog') ?>
</ul>
<!--mega menu end-->
</div>
</div>
</div>
</header>
<!--header end-->
<!--page title start-->
<section class="page-title pattern-bg ptb-50">
<div class="container">
<div class="row">
<div class="col-md-12">
<h2>Classic International</h2>
<span>Our Blog Page</span>
<ol class="breadcrumb">
<li><a href="home">Home</a></li>
<li><a href="index">Blog</a></li>
<li class="active">Blog View</li>
</ol>
</div>
</div>
</div>
</section>
<!--page title end-->
<!-- blog section start -->
<section class="blog-section section-padding">
<div class="container">
<div class="row">
<div class="col-md-8">
<?php
if (isset($blog) and $blog != FALSE) {
?>
<div class="posts-content single-post">
<article class="post-wrapper">
<header class="entry-header-wrapper clearfix">
<div class="entry-header">
<h2 class="entry-title"><?php echo $blog[0]->heading ?></h2>
<div class="entry-meta">
<ul class="list-inline">
<li>
<i class="fa fa-clock-o"></i><a href="#"><?php echo date('M d, Y',strtotime($blog[0]->date)) ?></a>
</li>
</ul>
</div>
<!-- .entry-meta -->
</div>
</header>
<!-- /.entry-header-wrapper -->
<div class="thumb-wrapper">
<img src="<?php echo $blog[0]->imgUrl?>"
class="img-responsive" alt="">
</div>
<!-- .post-thumb -->
<div class="entry-content">
<p><?php echo $blog[0]->content ?></p>
</div>
<!-- .entry-content -->
</article>
<!-- /.post-wrapper -->
</div><!-- /.posts-content -->
<?php
}
?>
</div><!-- /.col-md-8 -->
<div class="col-md-4">
<div class="tt-sidebar-wrapper" role="complementary">
<div class="widget widget_search">
<form role="search" method="get" class="search-form" >
<input type="text" class="form-control" value="" name="s" id="s" placeholder="Write any keywords">
<button type="submit"><i class="fa fa-search"></i></button>
</form>
</div><!-- /.widget_search -->
<div class="widget widget_tt_popular_post">
<div class="tt-popular-post border-bottom-tab">
<!-- Nav tabs -->
<ul class="nav nav-tabs">
<li class="active">
<a href="#tt-popular-post-tab1" data-toggle="tab" aria-expanded="true">Latest</a>
</li>
</ul>
<!-- Tab panes -->
<div class="tab-content">
<!-- latest post tab -->
<div id="tt-popular-post-tab1" class="tab-pane fade active in">
<?php
if (isset($blog_latest) and $blog_latest != FALSE) {
foreach ($blog_latest as $blog) {
?>
<div class="media">
<a class="media-left" href="<?php echo base_url() . 'blogView/'.$blog->id ?>">
<img
src="<?php echo $blog->imgUrl ?>"
alt="">
</a>
<div class="media-body">
<h4><a href="<?php echo base_url() . 'blogView/'.$blog->id ?>"><?php echo $blog->heading ?></a></h4>
</div>
<!-- /.media-body -->
</div> <!-- /.media -->
<?php
}
}?>
</div>
</div><!-- /.tab-content -->
</div><!-- /.tt-popular-post -->
</div><!-- /.widget_tt_popular_post -->
</div><!-- /.tt-sidebar-wrapper -->
</div><!-- /.col-md-4 -->
</div><!-- /.row -->
<nav class="single-post-navigation" role="navigation">
<div class="row">
<!-- Previous Post -->
<div class="col-xs-4">
<div class="previous-post-link">
<a class="waves-effect waves-light" href="#"><i class="fa fa-long-arrow-left"></i>Read Previous Post</a>
</div>
</div>
<!-- Back -->
<div class="col-xs-4">
<div class="previous-post-link">
<a class="waves-effect waves-light" href="blog"><i class="fa fa-home"></i>Back To Blog</a>
</div>
</div>
<!-- Next Post -->
<div class="col-xs-4">
<div class="next-post-link">
<a class="waves-effect waves-light" href="#">Read Next Post<i class="fa fa-long-arrow-right"></i></a>
</div>
</div>
</div> <!-- .row -->
</nav>
</div><!-- /.container -->
</section>
<!-- blog section end --><file_sep>/application/models/Slide_Image_Model.php
<?php
/**
* Created by PhpStorm.
* User: psybo-03
* Date: 25/4/17
* Time: 4:44 PM
*/
defined('BASEPATH') or exit ('No direct script access allowed');
require_once APPPATH . 'core/My_Model.php';
class Slide_Image_Model extends My_Model
{
protected $table = 'slide_images';
function __construct()
{
parent::__construct();
}
public function select($limit="", $order="")
{
$this->db->from($this->table);
if ($limit != "") {
$this->db->limit($limit);
}
if ($order != "") {
$this->db->order_by('id', $order);
}
$result = $this->db->get();
if ($result->num_rows() > 0) {
$data = $result->result();
foreach ($data as $value) {
if ($value->file_id != null) {
$query = $this->db->get_where('files', ['id' => $value->file_id]);
if ($query->num_rows() > 0) {
$file = $query->result();
$value->file_name = $file[0]->file_name;
$value->file_type = $file[0]->file_type;
$value->imgUrl = public_url() . 'uploads/' . $file[0]->file_name;
}
}
}
return $data;
}else
return FALSE;
}
public function select_where($where)
{
$this->db->from($this->table);
$this->db->where($where);
$result = $this->db->get();
if ($result->num_rows() > 0) {
$data = $result->result();
foreach ($data as $value) {
if ($value->file_id != null) {
$query = $this->db->get_where('files', ['id' => $value->file_id]);
if ($query->num_rows() > 0) {
$file = $query->result();
$value->file_name = $file[0]->file_name;
$value->file_type = $file[0]->file_type;
$value->imgUrl = public_url() . 'uploads/' . $file[0]->file_name;
$value->thumbImgUrl = public_url() . 'uploads/thumb/thumb_' . $file[0]->file_name;
}
}
}
return $data;
}else
return FALSE;
}
public function add($data)
{
return $this->insert($data);
}
public function edit($data, $id)
{
return $this->update($data, $id);
}
public function remove($id)
{
return $this->drop($id);
}
public function trunc()
{
return $this->truncate();
}
}
<file_sep>/application/models/Blog_Model.php
<?php
/**
* Created by PhpStorm.
* User: psybo-03
* Date: 25/4/17
* Time: 4:52 PM
*/
defined('BASEPATH') or exit ('No direct script access allowed');
require_once APPPATH . 'core/My_Model.php';
class Blog_Model extends My_Model
{
protected $table = 'blogs';
function __construct()
{
parent::__construct();
}
public function select($limit = "", $order = "")
{
// $this->db->select($this->fields);
$this->db->from($this->table);
if ($limit != "") {
$this->db->limit($limit);
}
if ($order != "") {
$this->db->order_by('id', $order);
}
$result = $this->db->get();
if ($result->num_rows() > 0) {
$data = $result->result();
foreach ($data as $value) {
if ($value->file_id != null) {
$query = $this->db->get_where('files', ['id' => $value->file_id]);
if ($query->num_rows() > 0) {
$file = $query->result();
$date = strtotime($value->date);
$value->day = date('d',$date);
$value->month = date('M',$date);
$value->file_name = $file[0]->file_name;
$value->file_type = $file[0]->file_type;
$value->imgUrl = public_url() . 'uploads/' . $file[0]->file_name;
$value->thumbImgUrl = public_url() . 'uploads/thumb/thumb_' . $file[0]->file_name;
}
}
}
return $data;
}else
return FALSE;
}
public function select_where(array $where)
{
$this->db->from($this->table);
$this->db->where($where);
$result = $this->db->get();
if ($result->num_rows() > 0) {
$data = $result->result();
foreach ($data as $value) {
if ($value->file_id != null) {
$query = $this->db->get_where('files', ['id' => $value->file_id]);
if ($query->num_rows() > 0) {
$file = $query->result();
$date = strtotime($value->date);
$value->day = date('d',$date);
$value->month = date('M',$date);
$value->file_name = $file[0]->file_name;
$value->file_type = $file[0]->file_type;
$value->imgUrl = public_url() . 'uploads/' . $file[0]->file_name;
$value->thumbImgUrl = public_url() . 'uploads/thumb/thumb_' . $file[0]->file_name;
}
}
}
return $data;
}else
return FALSE;
}
public function add($data)
{
return $this->insert($data);
}
public function edit($data, $id)
{
return $this->update($data, $id);
}
public function remove($id)
{
return $this->drop($id);
}
public function trunc()
{
return $this->truncate();
}
}
| f8e71048f081a7f2344d395f1745eb1d650c236b | [
"JavaScript",
"PHP"
]
| 29 | PHP | Noushid/nice-web | 8ce7b455b5a7558c95e17ae4a9e5f3319c4d37e1 | 104a269fea14544e4e3e3b9d867a0ee012749f91 | |
refs/heads/master | <repo_name>Shreedixi/VTiger_Project_Repo<file_sep>/VTiger_Project/src/main/java/com/crm/vtiger/GenericUtils/ListnerImplementation.java
package com.crm.vtiger.GenericUtils;
import java.io.File;
import java.io.IOException;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebDriverException;
import org.openqa.selenium.support.events.EventFiringWebDriver;
import org.testng.ITestContext;
import org.testng.ITestListener;
import org.testng.ITestResult;
import com.google.common.io.Files;
public class ListnerImplementation extends BaseClass implements ITestListener {
public void onTestStart(ITestResult result) {
}
public void onTestSuccess(ITestResult result) {
}
public void onTestFailure(ITestResult result) {
String testName=result.getMethod().getMethodName();
System.out.println(testName+"=====Execute & i m listening======");
EventFiringWebDriver eDriver=new EventFiringWebDriver(BaseClass.staticdriver);
//TakesScreenshot takescreenshot = (TakesScreenshot)BaseClass.staticdriver;
File sourceFile = eDriver.getScreenshotAs(OutputType.FILE);
// String screenshotpath = System.getProperty("user.dir")+"//screenshot//"
// +result.getMethod().getMethodName()+"_"+JavaUtility.getCurrentDate()+".PNG";
//// File dest=new File(screenshotpath);
try {
FileUtils.copyFile(sourceFile, new File("./screenshot/"+testName+".png"));
} catch (IOException e) {
e.printStackTrace();
}
}
public void onTestSkipped(ITestResult result) {
}
public void onTestFailedButWithinSuccessPercentage(ITestResult result) {
}
public void onTestFailedWithTimeout(ITestResult result) {
}
public void onStart(ITestContext context) {
}
public void onFinish(ITestContext context) {
}
}
| 9c91245d983ad2347d07e9a15af7ac272097ca16 | [
"Java"
]
| 1 | Java | Shreedixi/VTiger_Project_Repo | 4517f11eb482d37e7b28b7fcbbc5c2bbfacb98a9 | bb7c726021713ddd5f4e1cf1b0755b81d83179ba | |
refs/heads/master | <file_sep><?php
/**
* Initialise Taxonmies used for the MACS students pages
* i.e.: location, department, semester, level, delivery level
*
* posts_by_taxon() : return list of posts as classified by these txnms
*/
function location_init()
{
$name ='location';
$object_type = array('cs-course',
'maths-course',
'ams-course',
'person');
$args = array(
'label'=> 'Locations',
'hierarchical' => true,
'show_ui' => true,
'show_in_menu' => false,
'show_in_nav_menus' => true
);
register_taxonomy( $name, $object_type, $args);
}
add_action( 'init', 'location_init' );
function department_init()
{
$name ='department';
$object_type = 'person';
$args = array(
'label'=> 'Department',
'hierarchical' => true,
'show_ui' => true,
'show_in_menu' => false,
'show_in_nav_menus' => true
);
register_taxonomy( $name, $object_type, $args);
}
add_action( 'init', 'department_init' );
function semester_init()
{
$name ='semester';
$object_type = array('cs-course',
'maths-course',
'ams-course',
);
$args = array(
'label'=> 'Semester',
'hierarchical' => true,
'show_ui' => true,
'show_in_menu' => false,
'show_in_nav_menus' => true
);
register_taxonomy( $name, $object_type, $args);
}
add_action( 'init', 'semester_init' );
function level_init()
{
$name ='level';
$object_type = array('cs-course',
'maths-course',
'ams-course',
);
$args = array(
'label'=> 'SCQF Level',
'hierarchical' => true,
'show_ui' => true,
'show_in_menu' => false,
'show_in_nav_menus' => true
);
register_taxonomy( $name, $object_type, $args);
}
add_action( 'init', 'level_init' );
function delivery_level_init()
{
$name ='deliveryLevel';
$object_type = array('cs-course',
'maths-course',
'ams-course',
);
$args = array(
'label'=> 'Delivery Levels',
'hierarchical' => true,
'show_ui' => true,
'show_in_menu' => false,
'show_in_nav_menus' => true
);
register_taxonomy( $name, $object_type, $args);
}
add_action( 'init', 'delivery_level_init' );
function posts_by_taxon ( $atts )
{
$results = '<p>';
$query = array();
$query['posts_per_page'] = -1;
$query['tax_query'] = array();
$taxonomies = get_taxonomies();
if ( array_key_exists( 'debug', $atts ) )
{
$debug = ('true' === $atts['debug']);
} else {
$debug = false;
}
foreach ($taxonomies as $taxonomy)
{
if ( array_key_exists( $taxonomy, $atts ) )
{
if ($debug)
{
$results = $results.'Posts by taxon: '.$taxonomy.' = '.$atts[$taxonomy];
}
$tax_query = array(
'taxonomy' => $taxonomy,
'field' => 'name',
'terms' => $atts[$taxonomy]
);
$query['tax_query'][] = $tax_query;
}
}
if ( array_key_exists( 'type', $atts ) )
{
$query['post_type'] = $atts['type'];
} else {
$query['post_type'] = get_post_types();
}
if ( array_key_exists( 'orderby', $atts ) )
{
$query['orderby'] = $atts['orderby'];
if ( array_key_exists( 'order', $atts ) )
{
$query['order'] = strtoupper( $atts['order'] );
} else {
$query['order'] = 'ASC';
}
}
$posts_array = get_posts( $query );
$results = $results.'</p><ul>';
foreach ( $posts_array as $post )
{
$url = esc_url( get_permalink( $post->ID ) );
$title = $post->post_title;
$linkitem = '<li><a href="'.$url.'">'.$title.'</a></li>';
$results = $results.$linkitem;
}
$results = $results.'</ul>';
return $results;
}
add_shortcode( 'posts-by-taxon', 'posts_by_taxon' );
function macs_print_taxon( $taxonomy, $headword = NULL )
{
$taxon = implode( ', ', wp_get_post_terms( get_the_ID(),
$taxonomy,
array('fields'=>'names') )
);
if (NULL === $headword)
{
$headword = '<p><strong>'.ucfirst($taxonomy).': </strong>';
} else {
$headword = '<p><strong>'.$headword.': </strong>';
}
if ($taxon != '')
{
echo $headword.$taxon.'.</p>';
}
}
<file_sep><?php
/**
* Custom field functionality for student site
* Requires metabox plugin https://metabox.io
*/
/**
* Create custom post type for storing (links to) people's details
*/
add_action( 'init', 'macs_create_person_type' );
function macs_create_person_type() {
register_post_type( 'person',
array(
'labels' => array(
'name' => __( 'People' ),
'singular_name' => __( 'Person' ),
'add_new' => __( 'New person' ),
'add_new_item' => __( 'Add new person' ),
'edit_item' => __( 'Edit person' ),
'view_item' => __( 'View person' )
),
'public' => false,
'has_archive' => false,
'exclude_from_search' => true,
'show_ui' => true,
'rewrite' => array('slug' => 'person'),
'supports' => array('title', 'revisions' ),
'menu_position' => 22,
'menu_icon' => 'dashicons-admin-users'
)
);
}
function wpb_change_person_title_text( $title ){
$screen = get_current_screen();
if ( 'person' == $screen->post_type ) {
$title = 'Name';
}
return $title;
}
add_filter( 'enter_title_here', 'wpb_change_person_title_text' );
/**
* Register meta box for person metadata
*/
add_filter( 'rwmb_meta_boxes', 'macs_person_meta_boxes' );
function macs_person_meta_boxes( $meta_boxes ) {
$meta_boxes[] = array(
'title' => 'Person metadata',
'post_types' => 'person',
'fields' => array(
array(
'id' => 'staffDirURL',
'name' => 'Staff directory URL',
'type' => 'url',
'desc' => 'The URL for this person\'s page in the staff directory.'
),
array(
'id' => 'photoURL',
'name' => 'Photo URL',
'type' => 'url',
'desc' => 'The URL of the Profile Picture in the staff directory.'
),
)
);
return $meta_boxes;
}
/**
* Helper functions for printing out metadata fields
*
**/
function macs_print_person_link( $person_id ) {
$name = get_the_title( $person_id );
$url = esc_url( rwmb_meta( 'staffDirURL', array(), $person_id ) );
$location = implode(', ', wp_get_post_terms( $person_id,
'location',
array('fields' => 'names' )
) );
echo sprintf( '<a href="%s">%s</a> (%s)', $url, $name, $location);
}
function macs_print_person_img( $person_id ) {
$imgref = esc_url( rwmb_meta( 'photoURL', array(), $person_id ) );
if ($imgref != '') {
$name = get_the_title( $person_id );
$url = esc_url( rwmb_meta( 'staffDirURL', array(), $person_id ) );
echo sprintf( '<a href="%s"><img src="%s" alt="%s" class="MACSpersonimg"
style="width:135px;height:180px;"/></a>',
$url, $imgref, $name );
#Quick fix. Yes, the style info should go in the stylesheet
}
}
<file_sep><?php
/**
* The template used for displaying course info for all course types
* Called from single-*-course.php
*
* @package ThemeGrill
* @subpackage Accelerate
* @subpackage macs-student
* @since macs-student 1.0
*/
?>
<article id="post-<?php the_ID(); ?>" <?php post_class(); ?>>
<?php do_action( 'accelerate_before_post_content' ); ?>
<header class="entry-header">
<?php the_title( '<h1 class="entry-title">', '</h1>' ); ?>
</header>
<div class="entry-content clearfix">
<?php macs_print_course_leader_img(); ?>
<?php macs_print_course_leader(); ?>
<?php macs_print_course_aims(); ?>
<?php macs_print_course_summary(); ?>
<h2>Detailed Information</h2>
<?php macs_print_course_prereqs(); ?>
<?php macs_print_linked_courses(); ?>
<?php macs_print_taxon( 'location' ); ?>
<?php macs_print_taxon( 'semester' ); ?>
<?php macs_print_course_details(); ?>
<?php macs_print_course_contact_hours(); ?>
<?php macs_print_taxon( 'level', 'SCQF Level' ); ?>
<?php macs_print_course_scqf_credits(); ?>
<?php macs_print_boiler_text(); ?>
</div>
<?php do_action( 'accelerate_after_post_content' ); ?>
</article>
<file_sep><?php
add_action( 'wp_enqueue_scripts', 'theme_enqueue_styles' );
function theme_enqueue_styles() {
wp_enqueue_style( 'parent-style', get_template_directory_uri() .
'/style.css' );
}
@ini_set( 'upload_max_size' , '64M' );
@ini_set( 'post_max_size', '64M' );
@ini_set( 'max_execution_time', '300' );
add_action( 'widgets_init', 'my_register_sidebars' );
function macs_print_html_metadata($field_id, $lead_text)
{
if ( rwmb_meta( $field_id ) )
{
// to do: write style to support:
// echo '<h3 class="subhead">'.$lead_text.'</h3> '.rwmb_meta( $field_id );
echo '<p><strong>'.$lead_text.'</strong></p>'.rwmb_meta( $field_id );
}
}
include 'macs-course-type.php';
include 'macs-person-type.php';
include 'macs-taxonomies.php';
function my_register_sidebars() {
register_sidebar(array(
'id' => 'ams',
'name' => __('AMS_Sidebar'),
'description' => __('Sidebar used on AMS pages'),
'before_widget' => '<aside id="%1$s" class="widget %2$s">',
'after_widget' => '</aside>',
'before_title' => '<h3 class="widget-title"><span>',
'after_title' => '</span></h3>'
));
register_sidebar(array(
'id' => 'maths',
'name' => __('Maths_Sidebar'),
'description' => __('Sidebar used on Maths pages'),
'before_widget' => '<aside id="%1$s" class="widget %2$s">',
'after_widget' => '</aside>',
'before_title' => '<h3 class="widget-title"><span>',
'after_title' => '</span></h3>'
));
register_sidebar(array(
'id' => 'cs',
'name' => __('CS_Sidebar'),
'description' => __('Sidebar used on CS Pages'),
'before_widget' => '<aside id="%1$s" class="widget %2$s">',
'after_widget' => '</aside>',
'before_title' => '<h3 class="widget-title"><span>',
'after_title' => '</span></h3>'
));
register_sidebar(array(
'id' => 'edinburgh',
'name' => __('Edinburgh_Sidebar'),
'description' => __('Sidebar used on Edinburgh Pages'),
'before_widget' => '<aside id="%1$s" class="widget %2$s">',
'after_widget' => '</aside>',
'before_title' => '<h3 class="widget-title"><span>',
'after_title' => '</span></h3>'
));
register_sidebar(array(
'id' => 'dubai',
'name' => __('Dubai_Sidebar'),
'description' => __('Sidebar used on Dubai Pages'),
'before_widget' => '<aside id="%1$s" class="widget %2$s">',
'after_widget' => '</aside>',
'before_title' => '<h3 class="widget-title"><span>',
'after_title' => '</span></h3>'
));
register_sidebar(array(
'id' => 'malaysia',
'name' => __('Malaysia_Sidebar'),
'description' => __('Sidebar used on Malaysia Pages'),
'before_widget' => '<aside id="%1$s" class="widget %2$s">',
'after_widget' => '</aside>',
'before_title' => '<h3 class="widget-title"><span>',
'after_title' => '</span></h3>'
));
register_sidebar(array(
'id' => 'alp',
'name' => __('ALP_Sidebar'),
'description' => __('Sidebar used on ALP Pages'),
'before_widget' => '<aside id="%1$s" class="widget %2$s">',
'after_widget' => '</aside>',
'before_title' => '<h3 class="widget-title"><span>',
'after_title' => '</span></h3>'
));
register_sidebar(array(
'id' => 'pgr',
'name' => __('PGR_Sidebar'),
'description' => __('Sidebar used on PGR Pages'),
'before_widget' => '<aside id="%1$s" class="widget %2$s">',
'after_widget' => '</aside>',
'before_title' => '<h3 class="widget-title"><span>',
'after_title' => '</span></h3>'
));
}
?>
<file_sep><?php
/**
* Functions to support the Course type in MACS Students WP site.
* Requires metabox plugin https://metabox.io
*/
/**
* Create custom post types for courses per discipline
*/
add_action( 'init', 'macs_create_course_type' );
function macs_create_course_type() {
register_post_type( 'cs-course',
array(
'labels' => array(
'name' => __( 'CS Courses' ),
'singular_name' => __( 'CS Course' ),
'add_new' => __( 'New CS course' ),
'add_new_item' => __( 'Add new CS course' ),
'edit_item' => __( 'Edit CS course data' ),
'view_item' => __( 'View CS course data' )
),
'public' => true,
'has_archive' => false,
'rewrite' => array('slug' => 'cs/courses', 'with_front' => false),
'supports' => array('title', 'revisions' ),
'menu_position' => 20,
'capability_type' => 'page',
'hierachical' => true,
'taxonomies'=> array(),
'menu_position' => 20,
'menu_icon' => 'dashicons-admin-page'
)
);
register_post_type( 'maths-course',
array(
'labels' => array(
'name' => __( 'Maths Courses' ),
'singular_name' => __( 'Maths Course' ),
'add_new' => __( 'New Maths course' ),
'add_new_item' => __( 'Add new Maths course' ),
'edit_item' => __( 'Edit Maths course data' ),
'view_item' => __( 'View Maths course data' )
),
'public' => true,
'has_archive' => false,
'rewrite' => array('slug' => 'maths/courses'),
'supports' => array('title', 'revisions' ),
'menu_position' => 20,
'capability_type' => 'page',
'hierachical' => true,
'taxonomies'=> array(),
'menu_position' => 20,
'menu_icon' => 'dashicons-admin-page'
)
);
register_post_type( 'ams-course',
array(
'labels' => array(
'name' => __( 'AMS Courses' ),
'singular_name' => __( 'AMS Course' ),
'add_new' => __( 'New AMS course' ),
'add_new_item' => __( 'Add new AMS course' ),
'edit_item' => __( 'Edit AMS course data' ),
'view_item' => __( 'View AMS course data' )
),
'public' => true,
'has_archive' => false,
'rewrite' => array('slug' => 'ams/courses'),
'supports' => array('title', 'revisions' ),
'menu_position' => 20,
'capability_type' => 'page',
'hierachical' => true,
'taxonomies'=> array(),
'menu_position' => 20,
'menu_icon' => 'dashicons-admin-page'
)
);
}
function macs_rewrite_flush() {
macs_create_course_type();
flush_rewrite_rules();
}
add_action('after_switch_theme', 'macs_rewrite_flush');
function wpb_change_course_title_text( $title ){
$screen = get_current_screen();
if ( 'course' == $screen->post_type ) {
$title = 'Course code and title';
}
return $title;
}
add_filter( 'enter_title_here', 'wpb_change_course_title_text' );
add_filter( 'rwmb_meta_boxes', 'macs_courses_meta_boxes' );
function macs_courses_meta_boxes( $meta_boxes ) {
$meta_boxes[] = array(
'title' => 'Basic course metadata',
'post_types' => array('cs-course', 'ams-course', 'maths-course'),
'fields' => array(
array(
'id' => 'courseCode',
'name' => 'Course Code',
'type' => 'text',
'size' => '5',
'desc' => 'The course code, e.g. F29EG'
),
array(
'id' => 'courseLeader',
'name' => 'Course co-ordinator',
'type' => 'post',
'post_type' => 'person',
'field_type' => 'select_advanced',
'placeholder' => __( 'Select a person'),
'query_args' => array(
'post_status' => 'publish',
'posts_per_page' => - 1,
),
'clone' => 'true',
'desc' => 'Link to the entry for the course coordinator'
),
array(
'id' => 'courseSCQFcredits',
'name' => 'Course SCQF Credits',
'type' => 'text',
'size' => '3',
'desc' => 'The SCQF Level, e.g. 15'
),
array(
'id' => 'courseElective',
'name' => 'Course Elective',
'type' => 'radio',
'options' => array('true' => 'yes', 'false' => 'no'),
'desc' => 'Is this an Elective Course, Yes or No'
),
array(
'id' => 'coursePrerequisiteCourses',
'name' => 'Prerequisite Courses',
'type' => 'post',
'post_type' => array('cs-course', 'ams-course', 'maths-course'),
'field_type' => 'select_advanced',
'placeholder' => __( 'Select a course'),
'query_args' => array(
'post_status' => 'publish',
'posts_per_page' => - 1,
),
'clone' => 'true',
'desc' => 'Course Pre-requisites, e.g F27EG'
),
array(
'id' => 'coursePrerequisitesText',
'name' => 'Other Course Prerequisites',
'type' => 'text',
'desc' => 'Or equivalent for F27EG'
),
array(
'id' => 'courseLinkedCourses',
'name' => 'Course Linked Courses',
'type' => 'post',
'post_type' => array('cs-course', 'ams-course', 'maths-course'),
'field_type' => 'select_advanced',
'placeholder' => __( 'Select a course'),
'query_args' => array(
'post_status' => 'publish',
'posts_per_page' => - 1,
),
'clone' => 'true',
'desc' => 'Linked Course e.g F27EF'
),
array(
'id' => 'courseLinkedCoursesText',
'name' => 'Linked Courses notes',
'type' => 'text',
'desc' => 'e.g. synoptic courses'
),
array(
'id' => 'courseAims',
'name' => 'Course Aims',
'type' => 'wysiwyg',
'desc' => 'The course Aims',
'options' => array(
'media_buttons' => false,
'textarea_rows' => 6
)
),
array(
'id' => 'courseSyllabus',
'name' => 'Course Syllabus',
'type' => 'wysiwyg',
'desc' => 'The course Syllabus',
'options' => array(
'media_buttons' => false,
'textarea_rows' => 6
)
),
array(
'id' => 'courseLOSM',
'name' => 'Course Learning Outcomes: Subject Mastery',
'type' => 'wysiwyg',
'desc' => 'The course Learning Outcomes: Subject Mastery',
'options' => array(
'media_buttons' => false,
'textarea_rows' => 6
)
),
array(
'id' => 'courseLOPA',
'name' => 'Course Learning Outcomes: Personal Abilities',
'type' => 'wysiwyg',
'desc' => 'The course Learning Outcomes: Personal Abilities',
'options' => array(
'media_buttons' => false,
'textarea_rows' => 6
)
),
array(
'id' => 'courseAssessmentMethods',
'name' => 'Course Assessment Methods',
'type' => 'wysiwyg',
'desc' => 'The course Assessment (and Reassessment Methods), e.g Assessment: Examination: (weighting 70%) Coursework (weighting 30%); Re-assessment: Examination: (weighting 100%)',
'options' => array(
'media_buttons' => false,
'textarea_rows' => 6
)
),
),
);
$meta_boxes[] = array(
'title' => 'Extended course metadata (Maths & AMS)',
'post_types' => array('ams-course', 'maths-course'),
'fields' => array(
array(
'id' => 'courseSummary',
'name' => 'Course Summary',
'type' => 'wysiwyg',
'desc' => 'Provides a summary field (used by Maths and AMS)',
'options' => array(
'media_buttons' => false,
'textarea_rows' => 6
)
),
array(
'id' => 'courseDetailedAims',
'name' => 'Course Detailed Aims',
'type' => 'wysiwyg',
'desc' => 'Provides a more detailed aims field (used by Maths and AMS)',
'options' => array(
'media_buttons' => false,
'textarea_rows' => 6
)
),
array(
'id' => 'courseDetailedSyllabus',
'name' => 'Course Detailed Syllabus',
'type' => 'wysiwyg',
'desc' => 'Provides a more detailed syllabus field (used by Maths and AMS)',
'options' => array(
'media_buttons' => false,
'textarea_rows' => 6
)
),
array(
'id' => 'courseReadingList',
'name' => 'Course Reading List',
'type' => 'wysiwyg',
'desc' => 'Provides a reading list field (used by Maths and AMS)',
'options' => array(
'media_buttons' => false,
'textarea_rows' => 6
)
),
array(
'id' => 'courseDetailedLOs',
'name' => 'Course Detailed Learning Outcomes',
'type' => 'wysiwyg',
'desc' => 'Provides a more detailed Learning Outcomes field
(used by Maths and AMS)',
'options' => array(
'media_buttons' => false,
'textarea_rows' => 6
)
),
array(
'id' => 'courseContactHours',
'name' => 'Course Contact Hours',
'type' => 'text',
'desc' => 'The contact Hours for the course, (used by Maths)'
),
array(
'id' => 'suppressPDFLink',
'name' => 'Suppres pdf link',
'type' => 'checkbox',
'desc' => 'Do not automatically generate a link to a pdf with
more information about course (check this only if
you know such a file does not exist).',
'std' => 0
)
)
);
return $meta_boxes;
}
/**
* Helper functions for printing out metadata fields
*
**/
function macs_print_course_code( )
{
if ( rwmb_meta( 'courseCode' ) )
{
echo '<p><strong>Course code:</strong> '.rwmb_meta( 'courseCode' ).'</p>';
}
}
function macs_print_course_link( $course_id )
{
$name = get_the_title( $course_id );
$url = esc_url( get_permalink( $course_id ) );
echo sprintf( '<a href="%s">%s</a>', $url, $name );
}
function macs_print_course_leader( )
{
if ( implode( '', rwmb_meta( 'courseLeader' ) ) )
{
echo '<p><strong>Course co-ordinator(s):</strong> ';
$people = rwmb_meta( 'courseLeader' );
foreach ( $people as $person )
{
macs_print_person_link( $person );
if ($person === end( $people) )
{
echo '. ';
} else {
echo ', ';
}
}
echo '</p>';
}
}
function macs_print_course_leader_img( )
{
if ( implode( '', rwmb_meta( 'courseLeader' ) ) )
{
echo '<div class="alignright" >';
$people = rwmb_meta( 'courseLeader' );
foreach ( $people as $person )
{
macs_print_person_img( $person );
}
echo '</div>';
}
}
function macs_print_course_prereqs( )
{
if ( implode( '', rwmb_meta( 'coursePrerequisiteCourses' ) ) )
{
echo '<p><strong>Pre-requisite course(s):</strong> ';
$prereq_courses = rwmb_meta( 'coursePrerequisiteCourses' );
foreach ( $prereq_courses as $prereq )
{
macs_print_course_link( $prereq );
if ($prereq === end( $prereq_courses ) )
{
echo ' ';
} else {
echo ' & ';
}
}
if ( rwmb_meta( 'coursePrerequisitesText' ) )
{
echo ' '.rwmb_meta( 'coursePrerequisitesText' );
}
echo '.</p>';
}
elseif ( rwmb_meta( 'coursePrerequisitesText' ) )
{
echo '<p><strong>Pre-requisites:</strong> './/
rwmb_meta( 'coursePrerequisitesText' ).'.</p>';
}
else
{
echo '<p><strong>Pre-requisites:</strong> none.</p>';
}
}
function macs_print_linked_courses( )
{
if ( implode( '', rwmb_meta( 'courseLinkedCourses' ) ) )
{
echo '<p><strong>Linked course(s):</strong> ';
$linked_courses = rwmb_meta( 'courseLinkedCourses' );
foreach ( $linked_courses as $linked_course )
{
macs_print_course_link( $linked_course );
if ($linked_course === end( $linked_courses ) )
{
echo ' ';
} else {
echo ' & ';
}
}
if ( rwmb_meta( 'courseLinkedCoursesText' ) )
{
echo ' '.rwmb_meta( 'courseLinkedCoursesText' );
}
echo '.</p>';
}
elseif ( rwmb_meta( 'courseLinkedCoursesText' ) )
{
echo '<p><strong>Linked course(s):</strong> './/
rwmb_meta( 'courseLinkedCoursesText' ).'.</p>';
}
}
function macs_print_course_summary( )
{
if ( rwmb_meta( 'courseSummary' ) != '' ) {
macs_print_html_metadata('courseSummary', 'Summary:');
}
}
function macs_print_course_aims( )
{
if ( rwmb_meta( 'courseDetailedAims' ) != '' ) {
macs_print_html_metadata('courseDetailedAims', 'Aims:');
} else {
macs_print_html_metadata('courseAims', 'Aims:');
}
}
function print_html_course_info( )
{
if ( rwmb_meta( 'courseDetailedSyllabus' ) != '' ) {
macs_print_html_metadata('courseDetailedSyllabus', 'Syllabus:');
} else {
macs_print_html_metadata('courseSyllabus', 'Syllabus:');
}
if ( rwmb_meta( 'courseDetailedLOs' ) != '' ) {
macs_print_html_metadata('courseDetailedLOs', 'Learning Outcomes:');
} else {
macs_print_html_metadata('courseLOSM', 'Learning Outcomes: Subject Mastery');
macs_print_html_metadata('courseLOPA', 'Learning Outcomes: Personal Abilities');
}
if ( rwmb_meta( 'courseReadingList' ) != '' ) {
macs_print_html_metadata('courseReadingList', 'Reading list:');
}
macs_print_html_metadata('courseAssessmentMethods', 'Assessment Methods:');
}
function print_link_to_course_info_pdf( )
{
$base_url = 'http://www.ma.hw.ac.uk/maths/courseinfo/';
$course_code = rwmb_meta( 'courseCode' );
$semester = wp_get_post_terms( get_the_ID(),
'semester',
array('fields'=>'names') );
echo '<p><strong>Further information:</strong> ';
foreach ( $semester as $s )
{
$pdf_url = $base_url.$course_code.$s.'.pdf';
echo '<a href="'.$pdf_url.'">';
echo 'Syllabus, Number of lectures, Assessment, Learning Outcomes';
echo '</a>.<br />';
}
echo '</p>';
}
function macs_print_course_details( )
{
$post_type = get_post_type();
if ( 'maths-course' == $post_type )
{
if ( (0 == rwmb_meta( 'suppressPDFLink') )
&& ('' == rwmb_meta( 'courseDetailedSyllabus' ) )
&& ('' == rwmb_meta( 'courseDetailedLOs' ) )
)
{
print_link_to_course_info_pdf( );
} else {
print_html_course_info( );
}
} else {
print_html_course_info( );
}
}
function macs_print_course_contact_hours( )
{
if ( rwmb_meta( 'courseContactHours' ) != '' ) {
echo '<p><strong>Contact Hours:</strong> './/
rwmb_meta( 'courseContactHours' ).'.</p>';
}
}
function macs_print_course_scqf_credits( )
{
if ( rwmb_meta( 'courseSCQFcredits' ) != '' ) {
echo '<p><strong>Credits:</strong> './/
rwmb_meta( 'courseSCQFcredits' ).'.</p>';
}
}
function macs_print_boiler_text( )
{
if ('ams-course' == get_post_type() )
{
echo '<h2>Other Information</h2>';
echo '<p><strong>Help:</strong> If you have any problems or questions
regarding the course, you are encouraged to contact the lecturer</p>';
echo '<p><strong>VISION:</strong> further information and course materials
are available on <a href="http://vision.hw.ac.uk">VISION</a></p>';
}
}
<file_sep><?php
/**
* The Sidebar containing the main widget areas.
*
* @package ThemeGrill
* @subpackage Accelerate
* @since Accelerate 1.0
*/
?>
<div id="secondary">
<?php do_action( 'accelerate_before_sidebar' ); ?>
<?php
$post_type = get_post_type();
if( is_page_template( 'page-templates/contact.php' ) ) {
$sidebar = 'accelerate_contact_page_sidebar';
}
elseif( is_page_template( 'page-templates/ams.php' )
|| ( $post_type == 'ams-course' ) ) {
$sidebar = 'ams';
}
elseif( is_page_template( 'page-templates/maths.php' )
|| ( $post_type == 'maths-course' ) ) {
$sidebar = 'maths';
}
elseif( is_page_template( 'page-templates/cs.php' )
|| ( $post_type == 'cs-course' ) ) {
$sidebar = 'cs';
}
elseif( is_page_template( 'page-templates/edinburgh.php' ) ) {
$sidebar = 'edinburgh';
}
elseif( is_page_template( 'page-templates/dubai.php' ) ) {
$sidebar = 'dubai';
}
elseif( is_page_template( 'page-templates/malaysia.php' ) ) {
$sidebar = 'malaysia';
}
elseif( is_page_template( 'page-templates/alp.php' ) ) {
$sidebar = 'alp';
}
elseif( is_page_template( 'page-templates/pgr.php' ) ) {
$sidebar = 'pgr';
}
else {
$sidebar = 'accelerate_right_sidebar';
}
?>
<?php if ( ! dynamic_sidebar( $sidebar ) ) : ?>
<aside id="search" class="widget widget_search">
<?php get_search_form(); ?>
</aside>
<aside id="archives" class="widget">
<h3 class="widget-title"><span><?php _e( 'Archives', 'accelerate' ); ?></span></h3>
<ul>
<?php wp_get_archives( array( 'type' => 'monthly' ) ); ?>
</ul>
</aside>
<aside id="meta" class="widget">
<h3 class="widget-title"><span><?php _e( 'Meta', 'accelerate' ); ?></span></h3>
<ul>
<?php wp_register(); ?>
<li><?php wp_loginout(); ?></li>
<?php wp_meta(); ?>
</ul>
</aside>
<?php endif; ?>
<?php do_action( 'accelerate_after_sidebar' ); ?>
</div>
<file_sep><?php
/**
* The template used for displaying course info for all course types
* Called from single-*-course.php
*
* @package ThemeGrill
* @subpackage Accelerate
* @subpackage macs-student
* @since macs-student 1.0
*/
?>
<article id="post-<?php the_ID(); ?>" <?php post_class(); ?>>
<?php do_action( 'accelerate_before_post_content' ); ?>
<header class="entry-header">
<h2 class="entry-title">
<a href="<?php the_permalink(); ?>" title="<?php the_title_attribute();?>"><?php the_title(); ?></a>
</h2>
</header>
<div class="entry-content clearfix">
<?php macs_print_course_leader(); ?>
<?php macs_print_course_aims(); ?>
</div>
<?php do_action( 'accelerate_after_post_content' ); ?>
</article>
| 7a381d97532cddcd227a27c73352ed0aed19ac96 | [
"PHP"
]
| 7 | PHP | philbarker/macsstudent_wptheme | 929f1cc4c715c23f3bcf4e2ef6c2df61fd88116d | 8dffe8f8da251910e1d7d79dc1db09e1afd17732 | |
refs/heads/master | <repo_name>marinamcpeak/PaletteDrop<file_sep>/PaletteDrop/PaletteImageView.swift
//// PaletteImageView.swift
// Created: 2019-06-24
//
import Foundation
class PaletteImageView: UIViewController {
@IBOutlet weak var imageView: UIImageView!
var matcher:PaletteImageMatcher?
let feedbackGenerator = UINotificationFeedbackGenerator()
override func viewDidLoad() {
super.viewDidLoad()
showNextImage()
}
// We are willing to become first responder to get shake motion
override var canBecomeFirstResponder: Bool {
get {
return true
}
}
// Enable detection of shake motion
override func motionBegan(_ motion: UIEvent.EventSubtype, with event: UIEvent?) {
if motion == .motionShake {
showNextImage()
}
}
func showNextImage() {
print("Will Change Image")
feedbackGenerator.prepare()
guard let paletteImage = matcher?.nextImage() else {
feedbackGenerator.notificationOccurred(.warning)
return
}
guard let image = paletteImage.image else {
feedbackGenerator.notificationOccurred(.error)
return
}
if (paletteImage.height >= paletteImage.width) {
imageView.image = image;
} else {
imageView.image = image.rotate(radians: .pi/2)
}
imageView.setNeedsLayout();
feedbackGenerator.notificationOccurred(.success)
}
}
extension UIImage {
func rotate(radians: Float) -> UIImage? {
var newSize = CGRect(origin: CGPoint.zero, size: self.size).applying(CGAffineTransform(rotationAngle: CGFloat(radians))).size
// Trim off the extremely small float value to prevent core graphics from rounding it up
newSize.width = floor(newSize.width)
newSize.height = floor(newSize.height)
UIGraphicsBeginImageContextWithOptions(newSize, false, self.scale)
let context = UIGraphicsGetCurrentContext()!
// Move origin to middle
context.translateBy(x: newSize.width/2, y: newSize.height/2)
// Rotate around middle
context.rotate(by: CGFloat(radians))
// Draw the image at its center
self.draw(in: CGRect(x: -self.size.width/2, y: -self.size.height/2, width: self.size.width, height: self.size.height))
let newImage = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return newImage
}
}
<file_sep>/Podfile
# Uncomment the next line to define a global platform for your project
# platform :ios, '9.0'
target 'PaletteDrop' do
# Comment the next line if you don't want to use dynamic frameworks
use_frameworks!
# Pods for PaletteDrop
# Realm
pod 'Realm','~> 3.16'
target 'PaletteDropTests' do
inherit! :search_paths
# Pods for testing
end
target 'PaletteDropUITests' do
inherit! :search_paths
# Pods for testing
end
end
<file_sep>/PaletteDrop/Colors.swift
//// Colors.swift
// Created: 2019-06-22
//
import Foundation
class Colors {
static let names:[String:String] = [
"#000000": "black*",
"#000080": "navy*",
"#00008B": "blue 4 (darkblue)",
"#0000CD": "blue 3 (mediumblue)",
"#0000EE": "blue 2",
"#0000FF": "blue*",
"#006400": "darkgreen",
"#00688B": "deepskyblue 4",
"#008000": "green*",
"#008080": "teal*",
"#00868B": "turquoise 4",
"#008B00": "green 4",
"#008B45": "springgreen 3",
"#008B8B": "cyan 4 (darkcyan)",
"#009ACD": "deepskyblue 3",
"#00B2EE": "deepskyblue 2",
"#00BFFF": "deepskyblue 1 (deepskyblue)",
"#00C5CD": "turquoise 3",
"#00C78C": "turquoiseblue",
"#00C957": "emeraldgreen",
"#00CD00": "green 3",
"#00CD66": "springgreen 2",
"#00CDCD": "cyan 3",
"#00CED1": "darkturquoise",
"#00E5EE": "turquoise 2",
"#00EE00": "green 2",
"#00EE76": "springgreen 1",
"#00EEEE": "cyan 2",
"#00F5FF": "turquoise 1",
"#00FA9A": "mediumspringgreen",
"#00FF00": "green 1 (lime*)",
"#00FF7F": "springgreen",
"#00FFFF": "cyan / aqua*",
"#030303": "gray 1",
"#03A89E": "manganeseblue",
"#050505": "gray 2",
"#080808": "gray 3",
"#0A0A0A": "gray 4",
"#0D0D0D": "gray 5",
"#0F0F0F": "gray 6",
"#104E8B": "dodgerblue 4",
"#121212": "gray 7",
"#141414": "gray 8",
"#171717": "gray 9",
"#1874CD": "dodgerblue 3",
"#191970": "midnightblue",
"#1A1A1A": "gray 10",
"#1C1C1C": "gray 11",
"#1C86EE": "dodgerblue 2",
"#1E1E1E": "sgi gray 12",
"#1E90FF": "dodgerblue 1 (dodgerblue)",
"#1F1F1F": "gray 12",
"#20B2AA": "lightseagreen",
"#212121": "gray 13",
"#228B22": "forestgreen",
"#242424": "gray 14",
"#262626": "gray 15",
"#27408B": "royalblue 4",
"#282828": "sgi gray 16",
"#292421": "ivoryblack",
"#292929": "gray 16",
"#2B2B2B": "gray 17",
"#2E2E2E": "gray 18",
"#2E8B57": "seagreen 4 (seagreen)",
"#2F4F4F": "darkslategray",
"#303030": "gray 19",
"#308014": "sapgreen",
"#32CD32": "limegreen",
"#333333": "gray 20",
"#33A1C9": "peacock",
"#363636": "gray 21",
"#36648B": "steelblue 4",
"#383838": "gray 22",
"#388E8E": "sgi teal",
"#3A5FCD": "royalblue 3",
"#3B3B3B": "gray 23",
"#3CB371": "mediumseagreen",
"#3D3D3D": "gray 24",
"#3D59AB": "cobalt",
"#3D9140": "cobaltgreen",
"#404040": "gray 25",
"#40E0D0": "turquoise",
"#4169E1": "royalblue",
"#424242": "gray 26",
"#436EEE": "royalblue 2",
"#43CD80": "seagreen 3",
"#454545": "gray 27",
"#458B00": "chartreuse 4",
"#458B74": "aquamarine 4",
"#4682B4": "steelblue",
"#473C8B": "slateblue 4",
"#474747": "gray 28",
"#483D8B": "darkslateblue",
"#4876FF": "royalblue 1",
"#48D1CC": "mediumturquoise",
"#4A4A4A": "gray 29",
"#4A708B": "skyblue 4",
"#4B0082": "indigo",
"#4D4D4D": "gray 30",
"#4EEE94": "seagreen 2",
"#4F4F4F": "gray 31",
"#4F94CD": "steelblue 3",
"#515151": "sgi gray 32",
"#525252": "gray 32",
"#528B8B": "darkslategray 4",
"#53868B": "cadetblue 4",
"#545454": "gray 33",
"#548B54": "palegreen 4",
"#54FF9F": "seagreen 1",
"#551A8B": "purple 4",
"#555555": "sgi darkgray",
"#556B2F": "darkolivegreen",
"#575757": "gray 34",
"#595959": "gray 35",
"#5B5B5B": "sgi gray 36",
"#5C5C5C": "gray 36",
"#5CACEE": "steelblue 2",
"#5D478B": "mediumpurple 4",
"#5E2612": "sepia",
"#5E5E5E": "gray 37",
"#5F9EA0": "cadetblue",
"#607B8B": "lightskyblue 4",
"#616161": "gray 38",
"#636363": "gray 39",
"#63B8FF": "steelblue 1",
"#6495ED": "cornflowerblue",
"#666666": "gray 40",
"#668B8B": "paleturquoise 4",
"#66CD00": "chartreuse 3",
"#66CDAA": "aquamarine 3 (mediumaquamarine)",
"#68228B": "darkorchid 4",
"#68838B": "lightblue 4",
"#6959CD": "slateblue 3",
"#696969": "dimgray (gray 42)",
"#698B22": "olivedrab 4",
"#698B69": "darkseagreen 4",
"#6A5ACD": "slateblue",
"#6B6B6B": "gray 42",
"#6B8E23": "olivedrab",
"#6C7B8B": "slategray 4",
"#6CA6CD": "skyblue 3",
"#6E6E6E": "gray 43",
"#6E7B8B": "lightsteelblue 4",
"#6E8B3D": "darkolivegreen 4",
"#707070": "gray 44",
"#708090": "slategray",
"#7171C6": "sgi slateblue",
"#71C671": "sgi chartreuse",
"#737373": "gray 45",
"#757575": "gray 46",
"#76EE00": "chartreuse 2",
"#76EEC6": "aquamarine 2",
"#778899": "lightslategray",
"#787878": "gray 47",
"#79CDCD": "darkslategray 3",
"#7A378B": "mediumorchid 4",
"#7A67EE": "slateblue 2",
"#7A7A7A": "gray 48",
"#7A8B8B": "lightcyan 4",
"#7AC5CD": "cadetblue 3",
"#7B68EE": "mediumslateblue",
"#7CCD7C": "palegreen 3",
"#7CFC00": "lawngreen",
"#7D26CD": "purple 3",
"#7D7D7D": "gray 49",
"#7D9EC0": "sgi lightblue",
"#7EC0EE": "skyblue 2",
"#7F7F7F": "gray 50",
"#7FFF00": "chartreuse 1 (chartreuse)",
"#7FFFD4": "aquamarine 1 (aquamarine)",
"#800000": "maroon*",
"#800080": "purple*",
"#808000": "olive*",
"#808069": "warmgrey",
"#808080": "gray*",
"#808A87": "coldgrey",
"#828282": "gray 51",
"#836FFF": "slateblue 1",
"#838B83": "honeydew 4",
"#838B8B": "azure 4",
"#8470FF": "lightslateblue",
"#848484": "sgi gray 52",
"#858585": "gray 52",
"#872657": "raspberry",
"#878787": "gray 53",
"#87CEEB": "skyblue",
"#87CEFA": "lightskyblue",
"#87CEFF": "skyblue 1",
"#8968CD": "mediumpurple 3",
"#8A2BE2": "blueviolet",
"#8A3324": "burntumber",
"#8A360F": "burntsienna",
"#8A8A8A": "gray 54",
"#8B0000": "red 4 (darkred)",
"#8B008B": "magenta 4 (darkmagenta)",
"#8B0A50": "deeppink 4",
"#8B1A1A": "firebrick 4",
"#8B1C62": "maroon 4",
"#8B2252": "violetred 4",
"#8B2323": "brown 4",
"#8B2500": "orangered 4",
"#8B3626": "tomato 4",
"#8B3A3A": "indianred 4",
"#8B3A62": "hotpink 4",
"#8B3E2F": "coral 4",
"#8B4500": "darkorange 4",
"#8B4513": "chocolate 4 (saddlebrown)",
"#8B4726": "sienna 4",
"#8B475D": "palevioletred 4",
"#8B4789": "orchid 4",
"#8B4C39": "salmon 4",
"#8B5742": "lightsalmon 4",
"#8B5A00": "orange 4",
"#8B5A2B": "tan 4",
"#8B5F65": "lightpink 4",
"#8B636C": "pink 4",
"#8B6508": "darkgoldenrod 4",
"#8B668B": "plum 4",
"#8B6914": "goldenrod 4",
"#8B6969": "rosybrown 4",
"#8B7355": "burlywood 4",
"#8B7500": "gold 4",
"#8B7765": "peachpuff 4",
"#8B795E": "navajowhite 4",
"#8B7B8B": "thistle 4",
"#8B7D6B": "bisque 4",
"#8B7D7B": "mistyrose 4",
"#8B7E66": "wheat 4",
"#8B814C": "lightgoldenrod 4",
"#8B8378": "antiquewhite 4",
"#8B8386": "lavenderblush 4",
"#8B864E": "khaki 4",
"#8B8682": "seashell 4",
"#8B8878": "cornsilk 4",
"#8B8970": "lemonchiffon 4",
"#8B8989": "snow 4",
"#8B8B00": "yellow 4",
"#8B8B7A": "lightyellow 4",
"#8B8B83": "ivory 4",
"#8C8C8C": "gray 55",
"#8DB6CD": "lightskyblue 3",
"#8DEEEE": "darkslategray 2",
"#8E388E": "sgi beet",
"#8E8E38": "sgi olivedrab",
"#8E8E8E": "sgi gray 56",
"#8EE5EE": "cadetblue 2",
"#8F8F8F": "gray 56",
"#8FBC8F": "darkseagreen",
"#90EE90": "palegreen 2 (lightgreen)",
"#912CEE": "purple 2",
"#919191": "gray 57",
"#9370DB": "mediumpurple",
"#9400D3": "darkviolet",
"#949494": "gray 58",
"#969696": "gray 59",
"#96CDCD": "paleturquoise 3",
"#97FFFF": "darkslategray 1",
"#98F5FF": "cadetblue 1",
"#98FB98": "palegreen",
"#9932CC": "darkorchid",
"#999999": "gray 60",
"#9A32CD": "darkorchid 3",
"#9AC0CD": "lightblue 3",
"#9ACD32": "olivedrab 3 (yellowgreen)",
"#9AFF9A": "palegreen 1",
"#9B30FF": "purple 1",
"#9BCD9B": "darkseagreen 3",
"#9C661F": "brick",
"#9C9C9C": "gray 61",
"#9E9E9E": "gray 62",
"#9F79EE": "mediumpurple 2",
"#9FB6CD": "slategray 3",
"#A0522D": "sienna",
"#A1A1A1": "gray 63",
"#A2B5CD": "lightsteelblue 3",
"#A2CD5A": "darkolivegreen 3",
"#A3A3A3": "gray 64",
"#A4D3EE": "lightskyblue 2",
"#A52A2A": "brown",
"#A6A6A6": "gray 65",
"#A8A8A8": "gray 66",
"#A9A9A9": "darkgray",
"#AAAAAA": "sgi lightgray",
"#AB82FF": "mediumpurple 1",
"#ABABAB": "gray 67",
"#ADADAD": "gray 68",
"#ADD8E6": "lightblue",
"#ADFF2F": "greenyellow",
"#AEEEEE": "paleturquoise 2 (paleturquoise)",
"#B0171F": "indian red",
"#B0B0B0": "gray 69",
"#B0C4DE": "lightsteelblue",
"#B0E0E6": "powderblue",
"#B0E2FF": "lightskyblue 1",
"#B22222": "firebrick",
"#B23AEE": "darkorchid 2",
"#B2DFEE": "lightblue 2",
"#B3B3B3": "gray 70",
"#B3EE3A": "olivedrab 2",
"#B452CD": "mediumorchid 3",
"#B4CDCD": "lightcyan 3",
"#B4EEB4": "darkseagreen 2",
"#B5B5B5": "gray 71",
"#B7B7B7": "sgi gray 72",
"#B8860B": "darkgoldenrod",
"#B8B8B8": "gray 72",
"#B9D3EE": "slategray 2",
"#BA55D3": "mediumorchid",
"#BABABA": "gray 73",
"#BBFFFF": "paleturquoise 1",
"#BC8F8F": "rosybrown",
"#BCD2EE": "lightsteelblue 2",
"#BCEE68": "darkolivegreen 2",
"#BDB76B": "darkkhaki",
"#BDBDBD": "gray 74",
"#BDFCC9": "mint",
"#BF3EFF": "darkorchid 1",
"#BFBFBF": "gray 75",
"#BFEFFF": "lightblue 1",
"#C0C0C0": "silver*",
"#C0FF3E": "olivedrab 1",
"#C1C1C1": "sgi gray 76",
"#C1CDC1": "honeydew 3",
"#C1CDCD": "azure 3",
"#C1FFC1": "darkseagreen 1",
"#C2C2C2": "gray 76",
"#C4C4C4": "gray 77",
"#C5C1AA": "sgi brightgray",
"#C67171": "sgi salmon",
"#C6E2FF": "slategray 1",
"#C71585": "mediumvioletred",
"#C76114": "rawsienna",
"#C7C7C7": "gray 78",
"#C9C9C9": "gray 79",
"#CAE1FF": "lightsteelblue 1",
"#CAFF70": "darkolivegreen 1",
"#CCCCCC": "gray 80",
"#CD0000": "red 3",
"#CD00CD": "magenta 3",
"#CD1076": "deeppink 3",
"#CD2626": "firebrick 3",
"#CD2990": "maroon 3",
"#CD3278": "violetred 3",
"#CD3333": "brown 3",
"#CD3700": "orangered 3",
"#CD4F39": "tomato 3",
"#CD5555": "indianred 3",
"#CD5B45": "coral 3",
"#CD5C5C": "indianred",
"#CD6090": "hotpink 3",
"#CD6600": "darkorange 3",
"#CD661D": "chocolate 3",
"#CD6839": "sienna 3",
"#CD6889": "palevioletred 3",
"#CD69C9": "orchid 3",
"#CD7054": "salmon 3",
"#CD8162": "lightsalmon 3",
"#CD8500": "orange 3",
"#CD853F": "tan 3 (peru)",
"#CD8C95": "lightpink 3",
"#CD919E": "pink 3",
"#CD950C": "darkgoldenrod 3",
"#CD96CD": "plum 3",
"#CD9B1D": "goldenrod 3",
"#CD9B9B": "rosybrown 3",
"#CDAA7D": "burlywood 3",
"#CDAD00": "gold 3",
"#CDAF95": "peachpuff 3",
"#CDB38B": "navajowhite 3",
"#CDB5CD": "thistle 3",
"#CDB79E": "bisque 3",
"#CDB7B5": "mistyrose 3",
"#CDBA96": "wheat 3",
"#CDBE70": "lightgoldenrod 3",
"#CDC0B0": "antiquewhite 3",
"#CDC1C5": "lavenderblush 3",
"#CDC5BF": "seashell 3",
"#CDC673": "khaki 3",
"#CDC8B1": "cornsilk 3",
"#CDC9A5": "lemonchiffon 3",
"#CDC9C9": "snow 3",
"#CDCD00": "yellow 3",
"#CDCDB4": "lightyellow 3",
"#CDCDC1": "ivory 3",
"#CFCFCF": "gray 81",
"#D02090": "violetred",
"#D15FEE": "mediumorchid 2",
"#D1D1D1": "gray 82",
"#D1EEEE": "lightcyan 2",
"#D2691E": "chocolate",
"#D2B48C": "tan",
"#D3D3D3": "lightgrey",
"#D4D4D4": "gray 83",
"#D6D6D6": "gray 84",
"#D8BFD8": "thistle",
"#D9D9D9": "gray 85",
"#DA70D6": "orchid",
"#DAA520": "goldenrod",
"#DB7093": "palevioletred",
"#DBDBDB": "gray 86",
"#DC143C": "crimson",
"#DCDCDC": "gainsboro",
"#DDA0DD": "plum",
"#DEB887": "burlywood",
"#DEDEDE": "gray 87",
"#E066FF": "mediumorchid 1",
"#E0E0E0": "gray 88",
"#E0EEE0": "honeydew 2",
"#E0EEEE": "azure 2",
"#E0FFFF": "lightcyan 1 (lightcyan)",
"#E3A869": "melon",
"#E3CF57": "banana",
"#E3E3E3": "gray 89",
"#E5E5E5": "gray 90",
"#E6E6FA": "lavender",
"#E8E8E8": "gray 91",
"#E9967A": "darksalmon",
"#EAEAEA": "sgi gray 92",
"#EBEBEB": "gray 92",
"#ED9121": "carrot",
"#EDEDED": "gray 93",
"#EE0000": "red 2",
"#EE00EE": "magenta 2",
"#EE1289": "deeppink 2",
"#EE2C2C": "firebrick 2",
"#EE30A7": "maroon 2",
"#EE3A8C": "violetred 2",
"#EE3B3B": "brown 2",
"#EE4000": "orangered 2",
"#EE5C42": "tomato 2",
"#EE6363": "indianred 2",
"#EE6A50": "coral 2",
"#EE6AA7": "hotpink 2",
"#EE7600": "darkorange 2",
"#EE7621": "chocolate 2",
"#EE7942": "sienna 2",
"#EE799F": "palevioletred 2",
"#EE7AE9": "orchid 2",
"#EE8262": "salmon 2",
"#EE82EE": "violet",
"#EE9572": "lightsalmon 2",
"#EE9A00": "orange 2",
"#EE9A49": "tan 2",
"#EEA2AD": "lightpink 2",
"#EEA9B8": "pink 2",
"#EEAD0E": "darkgoldenrod 2",
"#EEAEEE": "plum 2",
"#EEB422": "goldenrod 2",
"#EEB4B4": "rosybrown 2",
"#EEC591": "burlywood 2",
"#EEC900": "gold 2",
"#EECBAD": "peachpuff 2",
"#EECFA1": "navajowhite 2",
"#EED2EE": "thistle 2",
"#EED5B7": "bisque 2",
"#EED5D2": "mistyrose 2",
"#EED8AE": "wheat 2",
"#EEDC82": "lightgoldenrod 2",
"#EEDFCC": "antiquewhite 2",
"#EEE0E5": "lavenderblush 2",
"#EEE5DE": "seashell 2",
"#EEE685": "khaki 2",
"#EEE8AA": "palegoldenrod",
"#EEE8CD": "cornsilk 2",
"#EEE9BF": "lemonchiffon 2",
"#EEE9E9": "snow 2",
"#EEEE00": "yellow 2",
"#EEEED1": "lightyellow 2",
"#EEEEE0": "ivory 2",
"#F08080": "lightcoral",
"#F0E68C": "khaki",
"#F0F0F0": "gray 94",
"#F0F8FF": "aliceblue",
"#F0FFF0": "honeydew 1 (honeydew)",
"#F0FFFF": "azure 1 (azure)",
"#F2F2F2": "gray 95",
"#F4A460": "sandybrown",
"#F4F4F4": "sgi gray 96",
"#F5DEB3": "wheat",
"#F5F5DC": "beige",
"#F5F5F5": "white smoke (gray 96)",
"#F5FFFA": "mintcream",
"#F7F7F7": "gray 97",
"#F8F8FF": "ghostwhite",
"#FA8072": "salmon",
"#FAEBD7": "antiquewhite",
"#FAF0E6": "linen",
"#FAFAD2": "lightgoldenrodyellow",
"#FAFAFA": "gray 98",
"#FCE6C9": "eggshell",
"#FCFCFC": "gray 99",
"#FDF5E6": "oldlace",
"#FF0000": "red 1 (red*)",
"#FF00FF": "magenta (fuchsia*)",
"#FF1493": "deeppink 1 (deeppink)",
"#FF3030": "firebrick 1",
"#FF34B3": "maroon 1",
"#FF3E96": "violetred 1",
"#FF4040": "brown 1",
"#FF4500": "orangered 1 (orangered)",
"#FF6103": "cadmiumorange",
"#FF6347": "tomato 1 (tomato)",
"#FF69B4": "hotpink",
"#FF6A6A": "indianred 1",
"#FF6EB4": "hotpink 1",
"#FF7256": "coral 1",
"#FF7D40": "flesh",
"#FF7F00": "darkorange 1",
"#FF7F24": "chocolate 1",
"#FF7F50": "coral",
"#FF8000": "orange",
"#FF8247": "sienna 1",
"#FF82AB": "palevioletred 1",
"#FF83FA": "orchid 1",
"#FF8C00": "darkorange",
"#FF8C69": "salmon 1",
"#FF9912": "cadmiumyellow",
"#FFA07A": "lightsalmon 1 (lightsalmon)",
"#FFA500": "orange 1 (orange)",
"#FFA54F": "tan 1",
"#FFAEB9": "lightpink 1",
"#FFB5C5": "pink 1",
"#FFB6C1": "lightpink",
"#FFB90F": "darkgoldenrod 1",
"#FFBBFF": "plum 1",
"#FFC0CB": "pink",
"#FFC125": "goldenrod 1",
"#FFC1C1": "rosybrown 1",
"#FFD39B": "burlywood 1",
"#FFD700": "gold 1 (gold)",
"#FFDAB9": "peachpuff 1 (peachpuff)",
"#FFDEAD": "navajowhite 1 (navajowhite)",
"#FFE1FF": "thistle 1",
"#FFE4B5": "moccasin",
"#FFE4C4": "bisque 1 (bisque)",
"#FFE4E1": "mistyrose 1 (mistyrose)",
"#FFE7BA": "wheat 1",
"#FFEBCD": "blanchedalmond",
"#FFEC8B": "lightgoldenrod 1",
"#FFEFD5": "papayawhip",
"#FFEFDB": "antiquewhite 1",
"#FFF0F5": "lavenderblush 1 (lavenderblush)",
"#FFF5EE": "seashell 1 (seashell)",
"#FFF68F": "khaki 1",
"#FFF8DC": "cornsilk 1 (cornsilk)",
"#FFFACD": "lemonchiffon 1 (lemonchiffon)",
"#FFFAF0": "floralwhite",
"#FFFAFA": "snow 1 (snow)",
"#FFFF00": "yellow 1 (yellow*)",
"#FFFFE0": "lightyellow 1 (lightyellow)",
"#FFFFF0": "ivory 1 (ivory)",
"#FFFFFF": "white*"
]
static func hexString(_ color:UIColor) -> String {
var red:CGFloat = 0
var blue:CGFloat = 0
var green:CGFloat = 0
var alpha:CGFloat = 0
color.getRed(&red, green: &green, blue: &blue, alpha: &alpha)
let colorVal:Int = (Int)(red*255)<<16 | (Int)(green*255)<<8 | (Int)(blue*255)<<0
return String(format:"#%06X", colorVal)
}
}
<file_sep>/PaletteDrop/Saved Palettes/SavedPaletteCell.swift
import UIKit
class SavedPaletteCell : UICollectionViewCell {
var colors = [UIColor]()
var savedPalettesView: SavedPaletteDesign?
override func draw(_ rect: CGRect) {
// PaletteDesign.drawBackground_MaskCanvas(frame: frame, resizing: PaletteDesign.ResizingBehavior.center);
}
override func awakeFromNib() {
super.awakeFromNib()
// view.backgroundColor = .yellow
}
override func layoutSubviews() {
if savedPalettesView == nil {
let view = SavedPaletteDesign(frame: bounds)
view.backgroundColor = UIColor(red: (41.0/255), green: (43.0/255), blue: (45.0/255), alpha: 1.0)
addSubview(view)
savedPalettesView = view
}
if colors.count > 0 {
savedPalettesView?.colors = colors;
savedPalettesView?.setNeedsDisplay();
}
}
}
<file_sep>/PaletteDrop/Colour Wheel/ColorWheel.swift
//// ColorWheel.swift
// Created: 2019-06-19
//
import UIKit
//source: https://stackoverflow.com/questions/12770181/how-to-get-the-pixel-color-on-touch
protocol ColorChange: AnyObject {
func colorChangedTo(changeColor: UIColor)
}
class ColorWheel: UIImageView {
weak var delegate:ColorChange?
var feedbackGenerator:UISelectionFeedbackGenerator?
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
// let touch = touches.first
// if let point = touch?.location(in: self) {
// let color = self.getPixelColorAtPoint(point: point)
// if let color = color {
// print(color)
// delegate?.colorChangedTo(changeColor: color)
// }
// }
self.alpha = 1
feedbackGenerator = UISelectionFeedbackGenerator()
feedbackGenerator?.prepare()
super.touchesBegan(touches, with: event)
}
override func touchesMoved(_ touches: Set<UITouch>, with event: UIEvent?) {
let touch = touches.first
if let point = touch?.location(in: self) {
if(self.point(inside: point, with: event)) {
let color = self.getPixelColorAtPoint(point: point)
if let color = color {
print(color)
delegate?.colorChangedTo(changeColor: color)
colorGeneratesHaptics(color)
}
}
}
super.touchesMoved(touches, with: event)
}
override func touchesEnded(_ touches: Set<UITouch>, with event: UIEvent?) {
feedbackGenerator = nil
UIView.animate(withDuration: 0.5, delay: 0, options: .curveEaseIn, animations: {
self.alpha = 0.3
}, completion: nil)
super.touchesEnded(touches, with: event)
}
func getPixelColorAtPoint(point:CGPoint) -> UIColor?{
let pixel = UnsafeMutablePointer<CUnsignedChar>.allocate(capacity: 4)
let colorSpace = CGColorSpaceCreateDeviceRGB()
let bitmapInfo = CGBitmapInfo(rawValue: CGImageAlphaInfo.premultipliedLast.rawValue)
let context = CGContext(data: pixel, width: 1, height: 1, bitsPerComponent: 8, bytesPerRow: 4, space: colorSpace, bitmapInfo: bitmapInfo.rawValue)
var color: UIColor? = nil
if let context = context {
context.translateBy(x: -point.x, y: -point.y)
self.layer.render(in: context)
color = UIColor(red: CGFloat(pixel[0])/255.0,
green: CGFloat(pixel[1])/255.0,
blue: CGFloat(pixel[2])/255.0,
alpha: CGFloat(pixel[3])/255.0)
pixel.deallocate()
}
return color
}
func colorGeneratesHaptics(_ color:UIColor) {
var red:CGFloat = 0
var blue:CGFloat = 0
var green:CGFloat = 0
var alpha:CGFloat = 0
color.getRed(&red, green: &green, blue: &blue, alpha: &alpha)
red = round(red * 10)
green = round(green * 10)
blue = round(blue * 10)
if red == blue || red == green || blue == green {
print("Haptics!!!")
feedbackGenerator?.selectionChanged()
feedbackGenerator?.prepare()
}
}
}
<file_sep>/PaletteDrop/PaletteExport.swift
//// Palette+Export.swift
// Created: 2019-06-23
//
import Foundation
class PaletteExport {
let palette:Palette
init(withPalette palette:Palette) {
self.palette = palette
}
func text() -> String {
var colorString = ""
for color in palette.colors {
colorString += Colors.hexString(color)
if color != palette.colors.last {
colorString += ", "
}
}
return colorString
}
func textWithPaletteName() -> String {
return palette.name + ": " + text()
}
func image(size:CGSize = CGSize(width: 256,height: 64)) -> UIImage? {
UIGraphicsBeginImageContext(size)
let width = size.width/CGFloat(palette.colors.count)
for i in 0..<palette.colors.count {
let baseColourPath = UIBezierPath(rect: CGRect(x: CGFloat(i) * width, y: 0, width: width, height: size.height))
palette.colors[i].setFill()
baseColourPath.fill()
}
let myImage = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return myImage
}
}
<file_sep>/PaletteDrop/Palettes/PaletteCell.swift
import UIKit
class PaletteCell : UITableViewCell {
var colors = [UIColor]()
var paletteView: PaletteDesignView?
override func draw(_ rect: CGRect) {
// PaletteDesign.drawBackground_MaskCanvas(frame: frame, resizing: PaletteDesign.ResizingBehavior.center);
}
override func awakeFromNib() {
super.awakeFromNib()
// view.backgroundColor = .yellow
}
override func layoutSubviews() {
if paletteView == nil {
let view = PaletteDesignView(frame: bounds)
view.backgroundColor = UIColor(red: (41.0/255), green: (43.0/255), blue: (45.0/255), alpha: 1.0)
addSubview(view)
paletteView = view
}
if colors.count > 0 {
paletteView?.colors = colors;
paletteView?.setNeedsDisplay();
}
}
// rgb(41,43,45)
func addPaletteView() {
}
}
<file_sep>/PaletteDrop/Colour Wheel/ColourWheelView.swift
//// ViewController.swift
// Created: 2019-06-19
//
import UIKit
class ColourWheelView: UIViewController, ColorChange {
@IBOutlet weak var colorWheel: ColorWheel!
@IBOutlet weak var selectedColourButton: UIButton!
let ioColorMindNamingService = IOColorMindNamingService.defaultInstance()
override func viewDidLoad() {
super.viewDidLoad()
// let button = UIButton(type: .custom)
// button.frame = CGRect(x: 160, y: 100, width: 50, height: 50)
// button.layer.cornerRadius = 0.5 * button.bounds.size.width
// button.clipsToBounds = true
// button.setImage(UIImage(named:"thumbsUp.png"), for: .normal)
// button.addTarget(self, action: #selector(thumbsUpButtonPressed), for: .touchUpInside)
// view.addSubview(button)
// selectedColourButton.layer.borderWidth = 7.
// selectedColourButton.frame = CGRect(50, 8, 120, 120)
// selectedColourButton.layer.cornerRadius = selectedColourButton.frame.width/2
// selectedColourButton.layer.masksToBounds = true
// colorWheel.delegate = self
selectedColourButton.frame = CGRect(x: 160, y: 160, width: 160, height: 160)
selectedColourButton.layer.cornerRadius = 0.5 * selectedColourButton.bounds.size.width
selectedColourButton.layer.masksToBounds = true
colorWheel.delegate = self
self.navigationItem.setHidesBackButton(true, animated:true);
}
// @objc dynamic public class func drawCanvas1(frame targetFrame: CGRect = CGRect(x: 0, y: 0, width: 150, height: 150)) {
// //// General Declarations
// let context = UIGraphicsGetCurrentContext()!
//
// //// Image Declarations
// let selectedColour = UIImage(named: "selectedColour.png")!
//
// //// Oval Drawing
// let ovalPath = UIBezierPath(ovalIn: CGRect(x: 0, y: 0, width: 150, height: 150))
// context.saveGState()
// ovalPath.addClip()
// context.scaleBy(x: 1, y: -1)
// context.draw(selectedColour.cgImage!, in: CGRect(x: 0, y: 0, width: selectedColour.size.width, height: selectedColour.size.height), byTiling: true)
// context.restoreGState()
//
// context.restoreGState()
//
// }
func colorChangedTo(changeColor: UIColor) {
selectedColourButton.backgroundColor = changeColor
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "paletteSegue" {
let palettesTableView = segue.destination as! PalettesTableView
palettesTableView.coreColor = selectedColourButton.backgroundColor
}
}
//Mark: Hide Navigation
// override func viewWillAppear(_ animated: Bool) {
// super.viewWillAppear(animated)
// navigationController?.setNavigationBarHidden(true, animated: animated)
// }
//
// override func viewWillDisappear(_ animated: Bool) {
// super.viewWillDisappear(animated)
// navigationController?.setNavigationBarHidden(false, animated: animated)
// }
}
<file_sep>/PaletteDrop/Generators.swift
//// Generators.swift
// Created: 2019-06-21
//
import Foundation
protocol PaletteViewerDelegate {
func didUpdatePalette(forTag: Int, palette: Palette);
}
class Generators : PaletteGeneratorDelegate {
var delegate:PaletteViewerDelegate? = nil;
var generators:[Int:PaletteGenerator] = [:]
var baseColor:UIColor = UIColor()
func generatorInstanceFromString(_ className: String) -> PaletteGenerator? {
/// get namespace
//let namespace = Bundle.main.infoDictionary!["CFBundleExecutable"] as! String;
/// get 'anyClass' with classname and namespace\(namespace).\(className)
guard let classType = NSClassFromString(className)
as? (NSObject).Type else {
return nil
}
let generator = classType.init()
if let generator = generator as? PaletteGenerator {
return generator
}
return nil
}
func register(generator className: String, withTag tag:Int, forPalette paletteName:String = "") {
if let generator = generatorInstanceFromString(className) {
generators[tag] = generator
generator.name = paletteName
generator.tag = tag
generator.delegate = self
}
}
func generateAllForColor(_ color:UIColor) {
for (_ , generator) in generators {
generator.baseColors = [color]
generator.generate()
}
}
func didGeneratePalette(forTag tag: Int) {
guard let generator = generators[tag], let delegate = self.delegate else {
return;
}
let palette = Palette(name:generator.name, andColors:generator.generatedPalette)
delegate.didUpdatePalette(forTag: tag, palette: palette)
}
}
<file_sep>/PaletteDrop/PaletteImageService.swift
//// PaletteImageService.swift
// Created: 2019-06-23
//
import Foundation
import UIKit
struct PaletteImage {
var image:UIImage? = nil
let url:URL?
let width:Int
let height:Int
let distance:Double
let title:String
let creator:String
let year:String
var palette = [[Int]]()
}
class PaletteImageMatcher {
var images = [PaletteImage]()
var enumeratedIndex:Int = 0;
var downloadIndex:Int = 0;
let defaultSession = URLSession(configuration: .default)
var dataTask: URLSessionDataTask?
//will be called from the main thread
func nextImage()->PaletteImage? {
for index in enumeratedIndex..<images.count {
if images[index].image != nil {
enumeratedIndex = index + 1
print("Sending image \(index)")
return images[index]
}
}
//Didn't find a match - rotate back to 0
for index in 0..<enumeratedIndex {
if images[index].image != nil {
enumeratedIndex = index + 1
print("[R] Sending image \(index)")
return images[index]
}
}
return nil
}
//will be called in background thread
func downloadNext() {
guard downloadIndex < images.count else {
return
}
dataTask?.cancel()
if let url = images[downloadIndex].url {
print("Downloading image \(url)")
dataTask = defaultSession.dataTask(with:url) {
data, response, error in
defer {
self.dataTask = nil
}
if let error = error {
print( "Image Download error: " + error.localizedDescription + "\n")
} else if let data = data,
let response = response as? HTTPURLResponse,
response.statusCode == 200 {
self.images[self.downloadIndex].image = UIImage.init(data:data)
self.downloadIndex+=1;
self.downloadNext()
// DispatchQueue.main.async {}
}
}
dataTask?.resume()
} else {
downloadNext()
}
}
func hasImages()->Bool {
return downloadIndex > 0
}
}
class PaletteImageService {
let defaultSession = URLSession(configuration: .default)
var dataTask: URLSessionDataTask?
func getMatchingImages(_ palette:Palette) -> PaletteImageMatcher? {
var paletteString:String = ""
for color in palette.colors {
var hexString = Colors.hexString(color).suffix(6).lowercased()
paletteString += hexString
if color != palette.colors.last {
paletteString.append("-")
}
}
var matcher = PaletteImageMatcher()
dataTask?.cancel()
let baseUrl = NSLocalizedString("googleURL", tableName: "Colors", bundle: Bundle.main, value: "", comment: "")
guard let url = URL(string:baseUrl + paletteString) else {
return nil
}
dataTask = defaultSession.dataTask(with: url) {
data, response, error in
defer { self.dataTask = nil }
// 5
if let error = error {
print ("Service Error: " + error.localizedDescription + "\n")
} else if let data = data,
let response = response as? HTTPURLResponse,
response.statusCode == 200 {
if let result=try? JSONSerialization.jsonObject(with: data.subdata(in: 6..<data.count), options: [.allowFragments]) as? [AnyObject] {
for imageInfo in result[0..<5] {
guard let info = imageInfo as? [String:AnyObject] else {
continue
}
let width = info["imageWidthPx"] as? Int ?? 0
let distance = info["distance"] as? Double ?? 0
let title = info["title"] as? String ?? ""
let height = info["imageHeightPx"] as? Int ?? 0
var url:URL?
if let imageUrl = info["imageUrl"] as? String {
url = URL(string:imageUrl)
}
let year = info["date"] as? String ?? ""
let creator = info["creator"] as? String ?? ""
var colorPalette = [[Int]]()
if let colors = info["palette"] as? [AnyObject] {
for color in colors {
if let color = color as? [String:Int] {
var rgb = [Int]()
for (_,v) in color {
rgb.append(v)
}
colorPalette.append(rgb)
}
}
}
let paletteImage = PaletteImage(image: nil, url: url, width: width, height: height, distance: distance, title: title, creator: creator, year:year, palette: colorPalette)
matcher.images.append(paletteImage)
}
matcher.downloadNext()
}
} else {
if let response = response as? HTTPURLResponse {
print("Failed to query:\(response.statusCode)")
}
}
}
dataTask?.resume()
return matcher
}
}
<file_sep>/PaletteDrop/Colours/ColourCell.swift
import UIKit
class ColourCell : UITableViewCell {
}
<file_sep>/PaletteDrop/Palettes/PaletteDesign.swift
import UIKit
class PaletteDesignView : UIView {
var colors = [
UIColor(red: 0.514, green: 0.612, blue: 0.847, alpha: 1.000),
UIColor(red: 0.082, green: 0.090, blue: 0.161, alpha: 1.000),
UIColor(red: 0.757, green: 0.541, blue: 0.769, alpha: 1.000),
UIColor(red: 0.643, green: 0.267, blue: 0.380, alpha: 1.000),
UIColor(red: 0.380, green: 0.314, blue: 0.506, alpha: 1.000)
];
var resizing: ResizingBehavior = .aspectFit
// Drawing Methods
override func draw(_ rect: CGRect) {
// General Declarations
let context = UIGraphicsGetCurrentContext()!
// Resize to Target Frame
context.saveGState()
let resizedFrame: CGRect = resizing.apply(rect: CGRect(x: 0, y: 0, width: 379, height: 104), target: frame)
context.translateBy(x: resizedFrame.minX, y: resizedFrame.minY)
context.scaleBy(x: resizedFrame.width / 379, y: resizedFrame.height / 104)
// Grey Background: Color Declarations
let strokeColor = UIColor(red: 0.208, green: 0.216, blue: 0.220, alpha: 1.000)
//let fillColor = UIColor(red: 0.514, green: 0.612, blue: 0.847, alpha: 1.000)
//let fillColor2 = UIColor(red: 0.082, green: 0.090, blue: 0.161, alpha: 1.000)
//let fillColor3 = UIColor(red: 0.757, green: 0.541, blue: 0.769, alpha: 1.000)
//let fillColor4 = UIColor(red: 0.643, green: 0.267, blue: 0.380, alpha: 1.000)
//let fillColor5 = UIColor(red: 0.380, green: 0.314, blue: 0.506, alpha: 1.000)
///First Colour: User selected colour
let baseColourPath = UIBezierPath(ovalIn: CGRect(x: 8, y: 8, width: 125, height: 88))
colors[0].setFill()
baseColourPath.fill()
// Second Colour
let lightestColourPath = UIBezierPath(ovalIn: CGRect(x: 74, y: 8, width: 117, height: 88))
colors[1].setFill()
lightestColourPath.fill()
// Third Colour
let midColour_OnePath = UIBezierPath(ovalIn: CGRect(x: 133, y: 8, width: 117, height: 88))
colors[2].setFill()
midColour_OnePath.fill()
// Fourth Colour
let midColour_TwoPath = UIBezierPath(ovalIn: CGRect(x: 197, y: 8, width: 111, height: 88))
colors[3].setFill()
midColour_TwoPath.fill()
// Fifth Colour
let darkestColourPath = UIBezierPath(ovalIn: CGRect(x: 258, y: 8, width: 113, height: 88))
colors[4].setFill()
darkestColourPath.fill()
// Paths
let pathPath = UIBezierPath(roundedRect: CGRect(x: 8, y: 8, width: 363, height: 88), cornerRadius: 44)
strokeColor.setStroke()
pathPath.lineWidth = 15
pathPath.stroke()
context.restoreGState()
}
@objc(PaletteSVGResizingBehavior)
public enum ResizingBehavior: Int {
case aspectFit /// The content is proportionally resized to fit into the target rectangle.
case aspectFill /// The content is proportionally resized to completely fill the target rectangle.
case stretch /// The content is stretched to match the entire target rectangle.
case center /// The content is centered in the target rectangle, but it is NOT resized.
public func apply(rect: CGRect, target: CGRect) -> CGRect {
if rect == target || target == CGRect.zero {
return rect
}
var scales = CGSize.zero
scales.width = abs(target.width / rect.width)
scales.height = abs(target.height / rect.height)
switch self {
case .aspectFit:
scales.width = min(scales.width, scales.height)
scales.height = scales.width
case .aspectFill:
scales.width = max(scales.width, scales.height)
scales.height = scales.width
case .stretch:
break
case .center:
scales.width = 1
scales.height = 1
}
var result = rect.standardized
result.size.width *= scales.width
result.size.height *= scales.height
result.origin.x = target.minX + (target.width - result.width) / 2
result.origin.y = target.minY + (target.height - result.height) / 2
return result
}
}
}
<file_sep>/PaletteDrop/Colours/ColoursTableView.swift
import UIKit
class ColoursTableView: UITableViewController {
var palette = Palette()
let paletteImageService = PaletteImageService();
var matcher:PaletteImageMatcher?
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
tableView.separatorStyle = .none
if palette.colors.count > 0 {
matcher = paletteImageService.getMatchingImages(palette)
}
}
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
let frame = self.view.safeAreaLayoutGuide.layoutFrame
return frame.height / 5
}
// Mark: Animation When Cells Appear
override func tableView(_ tableView: UITableView, willDisplay cell: UITableViewCell, forRowAt indexPath: IndexPath) {
// Add animations here
cell.alpha = 0
UIView.animate(
withDuration: 1.0,
delay: 0.1 * Double(indexPath.row),
options: [.curveEaseInOut],
animations: {
cell.alpha = 1
})
}
override func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return palette.colors.count
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "coloursCell", for: indexPath)
if palette.colors.count > 0 {
cell.backgroundColor = palette.colors[indexPath.row]
}
return cell
}
@IBAction func willStorePaletteInDatabase(_ sender: Any) {
PaletteStore.defaultInstance().save(palette)
let storyBoard : UIStoryboard = UIStoryboard(name: "Main", bundle:nil)
let savedPalettesCollectionView = storyBoard.instantiateViewController(withIdentifier: "savedPaletteCollectionView") as! SavedPalettesCollectionView
self.navigationController?.pushViewController(savedPalettesCollectionView, animated: true)
}
// We are willing to become first responder to get shake motion
override var canBecomeFirstResponder: Bool {
get {
return true
}
}
// Enable detection of shake motion
override func motionBegan(_ motion: UIEvent.EventSubtype, with event: UIEvent?) {
guard let matcher = self.matcher, matcher.hasImages() else {
return;
}
if motion == .motionShake {
let storyBoard : UIStoryboard = UIStoryboard(name: "Main", bundle:nil)
let paletteImageView = storyBoard.instantiateViewController(withIdentifier: "paletteImageView") as! PaletteImageView
paletteImageView.matcher = self.matcher
self.navigationController?
.pushViewController(paletteImageView, animated: true)
}
}
}
<file_sep>/PaletteDrop/Saved Palettes/SavedPalettesCollectionView.swift
import UIKit
class SavedPalettesCollectionView: UICollectionViewController, PaletteViewerDelegate {
func didUpdatePalette(forTag: Int, palette: Palette) {
palettes[forTag] = palette
collectionView.reloadData()
}
var generators:Generators?;
var coreColor:UIColor?
var palettes = [Palette]()
override func viewDidLoad() {
super.viewDidLoad()
palettes = PaletteStore.defaultInstance().loadAllPalettes()
}
override func numberOfSections(in collectionView: UICollectionView) -> Int {
return 1;
}
override func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return palettes.count;
}
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "savedPaletteCell", for: indexPath) as! SavedPaletteCell
if indexPath.row < palettes.count {
let palette = palettes[indexPath.row]
cell.colors = palette.colors
}
return cell;
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "coloursSegue-2" {
let colorsTableView = segue.destination as! ColoursTableView
if let segueCell = sender as? UICollectionViewCell {
if let index = self.collectionView.indexPath(for:segueCell),
index.row < palettes.count {
colorsTableView.palette = palettes[index.row]
}
}
}
}
@IBAction func onExportAction(_ sender: Any) {
let title = "[PaletteDrop]\t\n"
var activityItems = [Any]()
activityItems.append(title)
for palette in self.palettes {
let export = PaletteExport.init(withPalette: palette)
activityItems.append("\(export.text())\t\n")
activityItems.append(export.image() as Any)
}
let activityViewController:UIActivityViewController = UIActivityViewController(activityItems: activityItems, applicationActivities: nil)
//activityViewController.excludedActivityTypes = [ UIActivityTypeAddToReadingList, UIActivityTypePostToVimeo]
self.present(activityViewController, animated: true, completion: nil)
}
}
<file_sep>/PaletteDrop/Palettes/PalettesTableView.swift
import UIKit
class PalettesTableView: UITableViewController, PaletteViewerDelegate {
var generators:Generators?;
var coreColor:UIColor?
var palettes = [Palette]()
let ioColorMindNamingService = IOColorMindNamingService.defaultInstance()
override func viewDidLoad() {
super.viewDidLoad()
let placeHolderPalette = Palette(name: "HardCoded",
andColors: [UIColor.clear, UIColor.clear, UIColor.clear, UIColor.clear, UIColor.clear])
if let coreColor = self.coreColor {
generators = Generators()
generators!.delegate = self;
for i in 0..<ioColorMindNamingService.names.count {
palettes.append(placeHolderPalette)
generators!.register(generator: "IOColorMindGenerator", withTag: i, forPalette: ioColorMindNamingService.names[i])
}
generators!.generateAllForColor(coreColor)
}
// Do any additional setup after loading the view.
}
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
let frame = self.view.safeAreaLayoutGuide.layoutFrame
return frame.height / 5
}
func didUpdatePalette(forTag: Int, palette: Palette) {
palettes[forTag] = palette
tableView.reloadData()
}
// Mark: Animation When Cells Appear
override func tableView(_ tableView: UITableView, willDisplay cell: UITableViewCell, forRowAt indexPath: IndexPath) {
// Add animations here
cell.alpha = 0
UIView.animate(
withDuration: 1.0,
delay: 0.1 * Double(indexPath.row),
options: [.curveEaseInOut],
animations: {
cell.alpha = 1
})
}
override func numberOfSections(in tableView: UITableView) -> Int {
return 1;
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 5;
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "palettesCell", for: indexPath) as! PaletteCell
if indexPath.row < palettes.count {
let palette = palettes[indexPath.row]
cell.colors = palette.colors
}
return cell;
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "coloursSegue-1" {
let colorsTableView = segue.destination as! ColoursTableView
if let selectedIndex = self.tableView.indexPathForSelectedRow, selectedIndex.row < palettes.count {
colorsTableView.palette = palettes[selectedIndex.row]
}
}
}
}
| 9602df594892f34f7bd8e363e3cbdc55c00e3944 | [
"Swift",
"Ruby"
]
| 15 | Swift | marinamcpeak/PaletteDrop | fb58140134f68374d73e0d799126affda27dfe0a | d6f72e1c277d98e7b1a1d7940e960f3409e638ae | |
refs/heads/master | <repo_name>AshCoolman/tutorial-angular2-egghead<file_sep>/src/app/todo-service.ts
class TodoModel {
static STARTED:string = 'started';
static COMPLETED:string = 'completed';
public status : string = TodoModel.STARTED;
constructor (
public title : string = '',
public action : string = ''
) {
}
notStatus() {
return (this.status !== TodoModel.STARTED) ? TodoModel.STARTED : TodoModel.COMPLETED;
}
toggle() {
this.status = this.notStatus();
}
}
class TodoService {
public todos: TodoModel[] = [
new TodoModel('11', 'eleven'),
new TodoModel('12', 'twelve'),
new TodoModel('13', 'thirteen')
];
addTodo (value: TodoModel) {
this.todos.push(value);
}
}
export {TodoModel, TodoService}<file_sep>/src/app/has-filter.ts
import {Pipe} from "angular2/angular2"
@Pipe({
name: 'has',
pure: false
})
export class HasFilter {
transform(list, [fields, obj]) {
// let [title, action] = fields;
if (obj) {
let result = list.filter(function (item) {
return fields.some(function (field) {
return item[field].includes(obj[field]);
});
});
if (result.length !== 0) {
return result;
}
}
return list
}
}<file_sep>/src/app/filter-by.ts
import {Component, FORM_DIRECTIVES} from 'angular2/angular2';
@Component({
selector: 'filter-by',
directives: [ FORM_DIRECTIVES],
template: `
<form>
<input [(ng-model)]="title" placeholder="Filter title">
<input [(ng-model)]="action" placeholder="Filter action">
</form>
`
})
export class FilterBy {
public title :string;
public action : string;
constructor() {
}
}<file_sep>/src/app/todo-item-renderer.ts
import {Component, Input, NgClass} from 'angular2/angular2';
import {TodoModel} from './todo-service';
@Component({
selector: 'todo-item-render',
directives: [NgClass],
styles: [`
.${TodoModel.STARTED} { color: green }
.${TodoModel.COMPLETED} { text-decoration: line-through }
`
],
template: `
<div>
<span
[ng-class]="todo.status"
[(text-content)]="todo.title"
>
</span>
<span
[ng-class]="todo.status"
[(text-content)]="todo.action"
>
</span>
<button (click)="todo.toggle()">
{{'Set '+todo.notStatus()}}
</button>
</div>
`
})
export class TodoItemRenderer {
@Input('todo') todo:TodoModel;
}<file_sep>/src/app/todo-list.ts
import {Component, NgFor} from 'angular2/angular2';
import {TodoService} from './todo-service';
import {TodoItemRenderer} from './todo-item-renderer';
import {HasFilter} from './has-filter';
import {FilterBy} from './filter-by';
@Component({
selector: 'todo-list',
pipes: [HasFilter],
directives: [NgFor, FilterBy, TodoItemRenderer],
template: `
<div>
<filter-by #filter-by></filter-by> {{filterBy.title}}
<todo-item-render
*ng-for="
#todo of todoService.todos
| has:['title', 'action']:filterBy
"
[todo]="todo">
</todo-item-render>
</div>
`
})
// How would I bind to the text node? [(text-content)]="todo.title"
export class TodoList {
constructor(
public todoService:TodoService
) {
}
}<file_sep>/src/app/todo-input.ts
import {Component, FORM_DIRECTIVES} from 'angular2/angular2';
import {TodoService, TodoModel} from './todo-service';
@Component({
selector: 'todo-input',
directives: [FORM_DIRECTIVES],
template: `
<div>
<form (ng-submit)="title.focus() && doSubmit()">
<input
#title
type="text"
[(ng-model)]="todoModel.title"
placeholder="(title)"
>
<input
type="text"
[(ng-model)]="todoModel.action"
placeholder="(action)"
(keyup)="log($event)"
>
<button hidden type="submit">
Save
</button>
</form>
</div>
`
})
export class TodoInput {
public todoModel : TodoModel = new TodoModel();
constructor (
public todoService:TodoService
) {}
doSubmit () {
let {title, action} = this.todoModel;
if (title && action) {
this.todoService.addTodo(this.todoModel);
this.todoModel = new TodoModel();
} else {
console.log()
}
}
log (e) {
console.log.apply(console, Array.prototype.splice.apply(this, arguments))
}
}<file_sep>/src/app/app.ts
import {bootstrap, Component, View} from 'angular2/angular2';
import {TodoInput} from './todo-input';
import {TodoService, TodoModel} from './todo-service';
import {TodoList} from './todo-list';
@Component({
selector: ' app'
})
@View({
directives: [TodoInput, TodoList],
template: `
<div>
<h1>App</h1>
<todo-input></todo-input>
<todo-list></todo-list>
</div>
`
})
// decorators way to add behavior
class App {
}
bootstrap(App, [TodoService]); | ed96f24c71a7ab154f0a4710495ee1b7b6860f2b | [
"TypeScript"
]
| 7 | TypeScript | AshCoolman/tutorial-angular2-egghead | acf311bf17c5f07e4375618fa2e12ec359cad54a | de599326b4839aaf8f33cb428fc7d8a1e42b8d0b | |
refs/heads/master | <file_sep># -*- coding: utf-8 -*-
"""
Created on Wed Jun 6 14:41:03 2018
@author: spanier
"""
import random
# print random.randint(3, 9)
import math
#from myboolfuncs import
def Reshuma(N):
tup=()
for i in range(N):
T1= random.randint(3, 9)
tup += (T1,)
print(tup)
return tup
def MyGuess(N1):
myGuess=[]
for j in range(N1):
guess = input("Try to guess the numbers between 1-9! :")
myGuess.append(guess)
for i in myGuess:
print(i)
return myGuess
def nihushTest(r,g,num):
result=()
success=0
for i in range(num):
if r[i]==g[i]:
item=r[i]
result= result+item
success+=1
else:
result= result + ("X",)
p= success/num
percent= p*100
print(result, end=" ")
print (percent)
return
while True:
num = int(input("How many digits do you want to guess (press -1 to quit):"))
if num == -1:
break
else:
reshuma= Reshuma(num)
guessList= MyGuess(num)
nihushTest(reshuma,guessList,num)<file_sep># -*- coding: utf-8 -*-
"""
Created on Wed Jun 6 14:04:12 2018
@author: spanier
"""
def dictionary(L):
sumTuple=0
sumList=0
sumString=0
sumInt=0
sumFloat=0
for item in L:
if isinstance (item,tuple):
sumTuple+=1
if isinstance (item,list):
sumList+=1
if isinstance (item,str):
sumString+=1
if isinstance (item, int):
sumInt+=1
if isinstance (item, float):
sumFloat+=1
return {list : sumList, int : sumInt, float : sumFloat, str : sumString, tuple : sumTuple}<file_sep>"# Python-Structures"
<file_sep># -*- coding: utf-8 -*-
"""
Spyder Editor
This is a temporary script file.
"""
def TOlist(L):
lst=[]
for item in L:
if isinstance (item,tuple):
for value in item:
lst.append(value)
#print(lst)
return lst
def ToTuple(T):
tup=()
for item in T:
if isinstance (item,list):
T1=tuple(item)
tup= tup+T1
#print(tup)
return tup
def listOfStrings(LS):
L1=[]
for item in LS:
if isinstance (item,str):
for value in item:
if checkIfExist(LS, value):
L1.append(value)
#print(L1)
return L1
def listOfNums(LN):
L2=[]
for item in LN:
if isinstance (item, int or float):
if checkIfExist(LN, item):
L2.append(item)
#print(L2)
return L2
def checkIfExist(lst,val):
checkList=TOlist(lst)
checkTuple=ToTuple(lst)
for i in checkList:
if val==i:
return False
for j in checkTuple:
if val==j:
return False
return True
<file_sep># -*- coding: utf-8 -*-
"""
Created on Mon Jun 11 15:59:04 2018
@author: spanier
"""
def replaceSimaneyPisuk(mahrozet):
simaneyPisuk = ",.;:!?\t\n"
newMahrozet = ""
for c in mahrozet:
if c in simaneyPisuk:
newMahrozet += ' '
else:
newMahrozet += c
return newMahrozet
#
def countWords(fileObj):
D = dict([])
for line in fileObj:
wordsInLine = replaceSimaneyPisuk(line).split()
for word in wordsInLine:
if word not in D:
D[word] = 1
else:
D[word] += 1
return D
#
def prtWordCounts(D):
prtLines = 1
for word, count in D.items():
if prtLines != 10:
print(word, "was found", count, "times in the text.")
prtLines += 1
else:
while True:
ifBreak = input("Do you wish to stop printing? (Y, y, N or n)").upper()
if ifBreak == 'Y':
print ('The printing of the word counts was stopped.')
return
elif ifBreak == 'N':
prtLines = 1
break
#else:
# continue
else:
print ('All the word counts were printed')
return
#
#
# main program
#
if __name__ == "__main__":
fileName = input("Please, enter the text file to be processed: ")
# in this program I use exception handling, but the students may
# write their program assuming that the text file exists
try:
fileObj = open(fileName, "r")
D = countWords(fileObj)
prtWordCounts(D)
fileObj.close()
except FileNotFoundError:
print ("ERROR - file ", fileName, "not found!")
# | bad213bf41d179b6975f999d653d8a871c78045a | [
"Markdown",
"Python"
]
| 5 | Python | Ruth-Cohen/Python-Structures | 14fa03e8566ed00be38a9c5826cf0d16b45964e3 | d2f50d7500d36d0fc4d3b148aaa128ef685a46dd | |
refs/heads/main | <file_sep>import pygame as pg
from sys import exit
from random import randint, choice
from math import sqrt, pow, atan
from time import sleep, time, localtime, asctime
pg.init()
scr_wid = 1200
scr_hgt = 700
screen = pg.display.set_mode((scr_wid, scr_hgt))
pg.display.set_caption("Karlo's struggle 1")
title_icon = pg.image.load("yellow.png")
pg.display.set_icon(title_icon)
vol_bgm = 0.05
vol_click = 0.15
vol_Kill_bot = 0.35
vol_gameover = 0.35
pg.mixer.music.load('_bgm.ogg')
click_sound = pg.mixer.Sound('click.ogg')
kill_bot_sound = pg.mixer.Sound('kill_bot.ogg')
gameover_sound = pg.mixer.Sound('gameover.ogg')
pg.mixer.music.set_volume(vol_bgm)
click_sound.set_volume(vol_click)
kill_bot_sound.set_volume(vol_Kill_bot)
gameover_sound.set_volume(vol_gameover)
pg.mixer.music.play(-1)
music_on = True
sound_on = True
black = [0, 0, 0]
white = [255, 255, 255]
clock = pg.time.Clock()
chaser_wid = chaser_hgt = 35
player_wid = player_hgt = 40
tower_wid = tower_hgt = 70
Chaser_Player_r = 25
Tower_Player_r = 50
laser_range = 300
laser_width = 12
laser_kill_range = 20
die_wid = 70
die_hgt = 70
die = pg.image.load("star.png")
die = pg.transform.smoothscale(die, (die_wid, die_hgt))
score = 0
fps = 30
chaser_speed = 4
Gameover = False
H_page = False
A_page = False
St_page = False
SB_page = False
MnLp = False
min_dst = 40
bot_num = 8
gamemode = 'normal'
pos_yes_icon = [80, 300]
num_lst = []
def load_SB(file):
with open(file, 'r') as f:
f_lst = f.readlines()
SB = [
[int(f_lst[0][:-1]), f_lst[1][:-1]], [int(f_lst[2][:-1]), f_lst[3][:-1]],
[int(f_lst[4][:-1]), f_lst[5][:-1]], [int(f_lst[6][:-1]), f_lst[7][:-1]],
[int(f_lst[8][:-1]), f_lst[9][:-1]], [int(f_lst[10][:-1]), f_lst[11][:-1]],
[int(f_lst[12][:-1]), f_lst[13][:-1]], [int(f_lst[14][:-1]), f_lst[15][:-1]]
]
return SB
E_SB = load_SB(file='_e_sb.txt')
N_SB = load_SB(file='_n_sb.txt')
H_SB = load_SB(file='_h_sb.txt')
I_SB = load_SB(file='_i_sb.txt')
def save_all_SB():
def save_SB(file, SB):
SB = [
str(SB[0][0]), SB[0][1], str(SB[1][0]), SB[1][1],
str(SB[2][0]), SB[2][1], str(SB[3][0]), SB[3][1],
str(SB[4][0]), SB[4][1], str(SB[5][0]), SB[5][1],
str(SB[6][0]), SB[6][1], str(SB[7][0]), SB[7][1]
]
with open(file, 'w') as f:
for i in SB:
f.writelines(i)
f.write('\n')
save_SB('_e_sb.txt', E_SB)
save_SB('_n_sb.txt', N_SB)
save_SB('_h_sb.txt', H_SB)
save_SB('_i_sb.txt', I_SB)
def clear_all_SB():
global E_SB, N_SB, H_SB, I_SB
E_SB= [[0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0']]
N_SB= [[0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0']]
H_SB= [[0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0']]
I_SB= [[0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0'], [0, '0']]
def clear_SB(file):
with open(file, 'w') as f:
for i in range(16):
f.writelines('0')
f.write('\n')
clear_SB('_e_sb.txt')
clear_SB('_n_sb.txt')
clear_SB('_h_sb.txt')
clear_SB('_i_sb.txt')
class Me(object):
def __init__(self, pos=[500, 400]):
self.pos = pos
self.ctpos = [self.pos[0]+player_wid/2, self.pos[1]+tower_hgt/2]
self.player = pg.image.load("playerblue.png")
self.player = pg.transform.smoothscale(self.player, (player_wid, player_hgt))
self.W = False
self.A = False
self.S = False
self.D = False
self.a = 0.4
self.Uv = 0
self.Rv = 0
def event_response(self):
for event in pg.event.get():
if event.type == pg.QUIT:
save_all_SB()
pg.quit()
exit()
if event.type == pg.KEYDOWN:
if event.key == pg.K_w or event.key == pg.K_UP:
self.W = True
if event.key == pg.K_a or event.key == pg.K_LEFT:
self.A = True
if event.key == pg.K_s or event.key == pg.K_DOWN:
self.S = True
if event.key == pg.K_d or event.key == pg.K_RIGHT:
self.D = True
elif event.type == pg.KEYUP:
if event.key == pg.K_w or event.key == pg.K_UP:
self.W = False
if event.key == pg.K_a or event.key == pg.K_LEFT:
self.A = False
if event.key == pg.K_s or event.key == pg.K_DOWN:
self.S = False
if event.key == pg.K_d or event.key == pg.K_RIGHT:
self.D = False
if self.W == True:
self.Uv = self.Uv + self.a
if self.A == True:
self.Rv = self.Rv - self.a
if self.S == True:
self.Uv = self.Uv - self.a
if self.D == True:
self.Rv = self.Rv + self.a
self.pos = [self.pos[0]+self.Rv, self.pos[1]-self.Uv]
self.ctpos = [self.pos[0]+player_wid/2, self.pos[1]+tower_hgt/2]
def detect_out(self):
if self.pos[0] < -40 or self.pos[0] > scr_wid-player_wid+40 or self.pos[1] < -40 or\
self.pos[1] > scr_hgt-player_hgt+40:
global Gameover
Gameover = True
def rotate(self):
self.new_me = self.player
#pg.draw.line(screen, [0, 255, 0], self.ctpos, [self.ctpos[0], self.ctpos[1]-self.Uv*20], laser_width)
#pg.draw.line(screen, [255, 0, 0], self.ctpos, [self.ctpos[0]+self.Rv*20, (self.ctpos[1])], laser_width)
if not self.Uv == 0:
tanA = self.Rv/self.Uv
angle = atan(tanA)*180/3.14
if self.Uv > 0:
self.new_me = pg.transform.rotate(self.player, -angle)
else:
self.new_me = pg.transform.rotate(self.player, -angle+180)
else:
if self.Rv > 0:
self.new_me = pg.transform.rotate(self.player, -90)
else:
self.new_me = pg.transform.rotate(self.player, 90)
def draw(self):
screen.blit(self.new_me, self.pos)
def me_go(self):
me.event_response()
me.detect_out()
me.rotate()
me.draw()
me = Me()
class Tower(object):
def __init__(self, pos = [0, 0]):
self.pos = pos
self.tower = pg.image.load("_tower.png")
self.tower = pg.transform.smoothscale(self.tower, (tower_wid, tower_hgt))
self.ctpos = [self.pos[0]+tower_wid/2, self.pos[1]+tower_hgt/2]
def detect_player(self):
if sqrt(pow(me.pos[0]-self.pos[0], 2)+pow(me.pos[1]-self.pos[1], 2)) < Tower_Player_r:
global Gameover
Gameover = True
def laser(self):
if sqrt(pow(me.pos[0]-self.pos[0], 2)+pow(me.pos[1]-self.pos[1], 2)) < laser_range:
pg.draw.line(screen, [randint(0, 200), randint(0, 200), randint(0, 200)],
self.ctpos, me.ctpos, laser_width)
def draw(self):
screen.blit(self.tower, self.pos)
tower1 = Tower([scr_wid/4 - tower_wid/2 + 20, scr_hgt/4 - tower_hgt/2 + 20])
tower2 = Tower([3*scr_wid/4 - tower_wid/2 + 20, scr_hgt/4 - tower_hgt/2 - 20])
tower3 = Tower([3*scr_wid/4 - tower_wid/2 - 20, 3*scr_hgt/4 - tower_hgt/2 - 20])
tower4 = Tower([scr_wid/4 - tower_wid/2 - 20, 3*scr_hgt/4 - tower_hgt/2 + 20])
Towers = [tower1, tower2, tower3, tower4]
class Chaser(object):
def __init__(self, pos=[0, 0]):
self.pos = pos
self.ctpos = [self.pos[0]+chaser_wid/2, self.pos[1]+chaser_hgt/2]
self.edge_point = [self.pos, [self.pos[0]+chaser_wid, self.pos[1]], \
[self.pos[0]+chaser_wid, self.pos[1]+chaser_hgt], [self.pos[0], self.pos[1]+chaser_hgt]]
self.chaser = pg.image.load("_red.png")
self.chaser = pg.transform.smoothscale(self.chaser, (chaser_wid, chaser_hgt))
def chase(self):
x = self.pos[0]-me.pos[0]
y = self.pos[1]-me.pos[1]
l = sqrt(pow(x, 2) + pow(y, 2))
ratio = chaser_speed/l
x_mov = x*ratio
y_mov = y*ratio
self.pos[0] = self.pos[0] - x_mov
self.pos[1] = self.pos[1] - y_mov
self.ctpos = [self.pos[0]+chaser_wid/2, self.pos[1]+chaser_hgt/2]
self.edge_point = [self.pos, [self.pos[0]+chaser_wid, self.pos[1]], \
[self.pos[0]+chaser_wid, self.pos[1]+chaser_hgt], [self.pos[0], self.pos[1]+chaser_hgt]]
def detect_player(self):
if sqrt(pow(me.pos[0]-self.pos[0], 2)+pow(me.pos[1]-self.pos[1], 2)) < Chaser_Player_r:
global Gameover
Gameover = True
def rotate(self):
if not (me.pos[1]-self.pos[1]) == 0:
tanA = (me.pos[0]-self.pos[0])/(me.pos[1]-self.pos[1])
angle = atan(tanA)*180/3.14
if self.pos[1] > me.pos[1]:
self.new_chaser = pg.transform.rotate(self.chaser, angle)
else:
self.new_chaser = pg.transform.rotate(self.chaser, angle+180)
else:
if self.pos[0] > me.pos[0]:
self.new_chaser = pg.transform.rotate(self.chaser, 90)
else:
self.new_chaser = pg.transform.rotate(self.chaser, -90)
def draw(self):
screen.blit(self.new_chaser, self.pos)
class Txt_info(object):
def __init__(self, style = 'consolas', color = [230, 230, 230]):
self.style = style
self.color = color
self.bg_color = black
def draw_score_board(self, pos, size):
self.ft = pg.font.SysFont(self.style, size)
self.pos = pos
text = self.ft.render('score:{s}'.format(s=score), True, self.color, self.bg_color)
screen.blit(text, self.pos)
def draw_info(self, info, pos, size):
self.ft = pg.font.SysFont(self.style, size)
self.size = size
self.pos = pos
text = self.ft.render(info, True, self.color, self.bg_color)
screen.blit(text, self.pos)
score_board = Txt_info()
common_txt = Txt_info()
def tower_go():
for each_tower in Towers:
each_tower.detect_player()
each_tower.laser()
each_tower.draw()
def Bubble_Sort(lst):
for freq in range(1, len(lst)):
for each_elmt in range(0, len(lst)-1):
if lst[each_elmt] < lst[each_elmt+1]:
lst[each_elmt], lst[each_elmt+1]=lst[each_elmt+1], lst[each_elmt]
return lst
def refresh_SB():
global E_SB, N_SB, H_SB, I_SB
def refresh_one_SB(SB, mod):
global num_lst
if gamemode == mod:
num_lst = [SB[0][0], SB[1][0], SB[2][0], SB[3][0], SB[4][0], SB[5][0], SB[6][0], SB[7][0]]
if score > min(num_lst):
SB[7] = [score, asctime(localtime(time()))]
Bubble_Sort(SB)
refresh_one_SB(E_SB, 'easy')
refresh_one_SB(N_SB, 'normal')
refresh_one_SB(H_SB, 'hard')
refresh_one_SB(I_SB, 'hell')
def main_loop():
global score, MnLp
score = 0
timer = 0
Chasers = []
Chasers_ctpos = []
me.pos = [500, 400]
me.Rv = me.Uv = 0
for i in range(bot_num):
chaser = Chaser(choice([[randint(0, scr_wid), scr_hgt], [randint(0, scr_wid), -50], \
[-30, randint(0, scr_hgt)], [scr_wid, randint(0, scr_hgt)]]))
Chasers.append(chaser)
Chasers_ctpos.append(chaser.ctpos)
me.W = me.A = me.S = me.D = False
def end_page():
global Gameover, MnLp
me.Rv = 0
me.Uv = 0
common_txt.draw_info('Game Over!', pos = [500, 160], size = 30)
common_txt.draw_info('Your score is {s} :) mode:{m}'
.format(s=score, m=gamemode), pos = [330, 260], size = 30)
common_txt.draw_info('Restart (R)', pos = [500, 360], size = 30)
common_txt.draw_info('Main menu (M)', pos = [490, 460], size = 30)
common_txt.draw_info('Quit (Q)', pos = [520, 560], size = 30)
for event in pg.event.get():
if event.type == pg.QUIT:
save_all_SB()
pg.quit()
exit()
if event.type == pg.KEYDOWN:
if event.key == pg.K_q:
click_sound.play()
save_all_SB()
pg.quit()
exit()
if event.key == pg.K_r:
click_sound.play()
Gameover = False
if event.key == pg.K_m:
click_sound.play()
Gameover = False
MnLp = False
pg.display.update()
screen.fill(black)
clock.tick(fps)
while True:
score_board.draw_score_board([0, 0], size = 26)
common_txt.draw_info('{mod}'.format(mod = gamemode), pos = [150, 0], size = 26)
timer = timer + 1
if timer > 15:
timer = 0
score = score + 1
tower_go()
#####
t = 0
for each_bot in Chasers:
for EACH_TOWER in Towers:
if sqrt(pow(EACH_TOWER.pos[0]-each_bot.pos[0], 2) +\
pow(EACH_TOWER.pos[1]-each_bot.pos[1], 2)) < Tower_Player_r:
ex = EACH_TOWER.pos[0] - each_bot.pos[0]
ey = EACH_TOWER.pos[1] - each_bot.pos[1]
e_ratio = 8 / Tower_Player_r
x_back = ex*e_ratio
y_back = ey*e_ratio
each_bot.pos[0] = each_bot.pos[0] - x_back
each_bot.pos[1] = each_bot.pos[1] - y_back
x1 = me.pos[0]
y1 = me.pos[1]
x2 = EACH_TOWER.pos[0]
y2 = EACH_TOWER.pos[1]
x3 = each_bot.pos[0]
y3 = each_bot.pos[1]
if not x1-x2 == 0:
k = (y1-y2)/(x1-x2)
b = y1 - k*x1
d = sqrt(pow(-k*x3+y3-b, 2))/sqrt(pow(k, 2)+1)
D_pt = sqrt(pow(me.pos[0]-EACH_TOWER.pos[0], 2)+pow(me.pos[1]-EACH_TOWER.pos[1], 2))
D_bt = sqrt(pow(each_bot.pos[0]-EACH_TOWER.pos[0], 2)+pow(each_bot.pos[1]-EACH_TOWER.pos[1], 2))
D_pb = sqrt(pow(me.pos[0]-each_bot.pos[0], 2)+pow(me.pos[1]-each_bot.pos[1], 2))
if d < laser_kill_range and D_pt > D_bt and D_pt < laser_range and D_pb < D_pt:
score = score + 10
die_pos = [each_bot.pos[0]-13, each_bot.pos[1]-12]
kill_bot_sound.play()
each_bot.pos = choice([[randint(0, scr_wid), scr_hgt], [randint(0, scr_wid), -50], \
[-30, randint(0, scr_hgt)], [scr_wid, randint(0, scr_hgt)]])
screen.blit(die, die_pos)
Chasers_ctpos.pop(t)
for other_ctpos in Chasers_ctpos:
if other_ctpos[0]-each_bot.ctpos[0] == other_ctpos[1]-each_bot.ctpos[1] == 0:
each_bot.ctpos[0] = each_bot.ctpos[0] - 1
each_bot.ctpos[1] = each_bot.ctpos[1] - 1
ex = other_ctpos[0]-each_bot.ctpos[0]
ey = other_ctpos[1]-each_bot.ctpos[1]
dst = sqrt(pow(ex, 2)+pow(ey, 2))
if dst < min_dst:
e_ratio = 2/dst
x_back = ex*e_ratio
y_back = ey*e_ratio
each_bot.pos[0] = each_bot.pos[0] - x_back
each_bot.pos[1] = each_bot.pos[1] - y_back
Chasers_ctpos.insert(t, each_bot.ctpos)
t = t + 1
each_bot.chase()
each_bot.rotate()
each_bot.draw()
each_bot.detect_player()
#####
me.me_go()
pg.display.update()
screen.fill(black)
clock.tick(fps)
if Gameover == True:
gameover_sound.play()
refresh_SB()
for i in range(10):
sleep(0.1)
tower_go()
pg.display.update()
screen.fill([randint(0, 100), 0, 0])
break
while Gameover == True:
end_page()
def Start_page():
global MnLp, St_page, SB_page, A_page, H_page
title = pg.image.load("_title.png")
title = pg.transform.smoothscale(title, (scr_wid, 370))
screen.blit(title, [0, 0])
common_txt.draw_info('difficulty:{mod}'.format(mod = gamemode), pos = [10, scr_hgt-30], size = 26)
common_txt.draw_info('Play (P)', pos = [520, 370], size = 30)
common_txt.draw_info('settings (T)', pos = [480, 420], size = 30)
common_txt.draw_info('score board (S)', pos = [460, 470], size = 30)
common_txt.draw_info('Author (A)', pos = [500, 520], size = 30)
common_txt.draw_info('Help (H)', pos = [520, 570], size = 30)
common_txt.draw_info('Quit (Q)', pos = [520, 620], size = 30)
for event in pg.event.get():
if event.type == pg.QUIT:
save_all_SB()
pg.quit()
exit()
if event.type == pg.KEYDOWN:
click_sound.play()
if event.key == pg.K_p:
MnLp = True
if event.key == pg.K_t:
St_page = True
if event.key == pg.K_s:
SB_page = True
if event.key == pg.K_a:
A_page = True
if event.key == pg.K_h:
H_page = True
if event.key == pg.K_q:
save_all_SB()
pg.quit()
exit()
pg.display.update()
screen.fill(black)
clock.tick(fps)
def Help_page():
global H_page
common_txt.draw_info('*Use W, A, S, D to control Karlo', pos = [100, 200], size = 30)
common_txt.draw_info('*Avoid borders, enemies and laser towers', pos = [100, 250], size = 30)
common_txt.draw_info('*Get close to laser towers to activate destructive laser', pos = [100, 300], size = 30)
common_txt.draw_info('*Use laser to kill enemies and get points', pos = [100, 350], size = 30)
common_txt.draw_info('*Points will increase automatically as long as you are alive', pos = [100, 400], size = 30)
common_txt.draw_info('*ONE LIFE only, good luck :)', pos = [100, 450], size = 30)
common_txt.draw_info('Main menu (M)', pos = [100, 550], size = 30)
for event in pg.event.get():
if event.type == pg.QUIT:
save_all_SB()
pg.quit()
exit()
if event.type == pg.KEYDOWN:
click_sound.play()
if event.key == pg.K_m:
H_page = False
pg.display.update()
screen.fill(black)
clock.tick(fps)
def Author_page():
global A_page
common_txt.draw_info('Program: Jiamin', pos = [300, 200], size = 30)
common_txt.draw_info('Art&Sound: Jiamin', pos = [300, 250], size = 30)
common_txt.draw_info('Music: Motion Focus Music', pos = [300, 300], size = 30)
common_txt.draw_info('Test: Jiamin', pos = [300, 350], size = 30)
common_txt.draw_info('Thanks for paying attention to this game, if having any idea or suggestion, ', \
pos = [200, 400], size = 20)
common_txt.draw_info('contact us through the email:', pos = [200, 430], size = 20)
common_txt.draw_info('<EMAIL>', pos = [300, 480], size = 20)
common_txt.draw_info('Main menu (M)', pos = [300, 550], size = 30)
for event in pg.event.get():
if event.type == pg.QUIT:
save_all_SB()
pg.quit()
exit()
if event.type == pg.KEYDOWN:
click_sound.play()
if event.key == pg.K_m:
A_page = False
pg.display.update()
screen.fill(black)
clock.tick(fps)
def settings_page():
global fps, bot_num, St_page, gamemode, pos_yes_icon, chaser_speed, music_on, sound_on, vol_click, vol_Kill_bot, vol_gameover
if music_on == True:
status_M = 'on'
else:
status_M = 'off'
if sound_on == True:
status_S = 'on'
else:
status_S = 'off'
yes_icon = pg.image.load("yes_icon.png")
yes_icon = pg.transform.smoothscale(yes_icon, (30, 30))
screen.blit(yes_icon, pos_yes_icon)
common_txt.draw_info('difficulties:', pos = [120, 200], size = 30)
common_txt.draw_info('easy (E) :few of boring enemies', pos = [120, 250], size = 30)
common_txt.draw_info('normal (N) :normal numbers of normal enemies', pos = [120, 300], size = 30)
common_txt.draw_info('hard (H) :more of faster enemies', pos = [120, 350], size = 30)
common_txt.draw_info('hell(I) :large numbers of very challenging enemies', pos = [120, 400], size = 30)
common_txt.draw_info('music (C): {M}'.format(M = status_M), pos = [150, 500], size = 25)
common_txt.draw_info('sound (S): {S}'.format(S = status_S), pos = [150, 550], size = 25)
common_txt.draw_info('Main menu (M)', pos = [120, 650], size = 30)
for event in pg.event.get():
if event.type == pg.QUIT:
save_all_SB()
pg.quit()
exit()
if event.type == pg.KEYDOWN:
click_sound.play()
if event.key == pg.K_m:
St_page = False
if event.key == pg.K_e:
bot_num = 6
chaser_speed = 3
gamemode = 'easy'
pos_yes_icon = [80, 250]
if event.key == pg.K_n:
bot_num = 8
chaser_speed = 4
gamemode = 'normal'
pos_yes_icon = [80, 300]
if event.key == pg.K_h:
bot_num = 12
chaser_speed = 4.5
gamemode = 'hard'
pos_yes_icon = [80, 350]
if event.key == pg.K_i:
bot_num = 16
chaser_speed = 5
gamemode = 'hell'
pos_yes_icon = [80, 400]
if event.key == pg.K_c:
if music_on == True:
music_on = False
pg.mixer.music.pause()
else:
music_on = True
pg.mixer.music.unpause()
if event.key == pg.K_s:
if sound_on == True:
sound_on = False
vol_click = vol_Kill_bot = vol_gameover = 0
click_sound.set_volume(vol_click)
kill_bot_sound.set_volume(vol_Kill_bot)
gameover_sound.set_volume(vol_gameover)
else:
sound_on = True
vol_click = 0.15
vol_Kill_bot = 0.35
vol_gameover = 0.35
click_sound.set_volume(vol_click)
kill_bot_sound.set_volume(vol_Kill_bot)
gameover_sound.set_volume(vol_gameover)
pg.display.update()
screen.fill(black)
clock.tick(fps)
def score_board_page():
global SB_page, E_SB, N_SB, H_SB, I_SB
def draw_all_SB(title_size = 25, font_size = 14, space = 60):
def draw_one_SB(SB_name, line_x, start_y, SB):
common_txt.draw_info(SB_name, pos = [line_x, start_y], size = title_size)
for i in range(8):
common_txt.draw_info('{a}:{e}'.format(a=i+1, e=SB[i]), pos=[line_x, start_y+space*(i+1)], size=font_size)
draw_one_SB('easy', 0, 10, E_SB)
draw_one_SB('normal', scr_wid/4, 10, N_SB)
draw_one_SB('hard', scr_wid*2/4, 10, H_SB)
draw_one_SB('hell', scr_wid*3/4, 10, I_SB)
draw_all_SB()
common_txt.draw_info('Main menu (M)', pos = [490, scr_hgt-150], size = 30)
common_txt.draw_info('Clear score board (X)', pos = [0, scr_hgt-50], size = 18)
common_txt.draw_info('Warning: ALL score data will be delated PERMANENTLY', pos = [0, scr_hgt-30], size = 18)
for event in pg.event.get():
if event.type == pg.QUIT:
save_all_SB()
pg.quit()
exit()
if event.type == pg.KEYDOWN:
click_sound.play()
if event.key == pg.K_m:
SB_page = False
if event.key == pg.K_x:
clear_all_SB()
pg.display.update()
screen.fill(black)
clock.tick(fps)
def game_run():
global score, Gameover, MnLp
while True:
Start_page()
while MnLp == True:
main_loop()
while A_page == True:
Author_page()
while H_page == True:
Help_page()
while St_page == True:
settings_page()
while SB_page == True:
score_board_page()
game_run()
<file_sep># Karlo1-demo
This is a self made game demo which was finished in late 2019, under the purpose of learning python basis and getting familiar with the basic programming operations & concepts.
The demo project is written in python and all its game funtions are implemented by the module of pygame.
In this 2d arcade-like game, player needs to control the blue spaceship to survive as long as possible and use the laser generated from white towers to kill aggressive red enemies.
| 03cfc93adb9dc807ca28e1b9b02d1a646c42fed9 | [
"Markdown",
"Python"
]
| 2 | Python | fccm/Karlo1-demo | 802a9110e7796694b299c71bb8ba15a723549170 | 53d8ad2345a39b96d7710aac3ad2a5db152faa23 | |
refs/heads/master | <repo_name>jmuhammed/Selenium-<file_sep>/TestHide/src/com/pack/qatar/TestQatar.java
//package com.pack.qatar;
import java.util.List;
import org.openqa.selenium.*;
import org.openqa.selenium.firefox.FirefoxDriver;
public class TestQatar {
public void testdrop() throws Exception{
WebDriver driver=new FirefoxDriver();
JavascriptExecutor jex=(JavascriptExecutor)driver;
driver.get("http://www.qatarairways.com");
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
List <WebElement> wtabs=driver.findElements(By.id("tabContainerDIV_Home").id("flighttab_wrapper").className("darkpurple_tabs"));
System.out.println(wtabs.toString());
driver.findElement(By.className("darkpurple_tabs"));
Thread.sleep(3000);
/*Boolean result=(Boolean) jex.executeScript("$(document).ready()");
System.out.println(result);*/
jex.executeScript(" $(\"[title=Click to search our timetables]\").click();");
WebElement text=driver.findElement(By.id("TFromTemp"));
text.click();
text.sendKeys("maa");
Thread.sleep(3000);
jex.executeScript("$(\".ui-menu-item\").click();");
WebElement textdest=driver.findElement(By.id("TToTemp"));
textdest.click();
Thread.sleep(3000);
textdest.sendKeys("qat");
Thread.sleep(3000);
jex.executeScript("$(\".ui-menu-item\").click();");
Thread.sleep(3000);
jex.executeScript("$('#timeTableResult').click();");
}
public static void main(String[] args) throws Exception {
TestQatar tq=new TestQatar();
tq.testdrop();
}
}
| f2c662cafa49df237deb3ee0a607b3b16ed99079 | [
"Java"
]
| 1 | Java | jmuhammed/Selenium- | 36bfad6646a4b3dd1e7ff7579d3b3b927f4db318 | 3cc06885039ab8435bfe53769a7d6143ae5705a9 | |
refs/heads/master | <file_sep>import './Footer.scss';
export function Footer() {
return (
<div className="footer-container">
<div className="footer">
<p className="footer__text">© 2020 Travelize</p>
<p className="footer__text">Privacy Policy</p>
<p className="footer__text">Terms and conditions</p>
</div>
</div>
);
}
<file_sep>import './App.scss';
import { Navigation } from './components/Navigation/Navigation';
import { About } from './components/About';
import { Blog } from './components/Blog';
import { Home } from './components/Home';
import { Footer } from './components/Footer/Footer';
import {
BrowserRouter as Router,
Switch,
Route
} from "react-router-dom";
function App() {
return (
<Router>
<div className="app">
<Navigation />
<Switch>
<Route path="/blog">
<Blog />
</Route>
<Route path="/about">
<About />
</Route>
<Route path="/">
<Home />
</Route>
</Switch>
<Footer />
</div>
</Router>
);
}
export default App;
<file_sep>import React from 'react';
export function Home() {
const style = {
fontSize: 40,
gridRow: '2 / 3',
gridColumn: '2 / 3',
textAlign: 'center',
margin: '3rem auto'
};
return (
<div style={style}>
..Under Construction..
</div>
);
}
<file_sep>import './About.scss';
import React, { Fragment } from 'react';
export function About() {
return (
<Fragment>
<div className="hero-image"></div>
<div className="main">
<div className="main__image"></div>
<div className="main__content">
<h2 className="main__content__title">A little about me</h2>
<p className="main__content__text">
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nulla porttitor felis in lorem volutpat lacinia. Ut eget lacus ac est dapibus tempus. Sed sit amet arcu vel lectus maximus faucibus. Maecenas nec bibendum nisi. Nunc quis purus at nisi ornare dignissim a non nisl. Ut at mauris elementum.</p>
<p className="main__content__text">
Vivamus ante turpis, aliquet vitae lobortis sit amet, euismod sed ex. Vestibulum ante ipsum primis in faucibus orci luctus et ultrices posuere cubilia curae; Mauris malesuada turpis eu aliquet laoreet. Ut eget tellus consequat, sodales nisl ut, interdum quam.</p>
<p className="main__content__text">
Suspendisse ullamcorper in tortor sit amet suscipit. Mauris efficitur neque sit amet quam luctus, id pharetra ex viverra. Mauris leo metus, lobortis id lorem et, dignissim imperdiet dolor.</p>
<p className="main__content__text">
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nulla porttitor felis in lorem volutpat lacinia. Ut eget lacus ac est dapibus tempus. Sed sit amet arcu vel lectus maximus faucibus. Maecenas nec bibendum nisi. Nunc quis purus at nisi ornare dignissim a non nisl. Ut at mauris elementum.</p>
<p className="main__content__text">
Etiam ex orci, suscipit vel imperdiet congue, tempor et est. Nunc diam mi, viverra non tempus et, lobortis quis odio. Curabitur eu nulla congue, tristique sem eu, sodales diam. Fusce faucibus ipsum sed eleifend rutrum. Cras eu sagittis justo. Etiam augue diam, blandit ut venenatis sit ame.</p>
<p className="main__content__text">
In euismod lorem et dolor mollis, eu elementum ligula ullamcorper. Vivamus eu erat sapien. Aenean imperdiet finibus orci sit amet aliquet. Praesent nec tempor elit. Maecenas vitae pellentesque urna, nec mattis lacus. Fusce vehicula dui ut erat ullamcorper, malesuada rhoncus leo dignissim. Nullam molestie ut turpis lacinia gravida.</p>
</div>
</div>
</Fragment>
);
}
| e7df7eaa87b0cc7ef1a064861512c0ec57ae280b | [
"JavaScript"
]
| 4 | JavaScript | sierramoore/timescale_assignment | 4e554a7f19b278b9084c6e2cb286f78758d7529e | 5ad2132b67acc9962aa0d8d9e9ca96c34b053029 | |
refs/heads/master | <file_sep>package br.com.gbd.apostiladesignpatterns.estrutural.bridge;
/*
Autor: <NAME>
Data: 08/08/2016, 15:52:58
Arquivo: Documento
*/
public interface Documento {
void geraArquivo();
}
<file_sep>package br.com.gbd.apostiladesignpatterns.estrutural.flyweight;
import java.util.HashMap;
import java.util.Map;
/*
Autor: <NAME>
Data: 09/08/2016, 09:03:40
Arquivo: TemaFlyweightFactory
*/
public class TemaFlyweightFactory {
private static Map<Class<? extends TemaFlyweight>, TemaFlyweight> temas = new HashMap<>();
public static final Class<TemaAsterisco> ASTERISCCO = TemaAsterisco.class;
public static final Class<TemaHifen> HIFEN = TemaHifen.class;
public static final Class<TemaGBD> GBD = TemaGBD.class;
public static TemaFlyweight getTema(Class<? extends TemaFlyweight> clazz){
if(!temas.containsKey(clazz)){
try{
temas.put(clazz, clazz.newInstance());
} catch(Exception e){
e.printStackTrace();
}
}
return temas.get(clazz);
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.comportamental.visitor;
import java.util.ArrayList;
import java.util.List;
/*
Autor: <NAME>
Data: 09/08/2016, 15:47:21
Arquivo: Departamento
*/
public class Departamento {
private String nome;
private List<Funcionario> funcionarios = new ArrayList<>();
public Departamento(String nome) {
this.nome = nome;
}
public String getNome(){
return this.nome;
}
public List<Funcionario> getFuncionarios(){
return funcionarios;
}
public void aceita(AtualizadorDeFuncionario atual){
for(Funcionario f : this.funcionarios){
f.aceita(atual);
}
}
}
<file_sep>package br.com.gbd.designpatterns.abstractFactory;
import br.com.gbd.apostiladesignpatterns.abstractFactory.Emissor;
/*
Autor: <NAME>
Data: 08/08/2016, 09:48:16
Arquivo: EmissorVisa
*/
public class EmissorVisa implements Emissor{
@Override
public void envia(String mensagem) {
System.out.println("Enviando a seguinte msg para a Visa: ");
System.out.println(mensagem);
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns;
import br.com.gbd.apostiladesignpatterns.comportamental.visitor.AtualizadorDeFuncionario;
import br.com.gbd.apostiladesignpatterns.comportamental.visitor.AtualizadorSalarial;
import br.com.gbd.apostiladesignpatterns.comportamental.visitor.Departamento;
import br.com.gbd.apostiladesignpatterns.comportamental.visitor.Funcionario;
import br.com.gbd.apostiladesignpatterns.comportamental.visitor.Gerente;
import br.com.gbd.apostiladesignpatterns.comportamental.visitor.Telefonista;
import java.util.ArrayList;
import java.util.List;
/*
Autor: <NAME>
Data: 09/08/2016, 16:03:17
Arquivo: Visitor
*/
public class Visitor {
public static void testaVisitor(){
List<Departamento> lista = new ArrayList<>();
Departamento departamento = new Departamento("Departamento1");
Gerente gerente = new Gerente("1234", "Gerente1", 2000);
Telefonista telefonista = new Telefonista(2, "Telefonista1", 1000);
departamento.getFuncionarios().add(gerente);
departamento.getFuncionarios().add(telefonista);
lista.add(departamento);
departamento = new Departamento("Departamento2");
gerente = new Gerente("1234", "Gerente2", 2000);
telefonista = new Telefonista(3, "Telefonista2", 1000);
departamento.getFuncionarios().add(gerente);
departamento.getFuncionarios().add(telefonista);
gerente = new Gerente("1234", "Gerente3", 2000);
departamento.getFuncionarios().add(gerente);
lista.add(departamento);
AtualizadorDeFuncionario atual = new AtualizadorSalarial();
for(Departamento d : lista){
d.aceita(atual);
}
for(Departamento d : lista){
for(Funcionario f : d.getFuncionarios()){
System.out.println("Nome: " + f.getNome() + " - Salário: " + f.getSalario());
}
}
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns;
import br.com.gbd.apostiladesignpatterns.criacao.builder.BBBoletoBuilder;
import br.com.gbd.apostiladesignpatterns.criacao.builder.Boleto;
import br.com.gbd.apostiladesignpatterns.criacao.builder.BoletoBuilder;
import br.com.gbd.apostiladesignpatterns.criacao.builder.GeradorDeBoleto;
/*
Autor: <NAME>
Data: 08/08/2016, 11:33:03
Arquivo: Bulder
*/
public class Builder {
public static void testaGeradorDeBoletos(){
BoletoBuilder boletoBuilder = new BBBoletoBuilder();
GeradorDeBoleto geradorDeBoleto = new GeradorDeBoleto(boletoBuilder);
Boleto boleto = geradorDeBoleto.geraBoleto();
System.out.println(boleto);
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.comportamental.state;
/*
Autor: <NAME>
Data: 09/08/2016, 15:00:57
Arquivo: Bandeira1
*/
public class Bandeira1 implements Bandeira{
@Override
public double calcularValorDaCorrida(double tempo, double distancia) {
return 5.0 + tempo * 1.5 + distancia * 1.7;
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.estrutural.bridge;
/*
Autor: <NAME>
Data: 08/08/2016, 16:01:07
Arquivo: GeradorDeArquivo
*/
public interface GeradorDeArquivo {
public void gera(String conteudo);
}
<file_sep>package br.com.gbd.apostiladesignpatterns.estrutural.adapter;
import java.text.SimpleDateFormat;
import java.util.Calendar;
/*
Autor: <NAME>
Data: 08/08/2016, 15:39:37
Arquivo: ControleDePontoNovo
*/
public class ControleDePontoNovo {
public void registra(Funcionario f, boolean entrada) {
Calendar calendar = Calendar.getInstance();
SimpleDateFormat sdf = new SimpleDateFormat("dd/MM/yyyy H:m:s");
String format = sdf.format(calendar.getTime());
if (entrada) {
System.out.println("Entrada: " + f.getNome() + " às " + format);
} else {
System.out.println("Saída: " + f.getNome() + " às " + format);
}
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.criacao.abstractFactory;
/*
Autor: <NAME>
Data: 08/08/2016, 11:48:50
Arquivo: ReceptorCreator
*/
public class ReceptorCreator {
public static final int VISA = 0;
public static final int MASTERCARD = 1;
public Receptor create(int tipoDoReceptor){
if(tipoDoReceptor == EmissorCreator.VISA){
return new ReceptorVisa();
} else if (tipoDoReceptor == EmissorCreator.MASTERCARD){
return new ReceptorMatercard();
} else {
throw new IllegalArgumentException("Tipo de emissor não suportado");
}
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.comportamental.command;
/*
Autor: <NAME>
Data: 09/08/2016, 12:30:19
Arquivo: Comando
*/
public interface Comando {
void executa();
}
<file_sep>package br.com.gbd.apostiladesignpatterns.criacao.abstractFactory;
/*
Autor: <NAME>
Data: 08/08/2016, 09:35:22
Arquivo: comunicadorFactory
*/
public interface ComunicadorFactory {
Emissor createEmissor();
Receptor createReceptor();
}
<file_sep>package br.com.gbd.apostiladesignpatterns.comportamental.command;
import java.util.ArrayList;
import java.util.List;
/*
Autor: <NAME>
Data: 09/08/2016, 13:19:06
Arquivo: ListaDeComandos
*/
public class ListaDeComandos {
private List<Comando> comandos = new ArrayList<>();
public void adiciona(Comando comando){
this.comandos.add(comando);
}
public void executa(){
for(Comando comando : this.comandos){
comando.executa();
}
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.criacao.abstractFactory;
import br.com.gbd.apostiladesignpatterns.criacao.abstractFactory.Emissor;
/*
Autor: <NAME>
Data: 08/08/2016, 09:49:19
Arquivo: EmissorMastercard
*/
public class EmissorMastercard implements Emissor{
@Override
public void envia(String mensagem) {
System.out.println("Enviando a seguinte msg para a Mastercard: ");
System.out.println(mensagem);
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns;
import br.com.gbd.apostiladesignpatterns.comportamental.iterator.ListaDeNomes;
/*
Autor: <NAME>
Data: 09/08/2016, 13:42:48
Arquivo: Iterator
*/
public class Iterator {
public static void testaIterator(){
String[] nomes = new String[4];
nomes[0] = "José";
nomes[1] = "Carlos";
nomes[2] = "Freitas";
nomes[3] = "Henrique";
ListaDeNomes listaDeNomes = new ListaDeNomes(nomes);
//BUGADO
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.comportamental.command;
/*
Autor: <NAME>
Data: 09/08/2016, 12:26:38
Arquivo: Player
*/
public class Player {
public void play(String filename) throws InterruptedException {
System.out.println("Tocando arquivo: " + filename);
long duracao = (long)(Math.random()*2000);
System.out.println("Duração (s): " + duracao/1000.0);
Thread.sleep(duracao);
System.out.println("FIM");
}
public void increaseVol(int levels){
System.out.println("Aumentando o volume em: " + levels);
}
public void decreaseVol(int levels){
System.out.println("Diminuindo o volume em: " + levels);
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.estrutural.decorator;
/*
Autor: <NAME>
Data: 08/08/2016, 16:58:58
Arquivo: Emissor
*/
public interface Emissor {
void envia(String msg);
}
<file_sep>package br.com.gbd.apostiladesignpatterns.criacao.objectPool;
import java.util.ArrayList;
import java.util.List;
/*
Autor: <NAME>
Data: 08/08/2016, 15:13:21
Arquivo: FuncionarioPool
*/
public class FuncionarioPool implements Pool<Funcionario>{
private List<Funcionario> funcionarios;
public FuncionarioPool() {
this.funcionarios = new ArrayList<>();
this.funcionarios.add(new Funcionario("<NAME>"));
this.funcionarios.add(new Funcionario("Tadeu"));
this.funcionarios.add(new Funcionario("Henrique"));
}
@Override
public Funcionario acquire() {
if(this.funcionarios.size()>0){
return this.funcionarios.remove(0);
} else {
return null;
}
}
@Override
public void release(Funcionario funcionario) {
this.funcionarios.add(funcionario);
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.estrutural.facade;
import java.text.SimpleDateFormat;
import java.util.Calendar;
/*
Autor: <NAME>
Data: 09/08/2016, 08:46:20
Arquivo: PosVenda
*/
public class PosVenda {
public void agendaContato(String cliente, String produto){
Calendar calendar = Calendar.getInstance();
calendar.add(Calendar.DATE, 30);
SimpleDateFormat sdf = new SimpleDateFormat("dd/MM/yyyy");
String format = sdf.format(calendar.getTime());
System.out.println("Entrar em contato com " + cliente + " sobre o produto " + produto + " no dia " + format);
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.comportamental.observer;
/*
Autor: <NAME>
Data: 09/08/2016, 14:43:45
Arquivo: Corretora
*/
public class Corretora implements AcaoObserver{
private String nome;
public Corretora(String nome) {
this.nome = nome;
}
@Override
public void notificaAlteracao(Acao acao) {
System.out.println("Corretora " + this.nome + " sendo notificada: ");
System.out.println("A ação " + acao.getCodigo() + " teve o seu valor alterado para " + acao.getValor());
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns;
import br.com.gbd.apostiladesignpatterns.estrutural.bridge.GeradorDeArquivo;
import br.com.gbd.apostiladesignpatterns.estrutural.bridge.GeradorDeArquivoTXT;
import br.com.gbd.apostiladesignpatterns.estrutural.bridge.Recibo;
/*
Autor: <NAME>
Data: 08/08/2016, 16:09:50
Arquivo: Bridge
*/
public class Bridge {
public static void testaBridge(){
GeradorDeArquivo geradorDeArquivoTXT = new GeradorDeArquivoTXT();
Recibo recibo = new Recibo("Treinamento Design Patterns", "<NAME>", 1000, geradorDeArquivoTXT);
recibo.geraArquivo();
}
}
<file_sep>package br.com.gbd.designpatterns.abstractFactory;
import br.com.gbd.apostiladesignpatterns.abstractFactory.Emissor;
/*
Autor: <NAME>
Data: 08/08/2016, 09:52:10
Arquivo: EmissorCreator
*/
public class EmissorCreator {
public static final int VISA = 0;
public static final int MASTERCARD = 1;
public Emissor create(int tipoDoEmissor){
if(tipoDoEmissor == EmissorCreator.VISA){
return new EmissorVisa();
} else if (tipoDoEmissor == EmissorCreator.MASTERCARD){
return new EmissorMastercard();
} else {
throw new IllegalArgumentException("Tipo de emissor não suportado");
}
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.estrutural.decorator;
/*
Autor: <NAME>
Data: 08/08/2016, 16:59:33
Arquivo: EmissorBasico
*/
public class EmissorBasico implements Emissor{
@Override
public void envia(String msg) {
System.out.println("Enviando uma mensagem: ");
System.out.println(msg);
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.comportamental.strategy;
import java.util.List;
/*
Autor: <NAME>
Data: 09/08/2016, 15:11:25
Arquivo: Sorter
*/
public interface Sorter {
<T extends Comparable<? super T>> List<T> sort(List<T> list);
}
<file_sep>package br.com.gbd.apostiladesignpatterns;
import br.com.gbd.apostiladesignpatterns.estrutural.composicao.Caminho;
import br.com.gbd.apostiladesignpatterns.estrutural.composicao.Trecho;
import br.com.gbd.apostiladesignpatterns.estrutural.composicao.TrechoAndando;
import br.com.gbd.apostiladesignpatterns.estrutural.composicao.TrechoDeCarro;
/*
Autor: <NAME>
Data: 08/08/2016, 16:46:28
Arquivo: Composicao
*/
public class Composicao {
public static void testaComposicao(){
Trecho trecho1 = new TrechoAndando("Vá até a rua A.", 500);
Trecho trecho2 = new TrechoDeCarro("Vá até o cruzamento B.", 1500);
Trecho trecho3 = new TrechoDeCarro("Vá até o cruzamento C.", 2000);
Caminho caminho1 = new Caminho();
caminho1.adiciona(trecho1);
caminho1.adiciona(trecho2);
System.out.println("Caminho1: ");
caminho1.imprime();
Caminho caminho2 = new Caminho();
caminho2.adiciona(caminho1);
caminho2.adiciona(trecho3);
System.out.println("---------------------------");
System.out.println("Caminho 2: ");
caminho2.imprime();
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.criacao.factoryMethod;
/*
Autor: <NAME>
Data: 08/08/2016, 09:01:15
Arquivo: EmissorEmail
*/
public class EmissorEmail implements Emissor{
public void enviar(String mensagem){
System.out.println("Enviando msg por e-mail");
System.out.println(mensagem);
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns;
import br.com.gbd.apostiladesignpatterns.criacao.factoryMethod.Emissor;
import br.com.gbd.apostiladesignpatterns.criacao.factoryMethod.EmissorCreator;
/*
Autor: <NAME>
Data: 08/08/2016, 10:45:16
Arquivo: FactoryMethod
*/
public class FactoryMethod {
public static void testa(){
String mensagem = "Olá";
EmissorCreator creator = new EmissorCreator();
Emissor emissor = creator.create(EmissorCreator.SMS);
emissor.enviar(mensagem);
emissor = creator.create(EmissorCreator.EMAIL);
emissor.enviar(mensagem);
emissor = creator.create(EmissorCreator.JMS);
emissor.enviar(mensagem);
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.criacao.multion;
import java.awt.Color;
import java.util.HashMap;
import java.util.Map;
/*
Autor: <NAME>
Data: 08/08/2016, 14:56:51
Arquivo: T
*/
public class Tema {
private String nome;
private Color corDoFundo;
private Color corDaFonte;
private static Map<String, Tema> temas = new HashMap<>();
public static final String SKY = "Sky";
public static final String FIRE = "Fire";
static {
Tema tema1 = new Tema();
tema1.setNome(Tema.SKY);
tema1.setCorDoFundo(Color.BLUE);
tema1.setCorDaFonte((Color.BLACK));
Tema tema2 = new Tema();
tema1.setNome(Tema.FIRE);
tema1.setCorDoFundo(Color.RED);
tema1.setCorDaFonte((Color.WHITE));
temas.put(tema1.getNome(), tema1);
temas.put(tema2.getNome(), tema2);
}
private Tema() {
}
public static Tema getInstance(String nomeDoTema){
return Tema.temas.get(nomeDoTema);
}
public String getNome() {
return nome;
}
public void setNome(String nome) {
this.nome = nome;
}
public Color getCorDoFundo() {
return corDoFundo;
}
public void setCorDoFundo(Color corDoFundo) {
this.corDoFundo = corDoFundo;
}
public Color getCorDaFonte() {
return corDaFonte;
}
public void setCorDaFonte(Color corDaFonte) {
this.corDaFonte = corDaFonte;
}
public static Map<String, Tema> getTemas() {
return temas;
}
public static void setTemas(Map<String, Tema> temas) {
Tema.temas = temas;
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns;
import br.com.gbd.apostiladesignpatterns.comportamental.mediator.CentralDeTaxi;
import br.com.gbd.apostiladesignpatterns.comportamental.mediator.Passageiro;
import br.com.gbd.apostiladesignpatterns.comportamental.mediator.Taxi;
/*
Autor: <NAME>
Data: 09/08/2016, 14:23:19
Arquivo: Mediator
*/
public class Mediator {
public static void testaMediator() {
CentralDeTaxi central = new CentralDeTaxi();
Passageiro p1 = new Passageiro("José", central);
Passageiro p2 = new Passageiro("Carlos", central);
Passageiro p3 = new Passageiro("Freitas", central);
Taxi t1 = new Taxi(1, central);
central.adicionaTaxiDisponivel(t1);
Taxi t2 = new Taxi(2, central);
central.adicionaTaxiDisponivel(t2);
new Thread(p1).start();
new Thread(p2).start();
new Thread(p3).start();
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.comportamental.state;
/*
Autor: <NAME>
Data: 09/08/2016, 14:58:56
Arquivo: Taximetro
*/
public class Taximetro {
private Bandeira bandeira;
public Taximetro(Bandeira bandeira){
this.bandeira = bandeira;
}
public void setBandeira(Bandeira bandeira){
this.bandeira = bandeira;
}
public double calculaValorDaCorrida(double tempo, double distancia){
return this.bandeira.calcularValorDaCorrida(tempo, distancia);
}
}
<file_sep>package br.com.gbd.apostiladesignpatterns.builder;
import java.util.Calendar;
/*
Autor: <NAME>
Data: 08/08/2016, 11:07:18
Arquivo: Boleto
*/
public interface Boleto {
String getSacado();
String getCedente();
double getValor();
Calendar getVencimento();
int getNossoNumero();
String toString();
}
| 3b5a2ce0dba57e3ab8243fffe373b42ec5d470aa | [
"Java"
]
| 31 | Java | jcfreitas2000/designPatterns | 8c7f11bab9d83059ee89756eea41be1164dbc51a | f20a17f5047c49c3b9821b54617fdc69beff826a | |
refs/heads/master | <repo_name>easyCZ/hacker-news-react-native<file_sep>/app/api/HackerNews.js
import {fetch} from 'fetch';
let HACKER_NEWS_API_URL = "https://hacker-news.firebaseio.com";
let TOP_STORIES = '/v0/topstories.json';
let STORY = '/v0/item/';
class HackerNews {
static topStories() {
return fetch(HACKER_NEWS_API_URL + TOP_STORIES);
}
static article(id) {
return fetch(HACKER_NEWS_API_URL + STORY + id + '.json');
}
}
export default HackerNews;
<file_sep>/app/articles/ArticleListStyleSheet.js
import {StyleSheet} from 'react-native';
import {vw, vh, vmin, vmax} from 'react-native-viewport-units';
let ArticleListStyleSheet = StyleSheet.create({
container: {
marginTop: 54,
flex: 1,
backgroundColor: 'lightgrey'
}
});
export default ArticleListStyleSheet;
<file_sep>/app/Router.js
import React, {NavigatorIOS, StyleSheet} from 'react-native';
import ArticleList from './articles/ArticleList';
import ArticleActions from './articles/ArticleActions';
let styles = StyleSheet.create({
navigator: {
flex: 1
}
});
class Router extends React.Component {
render() {
let initialRoute = {
title: 'HN - Top Stories',
component: ArticleList,
rightButtonTitle: 'Refresh',
onRightButtonPress: () => ArticleActions.getArticles()
};
return (
<NavigatorIOS
style={styles.navigator}
initialRoute={initialRoute}
barTintColor="#ff6600"
/>
);
}
}
module.exports = Router;
<file_sep>/app/articles/ArticleList.js
import React, {ListView, Text} from 'react-native';
import ArticleListStyleSheet from './ArticleListStyleSheet';
import HackerNews from '../api/HackerNews';
import ArticleStore from './ArticleStore';
import ArticleActions from './ArticleActions';
import ArticleRow from './ArticleRow';
class ArticleList extends React.Component {
constructor(props) {
super(props);
this.articlesDataSource = new ListView.DataSource({
rowHasChanged: (r1, r2) => r1 !== r2
});
}
componentWillMount() {
this.updateArticles();
}
componentDidMount() {
ArticleStore.addArticlesChangeListener(this.updateArticles.bind(this));
ArticleActions.getArticles();
}
componentWillUnmount() {
ArticleStore.removeArticlesChangeListener(this.updateArticles.bind(this));
}
updateArticles() {
console.log('Update articles', ArticleStore.getArticles());
this.setState({
articles: this.articlesDataSource.cloneWithRows(
ArticleStore.getArticles().slice(0, 10))
});
}
renderRow(rowData, sectionID, rowID, highlightRow) {
console.log('highlight', rowData, sectionID, rowID, highlightRow);
return (
<ArticleRow
articleId={rowData}
highlightRowFn={highlightRow} />
);
}
render() {
if (!this.state) return (<Text>Loading...</Text>);
return (
<ListView
dataSource={this.state.articles}
initialListSize={10}
renderRow={this.renderRow} />
)
}
}
module.exports = ArticleList;
<file_sep>/app/articles/ArticleRowStyleSheet.js
import {StyleSheet} from 'react-native';
import {vw, vh, vmin, vmax} from 'react-native-viewport-units';
let ArticleRowStyleSheet = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'row',
height: 10 * vh,
width: 100 * vw,
alignItems: 'center',
padding: 5,
borderColor: 'orange'
// justifyContent: 'center',
// alignSelf: 'center'
},
score: {
width: 5 * vw,
height: 5 * vw,
backgroundColor: 'white'
}
});
export default ArticleRowStyleSheet;
<file_sep>/app/articles/ArticleStore.js
import AppDispatcher from '../AppDispatcher';
import events from 'events';
let _articles = [];
let _articleDetails = {};
class ArticleStore extends events.EventEmitter {
constructor() {
super();
}
getArticles() {
return _articles;
}
setArticles(articles) {
_articles = articles;
}
emitArticlesChange() {
this.emit('ARTICLES_CHANGE', this.getArticles());
}
addArticlesChangeListener(callback) {
this.on('ARTICLES_CHANGE', callback);
}
removeArticlesChangeListener(callback) {
this.removeListener('ARTICLES_CHANGE', callback);
}
getArticle(id) {
return _articleDetails[id];
}
setArticle(id, article) {
_articleDetails[id] = article;
}
emitArticleChange(id) {
this.emit('ARTICLE_CHANGE:' + id, this.getArticle(id))
}
addArticleChangeListener(id, callback) {
this.on('ARTICLE_CHANGE:' + id, callback);
}
removeArticleChangeListener(id, callback) {
this.removeListener('ARTICLE_CHANGE:' + id, callback);
}
}
let articleStore = new ArticleStore();
AppDispatcher.register( ({action, source}) => {
let {actionType, data} = action;
switch (actionType) {
case 'TOP_STORIES:LOADED':
articleStore.setArticles(data);
articleStore.emitArticlesChange();
break;
case 'TOP_STORY:LOADED':
articleStore.setArticle(data.id, data);
articleStore.emitArticleChange(data.id);
break;
default:
return true;
}
});
export default articleStore;
<file_sep>/app/articles/ArticleActions.js
import HackerNews from '../api/HackerNews';
import AppDispatcher from '../AppDispatcher';
let _onStoriesReceive = (stories) => {
console.log('Received stories', stories);
AppDispatcher.handleView({
actionType: 'TOP_STORIES:LOADED',
data: stories
});
}
let _onStoriesFail = (error) => {
console.log('Failed to get stories', error);
AppDispatcher.handleView({
actionType: 'TOP_STORIES:FAILED',
data: error
});
}
let _onStoryReceive = (story) => {
console.log('Received story', story.id, story);
AppDispatcher.handleView({
actionType: 'TOP_STORY:LOADED',
data: story
});
}
let _onStoryFail = (id, error) => {
console.log('Failed to fetch story #' + id, error);
AppDispatcher.handleView({
actionType: 'TOP_STORY:FAILED',
data: error
});
}
class ArticleActions {
static getArticles() {
HackerNews
.topStories()
.then(r => r.json(), e => _onStoriesFail(e))
.then(stories => _onStoriesReceive(stories));
}
static getArticle(id) {
HackerNews
.article(id)
.then(r => r.json(), e => _onStoryFail(id, e))
.then(story => _onStoryReceive(story));
}
}
export default ArticleActions;
<file_sep>/README.md
# hacker-news-react-native
React Native implementation of a Hacker News reader
as dasas das
<file_sep>/index.ios.js
'use strict';
var React = require('react-native');
var {AppRegistry} = React;
var HackerNews = require('./dist/App');
AppRegistry.registerComponent('HackerNewsRCT', () => HackerNews);
| 506f10fb756d154b181b50b8cc892a465802c5f2 | [
"JavaScript",
"Markdown"
]
| 9 | JavaScript | easyCZ/hacker-news-react-native | b4cc799376aebca7ee507e71849fc05095b208c6 | 98bdf72935b0b525d6f3e976e8e540f1a77936e0 | |
refs/heads/master | <file_sep>package Amazon;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.Select;
import org.testng.annotations.AfterMethod;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.Test;
public class amazon {
WebDriver driver;
@BeforeMethod
public void HpLappy() throws InterruptedException{
System.setProperty("webdriver.chrome.driver","D:\\\\Testing\\\\chromedriver.exe");
driver=new ChromeDriver();
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
driver.get("http://www.amazon.in");
try{
Thread.sleep(1000);
}catch(InterruptedException e){
e.printStackTrace();
}
}
@Test(priority=1)
public void Login() throws InterruptedException
{
driver.findElement(By.xpath("//span[contains(text(),'Hello, Sign in')]")).click();
Thread.sleep(4000);
driver.findElement(By.xpath("//input[@id='ap_email']")).sendKeys("<PASSWORD>",Keys.ENTER);
Thread.sleep(4000);
driver.findElement(By.xpath("//input[@id='ap_password']")).sendKeys("<PASSWORD>",Keys.ENTER);
Thread.sleep(4000);
driver.findElement(By.xpath("//input[@name='option' and @type='radio' and @value='sms']")).click();
driver.findElement(By.xpath("//input[@id='continue']")).click();
}
@Test(priority=2)
public void SearchItemandSelect() throws InterruptedException
{
driver.findElement(By.xpath("//input[@id='twotabsearchtextbox']")).sendKeys("t-shirts for men"+Keys.ENTER);
Thread.sleep(4000);
driver.findElement(By.xpath("//div[@class='sb_1LbkwDuT']//div[2]//div[1]//a[1]")).click();
Thread.sleep(6000);
Select dropdown=new Select(driver.findElement(By.xpath("//select[@name='dropdown_selected_size_name']")));
dropdown.selectByVisibleText("L");
Thread.sleep(4000);
driver.findElement(By.xpath("//input[@name='submit.buy-now']")).click();
Thread.sleep(4000);
}
@Test(priority=3)
public void PaymentThroughDebitCard() throws InterruptedException
{
driver.findElement(By.xpath("//input[@value='SelectableAddCreditCard']")).click();
Thread.sleep(2000);
driver.findElement(By.xpath("//input[@value='suneel']")).sendKeys("");
Thread.sleep(2000);
driver.findElement(By.xpath("//input[@name='addCreditCardNumber']")).sendKeys("918218025151");
Thread.sleep(2000);
Select dropdown1=new Select(driver.findElement(By.xpath("//span[@id='pp-Bf3YVu-81']//span[@class='a-button-text a-declarative']")));
dropdown1.selectByVisibleText("10");
Thread.sleep(2000);
Select dropdownYear=new Select(driver.findElement(By.xpath("//span[@id='pp-Bf3YVu-82']//span[@class='a-button-text a-declarative']")));
dropdownYear.selectByVisibleText("2019");
Thread.sleep(2000);
driver.findElement(By.xpath("//input[@name='ppw-widgetEvent:AddCreditCardEvent']")).click();
}
@Test(priority=4)
public void PaymentThroughNetBanking() throws InterruptedException
{
driver.findElement(By.xpath("//input[@type='radio' and @value='instrumentId=NetBanking&isExpired=false&paymentMethod=NB&tfxEligible=false']")).click();
Thread.sleep(2000);
Select drop=new Select(driver.findElement(By.xpath(".//span[@class='a-button a-button-dropdown']")));
drop.selectByVisibleText("HDFC Bank");
Thread.sleep(2000);
}
@AfterMethod
public void logout()
{
driver.close();
}
}
<file_sep>package Flipkart;
import java.util.ArrayList;
import java.util.concurrent.TimeUnit;
import org.eclipse.jdt.internal.compiler.ast.ThrowStatement;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.Select;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.Test;
public class Flipkart {
WebDriver driver;
@BeforeMethod
public void Shopping() throws InterruptedException{
System.setProperty("webdriver.chrome.driver","D:\\\\Testing\\\\chromedriver.exe");
driver=new ChromeDriver();
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
driver.get("https://www.flipkart.com/");
try{
Thread.sleep(1000);
}catch(InterruptedException e){
e.printStackTrace();
}
}
@Test
public void Login() throws InterruptedException
{
driver.findElement(By.xpath("//input[@class='_2zrpKA _1dBPDZ']")).sendKeys("8218025151");
Thread.sleep(4000);
driver.findElement(By.xpath("//input[@class='_2zrpKA _3v41xv _1dBPDZ']")).sendKeys("<PASSWORD>",Keys.ENTER);
Thread.sleep(4000);
driver.findElement(By.xpath("//input[@placeholder='Search for products, brands and more']")).sendKeys("i phone 11 pro",Keys.ENTER);
Thread.sleep(4000);
driver.findElement(By.xpath("//div[contains(@class,'t-0M7P _2doH3V')]//div[3]//div[1]//div[1]//div[1]//a[1]//div[1]//div[1]//div[1]//div[1]//img[1]")).click();
Thread.sleep(4000);
ArrayList<String> windowTabs=new ArrayList<String>(driver.getWindowHandles());
driver.switchTo().window(windowTabs.get(0));
Thread.sleep(4000);
driver.switchTo().window(windowTabs.get(1));
Thread.sleep(4000);
driver.findElement(By.xpath("//a[@class='_1TJldG _3c2Xi9']")).click();
Thread.sleep(4000);
driver.findElement(By.xpath("//button[@class='_2AkmmA _2Npkh4 _2kuvG8 _7UHT_c']")).click();
Thread.sleep(4000);
driver.findElement(By.xpath("//button[@class='_2AkmmA _I6-pD _7UHT_c']")).click();
Thread.sleep(4000);
driver.findElement(By.xpath("//button[@class='_2AkmmA _2Q4i61 _7UHT_c']")).click();
Thread.sleep(4000);
driver.findElement(By.xpath("//label[@class='_8J-bZE _3C6tOa _1syowc _2i24Q8 _1Icwrf']//div[@class='_6ATDKp']")).click();
Thread.sleep(4000);
driver.findElement(By.xpath("//input[@name='cardNumber']")).sendKeys("25464645878455");
Thread.sleep(4000);
Select dd1=new Select(driver.findElement(By.xpath("//select[@name='month']")));
dd1.selectByVisibleText("02");
Thread.sleep(4000);
Select dd2=new Select(driver.findElement(By.xpath("//select[@name='year']")));
dd2.selectByVisibleText("22");
Thread.sleep(4000);
driver.findElement(By.xpath("//input[@name='cvv']")).sendKeys("214");
driver.findElement(By.xpath("//button[@class='_2AkmmA wbv91z _7UHT_c']")).click();
}
}
| 04eba393983310b2e45ee380b02e7eced4d67057 | [
"Java"
]
| 2 | Java | Suneel12345/E-commerceProject | c2a726881bc0b808c044e2b8692e9d5786c4b22a | 45b42603e1cd0b8e51b1d31b873320fa216a4ab1 | |
refs/heads/master | <repo_name>irineu/eight-protocol<file_sep>/examples/echo/echoServer.js
////////////////////////////////////////
//IT WILL NOT WORK WITH TELNET CLIENT!!!
////////////////////////////////////////
import constants from "../helpers/constants.js";
import protocol from "../../index.js";
var server = new protocol.server(constants.port);
server.on('server_listening', function(){
console.log("Server is up! Now waiting for connections");
});
server.on('client_connected', function(socketClient){
console.log("NEW CLIENT CONNECTED! \t id:"+socketClient.id+" origin:"+socketClient.address().address);
});
server.on('client_close', function(socketClient){
console.log("CLIENT DISCONNECTED! \t id:"+socketClient.id);
});
server.on("data", function(socketClient, header, dataBuffer){
console.log("MESSAGE RECEIVED! \t id:"+socketClient.id+" message:"+dataBuffer.toString());
});<file_sep>/examples/chat/client.js
import protocol from "../../index.js";
import readline from 'readline';
var client = new protocol.client("0.0.0.0", 7890);
//this is a way for easily read lines, see more at: https://nodejs.org/api/readline.html
let rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
client.on("client_connected", function(socket){
console.log("Connected on server!");
});
client.on("client_end", function(socket){
//exit when lost connectio with the server
process.exit(0);
});
client.on("data", function(socket, header, dataBuffer){
//Based in a property (transaction) on header, we can handle de message:
switch(header.transaction){
case "ID":
rl.question('Type your name: ', (answer) => {
protocol.send(socket, {transaction : "ID", date : Date.now()}, answer);
});
break;
case "WELCOME":
//start asking for messages to send
recursiveInput();
break;
case "MESSAGE":
//print a message
console.log(dataBuffer.toString());
break;
case "ERROR":
console.log("SERVER ERROR: " +dataBuffer.toString());
process.exit(1);
break;
default:
console.log("Unrecognized transaction "+ header.transaction);
break;
}
});
function recursiveInput(){
rl.question('', (answer) => {
if(answer)
protocol.send(client.socket, {transaction : "MESSAGE", date : Date.now()}, answer);
recursiveInput();
});
}<file_sep>/README.md
# eight-protocol [](https://travis-ci.org/irineu/eight-protocol)
NodeJS socket communication lib over a protocol with 8 bytes descriptor
### Deprecation disclaimer:
This project will not be longer updated. Hachi NIO Protocol works like the 8-protocol but will have much more features. Please check: [https://github.com/irineu/hachi-nio-js](https://github.com/irineu/hachi-nio-js)
## Objective
When you test a simple socket communication, in the more of times everything works fine, but when your project grow up, after publish and test with remote and bad connections, you will get some problems with truncated messages or messages with extra data (part of the next message). It is normal. You will need create a protocol to handle that situation, maybe implement a header or a terminator (bad ideia), maybe you are already frustated with that notice.
The eight protocol is an implementation plug-and-play of a transparent protocol for handle those situations for you, and the most important: it does not change your code too much.
The message transfered is distributed in two sections:
##### Header
A Key/Value object to identify the message (like: transaction, id, token, type, etc...) like an HTTP header. It will be transformed into a JSON.
##### Body
Binary Data, you can pass anything here (Strings, Serialized Objects, Images, Files, etc...) you can use the header do describe the content of the message.
## Quick Start
##### Server
```javascript
var protocol = require("eight-protocol");
var server = new protocol.server(7890);
server.on('server_listening', function(){
console.log("Server ir up! Now waiting for connections");
});
server.on('client_connected', function(socketClient){
console.log("NEW CLIENT CONNECTED!");
});
server.on("data", function(socketClient, header, dataBuffer){
console.log("MESSAGE RECEIVED!",header,dataBuffer.toString());
});
server.on('client_close', function(socketClient){
console.log("CLIENT DISCONNECTED!");
});
```
##### Client
```javascript
var protocol = require("eight-protocol");
var client = new protocol.client("0.0.0.0", 7890);
client.on("client_connected", function(socket){
console.log("Connected on the server");
//Send message
protocol.send(socket, {transaction : "GREETINGS"}, "Hello World!");
})
```
## API
`protocol.send(socket, header, buffer, [callback]);`
Send a message for the given socket.
* Socket - The nodejs socket from the new connection callback
* Header - Key/Value object, it will be converted internally to a JSON String
* Buffer - Your data, must be a buffer or String
* Callback (optional) - A callback function with and error parameter if it have
### Server
`new protocol.server(port, [debug]);`
Instantiate a new server and try to start listen imediatly
* port - must be an integer, it will be the respective TCP port to listen
* debug (optional) - must be a boolean, if `true`, the lib will print some informations like message size, incoming bytes, outcoming bytes, etc.
##### Events
| Event name | Description |
|------------------|---------------------------------------------------------------------------------------------------------------|
| client_connected | callback(clientSocket : Socket) - When a new client connection is established on the server |
| client_close | callback(clientSocket : Socket, hadError : Boolean)When a client disconnect from the server |
| client_end | callback(clientSocket : Socket) - When a client connection ends |
| client_timeout | callback(clientSocket : Socket) - When a client timeout |
| client_error | callback(clientSocket : Socket, error : Object) - When client socket gives an error |
| data | callback(clientSocket : Socket, header : Object , dataBuffer : Buffer) - When receive a message from a client |
| server_error | callback(error : Object) - When the server gives an error |
| server_listening | callback() - When the server start listening |
### Client
`new protocol.client(address, port, timeout, debug)`
Instantiate a new client and try connect imediatly
* Address - Must me and string, should be the remote ip address
* port - must be an integer, it will be the respective TCP port to listen
* timeout (optional) - must be an integer, will set the socket timeout (see more at https://nodejs.org/api/net.html#net_socket_settimeout_timeout_callback)
* debug (optional) - must be a boolean, if true, the lib will print some informations like message size, incoming bytes, outcoming bytes, etc.
##### Events
| Event name | Description |
|------------------|---------------------------------------------------------------------------------------------------------------|
| client_connected | callback(clientSocket : Socket) - When connection is established on the server |
| client_close | callback(clientSocket : Socket, hadError : Boolean) When disconnect from the server |
| client_end | callback(clientSocket : Socket) - When connection ends |
| client_timeout | callback(clientSocket : Socket) - When connection timedout |
| client_error | callback(clientSocket : Socket, error : Object) - When client socket gives an error |
| data | callback(clientSocket : Socket, header : Object , dataBuffer : Buffer) - When receive a message from a Server |
<file_sep>/test/test.js
var assert = require('assert');
var protocol = require('./../index');
describe("Client/Server", function(){
var server = {
instance : null,
clients : []
}
var client;
var serverPort = 7890;
describe('#server:listen()', function() {
it('should start server', function(done) {
server.instance = new protocol.server(serverPort, false);
server.instance.once('server_listening', function(){
done();
});
});
});
describe('#client:connect()', function() {
it('should connect on server', function(done) {
client = new protocol.client("0.0.0.0", serverPort);
server.instance.once("client_connected", function(socket){
server.clients.push(socket);
})
client.once('client_connected', function(socket){
done();
});
});
});
describe('#client:send/server:recv()', function() {
it('should send a message for the server', function(done) {
var _header = {key1: "put", key2: "any", key3: "data"};
var _data = "It will be a binary data client";
server.instance.once("data", function(socket, header, dataBuffer){
assert.equal(dataBuffer, _data);
assert.equal(header.key1, _header.key1);
assert.equal(header.key2, _header.key2);
done();
})
protocol.send(client.socket,_header,_data);
});
});
describe('#client:recv/server:send()', function() {
it('should receive a message from the server', function(done) {
var _header = {key1: "put 1", key2: "any 2", key3: "data 3"};
var _data = "It will be a binary data from server";
client.once("data", function(socket, header, dataBuffer){
assert.equal(dataBuffer, _data);
assert.equal(header.key1, _header.key1);
assert.equal(header.key2, _header.key2);assert.equal(dataBuffer,_data);
done();
});
protocol.send(server.clients[0],_header,_data);
});
});
describe('#server:onDisconnect()', function() {
it('should reconize when a client disconnect', function(done) {
var _header = {key1: "put 1", key2: "any 2", key3: "data 3"};
var _data = "It will be a binary data from server";
server.instance.once("client_close", function(socket, header, dataBuffer){
assert.equal(server.clients[0].id, socket.id);
});
server.instance.once("client_end", function(socket, header, dataBuffer){
assert.equal(server.clients[0].id, socket.id);
done();
});
client.socket.end();
});
});
describe('#client:onDisconnect()', function() {
it('should reconize when the server disconnect a client', function(done) {
this.timeout(1000);
client = new protocol.client("0.0.0.0", serverPort);
server.instance.once("client_connected", function(socket){
server.clients.push(socket);
setTimeout(function(){
socket.end();
},200);
})
client.once('client_connected', function(socket){
client.once("client_end", function(){
done();
});
});
});
});
describe('#server:onDisconnect()', function() {
it('should reconize when a client disconnect from the server', function(done) {
this.timeout(5000);
server.instance.once("client_connected", function(socket){
var s = socket;
server.instance.once("client_end", function(socket){
assert.equal(socket.id, s.id);
done();
});
});
client = new protocol.client("0.0.0.0", serverPort);
client.once('client_connected', function(socket){
setTimeout(function(){
socket.end();
},200);
});
});
});
});
| 9e60938ffffb0f6a75d6909536b5eac40d61aeb3 | [
"JavaScript",
"Markdown"
]
| 4 | JavaScript | irineu/eight-protocol | 8116dc15bafa1b58be037d26c127155104f2c23f | b8cd097b2b52ec01b991719affcb07167d72ca04 | |
refs/heads/master | <repo_name>picoauth/picoauth<file_sep>/src/Security/Password/Encoder/Plaintext.php
<?php
namespace PicoAuth\Security\Password\Encoder;
/**
* Plaintext encoder
*
* Included mainly for unit testing, usage for any password storage
* is discouraged.
*/
class Plaintext implements PasswordEncoderInterface
{
/**
* The maximum length
*/
const MAX_LEN = 4096;
/**
* Case sensitivity option
*
* @var bool
*/
protected $ignoreCase = false;
public function __construct(array $options = [])
{
if (isset($options['ignoreCase'])) {
if (!is_bool($options['ignoreCase'])) {
throw new \InvalidArgumentException('Parameter ignoreCase must be a boolean.');
}
$this->ignoreCase = $options['ignoreCase'];
}
}
/**
* @inheritdoc
*/
public function encode($rawPassword)
{
if (strlen($rawPassword) > self::MAX_LEN) {
throw new EncoderException("Invalid length, maximum is ".self::MAX_LEN.".");
}
return $rawPassword;
}
/**
* @inheritdoc
*/
public function isValid($encodedPassword, $rawPassword)
{
if (strlen($rawPassword) > self::MAX_LEN) {
return false;
}
if ($this->ignoreCase) {
return hash_equals(strtolower($encodedPassword), strtolower($rawPassword));
} else {
return hash_equals($encodedPassword, $rawPassword);
}
}
/**
* @inheritdoc
*/
public function needsRehash($encodedPassword)
{
return false;
}
/**
* @inheritdoc
*/
public function getMaxAllowedLen()
{
return self::MAX_LEN;
}
}
<file_sep>/src/Storage/Interfaces/LocalAuthStorageInterface.php
<?php
namespace PicoAuth\Storage\Interfaces;
/**
* Interface for accessing data of local users (LocalAuth module)
*/
interface LocalAuthStorageInterface
{
/**
* Get configuration array
*
* @return array
*/
public function getConfiguration();
/**
* Retrieve user data
*
* @param string $name user identifier
* @return array|null Userdata or null if the user doesn't exist
*/
public function getUserByName($name);
/**
* Retrieve user data by email
*
* The returned userdata array must contain an additional 'name' key.
*
* @param string $email email
* @return array|null Userdata or null if the user doesn't exist
*/
public function getUserByEmail($email);
/**
* Save user
*
* @param string $id User identifier
* @param array $userdata Userdata array
* @throws \Exception On save failure
*/
public function saveUser($id, $userdata);
/**
* Save reset token
*
* @param string $id
* @param array $data
*/
public function saveResetToken($id, $data);
/**
* Get reset token by id
*
* The token must be retrievable only once. The method
* deletes the token entry from the storage on retrieval.
*
* @param string $id Token ID
* @return array|null Token array or null if not found
*/
public function getResetToken($id);
/**
* Username validation
*
* Check if the name is valid for this storage type
* If not, an exception is thrown with explanation message
*
* @param string $name Name to be checked
* @throws \RuntimeException On failure
*/
public function checkValidName($name);
/**
* Return the number of registered users
*
* @return int Users count
*/
public function getUsersCount();
}
<file_sep>/src/Storage/File/FileReader.php
<?php
namespace PicoAuth\Storage\File;
/**
* File reader
*
* Respects the locks while reading
*/
class FileReader extends File
{
const OPEN_MODE = 'r';
/**
* Opens the file for reading
*
* Obtains a shared lock for the file
* @return void
* @throws \RuntimeException On read/lock error
*/
public function open()
{
if ($this->isOpened()) {
return;
}
if (!file_exists($this->filePath)) {
throw new \RuntimeException($this->filePath . " does not exist");
}
$this->handle = @fopen($this->filePath, self::OPEN_MODE);
if ($this->handle === false) {
throw new \RuntimeException("Could not open file for reading: " . $this->filePath);
}
if (!$this->lock(LOCK_SH)) {
$this->close();
throw new \RuntimeException("Could not aquire a shared lock for " . $this->filePath);
}
}
/**
* Reads contents of the opened file
*
* @return string Read data
* @throws \RuntimeException On read error
*/
public function read()
{
$this->open();
// Better performance than fread() from the existing handle
// but it doesn't respect flock
$data = file_get_contents($this->filePath);
if ($data === false) {
throw new \RuntimeException("Could not read from file " . $this->filePath);
}
return $data;
}
}
<file_sep>/tests/PicoAuthTest.php
<?php
namespace PicoAuth;
class PicoAuthTest extends BaseTestCase
{
/**
* PicoAuth plugin instance
* @var \PicoAuth
*/
protected $picoAuth;
protected function setUp()
{
$picoMock = $this->getMockBuilder('\Pico')
->disableOriginalConstructor()
->getMock();
$this->picoAuth = new \PicoAuth($picoMock);
}
public function testGetPlugin()
{
$p = $this->picoAuth->getPlugin();
$this->assertTrue($p instanceof \PicoAuth\PicoAuthPlugin);
}
public function testHandleEvent()
{
$mock = $this->createMock(\PicoAuth\PicoAuthPlugin::class);
$mock->expects($this->once())
->method('handleEvent')
->with("onTest", [1,2,3]);
self::set($this->picoAuth, $mock, 'picoAuthPlugin');
self::set($this->picoAuth, true, 'enabled');
$this->picoAuth->handleEvent("onTest", [1,2,3]);
self::set($this->picoAuth, false, 'enabled');
$this->picoAuth->handleEvent("onSecondTest", [4,5,6]);
}
}
<file_sep>/content/pwreset.md
---
Title: Password reset
Robots: noindex,nofollow
Template: password
---
<file_sep>/src/Utils.php
<?php
namespace PicoAuth;
/**
* PicoAuth helper methods.
*/
final class Utils
{
/**
* Searches the url for a query parameter of a given key.
* @param string $url
* @param string $key
* @return mixed
*/
public static function getRefererQueryParam($url, $key)
{
if (!$url) {
return null;
}
$query = [];
parse_str(parse_url($url, PHP_URL_QUERY), $query);
if (isset($query[$key])) {
return $query[$key];
}
return null;
}
/**
* Validates a non-empty Pico url param.
* @param string $url
* @return bool
*/
public static function isValidPageId($url)
{
return 1 === preg_match("/^(?:[a-zA-Z0-9_-]+\/)*[a-zA-Z0-9_-]+$/", $url);
}
}
<file_sep>/tests/BaseTestCase.php
<?php
namespace PicoAuth;
use PHPUnit\Framework\TestCase;
abstract class BaseTestCase extends TestCase
{
/**
* Allows testing private/protected methods
* @param instance $entity
* @param string $name
* @return ReflectionMethod
*/
public static function getMethod($entity, $name)
{
$class = new \ReflectionClass($entity);
$method = $class->getMethod($name);
$method->setAccessible(true);
return $method;
}
/**
* Sets property value
* @param mixed $entity
* @param mixed $value
* @param string $propertyName
*/
public static function set($entity, $value, $propertyName)
{
$class = new \ReflectionClass($entity);
$property = $class->getProperty($propertyName);
$property->setAccessible(true);
$property->setValue($entity, $value);
}
/**
* Gets property value
* @param mixed $entity
* @param string $propertyName
* @return mixed the value
*/
public static function get($entity, $propertyName)
{
$class = new \ReflectionClass($entity);
$property = $class->getProperty($propertyName);
$property->setAccessible(true);
return $property->getValue($entity);
}
}
<file_sep>/content/locked.md
---
Title: Locked content
Robots: noindex,nofollow
Template: lock
---
# Locked content
Enter a secret key to unlock the page contents:
<file_sep>/src/Storage/OAuthFileStorage.php
<?php
namespace PicoAuth\Storage;
use PicoAuth\Storage\Interfaces\OAuthStorageInterface;
use PicoAuth\Cache\NullCache;
use Psr\SimpleCache\CacheInterface;
use PicoAuth\Storage\Configurator\OAuthConfigurator;
/**
* File storage for OAuth
*/
class OAuthFileStorage extends FileStorage implements OAuthStorageInterface
{
/**
* Configuration file name
*/
const CONFIG_FILE = 'PicoAuth/OAuth.yml';
public function __construct($dir, CacheInterface $cache = null)
{
$this->dir = $dir;
$this->cache = ($cache!==null) ? $cache : new NullCache;
$this->configurator = new OAuthConfigurator;
}
/**
* @inheritdoc
*/
public function getProviderByName($name)
{
$this->readConfiguration();
return $this->config['providers'][$name];
}
/**
* @inheritdoc
*/
public function getProviderNames()
{
$this->readConfiguration();
$providers = $this->config['providers'];
if (is_array($providers)) {
return array_keys($providers);
} else {
return null;
}
}
}
<file_sep>/src/PicoAuthInterface.php
<?php
namespace PicoAuth;
/**
* PicoAuth public API accessible to authentication/authorization modules.
* @version 1.0
*/
interface PicoAuthInterface
{
/**
* PicoAuth API version
* @var int
*/
const PICOAUTH_API_VERSION = 1;
/**
* Get PicoAuth's dependency container
* @return \League\Container\Container
*/
public function getContainer();
/**
* Get a value from the plugin configuration
* @param string $key The configuration key to return
* @param mixed $default Default value if the key is not set
* @return mixed
*/
public function getAuthConfig($key, $default = null);
/**
* Get the current user.
* Returns user instance even if the user is not authenticated.
* @return \PicoAuth\User
*/
public function getUser();
/**
* Return full path of plugin's installation directory.
* @return string absolute path of plugin location
*/
public function getPluginPath();
/**
* Returns URL of the default plugin theme.
* Used from the default twig templates to load js/css resources.
* @return string
*/
public function getDefaultThemeUrl();
/**
* Get module by name.
* If the module does not exist or is not enabled, the method returns null.
* @param string $name Module name
* @return null|\PicoAuth\Module\AbstractAuthModule
*/
public function getModule($name);
/**
* Get flash messages for this request.
* @return array
*/
public function getFlashes();
/**
* Get Pico instance.
* @see AbstractPicoPlugin::getPico()
* @return \Pico
*/
public function getPico();
/**
* Set user instance.
* @param \PicoAuth\User $user User instance
*/
public function setUser($user);
/**
* Change requested page id.
* The change can be propagated to Pico only if the call is made
* from a place originating from \PicoAuth::onRequestUrl().
* @see \PicoAuth::onRequestUrl()
* @param string $url Pico page id to be set
*/
public function setRequestUrl($url);
/**
* Set the requested file.
* @param string $file Path to the loaded .md file
*/
public function setRequestFile($file);
/**
* Adds a new value to plugin's Twig variables.
* Example: if a key "foo" is registered, it can be later displayed in
* the template under {{ auth.vars.foo }}
* @param string $key Output key
* @param mixed $value Output value
*/
public function addOutput($key, $value);
/**
* Adds an always allowed page.
* This url will always be available no matter which authorization modules
* are active and how are they configured. Useful for authentication modules
* to allow access to routes that are needed for authentication
* (e.g. login pages, registration, password reset pages, etc.)
* Modules need to register these routes before denyAccessIfRestricted event.
* @param string $url Pico page url to be always allowed
*/
public function addAllowed($url);
/**
* Performs CSRF validation.
* @see \PicoAuth\Security\CSRF
* @param string $token Token to be validated
* @param null|string $action Optional action the token is associated with
* @return boolean true if the token is valid, false otherwise
*/
public function isValidCSRF($token, $action = null);
/**
* After-login procedure.
* Called by any authentication module after successful login. Migrates session,
* logs the login, invalidates csrf tokens.
*/
public function afterLogin();
/**
* Redirects to page ID.
* Calls exit(), therefore no further code is executed after the call.
* Can be used also for a generic redirect by setting picoOnly to false.
* @param string $url Pico page id
* @param null|string $query Optional HTTP query params to append
* @param bool $picoOnly true=local scope redirect only, false=any url
*/
public function redirectToPage($url, $query = null, $picoOnly = true);
/**
* Redirects to the login page.
* If the Request instance is given, the method will try to extract an "afterLogin"
* query param from the referer header, and if it is a valid Pico page id
* it will add it to the redirect URL.
* Same as {@see PicoAuthInterface::redirectToPage()}, this method is terminal.
* @param null|string $query HTTP query params to append
* @param null|\Symfony\Component\HttpFoundation\Request $httpRequest The current request
*/
public function redirectToLogin($query = null, $httpRequest = null);
}
<file_sep>/content/register.md
---
Title: Registration
Robots: noindex,nofollow
Template: register
---
<file_sep>/src/Security/Password/Password.php
<?php
namespace PicoAuth\Security\Password;
/**
* The class for Password manipulation
*
* Prevents password disclosure in a stack trace if "display_errors"
* is enabled and an unhandled exception occurs.
*/
class Password
{
private $value;
/**
* Create Password instance from string
* @param string $string
*/
public function __construct($string)
{
$this->value = $string;
}
/**
* Get Password value
* @return string
*/
public function __toString()
{
return $this->value;
}
/**
* Get Password value
* @return string
*/
public function get()
{
return $this->value;
}
/**
* Prevent var_dump() of Password value
* @return array
*/
public function __debugInfo()
{
return [
'value' => '*hidden*',
];
}
}
<file_sep>/src/Storage/Configurator/LocalAuthConfigurator.php
<?php
namespace PicoAuth\Storage\Configurator;
use PicoAuth\Storage\Configurator\AbstractConfigurator;
use PicoAuth\Storage\Configurator\ConfigurationException;
/**
* The configurator for LocalAuth
*/
class LocalAuthConfigurator extends AbstractConfigurator
{
/**
* @inheritdoc
*/
public function validate($rawConfig)
{
$config = $this->applyDefaults($rawConfig, $this->getDefault());
$this->validateGlobalOptions($config);
$this->validateLoginSection($config);
$this->validateAccountEditSection($config);
$this->validatePasswordResetSection($config);
$this->validateRegistrationSection($config);
$this->validateUsersSection($config);
return $config;
}
/**
* The default configuration must contain all keys that are required.
* @return array
*/
protected function getDefault()
{
return array(
"encoder" => "bcrypt",
"login" => array(
"passwordRehash" => false
),
"accountEdit" => array(
"enabled" => false,
),
"passwordReset" => array(
"enabled" => false,
"emailMessage" => "Hello,\n\nVisit the link to reset your password "
. "to %site_title%.\n\nReset URL:\n%url%",
"emailSubject" => "%site_title% - Password Reset",
"tokenIdLen" => 10,
"tokenLen" => 50,
"tokenValidity" => 7200,
"resetTimeout" => 1800,
),
"registration" => array(
"enabled" => false,
"maxUsers" => 10000,
"nameLenMin" => 3,
"nameLenMax" => 20,
),
);
}
public function validateGlobalOptions($config)
{
$this->assertString($config, "encoder");
}
public function validateLoginSection($config)
{
$section = $config["login"];
$this->assertBool($section, "passwordRehash");
}
public function validateAccountEditSection($config)
{
$section = $config["accountEdit"];
$this->assertBool($section, "enabled");
}
public function validatePasswordResetSection($config)
{
$section = $config["passwordReset"];
$this->assertBool($section, "enabled")
->assertStringContaining($section, "emailMessage", "%url%")
->assertString($section, "emailSubject")
->assertInteger($section, "tokenIdLen", 4, 128)
->assertInteger($section, "tokenLen", 4, 1024)
->assertInteger($section, "tokenValidity", 60)
->assertInteger($section, "resetTimeout", 60);
}
public function validateRegistrationSection($config)
{
$section = $config["registration"];
$this->assertBool($section, "enabled")
->assertInteger($section, "maxUsers", 0)
->assertInteger($section, "nameLenMin", 1)
->assertInteger($section, "nameLenMax", 1, 200)
->assertGreaterThan($section, "nameLenMax", "nameLenMin");
}
/**
* Validates the users section of the configuration
*
* May have side effects in the configuration array,
* if some usernames are not defined in lowercase
*
* @param array $config Configuration reference
* @throws ConfigurationException On a failed assertation
*/
public function validateUsersSection(&$config)
{
if (!isset($config["users"])) {
// No users are specified in the configuration file
return;
}
$this->assertArray($config, "users");
foreach ($config["users"] as $username => $userData) {
$this->assertUsername($username, $config);
try {
$this->validateUserData($userData);
} catch (ConfigurationException $e) {
$e->addBeforeMessage("Invalid userdata for $username:");
throw $e;
}
// Assure case insensitivity of username indexing
$lowercaseName = strtolower($username);
if ($username !== $lowercaseName) {
if (!isset($config["users"][$lowercaseName])) {
$config["users"][$lowercaseName] = $userData;
unset($config["users"][$username]);
} else {
throw new ConfigurationException("User $username is defined multiple times.");
}
}
}
}
/**
* Validates all user parameters
*
* @param array $userData Userdata array
* @throws ConfigurationException On a failed assertation
*/
public function validateUserData($userData)
{
$this->assertRequired($userData, "pwhash");
$this->assertString($userData, "pwhash");
// All remaining options are optional
$this->assertString($userData, "email");
$this->assertArray($userData, "attributes");
$this->assertString($userData, "encoder");
$this->assertBool($userData, "pwreset");
$this->assertArrayOfStrings($userData, "groups");
$this->assertString($userData, "displayName");
}
/**
* Asserts a valid username format
*
* @param string $username Username being checked
* @param array $config The configuration array
* @throws ConfigurationException On a failed assertation
*/
public function assertUsername($username, $config)
{
if (!is_string($username)) {
throw new ConfigurationException("Username $username must be a string.");
}
$len = strlen($username);
$minLen=$config["registration"]["nameLenMin"];
$maxLen=$config["registration"]["nameLenMax"];
if ($len < $minLen || $len > $maxLen) {
throw new ConfigurationException(
sprintf("Length of a username $username must be between %d-%d characters.", $minLen, $maxLen)
);
}
if (!$this->checkValidNameFormat($username)) {
throw new ConfigurationException("Username $username contains invalid character/s.");
}
}
/**
* Checks a valid username format
*
* @param string $name file name to be checked
* @return bool true if the name format is accepted
*/
public function checkValidNameFormat($name)
{
return preg_match('/^[a-z0-9_\-]+$/i', $name) === 1;
}
}
<file_sep>/tests/Module/Generic/InstallerTest.php
<?php
namespace PicoAuth\Module\Generic;
use PicoAuth\Session\SessionInterface;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\HttpFoundation\ParameterBag;
use PicoAuth\PicoAuthInterface;
class InstallerTest extends \PicoAuth\BaseTestCase
{
protected $installer;
protected function setUp()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$this->installer = new Installer($picoAuth);
}
public function testOnPicoRequestAtPicoAuth()
{
$pico = $this->createMock(\Pico::class);
$pico->expects($this->exactly(3))
->method("getBaseUrl")
->willReturn("/");
$pico->expects($this->exactly(3))
->method("getConfig")
->willReturn("");
$pico->expects($this->exactly(2))
->method("getConfigDir")
->willReturn("/config");
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method("getPluginPath")
->willReturn("");
$picoAuth->expects($this->once())
->method("setRequestFile")
->with("/content/install.md");
$picoAuth->expects($this->exactly(3))
->method("getPico")
->willReturn($pico);
$picoAuth->expects($this->exactly(4))
->method("addOutput");
//not used
$request = $this->createMock(Request::class);
$request->request = $this->createMock(ParameterBag::class);
$installer = new Installer($picoAuth);
$installer->onPicoRequest("PicoAuth", $request);
}
public function testOnPicoRequestAtPicoAuthModules()
{
$pico = $this->createMock(\Pico::class);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method("getPluginPath")
->willReturn("");
$picoAuth->expects($this->once())
->method("setRequestFile")
->with("/content/install.md");
$picoAuth->expects($this->exactly(1))
->method("addOutput");
//not used
$request = $this->createMock(Request::class);
$request->request = $this->createMock(ParameterBag::class);
$installer = new Installer($picoAuth);
$installer->onPicoRequest("PicoAuth/modules", $request);
}
public function testGetName()
{
$this->assertEquals('installer', $this->installer->getName());
}
}
<file_sep>/src/Module/Authentication/OAuth.php
<?php
namespace PicoAuth\Module\Authentication;
use League\OAuth2\Client\Provider\Exception\IdentityProviderException;
use League\OAuth2\Client\Tool\ArrayAccessorTrait;
use League\OAuth2\Client\Provider\AbstractProvider;
use PicoAuth\Log\LoggerTrait;
use PicoAuth\Module\AbstractAuthModule;
use PicoAuth\Storage\Interfaces\OAuthStorageInterface;
use PicoAuth\Session\SessionInterface;
use PicoAuth\PicoAuthInterface;
use PicoAuth\User;
use PicoAuth\Utils;
use Psr\Log\LoggerAwareInterface;
use Symfony\Component\HttpFoundation\Request;
/**
* OAuth 2.0 authentication
*
* Uses authorization code grant
*/
class OAuth extends AbstractAuthModule implements LoggerAwareInterface
{
use LoggerTrait;
use ArrayAccessorTrait;
const LOGIN_CSRF_ACTION = 'OAuth';
/**
* Instance of the plugin
*
* @var PicoAuthInterface
*/
protected $picoAuth;
/**
* Session manager
*
* @var SessionInterface
*/
protected $session;
/**
* Configuration storage
*
* @var OAuthStorageInterface
*/
protected $storage;
/**
* Configuration array
*
* @var array
*/
protected $config;
/**
* OAuth 2.0 provider used
*
* @var AbstractProvider
*/
protected $provider;
/**
* Configuration array for the provider
*
* @var array
*/
protected $providerConfig;
public function __construct(
PicoAuthInterface $picoAuth,
SessionInterface $session,
OAuthStorageInterface $storage
) {
$this->picoAuth = $picoAuth;
$this->session = $session;
$this->storage = $storage;
$this->config = $this->storage->getConfiguration();
}
/**
* @inheritdoc
*/
public function getName()
{
return 'OAuth';
}
/**
* Can be used from twig template when dynamically compositing a login page
* @return OAuthStorageInterface
*/
public function getStorage()
{
return $this->storage;
}
/**
* @inheritdoc
*/
public function onPicoRequest($url, Request $httpRequest)
{
$post = $httpRequest->request;
//SSO login submission
if ($url === "login" && $post->has("oauth")) {
//CSRF validation
if (!$this->picoAuth->isValidCSRF($post->get("csrf_token"), self::LOGIN_CSRF_ACTION)) {
$this->picoAuth->redirectToLogin(null, $httpRequest);
}
$provider = $post->get("oauth");
// Find the requested provider (case sensitive)
$providerConfig = $this->storage->getProviderByName($provider);
if (!$providerConfig) {
$this->session->addFlash("error", "Requested provider is not available.");
$this->picoAuth->redirectToLogin(null, $httpRequest);
}
$this->initProvider($providerConfig);
$this->session->set("provider", $provider);
$this->saveAfterLogin($httpRequest);
// Starts with retreiving the OAuth authorization code
$this->startAuthentication();
} elseif ($url === $this->config["callbackPage"] && $this->isValidCallback($httpRequest)) {
// Request on the SSO endpoint
$provider = $this->session->get("provider");
$this->session->remove("provider");
$providerConfig = $this->storage->getProviderByName($provider);
if (!$providerConfig) {
$this->session->remove("oauth2state");
throw new \RuntimeException("Provider removed during auth process.");
}
$this->initProvider($providerConfig);
$this->finishAuthentication($httpRequest);
}
}
/**
* Initializes an instance of the provider from the configuration
*
* @param array $providerConfig Configuration array of the selected provider
* @throws \RuntimeException If the provider is not resolvable
*/
protected function initProvider($providerConfig)
{
$providerClass = $providerConfig['provider'];
$options = $providerConfig['options'];
if (!isset($options['redirectUri'])) {
// Set OAuth 2.0 callback page from the configuration
$options['redirectUri'] = $this->picoAuth->getPico()->getPageUrl($this->config["callbackPage"]);
}
if (!class_exists($providerClass)) {
throw new \RuntimeException("Provider class $providerClass does not exist.");
}
if (!is_subclass_of($providerClass, AbstractProvider::class, true)) {
throw new \RuntimeException("Class $providerClass is not a League\OAuth2 provider.");
}
$this->provider = new $providerClass($options);
$this->providerConfig = $providerConfig;
}
/**
* Starts the OAuth 2.0 process
*
* Redirects to the authorization URL of the selected provider
*/
protected function startAuthentication()
{
$authorizationUrl = $this->provider->getAuthorizationUrl();
$this->session->migrate(true);
$this->session->set("oauth2state", $this->provider->getState());
// The final redirect, halts the script
$this->picoAuth->redirectToPage($authorizationUrl, null, false);
}
/**
* Finishes the OAuth 2.0 process
*
* Handles the authorization code response to the authorization request
* Expects that the Request contains a valid callback, which should
* be checked by {@see OAuth::isValidCallback()}.
*
* @param Request $httpRequest
*/
protected function finishAuthentication(Request $httpRequest)
{
$sessionCode = $this->session->get("oauth2state");
$this->session->remove("oauth2state");
// Check that the state from OAuth response matches the one in the session
if ($httpRequest->query->get("state") !== $sessionCode) {
$this->onStateMismatch();
}
// Returns one of https://tools.ietf.org/html/rfc6749#section-4.1.2.1
if ($httpRequest->query->has("error")) {
$this->onOAuthError($httpRequest->query->get("error"));
}
// Error not set, but code not present (not an RFC complaint response)
if (!$httpRequest->query->has("code")) {
$this->onOAuthError("no_code");
}
try {
$accessToken = $this->provider->getAccessToken('authorization_code', [
'code' => $httpRequest->query->get("code"),
]);
$resourceOwner = $this->provider->getResourceOwner($accessToken);
$this->saveLoginInfo($resourceOwner);
} catch (IdentityProviderException $e) {
$this->onOauthResourceError($e);
}
}
/**
* Gets an attribute from the resource owner
*
* @param string $name Attribute name
* @param \League\OAuth2\Client\Provider\ResourceOwnerInterface $resourceOwner Resource owner instance
* @return mixed The retrieved value
*/
protected function getResourceAttribute($name, $resourceOwner)
{
// Call resource owner getter first
$method = "get" . $name;
if (is_callable(array($resourceOwner, $method))) {
$res = $resourceOwner->$method();
return $res;
} else {
$resourceArray = $resourceOwner->toArray();
$res = $this->getValueByKey($resourceArray, $name);
return $res;
}
}
/**
* Saves the information from the ResourceOwner
*
* @param \League\OAuth2\Client\Provider\ResourceOwnerInterface $resourceOwner
*/
protected function saveLoginInfo($resourceOwner)
{
// Initialize the user
$u = new User();
$u->setAuthenticated(true);
$u->setAuthenticator($this->getName());
// Get user id from the Resource Owner
$attrMap = $this->providerConfig['attributeMap'];
$userIdAttr = $attrMap['userId'];
$userId = $this->getResourceAttribute($userIdAttr, $resourceOwner);
$u->setId($userId);
unset($attrMap['userId']);
// Get display name from the Resource Owner (if configured)
if (isset($attrMap['displayName'])) {
$name = $this->getResourceAttribute($attrMap['displayName'], $resourceOwner);
$u->setDisplayName($name);
unset($attrMap['displayName']);
}
// Retrieve all other custom attributes from the attributeMap
foreach ($attrMap as $mapKey => $mapValue) {
$value = $this->getResourceAttribute($mapValue, $resourceOwner);
$u->setAttribute($mapKey, $value);
}
// Set default droups and default attributes
$u->setGroups($this->providerConfig['default']['groups']);
foreach ($this->providerConfig['default']['attributes'] as $key => $value) {
if (null === $u->getAttribute($key)) {
$u->setAttribute($key, $value);
}
}
$this->picoAuth->setUser($u);
$this->picoAuth->afterLogin();
}
/**
* Saves the afterLogin parameter
*
* @param Request $httpRequest
*/
protected function saveAfterLogin(Request $httpRequest)
{
$referer = $httpRequest->headers->get("referer", null, true);
$afterLogin = Utils::getRefererQueryParam($referer, "afterLogin");
if ($afterLogin && Utils::isValidPageId($afterLogin)) {
$this->session->set("afterLogin", $afterLogin);
}
}
/**
* Checks if the request and session have all the required fields for a OAuth 2.0 callback
*
* - The session must have "provider" name which is the provider the callback
* would be returned from.
* - The request must have "state" query param as a CSRF prevention.
* - The session must have "oauth2state" which must be a string
* a must be non-empty.
*
* @param Request $httpRequest
* @return bool true if the required fields are present, false otherwise
*/
protected function isValidCallback(Request $httpRequest)
{
return $this->session->has("provider")
&& $httpRequest->query->has("state")
&& $this->session->has("oauth2state")
&& is_string($this->session->get("oauth2state"))
&& (strlen($this->session->get("oauth2state")) > 0);
}
/**
* Logs invalid state in the OAuth 2.0 response
*/
protected function onStateMismatch()
{
$this->logger->warning(
"OAuth2 response state mismatch: provider: {provider} from {addr}",
array(
"provider" => get_class($this->provider),
"addr" => $_SERVER['REMOTE_ADDR']
)
);
$this->session->remove("oauth2state");
$this->session->addFlash("error", "Invalid OAuth response.");
$this->picoAuth->redirectToLogin();
}
/**
* On an OAuth error
*
* @param string $errorCode
*/
protected function onOAuthError($errorCode)
{
$errorCode = strlen($errorCode > 100) ? substr($errorCode, 0, 100) : $errorCode;
$this->logger->notice(
"OAuth2 error response: code {code}, provider {provider}",
array(
"code" => $errorCode,
"provider" => get_class($this->provider),
)
);
$this->session->addFlash("error", "The provider returned an error ($errorCode)");
$this->picoAuth->redirectToLogin();
}
/**
* On a Resource owner error
*
* @param IdentityProviderException $e
*/
protected function onOauthResourceError(IdentityProviderException $e)
{
$this->logger->critical(
"OAuth2 IdentityProviderException: {e}, provider {provider}",
array(
"e" => $e->getMessage(),
"provider" => get_class($this->provider),
)
);
$this->session->addFlash("error", "Failed to get an access token or user details.");
$this->picoAuth->redirectToLogin();
}
}
<file_sep>/tests/Storage/Configurator/PageLockConfiguratorTest.php
<?php
namespace PicoAuth\Storage\Configurator;
use PHPUnit\Framework\TestCase;
class PageLockConfiguratorTest extends TestCase
{
protected $configurator;
protected function setUp()
{
$this->configurator = new PageLockConfigurator;
}
/**
* @dataProvider badConfigurations
*/
public function testValidationErrors($rawConfig)
{
$this->expectException(ConfigurationException::class);
$this->configurator->validate($rawConfig);
}
public function badConfigurations()
{
return [
[["encoder"=>1]],
[["locks"=>[1=>["key"=>"a"]]]],
[["locks"=>[""=>["key"=>"a"]]]],
[["locks"=>["a"=>1]]],
[["locks"=>["a"=>["nokey"=>"a"]]]],
[["locks"=>["a"=>["key"=>1]]]],
[["locks"=>["a"=>["key"=>"1","encoder"=>1]]]],
[["locks"=>["a"=>["key"=>"1","file"=>1]]]],
[["urls"=>1]],
[["urls"=>[1=>[]]]],
[["urls"=>[""=>[]]]],
[["urls"=>["a"=>[]]]],
[["urls"=>["a"=>["lock"=>1]]]],
[["urls"=>["a"=>["lock"=>"1","recursive"=>1]]]],
];
}
public function testValidation()
{
$res = $this->configurator->validate([
"locks" => [
"a" => ["key"=>"a","file"=>"a","encoder"=>"plain"]
],
"urls" => [
"test" => ["lock"=>"1","recursive"=>true]
]
]);
$this->assertArrayHasKey("encoder", $res);
}
}
<file_sep>/tests/UtilsTest.php
<?php
namespace PicoAuth;
use PHPUnit\Framework\TestCase;
/**
* PicoAuth plugin tests
*/
class UtilsTest extends TestCase
{
/**
* @dataProvider queryProvider
*/
public function testGetRefererQueryParam($url, $key, $expected)
{
$this->assertEquals($expected, Utils::getRefererQueryParam($url, $key));
}
public function queryProvider()
{
return [
[null, null, null],
["https://test.xyz?a=1", "a", "1"],
["https://test.xyz?a=1&b=2", "b", "2"],
["https://", "b", null],
["https://test.xyz?a=1&b=2", null, null],
["aaa", "b", null],
];
}
/**
* @dataProvider pageProvider
*/
public function testIsValidPageId($url, $expected)
{
$this->assertEquals($expected, Utils::isValidPageId($url));
}
public function pageProvider()
{
return [
[null, false],
["", false],
["index", true],
["pAge-Name0_", true],
["a?", false],
["@^", false],
];
}
}
<file_sep>/src/Storage/FileStorage.php
<?php
namespace PicoAuth\Storage;
use PicoAuth\Storage\Configurator\ConfigurationException;
/**
* Base File Storage
*/
class FileStorage
{
/**
* Configuration file location, redefined in subclasses
*/
const CONFIG_FILE = '';
/**
* The configuration array
* @var array
*/
protected $config;
/**
* The Configurator instance
* @var null|Configurator\AbstractConfigurator
*/
protected $configurator;
/**
* Cache implementation
* @var \Psr\SimpleCache\CacheInterface
*/
protected $cache;
/**
* Base directory
* @var string
*/
protected $dir;
/**
* The last error message
* @var string
*/
protected static $lastError;
/**
* Reads contents of a file to a string
*
* Acquires blocking shared lock for the file.
*
* @param string $fileName Name of the file to read.
* @param array $options Options for the Reader instance
* @return string|bool String with the file contents on success, FALSE otherwise.
*/
public static function readFile($fileName, $options = [])
{
$reader = new File\FileReader($fileName, $options);
$success = true;
$contents = null;
try {
$reader->open();
$contents = $reader->read();
} catch (\RuntimeException $e) {
self::$lastError = $e->getMessage();
$success = false;
}
try {
$reader->close();
} catch (\RuntimeException $e) {
self::$lastError = $e->getMessage();
$success = false;
}
return ($success) ? $contents : false;
}
/**
* Writes data to a file
*
* @param string $fileName Name of the file
* @param string $data File contents to write
* @param array $options Options for the Writer instance
* @return bool Was the write operation successful
*/
public static function writeFile($fileName, $data, $options = [])
{
$writer = new File\FileWriter($fileName, $options);
$isSuccess = true;
$written = 0;
try {
$writer->open();
$written = $writer->write($data);
} catch (\RuntimeException $e) {
self::$lastError = $e->getMessage();
$isSuccess = false;
}
try {
$writer->close();
} catch (\RuntimeException $e) {
self::$lastError = $e->getMessage();
$isSuccess = false;
}
return $isSuccess;
}
/**
* Prepares path
*
* Check if $path relative to $basePath exists and is writable.
* Attempts to create it if it does not exist. Warning is supressed
* in case of mkdir failure.
*
* @param string $basePath
* @param string $path
*/
public static function preparePath($basePath, $path)
{
$basePath = rtrim($basePath, '/');
$path = ltrim($path, '/');
$fullPath = $basePath . '/' . $path;
if (file_exists($fullPath)) {
if (!is_dir($fullPath)) {
throw new \RuntimeException("Cannot create a directory, regular file already exists: {$path}.");
}
if (!is_writable($fullPath)) {
throw new \RuntimeException("Directory is not writable: {$path}.");
}
} else {
$res=@mkdir($fullPath, 0770, true);
if (!$res) {
throw new \RuntimeException("Unable to create a directory: {$path}.");
}
}
}
/**
* Get item by URL
*
* Find an item that applies to a given url
* Url keys must begin with a /
*
* @param array|null $items
* @param string $url
* @return array|null
*/
public static function getItemByUrl($items, $url)
{
if (!isset($items)) {
return null;
}
// Check for the exact rule
if (array_key_exists("/" . $url, $items)) {
return $items["/" . $url];
}
$urlParts = explode("/", trim($url, "/"));
$urlPartsLen = count($urlParts);
while ($urlPartsLen > 0) {
unset($urlParts[--$urlPartsLen]);
$subUrl = "/" . join("/", $urlParts);
// Use the higher level rule, if it doesn't have deactivated recursive application
if (array_key_exists($subUrl, $items)
&& (!isset($items[$subUrl]["recursive"])
|| $items[$subUrl]["recursive"]===true)) {
return $items[$subUrl];
}
}
return null;
}
/**
* Returns the configuration
*
* Reads the configuration if it hasn't been already read.
*
* @return Array
*/
public function getConfiguration()
{
$this->readConfiguration();
return $this->config;
}
/**
* Configuration validation
*
* Calls the specific configurator instance, which validates the user
* provided configuration and adds default values.
*
* @param mixed $userConfig Anything read from the configuration file
* @return array Modified configuration (added defaults)
* @throws \RuntimeException Missing configurator
* @throws ConfigurationException Validation error
*/
protected function validateConfiguration($userConfig = array())
{
if (!$this->configurator) {
throw new \RuntimeException("Configurator class is not set.");
}
try {
return $this->configurator->validate($userConfig);
} catch (ConfigurationException $e) {
$e->addBeforeMessage("Configuration error in ".static::CONFIG_FILE.":");
throw $e;
}
}
/**
* Initializes the configuration array
*
* If the file exists, tries to read from the cache first, if the cache
* has the data, but the file is newer, reads the file and renews the cache.
*
* md5 is used as a cache key, because some cache providers may not accept
* characters present in the file path.
* Collisions or reversibility do not present security concerns.
*
* @throws \RuntimeException On file read error
*/
protected function readConfiguration()
{
// Return if already loaded
if (is_array($this->config)) {
return;
}
$fileName = $this->dir . static::CONFIG_FILE;
// Abort if the file doesn't exist
if (!file_exists($fileName)) {
$this->config = $this->validateConfiguration();
return;
}
$modifyTime = filemtime($fileName);
if (false === $modifyTime) {
throw new \RuntimeException("Unable to get mtime of the configuration file.");
}
// Check if the configuration is in cache
$cacheKey = md5($fileName);
if ($this->cache->has($cacheKey)) {
$this->config=$this->cache->get($cacheKey);
// Cached file is up to date
if ($this->config["_mtime"] === $modifyTime) {
return;
}
}
if (($yaml = self::readFile($fileName)) !== false) {
$config = \Symfony\Component\Yaml\Yaml::parse($yaml);
$this->config = $this->validateConfiguration($config);
} else {
throw new \RuntimeException("Unable to read configuration file.");
}
// Save to cache with updated modify-time
$storedConfig = $this->config;
$storedConfig["_mtime"]= $modifyTime;
$this->cache->set($cacheKey, $storedConfig);
}
}
<file_sep>/src/Module/Authentication/LocalAuth/PasswordReset.php
<?php
namespace PicoAuth\Module\Authentication\LocalAuth;
use PicoAuth\Log\LoggerTrait;
use PicoAuth\Mail\MailerInterface;
use PicoAuth\Storage\Interfaces\LocalAuthStorageInterface;
use PicoAuth\Security\Password\Password;
use PicoAuth\Security\RateLimiting\RateLimitInterface;
use PicoAuth\Session\SessionInterface;
use PicoAuth\PicoAuthInterface;
use Psr\Log\LoggerAwareInterface;
use Symfony\Component\HttpFoundation\Request;
/**
* PasswordReset component for LocalAuth
*/
class PasswordReset implements LoggerAwareInterface
{
use LoggerTrait;
/**
* Mailer instance
*
* @see PasswordReset::setsetMailer()
* @var \PicoAuth\Mail\MailerInterface
*/
protected $mailer;
/**
* The password reser request
*
* @var Request
*/
protected $httpRequest;
/**
* The password reset configuration array
*
* @var array
*/
protected $config;
/**
* Instance of a rate limiter
*
* @var RateLimitInterface
*/
protected $limit;
/**
* Instance of the plugin
*
* @var PicoAuthInterface
*/
protected $picoAuth;
/**
* Session manager
*
* @var SessionInterface
*/
protected $session;
/**
* Configuration storage
*
* @var LocalAuthStorageInterface
*/
protected $storage;
public function __construct(
PicoAuthInterface $picoAuth,
SessionInterface $session,
LocalAuthStorageInterface $storage,
RateLimitInterface $limit
) {
$this->picoAuth = $picoAuth;
$this->session = $session;
$this->storage = $storage;
$this->limit = $limit;
}
/**
* Sets a Mailer instance
*
* @param MailerInterface $mailer
* @return $this
*/
public function setMailer(MailerInterface $mailer)
{
$this->mailer = $mailer;
return $this;
}
/**
* Sets the LocalAuth configuration array
*
* @param array $config
* @return $this
*/
public function setConfig(array $config)
{
$this->config = $config["passwordReset"];
return $this;
}
/**
* On a password reset request
*
* @return void
*/
public function handlePasswordReset(Request $httpRequest)
{
$this->httpRequest = $httpRequest;
// Check if a valid reset link is present
$this->checkResetLink();
// Check if the user already has a password reset session
$resetData = $this->session->get("pwreset");
if ($resetData === null) {
$this->beginPasswordReset();
} else {
$this->finishPasswordReset($resetData);
}
}
/**
* The first stage of a password reset process
*
* Shows a form to request a password reset and processes
* its submission (sends password reset link on success)
*
* @return void
*/
protected function beginPasswordReset()
{
// Check if password reset is enabled
if (!$this->config["enabled"]) {
return;
}
$this->picoAuth->addAllowed("password_reset");
$this->picoAuth->setRequestFile($this->picoAuth->getPluginPath() . '/content/pwbeginreset.md');
if (count($this->session->getFlash('_pwresetsent'))) {
$this->picoAuth->addOutput("resetSent", true);
return;
}
$post = $this->httpRequest->request;
if ($post->has("reset_email")) {
// CSRF validation
if (!$this->picoAuth->isValidCSRF($post->get("csrf_token"))) {
$this->picoAuth->redirectToPage("password_reset");
}
$email = trim($post->get("reset_email"));
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
$this->session->addFlash("error", "Email address does not have a valid format.");
$this->picoAuth->redirectToPage("password_reset");
}
// Check if the action is not rate limited
if (!$this->limit->action("passwordReset", true, array("email" => $email))) {
$this->session->addFlash("error", $this->limit->getError());
$this->picoAuth->redirectToPage("password_reset");
}
if ($userData = $this->storage->getUserByEmail($email)) {
$this->sendResetMail($userData);
}
// Always display a message with success
$this->session->addFlash("_pwresetsent", true);
$this->session->addFlash("success", "Reset link sent via email.");
$this->picoAuth->redirectToPage("password_reset");
}
}
/**
* The second stage of a password reset process
*
* Shows a password reset form and processes its submission
*
* @param array $resetData The resetData array with a fixed structure defined
* in {@see PasswordReset::startPasswordResetSession()}
*/
protected function finishPasswordReset(array $resetData)
{
if (time() > $resetData['validity']) {
$this->session->remove("pwreset");
$this->session->addFlash("error", "Page validity expired, please try again.");
$this->picoAuth->redirectToLogin();
}
$this->picoAuth->addOutput("isReset", true);
$this->picoAuth->setRequestFile($this->picoAuth->getPluginPath() . '/content/pwreset.md');
// Check the form submission
$post = $this->httpRequest->request;
if ($post->has("new_password")
&& $post->has("new_password_repeat")
) {
$newPassword = new Password($post->get("new_password"));
$newPasswordRepeat = new Password($post->get("new_password_repeat"));
$username = $resetData['user'];
// CSRF validation
if (!$this->picoAuth->isValidCSRF($post->get("csrf_token"))) {
$this->picoAuth->redirectToPage("password_reset");
}
if ($newPassword->get() !== $newPasswordRepeat->get()) {
$this->session->addFlash("error", "The passwords do not match.");
$this->picoAuth->redirectToPage("password_reset");
}
// Check password policy
$localAuth = $this->picoAuth->getContainer()->get('LocalAuth');
if (!$localAuth->checkPasswordPolicy($newPassword)) {
$this->picoAuth->redirectToPage("password_reset");
}
// Remove pwreset record on successful submission
$this->session->remove("pwreset");
// Save the new userdata
$userData = $this->storage->getUserByName($username);
$localAuth->userDataEncodePassword($userData, $newPassword);
$this->storage->saveUser($username, $userData);
$this->logPasswordReset($username);
$localAuth->login($username, $userData);
$this->picoAuth->afterLogin();
}
}
/**
* Validates a password reset link
*
* If a valid password reset link was provided, starts a password reset
* session and redirects to a password change form
*
* @return void
*/
protected function checkResetLink()
{
// Check if the reset links are enabled, if token is present,
// if it has a valid format and length
if (!$this->config["enabled"]
|| !($token = $this->httpRequest->query->get("confirm", false))
|| !preg_match("/^[a-f0-9]+$/", $token)
|| strlen($token) !== 2*($this->config["tokenIdLen"]+$this->config["tokenLen"])) {
return;
}
// Delete the active password reset session, if set
$this->session->remove("pwreset");
// Split token parts
$tokenId = substr($token, 0, 2 * $this->config["tokenIdLen"]);
$verifier = substr($token, 2 * $this->config["tokenIdLen"]);
// Validate token timeout
$tokenData = $this->storage->getResetToken($tokenId);
// Token not found or expired
if (!$tokenData || time() > $tokenData['valid']) {
$this->session->addFlash("error", "Reset link has expired.");
$this->getLogger()->warning("Bad reset token {t} from {addr}", [$token, $_SERVER['REMOTE_ADDR']]);
$this->picoAuth->redirectToPage("password_reset");
}
if (hash_equals($tokenData['token'], hash('sha256', $verifier))) {
$this->session->addFlash("success", "Please set a new password.");
$this->startPasswordResetSession($tokenData['user']);
$this->logResetLinkVisit($tokenData);
$this->picoAuth->redirectToPage("password_reset");
}
}
/**
* Starts a password reset session
*
* A password reset session is active when session key pwreset is present,
* which contains user identifier of the user who the reset session is valid
* for and an expiration date after which the password session cannot be used
* to change a password.
*
* @param string $user The user identifier
*/
public function startPasswordResetSession($user)
{
$this->session->migrate(true);
$this->session->set("pwreset", array(
'user' => $user,
'validity' => time() + $this->config["resetTimeout"]
));
}
protected function createResetToken($username)
{
// Get the reset token
$tokenId = bin2hex(random_bytes($this->config["tokenIdLen"]));
$verifier = bin2hex(random_bytes($this->config["tokenLen"]));
$url = $this->picoAuth->getPico()->getPageUrl("password_reset", array(
'confirm' => $tokenId . $verifier
));
$tokenData = array(
'token' => hash('sha256', $verifier),
'user' => $username,
'valid' => time() + $this->config["tokenValidity"]
);
$this->storage->saveResetToken($tokenId, $tokenData);
return $url;
}
/**
* Sends a password reset link
*
* @param array $userData Userdata array of an existing user
* @return void
*/
protected function sendResetMail($userData)
{
if (!$this->mailer) {
$this->getLogger()->critical("Sending mail but no mailer is set!");
return;
}
$url = $this->createResetToken($userData['name']);
// Replaces Pico-specific placeholders (like %site_title%)
$message = $this->picoAuth->getPico()->substituteFileContent($this->config["emailMessage"]);
$subject = $this->picoAuth->getPico()->substituteFileContent($this->config["emailSubject"]);
// Replaces placeholders in the configured email message template
$message = str_replace("%url%", $url, $message);
$message = str_replace("%username%", $userData['name'], $message);
$this->mailer->setup();
$this->mailer->setTo($userData['email']);
$this->mailer->setSubject($subject);
$this->mailer->setBody($message);
if (!$this->mailer->send()) {
$this->getLogger()->critical("Mailer error: {e}", ["e" => $this->mailer->getError()]);
} else {
$this->getLogger()->info("PwReset email sent to {email}", ["email" => $userData['email']]);
}
}
/**
* Logs a valid reset link visit
*
* @param array $tokenData Reset token data array
*/
protected function logResetLinkVisit(array $tokenData)
{
$this->getLogger()->info(
"Valid pwReset link for {name} visited by {addr}",
array(
"name" => $tokenData['user'],
"addr" => $_SERVER['REMOTE_ADDR'],
)
);
}
/**
* Logs a completed password reset
*
* @param string $username
*/
protected function logPasswordReset($username)
{
$this->getLogger()->info(
"Completed password reset for {name} by {addr}",
array(
"name" => $username,
"addr" => $_SERVER['REMOTE_ADDR'],
)
);
}
}
<file_sep>/tests/Session/SymfonySessionTest.php
<?php
namespace PicoAuth\Session;
use Symfony\Component\HttpFoundation\Session\Session;
use Symfony\Component\HttpFoundation\ParameterBag;
use Symfony\Component\HttpFoundation\Session\Storage\MockArraySessionStorage;
class SymfonySessionTest extends \PicoAuth\BaseTestCase
{
protected $session;
protected function setUp()
{
$storage = new MockArraySessionStorage();
$this->session = new SymfonySession($storage);
}
public function testClear()
{
$symfonySession = $this->createMock(Session::class);
$symfonySession->expects($this->once())
->method('clear');
self::set($this->session, $symfonySession, 'session');
$this->session->clear();
}
public function testInvalidate()
{
$symfonySession = $this->createMock(Session::class);
$symfonySession->expects($this->once())
->method('invalidate')
->with(321)
->willReturn(123);
self::set($this->session, $symfonySession, 'session');
$res = $this->session->invalidate(321);
$this->assertEquals(123, $res);
}
public function testMigrate()
{
$symfonySession = $this->createMock(Session::class);
$symfonySession->expects($this->once())
->method('migrate')
->with(true, 123)
->willReturn(123);
self::set($this->session, $symfonySession, 'session');
$res = $this->session->migrate(true, 123);
$this->assertEquals(123, $res);
}
public function testGetFlash()
{
$parameterBag = $this->createMock(ParameterBag::class);
$parameterBag->expects($this->once())
->method('get')
->with("key", array(1))
->willReturn(array("test"));
$symfonySession = $this->createMock(Session::class);
$symfonySession->expects($this->once())
->method('getFlashBag')
->willReturn($parameterBag);
self::set($this->session, $symfonySession, 'session');
$res = $this->session->getFlash("key", array(1));
$this->assertEquals(array("test"), $res);
}
}
<file_sep>/tests/PicoAuthPluginTest.php
<?php
namespace PicoAuth;
use Psr\Log\LoggerInterface;
use Symfony\Component\HttpFoundation\Session\Storage\MockArraySessionStorage;
/**
* PicoAuth plugin tests
*/
class PicoAuthPluginTest extends BaseTestCase
{
/**
* PicoAuth plugin instance
* @var PicoAuthPlugin
*/
protected $picoAuth;
/**
* Session
* @var \PicoAuth\Session\SessionInterface
*/
protected $session;
protected function setUp()
{
$storage = new MockArraySessionStorage();
$this->session = new Session\SymfonySession($storage);
$picoMock = $this->getMockBuilder('\Pico')
->disableOriginalConstructor()
->getMock();
$this->picoAuth = new PicoAuthPlugin($picoMock);
self::set($this->picoAuth, $this->session, 'session');
}
public function testIsValidCSRF()
{
$csrf = $this->createMock(Security\CSRF::class);
$csrf->expects($this->once())
->method('checkToken')
->with('valid')
->willReturn(true);
self::set($this->picoAuth, $csrf, 'csrf');
$this->assertTrue($this->picoAuth->isValidCSRF('valid'));
}
public function testIsInvalidCSRF()
{
$csrf = $this->createMock(Security\CSRF::class);
$csrf->expects($this->once())
->method('checkToken')
->with('invalid')
->willReturn(false);
self::set($this->picoAuth, $csrf, 'csrf');
$logger = $this->createMock(LoggerInterface::class);
$logger->expects($this->once())
->method('warning')->willReturnSelf();
self::set($this->picoAuth, $logger, 'logger');
$_SERVER["REMOTE_ADDR"] = 'localhost';
$this->assertFalse($this->picoAuth->isValidCSRF('invalid'));
}
public function testAfterLogin()
{
$session = $this->createMock(Session\SessionInterface::class);
$session->expects($this->once())
->method("migrate")
->with(true);
$session->expects($this->once())
->method("set")
->with('user', $this->anything());
$session->expects($this->once())
->method("has")
->with('afterLogin')
->willReturn(true);
$picoAuth = $this->getMockBuilder(PicoAuthPlugin::class)
->disableOriginalConstructor()
->setMethodsExcept(['afterLogin','setUser'])
->getMock();
$csrf = $this->createMock(Security\CSRF::class);
$csrf->expects($this->once())
->method('removeTokens');
$logger = $this->createMock(LoggerInterface::class);
$logger->expects($this->once())
->method('info')->willReturnSelf();
$user = $this->createMock(User::class);
$user->expects($this->once())
->method('getId');
$user->expects($this->once())
->method('getDisplayName');
$user->expects($this->once())
->method('getAuthenticator');
$r=\Symfony\Component\HttpFoundation\Request::create('/');
self::set($picoAuth, $csrf, 'csrf');
self::set($picoAuth, $session, 'session');
self::set($picoAuth, $logger, 'logger');
self::set($picoAuth, $r, 'request');
$picoAuth->setUser($user);
$picoAuth->afterLogin();
}
public function testSetUser()
{
$user = new User;
$this->picoAuth->setUser($user);
$this->assertSame($this->picoAuth->getUser(), $user);
$groups = $user->getGroups();
$this->assertFalse(array_search("default", $groups));
$user = new User;
$user->setAuthenticated(true);
$this->picoAuth->setUser($user);
$groups = $user->getGroups();
// Authenticated user should be gained the default group
$this->assertNotFalse(array_search("default", $groups));
}
public function testGetModule()
{
self::set($this->picoAuth, array('Name'=>1), 'modules');
$this->assertEquals(1, $this->picoAuth->getModule('Name'));
self::set($this->picoAuth, array(), 'modules');
$this->assertNull($this->picoAuth->getModule('Name'));
$this->assertNull($this->picoAuth->getModule(null));
}
public function testGetPico()
{
$picoMock = $this->getMockBuilder('\Pico')
->disableOriginalConstructor()
->getMock();
$picoAuth = new PicoAuthPlugin($picoMock);
$this->assertSame($picoMock, $picoAuth->getPico());
}
public function testGetDefaultThemeUrl()
{
$picoMock = $this->createMock(\Pico::class);
$picoMock->expects($this->once())
->method("getBaseUrl")
->willReturn("/");
$picoAuth = new PicoAuthPlugin($picoMock);
$this->assertSame("/plugins/PicoAuth/theme", $picoAuth->getDefaultThemeUrl());
}
public function testSetRequestFile()
{
$picoMock = $this->createMock(\Pico::class);
$picoAuth = new PicoAuthPlugin($picoMock);
$picoAuth->setRequestFile("/sub/page.md");
$reqFile=self::get($picoAuth, "requestFile");
$this->assertSame("/sub/page.md", $reqFile);
}
public function testSetRequestUrl()
{
$picoMock = $this->createMock(\Pico::class);
$picoMock->expects($this->once())
->method("resolveFilePath")
->with("sub/page")
->willReturn("/sub/page.md");
$picoAuth = new PicoAuthPlugin($picoMock);
$picoAuth->setRequestUrl("sub/page");
$reqUrl=self::get($picoAuth, "requestUrl");
$this->assertSame("sub/page", $reqUrl);
$reqFile=self::get($picoAuth, "requestFile");
$this->assertSame("/sub/page.md", $reqFile);
}
public function testGetFlashes()
{
$session = $this->createMock(Session\SessionInterface::class);
$session->expects($this->exactly(2))
->method("getFlash")
->willReturn(["test"]);
$picoMock = $this->getMockBuilder('\Pico')
->disableOriginalConstructor()
->getMock();
$picoAuth = new PicoAuthPlugin($picoMock);
self::set($picoAuth, $session, 'session');
$res=$picoAuth->getFlashes();
$this->assertTrue(count($res)==2);
}
public function testOnConfigLoaded()
{
$picoAuth = $this->getMockBuilder(PicoAuthPlugin::class)
->disableOriginalConstructor()
->setMethods(['loadDefaultConfig','createContainer','initLogger'])
->getMock();
$picoAuth->expects($this->once())
->method("loadDefaultConfig");
$picoAuth->expects($this->once())
->method("createContainer");
$picoAuth->expects($this->once())
->method("initLogger");
$config=[];
$picoAuth->handleEvent("onConfigLoaded", [&$config]);
}
public function testOnRequestUrl()
{
$picoAuth = $this->getMockBuilder(PicoAuthPlugin::class)
->disableOriginalConstructor()
->setMethods(['init','triggerEvent','errorHandler'])
->getMock();
$picoAuth->expects($this->once())
->method("init");
$picoAuth->expects($this->once())
->method("triggerEvent")
->willThrowException(new \RuntimeException("e"));
$picoAuth->expects($this->once())
->method("errorHandler");
$url="index";
$picoAuth->handleEvent("onRequestUrl", [&$url]);
}
public function testAllowed()
{
$picoAuth = $this->getMockBuilder(PicoAuthPlugin::class)
->disableOriginalConstructor()
->setMethods(['triggerEvent'])
->getMock();
$picoAuth->expects($this->once())
->method("triggerEvent")
->with('denyAccessIfRestricted', $this->anything());
$file = "test.md";
self::set($picoAuth, $file, 'requestFile');
self::set($picoAuth, 'test', 'requestUrl');
// Will trigger denyAccessIfRestricted
$picoAuth->onRequestFile($file);
$picoAuth->addAllowed("test");
// Won't trigger denyAccessIfRestricted
$picoAuth->onRequestFile($file);
}
}
<file_sep>/tests/Security/Password/Encoder/Argon2iTest.php
<?php
namespace PicoAuth\Security\Password\Encoder;
use PHPUnit\Framework\TestCase;
class Argon2iTest extends TestCase
{
protected function setUp()
{
if (!defined('PASSWORD_ARGON2I')) {
$this->markTestSkipped(
'ARGON2I algorithm is not available in the test environment.'
);
}
}
public function testEncodeDecode()
{
$arg = new Argon2i();
$res = $arg->encode("Test");
$this->assertStringStartsWith("$", $res);
$this->assertTrue($arg->isValid($res, "Test"));
}
public function testLengthLimit()
{
$raw = str_repeat("r", Argon2i::MAX_LEN+1);
$arg = new Argon2i();
$this->assertEquals(Argon2i::MAX_LEN, $arg->getMaxAllowedLen());
$this->assertFalse($arg->isValid("0", $raw));
$this->expectException(EncoderException::class);
$arg->encode($raw);
}
public function testNeedsRehash()
{
$arg1 = new Argon2i(['time_cost'=>1]);
$res1 = $arg1->encode("BcryptTest");
$arg2 = new Argon2i(['cost'=>2]);
$this->assertTrue($arg2->needsRehash($res1));
}
}
<file_sep>/README.md
PicoAuth
====
PicoAuth is a plugin for Pico CMS 2.0 providing various means of authentication and authorization to pages.
[](https://travis-ci.org/picoauth/picoauth)
[](https://packagist.org/packages/picoauth/picoauth)
[](https://github.com/picoauth/picoauth/blob/master/LICENSE)
The functionality of the plugin is contained in independent modules that can be individually enabled and configured to suit the needs of the website owner. Simple description of the modules that are included in the plugin:
* **Local user accounts**
* Visitors can login to the site using accounts stored locally
* User accounts defined in a configuration file
* User registration *(optional)*
* Password reset function *(optional)*
* Users can change their password *(optional)*
* **Login using OAuth 2.0 services**
* Visitors can login to the site using a 3rd party service that supports the OAuth 2.0 protocol
* **Access control**
* Access control to Pico pages based on permissions of an authenticated user
* **Page locks**
* Can be used to display selected pages only after the correct page key phrase is entered (no authentication required)
The plugin provides security features like CSRF tokens on all forms, IP-subnet rate limiting on sensitive actions (login attempts, registrations, etc.), option to enable logging, session security configuration, configurable password policy, selectable password hashing algorithms and other security options. There are many options for an advanced configuration like - caching configuration files, password reset options, dependency injection configuration. PicoAuth contains a default theme for its pages (login, registration form, etc.), but can be integrated into any existing Pico template with only few changes. The plugin also supports other alternative authentication/authorization methods that can be added via external plugins.
## Documentation
[GitHub wiki](https://github.com/picoauth/picoauth/wiki) or [picoauth.github.io](https://picoauth.github.io/).
Contains an Installation guide, Full Feature Reference with a description of all configuration options, Security considerations and options for advanced settings.
Screenshots
-----------

Install
-------
PicoAuth requires **PHP 5.6.0+** The recommended way of installation is using composer (possible only if you installed Pico using [composer-installer](https://github.com/picocms/composer-installer)).
The plugin can be installed by issuing this command in the Pico root directory:
```
composer require picoauth/picoauth
```
Then visit `/PicoAuth` page (`?PicoAuth` if not using url-rewriting) in your Pico installation to view the installer, which will perform a basic security check of your Pico installation and will guide you through the rest of the installation (like selection of the modules you want to use).
*It is also possible to install the plugin without composer - by downloading the plugin archive from the [Release page](https://github.com/picoauth/picoauth/releases) and extracting it to your `plugins` directory. This is not recommended due to complicated updating, so use it only if composer cannot be used. This method of installation is more described in the [Documentation](#documentation).*
Configuration
-------------
The main plugin configuration is located in Pico's `config/config.yml`. Each enabled authentication/authorization module has its own configuration in the `config/PicoAuth` directory. Usually the file name corresponds to the module name (e.g. `LocalAuth.yml`, `OAuth.yml`, `PageACL.yml`, `PageLock.yml`). Refer to the **plugin [Documentation](#documentation) for the full configuration reference**. See project [picoauth/picoauth-examples](https://github.com/picoauth/picoauth-examples) for in-depth configuration examples.
### See also
* [picoauth/picoauth-theme](https://github.com/picoauth/picoauth-theme) - An example theme for Pico CMS using the PicoAuth plugin. Can serve as a template for customizing your own theme to work with PicoAuth. See the Demo: [https://picoauth.xyz](https://picoauth.xyz).
* [picoauth/picoauth-examples](https://github.com/picoauth/picoauth-examples) - in-depth configuration examples.
Getting help
------------
If you encounter a problem with the plugin that cannot be resolved by reading the corresponding section of the [Documentation](#documentation), you can create a new Issue. Please describe the problem as clear as possible.
<file_sep>/src/Storage/PageLockFileStorage.php
<?php
namespace PicoAuth\Storage;
use PicoAuth\Storage\Interfaces\PageLockStorageInterface;
use PicoAuth\Cache\NullCache;
use Psr\SimpleCache\CacheInterface;
use PicoAuth\Storage\Configurator\PageLockConfigurator;
/**
* File storage for PageLock
*/
class PageLockFileStorage extends FileStorage implements PageLockStorageInterface
{
/**
* Configuration file name
*/
const CONFIG_FILE = 'PicoAuth/PageLock.yml';
public function __construct($dir, CacheInterface $cache = null)
{
$this->dir = $dir;
$this->cache = ($cache!==null) ? $cache : new NullCache;
$this->configurator = new PageLockConfigurator;
}
/**
* @inheritdoc
*/
public function getLockById($lockId)
{
$this->readConfiguration();
return isset($this->config['locks'][$lockId]) ? $this->config['locks'][$lockId] : null;
}
/**
* @inheritdoc
*/
public function getLockByURL($url)
{
$this->readConfiguration();
$urlRecord = self::getItemByUrl($this->config['urls'], $url);
if ($urlRecord && isset($urlRecord["lock"])) {
$lockId = $urlRecord["lock"];
return $lockId;
} else {
return null;
}
}
}
<file_sep>/tests/Security/RateLimiting/NullRateLimitTest.php
<?php
namespace PicoAuth\Security\RateLimiting;
use PicoAuth\BaseTestCase;
class NullRateLimitTest extends BaseTestCase
{
public function testAction()
{
$limit = new NullRateLimit();
$this->assertTrue($limit->action("any-action"));
}
public function testGetError()
{
$limit = new NullRateLimit();
$this->assertNull($limit->getError());
}
}
<file_sep>/src/Storage/Configurator/RateLimitConfigurator.php
<?php
namespace PicoAuth\Storage\Configurator;
use PicoAuth\Storage\Configurator\AbstractConfigurator;
use PicoAuth\Storage\Configurator\ConfigurationException;
/**
* The configurator for RateLimit
*/
class RateLimitConfigurator extends AbstractConfigurator
{
/**
* @inheritdoc
*/
public function validate($rawConfig)
{
$config = $this->applyDefaults($rawConfig, $this->getDefault(), 1);
$this->validateGlobalOptions($config);
$this->validateActionsSection($config);
return $config;
}
/**
* The default configuration must contain all keys that are required.
* @return array
*/
protected function getDefault()
{
return array(
"cleanupProbability" => 25,
"actions" => array(
"login" => array(
"ip" => array(
"count" => 50,
"counterTimeout" => 43200,
"blockDuration" => 900,
"errorMsg" => "Amount of failed attempts exceeded, wait %min% minutes.",
),
"account" => array(
"count" => 10,
"counterTimeout" => 43200,
"blockDuration" => 900,
"errorMsg" => "Amount of failed attempts exceeded, wait %min% minutes.",
)
),
"passwordReset" => array(
"email" => array(
"count" => 2,
"counterTimeout" => 86400,
"blockDuration" => 86400,
"errorMsg" => "Maximum of %cnt% reset emails were sent, check your inbox.",
),
"ip" => array(
"count" => 10,
"counterTimeout" => 86400,
"blockDuration" => 86400,
"errorMsg" => "Amount of maximum submissions exceeded, wait %min% minutes.",
),
),
"registration" => array(
"ip" => array(
"count" => 2,
"blockDuration" => 86400,
"errorMsg" => "Amount of maximum submissions exceeded, wait %min% minutes.",
),
),
"pageLock" => array(
"ip" => array(
"count" => 10,
"blockDuration" => 1800,
),
),
)
);
}
public function validateGlobalOptions(&$config)
{
$this->assertInteger($config, "cleanupProbability", 0, 100);
}
public function validateActionsSection($config)
{
$this->assertArray($config, "actions");
foreach ($config["actions"] as $actionName => $limitMethods) {
if (!is_string($actionName) || $actionName=="") {
throw new ConfigurationException("Action identifier must be a string.");
}
$this->assertArray($config["actions"], $actionName);
try {
$this->validateLimitMethodsSection($limitMethods);
} catch (ConfigurationException $e) {
$e->addBeforeMessage("Invalid rateLimit data for $actionName:");
throw $e;
}
}
}
public function validateLimitMethodsSection(&$config)
{
foreach ($config as $methodName => $limitData) {
if (!is_string($methodName) || $methodName=="") {
throw new ConfigurationException("Limit method identifier must be a string.");
}
$this->assertArray($config, $methodName);
// Mandatory attributes
$this->assertRequired($limitData, "count");
$this->assertInteger($limitData, "count", 0);
$this->assertRequired($limitData, "blockDuration");
$this->assertInteger($limitData, "blockDuration", 0);
// Set counterTimeout to blockDuration if not specified
if (!isset($limitData["counterTimeout"])) {
$limitData["counterTimeout"] = $limitData["blockDuration"];
} else {
$this->assertInteger($limitData, "counterTimeout", 0);
}
// Optional attributes
$this->assertString($limitData, "errorMsg");
$this->assertInteger($limitData, "netmask_IPv4", 0, 32);
$this->assertInteger($limitData, "netmask_IPv6", 0, 128);
}
}
}
<file_sep>/theme/js/installer.js
$(document).ready(function() {
$('#repeatTest').show();
var inst=new Installer(url_check);
$('#repeatTest').click(function(){
inst.checkUrls().bind(inst);
});
inst.checkUrls();
});
//------ Response testing results constants -------
/**
* The returned response has a successful status code and contains
* most likely a text file (yaml/markup)
* @type {Number}
*/
var RESPONSE_OK_TEXT=1;
/**
* The returned response has a successful status code and contains HTML
* @type {Number}
*/
var RESPONSE_OK_HTML=2;
/**
* The response is 403 or 404 status code, which is expected for all
* of the performed pre-installation tests
* @type {Number}
*/
var RESPONSE_NA_EXPECTED=3;
/**
* The response does not have a successful status code, but it is not expected
* for the pre-installation tests
* @type {Number}
*/
var RESPONSE_NA_UNEXPECTED_STATUS=4;
/**
* No response received or other error while sending the request
* @type {Number}
*/
var NO_RESPONSE=5;
//-----------------------------------------------------
function Installer(urls){
this.urls=urls;
};
Installer.prototype.checkUrls = function(){
$('#dir_listing, #config_file, #content_file')
.text("Testing...")
.removeClass();
this.testRequest(this.urls.dir_listing, 'dir_listing');
this.testRequest(this.urls.config_file, 'config_file');
this.testRequest(this.urls.content_file, 'content_file');
};
Installer.prototype.showTestResult = function(testId, errId, message){
var elem=$('#'+testId);
var result;
switch(errId){
case RESPONSE_OK_TEXT:
result='critical';
break;
case RESPONSE_OK_HTML:
if(testId==='dir_listing'){
result='critical';
}else{
result='warning';
}
break;
case RESPONSE_NA_EXPECTED:
result='success';
break;
default:
result='warning';
break;
}
elem.html("<strong>"+result.toUpperCase()+"</strong> - "+message);
elem.addClass(result);
};
Installer.prototype.testRequest = function(url, testId){
var self=this;
$.ajax({
url: url,
dataType: "text",
crossDomain: false,
timeout: 5000,
cache: false,
testId: testId,
resCallback: function(errId, message){
self.showTestResult(this.testId, errId, message);
},
beforeSend: function( xhr ) {
xhr.overrideMimeType( "text/plain" );
},
success: function( data, textStatus, xhr ){
var isTextFile=true;
if(data.indexOf("<html") >= 0){
isTextFile=false;
}
if(isTextFile){
this.resCallback(RESPONSE_OK_TEXT,"Text file returned, HTTP "+xhr.status+".");
}else{
this.resCallback(RESPONSE_OK_HTML,"Returned successful response, HTTP "+xhr.status+".");
}
},
error: function( xhr, status, errorThrown ) {
if(typeof xhr.status !== "undefined"){
if(xhr.status===403 || xhr.status===404){
this.resCallback(RESPONSE_NA_EXPECTED,"Not accessible (returns HTTP "+xhr.status+").");
}else{
this.resCallback(RESPONSE_NA_UNEXPECTED_STATUS,"Returned "+xhr.status+", was expecting 403 or 404.");
}
}else{
this.resCallback(NO_RESPONSE,"No response received, "+errorThrown+".");
}
}
});
};
<file_sep>/src/Security/Password/Encoder/BCrypt.php
<?php
namespace PicoAuth\Security\Password\Encoder;
/**
* BCrypt
*/
class BCrypt implements PasswordEncoderInterface
{
/**
* The maximum allowed length
*
* The BCrypt algorithm does not use characters after this length.
*/
const MAX_LEN = 72;
/**
* The options array
*
* @var array
*/
protected $options;
public function __construct(array $options = [])
{
if (isset($options['cost'])) {
if ($options['cost'] < 4 || $options['cost'] > 31) {
throw new \InvalidArgumentException('Cost must be in the range of 4-31.');
}
}
$defaults = array(
'cost' => 10
);
$options += $defaults;
$this->options = $options;
}
/**
* @inheritdoc
*/
public function encode($rawPassword)
{
if (strlen($rawPassword) > self::MAX_LEN) {
throw new EncoderException("Invalid length, maximum is ".self::MAX_LEN.".");
}
return \password_hash($rawPassword, PASSWORD_BCRYPT, $this->options);
}
/**
* @inheritdoc
*/
public function isValid($encodedPassword, $rawPassword)
{
if (strlen($rawPassword) > self::MAX_LEN) {
return false;
}
return \password_verify($rawPassword, $encodedPassword);
}
/**
* @inheritdoc
*/
public function needsRehash($encodedPassword)
{
return password_needs_rehash($encodedPassword, PASSWORD_BCRYPT, $this->options);
}
/**
* @inheritdoc
*/
public function getMaxAllowedLen()
{
return self::MAX_LEN;
}
}
<file_sep>/tests/Storage/Configurator/PageACLConfiguratorTest.php
<?php
namespace PicoAuth\Storage\Configurator;
use PHPUnit\Framework\TestCase;
class PageACLConfiguratorTest extends TestCase
{
protected $configurator;
protected function setUp()
{
$this->configurator = new PageACLConfigurator;
}
/**
* @dataProvider badConfigurations
*/
public function testValidationErrors($rawConfig)
{
$this->expectException(ConfigurationException::class);
$this->configurator->validate($rawConfig);
}
public function badConfigurations()
{
return [
[["access"=>1]],
[["access"=>[1=>["users"=>"a"]]]],
[["access"=>[""=>["users"=>"a"]]]],
[["access"=>["users"=>1]]],
[["access"=>["groups"=>1]]],
[["access"=>[["a"=>["users"=>[1,2,3]]]]]],
[["access"=>[["a"=>["groups"=>[1,2,3]]]]]],
[["access"=>[["a"=>["recursive"=>1]]]]],
];
}
public function testValidation()
{
$res = $this->configurator->validate(null);
$this->assertArrayHasKey("access", $res);
$res = $this->configurator->validate([
"access" => [
"/a" => ["users"=>["a"]],
"/b" => ["groups"=>["a"]],
"/c" => ["users"=>[1],"groups"=>["a"]],
"/c" => ["users"=>["a"],"groups"=>["a"],"recursive"=>true],
],
]);
$this->assertArrayHasKey("access", $res);
}
}
<file_sep>/src/Module/Authentication/LocalAuth/Registration.php
<?php
namespace PicoAuth\Module\Authentication\LocalAuth;
use PicoAuth\Log\LoggerTrait;
use PicoAuth\Storage\Interfaces\LocalAuthStorageInterface;
use PicoAuth\Security\Password\Password;
use PicoAuth\Security\RateLimiting\RateLimitInterface;
use PicoAuth\Session\SessionInterface;
use PicoAuth\PicoAuthInterface;
use Psr\Log\LoggerAwareInterface;
use Symfony\Component\HttpFoundation\Request;
/**
* Registration component for LocalAuth
*/
class Registration implements LoggerAwareInterface
{
use LoggerTrait;
const REGISTER_CSRF_ACTION = 'register';
/**
* The password reser request
*
* @var Request
*/
protected $httpRequest;
/**
* The registration configuration array
*
* @var array
*/
protected $config;
/**
* Instance of a rate limiter
*
* @var RateLimitInterface
*/
protected $limit;
/**
* Instance of the plugin
*
* @var PicoAuthInterface
*/
protected $picoAuth;
/**
* Session manager
*
* @var SessionInterface
*/
protected $session;
/**
* Configuration storage
*
* @var LocalAuthStorageInterface
*/
protected $storage;
public function __construct(
PicoAuthInterface $picoAuth,
SessionInterface $session,
LocalAuthStorageInterface $storage,
RateLimitInterface $limit
) {
$this->picoAuth = $picoAuth;
$this->session = $session;
$this->storage = $storage;
$this->limit = $limit;
}
/**
* Sets the LocalAuth configuration array
*
* @param array $config
* @return $this
*/
public function setConfig(array $config)
{
$this->config = $config["registration"];
return $this;
}
/**
* On a registration request
*
* @return void
*/
public function handleRegistration(Request $httpRequest)
{
// Abort if disabled
if (!$this->config["enabled"]) {
return;
}
$user = $this->picoAuth->getUser();
if ($user->getAuthenticated()) {
$this->picoAuth->redirectToPage("index");
}
$this->picoAuth->addAllowed("register");
$this->picoAuth->setRequestFile($this->picoAuth->getPluginPath() . '/content/register.md');
// Check the form submission
$post = $httpRequest->request;
if ($post->has("username")
&& $post->has("email")
&& $post->has("password")
&& $post->has("password_repeat")
) {
// CSRF validation
if (!$this->picoAuth->isValidCSRF($post->get("csrf_token"), self::REGISTER_CSRF_ACTION)) {
$this->picoAuth->redirectToPage("register");
}
// Abort if a limit for maximum users is exceeded
$this->assertLimits();
// Registration fields
$reg = array(
"username" => strtolower(trim($post->get("username"))),
"email" => trim($post->get("email")),
"password" => <PASSWORD>($<PASSWORD>("<PASSWORD>")),
"passwordRepeat" => <PASSWORD>($post->get("password_repeat")),
);
$isValid = $this->validateRegistration($reg);
if ($isValid) {
// Check if the action is not rate limited
if (!$this->limit->action("registration")) {
$this->session->addFlash("error", $this->limit->getError());
$this->picoAuth->redirectToPage("register");
}
$this->logSuccessfulRegistration($reg);
$userData = array('email' => $reg["email"]);
$localAuth = $this->picoAuth->getContainer()->get('LocalAuth');
$localAuth->userDataEncodePassword($userData, $reg["password"]);
$this->storage->saveUser($reg["username"], $userData);
$this->session->addFlash("success", "Registration completed successfully, you can now log in.");
$this->picoAuth->redirectToLogin();
} else {
// Prefill the old values to the form
$this->session->addFlash("old", array(
'username' => $reg["username"],
'email' => $reg["email"]
));
// Redirect back and display errors
$this->picoAuth->redirectToPage("register");
}
}
}
/**
* Validates the submitted registration
*
* @param array $reg Registration array
* @return boolean true if the registration is valid and can be saved, false otherwise
*/
protected function validateRegistration(array $reg)
{
$isValid = true;
// Username format
try {
$this->storage->checkValidName($reg["username"]);
} catch (\RuntimeException $e) {
$isValid = false;
$this->session->addFlash("error", $e->getMessage());
}
// Username length
$min = $this->config["nameLenMin"];
$max = $this->config["nameLenMax"];
if (strlen($reg["username"]) < $min || strlen($reg["username"]) > $max) {
$isValid = false;
$this->session->addFlash(
"error",
sprintf("Length of a username must be between %d-%d characters.", $min, $max)
);
}
// Email format
if (!filter_var($reg["email"], FILTER_VALIDATE_EMAIL)) {
$isValid = false;
$this->session->addFlash("error", "Email address does not have a valid format.");
}
// Email unique
if (null !== $this->storage->getUserByEmail($reg["email"])) {
$isValid = false;
$this->session->addFlash("error", "This email is already in use.");
}
// Password repeat matches
if ($reg["password"]->get() !== $reg["passwordRepeat"]->get()) {
$isValid = false;
$this->session->addFlash("error", "The passwords do not match.");
}
// Check password policy
$localAuth = $this->picoAuth->getContainer()->get('LocalAuth');
if (!$localAuth->checkPasswordPolicy($reg["password"])) {
$isValid = false;
}
// Username unique
if ($this->storage->getUserByName($reg["username"]) !== null) {
$isValid = false;
$this->session->addFlash("error", "The username is already taken.");
}
return $isValid;
}
/**
* Logs successful registration
*
* @param array $reg Registration array
*/
protected function logSuccessfulRegistration(array $reg)
{
$this->getLogger()->info(
"New registration: {name} ({email}) from {addr}",
array(
"name" => $reg["username"],
"email" => $reg["email"],
"addr" => $_SERVER['REMOTE_ADDR']
)
);
// Log the amount of users on each 10% of the maximum capacity
$max = $this->config["maxUsers"];
$count = $this->storage->getUsersCount()+1;
if ($count % ceil($max/10) === 0) {
$percent = intval($count/ceil($max/100));
$this->getLogger()->warning(
"The amount of users has reached {percent} of the maximum capacity {max}.",
array(
"percent" => $percent,
"max" => $max
)
);
}
}
/**
* Aborts the registration if the limit of an amount of users is reached
*/
protected function assertLimits()
{
if ($this->storage->getUsersCount() >= $this->config["maxUsers"]) {
$this->session->addFlash("error", "New registrations are currently disabled.");
$this->picoAuth->redirectToPage("register");
}
}
}
<file_sep>/tests/Cache/NullCacheTest.php
<?php
namespace PicoAuth\Cache;
class NullCacheTest extends \PicoAuth\BaseTestCase
{
protected $nullCache;
protected function setUp()
{
$this->nullCache = new NullCache;
}
public function testClear()
{
$this->assertTrue($this->nullCache->clear());
}
public function testDelete()
{
$this->assertTrue($this->nullCache->delete("test"));
}
public function testDeleteMultiple()
{
$this->assertTrue($this->nullCache->deleteMultiple(["t1","t2"]));
}
public function testGet()
{
$this->assertNull($this->nullCache->get("test"));
$this->assertEquals(1, $this->nullCache->get("test", 1));
}
public function testGetMultiple()
{
$this->assertEquals(
["t1"=>null,"t2"=>null],
$this->nullCache->getMultiple(["t1","t2"])
);
$this->assertEquals(
["t1"=>1,"t2"=>1],
$this->nullCache->getMultiple(["t1","t2"], 1)
);
}
public function testHas()
{
$this->assertFalse($this->nullCache->has("test"));
}
public function testSet()
{
$this->assertFalse($this->nullCache->set("test", 1));
}
public function testSetMultiple()
{
$this->assertFalse($this->nullCache->setMultiple(["a"=>1,"b"=>2]));
}
}
<file_sep>/src/Module/Authentication/LocalAuth/EditAccount.php
<?php
namespace PicoAuth\Module\Authentication\LocalAuth;
use PicoAuth\Storage\Interfaces\LocalAuthStorageInterface;
use PicoAuth\Security\Password\Password;
use PicoAuth\Session\SessionInterface;
use PicoAuth\PicoAuthInterface;
use Symfony\Component\HttpFoundation\Request;
/**
* EditAccount component for LocalAuth
*/
class EditAccount
{
/**
* Configuration array for EditAccount
*
* @var array
*/
protected $config;
/**
* Instance of the plugin
*
* @var PicoAuthInterface
*/
protected $picoAuth;
/**
* Session manager
*
* @var SessionInterface
*/
protected $session;
/**
* Configuration storage
*
* @var LocalAuthStorageInterface
*/
protected $storage;
public function __construct(
PicoAuthInterface $picoAuth,
SessionInterface $session,
LocalAuthStorageInterface $storage
) {
$this->picoAuth = $picoAuth;
$this->session = $session;
$this->storage = $storage;
}
/**
* Sets the configuration array
* @param array $config
* @return $this
*/
public function setConfig(array $config)
{
$this->config = $config["accountEdit"];
return $this;
}
/**
* Account page request
*
* @param Request $httpRequest
* @return void
*/
public function handleAccountPage(Request $httpRequest)
{
// Check if the functionality is enabled by the configuration
if (!$this->config["enabled"]) {
return;
}
$user = $this->picoAuth->getUser();
$this->picoAuth->addAllowed("account");
$this->picoAuth->setRequestFile($this->picoAuth->getPluginPath() . '/content/account.md');
//check form submission
$post = $httpRequest->request;
if ($post->has("new_password")
&& $post->has("new_password_repeat")
&& $post->has("old_password")
) {
$newPassword = new Password($post->get("new_password"));
$newPasswordRepeat = new Password($post->get("new_password_repeat"));
$oldPassword = new Password($post->get("old_password"));
$username = $user->getId();
// CSRF validation
if (!$this->picoAuth->isValidCSRF($post->get("csrf_token"))) {
$this->picoAuth->redirectToPage("account");
}
if ($newPassword->get() !== $newPasswordRepeat->get()) {
$this->session->addFlash("error", "The passwords do not match.");
$this->picoAuth->redirectToPage("account");
}
// The current password check
$localAuth = $this->picoAuth->getContainer()->get('LocalAuth');
if (!$localAuth->loginAttempt($username, $oldPassword)) {
$this->session->addFlash("error", "The current password is incorrect");
$this->picoAuth->redirectToPage("account");
}
// Check password policy
if (!$localAuth->checkPasswordPolicy($newPassword)) {
$this->picoAuth->redirectToPage("account");
}
// Save user data
$userData = $this->storage->getUserByName($username);
$localAuth->userDataEncodePassword($userData, $newPassword);
$this->storage->saveUser($username, $userData);
$this->session->addFlash("success", "Password changed successfully.");
$this->picoAuth->redirectToPage("account");
}
}
}
<file_sep>/content/login.md
---
Title: Login
Robots: noindex,nofollow
Template: login
---
<file_sep>/src/Module/Authorization/PageLock.php
<?php
namespace PicoAuth\Module\Authorization;
use PicoAuth\Storage\Interfaces\PageLockStorageInterface;
use PicoAuth\Security\RateLimiting\RateLimitInterface;
use PicoAuth\Session\SessionInterface;
use Symfony\Component\HttpFoundation\Request;
use PicoAuth\PicoAuthInterface;
use PicoAuth\Module\AbstractAuthModule;
/**
* Basic authorization by page locking
*/
class PageLock extends AbstractAuthModule
{
const UNLOCK_CSRF_ACTION = 'unlock';
/**
* Instance of the plugin
*
* @var PicoAuthInterface
*/
protected $picoAuth;
/**
* Session manager
*
* @var SessionInterface
*/
protected $session;
/**
* Configuration storage
*
* @var PageLockStorageInterface
*/
protected $storage;
/**
* The rate limiter
*
* @var RateLimitInterface
*/
protected $limit;
/**
* The configuration array
*
* @var array
*/
protected $config;
public function __construct(
PicoAuthInterface $picoAuth,
SessionInterface $session,
PageLockStorageInterface $storage,
RateLimitInterface $limit
) {
$this->picoAuth = $picoAuth;
$this->session = $session;
$this->storage = $storage;
$this->limit = $limit;
$this->config = $this->storage->getConfiguration();
}
/**
* @inheritdoc
*/
public function getName()
{
return 'pageLock';
}
/**
* @inheritdoc
*/
public function onPicoRequest($url, Request $httpRequest)
{
$post = $httpRequest->request;
if ($post->has("page-key")) {
// CSRF validation
if (!$this->picoAuth->isValidCSRF($post->get("csrf_token"), self::UNLOCK_CSRF_ACTION)) {
$this->picoAuth->redirectToPage($url);
}
// Check if the action is not rate limited
if (!$this->limit->action("pageLock", false)) {
$this->session->addFlash("error", $this->limit->getError());
$this->picoAuth->redirectToPage($url);
}
$pageKey = $post->get("page-key");
$lockId = $this->storage->getLockByURL($url);
$lockData = $this->storage->getLockById($lockId);
$keyEncoder = $this->getKeyEncoder($lockData);
if ($keyEncoder->isValid($lockData["key"], $pageKey)) {
$unlocked = $this->session->get("unlocked", []);
$unlocked[] = $lockId;
$this->session->migrate(true);
$this->session->set("unlocked", $unlocked);
} else {
$this->session->addFlash("error", "The specified key is invalid");
$this->limit->action("pageLock", true);
}
$this->picoAuth->redirectToPage($url);
}
// Option to lock the unlocked pages, independent from the plugin's Logout
// (authenticated user stays in the session and must use Logout to destroy session)
if ($post->has("logout-locks") && $this->picoAuth->isValidCSRF($post->get("csrf_token"))) {
$this->session->migrate(true);
$this->session->set("unlocked", []);
}
// Add unlocked locks to the output, so the theme can show "Close session"
// if any locks are currently opened, that is optional
$this->picoAuth->addOutput("locks", $this->session->get("unlocked", []));
}
/**
* @inheritdoc
*/
public function checkAccess($url)
{
$lockId = $this->storage->getLockByURL($url);
if ($lockId) {
return $this->isUnlocked($lockId);
} else {
return true;
}
}
/**
* @inheritdoc
*/
public function denyAccessIfRestricted($url)
{
$lockId = $this->storage->getLockByURL($url);
if ($lockId && !$this->isUnlocked($lockId)) {
$lockData = $this->storage->getLockById($lockId);
$this->picoAuth->addOutput("unlock_action", $this->picoAuth->getPico()->getPageUrl($url));
if (isset($lockData['file'])) {
$contentDir = $this->picoAuth->getPico()->getConfig('content_dir');
$this->picoAuth->setRequestFile($contentDir . $lockData['file']);
} else {
$this->picoAuth->setRequestFile($this->picoAuth->getPluginPath() . '/content/locked.md');
}
header($_SERVER['SERVER_PROTOCOL'] . ' 403 Forbidden');
}
}
/**
* Returns whether the lock is unlocked
*
* @param string $lockId Lock identifier
* @return boolean true if unlocked, false otherwise
*/
protected function isUnlocked($lockId)
{
$unlocked = $this->session->get("unlocked");
if ($unlocked && in_array($lockId, $unlocked)) {
return true;
}
return false;
}
/**
* Returns encoder instance for the specified lock
*
* @param array $lockData Lock data array
* @return \PicoAuth\Security\Password\Encoder\PasswordEncoderInterface
* @throws \RuntimeException If the encoder is not resolvable
*/
protected function getKeyEncoder($lockData)
{
if (isset($lockData['encoder']) && is_string($lockData['encoder'])) {
$name = $lockData['encoder'];
} else {
$name = $this->config["encoder"];
}
try {
$instance = $this->picoAuth->getContainer()->get($name);
} catch (\Exception $e) {
throw new \RuntimeException("Specified PageLock encoder not resolvable.");
}
return $instance;
}
}
<file_sep>/tests/Storage/Configurator/PluginConfiguratorTest.php
<?php
namespace PicoAuth\Storage\Configurator;
use PHPUnit\Framework\TestCase;
class PluginConfiguratorTest extends TestCase
{
protected $configurator;
protected function setUp()
{
$this->configurator = new PluginConfigurator;
}
/**
* @dataProvider badConfigurations
*/
public function testValidationErrors($rawConfig)
{
$this->expectException(ConfigurationException::class);
$this->configurator->validate($rawConfig);
}
public function badConfigurations()
{
return [
[["authModules"=>1]],
[["authModules"=>null]],
[["afterLogin"=>1]],
[["afterLogout"=>1]],
[["alterPageArray"=>1]],
[["sessionInterval"=>-1]],
[["sessionInterval"=>true]],
[["sessionTimeout"=>-1]],
[["sessionTimeout"=>true]],
[["sessionIdle"=>-1]],
[["sessionIdle"=>true]],
[["rateLimit"=>1]],
[["debug"=>1]],
];
}
/**
* Tests only the important default values
*/
public function testDefaults()
{
$default = $this->configurator->validate([]);
$this->assertFalse($default["debug"]);
$this->assertEquals(
["Installer"],
$default["authModules"]
);
}
}
<file_sep>/src/Security/RateLimiting/NullRateLimit.php
<?php
namespace PicoAuth\Security\RateLimiting;
/**
* Null object of RateLimitInterface, used when rate limiting is disabled.
*/
class NullRateLimit implements RateLimitInterface
{
/**
* {@inheritdoc}
*/
public function action($actionName, $increment = true, $params = array())
{
return true;
}
/**
* {@inheritdoc}
*/
public function getError()
{
return null;
}
}
<file_sep>/src/Module/Generic/ExampleModule.php
<?php
namespace PicoAuth\Module\Generic;
use PicoAuth\Module\AbstractAuthModule;
use Symfony\Component\HttpFoundation\Request;
/**
* An example Auth module
*
* This module does nothing, it contains specifications of all
* available auth event methods triggered by Pico Auth plugin
*/
class ExampleModule extends AbstractAuthModule
{
/**
* Called on each request
*
* This is the main method of every module - contains
* form submission checks, custom routing based on the Url or
* the Request parameters or no operation.
*
* @param string|null $url Requested URL in Pico (page ID)
* @param Request $httpRequest The current HTTP request
*/
public function onPicoRequest($url, Request $httpRequest)
{
}
/**
* Checks accessibility for a give page URL
*
* Used for evaluating accessibility of a page (for example when removing
* inaccessible links from Pico page array), to perform an access denial,
* the module should also implement {@see ExampleModule::denyAccessIfRestricted()}
*
* @param string|null $url Pico page URL
* @return bool true is the site is accessible, false otherwise
*/
public function checkAccess($url)
{
return true;
}
/**
* Denies access if restricted
*
* Each authorization module is in control of how it will deny the access:
* It can be done by a redirect to a different
* page (while aborting execution of the current request)
* {@see PicoAuthPlugin::redirectToPage()} or {{@see PicoAuthPlugin::redirectToLogin()}},
* or by setting a different requestFile (the requested URL will be the same,
* but the original content will not be displayed) {@see PicoAuthPlugin::setRequestFile()}
* or {@see PicoAuthPlugin::setRequestUrl()}.
*
* @param string|null $url Pico page URL
*/
public function denyAccessIfRestricted($url)
{
}
/**
* Triggered after a login
*
* @param \PicoAuth\User $user User instance
*/
public function afterLogin(\PicoAuth\User $user)
{
}
/**
* Triggered after a logout
*
* Module can perform a custom after-logout redirect or perform
* a single sign out
*
* @param \PicoAuth\User $oldUser The previously logged in user
*/
public function afterLogout(\PicoAuth\User $oldUser)
{
}
/**
* Required from AbstractAuthModule
*/
public function getName()
{
return 'example';
}
}
<file_sep>/src/Security/RateLimiting/RateLimitInterface.php
<?php
namespace PicoAuth\Security\RateLimiting;
/**
* Rate Limit Interface
*/
interface RateLimitInterface
{
/**
* Registers an action and determines if it is rate limited or not
*
* @param string $actionName Identifier for the limited action
* @param bool $increment True if the action counter should be incremented
* (e.g. after unsuccessful login attempt)
* False if the counter should stay unchanged
* (e.g. before processing the login form)
* @param array $params Additional parameters specific to the action
* @return bool true if action is allowed, false if blocked
*/
public function action($actionName, $increment = true, $params = array());
/**
* Get error message for the last action() call that returned false
* @return string|null Message, null if there were no blocked actions
*/
public function getError();
}
<file_sep>/tests/Storage/Configurator/ConfigurationExceptionTest.php
<?php
namespace PicoAuth\Storage\Configurator;
use PHPUnit\Framework\TestCase;
class ConfigurationExceptionTest extends TestCase
{
public function testAddBeforeMessage()
{
$e = new ConfigurationException("test");
$e->addBeforeMessage("_");
$this->assertEquals("_ test", $e->getMessage());
}
public function testToString()
{
$e = new ConfigurationException("test");
$this->assertEquals("test", (string)$e);
}
}
<file_sep>/src/Storage/RateLimitFileStorage.php
<?php
namespace PicoAuth\Storage;
use PicoAuth\Storage\Interfaces\RateLimitStorageInterface;
use PicoAuth\Cache\NullCache;
use Psr\SimpleCache\CacheInterface;
use PicoAuth\Storage\Configurator\RateLimitConfigurator;
/**
* File storage for RateLimit
*/
class RateLimitFileStorage extends FileStorage implements RateLimitStorageInterface
{
/**
* Data directory
*/
const DATA_DIR = 'PicoAuth/data/';
/**
* Configuration file name
*/
const CONFIG_FILE = 'PicoAuth/RateLimit.yml';
/**
* Configuration of the limits
* @var array
*/
protected $limits = array();
/**
* Transaction files
* @var \PicoAuth\Storage\File\FileReader[]
*/
protected $tFiles = array();
public function __construct($dir, CacheInterface $cache = null)
{
$this->dir = $dir;
$this->cache = ($cache!==null) ? $cache : new NullCache;
$this->configurator = new RateLimitConfigurator;
}
/**
* @inheritdoc
*/
public function getLimitFor($action, $type, $key)
{
$id = $this->getId($action, $type);
$fileName = $this->dir . static::DATA_DIR . $id;
if (isset($this->tFiles[$id])) {
$file = $this->tFiles[$id]->read();
if (!$file) {
$this->limits[$id] = array();
} else {
$this->limits[$id] = unserialize($file);
}
} elseif (\file_exists($fileName)) {
if (($file = self::readFile($fileName)) === false) {
throw new \RuntimeException("Unable to read limit file.");
}
$this->limits[$id] = unserialize($file);
} else {
$this->limits[$id] = array();
}
return (isset($this->limits[$id][$key])) ? $this->limits[$id][$key] : null;
}
/**
* @inheritdoc
*/
public function updateLimitFor($action, $type, $key, $limitData)
{
$id = $this->getId($action, $type);
$this->limits[$id][$key] = $limitData;
}
/**
* @inheritdoc
*/
public function cleanup($action, $type, $config)
{
$id = $this->getId($action, $type);
$time = time();
$removed = 0;
foreach ($this->limits[$id] as $key => $limit) {
if ($time > $limit["ts"] + $config["counterTimeout"]) {
unset($this->limits[$id][$key]);
++$removed;
}
}
return $removed;
}
/**
* {@inheritdoc}
*
* Save must be part of an exclusive transaction.
*/
public function save($action, $type)
{
$id = $this->getId($action, $type);
$fileName = $this->dir . static::DATA_DIR . $id;
$file = serialize($this->limits[$id]);
// Write the updated records
if (isset($this->tFiles[$id]) && $this->tFiles[$id]->isOpened()) {
$writer = new \PicoAuth\Storage\File\FileWriter(
$fileName,
["handle"=>$this->tFiles[$id]->getHandle()]
);
$writer->write($file);
} else {
throw new \RuntimeException("Transaction file not opened.");
}
}
/**
* @inheritdoc
*/
public function transaction($action, $type, $state)
{
$id = $this->getId($action, $type);
switch ($state) {
// Start transaction
case self::TRANSACTION_BEGIN:
$this->openTransactionFile($id);
if (!$this->tFiles[$id]->lock(LOCK_EX)) {
throw new \RuntimeException("Could not lock the file.");
}
break;
// Commit
case self::TRANSACTION_END:
if (!isset($this->tFiles[$id])) {
throw new \RuntimeException("Transation is not openened, cannot close.");
}
$this->tFiles[$id]->unlock();
$this->tFiles[$id]->close();
break;
default:
throw new \InvalidArgumentException("Unexpected transaction argument.");
}
}
/**
* Opens the transaction file
*
* This file is opened for read and write operations and it's lock
* will stay active during the transaction calls started and ended with
* {@see RateLimitFileStorage::transaction()}
*
* @param string $id action-type file id
* @throws \RuntimeException On file open error
*/
private function openTransactionFile($id)
{
if (!isset($this->tFiles[$id])) {
self::preparePath($this->dir, self::DATA_DIR);
$fileName = $this->dir . static::DATA_DIR . $id;
$handle = @fopen($fileName, 'c+');
if ($handle === false) {
throw new \RuntimeException("Could not open file: " . $fileName);
}
$this->tFiles[$id] = new \PicoAuth\Storage\File\FileReader(
$fileName,
["handle"=>$handle]
);
}
}
/**
* Get a single identifier for action-type pair
*
* @param string $action
* @param string $type
* @return string
*/
protected function getId($action, $type)
{
return $action . "_" . $type;
}
}
<file_sep>/src/Session/SessionInterface.php
<?php
namespace PicoAuth\Session;
/**
* Simple session manager interface with a direct support for
* flash values.
*/
interface SessionInterface
{
/**
* Checks if a session key is defined.
* @param string $name Session attribute name
* @return bool Whether attribute exists
*/
public function has($name);
/**
* Retrieves a value from the session.
* @param string $name Session attribute name
* @param mixed $default Default if the attribute is not defined
* @return mixed Value
*/
public function get($name, $default = null);
/**
* Creates/replaces value of a session attribute with value.
* @param string $name Session attribute name
* @param mixed $value Inserted value
*/
public function set($name, $value);
/**
* Removes a session key with a given name.
* If the key doesn't exist the call does nothing.
* @param string $name
*/
public function remove($name);
/**
* Clears all session attributes and regenerates the session
* @param int $lifetime Cookie lifetime in seconds, null = unchanged
* @return bool Whether the invalidation was successful
*/
public function invalidate($lifetime = null);
/**
* Move the session to a different session id.
* @param bool $destroy Delete the old session
* @param int $lifetime Cookie lifetime in seconds, null = unchanged
*/
public function migrate($destroy = false, $lifetime = null);
/**
* Removes all attributes
*/
public function clear();
/**
* Adds a flash value to the session.
* The flash values should be available only in the next request
* using the getFlash() call.
* @param string $type Flash type (category)
* @param mixed $message Flash value
*/
public function addFlash($type, $message);
/**
* Gets flash values set in the previous request.
* @param string $type Flash type (category)
* @param array $default Default response if the category is not defined
* @return array
*/
public function getFlash($type, array $default = array());
}
<file_sep>/src/User.php
<?php
namespace PicoAuth;
/**
* The User entity.
*/
class User
{
/**
* Unique identifier of the user.
* @var null|string
*/
protected $id = null;
/**
* Optional displayable name.
* @var null|string
*/
protected $displayName = null;
/**
* Authentication flag.
* @var bool
*/
protected $authenticated = false;
/**
* Module name that was used to authenticate the user.
* @see PicoAuth\Module\AuthModule::getName()
* @var string|null Authenticator name
*/
protected $authenticator = null;
/**
* Groups the user is member of.
* @var string[]
*/
protected $groups = [];
/**
* User attributes.
* @var array
*/
protected $attributes = [];
/**
* Gets user's authenticated state.
* @return bool
*/
public function getAuthenticated()
{
return $this->authenticated;
}
/**
* Sets user's authenticated state.
* @param bool $v
* @return $this
*/
public function setAuthenticated($v)
{
if (!$v) {
$this->authenticator = null;
}
$this->authenticated = $v;
return $this;
}
/**
* Gets user's authenticator.
* Will return null if the user is not authenticated.
* @see User::authenticator
* @return null|string
*/
public function getAuthenticator()
{
return $this->authenticator;
}
/**
* Sets user's authenticator.
* @param string $name
* @return $this
*/
public function setAuthenticator($name)
{
$this->authenticator = $name;
return $this;
}
/**
* Gets user's id.
* @return string
*/
public function getId()
{
return $this->id;
}
/**
* Sets user's id.
* @param string $id
* @return $this
*/
public function setId($id)
{
$this->id = $id;
return $this;
}
/**
* Returns user's display name if available.
* @return string|null
*/
public function getDisplayName()
{
return $this->displayName;
}
/**
* Sets user's display name.
* @param string $displayName
* @return $this
*/
public function setDisplayName($displayName)
{
$this->displayName = $displayName;
return $this;
}
/**
* Gets user's groups.
* @return string[]
*/
public function getGroups()
{
return $this->groups;
}
/**
* Sets user's groups.
* @param string[] $groups
* @return $this
*/
public function setGroups($groups)
{
if (is_array($groups)) {
$this->groups = $groups;
}
return $this;
}
/**
* Adds group.
* @param string $group
*/
public function addGroup($group)
{
if (is_string($group)) {
$this->groups[] = $group;
}
}
/**
* Gets user's attribute if available.
* @param string $key
* @return mixed
*/
public function getAttribute($key)
{
return (isset($this->attributes[$key])) ? $this->attributes[$key] : null;
}
/**
* Sets user's attribute.
* @param string $key
* @param mixed $value
* @return $this
*/
public function setAttribute($key, $value)
{
$this->attributes[$key] = $value;
return $this;
}
}
<file_sep>/src/Storage/File/FileWriter.php
<?php
namespace PicoAuth\Storage\File;
/**
* File writer
*
* Opens the file with an exclusive access
*/
class FileWriter extends File
{
/**
* Mode the file will be opened with
*/
const OPEN_MODE = 'c+';
/**
* Will be set to true if write error/s occurred
* @var bool
*/
protected $writeErrors = false;
/**
* File name of the write backup file, if used
* @var string|null
*/
protected $bkFilePath = null;
/**
* Opens the file for writing
*
* Obtains an exclusive lock
* Creates a backup file if the corresponding class option is set
*
* @return void
* @throws \RuntimeException On open/lock error
*/
public function open()
{
if ($this->isOpened()) {
return;
}
$this->handle = @fopen($this->filePath, self::OPEN_MODE);
if ($this->handle === false) {
throw new \RuntimeException("Could not open file for writing: " . $this->filePath);
}
if (!$this->lock(LOCK_EX)) {
$this->close();
throw new \RuntimeException("Could not aquire an exclusive lock for " . $this->filePath);
}
if ($this->options["backup"]) {
$this->createBkFile();
}
$this->writeErrors = false;
}
/**
* Writes contents of the file
*
* @param string $data Data to write
* @throws \InvalidArgumentException data not a string
* @throws \RuntimeException Write error
*/
public function write($data)
{
if (!is_string($data)) {
throw new \InvalidArgumentException("The data is not a string.");
}
$this->open();
if (!ftruncate($this->handle, 0)) {
$this->writeErrors = true;
throw new \RuntimeException("Could not truncate file " . $this->filePath);
}
fseek($this->handle, 0);
$res = fwrite($this->handle, $data);
if (strlen($data) !== $res) {
$this->writeErrors = true;
throw new \RuntimeException("Could not write to file " . $this->filePath);
}
}
/**
* @inheritdoc
*/
public function close()
{
// Unlock and close the main file
parent::close();
// Remove the backup file if there were no write errors or errors
// when closing the file (above call would throw an exception)
$this->removeBkFile();
}
/**
* Creates a backup file before writing
*
* If there is no write permission for the directory the original file is in,
* the backup file is not created. That way this option can be disabled,
* if not considered as needed.
*
* @throws \RuntimeException On write error
*/
protected function createBkFile()
{
if (!is_writable(dirname($this->filePath))) {
return;
}
$this->bkFilePath = $this->filePath . '.' . date("y-m-d-H-i-s") . '.bak';
$bkHandle = @fopen($this->bkFilePath, 'x+');
if ($bkHandle === false) {
$this->close();
throw new \RuntimeException("Could not create a temporary file " . $this->bkFilePath);
}
$stat = fstat($this->handle);
if (stream_copy_to_stream($this->handle, $bkHandle) !== $stat['size']) {
$this->close();
throw new \RuntimeException("Could not create a copy of " . $this->filePath);
}
if (!fclose($bkHandle)) {
throw new \RuntimeException("Could not close a backup file " . $this->bkFilePath);
}
fseek($this->handle, 0);
}
/**
* Closes the backup file
*
* Removes it if the write operation to the original file ended successfully
*
* @return void
*/
protected function removeBkFile()
{
if (!$this->options["backup"]) {
return;
}
// Remove backup file if the write was successful
if (!$this->writeErrors && $this->bkFilePath) {
unlink($this->bkFilePath);
}
}
}
<file_sep>/PicoAuth.php
<?php
/**
* PicoAuth wrapper class
*
* The implementation of the plugin itself is in PicoAuthPlugin class.
* The purpose of this class is only to register the plugin's autoloader
* if it is not installed in Pico with pico-composer. After that is done,
* it relies all Pico events to the main class with
* an overridden {@see PicoAuth::handleEvent()} method.
*
* @see \PicoAuth\PicoAuthPlugin
*/
class PicoAuth extends AbstractPicoPlugin
{
/**
* Pico API version used by this plugin.
* @var int
*/
const API_VERSION = 2;
/**
* The main class of the plugin
* @var \PicoAuth\PicoAuthPlugin
*/
protected $picoAuthPlugin;
/**
* Constructs a new PicoAuth instance
*
* If PicoAuth is not installed as a pico-composer plugin, this will
* register plugin's own autoloader. This will only work if any shared
* dependencies between Pico's and PicoAuths's autoloader are
* are present in the same versions.
*
* @param \Pico $pico
*/
public function __construct(\Pico $pico)
{
parent::__construct($pico);
if (!class_exists('\PicoAuth\PicoAuthPlugin', true)) {
if (is_file(__DIR__ . '/vendor/autoload.php')) {
require_once __DIR__ . '/vendor/autoload.php';
} else {
die("PicoAuth is neither installed as a pico-composer plugin "
. "nor it has its own vendor/autoload.php.");
}
}
$this->picoAuthPlugin = new \PicoAuth\PicoAuthPlugin($pico);
}
/**
* Pico API events pass-through
*
* Pico plugin events are sent over to the main PicoAuthPlugin class.
* {@inheritdoc}
*
* @param string $eventName
* @param array $params
*/
public function handleEvent($eventName, array $params)
{
parent::handleEvent($eventName, $params);
if ($this->isEnabled()) {
$this->picoAuthPlugin->handleEvent($eventName, $params);
}
}
/**
* Gets the instance of the main class
*
* May be used if other plugins installed in Pico and depending on PicoAuth
* need to access to the PicoAuthPlugin class.
*
* @return \PicoAuth\PicoAuthPlugin
*/
public function getPlugin()
{
return $this->picoAuthPlugin;
}
}
<file_sep>/src/Security/Password/Policy/PasswordPolicy.php
<?php
namespace PicoAuth\Security\Password\Policy;
use PicoAuth\Security\Password\Password;
/**
* Class for enforcing password constraints
*
* Reference:
* Unicode graphemes regex: https://www.regular-expressions.info/unicode.html
*/
class PasswordPolicy implements PasswordPolicyInterface
{
protected $constraints;
protected $errors;
/**
* The minimum length in characters
*
* Unicode code point is counted as a single character (NIST recommendation)
*
* @param int $n
* @return $this
*/
public function minLength($n)
{
$this->constraints[] = (function (Password $str) use ($n) {
if (mb_strlen($str) < $n) {
return sprintf("Minimum password length is %d characters.", $n);
} else {
return true;
}
});
return $this;
}
/**
* The maximum length in characters
*
* Note: This option is provided only to allow prevention from DoS attacks
* using computationally intensive password hashing algorithm on very long inputs.
* It is not advised to limit the maximum length without a specific reason.
*
* @param int $n
* @return $this
*/
public function maxLength($n)
{
$this->constraints[] = (function (Password $str) use ($n) {
if (mb_strlen($str) > $n) {
return sprintf("Maximum password length is %d characters.", $n);
} else {
return true;
}
});
return $this;
}
/**
* The minimum amount of numeric characters
* @param int $n
* @return $this
*/
public function minNumbers($n)
{
$this->constraints[] = (function (Password $str) use ($n) {
if (preg_match_all("/\\p{N}/", $str) < $n) {
return sprintf("Password must contain at least %d numbers.", $n);
} else {
return true;
}
});
return $this;
}
/**
* The minimum amount of uppercase letters (an uppercase letter that
* has a lowercase variant, from any language)
* @param int $n
* @return $this
*/
public function minUppercase($n)
{
$this->constraints[] = (function (Password $str) use ($n) {
if (preg_match_all("/\\p{Lu}/", $str) < $n) {
return sprintf("Password must contain at least %d uppercase letters.", $n);
} else {
return true;
}
});
return $this;
}
/**
* The minimum amount of lowercase letters (a lowercase letter that has
* an uppercase variant, from any language)
* @param int $n
* @return $this
*/
public function minLowercase($n)
{
$this->constraints[] = (function (Password $str) use ($n) {
if (preg_match_all("/\\p{Ll}/", $str) < $n) {
return sprintf("Password must contain at least %d lowercase letters.", $n);
} else {
return true;
}
});
return $this;
}
/**
* Minimum number of special characters (any character other
* than letter or number)
*
* Matches also language specific letters
* Possible replacement: "/[\\p{M}\\p{Z}\\p{S}\\p{P}]/"
* But does not match e.g. "ˇ"
*
* @param int $n
* @return $this
*/
public function minSpecial($n)
{
$this->constraints[] = (function (Password $str) use ($n) {
if (preg_match_all("/[^A-Za-z0-9]/", $str) < $n) {
return sprintf("Password must contain at least %d special characters.", $n);
} else {
return true;
}
});
return $this;
}
/**
* Matches provided regular expression
*
* @param string $regexp Regular expression that must be matched
* @param string $message Error message on failure
* @return $this
* @throws \InvalidArgumentException
*/
public function matches($regexp, $message)
{
if (!is_string($regexp) || !is_string($message)) {
throw new \InvalidArgumentException("Both arguments must be string.");
}
$this->constraints[] = (function (Password $str) use ($regexp, $message) {
if (!preg_match($regexp, $str)) {
return $message;
} else {
return true;
}
});
return $this;
}
/**
* {@inheritdoc}
*/
public function check(Password $str)
{
$this->errors = array();
foreach ($this->constraints as $constraint) {
$res = $constraint($str);
if ($res !== true) {
$this->errors[] = $res;
}
}
return count($this->errors) === 0;
}
/**
* {@inheritdoc}
*/
public function getErrors()
{
return $this->errors;
}
}
<file_sep>/tests/Module/Authorization/PageLockTest.php
<?php
namespace PicoAuth\Module\Authorization;
use PicoAuth\Storage\Interfaces\PageLockStorageInterface;
use PicoAuth\Security\RateLimiting\RateLimitInterface;
use PicoAuth\Session\SessionInterface;
use PicoAuth\PicoAuthInterface;
use League\Container\Container;
class PageLockTest extends \PicoAuth\BaseTestCase
{
protected $pageLock;
protected $testContainer;
protected function setUp()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(PageLockStorageInterface::class);
$storage->expects($this->exactly(1))
->method('getConfiguration')
->willReturn(null);
$rateLimit = $this->createMock(RateLimitInterface::class);
$this->pageLock = new PageLock($picoAuth, $sess, $storage, $rateLimit);
$this->testContainer=new Container;
$this->testContainer->share('plain', 'PicoAuth\Security\Password\Encoder\Plaintext');
}
public function testCheckAccess()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$rateLimit = $this->createMock(RateLimitInterface::class);
$storage = $this->createMock(PageLockStorageInterface::class);
$storage->expects($this->exactly(3))
->method('getLockByURL')
->with("url")
->willReturnOnConsecutiveCalls("lock1", "lock2", null);
$sess = $this->createMock(SessionInterface::class);
$sess->expects($this->exactly(2))
->method('get')
->with("unlocked")
->willReturn(["lock1"]);
$pageLock = new PageLock($picoAuth, $sess, $storage, $rateLimit);
$this->assertTrue($pageLock->checkAccess("url"));
$this->assertFalse($pageLock->checkAccess("url"));
$this->assertTrue($pageLock->checkAccess("url"));
}
public function testGetKeyEncoder()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method("getContainer")
->willReturn($this->testContainer);
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(PageLockStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn(null);
$rateLimit = $this->createMock(RateLimitInterface::class);
$this->pageLock = new PageLock($picoAuth, $sess, $storage, $rateLimit);
$method=self::getMethod($this->pageLock, 'getKeyEncoder');
$method->invokeArgs($this->pageLock, [
["encoder" => "plain"]
]);
}
public function testGetName()
{
$this->assertEquals('pageLock', $this->pageLock->getName());
}
}
<file_sep>/src/Storage/Configurator/OAuthConfigurator.php
<?php
namespace PicoAuth\Storage\Configurator;
use PicoAuth\Storage\Configurator\AbstractConfigurator;
use PicoAuth\Storage\Configurator\ConfigurationException;
/**
* The configurator for OAuth
*/
class OAuthConfigurator extends AbstractConfigurator
{
/**
* @inheritdoc
*/
public function validate($rawConfig)
{
$config = $this->applyDefaults($rawConfig, $this->getDefault(), 1);
$this->validateGlobalOptions($config);
$this->validateProvidersSection($config);
return $config;
}
/**
* The default configuration must contain all keys that are required.
* @return array
*/
protected function getDefault()
{
return array(
"callbackPage" => "oauth_callback",
"providers" => [],
);
}
public function validateGlobalOptions(&$config)
{
$this->assertString($config, "callbackPage");
$config["callbackPage"]=trim($config["callbackPage"], "/");
}
public function validateProvidersSection(&$config)
{
// The 3 mandatory string values have default=0,
// so they won't be allowed unless they are set.
$requiredFileds = array(
"provider" => "\League\OAuth2\Client\Provider\GenericProvider",
"options" => array(
"clientId" => 0,
"clientSecret" => 0,
),
"attributeMap" => array(
"userId" => "id"
),
"default" => array(
"groups" => [],
"attributes" => []
)
);
foreach ($config["providers"] as $name => $rawProviderData) {
$this->assertProviderName($name);
$this->assertArray($config["providers"], $name);
$providerData = $config["providers"][$name] = $this->applyDefaults($rawProviderData, $requiredFileds, 2);
$this->assertString($providerData, "provider");
$this->assertString($providerData["options"], "clientId");
$this->assertString($providerData["options"], "clientSecret");
foreach ($providerData["attributeMap"] as $attrName => $mappedName) {
if (!is_string($attrName) || !is_string($mappedName)) {
throw new ConfigurationException("Provider attribute map can contain only strings.");
}
}
$this->assertArrayOfStrings($providerData["default"], "groups");
}
}
public function assertProviderName($name)
{
if (!is_string($name) || $name==="") {
throw new ConfigurationException("Provider name must be a non-empty string.");
}
}
}
<file_sep>/tests/Module/Authentication/LocalAuth/LocalAuthTest.php
<?php
namespace PicoAuth\Module\Authentication\LocalAuth;
use PicoAuth\Storage\Interfaces\LocalAuthStorageInterface;
use PicoAuth\Security\RateLimiting\RateLimitInterface;
use PicoAuth\Session\SessionInterface;
use PicoAuth\PicoAuthInterface;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\HttpFoundation\ParameterBag;
use League\Container\Container;
use PicoAuth\Storage\Configurator\LocalAuthConfigurator;
class LocalAuthTest extends \PicoAuth\BaseTestCase
{
protected $localAuth;
protected $configurator;
protected $testContainer;
protected function setUp()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$rateLimit = $this->createMock(RateLimitInterface::class);
$this->localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$this->testContainer=new Container;
$this->initTestContainer($this->testContainer);
$this->configurator = new LocalAuthConfigurator;
}
protected function initTestContainer(Container $container)
{
$shortEncoder = $this->createMock(\PicoAuth\Security\Password\Encoder\PasswordEncoderInterface::class);
$shortEncoder->expects($this->any())
->method('getMaxAllowedLen')
->willReturn(4);
$shortEncoder->expects($this->any())
->method('encode')
->willThrowException(new \PicoAuth\Security\Password\Encoder\EncoderException("Too long"));
$container->share('plain', 'PicoAuth\Security\Password\Encoder\Plaintext');
$container->share('plain2', 'PicoAuth\Security\Password\Encoder\Plaintext');
$container->share('shortPw', $shortEncoder);
}
public function testHandleLoginNoPostFileds()
{
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn([]);
$rateLimit = $this->createMock(RateLimitInterface::class);
$sess = $this->createMock(SessionInterface::class);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$request = $this->createMock(Request::class);
$request->request = $this->createMock(ParameterBag::class);
$localAuth->onPicoRequest("login", $request);
}
public function testHandleLoginInvalidCSRF()
{
$request = $this->createMock(Request::class);
$post = new ParameterBag(["username"=>"test","password"=>"<PASSWORD>"]);
$request->request = $post;
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn([]);
$rateLimit = $this->createMock(RateLimitInterface::class);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method('isValidCSRF')
->withAnyParameters()
->willReturn(false);
$picoAuth->expects($this->once())
->method("redirectToLogin")
->with($this->isNull(), $this->equalTo($request));
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$localAuth->onPicoRequest("login", $request);
}
public function testHandleLoginRateLimited()
{
$request = $this->createMock(Request::class);
$post = new ParameterBag(["username"=>"test","password"=>"<PASSWORD>"]);
$request->request = $post;
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn($this->configurator->validate([]));
// The user is rate limited
$rateLimit = $this->createMock(RateLimitInterface::class);
$rateLimit->expects($this->once())
->method("action")
->withAnyParameters()
->willReturn(false);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method('isValidCSRF')
->withAnyParameters()
->willReturn(true);
$picoAuth->expects($this->once())
->method("redirectToLogin")
->with($this->isNull(), $this->equalTo($request));
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$localAuth->onPicoRequest("login", $request);
}
public function testHandleLoginInvalid()
{
$request = $this->createMock(Request::class);
$request->request = new ParameterBag(["username"=>"testtt","password"=>"<PASSWORD>"]);
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn($this->configurator->validate(["registration" => ["nameLenMax"=>4]]));
// The user is rate limited
$rateLimit = $this->createMock(RateLimitInterface::class);
$rateLimit->expects($this->exactly(2))
->method("action")
->withAnyParameters()
->willReturn(true);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method('isValidCSRF')
->withAnyParameters()
->willReturn(true);
$picoAuth->expects($this->once())
->method("redirectToLogin")
->with($this->isNull(), $this->equalTo($request));
$localAuth = $this->getMockBuilder(LocalAuth::class)
->setConstructorArgs([$picoAuth, $sess, $storage, $rateLimit])
->setMethodsExcept(['onPicoRequest','handleLogin'])
->getMock();
$localAuth->expects($this->once())
->method('loginAttempt')
->willReturn(false);
$_SERVER["REMOTE_ADDR"] = '10.0.0.1';
$localAuth->onPicoRequest("login", $request);
}
public function testHandleLoginSuccessfulWithRehash()
{
$request = $this->createMock(Request::class);
$post = new ParameterBag(["username"=>"test","password"=>"<PASSWORD>"]);
$request->request = $post;
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
// User's encoder is plain, but the configuration uses plain2 => rehash
$storage->expects($this->once())
->method('getConfiguration')
->willReturn($this->configurator->validate(
["encoder" => "plain2","login" => ["passwordRehash"=>true]]
));
$storage->expects($this->exactly(3))
->method('getUserByName')
->with("test")
->willReturn(["pwhash"=>"test","encoder"=>"plain"]);
$storage->expects($this->once())
->method('saveUser')
->willReturn(true);
$rateLimit = $this->createMock(RateLimitInterface::class);
$rateLimit->expects($this->once())
->method("action")
->withAnyParameters()
->willReturn(true);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method('isValidCSRF')
->withAnyParameters()
->willReturn(true);
$picoAuth->expects($this->once())
->method("afterLogin");
$picoAuth->expects($this->exactly(2))
->method("getContainer")
->willReturn($this->testContainer);
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$localAuth->onPicoRequest("login", $request);
}
public function testGetName()
{
$this->assertEquals('localAuth', $this->localAuth->getName());
}
public function testGetConfig()
{
$this->assertEquals(self::get($this->localAuth, 'config'), $this->localAuth->getConfig());
}
public function testHandleAccountPageWhileNotAuthenticated()
{
$request = $this->createMock(Request::class);
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$rateLimit = $this->createMock(RateLimitInterface::class);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method('getUser')
->willReturn(new \PicoAuth\User);
$picoAuth->expects($this->once())
->method('redirectToLogin');
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$localAuth->onPicoRequest("account", $request);
}
public function testHandleAccountPageWhileAuthenticatedNotViaLocalAuth()
{
$request = $this->createMock(Request::class);
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$rateLimit = $this->createMock(RateLimitInterface::class);
$user = new \PicoAuth\User;
$user->setAuthenticated(true)
->setAuthenticator('OAuth');
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method('getUser')
->willReturn($user);
$picoAuth->expects($this->once())
->method('redirectToPage')
->with("index");
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$localAuth->onPicoRequest("account", $request);
}
public function testHandleAccountPage()
{
$request = $this->createMock(Request::class);
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn($this->configurator->validate([]));
$rateLimit = $this->createMock(RateLimitInterface::class);
$user = new \PicoAuth\User;
$user->setAuthenticated(true)
->setAuthenticator($this->localAuth->getName());
$editMock = $this->createMock(EditAccount::class);
$editMock->expects($this->once())
->method('setConfig')
->willReturnSelf();
$editMock->expects($this->once())
->method('handleAccountPage');
$container=new Container;
$container->share('EditAccount', $editMock);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method('getUser')
->willReturn($user);
$picoAuth->expects($this->once())
->method("getContainer")
->willReturn($container);
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$localAuth->onPicoRequest("account", $request);
}
public function testHandleRegistration()
{
$request = $this->createMock(Request::class);
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn($this->configurator->validate([]));
$rateLimit = $this->createMock(RateLimitInterface::class);
$registrationMock = $this->createMock(Registration::class);
$registrationMock->expects($this->once())
->method('setConfig')
->willReturnSelf();
$registrationMock->expects($this->once())
->method('handleRegistration');
$container=new Container;
$container->share('Registration', $registrationMock);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method("getContainer")
->willReturn($container);
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$localAuth->onPicoRequest("register", $request);
}
public function testHandlePasswordReset()
{
$request = $this->createMock(Request::class);
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn($this->configurator->validate([]));
$rateLimit = $this->createMock(RateLimitInterface::class);
$pwResetMock = $this->createMock(PasswordReset::class);
$pwResetMock->expects($this->once())
->method('setConfig')
->willReturnSelf();
$pwResetMock->expects($this->once())
->method('handlePasswordReset');
$container=new Container;
$container->share('PasswordReset', $pwResetMock);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method("getContainer")
->willReturn($container);
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$localAuth->onPicoRequest("password_reset", $request);
}
public function testUserDataEncodePasswordPwreset()
{
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn(["encoder" => "plain2","login" => ["passwordRehash"=>true]]);
$rateLimit = $this->createMock(RateLimitInterface::class);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method("getContainer")
->willReturn($this->testContainer);
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$userData = ["pwreset"=>true];
$localAuth->userDataEncodePassword($userData, new \PicoAuth\Security\Password\Password("test"));
$this->assertFalse(isset($userData["pwreset"]));
$this->assertEquals("test", $userData["pwhash"]);
}
public function testCheckPasswordPolicy()
{
$testedPw = new \PicoAuth\Security\Password\Password("test");
$testedPwTooLong = new \PicoAuth\Security\Password\Password("<PASSWORD>");
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn(["encoder" => "shortPw"]);
$rateLimit = $this->createMock(RateLimitInterface::class);
$policy = $this->createMock(\PicoAuth\Security\Password\Policy\PasswordPolicyInterface::class);
$policy->expects($this->exactly(2))
->method("check")
->withConsecutive($this->equalTo($testedPw), $this->equalTo($testedPwTooLong))
->willReturnOnConsecutiveCalls(false, true);
$policy->expects($this->once())
->method("getErrors")
->willReturn(["e1"]);
$this->testContainer->share('PasswordPolicy', $policy);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->exactly(4))
->method("getContainer")
->willReturn($this->testContainer);
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$localAuth->checkPasswordPolicy($testedPw);
$localAuth->checkPasswordPolicy($testedPwTooLong);
}
public function testNeedsPasswordRehash()
{
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->exactly(2))
->method('getConfiguration')
->willReturnOnConsecutiveCalls(
["encoder" => "plain","login" => ["passwordRehash"=>false]],
["encoder" => "plain","login" => ["passwordRehash"=>true]]
);
$rateLimit = $this->createMock(RateLimitInterface::class);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method("getContainer")
->willReturn($this->testContainer);
for ($i=0; $i<2; $i++) {
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$method=self::getMethod($localAuth, 'needsPasswordRehash');
$method->invokeArgs($localAuth, [
["pwhash"=>"test"]
]);
}
}
public function testAbortIfExpired()
{
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$rateLimit = $this->createMock(RateLimitInterface::class);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$pwResetMock = $this->createMock(PasswordReset::class);
$pwResetMock->expects($this->once())
->method('startPasswordResetSession')
->with("test");
$container=new Container;
$container->share('PasswordReset', $pwResetMock);
$picoAuth->expects($this->once())
->method("redirectToPage")
->with("password_reset");
$picoAuth->expects($this->once())
->method("getContainer")
->willReturn($container);
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$method=self::getMethod($localAuth, 'abortIfExpired');
$method->invokeArgs($localAuth, [
"test",
["pwreset"=>true]
]);
}
public function testPasswordRehash()
{
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn(["encoder" => "shortPw"]);
$storage->expects($this->once())
->method('getUserByName')
->with("testUser")
->willReturn(["pwhash"=>"teest1","encoder"=>"plain"]);
$rateLimit = $this->createMock(RateLimitInterface::class);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$pwResetMock = $this->createMock(PasswordReset::class);
$pwResetMock->expects($this->once())
->method('startPasswordResetSession')
->with("testUser");
$container=new Container;
$this->initTestContainer($container);
$container->share('PasswordReset', $pwResetMock);
$picoAuth->expects($this->once())
->method("redirectToPage")
->with("password_reset");
$picoAuth->expects($this->exactly(2))
->method("getContainer")
->willReturn($container);
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$method=self::getMethod($localAuth, 'passwordRehash');
$method->invokeArgs($localAuth, [
"testUser",
new \PicoAuth\Security\Password\Password("<PASSWORD>")
]);
}
/**
* @dataProvider nameProvider
*/
public function testIsValidUsername($name, $valid)
{
$sess = $this->createMock(SessionInterface::class);
$rateLimit = $this->createMock(RateLimitInterface::class);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$storage->expects($this->once())
->method('getConfiguration')
->willReturn($this->configurator->validate(
["registration" => ["nameLenMin"=>3,"nameLenMax"=>4]]
));
$storage->expects($this->any())
->method('checkValidName')
->willReturn(true);
$localAuth = new LocalAuth($picoAuth, $sess, $storage, $rateLimit);
$res=self::getMethod($localAuth, 'isValidUsername')->invokeArgs($localAuth, [$name]);
$this->assertSame($valid, $res);
}
public function nameProvider()
{
return [
[null,false],
[0,false],
["aa",false],
["aaa",true],
["aaaa",true],
["aaaaa",false]
];
}
public function testLogin()
{
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(LocalAuthStorageInterface::class);
$rateLimit = $this->createMock(RateLimitInterface::class);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method('setUser')
->withAnyParameters()
->willReturn(true);
$localAuth = $this->getMockBuilder(LocalAuth::class)
->setConstructorArgs([$picoAuth, $sess, $storage, $rateLimit])
->setMethods(['abortIfExpired'])
->getMock();
$localAuth->expects($this->once())
->method('abortIfExpired');
$localAuth->login("user", [
"groups"=>["g1"],"displayName"=>"User",
"attributes"=>["a"=>1]
]);
}
}
<file_sep>/tests/Security/Password/Policy/PasswordPolicyTest.php
<?php
namespace PicoAuth\Security\Password\Policy;
use PHPUnit\Framework\TestCase;
use PicoAuth\Security\Password\Password;
class PasswordPolicyTest extends TestCase
{
public function testMinLength()
{
$pw1 = new Password("test");
$pw2 = new Password("<PASSWORD>");
$pw3 = new Password("1🐱"); //cat = 4 bytes
$pw4 = new Password("<PASSWORD>🐱");
$policy = new PasswordPolicy();
$policy->minLength(5);
$this->assertFalse($policy->check($pw1));
$this->assertTrue($policy->check($pw2));
$this->assertFalse($policy->check($pw3));
$this->assertTrue($policy->check($pw4));
}
public function testMaxLength()
{
$pw1 = new Password("test");
$pw2 = new Password("<PASSWORD>");
$pw3 = new Password("1🐱");
$policy = new PasswordPolicy();
$policy->maxLength(4);
$this->assertTrue($policy->check($pw1));
$this->assertFalse($policy->check($pw2));
$this->assertTrue($policy->check($pw3));
}
public function testMinNumbers()
{
$pw1 = new Password("test");
$pw2 = new Password("<PASSWORD>");
$policy = new PasswordPolicy();
$policy->minNumbers(1);
$this->assertFalse($policy->check($pw1));
$this->assertTrue($policy->check($pw2));
}
public function testMinUppercase()
{
$pw1 = new Password("test");
$pw2 = new Password("<PASSWORD>");
$pw3 = new Password("<PASSWORD>");
$policy = new PasswordPolicy();
$policy->minUppercase(1);
$this->assertFalse($policy->check($pw1));
$this->assertTrue($policy->check($pw2));
$this->assertTrue($policy->check($pw3));
}
public function testMinLowercase()
{
$pw1 = new Password("TEST");
$pw2 = new Password("<PASSWORD>");
// \p{Ll} regex specifier is unable to match ř as lowercase
//$pw3 = new Password("<PASSWORD>ř");
$policy = new PasswordPolicy();
$policy->minLowercase(1);
$this->assertFalse($policy->check($pw1));
$this->assertTrue($policy->check($pw2));
}
public function testMinSpecial()
{
$pw1 = new Password("<PASSWORD>");
$pw2 = new Password("<PASSWORD>^");
$policy = new PasswordPolicy();
$policy->minSpecial(1);
$this->assertFalse($policy->check($pw1));
$this->assertTrue($policy->check($pw2));
}
public function testMatches()
{
$pw1 = new Password("test");
$pw2 = new Password("<PASSWORD>");
$policy = new PasswordPolicy();
$policy->matches('/.*1$/', 'explanation');
$this->assertFalse($policy->check($pw1));
$this->assertEquals(array('explanation'), $policy->getErrors());
$this->assertTrue($policy->check($pw2));
$this->expectException(\InvalidArgumentException::class);
$policy->matches(null, null);
}
}
<file_sep>/tests/Security/CSRFTest.php
<?php
namespace PicoAuth\Security;
use PHPUnit\Framework\TestCase;
use Symfony\Component\HttpFoundation\Session\Storage\MockArraySessionStorage;
class CSRFTest extends TestCase
{
protected $CSRF;
protected $session;
protected function setUp()
{
$storage = new MockArraySessionStorage();
$this->session = new \PicoAuth\Session\SymfonySession($storage);
$this->CSRF = new CSRF($this->session);
}
public function testTokenAttributes()
{
// Generic (unnamed) token
$this->CSRF->getToken();
$this->assertTrue($this->session->has('CSRF'));
$tokenStorage = $this->session->get('CSRF');
$this->assertArrayHasKey(CSRF::DEFAULT_SELECTOR, $tokenStorage);
$tokenData = $tokenStorage[CSRF::DEFAULT_SELECTOR];
// Token attributes
$this->assertArrayHasKey('time', $tokenData);
$this->assertArrayHasKey('token', $tokenData);
// Token time
$this->assertGreaterThanOrEqual($tokenData['time'], time());
}
public function testTokenFormat()
{
$token = $this->CSRF->getToken();
$this->assertEquals(2, count(explode(CSRF::TOKEN_DELIMTER, $token)));
}
/**
* @depends testTokenAttributes
*/
public function testTokenLength()
{
// Generic (unnamed) token
$this->CSRF->getToken();
$tokenData = $this->session->get('CSRF')[CSRF::DEFAULT_SELECTOR];
// Assert token length in bytes
$this->assertEquals(CSRF::TOKEN_SIZE, strlen($tokenData['token'])/2);
}
public function testGetToken()
{
$this->assertTrue(is_string($this->CSRF->getToken()));
// Two tokens are not same
$this->assertNotEquals($this->CSRF->getToken(), $this->CSRF->getToken());
$this->assertNotEquals($this->CSRF->getToken("a"), $this->CSRF->getToken("a"));
}
public function testCheckToken()
{
$token = $this->CSRF->getToken(null, false); //not reusable
$actionToken = $this->CSRF->getToken("action", true); //reusable
$this->assertFalse($this->CSRF->checkToken("test"));
$this->assertFalse($this->CSRF->checkToken("test", "123"));
$this->assertFalse($this->CSRF->checkToken("test", "action"));
// First validation
$this->assertTrue($this->CSRF->checkToken($token));
$this->assertTrue($this->CSRF->checkToken($actionToken, "action"));
// Second validation of the same token
$this->assertFalse($this->CSRF->checkToken($token));
$this->assertTrue($this->CSRF->checkToken($actionToken, "action"));
}
/**
* When a token is requested for the 2nd time, the time should be updated.
* @depends testTokenAttributes
*/
public function testTokenTimeUpdate()
{
$token = $this->CSRF->getToken('test');
$tokenStorage = $this->session->get('CSRF');
$tokenValue = $tokenStorage['test']['token'];
$newTime = ($tokenStorage['test']['time'] -= CSRF::TOKEN_VALIDITY/2);
// Save the token with altered time
$this->session->set('CSRF', $tokenStorage);
// Request the same token
$token2 = $this->CSRF->getToken('test');
$tokenStorage = $this->session->get('CSRF');
$tokenValue2 = $tokenStorage['test']['token'];
// Internal token value should remain unchanged
$this->assertEquals($tokenValue, $tokenValue2);
// Token time should be updated (2nd > 1st)
$this->assertGreaterThan($newTime, $tokenStorage['test']['time']);
// First token still valid
$this->assertTrue($this->CSRF->checkToken($token, 'test'));
}
public function testTokenExpiration()
{
$token = $this->CSRF->getToken('expireAction');
$tokenStorage = $this->session->get('CSRF');
// expire
$tokenStorage['expireAction']['time'] = 0;
// Save the token with altered time
$this->session->set('CSRF', $tokenStorage);
// Expired token will not validate
$this->assertFalse($this->CSRF->checkToken($token, 'expireAction'));
}
public function testRemoveTokens()
{
$token = $this->CSRF->getToken();
$this->CSRF->removeTokens();
$this->assertFalse($this->CSRF->checkToken($token));
}
}
<file_sep>/src/Storage/Configurator/PageLockConfigurator.php
<?php
namespace PicoAuth\Storage\Configurator;
use PicoAuth\Storage\Configurator\AbstractConfigurator;
use PicoAuth\Storage\Configurator\ConfigurationException;
/**
* The configurator for PageLock
*/
class PageLockConfigurator extends AbstractConfigurator
{
/**
* @inheritdoc
*/
public function validate($rawConfig)
{
$config = $this->applyDefaults($rawConfig, $this->getDefault(), 0);
$this->validateGlobalOptions($config);
$this->validateLocksSection($config);
$this->validateUrlsSection($config);
return $config;
}
/**
* The default configuration must contain all keys that are required.
* @return array
*/
protected function getDefault()
{
return array(
"encoder" => "bcrypt",
"locks" => [],
"urls" => [],
);
}
public function validateGlobalOptions(&$config)
{
$this->assertString($config, "encoder");
}
public function validateLocksSection($config)
{
$this->assertArray($config, "locks");
foreach ($config["locks"] as $lockId => $lockData) {
if (!is_string($lockId) || $lockId=="") {
throw new ConfigurationException("Lock identifier must be a string.");
}
$this->assertArray($config["locks"], $lockId);
// Mandatory attributes
$this->assertRequired($lockData, "key");
$this->assertString($lockData, "key");
// Optional attributes
$this->assertString($lockData, "encoder");
$this->assertString($lockData, "file");
}
}
public function validateUrlsSection(&$config)
{
$this->assertArray($config, "urls");
foreach ($config["urls"] as $pageUrl => $ruleData) {
if (!is_string($pageUrl) || $pageUrl=="") {
throw new ConfigurationException("Page URL must be a string.");
}
$this->assertArray($config["urls"], $pageUrl);
// Add leading /, remove trailing /
$this->standardizeUrlFormat($config["urls"], $pageUrl);
// Mandatory attributes
$this->assertRequired($ruleData, "lock");
$this->assertString($ruleData, "lock");
// Optional attributes
$this->assertBool($ruleData, "recursive");
}
}
}
<file_sep>/content/install.md
---
Title: Install PicoAuth
Robots: noindex,nofollow
Template: installer
---
# PicoAuth Installation
<file_sep>/content/logout.md
---
Title: Logout
Robots: noindex,nofollow
Template: logout
---
<file_sep>/tests/Security/Password/Encoder/PlaintextTest.php
<?php
namespace PicoAuth\Security\Password\Encoder;
use PHPUnit\Framework\TestCase;
class PlaintextTest extends TestCase
{
public function testConstruct()
{
$this->expectException(\InvalidArgumentException::class);
$p = new Plaintext(['ignoreCase'=>100]);
}
public function testEncodeDecode()
{
$ptext = new Plaintext();
$res = $ptext->encode("Test");
$this->assertEquals("Test", $res);
$this->assertFalse($ptext->isValid($res, "test"));
$this->assertTrue($ptext->isValid($res, "Test"));
}
public function testLengthLimit()
{
$raw = str_repeat("r", Plaintext::MAX_LEN+1);
$ptext = new Plaintext();
$this->assertEquals(Plaintext::MAX_LEN, $ptext->getMaxAllowedLen());
$this->assertFalse($ptext->isValid("0", $raw));
$this->expectException(EncoderException::class);
$ptext->encode($raw);
}
public function testNeedsRehash()
{
$ptext = new Plaintext();
$this->assertFalse($ptext->needsRehash('a'));
}
public function testIgnoreCase()
{
$ptext = new Plaintext(['ignoreCase'=>true]);
$res = $ptext->encode("Test");
$this->assertTrue($ptext->isValid($res, "test"));
$this->assertTrue($ptext->isValid($res, "TesT"));
}
}
<file_sep>/src/Mail/MailerInterface.php
<?php
namespace PicoAuth\Mail;
/**
* A simple Mailer Interface PicoAuth uses to send mail.
*/
interface MailerInterface
{
/**
* Initialize the mailer before sending mail
*/
public function setup();
/**
* Set email recipient
* @param string $mail
*/
public function setTo($mail);
/**
* Set email subject
* @param string $subject
*/
public function setSubject($subject);
/**
* Set email body
* @param string $body
*/
public function setBody($body);
/**
* Send email with the previous configuration
* @return bool true on success, false on failure
*/
public function send();
/**
* Gets error message if send() returned false
* @return string Error message
*/
public function getError();
}
<file_sep>/src/Log/LoggerTrait.php
<?php
namespace PicoAuth\Log;
use Psr\Log\LoggerInterface;
use Psr\Log\NullLogger;
/**
* Optional logger trait.
* By using this trait, a class can be injected with a logger instance.
*/
trait LoggerTrait
{
/**
* Logger instance.
* @var LoggerInterface
*/
protected $logger;
/**
* Sets logger.
* @param LoggerInterface $logger
*/
public function setLogger(LoggerInterface $logger)
{
$this->logger = $logger;
}
/**
* Gets logger.
* If the logger instance is not set beforehand, it is set to NullLogger and returned.
* @return LoggerInterface
*/
protected function getLogger()
{
if (!$this->logger) {
$this->logger = new NullLogger();
}
return $this->logger;
}
}
<file_sep>/src/Storage/Interfaces/PageACLStorageInterface.php
<?php
namespace PicoAuth\Storage\Interfaces;
/**
* Interface for accessing PageACL configuration
*/
interface PageACLStorageInterface
{
/**
* Get configuration array
*
* @return array
*/
public function getConfiguration();
/**
* Get rule by Pico page URL
*
* @param string $url Page url
* @return array|null Rule data array, null if not found
*/
public function getRuleByURL($url);
}
<file_sep>/content/error.md
---
Title: Internal error
Robots: noindex,nofollow
Template: error.500
---
<file_sep>/src/Storage/Configurator/ConfigurationException.php
<?php
namespace PicoAuth\Storage\Configurator;
/**
* Exception caused by a bad configuration
*/
class ConfigurationException extends \Exception
{
public function __construct($message, $code = 0, \Exception $previous = null)
{
parent::__construct($message, $code, $previous);
}
/**
* Adds a message before the current one
* @param string $msg
*/
public function addBeforeMessage($msg)
{
$this->message = $msg . " ". $this->message;
}
/**
* Converts to string
* @return string
*/
public function __toString()
{
return $this->message;
}
}
<file_sep>/src/Storage/PageACLFileStorage.php
<?php
namespace PicoAuth\Storage;
use PicoAuth\Storage\Interfaces\PageACLStorageInterface;
use PicoAuth\Cache\NullCache;
use Psr\SimpleCache\CacheInterface;
use PicoAuth\Storage\Configurator\PageACLConfigurator;
/**
* File storage for PageACL
*/
class PageACLFileStorage extends FileStorage implements PageACLStorageInterface
{
/**
* Configuration file name
*/
const CONFIG_FILE = 'PicoAuth/PageACL.yml';
/**
* @inheritdoc
*/
public function __construct($dir, CacheInterface $cache = null)
{
$this->dir = $dir;
$this->cache = ($cache!==null) ? $cache : new NullCache;
$this->configurator = new PageACLConfigurator;
}
/**
* @inheritdoc
*/
public function getRuleByURL($url)
{
$this->readConfiguration();
return self::getItemByUrl($this->config['access'], $url);
}
}
<file_sep>/content/account.md
---
Title: Password change
Robots: noindex,nofollow
Template: password
---
<file_sep>/src/Storage/File/File.php
<?php
namespace PicoAuth\Storage\File;
/**
* File
*
* Provides file locking methods and other generic methods over an opened file
*/
class File
{
/**
* Number of maximum repeat attempts to lock a file
*/
const LOCK_MAX_TRIES = 100;
/**
* Microseconds to wait before next lock try
*/
const LOCK_RETRY_WAIT = 20;
/**
* File resource
* @var mixed
*/
protected $handle;
/**
* File location
* @var string
*/
protected $filePath;
/**
* Options
* @var array
*/
protected $options;
/**
* Creates a file instance
*
* @param string $path File path
* @param array $options Options array
*/
public function __construct($path, $options = [])
{
$this->filePath = $path;
$this->options = $options;
// Apply default options
$this->options += array(
"blocking" => true,
"backup" => false
);
// If an opened file is specified
if (isset($this->options["handle"])) {
$this->handle = $this->options["handle"];
unset($this->options["handle"]);
if (!$this->isOpened()) {
throw new \InvalidArgumentException("The file must be opened.");
}
}
}
/**
* Checks if a file is opened
*
* @return bool
*/
public function isOpened()
{
return is_resource($this->handle);
}
/**
* Obtains a file lock
*
* @param int $lockType Lock type PHP constant
* @return boolean true on successful lock, false otherwise
*/
public function lock($lockType)
{
if (!$this->isOpened()) {
return false;
}
if ($this->options["blocking"]) {
return flock($this->handle, $lockType);
} else {
$tries = 0;
do {
if (flock($this->handle, $lockType | LOCK_NB)) {
return true;
} else {
++$tries;
usleep(self::LOCK_RETRY_WAIT);
}
} while ($tries < self::LOCK_MAX_TRIES);
return false;
}
}
/**
* Unlocks the file
*
* @throws \RuntimeException
*/
public function unlock()
{
if (!flock($this->handle, LOCK_UN)) {
throw new \RuntimeException("Could not unlock file");
}
}
/**
* Closes the file
*
* @return void
* @throws \RuntimeException
*/
public function close()
{
if (!$this->isOpened()) {
return;
}
$this->unlock();
if ($this->handle && !fclose($this->handle)) {
throw new \RuntimeException("Could not close file " . $this->filePath);
}
}
/**
* Returns the file handle
*
* @return resource|null
*/
public function getHandle()
{
return ($this->isOpened()) ? $this->handle : null;
}
}
<file_sep>/src/Session/SymfonySession.php
<?php
namespace PicoAuth\Session;
use Symfony\Component\HttpFoundation\Session\Flash\AutoExpireFlashBag;
use Symfony\Component\HttpFoundation\Session\Session;
use Symfony\Component\HttpFoundation\Session\Storage\NativeSessionStorage;
use Symfony\Component\HttpFoundation\Session\Storage\SessionStorageInterface;
/**
* Session manager implementation using HttpFoundation
*/
class SymfonySession implements SessionInterface
{
/**
* Symfony session instance
* @var Session
*/
protected $session;
public function __construct(SessionStorageInterface $storage = null)
{
$storage = $storage ?: new NativeSessionStorage();
$flashes = new AutoExpireFlashBag('_flash');
$this->session = new Session($storage, null, $flashes);
}
/**
* @inheritdoc
*/
public function has($name)
{
return $this->session->has($name);
}
/**
* @inheritdoc
*/
public function get($name, $default = null)
{
return $this->session->get($name, $default);
}
/**
* @inheritdoc
*/
public function set($name, $value)
{
$this->session->set($name, $value);
}
/**
* @inheritdoc
*/
public function remove($name)
{
$this->session->remove($name);
}
/**
* @inheritdoc
*/
public function invalidate($lifetime = null)
{
return $this->session->invalidate($lifetime);
}
/**
* @inheritdoc
*/
public function migrate($destroy = false, $lifetime = null)
{
return $this->session->migrate($destroy, $lifetime);
}
/**
* @inheritdoc
*/
public function clear()
{
$this->session->clear();
}
/**
* @inheritdoc
*/
public function addFlash($type, $message)
{
$this->session->getFlashBag()->add($type, $message);
}
/**
* @inheritdoc
*/
public function getFlash($type, array $default = array())
{
return $this->session->getFlashBag()->get($type, $default);
}
}
<file_sep>/src/Storage/Configurator/AbstractConfigurator.php
<?php
namespace PicoAuth\Storage\Configurator;
/**
* Abstract configurator for validation of configuration arrays
* and setting default values.
*/
abstract class AbstractConfigurator
{
/**
* Validates the configuration
*
* Performs assertations on all fields of the configuration array,
* adds default values for the fields that were not specified.
*
* @throws ConfigurationException On validation failure
* @param mixed $config Value read from the configuration file
* @return array Resulting configuration with default values
*/
abstract public function validate($config);
/**
* Assert the property is present
* @param array $config Array being validated
* @param string $key Inspected array key
* @return $this
* @throws ConfigurationException On a failed assertation
*/
public function assertRequired($config, $key)
{
if (!isset($config[$key])) {
throw new ConfigurationException("Property $key is required.");
}
return $this;
}
/**
* Assert the property is array or is not set
* @param array $config Array being validated
* @param string $key Inspected array key
* @return $this
* @throws ConfigurationException On a failed assertation
*/
public function assertArray($config, $key)
{
if (array_key_exists($key, $config) && !is_array($config[$key])) {
throw new ConfigurationException($key." section must be an array.");
}
return $this;
}
/**
* Assert the property is boolean or is not set
* @param array $config Array being validated
* @param string|int $key Inspected array key
* @return $this
* @throws ConfigurationException On a failed assertation
*/
public function assertBool($config, $key)
{
if (array_key_exists($key, $config) && !is_bool($config[$key])) {
throw new ConfigurationException($key." must be a boolean value.");
}
return $this;
}
/**
* Assert the property is integer or is not set
* @param array $config Array being validated
* @param string|int $key Inspected array key
* @param int $lowest The lowest accepted values
* @param int $highest The highest accepted value
* @return $this
* @throws ConfigurationException On a failed assertation
*/
public function assertInteger($config, $key, $lowest = null, $highest = null)
{
if (array_key_exists($key, $config)) {
if (!is_int($config[$key])) {
throw new ConfigurationException($key." must be an integer.");
}
if ($lowest !== null && $config[$key] < $lowest) {
throw new ConfigurationException($key." cannot be lower than ".$lowest);
}
if ($highest !== null && $config[$key] > $highest) {
throw new ConfigurationException($key." cannot be higher than ".$highest);
}
}
return $this;
}
/**
* Assert both properties are set and 1st is greater than second
* @param array $config Array being validated
* @param string|int $keyGreater Array key with greater value
* @param string|int $keyLower Array key with lower value
* @return $this
* @throws ConfigurationException On a failed assertation
*/
public function assertGreaterThan($config, $keyGreater, $keyLower)
{
if (!isset($config[$keyLower])
|| !isset($config[$keyGreater])
|| $config[$keyLower] >= $config[$keyGreater]) {
throw new ConfigurationException($keyGreater." must be greater than ".$keyLower);
}
return $this;
}
/**
* Assert the property is string or is not set
* @param array $config Array being validated
* @param string|int $key Inspected array key
* @return $this
* @throws ConfigurationException On a failed assertation
*/
public function assertString($config, $key)
{
if (array_key_exists($key, $config) && !is_string($config[$key])) {
throw new ConfigurationException($key." must be a string.");
}
return $this;
}
/**
* Assert a string contains given substring or is not set
* @param array $config Array being validated
* @param string|int $key Inspected array key
* @param string $searchedPart The string being searched
* @return $this
* @throws ConfigurationException On a failed assertation
*/
public function assertStringContaining($config, $key, $searchedPart)
{
$this->assertString($config, $key);
if (array_key_exists($key, $config) && strpos($config[$key], $searchedPart) === false) {
throw new ConfigurationException($key." must contain ".$searchedPart);
}
return $this;
}
/**
* Assert the value is array of strings or is not set
* @param array $config Array being validated
* @param string|int $key Inspected array key
* @return $this
* @throws ConfigurationException On a failed assertation
*/
public function assertArrayOfStrings($config, $key)
{
if (!array_key_exists($key, $config)) {
return $this;
}
if (!is_array($config[$key])) {
throw new ConfigurationException($key." section must be an array.");
}
foreach ($config[$key] as $value) {
if (!is_string($value)) {
throw new ConfigurationException("Values in the `{$key}` must be strings"
. gettype($value) . " found.");
} elseif ($value==="") {
throw new ConfigurationException("Empty string not allowed in `{$key}` array.");
}
}
return $this;
}
/**
* Assert the value is either integer within bounds or false
*
* Some configuration values can be either false (disabled)
* or have an integer value set if enabled
*
* @param array $config
* @param string|int $key Array being validated
* @param int $lowest The lowest accepted value
* @param int $highest The highest accepted value
* @return $this
* @throws ConfigurationException On a failed assertation
*/
public function assertIntOrFalse($config, $key, $lowest = null, $highest = null)
{
try {
$this->assertInteger($config, $key, $lowest, $highest);
} catch (ConfigurationException $e) {
if ($config[$key]!==false) {
throw new ConfigurationException(
"Key `{$key}` can be either false or a non-negative integer."
);
}
}
return $this;
}
/**
* Corrects URL index if it does not have a correct format
*
* Add a leading slash to the page URL if not present.
* And remove trailing slash from the end if present.
* If the change is made, the array key is moved inside the
* {$rules} array.
*
* @param array $rules Reference to $rules array
* @param string $pageUrl URL to be checked and corrected
*/
public function standardizeUrlFormat(&$rules, $pageUrl)
{
if (!is_string($pageUrl) || $pageUrl==="" || !is_array($rules) ||
!array_key_exists($pageUrl, $rules) ) {
return;
}
$oldIndex=$pageUrl;
if ($pageUrl[0] !== '/') {
$pageUrl='/'.$pageUrl;
}
$len=strlen($pageUrl);
if ($len>1 && $pageUrl[$len-1]==='/') {
$pageUrl= rtrim($pageUrl, '/');
}
if ($oldIndex!==$pageUrl) {
$rules[$pageUrl]=$rules[$oldIndex];
unset($rules[$oldIndex]);
}
}
/**
* Returns configuration merged with the default values
*
* Performs a union operation up to {$depth} level of sub-arrays.
*
* @param array|null $config The configuration array supplied by the user
* @param array $defaults The default configuration array
* @return array The resulting configuration array
*/
public function applyDefaults($config, array $defaults, $depth = 1)
{
if (!is_int($depth) || $depth < 0) {
throw new \InvalidArgumentException("Depth must be non-negative integer.");
}
if (!is_array($config)) {
return $defaults;
}
if ($depth === 0) {
$config += $defaults;
return $config;
}
foreach ($defaults as $key => $defaultValue) {
// Use the default value, if user's array is missing this key
if (!isset($config[$key])) {
$config[$key] = $defaultValue;
continue;
}
if (is_array($defaultValue)) {
if (is_array($config[$key])) {
$config[$key] = $this->applyDefaults($config[$key], $defaultValue, $depth-1);
} else {
throw new ConfigurationException("Configuration key "
.$key." expects an array, a scalar value found.");
}
} else {
if (is_array($config[$key])) {
throw new ConfigurationException("Configuration key "
.$key." expects scalar, an array found.");
}
}
}
return $config;
}
}
<file_sep>/tests/Security/Password/Encoder/BcryptTest.php
<?php
namespace PicoAuth\Security\Password\Encoder;
use PHPUnit\Framework\TestCase;
class BcryptTest extends TestCase
{
public function testConstruct()
{
$b1 = new BCrypt();
$this->expectException(\InvalidArgumentException::class);
$b2 = new BCrypt(['cost'=>100]);
}
public function testEncodeDecode()
{
$bcrypt = new BCrypt(['cost'=>4]);
$res = $bcrypt->encode("BcryptTest");
$this->assertStringStartsWith("$2", $res);
$this->assertTrue($bcrypt->isValid($res, "BcryptTest"));
}
public function testLengthLimit()
{
$raw = str_repeat("r", 73);
$bcrypt = new BCrypt(['cost'=>4]);
$this->assertEquals(72, $bcrypt->getMaxAllowedLen());
$this->assertFalse($bcrypt->isValid("0", $raw));
$this->expectException(EncoderException::class);
$bcrypt->encode($raw);
}
public function testNeedsRehash()
{
$bcrypt4 = new BCrypt(['cost'=>4]);
$res4 = $bcrypt4->encode("BcryptTest");
$bcrypt5 = new BCrypt(['cost'=>5]);
$this->assertTrue($bcrypt5->needsRehash($res4));
}
}
<file_sep>/src/Storage/Configurator/PageACLConfigurator.php
<?php
namespace PicoAuth\Storage\Configurator;
use PicoAuth\Storage\Configurator\AbstractConfigurator;
use PicoAuth\Storage\Configurator\ConfigurationException;
/**
* The configurator for PageACL
*/
class PageACLConfigurator extends AbstractConfigurator
{
/**
* @inheritdoc
*/
public function validate($rawConfig)
{
$config = $this->applyDefaults($rawConfig, $this->getDefault(), 0);
$this->validateAccessRules($config);
return $config;
}
/**
* The default configuration must contain all keys that are required.
* @return array
*/
protected function getDefault()
{
return array(
"access" => [],
);
}
protected function validateAccessRules(&$config)
{
$this->assertArray($config, "access");
foreach ($config["access"] as $pageUrl => $ruleData) {
if (!is_string($pageUrl) || $pageUrl=="") {
throw new ConfigurationException("Page URL must be a string.");
}
$this->assertArray($config["access"], $pageUrl);
// Add leading /, remove trailing /
$this->standardizeUrlFormat($config["access"], $pageUrl);
// Optional attributes
$this->assertBool($ruleData, "recursive");
$this->assertArrayOfStrings($ruleData, "users");
$this->assertArrayOfStrings($ruleData, "groups");
}
}
}
<file_sep>/src/Module/Authentication/LocalAuth/LocalAuth.php
<?php
namespace PicoAuth\Module\Authentication\LocalAuth;
use PicoAuth\Module\AbstractAuthModule;
use PicoAuth\Storage\Interfaces\LocalAuthStorageInterface;
use PicoAuth\Security\Password\Password;
use PicoAuth\Security\RateLimiting\RateLimitInterface;
use PicoAuth\Session\SessionInterface;
use PicoAuth\PicoAuthInterface;
use PicoAuth\User;
use Psr\Log\LoggerAwareInterface;
use PicoAuth\Log\LoggerTrait;
use Symfony\Component\HttpFoundation\Request;
/**
* Authentication with local user accounts
*/
class LocalAuth extends AbstractAuthModule implements LoggerAwareInterface
{
use LoggerTrait;
const LOGIN_CSRF_ACTION = 'login';
/**
* Instance of the plugin
*
* @var PicoAuthInterface
*/
protected $picoAuth;
/**
* Session manager
*
* @var SessionInterface
*/
protected $session;
/**
* Configuration storage
*
* @var LocalAuthStorageInterface
*/
protected $storage;
/**
* The Configuration array
*
* @var array
*/
protected $config;
/**
* The rate limiter
*
* @var RateLimitInterface
*/
protected $limit;
public function __construct(
PicoAuthInterface $picoAuth,
SessionInterface $session,
LocalAuthStorageInterface $storage,
RateLimitInterface $limit
) {
$this->picoAuth = $picoAuth;
$this->session = $session;
$this->storage = $storage;
$this->limit = $limit;
$this->config = $this->storage->getConfiguration();
}
/**
* @inheritdoc
*/
public function getName()
{
return 'localAuth';
}
/**
* @inheritdoc
*/
public function onPicoRequest($url, Request $httpRequest)
{
switch ($url) {
case "login":
$this->handleLogin($httpRequest);
break;
case "register":
$this->handleRegistration($httpRequest);
break;
case "account":
$this->handleAccountPage($httpRequest);
break;
case "password_reset":
$this->handlePasswordReset($httpRequest);
break;
}
}
/**
* Get LocalAuth configuration
*
* Can be used from twig template when dynamically compositing a login page
* (e.g. to find out which functions are enabled to show appropriate links)
*
* @return array
*/
public function getConfig()
{
return $this->config;
}
/**
* Handles a login submission
*
* @param Request $httpRequest Login request
* @return void
*/
protected function handleLogin(Request $httpRequest)
{
$post = $httpRequest->request;
if (!$post->has("username") || !$post->has("password")) {
return;
}
//CSRF validation
if (!$this->picoAuth->isValidCSRF($post->get("csrf_token"), self::LOGIN_CSRF_ACTION)) {
$this->picoAuth->redirectToLogin(null, $httpRequest);
return;
}
$username = strtolower(trim($post->get("username")));
$password = new Password($post->get("password"));
//Check if the login action is not rate limited
if (!$this->limit->action("login", false, array("name" => $username))) {
$this->session->addFlash("error", $this->limit->getError());
$this->picoAuth->redirectToLogin(null, $httpRequest);
return;
}
if (!$this->loginAttempt($username, $password)) {
$this->logInvalidLoginAttempt($username);
$this->limit->action("login", true, array("name" => $username));
$this->session->addFlash("error", "Invalid username or password");
$this->picoAuth->redirectToLogin(null, $httpRequest);
return;
} else {
$userData = $this->storage->getUserByName($username);
if ($this->needsPasswordRehash($userData)) {
$this->passwordRehash($username, $password);
}
$this->login($username, $userData);
$this->picoAuth->afterLogin();
}
}
/**
* Attempt a login with the specified credentials
*
* @param string $username Username
* @param Password $password <PASSWORD>
* @return bool Whether login was successful
*/
public function loginAttempt($username, Password $password)
{
$userData = $this->storage->getUserByName($username);
$encoder = $this->getPasswordEncoder($userData);
$dummy = bin2hex(\random_bytes(32));
$dummyHash = $encoder->encode($dummy);
if (!$userData) {
// The user doesn't exist, dummy call is performed to prevent time analysis
$encoder->isValid($dummyHash, $password);
return false;
}
return $encoder->isValid($userData['pwhash'], $password->get());
}
/**
* Logs the user in
*
* @param string $id User identifier
* @param array $userData User data array
*/
public function login($id, $userData)
{
$this->abortIfExpired($id, $userData);
$u = new User();
$u->setAuthenticated(true);
$u->setAuthenticator($this->getName());
$u->setId($id);
if (isset($userData['groups'])) {
$u->setGroups($userData['groups']);
}
if (isset($userData['displayName'])) {
$u->setDisplayName($userData['displayName']);
}
if (isset($userData['attributes'])) {
foreach ($userData['attributes'] as $key => $value) {
$u->setAttribute($key, $value);
}
}
$this->picoAuth->setUser($u);
}
/**
* Aborts the current request if a password reset is required
*
* Starts a password reset session and redirects to the
* password reset form.
*
* @param string $id User identifier
* @param array $userData User data array
*/
protected function abortIfExpired($id, $userData)
{
if (isset($userData['pwreset']) && $userData['pwreset']) {
$this->session->addFlash("error", "Please set a new password.");
$this->picoAuth->getContainer()->get('PasswordReset')->startPasswordResetSession($id);
$this->picoAuth->redirectToPage("password_reset");
}
}
/**
* Returns encoder instance for the specified user
*
* If the user data array is not specified, returns the default
* encoder instance.
*
* @param null|array $userData User data array
* @return \PicoAuth\Security\Password\Encoder\PasswordEncoderInterface
* @throws \RuntimeException If the encoder is not resolvable
*/
protected function getPasswordEncoder($userData = null)
{
if (isset($userData['encoder']) && is_string($userData['encoder'])) {
$name = $userData['encoder'];
} else {
$name = $this->config["encoder"];
}
$container = $this->picoAuth->getContainer();
if (!$container->has($name)) {
throw new \RuntimeException("Specified LocalAuth encoder is not resolvable.");
}
return $container->get($name);
}
/**
* Fills user-data array with the encoded password
*
* @param array $userData User data array
* @param Password $newPassword The password to be encoded
*/
public function userDataEncodePassword(&$userData, Password $newPassword)
{
$encoderName = $this->config["encoder"];
$encoder = $this->picoAuth->getContainer()->get($encoderName);
$userData['pwhash'] = $encoder->encode($newPassword->get());
$userData['encoder'] = $encoderName;
if (isset($userData['pwreset'])) {
unset($userData['pwreset']);
}
}
/**
* Validates the password policy constraints against the supplied password
*
* Additionally, a maximum length constraint is added based on the limitations
* of the current password encoder used for storage.
*
* @param Password $password Password string to be checked
* @return boolean true - passed, false otherwise
*/
public function checkPasswordPolicy(Password $password)
{
$result = true;
$policy = $this->picoAuth->getContainer()->get("PasswordPolicy");
$maxAllowedLen = $this->getPasswordEncoder()->getMaxAllowedLen();
if (is_int($maxAllowedLen) && strlen($password)>$maxAllowedLen) {
$this->session->addFlash("error", "Maximum length is {$maxAllowedLen}.");
$result = false;
}
if (!$policy->check($password)) {
$errors = $policy->getErrors();
foreach ($errors as $error) {
$this->session->addFlash("error", $error);
}
return false;
}
return $result;
}
/**
* Returns whether a rehash is needed
*
* @param array $userData User data array
* @return boolean true if needed, false otherwise
*/
protected function needsPasswordRehash(array $userData)
{
// Return if password rehashing is not enabled
if ($this->config["login"]["passwordRehash"] !== true) {
return false;
}
// Password hash is created using a different algorithm than default
if (isset($userData['encoder']) && $userData['encoder'] !== $this->config["encoder"]) {
return true;
}
$encoder = $this->getPasswordEncoder($userData);
// If password hash algorithm options have changed
return $encoder->needsRehash($userData['pwhash']);
}
/**
* Performs a password rehash
*
* @param string $username User identifier
* @param Password $password The <PASSWORD> be resaved
*/
protected function passwordRehash($username, Password $password)
{
$userData = $this->storage->getUserByName($username);
try {
$this->userDataEncodePassword($userData, $password);
} catch (\PicoAuth\Security\Password\Encoder\EncoderException $e) {
// The encoder was changed to one that is not able to store this password
$this->session->addFlash("error", "Please set a new password.");
$this->picoAuth->getContainer()->get('PasswordReset')->startPasswordResetSession($username);
$this->picoAuth->redirectToPage("password_reset");
}
$this->storage->saveUser($username, $userData);
}
/**
* Checks username validity
*
* @param string $name Username to be checked
* @return boolean true if the username is valid, false otherwise
*/
protected function isValidUsername($name)
{
if (!is_string($name)
|| !$this->storage->checkValidName($name)
|| strlen($name) < $this->config["registration"]["nameLenMin"]
|| strlen($name) > $this->config["registration"]["nameLenMax"]
) {
return false;
}
return true;
}
/**
* Logs an invalid login attempt
*
* @param array $name Username the attempt was for
*/
protected function logInvalidLoginAttempt($name)
{
// Trim logged name to the maximum allowed length
$max = $this->config["registration"]["nameLenMax"];
if (strlen($name)>$max) {
$max = substr($name, 0, $max) . " (trimmed)";
}
$this->getLogger()->notice(
"Invalid login attempt for {name} by {addr}",
array(
"name" => $name,
"addr" => $_SERVER['REMOTE_ADDR'],
)
);
}
/**
* On account page request
*
* @param Request $httpRequest
* @return void
*/
protected function handleAccountPage(Request $httpRequest)
{
$user = $this->picoAuth->getUser();
if (!$user->getAuthenticated()) {
$this->session->addFlash("error", "Login to access this page.");
$this->picoAuth->redirectToLogin();
return;
}
// Page for password editing available only to local accounts
if ($user->getAuthenticator() !== $this->getName()) {
$this->picoAuth->redirectToPage("index");
return;
}
$editAccount = $this->picoAuth->getContainer()->get('EditAccount');
$editAccount->setConfig($this->config)
->handleAccountPage($httpRequest);
}
/**
* On registration request
*
* @param Request $httpRequest
*/
protected function handleRegistration(Request $httpRequest)
{
$registration = $this->picoAuth->getContainer()->get('Registration');
$registration->setConfig($this->config)
->handleRegistration($httpRequest);
}
/**
* On password reset request
*
* @param Request $httpRequest
*/
protected function handlePasswordReset(Request $httpRequest)
{
$passwordReset = $this->picoAuth->getContainer()->get('PasswordReset');
$passwordReset->setConfig($this->config)
->handlePasswordReset($httpRequest);
}
}
<file_sep>/tests/Storage/Configurator/AbstractConfiguratorTest.php
<?php
namespace PicoAuth\Storage\Configurator;
use PHPUnit\Framework\TestCase;
class AbstractConfiguratorTest extends TestCase
{
/**
* The tested class name
*/
const FQCN = '\PicoAuth\Storage\Configurator\AbstractConfigurator';
public function testApplyDefaultsInvalidArguments()
{
$this->expectException(\InvalidArgumentException::class);
$stub = $this->getMockForAbstractClass(self::FQCN);
$stub->applyDefaults([], [], -1);
}
/**
* @dataProvider applyDefaultsProvider
*/
public function testApplyDefaults($config, array $defaults, $depth, $expected)
{
$stub = $this->getMockForAbstractClass(self::FQCN);
$this->assertEquals($expected, $stub->applyDefaults($config, $defaults, $depth));
}
public function applyDefaultsProvider()
{
return [
[null, ["a"], 0, ["a"]],
[["a"=>1], ["a"=>2], 0, ["a"=>1]],
[["b"=>1], ["a"=>2], 0, ["a"=>2,"b"=>1]],
[["a"=>["aa"=>0,"ab"=>1]], ["a"=>1], 0, ["a"=>["aa"=>0,"ab"=>1]]],
[["a"=>["aa"=>0,"ab"=>1]], ["a"=>[]], 1, ["a"=>["aa"=>0,"ab"=>1]]],
[["a"=>["aa"=>0]], ["a"=>["ab"=>1]], 1, ["a"=>["aa"=>0,"ab"=>1]]],
[["a"=>["b"=>["c"=>1]]], ["a"=>["b"=>["d"=>1]]], 2, ["a"=>["b"=>["c"=>1,"d"=>1]]]],
];
}
/**
* @dataProvider invalidApplyDefaultsProvider
*/
public function testInvalidApplyDefaults($config, array $defaults, $depth)
{
$this->expectException(ConfigurationException::class);
$stub = $this->getMockForAbstractClass(self::FQCN);
$stub->applyDefaults($config, $defaults, $depth);
}
public function invalidApplyDefaultsProvider()
{
return [
[["a"=>[]], ["a"=>1], 1],
[["a"=>1], ["a"=>[]], 1],
[["a"=>["aa"=>0,"ab"=>1]], ["a"=>["aa"=>[]]], 2],
];
}
public function testAssertRequired()
{
$this->expectException(ConfigurationException::class);
$stub = $this->getMockForAbstractClass(self::FQCN);
$stub->assertRequired([], "key");
}
/**
* @dataProvider notArrayOfStrings
*/
public function testAssertArrayOfStrings($val)
{
$this->expectException(ConfigurationException::class);
$stub = $this->getMockForAbstractClass(self::FQCN);
$arr=array(
"key"=>$val
);
$stub->assertArrayOfStrings($arr, "key");
}
public function notArrayOfStrings()
{
return [
[null],
[1],
[["a"=>1]],
[[1,2,3,4]],
[["a","b","c",1,"d"]],
];
}
/**
* @dataProvider notIntOrFalse
*/
public function testAssertIntOrFalse($val, $min = null, $max = null)
{
$this->expectException(ConfigurationException::class);
$stub = $this->getMockForAbstractClass(self::FQCN);
$arr=array(
"key"=>$val
);
$stub->assertIntOrFalse($arr, "key", $min, $max);
}
public function notIntOrFalse()
{
return [
[null],
[true],
["false"],
[["a"=>1]],
[0,1,2],
[3,1,2],
[true,1,2],
];
}
public function testStandardizeUrlFormat()
{
$stub = $this->getMockForAbstractClass(self::FQCN);
// Prepend a slash
$arr=["page"=>1];
$stub->standardizeUrlFormat($arr, "page");
$this->assertEquals(["/page"=>1], $arr);
// Remove the additional slash
$arr=["page/"=>1];
$stub->standardizeUrlFormat($arr, "page/");
$this->assertEquals(["/page"=>1], $arr);
$arr=false;
$stub->standardizeUrlFormat($arr, "page");
$this->assertEquals(false, $arr);
}
}
<file_sep>/src/Security/Password/Encoder/PasswordEncoderInterface.php
<?php
namespace PicoAuth\Security\Password\Encoder;
/**
* Interface for a password representation
*/
interface PasswordEncoderInterface
{
/**
* Returns representation of the raw password suitable for storage
* Generally, a password hashing algorithm is applied
* @throws EncoderException if the rawPassword cannot be processed
* @param string $rawPassword
* @return string Resulting representation
*/
public function encode($rawPassword);
/**
* Returns true if the supplied $rawPassword is valid
* @param string $encodedPassword
* @param string $rawPassword
* @return bool true if validated successfully
*/
public function isValid($encodedPassword, $rawPassword);
/**
* Returns if the password needs rehashing
*
* This is usually the case when algorithm options have changed since
* the creation of the original hash and therefore they may not meet
* the cost requirements.
* @param string $encodedPassword
* @return bool If the password needs to be rehashed
*/
public function needsRehash($encodedPassword);
/**
* Returns the maximum length of rawPassword that can be used with the encoder.
* This can be either algorithm limitation (Bcrypt limits to 72) or a protection
* against a DoS by allowing time-consuming computation of unnecessarily long
* raw strings.
* @return int|null Maximum allowed strlen, null if unlimited
*/
public function getMaxAllowedLen();
}
<file_sep>/src/Security/Password/Policy/PasswordPolicyInterface.php
<?php
namespace PicoAuth\Security\Password\Policy;
use PicoAuth\Security\Password\Password;
/**
* Interface for enforcing password constraints
*/
interface PasswordPolicyInterface
{
/**
* Checks validity of the given string against the constraints of the Policy
* @param Password $password
* @return bool
*/
public function check(Password $password);
/**
* Returns an array of errors from the last check() call
* @return string[]
*/
public function getErrors();
}
<file_sep>/src/Storage/RateLimitSqliteStorage.php
<?php
namespace PicoAuth\Storage;
use PicoAuth\Storage\Interfaces\RateLimitStorageInterface;
use PicoAuth\Cache\NullCache;
use Psr\SimpleCache\CacheInterface;
use PicoAuth\Storage\Configurator\RateLimitConfigurator;
/**
* Sqlite storage for rate limiting
*
* Still uses FileStorage for the configuration file,
* the rate limiting data are stored in Sqlite 3 database.
*/
class RateLimitSqliteStorage extends FileStorage implements RateLimitStorageInterface
{
/**
* Database location
*/
const DB_NAME = 'PicoAuth/data/ratelimit.db';
/**
* Configuration file name
*/
const CONFIG_FILE = 'PicoAuth/RateLimit.yml';
/**
* SQLite 3 Database instance
*
* @var \SQLite3|null
*/
protected $db = null;
/**
* Storage options
* @var array
*/
protected $options;
public function __construct($dir, CacheInterface $cache = null, array $options = [])
{
if (!extension_loaded("sqlite3")) {
throw new \RuntimeException("Extension sqlite3 is required for RateLimitSqliteStorage.");
}
$this->dir = $dir;
$this->cache = ($cache!==null) ? $cache : new NullCache;
$this->configurator = new RateLimitConfigurator;
// Set options and apply defaults
$this->options = $options;
$this->options += array(
"busyTimeout" => 10000
);
$bt = $this->options["busyTimeout"];
if (!is_int($bt) || $bt<0) {
throw new \InvalidArgumentException("Invalid busyTimeout value.");
}
}
/**
* @inheritdoc
*/
public function getLimitFor($action, $type, $key)
{
$stmt = $this->getStatement("SELECT ts, cnt FROM limits WHERE
action=:action AND type=:type AND entity=:key;");
$stmt->bindValue(':action', $action, SQLITE3_TEXT);
$stmt->bindValue(':type', $type, SQLITE3_TEXT);
$stmt->bindValue(':key', $key, SQLITE3_TEXT);
if (!($res = $stmt->execute())) {
throw new \RuntimeException("Could not execute getLimitFor statement.");
}
$arr = $res->fetchArray(SQLITE3_ASSOC);
$stmt->close();
return $arr ? $arr : null;
}
/**
* @inheritdoc
*/
public function updateLimitFor($action, $type, $key, $limitData)
{
$stmt = $this->getStatement("INSERT OR REPLACE INTO limits (action, type, entity, ts, cnt)
VALUES (:action, :type, :key, :ts, :cnt);");
$stmt->bindValue(':action', $action, SQLITE3_TEXT);
$stmt->bindValue(':type', $type, SQLITE3_TEXT);
$stmt->bindValue(':key', $key, SQLITE3_TEXT);
$stmt->bindValue(':ts', $limitData["ts"], SQLITE3_INTEGER);
$stmt->bindValue(':cnt', $limitData["cnt"], SQLITE3_INTEGER);
if (!($res = $stmt->execute())) {
throw new \RuntimeException("Could not execute updateLimitFor statement.");
}
$stmt->close();
}
/**
* @inheritdoc
*/
public function cleanup($action, $type, $config)
{
$stmt = $this->getStatement("DELETE FROM limits WHERE ts < :time;");
// Delete expired records
$threshold = time() - $config["counterTimeout"];
$stmt->bindValue(':time', $threshold, SQLITE3_INTEGER);
if (!($res = $stmt->execute())) {
throw new \RuntimeException("Could not execute cleanup statement.");
}
$stmt->close();
// Number of deleted rows
return $this->db->changes();
}
/**
* {@inheritdoc}
*
* In file storage, save() is called after all changes.
* In sqlite storage the changes are persisted directly, in the above
* method calls, so no action is needed.
*/
public function save($action, $type)
{
return;
}
/**
* @inheritdoc
*/
public function transaction($action, $type, $state)
{
switch ($state) {
// Start transaction
case self::TRANSACTION_BEGIN:
$this->openDatabase();
if (!$this->db->exec("BEGIN EXCLUSIVE TRANSACTION;")) {
throw new \RuntimeException("Could not begin transaction.");
}
break;
// Commit
case self::TRANSACTION_END:
if (!$this->db) {
throw new \InvalidArgumentException("Cannot end transaction in closed DB.");
}
if (!$this->db->exec("END TRANSACTION;")) {
throw new \RuntimeException("Could not commit transaction.");
}
// Close the database
if (!$this->db->close()) {
throw new \RuntimeException("Could not close the database.");
}
$this->db=null;
break;
default:
throw new \InvalidArgumentException("Unexpected transaction argument.");
}
}
/**
* Prepares a statement
*
* @param string $query
* @return \SQLite3Stmt
* @throws \RuntimeException
*/
protected function getStatement($query)
{
$this->openDatabase();
if (!($stmt = $this->db->prepare($query))) {
throw new \RuntimeException("Could not prepare statement.");
}
return $stmt;
}
/**
* Opens the database if not opened
*
* Also creates the schema if not created
*
* @return void
*/
protected function openDatabase()
{
// Return if already conntected
if ($this->db) {
return;
}
// Open or create the database file
$dbPath = $this->dir . self::DB_NAME;
if (!\file_exists($dbPath)) {
self::preparePath($this->dir, dirname(self::DB_NAME));
}
// Opens the database file (will throw \Exception on failure)
$this->db = new \SQLite3($dbPath, SQLITE3_OPEN_READWRITE | SQLITE3_OPEN_CREATE);
// Maximum waiting time
if (!$this->db->busyTimeout($this->options["busyTimeout"])) {
throw new \RuntimeException("Could not set busy timout.");
}
// Create schema if not exists
$res = $this->db->exec("
CREATE TABLE IF NOT EXISTS limits (
id INTEGER PRIMARY KEY,
action TEXT NOT NULL,
type TEXT NOT NULL,
entity TEXT NOT NULL,
ts INTEGER NOT NULL,
cnt INTEGER NOT NULL
);
CREATE UNIQUE INDEX IF NOT EXISTS limitIndex ON limits (action,type,entity);
CREATE INDEX IF NOT EXISTS tsIndex ON limits (ts);
");
if (!$res) {
throw new \RuntimeException("Could not create table schema.");
}
}
}
<file_sep>/content/pwbeginreset.md
---
Title: Password reset
Robots: noindex,nofollow
Template: password_begin_reset
---
Enter the email used to register the account and a password reset link will be sent to it.<file_sep>/src/Module/Authorization/PageACL.php
<?php
namespace PicoAuth\Module\Authorization;
use PicoAuth\Storage\Interfaces\PageACLStorageInterface;
use PicoAuth\Session\SessionInterface;
use PicoAuth\PicoAuthInterface;
use PicoAuth\Module\AbstractAuthModule;
/**
* Authorization based on pre-defined access rules
*/
class PageACL extends AbstractAuthModule
{
protected $runtimeRules = [];
/**
* Instance of the plugin
*
* @var PicoAuthInterface
*/
protected $picoAuth;
/**
* Session manager
*
* @var SessionInterface
*/
protected $session;
/**
* Configuration storage
*
* @var PageACLStorageInterface
*/
protected $storage;
public function __construct(
PicoAuthInterface $picoAuth,
SessionInterface $session,
PageACLStorageInterface $storage
) {
$this->picoAuth = $picoAuth;
$this->session = $session;
$this->storage = $storage;
}
/**
* @inheritdoc
*/
public function getName()
{
return 'pageACL';
}
/**
* @inheritdoc
*/
public function checkAccess($url)
{
$user = $this->picoAuth->getUser();
$id = $user->getId();
$rule = $this->storage->getRuleByURL($url);
if ($rule === null && count($this->runtimeRules)) {
$rule = \PicoAuth\Storage\FileStorage::getItemByUrl($this->runtimeRules, $url);
}
if ($rule !== null) {
if (isset($rule['users']) && in_array($id, $rule['users'])) {
return true;
}
if (isset($rule['groups'])) {
$groups = $user->getGroups();
foreach ($groups as $group) {
if (in_array($group, $rule['groups'])) {
return true;
}
}
}
return false;
}
return true;
}
/**
* @inheritdoc
*/
public function denyAccessIfRestricted($url)
{
if (!$this->checkAccess($url)) {
$user = $this->picoAuth->getUser();
if ($user->getAuthenticated()) {
// The user is logged in but does not have permissions to view the page
$this->picoAuth->setRequestUrl("403");
header($_SERVER['SERVER_PROTOCOL'] . ' 403 Forbidden');
} else {
// The user is not logged in -> redirect to login
$this->session->addFlash("error", "Login first to access this page");
$afterLogin = "afterLogin=" . $url;
$this->picoAuth->redirectToLogin($afterLogin);
}
}
}
/**
* Adds an additional ACL rule
*
* Can be used by other plugins to control access to certain pages
*
* @param string $url Url for which the rule applies
* @param array $rule Rule to be added
*/
public function addRule($url, $rule)
{
if (!is_string($url) || !is_array($rule)) {
throw new \InvalidArgumentException("addRule() expects a string and an array.");
}
$this->runtimeRules[$url] = $rule;
}
}
<file_sep>/tests/Module/AbstractAuthModuleTest.php
<?php
namespace PicoAuth\Module;
use PicoAuth\PicoAuthInterface;
class AbstractAuthModuleTest extends \PicoAuth\BaseTestCase
{
protected $abstractModule;
protected function setUp()
{
$this->abstractModule = $this->getMockBuilder(AbstractAuthModule::class)
->getMockForAbstractClass();
}
public function testHandleEvent()
{
$this->assertNull($this->abstractModule->handleEvent("nonExistent", []));
}
}
<file_sep>/src/Storage/Interfaces/RateLimitStorageInterface.php
<?php
namespace PicoAuth\Storage\Interfaces;
/**
* Interface for accessing RateLimit data
*/
interface RateLimitStorageInterface
{
/**
* Close storage transaction
*/
const TRANSACTION_END = 0;
/**
* Begin storage transaction
*/
const TRANSACTION_BEGIN = 1;
/**
* Get configuration array
*
* @return array
*/
public function getConfiguration();
/**
* Get limit data for action-type-identifier
*
* @param string $action E.g. "login"
* @param string $type E.g. "account"
* @param string $key E.g. "user1"
* @return array|null
* @throws \Exception On read error
*/
public function getLimitFor($action, $type, $key);
/**
* Update limit data
*
* @param string $action E.g. "login"
* @param string $type E.g. "account"
* @param string $key E.g. "user1"
* @param array $limitData New limit data array
*/
public function updateLimitFor($action, $type, $key, $limitData);
/**
* Clean expired records
*
* @param string $action
* @param string $type
* @param array $config Limit configuration
* @return int Number of removed records
*/
public function cleanup($action, $type, $config);
/**
* Save datafile for action-type
*
* @param string $action
* @param string $type
* @throws \Exception On save error
*/
public function save($action, $type);
/**
* Transaction control (TCL)
*
* Begins or ends an exclusive transaction. After the transaction begins,
* all subsequent calls to other storage methods must be executed atomically,
* until the transaction ends.
*
* @param string $action
* @param string $type
* @param int $state One of the TRANSACTION_ constants
*/
public function transaction($action, $type, $state);
}
<file_sep>/src/Storage/LocalAuthFileStorage.php
<?php
namespace PicoAuth\Storage;
use PicoAuth\Storage\Interfaces\LocalAuthStorageInterface;
use PicoAuth\Cache\NullCache;
use Psr\SimpleCache\CacheInterface;
use PicoAuth\Storage\Configurator\LocalAuthConfigurator;
use PicoAuth\Storage\File\FileWriter;
/**
* File storage for LocalAuth
*/
class LocalAuthFileStorage extends FileStorage implements LocalAuthStorageInterface
{
/**
* Configuration file name
*/
const CONFIG_FILE = 'PicoAuth/LocalAuth.yml';
/**
* Directory with user files
*/
const USERS_DIR = 'PicoAuth/users';
/**
* Extension of user files
*/
const USERFILE_EXT = '.yml';
/**
* Reset tokens filename
*/
const RESET_TOKENS = 'PicoAuth/data/reset_tokens.yml';
/**
* Already fetched users
*
* @var array
*/
protected $fetchedUsers = array();
/**
* Number of users
*
* @var int
*/
protected $usersCount;
/**
* The LocalAuthConfigurator instance
* @var Configurator\LocalAuthConfigurator
*/
protected $configurator;
public function __construct($dir, CacheInterface $cache = null)
{
$this->dir = $dir;
$this->cache = ($cache!==null) ? $cache : new NullCache;
$this->configurator = new LocalAuthConfigurator;
}
/**
* @inheritdoc
*/
public function getUserByName($name)
{
// Check if the user wasn't already fetched during this request
if (isset($this->fetchedUsers[$name])) {
return $this->fetchedUsers[$name];
}
// Validate correct username format before search
try {
$this->checkValidName($name);
} catch (\RuntimeException $e) {
return null;
}
// Search in user files
$userFileName = $this->dir . self::USERS_DIR . '/' . $name . self::USERFILE_EXT;
if (($yaml = self::readFile($userFileName)) !== false) {
$userData = \Symfony\Component\Yaml\Yaml::parse($yaml);
$this->configurator->validateUserData($userData);
$this->fetchedUsers[$name] = $userData;
return $userData;
}
// Search in the main configuration file
$this->readConfiguration();
if (isset($this->config['users']) && isset($this->config['users'][$name])) {
$this->fetchedUsers[$name] = $this->config['users'][$name];
return $this->config['users'][$name];
}
return null;
}
/**
* @inheritdoc
*/
public function getUserByEmail($email)
{
// Search in user files
$searchDir = $this->dir . self::USERS_DIR;
if (is_dir($searchDir)) {
$userFiles = $this->getDirFiles($searchDir);
foreach ($userFiles as $filename) {
$username = substr($filename, 0, -strlen(self::USERFILE_EXT));
$user = $this->getUserByName($username);
if (isset($user['email']) && $email === $user['email']) {
$user['name'] = $username;
return $user;
}
}
}
// Search in the main configuration file
if (isset($this->config['users'])) {
foreach ($this->config['users'] as $name => $userData) {
if (isset($userData['email']) && $email === $userData['email']) {
$userData['name'] = $name;
return $userData;
}
}
}
return null;
}
/**
* @inheritdoc
*/
public function saveUser($id, $userdata)
{
$dir = $this->dir . self::USERS_DIR;
self::preparePath($this->dir, self::USERS_DIR);
$yaml = \Symfony\Component\Yaml\Yaml::dump($userdata, 2, 2);
$userFileName = $dir . '/' . $id . self::USERFILE_EXT;
// Write the new user file
if ((self::writeFile($userFileName, $yaml, ["backup" => true]) === false)) {
throw new \RuntimeException("Unable to save the user data (". basename($userFileName).").");
}
// If exists, remove the user record from the main configuration file
// Needs write permission for users.yml
$this->readConfiguration();
if (isset($this->config['users']) && isset($this->config['users'][$id])) {
unset($this->config['users'][$id]);
$yaml = \Symfony\Component\Yaml\Yaml::dump($this->config, 3, 2);
$fileName = $this->dir . static::CONFIG_FILE;
if ((self::writeFile($fileName, $yaml, ["backup" => true]) === false)) {
throw new \RuntimeException("Unable to save new configuration (".static::CONFIG_FILE.").");
}
}
}
/**
* {@inheritdoc}
*
* When adding a new token entry, the file must be read first, the token
* added and then saved. An exclusive access to the token file must be held
* since the initial read in order to avoid lost writes.
*/
public function saveResetToken($id, $data)
{
$fileName = $this->dir . self::RESET_TOKENS;
$tokens = array();
$writer = new FileWriter($fileName);
// Get exclusive access and read the current tokens
try {
$writer->open();
$reader = new \PicoAuth\Storage\File\FileReader(
$fileName,
["handle"=>$writer->getHandle()]
);
$yaml = $reader->read();
$tokens = \Symfony\Component\Yaml\Yaml::parse($yaml);
} catch (\RuntimeException $e) {
// File doesn't exist, no write permission, read or parse error
// If not writeable, will fail in saveResetTokens
$tokens = array();
}
// Add the new token entry
$tokens[$id] = $data;
// Save the token file, while keeping the same file lock
$this->saveResetTokens($tokens, $writer);
}
/**
* Saves reset tokens
*
* @param array $tokens Tokens array
* @param FileWriter $writer Optional file writer to use
* @throws \RuntimeException On save error
*/
protected function saveResetTokens($tokens, FileWriter $writer = null)
{
// Before saving, remove all expired tokens
$time = time();
foreach ($tokens as $id => $token) {
if ($time > $token['valid']) {
unset($tokens[$id]);
}
}
$fileName = $this->dir . self::RESET_TOKENS;
$yaml = \Symfony\Component\Yaml\Yaml::dump($tokens, 1, 2);
if ($writer && $writer->isOpened()) {
// An exclusive lock is already held, then use the given writer instance
$writer->write($yaml); // Will throw on write error
} else {
self::preparePath($this->dir, dirname(self::RESET_TOKENS));
if ((self::writeFile($fileName, $yaml) === false)) {
throw new \RuntimeException("Unable to save token file (".self::RESET_TOKENS.").");
}
}
}
/**
* @inheritdoc
*/
public function getResetToken($id)
{
$fileName = $this->dir . self::RESET_TOKENS;
$tokens = array();
if (($yaml = self::readFile($fileName)) !== false) {
$tokens = \Symfony\Component\Yaml\Yaml::parse($yaml);
}
if (isset($tokens[$id])) {
$token = $tokens[$id];
unset($tokens[$id]); // Remove the token when retrieved
$this->saveResetTokens($tokens);
return $token;
} else {
return null;
}
}
/**
* @inheritdoc
*/
public function checkValidName($name)
{
if (!$this->configurator->checkValidNameFormat($name)) {
throw new \RuntimeException("A username can contain only alphanumeric characters.");
}
return true;
}
/**
* @inheritdoc
*/
public function getUsersCount()
{
if (!isset($this->usersCount)) {
try {
$files = $this->getDirFiles($this->dir . self::USERS_DIR);
$this->usersCount=count($files);
} catch (\RuntimeException $e) {
// Return 0 if the users directory cannot be listed (no users yet)
$this->usersCount=0;
}
}
return $this->usersCount;
}
/**
* Gets directory files
*
* @param string $searchDir Searched directory
* @return array File names
* @throws \RuntimeException On read error
*/
protected function getDirFiles($searchDir)
{
// Error state is handled by the excpetion, warning disabled
$files = @scandir($searchDir, SCANDIR_SORT_NONE);
if ($files === false) {
throw new \RuntimeException("Cannot list directory contents: {$searchDir}.");
}
return array_diff($files, array('..', '.'));
}
}
<file_sep>/src/Security/Password/Encoder/Argon2i.php
<?php
namespace PicoAuth\Security\Password\Encoder;
/**
* Argon2i
*
* PHP RFC: https://wiki.php.net/rfc/argon2_password_hash
*/
class Argon2i implements PasswordEncoderInterface
{
/**
* The maximum length
*
* Not a limitation of the algorithm, but allowing very long passwords
* could lead to DoS using the computation difficulty of the algorithm.
*/
const MAX_LEN = 4096;
/**
* The array with options
*
* @var array
*/
protected $options;
public function __construct(array $options = [])
{
if (!defined('PASSWORD_ARGON2I')) {
throw new \RuntimeException("ARGON2I is not supported.");
}
$defaults = [
'memory_cost' => PASSWORD_ARGON2_DEFAULT_MEMORY_COST,
'time_cost' => PASSWORD_ARGON2_DEFAULT_TIME_COST,
'threads' => PASSWORD_ARGON2_DEFAULT_THREADS
];
$options += $defaults;
$this->validateOptions($options);
$this->options = $options;
}
/**
* @inheritdoc
*/
public function encode($rawPassword)
{
if (strlen($rawPassword) > self::MAX_LEN) {
throw new EncoderException("Invalid length, maximum is ".self::MAX_LEN.".");
}
return password_hash($rawPassword, PASSWORD_ARGON2I, $this->options);
}
/**
* @inheritdoc
*/
public function isValid($encodedPassword, $rawPassword)
{
if (strlen($rawPassword) > self::MAX_LEN) {
return false;
}
return password_verify($rawPassword, $encodedPassword);
}
/**
* @inheritdoc
*/
public function needsRehash($encodedPassword)
{
return password_needs_rehash($encodedPassword, PASSWORD_ARGON2I, $this->options);
}
/**
* Validates algorithm options
*
* From the algorithm's reference:
* https://password-hashing.net/argon2-specs.pdf
*
* @param array $options
* @throws \InvalidArgumentException
*/
private function validateOptions($options)
{
if ($options['threads'] < 1) {
throw new \InvalidArgumentException('Number of threads must be positive.');
}
if ($options['time_cost'] < 1) {
throw new \InvalidArgumentException('Time cost must be positive.');
}
if ($options['memory_cost'] < $options['threads']*8) {
throw new \InvalidArgumentException('Memory cost must be number of kilobytes from 8*threads.');
}
}
/**
* @inheritdoc
*/
public function getMaxAllowedLen()
{
return self::MAX_LEN;
}
}
<file_sep>/tests/Storage/Configurator/LocalAuthConfiguratorTest.php
<?php
namespace PicoAuth\Storage\Configurator;
use PHPUnit\Framework\TestCase;
class LocalAuthConfiguratorTest extends TestCase
{
protected $configurator;
protected function setUp()
{
$this->configurator = new LocalAuthConfigurator;
}
/**
* @dataProvider badConfigurations
*/
public function testValidationErrors($rawConfig)
{
$this->expectException(ConfigurationException::class);
$this->configurator->validate($rawConfig);
}
public function badConfigurations()
{
return [
[["encoder"=>1]],
[["login"=>["passwordRehash"=>1]]],
[["accountEdit"=>["enabled"=>1]]],
[["passwordReset"=>["enabled"=>1]]],
[["passwordReset"=>["emailMessage"=>1]]],
[["passwordReset"=>["emailMessage"=>"noUrlPlaceholder"]]],
[["passwordReset"=>["emailSubject"=>1]]],
[["passwordReset"=>["tokenIdLen"=>"a"]]],
[["passwordReset"=>["tokenIdLen"=>-1]]],
[["passwordReset"=>["tokenIdLen"=>0]]],
[["passwordReset"=>["tokenIdLen"=>2000000]]],
[["passwordReset"=>["tokenLen"=>"a"]]],
[["passwordReset"=>["tokenLen"=>-1]]],
[["passwordReset"=>["tokenLen"=>1]]],
[["passwordReset"=>["tokenLen"=>2000000]]],
[["passwordReset"=>["tokenValidity"=>"a"]]],
[["passwordReset"=>["tokenValidity"=>0]]],
[["passwordReset"=>["resetTimeout"=>"a"]]],
[["passwordReset"=>["resetTimeout"=>0]]],
[["registration"=>["maxUsers"=>-1]]],
[["registration"=>["maxUsers"=>"25"]]],
[["registration"=>["nameLenMin"=>"a"]]],
[["registration"=>["nameLenMin"=>0]]],
[["registration"=>["nameLenMax"=>"a"]]],
[["registration"=>["nameLenMax"=>0]]],
[["registration"=>["nameLenMax"=>2000000]]],
[["registration"=>["nameLenMax"=>8,"nameLenMin"=>9]]],
[["users"=>1]],
[["users"=>["a."=>[]]]],
[["users"=>["aaaaa."=>[]]]],
[["users"=>[1=>[]]]],
[["users"=>["tester"=>[]]]],
[["users"=>["tester"=>["pwhash"=>1]]]],
[["users"=>["tester"=>["pwhash"=>"b","email"=>1]]]],
[["users"=>["tester"=>["pwhash"=>"c","attributes"=>1]]]],
[["users"=>["tester"=>["pwhash"=>"d","encoder"=>1]]]],
[["users"=>["tester"=>["pwhash"=>"e","pwreset"=>1]]]],
[["users"=>["tester"=>["pwhash"=>"f","groups"=>1]]]],
[["users"=>["tester"=>["pwhash"=>"g","groups"=>[1,"a",3]]]]],
[["users"=>["tester"=>["pwhash"=>"h"],"TESTER"=>["pwhash"=>"i"]]]],
[["users"=>["tester"=>["pwhash"=>"i","displayName"=>false]]]],
];
}
public function testValidation()
{
$res = $this->configurator->validate(null);
$this->assertArrayHasKey("encoder", $res);
$res = $this->configurator->validate([
"users" => [
"TeStEr" => ["pwhash"=>"i"],
],
]);
$this->assertArrayHasKey("users", $res);
$this->assertArrayHasKey("tester", $res["users"]);
}
}
<file_sep>/tests/UserTest.php
<?php
namespace PicoAuth;
use PHPUnit\Framework\TestCase;
/**
* PicoAuth plugin tests
*/
class UserTest extends TestCase
{
public function testDefault()
{
$u = new User();
$this->assertFalse($u->getAuthenticated());
}
public function testSetAuthenticated()
{
$u = new User();
$u->setAuthenticated(true);
$u->setAuthenticator("test");
$this->assertTrue($u->getAuthenticated());
$this->assertEquals("test", $u->getAuthenticator());
$u->setAuthenticated(false);
$this->assertEquals(null, $u->getAuthenticated());
}
public function testAttributes()
{
$u = new User();
$this->assertNull($u->getAttribute("a"));
$this->assertNull($u->getAttribute(null));
$u->setAttribute("a", 1);
$this->assertEquals(1, $u->getAttribute("a"));
}
public function testId()
{
$u = new User();
$u->setId("u1");
$this->assertEquals("u1", $u->getId());
}
public function testDisplayName()
{
$u = new User();
$this->assertNull($u->getDisplayName());
$u->setDisplayName("a");
$this->assertEquals("a", $u->getDisplayName());
}
public function testGroups()
{
$u = new User();
$u->setGroups(null);
$this->assertEquals([], $u->getGroups());
$u->setGroups(0);
$this->assertEquals([], $u->getGroups());
$u->setGroups("g0");
$this->assertEquals([], $u->getGroups());
$u->addGroup("g1");
$this->assertEquals(["g1"], $u->getGroups());
$u->addGroup("g2");
$this->assertEquals(["g1","g2"], $u->getGroups());
$u->setGroups(["g3"]);
$this->assertEquals(["g3"], $u->getGroups());
$u->addGroup(null);
$u->addGroup(3);
$u->addGroup(["g4"]);
$this->assertEquals(["g3"], $u->getGroups());
}
}
<file_sep>/src/Cache/NullCache.php
<?php
namespace PicoAuth\Cache;
use Psr\SimpleCache\CacheInterface;
/**
* NullCache to be used when a real instance is not injected.
* Makes null checking unnecessary on places when a cache is utilized.
*/
class NullCache implements CacheInterface
{
/**
* {@inheritdoc}
*/
public function clear()
{
return true;
}
/**
* {@inheritdoc}
*/
public function delete($key)
{
return true;
}
/**
* {@inheritdoc}
*/
public function deleteMultiple($keys)
{
return true;
}
/**
* {@inheritdoc}
*/
public function get($key, $default = null)
{
return $default;
}
/**
* {@inheritdoc}
*/
public function getMultiple($keys, $default = null)
{
$result = [];
foreach ($keys as $key) {
$result[$key] = $default;
}
return $result;
}
/**
* {@inheritdoc}
*/
public function has($key)
{
return false;
}
/**
* {@inheritdoc}
*/
public function set($key, $value, $ttl = null)
{
return false;
}
/**
* {@inheritdoc}
*/
public function setMultiple($values, $ttl = null)
{
return false;
}
}
<file_sep>/tests/Security/RateLimiting/RateLimitTest.php
<?php
namespace PicoAuth\Security\RateLimiting;
use Psr\Log\LoggerInterface;
use PicoAuth\BaseTestCase;
use PicoAuth\Storage\Configurator\RateLimitConfigurator;
use PicoAuth\Storage\Interfaces\RateLimitStorageInterface;
class RateLimitTest extends BaseTestCase
{
protected $limit;
protected $configurator;
protected $testConfig;
protected function setUp()
{
$sto = $this->createMock(RateLimitStorageInterface::class);
$this->limit = new RateLimit($sto);
$this->testConfig = array(
"count" => 1,
"blockDuration" => 10000,
"counterTimeout" =>20000,
"errorMsg" => "%cnt%"
);
$this->configurator = new RateLimitConfigurator;
}
/**
* @dataProvider ipProvider
*/
public function testGetSubnet($ip, $mask, $expected)
{
$getSubnet = self::getMethod($this->limit, 'getSubnet');
$this->assertEquals($expected, $getSubnet->invokeArgs($this->limit, [$ip, $mask]));
}
public function ipProvider()
{
return [
["10.0.0.1",32,"10.0.0.1/32"],
["10.0.0.3",31,"10.0.0.2/31"],
["10.0.0.7",30,"10.0.0.4/30"],
["10.0.0.15",29,"10.0.0.8/29"],
["10.0.255.255",17,"10.0.128.0/17"],
["10.11.12.13",24,"10.11.12.0/24"],
["10.11.12.13",8,"10.0.0.0/8"],
["10.11.12.13",8,"10.0.0.0/8"],
["ffff::",1,"8000::/1"],
["fd00:c2b6:b24b:be67:2827:688d:e6a1:6a3b",64,"2000:dead:beef:4dad::/64"],
["1::",0,"::/0"],
];
}
/**
* @dataProvider invalidSubnetArgumentsProvider
*/
public function testGetSubnetInvalidArguments($ip, $mask)
{
$this->expectException(\InvalidArgumentException::class);
$getSubnet = self::getMethod($this->limit, 'getSubnet');
$getSubnet->invokeArgs($this->limit, [$ip, $mask]);
}
public function invalidSubnetArgumentsProvider()
{
return [
// Invalid netmasks
["10.0.0.1","32"],
["10.0.0.1",null],
["10.0.0.1",33],
["10.0.0.1",-1],
["2000::",-1],
["2000::",129],
// Invalid IP
["2000:cg:",129],
["2000:::",24],
["10.0.0.0.1",24],
["10.0.0",24],
["10.0.0.",24],
[null,24],
[24,24],
];
}
public function testGetLimitFor()
{
$sto = $this->createMock(RateLimitStorageInterface::class);
$sto->expects($this->once())
->method("getLimitFor")
->with("a", "b", "c")
->willReturn(null);
$this->limit = new RateLimit($sto);
$method = self::getMethod($this->limit, 'getLimitFor');
$ret = $method->invokeArgs($this->limit, ["a","b","c"]);
$this->assertEquals(array("ts" => 0, "cnt" => 0), $ret);
}
public function testAction()
{
$this->assertTrue($this->limit->action("undefined-action"));
$sto = $this->createMock(RateLimitStorageInterface::class);
$sto->expects($this->once())
->method("getConfiguration")
->willReturn($this->configurator->validate([]));
$sto->expects($this->at(1))
->method("transaction");
$sto->expects($this->at(2))
->method("getLimitFor")
->with("login", "ip", "10.0.0.1/".RateLimit::DEFAULT_NETMASK_IPV4)
->willReturn(array("ts" => time(), "cnt" => 1000000));
$sto->expects($this->at(3))
->method("transaction");
$sto->expects($this->at(4))
->method("transaction");
$sto->expects($this->at(5))
->method("getLimitFor")
->with("login", "ip", "2000::/".RateLimit::DEFAULT_NETMASK_IPV6)
->willReturn(array("ts" => time(), "cnt" => 1000000));
$sto->expects($this->at(6))
->method("transaction");
$limit = new RateLimit($sto);
$_SERVER["REMOTE_ADDR"] = '10.0.0.1';
$this->assertFalse($limit->action("login"));
$_SERVER["REMOTE_ADDR"] = '2000::';
$this->assertFalse($limit->action("login"));
}
public function testIncrementCounter()
{
$sto = $this->createMock(RateLimitStorageInterface::class);
$sto->expects($this->once())
->method("getConfiguration")
->willReturn($this->configurator->validate([]));
$sto->expects($this->once())
->method("save")
->with("login", "ip")
->willReturn(true);
$sto->expects($this->once())
->method("updateLimitFor");
$sto->expects($this->once())
->method("getLimitFor")
->with("login", "ip", "10.0.0.1/32")
->willReturn(array("ts" => time(), "cnt" => 0));
$limit = new RateLimit($sto);
$logger = $this->createMock(LoggerInterface::class);
$logger->expects($this->exactly(1))
->method('notice')->willReturnSelf();
$limit->setLogger($logger);
$method = self::getMethod($limit, 'incrementCounter');
$method->invokeArgs($limit, ["login","ip","10.0.0.1/32",$this->testConfig]);
}
public function testIncrementCounterNoIncrement()
{
$sto = $this->createMock(RateLimitStorageInterface::class);
$sto->expects($this->never())
->method("save")
->with("login", "ip")
->willReturn(true);
$sto->expects($this->once())
->method("getLimitFor")
->with("login", "ip", "10.0.0.1/32")
->willReturn(array("ts" => time(), "cnt" => 1));
$limit = new RateLimit($sto);
$logger = $this->createMock(LoggerInterface::class);
$logger->expects($this->never())
->method('notice')->willReturnSelf();
$limit->setLogger($logger);
$method = self::getMethod($limit, 'incrementCounter');
$method->invokeArgs($limit, ["login", "ip", "10.0.0.1/32", $this->testConfig]);
}
public function testIncrementCounterResetCounter()
{
$sto = $this->createMock(RateLimitStorageInterface::class);
$sto->expects($this->once())
->method("save")
->with("login", "ip")
->willReturn(true);
$sto->expects($this->once())
->method("getLimitFor")
->with("login", "ip", "10.0.0.1/32")
->willReturn(array("ts" => 0, "cnt" => 10));
$limit = new RateLimit($sto);
$method = self::getMethod($limit, 'incrementCounter');
$method->invokeArgs($limit, ["login", "ip", "10.0.0.1/32", $this->testConfig]);
}
public function testSetErrorMessage()
{
$method = self::getMethod($this->limit, 'setErrorMessage');
$method->invokeArgs($this->limit, [null]);
$this->assertTrue(is_string($this->limit->getError()));
$method->invokeArgs($this->limit, [$this->testConfig]);
$this->assertEquals("1", $this->limit->getError());
}
public function testGetEntityId()
{
$method = self::getMethod($this->limit, 'getEntityId');
$this->assertNull($method->invokeArgs($this->limit, ["test",array()]));
$this->assertEquals(md5("t1"), $method->invokeArgs(
$this->limit,
["account",[],[
"name" => "t1"
]]
));
$this->assertEquals(md5("<EMAIL>"), $method->invokeArgs(
$this->limit,
["email",[],[
"email" => "<EMAIL>"
]]
));
}
}
<file_sep>/src/Storage/Interfaces/OAuthStorageInterface.php
<?php
namespace PicoAuth\Storage\Interfaces;
/**
* Interface for accessing OAuth configuration
*/
interface OAuthStorageInterface
{
/**
* Get provider configuration
*
* @param string $name Provider identifier
* @return array|null Provider configuration, null if not found
*/
public function getProviderByName($name);
/**
* Get supported providers
*
* @return string[]|null
*/
public function getConfiguration();
/**
* Get available providers
*
* Can be used in the login form to print all available providers
*
* @return string[] Array with provider identifiers
*/
public function getProviderNames();
}
<file_sep>/src/container.php
<?php
/**
* Default container definition for PicoAuth plugin.
*
* Don't edit the plugin's file - but create a copy in
* {PicoRoot}/config/PicoAuth/container.php
*
* If the container.php exists in the configuration directory it is used
* instead of the default one.
*/
use League\Container\Argument\RawArgument;
use League\Container\Container;
$container = new Container;
// Version of this file, so PicoAuth can detect possibly outdated definition
$container->share('Version', new RawArgument(10000));
// ****************** Configuration cache setup ********************************
// Any implementation of Psr\SimpleCache\CacheInterface
// e.g. from package cache/cache: $pool = new ApcuCachePool();
$pool = 'PicoAuth\Cache\NullCache';
$container->share('cache', $pool);
// ****************** Logger setup *********************************************
// Any implementation of \Psr\Log\LoggerInterface
// e.g. $log = new \Monolog\Logger('name');
$log = 'Psr\Log\NullLogger';
$container->share('logger', $log);
// ****************** Mail setup ***********************************************
// Any implementation of PicoAuth\Mail\MailerInterface
//include 'Mailer.php';
//$container->share('mailer','PicoAuth\Mail\Mailer');
// ****************** Password policy setup ************************************
// Specify constraints of the default policy
// Or provide an alternative implementation of PicoAuth\Security\Password\Policy\PasswordPolicyInterface
$container->share('PasswordPolicy', 'PicoAuth\Security\Password\Policy\PasswordPolicy')
->withMethodCall('minLength', [new RawArgument(8)]);
// ****************** Session management configuration *************************
// PicoAuth default session driver
$container->share('session', 'PicoAuth\Session\SymfonySession')
->withArgument('session.storage');
$container->share('session.storage', 'Symfony\Component\HttpFoundation\Session\Storage\NativeSessionStorage')
->withArgument(new RawArgument(array(
//"cookie_secure" => "0", // Set to "1" if using HTTPS
"cookie_lifetime" => "0", // Until user closes the browser
"gc_maxlifetime" => "7200", // Activity timeout 2hrs
"name" => "Pico", // Session cookie name
"cookie_httponly" => "1",
)));
// *****************************************************************************
// PicoAuth modules
$container->share('LocalAuth', 'PicoAuth\Module\Authentication\LocalAuth\LocalAuth')
->withArgument('PicoAuth')
->withArgument('session')
->withArgument('LocalAuth.storage')
->withArgument('RateLimit');
$container->share('OAuth', 'PicoAuth\Module\Authentication\OAuth')
->withArgument('PicoAuth')
->withArgument('session')
->withArgument('OAuth.storage')
->withMethodCall('setLogger', ['logger']);
$container->share('PageACL', 'PicoAuth\Module\Authorization\PageACL')
->withArgument('PicoAuth')
->withArgument('session')
->withArgument('PageACL.storage');
$container->share('PageLock', 'PicoAuth\Module\Authorization\PageLock')
->withArgument('PicoAuth')
->withArgument('session')
->withArgument('PageLock.storage')
->withArgument('RateLimit');
$container->share('Installer', 'PicoAuth\Module\Generic\Installer')
->withArgument('PicoAuth');
// Storage
$container->share('LocalAuth.storage', 'PicoAuth\Storage\LocalAuthFileStorage')
->withArgument('configDir')
->withArgument('cache');
$container->share('OAuth.storage', 'PicoAuth\Storage\OAuthFileStorage')
->withArgument('configDir')
->withArgument('cache');
$container->share('PageACL.storage', 'PicoAuth\Storage\PageACLFileStorage')
->withArgument('configDir')
->withArgument('cache');
$container->share('PageLock.storage', 'PicoAuth\Storage\PageLockFileStorage')
->withArgument('configDir')
->withArgument('cache');
$container->share('RateLimit.storage', 'PicoAuth\Storage\RateLimitFileStorage')
->withArgument('configDir')
->withArgument('cache');
// Password hashing options
$container->add('bcrypt', 'PicoAuth\Security\Password\Encoder\BCrypt');
$container->add('argon2i', 'PicoAuth\Security\Password\Encoder\Argon2i');
$container->add('plain', 'PicoAuth\Security\Password\Encoder\Plaintext');
// Rate limiting
$container->share('RateLimit', 'PicoAuth\Security\RateLimiting\RateLimit')
->withArgument('RateLimit.storage')
->withMethodCall('setLogger', ['logger']);
// LocalAuth extensions
$container->share('PasswordReset', 'PicoAuth\Module\Authentication\LocalAuth\PasswordReset')
->withArgument('PicoAuth')
->withArgument('session')
->withArgument('LocalAuth.storage')
->withArgument('RateLimit')
// ->withMethodCall('setMailer',['mailer'])
->withMethodCall('setLogger', ['logger']);
$container->share('Registration', 'PicoAuth\Module\Authentication\LocalAuth\Registration')
->withArgument('PicoAuth')
->withArgument('session')
->withArgument('LocalAuth.storage')
->withArgument('RateLimit')
->withMethodCall('setLogger', ['logger']);
$container->share('EditAccount', 'PicoAuth\Module\Authentication\LocalAuth\EditAccount')
->withArgument('PicoAuth')
->withArgument('session')
->withArgument('LocalAuth.storage');
return $container;
<file_sep>/tests/Module/Authentication/OAuthTest.php
<?php
namespace PicoAuth\Module\Authentication;
use PicoAuth\Storage\Interfaces\OAuthStorageInterface;
use PicoAuth\Session\SessionInterface;
use PicoAuth\PicoAuthInterface;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\HttpFoundation\ParameterBag;
use League\Container\Container;
use PicoAuth\Storage\Configurator\LocalAuthConfigurator;
use League\OAuth2\Client\Provider\Exception\IdentityProviderException;
use Symfony\Component\HttpFoundation\Session\Storage\MockArraySessionStorage;
class OAuthTest extends \PicoAuth\BaseTestCase
{
protected $oAuth;
protected $session;
protected function setUp()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$storage = new MockArraySessionStorage();
$this->session = new \PicoAuth\Session\SymfonySession($storage);
$storage = $this->createMock(OAuthStorageInterface::class);
$this->oAuth = new OAuth($picoAuth, $this->session, $storage);
}
private function getTestProvider()
{
return array(
"provider" => "\League\OAuth2\Client\Provider\GenericProvider",
"options" => array(
"clientId" => "t",
"clientSecret" => "t",
"urlAuthorize" => "https://test.com/authorize",
"urlAccessToken" => "https://test.com/oauth/token",
"urlResourceOwnerDetails" => "https://test.com/user",
),
"attributeMap" => array(
"userId" => "id",
"displayName" => "dispName",
"attr" => "attr",
),
"default" => array(
"groups" => ["testGroup","g2"],
"attributes" => ["img"=>"test.png"]
)
);
}
public function testGetName()
{
$this->assertEquals('OAuth', $this->oAuth->getName());
}
public function testGetStorage()
{
$this->assertEquals(self::get($this->oAuth, 'storage'), $this->oAuth->getStorage());
}
public function testOnPicoRequestLoginSubmission()
{
$pico = $this->createMock(\Pico::class);
$pico->expects($this->exactly(1))
->method("getPageUrl")
->willReturn("callback");
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method("isValidCSRF")
->willReturn(false);
$picoAuth->expects($this->once())
->method("redirectToLogin") //after the wrong CSRF
->withAnyParameters();
$picoAuth->expects($this->once())
->method("getPico")
->willReturn($pico);
$picoAuth->expects($this->once())
->method("redirectToPage")
->with(
$this->stringStartsWith($this->getTestProvider()["options"]["urlAuthorize"]),
null,
false
);
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(OAuthStorageInterface::class);
$storage->expects($this->once())
->method("getProviderByName")
->willReturn($this->getTestProvider());
$OAuth = new OAuth($picoAuth, $sess, $storage);
$request = $this->createMock(Request::class);
$request->request = new ParameterBag(["oauth"=>"test"]);
$request->headers = new ParameterBag(["referer"=>"http://test.com/?afterLogin=index"]);
$OAuth->handleEvent("onPicoRequest", ["login", $request]);
}
/**
* Request for provider that doesn't exist
*/
public function testOnPicoRequestBadProvider()
{
$pico = $this->createMock(\Pico::class);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->once())
->method("isValidCSRF")
->willReturn(true);
// Redirect call will abort the script, here simulated with exception
$picoAuth->expects($this->once())
->method("redirectToLogin")
->withAnyParameters()
->willThrowException(new \LogicException());
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(OAuthStorageInterface::class);
$storage->expects($this->once())
->method("getProviderByName")
->willReturn(null);
$OAuth = new OAuth($picoAuth, $sess, $storage);
$request = $this->createMock(Request::class);
$request->request = new ParameterBag(["oauth"=>"test"]);
$this->expectException(\LogicException::class);
$OAuth->onPicoRequest("login", $request);
}
public function testOnPicoRequestStateMismatch()
{
$pico = $this->createMock(\Pico::class);
$log = $this->createMock(\Psr\Log\LoggerInterface::class);
$log->expects($this->once())
->method("warning");
$picoAuth = $this->createMock(PicoAuthInterface::class);
$this->session->set("provider", "testProvider");
$this->session->set("oauth2state", "testState");
$storage = $this->createMock(OAuthStorageInterface::class);
$storage->expects($this->once())
->method("getConfiguration")
->willReturn([
"callbackPage" => "callback"
]);
$storage->expects($this->once())
->method("getProviderByName")
->with("testProvider")
->willReturn($this->getTestProvider());
$picoAuth->expects($this->any())
->method("getPico")
->willReturn($pico);
$picoAuth->expects($this->once())
->method("redirectToLogin")
->withAnyParameters()
->willThrowException(new \LogicException());
$OAuth = new OAuth($picoAuth, $this->session, $storage);
$OAuth->setLogger($log);
$_SERVER["REMOTE_ADDR"] = '10.0.0.1';
$request = $this->createMock(Request::class);
$request->request = new ParameterBag(["oauth"=>"test"]);
$request->query = new ParameterBag(["state"=>"testStateWrong"]);
$this->expectException(\LogicException::class);
$OAuth->onPicoRequest("callback", $request);
}
public function testOnPicoRequestCallbackProviderMissing()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$this->session->set("provider", "testProvider");
$this->session->set("oauth2state", "testState");
$storage = $this->createMock(OAuthStorageInterface::class);
$storage->expects($this->once())
->method("getConfiguration")
->willReturn([
"callbackPage" => "callback"
]);
$storage->expects($this->once())
->method("getProviderByName")
->with("testProvider")
->willReturn(null);
$OAuth = new OAuth($picoAuth, $this->session, $storage);
$request = $this->createMock(Request::class);
$request->request = new ParameterBag(["oauth"=>"test"]);
$request->query = new ParameterBag(["state"=>"testState"]);
$this->expectException(\RuntimeException::class);
$OAuth->onPicoRequest("callback", $request);
}
public function testInitProviderNonExistent()
{
$OAuth = $this->getMockBuilder(OAuth::class)
->setMethodsExcept(["initProvider"])
->disableOriginalConstructor()
->getMock();
$this->expectException(\RuntimeException::class);
$method=self::getMethod($OAuth, 'initProvider');
$method->invokeArgs($OAuth, [[
"provider" => "\Non\Existing\Provider",
"options" => ["redirectUri"=>""]
]]);
}
public function testInitProviderWrongClass()
{
$OAuth = $this->getMockBuilder(OAuth::class)
->setMethodsExcept(["initProvider"])
->disableOriginalConstructor()
->getMock();
$this->expectException(\RuntimeException::class);
$method=self::getMethod($OAuth, 'initProvider');
$method->invokeArgs($OAuth, [[
"provider" => self::class, //random class that is not a League\OAuth2 provider
"options" => ["redirectUri"=>""]
]]);
}
public function testLoggingEvents()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$storage = $this->createMock(OAuthStorageInterface::class);
$OAuth = new OAuth($picoAuth, $this->session, $storage);
$idpExc=new IdentityProviderException("test", 0, "");
self::set($OAuth, new self(), 'provider'); //any class, but must be set
$log = $this->createMock(\Psr\Log\LoggerInterface::class);
$log->expects($this->at(0))
->method("warning");
$log->expects($this->at(1))
->method("notice");
$log->expects($this->at(2))
->method("critical");
$OAuth->setLogger($log);
self::getMethod($OAuth, 'onStateMismatch')->invokeArgs($OAuth, []);
self::getMethod($OAuth, 'onOAuthError')->invokeArgs($OAuth, ["errCode"]);
self::getMethod($OAuth, 'onOauthResourceError')->invokeArgs($OAuth, [$idpExc]);
}
public function testSaveLoginInfo()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$storage = $this->createMock(OAuthStorageInterface::class);
$resourceOwner = $this->createMock(\League\OAuth2\Client\Provider\ResourceOwnerInterface::class);
$resourceOwner->expects($this->exactly(2))
->method("toArray")
->willReturnOnConsecutiveCalls(["dispName"=>"test"], ["attr"=>"attr value"]);
$picoAuth->expects($this->once())
->method("afterLogin");
$user = null;
$picoAuth->expects($this->once())
->method("setUser")
->with($this->callback(function ($subject) use (&$user) {
$user = $subject;
return true;
}));
$OAuth = new OAuth($picoAuth, $this->session, $storage);
self::set($OAuth, $this->getTestProvider(), 'providerConfig');
self::getMethod($OAuth, 'saveLoginInfo')->invokeArgs($OAuth, [$resourceOwner]);
$this->assertInstanceOf(\PicoAuth\User::class, $user);
$this->assertSame($this->getTestProvider()["default"]["groups"], $user->getGroups());
$this->assertEquals("test.png", $user->getAttribute("img"));
}
public function testFinishAuthentication()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$storage = $this->createMock(OAuthStorageInterface::class);
$provider = $this->createMock(\League\OAuth2\Client\Provider\GenericProvider::class);
$provider->expects($this->once())
->method("getAccessToken")
->willReturn($this->createMock(\League\OAuth2\Client\Token\AccessToken::class));
$provider->expects($this->once())
->method("getResourceOwner")
->willReturn($this->createMock(\League\OAuth2\Client\Provider\ResourceOwnerInterface::class));
$this->session->set("provider", "testProvider");
$this->session->set("oauth2state", "testState");
$OAuth = $this->getMockBuilder(OAuth::class)
->setMethods(['saveLoginInfo','onOAuthError'])
->setConstructorArgs([$picoAuth, $this->session, $storage])
->getMock();
$OAuth->expects($this->once())
->method("saveLoginInfo");
$request = $this->createMock(Request::class);
$request->request = new ParameterBag(["oauth"=>"test"]);
$request->query = new ParameterBag(["state"=>"testState", "code"=>"testCode"]);
self::set($OAuth, $provider, 'provider');
self::getMethod($OAuth, 'finishAuthentication')->invokeArgs($OAuth, [$request]);
}
public function testFinishAuthenticationErrors()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$storage = $this->createMock(OAuthStorageInterface::class);
$provider = $this->createMock(\League\OAuth2\Client\Provider\GenericProvider::class);
$provider->expects($this->once())
->method("getAccessToken")
->willThrowException(new IdentityProviderException("idpExc", 0, "'"));
$this->session->set("provider", "testProvider");
$this->session->set("oauth2state", "testState");
$OAuth = $this->getMockBuilder(OAuth::class)
->setMethods(['onOauthResourceError','onOAuthError'])
->setConstructorArgs([$picoAuth, $this->session, $storage])
->getMock();
$OAuth->expects($this->once())
->method("onOauthResourceError");
$request = $this->createMock(Request::class);
$request->request = new ParameterBag(["oauth"=>"test"]);
$request->query = new ParameterBag(["state"=>"testState", "error"=>"err"]);
self::set($OAuth, $provider, 'provider');
self::getMethod($OAuth, 'finishAuthentication')->invokeArgs($OAuth, [$request]);
}
}
<file_sep>/tests/Module/Authorization/PageACLTest.php
<?php
namespace PicoAuth\Module\Authorization;
use PicoAuth\Storage\Interfaces\PageACLStorageInterface;
use PicoAuth\Session\SessionInterface;
use PicoAuth\PicoAuthInterface;
use PicoAuth\Module\AuthModule;
class PageACLTest extends \PicoAuth\BaseTestCase
{
protected $pageACL;
protected function setUp()
{
$picoAuth = $this->createMock(PicoAuthInterface::class);
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(PageACLStorageInterface::class);
$this->pageACL = new PageACL($picoAuth, $sess, $storage);
}
/**
* @dataProvider accessTestProvider
*/
public function testCheckAccess($user, $rule, $expectedAccess)
{
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(PageACLStorageInterface::class);
$storage->expects($this->exactly(1))
->method('getRuleByURL')
->willReturn($rule);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->exactly(1))
->method('getUser')
->willReturn($user);
$pageACL = new PageACL($picoAuth, $sess, $storage);
$res = $pageACL->checkAccess("randomUrl");
$this->assertSame($expectedAccess, $res);
}
public function accessTestProvider()
{
$user = new \PicoAuth\User;
$user->setId("user1");
$user->addGroup("testGroup");
return [
[$user,[
"users" => null,
"groups" => null,
],false],
[$user,[
"users" => [],
"groups" => [],
],false],
[$user,[
"users" => ["test","u8","tester"],
"groups" => ["g1","group"],
],false],
// Match on username
[$user,[
"users" => ["test","u8","tester", "user1"],
"groups" => ["g1","group"],
],true],
// Match on group
[$user,[
"users" => ["test","u8","tester"],
"groups" => ["g1","group", "testGroup"],
],true],
// No rule exists => access allowed
[$user,null,true],
[$user,[],false],
];
}
public function testRuntimeRules()
{
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(PageACLStorageInterface::class);
$storage->expects($this->exactly(1))
->method('getRuleByURL')
->willReturn(null);
$user = new \PicoAuth\User;
$user->setId("user1");
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->exactly(1))
->method('getUser')
->willReturn($user);
$pageACL = new PageACL($picoAuth, $sess, $storage);
$pageACL->addRule("randomUrl", ["users"=>["user1"]]);
$res = $pageACL->checkAccess("randomUrl");
$this->assertTrue($res);
$this->expectException(\InvalidArgumentException::class);
$pageACL->addRule("randomUrl", 1);
}
public function testDenyAccessIfRestrictedNotAuthenticated()
{
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(PageACLStorageInterface::class);
$storage->expects($this->exactly(1))
->method('getRuleByURL')
->willReturn([]);
$user = new \PicoAuth\User;
$user->setId("user1");
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->exactly(2))
->method('getUser')
->willReturn($user);
$picoAuth->expects($this->exactly(1))
->method('redirectToLogin')
->with($this->equalTo("afterLogin=randomUrl"));
$pageACL = new PageACL($picoAuth, $sess, $storage);
$pageACL->denyAccessIfRestricted("randomUrl");
}
/**
* @runInSeparateProcess
*/
public function testDenyAccessIfRestrictedAuthenticatedButDenied()
{
$sess = $this->createMock(SessionInterface::class);
$storage = $this->createMock(PageACLStorageInterface::class);
$storage->expects($this->exactly(1))
->method('getRuleByURL')
->willReturn([]);
$user = new \PicoAuth\User;
$user->setId("user1");
$user->setAuthenticated(true);
$picoAuth = $this->createMock(PicoAuthInterface::class);
$picoAuth->expects($this->exactly(2))
->method('getUser')
->willReturn($user);
$picoAuth->expects($this->exactly(1))
->method('setRequestUrl')
->with("403");
$_SERVER['SERVER_PROTOCOL'] = "HTTP/1.1";
$pageACL = new PageACL($picoAuth, $sess, $storage);
$pageACL->denyAccessIfRestricted("randomUrl");
}
public function testGetName()
{
$this->assertEquals('pageACL', $this->pageACL->getName());
}
}
<file_sep>/tests/ContainerTest.php
<?php
namespace PicoAuth;
class ContainerTest extends \PicoAuth\BaseTestCase
{
protected $container;
protected function setUp()
{
$path = __DIR__ . '/../src/container.php';
$this->container = include $path;
}
public function testContainer()
{
$this->assertNotNull($this->container);
$this->assertTrue($this->container instanceof \League\Container\Container);
}
/**
* @dataProvider dependencyNamesProvider
*/
public function testContainerHas($name)
{
$this->assertTrue($this->container->has($name));
}
public function dependencyNamesProvider()
{
return [
["Version"],
["cache"],
["logger"],
["PasswordPolicy"],
["session"],
["session.storage"],
["Version"],
["LocalAuth"],
["OAuth"],
["PageACL"],
["PageLock"],
["Installer"],
["LocalAuth.storage"],
["OAuth.storage"],
["PageACL.storage"],
["PageLock.storage"],
["RateLimit.storage"],
["bcrypt"],
["argon2i"],
["plain"],
["RateLimit"],
["PasswordReset"],
["Registration"],
["EditAccount"],
];
}
}
<file_sep>/src/Module/AbstractAuthModule.php
<?php
namespace PicoAuth\Module;
/**
* Abstract class for plugin modules
*/
abstract class AbstractAuthModule
{
/**
* Handles an event
*
* @param string $eventName Event name
* @param array $params Event parameters
* @return mixed Call return value or NULL if not called
*/
public function handleEvent($eventName, array $params)
{
if (method_exists($this, $eventName)) {
return call_user_func_array(array($this, $eventName), $params);
}
return null;
}
/**
* Get module name
*
* @return string Module name
*/
abstract public function getName();
}
<file_sep>/src/Module/Generic/Installer.php
<?php
namespace PicoAuth\Module\Generic;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\HttpFoundation\ParameterBag;
use PicoAuth\PicoAuthInterface;
use PicoAuth\Module\AbstractAuthModule;
/**
* PicoAuth installation module
*/
class Installer extends AbstractAuthModule
{
const CONFIG_PLUGIN_KEY = 'PicoAuth';
const CONFIG_MODULES_KEY = 'authModules';
/**
* PicoAuth plugin
* @var PicoAuthInterface
*/
protected $picoAuth;
/**
* Modules supported by the installer script
* Container-registered service names
* @var array
*/
protected $modules = array(
"LocalAuth" => "LocalAuth",
"OAuth" => "OAuth",
"PageACL" => "PageACL",
"PageLock" => "PageLock"
);
/**
* Creates an installer
*
* @param PicoAuthInterface $picoAuth
*/
public function __construct(PicoAuthInterface $picoAuth)
{
$this->picoAuth = $picoAuth;
}
/**
* @inheritdoc
*/
public function getName()
{
return 'installer';
}
/**
* @inheritdoc
*/
public function onPicoRequest($url, Request $httpRequest)
{
$post = $httpRequest->request;
if ($url === "PicoAuth") {
$this->picoAuth->setRequestFile($this->picoAuth->getPluginPath() . '/content/install.md');
$this->checkServerConfiguration();
} elseif ($url === "PicoAuth/modules") {
$this->picoAuth->setRequestFile($this->picoAuth->getPluginPath() . '/content/install.md');
if ($post->has("generate")) {
$this->configGenerationAction($post);
} else {
$this->picoAuth->addOutput("installer_step", 1);
}
}
}
/**
* Gets absolute Urls for pre-installation test
*
* In order to perform checks that the server configuration does not
* allow public access to Pico configuration or content directory,
* the tested Urls are passed to Javascript, where response
* tests are performed using AJAX calls.
*
* The method assumes default locations of the config and content
* directories, as specified in the Pico's index.php file.
*/
protected function checkServerConfiguration()
{
$pico = $this->picoAuth->getPico();
// Pico config.yml file
$configDir = $pico->getBaseUrl() . basename($pico->getConfigDir());
$configFile = $configDir . "/config.yml";
// index.md file
$contentDir = $pico->getBaseUrl() . basename($pico->getConfig('content_dir'));
$indexFile = $contentDir . "/index" . $pico->getConfig('content_ext');
$urls = array(
'dir_listing' => $configDir,
'config_file' => $configFile,
'content_file' => $indexFile
);
$this->httpsTest();
$this->webRootDirsTest();
$this->picoAuth->addOutput("installer_urltest", $urls);
}
/**
* Checks if this Pico installation uses https
*
* The resulting boolean value is set as a template variable
* to be displayed by the installer.
*/
protected function httpsTest()
{
$pico = $this->picoAuth->getPico();
$url = $pico->getBaseUrl();
$res = (substr($url, 0, 8) === "https://");
$this->picoAuth->addOutput("installer_https", $res);
}
/**
* Checks content and config dir locations
*
* Ideally, both of these directories should be outside the web server
* root, which is Pico::getRootDir().
* Adds the result to the output variables for display in the template.
*/
protected function webRootDirsTest()
{
$pico = $this->picoAuth->getPico();
$webRoot = realpath($pico->getRootDir());
$configDir = realpath($pico->getConfigDir());
$contentDir = realpath($pico->getConfig('content_dir'));
// Webroot path must not be at the beginning of both of the paths
$config = (substr($configDir, 0, strlen($webRoot)) !== $webRoot);
$content = (substr($contentDir, 0, strlen($webRoot)) !== $webRoot);
$this->picoAuth->addOutput("installer_wr_config", $config);
$this->picoAuth->addOutput("installer_wr_content", $content);
}
/**
* Form submission requesting to generate the plugin configuration
*
* @param ParameterBag $post
*/
protected function configGenerationAction(ParameterBag $post)
{
//CSRF validation
if (!$this->picoAuth->isValidCSRF($post->get("csrf_token"))) {
// On a token mismatch the submission gets ignored
$this->picoAuth->addOutput("installer_step", 1);
return;
}
$this->picoAuth->addOutput("installer_step", 2);
$this->outputModulesConfiguration($post);
}
/**
* Creates a plugin configuration based on the selection
*
* @param ParameterBag $post
*/
protected function outputModulesConfiguration(ParameterBag $post)
{
$modulesClasses = array();
$modulesNames = array();
foreach ($this->modules as $key => $value) {
if ($post->has($key)) {
$modulesClasses[] = $value;
$modulesNames[] = $key;
}
}
$config = array(
self::CONFIG_PLUGIN_KEY => array(
self::CONFIG_MODULES_KEY => $modulesClasses
)
);
$yaml = \Symfony\Component\Yaml\Yaml::dump($config, 2, 4);
// Adds output to the template variables
$this->picoAuth->addOutput("installer_modules_config", $yaml);
$this->picoAuth->addOutput("installer_modules_names", $modulesNames);
}
}
<file_sep>/src/Storage/Interfaces/PageLockStorageInterface.php
<?php
namespace PicoAuth\Storage\Interfaces;
/**
* Interface for accessing PageLock configuration
*/
interface PageLockStorageInterface
{
/**
* Get configuration array
*
* @return array
*/
public function getConfiguration();
/**
* Get lock by its identifier
*
* @param string $lockId
* @return array|null Lock data array, null if not found
*/
public function getLockById($lockId);
/**
* Get lock by Pico page URL
*
* @param string $url Page url
* @return string LockID
*/
public function getLockByURL($url);
}
<file_sep>/src/Storage/Configurator/PluginConfigurator.php
<?php
namespace PicoAuth\Storage\Configurator;
use PicoAuth\Storage\Configurator\AbstractConfigurator;
/**
* The configurator for the plugin
*
* This is the generic part of the plugin's configuration contained in the Pico's
* configuration file (usually config.yml)
*/
class PluginConfigurator extends AbstractConfigurator
{
protected $config;
/**
* @inheritdoc
*/
public function validate($rawConfig)
{
$config = $this->applyDefaults($rawConfig, $this->getDefault(), 0);
$this->validateGlobalOptions($config);
return $config;
}
/**
* The default configuration contains all keys that are required.
* @return array
*/
protected function getDefault()
{
return array(
"authModules" => [
"Installer"
],
"afterLogin" => "index",
"afterLogout" => "index",
"alterPageArray" => false,
"sessionInterval" => 900,
"sessionTimeout" => 7200,
"sessionIdle" => 3600,
"rateLimit" => false,
"debug" => false,
);
}
public function validateGlobalOptions($config)
{
$this->assertArrayOfStrings($config, "authModules");
$this->assertString($config, "afterLogin");
$this->assertString($config, "afterLogout");
$this->assertBool($config, "alterPageArray");
$this->assertBool($config, "rateLimit");
$this->assertBool($config, "debug");
$this->assertIntOrFalse($config, "sessionInterval", 0);
$this->assertIntOrFalse($config, "sessionTimeout", 0);
$this->assertIntOrFalse($config, "sessionIdle", 0);
}
}
<file_sep>/tests/Storage/Configurator/OAuthConfiguratorTest.php
<?php
namespace PicoAuth\Storage\Configurator;
use PHPUnit\Framework\TestCase;
class OAuthConfiguratorTest extends TestCase
{
protected $configurator;
protected function setUp()
{
$this->configurator = new OAuthConfigurator;
}
/**
* @dataProvider badConfigurations
*/
public function testValidationErrors($rawConfig)
{
$this->expectException(ConfigurationException::class);
$this->configurator->validate($rawConfig);
}
public function badConfigurations()
{
return [
[["callbackPage"=>1]],
[["providers"=>[1=>1]]],
[["providers"=>["t"=>1]]],
[["providers"=>["t"=>[]]]],
[["providers"=>["t"=>["provider"=>""]]]],
[["providers"=>["t"=>["options"=>[]]]]],
[["providers"=>["t"=>["options"=>["clientId"=>1,"clientSecret"=>"a"]]]]],
[["providers"=>["t"=>[
"default"=>["groups"=>["a",1]],
"options"=>["clientId"=>"a","clientSecret"=>"a"]]]]],
[["providers"=>["t"=>[
"attributeMap"=>["a",1],
"options"=>["clientId"=>"a","clientSecret"=>"a"]]]]],
[["providers"=>["t"=>[
"attributeMap"=>[1,"a"],
"options"=>["clientId"=>"a","clientSecret"=>"a"]]]]],
];
}
public function testValidation()
{
$res = $this->configurator->validate(null);
$this->assertArrayHasKey("providers", $res);
$res = $this->configurator->validate(
["providers"=>["test"=>["options"=>["clientId"=>"a","clientSecret"=>"a"]]]]
);
$res = $this->configurator->validate(
["providers"=> [
"test"=>[
"provider" => "a",
"options"=>[
"clientId"=>"a",
"clientSecret"=>"a"
]
]
]]
);
$this->assertArrayHasKey("providers", $res);
}
}
<file_sep>/src/Security/RateLimiting/RateLimit.php
<?php
namespace PicoAuth\Security\RateLimiting;
use PicoAuth\Log\LoggerTrait;
use Psr\Log\LoggerAwareInterface;
use PicoAuth\Storage\Interfaces\RateLimitStorageInterface;
/**
* Rate Limiter
*/
class RateLimit implements RateLimitInterface, LoggerAwareInterface
{
use LoggerTrait;
/**
* Default net mask applied on IPv4 addresses
*/
const DEFAULT_NETMASK_IPV4 = 32;
/**
* Default net mask applied on IPv6 addresses
*/
const DEFAULT_NETMASK_IPV6 = 48;
/**
* Configuration of actions for rate limiting
*
* @var array
*/
protected $actions;
/**
* Rate limit configuration array
*
* @var array
*/
protected $config;
/**
* Storage instance
*
* @var RateLimitStorageInterface
*/
protected $storage;
/**
* An error message
*
* @see RateLimit::getError()
* @see RateLimit::setErrorMessage()
* @var string
*/
protected $errorMessage;
public function __construct(RateLimitStorageInterface $storage)
{
$this->storage = $storage;
$this->config = $this->storage->getConfiguration();
$this->actions = $this->config["actions"];
}
/**
* @inheritdoc
*/
public function action($actionName, $increment = true, $params = array())
{
// the action is not configured for rate limiting
if (!isset($this->actions[$actionName])) {
return true;
}
$configOptions = $this->actions[$actionName];
foreach ($configOptions as $blockType => $config) {
if (!($entityId = $this->getEntityId($blockType, $config, $params))) {
continue;
}
if ($increment) {
$limit = $this->incrementCounter($actionName, $blockType, $entityId, $config);
} else {
$limit = $this->getLimitFor($actionName, $blockType, $entityId);
}
// If the action is not allowed, other potential blockTypes are not evaluated
if (!$this->isAllowed($limit, $config)) {
$this->setErrorMessage($config);
return false;
}
}
return true;
}
/**
* @inheritdoc
*/
public function getError()
{
return $this->errorMessage;
}
/**
* Returns whether the entity is currently rate limited
*
* @param array $limit Limit data for the entity
* @param array $config Limit configuration
* @return boolean false if the entity is rate limited, true otherwise
*/
protected function isAllowed($limit, $config)
{
if ($limit["cnt"] >= $config["count"]) {
if (time() > $limit["ts"] + $config["blockDuration"]) {
return true;
} else {
return false;
}
} else {
return true;
}
}
/**
* Returns IP subnet string for the client
*
* The net mask applied to the IP is used from the configuration supplied
* to the method, if not present default net mask is used.
*
* @param array $config Limit configuration
* @return string IP subnet string
*/
protected function getIp($config)
{
$remoteAddr = $_SERVER['REMOTE_ADDR'];
if (filter_var($remoteAddr, FILTER_VALIDATE_IP, FILTER_FLAG_IPV4)) {
$netmask = (isset($config["netmask_IPv4"]))
? $config["netmask_IPv4"] : self::DEFAULT_NETMASK_IPV4;
} else {
$netmask = (isset($config["netmask_IPv6"]))
? $config["netmask_IPv6"] : self::DEFAULT_NETMASK_IPV6;
}
$ipSubnet = $this->getSubnet($remoteAddr, $netmask);
return $ipSubnet;
}
/**
* Applies a given netmask to an IP address
*
* Both IPv4 and IPv6 are supported and detected automatically (inet_pton).
*
* @param string $ip IP address string
* @param int $netmask IP netmask to be applied
* @return string IP string in format: IP/netmask
* @throws \InvalidArgumentException If the IP or netmask is not valid
*/
protected function getSubnet($ip, $netmask)
{
$binString = @inet_pton($ip);
if ($binString === false) {
throw new \InvalidArgumentException("Not a valid IP address.");
}
// Length of the IP in bytes (4 or 16)
$byteLen = mb_strlen($binString, "8bit");
if (!is_int($netmask) || $netmask < 0 || $netmask > $byteLen * 8) {
throw new \InvalidArgumentException("Not a valid netmask.");
}
for ($byte = $byteLen - 1; ($byte + 1) * 8 > $netmask; --$byte) {
// Bitlength of a mask for the current byte
$maskLen = min(8, ($byte + 1) * 8 - $netmask);
// Create the byte mask of maskLen bits
$mask = (~((1 << $maskLen) - 1)) & 0xff;
// Export byte as 'unsigned char' and apply the mask
$maskedByte = $mask & unpack('C', $binString[$byte])[1];
$binString[$byte] = pack('C', $maskedByte);
}
return inet_ntop($binString) . '/' . $netmask;
}
/**
* Gets an entity identifier
*
* Returns a string that will identify the entity
* in the rate limiting process. It can be a hash of a username/email,
* or IP subnet of a size defined by the configuration.
*
* md5 is applied on a user-supplied input (email, username),
* collisions or reversibility do not present security concerns.
*
* @param string $blockType Blocking type (account,email,ip)
* @param array $config Configuration array for the specific rate limit
* @param array $params Additional parameters from the action() call
* @return string Entity id
*/
protected function getEntityId($blockType, $config, $params = array())
{
$entityId = null;
if ($blockType === "account" && isset($params["name"])) {
$entityId = md5($params["name"]);
} elseif ($blockType === "email" && isset($params["email"])) {
$entityId = md5($params["email"]);
} elseif ($blockType === "ip") {
$entityId = $this->getIp($config);
}
return $entityId;
}
/**
* Retrieves a limit array for a given action,type,id
*
* @param string $actionName Action id (e.g. "login")
* @param string $blockType Block type (e.g. "ip")
* @param string $entityId Entity identifier
* @return array Limit data array
*/
protected function getLimitFor($actionName, $blockType, $entityId)
{
$limit = $this->storage->getLimitFor($actionName, $blockType, $entityId);
if ($limit === null) {
$limit = array("ts" => 0, "cnt" => 0);
}
return $limit;
}
/**
* Increments a counter for the specified limit
*
* @param string $actionName Action id
* @param string $blockType Block type
* @param string $entityId Entity identifier
* @param array $config Limit configuration array
* @return array Limit data array before the increment
*/
protected function incrementCounter($actionName, $blockType, $entityId, $config)
{
// Begin an exclusive transaction
$this->storage->transaction($actionName, $blockType, RateLimitStorageInterface::TRANSACTION_BEGIN);
$limit = $this->getLimitFor($actionName, $blockType, $entityId);
$time = time();
$resetCounter =
// Counter reset after specified timeout since the last action
($time > $limit["ts"] + $config["counterTimeout"]) ||
// Counter reset after blockout delay timeout
($limit["cnt"] >= $config["count"] && $time > $limit["ts"] + $config["blockDuration"]);
if ($resetCounter) {
$limit["cnt"] = 0;
}
$limitBeforeIncrement = $limit;
// The limit will be reached with this increment
if ($limit["cnt"] === $config["count"] - 1) {
$this->logRateLimitReached($actionName, $blockType, $entityId, $config);
}
++$limit["cnt"];
$limit["ts"] = $time;
// Update the limit if the entity is not blocked
if ($limit["cnt"] <= $config["count"]) {
$this->storage->updateLimitFor($actionName, $blockType, $entityId, $limit);
if (rand(0, 100) <= $this->config["cleanupProbability"]) {
$this->storage->cleanup($actionName, $blockType, $config);
}
$this->storage->save($actionName, $blockType);
}
// Close the transaction
$this->storage->transaction($actionName, $blockType, RateLimitStorageInterface::TRANSACTION_END);
// Returns the limit array before the increment
return $limitBeforeIncrement;
}
/**
* Logs that the rate limit was reached
*
* @param string $actionName
* @param string $blockType
* @param string $entityId
* @param array $config
*/
protected function logRateLimitReached($actionName, $blockType, $entityId, $config)
{
$this->getLogger()->notice(
"Rate limit of {cnt} reached: {action} for {entity} ({type}).",
array('cnt' => $config["count"], 'action' => $actionName, 'entity' => $entityId, 'type' => $blockType)
);
}
/**
* Sets the error message that will be retrievable via {@see RateLimit::getError()}
*
* @param array $config Limit configuration
*/
protected function setErrorMessage($config)
{
if (isset($config["errorMsg"])) {
$msg = $config["errorMsg"];
} else {
$msg = "Rate limit exceeded, wait %min% minutes.";
}
$replace = array(
"%min%" => intval(ceil($config["blockDuration"] / 60)),
"%cnt%" => $config["count"],
);
$this->errorMessage = str_replace(array_keys($replace), $replace, $msg);
}
}
<file_sep>/src/Security/CSRF.php
<?php
namespace PicoAuth\Security;
/**
* PicoAuth CSRF token manager
*
* The token consists of two concatenated parts:
* key and HMAC-SHA256(realToken,key)
*
* The realToken is stored in the session under the action identifier.
* Together with realToken there is also a token generation timestamp
* for evaluating expired tokens.
*
* The above method allows for different but still valid output strings,
* which makes it impossible to reveal using BREACH attack (if HTTP compression
* is used, and the site reflects user input) if it is not
* prevented by other means. [1]
*
* 1. <NAME>; <NAME>; <NAME>.
* BREACH: reviving the CRIME attack. Unpublished manuscript, 2013.
*/
class CSRF
{
/**
* Length of the random part of the token in bytes
*/
const TOKEN_SIZE = 20;
/**
* Default action index when generic token is requested
*/
const DEFAULT_SELECTOR = '_';
/**
* Character used to split token parts
*/
const TOKEN_DELIMTER = '.';
/**
* Seconds of token validity
*
* Used as a default value if not specified explicitely in {@see CSRF::checkToken()}
*/
const TOKEN_VALIDITY = 3600;
/**
* Session index for the CSRF manager
*/
const SESSION_KEY = 'CSRF';
/**
* Session manager
* @var \PicoAuth\Session\SessionInterface
*/
protected $session;
/**
* Constructs CSRF manager with the provided session storage
* @param \PicoAuth\Session\SessionInterface $session
*/
public function __construct(\PicoAuth\Session\SessionInterface $session)
{
$this->session = $session;
}
/**
* Retrieve a token for the specified action
* @param string $action An action the token is associated with
* @param bool $reuse Is the token valid for multiple submissions
* @return string Token string
*/
public function getToken($action = null, $reuse = true)
{
$tokenStorage = $this->session->get(self::SESSION_KEY, []);
$index = ($action) ? $action : self::DEFAULT_SELECTOR;
if (!isset($tokenStorage[$index])) {
$token = bin2hex(random_bytes(self::TOKEN_SIZE));
$tokenStorage[$index] = array(
'time' => time(),
'token' => $token
);
} else {
// Token already exists and is not expired
$token = $tokenStorage[$index]['token'];
// Update token time
$tokenStorage[$index]['time'] = time();
}
$tokenStorage[$index]['reuse'] = $reuse;
$key = bin2hex(random_bytes(self::TOKEN_SIZE));
$tokenHMAC = $this->tokenHMAC($token, $key);
$this->session->set(self::SESSION_KEY, $tokenStorage);
return $key . self::TOKEN_DELIMTER . $tokenHMAC;
}
/**
* Token validation
* @param string $string token string to be validated
* @param string $action action the validated token is associated with
* @return boolean true on successful validation
*/
public function checkToken($string, $action = null, $tokenValidity = null)
{
// Get token data from session
$index = ($action) ? $action : self::DEFAULT_SELECTOR;
$tokenStorage = $this->session->get(self::SESSION_KEY, []);
if (!isset($tokenStorage[$index])) {
return false;
}
$tokenData = $tokenStorage[$index];
// Check token expiration
if ($this->isExpired($tokenData, $tokenValidity)) {
$this->ivalidateToken($index, $tokenStorage);
return false;
}
// Check correct format of received token
$parts = explode(self::TOKEN_DELIMTER, $string);
if (count($parts) !== 2) {
return false;
}
// Validate the token
$trueToken = $tokenData['token'];
$key = $parts[0]; // A key used to create a keyed hash of the real token
$tokenHMAC = $parts[1]; // Keyed hash of the real token
$trueTokenHMAC = $this->tokenHMAC($trueToken, $key);
$isValid = \hash_equals($trueTokenHMAC, $tokenHMAC);
// Remove if it is a one-time token
if ($isValid && !$tokenData['reuse']) {
$this->ivalidateToken($index, $tokenStorage);
}
return $isValid;
}
/**
* Invalidates tokens for all actions
* Should be called after any authentication
*/
public function removeTokens()
{
$this->session->remove(self::SESSION_KEY);
}
/**
* Checks token time validity
* @param array $tokenData
* @return bool true if token is expired (is after validity)
*/
protected function isExpired(array $tokenData, $tokenValidity = null)
{
return time() >
$tokenData['time'] + (($tokenValidity!==null) ? $tokenValidity : self::TOKEN_VALIDITY);
}
/**
* The real token is used as a key in the hash_hmac to create a keyed
* hash of the $key
* @param string $token
* @param string $key
* @return string
*/
protected function tokenHMAC($token, $key)
{
return hash_hmac('sha256', $key, $token, false);
}
/**
* Invalidates the specific token by unsetting it from the tokenStorage
* array in the session.
* @param string $index Token index
* @param array $tokenStorage Token storage array with all tokens
*/
protected function ivalidateToken($index, array &$tokenStorage)
{
unset($tokenStorage[$index]);
$this->session->set(self::SESSION_KEY, $tokenStorage);
}
}
<file_sep>/src/PicoAuthPlugin.php
<?php
namespace PicoAuth;
use Symfony\Component\HttpFoundation\Request;
use PicoAuth\Storage\Configurator\PluginConfigurator;
use PicoAuth\Security\CSRF;
use PicoAuth\PicoAuthInterface;
use PicoAuth\User;
use PicoAuth\Utils;
/**
* The main PicoAuth plugin class
*
* @author <NAME>
* @link https://github.com/picoauth/picoauth
* @license http://opensource.org/licenses/MIT The MIT License
* @version 1.0
*/
class PicoAuthPlugin implements PicoAuthInterface
{
/**
* PicoAuth plugin version
* @var string
*/
const VERSION = '1.0.0';
/**
* PicoAuth version ID
* @var int
*/
const VERSION_ID = 10000;
/**
* The name of the plugin
* @var string
*/
const PLUGIN_NAME = 'PicoAuth';
/**
* Action name for the logout action
* @var string
*/
const LOGOUT_CSRF_ACTION = 'logout';
/**
* Session manager for the plugin
* @var Session\SessionInterface
*/
protected $session;
/**
* CSRF Token manager
* @var CSRF
*/
protected $csrf;
/**
* Dependency container of the plugin
* @var \League\Container\Container
*/
protected $container;
/**
* Optional instance of a logger
* @see PicoAuth::initLogger()
* @var \Psr\Log\LoggerInterface
*/
protected $logger;
/**
* The current user
* @see PicoAuthPlugin::getUser()
* @see PicoAuthPlugin::getUserFromSession()
* @var User
*/
protected $user;
/**
* The current request
* @var \Symfony\Component\HttpFoundation\Request
*/
protected $request;
/**
* Pico page id of the current request
* @var string
*/
protected $requestUrl;
/**
* Requested .md file that will be displayed
* @see PicoAuthPlugin::onRequestFile()
* @var null|string
*/
protected $requestFile = null;
/**
* Plugin's configuration from Pico's main configuration file
* @var array
*/
protected $config;
/**
* Loaded PicoAuth modules
* @var Module\AbstractAuthModule[]
*/
protected $modules = array();
/**
* Output variables that will be accessible in Twig
* @var array
*/
protected $output = array();
/**
* Error flag, if true the plugin will display an error page
* @see PicoAuthPlugin::errorHandler()
* @var bool
*/
protected $errorOccurred = false;
/**
* Full path of the location of PicoAuth plugin
* @var string
*/
protected $pluginDir;
/**
* PicoCMS instance
* @var \Pico
*/
protected $pico;
/**
* Always allowed routes
* @see PicoAuthPlugin::addAllowed()
* @var string[]
*/
protected $alwaysAllowed = ["login", "logout"];
/**
* Creates the plugin
* @param \Pico $pico PicoCMS instance
*/
public function __construct(\Pico $pico)
{
$this->pico = $pico;
$this->pluginDir = dirname(__DIR__);
}
/**
* Handles an event that was triggered by Pico
*
* @param string $eventName Name of the Pico event
* @param array $params Event parameters
*/
public function handleEvent($eventName, array $params)
{
if (method_exists($this, $eventName)) {
call_user_func_array(array($this, $eventName), $params);
}
}
/**
* Triggers an auth event for all enabled Auth modules
*
* @param string $eventName
* @param array $params
*/
public function triggerEvent($eventName, array $params = array())
{
foreach ($this->modules as $module) {
$module->handleEvent($eventName, $params);
}
}
//-- Pico API registered methods ------------------------------------------
/**
* Pico API event - onConfigLoaded
*
* Validates plugin's configuration from the Pico's configuration file,
* fills it with default values, saves it to the config property.
* Initializes the plugin's dependency container.
*
* @param array $config CMS configuration
*/
public function onConfigLoaded(array &$config)
{
$config[self::PLUGIN_NAME] = $this->loadDefaultConfig($config);
$this->config = $config[self::PLUGIN_NAME];
$this->createContainer();
$this->initLogger();
}
/**
* Pico API event - onRequestUrl
*
* Runs active authentication and authorization modules.
*
* @param string $url Pico page id (e.g. "index" or "sub/page")
*/
public function onRequestUrl(&$url)
{
$this->requestUrl = $url;
try {
// Plugin initialization
$this->init();
// Check submissions in all modules and apply their routers
$this->triggerEvent('onPicoRequest', [$url, $this->request]);
} catch (\Exception $e) {
$this->errorHandler($e, $url);
}
if (!$this->errorOccurred) {
$this->authRoutes();
}
}
/**
* Pico API event - onRequestFile
*
* The plugin will change the requested file if the requested page
* is one of the plugin's pages or if it has been set by one of the plugin's
* modules in {@see PicoAuth::setRequestFile()}.
*
* @param string $file Reference to the file name Pico will load.
*/
public function onRequestFile(&$file)
{
// A special case for an error state of the plugin
if ($this->errorOccurred) {
$file = $this->requestFile;
return;
}
try {
// Resolve a normalized version of the url
$realUrl = ($this->requestFile) ? $this->requestUrl : $this->resolveRealUrl($file);
// Authorization
if (!in_array($realUrl, $this->alwaysAllowed, true)) {
$this->triggerEvent('denyAccessIfRestricted', [$realUrl]);
}
} catch (\Exception $e) {
$realUrl = (isset($realUrl)) ? $realUrl : "";
$this->errorHandler($e, $realUrl);
}
if ($this->requestFile) {
$file = $this->requestFile;
} else {
switch ($this->requestUrl) {
case 'login':
$file = $this->pluginDir . '/content/login.md';
break;
case 'logout':
$file = $this->pluginDir . '/content/logout.md';
break;
}
}
}
/**
* Pico API event - onPagesLoaded
*
* Removes pages that should not be displayed in the menus
* (for example the 403 page) from the Pico's page array.
* If "alterPageArray" plugin configuration is enabled, the pages with
* restricted access are also removed from the page array.
*
* @param array $pages Pico page array
*/
public function onPagesLoaded(array &$pages)
{
unset($pages["403"]);
if (!$this->config["alterPageArray"]) {
return;
}
// Erase all pages if an error occurred
if ($this->errorOccurred) {
$pages = array();
return;
}
foreach ($pages as $id => $page) {
try {
$allowed = $this->checkAccess($id);
} catch (\Exception $e) {
$this->errorHandler($e, $this->requestUrl);
$pages = array();
return;
}
if (!$allowed) {
unset($pages[$id]);
}
}
}
/**
* Pico API event - onTwigRegistered
*
* Registers CSRF functions in Pico's Twig environment.
*
* @param \Twig_Environment $twig Reference to the twig environment
*/
public function onTwigRegistered(&$twig)
{
// If a theme is not found, it will be searched for in PicoAuth/theme
$twig->getLoader()->addPath($this->pluginDir . '/theme');
$this_instance = $this;
$twig->addFunction(
new \Twig_SimpleFunction(
'csrf_token',
function ($action = null) use (&$this_instance) {
return $this_instance->csrf->getToken($action);
},
array('is_safe' => array('html'))
)
);
$twig->addFunction(
new \Twig_SimpleFunction(
'csrf_field',
function ($action = null) use (&$this_instance) {
return '<input type="hidden" name="csrf_token" value="'
. $this_instance->csrf->getToken($action)
. '">';
},
array('is_safe' => array('html'))
)
);
}
/**
* Pico API event
*
* Makes certain variables accessible in the twig templates
*
* @param string $templateName Name of the twig template file
* @param array $twigVariables Twig variables
*/
public function onPageRendering(&$templateName, array &$twigVariables)
{
$twigVariables['auth']['plugin'] = $this;
$twigVariables['auth']['vars'] = $this->output;
// Variables useful only in successful execution
if (!$this->errorOccurred) {
$twigVariables['auth']['user'] = $this->user;
// Previous form submission
$old = $this->session->getFlash('old');
if (count($old) && isset($old[0])) {
$twigVariables['auth']['old'] = $old[0];
}
}
}
//-- PicoAuthInterface public methods avaialble to Auth modules ------------
/**
* {@inheritdoc}
*/
public function getContainer()
{
return $this->container;
}
/**
* {@inheritdoc}
*/
public function getAuthConfig($key, $default = null)
{
return isset($this->config[$key]) ? $this->config[$key] : $default;
}
/**
* {@inheritdoc}
*/
public function getUser()
{
return $this->user;
}
/**
* {@inheritdoc}
*/
public function getPluginPath()
{
return $this->pluginDir;
}
/**
* {@inheritdoc}
*/
public function getDefaultThemeUrl()
{
return $this->pico->getBaseUrl() . 'plugins/PicoAuth/theme';
}
/**
* {@inheritdoc}
*/
public function getModule($name)
{
if (isset($this->modules[$name])) {
return $this->modules[$name];
}
return null;
}
/**
* {@inheritdoc}
*/
public function getFlashes()
{
$types = array("error", "success");
$result = array();
foreach ($types as $value) {
$flashesArr = $this->session->getFlash($value);
if (count($flashesArr)) {
$result[$value] = $flashesArr;
}
}
return $result;
}
/**
* {@inheritdoc}
*/
public function getPico()
{
return $this->pico;
}
/**
* {@inheritdoc}
*/
public function setUser($user)
{
if ($user->getAuthenticated()) {
$user->addGroup("default");
}
$this->user = $user;
}
/**
* {@inheritdoc}
*
* Will not take effect if invoked after onRequestFile Pico event.
* After that, the file is already read by Pico.
*/
public function setRequestUrl($url)
{
$this->requestUrl = $url;
$this->requestFile = $this->pico->resolveFilePath($url);
}
/**
* {@inheritdoc}
*/
public function setRequestFile($file)
{
$this->requestFile = $file;
}
/**
* {@inheritdoc}
*/
public function addOutput($key, $value)
{
$this->output[$key] = $value;
}
/**
* {@inheritdoc}
*/
public function addAllowed($url)
{
$this->alwaysAllowed[] = $url;
}
/**
* {@inheritdoc}
*/
public function isValidCSRF($token, $action = null)
{
if (!$this->csrf->checkToken($token, $action)) {
$this->logger->warning(
"CSRFt mismatch: for {action} from {addr}",
array(
"action" => $action,
"addr" => $_SERVER['REMOTE_ADDR']
)
);
$this->session->addFlash("error", "Invalid CSRF token, please try again.");
return false;
} else {
return true;
}
}
/**
* {@inheritdoc}
*/
public function afterLogin()
{
$this->csrf->removeTokens();
// Migrate session to a new ID to prevent fixation
$this->session->migrate(true);
// Set authentication information
$this->session->set('user', serialize($this->user));
$this->logger->info(
"Login: {id} ({name}) via {method} from {addr}",
array(
"id" => $this->user->getId(),
"name" => $this->user->getDisplayName(),
"method" => $this->user->getAuthenticator(),
"addr" => $_SERVER['REMOTE_ADDR']
)
);
$this->triggerEvent("afterLogin", [$this->user]);
$afterLogin = Utils::getRefererQueryParam($this->request->headers->get("referer"), "afterLogin");
if ($afterLogin && Utils::isValidPageId($afterLogin)) {
$this->redirectToPage($afterLogin);
} elseif ($this->session->has("afterLogin")) {
$page = $this->session->get("afterLogin");
$this->session->remove("afterLogin");
$this->redirectToPage($page);
}
//default redirect after login
$this->redirectToPage($this->config["afterLogin"]);
}
/**
* {@inheritdoc}
*/
public function redirectToPage($url, $query = null, $picoOnly = true)
{
$finalUrl = "/";
if ($picoOnly) {
$append = "";
if ($query) {
if (!is_string($query)) {
throw new \InvalidArgumentException("Query must be a string.");
}
$rewrite = $this->getPico()->isUrlRewritingEnabled();
$urlChar = ($rewrite) ? '?' : '&';
$append .= $urlChar . $query;
}
$finalUrl = $this->pico->getPageUrl($url) . $append;
} else {
$finalUrl = $url;
}
header('Location: ' . $finalUrl);
exit();
}
/**
* {@inheritdoc}
*/
public function redirectToLogin($query = null, $httpRequest = null)
{
/* Attempt to extract afterLogin param from the request referer.
* Login form submissions are sent to /login (without any GET query params)
* So in case of unsuccessful login attempt the afterLogin information would be lost.
*/
if ($httpRequest && $httpRequest->headers->has("referer")) {
$referer = $httpRequest->headers->get("referer");
$page = Utils::getRefererQueryParam($referer, "afterLogin");
if (Utils::isValidPageId($page)) {
$query .= ($query ? '&' : '') . "afterLogin=" . $page;
}
}
$this->redirectToPage("login", $query);
}
//-- Protected methods to PicoAuth -----------------------------------------
/**
* Resolves a given content file path to the pico url
*
* Used for obtaining a unique url for a page file. This is required
* for authorization as the $url pico provides in onRequest is not unique
* and can have many equivalents (e.g. /pico/?page vs /pico/?./page).
* Case sensitivity is the same as returned from realpath().
*
* The naming rules follow Pico-defined standards:
* /content/index.md => ""
* /content/sub/page.md => "sub/page"
* /content/sub/index.md => "sub"
*
* Example: pico content_dir is /var/pico/content
* then file name /var/pico/content/sub/page.md returns sub/page
*
* @param string $fileName Pico page file path (from onRequestFile event)
* @return string Resolved page url
* @throws \RuntimeException If the filepath cannot be resolved to a url
*/
protected function resolveRealUrl($fileName)
{
$fileNameClean = str_replace("\0", '', $fileName);
$realPath = realpath($fileNameClean);
if ($realPath === false) {
// the page doesn't exist or realpath failed
return $this->requestUrl;
}
// Get Pico content path and file extension
$contentPath = realpath($this->pico->getConfig('content_dir'));
$contentExt = $this->pico->getConfig('content_ext');
if (strpos($realPath, $contentPath) !== 0) {
// The file is not inside the content path (symbolic link)
throw new \RuntimeException("The plugin cannot be used with "
. "symbolic links inside the content directory.");
}
// Get a relative path of $realPath from inside the $contentPath and remove an extension
// len+1 to remove trailing path delimeter, which $contentPath doesn't have
$name = substr($realPath, strlen($contentPath)+1, -strlen($contentExt));
// Always use forward slashes
if (DIRECTORY_SEPARATOR !== '/') {
$name = str_replace(DIRECTORY_SEPARATOR, '/', $name);
}
// If the name ends with "/index", remove it, for the main page returns ""
if (strlen($name) >= 5 && 0 === substr_compare($name, "index", -5)) {
$name= rtrim(substr($name, 0, -5), '/');
}
return $name;
}
/**
* Initializes the main plugin components
*/
protected function init()
{
$this->loadModules();
$this->session = $this->container->get('session');
$this->csrf = new CSRF($this->session);
$this->user = $this->getUserFromSession();
$this->request = Request::createFromGlobals();
// Auto regenerate_id on specified intervals
$this->sessionTimeoutCheck("sessionInterval", "_migT", false);
// Enforce absolute maximum duration of a session
$this->sessionTimeoutCheck("sessionTimeout", "_start", true);
// Invalidate session if it is idle for too long
$this->sessionTimeoutCheck("sessionIdle", "_idle", true, true);
}
/**
* Checks the session timeouts
*
* Checks multiple session timeouts and applies the appropriate
* actions, see the usage in {@see PicoAuthPlugin::init()}
*
* @param string $configKey The configuration key containing the timeout value
* @param string $sessKey The session key with the deciding timestamp
* @param bool $clear If set to true, the session will be destroyed (invalidate)
* If set to false, the session will be migrated (change sessid)
* @param bool $alwaysUpdate If set to true, the timestamp in the session (under $sessKey)
* will be updated on every call, otherwise only on timeout
*/
protected function sessionTimeoutCheck($configKey, $sessKey, $clear, $alwaysUpdate = false)
{
if ($this->config[$configKey] !== false) {
$t = time();
if ($this->session->has($sessKey)) {
if ($this->session->get($sessKey) < $t - $this->config[$configKey]) {
if ($clear) {
$this->session->invalidate();
} else {
$this->session->migrate(true);
}
$this->session->set($sessKey, $t);
} elseif ($alwaysUpdate) {
$this->session->set($sessKey, $t);
}
} else {
$this->session->set($sessKey, $t);
}
}
}
/**
* Applies a default plugin configuration to the Pico config file.
*
* @param array $config Configuration of Pico CMS; plugin's specific
* configuration is under PLUGIN_NAME index.
*/
protected function loadDefaultConfig(array $config)
{
$configurator = new PluginConfigurator;
$validConfig = $configurator->validate(
isset($config[self::PLUGIN_NAME]) ? $config[self::PLUGIN_NAME] : null
);
return $validConfig;
}
/**
* Creates PicoAuth's dependency container instance.
*
* The plugin loads a default container definition from src/container.php
* If there is a container.php in plugin configuration directory it is used
* instead.
*
* @throws \RuntimeException if the user provided invalid container.php
*/
protected function createContainer()
{
$configDir = $this->pico->getConfigDir();
$userContainer = $configDir . "PicoAuth/container.php";
// If a user provided own container definiton, it is used
if (is_file($userContainer) && is_readable($userContainer)) {
$this->container = include $userContainer;
if ($this->container === false || !($this->container instanceof \League\Container\Container)) {
throw new \RuntimeException("The container.php does not return container instance.");
}
} else {
$this->container = include $this->pluginDir . '/src/container.php';
}
// Additional container entries
$this->container->share('configDir', new \League\Container\Argument\RawArgument($configDir));
$this->container->share('PicoAuth', $this);
if (!$this->config["rateLimit"]) {
$this->container->share('RateLimit', \PicoAuth\Security\RateLimiting\NullRateLimit::class);
}
}
/**
* Logger initialization
*/
protected function initLogger()
{
try {
$this->logger = $this->container->get("logger");
} catch (\League\Container\Exception\NotFoundException $e) {
$this->logger = new \Psr\Log\NullLogger();
}
}
/**
* Loads plugin's modules defined in the configuration
*
* @throws \RuntimeException if one of the modules could not be loaded
*/
protected function loadModules()
{
foreach ($this->config["authModules"] as $name) {
try {
$instance = $this->container->get($name);
} catch (\League\Container\Exception\NotFoundException $e) {
if (!class_exists($name)) {
throw new \RuntimeException("PicoAuth module not found: " . $name);
}
$instance = new $name;
}
if (!is_subclass_of($instance, Module\AbstractAuthModule::class, false)) {
throw new \RuntimeException("PicoAuth module class must inherit from AbstractAuthModule.");
}
$name = $instance->getName();
$this->modules[$name] = $instance;
}
}
/**
* Plugin routes that are always present
*/
protected function authRoutes()
{
switch ($this->requestUrl) {
case 'login':
// Redirect already authenticated user visiting login page
if ($this->user->getAuthenticated()) {
$this->redirectToPage($this->config["afterLogin"]);
}
break;
case 'logout':
// Redirect non authenticated user to login
if (!$this->user->getAuthenticated()) {
$this->redirectToLogin();
}
$this->checkLogoutSubmission();
break;
}
}
/**
* Checks the current request for logout action
*/
protected function checkLogoutSubmission()
{
$post = $this->request->request;
if ($post->has("logout")) {
if (!$this->isValidCSRF($post->get("csrf_token"), self::LOGOUT_CSRF_ACTION)) {
$this->redirectToPage("logout");
}
$this->logout();
}
}
/**
* Logs out the current user
*/
protected function logout()
{
$oldUser = $this->user;
$this->user = new User();
// Removes all session data (and invalidates all CSRF tokens)
$this->session->invalidate();
$this->triggerEvent("afterLogout", [$oldUser]);
// After logout redirect to main page
// If user was on restricted page, 403 would appear right after logout
$this->redirectToPage($this->config["afterLogout"]);
}
/**
* Checks access to a given Pico URL
*
* @param string $url Pico Page url
* @return bool True if the url is accessible, false otherwise
*/
protected function checkAccess($url)
{
foreach ($this->modules as $module) {
if (false === $module->handleEvent('checkAccess', [$url])) {
return false;
}
}
return true;
}
/**
* Returns the current user
*
* If there is no user in the session, a blank User instance is returned
*
* @return User
*/
protected function getUserFromSession()
{
if (!$this->session->has('user')) {
return new User();
} else {
return unserialize($this->session->get('user'));
}
}
/**
* Fatal error handler
*
* If an uncaught exception raises from the auth module, an error
* page will be rendered and HTTP 500 code returned.
* The error details are logged, and if "debug: true" is set in the plugin
* configuration, the error details are displayed also on the error page.
*
* @param \Exception $e Exception from the module
* @param string $url Pico page id that was being loaded
*/
protected function errorHandler(\Exception $e, $url = "")
{
$this->errorOccurred = true;
$this->requestFile = $this->pluginDir . '/content/error.md';
if ($this->config["debug"] === true) {
$this->addOutput("_exception", (string)$e);
}
$this->logger->critical(
"Exception on url '{url}': {e}",
array(
"url" => $url,
"e" => $e
)
);
header($_SERVER['SERVER_PROTOCOL'] . ' 500 Internal Server Error', true, 500);
// Change url to prevent other plugins that use url-based routing from
// changing the request file.
$this->requestUrl="500";
}
}
<file_sep>/tests/Security/Password/PasswordTest.php
<?php
namespace PicoAuth\Security\Password;
use PHPUnit\Framework\TestCase;
class PasswordTest extends TestCase
{
public function testToString()
{
$p = new Password("test");
$this->assertSame("test", (string)$p);
$this->assertSame("test", $p->get());
}
public function testDebugOutput()
{
$p = new Password('<PASSWORD>');
ob_start();
var_dump($p);
$result = ob_get_clean();
$this->assertFalse(strpos($result, '12345'));
$this->assertRegexp('/\*hidden\*/', $result);
}
}
| 7a6a16ad5ffd7d350e4493647a2b91338db54556 | [
"Markdown",
"JavaScript",
"PHP"
]
| 95 | PHP | picoauth/picoauth | 98b3865a89bbfa8076142926914d1fca497e749b | 30d4257566f767ee596e4ed3cdcfeab5751e63fa | |
refs/heads/master | <file_sep>package pl.taskmanager.model.dto;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import javax.persistence.Column;
import javax.validation.constraints.NotBlank;
import java.time.LocalDate;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class TaskDto {
@NotBlank(message = "Title must be not blank")
private String title;
@NotBlank(message = "Message must be not blank")
@Column(columnDefinition = "text")
private String message;
// @NotBlank
@Column(name = "task_interval")
private Integer interval;
}
<file_sep>DELETE FROM role;
INSERT INTO role VALUES (1,'ROLE_USER'),(2,'ROLE_ADMIN');<file_sep>package pl.taskmanager.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import pl.taskmanager.model.Project;
import pl.taskmanager.model.Task;
import pl.taskmanager.model.dto.ProjectDto;
import pl.taskmanager.model.dto.TaskDto;
import pl.taskmanager.service.ProjectService;
import java.time.LocalDate;
@RequestMapping("/rest")
@RestController
public class ProjectController {
private ProjectService projectService;
@Autowired
public ProjectController(ProjectService projectService) {
this.projectService = projectService;
}
@PostMapping("/project/{acronim}&{description}&{dateStart}&{dateStop}")
public Project createNewProject(
@PathVariable String acronim, @PathVariable String description,
@PathVariable @DateTimeFormat(pattern = "yyyy-MM-dd") LocalDate dateStart,
@PathVariable @DateTimeFormat(pattern = "yyyy-MM-dd") LocalDate dateStop
){
return projectService.createProject(new ProjectDto(acronim, description, dateStart,dateStop));
}
@PutMapping("/project/update/{project_id}&{dateStop}")
public Project changeProjectDeadline(
@PathVariable Long project_id,
@PathVariable @DateTimeFormat(pattern = "yyyy-MM-dd") LocalDate dateStop
){
return projectService.updateProjectStopDate(project_id,dateStop);
}
@PostMapping("/task/create/{title}&{message}&{dateStart}&{interval}&{project_id}")
public Task addTaskToProject(
@PathVariable String title,
@PathVariable String message,
@PathVariable Integer interval,
@PathVariable Long project_id){
return projectService.createTask(new TaskDto(
title,message,interval),project_id);
}
@DeleteMapping("/task/delete/{task_id}")
public String deleteTaskById(
@PathVariable Long task_id){
return "Usunięto: " + projectService.removeTask(task_id);
}
@DeleteMapping("/project/delete/{project_id}")
public String deleteProjectById(
@PathVariable Long project_id){
return "Usunięto " + projectService.removeProjectRecursively(project_id);
}
}
<file_sep>package pl.taskmanager.service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.stereotype.Component;
@Component
public class AutoMailingService {
@Autowired
public JavaMailSender javaMailSender;
public void sendSimpleMessage(
String to,
String subject,
String message)
{
SimpleMailMessage simpleMailMessage = new SimpleMailMessage();
simpleMailMessage.setTo(to);
simpleMailMessage.setSubject(subject);
simpleMailMessage.setText(message);
javaMailSender.send(simpleMailMessage);
}
}
<file_sep>package pl.taskmanager.service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import pl.taskmanager.model.Comment;
import pl.taskmanager.model.Project;
import pl.taskmanager.model.Task;
import pl.taskmanager.model.User;
import pl.taskmanager.model.dto.CommentDto;
import pl.taskmanager.model.dto.ProjectDto;
import pl.taskmanager.model.dto.TaskDto;
import pl.taskmanager.model.enums.TaskStatus;
import pl.taskmanager.repository.CommentRepository;
import pl.taskmanager.repository.ProjectRepository;
import pl.taskmanager.repository.TaskRepository;
import java.util.List;
import java.time.LocalDate;
import java.util.ListIterator;
import java.util.Optional;
@Service
public class ProjectService {
private ProjectRepository projectRepository;
private TaskRepository taskRepository;
private CommentRepository commentRepository;
@Autowired
public ProjectService(ProjectRepository projectRepository, TaskRepository taskRepository, CommentRepository commentRepository) {
this.projectRepository = projectRepository;
this.taskRepository = taskRepository;
this.commentRepository = commentRepository;
}
// utwórz projekt
public Project createProject(ProjectDto projectDto) {
return projectRepository.save(
new Project(
projectDto.getAcronim(),
projectDto.getDescription(),
projectDto.getDateStart(),
projectDto.getDateStop()));
}
// zmień datę końca projektu
public Project updateProjectStopDate(Long project_id, LocalDate dateStop) {
// pytamy o obiekt projektu po id
Optional<Project> projectToUpdate = projectRepository.findById(project_id);
if (projectToUpdate.isPresent()) {
Project project = projectToUpdate.get();
// zmieniamy deadline
project.setDateStop(dateStop);
// zapisujemy na tym samym obiekcie / update
return projectRepository.save(project);
}
return new Project();
}
// dodaj nowego taska do projektu
public Task createTask(TaskDto taskDto, Long project_id) {
// obiekt taska z przypisaniem do projektu
Task task = new Task(
taskDto.getTitle(),
taskDto.getMessage(),
LocalDate.now(),
taskDto.getInterval(),
projectRepository.getOne(project_id));
return taskRepository.save(task);
}
// usuń taska z projektu
public Task removeTask(Long task_id) {
// wyszukaj task po id
Task deletedTask = taskRepository.getOne(task_id);
// usuwam obiekt
taskRepository.delete(deletedTask);
// zwracam usunięty obiekt
return deletedTask;
}
// usunięcie projektu wraz z jego taskami
public Project removeProjectRecursively(Long project_id) {
Project deletedProject = projectRepository.getOne(project_id);
projectRepository.delete(deletedProject);
return deletedProject;
}
// metoda zwracająca wszystkie projekty
public List<Project> getAllProjects() {
return projectRepository.findAll();
}
// metoda zwaracjąca liczbę wszystkich tasków
public Long countTasks() {
return taskRepository.count();
}
// metoda zwracająca projekt po id
public Project getProjectById(Long project_id) {
return projectRepository.getOne(project_id);
}
public Task getTaskById(Long task_id) {
return taskRepository.getOne(task_id);
}
public void addUserToTask(User user, Long task_id) {
// wydobycie obiektu taska po id
Task task = taskRepository.getOne(task_id);
// dodanie obiektu User do listy users w Task
List<User> users = task.getUsers();
users.add(user);
task.setUsers(users);
// update taska
taskRepository.save(task);
}
public void deleteUserFromTaskUsersList(User user, Task task) {
List<User> users = task.getUsers();
users.remove(user);
task.setUsers(users);
taskRepository.save(task);
}
public void updateTaskStatusAndInterval(Long task_id, Integer interval, TaskStatus taskStatus) {
Task task = taskRepository.getOne(task_id);
task.setInterval(interval);
task.setTaskStatus(taskStatus);
taskRepository.save(task);
}
public List<Comment> getAllCommentsByTaskId(Long task_id) {
return commentRepository.findAllByTask(taskRepository.getOne(task_id));
}
public void createComment(CommentDto commentDto, Task task, String owner) {
Comment comment = new Comment();
comment.setContent(commentDto.getContent());
comment.setTask(task);
comment.setOwner(owner);
commentRepository.save(comment);
}
public Integer percentOfClosedTasks() {
Integer noClosed = taskRepository.countAllByTaskStatus(TaskStatus.CLOSED);
Integer noAll = Math.toIntExact(taskRepository.count());
System.out.println("CLOSED: " + noClosed);
System.out.println("ALL: " + noAll);
try {
Integer result = 100 * noClosed / noAll;
return result;
} catch (ArithmeticException e) {
return 0;
}
}
public Integer percentOfClosedTasksInProject(Long project_id) {
Project project = projectRepository.getOne(project_id);
List<Task> tasks = project.getTasks();
Integer noAll = tasks.size();
Integer noClosed = 0;
for (Task task : tasks) {
if (task.getTaskStatus() == TaskStatus.CLOSED) {
noClosed++;
}
}
System.out.println("CLOSED: " + noClosed);
System.out.println("ALL: " + noAll);
try {
Integer result = 100 * noClosed / noAll;
return result;
} catch (ArithmeticException e) {
return 0;
}
}
public Integer countAllComments(){
return (int) commentRepository.count();
}
public Integer countCommentsInProject(Long project_id){
List<Task> tasks = taskRepository.findAllByProject(projectRepository.getOne(project_id));
Integer no_comments = 0;
for (Task task : tasks) {
no_comments += countCommentsInTask(task.getTask_id());
}
return no_comments;
}
public Integer countCommentsInTask(Long task_id){
return commentRepository
.findAllByTask(taskRepository.getOne(task_id)).size();
}
public List<Task> getTasksInProject(Long project_id){
return taskRepository.findAllByProject(projectRepository.getOne(project_id));
}
}
<file_sep>package pl.taskmanager.model.dto;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import pl.taskmanager.model.User;
import javax.validation.constraints.NotBlank;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class ContactDto {
@NotBlank
private String content;
// @NotBlank
private String emailTo;
}
<file_sep>package pl.taskmanager.controller.frontEndControllers;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import pl.taskmanager.model.dto.UserDto;
import pl.taskmanager.service.UserService;
import javax.validation.Valid;
import java.security.NoSuchAlgorithmException;
@Controller
public class UserControllerFrontEnd {
UserService userService;
@Autowired
public UserControllerFrontEnd(UserService userService) {
this.userService = userService;
}
@GetMapping("/registrationConfirmed/{registration_hash}")
public String registrationConfirmed(
@PathVariable String registration_hash
){
System.out.println("hash: " + registration_hash);
userService.confirmedRegistration(registration_hash);
return "redirect:/login";
}
@GetMapping("/register")
public String register(Model model){
model.addAttribute("userDto",new UserDto());
model.addAttribute("password_repeat", "");
return "register";
}
@PostMapping("/register")
public String register(
@ModelAttribute @Valid UserDto userDto,
BindingResult bindingResult,
@ModelAttribute String password_repeat,
Model model
) throws NoSuchAlgorithmException {
// błędy formularza
if (bindingResult.hasErrors()){
return "register";
}
// porwnanie haseł
if (!userDto.getPassword().equals(userDto.getPassword_repeat())){
System.out.println(userDto.getPassword());
System.out.println(userDto.getPassword_repeat());
model.addAttribute("password_error", "different passwords!");
return "register";
}
// rejestracja
userService.addUser(userDto);
return "redirect:/projects";
}
}
<file_sep>package pl.taskmanager.model;
import com.fasterxml.jackson.annotation.JsonIgnore;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import javax.persistence.*;
import javax.validation.constraints.Email;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.Pattern;
import javax.validation.constraints.Size;
import java.time.LocalDateTime;
import java.util.HashSet;
import java.util.Set;
@Entity
@Table(name = "employee")
@Data // gettery i settery
@AllArgsConstructor // konstruktor z wsztystkimi agrumentami
@NoArgsConstructor // konstruktor domyślny
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long employee_id;
// dane wprowadzane przez użytkownika
@NotBlank // NN
private String name;
@NotBlank
private String lastname;
@Email
@NotBlank
private String email;
@NotBlank
@Size(min = 6)
// @Pattern(regexp = "[A-Z]{1,}")
private String password;
// konstruktor do rejestracji użytkownika
public User(@NotBlank String name, @NotBlank String lastname, @Email @NotBlank String email, @NotBlank @Size(min = 6) @Pattern(regexp = "[A-Z]{1,}") String password) {
this.name = name;
this.lastname = lastname;
this.email = email;
this.password = <PASSWORD>;
}
// dane generowane automatycznie
private LocalDateTime registration_datetime = LocalDateTime.now();
private Boolean isActivated = false;
private String confirmation;
// realacja n:m user to role
@ManyToMany
@JoinTable( // adnotacja złączająca tabele na posdstawie id
name = "employee_role",
joinColumns = @JoinColumn(name = "employee_id"),
inverseJoinColumns = @JoinColumn(name = "role_id")
)
@JsonIgnore
private Set<Role> roles = new HashSet<>();
// metoda do dodawania roli
public void addRole(Role role){
this.roles.add(role);
}
}
<file_sep>package pl.taskmanager.controller.frontEndControllers;
import org.springframework.boot.web.servlet.error.ErrorController;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class ErrorControllerFrontEnd implements ErrorController {
@Override
public String getErrorPath() {
return "/error";
}
@GetMapping("/error")
public String errorPage(){
return "404";
}
@GetMapping("loginError")
public String loginError(){
return "loginError";
}
}
<file_sep>package pl.taskmanager.service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.stereotype.Service;
import pl.taskmanager.model.Role;
import pl.taskmanager.model.User;
import pl.taskmanager.model.dto.UserDto;
import pl.taskmanager.repository.RoleRepository;
import pl.taskmanager.repository.UserRepository;
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.List;
@Service
public class UserService {
private UserRepository userRepository;
private RoleRepository roleRepository;
private AutoMailingService autoMailingService;
@Autowired
public UserService(UserRepository userRepository, RoleRepository roleRepository, AutoMailingService autoMailingService) {
this.userRepository = userRepository;
this.roleRepository = roleRepository;
this.autoMailingService = autoMailingService;
}
// wypisz wszystkich użytkowników
public List<User> getAllUsers(){
return userRepository.findAll();
}
@Autowired
private PasswordEncoder passwordEncoder;
// rejestracja użytkownika
public User addUser(UserDto user) throws NoSuchAlgorithmException {
// utwórz obiekt User
User registered_user = new User(
user.getName(),
user.getLastname(),
user.getEmail(),
passwordEncoder.encode(user.getPassword())); // zwraca hash hasła
registered_user.addRole(roleRepository.getOne(1L));
// /registrationConfirm/email=x
// /registrationConfirm/dsadef##!@#!@$!
registered_user.setConfirmation(shaEncoder("email="+user.getEmail()));
// link z potwierdzeniem
autoMailingService.sendSimpleMessage(
user.getEmail(),
"TASK MANAGER: confirm your registration",
"https://taskmanagerpub.herokuapp.com/registrationConfirmed/"+shaEncoder("email="+user.getEmail())
);
return userRepository.save(registered_user);
}
public String shaEncoder(String text) throws NoSuchAlgorithmException {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] encodedhash = digest.digest(text.getBytes(StandardCharsets.UTF_8));
String hash = "";
for (byte b : encodedhash) {
hash += b;
}
return hash.replaceAll("-","");
}
public void confirmedRegistration(String registration_hash){
User user = userRepository.findFirstByConfirmation(registration_hash);
if(user != null){
System.out.println("POTWIERDZONE");
user.setIsActivated(true);
userRepository.save(user);
}else{
System.out.println("NIC");
}
}
// logowanie użytkownika
public String loginUser(String email, String password){
User user = userRepository.findFirstByEmailAndPassword(email,password);
if(user == null){
return "błąd logowania";
}
return "zarejestrowano: " + user.toString();
}
// metoda zwracająca obiekt roli po id roli
public Role getRoleById(Long id){
return roleRepository.getOne(id);
}
// metoda pobierająca Usera po adresie email
public User getUserByEmail(String email){
return userRepository.findFirstByEmail(email);
}
public User getUserById(Long user_id){
return userRepository.getOne(user_id);
}
}
<file_sep>package pl.taskmanager.repository;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import pl.taskmanager.model.User;
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findFirstByEmailAndPassword(String email, String password);
User findFirstByEmail(String email);
User findFirstByConfirmation(String registration_confirm);
}
<file_sep>package pl.taskmanager.repository;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import pl.taskmanager.model.Project;
@Repository
public interface ProjectRepository extends JpaRepository<Project,Long> {
}
| 4a1fca0afbe719396c002a9d9900ebe29dccf556 | [
"Java",
"SQL"
]
| 12 | Java | MikiKru/spring_group | 2f44a4db568e27eb437edcc242131d39f0f45191 | 9ae6ac7f80763fe31fb287e281266f74f3724c3c | |
refs/heads/master | <repo_name>drozzy/anoGAN<file_sep>/run-bash.sh
docker build -t ml-31156-a1 .
docker run -it -v "$PWD":/usr/src/app/src --rm ml-31156-a1 bash<file_sep>/main.py
import numpy as np
import matplotlib.pyplot as plt
from keras.datasets import mnist
from tqdm import tqdm
import anogan
def main():
(X_train, y_train), (X_test, y_test) = mnist.load_data()
X_train = X_train.astype(np.float32)/255.
X_train = X_train.reshape(60000, 28, 28, 1)
Model_d, Model_g = anogan.train(32, X_train)
if __name__ == '__main__':
main()<file_sep>/requirements.txt
python-twitter==3.4.2
numpy==1.15.2
scipy==1.1.0
matplotlib==3.0.0
ipython==7.0.1
jupyter==1.0.0
pandas==0.23.4
sympy==1.3
nose==1.3.7
statsmodels==0.9.0
seaborn==0.9.0
scikit-learn==0.20.0
tensorflow==1.11.0
keras==2.2.2
tqdm==4.28.1
neat-python==0.92 | 4c52485d24ff23356570a48cc8aad6d6b1f08bf7 | [
"Python",
"Text",
"Shell"
]
| 3 | Shell | drozzy/anoGAN | 15b205aeaca8388b541654f60af87c8216076b64 | a08d56ca2887c4671424d9a53f9fb30718eb81bf | |
refs/heads/master | <file_sep>Rails.application.routes.draw do
devise_for :users, controllers: {
registrations: 'users/registrations',
sessions: 'users/sessions',
passwords: '<PASSWORD>',
confirmations: 'users/confirmations',
unlocks: 'users/unlocks',
}
devise_scope :user do
get 'signup', to: 'users/registrations#new'
get 'login', to: 'users/sessions#new'
get 'verify', to: 'users/registrations#verify'
get 'logout', to: 'users/sessions#destroy'
end
root to: 'users#index'
get 'home/index'
# For details on the DSL available within this file, see http://guides.rubyonrails.org/routing.html
end
| c61dd45f0386a7dc8d0bdc9d19ac0e4240222542 | [
"Ruby"
]
| 1 | Ruby | hiroshi-00/devise_rails_5 | 7a15a22a1207d9f35e56be9a0011717dde1f35a9 | 41a39c6b2de3ddbc884758193fd6afebac393977 | |
refs/heads/master | <repo_name>MichaelChansn/daemaged.compression<file_sep>/build-native-linux.sh
#!/bin/bash
PROJECT_ROOT=$(readlink -f $(dirname $0))
DEFAULT_CC=gcc-5
X86_SFX="-m32"
X64_SFX="-m64"
DEFAULT_FLAGS="-O3 -flto"
LIBLZO2DIR=extsrc/lzo2
LIBLZO2OUT=liblzo2
(cd $LIBLZO2DIR && git clean -fdx && \
cp $PROJECT_ROOT/native-cmakes/CMakeLists.lzo2.txt CMakeLists.txt && \
mkdir build && cd build && \
CC="$DEFAULT_CC $X86_SFX" cmake .. -DCMAKE_BUILD_TYPE=RELEASE -DCMAKE_C_FLAGS_RELEASE="$DEFAULT_FLAGS" && make -j4 &&
cp liblzo2.so $PROJECT_ROOT/$LIBLZO2OUT/x86)
(cd $LIBLZO2DIR && git clean -fdx && \
mkdir build && cd build && \
cp $PROJECT_ROOT/native-cmakes/CMakeLists.lzo2.txt CMakeLists.txt && \
CC="$DEFAULT_CC $X64_SFX" cmake .. -DCMAKE_BUILD_TYPE=RELEASE -DCMAKE_C_FLAGS_RELEASE="$DEFAULT_FLAGS" && make -j4 &&
cp liblzo2.so $PROJECT_ROOT/$LIBLZO2OUT/x64)
LIBBZ2DIR=extsrc/bzip2
LIBBZ2OUT=libbz2
(cd $LIBBZ2DIR && git clean -fdx && \
cp $PROJECT_ROOT/native-cmakes/CMakeLists.bzip2.txt CMakeLists.txt && \
CC="$DEFAULT_CC $X86_SFX" cmake . -DCMAKE_BUILD_TYPE=RELEASE -DCMAKE_C_FLAGS_RELEASE="$DEFAULT_FLAGS" && make -j4 &&
cp libbz2.so $PROJECT_ROOT/$LIBBZ2OUT/x86)
(cd $LIBBZ2DIR && git clean -fdx && \
cp $PROJECT_ROOT/native-cmakes/CMakeLists.bzip2.txt CMakeLists.txt && \
CC="$DEFAULT_CC $X64_SFX" cmake . -DCMAKE_BUILD_TYPE=RELEASE -DCMAKE_C_FLAGS_RELEASE="$DEFAULT_FLAGS" && make -j4 &&
cp libbz2.so $PROJECT_ROOT/$LIBBZ2OUT/x64)
LZ4DIR=extsrc/lz4/cmake_unofficial
LZ4OUT=liblz4
(cd $LZ4DIR && git clean -fdx && \
cp $PROJECT_ROOT/native-cmakes/CMakeLists.lz4.txt CMakeLists.txt && \
CC="$DEFAULT_CC $X86_SFX" cmake . -DCMAKE_BUILD_TYPE=RELEASE -DCMAKE_C_FLAGS_RELEASE="$DEFAULT_FLAGS" && make -j4 && \
cp liblz4.so $PROJECT_ROOT/$LZ4OUT/x86)
(cd $LZ4DIR && git clean -fdx && \
cp $PROJECT_ROOT/native-cmakes/CMakeLists.lz4.txt CMakeLists.txt && \
CC="$DEFAULT_CC $X64_SFX" cmake . -DCMAKE_BUILD_TYPE=RELEASE -DCMAKE_C_FLAGS_RELEASE="$DEFAULT_FLAGS" && make -j4 && \
cp liblz4.so $PROJECT_ROOT/$LZ4OUT/x64)
LIBZDIR=extsrc/zlib-ng
LIBZOUT=libz
(cd $LIBZDIR && git clean -fdx && \
CC="$DEFAULT_CC $X86_SFX" cmake . -DCMAKE_BUILD_TYPE=RELEASE -DCMAKE_C_FLAGS_RELEASE="$DEFAULT_FLAGS" && make -j4 && \
cp libz.so $PROJECT_ROOT/$LIBZOUT/x86)
(cd $LIBZDIR && git clean -fdx && \
CC="$DEFAULT_CC $X64_SFX" cmake . -DCMAKE_BUILD_TYPE=RELEASE -DCMAKE_C_FLAGS_RELEASE="$DEFAULT_FLAGS" && make -j4 && \
cp libz.so $PROJECT_ROOT/$LIBZOUT/x64)
LIBLZMADIR=extsrc/xz
LIBLZMAOUT=liblzma
(cd $LIBLZMADIR && git clean -fdx && \
./autogen.sh && CC="$DEFAULT_CC $X86_SFX" CFLAGS="$DEFAULT_FLAGS" ./configure && make V=0 -j4 && \
cp src/liblzma/.libs/liblzma.so $PROJECT_ROOT/$LIBLZMAOUT/x86)
(cd $LIBLZMADIR && git clean -fdx && \
./autogen.sh && CC="$DEFAULT_CC $X64_SFX" CFLAGS="$DEFAULT_FLAGS" ./configure && make V=0 -j4 && \
cp src/liblzma/.libs/liblzma.so $PROJECT_ROOT/$LIBLZMAOUT/x64)
for o in $LIBLZO2OUT $LZ4OUT $LIBZOUT $LIBLZMAOUT $LIBBZ2OUT; do strip --strip-unneeded $o/{x86,x64}/$o.so; done
| b4ca70f0243e5c0dca4237aa53cfb5f42a215c66 | [
"Shell"
]
| 1 | Shell | MichaelChansn/daemaged.compression | eeb3ab0a7053e845c116b07de753efc439ed2378 | 6d1bd22dbd519dfdf1cb314a1573d4908c3b31fd | |
refs/heads/master | <repo_name>VanMan123/games<file_sep>/HTML/paddle.js
class Paddle {
constructor() {
this.width = 150;
this.height = 30;
}
draw(ctx) {
}
}
<file_sep>/MagicWebsite-master/Instructions.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Instructions</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<div class="container">
<link rel="stylesheet" href="HREF2.css">
<style>
td{
color:red
}
</style>
<body style=background-color:black>
<div class="container-fluid">
<ul>
<li><a href="Main.html">Home</a></li>
<li><a href="Login.html">Login</a></li>
<li><a href="Create.html">Create an Account</a></li>
<li><a class="active" href="Instructions.html">Instructions</a></li>
</ul>
<style>
body, form, head{
background-color: black;
color:white
}
</style>
<h1 style=color:white>Instructions</h1>
<h4 style=color:aquamarine>
Magic may not seem like what it is. When you go behind
the scenes things get a lot more cooler. Ever thought about
those card tricks people may show you? They all have one basic
keypoint that helps the magic stay balanced. Here is an example;
If you are doing a card trick that includes guessing what a certain card is
without looking at it then you might have depend on another card to help.
One other good trick of magic is illusion magic. Illusion magic is magic that makes something look odd
but is really just a mind trick.
</h3>
<iframe width="560" height="315" src="https://www.youtube.com/embed/VWw_1-gEdLA" frameborder="0" allow="autoplay; encrypted-media" allowfullscreen></iframe>
<table class="table table-bordered table-hover" style=color:white>
<thead>
<tr>
<th>First Name</th>
<th>Last Name</th>
<th>Magic Trick Title</th>
</tr>
</thead>
<tbody>
<tr>
<td>Bhuvan</td>
<td>Kanna</td>
<td><a href="">The Amazing Card Illusion</a></td>
</tr>
<tr>
<td>Arnav</td>
<td>Sivakumar</td>
<td><a href="">Money Madness</a></td>
</tr>
<tr>
<td>Rishi</td>
<td>Sankar</td>
<td><a href="">Coin Magic</a></td>
</tr>
</tbody>
</table>
<form>
<input style="width: 300px; padding: 20px; cursor: pointer; box-shadow: 6px 6px 5px; #999; -webkit-box-shadow: 6px 6px 5px #999; -moz-box-shadow: 6px 6px 5px #999; font-weight: bold; background: LightBlue; color: #000; border-radius: 10px; border: 1px solid #999; font-size: 150%;" type="button" value="Click to post your trick" onclick="window.location.href='Post.html'" />
</form>
<h3 style=color:cyan>
Remember, sign up for Magic.com to post your tricks, and we will add your name to the list!!
</h3>
</div>
</body>
</html>
<file_sep>/MagicWebsite-master/README.md
# Magic Website
Magic Website<br>
<a href="Main.html">Click to go to the Main Page</a>
<br>
<a href="Login.html">Click to go to the Login</a>
<br>
<a href="Create.html">Click to go to the Sign Up</a>
<br>
<a href="Instruction.html">Click to go to the Instructions</a>
<br>
<a href="Post.html">Click to go to the Posting Page</a>
<embed name="Mysterious Music" src="Diabolus.mp3" loop="true" hidden="true" autostart="true">
| bff9c920170dbd6c25327d8cb79166f7f6006548 | [
"JavaScript",
"HTML",
"Markdown"
]
| 3 | JavaScript | VanMan123/games | a26006f4a5f070cc10a28d533d6b5c3e404453f0 | 594668076ccc138a813c892c470d9d3fad5df34a | |
refs/heads/master | <file_sep>package com.DAO;
import com.model.*;
import com.converter.*;
import java.util.ArrayList;
import java.util.List;
import org.bson.types.ObjectId;
import com.mongodb.BasicDBObjectBuilder;
import com.mongodb.DBCollection;
import com.mongodb.DBCursor;
import com.mongodb.DBObject;
import com.mongodb.MongoClient;
public class MongoDBIssueDAO {
private DBCollection col;
public MongoDBIssueDAO(MongoClient mongo) {
this.col = mongo.getDB("issuetracker").getCollection("Issues");
}
public Issue createIssue(Issue p) {
System.out.println("inside createIssue");
DBObject doc = IssueConverter.toDBObject(p);
this.col.insert(doc);
ObjectId id = (ObjectId) doc.get("_id");
p.setId(id.toString());
return p;
}
public void updateIssue(Issue p) {
DBObject query = BasicDBObjectBuilder.start()
.append("_id", new ObjectId(p.getId())).get();
this.col.update(query, IssueConverter.toDBObject(p));
}
public List<Issue> readAllIssue() {
System.out.println("inside read all Issue");
List<Issue> data = new ArrayList<Issue>();
DBCursor cursor = col.find();
while (cursor.hasNext()) {
DBObject doc = cursor.next();
Issue p = IssueConverter.toIssue(doc);
System.out.println(p.getId());
System.out.println(p.getDescription());
System.out.println(p.getStatus());
System.out.println(p.getSeverity());
data.add(p);
}
System.out.println("read all success");
return data;
}
public void deleteIssue(Issue p) {
DBObject query = BasicDBObjectBuilder.start()
.append("_id", new ObjectId(p.getId())).get();
this.col.remove(query);
}
public Issue readIssue(Issue p) {
DBObject query = BasicDBObjectBuilder.start()
.append("_id", new ObjectId(p.getId())).get();
DBObject data = this.col.findOne(query);
return IssueConverter.toIssue(data);
}
}
| cb5a72798b1a77a09a012e871a45126aa098a108 | [
"Java"
]
| 1 | Java | arunjagan/IssueTrackerWithMongoLab | d23c3d5d6f547d9c3a89beaa59db64c544bf06a6 | b8a5426eb35eec33417334c9ef7471967f40428f | |
refs/heads/master | <repo_name>tpohl/callmelater<file_sep>/lib/models/taskSchema.ts
import * as mongoose from 'mongoose';
const Schema = mongoose.Schema;
export const TaskSchema = new Schema({
url: {
type: String,
required: 'Provide an URL'
},
payload: {
type: String,
required: 'Provide some payload'
},
scheduled_date: {
type: Date,
required: 'Provide a scheduled date.'
},
created_date: {
type: Date,
default: Date.now
},
retry: {
type: Number,
default: 0
}
});
export const Task = mongoose.model('Task', TaskSchema);<file_sep>/start-callmelater-app.sh
docker stop callmelaterapp
docker rm callmelaterapp
#-p 127.0.0.1:80:80
docker run -d --name callmelaterapp --link callmelaterdb:mongo --restart always \
-e "NODE_ENV=production" \
-e "VIRTUAL_HOST=callmelater.pohl.rocks" -e "PORT=80" \
-e "LETSENCRYPT_HOST=callmelater.pohl.rocks" -e "LETSENCRYPT_EMAIL=<EMAIL>" \
-e "MONGODB_URI=mongodb://mongo/tasks" flightsweb<file_sep>/lib/scheduler.ts
import * as cron from "cron";
<file_sep>/Dockerfile
FROM node:8
WORKDIR /home/app
# Install Dependencies
ADD package.json /home/app/package.json
RUN npm install
# Make everything available for start
COPY . /home/app
# Install
RUN npm run build
# Set development environment as default
ENV NODE_ENV production
ENV PORT 80
EXPOSE 80
CMD ["npm", "start"]<file_sep>/lib/routes/taskRoutes.ts
import { Request, Response } from "express";
import { TaskController } from "./taskController";
export class Routes {
public taskController: TaskController = new TaskController();
public routes(app): void {
app.route('/')
.get((req: Request, res: Response) => {
res.status(200).send({
message: 'GET request successfulll!!!!'
})
})
// Contact
app.route('/tasks')
// GET endpoint
.get((req: Request, res: Response) => {
// Get all contacts
res.status(200).send({
message: 'GET request successfulll!!!!'
})
})
// POST endpoint
.post(this.taskController.createTask)
/*
.post((req: Request, res: Response) => {
// Create new contact
res.status(200).send({
message: 'POST request successfulll!!!!'
})
})
*/
// Contact detail
app.route('/tasks/:taskId')
// get specific contact
.get(this.taskController.getTaskWithID)
.put(this.taskController.scheduleTask)
.delete(this.taskController.deleteTask)
}
}<file_sep>/start-callmelater-db.sh
docker pull mongo
docker stop callmelaterdb
docker rm callmelaterdb
docker run -d -v /root/data/callmelaterdb:/data/db --name callmelaterdb mongo<file_sep>/lib/app.ts
import * as express from "express";
import * as bodyParser from "body-parser";
import { Routes } from "./routes/taskRoutes";
import * as mongoose from "mongoose";
import * as request from "request-promise-native";
import { CronJob } from "cron";
import { Task } from "./models/taskSchema";
const MONGODB_URI = process.env.MONGODB_URI = process.env.MONGODB_URI || 'mongodb://localhost/taskDb';
const tick = function () {
console.log('Cron Tick');
Task.find({})
.where('scheduled_date').lt(new Date())
.where('retry').lt(6)
.exec((err, taskArray) => {
taskArray.forEach(task => {
console.log('Found Task', task);
request({
method: 'POST',
uri: task.url,
body: task.payload,
json: false,
headers: {
'content-type': 'text/plain'
}
}).then((result) => {
console.log('Executed Task', task);
Task.remove({ _id: task._id }, (err, t2) => {
if (err) {
console.error('Could not delete task', task)
}
});
}, err => {
console.error('Cannot Execute Task', err, task);
task.retry = task.retry ? (task.retry + 1) : 1;
Task.findOneAndUpdate({ _id: task._id }, task, { new: true }, (err, tasknew) => {
if (err) {
console.error('Adding retry failed', err, task);
}
else {
console.log('Updated retry for task', tasknew.retry, task.retry, task._id);
}
});
});
});
});
}
class App {
public app: express.Application;
public routePrv: Routes = new Routes();
public mongoUrl: string = MONGODB_URI;
private cron;
constructor() {
this.app = express();
this.config();
this.routePrv.routes(this.app);
this.mongoSetup();
tick();
}
private config(): void {
this.app.use(bodyParser.json());
this.app.use(bodyParser.urlencoded({ extended: false }));
// Schedule the Cron
this.cron = new CronJob(`*/5 * * * *`, tick).start();
}
private mongoSetup(): void {
mongoose.Promise = global.Promise;
mongoose.connect(this.mongoUrl);
}
}
export default new App().app;<file_sep>/lib/routes/taskController.ts
import { Task } from '../models/taskSchema';
import { Request, Response } from 'express';
export class TaskController {
public createTask(req: Request, res: Response) {
let newTask = new Task(req.body);
newTask.save((err, task) => {
if (err) {
res.send(err);
}
console.log('Scheduled Task', task);
res.json(task);
});
}
public getAllTasks(req: Request, res: Response) {
Task.find({}, (err, task) => {
if (err) {
res.send(err);
}
res.json(task);
});
}
public getTaskWithID(req: Request, res: Response) {
Task.findById(req.params.taskId, (err, contact) => {
if (err) {
res.send(err);
}
res.json(contact);
});
}
public scheduleTask(req: Request, res: Response) {
Task.findOneAndUpdate({ _id: req.params.taskId }, req.body, { new: true }, (err, contact) => {
if (err) {
res.send(err);
}
res.json(contact);
});
}
public deleteTask(req: Request, res: Response) {
Task.remove({ _id: req.params.taskId }, (err, contact) => {
if (err) {
res.send(err);
}
res.json({ message: 'Successfully deleted Task!' });
});
}
}
<file_sep>/build.sh
docker build -t callmelaterapp . | b2b2cea310f590fc93476e46d5aebaa455304b2a | [
"TypeScript",
"Dockerfile",
"Shell"
]
| 9 | TypeScript | tpohl/callmelater | c79baf26c1c90824bb8f2d7935db902dba13c379 | 3db18742c36dde7b1402209fd198a2effacffe63 | |
refs/heads/master | <file_sep>// no jquery, since this is injected into an uncertain environment
(function() {
function supress(event) {
if(document.getElementById('shell-container') !== null &&
document.getElementById('shell-container').style.display === 'block')
{
//event.preventDefault();
event.stopPropagation();
//event.stopImmediatePropagation();
event.cancelBubble = true;
console.debug('[feedlyconsole/inject] supressing key');
return false;
} else {
console.debug('[feedlyconsole/inject] not supressing key');
return true;
}
}
target = document.getElementById('shell-panel') || document.getElementsByTagName('body')[0];
if(target.length === 0) {
console.error('[feedlyconsole/inject] no body found');
}
console.log('[feedlyconsole/inject] supressing %O', target);
target.addEventListener('keydown', supress);
target.addEventListener('keypress', supress);
})();
console.log('[feedlyconsole/inject] injected inject.js');
/* call from content script
function inject() {
// inject after readline is initilized
$(document).ready( function() {
var file = 'inject.js';
// supress keydown, keypress when console is active
console.debug("[feedlyconsole] injecting " + file);
var script = $('<script/>', {
src: chrome.extension.getURL(file),
type: 'text/javascript'
});
script.ready( function() {
console.debug("[feedlyconsole] loaded inject.js %O", script);
});
$('head').prepend(script);
});
}
inject()
}
<file_sep>#!/usr/bin/env python
import os
from pprint import pprint as pp
import json
import shutil
def main():
source = os.path.join(os.path.expanduser("~"), "icon")
destination = "icon"
# http://openiconlibrary.sourceforge.net/downloads.html
# Creative Common Package
print "source " + source
name = "utilities-terminal-5.png"
namepart, extpart = os.path.splitext(name)
svg = "%s.svg" % namepart
sizes = (16, 22, 32, 48, 128)
info = {}
for root, dirs, files in os.walk(source):
if svg in files:
source_path = os.path.join(root, svg)
destination_path = os.path.join(destination, svg)
info['svg'] = destination_path
shutil.copyfile(source_path,
destination_path)
if name in files:
path = root.split(os.sep)
size = path[-2].split('x')[0]
if int(size) in sizes:
newname = "%s_%s%s" % (namepart, size, extpart)
pp(newname)
source_path = os.path.join(root, name)
destination_path = os.path.join(destination, newname)
info[size] = destination_path
# todo: extract tags?
#tags = exifread.process_file(open(source_path, 'rb'))
#pp(tags)
shutil.copyfile(source_path,
destination_path)
sizes = [19, 38]
source = os.path.join(destination, svg)
for size in sizes:
filename = "%s_%i%s" % (namepart, size, extpart)
destination_path = os.path.join(destination, filename)
info[size] = destination_path
os.system("convert -resample %ix%i %s %s" % (size, size, source, destination_path))
namepart = open(os.path.join(destination, "icon_info.json"), 'w')
json_info = json.dumps(info, sort_keys=True, indent=4)
print json_info
namepart.write(json_info)
if __name__ == '__main__':
main()
<file_sep>feedlyconsole
=============
Embed feedly console (based on josh.js) in feedly web client
<a href="http://dougbeal.github.io/feedlyconsole/">Pages</a>
develoment
-----------
Cakefile and jeykll pull file list from manifest.json
<file_sep><!doctype html>
<html xmlns="http://www.w3.org/1999/html">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="chrome=1">
<title>{{ site.data.manifest.name }} {% if page.test %} Mocha Test Page {% else %} Demonsration Page {% endif %}</title>
<script type="text/javascript">
Josh = {Debug: true };
</script>
{% include dependencies.html %}
{% if page.test %}{% include test_dependencies.html %}
{% endif %}
</head>
<body>
<div id="feedlyconsole">
{% include feedlyconsole_fragment.html %}
</div>
<div>
<a href="https://github.com/dougbeal/feedlyconsole">{{ site.data.manifest.name }} - feedlyconsole on github</a>
</div>
<div id='mocha'>
</div>
</body>
</html>
<file_sep># requires current sandbox code in sandbox_client_secret.txt
client_secret_filename="sandbox_client_secret.txt"
if [ ! -e "$client_secret_filename" ]; then
echo "Client secret file $client_secret_filename required."
exit -1
fi
temp_dir=$(mktemp -dt "$0")
auth_response_file=$temp_dir/auth_response_file
token_response_file=$temp_dir/token_response_file
client_secret=$(cat $client_secret_filename)
(
# need -i 1 for 1 second delay, otherwise sent before browser make request?
printf "HTTP/1.1 200 OK\r\n\r\n <html>$(date)</html?>" | nc -v -v -i 1 -n -l 8080 > $auth_response_file
echo "nc exit code $?."
)&
child_pid=$!
#curl -v -v "http://localhost:8080"
#exit
open "http://sandbox.feedly.com/v3/auth/auth?response_type=code&client_id=sandbox&client_secret=$client_secret&redirect_uri=http://localhost:8080&scope=https://cloud.feedly.com/subscriptions&provider=google"
#sandbox doesn't bypass oauth - http://localhost/?code=...&state=
# GET /?code=...&state= HTTP/1.1
wait $child_pid
if [ ! -s $auth_response_file ]; then
echo "last exit code $?."
echo "failed to auth"
exit -1
fi
code=$(grep code $auth_response_file | sed 's/.*code=\([[:alnum:]]*\).*/\1/')
curl --verbose --data "code=$code&client_id=sandbox&client_secret=$client_secret&redirect_uri=http://localhost:8080&grant_type=authorization_code" http://sandbox.feedly.com/v3/auth/token > $token_response_file
#{"plan":"standard","access_token":"...:sandbox","refresh_token":"...:sandbox","expires_in":604800,"token_type":"Bearer","id":"<PASSWORD>"}macnboss:josh.js dougbeal$
extract=( "access_token" "refresh_token" "id")
for i in ${extract[@]}; do
declare ${i}=$(grep ${i} $token_response_file | sed "s/.*\"${i}\":\"\([^\"]*\)\".*/\1/")
done
curl --verbose --header "Authorization: OAuth $access_token" http://sandbox.feedly.com/v3/tags > $temp_dir/tags
curl --verbose --header "Authorization: OAuth $access_token" http://sandbox.feedly.com/v3/categories > $temp_dir/categories
curl --verbose --header "Authorization: OAuth $access_token" http://sandbox.feedly.com/v3/subscriptions > $temp_dir/subscriptions
<file_sep>Josh = Josh || {};
Josh.Debug = true;
Josh.config = {
history: new Josh.History(),
console: window.console,
killring: new Josh.KillRing(),
readline: null,
shell: null,
pathhandler: null
};
Josh.config.readline = new Josh.ReadLine(Josh.config);
Josh.config.shell = new Josh.Shell(Josh.config);
// `Josh.PathHandler` is attached to `Josh.Shell` to provide basic file system navigation.
Josh.config.pathhandler = new Josh.PathHandler(Josh.config.shell, {console: Josh.config.console});
console.log("[feedlyconsole] loading %O", Josh);
////////////////////////////////////////////////////////////
// based on josh.js:gh-pages githubconsole
(function(root, $, _) {
Josh.FeedlyConsole = (function(root, $, _) {
// Enable console debugging, when Josh.Debug is set and there is a console object on the document root.
var _console = (Josh.Debug && window.console) ? window.console : {
log: function() {
},
debug:function() {
}
};
// Console State
// =============
//
// `_self` contains all state variables for the console's operation
var _self = {
shell: Josh.config.shell,
api_version: "v3/",
api: "unset",
OAuth: "",
ui: {},
root_commands: {}
};
_self.pathhandler = Josh.config.pathhandler;
// Custom Templates
// ================
// `Josh.Shell` uses *Underscore* templates for rendering output to the shell. This console overrides some and adds a couple of new ones for its own commands.
//
// **templates.prompt**
// Override of the default prompt to provide a multi-line prompt of the current user, repo and path and branch.
_self.shell.templates.prompt = _.template("<strong><%= node.path %> $</strong>");
// **templates.ls**
// Override of the pathhandler ls template to create a multi-column listing.
_self.shell.templates.ls = _.template("<ul class='widelist'><% _.each(nodes, function(node) { %><li><%- node.name %></li><% }); %></ul><div class='clear'/>");
// **templates.not_found**
// Override of the pathhandler *not_found* template, since we will throw *not_found* if you try to access a valid file. This is done for the simplicity of the tutorial.
_self.shell.templates.not_found = _.template("<div><%=cmd%>: <%=path%>: No such directory</div>");
//**templates.rateLimitTemplate**
// rate limiting will be added later to feedly api
_self.shell.templates.rateLimitTemplate = _.template("<%=remaining%>/<%=limit%>");
_self.shell.templates.default_template = _.template("<div><%= JSON.stringify(data) %></div>");
//**templates.profile**
// user information
_self.shell.templates.profile = _.template("<div class='userinfo'>" +
"<img src='<%=profile.picture%>' style='float:right;'/>" +
"<table>" +
"<tr><td><strong>Id:</strong></td><td><%=profile.id %></td></tr>" +
"<tr><td><strong>Email:</strong></td><td><%=profile.email %></td></tr>" +
"<tr><td><strong>Name:</strong></td><td><%=profile.fullName %></td></tr>" +
"</table>" +
"</div>"
);
// Adding Commands to the Console
// ==============================
//<section id='cmd.user'/>
function buildExecCommandHandler(command_name) {
return {
// `exec` handles the execution of the command.
exec: function(cmd, args, callback) {
get(command_name, null, function(data) {
if(!data) {
return err("api request failed to get data");
}
var template = _self.shell.templates[command_name] || _self.shell.templates.default_template;
var template_args = {};
template_args[command_name] = template_args['data'] = data;
_console.debug("[Josh.FeedlyConsole] data %O cmd %O args %O", data, cmd, args);
return callback(template(template_args));
});
}
};
}
var simple_commands = ['profile',
'tags',
'subscriptions',
'preferences',
'categories',
'topics',
//'opml'
];
function addCommandHandler(name, map) {
_self.shell.setCommandHandler(name, map);
_self.root_commands[name] = map;
}
_.each( simple_commands, function(command) {
addCommandHandler(command, buildExecCommandHandler(command));
});
_self.root_commands.tags.help = "help here";
//<section id='onNewPrompt'/>
// This attaches a custom prompt render to the shell.
_self.shell.onNewPrompt(function(callback) {
callback(_self.shell.templates.prompt({self: _self, node: _self.pathhandler.current}));
});
// Wiring up PathHandler
// =====================
//<section id='getNode'/>
// getNode
// -------
// `getNode` is required by `Josh.PathHandler` to provide filesystem behavior. Given a path, it is expected to return
// a pathnode or null;
_self.pathhandler.getNode = function(path, callback) {
_console.debug("[Josh.FeedlyConsole] looking for node at %s.", path);
// If the given path is empty, just return the current pathnode.
if(!path) {
return callback(_self.pathhandler.current);
}
var parts = getPathParts(path);
// If the first part of path parts isn't empty, the path is a relative path, which can be turned into an
// *absolutish* path by pre-pending the parts of the current pathnode.
if(parts[0] !== '') {
parts = getPathParts(_self.pathhandler.current.path).concat(parts);
}
// At this point the path is *absolutish*, i.e. looks absolute, but all `.` and `..` mentions need to removed and
// resolved before it is truly absolute.
var resolved = [];
_.each(parts, function(x) {
if(x === '.') {
return;
}
if(x === '..') {
resolved.pop();
} else {
resolved.push(x);
}
});
var absolute = resolved.join('/');
_console.debug("[Josh.FeedlyConsole]path to fetch: " + absolute);
// if get getDir returns false, use same node
return getDir(absolute, callback) || self.node;
};
//<section id='getChildNodes'/>
// getChildNodes
// -------------
// `getChildNodes` is the second function implementation required for `Josh.PathHandler`. Given a pathnode, it returns
// a list of child pathnodes. This is used by `Tab` completion to resolve a partial path, after first resolving the
// nearest parent node using `getNode
_self.pathhandler.getChildNodes = function(node, callback) {
// If the given node is a file node, no further work is required.
if(node.isFile) {
_console.debug("[Josh.FeedlyConsole] it's a file, no children %O", node);
return callback();
}
// Otherwise, if the child nodes have already been initialized, which is done lazily, return them.
if(node.children) {
_console.debug("[Josh.FeedlyConsole] got children, let's turn them into nodes %O", node);
return callback(makeNodes(node.children));
}
// Finally, use `getDir` to fetch and populate the child nodes.
_console.debug("[Josh.FeedlyConsole] no children, fetch them %O", node);
return getDir(node.path, function(detailNode) {
node.children = detailNode.children;
callback(node.children);
});
};
// Supporting Functions
// ====================
//<section id='get'/>
// get
// ---
// This function is responsible for all API requests, given a partial API path, `resource`, and an query argument object,
// `args`.
function get(resource, args, callback) {
var url = _self.api + resource;
var cache = _self[resource];
if(cache) {
return callback(cache);
}
function cacheCallback(value) {
_self[resource] = value;
callback(value);
}
if(chrome.extension === undefined) {
// not embedded, demo mode
switch(resource) {
case 'tags':
return cacheCallback([
{
"id": "user/c805fcbf-3acf-4302-a97e-d82f9d7c897f/tag/global.saved"
},
{
"id": "user/c805fcbf-3acf-4302-a97e-d82f9d7c897f/tag/tech",
"label": "tech"
},
{
"id": "user/c805fcbf-3acf-4302-a97e-d82f9d7c897f/tag/inspiration",
"label": "inspiration"
}
]);
case 'subscriptions':
return cacheCallback([
{
"id": "feed/http://feeds.feedburner.com/design-milk",
"title": "Design Milk",
"categories": [
{
"id": "user/c805fcbf-3acf-4302-a97e-d82f9d7c897f/category/design",
"label": "design"
},
{
"id": "user/c805fcbf-3acf-4302-a97e-d82f9d7c897f/category/global.must",
"label": "must reads"
}
],
"sortid": "26152F8F",
"updated": 1367539068016,
"website": "http://design-milk.com"
},
{
"id": "feed/http://5secondrule.typepad.com/my_weblog/atom.xml",
"title": "5 second rule",
"categories": [
],
"sortid": "26152F8F",
"updated": 1367539068016,
"website": "http://5secondrule.typepad.com/my_weblog/"
},
{
"id": "feed/http://feed.500px.com/500px-editors",
"title": "500px: Editors' Choice",
"categories": [
{
"id": "user/c805fcbf-3acf-4302-a97e-d82f9d7c897f/category/photography",
"label": "photography"
}
],
"sortid": "26152F8F",
"updated": 1367539068016,
"website": "http://500px.com/editors"
}
]);
case 'profile':
return cacheCallback({
"id": "c805fcbf-3acf-4302-a97e-d82f9d7c897f",
"email": "<EMAIL>",
"givenName": "Jim",
"familyName": "Smith",
"picture": "img/download.jpeg",
"gender": "male",
"locale": "en",
"reader": "9080770707070700",
"google": "115562565652656565656",
"twitter": "jimsmith",
"facebook": "",
"wave": "2013.7"
});
case 'categories':
return cacheCallback([
{
"id": "user/c805fcbf-3acf-4302-a97e-d82f9d7c897f/category/tech",
"label": "tech"
},
{
"id": "user/c805fcbf-3acf-4302-a97e-d82f9d7c897f/category/design",
"label": "design"
}
]);
case 'topics':
return cacheCallback([
{
"id": "user/c805fcbf-3acf-4302-a97e-d82f9d7c897f/topic/arduino",
"interest": "high",
"updated": 1367539068016,
"created": 1367539068016
},
{
"id": "user/c805fcbf-3acf-4302-a97e-d82f9d7c897f/topic/rock climbing",
"interest": "low",
"updated": 1367539068016,
"created": 1367539068016
}
]);
case 'preferences':
return cacheCallback({
"autoMarkAsReadOnSelect": "50",
"category/reviews/entryOverviewSize": "0",
"subscription/feed/http://feeds.engadget.com/weblogsinc/engadget/entryOverviewSize": "4",
"subscription/feed/http://www.yatzer.com/feed/index.php/hideReadArticlesFilter": "off",
"category/photography/entryOverviewSize": "6",
"subscription/feed/http://feeds.feedburner.com/venturebeat/entryOverviewSize.mobile": "1"
});
}
} else {
if(args) {
url += "?" + _.map(args,function(v, k) {
return k + "=" + v;
}).join("&");
}
_console.debug("[Josh.FeedlyConsole] fetching %s.", url);
var request = {
url: url,
dataType: 'json',
headers: { "Authorization": "OAuth " + _self.OAuth },
xhrFields: {
withCredentials: true
}
};
$.ajax(request).done(function(response, status, xhr) {
// Every response from the API includes rate limiting headers, as well as an indicator injected by the API proxy
// whether the request was done with authentication. Both are used to display request rate information and a
// link to authenticate, if required.
var ratelimit = {
remaining: parseInt(xhr.getResponseHeader("X-RateLimit-Remaining")),
limit: parseInt(xhr.getResponseHeader("X-RateLimit-Limit")),
authenticated: xhr.getResponseHeader('Authenticated') === 'true'
};
$('#ratelimit').html(_self.shell.templates.rateLimitTemplate(ratelimit));
if(ratelimit.remaining === 0) {
alert("Whoops, you've hit the github rate limit. You'll need to authenticate to continue");
_self.shell.deactivate();
return null;
}
// For simplicity, this tutorial trivially deals with request failures by just returning null from this function
// via the callback.
if(status !== 'success') {
return callback();
}
return cacheCallback(response);
});
}
}
//<section id='initialize'/>
// initalize
// --------------
// This function sets the node
function initialize(evt) {//err, callback) {
insertShellUI();
return getDir("/", function(node) {
if(!node) {
return err("could not initialize root directory");
}
_self.pathhandler.current = node;
_self.root = node;
// return feedlyconsole.ready(function() {
// });
});
}
function insertCSSLink(name) {
// insert css into head
$('head').append( $('<link/>', {
rel: "stylesheet",
type: "text/css",
href: chrome.extension.getURL(name)
}));
}
function doInsertShellUI() {
observer.disconnect();
var file = "feedlyconsole.html";
_console.debug("[feedlyconsole] injecting %s.", file);
insertCSSLink("stylesheets/styles.css");
//insertCSSLink("stylesheets/source-code-pro.css");
insertCSSLink("stylesheets/jquery-ui.css");
insertCSSLink("feedlyconsole.css");
var feedlyconsole = $('<div/>', {
'id': 'feedlyconsole'
}
).load(chrome.extension.getURL(file), function() {
_console.log("[feedlyconsole] loaded %s %O readline.attach %O.", file, $('#feedlyconsole'), this);
Josh.config.readline.attach($('#shell-panel').get(0));
initializeUI();
});
$('body').prepend(feedlyconsole);
}
function mutationHandler (mutationRecords) {
_found = false;
mutationRecords.forEach ( function (mutation) {
var target = mutation.target;
if( target.id === 'box' ) {
var type = mutation.type;
var name = mutation.attributeName;
var attr = target.attributes.getNamedItem(name);
var value = "";
if( attr !== null ) {
value = attr.value;
}
_console.debug( "[feedlyconsole/observer] %s: [%s]=%s on %O", type, name, value, target);
// not sure if wide will always be set, so trigger on the next mod
if( !_found &&
((name === 'class' &&
value.indexOf("wide") != -1 ) ||
(name === '_pageid' &&
value.indexOf("rot21") != -1 )))
{
_console.debug("[feedlyconsole] mutation observer end %O", observer);
_found = true;
doInsertShellUI();
// found what we were looking for
return null;
}
}
});
}
observer = new MutationObserver (mutationHandler);
function insertShellUI() {
if( $('#feedlyconsole').length === 0 ) {
_console.debug("[feedlyconsole] mutation observer start");
var target = document;
var config = {
attributes: true,
subtree: true
};
_console.debug(target);
_console.debug(observer);
_console.debug(config);
observer.observe(target, config);
}
}
//<section id='getDir'/>
// getDir
// ------
// This function function fetches the directory listing for a path on a given repo and branch.
function getDir(path, callback) {
var node;
var name;
// remove trailing '/' for API requests.
if(path && path.length > 1 && path[path.length - 1] === '/') {
path = path.substr(0, path.length - 1);
}
if(!path || (path.length == 1 && path === '/')) {
// item 0, root, each command a subdir
name = '/';
node = {
name: '/',
path: path,
children: makeRootNodes()
};
_console.debug("[Josh.FeedlyConsole] root node %O.", node);
return callback(node);
} else {
var parts = getPathParts(path);
// leading '/' produces empty item
if(parts[0] === "") {
parts = parts.slice(1);
}
name = parts[0];
var handler = _self.root_commands[name];
if(handler === undefined) {
return callback();
} else if(parts.length == 1) {
// item 1, commands
node = {
name: name,
path: path,
children: null
};
// implicitly call command as part of path
var command = handler.exec;
command("", "", function(map) {
var json = _self[name];
_console.debug("[Josh.FeedlyConsole] json to nodes %O.", json);
node.children = makeJSONNodes(path, json, name);
return callback(node);
});
} else {
// item 2+, details
_console.debug("[Josh.FeedlyConsole] not implemented, path: %s, name %s", path, name);
get("streams/" );
return callback(undefined)
}
}
}
//<section id='getPathParts'/>
// getPathParts
// ------------
// This function splits a path on `/` and removes any empty trailing element.
function getPathParts(path) {
var parts = path.split("/");
if(parts[parts.length - 1] === '') {
return parts.slice(0, parts.length - 1);
}
return parts;
}
//<section id='makeNodes'/>
// makeNodes
// ---------
// This function builds child pathnodes from the directory information returned by getDir.
function makeNodes(children) {
_console.debug("[Josh.FeedlyConsole] makeNodes %O.", children);
return _.map(children, function(node) {
return {
name: node.name,
path: "/" + node.path,
isFile: node.type === 'leaf'
};
});
}
function makeJSONNodes(path, children, type) {
if( _.isArray( children ) ) {
var nodes = _.map(children, function(item) {
var name = item.label || item.title || item.id;
return $.extend( { name: name,
path: path + '/' + name,
isFile: type === 'leaf'
}, item );
});
return nodes;
} else {
return _.map(children, function(value, key) {
var name = [key, value].join(':');
return {
name: name,
path: path + '/' + name,
isFile: type === 'leaf'
};
});
}
}
function makeRootNodes() {
return _.map(_self.root_commands, function(value, key, list) {
return {
name: key,
path: "/" + key,
type: 'command',
isFile: 'command' === 'leaf'
};
});
}
// UI setup and initialization
// ===========================
//<section id='initializationError'/>
// initializationError
// -------------------
// This function is a lazy way with giving up if some request failed during intialization, forcing the user
// to reload to retry.
function initializationError(context, msg) {
_console.error("[%s] failed to initialize: %s.", context, msg);
//alert("unable to initialize shell. Encountered a problem talking to github api. Try reloading the page");
}
//<section id='initializeUI'/>
// intializeUI
// -----------
// After a current user and repo have been set, this function initializes the UI state to allow the shell to be
// shown and hidden.
function initializeUI() {
_console.log("[Josh.FeedlyConsole] initializeUI.");
// We grab the `consoletab` and wire up hover behavior for it.
var $consoletab = $('#consoletab');
if( $consoletab.length === 0 ) {
console.error('failed to find %s', $consoletab.selector);
}
$consoletab.hover(function() {
$consoletab.addClass('consoletab-hover');
$consoletab.removeClass('consoletab');
}, function() {
$consoletab.removeClass('consoletab-hover');
$consoletab.addClass('consoletab');
});
// We also wire up a click handler to show the console to the `consoletab`.
$consoletab.click(function() {
activateAndShow();
});
var $consolePanel = $('#shell-container');
$consolePanel.resizable({ handles: "s"});
$(document).on( 'keypress', (function(event) {
if(_self.shell.isActive()) {
return;
}
if(event.keyCode == 126) {
event.preventDefault();
activateAndShow();
}
}));
function toggleActivateAndShow() {
if(_self.shell.isActive()) {
hideAndDeactivate();
} else {
activateAndShow();
}
}
function activateAndShow() {
$consoletab.slideUp();
_self.shell.activate();
$consolePanel.slideDown();
$consolePanel.focus();
}
function hideAndDeactivate() {
_self.shell.deactivate();
$consolePanel.slideUp();
$consolePanel.blur();
$consoletab.slideDown();
}
_self.ui.toggleActivateAndShow = toggleActivateAndShow;
_self.ui.activateAndShow = activateAndShow;
_self.ui.hideAndDeactivate = hideAndDeactivate;
_self.shell.onEOT(hideAndDeactivate);
_self.shell.onCancel(hideAndDeactivate);
}
_console.log("[Josh.FeedlyConsole] initialize.");
initialize();
// wire up pageAction to toggle console
// kind of a mess, but we only want to create one listener,
// but initializeUI can be called multiple times because
// feedly will blow away console that are added to early
if(chrome.runtime !== undefined) { // when in extension
chrome.runtime.onMessage.addListener(
function(request, sender, sendResponse) {
_console.debug("[feedlyconsole] msg: %s.", request.msg);
if ( request.action === "icon_active" ) {
var url_array = request.url.split("/");
var url = url_array[0] + "//" + url_array[2] + "/" + _self.api_version;
_console.debug("[feedlyconsole] set api url: %s.", url);
_self.api = url;
} else if ( request.action === "toggle_console" ) {
if(_self.ui.toggleActivateAndShow === undefined) {
window.console.warn("[feedlyconsole] ui not yet ready");
} else {
_self.ui.toggleActivateAndShow();
}
} else if ( request.action === "cookie_feedlytoken" ) {
_self.OAuth = request.feedlytoken;
_console.debug("[feedlyconsole] token %s...",
_self.OAuth.slice(0,8) );
} else {
_console.debug("[feedlyconsole] unknown action %s request %O.",
request.action, request);
}
sendResponse({ "action": "ack" });
});
} else {
// not extension
$(document).ready(function() {
initializeUI();
_self.ui.activateAndShow();
});
}
return _self;
})(root, $, _);
})(this, $, _);
console.log("[feedlyconsole] loaded %O.", Josh);
<file_sep>#!/bin/bash
function copy_chmod() {
for filename in ${filenames[@]}; do
file=${filename}.${ext}
cp -vf $source_dir/$file .
done
}
(
mkdir -p javascript
cd javascript
filenames=( "readline" "history" "killring" "pathhandler" "shell" )
source_dir=../../josh.js/js
ext="js"
copy_chmod
filenames=( "optparse" )
source_dir=../../josh.js/ext/optparse-js/lib
copy_chmod
if [ ! -e jquery.min.js ]; then
curl -qO "http://ajax.googleapis.com/ajax/libs/jquery/1.9.0/jquery.js"
curl -qO "http://ajax.googleapis.com/ajax/libs/jquery/1.9.0/jquery.min.js"
curl -qO "http://ajax.googleapis.com/ajax/libs/jquery/1.9.0/jquery.min.map"
fi
if [ ! -e jquery-ui.min.js ]; then
curl -qO "http://ajax.googleapis.com/ajax/libs/jqueryui/1.9.2/jquery-ui.min.js"
curl -qO "http://ajax.googleapis.com/ajax/libs/jqueryui/1.9.2/jquery-ui.min.map"
fi
if [ ! -e underscore-min.js ]; then
curl -qO "http://cdnjs.cloudflare.com/ajax/libs/underscore.js/1.4.2/underscore-min.js"
fi
chmod a-w *
)
(
mkdir -p stylesheets
cd stylesheets
if [ ! -e source-code-pro.css ]; then
curl -q "http://fonts.googleapis.com/css?family=Source+Code+Pro" > source-code-pro.css
fi
if [ ! -e jquery-ui.css ]; then
curl -qO "http://code.jquery.com/ui/1.9.2/themes/base/jquery-ui.css"
fi
chmod a-w *
)
| a85ce9c947a6c33c8aa8a37f93232bd88f56b863 | [
"HTML",
"JavaScript",
"Markdown",
"Python",
"Shell"
]
| 7 | JavaScript | dougbeal/feedlyconsole | a9aa61c67b50ab94f2dc7c18ad42ad5abf2ecca5 | f53a5ae9196a50992c9167f2d9b7ac68550bfefb | |
refs/heads/master | <file_sep>class AddAvatarVkLgToUsers < ActiveRecord::Migration
def change
add_column :users, :avatar_vk_lg, :string
end
end
<file_sep># (с) goodprogrammer.ru
#
# Модель Пользователя
class User < ActiveRecord::Base
# добавляем к юзеру функции Девайза, перечисляем конкретные наборы функций
devise :database_authenticatable, :registerable,
:recoverable, :rememberable, :validatable,
:omniauthable, :omniauth_providers => [:vkontakte]
# юзер может создавать много событий
has_many :events, dependent: :destroy
has_many :comments, dependent: :destroy
has_many :subscriptions, dependent: :destroy
validates_associated :subscriptions
# имя юзера должно быть, и не длиннее 35 букв
validates :name, presence: true, length: {maximum: 35}
# при создании нового юзера (create), перед валидацией объекта выполнить метод set_name
before_validation :set_name, on: :create
after_commit :link_subscriptions, on: :create
# Добавляем аплоадер аватарок, чтобы заработал carrierwave
mount_uploader :avatar, AvatarUploader
private
# задаем юзеру случайное имя, если оно пустое
def set_name
self.name = "Товарисч №#{rand(777)}" if self.name.blank?
end
def link_subscriptions
Subscription.where(user_id: nil, user_email: self.email).update_all(user_id: self.id)
end
def self.find_for_vkontakte_oauth(access_token)
# Как выглядит объект access_token можно посмотреть на странице гема
# https://github.com/mamantoha/omniauth-vkontakte#authentication-hash
# Мы достаем из этого объекта url и provider, вместе они формируют
# уникального пользователя
url = access_token.info.urls.Vkontakte
provider = access_token.provider
# Ищем таких пользователей методом where, а методом
# first_or_create! мы либо выбираем первого (если такой нашелся)
# либо создаем нового с такими параметрами (url, provider),
# к этому юзеру в случае создания также будет применен блок
where(url: url, provider: provider).first_or_create! do |user|
# В блоке мы прописываем пользователю имя, которое получили от ВКонтатке
user.name = access_token.info.name
# Формируем email из id пользователя ВКонтакте
user.email = <EMAIL>"
# И генерируем ему случайный надежный парроль
# пользоваться им никто не будет, но формально по нему можно войти
user.password = <PASSWORD>token[0,20]
user.avatar_vk = access_token.info.image
user.avatar_vk_lg = access_token.extra.raw_info.photo_big
end
end
end
<file_sep>class ApplicationMailer < ActionMailer::Base
default from: "bbq24"
layout 'mailer'
end
<file_sep>class AddAvatarVkToUsers < ActiveRecord::Migration
def change
add_column :users, :avatar_vk, :string
end
end
| 922747c52c7078e473b8ebd78fb1020d0c5d85d6 | [
"Ruby"
]
| 4 | Ruby | Dmitry-Oksentyuk/bbq | f6992da763becd68ca89c0327faae8b148ded54d | 0cd3b20d4939fb049f1a6bb7f598628545570130 | |
refs/heads/master | <repo_name>milenig/Outlook-LPage<file_sep>/README.md
# Outlook-LPage
Projekt, kterým bych si pochlubil je úvodní stránka Microsoft Outlooku kterou jsem přepsal svým způsobem a vytvořil animace na základě Microsoftové prezentace. Tento projekt byl vytvořen z důvodu poukázat mé schopnosti vytvořit moderní, interaktivní stránky na základě již daného uživatelského rozhraní a prototypu.
<file_sep>/script.js
function scrollFunction() {
if (document.body.scrollTop > 120 || document.documentElement.scrollTop > 120) {
document.querySelector("header").style.position = "fixed";
document.querySelector(".hideOnScroll").style.display = "none";
document.querySelectorAll(".showOnScroll").forEach(function(val) {
val.style.display = 'inline';
});
document.querySelector("header").style.backgroundColor = "white";
document.querySelector("header").style.boxShadow = "0 2px 60px 14px rgba(0,0,0,0.13)";
document.querySelector("header").style.animation = "header 0.4s forwards linear";
document.querySelector(".button--outline").classList += " button--header";
document.querySelector(".button--secondary").classList += " button--header";
} else {
document.querySelector("header").style.position = "absolute";
document.querySelector(".hideOnScroll").style.display = "inline";
document.querySelectorAll(".showOnScroll").forEach(function(val) {
val.style.display = 'none';
});
document.querySelector("header").style.backgroundColor = "rgba(255, 255, 255, 0.2)";
document.querySelector("header").style.boxShadow = "0 2px 60px 14px rgba(0,0,0,0)";
document.querySelector("header").style.animation = "none";
document.querySelector(".button--outline").classList.remove("button--header");
document.querySelector(".button--secondary").classList.remove("button--header");
}
}
function openTab(e, tabName) {
let tabcontent, tablinks;
tabcontent = document.getElementsByClassName("tabcontent");
tablinks = document.getElementsByClassName("tablinks");
//hide all tabs
for( let i=0; i < tabcontent.length; i++){
tabcontent[i].style.display = "none";
}
//remove style from active button
for( let i=0; i < tablinks.length; i++){
tablinks[i].className = tablinks[i].className.replace(" active", "");
}
//show tab and style button
document.getElementById(tabName).style.display = "block";
e.currentTarget.className += " active";
}
document.getElementById("defaultOpen").click();
$(document).ready(function(){
$(".owl-carousel").owlCarousel({
items: 2,
loop: true,
nav: false,
center: true,
autoplay: true,
animateIn: true,
smartSpeed: 1000
});
});
function hidepopup(){
document.querySelector('.noResponsive-popup').style.display = "none";
} | f2315009de7408e16acfdab3e1d491d9f4f4a428 | [
"Markdown",
"JavaScript"
]
| 2 | Markdown | milenig/Outlook-LPage | 27162fc2e535f40de9218331550e5598db5199f5 | d44ee700c18f57d09685abb095f695771d5a2e2b | |
refs/heads/master | <repo_name>programadormovel/AppOracao<file_sep>/settings.gradle
include ':app'
rootProject.name = "AppOracao"<file_sep>/app/src/main/java/br/com/itb/pra3/apporacao/ui/home/HomeViewModel.java
package br.com.itb.pra3.apporacao.ui.home;
import androidx.lifecycle.LiveData;
import androidx.lifecycle.MutableLiveData;
import androidx.lifecycle.ViewModel;
import br.com.itb.pra3.apporacao.ObjetoEstatico;
public class HomeViewModel extends ViewModel {
// Objeto LiveData
private MutableLiveData<String> mText;
// Método construtor do ViewModel
public HomeViewModel() {
// Construção do objeto LiveData
mText = new MutableLiveData<>();
// Valor do objeto LiveData
// Aqui podemos capturar os dados do banco de dados, por exemplo
mText.setValue("texto");
}
// Método de recuperação dos dados inseridos no LiveData
public LiveData<String> getText() {
return mText;
}
}<file_sep>/app/src/main/java/br/com/itb/pra3/apporacao/ui/OracaoFragment.java
package br.com.itb.pra3.apporacao.ui;
import androidx.lifecycle.ViewModelProvider;
import android.os.Bundle;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
import androidx.navigation.fragment.NavHostFragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import br.com.itb.pra3.apporacao.R;
public class OracaoFragment extends Fragment {
private OracaoViewModel mViewModel;
public static OracaoFragment newInstance() {
return new OracaoFragment();
}
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container,
@Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.oracao_fragment, container, false);
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
mViewModel = new ViewModelProvider(this).get(OracaoViewModel.class);
// TODO: Use the ViewModel
}
public void onViewCreated(@NonNull View view, Bundle savedInstanceState) {
super.onViewCreated(view, savedInstanceState);
// Método de acionamento do botão
view.findViewById(R.id.btnBuscarOracao).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Navegar para a janela de fragmento da Oração Selecionada
NavHostFragment.findNavController(OracaoFragment.this)
.navigate(R.id.action_navigation_oracao_to_oracaoEscolhidaFragment);
}
});
}
}<file_sep>/app/src/main/java/br/com/itb/pra3/apporacao/ui/home/HomeFragment.java
package br.com.itb.pra3.apporacao.ui.home;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.EditText;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
import androidx.lifecycle.Observer;
import androidx.lifecycle.ViewModelProvider;
import androidx.lifecycle.ViewModelProviders;
import br.com.itb.pra3.apporacao.ObjetoEstatico;
import br.com.itb.pra3.apporacao.R;
public class HomeFragment extends Fragment {
// Objeto View Model
private HomeViewModel homeViewModel;
private EditText caixaDeTexto;
// Método de criação do layout em tempo de execução
public View onCreateView(@NonNull LayoutInflater inflater,
ViewGroup container, Bundle savedInstanceState) {
// Construção do objeto View Model
homeViewModel = new ViewModelProvider(this)
.get(HomeViewModel.class);
// Definição do layout de Fragmento que será controlado pela classe Java
View root = inflater.inflate(R.layout.fragment_home, container, false);
// Objeto label
final TextView textView = root.findViewById(R.id.text_home);
// Método observador, garante a persistência dos dados da caixa de texto
homeViewModel.getText().observe(getViewLifecycleOwner(), new Observer<String>() {
@Override
public void onChanged(@Nullable String s) {
textView.setText(s);
}
});
// Vínculo do objeto do tipo EditText com o objeto da janela (Fragmento)
caixaDeTexto = root.findViewById(R.id.edtTexto);
return root;
}
} | 01f5978f41ef694ac71d0af24afbbedfbc5e192e | [
"Java",
"Gradle"
]
| 4 | Gradle | programadormovel/AppOracao | 9784a477634a3092269ed699b60f2e78837c2e61 | c983ed8e4194a1384ba06448a4c52278911f1e27 | |
refs/heads/master | <file_sep>import React from 'react';
import { NavLink } from 'react-router-dom';
import { connect } from 'react-redux';
import { bindActionCreators } from 'redux';
import Constants from '../Constants';
class Header extends React.Component {
logout() {
this.props.logoutA();
}
render() {
let loggedIn = '';
if(this.props.authData.username) {
loggedIn = <li><NavLink to={Constants.ROUTE_THINGS_ALL}><span className="glyphicon glyphicon-user"></span> {this.props.authData.username} </NavLink></li>
}
let loginLink = '';
let logoutLink = '';
if(this.props.authData.username) {
logoutLink = <li><NavLink to={Constants.ROUTE_THINGS_ALL} onClick={this.logout.bind(this)}><span className="glyphicon glyphicon-log-out"></span> Logout</NavLink></li>
} else {
loginLink = <li><NavLink to={Constants.ROUTE_LOGIN}><span className="glyphicon glyphicon-log-in"></span> Login</NavLink></li>
}
let homeClassName = this.props.authData.loadingData ? 'glyphicon glyphicon-grain loading-data' : 'glyphicon glyphicon-grain';
return (<header>
<nav className="navbar navbar-default">
<div className="container-fluid">
<div className="navbar-header">
<button type="button" className="navbar-toggle" data-toggle="collapse" data-target="#myNavbar">
<span className="icon-bar"></span>
<span className="icon-bar"></span>
<span className="icon-bar"></span>
</button>
<NavLink className="navbar-brand" to={Constants.ROUTE_THINGS_ALL}>Bozo's playground <span className={homeClassName}></span></NavLink>
</div>
<div className="collapse navbar-collapse" id="myNavbar">
<ul className="nav navbar-nav">
<li><NavLink exact activeClassName='active' to={Constants.ROUTE_THINGS_ALL}>All things</NavLink></li>
<li><NavLink exact activeClassName='active' to={Constants.ROUTE_THING_CREATE}>Create new thing</NavLink></li>
</ul>
<ul className="nav navbar-nav navbar-right">
{loggedIn}
{loginLink}
{logoutLink}
</ul>
</div>
</div>
</nav>
</header>)
}
};
const logoutA = () => {
return {type: 'SET_USERNAME', payload: ''};
}
const mapStateToProps = (state) => {
return {
authData: state.authR.authData
};
};
const mapDispatchToProps = (dispatch) => {
return bindActionCreators({
logoutA : logoutA
}, dispatch);
};
export default connect(mapStateToProps, mapDispatchToProps)(Header);<file_sep>import React from 'react';
import { Route, Switch } from 'react-router-dom';
import { Things } from "../components/Things"
import Thing from "../components/Thing"
import { Login } from "../components/Login"
import AuthenticatedRoute from "./AuthenticatedRoute"
import Constants from '../Constants';
const Main = () => {
return (
<main>
<Switch>
<Route exact path={Constants.ROUTE_THINGS_ALL} component={Things} />
<Route exact path={Constants.ROUTE_THING_VIEW} component={Thing} />
<AuthenticatedRoute path={Constants.ROUTE_THING_CREATE} exact component={Thing} />
<AuthenticatedRoute path={Constants.ROUTE_THING_EDIT} exact component={Thing} />
<Route exact path={Constants.ROUTE_LOGIN} component={Login} />
<Route path="*" component={Things} />
</Switch>
</main>
)
};
export default Main;<file_sep>
# reactjs-boilerplate
Boilerplate code for ReactJS + Redux + Less + Controlled form + AJAX + HTTP request interceptor + Router v4 + Authentication
## Demo app
[Demo](http://www.srpskibre.com/react)
## Purpose
Boilerplate code for
- ReactJS + Redux
- Less -> CSS
- Controlled form
- AJAX with Axios + HTTP request interceptor
- Router v4 + 2 ways to redirect to another route
- User authentication
with the latest versions of all dependencies (March 16, 2018)
<file_sep>const Constants = {
ROUTE_PUBLIC: '/public',
ROUTE_PRIVATE: '/private',
ROUTE_LOGIN: '/login',
ROUTE_THING_VIEW: '/thing/view/:thingid',
ROUTE_THING_CREATE: '/thing/create',
ROUTE_THING_EDIT: '/thing/edit/:thingid',
ROUTE_THINGS_ALL: '/things',
API_URL: ''
};
export default Constants;<file_sep>import React, { Component } from 'react';
import './App.css';
import x from './assets/x.jpg';
import Main from './layout/Main';
import Header from './layout/Header';
class App extends Component {
render() {
return (
<div>
<Header />
<div className="container">
<Main />
</div>
{/* <br /><br /><img src={x} alt='x' width='600px' /> */}
</div>
);
}
}
export default App;
<file_sep>import React from 'react';
import { connect } from 'react-redux';
import { bindActionCreators } from 'redux';
import Constants from '../Constants';
import { loadAllThings } from "./Things"
class Thing extends React.Component {
constructor(props) {
super(props);
this.state = {
thingsData : {
things : [],
thingForm : {
id : null,
name : '',
surname : ''
}
}
}
}
componentDidMount() {
if(this.props.thingsData.things.length === 0) {
this.props.loadAllThings(this.props.history);
} else {
this.prepareThingToEdit();
}
}
componentWillReceiveProps(nextProps) {
this.prepareThingToEdit();
}
getThingIdParam() {
if(this.props.match.params) {
return parseInt(this.props.match.params.thingid, 10);
} else {
return null;
}
}
prepareThingToEdit() {
let thingToEdit = {
id : null,
name : '',
surname : ''
}
const thingId = this.getThingIdParam();
if(thingId) {
const allThings = this.props.thingsData.things;
const thingToEditIndex = allThings.findIndex(thing => thing.id === thingId);
const tte = allThings[thingToEditIndex];
if(tte) {
thingToEdit = tte;
}
}
this.setState({
thingsData : {
...this.props.thingsData,
thingForm : thingToEdit
}
});
}
onChange(e) {
this.setState({
thingsData : {
...this.state.thingsData,
thingForm : {
...this.state.thingsData.thingForm,
[e.target.name] : e.target.value
}
}
});
}
onSubmit(e) {
e.preventDefault();
if(this.state.thingsData.thingForm.name.trim() && this.state.thingsData.thingForm.surname.trim()) {
this.props.submitThing(this.state.thingsData.thingForm);
this.redirectTo(Constants.ROUTE_THINGS_ALL);
}
}
redirectTo(route) {
this.props.history.push(route);
}
render() {
if(this.props.match.path === Constants.ROUTE_THING_VIEW) {
return (<div>
<h1>View thing</h1>
<div className="row">
<div className="col-md-6">
<table className="table table-hover">
<tbody>
<tr>
<td><b>ID</b></td>
<td>{this.state.thingsData.thingForm.id}</td>
</tr>
<tr>
<td><b>Name</b></td>
<td>{this.state.thingsData.thingForm.name}</td>
</tr>
<tr>
<td><b>Surname</b></td>
<td>{this.state.thingsData.thingForm.surname}</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>)
}
return (<div>
<h1>{this.props.match.path === Constants.ROUTE_THING_CREATE ? 'Create' : 'Edit'} thing</h1>
<div className="row">
<div className="col-md-6">
<form onSubmit={this.onSubmit.bind(this)} >
<div className="form-group">
<label htmlFor="name">Name :</label>
<input type="text" className="form-control" id="name" name="name" value={this.state.thingsData.thingForm.name} onChange={this.onChange.bind(this)} />
</div>
<div className="form-group">
<label htmlFor="name">Surname :</label>
<input type="text" className="form-control" id="surname" name="surname" value={this.state.thingsData.thingForm.surname} onChange={this.onChange.bind(this)} />
</div>
<button type="submit" className="btn btn-primary">Save</button>
</form>
</div>
</div>
</div>)
}
};
const submitThing = (thing) => {
return {type: 'CREATE_EDIT_THING', payload: thing};
};
const mapStateToProps = (state) => {
return {
authData: state.authR.authData,
thingsData: state.thingsR.thingsData
};
};
const mapDispatchToProps = (dispatch) => {
return bindActionCreators({
submitThing: submitThing,
loadAllThings: loadAllThings
}, dispatch);
};
export default connect(mapStateToProps, mapDispatchToProps)(Thing);
<file_sep>import React from 'react';
import { connect } from 'react-redux';
import { bindActionCreators } from 'redux';
import axios from "axios";
import { Link } from 'react-router-dom';
import Constants from '../Constants';
import { interceptAjaxCalls } from "../Interceptor"
class ThingsC extends React.Component {
constructor(props) {
super(props);
this.state = {
}
}
componentDidMount() {
if(this.props.thingsData.things.length === 0) {
this.props.loadAllThings(this.props.history);
}
}
render() {
const thingsTable = this.props.thingsData.things.map(thing => <tr key={thing.id}>
<td>{thing.id}</td>
<td>{thing.name} {thing.surname}</td>
<td className="right-float">
<Link to={`/thing/view/${thing.id}`}><span className="glyphicon glyphicon-list" title="View thing" ></span></Link>
<Link to={`/thing/edit/${thing.id}`}><span className="glyphicon glyphicon-edit" title="Edit thing" ></span></Link>
</td>
</tr>);
return (<div>
<h1>All things</h1>
<div className="row">
<div className="col-md-6">
<table className="table">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th> </th>
</tr>
</thead>
<tbody>
{thingsTable}
</tbody>
</table>
</div>
</div>
</div>)
}
};
const loadAllThingsAction = (hist) => {
return function(dispatch) {
dispatch(interceptAjaxCalls(axios, hist));
dispatch({type: "SET_LOADING", payload: true});
axios.get(Constants.API_URL + '/things.json', {
}).then((response) => {
dispatch({type: "ADD_THINGS", payload: response.data.things});
dispatch({type: "SET_LOADING", payload: false});
})
.catch((err) => {
console.log(err);
dispatch({type: "SET_LOADING", payload: false});
})
}
}
const mapStateToProps = (state) => {
return {
authData: state.authR.authData,
thingsData: state.thingsR.thingsData
};
};
const mapDispatchToProps = (dispatch) => {
return bindActionCreators({
loadAllThings : loadAllThingsAction
}, dispatch);
};
export const Things = connect(mapStateToProps, mapDispatchToProps)(ThingsC);
export const loadAllThings = loadAllThingsAction;
<file_sep>
export default function reducer(state=
{
authData: {
username : null,
redirectedFrom : null,
loadingData : false
}
}, action) {
switch (action.type) {
case "SET_USERNAME": {
return {...state,
authData: {
...state.authData,
username: action.payload
}
}
}
case "SET_REDIRECTED_FROM": {
return {...state,
authData: {
...state.authData,
redirectedFrom: action.payload
}
}
}
case "SET_LOADING": {
return {...state,
authData: {
...state.authData,
loadingData: action.payload
}
}
}
default : {
return {...state};
}
}
}<file_sep>import React from "react";
import { connect } from 'react-redux';
import { bindActionCreators } from 'redux';
import { Redirect } from "react-router-dom";
class LoginC extends React.Component {
constructor(props) {
super(props);
this.state = {
username: ''
}
}
onChange(e) {
this.setState({ [e.target.name] : e.target.value });
}
onSubmit(e) {
e.preventDefault();
if(this.state.username) {
this.props.saveUsername(this.state);
}
}
componentWillUnmount() {
if(this.props.authData.username && this.props.authData.redirectedFrom) {
this.props.setRedirectedFrom(null);
}
}
render() {
if(this.props.authData.username) {
if(this.props.authData.redirectedFrom) {
return <Redirect to={this.props.authData.redirectedFrom} />;
} else {
return <Redirect to={'/'}/>;
}
}
return (<div className="row">
<div className="col-sm-4 col-md-4 offset-sm-4 offset-md-4">
<form onSubmit={this.onSubmit.bind(this)}>
<p>Please login!</p>
<div className="form-group">
<label htmlFor="username">Your name : </label>
<input type="username" className="form-control" id="username" name="username" value={this.state.username} onChange={this.onChange.bind(this)} placeholder="Just enter some name" />
</div>
<button type="submit" className="btn btn-default">Login</button>
</form>
</div>
</div>)
}
}
const saveUsername = (st) => {
return {type: 'SET_USERNAME', payload: st.username};
}
const setRedirectedFromAction = (redirectedFrom) => {
return {type: 'SET_REDIRECTED_FROM', payload: redirectedFrom};
}
const mapStateToProps = (state) => {
return {
authData: state.authR.authData
};
};
const mapDispatchToProps = (dispatch) => {
return bindActionCreators({
saveUsername : saveUsername,
setRedirectedFrom : setRedirectedFromAction,
}, dispatch);
};
export const Login = connect(mapStateToProps, mapDispatchToProps)(LoginC);
export const setRedirectedFrom = setRedirectedFromAction;
<file_sep>import { combineReducers } from "redux"
import authR from "./AuthReducer"
import thingsR from "./ThingsReducer"
export default combineReducers({
authR,
thingsR
})
| fdb59eff28d12dc756f51f9916fa24560382f472 | [
"JavaScript",
"Markdown"
]
| 10 | JavaScript | bozojovicic/reactjs-boilerplate | 7e14a3b710454763bee8594b49fb5ec70715a631 | b949983ae54a0c26b08a40c3e500325bdf52c1af | |
refs/heads/master | <file_sep># %%
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
from nltk.tokenize import word_tokenize, sent_tokenize
pd.set_option('display.max_column', None)
pd.set_option('display.max_rows', None)
pd.set_option('display.max_seq_items', None)
pd.set_option('display.max_colwidth', 10)
pd.set_option('expand_frame_repr', True)
df = pd.read_csv("/Users/developer/Desktop/debate_2018_cdmexico_muestra.csv")
df.shape
row = df.head(10)['Text']
count = 0
amloWords = ["peje", "amlo", "andres", "manuel", "lopez", "obrador","morena"]
broncoWords = ["bronco", "<NAME>"]
meadeWords = ["meade", "antonio","meade", "pri"]
anayaWords = ["anaya", "ricardo", "pan"]
amloTweets = []
broncoTweets = []
meadeTweets = []
anayaTweets = []
for x in row:
print(str(count) + ".- " + str(word_tokenize(row[count])))
if any(word in row[count].lower() for word in amloWords):
amloTweets.append(row[count])
if any(word in row[count].lower() for word in broncoWords):
broncoTweets.append(row[count])
if any(word in row[count].lower() for word in meadeWords):
meadeTweets.append(row[count])
if any(word in row[count].lower() for word in anayaWords):
anayaTweets.append(row[count])
count += 1
| 3bcfc8545b2de320151b86439bf98fa9e0939c14 | [
"Python"
]
| 1 | Python | charlitosbin/NLP | 8711a41290e21dff05885ede47ff17c2973f9015 | bf0205cec429454d58d5ce1177de99e23e449d53 | |
refs/heads/master | <repo_name>zeanamansell/BudgetOverview<file_sep>/src/test/java/ChromeBankStatement.java
import bank.CSVStatements.ProcessBankStatement;
import bank.CSVStatements.ReadAnzStatement;
import bank.CSVStatements.ReadMasterFile;
import io.github.bonigarcia.wdm.WebDriverManager;
import org.junit.After;
import org.junit.Before;
import org.junit.BeforeClass;
import org.junit.Test;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.support.PageFactory;
import web.ANZ.AnzAccountPage;
import web.ANZ.AnzHomePage;
import web.ANZ.AnzLogInPage;
import web.ANZ.AnzUserHomePage;
import java.util.List;
import static org.hamcrest.CoreMatchers.not;
public class ChromeBankStatement {
private WebDriver driver;
private AnzHomePage anzHomePage;
private AnzLogInPage anzLogInPage;
private AnzUserHomePage anzUserHomePage;
private AnzAccountPage anzAccountPage;
private ReadAnzStatement readAnzStatement;
private ReadMasterFile readMasterFile;
private ProcessBankStatement processBankStatement;
private String statementMonth;
private String statementYear;
private static ChromeOptions chromeOptions;
@BeforeClass
public static void setUpClass() {
WebDriverManager.chromedriver().setup();
}
@Before
public void setUpBrowser() {
driver = new ChromeDriver();
this.anzHomePage = PageFactory.initElements(this.driver, AnzHomePage.class);
}
@After
public void tearDownBrowser() {
if (driver != null) {
driver.quit();
}
}
@Test
public void getBudgetOutline() throws InterruptedException {
getAnzBankStatement();
getBudgetOverview();
}
public void getBudgetOverview() {
readAnzStatement = new ReadAnzStatement(statementMonth, statementYear);
List<String> descriptionValues = readAnzStatement.getDescriptionValues();
List<String> amountValues = readAnzStatement.getAmountValues();
readMasterFile = new ReadMasterFile().invoke();
List<String> masterDescriptionValues = readMasterFile.getMasterDescriptionValues();
List<String> masterCategoryValues = readMasterFile.getMasterCategoryValues();
processBankStatement = new ProcessBankStatement(descriptionValues, amountValues, masterDescriptionValues, masterCategoryValues).invoke();
List<String> monthlyCategories = processBankStatement.getMonthlyCategories();
List<Float> monthlySpend = processBankStatement.getMonthlySpend();
printBudgetOutline(monthlyCategories, monthlySpend);
}
public void getAnzBankStatement() throws InterruptedException {
String userCustomerNumber = "82816810";
String customerNumber = "BC7C8EDC9A";
String statementMonth = "March";
String statementYear = "2019";
anzLogInPage = anzHomePage.goToLoginPage();
anzLogInPage.enterLoginDetails(userCustomerNumber, customerNumber);
anzUserHomePage = anzLogInPage.logIn();
anzAccountPage = anzUserHomePage.goToAccount();
anzAccountPage.exportCSVStatement(statementMonth, statementYear);
anzAccountPage.logOut();
}
public void printBudgetOutline(List<String> monthlyCategories, List<Float> monthlySpend) {
System.out.println("Overview of spending for March 2019: ");
for (int i = 0; i < monthlyCategories.size(); i++) {
System.out.println(monthlyCategories.get(i) + ": " + monthlySpend.get(i));
}
}
}<file_sep>/src/main/java/web/ANZ/AnzAccountPage.java
package web.ANZ;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.Select;
import org.openqa.selenium.support.ui.WebDriverWait;
public class AnzAccountPage {
private WebDriver driver;
private WebDriverWait wait;
public AnzAccountPage (WebDriver driver) {
this.driver = driver;
this.wait = new WebDriverWait(this.driver, 10);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("transaction-search-input"))); //Waits until search bar has loaded
}
public void exportCSVStatement(String statementMonth, String statementYear) throws InterruptedException {
openExportParams();
String statementDate = statementMonth + " " + statementYear;
selectExportParams(statementDate);
downloadCSVStatement();
Thread.sleep(5000);
}
public void openExportParams() {
//Export CSV statements
WebElement exportSettingsButton = driver.findElement(By.id("transactions-export-panel-toggle"));
exportSettingsButton.click();
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("transaction-export-submit")));
// Thread.sleep(3000);
}
public void selectExportParams(String statementMonth) {
Select dateRangeDropbox = new Select(driver.findElement(By.name("date-range")));
dateRangeDropbox.selectByVisibleText(statementMonth);
Select fileFormat = new Select(driver.findElement(By.id("transactions-export-format")));
fileFormat.selectByVisibleText("CSV - Comma Separated Values");
}
public void downloadCSVStatement() {
WebElement exportButton = driver.findElement(By.id("transaction-export-submit"));
exportButton.click();
}
public void logOut() {
//Logoff
WebElement logOffButton = driver.findElement(By.id("logout"));
logOffButton.click();
}
}
<file_sep>/src/main/java/web/ANZ/AnzHomePage.java
package web.ANZ;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.PageFactory;
public class AnzHomePage {
private WebDriver driver;
public AnzHomePage (WebDriver driver) {
this.driver = driver;
this.driver.get("https://www.anz.co.nz/personal/");
}
public AnzLogInPage goToLoginPage() {
// Click login button
WebElement loginButton = this.driver.findElement(By.id("skip_logon"));
loginButton.click();
return PageFactory.initElements(this.driver, AnzLogInPage.class);
}
}
| b7ebebef748016b073fb9a2d2ebfe68465b3156a | [
"Java"
]
| 3 | Java | zeanamansell/BudgetOverview | b498c82ed4629865bf653f4f601c7ed0636d5446 | 4b63740b70778bb84d057d85b3e3dc1bd1ca12dd | |
refs/heads/master | <file_sep>package org.firstinspires.ftc.teamcode.kickoff2018;
import com.qualcomm.robotcore.eventloop.opmode.LinearOpMode;
public class TeleOp extends LinearOpMode{
private RobotClass robot;
@Override
public void runOpMode() throws InterruptedException {
robot = new RobotClass(this);
waitForStart();
while(opModeIsActive()){
robot.drive.power(-gamepad1.left_stick_y, - gamepad1.right_stick_y);
if(gamepad1.a)
robot.intake.setState(Intake.State.IN);
else if (gamepad1.b)
robot.intake.setState(Intake.State.OUT);
else if (gamepad1.x)
robot.intake.setState(Intake.State.STOP);
if (gamepad1.dpad_up)
robot.arm.setPosition(Arm.Position.HIGH_GOAL);
else if (gamepad1.dpad_right)
robot.arm.setPosition(Arm.Position.LOW_GOAL);
else if (gamepad1.dpad_down)
robot.arm.setPosition(Arm.Position.LOADING);
}
}
}
<file_sep>package org.firstinspires.ftc.teamcode.kickoff2018;
import com.qualcomm.robotcore.eventloop.opmode.LinearOpMode;
public class RobotClass {
public final Drive drive;
public final Intake intake;
public final Arm arm;
private final LinearOpMode opMode;
public RobotClass(LinearOpMode opMode){
this.opMode = opMode;
drive = new Drive(opMode.hardwareMap);
intake = new Intake(opMode.hardwareMap);
arm = new Arm(opMode.hardwareMap);
}
}
| db1992b38139e96c81bc0d33e2d6525f1a40b94e | [
"Java"
]
| 2 | Java | RechargedGreen/kickoff2018 | b9fb4bcdf8a23f0b517e5ccaf840c453e24603ea | fe8817f00d4c91794eec3025ff46e9c8e5b1eea6 | |
refs/heads/master | <repo_name>monisoi/nlp_api_for_aituber<file_sep>/README.md
# NLP API for AItuber
AItuber向けの様々な自然言語処理APIを追加していく予定です。
## 動作確認環境
- keras v2.3.0
- tensorflow v1.14.0
## /analyze_sentiment
感情分析です。
以下の手法を使わせていただいています。
[Twitter Sentiment Analysis](https://www.kaggle.com/paoloripamonti/twitter-sentiment-analysis)
学習させたファイルを `/models` に格納してください。<file_sep>/conversation_word_level.py
#!/usr/bin/env python
# -*- coding: utf-8 -*-
import numpy as np
import re
import pickle
from tensorflow.keras.models import Model, load_model
from tensorflow.keras.layers import Input
from tensorflow.keras.preprocessing.sequence import pad_sequences
SEQUENCE_LENGTH = 30
MAX_OUTPUT_LENGTH = 100
def clean_text(text):
text = text.lower()
text = re.sub(r"i'm", "i am", text)
text = re.sub(r"he's", "he is", text)
text = re.sub(r"she's", "she is", text)
text = re.sub(r"it's", "it is", text)
text = re.sub(r"that's", "that is", text)
text = re.sub(r"what's", "that is", text)
text = re.sub(r"where's", "where is", text)
text = re.sub(r"how's", "how is", text)
text = re.sub(r"\'ll", " will", text)
text = re.sub(r"\'ve", " have", text)
text = re.sub(r"\'re", " are", text)
text = re.sub(r"\'d", " would", text)
text = re.sub(r"\'re", " are", text)
text = re.sub(r"won't", "will not", text)
text = re.sub(r"can't", "cannot", text)
text = re.sub(r"n't", " not", text)
text = re.sub(r"n'", "ng", text)
text = re.sub(r"'bout", "about", text)
text = re.sub(r"'til", "until", text)
text = re.sub(r"[-()\"'#/@;:<>{}`+=~|.!?,]", "", text)
return text
class Conversation():
def __init__(self, tokenizer, model):
self.tokenizer, self.word2index, self.index2word = self.load_tokenizer(tokenizer)
self.model = load_model(model)
self.encoder_model, self.decoder_model = self.create_models()
def load_tokenizer(self, file_name):
with open(file_name, 'rb') as handle:
tokenizer = pickle.load(handle)
word2index = tokenizer.word_index
index2word = dict(map(reversed, word2index.items()))
return tokenizer, word2index, index2word
def create_models(self):
HIDDEN_DIM = 256
# encoder
encoder_inputs = self.model.input[0]
_, *encoder_states = self.model.layers[4].output
encoder_model = Model(encoder_inputs, encoder_states)
# decoder
decoder_inputs = Input(shape=(1,))
decoder_embedding_layer = self.model.layers[3]
decoder_embedded = decoder_embedding_layer(decoder_inputs)
decoder_lstm_layer = self.model.layers[5]
decoder_states_inputs = [Input(shape=(HIDDEN_DIM,)), Input(shape=(HIDDEN_DIM,))]
decoder_lstm, *decoder_states = decoder_lstm_layer(
decoder_embedded,
initial_state=decoder_states_inputs)
decoder_dense_layer = self.model.layers[6]
decoder_outputs = decoder_dense_layer(decoder_lstm)
decoder_model = Model(
[decoder_inputs] + decoder_states_inputs,
[decoder_outputs] + decoder_states)
return encoder_model, decoder_model
def decode_sequence(self, input_seq):
formated_input_seq = '<s> {} </s>'.format(clean_text(input_seq))
tokenized_input_seq = pad_sequences(
self.tokenizer.texts_to_sequences([formated_input_seq]),
padding='post',
maxlen=SEQUENCE_LENGTH)
bos = [self.word2index['<s>']]
eos = [self.word2index['</s>']]
states = self.encoder_model.predict(tokenized_input_seq)
target = np.array(bos)
output_seq = [bos[0]]
for i in range(MAX_OUTPUT_LENGTH):
tokens, *states = self.decoder_model.predict([target] + states)
output_index = [np.argmax(tokens[0, -1, :])]
output_seq += output_index
if output_index == eos:
break
target = np.array(output_index)
output_seq = ' '.join([self.index2word[i] for i in output_seq if i not in bos + eos])
return output_seq
def reply(self, input_seq):
output_seq = self.decode_sequence(input_seq)
response = {
"input_seq": input_seq,
"output_seq": output_seq,
}
return response
<file_sep>/conversation_char_level.py
#!/usr/bin/env python
# -*- coding: utf-8 -*-
import pandas as pd
import numpy as np
from keras.layers import Dense, LSTM, Input
from keras.models import Model
from keras.models import load_model
latent_dim = 256
num_encoder_tokens = 37
num_decoder_tokens = 39
max_decoder_seq_length = 301
input_token_index = {' ': 0, '0': 1, '1': 2, '2': 3, '3': 4, '4': 5, '5': 6, '6': 7, '7': 8,
'8': 9, '9': 10, 'a': 11, 'b': 12, 'c': 13, 'd': 14, 'e': 15, 'f': 16,
'g': 17, 'h': 18, 'i': 19, 'j': 20, 'k': 21, 'l': 22, 'm': 23, 'n': 24,
'o': 25, 'p': 26, 'q': 27, 'r': 28, 's': 29, 't': 30, 'u': 31, 'v': 32,
'w': 33, 'x': 34, 'y': 35, 'z': 36}
target_token_index = {'\t': 0, '\n': 1, ' ': 2, '0': 3, '1': 4, '2': 5, '3': 6, '4': 7, '5': 8,
'6': 9, '7': 10, '8': 11, '9': 12, 'a': 13, 'b': 14, 'c': 15, 'd': 16,
'e': 17, 'f': 18, 'g': 19, 'h': 20, 'i': 21, 'j': 22, 'k': 23, 'l': 24,
'm': 25, 'n': 26, 'o': 27, 'p': 28, 'q': 29, 'r': 30, 's': 31, 't': 32,
'u': 33, 'v': 34, 'w': 35, 'x': 36, 'y': 37, 'z': 38}
reverse_input_char_index = dict(
(i, char) for char, i in input_token_index.items())
reverse_target_char_index = dict(
(i, char) for char, i in target_token_index.items())
class Conversation():
def __init__(self, model):
self.model = load_model(model)
self.encoder_model = self.create_encoder()
self.decoder_model = self.create_decoder()
def create_encoder(self):
encoder_inputs = self.model.input[0] # input_1
encoder_outputs, state_h, state_c = self.model.layers[2].output
encoder_states = [state_h, state_c]
return Model(encoder_inputs, encoder_states)
def create_decoder(self):
decoder_inputs = self.model.input[1] # input_2
decoder_state_input_h = Input(shape=(latent_dim,), name='input_3')
decoder_state_input_c = Input(shape=(latent_dim,), name='input_4')
decoder_states_inputs = [decoder_state_input_h, decoder_state_input_c]
decoder_lstm = self.model.layers[3]
decoder_outputs, state_h, state_c = decoder_lstm(
decoder_inputs, initial_state=decoder_states_inputs)
decoder_states = [state_h, state_c]
decoder_dense = self.model.layers[4]
decoder_outputs = decoder_dense(decoder_outputs)
return Model(
[decoder_inputs] + decoder_states_inputs,
[decoder_outputs] + decoder_states)
def decode_sequence(self, input_seq):
states_value = self.encoder_model.predict(input_seq)
target_seq = np.zeros((1, 1, num_decoder_tokens))
target_seq[0, 0, target_token_index['\t']] = 1.
stop_condition = False
decoded_sentence = ''
while not stop_condition:
output_tokens, h, c = self.decoder_model.predict(
[target_seq] + states_value)
sampled_token_index = np.argmax(output_tokens[0, -1, :])
sampled_char = reverse_target_char_index[sampled_token_index]
decoded_sentence += sampled_char
if (sampled_char == '\n' or
len(decoded_sentence) > max_decoder_seq_length):
stop_condition = True
target_seq = np.zeros((1, 1, num_decoder_tokens))
target_seq[0, 0, sampled_token_index] = 1.
states_value = [h, c]
return decoded_sentence
def reply(self, input_seq):
onehot_input_seq = np.zeros(
(1, len(input_seq), num_encoder_tokens), dtype='float32')
for i, char in enumerate(input_seq):
onehot_input_seq[0, i, input_token_index[char]] = 1.
response = {
"input_seq": input_seq,
"output_seq": self.decode_sequence(onehot_input_seq),
}
return response
<file_sep>/main.py
#!/usr/bin/env python
# -*- coding: utf-8 -*-
from flask import Flask, request, jsonify
from sentiment_analysis import SentimentAnalysis
# from conversation_char_level import Conversation
from conversation_word_level import Conversation
SA_TOKENIZER = './models/sa_tokenizer.pkl'
SA_MODEL = './models/sa_model.h5'
CONV_TOKENIZER = './models/conv_tokenizer.pkl'
CONV_MODEL = './models/conv_model.h5'
app = Flask(__name__)
@app.before_first_request
def _load_model():
global sentiment_analysis
global conversation
sentiment_analysis = SentimentAnalysis(SA_TOKENIZER, SA_MODEL)
conversation = Conversation(CONV_TOKENIZER, CONV_MODEL)
@app.route('/')
def hello():
"""Return a friendly HTTP greeting."""
return 'Hello World!'
@app.route('/analyze_sentiment')
def analyze_sentiment():
if not sentiment_analysis:
_load_model()
if not sentiment_analysis:
return 'Sentiment Analysis Model not found.'
text = request.args.get('text')
result = sentiment_analysis.predict(text)
# result example
# {'label': 'NEGATIVE',
# 'score': 0.010753681883215904,
# 'elapsed_time': 0.26644086837768555}
return jsonify({
'status': 'OK',
'result': result
})
@app.route('/talk')
def talk():
if not conversation:
_load_model()
if not conversation:
return 'Conversation Model not found.'
text = request.args.get('text')
result = conversation.reply(text)
# result example
# {'input_seq': 'how are you',
# 'output_seq': 'i am fine'}
return jsonify({
'status': 'OK',
'result': result
})
if __name__ == '__main__':
# to solve a problem. see this issue.
# https://github.com/keras-team/keras/issues/13353
app.run(host='0.0.0.0', port=5000, threaded=False)
<file_sep>/sentiment_analysis.py
#!/usr/bin/env python
# -*- coding: utf-8 -*-
from tensorflow.keras.preprocessing.sequence import pad_sequences
from tensorflow.keras.models import load_model
import time
import pickle
SEQUENCE_LENGTH = 300
POSITIVE = "POSITIVE"
NEGATIVE = "NEGATIVE"
NEUTRAL = "NEUTRAL"
SENTIMENT_THRESHOLDS = (0.4, 0.7)
class SentimentAnalysis():
def __init__(self, tokenizer, model):
with open(tokenizer, 'rb') as handle:
self.tokenizer = pickle.load(handle)
self.model = load_model(model)
def decode_sentiment(self, score):
label = NEUTRAL
if score <= SENTIMENT_THRESHOLDS[0]:
label = NEGATIVE
elif score >= SENTIMENT_THRESHOLDS[1]:
label = POSITIVE
return label
def predict(self, text):
start_at = time.time()
x_test = pad_sequences(self.tokenizer.texts_to_sequences(
[text]), maxlen=SEQUENCE_LENGTH)
score = self.model.predict([x_test])[0]
label = self.decode_sentiment(score)
return {"label": label, "score": float(score),
"elapsed_time": time.time() - start_at}
| 64a8c5993128fe0bfc2c533c3c7cad631c6f2af3 | [
"Markdown",
"Python"
]
| 5 | Markdown | monisoi/nlp_api_for_aituber | e7e27836bbe0a419b4f7298164c8553940fccdac | 41977cae741ee779ce13da727e4476766675adc4 | |
refs/heads/master | <file_sep>cmake_minimum_required(VERSION 3.5)
project(webcam)
add_library(${PROJECT_NAME}
src/VideoFormat.hpp
src/VideoFormat.cpp
)
target_include_directories(${PROJECT_NAME} PUBLIC
$<BUILD_INTERFACE:${CMAKE_CURRENT_SOURCE_DIR}/src>
)
set_target_properties(${PROJECT_NAME} PROPERTIES FOLDER library)
<file_sep>#pragma once
#include <iosfwd>
namespace cam {
struct VideoFormat {
unsigned width;
unsigned height;
unsigned framerate;
};
bool operator==(const VideoFormat& l, const VideoFormat& r);
bool operator!=(const VideoFormat& l, const VideoFormat& r);
std::ostream& operator<<(std::ostream& oss, const VideoFormat& format);
}<file_sep>#include "VideoFormat.hpp"
#include <iostream>
namespace cam {
bool operator==(const VideoFormat & l, const VideoFormat & r) {
return l.width == r.width && l.height == r.height && l.framerate == r.framerate;
}
bool operator!=(const VideoFormat & l, const VideoFormat & r) {
return !(l == r);
}
std::ostream& operator<<(std::ostream& oss, const VideoFormat& format) {
return oss << format.width << "x" << format.height << "@" << format.framerate << "fps";
}
}<file_sep>cmake_minimum_required(VERSION 3.5)
project(webcam_solution)
add_subdirectory(webcam)
set(BUILD_TESTS on)
if (BUILD_TESTS)
add_subdirectory(test)
set(CMAKE_MODULE_PATH ${CMAKE_CURRENT_SOURCE_DIR}/.travis/cmake)
if (CMAKE_BUILD_TYPE STREQUAL "Coverage")
include(CodeCoverage)
setup_target_for_coverage(webcam_coverage webcam_tests coverage)
SET(CMAKE_CXX_FLAGS "-g -O0 -fprofile-arcs -ftest-coverage")
SET(CMAKE_C_FLAGS "-g -O0 -fprofile-arcs -ftest-coverage")
endif() #CMAKE_BUILD_TYPE STREQUAL "Coverage"
endif()
<file_sep>cmake_minimum_required(VERSION 3.5)
project(webcam_tests)
set(gtest_force_shared_crt ON CACHE BOOL "Always use msvcrt.dll" FORCE)
add_subdirectory(external/googletest)
set_target_properties(gtest PROPERTIES FOLDER gtest)
set_target_properties(gmock PROPERTIES FOLDER gtest)
set_target_properties(gtest_main PROPERTIES FOLDER gtest)
set_target_properties(gmock_main PROPERTIES FOLDER gtest)
add_executable(${PROJECT_NAME}
src/video_format_tests.cpp
)
target_link_libraries(${PROJECT_NAME} gtest gmock gtest_main webcam)
set_target_properties(${PROJECT_NAME} PROPERTIES FOLDER tests)
<file_sep>#pragma once
#include <sstream>
#include <gtest/gtest.h>
#include <VideoFormat.hpp>
TEST(VideoFormat, EqualityOperator) {
cam::VideoFormat a = {1280, 720, 30};
cam::VideoFormat b = {1280, 720, 30};
ASSERT_EQ(a, b);
}
TEST(VideoFormat, NotEqualsOperator) {
cam::VideoFormat c = {1280, 720, 30};
cam::VideoFormat d = {1280, 1280, 30};
ASSERT_NE(c, d);
}
TEST(VideoFormat, TestStreamOperator) {
cam::VideoFormat c = { 1280, 720, 30 };
std::ostringstream oss;
oss << c;
ASSERT_EQ(oss.str(), "1280x720@30fps");
} | 076d1fe42161bec675649383db00e0c9493babe1 | [
"CMake",
"C++"
]
| 6 | CMake | augustjd/webcam_ci | 09d8252e5b1971f355cb5e397ab930d4ac4e0861 | 37259820b7c274077b1cc5c0717ece8602b3d020 | |
refs/heads/master | <file_sep>package com.baidu.springboothibernate.repository;
import com.baidu.springboothibernate.domin.Users;
import org.springframework.data.jpa.repository.JpaRepository;
public interface UsersRepository extends JpaRepository<Users,Integer> {
}
<file_sep>package com.baidu.springboothibernate.controller;
import com.baidu.springboothibernate.domin.Users;
import com.baidu.springboothibernate.service.UsersService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class UsersController {
@Autowired
private UsersService usersService;
@RequestMapping("/find")
public Users findone(Integer id){
return usersService.findById(id);
}
@RequestMapping("/all")
public List<Users> findAll(){
return usersService.findAll();
}
@RequestMapping("/save")
public String save(Users users){
Users save = this.usersService.save(users);
if(save!=null){
return "成功";
}else{
return "失败";
}
}
@RequestMapping("/delete")
public String delete(Integer id){
Users user = usersService.findById(id);
this.usersService.delete(user);
return "成功";
}
@RequestMapping("/sort")
public List<Users> sort(){
return this.usersService.findAllSolrDesc();
}
@RequestMapping("/page")
public List<Users> page(){
return this.usersService.fingpageable();
}
}
<file_sep>package com.baidu.springboothibernate.domin;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import javax.persistence.*;
import java.io.Serializable;
@Entity
@Table(name = "users")
@JsonIgnoreProperties(value="hibernateLazyInitializer")
public class Users implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name="id")
private Integer id;
@Column(name="username")
private String username;
@Column(name="password")
private String password;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return <PASSWORD>;
}
public void setPassword(String password) {
this.password = <PASSWORD>;
}
@Override
public String toString() {
return "Users{" +
"id=" + id +
", username='" + username + '\'' +
", password='" + <PASSWORD> + '\'' +
'}';
}
}
| 8393e910d0e68adc4caa4ee8e4f478e06be3967d | [
"Java"
]
| 3 | Java | sxy2550101290/Test | 05919f32310c6b62ed63fb5d815ba8e5f56d8279 | e2362dc33989c610a0ef680eedb9b0d0c3fa463f | |
refs/heads/master | <file_sep>import '../css/style.css';
// class Client {
// constructor(firstName , lastName){
// this.firstName = firstName;
// this.lastName = lastName;
// }
// displayName(){
// return `Name is ${this.firstName} ${this.lastName}`;
// }
// }
// let client = new Client("Ivan", "Ivanov");
// console.log(client.displayName());
console.log("Index js");
console.log("New..");
const clients = ["Client1", "Client2", "Client3", "Client4", "Client5", "Client6"];
let html = "";
clients.forEach(client => {
html += `
<li>${client}</li>
`;
});
document.querySelector('#clients').innerHTML = html;
| 0e420ac6fb621ea9215a8751050180d799a3d319 | [
"JavaScript"
]
| 1 | JavaScript | ivanstoykovivanov-telerik/WEBPACK_Starter_template | 4a49aeb015e73939fc4a73e019fcbb618908c9a0 | e09900f416c30a50f206385e5e3c836503a9d7bb | |
refs/heads/master | <file_sep>$document.ready( function jj() {
$('#fileupload').fileupload({
dataType: 'json',
url: 'UploadFiles',
autoUpload: true,
done: function (e, data) {
alert("ssss");
$('.file_name').html(data.result.name);
$('.file_type').html(data.result.type);
$('.file_size').html(data.result.size);
}
});
});
$document.ready( $('#pst').click(function (e) {
e.preventDefault();
var status = document.getElementById("status").value;
alert("OOOOOOO");
if (status == "") {
return;
}
var today = new Date();
h = today.getHours();
if (h < 10) {
h = "0" + h;
}
else if (h > 12) {
h = h - 12;
}
m = today.getMinutes();
if (m < 10) {
m = "0" + m;
}
var id = null;
var a = $(this).data('id');
$.getJSON("/Home/Update_Status?status=" + status, function (data) {
if (data) {
id = data;
alert(id);
var chk = '<div id=' + id + ' ><div class="user-block"> <img class="img-circle img-bordered-sm" src="/Images/' + a + '" alt="user image"><span class="username"><a href="#"><NAME> Jr.</a><a href="#" class="pull-right btn-box-tool"><i class="fa fa-times"></i></a></span><span class="description">Shared publicly - ' + h + ':' + m + ' today</span>';
chk = chk + "</div><p >" + status + "</p></div>";
chk = chk + '<ul class="list-inline">';
chk = chk + '<li><a href="#" class="link-black text-sm"><i class="fa fa-share margin-r-5"></i> Share</a></li>';
chk = chk + '<li><a href="#" class="link-black text-sm"><i class="fa fa-thumbs-o-up margin-r-5"></i> Like</a></li>';
chk = chk + '<li class="pull-right"><a href="#" class="link-black text-sm"><i class="fa fa-comments-o margin-r-5"></i> Comments (5)</a></li></ul>';
chk = chk + '<div id="' + id + '"><input class="form-control input-sm" onKeyDown="key()" id="bttn" type="text" placeholder="Type a comment"></div></div>';
$("#post1").prepend(chk);
$("#status").val("");
$("button").closest("div").attr("id");
alert("OOOOOOO");
}
else {
}
});
}));
function key() {
document.getElementById('bttn').onkeydown = function (event) {
if (event.keyCode == 13) {
$("bttn").closest("div").attr("id");
}
}
} | 0a71dd7a49316fa441a58eb01caafb98c539c36b | [
"JavaScript"
]
| 1 | JavaScript | bcsf12a525/locale | 0d0e0dbf9d196d9d68af8db889eb68a38caa7681 | 78ac113c59ca9bb34d4d24050da97700d840746f | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
using System.Xml;
using System.Xml.Serialization;
namespace FormPartie1
{
static class Program
{
/// <summary>
/// Point d'entrée principal de l'application.
/// </summary>
[STAThread]
static void Main()
{
/*Question q = new Question("titre", "rep1", "rep2");
XmlSerializer xs = new XmlSerializer(typeof(Question));
using (StreamWriter wr = new StreamWriter("question.xml"))
{
xs.Serialize(wr, q);
}*/
//liste des questions et leurs réponses associées
List<Question> questions = new List<Question>();
questions.Add(new Question("Enoncé 1", " ", " "));
questions.Add(new Question("Enoncé 2", " ", " " ));
//pour la sérialisation
StreamWriter writer = new StreamWriter("questions.xml");
new XmlSerializer(typeof(List<Question>)).Serialize(writer, questions);
writer.Close();
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new MainForm());
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Partie_1
{
public class Reponse
{
public string Rep { get; set; }
public Reponse() { }
public override string ToString()
{
return Rep.ToString();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
using System.Xml;
using System.Xml.Serialization;
namespace Partie_1
{
static class Program
{
/// <summary>
/// Point d'entrée principal de l'application.
/// </summary>
[STAThread]
static void Main()
{
//créer des listes vide
List<Question> listeQuestions = new List<Question>();
List<Reponse> listeReponses = new List<Reponse>();
List<BonneReponse> listeBonne = new List<BonneReponse>();
// listeQuestions.Add(new Question() { Intitule = "bla" });
// listeQuestions.Add(new Question() { Intitule = "Première question" }); //ajoute un élément dans la liste
// listeQuestions.Add(new Question() { Intitule = "Seconde question" });
// listeReponses.Add(new Reponse() { Rep = "" });
// listeReponses.Add(new Reponse() { Rep = "Bonne réponse question 1" });
// listeReponses.Add(new Reponse() { Rep = "réponse 2" });
// listeBonne.Add(new BonneReponse() { BonneRep = "" });
// listeBonne.Add(new BonneReponse() { BonneRep = "Bonne réponse question 1" });
//permet de vérifier si la listeQuestion fonctionne
/* foreach (Question q in listeQuestions)
Console.WriteLine(q);
*/
//pour la sérialisation
/* StreamWriter writerQuestion = new StreamWriter("questions.xml"); //créer un fichier xml
new XmlSerializer(typeof(List<Question>)).Serialize(writerQuestion, listeQuestions); //entre la liste des questions dans le fichier xml
writerQuestion.Close(); //arrête d'écrire dans le fichier xml
StreamWriter writerReponse = new StreamWriter("reponses.xml");
new XmlSerializer(typeof(List<Reponse>)).Serialize(writerReponse, listeReponses);
writerReponse.Close();
StreamWriter writerBonne = new StreamWriter("bonneReponses.xml");
new XmlSerializer(typeof(List<BonneReponse>)).Serialize(writerBonne, listeBonne);
writerBonne.Close();
*/
//pour la désérialisation
XmlSerializer xs = new XmlSerializer(typeof(List<Question>));
using (Stream ins = File.Open("questions.xml", FileMode.Open))
foreach (Question o in (List<Question>)xs.Deserialize(ins))
listeQuestions.Add(o);
XmlSerializer xsRep = new XmlSerializer(typeof(List<Reponse>));
using (Stream insRep = File.Open("reponses.xml", FileMode.Open))
foreach (Reponse r in (List<Reponse>)xsRep.Deserialize(insRep))
listeReponses.Add(r);
XmlSerializer xsBonne = new XmlSerializer(typeof(List<BonneReponse>));
using (Stream insBonne = File.Open("bonneReponses.xml", FileMode.Open))
foreach (BonneReponse b in (List<BonneReponse>)xsBonne.Deserialize(insBonne))
listeBonne.Add(b);
//permet de vérifier que la désérialisation fonctionne
/*
foreach (Question q in listeQuestions)
Console.WriteLine(q);
*/
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new Form1(listeQuestions, listeReponses, listeBonne));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace FormPartie1
{
static class Program
{
/// <summary>
/// Point d'entrée principal de l'application.
/// </summary>
[STAThread]
static void Main()
{
Question qu = new Question("titre", "rep1", "rep2", "rep3", "rep4", "rep5", "rep6");
qu.SerializeQuestion();
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new FPartie1());
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace FormPartie1
{
public partial class FPartie1 : Form
{
public static int compteurQ = 1;
public static int cptPage = 1;
public FPartie1()
{
InitializeComponent();
}
private void EcritureQuestion()
{
string name = "LQuestion" + compteurQ.ToString();
this.LQuestion1.Text = name;
compteurQ++;
}
private void radioButton1_CheckedChanged(object sender, EventArgs e)
{
}
private void radioButton2_CheckedChanged(object sender, EventArgs e)
{
}
private void radioButton3_CheckedChanged(object sender, EventArgs e)
{
}
private void BSuivant_Click(object sender, EventArgs e)
{
cptPage++;
//mettre les questions suivantes
if (cptPage == 7)
{
this.BSuivant.Text = "Terminer";
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
using System.Xml;
using System.Xml.Serialization;
namespace FormPartie1
{
class Question
{
private static int compteur;
private int id;
private string intitule;
private string rep1;
private string rep2;
private string rep3;
private string rep4;
private string rep5;
private string rep6;
public static StreamWriter wr = new StreamWriter("questions.xml");
public static StreamReader re = new StreamReader("questions.xml");
public static XmlSerializer xs = new XmlSerializer(typeof(Question));
public Question(string intitule, string rep1, string rep2, string rep3,string rep4, string rep5, string rep6)
{
compteur++;
this.id=compteur;
this.intitule = intitule;
this.rep1 = rep1;
this.rep2 = rep2;
this.rep3 = rep3;
this.rep4 = rep4;
this.rep5 = rep5;
this.rep6 = rep6;
}
public void SerializeQuestion()
{
using (wr)
{
xs.Serialize(wr, this);
}
}
public void DeserializeQuestion()
{
using (re)
{
Question q = xs.Deserialize(re) as Question ;
}
}
public void choixQuestion()
{
Random r = new Random();
int n = r.Next(compteur);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Pluscourtchemin
{
public partial class Choix : Form
{
public Choix()
{
InitializeComponent();
}
private void buttonQuestionsCours_Click(object sender, EventArgs e)
{
Application.Run(new Dijkstra());
}
private void buttonAlgoDijkstra_Click(object sender, EventArgs e)
{
Application.Run(new Arbre());
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
using System.Windows.Forms;
namespace Pluscourtchemin
{
public class SearchTreeALaMain : SearchTree
{
private TreeView arbre = new TreeView();
private Button Verification;
private void AjouterNoeudOuvert(GenericNode N2)
{
L_Ouverts.Add(N2);
}
private void EnleverNoeudOuvert(GenericNode N2)
{
L_Ouverts.Remove(N2);
}
private void AjouterNoeudFerme(GenericNode N2)
{
L_Fermes.Add(N2);
}
private void verificationOuverts(SearchTree s)
{
foreach (GenericNode noeud in s.L_Ouverts)
{
}
}
private void verificationFermes(SearchTree s)
{
foreach (GenericNode noeud in s.L_Fermes)
{
}
}
private List<TreeNode> GetNoeuds (TreeView a)
{
List<TreeNode> noeuds = new List<TreeNode>();
for (int i=0;i < a.GetNodeCount(true); i++)
{
noeuds.Add(a.GetNodeAt(i,i));
}
return noeuds;
}
private void ajouterNoeudSurArbre(TreeNode noeud, TreeNode noeudPere)
{
arbre.BeginUpdate();
foreach (TreeNode n in this.GetNoeuds(arbre))
{
}
arbre.EndUpdate();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
using System.Xml;
using System.Xml.Serialization;
namespace FormPartie1
{
public class Question
{
private static int compteur;
private int id { get; set; }
public string intitule { get; set; }
public string rep1 { get; set; }
public string rep2 { get; set; }
public Question() { }
public Question(string intitule, string rep1, string rep2)
{
compteur++;
this.id=compteur;
this.intitule = intitule;
this.rep1 = rep1;
this.rep2 = rep2;
}
public void choixQuestion()
{
Random r = new Random();
int n = r.Next(compteur);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace FormPartie1
{
public partial class MainForm : Form
{
private List<Question> _questions;
public MainForm()
{
InitializeComponent();
_questions = new List<Question>();
_questions.Add(new Question("Q1", "Matt", "Québec"));
}
private void btn_commencer_Click(object sender, EventArgs e)
{
QuestionForm f2 = new QuestionForm(_questions);
f2.Show();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Pluscourtchemin
{
public partial class Arbre : Form
{
TreeView a = new TreeView();
public Arbre()
{
InitializeComponent();
}
private void button7_Click(object sender, EventArgs e) //bouton pour ajouter un noeud
{
}
private void button8_Click(object sender, EventArgs e) //bouton pour vérification
{
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Partie_1
{
public partial class Form1 : Form
{
private List<Question> _questions;
private List<Reponse> _reponses;
private List<BonneReponse> _bonnes;
private int points;
// public bool Checked { get; set; }
public Form1() { }
public Form1(List<Question> questions, List<Reponse> reponses, List<BonneReponse> bonnes)
{
InitializeComponent();
_questions = questions;
_reponses = reponses;
_bonnes = bonnes;
//tbQuestion.Text = "Bienvenue sur votre test d'IA !!";
}
private void groupBox1_Load(object sender, EventArgs e)
{
}
private void Btn_Click(object sender, EventArgs e)
{
/* foreach (Question q in _questions)
{*/
for(int i=0; i<6; i++)
{
tbQuestion.Text = _questions[i].ToString();
for(int j=0; j<8; j=j+4)
{
rbRep1.Text = _reponses[j].ToString();
rbRep2.Text = _reponses[j+1].ToString();
rbRep3.Text = _reponses[j+2].ToString();
rbRep4.Text = _reponses[j+3].ToString();
}
}
// tbQuestion.Text = _questions[1].ToString();
// rbRep1.Text = _reponses[1].ToString();
// rbRep2.Text = _reponses[2].ToString();
// rbRep3.Text = _reponses[3].ToString();
// rbRep4.Text = _reponses[4].ToString();
}
private void BtnVerifier_Click(object sender, EventArgs e)
{
string repFinale = (rbRep1.Checked) ? rbRep1.Text : rbRep2.Text;
if (repFinale == _bonnes[0].ToString())
{
MessageBox.Show("Bonne réponse ! ");
points++;
}
}
/* private void rbRep1_CheckedChanged(object sender, EventArgs e)
{
// Executed when any radio button is changed.
// ... It is wired up to every single radio button.
// Search for first radio button in GroupBox.
string result1 = null;
foreach (Control control in this.groupBox1.Controls)
{
if (control is RadioButton)
{
RadioButton radio = control as RadioButton;
if (radio.Checked)
{
result1 = radio.Text;
}
}
}
this.Text = result1;
}*/
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Partie_1
{
public class BonneReponse
{
public string BonneRep { get; set; }
public BonneReponse() { }
public override string ToString()
{
return BonneRep.ToString();
}
}
}
<file_sep># ProjetIA
Ceci est le projet de <NAME>, <NAME> et <NAME> dans le module d'intelligence artificielle de 2A.
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
namespace Pluscourtchemin
{
public partial class Dijkstra : Form
{
static public double[,] matrice;
static public int nbnodes = 10;
static public int numinitial;
static public int numfinal;
static public SearchTreeALaMain m = new SearchTreeALaMain();
static public SearchTree g = new SearchTree();
static private int compteurCorrectsNodes;
static private int compteurCorrectsNodesObjectif;
static private bool check;
static private List<string> fermesMain = new List<string>();
static private List<string> ouvertsMain = new List<string>();
public Dijkstra()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
matrice = new double[nbnodes, nbnodes];
for (int i = 0; i < nbnodes; i++)
for (int j = 0; j < nbnodes; j++)
matrice[i, j] = -1;
matrice[0, 1] = 3; matrice[1, 0] = 3;
matrice[0, 2] = 5; matrice[2, 0] = 5;
matrice[0, 3] = 7; matrice[3, 0] = 7;
matrice[1, 4] = 8; matrice[4, 1] = 8;
matrice[2, 4] = 3; matrice[4, 2] = 3;
matrice[4, 5] = 7; matrice[5, 4] = 7;
matrice[5, 6] = 4; matrice[6, 5] = 4;
}
private void button2_Click(object sender, EventArgs e)
{
numinitial = Convert.ToInt32(textBox1.Text);
numfinal = Convert.ToInt32(textBox2.Text);
SearchTree g = new SearchTree();
Node2 N0 = new Node2();
N0.numero = numinitial;
List<GenericNode> solution = g.RechercheSolutionAEtoile(N0);
Node2 N1 = N0;
for (int i = 1; i < solution.Count; i++)
{
Node2 N2 = (Node2)solution[i];
listBox1.Items.Add(Convert.ToString(N1.numero)
+ "--->" + Convert.ToString(N2.numero)
+ " : " + Convert.ToString(matrice[N1.numero, N2.numero]));
N1 = N2;
}
g.GetSearchTree(treeView1);
}
private void button3_Click(object sender, EventArgs e)
{
StreamReader monStreamReader = new StreamReader("graphe1.txt");
// Lecture du fichier avec un while, évidemment !
// 1ère ligne : "nombre de noeuds du graphe
string ligne = monStreamReader.ReadLine();
int i = 0;
while (ligne[i] != ':') i++;
string strnbnoeuds = "";
i++; // On dépasse le ":"
while (ligne[i] == ' ') i++; // on saute les blancs éventuels
while (i < ligne.Length) { strnbnoeuds = strnbnoeuds + ligne[i];
i++;
}
nbnodes = Convert.ToInt32(strnbnoeuds);
matrice = new double[nbnodes, nbnodes];
for (i = 0; i < nbnodes; i++)
for (int j = 0; j < nbnodes; j++)
matrice[i, j] = -1;
// Ensuite on a ls tructure suivante :
// arc : n°noeud départ n°noeud arrivée valeur
// exemple 4 :
ligne = monStreamReader.ReadLine();
while (ligne != null)
{
i = 0;
while (ligne[i] != ':') i++;
i++; // on passe le :
while (ligne[i] == ' ') i++; // on saute les blancs éventuels
string strN1 = "";
while (ligne[i] != ' ')
{
strN1 = strN1 + ligne[i];
i++;
}
int N1 = Convert.ToInt32(strN1);
// On saute les blancs éventuels
while (ligne[i] == ' ') i++;
string strN2 = "";
while (ligne[i] != ' ')
{
strN2 = strN2 + ligne[i];
i++;
}
int N2 = Convert.ToInt32(strN2);
// On saute les blancs éventuels
while (ligne[i] == ' ') i++;
string strVal = "";
while ((i < ligne.Length) && (ligne[i] != ' '))
{
strVal = strVal + ligne[i];
i++;
}
double val = Convert.ToDouble(strVal);
matrice[N1, N2] = val;
matrice[N2, N1] = val;
listBoxgraphe.Items.Add(Convert.ToString(N1)
+ "--->" + Convert.ToString(N2)
+ " : " + Convert.ToString(matrice[N1, N2]));
ligne = monStreamReader.ReadLine();
}
// Fermeture du StreamReader (obligatoire)
monStreamReader.Close();
}
//fonction qui transforme une liste de noeuds en liste de string
private List<string> transfoListesNoeudsEnChar(List<GenericNode> liste)
{
List<string> transfo = new List<string>();
for (int i = 0; i < liste.Count; i++)
{
transfo[i] = liste[i].ToString();
}
return transfo;
}
//bouton qui valide l'envoi des ouverts (verifie que l'utiliateur ne rentre pas n'importe quoi (a corrriger)
private void button4_Click(object sender, EventArgs e)
{
/* try
{
char ferme = Convert.ToChar(textBox3);
textBox3.Text = "";
}
catch
{
MessageBox.Show("Entrez quelque chose de correct svp");
textBox3.Text = "";
}
*/
}
//bouton qui valide l'envoi des ouverts (verifie que l'utiliateur ne rentre pas n'importe quoi (a corriger)
private void button5_Click(object sender, EventArgs e)
{
try
{
char ferme = Convert.ToChar(textBox3);
textBox3.Text = "";
}
catch
{
MessageBox.Show("Entrez quelque chose de correct svp");
textBox4.Text = "";
}
}
//bouton pour enlever un noeud
private void button7_Click(object sender, EventArgs e)
{
treeView2.SelectedNode.Remove();
}
//bouton qui change le texte d'un noeud
private void buttonEdition_Click(object sender, EventArgs e)
{
treeView2.SelectedNode.Text = textboxNoeudAjoute.Text;
}
//bouton qui lance la comparaison des deux arbres
private void buttonVerif_Click(object sender, EventArgs e)
{
CheckRecursive();
if((compteurCorrectsNodes == compteurCorrectsNodesObjectif) && (compteurCorrectsNodes != 0))
{
MessageBox.Show("Bien joué!");
}
}
//Fonction qui sert à comparer les deux arbres
private void CheckRecursive()
{
TreeNodeCollection nodes = treeView1.Nodes;
TreeNodeCollection nodesToCheck = treeView2.Nodes;
if(treeView1.GetNodeCount(false) != treeView2.GetNodeCount(false))
{
MessageBox.Show("Erreur");
}
else
{
for (int i = 0; i < nodes.Count; i++)
{
SubRecursive(nodes[i], nodesToCheck[i]);
}
}
}
/* Appelée par CheckRecursive */
private void SubRecursive(TreeNode treeNodeAlgo, TreeNode treeNodeToCheck)
{
if (treeNodeAlgo.Text == treeNodeToCheck.Text) { compteurCorrectsNodes++; }
compteurCorrectsNodesObjectif++;
if (treeNodeAlgo.Nodes.Count== treeNodeToCheck.Nodes.Count)
{
for (int j = 0; j < treeNodeAlgo.Nodes.Count; j++)
{
SubRecursive(treeNodeAlgo.Nodes[j], treeNodeToCheck.Nodes[j]);
}
}
else
{
MessageBox.Show("Aie, je crois que tu t'es trompé3..");
}
}
//ajouter un noeud à l'arbre
private void button9_Click(object sender, EventArgs e)
{
TreeNode node = new TreeNode(textboxNoeudAjoute.Text);
treeView2.Nodes.Add(node);
}
//ajouter un noeud fils à un noeud
private void button10_Click(object sender, EventArgs e)
{
string a = comboBox1.SelectedItem.ToString();
TreeNode node = new TreeNode(a);
if (a !="") {treeView2.SelectedNode.Nodes.Add(node); }
else {treeView2.Nodes.Add("nouveau noeud");}
}
private void textBox3_TextChanged(object sender, EventArgs e) //text box où on rentre les fermés
{
//permet de tester que la saisie soit bien un caractère alphabétique (les espaces et les virgules sont autorisés)
string text = textBox3.Text;
for (int i = 0; i < text.Length; i++)
{
if ((!char.IsLetter(text[i])) && (text[i] != ',') && (text[i] != ' '))
{
MessageBox.Show("Entrez quelque chose de correcte svp");
Console.Beep();
break;
}
else
{
MessageBox.Show("Prêt");
}
}
}
private void textBox4_TextChanged(object sender, EventArgs e) //text box où on rentre les ouverts
{
//permet de tester que la saisie soit bien un caractère alphabétique (les espaces et les virgules sont autorisés)
string text = textBox4.Text;
for (int i = 0; i < text.Length; i++)
{
if ((!char.IsLetter(text[i])) && (text[i] != ',') && (text[i] != ' '))
{
MessageBox.Show("Entrez quelque chose de correcte svp");
Console.Beep();
break;
}
else
{
MessageBox.Show("Prêt");
}
}
}
//bouton qui sert à lancer la comparaison
private void button6_Click(object sender, EventArgs e)
{
//On remplit la liste de fermés
string s = textBox3.Text;
string[] noeuds = s.Split(',');
foreach (string noeud in noeuds)
{
fermesMain.Add(noeud);
}
/*//On remplit la liste de fermés
string s = textBox3.Text;
string[] noeuds = s.Split(',');
foreach (string noeud in noeuds)
{
fermesMain.Add(noeud);
}*/
//On remplit la liste des ouverts
string t = textBox4.Text;
string[] noeudsO = t.Split(',');
foreach (string noeudO in noeudsO)
{
ouvertsMain.Add(noeudO);
}
Compare();
}
private void Compare() //Méthode de comparaison des listes des ouverts et fermes saisies et existantes entre elles
{
//comparaison de la liste des fermes existants avec la liste des fermes saisis
for (int i=0; i < g.L_Fermes.Count;i++)
{
if(g.L_Fermes[i].ToString() == fermesMain[i])
{
MessageBox.Show("Ok");
}
i++;
}
//comparaison de la liste des ouverts existants avec la liste des ouverts saisis
for (int j = 0; j < g.L_Ouverts.Count; j++)
{
if(g.L_Ouverts[j].ToString() == ouvertsMain[j])
{
MessageBox.Show("Pas OK");
}
}
}
//boucle qui parcourt les deux listes et test pour correspondance
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
using System.Xml;
using System.Xml.Serialization;
namespace Test
{
static class Program
{
/// <summary>
/// Point d'entrée principal de l'application.
/// </summary>
[STAThread]
static void Main()
{
List<Question> listeQuestions = new List<Question>(); //créer une liste de question vide
listeQuestions.Add(new Question() { Intitule = "Première question" }); //ajoute un élément dans la liste
//permet de vérifier si la listeQuestion fonctionne
/* foreach (Question q in listeQuestions)
Console.WriteLine(q);
*/
//pour la sérialisation
/* StreamWriter writer = new StreamWriter("questions.xml"); //créer un fichier xml
new XmlSerializer(typeof(List<Question>)).Serialize(writer, listeQuestions); //entre la liste des questions dans le fichier xml
writer.Close(); //arrête d'écrire dans le fichier xml
*/
//pour la désérialisation
XmlSerializer xs = new XmlSerializer(typeof(List<Question>));
using (Stream ins = File.Open("questions.xml", FileMode.Open))
foreach (Question o in (List<Question>)xs.Deserialize(ins))
listeQuestions.Add(o);
//permet de vérifier que la désérialisation fonctionne
/*
foreach (Question q in listeQuestions)
Console.WriteLine(q);
*/
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new Form1(listeQuestions));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
namespace Pluscourtchemin
{
public partial class Dijkstra : Form
{
static public double[,] matrice;
static public int nbnodes = 10;
static public int numinitial;
static public int numfinal;
static public SearchTree g = new SearchTree();
static private int compteurCorrectsNodes;
static private int compteurCorrectsNodesObjectif;
static private int compteurEtapes = 1;
public Dijkstra()
{
InitializeComponent();
Initialisation();
}
private void Initialisation()
{
matrice = new double[nbnodes, nbnodes];
for (int i = 0; i < nbnodes; i++)
for (int j = 0; j < nbnodes; j++)
matrice[i, j] = -1;
matrice[0, 1] = 3; matrice[1, 0] = 3;
matrice[0, 2] = 5; matrice[2, 0] = 5;
matrice[0, 3] = 7; matrice[3, 0] = 7;
matrice[1, 4] = 8; matrice[4, 1] = 8;
matrice[2, 4] = 3; matrice[4, 2] = 3;
matrice[4, 5] = 7; matrice[5, 4] = 7;
matrice[5, 6] = 4; matrice[6, 5] = 4;
numinitial = Convert.ToInt32(textBox1.Text);
numfinal = Convert.ToInt32(textBox2.Text);
Node2 N0 = new Node2();
N0.numero = numinitial;
List<GenericNode> solution = g.RechercheSolutionAEtoile(N0);
Node2 N1 = N0;
//affichage
for (int i = 1; i < solution.Count; i++)
{
Node2 N2 = (Node2)solution[i];
listBox1.Items.Add(Convert.ToString(N1.numero)
+ "--->" + Convert.ToString(N2.numero)
+ " : " + Convert.ToString(matrice[N1.numero, N2.numero]));
N1 = N2;
}
}
private void button2_Click(object sender, EventArgs e)
{
}
private void button3_Click(object sender, EventArgs e)
{
StreamReader monStreamReader = new StreamReader("graphe1.txt");
// Lecture du fichier avec un while, évidemment !
// 1ère ligne : nombre de noeuds du graphe
string ligne = monStreamReader.ReadLine();
int i = 0;
while (ligne[i] != ':') i++;
string strnbnoeuds = "";
i++; // On dépasse le ":"
while (ligne[i] == ' ') i++; // on saute les blancs éventuels
while (i < ligne.Length) { strnbnoeuds = strnbnoeuds + ligne[i];
i++;
}
nbnodes = Convert.ToInt32(strnbnoeuds);
matrice = new double[nbnodes, nbnodes];
for (i = 0; i < nbnodes; i++)
for (int j = 0; j < nbnodes; j++)
matrice[i, j] = -1;
// Ensuite on a la structure suivante :
// arc : n°noeud départ n°noeud arrivée valeur
// exemple 4 :
ligne = monStreamReader.ReadLine();
while (ligne != null)
{
i = 0;
while (ligne[i] != ':') i++;
i++; // on passe le :
while (ligne[i] == ' ') i++; // on saute les blancs éventuels
string strN1 = "";
while (ligne[i] != ' ')
{
strN1 = strN1 + ligne[i];
i++;
}
int N1 = Convert.ToInt32(strN1);
// On saute les blancs éventuels
while (ligne[i] == ' ') i++;
string strN2 = "";
while (ligne[i] != ' ')
{
strN2 = strN2 + ligne[i];
i++;
}
int N2 = Convert.ToInt32(strN2);
// On saute les blancs éventuels
while (ligne[i] == ' ') i++;
string strVal = "";
while ((i < ligne.Length) && (ligne[i] !=' '))
{
strVal = strVal + ligne[i];
i++;
}
double val = Convert.ToDouble(strVal);
matrice[N1, N2] = val;
matrice[N2, N1] = val;
listBoxgraphe.Items.Add(Convert.ToString(N1)
+ "--->" + Convert.ToString(N2)
+ " : " + Convert.ToString(matrice[N1, N2]));
ligne = monStreamReader.ReadLine();
}
// Fermeture du StreamReader (obligatoire)
monStreamReader.Close();
}
//fonction qui transforme une liste de noeuds en liste de string
private List<string> transfoListesNoeudsEnString(List<GenericNode> liste)
{
List < string > transfo = new List<string>();
foreach(GenericNode node in liste)
{
transfo.Add(node.ToString());
}
return transfo;
}
//bouton pour enlever un noeud
private void button7_Click(object sender, EventArgs e)
{
treeView2.SelectedNode.Remove();
}
//bouton qui change le texte d'un noeud
private void buttonEdition_Click(object sender, EventArgs e)
{
treeView2.SelectedNode.Text = textboxNoeudAjoute.Text;
}
//bouton qui lance la comparaison des deux arbres
private void buttonVerif_Click(object sender, EventArgs e)
{
g.GetSearchTree(treeView1);
CheckRecursive();
if((compteurCorrectsNodes == compteurCorrectsNodesObjectif) && (compteurCorrectsNodes != 0))
{
MessageBox.Show("Bien joué!");
}
}
//Fonction qui sert à comparer les deux arbres
private void CheckRecursive()
{
TreeNodeCollection nodes = treeView1.Nodes;
TreeNodeCollection nodesToCheck = treeView2.Nodes;
if(treeView1.GetNodeCount(false) != treeView2.GetNodeCount(false))
{
MessageBox.Show("Erreur");
}
else
{
for (int i = 0; i < nodes.Count; i++)
{
SubRecursive(nodes[i], nodesToCheck[i]);
}
}
}
/* Appelée par CheckRecursive */
private void SubRecursive(TreeNode treeNodeAlgo, TreeNode treeNodeToCheck)
{
if (treeNodeAlgo.Text == treeNodeToCheck.Text) { compteurCorrectsNodes++; }
compteurCorrectsNodesObjectif++;
if (treeNodeAlgo.Nodes.Count== treeNodeToCheck.Nodes.Count)
{
for (int j = 0; j < treeNodeAlgo.Nodes.Count; j++)
{
SubRecursive(treeNodeAlgo.Nodes[j], treeNodeToCheck.Nodes[j]);
}
}
else
{
MessageBox.Show("Aie, je crois que tu t'es trompé3..");
}
}
//ajouter un noeud à l'arbre
private void button9_Click(object sender, EventArgs e)
{
TreeNode node = new TreeNode(textboxNoeudAjoute.Text);
treeView2.Nodes.Add(node);
}
//ajouter un noeud fils à un noeud
private void button10_Click(object sender, EventArgs e)
{
string a = comboBox1.SelectedItem.ToString();
TreeNode node = new TreeNode(a);
if (a !="") {treeView2.SelectedNode.Nodes.Add(node); }
else {treeView2.Nodes.Add("nouveau noeud");}
}
private void verificationListes(List<string> Liste1, List<string> Liste2)
{
bool resultat = false;
if (Liste1.Count == Liste2.Count)
{
MessageBox.Show("On rentre ici L239");
for(int i = 0; i< Liste1.Count; i++)
{
MessageBox.Show("Les longueurs des listes sont de " + Liste2.Count);
MessageBox.Show(Liste1[i] + " " + Liste2[i]);
}
for(int i = 0; i < Liste1.Count; i++)
{
if (Liste1[i] == Liste2[i])
{
resultat = true;
}
else
{
resultat = false;
break;
}
}
}
if (resultat == true)
{
MessageBox.Show("C'est Ok!");
}
else
{
MessageBox.Show("Il y a des erreurs.");
}
}
//bouton qui sert à lancer la comparaison
private void button6_Click(object sender, EventArgs e)
{
List<string> fermesMain = new List<string>();
List<string> ouvertsMain = new List<string>();
//On remplit la liste de fermés
string s = textBox3.Text;
string[] noeuds1 = s.Split(',');
foreach (string noeud in noeuds1)
{
fermesMain.Add(noeud);
}
//On remplit la liste des ouverts
string t = textBox4.Text;
string[] noeuds2 = t.Split(',');
foreach (string noeud in noeuds2)
{
ouvertsMain.Add(noeud);
}
MessageBox.Show("Nombre de fermes rentrés à la main : "+fermesMain.Count + "\n Nombre de fermes de l'algo"+ g.liste_chaqueEtape_Fermes[compteurEtapes].Count);
MessageBox.Show("Nombre d'ouverts rentrés à la main : "+ouvertsMain.Count + "\n Nombre d ouverts de l'algo" + g.liste_chaqueEtape_Ouverts[compteurEtapes].Count);
List<string> fermesAlgoEnString = transfoListesNoeudsEnString(g.liste_chaqueEtape_Fermes [compteurEtapes]);
List<string> ouvertsAlgoEnString = transfoListesNoeudsEnString(g.liste_chaqueEtape_Ouverts [compteurEtapes]);
compteurEtapes++;
verificationListes(fermesMain, fermesAlgoEnString);
verificationListes(ouvertsMain, ouvertsAlgoEnString);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace FormPartie1
{
public partial class QuestionForm : Form
{
public static int compteurQ = 1;
public static int cptPage = 1;
private List<Question> _questions;
public QuestionForm(List<Question> questions)
{
InitializeComponent();
_questions = questions;
}
private void EcritureQuestion() //cette fonction doit être dans la classe Question, puis on l'appelle dans
//le Click suivant et dans initialise components
{
string name = "LQuestion" + compteurQ.ToString();
this.LQuestion1.Text = name;
compteurQ++;
}
private void btn_precedent_Click(object sender, EventArgs e)
{
cptPage--;
//retourner à la question précedente
}
private void btn_envoyer_Click(object sender, EventArgs e)
{
string message = "Voulez-vous vraiment Valider et Quitter ?";
if (MessageBox.Show(message, "Application Exit", MessageBoxButtons.YesNo) == DialogResult.Yes)
{
//envoyer les infos pour les sérialiser
Application.Exit();
}
}
private void btn_suivant_Click(object sender, EventArgs e)
{
cptPage++;
//mettre les questions suivantes
if (cptPage == 4)
{
btn_suivant.Text = "Fini!";
}
}
}
}
| 5f349f8fa68224a82f200fb03b68c0f52f4f7e2b | [
"Markdown",
"C#"
]
| 18 | C# | lucasdut/ProjetIA | d34d7d07a24fd62e94008575a1338a5cf061cf9f | c4d131f824a235e8ae28a3c684ea7460cd28dba9 | |
refs/heads/master | <file_sep>class Statement < ApplicationRecord
has_many :data
validates :statement, presence: true, uniqueness: true
end
<file_sep>class AddIndexToYearQuarters < ActiveRecord::Migration[5.2]
def change
add_index :year_quarters, [:year_id, :quarter_id], unique: true
end
end
<file_sep>class Quarter < ApplicationRecord
has_many :data
has_many :year_quarters
has_many :years, through: :year_quarters
validates :quarter, presence: true, uniqueness: true
end
<file_sep>class Datum < ApplicationRecord
belongs_to :statement
belongs_to :year
belongs_to :quarter
belongs_to :company
belongs_to :page
validates_associated :statement
validates_associated :year
validates_associated :quarter
validates_associated :company
validates_associated :page
end
<file_sep>class CreateYears < ActiveRecord::Migration[5.2]
def change
create_table :years do |t|
t.column :year, 'char(2)', null: false
t.index :year, unique: true
end
end
end
<file_sep>class CreateData < ActiveRecord::Migration[5.2]
def change
create_table :data do |t|
t.integer :row_index, null: false
t.integer :column_index, null: false
t.decimal :amount, null: false
t.references :statement, foreign_key: true
t.references :year, foreign_key: true
t.references :quarter, foreign_key: true
t.references :company, foreign_key: true
t.references :page, foreign_key: true
end
end
end
<file_sep># This file should contain all the record creation needed to seed the database with its default values.
# The data can then be loaded with the rails db:seed command (or created alongside the database with db:setup).
#
# Examples:
#
# movies = Movie.create([{ name: 'Star Wars' }, { name: 'Lord of the Rings' }])
# Character.create(name: 'Luke', movie: movies.first)
require 'csv'
path = "app/data/"
start = Time.now
# helpers
def fast_insert(insert_model, column, values)
values -= insert_model.pluck(column)
insert_values = values.map{|value| "(#{value})"}.join(",")
return if insert_values.empty?
sql = "INSERT INTO #{insert_model.table_name} (#{column}) VALUES #{insert_values}"
ActiveRecord::Base.connection.execute(sql)
end
def fast_insert_multiple(insert_model, unique_column, values)
conn = ActiveRecord::Base.connection
existing_values = insert_model.pluck(unique_column)
columns = values.first.map{ |k,v| k.to_s }.join(",")
values.delete_if{ |item| existing_values.include?(item[unique_column.to_sym]) }
values.map{ |item| "(#{item.map{ |k,v| conn.quote(v) }.join(',')})" }.join(",")
insert_values = values.map{ |item| "(#{item.map{ |k,v| conn.quote(v) }.join(',')})" }.join(",")
return if insert_values.empty?
sql = "INSERT INTO #{insert_model.table_name} (#{columns}) VALUES #{insert_values}"
conn.execute(sql)
end
def fast_insert_data(values)
conn = ActiveRecord::Base.connection
insert_model = Datum
insert_model.delete_all
columns = values.first.map{ |k,v| k.to_s }.join(",")
values.map{ |item| "(#{item.map{ |k,v| conn.quote(v) }.join(',')})" }.join(",")
insert_values = values.map{ |item| "(#{item.map{ |k,v| conn.quote(v) }.join(',')})" }.join(",")
return if insert_values.empty?
sql = "INSERT INTO #{insert_model.table_name} (#{columns}) VALUES #{insert_values}"
ActiveRecord::Base.connection.execute(sql)
# p sql
end
def create_lookup_hash(model, lookup_column )
result = Hash.new
model.pluck(lookup_column, :id).each{ |k,v| result[k]=v }
return result
end
# static data definitions
years = ("10".."18").to_a
quarters = ("1".."4").to_a
statements = [
{ name: "Canadian P&C Companies", code: "10" },
{ name: "Foreign P&C Companies", code: "20" },
]
pages = [
{ code: "6020", name: "Consolidated Premiums and Claims" },
{ code: "6021", name: "Claims Incurred - Discounted" },
{ code: "6030", name: "Claims and Adjustment Expenses - Paid, Current Year and Unpaid, Current and Prior Year" },
]
# parse data from files
# companies
companies = []
years.each { |year|
filename = "#{path}20#{year} P&C Companies.csv"
if File.exist?(filename) then
count = %x{wc -l '#{filename}'}.split.first.to_i
p "#{filename} company records found: " + count.to_s
CSV.foreach(filename, :headers => true, encoding:'iso-8859-1:utf-8') { |row|
companies.push({ code: row.field('Code'), name: row.field('Company') })
}
end
}
# database insertions
fast_insert(Year, "year", years)
fast_insert(Quarter, "quarter", quarters)
fast_insert_multiple(Statement, "code", statements)
fast_insert_multiple(Company, "code", companies)
fast_insert_multiple(Page, "code", pages)
# build data hashes
hash_years = create_lookup_hash(Year, :year)
hash_quarters = create_lookup_hash(Quarter, :quarter)
hash_statements = create_lookup_hash(Statement, :code)
hash_companies = create_lookup_hash(Company, :code)
hash_pages = create_lookup_hash(Page, :code)
# parse financial data
# TODO: set up and parse data for all pages, currently only using data for:
# 60.20, 60.21, 60.30
slice_map = [2,2,1,4,4,2,2,16]
schema = [
{statement_id: hash_statements},
{year_id: hash_years},
{quarter_id: hash_quarters},
{company_id: hash_companies},
{page_id: hash_pages},
{row_index: ''},
{column_index: ''},
{amount: ''}
]
def parse_data_line(line, slice_map)
count = 0
result = []
slice_map.each { |value|
result << line.slice(count, value)
count += value
}
return result
end
def format_data_line(line, schema)
result = Hash.new
schema.each_with_index { |schem,index|
line_value = line[index]
schema_hash = schem.first[1]
schema_key = schem.first[0]
lookup = schema_hash[line_value] || line_value
if schema_key == :value then
lookup = lookup.to_d
elsif schema_key == :row || schema_key == :column then
lookup = lookup.to_i
end
result[schema_key] = lookup
}
return result
end
restricted_pages = Page.pluck(:code)
skip_companies = ["D850"]
data_lines = []
total_records_searched = 0
Year.pluck(:year).each { |year|
Quarter.pluck(:quarter).each { |quarter|
Statement.pluck(:code).each { |statement|
statement = statement.slice(0)
filename = "#{path}20#{year}#{quarter}-#{statement}-pc.txt"
if File.exist?(filename) then
count = %x{wc -l '#{filename}'}.split.first.to_i
p "#{filename} data records found: " + count.to_s
total_records_searched += count
File.open(filename).each do |line|
# only parse lines for set up pages right now
page = line.slice(9,4)
company = line.slice(5,4)
if restricted_pages.include?(page) && !skip_companies.include?(company) then
parsed = parse_data_line(line, slice_map)
formatted = format_data_line(parsed, schema)
data_lines << formatted
end
end
end
}
}
}
fast_insert_data(data_lines)
p "TOTAL PARSED DATA LINES: #{data_lines.count} / #{total_records_searched}"
finish = Time.now
diff = finish - start
p "TOTAL TIME TAKEN: #{diff} seconds"<file_sep>class CreatePages < ActiveRecord::Migration[5.2]
def change
create_table :pages do |t|
t.string :name, null: false
t.column :code, 'char(4)', null: false, unique: true
end
end
end
<file_sep># Balsam API
Balsam provides a way to query, visualize and analyze financial data for Canadian property and casualty insurers.
## Concept
There is a wealth of publicly available financial data that has been filed with OSFI. By exposing this data to fast, modern means of analysis it is hoped that new insights into risk management can be found.
## Concerns
- Rails ActiveRecord does not support certain database features like composite keys.
- As the analysis aspect grows more complex this may impact performance.
- Must maintain detailed ERDs and documentation on data consumption in case migration to a different backend is necessary.
## Roadmap
1. Single Financial Statement Mapping (60.20 Consolidated Premiums and Claims)
a. Create a template to display the data as a financial statement
b. Generate year-specific schema
c. Parse, record, index and map the raw data to the template
2. Multiple Mapping
## Schema
1. Tables
a. Statement
i. Name (Domestic, Foreign)
b. Year
c. Quarter
d. Company
i. Name
ii. Code
e. Page
i. Code
ii. Name
f. Data
i. Row
ii. Column
iii. Value
iv. FK for all of the above<file_sep>class Year < ApplicationRecord
has_many :data
has_many :year_quarters
has_many :quarters, through: :year_quarters
validates :year, presence: true, uniqueness: true
end
<file_sep>class CreateQuarters < ActiveRecord::Migration[5.2]
def change
create_table :quarters do |t|
t.column :quarter, 'char(1)', null: false
t.index :quarter, unique: true
end
end
end
<file_sep>class YearQuarter < ApplicationRecord
belongs_to :year
belongs_to :quarter
validates :quarter, presence: true, uniqueness: { scope: :year, message: "no duplicate quarters per year" }
validates :year, presence: true
end
<file_sep>class CreateCompanies < ActiveRecord::Migration[5.2]
def change
create_table :companies do |t|
t.string :name, null: false
t.column :code, 'char(4)', null: false
t.index :code, unique: true
end
end
end
<file_sep>class CreateStatements < ActiveRecord::Migration[5.2]
def change
create_table :statements do |t|
t.string :name, null: false
t.column :code, 'char(2)'
t.index :code, unique: true
end
end
end
<file_sep>class CreateYearQuarters < ActiveRecord::Migration[5.2]
def change
create_table :year_quarters do |t|
t.references :year, foreign_key: true, null: false
t.references :quarter, foreign_key: true, null: false
end
end
end
| 5460fe1c5570317d641447c27763ff04bc9d0a24 | [
"Markdown",
"Ruby"
]
| 15 | Ruby | jasontau/osfi-analyzer | 0b14371bf423bf07b0d3b2ac4a26b338db650f38 | eb87e15f7b677cb8a9ecd6a0b94e7d742ce077b4 | |
refs/heads/master | <file_sep>function fetchMovies(title){
// 대기(pending): 이행하거나 거부되지 않은 초기 상태.
const OMDB_API_KEY = '7035c60c123'
return new Promise(async(resolve, reject) => {
try{
const res = await axios.get(`https://omdbapi.com?apikey=${OMDB_API_KEY}&s=${title}`)
// 이행(fulfilled): 연산이 성공적으로 완료됨.
resolve(res)
}catch(error){
console.log(error.message)
// 거부(rejected): 연산이 실패함.
reject('error!!')
}
})
}
async function test(){
try{
const res = await fetchMovies('frozen')
console.log(res)
}catch(error){
console.log(error)
}
}
test()
function hello(){
fetchMovies('jobs')
.then(res => console.log(res))
.catch((error) => {console.log(error)})
}
hello() | 7582261275bff55c305d993d18654a9b51352a66 | [
"JavaScript"
]
| 1 | JavaScript | 2Gyoon/promise_pr | 370b88fd3e85b946dccc94dc69542c60d5a512f2 | 5a05648b8206f1634802cf5b5c8df588e8d64602 | |
refs/heads/master | <file_sep>#!/bin/bash
# run the elm-live dev server with hot reloading
# point to dev-server/index.html
elm-live /app/dev-server/ButtonRender.elm \
--output=elm.js \
--port=4000 \
--host=0.0.0.0 \
--open --pushstate
<file_sep>#!/bin/bash
# runs shell in app container
NODE_ENV=${1:-local}
echo "Running with NODE_ENV=$NODE_ENV"
# stop and remove the containers if they are running
stop_and_remove_container()
{
docker stop elm-buttons
docker rm elm-buttons
}
stop_and_remove_container || true
# run the elm-buttons container
docker run \
--rm \
-v $(pwd)/src:/app/src/ \
-v $(pwd)/dev-server:/app/dev-server/ \
-e NODE_ENV=$NODE_ENV \
--publish 4000:4000 \
--publish 35729:35729 \
--entrypoint=/bin/bash \
-ti elm-buttons
<file_sep>#!/bin/bash
docker build -t elm-buttons .
<file_sep>FROM node:7.2.0
MAINTAINER <NAME> <<EMAIL>>
RUN npm install -g [email protected] \
&& npm install -g [email protected] \
&& npm install -g [email protected]
# Add project code
ADD . /app
WORKDIR /app
ADD elm-package.json /app/elm-package.json
RUN elm-package install --yes
EXPOSE 4000 4000
#live-reload
EXPOSE 35729 35729
<file_sep># elm-buttons
my goal is to produce well-designed, reusable button components written for elm applications
## setup
requirements:
* docker must be installed
* linux terminal must be available
run the dev-server with hot-reloading:
1. build the docker container (in a linux shell)
* `cd elm-buttons`
* `./docker/build-container.sh`
2. run the development server (in a linux shell)
* `./docker/dev-server.sh`
3. open up `localhost:4000` in a web browser
Once the dev-server is running, you can play around with source files in `elm-buttons/src`. Changes will automatically trigger a re-compile and browser refresh.
<file_sep>#!/bin/bash
# runs webpack in app container
NODE_ENV=${1:-development}
echo "Running with NODE_ENV=$NODE_ENV"
# stop and remove the containers if they are running
stop_and_remove_container()
{
docker stop elm-buttons
docker rm elm-buttons
}
stop_and_remove_container || true
# run the workbench container
docker run \
-v $(pwd)/src:/app/src/ \
-v $(pwd)/dev-server:/app/dev-server/ \
-e NODE_ENV=$NODE_ENV \
--name=elm-buttons \
--publish 4000:4000 \
--publish 35729:35729 \
--entrypoint=/app/entrypoints/dev-server.sh \
-t elm-buttons
| 53ea3292e047a3ccbd4cdb4670e441df132fec49 | [
"Markdown",
"Dockerfile",
"Shell"
]
| 6 | Shell | mac-s-g/elm-buttons | 2b802fdaba32ea4f09132592dae1db1cfff829e3 | 9f82cac9784ba71ad466c0d5df6d8d70335f9591 | |
refs/heads/master | <file_sep>#模拟数据
1. `mockData`模拟数据文件夹,可在该文件夹下创建多个json数据
2. `src/server.js`服务启动文件,可修改服务端口
监听端口 `prot`可随意修改
>```javascript
>const port = 22222
>app.listen(port, () =>
> console.log(`本机服务已启动>>> http://${getIP()}:${port}`)
>);
>```
3. 启动项目
```shell
# 同步依赖
npm install
# 启动项目
npm run serve
```
4. 数据请求访问连接该项目ip和端口,访问需要模拟数据的文件名即可
`server.js`已对`method`做定向,目前只测试到`get`、`post`请求方法可用
axios框架示例
>```javascript
>axios.get('/data.json')
>axios.post('/data.json')
>```# mock
# mock
<file_sep>function cross (app) {
/*跨域解决*/
app.all('*', function (req, res, next) {
res.header('Access-Control-Allow-Origin', req.headers.origin);//获取请求源 这样所有请求就都有访问权限了
res.header('Access-Control-Allow-Credentials', true);
res.header('Access-Control-Allow-Headers', 'Content-Type,Content-Length, Authorization, Accept,X-Requested-With')
res.header('Access-Control-Allow-Methods', 'PUT,POST,GET,DELETE,OPTIONS');
res.header('Content-Type', 'application/json;charset=utf-8');
next()
});
}
module.exports = cross<file_sep>const path = require('path');
const getIP = require('./utils/getIP');
const express = require('express');
const app = new express();
const cross = require('./utils/cross');
/*跨域*/
cross(app);
/*对method做定向*/
app.use((req, res, next) => (req.method = 'GET') && next());
/*静态文件*/
app.use('/', express.static(path.resolve(__dirname, '..', './mockData')));
/*监听端口*/
const port = 22222;
app.listen(port, () =>
console.log(`本机服务已启动>>> http://${getIP()}:${port}`)
); | 89dc1476414ee1dc9fb185407fcba6f7aff3b881 | [
"Markdown",
"JavaScript"
]
| 3 | Markdown | kang995/mock | 1f0a313f82f9fb38d53679299fdbd2c38530f4ca | f5e8c0c92da1b5fe2ba5f94517870207f26538bd | |
refs/heads/master | <repo_name>GoreLab/sorghum-multi-trait<file_sep>/codes/bayesian_network_analysis.py
#------------------------------------------------Modules-----------------------------------------------------#
# Load libraries:
import pandas as pd
import numpy as np
import os
import pickle
import re
import pystan as ps
import argparse
parser = argparse.ArgumentParser()
#-----------------------------------------Adding flags to the code-------------------------------------------#
# Get flags:
parser.add_argument("-y", "--y", dest = "y", default = "error", help="Name of the file with the phenotypes")
parser.add_argument("-x", "--x", dest = "x", default = 'error', help="Name of the file with the features")
parser.add_argument("-model", "--model", dest = "model", default = "BN", help="Name of the model that can be: 'BN' or 'PBN', or 'DBN'")
parser.add_argument("-rpath", "--rpath", dest = "rpath", help="The path of the repository")
parser.add_argument("-opath", "--opath", dest = "opath", help="The path of the folder with general outputs")
parser.add_argument("-cvpath", "--cvpath", dest = "cvpath", help="The path of the folder to receive outputs of the step of cross-validation analysis")
# Parse the paths:
args = parser.parse_args()
# Subset arguments:
y = args.y
x = args.x
model = args.model
REPO_PATH = args.rpath
OUT_PATH = args.opath
CV_OUT_PATH = args.cvpath
#---------------------------------------------Loading data---------------------------------------------------#
# Set the directory to store processed data:
os.chdir(OUT_PATH + "/processed_data")
# Get each trait file names:
if model=='PBN':
y_0 = y.split("&")[0]
y_1 = y.split("&")[1]
x_0 = x.split("&")[0]
x_1 = x.split("&")[1]
# Load data:
if (model=='BN') or bool(re.search('DBN', model)):
# Reading adjusted means:
y = pd.read_csv(args.y, index_col=0)
# Reading feature matrix:
X = pd.read_csv(args.x, index_col=0)
# Load data:
if model=='PBN':
# Reading adjusted means for both traits:
y_0 = pd.read_csv(y_0, index_col=0)
y_1 = pd.read_csv(y_1, index_col=0)
# Reading feature matrix for both traits:
X_0 = pd.read_csv(x_0, index_col=0)
X_1 = pd.read_csv(x_1, index_col=0)
#------------------------------------------Data input for stan-----------------------------------------------#
if model == 'BN':
# Create an empty list to receive the dictionaries:
dict_stan = []
# Subset time indexes and groups:
index = X.iloc[:,0].values
group = X.iloc[:,0].unique()
# Drop the first column used just for mapping:
X = X.drop(X.columns[0], axis=1)
# Build dictionaries:
for t in group:
dict_stan.append(dict(p_z = X[index==t].shape[1],
n = X[index==t].shape[0],
Z = X[index==t],
y = y[index==t].values.flatten(),
phi = y[index==t].max().values[0]*10))
if model == 'PBN':
# Create an empty list to receive the dictionaries:
dict_stan = []
# Subset time indexes and groups:
index1 = X_1.iloc[:,0].values
index0 = X_0.iloc[:,0].values
group1 = X_1.iloc[:,0].unique()
group0 = X_0.iloc[:,0].unique()
# Drop the first column used just for mapping:
X_0 = X_0.drop(X_0.columns[0], axis=1)
X_1 = X_1.drop(X_1.columns[0], axis=1)
# Case where the first input file have measures over time:
if len(group1)==1:
# Build dictionaries:
for t in group0:
dict_stan.append(dict(p_z = X_0.shape[1],
n_0 = X_0[index0==t].shape[0],
Z_0 = X_0[index0==t],
y_0 = y_0[index0==t].values.flatten(),
n_1 = X_1.shape[0],
Z_1 = X_1,
y_1 = y_1.values.flatten(),
phi = pd.concat([y_0[index0==t], y_1], axis=0).max().values[0]*10))
# Case where the second input file have measures over time:
if len(group0)==1:
# Build dictionaries:
for t in group1:
dict_stan.append(dict(p_z = X_0.shape[1],
n_0 = X_0.shape[0],
Z_0 = X_0,
y_0 = y_0.values.flatten(),
n_1 = X_1[index1==t].shape[0],
Z_1 = X_1[index1==t],
y_1 = y_1[index1==t].values.flatten(),
phi = pd.concat([y_0, y_1[index1==t]], axis=0).max().values[0]*10))
# Case where all input files have measures over time:
if (len(group0)!=1) & (len(group1)!=1):
# Build dictionaries:
for t0, t1 in zip(group0, group1):
dict_stan.append(dict(p_z = X_0.shape[1],
n_0 = X_0[index0==t0].shape[0],
Z_0 = X_0[index0==t0],
y_0 = y_0[index0==t0].values.flatten(),
n_1 = X_1[index1==t1].shape[0],
Z_1 = X_1[index1==t1],
y_1 = y_1[index1==t1].values.flatten(),
phi = pd.concat([y_0[index0==t0], y_1[index1==t1]], axis=0).max().values[0]*10))
if model == 'DBN-0~1':
# Subset time indexes and groups:
index = X.iloc[:,0].values
group = X.iloc[:,0].unique()
# Subset the time covariate and the features:
Z = X.drop(X.columns[0], axis=1)
# Build dictionaries:
dict_stan = dict(p_z = Z[index==group[0]].shape[1],
p_res = len(group),
n_0 = Z[index==group[0]].shape[0],
Z_0 = Z[index==group[0]],
y_0 = y[index==group[0]].values.flatten(),
n_1 = Z[index==group[1]].shape[0],
Z_1 = Z[index==group[1]],
y_1 = y[index==group[1]].values.flatten(),
phi = y.max().values[0]*10)
if model == 'DBN-0~2':
# Subset time indexes and groups:
index = X.iloc[:,0].values
group = X.iloc[:,0].unique()
# Subset the time covariate and the features:
Z = X.drop(X.columns[0], axis=1)
# Build dictionaries:
dict_stan = dict(p_z = Z[index==group[0]].shape[1],
p_res = len(group),
n_0 = Z[index==group[0]].shape[0],
Z_0 = Z[index==group[0]],
y_0 = y[index==group[0]].values.flatten(),
n_1 = Z[index==group[1]].shape[0],
Z_1 = Z[index==group[1]],
y_1 = y[index==group[1]].values.flatten(),
n_2 = Z[index==group[2]].shape[0],
Z_2 = Z[index==group[2]],
y_2 = y[index==group[2]].values.flatten(),
phi = y.max().values[0]*10)
if model == 'DBN-0~3':
# Subset time indexes and groups:
index = X.iloc[:,0].values
group = X.iloc[:,0].unique()
# Subset the time covariate and the features:
Z = X.drop(X.columns[0], axis=1)
# Build dictionaries:
dict_stan = dict(p_z = Z[index==group[0]].shape[1],
p_res = len(group),
n_0 = Z[index==group[0]].shape[0],
Z_0 = Z[index==group[0]],
y_0 = y[index==group[0]].values.flatten(),
n_1 = Z[index==group[1]].shape[0],
Z_1 = Z[index==group[1]],
y_1 = y[index==group[1]].values.flatten(),
n_2 = Z[index==group[2]].shape[0],
Z_2 = Z[index==group[2]],
y_2 = y[index==group[2]].values.flatten(),
n_3 = Z[index==group[3]].shape[0],
Z_3 = Z[index==group[3]],
y_3 = y[index==group[3]].values.flatten(),
phi = y.max().values[0]*10)
if model == 'DBN-0~4':
# Subset time indexes and groups:
index = X.iloc[:,0].values
group = X.iloc[:,0].unique()
# Subset the time covariate and the features:
Z = X.drop(X.columns[0], axis=1)
# Build dictionaries:
dict_stan = dict(p_z = Z[index==group[0]].shape[1],
p_res = len(group),
n_0 = Z[index==group[0]].shape[0],
Z_0 = Z[index==group[0]],
y_0 = y[index==group[0]].values.flatten(),
n_1 = Z[index==group[1]].shape[0],
Z_1 = Z[index==group[1]],
y_1 = y[index==group[1]].values.flatten(),
n_2 = Z[index==group[2]].shape[0],
Z_2 = Z[index==group[2]],
y_2 = y[index==group[2]].values.flatten(),
n_3 = Z[index==group[3]].shape[0],
Z_3 = Z[index==group[3]],
y_3 = y[index==group[3]].values.flatten(),
n_4 = Z[index==group[4]].shape[0],
Z_4 = Z[index==group[4]],
y_4 = y[index==group[4]].values.flatten(),
phi = y.max().values[0]*10)
if model == 'DBN-0~5':
# Subset time indexes and groups:
index = X.iloc[:,0].values
group = X.iloc[:,0].unique()
# Subset the time covariate and the features:
Z = X.drop(X.columns[0], axis=1)
# Build dictionaries:
dict_stan = dict(p_z = Z[index==group[0]].shape[1],
p_res = len(group),
n_0 = Z[index==group[0]].shape[0],
Z_0 = Z[index==group[0]],
y_0 = y[index==group[0]].values.flatten(),
n_1 = Z[index==group[1]].shape[0],
Z_1 = Z[index==group[1]],
y_1 = y[index==group[1]].values.flatten(),
n_2 = Z[index==group[2]].shape[0],
Z_2 = Z[index==group[2]],
y_2 = y[index==group[2]].values.flatten(),
n_3 = Z[index==group[3]].shape[0],
Z_3 = Z[index==group[3]],
y_3 = y[index==group[3]].values.flatten(),
n_4 = Z[index==group[4]].shape[0],
Z_4 = Z[index==group[4]],
y_4 = y[index==group[4]].values.flatten(),
n_5 = Z[index==group[5]].shape[0],
Z_5 = Z[index==group[5]],
y_5 = y[index==group[5]].values.flatten(),
phi = y.max().values[0]*10)
if model == 'DBN-0~6':
# Subset time indexes and groups:
index = X.iloc[:,0].values
group = X.iloc[:,0].unique()
# Subset the time covariate and the features:
Z = X.drop(X.columns[0], axis=1)
# Build dictionaries:
dict_stan = dict(p_z = Z[index==group[0]].shape[1],
p_res = len(group),
n_0 = Z[index==group[0]].shape[0],
Z_0 = Z[index==group[0]],
y_0 = y[index==group[0]].values.flatten(),
n_1 = Z[index==group[1]].shape[0],
Z_1 = Z[index==group[1]],
y_1 = y[index==group[1]].values.flatten(),
n_2 = Z[index==group[2]].shape[0],
Z_2 = Z[index==group[2]],
y_2 = y[index==group[2]].values.flatten(),
n_3 = Z[index==group[3]].shape[0],
Z_3 = Z[index==group[3]],
y_3 = y[index==group[3]].values.flatten(),
n_4 = Z[index==group[4]].shape[0],
Z_4 = Z[index==group[4]],
y_4 = y[index==group[4]].values.flatten(),
n_5 = Z[index==group[5]].shape[0],
Z_5 = Z[index==group[5]],
y_5 = y[index==group[5]].values.flatten(),
n_6 = Z[index==group[6]].shape[0],
Z_6 = Z[index==group[6]],
y_6 = y[index==group[6]].values.flatten(),
phi = y.max().values[0]*10)
#--------------------------------------Running the Bayesian Network------------------------------------------#
# Set directory:
os.chdir(REPO_PATH + "/codes")
# For running the Bayesian Network model:s
if model == 'BN':
# Compile the Bayesian Network:
model_stan = ps.StanModel(file='bayesian_network.stan')
# Create an empty list:
fit = []
# Fit the model:
for t in range(len(group)):
fit.append(model_stan.sampling(data=dict_stan[t], chains=4, iter=400))
# Run the Pleiotropic Bayesian Network model:
if model == 'PBN':
# Compile the Pleiotropic Bayesian Network:
model_stan = ps.StanModel(file='pleiotropic_bayesian_network.stan')
# Create an empty list:
fit = []
# Fit the model:
if len(group1)==1:
for t in range(len(group0)):
fit.append(model_stan.sampling(data=dict_stan[t], chains=4, iter=400))
if len(group0)==1:
for t in range(len(group1)):
fit.append(model_stan.sampling(data=dict_stan[t], chains=4, iter=400))
if (len(group0)!=1) & (len(group1)!=1):
for t in range(len(group0)):
fit.append(model_stan.sampling(data=dict_stan[t], chains=4, iter=400))
# Compile the DBN model:
if model == 'DBN-0~1':
model_stan = ps.StanModel(file='dynamic_bayesian_network_0_1.stan')
# Fit the model:
fit = model_stan.sampling(data=dict_stan, chains=4, iter=400)
# Compile the DBN model:
if model == 'DBN-0~2':
model_stan = ps.StanModel(file='dynamic_bayesian_network_0_2.stan')
# Fit the model:
fit = model_stan.sampling(data=dict_stan, chains=4, iter=400)
# Compile the DBN model:
if model == 'DBN-0~3':
model_stan = ps.StanModel(file='dynamic_bayesian_network_0_3.stan')
# Fit the model:
fit = model_stan.sampling(data=dict_stan, chains=4, iter=400)
# Compile the DBN model:
if model == 'DBN-0~4':
model_stan = ps.StanModel(file='dynamic_bayesian_network_0_4.stan')
# Fit the model:
fit = model_stan.sampling(data=dict_stan, chains=4, iter=400)
# Compile the DBN model:
if model == 'DBN-0~5':
model_stan = ps.StanModel(file='dynamic_bayesian_network_0_5.stan')
# Fit the model:
fit = model_stan.sampling(data=dict_stan, chains=4, iter=400)
# Compile the DBN model:
if model == 'DBN-0~6':
model_stan = ps.StanModel(file='dynamic_bayesian_network_0_6.stan')
# Fit the model:
fit = model_stan.sampling(data=dict_stan, chains=4, iter=400)
#---------------------------------Saving outputs from the Bayesian Network-----------------------------------#
# Set directory:
os.chdir(CV_OUT_PATH)
# Save stan fit object and model for the BN:
if model == 'BN':
for t in range(len(group)):
with open('output_' + model.lower() + '_fit_' + str(t) + '.pkl', 'wb') as f:
pickle.dump({'model' : model_stan, 'fit' : fit[t]}, f, protocol=-1)
# Save stan fit object and model for the PBN:
if model == 'PBN':
if len(group1)==1:
for t in range(len(group0)):
with open('output_' + model.lower() + '_fit_' + str(t) + '.pkl', 'wb') as f:
pickle.dump({'model' : model_stan, 'fit' : fit[t]}, f, protocol=-1)
if len(group0)==1:
for t in range(len(group1)):
with open('output_' + model.lower() + '_fit_' + str(t) + '.pkl', 'wb') as f:
pickle.dump({'model' : model_stan, 'fit' : fit[t]}, f, protocol=-1)
if (len(group0)!=1) & (len(group1)!=1):
for t in range(len(group0)):
with open('output_' + model.lower() + '_fit_' + str(t) + '.pkl', 'wb') as f:
pickle.dump({'model' : model_stan, 'fit' : fit[t]}, f, protocol=-1)
# Save stan fit object and model for the DBN:
if bool(re.search('DBN', model)):
with open('output_' + model.lower() + '.pkl', 'wb') as f:
pickle.dump({'model' : model_stan, 'fit' : fit}, f, protocol=-1)
<file_sep>/codes/genomic_prediction_results.py
#------------------------------------------------Modules-----------------------------------------------------#
# Load libraries:
import pandas as pd
import numpy as np
import os
import pickle
import argparse
from scipy.stats.stats import pearsonr
import matplotlib
import matplotlib.pyplot as plt
import seaborn as sns
parser = argparse.ArgumentParser()
# Turn off interactive mode:
plt.ioff()
#-------------------------------------------Add flags to the code--------------------------------------------#
# Get flags:
parser.add_argument("-rpath", "--rpath", dest = "rpath", help="The path of the repository")
parser.add_argument("-opath", "--opath", dest = "opath", help="The path of the folder with general outputs")
# Parse the paths:
args = parser.parse_args()
# Subset arguments:
REPO_PATH = args.rpath
OUT_PATH = args.opath
# REPO_PATH = '/workdir/jp2476/sorghum-multi-trait'
# OUT_PATH = '/workdir/jp2476/output_sorghum-multi-trait'
#-----------------------------------------Read train and test data-------------------------------------------#
# Type of forward-chaining cross-validation (fcv) schemes:
fcv_type = ['fcv-30~45', 'fcv-30~60', 'fcv-30~75', 'fcv-30~90', 'fcv-30~105',
'fcv-30~only', 'fcv-45~only', 'fcv-60~only', 'fcv-75~only', 'fcv-90~only', 'fcv-105~only']
# Create a list of the 5-fold cross-validation (cv5f) folds:
cv5f_fold = ['k0', 'k1', 'k2', 'k3', 'k4']
# Create a list with the traits set:
trait_set = ['drymass', 'height']
# Initialize list to receive the outputs:
y = dict()
X = dict()
# Set the directory to store processed data:
os.chdir(OUT_PATH + "/processed_data")
# Read cv5f files:
for s in range(len(trait_set)):
for j in range(len(cv5f_fold)):
# Create the suffix of the file name for the cv5f data case:
index = 'cv5f_' + trait_set[s] + '_' + cv5f_fold[j] + '_tst'
# Read data:
y[index] = pd.read_csv('y_' + index + '.csv', header = 0, index_col=0)
X[index] = pd.read_csv('x_' + index + '.csv', header = 0, index_col=0)
# Read fcv files for height:
for t in range(len(fcv_type)):
for j in range(len(cv5f_fold)):
# Create the suffix of the file name for the fcv data case:
index = fcv_type[t] + '_height_tst'
# Read data:
y[index] = pd.read_csv('y_' + index + '.csv', header = 0, index_col=0)
X[index] = pd.read_csv('x_' + index + '.csv', header = 0, index_col=0)
#----------------------------Organize data into a new format for easy subsetting-----------------------------#
# Read adjusted means:
df = pd.read_csv("adjusted_means.csv", index_col=0)
df.dap = df.dap.fillna(0).astype(int)
# Different DAP measures:
dap_group = ['30', '45', '60', '75', '90', '105', '120']
# Initialize dictionary to receive observations for cv5f analysis:
y_obs_cv5f = dict()
# Store observations for height stratified by modelling scenario for cv5f analysis:
for d in dap_group:
y_obs_cv5f['cv5f_height_dap:' + d] = df.y_hat[(df.trait=='height') & (df.dap==int(d))]
# Store observations for drymass stratified by modelling scenario for cv5f analysis:
y_obs_cv5f['cv5f_drymass'] = df.y_hat[df.trait=='drymass']
# Initialize dictionary to receive observations for fcv analysis:
y_obs_fcv = dict()
# Store observations for height stratified by modelling scenario for fcv analysis:
dap_group = ['30', '45', '60', '75', '90', '105']
for d in dap_group:
y_obs_fcv['fcv_height_for!trained!on:' + d] = y['fcv-' + d + '~only_height_tst']
# Store observations for height stratified by modelling scenario for fcv analysis:
dap_group = ['30~45', '30~60', '30~75', '30~90', '30~105']
for d in dap_group:
y_obs_fcv['fcv_height_for!trained!on:' + d] = y['fcv-' + d + '_height_tst']
#--------------------------------Compute predictions for the cv5f scheme-------------------------------------#
# Initialize list to receive the predictions:
y_pred_cv5f = dict()
# Different DAP measures:
dap_group = ['30', '45', '60', '75', '90', '105', '120']
# Compute predictions for the BN model:
for s in trait_set:
# Compute predictions for drymass:
if s=='drymass':
for j in cv5f_fold:
# Set the directory:
os.chdir(OUT_PATH + '/cv/BN/cv5f/drymass/' + j)
# Load stan fit object and model:
with open("output_bn_fit_0.pkl", "rb") as f:
data_dict = pickle.load(f)
# Index the fit object and model
out = data_dict['fit'].extract()
# Index and subsetting the feature matrix:
index1 = 'cv5f_drymass_' + j + '_tst'
index2 = 'bn_cv5f_drymass'
X_tmp = X[index1].drop(X[index1].columns[0], axis=1)
# Create the indexes for creating a data frame to receive predictions:
index = ["s_" + str(i) for i in range(out['mu'].size)]
col_name = X_tmp.index
# Initialize a matrix to receive the posterior predictions:
tmp = pd.DataFrame(index=index, columns=col_name)
# Compute predictions:
for sim in range(out['mu'].size):
# Subset parameters:
mu = out['mu'][sim]
alpha = out['alpha'][sim,:]
# Prediction:
tmp.iloc[sim] = (mu + X_tmp.dot(alpha)).values
# Store prediction:
if j=='k0':
y_pred_cv5f[index2] = tmp
if j!='k0':
y_pred_cv5f[index2] = pd.concat([y_pred_cv5f[index2], tmp], axis=1)
# Compute predictions for height:
if s=='height':
for d in range(len(dap_group)):
for j in cv5f_fold:
# Set the directory:
os.chdir(OUT_PATH + '/cv/BN/cv5f/height/' + j)
# Load stan fit object and model:
with open("output_bn_fit_" + str(d) + ".pkl", "rb") as f:
data_dict = pickle.load(f)
# Index the fit object and model
out = data_dict['fit'].extract()
# Index and subsetting the feature matrix:
index1 = 'cv5f_height_' + j + '_tst'
index2 = 'bn_cv5f_height_trained!on!dap:' + dap_group[d]
X_tmp = X[index1][X[index1].iloc[:,0] == int(dap_group[d])]
X_tmp = X_tmp.drop(X_tmp.columns[0], axis=1)
# Create the indexes for creating a data frame to receive predictions:
index = ["s_" + str(i) for i in range(out['mu'].size)]
col_name = X_tmp.index
# Initialize a matrix to receive the posterior predictions:
tmp = pd.DataFrame(index=index, columns=col_name)
# Compute predictions:
for sim in range(out['mu'].size):
# Subset parameters:
mu = out['mu'][sim]
alpha = out['alpha'][sim,:]
# Prediction:
tmp.iloc[sim] = (mu + X_tmp.dot(alpha)).values
# Store prediction:
if j=='k0':
y_pred_cv5f[index2] = tmp
if j!='k0':
y_pred_cv5f[index2] = pd.concat([y_pred_cv5f[index2], tmp], axis=1)
# Compute predictions for the PBN model:
for d in range(len(dap_group)):
for j in cv5f_fold:
# Set the directory:
os.chdir(OUT_PATH + '/cv/PBN/cv5f/drymass-height/' + j)
# Load stan fit object and model:
with open("output_pbn_fit_" + str(d) + ".pkl", "rb") as f:
data_dict = pickle.load(f)
# Index the fit object and model
out = data_dict['fit'].extract()
# Index and subset the feature matrix:
index1_0 = 'cv5f_drymass_' + j + '_tst'
index1_1 = 'cv5f_height_' + j + '_tst'
index2_0 = 'pbn_cv5f_drymass_trained!on!dap:' + dap_group[d]
index2_1 = 'pbn_cv5f_height_trained!on!dap:' + dap_group[d]
X_tmp_0 = X[index1_0]
X_tmp_0 = X_tmp_0.drop(X_tmp_0.columns[0], axis=1)
X_tmp_1 = X[index1_1][X[index1_1].iloc[:,0] == int(dap_group[d])]
X_tmp_1 = X_tmp_1.drop(X_tmp_1.columns[0], axis=1)
# Create the indexes for creating a data frame to receive predictions:
index = ["s_" + str(i) for i in range(out['mu_0'].size)]
col_name = X_tmp_0.index
# Initialize a matrix to receive the posterior predictions:
tmp_0 = pd.DataFrame(index=index, columns=col_name)
# Create the indexes for creating a data frame to receive predictions:
index = ["s_" + str(i) for i in range(out['mu_0'].size)]
col_name = X_tmp_1.index
# Initialize a matrix to receive the posterior predictions:
tmp_1 = pd.DataFrame(index=index, columns=col_name)
# Compute predictions:
for sim in range(out['mu_0'].size):
# Subset parameters:
mu_0 = out['mu_0'][sim]
mu_1 = out['mu_1'][sim]
alpha_0 = out['alpha_0'][sim,:]
alpha_1 = out['alpha_1'][sim,:]
eta_0 = out['eta_0'][sim,:]
eta_1 = out['eta_1'][sim,:]
# Prediction:
tmp_0.iloc[sim] = (mu_0 + X_tmp_0.dot(alpha_0 + eta_0)).values
tmp_1.iloc[sim] = (mu_1 + X_tmp_1.dot(alpha_1 + eta_1)).values
# Store prediction:
if j=='k0':
y_pred_cv5f[index2_0] = tmp_0
y_pred_cv5f[index2_1] = tmp_1
if j!='k0':
y_pred_cv5f[index2_0] = pd.concat([y_pred_cv5f[index2_0], tmp_0], axis=1)
y_pred_cv5f[index2_1] = pd.concat([y_pred_cv5f[index2_1], tmp_1], axis=1)
# List drymass predictions for ensambling:
tmp = []
for i in range(len(dap_group)):
tmp.append(y_pred_cv5f['pbn_cv5f_drymass_trained!on!dap:' + dap_group[i]])
# Ensamble predictions for drymass:
y_pred_cv5f['pbn_cv5f_drymass_ensambled'] = (tmp[0] + tmp[1] + tmp[2] + tmp[3] + tmp[4] + tmp[5] + tmp[6]) / 7
# Remove predictions that will not be used anymore:
for d in range(len(dap_group)):
y_pred_cv5f.pop('pbn_cv5f_drymass_trained!on!dap:' + dap_group[d])
# Compute predictions for the DBN model:
for j in cv5f_fold:
# Set the directory:
os.chdir(OUT_PATH + '/cv/DBN/cv5f/height/' + j)
# Load stan fit object and model:
with open("output_dbn-0~6.pkl", "rb") as f:
data_dict = pickle.load(f)
# Index the fit object and model
out = data_dict['fit'].extract()
for d in range(len(dap_group)):
# Index and subset the feature matrix:
index1 = 'cv5f_height_' + j + '_tst'
index2 = 'dbn_cv5f_height_trained!on!dap:' + dap_group[d]
X_tmp = X[index1][X[index1].iloc[:,0] == int(dap_group[d])]
Z_tmp = X_tmp.drop(X_tmp.columns[0], axis=1)
# Create the indexes for creating a data frame to receive predictions:
index = ["s_" + str(i) for i in range(out['mu_' + str(d)].size)]
col_name = X_tmp.index
# Initialize a matrix to receive the posterior predictions:
tmp = pd.DataFrame(index=index, columns=col_name)
# Compute predictions:
for sim in range(out['mu_0'].size):
# Subset parameters:
mu = out['mu_' + str(d)][sim]
alpha = out['alpha_' + str(d)][sim,:]
# Prediction:
tmp.iloc[sim] = (mu + Z_tmp.dot(alpha)).values
# Store prediction:
if j=='k0':
y_pred_cv5f[index2] = tmp
if j!='k0':
y_pred_cv5f[index2] = pd.concat([y_pred_cv5f[index2], tmp], axis=1)
#--------------------------------Computing predictions for the fcv scheme------------------------------------#
# Initialize list to receive the predictions:
y_pred_fcv = dict()
# Initialize list to receive the expectaions:
expect_fcv = dict()
# Different DAP measures:
dap_group = ['30', '45', '60', '75', '90', '105']
# Compute predictions for the BN model:
for d in range(len(dap_group)):
# Set the directory:
os.chdir(OUT_PATH + '/cv/BN/fcv-' + dap_group[d] + '~only/height/')
# Load stan fit object and model:
with open("output_bn_fit_0.pkl", "rb") as f:
data_dict = pickle.load(f)
# Index the fit object and model
out = data_dict['fit'].extract()
# Index and subset the feature matrix:
index1 = 'fcv-' + dap_group[d] + '~only_height_tst'
index2 = 'bn_fcv_height_trained!on!dap:' + dap_group[d]
X_tmp = X[index1].drop(X[index1].columns[0], axis=1)
# Create the indexes for creating a data frame to receive predictions:
index = ["s_" + str(i) for i in range(out['mu'].size)]
col_name = X_tmp.index
# Initialize a matrix to receive the posterior predictions:
tmp = pd.DataFrame(index=index, columns=col_name)
# Compute predictions:
for sim in range(out['mu'].size):
# Subset parameters:
mu = out['mu'][sim]
alpha = out['alpha'][sim,:]
# Prediction:
tmp.iloc[sim] = (mu + X_tmp.dot(alpha)).values
# Store predictions:
y_pred_fcv[index2] = tmp
# Store expectations:
expect_fcv[index2] = pd.DataFrame(out['expectation'], index=index, columns=df.index[df.dap==int(dap_group[d])])
# Compute predictions for the PBN model:
for d in range(len(dap_group)):
# Set the directory:
os.chdir(OUT_PATH + '/cv/PBN/fcv-' + dap_group[d] + '~only/drymass-height/')
# Load stan fit object and model:
with open("output_pbn_fit_0.pkl", "rb") as f:
data_dict = pickle.load(f)
# Index the fitted object and model
out = data_dict['fit'].extract()
# Index and subset the feature matrix:
index1 = 'fcv-' + dap_group[d] + '~only_height_tst'
index2 = 'pbn_fcv_height_trained!on!dap:' + dap_group[d]
X_tmp = X[index1].drop(X[index1].columns[0], axis=1)
# Create the indexes for creating a data frame to receive predictions:
index = ["s_" + str(i) for i in range(out['mu_1'].size)]
col_name = X_tmp.index
# Initialize a matrix to receive the posterior predictions:
tmp = pd.DataFrame(index=index, columns=col_name)
# Compute predictions:
for sim in range(out['mu_1'].size):
# Subset parameters:
mu = out['mu_1'][sim]
alpha = out['alpha_1'][sim,:]
eta = out['eta_1'][sim,:]
# Prediction:
tmp.iloc[sim] = (mu + X_tmp.dot(alpha + eta)).values
# Store predictions:
y_pred_fcv[index2] = tmp
# Store expectations:
expect_fcv[index2] = pd.DataFrame(out['expectation_1'], index=index, columns=df.index[df.dap==int(dap_group[d])])
# Different DAP measures:
fcv_type = ['fcv-30~45', 'fcv-30~60', 'fcv-30~75', 'fcv-30~90', 'fcv-30~105']
dap_index = ['0~1', '0~2', '0~3', '0~4', '0~5']
# Compute predictions for the DBN model:
for c in range(len(fcv_type)):
# Set the directory:
os.chdir(OUT_PATH + '/cv/DBN/' + fcv_type[c] + '/height/')
# Load stan fit object and model:
with open("output_dbn-" + dap_index[c] + ".pkl", "rb") as f:
data_dict = pickle.load(f)
# Index the fit object and model
out = data_dict['fit'].extract()
# Get the last time point used for training:
upper = dap_index[c].split('~')[1]
# Index and subset the feature matrix:
index1 = fcv_type[c] +'_height_tst'
index2 = 'dbn_fcv_height_trained!on!dap:' + fcv_type[c].split('-')[1]
Z_tmp = X[index1].drop(X[index1].columns[0], axis=1)
# Create the indexes for creating a data frame to receive predictions:
index = ["s_" + str(i) for i in range(out['mu_0'].size)]
col_name = Z_tmp.index
# Initialize a matrix to receive the posterior predictions:
tmp = pd.DataFrame(index=index, columns=col_name)
# Compute predictions:
for sim in range(out['mu_0'].size):
# Subset parameters:
mu = out['mu_' + upper][sim]
alpha = out['alpha_' + upper][sim,:]
# Prediction:
tmp.iloc[sim] = (mu + Z_tmp.dot(alpha)).values
# Prediction:
y_pred_fcv[index2] = tmp
# Store expectations:
expect_fcv[index2] = pd.DataFrame(out['expectation_' + upper], index=index, columns=df.index[df.dap==int(dap_group[int(upper)])])
#--------------------Compute prediction accuracies for the cv5f scheme (table and figures)--------------------#
# Different models:
models = ['BN', 'PBN', 'DBN', 'MTi-GBLUP', 'MTr-GBLUP']
# Different DAP measures:
dap_group = ['30', '45', '60', '75', '90', '105', '120']
# Number of Monte Carlo simulations:
n_sim = 800
# Initialize table to store prediction accuracy based on the posterior mean of the predictions from the Bayesian models:
cv5f_table_mean = pd.DataFrame(index = ['DB'] + ['PH_' + str(i) for i in dap_group], columns=models)
# Models for computing predictive accuracy:
models = ['BN', 'PBN', 'DBN']
# Accuracies based on the posterior means of the predictions for cv5f related to the height prediction:
for m in models:
for d in dap_group:
index = y_pred_cv5f[m.lower() + '_cv5f_height_trained!on!dap:' + d].columns
cor_tmp = np.round(pearsonr(y_pred_cv5f[m.lower() + '_cv5f_height_trained!on!dap:' + d].mean(axis=0), y_obs_cv5f['cv5f_height_dap:' + d][index])[0],2)
cv5f_table_mean.loc['PH_' + str(d), m] = cor_tmp
# Prediction accuracies based on the posterior means of the predictions for cv5f related to dry biomass prediction:
index = y_pred_cv5f['bn_cv5f_drymass'].columns
cv5f_table_mean.loc['DB', 'BN'] = np.round(pearsonr(y_pred_cv5f['bn_cv5f_drymass'].mean(axis=0), y_obs_cv5f['cv5f_drymass'][index])[0],2)
index = y_pred_cv5f['pbn_cv5f_drymass_ensambled'].columns
cv5f_table_mean.loc['DB', 'PBN'] = np.round(pearsonr(y_pred_cv5f['pbn_cv5f_drymass_ensambled'].mean(axis=0), y_obs_cv5f['cv5f_drymass'][index])[0],2)
# Initialize table to store the standard deviation of the prediction accuracies based on the samples of the posterior distribution of the predictions obtained by the Bayesian models:
cv5f_table_std = pd.DataFrame(index = ['DB'] + ['PH_' + str(i) for i in dap_group], columns=models)
# Standard deviation of the prediction accuracies based on the samples of the posterior distribution of the predictions obtained by the Bayesian models for height:
for m in models:
# Vector to store the predictive accuracy of each posterior draw:
cor_tmp = pd.DataFrame(index=["s_" + str(i) for i in range(n_sim)], columns=['acc'])
# To compute the standard deviation of the accuracies in the posterior level:
for d in dap_group:
index1 = y_pred_cv5f[m.lower() + '_cv5f_height_trained!on!dap:' + d].columns
index2 = y_pred_cv5f[m.lower() + '_cv5f_height_trained!on!dap:' + d].index
for s in index2:
cor_tmp.loc[s] = pearsonr(y_pred_cv5f[m.lower() + '_cv5f_height_trained!on!dap:' + d].loc[s], y_obs_cv5f['cv5f_height_dap:' + d][index1])[0]
print('Model: {}, DAP: {}, Simulation: {}'.format(m.upper(), d, s))
# Printing the standard deviation of the predictive accuracies:
cv5f_table_std.loc['PH_' + str(d), m] = float(np.round(cor_tmp.std(axis=0),3))
# Different models:
models = ['bn_biomass', 'pbn_biomass']
# Standard deviation of the prediction accuracies based on the samples of the posterior distribution of the predictions obtained by the Bayesian models for dry biomass:
for m in models:
# Vector to store the predictive accuracy of each posterior draw:
cor_tmp = pd.DataFrame(index=["s_" + str(i) for i in range(n_sim)], columns=['acc'])
# To compute the standard deviation of the accuracies in the posterior level:
for d in dap_group:
if m=='bn_biomass':
index1 = y_pred_cv5f['bn_cv5f_drymass'].columns
index2 = y_pred_cv5f['bn_cv5f_drymass'].index
if m=='pbn_biomass':
index1 = y_pred_cv5f['pbn_cv5f_drymass_ensambled'].columns
index2 = y_pred_cv5f['pbn_cv5f_drymass_ensambled'].index
for s in index2:
if m=='bn_biomass':
cor_tmp.loc[s] = pearsonr(y_pred_cv5f['bn_cv5f_drymass'].loc[s], y_obs_cv5f['cv5f_drymass'][index1])[0]
if m=='pbn_biomass':
cor_tmp.loc[s] = pearsonr(y_pred_cv5f['pbn_cv5f_drymass_ensambled'].loc[s], y_obs_cv5f['cv5f_drymass'][index1])[0]
print('Model: {}, Simulation: {}'.format(m.upper(), s))
if m=='bn_biomass':
# Printing the standard deviation of the predictive accuracies:
cv5f_table_std.loc['DB', 'BN'] = float(np.round(cor_tmp.std(axis=0),3))
if m=='pbn_biomass':
# Printing the standard deviation of the predictive accuracies:
cv5f_table_std.loc['DB', 'PBN'] = float(np.round(cor_tmp.std(axis=0),3))
#-----------------------------Compute prediction accuracies for the fcv scheme--------------------------------#
# Store into a list different DAP values:
dap_group = ['30', '45', '60', '75', '90', '105', '120']
# List of models to use:
model_set = ['bn', 'pbn']
# Dictionary to receive the accuracy matrices:
cor_dict = dict()
# Compute correlation for the Baysian Network and Pleiotropic Bayesian Network model under fcv scheme:
for k in model_set:
# Create an empty correlation matrix:
cor_tmp = np.empty([len(dap_group)]*2)
cor_tmp[:] = np.nan
for i in range(len(dap_group[:-1])):
# Subset predictions for correlation computation:
y_pred_tmp = y_pred_fcv[k + '_fcv_height_trained!on!dap:' + dap_group[i]].mean(axis=0)
y_obs_tmp = y_obs_fcv['fcv_height_for!trained!on:' + dap_group[i]].y_hat
for j in range(len(dap_group)):
# Conditional to compute correlation just forward in time:
if (j>i):
# Subset indexes for subsetting the data:
subset = df[df.dap==int(dap_group[j])].index
# Build correlation matrix for the Bayesian Network model:
cor_tmp[i, j] = np.round(pearsonr(y_pred_tmp[subset], y_obs_tmp[subset])[0],4)
# Store the computed correlation matrix for the Bayesian network model under fcv scheme:
cor_dict['fcv_' + k] = cor_tmp
# Store into a list different DAP values intervals:
dap_group1 = ['30~45', '30~60', '30~75', '30~90', '30~105']
dap_group2 = ['30', '45', '60', '75', '90', '105', '120']
# Create an empty correlation matrix:
cor_tmp = np.empty([len(dap_group)]*2)
cor_tmp[:] = np.nan
# Compute correlation for the Dynamic Bayesian Network model under fcv scheme:
for i in range(len(dap_group1)):
# Subset predictions for correlation computation:
y_pred_tmp = y_pred_fcv['dbn_fcv_height_trained!on!dap:' + dap_group1[i]].mean(axis=0)
y_obs_tmp = y_obs_fcv['fcv_height_for!trained!on:' + dap_group1[i]].y_hat
for j in range(len(dap_group2)):
# Get the upper bound of the interval:
upper = int(dap_group1[i].split('~')[1])
# Conditional to compute correlation just forward in time:
if (int(dap_group2[j])>upper):
# Subset indexes for subsetting the data:
subset = df[df.dap==int(dap_group2[j])].index
# Build correlation matrix for the Bayesian Network model:
cor_tmp[i, j] = np.round(pearsonr(y_pred_tmp[subset], y_obs_tmp[subset])[0],4)
# Store the computed correlation matrix for the Bayesian network model under fcv scheme:
cor_dict['fcv_dbn'] = cor_tmp
# Builda mask just to filter accuracies common across models:
mask = np.isnan(cor_dict['fcv_dbn'])
# Eliminate values not shared across all models:
cor_dict['fcv_bn'][mask] = np.nan
cor_dict['fcv_pbn'][mask] = np.nan
# Eliminate rows and columns without any correlation value:
cor_dict['fcv_bn'] = cor_dict['fcv_bn'][0:5,2:7]
cor_dict['fcv_pbn'] = cor_dict['fcv_pbn'][0:5,2:7]
cor_dict['fcv_dbn'] = cor_dict['fcv_dbn'][0:5,2:7]
# Print predictive accuracies
print(cor_dict['fcv_bn'])
print(cor_dict['fcv_pbn'])
print(cor_dict['fcv_dbn'])
# Set directory:
os.chdir(REPO_PATH + "/figures")
# List of models to use:
model_set = ['bn', 'pbn', 'dbn']
# Generate accuracy heatmaps:
for i in model_set:
# Labels for plotting the heatmap for the Pleiotropic Bayesian Network or Bayesian Network:
if (i=='bn') | (i=='pbn'):
labels_axis0 = ['DAP 45*', 'DAP 60*', 'DAP 75*', 'DAP 90*', 'DAP 105*']
# Labels for plotting the heatmap for the Dynamic Bayesian model:
if i=='dbn':
labels_axis0 = ['DAP 30:45*', 'DAP 30:60*', 'DAP 30:75*', 'DAP 30:90*', 'DAP 30:105*']
# Labels for plotting the heatmap:
labels_axis1 = ['DAP 60', 'DAP 75', 'DAP 90', 'DAP 105', 'DAP 120']
# Heat map of the adjusted means across traits:
heat = sns.heatmap(np.flip(np.flip(cor_dict['fcv_' + i],axis=1), axis=0),
linewidths=0.25,
cmap='YlOrBr',
vmin=0.3,
vmax=1,
annot=True,
annot_kws={"size": 18},
xticklabels=labels_axis0 ,
yticklabels=labels_axis1)
heat.set_ylabel('')
heat.set_xlabel('')
plt.xticks(rotation=25)
plt.yticks(rotation=45)
plt.savefig("heatplot_fcv_" + i + "_accuracy.pdf", dpi=350)
plt.savefig("heatplot_fcv_" + i + "_accuracy.png", dpi=350)
plt.clf()
#------------------------------Compute coincidence index based on lines (CIL)---------------------------------#
# Dictionary to receive CIL:
cil_dict = dict()
# Store into a list different DAP values intervals:
dap_group1 = ['30~45', '30~60', '30~75', '30~90', '30~105']
dap_group2 = ['30', '45', '60', '75', '90', '105', '120']
# Compute CIL for the Dynamic Bayesian network model (best Bayesian model on the fcv):
for i in range(len(dap_group1)):
# Subset predictions for correlation computation:
y_pred_tmp = y_pred_fcv['dbn_fcv_height_trained!on!dap:' + dap_group1[i]]
y_obs_tmp = y_obs_fcv['fcv_height_for!trained!on:' + dap_group1[i]].y_hat
for j in range(len(dap_group2)):
# Get the upper bound of the interval:
upper = int(dap_group1[i].split('~')[1])
# Conditional to compute correlation just forward in time:
if (int(dap_group2[j])>upper):
# Subset indexes for subsetting the data:
subset = df[df.dap==int(dap_group2[j])].index
# Get the number of selected individuals for 20% selection intensity:
n_selected = int(y_obs_tmp[subset].size * 0.2)
# Build the indexes for computing the CIL:
top_rank_obs = np.argsort(y_obs_tmp[subset])[::-1][0:n_selected]
# Vector for storing the indicators:
ind_vec = pd.DataFrame(index=y_pred_tmp[subset].index, columns=y_pred_tmp[subset].columns)
# Build CIL matrix for the Bayesian Network model:
for sim in range(y_pred_tmp.shape[0]):
# Top predicted lines:
top_rank_pred = np.argsort(y_pred_tmp[subset].iloc[sim])[::-1][0:n_selected]
# Top indicator:
ind_tmp = top_rank_pred.isin(top_rank_obs)
ind_vec.loc['s_' + str(sim), ind_tmp.index] = ind_tmp
# Index to store CIL into dictionary:
index = 'dbn_' + dap_group1[i] + '_' + dap_group2[j]
# Compute CIL:
cil_dict[index]=ind_vec.mean(axis=0)
print('Model: dbn, DAP_i: {}, DAP_j: {}'.format(dap_group1[i], dap_group2[j]))
# Set directory:
os.chdir(REPO_PATH + "/figures")
# DAP groups used for plotting
dap_group = ['45', '60', '75', '90', '105']
# Generating panel plot:
for i in range(len(dap_group)):
# Subset CIL for plotting:
cil=cil_dict['dbn_30~' + dap_group[i] + '_120']
# Remove entries from other time points with nan:
cil = cil[~np.isnan(cil)]
# Get the order of the first slice used to train, to plot the order of the other slices:
if i==0:
# Get the order decreasing of the CIL:
order_index = np.argsort(cil)[::-1]
# Individuais displaying CIL higher then 80%:
mask = cil.iloc[order_index] > 0.5
# Subset CIL for plotting:
p1 = cil.iloc[order_index][mask].plot.barh(color='red', figsize=(10,12))
p1.set(yticklabels=df.id_gbs[mask.index])
p1.tick_params(axis='y', labelsize=5)
p1.tick_params(axis='x', labelsize=12)
plt.xlabel('Top 20% posterior coincidence index based on lines', fontsize=20)
plt.ylabel('Sorghum lines', fontsize=20)
plt.xlim(0.5, 1)
plt.savefig('cilplot_fcv_' + 'dbn_30~' + dap_group[i] + '_120.pdf', dpi=350)
plt.savefig('cilplot_fcv_' + 'dbn_30~' + dap_group[i] + '_120.png', dpi=350)
plt.clf()
#----Compute coincidence index for dry biomass indirect selection using height adjusted means time series-----#
# Store into a list different DAP values:
dap_group = ['30', '45', '60', '75', '90', '105', '120']
# List of models to use:
model_set = ['bn', 'pbn']
# Compute coincidence index for the Bayesian network and Pleiotropic Bayesian Network model:
for k in model_set:
for i in range(len(dap_group[:-1])):
# Subset expectations and observations for coincidence index computation:
expect_tmp = expect_fcv[k + '_fcv_height_trained!on!dap:' + dap_group[i]].copy(deep=True)
# Get the name of the lines from the entries of the expectation:
expect_tmp.columns = df.loc[expect_tmp.columns].id_gbs
# Subset data frame:
df_tmp = df[df.trait=='drymass']
df_tmp.index = df_tmp.id_gbs
# Subset observations:
y_obs_tmp = df_tmp.y_hat[expect_tmp.columns]
# Get the number of selected individuals for 20% selection intensity:
n_selected = int(y_obs_tmp.size * 0.2)
# Build the indexes for computing the coincidence index:
top_rank_obs = np.argsort(y_obs_tmp)[::-1][0:n_selected]
# Vector for coincidence indexes:
ci_post_tmp = pd.DataFrame(index=expect_tmp.index, columns=['ci'])
# Compute the coincidence index:
for sim in range(ci_post_tmp.shape[0]):
# Build the indexes for computing the coincidence index:
top_rank_pred = np.argsort(expect_tmp.loc['s_' + str(sim)])[::-1][0:n_selected]
# Compute coincidence index in the top 20% or not:
ci_post_tmp.loc['s_' + str(sim)] = top_rank_pred.isin(top_rank_obs).mean(axis=0)
if (k==model_set[0]) & (i==0):
# Store the coincidence index:
ci_post = pd.DataFrame(columns=['post', 'model', 'dap'])
ci_post['model'] = np.repeat(k.upper(), ci_post_tmp.shape[0])
ci_post['dap'] = np.repeat((dap_group[i] + '*'), ci_post_tmp.shape[0])
ci_post['post'] = ci_post_tmp.ci.values
else:
# Store the coincidence index:
tmp1 = np.repeat(k.upper(), ci_post_tmp.shape[0])
tmp2 = np.repeat((dap_group[i] + '*'), ci_post_tmp.shape[0])
tmp = pd.DataFrame({'post': ci_post_tmp.ci.values, 'model': tmp1, 'dap': tmp2})
ci_post = pd.concat([ci_post, tmp], axis=0)
print('Model: {}, DAP_i: {}'.format(k, dap_group[i]))
# Store into a list different DAP values interv
dap_group = ['30~45', '30~60', '30~75', '30~90', '30~105']
# Compute coincidence index for the Dynamic Bayesian network:
for i in range(len(dap_group)):
# Subset expectations:
expect_tmp = expect_fcv['dbn_fcv_height_trained!on!dap:' + dap_group[i]].copy(deep=True)
# Get the name of the lines from the entries of the expectation:
expect_tmp.columns = df.loc[expect_tmp.columns].id_gbs
# Sub set data frame:
df_tmp = df[df.trait=='drymass']
df_tmp.index = df_tmp.id_gbs
# Subset observations:
y_obs_tmp = df_tmp.y_hat[expect_tmp.columns]
# Get the upper bound of the interval:
upper = int(dap_group[i].split('~')[1])
# Get the number of selected individuals for 20% selection intensity:
n_selected = int(y_obs_tmp.size * 0.2)
# Build the indexes for computing the coincidence index:
top_rank_obs = np.argsort(y_obs_tmp)[::-1][0:n_selected]
# Vector for coincidence indexes:
ci_post_tmp = pd.DataFrame(index=expect_tmp.index, columns=['ci'])
# Compute the coincidence index:
for sim in range(ci_post_tmp.shape[0]):
# Build the indexes for computing the coincidence index:
top_rank_pred = np.argsort(expect_tmp.loc['s_' + str(sim)])[::-1][0:n_selected]
# Compute coincidence index in the top 20% or not:
ci_post_tmp.iloc[sim] = top_rank_pred.isin(top_rank_obs).mean(axis=0)
# Store the coincidence index:
tmp1 = np.repeat('DBN', ci_post_tmp.shape[0])
tmp2 = np.repeat((str(upper) + '*'), ci_post_tmp.shape[0])
tmp = pd.DataFrame({'post': ci_post_tmp.ci.values, 'model': tmp1, 'dap': tmp2})
ci_post = pd.concat([ci_post, tmp], axis=0)
print('Model: dbn, DAP_i: {}'.format(dap_group[i]))
# Change data type:
ci_post['post'] = ci_post['post'].astype(float)
# Change labels:
ci_post.columns = ['Coincidence index posterior values', 'Model', 'Days after planting']
# Set directory:
os.chdir(REPO_PATH + "/figures")
# Plot coincidence indexes:
plt.figure(figsize=(20,15))
with sns.plotting_context(font_scale=1):
ax = sns.violinplot(x='Days after planting',
y='Coincidence index posterior values',
data=ci_post,
hue='Model')
plt.ylim(0.12, 0.4)
ax.tick_params(labelsize=30)
plt.xlabel('Days after planting', fontsize=40)
plt.ylabel( 'Coincidence index posterior values', fontsize=40)
plt.legend(fontsize='xx-large', title_fontsize=40)
plt.savefig("ci_plot.pdf", dpi=350)
plt.savefig("ci_plot.png", dpi=350)
plt.clf()
#--------------------------Accuracy heatmap for the multivariate linear mixed model--------------------------#
# Set directory:
os.chdir(REPO_PATH + "/tables")
# Initialize a dictionary to receive the accuracies:
gblup_dict = dict()
# Load predictive accuracies of the GBLUP models and store on the final table:
gblup_dict['MTi-GBLUP_cv5f'] = pd.read_csv('acc_MTi-GBLUP_cv5f.csv', header = 0, index_col=0)
gblup_dict['MTr-GBLUP_cv5f'] = pd.read_csv('acc_MTr-GBLUP_cv5f.csv', header = 0, index_col=0)
cv5f_table_mean['MTi-GBLUP'][gblup_dict['MTi-GBLUP_cv5f'].index] = np.round(gblup_dict['MTi-GBLUP_cv5f'].values.flatten(),2)
cv5f_table_mean['MTr-GBLUP'][gblup_dict['MTr-GBLUP_cv5f'].index] = np.round(gblup_dict['MTr-GBLUP_cv5f'].values.flatten(),2)
# Load correlation matrices:
gblup_dict['MTi-GBLUP_fcv'] = pd.read_csv('acc_MTi-GBLUP_fcv.csv', header = 0, index_col=0)
gblup_dict['MTr-GBLUP_fcv'] = pd.read_csv('acc_MTr-GBLUP_fcv.csv', header = 0, index_col=0)
# List of models to use:
model_set = ['MTi-GBLUP', 'MTr-GBLUP']
# Set directory:
os.chdir(REPO_PATH + "/figures")
# Generate accuracy heatmaps:
for i in range(len(model_set)):
# Labels for plotting the heatmap for the Dynamic Bayesian model:
labels_axis0 = ['DAP 30:45*', 'DAP 30:60*', 'DAP 30:75*', 'DAP 30:90*', 'DAP 30:105*']
# Labels for plotting the heatmap:
labels_axis1 = ['DAP 60', 'DAP 75', 'DAP 90', 'DAP 105', 'DAP 120']
# Heat map of the adjusted means across traits:
heat = sns.heatmap(np.round(np.array(gblup_dict[model_set[i] + '_fcv']),4),
linewidths=0.25,
cmap='YlOrBr',
vmin=0.3,
vmax=1,
annot=True,
annot_kws={"size": 18},
xticklabels=labels_axis0,
yticklabels=labels_axis1)
heat.set_ylabel('')
heat.set_xlabel('')
plt.xticks(rotation=25)
plt.yticks(rotation=45)
plt.savefig("heatplot_fcv_" + model_set[i] + "_accuracy.pdf", dpi=350)
plt.savefig("heatplot_fcv_" + model_set[i] + "_accuracy.png", dpi=350)
plt.clf()
# Set directory:
os.chdir(REPO_PATH + "/tables")
# Save tables with the results from the cv5f:
cv5f_table_mean.to_csv("cv5f_accuracy_table_mean_all_genomic_prediction_models.csv")
cv5f_table_std.to_csv("cv5f_accuracy_table_std_bayesian_genomic_prediction_models.csv")
###############################################################
# OUT_PATH = '/workdir/jp2476/output_sorghum-multi-trait'
# # Set the directory to store processed data:
# os.chdir(OUT_PATH + "/processed_data")
# data = [y, X, y_pred_cv5f, y_pred_fcv, y_obs_cv5f, y_obs_fcv, cil_dict, expect_fcv, df]
# np.savez('mtrait_results.npz', data)
# # Loading data:
# os.chdir(OUT_PATH + "/processed_data")
# container = np.load('mtrait_results.npz', allow_pickle=True)
# data = [container[key] for key in container]
# y = data[0][0]
# X = data[0][1]
# y_pred_cv5f = data[0][2]
# y_pred_fcv = data[0][3]
# y_obs_cv5f = data[0][4]
# y_obs_fcv = data[0][5]
# cil_dict = data[0][6]
# expect_fcv = data[0][7]
# df = data[0][8]
<file_sep>/codes/create_list_files_names_cross-validation.sh
#!/bin/bash
# Reading the flag:
while getopts ":o:" opt; do
case $opt in
o)
OUT_PATH=$OPTARG;
;;
\?)
echo "Invalid option: -$OPTARG" >&2
exit 1
;;
:)
echo "Option -$OPTARG requires an argument." >&2
exit 1
;;
esac
done
# Set directory where the data is:
cd ${OUT_PATH}/processed_data
# List training data files names related to the 5-fold cross-validation scheme (cv5f) scheme and storing it for latter usage:
ls y*cv5f*trn* > y_cv5f_bn_trn_files.txt
ls x*cv5f*trn* > x_cv5f_bn_trn_files.txt
ls y*cv5f*height*trn* > y_cv5f_dbn_trn_files.txt
ls x*cv5f*height*trn* > x_cv5f_dbn_trn_files.txt
# List training files names related to the cv5f scheme and storing it for latter usage on PBN model
grep "drymass" y_cv5f_bn_trn_files.txt > tmp1.txt
grep "height" y_cv5f_bn_trn_files.txt > tmp2.txt
paste -d'&' tmp1.txt tmp2.txt > y_cv5f_pbn_trn_files.txt
grep "drymass" x_cv5f_bn_trn_files.txt > tmp1.txt
grep "height" x_cv5f_bn_trn_files.txt > tmp2.txt
paste -d'&' tmp1.txt tmp2.txt > x_cv5f_pbn_trn_files.txt
rm tmp1.txt tmp2.txt
# List training phenotypic data files names related to the forward-chaining cross-validation (fcv) scheme and storing it for latter usage (BN):
ls y*fcv*only*trn* > y_fcv_bn_trn_files.txt
ls x*fcv*only*trn* > x_fcv_bn_trn_files.txt
# List training phenotypic data files names related to the fcv scheme and storing it for latter usage (PBN):
echo 'y_fcv_drymass_trn.csv' > tmp1.txt
echo 'x_fcv_drymass_trn.csv' > tmp2.txt
for i in $(seq 1 5); do
echo 'y_fcv_drymass_trn.csv' >> tmp1.txt
echo 'x_fcv_drymass_trn.csv' >> tmp2.txt
done;
paste -d'&' tmp1.txt y_fcv_bn_trn_files.txt > y_fcv_pbn_trn_files.txt
paste -d'&' tmp2.txt x_fcv_bn_trn_files.txt > x_fcv_pbn_trn_files.txt
rm tmp1.txt tmp2.txt
# List training data files names related to the fcv scheme and storing it for latter usage (DBN):
ls y*fcv*height*trn* > y_fcv_dbn_trn_files.txt
ls x*fcv*height*trn* > x_fcv_dbn_trn_files.txt
sed -i '/only/d' y_fcv_dbn_trn_files.txt
sed -i '/only/d' x_fcv_dbn_trn_files.txt
# Create a text file to store the different types of Dynamic Bayesian network models for latter usage (DBN);
echo "DBN-0~5" > dbn_models_fcv_list.txt
echo "DBN-0~1" >> dbn_models_fcv_list.txt
echo "DBN-0~2" >> dbn_models_fcv_list.txt
echo "DBN-0~3" >> dbn_models_fcv_list.txt
echo "DBN-0~4" >> dbn_models_fcv_list.txt
# List of files to get train data index into R to perform Multivariate GBLUP models analysis in R:
cat y_cv5f_dbn_trn_files.txt > cv5f_mti-gblup_files.txt
cat y_cv5f_pbn_trn_files.txt > cv5f_mtr-gblup_files.txt
cat y_fcv_dbn_trn_files.txt > fcv_mti-gblup_files.txt
echo 'y_fcv_drymass_trn.csv' > tmp1.txt
for i in $(seq 1 4); do
echo 'y_fcv_drymass_trn.csv' >> tmp1.txt
done;
paste -d'&' tmp1.txt y_fcv_dbn_trn_files.txt > fcv_mtr-gblup_files.txt
rm tmp1.txt
# Create a text file to store the different types of Multi Time GBLUP (MTi-GBLUP) models for latter usage;
echo "MTi-GBLUP-0~5" > mti-gblup_models_fcv_list.txt
echo "MTi-GBLUP-0~1" >> mti-gblup_models_fcv_list.txt
echo "MTi-GBLUP-0~2" >> mti-gblup_models_fcv_list.txt
echo "MTi-GBLUP-0~3" >> mti-gblup_models_fcv_list.txt
echo "MTi-GBLUP-0~4" >> mti-gblup_models_fcv_list.txt
# Create a text file to store the different types of Multi Trait GBLUP (MTr-GBLUP) models for latter usage;
echo "MTr-GBLUP-0~5" > mtr-gblup_models_fcv_list.txt
echo "MTr-GBLUP-0~1" >> mtr-gblup_models_fcv_list.txt
echo "MTr-GBLUP-0~2" >> mtr-gblup_models_fcv_list.txt
echo "MTr-GBLUP-0~3" >> mtr-gblup_models_fcv_list.txt
echo "MTr-GBLUP-0~4" >> mtr-gblup_models_fcv_list.txt
<file_sep>/codes/process_emmreml_output.R
#-------------------------------------------Loading libraries------------------------------------------------#
# Libraries to manipulate data:
library(optparse)
library(data.table)
library(stringr)
library(magrittr)
library(EMMREML)
library(tidyr)
#-----------------------------------------Adding flags to the code-------------------------------------------#
# Set the flags:
option_list = list(
make_option(c("-r", "--rpath"), type="character", default=NULL,
help="The path of the repository", metavar="character"),
make_option(c("-o", "--opath"), type="character", default=NULL,
help="The path of the folder to receive outputs", metavar="character")
)
# Parse the arguments:
opt_parser = OptionParser(option_list=option_list)
args = parse_args(opt_parser)
# Subset arguments:
REPO_PATH = args$rpath
OUT_PATH = args$opath
# REPO_PATH = '/workdir/jp2476/sorghum-multi-trait'
# OUT_PATH = '/workdir/jp2476/output_sorghum-multi-trait'
#--------------Load outputs, predict and compute accuracies from 5-fold cross-validation (cv5f)-------------------#
# Types of cross-validation:
cv_type = paste0('k', 0:4)
# Initialize list:
y_pred_lst = list()
# Create a variable to store part of the directory:
PARTIAL_PATH = '/cv/MTi-GBLUP/cv5f/height'
# Read cv5f outputs from the Multiple Time Linear Mixed model:
for (i in 1:length(cv_type)) {
# Set the directory:
setwd(paste0(OUT_PATH, PARTIAL_PATH , '/', cv_type[i]))
# Load the entire workspace:
load("output_mixed_models.RData")
# Naming the columns of the genomic estimated breeding values output:
colnames(out$Gpred) = as.character(df_melt$id_gbs)
rownames(out$Gpred) = rownames(Y)
# Broadcasting the mean:
mu = matrix(rep(out$Bhat,each=ncol(out$Gpred[,id_tst])), ncol=ncol(out$Gpred[,id_tst]), byrow=TRUE)
# Subset predictions:
y_pred_tmp = t(mu) + t(out$Gpred[,id_tst])
if (i==1) {
# Subset predictions:
y_pred_lst[['MTi-GBLUP']][['cv5f']] = y_pred_tmp
}
else {
# Subset predictions and update the prediction matrix:
y_pred_lst[['MTi-GBLUP']][['cv5f']] = rbind(y_pred_lst[['MTi-GBLUP']][['cv5f']], y_pred_tmp)
}
print(paste0("We are at the k: ", i))
}
# Initialize a list:
acc_lst = list()
# Saving predictive accuracy of the cv5f for the MTi-GBLUP:
index = df_melt$id_gbs %>% droplevels %>% as.character
acc_lst[['MTi-GBLUP']][['cv5f']] = diag(cor(y_pred_lst[['MTi-GBLUP']][['cv5f']][index,], t(Y)[index,]))
# Create a variable to store part of the directory:
PARTIAL_PATH = '/cv/MTr-GBLUP/cv5f/drymass-height/'
# Read cv5f outputs from the Multiple Time Linear Mixed model:
for (i in 1:length(cv_type)) {
# Set the directory:
setwd(paste0(OUT_PATH, PARTIAL_PATH , '/', cv_type[i]))
# Load the entire workspace:
load("output_mixed_models.RData")
# Naming the columns of the genomic estimated breeding values output:
colnames(out$Gpred) = as.character(df_melt$id_gbs)
rownames(out$Gpred) = rownames(Y)
# Broadcasting the mean:
mu = matrix(rep(out$Bhat,each=ncol(out$Gpred[,id_tst])), ncol=ncol(out$Gpred[,id_tst]), byrow=TRUE)
# Subset predictions:
y_pred_tmp = t(mu) + t(out$Gpred[,id_tst])
if (i==1) {
# Subset predictions:
y_pred_lst[['MTr-GBLUP']][['cv5f']] = y_pred_tmp
}
else {
# Subset predictions and update the prediction matrix:
y_pred_lst[['MTr-GBLUP']][['cv5f']] = rbind(y_pred_lst[['MTr-GBLUP']][['cv5f']], y_pred_tmp)
}
print(paste0("We are at the k: ", i))
}
# Saving predictive accuracy of the cv5f for the Mr-GBLUP:
index = df_melt$id_gbs %>% droplevels %>% as.character
acc_lst[['MTr-GBLUP']][['cv5f']] = diag(cor(y_pred_lst[['MTr-GBLUP']][['cv5f']][index,], t(Y)[index,]))
#----------Load outputs, predict and compute accuracies from forward chaining cross-validation (fcv)--------------#
# Create a variable to store part of the directory:
PARTIAL_PATH = '/cv/MTi-GBLUP'
# Types of cross-validation:
cv_type = paste0('fcv-30~', seq(45,105,15))
# Time points to correlate:
dap = seq(30,120,15)
# Read CV1 outputs from the Multiple Time Linear Mixed model:
for (i in 1:length(cv_type)) {
# Set the directory:
setwd(paste0(OUT_PATH, PARTIAL_PATH, '/', cv_type[i], '/height/'))
# Load the entire workspace:
load("output_mixed_models.RData")
# Naming the columns of the genomic estimated breeding values output:
colnames(out$Gpred) = as.character(df_melt$id_gbs)
rownames(out$Gpred) = rownames(Y)
# Get the upper bound of the time inverval used for training:
upper = str_split(cv_type[i], '~', simplify = TRUE)[1,2] %>% as.character
# Get a mask of the time points only used for training:
mask = str_detect(rownames(out$Gpred), upper)
# Broadcast the mean and subset just the one related to the last time point:
mu = matrix(rep(out$Bhat[mask,],each=ncol(out$Gpred)), ncol=ncol(out$Gpred), byrow=TRUE) %>% data.matrix
# Compute predictions:
y_pred_lst[['MTi-GBLUP']][[cv_type[i]]] = mu + t(out$Gpred[mask,])
# Initialize a matrix to receive the accuracies:
if (i == 1) {
acc_matrix = matrix(NA, length(dap), length(dap))
rownames(acc_matrix) = dap
colnames(acc_matrix) = dap
}
# Intervals to be subset to compute accuracies
begin = which(dap==upper)+1
end = length(dap)
# Compute accuracies:
for (j in dap[begin:end]){
# Subset observde data:
y_tmp = df[(df$trait=='height') & (df$dap==j),]$y_hat
names(y_tmp) = df[(df$trait=='height') & (df$dap==j),]$id
# Get inbred line names to correlate in the right pair:
index = colnames(y_pred_lst[['MTi-GBLUP']][[cv_type[i]]])
# Compute predictive accuraces:
acc_matrix[as.character(j), upper] = cor(c(y_pred_lst[['MTi-GBLUP']][[cv_type[i]]]), c(y_tmp[index]))
}
print(paste0("We are at the cross-validation: ", cv_type[i]))
}
# Saving predictive accuracy of the fcv for the MTi-GBLUP:
acc_lst[['MTi-GBLUP']][['fcv']] = acc_matrix[3:nrow(acc_matrix), 2:(nrow(acc_matrix)-1)]
# Create a variable to store part of the directory:
PARTIAL_PATH = '/cv/MTr-GBLUP'
# Types of cross-validation:
cv_type = paste0('fcv-30~', seq(45,105,15))
# Time points to correlate:
dap = seq(30,120,15)
# Read CV1 outputs from the Multiple Time Linear Mixed model:
for (i in 1:length(cv_type)) {
# Set the directory:
setwd(paste0(OUT_PATH, PARTIAL_PATH, '/', cv_type[i], '/drymass-height/'))
# Load the entire workspace:
load("output_mixed_models.RData")
# Naming the columns of the genomic estimated breeding values output:
colnames(out$Gpred) = as.character(df_melt$id_gbs)
rownames(out$Gpred) = rownames(Y)
# Get the upper bound of the time inverval used for training:
upper = str_split(cv_type[i], '~', simplify = TRUE)[1,2] %>% as.character
# Get a mask of the time points only used for training:
mask = str_detect(rownames(out$Gpred), upper)
# Broadcast the mean and subset just the one related to the last time point:
mu = matrix(rep(out$Bhat[mask,],each=ncol(out$Gpred)), ncol=ncol(out$Gpred), byrow=TRUE) %>% data.matrix
# Compute predictions:
y_pred_lst[['MTr-GBLUP']][[cv_type[i]]] = mu + t(out$Gpred[mask,])
# Initialize a matrix to receive the accuracies:
if (i == 1) {
acc_matrix = matrix(NA, length(dap), length(dap))
rownames(acc_matrix) = dap
colnames(acc_matrix) = dap
}
# Intervals to be subset to compute accuracies
begin = which(dap==upper)+1
end = length(dap)
# Compute accuracies:
for (j in dap[begin:end]){
# Subset observde data:
y_tmp = df[(df$trait=='height') & (df$dap==j),]$y_hat
names(y_tmp) = df[(df$trait=='height') & (df$dap==j),]$id
# Subset of observed inbred lines for both drymass and height:
subset = names(y_tmp) %in% colnames(y_pred_lst[['MTr-GBLUP']][[cv_type[i]]])
y_tmp = y_tmp[subset]
# Get inbred line names to correlate in the right pair:
index = colnames(y_pred_lst[['MTr-GBLUP']][[cv_type[i]]])
# Compute predictive accuraces:
acc_matrix[as.character(j), upper] = cor(c(y_pred_lst[['MTr-GBLUP']][[cv_type[i]]]), c(y_tmp[index]))
}
print(paste0("We are at the cross-validation: ", cv_type[i]))
}
# Saving predictive accuracy of the fcv for the MTi-GBLUP:
acc_lst[['MTr-GBLUP']][['fcv']] = acc_matrix[3:nrow(acc_matrix), 2:(nrow(acc_matrix)-1)]
# Set of models to compute the coincidence index:
model_set = c('MTi-GBLUP', 'MTr-GBLUP')
# Set of upper time points:
upper_set = seq(45,105,15)
# Create a vector to store the coincidence indexes:
ci_tmp = rep(NA, length(upper_set))
names(ci_tmp) = paste0('fcv-30~', upper_set)
# Initialize a list to store results:
ci_lst = list()
for (m in model_set) {
for (i in upper_set) {
# Subset the expectation of plant height for the i^th DAP:
yhat_height = y_pred_lst[[m]][[paste0('fcv-30~', i)]]
tmp = yhat_height %>% colnames
yhat_height = c(yhat_height)
names(yhat_height) = tmp
# Subset adjusted mean for drymass related:
yhat_drymass = df[df$trait=='drymass',]$y_hat
names(yhat_drymass) = df[df$trait=='drymass',]$id %>% as.character
# Number of individuals selected to order genotypes:
n_selected = as.integer(length(yhat_drymass) * 0.2)
# Get the index of the top observed selected inbred lines for dry mass:
top_lines_obs = yhat_drymass[order(yhat_drymass, decreasing=TRUE)][1:n_selected] %>% names
# Get the index of the predicted to be best selected inbred lines for height:
top_lines_pred = yhat_height[order(yhat_height, decreasing=TRUE)][1:n_selected] %>% names
# Compute the coincidence index:
ci_tmp[paste0('fcv-30~', i)] = mean(top_lines_pred %in% top_lines_obs)
}
# Store the coincidence indexes in a list:
ci_lst[[m]] = ci_tmp
}
# Rename entries of the cv5f accuracy:
names(acc_lst[['MTi-GBLUP']][['cv5f']]) = paste0('PH_', seq(30,120,15))
names(acc_lst[['MTr-GBLUP']][['cv5f']]) = c('DB', paste0('PH_', seq(30,120,15)))
# Set directory:
setwd(paste0(REPO_PATH, "/tables"))
# Write accuracy files:
for (i in names(acc_lst[["MTi-GBLUP"]])) write.csv(acc_lst[["MTi-GBLUP"]][[i]], file=paste0("acc_MTi-GBLUP_", i, ".csv"))
for (i in names(acc_lst[["MTr-GBLUP"]])) write.csv(acc_lst[["MTr-GBLUP"]][[i]], file=paste0("acc_MTr-GBLUP_", i, ".csv"))
# Write coincidence indexes:
for (i in names(ci_lst)) write.csv(ci_lst[[i]], file=paste0('coincidence_index_', i, '.csv'))
<file_sep>/README.md
# **Novel Bayesian Networks for Genomic Prediction of Developmental Traits in Biomass Sorghum**



<p align="center"><a href="https://github.com/GoreLab/sorghum-multi-trait/blob/master/edited_figures/figure_3_forward_cross_validation_figures/inkscape/heatplot_fcv_accuracy.png"><img src="https://github.com/GoreLab/sorghum-multi-trait/blob/master/edited_figures/figure_3_forward_cross_validation_figures/inkscape/heatplot_fcv_accuracy.png" width="555" height="328"/></a>
# **Abstract**
The ability to connect genetic information between traits over time allow Bayesian networks to offer a powerful probabilistic framework to construct genomic prediction models. In this study, we phenotyped a diversity panel of 869 biomass sorghum ($Sorghum$ $bicolor$ (L.) Moench] lines, which had been genotyped with 100,435 SNP markers, for plant height (PH) with biweekly measurements from 30 to 120 days after planting (DAP) and for end-of-season dry biomass yield (DBY) in four environments. We evaluated five genomic prediction models: Bayesian network (BN), Pleiotropic Bayesian network (PBN), Dynamic Bayesian network (DBN), multi-trait GBLUP (MTr-GBLUP), and multi-time GBLUP (MTi-GBLUP) models. In 5-fold cross-validation, prediction accuracies ranged from 0.48 (PBN) to 0.51 (MTr-GBLUP) for DBY and from 0.47 (DBN, DAP120) to 0.74 (MTi-GBLUP, DAP60) for PH. Forward-chaining cross-validation further improved prediction accuracies (36.4-52.4\%) of the DBN, MTi-GBLUP and MTr-GBLUP models for PH (training slice: 30-45 DAP) relative to the BN and PBN models. Coincidence indices (target: biomass, secondary: PH) and a coincidence index based on lines (PH time series) showed that the ranking of lines by PH changed minimally after 45 DAP. These results suggest a two-level indirect selection method for PH at harvest (first-level target trait) and DBY (second-level target trait) could be conducted earlier in the season based on ranking of lines by PH at 45 DAP (secondary trait). With the advance of high-throughput phenotyping technologies, our proposed two-level indirect selection framework could be valuable for enhancing genetic gain per unit of time when selecting on developmental traits.
# **Guidelines**
Instructions to reproduce the project, codes description, and data availability on the file `README.txt`.
<file_sep>/codes/create_directories.sh
#!/bin/bash
# Reading the flag:
while getopts ":p:" opt; do
case $opt in
p)
ROOT_PATH=$OPTARG;
;;
\?)
echo "Invalid option: -$OPTARG" >&2
exit 1
;;
:)
echo "Option -$OPTARG requires an argument." >&2
exit 1
;;
esac
done
# Create the folder for receiving the outputs of the codes:
mkdir ${ROOT_PATH}/output_sorghum-multi-trait;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/processed_data;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/heritabilities;
# Create CV directories on Bayesian network (BN) output folder:
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/height/k0;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/height/k1;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/height/k2;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/height/k3;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/height/k4;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/drymass;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/drymass/k0;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/drymass/k1;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/drymass/k2;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/drymass/k3;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/cv5f/drymass/k4;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-30~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-30~only/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-45~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-45~only/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-60~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-60~only/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-75~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-75~only/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-90~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-90~only/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-105~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/BN/fcv-105~only/height;
# Create CV directories on Pleiotropic Bayesian network (PBN) output folder:
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/cv5f;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/cv5f/drymass-height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/cv5f/drymass-height/k0;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/cv5f/drymass-height/k1;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/cv5f/drymass-height/k2;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/cv5f/drymass-height/k3;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/cv5f/drymass-height/k4;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-30~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-30~only/drymass-height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-45~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-45~only/drymass-height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-60~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-60~only/drymass-height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-75~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-75~only/drymass-height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-90~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-90~only/drymass-height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-105~only;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/PBN/fcv-105~only/drymass-height;
# Create CV directories on Dynamic Bayesian network (DBN) output folder:
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/cv5f;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/cv5f/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/cv5f/height/k0;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/cv5f/height/k1;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/cv5f/height/k2;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/cv5f/height/k3;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/cv5f/height/k4;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/fcv-30~45;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/fcv-30~45/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/fcv-30~60;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/fcv-30~60/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/fcv-30~75;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/fcv-30~75/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/fcv-30~90;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/fcv-30~90/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/fcv-30~105;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/DBN/fcv-30~105/height;
# Create CV directories for the Multiple Time Linear Mixed model (MTi-GBLUP) output folder:
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/cv5f;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/cv5f/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/cv5f/height/k0;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/cv5f/height/k1;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/cv5f/height/k2;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/cv5f/height/k3;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/cv5f/height/k4;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/fcv-30~45;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/fcv-30~45/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/fcv-30~60;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/fcv-30~60/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/fcv-30~75;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/fcv-30~75/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/fcv-30~90;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/fcv-30~90/height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/fcv-30~105;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTi-GBLUP/fcv-30~105/height;
# Create CV directories for the Multiple Trait Linear Mixed model (MTr-GBLUP) output folder:
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/cv5f;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/cv5f/drymass-height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/cv5f/drymass-height/k0;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/cv5f/drymass-height/k1;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/cv5f/drymass-height/k2;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/cv5f/drymass-height/k3;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/cv5f/drymass-height/k4;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/fcv-30~45;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/fcv-30~45/drymass-height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/fcv-30~60;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/fcv-30~60/drymass-height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/fcv-30~75;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/fcv-30~75/drymass-height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/fcv-30~90;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/fcv-30~90/drymass-height;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/fcv-30~105;
mkdir ${ROOT_PATH}/output_sorghum-multi-trait/cv/MTr-GBLUP/fcv-30~105/drymass-height;
<file_sep>/codes/create_figures_phenotypic_data_analysis.py
#------------------------------------------------Modules-----------------------------------------------------#
# Import python modules
import os
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
import seaborn as sns
import argparse
parser = argparse.ArgumentParser()
# Turn off interactive mode:
plt.ioff()
#-----------------------------------------Adding flags to the code-------------------------------------------#
# Get flags:
parser.add_argument("-rpath", "--rpath", dest = "rpath", help="The path of the repository")
parser.add_argument("-opath", "--opath", dest = "opath", help="The path of the folder to receive outputs")
# Parse the paths:
args = parser.parse_args()
# Subset arguments:
REPO_PATH = args.rpath
OUT_PATH = args.opath
# REPO_PATH = '/workdir/jp2476/sorghum-multi-trait'
# OUT_PATH = '/workdir/jp2476/output_sorghum-multi-trait'
#-----------------------------------------------Load data----------------------------------------------------#
# Set directory:
os.chdir(REPO_PATH + "/clean_repository/codes")
# Import functions:
from functions import *
# Set the directory to store processed data:
os.chdir(OUT_PATH + "/processed_data")
# Read adjusted means:
df = pd.read_csv("adjusted_means.csv", index_col=0)
# Change class of the dap:
df.dap = df.dap.fillna(0).astype(int)
#--------------------------------------------Generate Figures------------------------------------------------#
# Set directory:
os.chdir(REPO_PATH + "/clean_repository/figures")
# Add a new column in the data frame for plotting:
tmp = df.pivot(index='id_gbs', columns='dap', values='y_hat')
# Labels for plotting the heatmap:
labels = ["Dry biomass",
"Height DAP 30",
"Height DAP 45",
"Height DAP 60",
"Height DAP 75",
"Height DAP 90",
"Height DAP 105",
"Height DAP 120"]
# Heat map of the adjusted means across traits:
heat = sns.heatmap(tmp.corr(),
linewidths=0.25,
cmap='YlOrBr',
vmin=0.09,
vmax=1,
annot=True,
annot_kws={"size": 12},
xticklabels=labels ,
yticklabels=labels)
heat.set_ylabel('')
heat.set_xlabel('')
heat.tick_params(labelsize=7.6)
plt.xticks(rotation=25)
plt.yticks(rotation=45)
plt.savefig("heatplot_traits_adjusted_means.pdf", dpi=350)
plt.savefig("heatplot_traits_adjusted_means.png", dpi=350)
plt.clf()
# Density plot of the adjusted means from dry mass:
den_dm = sns.kdeplot(df.y_hat[df.trait=="drymass"], bw=1, shade=True, legend=False)
den_dm.set_ylabel('Density')
den_dm.set_xlabel('Biomass (t/ha)')
den_dm.get_lines()[0].set_color('#006d2c')
x = den_dm.get_lines()[0].get_data()[0]
y = den_dm.get_lines()[0].get_data()[1]
plt.fill_between(x,y, color='#006d2c').set_alpha(.25)
plt.savefig("denplot_drymass_adjusted_means.pdf", dpi=350)
plt.savefig("denplot_drymass_adjusted_means.png", dpi=350)
plt.clf()
# Box plot of the adjusted means from height measures:
box_ph = sns.boxplot(x='dap', y='y_hat',
data=df[df.trait=="height"])
box_ph.set_ylabel('Height (cm)')
box_ph.set_xlabel('Days after Planting')
colors = ['#fee391', '#fec44f', '#fe9929', '#ec7014', '#cc4c02', '#993404', '#662506']
for i in range(1,len(colors)):
box_ph.artists[i].set_facecolor(colors[i])
plt.savefig("boxplot_height_adjusted_means.pdf", dpi=350)
plt.savefig("boxplot_height_adjusted_means.png", dpi=350)
plt.clf()
# Set directory:
os.chdir(OUT_PATH + "/heritabilities")
# Read heritability values:
h2_table = pd.read_csv('heritabilities.csv', index_col=0)
# Add new labels to the h2_table for plotting:
h2_table['trait'] = labels
h2_table
# Add colors to be ploted:
h2_table['colors'] = ['#006d2c', '#fe9929', '#ec7014', '#cc4c02', '#993404', '#662506', '#fee391', '#fec44f']
# Reset index:
h2_table = h2_table.reset_index(drop=True)
# Set directory:
os.chdir(REPO_PATH + "/clean_repository/figures")
# Plot heritabilities:
bar_obj=plt.bar(h2_table['trait'].tolist(), h2_table['h2'].tolist(),
yerr = h2_table['se'].tolist(),
align='center',
alpha=1,
color= h2_table['colors'].tolist()
)
plt.tick_params(labelsize=7.6)
plt.xticks(h2_table['trait'].tolist())
plt.xticks(rotation=25)
plt.xlabel('Traits')
plt.ylabel('Broad-sense Heritability')
plt.savefig("barplot_heritabilities.pdf", dpi=350)
plt.savefig("barplot_heritabilities.png", dpi=350)
plt.clf()
<file_sep>/codes/phenotypic_data_analysis.R
#-------------------------------------------Loading libraries------------------------------------------------#
# Import python libraries:
library(optparse)
library(data.table)
library(magrittr)
library(stringr)
#-----------------------------------------Adding flags to the code-------------------------------------------#
# Set the flags:
option_list = list(
make_option(c("-a", "--asremlpath"), type="character", default=NULL,
help="The path of the folder with asreml license", metavar="character"),
make_option(c("-o", "--opath"), type="character", default=NULL,
help="The path of the folder to receive outputs", metavar="character")
)
# Parse the arguments:
opt_parser = OptionParser(option_list=option_list)
args = parse_args(opt_parser)
# Subset arguments:
OUT_PATH = args$opath
ASREML_PATH = args$asremlpath
# OUT_PATH = '/workdir/jp2476/output_sorghum-multi-trait'
# OUT_PATH = '/home/jhonathan/Documents/output_sorghum-multi-trait'
# ASREML_PATH = '/workdir/jp2476/asreml'
#---------------------------------------------Load asreml----------------------------------------------------#
# Load asreml:
setwd(ASREML_PATH)
library(asreml)
asreml.lic(license = "asreml.lic", install = TRUE)
#---------------------------------------------Loading data---------------------------------------------------#
# Set the directory:
setwd(paste0(OUT_PATH, '/processed_data'))
# Load the data:
df = fread('df.csv', header=T)[,-1]
# Change classes:
df$id_gbs = df$id_gbs %>% as.factor
df$name2 = df$name2 %>% as.factor
df$block = df$block %>% as.factor
df$loc = df$loc %>% as.factor
df$year = df$year %>% as.factor
df$trait = df$trait %>% as.factor
df$dap = df$dap %>% as.integer
df$drymass = df$drymass %>% as.numeric
df$height = df$height %>% as.numeric
# Get the group levels of the day after plantting (DAP) measures:
dap_groups = df$dap %>% unique %>% na.omit %>% as.integer
# Create a new column combing location and years:
df$env <- paste0(as.character(df$loc), "_",as.character(df$year))
df$env <- df$env %>% as.factor()
#------------------------------------------Biomass data analysis---------------------------------------------#
# Initialize a list to receive the first stage results:
fit = list()
g = list()
# Index for mapping the results:
index = "drymass"
# Subset part of the data set:
df_tmp = df[df$trait == "DM"]
# Drop levels not present in the data subset:
df_tmp$name2 = df_tmp$name2 %>% droplevels
df_tmp$block = df_tmp$block %>% droplevels
df_tmp$env = df_tmp$env %>% droplevels
fit[[index]] = asreml(drymass~ 1 + name2,
random = ~ block:env + env + name2:env,
na.method.Y = "include",
control = asreml.control(
maxiter = 200),
data = df_tmp)
# Extract fixed effects:
fixed_eff = summary(fit[[index]], all=T)$coef.fi[,1]
# Store the corrected mean:
g[[index]] = fixed_eff[length(fixed_eff)] + fixed_eff[-length(fixed_eff)]
#------------------------------------------Height data analysis----------------------------------------------#
for (i in dap_groups) {
# Index for mapping the results:
index = "height_" %>% paste0(i)
# Subsetting part of the data set:
df_tmp = df[(df$trait == "PH" & df$dap == i)]
# Dropping levels not present in the data subset:
df_tmp$name2 = df_tmp$name2 %>% droplevels
df_tmp$block = df_tmp$block %>% droplevels
df_tmp$env = df_tmp$env %>% droplevels
fit[[index]] = asreml(height~ 1 + name2,
random = ~ block:env + env + name2:env,
na.method.Y = "include",
control = asreml.control(
maxiter = 200),
data = df_tmp)
# Extract fixed effects:
fixed_eff = summary(fit[[index]], all=T)$coef.fi[,1]
# Store the corrected mean:
g[[index]] = fixed_eff[length(fixed_eff)] + fixed_eff[-length(fixed_eff)]
# Printing current analysis:
print(paste0("Analysis ", index, " done!"))
}
# Initialize a new data frame:
df_tmp = data.frame()
# Transform list into data frame:
for (i in names(g)) {
if (i=='drymass') {
df_tmp = data.frame(name2 = str_split(names(g[[i]]), pattern="name2_", simplify = TRUE)[,2],
y_hat = unname(g[[i]]),
trait = rep('drymass', length(g[[i]])),
dap = rep("NA", length(g[[i]])))
df_updated = df_tmp
}
if (i!='drymass') {
df_tmp = data.frame(name2 = str_split(names(g[[i]]), pattern="name2_", simplify = TRUE)[,2],
y_hat = unname(g[[i]]),
trait = rep('height', length(g[[i]])),
dap = rep(str_split(i, pattern="_", simplify = TRUE)[,2], length(g[[i]])))
df_updated = rbind(df_updated, df_tmp)
}
}
# Read bin matrix:
W_bin = read.csv('W_bin.csv', row.names=1)
# Get the ID:
id = df[!duplicated(df[,c('name2', 'id_gbs')])][,c('name2', 'id_gbs')]
# Subset line names that were phenotyped and genotyped:
id = id[id$id_gbs %in% rownames(W_bin),]
# Subset adjusted means of the lines that were phenotyped and genotyped:
df_updated = df_updated[df_updated$name2 %in% id$name2,]
# Add column mapping the ID of the GBS:
df_updated= merge(df_updated, id, by='name2', sort=F)
# Dropping levels:
df_updated$id_gbs = df_updated$id_gbs %>% droplevels
# Reodering the name of the data frame and eliminating the name2 identifier:
df_updated = df_updated[,c("id_gbs", "y_hat", "trait", "dap")]
df_updated = df_updated[order(df_updated$trait),]
df_updated = df_updated[order(df_updated$dap),]
#---------------------------------------------Saving outputs-------------------------------------------------#
# Set directory:
setwd(paste0(OUT_PATH, '/processed_data'))
# Save adjusted means:
write.csv(df_updated, file="adjusted_means.csv")
<file_sep>/codes/functions.py
#----------------------------------------------Functions-----------------------------------------------------#
# Loading libraries:
import numpy
import os
import pandas
# Build the Cockerham's model (2, 1 and 0 centered marker scores):
def W_model(x):
# Get allelic frequencies:
p = (numpy.mean(x, axis=0)/2).values.reshape(1,(x.shape[1]))
# Build Cokerham's model:
W = x - 2*numpy.repeat(p, repeats= x.shape[0], axis=0)
return(W)
# Construct the artificial bins:
def get_bin(x, n_bin, method):
# Generate batches
batches = numpy.array_split(numpy.arange(x.shape[1]), n_bin)
# Initialize the binned matrix:
W_bin = pandas.DataFrame(index=x.index, columns=map('bin_{}'.format, range(n_bin)))
e_bin = []
if method=='pca':
for i in range(n_bin):
# Compute SVD of the matrix bin:
u,s,v = numpy.linalg.svd(x.iloc[:,batches[i]], full_matrices=False)
# Compute the first principal component and adding to the binned matrix:
W_bin['bin_' + str(i)] = numpy.dot(u[:,:1], numpy.diag(s[:1]))
e_bin.append(s[0]/s.sum())
return([W_bin, e_bin, batches])
if method=='average':
for i in range(n_bin):
# Compute the mean across the columns and adding to the binned matrix:
W_bin['bin_' + str(i)] = x.iloc[:,batches[i]].mean(axis=1)
return([W_bin, batches])
<file_sep>/codes/process_raw_data.py
#------------------------------------------------Modules-----------------------------------------------------#
# Import python modules
import os
import numpy as np
import pandas as pd
import argparse
parser = argparse.ArgumentParser()
#-----------------------------------------Adding flags to the code-------------------------------------------#
# Get flags:
parser.add_argument("-dpath", "--dpath", dest = "dpath", help="The path of the folder with the raw data")
parser.add_argument("-rpath", "--rpath", dest = "rpath", help="The path of the repository")
parser.add_argument("-opath", "--opath", dest = "opath", help="The path of the folder to receive outputs (processed data)")
# Parse the paths:
args = parser.parse_args()
# Subset arguments:
DATA_PATH = args.dpath
REPO_PATH = args.rpath
OUT_PATH = args.opath
# DATA_PATH = '/workdir/jp2476/raw_data_sorghum-multi-trait'
# REPO_PATH = '/workdir/jp2476/sorghum-multi-trait'
# OUT_PATH = '/workdir/jp2476/output_sorghum-multi-trait'
#--------------------------------------------Processing data-------------------------------------------------#
# Set directory:
os.chdir(REPO_PATH + "/clean_repository/codes")
# Import functions:
from functions import *
# Set directory:
os.chdir(DATA_PATH + "/raw_data")
# Number of data frames:
n_df = 4
# Create an empty data frame:
df = []
# Load data:
df.append(pd.read_csv("Biomass_2016.csv", index_col=0))
df.append(pd.read_csv("Biomass_SF2017.csv", index_col=0))
df.append(pd.read_csv("heights_2016.csv", index_col=0))
df.append(pd.read_csv("heights_SF2017.csv", index_col=0))
# Get names of the data frame variables:
tmp = []
for i in range(n_df): tmp.append(list(df[i]))
# Rename the columns of the data frames:
df[0] = df[0].rename(index=str, columns={tmp[0][0]: 'loc',
tmp[0][1]: 'plot',
tmp[0][2]: 'name1',
tmp[0][3]: 'name2',
tmp[0][4]: 'set',
tmp[0][5]: 'block',
tmp[0][6]: 'drymass'})
df[1] = df[1].rename(index=str, columns={tmp[1][0]: 'plot',
tmp[1][1]: 'name1',
tmp[1][2]: 'name2',
tmp[1][3]: 'loc',
tmp[1][4]: 'set',
tmp[1][5]: 'block',
tmp[1][6]: 'drymass'})
df[2] = df[2].rename(index=str, columns={tmp[2][0]: 'plot',
tmp[2][1]: 'name1',
tmp[2][2]: 'name2'})
df[3] = df[3].rename(index=str, columns={tmp[3][0]: 'plot',
tmp[3][1]: 'name1',
tmp[3][2]: 'name2',
tmp[3][3]: 'taxa',
tmp[3][4]: 'year',
tmp[3][5]: 'loc',
tmp[3][6]: 'set',
tmp[3][7]: 'block'})
# Add column mapping traits to the df:
df[0] = df[0].assign(trait=pd.Series(np.repeat('biomass', df[0].shape[0])).values)
df[1] = df[1].assign(trait=pd.Series(np.repeat('biomass', df[1].shape[0])).values)
df[2] = df[2].assign(trait=pd.Series(np.repeat('height', df[2].shape[0])).values)
df[3] = df[3].assign(trait=pd.Series(np.repeat('height', df[3].shape[0])).values)
# Add columns mapping years to the df:
df[0] = df[0].assign(year=pd.Series(np.repeat('16', df[0].shape[0])).values)
df[1] = df[1].assign(year=pd.Series(np.repeat('17', df[1].shape[0])).values)
df[2] = df[2].assign(year=pd.Series(np.repeat('16', df[2].shape[0])).values)
# Load inbred lines ID:
os.chdir(DATA_PATH + "/raw_data")
line_names = pd.read_csv("genotype_names_corrected.csv")
# Rename columns:
line_names = line_names.rename(index=str, columns={'Name2': 'name2'})
# Subset columns:
line_names = line_names[['name2', 'taxa']]
# Inclusion of the identifiers to the data frame:
for i in range(len(df)):
df[i] = pd.merge(df[i], line_names, on='name2', how='left')
if i==3:
df[i] = df[i].drop('taxa_x', axis=1)
df[i] = df[i].rename(index=str, columns={'taxa_y': 'id_gbs'})
else:
df[i] = df[i].rename(index=str, columns={'taxa': 'id_gbs'})
# df[i] = df[i].loc[~df[i].id_gbs.isnull(),]
# Index of for selecting columns of the df mapping the design related to biomass collected on 2016:
tmp = ['plot','loc', 'set', 'block', 'range', 'row']
# Inclusion of the design variables to the combination height/2016
df[2] = pd.merge(df[2], df[0].loc[:,tmp], on='plot', how='left')
# Remove checks row from data not related to this experiment project:
for i in range(len(df)):
df[i].block = df[i].block.astype(str)
df[i] = df[i].loc[~(df[i]['set']=='CHK_STRP'),].copy(deep=True)
# Combine data frames into a unique data frame:
df = pd.concat(df, sort=False, axis=0)
# Change the index of the data frame:
df.index = np.arange(df.shape[0])
# Drop identifiers that will not be used:
df = df.drop(['name1'], axis=1)
# Load marker matrix:
M = pd.read_csv("gbs.csv")
# Load marker matrix:
loci_info = pd.read_csv("gbs_info.csv", index_col=0)
# Intersection between IDs:
mask = ~df['id_gbs'].isnull()
line_names = np.intersect1d(np.unique(df['id_gbs'][mask].astype(str)), list(M))
# Ordering lines:
M = M.loc[:, line_names]
# Function to build the Cockerham's model:
W = W_model(x=M.transpose())
# Build the bin matrix:
tmp = get_bin(x=W, n_bin=1000, method='pca')
W_bin = tmp[0]
W_bin.index = line_names
# Store the position of the bins into the genome:
bin_map = tmp[2]
# Add a new column for the data frame with the loci positions:
loci_info['bin'] = np.repeat(np.nan, loci_info.shape[0])
# Add the bins names for the bin column mapping its position:
for i in range(len(bin_map)):
loci_info['bin'].iloc[bin_map[i]] = np.repeat(["bin_" + str(i)], bin_map[i].size)
# Name of the height covariates (columns that will be melt):
tmp = []
tmp.append(list(map('h{}'.format, range(1,8))))
# Name of all covariates except heights (variables that will be unaffected):
tmp.append(np.setdiff1d(list(df), tmp[0]))
# Change the shape of the data frame, and adding a new column mapping the days after planting (DAP)
df = pd.melt(df, id_vars=tmp[1], value_vars=tmp[0], var_name="dap", value_name="height")
# Replace categories by the values:
df['dap'] = df['dap'].replace(tmp[0], range(30, 120+15,15))
# Replace codification of locations:
df['loc'] = df['loc'].replace(['16EF', '16FF'], ['EF', 'FF'])
# Change data type of the DAP:
df.dap = df.dap.astype(object)
# Remove the DAP values from biomass, DAP values were taken only for plant height:
index = ((df.trait == 'biomass') & (df.dap == 120)) | (df.trait == 'height')
df = df[index]
# Remove the DAP of 120 for biomass, it is not a right feature of biomass, actually it was collected in the end of the season:
index = (df.trait == 'biomass') & (df.dap == 120)
df.dap[index] = np.nan
# Remove the range column, it will not be used into the analysis:
df = df.drop('range', axis=1)
# Get just the features used in the paper:
df = df[['name2', 'id_gbs', 'block', 'loc', 'year', 'trait', 'dap', 'drymass', 'height']]
# Changing traits codification:
df.trait[df.trait == 'biomass'] = 'DM'
df.trait[df.trait == 'height'] = 'PH'
# Changing the indexes of the data frame:
df.index = range(df.shape[0])
# Changing the data type:
df['year'] = df['year'].astype(str)
df['drymass'] = df['drymass'].astype(str)
# Remove duplicate rows:
df = df.drop_duplicates()
# Changing the data type:
df['drymass'] = df['drymass'].astype(float)
## Writing into the disk the cleaned data:
# Set the directory to store processed data:
os.chdir(OUT_PATH + "/processed_data")
# Writing the full data frame with phenotypic data and IDs:
df.to_csv("df.csv")
# Writing the bin info:
loci_info.to_csv("loci_info.csv")
# Writing the genomic binned matrix under Cockerham's model:
W_bin.to_csv("W_bin.csv")
# Writing the full marker matrix:
M.to_csv("M.csv")
<file_sep>/codes/heritability_phenotypic_data_analysis.R
#-------------------------------------------Loading libraries------------------------------------------------#
# Import python libraries:
library(optparse)
library(data.table)
library(magrittr)
library(stringr)
#-----------------------------------------Adding flags to the code-------------------------------------------#
# Set the flags:
option_list = list(
make_option(c("-a", "--asremlpath"), type="character", default=NULL,
help="The path of the folder with asreml license", metavar="character"),
make_option(c("-o", "--opath"), type="character", default=NULL,
help="The path of the folder to receive outputs", metavar="character")
)
# Parse the arguments:
opt_parser = OptionParser(option_list=option_list)
args = parse_args(opt_parser)
# Subset arguments:
OUT_PATH = args$opath
ASREML_PATH = args$asremlpath
# OUT_PATH = '/workdir/jp2476/output_sorghum-multi-trait'
# OUT_PATH = '/home/jhonathan/Documents/output_sorghum-multi-trait'
# ASREML_PATH = '/workdir/jp2476/asreml'
#--------------------------------Load asreml and function for heritability-------------------------------------#
# Load asreml:
setwd(ASREML_PATH)
library(asreml)
asreml.lic(license = "asreml.lic", install = TRUE)
# Function to get the heritability and its standard deviation estimates with asreml v3.0:
get_h2 <- function(asreml_obj, n_env, n_plot) {
# Re-create the output from a basic, univariate model in asreml-R:
asrMod <- list(gammas = asreml_obj$gammas, gammas.type = asreml_obj$gammas.type, ai = asreml_obj$ai)
# Name objects:
names(asrMod[[1]]) <- names(asrMod[[2]]) <- names(asreml_obj$gammas)
# Compute the heritability and its standard deviation:
#---Observation: V4/n_env is the var_GxE/#environment and V5/#plot is the var_E/#plot
formula = eval(parse(text=paste0('h2 ~ V3 / (V3 + V4/', n_env, '+ V5/', n_plot, ')')))
return(nadiv:::pin(asrMod, formula))
}
#----------------------------------------------Load data-----------------------------------------------------#
# Set the directory:
setwd(paste0(OUT_PATH, '/processed_data'))
# Load the data:
df = fread('df.csv', header=T)[,-1]
# Change classes:
df$id_gbs = df$id_gbs %>% as.factor
df$name2 = df$name2 %>% as.factor
df$block = df$block %>% as.factor
df$loc = df$loc %>% as.factor
df$year = df$year %>% as.factor
df$trait = df$trait %>% as.factor
df$dap = df$dap %>% as.integer
df$drymass = df$drymass %>% as.numeric
df$height = df$height %>% as.numeric
# Get the group levels of the day after plantting (DAP) measures:
dap_groups = df$dap %>% unique %>% na.omit %>% as.integer
# Create a new column combing location and years:
df$env <- paste0(as.character(df$loc), "_",as.character(df$year))
df$env <- df$env %>% as.factor()
# Creating a list to receive the first stage results:
fit = list()
# Create a table to receive the heritabilities:
h2_comp = data.frame(trait=c('DM', paste0('PH_', unique(df$dap[!is.na(df$dap)]))), n_env=NA, n_plot=NA, h2=NA, se=NA)
#--------Compute the harmonic means of the number of environments and plots the lines were evaluated---------#
# Compute the harmonic mean of the number of environments in which each line was observed
#--> and the harmonic mean of the total number of plots in which each line was observed:
for (t in as.character(h2_comp$trait)) {
# Mask to subset the data of the trait:
if (t == 'DM') {
mask = df$trait=='DM' & (!is.na(df$drymass))
}
if (str_detect(t, 'PH')) {
mask = df$trait=='PH' & df$dap == str_split(t, pattern="_", simplify = TRUE)[,2] & (!is.na(df$height))
}
# Subset the data:
df_tmp = df[mask]
# Remove hybrids from the data:
mask = !(df_tmp$name2 %in% c('Pacesetter', 'SPX'))
df_tmp = df_tmp[mask]
for (i in unique(as.character(df_tmp$name2))) {
# Subset the line:
mask = df_tmp$name2==i
# Count the number of environments and plots the line i was evaluated:
n_env_tmp = c(length(unique(as.character(df_tmp[mask, ]$env))))
n_plot_tmp = c(nrow(df_tmp[mask, ]))
names(n_env_tmp) = i
names(n_plot_tmp) = i
# Stack numbers in the vector:
if (i==unique(as.character(df_tmp$name2))[1]) {
n_env = n_env_tmp
n_plot = n_plot_tmp
}
else {
n_env = c(n_env, n_env_tmp)
n_plot = c(n_plot, n_plot_tmp)
}
}
# Compute the harmonic mean of the number of environments and plots lines were evaluated:
h2_comp[h2_comp$trait==t, 'n_env'] = 1/mean(1/n_env)
h2_comp[h2_comp$trait==t, 'n_plot'] = 1/mean(1/n_plot)
}
#-----------------------------------------Biomass data analysis------------------------------------------------#
# Index for mapping the results:
index = "drymass"
# Subsetting part of the data set:
df_tmp = df[df$trait == "DM"]
# Dropping levels not present in the data subset:
df_tmp$id_gbs = df_tmp$id_gbs %>% droplevels
df_tmp$block = df_tmp$block %>% droplevels
df_tmp$env = df_tmp$env %>% droplevels
# Getting number of levels:
n_env = df_tmp$env %>% nlevels
n_rep = df_tmp$block %>% nlevels
# Fitting the model:
fit[[index]] = asreml(drymass~ 1 + id_gbs,
random = ~ id_gbs + env + block:env + id_gbs:env,
na.method.Y = "include",
control = asreml.control(
maxiter = 200),
data = df_tmp)
# Compute the heritability and its standard deviation:
h2_comp[h2_comp$trait == 'DM', c('h2','se')] = round(get_h2(fit[[index]],
n_env=h2_comp$n_env[h2_comp$trait == 'DM'],
n_plot=h2_comp$n_plot[h2_comp$trait == 'DM']), 4)
#------------------------------------------Height data analysis------------------------------------------------#
for (index in as.character(h2_comp$trait)[-1]) {
# Subsetting part of the data set:
mask = df$trait=='PH' & df$dap == str_split(index, pattern="_", simplify = TRUE)[,2]
df_tmp = df[mask]
# Dropping levels not present in the data subset:
df_tmp$id_gbs = df_tmp$id_gbs %>% droplevels
df_tmp$block = df_tmp$block %>% droplevels
df_tmp$env = df_tmp$env %>% droplevels
# Getting number of levels:
n_env = df_tmp$env %>% nlevels
n_rep = df_tmp$block %>% nlevels
fit[[index]] = asreml(height ~ 1 + id_gbs,
random = ~ id_gbs + block:env + env + id_gbs:env,
na.method.Y = "include",
control = asreml.control(
maxiter = 200),
data = df_tmp)
# Compute the heritability and its standard deviation:
h2_comp[h2_comp$trait == index, c('h2','se')] = round(get_h2(fit[[index]],
n_env=h2_comp$n_env[h2_comp$trait == index],
n_plot=h2_comp$n_plot[h2_comp$trait == index]), 4)
# Printing current analysis:
print(paste0("Analysis ", index, " done!"))
}
# Set the directory:
setwd(paste0(OUT_PATH, '/heritabilities'))
# Save adjusted means:
write.csv(h2_comp, file="heritabilities.csv")
<file_sep>/codes/split_data_cross_validation.py
#------------------------------------------------Modules-----------------------------------------------------#
# Load libraries:
import pandas as pd
import numpy as np
import os
from sklearn.model_selection import KFold
import argparse
from itertools import chain
parser = argparse.ArgumentParser()
#-----------------------------------------Adding flags to the code-------------------------------------------#
# Get flags:
parser.add_argument("-dpath", "--dpath", dest = "dpath", help="The path of the folder with the raw data")
parser.add_argument("-opath", "--opath", dest = "opath", help="The path of the folder to receive outputs")
# Parse the paths:
args = parser.parse_args()
# Subset arguments:
DATA_PATH = args.dpath
OUT_PATH = args.opath
# DATA_PATH = '/workdir/jp2476/raw_data_sorghum-multi-trait'
# OUT_PATH = '/workdir/jp2476/output_sorghum-multi-trait'
#------------------------------------Load Adjusted Means and plotting----------------------------------------#
# Set the directory to store processed data:
os.chdir(OUT_PATH + "/processed_data")
# Read adjusted means:
df = pd.read_csv("adjusted_means.csv", index_col=0)
# Change class of the dap:
df.dap = df.dap.fillna(0).astype(int)
# Read marker binned matrix:
W_bin = pd.read_csv("W_bin.csv", header = 0, index_col=0)
# Set the directory to store processed data:
os.chdir(DATA_PATH + "/raw_data")
# Read the file with the annotation of the population structure of the lines:
pop_struc = pd.read_csv("population_structure.txt", header = 0, sep='\t')
# Read the file with the annotation of the population structure of the lines:
pop_struc = pd.read_csv("population_structure.txt", header = 0, sep='\t')
pop_struc = pop_struc.rename(columns={'ID':'id_gbs', 'CATEGORY':'id_pop'})
#----------------------------Split data into groups for 5th-fold cross-validation----------------------------#
# Initialize dictionary to receive index stratified by population type:
pop_folds = dict()
# Number of folds:
n_fold = 5
# Initialize lists:
for counter in range(n_fold):
pop_folds['k' + str(counter)] = []
# Split the data into subsets stratified by population:
for pop in pop_struc.id_pop.unique():
counter=0
# Create five folds:
kf = KFold(n_splits=n_fold, shuffle=True, random_state=(1234+counter))
# Get the splits:
index = kf.split(pop_struc.id_gbs[pop_struc.id_pop == pop])
# Get the indexes:
for _, fold in index:
pop_folds['k' + str(counter)].append(pop_struc.id_gbs[pop_struc.id_pop == pop].iloc[fold].tolist())
counter+=1
# Unnest the lists:
for counter in range(n_fold):
pop_folds['k' + str(counter)] = list(chain.from_iterable(pop_folds['k' + str(counter)]))
# Initialize lists to receive the random indexes:
trn_index = []
tst_index = []
# Build sets:
trn_index.append(pop_folds['k0'] + pop_folds['k1'] + pop_folds['k2'] + pop_folds['k3'])
tst_index.append(pop_folds['k4'])
trn_index.append(pop_folds['k0'] + pop_folds['k1'] + pop_folds['k2'] + pop_folds['k4'])
tst_index.append(pop_folds['k3'])
trn_index.append(pop_folds['k0'] + pop_folds['k1'] + pop_folds['k3'] + pop_folds['k4'])
tst_index.append(pop_folds['k2'])
trn_index.append(pop_folds['k0'] + pop_folds['k2'] + pop_folds['k3'] + pop_folds['k4'])
tst_index.append(pop_folds['k1'])
trn_index.append(pop_folds['k1'] + pop_folds['k2'] + pop_folds['k3'] + pop_folds['k4'])
tst_index.append(pop_folds['k0'])
# Create dictionary with the data from the first cross-validation scheme:
y = dict()
X = dict()
# Build the response vector with all entries:
y_all = df.y_hat
# Build the feature matrix using all data set entries:
X_all = pd.get_dummies(df['id_gbs'])
X_all = X_all.dot(W_bin.loc[X_all.columns])
X_all = pd.concat([df.dap, X_all], axis=1)
# Create different sets:
sets = ['trn', 'tst']
# Build the sets of the data for the 5-fold cross-validation scheme:
for s in sets:
for t in df.trait.unique():
for i in range(n_fold):
# Key index for building the dictionary:
key_index = 'cv5f_' + t + '_k' + str(i) + '_' + s
if s == 'trn':
# Logical vector for indexation:
index = df.id_gbs.isin(trn_index[i]) & (df.trait==t)
if s == 'tst':
# Logical vector for indexation:
index = df.id_gbs.isin(tst_index[i]) & (df.trait==t)
# Build the response vector for the subset of data:
y[key_index] = df.y_hat[index]
# Build feature matrix for the subset of data:
X[key_index] = X_all[index]
if t == 'drymass':
X[key_index] = X[key_index].replace(to_replace=0, value=1) \
.rename(columns={'dap': 'mu_index'})
# Name of the forward-chaining cross-validation schemes:
fcv_types = ['fcv-30~45', 'fcv-30~60', 'fcv-30~75', 'fcv-30~90', 'fcv-30~105']
# Build the sets of the data for the forward-chaining cross-validation schemes for the DBN model:
for c in fcv_types:
for s in sets:
# Key index for building the dictionary:
key_index = c + '_height_' + s
# Get the upper index of the dap:
upper_index = int(c.split('~')[1])
if s == 'trn':
# Logical vector for indexation:
index = (df.trait=='height') & (df.dap<=upper_index)
if s == 'tst':
# Logical vector for indexation:
index = (df.trait=='height') & (df.dap>upper_index)
# Build the response vector for the subset of data:
y[key_index] = df.y_hat[index]
# Build feature matrix for the subset of data:
X[key_index] = X_all[index]
# Get DAP groups:
dap_group = df.dap.unique()[1:7]
# Build the sets of the data for the forward-chaining cross-validation schemes for the PBN and BN models using all data:
for d in dap_group:
for s in sets:
# Key index for mapping data into dictionary:
key_index = 'fcv-' + str(d) + '~only' + '_height_' + s
if s == 'trn':
# Logical vector for indexation:
index = (df.trait=='height') & (df.dap==d)
if s == 'tst':
# Logical vector for indexation:
index = (df.trait=='height') & (df.dap!=d)
# Build the response vector for the subset of data:
y[key_index] = df.y_hat[index]
# Build feature matrix for the subset of data:
X[key_index] = X_all[index]
# Create the sets only for the PBN model with drymass data together with height data:
key_index = 'fcv_drymass_trn'
y[key_index] = df.y_hat[df.trait=='drymass']
X[key_index] = X_all[df.trait=='drymass']
#------------------------------------Save different subsets of the data--------------------------------------#
# Set the directory to store processed data:
os.chdir(OUT_PATH + "/processed_data")
# Save cross-validation data:
for i in y.keys():
y[i].to_csv('y_' + i + '.csv', header='y_hat')
X[i].to_csv('x_' + i + '.csv')
<file_sep>/codes/Population_structure.Rmd
---
title: "Population structure analysis"
output:
pdf_document: default
html_notebook: default
---
# POPULATION STRUCTURE THROUGH PCA
## Calculating PCAs
```{r}
#source("http://bioconductor.org/biocLite.R")
#biocLite("gdsfmt")
#biocLite("SNPRelate")
library(gdsfmt)
library(SNPRelate)
library(dplyr)
library(magrittr)
library(tidyr)
# Get the path of the plink files
bed.fn <- "/workdir/share/Jhonathan_tmp/Exact/FINAL.bed"
fam.fn <- "/workdir/share/Jhonathan_tmp/Exact/FINAL.fam"
bim.fn <- "/workdir/share/Jhonathan_tmp/Exact/FINAL.bim"
# Build the gdsdb
snpgdsBED2GDS(bed.fn, fam.fn, bim.fn,out.gdsfn ="final.gds")
snpgdsSummary("final.gds")
genofile <- snpgdsOpen("final.gds")
# Getting the PCA object
pca <- snpgdsPCA(genofile, num.thread=2)
# Calculate the percentage of variance explained by the first 10 PC
pc.percent <- pca$varprop*100
head(round(pc.percent, 2))
```
## Coloring by Individuals with a known Race assignment
```{r}
tab <- data.frame(sample.id = pca$sample.id,
EV1 = pca$eigenvect[,1], # the first eigenvector
EV2 = pca$eigenvect[,2], # the second eigenvector
EV3 = pca$eigenvect[,3],
EV4 = pca$eigenvect[,4],
EV5 = pca$eigenvect[,5],
stringsAsFactors = FALSE)
colnames(tab) <- c("ID", "EV1", "EV2", "EV3", "EV4", "EV5")
write.table(tab, file = "/workdir/share/Jhonathan_tmp/Exact/EVs", row.names = F, col.names = F, sep = "\t", quote = F)
SIMPLEID <- read.delim("/workdir/share/Jhonathan_tmp/Exact/EVs_IDs", stringsAsFactors = F, header = F)
tab$ID <- SIMPLEID$V1
POPS <- read.delim("/workdir/share/Jhonathan_tmp/Exact/BN_str.4.meanQ", stringsAsFactors = F, header = F) %>%
`colnames<-`(c("POP1", "POP2", "POP3", "POP4"))
metadata <- cbind(tab, POPS)
#Load the metadata
Races <- read.delim("/workdir/share/Jhonathan_tmp/metadata/meta", stringsAsFactors = F) %>%
select(ID, RACE)
metadata2 <- metadata %>%
left_join(Races, by="ID") %>%
distinct %>%
mutate(RACE= case_when( is.na(RACE) ~ "NA",
RACE == "PCA outlier" ~ "NA",
TRUE ~ RACE))
library(ggplot2)
library(wesanderson)
library(patchwork)
fill <- wes_palette("IsleofDogs1")
pal <- wes_palette("Zissou1", 5, type = "continuous")
p1 <- ggplot(metadata2, aes(EV1, EV2)) +
geom_point(aes(colour = factor(RACE)), alpha =0.5, shape=19) +
#scale_color_manual(values=c("red", "blue", fill[1], fill[2], fill[3], fill[4])) +
scale_color_manual(values = c("black", pal[1], pal[1], pal[3], pal[3], "olivedrab4", "olivedrab4", "olivedrab4", "red", "lightgrey")) +
ylab("PC 2 (4.20%)") +
xlab("PC 1 (5.59%)") +
theme(legend.position="right", legend.direction="vertical",
legend.title = element_blank()) +
theme(axis.line.x = element_line(size=1, colour = "black"),
axis.line.y = element_line(size=1, colour = "black"),
panel.grid.major = element_line(colour = "#d3d3d3"), panel.grid.minor = element_blank(),
panel.border = element_blank(), panel.background = element_blank()) +
theme(plot.title = element_text(size = 14, family = "Tahoma", face = "bold"),
text=element_text(family="Tahoma"),
axis.text.x=element_text(colour="black", size = 10),
axis.text.y=element_text(colour="black", size = 10),
legend.key=element_rect(fill="white", colour="white"))
p1
```
## Coloring by the subpopulations estimated using fastStructure
```{r}
metadata3 <- metadata2 %>%
mutate(K= case_when( POP1 > POP2 & POP1 > POP3 & POP1 >POP4 ~ "POP1",
POP2 > POP1 & POP2 > POP3 & POP2 >POP4 ~ "POP2",
POP3 > POP1 & POP3 > POP2 & POP3 >POP4 ~ "POP3",
POP4 > POP1 & POP4 > POP2 & POP4 >POP3 ~ "POP4",
TRUE ~ "MIX"))
p2 <- ggplot(metadata3, aes(EV1, EV2)) +
geom_point(aes(colour = factor(K)), alpha =0.5, shape=19) +
#scale_color_manual(values=c("red", "blue", fill[1], fill[2], fill[3], fill[4])) +
scale_color_manual(values = c("black", "red", "green", "cyan", "purple")) +
ylab("PC 2 (4.20%)") +
xlab("PC 1 (5.59%)") +
theme(legend.position="right", legend.direction="vertical",
legend.title = element_blank()) +
theme(axis.line.x = element_line(size=1, colour = "black"),
axis.line.y = element_line(size=1, colour = "black"),
panel.grid.major = element_line(colour = "#d3d3d3"), panel.grid.minor = element_blank(),
panel.border = element_blank(), panel.background = element_blank()) +
theme(plot.title = element_text(size = 14, family = "Tahoma", face = "bold"),
text=element_text(family="Tahoma"),
axis.text.x=element_text(colour="black", size = 10),
axis.text.y=element_text(colour="black", size = 10),
legend.key=element_rect(fill="white", colour="white"))
p2
```
## Exporting the subpopulation assignmentas a table:
```{r}
metadata4 <- metadata3 %>%
mutate(PERC= case_when( K == "POP1" ~ POP1,
K == "POP2" ~ POP2,
K == "POP3" ~ POP3,
K == "POP4" ~ POP4,
K == "MIX" ~ 0.25))
metadata5 <- metadata4 %>%
mutate(CATEGORY = case_when(PERC < 0.8 ~ "ADMIX",
TRUE ~ K)) %>%
select(ID, CATEGORY)
write.table(x = metadata5, file="./Exact/Structure_groups_extended_simple.txt", quote = F, row.names = F, col.names = T, sep = "\t")
```
<file_sep>/codes/mixed_model_analysis.R
#-------------------------------------------Loading libraries------------------------------------------------#
# Libraries to manipulate data:
library(optparse)
library(data.table)
library(stringr)
library(magrittr)
library(EMMREML)
library(rrBLUP)
library(tidyr)
#-----------------------------------------Adding flags to the code-------------------------------------------#
# Set the flags:
option_list = list(
make_option(c("-y", "--y"), type="character", default=NULL,
help="Name of the file with the phenotypes", metavar="character"),
make_option(c("-m", "--model"), type="character", default=NULL,
help="Specify the model", metavar="character"),
make_option(c("-o", "--opath"), type="character", default=NULL,
help="The path of the folder to receive outputs", metavar="character"),
make_option(c("-c", "--cvpath"), type="character", default=NULL,
help="The path of the folder to receive outputs of the step of cross-validation analysis", metavar="character")
)
# Parse the arguments:
opt_parser = OptionParser(option_list=option_list)
args = parse_args(opt_parser)
# Subset arguments:
y = args$y
model = args$model
OUT_PATH = args$opath
CV_OUT_PATH = args$cvpath
# y = 'y_fcv-30~105_height_trn.csv'
# model = 'MTi-GBLUP-0~5'
# OUT_PATH = '/workdir/jp2476/output_sorghum-multi-trait'
# CV_OUT_PATH = '/workdir/jp2476/output_sorghum-multi-trait/cv/MTi-GBLUP/fcv-30~105/heightUP/fcv-30~60/drymass-height'
#---------------------------------Define function for spectral decomposition---------------------------------#
# Function to fix negative eigenvalues from the kinship matrix (K):
spec_dec <-function(K) {
# Eigenvalue decomposition of the matrix:
E=eigen(K)
# Create a diagonal matrix for receiving the eigenvalues:
Dg= diag(E$values)
# Identify eigenvalues lower then a small constant and pick the lowest one:
for (i in 1:nrow(Dg)) {
if (Dg[i,i]<1e-4) {
Dg[i,i]=Dg[(i-1),(i-1)]-0.01*Dg[(i-1),(i-1)]
}
}
# Create a matrix of the eigen vectors:
C=matrix(E$vectors,nrow(K),ncol(K))
# Reconstruct the marker matrix:
K = C%*%Dg%*%t(C)
# Return the transformed matrix:
return(K)
}
#-----------------------------------------------Load data----------------------------------------------------#
# Load train data just to get the indexes of the train, and test sets, used in the Bayesian Networks analysis:
# Set the directory:
setwd(paste0(OUT_PATH, '/processed_data'))
if (str_detect(model, 'MTr')) {
# Subset file names per trait:
y = str_split(y, "&", simplify = TRUE)[1,1]
}
# Read adjusted means:
y = fread(y, header=TRUE) %>% data.matrix
rownames(y) = y[,1]
y = y[,-1]
# Read the whole marker matrix:
M = fread('M.csv', header=TRUE)[,-1] %>% data.matrix %>% t(.)
# Load data frame with adjusted means to be processed and used on EMMREML for mixed model analysis:
df = fread('adjusted_means.csv', header=TRUE) %>% data.frame
rownames(df) = df[,1]
df = df[,-1]
df$id_gbs = df$id_gbs %>% as.factor %>% droplevels
df$trait = df$trait %>% as.factor %>% droplevels
df$dap = df$dap %>% as.factor %>% droplevels
# Get the indexes of train set:
index = names(y)
# Get the inbred lines ID for train set:
id_trn = df[index,]$id_gbs %>% as.character %>% unique
# Get the indexes of test set:
id_tst = df$id_gbs %>% as.character %>% unique %>% setdiff(., id_trn)
#-------------------------------------Prepare data format for EMMREML----------------------------------------#
# Create the relationship matrix:
A = A.mat(M-1)
rownames(A) = rownames(M)
colnames(A) = rownames(M)
# Change the names of the columns:
colnames(df) = c("id", "y_hat", "trait", "dap")
# Conditional based on models:
if (str_detect(model, 'MTi')) {
# Vector with the time points:
time = seq(30, 120, 15)
# Subset just time points used for training:
upper = str_split(model, '~', simplify = TRUE)[1,2] %>% as.numeric
# Change DAP class on the data frame:
df$dap = df$dap %>% as.character
# Subset data frame:
df_tmp = df[!(df$dap=='NA'),]
df_tmp = df_tmp[as.numeric(df_tmp$dap) <= time[upper+1],]
# Melting the data frame:
df_melt = df_tmp %>% spread(key = dap, value=y_hat)
# Subset the desired columns:
df_melt = df_melt[,c('id', as.character(seq(30, time[upper+1], by=15)))]
# Change row and column names:
rownames(df_melt) = df_melt$id
colnames(df_melt) = c('id_gbs', paste0('h', seq(30, time[upper+1], by=15)))
}
if (str_detect(model, 'MTr')) {
# Substitute the NA for a drymass for melting all traits together:
df$dap = df$dap %>% as.character
mask = df$dap == 'NA'
df[mask,]$dap = 'drymass'
# Vector with the time points:
time = seq(30, 120, 15)
# Subset just time points used for training:
upper = str_split(model, '~', simplify = TRUE)[1,2] %>% as.numeric
# Subset columns:
df_tmp = df[,colnames(df)!='trait']
# Melting the data frame:
df_melt = df_tmp %>% spread(key = dap, value=y_hat)
# Subset the desired columns:
df_melt = df_melt[,c('id','drymass', as.character(seq(30,120,by=15)))]
# Select height data used for train:
tmp = time[time <= time[upper+1]]
df_melt = df_melt[, c('id','drymass', tmp)]
rownames(df_melt) = df_melt$id
colnames(df_melt) = c('id_gbs','dm', paste0('h', seq(30, time[upper+1], by=15)))
mask = !is.na(df_melt$dm)
df_melt = df_melt[mask,]
mask = rownames(A) %in% df_melt$id_gbs
A = A[mask, mask]
}
# Reordering data frame:
df_melt = df_melt[rownames(A),] %>% droplevels
rownames(df_melt) = 1:nrow(df_melt)
# Changing the order of the factors:
df_melt$id_gbs = factor(df_melt$id_gbs, levels = c(as.character(df_melt$id_gbs)))
# Subset only individuals phenotyped and genotyped:
df_melt = df_melt[df_melt$id_gbs %in% rownames(A),]
# Reoder the relationship matrix as in the data frame:
index = as.character(df_melt$id_gbs)
A = A[index, index]
# Build design matrix of fixed effects:
n = nrow(df_melt)
X = matrix(1,nrow=1,ncol=n)
colnames(X) = df_melt$id_gbs %>% as.character
# Build design matrix of random effects:
Z = model.matrix(~ -1 + df_melt$id_gbs)
rownames(Z) = df_melt$id_gbs %>% as.character
colnames(Z) = df_melt$id_gbs %>% as.character
if (str_detect(model, 'MTi')) {
# Preparing matrix with the response variable:
select = paste0('h', seq(30,time[upper+1],15))
Y = t(df_melt[, select])
colnames(Y) = df_melt$id_gbs %>% as.character
}
if (str_detect(model, 'MTr')) {
# Preparing matrix with the response variable:
select = c('dm', paste0('h', seq(30,time[upper+1],15)))
Y = t(df_melt[, select])
colnames(Y) = df_melt$id_gbs %>% as.character
# Update train and test set ID:
id_trn = id_trn[id_trn %in% df_melt$id_gbs]
id_tst = id_tst[id_tst %in% df_melt$id_gbs]
}
# Fit the multivariate linear mixed model:
out <- emmremlMultivariate(Y = Y[,id_trn],
X = X[,id_trn],
Z = Z[,id_trn],
K = spec_dec(A),
tolpar = 1e-4,
varBhat = FALSE, varGhat = FALSE, PEVGhat = FALSE, test = FALSE)
# Save output:
setwd(CV_OUT_PATH)
save.image("output_mixed_models.RData")
<file_sep>/codes/generate_2pc_barplot.py
#------------------------------------------------Modules-----------------------------------------------------#
# Import python modules
import os
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
import argparse
parser = argparse.ArgumentParser()
#-----------------------------------------Adding flags to the code-------------------------------------------#
# Get flags:
parser.add_argument("-dpath", "--dpath", dest = "dpath", help="The path of the folder with the raw data")
parser.add_argument("-rpath", "--rpath", dest = "rpath", help="The path of the repository")
parser.add_argument("-opath", "--opath", dest = "opath", help="The path of the folder to receive outputs (processed data)")
# Parse the paths:
args = parser.parse_args()
# Subset arguments:
# DATA_PATH = args.dpath
# REPO_PATH = args.rpath
# OUT_PATH = args.opath
DATA_PATH = '/workdir/jp2476/raw_data_sorghum-multi-trait'
REPO_PATH = '/workdir/jp2476/sorghum-multi-trait'
OUT_PATH = '/workdir/jp2476/output_sorghum-multi-trait'
#----------------------------Generate histogram with percentage explained by the PCs-------------------------#
# Set directory:
os.chdir(DATA_PATH + "/raw_data")
# Load marker matrix:
M = pd.read_csv("gbs.csv")
# Load inbred lines ID:
line_names = pd.read_csv("genotype_names_corrected.csv")
# Load marker matrix:
loci_info = pd.read_csv("gbs_info.csv", index_col=0)
# Set the directory to store processed data:
os.chdir(OUT_PATH + "/processed_data")
# Read adjusted means:
df = pd.read_csv("adjusted_means.csv", index_col=0)
# Intersection between IDs:
mask = ~df['id_gbs'].isnull()
line_names = np.intersect1d(np.unique(df['id_gbs'][mask].astype(str)), list(M))
# Ordering lines:
M = M.loc[:, line_names]
# Function to construct the bins:
def get_perc_pca(x, n_bin):
# Generating batches
batches = np.array_split(np.arange(x.shape[1]), n_bin)
# Initializing the binned matrix:
e_bin = pd.DataFrame(index=map('bin_{}'.format, range(n_bin)), columns=['pca1', 'pca2'])
for i in range(n_bin):
# Computing SVD of the matrix bin:
u,s,v = np.linalg.svd(x.iloc[:,batches[i]], full_matrices=False)
# Computing the first principal component and adding to the binned matrix:
e_bin.loc['bin_' + str(i)]['pca1'] = (s[0]/s.sum())*100
e_bin.loc['bin_' + str(i)]['pca2'] = (s[1]/s.sum())*100
return e_bin
# Get percent explained by each bin:
perc_pca = get_perc_pca(M.transpose(), n_bin = 1000)
# Store index into a column:
perc_pca['Bin'] = perc_pca.index
# Melt data frame:
perc_pca = pd.melt(perc_pca, id_vars = 'Bin', var_name='pcs', value_name='perc')
# Change codification:
mask = perc_pca.pcs == 'pca1'
perc_pca.pcs[mask] = '1st PCA'
mask = perc_pca.pcs == 'pca2'
perc_pca.pcs[mask] = '2nd PCA'
perc_pca.columns = ['Bin', 'Principal Component', 'Percent explained (%)']
# Generate barplot with the percent explained by each bin:
sns.set(style="whitegrid")
# Draw a nested barplot to show survival for class and sex
g = sns.barplot(x="Bin", y='Percent explained (%)', hue='Principal Component', data=perc_pca)
g.despine(left=True)
# g.set_ylabels("survival probability")
# Set directory:
os.chdir(REPO_PATH + "/figures")
plt.savefig("perc_pca_expl.pdf", dpi=350)
plt.savefig("perc_pca_expl.png", dpi=350)
plt.clf()
| 51f7492d59d60aca44a8e22a6465f80d12c12eff | [
"Markdown",
"Python",
"R",
"RMarkdown",
"Shell"
]
| 15 | Python | GoreLab/sorghum-multi-trait | f89065801fe5e49c8cf7da116fdb63ee0c4bffb7 | 068528b88f516baf25a8b3d95e318371b86ea850 | |
refs/heads/master | <repo_name>tomtobac/llmm--prueba-preexa<file_sep>/firstPage.js
// https://developer.mozilla.org/es/docs/Web/API/GlobalEventHandlers/onload
window.onload = function() {
var titles = document.querySelectorAll('.smallTitle');
titles.forEach(function(title) {
title.addEventListener('click', function(e) {
var target = e.target;
// https://developer.mozilla.org/en-US/docs/Web/API/NonDocumentTypeChildNode/nextElementSibling
target.nextElementSibling.classList.toggle('u-display-none');
});
});
}
<file_sep>/README.md
llmm--prueba-preexa
| 2f83fac6633e104e5fd932b943e469acf4e13695 | [
"JavaScript",
"Markdown"
]
| 2 | JavaScript | tomtobac/llmm--prueba-preexa | fdc8c8ee65c1225d9c20b82bf49b928bfbdba982 | b6b75a40b1dc77991c9a2e85f6dfe9939539ea93 | |
refs/heads/master | <file_sep>import React, { Component } from 'react';
import { Container, Header, Content, Card, CardItem, Text, Body ,Left, Right, Title,Button,Icon, } from 'native-base';
import { Alert} from 'react-native'
import { connect } from 'react-redux'
import { getColor,postKategori } from '../public/redux/actions/kategori'
import Data from '../Data/Note'
class Register extends Component {
constructor(props) {
super(props)
this.state = {
data: props.navigation.getParam('data'),
}
}
delete =() => {
Data.splice( (this.state.data.id_note - 1) , 1);
Alert.alert(
'Alert Title',
'My Alert Msg',
[
{text: 'Ask me later', onPress: () => console.log('Ask me later pressed')},
{
text: 'Cancel',
onPress: () => console.log('Cancel Pressed'),
style: 'cancel',
},
{text: 'OK', onPress: () => this.props.navigation.goBack()},
],
{cancelable: false},
);
}
render() {
return (
<Container>
<Header>
<Left>
<Button onPress={() => this.props.navigation.goBack()} transparent>
<Icon name='arrow-back' />
</Button>
</Left>
<Body>
<Title><Text>detail note</Text></Title>
</Body>
<Right>
<Button onPress={() => this.delete()} transparent>
<Icon name='trash' />
</Button>
</Right>
</Header>
<Content>
<Card>
<CardItem header>
<Text>{this.state.data.title}</Text>
</CardItem>
<CardItem>
<Body>
<Text>
{this.state.data.text}
</Text>
</Body>
</CardItem>
<CardItem footer>
<Text>{this.state.data.category_name}</Text>
</CardItem>
</Card>
</Content>
</Container>
);
}
}
const mapStateToProps = state => {
return {
kategoriP: state.reKategori.ListKategori.result
}
}
export default connect(mapStateToProps)(Register)<file_sep>import axios from 'axios';
import URL from "../URL";
// let URL = 'http://192.168.6.169:5000'
//let URL = 'http://localhost:5000'
/////////////////////////////////////////////
export const getNote = () => {
return {
type: 'GET_NOTE',
payload: axios.get(URL+'/note'),
};
};
/////////////////////////////////////////////
export const postNote = (data) => {
return {
type: "POST_NOTE",
payload: axios.post(URL+'/note', data,{})
};
};
/////////////////////////////////////////////
export const deleteNote = (param) =>{
return{
type: 'DELETE_NOTE',
payload: axios.delete(URL +`/note/${param}`)
}
}
/////////////////////////////////////////////
export const getNote1 = (bookid) => {
console.log("book id: " + bookid)
return {
type: 'GET_NOTE1',
payload: axios.get(URL +`/note/byCategory/${bookid}`)
}
}
/////////////////////////////////////////////
export const updateNote = (bookid, data) => {
return {
type: 'UPDATE_NOTE',
payload: axios.patch(URL +`/note/${bookid}`, data)
}
}<file_sep>import React, { Component } from 'react'
import { TouchableOpacity, Image, View, AsyncStorage as storage, ActivityIndicator, StyleSheet, FlatList, RefreshControl } from 'react-native'
import { FlatGrid } from 'react-native-super-grid';
import { Button, Item, Card, CardItem, Icon, Fab, Text, Container, Header, Left, Body, Right, Title, Subtitle } from 'native-base';
import DonateBook from './AddNote'
import Data from '../Data/Note'
//import redux
import { connect } from 'react-redux'
class Home extends Component {
constructor(props) {
super(props)
this.initData = Data;
this.state = {
data: this.initData,
isLoading: false,
noteS: [],
iduser: '',
name: '',
refreshing: false,
}
}
pullDownRefresh = () => {
this.setState({ isLoading: true })
setTimeout(() => {
this.setState({data: Data, isLoading: false })
}, 500)
}
_onRefresh = () => {
this.setState({refreshing: true});
fetchData().then(() => {
this.setState({refreshing: false});
});
}
render() {
console.log('object', this.state.noteS)
return (
<Container >
<Header>
<Left>
<Button transparent>
<Icon name='person' />
</Button>
</Left>
<Body>
<Title>Title</Title>
<Subtitle>Subtitle</Subtitle>
</Body>
<Right>
<Button transparent>
<Icon name='funnel' />
</Button>
</Right>
</Header>
<View style={styles.FlatList}>
<FlatList
refreshing={this.state.isLoading}
onRefresh={this.pullDownRefresh}
data={this.state.data}
numColumns={2}
keyExtractor={item => item.id_item}
renderItem={({ item, index }) => {
return (
<TouchableOpacity onPress={()=> this.props.navigation.navigate('Detail', { data: item })} >
<Card style={{width:170}}>
<CardItem style={{backgroundColor:`${item.color}`}} header>
<Text>{item.title}</Text>
</CardItem>
<CardItem style={{backgroundColor:`${item.color}`}}>
<Body>
<Text>
{item.text}
</Text>
</Body>
</CardItem>
<CardItem style={{backgroundColor:`${item.color}`}} footer>
<Text> {item.category_name}</Text>
</CardItem>
</Card>
</TouchableOpacity>
);
}}
/>
</View>
<Fab style={{ backgroundColor: '#5067FF' }} onPress={() => this.props.navigation.navigate('AddNote')}>
<Icon name="add" />
</Fab>
</Container>
)
}
}
const mapStateToProps = state => {
return {
noteP: state.reNote.ListNote.result,
}
}
export default connect(mapStateToProps)(Home)
const styles = StyleSheet.create({
header: {
alignItems: "center",
justifyContent: "center",
backgroundColor: "#fff",
height: 60
},
text: {
fontSize: 30
},
Borrowed: {
fontSize: 10,
color: "white",
textAlign: "center",
backgroundColor: "grey",
borderRadius: 10,
paddingTop: 2,
justifyContent: "center",
position: "absolute",
zIndex: 1,
width: 60,
height: 20,
marginTop: 192
},
image: {
width: 170,
height: 211,
borderRadius: 10
},
searchBar: {
zIndex: 1,
backgroundColor: "#fff",
borderBottomColor: "transparent",
shadowColor: "#000",
shadowOffset: {
width: 0,
height: 5
},
shadowOpacity: 0.34,
shadowRadius: 6.27,
elevation: 5,
marginTop: 15,
alignSelf: "center",
marginRight: 0,
height: 38,
width: 307,
position: "absolute",
borderRadius: 20
},
FlatList: {
alignItems: "center",
display: "flex",
justifyContent: "center"
},
item: {
backgroundColor: "black",
margin: 15,
borderRadius: 8,
elevation: 6,
width: 145,
height: 215
}
});
<file_sep>const initialState = {
ListNote: [],
isLoading: false,
isFulfilled: false,
isRejected: false,
};
const buku = (state = initialState, action) => {
switch (action.type) {
/////////////////////////////////////////////////////
case 'GET_NOTE_PENDING':
return {
...state,
isLoading: true,
isFulfilled: false,
isRejected: false,
};
case 'GET_NOTE_REJECTED':
return {
...state,
isLoading: false,
isRejected: true,
};
case 'GET_NOTE_FULFILLED':
return {
...state,
isLoading: false,
isFulfilled: true,
ListNote: action.payload.data,
};
/////get limit////////////////////////////////////////////////
case 'GET_NOTE_LIMIT_PENDING':
return {
...state,
isLoading: true,
isFulfilled: false,
isRejected: false,
};
case 'GET_NOTE_LIMIT_REJECTED':
return {
...state,
isLoading: false,
isRejected: true,
};
case 'GET_NOTE_LIMIT_FULFILLED':
return {
...state,
isLoading: false,
isFulfilled: true,
ListNote: action.payload.data,
};
///////////POST////////////////////////////////////////////
case "POST_NOTE_PENDING":
return {
...state,
isLoading: true,
isRejected: false,
isFulfilled: false
};
case "POST_NOTE_REJECTED":
return {
...state,
isLoading: false,
isRejected: true
};
case "POST_NOTE_FULFILLED":
return {
...state,
isLoading: false,
isFulfilled: true,
ListNote: action.payload.data
};
/////////////GET1//////////////////////////////////////////
case 'GET_NOTE1_PENDING': // in case when loading post data
return {
...state,
isLoading: true,
isFulFilled: false,
isRejected: false
}
case 'GET_NOTE1_REJECTED': // in case error network/else
return {
...state,
isLoading: false,
isRejected: true,
}
case 'GET_NOTE1_FULFILLED': // in case successfuly post data
return {
...state,
isLoading: false,
isFulFilled: true,
ListNote: action.payload.data,
}
///////////////DELETE////////////////////////////////////////
case 'DELETE_NOTE_PENDING': // in case when loading post data
return {
...state,
isLoading: true,
isFulFilled: false,
isRejected: false
}
case 'DELETE_NOTE_REJECTED': // in case error network/else
return {
...state,
isLoading: false,
isRejected: true,
}
case 'DELETE_NOTE_FULFILLED': // in case successfuly post data
return {
...state,
isLoading: false,
isFulFilled: true,
ListNote: [state.ListNote, action.payload.data[0]],
}
//////////////UPDATE/////////////////////////////////////////
case 'UPDATE_NOTE_PENDING': // in case when loading post data
return {
...state,
isLoading: true,
isFulFilled: false,
isRejected: false
}
case 'UPDATE_NOTE_REJECTED': // in case error network/else
return {
...state,
isLoading: false,
isRejected: true,
}
case 'UPDATE_NOTE_FULFILLED': // in case successfuly post data
return {
...state,
isLoading: false,
isFulFilled: true,
ListNote: [state.ListNote, action.payload.data[0]],
}
default:
return state;
}
};
export default buku;
<file_sep>export default Data = [
{
id_category: 1,
category_name: 'personal',
icon: 'https://thegorbalsla.com/wp-content/uploads/2019/07/Hama-dan-Penyakit-Ikan-Kerapu-700x700.jpg',
id_color: "#ed5565",
},
{
id_category: 2,
category_name: 'Work',
icon: 'https://ecs7.tokopedia.net/img/cache/700/product-1/2018/2/21/0/0_fdcb14bf-83e9-473e-86b6-c84e31656cfe_800_800.jpg',
id_color: "#00FFFF",
},
{
id_category: 3,
category_name: 'shoping',
icon: 'https://ecs7.tokopedia.net/img/cache/700/product-1/2017/8/3/9244288/9244288_02cf5a39-7533-4bfa-93bf-d3d944f3548f_850_864.jpg',
id_color: "#ebe134",
},
];<file_sep>import React, { Component } from 'react';
import { FlatList, StyleSheet } from "react-native";
import { Container, Header, Content, List, ListItem, Thumbnail, Text, Left, Body, Title, Button } from 'native-base';
import { connect } from 'react-redux'
import { getKategory } from '../public/redux/actions/kategori'
import { withNavigation } from 'react-navigation';
import Data from '../Data/Kategori'
class Register extends Component {
constructor(props) {
super(props)
this.initData = Data;
this.state = {
data: this.initData,
text: '',
title:'',
id_category: '',
selected: undefined,
kategoriS:[]
}
}
// componentDidMount = async () => {
// await this.props.dispatch( getKategory() )
// this.setState({ kategoriS: this.props.kategoriP })
// }
render() {
return (
<Container>
<Header>
<Body>
<Title>kategori</Title>
</Body>
</Header>
<Content>
<List>
<FlatList
data={this.state.data}
renderItem={({ item: rowData }) => {
return (
<ListItem onPress={() => this.props.navigation.navigate('CategoryHome', { idCat: rowData.category_name })} thumbnail>
<Left>
<Thumbnail square source={{ uri: `${rowData.icon}` }} />
</Left>
<Body>
<Text>{rowData.category_name}</Text>
</Body>
</ListItem>
);
}}
keyExtractor={(item, index) => index}
/>
</List>
</Content>
<ListItem style={{top:'0%'}} onPress={() => { this.props.navigation.navigate('AddCategory') }} thumbnail>
<Left>
<Thumbnail square source={{ uri: `http://pluspng.com/img-png/a-plus-logo-vector-png-free-vector-plus-icon-png-560.png` }} />
</Left>
<Body >
<Text>Add categroy</Text>
</Body>
</ListItem>
</Container>
);
}
}
const mapStateToProps = state => {
return {
kategoriP: state.reKategori.ListKategori.result
}
}
export default connect(mapStateToProps)(withNavigation(Register))
| 40fd3dae770fc883bedc840b9104151509b2a530 | [
"JavaScript"
]
| 6 | JavaScript | kampretosjr/RN-Test-todoList | 8d0f2691df6918729f7f7b66779c8db40b7b9f99 | 85b5862539cc4759f47e6314468d05306de4aa12 | |
refs/heads/master | <file_sep>#!/bin/sh
#
# ------------------------------------------------------------------------------
# ISC License http://opensource.org/licenses/isc-license.txt
# ------------------------------------------------------------------------------
# Copyright (c) 2017, <NAME> <<EMAIL>>
#
# Permission to use, copy, modify, and/or distribute this software for any
# purpose with or without fee is hereby granted, provided that the above
# copyright notice and this permission notice appear in all copies.
#
# THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
# WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
# MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
# ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
# WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
# ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
# OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
VERSION=1.0
set -e
[ -z "$1" ] && {
echo "Version $VERSION"
echo "Usage:";
echo " ./$(basename $0) <your>.ovpn"
exit 1;
}
NAME=$(basename $1)
NAME="openvpn-${NAME%.*}"
FILE=$(realpath $1)
# container exists
[ $(docker ps -aqf "name=$NAME" | grep -cE '.+') != 0 ] || {
docker create --name $NAME -itv $FILE:/config.ovpn \
--net=host --cap-add=NET_ADMIN --device /dev/net/tun \
kylemanna/openvpn openvpn --config /config.ovpn;
EXIT_CODE=$?;
[ $EXIT_CODE = 0 ] || { exit $EXIT_CODE; }
}
# toggle container
[ $(docker ps -qf "name=$NAME" | grep -cE '.+') != 0 ] && {
echo "Your IP is: $(dig +short myip.opendns.com @resolver1.opendns.com)"
echo -n "Stopping "
docker stop $NAME;
EXIT_CODE=$?
docker ps -af "name=$NAME";
for i in $(seq 3); do echo -n '.'; sleep 1; done
echo
echo "Your IP is: $(dig +short myip.opendns.com @resolver1.opendns.com)"
exit $EXIT_CODE;
} || {
echo "Your IP is: $(dig +short myip.opendns.com @resolver1.opendns.com)"
echo -n "Starting "
docker start $NAME;
EXIT_CODE=$?
docker ps -af "name=$NAME";
for i in $(seq 4); do echo -n '.'; sleep 1; done
echo
echo "Your IP is: $(dig +short myip.opendns.com @resolver1.opendns.com)"
exit $EXIT_CODE;
}
<file_sep># FIXME
https://community.openvpn.net/openvpn/wiki/SWEET32
docker run --rm -it kylemanna/openvpn sh
# where?
--cipher AES-256-CBC
Answer
https://github.com/kylemanna/docker-openvpn/issues/163#issuecomment-277008738
# Server
([Run client](#client) see bottom)
**Note:** the cert passphrase you will find in the admin.kdbx
## Setup (only once)
```shell
$ OVPN_DATA="ovpn-data"
$ docker volume create --name $OVPN_DATA
$ docker run -v $OVPN_DATA:/etc/openvpn --rm kylemanna/openvpn \
ovpn_genconfig -u udp://heisenberg.aptly.de
$ docker run -v $OVPN_DATA:/etc/openvpn --rm -it kylemanna/openvpn ovpn_initpki
```
### Create & run OpenVPN server
```shell
$ docker run -v $OVPN_DATA:/etc/openvpn -d -p 1194:1194/udp --cap-add=NET_ADMIN \
--name openvpn kylemanna/openvpn
```
## Start/stop OpenVPN server
```shell
$ docker start openvpn
$ docker stop openvpn
```
## Create clients (example!)
```shell
$ for CLIENTNAME in lschr mthielcke dsteiner; do \
docker run -v $OVPN_DATA:/etc/openvpn --rm -it kylemanna/openvpn easyrsa build-client-full $CLIENTNAME nopass; \
docker run -v $OVPN_DATA:/etc/openvpn --rm kylemanna/openvpn ovpn_getclient $CLIENTNAME > $CLIENTNAME.ovpn; \
done
```
<a id="client"></a>
# Run client (Linux, your local machine)
```shell
$ sudo openvpn --config dsteiner.ovpn
$ sudo openvpn --config dsteiner.ovpn --daemon # background
```
| 309a2ff9682893d59500ca18a17aeea160874283 | [
"Markdown",
"Shell"
]
| 2 | Shell | DittmarSteiner/openvpn-docker | 5cc4ae17d3d2aee5f4bb57f56c50ab4ca4cc8e22 | 10f44ab0e6a8d19c5847c67ba9306dada2315ee1 | |
refs/heads/master | <file_sep>from tkinter import *
import subprocess
import os
from openpyxl import Workbook
from openpyxl.worksheet.datavalidation import DataValidation
import time
#import xlsxwriter
#def select():
# sel = "Value = " + str(v.get())
# label.config(text = sel)
def ver(value):
print(value)
root = Tk()
scl = Scale( root,from_=1,to=20,
tickinterval=2,
length=400,
resolution=1,
showvalue=NO,
orient=HORIZONTAL,
command=ver,
label="Velocidad del Puntero"
)
scl.pack(expand=YES, fill=Y)
def velocidad():
wb = Workbook()
sheet = wb.active
sheet['A1'] = 87
sheet['A2'] = "Devansh"
sheet['A3'] = 41.80
sheet['A4'] = 10
now = time.strftime("%x")
sheet['A5'] = now
wb.save('C:\\Users\\cesar\\Desktop\\python\\sample_file.xlsx')
file = open('C:\\Users\\cesar\\Desktop\\python\\archivo.sh', 'w')
file.write("#!/bin/sh" + os.linesep)
file.write("xset m " + str( scl.get())+ "1")
file.close()
subprocess.run(["chmod", "+x", 'C:\\Users\\cesar\\Desktop\\python\\archivo.sh'])
subprocess.run(['C:\\Users\\cesar\\Desktop\\python\\archivo.sh'])
aplicar = Button(
root,
text="Aplicar",
command=velocidad
)
aplicar.pack()
root.mainloop()<file_sep>#!/bin/sh
xset m 71<file_sep>from tkinter import *
raiz=Tk()
raiz.title("Bioalgo")
raiz.resizable(1,1)
#raiz.geometry("650x650")
raiz.config(bg="blue")
miframe=Frame()
miframe.pack( )
miframe.config(bg="red")
miframe.config(width="650",height="650")
raiz.mainloop() | b422014f7ec6d21b369497f2fb56b40c5a3dfbca | [
"Python",
"Shell"
]
| 3 | Python | CesarChavez96/proyecto_python_bio | bd819586cc4fcee6f3a4d58b2045ef96bebb1c68 | 47c3358da8dd61243b77c7ac8ab8b4166868a4cf | |
refs/heads/master | <repo_name>KBriscoe/WindController<file_sep>/controller.ino
#include <bluefruit.h>
#include <time.h>
#include <Adafruit_NeoPixel.h>
#include <cQueue.h>
#ifdef __AVR__
#include <avr/power.h>
#endif
#define PIN 30
#define ANIMATION_PERIOD_MS 300
class timer {
private:
unsigned long begTime;
bool running = false;
unsigned long end = 0;
public:
void start() {
begTime = clock();
running = true;
}
unsigned long elapsedTime() {
return ((unsigned long) clock() - begTime) / CLOCKS_PER_SEC;
}
bool isTimeout(unsigned long seconds) {
return seconds >= elapsedTime();
}
void stop() {
if(running) {
end = (unsigned long) clock();
running = false;
}
}
};
// Parameter 1 = number of pixels in strip
// Parameter 2 = Arduino pin number (most are valid)
// Parameter 3 = pixel type flags, add together as needed:
// NEO_KHZ800 800 KHz bitstream (most NeoPixel products w/WS2812 LEDs)
// NEO_KHZ400 400 KHz (classic 'v1' (not v2) FLORA pixels, WS2811 drivers)
// NEO_GRB Pixels are wired for GRB bitstream (most NeoPixel products)
// NEO_RGB Pixels are wired for RGB bitstream (v1 FLORA pixels, not v2)
// NEO_RGBW Pixels are wired for RGBW bitstream (NeoPixel RGBW products)
Adafruit_NeoPixel strip = Adafruit_NeoPixel(11, PIN, NEO_GRB + NEO_KHZ800);
BLEUart bleuart;
// Function prototypes for packetparser.cpp
uint8_t readPacket (BLEUart *ble_uart, uint16_t timeout);
float parsefloat (uint8_t *buffer);
void printHex (const uint8_t * data, const uint32_t numBytes);
// Packet buffer
extern uint8_t packetbuffer[];
int r;
int g;
int b;
//New Queue Item
int newLevel;
int newTime;
//Timer
timer t;
int deltaT;
int wTime;
int hTime;
int tTime;
//Level
int wLevel;
int hLevel;
int tLevel;
//bool
bool isTime;
bool initialLoop = true;
bool hasPoppedW;
bool hasPoppedH;
bool hasPoppedT;
//Settings
int currentMode = 3;
int defaultMode;
int weather[9];
int temperature[30];
int humidity[15];
typedef struct strItem {
int level;
int timeVal;
} Item;
//Queue counter
int wCount = 0;
int hCount = 0;
int tCount = 0;
Queue_t wSchedule; // Weather Queue declaration
Queue_t hSchedule; // Humidity Queue declaration
Queue_t tSchedule; // Temperature Queue declaration
void setup(void)
{
Serial.begin(19200);
Serial.println(F("Adafruit Bluefruit52 WIND Control"));
strip.begin();
strip.show();
Bluefruit.begin();
// Set max power. Accepted values are: -40, -30, -20, -16, -12, -8, -4, 0, 4
Bluefruit.setTxPower(4);
Bluefruit.setName("WIND Bracelet");
q_init(&wSchedule, sizeof(Item), 10, FIFO, true);
q_init(&hSchedule, sizeof(Item), 10, FIFO, true);
q_init(&tSchedule, sizeof(Item), 10, FIFO, true);
// Configure and start the BLE Uart service
bleuart.begin();
// Set up and start advertising
startAdv();
}
void startAdv(void)
{
// Advertising packet
Bluefruit.Advertising.addFlags(BLE_GAP_ADV_FLAGS_LE_ONLY_GENERAL_DISC_MODE);
Bluefruit.Advertising.addTxPower();
// Include the BLE UART (AKA 'NUS') 128-bit UUID
Bluefruit.Advertising.addService(bleuart);
// Secondary Scan Response packet (optional)
// Since there is no room for 'Name' in Advertising packet
Bluefruit.ScanResponse.addName();
/* Start Advertising
* - Enable auto advertising if disconnected
* - Interval: fast mode = 20 ms, slow mode = 152.5 ms
* - Timeout for fast mode is 30 seconds
* - Start(timeout) with timeout = 0 will advertise forever (until connected)
*
* For recommended advertising interval
* https://developer.apple.com/library/content/qa/qa1931/_index.html
*/
Bluefruit.Advertising.restartOnDisconnect(true);
Bluefruit.Advertising.setInterval(32, 244); // in unit of 0.625 ms
Bluefruit.Advertising.setFastTimeout(30); // number of seconds in fast mode
Bluefruit.Advertising.start(0); // 0 = Don't stop advertising after n seconds
}
/**************************************************************************/
/*!
@brief Constantly poll for new command or response data
*/
/**************************************************************************/
void loop() {
//Start timer if schedule Item exists
if (initialLoop){
t.start();
initialLoop = false;
isTime = true;
popAllSchedule();
Serial.print("TIME START");
}
//Calculate Time change on all popped queue items and set RGB
calcTime();
//Change to correct mode if new mode packet came last loop
getMode();
//Display correct values for this loop
animatePixels(strip, r, g, b, currentMode);
// Wait for new data to arrive
uint8_t len = readPacket(&bleuart, 500);
if (len == 0) return;
// Parse data
if (packetbuffer[1] == 'M') {
Serial.print("PARSE M");
defaultMode = packetbuffer[2];
}else if (packetbuffer[1] == 'S'){
Serial.print("PARSE S");
//Handle adding item to correct queue
switch(packetbuffer[2]){
//Weather Schedule Item
case 0: newLevel = packetbuffer[3];
newTime = packetbuffer[4];
Item wItem;
wItem.level = newLevel;
wItem.timeVal = newTime * 60;
q_push(&wSchedule, &wItem);
hasPoppedW = true;
break;
//Humidity Schedule Item
case 1: newLevel = packetbuffer[3];
newTime = packetbuffer[4];
Item hItem;
hItem.level = newLevel;
hItem.timeVal = newTime * 60;
q_push(&hSchedule, &hItem);
hasPoppedH = true;
break;
//Temperature Schedule Item
case 2: newLevel = packetbuffer[3];
newTime = packetbuffer[4];
Item tItem;
tItem.level = newLevel;
tItem.timeVal = newTime * 60;
q_push(&tSchedule, &tItem);
hasPoppedT = true;
break;
}
}else if (packetbuffer[1] == 'W') {
Serial.print("PARSE W SETTING");
switch(packetbuffer[2]){
case '0': weather[0] = packetbuffer[3];
weather[1] = packetbuffer[4];
weather[2] = packetbuffer[5];
Serial.print("WROTE SUNNY ");
break;
case '1': weather[3] = packetbuffer[3];
weather[4] = packetbuffer[4];
weather[5] = packetbuffer[5];
break;
case '2': weather[6] = packetbuffer[3];
weather[7] = packetbuffer[4];
weather[8] = packetbuffer[5];
break;
}
}else if (packetbuffer[1] == 'H') {
Serial.print("PARSE H SETTING");
switch(packetbuffer[2]){
case '0': humidity[0] = packetbuffer[3];
humidity[1] = packetbuffer[4];
humidity[2] = packetbuffer[5];
break;
case '1': humidity[3] = packetbuffer[3];
humidity[4] = packetbuffer[4];
humidity[5] = packetbuffer[5];
break;
case '2': humidity[6] = packetbuffer[3];
humidity[7] = packetbuffer[4];
humidity[8] = packetbuffer[5];
break;
case '3': humidity[9] = packetbuffer[3];
humidity[10] = packetbuffer[4];
humidity[11] = packetbuffer[5];
break;
case '4': humidity[12] = packetbuffer[3];
humidity[13] = packetbuffer[4];
humidity[14] = packetbuffer[5];
break;
}
}else if (packetbuffer[1]== 'T') {
Serial.print("PARSE T SETTING");
switch(packetbuffer[2]){
case '0': temperature[0] = packetbuffer[3];
temperature[1] = packetbuffer[4];
temperature[2] = packetbuffer[5];
break;
case '1': temperature[3] = packetbuffer[3];
temperature[4] = packetbuffer[4];
temperature[5] = packetbuffer[5];
break;
case '2': temperature[6] = packetbuffer[3];
temperature[7] = packetbuffer[4];
temperature[8] = packetbuffer[5];
break;
case '3': temperature[9] = packetbuffer[3];
temperature[10] = packetbuffer[4];
temperature[11] = packetbuffer[5];
break;
case '4': temperature[12] = packetbuffer[3];
temperature[13] = packetbuffer[4];
temperature[14] = packetbuffer[5];
break;
case '5': temperature[15] = packetbuffer[3];
temperature[16] = packetbuffer[4];
temperature[17] = packetbuffer[5];
break;
case '6': temperature[18] = packetbuffer[3];
temperature[19] = packetbuffer[4];
temperature[20] = packetbuffer[5];
break;
case '7': temperature[21] = packetbuffer[3];
temperature[22] = packetbuffer[4];
temperature[23] = packetbuffer[5];
break;
case '8': temperature[24] = packetbuffer[3];
temperature[25] = packetbuffer[4];
temperature[26] = packetbuffer[5];
break;
case '9': temperature[27] = packetbuffer[3];
temperature[28] = packetbuffer[4];
temperature[29] = packetbuffer[5];
break;
}
}
}
void calcTime() {
deltaT = t.elapsedTime() + 3;
if(hasPoppedW == true){
//Weather time calc
wTime = wTime - deltaT;
Serial.print(wTime);
Serial.print(" Time left minus ");
Serial.print(deltaT);
if(wTime <= 0){
hasPoppedW = false;
Serial.print("0 time popping next");
popNextW();
}else Serial.print("Time still valid");
}if(hasPoppedH == true){
//Humidity time calc
hTime = hTime - deltaT;
if(hTime <= 0){
hasPoppedH = false;
Serial.print("0 time popping next");
popNextH();
}else Serial.print("Not 0 time ");
}if(hasPoppedT == true){
//Temperature time calc
tTime = tTime - deltaT;
if(tTime <= 0){
hasPoppedT = false;
Serial.print("0 time popping next");
popNextT();
}else Serial.print("Not 0 time ");
}
}
void popNextW(){
if(q_nbRecs(&wSchedule) > 0){
Serial.print("Queue not empty");
Item currentWItem;
q_pop(&wSchedule, ¤tWItem);
wLevel = currentWItem.level;
wTime = currentWItem.timeVal;
hasPoppedW = true;
}else defaultMode = 3;
}
void popNextH(){
if(q_nbRecs(&hSchedule) > 0){
Item currentHItem;
q_pop(&hSchedule, ¤tHItem);
hLevel = currentHItem.level;
hTime = currentHItem.timeVal;
hasPoppedH = true;
}else defaultMode = 3;
}
void popNextT(){
if(q_nbRecs(&tSchedule) > 0){
Item currentTItem;
q_pop(&tSchedule, ¤tTItem);
tLevel = currentTItem.level;
tTime = currentTItem.timeVal;
hasPoppedT = true;
}else defaultMode = 3;
}
int getMode() {
currentMode = defaultMode;
return currentMode;
}
void popAllSchedule(){
popNextW();
popNextH();
popNextT();
if (!hasPoppedW && !hasPoppedH && !hasPoppedT) defaultMode = 3;
}
void animatePixels(Adafruit_NeoPixel& strip, uint8_t r, uint8_t g, uint8_t b, int currentMode) {
switch (currentMode){
case 0 : //weather
if(hasPoppedW){
r = weather[(wLevel * 3)];
g = weather[(wLevel * 3) + 1];
b = weather[(wLevel * 3) + 2];
colorWipe(strip.Color(r, g, b), 500);
}
break;
case 1 : //humidity
if(hasPoppedH){
r = humidity[hLevel * 3];
g = humidity[(hLevel * 3) + 1];
b = humidity[(hLevel * 3) + 2];
colorWipe(strip.Color(r, g, b), 500);
Serial.print("Animate Humidity");
}
break;
case 2 : //temperature
if(hasPoppedT){
r = temperature[tLevel * 3];
g = temperature[(tLevel * 3) + 1];
b = temperature[(tLevel * 3) + 2];
colorWipe(strip.Color(r, g, b), 500);
Serial.print("Animate Temperature");
}
break;
//Standby
case 3 : colorWipe(strip.Color(255, 255, 255), 500);
break;
}
}
void rainbow(uint8_t wait) {
uint16_t i, j;
for(j=0; j<256; j++) {
for(i=0; i<strip.numPixels(); i++) {
strip.setPixelColor(i, Wheel((i+j) & 255));
}
strip.show();
delay(wait);
}
}
// The colours are a transition r - g - b - back to r.
uint32_t Wheel(byte WheelPos) {
WheelPos = 255 - WheelPos;
if(WheelPos < 85) {
return strip.Color(255 - WheelPos * 3, 0, WheelPos * 3);
}
if(WheelPos < 170) {
WheelPos -= 85;
return strip.Color(0, WheelPos * 3, 255 - WheelPos * 3);
}
WheelPos -= 170;
return strip.Color(WheelPos * 3, 255 - WheelPos * 3, 0);
}
void changeColor(uint32_t c) {
for(uint16_t i=0; i<strip.numPixels(); i++) {
strip.setPixelColor(i, c);
}
strip.show();
}
// Fill the dots one after the other with a color
void colorWipe(uint32_t c, uint8_t wait) {
for(uint16_t i=0; i<strip.numPixels(); i++) {
strip.setPixelColor(i, c);
strip.show();
delay(wait);
}
} | 6b34e1151b3c05cb2126900a33670694d3677895 | [
"C++"
]
| 1 | C++ | KBriscoe/WindController | ef0c06a82c0d19edbd0ca0d14703a0c1513165c5 | d52254eb42ce86ac81951e605210bace20521bfa | |
refs/heads/main | <file_sep><?
$MESS ['MAIN_FEEDBACK_COMPONENT_NAME'] = "Форма обратной связи AVION";
$MESS ['MAIN_FEEDBACK_COMPONENT_DESCR'] = "Форма для отправки сообщения с сайта на E-mail";
?><file_sep><?
$aMenuLinks = Array(
Array(
"О компании",
"/about/",
Array(),
Array(),
""
),
Array(
"Сервис",
"/service/",
Array(),
Array(),
""
),
Array(
"Новинки",
"/novelty/",
Array(),
Array(),
""
),
Array(
"Новости",
"/news/",
Array(),
Array(),
""
)
);
?><file_sep><?
$sSectionName = "О компании";
$arDirProperties = [
];
<file_sep>document.addEventListener('DOMContentLoaded', () => {
document.querySelector('.header-mobile__burger').addEventListener('click', () => {
document.querySelectorAll('.header-mobile__nav, .header-mobile__burger')
.forEach(elem => elem.classList.toggle('active'));
document.body.classList.toggle('block');
})
document.querySelector('.header-bot__navigation_btn').addEventListener('click', () => {
const category = document.querySelector('.header-catalog');
category.classList.toggle('active')
})
document.querySelectorAll('.header-mobile__list_btnNav, .header-bot__navigation_btn').forEach((elem) =>{
elem.addEventListener('click', (event) => {
const currentElem = event.currentTarget.parentElement;
currentElem.classList.toggle('active')
})
})
document.querySelectorAll('.button-callback').forEach((elem) => {
elem.addEventListener('click', () => document.querySelector('#callback-form').classList.add('active'))
})
document.querySelectorAll('.callback-form__close').forEach((elem) => {
elem.addEventListener('click', event => event.currentTarget.parentElement.parentElement.classList.remove('active'))
})
document.querySelectorAll('.basket_btn').forEach((elem) => {
elem.addEventListener('click', (event) => {
const currentElem = event.currentTarget;
currentElem.classList.toggle('active')
})
})
document.querySelectorAll('.header-bot__search_input').forEach(el => {
el.addEventListener('focus', (event) => {
const curentElem = event.currentTarget;
curentElem.parentElement.classList.add('active');
})
el.addEventListener('blur', (event) => {
const curentElem = event.currentTarget;
if(!curentElem.value) {
curentElem.parentElement.classList.remove('active');
}
})
})
})
const swiperBannerAd = new Swiper ('.slider-banner-ad', {
pagination: {
el: '.banner-pagination',
clickable: true,
},
navigation: {
nextEl: '.banner-button-next',
prevEl: '.banner-button-prev',
},
});
const swiperSliderSell = new Swiper ('.slider-banner-sell', {
slidesPerView: 1.2,
spaceBetween: 15,
navigation: {
nextEl: '.sell-button-next',
prevEl: '.sell-button-prev',
},
pagination: {
el: '.sell-pagination',
clickable: true,
},
breakpoints: {
576: {
slidesPerView: 4,
}
}
});
const swiperSliderSection = new Swiper ('.slider-banner-section', {
slidesPerView: 1.2,
spaceBetween: 15,
pagination: {
el: '.section-pagination',
clickable: true,
},
navigation: {
nextEl: '.section-button-next',
prevEl: '.section-button-prev',
},
breakpoints: {
576: {
slidesPerView: 4,
}
}
});
const productImgSmall = new Swiper ('.banner-product__small', {
slidesPerView: 4,
watchOverflow: true,
});
const productImgBig = new Swiper ('.banner-product__big', {
effect: "fade",
thumbs: {
swiper: productImgSmall,
},
pagination: {
el: '.banner-product-pagination',
clickable: true,
},
});<file_sep><?
$sSectionName = "Новинки";
$arDirProperties = [
];
<file_sep>document.addEventListener('DOMContentLoaded', () => {
if(window['avion_main_feedback_loaded']) {
return;
}
window['avion_main_feedback_loaded'] = true;
const avionMainFeedback_result = (formElement, result) => {
console.log(result);
formElement.querySelectorAll('#feedback-form__title, .feedback-form__download, .feedback-form__subtitle, .feedback-form__file, button, .feedback-form__input-name').forEach(el => el.removeAttribute('style'))
formElement.querySelector('#feedback-form__sending').setAttribute('style', 'display:none');
if(result && result.success) {
document.querySelector('#callback-result').classList.add('active');
formElement.querySelector('input[type="file"]').dispatchEvent(new Event('recalc'));
} else {
alert(result.error || 'Произошла неизвестная ошибка, попробуйте позднее')
}
}
const handlerFunction = event => {
event.stopPropagation();
event.preventDefault();
switch (event.type) {
case 'dragover':
event.currentTarget.setAttribute('style', 'border-color:#62BB46');
break;
case 'dragleave':
event.currentTarget.removeAttribute('style');
break;
case 'drop':
event.currentTarget.removeAttribute('style');
const fileInput = event.currentTarget.parentElement.querySelector('input[type="file"]');
fileInput.addFiles(event.dataTransfer.files);
break;
}
};
document.querySelectorAll('.feedback-form__file').forEach(dropArea => {
let fileCounter = 0;
dropArea.buff = new ClipboardEvent("").clipboardData || new DataTransfer();
const formElement = dropArea.parentElement;
formElement.addEventListener('submit', async event => {
event.stopPropagation();
event.preventDefault();
const formData = new FormData(event.currentTarget);
try {
event.currentTarget.querySelectorAll('.feedback-form__download, #feedback-form__title, .feedback-form__subtitle, .feedback-form__file, button, .feedback-form__input-name').forEach(el => el.setAttribute('style', 'display:none'))
event.currentTarget.querySelector('#feedback-form__sending').removeAttribute('style');
const response = await fetch(event.currentTarget.getAttribute('action'), {
method: 'POST',
body: formData
});
avionMainFeedback_result(formElement, await response.json())
} catch (error) {
avionMainFeedback_result(formElement, {error: 'Произошла неизвестная ошибка, попробуйте позднее', success: false});
}
})
const fileInput = formElement.querySelector('input[type="file"]');
fileInput.addFiles = function (files) {
for (let i = 0, len = files.length; i < len; i++) {
fileCounter++;
files[i].id = fileCounter;
dropArea.buff.items.add(files[i])
}
this.files = dropArea.buff.files;
this.dispatchEvent(new Event('recalc'));
}
fileInput.removeFile = function (file_id) {
for (let i = 0, len = dropArea.buff.items.length; i < len; i++) {
if (file_id === dropArea.buff.items[i].id) {
dropArea.buff.items.remove(files[i])
}
}
this.files = dropArea.buff.files;
this.dispatchEvent(new Event('recalc'));
}
fileInput.addEventListener('change', function () {
fileInput.addFiles(this.files);
});
fileInput.addEventListener('recalc', (event) => {
[...event.currentTarget.files].forEach(file => {
if (document.querySelector(`#uploadedFile${file.id}`)) {
return;
}
const error = file.type.search(/^image\/*/) === -1 ? 'Загрузить можно только фографии' : null;
dropArea.insertAdjacentHTML('afterend', `
<div class="feedback-form__download ${error ? 'error' : 'finish'}">
<div class="feedback-form__download_content">
<div class="feedback-form__download_icon">
<svg width="24" height="24" viewBox="0 0 24 24" fill="none">
<path d="M6 1.5H13.5V3H6C5.60218 3 5.22064 3.15804 4.93934 3.43934C4.65804 3.72064 4.5 4.10218 4.5 4.5V19.5C4.5 19.8978 4.65804 20.2794 4.93934 20.5607C5.22064 20.842 5.60218 21 6 21H18C18.3978 21 18.7794 20.842 19.0607 20.5607C19.342 20.2794 19.5 19.8978 19.5 19.5V9H21V19.5C21 20.2956 20.6839 21.0587 20.1213 21.6213C19.5587 22.1839 18.7956 22.5 18 22.5H6C5.20435 22.5 4.44129 22.1839 3.87868 21.6213C3.31607 21.0587 3 20.2956 3 19.5V4.5C3 3.70435 3.31607 2.94129 3.87868 2.37868C4.44129 1.81607 5.20435 1.5 6 1.5Z"
fill="currentColor"></path>
<path d="M13.5 6.75V1.5L21 9H15.75C15.1533 9 14.581 8.76295 14.159 8.34099C13.7371 7.91903 13.5 7.34674 13.5 6.75Z"
fill="currentColor"></path>
</svg>
</div>
<div class="feedback-form__download_loading">
<div class="feedback-form__download_filename">
<div class="feedback-form__download_name">${file.name}</div>
<div class="feedback-form__download_number">${error ? '0' : '100'}%</div>
</div>
<div class="feedback-form__download_line"></div>
<div class="feedback-form__download_error">${error}</div>
</div>
</div>
<button class="feedback-form__download_del" id="uploadedFile${file.id}"></button>
</div>`);
document.querySelector(`#uploadedFile${file.id}`).addEventListener('click', event => {
event.stopPropagation();
event.preventDefault();
fileInput.removeFile(Number(event.currentTarget.id.replace('uploadedFile', '')))
event.currentTarget.parentElement.remove();
});
});
}, false)
dropArea.addEventListener('click', () => fileInput.click(), false)
dropArea.addEventListener('dragenter', handlerFunction, false)
dropArea.addEventListener('dragleave', handlerFunction, false)
dropArea.addEventListener('dragover', handlerFunction, false)
dropArea.addEventListener('drop', handlerFunction, false)
});
})<file_sep><?
if(!defined("B_PROLOG_INCLUDED") || B_PROLOG_INCLUDED!==true)die();
/** @var array $arParams */
/** @var array $arResult */
/** @global CMain $APPLICATION */
/** @global CUser $USER */
/** @global CDatabase $DB */
/** @var CBitrixComponentTemplate $this */
/** @var string $templateName */
/** @var string $templateFile */
/** @var string $templateFolder */
/** @var string $componentPath */
/** @var CBitrixComponent $component */
$this->setFrameMode(true);
?>
<form class="header-bot__search" action="<?=$arResult["FORM_ACTION"]?>">
<?if($arParams["USE_SUGGEST"] === "Y"):?>
<?$APPLICATION->IncludeComponent(
"bitrix:search.suggest.input",
"",
array(
"NAME" => "q",
"VALUE" => "",
"INPUT_SIZE" => 15,
"DROPDOWN_SIZE" => 10,
),
$component, array("HIDE_ICONS" => "Y")
);?>
<?else:?>
<input type="text" id="search-text"/>
<label for="search-text">Название или номер...</label>
<?endif;?>
<button class="header-bot__search_icon" name="s" type="submit" value="<?=GetMessage("BSF_T_SEARCH_BUTTON");?>">
<svg width="16" height="16" viewBox="0 0 16 16" fill="none">
<path fill-rule="evenodd" clip-rule="evenodd" d="M10.4422 10.442C10.5351 10.349 10.6454 10.2752 10.7667 10.2249C10.8881 10.1746 11.0183 10.1487 11.1497 10.1487C11.2811 10.1487 11.4112 10.1746 11.5326 10.2249C11.654 10.2752 11.7643 10.349 11.8572 10.442L15.7072 14.292C15.8948 14.4795 16.0003 14.7338 16.0004 14.9991C16.0005 15.2644 15.8952 15.5188 15.7077 15.7065C15.5202 15.8941 15.2658 15.9996 15.0005 15.9997C14.7353 15.9998 14.4808 15.8945 14.2932 15.707L10.4432 11.857C10.3502 11.7641 10.2765 11.6538 10.2261 11.5324C10.1758 11.411 10.1499 11.2809 10.1499 11.1495C10.1499 11.0181 10.1758 10.8879 10.2261 10.7665C10.2765 10.6451 10.3502 10.5348 10.4432 10.442H10.4422Z" fill="#00AEEF"/>
<path fill-rule="evenodd" clip-rule="evenodd" d="M6.5 12C7.22227 12 7.93747 11.8577 8.60476 11.5813C9.27205 11.3049 9.87837 10.8998 10.3891 10.3891C10.8998 9.87837 11.3049 9.27205 11.5813 8.60476C11.8577 7.93747 12 7.22227 12 6.5C12 5.77773 11.8577 5.06253 11.5813 4.39524C11.3049 3.72795 10.8998 3.12163 10.3891 2.61091C9.87837 2.10019 9.27205 1.69506 8.60476 1.41866C7.93747 1.14226 7.22227 1 6.5 1C5.04131 1 3.64236 1.57946 2.61091 2.61091C1.57946 3.64236 1 5.04131 1 6.5C1 7.95869 1.57946 9.35764 2.61091 10.3891C3.64236 11.4205 5.04131 12 6.5 12ZM13 6.5C13 8.22391 12.3152 9.87721 11.0962 11.0962C9.87721 12.3152 8.22391 13 6.5 13C4.77609 13 3.12279 12.3152 1.90381 11.0962C0.684819 9.87721 0 8.22391 0 6.5C0 4.77609 0.684819 3.12279 1.90381 1.90381C3.12279 0.684819 4.77609 0 6.5 0C8.22391 0 9.87721 0.684819 11.0962 1.90381C12.3152 3.12279 13 4.77609 13 6.5Z" fill="currentColor"/>
</svg>
</button>
</form>
<!-- // -->
<?/*
<div class="search-form">
<form action="<?=$arResult["FORM_ACTION"]?>">
<table border="0" cellspacing="0" cellpadding="2" align="center">
<tr>
<td align="center">
<?if($arParams["USE_SUGGEST"] === "Y"):?>
<?$APPLICATION->IncludeComponent(
"bitrix:search.suggest.input",
"",
array(
"NAME" => "q",
"VALUE" => "",
"INPUT_SIZE" => 15,
"DROPDOWN_SIZE" => 10,
),
$component, array("HIDE_ICONS" => "Y")
);?>
<?else:?>
<input type="text" name="q" value="" size="15" maxlength="50" />
<?endif;?>
</td>
</tr>
<tr>
<td align="right">
<input name="s" type="submit" value="<?=GetMessage("BSF_T_SEARCH_BUTTON");?>" />
</td>
</tr>
</table>
</form>
</div>
*/?><file_sep><p>© 2020-2021 ООО «Авион»</p><file_sep><?
$sSectionName = "Сервис";
$arDirProperties = [
];
<file_sep><?
$MESS ['MENU_DOT_DEFAULT_NAME'] = "Меню внизу (в футере) по умолчанию";
$MESS ['MENU_DOT_DEFAULT_DESC'] = "Нижнее меню по умолчанию";
?><file_sep><?php
if (!defined("B_PROLOG_INCLUDED") || B_PROLOG_INCLUDED !== true) die();
/**
* Bitrix vars
*
* @var array $arParams
* @var array $arResult
* @var CBitrixComponent $this
* @global CMain $APPLICATION
* @global CUser $USER
*/
$arResult["PARAMS_HASH"] = md5(serialize($arParams) . $this->GetTemplateName());
$arParams["USE_CAPTCHA"] = (($arParams["USE_CAPTCHA"] != "N" && !$USER->IsAuthorized()) ? "Y" : "N");
$arParams["EVENT_NAME"] = trim($arParams["EVENT_NAME"]);
if ($arParams["EVENT_NAME"] == '')
$arParams["EVENT_NAME"] = "FEEDBACK_FORM";
$arParams["EMAIL_TO"] = trim($arParams["EMAIL_TO"]);
if ($arParams["EMAIL_TO"] == '')
$arParams["EMAIL_TO"] = COption::GetOptionString("main", "email_from");
$arParams["OK_TEXT"] = trim($arParams["OK_TEXT"]);
if ($arParams["OK_TEXT"] == '')
$arParams["OK_TEXT"] = GetMessage("MF_OK_MESSAGE");
if (empty($arResult["ERROR_MESSAGE"])) {
if ($USER->IsAuthorized()) {
$arResult["AUTHOR_NAME"] = $USER->GetFormattedName(false);
} else {
if ($_SESSION["MF_NAME"] <> '')
$arResult["AUTHOR_NAME"] = htmlspecialcharsbx($_SESSION["MF_NAME"]);
if ($_SESSION["MF_PHONE"] <> '')
$arResult["AUTHOR_PHONE"] = htmlspecialcharsbx($_SESSION["MF_PHONE"]);
}
}
if ($arParams["USE_CAPTCHA"] == "Y")
$arResult["capCode"] = htmlspecialcharsbx($APPLICATION->CaptchaGetCode());
$this->IncludeComponentTemplate();
<file_sep><?
$sSectionName = "Включаемые области";
$arDirProperties = [
];
<file_sep><span>+7 (495) 000-00-00</span><file_sep><?
define('PUBLIC_AJAX_MODE', true);
require_once($_SERVER["DOCUMENT_ROOT"] . "/bitrix/modules/main/include/prolog_before.php");
/**
* Bitrix vars
*
* @var array $arParams
*/
CModule::IncludeModule('iblock');
print_r($arParams);
$response = ['error' => 'Произошла неизвестная ошибка, попробуйте позднее', 'success' => false];
if ($_SERVER["REQUEST_METHOD"] == "POST") {
if (check_bitrix_sessid()) {
do { //THIS TRICK FROR USE "break";
if (mb_strlen($_POST["user_name"]) <= 1) {
$response['error'] = "Укажите ваше имя.";
break;
} elseif (mb_strlen($_POST["user_phone"]) <= 1) {
$response['error'] = "Укажите телефон, на который ожидаете ответ.";
break;
}
if ($arParams["USE_CAPTCHA"] == "Y") {
$captcha_code = $_POST["captcha_sid"];
$captcha_word = $_POST["captcha_word"];
$cpt = new CCaptcha();
$captchaPass = COption::GetOptionString("main", "captcha_password", "");
if ($captcha_word <> '' && $captcha_code <> '') {
if (!$cpt->CheckCodeCrypt($captcha_word, $captcha_code, $captchaPass)) {
$response['error'] = GetMessage("MF_CAPTCHA_WRONG");
break;
}
} else {
$response['error'] = GetMessage("MF_CAPTHCA_EMPTY");
break;
}
}
function RemapFilesArray($name, $type, $tmp_name, $error, $size)
{
return [
'name' => $name,
'type' => $type,
'tmp_name' => $tmp_name,
'error' => $error,
'size' => $size,
];
}
$files = array_map('RemapFilesArray',
(array)$_FILES['file']['name'],
(array)$_FILES['file']['type'],
(array)$_FILES['file']['tmp_name'],
(array)$_FILES['file']['error'],
(array)$_FILES['file']['size']
);
$arFields = [
"IBLOCK_ID" => 4,
"NAME" => $_POST["user_name"],
"PROPERTY_VALUES" => [
"PHONE" => $_POST["user_phone"],
"FILES" => $files
]
];
$cIBlockElement = new CIBlockElement;
$cIBlockElement->Add($arFields);
$response = ['error' => null, 'success' => true];
/*if (!empty($arParams["EVENT_MESSAGE_ID"])) {
foreach ($arParams["EVENT_MESSAGE_ID"] as $v)
if (intval($v) > 0)
CEvent::Send($arParams["EVENT_NAME"], SITE_ID, $arFields, "N", intval($v));
} else {
CEvent::Send($arParams["EVENT_NAME"], SITE_ID, $arFields);
}*/
} while (false);
}
}
header('Content-Type: application/json');
echo json_encode($response);
die();
?><file_sep><?
$sSectionName = "Авторизация / Регистрация";
$arDirProperties = [
];
<file_sep><?
$sSectionName = "Поиск";
$arDirProperties = [
];
<file_sep><?
$sSectionName = "Новости";
$arDirProperties = [
];
<file_sep><?
define('NEED_AUTH',true);
require($_SERVER["DOCUMENT_ROOT"]."/bitrix/header.php");
$APPLICATION->SetTitle("Авторизация / Регистрация");
if ($USER->IsAuthorized()) {
LocalRedirect(SITE_DIR.'personal/');
}
?>
<div class="container">
<?$logout = $APPLICATION->GetCurPageParam(
'logout=yes',
[
'login',
'logout',
'register',
'forgot_password',
'change_password'
]
);?>
</div>
<?
require($_SERVER["DOCUMENT_ROOT"]."/bitrix/footer.php");
?><file_sep># avion
# sitemap
```
``` | eaa2c823e1bb9599ca1687d220ae3a8817a3159e | [
"JavaScript",
"Markdown",
"PHP"
]
| 19 | PHP | cesnakas/avionagro.ru | a6b76e079d05947fa25cfb9177e23637e1e364ea | f1077f2d968abeeed26a336b0594736ac9068a57 | |
refs/heads/master | <repo_name>abdeltiflouardi/bsound<file_sep>/nbproject/private/private.properties
copy.src.files=false
copy.src.target=/var/www/bsound
index.file=index.php
remote.connection=serv
remote.directory=/bsound
remote.upload=MANUALLY
run.as=REMOTE
url=http://bsound.trouvez-services.com/
<file_sep>/application/models/AddVideoForm.php
<?php
class Application_Model_AddVideoForm extends Zend_Form
{
public function init(){
$this->setMethod("post");
$video = $this->createElement('text', 'video');
$video ->setRequired(true)
->setLabel("Video");
$thumb = $this->createElement('text', 'thumb');
$thumb->setLabel("Thumb")
->setRequired(true);
// Ajout des éléments au formulaire
$this->addElement($video)
->addElement($thumb)
->addElement('submit', 'add', array('label' => 'add'));
}
}
<file_sep>/application/controllers/IndexController.php
<?php
class IndexController extends Zend_Controller_Action
{
public function init()
{
/* Initialize action controller here */
}
public function indexAction()
{
// action body
$v= new Application_Model_DbTable_Video();
$this->view->list = $v->fetchAll()->toArray();
}
}
| cbc7de6292e71a160baf83c3cb4c63f5e155bf0b | [
"PHP",
"INI"
]
| 3 | INI | abdeltiflouardi/bsound | 74eb050b6f02519f56b2615d6c62cf76dd647364 | 2b51f23f46b3a9c183c96e2266c8154b7bc10703 | |
refs/heads/master | <repo_name>jasilver99/final_project_2.0<file_sep>/README.md
# final_project_2.0
<file_sep>/config.ru
require 'sinatra'
require './application_controller.rb'
run MyApp<file_sep>/application_controller.rb
require 'bundler'
require 'twilio-ruby'
Bundler.require
require './models/model.rb'
class MyApp < Sinatra::Base
get'/' do
erb :index
end
post '/results' do
@choice = params[:compliment]
@number = params[:number]
@name = params[:name]
@name2 = params[:name2]
@callback_number = params[:callback_number]
@include_link = params[:include_link]
# @message = compliment(@choice)
@message1 = Message.new(@choice)
@message = @message1.pick_comp
@message1.send_message(@message, @name, @number, @name2, @callback_number, @include_link)
# send_message(@message, @name, @number, @name2, @callback_number, @include_link)
erb :results
end
end<file_sep>/models/model.rb
require 'twilio-ruby'
class Message
attr_reader :choice
def initialize(choice)
@choice = choice
@outfit = ["You killed the outfit game today. Chic, trendy, and shoes were on point.", "You just put fashion police out of business because your outfits have been so on point."]
@hair = ["Wow, love the new haircut! <NAME> will definitely be sporting it soon.", "You are going to be a liscensed trendsetter after showing up with that hairdo"]
@shoes = ["You’re shoes made me look like a peasant today! You have amazing taste!", "I would give up comfort for style any day if my shoes looked as good as yours did today." ]
@smile = ["Those pearly whites almost blinded me today! Nice smile!", "Hit me up with your dentist’s number because your smile just lit up the room"]
@sports = ["You’ve got mad skills! I wish I could be as athletic as you!", "You made <NAME> look uncoordidnated today with your skills at the sports field today."]
@musical = ["You’re voice puts Beyonce to shame! Keep up the amazing vocals and any recording studio would want you!", "Your musical talent is unparralleled! You should give YoYo Ma a lesson!"]
@intellectual = ["You are indubitably cerebral, phrenic, and erudite. You have the brains to make anything happen.", "Einstein would benefit from a class with you. Your intellect is unparalleled!"]
@charisma = ["Be more amicable, I dare you! People want to be around you because you are such a fun and charismatic person!", "Your charisma is out of control! Everyone feels imporant and valued around you!"]
@optimism = ["The glass is overflowing near you! Your positive energy and optimism is infectious!", "You should start filling up other people’s glasses because you are full of optimism!"]
@enthusiasm = ["You love everything you do and it makes other people happier around you! Keep up the energy and enthusiasm!", "Your passion and enthusiasm for everything you do is infectious!"]
@humor = ["I cannot stop laughing when I am with you! You are so funny that you always put people in a better mood!", "You should be a comedian because you crack me up like an egg!"]
@trustworthiness = ["I value that I can tell you anything and know my secret is safe. Few people are as trustworthy as you are.", "I have never felt more comfortable sharing secrets with somebody. You are one of the most trustworthy friends I have."]
end
def pick_comp
if @choice == "Outfit"
rand_selection(@outfit)
elsif @choice == "Hair"
rand_selection(@hair)
elsif @choice == "Shoes"
rand_selection(@shoes)
elsif @choice == "Smile"
rand_selection(@smile)
elsif @choice == "Sports"
rand_selection(@sports)
elsif @choice == "Musical"
rand_selection(@musical)
elsif @choice == "Intellectual"
rand_selection(@intellectual)
elsif @choice == "Charisma"
rand_selection(@charisma)
elsif @choice == "Optimism"
rand_selection(@optimism)
elsif @choice == "Enthusiasm"
rand_selection(@enthusiasm)
elsif @choice == "Humor"
rand_selection(@humor)
elsif @choice == "Trustworthiness"
rand_selection(@trustworthiness)
else
end
end
def rand_selection(choices)
array_length = (choices.length - 1)
random = rand(0..choices.length)
return choices[random]
end
def send_message(message, name, num, name2, callback_number, include_link)
if callback_number == ""
body = "Hey #{name},\n#{message}\nFrom,\n#{name2}"
else
body = "Hey #{name},\n#{message}\nFrom,\n#{name2} (#{callback_number})"
end
if include_link == "include_link"
link = "\nSent with FMS https://goo.gl/ML7AzI"
else
link = ""
end
account_sid = "ACed3ed813257f8acedfce46a695216257"
auth_token = "<PASSWORD>"
@client = Twilio::REST::Client.new(account_sid, auth_token)
@client.account.messages.create(
from: '+14342605034', # this is the Flatiron School's Twilio number
to: num,
body: "#{body}#{link}"
)
end
end
<file_sep>/.bundle/gems/twilio-ruby-4.2.1/lib/twilio-ruby/rest/task_router/workers.rb
module Twilio
module REST
module TaskRouter
class Workers < Twilio::REST::NextGenListResource
include Twilio::REST::TaskRouter::Statistics
end
class Worker < InstanceResource
include Twilio::REST::TaskRouter::Statistics
end
end
end
end
| b526fa0b61512842b96809faabd0283f28799f40 | [
"Markdown",
"Ruby"
]
| 5 | Markdown | jasilver99/final_project_2.0 | eb05e3f4912d72b58fac9d1f27c0c92e1faca128 | eeb01b18c4fd7772ea5be6f2ad66c2191e52767c | |
refs/heads/master | <repo_name>FarazKhalidZaki/tplmaps3d<file_sep>/core/src/data/mvtSource.cpp
#include <util/geom.h>
#include "mvtSource.h"
#include "util/mapProjection.h"
#include "tileData.h"
#include "tile/tileID.h"
#include "tile/tile.h"
#include "tile/tileTask.h"
#include "util/pbfParser.h"
#include "platform.h"
namespace Tangram {
MVTSource::MVTSource(const std::string& _name, const std::string& _urlTemplate, int32_t _maxZoom) :
DataSource(_name, _urlTemplate, _maxZoom) {
}
std::shared_ptr<TileData> MVTSource::parse(const TileTask& _task, const MapProjection& _projection) const {
auto tileData = std::make_shared<TileData>();
auto& task = static_cast<const DownloadTileTask&>(_task);
protobuf::message item(task.rawTileData->data(), task.rawTileData->size());
BoundingBox tileBounds(_projection.TileBounds(task.tileId()));
glm::dvec2 tileOrigin = {tileBounds.min.x, tileBounds.max.y*-1.0};
double tileInverseScale = 1.0 / tileBounds.width();
PbfParser::ParserContext ctx(m_id);
const auto projFn = [&](glm::dvec2 _lonLat){
glm::dvec2 tmp = _projection.LonLatToMeters(_lonLat);
return Point {
(tmp.x - tileOrigin.x) * tileInverseScale,
(tmp.y - tileOrigin.y) * tileInverseScale,
0
};
};
while(item.next()) {
if(item.tag == 3) {
tileData->layers.push_back(PbfParser::getLayer(ctx, item.getMessage(), projFn));
} else {
item.skip();
}
}
return tileData;
}
}
<file_sep>/core/src/AxisAlignmentBoundingBox.hpp
//
// AxisAlignmentBoundingBox.hpp
// tangram
//
// Created by <NAME> on 22/06/2016.
//
//
#ifndef AxisAlignmentBoundingBox_hpp
#define AxisAlignmentBoundingBox_hpp
#include <stdio.h>
struct PointD {
double x;
double y;
PointD():x(0.0),y(0.0){}
PointD(double x, double y):x(x),y(y) {}
};
struct ScreenSize {
double x;
double y;
ScreenSize(double x, double y): x(x), y(y) {};
};
class AxisAlignmentBoundingBox {
PointD min;
PointD max;
public:
AxisAlignmentBoundingBox();
void setCenter(PointD);
PointD getCenter();
void setWidth(double);
double getWidth();
void setHeight(double);
double getHeight();
void expandTo(double x, double y);
};
#endif /* AxisAlignmentBoundingBox_hpp */
<file_sep>/core/src/tangram.cpp
#include "tangram.h"
#include "platform.h"
#include "scene/scene.h"
#include "scene/sceneLoader.h"
#include "style/material.h"
#include "style/style.h"
#include "labels/labels.h"
#include "text/fontContext.h"
#include "tile/tileManager.h"
#include "tile/tile.h"
#include "gl/error.h"
#include "gl/shaderProgram.h"
#include "gl/renderState.h"
#include "gl/primitives.h"
#include "marker/marker.h"
#include "marker/markerManager.h"
#include "util/asyncWorker.h"
#include "util/inputHandler.h"
#include "tile/tileCache.h"
#include "util/fastmap.h"
#include "view/view.h"
#include "data/clientGeoJsonSource.h"
#include "gl.h"
#include "gl/hardware.h"
#include "util/ease.h"
#include "util/jobQueue.h"
#include "debug/textDisplay.h"
#include "debug/frameInfo.h"
#include <cmath>
#include <bitset>
namespace Tangram {
const static size_t MAX_WORKERS = 2;
enum class EaseField { position, zoom, rotation, tilt };
class Map::Impl {
public:
void setScene(std::shared_ptr<Scene>& _scene);
void setEase(EaseField _f, Ease _e);
void clearEase(EaseField _f);
void setPositionNow(double _lon, double _lat);
void setZoomNow(float _z);
void setRotationNow(float _radians);
void setTiltNow(float _radians);
void setPixelScale(float _pixelsPerPoint);
std::mutex tilesMutex;
std::mutex sceneMutex;
RenderState renderState;
JobQueue jobQueue;
View view;
Labels labels;
AsyncWorker asyncWorker;
InputHandler inputHandler{view};
TileWorker tileWorker{MAX_WORKERS};
TileManager tileManager{tileWorker};
MarkerManager markerManager;
std::vector<SceneUpdate> sceneUpdates;
std::array<Ease, 4> eases;
std::shared_ptr<Scene> scene = std::make_shared<Scene>();
std::shared_ptr<Scene> nextScene = nullptr;
bool cacheGlState;
};
void Map::Impl::setEase(EaseField _f, Ease _e) {
eases[static_cast<size_t>(_f)] = _e;
requestRender();
}
void Map::Impl::clearEase(EaseField _f) {
static Ease none = {};
eases[static_cast<size_t>(_f)] = none;
}
static std::bitset<8> g_flags = 0;
Map::Map() {
impl.reset(new Impl());
}
Map::~Map() {
// The unique_ptr to Impl will be automatically destroyed when Map is destroyed.
TextDisplay::Instance().deinit();
Primitives::deinit();
}
void Map::Impl::setScene(std::shared_ptr<Scene>& _scene) {
{
std::lock_guard<std::mutex> lock(sceneMutex);
scene = _scene;
}
scene->setPixelScale(view.pixelScale());
auto& camera = scene->camera();
view.setCameraType(camera.type);
switch (camera.type) {
case CameraType::perspective:
view.setVanishingPoint(camera.vanishingPoint.x, camera.vanishingPoint.y);
if (camera.fovStops) {
view.setFieldOfViewStops(camera.fovStops);
} else {
view.setFieldOfView(camera.fieldOfView);
}
break;
case CameraType::isometric:
view.setObliqueAxis(camera.obliqueAxis.x, camera.obliqueAxis.y);
break;
case CameraType::flat:
break;
}
if (camera.maxTiltStops) {
view.setMaxPitchStops(camera.maxTiltStops);
} else {
view.setMaxPitch(camera.maxTilt);
}
if (scene->useScenePosition) {
glm::dvec2 projPos = view.getMapProjection().LonLatToMeters(scene->startPosition);
view.setPosition(projPos.x, projPos.y);
view.setZoom(scene->startZoom);
}
inputHandler.setView(view);
tileManager.setDataSources(_scene->dataSources());
tileWorker.setScene(_scene);
markerManager.setScene(_scene);
setPixelScale(view.pixelScale());
bool animated = scene->animated() == Scene::animate::yes;
if (scene->animated() == Scene::animate::none) {
for (const auto& style : scene->styles()) {
animated |= style->isAnimated();
}
}
if (animated != isContinuousRendering()) {
setContinuousRendering(animated);
}
}
void Map::loadScene(const char* _scenePath, bool _useScenePosition) {
LOG("Loading scene file: %s", _scenePath);
// Copy old scene
auto scene = std::make_shared<Scene>(_scenePath);
scene->useScenePosition = _useScenePosition;
if (SceneLoader::loadScene(scene)) {
impl->setScene(scene);
}
}
void Map::loadSceneAsync(const char* _scenePath, bool _useScenePosition, MapReady _platformCallback) {
LOG("Loading scene file (async): %s", _scenePath);
{
std::lock_guard<std::mutex> lock(impl->sceneMutex);
impl->sceneUpdates.clear();
impl->nextScene = std::make_shared<Scene>(_scenePath);
impl->nextScene->useScenePosition = _useScenePosition;
}
runAsyncTask([scene = impl->nextScene, _platformCallback, &jobQueue = impl->jobQueue, this](){
bool ok = SceneLoader::loadScene(scene);
jobQueue.add([scene, ok, _platformCallback, this]() {
{
std::lock_guard<std::mutex> lock(impl->sceneMutex);
if (scene == impl->nextScene) {
impl->nextScene.reset();
} else { return; }
}
if (ok) {
auto s = scene;
impl->setScene(s);
applySceneUpdates();
if (_platformCallback) { _platformCallback(); }
}
});
});
}
void Map::queueSceneUpdate(const char* _path, const char* _value) {
std::lock_guard<std::mutex> lock(impl->sceneMutex);
impl->sceneUpdates.push_back({_path, _value});
}
void Map::applySceneUpdates() {
LOG("Applying %d scene updates", impl->sceneUpdates.size());
if (impl->nextScene) {
// Changes are automatically applied once the scene is loaded
return;
}
std::vector<SceneUpdate> updates;
{
std::lock_guard<std::mutex> lock(impl->sceneMutex);
if (impl->sceneUpdates.empty()) { return; }
impl->nextScene = std::make_shared<Scene>(*impl->scene);
impl->nextScene->useScenePosition = false;
updates = impl->sceneUpdates;
impl->sceneUpdates.clear();
}
runAsyncTask([scene = impl->nextScene, updates = std::move(updates), &jobQueue = impl->jobQueue, this](){
SceneLoader::applyUpdates(scene->config(), updates);
bool ok = SceneLoader::applyConfig(scene->config(), scene);
jobQueue.add([scene, ok, this]() {
if (scene == impl->nextScene) {
std::lock_guard<std::mutex> lock(impl->sceneMutex);
impl->nextScene.reset();
} else { return; }
if (ok) {
auto s = scene;
impl->setScene(s);
applySceneUpdates();
}
});
});
}
void Map::resize(int _newWidth, int _newHeight) {
LOGS("resize: %d x %d", _newWidth, _newHeight);
LOG("resize: %d x %d", _newWidth, _newHeight);
glViewport(0, 0, _newWidth, _newHeight);
impl->view.setSize(_newWidth, _newHeight);
Primitives::setResolution(impl->renderState, _newWidth, _newHeight);
}
bool Map::update(float _dt) {
// Wait until font resources are fully loaded
if (impl->scene->fontContext()->resourceLoad > 0) {
return false;
}
FrameInfo::beginUpdate();
impl->jobQueue.runJobs();
impl->scene->updateTime(_dt);
bool viewComplete = true;
bool markersNeedUpdate = false;
for (auto& ease : impl->eases) {
if (!ease.finished()) {
ease.update(_dt);
viewComplete = false;
}
}
impl->inputHandler.update(_dt);
impl->view.update();
impl->markerManager.update(static_cast<int>(impl->view.getZoom()));
for (const auto& style : impl->scene->styles()) {
style->onBeginUpdate();
}
{
std::lock_guard<std::mutex> lock(impl->tilesMutex);
ViewState viewState {
impl->view.getMapProjection(),
impl->view.changedOnLastUpdate(),
glm::dvec2{ impl->view.getPosition().x, -impl->view.getPosition().y },
impl->view.getZoom()
};
impl->tileManager.updateTileSets(viewState, impl->view.getVisibleTiles());
auto& tiles = impl->tileManager.getVisibleTiles();
auto& markers = impl->markerManager.markers();
for (const auto& marker : markers) {
marker->update(_dt, impl->view);
markersNeedUpdate |= marker->isEasing();
}
if (impl->view.changedOnLastUpdate() ||
impl->tileManager.hasTileSetChanged()) {
for (const auto& tile : tiles) {
tile->update(_dt, impl->view);
}
impl->labels.updateLabelSet(impl->view, _dt, impl->scene->styles(), tiles, markers,
*impl->tileManager.getTileCache());
} else {
impl->labels.updateLabels(impl->view, _dt, impl->scene->styles(), tiles, markers);
}
}
FrameInfo::endUpdate();
bool viewChanged = impl->view.changedOnLastUpdate();
bool tilesChanged = impl->tileManager.hasTileSetChanged();
bool tilesLoading = impl->tileManager.hasLoadingTiles();
bool labelsNeedUpdate = impl->labels.needUpdate();
bool resourceLoading = (impl->scene->resourceLoad > 0);
bool nextScene = bool(impl->nextScene);
if (viewChanged || tilesChanged || tilesLoading || labelsNeedUpdate || resourceLoading || nextScene) {
viewComplete = false;
}
// Request render if labels are in fading states or markers are easing.
if (labelsNeedUpdate || markersNeedUpdate) { requestRender(); }
return viewComplete;
}
void Map::render() {
FrameInfo::beginFrame();
// Invalidate render states for new frame
if (!impl->cacheGlState) {
impl->renderState.invalidate();
}
// Run render-thread tasks
impl->renderState.jobQueue.runJobs();
// Set up openGL for new frame
impl->renderState.depthMask(GL_TRUE);
auto& color = impl->scene->background();
impl->renderState.clearColor(color.r / 255.f, color.g / 255.f, color.b / 255.f, color.a / 255.f);
GL_CHECK(glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT));
for (const auto& style : impl->scene->styles()) {
style->onBeginFrame(impl->renderState);
}
{
std::lock_guard<std::mutex> lock(impl->tilesMutex);
// Loop over all styles
for (const auto& style : impl->scene->styles()) {
style->onBeginDrawFrame(impl->renderState, impl->view, *(impl->scene));
// Loop over all tiles in m_tileSet
for (const auto& tile : impl->tileManager.getVisibleTiles()) {
style->draw(impl->renderState, *tile);
}
for (const auto& marker : impl->markerManager.markers()) {
style->draw(impl->renderState, *marker);
}
style->onEndDrawFrame();
}
}
impl->labels.drawDebug(impl->renderState, impl->view);
FrameInfo::draw(impl->renderState, impl->view, impl->tileManager);
}
int Map::getViewportHeight() {
return impl->view.getHeight();
}
int Map::getViewportWidth() {
return impl->view.getWidth();
}
float Map::getPixelScale() {
return impl->view.pixelScale();
}
void Map::captureSnapshot(unsigned int* _data) {
GL_CHECK(glReadPixels(0, 0, impl->view.getWidth(), impl->view.getHeight(), GL_RGBA, GL_UNSIGNED_BYTE, (GLvoid*)_data));
}
void Map::Impl::setPositionNow(double _lon, double _lat) {
glm::dvec2 meters = view.getMapProjection().LonLatToMeters({ _lon, _lat});
view.setPosition(meters.x, meters.y);
inputHandler.cancelFling();
requestRender();
}
void Map::setPosition(double _lon, double _lat) {
impl->setPositionNow(_lon, _lat);
impl->clearEase(EaseField::position);
}
void Map::setPositionEased(double _lon, double _lat, float _duration, EaseType _e) {
double lon_start, lat_start;
getPosition(lon_start, lat_start);
auto cb = [=](float t) { impl->setPositionNow(ease(lon_start, _lon, t, _e), ease(lat_start, _lat, t, _e)); };
impl->setEase(EaseField::position, { _duration, cb });
}
void Map::getPosition(double& _lon, double& _lat) {
glm::dvec2 meters(impl->view.getPosition().x, impl->view.getPosition().y);
glm::dvec2 degrees = impl->view.getMapProjection().MetersToLonLat(meters);
_lon = degrees.x;
_lat = degrees.y;
}
void Map::Impl::setZoomNow(float _z) {
view.setZoom(_z);
inputHandler.cancelFling();
requestRender();
}
void Map::setZoom(float _z) {
impl->setZoomNow(_z);
impl->clearEase(EaseField::zoom);
}
void Map::setZoomEased(float _z, float _duration, EaseType _e) {
float z_start = getZoom();
auto cb = [=](float t) { impl->setZoomNow(ease(z_start, _z, t, _e)); };
impl->setEase(EaseField::zoom, { _duration, cb });
}
float Map::getZoom() {
return impl->view.getZoom();
}
void Map::Impl::setRotationNow(float _radians) {
view.setRoll(_radians);
requestRender();
}
void Map::setRotation(float _radians) {
impl->setRotationNow(_radians);
impl->clearEase(EaseField::rotation);
}
void Map::setRotationEased(float _radians, float _duration, EaseType _e) {
float radians_start = getRotation();
// Ease over the smallest angular distance needed
float radians_delta = glm::mod(_radians - radians_start, (float)TWO_PI);
if (radians_delta > PI) { radians_delta -= TWO_PI; }
_radians = radians_start + radians_delta;
auto cb = [=](float t) { impl->setRotationNow(ease(radians_start, _radians, t, _e)); };
impl->setEase(EaseField::rotation, { _duration, cb });
}
float Map::getRotation() {
return impl->view.getRoll();
}
void Map::Impl::setTiltNow(float _radians) {
view.setPitch(_radians);
requestRender();
}
void Map::setTilt(float _radians) {
impl->setTiltNow(_radians);
impl->clearEase(EaseField::tilt);
}
void Map::setTiltEased(float _radians, float _duration, EaseType _e) {
float tilt_start = getTilt();
auto cb = [=](float t) { impl->setTiltNow(ease(tilt_start, _radians, t, _e)); };
impl->setEase(EaseField::tilt, { _duration, cb });
}
float Map::getTilt() {
return impl->view.getPitch();
}
bool Map::screenPositionToLngLat(double _x, double _y, double* _lng, double* _lat) {
double intersection = impl->view.screenToGroundPlane(_x, _y);
glm::dvec3 eye = impl->view.getPosition();
glm::dvec2 meters(_x + eye.x, _y + eye.y);
glm::dvec2 lngLat = impl->view.getMapProjection().MetersToLonLat(meters);
*_lng = lngLat.x;
*_lat = lngLat.y;
return (intersection >= 0);
}
bool Map::lngLatToScreenPosition(double _lng, double _lat, double* _x, double* _y) {
bool clipped = false;
glm::vec2 screenCoords = impl->view.lonLatToScreenPosition(_lng, _lat, clipped);
*_x = screenCoords.x;
*_y = screenCoords.y;
float width = impl->view.getWidth();
float height = impl->view.getHeight();
bool withinViewport = *_x >= 0. && *_x <= width && *_y >= 0. && *_y <= height;
return !clipped && withinViewport;
}
void Map::setPixelScale(float _pixelsPerPoint) {
impl->setPixelScale(_pixelsPerPoint);
}
void Map::Impl::setPixelScale(float _pixelsPerPoint) {
view.setPixelScale(_pixelsPerPoint);
scene->setPixelScale(_pixelsPerPoint);
for (auto& style : scene->styles()) {
style->setPixelScale(_pixelsPerPoint);
}
}
void Map::setCameraType(int _type) {
impl->view.setCameraType(static_cast<CameraType>(_type));
requestRender();
}
int Map::getCameraType() {
return static_cast<int>(impl->view.cameraType());
}
void Map::addDataSource(std::shared_ptr<DataSource> _source) {
std::lock_guard<std::mutex> lock(impl->tilesMutex);
impl->tileManager.addClientDataSource(_source);
}
bool Map::removeDataSource(DataSource& source) {
std::lock_guard<std::mutex> lock(impl->tilesMutex);
return impl->tileManager.removeClientDataSource(source);
}
void Map::clearDataSource(DataSource& _source, bool _data, bool _tiles) {
std::lock_guard<std::mutex> lock(impl->tilesMutex);
if (_tiles) { impl->tileManager.clearTileSet(_source.id()); }
if (_data) { _source.clearData(); }
requestRender();
}
MarkerID Map::markerAdd() {
return impl->markerManager.add();
}
bool Map::markerRemove(MarkerID _marker) {
bool success = impl->markerManager.remove(_marker);
requestRender();
return success;
}
bool Map::markerSetPoint(MarkerID _marker, LngLat _lngLat) {
bool success = impl->markerManager.setPoint(_marker, _lngLat);
requestRender();
return success;
}
bool Map::markerSetPointEased(MarkerID _marker, LngLat _lngLat, float _duration, EaseType ease) {
bool success = impl->markerManager.setPointEased(_marker, _lngLat, _duration, ease);
requestRender();
return success;
}
bool Map::markerSetPolyline(MarkerID _marker, LngLat* _coordinates, int _count) {
bool success = impl->markerManager.setPolyline(_marker, _coordinates, _count);
requestRender();
return success;
}
bool Map::markerSetPolygon(MarkerID _marker, LngLat* _coordinates, int* _counts, int _rings) {
bool success = impl->markerManager.setPolygon(_marker, _coordinates, _counts, _rings);
requestRender();
return success;
}
bool Map::markerSetStyling(MarkerID _marker, const char* _styling) {
bool success = impl->markerManager.setStyling(_marker, _styling);
requestRender();
return success;
}
bool Map::markerSetVisible(MarkerID _marker, bool _visible) {
bool success = impl->markerManager.setVisible(_marker, _visible);
requestRender();
return success;
}
void Map::markerRemoveAll() {
impl->markerManager.removeAll();
requestRender();
}
void Map::handleTapGesture(float _posX, float _posY) {
impl->inputHandler.handleTapGesture(_posX, _posY);
}
void Map::handleDoubleTapGesture(float _posX, float _posY) {
impl->inputHandler.handleDoubleTapGesture(_posX, _posY);
}
void Map::handlePanGesture(float _startX, float _startY, float _endX, float _endY) {
impl->inputHandler.handlePanGesture(_startX, _startY, _endX, _endY);
}
void Map::handleFlingGesture(float _posX, float _posY, float _velocityX, float _velocityY) {
impl->inputHandler.handleFlingGesture(_posX, _posY, _velocityX, _velocityY);
}
void Map::handlePinchGesture(float _posX, float _posY, float _scale, float _velocity) {
impl->inputHandler.handlePinchGesture(_posX, _posY, _scale, _velocity);
}
void Map::handleRotateGesture(float _posX, float _posY, float _radians) {
impl->inputHandler.handleRotateGesture(_posX, _posY, _radians);
}
void Map::handleShoveGesture(float _distance) {
impl->inputHandler.handleShoveGesture(_distance);
}
void Map::setupGL() {
LOG("setup GL");
impl->tileManager.clearTileSets();
// Reconfigure the render states. Increases context 'generation'.
// The OpenGL context has been destroyed since the last time resources were
// created, so we invalidate all data that depends on OpenGL object handles.
impl->renderState.increaseGeneration();
impl->renderState.invalidate();
// Set default primitive render color
Primitives::setColor(impl->renderState, 0xffffff);
// Load GL extensions and capabilities
Hardware::loadExtensions();
Hardware::loadCapabilities();
Hardware::printAvailableExtensions();
}
void Map::useCachedGlState(bool _useCache) {
impl->cacheGlState = _useCache;
}
const std::vector<TouchItem>& Map::pickFeaturesAt(float _x, float _y) {
return impl->labels.getFeaturesAtPoint(impl->view, 0, impl->scene->styles(),
impl->tileManager.getVisibleTiles(),
_x, _y);
}
void Map::runAsyncTask(std::function<void()> _task) {
impl->asyncWorker.enqueue(std::move(_task));
}
void Map::zoomToShowSegment(std::vector<Tangram::LngLat> route, ScreenSize screenSize, float tilt,float rotation, float ratio) {
// Make sure we have some points to work with
if (route.empty()) {
return;
}
setRotation(0);
setTilt(tilt);
// Determine the smallest axis-aligned box that contains the route longitude and latitude
auto start = route.front();
//auto finish = route.back();
AxisAlignmentBoundingBox routeBounds;
routeBounds.setCenter(PointD(start.longitude, start.latitude));
for (auto p: route) {
routeBounds.expandTo(p.longitude, p.latitude);
}
// Add some padding to the box
double padding = 0.10;
routeBounds.setWidth((1.0 + padding) * routeBounds.getWidth());
routeBounds.setHeight((1.0 + padding) * routeBounds.getHeight());
// Determine the bounds of the current view in longitude and latitude
float pixleScale = impl->view. pixelScale();
ScreenSize screen = ScreenSize(screenSize.x*pixleScale, screenSize.y*pixleScale);
float upperOffset = ratio;
float lowerOffset = 1-ratio;
LOG("UpperOffset = %f",upperOffset);
LOG("UpperOffset = %f",lowerOffset);
// Take half of the y dimension to allow space for other UI elements
double x = 0.0;
double y = screen.y * upperOffset;
double *lng = new double;
double *lat = new double;
screenPositionToLngLat(x, y, lng, lat);
LngLat viewMin(*lng, *lat);
x = screen.x;
y = screen.y * lowerOffset;
screenPositionToLngLat(x, y, lng, lat);
LngLat viewMax(*lng, *lat);
// Determine the amount of re-scaling needed to view the route
double scaleX = routeBounds.getWidth() / std::abs(viewMax.longitude - viewMin.longitude);
double scaleY = routeBounds.getHeight() / std::abs(viewMax.latitude - viewMin.latitude);
double zoomDelta = -std::log(std::max(scaleX, scaleY)) / std::log(2.0);
// Update map position and zoom
float z = getZoom() + zoomDelta;
Map::setZoomEased(z,1.0f);
//if (getZoom() == z) {
Map::setPositionEased(routeBounds.getCenter().x, routeBounds.getCenter().y,1.0f);
//}
setRotation(rotation);
}
double degreesToRadians(double degrees) {
return degrees * M_PI / 180.0;
}
double radiansToDegrees(double radians) {
return radians * 180.0/M_PI;
}
float Map::rotationForSegment(std::vector<Tangram::LngLat> segment,float azimuth) {
LOG("azmith %f",azimuth);
if (azimuth<0) //Degrees
azimuth+=(float) 360.0;
azimuth = 0.0;
auto point1 = segment.front();
auto point2 = segment.back();
double lat1 = degreesToRadians(point1.latitude);
double lon1 = degreesToRadians(point1.longitude);
double lat2 = degreesToRadians(point2.latitude);
double lon2 = degreesToRadians(point2.longitude);
double dLon = lon2 - lon1;
double y = sin(dLon) * cos(lat2);
double x = cos(lat1) * sin(lat2) - sin(lat1) * cos(lat2) * cos(dLon);
double radiansBearing = atan2(y, x);
//LOG("radianBearing %f",radiansBearing);
if(radiansBearing < 0.0)
radiansBearing += 2*M_PI;
float bearTo = radiansToDegrees(radiansBearing);
LOG("Bearing %f",bearTo);
float rotation = bearTo - azimuth;
LOG("Rotation %f",rotation);
if (rotation < 0) {
rotation = rotation + 360;
}
rotation = 360-rotation;
LOG("Final Rotation %f",rotation);
rotation = degreesToRadians(rotation);
setRotationEased(rotation,1.0f);
return rotation;
}
void setDebugFlag(DebugFlags _flag, bool _on) {
g_flags.set(_flag, _on);
// m_view->setZoom(m_view->getZoom()); // Force the view to refresh
}
bool getDebugFlag(DebugFlags _flag) {
return g_flags.test(_flag);
}
void toggleDebugFlag(DebugFlags _flag) {
g_flags.flip(_flag);
// m_view->setZoom(m_view->getZoom()); // Force the view to refresh
// Rebuild tiles for debug modes that needs it
// if (_flag == DebugFlags::proxy_colors
// || _flag == DebugFlags::draw_all_labels
// || _flag == DebugFlags::tile_bounds
// || _flag == DebugFlags::tile_infos) {
// if (m_tileManager) {
// std::lock_guard<std::mutex> lock(m_tilesMutex);
// m_tileManager->clearTileSets();
// }
// }
}
double distanceBetweenUserAndSegment(std::vector<Tangram::LngLat> segment, Tangram::LngLat location) {
double minDistanceCalculated = -1;
for (size_t it = 0; it != segment.size()-1; it++) {
Tangram::LngLat first = segment.at(it);
Tangram::LngLat second = segment.at(it+1);
double pointLnA[] = {first.latitude, first.longitude};
double pointLnB[] = {second.latitude, second.longitude};
double pointUser[] = {location.latitude, location.longitude};
MathUtils mathUtil;
double distancePartialSegment = mathUtil.lineToPointDistance2D(pointLnA, pointLnB, pointUser, true);
distancePartialSegment *= 100000; // Conversion into meters
if (it == 0) {
minDistanceCalculated = distancePartialSegment;
} else {
if (distancePartialSegment < minDistanceCalculated)
minDistanceCalculated = distancePartialSegment;
}
}
return minDistanceCalculated;
}
}
<file_sep>/core/src/MathUtils.hpp
//
// MathUtils.hpp
// tangram
//
// Created by <NAME> on 28/06/2016.
//
//
#ifndef MathUtils_hpp
#define MathUtils_hpp
#include <stdio.h>
#include "math.h"
#include "cmath"
#include "tangram.h"
namespace Tangram {
class MathUtils {
public:
double dotProduct(double pointA[], double pointB[], double pointC[]);
double crossProduct(double pointA[], double pointB[], double pointC[]);
double distance(double pointA[], double pointB[]);
double lineToPointDistance2D(double pointA[], double pointB[], double pointC[], bool isSegment);
};
}
#endif /* MathUtils_hpp */
<file_sep>/core/src/MathUtils.cpp
//
// MathUtils.cpp
// tangram
//
// Created by <NAME> on 28/06/2016.
//
//
#include "MathUtils.hpp"
namespace Tangram {
double MathUtils::dotProduct(double *pointA, double *pointB, double *pointC) {
double AB[2];
double BC[2];
AB[0] = pointB[0] - pointA[0];
AB[1] = pointB[1] - pointA[1];
BC[0] = pointC[0] - pointB[0];
BC[1] = pointC[1] - pointB[1];
//double dot = AB[0] * BC[0] + AB[1] * BC[1];
return AB[0] * BC[0] + AB[1] * BC[1];
}
double MathUtils::crossProduct(double *pointA, double *pointB, double *pointC) {
double AB[2];
double AC[2];
AB[0] = pointB[0] - pointA[0];
AB[1] = pointB[1] - pointA[1];
AC[0] = pointC[0] - pointA[0];
AC[1] = pointC[1] - pointA[1];
//double cross = AB[0] * AC[1] - AB[1] * AC[0];
return AB[0] * AC[1] - AB[1] * AC[0];
}
double MathUtils::distance(double *pointA, double *pointB) {
double d1 = pointA[0] - pointB[0];
double d2 = pointA[1] - pointB[1];
return sqrt(d1 * d1 + d2 * d2);
}
double MathUtils::lineToPointDistance2D(double *pointA, double *pointB, double *pointC, bool isSegment) {
double dist = crossProduct(pointA, pointB, pointC) / distance(pointA, pointB);
if (isSegment) {
double dot1 = dotProduct(pointA, pointB, pointC);
if (dot1 > 0)
return distance(pointB, pointC);
double dot2 = dotProduct(pointB, pointA, pointC);
if (dot2 > 0)
return distance(pointA, pointC);
}
return std::abs(dist);
}
};
<file_sep>/core/src/AxisAlignmentBoundingBox.cpp
//
// AxisAlignmentBoundingBox.cpp
// tangram
//
// Created by <NAME> on 22/06/2016.
//
//
#include <algorithm>
#include "AxisAlignmentBoundingBox.hpp"
///Setters and Getters
AxisAlignmentBoundingBox::AxisAlignmentBoundingBox() {
min = PointD();
max = PointD();
}
//Center
void AxisAlignmentBoundingBox::setCenter(PointD c) {
double hw = 0.5 * getWidth();
double hh = 0.5 * getHeight();
max = PointD(c.x + hw, c.y + hh);
min = PointD(c.x - hw, c.y - hh);
}
PointD AxisAlignmentBoundingBox::getCenter() {
return PointD(0.5 * (max.x + min.x), 0.5 * (max.y + min.y));
}
//Width
void AxisAlignmentBoundingBox::setWidth(double w) {
PointD c = getCenter();
max.x = c.x + 0.5 * w;
min.x = c.x - 0.5 * w;
}
double AxisAlignmentBoundingBox::getWidth() {
return max.x - min.x;
}
//Height
void AxisAlignmentBoundingBox::setHeight(double h) {
PointD c = getCenter();
max.y = c.y + 0.5 * h;
min.y = c.y - 0.5 * h;
}
double AxisAlignmentBoundingBox::getHeight() {
return max.y - min.y;
}
///
void AxisAlignmentBoundingBox::expandTo(double x, double y) {
max.x = std::max(max.x, x);
max.y = std::max(max.y, y);
min.x = std::min(min.x, x);
min.y = std::min(min.y, y);
}
| 98bd896f9568afd71e7606a528b0deb7d9d706fc | [
"C++"
]
| 6 | C++ | FarazKhalidZaki/tplmaps3d | f30451b2e704e78dc54d4213411eae7991f1e2d1 | 6d001446e15d6a9fef309dd7e0019914b909d0b7 | |
refs/heads/master | <repo_name>LunaticFrindge064/esame<file_sep>/test.js
var assert = require('assert');
var list = require('./index.js');
describe('TEST TO DO LIST', function () {
it('add work, value ID Creator, Assignee and lenght todo',
function () {
list.ins({ idCr: 3, idAs: 4, activity: "Wash the car" });
assert.equal(list.allTodo()[1].idCr, 3);
assert.equal(list.allTodo()[1].idAs, 4);
assert.equal(list.allTodo().length, 2);
});
it('id exist, set true',
function () {
assert.equal(list.completed(1),"Update True");
});
it('id exist, set false',
function () {
assert.equal(list.notCompleted(2),"Update False");
});
it('all true, lenght 1',
function () {
assert.equal(list.allTrue().length,1);
});
it('all false, lenght 1',
function () {
assert.equal(list.allFalse().length,1);
});
it('add users, lenght 2',
function () {
list.addUsers({ idUsers: 3, Name: "Giuseppe" });
assert.equal(list.allUsers().length,3);
});
it('list todo of a single users, lenght 2',
function () {
assert.equal(list.todoUser(3).length,1);
});
it('id exist, del work, lenght 1',
function () {
list.del(1);
assert.equal(list.allTodo().length, 1);
});
});
| c7508987f26be8072e32b4339aaae72e4bd23bd4 | [
"JavaScript"
]
| 1 | JavaScript | LunaticFrindge064/esame | 7580f56c332b030b8a59a54af9dd39ec85732dcf | de76c35e9a2af02b066c040f4c7b44f73c074c22 | |
refs/heads/master | <repo_name>nulab/backlog4j<file_sep>/build.gradle
buildscript {
repositories {
mavenCentral()
}
}
plugins {
id 'java-library'
id 'maven-publish'
id 'signing'
}
sourceCompatibility = 1.8
targetCompatibility = 1.8
group = 'com.nulab-inc'
archivesBaseName = 'backlog4j'
version = '2.5.4-SNAPSHOT'
repositories {
maven {
url 'https://repo1.maven.org/maven2/'
}
}
dependencies {
testImplementation 'org.junit.jupiter:junit-jupiter:5.9.0'
testImplementation 'org.hamcrest:hamcrest-library:2.2'
testImplementation 'org.exparity:hamcrest-date:2.0.8'
testImplementation 'ch.qos.logback:logback-classic:1.2.11'
implementation 'org.apache.commons:commons-lang3:3.12.0'
implementation 'com.fasterxml.jackson.core:jackson-core:2.13.3'
implementation 'com.fasterxml.jackson.core:jackson-annotations:2.13.3'
implementation 'com.fasterxml.jackson.core:jackson-databind:2.13.3'
implementation 'org.slf4j:slf4j-api:1.7.36'
}
java {
compileJava.options.encoding = 'UTF-8'
compileTestJava.options.encoding = 'UTF-8'
withSourcesJar()
withJavadocJar()
}
test {
useJUnitPlatform()
}
javadoc {
options.locale = 'en_US'
options.encoding = 'UTF-8'
options.addStringOption('Xdoclint:none', '-quiet')
title = 'Backlog4j ' + version + ' API'
}
jar {
manifest {
attributes('Implementation-Title': archivesBaseName,
'Implementation-Version': archiveVersion,
'Automatic-Module-Name': archiveBaseName)
}
}
configurations {
deployerJars
}
publishing {
publications {
mavenJava(MavenPublication) {
from components.java
pom {
name = 'Backlog4j'
description = 'Backlog4j is a Backlog binding library for Java.'
url = 'https://github.com/nulab/backlog4j'
scm {
connection = 'https://github.com/nulab/backlog4j.git'
developerConnection = 'https://github.com/nulab/backlog4j.git'
url = 'https://github.com/nulab/backlog4j.git'
}
licenses {
license {
name = 'MIT License'
url = 'http://www.opensource.org/licenses/mit-license.php'
}
}
developers {
developer {
id = 'uchida'
name = '<NAME>'
email = '<EMAIL>'
}
}
}
}
}
repositories {
maven {
credentials {
username = findProperty("ossrhUsername") ?: System.getenv("OSSRH_USERNAME")
password = findProperty("ossrhPassword") ?: System.getenv("OSSRH_PASSWORD")
}
if (project.version.endsWith("-SNAPSHOT")) {
url = "https://oss.sonatype.org/content/repositories/snapshots"
} else {
url = "https://oss.sonatype.org/service/local/staging/deploy/maven2"
}
}
}
}
signing {
sign publishing.publications.mavenJava
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/InternalFactoryJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.nulabinc.backlog4j.*;
import com.nulabinc.backlog4j.auth.AccessToken;
import com.nulabinc.backlog4j.http.BacklogHttpResponse;
import com.nulabinc.backlog4j.internal.InternalFactory;
import com.nulabinc.backlog4j.internal.json.activities.ActivityJSONImpl;
import com.nulabinc.backlog4j.internal.json.auth.AccessTokenJSONImpl;
import com.nulabinc.backlog4j.internal.json.customFields.CustomFieldSettingJSONImpl;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class InternalFactoryJSONImpl implements InternalFactory {
@Override
public AccessToken createAccessToken(BacklogHttpResponse res) throws BacklogException {
return createAccessToken(res.asString());
}
public AccessToken createAccessToken(String resStr) throws BacklogException {
return createObject(resStr, AccessToken.class, AccessTokenJSONImpl.class);
}
@Override
public Space createSpace(BacklogHttpResponse res) throws BacklogException {
return createSpace(res.asString());
}
public Space createSpace(String resStr) throws BacklogException {
return createObject(resStr, Space.class, SpaceJSONImpl.class);
}
@Override
public SpaceNotification createSpaceNotification(BacklogHttpResponse res) throws BacklogException {
return createSpaceNotification(res.asString());
}
public SpaceNotification createSpaceNotification(String resStr) throws BacklogException {
return createObject(resStr, SpaceNotification.class, SpaceNotificationJSONImpl.class);
}
@Override
public DiskUsage createDiskUsage(BacklogHttpResponse res) throws BacklogException {
return createDiskUsage(res.asString());
}
public DiskUsage createDiskUsage(String resStr) throws BacklogException {
return createObject(resStr, DiskUsage.class, DiskUsageJSONImpl.class);
}
@Override
public DiskUsageDetail createDiskUsageDetail(BacklogHttpResponse res) throws BacklogException {
return createDiskUsageDetail(res.asString());
}
public DiskUsageDetail createDiskUsageDetail(String resStr) throws BacklogException {
return createObject(resStr, DiskUsageDetail.class, DiskUsageDetailJSONImpl.class);
}
@Override
public ResponseList<Project> createProjectList(BacklogHttpResponse res) throws BacklogException {
return createProjectList(res.asString());
}
public ResponseList<Project> createProjectList(String resStr) throws BacklogException {
return createObjectList(resStr, Project.class, ProjectJSONImpl[].class);
}
@Override
public ProjectWithVCS createProject(BacklogHttpResponse res) throws BacklogException {
return createProject(res.asString());
}
public ProjectWithVCS createProject(String resStr) throws BacklogException {
return createObject(resStr, ProjectWithVCS.class, ProjectWithVCSJSONImpl.class);
}
@Override
public ResponseList<Activity> createActivityList(BacklogHttpResponse res) throws BacklogException {
return createActivityList(res.asString());
}
public ResponseList<Activity> createActivityList(String resStr) throws BacklogException {
return createObjectList(resStr, Activity.class, ActivityJSONImpl[].class);
}
@Override
public Activity createActivity(BacklogHttpResponse res) throws BacklogException {
return createActivity(res.asString());
}
public Activity createActivity(String resStr) throws BacklogException {
return createObject(resStr, Activity.class, ActivityJSONImpl.class);
}
@Override
public ResponseList<Issue> createIssueList(BacklogHttpResponse res) throws BacklogException {
return createIssueList(res.asString());
}
public ResponseList<Issue> createIssueList(String resStr) throws BacklogException {
return createObjectList(resStr, Issue.class, IssueJSONImpl[].class);
}
@Override
public ResponseList<ViewedIssue> createViewedIssueList(BacklogHttpResponse res) throws BacklogException {
return createViewedIssueList(res.asString());
}
public ResponseList<ViewedIssue> createViewedIssueList(String resStr) throws BacklogException {
return createObjectList(resStr, ViewedIssue.class, ViewedIssueJSONImpl[].class);
}
@Override
public ResponseList<ViewedProject> createViewedProjectList(BacklogHttpResponse res) throws BacklogException {
return createViewedProjectList(res.asString());
}
public ResponseList<ViewedProject> createViewedProjectList(String resStr) throws BacklogException {
return createObjectList(resStr, ViewedProject.class, ViewedProjectJSONImpl[].class);
}
@Override
public ResponseList<ViewedWiki> createViewedWikiList(BacklogHttpResponse res) throws BacklogException {
return createViewedWikiList(res.asString());
}
public ResponseList<ViewedWiki> createViewedWikiList(String resStr) throws BacklogException {
return createObjectList(resStr, ViewedWiki.class, ViewedWikiJSONImpl[].class);
}
@Override
public Issue createIssue(BacklogHttpResponse res) throws BacklogException {
return createIssue(res.asString());
}
public Issue createIssue(String resStr) throws BacklogException {
return createObject(resStr, Issue.class, IssueJSONImpl.class);
}
@Override
public Issue importIssue(BacklogHttpResponse res) throws BacklogException {
return importIssue(res.asString());
}
public Issue importIssue(String resStr) throws BacklogException {
return createObject(resStr, Issue.class, IssueJSONImpl.class);
}
@Override
public IssueComment createIssueComment(BacklogHttpResponse res) throws BacklogException {
return createIssueComment(res.asString());
}
public IssueComment createIssueComment(String resStr) throws BacklogException {
return createObject(resStr, IssueComment.class, IssueCommentJSONImpl.class);
}
@Override
public ResponseList<IssueComment> createIssueCommentList(BacklogHttpResponse res) throws BacklogException {
return createIssueCommentList(res.asString());
}
public ResponseList<IssueComment> createIssueCommentList(String resStr) throws BacklogException {
return createObjectList(resStr, IssueComment.class, IssueCommentJSONImpl[].class);
}
@Override
public User createUser(BacklogHttpResponse res) throws BacklogException {
return createUser(res.asString());
}
public User createUser(String resStr) throws BacklogException {
return createObject(resStr, User.class, UserJSONImpl.class);
}
@Override
public ResponseList<User> createUserList(BacklogHttpResponse res) throws BacklogException {
return createUserList(res.asString());
}
public ResponseList<User> createUserList(String resStr) throws BacklogException {
return createObjectList(resStr, User.class, UserJSONImpl[].class);
}
@Override
public IssueType createIssueType(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), IssueType.class, IssueTypeJSONImpl.class);
}
public IssueType createIssueType(String resStr) throws BacklogException {
return createObject(resStr, IssueType.class, IssueTypeJSONImpl.class);
}
@Override
public ResponseList<IssueType> createIssueTypeList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), IssueType.class, IssueTypeJSONImpl[].class);
}
public ResponseList<IssueType> createIssueTypeList(String resStr) throws BacklogException {
return createObjectList(resStr, IssueType.class, IssueTypeJSONImpl[].class);
}
@Override
public Category createCategory(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), Category.class, CategoryJSONImpl.class);
}
public Category createCategory(String resStr) throws BacklogException {
return createObject(resStr, Category.class, CategoryJSONImpl.class);
}
@Override
public ResponseList<Category> createCategoryList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Category.class, CategoryJSONImpl[].class);
}
public ResponseList<Category> createCategoryList(String resStr) throws BacklogException {
return createObjectList(resStr, Category.class, CategoryJSONImpl[].class);
}
@Override
public CustomFieldSetting createCustomField(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), CustomFieldSetting.class, CustomFieldSettingJSONImpl.class);
}
public CustomFieldSetting createCustomField(String resStr) throws BacklogException {
return createObject(resStr, CustomFieldSetting.class, CustomFieldSettingJSONImpl.class);
}
@Override
public ResponseList<CustomFieldSetting> createCustomFieldList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), CustomFieldSetting.class, CustomFieldSettingJSONImpl[].class);
}
public ResponseList<CustomFieldSetting> createCustomFieldList(String resStr) throws BacklogException {
return createObjectList(resStr, CustomFieldSetting.class, CustomFieldSettingJSONImpl[].class);
}
@Override
public ResponseList<Priority> createPriorityList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Priority.class, PriorityJSONImpl[].class);
}
public ResponseList<Priority> createPriorityList(String resStr) throws BacklogException {
return createObjectList(resStr, Priority.class, PriorityJSONImpl[].class);
}
@Override
public ResponseList<Resolution> createResolutionList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Resolution.class, ResolutionJSONImpl[].class);
}
public ResponseList<Resolution> createResolutionList(String resStr) throws BacklogException {
return createObjectList(resStr, Resolution.class, ResolutionJSONImpl[].class);
}
@Override
public ResponseList<Status> createStatusList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Status.class, StatusJSONImpl[].class);
}
@Override
public Status createStatus(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), Status.class, StatusJSONImpl.class);
}
public ResponseList<Status> createStatusList(String resStr) throws BacklogException {
return createObjectList(resStr, Status.class, StatusJSONImpl[].class);
}
@Override
public Star createStar(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), Star.class, StarJSONImpl.class);
}
public Star createStar(String resStr) throws BacklogException {
return createObject(resStr, Star.class, StarJSONImpl.class);
}
@Override
public ResponseList<Star> createStarList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Star.class, StarJSONImpl[].class);
}
public ResponseList<Star> createStarList(String resStr) throws BacklogException {
return createObjectList(resStr, Star.class, StarJSONImpl[].class);
}
@Override
public Count createCount(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), Count.class, CountJSONImpl.class);
}
public Count createCount(String resStr) throws BacklogException {
return createObject(resStr, Count.class, CountJSONImpl.class);
}
@Override
public Version createVersion(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), Version.class, VersionJSONImpl.class);
}
public Version createVersion(String resStr) throws BacklogException {
return createObject(resStr, Version.class, VersionJSONImpl.class);
}
@Override
public ResponseList<Version> createVersionList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Version.class, VersionJSONImpl[].class);
}
public ResponseList<Version> createVersionList(String resStr) throws BacklogException {
return createObjectList(resStr, Version.class, VersionJSONImpl[].class);
}
@Override
public Milestone createMilestone(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), Milestone.class, MilestoneJSONImpl.class);
}
public Milestone createMilestone(String resStr) throws BacklogException {
return createObject(resStr, Milestone.class, MilestoneJSONImpl.class);
}
@Override
public ResponseList<Milestone> createMilestoneList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Milestone.class, MilestoneJSONImpl[].class);
}
public ResponseList<Milestone> createMilestoneList(String resStr) throws BacklogException {
return createObjectList(resStr, Milestone.class, MilestoneJSONImpl[].class);
}
@Override
public Wiki createWiki(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), Wiki.class, WikiJSONImpl.class);
}
public Wiki createWiki(String resStr) throws BacklogException {
return createObject(resStr, Wiki.class, WikiJSONImpl.class);
}
@Override
public Wiki importWiki(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), Wiki.class, WikiJSONImpl.class);
}
public Wiki importWiki(String resStr) throws BacklogException {
return createObject(resStr, Wiki.class, WikiJSONImpl.class);
}
@Override
public ResponseList<Wiki> createWikiList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Wiki.class, WikiJSONImpl[].class);
}
public ResponseList<Wiki> createWikiList(String resStr) throws BacklogException {
return createObjectList(resStr, Wiki.class, WikiJSONImpl[].class);
}
@Override
public WikiTag createWikiTag(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), WikiTag.class, WikiTagJSONImpl.class);
}
public WikiTag createWikiTag(String resStr) throws BacklogException {
return createObject(resStr, WikiTag.class, WikiTagJSONImpl.class);
}
@Override
public ResponseList<WikiTag> createWikiTagList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), WikiTag.class, WikiTagJSONImpl[].class);
}
public ResponseList<WikiTag> createWikiTagList(String resStr) throws BacklogException {
return createObjectList(resStr, WikiTag.class, WikiTagJSONImpl[].class);
}
@Override
public WikiHistory createWikiHistory(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), WikiHistory.class, WikiHistoryJSONImpl.class);
}
public WikiHistory createWikiHistory(String resStr) throws BacklogException {
return createObject(resStr, WikiHistory.class, WikiHistoryJSONImpl.class);
}
@Override
public ResponseList<WikiHistory> createWikiHistoryList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), WikiHistory.class, WikiHistoryJSONImpl[].class);
}
public ResponseList<WikiHistory> createWikiHistoryList(String resStr) throws BacklogException {
return createObjectList(resStr, WikiHistory.class, WikiHistoryJSONImpl[].class);
}
@Override
public ResponseList<Notification> createNotificationList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Notification.class, NotificationJSONImpl[].class);
}
public ResponseList<Notification> createNotificationList(String resStr) throws BacklogException {
return createObjectList(resStr, Notification.class, NotificationJSONImpl[].class);
}
@Override
public Repository createRepository(BacklogHttpResponse res) throws BacklogException {
return createRepository(res.asString());
}
public Repository createRepository(String resStr) throws BacklogException {
return createObject(resStr, Repository.class, RepositoryJSONImpl.class);
}
@Override
public ResponseList<Repository> createRepositoryList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Repository.class, RepositoryJSONImpl[].class);
}
@Override
public PullRequest createPullRequest(BacklogHttpResponse res) throws BacklogException {
return createPullRequest(res.asString());
}
public PullRequest createPullRequest(String resStr) throws BacklogException {
return createObject(resStr, PullRequest.class, PullRequestJSONImpl.class);
}
public ResponseList<Repository> createRepositoryList(String resStr) throws BacklogException {
return createObjectList(resStr, Repository.class, RepositoryJSONImpl[].class);
}
@Override
public ResponseList<PullRequest> createPullRequestList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), PullRequest.class, PullRequestJSONImpl[].class);
}
public ResponseList<PullRequest> createPullRequestList(String resStr) throws BacklogException {
return createObjectList(resStr, PullRequest.class, PullRequestJSONImpl[].class);
}
@Override
public PullRequestComment createPullRequestComment(BacklogHttpResponse res) throws BacklogException {
return createPullRequestComment(res.asString());
}
public PullRequestComment createPullRequestComment(String resStr) throws BacklogException {
return createObject(resStr, PullRequestComment.class, PullRequestCommentJSONImpl.class);
}
@Override
public ResponseList<PullRequestComment> createPullRequestCommentList(BacklogHttpResponse res) throws BacklogException {
return createPullRequestCommentList(res.asString());
}
public ResponseList<PullRequestComment> createPullRequestCommentList(String resStr) throws BacklogException {
return createObjectList(resStr, PullRequestComment.class, PullRequestCommentJSONImpl[].class);
}
@Override
public SharedFile createSharedFile(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), SharedFile.class, SharedFileJSONImpl.class);
}
public SharedFile createSharedFile(String resStr) throws BacklogException {
return createObject(resStr, SharedFile.class, SharedFileJSONImpl.class);
}
@Override
public ResponseList<SharedFile> createSharedFileList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), SharedFile.class, SharedFileJSONImpl[].class);
}
public ResponseList<SharedFile> createSharedFileList(String resStr) throws BacklogException {
return createObjectList(resStr, SharedFile.class, SharedFileJSONImpl[].class);
}
@Override
public Attachment createAttachment(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), Attachment.class, AttachmentJSONImpl.class);
}
public Attachment createAttachment(String resStr) throws BacklogException {
return createObject(resStr, Attachment.class, AttachmentJSONImpl.class);
}
@Override
public Group createGroup(BacklogHttpResponse res) throws BacklogException {
return createGroup(res.asString());
}
public Group createGroup(String resStr) throws BacklogException {
return createObject(resStr, Group.class, GroupJSONImpl.class);
}
@Override
public ResponseList<Group> createGroupList(BacklogHttpResponse res) throws BacklogException {
return createGroupList(res.asString());
}
public ResponseList<Group> createGroupList(String resStr) throws BacklogException {
return createObjectList(resStr, Group.class, GroupJSONImpl[].class);
}
@Override
public ResponseList<Attachment> createAttachmentList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Attachment.class, AttachmentJSONImpl[].class);
}
public ResponseList<Attachment> createAttachmentList(String resStr) throws BacklogException {
return createObjectList(resStr, Attachment.class, AttachmentJSONImpl[].class);
}
@Override
public ResponseList<Webhook> createWebhookList(BacklogHttpResponse res) throws BacklogException {
return createWebhookList(res.asString());
}
public ResponseList<Webhook> createWebhookList(String resStr) throws BacklogException {
return createObjectList(resStr, Webhook.class, WebhookJSONImpl[].class);
}
@Override
public Webhook createWebhook(BacklogHttpResponse res) throws BacklogException {
return createObject(res.asString(), Webhook.class, WebhookJSONImpl.class);
}
public Webhook createWebhook(String resStr) throws BacklogException {
return createObject(resStr, Webhook.class, WebhookJSONImpl.class);
}
@Override
public Watch createWatch(BacklogHttpResponse res) throws BacklogException {
return createWatch(res.asString());
}
@Override
public ResponseList<Watch> createWatchList(BacklogHttpResponse res) throws BacklogException {
return createObjectList(res.asString(), Watch.class, WatchJSONImpl[].class);
}
public Watch createWatch(String resStr) throws BacklogException {
return createObject(resStr, Watch.class, WatchJSONImpl.class);
}
private <T1 , T2 extends T1> ResponseList<T1> createObjectList(String content, Class<T1> clazz1, Class<T2[]> clazz2) throws BacklogException {
T1[] arrays = Jackson.fromJsonString(content, clazz2);
ResponseList<T1> list = new ResponseListImpl<T1>();
for (int i = 0; i < arrays.length; i++) {
list.add(arrays[i]);
}
return list;
}
private <T1 , T2 extends T1> T1 createObject(String content, Class<T1> clazz1, Class<T2> clazz2) throws BacklogException {
T1 obj = Jackson.fromJsonString(content, clazz2);
return obj;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/ChangeJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.nulabinc.backlog4j.Change;
import com.nulabinc.backlog4j.User;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class ChangeJSONImpl implements Change {
private String field;
@JsonProperty("new_value")
private String newValue;
@JsonProperty("old_value")
private String oldValue;
private String type;
@Override
public String getField() {
return field;
}
@Override
public String getNewValue() {
return newValue;
}
@Override
public String getOldValue() {
return oldValue;
}
@Override
public String getType() {
return type;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
ChangeJSONImpl rhs = (ChangeJSONImpl) obj;
return new EqualsBuilder()
.append(this.field, rhs.field)
.append(this.newValue, rhs.newValue)
.append(this.oldValue, rhs.oldValue)
.append(this.type, rhs.type)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(field)
.append(newValue)
.append(oldValue)
.append(type)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("field", field)
.append("newValue", newValue)
.append("oldValue", oldValue)
.append("type", type)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/BacklogAPIError.java
package com.nulabinc.backlog4j;
import java.util.Arrays;
import java.util.List;
/**
* The error class for Backlog exception.
* Contains the error massages.
*
* @author nulab-inc
*/
public class BacklogAPIError {
private BacklogAPIErrorMessage[] errors;
public List<BacklogAPIErrorMessage> getErrors() {
return Arrays.asList(errors);
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/internal/json/CategoryJSONImplTest.java
package com.nulabinc.backlog4j.internal.json;
import com.nulabinc.backlog4j.Category;
import com.nulabinc.backlog4j.ResponseList;
import org.junit.jupiter.api.Test;
import java.io.IOException;
import static org.junit.jupiter.api.Assertions.assertEquals;
/**
* @author nulab-inc
*/
public class CategoryJSONImplTest extends AbstractJSONImplTest {
@Test
public void createCategoryListTest() throws IOException {
String fileContentStr = getJsonString("json/categories.json");
ResponseList<Category> versions = factory.createCategoryList(fileContentStr);
assertEquals(4, versions.size());
Category version = versions.get(0);
assertEquals(1073967643, version.getId());
assertEquals("検討", version.getName());
version = versions.get(1);
assertEquals(1073967645, version.getId());
assertEquals("障害", version.getName());
version = versions.get(2);
assertEquals(1073967646, version.getId());
assertEquals("懸念", version.getName());
version = versions.get(3);
assertEquals(1073967647, version.getId());
assertEquals("企画", version.getName());
}
@Test
public void createCategoryTest() throws IOException {
String fileContentStr = getJsonString("json/category.json");
Category category = factory.createCategory(fileContentStr);
assertEquals(1073967641, category.getId());
assertEquals("検討", category.getName());
}
@Test
public void equalsTest() throws IOException {
String fileContentStr = getJsonString("json/category.json");
Category category1 = factory.createCategory(fileContentStr);
Category category2 = factory.createCategory(fileContentStr);
assertEquals(category1, category2);
}
@Test
public void hashCodeTest() throws IOException {
String fileContentStr = getJsonString("json/category.json");
Category category1 = factory.createCategory(fileContentStr);
Category category2 = factory.createCategory(fileContentStr);
assertEquals(category1.hashCode(), category2.hashCode());
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/WebhookMethods.java
package com.nulabinc.backlog4j.api;
import com.nulabinc.backlog4j.BacklogException;
import com.nulabinc.backlog4j.ResponseList;
import com.nulabinc.backlog4j.Webhook;
import com.nulabinc.backlog4j.api.option.CreateWebhookParams;
import com.nulabinc.backlog4j.api.option.UpdateWebhookParams;
/**
* Executes Backlog Webhook APIs.
*
* @author nulab-inc
*/
public interface WebhookMethods {
/**
* Returns all the webhooks.
*
* @param projectIdOrKey the project key
* @return the webhooks in a list.
* @throws BacklogException
*/
ResponseList<Webhook> getWebhooks(Object projectIdOrKey) throws BacklogException;
/**
* Create a webhook.
*
* @param params the creating webhook parameters.
* @return the created webhook
* @throws com.nulabinc.backlog4j.BacklogException
*/
Webhook createWebhook(CreateWebhookParams params) throws BacklogException;
/**
* Returns the webhook.
*
* @param projectIdOrKey the project identifier
* @param webhookId the webhook identifier
* @return the Webhook
* @throws com.nulabinc.backlog4j.BacklogException
*/
Webhook getWebhook(Object projectIdOrKey, Object webhookId) throws BacklogException;
/**
* Updates the existing webhook.
*
* @param params the updating webhook parameters
* @return the updated Webhook
* @throws com.nulabinc.backlog4j.BacklogException
*/
Webhook updateWebhook(UpdateWebhookParams params) throws BacklogException;
/**
* Deletes the existing webhook.
*
* @param projectIdOrKey the project key
* @param webhookId the webhook identifier
* @return the deleted webhook
* @throws com.nulabinc.backlog4j.BacklogException
*/
Webhook deleteWebhook(Object projectIdOrKey, Object webhookId) throws BacklogException;
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/AddIssueCommentNotificationParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.BacklogAPIException;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for add category API.
*
* @author nulab-inc
*/
public class AddIssueCommentNotificationParams extends PostParams {
private Object issueIdOrKey;
private Object commentId;
/**
* Constructor
*
* @param issueIdOrKey the issue identifier
* @param commentId the comment identifier
* @param notifiedUserIds the user identifiers for notification
*/
public AddIssueCommentNotificationParams(Object issueIdOrKey, Object commentId, List notifiedUserIds) {
this.issueIdOrKey = issueIdOrKey;
this.commentId = commentId;
for (Object userId : notifiedUserIds) {
parameters.add(new NameValuePair("notifiedUserId[]", String.valueOf(userId)));
}
}
/**
* Returns the comment identifier.
*
* @return comment id
*/
public String getCommentId() {
return commentId.toString();
}
/**
* Returns the issue identifier string.
*
* @return issue id or project key
*/
public String getIssueIdOrKeyString() {
return issueIdOrKey.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Version.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog version data.
*
* @author nulab-inc
*/
public interface Version {
long getId();
String getIdAsString();
long getProjectId();
String getProjectIdAsString();
String getName();
String getDescription();
Date getStartDate();
Date getReleaseDueDate();
Boolean getArchived();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/activities/NotificationAddedContent.java
package com.nulabinc.backlog4j.internal.json.activities;
/**
* @author nulab-inc
*/
public class NotificationAddedContent extends IssueCommentedContent {
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/api/option/AbstractParamsTest.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* @author nulab-inc
*/
public abstract class AbstractParamsTest {
protected boolean existsOneKeyValue(List<NameValuePair> parameters,
String key, String value) {
int count = 0;
for (NameValuePair pair : parameters) {
if (pair.getName().equals(key)
&& pair.getValue().equals(value))
count++;
}
return count == 1;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/SharedFile.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog shared file.
*
* @author nulab-inc
*/
public interface SharedFile {
long getId();
String getIdAsString();
String getType();
String getName();
String getDir();
long getSize();
User getCreatedUser();
Date getCreated();
User getUpdatedUser();
Date getUpdated();
boolean isImage();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/customFields/DateCustomFieldSetting.java
package com.nulabinc.backlog4j.internal.json.customFields;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.nulabinc.backlog4j.CustomFieldListItemSetting;
import com.nulabinc.backlog4j.internal.json.JacksonCustomDateDeserializer;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.List;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class DateCustomFieldSetting extends CustomFieldSettingJSONImpl {
public enum InitialValueType {
Today(1), TodayPlusShiftDays(2), FixedDate(3);
InitialValueType(int intValue) {
this.intValue = intValue;
}
public int getIntValue() {
return intValue;
}
public static InitialValueType valueOf(final int anIntValue) {
for (InitialValueType d : values()) {
if (d.getIntValue() == anIntValue) {
return d;
}
}
return null;
}
private int intValue;
}
private int typeId = 4;
private long[] applicableIssueTypes;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private java.util.Date min;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private java.util.Date max;
private DateValueSetting initialDate;
@Override
public int getFieldTypeId() {
return typeId;
}
@Override
public FieldType getFieldType() {
return FieldType.valueOf(typeId);
}
@Override
public long[] getApplicableIssueTypes() {
return applicableIssueTypes;
}
@Override
public List<? extends CustomFieldListItemSetting> getItems() {
return null;
}
public java.util.Date getMin() {
return min;
}
public java.util.Date getMax() {
return max;
}
public DateValueSetting getInitialDate() {
return initialDate;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
DateCustomFieldSetting rhs = (DateCustomFieldSetting) obj;
return new EqualsBuilder()
.append(getId(), rhs.getId())
.append(getName(), rhs.getName())
.append(this.typeId, rhs.typeId)
.append(this.applicableIssueTypes, rhs.applicableIssueTypes)
.append(this.min, rhs.min)
.append(this.max, rhs.max)
.append(this.initialDate, rhs.initialDate)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(getId())
.append(getName())
.append(typeId)
.append(applicableIssueTypes)
.append(min)
.append(max)
.append(initialDate)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", getId())
.append("name", getName())
.append("typeId", typeId)
.append("applicableIssueTypes", applicableIssueTypes)
.append("min", min)
.append("max", max)
.append("initialDate", initialDate)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Status.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog status data.
*
* @author nulab-inc
*/
public interface Status {
int getId();
String getIdAsString();
String getName();
Issue.StatusType getStatusType();
Project.CustomStatusColor getColor();
long getProjectId();
String getProjectIdAsString();
int getDisplayOrder();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/UserJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.nulabinc.backlog4j.User;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.Date;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class UserJSONImpl implements User {
private long id;
private String name;
private String userId;
private int roleType;
private String lang;
private String mailAddress;
private Date lastLoginTime;
@Override
public long getId() {
return this.id;
}
@Override
public String getIdAsString() {
return String.valueOf(this.id);
}
@Override
public String getName() {
return this.name;
}
@Override
public RoleType getRoleType() {
return RoleType.valueOf(roleType);
}
@Override
public String getLang() {
return this.lang;
}
@Override
public String getMailAddress() {
return this.mailAddress;
}
@Override
public Date getLastLoginTime() {
return this.lastLoginTime;
}
@Override
public String getUserId() {
return userId;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
UserJSONImpl rhs = (UserJSONImpl) obj;
return new EqualsBuilder()
.append(this.id, rhs.id)
.append(this.name, rhs.name)
.append(this.userId, rhs.userId)
.append(this.roleType, rhs.roleType)
.append(this.lang, rhs.lang)
.append(this.mailAddress, rhs.mailAddress)
.append(this.lastLoginTime, rhs.lastLoginTime)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(id)
.append(name)
.append(userId)
.append(roleType)
.append(lang)
.append(mailAddress)
.append(lastLoginTime)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", id)
.append("name", name)
.append("userId", userId)
.append("roleType", roleType)
.append("lang", lang)
.append("mailAddress", mailAddress)
.append("lastLoginTime", lastLoginTime)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/BacklogAPIException.java
package com.nulabinc.backlog4j;
import com.nulabinc.backlog4j.http.BacklogHttpResponse;
import com.nulabinc.backlog4j.internal.json.Jackson;
/**
* Exception thrown when a api response contains error.
*
* @author nulab-inc
*/
public class BacklogAPIException extends BacklogException {
public enum ErrorType {
Undefined(-1),
InternalError(1), LicenceError(2), LicenceExpiredError(3), AccessDeniedError(4),
UnauthorizedOperationError(5), NoResourceError(6), InvalidRequestError(7),
SpaceOverCapacityError(8), ResourceOverflowError(9), TooLargeFileError(10),
AuthenticationError(11);
ErrorType(int intValue) {
this.intValue = intValue;
}
public int getIntValue() {
return intValue;
}
public static ErrorType valueOf(final int anIntValue) {
for (ErrorType d : values()) {
if (d.getIntValue() == anIntValue) {
return d;
}
}
return Undefined;
}
private int intValue;
}
protected BacklogHttpResponse response;
private int statusCode = -1;
private BacklogAPIError backlogAPIError;
public BacklogAPIException(String message, Throwable cause) {
super(message, cause);
decode(message);
}
public BacklogAPIException(String message, Exception cause, int statusCode) {
this(message, cause);
decode(message);
this.statusCode = statusCode;
}
public BacklogAPIException(String message, BacklogHttpResponse response) {
this(message);
decode(response.asString());
this.response = response;
this.statusCode = response.getStatusCode();
}
public BacklogAPIException(String s) {
super(s);
}
public BacklogAPIException(Throwable throwable) {
super(throwable);
}
@Override
public String getMessage() {
StringBuilder value = new StringBuilder();
value.append(super.getMessage());
if(statusCode > 0){
value.append("\n");
value.append("status code - ").append(statusCode);
}
if (backlogAPIError != null) {
for (BacklogAPIErrorMessage errorMessage: backlogAPIError.getErrors()) {
value.append("\n");
value.append("message - ").append(errorMessage.getMessage()).append("\n");
value.append("code - ").append(errorMessage.getCode()).append("\n");
String info = errorMessage.getErrorInfo();
if (info != null && info.length() > 0) {
value.append("errorInfo - ").append(info).append("\n");
}
String moreInfo = errorMessage.getMoreInfo();
if (moreInfo != null && moreInfo.length() > 0) {
value.append("moreInfo - ").append(moreInfo).append("\n");
}
}
}
return value.toString();
}
public BacklogAPIError getBacklogAPIError() {
return backlogAPIError;
}
@Override
public int getStatusCode() {
return this.statusCode;
}
protected void decode(String str) {
if (str != null && str.startsWith("{")) {
backlogAPIError = Jackson.fromJsonString(str, BacklogAPIError.class);
}
}
public ErrorType getErrorType(){
if (backlogAPIError != null) {
for (BacklogAPIErrorMessage errorMessage: backlogAPIError.getErrors()) {
return ErrorType.valueOf(errorMessage.getCode());
}
}
return null;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Icon.java
package com.nulabinc.backlog4j;
import java.io.InputStream;
/**
* The interface for Backlog icon file data.
*
* @author nulab-inc
*/
public interface Icon {
String getFilename();
InputStream getContent();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/package-info.java
/**
* Implementation classes for internal usage.
*/
package com.nulabinc.backlog4j.internal;<file_sep>/src/main/java/com/nulabinc/backlog4j/NotificationInfo.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog notificationInfo data.
*
* @author nulab-inc
*/
public interface NotificationInfo {
String getType();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/QueryParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for query.
*
* @author nulab-inc
*/
public class QueryParams extends GetParams{
public enum Order {
Asc("asc"),
Desc("desc");
Order(String value) {
this.value = value;
}
public String getStrValue() {
return value;
}
public static Order strValueOf(final String anValue) {
for (Order d : values()) {
if (d.getStrValue().equals(anValue)) {
return d;
}
}
return null;
}
private String value;
}
public QueryParams minId(Object minId) {
parameters.add(new NameValuePair("minId", minId.toString()));
return this;
}
public QueryParams maxId(Object maxId) {
parameters.add(new NameValuePair("maxId", maxId.toString()));
return this;
}
public QueryParams count(int count) {
parameters.add(new NameValuePair("count", String.valueOf(count)));
return this;
}
public QueryParams count(long count) {
parameters.add(new NameValuePair("count", String.valueOf(count)));
return this;
}
public QueryParams order(Order order) {
parameters.add(new NameValuePair("order", order.getStrValue()));
return this;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/AddTextCustomFieldParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.CustomFieldSetting;
/**
* Parameters for add text type custom field API.
*
* @author nulab-inc
*/
public class AddTextCustomFieldParams extends AddCustomFieldParams {
public AddTextCustomFieldParams(Object projectIdOrKey, String name) {
super(projectIdOrKey, CustomFieldSetting.FieldType.Text, name);
}
}
<file_sep>/README.md
# Backlog4j 
Backlog4j is a Backlog binding library for Java.
(英語の下に日本文が記載されています)
<img src="https://raw.githubusercontent.com/nulab/backlog4j/master/Backlog_logo.png" width='200'>
* Backlog
* [https://backlog.com/](https://backlog.com/)
* [https://backlog.com/ja/](https://backlog.com/ja/)
* Backlog API version 2
* [https://developer.nulab.com/docs/backlog/#](https://developer.nulab.com/docs/backlog/#)
* [https://developer.nulab.com/ja/docs/backlog/#](https://developer.nulab.com/ja/docs/backlog/#)
## Updates
* 2023/07/27 2.5.3 released
* 2022/09/15 2.5.2 released
* 2022/08/24 2.5.1 released
* 2022/04/27 2.5.0 released
* 2021/06/10 2.4.4 released
https://github.com/nulab/backlog4j/releases
## How to Install
### gradle
'com.nulab-inc:backlog4j:2.5.3'
### maven
<dependency>
<groupId>com.nulab-inc</groupId>
<artifactId>backlog4j</artifactId>
<version>2.5.3</version>
</dependency>
## How to use
Make BacklogConfigure with your space id and your api key.
# If your space is in backlog.com
BacklogConfigure configure = new BacklogComConfigure("yourSpaceId").apiKey("yourApiKey");
# If your space is in backlogtool.com
BacklogConfigure configure = new BacklogToolConfigure("yourSpaceId").apiKey("yourApiKey");
# If your space is in backlog.jp
BacklogConfigure configure = new BacklogJpConfigure("yourSpaceId").apiKey("yourApiKey");
And get the BacklogClient.
BacklogClient backlog = new BacklogClientFactory(configure).newClient();
Then call Backlog API method. Enjoy Backlog API!
Project project = backlog.getProject("PROJECT-KEY");
## Documents
* javadoc
* http://nulab.github.io/backlog4j/javadoc/
## License
MIT License
* http://www.opensource.org/licenses/mit-license.php
## Requires
* Java 1.8
# Backlog4j とは
Backlog4j は Backlog API (https://developer.nulab.com/ja/docs/backlog/#) に簡単にアクセスするためのJavaクライアントライブラリです。
## インストール
### gradle を利用する場合
'com.nulab-inc:backlog4j:2.5.3'
### maven を利用する場合
<dependency>
<groupId>com.nulab-inc</groupId>
<artifactId>backlog4j</artifactId>
<version>2.5.3</version>
</dependency>
## 使い方
基本的な使い方は以下の 2 ステップとなります。
* BacklogConfigure を設定して BacklogClient オブジェクトを生成
* Backlog API に従ったメソッド呼び出し
以下の例では スペースIDとAPIキーを用いて BacklogConfigure を設定しています。
# あなたのスペースが backlog.com 内にある場合
BacklogConfigure configure = new BacklogComConfigure("yourSpaceId").apiKey("yourApiKey");
# あなたのスペースが backlog.jp 内にある場合
BacklogConfigure configure = new BacklogJpConfigure("yourSpaceId").apiKey("yourApiKey");
# あなたのスペースが backlogtool.com 内にある場合
BacklogConfigure configure = new BacklogToolConfigure("yourSpaceId").apiKey("yourApiKey");
続いて BacklogClient を生成します。
BacklogClient backlog = new BacklogClientFactory(configure).newClient();
そして、取得したいプロジェクトのキーを指定し、プロジェクト情報をAPI経由で取得します。
Project project = backlog.getProject("PROJECT-KEY");
## Android でご使用の場合
proguard-rules に以下を追記して難読化の対象外とすることを推奨します。
#Backlog4j
-keep public class com.nulabinc.backlog4j.** {*;}
#Jackson(Backlog4j is using jackson)
-keepattributes *Annotation*,EnclosingMethod, Signature, InnerClasses
-keepnames class com.fasterxml.jackson.** { *; }
-dontwarn com.fasterxml.jackson.databind.**
<file_sep>/src/main/java/com/nulabinc/backlog4j/DiskUsageDetail.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog disk usage data.
*
* @author nulab-inc
*/
public interface DiskUsageDetail {
long getProjectId();
long getIssue();
long getWiki();
long getFile();
long getSubversion();
long getGit();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/PullRequestComment.java
package com.nulabinc.backlog4j;
import java.util.Date;
import java.util.List;
/**
* Created by yuhkim on 2015/05/18.
*/
public interface PullRequestComment {
long getId();
String getIdAsString();
String getContent();
List<ChangeLog> getChangeLog();
User getCreatedUser();
Date getCreated();
Date getUpdated();
List<Star> getStars();
List<Notification> getNotifications();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/AddSingleListCustomFieldParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.CustomFieldSetting;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for add single list type custom field API.
*
* @author nulab-inc
*/
public class AddSingleListCustomFieldParams extends AddCustomFieldParams {
public AddSingleListCustomFieldParams(Object projectIdOrKey, String name) {
super(projectIdOrKey, CustomFieldSetting.FieldType.SingleList, name);
}
public AddSingleListCustomFieldParams items(List<String> items) {
for (String item : items) {
parameters.add(new NameValuePair("items[]", item));
}
return this;
}
public AddSingleListCustomFieldParams allowInput(boolean allowInput) {
parameters.add(new NameValuePair("allowInput", String.valueOf(allowInput)));
return this;
}
public AddSingleListCustomFieldParams allowAddItem(boolean allowAddItem) {
parameters.add(new NameValuePair("allowAddItem", String.valueOf(allowAddItem)));
return this;
}
@Override
public AddSingleListCustomFieldParams applicableIssueTypes(List<Long> applicableIssueTypes) {
return (AddSingleListCustomFieldParams)super.applicableIssueTypes(applicableIssueTypes);
}
@Override
public AddSingleListCustomFieldParams description(String description) {
return (AddSingleListCustomFieldParams)super.description(description);
}
@Override
public AddSingleListCustomFieldParams required(boolean required) {
return (AddSingleListCustomFieldParams)super.required(required);
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/AddStatusParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.Project;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for add status API.
*
* @author nulab-inc
*/
public class AddStatusParams extends PostParams {
private Object projectIdOrKey;
public AddStatusParams(Object projectIdOrKey, String name, Project.CustomStatusColor color) {
this.projectIdOrKey = projectIdOrKey;
parameters.add(new NameValuePair("name", name));
parameters.add(new NameValuePair("color", color.getStrValue()));
}
public String getProjectIdOrKeyString() {
return projectIdOrKey.toString();
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/internal/json/IssueCommentJSONImplTest.java
package com.nulabinc.backlog4j.internal.json;
import com.nulabinc.backlog4j.AttachmentInfo;
import com.nulabinc.backlog4j.ChangeLog;
import com.nulabinc.backlog4j.IssueComment;
import com.nulabinc.backlog4j.ResponseList;
import org.junit.jupiter.api.Test;
import java.io.IOException;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertEquals;
/**
* @author nulab-inc
*/
public class IssueCommentJSONImplTest extends AbstractJSONImplTest {
@Test
public void createIssueCommentListTest() throws IOException {
String fileContentStr = getJsonString("json/issue_comments.json");
ResponseList<IssueComment> comments = factory.createIssueCommentList(fileContentStr);
assertEquals(2, comments.size());
IssueComment comment = comments.get(0);
assertEquals(1102837675, comment.getId());
List<ChangeLog> logs = comment.getChangeLog();
ChangeLog log = logs.get(0);
assertEquals("*銀行振込:30件", log.getNewValue());
AttachmentInfo attachmentInfo = log.getAttachmentInfo();
assertEquals(1123344555, attachmentInfo.getId());
comment = comments.get(1);
assertEquals(1102836944, comment.getId());
}
@Test
public void createIssueCommentTest() throws IOException {
String fileContentStr = getJsonString("json/issue_comment.json");
IssueComment issueType = factory.createIssueComment(fileContentStr);
assertEquals(1102837675, issueType.getId());
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/customFields/ListItemSetting.java
package com.nulabinc.backlog4j.internal.json.customFields;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.nulabinc.backlog4j.CustomFieldListItemSetting;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class ListItemSetting implements CustomFieldListItemSetting {
private long id;
private String name;
private Boolean allowInput;
private int displayOrder;
public long getId() {
return id;
}
public String getName() {
return name;
}
public Boolean getAllowInput() {
return allowInput;
}
public int getDisplayOrder() {
return displayOrder;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
ListItemSetting rhs = (ListItemSetting) obj;
return new EqualsBuilder()
.append(this.id, rhs.id)
.append(this.name, rhs.name)
.append(this.allowInput, rhs.allowInput)
.append(this.displayOrder, rhs.displayOrder)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(id)
.append(name)
.append(allowInput)
.append(displayOrder)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", id)
.append("name", name)
.append("allowInput", allowInput)
.append("displayOrder", displayOrder)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/customFields/CustomFieldSettingJSONImpl.java
package com.nulabinc.backlog4j.internal.json.customFields;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.nulabinc.backlog4j.CustomField;
import com.nulabinc.backlog4j.CustomFieldSetting;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonTypeInfo(
use = JsonTypeInfo.Id.NAME,
include = JsonTypeInfo.As.PROPERTY,
property = "typeId")
@JsonSubTypes({
@JsonSubTypes.Type(value = TextCustomFieldSetting.class, name = "1"),
@JsonSubTypes.Type(value = TextAreaCustomFieldSetting.class, name = "2"),
@JsonSubTypes.Type(value = NumericCustomFieldSetting.class, name = "3"),
@JsonSubTypes.Type(value = DateCustomFieldSetting.class, name = "4"),
@JsonSubTypes.Type(value = SingleListCustomFieldSetting.class, name = "5"),
@JsonSubTypes.Type(value = MultipleListCustomFieldSetting.class, name = "6"),
@JsonSubTypes.Type(value = CheckBoxCustomFieldSetting.class, name = "7"),
@JsonSubTypes.Type(value = RadioCustomFieldSetting.class, name = "8") })
public abstract class CustomFieldSettingJSONImpl implements CustomFieldSetting {
private long id;
private int typeId;
private String name;
private long[] applicableIssueTypes;
private String description;
private Boolean required;
@Override
public long getId() {
return id;
}
@Override
public String getIdAsString() {
return String.valueOf(this.id);
}
@Override
public String getName() {
return name;
}
@Override
public long[] getApplicableIssueTypes() {
return applicableIssueTypes;
}
@Override
public String getDescription() {
return description;
}
@Override
public boolean isRequired() {
return required;
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", id)
.append("typeId", typeId)
.append("name", name)
.append("applicableIssueTypes", applicableIssueTypes)
.append("description", description)
.append("required", required)
.toString();
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
CustomFieldSettingJSONImpl rhs = (CustomFieldSettingJSONImpl) obj;
return new EqualsBuilder()
.append(this.id, rhs.id)
.append(this.typeId, rhs.typeId)
.append(this.name, rhs.name)
.append(this.applicableIssueTypes, rhs.applicableIssueTypes)
.append(this.description, rhs.description)
.append(this.required, rhs.required)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(id)
.append(typeId)
.append(name)
.append(applicableIssueTypes)
.append(description)
.append(required)
.toHashCode();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/ViewedWiki.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog viewed wiki data.
*
* @author nulab-inc
*/
public interface ViewedWiki {
Wiki getPage();
Date getUpdated();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdateWikiParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for update wiki page API.
*
* @author nulab-inc
*/
public class UpdateWikiParams extends PatchParams {
private Object wikiId;
public UpdateWikiParams(Object wikiId) {
this.wikiId = wikiId;
}
public String getWikiId() {
return wikiId.toString();
}
public UpdateWikiParams name(String name) {
parameters.add(new NameValuePair("name", name));
return this;
}
public UpdateWikiParams content(String content) {
parameters.add(new NameValuePair("content", content));
return this;
}
public UpdateWikiParams mailNotify(boolean mailNotify) {
parameters.add(new NameValuePair("mailNotify", String.valueOf(mailNotify)));
return this;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/AddIssueCommentParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.BacklogAPIException;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for add issue comment API.
*
* @author nulab-inc
*/
public class AddIssueCommentParams extends PostParams {
private Object issueIdOrKey;
/**
* Constructor
*
* @param issueIdOrKey the issue identifier
* @param content the comment content
*/
public AddIssueCommentParams(Object issueIdOrKey, String content) {
this.issueIdOrKey = issueIdOrKey;
parameters.add(new NameValuePair("content", content));
}
/**
* Returns the issue identifier string.
*
* @return issue id or issue key
*/
public String getIssueIdOrKeyString() {
return issueIdOrKey.toString();
}
/**
* Sets the notified users.
*
* @param notifiedUserIds the notified user identifiers
* @return AddIssueCommentParams instance
*/
public AddIssueCommentParams notifiedUserIds(List notifiedUserIds) {
for (Object notifiedUserId : notifiedUserIds) {
parameters.add(new NameValuePair("notifiedUserId[]", notifiedUserId.toString()));
}
return this;
}
/**
* Sets the attachment files.
*
* @param attachmentIds the notified file identifiers
* @return AddIssueCommentParams instance
*/
public AddIssueCommentParams attachmentIds(List attachmentIds) {
for (Object attachmentId : attachmentIds) {
parameters.add(new NameValuePair("attachmentId[]", attachmentId.toString()));
}
return this;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/GroupProjectActivity.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog group project activity data.
*
* @author nulab-inc
*/
public interface GroupProjectActivity {
long getId();
String getIdAsString();
Activity.Type getType();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/http/BacklogHttpResponse.java
package com.nulabinc.backlog4j.http;
import java.io.InputStream;
import java.util.Date;
/**
* @author nulab-inc
*/
public interface BacklogHttpResponse {
int getStatusCode();
int getRateLimitLimit();
int getRateLimitRemaining();
Date getRateLimitResetDate();
String getRateLimitReset();
InputStream asInputStream();
String asString();
String getFileNameFromContentDisposition();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/AttachmentInfo.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog attachment file.
*
* @author nulab-inc
*/
public interface AttachmentInfo {
long getId();
String getIdAsString();
String getName();
boolean isImage();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/http/MimeHelper.java
package com.nulabinc.backlog4j.internal.http;
import java.io.ByteArrayOutputStream;
import java.nio.charset.Charset;
import java.nio.charset.UnsupportedCharsetException;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
public final class MimeHelper {
private static final String DISPOSITION_FILENAME = "filename";
private static final String MIME_SPECIALS = "()<>@,;:\\\"/[]?=" + "\t ";
private static final String WHITE = " \t\n\r";
private static final char[] HEX_DIGITS = "0123456789ABCDEF".toCharArray();
private static final byte[] HEX_DECODE = new byte[0x80];
static {
for (int i = 0; i < HEX_DIGITS.length; i++) {
HEX_DECODE[HEX_DIGITS[i]] = (byte) i;
HEX_DECODE[Character.toLowerCase(HEX_DIGITS[i])] = (byte) i;
}
}
private MimeHelper() {
}
/**
* Decodes a filename from the Content-Disposition header value according to
* RFC 2183 and RFC 2231.
* <p/>
* See <a href="http://tools.ietf.org/html/rfc2231">RFC 2231</a> for
* details.
*
* @param value the header value to decode
* @return the filename
*/
public static String decodeContentDispositionFilename(String value) {
Map<String, String> params = new HashMap<String, String>();
decodeContentDisposition(value, params);
return params.get(DISPOSITION_FILENAME);
}
/**
* Decodes the Content-Disposition header value according to RFC 2183 and
* RFC 2231.
* <p/>
* Does not deal with continuation lines.
* <p/>
* See <a href="http://tools.ietf.org/html/rfc2231">RFC 2231</a> for
* details.
*
* @param value the header value to decode
* @param params the map of parameters to fill
* @return the disposition
*/
public static String decodeContentDisposition(String value, Map<String, String> params) {
try {
HeaderTokenizer tokenizer = new HeaderTokenizer(value);
// get the first token, which must be an ATOM
Token token = tokenizer.next();
if (token.getType() != Token.ATOM) {
return null;
}
String disposition = token.getValue();
// value ignored in this method
// the remainder is the parameters
String remainder = tokenizer.getRemainder();
if (remainder != null) {
getParameters(remainder, params);
}
return disposition;
} catch (ParseException e) {
return null;
}
}
protected static class ParseException extends Exception {
private static final long serialVersionUID = 1L;
public ParseException() {
super();
}
public ParseException(String message) {
super(message);
}
}
/*
* From geronimo-javamail_1.4_spec-1.7.1. Token
*/
protected static class Token {
// Constant values from J2SE 1.4 API Docs (Constant values)
public static final int ATOM = -1;
public static final int COMMENT = -3;
public static final int EOF = -4;
public static final int QUOTEDSTRING = -2;
private final int type;
private final String value;
public Token(int type, String value) {
this.type = type;
this.value = value;
}
public int getType() {
return type;
}
public String getValue() {
return value;
}
}
/*
* Tweaked from geronimo-javamail_1.4_spec-1.7.1. HeaderTokenizer
*/
protected static class HeaderTokenizer {
private static final Token EOF = new Token(Token.EOF, null);
private final String header;
private final String delimiters;
private final boolean skipComments;
private int pos;
public HeaderTokenizer(String header) {
this(header, MIME_SPECIALS, true);
}
protected HeaderTokenizer(String header, String delimiters, boolean skipComments) {
this.header = header;
this.delimiters = delimiters;
this.skipComments = skipComments;
}
public String getRemainder() {
return header.substring(pos);
}
public Token next() throws ParseException {
return readToken();
}
/**
* Read an ATOM token from the parsed header.
*
* @return A token containing the value of the atom token.
*/
private Token readAtomicToken() {
// skip to next delimiter
int start = pos;
while (++pos < header.length()) {
// break on the first non-atom character.
char ch = header.charAt(pos);
if (delimiters.indexOf(header.charAt(pos)) != -1 || ch < 32 || ch >= 127) {
break;
}
}
return new Token(Token.ATOM, header.substring(start, pos));
}
/**
* Read the next token from the header.
*
* @return The next token from the header. White space is skipped, and
* comment tokens are also skipped if indicated.
*/
private Token readToken() throws ParseException {
if (pos >= header.length()) {
return EOF;
} else {
char c = header.charAt(pos);
// comment token...read and skip over this
if (c == '(') {
Token comment = readComment();
if (skipComments) {
return readToken();
} else {
return comment;
}
// quoted literal
} else if (c == '\"') {
return readQuotedString();
// white space, eat this and find a real token.
} else if (WHITE.indexOf(c) != -1) {
eatWhiteSpace();
return readToken();
// either a CTL or special. These characters have a
// self-defining token type.
} else if (c < 32 || c >= 127 || delimiters.indexOf(c) != -1) {
pos++;
return new Token((int) c, String.valueOf(c));
} else {
// start of an atom, parse it off.
return readAtomicToken();
}
}
}
/**
* Extract a substring from the header string and apply any
* escaping/folding rules to the string.
*
* @param start The starting offset in the header.
* @param end The header end offset + 1.
* @return The processed string value.
*/
private String getEscapedValue(int start, int end) throws ParseException {
StringBuilder value = new StringBuilder();
for (int i = start; i < end; i++) {
char ch = header.charAt(i);
// is this an escape character?
if (ch == '\\') {
i++;
if (i == end) {
throw new ParseException("Invalid escape character");
}
value.append(header.charAt(i));
} else if (ch == '\r') {
// line breaks are ignored, except for naked '\n'
// characters, which are consider parts of linear
// whitespace.
// see if this is a CRLF sequence, and skip the second if it
// is.
if (i < end - 1 && header.charAt(i + 1) == '\n') {
i++;
}
} else {
// just append the ch value.
value.append(ch);
}
}
return value.toString();
}
/**
* Read a comment from the header, applying nesting and escape rules to
* the content.
*
* @return A comment token with the token value.
*/
private Token readComment() throws ParseException {
int start = pos + 1;
int nesting = 1;
boolean requiresEscaping = false;
// skip to end of comment/string
while (++pos < header.length()) {
char ch = header.charAt(pos);
if (ch == ')') {
nesting--;
if (nesting == 0) {
break;
}
} else if (ch == '(') {
nesting++;
} else if (ch == '\\') {
pos++;
requiresEscaping = true;
} else if (ch == '\r') {
// we need to process line breaks also
requiresEscaping = true;
}
}
if (nesting != 0) {
throw new ParseException("Unbalanced comments");
}
String value;
if (requiresEscaping) {
value = getEscapedValue(start, pos);
} else {
value = header.substring(start, pos++);
}
return new Token(Token.COMMENT, value);
}
/**
* Parse out a quoted string from the header, applying escaping rules to
* the value.
*
* @return The QUOTEDSTRING token with the value.
* @throws ParseException
*/
private Token readQuotedString() throws ParseException {
int start = pos + 1;
boolean requiresEscaping = false;
// skip to end of comment/string
while (++pos < header.length()) {
char ch = header.charAt(pos);
if (ch == '"') {
String value;
if (requiresEscaping) {
value = getEscapedValue(start, pos++);
} else {
value = header.substring(start, pos++);
}
return new Token(Token.QUOTEDSTRING, value);
} else if (ch == '\\') {
pos++;
requiresEscaping = true;
} else if (ch == '\r') {
// we need to process line breaks also
requiresEscaping = true;
}
}
throw new ParseException("Missing '\"'");
}
/**
* Skip white space in the token string.
*/
private void eatWhiteSpace() {
// skip to end of whitespace
while (++pos < header.length() && WHITE.indexOf(header.charAt(pos)) != -1) {
// just read
}
}
}
/*
* Tweaked from geronimo-javamail_1.4_spec-1.7.1. ParameterList
*/
protected static Map<String, String> getParameters(String list, Map<String, String> params) throws ParseException {
HeaderTokenizer tokenizer = new HeaderTokenizer(list);
while (true) {
Token token = tokenizer.next();
switch (token.getType()) {
case Token.EOF:
// the EOF token terminates parsing.
return params;
case ';':
// each new parameter is separated by a semicolon, including
// the first, which separates
// the parameters from the main part of the header.
// the next token needs to be a parameter name
token = tokenizer.next();
// allow a trailing semicolon on the parameters.
if (token.getType() == Token.EOF) {
return params;
}
if (token.getType() != Token.ATOM) {
throw new ParseException("Invalid parameter name: " + token.getValue());
}
// get the parameter name as a lower case version for better
// mapping.
String name = token.getValue().toLowerCase(Locale.ENGLISH);
token = tokenizer.next();
// parameters are name=value, so we must have the "=" here.
if (token.getType() != '=') {
throw new ParseException("Missing '='");
}
// now the value, which may be an atom or a literal
token = tokenizer.next();
if (token.getType() != Token.ATOM && token.getType() != Token.QUOTEDSTRING) {
throw new ParseException("Invalid parameter value: " + token.getValue());
}
String value = token.getValue();
// we might have to do some additional decoding. A name that
// ends with "*" is marked as being encoded, so if requested, we
// decode the value.
if (name.endsWith("*")) {
name = name.substring(0, name.length() - 1);
value = decodeRFC2231value(value);
}
params.put(name, value);
break;
default:
throw new ParseException("Missing ';'");
}
}
}
protected static String decodeRFC2231value(String value) {
int q1 = value.indexOf('\'');
if (q1 == -1) {
// missing charset
return value;
}
String mimeCharset = value.substring(0, q1);
int q2 = value.indexOf('\'', q1 + 1);
if (q2 == -1) {
// missing language
return value;
}
byte[] bytes = fromHex(value.substring(q2 + 1));
try {
return new String(bytes, Charset.forName(mimeCharset));
} catch (UnsupportedCharsetException e) {
// incorrect encoding
return value;
}
}
protected static byte[] fromHex(String data) {
ByteArrayOutputStream out = new ByteArrayOutputStream(data.length());
for (int i = 0; i < data.length(); ) {
char c = data.charAt(i++);
if (c == '%') {
if (i > data.length() - 2) {
break; // unterminated sequence
}
byte b1 = HEX_DECODE[data.charAt(i++) & 0x7f];
byte b2 = HEX_DECODE[data.charAt(i++) & 0x7f];
out.write((b1 << 4) | b2);
} else {
out.write((byte) c);
}
}
return out.toByteArray();
}
}<file_sep>/src/main/java/com/nulabinc/backlog4j/IssueType.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog issue type data.
*
* @author nulab-inc
*/
public interface IssueType {
long getId();
String getIdAsString();
long getProjectId();
String getProjectIdAsString();
String getName();
Project.IssueTypeColor getColor();
String getTemplateSummary();
String getTemplateDescription();
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/api/option/AddCustomFieldParamsTest.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
import com.nulabinc.backlog4j.internal.json.customFields.DateCustomFieldSetting;
import org.junit.jupiter.api.Test;
import java.util.Arrays;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertEquals;
/**
* @author nulab-inc
*/
public class AddCustomFieldParamsTest {
@Test
public void AddTextCustomFieldParamsTest() {
// when
AddTextCustomFieldParams params = new AddTextCustomFieldParams(
"PRJ", "TextCustomField");
params.description("TextCustomFieldです").required(true);
// then
List<NameValuePair> parameters = params.getParamList();
assertEquals(4, parameters.size());
NameValuePair pair = parameters.get(0);
assertEquals("typeId", pair.getName());
assertEquals("1", pair.getValue());
pair = parameters.get(1);
assertEquals("name", pair.getName());
assertEquals("TextCustomField", pair.getValue());
pair = parameters.get(2);
assertEquals("description", pair.getName());
assertEquals("TextCustomFieldです", pair.getValue());
pair = parameters.get(3);
assertEquals("required", pair.getName());
assertEquals("true", pair.getValue());
}
@Test
public void AddTextAreaCustomFieldParamsTest() {
// when
AddTextAreaCustomFieldParams params = new AddTextAreaCustomFieldParams(
"PRJ", "TextAreaCustomField");
params.description("TextCustomFieldです").required(true);
// then
List<NameValuePair> parameters = params.getParamList();
assertEquals(4, parameters.size());
NameValuePair pair = parameters.get(0);
assertEquals("typeId", pair.getName());
assertEquals("2", pair.getValue());
pair = parameters.get(1);
assertEquals("name", pair.getName());
assertEquals("TextAreaCustomField", pair.getValue());
pair = parameters.get(2);
assertEquals("description", pair.getName());
assertEquals("TextCustomFieldです", pair.getValue());
pair = parameters.get(3);
assertEquals("required", pair.getName());
assertEquals("true", pair.getValue());
}
@Test
public void AddNumericCustomFieldParamsTest() {
// when
AddNumericCustomFieldParams params = new AddNumericCustomFieldParams(
"PRJ", "NumericCustomField");
params.description("TextCustomFieldです").required(true).min(10).max(121212L).initialValue(21);
// then
List<NameValuePair> parameters = params.getParamList();
assertEquals(7, parameters.size());
NameValuePair pair = parameters.get(0);
assertEquals("typeId", pair.getName());
assertEquals("3", pair.getValue());
pair = parameters.get(1);
assertEquals("name", pair.getName());
assertEquals("NumericCustomField", pair.getValue());
pair = parameters.get(2);
assertEquals("description", pair.getName());
assertEquals("TextCustomFieldです", pair.getValue());
pair = parameters.get(3);
assertEquals("required", pair.getName());
assertEquals("true", pair.getValue());
pair = parameters.get(4);
assertEquals("min", pair.getName());
assertEquals("10.0", pair.getValue());
pair = parameters.get(5);
assertEquals("max", pair.getName());
assertEquals("121212.0", pair.getValue());
pair = parameters.get(6);
assertEquals("initialValue", pair.getName());
assertEquals("21.0", pair.getValue());
}
@Test
public void AddDateCustomFieldParamsTest() {
// when
AddDateCustomFieldParams params = new AddDateCustomFieldParams(
"PRJ", "DateCustomField");
params.description("DateCustomFieldです").required(true)
.initialValueType(DateCustomFieldSetting.InitialValueType.TodayPlusShiftDays)
.initialShift(2);
// then
List<NameValuePair> parameters = params.getParamList();
assertEquals(6, parameters.size());
NameValuePair pair = parameters.get(0);
assertEquals("typeId", pair.getName());
assertEquals("4", pair.getValue());
pair = parameters.get(1);
assertEquals("name", pair.getName());
assertEquals("DateCustomField", pair.getValue());
pair = parameters.get(2);
assertEquals("description", pair.getName());
assertEquals("DateCustomFieldです", pair.getValue());
pair = parameters.get(3);
assertEquals("required", pair.getName());
assertEquals("true", pair.getValue());
pair = parameters.get(4);
assertEquals("initialValueType", pair.getName());
assertEquals("2", pair.getValue());
pair = parameters.get(5);
assertEquals("initialShift", pair.getName());
assertEquals("2", pair.getValue());
}
@Test
public void AddSingleListCustomFieldParamsTest() {
// when
AddSingleListCustomFieldParams params = new AddSingleListCustomFieldParams(
"PRJ", "SingleListCustomField");
params.description("SingleListCustomFieldです").required(true)
.allowInput(false)
.allowAddItem(true)
.items(Arrays.asList("卵", "牛乳", "みかん"));
// then
List<NameValuePair> parameters = params.getParamList();
assertEquals(9, parameters.size());
NameValuePair pair = parameters.get(0);
assertEquals("typeId", pair.getName());
assertEquals("5", pair.getValue());
pair = parameters.get(1);
assertEquals("name", pair.getName());
assertEquals("SingleListCustomField", pair.getValue());
pair = parameters.get(2);
assertEquals("description", pair.getName());
assertEquals("SingleListCustomFieldです", pair.getValue());
pair = parameters.get(3);
assertEquals("required", pair.getName());
assertEquals("true", pair.getValue());
pair = parameters.get(4);
assertEquals("allowInput", pair.getName());
assertEquals("false", pair.getValue());
pair = parameters.get(5);
assertEquals("allowAddItem", pair.getName());
assertEquals("true", pair.getValue());
pair = parameters.get(6);
assertEquals("items[]", pair.getName());
assertEquals("卵", pair.getValue());
pair = parameters.get(7);
assertEquals("items[]", pair.getName());
assertEquals("牛乳", pair.getValue());
pair = parameters.get(8);
assertEquals("items[]", pair.getName());
assertEquals("みかん", pair.getValue());
}
@Test
public void AddMultipleListCustomFieldParamsTest() {
// when
AddMultipleListCustomFieldParams params = new AddMultipleListCustomFieldParams(
"PRJ", "MultipleListCustomField");
params.description("MultipleListCustomFieldです").required(true)
.allowInput(false)
.allowAddItem(true)
.items(Arrays.asList("卵", "牛乳", "みかん"));
// then
List<NameValuePair> parameters = params.getParamList();
assertEquals(9, parameters.size());
NameValuePair pair = parameters.get(0);
assertEquals("typeId", pair.getName());
assertEquals("6", pair.getValue());
pair = parameters.get(1);
assertEquals("name", pair.getName());
assertEquals("MultipleListCustomField", pair.getValue());
pair = parameters.get(2);
assertEquals("description", pair.getName());
assertEquals("MultipleListCustomFieldです", pair.getValue());
pair = parameters.get(3);
assertEquals("required", pair.getName());
assertEquals("true", pair.getValue());
pair = parameters.get(4);
assertEquals("allowInput", pair.getName());
assertEquals("false", pair.getValue());
pair = parameters.get(5);
assertEquals("allowAddItem", pair.getName());
assertEquals("true", pair.getValue());
pair = parameters.get(6);
assertEquals("items[]", pair.getName());
assertEquals("卵", pair.getValue());
pair = parameters.get(7);
assertEquals("items[]", pair.getName());
assertEquals("牛乳", pair.getValue());
pair = parameters.get(8);
assertEquals("items[]", pair.getName());
assertEquals("みかん", pair.getValue());
}
@Test
public void AddCheckBoxCustomFieldParamsTest() {
// when
AddCheckBoxCustomFieldParams params = new AddCheckBoxCustomFieldParams(
"PRJ", "CheckBoxCustomField");
params.description("CheckBoxCustomFieldです").required(true)
.allowInput(false)
.allowAddItem(true)
.items(Arrays.asList("卵", "牛乳", "みかん"));
// then
List<NameValuePair> parameters = params.getParamList();
assertEquals(9, parameters.size());
NameValuePair pair = parameters.get(0);
assertEquals("typeId", pair.getName());
assertEquals("7", pair.getValue());
pair = parameters.get(1);
assertEquals("name", pair.getName());
assertEquals("CheckBoxCustomField", pair.getValue());
pair = parameters.get(2);
assertEquals("description", pair.getName());
assertEquals("CheckBoxCustomFieldです", pair.getValue());
pair = parameters.get(3);
assertEquals("required", pair.getName());
assertEquals("true", pair.getValue());
pair = parameters.get(4);
assertEquals("allowInput", pair.getName());
assertEquals("false", pair.getValue());
pair = parameters.get(5);
assertEquals("allowAddItem", pair.getName());
assertEquals("true", pair.getValue());
pair = parameters.get(6);
assertEquals("items[]", pair.getName());
assertEquals("卵", pair.getValue());
pair = parameters.get(7);
assertEquals("items[]", pair.getName());
assertEquals("牛乳", pair.getValue());
pair = parameters.get(8);
assertEquals("items[]", pair.getName());
assertEquals("みかん", pair.getValue());
}
@Test
public void AddRadioCustomFieldParamsTest() {
// when
AddRadioCustomFieldParams params = new AddRadioCustomFieldParams(
"PRJ", "RadioCustomField");
params.description("RadioCustomFieldです").required(true)
.allowInput(false)
.allowAddItem(true)
.items(Arrays.asList("卵", "牛乳", "みかん"));
// then
List<NameValuePair> parameters = params.getParamList();
assertEquals(9, parameters.size());
NameValuePair pair = parameters.get(0);
assertEquals("typeId", pair.getName());
assertEquals("8", pair.getValue());
pair = parameters.get(1);
assertEquals("name", pair.getName());
assertEquals("RadioCustomField", pair.getValue());
pair = parameters.get(2);
assertEquals("description", pair.getName());
assertEquals("RadioCustomFieldです", pair.getValue());
pair = parameters.get(3);
assertEquals("required", pair.getName());
assertEquals("true", pair.getValue());
pair = parameters.get(4);
assertEquals("allowInput", pair.getName());
assertEquals("false", pair.getValue());
pair = parameters.get(5);
assertEquals("allowAddItem", pair.getName());
assertEquals("true", pair.getValue());
pair = parameters.get(6);
assertEquals("items[]", pair.getName());
assertEquals("卵", pair.getValue());
pair = parameters.get(7);
assertEquals("items[]", pair.getName());
assertEquals("牛乳", pair.getValue());
pair = parameters.get(8);
assertEquals("items[]", pair.getName());
assertEquals("みかん", pair.getValue());
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/api/option/UpdateGroupParamsTest.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
import org.junit.jupiter.api.Test;
import java.util.Arrays;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertTrue;
/**
* @author nulab-inc
*/
public class UpdateGroupParamsTest extends AbstractParamsTest {
@Test
public void createParamTest() {
// when
UpdateGroupParams params = new UpdateGroupParams(1000000001L);
params.name("group 1")
.members(Arrays.asList(2000000001L, 2000000002L));
// then
assertEquals("1000000001", params.getGroupId());
List<NameValuePair> parameters = params.getParamList();
assertEquals(3, parameters.size());
assertTrue(existsOneKeyValue(parameters, "name", "group 1"));
assertTrue(existsOneKeyValue(parameters, "members[]", "2000000001"));
assertTrue(existsOneKeyValue(parameters, "members[]", "2000000002"));
}
@Test
public void createParamWithStringIdTest() {
// when
UpdateGroupParams params = new UpdateGroupParams("1000000001");
params.name("group 2")
.members(Arrays.asList("2000000001", "2000000002"));
// then
assertEquals("1000000001", params.getGroupId());
List<NameValuePair> parameters = params.getParamList();
assertEquals(3, parameters.size());
assertTrue(existsOneKeyValue(parameters, "name", "group 2"));
assertTrue(existsOneKeyValue(parameters, "members[]", "2000000001"));
assertTrue(existsOneKeyValue(parameters, "members[]", "2000000002"));
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/AddDateCustomFieldParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.CustomFieldSetting;
import com.nulabinc.backlog4j.internal.json.customFields.DateCustomFieldSetting;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for add date type custom field API.
*
* @author nulab-inc
*/
public class AddDateCustomFieldParams extends AddCustomFieldParams {
public AddDateCustomFieldParams(Object projectIdOrKey, String name) {
super(projectIdOrKey, CustomFieldSetting.FieldType.Date, name);
}
public AddDateCustomFieldParams min(String min) {
parameters.add(new NameValuePair("min", min));
return this;
}
public AddDateCustomFieldParams max(String max) {
parameters.add(new NameValuePair("max", max));
return this;
}
public AddDateCustomFieldParams initialValueType(DateCustomFieldSetting.InitialValueType initialValueType) {
parameters.add(new NameValuePair("initialValueType", String.valueOf(initialValueType.getIntValue())));
return this;
}
public AddDateCustomFieldParams initialDate(String initialDate) {
parameters.add(new NameValuePair("initialDate", initialDate));
return this;
}
public AddDateCustomFieldParams initialShift(int initialShift) {
parameters.add(new NameValuePair("initialShift", String.valueOf(initialShift)));
return this;
}
@Override
public AddDateCustomFieldParams applicableIssueTypes(List<Long> applicableIssueTypes) {
return (AddDateCustomFieldParams) super.applicableIssueTypes(applicableIssueTypes);
}
@Override
public AddDateCustomFieldParams description(String description) {
return (AddDateCustomFieldParams) super.description(description);
}
@Override
public AddDateCustomFieldParams required(boolean required) {
return (AddDateCustomFieldParams) super.required(required);
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/GetParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.ArrayList;
import java.util.List;
/**
* Parameters for get request.
*
* @author nulab-inc
*/
public abstract class GetParams {
protected List<NameValuePair> parameters = new ArrayList<NameValuePair>();
public List<NameValuePair> getParamList() {
return parameters;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Repository.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog repository data.
*
* @author nulab-inc
*/
public interface Repository {
long getId();
String getIdAsString();
long getProjectId();
String getProjectIdAsString();
String getName();
String getDescription();
String getHookUrl();
String getHttpUrl();
String getSshUrl();
long getDisplayOrder();
Date getPushedAt();
User getCreatedUser();
Date getCreated();
User getUpdatedUser();
Date getUpdated();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Star.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog star data.
*
* @author nulab-inc
*/
public interface Star {
long getId();
String getIdAsString();
String getComment();
String getUrl();
String getTitle();
User getPresenter();
Date getCreated();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Comment.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog comment data.
*
* @author nulab-inc
*/
public interface Comment {
long getId();
String getIdAsString();
String getContent();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/WebhookJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.nulabinc.backlog4j.Activity;
import com.nulabinc.backlog4j.User;
import com.nulabinc.backlog4j.Webhook;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class WebhookJSONImpl implements Webhook {
private long id;
private String name;
private String description;
private String hookUrl;
private boolean allEvent;
private int[] activityTypeIds;
@JsonDeserialize(as=UserJSONImpl.class)
private User createdUser;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date created;
@JsonDeserialize(as=UserJSONImpl.class)
private User updatedUser;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date updated;
@Override
public long getId() {
return this.id;
}
@Override
public String getIdAsString() {
return String.valueOf(this.id);
}
@Override
public String getName() {
return this.name;
}
@Override
public String getDescription() {
return this.description;
}
@Override
public String getHookUrl() {
return this.hookUrl;
}
@Override
public boolean isAllEvent() {
return this.allEvent;
}
@Override
public List<Activity.Type> getActivityTypeIds() {
if(activityTypeIds==null){
return null;
}
List<Activity.Type> typeIds = new ArrayList<Activity.Type>();
for(int activityTypeId : activityTypeIds){
typeIds.add(Activity.Type.valueOf(activityTypeId));
}
return typeIds;
}
@Override
public User getCreatedUser() {
return createdUser;
}
@Override
public Date getCreated() {
return created;
}
@Override
public User getUpdatedUser() {
return updatedUser;
}
@Override
public Date getUpdated() {
return updated;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
WebhookJSONImpl rhs = (WebhookJSONImpl) obj;
return new EqualsBuilder()
.append(this.id, rhs.id)
.append(this.name, rhs.name)
.append(this.description, rhs.description)
.append(this.hookUrl, rhs.hookUrl)
.append(this.allEvent, rhs.allEvent)
.append(this.activityTypeIds, rhs.activityTypeIds)
.append(this.createdUser, rhs.createdUser)
.append(this.created, rhs.created)
.append(this.updatedUser, rhs.updatedUser)
.append(this.updated, rhs.updated)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(id)
.append(name)
.append(description)
.append(hookUrl)
.append(allEvent)
.append(activityTypeIds)
.append(createdUser)
.append(created)
.append(updatedUser)
.append(updated)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", id)
.append("name", name)
.append("description", description)
.append("hookUrl", hookUrl)
.append("allEvent", allEvent)
.append("activityTypeIds", activityTypeIds)
.append("createdUser", createdUser)
.append("created", created)
.append("updatedUser", updatedUser)
.append("updated", updated)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Link.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog link data.
*
* @author nulab-inc
*/
public interface Link {
long getId();
String getIdAsString();
long getKeyId();
String getKeyIdAsString();
String getTitle();
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/api/option/UpdateIssueParamsTest.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.Issue;
import com.nulabinc.backlog4j.http.NameValuePair;
import org.junit.jupiter.api.Test;
import java.math.BigDecimal;
import java.util.Arrays;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertTrue;
/**
* @author nulab-inc
*/
public class UpdateIssueParamsTest extends AbstractParamsTest {
@Test
public void createParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.summary("test issue 1")
.issueTypeId(2000000001L)
.priority(Issue.PriorityType.Normal)
.parentIssueId(3000000001L)
.description("This is a test issue.")
.startDate("2014-08-01")
.dueDate("2014-12-01")
.estimatedHours(16)
.actualHours(12.5f)
.assigneeId(4000000001L)
.categoryIds(Arrays.asList(5000000001L, 5000000002L))
.versionIds(Arrays.asList(6000000001L, 6000000002L))
.milestoneIds(Arrays.asList(7000000001L, 7000000002L))
.notifiedUserIds(Arrays.asList(8000000001L, 8000000002L))
.attachmentIds(Arrays.asList(9000000001L, 9000000002L))
.comment("issue comment")
.status(Issue.StatusType.Resolved)
.resolution(Issue.ResolutionType.Fixed);
// then
assertEquals("1000000001", params.getIssueIdOrKeyString());
List<NameValuePair> parameters = params.getParamList();
assertEquals(23, parameters.size());
assertTrue(existsOneKeyValue(parameters, "summary", "test issue 1"));
assertTrue(existsOneKeyValue(parameters, "issueTypeId", "2000000001"));
assertTrue(existsOneKeyValue(parameters, "priorityId", "3"));
assertTrue(existsOneKeyValue(parameters, "parentIssueId", "3000000001"));
assertTrue(existsOneKeyValue(parameters, "description", "This is a test issue."));
assertTrue(existsOneKeyValue(parameters, "startDate", "2014-08-01"));
assertTrue(existsOneKeyValue(parameters, "dueDate", "2014-12-01"));
assertTrue(existsOneKeyValue(parameters, "estimatedHours", "16"));
assertTrue(existsOneKeyValue(parameters, "actualHours", "12.5"));
assertTrue(existsOneKeyValue(parameters, "assigneeId", "4000000001"));
assertTrue(existsOneKeyValue(parameters, "categoryId[]", "5000000001"));
assertTrue(existsOneKeyValue(parameters, "categoryId[]", "5000000002"));
assertTrue(existsOneKeyValue(parameters, "versionId[]", "6000000001"));
assertTrue(existsOneKeyValue(parameters, "versionId[]", "6000000002"));
assertTrue(existsOneKeyValue(parameters, "milestoneId[]", "7000000001"));
assertTrue(existsOneKeyValue(parameters, "milestoneId[]", "7000000002"));
assertTrue(existsOneKeyValue(parameters, "notifiedUserId[]", "8000000001"));
assertTrue(existsOneKeyValue(parameters, "notifiedUserId[]", "8000000002"));
assertTrue(existsOneKeyValue(parameters, "attachmentId[]", "9000000001"));
assertTrue(existsOneKeyValue(parameters, "attachmentId[]", "9000000002"));
assertTrue(existsOneKeyValue(parameters, "comment", "issue comment"));
assertTrue(existsOneKeyValue(parameters, "statusId", "3"));
assertTrue(existsOneKeyValue(parameters, "resolutionId", "0"));
}
@Test
public void createParamEmptySetTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.resolution(null).assigneeId(0L)
.dueDate(null).startDate(null)
.categoryIds(null).versionIds(null).milestoneIds(null)
.estimatedHours(null).actualHours(null);
List<NameValuePair> parameters = params.getParamList();
assertTrue(existsOneKeyValue(parameters, "resolutionId", ""));
assertTrue(existsOneKeyValue(parameters, "assigneeId", ""));
assertTrue(existsOneKeyValue(parameters, "dueDate", ""));
assertTrue(existsOneKeyValue(parameters, "startDate", ""));
assertTrue(existsOneKeyValue(parameters, "categoryId[]", ""));
assertTrue(existsOneKeyValue(parameters, "versionId[]", ""));
assertTrue(existsOneKeyValue(parameters, "milestoneId[]", ""));
assertTrue(existsOneKeyValue(parameters, "estimatedHours", ""));
assertTrue(existsOneKeyValue(parameters, "actualHours", ""));
}
@Test
public void createTextCustomFieldParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.textCustomFields(Arrays.asList(
new CustomFiledValue(3000000001L, "egg"),
new CustomFiledValue(3000000002L, "rice")));
params.textCustomField(new CustomFiledValue(3000000003L, "tea"));
// then
List<NameValuePair> parameters = params.getParamList();
assertTrue(existsOneKeyValue(parameters, "customField_3000000001", "egg"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000002", "rice"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000003", "tea"));
}
@Test
public void createTextAreaCustomFieldParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.textAreaCustomFields(Arrays.asList(
new CustomFiledValue(3000000001L, "egg"),
new CustomFiledValue(3000000002L, "rice")));
params.textAreaCustomField(new CustomFiledValue(3000000003L, "tea"));
// then
List<NameValuePair> parameters = params.getParamList();
assertTrue(existsOneKeyValue(parameters, "customField_3000000001", "egg"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000002", "rice"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000003", "tea"));
}
@Test
public void createNumericCustomFieldParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.numericCustomFields(Arrays.asList(
new CustomFiledValue(3000000001L, -111f),
new CustomFiledValue(3000000002L, 5555.121f)));
params.numericCustomField(new CustomFiledValue(3000000003L, 123.61f));
params.numericCustomField(new CustomFiledValue(3000000004L, 123.67777));
// then
List<NameValuePair> parameters = params.getParamList();
assertTrue(existsOneKeyValue(parameters, "customField_3000000001", "-111.0"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000002", "5555.121"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000003", "123.61"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000004", "123.67777"));
}
@Test
public void createDateCustomFieldParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.dateCustomFields(Arrays.asList(
new CustomFiledValue(3000000001L, "2008-10-01"),
new CustomFiledValue(3000000002L, "2008-10-02")));
params.dateCustomField(new CustomFiledValue(3000000003L, "2008-10-10"));
// then
List<NameValuePair> parameters = params.getParamList();
assertTrue(existsOneKeyValue(parameters, "customField_3000000001", "2008-10-01"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000002", "2008-10-02"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000003", "2008-10-10"));
}
@Test
public void createSingleListCustomFieldParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.singleListCustomFields(Arrays.asList(
new CustomFiledItem(3000000001L, 4000000001L),
new CustomFiledItem(3000000002L, 4000000002L)));
params.singleListCustomField(new CustomFiledItem(3000000003L, 4000000003L));
// then
List<NameValuePair> parameters = params.getParamList();
assertTrue(existsOneKeyValue(parameters, "customField_3000000001", "4000000001"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000002", "4000000002"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000003", "4000000003"));
}
@Test
public void createRadioCustomFieldParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.radioCustomFields(Arrays.asList(
new CustomFiledItem(3000000001L, 4000000001L),
new CustomFiledItem(3000000002L, 4000000002L)));
params.radioCustomField(new CustomFiledItem(3000000003L, 4000000003L));
// then
List<NameValuePair> parameters = params.getParamList();
assertTrue(existsOneKeyValue(parameters, "customField_3000000001", "4000000001"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000002", "4000000002"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000003", "4000000003"));
}
@Test
public void createMultipleListCustomFieldParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.multipleListCustomFields(Arrays.asList(
new CustomFiledItems(3000000001L, Arrays.asList(4000000001L, 4000000002L)),
new CustomFiledItems(3000000002L, Arrays.asList(4000000003L, 4000000004L))));
params.multipleListCustomField(new CustomFiledItems(3000000003L, Arrays.asList(4000000005L, 4000000006L)));
// then
List<NameValuePair> parameters = params.getParamList();
assertTrue(existsOneKeyValue(parameters, "customField_3000000001", "4000000001"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000001", "4000000002"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000002", "4000000003"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000002", "4000000004"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000003", "4000000005"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000003", "4000000006"));
}
@Test
public void createCheckBoxListCustomFieldParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.checkBoxCustomFields(Arrays.asList(
new CustomFiledItems(3000000001L, Arrays.asList(4000000001L, 4000000002L)),
new CustomFiledItems(3000000002L, Arrays.asList(4000000003L, 4000000004L))));
params.checkBoxCustomField(new CustomFiledItems(3000000003L, Arrays.asList(4000000005L, 4000000006L)));
// then
List<NameValuePair> parameters = params.getParamList();
assertTrue(existsOneKeyValue(parameters, "customField_3000000001", "4000000001"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000001", "4000000002"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000002", "4000000003"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000002", "4000000004"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000003", "4000000005"));
assertTrue(existsOneKeyValue(parameters, "customField_3000000003", "4000000006"));
}
@Test
public void createIntHoursParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.estimatedHours(16)
.actualHours(12);
// then
assertEquals("1000000001", params.getIssueIdOrKeyString());
List<NameValuePair> parameters = params.getParamList();
assertEquals(2, parameters.size());
assertTrue(existsOneKeyValue(parameters, "estimatedHours", "16"));
assertTrue(existsOneKeyValue(parameters, "actualHours", "12"));
}
@Test
public void createFloatHoursParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.estimatedHours(16.00f)
.actualHours(12.12f);
// then
assertEquals("1000000001", params.getIssueIdOrKeyString());
List<NameValuePair> parameters = params.getParamList();
assertEquals(2, parameters.size());
assertTrue(existsOneKeyValue(parameters, "estimatedHours", "16.0"));
assertTrue(existsOneKeyValue(parameters, "actualHours", "12.12"));
}
@Test
public void createBigDecimalHoursParamTest() {
// when
UpdateIssueParams params = new UpdateIssueParams(1000000001L);
params.estimatedHours(new BigDecimal("16.00"))
.actualHours(new BigDecimal("12.12345"));
// then
assertEquals("1000000001", params.getIssueIdOrKeyString());
List<NameValuePair> parameters = params.getParamList();
assertEquals(2, parameters.size());
assertTrue(existsOneKeyValue(parameters, "estimatedHours", "16.00"));
assertTrue(existsOneKeyValue(parameters, "actualHours", "12.12"));
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/ViewedWikiJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.nulabinc.backlog4j.User;
import com.nulabinc.backlog4j.ViewedWiki;
import com.nulabinc.backlog4j.Wiki;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.Date;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class ViewedWikiJSONImpl implements ViewedWiki {
@JsonDeserialize(as = WikiJSONImpl.class)
private Wiki page;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date updated;
@Override
public Wiki getPage() {
return page;
}
@Override
public Date getUpdated() {
return updated;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
ViewedWikiJSONImpl rhs = (ViewedWikiJSONImpl) obj;
return new EqualsBuilder()
.append(this.page, rhs.page)
.append(this.updated, rhs.updated)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(page)
.append(updated)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("page", page)
.append("updated", updated)
.toString();
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/internal/json/PullRequestCommentJSONImplTest.java
package com.nulabinc.backlog4j.internal.json;
import com.nulabinc.backlog4j.AttachmentInfo;
import com.nulabinc.backlog4j.ChangeLog;
import com.nulabinc.backlog4j.Notification;
import com.nulabinc.backlog4j.PullRequestComment;
import com.nulabinc.backlog4j.ResponseList;
import com.nulabinc.backlog4j.Star;
import com.nulabinc.backlog4j.User;
import org.exparity.hamcrest.date.DateMatchers;
import org.junit.jupiter.api.Test;
import java.io.IOException;
import java.util.Calendar;
import java.util.List;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.junit.jupiter.api.Assertions.assertEquals;
/**
* @author nulab-inc
*/
public class PullRequestCommentJSONImplTest extends AbstractJSONImplTest {
@Test
public void createPullRequestCommentListTest() throws IOException {
String fileContentStr = getJsonString("json/pull_request_comments.json");
ResponseList<PullRequestComment> pullRequestComments = factory.createPullRequestCommentList(fileContentStr);
assertEquals(1, pullRequestComments.size());
PullRequestComment pullRequestComment = pullRequestComments.get(0);
assertEquals(12345, pullRequestComment.getId());
assertEquals("test comment\"(greenpepper)\"\"(Cacoo)\"", pullRequestComment.getContent());
List<ChangeLog> logs = pullRequestComment.getChangeLog();
ChangeLog log = logs.get(0);
assertEquals("*銀行振込:30件", log.getNewValue());
AttachmentInfo attachmentInfo = log.getAttachmentInfo();
assertEquals(1123344555, attachmentInfo.getId());
List<Star> stars = pullRequestComment.getStars();
assertEquals(1, stars.size());
List<Notification> notifications = pullRequestComment.getNotifications();
assertEquals(0, notifications.size());
User user = pullRequestComment.getCreatedUser();
assertEquals(11111111, user.getId());
Calendar calendar = Calendar.getInstance();
calendar.set(2015, Calendar.JULY, 16, 0, 0, 0);
assertThat(calendar.getTime(), DateMatchers.sameDay(pullRequestComment.getCreated()));
// User updateUser = pullRequestComment.getUpdatedUser();
// assertEquals(2, updateUser.getId());
calendar = Calendar.getInstance();
calendar.set(2015, Calendar.JULY, 16, 0, 0, 0);
assertThat(calendar.getTime(), DateMatchers.sameDay(pullRequestComment.getUpdated()));
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/PullRequestQueryParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.PullRequest;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for pull request query.
*
* @author nulab-inc
*/
public class PullRequestQueryParams extends QueryParams {
public PullRequestQueryParams statusType(List<PullRequest.StatusType> statusType) {
for (PullRequest.StatusType type : statusType) {
parameters.add(new NameValuePair("statusId[]", String.valueOf(type.getIntValue())));
}
return this;
}
public PullRequestQueryParams assigneeIds(List assigneeIds) {
for (Object assigneeId : assigneeIds) {
parameters.add(new NameValuePair("assigneeId[]", assigneeId.toString()));
}
return this;
}
public PullRequestQueryParams issueIds(List issueIds) {
for (Object issueId : issueIds) {
parameters.add(new NameValuePair("issueId[]", issueId.toString()));
}
return this;
}
public PullRequestQueryParams createdUserIds(List createdUserIds) {
for (Object createdUserId : createdUserIds) {
parameters.add(new NameValuePair("createdUserId[]", createdUserId.toString()));
}
return this;
}
@Override
public PullRequestQueryParams minId(Object minId) {
return (PullRequestQueryParams) super.minId(minId);
}
@Override
public PullRequestQueryParams maxId(Object maxId) {
return (PullRequestQueryParams) super.maxId(maxId);
}
@Override
public PullRequestQueryParams count(long count) {
return (PullRequestQueryParams) super.count(count);
}
@Override
public PullRequestQueryParams count(int count) {
return (PullRequestQueryParams) super.count(count);
}
@Override
public PullRequestQueryParams order(Order order) {
return (PullRequestQueryParams) super.order(order);
}
public PullRequestQueryParams offset(long offset) {
parameters.add(new NameValuePair("offset", String.valueOf(offset)));
return this;
}
public PullRequestQueryParams offset(int offset) {
parameters.add(new NameValuePair("offset", String.valueOf(offset)));
return this;
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/internal/json/ActivityJSONImplTest.java
package com.nulabinc.backlog4j.internal.json;
import com.nulabinc.backlog4j.Activity;
import com.nulabinc.backlog4j.Attachment;
import com.nulabinc.backlog4j.Change;
import com.nulabinc.backlog4j.Comment;
import com.nulabinc.backlog4j.GroupProjectActivity;
import com.nulabinc.backlog4j.Project;
import com.nulabinc.backlog4j.ResponseList;
import com.nulabinc.backlog4j.User;
import com.nulabinc.backlog4j.internal.json.activities.IssueCommentedActivity;
import com.nulabinc.backlog4j.internal.json.activities.IssueCommentedContent;
import com.nulabinc.backlog4j.internal.json.activities.IssueCreatedActivity;
import com.nulabinc.backlog4j.internal.json.activities.IssueCreatedContent;
import com.nulabinc.backlog4j.internal.json.activities.IssueUpdatedActivity;
import com.nulabinc.backlog4j.internal.json.activities.IssueUpdatedContent;
import com.nulabinc.backlog4j.internal.json.activities.ProjectUserAddedActivity;
import com.nulabinc.backlog4j.internal.json.activities.ProjectUserAddedContent;
import com.nulabinc.backlog4j.internal.json.activities.ProjectUserRemovedActivity;
import com.nulabinc.backlog4j.internal.json.activities.ProjectUserRemovedContent;
import com.nulabinc.backlog4j.internal.json.activities.PullRequestAddedActivity;
import com.nulabinc.backlog4j.internal.json.activities.PullRequestCommentedActivity;
import com.nulabinc.backlog4j.internal.json.activities.PullRequestContent;
import com.nulabinc.backlog4j.internal.json.activities.PullRequestUpdatedActivity;
import com.nulabinc.backlog4j.internal.json.activities.WikiCreatedActivity;
import com.nulabinc.backlog4j.internal.json.activities.WikiCreatedContent;
import com.nulabinc.backlog4j.internal.json.activities.WikiUpdatedActivity;
import com.nulabinc.backlog4j.internal.json.activities.WikiUpdatedContent;
import org.exparity.hamcrest.date.DateMatchers;
import org.junit.jupiter.api.Test;
import java.io.IOException;
import java.util.Calendar;
import java.util.List;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertFalse;
import static org.junit.jupiter.api.Assertions.assertNull;
import static org.junit.jupiter.api.Assertions.assertTrue;
/**
* Created by yuh kim on 2014/07/23.
*/
public class ActivityJSONImplTest extends AbstractJSONImplTest {
@Test
public void createActivityIssueCreatedTest() throws IOException {
String fileContentStr = getJsonString("json/activity_issue_created.json");
Activity activity = factory.createActivity(fileContentStr);
System.out.println(activity.toString());
assertEquals(Activity.Type.IssueCreated, activity.getType());
IssueCreatedActivity issueCreated = (IssueCreatedActivity) activity;
assertEquals(624929, issueCreated.getId());
Project project = issueCreated.getProject();
assertEquals(1073761031, project.getId());
assertEquals("TEST_PRJ1407208757143", project.getProjectKey());
assertEquals("テストプロジェクト", project.getName());
assertFalse(project.isChartEnabled());
assertTrue(project.isSubtaskingEnabled());
assertEquals(Project.TextFormattingRule.Backlog, project.getTextFormattingRule());
assertFalse(project.isArchived());
IssueCreatedContent content = issueCreated.getContent();
assertEquals(1073803919, content.getId());
assertEquals(1, content.getKeyId());
assertEquals("課題作成", content.getSummary());
assertEquals("テスト", content.getDescription());
User user = issueCreated.getCreatedUser();
assertEquals(1073751781, user.getId());
assertEquals("test_admin", user.getUserId());
assertEquals("あどみにさん", user.getName());
assertEquals(User.RoleType.Admin, user.getRoleType());
assertNull(user.getLang());
assertEquals("<EMAIL>", user.getMailAddress());
Calendar calendar = Calendar.getInstance();
calendar.set(2014, Calendar.AUGUST, 5, 5, 50, 59);
assertThat(calendar.getTime(), DateMatchers.sameDay(issueCreated.getCreated()));
}
@Test
public void createActivityIssueUpdatedTest() throws IOException {
String fileContentStr = getJsonString("json/activity_issue_updated.json");
Activity activity = factory.createActivity(fileContentStr);
System.out.println(activity.toString());
assertEquals(Activity.Type.IssueUpdated, activity.getType());
IssueUpdatedActivity issueUpdated = (IssueUpdatedActivity) activity;
assertEquals(39293172, issueUpdated.getId());
IssueUpdatedContent content = issueUpdated.getContent();
assertEquals(1079097008, content.getId());
assertEquals(1, content.getKeyId());
assertEquals("課題追加テスト", content.getSummary());
assertEquals("", content.getDescription());
Comment comment = content.getComment();
assertEquals(1102443987, comment.getId());
assertEquals("コメント時ファイル添付テスト", comment.getContent());
List<Attachment> attachments = content.getAttachments();
assert attachments.size() > 0;
Attachment attachment = attachments.get(0);
assertEquals(1076499483, attachment.getId());
assertEquals(attachment.getName(), "twoshot.JPG");
assertEquals(86513, attachment.getSize());
List<Change> changes = content.getChanges();
assert changes.size() > 0;
Change change = changes.get(0);
assertEquals("attachment", change.getField());
assertEquals("twoshot.JPG", change.getNewValue());
assertEquals("", change.getOldValue());
assertEquals("standard", change.getType());
}
@Test
public void createActivityIssueCommentedTest() throws IOException {
String fileContentStr = getJsonString("json/activity_issue_commented.json");
Activity activity = factory.createActivity(fileContentStr);
System.out.println(activity.toString());
assertEquals(Activity.Type.IssueCommented, activity.getType());
IssueCommentedActivity issueCommented = (IssueCommentedActivity) activity;
assertEquals(39261460, issueCommented.getId());
IssueCommentedContent content = issueCommented.getContent();
assertEquals(1079097008, content.getId());
assertEquals(1, content.getKeyId());
assertEquals("課題追加テスト", content.getSummary());
assertEquals("", content.getDescription());
Comment comment = content.getComment();
assertEquals(1102419188, comment.getId());
assertEquals("コメント時ファイル添付テスト", comment.getContent());
}
@Test
public void createActivityWikiCreatedTest() throws IOException {
String fileContentStr = getJsonString("json/activity_wiki_created.json");
Activity activity = factory.createActivity(fileContentStr);
System.out.println(activity.toString());
assertEquals(Activity.Type.WikiCreated, activity.getType());
WikiCreatedActivity wikiCreated = (WikiCreatedActivity) activity;
assertEquals(624763, wikiCreated.getId());
WikiCreatedContent content = wikiCreated.getContent();
assertEquals(1073768599, content.getId());
assertEquals("テスト", content.getName());
assertEquals("ああああ", content.getContent());
}
@Test
public void createActivityWikiUpdatedTest() throws IOException {
String fileContentStr = getJsonString("json/activity_wiki_updated.json");
Activity activity = factory.createActivity(fileContentStr);
System.out.println(activity.toString());
assertEquals(Activity.Type.WikiUpdated, activity.getType());
WikiUpdatedActivity wikiUpdated = (WikiUpdatedActivity) activity;
assertEquals(624764, wikiUpdated.getId());
WikiUpdatedContent content = wikiUpdated.getContent();
assertEquals(1073768599, content.getId());
assertEquals("テスト", content.getName());
assertEquals("ああああ", content.getContent());
List<Attachment> attachments = content.getAttachments();
assertEquals(18105, attachments.get(0).getId());
assertEquals("backlog_icon.png", attachments.get(0).getName());
assertEquals(5050, attachments.get(0).getSize());
}
@Test
public void createActivityProjectUserAddedTest() throws IOException {
String fileContentStr = getJsonString("json/activity_project_user_added.json");
Activity activity = factory.createActivity(fileContentStr);
System.out.println(activity.toString());
assertEquals(Activity.Type.ProjectUserAdded, activity.getType());
ProjectUserAddedActivity projectUserAdded = (ProjectUserAddedActivity) activity;
assertEquals(623680, projectUserAdded.getId());
ProjectUserAddedContent content = projectUserAdded.getContent();
List<User> users = content.getUsers();
assertEquals(1073751782, users.get(0).getId());
List<GroupProjectActivity> groupProjectActivities = content.getGroupProjectActivities();
assertEquals(623680, groupProjectActivities.get(0).getId());
assertEquals(Activity.Type.ProjectUserAdded, groupProjectActivities.get(0).getType());
}
@Test
public void createActivityProjectUserRemovedTest() throws IOException {
String fileContentStr = getJsonString("json/activity_project_user_removed.json");
Activity activity = factory.createActivity(fileContentStr);
System.out.println(activity.toString());
assertEquals(Activity.Type.ProjectUserRemoved, activity.getType());
ProjectUserRemovedActivity projectUserRemoved = (ProjectUserRemovedActivity) activity;
assertEquals(623559, projectUserRemoved.getId());
ProjectUserRemovedContent content = projectUserRemoved.getContent();
List<User> users = content.getUsers();
assertEquals(1073936985, users.get(0).getId());
assertEquals("test_admin", users.get(0).getUserId());
assertEquals(1073936985, users.get(0).getId());
List<GroupProjectActivity> groupProjectActivities = content.getGroupProjectActivities();
assertEquals(623554, groupProjectActivities.get(0).getId());
assertEquals(Activity.Type.ProjectUserRemoved, groupProjectActivities.get(0).getType());
assertEquals("よろしく", content.getComment());
}
@Test
public void createActivityPullRequestAddedTest() throws IOException {
String fileContentStr = getJsonString("json/activity_pull_request_added.json");
Activity activity = factory.createActivity(fileContentStr);
System.out.println(activity.toString());
assertEquals(Activity.Type.PullRequestAdded, activity.getType());
PullRequestAddedActivity pullRequestAdded = (PullRequestAddedActivity) activity;
assertEquals(623559, pullRequestAdded.getId());
PullRequestContent content = pullRequestAdded.getContent();
assertEquals(148, content.getId());
assertEquals(112, content.getNumber());
assertEquals("アイコンとトグルのデザイン最適化", content.getSummary());
assertEquals("マージおねがいします!", content.getDescription());
assertNull(content.getComment());
assertTrue(content.getChanges().isEmpty());
assertEquals(77777777, content.getIssue().getId());
}
@Test
public void createActivityPullRequestUpdatedTest() throws IOException {
String fileContentStr = getJsonString("json/activity_pull_request_updated.json");
Activity activity = factory.createActivity(fileContentStr);
System.out.println(activity.toString());
assertEquals(Activity.Type.PullRequestUpdated, activity.getType());
PullRequestUpdatedActivity pullRequestUpdated = (PullRequestUpdatedActivity) activity;
assertEquals(121212, pullRequestUpdated.getId());
PullRequestContent content = pullRequestUpdated.getContent();
assertEquals(148, content.getId());
assertEquals(112, content.getNumber());
assertEquals("デザイン最適化", content.getSummary());
assertEquals("マージおねがいします!", content.getDescription());
assertEquals(579, content.getComment().getId());
assertEquals("", content.getComment().getContent());
assertEquals("status", content.getChanges().get(0).getField());
assertEquals("3", content.getChanges().get(0).getNewValue());
assertEquals("1", content.getChanges().get(0).getOldValue());
}
@Test
public void createActivityPullRequestCommentedTest() throws IOException {
String fileContentStr = getJsonString("json/activity_pull_request_commented.json");
Activity activity = factory.createActivity(fileContentStr);
System.out.println(activity.toString());
assertEquals(Activity.Type.PullRequestCommented, activity.getType());
PullRequestCommentedActivity pullRequestCommented = (PullRequestCommentedActivity) activity;
assertEquals(3333333, pullRequestCommented.getId());
PullRequestContent content = pullRequestCommented.getContent();
assertEquals(146, content.getId());
assertEquals(111, content.getNumber());
assertEquals("統合", content.getSummary());
assertEquals("レビューおねがいします", content.getDescription());
assertEquals(576, content.getComment().getId());
assertEquals("マージしやしたー", content.getComment().getContent());
assertEquals(0, content.getChanges().size());
}
@Test
public void createActivityListTest() throws IOException {
String fileContentStr = getJsonString("json/activities.json");
ResponseList<Activity> list = factory.createActivityList(fileContentStr);
System.out.println(list.toString());
}
@Test
public void equalsTest() throws IOException {
String fileContentStr = getJsonString("json/activity_issue_created.json");
Activity activity1 = factory.createActivity(fileContentStr);
Activity activity2 = factory.createActivity(fileContentStr);
assertEquals(activity1, activity2);
}
@Test
public void hashCodeTest() throws IOException {
String fileContentStr = getJsonString("json/activity_issue_created.json");
Activity activity1 = factory.createActivity(fileContentStr);
Activity activity2 = factory.createActivity(fileContentStr);
assertEquals(activity1.hashCode(), activity2.hashCode());
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Group.java
package com.nulabinc.backlog4j;
import java.util.Date;
import java.util.List;
/**
* The interface for Backlog group data.
*
* @author nulab-inc
*/
public interface Group {
long getId();
String getIdAsString();
String getName();
List<User> getMembers();
long getDisplayOrder();
User getCreatedUser();
Date getCreated();
User getUpdatedUser();
Date getUpdated();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/BacklogClientBase.java
package com.nulabinc.backlog4j;
import com.nulabinc.backlog4j.api.option.*;
import com.nulabinc.backlog4j.auth.AccessToken;
import com.nulabinc.backlog4j.auth.OAuthSupport;
import com.nulabinc.backlog4j.conf.BacklogConfigure;
import com.nulabinc.backlog4j.http.BacklogHttpClient;
import com.nulabinc.backlog4j.http.BacklogHttpClientImpl;
import com.nulabinc.backlog4j.http.BacklogHttpResponse;
import com.nulabinc.backlog4j.http.NameValuePair;
import com.nulabinc.backlog4j.internal.InternalFactory;
import com.nulabinc.backlog4j.internal.json.InternalFactoryJSONImpl;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* Base of BacklogClient.
*
* @author nulab-inc
*/
public abstract class BacklogClientBase{
private final Logger logger = LoggerFactory.getLogger(BacklogClientBase.class);
protected BacklogHttpClient httpClient;
protected BacklogConfigure configure;
protected InternalFactory factory = new InternalFactoryJSONImpl();
protected OAuthSupport oAuthSupport;
protected BacklogEndPointSupport backlogEndPointSupport;
public BacklogClientBase(BacklogConfigure configure) {
this.configure = configure;
this.httpClient = new BacklogHttpClientImpl();
this.backlogEndPointSupport = new BacklogEndPointSupport(configure);
configureHttpClient();
}
public BacklogClientBase(BacklogConfigure configure, BacklogHttpClient httpClient) {
this.configure = configure;
this.httpClient = httpClient;
this.backlogEndPointSupport = new BacklogEndPointSupport(configure);
configureHttpClient();
}
public void setOAuthSupport(OAuthSupport oAuthSupport) {
this.oAuthSupport = oAuthSupport;
}
private void configureHttpClient() {
if (this.configure.getApiKey() != null) {
httpClient.setApiKey(this.configure.getApiKey());
} else if (this.configure.getAccessToken() != null) {
httpClient.setBearerToken(this.configure.getAccessToken().getToken());
} else {
throw new BacklogAPIException("ApiKey or AccessToken must not be null");
}
httpClient.setReadTimeout(this.configure.getReadTimeout());
httpClient.setConnectionTimeout(this.configure.getConnectionTimeout());
}
protected BacklogHttpResponse get(String endpoint) throws BacklogException {
return this.get(endpoint, null, null);
}
protected BacklogHttpResponse get(String endpoint, GetParams getParams) throws BacklogException {
return this.get(endpoint, getParams, null);
}
protected BacklogHttpResponse get(String endpoint, QueryParams queryParams) throws BacklogException {
return this.get(endpoint, null, queryParams);
}
protected BacklogHttpResponse get(String endpoint, GetParams getParams, QueryParams queryParams) throws BacklogException {
BacklogHttpResponse ires = httpClient.get(endpoint, getParams, queryParams);
if (needTokenRefresh(ires)) {
refreshToken();
ires = httpClient.get(endpoint, getParams, queryParams);
}
loggingResponse(endpoint, ires);
checkError(ires);
return ires;
}
protected BacklogHttpResponse post(String endpoint) throws BacklogException {
return this.post(endpoint, new ArrayList<NameValuePair>(), new ArrayList<NameValuePair>());
}
protected BacklogHttpResponse post(String endpoint, PostParams postParams) throws BacklogException {
return this.post(endpoint, postParams.getParamList(), new ArrayList<NameValuePair>());
}
protected BacklogHttpResponse post(String endpoint, List<NameValuePair> parameters, List<NameValuePair> headers) throws BacklogException {
BacklogHttpResponse ires = httpClient.post(endpoint, parameters, headers);
if (needTokenRefresh(ires)) {
refreshToken();
ires = httpClient.post(endpoint, parameters, headers);
}
loggingResponse(endpoint, ires);
checkError(ires);
return ires;
}
protected BacklogHttpResponse patch(String endpoint, PatchParams postParams) throws BacklogException {
return this.patch(endpoint, postParams.getParamList(), new ArrayList<NameValuePair>());
}
protected BacklogHttpResponse patch(String endpoint, List<NameValuePair> parameters, List<NameValuePair> headers) throws BacklogException {
BacklogHttpResponse ires = httpClient.patch(endpoint, parameters, headers);
if (needTokenRefresh(ires)) {
refreshToken();
ires = httpClient.patch(endpoint, parameters, headers);
}
loggingResponse(endpoint, ires);
checkError(ires);
return ires;
}
protected BacklogHttpResponse put(String endpoint, List<NameValuePair> parameters) throws BacklogException {
BacklogHttpResponse ires = httpClient.put(endpoint, parameters);
if (needTokenRefresh(ires)) {
refreshToken();
ires = httpClient.put(endpoint, parameters);
}
loggingResponse(endpoint, ires);
checkError(ires);
return ires;
}
protected BacklogHttpResponse delete(String endpoint) throws BacklogException {
return this.delete(endpoint, new ArrayList<NameValuePair>());
}
protected BacklogHttpResponse delete(String endpoint, DeleteParams deleteParams) throws BacklogException {
return this.delete(endpoint, deleteParams.getParamList());
}
protected BacklogHttpResponse delete(String endpoint, NameValuePair param) throws BacklogException {
List<NameValuePair> params = new ArrayList<NameValuePair>();
if (param != null) {
params.add(param);
}
return this.delete(endpoint, params);
}
protected BacklogHttpResponse delete(String endpoint, List<NameValuePair> parameters) throws BacklogException {
BacklogHttpResponse ires = httpClient.delete(endpoint, parameters);
if (needTokenRefresh(ires)) {
refreshToken();
ires = httpClient.delete(endpoint, parameters);
}
loggingResponse(endpoint, ires);
checkError(ires);
return ires;
}
protected BacklogHttpResponse postMultiPart(String endpoint, Map<String, Object> parameters) throws BacklogException {
BacklogHttpResponse ires = httpClient.postMultiPart(endpoint, parameters);
if (needTokenRefresh(ires)) {
refreshToken();
ires = httpClient.postMultiPart(endpoint, parameters);
}
loggingResponse(endpoint, ires);
checkError(ires);
return ires;
}
private void loggingResponse(String endpoint, BacklogHttpResponse ires) {
logger.info("status code:" + ires.getStatusCode() + " url:" + endpoint);
}
private String getRateLimitResetFormatedDate(BacklogHttpResponse ires) {
if (ires.getRateLimitResetDate() == null) {
return "";
}
SimpleDateFormat sdf = new SimpleDateFormat("yyyy/MM/dd HH:mm:ss");
return sdf.format(ires.getRateLimitResetDate());
}
protected String buildEndpoint(String connection) {
StringBuilder url = new StringBuilder()
.append(configure.getRestBaseURL())
.append(connection == null ? "" : "/" + connection);
return url.toString();
}
private void checkError(BacklogHttpResponse ires) {
if (ires.getStatusCode() != 200 &&
ires.getStatusCode() != 201 &&
ires.getStatusCode() != 202 &&
ires.getStatusCode() != 203 &&
ires.getStatusCode() != 204) {
if (ires.getStatusCode() == 429) {
throw new BacklogAPIException("The API usage limit has been exceeded, and will be available again from " + getRateLimitResetFormatedDate(ires) + ".", ires);
} else {
throw new BacklogAPIException("backlog api request failed.", ires);
}
}
}
private boolean needTokenRefresh(BacklogHttpResponse ires) {
return (ires.getStatusCode() == 401 ||
ires.getStatusCode() == 0) && // for android bug
configure.getApiKey() == null &&
configure.getAccessToken() != null;
}
private void refreshToken() {
AccessToken accessToken = oAuthSupport.refreshOAuthAccessToken();
configure.accessToken(accessToken);
configureHttpClient();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdateStatusParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.Project;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for update status API.
*
* @author nulab-inc
*/
public class UpdateStatusParams extends PatchParams {
private Object projectIdOrKey;
private Object statusId;
public UpdateStatusParams(Object projectIdOrKey, Object statusId, String name, Project.CustomStatusColor color) {
this.projectIdOrKey = projectIdOrKey;
this.statusId = statusId;
parameters.add(new NameValuePair("name", name));
parameters.add(new NameValuePair("color", color.getStrValue()));
}
public String getProjectIdOrKeyString() {
return projectIdOrKey.toString();
}
public String getStatusId() {
return statusId.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Activity.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog activity data.
*
* @author nulab-inc
*/
public interface Activity {
enum Type {
Undefined(-1), IssueCreated(1), IssueUpdated(2), IssueCommented(3), IssueDeleted(4),
WikiCreated(5), WikiUpdated(6), WikiDeleted(7),
FileAdded(8), FileUpdated(9), FileDeleted(10),
SvnCommitted(11), GitPushed(12), GitRepositoryCreated(13),
IssueMultiUpdated(14), ProjectUserAdded(15), ProjectUserRemoved(16), NotifyAdded(17),
PullRequestAdded(18), PullRequestUpdated(19), PullRequestCommented(20), PullRequestMerged(21);
Type(int intValue) {
this.intValue = intValue;
}
public int getIntValue() {
return intValue;
}
public static Type valueOf(final int anIntValue) {
for (Type d : values()) {
if (d.getIntValue() == anIntValue) {
return d;
}
}
return Undefined;
}
private int intValue;
}
long getId();
String getIdAsString();
Project getProject();
Type getType();
Content getContent();
User getCreatedUser();
Date getCreated();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/ProjectWithVCSJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.nulabinc.backlog4j.ProjectWithVCS;
import org.apache.commons.lang3.builder.ToStringBuilder;
@JsonIgnoreProperties(ignoreUnknown = true)
public class ProjectWithVCSJSONImpl extends ProjectJSONImpl implements ProjectWithVCS {
private boolean useSubversion;
private boolean useGit;
@Override
public boolean getUseSubversion() { return useSubversion; }
@Override
public boolean getUseGit() { return useGit; }
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
ProjectWithVCSJSONImpl rhs = (ProjectWithVCSJSONImpl) obj;
return super.createEqualsBuilder(rhs)
.append(this.useSubversion, rhs.useSubversion)
.append(this.useGit, rhs.useGit)
.isEquals();
}
@Override
public int hashCode() {
return super.createHashCodeBuilder()
.append(useSubversion)
.append(useGit)
.toHashCode();
}
@Override
public String toString() {
return super.createToStringBuilder()
.append("useSubversion", useSubversion)
.append("useGit", useGit)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/auth/BacklogOAuthSupport.java
package com.nulabinc.backlog4j.auth;
import com.nulabinc.backlog4j.BacklogAPIException;
import com.nulabinc.backlog4j.BacklogAuthException;
import com.nulabinc.backlog4j.BacklogException;
import com.nulabinc.backlog4j.conf.BacklogConfigure;
import com.nulabinc.backlog4j.http.BacklogHttpClient;
import com.nulabinc.backlog4j.http.BacklogHttpClientImpl;
import com.nulabinc.backlog4j.http.BacklogHttpResponse;
import com.nulabinc.backlog4j.http.NameValuePair;
import com.nulabinc.backlog4j.internal.InternalFactory;
import com.nulabinc.backlog4j.internal.json.InternalFactoryJSONImpl;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.List;
/**
* @author nulab-inc
*/
public class BacklogOAuthSupport implements OAuthSupport {
private String clientId;
private String clientSecret;
private String redirectUrl;
private BacklogConfigure configure;
private BacklogHttpClient httpClient;
private InternalFactory factory = new InternalFactoryJSONImpl();
private OnAccessTokenRefreshListener listener;
public BacklogOAuthSupport(BacklogConfigure configure) {
this.configure = configure;
this.httpClient = new BacklogHttpClientImpl();
init();
}
public BacklogOAuthSupport(BacklogConfigure configure, BacklogHttpClient httpClient) {
this.configure = configure;
this.httpClient = httpClient;
init();
}
private void init() {
httpClient.setReadTimeout(this.configure.getReadTimeout());
httpClient.setConnectionTimeout(this.configure.getConnectionTimeout());
}
public void setOAuthClientId(String clientId, String clientSecret) {
this.clientId = clientId;
this.clientSecret = clientSecret;
}
@Override
public void setOAuthRedirectUrl(String redirectUrl) {
this.redirectUrl = redirectUrl;
}
@Override
public String getOAuthAuthorizationURL() throws BacklogException {
String url = configure.getOAuthAuthorizationURL() +
"?client_id=" + this.clientId +
"&response_type=" + "code";
if (redirectUrl != null) {
try {
url += "&redirect_uri=" + URLEncoder.encode(this.redirectUrl, "UTF-8");
} catch (UnsupportedEncodingException e) {
throw new BacklogAPIException(e);
}
}
return url;
}
@Override
public AccessToken getOAuthAccessToken(String oauthCode) throws BacklogException {
if (oauthCode == null) {
throw new IllegalArgumentException("oauthCode must not be null");
}
BacklogHttpResponse httpResponse = getAccessTokenResponse(oauthCode);
checkError(httpResponse);
return factory.createAccessToken(httpResponse);
}
@Override
public AccessToken refreshOAuthAccessToken() throws BacklogException {
if (configure.getAccessToken() == null) {
throw new IllegalArgumentException("AccessToken must not be null");
}
BacklogHttpResponse httpResponse = getRefreshTokenResponse();
checkError(httpResponse);
AccessToken accessToken = factory.createAccessToken(httpResponse);
configure.accessToken(accessToken);
listener.onAccessTokenRefresh(accessToken);
return accessToken;
}
@Override
public void setOnAccessTokenRefreshListener(OnAccessTokenRefreshListener listener) {
this.listener = listener;
}
private BacklogHttpResponse getAccessTokenResponse(String oauthCode) throws BacklogException {
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new NameValuePair("code", oauthCode));
params.add(new NameValuePair("client_id", clientId));
params.add(new NameValuePair("client_secret", clientSecret));
params.add(new NameValuePair("redirect_uri", redirectUrl));
params.add(new NameValuePair("grant_type", "authorization_code"));
BacklogHttpResponse ires = httpClient.post(configure.getOAuthAccessTokenURL(), params, new ArrayList<NameValuePair>());
checkError(ires);
return ires;
}
private BacklogHttpResponse getRefreshTokenResponse() throws BacklogException {
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new NameValuePair("client_id", clientId));
params.add(new NameValuePair("client_secret", clientSecret));
params.add(new NameValuePair("refresh_token", configure.getAccessToken().getRefresh()));
params.add(new NameValuePair("grant_type", "refresh_token"));
BacklogHttpResponse ires = httpClient.post(configure.getOAuthAccessTokenURL(), params, new ArrayList<NameValuePair>());
checkError(ires);
return ires;
}
private void checkError(BacklogHttpResponse ires) {
if (ires.getStatusCode() != 200 &&
ires.getStatusCode() != 201) {
throw new BacklogAuthException("backlog oauth request failed.", ires);
}
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/PostedAttachment.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog posted attachment file.
*
* @author nulab-inc
*/
public interface PostedAttachment {
long getId();
String getIdAsString();
String getName();
long getSize();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/ResponseList.java
package com.nulabinc.backlog4j;
import java.util.List;
/**
* The interface for response list.
*
* @author nulab-inc
*/
public interface ResponseList<T> extends List<T> {
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdateTextCustomFieldParams.java
package com.nulabinc.backlog4j.api.option;
/**
* Parameters for update text type custom field API.
*
* @author nulab-inc
*/
public class UpdateTextCustomFieldParams extends UpdateCustomFieldParams {
public UpdateTextCustomFieldParams(Object projectIdOrKey, long customFiledId) {
super(projectIdOrKey, customFiledId);
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdateTextAreaCustomFieldParams.java
package com.nulabinc.backlog4j.api.option;
/**
* Parameters for update text area type custom field API.
*
* @author nulab-inc
*/
public class UpdateTextAreaCustomFieldParams extends UpdateCustomFieldParams {
public UpdateTextAreaCustomFieldParams(Object projectIdOrKey, long customFiledId) {
super(projectIdOrKey, customFiledId);
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/ViewedProject.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog viewed project data.
*
* @author nulab-inc
*/
public interface ViewedProject {
Project getProject();
Date getUpdated();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdateIssueCommentParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for update issue comment API.
*
* @author nulab-inc
*/
public class UpdateIssueCommentParams extends PatchParams {
private Object issueIdOrKey;
private Object commentId;
public UpdateIssueCommentParams(Object issueIdOrKey, Object commentId, String content) {
this.issueIdOrKey = issueIdOrKey;
this.commentId = commentId;
parameters.add(new NameValuePair("content", content));
}
public String getCommentId() {
return commentId.toString();
}
public String getIssueIdOrKeyString() {
return issueIdOrKey.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdateGroupParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for update group API.
*
* @author nulab-inc
*/
public class UpdateGroupParams extends PatchParams {
private String groupId;
public UpdateGroupParams(Object groupId){
this.groupId = groupId.toString();
}
public UpdateGroupParams name(String name) {
parameters.add(new NameValuePair("name", name));
return this;
}
public UpdateGroupParams members(List members) {
for (Object member : members) {
parameters.add(new NameValuePair("members[]", member.toString()));
}
return this;
}
public String getGroupId() {
return groupId;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/AddCategoryParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for add category API.
*
* @author nulab-inc
*/
public class AddCategoryParams extends PostParams {
private Object projectIdOrKey;
/**
* Constructor
*
* @param projectIdOrKey the project identifier
* @param name the category name
*/
public AddCategoryParams(Object projectIdOrKey, String name) {
this.projectIdOrKey = projectIdOrKey;
parameters.add(new NameValuePair("name", name));
}
/**
* Returns the project identifier string.
*
* @return project id or project key
*/
public String getProjectIdOrKeyString() {
return projectIdOrKey.toString();
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/internal/json/UserJSONImplTest.java
package com.nulabinc.backlog4j.internal.json;
import com.nulabinc.backlog4j.ResponseList;
import com.nulabinc.backlog4j.User;
import org.exparity.hamcrest.date.DateMatchers;
import org.junit.jupiter.api.Test;
import java.io.IOException;
import java.util.Calendar;
import java.util.Date;
import java.util.TimeZone;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNull;
/**
* @author nulab-inc
*/
public class UserJSONImplTest extends AbstractJSONImplTest {
@Test
public void createUserListTest() throws IOException {
String fileContentStr = getJsonString("json/users.json");
ResponseList<User> users = factory.createUserList(fileContentStr);
assertEquals(2, users.size());
User user = users.get(0);
assertEquals(1073910170, user.getId());
assertEquals("test1", user.getName());
assertEquals(User.RoleType.Admin, user.getRoleType());
assertNull(user.getLang());
assertEquals("<EMAIL>", user.getMailAddress());
Calendar calendar = Calendar.getInstance(TimeZone.getTimeZone("UTC"));
calendar.set(2022, Calendar.SEPTEMBER, 7, 11, 33, 45);
calendar.set(Calendar.MILLISECOND, 0);
assertEquals(calendar.getTime(), user.getLastLoginTime());
user = users.get(1);
assertEquals(1073910171, user.getId());
assertEquals("test2", user.getName());
assertEquals(User.RoleType.User, user.getRoleType());
assertEquals("ja", user.getLang());
assertEquals("<EMAIL>", user.getMailAddress());
assertNull(user.getLastLoginTime());
}
@Test
public void createUserTest() throws IOException {
String fileContentStr = getJsonString("json/user.json");
User user = factory.createUser(fileContentStr);
assertEquals(1073936936, user.getId());
assertEquals("test", user.getName());
assertEquals(User.RoleType.Admin, user.getRoleType());
assertNull(user.getLang());
assertEquals("<EMAIL>", user.getMailAddress());
}
@Test
public void equalsTest() throws IOException {
String fileContentStr = getJsonString("json/user.json");
User user1 = factory.createUser(fileContentStr);
User user2 = factory.createUser(fileContentStr);
assertEquals(user1, user2);
}
@Test
public void hashCodeTest() throws IOException {
String fileContentStr = getJsonString("json/user.json");
User user1 = factory.createUser(fileContentStr);
User user2 = factory.createUser(fileContentStr);
assertEquals(user1.hashCode(), user2.hashCode());
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/api/option/UpdateWebhookParamsTest.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.Activity;
import com.nulabinc.backlog4j.http.NameValuePair;
import org.junit.jupiter.api.Test;
import java.util.Arrays;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertTrue;
/**
* @author nulab-inc
*/
public class UpdateWebhookParamsTest extends AbstractParamsTest {
@Test
public void createParamTest() {
// when
long projectId = 1000001L;
long webhookId = 1L;
String name = "name";
String hookUrl = "hookurl";
UpdateWebhookParams params = new UpdateWebhookParams(projectId, webhookId);
params.name(name)
.hookUrl(hookUrl)
.description("description")
.activityTypeIds(Arrays.asList(Activity.Type.IssueCreated, Activity.Type.IssueUpdated));
// then
List<NameValuePair> parameters = params.getParamList();
assertEquals(5, parameters.size());
assertTrue(existsOneKeyValue(parameters, "name", name));
assertTrue(existsOneKeyValue(parameters, "hookUrl", hookUrl));
assertTrue(existsOneKeyValue(parameters, "description", "description"));
assertTrue(existsOneKeyValue(parameters, "activityTypeIds[]", "1"));
assertTrue(existsOneKeyValue(parameters, "activityTypeIds[]", "2"));
assertEquals(String.valueOf(projectId), params.getProjectIdOrKeyString());
assertEquals("1", params.getWebhookId());
}
@Test
public void createParam_AllEvent_Test() {
// when
String projectKey = "PROJECT";
long webhookId = 1L;
UpdateWebhookParams params = new UpdateWebhookParams(projectKey, webhookId);
params.allEvent(true);
// then
List<NameValuePair> parameters = params.getParamList();
assertEquals(1, parameters.size());
assertTrue(existsOneKeyValue(parameters, "allEvent", "true"));
assertEquals(projectKey, params.getProjectIdOrKeyString());
assertEquals("1", params.getWebhookId());
}
@Test
public void createParam_SetNull_Test() {
// when
long projectId = 1000001L;
long webhookId = 1L;
UpdateWebhookParams params = new UpdateWebhookParams(projectId, webhookId);
params.name(null)
.hookUrl(null)
.description(null);
// then
List<NameValuePair> parameters = params.getParamList();
assertEquals(3, parameters.size());
assertTrue(existsOneKeyValue(parameters, "name", ""));
assertTrue(existsOneKeyValue(parameters, "hookUrl", ""));
assertTrue(existsOneKeyValue(parameters, "description", ""));
assertEquals(String.valueOf(projectId), params.getProjectIdOrKeyString());
assertEquals("1", params.getWebhookId());
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdateWebhookParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.Activity;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for update webhook API.
*
* @author nulab-inc
*/
public class UpdateWebhookParams extends PatchParams {
private Object projectIdOrKey;
private Object webhookId;
public UpdateWebhookParams(Object projectIdOrKey, long webhookId) {
this.projectIdOrKey = projectIdOrKey;
this.webhookId = webhookId;
}
public String getProjectIdOrKeyString() {
return projectIdOrKey.toString();
}
public String getWebhookId() {
return webhookId.toString();
}
public UpdateWebhookParams name(String name) {
String value = (name==null) ? "" : name;
parameters.add(new NameValuePair("name", value));
return this;
}
public UpdateWebhookParams description(String description) {
String value = (description==null) ? "" : description;
parameters.add(new NameValuePair("description", value));
return this;
}
public UpdateWebhookParams hookUrl(String hookUrl) {
String value = (hookUrl==null) ? "" : hookUrl;
parameters.add(new NameValuePair("hookUrl", value));
return this;
}
public UpdateWebhookParams allEvent(boolean allEvent) {
parameters.add(new NameValuePair("allEvent", String.valueOf(allEvent)));
return this;
}
public UpdateWebhookParams activityTypeIds(List<Activity.Type> activityTypeIds) {
if (activityTypeIds != null) {
for (Activity.Type type : activityTypeIds) {
parameters.add(new NameValuePair("activityTypeIds[]", String.valueOf(type.getIntValue())));
}
}
return this;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/GetWikiTagsParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for get wiki page's tag API.
*
* @author nulab-inc
*/
public class GetWikiTagsParams extends GetParams {
public GetWikiTagsParams(Object projectKey) {
parameters.add(new NameValuePair("projectIdOrKey", projectKey.toString()));
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/conf/BacklogComConfigureTest.java
package com.nulabinc.backlog4j.conf;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
/**
* @author nulab-inc
*/
public class BacklogComConfigureTest {
@Test
public void getOAuthAuthorizationURLTest() throws Exception {
// when
String testSpace = "test";
BacklogConfigure configure = new BacklogComConfigure(testSpace);
// then
String expected = "https://" + testSpace + ".backlog.com/api/v2";
assertEquals(expected, configure.getRestBaseURL());
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/SpaceJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.nulabinc.backlog4j.SharedFile;
import com.nulabinc.backlog4j.Space;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.Date;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class SpaceJSONImpl implements Space {
private String spaceKey;
private String name;
private long ownerId;
private String lang;
private String timezone;
private String reportSendTime;
private String textFormattingRule;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date updated;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date created;
@Override
public String getSpaceKey() {
return spaceKey;
}
@Override
public String getName() {
return name;
}
@Override
public long getOwnerId() {
return ownerId;
}
@Override
public String getOwnerIdAsString() {
return String.valueOf(this.ownerId);
}
@Override
public String getLang() {
return lang;
}
@Override
public String getTimezone() {
return timezone;
}
@Override
public String getReportSendTime() {
return reportSendTime;
}
@Override
public String getTextFormattingRule() {
return textFormattingRule;
}
@Override
public Date getUpdated() {
return updated;
}
@Override
public Date getCreated() {
return created;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
SpaceJSONImpl rhs = (SpaceJSONImpl) obj;
return new EqualsBuilder()
.append(this.spaceKey, rhs.spaceKey)
.append(this.name, rhs.name)
.append(this.ownerId, rhs.ownerId)
.append(this.lang, rhs.lang)
.append(this.timezone, rhs.timezone)
.append(this.reportSendTime, rhs.reportSendTime)
.append(this.textFormattingRule, rhs.textFormattingRule)
.append(this.updated, rhs.updated)
.append(this.created, rhs.created)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(spaceKey)
.append(name)
.append(ownerId)
.append(lang)
.append(timezone)
.append(reportSendTime)
.append(textFormattingRule)
.append(updated)
.append(created)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("spaceKey", spaceKey)
.append("name", name)
.append("ownerId", ownerId)
.append("lang", lang)
.append("timezone", timezone)
.append("reportSendTime", reportSendTime)
.append("textFormattingRule", textFormattingRule)
.append("updated", updated)
.append("created", created)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdateDateCustomFieldParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.internal.json.customFields.DateCustomFieldSetting;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for update date type custom field API.
*
* @author nulab-inc
*/
public class UpdateDateCustomFieldParams extends UpdateCustomFieldParams {
public UpdateDateCustomFieldParams(Object projectIdOrKey, long customFiledId) {
super(projectIdOrKey, customFiledId);
}
public UpdateDateCustomFieldParams min(String min) {
parameters.add(new NameValuePair("min", min));
return this;
}
public UpdateDateCustomFieldParams max(String max) {
parameters.add(new NameValuePair("max", max));
return this;
}
public UpdateDateCustomFieldParams initialValueType(DateCustomFieldSetting.InitialValueType initialValueType) {
parameters.add(new NameValuePair("initialValueType", String.valueOf(initialValueType.getIntValue())));
return this;
}
public UpdateDateCustomFieldParams initialDate(String initialDate) {
parameters.add(new NameValuePair("initialDate", initialDate));
return this;
}
public UpdateDateCustomFieldParams initialShift(int initialShift) {
parameters.add(new NameValuePair("initialShift", String.valueOf(initialShift)));
return this;
}
@Override
public UpdateDateCustomFieldParams applicableIssueTypes(List<Long> applicableIssueTypes) {
return (UpdateDateCustomFieldParams) super.applicableIssueTypes(applicableIssueTypes);
}
@Override
public UpdateDateCustomFieldParams description(String description) {
return (UpdateDateCustomFieldParams) super.description(description);
}
@Override
public UpdateDateCustomFieldParams required(boolean required) {
return (UpdateDateCustomFieldParams) super.required(required);
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Watch.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog Watch data.
*/
public interface Watch {
long getId();
String getIdAsString();
boolean getAlreadyRead();
String getAlreadyReadAsString();
String getNote();
String getType();
Issue getIssue();
Date getCreated();
Date getUpdated();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/BacklogAPIErrorMessage.java
package com.nulabinc.backlog4j;
/**
* The error message class for Backlog exception.
*
* @author nulab-inc
*/
public class BacklogAPIErrorMessage {
private String message;
private int code;
private String errorInfo;
private String moreInfo;
public String getMessage() {
return message;
}
public int getCode() {
return code;
}
public String getErrorInfo() {
return errorInfo;
}
public String getMoreInfo() {
return moreInfo;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/InternalFactory.java
package com.nulabinc.backlog4j.internal;
import com.nulabinc.backlog4j.*;
import com.nulabinc.backlog4j.auth.AccessToken;
import com.nulabinc.backlog4j.http.BacklogHttpResponse;
/**
* @author nulab-inc
*/
public interface InternalFactory {
AccessToken createAccessToken(BacklogHttpResponse res) throws BacklogException;
Space createSpace(BacklogHttpResponse res) throws BacklogException;
SpaceNotification createSpaceNotification(BacklogHttpResponse res) throws BacklogException;
DiskUsage createDiskUsage(BacklogHttpResponse res) throws BacklogException;
DiskUsageDetail createDiskUsageDetail(BacklogHttpResponse res) throws BacklogException;
ResponseList<Project> createProjectList(BacklogHttpResponse res) throws BacklogException;
ProjectWithVCS createProject(BacklogHttpResponse res) throws BacklogException;
ResponseList<Activity> createActivityList(BacklogHttpResponse res) throws BacklogException;
Activity createActivity(BacklogHttpResponse res) throws BacklogException;
ResponseList<Issue> createIssueList(BacklogHttpResponse res) throws BacklogException;
Issue createIssue(BacklogHttpResponse res) throws BacklogException;
Issue importIssue(BacklogHttpResponse res) throws BacklogException;
ResponseList<IssueComment> createIssueCommentList(BacklogHttpResponse res) throws BacklogException;
IssueComment createIssueComment(BacklogHttpResponse res) throws BacklogException;
ResponseList<User> createUserList(BacklogHttpResponse res) throws BacklogException;
User createUser(BacklogHttpResponse res) throws BacklogException;
ResponseList<IssueType> createIssueTypeList(BacklogHttpResponse res) throws BacklogException;
IssueType createIssueType(BacklogHttpResponse res) throws BacklogException;
ResponseList<Category> createCategoryList(BacklogHttpResponse res) throws BacklogException;
Category createCategory(BacklogHttpResponse res) throws BacklogException;
ResponseList<CustomFieldSetting> createCustomFieldList(BacklogHttpResponse res) throws BacklogException;
CustomFieldSetting createCustomField(BacklogHttpResponse res) throws BacklogException;
ResponseList<Priority> createPriorityList(BacklogHttpResponse res) throws BacklogException;
ResponseList<Resolution> createResolutionList(BacklogHttpResponse res) throws BacklogException;
ResponseList<Status> createStatusList(BacklogHttpResponse res) throws BacklogException;
Status createStatus(BacklogHttpResponse res) throws BacklogException;
ResponseList<Star> createStarList(BacklogHttpResponse res) throws BacklogException;
Star createStar(BacklogHttpResponse res) throws BacklogException;
Count createCount(BacklogHttpResponse res) throws BacklogException;
ResponseList<Version> createVersionList(BacklogHttpResponse res) throws BacklogException;
Version createVersion(BacklogHttpResponse res) throws BacklogException;
ResponseList<Milestone> createMilestoneList(BacklogHttpResponse res) throws BacklogException;
Milestone createMilestone(BacklogHttpResponse res) throws BacklogException;
Wiki createWiki(BacklogHttpResponse res) throws BacklogException;
Wiki importWiki(BacklogHttpResponse res) throws BacklogException;
ResponseList<Wiki> createWikiList(BacklogHttpResponse res) throws BacklogException;
ResponseList<WikiTag> createWikiTagList(BacklogHttpResponse res) throws BacklogException;
WikiHistory createWikiHistory(BacklogHttpResponse res) throws BacklogException;
WikiTag createWikiTag(BacklogHttpResponse res) throws BacklogException;
ResponseList<WikiHistory> createWikiHistoryList(BacklogHttpResponse res) throws BacklogException;
ResponseList<Notification> createNotificationList(BacklogHttpResponse res) throws BacklogException;
Repository createRepository(BacklogHttpResponse res) throws BacklogException;
ResponseList<Repository> createRepositoryList(BacklogHttpResponse res) throws BacklogException;
PullRequest createPullRequest(BacklogHttpResponse res) throws BacklogException;
ResponseList<PullRequest> createPullRequestList(BacklogHttpResponse res) throws BacklogException;
PullRequestComment createPullRequestComment(BacklogHttpResponse res) throws BacklogException;
ResponseList<PullRequestComment> createPullRequestCommentList(BacklogHttpResponse res) throws BacklogException;
ResponseList<ViewedIssue> createViewedIssueList(BacklogHttpResponse res) throws BacklogException;
ResponseList<ViewedProject> createViewedProjectList(BacklogHttpResponse res) throws BacklogException;
ResponseList<ViewedWiki> createViewedWikiList(BacklogHttpResponse res) throws BacklogException;
ResponseList<SharedFile> createSharedFileList(BacklogHttpResponse res) throws BacklogException;
SharedFile createSharedFile(BacklogHttpResponse res) throws BacklogException;
ResponseList<Attachment> createAttachmentList(BacklogHttpResponse res) throws BacklogException;
Attachment createAttachment(BacklogHttpResponse res) throws BacklogException;
Group createGroup(BacklogHttpResponse res) throws BacklogException;
ResponseList<Group> createGroupList(BacklogHttpResponse res) throws BacklogException;
ResponseList<Webhook> createWebhookList(BacklogHttpResponse res) throws BacklogException;
Webhook createWebhook(BacklogHttpResponse res) throws BacklogException;
Watch createWatch(BacklogHttpResponse res) throws BacklogException;
ResponseList<Watch> createWatchList(BacklogHttpResponse res) throws BacklogException;
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/CreateUserParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.User;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for create user API.
*
* @author nulab-inc
*/
public class CreateUserParams extends PostParams {
public CreateUserParams(String userId, String password,
String name, String mailAddress,
User.RoleType roleType){
parameters.add(new NameValuePair("userId", userId));
parameters.add(new NameValuePair("password", <PASSWORD>));
parameters.add(new NameValuePair("name", name));
parameters.add(new NameValuePair("mailAddress", mailAddress));
parameters.add(new NameValuePair("roleType", String.valueOf(roleType.getIntValue())));
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/conf/BacklogPackageConfigure.java
package com.nulabinc.backlog4j.conf;
import java.net.MalformedURLException;
/**
* @author nulab-inc
*/
public class BacklogPackageConfigure extends BacklogConfigure {
private String url;
public BacklogPackageConfigure(String url) throws MalformedURLException {
if (url == null) {
throw new IllegalArgumentException("url must not be null");
}
if (url.endsWith("/")) {
this.url = url.substring(0, url.length() -1);
} else {
this.url = url;
}
}
@Override
public String getOAuthAuthorizationURL() {
return this.url + "/OAuth2AccessRequest.action";
}
@Override
public String getOAuthAccessTokenURL() {
return this.url + "/api/v2/oauth2/token";
}
@Override
public String getRestBaseURL() {
return this.url + "/api/v2";
}
@Override
public String getWebAppBaseURL() {
return this.url;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/IssueTypeJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
/**
* @author nulab-inc
*/
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.nulabinc.backlog4j.IssueType;
import com.nulabinc.backlog4j.Project;
import com.nulabinc.backlog4j.User;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class IssueTypeJSONImpl implements IssueType {
private long id;
private long projectId;
private String name;
private String color;
private String templateSummary;
private String templateDescription;
@Override
public long getId() {
return this.id;
}
@Override
public String getIdAsString() {
return String.valueOf(this.id);
}
@Override
public long getProjectId() {
return this.projectId;
}
@Override
public String getProjectIdAsString() {
return String.valueOf(this.projectId);
}
@Override
public String getName() {
return this.name;
}
@Override
public Project.IssueTypeColor getColor() {
return Project.IssueTypeColor.strValueOf(this.color);
}
@Override
public String getTemplateSummary() { return this.templateSummary; }
@Override
public String getTemplateDescription() { return this.templateDescription; }
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
IssueTypeJSONImpl rhs = (IssueTypeJSONImpl) obj;
return new EqualsBuilder()
.append(this.id, rhs.id)
.append(this.projectId, rhs.projectId)
.append(this.name, rhs.name)
.append(this.color, rhs.color)
.append(this.templateSummary, rhs.templateSummary)
.append(this.templateDescription, rhs.templateDescription)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(id)
.append(projectId)
.append(name)
.append(color)
.append(templateSummary)
.append(templateDescription)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", id)
.append("projectId", projectId)
.append("name", name)
.append("color", color)
.append("templateSummary", templateSummary)
.append("templateDescription", templateDescription)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/AttachmentData.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog attachment file data.
*
* @author nulab-inc
*/
public interface AttachmentData extends FileData{
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/AddWikiAttachmentParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for add attachment file to wiki page's API.
*
* @author nulab-inc
*/
public class AddWikiAttachmentParams extends PostParams {
private Object wikiId;
public AddWikiAttachmentParams(Object wikiId, List attachmentIds){
this.wikiId = wikiId;
for (Object attachmentId : attachmentIds) {
parameters.add(new NameValuePair("attachmentId[]", String.valueOf(attachmentId)));
}
}
public String getWikiId() {
return this.wikiId.toString();
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/internal/json/WebhookJSONImplTest.java
package com.nulabinc.backlog4j.internal.json;
import com.nulabinc.backlog4j.Activity;
import com.nulabinc.backlog4j.ResponseList;
import com.nulabinc.backlog4j.User;
import com.nulabinc.backlog4j.Webhook;
import org.apache.commons.lang3.time.DateFormatUtils;
import org.junit.jupiter.api.Test;
import java.io.IOException;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertFalse;
import static org.junit.jupiter.api.Assertions.assertNotNull;
import static org.junit.jupiter.api.Assertions.assertTrue;
/**
* @author nulab-inc
*/
public class WebhookJSONImplTest extends AbstractJSONImplTest {
@Test
public void createWebhookListTest() throws IOException {
String fileContentStr = getJsonString("json/webhooks.json");
ResponseList<Webhook> webhooks = factory.createWebhookList(fileContentStr);
assertEquals(2, webhooks.size());
Webhook webhook = webhooks.get(0);
assertEquals(1079196895, webhook.getId());
assertEquals("webhook", webhook.getName());
assertEquals("", webhook.getDescription());
assertEquals("http://nulab.test/", webhook.getHookUrl());
assertFalse(webhook.isAllEvent());
assertNotNull(webhook.getActivityTypeIds());
assertEquals(5, webhook.getActivityTypeIds().size());
List<Activity.Type> activityTypeIds = webhook.getActivityTypeIds();
assertEquals(Activity.Type.IssueCreated, activityTypeIds.get(0));
assertEquals(Activity.Type.IssueUpdated, activityTypeIds.get(1));
assertEquals(Activity.Type.IssueCommented, activityTypeIds.get(2));
assertEquals(Activity.Type.IssueDeleted, activityTypeIds.get(3));
assertEquals(Activity.Type.WikiCreated, activityTypeIds.get(4));
assertEquals("2014-11-30 01:22:21", DateFormatUtils.formatUTC(webhook.getCreated(), "yyyy-MM-dd HH:mm:ss"));
assertNotNull(webhook.getCreatedUser());
assertEquals(1, webhook.getCreatedUser().getId());
assertEquals("admin", webhook.getCreatedUser().getUserId());
assertEquals("admin", webhook.getCreatedUser().getName());
assertEquals(User.RoleType.Admin, webhook.getCreatedUser().getRoleType());
assertEquals("ja", webhook.getCreatedUser().getLang());
assertEquals("<EMAIL>", webhook.getCreatedUser().getMailAddress());
assertEquals("2015-02-13 17:36:59", DateFormatUtils.formatUTC(webhook.getUpdated(), "yyyy-MM-dd HH:mm:ss"));
assertNotNull(webhook.getUpdatedUser());
assertEquals(2, webhook.getUpdatedUser().getId());
assertEquals("developer", webhook.getUpdatedUser().getUserId());
assertEquals("developer", webhook.getUpdatedUser().getName());
assertEquals(User.RoleType.User, webhook.getUpdatedUser().getRoleType());
assertEquals("en", webhook.getUpdatedUser().getLang());
assertEquals("<EMAIL>", webhook.getUpdatedUser().getMailAddress());
webhook = webhooks.get(1);
assertEquals(1079097008, webhook.getId());
assertEquals("webhook2", webhook.getName());
assertEquals("this is description", webhook.getDescription());
assertEquals("http://nulab.test/", webhook.getHookUrl());
assertTrue(webhook.isAllEvent());
assertNotNull(webhook.getActivityTypeIds());
assertEquals(0, webhook.getActivityTypeIds().size());
}
@Test
public void createWebhookTest() throws IOException {
String fileContentStr = getJsonString("json/webhook.json");
Webhook webhook = factory.createWebhook(fileContentStr);
assertEquals(1079196895, webhook.getId());
assertEquals("webhook", webhook.getName());
assertEquals("", webhook.getDescription());
assertEquals("http://nulab.test/", webhook.getHookUrl());
assertFalse(webhook.isAllEvent());
assertNotNull(webhook.getActivityTypeIds());
assertEquals(5, webhook.getActivityTypeIds().size());
List<Activity.Type> activityTypeIds = webhook.getActivityTypeIds();
assertEquals(Activity.Type.IssueCreated, activityTypeIds.get(0));
assertEquals(Activity.Type.IssueUpdated, activityTypeIds.get(1));
assertEquals(Activity.Type.IssueCommented, activityTypeIds.get(2));
assertEquals(Activity.Type.IssueDeleted, activityTypeIds.get(3));
assertEquals(Activity.Type.WikiCreated, activityTypeIds.get(4));
assertEquals("2014-11-30 01:22:21", DateFormatUtils.formatUTC(webhook.getCreated(), "yyyy-MM-dd HH:mm:ss"));
assertNotNull(webhook.getCreatedUser());
assertEquals(1, webhook.getCreatedUser().getId());
assertEquals("admin", webhook.getCreatedUser().getUserId());
assertEquals("admin", webhook.getCreatedUser().getName());
assertEquals(User.RoleType.Admin, webhook.getCreatedUser().getRoleType());
assertEquals("ja", webhook.getCreatedUser().getLang());
assertEquals("<EMAIL>", webhook.getCreatedUser().getMailAddress());
assertEquals("2014-11-30 01:22:21", DateFormatUtils.formatUTC(webhook.getUpdated(), "yyyy-MM-dd HH:mm:ss"));
assertNotNull(webhook.getUpdatedUser());
assertEquals(1, webhook.getUpdatedUser().getId());
assertEquals("admin", webhook.getUpdatedUser().getUserId());
assertEquals("admin", webhook.getUpdatedUser().getName());
assertEquals(User.RoleType.Admin, webhook.getUpdatedUser().getRoleType());
assertEquals("ja", webhook.getUpdatedUser().getLang());
assertEquals("<EMAIL>", webhook.getUpdatedUser().getMailAddress());
}
@Test
public void equalsTest1() throws IOException {
String fileContentStr = getJsonString("json/webhook.json");
Webhook webhook1 = factory.createWebhook(fileContentStr);
Webhook webhook2 = factory.createWebhook(fileContentStr);
assertEquals(webhook1, webhook2);
}
@Test
public void hashCodeTest1() throws IOException {
String fileContentStr = getJsonString("json/webhook.json");
Webhook webhook1 = factory.createWebhook(fileContentStr);
Webhook webhook2 = factory.createWebhook(fileContentStr);
assertEquals(webhook1.hashCode(), webhook2.hashCode());
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdateWatchParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for update watch API.
*
* @author nulab-inc
*/
public class UpdateWatchParams extends PatchParams {
private Object watchingId;
public UpdateWatchParams(Object watchingId) {
this.watchingId = watchingId;
}
public String getWatchingIdString() {
return watchingId.toString();
}
public UpdateWatchParams note(String note) {
parameters.add(new NameValuePair("note", String.valueOf(note)));
return this;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/DiskUsageJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.nulabinc.backlog4j.DiskUsage;
import com.nulabinc.backlog4j.DiskUsageDetail;
import com.nulabinc.backlog4j.User;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.Arrays;
import java.util.List;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class DiskUsageJSONImpl implements DiskUsage {
private long capacity;
private long issue;
private long wiki;
private long file;
private long subversion;
private long git;
@JsonDeserialize(as = DiskUsageDetailJSONImpl[].class)
private DiskUsageDetail[] details;
@Override
public long getCapacity() {
return capacity;
}
@Override
public long getIssue() {
return issue;
}
@Override
public long getWiki() {
return wiki;
}
@Override
public long getFile() {
return file;
}
@Override
public long getSubversion() {
return subversion;
}
@Override
public long getGit() {
return git;
}
@Override
public List<DiskUsageDetail> getDetails() {
return Arrays.asList(details);
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
DiskUsageJSONImpl rhs = (DiskUsageJSONImpl) obj;
return new EqualsBuilder()
.append(this.capacity, rhs.capacity)
.append(this.issue, rhs.issue)
.append(this.wiki, rhs.wiki)
.append(this.file, rhs.file)
.append(this.subversion, rhs.subversion)
.append(this.git, rhs.git)
.append(this.details, rhs.details)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(capacity)
.append(issue)
.append(wiki)
.append(file)
.append(subversion)
.append(git)
.append(details)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("capacity", capacity)
.append("issue", issue)
.append("wiki", wiki)
.append("file", file)
.append("subversion", subversion)
.append("git", git)
.append("details", details)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/IssueJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.nulabinc.backlog4j.*;
import com.nulabinc.backlog4j.internal.json.customFields.CustomFieldJSONImpl;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.math.BigDecimal;
import java.util.*;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class IssueJSONImpl implements Issue {
private int id;
private String issueKey;
private int keyId;
private int projectId;
@JsonDeserialize(as = IssueTypeJSONImpl.class)
private IssueType issueType;
private String summary;
private String description;
@JsonDeserialize(as = ResolutionJSONImpl.class)
private Resolution resolution;
@JsonDeserialize(as = PriorityJSONImpl.class)
private Priority priority;
@JsonDeserialize(as = StatusJSONImpl.class)
private Status status;
@JsonDeserialize(as = UserJSONImpl.class)
private User assignee;
@JsonDeserialize(as = CategoryJSONImpl[].class)
private Category[] category;
@JsonDeserialize(as = VersionJSONImpl[].class)
private Version[] versions;
@JsonDeserialize(as = MilestoneJSONImpl[].class)
private Milestone[] milestone;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date startDate;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date dueDate;
@JsonDeserialize(using = JacksonCustomBigdecimalDeserializer.class)
private BigDecimal estimatedHours;
@JsonDeserialize(using = JacksonCustomBigdecimalDeserializer.class)
private BigDecimal actualHours;
private long parentIssueId;
@JsonDeserialize(as = UserJSONImpl.class)
private User createdUser;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date created;
@JsonDeserialize(as = UserJSONImpl.class)
private User updatedUser;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date updated;
@JsonDeserialize(as = CustomFieldJSONImpl[].class)
private CustomField[] customFields;
@JsonDeserialize(as = AttachmentJSONImpl[].class)
private Attachment[] attachments;
@JsonDeserialize(as = SharedFileJSONImpl[].class)
private SharedFile[] sharedFiles;
@JsonDeserialize(as = StarJSONImpl[].class)
private Star[] stars;
@Override
public long getId() {
return this.id;
}
@Override
public String getIdAsString() {
return String.valueOf(this.id);
}
@Override
public String getIssueKey() {
return this.issueKey;
}
@Override
public long getKeyId() {
return this.keyId;
}
@Override
public String getKeyIdAsString() {
return String.valueOf(this.keyId);
}
@Override
public long getProjectId() {
return this.projectId;
}
@Override
public String getProjectIdAsString() {
return String.valueOf(this.projectId);
}
@Override
public IssueType getIssueType() {
return this.issueType;
}
@Override
public String getSummary() {
return this.summary;
}
@Override
public String getDescription() {
return this.description;
}
@Override
public Resolution getResolution() {
return resolution;
}
@Override
public Priority getPriority() {
return priority;
}
@Override
public Status getStatus() {
return status;
}
@Override
public User getAssignee() {
return assignee;
}
@Override
public List<Category> getCategory() {
if(category == null || category.length == 0){
return Collections.emptyList();
}
return Arrays.asList(category);
}
@Override
public List<Version> getVersions() {
if(versions == null || versions.length == 0){
return Collections.emptyList();
}
return Arrays.asList(versions);
}
@Override
public List<Milestone> getMilestone() {
if(milestone == null || milestone.length == 0){
return Collections.emptyList();
}
return Arrays.asList(milestone);
}
@Override
public Date getStartDate() {
return startDate;
}
@Override
public Date getDueDate() {
return dueDate;
}
@Override
public BigDecimal getEstimatedHours() {
return estimatedHours;
}
@Override
public BigDecimal getActualHours() {
return actualHours;
}
@Override
public long getParentIssueId() {
return parentIssueId;
}
@Override
public User getCreatedUser() {
return createdUser;
}
@Override
public Date getCreated() {
return created;
}
@Override
public User getUpdatedUser() {
return updatedUser;
}
@Override
public Date getUpdated() {
return updated;
}
@Override
public List<CustomField> getCustomFields() {
if(customFields == null || customFields.length == 0){
return Collections.emptyList();
}
return Arrays.asList(customFields);
}
@Override
public List<Attachment> getAttachments() {
if(attachments == null || attachments.length == 0){
return Collections.emptyList();
}
return Arrays.asList(attachments);
}
@Override
public List<SharedFile> getSharedFiles() {
if(sharedFiles == null || sharedFiles.length == 0){
return Collections.emptyList();
}
return Arrays.asList(sharedFiles);
}
@Override
public List<Star> getStars() {
if(stars == null || stars.length == 0){
return Collections.emptyList();
}
return Arrays.asList(stars);
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
IssueJSONImpl rhs = (IssueJSONImpl) obj;
return new EqualsBuilder()
.append(this.id, rhs.id)
.append(this.issueKey, rhs.issueKey)
.append(this.keyId, rhs.keyId)
.append(this.projectId, rhs.projectId)
.append(this.issueType, rhs.issueType)
.append(this.summary, rhs.summary)
.append(this.description, rhs.description)
.append(this.resolution, rhs.resolution)
.append(this.priority, rhs.priority)
.append(this.status, rhs.status)
.append(this.assignee, rhs.assignee)
.append(this.category, rhs.category)
.append(this.versions, rhs.versions)
.append(this.milestone, rhs.milestone)
.append(this.startDate, rhs.startDate)
.append(this.dueDate, rhs.dueDate)
.append(this.estimatedHours, rhs.estimatedHours)
.append(this.actualHours, rhs.actualHours)
.append(this.parentIssueId, rhs.parentIssueId)
.append(this.createdUser, rhs.createdUser)
.append(this.created, rhs.created)
.append(this.updatedUser, rhs.updatedUser)
.append(this.updated, rhs.updated)
.append(this.customFields, rhs.customFields)
.append(this.attachments, rhs.attachments)
.append(this.sharedFiles, rhs.sharedFiles)
.append(this.stars, rhs.stars)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(id)
.append(issueKey)
.append(keyId)
.append(projectId)
.append(issueType)
.append(summary)
.append(description)
.append(resolution)
.append(priority)
.append(status)
.append(assignee)
.append(category)
.append(versions)
.append(milestone)
.append(startDate)
.append(dueDate)
.append(estimatedHours)
.append(actualHours)
.append(parentIssueId)
.append(createdUser)
.append(created)
.append(updatedUser)
.append(updated)
.append(customFields)
.append(attachments)
.append(sharedFiles)
.append(stars)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", id)
.append("issueKey", issueKey)
.append("keyId", keyId)
.append("projectId", projectId)
.append("issueType", issueType)
.append("summary", summary)
.append("description", description)
.append("resolution", resolution)
.append("priority", priority)
.append("status", status)
.append("assignee", assignee)
.append("category", category)
.append("versions", versions)
.append("milestone", milestone)
.append("startDate", startDate)
.append("dueDate", dueDate)
.append("estimatedHours", estimatedHours)
.append("actualHours", actualHours)
.append("parentIssueId", parentIssueId)
.append("createdUser", createdUser)
.append("created", created)
.append("updatedUser", updatedUser)
.append("updated", updated)
.append("customFields", customFields)
.append("attachments", attachments)
.append("sharedFiles", sharedFiles)
.append("stars", stars)
.toString();
}
}<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/CreateWikiParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for create wiki API.
*
* @author nulab-inc
*/
public class CreateWikiParams extends PostParams {
public CreateWikiParams(Object projectId, String name, String content) {
parameters.add(new NameValuePair("projectId", projectId.toString()));
parameters.add(new NameValuePair("name", name));
parameters.add(new NameValuePair("content", content));
}
public CreateWikiParams mailNotify(boolean mailNotify) {
parameters.add(new NameValuePair("mailNotify", String.valueOf(mailNotify)));
return this;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/AddIssueTypeParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.BacklogAPIException;
import com.nulabinc.backlog4j.Project;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for add issue type API.
*
* @author nulab-inc
*/
public class AddIssueTypeParams extends PostParams {
private Object projectIdOrKey;
public AddIssueTypeParams(Object projectIdOrKey, String name, Project.IssueTypeColor color){
parameters.add(new NameValuePair("name", name));
parameters.add(new NameValuePair("color", color.getStrValue()));
this.projectIdOrKey = projectIdOrKey;
}
public String getProjectIdOrKeyString() {
return projectIdOrKey.toString();
}
public AddIssueTypeParams templateSummary(String templateSummary) {
parameters.add(new NameValuePair("templateSummary", templateSummary));
return this;
}
public AddIssueTypeParams templateDescription(String templateDescription) {
parameters.add(new NameValuePair("templateDescription", templateDescription));
return this;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/DiskUsageDetailJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.nulabinc.backlog4j.DiskUsageDetail;
import com.nulabinc.backlog4j.User;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class DiskUsageDetailJSONImpl implements DiskUsageDetail {
private long projectId;
private long issue;
private long wiki;
private long file;
private long subversion;
private long git;
@Override
public long getProjectId() {
return projectId;
}
@Override
public long getIssue() {
return issue;
}
@Override
public long getWiki() {
return wiki;
}
@Override
public long getFile() {
return file;
}
@Override
public long getSubversion() {
return subversion;
}
@Override
public long getGit() {
return git;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
DiskUsageDetailJSONImpl rhs = (DiskUsageDetailJSONImpl) obj;
return new EqualsBuilder()
.append(this.projectId, rhs.projectId)
.append(this.issue, rhs.issue)
.append(this.wiki, rhs.wiki)
.append(this.file, rhs.file)
.append(this.subversion, rhs.subversion)
.append(this.git, rhs.git)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(projectId)
.append(issue)
.append(wiki)
.append(file)
.append(subversion)
.append(git)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("projectId", projectId)
.append("issue", issue)
.append("wiki", wiki)
.append("file", file)
.append("subversion", subversion)
.append("git", git)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/SharedFileData.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog activity shared file data.
*
* @author nulab-inc
*/
public interface SharedFileData extends FileData{
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/GitMethods.java
package com.nulabinc.backlog4j.api;
import com.nulabinc.backlog4j.BacklogException;
import com.nulabinc.backlog4j.Repository;
import com.nulabinc.backlog4j.ResponseList;
/**
* Executes Backlog Git APIs.
*
* @author nulab-inc
*/
public interface GitMethods {
/**
* Returns the git repositories in the project.
*
* @param projectIdOrKey the project identifier
* @return the git repositories in a list.
* @throws BacklogException
*/
ResponseList<Repository> getGitRepositories(Object projectIdOrKey) throws BacklogException;
/**
* Returns the git repository.
*
* @param projectIdOrKey the project identifier
* @param repoIdOrName the repository name
* @return the git repository.
* @throws BacklogException
*/
Repository getGitRepository(Object projectIdOrKey, Object repoIdOrName) throws BacklogException;
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdatePullRequestParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for update pull request API.
*
* @author nulab-inc
*/
public class UpdatePullRequestParams extends PatchParams {
private Object projectIdOrKey;
private Object repoIdOrName;
private Object number;
/**
* Constructor
*
* @param projectIdOrKey the project identifier
* @param repoIdOrName the repository identifier
* @param number the pull request title
*/
public UpdatePullRequestParams(Object projectIdOrKey, Object repoIdOrName, Object number) {
this.projectIdOrKey = projectIdOrKey;
this.repoIdOrName = repoIdOrName;
this.number = number;
}
public String getProjectIdOrKeyString() {
return projectIdOrKey.toString();
}
public String getRepoIdOrNameString() {
return repoIdOrName.toString();
}
public String getNumber() {
return this.number.toString();
}
/**
* Sets the summary.
*
* @param summary the pull request description
* @return UpdatePullRequestParams instance
*/
public UpdatePullRequestParams summary(String summary) {
parameters.add(new NameValuePair("summary", summary));
return this;
}
/**
* Sets the description.
*
* @param description the pull request description
* @return UpdatePullRequestParams instance
*/
public UpdatePullRequestParams description(String description) {
parameters.add(new NameValuePair("description", description));
return this;
}
/**
* Sets the base parameter.
*
* @param base the base branch name
* @return UpdatePullRequestParams instance
*/
public UpdatePullRequestParams base(String base) {
parameters.add(new NameValuePair("base", base));
return this;
}
/**
* Sets the branch parameter.
*
* @param branch the branch name merge to
* @return UpdatePullRequestParams instance
*/
public UpdatePullRequestParams branch(String branch) {
parameters.add(new NameValuePair("branch", branch));
return this;
}
/**
* Sets the related issue parameter.
*
* @param issueId the isssue identifier
* @return UpdatePullRequestParams instance
*/
public UpdatePullRequestParams issueId(long issueId) {
parameters.add(new NameValuePair("issueId", String.valueOf(issueId)));
return this;
}
/**
* Sets the pull request assignee user.
*
* @param assigneeId the assignee user id
* @return UpdatePullRequestParams instance
*/
public UpdatePullRequestParams assigneeId(long assigneeId) {
parameters.add(new NameValuePair("assigneeId", String.valueOf(assigneeId)));
return this;
}
/**
* Sets the pull request notified users.
*
* @param notifiedUserIds notified user identifiers
* @return UpdatePullRequestParams instance
*/
public UpdatePullRequestParams notifiedUserIds(List<Long> notifiedUserIds) {
for (Long notifiedUserId : notifiedUserIds) {
parameters.add(new NameValuePair("notifiedUserId[]", notifiedUserId.toString()));
}
return this;
}
/**
* Sets the pull request attachment files.
*
* @param attachmentIds the attachment file identifiers
* @return UpdatePullRequestParams instance
*/
public UpdatePullRequestParams attachmentIds(List<Long> attachmentIds) {
for (Long attachmentId : attachmentIds) {
parameters.add(new NameValuePair("attachmentId[]", attachmentId.toString()));
}
return this;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/ViewedIssue.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog viewed issue data.
*
* @author nulab-inc
*/
public interface ViewedIssue {
Issue getIssue();
Date getUpdated();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/SpaceNotification.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog space notification data.
*
* @author nulab-inc
*/
public interface SpaceNotification {
String getContent();
Date getUpdated();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/BacklogAuthErrorMessage.java
package com.nulabinc.backlog4j;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* The error message class for Backlog auth exception.
*
* @author nulab-inc
*/
public class BacklogAuthErrorMessage {
private String error;
@JsonProperty("error_description")
private String description;
public String getError() {
return error;
}
public String getDescription() {
return description;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/AddCustomFieldParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.BacklogAPIException;
import com.nulabinc.backlog4j.CustomFieldSetting;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for add custom field API.
*
* @author nulab-inc
*/
public abstract class AddCustomFieldParams extends PostParams {
private Object projectIdOrKey;
public AddCustomFieldParams(Object projectIdOrKey, CustomFieldSetting.FieldType fieldType, String name){
this.projectIdOrKey = projectIdOrKey;
parameters.add(new NameValuePair("typeId", String.valueOf(fieldType.getIntValue())));
parameters.add(new NameValuePair("name", name));
}
public String getProjectIdOrKeyString() {
return projectIdOrKey.toString();
}
public AddCustomFieldParams applicableIssueTypes(List<Long> applicableIssueTypes) {
for (Long applicableIssueType :applicableIssueTypes) {
parameters.add(new NameValuePair("applicableIssueTypes[]", String.valueOf(applicableIssueType)));
}
return this;
}
public AddCustomFieldParams description(String description) {
parameters.add(new NameValuePair("description", description));
return this;
}
public AddCustomFieldParams required(boolean required) {
parameters.add(new NameValuePair("required", String.valueOf(required)));
return this;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/auth/OnAccessTokenRefreshListener.java
package com.nulabinc.backlog4j.auth;
/**
* @author nulab-inc
*/
public class OnAccessTokenRefreshListener {
public void onAccessTokenRefresh(AccessToken accessToken){
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/CreateGroupParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for create group API.
*
* @author nulab-inc
*/
public class CreateGroupParams extends PostParams {
public CreateGroupParams(String name){
parameters.add(new NameValuePair("name", name));
}
public CreateGroupParams members(List members) {
for (Object member : members) {
parameters.add(new NameValuePair("members[]", member.toString()));
}
return this;
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/internal/json/WikiJSONImplTest.java
package com.nulabinc.backlog4j.internal.json;
import com.nulabinc.backlog4j.Attachment;
import com.nulabinc.backlog4j.ResponseList;
import com.nulabinc.backlog4j.SharedFile;
import com.nulabinc.backlog4j.Star;
import com.nulabinc.backlog4j.User;
import com.nulabinc.backlog4j.Wiki;
import com.nulabinc.backlog4j.WikiTag;
import org.exparity.hamcrest.date.DateMatchers;
import org.junit.jupiter.api.Test;
import java.io.IOException;
import java.util.Calendar;
import java.util.List;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNull;
/**
* @author nulab-inc
*/
public class WikiJSONImplTest extends AbstractJSONImplTest {
@Test
public void createWikiListTest() throws IOException {
String fileContentStr = getJsonString("json/wikis.json");
ResponseList<Wiki> wikis = factory.createWikiList(fileContentStr);
assertEquals(2, wikis.size());
}
@Test
public void createWikiTest() throws IOException {
String fileContentStr = getJsonString("json/wiki.json");
Wiki wiki = factory.createWiki(fileContentStr);
assertEquals(1073768473, wiki.getId());
assertEquals(1073760709, wiki.getProjectId());
assertEquals("テストwiki", wiki.getName());
assertEquals("テスト用wikiです", wiki.getContent());
List<WikiTag> tags = wiki.getTags();
assertEquals(2, tags.size());
assertEquals(4677, tags.get(0).getId());
assertEquals("tag1", tags.get(0).getName());
List<Attachment> attachments = wiki.getAttachments();
assertEquals(1, attachments.size());
List<SharedFile> sharedFiles = wiki.getSharedFiles();
assertEquals(1, sharedFiles.size());
List<Star> stars = wiki.getStars();
assertEquals(2, stars.size());
User user = wiki.getCreatedUser();
assertEquals(1073751781, user.getId());
assertEquals("test_admin", user.getUserId());
assertEquals("あどみにさん", user.getName());
assertEquals(User.RoleType.Admin, user.getRoleType());
assertNull(user.getLang());
assertEquals("<EMAIL>", user.getMailAddress());
Calendar calendar = Calendar.getInstance();
calendar.set(2014, Calendar.AUGUST, 5, 0, 59, 45);
assertThat(calendar.getTime(), DateMatchers.sameDay(wiki.getCreated()));
User updateUser = wiki.getUpdatedUser();
assertEquals(1073751782, updateUser.getId());
assertEquals("test_admin2", updateUser.getUserId());
assertEquals("あどみにさん2", updateUser.getName());
assertEquals(User.RoleType.Admin, updateUser.getRoleType());
assertNull(updateUser.getLang());
assertEquals("<EMAIL>", updateUser.getMailAddress());
calendar = Calendar.getInstance();
calendar.set(2014, Calendar.AUGUST, 5, 1, 0, 11);
assertThat(calendar.getTime(), DateMatchers.sameDay(wiki.getUpdated()));
}
@Test
public void equalsTest() throws IOException {
String fileContentStr = getJsonString("json/wiki.json");
Wiki wiki1 = factory.createWiki(fileContentStr);
Wiki wiki2 = factory.createWiki(fileContentStr);
assertEquals(wiki1, wiki2);
}
@Test
public void hashCodeTest() throws IOException {
String fileContentStr = getJsonString("json/wiki.json");
Wiki wiki1 = factory.createWiki(fileContentStr);
Wiki wiki2 = factory.createWiki(fileContentStr);
assertEquals(wiki1.hashCode(), wiki2.hashCode());
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/internal/json/PullRequestJSONImplTest.java
package com.nulabinc.backlog4j.internal.json;
import com.nulabinc.backlog4j.Issue;
import com.nulabinc.backlog4j.PullRequest;
import com.nulabinc.backlog4j.PullRequestStatus;
import com.nulabinc.backlog4j.ResponseList;
import com.nulabinc.backlog4j.User;
import org.exparity.hamcrest.date.DateMatchers;
import org.junit.jupiter.api.Test;
import java.io.IOException;
import java.util.Calendar;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.junit.jupiter.api.Assertions.assertEquals;
/**
* @author nulab-inc
*/
public class PullRequestJSONImplTest extends AbstractJSONImplTest {
@Test
public void createPullRequestListTest() throws IOException {
String fileContentStr = getJsonString("json/pull_requests.json");
ResponseList<PullRequest> pullRequests = factory.createPullRequestList(fileContentStr);
assertEquals(1, pullRequests.size());
PullRequest pullRequest = pullRequests.get(0);
assertEquals(2, pullRequest.getId());
assertEquals(3, pullRequest.getProjectId());
assertEquals(4, pullRequest.getRepositoryId());
assertEquals(1, pullRequest.getNumber());
assertEquals("test", pullRequest.getSummary());
assertEquals("test data", pullRequest.getDescription());
assertEquals("master", pullRequest.getBase());
assertEquals("develop", pullRequest.getBranch());
PullRequestStatus pullRequestStatus = pullRequest.getStatus();
assertEquals(1, pullRequestStatus.getId());
assertEquals("Open", pullRequestStatus.getName());
User assignee = pullRequest.getAssignee();
assertEquals(5, assignee.getId());
Issue issue = pullRequest.getIssue();
assertEquals(31, issue.getId());
// todo add test
/*
"mergeCommit": null,
"baseCommit": null,
"branchCommit": null,
"closeAt": null,
"mergeAt": null,
*/
User user = pullRequest.getCreatedUser();
assertEquals(1, user.getId());
Calendar calendar = Calendar.getInstance();
calendar.set(2015, Calendar.APRIL, 23, 0, 0, 0);
assertThat(calendar.getTime(), DateMatchers.sameDay(pullRequest.getCreated()));
User updateUser = pullRequest.getUpdatedUser();
assertEquals(2, updateUser.getId());
calendar = Calendar.getInstance();
calendar.set(2015, Calendar.APRIL, 24, 0, 0, 0);
assertThat(calendar.getTime(), DateMatchers.sameDay(pullRequest.getUpdated()));
assertEquals(1, pullRequest.getAttachments().size());
assertEquals(2, pullRequest.getStars().size());
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/GetWatchesParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for get watch API.
*
* @author nulab-inc
*/
public class GetWatchesParams extends GetParams {
public GetWatchesParams alreadyRead(boolean alreadyRead) {
parameters.add(new NameValuePair("alreadyRead", String.valueOf(alreadyRead)));
return this;
}
public GetWatchesParams resourceAlreadyRead(boolean resourceAlreadyRead) {
parameters.add(new NameValuePair("resourceAlreadyRead", String.valueOf(resourceAlreadyRead)));
return this;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/GroupMethods.java
package com.nulabinc.backlog4j.api;
import com.nulabinc.backlog4j.BacklogException;
import com.nulabinc.backlog4j.Group;
import com.nulabinc.backlog4j.ResponseList;
import com.nulabinc.backlog4j.api.option.CreateGroupParams;
import com.nulabinc.backlog4j.api.option.OffsetParams;
import com.nulabinc.backlog4j.api.option.UpdateGroupParams;
/**
* Executes Backlog Group APIs.
*
* @author nulab-inc
*/
public interface GroupMethods {
/**
* Returns all the groups.
*
* @return the groups in a list.
* @throws BacklogException
*/
ResponseList<Group> getGroups() throws BacklogException;
/**
* Returns all the groups.
*
* @param params the offset parameters
* @return the groups in a list.
* @throws BacklogException
*/
ResponseList<Group> getGroups(OffsetParams params) throws BacklogException;
/**
* Creates a group.
*
* @param params the group creating parameters
* @return the created Group
* @throws BacklogException
*/
Group createGroup(CreateGroupParams params) throws BacklogException;
/**
* Returns the groups identified by the group's id.
*
* @param groupId the group identifier
* @return the Group.
* @throws BacklogException
*/
Group getGroup(Object groupId) throws BacklogException;
/**
* Updates the existing group.
*
* @param params the group updating parameters
* @return the updated Group.
* @throws BacklogException
*/
Group updateGroup(UpdateGroupParams params) throws BacklogException;
/**
* Deletes the existing groups.
*
* @param groupId the group identifier
* @return the deleted Group.
* @throws BacklogException
*/
Group deleteGroup(Object groupId) throws BacklogException;
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/CountJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
/**
* @author nulab-inc
*/
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.nulabinc.backlog4j.Count;
import com.nulabinc.backlog4j.User;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class CountJSONImpl implements Count {
private int count;
public int getCount() {
return count;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
CountJSONImpl rhs = (CountJSONImpl) obj;
return new EqualsBuilder()
.append(this.count, rhs.count)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(count)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("count", count)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/WikiHistory.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog Wiki page history data.
*
* @author nulab-inc
*/
public interface WikiHistory {
long getPageId();
String getPageIdAsString();
int getVersion();
String getName();
String getContent();
User getCreatedUser();
Date getCreated();
User getUpdatedUser();
Date getUpdated();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Resolution.java
package com.nulabinc.backlog4j;
/**
* The interface for Backlog resolution data.
*
* @author nulab-inc
*/
public interface Resolution {
long getId();
String getIdAsString();
String getName();
Issue.ResolutionType getResolutionType();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/ResolutionMethods.java
package com.nulabinc.backlog4j.api;
import com.nulabinc.backlog4j.BacklogException;
import com.nulabinc.backlog4j.Resolution;
import com.nulabinc.backlog4j.ResponseList;
/**
* Executes Backlog Resolution APIs.
*
* @author nulab-inc
*/
public interface ResolutionMethods {
/**
* Returns the resolutions.
*
* @return the resolutions in a list
* @throws BacklogException
*/
ResponseList<Resolution> getResolutions() throws BacklogException;
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/GetNotificationCountParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for get notification API.
*
* @author nulab-inc
*/
public class GetNotificationCountParams extends GetParams {
public GetNotificationCountParams alreadyRead(boolean alreadyRead) {
parameters.add(new NameValuePair("alreadyRead", String.valueOf(alreadyRead)));
return this;
}
public GetNotificationCountParams resourceAlreadyRead(boolean resourceAlreadyRead) {
parameters.add(new NameValuePair("resourceAlreadyRead", String.valueOf(resourceAlreadyRead)));
return this;
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/WikiMethods.java
package com.nulabinc.backlog4j.api;
import com.nulabinc.backlog4j.*;
import com.nulabinc.backlog4j.api.option.*;
import java.util.List;
/**
* Executes Backlog Wiki APIs.
*
* @author nulab-inc
*/
public interface WikiMethods {
/**
* Returns Wiki pages in the project.
*
* @param projectIdOrKey the project identifier
* @return the Wiki pages in a list
*/
ResponseList<Wiki> getWikis(Object projectIdOrKey);
/**
* Returns Wiki pages in the project.
*
* @param params the finding wiki parameters
* @return the Wiki pages in a list
*/
ResponseList<Wiki> getWikis(GetWikisParams params);
/**
* Returns Wiki pages count.
*
* @param projectIdOrKey the project identifier
* @return the Wiki pages count
*/
int getWikiCount(Object projectIdOrKey);
/**
* Returns Wiki page's tags in the project.
*
* @param projectIdOrKey the project identifier
* @return the Wiki page's tags in a list
*/
ResponseList<WikiTag> getWikiTags(Object projectIdOrKey);
/**
* Create a Wiki page in the project.
*
* @param params the creating Wiki page parameters
* @return the created Wiki page
*/
Wiki createWiki(CreateWikiParams params);
/**
* Returns the Wiki page.
*
* @param wikiId the Wiki page identifier
* @return the Wiki page
*/
Wiki getWiki(Object wikiId);
/**
* Updates an existing Wiki page in the project.
*
* @param params the updating Wiki page parameters
* @return the updated Wiki page
*/
Wiki updateWiki(UpdateWikiParams params);
/**
* Deletes the Wiki page.
*
* @param wikiId the Wiki page identifier
* @param mailNotify
* @return the deleted Wiki page
*/
Wiki deleteWiki(Object wikiId, boolean mailNotify);
// Wiki添付ファイル一覧の取得
/**
* Returns the Wiki page's attachment files.
*
* @param wikiId the Wiki page identifier
* @return the Wiki page's attachment files in a list
*/
ResponseList<Attachment> getWikiAttachments(Object wikiId);
/**
* Attaches the files to the Wiki page.
*
* @param params the Wiki page's attachment parameters
* @return the added Wiki page's attachment file
*/
ResponseList<Attachment> addWikiAttachment(AddWikiAttachmentParams params);
/**
* Downloads the Wiki page's attachment file.
*
* @param wikiId the Wiki page identifier
* @param attachmentId the attachment file identifier
* @return downloaded file data
*/
AttachmentData downloadWikiAttachment(Object wikiId, Object attachmentId);
/**
* Deletes the Wiki page's attachment file
*
* @param wikiId the Wiki page identifier
* @param attachmentId the attachment file identifier
* @return deleted Wiki page's attachment file
*/
Attachment deleteWikiAttachment(Object wikiId, Object attachmentId);
/**
* Returns the Wiki page's shared files.
*
* @param wikiId the Wiki page identifier
* @return the Wiki page's shared files in a list
*/
ResponseList<SharedFile> getWikiSharedFiles(Object wikiId);
/**
* Links the shared files to Wiki.
*
* @param wikiId the Wiki page identifier
* @param fileIds the shared file identifiers
* @return the linked shared files
*/
ResponseList<SharedFile> linkWikiSharedFile(Object wikiId, List fileIds);
/**
* Removes link to shared Files from the Wiki.
*
* @param wikiId the Wiki page identifier
* @param fileId the shared file identifier
* @return the removed link shared file
*/
SharedFile unlinkWikiSharedFile(Object wikiId, Object fileId);
/**
* Returns history of the Wiki page.
*
* @param wikiId the Wiki page identifier
* @return the wiki histories in a list
*/
ResponseList<WikiHistory> getWikiHistories(Object wikiId);
/**
* Returns history of the Wiki page.
*
* @param wikiId the Wiki page identifier
* @param queryParams the query parameters
* @return the wiki histories in a list
*/
ResponseList<WikiHistory> getWikiHistories(Object wikiId, QueryParams queryParams);
/**
* Returns list of stars received on the Wiki page.
*
* @param wikiId the Wiki page identifier
* @return the wiki stars in a list
*/
ResponseList<Star> getWikiStars(Object wikiId);
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/customFields/NumericCustomField.java
package com.nulabinc.backlog4j.internal.json.customFields;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.nulabinc.backlog4j.internal.json.JacksonCustomBigdecimalDeserializer;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.math.BigDecimal;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class NumericCustomField extends CustomFieldJSONImpl {
private int fieldTypeId = 3;
@JsonDeserialize(using = JacksonCustomBigdecimalDeserializer.class)
private BigDecimal value;
@Override
public int getFieldTypeId() {
return fieldTypeId;
}
@Override
public FieldType getFieldType() {
return FieldType.valueOf(fieldTypeId);
}
public BigDecimal getValue() {
return value;
}
public float getFloatValue() {
return value.floatValue();
}
public int getIntValue() {
return value.intValue();
}
public long getLongValue() {
return value.longValue();
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
NumericCustomField rhs = (NumericCustomField) obj;
return new EqualsBuilder()
.append(getId(), rhs.getId())
.append(getName(), rhs.getName())
.append(this.fieldTypeId, rhs.fieldTypeId)
.append(this.value, rhs.value)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(getId())
.append(getName())
.append(fieldTypeId)
.append(value)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", getId())
.append("name", getName())
.append("fieldTypeId", fieldTypeId)
.append("value", value)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/GetRepositoriesParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
/**
* Parameters for get repositories API.
*
* @author nulab-inc
*/
public class GetRepositoriesParams extends GetParams {
public GetRepositoriesParams(long projectId) {
parameters.add(new NameValuePair("projectIdOrKey", String.valueOf(projectId)));
}
public GetRepositoriesParams(String projectKey) {
parameters.add(new NameValuePair("projectIdOrKey", projectKey));
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/PriorityMethods.java
package com.nulabinc.backlog4j.api;
import com.nulabinc.backlog4j.BacklogException;
import com.nulabinc.backlog4j.Priority;
import com.nulabinc.backlog4j.ResponseList;
/**
* Executes Backlog Priority APIs.
*
* @author nulab-inc
*/
public interface PriorityMethods {
/**
* Returns the priorities.
*
* @return the priorities in a list.
* @throws BacklogException
*/
ResponseList<Priority> getPriorities() throws BacklogException;
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/ViewedProjectJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.nulabinc.backlog4j.Project;
import com.nulabinc.backlog4j.User;
import com.nulabinc.backlog4j.ViewedProject;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.Date;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class ViewedProjectJSONImpl implements ViewedProject {
@JsonDeserialize(as = ProjectJSONImpl.class)
private Project project;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date updated;
@Override
public Project getProject() {
return project;
}
@Override
public Date getUpdated() {
return updated;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
ViewedProjectJSONImpl rhs = (ViewedProjectJSONImpl) obj;
return new EqualsBuilder()
.append(this.project, rhs.project)
.append(this.updated, rhs.updated)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(project)
.append(updated)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("project", project)
.append("updated", updated)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Webhook.java
package com.nulabinc.backlog4j;
import java.util.Date;
import java.util.List;
/**
* The interface for Backlog webhook data.
*
* @author nulab-inc
*/
public interface Webhook {
long getId();
String getIdAsString();
String getName();
String getDescription();
String getHookUrl();
boolean isAllEvent();
List<Activity.Type> getActivityTypeIds();
User getCreatedUser();
Date getCreated();
User getUpdatedUser();
Date getUpdated();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdateOrderOfStatusParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for updates order about status API.
*
* @author nulab-inc
*/
public class UpdateOrderOfStatusParams extends PatchParams {
private Object projectIdOrKey;
public UpdateOrderOfStatusParams(Object projectIdOrKey, List statusIds) {
this.projectIdOrKey = projectIdOrKey;
for (Object statusId : statusIds) {
parameters.add(new NameValuePair("statusId[]", statusId.toString()));
}
}
public String getProjectIdOrKeyString() {
return projectIdOrKey.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/activities/GitPushedContent.java
package com.nulabinc.backlog4j.internal.json.activities;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.nulabinc.backlog4j.Content;
import com.nulabinc.backlog4j.Repository;
import com.nulabinc.backlog4j.Revision;
import com.nulabinc.backlog4j.internal.json.RepositoryJSONImpl;
import com.nulabinc.backlog4j.internal.json.RevisionJSONImpl;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.Arrays;
import java.util.List;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class GitPushedContent extends Content {
@JsonProperty("change_type")
private String changeType;
private String ref;
@JsonProperty("revision_type")
private String revisionType;
@JsonDeserialize(as=RepositoryJSONImpl.class)
private Repository repository;
@JsonDeserialize(as=RevisionJSONImpl[].class)
private Revision[] revisions;
@JsonProperty("revision_count")
private long revisionCount;
public String getChangeType() {
return changeType;
}
public String getRef() {
return ref;
}
public String getRevisionType() {
return revisionType;
}
public Repository getRepository() {
return repository;
}
public List<Revision> getRevisions() {
return Arrays.asList(revisions);
}
public long getRevisionCount() {
return revisionCount;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
GitPushedContent rhs = (GitPushedContent) obj;
return new EqualsBuilder()
.append(this.changeType, rhs.changeType)
.append(this.ref, rhs.ref)
.append(this.revisionType, rhs.revisionType)
.append(this.repository, rhs.repository)
.append(this.revisions, rhs.revisions)
.append(this.revisionCount, rhs.revisionCount)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(changeType)
.append(ref)
.append(revisionType)
.append(repository)
.append(revisions)
.append(revisionCount)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("changeType", changeType)
.append("ref", ref)
.append("revisionType", revisionType)
.append("repository", repository)
.append("revisions", revisions)
.append("revisionCount", revisionCount)
.toString();
}
}
<file_sep>/src/test/java/com/nulabinc/backlog4j/internal/json/AccessTokenJSONImplTest.java
package com.nulabinc.backlog4j.internal.json;
import com.nulabinc.backlog4j.auth.AccessToken;
import org.junit.jupiter.api.Test;
import java.io.IOException;
import static org.junit.jupiter.api.Assertions.assertEquals;
/**
* @author nulab-inc
*/
public class AccessTokenJSONImplTest extends AbstractJSONImplTest {
@Test
public void createAccessTokenTest() throws IOException {
String fileContentStr = getJsonString("json/access_token.json");
AccessToken accessToken = factory.createAccessToken(fileContentStr);
assertEquals("Bearer", accessToken.getType());
assertEquals("<KEY>", accessToken.getToken());
assertEquals(3600, accessToken.getExpires().longValue());
assertEquals("<KEY>", accessToken.getRefresh());
}
@Test
public void equalsTest() throws IOException {
String fileContentStr = getJsonString("json/access_token.json");
AccessToken accessToken1 = factory.createAccessToken(fileContentStr);
AccessToken accessToken2 = factory.createAccessToken(fileContentStr);
assertEquals(accessToken1, accessToken2);
}
@Test
public void hashCodeTest() throws IOException {
String fileContentStr = getJsonString("json/access_token.json");
AccessToken accessToken1 = factory.createAccessToken(fileContentStr);
AccessToken accessToken2 = factory.createAccessToken(fileContentStr);
assertEquals(accessToken1.hashCode(), accessToken2.hashCode());
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/Attachment.java
package com.nulabinc.backlog4j;
import java.util.Date;
/**
* The interface for Backlog attachment file.
*
* @author nulab-inc
*/
public interface Attachment {
long getId();
String getIdAsString();
String getName();
long getSize();
User getCreatedUser();
Date getCreated();
boolean isImage();
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/internal/json/SharedFileJSONImpl.java
package com.nulabinc.backlog4j.internal.json;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.nulabinc.backlog4j.Revision;
import com.nulabinc.backlog4j.SharedFile;
import com.nulabinc.backlog4j.User;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.Date;
/**
* @author nulab-inc
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class SharedFileJSONImpl implements SharedFile {
private long id;
private String type;
private String name;
private String dir;
private long size;
@JsonDeserialize(as=UserJSONImpl.class)
private User createdUser;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date created;
@JsonDeserialize(as=UserJSONImpl.class)
private User updatedUser;
@JsonDeserialize(using = JacksonCustomDateDeserializer.class)
private Date updated;
@Override
public long getId() {
return id;
}
@Override
public String getIdAsString() {
return String.valueOf(this.id);
}
@Override
public String getType() {
return type;
}
@Override
public String getName() {
return name;
}
@Override
public boolean isImage() {
String lowerCase = name.toLowerCase();
return (lowerCase.endsWith(".jpg") ||
lowerCase.endsWith(".jpeg") ||
lowerCase.endsWith(".png") ||
lowerCase.endsWith(".gif"));
}
@Override
public String getDir() {
return dir;
}
@Override
public long getSize() {
return size;
}
@Override
public User getCreatedUser() {
return createdUser;
}
@Override
public Date getCreated() {
return created;
}
@Override
public User getUpdatedUser() {
return updatedUser;
}
@Override
public Date getUpdated() {
return updated;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
SharedFileJSONImpl rhs = (SharedFileJSONImpl) obj;
return new EqualsBuilder()
.append(this.id, rhs.id)
.append(this.type, rhs.type)
.append(this.name, rhs.name)
.append(this.dir, rhs.dir)
.append(this.size, rhs.size)
.append(this.createdUser, rhs.createdUser)
.append(this.created, rhs.created)
.append(this.updatedUser, rhs.updatedUser)
.append(this.updated, rhs.updated)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(id)
.append(type)
.append(name)
.append(dir)
.append(size)
.append(createdUser)
.append(created)
.append(updatedUser)
.append(updated)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", id)
.append("type", type)
.append("name", name)
.append("dir", dir)
.append("size", size)
.append("createdUser", createdUser)
.append("created", created)
.append("updatedUser", updatedUser)
.append("updated", updated)
.toString();
}
}
<file_sep>/src/main/java/com/nulabinc/backlog4j/api/option/UpdateCheckBoxCustomFieldParams.java
package com.nulabinc.backlog4j.api.option;
import com.nulabinc.backlog4j.http.NameValuePair;
import java.util.List;
/**
* Parameters for update checkbox type custom field API.
*
* @author nulab-inc
*/
public class UpdateCheckBoxCustomFieldParams extends UpdateCustomFieldParams {
public UpdateCheckBoxCustomFieldParams(Object projectIdOrKey, Object customFiledId) {
super(projectIdOrKey, customFiledId);
}
public UpdateCheckBoxCustomFieldParams items(List<String> items) {
if(items != null && items.size() > 0) {
for (String item : items) {
parameters.add(new NameValuePair("items[]", item));
}
}else{
parameters.add(new NameValuePair("items[]", ""));
}
return this;
}
public UpdateCheckBoxCustomFieldParams allowInput(boolean allowInput) {
parameters.add(new NameValuePair("allowInput", String.valueOf(allowInput)));
return this;
}
public UpdateCheckBoxCustomFieldParams allowAddItem(boolean allowAddItem) {
parameters.add(new NameValuePair("allowAddItem", String.valueOf(allowAddItem)));
return this;
}
@Override
public UpdateCheckBoxCustomFieldParams applicableIssueTypes(List<Long> applicableIssueTypes) {
return (UpdateCheckBoxCustomFieldParams)super.applicableIssueTypes(applicableIssueTypes);
}
@Override
public UpdateCheckBoxCustomFieldParams description(String description) {
return (UpdateCheckBoxCustomFieldParams)super.description(description);
}
@Override
public UpdateCheckBoxCustomFieldParams required(boolean required) {
return (UpdateCheckBoxCustomFieldParams)super.required(required);
}
}
| 3cd21e5a20d94df4b601e306b9efa42f207ec9d7 | [
"Markdown",
"Java",
"Gradle"
]
| 116 | Gradle | nulab/backlog4j | 21d1a60ffc3cb0ef6e132fe54a8f8508e91f40fc | 9209b66b1120ad30066ecab65dcb875695cdbbe6 | |
refs/heads/master | <repo_name>erikterwiel/FaceLock<file_sep>/android/app/src/main/java/erikterwiel/phoneprotection/Activities/SettingsActivity.java
package erikterwiel.phoneprotection.Activities;
import android.content.Context;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
import android.widget.SeekBar;
import android.widget.Switch;
import android.widget.TextView;
import android.widget.Toast;
import erikterwiel.phoneprotection.R;
public class SettingsActivity extends AppCompatActivity {
private static final String TAG = "SettingsActivity.java";
SharedPreferences mDatabase;
SharedPreferences.Editor mDatabaseEditor;
private MenuItem mDone;
private SeekBar mScanBar;
private TextView mScanDisplay;
private SeekBar mSafeBar;
private TextView mSafeDisplay;
private Switch mSirenToggle;
private Switch mMaxToggle;
@Override
protected void onCreate(Bundle savedInstanceState) {
Log.i(TAG, "onCreate() called");
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_settings);
mDatabase = getSharedPreferences("settings", Context.MODE_PRIVATE);
mDatabaseEditor = mDatabase.edit();
mScanBar = findViewById(R.id.settings_scan_frequency_bar);
mScanDisplay = findViewById(R.id.settings_scan_frequency_display);
mSafeBar = findViewById(R.id.settings_safe_mode_duration_bar);
mSafeDisplay = findViewById(R.id.settings_safe_mode_duration_display);
mSirenToggle = findViewById(R.id.settings_siren_toggle);
mMaxToggle = findViewById(R.id.settings_max_volume_toggle);
mScanBar.setProgress((int) (Math.sqrt(mDatabase.getInt("scan_frequency", 10000) / 1000 - 10) / 0.298329));
mScanDisplay.setText(((int) Math.pow(0.298329 * mScanBar.getProgress(), 2) + 10) + "s");
mScanBar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int i, boolean b) {
int frequency = (int) (Math.pow(0.298329 * i, 2)) + 10;
mDatabaseEditor.putInt("scan_frequency", frequency * 1000);
mScanDisplay.setText(frequency + "s");
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {}
});
mSafeBar.setProgress((int) ((mDatabase.getInt("safe_duration", 60000) / 60 / 1000 - 1) / 0.59));
mSafeDisplay.setText(((int) (0.59 * mSafeBar.getProgress()) + 1) + "m");
mSafeBar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int i, boolean b) {
int frequency = (int) (0.59 * i) + 1;
mDatabaseEditor.putInt("safe_duration", frequency * 60 * 1000);
mSafeDisplay.setText(frequency + "m");
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {}
});
mSirenToggle.setChecked(mDatabase.getBoolean("siren", false));
mSirenToggle.setOnCheckedChangeListener((compoundButton, isChecked) -> {
mDatabaseEditor.putBoolean("siren", isChecked);
});
mMaxToggle.setChecked(mDatabase.getBoolean("max", false));
mMaxToggle.setOnCheckedChangeListener((compoundButton, isChecked) -> {
mDatabaseEditor.putBoolean("max", isChecked);
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater menuInflater = getMenuInflater();
menuInflater.inflate(R.menu.settings_done, menu);
mDone = menu.findItem(R.id.settings_done);
mDone.setOnMenuItemClickListener((menuItem) -> {
mDatabaseEditor.apply();
Log.i(TAG, "Saved scan_frequency = " + mDatabase.getInt("scan_frequency", 0));
Toast.makeText(SettingsActivity.this, "Settings saved", Toast.LENGTH_LONG).show();
finish();
return false;
});
return super.onCreateOptionsMenu(menu);
}
}
<file_sep>/README.md
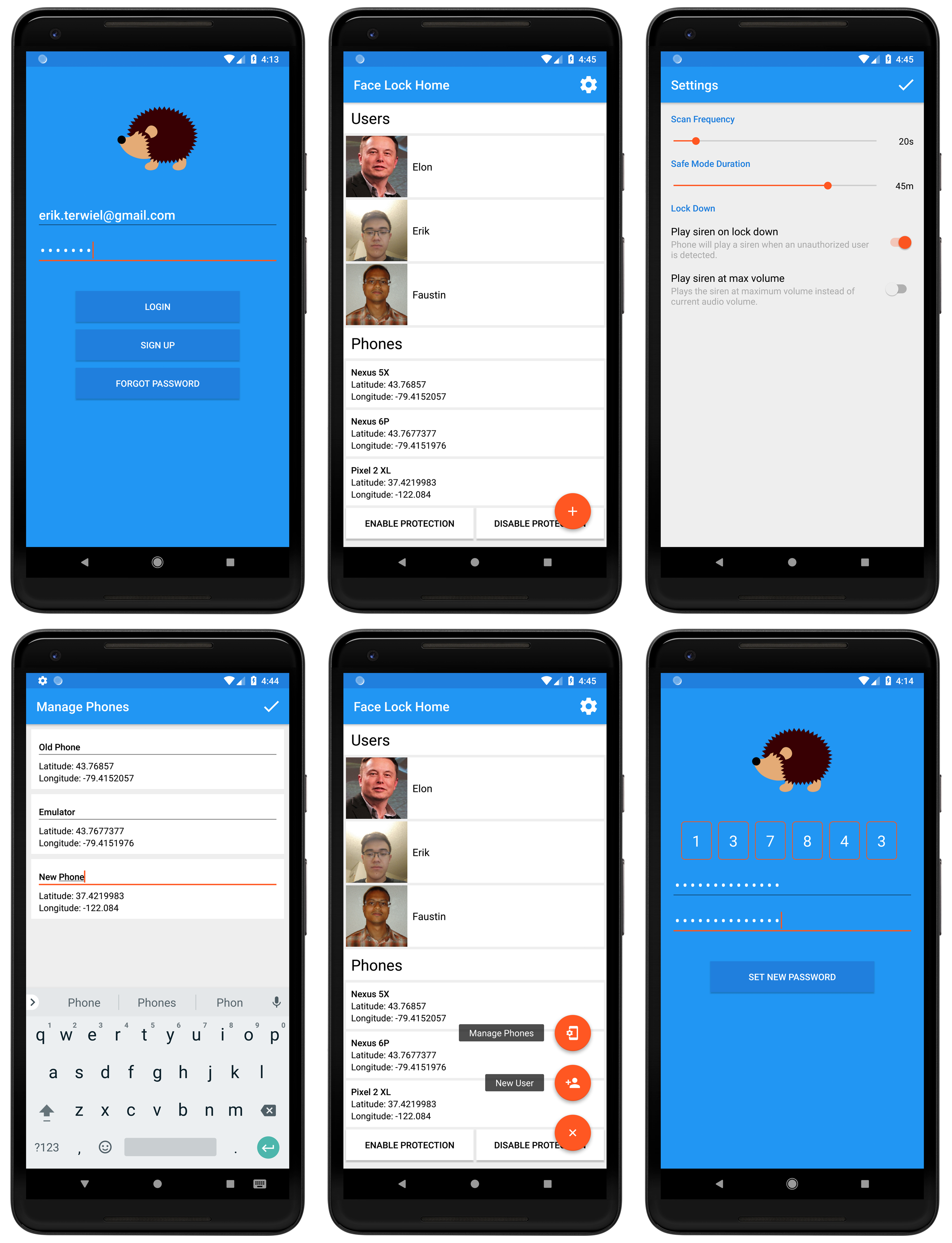
# Face Lock
Face Lock is an Android app/service uses facial recognition to lock the device when an unauthorized face is using it. The faces of close ones can be added to the accepted list and the feature can be toggled on and off to provide our users with utmost versatility for their unique needs. Further, if the phone has been stolen and locked, the user will immediately receive an email notification and will be able to log into our companion website for further support. On the website, users will be able to access their phone's location services to track down the phone and see updated photos taken by the front camera of the potential thief. Not only does this technology protect data and increase the chances of reclaiming a stolen phone, its proliferation will discourage phone theft.
Face Lock was started at HackPrinceton Fall 2017 and can be found on Devpost [here.](https://devpost.com/software/swiper-no-swiping)
Our project represented a unique challenge of having equal importance in web and mobile development - which were integrated through Amazon Web Services. On a set background task interval, Face Lock uses the front facing camera and AWS Rekognition to check if the user is authorized to use the device. If the user is unauthorized, their photo is uploaded to AWS S3 and sent to the device's owner through text and email with AWS SNS. Simultaniously, Face Lock begins rapid location tracking and uploads the phone's coordinates to DynamoDB.
This data stored on S3 and DynamoDB is then pulled down to our Node.js + EJS web app where the user can track their devices on our embedded Bing Maps and can view all previously identified intruders.
The website is currently being updated to an Angular + Node.js app but the older version can be viewed from our previous release.
<file_sep>/android/app/src/main/java/erikterwiel/phoneprotection/Activities/ForgotActivity.java
package erikterwiel.phoneprotection.Activities;
import android.os.AsyncTask;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.text.Editable;
import android.text.TextWatcher;
import android.util.Log;
import android.view.View;
import android.view.inputmethod.InputMethodManager;
import android.widget.Button;
import android.widget.EditText;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.Toast;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.CognitoUser;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.CognitoUserPool;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.continuations.ForgotPasswordContinuation;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.handlers.ForgotPasswordHandler;
import erikterwiel.phoneprotection.R;
import static erikterwiel.phoneprotection.Keys.CognitoKeys.CLIENT_ID;
import static erikterwiel.phoneprotection.Keys.CognitoKeys.CLIENT_SECRET;
import static erikterwiel.phoneprotection.Keys.CognitoKeys.POOL_ID;
public class ForgotActivity extends AppCompatActivity {
private static final String TAG = "ForgotActivity.java";
private EditText mEmail;
private Button mSubmit;
private LinearLayout mCode;
private TextView[] mCodeDigits = new TextView[6];
private EditText mCodeInput;
private EditText mPassword;
private EditText mConfirm;
private Button mSet;
private String mResult;
private boolean mSuccess = false;
private CognitoUser mCognitoUser;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_forgot);
getSupportActionBar().hide();
mEmail = findViewById(R.id.forgot_email);
mSubmit = findViewById(R.id.forgot_reset);
mCode = findViewById(R.id.forgot_code);
mCodeDigits[0] = findViewById(R.id.forgot_code0);
mCodeDigits[1] = findViewById(R.id.forgot_code1);
mCodeDigits[2] = findViewById(R.id.forgot_code2);
mCodeDigits[3] = findViewById(R.id.forgot_code3);
mCodeDigits[4] = findViewById(R.id.forgot_code4);
mCodeDigits[5] = findViewById(R.id.forgot_code5);
mCodeInput = findViewById(R.id.forgot_code_input);
mPassword = findViewById(R.id.forgot_password);
mConfirm = findViewById(R.id.forgot_confirm);
mSet = findViewById(R.id.forgot_set);
mSubmit.setOnClickListener(view -> new RequestNewPassword().execute());
mCode.setOnClickListener(view -> {
mCodeInput.requestFocus();
InputMethodManager imm = (InputMethodManager) getSystemService(INPUT_METHOD_SERVICE);
imm.showSoftInput(mCodeInput, InputMethodManager.SHOW_IMPLICIT);
});
mCodeInput.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence charSequence, int i, int i1, int i2) {}
@Override
public void onTextChanged(CharSequence input, int i, int i1, int i2) {
if (i2 == 1) {
mCodeDigits[i].setText(Character.toString(mCodeInput.getText().toString().charAt(i)));
} else {
mCodeDigits[i].setText("");
}
}
@Override
public void afterTextChanged(Editable editable) {}
});
mConfirm.setOnEditorActionListener((textView, i, keyEvent) -> {
mSet.performClick();
return true;
});
mSet.setOnClickListener(view -> {
if (mPassword.getText().toString().equals(mConfirm.getText().toString())) {
new SetPassword().execute();
} else {
Toast.makeText(
ForgotActivity.this,
"Entered password does not match confirmation.",
Toast.LENGTH_LONG).show();
}
});
}
private class RequestNewPassword extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... inputs) {
ClientConfiguration clientConfiguration = new ClientConfiguration();
CognitoUserPool userPool = new CognitoUserPool(
ForgotActivity.this, POOL_ID, CLIENT_ID, CLIENT_SECRET, clientConfiguration);
mCognitoUser = userPool.getUser(mEmail.getText().toString());
mCognitoUser.forgotPassword(new ForgotPasswordHandler() {
@Override
public void onSuccess() {}
@Override
public void getResetCode(ForgotPasswordContinuation continuation) {
mResult = "A verification code has been sent to your email to reset your password.";
mSuccess = true;
}
@Override
public void onFailure(Exception exception) {
mResult = exception.getMessage().split("\\(")[0];
}
});
return null;
}
@Override
protected void onPostExecute(Void aVoid) {
if (mSuccess) {
mSuccess = false;
mEmail.setVisibility(View.GONE);
mSubmit.setVisibility(View.GONE);
mCode.setVisibility(View.VISIBLE);
mPassword.setVisibility(View.VISIBLE);
mConfirm.setVisibility(View.VISIBLE);
mSet.setVisibility(View.VISIBLE);
}
Toast.makeText(ForgotActivity.this, mResult, Toast.LENGTH_LONG).show();
}
}
private class SetPassword extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... voids) {
mCognitoUser.confirmPassword(
mCodeInput.getText().toString(),
mPassword.getText().toString(),
new ForgotPasswordHandler() {
@Override
public void onSuccess() {
mResult = "Your password has been reset successfully.";
mSuccess = true;
}
@Override
public void getResetCode(ForgotPasswordContinuation continuation) { }
@Override
public void onFailure(Exception exception) {
mResult = exception.getMessage().split("\\(")[0];
}
});
return null;
}
@Override
protected void onPostExecute(Void aVoid) {
Toast.makeText(ForgotActivity.this, mResult, Toast.LENGTH_LONG).show();
if (mSuccess) finish();
}
}
}
<file_sep>/android/app/src/main/java/erikterwiel/phoneprotection/Receivers/DetectionReceiver.java
package erikterwiel.phoneprotection.Receivers;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.util.Log;
import erikterwiel.phoneprotection.Services.DetectionService;
public class DetectionReceiver extends BroadcastReceiver {
private static final String TAG = "DetectionReceiver.java";
@Override
public void onReceive(Context context, Intent intent) {
Log.i(TAG, "onReceive() called");
Intent serviceIntent = new Intent(context, DetectionService.class);
serviceIntent.putExtra("size", intent.getIntExtra("size", 0));
for (int i = 0; i < intent.getIntExtra("size", 0); i++) {
serviceIntent.putExtra("user" + i, intent.getStringExtra("user" + i));
serviceIntent.putExtra("bucketfiles" + i, intent.getStringExtra("bucketfiles" + i));
}
serviceIntent.putExtra("username", intent.getStringExtra("username"));
context.startService(serviceIntent);
}
}
<file_sep>/android/app/src/main/java/erikterwiel/phoneprotection/Singletons/Rekognition.java
package erikterwiel.phoneprotection.Singletons;
import android.content.Context;
import com.amazonaws.auth.AWSCredentialsProvider;
import com.amazonaws.auth.CognitoCachingCredentialsProvider;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.rekognition.AmazonRekognitionClient;
import static erikterwiel.phoneprotection.Keys.DynamoDBKeys.POOL_ID_UNAUTH;
import static erikterwiel.phoneprotection.Keys.DynamoDBKeys.POOL_REGION;
public class Rekognition {
private static Rekognition instance;
private AmazonRekognitionClient rekognitionClient;
private Rekognition(Context context) {
AWSCredentialsProvider credentialsProvider = new CognitoCachingCredentialsProvider(
context,
POOL_ID_UNAUTH,
Regions.fromName(POOL_REGION));
rekognitionClient = new AmazonRekognitionClient(credentialsProvider);
}
public static void init(Context context) {
if (instance == null) {
context = context.getApplicationContext();
instance = new Rekognition(context);
}
}
public static Rekognition getInstance() {
return instance;
}
public AmazonRekognitionClient getRekognitionClient() {
return rekognitionClient;
}
}
<file_sep>/android/app/src/main/java/erikterwiel/phoneprotection/Activities/AddPhoneActivity.java
package erikterwiel.phoneprotection.Activities;
import android.location.Location;
import android.os.AsyncTask;
import android.provider.Settings;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import com.amazonaws.mobileconnectors.dynamodbv2.dynamodbmapper.DynamoDBMapper;
import com.google.android.gms.location.FusedLocationProviderClient;
import com.google.android.gms.location.LocationServices;
import erikterwiel.phoneprotection.R;
import erikterwiel.phoneprotection.Singletons.DynamoDB;
import erikterwiel.phoneprotection.Account;
public class AddPhoneActivity extends AppCompatActivity {
private static final String TAG = "AddPhoneActivity.java";
private DynamoDBMapper mMapper;
private EditText mName;
private Button mAdd;
private Location mLocation;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_add_phone);
mMapper = DynamoDB.getInstance().getMapper();
mName = findViewById(R.id.phone_name);
mAdd = findViewById(R.id.phone_add_phone);
mAdd.setOnClickListener(view -> {
if (mName.getText().toString().equals("")) {
Toast.makeText(this, "Your phone must have a name!", Toast.LENGTH_LONG).show();
} else {
new UploadPhone().execute();
}
});
}
private class UploadPhone extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... inputs) {
try {
FusedLocationProviderClient locationClient = LocationServices.getFusedLocationProviderClient(AddPhoneActivity.this);
locationClient.getLastLocation().addOnSuccessListener(AddPhoneActivity.this, location -> {
if (location != null) {
mLocation = location;
} else {
mLocation = new Location("Default");
mLocation.setLatitude(37.421984);
mLocation.setLongitude(-122.084152);
}
});
} catch (SecurityException ex) {
ex.printStackTrace();
}
Account account = mMapper.load(Account.class, getIntent().getStringExtra("username"));
if (account == null) {
account = new Account();
account.setUsername(getIntent().getStringExtra("username"));
}
account.addUnique(Settings.Secure.getString(getApplicationContext().getContentResolver(), Settings.Secure.ANDROID_ID));
account.addName(mName.getText().toString());
account.addLatitude(mLocation.getLatitude());
account.addLongitude(mLocation.getLongitude());
mMapper.save(account);
finish();
return null;
}
}
}<file_sep>/android/app/src/main/java/erikterwiel/phoneprotection/Activities/LoginActivity.java
package erikterwiel.phoneprotection.Activities;
import android.Manifest;
import android.app.admin.DevicePolicyManager;
import android.content.ComponentName;
import android.content.Context;
import android.content.Intent;
import android.content.SharedPreferences;
import android.provider.Settings;
import android.support.annotation.NonNull;
import android.support.v4.app.ActivityCompat;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.inputmethod.EditorInfo;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.CognitoDevice;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.CognitoUser;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.CognitoUserPool;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.CognitoUserSession;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.continuations.AuthenticationContinuation;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.continuations.AuthenticationDetails;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.continuations.ChallengeContinuation;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.continuations.MultiFactorAuthenticationContinuation;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.handlers.AuthenticationHandler;
import java.util.List;
import erikterwiel.phoneprotection.MyAdminReceiver;
import erikterwiel.phoneprotection.R;
import static android.content.pm.PackageManager.PERMISSION_DENIED;
import static erikterwiel.phoneprotection.Keys.CognitoKeys.POOL_ID;
import static erikterwiel.phoneprotection.Keys.CognitoKeys.CLIENT_ID;
import static erikterwiel.phoneprotection.Keys.CognitoKeys.CLIENT_SECRET;
public class LoginActivity extends AppCompatActivity {
private static final String TAG = "LoginActivity.java";
private static final int REQUEST_PERMISSION = 100;
private static final int REQUEST_ADMIN = 105;
private CognitoUserPool mUserPool;
private CognitoUser mCognitoUser;
private CognitoUserSession mUserSession;
private EditText mEmail;
private EditText mPassword;
private Button mLogin;
private Button mRegister;
private Button mForgot;
private SharedPreferences mMemory;
@Override
protected void onCreate(Bundle savedInstanceState) {
Log.i(TAG, "onCreate() called");
setTheme(R.style.AppTheme);
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
getSupportActionBar().hide();
Log.i(TAG, "Unique ID: " + Settings.Secure.getString(this.getContentResolver(), Settings.Secure.ANDROID_ID));
DevicePolicyManager devicePolicyManager =
(DevicePolicyManager) getSystemService(DEVICE_POLICY_SERVICE);
List<ComponentName> admins = devicePolicyManager.getActiveAdmins();
boolean isAdminApp = false;
for (ComponentName name : admins) {
if (name.getClassName().equals("erikterwiel.phoneprotection.MyAdminReceiver")) {
isAdminApp = true;
break;
}
}
if (!isAdminApp) {
ComponentName compName = new ComponentName(this, MyAdminReceiver.class);
Intent adminIntent = new Intent(DevicePolicyManager.ACTION_ADD_DEVICE_ADMIN);
adminIntent.putExtra(DevicePolicyManager.EXTRA_DEVICE_ADMIN, compName);
adminIntent.putExtra(DevicePolicyManager.EXTRA_ADD_EXPLANATION,
"Admin app privilege needed to lock the screen on lock down.");
startActivityForResult(adminIntent, REQUEST_ADMIN);
}
mEmail = findViewById(R.id.login_email);
mPassword = findViewById(R.id.login_password);
mLogin = findViewById(R.id.login_login);
mRegister = findViewById(R.id.login_register);
mForgot = findViewById(R.id.login_forgot);
mMemory = getSharedPreferences("memory", Context.MODE_PRIVATE);
if (mMemory.contains("email")) {
mEmail.setText(mMemory.getString("email", null));
mPassword.requestFocus();
}
ClientConfiguration clientConfiguration = new ClientConfiguration();
mUserPool = new CognitoUserPool(
this, POOL_ID, CLIENT_ID, CLIENT_SECRET, clientConfiguration);
mLogin.setOnClickListener(view -> {
mCognitoUser = mUserPool.getUser();
AuthenticationHandler handler = new AuthenticationHandler() {
@Override
public void onSuccess(CognitoUserSession userSession, CognitoDevice newDevice) {
ActivityCompat.requestPermissions(
LoginActivity.this,
new String[] {
Manifest.permission.ACCESS_COARSE_LOCATION,
Manifest.permission.ACCESS_FINE_LOCATION,
Manifest.permission.READ_EXTERNAL_STORAGE,
Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.CAMERA,
Manifest.permission.READ_PHONE_STATE},
REQUEST_PERMISSION);
mUserSession = userSession;
}
@Override
public void onFailure(Exception exception) {
Toast.makeText(
LoginActivity.this,
exception.getMessage().split("\\(")[0],
Toast.LENGTH_LONG).show();
}
@Override
public void getAuthenticationDetails(AuthenticationContinuation continuation, String userId) {
AuthenticationDetails authDetails = new AuthenticationDetails(
mEmail.getText().toString() ,
mPassword.getText().toString(),
null
);
continuation.setAuthenticationDetails(authDetails);
continuation.continueTask();
}
@Override
public void getMFACode(MultiFactorAuthenticationContinuation continuation) {}
@Override
public void authenticationChallenge(ChallengeContinuation continuation) {}
};
mCognitoUser.getSessionInBackground(handler);
});
mPassword.setOnEditorActionListener((textView, actionID, keyEvent) -> {
if (actionID == EditorInfo.IME_ACTION_SEND) {
Log.i(TAG, "Attempting to perform a click");
mLogin.performClick();
return true;
}
return false;
});
mRegister.setOnClickListener(view -> {
Intent registerIntent = new Intent(LoginActivity.this, RegisterActivity.class);
startActivity(registerIntent);
});
mForgot.setOnClickListener(view -> {
Intent forgotIntent = new Intent(LoginActivity.this, ForgotActivity.class);
startActivity(forgotIntent);
});
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
for (int i = 0; i < permissions.length; i++) {
if (!permissions[i].equals("android.permission.READ_PHONE_STATE") && grantResults[i] == PERMISSION_DENIED) {
Toast.makeText(
LoginActivity.this,
"You must allow all permissions to use Face Lock.",
Toast.LENGTH_LONG).show();
return;
}
}
Toast.makeText(
LoginActivity.this,
"Authentication successful, loading...",
Toast.LENGTH_LONG).show();
Intent homeIntent = new Intent(LoginActivity.this, HomeActivity.class);
homeIntent.putExtra("username", mUserSession.getUsername());
homeIntent.putExtra("email", mEmail.getText().toString());
startActivity(homeIntent);
}
@Override
protected void onStop() {
super.onStop();
Log.i(TAG, "onStop() called");
SharedPreferences.Editor memoryEditor = mMemory.edit();
memoryEditor.putString("email", mEmail.getText().toString());
memoryEditor.apply();
}
}
<file_sep>/android/app/src/main/java/erikterwiel/phoneprotection/Services/TrackerService.java
package erikterwiel.phoneprotection.Services;
import android.app.Notification;
import android.app.Service;
import android.content.Intent;
import android.os.AsyncTask;
import android.os.IBinder;
import android.support.annotation.Nullable;
import android.util.Log;
import com.amazonaws.auth.CognitoCachingCredentialsProvider;
import com.amazonaws.mobileconnectors.dynamodbv2.dynamodbmapper.DynamoDBMapper;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClient;
import com.google.android.gms.location.FusedLocationProviderClient;
import com.google.android.gms.location.LocationServices;
import java.util.Timer;
import erikterwiel.phoneprotection.R;
import erikterwiel.phoneprotection.Account;
public class TrackerService extends Service {
private static final String TAG = "TrackerService.java";
private static final String POOL_ID_UNAUTH = "us-east-1:d2040261-6a0f-4cba-af96-8ead1b66ec38";
private static final int NOTIFICATION_ID = 104;
private AmazonDynamoDBClient mDDBClient;
private DynamoDBMapper mMapper;
private Notification mNotification;
private FusedLocationProviderClient mFusedLocationClient;
private Intent mIntent;
private Account mPhone;
private Timer mTimer;
private int mRunCounter;
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Log.i(TAG, "onStartCommand() called");
mNotification = new Notification.Builder(this)
.setSmallIcon(R.drawable.ic_security_black_48dp)
.setContentTitle("Phone Protection")
.setContentText("Monitoring phone users")
.setCategory(Notification.CATEGORY_STATUS)
.setPriority(Notification.PRIORITY_MIN)
.setAutoCancel(false)
.setOngoing(true)
.build();
startForeground(NOTIFICATION_ID, mNotification);
LocationServices.getFusedLocationProviderClient(this);
CognitoCachingCredentialsProvider credentialsProvider = new CognitoCachingCredentialsProvider(
getApplicationContext(),
POOL_ID_UNAUTH,
Regions.US_EAST_1);
mDDBClient = new AmazonDynamoDBClient(credentialsProvider);
mMapper = new DynamoDBMapper(mDDBClient);
mIntent = intent;
mFusedLocationClient = LocationServices.getFusedLocationProviderClient(this);
new DownloadPhone().execute();
try {
Thread.sleep(1500);
} catch (Exception ex) {}
new UpdatePhone().execute();
mTimer = new Timer();
// mTimer.scheduleAtFixedRate(new TimerTask() {
// @Override
// public void run() {
// try {
// mFusedLocationClient.getLastLocation().addOnSuccessListener(new OnSuccessListener<Location>() {
// @Override
// public void onSuccess(Location location) {
// if (location != null) {
// Log.i(TAG, "Latitude: " + location.getLatitude());
// Log.i(TAG, "Longitude: " + location.getLongitude());
// mPhone.setLatitudes(location.getLatitude());
// mPhone.setLongitudes(location.getLongitude());
// }
// }
// });
// } catch (SecurityException ex) {
// ex.printStackTrace();
// }
// new UpdatePhone().execute();
// mRunCounter += 1;
// if (mRunCounter == 20) onDestroy();
// }
// }, 0, 15000);
return START_STICKY;
}
private class DownloadPhone extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... inputs) {
mPhone = mMapper.load(Account.class, mIntent.getStringExtra("username"));
return null;
}
}
private class UpdatePhone extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... voids) {
Log.i(TAG, "doInBackground() called");
mMapper.save(mPhone);
return null;
}
}
@Override
public void onDestroy() {
super.onDestroy();
Log.i(TAG, "onDestroy() called");
mTimer.cancel();
mTimer.purge();
stopForeground(true);
}
@Nullable
@Override
public IBinder onBind(Intent intent) {
return null;
}
}
<file_sep>/android/app/src/main/java/erikterwiel/phoneprotection/Activities/RegisterActivity.java
package erikterwiel.phoneprotection.Activities;
import android.os.AsyncTask;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.auth.AWSCredentialsProvider;
import com.amazonaws.auth.CognitoCachingCredentialsProvider;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.CognitoUser;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.CognitoUserAttributes;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.CognitoUserCodeDeliveryDetails;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.CognitoUserPool;
import com.amazonaws.mobileconnectors.cognitoidentityprovider.handlers.SignUpHandler;
import com.amazonaws.regions.Region;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.sns.AmazonSNSClient;
import erikterwiel.phoneprotection.R;
import static erikterwiel.phoneprotection.Keys.CognitoKeys.CLIENT_ID;
import static erikterwiel.phoneprotection.Keys.CognitoKeys.CLIENT_SECRET;
import static erikterwiel.phoneprotection.Keys.CognitoKeys.POOL_ID;
import static erikterwiel.phoneprotection.Keys.DynamoDBKeys.POOL_ID_UNAUTH;
import static erikterwiel.phoneprotection.Keys.DynamoDBKeys.POOL_REGION;
import static erikterwiel.phoneprotection.Keys.SNSKeys.ACCOUNT;
import static erikterwiel.phoneprotection.Keys.SNSKeys.REGION;
public class RegisterActivity extends AppCompatActivity {
private static final String TAG = "RegisterActivity.java";
private CognitoUserPool mUserPool;
private MenuItem mDone;
private EditText mEmail;
private EditText mPassword;
private EditText mConfirm;
private Button mRegister;
@Override
protected void onCreate(Bundle savedInstanceState) {
Log.i(TAG, "onCreate() called");
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_register);
getSupportActionBar().hide();
ClientConfiguration clientConfiguration = new ClientConfiguration();
mUserPool = new CognitoUserPool(
this, POOL_ID, CLIENT_ID, CLIENT_SECRET, clientConfiguration);
mEmail = findViewById(R.id.register_email);
mPassword = findViewById(R.id.register_password);
mRegister = findViewById(R.id.register_register);
mConfirm = findViewById(R.id.register_confirm);
mRegister.setOnClickListener(view -> {
if (mPassword.getText().toString().equals(mConfirm.getText().toString())) {
SignUpHandler signupCallback = new SignUpHandler() {
@Override
public void onSuccess(CognitoUser user, boolean signUpConfirmationState, CognitoUserCodeDeliveryDetails cognitoUserCodeDeliveryDetails) {
new RegisterNotifications().execute();
}
@Override
public void onFailure(Exception exception) {
Toast.makeText(RegisterActivity.this,
exception.getClass().toString().split(" ")[1].split("\\.")[5],
Toast.LENGTH_LONG).show();
}
};
CognitoUserAttributes userAttributes = new CognitoUserAttributes();
mUserPool.signUpInBackground(mEmail.getText().toString(),
mPassword.getText().toString(),
userAttributes, null, signupCallback);
} else {
Toast.makeText(
RegisterActivity.this,
"Entered password does not match confirmation.",
Toast.LENGTH_LONG).show();
}
});
}
private class RegisterNotifications extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... inputs) {
AWSCredentialsProvider credentialsProvider = new CognitoCachingCredentialsProvider(
RegisterActivity.this,
POOL_ID_UNAUTH,
Regions.fromName(POOL_REGION));
AmazonSNSClient snsClient = new AmazonSNSClient(credentialsProvider);
snsClient.setRegion(Region.getRegion(Regions.US_EAST_1));
String topicName = mEmail.getText().toString();
topicName = topicName.replaceAll("\\.", "");
topicName = topicName.replaceAll("@", "");
topicName = topicName.replaceAll(":", "");
snsClient.createTopic(topicName);
snsClient.subscribe(
"arn:aws:sns:" + REGION + ":" + ACCOUNT + ":" + topicName,
"email", mEmail.getText().toString());
return null;
}
@Override
protected void onPostExecute(Void result) {
Toast.makeText(RegisterActivity.this,
"Registration successful, please check email for two verification links. " +
"One is to verify your email, the other is receive pictures of phone intruders",
Toast.LENGTH_LONG).show();
finish();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater menuInflater = getMenuInflater();
menuInflater.inflate(R.menu.register_done, menu);
mDone = menu.findItem(R.id.register_done);
mDone.setOnMenuItemClickListener(new MenuItem.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem menuItem) {
finish();
return false;
}
});
return super.onCreateOptionsMenu(menu);
}
}
<file_sep>/android/app/src/main/java/erikterwiel/phoneprotection/Singletons/DynamoDB.java
package erikterwiel.phoneprotection.Singletons;
import android.content.Context;
import com.amazonaws.auth.CognitoCachingCredentialsProvider;
import com.amazonaws.mobileconnectors.dynamodbv2.dynamodbmapper.DynamoDBMapper;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClient;
import static erikterwiel.phoneprotection.Keys.DynamoDBKeys.POOL_ID_UNAUTH;
import static erikterwiel.phoneprotection.Keys.DynamoDBKeys.POOL_REGION;
public class DynamoDB {
private static DynamoDB instance;
private DynamoDBMapper mapper;
private DynamoDB(Context context) {
CognitoCachingCredentialsProvider credentialsProvider = new CognitoCachingCredentialsProvider(
context,
POOL_ID_UNAUTH,
Regions.fromName(POOL_REGION));
AmazonDynamoDBClient DDBClient = new AmazonDynamoDBClient(credentialsProvider);
mapper = new DynamoDBMapper(DDBClient);
}
public static void init(Context context) {
if (instance == null) {
context = context.getApplicationContext();
instance = new DynamoDB(context);
}
}
public static DynamoDB getInstance() {
return instance;
}
public DynamoDBMapper getMapper() {
return mapper;
}
}
| 28c247a835666074b6d79c8318c7ed70179dcea6 | [
"Markdown",
"Java"
]
| 10 | Java | erikterwiel/FaceLock | f68d35568fedc01699b9236b17beb8eaea50d6b5 | ae33cb5b09dc03aa558f62b5500cecfd10b84e53 | |
refs/heads/master | <file_sep><?php
header("Content-Type: text/css");
header("X-Content-Type-Options: nosniff");
$iBaseXDp = 0;
$iBaseYDp = 0;
$jsonBackground = file_get_contents("../background/Cityscape.json");
$jsonBackground = json_decode($jsonBackground, true);
$iTilesWide = $jsonBackground["tileswide"];
//$iWidthDp = $jsonBackground["tilewidth"];
$iWidthDp = 100 / $iTilesWide;
$iTilesHigh = $jsonBackground["tileshigh"];
//$iHeightDp = $jsonBackground["tileheight"];
$iHeightDp = 100 / $iTilesHigh;
// I guess map tiles to filenames
$aTiles = array();
$aLayer = $jsonBackground["layers"][0]["tiles"];
foreach ($aLayer as $iTile => $aTile)
{
$aTileCss = array();
$aTileCss["position-x"] = $aTile["x"] * $iWidthDp + $iBaseXDp;
$aTileCss["position-y"] = $aTile["y"] * $iHeightDp + $iBaseYDp;
$aTileCss["size-x"] = $iWidthDp;
$aTileCss["size-y"] = $iHeightDp;
$iTileType = $aTile["tile"];
$strTileURL =
"/background/Cityscape_" .
sprintf("%'.02d", $iTileType) .
".png";
$strTileURL = "url(" . $strTileURL . ")";
$aTileCss["url"] = $strTileURL;
// Repeat the extreme tile per row.
if ($aTile["x"] == 0)
{
$aTileCss["repeat"] = "repeat-x";
}
else
{
$aTileCss["repeat"] = "no-repeat";
}
$aTiles[$iTile] = $aTileCss;
}
$aTiles = array_reverse($aTiles);
$aBackgroundTile = array_shift($aTiles);
// Do this to force repeating the rest of the way. Looks ugly sometimes
//$aBackgroundTile["repeat"] = "repeat";
array_push($aTiles, $aBackgroundTile);
$aCss = array();
$aCss["urls"] = "";
$aCss["positions"] = "";
$aCss["sizes"] = "";
$aCss["position-x"] = "";
$aCss["size-x"] = "";
$aCss["position-y"] = "";
$aCss["size-y"] = "";
$aCss["repeats"] = "";
$i = 0;
$strUnit = "vmin";
foreach ($aTiles as $aTile)
{
$aCss["urls"] .= $aTile["url"] . ", ";
$aCss["positions"] .=
$aTile["position-x"] . "$strUnit " .
$aTile["position-y"] . "$strUnit, ";
$aCss["sizes"] .=
$aTile["size-x"] . "$strUnit " .
$aTile["size-y"] . "$strUnit, ";
$aCss["position-x"] .=
$aTile["position-x"] . "$strUnit, ";
$aCss["position-y"] .=
$aTile["position-y"] . "$strUnit, ";
$aCss["size-x"] .=
$aTile["size-x"] . "$strUnit, ";
$aCss["size-y"] .=
$aTile["size-y"] . "$strUnit, ";
$aCss["repeats"] .= $aTile["repeat"] . ", ";
}
foreach ($aCss as &$strProperty)
{
$strProperty = rtrim($strProperty, ", ");
unset($strProperty);
}
$strUrls = $aCss["urls"];
$strPositions = $aCss["positions"];
$strSizes = $aCss["sizes"];
$strPositionX = $aCss["position-x"];
$strSizeX = $aCss["size-x"];
$strPositionY = $aCss["position-y"];
$strSizeY = $aCss["size-y"];
$strRepeats = $aCss["repeats"];
$strHeight = $iTilesHigh * $iHeightDp . "$strUnit";
echo <<<CSS
.footer {
position: absolute;
bottom: 0;
width: 100%;
/* Set the fixed height of the footer here */
height: 65vmin;
/*background-color: #f5f5f5;*/
background:
$strUrls;
background-position-x:
$strPositionX;
background-position-y:
$strPositionY;
background-size:
$strSizes;
background-repeat:
$strRepeats;
}
CSS;
| 4d904b7822746a21f9bb5b3f9b8abe81d0861ba2 | [
"PHP"
]
| 1 | PHP | vRPGmatch/techthetech | be13c83b7e4609de48b02d6515095217db30a3df | 7ef0927f481ed527dc17efa8b17d0ba10bca7005 | |
refs/heads/master | <repo_name>clockknock/CRM<file_sep>/src/org/itheima/crm/domain/PageBean.kt
package org.itheima.crm.domain
import org.itheima.crm.dao.impl.CustomerDaoImpl
/**
* Created by 钟未鸣 on 2017/9/11 .
*/
//data class PageBean(var pageSize:Int?,var currentPage:Int?,var totalPage:Int?,var datas:List<T>)
class PageBean<T> {
var currentPage: Int = 0// 当前页:1开始
var totalPage: Int = 0// 总页数
var totalCount: Long = 0// 总条数
var pageSize: Int = 0// 每页显示的条数
var datas: List<T>? = null
override fun toString(): String {
return "PageBean(currentPage=$currentPage, totalPage=$totalPage, totalCount=$totalCount, pageSize=$pageSize, datas=$datas)"
}
}
<file_sep>/src/org/itheima/crm/dao/LinkmanDao.kt
package org.itheima.crm.dao
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.domain.Linkman
/**
* Created by 钟未鸣 on 2017/9/13 .
*/
interface LinkmanDao {
fun save(linkman: Linkman)
fun totalCount(criteria: DetachedCriteria): Long
fun findList(criteria: DetachedCriteria, start: Int, pageSize: Int): List<Linkman>
}<file_sep>/src/org/itheima/crm/utils/UploadUtil.kt
package org.itheima.crm.utils
import java.util.*
/**
* Created by 钟未鸣 on 2017/9/11 .
*/
object UploadUtil{
fun genUploadPath(imageUpLoadFileName: String):String{
val sb = StringBuffer()
val calendar = Calendar.getInstance()
sb.append("/")
sb.append(calendar.get(Calendar.YEAR))
sb.append("/")
sb.append(calendar.get(Calendar.MONTH)+1)
sb.append("/")
sb.append(calendar.get(Calendar.DAY_OF_MONTH))
sb.append("/")
sb.append(calendar.get(Calendar.HOUR_OF_DAY))
sb.append("/")
val suffix=imageUpLoadFileName.substring(imageUpLoadFileName.lastIndexOf("."))
sb.append(UUID.randomUUID())
sb.append(suffix)
return sb.toString().replace("-","")
// return sb.toString()
}
}<file_sep>/src/org/itheima/crm/dao/impl/DictDaoImpl.kt
package org.itheima.crm.dao.impl
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.dao.DictDao
import org.itheima.crm.domain.BaseDict
import org.springframework.orm.hibernate5.support.HibernateDaoSupport
/**
* Created by 钟未鸣 on 2017/9/10 .
*/
class DictDaoImpl : DictDao,HibernateDaoSupport() {
override fun findList(criteria: DetachedCriteria): List<BaseDict> {
@Suppress("UNCHECKED_CAST")
return hibernateTemplate.findByCriteria(criteria) as List<BaseDict>
}
override fun save(dict: BaseDict) {
hibernateTemplate.save(dict)
}
}<file_sep>/src/org/itheima/crm/web/interceptor/LoginInterceptor.kt
package org.itheima.crm.web.interceptor
import com.opensymphony.xwork2.ActionInvocation
import com.opensymphony.xwork2.interceptor.AbstractInterceptor
import org.apache.struts2.ServletActionContext
import org.itheima.crm.web.action.UserAction
/**
* Created by 钟未鸣 on 2017/9/10 .
* 登录拦截器
*/
class LoginInterceptor: AbstractInterceptor(){
private val NEEDLOGIN="needLogin"
override fun intercept(invocation: ActionInvocation?): String {
//如果是UserAction,放行
val action = invocation?.action
if(action is UserAction){
return invocation.invoke()
}
val user = ServletActionContext.getRequest().session.getAttribute("user")
if(user !=null){
//已经登录,放行
if (invocation != null) {
return invocation.invoke()
}
}
return NEEDLOGIN
}
}<file_sep>/src/org/itheima/crm/service/LinkmanService.kt
package org.itheima.crm.service
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.domain.Linkman
import org.itheima.crm.domain.PageBean
/**
* Created by 钟未鸣 on 2017/9/13 .
*/
interface LinkmanService {
fun save(linkman: Linkman)
fun findPage(criteria: DetachedCriteria, currentPage: Int, pageSize: Int): PageBean<Linkman>
}<file_sep>/src/org/itheima/crm/web/action/UserAction.kt
package org.itheima.crm.web.action
import cn.dsna.util.images.ValidateCode
import com.opensymphony.xwork2.ActionSupport
import com.opensymphony.xwork2.ModelDriven
import org.apache.struts2.interceptor.ServletRequestAware
import org.itheima.crm.domain.User
import org.itheima.crm.service.UserService
import org.springframework.util.StringUtils
import java.io.ByteArrayInputStream
import java.io.ByteArrayOutputStream
import java.io.InputStream
import javax.servlet.http.HttpServletRequest
/**
* Created by 钟未鸣 on 2017/9/8 .
* userAction
*/
class UserAction : ActionSupport(), ModelDriven<User>, ServletRequestAware {
private val LOGIN_SUCCESS = "loginSuccess"
private val LOGIN_ERROR = "loginError"
private val VALIDATECODE_SUCCESS = "validateCodeSuccess"
private val LOGOUT_SUCCESS = "logoutSuccess"
private var request: HttpServletRequest? = null
override fun setServletRequest(request: HttpServletRequest?) {
this.request = request
}
private var imageStream: InputStream? = null
@Suppress("unused")
fun getImageStream(): InputStream? {
return imageStream
}
private var user: User? = null
private var userService: UserService? = null
fun setUserService(service: UserService) {
this.userService = service
}
override fun getModel(): User {
if (user == null) {
user = User()
}
return user as User
}
@Suppress("unused")
fun do_login(): String {
if (StringUtils.isEmpty(user!!.userCode)) {
addFieldError("userCode", "用户名不能为空")
return LOGIN_ERROR
}
if (StringUtils.isEmpty(user!!.userPassword)) {
addFieldError("userPassword", "密码不能为空")
return LOGIN_ERROR
}
if (StringUtils.isEmpty(user!!.validateCode)) {
addFieldError("validateCode", "验证码不能为空")
return LOGIN_ERROR
}
/* TODO 先不填验证码了
val validateCode = request!!.session.getAttribute("validateCode") as String
if(StringUtils.isEmpty(validateCode)){
addFieldError("validateCode","无法刷新验证码")
return LOGIN_ERROR
}
if(!validateCode.equals(user!!.validateCode,true)){
addFieldError("validateCode","验证码错误")
return LOGIN_ERROR
}*/
val loginUser: User = userService?.login(user!!) ?: return LOGIN_ERROR
val session = request!!.session
session.setAttribute("user", loginUser)
return LOGIN_SUCCESS
}
@Suppress("unused")
fun do_validateCode(): String {
val code = ValidateCode(130, 28, 4, 4)
request!!.session.setAttribute("validateCode", code.code)
imageStream = code.getInputStream()
return VALIDATECODE_SUCCESS
}
@Suppress("unused")
fun do_logout(): String {
request!!.session.removeAttribute("user")
return LOGOUT_SUCCESS
}
}
fun ValidateCode.getInputStream(): InputStream? {
var baos: ByteArrayOutputStream? = null
var bais: ByteArrayInputStream? = null
try {
baos = ByteArrayOutputStream()
this.write(baos)
bais = ByteArrayInputStream(baos.toByteArray())
} catch (e: Exception) {
e.printStackTrace()
} finally {
if (baos != null) {
try {
baos.close()
} catch (e: Exception) {
e.printStackTrace()
}
@Suppress("UNUSED_VALUE")
baos = null
}
}
return bais
}<file_sep>/src/org/itheima/crm/service/UserService.kt
package org.itheima.crm.service
import org.itheima.crm.domain.User
/**
* Created by 钟未鸣 on 2017/9/8 .
*/
interface UserService{
fun save(user: User)
fun login(user: User): User ?
}<file_sep>/src/org/itheima/crm/web/action/CustomerAction.kt
package org.itheima.crm.web.action
import com.opensymphony.xwork2.ActionContext
import com.opensymphony.xwork2.ActionSupport
import com.opensymphony.xwork2.ModelDriven
import org.hibernate.criterion.DetachedCriteria
import org.hibernate.criterion.Restrictions
import org.itheima.crm.domain.BaseDict
import org.itheima.crm.domain.Customer
import org.itheima.crm.service.CustomerService
import org.itheima.crm.service.DictService
import org.itheima.crm.utils.PropertyPlaceholder
import org.itheima.crm.utils.UploadUtil
import org.springframework.util.StringUtils
import java.io.File
/**
* Created by 钟未鸣 on 2017/9/10 .
*/
class CustomerAction : ActionSupport(), ModelDriven<Customer> {
private val SAVESUCCESS = "saveSuccess"
private val SAVEERROR = "saveError"
private val RESULTDICTSUCCESS = "resultDictSuccess"
private val LISTSUCCESS = "listSuccess"
private val EDITSUCCESS = "editSuccess"
private val UPDATESUCCESS = "updateSuccess"
private val UPDATEERROR = "updateError"
private val DELETESUCCESS = "deleteSuccess"
private var imageUpLoad: File? = null
private var imageUpLoadContentType: String? = null
private var imageUpLoadFileName: String? = null
private var imgDir: String? = PropertyPlaceholder.getProperty("file.upload.dir")
@Suppress("unused")
fun setImageUpLoad(imageUpLoad: File) {
this.imageUpLoad = imageUpLoad
}
@Suppress("unused")
fun setImageUpLoadContentType(contentType: String) {
this.imageUpLoadContentType = contentType
}
@Suppress("unused")
fun setImageUpLoadFileName(fileName: String) {
this.imageUpLoadFileName = fileName
}
private var dicts: ArrayList<*>? = null
private fun setDicts(dicts: ArrayList<*>) {
this.dicts = dicts
}
@Suppress("unused")
fun getDicts(): ArrayList<*>? {
return dicts
}
private var dictTypeCode: String? = null
@Suppress("unused")
fun setDictTypeCode(code: String) {
dictTypeCode = code
}
private var currentPage: Int = 1
@Suppress("unused")
fun setCurrentPage(currentPage: Int) {
this.currentPage = currentPage
}
private var pageSize: Int = 5
@Suppress("unused")
fun setPageSize(pageSize: Int) {
this.pageSize = pageSize
}
private var customer: Customer? = null
override fun getModel(): Customer {
if (customer == null) {
customer = Customer()
}
return customer as Customer
}
private var customerService: CustomerService? = null
fun setCustomerService(customerService: CustomerService) {
this.customerService = customerService
}
private var dictService: DictService? = null
fun setDictService(dictService: DictService) {
this.dictService = dictService
}
override fun hasErrors(): Boolean {
return false
}
/**
* Action
* 操
* 作
*/
@Suppress("unused")
fun do_save(): String {
if (checkCustomerDataHasError(customer!!)) {
return SAVEERROR
}
//处理文件上传
if (StringUtils.isEmpty(imageUpLoadFileName)) {
addActionError("未选择资质图片")
} else {
uploadImg()
}
customerService!!.saveCustomer(customer!!)
return SAVESUCCESS
}
@Suppress("unused")
fun do_dict(): String {
try {
val criteria = DetachedCriteria.forClass(BaseDict::class.java)
criteria.add(Restrictions.eq("dictTypeCode", dictTypeCode))
val findList = dictService!!.findList(criteria)
setDicts(findList as ArrayList<BaseDict>)
} catch (e: Exception) {
e.printStackTrace()
}
return RESULTDICTSUCCESS
}
@Suppress("unused")
fun do_list(): String {
val stack = ActionContext.getContext().valueStack
var criteria = DetachedCriteria.forClass(Customer::class.java)
criteria = handleQueryCriteria(customer!!, criteria)
val pageBean = customerService!!.findBean(criteria, currentPage, pageSize)
stack.push(pageBean)
return LISTSUCCESS
}
/**
* 通过id获取customer数据进入edit界面
*/
@Suppress("unused")
fun do_edit(): String {
val findCust: Customer = customerService!!.findById(customer!!.custId!!)
val stack = ActionContext.getContext().valueStack
stack.push(findCust)
return EDITSUCCESS
}
@Suppress("unused")
fun do_update(): String {
val hasError = checkCustomerDataHasError(customer!!)
if (hasError) {
return UPDATEERROR
}
if(imageUpLoad==null){
addActionError("图片不能为空!")
return UPDATEERROR
}
uploadImg()
customerService!!.update(customer!!)
return UPDATESUCCESS
}
@Suppress("unused")
fun do_delete(): String{
val find = customerService!!.findById(customer!!.custId!!)
customerService!!.delete(find)
/*不删图片
val image = File(imgDir, find.cstImage)
if(image.exists()){
val delete = image.delete()
println("delete:$delete")
}*/
return DELETESUCCESS
}
/**
* 抽
* 取
* 的
* 方
* 法
*/
/**
* 校验customer的数据有没有错误,以决定返回resultType
* @return true 有错误 false没错误
*/
private fun checkCustomerDataHasError(customer: Customer): Boolean {
if (StringUtils.isEmpty(customer.custName)) {
addActionError("客户名不能为空")
return true
}
if (StringUtils.isEmpty(customer.custPhone)) {
addActionError("固定电话不能为空")
return true
}
if (StringUtils.isEmpty(customer.custMobile)) {
addActionError("移动电话不能为空")
return true
}
//cstLevel为空或其id为0
if (customer.cstLevel == null || customer.cstLevel?.dictId ==
0L) {
addActionError("客户级别不能为空")
return true
}
//cstIndustry为空或其id为0
if (customer.custIndustry == null || customer.custIndustry?.dictId ==
0L) {
addActionError("所属行业不能为空")
return true
}
//cstSource为空或其id为0
if (customer.custSource == null || customer.custSource?.dictId ==
0L) {
addActionError("信息来源不能为空")
return true
}
return false
}
/**
* 校验customer的数据添加criteria
* @return 添加了新约束的criteria
*/
private fun handleQueryCriteria(customer: Customer, criteria: DetachedCriteria): DetachedCriteria {
if (!StringUtils.isEmpty(customer.custName)) {
criteria.add(Restrictions.like("custName", "%${customer.custName}%"))
}
if (!StringUtils.isEmpty(customer.custPhone)) {
criteria.add(Restrictions.like("custPhone", "%${customer.custPhone}%"))
}
if (!StringUtils.isEmpty(customer.custMobile)) {
criteria.add(Restrictions.like("custMobile", "%${customer.custMobile}%"))
}
//cstLevel为空或其id为0
if (customer.cstLevel != null && customer.cstLevel?.dictId != 0L) {
criteria.add(Restrictions.eq("cstLevel.dictId", customer.cstLevel!!.dictId))
}
//cstIndustry为空或其id为0
if (customer.custIndustry != null && customer.custIndustry?.dictId !=
0L) {
criteria.add(Restrictions.eq("custIndustry.dictId", customer.custIndustry!!.dictId))
}
//cstSource为空或其id为0
if (customer.custSource != null && customer.custSource?.dictId !=
0L) {
criteria.add(Restrictions.eq("custSource.dictId", customer.custSource!!.dictId))
}
return criteria
}
private fun uploadImg() {
val subPath = UploadUtil.genUploadPath(imageUpLoadFileName!!)
imgDir += subPath
val file = File(imgDir)
if (!file.exists()) {
file.mkdirs()
}
imageUpLoad?.copyTo(file, true)
customer!!.cstImage = subPath
}
}<file_sep>/src/org/itheima/crm/service/impl/CustomerServiceImpl.kt
package org.itheima.crm.service.impl
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.dao.CustomerDao
import org.itheima.crm.dao.impl.CustomerDaoImpl
import org.itheima.crm.domain.Customer
import org.itheima.crm.domain.PageBean
import org.itheima.crm.service.CustomerService
import org.springframework.beans.factory.annotation.Autowired
import org.springframework.transaction.annotation.Transactional
/**
* Created by 钟未鸣 on 2017/9/10 .
*/
@Transactional
open class CustomerServiceImpl : CustomerService {
override fun findList(): ArrayList<Customer> {
val criteria = DetachedCriteria.forClass(Customer::class.java)
return customerDao.findList(criteria,0,0) as ArrayList<Customer>
}
// private var customerDao: CustomerDao? = null
override fun delete(customer: Customer) {
customerDao.delete(customer)
}
fun setCustomerDao(customerDao: CustomerDao){
this.customerDao =customerDao
}
override fun update(customer: Customer) {
customerDao.update(customer)
}
override fun findById(custId: Long): Customer {
return customerDao.findById(custId)
}
override fun findBean(criteria: DetachedCriteria, currentPage: Int, pageSize: Int): PageBean<Customer> {
val pageBean = PageBean<Customer>()
val totalCount = customerDao.totalCount(criteria)
criteria.setProjection(null)
val start = (currentPage - 1) * pageSize
pageBean.datas = customerDao.findList(criteria,start, pageSize)
pageBean.totalCount = totalCount
pageBean.currentPage = currentPage
pageBean.pageSize = pageSize
pageBean.totalPage = (totalCount * 1.0 /pageSize).toInt()
return pageBean
}
override fun totalCount(criteria: DetachedCriteria): Long {
return customerDao.totalCount(criteria)
}
// override fun findList(criteria: DetachedCriteria): List<Customer> {
// return customerDao?.findList(criteria)!!
// }
override fun saveCustomer(customer: Customer) {
println(customer)
customerDao.saveCustomer(customer)
}
private lateinit var customerDao: CustomerDao
}<file_sep>/src/org/itheima/crm/dao/CustomerDao.kt
package org.itheima.crm.dao
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.domain.Customer
/**
* Created by 钟未鸣 on 2017/9/10 .
*/
interface CustomerDao {
fun saveCustomer(customer: Customer)
fun findList(criteria: DetachedCriteria, start: Int, end: Int): List<Customer>
fun totalCount(criteria: DetachedCriteria): Long
fun findById(custId: Long): Customer
fun update(customer: Customer)
fun delete(customer: Customer)
}<file_sep>/web/js/addOptions.js
/**
* Created by zhong on 2017/9/13.
*/
function addOptions(typeCode, selectId) {
var url = "${pageContext.request.contextPath}/customer/dict.action";
var data = {"dictTypeCode": typeCode};
var callback = function (dicts) {
$(dicts).each(function (i, dict) {
var idSelector = "#" + selectId;
var idData = "#" + selectId + "_data";
idData = $(idData).attr("data");
console.log(idData);
//处理数据回显的判断
if (idData == dict.dictId) {
$(idSelector).append("<option value='" + dict.dictId +
"' selected='selected'>" +
dict.dictItemName +
"</option>")
} else {
$(idSelector).append("<option value='" + dict.dictId + "' >" +
dict.dictItemName +
"</option>")
}
})
};
var type = "json";
$.post(url, data, callback, type)
}
<file_sep>/README.md
# CRM
基于黑马程序员JavaEE课程的CRM小练习
<file_sep>/src/org/itheima/crm/domain/BaseDict.kt
package org.itheima.crm.domain
/**
* Created by 钟未鸣 on 2017/9/10 .
private long dictId;
private String dictTypeCode;
private String dictTypeName;
private String dictItemName;
private String dictItemCode;
private Integer dictSort;
private Integer dictState;
private String dictComment;
*/
data class BaseDict(var dictId: Long?, var dictTypeCode: String?, var dictTypeName: String?, var
dictItemName: String?,
var dictItemCode: String?, var dictSort: Int?, var dictState: Int?, var
dictComment: String?) {
constructor() : this(null, null, null, null, null, null, null, null)
}<file_sep>/src/org/itheima/crm/utils/PropertyPlaceHolder.kt
package org.itheima.crm.utils
import org.springframework.beans.BeansException
import org.springframework.beans.factory.config.ConfigurableListableBeanFactory
import org.springframework.beans.factory.config.PropertyPlaceholderConfigurer
import java.util.*
import java.util.HashMap
/**
* Created by 钟未鸣 on 2017/9/11 .
*/
object PropertyPlaceholder : PropertyPlaceholderConfigurer() {
private var propertyMap: Map<String, String>? = null
override fun processProperties(beanFactoryToProcess: ConfigurableListableBeanFactory, props: Properties) {
super.processProperties(beanFactoryToProcess, props)
propertyMap = HashMap()
for ((key,value) in props) {
(propertyMap as HashMap<String, String>).put(key as String, value as String)
}
}
// static method for accessing context properties
fun getProperty(name: String): String {
return propertyMap!![name]!!
}
}<file_sep>/src/org/itheima/crm/service/CustomerService.kt
package org.itheima.crm.service
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.domain.Customer
import org.itheima.crm.domain.PageBean
/**
* Created by 钟未鸣 on 2017/9/10 .
*/
interface CustomerService {
fun saveCustomer(customer: Customer)
// fun findList(criteria: DetachedCriteria): List<Customer>
fun totalCount(criteria: DetachedCriteria): Long
fun findBean(criteria: DetachedCriteria, currentPage: Int, pageSize: Int): PageBean<Customer>
fun findById(custId: Long): Customer
fun update(customer: Customer)
fun delete(customer: Customer)
fun findList(): ArrayList<Customer>
}<file_sep>/src/org/itheima/crm/service/impl/DictServiceImpl.kt
package org.itheima.crm.service.impl
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.dao.DictDao
import org.itheima.crm.domain.BaseDict
import org.itheima.crm.service.DictService
import org.springframework.transaction.annotation.Transactional
/**
* Created by 钟未鸣 on 2017/9/10 .
*/
@Transactional
open class DictServiceImpl : DictService{
private var dictDao : DictDao?= null
fun setDictDao(dictDao: DictDao){
this.dictDao = dictDao
}
override fun save(dict: BaseDict) {
dictDao?.save(dict)
}
override fun findList(criteria: DetachedCriteria): List<BaseDict>? {
return dictDao?.findList(criteria)
}
}<file_sep>/src/org/itheima/crm/dao/impl/CustomerDaoImpl.kt
package org.itheima.crm.dao.impl
import org.hibernate.criterion.DetachedCriteria
import org.hibernate.criterion.Projections
import org.itheima.crm.dao.CustomerDao
import org.itheima.crm.domain.Customer
import org.springframework.orm.hibernate5.support.HibernateDaoSupport
/**
* Created by 钟未鸣 on 2017/9/10 .
*/
class CustomerDaoImpl : CustomerDao,HibernateDaoSupport() {
override fun delete(customer: Customer) {
hibernateTemplate.delete(customer)
}
override fun update(customer: Customer) {
hibernateTemplate.update(customer)
}
override fun findById(custId: Long): Customer {
return hibernateTemplate.get(Customer::class.java,custId)
}
override fun totalCount(criteria: DetachedCriteria): Long {
criteria.setProjection(Projections.rowCount())
return hibernateTemplate.findByCriteria(criteria)[0] as Long
}
override fun findList(criteria: DetachedCriteria, start: Int, end: Int): List<Customer> {
@Suppress("UNCHECKED_CAST")
if(start==0 && end ==0){
return hibernateTemplate.findByCriteria(criteria) as List<Customer>
}
return hibernateTemplate.findByCriteria(criteria,start,end) as List<Customer>
}
override fun saveCustomer(customer: Customer) {
hibernateTemplate.save(customer)
}
}<file_sep>/src/org/itheima/crm/service/DictService.kt
package org.itheima.crm.service
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.domain.BaseDict
/**
* Created by 钟未鸣 on 2017/9/10 .
*/
interface DictService{
fun save(dict: BaseDict)
fun findList(criteria: DetachedCriteria) : List<BaseDict>?
}<file_sep>/src/test/itheima/crm/TestLinkman.kt
package test.itheima.crm
import org.itheima.crm.domain.Linkman
import org.itheima.crm.service.CustomerService
import org.itheima.crm.service.LinkmanService
import org.itheima.crm.service.impl.CustomerServiceImpl
import org.itheima.crm.service.impl.LinkmanServiceImpl
import org.junit.Test
import org.junit.runner.RunWith
import org.springframework.beans.factory.annotation.Autowired
import org.springframework.test.context.ContextConfiguration
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner
/**
* Created by 钟未鸣 on 2017/9/13 .
*/
@RunWith(SpringJUnit4ClassRunner::class)
@ContextConfiguration("classpath:applicationContext.xml")
class TestLinkman{
@Autowired lateinit var linkmanService: LinkmanService
@Autowired lateinit var customerService: CustomerService
@Test
fun testSave(){
val findById = customerService.findById(11)
val linkman = Linkman()
linkman.customer = findById
linkman.lkmMobile="133"
linkman.lkmName="zs"
linkman.lkmEmail="<EMAIL>"
linkmanService.save(linkman)
}
}
<file_sep>/src/org/itheima/crm/service/impl/LinkmanServiceImpl.kt
package org.itheima.crm.service.impl
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.dao.LinkmanDao
import org.itheima.crm.dao.LinkmanDaoImpl
import org.itheima.crm.domain.Linkman
import org.itheima.crm.domain.PageBean
import org.itheima.crm.service.LinkmanService
import org.springframework.beans.factory.annotation.Autowired
import org.springframework.transaction.annotation.Transactional
/**
* Created by 钟未鸣 on 2017/9/13 .
*/
@Transactional
open class LinkmanServiceImpl : LinkmanService{
override fun findPage(criteria: DetachedCriteria, currentPage: Int, pageSize: Int): PageBean<Linkman> {
val pageBean = PageBean<Linkman>()
pageBean.pageSize =pageSize
pageBean.currentPage = currentPage
val totalCount = linkmanDao.totalCount(criteria)
pageBean.totalCount = totalCount
val start = (currentPage -1) * pageSize
pageBean.datas = linkmanDao.findList(criteria,start,pageSize)
pageBean.totalPage = (totalCount * 1.0 / pageSize).toInt()
return pageBean
}
override fun save(linkman: Linkman) {
linkmanDao.save(linkman)
}
@Autowired lateinit var linkmanDao: LinkmanDao
}<file_sep>/src/org/itheima/crm/dao/DictDao.kt
package org.itheima.crm.dao
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.domain.BaseDict
/**
* Created by 钟未鸣 on 2017/9/10 .
*/
interface DictDao{
fun save(dict: BaseDict)
fun findList(criteria: DetachedCriteria) : List<BaseDict>
}<file_sep>/src/org/itheima/crm/domain/Customer.kt
package org.itheima.crm.domain
/**
* Created by 钟未鸣 on 2017/9/10 .
private long custId;
private String custName;
private String custPhone;
private String custMobile;
private String cstImage;
private BaseDict custSource;
private BaseDict custIndustry;
private BaseDict cstLevel;
*/
data class Customer(var custId: Long?, var custName: String?, var custPhone: String?, var
custMobile:
String?, var cstImage: String?, var custSource: BaseDict?, var custIndustry: BaseDict?, var
cstLevel: BaseDict?) {
constructor() : this(null, null, null, null, null, null, null, null)
}<file_sep>/src/test/itheima/crm/TestCRM.kt
package test.itheima.crm
import org.itheima.crm.domain.User
import org.itheima.crm.service.UserService
import org.itheima.crm.utils.MD5Util
import org.junit.Test
import org.junit.runner.RunWith
import org.springframework.beans.factory.annotation.Autowired
import org.springframework.test.context.ContextConfiguration
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner
/**
* Created by 钟未鸣 on 2017/9/8.
*/
@RunWith(SpringJUnit4ClassRunner::class)
@ContextConfiguration("classpath:applicationContext.xml")
class TestCRM {
@Autowired
private var userService:UserService? = null
@Test fun testAdd(){
val user=User(null,"admin","admin","123",1,null)
userService!!.save(user)
}
@Test fun testMd5(){
val md5 = MD5Util.deafultMd5("123")
println(md5)
}
@Test fun testLogin(){
val user = User()
user.userCode="admin"
user.userPassword="123"
val loginUser = userService?.login(user)
println(loginUser)
}
@Test fun testStringEquals(){
var s1 = "1a"
var s2 = "1A"
println(s1.equals(s2,true))
}
}<file_sep>/src/org/itheima/crm/utils/MD5Util.kt
package org.itheima.crm.utils
import org.springframework.util.DigestUtils
/**
* Created by 钟未鸣 on 2017/9/8 .
*/
object MD5Util{
fun deafultMd5(msg: String): String{
return md5(msg,5)
}
fun md5(msg: String): String {
return DigestUtils.md5DigestAsHex(msg.byteInputStream())
}
/**
* 加密多次
*
* @param password
* @param count
* @return
*/
private fun md5(password: String, count: Int): String {
@Suppress("NAME_SHADOWING")
var password = <PASSWORD>
for (i in 0 until count) {
password = md5(password)
}
return password
}
}<file_sep>/src/org/itheima/crm/dao/impl/UserDaoImpl.kt
package org.itheima.crm.dao.impl
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.dao.UserDao
import org.itheima.crm.domain.User
import org.itheima.crm.utils.MD5Util
import org.springframework.orm.hibernate5.support.HibernateDaoSupport
import org.springframework.transaction.annotation.Transactional
/**
* Created by 钟未鸣 on 2017/9/8 .
*/
open class UserDaoImpl : UserDao,HibernateDaoSupport() {
override fun login(criteria: DetachedCriteria): MutableList<*>? {
return hibernateTemplate.findByCriteria(criteria)
}
override fun save(user: User) {
user.userPassword=MD5Util.<PASSWORD>(user.userPassword!!)
hibernateTemplate.save(user)
}
}<file_sep>/src/test/itheima/crm/TestBaseDictService.kt
package test.itheima.crm
import org.hibernate.Criteria
import org.hibernate.criterion.DetachedCriteria
import org.hibernate.criterion.Restrictions
import org.junit.Test
import org.junit.runner.RunWith
import org.springframework.test.context.ContextConfiguration
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner
import org.itheima.crm.domain.BaseDict
import org.itheima.crm.service.DictService
import org.springframework.beans.factory.annotation.Autowired
/**
* Created by 钟未鸣 on 2017/9/10 .
*/
@RunWith(SpringJUnit4ClassRunner::class)
@ContextConfiguration("classpath:applicationContext.xml")
class TestBaseDictService{
@Autowired
private var service: DictService?=null
@Test fun testFindList(){
val criteria = DetachedCriteria.forClass(BaseDict::class.java)
criteria.add(Restrictions.eq("dictTypeCode","001"))
val list = service!!.findList(criteria)
if (list != null) {
for(dict in list){
println(dict)
}
}
}
@Test fun testSave(){
service!!.save(BaseDict(null, "001", "客户行业", "教育培训 ", null, 1, 1, null))
service!!.save(BaseDict(null, "001", "客户行业", "电子商务", null, 2, 1, null))
service!!.save(BaseDict(null, "001", "客户行业", "对外贸易", null, 3, 1, null))
service!!.save(BaseDict(null, "001", "客户行业", "酒店旅游", null, 4, 1, null))
service!!.save(BaseDict(null, "001", "客户行业", "房地产", null, 5, 1, null))
service!!.save(BaseDict(null, "002", "客户信息来源", "电话营销", null, 1, 1, null))
service!!.save(BaseDict(null, "002", "客户信息来源", "网络营销", null, 2, 1, null))
service!!.save(BaseDict(null, "003", "公司性质", "合资", null, 1, 1, null))
service!!.save(BaseDict(null, "003", "公司性质", "国企", null, 2, 1, null))
service!!.save(BaseDict(null, "003", "公司性质", "民企", null, 3, 1, null))
service!!.save(BaseDict(null, "004", "年营业额", "1-10万", null, 1, 1, null))
service!!.save(BaseDict(null, "004", "年营业额", "10-20万", null, 2, 1, null))
service!!.save(BaseDict(null, "004", "年营业额", "20-50万", null, 3, 1, null))
service!!.save(BaseDict(null, "004", "年营业额", "50-100万", null, 4, 1, null))
service!!.save(BaseDict(null, "004", "年营业额", "100-500万", null, 5, 1, null))
service!!.save(BaseDict(null, "004", "年营业额", "500-1000万", null, 6, 1, null))
service!!.save(BaseDict(null, "005", "客户状态", "基础客户", null, 1, 1, null))
service!!.save(BaseDict(null, "005", "客户状态", "潜在客户", null, 2, 1, null))
service!!.save(BaseDict(null, "005", "客户状态", "成功客户", null, 3, 1, null))
service!!.save(BaseDict(null, "005", "客户状态", "无效客户", null, 4, 1, null))
service!!.save(BaseDict(null, "006", "客户级别", "普通客户", null, 1, 1, null))
service!!.save(BaseDict(null, "006", "客户级别", "VIP客户", null, 2, 1, null))
service!!.save(BaseDict(null, "007", "商机状态", "意向客户", null, 1, 1, null))
service!!.save(BaseDict(null, "007", "商机状态", "初步沟通", null, 2, 1, null))
service!!.save(BaseDict(null, "007", "商机状态", "深度沟通", null, 3, 1, null))
service!!.save(BaseDict(null, "007", "商机状态", "签订合同", null, 4, 1, null))
service!!.save(BaseDict(null, "008", "商机类型", "新业务", null, 1, 1, null))
service!!.save(BaseDict(null, "008", "商机类型", "现有业务", null, 2, 1, null))
service!!.save(BaseDict(null, "009", "商机来源", "电话营销", null, 1, 1, null))
service!!.save(BaseDict(null, "009", "商机来源", "网络营销", null, 2, 1, null))
service!!.save(BaseDict(null, "009", "商机来源", "推广活动", null, 3, 1, null))
}
}<file_sep>/src/org/itheima/crm/web/action/LinkmanAction.kt
package org.itheima.crm.web.action
import com.opensymphony.xwork2.ActionContext
import com.opensymphony.xwork2.ActionSupport
import com.opensymphony.xwork2.ModelDriven
import org.hibernate.criterion.DetachedCriteria
import org.hibernate.criterion.Restrictions
import org.itheima.crm.domain.Linkman
import org.itheima.crm.service.CustomerService
import org.itheima.crm.service.LinkmanService
import org.springframework.beans.factory.annotation.Autowired
/**
* Created by 钟未鸣 on 2017/9/13 .
*/
class LinkmanAction : ActionSupport(), ModelDriven<Linkman> {
private var currentPage = 1
private var pageSize = 10
var linkman: Linkman? = null
override fun getModel(): Linkman {
if (linkman == null) {
linkman = Linkman()
}
return linkman as Linkman
}
private val LISTSUCCESS = "listSuccess"
private val ADDSUCCESS = "addSuccess"
private val SAVESUCCESS = "saveSuccess"
private val SAVEERROR = "saveError"
override fun hasErrors(): Boolean {
return false
}
@Suppress("unused")
fun do_add(): String {
val customers = customerService.findList()
val stack = ActionContext.getContext().valueStack
stack.set("customers", customers)
return ADDSUCCESS
}
@Suppress("unused")
fun do_list(): String {
val criteria = DetachedCriteria.forClass(Linkman::class.java)
//增加筛选条件
criteria.addRestrictions(linkman!!)
val pageBean =linkmanService.findPage(criteria, currentPage, pageSize)
val stack = ActionContext.getContext().valueStack
stack.push(pageBean)
return LISTSUCCESS
}
@Suppress("unused")
fun do_save(): String {
val hasError: Boolean = checkLinkmanDataHasError()
if (hasError) {
return SAVEERROR
}
linkmanService.save(linkman!!)
return SAVESUCCESS
}
/**
* @return true:传过来的数据不齐全 false:传过来数据齐全
*/
private fun checkLinkmanDataHasError(): Boolean {
if (linkman!!.customer!!.custId == 0L) {
addActionError("所属客户不能为空!")
return true
}
if (linkman!!.lkmName.isNullOrEmpty()) {
addActionError("联系人姓名不能为空!")
return true
}
if (linkman!!.lkmPhone.isNullOrEmpty()) {
addActionError("联系人办公电话不能为空!")
return true
}
if (linkman!!.lkmMobile.isNullOrEmpty()) {
addActionError("联系人手机不能为空!")
return true
}
if (linkman!!.lkmEmail.isNullOrEmpty()) {
addActionError("联系人邮箱不能为空!")
return true
}
if (linkman!!.lkmQq.isNullOrEmpty()) {
addActionError("联系人QQ不能为空!")
return true
}
if (linkman!!.lkmPosition.isNullOrEmpty()) {
addActionError("联系人职位不能为空!")
return true
}
if (linkman!!.lkmComment.isNullOrEmpty()) {
addActionError("联系人备注不能为空!")
return true
}
return false
}
@Autowired private lateinit var linkmanService: LinkmanService
@Autowired private lateinit var customerService: CustomerService
}
private fun DetachedCriteria.addRestrictions(linkman: Linkman) {
val name = linkman.lkmName
if(!name.isNullOrEmpty()){
this.add(Restrictions.like("lkmName","%$name%"))
}
val lkmGender=linkman.lkmGender
if(lkmGender!=0 && lkmGender!=null){
this.add(Restrictions.eq("lkmGender",lkmGender))
}
val phone= linkman.lkmPhone
if(!phone.isNullOrEmpty()){
this.add(Restrictions.like("lkmPhone","%$phone%"))
}
val mobile= linkman.lkmMobile
if(!mobile.isNullOrEmpty()){
this.add(Restrictions.like("lkmMobile","%$mobile%"))
}
val email = linkman.lkmEmail
if(!email.isNullOrEmpty()){
this.add(Restrictions.like("lkmEmail","%$email%"))
}
val qq = linkman.lkmQq
if(!qq.isNullOrEmpty()){
this.add(Restrictions.like("lkmQq","%$qq%"))
}
}
<file_sep>/src/org/itheima/crm/dao/LinkmanDaoImpl.kt
package org.itheima.crm.dao
import org.hibernate.criterion.DetachedCriteria
import org.hibernate.criterion.Projections
import org.itheima.crm.domain.Linkman
import org.springframework.orm.hibernate5.support.HibernateDaoSupport
/**
* Created by 钟未鸣 on 2017/9/13 .
*/
class LinkmanDaoImpl : LinkmanDao,HibernateDaoSupport(){
override fun findList(criteria: DetachedCriteria, start: Int, pageSize: Int): List<Linkman> {
criteria.setProjection(null)
@Suppress("UNCHECKED_CAST")
return hibernateTemplate.findByCriteria(criteria,start,pageSize) as List<Linkman>
}
override fun totalCount(criteria: DetachedCriteria): Long {
criteria.setProjection(Projections.rowCount())
return hibernateTemplate.findByCriteria(criteria)[0] as Long
}
override fun save(linkman: Linkman) {
hibernateTemplate.save(linkman)
}
}<file_sep>/src/org/itheima/crm/service/impl/UserServiceImpl.kt
package org.itheima.crm.service.impl
import org.hibernate.criterion.DetachedCriteria
import org.hibernate.criterion.Restrictions
import org.itheima.crm.dao.UserDao
import org.itheima.crm.domain.User
import org.itheima.crm.service.UserService
import org.itheima.crm.utils.MD5Util
import org.springframework.transaction.annotation.Transactional
/**
* Created by 钟未鸣 on 2017/9/8 .
*/
@Transactional
open class UserServiceImpl :UserService {
private var userDao: UserDao? =null
fun setUserDao(userDao: UserDao){
this.userDao = userDao
}
override fun save(user: User) {
userDao?.save(user)
}
override fun login(user: User): User? {
val criteria = DetachedCriteria.forClass(User::class.java)
user.userPassword=MD5Util.deafultMd5(user.userPassword!!)
criteria.add(Restrictions.eq("userCode",user.userCode))
criteria.add(Restrictions.eq("userPassword",user.userPassword))
val users = userDao?.login(criteria)
if(users==null || users.size==0 ){
return null
}
return users[0] as User?
}
}
<file_sep>/src/org/itheima/crm/domain/User.kt
package org.itheima.crm.domain
/**
* Created by 钟未鸣 on 2017/9/8.
*/
data class User(var userId: Long?, var userCode: String?, var userName: String?,
var userPassword: String?, var userState: Int?,var validateCode: String?) {
constructor() :this(null,null,null,null,null,null)
}<file_sep>/src/org/itheima/crm/dao/UserDao.kt
package org.itheima.crm.dao
import org.hibernate.criterion.DetachedCriteria
import org.itheima.crm.domain.User
/**
* Created by 钟未鸣 on 2017/9/8 .
*/
interface UserDao {
fun save(user: User)
fun login(criteria: DetachedCriteria): MutableList<*>?
}<file_sep>/src/org/itheima/crm/domain/Linkman.kt
package org.itheima.crm.domain
/**
* Created by 钟未鸣 on 2017/9/13 .
*/
class Linkman {
var lkmId: Long = 0
var lkmName: String? = null
var lkmGender: Int? = null
var lkmPhone: String? = null
var lkmMobile: String? = null
var lkmEmail: String? = null
var lkmQq: String? = null
var lkmPosition: String? = null
var lkmComment: String? = null
var customer:Customer? =null
override fun toString(): String {
return "Linkman(lkmId=$lkmId, lkmName=$lkmName, lkmGender=$lkmGender, lkmPhone=$lkmPhone, lkmMobile=$lkmMobile, lkmEmail=$lkmEmail, lkmQq=$lkmQq, lkmPosition=$lkmPosition, lkmComment=$lkmComment)"
}
}
| 71999e458b90f12ed3cf165142822f8348264ea9 | [
"JavaScript",
"Kotlin",
"Markdown"
]
| 33 | Kotlin | clockknock/CRM | c533c222f9abb0f1db19b9db76221d533f1bb586 | 9ffe63c40a70706b6d92a59f2889bee9caede3ad | |
refs/heads/main | <repo_name>haseeb67/rydeluxury.github.io<file_sep>/help.html
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>help</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" href="assets/favicon.svg" type="image/svg" sizes="24x24">
<title>Luxury</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"
integrity="<KEY>" crossorigin="anonymous">
<link href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet"
integrity="<KEY>" crossorigin="anonymous">
<link rel="stylesheet" href="https://pro.fontawesome.com/releases/v5.10.0/css/all.css" />
<!-- Animation link -->
<link href="https://unpkg.com/[email protected]/dist/aos.css" rel="stylesheet">
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<div class="s_container">
<!-- header start -->
<nav class="main-menu navbar fixed-top navbar-expand-lg bg-white">
<a class="navbar-brand" href="index.html">
<img src="./assets/img/home/logo.svg" alt="img">
</a>
<div class="navbar-collapse" id="navbarNavDropdown">
<ul class="show-hide-nav navbar-nav justify-content-center">
<li class="nav-item ">
<a class="nav-link " href="services.html">
<img class="hovericon" src="assets/services.svg">
<img class="hovericon1" src="assets/services_color.svg">
Service </a>
</li>
<!-- <li class="nav-item">
<a class="nav-link" href="#">
<img class="hovericon" src="assets/img/home/Join as Partner.svg">
<img class="hovericon1" src="assets/join_as_color.svg" alt="">
Join as Partner</a>
</li> -->
<li class="nav-item">
<a class="nav-link" href="help.html">
<img class="hovericon" src="assets/img/home/help.svg">
<img class="hovericon1" src="assets/help_color.svg">
Help</a>
</li>
<li class="nav-item">
<a class="nav-link" href="contactus.html">
<img class="hovericon" src="assets/contact-us.svg">
<img class="hovericon1" src="assets/contact_us_color.svg">
Contact Us</a>
</li>
</ul>
<div class="nav-btns d-flex align-items-center w-20 justify-content-end">
<!-- <button class="btn btn-primary mr-2 ">
<img src="assets/login.svg" alt="">
Sign in
</button> -->
<button class="btn lan">
<img src="assets/lan.svg" alt="">
EN
</button>
</div>
<button id="bars-btn" class="toggle-bar">
<i class="fa fa-bars"></i>
</button>
</div>
</nav>
<!-- header end -->
<!-- Start Services page -->
<!-- Strat Banner -->
<div class="Services-banner Privacy-banner">
<div class="container">
<h5>Help</h5>
<h1>Having Trouble?<br>
We're Here To Help</h1>
<div class="help-search-bar">
<select class="form-control" id="exampleFormControlSelect1">
<option selected="">Ride</option>
<option>2</option>
<option>3</option>
<option>4</option>
<option>5</option>
</select>
<input type="text" placeholder="Describe your issue">
<button>Search</button>
</div>
</div>
</div>
<div class="brack-line m-t-custom"></div>
<div class="container help-content privacy-content">
<div class="nav flex-column nav-pills" id="v-pills-tab" role="tablist" aria-orientation="vertical">
<a class="nav-link active" id="v-pills-home-tab" data-toggle="pill" href="#v-pills-home" role="tab"
aria-controls="v-pills-home" aria-selected="true">Ride</a>
<a class="nav-link" id="v-pills-profile-tab" data-toggle="pill" href="#v-pills-profile" role="tab"
aria-controls="v-pills-profile" aria-selected="false">Partner</a>
<a class="nav-link" id="v-pills-messages-tab" data-toggle="pill" href="#v-pills-messages" role="tab"
aria-controls="v-pills-messages" aria-selected="false">Booking</a>
<a class="nav-link" id="v-pills-settings-tab" data-toggle="pill" href="#v-pills-settings" role="tab"
aria-controls="v-pills-settings" aria-selected="false">Payments & receipts</a>
<a class="nav-link" id="v-safty-tab" data-toggle="pill" href="#v-safty" role="tab" aria-controls="v-safty"
aria-selected="false">Safety & Security</a>
</div>
<div class="tab-content" id="v-pills-tabContent">
<div class="tab-pane fade show active" id="v-pills-home" role="tabpanel" aria-labelledby="v-pills-home-tab">
<h1>Group & Event Transportation </h1>
<div class="accordion" id="accordionExample">
<div class="card">
<div class="card-header" id="headingOne">
<h5 class="mb-0">
<button type="button" class="btn btn-link" data-toggle="collapse" data-target="#collapseOne"> Trip
issues and refunds <i class="fa fa-long-arrow-right"></i> </button>
</h5>
</div>
<div id="collapseOne" class="collapse" aria-labelledby="headingOne" data-parent="#accordionExample">
<div class="card-body">
<p>HTML stands for HyperText Markup Language. HTML is the standard markup language for describing the
structure of web pages. <a href="https://www.tutorialrepublic.com/html-tutorial/"
target="_blank">Learn more.</a></p>
</div>
</div>
</div>
<div class="card">
<div class="card-header" id="heading2">
<h5 class="mb-0">
<button type="button" class="btn btn-link" data-toggle="collapse" data-target="#collapse2"> Booking a
Ride <i class="fa fa-long-arrow-right"></i> </button>
</h5>
</div>
<div id="collapse2" class="collapse show" aria-labelledby="heading2" data-parent="#accordionExample">
<div class="card-body">
<p>But I must explain to you how all this mistaken idea of denouncing pleasure and praising pain was
born and I will give you a complete account of the system, and expound the actual teachings of the
great explorer of the truth, the master-builder of human happiness. No one rejects, dislikes, or
avoids pleasure itself, because it is pleasure, but because those who do not know how to pursue
pleasure rationally encounter consequences that are extremely painful.
Nor again is there anyone who loves or pursues or desires to obtain pain of itself, because it is
pain, but because occasionally circumstances occur in which toil and pain can procure him some great
pleasure. To take a trivial example, which of us ever undertakes laborious physical exercise, except
to obtain some advantage.</p>
<p>But I must explain to you how all this mistaken idea of denouncing pleasure and praising pain was
born and I will give you a complete account of the system, and expound the actual teachings of the
great explorer of the truth.</p>
<h4>Where can I get some</h4>
<p>But I must explain to you how all this mistaken idea of denouncing pleasure and praising pain was
born and I will give you a complete account of the system, and expound the actual teachings of the
great explorer of the truth, the master-builder of human happiness. No one rejects, dislikes, or
avoids pleasure itself, because it is pleasure, but because those who do not know how to pursue
pleasure rationally encounter consequences that are extremely painful.</p>
<p>But I must explain to you how all this mistaken idea of denouncing pleasure and praising pain was
born and I will give you a complete account of the system, and expound the actual teachings of the
great explorer .</p>
<p>But I must explain to you how all this mistaken idea of denouncing pleasure and praising pain was
born and I will give you a complete account of the system, and expound the actual teachings of the
great explorer of the truth, the master-builder of human happiness. No one rejects, dislikes, or
avoids pleasure itself, because it is pleasure, but because those who do not know how to pursue
pleasure rationally encounter consequences that are extremely painful.</p>
</div>
</div>
</div>
<div class="card">
<div class="card-header" id="heading3">
<h5 class="mb-0">
<button type="button" class="btn btn-link" data-toggle="collapse" data-target="#collapse3">More <i
class="fa fa-long-arrow-right"></i> </button>
</h5>
</div>
<div id="collapse3" class="collapse" aria-labelledby="heading3" data-parent="#accordionExample">
<div class="card-body">
<p>HTML stands for HyperText Markup Language. HTML is the standard markup language for describing the
structure of web pages. <a href="https://www.tutorialrepublic.com/html-tutorial/"
target="_blank">Learn more.</a></p>
</div>
</div>
</div>
<div class="card">
<div class="card-header" id="heading4">
<h5 class="mb-0">
<button type="button" class="btn btn-link" data-toggle="collapse" data-target="#collapse4"> A Guide to
Ryde Luxury<i class="fa fa-long-arrow-right"></i> </button>
</h5>
</div>
<div id="collapse4" class="collapse" aria-labelledby="heading4" data-parent="#accordionExample">
<div class="card-body">
<p>HTML stands for HyperText Markup Language. HTML is the standard markup language for describing the
structure of web pages. <a href="https://www.tutorialrepublic.com/html-tutorial/"
target="_blank">Learn more.</a></p>
</div>
</div>
</div>
<div class="card">
<div class="card-header" id="heading4">
<h5 class="mb-0">
<button type="button" class="btn btn-link" data-toggle="collapse" data-target="#collapse4"> Sign Up <i
class="fa fa-long-arrow-right"></i> </button>
</h5>
</div>
<div id="collapse4" class="collapse" aria-labelledby="heading4" data-parent="#accordionExample">
<div class="card-body">
<p>HTML stands for HyperText Markup Language. HTML is the standard markup language for describing the
structure of web pages. <a href="https://www.tutorialrepublic.com/html-tutorial/"
target="_blank">Learn more.</a></p>
</div>
</div>
</div>
</div>
</div>
<div class="tab-pane fade" id="v-pills-profile" role="tabpanel" aria-labelledby="v-pills-profile-tab">Partner
</div>
<div class="tab-pane fade" id="v-pills-messages" role="tabpanel" aria-labelledby="v-pills-messages-tab">Booking
</div>
<div class="tab-pane fade" id="v-pills-settings" role="tabpanel" aria-labelledby="v-pills-settings-tab">Payments
& receipts</div>
<div class="tab-pane fade" id="v-safty" role="tabpanel" aria-labelledby="v-safty-tab">Safety & Security</div>
</div>
</div>
<div class="brack-line m-t-custom"></div>
<!-- Start Servces on row -->
<div class="container">
<div class="row">
<div class="col-lg-4 col-sm-4 col-xs-12 ser-community" data-aos="zoom-in" data-aos-delay="200"
data-aos-duration="800">
<img src="assets/NoPath - Copy (36)@2x.jpg" alt="">
<div class="">
<h6>Community</h6>
<h5>host wants everyone to try tesla</h5>
<span>December 2, 2020</span>
</div>
</div>
<div class="col-lg-4 col-sm-4 col-xs-12 ser-community" data-aos="zoom-in" data-aos-delay="400"
data-aos-duration="800">
<img src="assets/NoPath - Copy (43)@2x.jpg" alt="">
<div class="">
<h6>Community</h6>
<h5>Channel a Beyoncé moment at your
next spa trip</h5>
<span>December 2, 2020</span>
</div>
</div>
<div class="col-lg-4 col-sm-4 col-xs-12 ser-community" data-aos="zoom-in" data-aos-delay="600"
data-aos-duration="800">
<img src="assets/NoPath - Copy (44)@2x.jpg" alt="">
<div class="">
<h6>Community</h6>
<h5>US Airmen surprise squadron
commander</h5>
<span>December 2, 2020</span>
</div>
</div>
</div>
</div>
<!-- Start Servces on row -->
<!-- Strat Your Luxury Ride Is One Click Away -->
<div class="YLR-box container">
<div class="YLR row">
<div class="col-lg-6" data-aos="fade-right" data-aos-delay="300" data-aos-duration="800">
<img class="w-100" src="assets/[email protected]" alt="">
</div>
<div class="col-lg-6 YLR-details" data-aos="fade-left" data-aos-delay="300" data-aos-duration="800">
<h1>Your Luxury Ride Is One <br> Click Away</h1>
<p>Easily Book, Change, Or Cancel Rides On The Go.</p>
<button onclick="window.location.href='booknow.html';">Book Now</button>
</div>
</div>
</div>
<!-- End Your Luxury Ride Is One Click Away -->
</div>
<!-- End Services on colum -->
<!-- End Services page -->
<!-- Strat Footer -->
<div class="container-fuild footer-main">
<div class="footer-inner">
<div class="footer-header">
<div class="footer-logo">
<img src="assets/footer-logo.svg" alt="">
</div>
<div class="footer-app-btn">
<img class="mr-3" src="assets/footer-play-store.svg" alt="">
<img src="assets/footer-google-play.svg" alt="">
</div>
</div>
<div class="footer-content row">
<div class="col-lg-4 col-sm-6">
<ul>
<li>
<h3>Company</h3>
</li>
<li>
<a href="aboutus.html">About Us</a>
</li>
<!-- <li>
<a href="#">Career</a>
</li>
<li>
<a href="">Press</a>
</li>
<li>
<a href="blog.html">Blog</a>
</li> -->
<li>
<a href="services.html">Service</a>
</li>
</ul>
</div>
<div class="col-lg-4 col-sm-6">
<ul>
<li>
<h3>Resource</h3>
</li>
<!-- <li>
<a href="">Download</a>
</li> -->
<li>
<a href="help.html">Help Center</a>
</li>
<!-- <li>
<a href="">Guides</a>
</li> -->
<li>
<a href="contactus.html">Partner</a>
</li>
<li>
<a href="contactus.html">Developers</a>
</li>
</ul>
</div>
<!-- <div class="col-lg-3 col-sm-6">
<ul>
<li>
<h3>Locations</h3>
</li>
<li>
<a href="">USA (EN)</a>
</li>
<li>
<a href="#">Canada (EN)</a>
</li>
<li>
<a href="#">Canada (FR)</a>
</li>
<li>
<a href="">UK (EN)</a>
</li>
</ul>
</div> -->
<div class="col-lg-4 col-sm-6">
<h3 class="footer-blog">Blogs</h3>
<img class="w-100 mt-4" src="assets/img/home/NoPath - [email protected]" alt="">
<div class="footer-b-detial">
<h6>Community</h6>
<h3>
What To Expect From A Professional <br>
Chauffeur
</h3>
<span>December 2, 2020</span>
</div>
</div>
<div class="social-icons col-lg-12">
<ul>
<li>
<a href="">
<i class="fab fa-facebook-square" aria-hidden="true"></i>
</a>
</li>
<li>
<a href="">
<i class="fab fa-twitter" aria-hidden="true"></i>
</a>
</li>
<li>
<a href="">
<i class="fab fa-youtube" aria-hidden="true"></i>
</a>
</li>
<li>
<a href="">
<i class="fab fa-linkedin" aria-hidden="true"></i>
</a>
</li>
<li>
<a href="">
<i class="fab fa-instagram" aria-hidden="true"></i>
</a>
</li>
</ul>
</div>
</div>
</div>
</div>
<div class="copy-rightbar">
<p>©2020 ryde Luxury</p>
<ul>
<li>
<a href="terms-condition.html">
Terms
</a>
</li>
<li>
<a href="privacy-policy.html">
Privacy
</a>
</li>
<li>
<a href="#">
Sitemap
</a>
</li>
</ul>
</div>
<!-- End Footer -->
<script src="https://code.jquery.com/jquery-3.2.1.slim.min.js"
integrity="<KEY>" crossorigin="anonymous">
</script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js"
integrity="<KEY>" crossorigin="anonymous">
</script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"
integrity="<KEY>" crossorigin="anonymous">
</script>
<!-- Animation -->
<script src="https://unpkg.com/[email protected]/dist/aos.js"></script>
<script>
AOS.init();
</script>
<!-- Animation -->
<script>
$(document).ready(function () {
$("#bars-btn").click(function () {
$(".show-hide-nav").toggle();
});
});
</script>
<script>
$(document).ready(function () {
// Add minus icon for collapse element which is open by default
$(".collapse.show").each(function () {
$(this).prev(".card-header").find(".fa").addClass("fa-long-arrow-up").removeClass(
"fa-long-arrow-right");
});
// Toggle plus minus icon on show hide of collapse element
$(".collapse").on('show.bs.collapse', function () {
$(this).prev(".card-header").find(".fa").removeClass("fa-long-arrow-right").addClass(
"fa-long-arrow-up");
}).on('hide.bs.collapse', function () {
$(this).prev(".card-header").find(".fa").removeClass("fa-long-arrow-up").addClass(
"fa-long-arrow-right");
});
});
</script>
</body>
</html>
<file_sep>/animation/003f9318d868c4b20255a585da2bbff28e6ea649.ccd63acade508110fdd9.js
(window.webpackJsonp_N_E = window.webpackJsonp_N_E || []).push([[9], {
"+jk2": function(t, e, i) {
"use strict";
function n(t) {
if (void 0 === t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return t
}
function r(t, e) {
t.prototype = Object.create(e.prototype),
t.prototype.constructor = t,
t.__proto__ = e
}
i.d(e, "a", (function() {
return Cn
}
));
var o, a, s, c, l, p, u, d, f = {
autoSleep: 120,
force3D: "auto",
nullTargetWarn: 1,
units: {
lineHeight: ""
}
}, b = {
duration: .5,
overwrite: !1,
delay: 0
}, O = 1e8, h = 1e-8, j = 2 * Math.PI, C = j / 4, y = 0, v = Math.sqrt, x = Math.cos, m = Math.sin, g = function(t) {
return "string" === typeof t
}, _ = function(t) {
return "function" === typeof t
}, w = function(t) {
return "number" === typeof t
}, F = function(t) {
return "undefined" === typeof t
}, U = function(t) {
return "object" === typeof t
}, S = function(t) {
return !1 !== t
}, M = function() {
return "undefined" !== typeof window
}, k = function(t) {
return _(t) || g(t)
}, G = "function" === typeof ArrayBuffer && ArrayBuffer.isView || function() {}
, P = Array.isArray, Z = /(?:-?\.?\d|\.)+/gi, T = /[-+=.]*\d+[.e\-+]*\d*[e\-\+]*\d*/g, I = /[-+=.]*\d+[.e-]*\d*[a-z%]*/g, E = /[-+=.]*\d+(?:\.|e-|e)*\d*/gi, D = /[+-]=-?[\.\d]+/, A = /[#\-+.]*\b[a-z\d-=+%.]+/gi, L = {}, z = {}, B = function(t) {
return (z = ft(t, L)) && Je
}, R = function(t, e) {
return console.warn("Invalid property", t, "set to", e, "Missing plugin? gsap.registerPlugin()")
}, W = function(t, e) {
return !e && console.warn(t)
}, V = function(t, e) {
return t && (L[t] = e) && z && (z[t] = e) || L
}, N = function() {
return 0
}, H = {}, q = [], Y = {}, X = {}, Q = {}, J = 30, K = [], $ = "", tt = function(t) {
var e, i, n = t[0];
if (U(n) || _(n) || (t = [t]),
!(e = (n._gsap || {}).harness)) {
for (i = K.length; i-- && !K[i].targetTest(n); )
;
e = K[i]
}
for (i = t.length; i--; )
t[i] && (t[i]._gsap || (t[i]._gsap = new me(t[i],e))) || t.splice(i, 1);
return t
}, et = function(t) {
return t._gsap || tt(Bt(t))[0]._gsap
}, it = function(t, e, i) {
return (i = t[e]) && _(i) ? t[e]() : F(i) && t.getAttribute && t.getAttribute(e) || i
}, nt = function(t, e) {
return (t = t.split(",")).forEach(e) || t
}, rt = function(t) {
return Math.round(1e5 * t) / 1e5 || 0
}, ot = function(t, e) {
for (var i = e.length, n = 0; t.indexOf(e[n]) < 0 && ++n < i; )
;
return n < i
}, at = function(t, e, i) {
var n, r = w(t[1]), o = (r ? 2 : 1) + (e < 2 ? 0 : 1), a = t[o];
if (r && (a.duration = t[1]),
a.parent = i,
e) {
for (n = a; i && !("immediateRender"in n); )
n = i.vars.defaults || {},
i = S(i.vars.inherit) && i.parent;
a.immediateRender = S(n.immediateRender),
e < 2 ? a.runBackwards = 1 : a.startAt = t[o - 1]
}
return a
}, st = function() {
var t, e, i = q.length, n = q.slice(0);
for (Y = {},
q.length = 0,
t = 0; t < i; t++)
(e = n[t]) && e._lazy && (e.render(e._lazy[0], e._lazy[1], !0)._lazy = 0)
}, ct = function(t, e, i, n) {
q.length && st(),
t.render(e, i, n),
q.length && st()
}, lt = function(t) {
var e = parseFloat(t);
return (e || 0 === e) && (t + "").match(A).length < 2 ? e : g(t) ? t.trim() : t
}, pt = function(t) {
return t
}, ut = function(t, e) {
for (var i in e)
i in t || (t[i] = e[i]);
return t
}, dt = function(t, e) {
for (var i in e)
i in t || "duration" === i || "ease" === i || (t[i] = e[i])
}, ft = function(t, e) {
for (var i in e)
t[i] = e[i];
return t
}, bt = function t(e, i) {
for (var n in i)
e[n] = U(i[n]) ? t(e[n] || (e[n] = {}), i[n]) : i[n];
return e
}, Ot = function(t, e) {
var i, n = {};
for (i in t)
i in e || (n[i] = t[i]);
return n
}, ht = function(t) {
var e = t.parent || o
, i = t.keyframes ? dt : ut;
if (S(t.inherit))
for (; e; )
i(t, e.vars.defaults),
e = e.parent || e._dp;
return t
}, jt = function(t, e, i, n) {
void 0 === i && (i = "_first"),
void 0 === n && (n = "_last");
var r = e._prev
, o = e._next;
r ? r._next = o : t[i] === e && (t[i] = o),
o ? o._prev = r : t[n] === e && (t[n] = r),
e._next = e._prev = e.parent = null
}, Ct = function(t, e) {
t.parent && (!e || t.parent.autoRemoveChildren) && t.parent.remove(t),
t._act = 0
}, yt = function(t, e) {
if (t && (!e || e._end > t._dur || e._start < 0))
for (var i = t; i; )
i._dirty = 1,
i = i.parent;
return t
}, vt = function(t) {
for (var e = t.parent; e && e.parent; )
e._dirty = 1,
e.totalDuration(),
e = e.parent;
return t
}, xt = function t(e) {
return !e || e._ts && t(e.parent)
}, mt = function(t) {
return t._repeat ? gt(t._tTime, t = t.duration() + t._rDelay) * t : 0
}, gt = function(t, e) {
return (t /= e) && ~~t === t ? ~~t - 1 : ~~t
}, _t = function(t, e) {
return (t - e._start) * e._ts + (e._ts >= 0 ? 0 : e._dirty ? e.totalDuration() : e._tDur)
}, wt = function(t) {
return t._end = rt(t._start + (t._tDur / Math.abs(t._ts || t._rts || h) || 0))
}, Ft = function(t, e) {
var i = t._dp;
return i && i.smoothChildTiming && t._ts && (t._start = rt(t._dp._time - (t._ts > 0 ? e / t._ts : ((t._dirty ? t.totalDuration() : t._tDur) - e) / -t._ts)),
wt(t),
i._dirty || yt(i, t)),
t
}, Ut = function(t, e) {
var i;
if ((e._time || e._initted && !e._dur) && (i = _t(t.rawTime(), e),
(!e._dur || Et(0, e.totalDuration(), i) - e._tTime > h) && e.render(i, !0)),
yt(t, e)._dp && t._initted && t._time >= t._dur && t._ts) {
if (t._dur < t.duration())
for (i = t; i._dp; )
i.rawTime() >= 0 && i.totalTime(i._tTime),
i = i._dp;
t._zTime = -1e-8
}
}, St = function(t, e, i, n) {
return e.parent && Ct(e),
e._start = rt(i + e._delay),
e._end = rt(e._start + (e.totalDuration() / Math.abs(e.timeScale()) || 0)),
function(t, e, i, n, r) {
void 0 === i && (i = "_first"),
void 0 === n && (n = "_last");
var o, a = t[n];
if (r)
for (o = e[r]; a && a[r] > o; )
a = a._prev;
a ? (e._next = a._next,
a._next = e) : (e._next = t[i],
t[i] = e),
e._next ? e._next._prev = e : t[n] = e,
e._prev = a,
e.parent = e._dp = t
}(t, e, "_first", "_last", t._sort ? "_start" : 0),
t._recent = e,
n || Ut(t, e),
t
}, Mt = function(t, e) {
return (L.ScrollTrigger || R("scrollTrigger", e)) && L.ScrollTrigger.create(e, t)
}, kt = function(t, e, i, n) {
return Me(t, e),
t._initted ? !i && t._pt && (t._dur && !1 !== t.vars.lazy || !t._dur && t.vars.lazy) && p !== le.frame ? (q.push(t),
t._lazy = [e, n],
1) : void 0 : 1
}, Gt = function(t, e, i, n) {
var r = t._repeat
, o = rt(e) || 0
, a = t._tTime / t._tDur;
return a && !n && (t._time *= o / t._dur),
t._dur = o,
t._tDur = r ? r < 0 ? 1e10 : rt(o * (r + 1) + t._rDelay * r) : o,
a && !n ? Ft(t, t._tTime = t._tDur * a) : t.parent && wt(t),
i || yt(t.parent, t),
t
}, Pt = function(t) {
return t instanceof _e ? yt(t) : Gt(t, t._dur)
}, Zt = {
_start: 0,
endTime: N
}, Tt = function t(e, i) {
var n, r, o = e.labels, a = e._recent || Zt, s = e.duration() >= O ? a.endTime(!1) : e._dur;
return g(i) && (isNaN(i) || i in o) ? "<" === (n = i.charAt(0)) || ">" === n ? ("<" === n ? a._start : a.endTime(a._repeat >= 0)) + (parseFloat(i.substr(1)) || 0) : (n = i.indexOf("=")) < 0 ? (i in o || (o[i] = s),
o[i]) : (r = +(i.charAt(n - 1) + i.substr(n + 1)),
n > 1 ? t(e, i.substr(0, n - 1)) + r : s + r) : null == i ? s : +i
}, It = function(t, e) {
return t || 0 === t ? e(t) : e
}, Et = function(t, e, i) {
return i < t ? t : i > e ? e : i
}, Dt = function(t) {
return (t = (t + "").substr((parseFloat(t) + "").length)) && isNaN(t) ? t : ""
}, At = [].slice, Lt = function(t, e) {
return t && U(t) && "length"in t && (!e && !t.length || t.length - 1 in t && U(t[0])) && !t.nodeType && t !== a
}, zt = function(t, e, i) {
return void 0 === i && (i = []),
t.forEach((function(t) {
var n;
return g(t) && !e || Lt(t, 1) ? (n = i).push.apply(n, Bt(t)) : i.push(t)
}
)) || i
}, Bt = function(t, e) {
return !g(t) || e || !s && pe() ? P(t) ? zt(t, e) : Lt(t) ? At.call(t, 0) : t ? [t] : [] : At.call(c.querySelectorAll(t), 0)
}, Rt = function(t) {
return t.sort((function() {
return .5 - Math.random()
}
))
}, Wt = function(t) {
if (_(t))
return t;
var e = U(t) ? t : {
each: t
}
, i = je(e.ease)
, n = e.from || 0
, r = parseFloat(e.base) || 0
, o = {}
, a = n > 0 && n < 1
, s = isNaN(n) || a
, c = e.axis
, l = n
, p = n;
return g(n) ? l = p = {
center: .5,
edges: .5,
end: 1
}[n] || 0 : !a && s && (l = n[0],
p = n[1]),
function(t, a, u) {
var d, f, b, h, j, C, y, x, m, g = (u || e).length, _ = o[g];
if (!_) {
if (!(m = "auto" === e.grid ? 0 : (e.grid || [1, O])[1])) {
for (y = -O; y < (y = u[m++].getBoundingClientRect().left) && m < g; )
;
m--
}
for (_ = o[g] = [],
d = s ? Math.min(m, g) * l - .5 : n % m,
f = s ? g * p / m - .5 : n / m | 0,
y = 0,
x = O,
C = 0; C < g; C++)
b = C % m - d,
h = f - (C / m | 0),
_[C] = j = c ? Math.abs("y" === c ? h : b) : v(b * b + h * h),
j > y && (y = j),
j < x && (x = j);
"random" === n && Rt(_),
_.max = y - x,
_.min = x,
_.v = g = (parseFloat(e.amount) || parseFloat(e.each) * (m > g ? g - 1 : c ? "y" === c ? g / m : m : Math.max(m, g / m)) || 0) * ("edges" === n ? -1 : 1),
_.b = g < 0 ? r - g : r,
_.u = Dt(e.amount || e.each) || 0,
i = i && g < 0 ? Oe(i) : i
}
return g = (_[t] - _.min) / _.max || 0,
rt(_.b + (i ? i(g) : g) * _.v) + _.u
}
}, Vt = function(t) {
var e = t < 1 ? Math.pow(10, (t + "").length - 2) : 1;
return function(i) {
return Math.floor(Math.round(parseFloat(i) / t) * t * e) / e + (w(i) ? 0 : Dt(i))
}
}, Nt = function(t, e) {
var i, n, r = P(t);
return !r && U(t) && (i = r = t.radius || O,
t.values ? (t = Bt(t.values),
(n = !w(t[0])) && (i *= i)) : t = Vt(t.increment)),
It(e, r ? _(t) ? function(e) {
return n = t(e),
Math.abs(n - e) <= i ? n : e
}
: function(e) {
for (var r, o, a = parseFloat(n ? e.x : e), s = parseFloat(n ? e.y : 0), c = O, l = 0, p = t.length; p--; )
(r = n ? (r = t[p].x - a) * r + (o = t[p].y - s) * o : Math.abs(t[p] - a)) < c && (c = r,
l = p);
return l = !i || c <= i ? t[l] : e,
n || l === e || w(e) ? l : l + Dt(e)
}
: Vt(t))
}, Ht = function(t, e, i, n) {
return It(P(t) ? !e : !0 === i ? !!(i = 0) : !n, (function() {
return P(t) ? t[~~(Math.random() * t.length)] : (i = i || 1e-5) && (n = i < 1 ? Math.pow(10, (i + "").length - 2) : 1) && Math.floor(Math.round((t + Math.random() * (e - t)) / i) * i * n) / n
}
))
}, qt = function(t, e, i) {
return It(i, (function(i) {
return t[~~e(i)]
}
))
}, Yt = function(t) {
for (var e, i, n, r, o = 0, a = ""; ~(e = t.indexOf("random(", o)); )
n = t.indexOf(")", e),
r = "[" === t.charAt(e + 7),
i = t.substr(e + 7, n - e - 7).match(r ? A : Z),
a += t.substr(o, e - o) + Ht(r ? i : +i[0], r ? 0 : +i[1], +i[2] || 1e-5),
o = n + 1;
return a + t.substr(o, t.length - o)
}, Xt = function(t, e, i, n, r) {
var o = e - t
, a = n - i;
return It(r, (function(e) {
return i + ((e - t) / o * a || 0)
}
))
}, Qt = function(t, e, i) {
var n, r, o, a = t.labels, s = O;
for (n in a)
(r = a[n] - e) < 0 === !!i && r && s > (r = Math.abs(r)) && (o = n,
s = r);
return o
}, Jt = function(t, e, i) {
var n, r, o = t.vars, a = o[e];
if (a)
return n = o[e + "Params"],
r = o.callbackScope || t,
i && q.length && st(),
n ? a.apply(r, n) : a.call(r)
}, Kt = function(t) {
return Ct(t),
t.progress() < 1 && Jt(t, "onInterrupt"),
t
}, $t = function(t) {
var e = (t = !t.name && t.default || t).name
, i = _(t)
, n = e && !i && t.init ? function() {
this._props = []
}
: t
, r = {
init: N,
render: Re,
add: Ue,
kill: Ve,
modifier: We,
rawVars: 0
}
, o = {
targetTest: 0,
get: 0,
getSetter: Ae,
aliases: {},
register: 0
};
if (pe(),
t !== n) {
if (X[e])
return;
ut(n, ut(Ot(t, r), o)),
ft(n.prototype, ft(r, Ot(t, o))),
X[n.prop = e] = n,
t.targetTest && (K.push(n),
H[e] = 1),
e = ("css" === e ? "CSS" : e.charAt(0).toUpperCase() + e.substr(1)) + "Plugin"
}
V(e, n),
t.register && t.register(Je, n, qe)
}, te = 255, ee = {
aqua: [0, te, te],
lime: [0, te, 0],
silver: [192, 192, 192],
black: [0, 0, 0],
maroon: [128, 0, 0],
teal: [0, 128, 128],
blue: [0, 0, te],
navy: [0, 0, 128],
white: [te, te, te],
olive: [128, 128, 0],
yellow: [te, te, 0],
orange: [te, 165, 0],
gray: [128, 128, 128],
purple: [128, 0, 128],
green: [0, 128, 0],
red: [te, 0, 0],
pink: [te, 192, 203],
cyan: [0, te, te],
transparent: [te, te, te, 0]
}, ie = function(t, e, i) {
return (6 * (t = t < 0 ? t + 1 : t > 1 ? t - 1 : t) < 1 ? e + (i - e) * t * 6 : t < .5 ? i : 3 * t < 2 ? e + (i - e) * (2 / 3 - t) * 6 : e) * te + .5 | 0
}, ne = function(t, e, i) {
var n, r, o, a, s, c, l, p, u, d, f = t ? w(t) ? [t >> 16, t >> 8 & te, t & te] : 0 : ee.black;
if (!f) {
if ("," === t.substr(-1) && (t = t.substr(0, t.length - 1)),
ee[t])
f = ee[t];
else if ("#" === t.charAt(0))
4 === t.length && (n = t.charAt(1),
r = t.charAt(2),
o = t.charAt(3),
t = "#" + n + n + r + r + o + o),
f = [(t = parseInt(t.substr(1), 16)) >> 16, t >> 8 & te, t & te];
else if ("hsl" === t.substr(0, 3))
if (f = d = t.match(Z),
e) {
if (~t.indexOf("="))
return f = t.match(T),
i && f.length < 4 && (f[3] = 1),
f
} else
a = +f[0] % 360 / 360,
s = +f[1] / 100,
n = 2 * (c = +f[2] / 100) - (r = c <= .5 ? c * (s + 1) : c + s - c * s),
f.length > 3 && (f[3] *= 1),
f[0] = ie(a + 1 / 3, n, r),
f[1] = ie(a, n, r),
f[2] = ie(a - 1 / 3, n, r);
else
f = t.match(Z) || ee.transparent;
f = f.map(Number)
}
return e && !d && (n = f[0] / te,
r = f[1] / te,
o = f[2] / te,
c = ((l = Math.max(n, r, o)) + (p = Math.min(n, r, o))) / 2,
l === p ? a = s = 0 : (u = l - p,
s = c > .5 ? u / (2 - l - p) : u / (l + p),
a = l === n ? (r - o) / u + (r < o ? 6 : 0) : l === r ? (o - n) / u + 2 : (n - r) / u + 4,
a *= 60),
f[0] = ~~(a + .5),
f[1] = ~~(100 * s + .5),
f[2] = ~~(100 * c + .5)),
i && f.length < 4 && (f[3] = 1),
f
}, re = function(t) {
var e = []
, i = []
, n = -1;
return t.split(ae).forEach((function(t) {
var r = t.match(I) || [];
e.push.apply(e, r),
i.push(n += r.length + 1)
}
)),
e.c = i,
e
}, oe = function(t, e, i) {
var n, r, o, a, s = "", c = (t + s).match(ae), l = e ? "hsla(" : "rgba(", p = 0;
if (!c)
return t;
if (c = c.map((function(t) {
return (t = ne(t, e, 1)) && l + (e ? t[0] + "," + t[1] + "%," + t[2] + "%," + t[3] : t.join(",")) + ")"
}
)),
i && (o = re(t),
(n = i.c).join(s) !== o.c.join(s)))
for (a = (r = t.replace(ae, "1").split(I)).length - 1; p < a; p++)
s += r[p] + (~n.indexOf(p) ? c.shift() || l + "0,0,0,0)" : (o.length ? o : c.length ? c : i).shift());
if (!r)
for (a = (r = t.split(ae)).length - 1; p < a; p++)
s += r[p] + c[p];
return s + r[a]
}, ae = function() {
var t, e = "(?:\\b(?:(?:rgb|rgba|hsl|hsla)\\(.+?\\))|\\B#(?:[0-9a-f]{3}){1,2}\\b";
for (t in ee)
e += "|" + t + "\\b";
return new RegExp(e + ")","gi")
}(), se = /hsl[a]?\(/, ce = function(t) {
var e, i = t.join(" ");
if (ae.lastIndex = 0,
ae.test(i))
return e = se.test(i),
t[1] = oe(t[1], e),
t[0] = oe(t[0], e, re(t[1])),
!0
}, le = function() {
var t, e, i, n, r, o, p = Date.now, u = 500, f = 33, b = p(), O = b, h = 1e3 / 240, j = h, C = [], y = function i(a) {
var s, c, l, d, y = p() - O, v = !0 === a;
if (y > u && (b += y - f),
((s = (l = (O += y) - b) - j) > 0 || v) && (d = ++n.frame,
r = l - 1e3 * n.time,
n.time = l /= 1e3,
j += s + (s >= h ? 4 : h - s),
c = 1),
v || (t = e(i)),
c)
for (o = 0; o < C.length; o++)
C[o](l, r, d, a)
};
return n = {
time: 0,
frame: 0,
tick: function() {
y(!0)
},
deltaRatio: function(t) {
return r / (1e3 / (t || 60))
},
wake: function() {
l && (!s && M() && (a = s = window,
c = a.document || {},
L.gsap = Je,
(a.gsapVersions || (a.gsapVersions = [])).push(Je.version),
B(z || a.GreenSockGlobals || !a.gsap && a || {}),
i = a.requestAnimationFrame),
t && n.sleep(),
e = i || function(t) {
return setTimeout(t, j - 1e3 * n.time + 1 | 0)
}
,
d = 1,
y(2))
},
sleep: function() {
(i ? a.cancelAnimationFrame : clearTimeout)(t),
d = 0,
e = N
},
lagSmoothing: function(t, e) {
u = t || 1e8,
f = Math.min(e, u, 0)
},
fps: function(t) {
h = 1e3 / (t || 240),
j = 1e3 * n.time + h
},
add: function(t) {
C.indexOf(t) < 0 && C.push(t),
pe()
},
remove: function(t) {
var e;
~(e = C.indexOf(t)) && C.splice(e, 1) && o >= e && o--
},
_listeners: C
}
}(), pe = function() {
return !d && le.wake()
}, ue = {}, de = /^[\d.\-M][\d.\-,\s]/, fe = /["']/g, be = function(t) {
for (var e, i, n, r = {}, o = t.substr(1, t.length - 3).split(":"), a = o[0], s = 1, c = o.length; s < c; s++)
i = o[s],
e = s !== c - 1 ? i.lastIndexOf(",") : i.length,
n = i.substr(0, e),
r[a] = isNaN(n) ? n.replace(fe, "").trim() : +n,
a = i.substr(e + 1).trim();
return r
}, Oe = function(t) {
return function(e) {
return 1 - t(1 - e)
}
}, he = function t(e, i) {
for (var n, r = e._first; r; )
r instanceof _e ? t(r, i) : !r.vars.yoyoEase || r._yoyo && r._repeat || r._yoyo === i || (r.timeline ? t(r.timeline, i) : (n = r._ease,
r._ease = r._yEase,
r._yEase = n,
r._yoyo = i)),
r = r._next
}, je = function(t, e) {
return t && (_(t) ? t : ue[t] || function(t) {
var e = (t + "").split("(")
, i = ue[e[0]];
return i && e.length > 1 && i.config ? i.config.apply(null, ~t.indexOf("{") ? [be(e[1])] : function(t) {
var e = t.indexOf("(") + 1
, i = t.indexOf(")")
, n = t.indexOf("(", e);
return t.substring(e, ~n && n < i ? t.indexOf(")", i + 1) : i)
}(t).split(",").map(lt)) : ue._CE && de.test(t) ? ue._CE("", t) : i
}(t)) || e
}, Ce = function(t, e, i, n) {
void 0 === i && (i = function(t) {
return 1 - e(1 - t)
}
),
void 0 === n && (n = function(t) {
return t < .5 ? e(2 * t) / 2 : 1 - e(2 * (1 - t)) / 2
}
);
var r, o = {
easeIn: e,
easeOut: i,
easeInOut: n
};
return nt(t, (function(t) {
for (var e in ue[t] = L[t] = o,
ue[r = t.toLowerCase()] = i,
o)
ue[r + ("easeIn" === e ? ".in" : "easeOut" === e ? ".out" : ".inOut")] = ue[t + "." + e] = o[e]
}
)),
o
}, ye = function(t) {
return function(e) {
return e < .5 ? (1 - t(1 - 2 * e)) / 2 : .5 + t(2 * (e - .5)) / 2
}
}, ve = function t(e, i, n) {
var r = i >= 1 ? i : 1
, o = (n || (e ? .3 : .45)) / (i < 1 ? i : 1)
, a = o / j * (Math.asin(1 / r) || 0)
, s = function(t) {
return 1 === t ? 1 : r * Math.pow(2, -10 * t) * m((t - a) * o) + 1
}
, c = "out" === e ? s : "in" === e ? function(t) {
return 1 - s(1 - t)
}
: ye(s);
return o = j / o,
c.config = function(i, n) {
return t(e, i, n)
}
,
c
}, xe = function t(e, i) {
void 0 === i && (i = 1.70158);
var n = function(t) {
return t ? --t * t * ((i + 1) * t + i) + 1 : 0
}
, r = "out" === e ? n : "in" === e ? function(t) {
return 1 - n(1 - t)
}
: ye(n);
return r.config = function(i) {
return t(e, i)
}
,
r
};
nt("Linear,Quad,Cubic,Quart,Quint,Strong", (function(t, e) {
var i = e < 5 ? e + 1 : e;
Ce(t + ",Power" + (i - 1), e ? function(t) {
return Math.pow(t, i)
}
: function(t) {
return t
}
, (function(t) {
return 1 - Math.pow(1 - t, i)
}
), (function(t) {
return t < .5 ? Math.pow(2 * t, i) / 2 : 1 - Math.pow(2 * (1 - t), i) / 2
}
))
}
)),
ue.Linear.easeNone = ue.none = ue.Linear.easeIn,
Ce("Elastic", ve("in"), ve("out"), ve()),
function(t, e) {
var i = 1 / e
, n = function(n) {
return n < i ? t * n * n : n < .7272727272727273 ? t * Math.pow(n - 1.5 / e, 2) + .75 : n < .9090909090909092 ? t * (n -= 2.25 / e) * n + .9375 : t * Math.pow(n - 2.625 / e, 2) + .984375
};
Ce("Bounce", (function(t) {
return 1 - n(1 - t)
}
), n)
}(7.5625, 2.75),
Ce("Expo", (function(t) {
return t ? Math.pow(2, 10 * (t - 1)) : 0
}
)),
Ce("Circ", (function(t) {
return -(v(1 - t * t) - 1)
}
)),
Ce("Sine", (function(t) {
return 1 === t ? 1 : 1 - x(t * C)
}
)),
Ce("Back", xe("in"), xe("out"), xe()),
ue.SteppedEase = ue.steps = L.SteppedEase = {
config: function(t, e) {
void 0 === t && (t = 1);
var i = 1 / t
, n = t + (e ? 0 : 1)
, r = e ? 1 : 0;
return function(t) {
return ((n * Et(0, .99999999, t) | 0) + r) * i
}
}
},
b.ease = ue["quad.out"],
nt("onComplete,onUpdate,onStart,onRepeat,onReverseComplete,onInterrupt", (function(t) {
return $ += t + "," + t + "Params,"
}
));
var me = function(t, e) {
this.id = y++,
t._gsap = this,
this.target = t,
this.harness = e,
this.get = e ? e.get : it,
this.set = e ? e.getSetter : Ae
}
, ge = function() {
function t(t, e) {
var i = t.parent || o;
this.vars = t,
this._delay = +t.delay || 0,
(this._repeat = t.repeat || 0) && (this._rDelay = t.repeatDelay || 0,
this._yoyo = !!t.yoyo || !!t.yoyoEase),
this._ts = 1,
Gt(this, +t.duration, 1, 1),
this.data = t.data,
d || le.wake(),
i && St(i, this, e || 0 === e ? e : i._time, 1),
t.reversed && this.reverse(),
t.paused && this.paused(!0)
}
var e = t.prototype;
return e.delay = function(t) {
return t || 0 === t ? (this.parent && this.parent.smoothChildTiming && this.startTime(this._start + t - this._delay),
this._delay = t,
this) : this._delay
}
,
e.duration = function(t) {
return arguments.length ? this.totalDuration(this._repeat > 0 ? t + (t + this._rDelay) * this._repeat : t) : this.totalDuration() && this._dur
}
,
e.totalDuration = function(t) {
return arguments.length ? (this._dirty = 0,
Gt(this, this._repeat < 0 ? t : (t - this._repeat * this._rDelay) / (this._repeat + 1))) : this._tDur
}
,
e.totalTime = function(t, e) {
if (pe(),
!arguments.length)
return this._tTime;
var i = this._dp;
if (i && i.smoothChildTiming && this._ts) {
for (Ft(this, t); i.parent; )
i.parent._time !== i._start + (i._ts >= 0 ? i._tTime / i._ts : (i.totalDuration() - i._tTime) / -i._ts) && i.totalTime(i._tTime, !0),
i = i.parent;
!this.parent && this._dp.autoRemoveChildren && (this._ts > 0 && t < this._tDur || this._ts < 0 && t > 0 || !this._tDur && !t) && St(this._dp, this, this._start - this._delay)
}
return (this._tTime !== t || !this._dur && !e || this._initted && Math.abs(this._zTime) === h || !t && !this._initted && (this.add || this._ptLookup)) && (this._ts || (this._pTime = t),
ct(this, t, e)),
this
}
,
e.time = function(t, e) {
return arguments.length ? this.totalTime(Math.min(this.totalDuration(), t + mt(this)) % this._dur || (t ? this._dur : 0), e) : this._time
}
,
e.totalProgress = function(t, e) {
return arguments.length ? this.totalTime(this.totalDuration() * t, e) : this.totalDuration() ? Math.min(1, this._tTime / this._tDur) : this.ratio
}
,
e.progress = function(t, e) {
return arguments.length ? this.totalTime(this.duration() * (!this._yoyo || 1 & this.iteration() ? t : 1 - t) + mt(this), e) : this.duration() ? Math.min(1, this._time / this._dur) : this.ratio
}
,
e.iteration = function(t, e) {
var i = this.duration() + this._rDelay;
return arguments.length ? this.totalTime(this._time + (t - 1) * i, e) : this._repeat ? gt(this._tTime, i) + 1 : 1
}
,
e.timeScale = function(t) {
if (!arguments.length)
return -1e-8 === this._rts ? 0 : this._rts;
if (this._rts === t)
return this;
var e = this.parent && this._ts ? _t(this.parent._time, this) : this._tTime;
return this._rts = +t || 0,
this._ts = this._ps || -1e-8 === t ? 0 : this._rts,
vt(this.totalTime(Et(-this._delay, this._tDur, e), !0))
}
,
e.paused = function(t) {
return arguments.length ? (this._ps !== t && (this._ps = t,
t ? (this._pTime = this._tTime || Math.max(-this._delay, this.rawTime()),
this._ts = this._act = 0) : (pe(),
this._ts = this._rts,
this.totalTime(this.parent && !this.parent.smoothChildTiming ? this.rawTime() : this._tTime || this._pTime, 1 === this.progress() && (this._tTime -= h) && Math.abs(this._zTime) !== h))),
this) : this._ps
}
,
e.startTime = function(t) {
if (arguments.length) {
this._start = t;
var e = this.parent || this._dp;
return e && (e._sort || !this.parent) && St(e, this, t - this._delay),
this
}
return this._start
}
,
e.endTime = function(t) {
return this._start + (S(t) ? this.totalDuration() : this.duration()) / Math.abs(this._ts)
}
,
e.rawTime = function(t) {
var e = this.parent || this._dp;
return e ? t && (!this._ts || this._repeat && this._time && this.totalProgress() < 1) ? this._tTime % (this._dur + this._rDelay) : this._ts ? _t(e.rawTime(t), this) : this._tTime : this._tTime
}
,
e.globalTime = function(t) {
for (var e = this, i = arguments.length ? t : e.rawTime(); e; )
i = e._start + i / (e._ts || 1),
e = e._dp;
return i
}
,
e.repeat = function(t) {
return arguments.length ? (this._repeat = t,
Pt(this)) : this._repeat
}
,
e.repeatDelay = function(t) {
return arguments.length ? (this._rDelay = t,
Pt(this)) : this._rDelay
}
,
e.yoyo = function(t) {
return arguments.length ? (this._yoyo = t,
this) : this._yoyo
}
,
e.seek = function(t, e) {
return this.totalTime(Tt(this, t), S(e))
}
,
e.restart = function(t, e) {
return this.play().totalTime(t ? -this._delay : 0, S(e))
}
,
e.play = function(t, e) {
return null != t && this.seek(t, e),
this.reversed(!1).paused(!1)
}
,
e.reverse = function(t, e) {
return null != t && this.seek(t || this.totalDuration(), e),
this.reversed(!0).paused(!1)
}
,
e.pause = function(t, e) {
return null != t && this.seek(t, e),
this.paused(!0)
}
,
e.resume = function() {
return this.paused(!1)
}
,
e.reversed = function(t) {
return arguments.length ? (!!t !== this.reversed() && this.timeScale(-this._rts || (t ? -1e-8 : 0)),
this) : this._rts < 0
}
,
e.invalidate = function() {
return this._initted = 0,
this._zTime = -1e-8,
this
}
,
e.isActive = function() {
var t, e = this.parent || this._dp, i = this._start;
return !(e && !(this._ts && this._initted && e.isActive() && (t = e.rawTime(!0)) >= i && t < this.endTime(!0) - h))
}
,
e.eventCallback = function(t, e, i) {
var n = this.vars;
return arguments.length > 1 ? (e ? (n[t] = e,
i && (n[t + "Params"] = i),
"onUpdate" === t && (this._onUpdate = e)) : delete n[t],
this) : n[t]
}
,
e.then = function(t) {
var e = this;
return new Promise((function(i) {
var n = _(t) ? t : pt
, r = function() {
var t = e.then;
e.then = null,
_(n) && (n = n(e)) && (n.then || n === e) && (e.then = t),
i(n),
e.then = t
};
e._initted && 1 === e.totalProgress() && e._ts >= 0 || !e._tTime && e._ts < 0 ? r() : e._prom = r
}
))
}
,
e.kill = function() {
Kt(this)
}
,
t
}();
ut(ge.prototype, {
_time: 0,
_start: 0,
_end: 0,
_tTime: 0,
_tDur: 0,
_dirty: 0,
_repeat: 0,
_yoyo: !1,
parent: null,
_initted: !1,
_rDelay: 0,
_ts: 1,
_dp: 0,
ratio: 0,
_zTime: -1e-8,
_prom: 0,
_ps: !1,
_rts: 1
});
var _e = function(t) {
function e(e, i) {
var r;
return void 0 === e && (e = {}),
(r = t.call(this, e, i) || this).labels = {},
r.smoothChildTiming = !!e.smoothChildTiming,
r.autoRemoveChildren = !!e.autoRemoveChildren,
r._sort = S(e.sortChildren),
r.parent && Ut(r.parent, n(r)),
e.scrollTrigger && Mt(n(r), e.scrollTrigger),
r
}
r(e, t);
var i = e.prototype;
return i.to = function(t, e, i) {
return new Ze(t,at(arguments, 0, this),Tt(this, w(e) ? arguments[3] : i)),
this
}
,
i.from = function(t, e, i) {
return new Ze(t,at(arguments, 1, this),Tt(this, w(e) ? arguments[3] : i)),
this
}
,
i.fromTo = function(t, e, i, n) {
return new Ze(t,at(arguments, 2, this),Tt(this, w(e) ? arguments[4] : n)),
this
}
,
i.set = function(t, e, i) {
return e.duration = 0,
e.parent = this,
ht(e).repeatDelay || (e.repeat = 0),
e.immediateRender = !!e.immediateRender,
new Ze(t,e,Tt(this, i),1),
this
}
,
i.call = function(t, e, i) {
return St(this, Ze.delayedCall(0, t, e), Tt(this, i))
}
,
i.staggerTo = function(t, e, i, n, r, o, a) {
return i.duration = e,
i.stagger = i.stagger || n,
i.onComplete = o,
i.onCompleteParams = a,
i.parent = this,
new Ze(t,i,Tt(this, r)),
this
}
,
i.staggerFrom = function(t, e, i, n, r, o, a) {
return i.runBackwards = 1,
ht(i).immediateRender = S(i.immediateRender),
this.staggerTo(t, e, i, n, r, o, a)
}
,
i.staggerFromTo = function(t, e, i, n, r, o, a, s) {
return n.startAt = i,
ht(n).immediateRender = S(n.immediateRender),
this.staggerTo(t, e, n, r, o, a, s)
}
,
i.render = function(t, e, i) {
var n, r, a, s, c, l, p, u, d, f, b, O, j = this._time, C = this._dirty ? this.totalDuration() : this._tDur, y = this._dur, v = this !== o && t > C - h && t >= 0 ? C : t < h ? 0 : t, x = this._zTime < 0 !== t < 0 && (this._initted || !y);
if (v !== this._tTime || i || x) {
if (j !== this._time && y && (v += this._time - j,
t += this._time - j),
n = v,
d = this._start,
l = !(u = this._ts),
x && (y || (j = this._zTime),
(t || !e) && (this._zTime = t)),
this._repeat && (b = this._yoyo,
c = y + this._rDelay,
n = rt(v % c),
v === C ? (s = this._repeat,
n = y) : ((s = ~~(v / c)) && s === v / c && (n = y,
s--),
n > y && (n = y)),
f = gt(this._tTime, c),
!j && this._tTime && f !== s && (f = s),
b && 1 & s && (n = y - n,
O = 1),
s !== f && !this._lock)) {
var m = b && 1 & f
, g = m === (b && 1 & s);
if (s < f && (m = !m),
j = m ? 0 : y,
this._lock = 1,
this.render(j || (O ? 0 : rt(s * c)), e, !y)._lock = 0,
!e && this.parent && Jt(this, "onRepeat"),
this.vars.repeatRefresh && !O && (this.invalidate()._lock = 1),
j !== this._time || l !== !this._ts)
return this;
if (y = this._dur,
C = this._tDur,
g && (this._lock = 2,
j = m ? y : -1e-4,
this.render(j, !0),
this.vars.repeatRefresh && !O && this.invalidate()),
this._lock = 0,
!this._ts && !l)
return this;
he(this, O)
}
if (this._hasPause && !this._forcing && this._lock < 2 && (p = function(t, e, i) {
var n;
if (i > e)
for (n = t._first; n && n._start <= i; ) {
if (!n._dur && "isPause" === n.data && n._start > e)
return n;
n = n._next
}
else
for (n = t._last; n && n._start >= i; ) {
if (!n._dur && "isPause" === n.data && n._start < e)
return n;
n = n._prev
}
}(this, rt(j), rt(n))) && (v -= n - (n = p._start)),
this._tTime = v,
this._time = n,
this._act = !u,
this._initted || (this._onUpdate = this.vars.onUpdate,
this._initted = 1,
this._zTime = t),
!j && n && !e && Jt(this, "onStart"),
n >= j && t >= 0)
for (r = this._first; r; ) {
if (a = r._next,
(r._act || n >= r._start) && r._ts && p !== r) {
if (r.parent !== this)
return this.render(t, e, i);
if (r.render(r._ts > 0 ? (n - r._start) * r._ts : (r._dirty ? r.totalDuration() : r._tDur) + (n - r._start) * r._ts, e, i),
n !== this._time || !this._ts && !l) {
p = 0,
a && (v += this._zTime = -1e-8);
break
}
}
r = a
}
else {
r = this._last;
for (var _ = t < 0 ? t : n; r; ) {
if (a = r._prev,
(r._act || _ <= r._end) && r._ts && p !== r) {
if (r.parent !== this)
return this.render(t, e, i);
if (r.render(r._ts > 0 ? (_ - r._start) * r._ts : (r._dirty ? r.totalDuration() : r._tDur) + (_ - r._start) * r._ts, e, i),
n !== this._time || !this._ts && !l) {
p = 0,
a && (v += this._zTime = _ ? -1e-8 : h);
break
}
}
r = a
}
}
if (p && !e && (this.pause(),
p.render(n >= j ? 0 : -1e-8)._zTime = n >= j ? 1 : -1,
this._ts))
return this._start = d,
wt(this),
this.render(t, e, i);
this._onUpdate && !e && Jt(this, "onUpdate", !0),
(v === C && C >= this.totalDuration() || !v && j) && (d !== this._start && Math.abs(u) === Math.abs(this._ts) || this._lock || ((t || !y) && (v === C && this._ts > 0 || !v && this._ts < 0) && Ct(this, 1),
e || t < 0 && !j || !v && !j || (Jt(this, v === C ? "onComplete" : "onReverseComplete", !0),
this._prom && !(v < C && this.timeScale() > 0) && this._prom())))
}
return this
}
,
i.add = function(t, e) {
var i = this;
if (w(e) || (e = Tt(this, e)),
!(t instanceof ge)) {
if (P(t))
return t.forEach((function(t) {
return i.add(t, e)
}
)),
this;
if (g(t))
return this.addLabel(t, e);
if (!_(t))
return this;
t = Ze.delayedCall(0, t)
}
return this !== t ? St(this, t, e) : this
}
,
i.getChildren = function(t, e, i, n) {
void 0 === t && (t = !0),
void 0 === e && (e = !0),
void 0 === i && (i = !0),
void 0 === n && (n = -O);
for (var r = [], o = this._first; o; )
o._start >= n && (o instanceof Ze ? e && r.push(o) : (i && r.push(o),
t && r.push.apply(r, o.getChildren(!0, e, i)))),
o = o._next;
return r
}
,
i.getById = function(t) {
for (var e = this.getChildren(1, 1, 1), i = e.length; i--; )
if (e[i].vars.id === t)
return e[i]
}
,
i.remove = function(t) {
return g(t) ? this.removeLabel(t) : _(t) ? this.killTweensOf(t) : (jt(this, t),
t === this._recent && (this._recent = this._last),
yt(this))
}
,
i.totalTime = function(e, i) {
return arguments.length ? (this._forcing = 1,
!this._dp && this._ts && (this._start = rt(le.time - (this._ts > 0 ? e / this._ts : (this.totalDuration() - e) / -this._ts))),
t.prototype.totalTime.call(this, e, i),
this._forcing = 0,
this) : this._tTime
}
,
i.addLabel = function(t, e) {
return this.labels[t] = Tt(this, e),
this
}
,
i.removeLabel = function(t) {
return delete this.labels[t],
this
}
,
i.addPause = function(t, e, i) {
var n = Ze.delayedCall(0, e || N, i);
return n.data = "isPause",
this._hasPause = 1,
St(this, n, Tt(this, t))
}
,
i.removePause = function(t) {
var e = this._first;
for (t = Tt(this, t); e; )
e._start === t && "isPause" === e.data && Ct(e),
e = e._next
}
,
i.killTweensOf = function(t, e, i) {
for (var n = this.getTweensOf(t, i), r = n.length; r--; )
we !== n[r] && n[r].kill(t, e);
return this
}
,
i.getTweensOf = function(t, e) {
for (var i, n = [], r = Bt(t), o = this._first, a = w(e); o; )
o instanceof Ze ? ot(o._targets, r) && (a ? (!we || o._initted && o._ts) && o.globalTime(0) <= e && o.globalTime(o.totalDuration()) > e : !e || o.isActive()) && n.push(o) : (i = o.getTweensOf(r, e)).length && n.push.apply(n, i),
o = o._next;
return n
}
,
i.tweenTo = function(t, e) {
e = e || {};
var i = this
, n = Tt(i, t)
, r = e
, o = r.startAt
, a = r.onStart
, s = r.onStartParams
, c = Ze.to(i, ut(e, {
ease: "none",
lazy: !1,
time: n,
overwrite: "auto",
duration: e.duration || Math.abs((n - (o && "time"in o ? o.time : i._time)) / i.timeScale()) || h,
onStart: function() {
i.pause();
var t = e.duration || Math.abs((n - i._time) / i.timeScale());
c._dur !== t && Gt(c, t, 0, 1).render(c._time, !0, !0),
a && a.apply(c, s || [])
}
}));
return c
}
,
i.tweenFromTo = function(t, e, i) {
return this.tweenTo(e, ut({
startAt: {
time: Tt(this, t)
}
}, i))
}
,
i.recent = function() {
return this._recent
}
,
i.nextLabel = function(t) {
return void 0 === t && (t = this._time),
Qt(this, Tt(this, t))
}
,
i.previousLabel = function(t) {
return void 0 === t && (t = this._time),
Qt(this, Tt(this, t), 1)
}
,
i.currentLabel = function(t) {
return arguments.length ? this.seek(t, !0) : this.previousLabel(this._time + h)
}
,
i.shiftChildren = function(t, e, i) {
void 0 === i && (i = 0);
for (var n, r = this._first, o = this.labels; r; )
r._start >= i && (r._start += t,
r._end += t),
r = r._next;
if (e)
for (n in o)
o[n] >= i && (o[n] += t);
return yt(this)
}
,
i.invalidate = function() {
var e = this._first;
for (this._lock = 0; e; )
e.invalidate(),
e = e._next;
return t.prototype.invalidate.call(this)
}
,
i.clear = function(t) {
void 0 === t && (t = !0);
for (var e, i = this._first; i; )
e = i._next,
this.remove(i),
i = e;
return this._time = this._tTime = this._pTime = 0,
t && (this.labels = {}),
yt(this)
}
,
i.totalDuration = function(t) {
var e, i, n, r = 0, a = this, s = a._last, c = O;
if (arguments.length)
return a.timeScale((a._repeat < 0 ? a.duration() : a.totalDuration()) / (a.reversed() ? -t : t));
if (a._dirty) {
for (n = a.parent; s; )
e = s._prev,
s._dirty && s.totalDuration(),
(i = s._start) > c && a._sort && s._ts && !a._lock ? (a._lock = 1,
St(a, s, i - s._delay, 1)._lock = 0) : c = i,
i < 0 && s._ts && (r -= i,
(!n && !a._dp || n && n.smoothChildTiming) && (a._start += i / a._ts,
a._time -= i,
a._tTime -= i),
a.shiftChildren(-i, !1, -Infinity),
c = 0),
s._end > r && s._ts && (r = s._end),
s = e;
Gt(a, a === o && a._time > r ? a._time : r, 1, 1),
a._dirty = 0
}
return a._tDur
}
,
e.updateRoot = function(t) {
if (o._ts && (ct(o, _t(t, o)),
p = le.frame),
le.frame >= J) {
J += f.autoSleep || 120;
var e = o._first;
if ((!e || !e._ts) && f.autoSleep && le._listeners.length < 2) {
for (; e && !e._ts; )
e = e._next;
e || le.sleep()
}
}
}
,
e
}(ge);
ut(_e.prototype, {
_lock: 0,
_hasPause: 0,
_forcing: 0
});
var we, Fe = function(t, e, i, n, r, o, a) {
var s, c, l, p, u, d, f, b, O = new qe(this._pt,t,e,0,1,Be,null,r), h = 0, j = 0;
for (O.b = i,
O.e = n,
i += "",
(f = ~(n += "").indexOf("random(")) && (n = Yt(n)),
o && (o(b = [i, n], t, e),
i = b[0],
n = b[1]),
c = i.match(E) || []; s = E.exec(n); )
p = s[0],
u = n.substring(h, s.index),
l ? l = (l + 1) % 5 : "rgba(" === u.substr(-5) && (l = 1),
p !== c[j++] && (d = parseFloat(c[j - 1]) || 0,
O._pt = {
_next: O._pt,
p: u || 1 === j ? u : ",",
s: d,
c: "=" === p.charAt(1) ? parseFloat(p.substr(2)) * ("-" === p.charAt(0) ? -1 : 1) : parseFloat(p) - d,
m: l && l < 4 ? Math.round : 0
},
h = E.lastIndex);
return O.c = h < n.length ? n.substring(h, n.length) : "",
O.fp = a,
(D.test(n) || f) && (O.e = 0),
this._pt = O,
O
}, Ue = function(t, e, i, n, r, o, a, s, c) {
_(n) && (n = n(r || 0, t, o));
var l, p = t[e], u = "get" !== i ? i : _(p) ? c ? t[e.indexOf("set") || !_(t["get" + e.substr(3)]) ? e : "get" + e.substr(3)](c) : t[e]() : p, d = _(p) ? c ? Ee : Ie : Te;
if (g(n) && (~n.indexOf("random(") && (n = Yt(n)),
"=" === n.charAt(1) && (n = parseFloat(u) + parseFloat(n.substr(2)) * ("-" === n.charAt(0) ? -1 : 1) + (Dt(u) || 0))),
u !== n)
return isNaN(u * n) ? (!p && !(e in t) && R(e, n),
Fe.call(this, t, e, u, n, d, s || f.stringFilter, c)) : (l = new qe(this._pt,t,e,+u || 0,n - (u || 0),"boolean" === typeof p ? ze : Le,0,d),
c && (l.fp = c),
a && l.modifier(a, this, t),
this._pt = l)
}, Se = function(t, e, i, n, r, o) {
var a, s, c, l;
if (X[t] && !1 !== (a = new X[t]).init(r, a.rawVars ? e[t] : function(t, e, i, n, r) {
if (_(t) && (t = ke(t, r, e, i, n)),
!U(t) || t.style && t.nodeType || P(t) || G(t))
return g(t) ? ke(t, r, e, i, n) : t;
var o, a = {};
for (o in t)
a[o] = ke(t[o], r, e, i, n);
return a
}(e[t], n, r, o, i), i, n, o) && (i._pt = s = new qe(i._pt,r,t,0,1,a.render,a,0,a.priority),
i !== u))
for (c = i._ptLookup[i._targets.indexOf(r)],
l = a._props.length; l--; )
c[a._props[l]] = s;
return a
}, Me = function t(e, i) {
var n, r, a, s, c, l, p, u, d, f, O, j, C, y = e.vars, v = y.ease, x = y.startAt, m = y.immediateRender, g = y.lazy, _ = y.onUpdate, w = y.onUpdateParams, F = y.callbackScope, U = y.runBackwards, M = y.yoyoEase, k = y.keyframes, G = y.autoRevert, P = e._dur, Z = e._startAt, T = e._targets, I = e.parent, E = I && "nested" === I.data ? I.parent._targets : T, D = "auto" === e._overwrite, A = e.timeline;
if (A && (!k || !v) && (v = "none"),
e._ease = je(v, b.ease),
e._yEase = M ? Oe(je(!0 === M ? v : M, b.ease)) : 0,
M && e._yoyo && !e._repeat && (M = e._yEase,
e._yEase = e._ease,
e._ease = M),
!A) {
if (j = (u = T[0] ? et(T[0]).harness : 0) && y[u.prop],
n = Ot(y, H),
Z && Z.render(-1, !0).kill(),
x) {
if (Ct(e._startAt = Ze.set(T, ut({
data: "isStart",
overwrite: !1,
parent: I,
immediateRender: !0,
lazy: S(g),
startAt: null,
delay: 0,
onUpdate: _,
onUpdateParams: w,
callbackScope: F,
stagger: 0
}, x))),
m)
if (i > 0)
G || (e._startAt = 0);
else if (P && !(i < 0 && Z))
return void (i && (e._zTime = i))
} else if (U && P)
if (Z)
!G && (e._startAt = 0);
else if (i && (m = !1),
a = ut({
overwrite: !1,
data: "isFromStart",
lazy: m && S(g),
immediateRender: m,
stagger: 0,
parent: I
}, n),
j && (a[u.prop] = j),
Ct(e._startAt = Ze.set(T, a)),
m) {
if (!i)
return
} else
t(e._startAt, h);
for (e._pt = 0,
g = P && S(g) || g && !P,
r = 0; r < T.length; r++) {
if (p = (c = T[r])._gsap || tt(T)[r]._gsap,
e._ptLookup[r] = f = {},
Y[p.id] && q.length && st(),
O = E === T ? r : E.indexOf(c),
u && !1 !== (d = new u).init(c, j || n, e, O, E) && (e._pt = s = new qe(e._pt,c,d.name,0,1,d.render,d,0,d.priority),
d._props.forEach((function(t) {
f[t] = s
}
)),
d.priority && (l = 1)),
!u || j)
for (a in n)
X[a] && (d = Se(a, n, e, O, c, E)) ? d.priority && (l = 1) : f[a] = s = Ue.call(e, c, a, "get", n[a], O, E, 0, y.stringFilter);
e._op && e._op[r] && e.kill(c, e._op[r]),
D && e._pt && (we = e,
o.killTweensOf(c, f, e.globalTime(0)),
C = !e.parent,
we = 0),
e._pt && g && (Y[p.id] = 1)
}
l && He(e),
e._onInit && e._onInit(e)
}
e._from = !A && !!y.runBackwards,
e._onUpdate = _,
e._initted = (!e._op || e._pt) && !C
}, ke = function(t, e, i, n, r) {
return _(t) ? t.call(e, i, n, r) : g(t) && ~t.indexOf("random(") ? Yt(t) : t
}, Ge = $ + "repeat,repeatDelay,yoyo,repeatRefresh,yoyoEase", Pe = (Ge + ",id,stagger,delay,duration,paused,scrollTrigger").split(","), Ze = function(t) {
function e(e, i, r, a) {
var s;
"number" === typeof i && (r.duration = i,
i = r,
r = null);
var c, l, p, u, d, b, O, h, j = (s = t.call(this, a ? i : ht(i), r) || this).vars, C = j.duration, y = j.delay, v = j.immediateRender, x = j.stagger, m = j.overwrite, g = j.keyframes, _ = j.defaults, F = j.scrollTrigger, M = j.yoyoEase, Z = s.parent, T = (P(e) || G(e) ? w(e[0]) : "length"in i) ? [e] : Bt(e);
if (s._targets = T.length ? tt(T) : W("GSAP target " + e + " not found. https://greensock.com", !f.nullTargetWarn) || [],
s._ptLookup = [],
s._overwrite = m,
g || x || k(C) || k(y)) {
if (i = s.vars,
(c = s.timeline = new _e({
data: "nested",
defaults: _ || {}
})).kill(),
c.parent = n(s),
g)
ut(c.vars.defaults, {
ease: "none"
}),
g.forEach((function(t) {
return c.to(T, t, ">")
}
));
else {
if (u = T.length,
O = x ? Wt(x) : N,
U(x))
for (d in x)
~Ge.indexOf(d) && (h || (h = {}),
h[d] = x[d]);
for (l = 0; l < u; l++) {
for (d in p = {},
i)
Pe.indexOf(d) < 0 && (p[d] = i[d]);
p.stagger = 0,
M && (p.yoyoEase = M),
h && ft(p, h),
b = T[l],
p.duration = +ke(C, n(s), l, b, T),
p.delay = (+ke(y, n(s), l, b, T) || 0) - s._delay,
!x && 1 === u && p.delay && (s._delay = y = p.delay,
s._start += y,
p.delay = 0),
c.to(b, p, O(l, b, T))
}
c.duration() ? C = y = 0 : s.timeline = 0
}
C || s.duration(C = c.duration())
} else
s.timeline = 0;
return !0 === m && (we = n(s),
o.killTweensOf(T),
we = 0),
Z && Ut(Z, n(s)),
(v || !C && !g && s._start === rt(Z._time) && S(v) && xt(n(s)) && "nested" !== Z.data) && (s._tTime = -1e-8,
s.render(Math.max(0, -y))),
F && Mt(n(s), F),
s
}
r(e, t);
var i = e.prototype;
return i.render = function(t, e, i) {
var n, r, o, a, s, c, l, p, u, d = this._time, f = this._tDur, b = this._dur, O = t > f - h && t >= 0 ? f : t < h ? 0 : t;
if (b) {
if (O !== this._tTime || !t || i || this._startAt && this._zTime < 0 !== t < 0) {
if (n = O,
p = this.timeline,
this._repeat) {
if (a = b + this._rDelay,
n = rt(O % a),
O === f ? (o = this._repeat,
n = b) : ((o = ~~(O / a)) && o === O / a && (n = b,
o--),
n > b && (n = b)),
(c = this._yoyo && 1 & o) && (u = this._yEase,
n = b - n),
s = gt(this._tTime, a),
n === d && !i && this._initted)
return this;
o !== s && (p && this._yEase && he(p, c),
!this.vars.repeatRefresh || c || this._lock || (this._lock = i = 1,
this.render(rt(a * o), !0).invalidate()._lock = 0))
}
if (!this._initted) {
if (kt(this, t < 0 ? t : n, i, e))
return this._tTime = 0,
this;
if (b !== this._dur)
return this.render(t, e, i)
}
for (this._tTime = O,
this._time = n,
!this._act && this._ts && (this._act = 1,
this._lazy = 0),
this.ratio = l = (u || this._ease)(n / b),
this._from && (this.ratio = l = 1 - l),
n && !d && !e && Jt(this, "onStart"),
r = this._pt; r; )
r.r(l, r.d),
r = r._next;
p && p.render(t < 0 ? t : !n && c ? -1e-8 : p._dur * l, e, i) || this._startAt && (this._zTime = t),
this._onUpdate && !e && (t < 0 && this._startAt && this._startAt.render(t, !0, i),
Jt(this, "onUpdate")),
this._repeat && o !== s && this.vars.onRepeat && !e && this.parent && Jt(this, "onRepeat"),
O !== this._tDur && O || this._tTime !== O || (t < 0 && this._startAt && !this._onUpdate && this._startAt.render(t, !0, !0),
(t || !b) && (O === this._tDur && this._ts > 0 || !O && this._ts < 0) && Ct(this, 1),
e || t < 0 && !d || !O && !d || (Jt(this, O === f ? "onComplete" : "onReverseComplete", !0),
this._prom && !(O < f && this.timeScale() > 0) && this._prom()))
}
} else
!function(t, e, i, n) {
var r, o, a = t.ratio, s = e < 0 || !e && a && !t._start && t._zTime > h && !t._dp._lock || (t._ts < 0 || t._dp._ts < 0) && "isFromStart" !== t.data && "isStart" !== t.data ? 0 : 1, c = t._rDelay, l = 0;
if (c && t._repeat && (l = Et(0, t._tDur, e),
gt(l, c) !== (o = gt(t._tTime, c)) && (a = 1 - s,
t.vars.repeatRefresh && t._initted && t.invalidate())),
s !== a || n || t._zTime === h || !e && t._zTime) {
if (!t._initted && kt(t, e, n, i))
return;
for (o = t._zTime,
t._zTime = e || (i ? h : 0),
i || (i = e && !o),
t.ratio = s,
t._from && (s = 1 - s),
t._time = 0,
t._tTime = l,
i || Jt(t, "onStart"),
r = t._pt; r; )
r.r(s, r.d),
r = r._next;
t._startAt && e < 0 && t._startAt.render(e, !0, !0),
t._onUpdate && !i && Jt(t, "onUpdate"),
l && t._repeat && !i && t.parent && Jt(t, "onRepeat"),
(e >= t._tDur || e < 0) && t.ratio === s && (s && Ct(t, 1),
i || (Jt(t, s ? "onComplete" : "onReverseComplete", !0),
t._prom && t._prom()))
} else
t._zTime || (t._zTime = e)
}(this, t, e, i);
return this
}
,
i.targets = function() {
return this._targets
}
,
i.invalidate = function() {
return this._pt = this._op = this._startAt = this._onUpdate = this._act = this._lazy = 0,
this._ptLookup = [],
this.timeline && this.timeline.invalidate(),
t.prototype.invalidate.call(this)
}
,
i.kill = function(t, e) {
if (void 0 === e && (e = "all"),
!t && (!e || "all" === e) && (this._lazy = 0,
this.parent))
return Kt(this);
if (this.timeline) {
var i = this.timeline.totalDuration();
return this.timeline.killTweensOf(t, e, we && !0 !== we.vars.overwrite)._first || Kt(this),
this.parent && i !== this.timeline.totalDuration() && Gt(this, this._dur * this.timeline._tDur / i, 0, 1),
this
}
var n, r, o, a, s, c, l, p = this._targets, u = t ? Bt(t) : p, d = this._ptLookup, f = this._pt;
if ((!e || "all" === e) && function(t, e) {
for (var i = t.length, n = i === e.length; n && i-- && t[i] === e[i]; )
;
return i < 0
}(p, u))
return "all" === e && (this._pt = 0),
Kt(this);
for (n = this._op = this._op || [],
"all" !== e && (g(e) && (s = {},
nt(e, (function(t) {
return s[t] = 1
}
)),
e = s),
e = function(t, e) {
var i, n, r, o, a = t[0] ? et(t[0]).harness : 0, s = a && a.aliases;
if (!s)
return e;
for (n in i = ft({}, e),
s)
if (n in i)
for (r = (o = s[n].split(",")).length; r--; )
i[o[r]] = i[n];
return i
}(p, e)),
l = p.length; l--; )
if (~u.indexOf(p[l]))
for (s in r = d[l],
"all" === e ? (n[l] = e,
a = r,
o = {}) : (o = n[l] = n[l] || {},
a = e),
a)
(c = r && r[s]) && ("kill"in c.d && !0 !== c.d.kill(s) || jt(this, c, "_pt"),
delete r[s]),
"all" !== o && (o[s] = 1);
return this._initted && !this._pt && f && Kt(this),
this
}
,
e.to = function(t, i) {
return new e(t,i,arguments[2])
}
,
e.from = function(t, i) {
return new e(t,at(arguments, 1))
}
,
e.delayedCall = function(t, i, n, r) {
return new e(i,0,{
immediateRender: !1,
lazy: !1,
overwrite: !1,
delay: t,
onComplete: i,
onReverseComplete: i,
onCompleteParams: n,
onReverseCompleteParams: n,
callbackScope: r
})
}
,
e.fromTo = function(t, i, n) {
return new e(t,at(arguments, 2))
}
,
e.set = function(t, i) {
return i.duration = 0,
i.repeatDelay || (i.repeat = 0),
new e(t,i)
}
,
e.killTweensOf = function(t, e, i) {
return o.killTweensOf(t, e, i)
}
,
e
}(ge);
ut(Ze.prototype, {
_targets: [],
_lazy: 0,
_startAt: 0,
_op: 0,
_onInit: 0
}),
nt("staggerTo,staggerFrom,staggerFromTo", (function(t) {
Ze[t] = function() {
var e = new _e
, i = At.call(arguments, 0);
return i.splice("staggerFromTo" === t ? 5 : 4, 0, 0),
e[t].apply(e, i)
}
}
));
var Te = function(t, e, i) {
return t[e] = i
}
, Ie = function(t, e, i) {
return t[e](i)
}
, Ee = function(t, e, i, n) {
return t[e](n.fp, i)
}
, De = function(t, e, i) {
return t.setAttribute(e, i)
}
, Ae = function(t, e) {
return _(t[e]) ? Ie : F(t[e]) && t.setAttribute ? De : Te
}
, Le = function(t, e) {
return e.set(e.t, e.p, Math.round(1e4 * (e.s + e.c * t)) / 1e4, e)
}
, ze = function(t, e) {
return e.set(e.t, e.p, !!(e.s + e.c * t), e)
}
, Be = function(t, e) {
var i = e._pt
, n = "";
if (!t && e.b)
n = e.b;
else if (1 === t && e.e)
n = e.e;
else {
for (; i; )
n = i.p + (i.m ? i.m(i.s + i.c * t) : Math.round(1e4 * (i.s + i.c * t)) / 1e4) + n,
i = i._next;
n += e.c
}
e.set(e.t, e.p, n, e)
}
, Re = function(t, e) {
for (var i = e._pt; i; )
i.r(t, i.d),
i = i._next
}
, We = function(t, e, i, n) {
for (var r, o = this._pt; o; )
r = o._next,
o.p === n && o.modifier(t, e, i),
o = r
}
, Ve = function(t) {
for (var e, i, n = this._pt; n; )
i = n._next,
n.p === t && !n.op || n.op === t ? jt(this, n, "_pt") : n.dep || (e = 1),
n = i;
return !e
}
, Ne = function(t, e, i, n) {
n.mSet(t, e, n.m.call(n.tween, i, n.mt), n)
}
, He = function(t) {
for (var e, i, n, r, o = t._pt; o; ) {
for (e = o._next,
i = n; i && i.pr > o.pr; )
i = i._next;
(o._prev = i ? i._prev : r) ? o._prev._next = o : n = o,
(o._next = i) ? i._prev = o : r = o,
o = e
}
t._pt = n
}
, qe = function() {
function t(t, e, i, n, r, o, a, s, c) {
this.t = e,
this.s = n,
this.c = r,
this.p = i,
this.r = o || Le,
this.d = a || this,
this.set = s || Te,
this.pr = c || 0,
this._next = t,
t && (t._prev = this)
}
return t.prototype.modifier = function(t, e, i) {
this.mSet = this.mSet || this.set,
this.set = Ne,
this.m = t,
this.mt = i,
this.tween = e
}
,
t
}();
nt($ + "parent,duration,ease,delay,overwrite,runBackwards,startAt,yoyo,immediateRender,repeat,repeatDelay,data,paused,reversed,lazy,callbackScope,stringFilter,id,yoyoEase,stagger,inherit,repeatRefresh,keyframes,autoRevert,scrollTrigger", (function(t) {
return H[t] = 1
}
)),
L.TweenMax = L.TweenLite = Ze,
L.TimelineLite = L.TimelineMax = _e,
o = new _e({
sortChildren: !1,
defaults: b,
autoRemoveChildren: !0,
id: "root",
smoothChildTiming: !0
}),
f.stringFilter = ce;
var Ye = {
registerPlugin: function() {
for (var t = arguments.length, e = new Array(t), i = 0; i < t; i++)
e[i] = arguments[i];
e.forEach((function(t) {
return $t(t)
}
))
},
timeline: function(t) {
return new _e(t)
},
getTweensOf: function(t, e) {
return o.getTweensOf(t, e)
},
getProperty: function(t, e, i, n) {
g(t) && (t = Bt(t)[0]);
var r = et(t || {}).get
, o = i ? pt : lt;
return "native" === i && (i = ""),
t ? e ? o((X[e] && X[e].get || r)(t, e, i, n)) : function(e, i, n) {
return o((X[e] && X[e].get || r)(t, e, i, n))
}
: t
},
quickSetter: function(t, e, i) {
if ((t = Bt(t)).length > 1) {
var n = t.map((function(t) {
return Je.quickSetter(t, e, i)
}
))
, r = n.length;
return function(t) {
for (var e = r; e--; )
n[e](t)
}
}
t = t[0] || {};
var o = X[e]
, a = et(t)
, s = a.harness && (a.harness.aliases || {})[e] || e
, c = o ? function(e) {
var n = new o;
u._pt = 0,
n.init(t, i ? e + i : e, u, 0, [t]),
n.render(1, n),
u._pt && Re(1, u)
}
: a.set(t, s);
return o ? c : function(e) {
return c(t, s, i ? e + i : e, a, 1)
}
},
isTweening: function(t) {
return o.getTweensOf(t, !0).length > 0
},
defaults: function(t) {
return t && t.ease && (t.ease = je(t.ease, b.ease)),
bt(b, t || {})
},
config: function(t) {
return bt(f, t || {})
},
registerEffect: function(t) {
var e = t.name
, i = t.effect
, n = t.plugins
, r = t.defaults
, o = t.extendTimeline;
(n || "").split(",").forEach((function(t) {
return t && !X[t] && !L[t] && W(e + " effect requires " + t + " plugin.")
}
)),
Q[e] = function(t, e, n) {
return i(Bt(t), ut(e || {}, r), n)
}
,
o && (_e.prototype[e] = function(t, i, n) {
return this.add(Q[e](t, U(i) ? i : (n = i) && {}, this), n)
}
)
},
registerEase: function(t, e) {
ue[t] = je(e)
},
parseEase: function(t, e) {
return arguments.length ? je(t, e) : ue
},
getById: function(t) {
return o.getById(t)
},
exportRoot: function(t, e) {
void 0 === t && (t = {});
var i, n, r = new _e(t);
for (r.smoothChildTiming = S(t.smoothChildTiming),
o.remove(r),
r._dp = 0,
r._time = r._tTime = o._time,
i = o._first; i; )
n = i._next,
!e && !i._dur && i instanceof Ze && i.vars.onComplete === i._targets[0] || St(r, i, i._start - i._delay),
i = n;
return St(o, r, 0),
r
},
utils: {
wrap: function t(e, i, n) {
var r = i - e;
return P(e) ? qt(e, t(0, e.length), i) : It(n, (function(t) {
return (r + (t - e) % r) % r + e
}
))
},
wrapYoyo: function t(e, i, n) {
var r = i - e
, o = 2 * r;
return P(e) ? qt(e, t(0, e.length - 1), i) : It(n, (function(t) {
return e + ((t = (o + (t - e) % o) % o || 0) > r ? o - t : t)
}
))
},
distribute: Wt,
random: Ht,
snap: Nt,
normalize: function(t, e, i) {
return Xt(t, e, 0, 1, i)
},
getUnit: Dt,
clamp: function(t, e, i) {
return It(i, (function(i) {
return Et(t, e, i)
}
))
},
splitColor: ne,
toArray: Bt,
mapRange: Xt,
pipe: function() {
for (var t = arguments.length, e = new Array(t), i = 0; i < t; i++)
e[i] = arguments[i];
return function(t) {
return e.reduce((function(t, e) {
return e(t)
}
), t)
}
},
unitize: function(t, e) {
return function(i) {
return t(parseFloat(i)) + (e || Dt(i))
}
},
interpolate: function t(e, i, n, r) {
var o = isNaN(e + i) ? 0 : function(t) {
return (1 - t) * e + t * i
}
;
if (!o) {
var a, s, c, l, p, u = g(e), d = {};
if (!0 === n && (r = 1) && (n = null),
u)
e = {
p: e
},
i = {
p: i
};
else if (P(e) && !P(i)) {
for (c = [],
l = e.length,
p = l - 2,
s = 1; s < l; s++)
c.push(t(e[s - 1], e[s]));
l--,
o = function(t) {
t *= l;
var e = Math.min(p, ~~t);
return c[e](t - e)
}
,
n = i
} else
r || (e = ft(P(e) ? [] : {}, e));
if (!c) {
for (a in i)
Ue.call(d, e, a, "get", i[a]);
o = function(t) {
return Re(t, d) || (u ? e.p : e)
}
}
}
return It(n, o)
},
shuffle: Rt
},
install: B,
effects: Q,
ticker: le,
updateRoot: _e.updateRoot,
plugins: X,
globalTimeline: o,
core: {
PropTween: qe,
globals: V,
Tween: Ze,
Timeline: _e,
Animation: ge,
getCache: et,
_removeLinkedListItem: jt
}
};
nt("to,from,fromTo,delayedCall,set,killTweensOf", (function(t) {
return Ye[t] = Ze[t]
}
)),
le.add(_e.updateRoot),
u = Ye.to({}, {
duration: 0
});
var Xe = function(t, e) {
for (var i = t._pt; i && i.p !== e && i.op !== e && i.fp !== e; )
i = i._next;
return i
}
, Qe = function(t, e) {
return {
name: t,
rawVars: 1,
init: function(t, i, n) {
n._onInit = function(t) {
var n, r;
if (g(i) && (n = {},
nt(i, (function(t) {
return n[t] = 1
}
)),
i = n),
e) {
for (r in n = {},
i)
n[r] = e(i[r]);
i = n
}
!function(t, e) {
var i, n, r, o = t._targets;
for (i in e)
for (n = o.length; n--; )
(r = t._ptLookup[n][i]) && (r = r.d) && (r._pt && (r = Xe(r, i)),
r && r.modifier && r.modifier(e[i], t, o[n], i))
}(t, i)
}
}
}
}
, Je = Ye.registerPlugin({
name: "attr",
init: function(t, e, i, n, r) {
var o, a;
for (o in e)
(a = this.add(t, "setAttribute", (t.getAttribute(o) || 0) + "", e[o], n, r, 0, 0, o)) && (a.op = o),
this._props.push(o)
}
}, {
name: "endArray",
init: function(t, e) {
for (var i = e.length; i--; )
this.add(t, i, t[i] || 0, e[i])
}
}, Qe("roundProps", Vt), Qe("modifiers"), Qe("snap", Nt)) || Ye;
Ze.version = _e.version = Je.version = "3.5.1",
l = 1,
M() && pe();
ue.Power0,
ue.Power1,
ue.Power2,
ue.Power3,
ue.Power4,
ue.Linear,
ue.Quad,
ue.Cubic,
ue.Quart,
ue.Quint,
ue.Strong,
ue.Elastic,
ue.Back,
ue.SteppedEase,
ue.Bounce,
ue.Sine,
ue.Expo,
ue.Circ;
var Ke, $e, ti, ei, ii, ni, ri, oi, ai = {}, si = 180 / Math.PI, ci = Math.PI / 180, li = Math.atan2, pi = /([A-Z])/g, ui = /(?:left|right|width|margin|padding|x)/i, di = /[\s,\(]\S/, fi = {
autoAlpha: "opacity,visibility",
scale: "scaleX,scaleY",
alpha: "opacity"
}, bi = function(t, e) {
return e.set(e.t, e.p, Math.round(1e4 * (e.s + e.c * t)) / 1e4 + e.u, e)
}, Oi = function(t, e) {
return e.set(e.t, e.p, 1 === t ? e.e : Math.round(1e4 * (e.s + e.c * t)) / 1e4 + e.u, e)
}, hi = function(t, e) {
return e.set(e.t, e.p, t ? Math.round(1e4 * (e.s + e.c * t)) / 1e4 + e.u : e.b, e)
}, ji = function(t, e) {
var i = e.s + e.c * t;
e.set(e.t, e.p, ~~(i + (i < 0 ? -.5 : .5)) + e.u, e)
}, Ci = function(t, e) {
return e.set(e.t, e.p, t ? e.e : e.b, e)
}, yi = function(t, e) {
return e.set(e.t, e.p, 1 !== t ? e.b : e.e, e)
}, vi = function(t, e, i) {
return t.style[e] = i
}, xi = function(t, e, i) {
return t.style.setProperty(e, i)
}, mi = function(t, e, i) {
return t._gsap[e] = i
}, gi = function(t, e, i) {
return t._gsap.scaleX = t._gsap.scaleY = i
}, _i = function(t, e, i, n, r) {
var o = t._gsap;
o.scaleX = o.scaleY = i,
o.renderTransform(r, o)
}, wi = function(t, e, i, n, r) {
var o = t._gsap;
o[e] = i,
o.renderTransform(r, o)
}, Fi = "transform", Ui = Fi + "Origin", Si = function(t, e) {
var i = $e.createElementNS ? $e.createElementNS((e || "http://www.w3.org/1999/xhtml").replace(/^https/, "http"), t) : $e.createElement(t);
return i.style ? i : $e.createElement(t)
}, Mi = function t(e, i, n) {
var r = getComputedStyle(e);
return r[i] || r.getPropertyValue(i.replace(pi, "-$1").toLowerCase()) || r.getPropertyValue(i) || !n && t(e, Gi(i) || i, 1) || ""
}, ki = "O,Moz,ms,Ms,Webkit".split(","), Gi = function(t, e, i) {
var n = (e || ii).style
, r = 5;
if (t in n && !i)
return t;
for (t = t.charAt(0).toUpperCase() + t.substr(1); r-- && !(ki[r] + t in n); )
;
return r < 0 ? null : (3 === r ? "ms" : r >= 0 ? ki[r] : "") + t
}, Pi = function() {
"undefined" !== typeof window && window.document && (Ke = window,
$e = Ke.document,
ti = $e.documentElement,
ii = Si("div") || {
style: {}
},
ni = Si("div"),
Fi = Gi(Fi),
Ui = Fi + "Origin",
ii.style.cssText = "border-width:0;line-height:0;position:absolute;padding:0",
oi = !!Gi("perspective"),
ei = 1)
}, Zi = function t(e) {
var i, n = Si("svg", this.ownerSVGElement && this.ownerSVGElement.getAttribute("xmlns") || "http://www.w3.org/2000/svg"), r = this.parentNode, o = this.nextSibling, a = this.style.cssText;
if (ti.appendChild(n),
n.appendChild(this),
this.style.display = "block",
e)
try {
i = this.getBBox(),
this._gsapBBox = this.getBBox,
this.getBBox = t
} catch (s) {}
else
this._gsapBBox && (i = this._gsapBBox());
return r && (o ? r.insertBefore(this, o) : r.appendChild(this)),
ti.removeChild(n),
this.style.cssText = a,
i
}, Ti = function(t, e) {
for (var i = e.length; i--; )
if (t.hasAttribute(e[i]))
return t.getAttribute(e[i])
}, Ii = function(t) {
var e;
try {
e = t.getBBox()
} catch (i) {
e = Zi.call(t, !0)
}
return e && (e.width || e.height) || t.getBBox === Zi || (e = Zi.call(t, !0)),
!e || e.width || e.x || e.y ? e : {
x: +Ti(t, ["x", "cx", "x1"]) || 0,
y: +Ti(t, ["y", "cy", "y1"]) || 0,
width: 0,
height: 0
}
}, Ei = function(t) {
return !(!t.getCTM || t.parentNode && !t.ownerSVGElement || !Ii(t))
}, Di = function(t, e) {
if (e) {
var i = t.style;
e in ai && e !== Ui && (e = Fi),
i.removeProperty ? ("ms" !== e.substr(0, 2) && "webkit" !== e.substr(0, 6) || (e = "-" + e),
i.removeProperty(e.replace(pi, "-$1").toLowerCase())) : i.removeAttribute(e)
}
}, Ai = function(t, e, i, n, r, o) {
var a = new qe(t._pt,e,i,0,1,o ? yi : Ci);
return t._pt = a,
a.b = n,
a.e = r,
t._props.push(i),
a
}, Li = {
deg: 1,
rad: 1,
turn: 1
}, zi = function t(e, i, n, r) {
var o, a, s, c, l = parseFloat(n) || 0, p = (n + "").trim().substr((l + "").length) || "px", u = ii.style, d = ui.test(i), f = "svg" === e.tagName.toLowerCase(), b = (f ? "client" : "offset") + (d ? "Width" : "Height"), O = 100, h = "px" === r, j = "%" === r;
return r === p || !l || Li[r] || Li[p] ? l : ("px" !== p && !h && (l = t(e, i, n, "px")),
c = e.getCTM && Ei(e),
j && (ai[i] || ~i.indexOf("adius")) ? rt(l / (c ? e.getBBox()[d ? "width" : "height"] : e[b]) * O) : (u[d ? "width" : "height"] = O + (h ? p : r),
a = ~i.indexOf("adius") || "em" === r && e.appendChild && !f ? e : e.parentNode,
c && (a = (e.ownerSVGElement || {}).parentNode),
a && a !== $e && a.appendChild || (a = $e.body),
(s = a._gsap) && j && s.width && d && s.time === le.time ? rt(l / s.width * O) : ((j || "%" === p) && (u.position = Mi(e, "position")),
a === e && (u.position = "static"),
a.appendChild(ii),
o = ii[b],
a.removeChild(ii),
u.position = "absolute",
d && j && ((s = et(a)).time = le.time,
s.width = a[b]),
rt(h ? o * l / O : o && l ? O / o * l : 0))))
}, Bi = function(t, e, i, n) {
var r;
return ei || Pi(),
e in fi && "transform" !== e && ~(e = fi[e]).indexOf(",") && (e = e.split(",")[0]),
ai[e] && "transform" !== e ? (r = $i(t, n),
r = "transformOrigin" !== e ? r[e] : tn(Mi(t, Ui)) + " " + r.zOrigin + "px") : (!(r = t.style[e]) || "auto" === r || n || ~(r + "").indexOf("calc(")) && (r = Hi[e] && Hi[e](t, e, i) || Mi(t, e) || it(t, e) || ("opacity" === e ? 1 : 0)),
i && !~(r + "").indexOf(" ") ? zi(t, e, r, i) + i : r
}, Ri = function(t, e, i, n) {
if (!i || "none" === i) {
var r = Gi(e, t, 1)
, o = r && Mi(t, r, 1);
o && o !== i ? (e = r,
i = o) : "borderColor" === e && (i = Mi(t, "borderTopColor"))
}
var a, s, c, l, p, u, d, b, O, h, j, C, y = new qe(this._pt,t.style,e,0,1,Be), v = 0, x = 0;
if (y.b = i,
y.e = n,
i += "",
"auto" === (n += "") && (t.style[e] = n,
n = Mi(t, e) || n,
t.style[e] = i),
ce(a = [i, n]),
n = a[1],
c = (i = a[0]).match(I) || [],
(n.match(I) || []).length) {
for (; s = I.exec(n); )
d = s[0],
O = n.substring(v, s.index),
p ? p = (p + 1) % 5 : "rgba(" !== O.substr(-5) && "hsla(" !== O.substr(-5) || (p = 1),
d !== (u = c[x++] || "") && (l = parseFloat(u) || 0,
j = u.substr((l + "").length),
(C = "=" === d.charAt(1) ? +(d.charAt(0) + "1") : 0) && (d = d.substr(2)),
b = parseFloat(d),
h = d.substr((b + "").length),
v = I.lastIndex - h.length,
h || (h = h || f.units[e] || j,
v === n.length && (n += h,
y.e += h)),
j !== h && (l = zi(t, e, u, h) || 0),
y._pt = {
_next: y._pt,
p: O || 1 === x ? O : ",",
s: l,
c: C ? C * b : b - l,
m: p && p < 4 ? Math.round : 0
});
y.c = v < n.length ? n.substring(v, n.length) : ""
} else
y.r = "display" === e && "none" === n ? yi : Ci;
return D.test(n) && (y.e = 0),
this._pt = y,
y
}, Wi = {
top: "0%",
bottom: "100%",
left: "0%",
right: "100%",
center: "50%"
}, Vi = function(t) {
var e = t.split(" ")
, i = e[0]
, n = e[1] || "50%";
return "top" !== i && "bottom" !== i && "left" !== n && "right" !== n || (t = i,
i = n,
n = t),
e[0] = Wi[i] || i,
e[1] = Wi[n] || n,
e.join(" ")
}, Ni = function(t, e) {
if (e.tween && e.tween._time === e.tween._dur) {
var i, n, r, o = e.t, a = o.style, s = e.u, c = o._gsap;
if ("all" === s || !0 === s)
a.cssText = "",
n = 1;
else
for (r = (s = s.split(",")).length; --r > -1; )
i = s[r],
ai[i] && (n = 1,
i = "transformOrigin" === i ? Ui : Fi),
Di(o, i);
n && (Di(o, Fi),
c && (c.svg && o.removeAttribute("transform"),
$i(o, 1),
c.uncache = 1))
}
}, Hi = {
clearProps: function(t, e, i, n, r) {
if ("isFromStart" !== r.data) {
var o = t._pt = new qe(t._pt,e,i,0,0,Ni);
return o.u = n,
o.pr = -10,
o.tween = r,
t._props.push(i),
1
}
}
}, qi = [1, 0, 0, 1, 0, 0], Yi = {}, Xi = function(t) {
return "matrix(1, 0, 0, 1, 0, 0)" === t || "none" === t || !t
}, Qi = function(t) {
var e = Mi(t, Fi);
return Xi(e) ? qi : e.substr(7).match(T).map(rt)
}, Ji = function(t, e) {
var i, n, r, o, a = t._gsap || et(t), s = t.style, c = Qi(t);
return a.svg && t.getAttribute("transform") ? "1,0,0,1,0,0" === (c = [(r = t.transform.baseVal.consolidate().matrix).a, r.b, r.c, r.d, r.e, r.f]).join(",") ? qi : c : (c !== qi || t.offsetParent || t === ti || a.svg || (r = s.display,
s.display = "block",
(i = t.parentNode) && t.offsetParent || (o = 1,
n = t.nextSibling,
ti.appendChild(t)),
c = Qi(t),
r ? s.display = r : Di(t, "display"),
o && (n ? i.insertBefore(t, n) : i ? i.appendChild(t) : ti.removeChild(t))),
e && c.length > 6 ? [c[0], c[1], c[4], c[5], c[12], c[13]] : c)
}, Ki = function(t, e, i, n, r, o) {
var a, s, c, l = t._gsap, p = r || Ji(t, !0), u = l.xOrigin || 0, d = l.yOrigin || 0, f = l.xOffset || 0, b = l.yOffset || 0, O = p[0], h = p[1], j = p[2], C = p[3], y = p[4], v = p[5], x = e.split(" "), m = parseFloat(x[0]) || 0, g = parseFloat(x[1]) || 0;
i ? p !== qi && (s = O * C - h * j) && (c = m * (-h / s) + g * (O / s) - (O * v - h * y) / s,
m = m * (C / s) + g * (-j / s) + (j * v - C * y) / s,
g = c) : (m = (a = Ii(t)).x + (~x[0].indexOf("%") ? m / 100 * a.width : m),
g = a.y + (~(x[1] || x[0]).indexOf("%") ? g / 100 * a.height : g)),
n || !1 !== n && l.smooth ? (y = m - u,
v = g - d,
l.xOffset = f + (y * O + v * j) - y,
l.yOffset = b + (y * h + v * C) - v) : l.xOffset = l.yOffset = 0,
l.xOrigin = m,
l.yOrigin = g,
l.smooth = !!n,
l.origin = e,
l.originIsAbsolute = !!i,
t.style[Ui] = "0px 0px",
o && (Ai(o, l, "xOrigin", u, m),
Ai(o, l, "yOrigin", d, g),
Ai(o, l, "xOffset", f, l.xOffset),
Ai(o, l, "yOffset", b, l.yOffset)),
t.setAttribute("data-svg-origin", m + " " + g)
}, $i = function(t, e) {
var i = t._gsap || new me(t);
if ("x"in i && !e && !i.uncache)
return i;
var n, r, o, a, s, c, l, p, u, d, b, O, h, j, C, y, v, x, m, g, _, w, F, U, S, M, k, G, P, Z, T, I, E = t.style, D = i.scaleX < 0, A = "px", L = "deg", z = Mi(t, Ui) || "0";
return n = r = o = c = l = p = u = d = b = 0,
a = s = 1,
i.svg = !(!t.getCTM || !Ei(t)),
j = Ji(t, i.svg),
i.svg && (U = !i.uncache && t.getAttribute("data-svg-origin"),
Ki(t, U || z, !!U || i.originIsAbsolute, !1 !== i.smooth, j)),
O = i.xOrigin || 0,
h = i.yOrigin || 0,
j !== qi && (x = j[0],
m = j[1],
g = j[2],
_ = j[3],
n = w = j[4],
r = F = j[5],
6 === j.length ? (a = Math.sqrt(x * x + m * m),
s = Math.sqrt(_ * _ + g * g),
c = x || m ? li(m, x) * si : 0,
(u = g || _ ? li(g, _) * si + c : 0) && (s *= Math.cos(u * ci)),
i.svg && (n -= O - (O * x + h * g),
r -= h - (O * m + h * _))) : (I = j[6],
Z = j[7],
k = j[8],
G = j[9],
P = j[10],
T = j[11],
n = j[12],
r = j[13],
o = j[14],
l = (C = li(I, P)) * si,
C && (U = w * (y = Math.cos(-C)) + k * (v = Math.sin(-C)),
S = F * y + G * v,
M = I * y + P * v,
k = w * -v + k * y,
G = F * -v + G * y,
P = I * -v + P * y,
T = Z * -v + T * y,
w = U,
F = S,
I = M),
p = (C = li(-g, P)) * si,
C && (y = Math.cos(-C),
T = _ * (v = Math.sin(-C)) + T * y,
x = U = x * y - k * v,
m = S = m * y - G * v,
g = M = g * y - P * v),
c = (C = li(m, x)) * si,
C && (U = x * (y = Math.cos(C)) + m * (v = Math.sin(C)),
S = w * y + F * v,
m = m * y - x * v,
F = F * y - w * v,
x = U,
w = S),
l && Math.abs(l) + Math.abs(c) > 359.9 && (l = c = 0,
p = 180 - p),
a = rt(Math.sqrt(x * x + m * m + g * g)),
s = rt(Math.sqrt(F * F + I * I)),
C = li(w, F),
u = Math.abs(C) > 2e-4 ? C * si : 0,
b = T ? 1 / (T < 0 ? -T : T) : 0),
i.svg && (U = t.getAttribute("transform"),
i.forceCSS = t.setAttribute("transform", "") || !Xi(Mi(t, Fi)),
U && t.setAttribute("transform", U))),
Math.abs(u) > 90 && Math.abs(u) < 270 && (D ? (a *= -1,
u += c <= 0 ? 180 : -180,
c += c <= 0 ? 180 : -180) : (s *= -1,
u += u <= 0 ? 180 : -180)),
i.x = ((i.xPercent = n && Math.round(t.offsetWidth / 2) === Math.round(-n) ? -50 : 0) ? 0 : n) + A,
i.y = ((i.yPercent = r && Math.round(t.offsetHeight / 2) === Math.round(-r) ? -50 : 0) ? 0 : r) + A,
i.z = o + A,
i.scaleX = rt(a),
i.scaleY = rt(s),
i.rotation = rt(c) + L,
i.rotationX = rt(l) + L,
i.rotationY = rt(p) + L,
i.skewX = u + L,
i.skewY = d + L,
i.transformPerspective = b + A,
(i.zOrigin = parseFloat(z.split(" ")[2]) || 0) && (E[Ui] = tn(z)),
i.xOffset = i.yOffset = 0,
i.force3D = f.force3D,
i.renderTransform = i.svg ? cn : oi ? sn : nn,
i.uncache = 0,
i
}, tn = function(t) {
return (t = t.split(" "))[0] + " " + t[1]
}, en = function(t, e, i) {
var n = Dt(e);
return rt(parseFloat(e) + parseFloat(zi(t, "x", i + "px", n))) + n
}, nn = function(t, e) {
e.z = "0px",
e.rotationY = e.rotationX = "0deg",
e.force3D = 0,
sn(t, e)
}, rn = "0deg", on = "0px", an = ") ", sn = function(t, e) {
var i = e || this
, n = i.xPercent
, r = i.yPercent
, o = i.x
, a = i.y
, s = i.z
, c = i.rotation
, l = i.rotationY
, p = i.rotationX
, u = i.skewX
, d = i.skewY
, f = i.scaleX
, b = i.scaleY
, O = i.transformPerspective
, h = i.force3D
, j = i.target
, C = i.zOrigin
, y = ""
, v = "auto" === h && t && 1 !== t || !0 === h;
if (C && (p !== rn || l !== rn)) {
var x, m = parseFloat(l) * ci, g = Math.sin(m), _ = Math.cos(m);
m = parseFloat(p) * ci,
x = Math.cos(m),
o = en(j, o, g * x * -C),
a = en(j, a, -Math.sin(m) * -C),
s = en(j, s, _ * x * -C + C)
}
O !== on && (y += "perspective(" + O + an),
(n || r) && (y += "translate(" + n + "%, " + r + "%) "),
(v || o !== on || a !== on || s !== on) && (y += s !== on || v ? "translate3d(" + o + ", " + a + ", " + s + ") " : "translate(" + o + ", " + a + an),
c !== rn && (y += "rotate(" + c + an),
l !== rn && (y += "rotateY(" + l + an),
p !== rn && (y += "rotateX(" + p + an),
u === rn && d === rn || (y += "skew(" + u + ", " + d + an),
1 === f && 1 === b || (y += "scale(" + f + ", " + b + an),
j.style[Fi] = y || "translate(0, 0)"
}, cn = function(t, e) {
var i, n, r, o, a, s = e || this, c = s.xPercent, l = s.yPercent, p = s.x, u = s.y, d = s.rotation, f = s.skewX, b = s.skewY, O = s.scaleX, h = s.scaleY, j = s.target, C = s.xOrigin, y = s.yOrigin, v = s.xOffset, x = s.yOffset, m = s.forceCSS, g = parseFloat(p), _ = parseFloat(u);
d = parseFloat(d),
f = parseFloat(f),
(b = parseFloat(b)) && (f += b = parseFloat(b),
d += b),
d || f ? (d *= ci,
f *= ci,
i = Math.cos(d) * O,
n = Math.sin(d) * O,
r = Math.sin(d - f) * -h,
o = Math.cos(d - f) * h,
f && (b *= ci,
a = Math.tan(f - b),
r *= a = Math.sqrt(1 + a * a),
o *= a,
b && (a = Math.tan(b),
i *= a = Math.sqrt(1 + a * a),
n *= a)),
i = rt(i),
n = rt(n),
r = rt(r),
o = rt(o)) : (i = O,
o = h,
n = r = 0),
(g && !~(p + "").indexOf("px") || _ && !~(u + "").indexOf("px")) && (g = zi(j, "x", p, "px"),
_ = zi(j, "y", u, "px")),
(C || y || v || x) && (g = rt(g + C - (C * i + y * r) + v),
_ = rt(_ + y - (C * n + y * o) + x)),
(c || l) && (a = j.getBBox(),
g = rt(g + c / 100 * a.width),
_ = rt(_ + l / 100 * a.height)),
a = "matrix(" + i + "," + n + "," + r + "," + o + "," + g + "," + _ + ")",
j.setAttribute("transform", a),
m && (j.style[Fi] = a)
}, ln = function(t, e, i, n, r, o) {
var a, s, c = 360, l = g(r), p = parseFloat(r) * (l && ~r.indexOf("rad") ? si : 1), u = o ? p * o : p - n, d = n + u + "deg";
return l && ("short" === (a = r.split("_")[1]) && (u %= c) !== u % 180 && (u += u < 0 ? c : -360),
"cw" === a && u < 0 ? u = (u + 36e9) % c - ~~(u / c) * c : "ccw" === a && u > 0 && (u = (u - 36e9) % c - ~~(u / c) * c)),
t._pt = s = new qe(t._pt,e,i,n,u,Oi),
s.e = d,
s.u = "deg",
t._props.push(i),
s
}, pn = function(t, e, i) {
var n, r, o, a, s, c, l, p = ni.style, u = i._gsap;
for (r in p.cssText = getComputedStyle(i).cssText + ";position:absolute;display:block;",
p[Fi] = e,
$e.body.appendChild(ni),
n = $i(ni, 1),
ai)
(o = u[r]) !== (a = n[r]) && "perspective,force3D,transformOrigin,svgOrigin".indexOf(r) < 0 && (s = Dt(o) !== (l = Dt(a)) ? zi(i, r, o, l) : parseFloat(o),
c = parseFloat(a),
t._pt = new qe(t._pt,u,r,s,c - s,bi),
t._pt.u = l || 0,
t._props.push(r));
$e.body.removeChild(ni)
};
nt("padding,margin,Width,Radius", (function(t, e) {
var i = "Top"
, n = "Right"
, r = "Bottom"
, o = "Left"
, a = (e < 3 ? [i, n, r, o] : [i + o, i + n, r + n, r + o]).map((function(i) {
return e < 2 ? t + i : "border" + i + t
}
));
Hi[e > 1 ? "border" + t : t] = function(t, e, i, n, r) {
var o, s;
if (arguments.length < 4)
return o = a.map((function(e) {
return Bi(t, e, i)
}
)),
5 === (s = o.join(" ")).split(o[0]).length ? o[0] : s;
o = (n + "").split(" "),
s = {},
a.forEach((function(t, e) {
return s[t] = o[e] = o[e] || o[(e - 1) / 2 | 0]
}
)),
t.init(e, s, r)
}
}
));
var un = {
name: "css",
register: Pi,
targetTest: function(t) {
return t.style && t.nodeType
},
init: function(t, e, i, n, r) {
var o, a, s, c, l, p, u, d, b, O, h, j, C, y, v, x = this._props, m = t.style;
for (u in ei || Pi(),
e)
if ("autoRound" !== u && (a = e[u],
!X[u] || !Se(u, e, i, n, t, r)))
if (l = typeof a,
p = Hi[u],
"function" === l && (l = typeof (a = a.call(i, n, t, r))),
"string" === l && ~a.indexOf("random(") && (a = Yt(a)),
p)
p(this, t, u, a, i) && (v = 1);
else if ("--" === u.substr(0, 2))
this.add(m, "setProperty", getComputedStyle(t).getPropertyValue(u) + "", a + "", n, r, 0, 0, u);
else if ("undefined" !== l) {
if (o = Bi(t, u),
c = parseFloat(o),
(O = "string" === l && "=" === a.charAt(1) ? +(a.charAt(0) + "1") : 0) && (a = a.substr(2)),
s = parseFloat(a),
u in fi && ("autoAlpha" === u && (1 === c && "hidden" === Bi(t, "visibility") && s && (c = 0),
Ai(this, m, "visibility", c ? "inherit" : "hidden", s ? "inherit" : "hidden", !s)),
"scale" !== u && "transform" !== u && ~(u = fi[u]).indexOf(",") && (u = u.split(",")[0])),
h = u in ai)
if (j || ((C = t._gsap).renderTransform || $i(t),
y = !1 !== e.smoothOrigin && C.smooth,
(j = this._pt = new qe(this._pt,m,Fi,0,1,C.renderTransform,C,0,-1)).dep = 1),
"scale" === u)
this._pt = new qe(this._pt,C,"scaleY",C.scaleY,O ? O * s : s - C.scaleY),
x.push("scaleY", u),
u += "X";
else {
if ("transformOrigin" === u) {
a = Vi(a),
C.svg ? Ki(t, a, 0, y, 0, this) : ((b = parseFloat(a.split(" ")[2]) || 0) !== C.zOrigin && Ai(this, C, "zOrigin", C.zOrigin, b),
Ai(this, m, u, tn(o), tn(a)));
continue
}
if ("svgOrigin" === u) {
Ki(t, a, 1, y, 0, this);
continue
}
if (u in Yi) {
ln(this, C, u, c, a, O);
continue
}
if ("smoothOrigin" === u) {
Ai(this, C, "smooth", C.smooth, a);
continue
}
if ("force3D" === u) {
C[u] = a;
continue
}
if ("transform" === u) {
pn(this, a, t);
continue
}
}
else
u in m || (u = Gi(u) || u);
if (h || (s || 0 === s) && (c || 0 === c) && !di.test(a) && u in m)
s || (s = 0),
(d = (o + "").substr((c + "").length)) !== (b = Dt(a) || (u in f.units ? f.units[u] : d)) && (c = zi(t, u, o, b)),
this._pt = new qe(this._pt,h ? C : m,u,c,O ? O * s : s - c,"px" !== b || !1 === e.autoRound || h ? bi : ji),
this._pt.u = b || 0,
d !== b && (this._pt.b = o,
this._pt.r = hi);
else if (u in m)
Ri.call(this, t, u, o, a);
else {
if (!(u in t)) {
R(u, a);
continue
}
this.add(t, u, t[u], a, n, r)
}
x.push(u)
}
v && He(this)
},
get: Bi,
aliases: fi,
getSetter: function(t, e, i) {
var n = fi[e];
return n && n.indexOf(",") < 0 && (e = n),
e in ai && e !== Ui && (t._gsap.x || Bi(t, "x")) ? i && ri === i ? "scale" === e ? gi : mi : (ri = i || {}) && ("scale" === e ? _i : wi) : t.style && !F(t.style[e]) ? vi : ~e.indexOf("-") ? xi : Ae(t, e)
},
core: {
_removeProperty: Di,
_getMatrix: Ji
}
};
Je.utils.checkPrefix = Gi,
function(t, e, i, n) {
var r = nt(t + "," + e + ",transform,transformOrigin,svgOrigin,force3D,smoothOrigin,transformPerspective", (function(t) {
ai[t] = 1
}
));
nt(e, (function(t) {
f.units[t] = "deg",
Yi[t] = 1
}
)),
fi[r[13]] = t + "," + e,
nt("0:translateX,1:translateY,2:translateZ,8:rotate,8:rotationZ,8:rotateZ,9:rotateX,10:rotateY", (function(t) {
var e = t.split(":");
fi[e[1]] = r[e[0]]
}
))
}("x,y,z,scale,scaleX,scaleY,xPercent,yPercent", "rotation,rotationX,rotationY,skewX,skewY"),
nt("x,y,z,top,right,bottom,left,width,height,fontSize,padding,margin,perspective", (function(t) {
f.units[t] = "px"
}
)),
Je.registerPlugin(un);
var dn = Je.registerPlugin(un) || Je
, fn = (dn.core.Tween,
i("shcM"))
, bn = i("q1tI");
dn.registerPlugin(fn.DrawSVGPlugin);
var On = 3 * (1 / ((1 + Math.sqrt(5)) / 2))
, hn = On / 3
, jn = function(t) {
return 0 === t ? t : On / t
}
, Cn = function() {
var t = arguments.length > 0 && void 0 !== arguments[0] ? arguments[0] : ".path"
, e = Object(bn.useRef)(null);
return Object(bn.useEffect)((function() {
if (e.current) {
var i = e.current.querySelectorAll(t)
, n = [];
return i.forEach((function(t, e) {
var i = dn.timeline({
delay: jn(e),
repeat: -1,
repeatDelay: hn,
repeatRefresh: !0,
defaults: {
duration: On,
ease: "slow(0.3, 0.4, false)"
}
});
i.fromTo(t, {
attr: {
x1: "random(30, 400, 32)",
x2: "random(600, 1000, 16)"
},
autoAlpha: 0,
drawSVG: "0%",
xPercent: "random(-500, -100, 80)"
}, {
autoAlpha: 1,
drawSVG: "100%",
xPercent: "random(500, 200, 100)"
}),
i.to(t, {
duration: On / 2,
autoAlpha: 0
}, ">-0.1"),
n.push(i)
}
)),
function() {
n.forEach((function(t) {
return t.clear()
}
))
}
}
}
), [e, t]),
e
}
},
"/3Dz": function(t, e, i) {
"use strict";
var n = i("wx14")
, r = i("rePB")
, o = i("Ff2n")
, a = i("q1tI")
, s = i.n(a)
, c = i("2A+t");
s.a.createElement;
function l(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function p(t) {
for (var e = 1; e < arguments.length; e++) {
var i = null != arguments[e] ? arguments[e] : {};
e % 2 ? l(Object(i), !0).forEach((function(e) {
Object(r.a)(t, e, i[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(i)) : l(Object(i)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(i, e))
}
))
}
return t
}
var u = "#0047FF"
, d = "#0047FF"
, f = "#000000"
, b = "#000000"
, O = Object(a.forwardRef)((function(t, e) {
var i = t.pushSx
, r = Object(o.a)(t, ["pushSx"]);
return Object(c.c)("svg", Object(n.a)({
viewBox: "0 0 1896 110",
fill: "none",
xmlns: "http://www.w3.org/2000/svg"
}, r, {
ref: e,
sx: p({
minWidth: "1400px"
}, i)
}), Object(c.c)("g", {
clipPath: "url(#clip0)"
}, Object(c.c)("rect", {
width: "1896",
height: "110",
fill: "white"
}), Object(c.c)("line", {
className: "path",
sx: {
opacity: 0
},
x1: "581",
y1: "37.5",
x2: "961",
y2: "37.5",
stroke: "url(#paint1_linear)",
strokeWidth: "3"
}), Object(c.c)("line", {
className: "path",
sx: {
opacity: 0
},
x1: "1197",
y1: "71.75",
x2: "1372",
y2: "71.75",
stroke: "url(#paint2_linear)",
strokeWidth: "3"
}), Object(c.c)("line", {
className: "path",
sx: {
opacity: 0
},
x1: "342",
y1: "71.75",
x2: "790",
y2: "71.75",
stroke: "url(#paint3_linear)",
strokeWidth: "3"
}), Object(c.c)("line", {
className: "path",
sx: {
opacity: 0
},
x1: "889",
y1: "106",
x2: "1098",
y2: "106",
stroke: "url(#paint0_linear)",
strokeWidth: "3"
}), Object(c.c)("g", {
id: "pattern"
}, Object(c.c)("path", {
d: "M1437.37 106.006C1437.37 105.279 1437.96 104.69 1438.68 104.69C1439.41 104.69 1440 105.279 1440 106.006C1440 106.733 1439.41 107.323 1438.68 107.323C1437.96 107.323 1437.37 106.733 1437.37 106.006Z",
fill: "url(#paint4_linear)"
}), Object(c.c)("path", {
d: "M1403.14 106.006C1403.14 105.279 1403.73 104.69 1404.46 104.69C1405.19 104.69 1405.78 105.279 1405.78 106.006C1405.78 106.733 1405.19 107.323 1404.46 107.323C1403.73 107.323 1403.14 106.733 1403.14 106.006Z",
fill: "url(#paint5_linear)"
}), Object(c.c)("path", {
d: "M1368.92 106.006C1368.92 105.279 1369.51 104.69 1370.24 104.69C1370.96 104.69 1371.55 105.279 1371.55 106.006C1371.55 106.733 1370.96 107.323 1370.24 107.323C1369.51 107.323 1368.92 106.733 1368.92 106.006Z",
fill: "url(#paint6_linear)"
}), Object(c.c)("path", {
d: "M1334.7 106.006C1334.7 105.279 1335.29 104.69 1336.01 104.69C1336.74 104.69 1337.33 105.279 1337.33 106.006C1337.33 106.733 1336.74 107.323 1336.01 107.323C1335.29 107.323 1334.7 106.733 1334.7 106.006Z",
fill: "url(#paint7_linear)"
}), Object(c.c)("path", {
d: "M1300.48 106.006C1300.48 105.279 1301.06 104.69 1301.79 104.69C1302.52 104.69 1303.11 105.279 1303.11 106.006C1303.11 106.733 1302.52 107.323 1301.79 107.323C1301.06 107.323 1300.48 106.733 1300.48 106.006Z",
fill: "url(#paint8_linear)"
}), Object(c.c)("path", {
d: "M1266.25 106.006C1266.25 105.279 1266.84 104.69 1267.57 104.69C1268.3 104.69 1268.88 105.279 1268.88 106.006C1268.88 106.733 1268.3 107.323 1267.57 107.323C1266.84 107.323 1266.25 106.733 1266.25 106.006Z",
fill: "url(#paint9_linear)"
}), Object(c.c)("path", {
d: "M1232.03 106.006C1232.03 105.279 1232.62 104.69 1233.35 104.69C1234.07 104.69 1234.66 105.279 1234.66 106.006C1234.66 106.733 1234.07 107.323 1233.35 107.323C1232.62 107.323 1232.03 106.733 1232.03 106.006Z",
fill: "url(#paint10_linear)"
}), Object(c.c)("path", {
d: "M1197.81 106.006C1197.81 105.279 1198.4 104.69 1199.12 104.69C1199.85 104.69 1200.44 105.279 1200.44 106.006C1200.44 106.733 1199.85 107.323 1199.12 107.323C1198.4 107.323 1197.81 106.733 1197.81 106.006Z",
fill: "url(#paint11_linear)"
}), Object(c.c)("path", {
d: "M1163.58 106.006C1163.58 105.279 1164.17 104.69 1164.9 104.69C1165.63 104.69 1166.22 105.279 1166.22 106.006C1166.22 106.733 1165.63 107.323 1164.9 107.323C1164.17 107.323 1163.58 106.733 1163.58 106.006Z",
fill: "url(#paint12_linear)"
}), Object(c.c)("path", {
d: "M1129.36 106.006C1129.36 105.279 1129.95 104.69 1130.68 104.69C1131.4 104.69 1131.99 105.279 1131.99 106.006C1131.99 106.733 1131.4 107.323 1130.68 107.323C1129.95 107.323 1129.36 106.733 1129.36 106.006Z",
fill: "url(#paint13_linear)"
}), Object(c.c)("path", {
d: "M1095.14 106.006C1095.14 105.279 1095.73 104.69 1096.45 104.69C1097.18 104.69 1097.77 105.279 1097.77 106.006C1097.77 106.733 1097.18 107.323 1096.45 107.323C1095.73 107.323 1095.14 106.733 1095.14 106.006Z",
fill: "url(#paint14_linear)"
}), Object(c.c)("path", {
d: "M1060.91 106.006C1060.91 105.279 1061.5 104.69 1062.23 104.69C1062.96 104.69 1063.55 105.279 1063.55 106.006C1063.55 106.733 1062.96 107.323 1062.23 107.323C1061.5 107.323 1060.91 106.733 1060.91 106.006Z",
fill: "url(#paint15_linear)"
}), Object(c.c)("path", {
d: "M1026.69 106.006C1026.69 105.279 1027.28 104.69 1028.01 104.69C1028.73 104.69 1029.32 105.279 1029.32 106.006C1029.32 106.733 1028.73 107.323 1028.01 107.323C1027.28 107.323 1026.69 106.733 1026.69 106.006Z",
fill: "url(#paint16_linear)"
}), Object(c.c)("path", {
d: "M992.468 106.006C992.468 105.279 993.057 104.69 993.784 104.69C994.511 104.69 995.101 105.279 995.101 106.006C995.101 106.733 994.511 107.323 993.784 107.323C993.057 107.323 992.468 106.733 992.468 106.006Z",
fill: "url(#paint17_linear)"
}), Object(c.c)("path", {
d: "M958.245 106.006C958.245 105.279 958.834 104.69 959.561 104.69C960.288 104.69 960.878 105.279 960.878 106.006C960.878 106.733 960.288 107.323 959.561 107.323C958.834 107.323 958.245 106.733 958.245 106.006Z",
fill: "url(#paint18_linear)"
}), Object(c.c)("path", {
d: "M924.022 106.006C924.022 105.279 924.611 104.69 925.338 104.69C926.065 104.69 926.654 105.279 926.654 106.006C926.654 106.733 926.065 107.323 925.338 107.323C924.611 107.323 924.022 106.733 924.022 106.006Z",
fill: "url(#paint19_linear)"
}), Object(c.c)("path", {
d: "M889.799 106.006C889.799 105.279 890.388 104.69 891.115 104.69C891.842 104.69 892.431 105.279 892.431 106.006C892.431 106.733 891.842 107.323 891.115 107.323C890.388 107.323 889.799 106.733 889.799 106.006Z",
fill: "url(#paint20_linear)"
}), Object(c.c)("path", {
d: "M855.576 106.006C855.576 105.279 856.165 104.69 856.892 104.69C857.619 104.69 858.208 105.279 858.208 106.006C858.208 106.733 857.619 107.323 856.892 107.323C856.165 107.323 855.576 106.733 855.576 106.006Z",
fill: "url(#paint21_linear)"
}), Object(c.c)("path", {
d: "M821.353 106.006C821.353 105.279 821.942 104.69 822.669 104.69C823.396 104.69 823.985 105.279 823.985 106.006C823.985 106.733 823.396 107.323 822.669 107.323C821.942 107.323 821.353 106.733 821.353 106.006Z",
fill: "url(#paint22_linear)"
}), Object(c.c)("path", {
d: "M787.13 106.006C787.13 105.279 787.719 104.69 788.446 104.69C789.173 104.69 789.762 105.279 789.762 106.006C789.762 106.733 789.173 107.323 788.446 107.323C787.719 107.323 787.13 106.733 787.13 106.006Z",
fill: "url(#paint23_linear)"
}), Object(c.c)("path", {
d: "M752.907 106.006C752.907 105.279 753.496 104.69 754.223 104.69C754.95 104.69 755.539 105.279 755.539 106.006C755.539 106.733 754.95 107.323 754.223 107.323C753.496 107.323 752.907 106.733 752.907 106.006Z",
fill: "url(#paint24_linear)"
}), Object(c.c)("path", {
d: "M718.684 106.006C718.684 105.279 719.273 104.69 720 104.69C720.727 104.69 721.316 105.279 721.316 106.006C721.316 106.733 720.727 107.323 720 107.323C719.273 107.323 718.684 106.733 718.684 106.006Z",
fill: "url(#paint25_linear)"
}), Object(c.c)("path", {
d: "M684.461 106.006C684.461 105.279 685.05 104.69 685.777 104.69C686.504 104.69 687.093 105.279 687.093 106.006C687.093 106.733 686.504 107.323 685.777 107.323C685.05 107.323 684.461 106.733 684.461 106.006Z",
fill: "url(#paint26_linear)"
}), Object(c.c)("path", {
d: "M650.238 106.006C650.238 105.279 650.827 104.69 651.554 104.69C652.281 104.69 652.87 105.279 652.87 106.006C652.87 106.733 652.281 107.323 651.554 107.323C650.827 107.323 650.238 106.733 650.238 106.006Z",
fill: "url(#paint27_linear)"
}), Object(c.c)("path", {
d: "M616.015 106.006C616.015 105.279 616.604 104.69 617.331 104.69C618.058 104.69 618.647 105.279 618.647 106.006C618.647 106.733 618.058 107.323 617.331 107.323C616.604 107.323 616.015 106.733 616.015 106.006Z",
fill: "url(#paint28_linear)"
}), Object(c.c)("path", {
d: "M581.792 106.006C581.792 105.279 582.381 104.69 583.108 104.69C583.835 104.69 584.424 105.279 584.424 106.006C584.424 106.733 583.835 107.323 583.108 107.323C582.381 107.323 581.792 106.733 581.792 106.006Z",
fill: "url(#paint29_linear)"
}), Object(c.c)("path", {
d: "M547.569 106.006C547.569 105.279 548.158 104.69 548.885 104.69C549.612 104.69 550.201 105.279 550.201 106.006C550.201 106.733 549.612 107.323 548.885 107.323C548.158 107.323 547.569 106.733 547.569 106.006Z",
fill: "url(#paint30_linear)"
}), Object(c.c)("path", {
d: "M513.345 106.006C513.345 105.279 513.935 104.69 514.662 104.69C515.389 104.69 515.978 105.279 515.978 106.006C515.978 106.733 515.389 107.323 514.662 107.323C513.935 107.323 513.345 106.733 513.345 106.006Z",
fill: "url(#paint31_linear)"
}), Object(c.c)("path", {
d: "M479.123 106.006C479.123 105.279 479.712 104.69 480.439 104.69C481.166 104.69 481.755 105.279 481.755 106.006C481.755 106.733 481.166 107.323 480.439 107.323C479.712 107.323 479.123 106.733 479.123 106.006Z",
fill: "url(#paint32_linear)"
}), Object(c.c)("path", {
d: "M444.899 106.006C444.899 105.279 445.489 104.69 446.216 104.69C446.943 104.69 447.532 105.279 447.532 106.006C447.532 106.733 446.943 107.323 446.216 107.323C445.489 107.323 444.899 106.733 444.899 106.006Z",
fill: "url(#paint33_linear)"
}), Object(c.c)("path", {
d: "M410.676 106.006C410.676 105.279 411.266 104.69 411.993 104.69C412.72 104.69 413.309 105.279 413.309 106.006C413.309 106.733 412.72 107.323 411.993 107.323C411.266 107.323 410.676 106.733 410.676 106.006Z",
fill: "url(#paint34_linear)"
}), Object(c.c)("path", {
d: "M376.453 106.006C376.453 105.279 377.043 104.69 377.77 104.69C378.497 104.69 379.086 105.279 379.086 106.006C379.086 106.733 378.497 107.323 377.77 107.323C377.043 107.323 376.453 106.733 376.453 106.006Z",
fill: "url(#paint35_linear)"
}), Object(c.c)("path", {
d: "M342.23 106.006C342.23 105.279 342.82 104.69 343.547 104.69C344.274 104.69 344.863 105.279 344.863 106.006C344.863 106.733 344.274 107.323 343.547 107.323C342.82 107.323 342.23 106.733 342.23 106.006Z",
fill: "url(#paint36_linear)"
}), Object(c.c)("path", {
d: "M308.007 106.006C308.007 105.279 308.597 104.69 309.324 104.69C310.051 104.69 310.64 105.279 310.64 106.006C310.64 106.733 310.051 107.323 309.324 107.323C308.597 107.323 308.007 106.733 308.007 106.006Z",
fill: "url(#paint37_linear)"
}), Object(c.c)("path", {
d: "M273.784 106.006C273.784 105.279 274.374 104.69 275.101 104.69C275.828 104.69 276.417 105.279 276.417 106.006C276.417 106.733 275.828 107.323 275.101 107.323C274.374 107.323 273.784 106.733 273.784 106.006Z",
fill: "url(#paint38_linear)"
}), Object(c.c)("path", {
d: "M239.561 106.006C239.561 105.279 240.15 104.69 240.877 104.69C241.604 104.69 242.194 105.279 242.194 106.006C242.194 106.733 241.604 107.323 240.877 107.323C240.15 107.323 239.561 106.733 239.561 106.006Z",
fill: "url(#paint39_linear)"
}), Object(c.c)("path", {
d: "M205.338 106.006C205.338 105.279 205.927 104.69 206.654 104.69C207.381 104.69 207.971 105.279 207.971 106.006C207.971 106.733 207.381 107.323 206.654 107.323C205.927 107.323 205.338 106.733 205.338 106.006Z",
fill: "url(#paint40_linear)"
}), Object(c.c)("path", {
d: "M171.115 106.006C171.115 105.279 171.704 104.69 172.431 104.69C173.158 104.69 173.748 105.279 173.748 106.006C173.748 106.733 173.158 107.323 172.431 107.323C171.704 107.323 171.115 106.733 171.115 106.006Z",
fill: "url(#paint41_linear)"
}), Object(c.c)("path", {
d: "M136.892 106.006C136.892 105.279 137.481 104.69 138.208 104.69C138.935 104.69 139.525 105.279 139.525 106.006C139.525 106.733 138.935 107.323 138.208 107.323C137.481 107.323 136.892 106.733 136.892 106.006Z",
fill: "url(#paint42_linear)"
}), Object(c.c)("path", {
d: "M102.669 106.006C102.669 105.279 103.258 104.69 103.985 104.69C104.712 104.69 105.302 105.279 105.302 106.006C105.302 106.733 104.712 107.323 103.985 107.323C103.258 107.323 102.669 106.733 102.669 106.006Z",
fill: "url(#paint43_linear)"
}), Object(c.c)("path", {
d: "M68.446 106.006C68.446 105.279 69.0354 104.69 69.7623 104.69C70.4893 104.69 71.0786 105.279 71.0786 106.006C71.0786 106.733 70.4893 107.323 69.7623 107.323C69.0354 107.323 68.446 106.733 68.446 106.006Z",
fill: "url(#paint44_linear)"
}), Object(c.c)("path", {
d: "M34.223 106.006C34.223 105.279 34.8124 104.69 35.5393 104.69C36.2663 104.69 36.8556 105.279 36.8556 106.006C36.8556 106.733 36.2663 107.323 35.5393 107.323C34.8124 107.323 34.223 106.733 34.223 106.006Z",
fill: "url(#paint45_linear)"
}), Object(c.c)("path", {
d: "M1.15095e-07 106.006C1.7866e-07 105.279 0.589328 104.69 1.31628 104.69C2.04324 104.69 2.63257 105.279 2.63257 106.006C2.63257 106.733 2.04324 107.323 1.31628 107.323C0.589328 107.323 5.15297e-08 106.733 1.15095e-07 106.006Z",
fill: "url(#paint46_linear)"
}), Object(c.c)("path", {
d: "M1437.37 71.7763C1437.37 71.0492 1437.96 70.4598 1438.68 70.4598C1439.41 70.4598 1440 71.0492 1440 71.7763C1440 72.5034 1439.41 73.0929 1438.68 73.0929C1437.96 73.0929 1437.37 72.5034 1437.37 71.7763Z",
fill: "url(#paint47_linear)"
}), Object(c.c)("path", {
d: "M1403.14 71.7763C1403.14 71.0492 1403.73 70.4598 1404.46 70.4598C1405.19 70.4598 1405.78 71.0492 1405.78 71.7763C1405.78 72.5034 1405.19 73.0929 1404.46 73.0929C1403.73 73.0929 1403.14 72.5034 1403.14 71.7763Z",
fill: "url(#paint48_linear)"
}), Object(c.c)("path", {
d: "M1368.92 71.7763C1368.92 71.0492 1369.51 70.4598 1370.24 70.4598C1370.96 70.4598 1371.55 71.0492 1371.55 71.7763C1371.55 72.5034 1370.96 73.0929 1370.24 73.0929C1369.51 73.0929 1368.92 72.5034 1368.92 71.7763Z",
fill: "url(#paint49_linear)"
}), Object(c.c)("path", {
d: "M1334.7 71.7763C1334.7 71.0492 1335.29 70.4598 1336.01 70.4598C1336.74 70.4598 1337.33 71.0492 1337.33 71.7763C1337.33 72.5034 1336.74 73.0929 1336.01 73.0929C1335.29 73.0929 1334.7 72.5034 1334.7 71.7763Z",
fill: "url(#paint50_linear)"
}), Object(c.c)("path", {
d: "M1300.48 71.7763C1300.48 71.0492 1301.06 70.4598 1301.79 70.4598C1302.52 70.4598 1303.11 71.0492 1303.11 71.7763C1303.11 72.5034 1302.52 73.0929 1301.79 73.0929C1301.06 73.0929 1300.48 72.5034 1300.48 71.7763Z",
fill: "url(#paint51_linear)"
}), Object(c.c)("path", {
d: "M1266.25 71.7763C1266.25 71.0492 1266.84 70.4598 1267.57 70.4598C1268.3 70.4598 1268.88 71.0492 1268.88 71.7763C1268.88 72.5034 1268.3 73.0929 1267.57 73.0929C1266.84 73.0929 1266.25 72.5034 1266.25 71.7763Z",
fill: "url(#paint52_linear)"
}), Object(c.c)("path", {
d: "M1232.03 71.7763C1232.03 71.0492 1232.62 70.4598 1233.35 70.4598C1234.07 70.4598 1234.66 71.0492 1234.66 71.7763C1234.66 72.5034 1234.07 73.0929 1233.35 73.0929C1232.62 73.0929 1232.03 72.5034 1232.03 71.7763Z",
fill: "url(#paint53_linear)"
}), Object(c.c)("path", {
d: "M1197.81 71.7763C1197.81 71.0492 1198.4 70.4598 1199.12 70.4598C1199.85 70.4598 1200.44 71.0492 1200.44 71.7763C1200.44 72.5034 1199.85 73.0929 1199.12 73.0929C1198.4 73.0929 1197.81 72.5034 1197.81 71.7763Z",
fill: "url(#paint54_linear)"
}), Object(c.c)("path", {
d: "M1163.58 71.7763C1163.58 71.0492 1164.17 70.4598 1164.9 70.4598C1165.63 70.4598 1166.22 71.0492 1166.22 71.7763C1166.22 72.5034 1165.63 73.0929 1164.9 73.0929C1164.17 73.0929 1163.58 72.5034 1163.58 71.7763Z",
fill: "url(#paint55_linear)"
}), Object(c.c)("path", {
d: "M1129.36 71.7763C1129.36 71.0492 1129.95 70.4598 1130.68 70.4598C1131.4 70.4598 1131.99 71.0492 1131.99 71.7763C1131.99 72.5034 1131.4 73.0928 1130.68 73.0928C1129.95 73.0928 1129.36 72.5034 1129.36 71.7763Z",
fill: "url(#paint56_linear)"
}), Object(c.c)("path", {
d: "M1095.14 71.7763C1095.14 71.0492 1095.73 70.4598 1096.45 70.4598C1097.18 70.4598 1097.77 71.0492 1097.77 71.7763C1097.77 72.5034 1097.18 73.0928 1096.45 73.0928C1095.73 73.0928 1095.14 72.5034 1095.14 71.7763Z",
fill: "url(#paint57_linear)"
}), Object(c.c)("path", {
d: "M1060.91 71.7763C1060.91 71.0492 1061.5 70.4598 1062.23 70.4598C1062.96 70.4598 1063.55 71.0492 1063.55 71.7763C1063.55 72.5034 1062.96 73.0928 1062.23 73.0928C1061.5 73.0928 1060.91 72.5034 1060.91 71.7763Z",
fill: "url(#paint58_linear)"
}), Object(c.c)("path", {
d: "M1026.69 71.7763C1026.69 71.0492 1027.28 70.4598 1028.01 70.4598C1028.73 70.4598 1029.32 71.0492 1029.32 71.7763C1029.32 72.5034 1028.73 73.0928 1028.01 73.0928C1027.28 73.0928 1026.69 72.5034 1026.69 71.7763Z",
fill: "url(#paint59_linear)"
}), Object(c.c)("path", {
d: "M992.468 71.7763C992.468 71.0492 993.057 70.4598 993.784 70.4598C994.511 70.4598 995.101 71.0492 995.101 71.7763C995.101 72.5034 994.511 73.0928 993.784 73.0928C993.057 73.0928 992.468 72.5034 992.468 71.7763Z",
fill: "url(#paint60_linear)"
}), Object(c.c)("path", {
d: "M958.245 71.7763C958.245 71.0492 958.834 70.4598 959.561 70.4598C960.288 70.4598 960.878 71.0492 960.878 71.7763C960.878 72.5034 960.288 73.0928 959.561 73.0928C958.834 73.0928 958.245 72.5034 958.245 71.7763Z",
fill: "url(#paint61_linear)"
}), Object(c.c)("path", {
d: "M924.022 71.7763C924.022 71.0492 924.611 70.4598 925.338 70.4598C926.065 70.4598 926.654 71.0492 926.654 71.7763C926.654 72.5034 926.065 73.0928 925.338 73.0928C924.611 73.0928 924.022 72.5034 924.022 71.7763Z",
fill: "url(#paint62_linear)"
}), Object(c.c)("path", {
d: "M889.799 71.7763C889.799 71.0492 890.388 70.4598 891.115 70.4598C891.842 70.4598 892.431 71.0492 892.431 71.7763C892.431 72.5034 891.842 73.0928 891.115 73.0928C890.388 73.0928 889.799 72.5034 889.799 71.7763Z",
fill: "url(#paint63_linear)"
}), Object(c.c)("path", {
d: "M855.576 71.7763C855.576 71.0492 856.165 70.4598 856.892 70.4598C857.619 70.4598 858.208 71.0492 858.208 71.7763C858.208 72.5034 857.619 73.0928 856.892 73.0928C856.165 73.0928 855.576 72.5034 855.576 71.7763Z",
fill: "url(#paint64_linear)"
}), Object(c.c)("path", {
d: "M821.353 71.7763C821.353 71.0492 821.942 70.4598 822.669 70.4598C823.396 70.4598 823.985 71.0492 823.985 71.7763C823.985 72.5034 823.396 73.0928 822.669 73.0928C821.942 73.0928 821.353 72.5034 821.353 71.7763Z",
fill: "url(#paint65_linear)"
}), Object(c.c)("path", {
d: "M787.13 71.7763C787.13 71.0492 787.719 70.4598 788.446 70.4598C789.173 70.4598 789.762 71.0492 789.762 71.7763C789.762 72.5034 789.173 73.0928 788.446 73.0928C787.719 73.0928 787.13 72.5034 787.13 71.7763Z",
fill: "url(#paint66_linear)"
}), Object(c.c)("path", {
d: "M752.907 71.7763C752.907 71.0492 753.496 70.4598 754.223 70.4598C754.95 70.4598 755.539 71.0492 755.539 71.7763C755.539 72.5034 754.95 73.0928 754.223 73.0928C753.496 73.0928 752.907 72.5034 752.907 71.7763Z",
fill: "url(#paint67_linear)"
}), Object(c.c)("path", {
d: "M718.684 71.7763C718.684 71.0492 719.273 70.4597 720 70.4597C720.727 70.4597 721.316 71.0492 721.316 71.7763C721.316 72.5034 720.727 73.0928 720 73.0928C719.273 73.0928 718.684 72.5034 718.684 71.7763Z",
fill: "url(#paint68_linear)"
}), Object(c.c)("path", {
d: "M684.461 71.7763C684.461 71.0492 685.05 70.4597 685.777 70.4597C686.504 70.4597 687.093 71.0492 687.093 71.7763C687.093 72.5034 686.504 73.0928 685.777 73.0928C685.05 73.0928 684.461 72.5034 684.461 71.7763Z",
fill: "url(#paint69_linear)"
}), Object(c.c)("path", {
d: "M650.238 71.7763C650.238 71.0492 650.827 70.4597 651.554 70.4597C652.281 70.4597 652.87 71.0492 652.87 71.7763C652.87 72.5034 652.281 73.0928 651.554 73.0928C650.827 73.0928 650.238 72.5034 650.238 71.7763Z",
fill: "url(#paint70_linear)"
}), Object(c.c)("path", {
d: "M616.015 71.7763C616.015 71.0492 616.604 70.4597 617.331 70.4597C618.058 70.4597 618.647 71.0492 618.647 71.7763C618.647 72.5034 618.058 73.0928 617.331 73.0928C616.604 73.0928 616.015 72.5034 616.015 71.7763Z",
fill: "url(#paint71_linear)"
}), Object(c.c)("path", {
d: "M581.792 71.7763C581.792 71.0492 582.381 70.4597 583.108 70.4597C583.835 70.4597 584.424 71.0492 584.424 71.7763C584.424 72.5034 583.835 73.0928 583.108 73.0928C582.381 73.0928 581.792 72.5034 581.792 71.7763Z",
fill: "url(#paint72_linear)"
}), Object(c.c)("path", {
d: "M547.569 71.7763C547.569 71.0492 548.158 70.4597 548.885 70.4597C549.612 70.4597 550.201 71.0492 550.201 71.7763C550.201 72.5034 549.612 73.0928 548.885 73.0928C548.158 73.0928 547.569 72.5034 547.569 71.7763Z",
fill: "url(#paint73_linear)"
}), Object(c.c)("path", {
d: "M513.345 71.7763C513.345 71.0492 513.935 70.4597 514.662 70.4597C515.389 70.4597 515.978 71.0492 515.978 71.7763C515.978 72.5034 515.389 73.0928 514.662 73.0928C513.935 73.0928 513.345 72.5034 513.345 71.7763Z",
fill: "url(#paint74_linear)"
}), Object(c.c)("path", {
d: "M479.123 71.7763C479.123 71.0492 479.712 70.4597 480.439 70.4597C481.166 70.4597 481.755 71.0492 481.755 71.7763C481.755 72.5034 481.166 73.0928 480.439 73.0928C479.712 73.0928 479.123 72.5034 479.123 71.7763Z",
fill: "url(#paint75_linear)"
}), Object(c.c)("path", {
d: "M444.899 71.7763C444.899 71.0492 445.489 70.4597 446.216 70.4597C446.943 70.4597 447.532 71.0492 447.532 71.7763C447.532 72.5034 446.943 73.0928 446.216 73.0928C445.489 73.0928 444.899 72.5034 444.899 71.7763Z",
fill: "url(#paint76_linear)"
}), Object(c.c)("path", {
d: "M410.676 71.7763C410.676 71.0492 411.266 70.4597 411.993 70.4597C412.72 70.4597 413.309 71.0492 413.309 71.7763C413.309 72.5034 412.72 73.0928 411.993 73.0928C411.266 73.0928 410.676 72.5034 410.676 71.7763Z",
fill: "url(#paint77_linear)"
}), Object(c.c)("path", {
d: "M376.453 71.7762C376.453 71.0491 377.043 70.4597 377.77 70.4597C378.497 70.4597 379.086 71.0491 379.086 71.7762C379.086 72.5034 378.497 73.0928 377.77 73.0928C377.043 73.0928 376.453 72.5034 376.453 71.7762Z",
fill: "url(#paint78_linear)"
}), Object(c.c)("path", {
d: "M342.23 71.7762C342.23 71.0491 342.82 70.4597 343.547 70.4597C344.274 70.4597 344.863 71.0491 344.863 71.7762C344.863 72.5033 344.274 73.0928 343.547 73.0928C342.82 73.0928 342.23 72.5033 342.23 71.7762Z",
fill: "url(#paint79_linear)"
}), Object(c.c)("path", {
d: "M308.007 71.7762C308.007 71.0491 308.597 70.4597 309.324 70.4597C310.051 70.4597 310.64 71.0491 310.64 71.7762C310.64 72.5033 310.051 73.0928 309.324 73.0928C308.597 73.0928 308.007 72.5033 308.007 71.7762Z",
fill: "url(#paint80_linear)"
}), Object(c.c)("path", {
d: "M273.784 71.7762C273.784 71.0491 274.374 70.4597 275.101 70.4597C275.828 70.4597 276.417 71.0491 276.417 71.7762C276.417 72.5033 275.828 73.0928 275.101 73.0928C274.374 73.0928 273.784 72.5033 273.784 71.7762Z",
fill: "url(#paint81_linear)"
}), Object(c.c)("path", {
d: "M239.561 71.7762C239.561 71.0491 240.15 70.4597 240.877 70.4597C241.604 70.4597 242.194 71.0491 242.194 71.7762C242.194 72.5033 241.604 73.0928 240.877 73.0928C240.15 73.0928 239.561 72.5033 239.561 71.7762Z",
fill: "url(#paint82_linear)"
}), Object(c.c)("path", {
d: "M205.338 71.7762C205.338 71.0491 205.927 70.4597 206.654 70.4597C207.381 70.4597 207.971 71.0491 207.971 71.7762C207.971 72.5033 207.381 73.0928 206.654 73.0928C205.927 73.0928 205.338 72.5033 205.338 71.7762Z",
fill: "url(#paint83_linear)"
}), Object(c.c)("path", {
d: "M171.115 71.7762C171.115 71.0491 171.704 70.4597 172.431 70.4597C173.158 70.4597 173.748 71.0491 173.748 71.7762C173.748 72.5033 173.158 73.0928 172.431 73.0928C171.704 73.0928 171.115 72.5033 171.115 71.7762Z",
fill: "url(#paint84_linear)"
}), Object(c.c)("path", {
d: "M136.892 71.7762C136.892 71.0491 137.481 70.4597 138.208 70.4597C138.935 70.4597 139.525 71.0491 139.525 71.7762C139.525 72.5033 138.935 73.0928 138.208 73.0928C137.481 73.0928 136.892 72.5033 136.892 71.7762Z",
fill: "url(#paint85_linear)"
}), Object(c.c)("path", {
d: "M102.669 71.7762C102.669 71.0491 103.258 70.4597 103.985 70.4597C104.712 70.4597 105.302 71.0491 105.302 71.7762C105.302 72.5033 104.712 73.0928 103.985 73.0928C103.258 73.0928 102.669 72.5033 102.669 71.7762Z",
fill: "url(#paint86_linear)"
}), Object(c.c)("path", {
d: "M68.446 71.7762C68.446 71.0491 69.0354 70.4597 69.7623 70.4597C70.4893 70.4597 71.0786 71.0491 71.0786 71.7762C71.0786 72.5033 70.4893 73.0928 69.7623 73.0928C69.0354 73.0928 68.446 72.5033 68.446 71.7762Z",
fill: "url(#paint87_linear)"
}), Object(c.c)("path", {
d: "M34.223 71.7762C34.223 71.0491 34.8124 70.4597 35.5393 70.4597C36.2663 70.4597 36.8556 71.0491 36.8556 71.7762C36.8556 72.5033 36.2663 73.0928 35.5393 73.0928C34.8124 73.0928 34.223 72.5033 34.223 71.7762Z",
fill: "url(#paint88_linear)"
}), Object(c.c)("path", {
d: "M3.10756e-06 71.7762C3.17113e-06 71.0491 0.589331 70.4597 1.31629 70.4597C2.04324 70.4597 2.63257 71.0491 2.63257 71.7762C2.63257 72.5033 2.04324 73.0927 1.31629 73.0927C0.589331 73.0927 3.044e-06 72.5033 3.10756e-06 71.7762Z",
fill: "url(#paint89_linear)"
}), Object(c.c)("path", {
d: "M1437.37 37.5465C1437.37 36.8194 1437.96 36.23 1438.68 36.23C1439.41 36.23 1440 36.8194 1440 37.5465C1440 38.2736 1439.41 38.863 1438.68 38.863C1437.96 38.863 1437.37 38.2736 1437.37 37.5465Z",
fill: "url(#paint90_linear)"
}), Object(c.c)("path", {
d: "M1403.14 37.5465C1403.14 36.8194 1403.73 36.23 1404.46 36.23C1405.19 36.23 1405.78 36.8194 1405.78 37.5465C1405.78 38.2736 1405.19 38.863 1404.46 38.863C1403.73 38.863 1403.14 38.2736 1403.14 37.5465Z",
fill: "url(#paint91_linear)"
}), Object(c.c)("path", {
d: "M1368.92 37.5465C1368.92 36.8194 1369.51 36.23 1370.24 36.23C1370.96 36.23 1371.55 36.8194 1371.55 37.5465C1371.55 38.2736 1370.96 38.863 1370.24 38.863C1369.51 38.863 1368.92 38.2736 1368.92 37.5465Z",
fill: "url(#paint92_linear)"
}), Object(c.c)("path", {
d: "M1334.7 37.5465C1334.7 36.8194 1335.29 36.23 1336.01 36.23C1336.74 36.23 1337.33 36.8194 1337.33 37.5465C1337.33 38.2736 1336.74 38.863 1336.01 38.863C1335.29 38.863 1334.7 38.2736 1334.7 37.5465Z",
fill: "url(#paint93_linear)"
}), Object(c.c)("path", {
d: "M1300.48 37.5465C1300.48 36.8194 1301.06 36.23 1301.79 36.23C1302.52 36.23 1303.11 36.8194 1303.11 37.5465C1303.11 38.2736 1302.52 38.863 1301.79 38.863C1301.06 38.863 1300.48 38.2736 1300.48 37.5465Z",
fill: "url(#paint94_linear)"
}), Object(c.c)("path", {
d: "M1266.25 37.5465C1266.25 36.8194 1266.84 36.23 1267.57 36.23C1268.3 36.23 1268.88 36.8194 1268.88 37.5465C1268.88 38.2736 1268.3 38.863 1267.57 38.863C1266.84 38.863 1266.25 38.2736 1266.25 37.5465Z",
fill: "url(#paint95_linear)"
}), Object(c.c)("path", {
d: "M1232.03 37.5465C1232.03 36.8194 1232.62 36.23 1233.35 36.23C1234.07 36.23 1234.66 36.8194 1234.66 37.5465C1234.66 38.2736 1234.07 38.863 1233.35 38.863C1232.62 38.863 1232.03 38.2736 1232.03 37.5465Z",
fill: "url(#paint96_linear)"
}), Object(c.c)("path", {
d: "M1197.81 37.5465C1197.81 36.8194 1198.4 36.2299 1199.12 36.23C1199.85 36.23 1200.44 36.8194 1200.44 37.5465C1200.44 38.2736 1199.85 38.863 1199.12 38.863C1198.4 38.863 1197.81 38.2736 1197.81 37.5465Z",
fill: "url(#paint97_linear)"
}), Object(c.c)("path", {
d: "M1163.58 37.5465C1163.58 36.8194 1164.17 36.2299 1164.9 36.2299C1165.63 36.2299 1166.22 36.8194 1166.22 37.5465C1166.22 38.2736 1165.63 38.863 1164.9 38.863C1164.17 38.863 1163.58 38.2736 1163.58 37.5465Z",
fill: "url(#paint98_linear)"
}), Object(c.c)("path", {
d: "M1129.36 37.5465C1129.36 36.8194 1129.95 36.2299 1130.68 36.2299C1131.4 36.2299 1131.99 36.8194 1131.99 37.5465C1131.99 38.2736 1131.4 38.863 1130.68 38.863C1129.95 38.863 1129.36 38.2736 1129.36 37.5465Z",
fill: "url(#paint99_linear)"
}), Object(c.c)("path", {
d: "M1095.14 37.5465C1095.14 36.8194 1095.73 36.2299 1096.45 36.2299C1097.18 36.2299 1097.77 36.8194 1097.77 37.5465C1097.77 38.2736 1097.18 38.863 1096.45 38.863C1095.73 38.863 1095.14 38.2736 1095.14 37.5465Z",
fill: "url(#paint100_linear)"
}), Object(c.c)("path", {
d: "M1060.91 37.5465C1060.91 36.8194 1061.5 36.2299 1062.23 36.2299C1062.96 36.2299 1063.55 36.8194 1063.55 37.5465C1063.55 38.2736 1062.96 38.863 1062.23 38.863C1061.5 38.863 1060.91 38.2736 1060.91 37.5465Z",
fill: "url(#paint101_linear)"
}), Object(c.c)("path", {
d: "M1026.69 37.5465C1026.69 36.8194 1027.28 36.2299 1028.01 36.2299C1028.73 36.2299 1029.32 36.8194 1029.32 37.5465C1029.32 38.2736 1028.73 38.863 1028.01 38.863C1027.28 38.863 1026.69 38.2736 1026.69 37.5465Z",
fill: "url(#paint102_linear)"
}), Object(c.c)("path", {
d: "M992.468 37.5465C992.468 36.8194 993.057 36.2299 993.784 36.2299C994.511 36.2299 995.101 36.8194 995.101 37.5465C995.101 38.2736 994.511 38.863 993.784 38.863C993.057 38.863 992.468 38.2736 992.468 37.5465Z",
fill: "url(#paint103_linear)"
}), Object(c.c)("path", {
d: "M958.245 37.5465C958.245 36.8194 958.834 36.2299 959.561 36.2299C960.288 36.2299 960.878 36.8194 960.878 37.5465C960.878 38.2736 960.288 38.863 959.561 38.863C958.834 38.863 958.245 38.2736 958.245 37.5465Z",
fill: "url(#paint104_linear)"
}), Object(c.c)("path", {
d: "M924.022 37.5465C924.022 36.8194 924.611 36.2299 925.338 36.2299C926.065 36.2299 926.654 36.8194 926.654 37.5465C926.654 38.2736 926.065 38.863 925.338 38.863C924.611 38.863 924.022 38.2736 924.022 37.5465Z",
fill: "url(#paint105_linear)"
}), Object(c.c)("path", {
d: "M889.799 37.5465C889.799 36.8194 890.388 36.2299 891.115 36.2299C891.842 36.2299 892.431 36.8194 892.431 37.5465C892.431 38.2736 891.842 38.863 891.115 38.863C890.388 38.863 889.799 38.2736 889.799 37.5465Z",
fill: "url(#paint106_linear)"
}), Object(c.c)("path", {
d: "M855.576 37.5465C855.576 36.8194 856.165 36.2299 856.892 36.2299C857.619 36.2299 858.208 36.8194 858.208 37.5465C858.208 38.2736 857.619 38.863 856.892 38.863C856.165 38.863 855.576 38.2736 855.576 37.5465Z",
fill: "url(#paint107_linear)"
}), Object(c.c)("path", {
d: "M821.353 37.5465C821.353 36.8194 821.942 36.2299 822.669 36.2299C823.396 36.2299 823.985 36.8194 823.985 37.5465C823.985 38.2736 823.396 38.863 822.669 38.863C821.942 38.863 821.353 38.2736 821.353 37.5465Z",
fill: "url(#paint108_linear)"
}), Object(c.c)("path", {
d: "M787.13 37.5464C787.13 36.8193 787.719 36.2299 788.446 36.2299C789.173 36.2299 789.762 36.8193 789.762 37.5465C789.762 38.2736 789.173 38.863 788.446 38.863C787.719 38.863 787.13 38.2736 787.13 37.5464Z",
fill: "url(#paint109_linear)"
}), Object(c.c)("path", {
d: "M752.907 37.5464C752.907 36.8193 753.496 36.2299 754.223 36.2299C754.95 36.2299 755.539 36.8193 755.539 37.5464C755.539 38.2735 754.95 38.863 754.223 38.863C753.496 38.863 752.907 38.2735 752.907 37.5464Z",
fill: "url(#paint110_linear)"
}), Object(c.c)("path", {
d: "M718.684 37.5464C718.684 36.8193 719.273 36.2299 720 36.2299C720.727 36.2299 721.316 36.8193 721.316 37.5464C721.316 38.2735 720.727 38.863 720 38.863C719.273 38.863 718.684 38.2735 718.684 37.5464Z",
fill: "url(#paint111_linear)"
}), Object(c.c)("path", {
d: "M684.461 37.5464C684.461 36.8193 685.05 36.2299 685.777 36.2299C686.504 36.2299 687.093 36.8193 687.093 37.5464C687.093 38.2735 686.504 38.863 685.777 38.863C685.05 38.863 684.461 38.2735 684.461 37.5464Z",
fill: "url(#paint112_linear)"
}), Object(c.c)("path", {
d: "M650.238 37.5464C650.238 36.8193 650.827 36.2299 651.554 36.2299C652.281 36.2299 652.87 36.8193 652.87 37.5464C652.87 38.2735 652.281 38.863 651.554 38.863C650.827 38.863 650.238 38.2735 650.238 37.5464Z",
fill: "url(#paint113_linear)"
}), Object(c.c)("path", {
d: "M616.015 37.5464C616.015 36.8193 616.604 36.2299 617.331 36.2299C618.058 36.2299 618.647 36.8193 618.647 37.5464C618.647 38.2735 618.058 38.863 617.331 38.863C616.604 38.863 616.015 38.2735 616.015 37.5464Z",
fill: "url(#paint114_linear)"
}), Object(c.c)("path", {
d: "M581.792 37.5464C581.792 36.8193 582.381 36.2299 583.108 36.2299C583.835 36.2299 584.424 36.8193 584.424 37.5464C584.424 38.2735 583.835 38.863 583.108 38.863C582.381 38.863 581.792 38.2735 581.792 37.5464Z",
fill: "url(#paint115_linear)"
}), Object(c.c)("path", {
d: "M547.569 37.5464C547.569 36.8193 548.158 36.2299 548.885 36.2299C549.612 36.2299 550.201 36.8193 550.201 37.5464C550.201 38.2735 549.612 38.863 548.885 38.863C548.158 38.863 547.569 38.2735 547.569 37.5464Z",
fill: "url(#paint116_linear)"
}), Object(c.c)("path", {
d: "M513.345 37.5464C513.345 36.8193 513.935 36.2299 514.662 36.2299C515.389 36.2299 515.978 36.8193 515.978 37.5464C515.978 38.2735 515.389 38.863 514.662 38.863C513.935 38.863 513.345 38.2735 513.345 37.5464Z",
fill: "url(#paint117_linear)"
}), Object(c.c)("path", {
d: "M479.123 37.5464C479.123 36.8193 479.712 36.2299 480.439 36.2299C481.166 36.2299 481.755 36.8193 481.755 37.5464C481.755 38.2735 481.166 38.863 480.439 38.863C479.712 38.863 479.123 38.2735 479.123 37.5464Z",
fill: "url(#paint118_linear)"
}), Object(c.c)("path", {
d: "M444.899 37.5464C444.899 36.8193 445.489 36.2299 446.216 36.2299C446.943 36.2299 447.532 36.8193 447.532 37.5464C447.532 38.2735 446.943 38.8629 446.216 38.8629C445.489 38.8629 444.899 38.2735 444.899 37.5464Z",
fill: "url(#paint119_linear)"
}), Object(c.c)("path", {
d: "M410.676 37.5464C410.676 36.8193 411.266 36.2299 411.993 36.2299C412.72 36.2299 413.309 36.8193 413.309 37.5464C413.309 38.2735 412.72 38.8629 411.993 38.8629C411.266 38.8629 410.676 38.2735 410.676 37.5464Z",
fill: "url(#paint120_linear)"
}), Object(c.c)("path", {
d: "M376.453 37.5464C376.453 36.8193 377.043 36.2299 377.77 36.2299C378.497 36.2299 379.086 36.8193 379.086 37.5464C379.086 38.2735 378.497 38.8629 377.77 38.8629C377.043 38.8629 376.453 38.2735 376.453 37.5464Z",
fill: "url(#paint121_linear)"
}), Object(c.c)("path", {
d: "M342.23 37.5464C342.23 36.8193 342.82 36.2299 343.547 36.2299C344.274 36.2299 344.863 36.8193 344.863 37.5464C344.863 38.2735 344.274 38.8629 343.547 38.8629C342.82 38.8629 342.23 38.2735 342.23 37.5464Z",
fill: "url(#paint122_linear)"
}), Object(c.c)("path", {
d: "M308.007 37.5464C308.007 36.8193 308.597 36.2299 309.324 36.2299C310.051 36.2299 310.64 36.8193 310.64 37.5464C310.64 38.2735 310.051 38.8629 309.324 38.8629C308.597 38.8629 308.007 38.2735 308.007 37.5464Z",
fill: "url(#paint123_linear)"
}), Object(c.c)("path", {
d: "M273.784 37.5464C273.784 36.8193 274.374 36.2299 275.101 36.2299C275.828 36.2299 276.417 36.8193 276.417 37.5464C276.417 38.2735 275.828 38.8629 275.101 38.8629C274.374 38.8629 273.784 38.2735 273.784 37.5464Z",
fill: "url(#paint124_linear)"
}), Object(c.c)("path", {
d: "M239.561 37.5464C239.561 36.8193 240.15 36.2299 240.877 36.2299C241.604 36.2299 242.194 36.8193 242.194 37.5464C242.194 38.2735 241.604 38.8629 240.877 38.8629C240.15 38.8629 239.561 38.2735 239.561 37.5464Z",
fill: "url(#paint125_linear)"
}), Object(c.c)("path", {
d: "M205.338 37.5464C205.338 36.8193 205.927 36.2299 206.654 36.2299C207.381 36.2299 207.971 36.8193 207.971 37.5464C207.971 38.2735 207.381 38.8629 206.654 38.8629C205.927 38.8629 205.338 38.2735 205.338 37.5464Z",
fill: "url(#paint126_linear)"
}), Object(c.c)("path", {
d: "M171.115 37.5464C171.115 36.8193 171.704 36.2299 172.431 36.2299C173.158 36.2299 173.748 36.8193 173.748 37.5464C173.748 38.2735 173.158 38.8629 172.431 38.8629C171.704 38.8629 171.115 38.2735 171.115 37.5464Z",
fill: "url(#paint127_linear)"
}), Object(c.c)("path", {
d: "M136.892 37.5464C136.892 36.8193 137.481 36.2299 138.208 36.2299C138.935 36.2299 139.525 36.8193 139.525 37.5464C139.525 38.2735 138.935 38.8629 138.208 38.8629C137.481 38.8629 136.892 38.2735 136.892 37.5464Z",
fill: "url(#paint128_linear)"
}), Object(c.c)("path", {
d: "M102.669 37.5464C102.669 36.8193 103.258 36.2299 103.985 36.2299C104.712 36.2299 105.302 36.8193 105.302 37.5464C105.302 38.2735 104.712 38.8629 103.985 38.8629C103.258 38.8629 102.669 38.2735 102.669 37.5464Z",
fill: "url(#paint129_linear)"
}), Object(c.c)("path", {
d: "M68.4461 37.5464C68.4461 36.8193 69.0354 36.2299 69.7623 36.2299C70.4893 36.2299 71.0786 36.8193 71.0786 37.5464C71.0786 38.2735 70.4893 38.8629 69.7623 38.8629C69.0354 38.8629 68.4461 38.2735 68.4461 37.5464Z",
fill: "url(#paint130_linear)"
}), Object(c.c)("path", {
d: "M34.223 37.5464C34.223 36.8193 34.8124 36.2298 35.5393 36.2298C36.2663 36.2298 36.8556 36.8193 36.8556 37.5464C36.8556 38.2735 36.2663 38.8629 35.5393 38.8629C34.8124 38.8629 34.223 38.2735 34.223 37.5464Z",
fill: "url(#paint131_linear)"
}), Object(c.c)("path", {
d: "M6.10003e-06 37.5464C6.16359e-06 36.8193 0.589334 36.2298 1.31629 36.2298C2.04325 36.2298 2.63257 36.8193 2.63257 37.5464C2.63257 38.2735 2.04325 38.8629 1.31629 38.8629C0.589334 38.8629 6.03646e-06 38.2735 6.10003e-06 37.5464Z",
fill: "url(#paint132_linear)"
}), Object(c.c)("path", {
d: "M1437.37 3.31666C1437.37 2.58956 1437.96 2.00014 1438.68 2.00014C1439.41 2.00014 1440 2.58956 1440 3.31666C1440 4.04376 1439.41 4.6332 1438.68 4.6332C1437.96 4.6332 1437.37 4.04376 1437.37 3.31666Z",
fill: "url(#paint133_linear)"
}), Object(c.c)("path", {
d: "M1403.14 3.31666C1403.14 2.58956 1403.73 2.00013 1404.46 2.00013C1405.19 2.00013 1405.78 2.58956 1405.78 3.31666C1405.78 4.04376 1405.19 4.6332 1404.46 4.6332C1403.73 4.6332 1403.14 4.04376 1403.14 3.31666Z",
fill: "url(#paint134_linear)"
}), Object(c.c)("path", {
d: "M1368.92 3.31666C1368.92 2.58956 1369.51 2.00013 1370.24 2.00013C1370.96 2.00013 1371.55 2.58956 1371.55 3.31666C1371.55 4.04376 1370.96 4.63319 1370.24 4.63319C1369.51 4.63319 1368.92 4.04376 1368.92 3.31666Z",
fill: "url(#paint135_linear)"
}), Object(c.c)("path", {
d: "M1334.7 3.31665C1334.7 2.58955 1335.29 2.00013 1336.01 2.00013C1336.74 2.00013 1337.33 2.58955 1337.33 3.31665C1337.33 4.04376 1336.74 4.63319 1336.01 4.63319C1335.29 4.63319 1334.7 4.04376 1334.7 3.31665Z",
fill: "url(#paint136_linear)"
}), Object(c.c)("path", {
d: "M1300.48 3.31665C1300.48 2.58955 1301.06 2.00012 1301.79 2.00012C1302.52 2.00012 1303.11 2.58955 1303.11 3.31665C1303.11 4.04375 1302.52 4.63319 1301.79 4.63319C1301.06 4.63319 1300.48 4.04375 1300.48 3.31665Z",
fill: "url(#paint137_linear)"
}), Object(c.c)("path", {
d: "M1266.25 3.31665C1266.25 2.58955 1266.84 2.00012 1267.57 2.00012C1268.3 2.00012 1268.88 2.58955 1268.88 3.31665C1268.88 4.04375 1268.3 4.63318 1267.57 4.63318C1266.84 4.63318 1266.25 4.04375 1266.25 3.31665Z",
fill: "url(#paint138_linear)"
}), Object(c.c)("path", {
d: "M1232.03 3.31665C1232.03 2.58955 1232.62 2.00012 1233.35 2.00012C1234.07 2.00012 1234.66 2.58955 1234.66 3.31665C1234.66 4.04375 1234.07 4.63318 1233.35 4.63318C1232.62 4.63318 1232.03 4.04375 1232.03 3.31665Z",
fill: "url(#paint139_linear)"
}), Object(c.c)("path", {
d: "M1197.81 3.31664C1197.81 2.58954 1198.4 2.00011 1199.12 2.00011C1199.85 2.00011 1200.44 2.58954 1200.44 3.31664C1200.44 4.04374 1199.85 4.63318 1199.12 4.63318C1198.4 4.63318 1197.81 4.04374 1197.81 3.31664Z",
fill: "url(#paint140_linear)"
}), Object(c.c)("path", {
d: "M1163.58 3.31664C1163.58 2.58954 1164.17 2.00011 1164.9 2.00011C1165.63 2.00011 1166.22 2.58954 1166.22 3.31664C1166.22 4.04374 1165.63 4.63318 1164.9 4.63318C1164.17 4.63318 1163.58 4.04374 1163.58 3.31664Z",
fill: "url(#paint141_linear)"
}), Object(c.c)("path", {
d: "M1129.36 3.31664C1129.36 2.58954 1129.95 2.00011 1130.68 2.00011C1131.4 2.00011 1131.99 2.58954 1131.99 3.31664C1131.99 4.04374 1131.4 4.63317 1130.68 4.63317C1129.95 4.63317 1129.36 4.04374 1129.36 3.31664Z",
fill: "url(#paint142_linear)"
}), Object(c.c)("path", {
d: "M1095.14 3.31663C1095.14 2.58953 1095.73 2.00011 1096.45 2.00011C1097.18 2.00011 1097.77 2.58953 1097.77 3.31663C1097.77 4.04373 1097.18 4.63317 1096.45 4.63317C1095.73 4.63317 1095.14 4.04373 1095.14 3.31663Z",
fill: "url(#paint143_linear)"
}), Object(c.c)("path", {
d: "M1060.91 3.31663C1060.91 2.58953 1061.5 2.0001 1062.23 2.0001C1062.96 2.0001 1063.55 2.58953 1063.55 3.31663C1063.55 4.04373 1062.96 4.63317 1062.23 4.63317C1061.5 4.63317 1060.91 4.04373 1060.91 3.31663Z",
fill: "url(#paint144_linear)"
}), Object(c.c)("path", {
d: "M1026.69 3.31663C1026.69 2.58953 1027.28 2.0001 1028.01 2.0001C1028.73 2.0001 1029.32 2.58953 1029.32 3.31663C1029.32 4.04373 1028.73 4.63316 1028.01 4.63316C1027.28 4.63316 1026.69 4.04373 1026.69 3.31663Z",
fill: "url(#paint145_linear)"
}), Object(c.c)("path", {
d: "M992.468 3.31662C992.468 2.58952 993.057 2.0001 993.784 2.0001C994.511 2.0001 995.101 2.58952 995.101 3.31663C995.101 4.04373 994.511 4.63316 993.784 4.63316C993.057 4.63316 992.468 4.04373 992.468 3.31662Z",
fill: "url(#paint146_linear)"
}), Object(c.c)("path", {
d: "M958.245 3.31662C958.245 2.58952 958.834 2.00009 959.561 2.00009C960.288 2.00009 960.878 2.58952 960.878 3.31662C960.878 4.04372 960.288 4.63316 959.561 4.63316C958.834 4.63316 958.245 4.04372 958.245 3.31662Z",
fill: "url(#paint147_linear)"
}), Object(c.c)("path", {
d: "M924.022 3.31662C924.022 2.58952 924.611 2.00009 925.338 2.00009C926.065 2.00009 926.654 2.58952 926.654 3.31662C926.654 4.04372 926.065 4.63315 925.338 4.63315C924.611 4.63315 924.022 4.04372 924.022 3.31662Z",
fill: "url(#paint148_linear)"
}), Object(c.c)("path", {
d: "M889.799 3.31662C889.799 2.58952 890.388 2.00009 891.115 2.00009C891.842 2.00009 892.431 2.58952 892.431 3.31662C892.431 4.04372 891.842 4.63315 891.115 4.63315C890.388 4.63315 889.799 4.04372 889.799 3.31662Z",
fill: "url(#paint149_linear)"
}), Object(c.c)("path", {
d: "M855.576 3.31661C855.576 2.58951 856.165 2.00008 856.892 2.00008C857.619 2.00008 858.208 2.58951 858.208 3.31661C858.208 4.04371 857.619 4.63315 856.892 4.63315C856.165 4.63315 855.576 4.04371 855.576 3.31661Z",
fill: "url(#paint150_linear)"
}), Object(c.c)("path", {
d: "M821.353 3.31661C821.353 2.58951 821.942 2.00008 822.669 2.00008C823.396 2.00008 823.985 2.58951 823.985 3.31661C823.985 4.04371 823.396 4.63315 822.669 4.63315C821.942 4.63315 821.353 4.04371 821.353 3.31661Z",
fill: "url(#paint151_linear)"
}), Object(c.c)("path", {
d: "M787.13 3.31661C787.13 2.58951 787.719 2.00008 788.446 2.00008C789.173 2.00008 789.762 2.58951 789.762 3.31661C789.762 4.04371 789.173 4.63314 788.446 4.63314C787.719 4.63314 787.13 4.04371 787.13 3.31661Z",
fill: "url(#paint152_linear)"
}), Object(c.c)("path", {
d: "M752.907 3.3166C752.907 2.5895 753.496 2.00008 754.223 2.00008C754.95 2.00008 755.539 2.5895 755.539 3.3166C755.539 4.0437 754.95 4.63314 754.223 4.63314C753.496 4.63314 752.907 4.0437 752.907 3.3166Z",
fill: "url(#paint153_linear)"
}), Object(c.c)("path", {
d: "M718.684 3.3166C718.684 2.5895 719.273 2.00007 720 2.00007C720.727 2.00007 721.316 2.5895 721.316 3.3166C721.316 4.0437 720.727 4.63314 720 4.63314C719.273 4.63314 718.684 4.0437 718.684 3.3166Z",
fill: "url(#paint154_linear)"
}), Object(c.c)("path", {
d: "M684.461 3.3166C684.461 2.5895 685.05 2.00007 685.777 2.00007C686.504 2.00007 687.093 2.5895 687.093 3.3166C687.093 4.0437 686.504 4.63313 685.777 4.63313C685.05 4.63313 684.461 4.0437 684.461 3.3166Z",
fill: "url(#paint155_linear)"
}), Object(c.c)("path", {
d: "M650.238 3.31659C650.238 2.58949 650.827 2.00007 651.554 2.00007C652.281 2.00007 652.87 2.58949 652.87 3.3166C652.87 4.0437 652.281 4.63313 651.554 4.63313C650.827 4.63313 650.238 4.0437 650.238 3.31659Z",
fill: "url(#paint156_linear)"
}), Object(c.c)("path", {
d: "M616.015 3.31659C616.015 2.58949 616.604 2.00006 617.331 2.00006C618.058 2.00006 618.647 2.58949 618.647 3.31659C618.647 4.04369 618.058 4.63313 617.331 4.63313C616.604 4.63313 616.015 4.04369 616.015 3.31659Z",
fill: "url(#paint157_linear)"
}), Object(c.c)("path", {
d: "M581.792 3.31659C581.792 2.58949 582.381 2.00006 583.108 2.00006C583.835 2.00006 584.424 2.58949 584.424 3.31659C584.424 4.04369 583.835 4.63313 583.108 4.63312C582.381 4.63312 581.792 4.04369 581.792 3.31659Z",
fill: "url(#paint158_linear)"
}), Object(c.c)("path", {
d: "M547.569 3.31659C547.569 2.58949 548.158 2.00006 548.885 2.00006C549.612 2.00006 550.201 2.58949 550.201 3.31659C550.201 4.04369 549.612 4.63312 548.885 4.63312C548.158 4.63312 547.569 4.04369 547.569 3.31659Z",
fill: "url(#paint159_linear)"
}), Object(c.c)("path", {
d: "M513.345 3.31658C513.345 2.58948 513.935 2.00005 514.662 2.00005C515.389 2.00005 515.978 2.58948 515.978 3.31658C515.978 4.04368 515.389 4.63312 514.662 4.63312C513.935 4.63312 513.345 4.04368 513.345 3.31658Z",
fill: "url(#paint160_linear)"
}), Object(c.c)("path", {
d: "M479.123 3.31658C479.123 2.58948 479.712 2.00005 480.439 2.00005C481.166 2.00005 481.755 2.58948 481.755 3.31658C481.755 4.04368 481.166 4.63312 480.439 4.63312C479.712 4.63312 479.123 4.04368 479.123 3.31658Z",
fill: "url(#paint161_linear)"
}), Object(c.c)("path", {
d: "M444.899 3.31658C444.899 2.58948 445.489 2.00005 446.216 2.00005C446.943 2.00005 447.532 2.58948 447.532 3.31658C447.532 4.04368 446.943 4.63311 446.216 4.63311C445.489 4.63311 444.899 4.04368 444.899 3.31658Z",
fill: "url(#paint162_linear)"
}), Object(c.c)("path", {
d: "M410.676 3.31657C410.676 2.58947 411.266 2.00005 411.993 2.00005C412.72 2.00005 413.309 2.58947 413.309 3.31657C413.309 4.04367 412.72 4.63311 411.993 4.63311C411.266 4.63311 410.676 4.04367 410.676 3.31657Z",
fill: "url(#paint163_linear)"
}), Object(c.c)("path", {
d: "M376.453 3.31657C376.453 2.58947 377.043 2.00004 377.77 2.00004C378.497 2.00004 379.086 2.58947 379.086 3.31657C379.086 4.04367 378.497 4.63311 377.77 4.63311C377.043 4.63311 376.453 4.04367 376.453 3.31657Z",
fill: "url(#paint164_linear)"
}), Object(c.c)("path", {
d: "M342.23 3.31657C342.23 2.58947 342.82 2.00004 343.547 2.00004C344.274 2.00004 344.863 2.58947 344.863 3.31657C344.863 4.04367 344.274 4.6331 343.547 4.6331C342.82 4.6331 342.23 4.04367 342.23 3.31657Z",
fill: "url(#paint165_linear)"
}), Object(c.c)("path", {
d: "M308.007 3.31656C308.007 2.58946 308.597 2.00004 309.324 2.00004C310.051 2.00004 310.64 2.58946 310.64 3.31657C310.64 4.04367 310.051 4.6331 309.324 4.6331C308.597 4.6331 308.007 4.04367 308.007 3.31656Z",
fill: "url(#paint166_linear)"
}), Object(c.c)("path", {
d: "M273.784 3.31656C273.784 2.58946 274.374 2.00003 275.101 2.00003C275.828 2.00003 276.417 2.58946 276.417 3.31656C276.417 4.04366 275.828 4.6331 275.101 4.6331C274.374 4.6331 273.784 4.04366 273.784 3.31656Z",
fill: "url(#paint167_linear)"
}), Object(c.c)("path", {
d: "M239.561 3.31656C239.561 2.58946 240.15 2.00003 240.877 2.00003C241.604 2.00003 242.194 2.58946 242.194 3.31656C242.194 4.04366 241.604 4.6331 240.877 4.6331C240.15 4.63309 239.561 4.04366 239.561 3.31656Z",
fill: "url(#paint168_linear)"
}), Object(c.c)("path", {
d: "M205.338 3.31656C205.338 2.58946 205.927 2.00003 206.654 2.00003C207.381 2.00003 207.971 2.58946 207.971 3.31656C207.971 4.04366 207.381 4.63309 206.654 4.63309C205.927 4.63309 205.338 4.04366 205.338 3.31656Z",
fill: "url(#paint169_linear)"
}), Object(c.c)("path", {
d: "M171.115 3.31655C171.115 2.58945 171.704 2.00002 172.431 2.00002C173.158 2.00002 173.748 2.58945 173.748 3.31655C173.748 4.04365 173.158 4.63309 172.431 4.63309C171.704 4.63309 171.115 4.04365 171.115 3.31655Z",
fill: "url(#paint170_linear)"
}), Object(c.c)("path", {
d: "M136.892 3.31655C136.892 2.58945 137.481 2.00002 138.208 2.00002C138.935 2.00002 139.525 2.58945 139.525 3.31655C139.525 4.04365 138.935 4.63309 138.208 4.63309C137.481 4.63309 136.892 4.04365 136.892 3.31655Z",
fill: "url(#paint171_linear)"
}), Object(c.c)("path", {
d: "M102.669 3.31655C102.669 2.58945 103.258 2.00002 103.985 2.00002C104.712 2.00002 105.302 2.58945 105.302 3.31655C105.302 4.04365 104.712 4.63308 103.985 4.63308C103.258 4.63308 102.669 4.04365 102.669 3.31655Z",
fill: "url(#paint172_linear)"
}), Object(c.c)("path", {
d: "M68.4461 3.31654C68.4461 2.58944 69.0354 2.00002 69.7623 2.00002C70.4893 2.00002 71.0786 2.58944 71.0786 3.31654C71.0786 4.04364 70.4893 4.63308 69.7623 4.63308C69.0354 4.63308 68.4461 4.04364 68.4461 3.31654Z",
fill: "url(#paint173_linear)"
}), Object(c.c)("path", {
d: "M34.223 3.31654C34.223 2.58944 34.8124 2.00001 35.5393 2.00001C36.2663 2.00001 36.8556 2.58944 36.8556 3.31654C36.8556 4.04364 36.2663 4.63308 35.5393 4.63308C34.8124 4.63308 34.223 4.04364 34.223 3.31654Z",
fill: "url(#paint174_linear)"
}), Object(c.c)("path", {
d: "M9.0925e-06 3.31654C9.15606e-06 2.58944 0.589337 2.00001 1.31629 2.00001C2.04325 2.00001 2.63258 2.58944 2.63258 3.31654C2.63258 4.04364 2.04325 4.63307 1.31629 4.63307C0.589337 4.63307 9.02893e-06 4.04364 9.0925e-06 3.31654Z",
fill: "url(#paint175_linear)"
}), Object(c.c)("path", {
d: "M1507.37 106.006C1507.37 105.279 1507.96 104.69 1508.68 104.69C1509.41 104.69 1510 105.279 1510 106.006C1510 106.733 1509.41 107.323 1508.68 107.323C1507.96 107.323 1507.37 106.733 1507.37 106.006Z",
fill: "url(#paint176_linear)"
}), Object(c.c)("path", {
d: "M1473.14 106.006C1473.14 105.279 1473.73 104.69 1474.46 104.69C1475.19 104.69 1475.78 105.279 1475.78 106.006C1475.78 106.733 1475.19 107.323 1474.46 107.323C1473.73 107.323 1473.14 106.733 1473.14 106.006Z",
fill: "url(#paint177_linear)"
}), Object(c.c)("path", {
d: "M1507.37 71.7763C1507.37 71.0492 1507.96 70.4598 1508.68 70.4598C1509.41 70.4598 1510 71.0492 1510 71.7763C1510 72.5034 1509.41 73.0929 1508.68 73.0929C1507.96 73.0929 1507.37 72.5034 1507.37 71.7763Z",
fill: "url(#paint178_linear)"
}), Object(c.c)("path", {
d: "M1473.14 71.7763C1473.14 71.0492 1473.73 70.4598 1474.46 70.4598C1475.19 70.4598 1475.78 71.0492 1475.78 71.7763C1475.78 72.5034 1475.19 73.0929 1474.46 73.0929C1473.73 73.0929 1473.14 72.5034 1473.14 71.7763Z",
fill: "url(#paint179_linear)"
}), Object(c.c)("path", {
d: "M1507.37 37.5465C1507.37 36.8194 1507.96 36.23 1508.68 36.23C1509.41 36.23 1510 36.8194 1510 37.5465C1510 38.2736 1509.41 38.863 1508.68 38.863C1507.96 38.863 1507.37 38.2736 1507.37 37.5465Z",
fill: "url(#paint180_linear)"
}), Object(c.c)("path", {
d: "M1473.14 37.5465C1473.14 36.8194 1473.73 36.23 1474.46 36.23C1475.19 36.23 1475.78 36.8194 1475.78 37.5465C1475.78 38.2736 1475.19 38.863 1474.46 38.863C1473.73 38.863 1473.14 38.2736 1473.14 37.5465Z",
fill: "url(#paint181_linear)"
}), Object(c.c)("path", {
d: "M1507.37 3.31667C1507.37 2.58957 1507.96 2.00014 1508.68 2.00014C1509.41 2.00014 1510 2.58957 1510 3.31667C1510 4.04377 1509.41 4.63321 1508.68 4.63321C1507.96 4.63321 1507.37 4.04377 1507.37 3.31667Z",
fill: "url(#paint182_linear)"
}), Object(c.c)("path", {
d: "M1473.14 3.31667C1473.14 2.58957 1473.73 2.00014 1474.46 2.00014C1475.19 2.00014 1475.78 2.58957 1475.78 3.31667C1475.78 4.04377 1475.19 4.6332 1474.46 4.6332C1473.73 4.6332 1473.14 4.04377 1473.14 3.31667Z",
fill: "url(#paint183_linear)"
}), Object(c.c)("path", {
d: "M1577.37 106.006C1577.37 105.279 1577.96 104.69 1578.68 104.69C1579.41 104.69 1580 105.279 1580 106.006C1580 106.733 1579.41 107.323 1578.68 107.323C1577.96 107.323 1577.37 106.733 1577.37 106.006Z",
fill: "url(#paint184_linear)"
}), Object(c.c)("path", {
d: "M1543.14 106.006C1543.14 105.279 1543.73 104.69 1544.46 104.69C1545.19 104.69 1545.78 105.279 1545.78 106.006C1545.78 106.733 1545.19 107.323 1544.46 107.323C1543.73 107.323 1543.14 106.733 1543.14 106.006Z",
fill: "url(#paint185_linear)"
}), Object(c.c)("path", {
d: "M1577.37 71.7764C1577.37 71.0493 1577.96 70.4598 1578.68 70.4598C1579.41 70.4598 1580 71.0493 1580 71.7764C1580 72.5035 1579.41 73.0929 1578.68 73.0929C1577.96 73.0929 1577.37 72.5035 1577.37 71.7764Z",
fill: "url(#paint186_linear)"
}), Object(c.c)("path", {
d: "M1543.14 71.7764C1543.14 71.0493 1543.73 70.4598 1544.46 70.4598C1545.19 70.4598 1545.78 71.0493 1545.78 71.7764C1545.78 72.5035 1545.19 73.0929 1544.46 73.0929C1543.73 73.0929 1543.14 72.5035 1543.14 71.7764Z",
fill: "url(#paint187_linear)"
}), Object(c.c)("path", {
d: "M1577.37 37.5465C1577.37 36.8194 1577.96 36.23 1578.68 36.23C1579.41 36.23 1580 36.8194 1580 37.5465C1580 38.2736 1579.41 38.863 1578.68 38.863C1577.96 38.863 1577.37 38.2736 1577.37 37.5465Z",
fill: "url(#paint188_linear)"
}), Object(c.c)("path", {
d: "M1543.14 37.5465C1543.14 36.8194 1543.73 36.23 1544.46 36.23C1545.19 36.23 1545.78 36.8194 1545.78 37.5465C1545.78 38.2736 1545.19 38.863 1544.46 38.863C1543.73 38.863 1543.14 38.2736 1543.14 37.5465Z",
fill: "url(#paint189_linear)"
}), Object(c.c)("path", {
d: "M1577.37 3.31668C1577.37 2.58958 1577.96 2.00015 1578.68 2.00015C1579.41 2.00015 1580 2.58958 1580 3.31668C1580 4.04378 1579.41 4.63321 1578.68 4.63321C1577.96 4.63321 1577.37 4.04378 1577.37 3.31668Z",
fill: "url(#paint190_linear)"
}), Object(c.c)("path", {
d: "M1543.14 3.31667C1543.14 2.58957 1543.73 2.00014 1544.46 2.00014C1545.19 2.00014 1545.78 2.58957 1545.78 3.31667C1545.78 4.04377 1545.19 4.63321 1544.46 4.63321C1543.73 4.63321 1543.14 4.04377 1543.14 3.31667Z",
fill: "url(#paint191_linear)"
}), Object(c.c)("path", {
d: "M1647.37 106.006C1647.37 105.279 1647.96 104.69 1648.68 104.69C1649.41 104.69 1650 105.279 1650 106.006C1650 106.733 1649.41 107.323 1648.68 107.323C1647.96 107.323 1647.37 106.733 1647.37 106.006Z",
fill: "url(#paint192_linear)"
}), Object(c.c)("path", {
d: "M1613.14 106.006C1613.14 105.279 1613.73 104.69 1614.46 104.69C1615.19 104.69 1615.78 105.279 1615.78 106.006C1615.78 106.733 1615.19 107.323 1614.46 107.323C1613.73 107.323 1613.14 106.733 1613.14 106.006Z",
fill: "url(#paint193_linear)"
}), Object(c.c)("path", {
d: "M1647.37 71.7764C1647.37 71.0493 1647.96 70.4598 1648.68 70.4598C1649.41 70.4598 1650 71.0493 1650 71.7764C1650 72.5035 1649.41 73.0929 1648.68 73.0929C1647.96 73.0929 1647.37 72.5035 1647.37 71.7764Z",
fill: "url(#paint194_linear)"
}), Object(c.c)("path", {
d: "M1613.14 71.7764C1613.14 71.0493 1613.73 70.4598 1614.46 70.4598C1615.19 70.4598 1615.78 71.0493 1615.78 71.7764C1615.78 72.5035 1615.19 73.0929 1614.46 73.0929C1613.73 73.0929 1613.14 72.5035 1613.14 71.7764Z",
fill: "url(#paint195_linear)"
}), Object(c.c)("path", {
d: "M1647.37 37.5465C1647.37 36.8194 1647.96 36.23 1648.68 36.23C1649.41 36.23 1650 36.8194 1650 37.5465C1650 38.2736 1649.41 38.8631 1648.68 38.8631C1647.96 38.8631 1647.37 38.2736 1647.37 37.5465Z",
fill: "url(#paint196_linear)"
}), Object(c.c)("path", {
d: "M1613.14 37.5465C1613.14 36.8194 1613.73 36.23 1614.46 36.23C1615.19 36.23 1615.78 36.8194 1615.78 37.5465C1615.78 38.2736 1615.19 38.8631 1614.46 38.8631C1613.73 38.8631 1613.14 38.2736 1613.14 37.5465Z",
fill: "url(#paint197_linear)"
}), Object(c.c)("path", {
d: "M1647.37 3.31668C1647.37 2.58958 1647.96 2.00015 1648.68 2.00015C1649.41 2.00015 1650 2.58958 1650 3.31668C1650 4.04378 1649.41 4.63322 1648.68 4.63322C1647.96 4.63322 1647.37 4.04378 1647.37 3.31668Z",
fill: "url(#paint198_linear)"
}), Object(c.c)("path", {
d: "M1613.14 3.31668C1613.14 2.58958 1613.73 2.00015 1614.46 2.00015C1615.19 2.00015 1615.78 2.58958 1615.78 3.31668C1615.78 4.04378 1615.19 4.63322 1614.46 4.63322C1613.73 4.63322 1613.14 4.04378 1613.14 3.31668Z",
fill: "url(#paint199_linear)"
}), Object(c.c)("path", {
d: "M1717.37 106.006C1717.37 105.279 1717.96 104.69 1718.68 104.69C1719.41 104.69 1720 105.279 1720 106.006C1720 106.733 1719.41 107.323 1718.68 107.323C1717.96 107.323 1717.37 106.733 1717.37 106.006Z",
fill: "url(#paint200_linear)"
}), Object(c.c)("path", {
d: "M1683.14 106.006C1683.14 105.279 1683.73 104.69 1684.46 104.69C1685.19 104.69 1685.78 105.279 1685.78 106.006C1685.78 106.733 1685.19 107.323 1684.46 107.323C1683.73 107.323 1683.14 106.733 1683.14 106.006Z",
fill: "url(#paint201_linear)"
}), Object(c.c)("path", {
d: "M1717.37 71.7764C1717.37 71.0493 1717.96 70.4598 1718.68 70.4598C1719.41 70.4598 1720 71.0493 1720 71.7764C1720 72.5035 1719.41 73.0929 1718.68 73.0929C1717.96 73.0929 1717.37 72.5035 1717.37 71.7764Z",
fill: "url(#paint202_linear)"
}), Object(c.c)("path", {
d: "M1683.14 71.7764C1683.14 71.0493 1683.73 70.4598 1684.46 70.4598C1685.19 70.4598 1685.78 71.0493 1685.78 71.7764C1685.78 72.5035 1685.19 73.0929 1684.46 73.0929C1683.73 73.0929 1683.14 72.5035 1683.14 71.7764Z",
fill: "url(#paint203_linear)"
}), Object(c.c)("path", {
d: "M1717.37 37.5465C1717.37 36.8194 1717.96 36.23 1718.68 36.23C1719.41 36.23 1720 36.8194 1720 37.5465C1720 38.2736 1719.41 38.8631 1718.68 38.8631C1717.96 38.8631 1717.37 38.2736 1717.37 37.5465Z",
fill: "url(#paint204_linear)"
}), Object(c.c)("path", {
d: "M1683.14 37.5465C1683.14 36.8194 1683.73 36.23 1684.46 36.23C1685.19 36.23 1685.78 36.8194 1685.78 37.5465C1685.78 38.2736 1685.19 38.8631 1684.46 38.8631C1683.73 38.8631 1683.14 38.2736 1683.14 37.5465Z",
fill: "url(#paint205_linear)"
}), Object(c.c)("path", {
d: "M1717.37 3.31669C1717.37 2.58959 1717.96 2.00016 1718.68 2.00016C1719.41 2.00016 1720 2.58959 1720 3.31669C1720 4.04379 1719.41 4.63322 1718.68 4.63322C1717.96 4.63322 1717.37 4.04379 1717.37 3.31669Z",
fill: "url(#paint206_linear)"
}), Object(c.c)("path", {
d: "M1683.14 3.31669C1683.14 2.58958 1683.73 2.00016 1684.46 2.00016C1685.19 2.00016 1685.78 2.58958 1685.78 3.31669C1685.78 4.04379 1685.19 4.63322 1684.46 4.63322C1683.73 4.63322 1683.14 4.04379 1683.14 3.31669Z",
fill: "url(#paint207_linear)"
}), Object(c.c)("path", {
d: "M1787.37 106.006C1787.37 105.279 1787.96 104.69 1788.68 104.69C1789.41 104.69 1790 105.279 1790 106.006C1790 106.733 1789.41 107.323 1788.68 107.323C1787.96 107.323 1787.37 106.733 1787.37 106.006Z",
fill: "url(#paint208_linear)"
}), Object(c.c)("path", {
d: "M1753.14 106.006C1753.14 105.279 1753.73 104.69 1754.46 104.69C1755.19 104.69 1755.78 105.279 1755.78 106.006C1755.78 106.733 1755.19 107.323 1754.46 107.323C1753.73 107.323 1753.14 106.733 1753.14 106.006Z",
fill: "url(#paint209_linear)"
}), Object(c.c)("path", {
d: "M1787.37 71.7764C1787.37 71.0493 1787.96 70.4598 1788.68 70.4598C1789.41 70.4598 1790 71.0493 1790 71.7764C1790 72.5035 1789.41 73.0929 1788.68 73.0929C1787.96 73.0929 1787.37 72.5035 1787.37 71.7764Z",
fill: "url(#paint210_linear)"
}), Object(c.c)("path", {
d: "M1753.14 71.7764C1753.14 71.0493 1753.73 70.4598 1754.46 70.4598C1755.19 70.4598 1755.78 71.0493 1755.78 71.7764C1755.78 72.5035 1755.19 73.0929 1754.46 73.0929C1753.73 73.0929 1753.14 72.5035 1753.14 71.7764Z",
fill: "url(#paint211_linear)"
}), Object(c.c)("path", {
d: "M1787.37 37.5465C1787.37 36.8194 1787.96 36.23 1788.68 36.23C1789.41 36.23 1790 36.8194 1790 37.5465C1790 38.2736 1789.41 38.8631 1788.68 38.8631C1787.96 38.8631 1787.37 38.2736 1787.37 37.5465Z",
fill: "url(#paint212_linear)"
}), Object(c.c)("path", {
d: "M1753.14 37.5465C1753.14 36.8194 1753.73 36.23 1754.46 36.23C1755.19 36.23 1755.78 36.8194 1755.78 37.5465C1755.78 38.2736 1755.19 38.8631 1754.46 38.8631C1753.73 38.8631 1753.14 38.2736 1753.14 37.5465Z",
fill: "url(#paint213_linear)"
}), Object(c.c)("path", {
d: "M1787.37 3.31669C1787.37 2.58959 1787.96 2.00017 1788.68 2.00017C1789.41 2.00017 1790 2.58959 1790 3.31669C1790 4.0438 1789.41 4.63323 1788.68 4.63323C1787.96 4.63323 1787.37 4.0438 1787.37 3.31669Z",
fill: "url(#paint214_linear)"
}), Object(c.c)("path", {
d: "M1753.14 3.31669C1753.14 2.58959 1753.73 2.00016 1754.46 2.00016C1755.19 2.00016 1755.78 2.58959 1755.78 3.31669C1755.78 4.04379 1755.19 4.63323 1754.46 4.63323C1753.73 4.63323 1753.14 4.04379 1753.14 3.31669Z",
fill: "url(#paint215_linear)"
}), Object(c.c)("path", {
d: "M1823.37 106.006C1823.37 105.279 1823.96 104.69 1824.68 104.69C1825.41 104.69 1826 105.279 1826 106.006C1826 106.733 1825.41 107.323 1824.68 107.323C1823.96 107.323 1823.37 106.733 1823.37 106.006Z",
fill: "url(#paint216_linear)"
}), Object(c.c)("path", {
d: "M1823.37 71.7764C1823.37 71.0493 1823.96 70.4598 1824.68 70.4598C1825.41 70.4598 1826 71.0493 1826 71.7764C1826 72.5035 1825.41 73.0929 1824.68 73.0929C1823.96 73.0929 1823.37 72.5035 1823.37 71.7764Z",
fill: "url(#paint217_linear)"
}), Object(c.c)("path", {
d: "M1823.37 37.5465C1823.37 36.8194 1823.96 36.23 1824.68 36.23C1825.41 36.23 1826 36.8194 1826 37.5465C1826 38.2736 1825.41 38.8631 1824.68 38.8631C1823.96 38.8631 1823.37 38.2736 1823.37 37.5465Z",
fill: "url(#paint218_linear)"
}), Object(c.c)("path", {
d: "M1823.37 3.3167C1823.37 2.5896 1823.96 2.00017 1824.68 2.00017C1825.41 2.00017 1826 2.5896 1826 3.3167C1826 4.0438 1825.41 4.63323 1824.68 4.63323C1823.96 4.63323 1823.37 4.0438 1823.37 3.3167Z",
fill: "url(#paint219_linear)"
}), Object(c.c)("path", {
d: "M1893.37 106.006C1893.37 105.279 1893.96 104.69 1894.68 104.69C1895.41 104.69 1896 105.279 1896 106.006C1896 106.733 1895.41 107.323 1894.68 107.323C1893.96 107.323 1893.37 106.733 1893.37 106.006Z",
fill: "url(#paint220_linear)"
}), Object(c.c)("path", {
d: "M1859.14 106.006C1859.14 105.279 1859.73 104.69 1860.46 104.69C1861.19 104.69 1861.78 105.279 1861.78 106.006C1861.78 106.733 1861.19 107.323 1860.46 107.323C1859.73 107.323 1859.14 106.733 1859.14 106.006Z",
fill: "url(#paint221_linear)"
}), Object(c.c)("path", {
d: "M1893.37 71.7764C1893.37 71.0493 1893.96 70.4599 1894.68 70.4599C1895.41 70.4599 1896 71.0493 1896 71.7764C1896 72.5035 1895.41 73.0929 1894.68 73.0929C1893.96 73.0929 1893.37 72.5035 1893.37 71.7764Z",
fill: "url(#paint222_linear)"
}), Object(c.c)("path", {
d: "M1859.14 71.7764C1859.14 71.0493 1859.73 70.4598 1860.46 70.4598C1861.19 70.4598 1861.78 71.0493 1861.78 71.7764C1861.78 72.5035 1861.19 73.0929 1860.46 73.0929C1859.73 73.0929 1859.14 72.5035 1859.14 71.7764Z",
fill: "url(#paint223_linear)"
}), Object(c.c)("path", {
d: "M1893.37 37.5465C1893.37 36.8194 1893.96 36.23 1894.68 36.23C1895.41 36.23 1896 36.8194 1896 37.5465C1896 38.2736 1895.41 38.8631 1894.68 38.8631C1893.96 38.8631 1893.37 38.2736 1893.37 37.5465Z",
fill: "url(#paint224_linear)"
}), Object(c.c)("path", {
d: "M1859.14 37.5465C1859.14 36.8194 1859.73 36.23 1860.46 36.23C1861.19 36.23 1861.78 36.8194 1861.78 37.5465C1861.78 38.2736 1861.19 38.8631 1860.46 38.8631C1859.73 38.8631 1859.14 38.2736 1859.14 37.5465Z",
fill: "url(#paint225_linear)"
}), Object(c.c)("path", {
d: "M1893.37 3.3167C1893.37 2.5896 1893.96 2.00018 1894.68 2.00018C1895.41 2.00018 1896 2.5896 1896 3.3167C1896 4.0438 1895.41 4.63324 1894.68 4.63324C1893.96 4.63324 1893.37 4.0438 1893.37 3.3167Z",
fill: "url(#paint226_linear)"
}), Object(c.c)("path", {
d: "M1859.14 3.3167C1859.14 2.5896 1859.73 2.00017 1860.46 2.00017C1861.19 2.00017 1861.78 2.5896 1861.78 3.3167C1861.78 4.0438 1861.19 4.63324 1860.46 4.63324C1859.73 4.63324 1859.14 4.0438 1859.14 3.3167Z",
fill: "url(#paint227_linear)"
}))), Object(c.c)("defs", null, Object(c.c)("linearGradient", {
id: "paint0_linear",
x1: "889",
y1: "108.086",
x2: "1022.77",
y2: "9.46871",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: u
}), Object(c.c)("stop", {
offset: "1",
stopColor: d
})), Object(c.c)("linearGradient", {
id: "paint1_linear",
x1: "581",
y1: "39.5862",
x2: "715.226",
y2: "-140.336",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: u
}), Object(c.c)("stop", {
offset: "1",
stopColor: d
})), Object(c.c)("linearGradient", {
id: "paint2_linear",
x1: "1197",
y1: "73.8362",
x2: "1322.18",
y2: "-3.43825",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: f
}), Object(c.c)("stop", {
offset: "1",
stopColor: b
})), Object(c.c)("linearGradient", {
id: "paint3_linear",
x1: "342",
y1: "73.8362",
x2: "468.546",
y2: "-126.145",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: f
}), Object(c.c)("stop", {
offset: "1",
stopColor: b
})), Object(c.c)("linearGradient", {
id: "paint4_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint5_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint6_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint7_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint8_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint9_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint10_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint11_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint12_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint13_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint14_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint15_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint16_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint17_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint18_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint19_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint20_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint21_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint22_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint23_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint24_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint25_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint26_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint27_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint28_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint29_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint30_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint31_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint32_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint33_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint34_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint35_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint36_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint37_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint38_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint39_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint40_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint41_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint42_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint43_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint44_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint45_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint46_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint47_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint48_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint49_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint50_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint51_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint52_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint53_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint54_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint55_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint56_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint57_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint58_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint59_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint60_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint61_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint62_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint63_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint64_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint65_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint66_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint67_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint68_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint69_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint70_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint71_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint72_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint73_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint74_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint75_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint76_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint77_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint78_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint79_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint80_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint81_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint82_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint83_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint84_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint85_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint86_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint87_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint88_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint89_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint90_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint91_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint92_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint93_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint94_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint95_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint96_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint97_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint98_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint99_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint100_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint101_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint102_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint103_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint104_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint105_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint106_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint107_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint108_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint109_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint110_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint111_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint112_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint113_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint114_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint115_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint116_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint117_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint118_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint119_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint120_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint121_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint122_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint123_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint124_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint125_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint126_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint127_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint128_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint129_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint130_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint131_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint132_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint133_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint134_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint135_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint136_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint137_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint138_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint139_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint140_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint141_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint142_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint143_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint144_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint145_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint146_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint147_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint148_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint149_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint150_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint151_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint152_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint153_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint154_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint155_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint156_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint157_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint158_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint159_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint160_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint161_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint162_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint163_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint164_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint165_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint166_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint167_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint168_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint169_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint170_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint171_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint172_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint173_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint174_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint175_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint176_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint177_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint178_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint179_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint180_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint181_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint182_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint183_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint184_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint185_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint186_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint187_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint188_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint189_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint190_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint191_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint192_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint193_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint194_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint195_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint196_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint197_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint198_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint199_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint200_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint201_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint202_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint203_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint204_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint205_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint206_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint207_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint208_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint209_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint210_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint211_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint212_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint213_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint214_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint215_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint216_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint217_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint218_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint219_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint220_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint221_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint222_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint223_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint224_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint225_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint226_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("linearGradient", {
id: "paint227_linear",
x1: "720.001",
y1: "107.323",
x2: "720.001",
y2: "-68.6774",
gradientUnits: "userSpaceOnUse"
}, Object(c.c)("stop", {
stopColor: "#2F2F2F"
}), Object(c.c)("stop", {
offset: "0.114583"
}), Object(c.c)("stop", {
offset: "0.817708",
stopColor: "white",
stopOpacity: "0"
})), Object(c.c)("clipPath", {
id: "clip0"
}, Object(c.c)("rect", {
width: "1896",
height: "110",
fill: "white"
}))))
}
));
e.a = O
},
CWDR: function(t, e, i) {
"use strict";
var n = i("wx14")
, r = i("rePB")
, o = i("q1tI")
, a = i.n(o)
, s = i("2A+t")
, c = i("It7/")
, l = i("G8A4");
a.a.createElement;
function p(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function u(t) {
for (var e = 1; e < arguments.length; e++) {
var i = null != arguments[e] ? arguments[e] : {};
e % 2 ? p(Object(i), !0).forEach((function(e) {
Object(r.a)(t, e, i[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(i)) : p(Object(i)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(i, e))
}
))
}
return t
}
var d = {
display: "inline-flex",
mx: 3,
width: "40px",
justifyContent: "center",
alignItems: "center",
opacity: .8,
transition: "opacity .15s",
"&:hover": {
opacity: 1
},
img: {
width: "40px"
}
};
e.a = function(t) {
var e = t.logosCollection
, i = t.pushSx;
return Object(s.c)("div", {
sx: i
}, Object(s.c)("div", {
sx: {
display: ["none", null, null, "flex"],
justifyContent: "center",
alignItems: "center",
mx: "auto",
flexWrap: "wrap",
mt: -4
}
}, null === e || void 0 === e ? void 0 : e.items.map((function(t) {
return Object(s.c)(l.a, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.name
}, t, {
pushSx: u(u({}, d), {}, {
mt: 4
})
}))
}
))), Object(s.c)(c.a, {
pushSx: {
display: ["block", null, null, "none"]
},
duration: 1
}, null === e || void 0 === e ? void 0 : e.items.map((function(t) {
return Object(s.c)(l.a, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.name
}, t, {
pushSx: u({}, d)
}))
}
))))
}
},
G8A4: function(t, e, i) {
"use strict";
var n = i("rePB")
, r = i("wx14")
, o = i("q1tI")
, a = i.n(o)
, s = i("2A+t")
, c = i("nJuf");
a.a.createElement;
function l(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function p(t) {
for (var e = 1; e < arguments.length; e++) {
var i = null != arguments[e] ? arguments[e] : {};
e % 2 ? l(Object(i), !0).forEach((function(e) {
Object(n.a)(t, e, i[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(i)) : l(Object(i)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(i, e))
}
))
}
return t
}
e.a = function(t) {
var e, i, n = t.name, o = t.image, a = t.action, l = t.pushSx;
return a ? Object(s.c)(c.a, Object(r.a)({}, a, {
sx: l
}), Object(s.c)("img", {
src: null !== (i = null === o || void 0 === o ? void 0 : o.url) && void 0 !== i ? i : "",
alt: "".concat(n, " Image"),
sx: {
objectFit: "contain"
}
})) : Object(s.c)("img", {
src: null !== (e = null === o || void 0 === o ? void 0 : o.url) && void 0 !== e ? e : "",
alt: "".concat(n, " Image"),
sx: p(p({}, l), {}, {
objectFit: "contain"
})
})
}
},
"It7/": function(t, e, i) {
"use strict";
var n = i("rePB")
, r = i("h4VS")
, o = i("q1tI")
, a = i.n(o)
, s = i("2A+t")
, c = i("qKvR")
, l = i("3eft");
a.a.createElement;
function p(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function u(t) {
for (var e = 1; e < arguments.length; e++) {
var i = null != arguments[e] ? arguments[e] : {};
e % 2 ? p(Object(i), !0).forEach((function(e) {
Object(n.a)(t, e, i[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(i)) : p(Object(i)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(i, e))
}
))
}
return t
}
function d() {
var t = Object(r.a)(["\nfrom {\n transform: translate3d(0, 0, 0);\n }\n to {\n transform: translate3d(-50%, 0, 0);\n }\n"]);
return d = function() {
return t
}
,
t
}
var f = Object(c.d)(d());
e.a = function(t) {
var e = t.duration
, i = void 0 === e ? 3 : e
, n = t.children
, r = t.pushSx
, a = t.pushItemSx
, c = t.isFullScreen
, p = void 0 === c || c
, d = t.numberOfCopies
, b = void 0 === d ? 2 : d
, O = t.left;
if (b % 2 !== 0 && l.b)
throw new Error("numberOfCopies must be even");
var h = i * o.Children.count(n) * b;
return Object(s.c)("div", {
sx: u({
overflow: "hidden",
whiteSpace: "nowrap",
position: "relative",
width: p ? "calc(var(--vw, 1vw) * 100)" : "100%",
left: p ? "50%" : "0",
transform: p ? "translateX(-50%)" : "0"
}, r)
}, Object(s.c)("div", {
sx: {
display: "inline-block",
whiteSpace: "nowrap",
animation: "".concat(f, " ").concat(h, "s linear infinite"),
animationDirection: O ? "reverse" : "normal"
}
}, Array.from({
length: b
}, (function(t, e) {
return e + 1
}
)).map((function(t) {
return Object(s.c)("div", {
key: "auto-slider-copy-".concat(t),
sx: u({
display: "inline-flex",
"& > div": {
display: "inline-block"
}
}, a)
}, n)
}
))))
}
},
iWaP: function(t, e, i) {
!function(t, e) {
"use strict";
function i(t, e, i) {
return e in t ? Object.defineProperty(t, e, {
value: i,
enumerable: !0,
configurable: !0,
writable: !0
}) : t[e] = i,
t
}
function n(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function r(t) {
for (var e = 1; e < arguments.length; e++) {
var r = null != arguments[e] ? arguments[e] : {};
e % 2 ? n(Object(r), !0).forEach((function(e) {
i(t, e, r[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(r)) : n(Object(r)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(r, e))
}
))
}
return t
}
function o(t, e) {
return function(t) {
if (Array.isArray(t))
return t
}(t) || function(t, e) {
if ("undefined" != typeof Symbol && Symbol.iterator in Object(t)) {
var i = []
, n = !0
, r = !1
, o = void 0;
try {
for (var a, s = t[Symbol.iterator](); !(n = (a = s.next()).done) && (i.push(a.value),
!e || i.length !== e); n = !0)
;
} catch (t) {
r = !0,
o = t
} finally {
try {
n || null == s.return || s.return()
} finally {
if (r)
throw o
}
}
return i
}
}(t, e) || s(t, e) || function() {
throw new TypeError("Invalid attempt to destructure non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.")
}()
}
function a(t) {
return function(t) {
if (Array.isArray(t))
return c(t)
}(t) || function(t) {
if ("undefined" != typeof Symbol && Symbol.iterator in Object(t))
return Array.from(t)
}(t) || s(t) || function() {
throw new TypeError("Invalid attempt to spread non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.")
}()
}
function s(t, e) {
if (t) {
if ("string" == typeof t)
return c(t, e);
var i = Object.prototype.toString.call(t).slice(8, -1);
return "Object" === i && t.constructor && (i = t.constructor.name),
"Map" === i || "Set" === i ? Array.from(t) : "Arguments" === i || /^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(i) ? c(t, e) : void 0
}
}
function c(t, e) {
(null == e || e > t.length) && (e = t.length);
for (var i = 0, n = new Array(e); i < e; i++)
n[i] = t[i];
return n
}
function l(t) {
var e, i, n, o, s, c, l, p, f, b, O, h, j, C, y, v, x, m, g, _, w, F, U, S, M, k, G, P, Z, T, I, E, D = arguments.length > 1 && void 0 !== arguments[1] ? arguments[1] : {}, A = "data-keen-slider-moves", L = "data-keen-slider-v", z = [], B = null, R = !1, W = !1, V = 0, N = [];
function H(t, e, i) {
var n = arguments.length > 3 && void 0 !== arguments[3] ? arguments[3] : {};
t.addEventListener(e, i, n),
z.push([t, e, i, n])
}
function q(t) {
if (m && g === J(t) && st()) {
var i = $(t).x;
if (!et(t) && S)
return X(t);
S && (Bt(),
_ = i,
e.setAttribute(A, !0),
S = !1),
t.cancelable && t.preventDefault(),
Zt(U(_ - i, qt), t.timeStamp),
_ = i
}
}
function Y(t) {
m || !st() || tt(t.target) || (m = !0,
S = !0,
g = J(t),
et(t),
dt(),
x = b,
_ = $(t).x,
Zt(0, t.timeStamp),
ot("dragStart"))
}
function X(t) {
m && g === J(t, !0) && st() && (e.removeAttribute(A),
m = !1,
Ot(),
ot("dragEnd"))
}
function Q(t) {
return t.changedTouches
}
function J(t) {
var e = arguments.length > 1 && void 0 !== arguments[1] && arguments[1] ? Q(t) : K(t);
return e ? e[0] ? e[0].identifier : "error" : "default"
}
function K(t) {
return t.targetTouches
}
function $(t) {
var e = K(t);
return {
x: pt() ? e ? e[0].screenY : t.pageY : e ? e[0].screenX : t.pageX,
timestamp: t.timeStamp
}
}
function tt(t) {
return t.hasAttribute(v.preventEvent)
}
function et(t) {
var e = K(t);
if (!e)
return !0;
var i = e[0]
, n = pt() ? i.clientY : i.clientX
, r = pt() ? i.clientX : i.clientY
, o = void 0 !== w && void 0 !== F && Math.abs(F - r) <= Math.abs(w - n);
return w = n,
F = r,
o
}
function it(t) {
st() && m && t.preventDefault()
}
function nt() {
H(window, "orientationchange", St),
H(window, "resize", (function() {
return Ut()
}
)),
H(e, "dragstart", (function(t) {
st() && t.preventDefault()
}
)),
H(e, "mousedown", Y),
H(e, "mousemove", q),
H(e, "mouseleave", X),
H(e, "mouseup", X),
H(e, "touchstart", Y, {
passive: !0
}),
H(e, "touchmove", q, {
passive: !1
}),
H(e, "touchend", X, {
passive: !0
}),
H(e, "touchcancel", X, {
passive: !0
}),
H(window, "wheel", it, {
passive: !1
})
}
function rt() {
z.forEach((function(t) {
t[0].removeEventListener(t[1], t[2], t[3])
}
)),
z = []
}
function ot(t) {
v[t] && v[t](qt)
}
function at() {
return v.centered
}
function st() {
return void 0 !== i ? i : v.controls
}
function ct() {
return v.loop
}
function lt() {
return !v.loop && v.rubberband
}
function pt() {
return !!v.vertical
}
function ut() {
M = window.requestAnimationFrame(ft)
}
function dt() {
M && (window.cancelAnimationFrame(M),
M = null),
k = null
}
function ft(t) {
k || (k = t);
var e = t - k
, i = bt(e);
if (e >= P)
return Zt(G - T, !1),
E ? E() : void ot("afterChange");
var n = Tt(i);
if (0 === n || ct() || lt() || I) {
if (0 !== n && lt() && !I)
return vt();
T += i,
Zt(i, !1),
ut()
} else
Zt(i - n, !1)
}
function bt(t) {
return G * Z(t / P) - T
}
function Ot() {
switch (ot("beforeChange"),
v.mode) {
case "free":
Ct();
break;
case "free-snap":
yt();
break;
case "snap":
default:
ht()
}
}
function ht() {
jt((1 === l && 0 !== O ? x : b) + Math.sign(O))
}
function jt(t, e) {
var i = arguments.length > 2 && void 0 !== arguments[2] ? arguments[2] : v.duration
, n = function(t) {
return 1 + --t * t * t * t * t
};
xt(At(t = Dt(t, arguments.length > 3 && void 0 !== arguments[3] && arguments[3], arguments.length > 4 && void 0 !== arguments[4] && arguments[4])), i, n, e)
}
function Ct() {
if (0 === j)
return !(!Tt(0) || ct()) && jt(b);
var t = v.friction / Math.pow(Math.abs(j), -.5);
xt(Math.pow(j, 2) / t * Math.sign(j), 6 * Math.abs(j / t), (function(t) {
return 1 - Math.pow(1 - t, 5)
}
))
}
function yt() {
if (0 === j)
return jt(b);
var t = v.friction / Math.pow(Math.abs(j), -.5)
, e = Math.pow(j, 2) / t * Math.sign(j)
, i = 6 * Math.abs(j / t)
, n = (V + e) / (c / l);
xt((-1 === O ? Math.floor(n) : Math.ceil(n)) * (c / l) - V, i, (function(t) {
return 1 - Math.pow(1 - t, 5)
}
))
}
function vt() {
if (dt(),
0 === j)
return jt(b, !0);
var t = .04 / Math.pow(Math.abs(j), -.5)
, e = Math.pow(j, 2) / t * Math.sign(j)
, i = function(t) {
return --t * t * t + 1
}
, n = j;
xt(e, 3 * Math.abs(n / t), i, !0, (function() {
xt(At(Dt(b)), 500, i, !0)
}
))
}
function xt(t, e, i, n, r) {
dt(),
G = t,
T = 0,
P = e,
Z = i,
I = n,
E = r,
k = null,
ut()
}
function mt(i) {
var n = u(t);
n.length && (e = n[0],
Ut(i),
nt(),
ot("mounted"))
}
function gt() {
var t, e = D.breakpoints || [];
for (var i in e)
window.matchMedia(i).matches && (t = i);
if (t === B)
return !0;
var n = (B = t) ? e[B] : D;
n.breakpoints && B && delete n.breakpoints,
v = r(r(r({}, Ht), D), n),
R = !0,
f = null,
Ft()
}
function _t(t) {
return "function" == typeof t ? t() : d(t, 1, Math.max(ct() ? n - 1 : n, 1))
}
function wt() {
gt(),
W = !0,
ot("created")
}
function Ft(t, e) {
t && (D = t),
e && (B = null),
Mt(),
mt(e)
}
function Ut(t) {
var i = window.innerWidth;
if (gt() && (i !== f || t)) {
f = i;
var r = v.slides;
"number" == typeof r ? (s = null,
n = r) : (s = u(r, e),
n = s ? s.length : 0);
var a = v.dragSpeed;
U = "function" == typeof a ? a : function(t) {
return t * a
}
,
c = pt() ? e.offsetHeight : e.offsetWidth,
l = _t(v.slidesPerView),
p = d(v.spacing, 0, c / (l - 1) - 1),
c += p,
o = at() ? (c / 2 - c / l / 2) / c : 0,
Gt();
var O = !W || R && v.resetSlide ? v.initial : b;
Nt(ct() ? O : It(O)),
pt() && e.setAttribute(L, !0),
R = !1
}
}
function St(t) {
Ut(),
setTimeout(Ut, 500),
setTimeout(Ut, 2e3)
}
function Mt() {
rt(),
Pt(),
e && e.hasAttribute(L) && e.removeAttribute(L),
ot("destroyed")
}
function kt() {
s && s.forEach((function(t, e) {
var i = C[e].distance * c - e * (c / l - p / l - p / l * (l - 1))
, n = pt() ? 0 : i
, r = pt() ? i : 0
, o = "translate3d(".concat(n, "px, ").concat(r, "px, 0)");
t.style.transform = o,
t.style["-webkit-transform"] = o
}
))
}
function Gt() {
s && s.forEach((function(t) {
var e = "calc(".concat(100 / l, "% - ").concat(p / l * (l - 1), "px)");
pt() ? (t.style["min-height"] = e,
t.style["max-height"] = e) : (t.style["min-width"] = e,
t.style["max-width"] = e)
}
))
}
function Pt() {
if (s) {
var t = ["transform", "-webkit-transform"];
t = [].concat(a(t), pt ? ["min-height", "max-height"] : ["min-width", "max-width"]),
s.forEach((function(e) {
t.forEach((function(t) {
e.style.removeProperty(t)
}
))
}
))
}
}
function Zt(t) {
var e = !(arguments.length > 1 && void 0 !== arguments[1]) || arguments[1];
zt(t, arguments.length > 2 && void 0 !== arguments[2] ? arguments[2] : Date.now()),
e && (t = Wt(t)),
V += t,
Rt()
}
function Tt(t) {
var e = c * (n - 1 * (at() ? 1 : l)) / l
, i = V + t;
return i > e ? i - e : i < 0 ? i : 0
}
function It(t) {
return d(t, 0, n - 1 - (at() ? 0 : l - 1))
}
function Et() {
var t = Math.abs(y)
, e = V < 0 ? 1 - t : t;
return {
direction: O,
progressTrack: e,
progressSlides: e * n / (n - 1),
positions: C,
position: V,
speed: j,
relativeSlide: (b % n + n) % n,
absoluteSlide: b,
size: n,
slidesPerView: l,
widthOrHeight: c
}
}
function Dt(t) {
var e = arguments.length > 1 && void 0 !== arguments[1] && arguments[1]
, i = arguments.length > 2 && void 0 !== arguments[2] && arguments[2];
return ct() ? e ? Lt(t, i) : t : It(t)
}
function At(t) {
return -(-c / l * t + V)
}
function Lt(t, e) {
var i = (b % n + n) % n
, r = i < (t = (t % n + n) % n) ? -i - n + t : -(i - t)
, o = i > t ? n - i + t : t - i
, a = e ? Math.abs(r) <= o ? r : o : t < i ? r : o;
return b + a
}
function zt(t, e) {
clearTimeout(h);
var i = Math.sign(t);
if (i !== O && Bt(),
O = i,
N.push({
distance: t,
time: e
}),
h = setTimeout((function() {
N = [],
j = 0
}
), 50),
(N = N.slice(-6)).length <= 1 || 0 === O)
return j = 0;
var n = N.slice(0, -1).reduce((function(t, e) {
return t + e.distance
}
), 0)
, r = N[N.length - 1].time
, o = N[0].time;
j = d(n / (r - o), -10, 10)
}
function Bt() {
N = []
}
function Rt() {
y = ct() ? V % (c * n / l) / (c * n / l) : V / (c * n / l),
Vt();
for (var t = [], e = 0; e < n; e++) {
var i = (1 / n * e - (y < 0 && ct() ? y + 1 : y)) * n / l + o;
ct() && (i += i > (n - 1) / l ? -n / l : i < -n / l + 1 ? n / l : 0);
var r = 1 / l
, a = i + r
, s = a < r ? a / r : a > 1 ? 1 - (a - 1) * l / 1 : 1;
t.push({
portion: s < 0 || s > 1 ? 0 : s,
distance: i
})
}
C = t,
kt(),
ot("move")
}
function Wt(t) {
if (ct())
return t;
var e, i = Tt(t);
return lt() ? 0 === i ? t : t * (e = i / c,
(1 - Math.abs(e)) * (1 - Math.abs(e))) : t - i
}
function Vt() {
var t = Math.round(V / (c / l));
t !== b && (b = t,
ot("slideChanged"))
}
function Nt(t) {
ot("beforeChange"),
Zt(At(t), !1),
ot("afterChange")
}
var Ht = {
centered: !1,
breakpoints: null,
controls: !0,
dragSpeed: 1,
friction: .0025,
loop: !1,
initial: 0,
duration: 500,
preventEvent: "data-keen-slider-pe",
slides: ".keen-slider__slide",
vertical: !1,
resetSlide: !1,
slidesPerView: 1,
spacing: 0,
mode: "snap",
rubberband: !0
}
, qt = {
controls: function(t) {
i = t
},
destroy: Mt,
refresh: function(t) {
Ft(t, !0)
},
next: function() {
jt(b + 1, !0)
},
prev: function() {
jt(b - 1, !0)
},
moveToSlide: function(t, e) {
jt(t, !0, e)
},
moveToSlideRelative: function(t) {
jt(t, !0, arguments.length > 2 ? arguments[2] : void 0, !0, arguments.length > 1 && void 0 !== arguments[1] && arguments[1])
},
resize: function() {
Ut(!0)
},
details: function() {
return Et()
}
};
return wt(),
qt
}
function p(t) {
return Array.prototype.slice.call(t)
}
function u(t) {
var e = arguments.length > 1 && void 0 !== arguments[1] ? arguments[1] : document;
return "function" == typeof t ? p(t()) : "string" == typeof t ? p(e.querySelectorAll(t)) : t instanceof HTMLElement != 0 ? [t] : t instanceof NodeList != 0 ? t : []
}
function d(t, e, i) {
return Math.min(Math.max(t, e), i)
}
Math.sign || (Math.sign = function(t) {
return (t > 0) - (t < 0) || +t
}
);
var f = function t(e, i) {
var n = Object.prototype.toString.call(e);
if (n !== Object.prototype.toString.call(i))
return !1;
if (["[object Array]", "[object Object]"].indexOf(n) < 0)
return !1;
var r = "[object Array]" === n ? e.length : Object.keys(e).length;
if (r !== ("[object Array]" === n ? i.length : Object.keys(i).length))
return !1;
var o = function(e, i) {
var n = Object.prototype.toString.call(e);
if (["[object Array]", "[object Object]"].indexOf(n) >= 0) {
if (!t(e, i))
return !1
} else {
if (n !== Object.prototype.toString.call(i))
return !1;
if ("[object Function]" === n) {
if (e.toString() !== i.toString())
return !1
} else if (e !== i)
return !1
}
};
if ("[object Array]" === n) {
for (var a = 0; a < r; a++)
if (!1 === o(e[a], i[a]))
return !1
} else
for (var s in e)
if (e.hasOwnProperty(s) && !1 === o(e[s], i[s]))
return !1;
return !0
};
t.default = l,
t.useKeenSlider = function() {
var t = arguments.length > 0 && void 0 !== arguments[0] ? arguments[0] : {}
, i = e.useRef()
, n = e.useRef();
function r(t) {
return f(n.current, t) || (n.current = t),
n.current
}
var a = o(e.useState(null), 2)
, s = a[0]
, c = a[1];
return e.useEffect((function() {
var t = new l(i.current,n.current);
return c(t),
function() {
t.destroy()
}
}
), [r(t)]),
[i, s]
}
,
Object.defineProperty(t, "__esModule", {
value: !0
})
}(e, i("q1tI"))
},
sKf7: function(t, e, i) {
"use strict";
i.d(e, "a", (function() {
return de
}
));
var n = i("wx14")
, r = i("q1tI")
, o = i.n(r)
, a = i("3eft")
, s = i("rePB")
, c = i("2A+t")
, l = i("izhR")
, p = i("35g/")
, u = i("N3lO")
, d = (o.a.createElement,
function(t) {
var e = t.blocks;
return Object(c.c)(l.d, {
sx: {
display: "flex",
justifyContent: "space-between",
p: 5,
flexDirection: ["column", null, "row"]
}
}, e.map((function(t, i) {
var n, o, a, s, d, f;
return Object(c.c)(r.Fragment, {
key: t.title
}, Object(c.c)("div", {
sx: {
textAlign: "center",
maxWidth: "lg",
mx: "auto"
}
}, Object(c.c)(l.i, {
sx: {
fontWeight: 600,
fontSize: 3,
mb: 3
}
}, t.title), Object(c.c)(l.o, {
variant: "small"
}, Object(p.a)(null === (n = t.subtitle) || void 0 === n ? void 0 : n.json)), Object(c.c)("div", {
sx: {
mb: [3, null, 5],
mt: 3
}
}, null === (o = t.listItems) || void 0 === o ? void 0 : o.map((function(e) {
var i = Object(u.a)(t.icon);
return Object(c.c)(l.o, {
key: e,
as: "span",
sx: {
display: "inline-flex",
alignItems: "center",
":not(:last-of-type)": {
mr: 3
}
},
variant: "small"
}, Object(c.c)("i", {
sx: {
mr: 2,
display: "inline-flex",
alignItems: "center",
justifyContent: "center",
color: t.isPositive ? "green" : "red"
}
}, i && Object(c.c)(i, null)), e)
}
))), Object(c.c)("div", {
sx: {
display: "flex",
alignItems: "center",
justifyContent: "center"
}
}, Object(c.c)("img", {
src: null !== (a = null === (s = t.media) || void 0 === s ? void 0 : s.url) && void 0 !== a ? a : "",
alt: null !== (d = null === (f = t.media) || void 0 === f ? void 0 : f.title) && void 0 !== d ? d : ""
}))), i !== e.length - 1 && Object(c.c)("div", {
sx: {
border: "dashed 1px",
borderColor: "primary",
borderImage: 'url("/images/misc/border.png") 2 round',
mx: [0, null, 40],
my: [40, null, 0]
}
}))
}
)))
}
)
, f = i("thNe")
, b = i("nJuf");
o.a.createElement;
function O(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function h(t) {
for (var e = 1; e < arguments.length; e++) {
var i = null != arguments[e] ? arguments[e] : {};
e % 2 ? O(Object(i), !0).forEach((function(e) {
Object(s.a)(t, e, i[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(i)) : O(Object(i)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(i, e))
}
))
}
return t
}
var j = function(t) {
var e, i = t.title, r = t.subtitle, o = t.actionsCollection, a = t.blocksCollection, s = t.anchorLinkId, l = null !== (e = null === a || void 0 === a ? void 0 : a.items.filter((function(t) {
return "ListBlock" === (null === t || void 0 === t ? void 0 : t.__typename)
}
))) && void 0 !== e ? e : [];
return Object(c.c)(f.a, {
anchorId: s,
title: {
text: i
},
subtitle: null === r || void 0 === r ? void 0 : r.json
}, Object(c.c)("div", {
sx: h({
display: "flex",
flexDirection: "column",
alignItems: "center"
}, i || null !== r && void 0 !== r && r.json ? void 0 : {
pt: 0
})
}, Object(c.c)(d, {
blocks: l.map((function(t, e) {
return h(h({}, t), {}, 0 === e ? {
isPositive: !1
} : {
isPositive: !0
})
}
))
}), (null === o || void 0 === o ? void 0 : o.items[0]) && Object(c.c)(b.a, Object(n.a)({
sx: {
display: "block",
mt: 4
},
variant: "accent",
arrow: !0
}, null === o || void 0 === o ? void 0 : o.items[0]))))
}
, C = i("oe78")
, y = i("GspY")
, v = (o.a.createElement,
function(t) {
var e, i, r, o, a = t.logo, s = t.quote, p = t.className, u = t.action, d = t.person;
return Object(c.c)(l.d, {
className: p,
sx: {
py: 48,
px: 40,
display: "flex",
flexDirection: "column",
justifyContent: "space-between"
}
}, Object(c.c)("div", null, Object(c.c)(l.o, {
variant: "small"
}, Object(c.c)(y.a, {
availableHeight: 100
}, null !== s && void 0 !== s && s.startsWith('"') ? null : '"', Object(C.a)(s), null !== s && void 0 !== s && s.endsWith('"') ? null : '"')), d && Object(c.c)(l.o, {
sx: {
mt: 20
}
}, null === d || void 0 === d ? void 0 : d.name, " - ", null === d || void 0 === d ? void 0 : d.position), u && Object(c.c)(b.a, Object(n.a)({
variant: "accent",
arrow: !0
}, u, {
sx: {
mt: 3
}
}))), Object(c.c)("div", {
sx: {
mt: 3,
width: "200px"
}
}, Object(c.c)("img", {
src: null !== (e = null === a || void 0 === a || null === (i = a.image) || void 0 === i ? void 0 : i.url) && void 0 !== e ? e : void 0,
alt: null !== (r = null === a || void 0 === a || null === (o = a.image) || void 0 === o ? void 0 : o.title) && void 0 !== r ? r : void 0
})))
}
)
, x = i("KQm4")
, m = i("ODXe")
, g = i("iWaP")
, _ = i("u9V9");
o.a.createElement;
function w(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function F(t) {
for (var e = 1; e < arguments.length; e++) {
var i = null != arguments[e] ? arguments[e] : {};
e % 2 ? w(Object(i), !0).forEach((function(e) {
Object(s.a)(t, e, i[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(i)) : w(Object(i)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(i, e))
}
))
}
return t
}
var U = function(t) {
var e = t.children
, i = t.config
, n = t.pushSx
, a = t.withArrowControls
, s = void 0 !== a && a
, p = t.slidesPerView
, u = Object(r.useState)(0)
, d = u[0]
, f = u[1]
, b = Object(r.useMemo)((function() {
if (Array.isArray(p)) {
var t = {};
return p.forEach((function(e, i) {
t["(min-width: ".concat(_.a.breakpoints[i], ")")] = {
slidesPerView: e
}
}
)),
t
}
}
), [p])
, O = Object(g.useKeenSlider)(F({
breakpoints: b,
slidesPerView: Array.isArray(p) ? p[0] : p,
duration: 1e3,
spacing: 20,
initial: 0,
slideChanged: function(t) {
f(t.details().relativeSlide)
}
}, i))
, h = Object(m.a)(O, 2)
, j = h[0]
, C = h[1];
return Object(c.c)(o.a.Fragment, null, Object(c.c)(l.b, {
className: "keen-slider",
ref: j,
sx: F({
position: "relative",
overflow: "visible",
width: "100%"
}, n)
}, r.Children.map(e, (function(t) {
return Object(r.isValidElement)(t) ? F(F({}, t), {}, {
props: F(F({}, t.props), {}, {
className: "".concat(t.props.className ? "".concat(t.props.className, " ") : "", "keen-slider__slide")
})
}) : t
}
))), C && s && Object(c.c)(l.b, {
sx: {
display: "flex",
alignItems: "center",
justifyContent: "center",
mt: 4
}
}, Object(c.c)(l.j, {
sx: {
variant: "links.accent",
"&:disabled": {
"&:hover": {
opacity: 1
}
}
},
onClick: null === C || void 0 === C ? void 0 : C.prev,
disabled: 0 === d
}, "<-"), Object(c.c)("div", null, Object(x.a)(Array(C.details().size).keys()).map((function(t) {
return Object(c.c)("button", {
key: t,
onClick: function() {
C.moveToSlideRelative(t)
},
sx: {
width: "10px",
height: "10px",
bg: d === t ? "primary" : "gray",
borderRadius: "full",
mx: "2",
"@media screen and (max-width: 395px)": {
mx: "6px"
},
"@media screen and (max-width: 350px)": {
mx: "1"
},
"&:focus": {
outline: "none"
}
}
})
}
))), Object(c.c)(l.j, {
sx: {
variant: "links.accent",
"&:disabled": {
"&:hover": {
opacity: 1
}
}
},
onClick: null === C || void 0 === C ? void 0 : C.next,
disabled: d === C.details().size - 1
}, "->")))
}
, S = o.a.createElement
, M = function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.actionsCollection
, s = t.anchorLinkId;
return S(l.b, {
sx: {
overflow: "hidden"
}
}, S(f.a, {
anchorId: s,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json,
pushSx: {
display: "flex",
flexDirection: "column",
alignItems: "center"
},
noContentDivider: !0
}, null === o || void 0 === o ? void 0 : o.items.map((function(t) {
return S(b.a, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.children
}, t, {
variant: "accent",
sx: {
":not(:last-of-type)": {
mr: 3
}
},
arrow: !0
}))
}
)), S(U, {
slidesPerView: [1, 1, 1.7],
config: {
centered: !0,
spacing: 60,
initial: 1
},
pushSx: {
pt: [4, null, 5]
},
withArrowControls: !0
}, null === r || void 0 === r ? void 0 : r.items.map((function(t, e) {
if ("Quote2" === (null === t || void 0 === t ? void 0 : t.__typename))
return S(v, Object(n.a)({
key: "".concat(null === t || void 0 === t ? void 0 : t.quote, "-").concat(e)
}, t));
if (a.b)
throw new Error("Unsupported block type, ".concat(null === t || void 0 === t ? void 0 : t.__typename));
return null
}
)))))
}
, k = i("It7/")
, G = i("G8A4");
o.a.createElement;
function P(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function Z(t) {
for (var e = 1; e < arguments.length; e++) {
var i = null != arguments[e] ? arguments[e] : {};
e % 2 ? P(Object(i), !0).forEach((function(e) {
Object(s.a)(t, e, i[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(i)) : P(Object(i)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(i, e))
}
))
}
return t
}
var T = {
display: "inline-flex",
mx: 4,
width: "40px",
justifyContent: "center",
alignItems: "center",
opacity: .8,
transition: "opacity .15s",
"&:hover": {
opacity: 1
},
img: {
width: "40px"
}
}
, I = function(t) {
var e = t.tagline
, i = t.logosCollection
, r = t.action;
return Object(c.c)("div", {
sx: {
textAlign: "center"
}
}, e && Object(c.c)(l.i, {
as: "h3",
sx: {
mb: 4
},
variant: "heading.3"
}, Object(C.a)(e)), Object(c.c)("div", {
sx: {
display: ["none", null, "flex"],
justifyContent: "center",
alignItems: "center",
mx: "auto",
flexWrap: "wrap",
mt: -4
}
}, null === i || void 0 === i ? void 0 : i.items.map((function(t) {
return Object(c.c)(G.a, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.name
}, t, {
pushSx: Z(Z({}, T), {}, {
mt: 4
})
}))
}
))), Object(c.c)(k.a, {
pushSx: {
display: ["block", null, "none"]
},
duration: 1
}, null === i || void 0 === i ? void 0 : i.items.map((function(t) {
return Object(c.c)(G.a, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.name
}, t, {
pushSx: T
}))
}
))), r && Object(c.c)(b.a, Object(n.a)({
variant: "accent",
sx: {
mt: 4,
display: "block"
},
arrow: !0
}, r)))
}
, E = (o.a.createElement,
function(t) {
var e = t.title
, i = t.subtitle
, r = t.actionsCollection
, o = t.icon
, a = Object(u.a)(o);
return Object(c.c)("div", null, Object(c.c)("div", {
sx: {
borderRadius: "full",
variant: "gradients.circleSoft",
color: "primary",
fontSize: 4,
width: "48px",
height: "48px",
display: "flex",
alignItems: "center",
justifyContent: "center"
}
}, a && Object(c.c)(a, null)), Object(c.c)(l.i, {
as: "h4",
variant: "heading.base",
sx: {
mb: "10px",
mt: 24
}
}, Object(C.a)(e)), Object(c.c)(l.o, {
variant: "small"
}, Object(p.a)(null === i || void 0 === i ? void 0 : i.json)), (null === r || void 0 === r ? void 0 : r.items[0]) && Object(c.c)(b.a, Object(n.a)({}, null === r || void 0 === r ? void 0 : r.items[0], {
variant: "accent",
sx: {
mt: 3
},
arrow: !0
})))
}
)
, D = o.a.createElement
, A = function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.logosCollection
, s = t.anchorLinkId;
return D(f.a, {
anchorId: s,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json,
smallContainer: !0
}, D(l.h, {
variant: "three",
gap: [4, 5],
sx: {
mx: "auto",
width: "fit-content",
mb: 6
}
}, null === r || void 0 === r ? void 0 : r.items.map((function(t) {
if ("SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename))
return D(E, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.title
}, t));
if (a.b)
throw new Error("Unsupported block type, ".concat(null === t || void 0 === t ? void 0 : t.__typename));
return null
}
))), o && D(I, o))
}
, L = (o.a.createElement,
function(t) {
var e, i, r = t.title, o = t.subtitle, a = t.media, s = t.actionsCollection, u = t.toTheRight, d = t.stripDown;
return Object(c.c)(l.h, {
columns: [1, null, "repeat(2, fit-content(50%))"],
gap: [4, null, 5, 6],
sx: {
width: "fit-content",
mx: "auto",
gridAutoFlow: "dense",
textAlign: ["center", null, "left"],
alignItems: "center"
}
}, Object(c.c)("div", {
sx: {
maxWidth: "md",
gridColumn: u ? ["1", null, "2"] : "1"
}
}, Object(c.c)(l.i, {
as: "h3",
variant: "heading.3",
sx: {
mb: 2
}
}, Object(C.a)(r)), Object(c.c)(l.o, {
variant: "small",
sx: {
mb: 3
}
}, Object(p.a)(null === o || void 0 === o ? void 0 : o.json)), null === s || void 0 === s ? void 0 : s.items.map((function(t) {
return Object(c.c)(b.a, Object(n.a)({}, t, {
key: null === t || void 0 === t ? void 0 : t.href,
sx: {
":not(:last-of-type)": {
mr: 3
}
},
variant: "accent",
arrow: !0
}))
}
))), Object(c.c)("div", {
sx: {
maxWidth: "100%",
height: d ? "" : "429px",
width: ["100%", null, "429px"],
backgroundImage: d ? "" : "url(/images/backgrounds/dots/circle.svg)",
backgroundPosition: "center",
backgroundSize: "cover",
display: "flex",
alignItems: "center",
justifyContent: "center"
}
}, Object(c.c)("img", {
src: null !== (e = null === a || void 0 === a ? void 0 : a.url) && void 0 !== e ? e : "",
alt: null !== (i = null === a || void 0 === a ? void 0 : a.title) && void 0 !== i ? i : "",
sx: {
width: "100%"
}
})))
}
)
, z = o.a.createElement
, B = function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.isFixed
, s = t.anchorLinkId;
return z(f.a, {
anchorId: s,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json
}, z(l.h, {
gap: 5
}, null === r || void 0 === r ? void 0 : r.items.map((function(t, e) {
if ("SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename))
return z(L, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.title
}, t, {
toTheRight: !!o || e % 2 === 0,
stripDown: o
}));
if (a.b)
throw new Error("Unsupported block type, ".concat(null === t || void 0 === t ? void 0 : t.__typename));
return null
}
))))
}
, R = i("jrSh")
, W = i("s4vu")
, V = i("/3Dz")
, N = i("+jk2")
, H = o.a.createElement
, q = function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.anchorLinkId
, s = Object(N.a)();
return H(f.a, {
anchorId: o,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json,
pushSx: {
pb: 0,
mb: "-100px"
},
smallContainer: !0
}, H(l.h, {
variant: "three"
}, null === r || void 0 === r ? void 0 : r.items.map((function(t) {
var e;
switch (null === t || void 0 === t ? void 0 : t.__typename) {
case "BlogPost":
return H(R.a, {
key: null === t || void 0 === t ? void 0 : t.slug,
title: t.title,
image: null !== (e = t.thumbnailImage) && void 0 !== e ? e : t.coverImage,
action: {
children: "Read More",
href: "https://fauna.com/blog/".concat(t.slug)
}
});
case "SectionBlock":
var i, r = null === (i = t.actionsCollection) || void 0 === i ? void 0 : i.items[0];
if (!r && a.b)
throw new Error("".concat(null === t || void 0 === t ? void 0 : t.__typename, " must have an action"));
return H(R.a, {
key: null === t || void 0 === t ? void 0 : t.title,
title: t.title,
image: t.media,
action: {
children: "Read More",
href: null === r || void 0 === r ? void 0 : r.href
}
});
case "MediaBlock":
if (!t.action && a.b)
throw new Error("".concat(null === t || void 0 === t ? void 0 : t.__typename, " must have an action"));
return H(R.a, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.title
}, t));
default:
if (a.b)
throw new Error("Unsupported block type, ".concat(null === t || void 0 === t ? void 0 : t.__typename));
return null
}
}
))), H(W.a, {
sx: {
zIndex: "behind"
}
}, H(V.a, {
ref: s
})))
}
, Y = i("Xw5J")
, X = (o.a.createElement,
function(t) {
var e = t.title
, i = t.subtitle
, r = t.actionsCollection
, o = t.media
, a = !(null === o || void 0 === o || !o.url);
return Object(c.c)(l.d, {
sx: {
px: 24,
pt: 3,
pb: 28
}
}, a ? Object(c.c)(Y.a, {
image: o,
ratio: 1,
width: 56
}) : null, Object(c.c)(l.i, {
as: "h4",
variant: "heading.base",
sx: {
mb: 2,
mt: 3
}
}, Object(C.a)(e)), Object(c.c)(l.o, {
variant: "small"
}, Object(p.a)(null === i || void 0 === i ? void 0 : i.json)), (null === r || void 0 === r ? void 0 : r.items[0]) && Object(c.c)(b.a, Object(n.a)({}, null === r || void 0 === r ? void 0 : r.items[0], {
variant: "accent",
sx: {
mt: 3
},
arrow: !0
})))
}
)
, Q = (o.a.createElement,
function(t) {
var e = t.blocksCollection
, i = t.title
, r = t.subtitle
, o = t.actionsCollection
, s = t.anchorLinkId;
return Object(c.c)(f.a, {
anchorId: s,
title: {
text: i
},
subtitle: null === r || void 0 === r ? void 0 : r.json
}, Object(c.c)(W.a, {
sx: {
background: "linear-gradient(180deg, rgba(255, 255, 255, 0) 0%, #FFFFFF 100%), #FAF5FF",
height: "200px",
mb: "-64px",
zIndex: "behind",
position: "absolute",
top: 0
}
}), Object(c.c)(l.h, {
variant: "three",
sx: {
mx: "auto",
width: "fit-content",
position: "relative",
variant: "layout.sectionContent"
}
}, Object(c.c)("div", {
sx: {
position: "absolute",
top: 0,
bottom: 0,
right: 0,
left: 0,
backgroundImage: "url(/images/backgrounds/features-grid.svg)",
backgroundSize: "contain",
backgroundRepeat: "no-repeat",
backgroundPosition: "center",
zIndex: "behind"
}
}), null === e || void 0 === e ? void 0 : e.items.map((function(t) {
if ("SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename))
return Object(c.c)(X, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.title
}, t));
if (a.b)
throw new Error("Unsupported block type, ".concat(null === t || void 0 === t ? void 0 : t.__typename));
return null
}
))), Object(c.c)("div", {
sx: {
mt: 4,
display: "flex",
justifyContent: "flex-end"
}
}, null === o || void 0 === o ? void 0 : o.items.map((function(t) {
return Object(c.c)(b.a, Object(n.a)({}, t, {
key: null === t || void 0 === t ? void 0 : t.href,
sx: {
":not(:last-of-type)": {
mr: 3
}
},
variant: "accent",
arrow: !0
}))
}
))))
}
)
, J = o.a.createElement
, K = function(t) {
var e = t.actions
, i = t.linkProps;
return e ? J("div", null, e.map((function(t, e) {
return J(b.a, Object(n.a)({}, t, {
key: e,
sx: {
":not(:last-of-type)": {
mr: 3
}
}
}, i))
}
))) : null
}
, $ = (o.a.createElement,
function(t) {
var e, i, n, r, o, a, s, p, u, d = t.title, b = t.subtitle, O = t.image, h = t.blocksCollection, j = t.actionsCollection, y = t.anchorLinkId, v = null === h || void 0 === h ? void 0 : h.items[0];
return Object(c.c)(l.b, {
sx: {
background: "linear-gradient(180deg, #FFFFFF 0%, #F9FAFF 100%)",
mb: "32px",
overflow: "hidden"
}
}, Object(c.c)(f.a, {
anchorId: y,
title: {
text: d
},
subtitle: null === b || void 0 === b ? void 0 : b.json,
smallContainer: !0
}, Object(c.c)(l.h, {
columns: ["1fr", null, "10fr 12fr"],
gap: [4, null, 5]
}, Object(c.c)("div", {
sx: {
position: "relative",
gridRow: [2, null, "unset"],
height: ["250px", null, "auto"]
}
}, Object(c.c)("img", {
sx: {
position: "absolute",
width: "100%",
borderRadius: "6px",
boxShadow: "0px 4px 20px rgba(15, 29, 47, 0.07)",
border: "1px solid",
borderColor: "border"
},
src: null !== (e = null === O || void 0 === O ? void 0 : O.url) && void 0 !== e ? e : "",
alt: null !== (i = null === O || void 0 === O ? void 0 : O.title) && void 0 !== i ? i : ""
})), "Quote2" === (null === v || void 0 === v ? void 0 : v.__typename) && Object(c.c)("div", {
sx: {
pt: 5
}
}, Object(c.c)("img", {
src: null !== (n = null === (r = v.person) || void 0 === r || null === (o = r.avatar) || void 0 === o ? void 0 : o.url) && void 0 !== n ? n : "",
alt: null !== (a = null === (s = v.person) || void 0 === s || null === (p = s.avatar) || void 0 === p ? void 0 : p.title) && void 0 !== a ? a : "",
sx: {
width: ["48px", "88px"],
height: ["48px", "88px"],
objectFit: "cover"
}
}), Object(c.c)(l.o, {
variant: "small",
sx: {
my: 3,
maxWidth: "2xl"
}
}, Object(C.a)(v.quote)), Object(c.c)(l.o, {
variant: "large",
sx: {
fontWeight: 600,
mb: 3
}
}, null === (u = v.person) || void 0 === u ? void 0 : u.name), Object(c.c)(K, {
actions: null === j || void 0 === j ? void 0 : j.items,
linkProps: {
variant: "accent",
arrow: !0
}
})))))
}
)
, tt = i("CWDR")
, et = o.a.createElement
, it = function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.logosCollection
, s = t.anchorLinkId;
return et(f.a, {
anchorId: s,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json,
smallContainer: !0
}, o && et(tt.a, Object(n.a)({}, o, {
pushSx: {
mb: 3
}
})), et(l.h, {
sx: {
variant: "layout.sectionContent"
},
variant: "three"
}, null === r || void 0 === r ? void 0 : r.items.map((function(t) {
switch (null === t || void 0 === t ? void 0 : t.__typename) {
case "SectionBlock":
var e, i = null === (e = t.actionsCollection) || void 0 === e ? void 0 : e.items[0];
return et(R.a, {
key: null === t || void 0 === t ? void 0 : t.title,
title: null === t || void 0 === t ? void 0 : t.title,
description: t.subtitle,
image: t.media,
action: i
});
default:
if (a.b)
throw new Error("Unsupported block type, ".concat(null === t || void 0 === t ? void 0 : t.__typename));
return null
}
}
))))
}
, nt = i("Ff2n")
, rt = o.a.createElement;
function ot(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function at(t) {
for (var e = 1; e < arguments.length; e++) {
var i = null != arguments[e] ? arguments[e] : {};
e % 2 ? ot(Object(i), !0).forEach((function(e) {
Object(s.a)(t, e, i[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(i)) : ot(Object(i)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(i, e))
}
))
}
return t
}
var st = Object(r.forwardRef)((function(t, e) {
var i = t.ratio
, r = t.width
, o = void 0 === r ? "100%" : r
, a = t.children
, s = t.pushSx
, c = Object(nt.a)(t, ["ratio", "width", "children", "pushSx"]);
return rt(l.b, {
sx: {
width: o
}
}, rt(l.b, Object(n.a)({}, c, {
ref: e,
sx: at({
position: "relative",
height: 0,
overflow: "hidden",
pb: "".concat(100 / i, "%")
}, s)
}), rt(l.b, {
sx: {
position: "absolute",
top: 0,
right: 0,
bottom: 0,
left: 0
}
}, a)))
}
))
, ct = (o.a.createElement,
function(t) {
var e, i, r = t.title, o = t.subtitle, a = t.media, s = t.actionsCollection, u = t.invert;
return Object(c.c)(l.h, {
columns: [1, null, "repeat(2, fit-content(50%))"],
gap: [4, null, null, 5],
sx: {
width: ["100%", null, "fit-content"],
mx: "auto",
gridAutoFlow: "dense",
alignItems: "center"
}
}, Object(c.c)("div", {
sx: {
gridColumn: u ? ["1", null, "2"] : "1",
height: "100%",
minHeight: "250px",
maxHeight: "450px",
width: ["100%", null, "469px"]
}
}, Object(c.c)(st, {
ratio: 497 / 300
}, Object(c.c)("div", {
sx: {
height: "100%",
width: "100%",
variant: "gradients.soft",
border: "1px solid",
borderColor: "border",
boxShadow: "card",
borderRadius: "md",
overflow: "hidden",
gridColumn: u ? ["1", null, "2"] : "1"
}
}, Object(c.c)("img", {
src: null !== (e = null === a || void 0 === a ? void 0 : a.url) && void 0 !== e ? e : "",
alt: null !== (i = null === a || void 0 === a ? void 0 : a.title) && void 0 !== i ? i : "",
sx: {
objectFit: "cover",
width: "100%",
height: "100%"
}
})))), Object(c.c)("div", {
sx: {
maxWidth: "md"
}
}, Object(c.c)(l.i, {
as: "h3",
variant: "heading.3",
sx: {
mb: 3
}
}, Object(C.a)(r)), Object(c.c)(l.o, {
variant: "small",
sx: {
mb: 3,
"p:not(:last-of-type)": {
mb: 3
}
}
}, Object(p.a)(null === o || void 0 === o ? void 0 : o.json)), null === s || void 0 === s ? void 0 : s.items.map((function(t) {
return Object(c.c)(b.a, Object(n.a)({}, t, {
key: null === t || void 0 === t ? void 0 : t.href,
sx: {
":not(:last-of-type)": {
mr: 3
}
},
variant: "accent",
arrow: !0
}))
}
))))
}
)
, lt = (o.a.createElement,
function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.anchorLinkId
, s = t.actionsCollection;
return Object(c.c)(f.a, {
anchorId: o,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json,
smallContainer: !0
}, Object(c.c)(l.h, {
columns: 1,
gap: 5
}, null === r || void 0 === r ? void 0 : r.items.map((function(t) {
if ("SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename))
return Object(c.c)(ct, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.title
}, t));
if (a.b)
throw new Error("Unsupported block type, ".concat(null === t || void 0 === t ? void 0 : t.__typename));
return null
}
))), Object(c.c)(l.g, {
sx: {
justifyContent: "flex-end",
mt: 4
}
}, null === s || void 0 === s ? void 0 : s.items.map((function(t) {
return Object(c.c)(b.a, Object(n.a)({}, t, {
key: null === t || void 0 === t ? void 0 : t.href,
sx: {
":not(:last-of-type)": {
mr: 3
}
},
variant: "accent",
arrow: !0
}))
}
))))
}
)
, pt = (o.a.createElement,
function(t) {
var e, i, r = t.title, o = t.subtitle, a = t.image, s = t.actionsCollection, u = t.anchorLinkId, d = null === s || void 0 === s ? void 0 : s.items[0];
return Object(c.c)(f.a, {
anchorId: u,
title: {
text: r
}
}, Object(c.c)(l.h, {
columns: [1, null, "12fr 10fr"],
gap: [4, null, 5]
}, Object(c.c)("img", {
src: null !== (e = null === a || void 0 === a ? void 0 : a.url) && void 0 !== e ? e : "",
alt: null !== (i = null === a || void 0 === a ? void 0 : a.title) && void 0 !== i ? i : ""
}), Object(c.c)("div", {
sx: {
maxWidth: "md",
mx: ["auto", null, "0"],
pt: [0, null, 5],
textAlign: ["center", null, "left"]
}
}, Object(c.c)(l.o, {
variant: "small",
sx: {
mb: 4
}
}, Object(p.a)(null === o || void 0 === o ? void 0 : o.json)), d && Object(c.c)(b.a, Object(n.a)({}, d, {
variant: "buttons.primary",
sx: {
":not(:last-of-type)": {
mr: 12
}
}
})))))
}
)
, ut = o.a.createElement
, dt = function(t) {
var e = t.title
, i = t.subtitle
, r = t.image
, o = t.actionsCollection;
return ut(l.b, {
sx: {
px: [3, 4],
zIndex: "general2",
position: "relative"
}
}, ut(l.e, {
sx: {
backgroundImage: "url(".concat(null === r || void 0 === r ? void 0 : r.url, ")"),
backgroundSize: "cover",
borderRadius: "xl",
p: ["32px", "56px", "90px"],
maxWidth: 900,
color: "background",
boxShadow: "0px 100px 80px rgba(0, 0, 0, 0.07), 0px 42px 33px rgba(0, 0, 0, 0.05), 0px 22px 17px rgba(0, 0, 0, 0.04), 0px 12px 10px rgba(0, 0, 0, 0.03), 0px 6px 5px rgba(0, 0, 0, 0.02), 0px 3px 3px rgba(0, 0, 0, 0.01)",
my: ["64px", null, "80px"],
textAlign: ["center", null, "left"]
}
}, ut(l.i, {
as: "h2",
sx: {
fontSize: [7, 8, 9]
}
}, Object(C.a)(e)), ut(l.o, {
sx: {
maxWidth: "xl",
lineHeight: "large",
mt: 2,
mb: "24px"
}
}, Object(p.a)(null === i || void 0 === i ? void 0 : i.json)), null === o || void 0 === o ? void 0 : o.items.map((function(t, e) {
if (!t)
return null;
var i = 0 === e;
return ut(b.a, Object(n.a)({
key: "".concat(null === t || void 0 === t ? void 0 : t.href, "-").concat(e),
variant: i ? "buttons.primary" : "buttons.secondary",
sx: i ? {
mr: "12px",
variant: "gradients.button"
} : {
color: "background"
}
}, t))
}
))))
}
, ft = o.a.createElement
, bt = function(t) {
var e = t.title
, i = t.subtitle
, r = t.image
, o = t.actionsCollection;
return ft(l.b, {
sx: {
px: [3, 4],
zIndex: "general2",
position: "relative"
}
}, ft(l.e, {
sx: {
backgroundImage: "url(".concat(null === r || void 0 === r ? void 0 : r.url, ")"),
backgroundSize: "cover",
borderRadius: "xl",
p: ["32px", "56px", "90px"],
maxWidth: 900,
color: "text",
boxShadow: "0px 100px 80px rgba(0, 0, 0, 0.07), 0px 42px 33px rgba(0, 0, 0, 0.05), 0px 22px 17px rgba(0, 0, 0, 0.04), 0px 12px 10px rgba(0, 0, 0, 0.03), 0px 6px 5px rgba(0, 0, 0, 0.02), 0px 3px 3px rgba(0, 0, 0, 0.01)",
my: ["64px", null, "80px"],
textAlign: ["center", null, "left"]
}
}, ft(l.i, {
as: "h2",
sx: {
fontSize: [7, 8, 9]
}
}, Object(C.a)(e)), ft(l.o, {
sx: {
maxWidth: "xl",
lineHeight: "large",
mt: 2,
mb: "24px"
}
}, Object(p.a)(null === i || void 0 === i ? void 0 : i.json)), ft(l.h, {
sx: {
display: ["grid", "block"]
}
}, null === o || void 0 === o ? void 0 : o.items.map((function(t, e) {
if (!t)
return null;
var i = 0 === e;
return ft(b.a, Object(n.a)({
key: "".concat(null === t || void 0 === t ? void 0 : t.href, "-").concat(e),
variant: i ? "buttons.primary" : "buttons.secondary",
sx: i ? {
mr: [0, "12px"],
variant: "gradients.button"
} : {}
}, t))
}
)))))
}
, Ot = o.a.createElement;
function ht(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function jt(t) {
for (var e = 1; e < arguments.length; e++) {
var i = null != arguments[e] ? arguments[e] : {};
e % 2 ? ht(Object(i), !0).forEach((function(e) {
Object(s.a)(t, e, i[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(i)) : ht(Object(i)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(i, e))
}
))
}
return t
}
var Ct = function(t) {
var e = t.title
, i = t.subtitle
, n = t.blocksCollection
, r = t.anchorLinkId
, o = null === n || void 0 === n ? void 0 : n.items[0];
if ("ListBlock" !== (null === o || void 0 === o ? void 0 : o.__typename)) {
if (a.b)
throw new Error("Block has to be of type ListBlock, ".concat(JSON.stringify(o, null, 2)));
return null
}
return Ot(f.a, {
anchorId: r,
title: {
text: e
}
}, Ot(l.h, {
sx: {
alignItems: "center"
},
variant: "two"
}, Ot(d, {
blocks: [jt(jt({}, o), {}, {
isPositive: !0
})]
}), Ot(l.b, {
sx: {
variant: "text.small",
p: {
mb: 24
}
}
}, Object(p.a)(null === i || void 0 === i ? void 0 : i.json))))
}
, yt = i("ewfY")
, vt = (o.a.createElement,
function(t) {
var e, i, r = t.name, o = t.position, a = t.bio, s = t.avatar, p = t.socialMedia;
return Object(c.c)(l.d, {
sx: {
p: 0
}
}, s && Object(c.c)(st, {
ratio: 1
}, Object(c.c)("img", {
src: null !== (e = null === s || void 0 === s ? void 0 : s.url) && void 0 !== e ? e : "",
alt: null !== (i = null === s || void 0 === s ? void 0 : s.title) && void 0 !== i ? i : "",
sx: {
width: "100%",
objectFit: "cover",
height: "100%"
}
})), Object(c.c)(l.b, {
sx: {
p: 24
}
}, Object(c.c)(l.i, {
as: "h4",
variant: "heading.base",
sx: {
color: "primary",
fontWeight: 700,
fontSize: "22px"
}
}, r), o && Object(c.c)(l.o, {
sx: {
mt: "8px"
}
}, o), a && Object(c.c)(l.o, {
variant: "small",
sx: {
mt: 3
}
}, a), p && Object(c.c)(yt.a, Object(n.a)({}, p, {
pushSx: {
mt: 3
}
}))))
}
)
, xt = o.a.createElement
, mt = function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.anchorLinkId;
return xt(f.a, {
anchorId: o,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json,
smallContainer: !0
}, xt(l.h, {
variant: "three"
}, null === r || void 0 === r ? void 0 : r.items.map((function(t) {
if ("Person" === (null === t || void 0 === t ? void 0 : t.__typename))
return xt(vt, Object(n.a)({
key: t.name
}, t))
}
))))
}
, gt = (o.a.createElement,
function(t) {
var e, i, n = t.name, r = t.position, o = t.avatar;
return Object(c.c)(l.d, {
sx: {
p: 0
}
}, o && Object(c.c)(st, {
ratio: 1
}, Object(c.c)("img", {
src: null !== (e = null === o || void 0 === o ? void 0 : o.url) && void 0 !== e ? e : "",
alt: null !== (i = null === o || void 0 === o ? void 0 : o.title) && void 0 !== i ? i : "",
sx: {
width: "100%",
objectFit: "cover",
height: "100%"
}
})), Object(c.c)(l.b, {
sx: {
p: 24
}
}, Object(c.c)(l.i, {
as: "h4",
variant: "heading.base",
sx: {
color: "text",
fontWeight: 700,
fontSize: "18px"
}
}, n), r && Object(c.c)(l.o, {
variant: "small",
sx: {
mt: "4px"
}
}, r)))
}
)
, _t = o.a.createElement
, wt = function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.anchorLinkId;
return _t(f.a, {
anchorId: o,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json,
smallContainer: !0
}, _t(l.h, {
variant: "four"
}, null === r || void 0 === r ? void 0 : r.items.map((function(t) {
if ("Person" === (null === t || void 0 === t ? void 0 : t.__typename))
return _t(gt, Object(n.a)({
key: t.name
}, t))
}
))))
}
, Ft = o.a.createElement
, Ut = function(t) {
var e, i = t.title, r = t.logosCollection, o = t.anchorLinkId;
return Ft(f.a, {
anchorId: o,
smallContainer: !0
}, Ft(l.b, {
sx: {
position: "absolute",
top: -100,
bottom: -100,
right: 0,
left: 0,
backgroundImage: "url(/images/backgrounds/features-grid.svg)",
backgroundSize: "contain",
backgroundRepeat: "no-repeat",
backgroundPosition: "center",
zIndex: "behind",
pointerEvents: "none"
}
}), Ft(l.d, {
sx: {
mx: "auto",
py: 5,
px: 5,
position: "relative"
}
}, Ft(l.i, {
as: "h2",
variant: "heading.2",
sx: {
position: "relative",
maxWidth: "748px",
mx: "auto",
textAlign: "center"
}
}, Object(C.a)(i)), Ft(l.b, {
variant: "layout.sectionContent",
sx: {
display: "flex",
justifyContent: "center",
flexWrap: "wrap",
mt: -3
}
}, null === r || void 0 === r || null === (e = r.logosCollection) || void 0 === e ? void 0 : e.items.map((function(t) {
return Ft(G.a, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.name
}, t, {
pushSx: {
mx: 4,
mt: 3
}
}))
}
)))))
}
, St = o.a.createElement
, Mt = function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.anchorLinkId;
return St(f.a, {
anchorId: o,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json,
smallContainer: !0
}, St(l.h, {
variant: "three"
}, null === r || void 0 === r ? void 0 : r.items.map((function(t) {
if ("Person" === (null === t || void 0 === t ? void 0 : t.__typename))
return St(gt, Object(n.a)({
key: t.name
}, t, {
avatar: null
}))
}
))))
}
, kt = (o.a.createElement,
function(t) {
var e, i, n = t.title, r = t.subtitle, o = t.media, a = t.actionsCollection, s = null === a || void 0 === a ? void 0 : a.items[0];
return Object(c.c)(b.a, {
href: s ? s.href : void 0,
as: s ? "a" : "div",
variant: "cards.primary",
sx: {
display: "block",
p: 0,
"&:hover": {
boxShadow: "cardHover"
}
}
}, o && Object(c.c)("img", {
src: null !== (e = null === o || void 0 === o ? void 0 : o.url) && void 0 !== e ? e : "",
alt: null !== (i = null === o || void 0 === o ? void 0 : o.title) && void 0 !== i ? i : "",
sx: {
height: "275px",
width: "100%",
objectFit: "cover"
}
}), Object(c.c)(l.b, {
sx: {
p: 24
}
}, Object(c.c)(l.i, {
as: "h4",
variant: "heading.base",
sx: {
mb: 3,
fontWeight: 600
}
}, n), Object(c.c)(l.o, {
variant: "small"
}, Object(p.a)(null === r || void 0 === r ? void 0 : r.json)), s && Object(c.c)(l.o, {
sx: {
color: "primary",
mt: 3
}
}, s.children, " ", "->")))
}
)
, Gt = (o.a.createElement,
function(t) {
var e, i = t.title, r = t.subtitle, o = t.actionsCollection, a = t.blocksCollection, s = t.anchorLinkId, p = null !== (e = null === a || void 0 === a ? void 0 : a.items.filter((function(t) {
return "SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename)
}
))) && void 0 !== e ? e : [];
return Object(c.c)(f.a, {
anchorId: s,
title: {
text: i
},
subtitle: null === r || void 0 === r ? void 0 : r.json,
smallContainer: !0
}, Object(c.c)(l.h, {
variant: "two"
}, p.map((function(t, e) {
return Object(c.c)(kt, Object(n.a)({}, t, {
key: "".concat(t.title, "-").concat(e)
}))
}
))), (null === o || void 0 === o ? void 0 : o.items[0]) && Object(c.c)(b.a, Object(n.a)({
sx: {
display: "block",
mt: 4
},
variant: "accent",
arrow: !0
}, null === o || void 0 === o ? void 0 : o.items[0])))
}
)
, Pt = (o.a.createElement,
function(t) {
var e = t.name
, i = t.bio
, r = t.avatar
, o = t.socialMedia;
return Object(c.c)(l.d, {
sx: {
p: 0,
display: "flex",
flexDirection: "column",
alignItems: "center",
textAlign: "center",
overflow: "visible",
mt: "56px"
}
}, r && Object(c.c)(Y.a, {
image: r,
ratio: 1,
pushImageSx: {
borderRadius: "full"
},
pushSx: {
width: "114px",
mt: "-56px"
}
}), Object(c.c)(l.b, {
sx: {
p: 24
}
}, Object(c.c)(l.i, {
as: "h4",
variant: "heading.base",
sx: {
fontWeight: 700,
fontSize: "22px"
}
}, e), i && Object(c.c)(l.o, {
variant: "small",
sx: {
mt: 3
}
}, i), o && Object(c.c)(yt.a, Object(n.a)({}, o, {
pushSx: {
mt: 3,
mx: "auto"
}
}))))
}
)
, Zt = (o.a.createElement,
function(t) {
var e, i = t.title, r = t.subtitle, o = t.actionsCollection, a = t.blocksCollection, s = t.anchorLinkId, p = null !== (e = null === a || void 0 === a ? void 0 : a.items.filter((function(t) {
return "Person" === (null === t || void 0 === t ? void 0 : t.__typename)
}
))) && void 0 !== e ? e : [];
return Object(c.c)(f.a, {
anchorId: s,
title: {
text: i
},
subtitle: null === r || void 0 === r ? void 0 : r.json
}, Object(c.c)(l.h, {
variant: "three"
}, p.map((function(t, e) {
return Object(c.c)(Pt, Object(n.a)({}, t, {
key: "".concat(t.name, "-").concat(e)
}))
}
))), (null === o || void 0 === o ? void 0 : o.items[0]) && Object(c.c)(b.a, Object(n.a)({
sx: {
display: "block",
mt: 4
},
variant: "accent",
arrow: !0
}, null === o || void 0 === o ? void 0 : o.items[0])))
}
)
, Tt = o.a.createElement
, It = function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.smallHeading
, s = t.anchorLinkId;
return Tt(f.a, {
anchorId: s,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json,
smallHeading: o
}, Tt(l.h, {
variant: "four"
}, null === r || void 0 === r ? void 0 : r.items.map((function(t, e) {
switch (null === t || void 0 === t ? void 0 : t.__typename) {
case "MediaBlock":
return Tt(R.a, Object(n.a)({
key: "".concat(null === t || void 0 === t ? void 0 : t.title, "-").concat(e)
}, t));
default:
if (a.b)
throw new Error("Unsupported block type, ".concat(null === t || void 0 === t ? void 0 : t.__typename));
return null
}
}
))))
}
, Et = i("Tgqd");
o.a.createElement;
function Dt(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function At(t) {
for (var e = 1; e < arguments.length; e++) {
var i = null != arguments[e] ? arguments[e] : {};
e % 2 ? Dt(Object(i), !0).forEach((function(e) {
Object(s.a)(t, e, i[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(i)) : Dt(Object(i)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(i, e))
}
))
}
return t
}
var Lt = function(t) {
var e = t.text
, i = t.userName
, n = t.userTag
, o = t.pushSx;
return Object(c.c)(l.d, {
sx: At({
width: "380px",
background: "white"
}, o)
}, Object(c.c)(l.g, {
sx: {
alignItems: "center",
justifyContent: "space-between",
mb: 3
}
}, Object(c.c)("div", null, Object(c.c)(l.o, {
variant: "small",
sx: {
fontWeight: 600,
lineHeight: 1,
mb: 2
}
}, i), Object(c.c)(l.o, {
variant: "small",
sx: {
lineHeight: 1
}
}, "@", n)), Object(c.c)("i", {
sx: {
fontSize: 6
}
}, Object(c.c)(Et.FiTwitter, {
fill: "#059FF5",
stroke: "#059FF5"
}))), Object(c.c)(l.o, {
sx: {
fontWeight: 500,
b: {
color: "#059FF5"
},
whiteSpace: "break-spaces"
}
}, function(t) {
return t ? t.split(" ").map((function(t, e) {
if (t.includes("@") || t.includes("#")) {
var i = t.split("\n");
return Object(c.c)(r.Fragment, {
key: e
}, e > 0 && " ", i.map((function(t, e) {
return t.includes("@") || t.includes("#") ? Object(c.c)(r.Fragment, {
key: e
}, e > 0 && Object(c.c)("br", null), Object(c.c)("b", null, t)) : Object(c.c)(r.Fragment, {
key: e
}, e > 0 && Object(c.c)("br", null), t)
}
)))
}
return Object(c.c)(r.Fragment, {
key: e
}, e > 0 && " ", t)
}
)) : ""
}(e)))
}
, zt = o.a.createElement
, Bt = function(t) {
var e, i = t.title, r = t.subtitle, o = t.actionsCollection, a = t.blocksCollection, s = t.anchorLinkId, c = null !== (e = null === a || void 0 === a ? void 0 : a.items.filter((function(t) {
return "Tweet" === (null === t || void 0 === t ? void 0 : t.__typename)
}
))) && void 0 !== e ? e : [], l = Math.floor(c.length / 2), p = [c.slice(0, l), c.slice(c.length / 2)];
return zt(f.a, {
anchorId: s,
title: {
text: i
},
subtitle: null === r || void 0 === r ? void 0 : r.json,
pushSx: {
pb: [0, 0]
}
}, p[0] && zt(k.a, {
pushItemSx: {
alignItems: "flex-end",
mb: 4
}
}, p[0].map((function(t, e) {
return zt(Lt, Object(n.a)({}, t, {
key: "".concat(t.text, "-").concat(e),
pushSx: {
mx: 3
}
}))
}
))), p[1] && zt(k.a, {
pushItemSx: {
alignItems: "flex-start"
},
pushSx: {
pb: ["64px", null, "80px"]
},
left: !0
}, p[1].map((function(t, e) {
return zt(Lt, Object(n.a)({}, t, {
key: "".concat(t.text, "-").concat(e),
pushSx: {
mx: 3
}
}))
}
))), (null === o || void 0 === o ? void 0 : o.items[0]) && zt(b.a, Object(n.a)({
sx: {
display: "block",
mt: 4
},
variant: "accent",
arrow: !0
}, null === o || void 0 === o ? void 0 : o.items[0])))
}
, Rt = (o.a.createElement,
function(t) {
var e = t.name
, i = t.description
, r = t.actionsCollection
, o = t.logo;
return Object(c.c)(l.d, {
sx: {
p: 0,
display: "flex",
flexDirection: "column"
}
}, o && Object(c.c)(l.b, {
sx: {
p: "32px",
bg: "background",
borderBottom: "1px solid",
borderColor: "border"
}
}, Object(c.c)(st, {
ratio: 368 / 131
}, Object(c.c)(G.a, Object(n.a)({}, o, {
pushSx: {
height: "100%",
display: "flex",
alignItems: "center",
justifyContent: "center"
}
})))), Object(c.c)(l.g, {
sx: {
flexDirection: "column",
justifyContent: "space-between",
height: "100%"
}
}, Object(c.c)(l.b, {
sx: {
p: 24,
pb: 0
}
}, Object(c.c)(l.i, {
as: "h4",
variant: "heading.base",
sx: {
fontWeight: 700,
fontSize: "22px",
mt: 3
}
}, e), i && Object(c.c)(l.o, {
variant: "small",
sx: {
mt: 3
}
}, Object(p.a)(i.json))), Object(c.c)(l.b, {
sx: {
p: 24,
pt: 0
}
}, null !== r && void 0 !== r && r.items.length ? Object(c.c)(l.g, {
sx: {
justifyContent: "flex-end",
mt: "24px"
}
}, r.items.map((function(t) {
return Object(c.c)(b.a, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.href
}, t, {
sx: {
":not(:last-of-type)": {
mr: 3
}
},
variant: "accent",
arrow: !0
}))
}
))) : null)))
}
)
, Wt = o.a.createElement
, Vt = function(t) {
var e = t.title
, i = t.subtitle
, o = t.blocksCollection
, a = t.logosCollection
, s = t.anchorLinkId
, c = Object(r.useMemo)((function() {
var t;
return null !== (t = null === o || void 0 === o ? void 0 : o.items.filter((function(t) {
return "Customer" === (null === t || void 0 === t ? void 0 : t.__typename)
}
))) && void 0 !== t ? t : []
}
), [o]);
return Wt(f.a, {
anchorId: s,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json,
pushSx: {
pt: 0
}
}, Wt(l.h, {
variant: "three",
sx: {
mx: "auto",
width: "fit-content",
rowGap: 5,
columnGap: 4
}
}, c.map((function(t) {
return Wt(Rt, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.name
}, t))
}
))), a && Wt(I, a))
}
, Nt = i("vMDD")
, Ht = o.a.createElement
, qt = function(t) {
var e, i = t.title, n = t.subtitle, r = t.blocksCollection, o = t.anchorLinkId, s = null === r || void 0 === r ? void 0 : r.items.find((function(t) {
return "MarkdownBody" === (null === t || void 0 === t ? void 0 : t.__typename)
}
));
if (!s && a.b)
throw new Error("Body not found.");
return Ht(f.a, {
anchorId: o,
title: {
text: i
},
subtitle: null === n || void 0 === n ? void 0 : n.json
}, Ht(l.e, {
variant: "blogPost",
pt: ["64px", null, "80px"]
}, Ht(Nt.a, {
source: null !== (e = null === s || void 0 === s ? void 0 : s.body) && void 0 !== e ? e : ""
})))
}
, Yt = (o.a.createElement,
function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.anchorLinkId;
return Object(c.c)(f.a, {
anchorId: o,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json
}, Object(c.c)(l.h, {
columns: 1,
gap: 5
}, null === r || void 0 === r ? void 0 : r.items.map((function(t, e) {
if ("SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename))
return Object(c.c)(ct, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.title
}, t, {
invert: e % 2 === 0
}));
if (a.b)
throw new Error("Unsupported block type, ".concat(null === t || void 0 === t ? void 0 : t.__typename));
return null
}
))))
}
)
, Xt = (o.a.createElement,
function(t) {
var e = t.title
, i = t.subtitle
, r = t.actionsCollection
, o = t.icon
, a = Object(u.a)(o);
return Object(c.c)(l.d, null, Object(c.c)("div", {
sx: {
color: "primary",
fontSize: 6,
mb: 3
}
}, a && Object(c.c)(a, null)), Object(c.c)("div", null, Object(c.c)(l.i, {
as: "h4",
variant: "heading.base",
sx: {
fontWeight: 400
}
}, Object(C.a)(e)), i && Object(c.c)(l.o, {
variant: "small",
sx: {
mt: "8px"
}
}, Object(p.a)(null === i || void 0 === i ? void 0 : i.json)), (null === r || void 0 === r ? void 0 : r.items[0]) && Object(c.c)(b.a, Object(n.a)({}, null === r || void 0 === r ? void 0 : r.items[0], {
variant: "accent",
sx: {
mt: 3
},
arrow: !0
}))))
}
)
, Qt = (o.a.createElement,
function(t) {
var e = t.blocksCollection
, i = t.title
, r = t.subtitle
, o = t.anchorLinkId;
return Object(c.c)(f.a, {
anchorId: o,
title: {
text: i
},
subtitle: null === r || void 0 === r ? void 0 : r.json
}, Object(c.c)(l.h, {
variant: "three",
sx: {
mx: "auto",
width: "fit-content",
position: "relative"
}
}, null === e || void 0 === e ? void 0 : e.items.map((function(t) {
if ("SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename))
return Object(c.c)(Xt, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.title
}, t));
if (a.b)
throw new Error("Unsupported block type, ".concat(null === t || void 0 === t ? void 0 : t.__typename));
return null
}
))))
}
)
, Jt = i("z27g")
, Kt = i.n(Jt)
, $t = o.a.createElement
, te = function() {
window.whr_embed(436189, {
detail: "titles",
base: "jobs",
zoom: "country",
grouping: "departments"
})
}
, ee = function(t) {
var e = t.title
, i = t.subtitle
, n = t.anchorLinkId;
Object(r.useEffect)((function() {
!function(t) {
var e = document.createElement("script");
e.async = !0,
e.onload = te,
e.src = t,
document.body.appendChild(e)
}("https://www.workable.com/assets/embed.js")
}
), []);
var o = Object(r.useCallback)((function(t) {
var e, i = t.target;
if (t.preventDefault(),
function(t) {
return "function" === typeof (null === t || void 0 === t ? void 0 : t.closest)
}(i)) {
var n = i.closest(".whr-item");
if (n) {
var r = null === (e = n.querySelector("a")) || void 0 === e ? void 0 : e.href;
r && window.open(r, "_blank")
}
}
}
), []);
return $t(f.a, {
anchorId: n,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json,
id: "job-listings"
}, $t("div", {
id: "whr_embed_hook",
className: Kt.a.container,
onClick: o
}))
}
, ie = (o.a.createElement,
function(t) {
var e = t.name
, i = t.description
, r = t.listItemsCollection
, o = t.action
, a = t.isFeatured
, s = t.priceLabel;
return Object(c.c)(l.d, {
sx: {
position: "relative",
":hover": {
boxShadow: "cardHover"
}
}
}, Object(c.c)("div", {
sx: {
position: "absolute",
top: 0,
left: 0,
bottom: 0,
right: 0,
variant: "gradients.button",
display: a ? "block" : "none"
}
}), Object(c.c)("div", {
sx: {
position: "absolute",
top: 1,
left: 1,
bottom: 1,
right: 1,
borderRadius: "md",
variant: "gradients.muted",
display: a ? "block" : "none"
}
}), Object(c.c)(l.g, {
sx: {
flexDirection: "column",
justifyContent: "space-between",
zIndex: "general",
position: "relative",
height: "100%",
textAlign: "center"
}
}, Object(c.c)("div", null, Object(c.c)(l.i, {
variant: "heading.base",
sx: {
fontWeight: 600,
mb: 3
}
}, e), Object(c.c)(l.o, {
variant: "small"
}, Object(p.a)(null === i || void 0 === i ? void 0 : i.json)), Object(c.c)(l.i, {
variant: "heading.base",
sx: {
fontSize: "24px",
my: 4
}
}, Object(C.a)(s)), o && Object(c.c)("div", null, Object(c.c)(b.a, Object(n.a)({}, o, {
variant: a ? "buttons.primary" : "buttons.outline",
sx: {
width: "100%"
}
}))), Object(c.c)("div", {
sx: {
textAlign: "left",
mt: 4
}
}, null === r || void 0 === r ? void 0 : r.items.map((function(t) {
var e;
return Object(c.c)("div", {
key: null === t || void 0 === t ? void 0 : t.title,
sx: {
mb: 3
}
}, Object(c.c)(l.o, {
sx: {
fontSize: 1,
fontWeight: 700,
mb: 1
}
}, null === t || void 0 === t ? void 0 : t.title), Object(c.c)("ul", null, null === t || void 0 === t || null === (e = t.items) || void 0 === e ? void 0 : e.map((function(t) {
return Object(c.c)("li", {
key: t,
sx: {
pl: "16px",
variant: "text.small",
lineHeight: "loose",
":not(:last-of-type)": {
mb: "4px"
},
"&::before": {
content: '"\u2022"',
color: "primary",
fontWeight: "bold",
display: "inline-block",
width: "16px",
ml: "-16px",
fontSize: "18px",
lineHeight: 0
}
}
}, Object(C.a)(t))
}
))))
}
))))))
}
)
, ne = (o.a.createElement,
function(t) {
var e, i, r, o = t.title, a = t.subtitle, s = t.blocksCollection, u = t.anchorLinkId, d = null !== (e = null === s || void 0 === s ? void 0 : s.items.filter((function(t) {
return "PricingPlan" === (null === t || void 0 === t ? void 0 : t.__typename)
}
))) && void 0 !== e ? e : [], O = null === s || void 0 === s ? void 0 : s.items.find((function(t) {
return "SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename)
}
));
return Object(c.c)(f.a, {
anchorId: u,
title: {
text: o
},
subtitle: null === a || void 0 === a ? void 0 : a.json,
pushSx: {
pt: "0 !important"
}
}, Object(c.c)(l.h, {
variant: "four",
sx: {
gridTemplateColumns: ["1fr", "1fr 1fr", null, "repeat(3, 1fr)", "repeat(4, 1fr)"]
}
}, d.map((function(t) {
return Object(c.c)(ie, Object(n.a)({
key: t.name
}, t))
}
))), O && Object(c.c)(l.d, {
sx: {
display: ["block", "flex"],
justifyContent: "space-between",
mt: 5
}
}, Object(c.c)(l.b, {
sx: {
display: ["block", "flex"],
alignItems: "center"
}
}, Object(c.c)(l.i, {
variant: "heading.base",
sx: {
fontWeight: 600,
fontSize: "24px",
minWidth: "fit-content"
}
}, O.title), Object(c.c)(l.o, {
sx: {
my: [3, 0],
mx: [0, 4]
}
}, Object(p.a)(null === (i = O.subtitle) || void 0 === i ? void 0 : i.json))), Object(c.c)(b.a, Object(n.a)({}, null === (r = O.actionsCollection) || void 0 === r ? void 0 : r.items[0], {
variant: "buttons.primary",
sx: {
minWidth: "fit-content"
}
}))), Object(c.c)(l.o, {
variant: "small",
sx: {
mt: 4
}
}, "* coming soon"))
}
)
, re = (o.a.createElement,
function(t) {
var e, i = t.title, r = t.subtitle, o = t.blocksCollection, a = t.anchorLinkId, s = t.actionsCollection, l = null !== (e = null === o || void 0 === o ? void 0 : o.items.filter((function(t) {
return "Column" === (null === t || void 0 === t ? void 0 : t.__typename)
}
))) && void 0 !== e ? e : [], u = l.reduce((function(t, e) {
var i, n, r = null !== (i = null === (n = e.rowsCollection) || void 0 === n ? void 0 : n.items.length) && void 0 !== i ? i : 0;
return t < r ? r : t
}
), 0);
return Object(c.c)(f.a, {
anchorId: a,
title: {
text: i
},
subtitle: null === r || void 0 === r ? void 0 : r.json,
noContentDivider: !0
}, Object(c.c)("div", {
sx: {
overflowX: "auto"
}
}, Object(c.c)("table", {
sx: {
variant: "table",
margin: "auto"
}
}, Object(c.c)("thead", null, Object(c.c)("tr", null, l.map((function(t) {
return Object(c.c)("th", {
key: t.head,
sx: {
width: t.widthPercentage ? "".concat(t.widthPercentage, "%") : void 0
}
}, t.head)
}
)))), Object(c.c)("tbody", null, Object(x.a)(Array(u).keys()).map((function(t) {
return Object(c.c)("tr", {
key: t
}, l.map((function(e) {
var i, n, r = null === (i = e.rowsCollection) || void 0 === i ? void 0 : i.items[t];
return Object(c.c)("td", {
key: "".concat(e.head, "-row-").concat(t)
}, Object(p.a)(null === r || void 0 === r || null === (n = r.text) || void 0 === n ? void 0 : n.json))
}
)))
}
))))), Object(c.c)("div", {
sx: {
mt: 4,
display: "flex",
justifyContent: "flex-end"
}
}, null === s || void 0 === s ? void 0 : s.items.map((function(t) {
return Object(c.c)(b.a, Object(n.a)({}, t, {
key: null === t || void 0 === t ? void 0 : t.href,
sx: {
":not(:last-of-type)": {
mr: 3
}
},
variant: "accent",
arrow: !0
}))
}
))))
}
)
, oe = i("jhz8");
o.a.createElement;
function ae(t, e) {
var i = Object.keys(t);
if (Object.getOwnPropertySymbols) {
var n = Object.getOwnPropertySymbols(t);
e && (n = n.filter((function(e) {
return Object.getOwnPropertyDescriptor(t, e).enumerable
}
))),
i.push.apply(i, n)
}
return i
}
function se(t) {
for (var e = 1; e < arguments.length; e++) {
var i = null != arguments[e] ? arguments[e] : {};
e % 2 ? ae(Object(i), !0).forEach((function(e) {
Object(s.a)(t, e, i[e])
}
)) : Object.getOwnPropertyDescriptors ? Object.defineProperties(t, Object.getOwnPropertyDescriptors(i)) : ae(Object(i)).forEach((function(e) {
Object.defineProperty(t, e, Object.getOwnPropertyDescriptor(i, e))
}
))
}
return t
}
var ce = function(t) {
var e = t.label
, i = t.id
, n = t.placeholder
, o = t.usageRate
, a = t.calculate
, p = t.value
, u = t.setValues
, d = Object(r.useState)("")
, f = d[0]
, b = d[1]
, O = Object(r.useCallback)((function(t) {
if (o && o[i]) {
var e = o[i];
if (e) {
b(t.currentTarget.value);
var n = function(t) {
return parseInt(t.replace(/\D/g, ""))
}(t.currentTarget.value);
u((function(t) {
return se(se({}, t), {}, Object(s.a)({}, i, a(isNaN(n) ? 0 : n, e)))
}
))
}
}
}
), [a, i, u, o]);
return Object(c.c)(l.h, {
columns: [1, "1fr fit-content(14px) ".concat(le)],
sx: {
alignItems: "end"
},
gap: [0, 4]
}, Object(c.c)("div", null, Object(c.c)(l.l, {
htmlFor: i
}, e), Object(c.c)(l.k, {
placeholder: n,
id: i,
name: i,
type: "number",
onChange: O,
value: f,
sx: {
":after": {
content: '"OPS"'
}
}
})), Object(c.c)(l.o, {
sx: {
height: "50px",
display: "flex",
alignItems: "center",
fontSize: 4,
justifyContent: "center"
}
}, "="), Object(c.c)("div", {
sx: {
fontSize: "18px",
fontWeight: 600,
display: "flex",
alignItems: "center",
justifyContent: "center",
height: "50px",
width: ["100%", le],
bg: "#DFE7F8",
color: "text",
borderRadius: "md",
px: 2
}
}, Object(c.c)("span", {
sx: {
maxWidth: "100%",
textOverflow: "ellipsis",
overflow: "hidden",
whiteSpace: "nowrap"
}
}, Object(oe.b)(p))))
}
, le = (o.a.createElement,
"220px")
, pe = 1e6
, ue = [{
label: "Monthly read operations",
id: "readOperationsMillion",
placeholder: "",
calculate: function(t, e) {
return t * (e / pe)
}
}, {
label: "Monthly write operations",
id: "writeOperationsMillion",
placeholder: "",
calculate: function(t, e) {
return t * (e / pe)
}
}, {
label: "Monthly compute operations",
id: "transactionalComputeOperationsMillion",
placeholder: "",
calculate: function(t, e) {
return t * (e / pe)
}
}, {
label: "Monthly data stored (GB)",
id: "storageGb",
placeholder: "",
calculate: function(t, e) {
return t * e
}
}];
var de, fe = function(t) {
var e, i, o = t.title, a = t.subtitle, s = t.blocksCollection, p = t.totalLabel, u = t.anchorLinkId, d = null !== (e = null === s || void 0 === s ? void 0 : s.items.filter((function(t) {
return "PricingPlan" === (null === t || void 0 === t ? void 0 : t.__typename)
}
))) && void 0 !== e ? e : [], b = Object(r.useState)(null !== (i = d[0].name) && void 0 !== i ? i : ""), O = b[0], h = b[1], j = Object(r.useState)({
readOperationsMillion: 0,
storageGb: 0,
transactionalComputeOperationsMillion: 0,
writeOperationsMillion: 0
}), C = j[0], y = j[1], v = d.find((function(t) {
return t.name === O
}
)), x = function(t, e) {
var i = Object.values(t).reduce((function(t, e) {
return t + e
}
), 0);
return e && i < e ? e : i
}(C, null === v || void 0 === v ? void 0 : v.creditsPerMonth), m = Object(r.useCallback)((function(t) {
h(t.target.value)
}
), []);
return Object(c.c)(f.a, {
anchorId: u,
title: {
text: o
},
subtitle: null === a || void 0 === a ? void 0 : a.json
}, Object(c.c)(l.d, {
sx: {
maxWidth: "900px",
mx: "auto"
}
}, Object(c.c)(l.g, {
sx: {
alignItems: "center",
justifyContent: ["space-between", "flex-start"]
}
}, Object(c.c)(l.o, {
sx: {
mr: 4
}
}, "Select plan"), Object(c.c)("div", null, Object(c.c)(l.n, {
onChange: m,
value: O,
sx: {
width: "155px"
}
}, d.map((function(t) {
return Object(c.c)("option", {
key: t.name,
value: t.name
}, t.name)
}
))))), Object(c.c)("div", {
sx: {
"> div:not(:last-of-type)": {
mb: 4
},
mt: 4,
mb: 5
}
}, ue.map((function(t) {
return Object(c.c)(ce, Object(n.a)({
key: t.id
}, t, {
value: C[t.id],
setValues: y,
usageRate: null === v || void 0 === v ? void 0 : v.usageRate,
placeholder: "0"
}))
}
))), Object(c.c)(l.g, {
sx: {
justifyContent: "flex-end",
alignItems: "center",
flexDirection: ["column-reverse", "row"]
}
}, Object(c.c)(l.o, {
variant: "small",
sx: {
mr: [0, 4],
mt: 2
}
}, p), Object(c.c)("div", {
sx: {
fontSize: "18px",
fontWeight: 600,
display: "flex",
alignItems: "center",
justifyContent: "center",
height: "50px",
width: ["100%", le],
borderRadius: "md",
border: "2px solid",
borderColor: "primary",
color: "text",
px: 2
}
}, Object(c.c)("span", {
sx: {
maxWidth: "100%",
textOverflow: "ellipsis",
overflow: "hidden",
whiteSpace: "nowrap"
}
}, Object(oe.b)(x))))))
}, be = (o.a.createElement,
function() {
return Object(c.c)("svg", {
width: "23",
height: "19",
viewBox: "0 0 23 19",
fill: "none",
xmlns: "http://www.w3.org/2000/svg"
}, Object(c.c)("path", {
d: "M0 18.3158H9.48996V9.17295H4.87678C4.87678 5.84828 5.4699 3.48264 8.89683 3.4187L8.106 0.157959C2.04298 0.157959 0.395415 3.86625 0.131805 11.2189C0.0659023 13.2649 0 15.7584 0 18.3158ZM13.51 18.3158H23V9.17295H18.3868C18.3868 5.84828 18.9799 3.48264 22.4068 3.4187L21.616 0.157959C15.553 0.157959 13.9054 3.86625 13.6418 11.2189C13.5759 13.2649 13.51 15.7584 13.51 18.3158Z",
fill: "#444444"
}))
}
), Oe = function(t) {
var e, i, n = t.quote, r = t.person, o = t.logo;
return Object(c.c)(l.d, {
sx: {
padding: ["24px 32px", "40px 56px"],
bg: "background",
borderBottom: "1px solid",
borderColor: "border",
boxShadow: "0px 3px 12px rgba(0, 0, 0, 0.04)",
borderRadius: "10px",
display: "flex",
flexDirection: "column",
justifyContent: "space-between",
width: "100%"
}
}, Object(c.c)("div", {
sx: {
width: "100%",
display: "flex",
flexDirection: "column",
mb: "25px"
}
}, Object(c.c)("i", {
sx: {
alignSelf: "center"
}
}, Object(c.c)(be, null)), Object(c.c)("p", {
sx: {
fontSize: "16px",
lineHeight: "25px",
width: "100%",
mt: "34px",
mb: "9px"
}
}, n), Object(c.c)("p", {
sx: {
fontSize: "14px",
fontWeight: "500",
lineHeight: "22px"
}
}, null === r || void 0 === r ? void 0 : r.name, ", ", null === r || void 0 === r ? void 0 : r.position)), Object(c.c)("img", {
src: null !== (e = null === o || void 0 === o || null === (i = o.image) || void 0 === i ? void 0 : i.url) && void 0 !== e ? e : "",
sx: {
height: "22px",
minWidth: "82px",
objectFit: "cover",
alignSelf: "flex-end"
}
}))
}, he = (o.a.createElement,
function(t) {
var e, i = t.title, n = t.subtitle, r = t.blocksCollection, o = t.anchorLinkId, a = null !== (e = null === r || void 0 === r ? void 0 : r.items.filter((function(t) {
return "Quote2" === (null === t || void 0 === t ? void 0 : t.__typename)
}
))) && void 0 !== e ? e : [];
return Object(c.c)(f.a, {
anchorId: o,
title: {
text: i
},
subtitle: null === n || void 0 === n ? void 0 : n.json
}, Object(c.c)(l.h, {
gap: [3, 4],
columns: ["1fr", "1fr", "1fr 1fr"],
sx: {
display: "grid",
width: "100%"
}
}, a.map((function(t, e) {
return Object(c.c)(Oe, {
key: e,
quote: t.quote,
person: t.person,
logo: t.logo
})
}
))))
}
), je = (o.a.createElement,
function() {
return Object(c.c)("svg", {
width: "14",
height: "13",
viewBox: "0 0 14 13",
fill: "none",
xmlns: "http://www.w3.org/2000/svg"
}, Object(c.c)("path", {
fillRule: "evenodd",
clipRule: "evenodd",
d: "M7.25459 0.257034C7.33586 0.175557 7.43241 0.110915 7.5387 0.0668087C7.64499 0.0227025 7.75894 0 7.87402 0C7.9891 0 8.10305 0.0227025 8.20934 0.0668087C8.31563 0.110915 8.41218 0.175557 8.49345 0.257034L13.7428 5.50642C13.8243 5.58769 13.889 5.68424 13.9331 5.79053C13.9772 5.89682 13.9999 6.01077 13.9999 6.12585C13.9999 6.24093 13.9772 6.35488 13.9331 6.46117C13.889 6.56746 13.8243 6.66401 13.7428 6.74528L8.49345 11.9947C8.32917 12.1589 8.10635 12.2512 7.87402 12.2512C7.64169 12.2512 7.41887 12.1589 7.25459 11.9947C7.09031 11.8304 6.99802 11.6076 6.99802 11.3752C6.99802 11.1429 7.09031 10.9201 7.25459 10.7558L11.8863 6.12585L7.25459 1.49589C7.17312 1.41462 7.10847 1.31807 7.06437 1.21178C7.02026 1.10549 6.99756 0.991541 6.99756 0.876462C6.99756 0.761382 7.02026 0.647433 7.06437 0.541142C7.10847 0.43485 7.17312 0.338304 7.25459 0.257034Z",
fill: "#3736CD"
}), Object(c.c)("path", {
fillRule: "evenodd",
clipRule: "evenodd",
d: "M0 6.12612C0 5.89408 0.0921767 5.67155 0.256252 5.50747C0.420327 5.3434 0.642861 5.25122 0.874898 5.25122H12.2486C12.4806 5.25122 12.7031 5.3434 12.8672 5.50747C13.0313 5.67155 13.1235 5.89408 13.1235 6.12612C13.1235 6.35816 13.0313 6.58069 12.8672 6.74477C12.7031 6.90884 12.4806 7.00102 12.2486 7.00102H0.874898C0.642861 7.00102 0.420327 6.90884 0.256252 6.74477C0.0921767 6.58069 0 6.35816 0 6.12612Z",
fill: "#3736CD"
}))
}
), Ce = function(t) {
var e, i = t.title, n = t.subtitle, r = t.blocksCollection, o = t.anchorLinkId, a = null !== (e = null === r || void 0 === r ? void 0 : r.items.filter((function(t) {
return "CallToAction" === (null === t || void 0 === t ? void 0 : t.__typename)
}
))) && void 0 !== e ? e : [];
return Object(c.c)(f.a, {
anchorId: o,
title: {
text: i
},
subtitle: null === n || void 0 === n ? void 0 : n.json
}, Object(c.c)("div", {
sx: {
display: "flex",
flexDirection: "column",
alignItems: "center",
width: "100%"
}
}, a.map((function(t, e) {
var i;
return Object(c.c)(l.d, {
key: e,
sx: {
bg: "background",
borderBottom: "1px solid",
borderColor: "border",
borderRadius: "4px",
boxShadow: "0px 3px 12px rgba(0, 0, 0, 0.04)",
height: ["auto", "76px"],
minHeight: "76px",
padding: "12px 30px 12px 35px",
width: "100%",
maxWidth: "850px",
mb: "10px",
cursor: "pointer",
display: "flex",
alignItems: "center",
transition: "all 0.3s",
":hover": {
boxShadow: "cardHover"
}
}
}, Object(c.c)("a", {
href: null !== (i = null === t || void 0 === t ? void 0 : t.href) && void 0 !== i ? i : "",
sx: {
display: "flex",
justifyContent: "space-between",
alignItems: "center",
width: "100%"
}
}, Object(c.c)("p", {
sx: {
fontSize: ["14px", "16px", "18px", "20px"],
mr: "15px"
}
}, t.children), Object(c.c)("i", null, Object(c.c)(je, null))))
}
))))
}, ye = (o.a.createElement,
function() {
return Object(c.c)("svg", {
sx: {
width: ["22px", "30px", "39px"],
height: ["18px", "24px", "31px"]
},
viewBox: "0 0 39 31",
fill: "none",
xmlns: "http://www.w3.org/2000/svg"
}, Object(c.c)("path", {
d: "M0 0.000175476H16.0917V15.5033H8.26935C8.26935 21.1409 9.27508 25.1522 15.086 25.2606L13.745 30.7897C3.46419 30.7897 0.670487 24.5017 0.223496 12.0341C0.111748 8.56486 0 4.33672 0 0.000175476ZM22.9083 0.000175476H39V15.5033H31.1777C31.1777 21.1409 32.1834 25.1522 37.9943 25.2606L36.6533 30.7897C26.3725 30.7897 23.5788 24.5017 23.1318 12.0341C23.0201 8.56486 22.9083 4.33672 22.9083 0.000175476Z",
fill: "black"
}))
}
), ve = function() {
return Object(c.c)("svg", {
sx: {
width: ["22px", "30px", "39px"],
height: ["18px", "24px", "31px"]
},
viewBox: "0 0 39 31",
fill: "none",
xmlns: "http://www.w3.org/2000/svg"
}, Object(c.c)("path", {
d: "M39 0.210503L22.9083 0.210501L22.9083 15.7137L30.7307 15.7137C30.7307 21.3512 29.7249 25.3625 23.914 25.4709L25.255 31C35.5358 31 38.3295 24.712 38.7765 12.2444C38.8883 8.77519 39 4.54705 39 0.210503ZM16.0917 0.210501L-1.63818e-05 0.210499L-1.77371e-05 15.7137L7.82233 15.7137C7.82233 21.3512 6.8166 25.3625 1.00571 25.4709L2.34669 31C12.6275 31 15.4212 24.712 15.8682 12.2444C15.9799 8.77519 16.0917 4.54705 16.0917 0.210501Z",
fill: "black"
}))
}, xe = function(t) {
var e, i = t.title, n = t.blocksCollection, r = t.anchorLinkId, o = "Quote2" === (null === n || void 0 === n || null === (e = n.items[0]) || void 0 === e ? void 0 : e.__typename) ? null === n || void 0 === n ? void 0 : n.items[0] : null;
return Object(c.c)(f.a, {
anchorId: r,
isHero: !0,
title: {
text: i
}
}, Object(c.c)("div", {
sx: {
display: "flex",
flexDirection: "column",
width: "100%",
alignItems: "center"
}
}, Object(c.c)("div", {
sx: {
width: "100%",
display: "flex",
justifyContent: "space-between",
alignItems: "center",
maxWidth: "915px"
}
}, Object(c.c)("div", {
sx: {
height: "1px",
background: "rgba(0, 0, 0, 0.3)",
width: "6.5%"
}
}), Object(c.c)(ye, null), Object(c.c)("div", {
sx: {
height: "1px",
background: "rgba(0, 0, 0, 0.3)",
width: "80%"
}
})), Object(c.c)(l.i, {
variant: "heading",
sx: {
fontSize: ["25px", "32px", "40px"],
lineHeight: "1.35",
fontStyle: "italic",
width: "100%",
maxWidth: "760px",
padding: ["0 10px", "0 15px", "0 20px"],
textAlign: "center",
margin: ["35px 0 35px 0", "45px 0 45px 0", "55px 0 55px 0"]
}
}, null === o || void 0 === o ? void 0 : o.quote), Object(c.c)("div", {
sx: {
width: "100%",
display: "flex",
justifyContent: "space-between",
alignItems: "center",
maxWidth: "915px"
}
}, Object(c.c)("div", {
sx: {
height: "1px",
background: "rgba(0, 0, 0, 0.5)",
width: "80%"
}
}), Object(c.c)(ve, null), Object(c.c)("div", {
sx: {
height: "1px",
background: "rgba(0, 0, 0, 0.5)",
width: "6.5%"
}
}))))
}, me = (o.a.createElement,
function(t) {
var e = t.className
, i = t.title
, n = t.content;
return Object(c.c)(l.d, {
sx: {
p: ["40px 20px 40px 30px", "50px 40px 60px 50px", "60px 50px 77px 60px"]
},
className: e
}, Object(c.c)("div", null, Object(c.c)("p", {
sx: {
fontFamily: "Acumin Pro",
fontWeight: 600,
fontSize: "18px",
mb: [2, 3],
lineHeight: 1.5
}
}, i), Object(c.c)("p", {
sx: {
fontSize: "14px",
fontWeight: 400,
lineHeight: 1.85,
fontFamily: "Acumin Pro"
}
}, n)))
}
), ge = (o.a.createElement,
function(t) {
var e, i = t.title, n = t.subtitle, o = t.blocksCollection, a = t.anchorLinkId, s = Object(r.useState)(0), u = s[0], d = s[1], b = Object(g.useKeenSlider)({
slidesPerView: 1.7,
duration: 1e3,
spacing: 60,
centered: !0,
initial: 1,
breakpoints: {
"(max-width: 1024px)": {
slidesPerView: 1.2
},
"(max-width: 640px)": {
slidesPerView: 1
}
},
slideChanged: function(t) {
d(t.details().relativeSlide)
}
}), O = Object(m.a)(b, 2), h = O[0], j = O[1];
return Object(c.c)(l.b, {
sx: {
overflow: "hidden"
}
}, Object(c.c)(f.a, {
anchorId: a,
subtitle: null === n || void 0 === n ? void 0 : n.json,
title: {
text: i
},
alignLeft: !0,
isHero: !0,
smallContainer: !0
}, Object(c.c)("div", {
sx: {
display: "grid",
gridTemplateColumns: ["1fr", "1fr 1fr", "1fr 1fr 1fr"],
gap: ["20px 0", "40px 60px", "40px 60px"]
}
}, null === o || void 0 === o || null === (e = o.items) || void 0 === e ? void 0 : e.map((function(t, e) {
var i, n, r, o;
return "SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename) ? Object(c.c)("div", {
key: e,
sx: {
display: "flex",
alignItems: "flex-start"
}
}, Object(c.c)("img", {
src: null !== (i = null === t || void 0 === t || null === (n = t.media) || void 0 === n ? void 0 : n.url) && void 0 !== i ? i : "",
alt: null !== (r = null === t || void 0 === t || null === (o = t.media) || void 0 === o ? void 0 : o.title) && void 0 !== r ? r : ""
}), Object(c.c)("p", {
onClick: function() {
j.moveToSlideRelative(e)
},
sx: {
fontWeight: 600,
fontSize: "16px",
fontFamily: "Acumin pro",
marginLeft: "15px",
marginTop: "-4px",
color: u === e ? "#9439F9" : "black",
cursor: "pointer"
}
}, null === t || void 0 === t ? void 0 : t.title)) : null
}
)))), Object(c.c)(f.a, null, Object(c.c)(l.b, {
className: "keen-slider",
ref: h,
sx: {
position: "relative",
overflow: "visible",
width: "100%"
}
}, null === o || void 0 === o ? void 0 : o.items.map((function(t, e) {
var i, n, r;
return "SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename) ? Object(c.c)(me, {
className: "keen-slider__slide",
key: e,
title: null !== (i = t.title) && void 0 !== i ? i : "",
content: null !== (n = Object(p.a)(null === (r = t.subtitle) || void 0 === r ? void 0 : r.json)) && void 0 !== n ? n : ""
}) : null
}
))), Object(c.c)(l.b, {
sx: {
display: "flex",
alignItems: "center",
justifyContent: "center",
mt: 4
}
}, Object(c.c)(l.j, {
sx: {
variant: "links.accent",
"&:disabled": {
"&:hover": {
opacity: 1
}
}
},
onClick: null === j || void 0 === j ? void 0 : j.prev,
disabled: 0 === u
}, "<-"), Object(c.c)("div", null, null === o || void 0 === o ? void 0 : o.items.map((function(t, e) {
return Object(c.c)("button", {
key: "SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename) ? t.title : e,
onClick: function() {
j.moveToSlideRelative(e)
},
sx: {
width: "10px",
height: "10px",
bg: u === e ? "primary" : "gray",
borderRadius: "full",
mx: "2",
"@media screen and (max-width: 395px)": {
mx: "6px"
},
"@media screen and (max-width: 350px)": {
mx: "1"
},
"&:focus": {
outline: "none"
}
}
})
}
))), Object(c.c)(l.j, {
sx: {
variant: "links.accent",
"&:disabled": {
"&:hover": {
opacity: 1
}
}
},
onClick: null === j || void 0 === j ? void 0 : j.next,
disabled: u === (null === j || void 0 === j ? void 0 : j.details().size) - 1
}, "->"))))
}
), _e = (o.a.createElement,
function(t) {
var e, i, r = t.title, o = t.subtitle, a = t.media, s = t.actionsCollection, u = t.invert;
return Object(c.c)(l.h, {
columns: [1, null, "repeat(2, fit-content(50%))"],
gap: [4, null, null, 5],
sx: {
mx: "auto",
gridAutoFlow: "dense",
alignItems: "center",
justifyContent: "center"
}
}, Object(c.c)("div", {
sx: {
gridColumn: u ? ["1", null, "2"] : "1",
height: "100%",
minHeight: "250px",
maxHeight: "450px",
width: ["100%", null, "525px"]
}
}, Object(c.c)(st, {
ratio: 497 / 300
}, Object(c.c)("div", {
sx: {
height: "100%",
width: "100%",
variant: "gradients.soft",
border: "1px solid",
borderColor: "border",
boxShadow: "card",
borderRadius: "md",
overflow: "hidden",
gridColumn: u ? ["1", null, "2"] : "1"
}
}, Object(c.c)("img", {
src: null !== (e = null === a || void 0 === a ? void 0 : a.url) && void 0 !== e ? e : "",
alt: null !== (i = null === a || void 0 === a ? void 0 : a.title) && void 0 !== i ? i : "",
sx: {
objectFit: "cover",
width: "100%",
height: "100%"
}
})))), Object(c.c)("div", null, Object(c.c)(l.i, {
as: "h3",
variant: "heading.3",
sx: {
mb: 3
}
}, Object(C.a)(r)), Object(c.c)(l.o, {
variant: "small",
sx: {
mb: 3,
"p:not(:last-of-type)": {
mb: 3
}
}
}, Object(p.a)(null === o || void 0 === o ? void 0 : o.json)), null === s || void 0 === s ? void 0 : s.items.map((function(t) {
return Object(c.c)(b.a, Object(n.a)({}, t, {
key: null === t || void 0 === t ? void 0 : t.href,
sx: {
":not(:last-of-type)": {
mr: 3
}
},
variant: "accent",
arrow: !0
}))
}
))))
}
), we = (o.a.createElement,
function(t) {
var e = t.title
, i = t.subtitle
, r = t.blocksCollection
, o = t.anchorLinkId
, s = t.actionsCollection;
return Object(c.c)(f.a, {
anchorId: o,
title: {
text: e
},
subtitle: null === i || void 0 === i ? void 0 : i.json
}, Object(c.c)(l.h, {
columns: 1,
gap: 5
}, null === r || void 0 === r ? void 0 : r.items.map((function(t) {
if ("SectionBlock" === (null === t || void 0 === t ? void 0 : t.__typename))
return Object(c.c)(_e, Object(n.a)({
key: null === t || void 0 === t ? void 0 : t.title
}, t));
if (a.b)
throw new Error("Unsupported block type, ".concat(null === t || void 0 === t ? void 0 : t.__typename));
return null
}
))), Object(c.c)(l.g, {
sx: {
justifyContent: "flex-end",
mt: 4
}
}, null === s || void 0 === s ? void 0 : s.items.map((function(t) {
return Object(c.c)(b.a, Object(n.a)({}, t, {
key: null === t || void 0 === t ? void 0 : t.href,
sx: {
":not(:last-of-type)": {
mr: 3
}
},
variant: "accent",
arrow: !0
}))
}
))))
}
), Fe = o.a.createElement;
!function(t) {
t.ZigZag = "Zig Zag",
t.CaseStudies = "Case Studies",
t.IconCardsGrid = "Icon Cards Grid",
t.ApplicationsComparison = "Applications Comparison",
t.NewsCards = "News Cards",
t.FeaturesGrid = "Features Grid",
t.VerifiedbyJepsen = "Verified by Jepsen",
t.DeveloperCards = "Developer Cards",
t.VerticalImages = "Vertical Images",
t.Regions = "Regions",
t.Prefooter = "Prefooter",
t.PrefooterLight = "Prefooter Light",
t.CompanyVision = "Company Vision",
t.TeamGrid = "Team Grid",
t.BoardMembersGrid = "Board Members Grid",
t.BoardMembersWithoutAvatarGrid = "Board Members Without Avatar Grid",
t.LogosCard = "Logos Card",
t.TwoBigCards = "Two Big Cards",
t.CommunityLeadersGrid = "Community Leaders Grid",
t.MediaBlocksGrid = "Media Blocks Grid",
t.TweetsSlider = "Tweets Slider",
t.CustomersGrid = "Customers Grid",
t.MarkdownBody = "Markdown Body",
t.CareerPerksGrid = "Career Perks Grid",
t.ZigZagCareers = "Zig Zag Careers",
t.JobListings = "Job Listings",
t.PricingPlans = "Pricing Plans",
t.Table = "Table",
t.PriceCalculator = "Price Calculator",
t.IllustrationImages = "Illustration Images",
t.TwoQuotes = "Two Quotes",
t.CardLinks = "Card Links",
t.OneBigQuote = "One Big Quote",
t.Principles = "Principles",
t.CareersSlider = "Careers Slider",
t.WideVerticalImages = "Wide Vertical Images"
}(de || (de = {}));
e.b = function(t) {
var e, i = t.section, r = t.smallHeading, o = t.generalData;
if (!i) {
if (a.b)
throw new Error("Section came out as null.");
return null
}
switch (i.type) {
case de.ZigZag:
return Fe(B, i);
case de.CaseStudies:
return Fe(M, i);
case de.IconCardsGrid:
return Fe(A, i);
case de.ApplicationsComparison:
return Fe(j, i);
case de.NewsCards:
return Fe(q, i);
case de.FeaturesGrid:
return Fe(Q, i);
case de.VerifiedbyJepsen:
return Fe($, i);
case de.DeveloperCards:
return Fe(it, i);
case de.VerticalImages:
return Fe(lt, i);
case de.Regions:
return Fe(pt, i);
case de.Prefooter:
return Fe(dt, i);
case de.PrefooterLight:
return Fe(bt, i);
case de.CompanyVision:
return Fe(Ct, i);
case de.TeamGrid:
return Fe(mt, i);
case de.BoardMembersGrid:
return Fe(wt, i);
case de.BoardMembersWithoutAvatarGrid:
return Fe(Mt, i);
case de.LogosCard:
return Fe(Ut, i);
case de.TwoBigCards:
return Fe(Gt, i);
case de.CommunityLeadersGrid:
return Fe(Zt, i);
case de.MediaBlocksGrid:
return Fe(It, Object(n.a)({}, i, {
smallHeading: r
}));
case de.TweetsSlider:
return Fe(Bt, i);
case de.CustomersGrid:
return Fe(Vt, i);
case de.MarkdownBody:
return Fe(qt, i);
case de.ZigZagCareers:
return Fe(Yt, i);
case de.CareerPerksGrid:
return Fe(Qt, i);
case de.JobListings:
return Fe(ee, i);
case de.PricingPlans:
return Fe(ne, i);
case de.Table:
return Fe(re, i);
case de.PriceCalculator:
return Fe(fe, Object(n.a)({}, i, {
totalLabel: null !== (e = null === o || void 0 === o ? void 0 : o.pricingPageTotalLabel) && void 0 !== e ? e : "Total"
}));
case de.IllustrationImages:
return Fe(B, Object(n.a)({}, i, {
isFixed: !0
}));
case de.TwoQuotes:
return Fe(he, i);
case de.CardLinks:
return Fe(Ce, i);
case de.OneBigQuote:
return Fe(xe, i);
case de.Principles:
return Fe(ge, i);
case de.WideVerticalImages:
return Fe(we, i);
default:
if (a.b)
throw new Error("Unsupported section type, ".concat(i.type, "."));
return null
}
}
},
shcM: function(t, e, i) {
!function(t) {
"use strict";
var e, i, n, r, o, a = function() {
return "undefined" !== typeof window
}, s = function() {
return e || a() && (e = window.gsap) && e.registerPlugin && e
}, c = /[-+=\.]*\d+[\.e\-\+]*\d*[e\-\+]*\d*/gi, l = {
rect: ["width", "height"],
circle: ["r", "r"],
ellipse: ["rx", "ry"],
line: ["x2", "y2"]
}, p = function(t) {
return Math.round(1e4 * t) / 1e4
}, u = function(t) {
return parseFloat(t || 0)
}, d = function(t, e) {
return u(t.getAttribute(e))
}, f = Math.sqrt, b = function(t, e, i, n, r, o) {
return f(Math.pow((u(i) - u(t)) * r, 2) + Math.pow((u(n) - u(e)) * o, 2))
}, O = function(t) {
return console.warn(t)
}, h = function(t) {
return "non-scaling-stroke" === t.getAttribute("vector-effect")
}, j = 1, C = function(t, e, i) {
var n, r, o = t.indexOf(" ");
return o < 0 ? (n = void 0 !== i ? i + "" : t,
r = t) : (n = t.substr(0, o),
r = t.substr(o + 1)),
(n = ~n.indexOf("%") ? u(n) / 100 * e : u(n)) > (r = ~r.indexOf("%") ? u(r) / 100 * e : u(r)) ? [r, n] : [n, r]
}, y = function(t) {
if (!(t = i(t)[0]))
return 0;
var e, n, r, o, a, s, p, u = t.tagName.toLowerCase(), j = t.style, C = 1, y = 1;
h(t) && (y = t.getScreenCTM(),
C = f(y.a * y.a + y.b * y.b),
y = f(y.d * y.d + y.c * y.c));
try {
n = t.getBBox()
} catch (w) {
O("Some browsers won't measure invisible elements (like display:none or masks inside defs).")
}
var v = n || {
x: 0,
y: 0,
width: 0,
height: 0
}
, x = v.x
, m = v.y
, g = v.width
, _ = v.height;
if (n && (g || _) || !l[u] || (g = d(t, l[u][0]),
_ = d(t, l[u][1]),
"rect" !== u && "line" !== u && (g *= 2,
_ *= 2),
"line" === u && (x = d(t, "x1"),
m = d(t, "y1"),
g = Math.abs(g - x),
_ = Math.abs(_ - m))),
"path" === u)
o = j.strokeDasharray,
j.strokeDasharray = "none",
e = t.getTotalLength() || 0,
C !== y && O("Warning: <path> length cannot be measured when vector-effect is non-scaling-stroke and the element isn't proportionally scaled."),
e *= (C + y) / 2,
j.strokeDasharray = o;
else if ("rect" === u)
e = 2 * g * C + 2 * _ * y;
else if ("line" === u)
e = b(x, m, x + g, m + _, C, y);
else if ("polyline" === u || "polygon" === u)
for (r = t.getAttribute("points").match(c) || [],
"polygon" === u && r.push(r[0], r[1]),
e = 0,
a = 2; a < r.length; a += 2)
e += b(r[a - 2], r[a - 1], r[a], r[a + 1], C, y) || 0;
else
"circle" !== u && "ellipse" !== u || (s = g / 2 * C,
p = _ / 2 * y,
e = Math.PI * (3 * (s + p) - f((3 * s + p) * (s + 3 * p))));
return e || 0
}, v = function(t, e) {
if (!(t = i(t)[0]))
return [0, 0];
e || (e = y(t) + 1);
var r = n.getComputedStyle(t)
, o = r.strokeDasharray || ""
, a = u(r.strokeDashoffset)
, s = o.indexOf(",");
return s < 0 && (s = o.indexOf(" ")),
(o = s < 0 ? e : u(o.substr(0, s)) || 1e-5) > e && (o = e),
[Math.max(0, -a), Math.max(0, o - a)]
}, x = function() {
a() && (n = window,
o = e = s(),
i = e.utils.toArray,
r = -1 !== ((n.navigator || {}).userAgent || "").indexOf("Edge"))
}, m = {
version: "3.5.1",
name: "drawSVG",
register: function(t) {
e = t,
x()
},
init: function(t, e, i, a, s) {
if (!t.getBBox)
return !1;
o || x();
var c, l, d, f, b = y(t) + 1;
return this._style = t.style,
this._target = t,
e + "" === "true" ? e = "0 100%" : e ? -1 === (e + "").indexOf(" ") && (e = "0 " + e) : e = "0 0",
c = v(t, b),
l = C(e, b, c[0]),
this._length = p(b + 10),
0 === c[0] && 0 === l[0] ? (d = Math.max(1e-5, l[1] - b),
this._dash = p(b + d),
this._offset = p(b - c[1] + d),
this._offsetPT = this.add(this, "_offset", this._offset, p(b - l[1] + d))) : (this._dash = p(c[1] - c[0]) || 1e-6,
this._offset = p(-c[0]),
this._dashPT = this.add(this, "_dash", this._dash, p(l[1] - l[0]) || 1e-5),
this._offsetPT = this.add(this, "_offset", this._offset, p(-l[0]))),
r && (f = n.getComputedStyle(t)).strokeLinecap !== f.strokeLinejoin && (l = u(f.strokeMiterlimit),
this.add(t.style, "strokeMiterlimit", l, l + .01)),
this._live = h(t) || ~(e + "").indexOf("live"),
this._props.push("drawSVG"),
j
},
render: function(t, e) {
var i, n, r, o, a = e._pt, s = e._style;
if (a) {
for (e._live && (i = y(e._target) + 11) !== e._length && (n = i / e._length,
e._length = i,
e._offsetPT.s *= n,
e._offsetPT.c *= n,
e._dashPT ? (e._dashPT.s *= n,
e._dashPT.c *= n) : e._dash *= n); a; )
a.r(t, a.d),
a = a._next;
r = e._dash,
o = e._offset,
i = e._length,
s.strokeDashoffset = e._offset,
1 !== t && t ? s.strokeDasharray = r + "px," + i + "px" : (r - o < .001 && i - r <= 10 && (s.strokeDashoffset = o + 1),
s.strokeDasharray = o < .001 && i - r <= 10 ? "none" : o === r ? "0px, 999999px" : r + "px," + i + "px")
}
},
getLength: y,
getPosition: v
};
s() && e.registerPlugin(m),
t.DrawSVGPlugin = m,
t.default = m,
Object.defineProperty(t, "__esModule", {
value: !0
})
}(e)
},
z27g: function(t, e, i) {
t.exports = {
container: "workable_container__3oTzf"
}
}
}]);
<file_sep>/terms-condition.html
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Terms & Condition</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" href="assets/favicon.svg" type="image/svg" sizes="24x24">
<title>Luxury</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"
integrity="<KEY>" crossorigin="anonymous">
<link href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet"
integrity="<KEY>" crossorigin="anonymous">
<link rel="stylesheet" href="https://pro.fontawesome.com/releases/v5.10.0/css/all.css"/>
<!-- Animation link -->
<link href="https://unpkg.com/[email protected]/dist/aos.css" rel="stylesheet">
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<div class="s_container">
<!-- header start -->
<nav class="main-menu navbar fixed-top navbar-expand-lg bg-white">
<a class="navbar-brand" href="index.html">
<img src="./assets/img/home/logo.svg" alt="img">
</a>
<div class="navbar-collapse" id="navbarNavDropdown">
<ul class="show-hide-nav navbar-nav justify-content-center">
<li class="nav-item ">
<a class="nav-link " href="services.html">
<img class="hovericon" src="assets/services.svg">
<img class="hovericon1" src="assets/services_color.svg">
Service </a>
</li>
<!-- <li class="nav-item">
<a class="nav-link" href="#">
<img class="hovericon" src="assets/img/home/Join as Partner.svg">
<img class="hovericon1" src="assets/join_as_color.svg" alt="">
Join as Partner</a>
</li> -->
<li class="nav-item">
<a class="nav-link" href="help.html">
<img class="hovericon" src="assets/img/home/help.svg">
<img class="hovericon1" src="assets/help_color.svg">
Help</a>
</li>
<li class="nav-item">
<a class="nav-link" href="contactus.html">
<img class="hovericon" src="assets/contact-us.svg">
<img class="hovericon1" src="assets/contact_us_color.svg">
Contact Us</a>
</li>
</ul>
<div class="nav-btns d-flex align-items-center w-20 justify-content-end">
<!-- <button class="btn btn-primary mr-2 ">
<img src="assets/login.svg" alt="">
Sign in
</button> -->
<button class="btn lan">
<img src="assets/lan.svg" alt="">
EN
</button>
</div>
<button id="bars-btn" class="toggle-bar">
<i class="fa fa-bars"></i>
</button>
</div>
</nav>
<!-- header end -->
<!-- Start Services page -->
<!-- Strat Banner -->
<div class="Services-banner Privacy-banner">
<div class="container">
<h5>Ryde Luxury</h5>
<h1>Terms And Conditions</h1>
<p>Last Updated: December 4Th, 2017</p>
</div>
</div>
<div class="brack-line m-t-custom"></div>
<div class="container privacy-content">
<h2>1. Contractual Relationship</h2>
<p>These Terms of Use (“Terms”) govern the access or use by you, an individual, from within any country in the world (excluding the United States and its territories and possessions and Mainland China) of applications, websites, content, products, and services (the “Services”) made available by Ryde Luxury B.V., a private limited liability company established in the Netherlands, having its offices at Mr. Treublaan 7, 1097 DP, Amsterdam, the Netherlands, registered at the Amsterdam Chamber of Commerce under number 56317441 (“Ryde luxury”).
</p>
<p>PLEASE READ THESE TERMS CAREFULLY BEFORE ACCESSING OR USING THE SERVICES.
</p>
<p>
Your access and use of the Services constitutes your agreement to be bound by these Terms, which establishes a contractual relationship between you and Ryde Luxury. If you do not agree to these Terms, you may not access or use the Services. These Terms expressly supersede prior agreements or arrangements with you. Ryde Luxury may immediately terminate these Terms or any Services with respect to you, or generally cease offering or deny access to the Services or any portion thereof, at any time for any reason.
</p>
<p>
Supplemental terms may apply to certain Services, such as policies for a particular event, activity or promotion, and such supplemental terms will be disclosed to you in connection with the applicable Services. Supplemental terms are in addition to, and shall be deemed a part of, the Terms for the purposes of the applicable Services. Supplemental terms shall prevail over these Terms in the event of a conflict with respect to the applicable Services.
</p>
<p>
Ryde Luxury may amend the Terms related to the Services from time to time. Amendments will be effective upon Uber’s posting of such updated Terms at this location or the amended policies or supplemental terms on the applicable Service. Your continued access or use of the Services after such posting constitutes your consent to be bound by the Terms, as amended.
</p>
<p>Our collection and use of personal information in connection with the Services is as provided in Uber’s Privacy Policy located at https://www.uber.com/privacy/notice. Ryde Luxury may provide to a claims processor or an insurer any necessary information (including your contact information) if there is a complaint, dispute or conflict, which may include an accident, involving you and a Third Party Provider (including a transportation network company driver) and such information or data is necessary to resolve the complaint, dispute or conflict.</p>
<h2>2. The Services</h2>
<p>These Terms of Use (“Terms”) govern the access or use by you, an individual, from within any country in the world (excluding the United States and its territories and possessions and Mainland China) of applications, websites, content, products, and services (the “Services”) made available by Ryde Luxury B.V., a private limited liability company established in the Netherlands, having its offices at Mr. Treublaan 7, 1097 DP, Amsterdam, the Netherlands, registered at the Amsterdam Chamber of Commerce under number 56317441 (“Ryde luxury”).
</p>
<p>PLEASE READ THESE TERMS CAREFULLY BEFORE ACCESSING OR USING THE SERVICES.
</p>
<h3>License.</h3>
<p>Your access and use of the Services constitutes your agreement to be bound by these Terms, which establishes a contractual relationship between you and Ryde Luxury. If you do not agree to these Terms, you may not access or use the Services. These Terms expressly supersede prior agreements or arrangements with you. Ryde Luxury may immediately terminate these Terms or any Services with respect to you, or generally cease offering or deny access to the Services or any portion thereof, at any time for any reason.
</p>
<h3>Restrictions.</h3>
<p>Your access and use of the Services constitutes your agreement to be bound by these Terms, which establishes a contractual relationship between you and Ryde Luxury. If you do not agree to these Terms, you may not access or use the Services. These Terms expressly supersede prior agreements or arrangements with you. Ryde Luxury may immediately terminate these Terms or any Services with respect to you, or generally cease offering or deny access to the Services or any portion thereof, at any time for any reason.
</p>
<h3>Provision of the Services.</h3>
<p>Your access and use of the Services constitutes your agreement to be bound by these Terms, which establishes a contractual relationship between you and Ryde Luxury. If you do not agree to these Terms, you may not access or use the Services. These Terms expressly supersede prior agreements or arrangements with you. Ryde Luxury may immediately terminate these Terms or any Services with respect to you, or generally cease offering or deny access to the Services or any portion thereof, at any time for any reason.
</p>
<h3>Third Party Services and Content.</h3>
<p>Your access and use of the Services constitutes your agreement to be bound by these Terms, which establishes a contractual relationship between you and Ryde Luxury. If you do not agree to these Terms, you may not access or use the Services. These Terms expressly supersede prior agreements or arrangements with you. Ryde Luxury may immediately terminate these Terms or any Services with respect to you, or generally cease offering or deny access to the Services or any portion thereof, at any time for any reason.
</p>
<h2>3. Your Use of the Services</h2>
<p>These Terms of Use (“Terms”) govern the access or use by you, an individual, from within any country in the world (excluding the United States and its territories and possessions and Mainland China) of applications, websites, content, products, and services (the “Services”) made available by Ryde Luxury B.V., a private limited liability company established in the Netherlands, having its offices at Mr. Treublaan 7, 1097 DP, Amsterdam, the Netherlands, registered at the Amsterdam Chamber of Commerce under number 56317441 (“Ryde luxury”).
</p>
<p>PLEASE READ THESE TERMS CAREFULLY BEFORE ACCESSING OR USING THE SERVICES.
</p>
<p>Your access and use of the Services constitutes your agreement to be bound by these Terms, which establishes a contractual relationship between you and Ryde Luxury. If you do not agree to these Terms, you may not access or use the Services. These Terms expressly supersede prior agreements or arrangements with you. Ryde Luxury may immediately terminate these Terms or any Services with respect to you, or generally cease offering or deny access to the Services or any portion thereof, at any time for any reason.</p>
<h2>4. Payment</h2>
<p>You understand that use of the Services may result in charges to you for the services or goods you receive from a Third Party Provider (“Charges ”). After you have received services or goods obtained through your use of the Service, Ryde luxury will facilitate your payment of the applicable Charges on behalf of the Third Party Provider as such Third Party Provider’s limited payment collection agent. Payment of the Charges in such manner shall be considered the same as payment made directly by you to the Third Party Provider. Charges will be inclusive of applicable taxes where required by law. Charges paid by you are final and non-refundable, unless otherwise determined by Ryde luxury. You retain the right to request lower Charges from a Third Party Provider for services or goods received by you from such Third Party Provider at the time you receive such services or goods. Ryde luxury will respond accordingly to any request from a Third Party Provider to modify the Charges for a particular service or good.
</p>
<p>All Charges are due immediately and payment will be facilitated by Uber using the preferred payment method designated in your Account, after which Ryde luxury will send you a receipt by email. If your primary Account payment method is determined to be expired, invalid or otherwise not able to be charged, you agree that Uber may, as the Third Party Provider’s limited payment collection agent, use a secondary payment method in your Account, if available.</p>
<p>As between you and Ryde luxury, Ryde luxury reserves the right to establish, remove and/or revise Charges for any or all services or goods obtained through the use of the Services at any time in Ryde luxury sole discretion. Further, you acknowledge and agree that Charges applicable in certain geographical areas may increase substantially during times of high demand. Ryde luxury will use reasonable efforts to inform you of Charges that may apply, provided that you will be responsible for Charges incurred under your Account regardless of your awareness of such Charges or the amounts thereof. Ryde luxury may from time to time provide certain users with promotional offers and discounts that may result in different amounts charged for the same or similar services or goods obtained through the use of the Services, and you agree that such promotional offers and discounts, unless also made available to you, shall have no bearing on your use of the Services or the Charges applied to you. You may elect to cancel your request for services or goods from a Third Party Provider at any time prior to such Third Party Provider’s arrival, in which case you may be charged a cancellation fee.
</p>
<p>This payment structure is intended to fully compensate the Third Party Provider for the services or goods provided. Except with respect to taxicab transportation services requested through the Application, Uber does not designate any portion of your payment as a tip or gratuity to the Third Party Provider. Any representation by Ryde luxury (on Ryde luxuryr’s website, in the Application, or in Ryde luxurys marketing materials) to the effect that tipping is “voluntary,” “not required,” and/or “included” in the payments you make for services or goods provided is not intended to suggest that Ryde luxury provides any additional amounts, beyond those described above, to the Third Party Provider. You understand and agree that, while you are free to provide additional payment as a gratuity to any Third Party Provider who provides you with services or goods obtained through the Service, you are under no obligation to do so. Gratuities are voluntary. After you have received services or goods obtained through the Service, you will have the opportunity to rate your experience and leave additional feedback about your Third Party Provider.</p>
</div>
<div class="brack-line m-t-custom"></div>
<!-- Start Servces on row -->
<div class="container">
<div class="row">
<div class="col-lg-4 col-sm-4 col-xs-12 ser-community" data-aos="zoom-in" data-aos-delay="200" data-aos-duration="800">
<img src="assets/NoPath - Copy (36)@2x.jpg" alt="">
<div class="">
<h6>Community</h6>
<h5>host wants everyone to try tesla</h5>
<span>December 2, 2020</span>
</div>
</div>
<div class="col-lg-4 col-sm-4 col-xs-12 ser-community" data-aos="zoom-in" data-aos-delay="400" data-aos-duration="800">
<img src="assets/NoPath - Copy (43)@2x.jpg" alt="">
<div class="">
<h6>Community</h6>
<h5>Channel a Beyoncé moment at your
next spa trip</h5>
<span>December 2, 2020</span>
</div>
</div>
<div class="col-lg-4 col-sm-4 col-xs-12 ser-community" data-aos="zoom-in" data-aos-delay="600" data-aos-duration="800">
<img src="assets/NoPath - Copy (44)@2x.jpg" alt="">
<div class="">
<h6>Community</h6>
<h5>US Airmen surprise squadron
commander</h5>
<span>December 2, 2020</span>
</div>
</div>
</div>
</div>
<!-- Start Servces on row -->
<!-- Strat Your Luxury Ride Is One Click Away -->
<div class="YLR-box container">
<div class="YLR row">
<div class="col-lg-6" data-aos="fade-right" data-aos-delay="300" data-aos-duration="800">
<img class="w-100" src="assets/[email protected]" alt="">
</div>
<div class="col-lg-6 YLR-details" data-aos="fade-left" data-aos-delay="300" data-aos-duration="800">
<h1>Your Luxury Ride Is One <br> Click Away</h1>
<p>Easily Book, Change, Or Cancel Rides On The Go.</p>
<button onclick="window.location.href='booknow.html';">Book Now</button>
</div>
</div>
</div>
<!-- End Your Luxury Ride Is One Click Away -->
</div>
<!-- End Services on colum -->
<!-- End Services page -->
<!-- Strat Footer -->
<div class="container-fuild footer-main">
<div class="footer-inner">
<div class="footer-header">
<div class="footer-logo">
<img src="assets/footer-logo.svg" alt="">
</div>
<div class="footer-app-btn">
<img class="mr-3" src="assets/footer-play-store.svg" alt="">
<img src="assets/footer-google-play.svg" alt="">
</div>
</div>
<div class="footer-content row">
<div class="col-lg-4 col-sm-6">
<ul>
<li>
<h3>Company</h3>
</li>
<li>
<a href="aboutus.html">About Us</a>
</li>
<!-- <li>
<a href="#">Career</a>
</li>
<li>
<a href="">Press</a>
</li>
<li>
<a href="blog.html">Blog</a>
</li> -->
<li>
<a href="services.html">Service</a>
</li>
</ul>
</div>
<div class="col-lg-4 col-sm-6">
<ul>
<li>
<h3>Resource</h3>
</li>
<!-- <li>
<a href="">Download</a>
</li> -->
<li>
<a href="help.html">Help Center</a>
</li>
<!-- <li>
<a href="">Guides</a>
</li> -->
<li>
<a href="contactus.html">Partner</a>
</li>
<li>
<a href="contactus.html">Developers</a>
</li>
</ul>
</div>
<!-- <div class="col-lg-3 col-sm-6">
<ul>
<li>
<h3>Locations</h3>
</li>
<li>
<a href="">USA (EN)</a>
</li>
<li>
<a href="#">Canada (EN)</a>
</li>
<li>
<a href="#">Canada (FR)</a>
</li>
<li>
<a href="">UK (EN)</a>
</li>
</ul>
</div> -->
<div class="col-lg-4 col-sm-6">
<h3 class="footer-blog">Blogs</h3>
<img class="w-100 mt-4" src="assets/img/home/NoPath - [email protected]" alt="">
<div class="footer-b-detial">
<h6>Community</h6>
<h3>
What To Expect From A Professional <br>
Chauffeur
</h3>
<span>December 2, 2020</span>
</div>
</div>
<div class="social-icons col-lg-12">
<ul>
<li>
<a href="">
<i class="fab fa-facebook-square" aria-hidden="true"></i>
</a>
</li>
<li>
<a href="">
<i class="fab fa-twitter" aria-hidden="true"></i>
</a>
</li>
<li>
<a href="">
<i class="fab fa-youtube" aria-hidden="true"></i>
</a>
</li>
<li>
<a href="">
<i class="fab fa-linkedin" aria-hidden="true"></i>
</a>
</li>
<li>
<a href="">
<i class="fab fa-instagram" aria-hidden="true"></i>
</a>
</li>
</ul>
</div>
</div>
</div>
</div>
<div class="copy-rightbar">
<p>©2020 ryde Luxury</p>
<ul>
<li>
<a href="terms-condition.html">
Terms
</a>
</li>
<li>
<a href="privacy-policy.html">
Privacy
</a>
</li>
<li>
<a href="#">
Sitemap
</a>
</li>
</ul>
</div>
<!-- End Footer -->
<script src="https://code.jquery.com/jquery-3.2.1.slim.min.js"
integrity="<KEY>" crossorigin="anonymous">
</script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js"
integrity="<KEY>" crossorigin="anonymous">
</script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"
integrity="<KEY>" crossorigin="anonymous">
</script>
<!-- Animation -->
<script src="https://unpkg.com/[email protected]/dist/aos.js"></script>
<script>
AOS.init();
</script>
<!-- Animation -->
<script>
$(document).ready(function(){
$("#bars-btn").click(function(){
$(".show-hide-nav").toggle();
});
});
</script>
</body>
</html>
| 06666653efd980f23d3a9024d47f7116f10fc2f8 | [
"JavaScript",
"HTML"
]
| 3 | HTML | haseeb67/rydeluxury.github.io | 69f9dbe7fca4e50cca1a37eaab6095dd85ed361f | e49bef3f20f1f6cd3e34f6ada22351aa517308d2 | |
refs/heads/master | <repo_name>battbeach/netdata<file_sep>/README.md
# netdata
Dockerfile for building and running a netdata deamon for your host instance.
Netdata monitors your server with thoughts of performance and memory usage, providing detailed insight into
very recent server metrics. It's nice, and now it's also dockerized.
More info about project: https://github.com/firehol/netdata
# Using
```
docker run -d --cap-add SYS_PTRACE \
-v /proc:/host/proc:ro \
-v /sys:/host/sys:ro \
-p 19999:19999 titpetric/netdata
```
Open a browser on http://server:19999/ and watch how your server is doing.
# Getting emails on alarms
Netdata supports forwarding alarms to an email address. You can set up sSMTP by setting the following ENV variables:
- SSMTP_TO - This is the address alarms will be delivered to.
- SSMTP_SERVER - This is your SMTP server. Defaults to smtp.gmail.com.
- SSMTP_PORT - This is the SMTP server port. Defaults to 587.
- SSMTP_USER - This is your username for the SMTP server.
- SSMTP_PASS - This is your password for the SMTP server. Use an app password if using Gmail.
- SSMTP_TLS - Use TLS for the connection. Defaults to YES.
- SSMTP_HOSTNAME - The hostname mail will come from. Defaults to localhost.
For example, using gmail:
```
-e SSMTP_TO=<EMAIL> -e SSMTP_USER=user -e SSMTP_PASS=<PASSWORD>
```
Alternatively, if you already have s sSMTP config, you can use that config with:
~~~
-v /path/to/config:/etc/ssmtp
~~~
See the following link for details on setting up sSMTP: [SSMTP - ArchWiki](https://wiki.archlinux.org/index.php/SSMTP)
# Monitoring docker container metrics
Netdata supports fetching container data from `docker.sock`. You can forward it to the netdata container with:
~~~
-v /var/run/docker.sock:/var/run/docker.sock
~~~
This will allow netdata to resolve container names.
> Note: forwarding docker.sock exposes the administrative docker API. If due to some security issue access has been obtained to the container, it will expose full docker API, allowing to stop, create or delete containers, as well as download new images in the host.
>
> TL;DR If you care about security, consider forwarding a secure docker socket with [docker-proxy-acl](https://github.com/titpetric/docker-proxy-acl)
# Monitoring docker notes on some systems (Debian jessie)
On debian jessie only 'cpu' and 'disk' metrics show up under individual docker containers. To get the memory metric, you will have to add `cgroup_enable=memory swapaccount=1` to `/etc/default/grub`, appending the `GRUB_CMDLINE_LINUX_DEFAULT` variable:
~~~
$ cat /etc/default/grub | grep GRUB_CMDLINE_LINUX_DEFAULT
GRUB_CMDLINE_LINUX_DEFAULT="quiet cgroup_enable=memory swapaccount=1"
~~~
After rebooting your linux instance, the memory accounting subsystem of the kernel will be enabled. Netdata will pick up additional metrics for the containers when it starts.
# Environment variables
It's possible to pass a NETDATA_PORT environment variable with -e, to start up netdata on a different port.
```
docker run -e NETDATA_PORT=80 [...]
```
# Some explanation is in order
Docker needs to run with the SYS_PTRACE capability. Without it, the mapped host/proc filesystem
is not fully readable to the netdata deamon, more specifically the "apps" plugin:
```
16-01-12 07:58:16: ERROR: apps.plugin: Cannot process /host/proc/1/io (errno 13, Permission denied)
```
See the following link for more details: [/proc/1/environ is unavailable in a container that is not priviledged](https://github.com/docker/docker/issues/6607)
# Limitations
In addition to the above requirements and limitations, monitoring the complete network interface list of
the host is not possible from within the Docker container. If you're running netdata and want to graph
all the interfaces available on the host, you will have to use `--net=host` mode.
See the following link for more details: [network interfaces missing when mounting proc inside a container](https://github.com/docker/docker/issues/13398)
# Additional notes
Netdata provides monitoring via a plugin architecture. This plugin supports many projects that don't
provide data over the `/proc` filesystem. When you're running netdata in the container, you will have
difficulty providing many of these paths to the netdata container.
What you do get (even with the docker version) is:
* Host CPU statististics
* Host Network I/O, QoS
* Host Disk I/O
* Applications monitoring
* Container surface metrics (cpu/disk per name)
You will not get detailed application metrics (mysql, etc.) from other containers or from the host if running netdata in a container. It may be possible to get *some* of those metrics, but it might not be easy, and most likely not worth it. For most detailed metrics, netdata needs to share the same environment as the application server it monitors. This means it would need to run either in the same container (not even remotely practical), or in the same virtual machine (no containers).
What I can tell you is that it's very stable, and snappy. Godspeed!
<file_sep>/Makefile
build:
docker build --rm --no-cache=true -t titpetric/netdata .
.PHONY: build<file_sep>/run.sh
#!/bin/bash
# fix permissions due to netdata running as root
chown root:root /usr/share/netdata/web/ -R
# set up ssmtp
if [[ $SSMTP_TO ]] && [[ $SSMTP_USER ]] && [[ $SSMTP_PASS ]]; then
cat << EOF > /etc/ssmtp/ssmtp.conf
root=$SSMTP_TO
mailhub=$SSMTP_SERVER:$SSMTP_PORT
AuthUser=$SSMTP_USER
AuthPass=$SSMTP_<PASSWORD>
UseSTARTTLS=$SSMTP_TLS
hostname=$SSMTP_HOSTNAME
FromLineOverride=NO
EOF
cat << EOF > /etc/ssmtp/revaliases
netdata:netdata@$SSMTP_HOSTNAME:$SSMTP_SERVER:$SSMTP_PORT
root:netdata@$SSMTP_HOSTNAME:$SSMTP_SERVER:$SSMTP_PORT
EOF
fi
# exec custom command
if [[ $# -gt 0 ]] ; then
exec "$@"
exit
fi
# main entrypoint
exec /usr/sbin/netdata -D -u root -s /host -p ${NETDATA_PORT}
| 1f5d4a1f862aa16c5126df91a1799cc3ef064717 | [
"Markdown",
"Makefile",
"Shell"
]
| 3 | Markdown | battbeach/netdata | 29f5e4dafba2944bcc16ffd718fe13a5ce6586ea | 2aeea674578aa881fade90b74bfaa32df2068f8e | |
refs/heads/master | <repo_name>mfrancetic/guess-the-celebrity<file_sep>/README.md
# guess-the-celebrity
"Guess the Celebrity" project for the Udemy's "The Complete Android Oreo Developer Course"
A game for guessing the top 100 celebrities from the website http://www.posh24.se/kandisar.
## App Screenshot
<img src="https://user-images.githubusercontent.com/33599053/68930734-4fdfb480-078f-11ea-91b4-ece9d9af30fe.png" width=30% height=30%>
<file_sep>/app/src/main/java/com/mfrancetic/guessthecelebrity/MainActivity.java
package com.mfrancetic.guessthecelebrity;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.AsyncTask;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.Toast;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.ArrayList;
import java.util.Random;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class MainActivity extends AppCompatActivity {
private ImageView imageView;
private String websiteContent;
private Pattern pattern;
private Matcher matcher;
private ArrayList<String> celebrityPhotoUrlList = new ArrayList<>();
private ArrayList<String> celebrityNameList = new ArrayList<>();
private int currentTask;
private Random random;
private Button button0;
private Button button1;
private Button button2;
private Button button3;
private int locationOfCorrectAnswer;
private ArrayList<String> answerNameList;
private static final String answerNameListTag = "answerNameList";
private static final String currentTaskTag = "currentTask";
private static final String websiteContentTag = "websiteContent";
private static final String celebrityPhotoUrlListTag = "celebrityPhotoUrlList";
private static final String celebrityNameListTag = "celebrityNameList";
private static final String locationOfCorrectAnswerTag = "locationOfCorrectAnswer";
private static final String photoUrlPattern = "<img src=\"(.*?)\"";
private static final String namePattern = "alt=\"(.*?)\"";
private static final String photoUrlFilter = ":profile/";
private static final String websiteUrl = "http://www.posh24.se/kandisar";
private int celebrityListBound;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageView = findViewById(R.id.celebrity_image_view);
button0 = findViewById(R.id.button0);
button1 = findViewById(R.id.button1);
button2 = findViewById(R.id.button2);
button3 = findViewById(R.id.button3);
if (savedInstanceState != null) {
currentTask = savedInstanceState.getInt(currentTaskTag);
websiteContent = savedInstanceState.getString(websiteContent);
celebrityPhotoUrlList = savedInstanceState.getStringArrayList(celebrityPhotoUrlListTag);
celebrityNameList = savedInstanceState.getStringArrayList(celebrityNameListTag);
if (celebrityNameList != null) {
celebrityListBound = celebrityNameList.size() - 1;
}
answerNameList = savedInstanceState.getStringArrayList(answerNameListTag);
locationOfCorrectAnswer = savedInstanceState.getInt(locationOfCorrectAnswerTag);
createNewCelebrityPhoto(currentTask);
populateAnswerList(answerNameList);
} else {
websiteContent = getWebsiteContent();
if (websiteContent != null) {
celebrityPhotoUrlList = getCelebrityPhotoUrlList(websiteContent);
celebrityNameList = getCelebrityNameList(websiteContent);
celebrityListBound = celebrityNameList.size() - 1;
currentTask = getCurrentTask();
createNewTask(currentTask);
}
}
}
public static class DownloadCelebrityImageTask extends AsyncTask<String, Void, Bitmap> {
@Override
protected Bitmap doInBackground(String... urls) {
URL url;
try {
url = new URL(urls[0]);
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.connect();
InputStream inputStream = urlConnection.getInputStream();
return BitmapFactory.decodeStream(inputStream);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
}
public static class DownloadWebsiteContentTask extends AsyncTask<String, Void, String> {
@Override
protected String doInBackground(String... urls) {
String result = "";
URL url;
try {
url = new URL(urls[0]);
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.connect();
InputStream inputStream = urlConnection.getInputStream();
InputStreamReader reader = new InputStreamReader(inputStream);
int data = reader.read();
while (data != -1) {
char current = (char) data;
result += current;
data = reader.read();
}
return result;
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
}
private void createNewTask(int taskCounter) {
createNewCelebrityPhoto(taskCounter);
createNewCelebrityNameList(taskCounter);
}
private String getWebsiteContent() {
DownloadWebsiteContentTask downloadWebsiteContentTask = new DownloadWebsiteContentTask();
String result = null;
try {
result = downloadWebsiteContentTask.execute(websiteUrl).get();
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
private ArrayList<String> getCelebrityPhotoUrlList(String websiteContent) {
pattern = Pattern.compile(photoUrlPattern);
String celebrityPhotoUrl;
ArrayList<String> celebrityPhotoUrlList = new ArrayList<>();
matcher = pattern.matcher(websiteContent);
while (matcher.find()) {
celebrityPhotoUrl = matcher.group(1);
if (celebrityPhotoUrl != null && celebrityPhotoUrl.contains(photoUrlFilter)) {
celebrityPhotoUrlList.add(celebrityPhotoUrl);
}
}
return celebrityPhotoUrlList;
}
private ArrayList<String> getCelebrityNameList(String websiteContent) {
pattern = Pattern.compile(namePattern);
String name;
ArrayList<String> celebrityNameList = new ArrayList<>();
matcher = pattern.matcher(websiteContent);
while (matcher.find()) {
name = matcher.group(1);
celebrityNameList.add(name);
}
return celebrityNameList;
}
private void createNewCelebrityPhoto(int taskCounter) {
String url = celebrityPhotoUrlList.get(taskCounter);
DownloadCelebrityImageTask downloadCelebrityImageTask = new DownloadCelebrityImageTask();
Bitmap bitmap;
try {
bitmap = downloadCelebrityImageTask.execute(url).get();
imageView.setImageBitmap(bitmap);
} catch (Exception e) {
e.printStackTrace();
}
}
private void createNewCelebrityNameList(int correctAnswer) {
String name;
answerNameList = new ArrayList<>();
random = new Random();
int wrongAnswer;
locationOfCorrectAnswer = random.nextInt(4);
for (int i = 0; i < 4; i++) {
if (i == locationOfCorrectAnswer) {
answerNameList.add(celebrityNameList.get(correctAnswer));
} else {
wrongAnswer = random.nextInt(celebrityListBound);
while (wrongAnswer == correctAnswer) {
wrongAnswer = random.nextInt(celebrityListBound);
}
name = celebrityNameList.get(wrongAnswer);
answerNameList.add(name);
}
}
populateAnswerList(answerNameList);
}
public void chooseAnswer(View view) {
int tag = Integer.parseInt(view.getTag().toString());
String toast;
if (isAnswerCorrect(tag)) {
toast = getString(R.string.answer_correct);
} else {
toast = getString(R.string.answer_wrong) + " " + celebrityNameList.get(currentTask);
}
Toast.makeText(this, toast, Toast.LENGTH_SHORT).show();
currentTask = getCurrentTask();
createNewTask(currentTask);
}
private boolean isAnswerCorrect(int tag) {
return tag == locationOfCorrectAnswer;
}
@Override
protected void onSaveInstanceState(@NonNull Bundle outState) {
outState.putInt(currentTaskTag, currentTask);
outState.putString(websiteContentTag, websiteContent);
outState.putStringArrayList(celebrityPhotoUrlListTag, celebrityPhotoUrlList);
outState.putStringArrayList(celebrityNameListTag, celebrityNameList);
outState.putStringArrayList(answerNameListTag, answerNameList);
outState.putInt(locationOfCorrectAnswerTag, locationOfCorrectAnswer);
super.onSaveInstanceState(outState);
}
private void populateAnswerList(ArrayList<String> nameList) {
button0.setText(nameList.get(0));
button1.setText(nameList.get(1));
button2.setText(nameList.get(2));
button3.setText(nameList.get(3));
}
private int getCurrentTask() {
random = new Random();
int newTask = random.nextInt(celebrityListBound);
while (newTask == currentTask) {
newTask = random.nextInt(celebrityListBound);
}
return newTask;
}
} | 0d06a923771948f7626b332dadee16b1db081597 | [
"Markdown",
"Java"
]
| 2 | Markdown | mfrancetic/guess-the-celebrity | 39b26d8cc5f26f1afc415e1fd27e54766710ef6f | ce72915ba313da34709cec324b45d23df23d851d | |
refs/heads/master | <file_sep>require('./index');
describe('required', () => {
const arr = [1, 2, 3, 2, 4, 1, 5, 1, 6];
const fn = (val => val % 3);
test('default', () => {
const a = arr.groupBy();
const b = new Map([
[1, [1, 1, 1]],
[2, [2, 2]],
[3, [3]],
[4, [4]],
[5, [5]],
[6, [6]],
]);
expect(a).toEqual(b);
});
test('custom', () => {
const a = arr.groupBy(fn);
const b = new Map([
[1, [1, 4, 1, 1]],
[2, [2, 2, 5]],
[0, [3, 6]],
]);
expect(a).toEqual(b);
});
});
describe('custom', () => {
const foo = () => {};
const bar = { a: 42 };
const arr = [
1, NaN, 2, 'foo', null, 'bar', 0b1,
'foo', NaN, undefined, null, 0, '', '',
foo, bar, bar, foo, undefined, 652,
];
const fn = (val => val % 3);
const err = 'Not a function';
test('null', () => {
expect(() => arr.groupBy(null)).toThrowError(err);
});
test('invalid', () => {
expect(() => arr.groupBy(42)).toThrowError(err);
});
test('default', () => {
const a = arr.groupBy();
const b = new Map([
[1, [1, 1]],
[NaN, [NaN, NaN]],
[2, [2]],
['foo', ['foo', 'foo']],
[null, [null, null]],
['bar', ['bar']],
[undefined, [undefined, undefined]],
[0, [0]],
['', ['', '']],
[foo, [foo, foo]],
[bar, [bar, bar]],
[652, [652]],
]);
expect(a).toEqual(b);
});
test('custom', () => {
const a = arr.groupBy(fn);
const b = new Map([
[1, [1, 1, 652]],
[NaN, [NaN, 'foo', 'bar', 'foo', NaN, undefined, foo, bar, bar, foo, undefined]],
[2, [2]],
[0, [null, null, 0, '', '']],
]);
expect(a).toEqual(b);
});
test('immutable', () => {
const a = arr;
arr.groupBy(fn);
expect(arr).toEqual(a);
});
});
<file_sep>const quaddouble = require('./index');
describe('required', () => {
test('(45568411115, 11223344) -> true', () => {
expect(quaddouble(45568411115, 11223344)).toBe(true);
});
test('(11112344445, 442253) -> true', () => {
expect(quaddouble(11112344445, 442253)).toBe(true);
});
test('(12222345, 123452) -> false', () => {
expect(quaddouble(12222345, 123452)).toBe(false);
});
test('(12345, 12345) -> false', () => {
expect(quaddouble(12345, 12345)).toBe(false);
});
});
describe('custom', () => {
test('(4556841.1115, 0.11223344) -> false', () => {
expect(quaddouble(4556841.1115, 0.11223344)).toBe(false);
});
test('(121122111222, 0) -> false', () => {
expect(quaddouble(121122111222, 0)).toBe(false);
});
test('(1 / 3, 33) -> true', () => {
expect(quaddouble(1 / 3, 33)).toBe(true);
});
test('(0b100001, 0b1001) -> false', () => {
expect(quaddouble(0b100001, 0b1001)).toBe(false);
});
});
<file_sep>import { GameStrategy, playMultipleTimes } from '.'
describe('default', () => {
test('change', () => {
const probabilityOnChange = playMultipleTimes(GameStrategy.CHANGE)
expect(probabilityOnChange).toBeCloseTo(2 / 3)
})
test('insist', () => {
const probabilityOnInsist = playMultipleTimes(GameStrategy.INSIST)
expect(probabilityOnInsist).toBeCloseTo(1 / 3)
})
})
<file_sep>class Item<TValue = unknown> {
// eslint-disable-next-line no-use-before-define
public next: Item<TValue> | undefined
public value: TValue
public constructor(value: TValue) {
this.next = undefined
this.value = value
}
}
export class List<TValue = unknown> {
private head: Item<TValue> | undefined
public constructor(size: number, generateValue: () => TValue) {
let i: number = size
let item: Item<TValue> | undefined
let tail: Item<TValue> | undefined
while (--i >= 0) {
item = new Item(generateValue())
if (tail) tail.next = item
else this.head = item
tail = item
}
}
public reverse(): this {
let current: Item<TValue> | undefined = this.head
let next: Item<TValue> | undefined
let prev: Item<TValue> | undefined
while (current) {
next = current.next
current.next = prev
prev = current
current = next
}
this.head = prev
return this
}
public values(): TValue[] {
const values: TValue[] = []
let item: Item<TValue> | undefined = this.head
while (item) {
values.push(item.value)
item = item.next
}
return values
}
}
<file_sep>class Heap {
private static heapify(a: number[]): void {
let swaps: number = 0
const parent = (i: number): number => {
if (i === 0) return 0
else {
return i % 2 === 0
? (i - 2) / 2 // i = 2p + 2
: (i - 1) / 2
} // i = 2p + 1
}
const swap = (a: number[], i1: number, i2: number): void => {
const v = a[i1]
a[i1] = a[i2]
a[i2] = v
swaps++
}
const bubble = (a: number[], i: number): void => {
while (true) {
const p = parent(i)
if (a[i] > a[p]) {
swap(a, i, p)
i = p
} else {
break
}
}
}
for (let i = 0, l = a.length; i < l; i++) {
bubble(a, i)
}
console.info(Math.round(swaps / a.length))
}
private readonly a: number[]
public constructor(a: number[]) {
const h: number[] = a.slice()
Heap.heapify(h)
this.a = h
}
public print(): void {
let l = 1
let i = 0
while (i < this.a.length) {
console.log(this.a.slice(i, i + l))
i += l
l *= 2
}
}
}
for (let i = 0; i < 8; i++) {
const numbers: number[] = new Array(Math.pow(10, i)).fill(0).map((_, i) => i)
const heap: Heap = new Heap(numbers)
// heap.print()
}
<file_sep>'use strict';
function look (graph, component, queue) {
var vertex, i;
while (queue.length) {
vertex = graph[queue.shift()];
vertex.component = component;
for (i = 0; i < vertex.length; i++) {
if (!graph[vertex[i]].component) queue.push(vertex[i]);
}
}
}
function check (graph) {
for (var i = 0; i < graph.length; i++) {
if (!graph[i].component) return i;
}
return null;
}
module.exports = function (graph) {
var start = 0;
var component = 1;
do {
look(graph, component, [start]);
start = check(graph);
component++;
} while (start);
return graph;
};
<file_sep>'use strict';
var list = require('./list');
var action = require('./' + (process.argv[2] || 'reverse'));
var len = Math.round(process.argv[3]) || 30;
var list1 = list(len);
list1.print();
var list2 = action(list1);
list2.print();
<file_sep>'use strict';
function RandomArray (length) {
for (var i = 0; i < length; i++) {
this.push(Math.round(Math.random() * length));
}
}
RandomArray.prototype = Object.create(Array.prototype);
RandomArray.prototype.constructor = RandomArray;
RandomArray.prototype.copy = function (arr) {
var i = arr.length;
while (--i >= 0) this[i] = arr[i];
return this;
};
RandomArray.prototype.print = function () {
if (this.length < 40) console.log(this.join(','));
return this;
};
RandomArray.prototype.swap = function (i, j) {
var val = this[i];
this[i] = this[j];
this[j] = val;
return this;
};
module.exports = RandomArray;
<file_sep>// https://leetcode.com/problems/add-two-numbers/
describe('', () => {
function foo(l1: number[], l2: number[]): number[] {
let sum: number
let next: number = 0
let i: number = 0
const result: number[] = []
while (l1[i] !== undefined || l2[i] !== undefined || next) {
sum = (l1[i] ?? 0) + (l2[i] ?? 0) + (next ?? 0)
result.push(sum % 10)
next = sum > 9 ? 1 : 0
i++
}
return result
}
test('', () => expect(foo([2, 4, 3], [5, 6, 4])).toEqual([7, 0, 8]))
test('', () => expect(foo([0], [0])).toEqual([0]))
test('', () => expect(foo([9, 9, 9, 9, 9, 9, 9], [9, 9, 9, 9])).toEqual([8, 9, 9, 9, 0, 0, 0, 1]))
})
<file_sep>describe('', () => {
enum Direction {
DOWN,
UP,
}
class Heap {
private static readonly Direction: typeof Direction = Direction
private static readonly getIndexChildren = (i: number): number[] => [2 * i + 1, 2 * i + 2]
private static readonly getIndexParent = (i: number): number =>
(i % 2 === 1 ? i - 1 : i - 2) / 2
private static heapifyParent(ns: number[], i: number, direction: Direction): void {
if (i < 0) return
let ilargest = i
const [ileft, iright] = Heap.getIndexChildren(i)
const imax = ns.length
if (ileft < imax && ns[ileft] > ns[ilargest]) ilargest = ileft
if (iright < imax && ns[iright] > ns[ilargest]) ilargest = iright
if (ilargest !== i) {
const tmp = ns[i]
ns[i] = ns[ilargest]
ns[ilargest] = tmp
Heap.heapifyParent(
ns,
direction === Heap.Direction.DOWN ? ilargest : Heap.getIndexParent(i),
direction,
)
}
}
private static print(ns: number[]): void {
for (let i = 0; i < ns.length; i = 2 * i + 1) {
console.log(ns.slice(i, 2 * i + 1))
}
}
//
private _ns: number[]
public constructor(ns: number[] = []) {
this._ns = []
for (const n of ns) this.push(n)
}
//
public getNs(): number[] {
return this._ns.slice()
}
public pop(): number | undefined {
const nfirst = this._ns[0]
const nlast = this._ns.pop()
if (this._ns.length !== 0 && nlast !== undefined) this._ns[0] = nlast
Heap.heapifyParent(this._ns, 0, Heap.Direction.DOWN)
return nfirst
}
public print(): void {
Heap.print(this._ns)
}
public push(n: number): void {
const length = this._ns.push(n)
Heap.heapifyParent(this._ns, Heap.getIndexParent(length - 1), Heap.Direction.UP)
}
}
test('', () => {
const h = new Heap()
for (let i = 1; i < 12; i++) h.push(i)
expect(h.getNs()).toEqual([11, 10, 6, 7, 9, 2, 5, 1, 4, 3, 8])
for (let i = 1; i < 12; i++) h.pop()
expect(h.getNs()).toEqual([])
})
})
<file_sep>const round = new RegExp(/\(\)/, 'g');
const square = new RegExp(/\[]/, 'g');
const curly = new RegExp(/\{\}/, 'g');
const empty = '';
module.exports = function validbraces(input) {
let str = input.slice(0);
let length = 0;
while (str.length !== length) {
length = str.length;
str = str
.replace(round, empty)
.replace(square, empty)
.replace(curly, empty);
}
return !length;
};
/*
* Примечание:
*
* O(n^2) конечно не очень, зато коротко и просто,
* и в требованиях к решению нет ничего кроме тестов.
*
* Быстрее будет стандартный скобочный алгоритм с использованием стека
* (push на открытии скобки, pop и проверка корректности на закрытии).
*/
<file_sep>import { List } from '.'
describe('default', () => {
const create = (size: number): List =>
new List(size, (): number => Math.floor(Math.random() * 10))
const verify = (list: List): void =>
expect(
list
.values()
.reverse()
.join(','),
).toEqual(
list
.reverse()
.values()
.join(','),
)
test('size 0', () => verify(create(0)))
test('size 1', () => verify(create(1)))
test('size 2', () => verify(create(2)))
test('size 4', () => verify(create(4)))
test('size 1024', () => verify(create(1024)))
})
<file_sep>// https://leetcode.com/problems/two-sum
describe('', () => {
function foo(nums: number[], sum: number): number[] {
const seen = new Map<number, number>()
for (let i = 0, l = nums.length; i < l; i++) {
const n = nums[i]
const complement = sum - n
if (seen.has(complement)) return [seen.get(complement)!, i]
else seen.set(n, i)
}
return []
}
test('', () => expect(foo([2, 7, 11, 15], 9)).toEqual([0, 1]))
test('', () => expect(foo([3, 2, 4], 6)).toEqual([1, 2]))
test('', () => expect(foo([3, 3], 6)).toEqual([0, 1]))
})
<file_sep>'use strict';
function look (graph, component, index) {
var vertex = graph[index];
if (!vertex.component) {
vertex.component = component;
for (var i = 0; i < vertex.length; i++) {
look(graph, component, vertex[i]);
}
}
}
function check (graph) {
for (var i = 0; i < graph.length; i++) {
if (!graph[i].component) return i;
}
return null;
}
module.exports = function (graph) {
var start = 0;
var component = 1;
do {
look(graph, component, start);
start = check(graph);
component++;
} while (start);
return graph;
};
<file_sep>function checker(num1, num2) {
const str1 = num1.toString();
const str2 = num2.toString();
return (digit) => {
const re4 = new RegExp(`${digit}{4}`);
const re2 = new RegExp(`${digit}{2}`);
return re4.test(str1) && re2.test(str2);
};
}
module.exports = function quaddouble(num1, num2) {
const check = checker(num1, num2);
for (let i = 0; i <= 9; i++) if (check(i)) return true;
return false;
};
/*
* Примечание:
* можно было бы смотреть по одной цифре в цикле только арифметикой,
* и сохранять найденные значения в хеш таблицу (можно в объект или массив),
* может быть немножко быстрее бы работало (хотя хз, это надо тестировать),
* но зато так код гораздо короче и поддерживаемее.
*/
<file_sep>// https://leetcode.com/problems/longest-substring-without-repeating-characters/
describe('', () => {
function foo(s: string): number {
let ileft = 0
let iright = 0
let char
let seen = new Set()
let result = 0
while (iright < s.length) {
char = s[iright]
if (!seen.has(char)) {
seen.add(char)
iright++
} else {
result = Math.max(result, iright - ileft)
seen = new Set()
ileft = iright
while (ileft - 1 >= 0 && s[ileft - 1] !== char) {
ileft--
seen.add(s[ileft])
}
}
}
return result
}
test('', () => expect(foo('abcabcbb')).toEqual(3))
test('', () => expect(foo('bbbbb')).toEqual(1))
test('', () => expect(foo('pwwkew')).toEqual(3))
})
<file_sep>'use strict';
function Node (index, next) {
this.i = index;
this.next = next || null;
}
Node.prototype.array = function () {
var node = this;
var array = [];
do {
array.push(node.i);
node = node.next;
} while (node);
return array;
};
Node.prototype.print = function () {
console.log.apply(console, this.array());
};
module.exports = function (size) {
var first, curr, last;
for (var i = 0; i < size; i++) {
curr = new Node(i);
if (!first) first = curr;
if (last) last.next = curr;
last = curr;
}
return first;
};
<file_sep>const validbraces = require('./index');
describe('required', () => {
test('() -> true', () => {
expect(validbraces('()')).toEqual(true);
});
test('[) -> false', () => {
expect(validbraces('[)')).toEqual(false);
});
test('{}[]() -> true', () => {
expect(validbraces('{}[]()')).toEqual(true);
});
test('([{}]) -> true', () => {
expect(validbraces('([{}])')).toEqual(true);
});
test('())({}}{()][][ -> false', () => {
expect(validbraces('())({}}{()][][')).toEqual(false);
});
});
describe('custom', () => {
test('(() -> false', () => {
expect(validbraces('(()')).toEqual(false);
});
test('([)] -> false', () => {
expect(validbraces('([)]')).toEqual(false);
});
test('{{}[[]}] -> false', () => {
expect(validbraces('{{}[[]}]')).toEqual(false);
});
test('([{}()]({})([])) -> true', () => {
expect(validbraces('([{}()]({})([]))')).toEqual(true);
});
test('((((((()))))))) -> false', () => {
expect(validbraces('((((((())))))))')).toEqual(false);
});
});
<file_sep>'use strict';
var MAX = 1000;
function random (max) {
return Math.round(Math.random() * max);
}
function gcd (min, max) {
var guess = max % min;
if (min % guess === 0) return guess;
else return gcd(guess, min);
}
var a = random(MAX);
var b = random(MAX);
console.log('(' + a + ', ' + b + ') -> ' + gcd(Math.min(a, b), Math.max(a, b)));
<file_sep>'use strict';
function reverse (start) {
var prev, next, curr = start;
while (curr) {
next = curr.next;
curr.next = prev;
prev = curr;
curr = next;
}
return prev;
}
module.exports = reverse;
<file_sep>/*
Given an integer number N > 0, return a number of ways to get N chicken nuggets from McDonalds, if they only sell packs of 6, 9 and 20, or undefined if it's not possible.
*/
// 18:15 -> + implementation 18:25 -> + debug 18:35 -> + details 18:50 -> + deduplicate 19:00
const numberOfWays = ((): ((n: number) => void) => {
const availablePackSizes: number[] = [7, 9, 20]
const alreadyCalculatedAnswers: Array<Array<Array<number | undefined>>> = [[[]]]
// eslint-disable-next-line max-lines-per-function
return (n: number): Array<Array<number | undefined>> => {
const highestAlreadyCalculatedIndex: number = alreadyCalculatedAnswers.length
if (n < highestAlreadyCalculatedIndex) return alreadyCalculatedAnswers[n]
else {
for (let i = highestAlreadyCalculatedIndex; i <= n; i++) {
availablePackSizes.forEach((packSize: number): void => {
const indexBeforeAddingTheCurrentPack: number = i - packSize
if (
indexBeforeAddingTheCurrentPack >= 0 &&
// eslint-disable-next-line @typescript-eslint/no-unnecessary-condition
alreadyCalculatedAnswers[indexBeforeAddingTheCurrentPack] !== undefined
) {
if (!alreadyCalculatedAnswers[i]) alreadyCalculatedAnswers[i] = []
alreadyCalculatedAnswers[i].push(
...alreadyCalculatedAnswers[indexBeforeAddingTheCurrentPack].map((answer) => [
...answer,
packSize,
]),
)
}
})
}
if (alreadyCalculatedAnswers[n]) {
for (const answer of alreadyCalculatedAnswers[n]) {
answer.sort((a, b) => (a && b ? a - b : 0))
}
alreadyCalculatedAnswers[n] = Array.from(
new Map(
alreadyCalculatedAnswers[n].map((answer: Array<number | undefined>) => {
return [answer.join(','), answer]
}),
).values(),
)
}
return alreadyCalculatedAnswers[n]
}
}
})()
for (let n = 0; n <= 50; n++) {
console.log(n, ' -> ', numberOfWays(n))
}
<file_sep>'use strict';
var RandomArray = require('./RandomArray');
var sort = require('./' + (process.argv[2] || 'quick'));
var len = Math.round(process.argv[3]) || 1000;
console.time('1000 iterations');
for (var i = 0; i < 1000; i++) sort(new RandomArray(len));
console.timeEnd('1000 iterations');
<file_sep>'use strict';
function partition (arr, lo, hi) {
var i = lo;
var pi = hi;
var pv = arr[hi];
while (i < pi) {
if (arr[i] < pv) i++;
else {
arr.swap(i, pi - 1);
arr.swap(pi - 1, pi);
pi--;
}
}
return pi;
}
function sort (arr, lo, hi) {
if (lo < hi) {
var p = partition(arr, lo, hi);
sort(arr, lo, p - 1);
sort(arr, p + 1, hi);
}
}
module.exports = function (arr) {
sort(arr, 0, arr.length - 1);
return arr;
};
<file_sep>export enum GameStrategy {
CHANGE,
INSIST,
}
const defaultNumberOfBoxes: number = 3
const defaultNumberOfPlays: number = 1e5
const getRandomBox = (numberOfBoxes: number): number => {
return Math.floor(Math.random() * numberOfBoxes)
}
const getRandomBoxExcept = (numberOfBoxes: number, ...forbiddenBoxes: number[]): number => {
let box: number
do {
box = getRandomBox(numberOfBoxes)
} while (forbiddenBoxes.includes(box))
return box
}
const playOnce = (gameStrategy: GameStrategy, numberOfBoxes: number): boolean => {
const prizeBox: number = getRandomBox(numberOfBoxes)
const firstGuessBox: number = getRandomBox(numberOfBoxes)
const emptyBox: number = getRandomBoxExcept(numberOfBoxes, prizeBox, firstGuessBox)
const secondGuessBox: number =
gameStrategy === GameStrategy.CHANGE
? getRandomBoxExcept(numberOfBoxes, firstGuessBox, emptyBox)
: firstGuessBox
return secondGuessBox === prizeBox
}
export const playMultipleTimes = (
gameStrategy: GameStrategy,
numberOfBoxes: number = defaultNumberOfBoxes,
numberOfPlays: number = defaultNumberOfPlays,
): number => {
let score: number = 0
let i: number = numberOfPlays
// eslint-disable-next-line @typescript-eslint/no-magic-numbers
while (--i >= 0) score += playOnce(gameStrategy, numberOfBoxes) ? 1 : 0
return score / numberOfPlays
}
<file_sep>'use strict';
var DENSITY = 0.15;
function Graph (size) {
var i, j;
var edge, edgescount;
// generate vertices
for (i = 0; i < size; i++) this.push([]);
// generate edges
for (i = 0; i < size; i++) {
edgescount = Math.floor(Math.random() * size * DENSITY);
for (edge = 0; edge < edgescount; edge++) {
j = Math.floor(Math.random() * size);
if (j !== i) {
if (this[i].indexOf(j) === -1) this[i].push(j);
if (this[j].indexOf(i) === -1) this[j].push(i);
}
}
}
// sort edges
for (i = 0; i < size; i++)
this[i].sort(function (a, b) {
return (a - b);
});
}
Graph.prototype = Object.create(Array.prototype);
Graph.prototype.constructor = Graph;
Graph.prototype.print = function () {
console.log(this);
return this;
};
module.exports = Graph;
<file_sep>describe('', () => {
function f() {
return 42
}
test('', () => expect(f()).toEqual(42))
})
| f72007244aec067a34dd92db42606e19485abbbd | [
"JavaScript",
"TypeScript"
]
| 26 | JavaScript | uqee/empl-interviews | 47f83c878dbecd1c80712a6c0983a497a4568e59 | c3c9df2f181993af9b19b3d0f6dafea9f1dbb32e | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace SinglyLinkedLists
{
public class SinglyLinkedList
{
private SinglyLinkedListNode LastNode { get; set; }
public SinglyLinkedListNode FirstNode { get; set; }
public SinglyLinkedList list { get; set; }
public SinglyLinkedList()
{
// NOTE: This constructor isn't necessary, once you've implemented the constructor below.
}
public override string ToString()
{
if (this.First() == null)
{
// If list is empty, return string representation of empty array
return "{ }";
}
else
{
var list_as_array = this.ToArray();
var strBuilder = new StringBuilder();
strBuilder.Append("{ ");
int length = list_as_array.Length;
for (int i = 0; i <= length - 1; i++)
{
if (i == length - 1)
{
strBuilder.Append("\"" + list_as_array[i] + "\"");
}
else
{
strBuilder.Append("\"" + list_as_array[i] + "\", ");
}
}
strBuilder.Append(" }");
return strBuilder.ToString();
}
}
// READ: http://msdn.microsoft.com/en-us/library/aa691335(v=vs.71).aspx
public SinglyLinkedList(params object[] values)
{
if (values.Length == 0)
{
throw new ArgumentException();
}
for (int i = 0; i < values.Length; i++)
{
AddLast(values[i].ToString());
}
}
// READ: http://msdn.microsoft.com/en-us/library/6x16t2tx.aspx
public string this[int i]
{
get
{
return this.ElementAt(i);
}
set
{
var placeholder = new SinglyLinkedList();
for (int j = 0; j < this.Count(); j++)
{
if (i == j)
{
placeholder.AddLast(value);
}
else
{
placeholder.AddLast(this.ElementAt(j));
}
}
this.FirstNode = new SinglyLinkedListNode(placeholder.First());
for (int k = 1; k < placeholder.Count(); k++)
{
this.AddLast(placeholder.ElementAt(k));
}
}
}
public void AddAfter(string existingValue, string value)
{
int location = this.IndexOf(existingValue);
if (location == -1)
{
throw new ArgumentException();
}
var ref_list = new SinglyLinkedList();
for (int i = 0; i < this.Count(); i++)
{
ref_list.AddLast(this.ElementAt(i));
if (location == i) // Find the correct position to add the new node value
{
ref_list.AddLast(value);
}
}
this.FirstNode = new SinglyLinkedListNode(ref_list.First()); //
for (int j = 1; j < ref_list.Count(); j++)
{
this.AddLast(ref_list.ElementAt(j));
}
}
public void AddFirst(string value)
{
SinglyLinkedListNode new_node = new SinglyLinkedListNode(value);
if (First() == null)
{
FirstNode = new_node;
}
else
{
var currentFirstNode = FirstNode;
new_node.Next = currentFirstNode;
FirstNode = new_node;
}
}
public void AddLast(string value)
{
SinglyLinkedListNode new_node = new SinglyLinkedListNode(value);
if (First() == null)
{
FirstNode = new_node;
}
else
{
SinglyLinkedListNode ref_node = FirstNode;
while (!ref_node.IsLast())
{
ref_node = ref_node.Next;
}
ref_node.Next = new_node;
}
}
// NOTE: There is more than one way to accomplish this. One is O(n). The other is O(1).
public int Count()
{
if (this.First() == null)
{
return 0;
}
else
{
int list_length = 1;
var ref_node = FirstNode;
// Complexity at this point is O(n)
// What would O(1) implementation look like?
while (!ref_node.IsLast())
{
ref_node = ref_node.Next;
list_length++;
}
return list_length;
}
}
public string ElementAt(int index)
{
SinglyLinkedListNode desired_node = FirstNode;
if (First() == null)
{
throw new ArgumentOutOfRangeException();
}
for (int i = 0; i < index; i++)
{
desired_node = desired_node.Next;
}
return desired_node.ToString();
}
public string First()
{
return FirstNode?.ToString();
}
public int IndexOf(string value)
{
int counter = 0;
var ref_node = FirstNode;
if (ref_node == null)
{
return -1;
}
while (!(ref_node.Value == value))
{
if (ref_node.Next == null)
{
return -1;
}
ref_node = ref_node.Next;
counter++;
}
return counter;
}
public bool IsSorted()
{
if (this.First() != null)
{
for (int i = 0; i < this.Count()-1; i++)
{
if (String.Compare(this.ElementAt(i), this.ElementAt(i+1), StringComparison.CurrentCulture) == 1)
{
return false;
}
}
}
return true;
}
// HINT 1: You can extract this functionality (finding the last item in the list) from a method you've already written!
// HINT 2: I suggest writing a private helper method LastNode()
// HINT 3: If you highlight code and right click, you can use the refactor menu to extract a method for you...
public string Last()
{
LastNode = FirstNode;
if (LastNode == null)
{
return null;
}
else
{
while (!LastNode.IsLast())
{
LastNode = LastNode.Next;
}
return LastNode.ToString();
}
}
public string ToString(SinglyLinkedListNode[] values)
{
for (int i = 0; i < values.Length; i++)
{
values[i].ToString();
}
return values.ToString();
}
public void Remove(string value)
{
int location = this.IndexOf(value);
var placeholder = new SinglyLinkedList();
for (int i = 0; i < this.Count(); i ++)
{
if (i != location)
{
placeholder.AddLast(ElementAt(i));
}
}
this.FirstNode = new SinglyLinkedListNode(placeholder.First());
// Assign FirstNode to be the new list.
for (int j = 1; j < placeholder.Count(); j++)
{
// Then add the remaining values in the list.
this.AddLast(placeholder.ElementAt(j));
}
}
public void Sort()
{
int length = this.Count();
if (length < 2)
{
return;
}
else
{
while (!this.IsSorted())
{
var ref_node = this.FirstNode;
var next_node = ref_node.Next;
for (int i = 1; i < length; i++)
{
if (ref_node.Value.CompareTo(next_node.Value) > 0)
{
var temp = ref_node.Next.Value;
next_node.Value = ref_node.Value;
ref_node.Value = temp;
}
ref_node = ref_node.Next;
next_node = next_node.Next;
}
// Decrement the length of the list to be sorted, since elements are already sorted.
length--;
}
}
}
public string[] ToArray()
{
string[] empty_array = new string[] { };
string[] new_array = new string[this.Count()];
if (this.First() == null)
{
return empty_array;
}
else
{
for (int i = 0; i < this.Count(); i++)
{
new_array[i] = this.ElementAt(i);
}
return new_array;
}
}
}
}
| 5393cd7d7876317e3f7d1a2cc4b4ff622405fd7a | [
"C#"
]
| 1 | C# | kirkwgraves/tdl-linkedlist | c8208c1b2e1d9989eab61e73e0bae93bd98ba189 | 71e5be03a69f8a17a216a173070d75903b9c0d94 | |
refs/heads/master | <file_sep>import org.testng.Assert;
import org.testng.annotations.Test;
public class TriangleTest {
@Test
public void equilateralTest() {
Assert.assertEquals(Triangle.function("3", "3", "3"), "3 - Equilateral равносторонний");
Assert.assertEquals(Triangle.function("6", "6", "6"), "3 - Equilateral равносторонний");
Assert.assertEquals(Triangle.function("2", "2", "2"), "3 - Equilateral равносторонний");
}
@Test
public void isoscelesTest() {
Assert.assertEquals(Triangle.function("5", "5", "3"), "2 - Isosceles равнобедренный");
Assert.assertEquals(Triangle.function("3", "5", "3"), "2 - Isosceles равнобедренный");
}
@Test
public void erorTest() {
Assert.assertEquals(Triangle.function("5", "0", "3"), "4 - Error");
Assert.assertEquals(Triangle.function("5", "shyjcg", "3"), "4 - Error");
Assert.assertEquals(Triangle.function("-5", "4", "3"), "4 - Error");
Assert.assertEquals(Triangle.function("5", "0", "0"), "4 - Error");
Assert.assertEquals(Triangle.function("5", "0", "dfh"), "4 - Error");
Assert.assertEquals(Triangle.function("5.0", "0", "dfh"), "4 - Error");
Assert.assertEquals(Triangle.function("null", "4", "7"), "4 - Error");
}
}
<file_sep>import java.util.Scanner;
public class Triangle {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("Enter some a: ");
String a1 = in.nextLine();
System.out.print("Enter some b: ");
String b1 = in.nextLine();
System.out.print("Enter some c: ");
String c1 = in.nextLine();
System.out.println("Your input is a:" + a1 + " b:" + b1 + " c:" + c1);
String result = function(a1, b1, c1);
System.out.print(result);
}
public static String function(String a1, String b1, String c1) {
int a = 0;
int b = 0;
int c = 0;
try {
a = Integer.parseInt(a1);
b = Integer.parseInt(b1);
c = Integer.parseInt(c1);
} catch (NumberFormatException e) {
return "4 - Error";
}
if ((a<=0) || (b<=0) || (c<=0)) return "4 - Error";
if ((a==b) && (a==c)) return "3 - Equilateral равносторонний"; else
if ((a==b) || (a==c) || (c==b)) return "2 - Isosceles равнобедренный";
else return "1 - Scalene Неравносторонний";
}
}
| 3c73da4c6cb2538731339e85a70b41afe9dea17e | [
"Java"
]
| 2 | Java | ktulxy/SomeTest | 94d6c15620c8ae75030a69b898acfa36b775bd2a | 074678a8ddcfb9e8df5562fffc0be87b41ac7b0c | |
refs/heads/master | <file_sep>#!/bin/bash
var_file='demo-redhat-variables-scott.json'
packer_file='demo-redhat.json'
function capture_var() {
val=$(cat $var_file | jq .$1 | tail -c +2 | head -c -2)
echo $val
}
service_principal_client_id=$(capture_var service_principal_client_id)
service_principal_client_secret=$(capture_var service_principal_client_secret)
azure_ad_tenant_id=$(capture_var azure_ad_tenant_id)
azure_subscription_id=$(capture_var azure_subscription_id)
resource_group_name=$(capture_var resource_group_name)
resource_group_location=$(capture_var resource_group_location)
azure_storage_account=$(capture_var azure_storage_account)
echo "Logging in"
az login --service-principal -u $service_principal_client_id -p $service_principal_client_secret --tenant $azure_ad_tenant_id
# Check if the needed resource group has been created
echo "Making sure that the resource group $resource_group_name exists"
if [[ "false" == $(az group exists -n $resource_group_name) ]]; then
echo "Creating resource group"
az group create -l $resource_group_location -n $resource_group_name
fi
if [[ "true" == $(az storage account check-name --name $azure_storage_account --query nameAvailable) ]]; then
echo "Creating storage account"
az storage account create \
-n $azure_storage_account \
-g $resource_group_name \
-l $resource_group_location \
--sku Standard_LRS
fi
echo "Building packer image"
packer build -var-file=$var_file -force $packer_file
az logout
<file_sep>#!/bin/bash
echo "Running deploy.sh"
# Setup error handling
tempfiles=( )
cleanup() {
rm -f "${tempfiles[@]}"
}
trap cleanup 0
error() {
local parent_lineno="$1"
local message="$2"
local code="${3:-1}"
if [[ -n "$message" ]] ; then
echo "Error on or near line ${parent_lineno}: ${message}; exiting with status ${code}"
else
echo "Error on or near line ${parent_lineno}; exiting with status ${code}"
fi
exit "${code}"
}
trap 'error ${LINENO}' ERR
echo "Checking terraform"
if [[ ! -d ".terraform" ]]; then
terraform init
fi
# Load the config
echo "Loading config"
. variables.conf
resource_group_name=$(echo $base_name)rg
# Login as the service principal
echo "Getting the connection string"
#az login --service-principal -u $service_principal_id -p $service_principal_secret --tenant $azure_ad_tenant_id
az account set -s $azure_subscription_id
storage_connection_string=$(az storage account show-connection-string --name $vhd_storage_account --query connectionString --output tsv)
# Pull the most recent VHD
echo "Getting the most recent VHD"
latest_vhd=$(az storage blob list --account-name $vhd_storage_account \
--container-name $vhd_storage_container \
--auth-mode key \
--connection-string $storage_connection_string \
--prefix $vhd_path \
--query '[].{name: name, create: properties.creationTime}' --output tsv \
| grep -F '.vhd' \
| sort -k2 -r \
| head -n 1 \
| cut -f1)
echo "Found $latest_vhd"
# Generate a SAS URI which expires 1 month from today
echo "Generating SAS URI"
expiry_date=$(date -d "+1 month" --iso-8601)
source_vhd=$(az storage blob generate-sas --account-name $vhd_storage_account \
--container-name $vhd_storage_container \
--auth-mode key \
--connection-string $storage_connection_string \
--name $latest_vhd \
--permissions r \
--expiry $expiry_date \
--full-uri \
--output tsv)
if [[ $(az vm list -g $resource_group_name --query '[].name' --output tsv | wc -l) != "0" ]]; then
echo "Deleting vm $(echo $base_name)vm"
az vm delete --name $(echo $base_name)vm \
--resource-group $resource_group_name \
--yes
echo "Deleting VHD images"
vhds_storage_connection_string=$(az storage account show-connection-string --name $stg_account_name --query connectionString --output tsv)
az storage container delete --name vhds --connection-string $vhds_storage_connection_string
echo "Pausing for 60s. When deleting a container, the system can take a bit to fully remove the resource.."
for i in {1..60}
do
echo -ne "$i.."\\r
sleep 1s
done
fi
terraform apply -auto-approve -var="azure_ad_tenant_id=$azure_ad_tenant_id" \
-var="azure_subscription_id=$azure_subscription_id" \
-var="base_name=$base_name" \
-var="location=$resource_group_location" \
-var="source_vhd=$source_vhd" \
-var="stg_account_name=$stg_account_name"
echo "Creating VM"
az vm create --name $(echo $base_name)vm \
--resource-group $resource_group_name \
--os-type Windows \
--use-unmanaged-disk \
--admin-username $admin_username \
--admin-password $<PASSWORD> \
--image "https://$stg_account_name.blob.core.windows.net/vhd/$base_name.vhd" \
--nics $(echo $base_name)Nic \
--storage-account $stg_account_name \
--storage-container-name vhds \
--size $vm_sku
#az logout<file_sep>#!/bin/bash
# Run this script as root via sudo. If you don't, most of the script fails.
# This script makes the following assumptions:
# 1. The VHD starts with 2 partitions on /dev/sda
# 2. The os-disk-size was set to 128 GB
# 3. The LVM should use all remaining space (~95GB)
#
# So, if you use this script, validate these assumptions. If you use
# the script blindly, bad things may happen.
# set the directory to map all the new space to
map_dir=/usr/mydata
# This bit assumes that the empty space is on sda3. If that's wrong,
# change the device_id.
device_id=/dev/sda
device_num=3
device_path=$(echo $device_id$device_num)
# First, determine what the last block is on the device.
last_block=$(fdisk -l $device_id | grep 'sda2' | awk '{ print $3 }')
# Create the string to start the new partition on the next
# blank spot.
new_block=$(($last_block + 1))
new_block_string=$(echo "$new_block"s)
# Create the partition
parted -a optimal -s $device_id mkpart primary $new_block_string 100%
# Set the partition as an LVM volume
parted $device_id set $device_num lvm on
# Create the physical volume
pvcreate $device_path
# Extend rootvg to this volume
# This bit exercises assumption 1
vgextend rootvg $device_path
lvcreate -L 95GB -n mydatalv rootvg
# Set the file system
mkfs -t ext4 /dev/rootvg/mydatalv
# Create the mount point
mkdir $map_dir
# Note: echoing directly to /etc/fstab did not work, so we do it locally.
cp /etc/fstab .
chmod 666 fstab
# Edit the fstab file so that things mount every time.
echo -e "/dev/mapper/rootvg-mydatalv\t$map_dir\text4\tdefaults\t0 0" >> fstab
# Copy the edits back
chmod 644 fstab
cp fstab /etc/fstab
rm fstab
# Extra, if needed: make sure the LVM is mounted, it will auto-mount on reboot
mount $map_dir<file_sep>last_block=$(sudo fdisk -l /dev/sda | grep 'sda2' | awk '{ print $3 }')
# Create the string to start the new partition on the next
# blank spot.
new_block=$(($last_block + 1))
new_block_string=$(echo "$new_block"s)
# Create the partition
sudo parted -a optimal -s /dev/sda mkpart primary $new_block_string 100%
# Set the partition as an LVM volume
sudo parted /dev/sda set 3 lvm on
# Create the physical volume
sudo pvcreate /dev/sda3
# Extend rootvg to this volume
sudo vgextend rootvg /dev/sda3
sudo lvcreate -L 95GB -n fioranolv rootvg
# Set the file system
sudo mkfs -t ext4 /dev/rootvg/fioranolv
# Create the mount point
sudo mkdir /var/fiorano
# Note: echoing directly to /etc/fstab did not work, so we do it locally.
sudo cp /etc/fstab .
sudo chmod 666 fstab
# Edit the fstab file so that things mount every time.
sudo echo -e "/dev/mapper/rootvg-fioranolv\t/var/fiorano\text4\tdefaults\t0 0" >> fstab
# Copy the edits back
sudo chmod 644 fstab
sudo cp fstab /etc/fstab
sudo rm fstab
# Extra, if needed: make sure the LVM is mounted, it will auto-mount on reboot
sudo mount /var/fiorano<file_sep># Requirements
Must have Azure CLI and jq installed.
* Azure CLI: https://docs.microsoft.com/en-us/cli/azure/install-azure-cli-apt?view=azure-cli-latest
* jq: https://stedolan.github.io/jq/download/
Next, login to your Azure account:
>az login
>az account set -s <subscription id>
Now, create a Service Principal to handle the login (and capture the output for this command! You can't retrieve the generated password later). Documentation for this is found at https://docs.microsoft.com/cli/azure/create-an-azure-service-principal-azure-cli?view=azure-cli-latest:
>az ad sp create-for-rbac --name ServicePrincipalName
From the output of the previous step, update demo-centos-variables.json such that the variables are appropriate for your environment.
Finally, run packvm.sh. This will create the VM as you require.
You can now adapt the packer file, demo-centos.json, to your requirements.
# Updating the base image:
The following command lists the CentOS images published by OpenLogic:
>az vm image list --location centralus --publisher OpenLogic --all
Finally, when updating an existing VM offer on Azure, follow [these guidelines](https://docs.microsoft.com/azure/marketplace/cloud-partner-portal/virtual-machine/cpp-update-existing-offer#common-update-operations).<file_sep>#!/bin/bash
# Run this script as root via sudo. If you don't, most of the script fails.
# This script makes the following assumptions:
# Set a label on the device
# TODO: discover the device, don't hard code it. This flows
# through the rest of the script. Reason: a data disk may sometimes attach
# as /dev/sda or other letters too. The assumptions in this sample are bad
# for production.
parted /dev/sdc mklabel msdos
# Create the partition
parted -a optimal -s /dev/sdc mkpart primary 1 100%
# Set the partition as an LVM volume
parted /dev/sdc set 1 lvm on
# Create the physical volume
pvcreate /dev/sdc1
# Extend rootvg to this volume
vgextend rootvg /dev/sdc1
lvcreate -L 127GB -n datavol rootvg
# Set the file system
mkfs -t ext4 /dev/rootvg/datavol
# Create the mount point
mkdir /var/datavol
# Note: echoing directly to /etc/fstab did not work, so we do it locally.
cp /etc/fstab .
chmod 666 fstab
# Get blkid of rootvg-datavols
datavol=$(blkid | grep 'rootvg-datavol')
startuuid=$(echo $datavol | \grep -aob '"' | head -n1 | cut -d: -f1)
enduuid=$(echo $datavol | \grep -aob '"' | head -n2 | tail -n1 | cut -d: -f1)
let "startuuid = $startuuid +1"
let "enduuid = $enduuid - $startuuid"
blockid=$(echo ${datavol:$startuuid:$enduuid})
# Edit the fstab file so that things mount every time.
echo -e "UUID=$blockid\t/var/datavol\text4\tdefaults,nofail\t1" >> fstab
# Copy the edits back
chmod 644 fstab
cp fstab /etc/fstab
rm fstab
# Extra, if needed: make sure the LVM is mounted, it will auto-mount on reboot
mount /var/datavol
| 56e58cdd593ff58e399ba8bbef6acdfabfb724c0 | [
"Markdown",
"Shell"
]
| 6 | Shell | scseely/PackerAzureDemo | 801a0d279723cfd2d4ff4851ccac7ca5ed9591a8 | c45156e31e41b903d977a3fa2e31ee9b538525fb | |
refs/heads/master | <repo_name>lawtonB/adventureGame<file_sep>/spec/specs.js
describe("enterCommand", function() {
if(document.getElementById('textbox') != null) {
var str =document.getElementById('textbox').val;
}
else {
var str = null;
}
})
<file_sep>/README.md
# _program_
#### _Introduction: ,date_
#### By _**<NAME>**, **<NAME>** , **<NAME>**, **Lawton**_
## Description:
_This is a word based game that uses objects to represent rooms and allows a player to move from one room to another room by inputting different commmands.
## Setup/Installation Requirements
*_Clone this repository from https://github.com/Whi7ee/*repo-name*
*_Open project folder, open index.html to view; modern browsers only_
## Known Bugs
_No known bugs_
## Support and contact details
_Contact me about any: Questions, Suggestions, or Bugs through GitHub comments_
## Technologies Used
* HTML
* CSS
* JS
(mocha and chai ; spec-runner)
### License
*MIT License*
Copyright (c) 2016 **_<NAME>_**
| 32719a66bda01bef952a0905dc3edb82a097b16e | [
"JavaScript",
"Markdown"
]
| 2 | JavaScript | lawtonB/adventureGame | 6585a174831cd1db6a5f1487340f2d85d180ca14 | c315e6e64949779ac6ec68f652ba5e29c9a3893b | |
refs/heads/master | <file_sep>#!/usr/bin/env python
# coding: utf-8
# In[1]:
import math
import time
# In[2]:
def iteration (n) :
#Reverse number and add
r=0
d = math.floor(math.log(n,10))+1
for i in range(1,d+1) :
r+=(n%10**i-n%10**(i-1))*10**(d-2*i+1)
return int(n+r)
def is_palin (n) :
#Checks if the number is palindromic
palin = True
s = str(n)
l = len(s)
r = math.floor(l/2)
for i in range(r):
if s[i] != s[l-i-1] :
palin = False
return (palin)
def test (n) :
#Checks if the number if Lychrel i.e. doesn't produce a palindrome below 51 iterations
p = n
not_found = True
c = 0
while not_found and c<51 :
p = iteration(p)
not_found = not is_palin(p)
c+=1
return not_found
# In[3]:
St = time.time()
Lychrel = []
for n in range(1,10001) :
if test(n) : Lychrel.append(n)
Et = time.time() - St
print(len(Lychrel), Et)
# In[4]:
Lychrel
# In[ ]:
# In[ ]:
| 540c4d9c7dcb8a4263c714d6d0343fc2932da9bd | [
"Python"
]
| 1 | Python | rodrigojimc/ProjectEuler | 7d890ccaa8c9925c47cb23336aa8b4accd621748 | 5dfa54afa25a3c145d559a6f8eb91643bb65cc85 | |
refs/heads/master | <repo_name>Bulbul017/Online-Admission-System<file_sep>/README.md
# Online-Admission-System
It's a simple JAVA project which deals with online registration procedures for a university admission seeking student and after updating all the necessary informations, offers the student with an admit-card.
# Features
- This project has been developed using JAVA.
- It's a multi threaded project.
- Socket programming has been used.
- Any number of student can apply for the registration at any time.
- User friendly GUI.
- The design of the project is based on Client-Server module of networking.
# Installation
- Platform : Windows,Linux.
- Language : JAVA (JDK 1.8).
- Tool : Netbeans IDE,Scene builder.
- Application type : JAVA FXML application.
<file_sep>/Admission System/AdmissionSystem/src/admissionsystem/FXMLDocumentController.java
package admissionsystem;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.net.InetAddress;
import java.net.Socket;
import java.net.URL;
import java.util.ResourceBundle;
import java.util.Scanner;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.fxml.FXMLLoader;
import javafx.fxml.Initializable;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.Tab;
import javafx.scene.control.TextField;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
/**
*
* @author ac
*/
public class FXMLDocumentController implements Initializable {
@FXML
private Button submitButton;
@FXML
private Label errorInHscRoll;
@FXML
private TextField hscRollTextField;
private String hscRoll;
@FXML
private Label errorInSscRoll;
@FXML
private TextField sscRollTextField;
private String sscRoll;
@FXML
private TextField hscPassingYearTextField;
private String hscPassingYear;
private String board;
@FXML
private TextField serverTextField;
private String serverAdress;
@FXML
private Tab ar;
@FXML
private TextField checkAdmitCardRoll;
@FXML
private Label ipAddressLabel;
//Submit button action
@FXML
private void submitButtonOnAction(ActionEvent event)throws Exception {
int flag1 = 1; //initialization
int flag2 = 1; //initialization
this.hscRoll = this.hscRollTextField.getText();
System.out.println(this.hscRoll);
//Checking validity of hsc roll
try{
this.errorInHscRoll.setText("") ;
for (int i=0; i < this.hscRoll.length(); i++){
//if invalid
if(this.hscRoll.charAt(i)<48 && this.hscRoll.charAt(i)>57){
flag1 = 0;
throw new Exception();
}
}
this.errorInHscRoll.setText("") ;
}catch(Exception e){
flag1 = 0;
//Showing the invalid message
this.errorInHscRoll.setText("HSC Roll isn't correct") ;
}
try{
if(this.hscRoll.length()!=6){
flag1 = 0;
throw new Exception();
}
}catch(Exception e){
flag1 = 0;
this.errorInHscRoll.setText("HSC Roll isn't correct") ;
}
this.sscRoll = this.sscRollTextField.getText();
System.out.println(this.sscRoll);
//Checking validity of hsc roll
try{
this.errorInSscRoll.setText("") ;
for (int i=0; i < this.sscRoll.length(); i++){
if(this.sscRoll.charAt(i)<48 && this.sscRoll.charAt(i)>57){
flag2 = 0;
throw new Exception();
}
}
this.errorInSscRoll.setText("") ;
}catch(Exception e){
flag2 = 0;
//showing error message
this.errorInSscRoll.setText("SSC Roll isn't correct") ;
}
try{
if(this.sscRoll.length()!=6){
flag2 = 0;
throw new Exception();
}
}catch(Exception e){
flag2 = 0;
this.errorInSscRoll.setText("SSC Roll isn't correct") ;
}
this.hscPassingYear = this.hscPassingYearTextField.getText();
System.out.println(this.hscPassingYear);
System.out.println(this.board);
this.serverAdress = this.serverTextField.getText();
System.out.println(this.serverAdress);
//If valid then create student object
if (flag1 == 1 && flag2 == 1){
System.out.println("All is well");
Student student = new Student();
student.setHSCRoll(this.hscRoll);
student.setHSCPassingYear(this.hscPassingYear);
student.setSSCRoll(this.sscRoll);
student.setBoard(this.board);
System.out.println(student);
//connect to server
this.connect();
System.out.println("I have come out of cliet class");
}
}
@FXML
private void barisalBoardAction(ActionEvent event)throws Exception{
this.board = "Barisal";
}
@FXML
private void dinajpurBoardAction(ActionEvent event)throws Exception{
this.board = "Dinajpur";
}
@FXML
private void dhakaBoardAction(ActionEvent event)throws Exception{
this.board = "Dhaka";
}
@FXML
private void rajshahiBoardAction(ActionEvent event)throws Exception{
this.board = "Rajshahi";
}
@FXML
private void jessoreBoardAction(ActionEvent event)throws Exception{
this.board = "Jessore";
}
@FXML
private void sylhetBoardAction(ActionEvent event)throws Exception{
this.board = "Sylhet";
}
@FXML
private void chittagongBoardAction(ActionEvent event)throws Exception{
this.board = "Chittagong";
}
@Override
public void initialize(URL url, ResourceBundle rb) {
// TODO
}
//To connect with the server
public void connect() throws IOException
{
try
{
InetAddress ip = InetAddress.getByName("localhost");
// establish the connection with server port 5056
//Socket s = new Socket(this.serverAdress, 5056);
Socket s = new Socket(ip, 5056);
// obtaining input and out streams
DataInputStream dis = new DataInputStream(s.getInputStream());
DataOutputStream dos = new DataOutputStream(s.getOutputStream());
//Client sending information to server
dos.writeUTF(this.hscRoll);
dos.writeUTF(this.hscPassingYear);
dos.writeUTF(this.board);
dos.writeUTF(this.sscRoll);
System.out.println("Just sent.....");
String messageFromServer;
messageFromServer = dis.readUTF();
if(messageFromServer.equals("New")){
System.out.println("New Student");
Parent root = FXMLLoader.load(getClass().getResource("UserInfo.fxml"));
Scene scene = new Scene(root);
Stage stage = new Stage();
stage.setScene(scene);
stage.show();
}
else if(messageFromServer.equals("Old")){
System.out.println("Old Student");
Parent root = FXMLLoader.load(getClass().getResource("AlreadyRegistered.fxml"));
Scene scene = new Scene(root);
Stage stage = new Stage();
stage.setScene(scene);
stage.show();
}
// closing resources
dos.writeUTF("Exit");
s.close();
System.out.println("Connection closed");
dis.close();
dos.close();
}catch(Exception e){
e.printStackTrace();
}
}
@FXML
private void checkSubmitOnAction(ActionEvent event) throws IOException {
File file = new File("AdmitCardRoll.txt");
if(!file.exists()){
file.createNewFile();
}
FileWriter fw1 = new FileWriter(file);
fw1.write(this.checkAdmitCardRoll.getText());
fw1.close();
Parent root = FXMLLoader.load(getClass().getResource("AdmitCard.fxml"));
Scene scene = new Scene(root);
Stage stage = new Stage();
stage.setScene(scene);
stage.show();
}
}
<file_sep>/Admission System/Server/src/server/ClientHandler.java
package server;
import java.io.*;
import java.text.*;
import java.util.*;
import java.net.*;
// ClientHandler class
class ClientHandler extends Thread
{
final DataInputStream dis;
final DataOutputStream dos;
final Socket s;
private String HSCroll;
private String HSCyear;
private String board;
private String SSCroll;
// Constructor
public ClientHandler(Socket s, DataInputStream dis, DataOutputStream dos)
{
this.s = s;
this.dis = dis;
this.dos = dos;
}
@Override
public void run()
{
String received;
String toreturn;
try {
StudentWithServer student = new StudentWithServer();
//Getting information from client
received = dis.readUTF();
student.setHSCRoll(received);
received = dis.readUTF();
student.setHSCPassingYear(received);
received = dis.readUTF();
student.setBoard(received);
received = dis.readUTF();
student.setSSCRoll(received);
System.out.println(student);
//dos.writeUTF("New");
if(student.hscRollCheck()==0){
dos.writeUTF("New");
}
else if(student.hscRollCheck()==1){
dos.writeUTF("Old");
}
//student.StoreInFile(registrationNumber, admitCardRoll);
received = dis.readUTF();
if(received.equals("Exit"))
{
this.s.close();
System.out.println("Connection closed");
try
{
//close the strem
this.dis.close();
this.dos.close();
}catch(IOException e){
e.printStackTrace();
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
<file_sep>/Admission System/ServerTwo/src/servertwo/ClientHandler.java
package servertwo;
import java.io.*;
import java.text.*;
import java.util.*;
import java.net.*;
import java.util.logging.Level;
import java.util.logging.Logger;
// ClientHandler class
class ClientHandler extends Thread
{
final DataInputStream dis;
final DataOutputStream dos;
final Socket s;
private int registrationNumber;
private int admitCardRoll;
private String name;
private String contactNo ;
private String email;
private String birthDate;
private String unit;
private String allocatedSeat;
// Constructor
public ClientHandler(Socket s, DataInputStream dis, DataOutputStream dos,int registrationNumber,int admitCardRoll,String allocatedSeat)
{
this.s = s;
this.dis = dis;
this.dos = dos;
this.registrationNumber = registrationNumber;
this.admitCardRoll = admitCardRoll;
this.allocatedSeat = allocatedSeat;
}
@Override
public void run(){
try{
String received;
StudentWithServerTwo student = new StudentWithServerTwo();
received = dis.readUTF();
student.setName(received);
received = dis.readUTF();
student.setContactNo(received);
received = dis.readUTF();
student.setEmail(received);
received = dis.readUTF();
student.setBirthDate(received);
received = dis.readUTF();
student.setUnit(received);
String registrationNumber = Integer.toString(this.registrationNumber);
student.setRegistrationNumber(registrationNumber);
String admitCardRoll = Integer.toString(this.admitCardRoll);
student.setAdmitCardRoll(admitCardRoll);
dos.writeUTF(registrationNumber);
dos.writeUTF(admitCardRoll);
dos.writeUTF(this.allocatedSeat);
System.out.println(student);
student.storeInFile();
received = dis.readUTF();
if(received.equals("Exit"))
{
this.s.close();
System.out.println("Connection closed");
try
{
//close the strem
this.dis.close();
this.dos.close();
}catch(IOException e){
e.printStackTrace();
}
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
<file_sep>/Admission System/Server/src/server/StudentWithServer.java
package server;
/**
*
* @author ac
*/
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
/**
*
* @author <NAME>, Md. <NAME>, <NAME>
*/
public class StudentWithServer {
private String HSCRoll;
private String HSCPassingYear;
private String board;
private String SSCRoll;
private String registrationNumber;
private String admitCardRoll;
StudentWithServer() {
}
//Checking the validity of HSC roll
public int hscRollCheck() throws IOException{
String fileName = this.getHSCRoll() + ".txt";
File file = new File(fileName);
if(!file.exists()){
file.createNewFile();
return 0;
}
else return 1;
}
/**
* @return the HSCRoll
*/
public String getHSCRoll() {
return HSCRoll;
}
/**
* @param HSCRoll the HSCRoll to set
*/
public void setHSCRoll(String HSCRoll) {
this.HSCRoll = HSCRoll;
}
/**
* @return the HSCPassingYear
*/
public String getHSCPassingYear() {
return HSCPassingYear;
}
/**
* @param HSCPassingYear the HSCPassingYear to set
*/
public void setHSCPassingYear(String HSCPassingYear) {
this.HSCPassingYear = HSCPassingYear;
}
/**
* @return the board
*/
public String getBoard() {
return board;
}
/**
* @param board the board to set
*/
public void setBoard(String board) {
this.board = board;
}
/**
* @return the SSCRoll
*/
public String getSSCRoll() {
return SSCRoll;
}
/**
* @param SSCRoll the SSCRoll to set
*/
public void setSSCRoll(String SSCRoll) {
this.SSCRoll = SSCRoll;
}
/**
* @return the registrationNumber
*/
public String getRegistrationNumber() {
return registrationNumber;
}
/**
* @param registrationNumber the registrationNumber to set
*/
public void setRegistrationNumber(String registrationNumber) {
this.registrationNumber = registrationNumber;
}
/**
* @return the admitCardRoll
*/
public String getAdmitCardRoll() {
return admitCardRoll;
}
/**
* @param admitCardRoll the admitCardRoll to set
*/
public void setAdmitCardRoll(String admitCardRoll) {
this.admitCardRoll = admitCardRoll;
}
}
<file_sep>/Admission System/AdmissionSystem/src/admissionsystem/AdmitCardController.java
package admissionsystem;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.net.URL;
import java.util.ResourceBundle;
import java.util.Scanner;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
/**
* FXML Controller class
*
* @author <NAME> & Md. <NAME> & <NAME>
*/
public class AdmitCardController implements Initializable {
@FXML
private TextField admissionRollTextField;
@FXML
private TextField registrationTextField;
@FXML
private TextField nameTextField;
@FXML
private TextField emailTextField;
@FXML
private TextField contactNoTextField;
@FXML
private TextField unitTextField;
@FXML
private Button downloadButton;
@FXML
private Button previewButton;
private String readFromFile;
private String readFromFile2;
private String fileName;
private File file;
private FileWriter fw;
private Scanner sc;
@FXML
private TextField seatTextField;
/**
* Initializes the controller class.
*/
@Override
public void initialize(URL url, ResourceBundle rb) {
// TODO
}
@FXML
private void downloadButtonOnAction(ActionEvent event) {
}
//Preview button action
@FXML
private void previewButtonOnAction(ActionEvent event) throws IOException {
//Reading the admit roll of the student from file AdmitCardRoll.txt
this.file = new File("AdmitCardRoll.txt");
if(!this.file.exists()){
this.file.createNewFile();
}
this.sc = new Scanner(this.file);
this.readFromFile = sc.nextLine();
//Getting information of the student from files
this.fileName = this.readFromFile + "Name" + ".txt";
this.file = new File(fileName);
if(!this.file.exists()){
this.file.createNewFile();
}
this.sc = new Scanner(this.file);
this.readFromFile2 = sc.nextLine();
this.nameTextField.setText(this.readFromFile2);
this.fileName = this.readFromFile + "ContactNo" + ".txt";
this.file = new File(fileName);
if(!this.file.exists()){
this.file.createNewFile();
}
this.sc = new Scanner(this.file);
this.readFromFile2 = sc.nextLine();
this.contactNoTextField.setText(this.readFromFile2);
this.fileName = this.readFromFile + "Email" + ".txt";
this.file = new File(fileName);
if(!this.file.exists()){
this.file.createNewFile();
}
this.sc = new Scanner(this.file);
this.readFromFile2 = sc.nextLine();
this.emailTextField.setText(this.readFromFile2);
this.fileName = this.readFromFile + "Unit" + ".txt";
this.file = new File(fileName);
if(!this.file.exists()){
this.file.createNewFile();
}
this.sc = new Scanner(this.file);
this.readFromFile2 = sc.nextLine();
this.unitTextField.setText(this.readFromFile2);
this.fileName = this.readFromFile + "Registration" + ".txt";
this.file = new File(fileName);
if(!this.file.exists()){
this.file.createNewFile();
}
this.sc = new Scanner(this.file);
this.readFromFile2 = sc.nextLine();
this.registrationTextField.setText(this.readFromFile2);
this.fileName = this.readFromFile + "Admit" + ".txt";
this.file = new File(fileName);
if(!this.file.exists()){
this.file.createNewFile();
}
this.sc = new Scanner(this.file);
this.readFromFile2 = sc.nextLine();
this.admissionRollTextField.setText(this.readFromFile2);
this.fileName = this.readFromFile + "Seat" + ".txt";
this.file = new File(fileName);
if(!this.file.exists()){
this.file.createNewFile();
}
this.sc = new Scanner(this.file);
this.readFromFile2 = sc.nextLine();
this.seatTextField.setText(this.readFromFile2);
}
}
| 29a7102c7f261bbb94b298d32aca645078d617c5 | [
"Markdown",
"Java"
]
| 6 | Markdown | Bulbul017/Online-Admission-System | 8a517cf3913b66f8bc35073767f6b52842226122 | c34da3563d732a9287336fdf11b1cd1bae49f1eb | |
refs/heads/master | <repo_name>abiaoLHB/LHBExtension-Swift<file_sep>/LHBExtension-Swift/LHBExtension-Swift/AppDelegate.swift
//
// AppDelegate.swift
// LHBExtension-Swift
//
// Created by LHB on 16/8/15.
// Copyright © 2016年 LHB. All rights reserved.
//
import UIKit
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool {
LHBPrint("吃饭了吗")
return true
}
}
///全局函数,自定义打印
/*
#if DEBUG
#endif
配置:Build Settings - 搜索 swift fl -固定格式写 -D (一般命名DEBUG)
*/
func LHBPrint<T>(message : T,className : String = #file,funcName : String = #function,lineNum : Int = #line) -> Void{
#if DEBUG
let className = (className as NSString).lastPathComponent.stringByReplacingOccurrencesOfString(".swift", withString:"")
//print("该类:\(className)---该方法:\(funcName)---行数:\(lineNum)---打印信息:\(message)")
print("类名:\(className)---行数:\(lineNum)---打印信息:\(message)")
#endif
}<file_sep>/README.md
# LHBExtension-Swift
swift里面常用的工具
| a25291efe032eff153808460ed877164b88dbf45 | [
"Swift",
"Markdown"
]
| 2 | Swift | abiaoLHB/LHBExtension-Swift | e727d3c8283c5f7baa3b92217b27631ff3c2b79a | e133c0d40203bd4ff45778d5ccf20c6d769c93d0 | |
refs/heads/master | <file_sep>package main
import (
"github.com/mediocregopher/radix.v2/pool"
"github.com/stretchr/testify/assert"
"net/http"
"net/http/httptest"
"testing"
)
func beforeTest() chan Competitor {
statsPool, _ = pool.New("tcp", "localhost:6379", 10)
conn, _ := statsPool.Get()
defer statsPool.Put(conn)
conn.Cmd("DEL", "user-tester1")
conn.Cmd("DEL", "user-tester2")
conn.Cmd("ZREM", "rps-wins", "tester1", "tester2")
conn.Cmd("ZREM", "rps-losses", "tester1", "tester2")
conn.Cmd("ZREM", "rps-draws", "tester1", "tester2")
compChan := make(chan Competitor)
go matchmaker(compChan)
return compChan
}
func TestPlayerTwoWins(t *testing.T) {
compChan := beforeTest()
conn, _ := statsPool.Get()
defer statsPool.Put(conn)
rpsHandle1 := rpsHandler(Rock, compChan)
rpsHandle2 := rpsHandler(Paper, compChan)
req1, _ := http.NewRequest("GET", "/rock?iam=tester1", nil)
req2, _ := http.NewRequest("GET", "/paper?iam=tester2", nil)
w1 := httptest.NewRecorder()
w2 := httptest.NewRecorder()
w1Chan := make(chan bool)
go func() {
rpsHandle1.ServeHTTP(w1, req1)
w1Chan <- true
}()
rpsHandle2.ServeHTTP(w2, req2)
<-w1Chan
r := conn.Cmd("HMGET", "user-tester2", "wins", "losses")
elems, _ := r.Array()
wins, _ := elems[0].Int()
losses, _ := elems[1].Int()
assert.Equal(t, wins, 1)
assert.Equal(t, losses, 0)
assert.Equal(t, w2.Body.String(), "Congrats, tester2, you won!")
r = conn.Cmd("HMGET", "user-tester1", "wins", "losses")
elems, _ = r.Array()
wins, _ = elems[0].Int()
losses, _ = elems[1].Int()
assert.Equal(t, wins, 0)
assert.Equal(t, losses, 1)
assert.Equal(t, w1.Body.String(), "Sorry, tester1, you lost!")
}
func TestPlayerOneWins(t *testing.T) {
compChan := beforeTest()
conn, _ := statsPool.Get()
defer statsPool.Put(conn)
rpsHandle1 := rpsHandler(Rock, compChan)
rpsHandle2 := rpsHandler(Scissors, compChan)
req1, _ := http.NewRequest("GET", "/rock?iam=tester1", nil)
req2, _ := http.NewRequest("GET", "/scissors?iam=tester2", nil)
w1 := httptest.NewRecorder()
w2 := httptest.NewRecorder()
w1Chan := make(chan bool)
go func() {
rpsHandle1.ServeHTTP(w1, req1)
w1Chan <- true
}()
rpsHandle2.ServeHTTP(w2, req2)
<-w1Chan
r := conn.Cmd("HMGET", "user-tester2", "wins", "losses")
elems, _ := r.Array()
wins, _ := elems[0].Int()
losses, _ := elems[1].Int()
assert.Equal(t, wins, 0)
assert.Equal(t, losses, 1)
assert.Equal(t, w2.Body.String(), "Sorry, tester2, you lost!")
r = conn.Cmd("HMGET", "user-tester1", "wins", "losses")
elems, _ = r.Array()
wins, _ = elems[0].Int()
losses, _ = elems[1].Int()
assert.Equal(t, wins, 1)
assert.Equal(t, losses, 0)
assert.Equal(t, w1.Body.String(), "Congrats, tester1, you won!")
}
func TestDraw(t *testing.T) {
compChan := beforeTest()
conn, _ := statsPool.Get()
defer statsPool.Put(conn)
rpsHandle1 := rpsHandler(Paper, compChan)
rpsHandle2 := rpsHandler(Paper, compChan)
req1, _ := http.NewRequest("GET", "/paper?iam=tester1", nil)
req2, _ := http.NewRequest("GET", "/paper?iam=tester2", nil)
w1 := httptest.NewRecorder()
w2 := httptest.NewRecorder()
w1Chan := make(chan bool)
go func() {
rpsHandle1.ServeHTTP(w1, req1)
w1Chan <- true
}()
rpsHandle2.ServeHTTP(w2, req2)
<-w1Chan
r := conn.Cmd("HMGET", "user-tester2", "wins", "losses")
elems, _ := r.Array()
wins, _ := elems[0].Int()
losses, _ := elems[1].Int()
assert.Equal(t, wins, 0)
assert.Equal(t, losses, 0)
assert.Equal(t, w2.Body.String(), "Well, tester2, you didn't lose, but you didn't win either. It was a draw!")
r = conn.Cmd("HMGET", "user-tester1", "wins", "losses")
elems, _ = r.Array()
wins, _ = elems[0].Int()
losses, _ = elems[1].Int()
assert.Equal(t, wins, 0)
assert.Equal(t, losses, 0)
assert.Equal(t, w1.Body.String(), "Well, tester1, you didn't lose, but you didn't win either. It was a draw!")
}
<file_sep>package main
import (
"fmt"
"github.com/levenlabs/go-llog"
"github.com/mediocregopher/lever"
"github.com/mediocregopher/radix.v2/pool"
"net/http"
)
const (
Rock = 0
Paper = 1
Scissors = 2
Win = 0
Lose = 1
Draw = 2
)
// Given weapon1 and weapon2 are each one of the consts Rock, Paper, or Scissors, get the result
// for weapon1 of a game between those two weapons via resultChart[weapon1][weapon2]
var resultsChart = [][]int{
[]int{Draw, Lose, Win},
[]int{Win, Draw, Lose},
[]int{Lose, Win, Draw},
}
// Slice for converting between the weapon consts and weapon strings
var weaponList = []string{"rock", "paper", "scissors"}
var statsPool *pool.Pool
// A Competitor holds the username and weapon chosen by a client, as well as a channel
// for informing the client of the result of their match
type Competitor struct {
Username string
Weapon int
ResultChannel chan int
}
// Returns a string representation of the Competitor
func (c *Competitor) String() string {
return fmt.Sprintf("{Username: %v, Weapon: %v}", c.Username, weaponList[c.Weapon])
}
// Given two weapons, return (result for the first weapon, result for the second weapon)
func compete(weapon1, weapon2 int) (int, int) {
return resultsChart[weapon1][weapon2], resultsChart[weapon2][weapon1]
}
// Given two usernames, and the first username's result, update the leaderboard, and
// return the username of the winner. If the result was a draw, returns ""
func updateStats(username1, username2 string, result1 int) string {
conn, err := statsPool.Get()
if err != nil {
llog.Error("Error getting connection from redis pool", llog.KV{"error": err})
}
var resultString string
switch result1 {
case Win:
resultString = username1
conn.Cmd("ZINCRBY", "rps-wins", 1, username1)
conn.Cmd("ZINCRBY", "rps-losses", 1, username2)
conn.Cmd("HINCRBY", "user-"+username1, "wins", 1)
conn.Cmd("HINCRBY", "user-"+username2, "losses", 1)
case Lose:
resultString = username2
conn.Cmd("ZINCRBY", "rps-losses", 1, username1)
conn.Cmd("ZINCRBY", "rps-wins", 1, username2)
conn.Cmd("HINCRBY", "user-"+username1, "losses", 1)
conn.Cmd("HINCRBY", "user-"+username2, "wins", 1)
default:
resultString = ""
conn.Cmd("ZINCRBY", "rps-draws", 1, username1)
conn.Cmd("ZINCRBY", "rps-draws", 1, username2)
}
statsPool.Put(conn)
return resultString
}
// Given a Competitor channel, matchmaker gets two Competitors from the channel, sends the
// Competitors' results to their respective ResultsChannel, and logs the match. This is repeated
// until the program is terminated.
func matchmaker(competitorChannel chan Competitor) {
for {
competitor1 := <-competitorChannel
competitor2 := <-competitorChannel
result1, result2 := compete(competitor1.Weapon, competitor2.Weapon)
resultString := updateStats(competitor1.Username, competitor2.Username, result1)
competitor1.ResultChannel <- result1
competitor2.ResultChannel <- result2
llog.Info("A grand battle has occured", llog.KV{
"Competitor 1": competitor1.String(),
"Competitor 2": competitor2.String(),
"Winner": resultString,
})
}
}
// Wrapper function for creating an endpoint for a weapon. If "iam" is defined in the query
// string, use its value as the Competitor's username. Otherwise use the client's ip.
// After a result is sent to the Competitor's ResultChannel, write an appropriate message
// to the client's ResponseWriter.
func rpsHandler(weapon int, competitorChannel chan Competitor) http.HandlerFunc {
return func(w http.ResponseWriter, r *http.Request) {
resultChannel := make(chan int)
query := r.URL.Query()
username := query.Get("iam")
if username == "" {
username = r.RemoteAddr
}
competitor := Competitor{username, weapon, resultChannel}
competitorChannel <- competitor
result := <-resultChannel
switch result {
case Win:
fmt.Fprintf(w, "Congrats, %s, you won!", username)
case Lose:
fmt.Fprintf(w, "Sorry, %s, you lost!", username)
default:
fmt.Fprintf(w, "Well, %s, you didn't lose, but you didn't win either. It was a draw!", username)
}
}
}
// Initializes redis pool, spins up a matchmaker routine, and creates the endpoints for the three weapons.
// When starting rps-go, you can specify the port to listen on via the --port tag.
// (e.g. ./rps-go --port 3000)
// Default port is 8080
func main() {
f := lever.New("rps-go", nil)
f.Add(lever.Param{Name: "--port", Default: "8080"})
f.Parse()
var err error
statsPool, err = pool.New("tcp", "localhost:6379", 10)
if err != nil {
llog.Error("Error getting redis pool", llog.KV{"error": err})
}
port, _ := f.ParamStr("--port")
competitorChannel := make(chan Competitor)
go matchmaker(competitorChannel)
http.HandleFunc("/rock", rpsHandler(Rock, competitorChannel))
http.HandleFunc("/paper", rpsHandler(Paper, competitorChannel))
http.HandleFunc("/scissors", rpsHandler(Scissors, competitorChannel))
http.ListenAndServe(":"+port, nil)
}
| aa4fd28929d405357eb91513e8d422a10891375e | [
"Go"
]
| 2 | Go | prestonmorgan/rps-go | c4d3a63f4d4c751a37e116e0084c26a45ca50afc | 764e3569d1b66a8a725208b4b5b272aa0e0ee9fe | |
refs/heads/master | <repo_name>lenage/language-coursescript<file_sep>/components/course-types.js
"use babel";
const COURSE_TYPES = {
DARWIN: { courseType: 1, apiType: 1, courseName: "DARWIN" },
LT: { courseType: 2, apiType: 2, courseName: "LT" },
PT: { courseType: 3, apiType: 3, courseName: "PT" },
OCC: { courseType: 4, apiType: 4, courseName: "OCC" },
BE: { courseType: 4, apiType: 5, courseName: "BE" },
PILOT: { courseType: 4, apiType: 6, courseName: "PILOT" },
PHONICS: { courseType: 5, apiType: 7, courseName: "PHONICS" },
BELL: { courseType: 6, apiType: 8, courseName: "BELL" },
TOURISM: { courseType: 1, apiType: 9, courseName: "TOURISM" },
// reuse TOURISM
ALIX_V2: { courseType: 1, apiType: 9, courseName: "TOURISM" },
LINGOCHAMP: { courseType: 7, apiType: 10, courseName: "LINGOCHAMP" },
"DARWIN BUSINESS": {
courseType: 1,
apiType: 9,
courseName: "DARWIN BUSINESS"
},
"DARWIN EXTENSION": { courseType: 1, apiType: 26, courseName: "DARWIN EXTENSION" },
"<NAME>IFI": { courseType: 1, apiType: 15, courseName: "<NAME>" },
"DARWIN HOMEWORK": {
courseType: 1,
apiType: 17,
courseName: "DARWIN HOMEWORK"
},
AIX: { courseType: 15, apiType: 18, courseName: "AIX" },
"SPROUT DAILY READERS": {
courseType: 16,
apiType: 19,
courseName: "SPROUT DAILY READERS"
},
"<NAME>": { courseType: 1, apiType: 20, courseName: "<NAME>" },
"SPROUT WORD COURSE": {
courseType: 17,
apiType: 21,
courseName: "SPROUT DAILY WORDS"
},
"SPROUT EXTENSION": {
courseType: 18,
apiType: 22,
courseName: "SPROUT EXTENSION"
},
"SPROUT GOODNIGHT": {
courseType: 18,
apiType: 23,
courseName: "SPROUT GOODNIGHT"
},
"OL SHORT VIDEO": { courseType: 19, apiType: 24, courseName: "OL SHORT VIDEO" },
"SPROUT COMPAT READERS": {
courseType: 20,
apiType: 28,
courseName: "SPROUT COMPATRDR",
},
"OL LISTENING": {
courseType: 1, apiType: 29, courseName: "OL LISTENING"
},
"LINGOME SPEAKING": {
courseType: 21, apiType: 27, courseName: "LINGOME CMS"
},
"SUPRA": {
courseType: 22, apiType: 30, courseName: "SUPRA"
},
"SPROUT GUIDANCE": {
courseType: 23, apiType: 34, courseName: "SPROUT GUIDANCE"
},
TOEIC: {
courseType: 24, apiType: 33, courseName: "TOEIC"
},
'SUBMARINE K12': { courseType: 1, apiType: 1, courseName: "SUBMARINE K12" },
INDUSTRY: {
courseType: 24, apiType: 33, courseName: "INDUSTRY",
},
};
export default COURSE_TYPES; | f988436d183b68faa7b0315c6d8280cda3060fda | [
"JavaScript"
]
| 1 | JavaScript | lenage/language-coursescript | 2eef9f3adbb276dc362e41a75005cf85b52d1b45 | 8fac9e1be76a4f65a21e754109409cc7bc25f391 | |
refs/heads/master | <file_sep>package com.sebamaty.easyuitests;
import android.support.test.espresso.Espresso;
import android.support.test.espresso.action.ViewActions;
import android.support.test.espresso.assertion.ViewAssertions;
import android.support.test.espresso.contrib.RecyclerViewActions;
import android.support.test.espresso.matcher.ViewMatchers;
import org.hamcrest.Matchers;
public class UiTestsHelper {
public static void clickOnView(int viewId) {
Espresso.onView(ViewMatchers.withId(viewId)).perform(ViewActions.click());
}
public static void clickOnViewWithText(String text) {
Espresso.onView(ViewMatchers.withText(text)).perform(ViewActions.click());
}
public static void scrollToAndClickOnView(int viewId) {
Espresso.onView(ViewMatchers.withId(viewId)).perform(ViewActions.scrollTo(), ViewActions.click());
}
public static void scrollToAndClickOnViewWithText(String text) {
Espresso.onView(ViewMatchers.withText(text)).perform(ViewActions.scrollTo(), ViewActions.click());
}
public static void checkIfViewIsDisplayed(int viewId) {
Espresso.onView(ViewMatchers.withId(viewId)).check(ViewAssertions.matches(ViewMatchers.isDisplayed()));
}
public static void checkIfViewIsNotDisplayed(int viewId) {
Espresso.onView(ViewMatchers.withId(viewId)).check(ViewAssertions.matches(Matchers.not(ViewMatchers.isDisplayed())));
}
public static void checkIfViewWithTextIsDisplayed(String text) {
Espresso.onView(ViewMatchers.withText(text)).check(ViewAssertions.matches(ViewMatchers.isDisplayed()));
}
public static void checkIfViewWithTextIsNotDisplayed(String text) {
Espresso.onView(ViewMatchers.withText(text)).check(ViewAssertions.matches(Matchers.not(ViewMatchers.isDisplayed())));
}
public static void assertTextOnViewWithId(int viewId, String text) {
Espresso.onView(ViewMatchers.withId(viewId)).check(ViewAssertions.matches(ViewMatchers.withText(text)));
}
public static void replaceTextOnView(int viewId, String text) {
Espresso.onView(ViewMatchers.withId(viewId)).perform(ViewActions.replaceText(text));
}
public static void clickOnItemInListView(int listViewId, int itemPosition) {
Espresso.onData(Matchers.anything())
.inAdapterView(ViewMatchers.withId(listViewId))
.atPosition(itemPosition)
.perform(ViewActions.click());
}
public static void assertTextOnListViewItem(String text) {
Espresso.onData(Matchers.allOf(Matchers.is(Matchers.instanceOf(String.class)), Matchers.is(text))).check(ViewAssertions.matches(ViewMatchers.withText(text)));
}
public static void assertTextOnRecyclerViewItem(int recyclerViewId, int position, int childViewId, String text) {
Espresso.onView(withRecyclerView(recyclerViewId)
.atPositionOnView(position, childViewId))
.check(ViewAssertions.matches(ViewMatchers.withText(text)));
}
public static void clickOnItemInRecyclerView(int recyclerViewId, int position) {
Espresso.onView(Matchers.allOf(ViewMatchers.withId(recyclerViewId), ViewMatchers.isCompletelyDisplayed()))
.perform(RecyclerViewActions.actionOnItemAtPosition(position, ViewActions.click()));
}
public static void scrollToPositionInRecyclerView(int recyclerViewId, int position) {
Espresso.onView(Matchers.allOf(ViewMatchers.withId(recyclerViewId), ViewMatchers.isCompletelyDisplayed()))
.perform(RecyclerViewActions.actionOnItemAtPosition(position, ViewActions.scrollTo()));
}
public static void clickOnItemChildInRecyclerView(int recyclerViewId, int position, int childViewId) {
Espresso.onView(Matchers.allOf(ViewMatchers.withId(recyclerViewId), ViewMatchers.isCompletelyDisplayed()))
.perform(RecyclerViewActions.actionOnItemAtPosition(position, ChildViewAction.clickChildViewWithId(childViewId)));
}
private static RecyclerViewMatcher withRecyclerView(final int recyclerViewId) {
return new RecyclerViewMatcher(recyclerViewId);
}
public static void isButtonSelected(int buttonId) {
Espresso.onView(ViewMatchers.withId(buttonId)).check(ViewAssertions.matches(ViewMatchers.isSelected()));
}
}
<file_sep># easy-ui-tests
A helper library for Android that reduces the boilerplate code when writing UI tests with Espresso.
# Usage example
Add the following code to a UI test class:
```java
@Rule
public ActivityTestRule<MainActivity> activityTestRule = new ActivityTestRule<>(MainActivity.class);
@Test
public void assertWelcomeText() throws Exception {
UiTestsHelper.assertTextOnViewWithId(R.id.welcome_text_view, "Hello World!");
}
```
# What can you test with it?
All methods below are static and void
```java
assertTextOnListViewItem(String text)
assertTextOnRecyclerViewItem(int recyclerViewId, int position, int childViewId, String text)
assertTextOnViewWithId(int viewId, String text)
checkIfViewIsDisplayed(int viewId)
checkIfViewIsNotDisplayed(int viewId)
checkIfViewWithTextIsDisplayed(String text)
checkIfViewWithTextIsNotDisplayed(String text)
clickOnItemChildInRecyclerView(int recyclerViewId, int position, int childViewId)
clickOnItemInListView(int listViewId, int itemPosition)
clickOnItemInRecyclerView(int recyclerViewId, int position)
clickOnView(int viewId)
clickOnViewWithText(String text)
isButtonSelected(int buttonId)
replaceTextOnView(int viewId, String text)
scrollToAndClickOnView(int viewId)
scrollToAndClickOnViewWithText(String text)
scrollToPositionInRecyclerView(int recyclerViewId, int position)
```
# Download
```gradle
androidTestCompile 'com.sebamaty:easyuitests:1.0.6'
```
Make sure you have added [JUnit][junit] dependency. Android Studio won't find @Rule annotation and ActivityTestRule class otherwise.
[junit]: https://github.com/junit-team/junit4/wiki/Use-with-Gradle
# License
```
Copyright 2016 <NAME>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
```
<file_sep>include ':app', ':easyuitests'
<file_sep>package com.sebamaty.easyuitestsexample;
import android.support.test.rule.ActivityTestRule;
import android.support.test.runner.AndroidJUnit4;
import com.sebamaty.easyuitests.UiTestsHelper;
import org.junit.Rule;
import org.junit.Test;
import org.junit.runner.RunWith;
@RunWith(AndroidJUnit4.class)
public class ExampleInstrumentedTest {
@Rule
public ActivityTestRule<MainActivity> activityTestRule = new ActivityTestRule<>(MainActivity.class);
@Test
public void assertWelcomeText() throws Exception {
UiTestsHelper.assertTextOnViewWithId(R.id.welcome_text_view, "Hello World!");
}
}
| a49223c65a7ea60f9029bbcd1d9290f785841d71 | [
"Markdown",
"Java",
"Gradle"
]
| 4 | Java | sebamaty/easy-android-ui-tests | d4f133c4fddcf6c7a23fabc5edd02ad99fa2e1b5 | fa56a8adb771d82c9ba3c53e03aa7e53febc28d3 | |
refs/heads/master | <repo_name>HackathonNicaragua2019/Uncover-Maps<file_sep>/UncoverMapsProject/Assets/Scripts/Animaciones.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Animaciones : MonoBehaviour
{
public GameObject letreroAzul1, letreroAzul2, letreroAzul3, letreroAzul4;
public GameObject letreroRojo1, letreroRojo2, letreroRojo3;
public GameObject letreroVerde1;
int contador = 0, contadorY = 0;
float velocidadGiro = -144f, limiteGiro = 180f;
bool test = false;
// Start is called before the first frame update
void Start()
{
componentesTransparentes();
inicializacionIconosGiro();
}
// Update is called once per frame
void Update()
{
if (contador < 60)
{
contador += 1;
}
else {
fadeTransition();
giroIconos();
}
}
public void componentesTransparentes()
{
letreroAzul1.GetComponent<Renderer>().material.color = new Color(
letreroAzul1.GetComponent<Renderer>().material.color.r,
letreroAzul1.GetComponent<Renderer>().material.color.g,
letreroAzul1.GetComponent<Renderer>().material.color.b,
0.0f);
letreroAzul2.GetComponent<Renderer>().material.color = new Color(
letreroAzul2.GetComponent<Renderer>().material.color.r,
letreroAzul2.GetComponent<Renderer>().material.color.g,
letreroAzul2.GetComponent<Renderer>().material.color.b,
0.0f);
letreroAzul3.GetComponent<Renderer>().material.color = new Color(
letreroAzul3.GetComponent<Renderer>().material.color.r,
letreroAzul3.GetComponent<Renderer>().material.color.g,
letreroAzul3.GetComponent<Renderer>().material.color.b,
0.0f);
letreroAzul4.GetComponent<Renderer>().material.color = new Color(
letreroAzul4.GetComponent<Renderer>().material.color.r,
letreroAzul4.GetComponent<Renderer>().material.color.g,
letreroAzul4.GetComponent<Renderer>().material.color.b,
0.0f);
letreroRojo1.GetComponent<Renderer>().material.color = new Color(
letreroRojo1.GetComponent<Renderer>().material.color.r,
letreroRojo1.GetComponent<Renderer>().material.color.g,
letreroRojo1.GetComponent<Renderer>().material.color.b,
0.0f);
letreroRojo2.GetComponent<Renderer>().material.color = new Color(
letreroRojo2.GetComponent<Renderer>().material.color.r,
letreroRojo2.GetComponent<Renderer>().material.color.g,
letreroRojo2.GetComponent<Renderer>().material.color.b,
0.0f);
letreroRojo3.GetComponent<Renderer>().material.color = new Color(
letreroRojo3.GetComponent<Renderer>().material.color.r,
letreroRojo3.GetComponent<Renderer>().material.color.g,
letreroRojo3.GetComponent<Renderer>().material.color.b,
0.0f);
letreroVerde1.GetComponent<Renderer>().material.color = new Color(
letreroVerde1.GetComponent<Renderer>().material.color.r,
letreroVerde1.GetComponent<Renderer>().material.color.g,
letreroVerde1.GetComponent<Renderer>().material.color.b,
0.0f);
}
public void fadeTransition()
{
if (letreroVerde1.GetComponent<Renderer>().material.color.a < 1f)
{
letreroAzul1.GetComponent<Renderer>().material.color = new Color(
letreroAzul1.GetComponent<Renderer>().material.color.r,
letreroAzul1.GetComponent<Renderer>().material.color.g,
letreroAzul1.GetComponent<Renderer>().material.color.b,
letreroAzul1.GetComponent<Renderer>().material.color.a + 0.03f);
letreroAzul2.GetComponent<Renderer>().material.color = new Color(
letreroAzul2.GetComponent<Renderer>().material.color.r,
letreroAzul2.GetComponent<Renderer>().material.color.g,
letreroAzul2.GetComponent<Renderer>().material.color.b,
letreroAzul2.GetComponent<Renderer>().material.color.a + 0.02f);
letreroAzul3.GetComponent<Renderer>().material.color = new Color(
letreroAzul3.GetComponent<Renderer>().material.color.r,
letreroAzul3.GetComponent<Renderer>().material.color.g,
letreroAzul3.GetComponent<Renderer>().material.color.b,
letreroAzul3.GetComponent<Renderer>().material.color.a + 0.015f);
letreroAzul4.GetComponent<Renderer>().material.color = new Color(
letreroAzul4.GetComponent<Renderer>().material.color.r,
letreroAzul4.GetComponent<Renderer>().material.color.g,
letreroAzul4.GetComponent<Renderer>().material.color.b,
letreroAzul4.GetComponent<Renderer>().material.color.a + 0.015f);
letreroRojo1.GetComponent<Renderer>().material.color = new Color(
letreroRojo1.GetComponent<Renderer>().material.color.r,
letreroRojo1.GetComponent<Renderer>().material.color.g,
letreroRojo1.GetComponent<Renderer>().material.color.b,
letreroRojo1.GetComponent<Renderer>().material.color.a + 0.014f);
letreroRojo2.GetComponent<Renderer>().material.color = new Color(
letreroRojo2.GetComponent<Renderer>().material.color.r,
letreroRojo2.GetComponent<Renderer>().material.color.g,
letreroRojo2.GetComponent<Renderer>().material.color.b,
letreroRojo2.GetComponent<Renderer>().material.color.a + 0.012f);
letreroRojo3.GetComponent<Renderer>().material.color = new Color(
letreroRojo3.GetComponent<Renderer>().material.color.r,
letreroRojo3.GetComponent<Renderer>().material.color.g,
letreroRojo3.GetComponent<Renderer>().material.color.b,
letreroRojo3.GetComponent<Renderer>().material.color.a + 0.010f);
letreroVerde1.GetComponent<Renderer>().material.color = new Color(
letreroVerde1.GetComponent<Renderer>().material.color.r,
letreroVerde1.GetComponent<Renderer>().material.color.g,
letreroVerde1.GetComponent<Renderer>().material.color.b,
letreroVerde1.GetComponent<Renderer>().material.color.a + 0.01f);
}
}
public void inicializacionIconosGiro()
{
letreroAzul1.transform.Rotate(new Vector3(0, 80f, 0));
letreroAzul2.transform.Rotate(new Vector3(0, 95f, 0));
letreroAzul3.transform.Rotate(new Vector3(0, 110f, 0));
letreroAzul4.transform.Rotate(new Vector3(0, 110f, 0));
letreroRojo1.transform.Rotate(new Vector3(0, 125f, 0));
letreroRojo2.transform.Rotate(new Vector3(0, 155f, 0));
letreroRojo3.transform.Rotate(new Vector3(0, 165f, 0));
letreroVerde1.transform.Rotate(new Vector3(0, 175f, 0));
}
public void giroIconos()
{
if (letreroAzul1.transform.localEulerAngles.y < limiteGiro)
{
letreroAzul1.transform.Rotate(new Vector3(0, velocidadGiro, 0) * Time.deltaTime);
}
if(letreroAzul2.transform.localEulerAngles.y < limiteGiro)
{
letreroAzul2.transform.Rotate(new Vector3(0, velocidadGiro, 0) * Time.deltaTime);
}
if(letreroAzul3.transform.localEulerAngles.y < limiteGiro)
{
letreroAzul3.transform.Rotate(new Vector3(0, velocidadGiro, 0) * Time.deltaTime);
}
if(letreroAzul4.transform.localEulerAngles.y < limiteGiro)
{
letreroAzul4.transform.Rotate(new Vector3(0, velocidadGiro, 0) * Time.deltaTime);
}
if(letreroRojo1.transform.localEulerAngles.y < limiteGiro)
{
letreroRojo1.transform.Rotate(new Vector3(0, velocidadGiro, 0) * Time.deltaTime);
}
if(letreroRojo2.transform.localEulerAngles.y < limiteGiro)
{
letreroRojo2.transform.Rotate(new Vector3(0, velocidadGiro, 0) * Time.deltaTime);
}
if(letreroRojo3.transform.localEulerAngles.y < limiteGiro)
{
letreroRojo3.transform.Rotate(new Vector3(0, velocidadGiro, 0) * Time.deltaTime);
}
if(letreroVerde1.transform.localEulerAngles.y < limiteGiro)
{
letreroVerde1.transform.Rotate(new Vector3(0, velocidadGiro, 0) * Time.deltaTime);
}
}
}
<file_sep>/UncoverMapsProject/Assets/Scripts/TouchLetreros.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.EventSystems;
public class TouchLetreros : MonoBehaviour
{
public GameObject letreroAzul1, letreroAzul2, letreroAzul3, letreroAzul4;
public GameObject letreroRojo1, letreroRojo2, letreroRojo3;
public GameObject letreroVerde1;
public GameObject panel1, panel2, panel3, panel4, panel5, panel6, panel7, panel8;
public GameObject btnCerrarP1;
bool test1, test2, test3, test4, test5, test6, test7, test8;
string nombreLetrero, cerrar;
float velocidadRotacion = -944, limiteGiro = 180;
int contadorY;
//// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
Touch();
if (test1)
{
Rotacion1();
}
else if (test2)
{
Rotacion2();
}
else if (test3)
{
Rotacion3();
}
else if (test4)
{
Rotacion4();
}
else if (test5)
{
Rotacion5();
}
else if (test6)
{
Rotacion6();
}
else if (test7)
{
Rotacion7();
}
else if (test8)
{
Rotacion8();
}
}
public void Touch()
{
if (Input.touchCount > 0 && Input.touches[0].phase == TouchPhase.Began && !panel1.activeInHierarchy)
{
Ray ray = Camera.main.ScreenPointToRay(Input.GetTouch(0).position);
RaycastHit hit;
if (Physics.Raycast(ray, out hit))
{
nombreLetrero = hit.transform.name;
switch (nombreLetrero)
{
case "PlanoTouch1":
test1 = true;
break;
case "PlanoTouch2":
test2 = true;
break;
case "PlanoTouch3":
test3 = true;
break;
case "PlanoTouch4":
test4 = true;
break;
case "PlanoTouch5":
test5 = true;
break;
case "PlanoTouch6":
test6 = true;
break;
case "PlanoTouch7":
test7 = true;
break;
case "PlanoTouch8":
test8 = true;
break;
}
}
}
}
public void Rotacion1()
{
if (letreroAzul1.transform.localEulerAngles.y < limiteGiro)
{
contadorY += 1;
}
if (contadorY <= 15)
{
letreroAzul1.transform.Rotate(new Vector3(0, velocidadRotacion, 0) * Time.deltaTime);
}
else
{
test1 = false;
contadorY = 0;
panel1.SetActive(true);
btnCerrarP1.SetActive(true);
}
}
public void Rotacion2()
{
if (letreroAzul2.transform.localEulerAngles.y < limiteGiro)
{
contadorY += 1;
}
if (contadorY <= 15)
{
letreroAzul2.transform.Rotate(new Vector3(0, velocidadRotacion, 0) * Time.deltaTime);
}
else
{
test2 = false;
contadorY = 0;
panel1.SetActive(true);
btnCerrarP1.SetActive(true);
}
}
public void Rotacion3()
{
if (letreroRojo1.transform.localEulerAngles.y < limiteGiro)
{
contadorY += 1;
}
if (contadorY <= 15)
{
letreroRojo1.transform.Rotate(new Vector3(0, velocidadRotacion, 0) * Time.deltaTime);
}
else
{
test3 = false;
contadorY = 0;
panel1.SetActive(true);
btnCerrarP1.SetActive(true);
}
}
public void Rotacion4()
{
if (letreroRojo2.transform.localEulerAngles.y < limiteGiro)
{
contadorY += 1;
}
if (contadorY <= 15)
{
letreroRojo2.transform.Rotate(new Vector3(0, velocidadRotacion, 0) * Time.deltaTime);
}
else
{
test4 = false;
contadorY = 0;
panel1.SetActive(true);
btnCerrarP1.SetActive(true);
}
}
public void Rotacion5()
{
if (letreroAzul3.transform.localEulerAngles.y < limiteGiro)
{
contadorY += 1;
}
if (contadorY <= 15)
{
letreroAzul3.transform.Rotate(new Vector3(0, velocidadRotacion, 0) * Time.deltaTime);
}
else
{
test5 = false;
contadorY = 0;
panel1.SetActive(true);
btnCerrarP1.SetActive(true);
}
}
public void Rotacion6()
{
if (letreroRojo3.transform.localEulerAngles.y < limiteGiro)
{
contadorY += 1;
}
if (contadorY <= 15)
{
letreroRojo3.transform.Rotate(new Vector3(0, velocidadRotacion, 0) * Time.deltaTime);
}
else
{
test6 = false;
contadorY = 0;
panel1.SetActive(true);
btnCerrarP1.SetActive(true);
}
}
public void Rotacion7()
{
if (letreroAzul4.transform.localEulerAngles.y < limiteGiro)
{
contadorY += 1;
}
if (contadorY <= 15)
{
letreroAzul4.transform.Rotate(new Vector3(0, velocidadRotacion, 0) * Time.deltaTime);
}
else
{
test7 = false;
contadorY = 0;
panel1.SetActive(true);
btnCerrarP1.SetActive(true);
}
}
public void Rotacion8()
{
if (letreroVerde1.transform.localEulerAngles.y < limiteGiro)
{
contadorY += 1;
}
if (contadorY <= 15)
{
letreroVerde1.transform.Rotate(new Vector3(0, velocidadRotacion, 0) * Time.deltaTime);
}
else
{
test8 = false;
contadorY = 0;
panel1.SetActive(true);
btnCerrarP1.SetActive(true);
}
}
}
<file_sep>/UncoverMapsProject/Assets/Scripts/btnCerrar.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.EventSystems;
public class btnCerrar : MonoBehaviour, IPointerDownHandler
{
public GameObject panelCerrar1, panelCerrar2, panelCerrar3, panelCerrar4, panelCerrar5, panelCerrar6, panelCerrar7, panelCerrar8;
void Start()
{
}
// Update is called once per frame
void Update()
{
}
public void OnPointerDown(PointerEventData eventData)
{
if ("btnCerrarP1".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
Debug.Log(gameObject.name);
panelCerrar1.SetActive(false);
}
if ("btnCerrarP1".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
Debug.Log(gameObject.name);
panelCerrar2.SetActive(false);
}
if ("btnCerrarP1".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
Debug.Log(gameObject.name);
panelCerrar3.SetActive(false);
}
if ("btnCerrarP1".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
Debug.Log(gameObject.name);
panelCerrar4.SetActive(false);
}
if ("btnCerrarP1".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
Debug.Log(gameObject.name);
panelCerrar5.SetActive(false);
}
if ("btnCerrarP1".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
Debug.Log(gameObject.name);
panelCerrar6.SetActive(false);
}
if ("btnCerrarP1".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
Debug.Log(gameObject.name);
panelCerrar7.SetActive(false);
}
if ("btnCerrarP1".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
Debug.Log(gameObject.name);
panelCerrar8.SetActive(false);
}
}
}
<file_sep>/UncoverMapsProject/Assets/Scripts/BtnCentral.cs
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.EventSystems;
public class BtnCentral : MonoBehaviour, IPointerDownHandler
{
bool transicion = false, clickBus = false, clickTelefono = false, clickComida = false, clickCampamento = false, clickBtnIzquierdo = false;
public GameObject btnBus, btnCampamento, btnComida, btnTelefono, btnMenu, btnMenuIzquierda, panelBus, panelTelefono, panelComida, panelCampamento;
float y1, y2, y3, y4, y, x, x1;
float velocidadMovimiento = 20;
string btnName;
// Start is called before the first frame update
void Start()
{
llenadoVariablesXYZ();
btnBus.transform.position = new Vector2(x, y);
btnCampamento.transform.position = new Vector2(x, y);
btnComida.transform.position = new Vector2(x, y);
btnTelefono.transform.position = new Vector2(x, y);
btnMenuIzquierda.transform.position = new Vector2(x, y);
}
// Update is called once per frame
void Update()
{
if (transicion)
{
despliegeMenu();
}
else
{
retraerMenu();
}
if (clickBus)
{
transicion = false;
btnBus.transform.SetAsLastSibling();
if (btnBus.transform.position.y <= btnMenuIzquierda.transform.position.y)
{
if (btnMenuIzquierda.transform.position.x > x1)
{
btnMenuIzquierda.transform.position = new Vector2(btnMenuIzquierda.transform.position.x - velocidadMovimiento, y);
}
else
{
panelBus.SetActive(true);
clickBus = false;
}
}
}
if (clickTelefono)
{
transicion = false;
btnTelefono.transform.SetAsLastSibling();
if (btnTelefono.transform.position.y <= btnMenuIzquierda.transform.position.y)
{
if (btnMenuIzquierda.transform.position.x > x1)
{
btnMenuIzquierda.transform.position = new Vector2(btnMenuIzquierda.transform.position.x - velocidadMovimiento, y);
}
else
{
panelTelefono.SetActive(true);
clickTelefono = false;
}
}
}
if (clickCampamento)
{
transicion = false;
btnCampamento.transform.SetAsLastSibling();
if (btnCampamento.transform.position.y <= btnMenuIzquierda.transform.position.y)
{
if (btnMenuIzquierda.transform.position.x > x1)
{
btnMenuIzquierda.transform.position = new Vector2(btnMenuIzquierda.transform.position.x - velocidadMovimiento, y);
}
else
{
panelCampamento.SetActive(true);
clickCampamento = false;
}
}
}
if (clickComida)
{
transicion = false;
btnComida.transform.SetAsLastSibling();
if (btnComida.transform.position.y <= btnMenuIzquierda.transform.position.y)
{
if (btnMenuIzquierda.transform.position.x > x1)
{
btnMenuIzquierda.transform.position = new Vector2(btnMenuIzquierda.transform.position.x - velocidadMovimiento, y);
}
else
{
panelComida.SetActive(true);
clickComida = false;
}
}
}
if (clickBtnIzquierdo)
{
if (btnMenuIzquierda.transform.position.x < x)
{
btnMenuIzquierda.transform.position = new Vector2(btnMenuIzquierda.transform.position.x + velocidadMovimiento, y);
}
else
{
panelBus.transform.position = new Vector2(panelBus.transform.position.x, panelBus.transform.position.y - 700);
panelTelefono.transform.position = new Vector2(panelTelefono.transform.position.x, panelTelefono.transform.position.y - 700);
panelCampamento.transform.position = new Vector2(panelCampamento.transform.position.x, panelCampamento.transform.position.y - 700);
panelComida.transform.position = new Vector2(panelComida.transform.position.x, panelComida.transform.position.y - 700);
panelBus.SetActive(false);
panelTelefono.SetActive(false);
panelCampamento.SetActive(false);
panelComida.SetActive(false);
clickBtnIzquierdo = false;
btnMenu.transform.SetAsLastSibling();
}
}
}
public void OnPointerDown(PointerEventData eventData)
{
if ("BtnCentral".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
if (transicion)
{
transicion = false;
}
else
{
transicion = true;
}
}
if ("BtnTransporte".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
if (!clickBus)
{
clickBus = true;
}
}
if ("BtnLlamadas".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
if (!clickTelefono)
{
clickTelefono = true;
}
}
if ("BtnRestaurante".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
if (!clickComida)
{
clickComida = true;
}
}
if ("BtnHospedaje".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
if (!clickCampamento)
{
clickCampamento = true;
}
}
if ("BtnRegresar".Equals(eventData.pointerCurrentRaycast.gameObject.name))
{
if (!clickBtnIzquierdo)
{
clickBtnIzquierdo = true;
}
}
}
public void llenadoVariablesXYZ()
{
x = btnMenu.transform.position.x;
y = btnMenu.transform.position.y;
x1 = btnMenuIzquierda.transform.position.x;
y1 = btnBus.transform.position.y;
y2 = btnCampamento.transform.position.y;
y3 = btnComida.transform.position.y;
y4 = btnTelefono.transform.position.y;
}
public void desplegarBotonInicioIzquierda()
{
if (btnMenuIzquierda.transform.position.x > x1)
{
btnMenuIzquierda.transform.position = new Vector2(btnMenuIzquierda.transform.position.x - velocidadMovimiento, y);
}
}
public void retraerBotonInicioIzquierda()
{
if (btnMenuIzquierda.transform.position.x < x)
{
btnMenuIzquierda.transform.position = new Vector2(btnMenuIzquierda.transform.position.x + velocidadMovimiento, y);
}
}
public void despliegeMenu()
{
if (btnBus.transform.position.y < y1)
{
btnBus.transform.position = new Vector2(btnMenu.transform.position.x, btnBus.transform.position.y + velocidadMovimiento);
}
if (btnCampamento.transform.position.y < y2)
{
btnCampamento.transform.position = new Vector2(btnMenu.transform.position.x, btnCampamento.transform.position.y + velocidadMovimiento);
}
if (btnComida.transform.position.y < y3)
{
btnComida.transform.position = new Vector2(btnMenu.transform.position.x, btnComida.transform.position.y + velocidadMovimiento);
}
if (btnTelefono.transform.position.y < y4)
{
btnTelefono.transform.position = new Vector2(btnMenu.transform.position.x, btnTelefono.transform.position.y + velocidadMovimiento);
}
}
public void retraerMenu()
{
if (btnBus.transform.position.y > y)
{
btnBus.transform.position = new Vector2(btnMenu.transform.position.x, btnBus.transform.position.y - velocidadMovimiento);
}
if (btnCampamento.transform.position.y > y)
{
btnCampamento.transform.position = new Vector2(btnMenu.transform.position.x, btnCampamento.transform.position.y - velocidadMovimiento);
}
if (btnComida.transform.position.y > y)
{
btnComida.transform.position = new Vector2(btnMenu.transform.position.x, btnComida.transform.position.y - velocidadMovimiento);
}
if (btnTelefono.transform.position.y > y)
{
btnTelefono.transform.position = new Vector2(btnMenu.transform.position.x, btnTelefono.transform.position.y - velocidadMovimiento);
}
}
}
<file_sep>/UncoverMapsProject/Assets/Scripts/ControlClima.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ControlClima : MonoBehaviour
{
public GameObject luna, sol, nubesClaras;
Animation animacionSol, animacionLuna, animacionNubesClaras;
public AnimationClip solIn, solOut, lunaIn, lunaOut, nubesClarasIn, nubesClarasOut;
int count = 0;
bool inSol = false, outSol = false, inLuna = false, outLuna = false, inNubes = false, outNubes = false;
// Start is called before the first frame update
void Start()
{
initialObject();
animacionSol = sol.AddComponent<Animation>();
animacionSol.AddClip(solIn, "solIN");
animacionSol.AddClip(solOut, "solOut");
animacionLuna = luna.AddComponent<Animation>();
animacionLuna.AddClip(lunaIn, "lunaIn");
animacionLuna.AddClip(lunaOut, "lunaOut");
animacionNubesClaras = nubesClaras.AddComponent<Animation>();
animacionNubesClaras.AddClip(nubesClarasIn, "nubesIn");
animacionNubesClaras.AddClip(nubesClarasOut, "nubesOut");
}
// Update is called once per frame
void Update()
{
count++;
if (count == 200)
{
Debug.Log("sol true");
inSol = true;
}
if (count == 600)
{
Debug.Log("luna true");
inLuna = true;
}
if (count == 1000)
{
Debug.Log("nubes true");
inNubes = true;
count = 0;
}
if (inSol)
{
inSol = false;
outSol = true;
animacionSol.Play("solIN");
}
if (outSol)
{
outSol = false;
animacionSol.Play("solOut");
}
if (inLuna)
{
inLuna = false;
outLuna = true;
animacionLuna.Play("lunaIn");
}
if (outLuna)
{
outLuna = false;
animacionLuna.Play("lunaOut");
}
if (inNubes)
{
inNubes = false;
outNubes = true;
animacionNubesClaras.Play("nubesIn");
}
if (outNubes)
{
outNubes = false;
animacionNubesClaras.Play("nubesOut");
}
}
public void initialObject()
{
luna.GetComponent<Renderer>().material.color = new Color(luna.GetComponent<Renderer>().material.color.r,
luna.GetComponent<Renderer>().material.color.g, luna.GetComponent<Renderer>().material.color.b, 0.0f);
sol.GetComponent<Renderer>().material.color = new Color(sol.GetComponent<Renderer>().material.color.r,
sol.GetComponent<Renderer>().material.color.g, sol.GetComponent<Renderer>().material.color.b, 0.0f);
nubesClaras.GetComponent<Renderer>().material.color = new Color(nubesClaras.GetComponent<Renderer>().material.color.r,
nubesClaras.GetComponent<Renderer>().material.color.g, nubesClaras.GetComponent<Renderer>().material.color.b, 0.0f);
}
}
| c6b1579f8753beac8cbb0ff793646b14fdb96ea2 | [
"C#"
]
| 5 | C# | HackathonNicaragua2019/Uncover-Maps | 018110a0d3f48cad8229213e1003f79bbaebea59 | 0e1113d1e817927529ec9568b9ef5f1a41db0c03 | |
refs/heads/master | <repo_name>floatflower/FFParser<file_sep>/src/tablerecord.h
#ifndef TABLERECORD_H
#define TABLERECORD_H
#include <QObject>
#include <QVector>
#include "rule.h"
class TableRecord : public QVector<Rule*>
{
public:
explicit TableRecord();
void findFirstSet();
QVector<QString> firstSet();
void findFollowSet();
QVector<QString> followSet();
void mergeFirstSet(QVector<QString> firstSet);
void mergeFollowSet(QVector<QString> followSet);
bool derivedLamda();
void setKey(QString key) { m_key = key; }
QString key() { return m_key; }
void setHasFollowSet(bool set) { m_hasFollowSet = set; }
bool hasFollowSet() { return m_hasFollowSet; }
signals:
public slots:
private:
bool m_hasFirset;
QVector<QString> m_firstSet;
QString m_key;
bool m_hasFollowSet;
QVector<QString> m_followSet;
};
#endif // TABLERECORD_H
<file_sep>/src/rule.cpp
#include "rule.h"
#include "table.h"
#include <QDebug>
Rule::Rule(QObject *parent)
: QObject(parent)
, m_derivedLamda(true)
, m_hasFirstSet(false)
{
}
void Rule::findFirstSet()
{
if (m_hasFirstSet) return;
m_hasFirstSet = true;
Table *table = Table::instance();
for (QVector<QString>::iterator it_derived = m_derived.begin();
it_derived != m_derived.end();
it_derived ++) {
if (table->isNonterminal(*it_derived)) {
QVector<QString> firstSet = table->firstSet(*it_derived);
mergeFirstSet(firstSet);
m_derivedLamda &= table->derivedLamda(*it_derived);
}
else {
if (*it_derived != "lamda") {
m_firstSet.push_back(*it_derived);
m_derivedLamda = false;
}
else {
m_derivedLamda = true;
}
break;
}
if (table->derivedLamda(*it_derived)) {
continue;
}
else {
break;
}
}
}
QVector<QString> Rule::firstSet()
{
findFirstSet();
return m_firstSet;
}
void Rule::findPredictSet()
{
for (QVector<QString>::iterator it_firstSet = m_firstSet.begin();
it_firstSet != m_firstSet.end();
it_firstSet ++) {
m_predictSet.push_back(*it_firstSet);
}
if (m_derivedLamda) {
for (QVector<QString>::iterator it_followSet = m_followSet.begin();
it_followSet != m_followSet.end();
it_followSet ++) {
m_predictSet.push_back(*it_followSet);
}
}
}
QVector<QString> Rule::predictSet()
{
return m_predictSet;
}
void Rule::mergeFirstSet(QVector<QString>& firstSet)
{
for (QVector<QString>::iterator it_firstSet = firstSet.begin();
it_firstSet != firstSet.end();
it_firstSet ++) {
if (m_firstSet.indexOf(*it_firstSet) == -1) {
m_firstSet.push_back(*it_firstSet);
}
}
}
void Rule::mergeFollowSet(QVector<QString> followSet)
{
for (QVector<QString>::iterator it_followSet = followSet.begin();
it_followSet != followSet.end();
it_followSet ++) {
if (m_followSet.indexOf(*it_followSet) == -1 && (*it_followSet) != "lamda") {
m_followSet.push_back(*it_followSet);
}
}
}
<file_sep>/src/main.cpp
#include <QCoreApplication>
#include "ffparser.h"
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
FFParser p;
p.run();
return 0;
}
<file_sep>/README.md
FFParser
===
[](https://travis-ci.org/floatflower/FFParser)
這個專案是為了編譯原理所開發的 LL(1) Parser,這個專案已經完成開發並完全符合老師要求,取得專題滿分。
This LL(1) Parser is the final project for the class "compiler", this project had been developed completely, and matched all requirement from professor.
## Notice
若需要使用此專案作為個人作業繳交,請先來信告知作者,
此外,若為其他教學用途,敬請使用並附上原始碼出處。
## Developer
+ Developer: FloatFlower.Huang
+ Email: <EMAIL>
+ Website: https://blog.floatflower.me
## How to use
### Compile this project
```
$ sudo add-apt-repository ppa:beineri/opt-qt562-trusty
$ sudo apt-get update
$ sudo apt-get install -qq qt5-qmake qt56base
$ source /opt/qt56/bin/qt56-env.sh
$ /opt/qt56/bin/qmake
$ make
```
### Execute
```
$ ./ffparser --cfg,-c <CFG_FILE>
--source,-s <SOURCE_CODE_PATH>
--output,-o <OUTPUT_FILE_PATH>
```
<file_sep>/src/lookaheadtable.h
#ifndef LOOKAHEADTABLE_H
#define LOOKAHEADTABLE_H
#include <QObject>
#include <QHash>
class LookAheadTable : public QObject
{
Q_OBJECT
public:
explicit LookAheadTable(QObject *parent = nullptr);
void addRule(QString nonterminal, QString terminal, int ruleNumber);
QHash<QString, QHash<QString, int>> table() { return m_lookAheadTable; }
int findRule(QString source, QString target);
signals:
public slots:
private:
QHash<QString, QHash<QString, int>> m_lookAheadTable;
};
#endif // LOOKAHEADTABLE_H
<file_sep>/src/rule.h
#ifndef RULE_H
#define RULE_H
#include <QObject>
#include <QString>
#include <QVector>
class Rule : public QObject
{
Q_OBJECT
public:
explicit Rule(QObject *parent = nullptr);
void setRuleNumber(int number) { m_ruleNumber = number; }
int ruleNumber() { return m_ruleNumber; }
void addDerived(QString derived) { m_derived.push_back(derived); }
QVector<QString> derived() { return m_derived; }
bool derivedLamda() { return m_derivedLamda; }
void findFirstSet();
QVector<QString> firstSet();
QVector<QString> followSet() { return m_followSet; }
void findPredictSet();
QVector<QString> predictSet();
void mergeFirstSet(QVector<QString> &firstSet);
void mergeFollowSet(QVector<QString> followSet);
signals:
public slots:
private:
int m_ruleNumber;
QVector<QString> m_derived;
QVector<QString> m_firstSet;
QVector<QString> m_followSet;
QVector<QString> m_predictSet;
bool m_derivedLamda;
bool m_hasFirstSet;
};
#endif // RULE_H
<file_sep>/src/tablerecord.cpp
#include "tablerecord.h"
#include <QDebug>
#include "table.h"
TableRecord::TableRecord()
: m_hasFirset(false)
, m_hasFollowSet(false)
{
}
void TableRecord::findFirstSet()
{
int tableSize = size();
for (int i = 0; i < tableSize; i ++) {
mergeFirstSet(at(i)->firstSet());
}
}
QVector<QString> TableRecord::firstSet()
{
if (!m_hasFirset) findFirstSet();
m_hasFirset = true;
return m_firstSet;
}
void TableRecord::findFollowSet()
{
//qDebug() << "Find Follow" << key();
Table *table = Table::instance();
//qDebug() << "Current finding is:" << table->currentFindingFollow();
for (TableRecord::iterator it_tableRecord = begin();
it_tableRecord != end();
it_tableRecord ++) {
// iterate all rule.
/**
* Iterator whole table to find who derive the "key()"
*/
//if (!(*it_tableRecord)->derivedLamda()) continue;
for (Table::iterator it_searchTable = table->begin();
it_searchTable != table->end();
it_searchTable ++) {
//qDebug() << "Searching Record..." << it_searchTable.key();
for (TableRecord::iterator it_searchTableRecord = (*it_searchTable)->begin();
it_searchTableRecord != (*it_searchTable)->end();
it_searchTableRecord ++) {
//qDebug() << "Searching Rule..." << (*it_searchTableRecord)->ruleNumber();
QVector<QString> derived = (*it_searchTableRecord)->derived();
int derivedSize = derived.size();
int derivedAmount = derived.count(key());
bool tmp_derivedLamda = false;
if (derivedAmount != 0) {
int thisDerived = 0;
for (int eachDerived = 1; eachDerived <= derivedAmount; eachDerived ++) {
int derivedIndex = derived.indexOf(key(), thisDerived);
thisDerived = derivedIndex + 1;
for (int i = derivedIndex + 1; i < derivedSize; i ++) {
//qDebug() << "Find first " << derived.at(i);
if (table->isNonterminal(derived.at(i))) {
QVector<QString> tmp_firstSet = table->firstSet(derived.at(i));
(*it_tableRecord)->mergeFollowSet(tmp_firstSet);
tmp_derivedLamda = table->derivedLamda(derived.at(i));
}
else {
QVector<QString> terminal;
terminal.push_back(derived.at(i));
(*it_tableRecord)->mergeFollowSet(terminal);
tmp_derivedLamda = false;
}
if (!tmp_derivedLamda) break;
}
if (derivedIndex + 1 == derivedSize) {
//qDebug() << "is last element";
tmp_derivedLamda = true;
}
if (tmp_derivedLamda
&& table->currentFindingFollow() != (*it_searchTable)->key()) {
//qDebug() << "get follow set" << (*it_searchTable)->key();
(*it_tableRecord)->mergeFollowSet(table->followSet((*it_searchTable)->key()));
}
}
}
}
}
}
}
QVector<QString> TableRecord::followSet()
{
if (!m_hasFollowSet) {
Table *table = Table::instance();
if (!table->m_currentFindingRecord.contains(key())) {
table->m_currentFindingRecord.push_back(key());
findFollowSet();
}
for (TableRecord::iterator it_tableRecord = begin();
it_tableRecord != end();
it_tableRecord ++) {
mergeFollowSet((*it_tableRecord)->followSet());
}
}
return m_followSet;
}
void TableRecord::mergeFirstSet(QVector<QString> firstSet)
{
for (QVector<QString>::iterator it_firstSet = firstSet.begin();
it_firstSet != firstSet.end();
it_firstSet ++) {
if (m_firstSet.indexOf(*it_firstSet) == -1) {
m_firstSet.push_back(*it_firstSet);
}
}
m_hasFirset = true;
}
void TableRecord::mergeFollowSet(QVector<QString> followSet)
{
for (QVector<QString>::iterator it_followSet = followSet.begin();
it_followSet != followSet.end();
it_followSet ++) {
if (m_followSet.indexOf(*it_followSet) == -1) {
m_followSet.push_back(*it_followSet);
}
}
}
bool TableRecord::derivedLamda()
{
bool result = false;
int tableSize = size();
for (int i = 0; i < tableSize; i ++) {
result |= at(i)->derivedLamda();
}
return result;
}
<file_sep>/src/sourcecodehandler.h
#ifndef SOURCECODEHANDLER_H
#define SOURCECODEHANDLER_H
#include <QObject>
#include <QVector>
#include <QStack>
class SourceCodeHandler : public QObject
{
Q_OBJECT
public:
explicit SourceCodeHandler(QObject *parent = nullptr);
QVector<int> process(QStringList line);
void setStartPoint(QString startPoint) { m_startPoint = startPoint; }
void showStackStatus();
QVector<int> ruleApplyOrder() { return m_ruleApplyOrder; }
bool isAccept() { return m_isAccept; }
signals:
public slots:
private:
QStack<QString> m_parseStack;
QString m_startPoint;
QVector<int> m_ruleApplyOrder;
bool m_isAccept;
};
#endif // SOURCECODEHANDLER_H
<file_sep>/src/table.cpp
#include "table.h"
#include <QDebug>
#include <iostream>
Table* Table::m_instance = nullptr;
Table::Table()
{
}
void Table::addRule(QString key, Rule* rule)
{
Table::iterator it_table = find(key);
m_ruleMap.insert(rule->ruleNumber(), rule);
if (it_table != end()) {
// Exist
(*it_table)->push_back(rule);
}
else {
// Not exist
TableRecord *r = new TableRecord;
r->push_back(rule);
r->setKey(key);
insert(key, r);
}
}
Rule *Table::rule(int ruleNumber)
{
QHash<int, Rule*>::iterator it_rule = m_ruleMap.find(ruleNumber);
if (it_rule != m_ruleMap.end()) {
return *it_rule;
}
return nullptr;
}
void Table::findFirstSet()
{
for (Table::iterator it_table = begin();
it_table != end();
it_table ++) {
(*it_table)->findFirstSet();
// qDebug() << "TableRecord" << it_table.key() << (*it_table)->firstSet();
}
}
QVector<QString> Table::firstSet(QString key)
{
Table::iterator it_table = find(key);
return (*it_table)->firstSet();
}
void Table::findFollowSet()
{
for (Table::iterator it_table = begin();
it_table != end();
it_table ++) {
m_currentFindingFollow = it_table.key();
m_currentFindingRecord.push_back(it_table.key());
(*it_table)->findFollowSet();
m_currentFindingRecord.clear();
}
}
QVector<QString> Table::followSet(QString key)
{
Table::iterator it_table = find(key);
return (*it_table)->followSet();
}
void Table::findPreictSet()
{
for (Table::iterator it_table = begin();
it_table != end();
it_table ++) {
for (TableRecord::iterator it_tableRecord = (*it_table)->begin();
it_tableRecord != (*it_table)->end();
it_tableRecord ++) {
(*it_tableRecord)->findPredictSet();
}
}
}
bool Table::derivedLamda(QString key)
{
Table::iterator it_table = find(key);
return (*it_table)->derivedLamda();
}
Table* Table::instance()
{
if (Table::m_instance == nullptr) {
Table::m_instance = new Table;
}
return Table::m_instance;
}
void Table::printTable()
{
for (Table::iterator it_table = begin();
it_table != end();
it_table ++) {
for (TableRecord::iterator it_tableRecord = (*it_table)->begin();
it_tableRecord != (*it_table)->end();
it_tableRecord ++) {
qDebug() << "Rule" << (*it_tableRecord)->ruleNumber() << ": \n"
<< "Derived:" << (*it_tableRecord)->derived() << "\n"
<< "First set: " << (*it_tableRecord)->firstSet() << "\n"
<< "Derived lamda: " << (*it_tableRecord)->derivedLamda() << "\n"
<< "Follow set: " << (*it_tableRecord)->followSet() << "\n"
<< "Predict set: " << (*it_tableRecord)->predictSet() << "\n";
}
}
for (Table::iterator it_table = begin();
it_table != end();
it_table ++) {
for (TableRecord::iterator it_tableRecord = (*it_table)->begin();
it_tableRecord != (*it_table)->end();
it_tableRecord ++) {
QVector<QString> m_derived = (*it_tableRecord)->derived();
for (QVector<QString>::iterator it_derived = m_derived.begin();
it_derived != m_derived.end();
it_derived ++) {
if (!isNonterminal(*it_derived) && (*it_derived) != "lamda") {
m_terminal.push_back(*it_derived);
}
}
}
}
std::cout << "LL1 Table: "<< std::endl;
for (Table::iterator it_table = begin();
it_table != end();
it_table ++) {
for (TableRecord::iterator it_tableRecord = (*it_table)->begin();
it_tableRecord != (*it_table)->end();
it_tableRecord ++) {
QVector<QString> predictSet = (*it_tableRecord)->predictSet();
for (QVector<QString>::iterator it_terminal = m_terminal.begin();
it_terminal != m_terminal.end();
it_terminal ++) {
if (predictSet.indexOf(*it_terminal) != -1) {
m_lookAheadTable.addRule((*it_table)->key(),
*it_terminal,
(*it_tableRecord)->ruleNumber());
}
}
}
}
std::cout << "\t\t";
for (QVector<QString>::iterator it_terminal = m_terminal.begin();
it_terminal != m_terminal.end();
it_terminal ++) {
std::cout << (*it_terminal).toStdString().c_str() << "\t";
}
std::cout << std::endl;
QHash<QString, QHash<QString, int>> lookAheadTable = m_lookAheadTable.table();
for (QHash<QString, QHash<QString, int>>::iterator it_lookAheadTable = lookAheadTable.begin();
it_lookAheadTable != lookAheadTable.end();
it_lookAheadTable ++) {
std::cout << it_lookAheadTable.key().toStdString().c_str() << "\t\t";
for (QVector<QString>::iterator it_terminal = m_terminal.begin();
it_terminal != m_terminal.end();
it_terminal ++) {
QHash<QString, int>::iterator it_row = (*it_lookAheadTable).find(*it_terminal);
if (it_row != (*it_lookAheadTable).end()) {
std::cout << *it_row << "\t";
}
else {
std::cout << "\t";
}
}
std::cout << std::endl;
}
}
void Table::addTerminal(QString terminal)
{
if (m_terminal.indexOf(terminal) == -1) {
m_terminal.push_back(terminal);
}
}
<file_sep>/src/ffparser.cpp
#include "ffparser.h"
#include <QCoreApplication>
#include <QDebug>
#include <iostream>
#include <QRegExp>
#include "rule.h"
#include "tablerecord.h"
#include "table.h"
#include "sourcecodehandler.h"
FFParser::FFParser(QObject *parent) : QObject(parent)
{
QCoreApplication *app = QCoreApplication::instance();
m_arguments = app->arguments();
for (QStringList::iterator it_arguments = m_arguments.begin();
it_arguments != m_arguments.end();
it_arguments ++) {
if ((*it_arguments) == "-o" || (*it_arguments) == "--output") {
m_outputFilename = *(it_arguments + 1);
}
else if ((*it_arguments) == "-s" || (*it_arguments) == "--source") {
m_sourceCodeFile.setFileName(*(it_arguments + 1));
}
else if ((*it_arguments) == "-c" || (*it_arguments) == "--cfg") {
m_cfgFile.setFileName(*(it_arguments + 1));
}
}
}
void FFParser::run()
{
if (!m_sourceCodeFile.exists()) {
qFatal("Source code file is required");
}
if (!m_cfgFile.exists()) {
qFatal("CFG file is required.");
}
buildTable();
Table *table = Table::instance();
table->findFirstSet();
table->findFollowSet();
table->findPreictSet();
table->printTable();
readSourceCodeLine();
for (QVector<QStringList>::iterator it_line = m_sourceCodeLine.begin();
it_line != m_sourceCodeLine.end();
it_line ++) {
SourceCodeHandler handler;
handler.setStartPoint(m_startPoint);
handler.process(*it_line);
QVector<int> result = handler.ruleApplyOrder();
for (QStringList::iterator it_rawLine = (*it_line).begin();
it_rawLine != (*it_line).end();
it_rawLine ++) {
std::cout << (*it_rawLine).toStdString().c_str() << " ";
}
for (QVector<int>::iterator it_result = result.begin();
it_result != result.end();
it_result ++) {
std::cout << (*it_result) << " ";
}
handler.isAccept() ? std::cout << std::endl : std::cout << "Error" << std::endl;
}
}
void FFParser::buildTable()
{
if (!m_cfgFile.open(QIODevice::ReadOnly | QIODevice::Text)) {
qFatal("CFG file cannot be opened.");
}
QString currentToken;
while (!m_cfgFile.atEnd()) {
QString line(m_cfgFile.readLine());
line = line.trimmed();
QStringList tokens = line.split(QRegExp("[ \t\r\n\f]"));
Rule *r = new Rule();
r->setRuleNumber(tokens.at(0).toInt());
if (tokens.at(0).toInt() == 1) {
m_startPoint = tokens.at(1);
}
if (tokens.at(1) != QString("|")) {
currentToken = tokens.at(1);
for (int i =3; i < tokens.size(); i ++) {
if (tokens.at(i) == "lamda") continue;
r->addDerived(tokens.at(i));
}
}
else {
for (int i =2; i < tokens.size(); i ++) {
if (tokens.at(i) == "lamda") continue;
r->addDerived(tokens.at(i));
}
}
Table *table = Table::instance();
table->addRule(currentToken, r);
}
}
void FFParser::readSourceCodeLine()
{
while(!m_sourceCodeFile.open(QIODevice::ReadOnly | QIODevice::Text)) {
qFatal("Source code file cannot be opened");
}
while(!m_sourceCodeFile.atEnd()) {
QString line(m_sourceCodeFile.readLine());
line = line.trimmed();
QStringList tokens = line.split(QRegExp("[ \t\r\n\f]"));
m_sourceCodeLine.push_back(tokens);
}
}
<file_sep>/src/sourcecodehandler.cpp
#include "sourcecodehandler.h"
#include "table.h"
#include "lookaheadtable.h"
#include <QDebug>
#include <iostream>
SourceCodeHandler::SourceCodeHandler(QObject *parent)
: QObject(parent)
, m_isAccept(false)
{
}
QVector<int> SourceCodeHandler::process(QStringList line)
{
QVector<int> result;
Table *table = Table::instance();
LookAheadTable *lookAheadTable = table->lookAheadTable();
m_parseStack.push(m_startPoint);
while(!m_parseStack.isEmpty() && line.size() != 0) {
if (table->isNonterminal(m_parseStack.top())) {
QString top = m_parseStack.top();
int ruleNumber = lookAheadTable->findRule(top, line.at(0));
if (ruleNumber == -1) { break; }
m_ruleApplyOrder.push_back(ruleNumber);
qDebug() << "Apply rule number" << ruleNumber;
Rule* applyRule = table->rule(ruleNumber);
QVector<QString> derived = applyRule->derived();
if (derived.size() > 0) {
m_parseStack.pop();
for (QVector<QString>::reverse_iterator rit_derived = derived.rbegin();
rit_derived != derived.rend();
rit_derived ++) {
m_parseStack.push_back(*rit_derived);
}
while( ((!m_parseStack.isEmpty())&&(line.size()!=0))
&&(m_parseStack.top() == line.at(0)) )
{
qDebug() << "matched" << m_parseStack.top();
m_parseStack.pop();
line.pop_front();
}
}
else {
m_parseStack.pop();
}
}
else {
if (m_parseStack.top() == line.at(0)) {
while( ((!m_parseStack.isEmpty())&&(line.size()!=0))
&&(m_parseStack.top() == line.at(0)) )
{
qDebug() << "matched!" << m_parseStack.top();
m_parseStack.pop();
line.pop_front();
}
}
else {
break;
}
}
}
if (m_parseStack.isEmpty() && line.size() == 0) {
qDebug() << "Accept\n";
m_isAccept = true;
}
else {
qDebug() << "Error\n";
m_isAccept = false;
}
return result;
}
void SourceCodeHandler::showStackStatus()
{
for (QStack<QString>::iterator it_parseStack = m_parseStack.begin();
it_parseStack != m_parseStack.end();
it_parseStack ++) {
std::cout << (*it_parseStack).toStdString().c_str() << " ";
}
std::cout << std::endl;
}
<file_sep>/src/lookaheadtable.cpp
#include "lookaheadtable.h"
LookAheadTable::LookAheadTable(QObject *parent) : QObject(parent)
{
}
void LookAheadTable::addRule(QString nonterminal, QString terminal, int ruleNumber)
{
QHash<QString, QHash<QString,int>>::iterator it_terminalMap
= m_lookAheadTable.find(nonterminal);
if (it_terminalMap != m_lookAheadTable.end()) {
(*it_terminalMap).insert(terminal, ruleNumber);
}
else {
QHash<QString, int> newRow;
newRow.insert(terminal, ruleNumber);
m_lookAheadTable.insert(nonterminal, newRow);
}
}
int LookAheadTable::findRule(QString source, QString target)
{
QHash<QString, QHash<QString,int>>::iterator it_terminalMap
= m_lookAheadTable.find(source);
QHash<QString, int>::iterator it_row = (*it_terminalMap).find(target);
if (it_row != (*it_terminalMap).end()) {
return *it_row;
}
else return -1;
}
<file_sep>/src/table.h
#ifndef TABLE_H
#define TABLE_H
#include <QObject>
#include <QHash>
#include <QString>
#include "tablerecord.h"
#include "rule.h"
#include "lookaheadtable.h"
class Table : public QHash<QString, TableRecord*>
{
public:
explicit Table();
void addRule(QString key, Rule* rule);
Rule* rule(int ruleNumber);
void findFirstSet();
void findFollowSet();
void findPreictSet();
QVector<QString> firstSet(QString key);
QVector<QString> followSet(QString key);
static Table* instance();
bool isNonterminal(QString key) { return (find(key) != end()); }
bool derivedLamda(QString key);
void printTable();
void addTerminal(QString terminal);
QString currentFindingFollow() { return m_currentFindingFollow; }
LookAheadTable* lookAheadTable() { return &m_lookAheadTable; }
QVector<QString> m_currentFindingRecord;
signals:
public slots:
private:
static Table* m_instance;
QVector<QString> m_terminal;
QHash<int, Rule*> m_ruleMap;
LookAheadTable m_lookAheadTable;
QString m_currentFindingFollow;
};
#endif // TABLE_H
<file_sep>/src/ffparser.h
#ifndef FFPARSER_H
#define FFPARSER_H
#include <QObject>
#include <QStringList>
#include <QFile>
#include "rule.h"
#include "table.h"
class FFParser : public QObject
{
Q_OBJECT
public:
explicit FFParser(QObject *parent = nullptr);
void run();
void buildTable();
void readSourceCodeLine();
signals:
public slots:
private:
QStringList m_arguments;
QString m_outputFilename;
QFile m_cfgFile;
QFile m_sourceCodeFile;
QVector<QStringList> m_sourceCodeLine;
QString m_startPoint;
};
#endif // FFPARSER_H
| 0966407a2017ffb8ed966427da02ba1c03ff5ae5 | [
"Markdown",
"C++"
]
| 14 | C++ | floatflower/FFParser | 772c087699c531075664030c982a854ece9338df | 956710d29764359eaf76c887a155422171d796cf | |
refs/heads/master | <repo_name>vishpitama/Algorithms-1-Lab<file_sep>/Assignment 10/17CS30038_10.c
#include<stdio.h>
#include<stdlib.h>
typedef struct _rwd
{
int start_time;
int service_time;
}reqWD;
typedef struct _rwd1
{
int start_time;
int service_time;
int id;
}reqWD1;
typedef struct _rsd
{
int service_time;
int target_time;
} reqSD;
typedef struct _rsd1
{
int service_time;
int target_time;
int id;
} reqSD1;
void merge1(reqWD1 a[],int low ,int mid,int high)
{
int i=low,j=mid+1;
int k=0;
reqWD1 *b=(reqWD1*)malloc((high-low+1)*sizeof(reqWD));
while(i<=mid&&j<=high)
{
if(a[i].start_time>a[j].start_time)
{
b[k]=a[j];
j++;
k++;
}
else
{
b[k]=a[i];
i++;
k++;
}
}
while(i<=mid)
{
b[k]=a[i];
i++;
k++;
}
while(j<=high)
{
b[k]=a[j];
j++;
k++;
}
for(i=low,k=0;i<=high;i++,k++)
{
a[i]=b[k];
}
return ;
}
void sort1(reqWD1 a[],int low,int high)
{
if(low<high)
{
int mid=(low+high)/2;
sort1(a,low,mid);
sort1(a,mid+1,high);
merge1(a,low,mid,high);
}
}
void fun1(reqWD1* A,int n)
{
reqWD1* B=(reqWD1*)malloc(sizeof(reqWD1)*n);
int *keeper=(int* )malloc(n*sizeof(int));//stores counter no of ith index
int i,j,index,max_count=1;
B[0]=A[0];
keeper[0]=0;
for(i=1;i<n;i++)
{
for(j=0;j<max_count;j++)
{
if(B[j].start_time+B[j].service_time<=A[i].start_time)
{
B[j]=A[i];
keeper[i]=j;
// count+++;
// maxcount++;
break;
}
else
continue;
}
if(j==max_count)
{
B[j]=A[i];
keeper[i]=max_count;
// count++;
max_count++;
}
}
/* for(int i=0;i<n;i++)
printf("%d\n",keeper[i]);*/
for(i=0;i<max_count;i++)
{
printf("counter %d::\n",i);
for(j=0;j<n;j++)
{
if(keeper[j]==i)
{
printf("%d\t%d\t%d\n",A[j].id,A[j].start_time,A[j].start_time+A[j].service_time);
}
}
}
return ;
}
void merge2(reqSD1 a[],int low ,int mid,int high)
{
int i=low,j=mid+1;
int k=0;
reqSD1 *b=(reqSD1*)malloc((high-low+1)*sizeof(reqWD));
while(i<=mid&&j<=high)
{
if(a[i].target_time>a[j].target_time)
{
b[k]=a[j];
j++;
k++;
}
else
{
b[k]=a[i];
i++;
k++;
}
}
while(i<=mid)
{
b[k]=a[i];
i++;
k++;
}
while(j<=high)
{
b[k]=a[j];
j++;
k++;
}
for(i=low,k=0;i<=high;i++,k++)
{
a[i]=b[k];
}
return ;
}
void sort2(reqSD1 a[],int low,int high)
{
if(low<high)
{
int mid=(low+high)/2;
sort2(a,low,mid);
sort2(a,mid+1,high);
merge2(a,low,mid,high);
}
}
void fun2(reqSD1* A,int n)
{
int start_time=0;
int end_time=0;
for(int i=0;i<n;i++)
{
start_time=end_time;
end_time=start_time+A[i].service_time;
printf("request %d:: ",A[i].id);
printf("%d %d\n",start_time,end_time);
}
return ;
}
int main()
{
int n;
printf("Enter the number of requests over weekday:\n");
scanf("%d",&n);
reqWD *rw=(reqWD*)malloc(n*sizeof(reqWD));
reqWD1* RW=(reqWD1*)malloc(n*sizeof(reqWD1));
printf("Enter the start time and length for each of the requests\n");
for(int i=0;i<n;i++)
{
printf("request no %d::",i);
scanf("%d%d",&RW[i].start_time,&RW[i].service_time);
RW[i].id=i;
rw[i].start_time=RW[i].start_time;
rw[i].service_time=RW[i].service_time;
}
sort1(RW,0,n-1);
/* for(int i=0;i<n;i++)
{
printf("%d: %d :: %d\n",RW[i].id,RW[i].start_time,RW[i].service_time);
}*/
fun1(RW,n);
int m;
printf("Enter the number of requests over Saturday:\n");
scanf("%d",&m);
reqSD *sw=(reqSD*)malloc(m*sizeof(reqSD));
reqSD1* SW=(reqSD1*)malloc(m*sizeof(reqSD1));
printf("Enter the length and target time for each of the requests\n");
for(int i=0;i<m;i++)
{
printf("request no %d::",i);
scanf("%d%d",&SW[i].service_time,&SW[i].target_time);
SW[i].id=i;
sw[i].service_time=SW[i].service_time;
sw[i].target_time=SW[i].target_time;
}
sort2(SW,0,m-1);
fun2(SW,m);
return 0;
}
<file_sep>/Assignment 12/17CS30038_12.c
#include<stdio.h>
#include<stdlib.h>
#include<limits.h>
typedef struct node_
{
int vno;
struct node_* next;
}node;
typedef struct _vertex
{
node *adjlist;
} vertex;
typedef vertex *graph;
void printlist(graph G,int v)
{
node* tmp;
for(int i=0;i<v;i++)
{
printf("vertex %d:",i);
tmp=G[i].adjlist;
while(tmp!=NULL)
{
printf("%d ",tmp->vno);
tmp=tmp->next;
}
printf("\n");
}
return ;
}
void dfsvisit(graph G,int *visit,int i,int rem)
{
visit[i]=1;
node* tmp=G[i].adjlist;
while(tmp!=NULL)
{
if(tmp->vno==rem)
{
tmp=tmp->next;
}
else
{
if(!visit[tmp->vno])
dfsvisit(G,visit,tmp->vno,rem);
tmp=tmp->next;
}
}
}
int DFSComp (graph G,int v, int rem)
{
int *visit=(int*)malloc(v*sizeof(int));
for(int i=0;i<v;i++)
visit[i]=0;
int t=0;
for(int i=0;i<v;i++)
{
if(i==rem)
continue;
else
{
if(visit[i]==0)
{
dfsvisit(G,visit,i,rem);
t++;
}
}
}
return t;
}
void findCritical (graph G,int v)
{
printf("Critical junctions using the simple algorithm:\n");
for(int i=0;i<v;i++)
{
int k=DFSComp (G,v, i);
if(k>1)
{
printf("vertex %d : %d components\n",i,k);
}
}
return ;
}
int dfsvisit2(graph G,int *visit,int* low,int* d,int* P,int i,int* time)
{
int t=INT_MAX;;
visit[i]=1;
d[i]=*time;
(*time)++;
node* tmp=G[i].adjlist,*tmp2;
tmp2=tmp;
while(tmp2!=NULL)
{
if(low[tmp2->vno]>d[i])
low[tmp2->vno]=d[i];
tmp2=tmp2->next;
}
while(tmp!=NULL)
{
if(!visit[tmp->vno])
{
P[tmp->vno]=i;
t=dfsvisit2(G,visit,low,d,P,tmp->vno,time);
if(t<low[i])
low[i]=t;
}
tmp=tmp->next;
}
return low[i];
}
void findCriticalFast (graph G,int v)
{
int *visit=(int*)malloc(v*sizeof(int));
int *low=(int*)malloc(v*sizeof(int));
int* d=(int*)malloc(v*sizeof(int));
int* P=(int*)malloc(v*sizeof(int));
for(int i=0;i<v;i++)
{
d[i]=INT_MAX;
low[i]=INT_MAX;
visit[i]=0;
P[i]=-1;
}
int time=1;
for(int i=0;i<v;i++)
{
if(visit[i]==0)
{
dfsvisit2(G,visit,low,d,P,i,&time);
}
}
int t=0;
for(int i=1;i<v;i++)
{
if(low[i]<d[P[i]])
printf("%d\t",i);
}
printf("\n");
return ;
}
int main()
{
int v,e,deg;
node *tmp;
printf("Enter the number of vertices and edges\n");
scanf("%d%d",&v,&e);
graph A=(graph)malloc(v*sizeof(vertex));
for(int i=0;i<v;i++)
{
A[i].adjlist=NULL;
}
printf("Enter the neighbors for each of the vertex\n");
for(int i=0;i<v;i++)
{
printf("enter the degree of vertex %d :\n",i);
scanf("%d",°);
printf("enter neighbours\n");
for(int j=0;j<deg;j++)
{
tmp=(node*)malloc(sizeof(node));
scanf("%d",&tmp->vno);
tmp->next=A[i].adjlist;
A[i].adjlist=tmp;
}
}
printlist(A,v);
findCritical (A, v);
findCriticalFast (A, v);
}
<file_sep>/Assignment 7/17CS30038_7.c
#include<stdio.h>
#include<stdlib.h>
#define MAX_SIZE 100
int parent(int i)
{
return i/2;
}
int left(int i)
{
return 2*i;
}
int right(int i)
{
return 2*i+1;
}
typedef struct _job
{
int jobId;
int startTime;
int jobLength;
int remLength;
} job;
typedef struct _heap
{
job list[MAX_SIZE];
int index_list[MAX_SIZE];
int numJobs;
} heap;
typedef struct _jobPair
{
int jobid_from;
int jobid_to;
} jobPair;
void initHeap(heap *H)
{
H->numJobs=0;
for(int i=1;i<MAX_SIZE;i++)
H->index_list[i]=-1;
}
void insertJob(heap *H,job j)
{
int x,i;
job temp;
int temp_index;
//printf("H->numJobs:%d",H->numJobs);
H->list[++(H->numJobs)]=j;
H->index_list[j.jobId]=H->numJobs;
i=H->numJobs;
//printf("i:%d",i);
while(parent(i)>0)
{
if(H->list[parent(i)].remLength > H->list[i].remLength )
{
temp=H->list[parent(i)];
H->list[parent(i)]=H->list[i];
H->list[i]=temp;
H->index_list[H->list[i].jobId]=i;
H->index_list[H->list[parent(i)].jobId]=parent(i);
i=parent(i);
}
else if( (H->list[parent(i)].remLength == H->list[i].remLength) && (H->list[parent(i)].jobId > H->list[i].jobId) )
{
temp=H->list[parent(i)];
H->list[parent(i)]=H->list[i];
H->list[i]=temp;
H->index_list[H->list[i].jobId]=i;
H->index_list[H->list[parent(i)].jobId]=parent(i);
i=parent(i);
}
else
break;
}
}
void min_heapify(heap *H,int i)
{
int smallest=i;
if ( (left(i)<=H->numJobs) && ( (H->list[left(i)].remLength < H->list[i].remLength) || ( (H->list[left(i)].remLength==H->list[i].remLength) && (H->list[left(i)].jobId<H->list[i].jobId) ) ) )
smallest=left(i);
if( (right(i)<=H->numJobs) && ( (H->list[right(i)].remLength < H->list[smallest].remLength) || ( (H->list[right(i)].remLength==H->list[smallest].remLength) && (H->list[right(i)].jobId<H->list[smallest].jobId) ) ) )
smallest=right(i);
if(smallest!=i)
{
job temp;
temp=H->list[smallest];
H->list[smallest]=H->list[i];
H->list[i]=temp;
H->index_list[H->list[smallest].jobId]=smallest;
H->index_list[H->list[i].jobId]=i;
min_heapify(H,smallest);
}
}
int extractMinJob(heap *H,job *j)
{
if(H->numJobs==0)
return -1;
else
{
*j=H->list[1];
H->list[1]=H->list[(H->numJobs)--];
H->index_list[H->list[1].jobId]=1;
min_heapify(H,1);
return 0;
}
}
void printHeap(heap *H,int n)
{
int i;
printf("\n JobId--startTime--jobLength--remLength--%d",H->numJobs);
for(i=1;i<=H->numJobs;i++)
{
printf("\n %d -- %d -- %d -- %d",H->list[i].jobId,H->list[i].startTime,H->list[i].jobLength,H->list[i].remLength);
}
printf("\nindex_list:JobId--index_location");
for(i=1;i<=n;i++)
{
printf("\n%d--%d\n",i,H->index_list[i]);
}
}
void bubble_sort(job joblist[],int n)
{
int i,j,flag;
job temp;
for(i=0;i<n-1;i++)
{
flag=0;
for(j=0;j<n-i-1;j++)
if(joblist[j].startTime>joblist[j+1].startTime)
{
flag=1;
temp=joblist[j];
joblist[j]=joblist[j+1];
joblist[j+1]=temp;
}
if(flag==0)
break;
}
}
void bubble_sort2(jobPair pairList[],int n)
{
int i,j,flag;
jobPair temp;
for(i=0;i<n-1;i++)
{
flag=0;
for(j=0;j<n-i-1;j++)
if(pairList[j].jobid_from>pairList[j+1].jobid_from)
{
flag=1;
temp=pairList[j];
pairList[j]=pairList[j+1];
pairList[j+1]=temp;
}
if(flag==0)
break;
}
}
void decreaseKey(heap *H,int jid)
{
//printf("K");
//printf("%d",H->list[H->index_list[jid]].remLength);
if(H->list[H->index_list[jid]].remLength==H->list[H->index_list[jid]].jobLength)
H->list[H->index_list[jid]].jobLength=H->list[H->index_list[jid]].jobLength/2;
H->list[H->index_list[jid]].remLength=(H->list[H->index_list[jid]].remLength)/2;
//printf("---%d",H->list[H->index_list[jid]].remLength);
}
void newScheduler(job joblist[], int n,jobPair pairList[],int m)
{
heap H;
initHeap(&H);
job current;
current.remLength=-1;
int time=0,i;
int index=0;
int job_flag=0;
float turn=0;
float number=0;
int pairList_index[MAX_SIZE];
int j=0;
for(i=1;i<=n;i++)
{
if(i==pairList[j].jobid_from)
{
pairList_index[i]=pairList[j].jobid_to;
j++;
}
else
pairList_index[i]=0;
}
//for(i=1;i<=n;i++)
//{
// printf("\n%d--%d",i,pairList_index[i]);
//}
printf("Jobs scheduled at each timestep are:");
do
{
if(joblist[index].startTime==time)
{
do
{
if(time==0)
insertJob(&H,joblist[index]);
else
{
if(joblist[index].remLength<current.remLength || (joblist[index].remLength==current.remLength && joblist[index].jobId<current.jobId) )
{
insertJob(&H,current);
current=joblist[index];
}
else
{
insertJob(&H,joblist[index]);
}
}
index++;
}while( index<n && joblist[index].startTime==joblist[index-1].startTime);
}
if(current.remLength==0)
{
//printf(" ->%d",current.jobId-1);
if(pairList_index[current.jobId]!=0)
{
//printf("-%d-",pairList_index[current.jobId]);
decreaseKey(&H,pairList_index[current.jobId]);
i=H.index_list[pairList_index[current.jobId]];
job temp;
while(parent(i)>0)
{
if(H.list[parent(i)].remLength > H.list[i].remLength )
{
temp=H.list[parent(i)];
H.list[parent(i)]=H.list[i];
H.list[i]=temp;
H.index_list[H.list[i].jobId]=i;
H.index_list[H.list[parent(i)].jobId]=parent(i);
i=parent(i);
}
else if( (H.list[parent(i)].remLength == H.list[i].remLength) && (H.list[parent(i)].jobId > H.list[i].jobId) )
{
temp=H.list[parent(i)];
H.list[parent(i)]=H.list[i];
H.list[i]=temp;
H.index_list[H.list[i].jobId]=i;
H.index_list[H.list[parent(i)].jobId]=parent(i);
i=parent(i);
}
else
break;
}
}
extractMinJob(&H,¤t);
//printf("--%d %d--",current.jobLength,current.remLength);
}
if(time==0)
{
extractMinJob(&H,¤t);
}
if(current.jobLength==current.remLength)
{
turn=turn+time-current.startTime;
}
current.remLength--;
time++;
printf(" %d ",current.jobId);
}while( current.remLength!=0 || H.numJobs!=0);
printf("\n Average Turnaround time is: %f",turn/n);
}
void main()
{
int n,i,m;
job temp;
job temp2;
printf("Enter number of jobs (n): ");
scanf("%d",&n);
job *joblist= (job*)malloc((n)*sizeof(job));
for(i=0;i<n;i++)
{
scanf("%d",&(joblist[i].jobId));
scanf(" %d",&(joblist[i].startTime));
scanf(" %d",&(joblist[i].jobLength));
joblist[i].remLength=joblist[i].jobLength;
}
bubble_sort(joblist,n);
printf("Input number of job-dependancies:");
scanf("%d",&m);
jobPair *pairList= (jobPair*)malloc((m)*sizeof(jobPair));
for(i=0;i<m;i++)
{
scanf("%d",&pairList[i].jobid_from);
scanf("%d",&pairList[i].jobid_to);
}
bubble_sort2(pairList,m);
newScheduler(joblist,n,pairList,m);
if(joblist!=NULL)
{
free(joblist);
joblist=NULL;
}
if(pairList!=NULL)
{
free(pairList);
pairList=NULL;
}
/*
printf("\nJobid_from,Jobid_to");
for(i=0;i<m;i++)
printf("\n%d--%d",pairList[i].jobid_from,pairList[i].jobid_to);
heap H;
initHeap(&H);
for(i=0;i<n;i++)
{
scanf("%d",&(temp.jobId));
scanf(" %d",&(temp.startTime));
scanf(" %d",&(temp.jobLength));
temp.remLength=temp.jobLength;
insertJob(&H,temp);
printf("\n \n");
printHeap(&H,n);
}
printf("\n \n");
extractMinJob(&H,&temp);
printf("\n %d -- %d -- %d -- %d",temp.jobId,temp.startTime,temp.jobLength,temp.remLength);
printf("\n \n");
printHeap(&H,n);
printf("\n \n");
extractMinJob(&H,&temp);
printf("\n %d -- %d -- %d -- %d",temp.jobId,temp.startTime,temp.jobLength,temp.remLength);
printf("\n \n");
printHeap(&H,n);*/
}
<file_sep>/Assignment 4/17CS30038_4.c
//<NAME>
//17CS10061
#include<stdio.h>
#include<stdlib.h>
typedef struct node
{
int value;
struct node *left;
struct node *right;
struct node *parent;
}NODE, *NODEPTR;
void preOrder(NODEPTR root)
{
if(root!=NULL)
{
printf(" %d ",root->value);
preOrder(root->left);
preOrder(root->right);
}
}
void inOrder(NODEPTR root)
{
if(root!=NULL)
{
inOrder(root->left);
printf(" %d ",root->value);
inOrder(root->right);
}
}
NODEPTR search(NODEPTR x,int k)
{
if(x==NULL)
return NULL;
else if ( x->value == k)
return x;
else if ( x->value > k)
return search(x->left,k);
else
return search(x->right,k);
}
NODEPTR right_rotate(NODEPTR root, NODEPTR x)
{
NODEPTR y=NULL;
y=x->left;
x->left=y->right;
if(y->right!=NULL)
y->right->parent=x;
y->parent=x->parent;
if(x->parent==NULL)
root=y;
else if(x->parent->left==x)
x->parent->left=y;
else
x->parent->right=y;
y->right=x;
x->parent=y;
return root;
}
NODEPTR left_rotate(NODEPTR root, NODEPTR x)
{
NODEPTR y=NULL;
y=x->right;
x->right=y->left;
if(y->left!=NULL)
y->left->parent=x;
y->parent=x->parent;
if(x->parent==NULL)
root=y;
else if(x->parent->right==x)
x->parent->right=y;
else
x->parent->left=y;
y->left=x;
x->parent=y;
return root;
}
NODEPTR makeRoot(NODEPTR root, NODEPTR N)
{
if(root->left==N)
root=right_rotate(root,root);
else if(root->right==N)
root=left_rotate(root,root);
return root;
}
NODEPTR sameOrientation(NODEPTR root, NODEPTR N)
{
if( ( N->parent->parent->left == N->parent) && (N->parent->left==N ) )
{
root=right_rotate(root,N->parent->parent);
root=right_rotate(root,N->parent);
}
else if ( ( N->parent->parent->right == N->parent) && (N->parent->right==N) )
{
root=left_rotate(root,N->parent->parent);
root=left_rotate(root,N->parent);
}
return root;
}
NODEPTR oppositeOrientation(NODEPTR root, NODEPTR N)
{
if( (N->parent->parent->left == N->parent) && (N->parent->right==N) )
{
root=left_rotate(root,N->parent);
root=right_rotate(root,N->parent);
}
else if( (N->parent->parent->right == N->parent) && (N->parent->left==N) )
{
root=right_rotate(root,N->parent);
root=left_rotate(root,N->parent);
}
return root;
}
NODEPTR lift_insert(NODEPTR root,NODEPTR N)
{
while(N!=root)
{
if(N->parent==root)
root=makeRoot(root,N);
else if ( ( ( N->parent->parent->left == N->parent) && (N->parent->left==N ) ) || ( ( N->parent->parent->right == N->parent) && (N->parent->right==N) ) )
root=sameOrientation(root,N);
else if ( ( ( N->parent->parent->left == N->parent) && (N->parent->right==N) ) || ( (N->parent->parent->right == N->parent) && (N->parent->left==N) ) )
root=oppositeOrientation(root,N);
}
return root;
}
NODEPTR insert(NODEPTR x,int key)
{
int input=key;
if (x==NULL)
{
x=(NODE*)malloc(sizeof(NODE));
x->value = input;
x->parent = NULL;
x->left=NULL;
x->right=NULL;
return x;
}
else
{
NODEPTR temp=x;
while(temp!=NULL)
{
if( temp->value > input ) //input < temp->value
{
if(temp->left == NULL)
{
temp->left=(NODE*)malloc(sizeof(NODE));
(temp->left)->value=input;
(temp->left)->parent = temp;
(temp->left)->left=NULL;
(temp->left)->right=NULL;
temp=temp->left;
break;
}
else
temp=temp->left;
}
else if( temp->value < input ) //input > x->value
{
if(temp->right == NULL)
{
temp->right=(NODE*)malloc(sizeof(NODE));
(temp->right)->value=input;
(temp->right)->parent = temp;
(temp->right)->left=NULL;
(temp->right)->right=NULL;
temp=temp->right;
break;
}
else
temp=temp->right;
}
}
x=lift_insert(x,temp);
return x;
}
}
NODEPTR leftmost(NODEPTR root)
{
while (root != NULL && root->left != NULL)
root = root->left;
return root;
}
NODEPTR rightmost(NODEPTR root)
{
while (root != NULL && root->right != NULL)
root = root->right;
return root;
}
NODEPTR successor(NODEPTR x)
{
if (x->right != NULL)
{
return leftmost(x->right);
}
if(x->right==NULL)
{
while(x==x->parent->right)
x=x->parent;
return x->parent;
}
}
NODEPTR delete(NODEPTR root, int key)
{
NODEPTR N;
N=search(root,key);
NODEPTR M=N->parent;
while(root!=NULL)
{
if(N->left==NULL && N->right==NULL)
{
if(N->parent==NULL)
return NULL;
NODEPTR temp=N->parent;
if(temp->left==N)
temp->left=NULL;
else
temp->right=NULL;
N->parent=NULL;
break;
}
else if(N->left==NULL)
{
NODEPTR temp=N->right;
N->right==NULL;
temp->parent=N->parent;
if(N->parent!=NULL)
{
if(temp->parent->left==N)
temp->parent->left=temp;
else
temp->parent->right=temp;
}
else
root=temp;
N->parent=NULL;
break;
}
else if(N->right==NULL)
{
NODEPTR temp=N->left;
N->left=NULL;
temp->parent=N->parent;
if(N->parent!=NULL)
{
if(temp->parent->left==N)
temp->parent->left=temp;
else
temp->parent->right=temp;
}
else
root=temp;
N->parent=NULL;
break;
}
else
{
NODEPTR temp=successor(N);
N->value=temp->value;
N=temp;
}
}
if(M!=NULL)
{
root=lift_insert(root,M);
}
return root;
}
void main()
{
int n,i,s,l;
NODEPTR root=NULL,current=NULL;
printf("Enter the number of insertions:");
scanf("%d",&n);
printf("Input Numbers to be inserted:");
for(i=0;i<n;i++)
{
scanf("%d",&s);
if(search(root,s)==NULL)
{
root=insert(root,s);
}
}
printf("\nPreorder traversal:");
preOrder(root);
printf("\nInorder traversal:");
inOrder(root);
printf("\nEnter the number of deletions:");
scanf("%d",&l);
for(i=0;i<l;i++)
{
printf("\nInput value to be deleted:");
scanf("%d",&s);
if(search(root,s)!=NULL)
{
root=delete(root,s);
printf("\nPreorder traversal:");
preOrder(root);
printf("\nInorder traversal:");
inOrder(root);
printf("\n");
}
else
printf("Number not found\n");
}
}
<file_sep>/Assignment 1/17CS30038_1.c
#include<stdio.h>
#include<stdlib.h>
#include<stdint.h>
struct node
{
int data;
struct node *xor;
};
struct node *xor(struct node *a,struct node *b)
{
return (struct node*) ((uintptr_t) a ^ (uintptr_t) b);
}
void trafte(struct node *head)
{
int i;
struct node* temp=NULL,*prev=NULL,*temp2;
temp=head;
printf("front to end traversal\n");
printf("%d\t",temp->data);
temp=temp->xor;
prev=head;
while(xor(temp->xor,prev))
{
printf("%d\t",temp->data);
temp2=prev;
prev=temp;
temp=xor(temp->xor,temp2);
}
printf("%d\n",temp->data);
}
int main()
{
int n;
scanf("%d",&n);
struct node * front=NULL,*end,*move,*temp,*prev,*temp2; ;
int i=0;
for(i=0;i<n;i++)
{
move=(struct node*)malloc(sizeof(struct node*));
move->data=rand()%100;
move->xor=NULL;
if(front==NULL)
{
front=move;
}
else
{
prev->xor=move;
}
prev=move;
}
end=move;
move=front;
prev=NULL;
for(int i=0;i<n-1;i++)
{
temp=move->xor;
temp2=move;
move->xor=xor(prev,temp);
move=temp;
prev=temp2;
}
move->xor=prev;
trafte(front);
return 0;
}
<file_sep>/Assignment 6/17CS30038_6.c
#include<stdio.h>
#include<stdlib.h>
void combine(int A[], int left, int middle, int right)
{
int i=left,j=middle+1,k=0;
int *b;
b=(int*)malloc(sizeof(int)*(right-left+1));
while(i<=middle&&j<=right)
{
if(A[i]<=A[j])
{
b[k]=A[i];
i++;
k++;
}
else
{
b[k]=A[j];
k++;
j++;
}
}
while(i<=middle)
{
b[k]=A[i];
i++;
k++;
}
while(j<=right)
{
b[k]=A[j];
k++;
j++;
}
for(i=left,k=0;i<=right;k++,i++)
{
A[i]=b[k];
}
free(b);
return;
}
void superbSorting(int A[], int sizeOfA)
{
int block=1;
int i,left,middle,right;
for(block=1;block<=sizeOfA;block*=2)
{
for(i=0;i<sizeOfA;i+=block*2)
{
left=i;
right=i+2*block-1;
if(right>=sizeOfA)
right=sizeOfA-1;
middle=left+block-1;
combine(A, left, middle, right);
}
}
}
int compare1(int X[],int sizeOfX,int x,int y)
{
int i,x_pos,y_pos;
for(i=0;i<sizeOfX;i++)
{
if(X[i]==x)
x_pos=i;
if(X[i]==y)
y_pos=i;
}
if(x_pos<y_pos)
return 1;
else
return -1;
}
void combine1(int A[], int left, int middle, int right, int B[], int sizeOfB)
{
int i=left,j=middle+1,k=0;
int *b;
b=(int*)malloc(sizeof(int)*(right-left+1));
while(i<=middle&&j<=right)
{
if(compare1(B,sizeOfB,A[i],A[j])==1)
{
b[k]=A[i];
i++;
k++;
}
else
{
b[k]=A[j];
k++;
j++;
}
}
while(i<=middle)
{
b[k]=A[i];
i++;
k++;
}
while(j<=right)
{
b[k]=A[j];
k++;
j++;
}
for(i=left,k=0;i<=right;k++,i++)
{
A[i]=b[k];
}
free(b);
return;
}
void superbSorting1(int A [], int sizeOfA, int B[], int sizeOfB)
{
int block=1;
int i,left,middle,right;
for(block=1;block<=sizeOfA;block*=2)
{
for(i=0;i<sizeOfA;i+=block*2)
{
left=i;
right=i+2*block-1;
if(right>=sizeOfA)
right=sizeOfA-1;
middle=left+block-1;
combine1(A, left, middle, right,B,sizeOfB);
}
}
}
void F_combine(int A[], int left, int middle, int right,int B[])
{
int i=left,j=middle+1,k=0;
int *b;
b=(int*)malloc(sizeof(int)*(right-left+1));
while(i<=middle&&j<=right)
{
if(B[A[i]]<B[A[j]])
{
b[k]=A[i];
i++;
k++;
}
else
{
b[k]=A[j];
k++;
j++;
}
}
while(i<=middle)
{
b[k]=A[i];
i++;
k++;
}
while(j<=right)
{
b[k]=A[j];
k++;
j++;
}
for(i=left,k=0;i<=right;k++,i++)
{
A[i]=b[k];
}
free(b);
return;
}
void F_sort(int A [], int sizeOfA, int B[])
{
int block=1;
int i,left,middle,right;
for(block=1;block<=sizeOfA;block*=2)
{
for(i=0;i<sizeOfA;i+=block*2)
{
left=i;
right=i+2*block-1;
if(right>=sizeOfA)
right=sizeOfA-1;
middle=left+block-1;
F_combine(A, left, middle, right,B);
}
}
}
int main()
{
int i,n,*A;
printf("Enter number of numbers in the array A :\n");
scanf("%d",&n);
printf("\n");
A=(int*)malloc(n*sizeof(int));
printf("Enter numbers in the arrayA:\n");
for(i=0;i<n;i++)
{
scanf("%d",&A[i]);
}
printf("\nnon decreasing order\n");
superbSorting(A,n);
for(i=0;i<n;i++)
{
printf("%d\t",A[i]);
}
printf("\n");
printf("\nArray conversion+++++++\n");
int n1,*A1,*B;
A1=(int*)malloc(n*sizeof(int));
printf("Enter numbers in the arrayA1:\n");
for(i=0;i<n;i++)
{
scanf("%d",&A1[i]);
}
printf("Enter number of numbers in the array B:\n");
scanf("%d",&n1);
printf("\nenter elements of B:\n");
B=(int*)malloc(n1*sizeof(int));
for(i=0;i<n1;i++)
{
scanf("%d",&B[i]);
}
printf("\nrearranged order\n");
superbSorting1(A1,n,B, n1);
for(i=0;i<n;i++)
{
printf("%d\t",A1[i]);
}
printf("\n");
free(A1);
free(B);
printf("\nFaster Algo++++++++++++++\n");
int *B1,tmp,m;
B1=(int*)malloc(sizeof(int)*10*n);
printf("re-enter A for faster algo:\n");
for(i=0;i<n;i++)
{
scanf("%d",&A[i]);
}
printf("Re-enter number of numbers in the array B for fast algo:\n");
scanf("%d",&m);
printf("\nenter elements of B:\n");
for(i=0;i<m;i++)
{
scanf("%d",&tmp);
B1[tmp]=i;
}
F_sort(A, n, B1);
printf("reaaranged A using faster algorithm:\n");
for(i=0;i<n;i++)
{
printf("%d\t",A[i]);
}
printf("\n");
free(A);
free(B1);
return 0;
}
<file_sep>/Assignment 8/17CS30038_8.c
#include<stdlib.h>
#include<stdio.h>
#include<math.h>
typedef struct score
{
int mscore;
int escore;
} score, *scorelist;
int distance(score a, score b)
{
//printf("\n%d--%d--%d--%d %d\n",a.mscore,a.escore,b.mscore,b.escore,(abs(a.mscore-b.mscore)+abs(a.escore-b.escore) ));
return (abs(a.mscore-b.mscore)+abs(a.escore-b.escore) );
}
void mergeM(score a[],int lower,int middle,int upper)
{
score b[10000],c[10000];
int i,j,k,h1,h2;
h1=0;
for(i=lower;i<=middle;i++)
b[h1++]=a[i];
h2=0;
for(i=middle+1;i<=upper;i++)
c[h2++]=a[i];
i=0;
j=0;
k=lower;
while(i<h1 && j<h2)
{
if(b[i].mscore>c[j].mscore)
a[k++]=c[j++];
else
a[k++]=b[i++];
}
while(i<h1)
a[k++]=b[i++];
while(j<h2)
a[k++]=c[j++];
}
void merge_sortM(score a[],int lower,int upper)
{
if(lower!=upper)
{
int middle=(lower+upper)/2;
merge_sortM(a,lower,middle);
merge_sortM(a,middle+1,upper);
mergeM(a,lower,middle,upper);
}
}
void mergeE(score a[],int lower,int middle,int upper)
{
score b[1000],c[1000];
int i,j,k,h1,h2;
h1=0;
for(i=lower;i<=middle;i++)
b[h1++]=a[i];
h2=0;
for(i=middle+1;i<=upper;i++)
c[h2++]=a[i];
i=0;
j=0;
k=lower;
while(i<h1 && j<h2)
{
if(b[i].escore>c[j].escore)
a[k++]=c[j++];
else
a[k++]=b[i++];
}
while(i<h1)
a[k++]=b[i++];
while(j<h2)
a[k++]=c[j++];
}
void merge_sortE(score a[],int lower,int upper)
{
if(lower!=upper)
{
int middle=(lower+upper)/2;
merge_sortE(a,lower,middle);
merge_sortE(a,middle+1,upper);
mergeE(a,lower,middle,upper);
}
}
int explore_strip(score strip[],int size,int current_minimum, score *score1, score *score2)
{
//printf("IN STRIP");
//printf("Size%d:",size);
int new_minimum=current_minimum;
int i,j;
//for(i=0;i<size;i++)
//{
// printf("\nstrip--%d--%d",strip[i].mscore,strip[i].escore);
//}
for(i=0;i<size;i++)
{
for(j=i+1; (j<size) && abs(strip[i].escore-strip[j].escore)<current_minimum;j++ )
{
if(distance(strip[j],strip[i])<new_minimum )
{
new_minimum=distance(strip[j],strip[i]);
(*score1)=strip[j];
(*score2)=strip[i];
}
}
}
//printf("Out of strip");
return new_minimum;
}
int closest_pair(scorelist T_M,scorelist T_E, int l, int r, score *score1, score *score2)
{
if((r-l)==1)
{
*score1=T_M[l];
*score2=T_M[r];
return distance(T_M[l],T_M[r]);
}
else if((r-l)==2)
{
int d1=distance(T_M[l],T_M[l+1]);
int d2=distance(T_M[l+1],T_M[l+2]);
int d3=distance(T_M[l],T_M[l+2]);
if(d1<d2 && d1<d3) //d1 is min
{
*score1=T_M[l];
*score2=T_M[l+1];
return d1;
}
else if(d2<d1 && d2<d3) //d2 is min
{
*score1=T_M[l+1];
*score2=T_M[l+2];
return d2;
}
else //d3 is min
{
*score1=T_M[l];
*score2=T_M[l+2];
return d3;
}
}
else
{
int mid=(l+r)/2;
score T_E_l[10000],T_E_r[10000];
int i,j=0,k=0;
for(i=0;i<=(r-l);i++)
{
if(T_E[i].mscore<=T_M[mid].mscore)
T_E_l[j++]=T_E[i];
else
T_E_r[k++]=T_E[i];
}
//printf("\nstart--->%d--%d--%d--%d<----",score1->mscore,score1->escore,score2->mscore,score2->escore);
score score3=(*score1);
score score4=(*score2);
int a=closest_pair(T_M,T_E_l,l,mid,score1,score2);
//printf("\nmid--->%d--%d--%d--%d<----",score1->mscore,score1->escore,score2->mscore,score2->escore);
int b=closest_pair(T_M,T_E_r,mid+1,r,&score3,&score4);
int minimum=a;
if(minimum>=b)
{
minimum=b;
(*score1)=score3;
(*score2)=score4;
}
//printf("\nend--->%d--%d--%d--%d<----",score1->mscore,score1->escore,score2->mscore,score2->escore);
score strip[10000];
j=0;
for(i=0;i<=(r-l);i++)
{
if(abs(T_E[i].mscore-T_M[mid].mscore)<minimum)
{
strip[j++]=T_E[i];
//printf("\nT_E--%d--%d",T_E[i].mscore,T_E[i].escore);
}
}
return explore_strip(strip,j,minimum,score1,score2);
}
}
int NearestProfiles(scorelist T,int n,score *score1,score *score2)
{
int i;
scorelist T_M,T_E;
T_M = (scorelist)malloc((n)*sizeof(score));
T_E = (scorelist)malloc((n)*sizeof(score));
for(i=0;i<n;i++)
{
T_M[i]=T[i];
T_E[i]=T[i];
}
merge_sortM(T_M,0,n-1);
merge_sortE(T_E,0,n-1);
return closest_pair(T_M,T_E,0,n-1,score1,score2);
}
int closest_cluster_pair(scorelist section1_M,scorelist section1_E,int l1,int r1, scorelist section2_M, scorelist section2_E,int l2,int r2,score *score1,score *score2)
{
if((r1-l1)==0)
{
int i;
int minimum=distance(section1_M[l1],section2_M[l2]);
*score1=section1_M[l1];
*score2=section2_M[l2];
for(i=l2+1;i<=r2;i++)
{
if(distance(section1_M[l1],section2_M[i])<minimum)
{
minimum=distance(section1_M[l1],section2_M[i]);
*score2=section1_M[l1];
*score1=section2_M[i];
}
}
return minimum;
}
else if((r2-l2)==0)
{
int i;
int minimum=distance(section2_M[l2],section1_M[l1]);
*score1=section2_M[l2];
*score2=section1_M[l1];
for(i=l1+1;i<=r1;i++)
{
if(distance(section2_M[l2],section1_M[i])<minimum)
{
minimum=distance(section2_M[l2],section1_M[i]);
*score1=section2_M[l2];
*score2=section1_M[i];
}
}
return minimum;
}
else
{
int mid1=(l1+r1)/2;
score section1_E_l[10000],section1_E_r[10000];
int i,j=0,k=0;
for(i=0;i<=(r1-l1);i++)
{
if(section1_E[i].mscore<=section1_M[mid1].mscore)
section1_E_l[j++]=section1_E[i];
else
section1_E_r[k++]=section1_E[i];
}
int mid2=(l2+r2)/2;
score section2_E_l[10000],section2_E_r[10000];
j=0;
k=0;
for(i=0;i<=(r2-l2);i++)
{
if(section2_E[i].mscore<=section2_M[mid2].mscore)
section2_E_l[j++]=section2_E[i];
else
section2_E_r[k++]=section2_E[i];
}
score score3=(*score1);
score score4=(*score2);
int a=closest_cluster_pair(section1_M,section1_E_l,l1,mid1,section2_M,section2_E_l,l2,mid2,score1,score2);
int b=closest_cluster_pair(section1_M,section1_E_r,mid1+1,r1,section2_M,section2_E_l,mid2+1,r2,&score3,&score4);
int minimum=a;
if(minimum>=b)
{
minimum=b;
(*score1)=score3;
(*score2)=score4;
}
/*
//printf("\nend--->%d--%d--%d--%d<----",score1->mscore,score1->escore,score2->mscore,score2->escore);
score strip[10000];
j=0;
for(i=0;i<=(r-l);i++)
{
if(abs(T_E[i].mscore-T_M[mid].mscore)<minimum)
{
strip[j++]=T_E[i];
//printf("\nT_E--%d--%d",T_E[i].mscore,T_E[i].escore);
}
}
return explore_strip(strip,j,minimum,score1,score2);*/
return minimum;
}
}
int ClusterDist(scorelist section1,int n, scorelist section2,int m,score *score1,score *score2)
{
int i;
scorelist section1_M,section1_E;
section1_M = (scorelist)malloc((n)*sizeof(score));
section1_E = (scorelist)malloc((n)*sizeof(score));
for(i=0;i<n;i++)
{
section1_M[i]=section1[i];
section1_E[i]=section1[i];
}
merge_sortM(section1_M,0,n-1);
merge_sortE(section1_E,0,n-1);
scorelist section2_M,section2_E;
section2_M = (scorelist)malloc((m)*sizeof(score));
section2_E = (scorelist)malloc((m)*sizeof(score));
for(i=0;i<n;i++)
{
section2_M[i]=section2[i];
section2_E[i]=section2[i];
}
merge_sortM(section2_M,0,n-1);
merge_sortE(section2_E,0,n-1);
return closest_cluster_pair(section1_M,section1_E,0,n-1,section2_M,section2_E,0,m-1,score1,score2);
}
int main()
{
score profile1,profile2;
score T1[10000],T2[10000];
int n,m,i;
printf("Enter number of students:");
scanf("%d",&n);
for(i=0;i<n;i++)
{
printf("Enter math and english scores:");
scanf("%d",&T1[i].mscore);
scanf("%d",&T1[i].escore);
}
int minimum=NearestProfiles(T1,n,&profile1,&profile2);
printf("\nClosest pair: (%d,%d) and (%d,%d)",profile1.mscore,profile1.escore,profile2.mscore,profile2.escore);
printf("\nDistance: %d",minimum);
printf("\n\n");
printf("Enter the size of the second cluster:");
scanf("%d",&m);
for(i=0;i<m;i++)
{
printf("Enter math and english scores:");
scanf("%d",&T2[i].mscore);
scanf("%d",&T2[i].escore);
}
minimum=ClusterDist(T1, n, T2,m,&profile1,&profile2);
printf("\nClosest pair: (%d,%d) and (%d,%d)",profile1.mscore,profile1.escore,profile2.mscore,profile2.escore);
printf("\nDistance: %d",minimum);
return 0;
}
<file_sep>/Assignment 3/17CS30038_3.c
#include <stdio.h>
#include<stdlib.h>
#include<string.h>
typedef struct treenode
{
char word[100];
struct treenode *leftchild;
struct treenode *rightchild;
struct treenode *parent;
} NODE, *NODEPTR;
NODEPTR insert(NODEPTR x)
{
char input[100];
printf("Input String:");
scanf("%s",input);
if (x==NULL)
{
x=(NODE*)malloc(sizeof(NODE));
strcpy(x->word,input);
x->parent = NULL;
x->leftchild=NULL;
x->rightchild==NULL;
return x;
}
else
{
NODEPTR temp=x;
while(temp!=NULL)
{
if( strcmp(temp->word,input) > 0 ) //input < temp->word
{
if(temp->leftchild == NULL)
{
temp->leftchild=(NODE*)malloc(sizeof(NODE));
strcpy((temp->leftchild)->word,input);
(temp->leftchild)->parent = temp;
(temp->leftchild)->leftchild=NULL;
(temp->leftchild)->rightchild==NULL;
return x;
}
else
temp=temp->leftchild;
}
else if( strcmp(temp->word,input) < 0 ) //input > x->word
{
if(temp->rightchild == NULL)
{
temp->rightchild=(NODE*)malloc(sizeof(NODE));
strcpy((temp->rightchild)->word,input);
(temp->rightchild)->parent = x;
(temp->rightchild)->leftchild=NULL;
(temp->rightchild)->rightchild==NULL;
return x;
}
else
temp=temp->rightchild;
}
}
}
}
void inorder(NODEPTR x)
{
if(x!=NULL)
{
inorder(x->leftchild);
printf("%s ",x->word);
inorder(x->rightchild);
}
}
int prefix( char A[100],char B[100]) //returns 1 if B is a prefix
{
int a,b,i;
a=strlen(A);
b=strlen(B);
if(b>a)
return 0;
else
{
for(i=0;i<b;i++)
{
if(A[i]!=B[i])
return 0;
}
return 1;
}
}
NODEPTR leftmost(NODEPTR root)
{
while (root != NULL && root->leftchild != NULL)
root = root->leftchild;
return root;
}
NODEPTR rightmost(NODEPTR root)
{
while (root != NULL && root->rightchild != NULL)
root = root->rightchild;
return root;
}
NODEPTR successor(NODEPTR x)
{
if (x->rightchild != NULL)
{
return leftmost(x->rightchild);
}
if(x->rightchild==NULL)
{
while(x==x->parent->rightchild)
x=x->parent;
return x->parent;
}
}
void find_extensions(NODEPTR root, char pattern[100])
{
while(root!=NULL)
{
if(prefix(root->word,pattern)==1)
{
if(root->leftchild==NULL)
{
printf(" %s",root->word);
root=root->rightchild;
}
else if(root->rightchild==NULL)
root=root->leftchild;
else
{
NODEPTR temp1,temp2;
temp1=root->leftchild;
temp2=root->leftchild;
while(temp1!=NULL && temp1->leftchild!=NULL)
temp1=temp1->leftchild;
while(temp2!=NULL && temp2->rightchild!=NULL)
temp2=temp2->rightchild;
while(temp1!=temp2)
{
if(prefix(temp1->word,pattern)==1)
printf(" %s",temp1->word);
temp1=successor(temp1);
}
printf(" %s",temp2->word);
printf(" %s",root->word);
root=root->rightchild;
}
}
else if ( strcmp(root->word,pattern)>0)
root=root->leftchild;
else
root=root->rightchild;
}
}
void main()
{
int n,i;
char input[100];
NODEPTR root=NULL;
printf("Enter the number of words:");
scanf("%d",&n);
for(i=0;i<n;i++)
{
root=insert(root);
}
inorder(root);
printf("\n\n");
find_extensions(root,"pag");
}
<file_sep>/Lab Test/even/9_17CS30038.c
//<NAME>
//17CS30038
//PC No-9
/*
subproblem: max probabilty with P persons in completing the project will be max of prob by p persons(n<=p<P) and multiplying by probability ratios of changing number of persons in any one of the component
let dp(n,p) be person on each component with total persons=p;
let maxp(p) store max prob by p persons
dp(n,p)=0 ...for all nXp where (p<N)
maxp(p)=0 ...for all nXp where (p<N)
dp(n,p)=1 ...when p=N as only one possible way of distributing min 1 perswon to a component
maxp(p)=prod(p[i][1]) ...when p=N
maxp(p)=max(max(maxp(p_i)*(p[i][dp(p_i)+p-p_i])/(p[i][p_i]) for(i=1 to n))for(N<=p_i<p))
let p_i_max and i_max be corresponding obtained values
dp(n,p)=dp(n,p_i_max) for(n !=i )
dp(n,p)=dp(n,p_i_max)+p_i-p_i_max for(n==i)
*/
#include<stdio.h>
#include<stdlib.h>
int main()
{
int N,P;
scanf("%d%d",&N,&P);
double **p;
p=(double**)malloc((N+1)*sizeof(double*));
for(int i=0;i<=N;i++)
{
p[i]=(double*)malloc((P+1)*sizeof(double));
}
for(int i=0;i<=N;i++)
{
for(int j=0;j<=P;j++)
{
if(i==0||j==0)
p[i][j]=0;
else
scanf("%lf",&p[i][j]);
}
}
int **dp;
dp=(int**)malloc((N+1)*sizeof(int*));
for(int i=0;i<=N;i++)
{
dp[i]=(int*)malloc((P+1)*sizeof(int));
}
double *maxp;
maxp=(double*)malloc((P+1)*sizeof(double));
for(int i=0;i<P;i++)
maxp[i]=1.0;
for(int i=1;i<=N;i++)
{
dp[i][N]=1;
maxp[N]=p[i][1]*maxp[N];
}
double max,tmp;
int indi,indk;
for(int j=N+1;j<=P;j++)
{
max=0;
for(int k=N;k<j;k++)
{
for(int i=1;i<=N;i++)
{
tmp=maxp[k]*(p[i][dp[i][k]+j-k])/(p[i][dp[i][k]]);
if(tmp>max)
{
max=tmp;
indi=i;
indk=k;
}
}
}
maxp[j]=max;
for(int i=1;i<=N;i++)
{
dp[i][j]=dp[i][indk];
}
dp[indi][j]+=j-indk;
}
printf("max success probability is:%lf\n",maxp[P]);
printf("assignment of people for max success probability:\n");
for(int i=1;i<=N;i++)
{
printf("counter %d::%d\n",i,dp[i][P]);
}
return 0;
}
<file_sep>/Lab Test/odd/odd.c
//NAME: <NAME>
//ROLL NO: 17CS10061
//PC NO: 71
/*
Definition of subproblem used:
I have defined DP[i][j] as the max success probability that a project has with i essential components and j number of people.
For storing the components I have used a 2D array 'comp'. comp[i][j] stores the number of people assigned to the ith essential component such that we can get the max success probability with i essential components and j number of people.
base cases:
DP[k][0]=0 for 1<=k<=N because its given p[k][0]=0
DP[1][j]=p[1][j] for 1<=j<=P as if there is only one essential component, all the j people available must be assigned to it (as p(k,j) values are non decreasing for increasing values of j)
comp[1][j]=j for 1<=j<=P as for only 1 task all people available must be set to that task
Recursive formulation:
for 2<=x<N, x<=y<=P :
if(x==y) : DP[x][y]=D[x-1][y-1]*a[x][1] (as all essential components must have one person each)
comp[x][y]=1 as xth essential task will be assigned 1 person
else : DP[x][z]=max(DP[x-1][y-z]*a[x][z]) where 1<=z<=(y-1) ( 1 as each essential component must have atleast 1 person)
comp[x][y]=tempz where tempz=value of z for which maximum is found above (as this means xth essential component must have tempz people)
Answer: maximum success probability: DP[N][P]
components: components printed using 2D array comp.
*/
#include<stdio.h>
#include<stdlib.h>
int main()
{
int N,P,j,k,x,y,z,i,tempz,res;
float q,max;
float a[100][100];
float DP[100][100];
int output[100];
int comp[100][100];
printf("\nEnter N:");
scanf(" %d",&N);
printf("\nEnter P:");
scanf(" %d",&P);
printf("\nEnter the probabilities:\n");
for(k=1;k<=N;k++)
for(j=1;j<=P;j++)
scanf(" %f",&a[k][j]);
//DP array initialized to 0
for(x=0;x<=N;x++)
for(y=0;y<=P;y++)
DP[x][y]=0;
for(k=1;k<=N;k++)
DP[k][0]=0;
for(j=1;j<=P;j++)
DP[1][j]=a[1][j];
for(x=2;x<=N;x++)
for(y=1;y<=P;y++)
{
comp[i][j]=0;
}
for(j=1;j<=P;j++)
{
comp[1][j]=j;
}
for(x=2;x<=N;x++)
for(y=x;y<=P;y++)
{
if(x==y)
{
DP[x][y]=DP[x-1][y-1]*a[x][1];
comp[x][y]=1;
}
else
{
max=0;
for(z=1;z<=(y-1);z++)
{
q=DP[x-1][y-z]*a[x][z];
if(q>max)
{
tempz=z;
max=q;
}
}
DP[x][y]=max;
comp[x][y]=tempz;
}
}
printf("\nThe maximum success probability is: %f\n",DP[N][P]);
printf("Assignment of people for max success probability:\n");
res=P;
for(x=N;x>0;x--)
{
output[x]=comp[x][res];
res=res-output[x];
}
for(i=1;i<=N;i++)
printf("Component %d: %d\n",i,output[i]);
return 0;
}
<file_sep>/Assignment 2/17CS30038_2.c
#include<stdio.h>
#include<stdlib.h>
typedef struct rm {
int hInd;
int vInd;
} room;
struct node
{
room data;
struct node *next;
};
typedef struct node node, *list;
typedef struct {
struct node *head ;
} STACK ;
void init(STACK *s)
{
s->head=NULL;
}
int isempty(STACK s)
{
if(s.head==NULL)
return 1;
else
return 0;
}
void push(STACK *s, room data)
{
struct node *temp;
temp=(struct node*)malloc(sizeof(struct node));
temp->data.hInd=data.hInd;
temp->data.vInd=data.vInd;
temp->next=s->head;
s->head=temp;
}
void pop(STACK *s);
void printmaze(int H[][20], int V[][21], int n)
{
int i,j;
for(i=0;i<4*n+1;i++)
for(j=0;j<4*n+1;j++)
{
if(i%4==0&&j%4==0)
{
printf("+");
}
if(i%4==0&&j%4!=0)
{
if(H[i/4][j/4]==0)
printf("-");
else
printf(" ");
}
if(i%4!=0&&j%4==0)
{
if(i%4==1||i%4==3)
printf(" ");
else
{
if(V[i/4][j/4]==0)
printf("|");
else
printf(" ");
}
}
if(i%4!=0&&j%4!=0)
{
printf(" ");
}
if(j==4*n)
printf("\n");
}
}
void createmaze(list maze[][20], int n, int H[][20], int V[][21])
{
int i,j;
struct node* temp;
for(i=0;i<n;i++)
for(j=0;j<n;j++)
{
maze[i][j]=NULL;
if(H[i][j]==1)
{
struct node* t;
t=(struct node*)malloc(sizeof(struct node));
t->data.hInd=i-1;
t->data.vInd=j;
if(maze[i][j]==NULL)
{ maze[i][j]=t;temp=t;}
else
{
temp->next=t;
temp=t;
}
}
if(H[i+1][j]==1)
{
struct node* t;
t=(struct node*)malloc(sizeof(struct node));
t->data.hInd=i+1;
t->data.vInd=j;
if(maze[i][j]==NULL)
{ maze[i][j]=t;temp=t;}
else
{
temp->next=t;
temp=t;
}
}
if(V[i][j]==1)
{
struct node* t;
t=(struct node*)malloc(sizeof(struct node));
t->data.hInd=i;
t->data.vInd=j-1;
if(maze[i][j]==NULL)
{ maze[i][j]=t;temp=t;}
else
{
temp->next=t;
temp=t;
}
}
if(V[i][j+1]==1)
{
struct node* t;
t=(struct node*)malloc(sizeof(struct node));
t->data.hInd=i;
t->data.vInd=j+1;
if(maze[i][j]==NULL)
{ maze[i][j]=t;temp=t;}
else
{
temp->next=t;
temp=t;
}
}
temp->next=NULL;
}
for(i=0;i<n;i++)
for(j=0;j<n;j++)
{
printf("(%d,%d)",i,j);
temp=maze[i][j];
if(temp!=NULL)
{printf("::-->");printf("(%d,%d)",temp->data.hInd,temp->data.vInd);}
while(temp->next!=NULL)
{
temp=temp->next;
printf("-->");
printf("(%d,%d)",temp->data.hInd,temp->data.vInd);
} printf("\n");
}
return;
}
int main()
{
<file_sep>/Assignment 13/17CS10061_13.c
#include<stdio.h>
#include<stdlib.h>
#include<limits.h>
typedef int** graph;
typedef struct queue
{
int n;
struct queue* next;
}que;
typedef struct Edge
{
int src, dest,weight;
}edge;
void merge(edge a[],int low ,int mid,int high)
{
int i=low,j=mid+1;
int k=0;
edge b[high-low+1];
while(i<=mid&&j<=high)
{
if(a[i].weight<a[j].weight)
{
b[k]=a[j];
j++;
k++;
}
else
{
b[k]=a[i];
i++;
k++;
}
}
while(i<=mid)
{
b[k]=a[i];
i++;
k++;
}
while(j<=high)
{
b[k]=a[j];
j++;
k++;
}
for(i=low,k=0;i<=high;i++,k++)
{
a[i]=b[k];
}
return ;
}
void mergesort(edge a[],int low,int high)
{
if(low<high)
{
int mid=(low+high)/2;
mergesort(a,low,mid);
mergesort(a,mid+1,high);
merge(a,low,mid,high);
}
}
que* enqueue(que *front,int n)
{
que* move=(que*)malloc(sizeof(que)),*temp;
move->n=n;
move->next=NULL;
if(front==NULL)
{
front =move;
return front ;
}
else
{
temp=front;
while(temp->next!=NULL)
temp=temp->next;
temp->next=move;
return front;
}
}
int first(que* front)
{
if(front!=NULL)
return front->n;
}
que* dequeue(que* front)
{
if(front!=NULL)
return front->next;
}
int isempty(que* front)
{
if(front==NULL)
return 0;
return 1;
}
int isConnectedUsingBFS(graph G,int n)
{
int *v=(int*)malloc(n*sizeof(int));
for(int i=0;i<n;i++)
v[i]=0;
v[0]=1;
que* Q=NULL;
Q=enqueue(Q,0);
while(isempty(Q))
{
int u=first(Q);
Q=dequeue(Q);
for(int j=0;j<n;j++)
{
if(G[u][j]>0 && v[j]==0)
{
Q=enqueue(Q,j);
v[j]=1;
}
}
}
for(int i=0;i<n;i++)
if(v[i]==0)
{ free(v);
return 0;
}
free(v);
return 1;
}
void findMST(graph G, int numberOfNodes)
{
int v=numberOfNodes,e=0;
edge ed[10000];
for(int i=0;i<v;i++)
{
for(int j=i;j<v;j++)
{
if(G[i][j]>0)
{
ed[e].src=i;
ed[e].dest=j;
ed[e].weight=G[i][j];
e++;
}
}
}
mergesort(ed,0,e-1);
for(int i=0;i<e;i++)
{
int t=G[ed[i].src][ed[i].dest];
G[ed[i].src][ed[i].dest]=0;
G[ed[i].dest][ed[i].src]=0;
if( isConnectedUsingBFS( G,v))
continue;
else
{
G[ed[i].src][ed[i].dest]=t;
G[ed[i].dest][ed[i].src]=t;
}
}
for(int i=0;i<v;i++)
{
printf("vertex %d : ",i);
for(int j=0;j<v ;j++)
if(G[i][j]>0)
printf("%d %d ",j,G[i][j]);
printf("\n");
}
}
int main()
{
int n,m,a,b,deg;
printf("Enter the number of nodes and edges\n");
scanf("%d%d",&n,&m);
graph A=(int**)malloc(n*sizeof(int*));
for(int i=0;i<n;i++)
{
A[i]=(int*)malloc(n*sizeof(int));
}
printf("Enter the neighbors for each of the vertex\n");
for(int i=0;i<n;i++)
{
for(int j=0;j<n;j++)
A[i][j]=0;
printf("enter the degree of vertex %d :\n",i);
scanf("%d",°);
printf("enter neighbours\n");
for(int j=0;j<deg;j++)
{
scanf("%d%d",&a,&b);
A[i][a]=b;
}
}
for(int i=0;i<n;i++)
{
printf("vertex %d : ",i);
for(int j=0;j<n ;j++)
if(A[i][j]>0)
printf("%d %d ",j,A[i][j]);
printf("\n");
}
printf("neughbours of required mst\n");
findMST( A, n) ;
for(int i=0;i<n;i++)
free(A[i]);
return 0;
}
<file_sep>/Assignment 11/17CS30038_11.c
#include<stdio.h>
#include<stdlib.h>
typedef struct
{
int subpartID;
int cost_per_day;
int duration;
} subpart_data;
typedef struct{
int predecessorID;
int successorID;
} dependency_info;
void merge(subpart_data a[],int lower,int middle,int upper)
{
subpart_data b[100],c[100];
int i,j,k,h1,h2;
h1=0;
for(i=lower;i<=middle;i++)
b[h1++]=a[i];
h2=0;
for(i=middle+1;i<=upper;i++)
c[h2++]=a[i];
i=0;
j=0;
k=lower;
while(i<h1 && j<h2)
{
if(b[i].cost_per_day*c[j].duration>c[j].cost_per_day*b[i].duration)
a[k++]=b[i++];
else
a[k++]=c[j++];
}
while(i<h1)
a[k++]=b[i++];
while(j<h2)
a[k++]=c[j++];
}
void merge_sort(subpart_data a[],int lower,int upper)
{
if(lower!=upper)
{
int middle=(lower+upper)/2;
merge_sort(a,lower,middle);
merge_sort(a,middle+1,upper);
merge(a,lower,middle,upper);
}
}
void print_schedule(subpart_data *A, int K)
{
merge_sort(A,0,K-1);//sort in descending based on cost/duration
int no_of_days=0;
int i,cost=0;
for(i=0;i<K;i++)
{
printf("%d ",A[i].subpartID);
no_of_days=no_of_days+A[i].duration;
cost=cost+no_of_days*A[i].cost_per_day;
}
printf("\nCost:%d",cost);
}
void print_schedule1(subpart_data *A,int K,dependency_info *B,int l)
{
int i,j,m,col,flag;
int number_of_chains;
int chain[100][100];
int dependent[100];
int high[100];
for(i=0;i<K;i++)
dependent[i]=0;
for(i=0;i<l;i++)
dependent[B[i].successorID-1]=1;
printf("\n");
for(i=0;i<K;i++)
printf("%d ",dependent[i]);
for(i=0;i<K;i++)
{
high[i]=0;
for(j=0;j<K;j++)
chain[i][j]=0;
}
j=0;
for(i=0;i<K;i++)
{
if(dependent[i]==0)
{
chain[j][0]=i+1;
col=0;
while(1)
{
flag=0;
for(m=0;m<l;m++)
if(B[m].predecessorID==chain[j][col])
{
chain[j][++col]=B[m].successorID;
flag=1;
break;
}
if(flag==0)
break;
}
high[j]=col+1;
j++;
}
}
number_of_chains=j;
for(i=0;i<K;i++)
{
printf("\n %d - ",high[i]);
for(j=0;j<K;j++)
printf("%d ",chain[i][j]);
}
printf("\n");
//merge number_of_chains chains
float max_value=0;
int max_index;
int r,c;
int output[100];
int output_index=0;
int low[100];
int duration_sum,cost_sum;
int active_chain[100];
for(i=0;i<number_of_chains;i++)
active_chain[i]=1;
for(i=0;i<number_of_chains;i++)
low[i]=0;
for(i=0;i<number_of_chains;i++)
high[i]--;
while(1)
{
max_value=0;
for(i=0;i<number_of_chains;i++)
{
if(active_chain[i]==1)
{
duration_sum=0;
cost_sum=0;
for(j=low[i];j<=high[i];j++)
{
duration_sum=duration_sum+A[chain[i][j]-1].duration;
cost_sum=cost_sum+A[chain[i][j]-1].cost_per_day;
if(max_value< ((float)cost_sum)/((float)duration_sum) )
{
max_value=((float)cost_sum)/((float)duration_sum);
r=i;
c=j;
}
}
}
}
for(m=low[r];m<=c;m++)
output[output_index++]=chain[r][m];
low[r]=c+1;
printf(" %d",output_index);
if(output_index==K)
break;
}
printf("\n Scheduled Index: ");
for(i=0;i<K;i++)
printf("%d ",output[i]);
int cost=0;
int no_of_days=0;
for(i=0;i<K;i++)
{
no_of_days=no_of_days+A[output[i]-1].duration;
cost=cost+no_of_days*A[output[i]-1].cost_per_day;
}
printf("\nCost:%d",cost);
}
int main()
{
subpart_data* A;
dependency_info* B;
int K,i,l;
printf("Input number of subparts (K) :");
scanf("%d",&K);
A = (subpart_data*)malloc((K)*sizeof(subpart_data));
for(i=0;i<K;i++)
{
A[i].subpartID=i+1;
scanf("%d",&A[i].duration);
}
for(i=0;i<K;i++)
scanf("%d",&A[i].cost_per_day);
print_schedule(A,K);
printf("\nInput number of dependencies (l) :");
scanf("%d",&l);
B = (dependency_info*)malloc((l)*sizeof(dependency_info));
for(i=0;i<l;i++)
{
scanf("%d",&B[i].predecessorID);
scanf("%d",&B[i].successorID);
}
print_schedule1(A,K,B,l);
return 0;
}
<file_sep>/README.md
# Algorithms-1-Lab
IIT KGP CS29003
2018 Autumn Semester
<file_sep>/Lab Test/even/9_17CS30038(1).c
//<NAME>
//17CS30038
//PC no=9
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#define max(a,b)(a>b?a:b)
int main()
{
char input1[100],input2[100],output[100];
int DP[100][100];
int i,j;
int m,n;
printf("enter m:");
scanf("%d",&m);
printf("enter n:");
scanf("%d",&n);
printf("enter A:");
scanf(" %s",input1);
printf("enter B:");
scanf(" %s",input2);
for(i=0;i<=strlen(input1);i++)
for(j=0;j<=strlen(input2);j++)
{
if(i==0 || j==0)
DP[i][j]=0;
else if(input1[i-1]==input2[j-1])
DP[i][j]=DP[i-1][j-1]+1;
else
DP[i][j]=max(DP[i][j-1],DP[i-1][j]);
}
int temp=DP[strlen(input1)][strlen(input2)];
printf("\nLength of LCS is %d",DP[strlen(input1)][strlen(input2)]);
return 0;
}
<file_sep>/Assignment 5/17CS30038_5.c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <string.h>
typedef struct _wordR
{
char word[100];
double x, y;
} wordR;
typedef struct _node
{
wordR w;
struct _node *next;
}node, *nodePointer;
typedef nodePointer **hashTable;
void insertH(hashTable H, int m, wordR a)
{
int X=floor(m*(a.x));
int Y=floor(m*(a.y));
nodePointer temp=NULL;
if(H[X][Y]==NULL)
{
H[X][Y]=(nodePointer)malloc(sizeof(node));
H[X][Y]->w=a;
H[X][Y]->next=NULL;
}
else
{
temp=H[X][Y];
while(temp->next!=NULL)
{
temp=temp->next;
}
temp->next=(nodePointer)malloc(sizeof(node));
temp->next->w=a;
temp->next->next=NULL;
}
}
void printH(hashTable H, int m,FILE* fp)
{
int i,j;
nodePointer temp=NULL;
printf("%d",m);
for(i=0;i<m;i++)
{
for(j=0;j<m;j++)
{
fprintf(fp,"\n[%d,%d]::(",i,j);
temp=H[i][j];
while(temp!=NULL)
{
fprintf(fp," %s ",temp->w.word);
temp=temp->next;
}
}
}
}
void findNN (hashTable H, int m, wordR a)
{
int X=floor(m*(a.x));
int Y=floor(m*(a.y));
wordR output;
nodePointer temp=NULL;
int flag=0;
double min=1000;
double dis=0;
int s,t,i,j;
i=X;
j=Y;
printf("--%d--%d--",i,j);
if(H[X][Y]!=NULL)
{
temp=H[X][Y];
while(temp!=NULL)
{
if(strcmp( (temp->w.word),a.word ) !=0)
{
dis=sqrt( ((temp->w.x)-a.x)*((temp->w.x)-a.x) + ((temp->w.x)-a.x)*((temp->w.x)-a.x) );
if(dis<min)
{
min=dis;
output=temp->w;
}
flag=1;
}
temp=temp->next;
}
if(flag==1)
{
printf("%s%lf%lf",output.word,output.x,output.y);
}
}
if(flag==0)
{
int k=1;
while(1)
{
for(s=i-k,t=j-k;t<=j+k;t++)
{
temp=H[s][t];
while(temp!=NULL)
{
dis=sqrt( ((temp->w.x)-a.x)*((temp->w.x)-a.x) + ((temp->w.x)-a.x)*((temp->w.x)-a.x) );
if(dis<min)
{
min=dis;
output=temp->w;
}
flag=1;
temp=temp->next;
}
}
for(s=i+k,t=j-k;t<=j+k;t++)
{
temp=H[s][t];
while(temp!=NULL)
{
dis=sqrt( ((temp->w.x)-a.x)*((temp->w.x)-a.x) + ((temp->w.x)-a.x)*((temp->w.x)-a.x) );
if(dis<min)
{
min=dis;
output=temp->w;
}
flag=1;
temp=temp->next;
}
}
for(t=j-k,s=i-k+1;s<i+k;s++)
{
temp=H[s][t];
while(temp!=NULL)
{
dis=sqrt( ((temp->w.x)-a.x)*((temp->w.x)-a.x) + ((temp->w.x)-a.x)*((temp->w.x)-a.x) );
if(dis<min)
{
min=dis;
output=temp->w;
}
flag=1;
temp=temp->next;
}
}
for(t=j+k,s=i-k+1;s<i+k;s++)
{
temp=H[s][t];
while(temp!=NULL)
{
dis=sqrt( ((temp->w.x)-a.x)*((temp->w.x)-a.x) + ((temp->w.x)-a.x)*((temp->w.x)-a.x) );
if(dis<min)
{
min=dis;
output=temp->w;
}
flag=1;
temp=temp->next;
}
}
if(flag==1)
{
printf("%s%lf%lf",output.word,output.x,output.y);
break;
}
}
}
}
int main()
{
FILE *inpf, *outf;
int n, i,j, m,k;
wordR w,a;
hashTable H;
inpf = fopen("input.txt","r");
if (inpf==NULL)
{
printf("Error opening input file input.txt\n");
return (-1);
}
outf = fopen("output.txt","w");
if (outf==NULL)
{
printf("Error creating output file output.txt\n");
return (-1);
}
fscanf(inpf,"%d",&n);
m=ceil(sqrt(n));
printf("%d--%d",n,m);
//creating dynamic array
H= (nodePointer **)malloc(m*sizeof(nodePointer*));
for(i=0;i<m;i++)
{
H[i]=(nodePointer*)malloc(m*sizeof(nodePointer));
for(j=0;j<m;j++)
H[i][j]=NULL;
}
for(i=0;i<n;i++)
{
fscanf(inpf,"%s%lf%lf",w.word,&w.x,&w.y);
insertH(H,m,w);
}
printH(H,m,outf);
printf("Input number of words to search for:");
scanf("%d",&k);
for(i=0;i<k;i++)
{
printf("Input Word");
scanf("%s%lf%lf",a.word,&a.x,&a.y);
findNN(H,m,a);
}
fclose(inpf);
fclose(outf);
}
| 344b4f32c2b9810e3cdb94768417bcb5de2f4e72 | [
"Markdown",
"C"
]
| 16 | C | vishpitama/Algorithms-1-Lab | c97cef8d29a21f059bcb4d8eb200747a3e8998f9 | a76b35afe078882a758ebc00629b6bf89a90ae0c | |
refs/heads/master | <repo_name>Akhlak-Hossain-Jim/demo-project-Zaynax-LTD<file_sep>/src/assets/components/Blog.js
import React from "react";
import "../styles/components/blog.scss";
import "../styles/components/boxModel.scss";
// blog image importation
import blogImg1 from "../media/posters/26801830821918.png";
import blogImg2 from "../media/posters/26824312324126.png";
import blogImg3 from "../media/posters/Hair-Care.png";
import blogImg4 from "../media/posters/Image 117.png";
function Blog() {
return (
<div className="blogSection grid_parent">
<h3>LATEST BLOGS</h3>
<p>
This is a place devoted to giving you deeper insight into the news,
<br />
trends, people and technology behind Bing.
</p>
<div className="flex">
<img src={blogImg1} alt="" />
<img src={blogImg2} alt="" />
<img src={blogImg3} alt="" />
<img src={blogImg4} alt="" />
</div>
<div className="flex">
<span className="indicators active"></span>
<span className="indicators"></span>
<span className="indicators"></span>
<span className="indicators"></span>
<span className="indicators"></span>
</div>
</div>
);
}
export default Blog;
<file_sep>/src/assets/components/Header.js
import React from "react";
import { Link } from "react-router-dom";
import "../styles/components/header.scss";
import "../styles/components/boxModel.scss";
import ArrowDropDownIcon from "@material-ui/icons/ArrowDropDown";
import searchIcon from "../media/social icons/loupe.png";
function Header() {
return (
<header>
<nav className="nav first__nav">
<Link className="items nav__items active">Homepage</Link>
<Link className="items nav__items second">All Product</Link>
</nav>
<nav className="nav flex secondery_nav">
<div className="showing_products">
Showing
<span>{/* {from}*/}1</span> - {/* {to} */}40 of{" "}
{/* {total products}*/}
80,068 products
</div>
<div className="filter_by flex">
<div className="resp_filter flex">
<div
className="item"
onClick={() => {
document.querySelector("#filters").classList.toggle("fly");
}}
>
Filter
</div>
</div>
<div className="filters flex" id="filters">
<div className="rexp">
<div className="refined_by flex">
<span>Refine by | </span> Price{" "}
<ArrowDropDownIcon />
</div>
<div className="short_by flex">
<span>Sort by | </span> Best Seller{" "}
<ArrowDropDownIcon />
</div>
</div>
</div>
<div className="search_bar flex">
<div style={{ flexBasis: 1 }}></div>
<div className="search flex">
<input type="text" placeholder="Search" id="searchInput" />
<div className="icon">
<img
src={searchIcon}
alt=""
onClick={() => {
document
.querySelector("#searchInput")
.classList.toggle("fly");
}}
/>
</div>
</div>
</div>
</div>
</nav>
</header>
);
}
export default Header;
<file_sep>/src/assets/components/ReactHelmet.js
import React from "react";
import { Helmet } from "react-helmet";
class ReactHelmet extends React.Component {
render() {
return (
<Helmet>
<meta charSet="utf-8" />
<title>{this.props.pageTitle}</title>
<link rel="canonical" href={this.props.cononical} />
</Helmet>
);
}
}
export default ReactHelmet;
<file_sep>/src/assets/components/Footer.js
import React from "react";
// style imports
import "../styles/components/footer.scss";
import "../styles/components/boxModel.scss";
// image logo imports
import footerIllastration from "../media/footer illastration.png";
import SendIcon from "@material-ui/icons/Send";
import facebookIcon from "../media/social icons/002-facebook-logo.png";
import tweeterIcon from "../media/social icons/001-twitter-logo-silhouette.png";
import linkedinIcon from "../media/social icons/004-linkedin-logo.png";
import instagramIcon from "../media/social icons/instagram (1).png";
import youtubeIcon from "../media/social icons/005-youtube.png";
import bkashIcon from "../media/vendor icons/BKash-bKash-Logo.png";
import nagadIcon from "../media/vendor icons/nagad-logo-7A70CCFEE0-seeklogo.com.png";
// import roketIcon from "../media/vendor icons/005-youtube.png";
import visaIcon from "../media/vendor icons/g4158.png";
import mastercardIcon from "../media/vendor icons/Mastercard-logo.png";
import amairicanExpressIcon from "../media/vendor icons/amex-logo-png.png";
import hotlinkeIcon from "../media/social icons/customer-service-2-line.png";
import callIcon from "../media/social icons/phone-line.png";
import emailIcon from "../media/social icons/mail-send-line.png";
function Footer() {
return (
<footer>
<div className="grid__parent">
<img
className="col_1_4 footer__illustration"
src={footerIllastration}
alt=""
/>
<div className="col_2_4 mid_section">
<section className="social_link_container flex">
<a href="null" className="social_links fa">
<img src={facebookIcon} alt="" className="social_link" />
</a>
<a href="null" className="social_links">
<img src={tweeterIcon} alt="" className="social_link" />
</a>
<a href="null" className="social_links">
<img src={linkedinIcon} alt="" className="social_link" />
</a>
<a href="null" className="social_links">
<img src={instagramIcon} alt="" className="social_link" />
</a>
<a href="null" className="social_links">
<img src={youtubeIcon} alt="" className="social_link" />
</a>
</section>
<section className="subscription">
<h3>SUBSCRIBE</h3>
<h6>Get the leatest news from zDrop</h6>
<form action="./action" className="flex">
<input
type="email"
name="email"
id="getEmail"
placeholder="Email address"
/>
<SendIcon role="button" className="submit_ico" />
</form>
<div className="quick_links flex">
<a href="null" className="link">
About zDrop
</a>
<a href="null" className="link">
FAQ & Support
</a>
<a href="null" className="link">
Term & Condition
</a>
<a href="null" className="link">
Privacy Policy
</a>
</div>
</section>
<section className="payment_partner flex">
<a href="null">
<img src={bkashIcon} alt="" />
</a>
<a href="null">
<img src={nagadIcon} alt="" />
</a>
<a href="null">
<img src={visaIcon} alt="" />
</a>
<a href="null">
<img src={mastercardIcon} alt="" />
</a>
<a href="null">
<img src={amairicanExpressIcon} alt="" />
</a>
{/* <a href="">
<img src="" alt="" />
</a> */}
</section>
</div>
<div className="col_3_4 right_sec flex">
<section className="contact">
<h3>CONTACT US</h3>
<div className="block flex">
<div className="img_con">
<img src={hotlinkeIcon} alt="" />
</div>
<div>
<h5>Hotline:</h5>
<br />
<h6>
<a href="tel:+8801929459195">
+8801929459195 (10am-10pm/Sat-Thur)
</a>
</h6>
</div>
</div>
<div className="block flex">
<div className="img_con">
<img src={callIcon} alt="" />
</div>
<div>
<h5>Whole Sales</h5>
<br />
<h6>
<a href="tel:+8801929459195">01929459195 (10am-12pm)</a>
</h6>
</div>
</div>
<div className="block flex">
<div className="img_con">
<img src={emailIcon} alt="" />
</div>
<div>
<h5>Email</h5>
<br />
<h6>
<a href="mailto:<EMAIL>">
<EMAIL>
</a>
</h6>
</div>
</div>
</section>
</div>
</div>
</footer>
);
}
export default Footer;
<file_sep>/src/assets/components/Promotions.js
import React from "react";
import "../styles/components/Promotion.scss";
import "../styles/components/boxModel.scss";
import FavoriteIcon from "@material-ui/icons/Favorite";
import TurnedInIcon from "@material-ui/icons/TurnedIn";
import SendIcon from "@material-ui/icons/Send";
import AccountBalanceWalletIcon from "@material-ui/icons/AccountBalanceWallet";
import SmsRoundedIcon from "@material-ui/icons/SmsRounded";
function Promotions() {
return (
<div className="promotions flex">
<div className="promotionBox">
<FavoriteIcon className="icon pink" />
<h4>Quality and Saving</h4>
<p>Comprehensive quality control and affordable prices</p>
</div>
<div className="promotionBox">
<TurnedInIcon className="icon blue" />
<h4>Global Brands</h4>
<p>Buy you favorite items from your favorite global brands</p>
</div>
<div className="promotionBox">
<SendIcon className="icon orange" />
<h4>Fast Delivery</h4>
<p>Fast and convenient door to door delivery</p>
</div>
<div className="promotionBox">
<AccountBalanceWalletIcon className="icon ass" />
<h4>Secure Payment</h4>
<p>Different secure payment methods</p>
</div>
<div className="promotionBox">
<SmsRoundedIcon className="icon green" />
<h4>Professional Service</h4>
<p>Efficient customer support from passionate team</p>
</div>
</div>
);
}
export default Promotions;
<file_sep>/src/App.js
import React from "react";
import { BrowserRouter as Router } from "react-router-dom";
import "./assets/styles/app.scss";
import Footer from "./assets/components/Footer";
import Header from "./assets/components/Header";
import ProductPage from "./assets/pages/ProductPage";
function App() {
return (
<div className="app">
<Router>
<Header />
<ProductPage />
<Footer />
</Router>
</div>
);
}
export default App;
| 3db23ce5d9b2b8c51072985f2b7a091919c0b6be | [
"JavaScript"
]
| 6 | JavaScript | Akhlak-Hossain-Jim/demo-project-Zaynax-LTD | 41ec1300b92bbbb524cddffe788c5c682cac8721 | e4269c9485dbd2962a249d626a80c41ca72c10b2 | |
refs/heads/master | <file_sep>package com.palmergames.bukkit.towny.event;
import com.palmergames.bukkit.towny.object.Resident;
import com.palmergames.bukkit.towny.object.Town;
import org.bukkit.event.Event;
import org.bukkit.event.HandlerList;
/**
* Author: <NAME> (Zren / Shade)
* Date: 5/23/12
*
* Fired after a resident has been added to a town.
*/
public class TownAddResidentEvent extends Event {
private static final HandlerList handlers = new HandlerList();
private Resident resident;
private Town town;
public TownAddResidentEvent(Resident resident, Town town) {
this.resident = resident;
this.town = town;
}
/**
*
* @return the resident who has joined a town.
*/
public Resident getResident() {
return resident;
}
/**
*
* @return the town the resident has just joined.
*/
public Town getTown() {
return town;
}
@Override
public HandlerList getHandlers() {
return handlers;
}
}
<file_sep>package com.palmergames.bukkit.towny.regen;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.Hashtable;
import java.util.List;
import java.util.Set;
import org.bukkit.Bukkit;
import org.bukkit.Chunk;
import org.bukkit.Material;
import org.bukkit.World;
import org.bukkit.block.Block;
import org.bukkit.block.BlockState;
import org.bukkit.block.CreatureSpawner;
import org.bukkit.block.Sign;
import org.bukkit.entity.Player;
import org.bukkit.inventory.InventoryHolder;
import com.palmergames.bukkit.towny.Towny;
import com.palmergames.bukkit.towny.TownyMessaging;
import com.palmergames.bukkit.towny.TownySettings;
import com.palmergames.bukkit.towny.exceptions.NotRegisteredException;
import com.palmergames.bukkit.towny.object.Coord;
import com.palmergames.bukkit.towny.object.Resident;
import com.palmergames.bukkit.towny.object.TownBlock;
import com.palmergames.bukkit.towny.object.TownyUniverse;
import com.palmergames.bukkit.towny.object.WorldCoord;
import com.palmergames.bukkit.towny.regen.block.BlockInventoryHolder;
import com.palmergames.bukkit.towny.regen.block.BlockLocation;
import com.palmergames.bukkit.towny.regen.block.BlockMobSpawner;
import com.palmergames.bukkit.towny.regen.block.BlockObject;
import com.palmergames.bukkit.towny.regen.block.BlockSign;
import com.palmergames.bukkit.towny.tasks.ProtectionRegenTask;
import com.palmergames.bukkit.util.BukkitTools;
/**
* @author ElgarL
*
*/
public class TownyRegenAPI {
//private static Towny plugin = null;
public static void initialize(Towny plugin) {
//TownyRegenAPI.plugin = plugin;
}
// table containing snapshot data of active reversions.
private static Hashtable<String, PlotBlockData> PlotChunks = new Hashtable<String, PlotBlockData>();
// List of all old plots still to be processed for Block removal
private static List<WorldCoord> deleteTownBlockIdQueue = new ArrayList<WorldCoord>();
// A list of worldCoords which are needing snapshots
private static List<WorldCoord> worldCoords = new ArrayList<WorldCoord>();
// A holder for each protection regen task
private static Hashtable<BlockLocation, ProtectionRegenTask> protectionRegenTasks = new Hashtable<BlockLocation, ProtectionRegenTask>();
// List of protection blocks placed to prevent blockPhysics.
private static Set<Block> protectionPlaceholders = new HashSet<Block>();
/**
* Add a TownBlocks WorldCoord for a snapshot to be taken.
*
* @param worldCoord
*/
public static void addWorldCoord(WorldCoord worldCoord) {
if (!worldCoords.contains(worldCoord))
worldCoords.add(worldCoord);
}
/**
* @return true if there are any TownBlocks to be processed.
*/
public static boolean hasWorldCoords() {
return worldCoords.size() != 0;
}
/**
* Check if this WorldCoord is waiting for a snapshot to be taken.
*
* @param worldCoord
* @return true if it's in the queue.
*/
public static boolean hasWorldCoord(WorldCoord worldCoord) {
return worldCoords.contains(worldCoord);
}
/**
* @return First WorldCoord to be processed.
*/
public static WorldCoord getWorldCoord() {
if (!worldCoords.isEmpty()) {
WorldCoord wc = worldCoords.get(0);
worldCoords.remove(0);
return wc;
}
return null;
}
/**
* @return the plotChunks which are being processed
*/
public static Hashtable<String, PlotBlockData> getPlotChunks() {
return PlotChunks;
}
/**
* @return true if there are any chunks being processed.
*/
public static boolean hasPlotChunks() {
return !PlotChunks.isEmpty();
}
/**
* @param plotChunks the plotChunks to set
*/
public static void setPlotChunks(Hashtable<String, PlotBlockData> plotChunks) {
PlotChunks = plotChunks;
}
/**
* Removes a Plot Chunk from the regeneration Hashtable
*
* @param plotChunk
*/
public static void deletePlotChunk(PlotBlockData plotChunk) {
if (PlotChunks.containsKey(getPlotKey(plotChunk))) {
PlotChunks.remove(getPlotKey(plotChunk));
TownyUniverse.getDataSource().saveRegenList();
}
}
/**
* Adds a Plot Chunk to the regeneration Hashtable
*
* @param plotChunk
* @param save
*/
public static void addPlotChunk(PlotBlockData plotChunk, boolean save) {
if (!PlotChunks.containsKey(getPlotKey(plotChunk))) {
//plotChunk.initialize();
PlotChunks.put(getPlotKey(plotChunk), plotChunk);
if (save)
TownyUniverse.getDataSource().saveRegenList();
}
}
/**
* Saves a Plot Chunk snapshot to the datasource
*
* @param plotChunk
*/
public static void addPlotChunkSnapshot(PlotBlockData plotChunk) {
if (TownyUniverse.getDataSource().loadPlotData(plotChunk.getWorldName(), plotChunk.getX(), plotChunk.getZ()) == null) {
TownyUniverse.getDataSource().savePlotData(plotChunk);
}
}
/**
* Deletes a Plot Chunk snapshot from the datasource
*
* @param plotChunk
*/
public static void deletePlotChunkSnapshot(PlotBlockData plotChunk) {
TownyUniverse.getDataSource().deletePlotData(plotChunk);
}
/**
* Loads a Plot Chunk snapshot from the datasource
*
* @param townBlock
*/
public static PlotBlockData getPlotChunkSnapshot(TownBlock townBlock) {
return TownyUniverse.getDataSource().loadPlotData(townBlock);
}
/**
* Gets a Plot Chunk from the regeneration Hashtable
*
* @param townBlock
*/
public static PlotBlockData getPlotChunk(TownBlock townBlock) {
if (PlotChunks.containsKey(getPlotKey(townBlock))) {
return PlotChunks.get(getPlotKey(townBlock));
}
return null;
}
private static String getPlotKey(PlotBlockData plotChunk) {
return "[" + plotChunk.getWorldName() + "|" + plotChunk.getX() + "|" + plotChunk.getZ() + "]";
}
public static String getPlotKey(TownBlock townBlock) {
return "[" + townBlock.getWorld().getName() + "|" + townBlock.getX() + "|" + townBlock.getZ() + "]";
}
/**
* Regenerate the chunk the player is stood in and store the block data so it can be undone later.
*
* @param player
*/
public static void regenChunk(Player player) {
try {
Coord coord = Coord.parseCoord(player);
World world = player.getWorld();
Chunk chunk = world.getChunkAt(player.getLocation());
int maxHeight = world.getMaxHeight();
Object[][][] snapshot = new Object[16][maxHeight][16];
for (int x = 0; x < 16; x++) {
for (int z = 0; z < 16; z++) {
for (int y = 0; y < maxHeight; y++) {
//Current block to save
BlockState state = chunk.getBlock(x, y, z).getState();
if (state instanceof org.bukkit.block.Sign) {
BlockSign sign = new BlockSign(state.getTypeId(), state.getData().getData(), ((org.bukkit.block.Sign) state).getLines());
sign.setLocation(state.getLocation());
snapshot[x][y][z] = sign;
} else if (state instanceof CreatureSpawner) {
BlockMobSpawner spawner = new BlockMobSpawner(((CreatureSpawner) state).getSpawnedType());
spawner.setLocation(state.getLocation());
spawner.setDelay(((CreatureSpawner) state).getDelay());
snapshot[x][y][z] = spawner;
} else if ((state instanceof InventoryHolder) && !(state instanceof Player)) {
BlockInventoryHolder holder = new BlockInventoryHolder(state.getTypeId(), state.getData().getData(), ((InventoryHolder) state).getInventory().getContents());
holder.setLocation(state.getLocation());
snapshot[x][y][z] = holder;
} else {
snapshot[x][y][z] = new BlockObject(state.getTypeId(), state.getData().getData(), state.getLocation());
}
}
}
}
TownyUniverse.getDataSource().getResident(player.getName()).addUndo(snapshot);
Bukkit.getWorld(player.getWorld().getName()).regenerateChunk(coord.getX(), coord.getZ());
} catch (NotRegisteredException e) {
// Failed to get resident
}
}
/**
* Restore the relevant chunk using the snapshot data stored in the resident
* object.
*
* @param snapshot
* @param resident
*/
public static void regenUndo(Object[][][] snapshot, Resident resident) {
BlockObject key = ((BlockObject) snapshot[0][0][0]);
World world = key.getLocation().getWorld();
Chunk chunk = key.getLocation().getChunk();
int maxHeight = world.getMaxHeight();
for (int x = 0; x < 16; x++) {
for (int z = 0; z < 16; z++) {
for (int y = 0; y < maxHeight; y++) {
// Snapshot data we need to update the world.
Object state = snapshot[x][y][z];
// The block we will be updating
Block block = chunk.getBlock(x, y, z);
if (state instanceof BlockSign) {
BlockSign signData = (BlockSign)state;
block.setTypeIdAndData(signData.getTypeId(), signData.getData(), false);
Sign sign = (Sign) block.getState();
int i = 0;
for (String line : signData.getLines())
sign.setLine(i++, line);
sign.update(true);
} else if (state instanceof BlockMobSpawner) {
BlockMobSpawner spawnerData = (BlockMobSpawner) state;
block.setTypeIdAndData(spawnerData.getTypeId(), spawnerData.getData(), false);
((CreatureSpawner) block.getState()).setSpawnedType(spawnerData.getSpawnedType());
((CreatureSpawner) block.getState()).setDelay(spawnerData.getDelay());
} else if ((state instanceof BlockInventoryHolder) && !(state instanceof Player)) {
BlockInventoryHolder containerData = (BlockInventoryHolder) state;
block.setTypeIdAndData(containerData.getTypeId(), containerData.getData(), false);
// Container to receive the inventory
InventoryHolder container = (InventoryHolder) block.getState();
// Contents we are respawning.
if (containerData.getItems().length > 0)
container.getInventory().setContents(containerData.getItems());
} else {
BlockObject blockData = (BlockObject) state;
block.setTypeIdAndData(blockData.getTypeId(), blockData.getData(), false);
}
}
}
}
TownyMessaging.sendMessage(BukkitTools.getServer().getPlayerExact(resident.getName()), TownySettings.getLangString("msg_undo_complete"));
}
/////////////////////////////////
/**
* @return true if there are any chunks being processed.
*/
public static boolean hasDeleteTownBlockIdQueue() {
return !deleteTownBlockIdQueue.isEmpty();
}
public static boolean isDeleteTownBlockIdQueue(WorldCoord plot) {
return deleteTownBlockIdQueue.contains(plot);
}
public static void addDeleteTownBlockIdQueue(WorldCoord plot) {
if (!deleteTownBlockIdQueue.contains(plot))
deleteTownBlockIdQueue.add(plot);
}
public static WorldCoord getDeleteTownBlockIdQueue() {
if (!deleteTownBlockIdQueue.isEmpty()) {
WorldCoord wc = deleteTownBlockIdQueue.get(0);
deleteTownBlockIdQueue.remove(0);
return wc;
}
return null;
}
/**
* Deletes all of a specified block type from a TownBlock
*
* @param worldCoord
*/
public static void doDeleteTownBlockIds(WorldCoord worldCoord) {
//Block block = null;
World world = null;
int plotSize = TownySettings.getTownBlockSize();
TownyMessaging.sendDebugMsg("Processing deleteTownBlockIds");
world = worldCoord.getBukkitWorld();
if (world != null) {
/*
* if
* (!world.isChunkLoaded(MinecraftTools.calcChunk(townBlock.getX()),
* MinecraftTools.calcChunk(townBlock.getZ())))
* return;
*/
int height = world.getMaxHeight() - 1;
int worldx = worldCoord.getX() * plotSize, worldz = worldCoord.getZ() * plotSize;
for (int z = 0; z < plotSize; z++)
for (int x = 0; x < plotSize; x++)
for (int y = height; y > 0; y--) { //Check from bottom up else minecraft won't remove doors
Block block = world.getBlockAt(worldx + x, y, worldz + z);
try {
if (worldCoord.getTownyWorld().isPlotManagementDeleteIds(block.getTypeId())) {
block.setType(Material.AIR);
}
} catch (NotRegisteredException e) {
// Not a registered world
}
block = null;
}
}
}
/**
* Deletes all of a specified block type from a TownBlock
*
* @param townBlock
* @param material
*/
public static void deleteTownBlockMaterial(TownBlock townBlock, int material) {
//Block block = null;
int plotSize = TownySettings.getTownBlockSize();
TownyMessaging.sendDebugMsg("Processing deleteTownBlockMaterial");
World world = BukkitTools.getServer().getWorld(townBlock.getWorld().getName());
if (world != null) {
/*
* if
* (!world.isChunkLoaded(MinecraftTools.calcChunk(townBlock.getX()),
* MinecraftTools.calcChunk(townBlock.getZ())))
* return;
*/
int height = world.getMaxHeight() - 1;
int worldx = townBlock.getX() * plotSize, worldz = townBlock.getZ() * plotSize;
for (int z = 0; z < plotSize; z++)
for (int x = 0; x < plotSize; x++)
for (int y = height; y > 0; y--) { //Check from bottom up else minecraft won't remove doors
Block block = world.getBlockAt(worldx + x, y, worldz + z);
if (block.getTypeId() == material) {
block.setType(Material.AIR);
}
block = null;
}
}
}
/*
* Protection Regen follows
*/
/**
* Does a task for this block already exist?
*
* @param blockLocation
* @return true if a task exists
*/
public static boolean hasProtectionRegenTask(BlockLocation blockLocation) {
for (BlockLocation location : protectionRegenTasks.keySet()) {
if (location.isLocation(blockLocation)) {
return true;
}
}
return false;
}
/**
* Fetch the relevant regen task for this block
*
* @param blockLocation
* @return the stored task, or null if there is none.
*/
public static ProtectionRegenTask GetProtectionRegenTask(BlockLocation blockLocation) {
for (BlockLocation location : protectionRegenTasks.keySet()) {
if (location.isLocation(blockLocation)) {
return protectionRegenTasks.get(location);
}
}
return null;
}
/**
* Add this task to the protection regen queue.
*
* @param task
*/
public static void addProtectionRegenTask(ProtectionRegenTask task) {
protectionRegenTasks.put(task.getBlockLocation(), task);
}
/**
* Remove this task form teh protection regen queue
*
* @param task
*/
public static void removeProtectionRegenTask(ProtectionRegenTask task) {
protectionRegenTasks.remove(task.getBlockLocation());
if (protectionRegenTasks.isEmpty())
protectionPlaceholders.clear();
}
/**
* Cancel all regenerating tasks and clear all queues.
*/
public static void cancelProtectionRegenTasks() {
for (ProtectionRegenTask task : protectionRegenTasks.values()) {
BukkitTools.getServer().getScheduler().cancelTask(task.getTaskId());
task.replaceProtections();
}
protectionRegenTasks.clear();
protectionPlaceholders.clear();
}
/**
* Is this a placholder block?
*
* @param block
* @return true if it is a placeholder
*/
public static boolean isPlaceholder(Block block) {
return protectionPlaceholders.contains(block);
}
/**
* Add this block as a placeholder (will be replaced when it's regeneration task occurs)
*
* @param block
*/
public static void addPlaceholder(Block block) {
protectionPlaceholders.add(block);
}
/**
* Remove this block from being tracked as a placeholder.
*
* @param block
*/
public static void removePlaceholder(Block block) {
protectionPlaceholders.remove(block);
}
}<file_sep>/**
*
*/
package com.palmergames.bukkit.towny.permissions;
import java.io.File;
import java.io.IOException;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.ListIterator;
import java.util.Map;
import java.util.Set;
import org.bukkit.configuration.MemorySection;
import org.bukkit.entity.Player;
import org.bukkit.permissions.Permission;
import org.bukkit.permissions.PermissionAttachment;
import org.bukkit.permissions.PermissionDefault;
import com.palmergames.bukkit.config.CommentedConfiguration;
import com.palmergames.bukkit.towny.Towny;
import com.palmergames.bukkit.towny.exceptions.NotRegisteredException;
import com.palmergames.bukkit.towny.object.Nation;
import com.palmergames.bukkit.towny.object.Resident;
import com.palmergames.bukkit.towny.object.Town;
import com.palmergames.bukkit.towny.object.TownyUniverse;
import com.palmergames.bukkit.towny.object.TownyWorld;
import com.palmergames.bukkit.util.BukkitTools;
import com.palmergames.util.FileMgmt;
/**
* @author ElgarL
*
*/
public class TownyPerms {
protected static LinkedHashMap<String, Permission> registeredPermissions = new LinkedHashMap<String, Permission>();
protected static HashMap<String, PermissionAttachment> attachments = new HashMap<String, PermissionAttachment>();
private static CommentedConfiguration perms;
private static Towny plugin;
public static void initialize(Towny plugin) {
TownyPerms.plugin = plugin;
}
private static Field permissions;
// Setup reflection (Thanks to Codename_B for the reflection source)
static {
try {
permissions = PermissionAttachment.class.getDeclaredField("permissions");
permissions.setAccessible(true);
} catch (SecurityException e) {
e.printStackTrace();
} catch (NoSuchFieldException e) {
e.printStackTrace();
}
}
/**
* Load the townyperms.yml file.
* If it doesn't exist create it from the resource file in the jar.
*
* @param filepath
* @param defaultRes
* @throws IOException
*/
public static void loadPerms(String filepath, String defaultRes) throws IOException {
String fullPath = filepath + FileMgmt.fileSeparator() + defaultRes;
File file = FileMgmt.unpackResourceFile(fullPath, defaultRes, defaultRes);
if (file != null) {
// read the (language).yml into memory
perms = new CommentedConfiguration(file);
perms.load();
}
/*
* Only do this once as we are really only interested in Towny perms.
*/
collectPermissions();
}
/**
* Register a specific residents permissions with Bukkit.
*
* @param resident
*/
public static void assignPermissions(Resident resident, Player player) {
PermissionAttachment playersAttachment= null;
if (resident == null) {
try {
resident = TownyUniverse.getDataSource().getResident(player.getName());
} catch (NotRegisteredException e) {
// failed to get resident
e.printStackTrace();
return;
}
} else {
player = BukkitTools.getPlayer(resident.getName());
}
/*
* Find the current attachment
* or create a new one (if the player is online)
*/
if ((player == null) || !player.isOnline()) {
attachments.remove(resident.getName());
return;
}
TownyWorld World = null;
try {
World = TownyUniverse.getDataSource().getWorld(player.getLocation().getWorld().getName());
} catch (NotRegisteredException e) {
// World not registered with Towny.
e.printStackTrace();
return;
}
if (attachments.containsKey(resident.getName())) {
playersAttachment = attachments.get(resident.getName());
} else
playersAttachment = BukkitTools.getPlayer(resident.getName()).addAttachment(plugin);
/*
* Set all our Towny default permissions using reflection else
* bukkit will perform a recalculation of perms for each addition.
*/
try {
@SuppressWarnings("unchecked")
Map<String, Boolean> orig = (Map<String, Boolean>) permissions.get(playersAttachment);
/*
* Clear the map (faster than removing the attachment and recalculating)
*/
orig.clear();
if (World.isUsingTowny()) {
/*
* Fill with the fresh perm nodes
*/
orig.putAll(TownyPerms.getResidentPerms(resident));
//System.out.print("Perms set for: " + resident.getName());
}
/*
* Tell bukkit to update it's permissions
*/
playersAttachment.getPermissible().recalculatePermissions();
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
/*
* Store the attachment for future reference
*/
attachments.put(resident.getName(), playersAttachment);
}
/**
* Should only be called when a player leaves the server.
*
* @param name
*/
public static void removeAttachment(String name) {
if (attachments.containsKey(name))
attachments.remove(name);
}
/**
* Update the permissions for all residents of a town (if online)
*
* @param town
*/
public static void updateTownPerms(Town town) {
for (Resident resident: town.getResidents())
assignPermissions(resident, null);
}
/**
* Update the permissions for all residents of a nation (if online)
*
* @param nation
*/
public static void updateNationPerms(Nation nation) {
for (Town town: nation.getTowns())
updateTownPerms(town);
}
/**
* Fetch a list of permission nodes
*
* @param path
* @return a List of permission nodes.
*/
private static List<String> getList(String path) {
if (perms.contains(path)) {
return perms.getStringList(path);
}
return null;
}
/**
* Returns a sorted map of this residents current permissions.
*
* @param resident
* @return a sorted Map of permission nodes
*/
public static LinkedHashMap<String, Boolean> getResidentPerms(Resident resident) {
Set<String> permList = new HashSet<String>();
// Start by adding the default perms everyone gets
permList.addAll(getDefault());
//Check for town membership
if (resident.hasTown()) {
permList.addAll(getTownDefault());
// Is Mayor?
if (resident.isMayor()) permList.addAll(getTownMayor());
//Add town ranks here
for (String rank: resident.getTownRanks()) {
permList.addAll(getTownRank(rank));
}
//Check for nation membership
if (resident.hasNation()) {
permList.addAll(getNationDefault());
// Is King?
if (resident.isKing()) permList.addAll(getNationKing());
//Add nation ranks here
for (String rank: resident.getNationRanks()) {
permList.addAll(getNationRank(rank));
}
}
}
List<String> playerPermArray = sort(new ArrayList<String>(permList));
LinkedHashMap<String, Boolean> newPerms = new LinkedHashMap<String, Boolean>();
Boolean value = false;
for (String permission : playerPermArray) {
value = (!permission.startsWith("-"));
newPerms.put((value ? permission : permission.substring(1)), value);
}
return newPerms;
}
public static void registerPermissionNodes() {
plugin.getServer().getScheduler().scheduleSyncDelayedTask(plugin, new
Runnable(){
@Override
public void run() {
Permission perm;
/*
* Register Town ranks
*/
for (String rank : getTownRanks()) {
perm = new
Permission(PermissionNodes.TOWNY_COMMAND_TOWN_RANK.getNode(rank),
"User can grant this town rank to others..",
PermissionDefault.FALSE, null);
perm.addParent(PermissionNodes.TOWNY_COMMAND_TOWN_RANK.getNode(), true);
}
/*
* Register Nation ranks
*/
for (String rank : getNationRanks()) {
perm = new
Permission(PermissionNodes.TOWNY_COMMAND_NATION_RANK.getNode(rank),
"User can grant this town rank to others..",
PermissionDefault.FALSE, null);
perm.addParent(PermissionNodes.TOWNY_COMMAND_NATION_RANK.getNode(), true);
}
}
},1);
}
/*
* Getter/Setters for TownyPerms
*/
/**
* Default permissions everyone gets
*
* @return a List of permissions
*/
public static List<String> getDefault() {
List<String> permsList = getList("nomad");
return (permsList == null)? new ArrayList<String>() : permsList;
}
/*
* Town permission section
*/
/**
* Fetch a list of all available town ranks
*
* @return a list of rank names.
*/
public static List<String> getTownRanks() {
return new ArrayList<String>(((MemorySection) perms.get("towns.ranks")).getKeys(false));
}
/**
* Default permissions everyone in a town gets
*
* @return a list of permissions
*/
public static List<String> getTownDefault() {
List<String> permsList = getList("towns.default");
return (permsList == null)? new ArrayList<String>() : permsList;
}
/**
* A town mayors permissions
*
* @return a list of permissions
*/
public static List<String> getTownMayor() {
List<String> permsList = getList("towns.mayor");
return (permsList == null)? new ArrayList<String>() : permsList;
}
/**
* Get a specific ranks permissions
*
* @param rank
* @return a List of permissions
*/
public static List<String> getTownRank(String rank) {
List<String> permsList = getList("towns.ranks." + rank.toLowerCase());
return (permsList == null)? new ArrayList<String>() : permsList;
}
/*
* Nation permission section
*/
/**
* Fetch a list of all available nation ranks
*
* @return a list of rank names.
*/
public static List<String> getNationRanks() {
return new ArrayList<String>(((MemorySection) perms.get("nations.ranks")).getKeys(false));
}
/**
* Default permissions everyone in a nation gets
*
* @return a List of permissions
*/
public static List<String> getNationDefault() {
List<String> permsList = getList("nations.default");
return (permsList == null)? new ArrayList<String>() : permsList;
}
/**
* A nations kings permissions
*
* @return a List of permissions
*/
public static List<String> getNationKing() {
List<String> permsList = getList("nations.king");
return (permsList == null)? new ArrayList<String>() : permsList;
}
/**
* Get a specific ranks permissions
*
* @param rank
* @return a List of Permissions
*/
public static List<String> getNationRank(String rank) {
List<String> permsList = getList("nations.ranks." + rank.toLowerCase());
return (permsList == null)? new ArrayList<String>() : permsList;
}
/*
* Permission utility functions taken from GroupManager (which I wrote anyway).
*/
/**
* Update the list of permissions registered with bukkit
*/
public static void collectPermissions() {
registeredPermissions.clear();
for (Permission perm : BukkitTools.getPluginManager().getPermissions()) {
registeredPermissions.put(perm.getName().toLowerCase(), perm);
}
}
/**
* Sort a permission node list by parent/child
*
* @param permList
* @return List sorted for priority
*/
private static List<String> sort(List<String> permList) {
List<String> result = new ArrayList<String>();
for (String key : permList) {
String a = key.charAt(0) == '-' ? key.substring(1) : key;
Map<String, Boolean> allchildren = getAllChildren(a, new HashSet<String>());
if (allchildren != null) {
ListIterator<String> itr = result.listIterator();
while (itr.hasNext()) {
String node = (String) itr.next();
String b = node.charAt(0) == '-' ? node.substring(1) : node;
// Insert the parent node before the child
if (allchildren.containsKey(b)) {
itr.set(key);
itr.add(node);
break;
}
}
}
if (!result.contains(key))
result.add(key);
}
return result;
}
/**
* Fetch all permissions which are registered with superperms.
* {can include child nodes)
*
* @param includeChildren
* @return List of all permission nodes
*/
public List<String> getAllRegisteredPermissions(boolean includeChildren) {
List<String> perms = new ArrayList<String>();
for (String key : registeredPermissions.keySet()) {
if (!perms.contains(key)) {
perms.add(key);
if (includeChildren) {
Map<String, Boolean> children = getAllChildren(key, new HashSet<String>());
if (children != null) {
for (String node : children.keySet())
if (!perms.contains(node))
perms.add(node);
}
}
}
}
return perms;
}
/**
* Returns a map of ALL child permissions registered with bukkit
* null is empty
*
* @param node
* @param playerPermArray current list of perms to check against for
* negations
* @return Map of child permissions
*/
public static Map<String, Boolean> getAllChildren(String node, Set<String> playerPermArray) {
LinkedList<String> stack = new LinkedList<String>();
Map<String, Boolean> alreadyVisited = new HashMap<String, Boolean>();
stack.push(node);
alreadyVisited.put(node, true);
while (!stack.isEmpty()) {
String now = stack.pop();
Map<String, Boolean> children = getChildren(now);
if ((children != null) && (!playerPermArray.contains("-" + now))) {
for (String childName : children.keySet()) {
if (!alreadyVisited.containsKey(childName)) {
stack.push(childName);
alreadyVisited.put(childName, children.get(childName));
}
}
}
}
alreadyVisited.remove(node);
if (!alreadyVisited.isEmpty())
return alreadyVisited;
return null;
}
/**
* Returns a map of the child permissions (1 node deep) as registered with
* Bukkit.
* null is empty
*
* @param node
* @return Map of child permissions
*/
public static Map<String, Boolean> getChildren(String node) {
Permission perm = registeredPermissions.get(node.toLowerCase());
if (perm == null)
return null;
return perm.getChildren();
}
}
<file_sep>package com.palmergames.bukkit.towny;
import ca.xshade.bukkit.questioner.Questioner;
import ca.xshade.questionmanager.Option;
import ca.xshade.questionmanager.Question;
import com.earth2me.essentials.Essentials;
import com.nijiko.permissions.PermissionHandler;
import com.palmergames.bukkit.towny.command.*;
import com.palmergames.bukkit.towny.exceptions.NotRegisteredException;
import com.palmergames.bukkit.towny.exceptions.TownyException;
import com.palmergames.bukkit.towny.listeners.*;
import com.palmergames.bukkit.towny.object.*;
import com.palmergames.bukkit.towny.permissions.*;
import com.palmergames.bukkit.towny.questioner.TownyQuestionTask;
import com.palmergames.bukkit.towny.regen.TownyRegenAPI;
import com.palmergames.bukkit.towny.utils.PlayerCacheUtil;
import com.palmergames.bukkit.towny.war.flagwar.TownyWar;
import com.palmergames.bukkit.towny.war.flagwar.listeners.TownyWarBlockListener;
import com.palmergames.bukkit.towny.war.flagwar.listeners.TownyWarCustomListener;
import com.palmergames.bukkit.towny.war.flagwar.listeners.TownyWarEntityListener;
import com.palmergames.bukkit.util.BukkitTools;
import com.palmergames.util.FileMgmt;
import com.palmergames.util.JavaUtil;
import com.palmergames.util.StringMgmt;
import org.bukkit.ChatColor;
import org.bukkit.World;
import org.bukkit.entity.Player;
import org.bukkit.plugin.Plugin;
import org.bukkit.plugin.PluginManager;
import org.bukkit.plugin.java.JavaPlugin;
import java.io.IOException;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Towny Plugin for Bukkit
*
* Website: http://code.google.com/a/eclipselabs.org/p/towny/ Source:
* http://code.google.com/a/eclipselabs.org/p/towny/source/browse/
*
* @author Shade, ElgarL
*/
public class Towny extends JavaPlugin {
private String version = "2.0.0";
public static PermissionHandler permissionHandler;
private final TownyPlayerListener playerListener = new TownyPlayerListener(this);
private final TownyBlockListener blockListener = new TownyBlockListener(this);
private final TownyCustomListener customListener = new TownyCustomListener(this);
private final TownyEntityListener entityListener = new TownyEntityListener(this);
private final TownyWeatherListener weatherListener = new TownyWeatherListener(this);
private final TownyEntityMonitorListener entityMonitorListener = new TownyEntityMonitorListener(this);
private final TownyWorldListener worldListener = new TownyWorldListener(this);
private final TownyWarBlockListener townyWarBlockListener = new TownyWarBlockListener(this);
private final TownyWarCustomListener townyWarCustomListener = new TownyWarCustomListener(this);
private final TownyWarEntityListener townyWarEntityListener = new TownyWarEntityListener(this);
private TownyUniverse townyUniverse;
private Map<String, PlayerCache> playerCache = Collections.synchronizedMap(new HashMap<String, PlayerCache>());
private Essentials essentials = null;
private boolean citizens2 = false;
private boolean error = false;
@Override
public void onEnable() {
System.out.println("==================== Towny ========================");
version = this.getDescription().getVersion();
townyUniverse = new TownyUniverse(this);
// Setup classes
BukkitTools.initialize(this);
TownyTimerHandler.initialize(this);
TownyEconomyHandler.initialize(this);
TownyFormatter.initialize(this);
TownyRegenAPI.initialize(this);
PlayerCacheUtil.initialize(this);
TownyPerms.initialize(this);
if (load()) {
// Setup bukkit command interfaces
getCommand("townyadmin").setExecutor(new TownyAdminCommand(this));
getCommand("townyworld").setExecutor(new TownyWorldCommand(this));
getCommand("resident").setExecutor(new ResidentCommand(this));
getCommand("towny").setExecutor(new TownyCommand(this));
getCommand("town").setExecutor(new TownCommand(this));
getCommand("nation").setExecutor(new NationCommand(this));
getCommand("plot").setExecutor(new PlotCommand(this));
TownyWar.onEnable();
if (TownySettings.isTownyUpdating(getVersion()))
update();
// Register all child permissions for ranks
TownyPerms.registerPermissionNodes();
}
registerEvents();
TownyLogger.log.info("=============================================================");
if (isError())
TownyLogger.log.info("[WARNING] - ***** SAFE MODE ***** " + version);
else
TownyLogger.log.info("[Towny] Version: " + version + " - Mod Enabled");
TownyLogger.log.info("=============================================================");
if (!isError()) {
// Re login anyone online. (In case of plugin reloading)
for (Player player : BukkitTools.getOnlinePlayers())
if (player != null)
try {
getTownyUniverse().onLogin(player);
} catch (TownyException x) {
TownyMessaging.sendErrorMsg(player, x.getMessage());
}
}
}
public void SetWorldFlags() {
for (Town town : TownyUniverse.getDataSource().getTowns()) {
TownyMessaging.sendDebugMsg("[Towny] Setting flags for: " + town.getName());
if (town.getWorld() == null) {
TownyLogger.log.warning("[Towny Error] Detected an error with the world files. Attempting to repair");
if (town.hasHomeBlock())
try {
TownyWorld world = town.getHomeBlock().getWorld();
if (!world.hasTown(town)) {
world.addTown(town);
TownyUniverse.getDataSource().saveTown(town);
TownyUniverse.getDataSource().saveWorld(world);
}
} catch (TownyException e) {
// Error fetching homeblock
TownyLogger.log.warning("[Towny Error] Failed get world data for: " + town.getName());
}
else
TownyLogger.log.warning("[Towny Error] No Homeblock - Failed to detect world for: " + town.getName());
}
}
}
@Override
public void onDisable() {
System.out.println("==============================================================");
if (TownyUniverse.getDataSource() != null && error == false)
TownyUniverse.getDataSource().saveQueues();
if (error == false)
TownyWar.onDisable();
if (TownyUniverse.isWarTime())
getTownyUniverse().getWarEvent().toggleEnd();
TownyTimerHandler.toggleTownyRepeatingTimer(false);
TownyTimerHandler.toggleDailyTimer(false);
TownyTimerHandler.toggleMobRemoval(false);
TownyTimerHandler.toggleHealthRegen(false);
TownyTimerHandler.toggleTeleportWarmup(false);
TownyRegenAPI.cancelProtectionRegenTasks();
playerCache.clear();
townyUniverse = null;
System.out.println("[Towny] Version: " + version + " - Mod Disabled");
System.out.println("=============================================================");
TownyLogger.shutDown();
}
public boolean load() {
Pattern pattern = Pattern.compile("-b(\\d*?)jnks", Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(getServer().getVersion());
//TownyEconomyHandler.setupEconomy();
if (!townyUniverse.loadSettings()) {
setError(true);
//getServer().getPluginManager().disablePlugin(this);
return false;
}
setupLogger();
if (TownySettings.isBypassVersionCheck()) {
TownyLogger.log.info("[Towny] Bypassing CraftBukkit Version check.");
} else {
int bukkitVer = TownySettings.getMinBukkitVersion();
if (!matcher.find() || matcher.group(1) == null) {
error = true;
TownyLogger.log.severe("[Towny Error] Unable to read CraftBukkit Version.");
TownyLogger.log.severe("[Towny Error] Towny requires version " + bukkitVer + " or higher.");
TownyLogger.log.severe("[Towny Error] Check your CraftBukkit version or set 'bypass_version_check' to true in the Towny config.");
getServer().getPluginManager().disablePlugin(this);
return false;
}
int curBuild = Integer.parseInt(matcher.group(1));
if (curBuild < bukkitVer) {
error = true;
TownyLogger.log.severe("[Towny Error] CraftBukkit Version (" + curBuild + ") is outdated! ");
TownyLogger.log.severe("[Towny Error] Towny requires version " + bukkitVer + " or higher.");
getServer().getPluginManager().disablePlugin(this);
return false;
}
}
//Coord.setCellSize(TownySettings.getTownBlockSize());
//TownyCommand.setUniverse(townyUniverse);
checkPlugins();
SetWorldFlags();
//make sure the timers are stopped for a reset
TownyTimerHandler.toggleTownyRepeatingTimer(false);
TownyTimerHandler.toggleDailyTimer(false);
TownyTimerHandler.toggleMobRemoval(false);
TownyTimerHandler.toggleHealthRegen(false);
TownyTimerHandler.toggleTeleportWarmup(false);
//Start timers
TownyTimerHandler.toggleTownyRepeatingTimer(true);
TownyTimerHandler.toggleDailyTimer(true);
TownyTimerHandler.toggleMobRemoval(true);
TownyTimerHandler.toggleHealthRegen(TownySettings.hasHealthRegen());
TownyTimerHandler.toggleTeleportWarmup(TownySettings.getTeleportWarmupTime() > 0);
resetCache();
return true;
}
private void checkPlugins() {
List<String> using = new ArrayList<String>();
Plugin test;
if (TownySettings.isUsingPermissions()) {
test = getServer().getPluginManager().getPlugin("GroupManager");
if (test != null) {
//groupManager = (GroupManager)test;
this.getTownyUniverse().setPermissionSource(new GroupManagerSource(this, test));
using.add(String.format("%s v%s", "GroupManager", test.getDescription().getVersion()));
} else {
test = getServer().getPluginManager().getPlugin("PermissionsEx");
if (test != null) {
//permissions = (PermissionsEX)test;
getTownyUniverse().setPermissionSource(new PEXSource(this, test));
using.add(String.format("%s v%s", "PermissionsEX", test.getDescription().getVersion()));
} else {
test = getServer().getPluginManager().getPlugin("bPermissions");
if (test != null) {
//permissions = (Permissions)test;
getTownyUniverse().setPermissionSource(new bPermsSource(this, test));
using.add(String.format("%s v%s", "bPermissions", test.getDescription().getVersion()));
} else {
test = getServer().getPluginManager().getPlugin("Permissions");
if (test != null) {
//permissions = (Permissions)test;
getTownyUniverse().setPermissionSource(new Perms3Source(this, test));
using.add(String.format("%s v%s", "Permissions", test.getDescription().getVersion()));
} else {
getTownyUniverse().setPermissionSource(new BukkitPermSource(this));
using.add("BukkitPermissions");
}
}
}
}
} else {
// Not using Permissions
getTownyUniverse().setPermissionSource(new NullPermSource(this));
}
if (TownySettings.isUsingEconomy()) {
if (TownyEconomyHandler.setupEconomy())
using.add(TownyEconomyHandler.getVersion());
else
TownyMessaging.sendErrorMsg("No compatible Economy plugins found. You need iConomy 5.01, or the vault/Register.jar with any of the supported eco systems.");
}
test = getServer().getPluginManager().getPlugin("Essentials");
if (test == null)
TownySettings.setUsingEssentials(false);
else if (TownySettings.isUsingEssentials()) {
this.essentials = (Essentials) test;
using.add(String.format("%s v%s", "Essentials", test.getDescription().getVersion()));
}
test = getServer().getPluginManager().getPlugin("Questioner");
if (test == null)
TownySettings.setUsingQuestioner(false);
else if (TownySettings.isUsingQuestioner())
using.add(String.format("%s v%s", "Questioner", test.getDescription().getVersion()));
/*
* Test for Citizens2 so we can avoid removing their NPC's
*/
test = getServer().getPluginManager().getPlugin("Citizens");
if (test != null) {
citizens2 = test.getDescription().getVersion().startsWith("2");
}
if (using.size() > 0)
TownyLogger.log.info("[Towny] Using: " + StringMgmt.join(using, ", "));
}
private void registerEvents() {
final PluginManager pluginManager = getServer().getPluginManager();
if (!isError()) {
// Have War Events get launched before regular events.
pluginManager.registerEvents(townyWarBlockListener, this);
pluginManager.registerEvents(townyWarEntityListener, this);
// Manage player deaths and death payments
pluginManager.registerEvents(entityMonitorListener, this);
pluginManager.registerEvents(weatherListener, this);
pluginManager.registerEvents(townyWarCustomListener, this);
pluginManager.registerEvents(customListener, this);
pluginManager.registerEvents(worldListener, this);
}
// Always register these events.
pluginManager.registerEvents(playerListener, this);
pluginManager.registerEvents(blockListener, this);
pluginManager.registerEvents(entityListener, this);
}
private void update() {
try {
List<String> changeLog = JavaUtil.readTextFromJar("/ChangeLog.txt");
boolean display = false;
TownyLogger.log.info("------------------------------------");
TownyLogger.log.info("[Towny] ChangeLog up until v" + getVersion());
String lastVersion = TownySettings.getLastRunVersion(getVersion());
for (String line : changeLog) { //TODO: crawl from the bottom, then past from that index.
if (line.startsWith("v" + lastVersion))
display = true;
if (display && line.replaceAll(" ", "").replaceAll("\t", "").length() > 0)
TownyLogger.log.info(line);
}
TownyLogger.log.info("------------------------------------");
} catch (IOException e) {
TownyMessaging.sendDebugMsg("Could not read ChangeLog.txt");
}
TownySettings.setLastRunVersion(getVersion());
}
/**
* Fetch the TownyUniverse instance
*
* @return TownyUniverse
*/
public TownyUniverse getTownyUniverse() {
return townyUniverse;
}
public String getVersion() {
return version;
}
/**
* @return the error
*/
public boolean isError() {
return error;
}
/**
* @param error the error to set
*/
protected void setError(boolean error) {
this.error = error;
}
// is permissions active
public boolean isPermissions() {
return TownySettings.isUsingPermissions();
}
// is Essentials active
public boolean isEssentials() {
return (TownySettings.isUsingEssentials() && (this.essentials != null));
}
// is Citizens2 active
public boolean isCitizens2() {
return citizens2;
}
/**
* @return Essentials object
* @throws TownyException
*/
public Essentials getEssentials() throws TownyException {
if (essentials == null)
throw new TownyException("Essentials is not installed, or not enabled!");
else
return essentials;
}
public World getServerWorld(String name) throws NotRegisteredException {
for (World world : BukkitTools.getWorlds())
if (world.getName().equals(name))
return world;
throw new NotRegisteredException(String.format("A world called '$%s' has not been registered.", name));
}
public boolean hasCache(Player player) {
return playerCache.containsKey(player.getName().toLowerCase());
}
public void newCache(Player player) {
try {
getTownyUniverse();
playerCache.put(player.getName().toLowerCase(), new PlayerCache(TownyUniverse.getDataSource().getWorld(player.getWorld().getName()), player));
} catch (NotRegisteredException e) {
TownyMessaging.sendErrorMsg(player, "Could not create permission cache for this world (" + player.getWorld().getName() + ".");
}
}
public void deleteCache(Player player) {
deleteCache(player.getName());
}
public void deleteCache(String name) {
playerCache.remove(name.toLowerCase());
}
/**
* Fetch the current players cache
* Creates a new one, if one doesn't exist.
*
* @param player
* @return the current (or new) cache for this player.
*/
public PlayerCache getCache(Player player) {
if (!hasCache(player)) {
newCache(player);
getCache(player).setLastTownBlock(new WorldCoord(player.getWorld().getName(), Coord.parseCoord(player)));
}
return playerCache.get(player.getName().toLowerCase());
}
/**
* Resets all Online player caches, retaining their location info.
*/
public void resetCache() {
for (Player player : BukkitTools.getOnlinePlayers())
if (player != null)
getCache(player).resetAndUpdate(new WorldCoord(player.getWorld().getName(), Coord.parseCoord(player))); //Automatically resets permissions.
}
/**
* Resets all Online player caches if their location equals this one
*/
public void updateCache(WorldCoord worldCoord) {
for (Player player : BukkitTools.getOnlinePlayers())
if (player != null)
if (Coord.parseCoord(player).equals(worldCoord))
getCache(player).resetAndUpdate(worldCoord); //Automatically resets permissions.
}
/**
* Resets all Online player caches if their location has changed
*/
public void updateCache() {
WorldCoord worldCoord = null;
for (Player player : BukkitTools.getOnlinePlayers()) {
if (player != null) {
worldCoord = new WorldCoord(player.getWorld().getName(), Coord.parseCoord(player));
PlayerCache cache = getCache(player);
if (cache.getLastTownBlock() != worldCoord)
cache.resetAndUpdate(worldCoord);
}
}
}
/**
* Resets a specific players cache if their location has changed
*
* @param player
*/
public void updateCache(Player player) {
WorldCoord worldCoord = new WorldCoord(player.getWorld().getName(), Coord.parseCoord(player));
PlayerCache cache = getCache(player);
if (cache.getLastTownBlock() != worldCoord)
cache.resetAndUpdate(worldCoord);
}
/**
* Resets a specific players cache
*
* @param player
*/
public void resetCache(Player player) {
getCache(player).resetAndUpdate(new WorldCoord(player.getWorld().getName(), Coord.parseCoord(player)));
}
public void setPlayerMode(Player player, String[] modes, boolean notify) {
if (player == null)
return;
try {
Resident resident = TownyUniverse.getDataSource().getResident(player.getName());
resident.setModes(modes, notify);
} catch (NotRegisteredException e) {
// Resident doesn't exist
}
}
/**
* Remove ALL current modes (and set the defaults)
*
* @param player
*/
public void removePlayerMode(Player player) {
try {
Resident resident = TownyUniverse.getDataSource().getResident(player.getName());
resident.clearModes();
} catch (NotRegisteredException e) {
// Resident doesn't exist
}
}
/**
* Fetch a list of all the players current modes.
*
* @param player
* @return list of modes
*/
public List<String> getPlayerMode(Player player) {
return getPlayerMode(player.getName());
}
public List<String> getPlayerMode(String name) {
try {
Resident resident = TownyUniverse.getDataSource().getResident(name);
return resident.getModes();
} catch (NotRegisteredException e) {
// Resident doesn't exist
return null;
}
}
/**
* Check if the player has a specific mode.
*
* @param player
* @param mode
* @return true if the mode is present.
*/
public boolean hasPlayerMode(Player player, String mode) {
return hasPlayerMode(player.getName(), mode);
}
public boolean hasPlayerMode(String name, String mode) {
try {
Resident resident = TownyUniverse.getDataSource().getResident(name);
return resident.hasMode(mode);
} catch (NotRegisteredException e) {
// Resident doesn't exist
return false;
}
}
public String getConfigPath() {
return getDataFolder().getPath() + FileMgmt.fileSeparator() + "settings" + FileMgmt.fileSeparator() + "config.yml";
}
public Object getSetting(String root) {
return TownySettings.getProperty(root);
}
public void log(String msg) {
if (TownySettings.isLogging())
TownyLogger.log.info(ChatColor.stripColor(msg));
}
public void setupLogger() {
TownyLogger.setup(getTownyUniverse().getRootFolder(), TownySettings.isAppendingToLog());
}
public void appendQuestion(Questioner questioner, Question question) throws Exception {
for (Option option : question.getOptions())
if (option.getReaction() instanceof TownyQuestionTask)
((TownyQuestionTask) option.getReaction()).setTowny(this);
questioner.appendQuestion(question);
}
public boolean parseOnOff(String s) throws Exception {
if (s.equalsIgnoreCase("on"))
return true;
else if (s.equalsIgnoreCase("off"))
return false;
else
throw new Exception(String.format(TownySettings.getLangString("msg_err_invalid_input"), " on/off."));
}
}
<file_sep>package com.palmergames.bukkit.towny.questioner;
import com.palmergames.bukkit.towny.object.Town;
public class TownQuestionTask extends TownyQuestionTask {
protected Town town;
public TownQuestionTask(Town town) {
this.town = town;
}
public Town getTown() {
return town;
}
@Override
public void run() {
}
}
<file_sep>package com.palmergames.bukkit.towny.tasks;
import java.util.TimerTask;
import com.palmergames.bukkit.towny.exceptions.NotRegisteredException;
import com.palmergames.bukkit.towny.object.TownyUniverse;
import com.palmergames.bukkit.towny.permissions.PermissionNodes;
import com.palmergames.bukkit.util.BukkitTools;
/**
* @author ElgarL
*
*/
public class SetDefaultModes extends TimerTask {
protected String name;
protected boolean notify;
public SetDefaultModes(String name, boolean notify) {
this.name = name;
this.notify = notify;
}
@Override
public void run() {
// Is the player still available
if (!BukkitTools.isOnline(name))
return;
//setup default modes
String[] modes = TownyUniverse.getPermissionSource().getPlayerPermissionStringNode(name, PermissionNodes.TOWNY_DEFAULT_MODES.getNode()).split(",");
try {
TownyUniverse.getDataSource().getResident(name).setModes(modes, notify);
} catch (NotRegisteredException e) {
// No resident by this name.
}
}
}<file_sep><?xml version="1.0" encoding="UTF-8"?>
<project name="Towny" default="jar" basedir=".">
<property name="build" value="bin"/>
<property file="build.properties"/>
<target name="clean">
<delete dir="${build}"/>
</target>
<target name="init" depends="clean">
<mkdir dir="${build}"/>
</target>
<target name="compile" depends="init">
<!-- Compile the java code -->
<javac srcdir="src" destdir="${build}" includeantruntime="false" target="1.6" source="1.6">
<classpath>
<pathelement location="${env.LIB}/bukkit.jar"/>
<pathelement location="${env.LIB}/bpermissions.jar"/>
<pathelement location="${env.LIB}/Essentials.jar"/>
<pathelement location="${env.LIB}/EssentialsGroupManager.jar"/>
<pathelement location="${env.LIB}/Permissions.jar"/>
<pathelement location="${env.LIB}/PermissionsEx.jar"/>
<pathelement location="${env.LIB}/PermissionsBukkit.jar"/>
<pathelement location="${env.LIB}/Register.jar"/>
<pathelement location="${env.LIB}/iConomy.jar"/>
<pathelement location="${env.LIB}/Questioner.jar"/>
<pathelement location="${env.LIB}/Vault.jar"/>
<pathelement location="${env.LIB}/citizensapi-2.0-SNAPSHOT.jar"/>
</classpath>
</javac>
</target>
<target name="jar" depends="compile">
<!-- Build the jar file -->
<jar basedir="${build}" destfile="${env.LIB}/Towny.jar">
<fileset dir="./src" includes="ChangeLog.txt"/>
<fileset dir="./src" includes="english.yml"/>
<fileset dir="./src" includes="german.yml"/>
<fileset dir="./src" includes="plugin.yml"/>
<fileset dir="./src" includes="spanish.yml"/>
<fileset dir="./src" includes="townyperms.yml"/>
<fileset dir="./src" includes="ToDo.txt"/>
</jar>
</target>
</project> | c3648c4c40ad81060b4d0d719bd1c52f9695a371 | [
"Java",
"Ant Build System"
]
| 7 | Java | Zren/Towny-ElgarL | e7691c80588cd85e1b2eb4273bc65162f71c667e | 110e5b51af8c8788e23acae72c1d50391a6653c5 | |
refs/heads/master | <repo_name>loqtaApp/loqtaOrders<file_sep>/getProductByCustomerOrOrder.php
<?php
include 'slackWebHooks/settings.php';
$data = json_decode($data, true);
$ordersURL = "{$mainURLEndPoint}/orders.json";
$customersURL = "{$mainURLEndPoint}/customers/search.json?query=";
$palpayOauthKey = '83c9c662d05a81a4b58d009fe792e7e953e26a6b';
$currentTime = date("Y/m/d h:i");
$dateToVeirifyToken = date("d/m/y H");
$tokenToVeirify = md5($palpayOauthKey . $dateToVeirifyToken);
//die($tokenToVeirify);
//die(sha1(uniqid("palpay_operations", true)));
$token = $_GET['tt'];
$retriveKeyValue = ($_GET['idd']);
$actionKey = ($_GET['pay']);
$point_of_sale = ($_GET['pos']);
$payment_amount = ($_GET['amount']);
$orderFilterUnPaidOnlyStatus = ($_GET['unpaid']);
$payment_method = ($_GET['method']) ? $_GET['method'] : 'palpay';
$payment_currency = ($_GET['currency']) ? $_GET['currency'] : 'ILS';
define('PAY_ACTION', 1);
define('CANCEL_ACTION', 2);
define('PALPAY_PAID_TAG', 'PAID_PAL_PAY');
define('PALPAY_TAG', PALPAY_PAID_TAG . ', ' . strtoupper($payment_currency));
$palpay_note = '"';
$palpay_note .= 'تم دفع';
$palpay_note .= "($payment_amount)";
$palpay_note .= "بعملة ال";
$palpay_note .= "($payment_currency)";
$palpay_note .= 'بتاريخ ';
$palpay_note .= "($currentTime)";
$palpay_note .= '"';
$payment_inc_amount['palpay'] = 2;
define('PALPAY_ORDER_NOTE', $palpay_note);
define('PALPAY_ORDER_CANCEL_NOTE', ' تم إلغاء دفع هذا الطلب بواسطة بال بي');
$ordersFilter = "financial_status=pending&fulfillment_status=unshipped&name=";
$note_palpay_elements[] = array('name' => 'palpay_paid_date', 'value' => date("Y/m/d h:i"));
$note_palpay_elements[] = array('name' => 'palpay_pos', 'value' => $point_of_sale);
$note_palpay_elements[] = array('name' => 'payment_amount', 'value' => $payment_amount);
$note_palpay_elements[] = array('name' => 'payment_currency', 'value' => $payment_currency);
//operations
define('LOOK_FOR_CUSTOMER_VIA_CUSTOMER_ID', 1);
define('LOOK_FOR_ORDER_VIA_CUSTOMER_ID', 2);
define('LOOK_FOR_ORDER_VIA_ORDER_ID', 3);
define('PAY_ORDER_VIA_ORDER_ID', 4);
define('CANCEL_ORDER_VIA_ORDER_ID', 5);
$resultMessages = array();
$resultOperations = array();
$resultOrders = array();
$resultStatus = true;
$customerInformation = array();
if ($tokenToVeirify != $token) {
$resultStatus = false;
$resultMessages[] = 'Invalid Token, Or Expired Token';
}
if ($resultStatus) {
function estimateOrderPriceInUSD($order) {
$exRate = $order['total_price'] / $order['total_price_usd'];
return $order["subtotal_price"] / $exRate;
}
function getOrdersByLink($link) {
$request = new Request($link);
$response = $request->execute();
if (array_key_exists('orders', $response) && sizeof($response["orders"]) > 0) {
return $response['orders'];
} else {
//no orders found
return false;
}
}
function filterOrders($order) {
global $orderFilterUnPaidOnlyStatus;
if ($orderFilterUnPaidOnlyStatus == true) {
if (array_key_exists('tags', $order) && $order['tags'] != '') {
if (strstr($order['tags'], PALPAY_PAID_TAG)) {
return false;
} else {
return true;
}
} else {
return true;
}
} else {
return true;
}
}
function getTransactionFees($price) {
global $payment_inc_amount, $payment_method;
return ceil(($payment_inc_amount[$payment_method] * $price) / 100);
}
function getTheFinalPriceWithTransactionFees($price) {
return ceil(getTransactionFees($price) + $price);
}
function getPreparedOrderInformation($order) {
global $payment_inc_amount, $payment_method;
$preparedOrder['order_number'] = $order['order_number'];
$preparedOrder["subtotal_price"] = getTheFinalPriceWithTransactionFees($order["subtotal_price"]);
$preparedOrder["subtotal_price_usd"] = estimateOrderPriceInUSD($order);
$itemTitles = '';
$itemsSize = sizeof($order["line_items"]);
$i = 0;
foreach ($order["line_items"] as $lineItem) {
$itemTitles .= $lineItem['title'] . ' - ' . $lineItem['price'] . ((($itemsSize - 1) == $i) ? '' : ',');
$i++;
}
$itemTitles .= "+ Transfer Price (" . getTransactionFees($order["subtotal_price"]) . ")";
$preparedOrder["line_items"] = $itemTitles;
$preparedOrder["address"] = ($order['shipping_address']["address1"] != null) ? $order['shipping_address']["address1"] : $order['customer']["default_address"]["address1"];
return $preparedOrder;
}
function updateOrderPalPay($requestedOrder, $postNoteData) {
global $mainURLEndPoint;
$shopifyParamsURL = $mainURLEndPoint . '/orders/' . $requestedOrder['id'] . ".json";
$request = new Request($shopifyParamsURL);
$request->setURL($shopifyParamsURL);
$request->setHeaders(array(
'Content-Type:application/json'
));
$request->setData(json_encode($postNoteData));
$request->setMethod('PUT');
return $request->execute();
}
$numberOfDigits = strlen((string) $retriveKeyValue);
if ($numberOfDigits < 8) {
//get orders path
$params = "?" . $ordersFilter . $retriveKeyValue;
$requestedOrder = '';
$orders = getOrdersByLink($ordersURL . $params);
foreach ($orders as $order) {
if ($order['order_number'] == $retriveKeyValue) {
$requestedOrder = $order;
}
}
if (!$orders || $requestedOrder == '' || !(filterOrders($requestedOrder))) {
$resultOperations[] = array(LOOK_FOR_ORDER_VIA_ORDER_ID => false);
$resultMessages[] = 'No orders found for "' . $retriveKeyValue . '"';
$resultStatus = false;
} else {
$resultOperations[] = array(LOOK_FOR_ORDER_VIA_ORDER_ID => true);
$resultMessages[] = 'Order found with number "' . $retriveKeyValue . '"';
$customerInformation["first_name"] = $orders[0]['customer']["first_name"];
$customerInformation["last_name"] = $orders[0]['customer']["last_name"];
$customerInformation["phone"] = ($orders[0]['customer']["phone"] != null) ? $orders[0]['customer']["phone"] : $orders[0]['customer']["default_address"]["phone"];
$preparedOrder = getPreparedOrderInformation($requestedOrder);
$resultOrders = [$preparedOrder];
if ($actionKey == PAY_ACTION) {
$postNoteData['order']['id'] = $requestedOrder['id'];
$postNoteData['order']['tags'] = (array_key_exists('tags', $requestedOrder) && $requestedOrder['tags'] != '') ? $requestedOrder['tags'] . ', ' . PALPAY_TAG : PALPAY_TAG;
if (array_key_exists('note_attributes', $requestedOrder) && is_array($requestedOrder['note_attributes'])) {
$note_attributes = $requestedOrder['note_attributes'];
foreach ($note_palpay_elements as $note_palpay_element)
array_push($note_attributes, $note_palpay_element);
} else {
$note_attributes = $note_palpay_elements;
}
$postNoteData ['order']['note_attributes'] = $note_attributes;
$postNoteData ['order']['note'] = (array_key_exists('note', $requestedOrder) && $requestedOrder['note'] != '') ? $requestedOrder['note'] . ' ' . PALPAY_ORDER_NOTE : PALPAY_ORDER_NOTE;
$shopifyResponse = updateOrderPalPay($requestedOrder, $postNoteData);
if (array_key_exists('order', $shopifyResponse) && $shopifyResponse['order']['id']) {
$resultMessages[] = 'Order "' . $retriveKeyValue . '" was paid Successfully';
$resultOperations[] = array(PAY_ORDER_VIA_ORDER_ID => true);
} else {
$resultMessages[] = 'Error in pay order "' . $retriveKeyValue . '"';
$resultOperations[] = array(PAY_ORDER_VIA_ORDER_ID => false);
$resultStatus = false;
}
}
if ($actionKey == CANCEL_ACTION) {
$tagsStr = str_replace(', ' . PALPAY_TAG, "", $requestedOrder['tags']);
$tagsStr = str_replace(PALPAY_TAG, "", $tagsStr);
$postNoteData['order']['tags'] = $tagsStr;
$postNoteData['order']['note'] = $requestedOrder['note'] . ' ' . PALPAY_ORDER_CANCEL_NOTE;
$note_attributes = $requestedOrder['note_attributes'];
array_push($note_attributes, array('name' => 'palpay_cancel_date', 'value' => date("Y/m/d h:i")));
$postNoteData['order']['note_attributes'] = $note_attributes;
$shopifyResponse = updateOrderPalPay($requestedOrder, $postNoteData);
if (array_key_exists('order', $shopifyResponse) && $shopifyResponse['order']['id']) {
$resultMessages[] = 'Order "' . $retriveKeyValue . '" was canceled Successfully';
$resultOperations[] = array(CANCEL_ORDER_VIA_ORDER_ID => true);
} else {
$resultMessages[] = 'Error in cancel order "' . $retriveKeyValue . '"';
$resultOperations[] = array(CANCEL_ORDER_VIA_ORDER_ID => false);
$resultStatus = false;
}
}
}
} else {
$email = $retriveKeyValue . '@loqta.ps';
$requestedCustomer = '';
//get customer path
$customersURL = $customersURL . 'email:' . $email . ';fields=id,email,phone';
$request = new Request($customersURL);
$response = $request->execute();
if (array_key_exists('customers', $response) && sizeof($response["customers"]) > 0) {
$resultOperations[] = array(LOOK_FOR_CUSTOMER_VIA_CUSTOMER_ID => true);
$customers = $response["customers"];
foreach ($customers as $customer) {
if ($customer['email'] == $email) {
$requestedCustomer = $customer;
}
}
$customerID = $requestedCustomer["id"];
$orders = getOrdersByLink($ordersURL . "?customer_id=" . $customerID . '&' . $ordersFilter);
if (!$orders || $requestedCustomer == '') {
$resultOperations[] = array(LOOK_FOR_ORDER_VIA_CUSTOMER_ID => false);
$resultMessages[] = 'No orders found for customer "' . $customer['first_name'] . '"';
} else {
$resultOperations[] = array(LOOK_FOR_ORDER_VIA_CUSTOMER_ID => true);
$preparedOrders = [];
foreach ($orders as $order) {
if (filterOrders($order)) {
$preparedOrders[] = getPreparedOrderInformation($order);
}
}
$customerInformation["first_name"] = $orders[0]['customer']["first_name"];
$customerInformation["last_name"] = $orders[0]['customer']["last_name"];
$customerInformation["phone"] = ($orders[0]['customer']["phone"] != null) ? $orders[0]['customer']["phone"] : $orders[0]['customer']["default_address"]["phone"];
$resultOrders = ($preparedOrders);
}
} else {
$resultOperations[] = array(LOOK_FOR_CUSTOMER_VIA_CUSTOMER_ID => false);
//no customer found
$resultMessages[] = 'No customer name found for id "' . $retriveKeyValue . '"';
}
}
}
$result_of_operations = array(
'orders' => $resultOrders,
'customer' => $customerInformation,
'operations' => $resultOperations,
'status' => $resultStatus,
'messages' => $resultMessages
);
die(json_encode($result_of_operations));
<file_sep>/firebase/nbproject/private/private.properties
index.file=index.php
url=http://localhost/firebase/
<file_sep>/slackWebHooks/request.php
<?php
class Request {
protected $url;
protected $headers = array();
protected $data = array();
protected $filePointer;
protected $method = 'GET';
protected $timeOut = 15;
function __construct($url, $method = 'GET', $data = NULL) {
$this->url = $url;
$this->data = $data;
$this->method = $method;
$this->headers = array(
'Accept: application/json',
'Content-Type: application/json',
);
}
public function setURL($url) {
$this->url = $url;
}
public function setData($data) {
$this->data = $data;
}
public function setMethod($method) {
$this->method = $method;
}
public function putHeaders(&$ch) {
$fp = fopen('php://temp/maxmemory:256000', 'w');
if (!$fp) {
die('could not open temp memory data');
}
fwrite($fp, $this->data);
fseek($fp, 0);
curl_setopt($ch, CURLOPT_PUT, 1);
curl_setopt($ch, CURLOPT_INFILE, $fp); // file pointer
curl_setopt($ch, CURLOPT_INFILESIZE, strlen($this->data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
}
public function getHeaders(&$ch) {
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
}
public function postHeaders(&$ch) {
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $this->data);
}
public function deleteHeaders(&$ch) {
}
public function setHeaders($headers) {
$this->headers = $headers;
}
public function execute($arrayResponse = true) {
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $this->url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $this->headers);
$methodHeaders = (strtolower($this->method) . 'Headers');
$this->$methodHeaders($ch);
curl_setopt($ch, CURLOPT_TIMEOUT, $this->timeOut);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 2);
$xml_response = curl_exec($ch);
curl_close($ch);
return ($arrayResponse) ? json_decode($xml_response, true) : $xml_response;
}
}
<file_sep>/firebase/index.php
<?php
header('Content-Type: application/json');
//initialize request to create order with wehook
require __DIR__.'/vendor/autoload.php';
use Kreait\Firebase\Factory;
use Kreait\Firebase\ServiceAccount;
use Kreait\Auth;
$data = json_decode(file_get_contents('php://input'),true);
$mainURL = "https://f3aa0d6659405ab34f9c0af85d0f2ef9:590b142f0e9922bd187703cd6729bae8@<EMAIL>.myshopify.com/admin/customers/".$data['customer']['id']."/metafields.json";
/***
* initliaze request
*/
$headers = array(
'Content-Type:application/json'
);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $mainURL);
curl_setopt($ch, CURLOPT_GET, true);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
$result = curl_exec($ch);
$serviceAccount = ServiceAccount::fromJsonFile(__DIR__.'/google-services.json');
$firebase = (new Factory)
->withServiceAccount($serviceAccount)
->create();
$auth = $firebase->getAuth();
try{
$users = $auth->createUserWithEmailAndPassword($data['customer']['email'], $result['metafields']['value']);
echo "true";
}catch(Exception $e){
echo "fasle";
}
?>
<file_sep>/slackWebHooks/slackwebhook.php
<?php
define('NO_DEFAULT_HEADERS', true);
include 'settings.php';
//initialize request to create order with wehook
//define the link for the slack (out Gaza or in Gaza)
$outGazaCarrier = 'CarrierMohammed';
date_default_timezone_set("Asia/Gaza");
$todayDate = date("Y/m/d h:i");
$orderStatus = '';
$orderNoteForShortLink = 'shortAdminLink';
$orderNoteShortLinkValue = '';
//////////////////slack
$channel = 'C6LB5HXD0';
$cancelledChannelID = 'C7WUEGNHG';
$text = '';
$fullfilledStatusReaction = ':racing_motorcycle:';
$paidStatusReaction = ':100:';
$cancelStatusReaction = ':x:';
$slackMSGTS = 'slack_message_id';
$token = base64_decode("<KEY>);
$tsMSG_ID = '';
$postMessageEndPoint = "https://slack.com/api/chat.postMessage";
$updatePostMessageEndPoint = "https://slack.com/api/chat.update";
//////////////////slack
//define the client source if possible
//https://github.com/firebase/quickstart-js/tree/master/messaging
$data = json_decode($data, true);
/// Get all the order information
$request = new Request($mainURLEndPoint . '/orders.json?ids=' . $data['id'] . '&status=any');
$response = $request->execute();
$order = $response['orders'][0];
//////////////////
$pushNotificationToken = '';
$pushNotificationTokenAttribute = 'PushNotificationToken';
$fcmEndPoint = "https://fcm.googleapis.com/fcm/send";
$webAPIKey = base64_decode('<KEY>');
$fullFillmentPushMSGTitle = ' تم خروج طلب رقم';
$fullFillmentPushMSGTitle .= $order['name'];
$fullFillmentPushMSGBody .= 'شكرا لتعاملك معنا';
$paidStatusPushMSGTitle = ' تم تأكيد إستلام طلب رقم';
$paidStatusPushMSGTitle .= $order['name'];
$paidStatusPushMSGBody = 'شكرا لتعاملك معنا';
$cancelPushMSGTitle = ' تم إلغاء طلب رقم';
$cancelPushMSGTitle .= $order['name'];
$cancelPushMSGBody .= 'شكرا لتعاملك معنا';
//////////////////
//
// check the order params
$notesArray = array();
foreach ($order['note_attributes'] as $note) {
//push notification token
$notesArray[$note['name']] = $note['value'];
if ($note['name'] == $pushNotificationTokenAttribute) {
$pushNotificationToken = $note['value'];
}
if ($note['name'] == $slackMSGTS) {
$tsMSG_ID = $note['value'];
}
if($note['name'] == $orderNoteForShortLink){
$orderNoteShortLinkValue = $note['value'];
}
}
$order = $response['orders'][0];
// google short link
if($orderNoteShortLinkValue == ''){
$request = new Request($googleShortURL);
$request->setMethod('POST');
$request->setData(json_encode(array('longUrl' => $shopifyOrderLink.$order['id'])));
$response = $request->execute();
$orderNoteShortLinkValue = $response['id'];
$notesArray[$orderNoteForShortLink] = $response['id'];
}
$slackMSG = $order['name'];
//define the action the we want to do (Reply, Post Message ..etc)
//if fullfilled
if (strpos(strtolower($order['tags']), strtolower($outGazaCarrier)) !== FALSE) {
//out side gaza channel
$channel = 'C6KU0SLSV';
}
if ($order['cancelled_at'] != '') { //update existing
$slackMSG = $slackMSG . ' ' . $cancelStatusReaction;
if ($pushNotificationToken != '') {
//send Push Notification
$notificationArr = Array(
'notification' => array(
'title' => $cancelPushMSGTitle,
'body' => $cancelPushMSGBody,
),
'to' => $pushNotificationToken,
);
}
$orderStatus = 'ملغي';
} elseif ($order['financial_status'] == 'paid') { //update existing
$slackMSG = $slackMSG . ' ' . $paidStatusReaction;
if ($pushNotificationToken != '') {
//send Push Notification
$notificationArr = Array(
'notification' => array(
'title' => $paidStatusPushMSGTitle,
'body' => $paidStatusPushMSGBody
),
'to' => $pushNotificationToken,
);
}
$orderStatus = 'مدفوع';
} elseif ($order['fulfillment_status'] == 'fulfilled') {
//get the carriar information
$orderStatus = 'شحن';
$slackMSG = $slackMSG . ' ' . $fullfilledStatusReaction;
//in Gaza channel
//get the total information
if ($pushNotificationToken != '') {
//send Push Notification
$notificationArr = Array(
'notification' => array(
'title' => $fullFillmentPushMSGTitle,
'body' => $fullFillmentPushMSGBody,
),
'to' => $pushNotificationToken,
);
}
}
$slackMSG.= ' ('.$order['customer']['first_name']. ' ' .$order['customer']['last_name'].')';
$slackMSG.= ' - ('.$order['subtotal_price'].')';
if($order['note'] != ''){
$slackMSG.= ' -- '.$order['note'];
}
if($orderNoteShortLinkValue != ''){
$slackMSG.= ' - '.$orderNoteShortLinkValue;
}
/**/
$slackMSGRequestArray = array();
$slackMSGRequestArray['channel'] = $channel;
$slackMSGRequestArray['text'] = $slackMSG;
$request->setHeaders(array(
'Content-Type:application/json',
'Authorization:Bearer ' . $token
));
$request->setMethod('POST');
if ($tsMSG_ID != '') { //slack message before
$slackMSGRequestArray['ts'] = $tsMSG_ID;
$request->setURL($updatePostMessageEndPoint);
$request->setData(json_encode($slackMSGRequestArray));
$slackResponse = $request->execute();
if(!array_key_exists('ts', $slackResponse)){
$tsMSG_ID = '';
}
}
if($tsMSG_ID == ''){//new slack message
if($orderStatus == 'ملغي'){
$slackMSGRequestArray['channel'] = $cancelledChannelID;
$channel = $cancelledChannelID;
}
$request->setURL($postMessageEndPoint);
$request->setData(json_encode($slackMSGRequestArray));
$slackResponse = $request->execute();
$tsMSG_ID = $slackResponse['ts'];
if($tsMSG_ID != ''){
$notesArray[$slackMSGTS] = $tsMSG_ID;
//we must update the order with the new message ID
$postNoteData ['order']['id'] = $order['id'];
$postNoteData ['order']['note_attributes'] = $notesArray;
$shopifyParamsURL = $mainURLEndPoint . '/orders/' . $order['id'] . ".json";
$request->setURL($shopifyParamsURL);
$request->setHeaders(array(
'Content-Type:application/json'
));
$request->setData(json_encode($postNoteData));
$request->setMethod('PUT');
$shopifyResponse = $request->execute();
}
}
//second message for the order status and date change
if($tsMSG_ID != ''){
$slackMSGRequestArray = array();
$slackMSGRequestArray['channel'] = $channel;
$slackMSGRequestArray['text'] = $orderStatus . ' - ' . $todayDate;
$slackMSGRequestArray['thread_ts'] = $tsMSG_ID;
$request->setHeaders(array(
'Content-Type:application/json',
'Authorization:Bearer ' . $token
));
$request->setMethod('POST');
$request->setURL($postMessageEndPoint);
$request->setData(json_encode($slackMSGRequestArray));
$request->execute();
}
// push notification to the client
if ($pushNotificationToken != '' && sizeof($notificationArr) > 0) {
//send the push notification
$request->setURL($fcmEndPoint);
$request->setHeaders(array(
'Content-Type:application/json',
'Authorization:key= ' . $webAPIKey,
'Host:fcm.googleapis.com'
));
$request->setData(json_encode($notificationArr));
$request->setMethod('POST');
$response = $request->execute();
}
//Post the message order with motocycle reaction
//if the order contain token for FCM
//Post fCM message about fullfillmenet status
//Post the message order
//Get channel, Messaage Ts
//Save it in the same order info
/*
$postNoteData ['order']['id'] = $postOrderInfo['Order_ID'];
$postNoteData ['order']['note_attributes']['slack_message_id'] = $rowData[0];
$shopifyParamsURL = $mainURLEndPoint.$orderDataSet['id'] . ".json";
*/
/***thread_ts
* initliaze request
$headers = array(
'Content-Type:application/json',
'Authorization:Bearer '.$token
);
$request = new Request($postMessageEndPoint, 'POST', $data);
$request->setHeaders($headers);
$result = $request->execute(false);
echo $result;
/**
* thread_ts
* {"channel":"C5R3RPLQZ","text":"I hope the tour went well, <NAME>.","attachments":[{"text":"Who wins the lifetime supply of chocolate?","fallback":"You could be telling the computer exactly what it can do with a lifetime supply of chocolate.","color":"#3AA3E3","attachment_type":"default","callback_id":"select_simple_1234","actions":[{"name":"winners_list","text":"Who should win?","type":"select","data_source":"users"}]}]}
*/<file_sep>/slackWebHooks/setOrderMetaFields.php
<?php
include 'settings.php';
$data = json_decode($data, true);
$mainURL = $mainURLEndPoint . "/orders/" . $data['id'] . "/metafields.json";
if ($data['cancelled_at'] != '') {
$key = 'cancel_date';
}
elseif ($data['financial_status'] == 'paid') {
$key = 'paid_date';
}
elseif ($data['fulfillment_status'] == 'fulfilled') {
$key = 'fulfill_date';
}
$metaFieldData = array(
'metafield' => array(
'namespace' => 'operations',
'key' => $key,
'value' => date('d/m/Y h:i:s A'),
"value_type" => "string"
)
);
$headers = array(
'Content-Type:application/json'
);
$request = new Request($mainURL, 'POST', json_encode($metaFieldData));
$request->setHeaders($headers);
$result = $request->execute(false);
echo $result;
<file_sep>/paid.php
<?php
header('Access-Control-Allow-Origin: *');
header('Access-Control-Allow-Methods: POST,OPTIONS');
header('Cache-Control: no-cache');
header('Pragma: no-cache');
//(0);
session_start();
require_once __DIR__ . '/lib/google/vendor/autoload.php';
define('OAUTH2_CLIENT_ID', '1089990018340-frdjldsicdgrbn7r637b63brstqj0fie.apps.googleusercontent.com');
define('OAUTH2_CLIENT_SECRET', '<KEY>');
$key = file_get_contents('token.txt');
$data = json_decode(file_get_contents('php://input'), true);
// Client init
$client = new Google_Client();
$client->setClientId(OAUTH2_CLIENT_ID);
$client->setAccessType('offline');
$client->setApprovalPrompt('force');
$client->setAccessToken($key);
$client->setClientSecret(OAUTH2_CLIENT_SECRET);
/**
* Check to see if our access token has expired. If so, get a new one and save it to file for future use.
*/
if ($client->isAccessTokenExpired()) {
$newToken = json_encode($client->getAccessToken());
$client->refreshToken($newToken->refresh_token);
file_put_contents('token.txt', json_encode($client->getAccessToken()));
}
$client->setScopes('https://www.googleapis.com/auth/spreadsheets');
// Define an object that will be used to make all API requests.
// Check if an auth token exists for the required scopes
$tokenSessionKey = 'token-' . $client->prepareScopes();
if (isset($_SESSION[$tokenSessionKey])) {
$client->setAccessToken($_SESSION[$tokenSessionKey]);
}
if ($client->getAccessToken()) {
$spreadsheetId = '1v0gHqEXScAqnBg9hudpGfINGyKVQUnS--Co0UVgfBkc';
$rowData = array();
/// read from Excel ordersbeforePaid sheet to get the custom data
$service = new Google_Service_Sheets($client);
$orderfoundFlag = false;
//check if in orders sheet
if (!$orderfoundFlag) {
$range = 'fullfilled!A:E';
$response = $service->spreadsheets_values->get($spreadsheetId, $range);
$values = $response->getValues();
$count = count($values) - 1;
$i = '';
if (count($values) == 0) {
} else {
for ($i = $count; $i >= 0; $i --) {
// Print columns A and E, which correspond to indices 0 and 4.
if ($values[$i][1] == $data['name']) {
$rowData = $values[$i];
break;
}
}
}
if (sizeof($rowData) > 0) {
///Delete the order from Orders Sheet and store it in Fullfilled sheet
$orderfoundFlag = true;
$requests[] = new Google_Service_Sheets_Request(array(
'deleteDimension' => array('range' => array(
'sheetId' => 497374135,
'dimension' => "ROWS",
'startIndex' => $i,
'endIndex' => ($i + 1),
)
)));
// Add additional requests (operations) ...
$batchUpdateRequest = new Google_Service_Sheets_BatchUpdateSpreadsheetRequest(array(
'requests' => $requests
));
$response = $service->spreadsheets->batchUpdate($spreadsheetId, $batchUpdateRequest);
}
} elseif (!$orderfoundFlag) {
//check if in orders sheet
$range = 'orders!A:E';
$response = $service->spreadsheets_values->get($spreadsheetId, $range);
$values = $response->getValues();
$count = count($values) - 1;
$i = '';
if (count($values) == 0) {
} else {
for ($i = $count; $i >= 0; $i --) {
// Print columns A and E, which correspond to indices 0 and 4.
if ($values[$i][1] == $data['name']) {
$rowData = $values[$i];
break;
}
}
}
if (sizeof($rowData) > 0) {
///Delete the order from Orders Sheet and store it in Fullfilled sheet
$orderfoundFlag = true;
$requests[] = new Google_Service_Sheets_Request(array(
'deleteDimension' => array('range' => array(
'sheetId' => 0,
'dimension' => "ROWS",
'startIndex' => $i,
'endIndex' => ($i + 1),
)
)));
// Add additional requests (operations) ...
$batchUpdateRequest = new Google_Service_Sheets_BatchUpdateSpreadsheetRequest(array(
'requests' => $requests
));
$response = $service->spreadsheets->batchUpdate($spreadsheetId, $batchUpdateRequest);
}
}
/////write on excel
$values = array(
array(
$data['id'],
$data['name'],
$data['customer']['first_name'] . ' ' . $data['customer']['last_name'],
$data['created_at'],
$data['total_price']
// Cell values ...
),
// Additional rows ...
);
$range = 'paid!A2:E';
$body = new Google_Service_Sheets_ValueRange(array(
'values' => $values
));
$params = array(
'valueInputOption' => "RAW"
);
$result = $service->spreadsheets_values->append($spreadsheetId, $range, $body, $params);
} elseif (OAUTH2_CLIENT_ID == 'REPLACE_ME') {
$OAUTH2_CLIENT_ID = OAUTH2_CLIENT_ID;
$htmlBody = <<<END
<h3>Client Credentials Required</h3>
<p>
You need to set <code>\$OAUTH2_CLIENT_ID</code> and
<code>\$OAUTH2_CLIENT_ID</code> before proceeding.
<p>
END;
} else {
// If the user hasn't authorized the app, initiate the OAuth flow
$state = mt_rand();
$client->setState($state);
$_SESSION['state'] = $state;
$authUrl = $client->createAuthUrl();
$htmlBody = <<<END
<h3>Authorization Required</h3>
<p>You need to <a href="$authUrl">authorize access</a> before proceeding.<p>
END;
}
?>
<file_sep>/loqtaSendContactEmail.php
<?php
header('Content-Type: application/json');
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
//die(sha1(uniqid("loqta_sendContactEmail", true)));
//f1e1f4421d57660a7fa3bafe7c569eb41899314c
include 'lib/phpmailer/PHPMailerAutoload.php';
error_reporting(0);
$loqtaContactKey = "f1e1f4421d57660a7fa3bafe7c569eb41899314c";
$dateToVeirifyToken = date("d/m/y H");
$tokenToVeirify = base64_encode($loqtaContactKey . $dateToVeirifyToken);
$token = $_GET['tt'];
$name = ($_POST['name']) ? $_POST['name'] : '';
$region = ($_POST['region']) ? $_POST['region'] : '';
$mobileNumber = ($_POST['mobileNumber']) ? $_POST['mobileNumber'] : '';
$email = ($_POST['email']) ? $_POST['email'] : '';
$reason = ($_POST['reason']) ? $_POST['reason'] : '';
$subject = ($_POST['subject']) ? $_POST['subject'] : '';
$message = ($_POST['message']) ? $_POST['message'] : '';
$subjectToSend = "Loqta Subject: ".$subject;
$formatedMessageToSend = "Name:".$name." <br><br> Region:".$region." <br><br> Mobile Number:".$mobileNumber." <br><br> Email:".$email."<br><br> Reason:".$reason."<br><br> Message:".$message." ";
if ($tokenToVeirify != $token) {
$resultStatus = false;
$resultMessages['status'] = false;
$resultMessages['message'] = 'Invalid Token, Or Expired Token';
echo json_encode($resultMessages);
}else{
//Load Composer's autoloader
$mail = new PHPMailer(); // Passing `true` enables exceptions
try {
// $mail->IsSMTP(); // telling the class to use SMTP
$mail->SMTPDebug = 0; // enables SMTP debug information (for testing)
// 1 = errors and messages
// 2 = messages only
$mail->SMTPAuth = true; // enable SMTP authentication
$mail->SMTPSecure = "tls";
$mail->Host = "smtp.gmail.com"; // SMTP server
$mail->Port = 587; // SMTP port
$mail->Username = "<EMAIL>"; // username
$passord = <PASSWORD>";
$mail->Password = $<PASSWORD>; // password
$mail->SetFrom('<EMAIL>', 'Loqta');
$mail->Subject = $subjectToSend;
$mail->MsgHTML($formatedMessageToSend);
//Recipients
$mail->AddAddress('<EMAIL>'); // Add a recipient
$mail->AddCC('<EMAIL>');
$mail->isHTML(true); // Set email format to HTML
$mail->Body = $formatedMessageToSend;
if($mail->send()){
$resultMessages['status'] = true;
$resultMessages['message'] = 'send!!';
echo json_encode($resultMessages);
}else{
$resultMessages['status'] = false;
$resultMessages['message'] = 'Message could not be sent. Mailer Error: '. $mail->ErrorInfo;
echo json_encode($resultMessages);
}
} catch (Exception $e) {
$resultMessages['status'] = false;
$resultMessages['message'] = 'Message could not be sent. Mailer Error: '. $mail->ErrorInfo;
echo json_encode($resultMessages);
}
}<file_sep>/slackWebHooks/settings.php
<?php
error_reporting(0);
include 'request.php';
$mainURLEndPoint = 'https://f3aa0d6659405ab34f9c0af85d0f2ef9:[email protected]/admin';
$shopifyOrderLink = 'https://loqta-ps.myshopify.com/admin/orders/';
$googleKey = '<KEY>';
$googleShortURL = "https://www.googleapis.com/urlshortener/v1/url?key=${googleKey}";
$data = file_get_contents('php://input');
$postOrderInfo = array();
if(!defined('NO_DEFAULT_HEADERS')){
header('Content-Type: application/json');
header('Accept: application/json');
}
date_default_timezone_set ( 'Asia/Gaza' );
| bcd1782ded86e995938d8f77078dbb1ee3ec37bf | [
"PHP",
"INI"
]
| 9 | PHP | loqtaApp/loqtaOrders | 6f82f69236414b938fa8a80e638d5ed8a97789e3 | 7740332e786941d0c9bce9e806846d1ecc360c76 | |
refs/heads/master | <file_sep># Python-CSV Data Analysis
Using python scripting to data mine two different CSV datasets and extract the useful data.
## PyBank
Analyze the dataset for a company budget (budget_data.csv) to discover:
* The total number of months included in the dataset
* The total net amount of "Profit/Losses" over the entire period
* The average change in "Profit/Losses" between months over the entire period
* The greatest increase in profits (date and amount) over the entire period
* The greatest decrease in losses (date and amount) over the entire period

## Pypoll
Use Python scripting to speed up the counting of the [election_data](PyPoll/Resources/election_data.csv) from a small town by calculating each of the following:
* The total number of votes cast
* A complete list of candidates who received votes
* The percentage of votes each candidate won
* The total number of votes each candidate won
* The winner of the election based on popular vote.

<file_sep># Import dependencies and open file path
import os
import csv
file = os.path.join("PyBank","Resources", "budget_data.csv")
with open(file, newline="") as csvfile:
csvreader = csv.reader(csvfile, delimiter=",")
next(csvreader)
# Create variables to hold values.
total_rev=0
prior_month_rev=0
total_monthly_change=0
max_monthly_rev_inc=0
max_monthly_rev_dec=0
# Loop throught each row/line of data in the input file (budget_csv).
for row in csvreader:
date = row[0]
total_rev += float(row[1])
# Calculate monthly revenue change.
# Skip first month since no previous month data.
if csvreader.line_num == 2:
monthly_change = 0
else:
monthly_change = float(row[1])-prior_month_rev
# Add monthly_change to total.
total_monthly_change += monthly_change
# While looping, find the greatest revenue increase/decrease
if monthly_change > max_monthly_rev_inc:
max_monthly_rev_inc = monthly_change
date_max_rev_inc = date
elif monthly_change < max_monthly_rev_dec:
max_monthly_rev_dec = monthly_change
date_max_rev_dec = date
# Update the prior month revenue variable.
prior_month_rev = float(row[1])
#Count up total months
total_months = int(csvreader.line_num-1)
#Print results
print("Financial Analysis")
print("----------------------------")
print("Total Months: " + str(total_months))
# In calculations and print statements below, various numeric values are cast to int in order to print a whole number
print("Total Revenue: $" + str(int(total_rev)))
print("Average Revenue Change $" + str(int(total_monthly_change/total_months)))
print("Greatest Increase in Revenue: " + date_max_rev_inc + " ($" +
str(int(max_monthly_rev_inc))+")")
print("Greatest Decrease in Revenue: " + date_max_rev_dec + " ($" +
str(int(max_monthly_rev_dec)) + ")" )
# Export a text file with the same results.
text_file = os.path.join("instructions","PyBank",'Resources', 'Financial_Analysis_1.txt')
with open(text_file, 'w') as f:
f.write("Financial Analysis\n")
f.write("----------------------------\n")
f.write("Total Months: " + str(total_months) + "\n")
f.write("Average Revenue Change $" + str(int(total_monthly_change/total_months)) + "\n")
f.write("Greatest Increase in Revenue: " + date_max_rev_inc + " ($" +
str(int(max_monthly_rev_inc)) + ")" + "\n")
f.write("Greatest Decrease in Revenue: " + date_max_rev_dec + " ($" +
str(int(max_monthly_rev_dec)) + ")" + "\n")<file_sep>#Import dependencies
import os
import csv
#Create variables
poll = {}
total_votes = 0
candidates = []
num_votes = []
vote_percent = []
winner_list = []
# Open and read csv file.
file = os.path.join("PyPoll","Resources", "election_data.csv")
with open(file, 'r') as csvfile:
csvread = csv.reader(csvfile)
next(csvread, None)
# Loops through rows, use column 3 as keys. Count votes for each candidate.
for row in csvread:
total_votes += 1
if row[2] in poll.keys():
poll[row[2]] = poll[row[2]] + 1
else:
poll[row[2]] = 1
for key, value in poll.items():
candidates.append(key)
num_votes.append(value)
# Calculate percentage of votes each candidate won.
for n in num_votes:
vote_percent.append(round(n/total_votes*100, 1))
# Zip candidates, num_votes, vote_percent into tuples.
clean_data = list(zip(candidates, num_votes, vote_percent))
# Calculate and declare winner.
for name in clean_data:
if max(num_votes) == name[1]:
winner_list.append(name[0])
winner = winner_list[0]
# In the event of a tie, print both names.
if len(winner_list) > 1:
for w in range(1, len(winner_list)):
winner = winner + " tied with " + winner_list[w]
# Create outputs text file
text_file = os.path.join('Output', 'election_results_' + str(file_num) +'.txt')
with open(text_file, 'w') as txtfile:
txtfile.writelines('Election Results \n------------------------- \nTotal Votes: ' + str(total_votes) +
'\n-------------------------\n')
for entry in clean_data:
txtfile.writelines(entry[0] + ": " + str(entry[2]) +'% (' + str(entry[1]) + ')\n')
txtfile.writelines('------------------------- \nWinner: ' + winner + '\n-------------------------')
# Print file to terminal
with open(output_file, 'r') as readfile:
print(readfile.read()) | 067d15b94235bc59a9f8145ea40b080558a5b534 | [
"Markdown",
"Python"
]
| 3 | Markdown | SamLingle/python-Scripting-challenge | 7a90d3e8f5fdb744fb06e9e12b784b190188b5cc | 1ce1a0309e84d0b772f5d6918bdcf19b2962f39b | |
refs/heads/master | <file_sep>const initialState = {
cartContents: [],
}
export default (state = initialState, action) => {
const {type, payload} = action;
switch(type){
case 'addToCart':
let updatedState = state.cartContents.push(payload);
return {...state, updatedState};
default:
return state;
}
}
export const addToCart = (chosenProduct) => {
return{
type: 'addToCart',
payload: chosenProduct
}
}<file_sep>import reducer, {swapActive} from './store/categories';
it('should have initial state', () => {
const state = reducer(undefined, {});
expect (state.activeCategory.displayName).toBe('Electronic Products');
})
it('should have be able to swap categories', () => {
const state = reducer(undefined, swapActive('food'));
expect (state.activeCategory.displayName).toBe('Artisanal Eats');
})<file_sep>import React from 'react'
export default props => (
<header>
<p>Our Store</p>
</header>
)<file_sep>// A <Products> component
// Displays a list of products associated with the selected category
import React, {useEffect} from 'react'
import {connect} from 'react-redux'
import {getProducts} from '../../store/products'
import {addToCart} from '../../store/cart';
const Products = props => {
useEffect(() => {
props.getProducts();
}, [])
return (
<section className="products">
{props.products.displayedProducts.map(product => {
console.log('Product for Cart', product);
return <li onClick={() => props.addToCart(product)}>{product.name}</li>
})}
</section>
)
}
const mapDispatchToProps = {addToCart, getProducts}
const mapStateToProps = state => ({
products: state.products,
cartContents: state.cart.cartContents,
})
export default connect(mapStateToProps, mapDispatchToProps)(Products);<file_sep>// State should contain a list of categories as well as the active category
// Each category should have a normalized name, display name, and a description
// Create an action that will trigger the reducer to change the active category
// Update the active category in the reducer when this action is dispatched
import axios from 'axios';
// Define initial state
const initialState = {
categories: {
// electronics: {displayName: 'Electronic Products', description: 'Devices to keep you connected'},
// food: {displayName: 'Artisanal Eats', description: 'Healthy, locally sourced ingredients'},
},
activeCategory: {
// displayName: '', description: ''
},
}
// Define reducer
export default (state = initialState, action) => {
const {type, payload} = action;
switch(type) {
case 'ChangeCategory':
return{...state, activeCategory: payload}
case 'GetCategories':
return {...state, categories: payload};
default:
return state;
}
}
// Define action creators
export const swapActive = (active) => {
return {
type: 'ChangeCategory',
payload: active
}
}
export function getCategories() {
return async function (dispatch){
const response = await axios.get('https://api-js401.herokuapp.com/api/v1/categories');
dispatch({
type: 'GetCategories',
payload: response.data.results
})
}
}<file_sep>import React from 'react'
export default props => (
<footer>
<p>© Our Store - 1(800)OUR-STORE</p>
</footer>
)<file_sep>// A <Categories> component
// Shows a list of all categories
// Dispatches an action when one is clicked to “activate” it
import React, {useEffect} from 'react'
import {connect} from 'react-redux'
import {swapActive, getCategories} from '../../store/categories'
const Categories = props => {
useEffect(() => {
props.getCategories();
}, [])
return (
<section className="category">
{console.log('Props at Category:', props)}
<h3>Current Category: {props.category.activeCategory.name}</h3>
<ul>
{Object.keys(props.category.categories).map(category => {
return <li onClick={() => props.swapActive(props.category.categories[category])}>{props.category.categories[category].name}</li>
})}
</ul>
</section>
)
}
const mapStateToProps = state => ({
category: state.categories,
})
const mapDispatchToProps = {swapActive, getCategories}
export default connect(mapStateToProps, mapDispatchToProps)(Categories); | 0996070faea4304c61b8ad237868a07e251fd200 | [
"JavaScript"
]
| 7 | JavaScript | jenniferchinzi-401-advanced-javascript/storefront | f34ce4fbb4c5e2d0d18d9342dc6bb99dced037ae | a0839b507649428d611e9536b7e491b527ab2f43 | |
HEAD | <file_sep>package com.mmall.service.Impl;
import com.google.common.collect.Lists;
import com.mmall.common.ServerResponse;
import com.mmall.service.FileService;
import com.mmall.util.FTPUtil;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
import java.util.UUID;
/**
*Author gaofeng <<EMAIL>>
*/
@Service
public class FileServiceImpl implements FileService{
Logger logger = LoggerFactory.getLogger(FileServiceImpl.class);
public String upload(MultipartFile file,String path){
String fileName = file.getOriginalFilename();
String extensionName = fileName.substring(fileName.lastIndexOf(".")+1);
String newFileName = UUID.randomUUID().toString()+extensionName;
logger.info("文件开始上传,上传文件的文件名:{},上传的路径:{},新文件名:{}",fileName,path,newFileName);
File fileDir = new File(path);
if (!fileDir.exists()){
fileDir.setWritable(true);
fileDir.mkdirs();
}
File fileTarget = new File(path,newFileName);
try {
//上传文件到Upload文件夹下
file.transferTo(fileTarget);
//然后再上传到FTP服务器上存储图片
FTPUtil.uploadFile(Lists.newArrayList(fileTarget));
//删除upload下的fileTarget文件
fileTarget.delete();
} catch (IOException e) {
logger.error("上传失败",e);
return null;
}
return fileTarget.getName();
}
}
<file_sep>package com.mmall.service;
import com.mmall.common.ServerResponse;
import com.mmall.pojo.Shipping;
import java.util.Map;
/**
*Author gaofeng <<EMAIL>>
*/
public interface ShippingService {
ServerResponse<Map> add(Integer userId, Shipping shipping);
ServerResponse delete(Integer userId,Integer shippingId);
ServerResponse update(Integer userId,Shipping shipping);
ServerResponse<Shipping> select(Integer userId,Integer shippingId);
}
<file_sep>package com.mmall.controller.backend;
import com.google.common.collect.Maps;
import com.mmall.common.Const;
import com.mmall.common.ResponseCode;
import com.mmall.common.ServerResponse;
import com.mmall.pojo.Product;
import com.mmall.pojo.User;
import com.mmall.service.FileService;
import com.mmall.service.IUserService;
import com.mmall.service.ProductService;
import com.mmall.util.PropertiesUtil;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.util.HashMap;
import java.util.Map;
/**
*Author gaofeng <<EMAIL>>
*/
@Controller
@RequestMapping("/manage/product")
public class ProductManageController {
@Autowired
private IUserService iUserService;
private ProductService productService;
private FileService fileService;
//后台新增或者更新产品接口
@RequestMapping("/save.do")
@ResponseBody
public ServerResponse addOrUpdateProduct(HttpSession httpSession, Product product){
User user = (User) httpSession.getAttribute(Const.CURRENT_USER);
if (user==null){
return ServerResponse.createByError(ResponseCode.NEED_LOGIN.getCode(),"用户未登录");
}
if (iUserService.checkAdmin(user).isSuccess()){
//添加业务逻辑
return productService.addOrUpdateProduct(product);
}else {
return ServerResponse.createByError("新增或者更新失败");
}
}
//后台管理员更新产品销售状态
@RequestMapping("set_sale_status.do")
@ResponseBody
public ServerResponse setSaleStatus(HttpSession httpSession, Integer productId,Integer status){
User user = (User) httpSession.getAttribute(Const.CURRENT_USER);
if (user==null){
return ServerResponse.createByError(ResponseCode.NEED_LOGIN.getCode(),"用户未登录");
}
if (iUserService.checkAdmin(user).isSuccess()){
//添加业务逻辑
return productService.setSaleStatus(productId,status);
}else {
return ServerResponse.createByError("无权限操作");
}
}
//获取商品详情
@RequestMapping("detail.do")
@ResponseBody
public ServerResponse setSaleStatus(HttpSession httpSession, Integer productId){
User user = (User) httpSession.getAttribute(Const.CURRENT_USER);
if (user==null){
return ServerResponse.createByError(ResponseCode.NEED_LOGIN.getCode(),"用户未登录");
}
if (iUserService.checkAdmin(user).isSuccess()){
//添加业务逻辑
return productService.manageProductDetail(productId);
}else {
return ServerResponse.createByError("无权限操作");
}
}
//产品list
@RequestMapping("list.do")
@ResponseBody
public ServerResponse getList(HttpSession httpSession, @RequestParam(value = "pageNum",defaultValue = "1") int pageNum,@RequestParam(value = "pageSize",defaultValue = "10") int pageSize){
User user = (User) httpSession.getAttribute(Const.CURRENT_USER);
if (user==null){
return ServerResponse.createByError(ResponseCode.NEED_LOGIN.getCode(),"用户未登录");
}
if (iUserService.checkAdmin(user).isSuccess()){
//添加业务逻辑
return productService.getProductList(pageNum,pageSize);
}else {
return ServerResponse.createByError("无权限操作");
}
}
//后台产品搜索接口
@RequestMapping("search.do")
@ResponseBody
public ServerResponse getList(HttpSession httpSession,String productName,Integer productId, @RequestParam(value = "pageNum",defaultValue = "1") int pageNum,@RequestParam(value = "pageSize",defaultValue = "10") int pageSize){
User user = (User) httpSession.getAttribute(Const.CURRENT_USER);
if (user==null){
return ServerResponse.createByError(ResponseCode.NEED_LOGIN.getCode(),"用户未登录");
}
if (iUserService.checkAdmin(user).isSuccess()){
//添加业务逻辑
return productService.searchProduct(productName,productId,pageNum,pageSize);
}else {
return ServerResponse.createByError("无权限操作");
}
}
//图片文件上传
@RequestMapping("upload.do")
@ResponseBody
public ServerResponse upload(HttpSession httpSession, MultipartFile file, HttpServletRequest request){
User user = (User) httpSession.getAttribute(Const.CURRENT_USER);
if (user==null){
return ServerResponse.createByError(ResponseCode.NEED_LOGIN.getCode(),"用户未登录");
}
if (iUserService.checkAdmin(user).isSuccess()){
//添加业务逻辑,返回图片的存储名称和它在服务器中的地址
String path = request.getSession().getServletContext().getRealPath("upload");
String fileTargetName = fileService.upload(file, path);
String url = PropertiesUtil.getProperty("ftp.server.http.prefix", "http://img.happymmall.com/") + fileTargetName;
HashMap<String, String > mapFile = Maps.newHashMap();
mapFile.put("uri",fileTargetName);
mapFile.put("url",url);
return ServerResponse.createBySuccess(mapFile);
}else {
return ServerResponse.createByError("无权限操作");
}
}
//富文本编辑器图片上传,由于前端使用simditor富文本,应该按照官方文档的返回格式返回(可以用map来接收),加上对应的响应头
@RequestMapping("richtext_img_upload.do")
@ResponseBody
public Map richtextImgUpload(HttpSession httpSession, MultipartFile file, HttpServletRequest request, HttpServletResponse response){
User user = (User) httpSession.getAttribute(Const.CURRENT_USER);
Map map = Maps.newHashMap();
if (user==null){
map.put("success","false");
map.put("msg","用户未登陆");
map.put("file_path","[real file path]");
return map;
}
if (iUserService.checkAdmin(user).isSuccess()){
//添加业务逻辑,返回图片的存储名称和它在服务器中的地址
String path = request.getSession().getServletContext().getRealPath("upload");
String fileTargetName = fileService.upload(file, path);
if (StringUtils.isBlank(fileTargetName)){
map.put("success","false");
map.put("msg","上传失败");
return map;
}
String url = PropertiesUtil.getProperty("ftp.server.http.prefix", "http://img.happymmall.com/") + fileTargetName;
map.put("success","true");
map.put("msg","上传成功");
map.put("file_path",url);
response.addHeader("Access-Control-Allow-Headers","X-File-Name");
return map;
}else {
map.put("success","false");
map.put("msg","无权限操作");
map.put("file_path","[real file path]");
return map;
}
}
}
<file_sep>package com.mmall.common;
import com.google.common.collect.Sets;
import java.util.Set;
/**
*Author gaofeng <<EMAIL>>
*/
public class Const {
public static final String CURRENT_USER = "currentUser";
public static final String EMAIL = "email";
public static final String USERNAME = "username";
public interface Role{
int ROLE_COSTEMER=0;//用户
int ROLE_ADMIN=1;//管理员
}
public interface CartCheck{
Integer CHECKED = 1;//已选择
Integer UN_CHECKED = 0;//未选择
String LIMIT_NUM_FAIL = "LIMIT_NUM_FAIL";
String LIMIT_NUM_SUCCESS = "LIMIT_NUM_SUCCESS";
}
public interface ProductListOrderBy{
Set<String> PRICE_ASC_DESC = Sets.newHashSet("price_desc","price_asc");
}
public enum ProductStatus{
ON_SALE("商品在架",1);
private String status;
private int num;
ProductStatus(String status,int num){
this.status = status;
this.num = num;
}
public String getStatus() {
return status;
}
public int getNum() {
return num;
}
}
}
<file_sep>package com.mmall.service.Impl;
import com.google.common.base.Splitter;
import com.google.common.collect.Lists;
import com.mmall.common.Const;
import com.mmall.common.ResponseCode;
import com.mmall.common.ServerResponse;
import com.mmall.dao.CartMapper;
import com.mmall.dao.ProductMapper;
import com.mmall.pojo.Cart;
import com.mmall.pojo.Product;
import com.mmall.pojo.User;
import com.mmall.service.CartService;
import com.mmall.util.BigDecimalUtil;
import com.mmall.vo.CartProductVo;
import com.mmall.vo.CartVo;
import org.apache.commons.collections.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.math.BigDecimal;
import java.util.List;
/**
*Author gaofeng <<EMAIL>>
*/
@Service
public class CartServiceImpl implements CartService{
@Autowired
private CartMapper cartMapper;
@Autowired
private ProductMapper productMapper;
//购物车添加商品
public ServerResponse<CartVo> add(Integer userId,Integer productId,Integer count){
if (productId==null || count==null){
return ServerResponse.createByError(ResponseCode.ILLEGAL_ARGUMENT.getCode(),ResponseCode.ILLEGAL_ARGUMENT.getDesc());
}
Cart cart = cartMapper.selectCart(userId, productId);
if (cart==null){
Cart newCart = new Cart();
newCart.setUserId(userId);
newCart.setProductId(productId);
newCart.setQuantity(count);
newCart.setChecked(Const.CartCheck.CHECKED);
cartMapper.insert(newCart);
}else {
count = count + cart.getQuantity();
cart.setQuantity(count);
cartMapper.updateByPrimaryKeySelective(cart);
}
CartVo cartVo = getCartVoLimit(userId);
return ServerResponse.createBySuccess(cartVo);
}
//更新购物车产品数量
public ServerResponse<CartVo> update(Integer userId,Integer productId,Integer count){
if (productId==null || count==null){
return ServerResponse.createByError(ResponseCode.ILLEGAL_ARGUMENT.getCode(),ResponseCode.ILLEGAL_ARGUMENT.getDesc());
}
Cart cart = cartMapper.selectCart(userId, productId);
if (cart!=null){
cart.setQuantity(count);
}
cartMapper.updateByPrimaryKeySelective(cart);
return this.getCartVo(userId);
}
//删除购物车里面的商品
public ServerResponse<CartVo> delete(Integer userId,String productIds){
if (StringUtils.isBlank(productIds)){
return ServerResponse.createByError(ResponseCode.ILLEGAL_ARGUMENT.getCode(),ResponseCode.ILLEGAL_ARGUMENT.getDesc());
}
List<String> list = Splitter.on(",").splitToList(productIds);
cartMapper.deleteByUserIdAndProductIds(userId,list);
return this.getCartVo(userId);
}
//查询购物车List
public ServerResponse<CartVo> list(Integer userId){
return this.list(userId);
}
//选择或者不选择购物车商品
public ServerResponse<CartVo> selectOrUnselect(Integer userId,Integer productId,Integer check){
cartMapper.updateCheck(userId, productId, check);
return this.list(userId);
}
//获取购物车产品数量
public ServerResponse<Integer> getProductCount(Integer userId){
if (userId==null){
return ServerResponse.createByError("0");
}
return ServerResponse.createBySuccess(cartMapper.getProductCount(userId));
}
//购物车操作通用功能方法
private ServerResponse<CartVo> getCartVo(Integer userId){
CartVo newCartVo = this.getCartVoLimit(userId);
return ServerResponse.createBySuccess(newCartVo);
}
private CartVo getCartVoLimit(Integer userId){
CartVo cartVo = new CartVo();
List<Cart> cartList = cartMapper.selectCartByUserId(userId);
List<CartProductVo> cartProductVoList = Lists.newArrayList();
BigDecimal cartTotalPrice = new BigDecimal("0");
if (CollectionUtils.isNotEmpty(cartList)){
for (Cart cartItem:cartList
) {
CartProductVo cartProductVo = new CartProductVo();
cartProductVo.setId(cartItem.getId());
cartProductVo.setUserId(cartItem.getUserId());
cartProductVo.setProductId(cartItem.getProductId());
Product product = productMapper.selectByPrimaryKey(cartItem.getProductId());
if (product!=null){
cartProductVo.setProductName(product.getName());
cartProductVo.setProductStatus(product.getStatus());
cartProductVo.setProductChecked(cartItem.getChecked());
cartProductVo.setProductMainImage(product.getMainImage());
cartProductVo.setProductSubtitle(product.getSubtitle());
cartProductVo.setProductPrice(product.getPrice());
cartProductVo.setProductStock(product.getStock());
if (product.getStock()>=cartItem.getQuantity()){
//库存充足
cartProductVo.setLimitQuantity(Const.CartCheck.LIMIT_NUM_SUCCESS);
cartProductVo.setQuantity(cartItem.getQuantity());
}else {
//库存不足时先在购物车更新有效库存
int canBuy = product.getStock();
Cart updateCartQuantity = new Cart();
updateCartQuantity.setId(cartItem.getId());
updateCartQuantity.setQuantity(canBuy);
cartMapper.updateByPrimaryKeySelective(updateCartQuantity);
cartProductVo.setLimitQuantity(Const.CartCheck.LIMIT_NUM_FAIL);
cartProductVo.setQuantity(product.getStock());
}
cartProductVo.setProductTotalPrice(BigDecimalUtil.mul(product.getPrice().doubleValue(),cartProductVo.getQuantity()));
if(cartItem.getChecked()==Const.CartCheck.CHECKED){
cartTotalPrice=BigDecimalUtil.add(cartProductVo.getProductTotalPrice().doubleValue(),cartTotalPrice.doubleValue());
}
}
cartProductVoList.add(cartProductVo);
}
}
cartVo.setCartTotalPrice(cartTotalPrice);
cartVo.setCartProductVoList(cartProductVoList);
cartVo.setAllChecked(this.getAllCheckedStatus(userId));
cartVo.setImageHost("ftp.server.http.prefix");
return cartVo;
}
private boolean getAllCheckedStatus(Integer userId){
if(userId == null){
return false;
}
return cartMapper.selectCartProductCheckedStatusByUserId(userId) == 0;
}
}
<file_sep>package com.mmall.service;
import com.mmall.common.ServerResponse;
import com.mmall.vo.CartVo;
/**
*Author gaofeng <<EMAIL>>
*/
public interface CartService {
ServerResponse<CartVo> add(Integer userId, Integer productId, Integer count);
ServerResponse<CartVo> update(Integer userId,Integer productId,Integer count);
ServerResponse<CartVo> delete(Integer userId,String productIds);
ServerResponse<CartVo> list(Integer userId);
ServerResponse<CartVo> selectOrUnselect(Integer userId,Integer productId,Integer check);
ServerResponse<Integer> getProductCount(Integer userId);
}
| 67e6cd52853ad1892a723021dad3850a6d07949e | [
"Java"
]
| 6 | Java | ARSblithe212/mmall | 2c1f7c48e06e2ede3ade6c5750382ae854c0ed2a | 6c39133ef409a7eef1178cb2b0575a2fef614ac1 | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace lab1
{
public interface IConverter
{
void Convert(List<Student> students, string outFile, List<string> header);
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.IO;
using System.Text;
using OfficeOpenXml;
namespace lab1
{
public class ExcelConverter : IConverter
{
/// <summary>
/// Create .xlsx file and write data there
/// </summary>
public void Convert(List<Student> students, string outFile, List<string> header)
{
FileInfo fi = new FileInfo(outFile + ".xlsx");
//Create a new ExcelPackage
using (ExcelPackage excelPackage = new ExcelPackage())
{
//Create the WorkSheet
ExcelWorksheet worksheet = excelPackage.Workbook.Worksheets.Add("Sheet 1");
for (int i = 0; i < header.Count; i++)
{
worksheet.Cells[1, i + 1].Value = header[i].ToString();
}
for (int i = 0, j = 0; i < students.Count; i++)
{
worksheet.Cells[i + 2, j + 1].Value = students[i].Name;
worksheet.Cells[i + 2, j + 2].Value = students[i].Surname;
worksheet.Cells[i + 2, j + 3].Value = students[i].Fathername;
foreach (Subject subject in students[i].Subjects)
{
worksheet.Cells[i + 2, j + 4].Value = subject.Mark;
j++;
}
worksheet.Cells[i + 2, header.Count + 1].Value = students[i].AverageMark;
j = 0;
}
int number = 0;
for (int z = 3, j = 4; z < students[0].Subjects.Count + 3; z++)
{
worksheet.Cells[students.Count + 2, j].Value = AverageForSubject(students, header[z]);
j++;
number = j;
}
worksheet.Cells[students.Count + 2, number].Value = AverageForAllGroup(students);
//Save your file
excelPackage.SaveAs(fi);
}
}
/// <summary>
/// Average for subject
/// </summary>
/// <param name="nameSubject"></param>
/// <returns></returns>
public double AverageForSubject(List<Student> students, string nameSubject)
{
int sum = 0;
foreach (Student student in students)
{
foreach (Subject subject in student.Subjects)
{
if (subject.Name.Equals(nameSubject))
{
sum += subject.Mark;
}
}
}
return Math.Round((double)sum / students.Count, 3);
}
/// <summary>
/// Average for all group and all subject
/// </summary>
/// <returns>one number(average mark)</returns>
public double AverageForAllGroup(List<Student> students)
{
double sum = 0;
foreach (Student student in students)
{
sum += student.AverageMark;
}
return Math.Round(sum / students.Count, 2);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.IO;
using System.Text;
using Newtonsoft.Json;
namespace lab1
{
public class JsonConverter : IConverter
{
public void Convert(List<Student> students, string outFile, List<string> header)
{
//open file stream
using (StreamWriter file = File.CreateText(outFile + ".json"))
{
JsonSerializer serializer = new JsonSerializer();
//serialize object directly into file stream
serializer.Serialize(file, students);
}
}
}
}
<file_sep>using System;
namespace lab1
{
class Program
{
static void Main(string[] args)
{
FileWorker excelAgregator = new FileWorker(args[0], args[1], args[2]);
foreach (Student student in excelAgregator.Students)
{
Console.WriteLine(student);
}
if (excelAgregator.Format == "Excel")
{
excelAgregator.Convert(new ExcelConverter());
}
else
{
excelAgregator.Convert(new JsonConverter());
}
Console.ReadKey();
}
}
}
<file_sep>using Newtonsoft.Json;
using OfficeOpenXml;
using System;
using System.Collections.Generic;
using System.IO;
using System.Text;
namespace lab1
{
public class FileWorker
{
public List<Student> Students = new List<Student>();
public List<string> Header = new List<string>();
public string Format;
public readonly string OutFile;
public readonly string NameFile;
/// <summary>
/// Constructor for ClassCollection
/// </summary>
/// <param name="nameFile"></param>
/// <param name="outFile"></param>
public FileWorker(string nameFile = "", string outFile = "", string format = "Excel")
{
OutFile = outFile;
NameFile = nameFile;
Format = format;
ReadFromFile();
}
public void ReadFromFile()
{
bool isHeader = true;
try
{
StreamReader streamReader = new StreamReader(NameFile + ".csv");
string str;
while ((str = streamReader.ReadLine()) != null)
{
if (isHeader)
{
CreateHeader(str);
isHeader = false;
}
else
{
try
{
Students.Add(CreateStudent(str));
}
catch (CsvLineException e)
{
Console.WriteLine("Ошибка " + e.Message);
Console.WriteLine($"Некорректная строка : {e.CsvStr}");
}
}
}
}
catch (FileNotFoundException)
{
Console.WriteLine("Файл " + NameFile + " не найден.");
}
}
public void Convert(IConverter converter)
{
converter.Convert(Students, OutFile, Header);
}
/// <summary>
/// Create header for sheet
/// </summary>
/// <param name="str"></param>
public void CreateHeader(string str)
{
string[] mass = str.Split(';');
foreach (var header in mass)
{
Header.Add(header);
}
}
/// <summary>
/// Factory for create student
/// </summary>
/// <returns>Student</returns>
public Student CreateStudent(string inputData)
{
string[] splitData = inputData.Split(';');
List<Subject> subjects = new List<Subject>();
for (int i = 3; i < splitData.Length; i++)
{
subjects.Add(new Subject() { Name = Header[i], Mark = Int32.Parse(splitData[i]) });
}
var student = new Student() { Name = splitData[0], Surname = splitData[1], Fathername = splitData[2], Subjects = subjects };
student.AverageMark = student.GetAverageMark();
return student;
}
}
}
| dfe34ecbc6a3f38d00f8b2553812be6463f96467 | [
"C#"
]
| 5 | C# | ArtemGontar/lab1GontarCore | 4dd2a303ed6569a921cdba425190d14a0f4fcd00 | 2573b2606f5a062558dc6c8de007e51ba9b184b1 | |
refs/heads/master | <file_sep>import React, { Component } from 'react';
import FlatButton from 'material-ui/FlatButton';
class UserLogin extends Component {
render() {
return (
<div>
<FlatButton label="Login"/>
<FlatButton label="Sign In"/>
</div>
);
}
}
export default UserLogin;
<file_sep>import React, { Component } from 'react';
import { Card, CardHeader, CardMedia, CardTitle } from 'material-ui/Card';
class Recipe extends Component {
render() {
return (
<Card>
<CardHeader>
<h3>Card Header</h3>
</CardHeader>
<CardMedia>
<img src="https://placehold.it/200?text=No+Image" alt=""/>
</CardMedia>
<CardTitle>
<p>Card Title</p>
</CardTitle>
</Card>
);
}
}
export default Recipe;
<file_sep>import React, { Component } from 'react';
import AppBar from 'material-ui/AppBar';
import UserLogin from '../components/UserLogin';
class Navbar extends Component {
render() {
return (
<AppBar iconElementRight={<UserLogin />}/>
);
}
}
export default Navbar;
<file_sep>import React, { Component } from 'react';
import MuiThemeProvider from 'material-ui/styles/MuiThemeProvider';
import { Grid, Row, Col } from 'react-flexbox-grid';
import injectTapEventPlugin from 'react-tap-event-plugin';
import Navbar from './Navbar';
import Sidebar from './Sidebar';
import SearchForm from './SearchForm';
import RecipesList from './RecipesList';
class MainPage extends Component {
constructor(props) {
super(props);
injectTapEventPlugin();
}
render() {
return (
<MuiThemeProvider>
<Grid fluid>
<Row>
<Col xs={12} md={12}>
<Navbar />
<Sidebar />
<SearchForm />
<RecipesList />
</Col>
</Row>
</Grid>
</MuiThemeProvider>
);
}
}
export default MainPage;
<file_sep>import { combineReducers } from 'redux';
import auth from './auth';
import search from './search';
import recepies from './recepies';
const ingridientsApp = combineReducers({
auth,
search,
recepies
})
export default ingridientsApp;
| 021a7c4ac846c536ea9a99351af4a65ade2fe91a | [
"JavaScript"
]
| 5 | JavaScript | andrei-sheina/ingredients-frontend | 21a892f0b3acd1999423bdfa45dff595d53fe393 | 496bd32531d3e4c90ab5d9dcce358e52905f012f | |
refs/heads/master | <file_sep>import React, { Component } from 'react';
class HotelComentsForm extends Component {
handleSubmit(e){
e.preventDefault();
let cal = Number(this.refs.calificacion.value);
console.log(cal)
if(cal == NaN){
alert('Calificacion debe ser un numero');
}else if(this.refs.calificacion.value<0 || this.refs.calificacion.value>5){
alert('Calificacion debe ser entre 1 y 5');
}else{
let comentario = {
nombre: this.refs.nombre.value,
correo: this.refs.correo.value,
comentario: this.refs.comentario.value,
calificacion: this.refs.calificacion.value
}
this.props.handleComentario(comentario);
}
}
render() {
return(
<div className='container'>
<form onSubmit={this.handleSubmit.bind(this)} className='form-inline'>
<div className='form-group'>
<label>Nombre</label>
<input type='text' className='form-control' ref='nombre'/>
</div>
<div className='form-group'>
<label>Correo</label>
<input type='textarea' className='form-control' ref='correo'/>
</div>
<div className='form-group'>
<label>Comentario</label>
<input type='text' className='form-control' ref='comentario'/>
</div>
<div className='form-group'>
<label>Calificacion</label>
<input type='text' className='form-control' ref='calificacion'/>
</div>
<input type='submit' className='btn btn-default'/>
</form>
</div>
);
}
}
export default HotelComentsForm;
<file_sep>import React, { Component } from 'react';
class HotelComents extends Component {
render() {
let comentarios = this.props.comentarios;
let comentArray = Object.values(comentarios);
let comentariosA = comentArray.map((comentario)=>{
return(
<div className='row'>
<ul className="list-group">
<li className="list-group-item">Nombre</li>
<li className="list-group-item">{comentario.nombre}</li>
<li className="list-group-item">Email</li>
<li className="list-group-item">{comentario.correo}</li>
<li className="list-group-item">Comentario</li>
<li className="list-group-item">{comentario.comentario}</li>
<li className="list-group-item">Calificacion</li>
<li className="list-group-item">{comentario.calificacion}</li>
</ul>
</div>
)
})
return (
<div className="col-sm-6 col-md-4">
{comentariosA}
</div>
);
}
}
export default HotelComents;
<file_sep>import React, { Component } from 'react';
class HotelAdd extends Component {
handleSubmit(e){
e.preventDefault();
let comentarios = [];
let hotel = {
nombre: this.refs.nombre.value,
descripcion: this.refs.descripcion.value,
ciudad: this.refs.ciudad.value,
imagen: this.refs.nombre.value + ".jpg",
uLatitud: this.refs.ulatitud.value,
uLongitud: this.refs.ulongitud.value,
costo: this.refs.costo.value,
comentarios: comentarios
};
let image = this.refs.image.files[0];
this.props.handleAdd(hotel, image);
this.props.changePrincipal();
}
render() {
return(
<div className='container'>
<form onSubmit={this.handleSubmit.bind(this)}>
<div className='form-group'>
<label>Nombre del hotel</label>
<input type='text' className='form-control' ref='nombre'/>
</div>
<div className='form-group'>
<label>Descripcion</label>
<input type='textarea' className='form-control' ref='descripcion'/>
</div>
<div className='form-group'>
<label>Ciudad</label>
<input type='text' className='form-control' ref='ciudad'/>
</div>
<div className='form-group'>
<label>Costo del hotel</label>
<input type='text' className='form-control' ref='costo'/>
</div>
<div className='form-group'>
<label>Latitud</label>
<input type='text' className='form-control' ref='ulatitud'/>
</div>
<div className='form-group'>
<label>Longitud</label>
<input type='text' className='form-control' ref='ulongitud'/>
</div>
<div className='form-group'>
<label>Subir foto del hotel</label>
<input type='file' ref='image' accept='image/*'/>
<p className='help-block'>Imagen para el banner del hotel</p>
</div>
<input type='submit' className='btn btn-default'/>
</form>
</div>
);
}
}
export default HotelAdd;
<file_sep>import {Map, InfoWindow, Marker, GoogleApiWrapper} from 'google-maps-react';
import React, { Component } from 'react';
export class MapContainer extends Component {
render() {
let style = this.props.style;
let latitud = this.props.latitud;
let longitud = this.props.longitud;
console.log(latitud);
return (
<div className="contenedor">
<Map google={this.props.google} initialCenter ={{
lat: latitud,
lng: longitud
}} style={style} zoom={14}>
<Marker onClick={this.onMarkerClick}
name={'Current location'} />
<InfoWindow onClose={this.onInfoWindowClose}>
<div>
<h1>{'this.state.selectedPlace.name'}</h1>
</div>
</InfoWindow>
</Map>
</div>
);
}
}
export default GoogleApiWrapper({
apiKey: ('<KEY>')
})(MapContainer) | 10ba338c95d4149eea64cf39ac09fdcf3dd784ab | [
"JavaScript"
]
| 4 | JavaScript | juseprada96/Taller2Web | e82c569b87d0ee00f2ba1868e53c9d52e1993133 | 18133bcd3aa2bcf2a01bc6f4ca792afaf3abe206 | |
refs/heads/master | <file_sep>import java.lang.IllegalStateException
import java.lang.RuntimeException
import kotlin.reflect.KClass
import kotlin.reflect.full.primaryConstructor
/**
* Di container.
* Used to retrieve instances and create subDi containers.
* Mark classes with @InjectClass annotation and your own scope annotation to
* automatically inject it to container with given scope.
* Constructor params of inject classes may be usual classes (not generics),
* Kotlin Lazy for lazy instances and Kotlin nullable types for optional injection.
*/
class DiContainer(
private val scope: KClass<*>,
bindings: Bindings.() -> Unit = {},
private val superDi: DiContainer? = null
) {
private val instances = hashMapOf<KClass<*>, Any?>()
private val depsProviders = DepsProviders(this)
private val callStackClasses = HashSet<KClass<*>>()
init {
val bootStrap = Bootstrap(this)
Bindings(depsProviders, bootStrap).bindings()
bootStrap.boot()
}
/**
* Get instance of given class.
*/
inline fun <reified T : Any> get(): T {
return get(T::class)!!
}
/**
* Get lazy instance of given class.
*/
inline fun <reified T : Any> getLazy(): Lazy<T> {
return getLazy(T::class)!!
}
/**
* Get optional instance of given class.
*/
inline fun <reified T : Any> getOptional(): T? {
return get(T::class, optional = true)
}
/**
* Get optional lazy instance of given class.
*/
inline fun <reified T : Any> getOptionalLazy(): Lazy<T>? {
return getLazy(T::class, optional = true)
}
fun <T : Any> getLazy(clazz: KClass<T>, optional: Boolean = false): Lazy<T>? {
if (!canProvide(clazz)) {
instances[clazz] = null
throwNotFoundExceptionIfNeeded(clazz, null, optional)
return null
}
return lazy { get(clazz)!! }
}
fun <T : Any> get(clazz: KClass<T>, optional: Boolean = false): T? {
if (clazz == Lazy::class) {
throw RuntimeException("Using get() for lazy type. Please use getLazy() instead")
}
if (instances.containsKey(clazz)) {
val instance = instances[clazz]
throwNotFoundExceptionIfNeeded(clazz, instance, optional)
return instance as T
}
verifyNoCircularDependencies(clazz) {
val newInstance = createInstance(clazz) ?: superDi?.get(clazz)
instances[clazz] = newInstance
throwNotFoundExceptionIfNeeded(clazz, newInstance, optional)
return newInstance
}
throw IllegalStateException("Cannot create instance due to internal error")
}
private fun throwNotFoundExceptionIfNeeded(clazz: KClass<*>, instance: Any?, optional: Boolean) {
if (instance == null && !optional) {
throw RuntimeException(
"No @InjectClass classes in scope ${scope.qualifiedName} " +
"and providers for class ${clazz.qualifiedName}. " +
"If you want to use optional injection, specify constructor param as nullable, " +
"or use getOptional()"
)
}
}
private inline fun verifyNoCircularDependencies(clazz: KClass<*>, block: () -> Unit) {
if (callStackClasses.contains(clazz)) {
throw RuntimeException("Circular dependency in class ${clazz.qualifiedName}")
}
callStackClasses.add(clazz)
try {
block()
} finally {
callStackClasses.clear()
}
}
private fun <T : Any> createInstance(clazz: KClass<T>): T? {
if (clazz == DiContainer::class) {
return this as T
}
if (depsProviders.hasProvider(clazz)) {
return depsProviders.instantiate(clazz) as T
}
if (annotatedForInject(clazz)) {
val primaryConstructor = clazz.primaryConstructor
?: throw RuntimeException("No primary constructor for class ${clazz.qualifiedName}")
val constructorArgumentsInstances = primaryConstructor.parameters.map {
val type = it.type
val argClass = type.classifier as KClass<*>
val isOptional = type.isMarkedNullable
if (argClass == Lazy::class) {
val lazyArgClass = type.arguments[0].type!!.classifier as KClass<*>
getLazy(lazyArgClass, optional = isOptional)
} else {
get(argClass, optional = isOptional)
}
}
return primaryConstructor.call(*constructorArgumentsInstances.toTypedArray())
}
return null
}
private fun annotatedForInject(clazz: KClass<*>): Boolean {
return clazz.hasAnnotation(InjectClass::class) && clazz.hasAnnotation(scope)
}
private fun canProvide(clazz: KClass<*>): Boolean {
return instances[clazz] != null || depsProviders.hasProvider(clazz)
|| annotatedForInject(clazz) && clazz.primaryConstructor != null || superDi?.canProvide(clazz) == true
}
/**
* Create subDi container that has access to all dependencies from parent di container.
* Scope of subDi must be different.
*/
inline fun <reified SubScope : Annotation> subDi(noinline bindings: Bindings.() -> Unit = {}): DiContainer {
return subDi(SubScope::class, bindings)
}
fun subDi(subScope: KClass<*>, bindings: Bindings.() -> Unit = {}): DiContainer {
if (this.scope == subScope) {
throw RuntimeException(
"Cannot create subDi with same scope, " +
"please, use different scope annotation"
)
}
return DiContainer(subScope, bindings, this)
}
}
private fun KClass<*>.hasAnnotation(annotationClass: KClass<*>): Boolean {
return annotations.any { it.annotationClass == annotationClass }
}
/**
* Holds registered providers for classes.
*/
class DepsProviders(
container: DiContainer
) {
private val provider = Provider(container)
private val registeredProviders = hashMapOf<KClass<*>, Provider.() -> Any>()
fun setProvider(clazz: KClass<*>, provider: Provider.() -> Any) {
if (clazz == Lazy::class) {
throw RuntimeException(
"Cannot set provider of lazy type. " +
"Please, set usual provider instead and use getLazy() " +
"or Lazy<> parameter in constructor"
)
}
registeredProviders[clazz] = provider
}
fun hasProvider(clazz: KClass<*>): Boolean {
return registeredProviders.containsKey(clazz)
}
fun instantiate(clazz: KClass<*>): Any {
if (!hasProvider(clazz)) {
throw RuntimeException("No provider for class ${clazz.qualifiedName}")
}
return registeredProviders[clazz]!!.invoke(provider)
}
}
/**
* Used to instantiate some classes immediately after container creation.
*/
class Bootstrap(private val container: DiContainer) {
var bootClasses: Array<out KClass<*>>? = null
fun boot() {
bootClasses?.forEach { container.get(it) }
}
}
<file_sep>plugins {
id 'org.jetbrains.kotlin.jvm' version '1.3.50'
}
group 'ak.container'
version '1.0-SNAPSHOT'
repositories {
mavenCentral()
}
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk8"
runtime group: 'org.jetbrains.kotlin', name: 'kotlin-reflect', version: '1.4.10'
compile "org.jetbrains.kotlin:kotlin-reflect:1.3.50"
compile group: 'javax.inject', name: 'javax.inject', version: '1'
}
compileKotlin {
kotlinOptions.jvmTarget = "1.8"
}
compileTestKotlin {
kotlinOptions.jvmTarget = "1.8"
}<file_sep>import kotlin.reflect.KClass
@Target(AnnotationTarget.CLASS)
annotation class InjectClass
/**
* Create di container instance for given scope.
* Scope is annotation used on classes with @InjectClass annotation.
* Container manages instances of classes from bound scope.
*/
inline fun <reified Scope : Annotation> di(noinline bindings: Bindings.() -> Unit = {}) =
DiContainer(Scope::class, bindings)
/**
* Dsl for creating instance bibdings.
*/
class Bindings(
private val depsProviders: DepsProviders,
private val bootStrap: Bootstrap
) {
/**
* Set instance provider.
*/
inline fun <reified T : Any> provide(noinline provider: Provider.() -> T) {
provide(T::class, provider)
}
fun provide(clazz: KClass<*>, provider: Provider.() -> Any) {
depsProviders.setProvider(clazz, provider)
}
/**
* Bind implementation to interface.
*/
inline fun <reified T : Any, reified Impl : T> bind() {
provide<T> { get<Impl>() }
}
/**
* Set classes to instantiate immediately after di creation.
*/
fun boot(vararg classes: KClass<*>) {
bootStrap.bootClasses = classes
}
}
/**
* Dsl for access container from provider function.
*/
class Provider(
private val container: DiContainer
) {
/**
* Get instance from container.
*/
inline fun <reified T : Any> get(): T {
return get(T::class)
}
fun <T : Any> get(clazz: KClass<T>): T {
return container.get(clazz)!!
}
/**
* Get lazy instance from container.
*/
inline fun <reified T : Any> getLazy(): Lazy<T> {
return getLazy(T::class)
}
fun <T : Any> getLazy(clazz: KClass<T>): Lazy<T> {
return container.getLazy(clazz)!!
}
/**
* Get optional instance from container.
*/
inline fun <reified T : Any> getOptional(): T? {
return getOptional(T::class)
}
fun <T : Any> getOptional(clazz: KClass<T>): T? {
return container.get(clazz, optional = true)
}
/**
* Get optional lazy instance from container.
*/
inline fun <reified T : Any> getOptionalLazy(): Lazy<T>? {
return getOptionalLazy(T::class)
}
fun <T : Any> getOptionalLazy(clazz: KClass<T>): Lazy<T>? {
return container.getLazy(clazz, optional = true)
}
}
<file_sep># KDI
Convenient di container for Kotlin
| db1ba66261ecd7394b10b34035d3f3755f70df6f | [
"Markdown",
"Kotlin",
"Gradle"
]
| 4 | Kotlin | AlexeyKozyakov/KDI | c71cc3f59d4c309c86ff43f3de3963cbfb44462a | 5f7593874a42a4718fd2dc36cb238da31f36ee7c | |
refs/heads/master | <file_sep>from __future__ import unicode_literals
import subprocess
from dagit.version import __version__
def test_version():
assert __version__
<file_sep>import os
import sys
from dagster import (
DependencyDefinition,
InputDefinition,
OutputDefinition,
PipelineDefinition,
RepositoryDefinition,
SolidDefinition,
check,
)
from dagster.cli.dynamic_loader import RepositoryContainer
from dagster.core.types.config import ALL_CONFIG_BUILTINS
from dagster.utils import script_relative_path
from dagster_pandas import DataFrame
from dagster_graphql.implementation.context import DagsterGraphQLContext
from dagster_graphql.implementation.pipeline_execution_manager import SynchronousExecutionManager
from dagster_graphql.implementation.pipeline_run_storage import PipelineRunStorage
from .setup import define_context, define_repository, execute_dagster_graphql
# This is needed to find production query in all cases
sys.path.insert(0, os.path.abspath(script_relative_path('.')))
from production_query import ( # pylint: disable=wrong-import-position,wrong-import-order
PRODUCTION_QUERY,
)
def test_enum_query():
ENUM_QUERY = '''{
pipeline(params: { name:"pipeline_with_enum_config" }){
name
configTypes {
__typename
name
... on EnumConfigType {
values
{
value
description
}
}
}
}
}
'''
result = execute_dagster_graphql(define_context(), ENUM_QUERY)
assert not result.errors
assert result.data
enum_type_data = None
for td in result.data['pipeline']['configTypes']:
if td['name'] == 'TestEnum':
enum_type_data = td
break
assert enum_type_data
assert enum_type_data['name'] == 'TestEnum'
assert enum_type_data['values'] == [
{'value': 'ENUM_VALUE_ONE', 'description': 'An enum value.'},
{'value': 'ENUM_VALUE_TWO', 'description': 'An enum value.'},
{'value': 'ENUM_VALUE_THREE', 'description': 'An enum value.'},
]
TYPE_RENDER_QUERY = '''
fragment innerInfo on ConfigType {
name
isList
isNullable
innerTypes {
name
}
... on CompositeConfigType {
fields {
name
configType {
name
}
isOptional
}
}
}
{
pipeline(params: { name: "more_complicated_nested_config" }) {
name
solids {
name
definition {
configDefinition {
configType {
...innerInfo
innerTypes {
...innerInfo
}
}
}
}
}
}
}
'''
def test_type_rendering():
result = execute_dagster_graphql(define_context(), TYPE_RENDER_QUERY)
assert not result.errors
assert result.data
def define_circular_dependency_pipeline():
return PipelineDefinition(
name='circular_dependency_pipeline',
solids=[
SolidDefinition(
name='csolid',
inputs=[InputDefinition('num', DataFrame)],
outputs=[OutputDefinition(DataFrame)],
transform_fn=lambda *_args: None,
)
],
dependencies={'csolid': {'num': DependencyDefinition('csolid')}},
)
def test_pipelines():
result = execute_dagster_graphql(define_context(), '{ pipelines { nodes { name } } }')
assert not result.errors
assert result.data
assert {p['name'] for p in result.data['pipelines']['nodes']} == {
p.name for p in define_repository().get_all_pipelines()
}
def test_pipelines_or_error():
result = execute_dagster_graphql(
define_context(), '{ pipelinesOrError { ... on PipelineConnection { nodes { name } } } } '
)
assert not result.errors
assert result.data
assert {p['name'] for p in result.data['pipelinesOrError']['nodes']} == {
p.name for p in define_repository().get_all_pipelines()
}
def test_pipelines_or_error_invalid():
repository = RepositoryDefinition(
name='test', pipeline_dict={'pipeline': define_circular_dependency_pipeline}
)
context = DagsterGraphQLContext(
RepositoryContainer(repository=repository),
PipelineRunStorage(),
execution_manager=SynchronousExecutionManager(),
)
result = execute_dagster_graphql(
context, '{ pipelinesOrError { ... on InvalidDefinitionError { message } } }'
)
msg = result.data['pipelinesOrError']['message']
assert "Circular reference detected in solid csolid" in msg
def test_pipeline_by_name():
result = execute_dagster_graphql(
define_context(),
'''
{
pipeline(params: {name: "pandas_hello_world_two"}) {
name
}
}''',
)
assert not result.errors
assert result.data
assert result.data['pipeline']['name'] == 'pandas_hello_world_two'
def test_pipeline_or_error_by_name():
result = execute_dagster_graphql(
define_context(),
'''
{
pipelineOrError(params: { name: "pandas_hello_world_two" }) {
... on Pipeline {
name
}
}
}''',
)
assert not result.errors
assert result.data
assert result.data['pipelineOrError']['name'] == 'pandas_hello_world_two'
def pipeline_named(result, name):
for pipeline_data in result.data['pipelines']['nodes']:
if pipeline_data['name'] == name:
return pipeline_data
check.failed('Did not find')
def has_config_type_with_key_prefix(pipeline_data, prefix):
for config_type_data in pipeline_data['configTypes']:
if config_type_data['key'].startswith(prefix):
return True
return False
def has_config_type(pipeline_data, name):
for config_type_data in pipeline_data['configTypes']:
if config_type_data['name'] == name:
return True
return False
def test_smoke_test_config_type_system():
result = execute_dagster_graphql(define_context(), ALL_CONFIG_TYPES_QUERY)
assert not result.errors
assert result.data
pipeline_data = pipeline_named(result, 'more_complicated_nested_config')
assert pipeline_data
assert has_config_type_with_key_prefix(pipeline_data, 'Dict.')
assert not has_config_type_with_key_prefix(pipeline_data, 'List.')
assert not has_config_type_with_key_prefix(pipeline_data, 'Nullable.')
for builtin_config_type in ALL_CONFIG_BUILTINS:
assert has_config_type(pipeline_data, builtin_config_type.name)
ALL_CONFIG_TYPES_QUERY = '''
fragment configTypeFragment on ConfigType {
__typename
key
name
description
isNullable
isList
isSelector
isBuiltin
isSystemGenerated
innerTypes {
key
name
description
... on CompositeConfigType {
fields {
name
isOptional
description
}
}
... on WrappingConfigType {
ofType { key }
}
}
... on EnumConfigType {
values {
value
description
}
}
... on CompositeConfigType {
fields {
name
isOptional
description
}
}
... on WrappingConfigType {
ofType { key }
}
}
{
pipelines {
nodes {
name
configTypes {
...configTypeFragment
}
}
}
}
'''
CONFIG_TYPE_QUERY = '''
query ConfigTypeQuery($pipelineName: String! $configTypeName: String!)
{
configTypeOrError(
pipelineName: $pipelineName
configTypeName: $configTypeName
) {
__typename
... on RegularConfigType {
name
}
... on CompositeConfigType {
name
innerTypes { key name }
fields { name configType { key name } }
}
... on EnumConfigType {
name
}
... on PipelineNotFoundError {
pipelineName
}
... on ConfigTypeNotFoundError {
pipeline { name }
configTypeName
}
}
}
'''
def test_config_type_or_error_query_success():
result = execute_dagster_graphql(
define_context(),
CONFIG_TYPE_QUERY,
{'pipelineName': 'pandas_hello_world', 'configTypeName': 'PandasHelloWorld.Environment'},
)
assert not result.errors
assert result.data
assert result.data['configTypeOrError']['__typename'] == 'CompositeConfigType'
assert result.data['configTypeOrError']['name'] == 'PandasHelloWorld.Environment'
def test_config_type_or_error_pipeline_not_found():
result = execute_dagster_graphql(
define_context(),
CONFIG_TYPE_QUERY,
{'pipelineName': 'nope', 'configTypeName': 'PandasHelloWorld.Environment'},
)
assert not result.errors
assert result.data
assert result.data['configTypeOrError']['__typename'] == 'PipelineNotFoundError'
assert result.data['configTypeOrError']['pipelineName'] == 'nope'
def test_config_type_or_error_type_not_found():
result = execute_dagster_graphql(
define_context(),
CONFIG_TYPE_QUERY,
{'pipelineName': 'pandas_hello_world', 'configTypeName': 'nope'},
)
assert not result.errors
assert result.data
assert result.data['configTypeOrError']['__typename'] == 'ConfigTypeNotFoundError'
assert result.data['configTypeOrError']['pipeline']['name'] == 'pandas_hello_world'
assert result.data['configTypeOrError']['configTypeName'] == 'nope'
def test_config_type_or_error_nested_complicated():
result = execute_dagster_graphql(
define_context(),
CONFIG_TYPE_QUERY,
{
'pipelineName': 'more_complicated_nested_config',
'configTypeName': (
'MoreComplicatedNestedConfig.SolidConfig.ASolidWithMultilayeredConfig'
),
},
)
assert not result.errors
assert result.data
assert result.data['configTypeOrError']['__typename'] == 'CompositeConfigType'
assert (
result.data['configTypeOrError']['name']
== 'MoreComplicatedNestedConfig.SolidConfig.ASolidWithMultilayeredConfig'
)
assert len(result.data['configTypeOrError']['innerTypes']) == 6
def test_production_query():
result = execute_dagster_graphql(define_context(), PRODUCTION_QUERY)
assert not result.errors
assert result.data
ALL_TYPES_QUERY = '''
{
pipelinesOrError {
__typename
... on PipelineConnection {
nodes {
runtimeTypes {
__typename
name
}
configTypes {
__typename
name
... on CompositeConfigType {
fields {
name
configType {
name
__typename
}
__typename
}
__typename
}
}
__typename
}
}
}
}
'''
def test_production_config_editor_query():
result = execute_dagster_graphql(define_context(), ALL_TYPES_QUERY)
assert not result.errors
assert result.data
| 080df7eb13fa0f8e08b5b2f5b3a4f003663c3fd6 | [
"Python"
]
| 2 | Python | zorrock/dagster | 714235ed4cc10b0689a1a3196daad6469f74ef15 | 60d08cd3781bbf7f50fc0c3afc7eb1e21d5cc2da | |
refs/heads/master | <file_sep>Last year Netflix released some simple guidelines for DIY socks that would pause your Netflix show/movie if you were detected to be sleeping. I thought this would be a great gift so I took the challenge. Due to the lack of information online, I figured I would share the solutions I have found to some problems encountered during my journey.
Here is the official guideline: https://makeit.netflix.com/
Note: The most difficult part of this tutorial is working with microelectronics. If you have zero experience with electronics or soldering, proceed with caution. The circuit schematic is complicated for beginners and it leaves out very important details that beginners will not account for. Additionally, this project is NOT cheap. There's a reason Netflix released this as a DIY project, it's not profitable. If you don't have spare parts laying around and need to buy everything required, this will easily run you $250. If you're okay with all this, check out my components list.
<file_sep>// Default code for Arduino board from Netflix using IR pulses for a Sony Televison
// ======================== LIBS ==============================
#include <avr/power.h>
#include <avr/sleep.h>
#include <Adafruit_NeoPixel.h>
// ======================== PINS ==============================
//neopixel
#define INDICATOR_PIN 8
#define SOFT_SWITCH_PIN 3
//accelerometer
#define x A3
#define y A4
#define z A5
#define IR_PIN 4
#define NUM_PULSES 60
int pulse_widths[NUM_PULSES][2] = {{4716,2416},{572,628},{568,628},{572,616},{580,1224},{572,1224},{568,620},{580,620},{572,1220},{576,1228},{568,1224},{572,616},{580,1224},{572,620},{576,620},{576,1220},{20308,2420},{572,628},{568,620},{576,624},{572,1220},{576,1220},{576,620},{576,624},{572,1220},{576,1220},{572,1220},{576,624},{572,1220},{576,624},{572,620},{576,1224},{20304,2416},{572,620},{576,620},{576,624},{572,1224},{572,1220},{576,624},{572,628},{568,1224},{568,1224},{572,1224},{572,624},{572,1224},{568,628},{572,620},{576,1216},{20360,2420},{568,620},{576,624},{572,628},{568,1224},{572,1224},{572,624},{568,624},{576,1216},{576,1216},{580,1224},{572,620}};
// ======================== VARS ==============================
Adafruit_NeoPixel indicator = Adafruit_NeoPixel(1, INDICATOR_PIN);
uint8_t colors[3][3] = {{1, 1, 1}, {1, 1, 1}, {1, 0, 0}};
uint8_t colorState = 255;
uint8_t colorPulseIncrement = -1;
volatile bool cpuSleepFlag = true;
unsigned long nextReadTime, windowTime, cpuAwoken, userReallyAsleepStart, indicatorPulseTime;
int indicatorPulseDelay = 5;
int readDelay = 50;
int windowDelay = 1500;
unsigned long userReallyAsleepDelay = 60000UL;
int threshold = 50;
int consecutiveThresholdTime = 60;
int userSleepState = 0;
bool userReallyAsleep = false;
bool newWindow = true;
int pxVal = 0;
int pyVal = 0;
int pzVal = 0;
int movementSum = 0;
int consecutivePossibleSleeps = 0;
// ======================== SETUP ==============================
void setup()
{
analogReference(EXTERNAL);
pinMode(SOFT_SWITCH_PIN, INPUT);
pinMode(IR_PIN, OUTPUT);
digitalWrite(IR_PIN, LOW);
nextReadTime = millis();
windowTime = nextReadTime;
indicator.begin();
indicator.setBrightness(50);
cpuSleepNow();
}
// ======================== LOOP ==============================
void loop() {
//everthing broken up into seperate functions to keep things simple.
if(millis() - indicatorPulseTime > indicatorPulseDelay){
indicatorPulseTime = millis();
indicatorHandler();
}
softSwitchHandler();
sleepHandler();
irHandler();
//new calculation window every windowDelay
if (millis() - windowTime > windowDelay) {
windowTime = millis();
newWindow = true;
}
//read accelerometer every readDelay amount of time
if (millis() - nextReadTime > readDelay) {
nextReadTime = millis();
accelerometerHandler();
}
}
// ======================== SOFT SWITCH ==============================
void softSwitchHandler(){
if(digitalRead(SOFT_SWITCH_PIN) == LOW && millis()-cpuAwoken > 1000){
//its held down.
cpuSleepNow();
}
}
// ======================== ACCELEROMETER ==============================
void accelerometerHandler() {
//read the accelerometer
int xVal = analogRead(x);
int yVal = analogRead(y);
int zVal = analogRead(z);
//if its a new calculation window
if (newWindow) {
//if the displacement is less than the threshold, then the user may be asleep.
if (movementSum < threshold) {
if(userSleepState !=2){
userSleepState = 1;
}
} else {
userSleepState = 0;
}
if (userSleepState >= 1) {
consecutivePossibleSleeps++;
if (consecutivePossibleSleeps > (consecutiveThresholdTime * ((float)1000/windowDelay))){
userSleepState = 2;
}
} else {
consecutivePossibleSleeps = 0;
}
pxVal = xVal;
pyVal = yVal;
pzVal = zVal;
movementSum = 0;
newWindow = false;
} else {
//compute magnitude changes
movementSum += abs(xVal - pxVal) + abs(yVal - pyVal) + abs(zVal - pzVal);
}
}
// ======================== USER SLEEP ==============================
void sleepHandler(){
if(userSleepState == 2){
if(millis() - userReallyAsleepStart > userReallyAsleepDelay){
userReallyAsleep = true;
}
}else{
userReallyAsleepStart = millis();
}
}
// ======================== INDICATOR ==============================
void indicatorHandler(){
//pulse the indicator for whichever color it is. can change what the pulse looks like here.
colorState+=colorPulseIncrement;
if(colorState < 1){
colorPulseIncrement = 1;
}else if(colorState >254){
colorPulseIncrement = -1;
}
indicator.setPixelColor(0,colorState * colors[userSleepState][0], colorState * colors[userSleepState][1], colorState * colors[userSleepState][2]);
indicator.show();
}
// ======================== CPU SLEEP ==============================
void cpuSleepNow() {
set_sleep_mode(SLEEP_MODE_PWR_DOWN);
sleep_enable();
delay(100);
//turn stuff off
indicator.setPixelColor(0,0,0,0);
indicator.show();
attachInterrupt(digitalPinToInterrupt(3),pinInterrupt, FALLING);
sleep_mode();
sleep_disable();
detachInterrupt(digitalPinToInterrupt(3));
}
void pinInterrupt()
{
cpuAwoken = millis();
reSetup();
}
//when the microcontroller is woken up, lets reset values
void reSetup(){
userReallyAsleep = false;
userSleepState = 0;
}
// ======================== IR ==============================
void irHandler(){
if(userReallyAsleep){
//shut down the indicator
indicator.setPixelColor(0,0,0,0);
indicator.show();
IR_transmit_pwr();
//repeat if desired. or add any other codes syou may want to handle. This is whatever your TV needs.
delay(500);
IR_transmit_pwr();
//put the microcontrolelr to sleep
cpuSleepNow();
}
}
//based on src from https://learn.adafruit.com/ir-sensor
void pulseIR(long microsecs) {
while (microsecs > 0) {
// 38 kHz is about 13 microseconds high and 13 microseconds low
digitalWrite(IR_PIN, HIGH); // this takes about 3 microseconds to happen
delayMicroseconds(10); // hang out for 10 microseconds
digitalWrite(IR_PIN, LOW); // this also takes about 3 microseconds
delayMicroseconds(10); // hang out for 10 microseconds
// so 26 microseconds altogether
microsecs -= 26;
}
}
void IR_transmit_pwr() {
for (int i = 0; i < NUM_PULSES; i++) {
delayMicroseconds(pulse_widths[i][0]);
pulseIR(pulse_widths[i][1]);
}
}
<file_sep><i>Note: Try to bundle everything you need in the lowest number of orders from the least amount of companies. Shipping electronics is expensive! Usually around $15 regardless of order size.</i>
Here is their official list, but there are some things are missing: https://makeit.netflix.com/netflix_socks_materials_list.pdf
<b>Prototyping and Building</b>
1. Breadboard
https://www.adafruit.com/products/239
2. Soldering Iron / Stand
https://www.adafruit.com/products/180 / https://www.adafruit.com/products/150
3. Wire for prototyping
https://www.adafruit.com/products/153
4. Wire for soldering
https://www.adafruit.com/products/1446
5. USB Micro
https://www.adafruit.com/products/592
<b>Actual Parts for the Sock</b>
1. Arduino Pro Trinket 3V
https://www.adafruit.com/products/2010
2. Pro Trinket Backpack
https://www.adafruit.com/product/2124
3. Lithium Ion Battery 3.7 v / 500 mAH
https://www.adafruit.com/products/1578
4. Flora RGB Neo Pixel
https://www.adafruit.com/products/1260
5. Accelerometer
https://www.adafruit.com/products/1120
6. Mosfet
https://www.adafruit.com/products/355
7. 0.1 uF Capacitors
https://www.adafruit.com/products/753
8. 10K Ohm Resistors
https://www.adafruit.com/products/2784
9. Switch
https://www.adafruit.com/products/1119
10. 1 Ohm Resistors
http://www.digikey.com/product-detail/en/CFM14JT1R00/S1QCT-ND/2617699
11. IR Emitter
http://www.digikey.com/product-search/en?keywords=1125-1235-ND
| 6b0b0d522379efa523b704a2070237163c7fe935 | [
"Markdown",
"C++"
]
| 3 | Markdown | chairmanreggie/Netflix-Socks | 91c993e0fd93e7f7d5e766121fe4d6e02abdbfb5 | bd6de858fca30511c4732bda0b933e801a5f6e6d | |
refs/heads/master | <repo_name>rajatkumar-96/java-problem-5<file_sep>/setSorter.java
import java.util.*;
class setSorter{
public static void main(String[] args) {
System.out.println("ENTER THE NUMBER OF STRINGS YOU WANT TO ADD");
TreeSet<String> treeSet= new TreeSet<String>();
Scanner input= new Scanner(System.in);
int num= input.nextInt();
for(int i=0;i<num;i++){
String str=input.next();
treeSet.add(str);
}
List<String> li=new ArrayList<String>(treeSet);
System.out.println(li);
}
}<file_sep>/arraylistreplace.java
import java.util.*;
import java.lang.*;
public class arraylistreplace{
public static void main(String[] args) {
ArrayList<String> newlist= new ArrayList<String>();
newlist.add("Apple");
newlist.add("Grape");
newlist.add("Melon");
newlist.add("Berry");
String replacer="Kiwi";
Scanner input= new Scanner(System.in);
System.out.println("Enter the index you want to replace:");
int index= input.nextInt();
newlist.set(index, replacer);
for(String str1:newlist){
System.out.println(str1);
}
}
} | 56d7ed48a52dcc40d495e4dd0e2c141a717650c7 | [
"Java"
]
| 2 | Java | rajatkumar-96/java-problem-5 | 5eacf8ac738a6c9d5078837e054f5027e9442cdf | 040186364eb5bd008723ff9d9bcf4bb2515da605 | |
refs/heads/master | <repo_name>zyimm/Api-Manager-Plus<file_sep>/framework/Db.php
<?php
namespace framework;
class Db
{
}<file_sep>/framework/Log.php
<?php
namespace framework;
class Log
{
}<file_sep>/framework/Error.php
<?php
/**
* Exception
*
*/
namespace framework;
use Exception;
use TypeError;
class Error
{
public static function register()
{
error_reporting(E_ALL);
set_error_handler([
__CLASS__,
'error'
]);
set_exception_handler([
__CLASS__,
'exception'
]);
register_shutdown_function([
__CLASS__,
'shutdown'
]);
}
public static function error($errno, $errstr, $errfile = '', $errline = 0, $errcontext = [])
{
$exception = new Exception($errno, $errstr, $errfile, $errline, $errcontext);
throw self;
}
public static function exception($error)
{
$error = new TypeError($error);
}
public static function shutdown()
{
if ($error = error_get_last()) {
$exception = new Exception($error['type'], $error['message'], $error['file'], $error['line']);
$this->exception($exception);
}
}
}<file_sep>/framework/Application.php
<?php
/**
* Application
*
* @author zhouyangyang 2017年7月13日上午11:51:39
*/
namespace framework;
use ArrayAccess;
class Application extends Bootstrap implements ArrayAccess
{
public static $app;
public static $starRunTime;
public static $endRunTime;
public function bootstrap()
{
self::$starRunTime = microtime(true);
self::$app = new self;
self::$app->run();
self::$endRunTime = microtime(true);
}
public function offsetGet($offset)
{}
public function offsetExists($offset)
{}
public function offsetUnset($offset)
{}
public function offsetSet($offset, $value)
{}
}<file_sep>/framework/Environment.php
<?php
namespace framework;
class Environment extends ServiceLocator
{
const VERSION = '1.0.1';
protected static function getVersion()
{
return self::VERSION;
}
public static function check()
{
}
}
<file_sep>/framework/ServiceLocator.php
<?php
namespace framework;
class ServiceLocator extends Component
{
public function has()
{}
public function set()
{}
public function get()
{}
public function bulid()
{}
}<file_sep>/framework/Cache.php
<?php
namespace framework;
class Cache
{
}<file_sep>/framework/Controller.php
<?php
namespace framework;
class Controller
{
}
<file_sep>/index.php
<?php
/**
* 入口文件
*/
defined('FRAMEWORK_PATH') || define('FRAMEWORK_PATH', dirname(__FILE__) . DS . 'framework' . DS);
defined('API_DEBUG') || define('API_DEBUG', true);
defined('DS') || define('DS', DIRECTORY_SEPARATOR);
defined('APP_PATH') || define('APP_PATH', dirname(__FILE__) . DS . 'Api' . DS);
defined('WEB_PATH') || define('WEB_PATH', dirname(__FILE__) . DS);
//load
require (FRAMEWORK_PATH . 'Loader.php');
//register
\framework\Loader::register();
//run framework
(new \framework\Application())->bootstrap();<file_sep>/framework/Request.php
<?php
namespace framework;
class Request
{
}<file_sep>/framework/Response.php
<?php
namespace framework;
class Response
{
}<file_sep>/framework/Object.php
<?php
namespace framework;
class Object{
}<file_sep>/framework/Helper.php
<?php
namespace framework;
class Helper
{
}<file_sep>/framework/Bootstrap.php
<?php
namespace framework;
class Bootstrap extends Environment
{
public function run()
{
Environment::check();
$this->start()->end();
}
public function start()
{
return $this;
}
public function end()
{
}
}<file_sep>/framework/Component.php
<?php
namespace framework;
class Component extends Object
{
// 用于保存单例Singleton对象,以对象类型为键
private $_singletons = [];
// 用于保存依赖的定义,以对象类型为键
private $_definitions = [];
// 用于保存构造函数的参数,以对象类型为键
private $_params = [];
// 用于缓存ReflectionClass对象,以类名或接口名为键
private $_reflections = [];
// 用于缓存依赖信息,以类名或接口名为键
private $_dependencies = [];
}<file_sep>/framework/Model.php
<?php
namespace framework;
class Model
{
}<file_sep>/framework/Loader.php
<?php
namespace framework;
class Loader
{
// 自动加载的文件
private static $autoloadFiles = [];
// 映射文件
private static $mapFiles = [];
public static $namespaceAlias;
public static $isWin;
// 自动加载
public static function autoload($class)
{
// 检测命名空间别名
if (!empty(self::$namespaceAlias)) {
$namespace = dirname($class);
if (isset(self::$namespaceAlias[$namespace])) {
$original = self::$namespaceAlias[$namespace] . '\\' . basename($class);
if (class_exists($original)) {
return class_alias($original, $class, false);
}
}
}
if ($file = self::includeFile($class)) {
// Win环境严格区分大小写
if (self::$isWin && pathinfo($file, PATHINFO_FILENAME) != pathinfo(realpath($file), PATHINFO_FILENAME)) {
return false;
}
include $file;
return true;
}
}
public static function register($autoload = '')
{
self::$isWin = (strpos(PHP_OS, 'WIN') !== false)?true:false;
// 注册系统自动加载
spl_autoload_register($autoload ?: 'framework\\Loader::autoload', true, true);
Error::register();
}
private static function includeFile($class)
{
if (!empty(self::$mapFiles[$class])) {
return self::$mapFiles[$class];
}
$class = strtr($class, '\\',DS).'.php';
if(current(explode(DS, $class)) == 'framework'){
return include dirname(FRAMEWORK_PATH).DS.$class;
}else{
return include APP_PATH.$class;
}
}
}<file_sep>/README.md
# Api-Manager-Plus
PHP 接口文档管理;PHP Api Document Management
# 特点 | 6010eef7bdabf3ebc5cc004c50f13e1e08b20661 | [
"Markdown",
"PHP"
]
| 18 | PHP | zyimm/Api-Manager-Plus | 0df1f8224b63c2da1029cc9fb52f23e69facc0a3 | 1f4a0a8ee8a8e8cf75be86b4f2ae17665e3b47fe | |
refs/heads/master | <file_sep>package com.hm.application.network;
import android.util.Log;
import com.android.volley.NetworkResponse;
import com.android.volley.Response;
import com.android.volley.Response.ErrorListener;
import com.android.volley.Response.Listener;
import com.android.volley.toolbox.HttpHeaderParser;
import com.android.volley.toolbox.JsonRequest;
import com.crashlytics.android.Crashlytics;
import org.json.JSONObject;
public class GetObjRequest extends JsonRequest<JSONObject> {
public GetObjRequest(String url, JSONObject jsonObject, Listener<JSONObject> listener, ErrorListener errorListener) {
super(Method.GET, url, jsonObject.toString(), listener, errorListener);
Log.d("HM_URL", " Get Obj Req: " + url + " : " + jsonObject.toString());
}
protected Response<JSONObject> parseNetworkResponse(NetworkResponse response) {
try {
Log.d("HM_URL", " Get Obj Resp: " + response.statusCode + " : " + response.networkTimeMs);
return Response.success(new JSONObject(new String(response.data, HttpHeaderParser.parseCharset(response.headers))), HttpHeaderParser.parseCacheHeaders(response));
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
return null;
}
}
}<file_sep>package com.hm.application.network;
import android.content.Context;
import com.android.volley.NetworkResponse;
import com.android.volley.ParseError;
import com.android.volley.Request;
import com.android.volley.Response;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.HttpHeaderParser;
import com.android.volley.toolbox.JsonObjectRequest;
import com.android.volley.toolbox.StringRequest;
import com.crashlytics.android.Crashlytics;
import org.json.JSONObject;
import java.util.HashMap;
import java.util.Map;
public class VolleyUtils {
String request;
public static void POST_METHOD(Context context, String url, final Map<String, String> getParams, final VolleyResponseListener listener) {
// Initialize a new StringRequest
StringRequest stringRequest = new StringRequest(
Request.Method.POST,
url,
new Response.Listener<String>() {
@Override
public void onResponse(String response) {
listener.onResponse(response);
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
listener.onError(error.toString());
}
})
{
/**
* Passing some request headers
* */
@Override
public Map<String, String> getHeaders() {
HashMap<String, String> headers = new HashMap<String, String>();
getParams.put("Content-Type", "application/json; charset=utf-8");
return headers;
}
};
// Access the RequestQueue through singleton class.
VolleySingleton.getInstance(context).addToRequestQueue(stringRequest, "TAG " + stringRequest);
}
public static void makeJsonObjectRequest(Context context, String url, final VolleyResponseListener listener) {
JsonObjectRequest jsonObjectRequest = new JsonObjectRequest
(url, null, new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
listener.onResponse(response);
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
listener.onError(error.toString());
}
}) {
@Override
protected Response<JSONObject> parseNetworkResponse(NetworkResponse response) {
try {
String jsonString = new String(response.data,
HttpHeaderParser.parseCharset(response.headers, PROTOCOL_CHARSET));
return Response.success(new JSONObject(jsonString),
HttpHeaderParser.parseCacheHeaders(response));
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
return Response.error(new ParseError(e));
}
}
};
// Access the RequestQueue through singleton class.
VolleySingleton.getInstance(context).addToRequestQueue(jsonObjectRequest);
}
}
<file_sep>package com.hm.application.activity;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.support.v7.app.AppCompatActivity;
import com.hm.application.R;
import com.hm.application.model.AppConstants;
import com.hm.application.model.AppDataStorage;
import com.hm.application.model.User;
import com.hm.application.services.MyFirebaseInstanceIDService;
import com.hm.application.user_data.LoginActivity;
public class SplashActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_splash);
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
AppDataStorage.getUserInfo(SplashActivity.this);
new MyFirebaseInstanceIDService().onTokenRefresh();
if (User.getUser(SplashActivity.this).getUid() != null) {
startActivity(new Intent(SplashActivity.this, MainHomeActivity.class));
} else {
startActivity(new Intent(SplashActivity.this, LoginActivity.class).putExtra(AppConstants.USERDATA, AppConstants.LOGIN));
}
finish();
}
}, 1000);
}
}<file_sep>package com.hm.application.fragments;
import android.os.Bundle;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.support.v4.app.Fragment;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import android.view.KeyEvent;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.view.inputmethod.EditorInfo;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
import com.android.volley.Request;
import com.android.volley.Response;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.StringRequest;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.adapter.DisplayReplyAdapter;
import com.hm.application.model.AppConstants;
import com.hm.application.model.User;
import com.hm.application.network.VolleySingleton;
import com.hm.application.utils.CommonFunctions;
import com.hm.application.utils.HmFonts;
import com.squareup.picasso.Picasso;
import org.json.JSONArray;
import org.json.JSONObject;
import java.util.HashMap;
import java.util.Map;
import de.hdodenhof.circleimageview.CircleImageView;
public class ReplyToCommentFragment extends Fragment {
public String commentId = null;
private RecyclerView mRv;
// private TextView mTvLikesData;
private EditText mEdtCmt;
private Button mBtnCmt;
private LinearLayout mLlAddCmt;
// private RelativeLayout mllCuCall;
private ImageView mIvProfilePic;
public ReplyToCommentFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(@NonNull LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_comment, container, false);
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
try {
toBindViews();
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private void checkInternetConnection() throws Error {
if (CommonFunctions.isOnline(getContext())) {
toDisplayReply();
} else {
CommonFunctions.toDisplayToast(getResources().getString(R.string.lbl_no_check_internet), getContext());
}
}
@NonNull
private void toBindViews() throws Error {
mRv = getActivity().findViewById(R.id.rvComments);
mRv.setNestedScrollingEnabled(false);
// mTvLikesData = getActivity().findViewById(R.id.txtCmtData);
mEdtCmt = getActivity().findViewById(R.id.edtCfPost);
mBtnCmt = getActivity().findViewById(R.id.btnCfSend);
mLlAddCmt = getActivity().findViewById(R.id.llAddCmt);
// mllCuCall = getActivity().findViewById(R.id.llCuCall);
// mllCuCall.setVisibility(View.VISIBLE);
mIvProfilePic = getActivity().findViewById(R.id.imgCf);
if (User.getUser(getContext()).getPicPath() != null) {
Picasso.with(getContext())
.load(AppConstants.URL + User.getUser(getContext()).getPicPath().replaceAll("\\s", "%20"))
.resize(200, 200)
.error(R.color.light2)
.placeholder(R.color.light)
.into(mIvProfilePic);
}
if (getArguments() != null) {
if (getArguments().getString(AppConstants.COMMENT_ID) != null) {
commentId = getArguments().getString(AppConstants.COMMENT_ID);
toAddComment(getArguments().getString("Data"));
checkInternetConnection();
} else {
CommonFunctions.toDisplayToast("No Reply", getContext());
}
} else {
CommonFunctions.toDisplayToast("No Reply", getContext());
}
mEdtCmt.setOnEditorActionListener(new EditText.OnEditorActionListener() {
@Override
public boolean onEditorAction(TextView v, int actionId, KeyEvent event) {
if (actionId == EditorInfo.IME_ACTION_DONE) {
toSubmitReply();
}
return false;
}
});
mBtnCmt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
toSubmitReply();
}
});
}
private void toAddComment(String data) {
try {
JSONObject obj = new JSONObject(data);
CircleImageView mIvCu;
TextView mTvCuName, mTvCuCmt, mTvCuTime, mTvCuLike, mTvCuReply;
mIvCu = getActivity().findViewById(R.id.imgCu);
if (!obj.isNull(getContext().getString(R.string.str_profile_pic))) {
if (obj.getString(getContext().getString(R.string.str_profile_pic)).toLowerCase().contains("upload")) {
Picasso.with(getContext()).load(AppConstants.URL + obj.getString(getContext().getString(R.string.str_profile_pic))).placeholder(R.color.light).error(R.color.light2).into(mIvCu);
} else {
Picasso.with(getContext()).load(obj.getString(getContext().getString(R.string.str_profile_pic))).placeholder(R.color.light).error(R.color.light2).into(mIvCu);
}
}
mTvCuName = getActivity().findViewById(R.id.txtCuName);
mTvCuName.setTypeface(HmFonts.getRobotoBold(getContext()));
if (!obj.isNull(getContext().getString(R.string.str_username_))) {
mTvCuName.setText(obj.getString(getContext().getString(R.string.str_username_)));
}
mTvCuCmt = getActivity().findViewById(R.id.txtCuCmt);
mTvCuCmt.setTypeface(HmFonts.getRobotoRegular(getContext()));
if (!obj.isNull(getContext().getString(R.string.str_comment_small))) {
mTvCuCmt.setText(obj.getString(getContext().getString(R.string.str_comment_small)));
}
mTvCuTime = getActivity().findViewById(R.id.txtCuTime);
mTvCuTime.setTypeface(HmFonts.getRobotoRegular(getContext()));
if (!obj.isNull(getContext().getString(R.string.str_time_small))) {
mTvCuTime.setText(CommonFunctions.toSetDate(obj.getString(getContext().getString(R.string.str_time_small))));
}
mTvCuLike = getActivity().findViewById(R.id.txtCuLike);
mTvCuLike.setTypeface(HmFonts.getRobotoRegular(getContext()));
if (!obj.isNull(getContext().getString(R.string.str_like_count))) {
mTvCuLike.setText(obj.getString(getContext().getString(R.string.str_like_count)) + " " + getContext().getString(R.string.str_like));
}
mTvCuReply = getActivity().findViewById(R.id.txtCuReply);
mTvCuReply.setTypeface(HmFonts.getRobotoRegular(getContext()));
if (!obj.isNull(getContext().getString(R.string.str_reply_count))) {
mTvCuReply.setText(obj.getString(getContext().getString(R.string.str_reply_count)) + " " + getContext().getString(R.string.str_reply));
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private void toSubmitReply() {
try {
if (mEdtCmt.getText().toString().trim().length() > 0) {
// MyPost.toReplyOnComment(getContext(), commentId, mEdtCmt.getText().toString().trim());
toDisplayReply();
mEdtCmt.setText("");
} else {
CommonFunctions.toDisplayToast("Empty", getContext());
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
public void toDisplayReply() {
try {
final View view = LayoutInflater.from(getContext()).inflate(R.layout.rv_layout, null, false);
if (view != null) {
VolleySingleton.getInstance(getContext())
.addToRequestQueue(
new StringRequest(Request.Method.POST,
AppConstants.URL + getContext().getString(R.string.str_like_share_comment) + getContext().getString(R.string.str_php),
new Response.Listener<String>() {
@Override
public void onResponse(String res) {
try {
if (res != null && res.length() > 0) {
Log.d("HmApp", "Reply Res " + res);
JSONArray array = new JSONArray(res);
if (array != null) {
if (array.length() > 0) {
LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(LinearLayout.LayoutParams.MATCH_PARENT, LinearLayout.LayoutParams.WRAP_CONTENT);
params.setMargins(80, 0, 0, 0);
mRv.setLayoutParams(params);
mRv.setLayoutManager(new LinearLayoutManager(getContext()));
mRv.hasFixedSize();
mRv.setNestedScrollingEnabled(false);
mRv.setAdapter(new DisplayReplyAdapter(getContext(), array));
} else {
CommonFunctions.toDisplayToast("No Reply", getContext());
}
} else {
CommonFunctions.toDisplayToast("No Reply", getContext());
}
} else {
CommonFunctions.toDisplayToast("No Reply", getContext());
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
error.printStackTrace();
}
}
) {
@Override
protected Map<String, String> getParams() {
Map<String, String> params = new HashMap<String, String>();
params.put(getContext().getString(R.string.str_action_), getContext().getString(R.string.str_fetch_reply_comment_));
params.put(getContext().getString(R.string.str_comment_id), commentId);
return params;
}
}
, getContext().getString(R.string.str_fetch_reply_comment_));
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
}<file_sep>package com.hm.application.utils.insta.utils;
import android.os.Environment;
public class FilePaths {
//"storage/emulated/0"
public String ROOT_DIR = Environment.getExternalStorageDirectory().getPath();
public String PICTURES = ROOT_DIR + "/Pictures";
public String DOWNLOADS = ROOT_DIR + "/Downloads";
public String MAIN = ROOT_DIR + "/";
public String DICM_CAMERA = ROOT_DIR + "/DCIM/camera";
public String CAMERA = ROOT_DIR + "/Camera";
public String STORIES = ROOT_DIR + "/Stories";
public String FIREBASE_STORY_STORAGE = "stories/users";
public String FIREBASE_IMAGE_STORAGE = "photos/users/";
}
<file_sep>package com.hm.application.fragments;
import android.content.Context;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.design.widget.TextInputEditText;
import android.support.design.widget.TextInputLayout;
import android.support.v4.app.Fragment;
import android.text.Editable;
import android.text.TextWatcher;
import android.util.Log;
import android.view.KeyEvent;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.view.inputmethod.EditorInfo;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
import com.android.volley.Request;
import com.android.volley.Response;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.StringRequest;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.activity.AccountSettingsActivity;
import com.hm.application.model.AppConstants;
import com.hm.application.model.User;
import com.hm.application.network.VolleySingleton;
import com.hm.application.utils.CommonFunctions;
import com.hm.application.utils.HmFonts;
import com.hm.application.utils.KeyBoard;
import org.json.JSONObject;
import java.util.HashMap;
import java.util.Map;
public class ChangePasswordFragment extends Fragment {
LinearLayout mLlChangePwdMain, mLlChangePwd, mLlChangePwdEdit;
TextInputLayout mTilCurrentPwd, mTilNewPwd, mTilConfirmPwd;
TextInputEditText mEdtCurrentPwd, mEdtNewPwd, mEdtConfirmPwd;
ImageView mIvChangePwdCancel;
TextView mTvLblChangePwd;
Button mBtnSubmit;
public ChangePasswordFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_change_password, container, false);
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
dataBinding();
}
private void dataBinding() {
try {
mIvChangePwdCancel = getActivity().findViewById(R.id.imgChangePwdCancel);
mBtnSubmit = getActivity().findViewById(R.id.btnSubmit);
mTvLblChangePwd = getActivity().findViewById(R.id.txtLblChangePwd);
mTvLblChangePwd.setTypeface(HmFonts.getRobotoBold(getContext()));
mLlChangePwdMain = getActivity().findViewById(R.id.llChangePwdMain);
mLlChangePwd = getActivity().findViewById(R.id.llChangePwd);
mLlChangePwdEdit = getActivity().findViewById(R.id.llChangePwdEdit);
mEdtCurrentPwd = getActivity().findViewById(R.id.edtCurrentPwd);
mEdtCurrentPwd.setTypeface(HmFonts.getRobotoRegular(getContext()));
mEdtNewPwd = getActivity().findViewById(R.id.edtNewPwd);
mEdtNewPwd.setTypeface(HmFonts.getRobotoRegular(getContext()));
mEdtConfirmPwd = getActivity().findViewById(R.id.edtConfirmPwd);
mEdtConfirmPwd.setTypeface(HmFonts.getRobotoRegular(getContext()));
mTilCurrentPwd = getActivity().findViewById(R.id.mTilCurrentPwd);
mTilCurrentPwd.setTypeface(HmFonts.getRobotoRegular(getContext()));
mTilNewPwd = getActivity().findViewById(R.id.mTilNewPwd);
mTilNewPwd.setTypeface(HmFonts.getRobotoRegular(getContext()));
mTilConfirmPwd = getActivity().findViewById(R.id.mTilConfirmPwd);
mTilConfirmPwd.setTypeface(HmFonts.getRobotoRegular(getContext()));
mIvChangePwdCancel.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
getActivity().getSupportFragmentManager().beginTransaction().remove(ChangePasswordFragment.this).commit();
}
});
mBtnSubmit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
KeyBoard.hideKeyboard(getActivity());
toSetNewPassword();
}
});
mEdtCurrentPwd.setOnEditorActionListener(new EditText.OnEditorActionListener() {
@Override
public boolean onEditorAction(TextView v, int actionId, KeyEvent event) {
if (actionId == EditorInfo.IME_ACTION_NEXT) {
if (isValidCurrentPassword()) {
mEdtNewPwd.requestFocus();
return true;
} else {
mEdtCurrentPwd.requestFocus();
return false;
}
} else {
return false;
}
}
});
mEdtNewPwd.setOnEditorActionListener(new EditText.OnEditorActionListener() {
@Override
public boolean onEditorAction(TextView v, int actionId, KeyEvent event) {
if (actionId == EditorInfo.IME_ACTION_NEXT) {
if (isValidNewPassword()) {
mEdtConfirmPwd.requestFocus();
return true;
} else {
mEdtNewPwd.requestFocus();
return false;
}
} else {
return false;
}
}
});
mEdtConfirmPwd.setOnEditorActionListener(new EditText.OnEditorActionListener() {
@Override
public boolean onEditorAction(TextView v, int actionId, KeyEvent event) {
if (actionId == EditorInfo.IME_ACTION_NEXT) {
if (isValidCnfPassword()) {
mBtnSubmit.requestFocus();
return true;
} else {
mEdtConfirmPwd.requestFocus();
return false;
}
} else {
return false;
}
}
});
mEdtCurrentPwd.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
isValidCurrentPassword();
}
@Override
public void afterTextChanged(Editable s) {
isValidCurrentPassword();
}
});
mEdtNewPwd.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
isValidNewPassword();
}
@Override
public void afterTextChanged(Editable s) {
isValidNewPassword();
}
});
mEdtConfirmPwd.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
isValidCnfPassword();
}
@Override
public void afterTextChanged(Editable s) {
isValidCnfPassword();
}
});
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
public boolean isValidCurrentPassword() {
if (mEdtCurrentPwd.getText().toString().trim().length() == 0) {
mTilCurrentPwd.setError(getString(R.string.str_field_cant_be_empty));
return false;
} else if (mEdtCurrentPwd.getText().toString().trim().length() < 8) {
mTilCurrentPwd.setError(getString(R.string.str_error_minimum_8));
return false;
} else if (mEdtCurrentPwd.getText().toString().trim().length() > 15) {
mTilCurrentPwd.setError(getString(R.string.str_error_maximum_15));
return false;
} else if (CommonFunctions.isValidPassword(mEdtCurrentPwd.getText().toString().trim())) {
mTilCurrentPwd.setError(getString(R.string.str_error_pswd));
return false;
} else {
mTilCurrentPwd.setError(null);
return true;
}
}
public boolean isValidNewPassword() {
if (mEdtNewPwd.getText().toString().trim().length() == 0) {
mTilNewPwd.setError(getString(R.string.str_field_cant_be_empty));
return false;
} else if (mEdtNewPwd.getText().toString().trim().length() < 8) {
mTilNewPwd.setError(getString(R.string.str_error_minimum_8));
return false;
} else if (mEdtNewPwd.getText().toString().trim().length() > 15) {
mTilNewPwd.setError(getString(R.string.str_error_maximum_15));
return false;
} else if (CommonFunctions.isValidPassword(mEdtNewPwd.getText().toString().trim())) {
mTilNewPwd.setError(getString(R.string.str_error_pswd));
return false;
} else {
mTilNewPwd.setError(null);
return true;
}
}
public boolean isValidCnfPassword() {
if (mEdtConfirmPwd.getText().toString().trim().length() == 0) {
mTilConfirmPwd.setError(getString(R.string.str_field_cant_be_empty));
return false;
} else if (mEdtConfirmPwd.getText().toString().trim().length() < 8) {
mTilConfirmPwd.setError(getString(R.string.str_error_minimum_8));
return false;
} else if (mEdtConfirmPwd.getText().toString().trim().length() > 15) {
mTilConfirmPwd.setError(getString(R.string.str_error_maximum_15));
return false;
} else if (CommonFunctions.isValidPassword(mEdtConfirmPwd.getText().toString().trim())) {
mTilConfirmPwd.setError(getString(R.string.str_error_pswd));
return false;
} else {
mTilConfirmPwd.setError(null);
return true;
}
}
public void toSetNewPassword(){
String oldPwd = mEdtCurrentPwd.getText().toString().trim();
String newPwd = mEdtNewPwd.getText().toString().trim();
String cnfPwd = mEdtConfirmPwd.getText().toString().trim();
if (!oldPwd.equals(newPwd)){
if (newPwd.equals(cnfPwd)){
toChangePassword(getContext());
}else {
mTilConfirmPwd.setError("Invalid password");
mTilNewPwd.setError("Invalid Password");
CommonFunctions.toDisplayToast("Password Don't Match !",getContext());
}
}else {
mTilCurrentPwd.setError("Invalid Password");
mTilNewPwd.setError("Invalid Password");
CommonFunctions.toDisplayToast("Current Password is Incorrect!",getContext());
}
}
public void toChangePassword(final Context context) {
try {
CommonFunctions.toCallLoader(context, "Loading");
VolleySingleton.getInstance(context)
.addToRequestQueue(
new StringRequest(
Request.Method.POST,
AppConstants.URL + context.getResources().getString(R.string.str_register_login) + context.getResources().getString(R.string.str_php),
new Response.Listener<String>() {
@Override
public void onResponse(String res) {
try {
CommonFunctions.toCloseLoader();
Log.d("HmApp", " Change Password: " + res);
if (res != null) {
JSONObject response = new JSONObject(res.trim());
if (response != null) {
Log.d("HmApp", "Change Password123:" + response);
if (!response.isNull("status")) {
if (response.getInt("status") == 0) {
CommonFunctions.toDisplayToast("Unable to Change Password", context);
} else if (response.getInt("status") == 1) {
CommonFunctions.toDisplayToast("Password Change Successfully", context);
getActivity().getSupportFragmentManager().beginTransaction().remove(ChangePasswordFragment.this).commit();
}
}
} else {
CommonFunctions.toDisplayToast("Unable to Change Password", context);
}
} else {
CommonFunctions.toDisplayToast("Unable to Change Password", context);
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
CommonFunctions.toCloseLoader();
}
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
error.printStackTrace();
CommonFunctions.toCloseLoader();
CommonFunctions.toDisplayToast("Unable to Change Password", context);
}
}
) {
@Override
protected Map<String, String> getParams() {
Map<String, String> params = new HashMap<String, String>();
params.put(context.getResources().getString(R.string.str_action_), context.getString(R.string.str_change_password_));
params.put(context.getString(R.string.str_uid), User.getUser(context).getUid());
params.put(context.getResources().getString(R.string.str_old_password),mEdtCurrentPwd.getText().toString().trim());
params.put(context.getResources().getString(R.string.str_new_password_), mEdtNewPwd.getText().toString().trim());
return params;
}
}
, context.getString(R.string.str_change_password_));
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
CommonFunctions.toCloseLoader();
}
}
}
<file_sep>package com.hm.application.adapter;
import android.annotation.SuppressLint;
import android.content.Context;
import android.support.annotation.NonNull;
import android.support.design.widget.TabLayout;
import android.support.v4.view.PagerAdapter;
import android.util.Log;
import android.view.GestureDetector;
import android.view.LayoutInflater;
import android.view.MotionEvent;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.common.MyPost;
import com.hm.application.fragments.UserTab1Fragment;
import com.hm.application.model.AppConstants;
import com.squareup.picasso.Picasso;
public class SlidingImageAdapter extends PagerAdapter {
private String[] images;
private String pos;
private Context context;
private TabLayout tabLayout;
private boolean status;
private UserTab1Fragment userTab1Fragment;
public SlidingImageAdapter(Context ctx, String[] img, String position, UserTab1Fragment userTab1Frg, TabLayout mTl) {
context = ctx;
images = img;
pos = position;
tabLayout = mTl;
userTab1Fragment = userTab1Frg;
}
@Override
public void destroyItem(@NonNull ViewGroup container, int position, @NonNull Object object) {
container.removeView((View) object);
}
@Override
public int getCount() {
return images.length;
}
@SuppressLint("ClickableViewAccessibility")
@NonNull
@Override
public Object instantiateItem(@NonNull ViewGroup view, final int position) {
try {
Log.d("Hmapp", "images " + images[position]);
View inflate = LayoutInflater.from(context).inflate(R.layout.single_image_view, view, false);
ImageView imageView = inflate.findViewById(R.id.image_single);
Picasso.with(context)
.load(AppConstants.URL + images[position].trim().replace("\\s", "%20"))
.into(imageView);
view.addView(inflate, 0);
// imageView.setOnClickListener(new View.OnClickListener() {
//// @Override
//// public void onClick(View v) {
//// if (userTab1Fragment != null) {
//// userTab1Fragment.toCallSinglePostData(Integer.parseInt(pos), "Multiple");
//// }
//// }
//// });
if (images.length > 1) {
tabLayout.setVisibility(View.VISIBLE);
} else {
tabLayout.setVisibility(View.GONE);
}
return inflate;
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
return null;
}
@Override
public boolean isViewFromObject(@NonNull View view, @NonNull Object object) {
return view.equals(object);
}
}<file_sep>package com.hm.application.fragments;
import android.content.Context;
import android.net.Uri;
import android.os.AsyncTask;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentTransaction;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.RelativeLayout;
import android.widget.TextView;
import com.android.volley.Request;
import com.android.volley.Response;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.StringRequest;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.adapter.NotificationAdapter;
import com.hm.application.model.AppConstants;
import com.hm.application.model.User;
import com.hm.application.network.VolleySingleton;
import com.hm.application.utils.CommonFunctions;
import com.hm.application.utils.HmFonts;
import org.json.JSONArray;
import org.json.JSONObject;
import java.util.HashMap;
import java.util.Map;
public class NotificationYouFragment extends Fragment {
Bundle bundle;
private RecyclerView mRvNfMain;
private RelativeLayout mRlFollowRequest;
private TextView mTvNfFollow, mTvNfYou, mTvFollowRequest, mTvFollow;
private OnFragmentInteractionListener mListener;
private String timelineId = null;
private JSONObject obj;
public NotificationYouFragment() {
// Required empty public constructor
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_notification_you, container, false);
}
@Override
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
checkInternetConnection();
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
private void checkInternetConnection() {
try {
if (CommonFunctions.isOnline(getContext())) {
new toGetNotification().execute();
mTvNfYou = getActivity().findViewById(R.id.tvNfYou);
mTvNfYou.setTypeface(HmFonts.getRobotoBold(getContext()));
mTvFollowRequest = getActivity().findViewById(R.id.tvFollowRequest);
mTvFollowRequest.setTypeface(HmFonts.getRobotoBold(getContext()));
mTvFollow = getActivity().findViewById(R.id.tvFollow);
mTvFollow.setTypeface(HmFonts.getRobotoRegular(getContext()));
mRlFollowRequest = getActivity().findViewById(R.id.rlFollowRequest);
mRlFollowRequest.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Main_FriendRequestFragment main = new Main_FriendRequestFragment();
getActivity()
.getSupportFragmentManager()
.beginTransaction()
.add(R.id.flHomeContainer, main)
.addToBackStack(main.getClass().getName())
.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN)
.commit();
}
});
} else {
CommonFunctions.toDisplayToast(getResources().getString(R.string.lbl_no_check_internet), getContext());
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
public interface OnFragmentInteractionListener {
void onFragmentInteraction(Uri uri);
}
private class toGetNotification extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... voids) {
try {
VolleySingleton.getInstance(getContext())
.addToRequestQueue(
new StringRequest(Request.Method.POST,
AppConstants.URL + getString(R.string.str_notification_uid) + getContext().getResources().getString(R.string.str_php),
new Response.Listener<String>() {
@Override
public void onResponse(String res) {
Log.d("HmApp", "notification:" + res);
try {
if (res != null && res.length() > 0) {
JSONArray array = new JSONArray(res.trim());
if (array != null) {
if (array.length() > 0) {
mRvNfMain = getActivity().findViewById(R.id.rvNfYou);
LinearLayoutManager llm = new LinearLayoutManager(getContext());
llm.setReverseLayout(true);
mRvNfMain.setLayoutManager(llm);
mRvNfMain.hasFixedSize();
mRvNfMain.setAdapter(new NotificationAdapter(getContext(), array));
} else {
CommonFunctions.toDisplayToast("No Data Found", getContext());
}
} else {
CommonFunctions.toDisplayToast("No Data Found", getContext());
}
} else {
CommonFunctions.toDisplayToast("No Data Found", getContext());
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
error.printStackTrace();
}
}
) {
@Override
protected Map<String, String> getParams() {
Map<String, String> params = new HashMap<String, String>();
params.put(getString(R.string.str_action_), getString(R.string.str_list_notification));
params.put(getString(R.string.str_uid), User.getUser(getContext()).getUid());
return params;
}
}
, getContext().getString(R.string.str_sent_notification));
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
return null;
}
}
}
<file_sep>package com.hm.application.services;
import android.util.Log;
import com.crashlytics.android.Crashlytics;
import com.google.firebase.iid.FirebaseInstanceId;
import com.google.firebase.iid.FirebaseInstanceIdService;
import com.hm.application.model.AppDataStorage;
import com.hm.application.model.User;
import java.util.zip.CRC32;
public class MyFirebaseInstanceIDService extends FirebaseInstanceIdService {
@Override
public void onTokenRefresh() {
try {
// Get updated InstanceID token.
String refreshedToken = FirebaseInstanceId.getInstance().getToken();
// If you want to send messages to this application instance or
// manage this apps subscriptions on the server side, send the
// Instance ID token to your app server.
Log.d("HmApp", "Refreshed token: " + refreshedToken);
User.getUser(this).setFcmToken(refreshedToken);
AppDataStorage.setUserInfo(this);
} catch (Exception| Error e){
e.printStackTrace();
Crashlytics.logException(e);
}
}
}<file_sep>package com.hm.application.adapter;
import android.content.Context;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.v4.content.ContextCompat;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.activity.SinglePostDataActivity;
import com.hm.application.model.AppConstants;
import com.squareup.picasso.Picasso;
import org.json.JSONArray;
public class UserTab21Adapter extends RecyclerView.Adapter<UserTab21Adapter.ViewHolder> {
private Context context;
private JSONArray array;
public UserTab21Adapter(Context ctx, JSONArray data) {
context = ctx;
array = data;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
return new ViewHolder(LayoutInflater.from(context).inflate(R.layout.album_layout, parent, false));
}
@Override
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
try {
if (!array.getJSONObject(position).isNull(context.getString(R.string.str_image_url))) {
Picasso.with(context)
.load(AppConstants.URL + array.getJSONObject(position).getString(context.getString(R.string.str_image_url)).replaceAll("\\s", "%20"))
.error(R.color.light2)
.placeholder(R.color.light)
.into(holder.mImgActPic);
} else {
holder.mImgActPic.setBackgroundColor(ContextCompat.getColor(context, R.color.light2));
}
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
@Override
public int getItemCount() {
return array == null ? 0 : array.length();
}
class ViewHolder extends RecyclerView.ViewHolder {
private ImageView mImgActPic;
private TextView mTxtAlbumName;
ViewHolder(View itemView) {
super(itemView);
mTxtAlbumName = itemView.findViewById(R.id.txtAlbumName);
mTxtAlbumName.setVisibility(View.GONE);
mImgActPic = itemView.findViewById(R.id.imgAlbumPic);
mImgActPic.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
context.startActivity(
new Intent(context, SinglePostDataActivity.class)
.putExtra(AppConstants.FROM, "Single")
.putExtra(AppConstants.BUNDLE, array.getJSONObject(getAdapterPosition()).toString()));
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
});
}
}
}<file_sep>package com.hm.application.classes;
import android.content.Context;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.LinearLayout;
import com.android.volley.Request;
import com.android.volley.Response;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.StringRequest;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.adapter.CommentsAdapter;
import com.hm.application.adapter.DisplayReplyAdapter;
import com.hm.application.model.AppConstants;
import com.hm.application.network.VolleySingleton;
import com.hm.application.utils.CommonFunctions;
import org.json.JSONArray;
import java.util.HashMap;
import java.util.Map;
public class Post {
static RecyclerView mRvCmt;
static LinearLayout mLlAddCmt;
static Context context;
static String timelineId;
public static void toDisplayComments(String timelineId_, RecyclerView mRvCmt_, Context context_, LinearLayout mLlAddCmt_) {
timelineId = timelineId_;
mRvCmt = mRvCmt_;
mLlAddCmt = mLlAddCmt_;
context = context_;
toDisplayComments();
}
private static void toDisplayComments() {// extends AsyncTask<Void, Void, Void> {
// @Override
// protected Void doInBackground(Void... voids) {
new Thread(new Runnable() {
@Override
public void run() {
try {
VolleySingleton.getInstance(context)
.addToRequestQueue(
new StringRequest(Request.Method.POST,
AppConstants.URL + context.getString(R.string.str_like_share_comment) + context.getString(R.string.str_php),
new Response.Listener<String>() {
@Override
public void onResponse(String res) {
try {
if (res != null && res.length()>0) {
Log.d("HmApp", "Comment Res: " + res);
JSONArray array = new JSONArray(res.trim());
if (array.length() > 0) {
if (mLlAddCmt.getChildCount() > 0) {
mLlAddCmt.removeAllViews();
}
LinearLayoutManager llm = new LinearLayoutManager(context);
// llm.setReverseLayout(true);
// llm.setStackFromEnd(true);
mRvCmt.setLayoutManager(llm);
mRvCmt.hasFixedSize();
mRvCmt.setVisibility(View.VISIBLE);
mRvCmt.setNestedScrollingEnabled(false);
mRvCmt.setAdapter(new CommentsAdapter(context, array));
// mRvCmt.smoothScrollToPosition(array.length() - 1);
} else {
CommonFunctions.toDisplayToast("No Comment", context);
}
} else {
CommonFunctions.toDisplayToast("No Comment", context);
}
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
error.printStackTrace();
}
}
) {
@Override
protected Map<String, String> getParams() {
Map<String, String> params = new HashMap<>();
params.put(context.getString(R.string.str_action_), context.getString(R.string.str_fetch_comment_));
params.put(context.getString(R.string.str_timeline_id_), timelineId);
// params.put(context.getString(R.string.str_timeline_id_), "102");
Log.d("hmapp", " comment fragment_timeline Api:" + timelineId);
return params;
}
}
, context.getString(R.string.str_fetch_comment_));
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
}).start();
// return null;
// }
}
public static void toDisplayReply(final String id, LinearLayout mLlCuReply, final Context context) {
try {
if (mLlCuReply.getChildCount() > 0) {
mLlCuReply.removeAllViews();
}
final View view = LayoutInflater.from(context).inflate(R.layout.rv_layout, null, false);
if (view != null) {
VolleySingleton.getInstance(context)
.addToRequestQueue(
new StringRequest(Request.Method.POST,
AppConstants.URL + context.getString(R.string.str_like_share_comment) + context.getString(R.string.str_php),
new Response.Listener<String>() {
@Override
public void onResponse(String res) {
try {
if (res != null && res.trim().length()>0){
Log.d("HmApp", "Reply Res " + res);
JSONArray array = new JSONArray(res);
if (array != null) {
if (array.length() > 0) {
RecyclerView mRv = view.findViewById(R.id.mRvCommon);
mRv.setLayoutManager(new LinearLayoutManager(context));
mRv.hasFixedSize();
mRv.setNestedScrollingEnabled(false);
mRv.setAdapter(new DisplayReplyAdapter(context, array));
} else {
CommonFunctions.toDisplayToast("No Reply", context);
}
} else {
CommonFunctions.toDisplayToast("No Reply", context);
}
} else {
CommonFunctions.toDisplayToast("No Reply", context);
}
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
error.printStackTrace();
}
}
) {
@Override
protected Map<String, String> getParams() {
Map<String, String> params = new HashMap<String, String>();
params.put(context.getString(R.string.str_action_), context.getString(R.string.str_fetch_reply_comment_));
params.put(context.getString(R.string.str_comment_id), id);
return params;
}
}
, context.getString(R.string.str_fetch_reply_comment_));
mLlCuReply.addView(view);
}
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
}<file_sep>package com.hm.application.fragments;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.database.Cursor;
import android.graphics.Bitmap;
import android.net.Uri;
import android.os.Bundle;
import android.provider.MediaStore;
import android.provider.MediaStore.MediaColumns;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.support.v4.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.CheckBox;
import android.widget.GridView;
import android.widget.ImageView;
import android.widget.RelativeLayout;
import android.widget.TextView;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.activity.NextActivity;
import com.hm.application.model.AppConstants;
import com.hm.application.utils.CommonFunctions;
import com.hm.application.utils.insta.utils.SquareImageView;
import com.nostra13.universalimageloader.core.ImageLoader;
import com.nostra13.universalimageloader.core.ImageLoaderConfiguration;
import com.nostra13.universalimageloader.core.assist.FailReason;
import com.nostra13.universalimageloader.core.listener.ImageLoadingListener;
import java.util.ArrayList;
public class GalleryFragment extends Fragment {
private GridView mGridView;
private ImageView mIvSelectImage;
private TextView mTvNextScreen;
private ArrayList<String> mMultiSelectImages, mAllImages;
private OnFragmentInteractionListener mListener;
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
public interface OnFragmentInteractionListener {
void onFragmentInteraction(Uri uri);
}
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = null;
try {
view = inflater.inflate(R.layout.fragment_gallery, container, false);
mIvSelectImage = view.findViewById(R.id.galleryImageView);
mGridView = view.findViewById(R.id.gridViewGallery);
mMultiSelectImages = new ArrayList<>();
mAllImages = new ArrayList<>();
mTvNextScreen = view.findViewById(R.id.tvNext);
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
return view;
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
try {
mAllImages = getImagesPath(getActivity());
int gridWidth = getResources().getDisplayMetrics().widthPixels;
int imageWidth = gridWidth / 3;
mGridView.setColumnWidth(imageWidth);
mTvNextScreen.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (mMultiSelectImages.size() == 0) {
CommonFunctions.toDisplayToast("Please select at least one image", getContext());
} else {
if (mMultiSelectImages.size() > 1) {
CommonFunctions.toDisplayToast("You've selected Total " + mMultiSelectImages.size() + " image(s).", getContext());
}
Intent intent = new Intent(getActivity(), NextActivity.class);
// intent.putExtra(getString(R.string.selected_image), mSelectedImage);
intent.putExtra("list", mMultiSelectImages);
startActivity(intent);
}
}
});
if (mAllImages != null && mAllImages.size() > 0) {
mGridView.setAdapter(new GridImageAdapter(getContext(), R.layout.layout_grid_imageview, mAllImages));
setImage(mAllImages.get(0), mIvSelectImage, AppConstants.Append);
} else {
CommonFunctions.toDisplayToast(" No Images ", getContext());
}
mGridView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
setImage(mAllImages.get(position), mIvSelectImage, AppConstants.Append);
}
});
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
public static ArrayList<String> getImagesPath(Activity activity) {
Uri uri;
ArrayList<String> listOfAllImages = new ArrayList<String>();
Cursor cursor;
int column_index_data;
String PathOfImage = null;
uri = android.provider.MediaStore.Images.Media.EXTERNAL_CONTENT_URI;
String[] projection = {MediaColumns.DATA,
MediaStore.Images.Media.BUCKET_DISPLAY_NAME};
cursor = activity.getContentResolver().query(uri, projection, null,
null, null);
column_index_data = cursor.getColumnIndexOrThrow(MediaColumns.DATA);
while (cursor.moveToNext()) {
PathOfImage = cursor.getString(column_index_data);
listOfAllImages.add(PathOfImage);
}
return listOfAllImages;
}
private void setImage(String imgURL, ImageView image, String append) {
ImageLoader imageLoader = ImageLoader.getInstance();
imageLoader.init(ImageLoaderConfiguration.createDefault(getContext()));
imageLoader.displayImage(append + imgURL, image, new ImageLoadingListener() {
@Override
public void onLoadingStarted(String imageUri, View view) {
}
@Override
public void onLoadingFailed(String imageUri, View view, FailReason failReason) {
}
@Override
public void onLoadingComplete(String imageUri, View view, Bitmap loadedImage) {
}
@Override
public void onLoadingCancelled(String imageUri, View view) {
}
});
}
public class GridImageAdapter extends ArrayAdapter<String> {
private Context context;
private int layoutResource;
private ArrayList<String> imgURLs;
public GridImageAdapter(Context context, int layoutResource, ArrayList<String> imgURLs) {
super(context, layoutResource, imgURLs);
this.context = context;
this.layoutResource = layoutResource;
this.imgURLs = imgURLs;
}
private class ViewHolder {
SquareImageView image;
CheckBox checkbox;
RelativeLayout relativeLayout;
int id;
}
@NonNull
@Override
public View getView(final int position, @Nullable View row, @NonNull ViewGroup parent) {
final ViewHolder holder;
View convertView = row;
if (convertView == null) {
LayoutInflater inflater = ((Activity) context).getLayoutInflater();
convertView = inflater.inflate(layoutResource, parent, false);
holder = new ViewHolder();
holder.image = convertView.findViewById(R.id.gridImageView);
holder.checkbox = convertView.findViewById(R.id.itemCheckBox);
holder.relativeLayout = convertView.findViewById(R.id.rl1);
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
holder.checkbox.setId(position);
holder.relativeLayout.setId(position);
holder.image.setId(position);
holder.relativeLayout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int id = v.getId();
if (holder.checkbox.isChecked()) {
holder.checkbox.setChecked(false);
if (mMultiSelectImages != null) {
mMultiSelectImages.remove(getItem(id));
}
} else {
holder.checkbox.setChecked(true);
if (mMultiSelectImages != null) {
mMultiSelectImages.add(getItem(id));
}
setImage(getItem(id), mIvSelectImage, AppConstants.Append);
}
}
});
holder.checkbox.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
toSetView(v);
}
});
ImageLoader imageLoader = ImageLoader.getInstance();
imageLoader.init(ImageLoaderConfiguration.createDefault(getContext()));
imageLoader.displayImage(AppConstants.Append + getItem(position), holder.image, new ImageLoadingListener() {
@Override
public void onLoadingStarted(String imageUri, View view) {
}
@Override
public void onLoadingFailed(String imageUri, View view, FailReason failReason) {
}
@Override
public void onLoadingComplete(String imageUri, View view, Bitmap loadedImage) {
}
@Override
public void onLoadingCancelled(String imageUri, View view) {
}
});
holder.checkbox.setChecked(mMultiSelectImages.contains(getItem(position)));
return convertView;
}
private void toSetView(View v) {
CheckBox cb = (CheckBox) v;
int id = cb.getId();
if (mMultiSelectImages.contains(getItem(id))) {
cb.setChecked(false);
if (mMultiSelectImages != null) {
mMultiSelectImages.remove(getItem(id));
}
} else {
cb.setChecked(true);
if (mMultiSelectImages != null) {
mMultiSelectImages.add(getItem(id));
}
setImage(getItem(id), mIvSelectImage, AppConstants.Append);
}
}
}
}<file_sep>apply plugin: 'com.android.application'
apply plugin: 'io.fabric'
apply plugin: 'com.google.firebase.firebase-perf'
android {
useLibrary 'org.apache.http.legacy'
compileSdkVersion 27
defaultConfig {
applicationId "com.hm.application"
minSdkVersion 16
targetSdkVersion 27
versionCode 1
versionName "1.0"
multiDexEnabled true
testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
debug {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
packagingOptions {
exclude 'META-INF/DEPENDENCIES'
exclude 'META-INF/LICENSE'
exclude 'META-INF/LICENSE.txt'
exclude 'META-INF/license.txt'
exclude 'META-INF/NOTICE'
exclude 'META-INF/NOTICE.txt'
exclude 'META-INF/notice.txt'
exclude 'META-INF/ASL2.0'
}
}
dependencies {
implementation fileTree(include: ['*.jar'], dir: 'libs')
implementation 'com.android.support:appcompat-v7:27.1.1'
implementation 'com.android.support:cardview-v7:27.1.1'
implementation 'com.android.support.constraint:constraint-layout:1.1.0'
implementation 'com.android.support:design:27.1.1'
implementation 'com.android.support:gridlayout-v7:27.1.1'
implementation 'com.android.support:mediarouter-v7:27.1.1'
implementation 'com.android.support:multidex:1.0.3'
implementation 'com.android.support:recyclerview-v7:27.1.1'
implementation 'com.android.support:support-v4:27.1.1'
implementation 'com.android.volley:volley:1.1.0'
implementation 'com.crashlytics.sdk.android:crashlytics:2.9.2'
implementation 'com.google.android.gms:play-services-analytics:15.0.2'
implementation 'com.google.android.gms:play-services-gcm:15.0.1'
implementation 'com.google.android.gms:play-services-location:15.0.1'
implementation 'com.google.android.gms:play-services-maps:15.0.1'
implementation 'com.google.android.gms:play-services-places:15.0.1'
implementation 'com.google.android.exoplayer:exoplayer:2.6.0'
implementation 'com.google.code.gson:gson:2.8.0'
implementation 'com.google.firebase:firebase-messaging:15.0.2'
implementation 'com.google.firebase:firebase-perf:15.1.0'
implementation 'com.github.ittianyu:BottomNavigationViewEx:1.1.9'
implementation 'com.github.eschao:android-ElasticListView:v1.0'
implementation 'com.github.bumptech.glide:glide:4.4.0'
implementation 'com.nostra13.universalimageloader:universal-image-loader:1.9.5'
implementation 'com.afollestad.material-dialogs:core:0.9.6.0'
implementation 'com.android.support:exifinterface:27.1.1'
implementation 'com.felipecsl.asymmetricgridview:library:2.0.1'
implementation 'com.squareup.picasso:picasso:2.5.2'
implementation 'de.hdodenhof:circleimageview:2.2.0'
implementation 'org.apache.httpcomponents:httpcore:4.3.2'
implementation 'org.apache.httpcomponents:httpmime:4.3'
implementation 'nl.psdcompany:duo-navigation-drawer:2.0.5'
implementation files('libs/aspectjrt-1.7.3 (1).jar')
implementation files('libs/aspectjrt-1.7.3 (1).jar')
annotationProcessor 'com.github.bumptech.glide:compiler:4.4.0'
testImplementation 'junit:junit:4.12'
androidTestImplementation 'com.android.support.test:runner:1.0.2'
androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2'
}
// Add to the bottom of the file
apply plugin: 'com.google.gms.google-services'
<file_sep>package com.hm.application.fragments;
import android.content.Context;
import android.net.Uri;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.design.widget.TabLayout;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentTransaction;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.model.AppConstants;
import com.hm.application.utils.CommonFunctions;
public class UserTab2Fragment extends Fragment {
Bundle bundle;
private TabLayout tabLayout;
private OnFragmentInteractionListener mListener;
public UserTab2Fragment() {
// Required empty public constructor
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_user_tab2, container, false);
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
checkInternetConnection();
tabLayout = getActivity().findViewById(R.id.tbUsersTab2);
replacePage(new UserTab21Fragment());
tabLayout.addOnTabSelectedListener(new TabLayout.OnTabSelectedListener() {
@Override
public void onTabSelected(TabLayout.Tab tab) {
switch (tab.getPosition()) {
case 0:
replacePage(new UserTab21Fragment());
break;
case 1:
replacePage(new UserTab22Fragment());
break;
case 2:
replacePage(new UserTab23Fragment());
break;
case 3:
replacePage(new UserTab24Fragment());
break;
default:
replacePage(new UserTab21Fragment());
break;
}
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {
}
@Override
public void onTabReselected(TabLayout.Tab tab) {
}
});
}
private void checkInternetConnection() {
try {
if (CommonFunctions.isOnline(getContext())) {
Log.d("HmApp", " agr fetch_timeline 1 " + getArguments());
if (getArguments() != null) {
bundle = new Bundle();
Log.d("HmApp", " agr2 " + getArguments().getBoolean("other_user"));
bundle.putBoolean("other_user2", getArguments().getBoolean("other_user"));
bundle.putString(AppConstants.F_UID, getArguments().getString("F_UID"));
bundle.putString("fetch_photos2", getArguments().getString("fetch_photos"));
bundle.putString("fetch_albums2", getArguments().getString("fetch_albums"));
}
} else {
CommonFunctions.toDisplayToast(getResources().getString(R.string.lbl_no_check_internet), getContext());
}
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
public void replacePage(Fragment fragment) {
fragment.setArguments(bundle);
getActivity().getSupportFragmentManager()
.beginTransaction()
.replace(R.id.flUsersTab2Container, fragment)
// .addToBackStack(fragment.getClass().getName())
.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN)
.commit();
}
public interface OnFragmentInteractionListener {
void onFragmentInteraction(Uri uri);
void toSetTitle(String s, boolean b);
}
}
<file_sep>package com.hm.application.utils.insta.utils;
import android.util.Log;
import java.io.File;
import java.io.FileFilter;
import java.util.ArrayList;
import java.util.Arrays;
public class FileSearch {
public static ArrayList<String> getDirectoryPaths(String directory) {
ArrayList<String> pathArray = new ArrayList<>();
File file = new File(directory);
File[] listfiles = file.listFiles();
for (int i = 0; i < listfiles.length; i++) {
Log.d("hmapp", " isdirectory " + listfiles[i] + " : " + (listfiles[i].isDirectory()&& file.listFiles(new ImageFileFilter()).length > 0)
+ " : " + listfiles[i].isDirectory()
// + " : " + Arrays.toString(file.listFiles())
+ " : " + (file.listFiles(new ImageFileFilter()).length > 0)
);
if (listfiles[i].isDirectory()&& file.listFiles(new ImageFileFilter()).length > 0) {
pathArray.add(listfiles[i].getAbsolutePath());
}
}
return pathArray;
}
/**
* Search a directory and return a list of all **files** contained inside
*
* @param directory
* @return
*/
public static ArrayList<String> getFilePaths(String directory) {
Log.d("hmapp Gallery", " getfilepaths : " + directory);
ArrayList<String> pathArray = new ArrayList<>();
File file = new File(directory);
Log.d("hmaPp Gallery", "listFiles : " + Arrays.toString(file.listFiles())) ;
File[] listfiles = file.listFiles();
for (int i = 0; i < listfiles.length; i++) {
if (listfiles[i].isFile()) {
Log.d("hmapp Gallery", " getabsolutepath "+ listfiles[i].getAbsolutePath());
pathArray.add(listfiles[i].getAbsolutePath());
}
}
return pathArray;
}
/**
* Checks the file to see if it has a compatible extension.
*/
private static boolean isImageFile(String filePath) {
return filePath.endsWith(".jpg") || filePath.endsWith(".png");
}
/**
* This can be used to filter files.
*/
private static class ImageFileFilter implements FileFilter {
@Override
public boolean accept(File file) {
Log.d("Hmapp Gallery", " ImageFileFilter : " + file.getAbsolutePath());
if (file.isDirectory()) {
return true;
}
else
return isImageFile(file.getAbsolutePath());
}
}
}
<file_sep>package com.hm.application.network;
import android.content.Context;
import android.util.Log;
import com.android.volley.Request;
import com.android.volley.RequestQueue;
import com.android.volley.RetryPolicy;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.Volley;
import com.crashlytics.android.Crashlytics;
public class VolleySingleton {
private static VolleySingleton mInstance;
private static Context mContext;
private RequestQueue mRequestQueue;
private VolleySingleton(Context context) {
mContext = context;
mRequestQueue = getRequestQueue();
}
public static synchronized VolleySingleton getInstance(Context context) {
if (mInstance == null) {
mInstance = new VolleySingleton(context);
}
return mInstance;
}
private RequestQueue getRequestQueue() {
if (mRequestQueue == null) {
mRequestQueue = Volley.newRequestQueue(mContext.getApplicationContext());
}
return mRequestQueue;
}
public <T> void addToRequestQueue(Request<T> req, String tag) {
try {
Log.d("HM_URL", " tag 1: " + tag + " : " + req.getUrl());
Log.d("HM_URL", " tag 2: " + new String(req.getBody(), "UTF-8"));
req.setRetryPolicy(new RetryPolicy() {
@Override
public int getCurrentTimeout() {
return 50000;
}
@Override
public int getCurrentRetryCount() {
return 50000;
}
@Override
public void retry(VolleyError error) {
error.printStackTrace();
}
});
getRequestQueue().add(req).setTag(tag);
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
public void cancelPendingRequests(Object tag) {
if (this.mRequestQueue != null) {
this.mRequestQueue.cancelAll(tag);
}
}
public <T> void addToRequestQueue(Request<T> request) {
getRequestQueue().add(request);
}
}<file_sep>package com.hm.application.activity;
import android.content.Context;
import android.content.Intent;
import android.graphics.drawable.ColorDrawable;
import android.os.Bundle;
import android.support.design.widget.TabLayout;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentTransaction;
import android.support.v4.content.ContextCompat;
import android.support.v4.view.ViewPager;
import android.support.v4.widget.NestedScrollView;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import android.view.KeyEvent;
import android.view.LayoutInflater;
import android.view.MenuItem;
import android.view.View;
import android.view.inputmethod.EditorInfo;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.FrameLayout;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.RelativeLayout;
import android.widget.TextView;
import com.android.volley.Request;
import com.android.volley.Response;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.StringRequest;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.adapter.SlidingImageAdapter;
import com.hm.application.classes.Post;
import com.hm.application.common.MyPost;
import com.hm.application.fragments.CommentFragment;
import com.hm.application.fragments.TimelineLikeListFragment;
import com.hm.application.model.AppConstants;
import com.hm.application.model.User;
import com.hm.application.network.VolleySingleton;
import com.hm.application.utils.CommonFunctions;
import com.hm.application.utils.HmFonts;
import com.squareup.picasso.Picasso;
import org.json.JSONArray;
import org.json.JSONObject;
import java.util.HashMap;
import java.util.Map;
import de.hdodenhof.circleimageview.CircleImageView;
public class SinglePostDataActivity extends AppCompatActivity {
private RelativeLayout mrr_header_file, mRrVpMain;
private CircleImageView mcircle_img;
private TextView mtxt_label, mtxt_time_ago, mtxtSpdPost, mTvTimeLineId;
private LinearLayout mLlSpdMain, mLlMainVpPost;
private TextView mtxtNo_like, mtxtNo_comment, mTvUserLikeName;
private FrameLayout mFlSpdHome;
private NestedScrollView mNsvSpdPost;
private CheckBox mChkPostLiked, mChkLike;
private LinearLayout mll_footer;
private ImageView mImgComment, mImgShare;
private ViewPager mVp;
private TabLayout mTl;
// Comments
private RecyclerView mRvCmt;
private Button mBtnCmt;
private LinearLayout mLlAddCmt, mllAddReply;
private ImageView mIvProfilePic;
private EditText mEdtCmt;
private TextView mTvCuReply;
private String timelineId = null, commentId = null;
private JSONObject obj;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_single_post_data);
try {
toSetTitle();
bindViews();
toSetData();
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private void toSetTitle() throws Error {
if (getSupportActionBar() != null) {
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowTitleEnabled(true);
getSupportActionBar().setHomeAsUpIndicator(ContextCompat.getDrawable(this, R.drawable.ic_back_black_24dp));
getSupportActionBar().setTitle(getResources().getString(R.string.app_name));
getSupportActionBar().setBackgroundDrawable(new ColorDrawable(getResources().getColor(R.color.white)));
}
}
private void bindViews() throws Error {
mNsvSpdPost = findViewById(R.id.nsvSpdPost);
mFlSpdHome = findViewById(R.id.flSpdHome);
mLlSpdMain = findViewById(R.id.llSpdMain);
mLlMainVpPost = findViewById(R.id.llMainVpPost);
mrr_header_file = findViewById(R.id.rrHeaderMain);
mRrVpMain = findViewById(R.id.rrVpMain);
mcircle_img = findViewById(R.id.circle_img);
mtxt_label = findViewById(R.id.txt_label);
mtxt_label.setTypeface(HmFonts.getRobotoMedium(SinglePostDataActivity.this));
mtxt_time_ago = findViewById(R.id.txt_time_ago);
mtxt_time_ago.setTypeface(HmFonts.getRobotoRegular(SinglePostDataActivity.this));
mtxtSpdPost = findViewById(R.id.txtSpdPost);
mtxtSpdPost.setTypeface(HmFonts.getRobotoMedium(SinglePostDataActivity.this));
mTvTimeLineId = findViewById(R.id.tvTimelineId);
mChkPostLiked = findViewById(R.id.chkPostLiked);
mChkLike = findViewById(R.id.chkLike);
mtxtNo_like = findViewById(R.id.txtNo_like);
mtxtNo_like.setTypeface(HmFonts.getRobotoRegular(SinglePostDataActivity.this));
mtxtNo_comment = findViewById(R.id.txtNo_comment);
mtxtNo_comment.setTypeface(HmFonts.getRobotoRegular(SinglePostDataActivity.this));
// mTvUserLikeName = findViewById(R.id.tvUserLikeName);
mll_footer = findViewById(R.id.llSdpFooter);
mImgComment = findViewById(R.id.imgComment);
mImgShare = findViewById(R.id.imgShare);
mVp = findViewById(R.id.vpMainPost);
mTl = findViewById(R.id.tlMainPost);
mLlAddCmt = findViewById(R.id.llAddCmtSPD);
mRvCmt = findViewById(R.id.rvCommentsSPD);
mRvCmt.setNestedScrollingEnabled(false);
mEdtCmt = findViewById(R.id.edtCfPost);
mBtnCmt = findViewById(R.id.btnCfSend);
mEdtCmt.setOnEditorActionListener(new EditText.OnEditorActionListener() {
@Override
public boolean onEditorAction(TextView v, int actionId, KeyEvent event) {
if (actionId == EditorInfo.IME_ACTION_DONE) {
toSetDataSending();
}
return false;
}
});
mBtnCmt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Log.d("hmapp", " comment submit spd: " + mEdtCmt.getText());
toSetDataSending();
}
});
mIvProfilePic = findViewById(R.id.imgCf);
if (User.getUser(this).getPicPath() != null) {
Picasso.with(this)
.load(AppConstants.URL + User.getUser(this).getPicPath().replaceAll("\\s", "%20"))
.resize(200, 200)
.error(R.color.light2)
.placeholder(R.color.light)
.into(mIvProfilePic);
}
}
private void toSetDataSending() {
try {
if (commentId != null) {
toSubmitReply();
} else {
toSubmitComment();
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private void toSubmitReply() {
try {
if (mEdtCmt.getText().toString().trim().length() > 0) {
MyPost.toReplyOnComment(this, commentId, mEdtCmt.getText().toString().trim(), mTvCuReply);
if (mllAddReply != null) {
toAddReply(mEdtCmt.getText().toString().trim(), mllAddReply);
}
mEdtCmt.setText("");
} else {
CommonFunctions.toDisplayToast("Empty", this);
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private void toSubmitComment() {
try {
if (mEdtCmt.getText().toString().trim().length() > 0) {
MyPost.toCommentOnPost(this, timelineId, mEdtCmt.getText().toString().trim(), mLlAddCmt);
toAddComment(mEdtCmt.getText().toString().trim(), mLlAddCmt);
mEdtCmt.setText("");
} else {
CommonFunctions.toDisplayToast("Empty", this);
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private void toAddComment(String data, LinearLayout mLlAddCmt) {
try {
View itemView = LayoutInflater.from(this).inflate(R.layout.comment_user, null);
if (itemView != null) {
Context context = this;
CircleImageView mIvCu;
TextView mTvCuName, mTvCuCmt, mTvCuTime, mTvCuLike, mTvCuReply;
mIvCu = itemView.findViewById(R.id.imgCu);
Picasso.with(context).load(AppConstants.URL + User.getUser(context).getPicPath().replaceAll("\\s", "%20"))
.error(R.color.light2)
.placeholder(R.color.light)
.into(mIvCu);
mTvCuName = itemView.findViewById(R.id.txtCuName);
mTvCuName.setTypeface(HmFonts.getRobotoRegular(context));
mTvCuName.setText(User.getUser(context).getUsername());
mTvCuCmt = itemView.findViewById(R.id.txtCuCmt);
mTvCuCmt.setTypeface(HmFonts.getRobotoRegular(context));
mTvCuCmt.setText(data);
mTvCuTime = itemView.findViewById(R.id.txtCuTime);
mTvCuTime.setTypeface(HmFonts.getRobotoRegular(context));
mTvCuLike = itemView.findViewById(R.id.txtCuLike);
mTvCuLike.setTypeface(HmFonts.getRobotoRegular(context));
mTvCuLike.setText("0 " + context.getString(R.string.str_like));
mTvCuReply = itemView.findViewById(R.id.txtCuReply);
mTvCuReply.setTypeface(HmFonts.getRobotoRegular(context));
mLlAddCmt.addView(itemView);
// new toDisplayComments().execute();
// toDisplayComments();
Post.toDisplayComments(timelineId, mRvCmt, this, mLlAddCmt);
mLlAddCmt.requestFocus();
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private void toAddReply(String data, LinearLayout mLlAddCmt) {
try {
View itemView = LayoutInflater.from(this).inflate(R.layout.comment_user, null);
if (itemView != null) {
Context context = this;
CircleImageView mIvCu;
TextView mTvCuName, mTvCuCmt, mTvCuTime, mTvCuLike, mTvCuReply;
mIvCu = itemView.findViewById(R.id.imgCu);
Picasso.with(context).load(AppConstants.URL + User.getUser(context).getPicPath().replaceAll("\\s", "%20"))
.error(R.color.light2)
.placeholder(R.color.light)
.into(mIvCu);
mTvCuName = itemView.findViewById(R.id.txtCuName);
mTvCuName.setTypeface(HmFonts.getRobotoRegular(context));
mTvCuName.setText(User.getUser(context).getUsername());
mTvCuCmt = itemView.findViewById(R.id.txtCuCmt);
mTvCuCmt.setTypeface(HmFonts.getRobotoRegular(context));
mTvCuCmt.setText(data);
mTvCuTime = itemView.findViewById(R.id.txtCuTime);
mTvCuTime.setTypeface(HmFonts.getRobotoRegular(context));
mTvCuLike = itemView.findViewById(R.id.txtCuLike);
mTvCuLike.setTypeface(HmFonts.getRobotoRegular(context));
mTvCuLike.setText("0 " + context.getString(R.string.str_like));
mTvCuReply = itemView.findViewById(R.id.txtCuReply);
mTvCuReply.setTypeface(HmFonts.getRobotoRegular(context));
mLlAddCmt.addView(itemView);
// new toDisplayComments().execute();
// toDisplayComments();
mLlAddCmt.requestFocus();
Post.toDisplayComments(timelineId, mRvCmt, this, mLlAddCmt);
mLlAddCmt.requestFocus();
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private void toSetData() {
try {
if (getIntent() != null) {
Log.d("HmApp", " singlePostData : " + getIntent());
if (getIntent().getStringExtra(AppConstants.BUNDLE) != null) {
obj = new JSONObject(getIntent().getStringExtra(AppConstants.BUNDLE));
if (getIntent().getStringExtra(AppConstants.TIMELINE_ID) != null) {
timelineId = getIntent().getStringExtra(AppConstants.TIMELINE_ID);
} else {
timelineId = obj.getString("timeline_id");
}
Log.d("HmApp", " SinglePost Timeline 1 " + timelineId);
toDisplayData(obj);
} else if (getIntent().getStringExtra(AppConstants.TIMELINE_ID) != null) {
timelineId = getIntent().getStringExtra(AppConstants.TIMELINE_ID);
Log.d("HmApp", " SinglePost Timeline 2 " + timelineId);
if (CommonFunctions.isOnline(SinglePostDataActivity.this)) {
toDisplayNotificationData();
} else {
CommonFunctions.toDisplayToast(getResources().getString(R.string.lbl_no_check_internet), SinglePostDataActivity.this);
}
}
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private void toCallCommentUi() {
try {
Bundle bundle = new Bundle();
Log.d("hmapp", " Spd Timeline1" + timelineId);
bundle.putString(AppConstants.TIMELINE_ID, obj.getString(getString(R.string.str_timeline_id_)));
Log.d("hmapp", " Spd Timeline2 " + timelineId);
CommentFragment cm = new CommentFragment();
cm.setArguments(bundle);
replaceMainHomePage(cm);
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
public void replaceMainHomePage(Fragment fragment) {
Log.d("Hmapp", " agr replaceTabData 1 bundle " + fragment.getArguments());
getSupportFragmentManager()
.beginTransaction()
.replace(R.id.flSpdHome, fragment)
// .addToBackStack(fragment.getClass().getName())
.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN)
.commit();
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case android.R.id.home:
onBackPressed();
break;
default:
break;
}
return super.onOptionsItemSelected(item);
}
public void toDisplayData(final JSONObject obj) throws Exception, Error {
Log.d("hmapp", " data " + obj);
if (!obj.isNull(getString(R.string.str_username_))) {
mtxt_label.setText(CommonFunctions.firstLetterCaps(obj.getString(getString(R.string.str_username_))));
} else {
mtxt_label.setText(CommonFunctions.firstLetterCaps(User.getUser(SinglePostDataActivity.this).getUsername()));
}
if (!obj.isNull(getString(R.string.str_post_small)) && obj.getString(getString(R.string.str_post_small)).trim().length() > 0) {
mtxtSpdPost.setVisibility(View.VISIBLE);
mtxtSpdPost.setText(User.getUser(SinglePostDataActivity.this).getUsername()+" : "+obj.getString(getString(R.string.str_post_small)));
} else if (!obj.isNull(getString(R.string.str_caption)) && obj.getString(getString(R.string.str_caption)).trim().length() > 0) {
mtxtSpdPost.setVisibility(View.VISIBLE);
mtxtSpdPost.setText(User.getUser(SinglePostDataActivity.this).getUsername()+" : "+obj.getString(getString(R.string.str_caption)));
} else if (!obj.isNull(getString(R.string.str_post_data)) && obj.getString(getString(R.string.str_post_data)).trim().length() > 0) {
mtxtSpdPost.setVisibility(View.VISIBLE);
mtxtSpdPost.setText(User.getUser(SinglePostDataActivity.this).getUsername()+" : "+obj.getString(getString(R.string.str_post_data)));
} else {
mtxtSpdPost.setVisibility(View.GONE);
}
if (!obj.isNull(getString(R.string.str_time))) {
mtxt_time_ago.setText(CommonFunctions.toSetDate(obj.getString(getString(R.string.str_time))));
}
if (!obj.isNull(getString(R.string.str_timeline_id_))) {
mTvTimeLineId.setText(obj.getString(getString(R.string.str_timeline_id_)));
}
// if (!obj.isNull(getString(R.string.str_friend_like))) {
// mTvUserLikeName.setText(obj.getString(getString(R.string.str_friend_like)));
// }
if (!obj.isNull(getString(R.string.str_like_count)) && !obj.getString(getString(R.string.str_like_count)).equals("0")) {
if (!obj.isNull(getString(R.string.str_friend_like))) {
mtxtNo_like.setVisibility(View.VISIBLE);
mtxtNo_like.setText("Liked by "+(obj.getString(getString(R.string.str_friend_like)) + " and " + obj.getString(getString(R.string.str_like_count)) + " others"));
}else if (!obj.isNull(getString(R.string.str_like_count))){
mtxtNo_like.setVisibility(View.VISIBLE);
mtxtNo_like.setText(obj.getString(getString(R.string.str_like_count)) + " likes");
}
}else {
mtxtNo_like.setVisibility(View.GONE);
}
if (!obj.isNull(getString(R.string.str_comment_count))) {
mtxtNo_comment.setText(obj.getString(getString(R.string.str_comment_count)) + " " + getString(R.string.str_comment));
}
// if (!obj.isNull(getString(R.string.str_share_count))) {
// mtxtNo_share.setText(obj.getString(getString(R.string.str_share_count)) + " " + getResources().getString(R.string.str_share));
// }
if (User.getUser(SinglePostDataActivity.this).getPicPath() != null) {
Picasso.with(SinglePostDataActivity.this)
.load(AppConstants.URL + User.getUser(SinglePostDataActivity.this).getPicPath().replaceAll("\\s", "%20"))
.error(R.color.light2)
.placeholder(R.color.light)
.resize(100, 100)
.into(mcircle_img);
}
if (!obj.isNull(getString(R.string.str_image_url))) {
mVp.setAdapter(
new SlidingImageAdapter(
SinglePostDataActivity.this,
obj.getString(getString(R.string.str_image_url)).split(","),
null,
null,
mTl)
);
if (obj.getString(getString(R.string.str_image_url)).split(",").length > 1) {
mTl.setupWithViewPager(mVp);
} else {
mTl.setVisibility(View.GONE);
}
} else if (!obj.isNull(getString(R.string.str_image))) {
mVp.setAdapter(
new SlidingImageAdapter(
SinglePostDataActivity.this,
obj.getString(getString(R.string.str_image)).split(","),
null,
null,
mTl)
);
mTl.setupWithViewPager(mVp);
} else {
mVp.setVisibility(View.GONE);
mTl.setVisibility(View.GONE);
}
mrr_header_file.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
startActivity(
new Intent(SinglePostDataActivity.this, UserInfoActivity.class)
.putExtra(AppConstants.F_UID, obj.getString("Uid")));
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
});
mImgShare.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
CommonFunctions
.toShareData(SinglePostDataActivity.this,
getString(R.string.app_name),
obj.getString(getString(R.string.str_caption)),
obj.getString(getString(R.string.str_timeline_id_)), null);
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
});
mChkLike.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
MyPost.toLikeUnlikePost(SinglePostDataActivity.this, obj.getString(getString(R.string.str_timeline_id_)), null, null, mChkPostLiked, mChkLike);
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
});
mtxtNo_like.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
Bundle bundle = new Bundle();
bundle.putString(AppConstants.TIMELINE_ID, obj.getString(getString(R.string.str_timeline_id_)));
TimelineLikeListFragment time = new TimelineLikeListFragment();
time.setArguments(bundle);
replaceMainHomePage(time);
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
});
mImgComment.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
toCallCommentUi();
}
});
mtxtNo_comment.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
toCallCommentUi();
}
});
// toDisplay comments Below
// Bundle bundle = new Bundle();
// bundle.putString(AppConstants.TIMELINE_ID, timelineId);
// CommentFragment cm = new CommentFragment();
// cm.setArguments(bundle);
// getSupportFragmentManager()
// .beginTransaction()
// .replace(R.id.flSpdComment, cm)
// .setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN)
// .commit();
Post.toDisplayComments(timelineId, mRvCmt, this, mLlAddCmt);
}
public void toDisplayNotificationData() {
try {
VolleySingleton.getInstance(SinglePostDataActivity.this)
.addToRequestQueue(
new StringRequest(Request.Method.POST,
AppConstants.URL + getString(R.string.str_notification_uid) + getString(R.string.str_php),
new Response.Listener<String>() {
@Override
public void onResponse(String res) {
try {
Log.d("HmApp", "notification:" + res);
if (res != null && res.trim().length()>0){
JSONArray array = new JSONArray(res.trim());
if (array != null) {
if (array.length() > 0) {
if (!array.isNull(0)) {
obj = array.getJSONObject(0);
toDisplayData(obj);
}
}
}
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
error.printStackTrace();
}
}
) {
@Override
protected Map<String, String> getParams() {
Map<String, String> params = new HashMap<>();
params.put(getString(R.string.str_action_), getString(R.string.str_notification_post));
params.put(getString(R.string.str_timeline_id_), timelineId);
Log.d("HmApp", "Notification timeline: " + timelineId);
return params;
}
}
, getString(R.string.str_notification_post));
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
}<file_sep>package com.hm.application.fragments;
import android.content.Context;
import android.content.Intent;
import android.net.Uri;
import android.os.AsyncTask;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.v4.app.Fragment;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.LinearLayout;
import android.widget.ProgressBar;
import com.android.volley.Request;
import com.android.volley.Response;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.StringRequest;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.activity.SinglePostDataActivity;
import com.hm.application.classes.UserTimelinePostNew;
import com.hm.application.model.AppConstants;
import com.hm.application.model.User;
import com.hm.application.network.VolleySingleton;
import com.hm.application.utils.CommonFunctions;
import org.json.JSONArray;
import java.util.HashMap;
import java.util.Map;
public class UserTab1Fragment extends Fragment {
private LinearLayout mLlPostMain;
private JSONArray array;
private String uid;
private ProgressBar mPb;
private OnFragmentInteractionListener mListener;
public UserTab1Fragment() {
// Required empty public constructor
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_user_tab1, container, false);
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
try {
mLlPostMain = getActivity().findViewById(R.id.llPostMain);
mPb = getActivity().findViewById(R.id.progressBarPM);
if (mLlPostMain.getChildCount() > 0) {
mLlPostMain.removeAllViews();
}
checkInternetConnection();
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private void checkInternetConnection() {
try {
uid = User.getUser(getContext()).getUid();
if (CommonFunctions.isOnline(getContext())) {
if (getArguments() != null) {
if (getArguments().getBoolean("other_user")) {
if (getArguments().getString("fetch_timeline") != null) {
toDisplayData(getArguments().getString("fetch_timeline"), getArguments().getString("name"));
} else if (getArguments().getString(AppConstants.F_UID) != null) {
uid = getArguments().getString(AppConstants.F_UID);
new toGetData().execute();
} else {
new toGetData().execute();
}
} else {
new toGetData().execute();
}
} else {
new toGetData().execute();
}
} else {
CommonFunctions.toDisplayToast(getResources().getString(R.string.lbl_no_check_internet), getContext());
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private void toDisplayData(String res, String name) {
try {
if (res != null && res.length() > 0) {
array = new JSONArray(res);
if (array != null) {
if (array.length() > 0) {
for (int i = 0; i < array.length(); i++) {
if (!array.getJSONObject(i).isNull(getString(R.string.str_activity_small))) {
if (array.getJSONObject(i).getString(getString(R.string.str_activity_small)).equals(getString(R.string.str_photo_small))) {
// UserTimeLinePost.toDisplayNormalPost(array.getJSONObject(i), getContext(), mLlPostMain, i, name, UserTab1Fragment.this);
UserTimelinePostNew.toDisplayPost(array.getJSONObject(i), getContext(), mLlPostMain, i, name, UserTab1Fragment.this);
// } else if (array.getJSONObject(i).getString(getString(R.string.str_activity_small)).equals(getString(R.string.str_post_small))) {
// UserTimeLinePost.toDisplayNormalPost(array.getJSONObject(i), getContext(), mLlPostMain, i, name, UserTab1Fragment.this);
} else if (array.getJSONObject(i).getString(getString(R.string.str_activity_small)).equals(getString(R.string.str_album_small))) {
// UserTimeLinePost.toDisplayPhotoPost(array.getJSONObject(i), getContext(), mLlPostMain, i, name, UserTab1Fragment.this);
UserTimelinePostNew.toDisplayPost(array.getJSONObject(i), getContext(), mLlPostMain, i, name, UserTab1Fragment.this);
// } else {
// UserTimeLinePost.toDisplayNormalPost(array.getJSONObject(i), getContext(), mLlPostMain, i, name, UserTab1Fragment.this);
}
}
}
} else {
CommonFunctions.toDisplayToast(getString(R.string.str_no_post_found), getContext());
}
} else {
CommonFunctions.toDisplayToast(getString(R.string.str_no_post_found), getContext());
}
} else {
CommonFunctions.toDisplayToast(getString(R.string.str_no_post_found), getContext());
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
public void toCallSinglePostData(int position, String from) {
try {
startActivity(new Intent(getContext(), SinglePostDataActivity.class)
.putExtra(AppConstants.FROM, from)
.putExtra(AppConstants.BUNDLE, array.getJSONObject(position).toString()));
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
public interface OnFragmentInteractionListener {
void onFragmentInteraction(Uri uri);
void toSetTitle(String s, boolean b);
}
private class toGetData extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... voids) {
try {
if (mLlPostMain.getChildCount() > 0) {
mLlPostMain.removeAllViews();
}
VolleySingleton.getInstance(getContext())
.addToRequestQueue(
new StringRequest(Request.Method.POST,
AppConstants.URL + getString(R.string.str_feed) + getString(R.string.str_php),
new Response.Listener<String>() {
@Override
public void onResponse(String response) {
Log.d("hmapp", " response : fetch_timeline " + response);
toDisplayData(response, User.getUser(getContext()).getUsername());
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
error.printStackTrace();
}
}
) {
@Override
protected Map<String, String> getParams() {
Map<String, String> params = new HashMap<String, String>();
params.put(getString(R.string.str_action_), getString(R.string.str_fetch_timeline_));
params.put(getString(R.string.str_uid), uid);
return params;
}
}
, getString(R.string.str_fetch_timeline_));
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
return null;
}
}
}<file_sep>package com.hm.application.services;
public interface SmsListener {
void messageReceived(String str);
}
<file_sep>package com.hm.application.adapter;
import android.content.Context;
import android.content.DialogInterface;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.v4.content.ContextCompat;
import android.support.v7.app.AlertDialog;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.RelativeLayout;
import android.widget.TextView;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.activity.UserInfoActivity;
import com.hm.application.common.MyFriendRequest;
import com.hm.application.model.AppConstants;
import com.hm.application.utils.CommonFunctions;
import com.hm.application.utils.HmFonts;
import com.squareup.picasso.Picasso;
import org.json.JSONArray;
import de.hdodenhof.circleimageview.CircleImageView;
public class UserFollowingListAdapter extends RecyclerView.Adapter<com.hm.application.adapter.UserFollowingListAdapter.ViewHolder> {
private Context context;
private JSONArray array;
public UserFollowingListAdapter(Context ctx, JSONArray data) {
context = ctx;
array = data;
}
@NonNull
@Override
public com.hm.application.adapter.UserFollowingListAdapter.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
return new com.hm.application.adapter.UserFollowingListAdapter.ViewHolder(LayoutInflater.from(context).inflate(R.layout.friend_request_item_layout, parent, false));
}
@Override
public void onBindViewHolder(@NonNull com.hm.application.adapter.UserFollowingListAdapter.ViewHolder holder, int position) {
try {
if (!array.getJSONObject(position).isNull(context.getString(R.string.str_name))) {
holder.mTvName.setText(array.getJSONObject(position).getString(context.getString(R.string.str_name)));
}
if (!array.getJSONObject(position).isNull(context.getString(R.string.str_mutual_friend_count))) {
holder.mTvData.setText(array.getJSONObject(position).getString(context.getString(R.string.str_mutual_friend_count)) + " " + context.getString(R.string.str_common_friends));
} else {
holder.mTvData.setText("0 Common Friends");
}
if (!array.getJSONObject(position).isNull(context.getString(R.string.str_profile_pic))) {
if (array.getJSONObject(position).getString(context.getString(R.string.str_profile_pic)).toLowerCase().contains("upload")) {
Picasso.with(context)
.load(AppConstants.URL + array.getJSONObject(position).getString(context.getString(R.string.str_profile_pic)).replaceAll("\\s", "%20"))
.placeholder(R.color.light)
.error(R.color.light)
.into(holder.mIvProfilePic);
} else {
Picasso.with(context)
.load(array.getJSONObject(position).getString(context.getString(R.string.str_profile_pic)).replaceAll("\\s", "%20"))
.placeholder(R.color.light)
.error(R.color.light)
.into(holder.mIvProfilePic);
}
}
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
@Override
public int getItemCount() {
return array == null ? 0 : array.length();
}
private void toAskConfirmUnFollow(final String f_uid, String f_name, final Button mBtnIgnore) throws Error {
try {
AlertDialog.Builder builder = new AlertDialog.Builder(context, R.style.MyAlertDialogTheme);
builder.setTitle(context.getString(R.string.str_unfollow) + "\n" + CommonFunctions.firstLetterCaps(f_name));
builder.setMessage(context.getString(R.string.str_msg_unfollow_friend) + " " + f_name);
builder.setCancelable(true);
builder.setPositiveButton(
context.getString(R.string.str_lbl_yes),
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
MyFriendRequest.toUnFriendRequest(context, f_uid, mBtnIgnore);
dialog.cancel();
}
});
builder.setNegativeButton(
context.getString(R.string.str_lbl_no),
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
dialog.cancel();
}
});
AlertDialog alert1 = builder.create();
alert1.show();
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
class ViewHolder extends RecyclerView.ViewHolder {
private CircleImageView mIvProfilePic;
private Button mBtnIgnore, mBtnConfirm;
private TextView mTvName, mTvData;
private RelativeLayout mRl;
ViewHolder(View itemView) {
super(itemView);
try {
mRl = itemView.findViewById(R.id.rr_friend_req);
mIvProfilePic = itemView.findViewById(R.id.imgFriendPic);
mBtnConfirm = itemView.findViewById(R.id.btnFrConfirm);
mBtnConfirm.setTypeface(HmFonts.getRobotoBold(context));
mBtnConfirm.setVisibility(View.GONE);
mBtnIgnore = itemView.findViewById(R.id.btnFrIgnore);
// mBtnIgnore.setText(context.getString(R.string.str_unfollow));
mBtnIgnore.setText(CommonFunctions.firstLetterCaps(context.getString(R.string.str_following_small)));
mBtnIgnore.setTextColor(ContextCompat.getColor(context, R.color.grey5));
mBtnIgnore.setBackground(ContextCompat.getDrawable(context, R.drawable.round_border_white));
mBtnIgnore.setTypeface(HmFonts.getRobotoBold(context));
mTvName = itemView.findViewById(R.id.txt_friend_name);
mTvName.setTypeface(HmFonts.getRobotoBold(context));
mTvData = itemView.findViewById(R.id.txt_friend_data);
mTvData.setTypeface(HmFonts.getRobotoRegular(context));
mTvName.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
context.startActivity(new Intent(context, UserInfoActivity.class).putExtra(AppConstants.F_UID, array.getJSONObject(getAdapterPosition()).getString(context.getString(R.string.str_uid))));
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
});
mRl.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
context.startActivity(new Intent(context, UserInfoActivity.class).putExtra(AppConstants.F_UID, array.getJSONObject(getAdapterPosition()).getString(context.getString(R.string.str_uid))));
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
});
mBtnIgnore.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
mBtnIgnore.setEnabled(false);
// if (mBtnIgnore.getText().toString().trim().equals(context.getString(R.string.str_unfollow))) {
if (mBtnIgnore.getText().toString().trim().equals(CommonFunctions.firstLetterCaps(context.getString(R.string.str_following_small)))) {
toAskConfirmUnFollow(array.getJSONObject(getAdapterPosition()).getString(context.getString(R.string.str_uid)), array.getJSONObject(getAdapterPosition()).getString(context.getString(R.string.str_name)), mBtnIgnore);
} else if (mBtnIgnore.getText().toString().trim().equals(context.getString(R.string.str_follow))) {
MyFriendRequest.toFollowFriendRequest(context, array.getJSONObject(getAdapterPosition()).getString(context.getString(R.string.str_uid)), mBtnIgnore);
}
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
});
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
}
}<file_sep>package com.hm.application.services;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.telephony.SmsMessage;
import com.crashlytics.android.Crashlytics;
public class SmsReceiver extends BroadcastReceiver {
private static SmsListener mListener;
public static void bindListener(SmsListener listener) {
mListener = listener;
}
public void onReceive(Context context, Intent intent) {
try {
Object[] pdus = (Object[]) intent.getExtras().get("pdus");
if (pdus != null) {
for (Object obj : pdus) {
SmsMessage smsMessage = SmsMessage.createFromPdu((byte[]) obj);
if (smsMessage.getDisplayOriginatingAddress().contains("SNSWAP")) {
String messageBody = smsMessage.getMessageBody();
if (messageBody != null) {
mListener.messageReceived(messageBody);
// } else {
// FirebaseCrash.log("SMSReceiver:1 " + smsMessage.getDisplayMessageBody() + "::" + smsMessage.getEmailBody() + "::" + smsMessage.getEmailFrom() + "::" + smsMessage.getPseudoSubject() + "::" + smsMessage.getMessageBody() + "::" + smsMessage.getStatus() + "::" + smsMessage.getServiceCenterAddress() + "::" + smsMessage.getOriginatingAddress() + "::" + smsMessage.getDisplayOriginatingAddress());
}
// } else {
// FirebaseCrash.log("SMSReceiver:2 " + smsMessage.getDisplayMessageBody() + "::" + smsMessage.getEmailBody() + "::" + smsMessage.getEmailFrom() + "::" + smsMessage.getPseudoSubject() + "::" + smsMessage.getMessageBody() + "::" + smsMessage.getStatus() + "::" + smsMessage.getServiceCenterAddress() + "::" + smsMessage.getOriginatingAddress() + "::" + smsMessage.getDisplayOriginatingAddress());
}
}
// return;
}
// FirebaseCrash.log("SMSReceiver:3 " + intent.getExtras());
// return;
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
}
<file_sep>package com.hm.application.fragments;
import android.os.AsyncTask;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.v4.app.Fragment;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import com.android.volley.Request;
import com.android.volley.Response;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.StringRequest;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.adapter.NotificationAdapter;
import com.hm.application.model.AppConstants;
import com.hm.application.model.User;
import com.hm.application.network.VolleySingleton;
import com.hm.application.utils.CommonFunctions;
import org.json.JSONArray;
import java.util.HashMap;
import java.util.Map;
public class NotificationFollowRequestFragment extends Fragment {
private RecyclerView mRvNfMain;
public NotificationFollowRequestFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_follow_request, container, false);
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
checkInternetConnection();
}
private void checkInternetConnection() {
try {
if (CommonFunctions.isOnline(getContext())) {
new toGetFollowNotification().execute();
} else {
CommonFunctions.toDisplayToast(getResources().getString(R.string.lbl_no_check_internet), getContext());
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
private class toGetFollowNotification extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... voids) {
try {
VolleySingleton.getInstance(getContext())
.addToRequestQueue(
new StringRequest(Request.Method.POST,
AppConstants.URL + getString(R.string.str_notification_uid) + getContext().getResources().getString(R.string.str_php),
new Response.Listener<String>() {
@Override
public void onResponse(String res) {
Log.d("HmApp", "notification:" + res);
try {
if (res != null && res.length() > 0) {
JSONArray array = new JSONArray(res.trim());
if (array != null) {
if (array.length() > 0) {
mRvNfMain = getActivity().findViewById(R.id.rvMNFR);
mRvNfMain.setLayoutManager(new LinearLayoutManager(getContext()));
mRvNfMain.hasFixedSize();
mRvNfMain.setAdapter(new NotificationAdapter(getContext(), array));
} else {
CommonFunctions.toDisplayToast("No Notification", getContext());
}
} else {
CommonFunctions.toDisplayToast("No Notification", getContext());
}
} else {
CommonFunctions.toDisplayToast("No Notification", getContext());
}
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
error.printStackTrace();
}
}
) {
@Override
protected Map<String, String> getParams() {
Map<String, String> params = new HashMap<String, String>();
params.put(getString(R.string.str_action_), getString(R.string.str_list_notification));
params.put(getString(R.string.str_uid), User.getUser(getContext()).getUid());
return params;
}
}
, getContext().getString(R.string.str_sent_notification));
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
}
return null;
}
}
}<file_sep>package com.hm.application.activity;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentTransaction;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.CompoundButton;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.RelativeLayout;
import android.widget.Switch;
import android.widget.TextView;
import android.widget.Toolbar;
import com.crashlytics.android.Crashlytics;
import com.hm.application.R;
import com.hm.application.classes.Common_Alert_box;
import com.hm.application.fragments.ChangePasswordFragment;
import com.hm.application.fragments.UserProfileEditFragment;
import com.hm.application.fragments.UserTab21Fragment;
import com.hm.application.model.User;
import com.hm.application.utils.CommonFunctions;
import com.hm.application.utils.HmFonts;
public class AccountSettingsActivity extends AppCompatActivity {
private RelativeLayout mRlAccSettingMain, mRlAccSettingTool, mRlAccSettingTb;
private LinearLayout mLlLblAccount, mLlLblSupport, mLlAccountSetting;
private Toolbar mProfileToolBar;
private ImageView mIvBackArrow;
private TextView mTvLblOption, mTvLblAccount, mTvPhotos, mTvPostLiked, mTvEditProf, mTvCngPassword, mTvBlockUser, mTvPrivateAcc, mTvLblSupport, mTvProblem, mTvHelpCenter, mTvAddAccount, mTvLogout;
private Switch mSwitchAccount;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_accountsettings);
try {
bindViews();
} catch (Exception | Error e) {
e.printStackTrace();
Crashlytics.logException(e);
} }
private void bindViews() throws Error {
mRlAccSettingMain = findViewById(R.id.rlAccSettingMain);
mRlAccSettingTool = findViewById(R.id.rlAccSettingTool);
mRlAccSettingTb = findViewById(R.id.rlAccSettingTb);
mLlLblAccount = findViewById(R.id.llLblAccount);
mLlLblSupport = findViewById(R.id.llLblSupport);
mLlAccountSetting = findViewById(R.id.llAccountSetting);
mIvBackArrow = findViewById(R.id.ivBackArrow);
mTvLblOption = findViewById(R.id.tvLblOption);
mTvLblOption.setTypeface(HmFonts.getRobotoBold(AccountSettingsActivity.this));
mTvPhotos = findViewById(R.id.tvPhotos);
mTvPhotos.setTypeface(HmFonts.getRobotoRegular(AccountSettingsActivity.this));
mTvLblAccount = findViewById(R.id.tvLblAccount);
mTvLblAccount.setTypeface(HmFonts.getRobotoBold(AccountSettingsActivity.this));
mTvPostLiked = findViewById(R.id.tvPostLiked);
mTvPostLiked.setTypeface(HmFonts.getRobotoRegular(AccountSettingsActivity.this));
mTvEditProf = findViewById(R.id.tvEditProf);
mTvEditProf.setTypeface(HmFonts.getRobotoRegular(AccountSettingsActivity.this));
mTvCngPassword = findViewById(R.id.tvCngPassword);
mTvCngPassword.setTypeface(HmFonts.getRobotoRegular(AccountSettingsActivity.this));
mTvBlockUser = findViewById(R.id.tvBlockUser);
mTvBlockUser.setTypeface(HmFonts.getRobotoRegular(AccountSettingsActivity.this));
mTvLblSupport = findViewById(R.id.tvLblSupport);
mTvLblSupport.setTypeface(HmFonts.getRobotoBold(AccountSettingsActivity.this));
mTvProblem = findViewById(R.id.tvProblem);
mTvProblem.setTypeface(HmFonts.getRobotoRegular(AccountSettingsActivity.this));
mTvHelpCenter = findViewById(R.id.tvHelpCenter);
mTvHelpCenter.setTypeface(HmFonts.getRobotoRegular(AccountSettingsActivity.this));
mTvAddAccount = findViewById(R.id.tvAddAccount);
mTvAddAccount.setTypeface(HmFonts.getRobotoRegular(AccountSettingsActivity.this));
mTvAddAccount.setVisibility(View.GONE);
mTvLogout = findViewById(R.id.tvLogout);
mTvLogout.setTypeface(HmFonts.getRobotoRegular(AccountSettingsActivity.this));
mSwitchAccount = findViewById(R.id.switchAccount);
mSwitchAccount.setTypeface(HmFonts.getRobotoBold(AccountSettingsActivity.this));
if (User.getUser(AccountSettingsActivity.this).getStatus() == 1) {
mSwitchAccount.setChecked(true);
} else {
mSwitchAccount.setChecked(false);
}
mSwitchAccount.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if (isChecked) {
Common_Alert_box.toPrivateAccount(AccountSettingsActivity.this, true);
} else {
Common_Alert_box.toPrivateAccount(AccountSettingsActivity.this, false);
}
}
});
mTvHelpCenter.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Common_Alert_box.toContactUs(AccountSettingsActivity.this);
}
});
mTvLogout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
CommonFunctions.toLogout(AccountSettingsActivity.this);
}
});
mTvEditProf.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
replaceMainHomePage(new UserProfileEditFragment());
}
});
mTvPhotos.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
replaceMainHomePage(new UserTab21Fragment());
}
});
mIvBackArrow.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
onBackPressed();
}
});
mTvCngPassword.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
replaceMainHomePage(new ChangePasswordFragment());
}
});
}
public void replaceMainHomePage(Fragment fragment) {
getSupportFragmentManager()
.beginTransaction()
.replace(R.id.rlAccSettingMain, fragment)
// .addToBackStack(fragment.getClass().getName())
.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN)
.commit();
}
}
<file_sep>package com.hm.application.fragments;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentTransaction;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import com.hm.application.R;
public class Main_HomeFragment extends Fragment {
public Main_HomeFragment() {
// Required empty public constructor
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_main_home, container, false);
}
@Override
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
}
public void replacePage(Fragment fragment) {
getActivity().getSupportFragmentManager()
.beginTransaction()
// .addToBackStack(fragment.getClass().getName())
.replace(R.id.flHomeContainer, fragment)
.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN)
.commit();
}
}<file_sep>org.gradle.jvmargs=-Xmx3000m
android.enableBuildCache=false<file_sep>package com.hm.application.model;
import android.content.Context;
import android.content.SharedPreferences.Editor;
import android.util.Log;
import com.crashlytics.android.Crashlytics;
import com.google.gson.Gson;
import com.hm.application.R;
import org.json.JSONObject;
//
public class AppDataStorage {
private static final String USER_INFO = "USER_INFO";
private static final String USER_INFO_PREFERS = "USER_INFO_PREFERS";
public static void getUserInfo(Context context) {
try {
String us = context.getSharedPreferences(USER_INFO_PREFERS, 0).getString(USER_INFO, null);
if (us != null) {
User user = User.getUser(context);
JSONObject userJSON = new JSONObject(us);
// {"dob":"1\/1\/1990","email":"swapnil","gender":"M","livesIn":"NSP","mobile":"123454","name":"<NAME>","notificationCount":0,"picPath":"uploads\/20\/profile_pics\/23-03-2018 16:28:24 PM_202879ad42dec8375e.jpg","referralCode":"123","uid":"20","username":"swapnil"}
Log.d("HmApp", " getUserInfo " + userJSON);
if (!userJSON.isNull(context.getString(R.string.str_uid))) {
user.setUid(userJSON.getString(context.getString(R.string.str_uid)));
}
if (!userJSON.isNull("email")) {
user.setEmail(userJSON.getString("email"));
}
if (!userJSON.isNull("name")) {
user.setName(userJSON.getString("name"));
}
if (!userJSON.isNull("username")) {
user.setUsername(userJSON.getString("username"));
}
if (!userJSON.isNull("mobile")) {
user.setMobile(userJSON.getString("mobile"));
}
if (!userJSON.isNull("dob")) {
user.setDob(userJSON.getString("dob"));
}
if (!userJSON.isNull("picPath")) {
user.setPicPath(userJSON.getString("picPath"));
}
if (!userJSON.isNull("livesIn")) {
user.setLivesIn(userJSON.getString("livesIn"));
}
if (!userJSON.isNull("referralCode")) {
user.setReferralCode(userJSON.getString("referralCode"));
}
if (!userJSON.isNull("gender")) {
user.setGender(userJSON.getString("gender"));
}
if (!userJSON.isNull("fromDest")) {
user.setFromDest(userJSON.getString("fromDest"));
}
if (!userJSON.isNull("relationStatus")) {
user.setRelationStatus(userJSON.getString("relationStatus"));
}
if (!userJSON.isNull("favQuote")) {
user.setFavQuote(userJSON.getString("favQuote"));
}
if (!userJSON.isNull("bio")) {
user.setBio(userJSON.getString("bio"));
}
if (!userJSON.isNull("fcmToken")) {
user.setFcmToken(userJSON.getString("fcmToken"));
}
if (!userJSON.isNull("notificationCount")) {
user.setNotificationCount(userJSON.getInt("notificationCount"));
}
}
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
public static void setUserInfo(Context context) {
try {
Editor editor = context.getSharedPreferences(USER_INFO_PREFERS, 0).edit();
Log.d("HmApp", " setUserInfo " + new JSONObject(new Gson().toJson(User.getUser(context))).toString());
editor.putString(USER_INFO, new JSONObject(new Gson().toJson(User.getUser(context))).toString());
editor.apply();
editor.commit();
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
}
}
}<file_sep>package com.hm.application.network;
import com.android.volley.NetworkResponse;
import com.android.volley.Request;
import com.android.volley.Response;
import com.android.volley.Response.ErrorListener;
import com.android.volley.Response.Listener;
import com.crashlytics.android.Crashlytics;
import org.apache.http.HttpEntity;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.HttpMultipartMode;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.FileBody;
import org.apache.http.util.CharsetUtils;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FilterOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class MultipartRequest extends Request<String> {
private MultipartEntityBuilder entity = MultipartEntityBuilder.create();
private long fileLength = 0;
private HttpEntity httpentity;
private File mFilePart;
private Listener<String> mListener;
private MultipartProgressListener multipartProgressListener;
private String token;
public MultipartRequest(String url, File file, String token, MultipartProgressListener proListener, ErrorListener errorListener, Listener<String> listener) {
super(1, url, errorListener);
this.mListener = listener;
this.mFilePart = file;
this.fileLength = file.length();
this.token = token;
this.multipartProgressListener = proListener;
this.entity.setMode(HttpMultipartMode.BROWSER_COMPATIBLE);
try {
this.entity.setCharset(CharsetUtils.get("UTF-8"));
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
buildMultipartEntity();
this.httpentity = this.entity.build();
}
buildMultipartEntity();
this.httpentity = this.entity.build();
}
private void buildMultipartEntity() {
this.entity.addTextBody("api_token", this.token);
this.entity.addPart("profile-picture", new FileBody(this.mFilePart, ContentType.create("image/*"), this.mFilePart.getName()));
}
public String getBodyContentType() {
return this.httpentity.getContentType().getValue();
}
public byte[] getBody() {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
try {
this.httpentity.writeTo(new CountingOutputStream(bos, this.fileLength, this.multipartProgressListener));
} catch (Exception|Error e) {
e.printStackTrace(); Crashlytics.logException(e);
return bos.toByteArray();
}
return bos.toByteArray();
}
protected Response<String> parseNetworkResponse(NetworkResponse response) {
try {
return Response.success(new String(response.data, "UTF-8"), getCacheEntry());
} catch (Exception | Error e) {
e.printStackTrace(); Crashlytics.logException(e);
return Response.success(new String(response.data), getCacheEntry());
}
}
protected void deliverResponse(String response) {
this.mListener.onResponse(response);
}
public interface MultipartProgressListener {
void transferred(long j, int i);
}
private static class CountingOutputStream extends FilterOutputStream {
private final MultipartProgressListener progListener;
private long fileLength;
private long transferred = 0;
public CountingOutputStream(OutputStream out, long fileLength, MultipartProgressListener listener) {
super(out);
this.fileLength = fileLength;
this.progListener = listener;
}
public void write(byte[] b, int off, int len) throws IOException {
this.out.write(b, off, len);
if (this.progListener != null) {
this.transferred += (long) len;
if (this.fileLength != 0) {
this.progListener.transferred(this.transferred, (int) ((this.transferred * 100) / this.fileLength));
}
}
}
public void write(int b) throws IOException {
this.out.write(b);
if (this.progListener != null) {
this.transferred++;
if (this.fileLength != 0) {
this.progListener.transferred(this.transferred, (int) ((this.transferred * 100) / this.fileLength));
}
}
}
}
}
/*
public class MultipartRequest extends Request<String> {
private MultipartEntityBuilder mBuilder = MultipartEntityBuilder.create();
private final Response.Listener<String> mListener;
private final File mImageFile;
protected Map<String, String> headers;
private String mBoundary;
private Map<String, String> mParams;
private String mFileFieldName;
private String mFilename;
private String mBodyContentType;
public void setBoundary(String boundary) {
this.mBoundary = boundary;
}
public MultipartRequest(String url, final Map<String, String> params, File imageFile, String filename, String fileFieldName, ErrorListener errorListener, Listener<String> listener) {
super(Method.POST, url, errorListener);
mListener = listener;
mImageFile = imageFile;
mParams = params;
mFileFieldName = fileFieldName;
mFilename = filename;
buildMultipartEntity();
}
@Override
public Map<String, String> getHeaders() throws AuthFailureError {
Map<String, String> headers = super.getHeaders();
if (headers == null || headers.equals(Collections.emptyMap())) {
headers = new HashMap<String, String>();
}
headers.put("Accept", "application/json");
headers.put("X-Requested-With", "XMLHTTPRequest");
headers.put("User-Agent", "KaliMessenger");
return headers;
}
private void buildMultipartEntity() {
for (Map.Entry<String, String> entry : mParams.entrySet()) {
mBuilder.addTextBody(entry.getKey(), entry.getValue());
}
mBuilder.addBinaryBody(mFileFieldName, mImageFile, ContentType.create("image/jpg"), mFilename);
mBuilder.setMode(HttpMultipartMode.BROWSER_COMPATIBLE);
}
@Override
public String getBodyContentType() {
return mBodyContentType;
}
@Override
public byte[] getBody() throws AuthFailureError {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
try {
HttpEntity entity = mBuilder.build();
mBodyContentType = entity.getContentType().getValue();
entity.writeTo(bos);
} catch (IOException e) {
VolleyLog.e("IOException writing to ByteArrayOutputStream bos, building the multipart request.");
}
return bos.toByteArray();
}
@Override
protected void deliverResponse(String response) {
mListener.onResponse(response);
}
@Override
protected Response<String> parseNetworkResponse(NetworkResponse response) {
String parsed;
try {
parsed = new String(response.data, HttpHeaderParser.parseCharset(response.headers));
} catch (UnsupportedEncodingException e) {
parsed = new String(response.data);
}
return Response.success(parsed, HttpHeaderParser.parseCacheHeaders(response));
}
}*/ | eb4c58648c2d971ff1086c600a2031d67525d75e | [
"Java",
"INI",
"Gradle"
]
| 27 | Java | dttminers/HM_android | bbfb10a27a823d41bc8344edb4178189676cbdb8 | c0c3fa08056273bbc6d196197cdbf815fb31a836 | |
refs/heads/master | <repo_name>miyao120725/react-webpack-ant-design<file_sep>/src/models/details.js
import React from 'react';
import { connect } from 'dva';
import './details.scss';
function IndexPage() {
return (
<div className="details-auto">
<div className="details-top">
<a href="javascript:void(0)" className="topBack"></a>
<div className="topCenter">4545122</div>
<div className="topRight">
<span className="btn-reply"></span>
<span className="btn-share"></span>
</div>
</div>
<div className="details-content">
<div className="content-top">
<h2>男子把嫂子和侄 子骗回家,3天没出门,警察冲进屋 使劲揉眼睛</h2>
<div className="content-top-user">
<img src="../assets/icon-07.png" alt=""/>
<div className="top-user-describe">
<h3>
来源:实时社会资讯
</h3>
<span>2018-02-28 18:28</span>
</div>
</div>
</div>
<div className="content-text"></div>
</div>
</div>
);
}
IndexPage.propTypes = {
};
export default connect()(IndexPage);
<file_sep>/src/models/header.js
import React from 'react';
// import { connect } from 'dva';
import style from'./home.scss';
class Header extends React.Component{
render(){
return (
<div className='navTop clearfix'>
<div className="nav-left fl">
<a href="javascript:;"></a>
</div>
<span className="nav-text">头条在线</span>
<div className="nav-right fr">
<a href="javascript:;"></a>
</div>
</div>
)
}
}
export default Header;
<file_sep>/README.md
# react-webpack-ant-design
关于react的后台管理系统架构,用webpack和ant design进行搭建
<file_sep>/src/models/content.js
import React from 'react';
import { connect } from 'dva';
import style from'./home.scss';
import Header from './header.js';
import { Link } from 'dva/router';
import http from '../axios-http.js';
import GLOBAL from '../common/global.js'
const Content=(props)=>{
let jsonObject=strJosn(props.name.imgjs);
return(
<section className="content-one">
<a href="javascript: void(0)" className="title">
<h3>{props.name.title}</h3>
</a>
<div className="post-info clearfix">
<a href="" className="post-man fl">
<img src={'//i1.mopimg.cn/head/'+props.name.userid+'/80x80'} alt=""/>
<span>{props.name.username}</span>
</a>
<div className="post-count fr">
<span className="post-view">
<i></i>
{props.name.readnum}
</span>
<span className="post-reply">
<i></i>
{props.name.replynum}
</span>
</div>
</div>
</section>
)
}
const ContentTwo=(props)=>{
let jsonObject=strJosn(props.name.imgjs);
// console.log(props.imgjs)
return(
<section className="content-one">
<Link to={'/details/'+props.name.readnum} className="title">
<h3>{props.name.title}</h3>
<div className="post-imgs img-preview">
<div className="img-wrap">
<img src={jsonObject[0].src} alt=""/>
</div>
<div className="img-wrap">
<img src={jsonObject[1].src} alt=""/>
</div>
<div className="img-wrap">
<img src={jsonObject[2].src} alt=""/>
</div>
</div>
</Link>
<div className="post-info clearfix">
<a href="" className="post-man fl">
<img src={'//i1.mopimg.cn/head/'+props.name.userid+'/80x80'} alt=""/>
<span>{props.name.username}</span>
</a>
<div className="post-count fr">
<span className="post-view">
<i></i>
{props.name.readnum}
</span>
<span className="post-reply">
<i></i>
{props.name.replynum}
</span>
</div>
</div>
</section>
)
}
const strJosn=(data)=>{
return JSON.parse(data)
}
const ContentThree=(props)=>{
let jsonObject=strJosn(props.name.imgjs);
// console.log(props)
return(
<section className="content-one single-img">
<a href="javascript: void(0)" className="title">
<h3>{props.name.title}</h3>
<div className="post-img img-preview">
<div className="img-wrap">
<img src={jsonObject[0].src} alt=""/>
</div>
</div>
</a>
<div className="post-info clearfix">
<a href="" className="post-man fl">
<img src={'//i1.mopimg.cn/head/'+props.name.userid+'/80x80'} alt=""/>
<span>{props.name.username}</span>
</a>
<div className="post-count fr">
<span className="post-view">
<i></i>
{props.name.readnum}
</span>
<span className="post-reply">
<i></i>
{props.name.replynum}
</span>
</div>
</div>
</section>
)
}
class Item extends React.Component{
render() {
// let {item: item} = this.props;
let { item } = this.props;
function setItem(item) {
let imgJs='';
if(item.imgjs.length>0){
imgJs=strJosn(item.imgjs);
if(imgJs===''||imgJs===null){
return <Content name={item}></Content>;
}else if(imgJs.length>3){
return <ContentTwo name={item}/>;
}else if(imgJs.length<3){
return <ContentThree name={item}/>;
}
}
}
return (
<div>
{
setItem(item)
}
</div>
)
}
}
class List extends React.Component{
render() {
console.log(this.props)
return (
<div>
{
this.props.listdata.map((item, i) => <Item item={item}/>)
}
</div>
)
}
}
class ContentIndex extends React.Component{
constructor(props){
super(props);
this.state ={
data:[],
listdata: [
{'url':'javascript:;','title':'推荐','id':'999999'},
{'url':'javascript:;','title':'热点','id':'100001'},
{'url':'javascript:;','title':'社会','id':'100002'},
{'url':'javascript:;','title':'娱乐','id':'100003'},
{'url':'javascript:;','title':'国际','id':'100004'},
{'url':'javascript:;','title':'时尚','id':'100005'},
{'url':'javascript:;','title':'汽车','id':'100006'},
{'url':'javascript:;','title':'体育','id':'100007'},
{'url':'javascript:;','title':'军事','id':'100008'},
{'url':'javascript:;','title':'故事','id':'100009'},
],
click:0,
width:'',
left:'',
};
this.fleg=true;
this.numpage=0;
this.navId="999999";
this.startCol="";
this.mirrorId="";
this.active=this.active.bind(this);
}
componentWillMount(){
console.log(11111111111)
// this.ajaxcon()
}
componentDidMount(){
let $this=this;
this.ajaxcon({pgNum:0,listId:'999999'});
this.scrollFun();
console.log(this.props.match.params.id)
}
scrollFun(){
let distance=600,_this=this;
window.addEventListener('scroll', function () {
let scrollTop=document.body.scrollTop || document.documentElement.scrollTop ,
screenHeight=window.innerHeight,
domHeight=document.body.clientHeight ;
if((domHeight-distance)<=(scrollTop+screenHeight)&&_this.fleg){
_this.fleg=false;
_this.numpage++;
_this.ajaxcon({pgNum:_this.numpage,listId:_this.navId})
console.log(22222222222)
}
})
}
ajaxcon(obj){
let _this=this;
http.listHttp({
pgnum: obj.pgNum,
colid: obj.listId,
pgsize: 20,
serialnum: '300000',
startcol: this.startCol||null,
mirrorid: this.mirrorId||null,
platform: 'wap',
qid: '01711',
uid: '15184108460509177',
}).then(res=>{
if(res.data.data.length>0){
let dataLength=res.data.data.length-1;
this.mirrorId=res.data.mirrorid;
this.startCol=res.data.data[dataLength].startcol;
if(_this.numpage>0){
_this.setState({data:_this.state.data.concat(res.data.data)});
}else{
_this.setState({data:res.data.data});
}
_this.fleg=true;
}
console.log(res.data.data)
})
}
// contdata(){
// let datas=this.state.data,
// contents=[];
// for(let i=0;i<datas.length;i++){
// if(datas[i].imgjs===''||datas[i].imgjs===null){
// contents.push(<Content name={datas[i]}></Content>);
// }else if(strJosn(datas[i].imgjs).length>3){
// contents.push(<ContentTwo name={datas[i]}/>);
// }else if(strJosn(datas[i].imgjs)<3){
// contents.push(<ContentThree name={datas[i]}/>);
// }
// }
// return contents
// }
active(item,i,ele){
this.setState({
click:i,
width:ele.target.offsetWidth
})
document.documentElement.scrollTop=0;
this.mirrorId=null;
this.startCol=null;
this.numpage=0;
let scrollDom=document.querySelector('.navTwo-left'),
bodyWidth=document.body.clientWidth,
iLeft=ele.target.offsetLeft,
iWidth=ele.target.offsetWidth;
if(iLeft>(bodyWidth/2)){
scrollDom.scrollLeft=iLeft-(bodyWidth-iWidth)/2;
this.setState({
left:iLeft
})
}else{
scrollDom.scrollLeft=0;
this.setState({
left:iLeft
})
}
// console.log(document.querySelector('.navTwo-left').scrollLeft=200)
let navData=this.state.listdata;
for(let i=0;i<navData.length;i++){
if(navData[i].title===item.title){
this.navId=navData[i].id;
this.ajaxcon({
pgNum:0,
listId:navData[i].id
})
}
}
}
render(){
return (
<div className="app-h5">
<div className="nav-flexd">
<Header/>
<div className="navTwo">
<div className="navTwo-left clearfix">
{
this.state.listdata.map((item,i) => <a href={item.url} onClick={this.active.bind(null, item, i)} className={this.state.click===i?'active':''}>{item.title}</a>)
}
<i className="tabs-line" style={{left:this.state.left,width:this.state.width}}></i>
</div>
<div className="navTwo-right">
<div></div>
<a href="javascript:void(0)">
<span></span>
</a>
</div>
</div>
</div>
<div className="content">
<List listdata={this.state.data} />
</div>
</div>
)
}
}
export default connect()(ContentIndex);
| 8dc6253053134932d5aa27c73d20101816d78360 | [
"JavaScript",
"Markdown"
]
| 4 | JavaScript | miyao120725/react-webpack-ant-design | 6aa6db11864516c20106ba405d7e8170a10df9d6 | 6158bfbf302f3929144a215345976795cf811720 | |
refs/heads/master | <file_sep>import Vue from 'vue';
import axios from 'axios';
import hmy from './hmy.js';
window.hmy = hmy;
const EMOJI_ABI = require('./emoji.sol.json');
const EMOJI_ADDRESS = 'one1w9fefdt20uzjtjkly88t57fr9pvpn04qufchep';
//'one1kml3y2emq6fmh6ea7vta5r33rwd09rku2z5mpl';
const EMOJI_URL = 'https://raw.githubusercontent.com/peekpi/nftymoji/master/emoji'
//const EMOJI_URL = '../emoji';
//const EMOJI_URL = 'emoji';
function fetchEmoji(url) {
return axios.get(url).then(rez => {
return rez.data;
});
}
let store = {
data: {
EMOJI_ADDRESS,
EMOJI_ABI,
contract: hmy.contract(EMOJI_ABI, EMOJI_ADDRESS),
txlist: [],
hmy,
},
async GetEmoji(name){
return await fetchEmoji(`${EMOJI_URL}/${name}.svg`);
},
async updateContact(){
await hmy.login();
this.data.contract = hmy.contract(EMOJI_ABI, hmy.hmySDK.crypto.fromBech32(EMOJI_ADDRESS));
return this.data.contract;
},
async txCommit(tx) {
await hmy.txSignSend(tx);
this.data.txlist.push(tx);
return tx.confirm(tx.id, 5)
}
}
Vue.prototype.$store = store;<file_sep>const isProduction = process.env.NODE_ENV === 'production';
module.exports = {
publicPath: isProduction
? '/onemoji/dist/'
: '/',
productionSourceMap: isProduction
? false : true,
} | 325557d6a8c138ec9a15d276c56e94887d683f38 | [
"JavaScript"
]
| 2 | JavaScript | forseti/onemoji | d59d4170f9b22e1d86859c1470cfa39142b931ab | 7b2d42265e21e3e3910a6f25b7d38691552a24c9 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.