branch_name
stringclasses 15
values | target
stringlengths 26
10.3M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
9
| num_files
int64 1
1.47k
| repo_language
stringclasses 34
values | repo_name
stringlengths 6
91
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
| input
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
refs/heads/master | <file_sep>package com.alankrita.fotos.model;
import java.util.List;
public class PhotoData {
private int page;
private List<Photos> photo;
public PhotoData(int page, List<Photos> photo) {
this.page = page;
this.photo = photo;
}
public int getPage() {
return page;
}
public void setPage(int page) {
this.page = page;
}
public List<Photos> getPhoto() {
return photo;
}
}
<file_sep>package com.alankrita.fotos.network;
import com.alankrita.fotos.Config;
import com.alankrita.fotos.model.PhotoResponse;
import retrofit2.Call;
import retrofit2.http.GET;
import retrofit2.http.Query;
public interface ApiService {
String FLICKR_API_KEY = "062a6c0c49e4de1d78497d13a7dbb360";
String query = "?method=flickr.photos.search&format=json&nojsoncallback=1&api_key="+FLICKR_API_KEY+"&per_page="+ Config.PAGE_SIZE;
@GET(query)
Call<PhotoResponse> fetchPhotos(@Query("page") long page, @Query("text") String searchText);
}
| 62c02c2818593899d40a971104a496d456710c5e | [
"Java"
] | 2 | Java | AlankritaShah/Fotos | b039386ddeda74e97aa816b4258ffdff7434af06 | cb611070d7b34418979ecf2f07caadf8ca7f7fbf | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.IO;
using System.Linq;
using System.Security.Cryptography;
using System.Text;
using System.Management;
using System.Net.Mime;
using System.Runtime.Serialization.Formatters.Binary;
namespace RegLib
{
/// <summary>
/// 加密解密类
/// </summary>
public class EncryptDecrypt
{
/// <summary>
/// 获得CUPID
/// </summary>
private static string GetCPUID()
{
try
{
ManagementClass mc = new ManagementClass("Win32_Processor");
ManagementObjectCollection moc = mc.GetInstances();
String strCpuID = null;
foreach (ManagementObject mo in moc)
{
strCpuID = mo.Properties["ProcessorId"].Value.ToString();
break;
}
return strCpuID;
}
catch
{
return "unknown";
}
}
/// <summary>
/// 获取主板ID
/// </summary>
private static string GetMotherBoardID()
{
try
{
ManagementClass mc = new ManagementClass("Win32_BaseBoard");
ManagementObjectCollection moc = mc.GetInstances();
string strID = null;
foreach (ManagementObject mo in moc)
{
strID = mo.Properties["SerialNumber"].Value.ToString();
break;
}
return strID;
}
catch
{
return "unknown";
}
}
/// <summary>
/// 获取网卡ID
/// </summary>
private static string GetMacID()
{
try
{
ManagementClass mc = new ManagementClass("win32_networkadapterconfiguration");
ManagementObjectCollection moc = mc.GetInstances();
string str = "";
foreach (ManagementObject mo in moc)
{
if ((bool)mo["ipenabled"] == true)
{
str = mo["macaddress"].ToString();
}
}
str = str.Replace(":", "");
return str;
}
catch (Exception)
{
return "unknown";
}
}
/// <summary>
/// 获得本机ID
/// </summary>
public static string GetID()
{
string strCPUID = GetCPUID();
string strMotherBoardID = GetMotherBoardID();
string strMacID = GetMacID();
string Temp = strCPUID + strMotherBoardID + strMacID;
Temp = MD5(Temp);
return Temp;
}
/// <summary>
/// MD5加密
/// </summary>
/// <param name="pToEncrypt">需要加密的字符串</param>
public static string MD5(string pToEncrypt)
{
byte[] result = Encoding.Default.GetBytes(pToEncrypt.Trim());
System.Security.Cryptography.MD5 md5 = new System.Security.Cryptography.MD5CryptoServiceProvider();
byte[] output = md5.ComputeHash(result);
return BitConverter.ToString(output).Replace("-", "").ToUpper();
}
/// <summary>
/// DES加密
/// </summary>
/// <param name="pToEncrypt">需要加密的字符串</param>
/// <param name="pKey">密匙,长度为8</param>
public static string DESEncrypt(string pToEncrypt, string pKey)
{
if (pKey.Length != 8)
{
return "Error";
}
try
{
DESCryptoServiceProvider des = new DESCryptoServiceProvider();
byte[] inputByteArray = Encoding.UTF8.GetBytes(pToEncrypt);
des.Key = Encoding.UTF8.GetBytes(pKey);
des.IV = Encoding.UTF8.GetBytes(pKey);
MemoryStream ms = new MemoryStream();
CryptoStream cs = new CryptoStream(ms, des.CreateEncryptor(), CryptoStreamMode.Write);
cs.Write(inputByteArray, 0, inputByteArray.Length);
cs.FlushFinalBlock();
StringBuilder ret = new StringBuilder();
foreach (byte b in ms.ToArray())
{
ret.AppendFormat("{0:X2}", b);
}
return ret.ToString();
}
catch (Exception)
{
return "Error";
}
}
/// <summary>
/// DES解密
/// </summary>
/// <param name="pToDecrypt">需要DES解密的字符串</param>
/// <param name="pKey">DES密匙</param>
public static string DESDecrypt(string pToDecrypt, string pKey)
{
if (pKey.Length != 8)
{
return "Error";
}
try
{
DESCryptoServiceProvider des = new DESCryptoServiceProvider();
byte[] inputByteArray = new byte[pToDecrypt.Length / 2];
for (int x = 0; x < pToDecrypt.Length / 2; x++)
{
int i = (Convert.ToInt32(pToDecrypt.Substring(x * 2, 2), 16));
inputByteArray[x] = (byte)i;
}
des.Key = ASCIIEncoding.UTF8.GetBytes(pKey);
des.IV = ASCIIEncoding.UTF8.GetBytes(pKey);
MemoryStream ms = new MemoryStream();
CryptoStream cs = new CryptoStream(ms, des.CreateDecryptor(), CryptoStreamMode.Write);
cs.Write(inputByteArray, 0, inputByteArray.Length);
cs.FlushFinalBlock();
return Encoding.Default.GetString(ms.ToArray());
}
catch (Exception)
{
return "Error";
}
}
/// <summary>
/// 生成注册文件
/// </summary>
/// <param name="RegInfo">注册信息</param>
/// <param name="path">导出路径</param>
public static bool ExportRegFile(clsRegInfo RegInfo, string path)
{
try
{
Stream fStream = new FileStream(Environment.GetEnvironmentVariable("TEMP",EnvironmentVariableTarget.Machine) + "\\tmpRegInfo.tmp", FileMode.Create, FileAccess.ReadWrite);
BinaryFormatter binFormat = new BinaryFormatter();//创建二进制序列化器
binFormat.Serialize(fStream, RegInfo);
fStream.Seek(0, SeekOrigin.Begin);
StringBuilder ret = new StringBuilder();
while (fStream.Position != fStream.Length)
{
ret.AppendFormat("{0:X2}", fStream.ReadByte());
}
string regInfo = ret.ToString();
fStream.Close();
File.Delete(Environment.GetEnvironmentVariable("TEMP", EnvironmentVariableTarget.Machine) + "\\tmpRegInfo.tmp");
string ID_8 = RegInfo.ID.Substring(0,8);
regInfo = ID_8 + regInfo;
regInfo = DESEncrypt(regInfo, ID_8);
regInfo = ID_8 + regInfo;
StreamWriter sw = new StreamWriter(path, false, Encoding.UTF8);
sw.Write(regInfo);
sw.Close();
return true;
}
catch (Exception)
{
return false;
}
}
/// <summary>
/// 读取注册文件
/// </summary>
/// <param name="path">文件路径</param>
public static clsRegInfo LoadRegFile(string path)
{
StreamReader sr = new StreamReader(path, Encoding.UTF8);
string regInfo = sr.ReadToEnd();
sr.Close();
string ID_8 = regInfo.Substring(0, 8);
regInfo = regInfo.Substring(8, regInfo.Length - 8);
regInfo = DESDecrypt(regInfo, ID_8);
if (regInfo.Substring(0, 8) != ID_8)
{
return null;
}
regInfo = regInfo.Substring(8, regInfo.Length - 8);
byte[] reg = new byte[regInfo.Length/2];
for (int i = 0; i < regInfo.Length/2; i++)
{
reg[i] = Convert.ToByte(regInfo.Substring(2*i, 2), 16);
}
BinaryFormatter binFormat = new BinaryFormatter();
Stream sm = new MemoryStream(reg);
clsRegInfo ret = (clsRegInfo)binFormat.Deserialize(sm);
sm.Close();
return ret;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using RegLib;
namespace Demo
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
if (!File.Exists(Application.StartupPath + "\\reg.key"))
{
clsPublic.isReg = false;
}
else
{
clsRegInfo info = RegLib.EncryptDecrypt.LoadRegFile(Application.StartupPath + "\\reg.key");
string localID = RegLib.EncryptDecrypt.GetID();
if (info == null || info.ID != localID)
{
clsPublic.isReg = false;
}
}
MessageBox.Show("软件未注册!","提示",MessageBoxButtons.OK,MessageBoxIcon.Warning);
label1.Visible = true;
}
private void 注册ToolStripMenuItem_Click(object sender, EventArgs e)
{
Form2 frmReg = new Form2();
frmReg.ShowDialog();
}
}
}
<file_sep>using System;
using System.Windows.Forms;
using Microsoft.Win32;
using System.Text;
using System.CodeDom.Compiler;
using Microsoft.CSharp;
using System.Reflection;
namespace test
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
CSharpCodeProvider privoder = new CSharpCodeProvider();
// 2.ICodeComplier
//ICodeCompiler objICodeCompiler = objCSharpCodePrivoder.CreateCompiler();
// 3.CompilerParameters
CompilerParameters options = new CompilerParameters();
//options.ReferencedAssemblies.Add("System.dll");
options.ReferencedAssemblies.Add("System.Windows.Forms.dll");
//options.GenerateExecutable = false;
options.GenerateInMemory = true;
// 4.CompilerResults
CompilerResults cr = privoder.CompileAssemblyFromSource(options, getCode());
if (cr.Errors.HasErrors)
{
Console.WriteLine("编译错误:");
foreach (CompilerError err in cr.Errors)
{
Console.WriteLine(err.ErrorText);
}
}
else
{
// 通过反射,调用HelloWorld的实例
Type DriverType = cr.CompiledAssembly.GetType("Driver");
DriverType.InvokeMember("OutPut",
BindingFlags.InvokeMethod | BindingFlags.Static | BindingFlags.Public,
null, null, new object[]{"abcde"});
//Assembly objAssembly = cr.CompiledAssembly;
//object objHelloWorld = objAssembly.CreateInstance("test.Driver");
//MethodInfo objMI = objHelloWorld.GetType().GetMethod("OutPut");
//objMI.Invoke(objHelloWorld, null);
}
}
private string getCode()
{
StringBuilder sb = new StringBuilder();
//DateTime tm = DateTime.Now;
sb.Append("using System;");
sb.Append("using System.Windows.Forms;");
//sb.Append("namespace test {");
sb.Append(" public static class Driver");
sb.Append(" {");
sb.Append(" public static void OutPut(string testStr)");
sb.Append(" {");
sb.Append(" DateTime tm = DateTime.Now;");
sb.Append(" MessageBox.Show(\"This is a test.\" + testStr + tm.ToString());");
sb.Append(" }");
sb.Append(" }");
//sb.Append("}");
return sb.ToString();
}
private string getCode2()
{
StringBuilder sb = new StringBuilder();
sb.Append("using System;");
sb.Append("using System.Windows.Forms;");
sb.Append("namespace test {");
sb.Append(" public class Driver");
sb.Append(" {");
sb.Append(" public void OutPut()");
sb.Append(" {");
sb.Append(" MessageBox.Show(\"This is a test.\");");
sb.Append(" }");
sb.Append(" }");
sb.Append("}");
return sb.ToString();
}
private void button2_Click(object sender, EventArgs e)
{
CSharpCodeProvider privoder = new CSharpCodeProvider();
CompilerParameters options = new CompilerParameters();
options.ReferencedAssemblies.Add("System.Windows.Forms.dll");
options.GenerateExecutable = false;
options.GenerateInMemory = true;
CompilerResults cr = privoder.CompileAssemblyFromSource(options, getCode2());
if (cr.Errors.HasErrors)
{
Console.WriteLine("编译错误:");
foreach (CompilerError err in cr.Errors)
{
Console.WriteLine(err.ErrorText);
}
}
else
{
// 通过反射,调用HelloWorld的实例
//Type DriverType = cr.CompiledAssembly.GetType("Driver");
//DriverType.InvokeMember("OutPut",
// BindingFlags.InvokeMethod | BindingFlags.Static | BindingFlags.Public,
// null, null, null);
Assembly objAssembly = cr.CompiledAssembly;
object objHelloWorld = objAssembly.CreateInstance("test.Driver");
MethodInfo objMI = objHelloWorld.GetType().GetMethod("OutPut");
objMI.Invoke(objHelloWorld, null);
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using RegLib;
namespace Demo
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
private void Form2_Load(object sender, EventArgs e)
{
label1.Visible = false;
if (!File.Exists(Application.StartupPath + "\\reg.key"))
{
button1.Enabled = true;
}
else
{
clsRegInfo info = RegLib.EncryptDecrypt.LoadRegFile(Application.StartupPath + "\\reg.key");
string localID = RegLib.EncryptDecrypt.GetID();
if (info == null || info.ID != localID)
{
button1.Enabled = true;
}
else
{
button1.Enabled = false;
label1.Visible = true;
DateTime dt = new DateTime(info.TimeTo);
label1.Text = "授权时间到:" + dt.ToString();
}
}
textBox1.Text = RegLib.EncryptDecrypt.GetID();
}
private void button1_Click(object sender, EventArgs e)
{
openFileDialog1.FileName = "reg.key";
openFileDialog1.Filter = "密匙文件|reg.key";
openFileDialog1.Title = "选择注册文件";
//openFileDialog1.ShowDialog();
if (DialogResult.OK == openFileDialog1.ShowDialog())
{
clsRegInfo info = RegLib.EncryptDecrypt.LoadRegFile(openFileDialog1.FileName);
string localID = RegLib.EncryptDecrypt.GetID();
if (info == null || info.ID != localID)
{
MessageBox.Show("注册文件非法!", "错误", MessageBoxButtons.OK, MessageBoxIcon.Stop);
clsPublic.isReg = false;
}
else
{
long timeNow = DateTime.Now.Ticks;
if (timeNow > info.TimeTo)
{
MessageBox.Show("注册文件已经失效!", "错误", MessageBoxButtons.OK, MessageBoxIcon.Stop);
clsPublic.isReg = false;
}
else
{
File.Copy(openFileDialog1.FileName, Application.StartupPath + "\\reg.key");
clsPublic.isReg = true;
label1.Text = "授权时间到:" + new DateTime(info.TimeTo);
MessageBox.Show("注册成功!", "信息", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Demo
{
public class clsPublic
{
public static bool isReg = false;
}
}
| 63604ed63428eb57ddb40d06e6cb179a48312767 | [
"C#"
] | 5 | C# | Levelangel/authority | 364b1b8aea0e053eaaa58a0a094a5e232db9aad4 | 1cee2ccc38eb2a2f747a7c4918566b3e8a6c38e1 | |
refs/heads/master | <file_sep>I can't figure out a cross-platform solution to expand relative path (possibly a symbolic link) to its absolute path.
So this utility.
```
$ normpath ../mytoken/solar.development.json
/Users/howard/p/qtum/qtumbook/examples/mytoken/solar.development.json
```
Install:
```
go get -u github.com/hayeah/normpath
```
See: https://unix.stackexchange.com/questions/36547/how-can-i-expand-a-relative-path-at-the-command-line-with-tab-completion
<file_sep>package main
import (
"fmt"
"log"
"os"
"path/filepath"
)
func help() {
fmt.Println(
`Usage: normpath <path>
Expand relative path to absolute path. Resolve symbolic links.
`)
os.Exit(1)
}
func main() {
if len(os.Args) < 2 {
fmt.Println("Invalid usage")
help()
}
pathname := os.Args[1]
abspath, err := filepath.Abs(pathname)
if err != nil {
log.Fatalln(err)
}
resolvedPath, err := filepath.EvalSymlinks(abspath)
if err != nil {
log.Fatalln(err)
}
fmt.Println(resolvedPath)
}
| 91d420da6c86295f843c8b1a8915fbbb0a29539e | [
"Markdown",
"Go"
] | 2 | Markdown | hayeah/normpath | 7fa77c0a57c5f869d5f28372f394667c057f9ca7 | 5d1bf3a48316e0432651e04a94dcafeba061cde2 | |
refs/heads/master | <repo_name>te4/HelpfulEndermen<file_sep>/src/me/te3/helpfulendermen/HelpfulEndermen.java
package me.te3.helpfulendermen;
import java.util.logging.Level;
import org.bukkit.Material;
import org.bukkit.block.Block;
import org.bukkit.World;
import org.bukkit.plugin.java.JavaPlugin;
import org.bukkit.event.Listener;
import org.bukkit.event.EventHandler;
import org.bukkit.event.entity.EntityChangeBlockEvent;
import org.bukkit.entity.EntityType;
import org.bukkit.entity.Enderman;
/**
*
* @author te3
*/
public class HelpfulEndermen extends JavaPlugin implements Listener {
JavaPlugin plugin = this;
public void onEnable() {
getServer().getPluginManager().registerEvents(this, this);
}
@EventHandler
public void onEndermanActivity(EntityChangeBlockEvent blockchange) {
Enderman enderman;
if (blockchange.getEntityType() == EntityType.ENDERMAN) {
enderman = (Enderman) blockchange.getEntity();
Material newmaterial = enderman.getCarriedMaterial().getItemType();
if (newmaterial == Material.DIRT || newmaterial == Material.GRASS) {
Block placed = blockchange.getBlock();
World world = placed.getWorld();
if (placed.getY() < world.getMaxHeight() - 1) {
world.getBlockAt(placed.getX(), placed.getY() + 1, placed.getZ()).setType(Material.SAPLING);
}
}
}
}
} | 1cadcfab91c0e1f249e59a5c7cfc58904ee8ca79 | [
"Java"
] | 1 | Java | te4/HelpfulEndermen | 13fc32a4ed07bdcb0b5fabe8a7875721cbe15426 | 7768e428d7676e81e470c3460cc50634fbdf4a3d | |
refs/heads/main | <repo_name>lora-aprs/LoRa_APRS_Digi<file_sep>/platformio.ini
[env]
platform = espressif32
framework = arduino
lib_ldf_mode = deep+
monitor_speed = 115200
lib_deps =
Adafruit GFX [email protected]
Adafruit SSD1306
APRS-Decoder-Lib
APRS-IS-Lib
LoRa
LoRa-APRS-Lib
NTPClient
ArduinoJson
AXP202X_Library
check_tool = cppcheck
check_flags =
cppcheck: --suppress=*:*.pio\* --inline-suppr
[env:heltec_wifi_lora_32_V1]
board = ttgo-lora32-v1
build_flags = -Werror -Wall -DHELTEC_WIFI_LORA_32_V1
[env:heltec_wifi_lora_32_V2]
board = ttgo-lora32-v1
build_flags = -Werror -Wall -DHELTEC_WIFI_LORA_32_V2
[env:ttgo-lora32-v1]
board = ttgo-lora32-v1
build_flags = -Werror -Wall -DTTGO_LORA32_V1
[env:ttgo-lora32-v2]
board = ttgo-lora32-v1
build_flags = -Werror -Wall -DTTGO_LORA32_V2
[env:ttgo-t-beam-v1]
board = ttgo-t-beam
build_flags = -Werror -Wall -DTTGO_T_Beam_V1_0
[env:ttgo-t-beam-v0_7]
board = ttgo-t-beam
build_flags = -Werror -Wall -DTTGO_T_Beam_V0_7
<file_sep>/src/display.h
#ifndef DISPLAY_H_
#define DISPLAY_H_
void setup_display();
void show_display(String header, int wait = 0);
void show_display(String header, String line1, int wait = 0);
void show_display(String header, String line1, String line2, int wait = 0);
void show_display(String header, String line1, String line2, String line3, int wait = 0);
void show_display(String header, String line1, String line2, String line3, String line4, int wait = 0);
void show_display(String header, String line1, String line2, String line3, String line4, String line5, int wait = 0);
#endif
<file_sep>/src/display.cpp
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include "pins.h"
#include "display.h"
#include "settings.h"
Adafruit_SSD1306 display(128, 64, &Wire, OLED_RST);
void setup_display()
{
pinMode(OLED_RST, OUTPUT);
digitalWrite(OLED_RST, LOW);
delay(20);
digitalWrite(OLED_RST, HIGH);
Wire.begin(OLED_SDA, OLED_SCL);
if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3c, false, false))
{
Serial.println("SSD1306 allocation failed");
while (1);
}
display.clearDisplay();
display.setTextColor(WHITE);
display.setTextSize(1);
display.setCursor(0,0);
display.print("LORA SENDER ");
display.ssd1306_command(SSD1306_SETCONTRAST);
display.ssd1306_command(1);
display.display();
}
void show_display(String header, int wait)
{
display.clearDisplay();
display.setTextColor(WHITE);
display.setTextSize(2);
display.setCursor(0,0);
display.println(header);
display.ssd1306_command(SSD1306_SETCONTRAST);
display.ssd1306_command(1);
display.display();
delay(wait);
}
void show_display(String header, String line1, int wait)
{
display.clearDisplay();
display.setTextColor(WHITE);
display.setTextSize(2);
display.setCursor(0,0);
display.println(header);
display.setTextSize(1);
display.setCursor(0,16);
display.println(line1);
display.ssd1306_command(SSD1306_SETCONTRAST);
display.ssd1306_command(1);
display.display();
delay(wait);
}
void show_display(String header, String line1, String line2, int wait)
{
display.clearDisplay();
display.setTextColor(WHITE);
display.setTextSize(2);
display.setCursor(0,0);
display.println(header);
display.setTextSize(1);
display.setCursor(0,16);
display.println(line1);
display.setCursor(0,26);
display.println(line2);
display.ssd1306_command(SSD1306_SETCONTRAST);
display.ssd1306_command(1);
display.display();
delay(wait);
}
void show_display(String header, String line1, String line2, String line3, int wait)
{
display.clearDisplay();
display.setTextColor(WHITE);
display.setTextSize(2);
display.setCursor(0,0);
display.println(header);
display.setTextSize(1);
display.setCursor(0,16);
display.println(line1);
display.setCursor(0,26);
display.println(line2);
display.setCursor(0,36);
display.println(line3);
display.ssd1306_command(SSD1306_SETCONTRAST);
display.ssd1306_command(1);
display.display();
delay(wait);
}
void show_display(String header, String line1, String line2, String line3, String line4, int wait)
{
display.clearDisplay();
display.setTextColor(WHITE);
display.setTextSize(2);
display.setCursor(0,0);
display.println(header);
display.setTextSize(1);
display.setCursor(0,16);
display.println(line1);
display.setCursor(0,26);
display.println(line2);
display.setCursor(0,36);
display.println(line3);
display.setCursor(0,46);
display.println(line4);
display.ssd1306_command(SSD1306_SETCONTRAST);
display.ssd1306_command(1);
display.display();
delay(wait);
}
void show_display(String header, String line1, String line2, String line3, String line4, String line5, int wait)
{
display.clearDisplay();
display.setTextColor(WHITE);
display.setTextSize(2);
display.setCursor(0,0);
display.println(header);
display.setTextSize(1);
display.setCursor(0,16);
display.println(line1);
display.setCursor(0,26);
display.println(line2);
display.setCursor(0,36);
display.println(line3);
display.setCursor(0,46);
display.println(line4);
display.setCursor(0,56);
display.println(line5);
display.ssd1306_command(SSD1306_SETCONTRAST);
display.ssd1306_command(1);
display.display();
delay(wait);
}
<file_sep>/src/LoRa_APRS_Digi.cpp
#include <map>
#include <Arduino.h>
#include <APRS-Decoder.h>
#include <LoRa_APRS.h>
#include "pins.h"
#include "settings.h"
#include "display.h"
#if defined(ARDUINO_T_Beam) && !defined(ARDUINO_T_Beam_V0_7)
#include "power_management.h"
PowerManagement powerManagement;
#endif
portMUX_TYPE timerMux = portMUX_INITIALIZER_UNLOCKED;
hw_timer_t * timer = NULL;
volatile uint secondsSinceLastTX = 0;
volatile uint secondsSinceStartup = 0;
LoRa_APRS lora_aprs;
String create_lat_aprs(double lat);
String create_long_aprs(double lng);
void setup_lora();
std::map<uint, std::shared_ptr<APRSMessage>> lastMessages;
void IRAM_ATTR onTimer()
{
portENTER_CRITICAL_ISR(&timerMux);
secondsSinceLastTX++;
secondsSinceStartup++;
portEXIT_CRITICAL_ISR(&timerMux);
}
// cppcheck-suppress unusedFunction
void setup()
{
Serial.begin(115200);
#if defined(ARDUINO_T_Beam) && !defined(ARDUINO_T_Beam_V0_7)
Wire.begin(SDA, SCL);
if (!powerManagement.begin(Wire))
{
Serial.println("LoRa-APRS / Init / AXP192 Begin PASS");
} else {
Serial.println("LoRa-APRS / Init / AXP192 Begin FAIL");
}
powerManagement.activateLoRa();
powerManagement.activateOLED();
powerManagement.deactivateGPS();
#endif
setup_display();
delay(500);
Serial.println("[INFO] LoRa APRS Digi by OE5BPA (<NAME>)");
show_display("OE5BPA", "LoRa APRS Digi", "by <NAME>", 2000);
setup_lora();
timer = timerBegin(0, 80, true);
timerAlarmWrite(timer, 1000000, true);
timerAttachInterrupt(timer, &onTimer, true);
timerAlarmEnable(timer);
delay(500);
}
// cppcheck-suppress unusedFunction
void loop()
{
static bool send_update = true;
if(secondsSinceLastTX >= (BEACON_TIMEOUT*60))
{
portENTER_CRITICAL(&timerMux);
secondsSinceLastTX -= (BEACON_TIMEOUT*60);
portEXIT_CRITICAL(&timerMux);
send_update = true;
}
if(send_update)
{
send_update = false;
std::shared_ptr<APRSMessage> msg = std::shared_ptr<APRSMessage>(new APRSMessage());
msg->setSource(CALL);
msg->setDestination("APLG0");
String lat = create_lat_aprs(BEACON_LAT);
String lng = create_long_aprs(BEACON_LNG);
msg->getAPRSBody()->setData(String("=") + lat + "R" + lng + "#" + BEACON_MESSAGE);
String data = msg->encode();
Serial.print(data);
show_display(CALL, "<< Beaconing myself >>", data);
lora_aprs.sendMessage(msg);
Serial.println("finished TXing...");
}
if(lora_aprs.hasMessage())
{
std::shared_ptr<APRSMessage> msg = lora_aprs.getMessage();
if(msg->getSource().indexOf(CALL) != -1)
{
Serial.print("Message already received as repeater: '");
Serial.print(msg->toString());
Serial.print("' with RSSI ");
Serial.print(lora_aprs.getMessageRssi());
Serial.print(" and SNR ");
Serial.println(lora_aprs.getMessageSnr());
return;
}
// lets try not to flood the LoRa frequency in limiting the same messages:
std::map<uint, std::shared_ptr<APRSMessage>>::iterator foundMsg = std::find_if(lastMessages.begin(), lastMessages.end(), [&](std::pair<const unsigned int, std::shared_ptr<APRSMessage> > & old_msg)
{
if(msg->getSource() == old_msg.second->getSource() &&
msg->getDestination() == old_msg.second->getDestination() &&
msg->getAPRSBody()->getData() == old_msg.second->getAPRSBody()->getData())
{
return true;
}
return false;
});
if(foundMsg == lastMessages.end())
{
show_display(CALL, "RSSI: " + String(lora_aprs.getMessageRssi()) + ", SNR: " + String(lora_aprs.getMessageSnr()), msg->toString(), 0);
Serial.print("Received packet '");
Serial.print(msg->toString());
Serial.print("' with RSSI ");
Serial.print(lora_aprs.getMessageRssi());
Serial.print(" and SNR ");
Serial.println(lora_aprs.getMessageSnr());
msg->setPath(String(CALL) + "*");
lora_aprs.sendMessage(msg);
lastMessages.insert({secondsSinceStartup, msg});
}
else
{
Serial.print("Message already received (timeout): '");
Serial.print(msg->toString());
Serial.print("' with RSSI ");
Serial.print(lora_aprs.getMessageRssi());
Serial.print(" and SNR ");
Serial.println(lora_aprs.getMessageSnr());
}
return;
}
for(std::map<uint, std::shared_ptr<APRSMessage>>::iterator iter = lastMessages.begin(); iter != lastMessages.end(); )
{
if(secondsSinceStartup >= iter->first + FORWARD_TIMEOUT*60)
{
iter = lastMessages.erase(iter);
}
else
{
iter++;
}
}
static int _secondsSinceLastTX = 0;
if(secondsSinceLastTX != _secondsSinceLastTX)
{
show_display(CALL, "Time to next beaconing: " + String((BEACON_TIMEOUT*60) - secondsSinceLastTX));
}
}
void setup_lora()
{
lora_aprs.tx_frequency = LORA_RX_FREQUENCY;
//lora_aprs.rx_frequency = LORA_TX_FREQUENCY; // for debugging
if (!lora_aprs.begin())
{
Serial.println("[ERROR] Starting LoRa failed!");
show_display("ERROR", "Starting LoRa failed!");
while (1);
}
Serial.println("[INFO] LoRa init done!");
show_display("INFO", "LoRa init done!", 2000);
}
String create_lat_aprs(double lat)
{
char str[20];
char n_s = 'N';
if(lat < 0)
{
n_s = 'S';
}
lat = std::abs(lat);
sprintf(str, "%02d%05.2f%c", (int)lat, (lat - (double)((int)lat)) * 60.0, n_s);
String lat_str(str);
return lat_str;
}
String create_long_aprs(double lng)
{
char str[20];
char e_w = 'E';
if(lng < 0)
{
e_w = 'W';
}
lng = std::abs(lng);
sprintf(str, "%03d%05.2f%c", (int)lng, (lng - (double)((int)lng)) * 60.0, e_w);
String lng_str(str);
return lng_str;
}
<file_sep>/README.md
**DON'T USE THIS CODE ANYMORE!**
If you want to have a LoRa Digi, use the main project: https://github.com/lora-aprs/LoRa_APRS_iGate
# LoRa APRS Digi
This is a LoRa APRS Digipeater which will work with TTGO LoRa32 PCBs. **This is an experimental Firmware!**
<file_sep>/src/pins.h
#ifndef PINS_H_
#define PINS_H_
#undef OLED_SDA
#undef OLED_SCL
#undef OLED_RST
#if defined(HELTEC_WIFI_LORA_32_V1) || defined(HELTEC_WIFI_LORA_32_V2) || defined(TTGO_LORA32_V1)
#define OLED_SDA 4
#define OLED_SCL 15
#define OLED_RST 16
#endif
#if defined(TTGO_LORA32_V2) || defined(TTGO_T_Beam_V0_7) || defined(TTGO_T_Beam_V1_0)
#define OLED_SDA 21
#define OLED_SCL 22
#define OLED_RST 16
#endif
#ifdef TRACKERD
#define OLED_SDA 5
#define OLED_SCL 4
#define OLED_RST 25
#endif
#endif
<file_sep>/src/settings.h
#ifndef SETTINGS_H_
#define SETTINGS_H_
#define CALL "NOCALL-15"
#define BEACON_MESSAGE "LoRa APRS Repeater Test"
#define BEACON_TIMEOUT 30
#define BEACON_LAT 00.000000
#define BEACON_LNG 00.000000
#define FORWARD_TIMEOUT 5
#endif
| 147709871a92a1a43b4460fe3d4690dab91e78cc | [
"Markdown",
"C",
"C++",
"INI"
] | 7 | INI | lora-aprs/LoRa_APRS_Digi | c0faabea5f7ac4c2bdf8a44d2d8f57c7e1e7e22f | 62dda2db282ca619506bdd564b8022bb07f2d31d | |
refs/heads/master | <repo_name>aidlpy/vue<file_sep>/src/router/index.js
import Vue from 'vue'
import Router from 'vue-router'
import ElementUI from 'element-ui'
import HelloWorld from '@/components/HelloWorld'
import HomePage from '@/components/Home'
import FirstPage from '@/components/FirstPage'
import SecondPage from '@/components/SecondPage'
Vue.use(Router)
Vue.use(ElementUI)
export default new Router({
routes: [
{
path: '/Hello',
name: 'HelloWorld',
component: HelloWorld
}, {
path: '/homePage',
name: 'HomePage',
component: HomePage
}, {
path: '/firstPage',
name: 'FirstPage',
component: FirstPage
}, {
path: '/secondPage',
name: 'SecondPage',
component: SecondPage
}
]
})
| e35deb2b6a2c821bee9b9a01e40f71f1017cbaa6 | [
"JavaScript"
] | 1 | JavaScript | aidlpy/vue | 1af9b769ede78f93a08a3913cdcb4aa216316f18 | 7030fc8f54a576aab769a4689dce6a0ed295f08d | |
refs/heads/master | <file_sep># [0.3.0](https://github.com/OpenCodeFoundation/eSchool/compare/v0.2.0...v0.3.0) (2020-08-01)
### Features
* **deployment:** Initial deployment script ([#178](https://github.com/OpenCodeFoundation/eSchool/issues/178)) ([5344488](https://github.com/OpenCodeFoundation/eSchool/commit/5344488dd5b13f81423ce4fce4cc256223957569)), closes [#146](https://github.com/OpenCodeFoundation/eSchool/issues/146)
* **enrolling:** add open telemetry integration ([eb751de](https://github.com/OpenCodeFoundation/eSchool/commit/eb751ded855a5923ef1f42707c9476e87e36a825))
* **enrolling:** publish docker image to docker hub ([#177](https://github.com/OpenCodeFoundation/eSchool/issues/177))Closes [#173](https://github.com/OpenCodeFoundation/eSchool/issues/173) ([43fb95c](https://github.com/OpenCodeFoundation/eSchool/commit/43fb95c3bb5039a2d47f2ed0b5e2fa496603c1d0))
* **enrolling:** update enrolling service to .NET 5.0 ([#186](https://github.com/OpenCodeFoundation/eSchool/issues/186)) ([a03d284](https://github.com/OpenCodeFoundation/eSchool/commit/a03d284d9ccdeb4127ce4377084557ffffb82120)), closes [#167](https://github.com/OpenCodeFoundation/eSchool/issues/167)
* **pipeline:** improve pipeline trigger ([60e7fb7](https://github.com/OpenCodeFoundation/eSchool/commit/60e7fb7efce0db773d6d8be3d8698c1ca3b0bad0))
## [0.2.0](https://github.com/OpenCodeFoundation/eSchool/compare/62af44a5c22bd198491bc95684b0a136f0a2b9cd...v0.2.0) (2020-07-01)
### Bug Fixes
* **enrolling:** change email address as required field ([e3da303](https://github.com/OpenCodeFoundation/eSchool/commit/e3da303c162a0a54158d5b8c07a919e37bd4ae44))
* **enrolling:** modify source path for code coverage ([#166](https://github.com/OpenCodeFoundation/eSchool/issues/166)) ([2845652](https://github.com/OpenCodeFoundation/eSchool/commit/284565244a4a278fbb3bbbb0a30d85f0f66ffc1f)), closes [#164](https://github.com/OpenCodeFoundation/eSchool/issues/164)
* **enrolling:** update NuGet packages ([#161](https://github.com/OpenCodeFoundation/eSchool/issues/161)) ([d4797b4](https://github.com/OpenCodeFoundation/eSchool/commit/d4797b4fb527ee2a664c037761cb0d123e44d03f))
* broken identity service removed ([62af44a](https://github.com/OpenCodeFoundation/eSchool/commit/62af44a5c22bd198491bc95684b0a136f0a2b9cd)), closes [#95](https://github.com/OpenCodeFoundation/eSchool/issues/95)
### Features
* add GitHub code scanning ([#165](https://github.com/OpenCodeFoundation/eSchool/issues/165)) ([0cbc451](https://github.com/OpenCodeFoundation/eSchool/commit/0cbc4519ae075f91a9d3d2af41af2755481d899e))
* **enrolling:** enable logging every command and query processing ([0ac94ec](https://github.com/OpenCodeFoundation/eSchool/commit/0ac94ec4aea4a4567db7487282e4daa267fafccb))
* **enrolling:** use mediatR to handle query ([70114d7](https://github.com/OpenCodeFoundation/eSchool/commit/70114d761aae5fcbe6f1ec9511c1578d46680e85))
* enable w3c trace context support ([#159](https://github.com/OpenCodeFoundation/eSchool/issues/159)) ([e1a69b8](https://github.com/OpenCodeFoundation/eSchool/commit/e1a69b8a8e95a84d60c988d42a0dd70ded6f06fb))
* **service-status:** use sql database to store service health status ([#157](https://github.com/OpenCodeFoundation/eSchool/issues/157)) ([43b1148](https://github.com/OpenCodeFoundation/eSchool/commit/43b114895dfc06a1efe053d8b3967429807853a2))
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Microsoft.EntityFrameworkCore;
using OpenCodeFoundation.ESchool.Services.Joining.Domain.AggregatesModel.JoinAggregate;
namespace OpenCodeFoundation.ESchool.Services.Joining.Infrastructure.Repositories
{
public class JoinRepository
: IJoinRepository
{
private readonly JoiningContext _context;
public JoinRepository(JoiningContext context)
{
_context = context;
}
public Join Add(Join join)
{
return _context.Joins
.Add(join)
.Entity;
}
public async Task<Join> FindByIdAsync(Guid id)
{
return await _context.Joins
.Where(e => e.Id == id)
.SingleOrDefaultAsync();
}
public Join Update(Join join)
{
return _context.Joins
.Update(join)
.Entity;
}
}
}
<file_sep>using System;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using OpenTelemetry.Context.Propagation;
using OpenTelemetry.Trace;
using OpenTelemetry.Trace.Samplers;
namespace OpenCodeFoundation.OpenTelemetry
{
public static class Extensions
{
private const string SectionName = "OpenTelemetry";
public static IServiceCollection AddOpenTelemetryIntegration(
this IServiceCollection services,
Action<OpenTelemetryOptions> options = null,
string sectionName = SectionName)
{
var openTelemetryOptions = services.GetOptions(sectionName, options);
if (openTelemetryOptions.Enabled)
{
ConfigureOpenTelemetry(services, openTelemetryOptions);
}
return services;
}
public static T GetOptions<T>(
this IServiceCollection services,
string sectionName,
Action<T> configure = null)
where T : IConfigurationOptions, new()
{
var provider = services.BuildServiceProvider();
var configuration = provider.GetRequiredService<IConfiguration>();
var options = new T();
configure?.Invoke(options);
configuration.GetSection(sectionName).Bind(options);
options.Validate();
return options;
}
private static void ConfigureOpenTelemetry(IServiceCollection services, OpenTelemetryOptions openTelemetryOptions)
{
services.AddOpenTelemetry(configure =>
{
ConfigureSampler(openTelemetryOptions, configure);
ConfigureInstrumentations(openTelemetryOptions, configure);
ConfigureExporters(openTelemetryOptions, configure);
});
}
private static void ConfigureSampler(OpenTelemetryOptions openTelemetryOptions, TracerProviderBuilder configure)
{
if (openTelemetryOptions.AlwaysOnSampler)
{
configure.SetSampler(new AlwaysOnSampler());
}
}
private static void ConfigureExporters(OpenTelemetryOptions openTelemetryOptions, TracerProviderBuilder configure)
{
if (openTelemetryOptions.Jaeger.Enabled)
{
configure.UseJaegerExporter(config =>
{
config.ServiceName = openTelemetryOptions.Jaeger.ServiceName;
config.AgentHost = openTelemetryOptions.Jaeger.Host;
config.AgentPort = openTelemetryOptions.Jaeger.Port;
});
}
}
private static void ConfigureInstrumentations(OpenTelemetryOptions openTelemetryOptions, TracerProviderBuilder configure)
{
configure.AddAspNetCoreInstrumentation(config =>
{
config.TextFormat = GetTextFormat(openTelemetryOptions);
});
configure.AddHttpClientInstrumentation(config =>
{
config.TextFormat = GetTextFormat(openTelemetryOptions);
});
configure.AddSqlClientDependencyInstrumentation();
}
private static ITextFormat GetTextFormat(OpenTelemetryOptions openTelemetryOptions)
=> openTelemetryOptions.Istio
? new B3Format()
: (ITextFormat)new TraceContextFormat();
}
}
<file_sep>using System;
using System.Threading.Tasks;
using OpenCodeFoundation.ESchool.Services.Joining.Domain.SeedWork;
namespace OpenCodeFoundation.ESchool.Services.Joining.Domain.AggregatesModel.JoinAggregate
{
public interface IJoinRepository
: IRepository<Join>
{
Join Add(Join join);
Join Update(Join join);
Task<Join> FindByIdAsync(Guid id);
}
}
<file_sep>using MediatR;
namespace OpenCodeFoundation.ESchool.Services.Joining.API.Application.Commands
{
public class JoinApplicationCommand
: IRequest<bool>
{
public string Name { get; set; }
public string Email { get; set; }
public string Mobile { get; set; }
}
}
<file_sep>#See https://aka.ms/containerfastmode to understand how Visual Studio uses this Dockerfile to build your images for faster debugging.
FROM mcr.microsoft.com/dotnet/core/aspnet:3.1-buster-slim AS base
WORKDIR /app
EXPOSE 80
EXPOSE 443
FROM mcr.microsoft.com/dotnet/core/sdk:3.1-buster AS build
WORKDIR /src
COPY ["src/Services/Joining/Joining.API/Joining.API.csproj", "src/Services/Joining/Joining.API/"]
COPY ["src/Services/Joining/Joining.Domain/Joining.Domain.csproj", "src/Services/Joining/Joining.Domain/"]
COPY ["src/Services/Joining/Joining.Infrastructure/Joining.Infrastructure.csproj", "src/Services/Joining/Joining.Infrastructure/"]
COPY ["src/Services/Joining/Joining.UnitTests/Joining.UnitTests.csproj", "src/Services/Joining/Joining.UnitTests/"]
COPY ["src/Services/Joining/Joining.FunctionalTests/Joining.FunctionalTests.csproj", "src/Services/Joining/Joining.FunctionalTests/"]
RUN dotnet restore "src/Services/Joining/Joining.API/Joining.API.csproj"
RUN dotnet restore "src/Services/Joining/Joining.UnitTests/Joining.UnitTests.csproj"
RUN dotnet restore "src/Services/Joining/Joining.FunctionalTests/Joining.FunctionalTests.csproj"
COPY . .
WORKDIR "/src/src/Services/Joining/Joining.API"
RUN dotnet build "Joining.API.csproj" -c Release -o /app/build
FROM build as unittest
WORKDIR /src/src/Services/Joining/Joining.UnitTests
FROM build as functionaltest
WORKDIR /src/src/Services/Joining/Joining.FunctionalTests
FROM build AS publish
RUN dotnet publish "Joining.API.csproj" -c Release -o /app/publish
FROM base AS final
WORKDIR /app
COPY --from=publish /app/publish .
ENTRYPOINT ["dotnet", "Joining.API.dll"]<file_sep>using Microsoft.EntityFrameworkCore;
using Microsoft.EntityFrameworkCore.Design;
using OpenCodeFoundation.ESchool.Services.Joining.Domain.AggregatesModel.JoinAggregate;
namespace OpenCodeFoundation.ESchool.Services.Joining.Infrastructure
{
public class JoiningContext
: DbContext
{
public JoiningContext(DbContextOptions<JoiningContext> options)
: base(options)
{
}
public DbSet<Join> Joins { get; set; }
}
public class JoiningContextFactory : IDesignTimeDbContextFactory<JoiningContext>
{
public JoiningContext CreateDbContext(string[] args)
{
var optionsBuilder = new DbContextOptionsBuilder<JoiningContext>()
.UseSqlServer("Server=.;Initial Catalog=OpenCodeFoundation.JoiningDb;Integrated Security=true");
return new JoiningContext(optionsBuilder.Options);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading;
using System.Threading.Tasks;
using MediatR;
using Microsoft.Extensions.Logging;
using OpenCodeFoundation.ESchool.Services.Joining.Domain.AggregatesModel.JoinAggregate;
using OpenCodeFoundation.ESchool.Services.Joining.Infrastructure;
namespace OpenCodeFoundation.ESchool.Services.Joining.API.Application.Commands
{
public class JoinApplicationCommandHandler
:IRequestHandler<JoinApplicationCommand, bool>
{
private readonly ILogger<JoinApplicationCommandHandler> _logger;
private readonly JoiningContext _context;
public JoinApplicationCommandHandler(
JoiningContext context,
ILogger<JoinApplicationCommandHandler> logger)
{
_context = context ?? throw new ArgumentNullException(nameof(context));
_logger = logger ?? throw new ArgumentNullException(nameof(logger));
}
public async Task<bool> Handle(JoinApplicationCommand command, CancellationToken cancellationToken)
{
var join = new Join(command.Name, command.Email, command.Mobile);
_context.Joins.Add(join);
await _context.SaveChangesAsync();
return true;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using MediatR;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Logging;
using OpenCodeFoundation.ESchool.Services.Joining.API.Application.Commands;
using OpenCodeFoundation.ESchool.Services.Joining.Domain.AggregatesModel.JoinAggregate;
using OpenCodeFoundation.ESchool.Services.Joining.Infrastructure;
namespace OpenCodeFoundation.ESchool.Services.Joining.API.Controllers
{
//[Route("api/[controller]")]
[ApiController]
[Route("[controller]")]
public class JoinController : ControllerBase
{
private readonly ILogger<JoinController> _logger;
private readonly IMediator _mediator;
private readonly JoiningContext _context;
public JoinController(
ILogger<JoinController> logger,
IMediator mediator,
JoiningContext context)
{
_logger = logger;
_mediator = mediator;
_context = context;
}
[HttpGet]
public async Task<List<Join>> Get()
{
_logger.LogInformation("Getting all Joins");
var joins = await _context.Joins.ToListAsync();
_logger.LogInformation("Total {NumberOfJoin} joins retrieved", joins.Count);
return joins;
}
[HttpPost]
public async Task<IActionResult> Post([FromBody] JoinApplicationCommand command)
{
_logger.LogInformation(
"Sending command: {CommandName} - ({@Command})",
command.GetType().Name,
command);
await _mediator.Send(command);
return Ok();
}
}
}
<file_sep>namespace OpenCodeFoundation.ESchool.Services.Joining.Domain.SeedWork
{
public interface IRepository<T>
where T : IAggregateRoot
{
}
}
| 2681b4b5a206d0edb93101928e82077aa1404efa | [
"Markdown",
"C#",
"Dockerfile"
] | 10 | Markdown | royalhouse/eSchool | e765e380836760923f7041fa94d5f52b9be8842b | d60f0d72c598cdda20c6beb87dc72962952a4e93 | |
refs/heads/main | <file_sep>const express = require ('express');
const router = express.Router ();
const Director = require ('../model/Director')
const mongoose = require ('mongoose')
// todo qidirsih ====
router.get('/', (req,res,next) =>{
const user = Director.find({});
user.then(data =>{
res.json(data)
}).catch(err =>{
console.log(err)
})
})
//todo get user ulimiz agregat ==
router.get ('/', (req, res, next) => {
//usersga kirasan
const user = Director.aggregate ([
{
$lookup: {
from: 'movies',//users mongo atlasdegi
localField: '_id',// userni idi bilan movies ni user_id bn bir hil boldi
foreignField: `user_id`,//Movies ni user_idsini ol uni kino kategoriyaga sol
as: 'kino',//uni kino qilib oladi
}
},
{
$unwind: {
path: `$kino`
//kino yozilgan joyga kiradi faqat shu tarafini korsatadi
}
},
{
//todo userni olib uni shu toigfalarini cqaradi
$group: {
_id: {
_id: `$_id`,
name: `$name`,
surname: `$surname`,
bio: `$bio`,
},
//todo || va usersni ichiga moviesni idisiga togri kelgan kino cconnectioni push qiladi
movies: {
$push: `$kino`
}
}
},
//qisqartma tutish
{
$project: {
_id: '$_id._id',
name: '$_id.name',
surname: '$_id.surname',
bio: '$_id.bio',
kino: '$kino'
}
}
])
user.then (data => {
res.json (data)
}).catch (err => {
console.log (err)
})
})
//todo idi orqali tutish
router.get ('/:us_id', (req, res, next) => {
//usersga kirasan
const user = Director.aggregate ([
{
$match: {
'_id': mongoose.Types.ObjectId (req.params.us_id)
}
},
{
$lookup: {
from: 'movies',//users mongo atlasdegi
localField: '_id',// userni idi bilan movies ni user_id bn bir hil boldi
foreignField: `user_id`,//Movies ni user_idsini ol uni kino kategoriyaga sol
as: 'kino',//uni kino qilib oladi
}
},
{
$unwind: {
path: `$kino`
//kino yozilgan joyga kiradi faqat shu tarafini korsatadi
}
},
{
//todo userni olib uni shu toigfalarini cqaradi
$group: {
_id: {
_id: `$_id`,
name: `$name`,
surname: `$surname`,
bio: `$bio`,
},
//todo || va usersni ichiga moviesni idisiga togri kelgan kino cconnectioni push qiladi
movies: {
$push: `$kino`
}
}
},
//qisqartma tutish
{
$project: {
_id: '$_id._id',
name: '$_id.name',
surname: '$_id.surname',
bio: '$_id.bio',
kino: '$kino'
}
}
])
user.then (data => {
res.json (data)
}).catch (err => {
console.log (err)
})
})
//todo|| malumot qoshish
router.post ('/', (req, res, next) => {
const user = new Director (req.body)
const promise = user.save ();
promise.then (data => {
res.json (data)
}).catch (err => {
console.log (err)
})
})
//todo || idi orqali yangilash
router.put ('/:us_id', (req, res) => {
const promise = Director.findByIdAndUpdate (req.params.us_id, req.body, {new: true});
promise.then(data =>{
res.json(data)
}).catch(err =>{
console.log(err)
})
})
//TODO || idi orqali ochirish - DELETE
router.delete ('/:us_id', (req, res) => {
const promise = Director.findByIdAndDelete (req.params.us_id);
promise.then(data =>{
res.json(data)
}).catch(err =>{
console.log(err)
})
})
//todo || top10 qidirish
router.get ('/:user_id/top10', (req, res, next) => {
//usersga kirasan
const user = Director.aggregate ([
{
//idisi shunga tori kelsa
$match: {
'_id': mongoose.Types.ObjectId (req.params.user_id)
}
},
{
$lookup: {
from: 'movies',//users mongo atlasdegi
localField: '_id',// userni idi bilan movies ni user_id bn bir hil boldi
foreignField: `user_id`,//Movies ni user_idsini ol uni kino kategoriyaga sol
as: 'kino',//uni kino qilib oladi
}
},
{
$unwind: {
path: `$kino`
//kino yozilgan joyga kiradi faqat shu tarafini korsatadi
}
},
{
//todo userni olib uni shu toigfalarini cqaradi
$group: {
_id: {
_id: `$_id`,
name: `$name`,
surname: `$surname`,
bio: `$bio`,
},
//todo || va usersni ichiga moviesni idisiga togri kelgan kino cconnectioni push qiladi
movies: {
$push: `$kino`
}
}
},
//qisqartma tutish
{
$project: {
_id: false,
kino: '$kino'
}
}
])
user.then (data => {
res.json (data)
}).catch (err => {
console.log (err)
})
})
module.exports = router
<file_sep>const express = require ('express');
const router = express.Router ();
const Movies = require ('../model/Movies')
/* GET home page. */
// router.get ('/', (req, res, next) => {
// res.render ('index', {title: 'Express'});
// });
//todo || --- malumotlar yaratish ---
router.post ('/', (req, res, next) => {
const movie = new Movies (req.body)
const promise = movie.save ();
promise.then (data => {
res.json (data)
}).catch (err => {
console.log (err)
})
});
//todo Get -- malumotlarni olish
router.get ('/', (req, res, next) => {
const promise = Movies.find ({})
promise.then (data => {
res.json (data)
}).catch (err => {
console.log (err)
})
});
//todo /api/movies/:id idi orqali tutish
//owatga yozgan idini cqaradi :id
router.get ('/:id', (req, res) => {
const promise = Movies.findById (req.params.id)
promise.then (data => {
res.json (data)
}).catch (err => {
console.log (err)
})
})
//todo || Put - yangilash ---
router.put ('/:id', (req, res) => {
const promise = Movies.findByIdAndUpdate (
req.params.id,
req.body);
promise.then (data => {
res.json (data)
}).catch (err => console.log (err)
)
})
//todo || udalit qilish ===
router.delete ('/:id', (req, res) => {
const promise = Movies.findByIdAndRemove (req.params.id);
promise.then (data => {
res.json (data)
}).catch (err => console.log (err)
)
})
//todo top 10 lani qidiring
router.get ('/top/top10', (req, res, next) => {
const promise = Movies.find ({}).limit (10).sort ({ovoz_ber: -1})
promise.then (data => {
res.json (data)
}).catch (err => {
console.log (err)
})
})
//todo || shu yildan shu yilgacham bogan <NAME>
router.get ('/yil/:start/:end', (res, req, next) => {
const {start, end} = req.params
//todo hama yilani olamiz
const promise = Movies.find ({
year: {'$gte': parseInt (start), '$lte': parseInt (end)}
})
promise.then (data => {
res.json (data)
}).catch (err => {
console.log (err)
})
})
module.exports = router;
<file_sep>const express = require ('express');
const router = express.Router();
const User = require ('../model/Users')
const bcrypt = require('bcryptjs')
const jwt = require('jsonwebtoken')
/* GET home page. */
router.get ('/', (req, res, next) => {
res.render ('index', {title: 'Express'});
})
router.post ('/register', (req, res) => {
//todo || posmanda yozgan kod tuWadi passwordga
const {username, password} = req.body;
//todo | passwordegi 123 hashga tushadi 10 talik uzunligi boladi
bcrypt.hash(password,10,(err,hash)=>{
const user = new User ({
username,
password:hash, //todo || passwordga hashlangan kodi yozadi
})
const promise = user.save ();
promise.then (data => res.json (data))
.catch (err => console.log (err))
})
})
//todo ============ auth ===================================
router.post ('/auth', (req, res) => {
const {username, password} = req.body;
//username ni user oz ichiga oladi
User.findOne({ username },(err,user)=>{
if (err)
throw err;
// todo : agar kevotgan user user bolmasa shuni chiqaradi
if (!user){
res.json({status:'Topilmadi idi ',msg:"Kirish Muffoqiyatsiz faqat admin kirish mumkun"})
}
//todo || else da agar user kirsa
else{
// parolni bizaga qaytarib beradi usernemi passwortini uni soliwtradi
bcrypt.compare(password,user.password).then((result) =>{
// agar kegan parol natori tersa
if(!result) {
res.json ({status: false, msg: "Foydalanuvchi paroli natori "})
}
//agar parol tori kelsa username chiqar
else{
const payload = {username}
// secret_key ni app.js dan olib keladi app.set dagi
const token= jwt.sign(payload,req.app.get('secret_key'),{
expiresIn:720 //12soat turadi kompyuterga hotira jonatadi
})
// res.jsonda uni tutvolamiz korsatgin
res.json({
status:true,
token // 12soatli tokeni koramiz
})
}
})
}
})
})
module.exports = router;
<file_sep>const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const UserSchema = new Schema({
name:{type:String,required:true},
surname:String,
bio:String,
createdAt:{
type:Date,
default:Date.now
}
})
module.exports = mongoose.model('user',UserSchema)
<file_sep># movieApi
movies
<file_sep>const jwt =require('jsonwebtoken')
//agar token kirtsek yoki postmandan
module.exports =(req,res,next) =>{
//agar token true bolsa shulardan oladi || yoki url adressdan req.query.token
const token = req.headers['x-acces-token'] || req.body.token || req.query.token
//agar token true bolsa tokeni raqamlarini tekshiradi
if(token){
// tepadegi tokenimiz bolsa toke ni tekwiradi || err -dec qaytaradi
jwt.verify(token, req.app.get('secret_key'),(err,dec) =>{
//agar hato bolsa
if(err){
res.json({status:false,msg:'Kira olmaysiz'})
}
else{
req.decoded = dec
next() //agar togri kelsa next ishlidi otqzvoradi app.jsga
}
})
}
//registratsiya dan otilmasa shuni qaytaradi
else{
res.json({status:false, msg:'token topilmadi '})
}
}
<file_sep>const chai = require ('chai');
const chaiHttp = require ('chai-http');
const should = chai.should ();
const server = require ('../../app') // app.jsdan userni get gacham oladi
chai.use (chaiHttp)
describe (`bosh sahifani testdan otqizdik`, () => {
it ('Get method orqali bosh sahifani tekishiruvdan otqazdik', (done) => {
chai.request (server)
.get ('/')
.end ((err, res) => {
res.should.have.status (200)
done ()
})
})
})
<file_sep>const mongoose = require('mongoose');
module.exports =() =>{
mongoose.connect('mongodb+srv://madaminov:iphone11@@cluster0.pnhzl.mongodb.net/test',
{useFindAndModify:true,useUnifiedTopology:true,useNewUrlParser:true,useCreateIndex:true})
const db = mongoose.connection;
db.on('open',() =>{
console.log('Mongo db ishga tushdi')
})
db.on('error',(err)=>{
console.log('Mongoda qayerdadur hatolik bor',err)
})
}
<file_sep>const mongoose = require('mongoose')
const Schema = mongoose.Schema;
const MoviesSchema = new Schema({
title: {
type:String,
required:true
},
category:String,
country:String,
year:Number,
user_id:Schema.Types.ObjectId,
ovoz_ber:Number,
createAt:{
type:Date,
default:Date.now,
}
})
module.exports = mongoose.model('movie',MoviesSchema)
| 3c99293e08872af38db062de6d9dffb5f09399a8 | [
"JavaScript",
"Markdown"
] | 9 | JavaScript | Doniyor-Mr/movieApi | 2521f16f82c99bbaf9e80989dff60cbeeedde09b | 68d748a9126e423b9ed8241dbb88f29b578f277a | |
refs/heads/main | <file_sep>package com.quypham.vdc.repo.weather
import com.quypham.vdc.api.RepoResponse
import com.quypham.vdc.data.WeatherForecastEntity
interface WeatherDataSource {
suspend fun query(city: String, daysOfForecast: Int): RepoResponse<WeatherForecastEntity>
}<file_sep>package com.quypham.vdc.di
import android.content.Context
import androidx.room.Room
import com.quypham.vdc.Constants
import com.quypham.vdc.api.ApiService
import com.quypham.vdc.api.ServiceGenerator
import com.quypham.vdc.database.AppDatabase
import com.quypham.vdc.utils.NetworkUtil
import dagger.Module
import dagger.Provides
import dagger.hilt.InstallIn
import dagger.hilt.android.components.ApplicationComponent
import dagger.hilt.android.qualifiers.ApplicationContext
import okhttp3.Interceptor
import javax.inject.Singleton
@InstallIn(ApplicationComponent::class)
@Module
class ApplicationModule {
@Singleton
@Provides
fun provideAppDatabase(@ApplicationContext context: Context): AppDatabase {
return Room.databaseBuilder(context, AppDatabase::class.java, Constants.APP_DATABASE_NAME)
.fallbackToDestructiveMigration()
.build()
}
@Singleton
@Provides
fun provideApiService(@ApplicationContext context: Context): ApiService {
val cacheInterceptor = Interceptor { chain ->
val requestBuilder = chain.request().newBuilder()
if (NetworkUtil.hasNetworkConnection(context))
//Get cache from last 5 mins in case internet is available
requestBuilder.header("Cache-Control", "public, max-age=" + (5 * 60)).build()
else
//Get cache from 2 days in case no internet is available
requestBuilder.header(
"Cache-Control",
"public, only-if-cached, max-stale=" + 60 * 60 * 24 * 7
).build()
chain.proceed(requestBuilder.build())
}
ServiceGenerator.setCache(context.cacheDir)
ServiceGenerator.setInterceptor(cacheInterceptor)
ServiceGenerator.setBaseUrl(Constants.BASE_URL)
return ServiceGenerator.createService(ApiService::class.java)
}
}<file_sep>package com.quypham.vdc.base
import androidx.appcompat.app.AppCompatActivity
import androidx.fragment.app.Fragment
import com.quypham.vdc.R
abstract class BaseActivity : AppCompatActivity() {
protected fun addFragment(fragment: Fragment, tag: String) {
addFragment(fragment, tag, R.id.container)
}
private fun addFragment(fragment: Fragment, tag: String?, frameId: Int) {
supportFragmentManager.beginTransaction()
.replace(frameId, fragment, tag)
.addToBackStack(tag)
.commitAllowingStateLoss()
}
}<file_sep>package com.quypham.vdc.domain
import android.util.Log
import com.quypham.vdc.UseCase
import com.quypham.vdc.api.RepoResponse
import com.quypham.vdc.data.QueryForecastParameter
import com.quypham.vdc.data.WeatherForecastEntity
import com.quypham.vdc.di.IoDispatcher
import com.quypham.vdc.repo.WeatherRepository
import kotlinx.coroutines.CoroutineDispatcher
import javax.inject.Inject
open class GetWeatherForecast @Inject constructor(private val mWeatherRepository: WeatherRepository,
@IoDispatcher dispatcher: CoroutineDispatcher) :
UseCase<QueryForecastParameter, RepoResponse<WeatherForecastEntity>>(dispatcher) {
companion object {
const val TAG = "GetWeatherForecast"
}
override suspend fun execute(parameters: QueryForecastParameter): RepoResponse<WeatherForecastEntity> {
Log.d(TAG,"GetWeatherForecast execute with parameter $parameters")
return mWeatherRepository.query(parameters.city, parameters.setting.daysOfForecast)
}
}<file_sep>package com.quypham.vdc.api
import android.util.Log
import com.google.gson.Gson
import com.quypham.vdc.Constants
import retrofit2.Response
import java.net.HttpURLConnection
import java.net.SocketTimeoutException
import java.net.UnknownHostException
suspend fun <T: Any?> handleRequest(requestFunc: suspend () -> Response<T>): RepoResponse<T> {
return try {
requestFunc.invoke().createRepoResponse()
} catch (exception: Exception) {
Log.d("XXX","handleRequest exception $exception")
when (exception) {
is SocketTimeoutException -> {
Failure(Constants.INTERNAL_HTTP_CLIENT_TIMEOUT, null, exception)
}
is UnknownHostException -> {
Failure(Constants.INTERNAL_HTTP_NO_INTERNET_CONNECTION, null, exception)
}
else -> {
Failure(Constants.INTERNAL_HTTP_UNKNOWN_ERROR, null, exception)
}
}
}
}
fun <T> Response<T>.createRepoResponse(): RepoResponse<T> {
let { response ->
Log.d("XXX","createRepoResponse response $response")
return try {
createSuccessResponse(response)
} catch (t: Throwable) {
createFailure(t)
}
}
}
private fun <T> createSuccessResponse(response: Response<T>): RepoResponse<T> {
return if (response.isSuccessful) {
val body = response.body()
var isFromCache = false
if (response.raw().cacheResponse != null) {
isFromCache = true
}
if (response.raw().networkResponse != null && response.raw().networkResponse!!.code != HttpURLConnection.HTTP_NOT_MODIFIED) {
isFromCache = false
}
Success(response = body, isFromCache = isFromCache)
} else {
try {
Log.d("XXX","createSuccessResponse error response $response")
val errorContent = response.errorBody()?.string() ?: response.message()
val serverError = Gson().fromJson(errorContent, ServerError::class.java)
Failure(response.code(), serverError, null)
} catch (exception: Exception) {
Failure(response.code(), null, null)
}
}
}
private fun <T> createFailure(throwable: Throwable): Failure<T> {
Log.d("XXX","createFailure $throwable")
return when (throwable) {
is SocketTimeoutException -> {
Failure(Constants.INTERNAL_HTTP_CLIENT_TIMEOUT, null, throwable)
}
is UnknownHostException -> {
Failure(Constants.INTERNAL_HTTP_NO_INTERNET_CONNECTION, null, throwable)
}
else -> {
Failure(Constants.INTERNAL_HTTP_UNKNOWN_ERROR, null, throwable)
}
}
}
<file_sep>package com.quypham.vdc.ui.weather
import android.os.Bundle
import android.util.Log
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import androidx.core.widget.doOnTextChanged
import androidx.fragment.app.viewModels
import com.quypham.vdc.base.BaseFragment
import com.quypham.vdc.databinding.FragmentWeatherInfoBinding
import com.quypham.vdc.ui.setting.SettingFragment
import dagger.hilt.android.AndroidEntryPoint
@AndroidEntryPoint
class WeatherInfoFragment : BaseFragment() {
companion object {
const val TAG = "MainFragment"
fun newInstance(): WeatherInfoFragment {
return WeatherInfoFragment()
}
}
private val mViewModel: WeatherInfoViewModel by viewModels()
private lateinit var mBinding: FragmentWeatherInfoBinding
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View {
mBinding = FragmentWeatherInfoBinding.inflate(layoutInflater, container, false)
return mBinding.root
}
override fun onActivityCreated(savedInstanceState: Bundle?) {
super.onActivityCreated(savedInstanceState)
mBinding.etCity.doOnTextChanged { text, _, _, _ ->
mBinding.rvWeathers.showLoadingView()
mViewModel.onQueryChange(text.toString())
}
mBinding.setting.setOnClickListener {
val settingFragment = SettingFragment()
settingFragment.show(childFragmentManager, settingFragment.tag)
}
mViewModel.weatherInfos.observe(viewLifecycleOwner, { response ->
Log.d(TAG, "onWeatherResponse $response")
if (response.isSuccess) {
mBinding.rvWeathers.showRecyclerView()
mBinding.rvWeathers.recyclerView.apply {
if (this.adapter == null) {
this.adapter = WeatherInfoAdapter()
}
(this.adapter as WeatherInfoAdapter).apply {
Log.d(TAG, "submit new list ${response.weatherInfoList}")
submitList(response.weatherInfoList)
}
}
} else {
mBinding.rvWeathers.showErrorView(response.errorMessage)
}
})
}
}<file_sep>package com.quypham.vdc.ui.setting
import androidx.hilt.lifecycle.ViewModelInject
import androidx.lifecycle.MutableLiveData
import androidx.lifecycle.ViewModel
import androidx.lifecycle.viewModelScope
import com.quypham.vdc.Constants
import com.quypham.vdc.data.TempUnit
import com.quypham.vdc.data.UserSetting
import com.quypham.vdc.di.AppScope
import com.quypham.vdc.domain.UpdateUserSetting
import com.quypham.vdc.repo.SettingRepository
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.launch
class SettingViewModel @ViewModelInject constructor(
private val settingRepository: SettingRepository,
private val updateUserSetting: UpdateUserSetting,
@AppScope private val appScope: CoroutineScope
) : ViewModel() {
companion object {
const val TAG = "SettingViewModel"
}
private val _userSetting = MutableLiveData<UserSetting?>()
init {
viewModelScope.launch {
_userSetting.value = settingRepository.getSetting()
}
}
val userSetting = _userSetting
fun updateSetting(daysForecast: Int? = null, unit: TempUnit? = null) {
val currentSetting =
_userSetting.value ?: UserSetting(Constants.DEFAULT_DAYS_FORECAST, TempUnit.KELVIN)
daysForecast?.let {
currentSetting.daysOfForecast = daysForecast
}
unit?.let {
currentSetting.tempUnit = unit
}
_userSetting.value = currentSetting
}
override fun onCleared() {
appScope.launch {
updateUserSetting.invoke(_userSetting.value ?: UserSetting(Constants.DEFAULT_DAYS_FORECAST, TempUnit.KELVIN))
}
super.onCleared()
}
}<file_sep>package com.quypham.vdc.repo
import androidx.lifecycle.LiveData
import com.quypham.vdc.Constants
import com.quypham.vdc.data.UserSetting
import com.quypham.vdc.repo.setting.SettingDataSource
import javax.inject.Inject
import javax.inject.Named
interface SettingRepository {
suspend fun getSetting(): UserSetting?
fun getSettingLive(): LiveData<UserSetting?>
suspend fun updateSetting(setting: UserSetting)
}
class SettingRepositoryImp @Inject constructor(@Named(Constants.LOCAL) private val localSource: SettingDataSource) : SettingRepository{
override suspend fun getSetting(): UserSetting? {
return localSource.getSetting()
}
override fun getSettingLive(): LiveData<UserSetting?> {
return localSource.getSettingLive()
}
override suspend fun updateSetting(setting: UserSetting) {
return localSource.updateSetting(setting)
}
}<file_sep>package com.quypham.vdc
object Constants {
//internal http error code
const val INTERNAL_HTTP_UNKNOWN_ERROR = 600
const val INTERNAL_HTTP_NO_INTERNET_CONNECTION = 601
const val INTERNAL_HTTP_CLIENT_TIMEOUT = 408
const val UNSATISFIABLE_REQUEST= 504
const val INTERNAL_HTTP_NO_RESULT = 602
//weather api base url
const val BASE_URL = "https://api.openweathermap.org/data/2.5/"
const val QUERY_APP_ID = "60c6fbeb4b93ac653c492ba806fc346d"
//database constant
const val APP_DATABASE_VERSION = 1
const val APP_DATABASE_NAME = "app.db"
const val MINIMUM_DAYS_FORECAST = 1
//tested on weather api, this could be larger but user will receive error anyway
const val MAXIMUM_DAYS_FORECAST = 17
const val DEFAULT_DAYS_FORECAST = 5
const val MINIMUM_CITY_CHARACTERS_ALLOW = 3
const val LOCAL = "local"
const val REMOTE = "remote"
}<file_sep># VDC-Weather-Forecast
Build Environment:
- Android Studio 4.1.1
- Build tools: 29
- Target SDK: 29
Summary:
This app is written in Kotlin with MVVM pattern and follow Android Architecture Component including:
- ViewModel
- LiveData & Flow
- View Binding
- Room
- Hilt
- Coroutines
Done:
- Query weather from Weather API and render on UI
- Ability to change setting including temperature unit and days of forecast
- Handling exception and show meaningful explaination on UI
- Caching mechanism based on HTTP Caching
Things could be improved:
- Add more caching mechanism on local database on both memory and file.
- Paging for list weather but WeatherAPI just return maximum of 17 records per city so this is not neccessary.
- Add custom style and themes so that we can changed app UI quickly.
- Accessibility support
<file_sep>package com.quypham.vdc.database
import androidx.lifecycle.LiveData
import androidx.room.*
import com.quypham.vdc.data.UserSetting
@Dao
interface UserSettingDao {
@Insert(onConflict = OnConflictStrategy.REPLACE)
suspend fun updateUserSetting(setting: UserSetting)
@Query("SELECT * FROM userSetting")
fun getUserSettingLive(): LiveData<UserSetting?>
@Query("SELECT * FROM userSetting")
suspend fun getUserSetting(): UserSetting?
}<file_sep>package com.quypham.vdc.api
import com.quypham.vdc.Constants
import com.quypham.vdc.data.WeatherForecastEntity
import retrofit2.Response
import retrofit2.http.GET
import retrofit2.http.Query
interface ApiService {
@GET("forecast/daily")
suspend fun queryForecastDaily(@Query("q") city: String,
@Query("cnt") daysOfForecast: Int,
@Query("appid") appId: String = Constants.QUERY_APP_ID): Response<WeatherForecastEntity?>
}<file_sep>package com.quypham.vdc.api
data class ServerError constructor(var cod: String, var message: String)<file_sep>include ':app'
rootProject.name = "VDC Apps"<file_sep>package com.quypham.vdc.data
data class QueryForecastParameter(val city: String, val setting: UserSetting)<file_sep>package com.quypham.vdc.database
import androidx.room.TypeConverter
import com.quypham.vdc.data.TempUnit
class TempUnitConverter {
@TypeConverter
fun fromTempUnitToString(tempUnit: TempUnit): String {
return tempUnit.name
}
@TypeConverter
fun fromStringToTempUnit(value: String): TempUnit {
return TempUnit.valueOf(value)
}
}<file_sep>package com.quypham.vdc.domain
import android.util.Log
import com.quypham.vdc.UseCase
import com.quypham.vdc.data.UserSetting
import com.quypham.vdc.di.IoDispatcher
import com.quypham.vdc.repo.SettingRepository
import kotlinx.coroutines.CoroutineDispatcher
import javax.inject.Inject
class UpdateUserSetting @Inject constructor(private val settingRepository: SettingRepository,
@IoDispatcher dispatcher: CoroutineDispatcher
) : UseCase<UserSetting, Any>(dispatcher) {
companion object {
const val TAG = "UpdateUserSetting"
}
override suspend fun execute(parameters: UserSetting): Any {
Log.d(TAG,"UpdateUserSetting execute")
settingRepository.updateSetting(parameters)
return Any()
}
}<file_sep>package com.quypham.vdc.repo.setting
import androidx.lifecycle.LiveData
import com.quypham.vdc.data.UserSetting
import com.quypham.vdc.database.AppDatabase
import javax.inject.Inject
class SettingLocalDataSource @Inject constructor(private val appDatabase: AppDatabase): SettingDataSource {
override fun getSettingLive(): LiveData<UserSetting?> {
return appDatabase.userSettingDao().getUserSettingLive()
}
override suspend fun getSetting(): UserSetting? {
return appDatabase.userSettingDao().getUserSetting()
}
override suspend fun updateSetting(setting: UserSetting) {
val currentSetting = appDatabase.userSettingDao().getUserSetting() ?: setting
currentSetting.daysOfForecast = setting.daysOfForecast
currentSetting.tempUnit = setting.tempUnit
return appDatabase.userSettingDao().updateUserSetting(currentSetting)
}
}<file_sep>package com.quypham.vdc.ui.setting
import android.os.Bundle
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import androidx.core.content.ContextCompat
import androidx.fragment.app.viewModels
import com.google.android.material.bottomsheet.BottomSheetDialogFragment
import com.quypham.vdc.Constants
import com.quypham.vdc.R
import com.quypham.vdc.data.TempUnit
import com.quypham.vdc.databinding.FragmentSettingBinding
import dagger.hilt.android.AndroidEntryPoint
@AndroidEntryPoint
class SettingFragment : BottomSheetDialogFragment() {
companion object {
fun newInstance(): SettingFragment {
return SettingFragment()
}
}
private val mViewModel: SettingViewModel by viewModels()
private lateinit var mBinding: FragmentSettingBinding
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View {
mBinding = FragmentSettingBinding.inflate(layoutInflater, container, false)
return mBinding.root
}
override fun onActivityCreated(savedInstanceState: Bundle?) {
super.onActivityCreated(savedInstanceState)
mBinding.tvImperial.setOnClickListener {
mViewModel.updateSetting(unit = TempUnit.IMPERIAL)
}
mBinding.tvMetric.setOnClickListener {
mViewModel.updateSetting(unit = TempUnit.METRIC)
}
mBinding.tvKelvin.setOnClickListener {
mViewModel.updateSetting(unit = TempUnit.KELVIN)
}
mBinding.npDaysForecast.apply {
this.minValue = Constants.MINIMUM_DAYS_FORECAST
this.maxValue = Constants.MAXIMUM_DAYS_FORECAST
this.setOnValueChangedListener { _, _, newVal ->
mViewModel.updateSetting(daysForecast = newVal)
}
}
mViewModel.userSetting.observe(viewLifecycleOwner, {
mBinding.npDaysForecast.value = it?.daysOfForecast ?: Constants.DEFAULT_DAYS_FORECAST
switchMetric(it?.tempUnit ?: TempUnit.KELVIN)
})
}
private fun switchMetric(unit: TempUnit) {
mBinding.apply {
this.tvKelvin.background = ContextCompat.getDrawable(requireContext(), R.drawable.outline_white_background)
this.tvImperial.background = ContextCompat.getDrawable(requireContext(), R.drawable.end_rounded_white_background)
this.tvMetric.background = ContextCompat.getDrawable(requireContext(), R.drawable.start_rounded_white_background)
this.tvMetric.setTextColor(ContextCompat.getColor(requireContext(),R.color.black))
this.tvImperial.setTextColor(ContextCompat.getColor(requireContext(),R.color.black))
this.tvKelvin.setTextColor(ContextCompat.getColor(requireContext(),R.color.black))
when (unit) {
TempUnit.METRIC -> {
this.tvMetric.background = ContextCompat.getDrawable(requireContext(), R.drawable.start_rounded_black_background)
this.tvMetric.setTextColor(ContextCompat.getColor(requireContext(),R.color.white))
}
TempUnit.IMPERIAL -> {
this.tvImperial.background = ContextCompat.getDrawable(requireContext(), R.drawable.end_rounded_black_background)
this.tvImperial.setTextColor(ContextCompat.getColor(requireContext(),R.color.white))
}
TempUnit.KELVIN -> {
this.tvKelvin.background = ContextCompat.getDrawable(requireContext(), R.drawable.outline_black_background)
this.tvKelvin.setTextColor(ContextCompat.getColor(requireContext(),R.color.white))
}
}
}
}
}<file_sep>package com.quypham.vdc.repo.weather
import com.quypham.vdc.api.RepoResponse
import com.quypham.vdc.data.WeatherForecastEntity
import javax.inject.Inject
import javax.inject.Singleton
@Singleton
class WeatherLocalDataSource @Inject constructor(): WeatherDataSource {
override suspend fun query(city: String, daysOfForecast: Int): RepoResponse<WeatherForecastEntity> {
throw Exception("not implemented")
}
}<file_sep>package com.quypham.vdc.ui.weather
import android.content.Context
import android.util.Log
import androidx.hilt.lifecycle.ViewModelInject
import androidx.lifecycle.*
import com.quypham.vdc.Constants
import com.quypham.vdc.Constants.DEFAULT_DAYS_FORECAST
import com.quypham.vdc.Constants.MINIMUM_CITY_CHARACTERS_ALLOW
import com.quypham.vdc.R
import com.quypham.vdc.api.Failure
import com.quypham.vdc.api.Success
import com.quypham.vdc.data
import com.quypham.vdc.data.QueryForecastParameter
import com.quypham.vdc.data.TempUnit
import com.quypham.vdc.data.UserSetting
import com.quypham.vdc.domain.GetWeatherForecast
import com.quypham.vdc.repo.SettingRepository
import com.quypham.vdc.utils.CommonUtils
import dagger.hilt.android.qualifiers.ApplicationContext
import kotlinx.coroutines.flow.collect
import kotlinx.coroutines.launch
class WeatherInfoViewModel @ViewModelInject constructor(
private val getWeatherForecast: GetWeatherForecast,
settingRepository: SettingRepository,
@ApplicationContext private val context: Context
) :
ViewModel() {
companion object {
const val TAG = "WeatherInfoViewModel"
}
private val _queryParameterLive = MutableLiveData<QueryForecastParameter>()
private val _settingLive = settingRepository.getSettingLive()
private var _currentSetting = UserSetting(DEFAULT_DAYS_FORECAST, TempUnit.KELVIN)
//display symbol
private val celsiusSymbol by lazy {
context.getString(R.string.unit_metric)
}
private val kelvinSymbol by lazy {
context.getString(R.string.unit_kelvin)
}
private val fahrenheitSymbol by lazy {
context.getString(R.string.unit_imperial)
}
private val _weatherQueryResponse =
_queryParameterLive.switchMap<QueryForecastParameter, WeatherUiResponse> {
liveData {
emit(
when {
it.city.isEmpty() -> {
WeatherUiResponse(
isSuccess = false,
errorMessage = context.getString(R.string.empty_city_query)
)
}
it.city.length < MINIMUM_CITY_CHARACTERS_ALLOW -> {
WeatherUiResponse(
isSuccess = false,
errorMessage = context.getString(R.string.invalid_city_name)
)
}
else -> {
val response = getWeatherForecast(it).data
Log.d(TAG, "getDailyForecast result $response")
when (response) {
is Success -> {
//convert weather model to ui expected model
if (response.response != null) {
if (response.response.list.isNotEmpty()) {
val correctUnitData =
response.response.list.map { weatherItem ->
val tempSymbol: String
val weatherDesc =
if (weatherItem.weather.isNotEmpty()) {
weatherItem.weather[0].description
} else {
context.getString(R.string.description_not_found)
}
val averageTemp =
(weatherItem.temp.max + weatherItem.temp.min) / 2
val correctAverageTempWithUnit =
when (it.setting.tempUnit) {
TempUnit.METRIC -> {
tempSymbol = celsiusSymbol
CommonUtils.kelvinToCelsius(
averageTemp
)
.toInt()
}
TempUnit.IMPERIAL -> {
tempSymbol = fahrenheitSymbol
CommonUtils.kelvinToFahrenheit(
averageTemp
).toInt()
}
else -> {
tempSymbol = kelvinSymbol
averageTemp.toInt()
}
}
WeatherInfo(
weatherItem.dt,
correctAverageTempWithUnit,
weatherItem.pressure,
weatherItem.humidity,
weatherDesc,
tempSymbol
)
}
WeatherUiResponse(
true,
weatherInfoList = correctUnitData
)
} else {
WeatherUiResponse(
isSuccess = false,
errorMessage = context.getString(R.string.empty_result_error)
)
}
} else {
WeatherUiResponse(
isSuccess = false,
errorMessage = context.getString(R.string.general_error)
)
}
}
is Failure -> {
val httpCodeErrorMessage = when (response.code) {
Constants.UNSATISFIABLE_REQUEST -> context.getString(R.string.no_internet_connection)
Constants.INTERNAL_HTTP_CLIENT_TIMEOUT -> context.getString(
R.string.network_timeout
)
else -> context.getString(R.string.general_error)
}
WeatherUiResponse(
isSuccess = false,
errorMessage = response.serverError?.message
?: httpCodeErrorMessage
)
}
else -> {
WeatherUiResponse(
isSuccess = false,
errorMessage = context.getString(R.string.general_error)
)
}
}
}
}
)
}
}
init {
viewModelScope.launch {
_settingLive.asFlow().collect { updatedSetting ->
if (updatedSetting == null) return@collect
//process data when setting is changed
_queryParameterLive.value?.let {
//day of forecast change, re query this city data
it.setting.daysOfForecast = updatedSetting.daysOfForecast
it.setting.tempUnit = updatedSetting.tempUnit
_queryParameterLive.postValue(it)
}
_currentSetting = updatedSetting
}
}
}
val weatherInfos: LiveData<WeatherUiResponse> = _weatherQueryResponse
fun onQueryChange(city: String) {
Log.d(TAG, "onQueryChange city $city")
_queryParameterLive.postValue(QueryForecastParameter(city, _currentSetting))
}
}
data class WeatherInfo constructor(
var timestamp: Long, var averageTemp: Int, var pressure: Int,
var humidity: Int, var description: String, var tempUnit: String
)
data class WeatherUiResponse(
val isSuccess: Boolean, val errorMessage: String = "",
val weatherInfoList: List<WeatherInfo>? = null
)
<file_sep>package com.quypham.vdc.api
import android.util.Log
import com.google.gson.Gson
import com.quypham.vdc.Constants
import retrofit2.Response
import java.net.HttpURLConnection
import java.net.SocketTimeoutException
import java.net.UnknownHostException
sealed class RepoResponse<T> {
companion object {
fun <T> create(error: Throwable): Failure<T> {
Log.d("XXX","handleRequest error $error")
return when (error) {
is SocketTimeoutException -> {
Failure(Constants.INTERNAL_HTTP_CLIENT_TIMEOUT, null, error)
}
is UnknownHostException -> {
Failure(Constants.INTERNAL_HTTP_NO_INTERNET_CONNECTION, null, error)
}
else -> {
Failure(Constants.INTERNAL_HTTP_UNKNOWN_ERROR, null, error)
}
}
}
fun <T> create(response: Response<T>): RepoResponse<T> {
return if (response.isSuccessful) {
val body = response.body()
var isFromCache = false
if (response.raw().cacheResponse != null) {
isFromCache = true
}
if (response.raw().networkResponse != null && response.raw().networkResponse!!.code != HttpURLConnection.HTTP_NOT_MODIFIED) {
isFromCache = false
}
Success(response = body, isFromCache = isFromCache)
} else {
Log.d("XXX","create exception $response")
try {
val errorContent = response.errorBody()?.string() ?: response.message()
val serverError = Gson().fromJson(errorContent, ServerError::class.java)
Failure(response.code(), serverError, null)
} catch (exception: Exception) {
Failure(response.code(), null, null)
}
}
}
}
}
data class Success<T>(val response: T?, val isFromCache: Boolean = false) : RepoResponse<T>()
data class Failure<T>(val code: Int, val serverError: ServerError?, val throwable: Throwable? = null, val errorItems: String? = null, val data : T? = null) : RepoResponse<T>()
<file_sep>package com.quypham.vdc.utils
import java.text.SimpleDateFormat
import java.util.*
object DateFormatUtils {
private var WEATHER_INFO_DATE_FORMAT: ThreadLocal<SimpleDateFormat> =
object : ThreadLocal<SimpleDateFormat>() {
override fun initialValue(): SimpleDateFormat {
return SimpleDateFormat("EEE,dd MMM yyyy", Locale.US)
}
}
fun toWeatherDateFormat(timestamp: Long): String {
val calendarInstance = Calendar.getInstance()
calendarInstance.timeInMillis = timestamp*1000
return WEATHER_INFO_DATE_FORMAT.get()?.format(calendarInstance.time) ?: "Weather format not found"
}
}<file_sep>package com.quypham.vdc.utils
object CommonUtils {
fun kelvinToCelsius(kelvinDegree: Double): Double {
return kelvinDegree - 273.15
}
fun kelvinToFahrenheit(kelvinDegree: Double): Double {
return (kelvinDegree - 273.15) * 1.8 + 32.0
}
}<file_sep>package com.quypham.vdc.repo.weather
import android.util.Log
import com.quypham.vdc.Constants
import com.quypham.vdc.api.*
import com.quypham.vdc.data.WeatherForecastEntity
import javax.inject.Inject
class WeatherRemoteDataSource @Inject constructor(private val mApiService: ApiService): WeatherDataSource {
companion object {
const val TAG = "WeatherRemoteDataSource"
}
override suspend fun query(city: String, daysOfForecast: Int): RepoResponse<WeatherForecastEntity> {
val response = handleRequest {
mApiService.queryForecastDaily(city, daysOfForecast)
}
Log.d(TAG,"query of $city in $daysOfForecast days response $response")
return when (response) {
is Success -> {
val weatherRemotes = response.response
if (weatherRemotes != null) {
Success(weatherRemotes)
} else {
Failure(Constants.INTERNAL_HTTP_NO_RESULT, null, null, null,null)
}
}
is Failure -> {
Failure(response.code, response.serverError, null, null,null)
}
}
}
}<file_sep>package com.quypham.vdc.ui
import android.os.Bundle
import com.quypham.vdc.R
import com.quypham.vdc.base.BaseActivity
import com.quypham.vdc.ui.weather.WeatherInfoFragment
import dagger.hilt.android.AndroidEntryPoint
@AndroidEntryPoint
class MainActivity: BaseActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.main_activity)
var infoFragment: WeatherInfoFragment? = supportFragmentManager.findFragmentByTag(WeatherInfoFragment.TAG) as WeatherInfoFragment?
if (infoFragment == null) { // first time
infoFragment = WeatherInfoFragment.newInstance()
}
addFragment(infoFragment, WeatherInfoFragment.TAG)
}
override fun onBackPressed() {}
}<file_sep>package com.quypham.vdc.repo.setting
import androidx.lifecycle.LiveData
import com.quypham.vdc.data.UserSetting
interface SettingDataSource {
fun getSettingLive(): LiveData<UserSetting?>
suspend fun getSetting(): UserSetting?
suspend fun updateSetting(setting: UserSetting)
}<file_sep>package com.quypham.vdc.api
import okhttp3.Cache
import okhttp3.Interceptor
import okhttp3.OkHttpClient
import okhttp3.logging.HttpLoggingInterceptor
import retrofit2.Retrofit
import retrofit2.adapter.rxjava2.RxJava2CallAdapterFactory
import retrofit2.converter.gson.GsonConverterFactory
import java.io.File
object ServiceGenerator {
private const val CACHE_SIZE: Long = 10 * 1024 * 1024 // 10 MB
private const val CACHE_FILE_NAME = "cacheResponse"
private val httpLoggingInterceptor = HttpLoggingInterceptor(HttpLoggingInterceptor.Logger.DEFAULT).apply {
this.level = HttpLoggingInterceptor.Level.BODY
}
private val mRetrofitBuilder = Retrofit.Builder().addConverterFactory(GsonConverterFactory.create())
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
private var mRetrofit: Retrofit? = null
private var mCache: Cache? = null
private val mHttpClientBuilder = OkHttpClient.Builder()
fun setBaseUrl(baseUrl: String) {
mRetrofit = mRetrofitBuilder.baseUrl(baseUrl)
.client(mHttpClientBuilder.build())
.build()
}
fun setInterceptor(interceptor: Interceptor) {
mHttpClientBuilder.interceptors().clear()
mHttpClientBuilder.addInterceptor(httpLoggingInterceptor)
if (!mHttpClientBuilder.interceptors().contains(interceptor)) {
mHttpClientBuilder.addInterceptor(interceptor)
}
mRetrofitBuilder.client(mHttpClientBuilder.build())
}
fun setCache(cacheDir: File) {
if (mCache == null) {
val file = File(cacheDir.absolutePath, CACHE_FILE_NAME)
if (!file.exists()) {
file.mkdir()
}
mCache = Cache(file, CACHE_SIZE)
}
mHttpClientBuilder.cache(mCache)
}
fun <S> createService(serviceClass: Class<S>): S = mRetrofit!!.create(serviceClass)
}<file_sep>plugins {
id 'com.android.application'
id 'kotlin-android'
id 'kotlin-kapt'
id 'dagger.hilt.android.plugin'
}
android {
compileSdkVersion 30
buildToolsVersion "30.0.2"
defaultConfig {
multiDexEnabled = true
applicationId "com.quypham.vdc"
minSdkVersion 16
targetSdkVersion 30
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildFeatures {
viewBinding true
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
kotlinOptions {
jvmTarget = '1.8'
}
}
dependencies {
implementation fileTree(include: ['*.jar'], dir: 'libs')
// android
implementation "androidx.multidex:multidex:$multidexVersion"
implementation "androidx.cardview:cardview:$supportLibraryVersion"
implementation "androidx.appcompat:appcompat:$androidXAppCompatVersion"
implementation "androidx.recyclerview:recyclerview:$recyclerViewVersion"
implementation "androidx.annotation:annotation:$supportLibraryVersion"
implementation "androidx.constraintlayout:constraintlayout:$constraintVersion"
implementation group: 'androidx.lifecycle', name: 'lifecycle-viewmodel-ktx', version: "$lifecycle_version"
implementation 'androidx.hilt:hilt-lifecycle-viewmodel:1.0.0-alpha02'
//hilt
implementation "com.google.dagger:hilt-android:2.28-alpha"
kapt "com.google.dagger:hilt-android-compiler:2.28-alpha"
implementation 'androidx.hilt:hilt-lifecycle-viewmodel:1.0.0-alpha02'
kapt 'androidx.hilt:hilt-compiler:1.0.0-alpha02'
annotationProcessor 'androidx.hilt:hilt-compiler:1.0.0-alpha02'
// google
implementation "com.google.android.gms:play-services-gcm:$playServiceGcm"
implementation "com.google.android.gms:play-services-auth:$playServiceAuth"
implementation "com.google.android.gms:play-services-maps:$playServiceMaps"
implementation "com.google.android.gms:play-services-fitness:$playServiceFitness"
implementation "com.google.android.gms:play-services-location:$playServiceLocation"
implementation "com.google.code.gson:gson:$gsonVersion"
// google recommend
implementation "com.squareup.retrofit2:retrofit:$retrofitVersion"
implementation "com.squareup.retrofit2:converter-gson:$retrofitVersion"
implementation "com.squareup.retrofit2:adapter-rxjava2:$retrofitVersion"
implementation "com.squareup.okhttp3:logging-interceptor:$loggingInterceptor"
implementation "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version"
kapt "com.google.dagger:dagger-compiler:$daggerVersion"
kapt "com.google.dagger:dagger-android-processor:$daggerVersion"
// Coroutines
implementation "org.jetbrains.kotlinx:kotlinx-coroutines-core:$coroutinesCoreVersion"
implementation "org.jetbrains.kotlinx:kotlinx-coroutines-android:$coroutinesAndroidVersion"
//Parceler
implementation 'com.squareup:otto:1.3.8'
implementation "org.parceler:parceler-api:$parcelerVersion"
annotationProcessor "org.parceler:parceler:$parcelerVersion"
//dagger
implementation "com.google.dagger:dagger:$daggerVersion"
implementation "com.google.dagger:dagger-android:$daggerVersion"
implementation "com.google.dagger:dagger-android-support:$daggerVersion"
kapt "com.google.dagger:dagger-compiler:$daggerVersion"
kapt "com.google.dagger:dagger-android-processor:$daggerVersion"
//jetpack
implementation "androidx.lifecycle:lifecycle-common-java8:$lifecycleVersion"
implementation 'androidx.paging:paging-runtime-ktx:2.1.2'
implementation "androidx.room:room-runtime:$room_version"
kapt "androidx.room:room-compiler:$room_version"
implementation "com.google.android.material:material:1.2.1"
//ktx
implementation "androidx.room:room-ktx:2.2.6"
implementation "androidx.fragment:fragment-ktx:$fragment_version"
implementation "androidx.lifecycle:lifecycle-viewmodel-ktx:$lifecycleVersion"
implementation "androidx.lifecycle:lifecycle-runtime-ktx:$lifecycleVersion"
implementation "androidx.lifecycle:lifecycle-livedata-ktx:$lifecycleVersion"
implementation "androidx.fragment:fragment-ktx:$fragment_version"
}
/**
* This line should be at the BOTTOM always
* */
apply plugin: 'com.google.gms.google-services'
<file_sep>// Top-level build file where you can add configuration options common to all sub-projects/modules.
buildscript {
ext.kotlin_version = "1.4.20"
ext.googleServicesVersion = '4.3.4'
ext.hiltVersion = "2.28-alpha"
repositories {
google()
jcenter()
}
dependencies {
classpath "com.android.tools.build:gradle:4.1.1"
classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version"
classpath "com.google.gms:google-services:$googleServicesVersion"
classpath "com.google.dagger:hilt-android-gradle-plugin:$hiltVersion"
// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files
}
}
allprojects {
repositories {
google()
jcenter()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
ext {
// Sdk and tools
minSdkVersion = 21
targetSdkVersion = 29
compileSdkVersion = 29
buildToolsVersion = '29.0.3'
gsonVersion = '2.8.6'
daggerVersion = '2.30.1'
multidexVersion = '2.0.1'
constraintVersion = '2.0.4'
androidXAppCompatVersion = '1.2.0-rc01'
playServiceGcm = '17.0.0'
playServiceAuth = '19.0.0'
playServiceMaps = '17.0.0'
playServiceFitness = '20.0.0'
playServiceLocation = '17.1.0'
firebaseMessagingVersion = '20.2.3'
supportLibraryVersion = '1.0.0'
recyclerViewVersion = '1.1.0'
materialDesignVersion = '1.1.0'
coroutinesCoreVersion = '1.3.7'
coroutinesAndroidVersion = '1.4.1'
retrofitVersion = '2.9.0'
lifecycle_version = '2.2.0'
room_version = '2.2.6'
parcelerVersion = '1.1.12'
fragment_version = '1.2.5'
loggingInterceptor = '4.9.0'
lifecycleVersion = '2.2.0'
hiltVersion = "2.28-alpha"
// google-services.json
googleServicesJson = 'google-services.json'
}
<file_sep>package com.quypham.vdc.data
class WeatherForecastEntity constructor(var city: WeatherCity,
var cod: String,
var message: Double,
var cnt: Int,
var list: List<WeatherDetail>)
class WeatherCity constructor(var id: Int,
var name: String,
var coord: Coordinate,
var country: String,
var population: Int,
var timezone: Int)
class Coordinate constructor(var lat: Double,
var lng: Double)
class WeatherDetail constructor(var dt: Long,
var sunrise: Long,
var sunset: Long,
var temp: WeatherTemp,
var feels_like: WeatherFeelLike,
var pressure: Int,
var humidity: Int,
var weather: List<WeatherDescription>,
var speed: Double,
var deg: Int,
var clouds: Int,
var pop: Double)
class WeatherTemp constructor(var day: Double,
var min: Double,
var max: Double,
var night: Double,
var eve: Double,
var mom: Double)
class WeatherFeelLike constructor(var day: Double,
var night: Double,
var eve: Double,
var mom: Double)
class WeatherDescription constructor(var id: Int, var main: String, var description: String, var icon: String)<file_sep>package com.quypham.vdc.data
import androidx.room.Entity
import androidx.room.PrimaryKey
import androidx.room.TypeConverters
import com.quypham.vdc.database.TempUnitConverter
@Entity(tableName = "userSetting")
@TypeConverters(TempUnitConverter::class)
data class UserSetting constructor(var daysOfForecast: Int, var tempUnit: TempUnit) {
@PrimaryKey(autoGenerate = true)
var id: Int = 0
}
enum class TempUnit {
KELVIN, METRIC, IMPERIAL
}<file_sep>package com.quypham.vdc.ui.weather
import android.content.Context
import android.view.LayoutInflater
import android.view.ViewGroup
import androidx.recyclerview.widget.DiffUtil
import androidx.recyclerview.widget.ListAdapter
import androidx.recyclerview.widget.RecyclerView
import com.quypham.vdc.R
import com.quypham.vdc.databinding.ItemWeatherBinding
import com.quypham.vdc.utils.DateFormatUtils
class WeatherInfoAdapter :
ListAdapter<WeatherInfo, WeatherInfoAdapter.WeatherInfoViewHolder>(WeatherDiff) {
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): WeatherInfoViewHolder {
return WeatherInfoViewHolder(
ItemWeatherBinding.inflate(
LayoutInflater.from(parent.context),
parent,
false
), parent.context
)
}
override fun onBindViewHolder(holder: WeatherInfoViewHolder, position: Int) {
holder.bindData(getItem(position))
}
class WeatherInfoViewHolder(
private val binding: ItemWeatherBinding,
private val context: Context
) : RecyclerView.ViewHolder(binding.root) {
fun bindData(weatherInfo: WeatherInfo) {
binding.tvDate.text = String.format(
context.getString(R.string.weather_date_format),
DateFormatUtils.toWeatherDateFormat(weatherInfo.timestamp)
)
binding.tvAvgTemp.text = String.format(
context.getString(R.string.weather_avg_temp_format),
weatherInfo.averageTemp,
weatherInfo.tempUnit
)
binding.tvPressure.text = String.format(
context.getString(R.string.weather_pressure_format),
weatherInfo.pressure
)
binding.tvHumidity.text = String.format(
context.getString(R.string.weather_humidity_format),
weatherInfo.humidity
)
binding.tvDescription.text = String.format(
context.getString(R.string.weather_description_format),
weatherInfo.description
)
}
}
}
object WeatherDiff : DiffUtil.ItemCallback<WeatherInfo>() {
override fun areItemsTheSame(oldItem: WeatherInfo, newItem: WeatherInfo): Boolean {
return oldItem.timestamp == newItem.timestamp
}
override fun areContentsTheSame(oldItem: WeatherInfo, newItem: WeatherInfo): Boolean {
return oldItem == newItem
}
}<file_sep>package com.quypham.vdc.di
import com.quypham.vdc.Constants
import com.quypham.vdc.api.ApiService
import com.quypham.vdc.database.AppDatabase
import com.quypham.vdc.repo.SettingRepository
import com.quypham.vdc.repo.SettingRepositoryImp
import com.quypham.vdc.repo.WeatherRepository
import com.quypham.vdc.repo.WeatherRepositoryImp
import com.quypham.vdc.repo.setting.SettingDataSource
import com.quypham.vdc.repo.setting.SettingLocalDataSource
import com.quypham.vdc.repo.weather.WeatherDataSource
import com.quypham.vdc.repo.weather.WeatherLocalDataSource
import com.quypham.vdc.repo.weather.WeatherRemoteDataSource
import dagger.Module
import dagger.Provides
import dagger.hilt.InstallIn
import dagger.hilt.android.components.ApplicationComponent
import javax.inject.Named
import javax.inject.Singleton
@InstallIn(ApplicationComponent::class)
@Module
class RepositoryModule {
@Singleton
@Provides
@Named(Constants.LOCAL)
fun provideLocalUserDataSource(): WeatherDataSource {
return WeatherLocalDataSource()
}
@Singleton
@Provides
@Named(Constants.REMOTE)
fun provideRemoteUserDataSource(apiService: ApiService): WeatherDataSource {
return WeatherRemoteDataSource(apiService)
}
@Singleton
@Provides
fun provideUserRepository(@Named(Constants.REMOTE) remoteSource: WeatherDataSource): WeatherRepository {
return WeatherRepositoryImp(remoteSource)
}
@Singleton
@Provides
@Named(Constants.LOCAL)
fun provideLocalSettingDataSource(appDatabase: AppDatabase): SettingDataSource {
return SettingLocalDataSource(appDatabase)
}
@Singleton
@Provides
fun provideSettingRepository(@Named(Constants.LOCAL) localSource: SettingDataSource): SettingRepository {
return SettingRepositoryImp(localSource)
}
}<file_sep>package com.quypham.vdc.repo
import android.util.Log
import com.quypham.vdc.Constants
import com.quypham.vdc.api.RepoResponse
import com.quypham.vdc.data.WeatherForecastEntity
import com.quypham.vdc.repo.weather.WeatherDataSource
import javax.inject.Inject
import javax.inject.Named
import javax.inject.Singleton
interface WeatherRepository {
suspend fun query(city: String, daysOfForecast: Int): RepoResponse<WeatherForecastEntity>
}
@Singleton
class WeatherRepositoryImp @Inject constructor(@Named(Constants.REMOTE) private val mWeatherRemoteDataSource: WeatherDataSource): WeatherRepository {
companion object {
const val TAG = "WeatherRepository"
}
override suspend fun query(city: String, daysOfForecast: Int): RepoResponse<WeatherForecastEntity> {
Log.d(TAG,"query with city $city days $daysOfForecast")
return mWeatherRemoteDataSource.query(city, daysOfForecast)
}
}<file_sep>package com.quypham.vdc.customview
import android.content.Context
import android.util.AttributeSet
import android.view.LayoutInflater
import android.view.View
import androidx.annotation.DrawableRes
import androidx.constraintlayout.widget.ConstraintLayout
import androidx.recyclerview.widget.RecyclerView
import com.quypham.vdc.R
import com.quypham.vdc.databinding.RecyclerErrorViewBinding
import com.quypham.vdc.databinding.RecyclerLoadingViewBinding
import com.quypham.vdc.databinding.StateRecyclerViewBinding
class StateRecyclerView constructor(
context: Context,
attrs: AttributeSet? = null,
defStyleAttr: Int = 0
) : ConstraintLayout(context, attrs, defStyleAttr) {
constructor(context: Context) : this(context, null, 0)
constructor(context: Context, attrs: AttributeSet) : this(context, attrs, 0)
private val mBinding: StateRecyclerViewBinding = StateRecyclerViewBinding.inflate(
LayoutInflater.from(context), this
)
private val mErrorViewBinding: RecyclerErrorViewBinding
private val mLoadingViewBinding: RecyclerLoadingViewBinding
val recyclerView: RecyclerView
get() = mBinding.recyclerView
var errorText: String = ""
set(value) {
field = value
mErrorViewBinding.tvErrorMessage.text = value
}
@DrawableRes
var errorIcon = 0
set(value) {
field = value
mErrorViewBinding.ivError.setImageResource(value)
}
init {
// inflate the layout
mErrorViewBinding = mBinding.errorView
mLoadingViewBinding = mBinding.loadingView
context.theme.obtainStyledAttributes(
attrs,
R.styleable.StateRecyclerView,
0,
0
).apply {
try {
errorText = getString(R.styleable.StateRecyclerView_errorText) ?: context.getString(
R.string.general_error
)
errorIcon = getResourceId(
R.styleable.StateRecyclerView_errorIcon,
R.drawable.baseline_error_outline_white_48
)
} finally {
recycle()
}
}
}
fun showRecyclerView() {
mLoadingViewBinding.root.visibility = View.GONE
mErrorViewBinding.root.visibility = View.GONE
recyclerView.visibility = View.VISIBLE
}
fun showErrorView(errorMess: String) {
errorText = errorMess
mLoadingViewBinding.root.visibility = View.GONE
recyclerView.visibility = View.GONE
mErrorViewBinding.root.visibility = View.VISIBLE
}
fun showLoadingView() {
mLoadingViewBinding.root.visibility = View.VISIBLE
mErrorViewBinding.root.visibility = View.GONE
}
}<file_sep>package com.quypham.vdc.database
import androidx.room.Database
import androidx.room.RoomDatabase
import com.quypham.vdc.Constants
import com.quypham.vdc.data.UserSetting
@Database(entities = [UserSetting::class], version = Constants.APP_DATABASE_VERSION, exportSchema = false)
abstract class AppDatabase: RoomDatabase() {
abstract fun userSettingDao(): UserSettingDao
} | cd17a5cdafcca5cfed964f9a540c0d89426d792b | [
"Markdown",
"Kotlin",
"Gradle"
] | 37 | Kotlin | alvis411/VDC-Weather-Forecast | d2896bbbe869cb744d36f207d995d2543cf0e6af | 961f3602ab044c11c6bdc46c3db03395d0eabfd2 | |
refs/heads/master | <file_sep>#!/bin/bash
# Usage: `./this-script <image name>`
#
# The image name should be of the form 'namespace:tag', e.g.
# 'yourname/yourproject:latest', see also https://docs.docker.com/docker-hub/builds/advanced/.
#
# If $IMAGE_NAME is defined as an environment variable, it takes precedent and the
# command line argument image name will be ignored.
# If neither is given, this script fails.
# In 2021, Docker retired its free automated builds. They now require a Pro plan.
# (We can thank abusive miners for that).
# To cope, and to not have to pay, these images are now built locally and pushed
# manually. Hence, this script now works for both local use as well as DockerHub use
# (should a Pro plan be available).
set -u
# For more info, see:
# https://docs.docker.com/docker-hub/builds/advanced/
# https://web.archive.org/web/20201002130055/https://dev.to/samuelea/automate-your-builds-on-docker-hub-by-writing-a-build-hook-script-13fp
BUILD_DATE=$(date --utc +%Y-%m-%dT%H:%M:%S%Z)
cut_tag_field() { echo "$DOCKER_TAG" | cut --delimiter="-" --fields="$1"; }
# Assign some (dynamic) default values if variables undefined.
# This is compatible with the DockerHub infrastructure where these are defined
# (see https://docs.docker.com/docker-hub/builds/advanced/) but also allows easy
# local execution.
: "${SOURCE_COMMIT:=$(git rev-parse HEAD)}" # https://stackoverflow.com/a/28085062
: "${DOCKER_TAG:=latest}" # https://stackoverflow.com/a/28085062
# This will fail if compiling locally where this env var is (probably) not defined,
# and no command line argument is given:
: "${IMAGE_NAME:=$1}" # https://stackoverflow.com/a/28085062
echo "Using Docker tag '$DOCKER_TAG'."
echo "Version control reference is '$SOURCE_COMMIT'."
echo "Building with image name '$IMAGE_NAME'."
if [[ "$DOCKER_TAG" == "latest" ]]
then
# Default OS:
BASE_OS="debian"
# `debian:latest` will get latest *stable*, which has between 1.5 to 2 years old,
# well-tested software. While that is suitable for servers etc., this Docker image
# does not need highest stability. More recent features are more important (e.g. for
# pandoc). Debian's `testing` branch sounds cutting-edge but is roughly 6 months old
# software, so should be stable enough here.
# This image's main component, TeXLive, is largely unaffected by this anyway.
OS_VERSION="testing"
# Installation script takes care of finding the appropriate version of TeXLive
# for the 'latest' tag:
TL_VERSION="$DOCKER_TAG"
else
# Otherwise, take versions as manually specified in build process.
#
# This assumes tags in the form: '<baseos>-<version>-texlive-<version>',
# e.g. 'debian-10-texlive-2019'
#
# E.g. 'debian'
BASE_OS=$(cut_tag_field 1)
# Version e.g. '10' for Debian, but can also be 'buster', see:
# https://wiki.debian.org/DebianReleases
# These are the available versions:
# https://hub.docker.com/_/debian?tab=tags
OS_VERSION=$(cut_tag_field 2)
# E.g. '2020':
TL_VERSION=$(cut_tag_field 4)
fi
docker build \
--build-arg VCS_REF="$SOURCE_COMMIT" \
--build-arg BUILD_DATE="$BUILD_DATE" \
--build-arg BASE_OS="$BASE_OS" \
--build-arg OS_VERSION="$OS_VERSION" \
--build-arg TL_VERSION="$TL_VERSION" \
--tag "$IMAGE_NAME" \
.
<file_sep># Docker image with custom, almost-full TeXLive distribution & various tools
[](https://hub.docker.com/r/alexpovel/latex)
Serves a lot of needs surrounding LaTeX file generation and handling.
For the rationale behind the installed Debian packages, see [below](#custom-tools).
To see how to build the image, also see [below](#building).
## Quick Intro
To use the image, you can use the [example](tests/minimal.tex) provided in this repository:
- Bash:
```bash
docker run \
--rm \
--volume $(pwd)/tests:/tex \
alexpovel/latex
```
- PowerShell:
```powershell
docker run `
--rm `
--volume ${PWD}/tests:/tex `
alexpovel/latex
```
The parts making up the command are:
- The last line is the location on [DockerHub](https://hub.docker.com/repository/docker/alexpovel/latex).
Without specifying a [*tag*](https://hub.docker.com/repository/docker/alexpovel/latex/tags?page=1),
the default `latest` is implied.
See [below](#historic-builds) for more options.
- The `--rm` option removes the container after a successful run.
This is generally desired since containers are not supposed to be stateful:
once their process terminates, the container terminates, and it can be considered junk.
- Providing a `--volume`, in this case [`tests/`](./tests/) in the current working directory,
is required for the container to find files to work on.
It has to be *mounted* to a location *inside* the container.
This has to be whatever the last `WORKDIR` instruction in the [Dockerfile](Dockerfile) is,
e.g. `/tex`.
Otherwise, you can always override the `WORKDIR` using the `--workdir` option.
This is the directory in which the Docker container's process works in and expects to
find files.
- Note that there is no command given, e.g. there is nothing *after* `alexpovel/latex`.
In this form, the container runs as an executable (just as if you ran `lualatex` or
similar commands), where the program to be executed is determined by the `ENTRYPOINT`
instruction in the [Dockerfile](Dockerfile).
For example, if the `ENTRYPOINT` is set to `latexmk`, running the above command will
execute `latexmk` in the container's context, without you having to specify it.
(`latexmk` is a recipe-like tool that automates LaTeX document compilation by running
`lualatex`, `biber` and whatever else required for compilation as many times as
needed for proper PDF output (so references like `??` in the PDF are resolved).
It does this by detecting that auxiliary files no longer change (steady-state).
The tool is best configured using its config file, `.latexmkrc`.)
Any options to the `ENTRYPOINT` executable are given at the end of the command, e.g.:
```bash
docker run \
--rm \
--volume $(pwd)/tests:/tex \
alexpovel/latex \
-c
```
to run, if `latexmk` is the `ENTRYPOINT`, the equivalent of `latexmk -c`
([cleaning auxiliary files](https://mg.readthedocs.io/latexmk.html#cleaning-up)).
To **overwrite** the `ENTRYPOINT`, e.g. because you want to run only `lualatex`,
use the `--entrypoint` option, e.g. `--entrypoint="lualatex"`.
Similarly, you can work inside of the container directly, e.g. for debugging, using
`--entrypoint="bash"`.
## Approach
This Dockerfile is based on a custom TeXLive installer using their
[`install-tl` tool](https://www.tug.org/texlive/doc/install-tl.html),
instead of
[Debian's `texlive-full`](https://packages.debian.org/search?searchon=names&keywords=texlive-full).
Other, smaller `texlive-` collections would be
[available](https://packages.debian.org/search?suite=default§ion=all&arch=any&searchon=names&keywords=texlive),
but TeXLive cannot install missing packages on-the-fly,
[unlike MiKTeX](https://miktex.org/howto/miktex-console/).
Therefore, images should come with all desirable packages in place; installing them after
the fact in running containers using [`tlmgr`](https://www.tug.org/texlive/tlmgr.html)
is the wrong approach (containers are only meant to be run; if they are incomplete,
modify the *image*, ordinarily by modifying the `Dockerfile`).
This approach has the following advantages:
- Using ["vanilla" TeXLive](https://www.tug.org/texlive/debian.html)
affords us the latest packages directly from the source, while the official
Debian package might lag behind a bit.
This is often not relevant, but has bitten me several times while working with the
latest packages.
- The installation can be adjusted better.
For example, *multiple GBs* of storage/image size are saved by omitting unneeded PDF
documentation files.
- We can install arbitrary TeXLive versions, as long as they are the
[current](https://tug.org/texlive/acquire-netinstall.html)
or an
archived (<ftp://tug.org/historic/systems/texlive/>)
version.
Otherwise, to obtain [older or even obsolete TeXLive installations](#historic-builds),
one would have to also obtain a corresponding Debian version.
This way, we can choose a somewhat recent Debian version and simply install an old
TeXLive into it.
Eligible archive versions are those year directories (`2019`, `2020`, ...) present
at the above FTP link that have a `tlnet-final` subdirectory.
This is (speculating here) a frozen, aka final, version, put online the day the *next*
major release goes live.
For example, version `2021` released on 2021-04-01 and `2020` received its `tlnet-final`
subdirectory that same day.
The `install-tl` tool is configured via a [*Profile* file](config/texlive.profile), see also
the [documentation](https://www.tug.org/texlive/doc/install-tl.html#PROFILES).
This enables unattended, pre-configured installs, as required for a Docker installation.
---
The (official?) [`texlive/texlive` image](https://hub.docker.com/r/texlive/texlive) follows
[the same approach](https://hub.docker.com/layers/texlive/texlive/latest/images/sha256-70fdbc1d9596c8eeb4a80c71a8eb3a5aeb63bed784112cbdb87f740e28de7a80?context=explore).
However, there are a bunch of things this Dockerfile does differently that warrant not
building `FROM` that image:
- a user-editable, thus more easily configurable [profile](config/texlive.profile).
This is mainly concerning the picked package *collections*. Unchecking (putting a `0`)
unused ones saves around 500MB at the time of writing.
- more elaborate support for [historic versions](#historic-builds)
- an installation procedure incorporating a proper `USER`
Things they do that do not happen here:
- verify download integrity using `gpg`
### Historic Builds
LaTeX is a slow world, and many documents/templates in circulation still rely on
outdated practices or packages.
This can be a huge hassle.
Maintaining an old LaTeX distribution next to a current one on the same host is
not fun.
This is complicated by the fact that (La)TeX seems to do things differently than pretty much
everything else.
For this, Docker is the perfect tool.
This image can be built (`docker build`) with different build `ARG`s, and the build
process will figure out the proper way to handle installation.
There is a [script](texlive.sh) to handle getting and installing TeXLive from the
proper location ([current](https://www.tug.org/texlive/acquire-netinstall.html) or
[archive](ftp://tug.org/historic/systems/texlive/)).
Refer to the [Dockerfile](Dockerfile) for the available `ARG`s (all `ARG` have a default).
These are handed to the build process via the
[`--build-arg` option](https://docs.docker.com/engine/reference/commandline/build/#options).
Note that for a *specific* TeXLive version to be picked, it needs to be present in their
[archives](ftp://tug.org/historic/systems/texlive/).
The *current* TeXLive is not present there (it's not historic), but is available under
the `latest` Docker tag.
As such, if for example `2020` is the *current* TeXLive, and the image is to be based on
Debian 10, there is *no* `debian-10-texlive-2020` tag.
You would obtain this using the `latest` tag.
As soon as TeXLive 2020 is superseded and consequently moved to the archives,
the former tag can become available.
To build an array of different versions automatically, DockerHub provides
[advanced options](https://docs.docker.com/docker-hub/builds/advanced/) in the form of
hooks, e.g. a [build hook](hooks/build).
These are bash scripts that override the default DockerHub build process.
At build time, DockerHub provides
[environment variables](https://docs.docker.com/docker-hub/builds/advanced/#environment-variables-for-building-and-testing)
which can be used in the build hook to forward these into the Dockerfile build process.
As such, by just specifying the image *tags* on DockerHub, we can build corresponding
images automatically (see also
[here](https://web.archive.org/web/20201005132636/https://dev.to/samuelea/automate-your-builds-on-docker-hub-by-writing-a-build-hook-script-13fp)).
For more info on this, see [below](#on-dockerhub).
The approximate [matching of Debian to TeXLive versions](https://www.tug.org/texlive/debian.html)
is (see also [here](https://www.debian.org/releases/) and [here](https://www.debian.org/distrib/archive).):
| Debian Codename | Debian Version | TeXLive Version |
| --------------- | :------------: | :-------------: |
| bullseye | 11 | 2020 |
| buster | 10 | 2018 |
| stretch | 9 | 2016 |
| jessie | 8 | 2014 |
| wheezy | 7 | 2012 |
| squeeze | 6.0 | 2009 |
| lenny | 5.0 | unknown |
| etch | 4.0 | unknown |
| sarge | 3.1 | unknown |
| woody | 3.0 | unknown |
| potato | 2.2 | unknown |
| slink | 2.1 | unknown |
| hamm | 2.0 | unknown |
This is only how the official Debian package is shipped.
These versions can be, to a narrow extend, freely mixed.
Using `install-tl`, older versions of TeXLive can be installed on modern Debian versions.
#### Issues
Using [*obsolete* Debian releases](https://www.debian.org/releases/) comes with a long
list of headaches.
As such, Debian versions do not reach too far back.
It does not seem worth the effort.
Instead, it seems much easier to install older TeXLive versions onto reasonably recent
Debians.
Issues I ran into are:
- `apt-get update` will fail if the original Debian repository is dead (Debian 6/TeXLive 2014):
```plaintext
W: Failed to fetch http://httpredir.debian.org/debian/dists/squeeze/main/binary-amd64/Packages.gz 404 Not Found
W: Failed to fetch http://httpredir.debian.org/debian/dists/squeeze-updates/main/binary-amd64/Packages.gz 404 Not Found
W: Failed to fetch http://httpredir.debian.org/debian/dists/squeeze-lts/main/binary-amd64/Packages.gz 404 Not Found
E: Some index files failed to download, they have been ignored, or old ones used instead.
```
As such, there needs to be a [dynamic way to update `/etc/apt/sources.list`](https://github.com/alexpovel/latex-extras-docker/blob/fa9452c236079a65563daff22767b2b637dd80c6/adjust_sources_list.sh)
if the Debian version to be used in an archived one, see also
[here](https://web.archive.org/web/20201007095943/https://www.prado.lt/using-old-debian-versions-in-your-sources-list).
- `RUN wget` (or `curl` etc.) via `HTTPS` will fail for older releases, e.g. GitHub
rejected the connection due to the outdated TLS version of the old release (Debian 6/TeXLive 2015):
```text
Connecting to github.com|172.16.31.10|:443... connected.
OpenSSL: error:1407742E:SSL routines:SSL23_GET_SERVER_HELLO:tlsv1 alert protocol version
```
- Downloading older releases requires using the [Debian archives](http://archive.debian.org/debian/).
This works fine, however a warning is issued (Debian 6/TeXLive 2014):
```plaintext
W: GPG error: http://archive.debian.org squeeze Release: The following signatures were invalid: KEYEXPIRED 1520281423 KEYEXPIRED 1501892461
```
Probably related to this, the installation then fails:
```plaintext
WARNING: The following packages cannot be authenticated!
libgdbm3 libssl0.9.8 wget libdb4.7 perl-modules perl openssl ca-certificates
E: There are problems and -y was used without --force-yes
```
According to `man apt-get`, `--force-yes` is both deprecated and absolutely not
recommended.
The correct course here is to `--allow-unauthenticated`, however this would also
affect the build process for modern versions, where authentication *did not* fail.
The official Debian archives are probably trustworthy, but this is still an issue.
- A more obscure issue is (Debian 7/TeXLive 2011):
```plaintext
The following packages have unmet dependencies:
perl : Depends: perl-base (= 5.14.2-21+deb7u3) but 5.14.2-21+deb7u6 is to be installed
E: Unable to correct problems, you have held broken packages.
```
While the error message itself is crystal-clear, debugging this is probably a nightmare.
- Tools like `pandoc`, which was released in [2006](https://pandoc.org/releases.html),
limit the earliest possible Debian version as long as the tool's installation is part
of the Dockerfile.
In this example, 2006 should in any case be early enough (if not, update your LaTeX
file to work with more recent versions, that is probably decidedly less work).
## Custom Tools
The auxiliary tools are (for the actual, up-to-date list, see the [Dockerfile](Dockerfile)):
- A *Java Runtime Environment* for [`bib2gls`](https://ctan.org/pkg/bib2gls) from the
[`glossaries-extra` package](https://www.ctan.org/pkg/glossaries-extra).
`bib2gls` takes in `*.bib` files with glossary, symbol, index and other definitions
and applies sorting, filtering etc.
For this, it requires Java.
- [`inkscape`](https://inkscape.org/) because the [`svg`](https://ctan.org/pkg/svg)
package needs it.
We only require the CLI, however there is no "no-GUI" version available.
Headless Inkscape is [currently in the making](https://web.archive.org/web/20201007100140/https://wiki.inkscape.org/wiki/index.php/Future_Architecture).
Using that package, required PDFs and PDF_TEXs are only generated at build-time
(on the server or locally) and treated as a cache.
As such, they can be discarded freely and are regenerated in the next compilation run,
using `svg`, which calls `inkscape`.
Therefore, the `svg` package gets rid of all the PDF/PDF_TEX intermediate junk and lets us
deal with the true source -- `*.svg` files -- directly.
These files are really [XML](https://en.wikipedia.org/wiki/Scalable_Vector_Graphics),
ergo text-based, ergo suitable for VCS like `git` (as opposed to binary PDFs).
Being an external tool, `svg`/`inkscape` also requires the `--shell-escape` option to
`lualatex` etc. for writing outside files.
- [`gnuplot`](http://www.gnuplot.info/) for `contour gnuplot` commands for `addplot3` in `pgfplots`.
So essentially, an external add-on for the magnificent `pgfplots` package.
For `gnuplot` to write computed results to a file for `pgfplots` to read,
`--shell-escape` is also required.
- [`pandoc`](https://pandoc.org/) as a very flexible, convenient markup conversion tool.
For example, it can convert Markdown (like this very [README](README.md)) to PDF
via LaTeX:
```bash
pandoc README.md --output=README.pdf # pandoc infers what to do from suffixes
```
The default output is usable, but not very pretty.
This is where *templates* come into play.
A very tidy and well-made such template is
[*Eisvogel*](https://github.com/Wandmalfarbe/pandoc-latex-template).
Its installation is not via a (Debian) package, so it has to be downloaded specifically.
For this, additional requirements are:
- `wget` to download the archive,
- `librsvg2-bin` for the `rsvg-convert` tool.
This is used by `pandoc` to convert SVGs when embedding them into the new PDF.
Note that `pandoc` and its *Eisvogel* template can draw
[metadata from a YAML header](https://pandoc.org/MANUAL.html#metadata-variables),
for example:
```yaml
---
title: "Title"
author: [Author]
date: "YYYY-MM-DD"
subject: "Subject"
keywords: [Keyword1, Keyword2]
lang: "en"
...
```
among other metadata variables.
*Eisvogel* uses it to fill the document with info, *e.g.* the PDF header and
footer.
`pandoc` is not required for LaTeX work, but is convenient to have at the ready for
various conversion jobs.
## Building
The proper way to access these images is via DockerHub.
### On DockerHub
This repository and its [Dockerfile](Dockerfile) are built into the corresponding image
continuously and made available on [DockerHub](https://hub.docker.com/repository/docker/alexpovel/latex).
There, the automated build process looks somewhat like:

The DockerHub build process combines these build rules with the [build hook](hooks/build)
to automatically build and tag images as specified by those rules.
For the currently available tags, see [here](https://hub.docker.com/repository/docker/alexpovel/latex/tags?page=1).
### Locally
The Dockerfile can of course also be built locally.
If you desire to use non-default `ARG`s, the build might look like:
```bash
export TL_VERSION="<version>"
export BASE_OS="<Linux distro name>"
export OS_VERSION="<version corresponding to that name, e.g. '8' or 'buster' (Debian)>"
docker build . \
--build-arg TL_VERSION=${TL_VERSION} \
--build-arg BASE_OS=${BASE_OS} \
--build-arg OS_VERSION=${OS_VERSION} \
--tag <some>/<name>:${BASE_OS}-${OS_VERSION}-texlive-${TL_VERSION}
```
Essentially, you would want to emulate what the [build hook](hooks/build) does.
This process can take a very long time, especially when downloading from the TeXLive/TUG
archives.
For developing/debugging, it is advisable to download the archive files once.
E.g. for TexLive 2014, you would want this directory: <ftp://tug.org/historic/systems/texlive/2014/tlnet-final/>.
Download in one go using:
```bash
wget --recursive --no-clobber ftp://tug.org/historic/systems/texlive/2014/tlnet-final
```
The `--no-clobber` option allows you to restart the download at will.
Then, work with the `--repository=/some/local/path` option of `install-tl`,
after [copying the archive directory into the image at build time](https://docs.docker.com/develop/develop-images/dockerfile_best-practices/#understand-build-context)
(see also [here](https://tex.stackexchange.com/a/374651/120853)).
Having a local copy circumvents [unstable connections](https://tex.stackexchange.com/q/370686/120853)
and minimizes unnecessary load onto TUG servers.
<file_sep># ARGs before the first FROM are global and usable in all stages
ARG BASE_OS=debian
ARG OS_VERSION=testing
# Image with layers as used by all succeeding steps
FROM ${BASE_OS}:${OS_VERSION} as base
# Use `apt-get` over just `apt`, see https://askubuntu.com/a/990838/978477.
# Also run `apt-get update` on every `RUN`, see:
# https://docs.docker.com/develop/develop-images/dockerfile_best-practices/#run
RUN apt-get update && \
apt-get install --yes --no-install-recommends \
# wget for `install-tl` script to download TeXLive, and other downloads.
wget \
# In a similar vein, `curl` is required by various tools, or is just very
# nice to have for various scripting tasks.
curl \
# wget/install-tl requires capability to check certificate validity.
# Without this, executing `install-tl` fails with:
#
# install-tl: TLPDB::from_file could not initialize from: https://<mirror>/pub/ctan/systems/texlive/tlnet/tlpkg/texlive.tlpdb
# install-tl: Maybe the repository setting should be changed.
# install-tl: More info: https://tug.org/texlive/acquire.html
#
# Using `install-tl -v`, found out that mirrors use HTTPS, for which the
# underlying `wget` (as used by `install-tl`) returns:
#
# ERROR: The certificate of '<mirror>' is not trusted.
# ERROR: The certificate of '<mirror>' doesn't have a known issuer.
#
# This is resolved by installing:
ca-certificates \
# Update Perl, otherwise: "Can't locate Pod/Usage.pm in @INC" in install-tl
# script; Perl is already installed, but do not use `upgrade`, see
# https://docs.docker.com/develop/develop-images/dockerfile_best-practices/#run
perl \
# Install `latexindent` Perl dependencies.
# Found these using this method: https://unix.stackexchange.com/a/506964/374985
# List of `latexindent` dependencies is here:
# https://latexindentpl.readthedocs.io/en/latest/appendices.html#linux,
# see also the helper script at
# https://github.com/cmhughes/latexindent.pl/blob/master/helper-scripts/latexindent-module-installer.pl
#
# Installing via Debian system packages because installing the modules via
# `cpanm` requires `gcc` and I wanted to avoid installing that (~200MB).
#
# YAML::Tiny:
libyaml-tiny-perl \
# File::HomeDir:
libfile-homedir-perl \
# Unicode:GCString:
libunicode-linebreak-perl \
# Log::Log4perl:
liblog-log4perl-perl \
# Log::Dispatch:
liblog-dispatch-perl \
# Usually, `latexmk` is THE tool to use to automate, in a `make`-like style,
# LaTeX (PDF) file generation. However, if that is not enough, the following
# will fill the gaps and cover all other use cases:
make \
# Get `envsubst` to replace environment variables in files with their actual
# values.
gettext-base \
# Using the LaTeX package `minted` for syntax highlighting of source code
# snippets. It's much more powerful than the alternative `listings` (which is
# pure TeX: no outside dependencies but limited functionality) but requires
# Python's `pygments` package:
python3 \
python3-pygments \
# Required to embed git metadata into PDF from within Docker container:
git
# The `minted` LaTeX package provides syntax highlighting using the Python `pygmentize`
# package. That package also installs a callable script, which `minted` uses, see
# https://tex.stackexchange.com/a/281152/120853.
# Therefore, `minted` primarily works by invoking `pygmentize` (or whatever it was
# overridden by using `\MintedPygmentize`). However, it requires `python` for other
# jobs, e.g. to remove leading whitespace for the `autogobble` function, see the
# "\minted@autogobble Remove common leading whitespace." line in the docs.
# It is invoked with `python -c`, but Debian only has `python3`. Therefore, alias
# `python` to invoke `python3`. Use `update-alternatives` because it's cooler than
# symbolic linking, and made for this purpose.
# If `python` is not available but `pygmentize` is, stuff like `autogobble` and
# `\inputminted`won't work but syntax highlighting will.
# See also https://stackoverflow.com/a/55351449/11477374
# Last argument is `priority`, whose value shouldn't matter since there's nothing else.
RUN update-alternatives --install /usr/bin/python python /usr/bin/python3 1
FROM base as downloads
# Cannot share ARGs over multiple stages, see also:
# https://github.com/moby/moby/issues/37345.
# Therefore, work in root (no so `WORKDIR`), so in later stages, the location of
# files copied from this stage does not have to be guessed/WET.
# Using an ARG with 'TEX' in the name, TeXLive will warn:
#
# ----------------------------------------------------------------------
# The following environment variables contain the string "tex"
# (case-independent). If you're doing anything but adding personal
# directories to the system paths, they may well cause trouble somewhere
# while running TeX. If you encounter problems, try unsetting them.
# Please ignore spurious matches unrelated to TeX.
# TEXPROFILE_FILE=texlive.profile
# ----------------------------------------------------------------------
#
# This also happens when the *value* contains 'TEX'.
# `ARG`s are only set during Docker image build-time, so this warning should be void.
ARG TL_VERSION=latest
ARG TL_INSTALL_ARCHIVE="install-tl-unx.tar.gz"
ARG EISVOGEL_ARCHIVE="Eisvogel.tar.gz"
ARG INSTALL_TL_DIR="install-tl"
COPY texlive.sh .
RUN \
# Get appropriate installer for the TeXLive version to be installed:
./texlive.sh get_installer ${TL_VERSION} && \
# Get Eisvogel LaTeX template for pandoc,
# see also #175 in that repo.
wget https://github.com/Wandmalfarbe/pandoc-latex-template/releases/latest/download/${EISVOGEL_ARCHIVE}
RUN \
mkdir ${INSTALL_TL_DIR} && \
# Save archive to predictable directory, in case its name ever changes; see
# https://unix.stackexchange.com/a/11019/374985.
# The archive comes with a name in the form of 'install-tl-YYYYMMDD' from the source,
# which is of course unpredictable.
tar --extract --file=${TL_INSTALL_ARCHIVE} --directory=${INSTALL_TL_DIR} --strip-components 1 && \
\
# Prepare Eisvogel pandoc template (yields `eisvogel.latex` among other things):
tar --extract --file=${EISVOGEL_ARCHIVE}
FROM base as main
# Metadata
ARG BUILD_DATE="n/a"
ARG VCS_REF="n/a"
ARG TL_VERSION="latest"
ARG TL_PROFILE="texlive.profile"
# Label according to http://label-schema.org/rc1/ to have some metadata in the image.
# This is important e.g. to know *when* an image was built. Depending on that, it can
# contain different software versions (even if the base image is specified as a fixed
# version).
LABEL \
maintainer="<NAME> <<EMAIL>>" \
org.label-schema.build-date=${BUILD_DATE} \
org.label-schema.description="TeXLive with most packages, JavaRE, Inkscape, pandoc and more" \
org.label-schema.url="https://collaborating.tuhh.de/alex/latex-git-cookbook" \
org.label-schema.vcs-url="https://github.com/alexpovel/latex-extras-docker" \
org.label-schema.vcs-ref=${VCS_REF} \
org.label-schema.schema-version="1.0"
# User to install and run LaTeX as.
# This is a security and convenience measure: by default, containers run as root.
# To work and compile PDFs using this container, you will need to map volumes into it
# from your host machine. Those bind-mounts will then be accessed as root from this
# container, and any generated files will also be owned by root. This is inconvenient at
# best and dangerous at worst.
# The generated user here will have IDs of 1000:1000. If your local user also has those
# (the case for single-user Debians etc.), your local user will already have correct
# ownership of all files generated by the user we create here.
ARG USER="tex"
RUN useradd --create-home ${USER}
ARG INSTALL_DIR="/install"
WORKDIR ${INSTALL_DIR}
# Copy custom file containing TeXLive installation instructions
COPY config/${TL_PROFILE} .
COPY --from=downloads /install-tl/ /texlive.sh ./
# Global wget config file, see the comments in that file for more info and the rationale.
# Location of that file depends on system, e.g.: https://askubuntu.com/a/368050
COPY config/.wgetrc /etc/wgetrc
# Move to where pandoc looks for templates, see https://pandoc.org/MANUAL.html#option--data-dir
COPY --from=downloads /eisvogel.latex /home/${USER}/.pandoc/templates/
# "In-place" `envsubst` run is a bit more involved, see also:
# https://stackoverflow.com/q/35078753/11477374.
RUN tmpfile=$(mktemp) && \
cp ${TL_PROFILE} tmpfile && \
echo "Using profile file (indent purely visual):" && \
cat ${TL_PROFILE} | envsubst | tee tmpfile | sed "s/^/\t/" && \
mv tmpfile ${TL_PROFILE}
# (Large) LaTeX layer
RUN ./texlive.sh install ${TL_VERSION}
# Remove no longer needed installation workdir.
# Cannot run this earlier because it would be recreated for any succeeding `RUN`
# instructions.
# Therefore, change `WORKDIR` first, then delete the old one.
WORKDIR /${USER}
RUN rm --recursive ${INSTALL_DIR}
# Layer with graphical and auxiliary tools
RUN apt-get update && \
apt-get install --yes --no-install-recommends \
# headless, 25% of normal size:
default-jre-headless \
# No headless inkscape available currently:
inkscape \
# nox (no X Window System): CLI version, 10% of normal size:
gnuplot-nox \
# For various conversion tasks, e.g. EPS -> PDF (for legacy support):
ghostscript
# Pandoc layer; not required for LaTeX compilation, but useful for document conversions
RUN apt-get update && \
apt-get install --yes --no-install-recommends \
# librsvg2 for 'rsvg-convert' used by pandoc to convert SVGs when embedding
# into PDF
librsvg2-bin \
pandoc
USER ${USER}
# Load font cache, has to be done on each compilation otherwise
# ("luaotfload | db : Font names database not found, generating new one.").
# If not found, e.g. TeXLive 2012 and earlier, simply skip it. Will return exit code
# 0 and allow the build to continue.
# Warning: This is USER-specific. If the current `USER` for which we run this is not
# the container user, the font will be regenerated for that new user.
RUN luaotfload-tool --update || echo "luaotfload-tool did not succeed, skipping."
# The default parameters to the entrypoint; overridden if any arguments are given to
# `docker run`.
# `lualatex` usage for `latexmk` implies PDF generation, otherwise DVI is generated.
CMD [ "--lualatex" ]
# Allow container to run as an executable; override with `--entrypoint`.
# Allows to simply `run` the image without specifying any executable.
# If `latexmk` is called without a file argument, it will run on all *.tex files found.
ENTRYPOINT [ "latexmk" ]
<file_sep>#!/bin/bash
# Script to fetch `install-tl` script from different sources, depending on argument
# given.
set -ueo pipefail
usage() {
echo "Usage: $0 get_installer|install latest|version (YYYY)"
}
check_path() {
# The following test assumes the most basic program, `tex`, is present, see also
# https://www.tug.org/texlive/doc/texlive-en/texlive-en.html#x1-380003.5
echo "Checking PATH and installation..."
if tex --version
then
echo "PATH and installation seem OK, exiting with success."
exit 0
else
echoerr "PATH or installation unhealthy, further action required..."
fi
}
if [[ $# != 2 ]]; then
echoerr "Unsuitable number of arguments given."
usage
# From /usr/include/sysexits.h
exit 64
fi
# From: https://stackoverflow.com/a/2990533/11477374
echoerr() { echo "$@" 1>&2; }
# Bind CLI arguments to explicit names:
ACTION=${1}
VERSION=${2}
# Download the `install-tl` script from the `tlnet-final` subdirectory, NOT
# from the parent directory. The latter contains an outdated, non-final `install-tl`
# script, causing this exact problem:
# https://tug.org/pipermail/tex-live/2017-June/040376.html
HISTORIC_URL="ftp://tug.org/historic/systems/texlive/${VERSION}/tlnet-final"
REGULAR_URL="http://mirror.ctan.org/systems/texlive/tlnet"
case ${ACTION} in
"get_installer")
if [[ ${VERSION} == "latest" ]]
then
# Get from default, current repository
GET_URL="${REGULAR_URL}/${TL_INSTALL_ARCHIVE}"
else
# Get from historic repository
GET_URL="${HISTORIC_URL}/${TL_INSTALL_ARCHIVE}"
fi
wget "$GET_URL"
;;
"install")
if [[ ${VERSION} == "latest" ]]
then
# Install using default, current repository.
# Install process/script documentation is here:
# https://www.tug.org/texlive/doc/texlive-en/texlive-en.html
perl install-tl \
--profile="$TL_PROFILE"
else
# Install using historic repository (`install-tl` script and repository
# versions need to match)
perl install-tl \
--profile="$TL_PROFILE" \
--repository="$HISTORIC_URL"
fi
# If automatic `install-tl` process has already adjusted PATH, we are happy.
check_path
echo "install-tl procedure did not adjust PATH automatically, trying other options..."
# `\d` class doesn't exist for basic `grep`, use `0-9`, which is much more
# portable. Finding the initial dir is very fast, but looking through everything
# else afterwards might take a while. Therefore, print and quit after first result.
# Path example: `/usr/local/texlive/2018/bin/x86_64-linux`
TEXLIVE_BIN_DIR=$(find / -type d -regextype grep -regex '.*/texlive/[0-9]\{4\}/bin/.*' -print -quit)
# -z test: string zero length?
if [ -z "$TEXLIVE_BIN_DIR" ]
then
echoerr "Expected TeXLive installation dir not found and TeXLive installation did not modify PATH automatically."
echoerr "Exiting."
exit 1
fi
echo "Found TeXLive binaries at $TEXLIVE_BIN_DIR"
echo "Trying native TeXLive symlinking using tlmgr..."
# To my amazement, `tlmgr path add` can fail but still link successfully. So
# check if PATH is OK despite that command failing.
"$TEXLIVE_BIN_DIR"/tlmgr path add || \
echoerr "Command borked, checking if it worked regardless..."
check_path
echoerr "Symlinking using tlmgr did not succeed, trying manual linking..."
SYMLINK_DESTINATION="/usr/local/bin"
# "String contains", see: https://stackoverflow.com/a/229606/11477374
if [[ ! ${PATH} == *${SYMLINK_DESTINATION}* ]]
then
# Should never get here, but make sure.
echoerr "Symlink destination ${SYMLINK_DESTINATION} not in PATH (${PATH}), exiting."
exit 1
fi
echo "Symlinking TeXLive binaries in ${TEXLIVE_BIN_DIR}"
echo "to a directory (${SYMLINK_DESTINATION}) found on PATH (${PATH})"
# Notice the slash and wildcard.
ln \
--symbolic \
--verbose \
--target-directory="$SYMLINK_DESTINATION" \
"$TEXLIVE_BIN_DIR"/*
check_path
echoerr "All attempts failed, exiting."
exit 1
;;
*)
echoerr "Input not understood."
usage
# From /usr/include/sysexits.h
exit 64
esac
| 8aeb39da0c3fb5ba047024103e8ce0febdcdc192 | [
"Markdown",
"Dockerfile",
"Shell"
] | 4 | Shell | alexpovel/latex-extras-docker | 19a65528ab636c3b4f2e5b18812353d90a86e264 | 3df1834706cac1dd27ecfa0f97f991fbf1524d22 | |
refs/heads/master | <file_sep>certifi==2020.4.5.1
cffi==1.14.0
chardet==3.0.4
cryptography==2.9
defusedxml==0.6.0
Django==2.0.5
idna==2.9
oauthlib==3.1.0
Pillow==5.1.0
pycparser==2.20
PyJWT==1.7.1
python3-openid==3.1.0
pytz==2019.3
requests==2.23.0
requests-oauthlib==1.3.0
six==1.14.0
social-auth-app-django==2.1.0
social-auth-core==3.3.3
urllib3==1.25.8
<file_sep># Generated by Django 2.0.5 on 2020-04-16 04:08
from django.conf import settings
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('contenttypes', '0002_remove_content_type_name'),
migrations.swappable_dependency(settings.AUTH_USER_MODEL),
('actions', '0002_auto_20200416_0931'),
]
operations = [
migrations.RenameModel(
old_name='Actions',
new_name='Action',
),
]
<file_sep>{% extends "base.html" %}
{% block title %}Reset your password {% endblock %}
{% block content%}
<h1> Reset your password </h1>
<p>We've emails your instructons for setting your password. </p>
<p>If you don't recieve an email, please make sure you've entered the
address you registered with</p>
{% endblock %} | aefdd64d432d98d3919ba1e92385a8d18aac4071 | [
"Python",
"Text",
"HTML"
] | 3 | Text | IndikaMaligaspe/bookmarks | 2c2815bbe0badad58fd0058109e80c7af0b9b995 | c23021261c4b11862726b9b69e57ab2c82329bc5 | |
refs/heads/master | <repo_name>x2449265627/elven<file_sep>/day4/two.php
<?php
class people{
public $name = '魏晓';
private $sex;
public function say(){
echo $this->name;
}
public function love($sex){
$this->sex = $sex;
if($this->sex == "男"){
echo "我要找女朋友";
die;
}
echo "我要找男朋友";
}
}
$people = new people();
$people->say();
$people->love('男');
?><file_sep>/day2/index.php
<?php
cLass math{
public function calSteps($n){
//台阶数量为1 或者 2 时候 直接返回当前值
if($n == 1){
return 1;
}
if($n == 2){
return 2;
}
//台阶数量大于1跟 2 返回前两项的和
return $this->calSteps($n-1)+$this->calSteps($n-2);
}
}
//青蛙跳 其实是一个菲比那切数列
$math = new math();
$res = $math->calSteps(5);
echo $res;
?><file_sep>/day4/index.php
<?php
class Math{
public function calFn($n,$m){
if($m<$n){
return false;
}
$count = 0;
$n_len = strlen($n);
$m_len = strlen($m);
for($i=$n;$i<=$m;$i++){
$len = strlen($i);
if($len == 1){
if($i%pow(10, $len) == 1){
$count++;
continue;
}
}
for ($j=$len; $j >=1 ; $j--) {
if($j == 2){
if($i/10 == 1){
$count++;
}
}
if($i%pow(10, $j) == 1){
$count++;
continue;
}
}
}
echo $count;
}
}
$math = new Math();
$math->calFn(1,13);
?><file_sep>/day12/index.php
<?php
class math{
public function NumberOf1($n){
$i=0;
while ($n >=1) {
if($n%2 == 1){
$i++;
}
$n = floor($n/2);
}
echo $i;
}
}
$math = new math();
$res = $math->NumberOf1(4);
?><file_sep>/day10/index.php
<?php
class math{
public function Find($target, $array){
$count_o = count($array);
if($target < $array[0][0]){
return false;
}
$count_t = count($array[$count_o-1]);
if($target > $array[$count_o-1][$count_t-1]){
return false;
}
foreach ($array as $k => $v) {
foreach ($v as $key => $value) {
if($value == $target){
return true;
}
}
}
}
}
$target = 7;
$array=[[1,2,8,9],[2,4,9,12],[4,7,10,13],[6,8,11,15]];
$math = new math();
$res = $math->Find($target, $array);
var_dump($res);
?><file_sep>/day6/index.php
<?php
class math{
public function FindNumsAppearOnce($array){
$arr = [];
$res = array_count_values($array);
foreach ($res as $k => $v) {
if($v == 1){
$arr[] = $k;
}
}
// foreach ($array as $k => $v) {
// if(in_array($v,$arr)){
// unset($arr[$v]);
// continue;
// }
// $arr[$v] = $v;
// }
return $arr;
}
}
$math = new math();
$array = [2,4,3,6,3,2,5,5,5];
$res = $math->FindNumsAppearOnce($array);
var_dump($res);
?><file_sep>/day5/index.php
<?php
class Math{
public static $num;
public function GetUglyNumber_Solution($index){
if($index == 1){
echo '是';
die;
}
if($index % 2 == 0){
$index = $index/2;
$this->GetUglyNumber_Solution($index);
die;
}
if($index % 3 == 0){
$index = $index/3;
$this->GetUglyNumber_Solution($index);
die;
}
if($index % 5 == 0){
$index = $index/5;
$this->GetUglyNumber_Solution($index);
die;
}
echo '不是';
}
}
$math = new Math();
$res = $math->GetUglyNumber_Solution(7);
echo $res;
?>
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
</body>
</html>
<script type="text/javascript"></script><file_sep>/day3/index.php
<?php
class Math{
public function getstr($arr = []){
$arrlen = count($arr);
for($i=0;$i<$arrlen;$i++){
if($arr[$i]%2 == 0){
$arr1[] = $arr[$i];
}else{
$arr2[] = $arr[$i];
}
}
$arr3 =array_merge($arr2,$arr1);
var_dump($arr3);
}
}
$arr = [1,2,3,4,5,6];
$math = new Math();
$res = $math->getstr($arr);
?><file_sep>/day1/index.php
<?php
class Math{
public function countstr($str=''){
if($str == ''){
return false;
die;
}
$strtoarr = str_split($str);
// var_dump($strtoarr);
foreach ($strtoarr as $key => $value) {
if(isset($arr[$value])){
$arr[$value]++;
}else{
$arr[$value] = 1;
}
if($arr[$value] >= 3 ){
return $value;
}
}
}
}
$str = 'Have you ever gone shopping and';
$math = new Math();
$res = $math->countstr($str);
echo $res;
?><file_sep>/day11/index.php
<?php
class math{
public function Combine($arr_A, $arr_B) {
$newArr =[];
$i =0;
$j = 0;
$count_a = count($arr_A);
$count_b = count($arr_B);
$count_c = ($count_a + $count_b) -1;
for($k=0;$k<=$count_c;$k++){
if($arr_A[$i] <= $arr_B[$j]){
echo $i;
if($i >= $count_a - 1){
echo $j;
$newArr[$k] = $arr_B[$j];
$j++;
break;
}
$newArr[$k] = $arr_A[$i];
$i++;
}else{
echo $j;
$newArr[$k] = $arr_B[$j];
$j++;
}
}
echo "<pre>";
var_dump($newArr);
}
}
$math = new math();
$arr_A=[1,3,6,9];
$arr_B=[2,4,5,8,20];
$res = $math->Combine($arr_A, $arr_B);
?><file_sep>/day8/index.php
<?php
class Math{
public static $sum;
public function Sum_Solution($n){
self::$sum += $n;
$n && $n + $this->Sum_Solution($n-1);
}
}
$math = new Math();
$math->Sum_Solution(5);
echo $math::$sum;
?><file_sep>/day9/index.php
<?php
$i = 0;
$j =1;
$x = $i++ + $j++;
echo $x;
?><file_sep>/day7/index.php
<?php
class Db{
private static $db;
private $yy = '22';
private function __construct(){
new PDO("mysql:dbname=dianshang;host=127.0.0.1",'root','root');
}
public static function getDb(){
$res = $this->yy;
var_dump($res);
if (!(self::$db instanceof Db)) {
// echo 1;die;
self::$db = new Db();
}
return self::$db;
}
private function __clone(){
}
}
$res = Db::getDB();
var_dump($res);
?> | f37f9526086bccc3fb75b8ce51175bf2d05dfddb | [
"PHP"
] | 13 | PHP | x2449265627/elven | 838ae4a3d59f41e0e988017319d56fdda2b7badf | 0061cb0feb07f18746a61014658d7ca68ced89e3 | |
refs/heads/master | <file_sep>/**
* WebSocket services, using Spring Websocket.
*/
package com.thedevbridge.videochat.web.websocket;
<file_sep>(function() {
'use strict';
angular
.module('videoChatApp')
.service('webRTCservice', service);
service.$inject = [];
function service () {
var callState = null;
const NO_CALL = 0;
const PROCESSING_CALL = 1;
const IN_CALL = 2;
return {
call:function(){
if(/*$scope.peerName == ""*/true){
//$scope.error = false;
}
setCallState(PROCESSING_CALL);
//showSpinner(videoInput,videoOutput);
var options = {
localVideo : videoInput,
remoteVideo : videoOutput,
onicecandidate : onIceCandidate,
onerror : onError
};
webRtcPeer = new kurentoUtils.WebRtcPeer.WebRtcPeerSendrecv(options,function(error){
if(error){
console.error(error);
}
webRtcPeer.generateOffer(onOfferCall);
});
},
sendMessage: function (message) {
var jsonMessage = JSON.stringify(message);
console.log('Sending message: ' + jsonMessage);
ws.send(jsonMessage);
},
incomingCall : function(message){
if(callState != NO_CALL){
var response = {
id : "incomingCall",
from : message.from,
callResponse : "reject",
message : "busy"
};
return sendMessage(response);
}
//setCallState(PROCESSING_CALL);
if(confirm('User ' + message.from + ' is calling you. Do you accept the call?')){
//showSpinner(videoInput,videoOutput);
from = message.from;
var options = {
localVideo : videoInput,
remoteVideo : videoOutput,
onicecandidate : onIceCandidate,
onerror : onError
};
webRtcPeer = new kurentoUtils.WebRtcPeer.WebRtcPeerSendrecv(options,
function(error){
if(error){
console.error(error);
}
webRtcPeer.generateOffer(incomingCall)
}
);
}else {
var response = {
id :"incomingCalldenied",
from : message.from,
callResponse : "reject",
message : " User declined"
};
sendMessage(response);
stop();
}
}
};
}
})();
<file_sep>(function() {
'use strict';
angular
.module('videoChatApp')
.controller('HomeController', HomeController);
HomeController.$inject = ['$scope', 'Principal','$state','$document'];
function HomeController ($scope, Principal,$state,$document) {
var vm = this;
vm.account = null;
vm.isAuthenticated = null;
vm.register = register;
$scope.$on('authenticationSuccess', function() {
getAccount();
});
getAccount();
function getAccount() {
Principal.identity().then(function(account) {
vm.account = account;
vm.isAuthenticated = Principal.isAuthenticated;
});
}
function register () {
$state.go('register');
}
var ws = new WebSocket("ws://"+location.host+"/call");
$scope.keydown = function(e){
if(e.keyCode == 13){
registername();
}
};
$scope.keycall = function(e){
if(e.keyCode == 13){
call();
}
};
$scope.error = true;
$scope.error1 = true;
$scope.disabled = false;
$scope.disabled1 = true;
$scope.disabled2 = true;
var videoInput = document.getElementById('videoInput');
var videoOutput = document.getElementById('videoOutput');
var webRtcPeer;
var response;
var callerMessage;
var from;
$('#name').focus();
var registerName = null;
var registerState = null;
const NOT_REGISTERED = 0;
const REGISTERING = 1;
const REGISTERED = 2;
var callState = null;
const NO_CALL = 0;
const PROCESSING_CALL = 1;
const IN_CALL = 2;
$scope.call=function(){
//webRTCservice.call();
call();
};
$scope.Stop=function(){
stop();
};
ws.onmessage = function(message){
var parsedmessage = JSON.parse(message.data);
console.info("we have just received a message: "+ message.data);
switch(parsedmessage.id){
case "registerResponse":{
registerResponse(parsedmessage);
break;
}
case "callResponse":{
callResponse(parsedmessage);
break;
}
case "incomingCall":{
//webRTCservice.incomingCall(parsedmessage);
incomingCall(parsedmessage);
break;
}
case "startCommunication":{
startCommunication(parsedmessage);
break;
}
case "stopCommunication":{
console.info("communication ended by remote peer ");
stop(true);
break;
}
case "iceCandidate":{
webRtcPeer.addIceCandidate(parsedmessage.candidate,function(error){
if(!error)return;
console.error("Error when adding candidate "+ error);
});
break;
}
default : {
console.error("Unreconized message: "+ parsedmessage);
}
}
};
/*function setRegisterState(nextState) {
switch (nextState) {
case 0:
register();
setCallState(0);
break;
case 1:
$scope.disabled = false;
break;
case 2:
$scope.disabled = false;
setCallState(0);
break;
default:
return;
}
registerState = nextState;
}
function setCallState(nextState) {
switch (nextState) {
case 0:
$scope.disabled2 = false;
//webRTCservice.call();
call();
$scope.disabled1 = false;
$scope.disabled2 = false;
break;
case 1:
$scope.disabled2 = false;
$scope.disabled1 = false;
break;
case 2:
$scope.disabled2 = true;
$scope.disabled1 = false;
stop();
break;
default:
return;
}
callState = nextState;
}*/
$scope.registername=function() {
var name = $scope.callerName;
if(name == null){
$scope.error1=false;
}else{
//setRegisterState(REGISTERING);
$scope.error1=true;
$scope.disabled2 = false;
$scope.disabled1 = false;
var message = {
id : 'register',
name : name
};
//webRTCservice.sendMessage(message);
sendMessage(message);
$('#peer').focus();
}
};
function onOfferCall(error, offerSdp) {
if (error)
return console.error('Error generating the offer');
console.log('Invoking SDP offer callback function');
var message = {
id : 'call',
from : $scope.callerName,
to : $scope.peerName,
sdpOffer : offerSdp
};
//webRTCservice.sendMessage(message);
sendMessage(message);
}
function stop(message) {
//setCallState(NO_CALL);
if (webRtcPeer) {
webRtcPeer.dispose();
webRtcPeer = null;
if (!message) {
var message = {
id : 'stop'
};
//webRTCservice.sendMessage(message);
sendMessage(message);
}
}
//hideSpinner(videoInput, videoOutput);
}
function onError() {
//setCallState(NO_CALL);
console.log("erreur");
if(error){
console.error(error);
stop();
}
}
function onIceCandidate(candidate) {
console.log("Local candidate" + JSON.stringify(candidate));
var message = {
id : 'onIceCandidate',
candidate : candidate
};
//webRTCservice.sendMessage(message);
sendMessage(message);
}
function resgisterResponse(message) {
if (message.response == 'accepted') {
//setRegisterState(REGISTERED);
console.log("accepted");
} else {
//setRegisterState(NOT_REGISTERED);
var errorMessage = message.message ? message.message
: 'Unknown reason for register rejection.';
console.log(errorMessage);
//alert('Error registering user. See console for further information.');
}
}
function callResponse(message) {
if (message.response != 'accepted') {
console.info('Call not accepted by peer. Closing call');
var errorMessage = message.message ? message.message
: 'Unknown reason for call rejection.';
console.log(errorMessage);
stop();
} else {
//setCallState(IN_CALL);
webRtcPeer.processAnswer(message.sdpAnswer, function(error) {
if (error)
return console.error(error);
});
}
}
function startCommunication(message) {
//setCallState(IN_CALL);
webRtcPeer.processAnswer(message.sdpAnswer, function(error) {
if (error){
return console.error(error);
}
});
}
function onOfferIncomingCall(error,offerSdp) {
if (error)
return console.error("Error generating the offer");
var response = {
id : 'incomingCallResponse',
from : from,
callResponse : 'accept',
sdpOffer : offerSdp
};
//webRTCservice.sendMessage(response);
sendMessage(response);
}
function call(){
if($scope.peerName == null){
$scope.error = false;
}else{
$scope.error = true;
//setCallState(PROCESSING_CALL);
//showSpinner(videoInput,videoOutput);
var pc_config = {
iceServers:[{url:"stun:stun.l.google.com:19302"},
{
url:"turn:numb.viagenie.ca:3478",
credential: "levoyageur",
username: "<EMAIL>"
}]
};
var options = {
localVideo : videoInput,
remoteVideo : videoOutput,
onicecandidate : onIceCandidate,
configuration :pc_config,
onerror : onError
};
webRtcPeer = new kurentoUtils.WebRtcPeer.WebRtcPeerSendrecv(options,function(error){
if(error){
console.error(error);
}
webRtcPeer.generateOffer(onOfferCall);
});
}
}
function sendMessage(message) {
var jsonMessage = JSON.stringify(message);
console.log('Sending message: ' + jsonMessage);
ws.send(jsonMessage);
}
function incomingCall(message){
if(0/*callState*/ != 0/*NO_CALL*/){
var response = {
id : "incomingCall",
from : message.from,
callResponse : "reject",
message : "busy"
};
return sendMessage(response);
}
//setCallState(PROCESSING_CALL);
if(confirm('User ' + message.from.toLowerCase() + ' is calling you. Do you accept the call?')){
//showSpinner(videoInput,videoOutput);
console.log("confirm call");
from = message.from;
var pc_config = {
iceServers:[{url:"stun:stun.l.google.com:19302"},
{
url:"turn:numb.viagenie.ca:3478",
credential: "levoyageur",
username: "<EMAIL>"
}]
};
var options = {
localVideo : videoInput,
remoteVideo : videoOutput,
onicecandidate : onIceCandidate,
configuration :pc_config,
onerror : onError
};
webRtcPeer = new kurentoUtils.WebRtcPeer.WebRtcPeerSendrecv(options,
function(error){
if(error){
console.error(error);
}
webRtcPeer.generateOffer(onOfferIncomingCall);
}
);
}else {
var response = {
id :"incomingCalldenied",
from : message.from,
callResponse : "reject",
message : " User declined"
};
sendMessage(response);
stop();
}
}
/*window.onload = function() {
console = new Console();
//setRegisterState(NOT_REGISTERED);
var drag = new Draggabilly($('#videoSmall'));
}*/
window.onbeforeunload = function() {
ws.close();
}
}
})();
<file_sep>/**
* Audit specific code.
*/
package com.thedevbridge.videochat.config.audit;
<file_sep>package com.thedevbridge.videochat.config;
import org.kurento.client.KurentoClient;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.socket.config.annotation.EnableWebSocket;
import org.springframework.web.socket.config.annotation.WebSocketConfigurer;
import org.springframework.web.socket.config.annotation.WebSocketHandlerRegistry;
import com.thedevbridge.videochat.OneToOneVideoChat.CallHandler;
import com.thedevbridge.videochat.OneToOneVideoChat.UserRegistry;
@Configuration
@EnableWebSocket
public class OneToOneCallSocketConfig implements WebSocketConfigurer {
final static String DEFAULT_KMS_WS_URI = "ws://localhost:8888/kurento";
@Bean
public CallHandler Handler(){
return new CallHandler();
}
@Bean
public UserRegistry registry(){
return new UserRegistry();
}
@Bean
public KurentoClient kurentoClient(){
return KurentoClient.create(System.getProperty("kms.url",
DEFAULT_KMS_WS_URI));
}
@Override
public void registerWebSocketHandlers(WebSocketHandlerRegistry registry){
registry.addHandler(Handler(), "/call");
}
}
<file_sep>/**
* Spring MVC REST controllers.
*/
package com.thedevbridge.videochat.web.rest;
<file_sep>package com.thedevbridge.videochat.repository;
import com.thedevbridge.videochat.domain.Authority;
import org.springframework.data.jpa.repository.JpaRepository;
/**
* Spring Data JPA repository for the Authority entity.
*/
public interface AuthorityRepository extends JpaRepository<Authority, String> {
}
| 84d3de118ee875abb4788a52e8133ea6bd25e9f6 | [
"JavaScript",
"Java"
] | 7 | Java | BulkSecurityGeneratorProject/laakariVideoChat | 5aa9d790ad1c767f361f4c4b55831a123d99917f | 3b83bb894d3c7daf5746a645b69105452c3b78bc | |
refs/heads/main | <repo_name>arielcortez/dotnet-webapi-boilerplate<file_sep>/src/Infrastructure/Persistence/Migrations/PostgreSQL/20210830212238_CleanMigrations.cs
using Microsoft.EntityFrameworkCore.Migrations;
namespace DN.WebApi.Infrastructure.Persistence.Migrations.PostgreSQL
{
public partial class CleanMigrations : Migration
{
protected override void Up(MigrationBuilder migrationBuilder)
{
}
protected override void Down(MigrationBuilder migrationBuilder)
{
}
}
}<file_sep>/src/Infrastructure/Persistence/Migrations/PostgreSQL/20210903002350_AuditTrail.cs
using System;
using Microsoft.EntityFrameworkCore.Migrations;
namespace DN.WebApi.Infrastructure.Persistence.Migrations.PostgreSQL
{
public partial class AuditTrail : Migration
{
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.CreateTable(
name: "AuditTrails",
columns: table => new
{
Id = table.Column<Guid>(type: "uuid", nullable: false),
UserId = table.Column<Guid>(type: "uuid", nullable: false),
Type = table.Column<string>(type: "text", nullable: true),
TableName = table.Column<string>(type: "text", nullable: true),
DateTime = table.Column<DateTime>(type: "timestamp without time zone", nullable: false),
OldValues = table.Column<string>(type: "text", nullable: true),
NewValues = table.Column<string>(type: "text", nullable: true),
AffectedColumns = table.Column<string>(type: "text", nullable: true),
PrimaryKey = table.Column<string>(type: "text", nullable: true)
},
constraints: table =>
{
table.PrimaryKey("PK_AuditTrails", x => x.Id);
});
}
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropTable(
name: "AuditTrails");
}
}
}
<file_sep>/src/Infrastructure/Persistence/Extensions/TenantDatabaseExtensions.cs
using DN.WebApi.Application.Settings;
using DN.WebApi.Domain.Constants;
using DN.WebApi.Infrastructure.Identity.Models;
using DN.WebApi.Infrastructure.Utilties;
using Hangfire;
using Hangfire.PostgreSql;
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Identity.EntityFrameworkCore;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Serilog;
namespace DN.WebApi.Infrastructure.Persistence.Extensions
{
public static class TenantDatabaseExtensions
{
private static readonly ILogger _logger = Log.ForContext(typeof(TenantDatabaseExtensions));
public static IServiceCollection PrepareTenantDatabases<T>(this IServiceCollection services, IConfiguration config)
where T : ApplicationDbContext
{
services.Configure<TenantSettings>(config.GetSection(nameof(TenantSettings)));
var options = services.GetOptions<TenantSettings>(nameof(TenantSettings));
var defaultConnectionString = options.Defaults?.ConnectionString;
var defaultDbProvider = options.Defaults?.DBProvider;
var tenants = options.Tenants;
foreach (var tenant in tenants)
{
string connectionString;
if (string.IsNullOrEmpty(tenant.ConnectionString))
{
connectionString = defaultConnectionString;
}
else
{
connectionString = tenant.ConnectionString;
}
if (defaultDbProvider.ToLower() == "postgresql")
{
services.AddDbContext<T>(m => m.UseNpgsql(e => e.MigrationsAssembly(typeof(T).Assembly.FullName)));
services.MigrateAndSeedIdentityData<T>(connectionString, tenant.TID, options);
services.AddHangfire(x => x.UsePostgreSqlStorage(defaultConnectionString));
}
}
return services;
}
private static IServiceCollection MigrateAndSeedIdentityData<T>(this IServiceCollection services, string connectionString, string tenantId, TenantSettings options)
where T : ApplicationDbContext
{
var tenant = options.Tenants.Where(a => a.TID == tenantId).FirstOrDefault();
using var scope = services.BuildServiceProvider().CreateScope();
var dbContext = scope.ServiceProvider.GetRequiredService<T>();
dbContext.Database.SetConnectionString(connectionString);
SeedRoles(tenantId, tenant, dbContext);
SeedTenantAdmins(tenantId, tenant, scope, dbContext);
if (dbContext.Database.GetPendingMigrations().Any())
{
dbContext.Database.Migrate();
_logger.Information($"{tenant.Name} : Migrations complete....");
}
return services;
}
#region Seeding
private static void SeedTenantAdmins<T>(string tenantId, Tenant tenant, IServiceScope scope, T dbContext)
where T : ApplicationDbContext
{
var adminUserName = $"{tenant.Name}.admin";
var superUser = new ApplicationUser
{
FirstName = tenant.Name,
LastName = "admin",
Email = tenant.AdminEmail,
UserName = adminUserName,
EmailConfirmed = true,
PhoneNumberConfirmed = true,
NormalizedEmail = tenant.AdminEmail.ToUpper(),
NormalizedUserName = adminUserName.ToUpper(),
IsActive = true,
TenantId = tenantId
};
if (!dbContext.Users.IgnoreQueryFilters().Any(u => u.Email == tenant.AdminEmail))
{
var password = new PasswordHasher<ApplicationUser>();
var hashed = password.HashPassword(superUser, UserConstants.DefaultPassword);
superUser.PasswordHash = hashed;
var userStore = new UserStore<ApplicationUser>(dbContext);
userStore.CreateAsync(superUser).Wait();
_logger.Information($"{tenant.Name} : Seeding Admin User {tenant.AdminEmail}....");
AssignRolesAsync(scope.ServiceProvider, superUser.Email, RoleConstants.Admin).Wait();
}
}
private static void SeedRoles<T>(string tenantId, Tenant tenant, T dbContext)
where T : ApplicationDbContext
{
foreach (string roleName in typeof(RoleConstants).GetAllPublicConstantValues<string>())
{
var roleStore = new RoleStore<ApplicationRole>(dbContext);
if (!dbContext.Roles.IgnoreQueryFilters().Any(r => r.Name == $"{tenant.Name}{roleName}"))
{
var role = new ApplicationRole(roleName, tenantId, $"{roleName} Role for {tenant.Name} Tenant");
roleStore.CreateAsync(role).Wait();
_logger.Information($"{tenant.Name} : Seeding {roleName} Role....");
}
}
}
public static async Task<IdentityResult> AssignRolesAsync(IServiceProvider services, string email, string role)
{
UserManager<ApplicationUser> userManager = services.GetService<UserManager<ApplicationUser>>();
var user = await userManager.Users.IgnoreQueryFilters().FirstOrDefaultAsync(u => u.Email.Equals(email));
var result = await userManager.AddToRoleAsync(user, role);
return result;
}
#endregion
public static T GetOptions<T>(this IServiceCollection services, string sectionName)
where T : new()
{
using var serviceProvider = services.BuildServiceProvider();
var configuration = serviceProvider.GetRequiredService<IConfiguration>();
var section = configuration.GetSection(sectionName);
var options = new T();
section.Bind(options);
return options;
}
}
}<file_sep>/src/Infrastructure/Persistence/Migrations/PostgreSQL/20210901191034_SoftDeleteSupport.cs
using System;
using Microsoft.EntityFrameworkCore.Migrations;
namespace DN.WebApi.Infrastructure.Persistence.Migrations.PostgreSQL
{
public partial class SoftDeleteSupport : Migration
{
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.AddColumn<Guid>(
name: "DeletedBy",
table: "Products",
type: "uuid",
nullable: true);
migrationBuilder.AddColumn<DateTime>(
name: "DeletedOn",
table: "Products",
type: "timestamp without time zone",
nullable: true);
}
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropColumn(
name: "DeletedBy",
table: "Products");
migrationBuilder.DropColumn(
name: "DeletedOn",
table: "Products");
}
}
} | 29959482a6df382032807d85b7d236fbcd4851cf | [
"C#"
] | 4 | C# | arielcortez/dotnet-webapi-boilerplate | da68b25fe50a7d022ed872a002efb80a053a1b78 | b95bbe9bf366c8ad4e0801be65bead08fdc7273b | |
refs/heads/master | <file_sep>import numpy as np
from scipy.spatial import ConvexHull
from fit_sphere_2_points import fit_sphere_2_points
def exact_min_bound_sphere_3D(array):
"""
Compute exact minimum bounding sphere of a 3D point cloud (or a
triangular surface mesh) using Welzl's algorithm.
- X : M-by-3 list of point co-ordinates or a triangular surface
mesh specified as a TriRep object.
- R : radius of the sphere.
- C : 1-by-3 vector specifying the centroid of the sphere.
- Xb : subset of X, listing K-by-3 list of point coordinates from
which R and C were computed. See function titled
'FitSphere2Points' for more info.
REREFERENCES:
[1] Welzl, E. (1991), 'Smallest enclosing disks (balls and ellipsoids)',
Lecture Notes in Computer Science, Vol. 555, pp. 359-370
Matlab code author: <NAME> (<EMAIL>)
Date: Dec.2014"""
# Get the convex hull of the point set
hull = ConvexHull(array)
hull_array = array[hull.vertices]
hull_array = np.unique(hull_array, axis=0)
print(len(hull_array))
# Randomly permute the point set
hull_array = np.random.permutation(hull_array)
if len(hull_array) <= 4:
R, C = fit_sphere_2_points(hull_array)
return R, C, hull_array
elif len(hull_array) < 1000:
try:
R, C, _ = B_min_sphere(hull_array, [])
# Coordiantes of the points used to compute parameters of the
# minimum bounding sphere
D = np.sum(np.square(hull_array - C), axis=1)
idx = np.argsort(D - R**2)
D = D[idx]
Xb = hull_array[idx[:5]]
D = D[:5]
Xb = Xb[D < 1E-6]
idx = np.argsort(Xb[:,0])
Xb = Xb[idx]
return R, C, Xb
except:
raise Exception
else:
M = len(hull_array)
dM = min([M // 4, 300])
# unnecessary ?
# res = M % dM
# n = np.ceil(M/dM)
# idx = dM * np.ones((1, n))
# if res > 0:
# idx[-1] = res
#
# if res <= 0.25 * dM:
# idx[n-2] = idx[n-2] + idx[n-1]
# idx = idx[:-1]
# n -= 1
hull_array = np.array_split(hull_array, dM)
Xb = np.empty([0, 3])
for i in range(len(hull_array)):
R, C, Xi = B_min_sphere(np.vstack([Xb, hull_array[i]]), [])
# 40 points closest to the sphere
D = np.abs(np.sqrt(np.sum((Xi - C)**2, axis=1)) - R)
idx = np.argsort(D, axis=0)
Xb = Xi[idx[:40]]
D = np.sort(D, axis=0)[:4]
print(Xb)
print(D)
print(np.where(D/R < 1e-3)[0])
Xb = np.take(Xb, np.where(D/R < 1e-3)[0], axis=0)
Xb = np.sort(Xb, axis=0)
print(Xb)
return R, C, Xb
def B_min_sphere(P, B):
eps = 1E-6
if len(B) == 4 or len(P) == 0:
R, C = fit_sphere_2_points(B) # fit sphere to boundary points
return R, C, P
# Remove the last (i.e., end) point, p, from the list
P_new = P[:-1].copy()
p = P[-1].copy()
# Check if p is on or inside the bounding sphere. If not, it must be
# part of the new boundary.
R, C, P_new = B_min_sphere(P_new, B)
if np.isnan(R) or np.isinf(R) or R < eps:
chk = True
else:
chk = np.linalg.norm(p - C) > (R + eps)
if chk:
if len(B) == 0:
B = np.array([p])
else:
B = np.array(np.insert(B, 0, p, axis=0))
R, C, _ = B_min_sphere(P_new, B)
P = np.insert(P_new.copy(), 0, p, axis=0)
return R, C, P
if __name__ == "__main__":
#array = np.random.rand(30, 3)
#R, C, hull_array = exact_min_bound_sphere_3D(array)
#P = np.random.rand(7, 3)
P = np.loadtxt("../tests/5000x3s.dat")
#R, C, P_new = B_min_sphere(P, np.array([]))
#print(R, C, P_new, sep="\n")
R, C, Xb = exact_min_bound_sphere_3D(P)
print(R, C, Xb, sep="\n")
<file_sep>import numpy as np
from scipy import linalg
def fit_sphere_2_points(array):
"""Fit a sphere to a set of 2, 3, or at most 4 points in 3D space. Note that
point configurations with 3 collinear or 4 coplanar points do not have
well-defined solutions (i.e., they lie on spheres with inf radius).
- X : M-by-3 array of point coordinates, where M<=4.
- R : radius of the sphere. R=Inf when the sphere is undefined, as
specified above.
- C : 1-by-3 vector specifying the centroid of the sphere.
C=nan(1,3) when the sphere is undefined, as specified above.
Matlab code author: <NAME> (<EMAIL>)
Date: Dec.2014"""
N = len(array)
if N > 4:
print('Input must a N-by-3 array of point coordinates, with N<=4')
return
# Empty set
elif N == 0:
R = np.nan
C = np.full(3, np.nan)
return R, C
# A single point
elif N == 1:
R = 0.
C = array[0]
return R, C
# Line segment
elif N == 2:
R = np.linalg.norm(array[1] - array[0]) / 2
C = np.mean(array, axis=0)
return R, C
else: # 3 or 4 points
# Remove duplicate vertices, if there are any
uniq, index = np.unique(array, axis=0, return_index=True)
array_nd = uniq[index.argsort()]
if not np.array_equal(array, array_nd):
print("found duplicate")
print(array_nd)
R, C = fit_sphere_2_points(array_nd)
return R, C
tol = 0.01 # collinearity/co-planarity threshold (in degrees)
if N == 3:
# Check for collinearity
D12 = array[1] - array[0]
D12 = D12 / np.linalg.norm(D12)
D13 = array[2] - array[0]
D13 = D13 / np.linalg.norm(D13)
chk = np.clip( np.abs(np.dot(D12, D13)) , 0., 1.)
if np.arccos(chk)/np.pi*180 < tol:
R = np.inf
C = np.full(3, np.nan)
return R, C
# Make plane formed by the points parallel with the xy-plane
n = np.cross(D13,D12)
n = n / np.linalg.norm(n)
##print("n", n)
r = np.cross(n, np.array([0, 0, 1]))
r = np.arccos(n[2]) * r / np.linalg.norm(r) # Euler rotation vector
##print("r", r)
Rmat = linalg.expm(np.array([
[0., -r[2], r[1]],
[r[2], 0., -r[0]],
[-r[1], r[0], 0.]
]))
##print("Rmat", Rmat)
#Xr = np.transpose(Rmat*np.transpose(array))
Xr = np.transpose( np.dot(Rmat, np.transpose(array)) )
##print("Xr", Xr)
# Circle centroid
x = Xr[:,:2]
A = 2 * (x[1:] - np.full(2, x[0]))
b = np.sum( (np.square(x[1:]) - np.square( np.full(2, x[0]) )), axis=1 )
C = np.transpose(ldivide(A, b))
# Circle radius
R = np.sqrt( np.sum(np.square(x[0] - C)) )
# Rotate centroid back into the original frame of reference
C = np.append(C, [np.mean(Xr[:,2])], axis=0)
C = np.transpose( np.dot(np.transpose(Rmat), C) )
return R, C
# If we got to this point then we have 4 unique, though possibly co-linear
# or co-planar points.
else:
# Check if the the points are co-linear
D12 = array[1] - array[0]
D12 = D12 / np.linalg.norm(D12)
D13 = array[2] - array[0]
D13 = D13 / np.linalg.norm(D13)
D14 = array[3] - array[0]
D14 = D14 / np.linalg.norm(D14)
chk1 = np.clip( np.abs(np.dot(D12, D13)) , 0., 1.)
chk2 = np.clip( np.abs(np.dot(D12, D14)) , 0., 1.)
if np.arccos(chk1)/np.pi*180 < tol or np.arccos(chk2)/np.pi*180 < tol:
R = np.inf
C = np.full(3, np.nan)
return R, C
# Check if the the points are co-planar
n1 = np.linalg.norm(np.cross(D12, D13))
n2 = np.linalg.norm(np.cross(D12, D14))
chk = np.clip( np.abs(np.dot(n1, n2)) , 0., 1.)
if np.arccos(chk)/np.pi*180 < tol:
R = np.inf
C = np.full(3, np.nan)
return R, C
# Centroid of the sphere
A = 2 * (array[1:] - np.full(len(array)-1, array[0]))
b = np.sum( (np.square(array[1:]) - np.square( np.full(len(array)-1, array[0]) )), axis=1 )
C = np.transpose(ldivide(A, b))
# Radius of the sphere
R = np.sqrt( np.sum(np.square(array[0] - C), axis=0) )
return R, C
def permute_dims(array, dims):
return np.transpose( np.expand_dims(array, axis=max(dims)), dims )
def ldivide(array, vector):
return np.linalg.solve(array, vector)
if __name__ == "__main__":
for i in range(1,6):
array = np.loadtxt("../tests/fit_sphere_2_points_{}.dat".format(i)).reshape((-1, 3))
print(i, array, sep="\n")
R, C = fit_sphere_2_points(array)
print(R, C, sep="\n")
| 28a976b4dcadb7f51a24650faa66c1b3ea129e71 | [
"Python"
] | 2 | Python | dj-boy/DGCNNPointClouds | df064df8c7fc4c45e13159626d6618d0d1300e88 | 76cbb54efddf2ffb57a5c744eec07e71b703ffa7 | |
refs/heads/master | <repo_name>daf78/CatchMeIfYouCan<file_sep>/src/org/academiadecodigo/variachis/expertCoders/grid/position/Position.java
package org.academiadecodigo.variachis.expertCoders.grid.position;
import org.academiadecodigo.variachis.expertCoders.grid.Grid;
public class Position {
private int row;
private int col;
private Grid grid;
public Position(int col, int row, Grid grid) {
this.col = col;
this.row = row;
this.grid = grid;
}
public enum Direction {
DOWN,
RIGHT,
LEFT;
}
public void setRowZero() {
this.row = 0;
}
public void setCol() {
this.col = ((int) (Math.random() * grid.getCols()));
}
public void move(Direction direction) {
switch (direction) {
case LEFT:
moveLeft();
break;
case RIGHT:
moveRight();
break;
case DOWN:
moveDown();
break;
}
}
private void moveRight() {
this.col++;
}
private void moveLeft() {
this.col--;
}
private void moveDown() {
this.row++;
}
public boolean equals(Position position) {
//positions plus five because item collides with grid at row 56/55
if(this.row == position.row + 5 && this.col == position.col){
return true;
}
if(this.row == position.row + 5 && this.col == position.col +1){
return true;
}
if(this.row == position.row + 5 && this.col == position.col +2){
return true;
}
if(this.row == position.row + 5 && this.col == position.col + 3){
return true;
}
if(this.row == position.row + 5 && this.col == position.col +4){
return true;
}
if(this.row == position.row + 5 && this.col == position.col +5){
return true;
}
if(this.row == position.row + 5 && this.col == position.col +6){
return true;
}
if(this.row == position.row + 5 && this.col == position.col +7){
return true;
}
if(this.row == position.row + 5 && this.col == position.col +8){
return true;
}
if(this.row == position.row + 5 && this.col == position.col -1){
return true;
}
if(this.row == position.row + 5 && this.col == position.col -2){
return true;
}
if(this.row == position.row + 5 && this.col == position.col -3){
return true;
}
if(this.row == position.row + 5 && this.col == position.col -4){
return true;
}
if(this.row == position.row + 5 && this.col == position.col -5){
return true;
}
if(this.row == position.row + 5 && this.col == position.col -6){
return true;
}
if(this.row == position.row + 5 && this.col == position.col -7){
return true;
}
if(this.row == position.row + 5 && this.col == position.col -8){
return true;
}
return false;
}
public int getRow() {
return row;
}
public int getCol() {
return col;
}
@Override
public String toString() {
return "Position{" +
"row=" + row +
", col=" + col +
'}';
}
}<file_sep>/src/org/academiadecodigo/variachis/expertCoders/GameLevel.java
package org.academiadecodigo.variachis.expertCoders;
import org.academiadecodigo.simplegraphics.pictures.Picture;
import org.academiadecodigo.variachis.expertCoders.grid.Grid;
import org.academiadecodigo.variachis.expertCoders.interfaces.Drawable;
public class GameLevel implements Drawable {
private Level actualLevel ;
private Picture picture;
public GameLevel() {
actualLevel = Level.ZERO;
picture = new Picture(Grid.PADDING, Grid.PADDING, actualLevel.pic);
}
@Override
public void draw() {
picture.load(actualLevel.getPic());
picture.draw();
}
public void setActualLevel(Level actualLevel) {
this.actualLevel = actualLevel;
}
public enum Level {
ZERO("inicial_screen.jpg"),
ONE("levelOne.jpg"),
TWO("salinha_screen_2_salinha.jpg"),
HELP("help.jpg"),
OVER("gameover_screen.jpg");
private String pic;
Level(String pic) {
this.pic = pic;
}
public String getPic() {
return pic;
}
}
public Level getActualLevel() {
return actualLevel;
}
}
<file_sep>/src/org/academiadecodigo/variachis/expertCoders/interfaces/Collidable.java
package org.academiadecodigo.variachis.expertCoders.interfaces;
import org.academiadecodigo.variachis.expertCoders.grid.position.Position;
public interface Collidable extends Drawable {
boolean checkCollision(Position position);
}
<file_sep>/src/org/academiadecodigo/variachis/expertCoders/AbstractCollidable.java
package org.academiadecodigo.variachis.expertCoders;
import org.academiadecodigo.variachis.expertCoders.grid.position.Position;
import org.academiadecodigo.variachis.expertCoders.interfaces.Collidable;
import org.academiadecodigo.variachis.expertCoders.grid.position.Position.Direction;
public abstract class AbstractCollidable implements Collidable {
private Position position;
public AbstractCollidable(Position position) {
this.position = position;
}
public Position getPosition() {
return position;
}
public abstract void draw();
protected void move(Direction direction) {
position.move(direction);
}
}
<file_sep>/src/org/academiadecodigo/variachis/expertCoders/item/ItemFactory.java
package org.academiadecodigo.variachis.expertCoders.item;
import org.academiadecodigo.variachis.expertCoders.grid.Grid;
import org.academiadecodigo.variachis.expertCoders.grid.position.PositionFactory;
public class ItemFactory {
//Create new items -- random values
public static Item getItem(Grid grid) {
return new Item(Item.random(), PositionFactory.getItemPosition(grid));
}
}
<file_sep>/README.md
# Catch If You Can!
some story here
## Developers
Developed @ <Academia de Código_> <br>
Created by Code Cadets:
<ul>
<li><NAME></li>
<li><NAME></li>
<li><NAME></li>
<li><NAME></li>
</ul>
## Concepts Used
Project developed during the 4th week of Academia de Código's 18th bootcamp.
<h4> Concepts learned until now:</h4>
<ul>
<li>Basic Javao</li>
<li>Java Build Process</li>
<li>Basic OOP</li>
<li>Composition</li>
<li>Inheritance</li>
<li>Polymorphism</li>
<li>Containers</li>
<li>Nested Classes</li>
<li>Simple Graphics Library</li>
</ul>
## Rules && Controles
<h4>Controls:</h4>
```python
<RIGHT> && <LEFT> arrows.
```
<h4>Rules:</h4>
```
You need to catch some good JAVA concepts that are represented as an image in the game.
In game you can check what are the representations of the concepts and also what are the stats that they give you.
If your levels reach a good score you will be moved into next level!
```
<file_sep>/src/org/academiadecodigo/variachis/expertCoders/grid/position/PositionFactory.java
package org.academiadecodigo.variachis.expertCoders.grid.position;
import org.academiadecodigo.variachis.expertCoders.grid.Grid;
/**
* A factory to create different positions for different items.
*/
public class PositionFactory {
public static Position getPlayerPosition(Grid grid) {
return new Position((grid.getCols() / 2), (grid.getRows()), grid);
}
public static Position getItemPosition(Grid grid) {
int randomCol = (int) (Math.random() * (grid.getCols() * Grid.CELLSIZE));
return new Position(randomCol - Grid.CELLSIZE, 0, grid);
}
}
<file_sep>/src/org/academiadecodigo/variachis/expertCoders/player/Player.java
package org.academiadecodigo.variachis.expertCoders.player;
import org.academiadecodigo.simplegraphics.pictures.Picture;
import org.academiadecodigo.variachis.expertCoders.AbstractCollidable;
import org.academiadecodigo.variachis.expertCoders.grid.Grid;
import org.academiadecodigo.variachis.expertCoders.grid.position.Position;
import org.academiadecodigo.variachis.expertCoders.item.Item;
public class Player extends AbstractCollidable {
private int knowledge = 50;
private int fun = 50;
private Picture playerPicture;
private Position[] positions = new Position[4]; // TODO: 11/10/2018
public Player(Position position) {
super(position);
playerPicture = new Picture(super.getPosition().getCol() * Grid.CELLSIZE + Grid.PADDING,
super.getPosition().getRow() * Grid.CELLSIZE + Grid.PADDING,
"filipe.png");
}
@Override
public boolean checkCollision(Position position) {
return getPosition().equals(position);
}
@Override
public void draw() {
playerPicture.translate(-playerPicture.getWidth(), -playerPicture.getHeight());
playerPicture.draw();
}
@Override
public void move(Position.Direction direction) {
super.move(direction);
int dx = direction == Position.Direction.RIGHT ? 1 : -1;
int diff = playerPicture.getX() + dx > Grid.PADDING ? dx : 0; // TODO: 11/10/2018
playerPicture.translate(diff * Grid.CELLSIZE, 0);
}
public boolean gameOver(){
return (getKnowledge() <= 0 || getFun() <= 0);
}
public void beingHit(Item item) {
switch (item.getType()) {
case CAP:
knowledge -= 20;
break;
case BEER:
fun += 20;
break;
case TREE:
knowledge += 20;
break;
case ABSTRACTION:
fun -= 20;
break;
case POLY:
int random = ((int) (Math.random() * 4));
switch (random){
case 0:
playerPicture.load("mestre_shorts.png");
playerPicture.draw();
break;
case 1:
playerPicture.load("sid.png");
playerPicture.draw();
break;
case 2:
playerPicture.load("filipe.png");
playerPicture.draw();
break;
case 3:
playerPicture.load("seringas.png");
playerPicture.draw();
break;
}
break;
}
}
public boolean nextlevel(){
return (getFun() >= 100 || getKnowledge() >= 100);
}
public int getFun() {
return fun;
}
public int getKnowledge() {
return knowledge;
}
public boolean isLeftOf(int cols) {
return getPosition().getCol() < cols;
}
public boolean isRightOf(int cols) {
return getPosition().getCol() > cols;
}
@Override
public String toString() {
return "Player{" +
"playerPicture=" + playerPicture +
'}';
}
}
| 5dd8fc58f956d6d810f9bdd04784d59e8ba1177a | [
"Markdown",
"Java"
] | 8 | Java | daf78/CatchMeIfYouCan | a74c4c279761888edf07e24f497205c4ea2a2fc7 | f0fb37cf7052aae1da2fe9c6165c2f1e9d4b35e8 | |
refs/heads/master | <file_sep>"# PhyCy"
<file_sep>import { Component } from '@angular/core';
import { NavController, NavParams } from 'ionic-angular';
/*
Generated class for the Humid page.
See http://ionicframework.com/docs/v2/components/#navigation for more info on
Ionic pages and navigation.
*/
@Component({
selector: 'page-humid',
templateUrl: 'humid.html'
})
export class HumidPage {
constructor(public navCtrl: NavController, public navParams: NavParams) {}
ionViewDidLoad() {
console.log('ionViewDidLoad HumidPage');
}
}
| 5e8e50d25789661f2dbd0450016a808435530afe | [
"Markdown",
"TypeScript"
] | 2 | Markdown | Wittowski/PhyCy | 0449c88f5a28b8b6afe6d8f3117d5ec2b92f20b3 | 2ae31587484c31ad82d49c7cddb15c1a651620ab | |
refs/heads/main | <file_sep>import datetime
from django.db import models
from django.utils.translation import ugettext_lazy as _
from parler.models import TranslatableModel, TranslatedFields
from django.contrib.auth.models import User
from django.utils import timezone
class Country(TranslatableModel):
translations = TranslatedFields(
name=models.CharField(max_length=40, verbose_name=_('Name'))
)
creation_date = models.DateTimeField(
default=timezone.now, verbose_name=_("Creation Date"))
class Meta:
verbose_name = _('Country')
verbose_name_plural = _('Countries')
def __str__(self):
return self.name
class City(TranslatableModel):
country = models.ForeignKey(
Country,
related_name='cities',
on_delete=models.CASCADE,
verbose_name=_('Country')
)
translations = TranslatedFields(
name=models.CharField(max_length=50, verbose_name=_('Name'))
)
class Meta:
verbose_name = _('City')
verbose_name_plural = _('Cities')
def __str__(self):
return self.name
class School(TranslatableModel):
translations = TranslatedFields(
name=models.CharField(max_length=200, verbose_name=_('Name'))
)
city = models.ForeignKey(
City, on_delete=models.CASCADE, verbose_name=_('City'))
class Meta:
verbose_name = _('School')
verbose_name_plural = _('Schools')
def __str__(self):
return self.name
class Profession(TranslatableModel):
translations = TranslatedFields(
name=models.CharField(max_length=50, verbose_name=_('Name')),
description=models.CharField(
max_length=200, default='', verbose_name=_('Description'))
)
class Meta:
verbose_name = _('Profession')
verbose_name_plural = _('Professions')
def __str__(self):
return self.name
class Professional(models.Model):
user = models.OneToOneField(
User, on_delete=models.CASCADE, verbose_name=_('User'))
city = models.ForeignKey(
City,
related_name=_('professionals'),
on_delete=models.CASCADE,
default='',
verbose_name=_('City')
)
professions = models.ManyToManyField(
Profession, verbose_name=_('Professions'))
phone = models.CharField(
max_length=9, default='', verbose_name=_('Phone'))
id_number = models.CharField(
max_length=11, default='', verbose_name=_('Id Number'))
id_image = models.ImageField(
null=False, blank=True, verbose_name=_('Id Image'))
status = models.BooleanField(default=True, verbose_name=_('Status'))
birthdate = models.DateField(
default=datetime.date.today, verbose_name=_('Birthdate'))
creation_date = models.DateTimeField(
default=timezone.now, verbose_name=_('Creation Date'))
profile_picture = models.ImageField(
null=True, blank=True, verbose_name=_('Profile Picture'))
class Meta:
verbose_name = _('Professional')
verbose_name_plural = _('Professionals')
def __str__(self):
return self.user.get_username()
class Curriculum(models.Model):
owner = models.OneToOneField(
Professional, on_delete=models.CASCADE, verbose_name=_('Owner'))
contract_price = models.IntegerField(
default=0, verbose_name=_('Contract price'))
score = models.IntegerField(default=5, verbose_name=_('Score'))
class Meta:
verbose_name = _('Curriculum')
verbose_name_plural = _('Curriculums')
def __str__(self):
return self.owner.user.get_username() + '-cv'
class Job(models.Model):
cv = models.ForeignKey(
Curriculum,
related_name=_('jobs'),
on_delete=models.CASCADE
)
profession = models.ForeignKey(
Profession, on_delete=models.CASCADE, verbose_name=_('Profession'))
description = models.TextField(
max_length=500, default='', verbose_name=_('Description'))
start_date = models.DateTimeField(
default=timezone.now, verbose_name=_('Start Date'))
finish_date = models.DateTimeField(
default=timezone.now, verbose_name=_('Finish Date'))
class Meta:
verbose_name = _('Job')
verbose_name_plural = _('Jobs')
def __str__(self):
return self.profession.name
class JobOffer(models.Model):
user = models.ForeignKey(
Professional,
related_name='job_offers',
on_delete=models.CASCADE,
verbose_name=_('User')
)
city = models.ForeignKey(
City,
related_name='job_offers',
on_delete=models.CASCADE, default='',
verbose_name=_('City')
)
description = models.CharField(
max_length=100, default='', verbose_name=_('Description'))
creation_date = models.DateTimeField(
default=timezone.now, verbose_name=_('Creation Date'))
status = models.BooleanField(default=True, verbose_name=_('Status'))
class Meta:
verbose_name = _('Job Offer')
verbose_name_plural = _('Job Offers')
class Employment(models.Model):
offer = models.ForeignKey(
JobOffer,
related_name='employments',
on_delete=models.CASCADE,
verbose_name=_('Offer')
)
profession = models.ForeignKey(
Profession, on_delete=models.CASCADE, verbose_name=_('Profession'))
description = models.TextField(
max_length=200, default='', verbose_name=_('Description'))
reward = models.FloatField(default=0, verbose_name=_('Reward'))
status = models.BooleanField(default=True, verbose_name=_('Status'))
class Meta:
verbose_name = _('Employment')
verbose_name_plural = _('Employments')
class Study(models.Model):
cv = models.ForeignKey(
Curriculum,
related_name='studies',
on_delete=models.CASCADE
)
school = models.ForeignKey(
School, on_delete=models.CASCADE, verbose_name=_('School')
)
profession = models.ForeignKey(
Profession, on_delete=models.CASCADE, verbose_name=_('Profession')
)
name = models.CharField(max_length=100, default='', verbose_name=_('Name'))
image = models.ImageField(null=False, blank=True, verbose_name=_('Image'))
class Meta:
verbose_name = _('Study')
verbose_name_plural = _('Studies')
class ChatRoom(models.Model):
users = models.ManyToManyField(Professional, verbose_name=_('Users'))
creation_date = models.DateTimeField(
default=timezone.now, verbose_name=_('Creation Date')
)
status = models.BooleanField(default=True, verbose_name=_('Status'))
class Meta:
verbose_name = _('Chat Room')
verbose_name_plural = _('Chat Rooms')
class Message(models.Model):
chat_room = models.ForeignKey(
ChatRoom, on_delete=models.CASCADE, verbose_name=_('Chat Room'))
user = models.ForeignKey(
Professional, on_delete=models.CASCADE, verbose_name=_('User'))
message = models.CharField(
max_length=400, verbose_name=_('Message'))
creation_date = models.DateTimeField(
default=timezone.now, verbose_name=_('Creation Date'))
class Meta:
verbose_name = _('Message')
verbose_name_plural = _('Messages')
<file_sep># Generated by Django 3.1.2 on 2020-11-30 19:19
import datetime
from django.db import migrations, models
import django.db.models.deletion
from django.utils.timezone import utc
class Migration(migrations.Migration):
dependencies = [
('work_day', '0009_auto_20201129_0123'),
]
operations = [
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 12, 1, 19, 19, 56, 57812, tzinfo=utc)),
),
migrations.CreateModel(
name='Study',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(default='', max_length=50)),
('cv', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.curriculum')),
('profession', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.profession')),
('school', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.school')),
],
),
]
<file_sep>from selenium import webdriver
from django.contrib.staticfiles.testing import StaticLiveServerTestCase
class SeleniumBaseTestCase(StaticLiveServerTestCase):
def setUp(self) -> None:
self.driver = webdriver.Chrome()
self.driver.implicitly_wait(2)
self.driver.maximize_window()
def tearDown(self) -> None:
self.driver.close()
<file_sep># Generated by Django 3.1.2 on 2020-11-27 00:16
from django.db import migrations, models
import django.db.models.deletion
import django.utils.timezone
import parler.models
class Migration(migrations.Migration):
dependencies = [
('work_day', '0001_initial'),
]
operations = [
migrations.CreateModel(
name='ChatRoom',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('creation_date', models.DateTimeField(default=django.utils.timezone.now)),
],
),
migrations.CreateModel(
name='Profession',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=50)),
],
bases=(parler.models.TranslatableModelMixin, models.Model),
),
migrations.RemoveField(
model_name='professional',
name='description',
),
migrations.AddField(
model_name='professional',
name='creation_date',
field=models.DateTimeField(default=django.utils.timezone.now),
),
migrations.AddField(
model_name='professional',
name='ruc',
field=models.CharField(default='00000000000', max_length=11),
),
migrations.CreateModel(
name='Message',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('message', models.CharField(max_length=400)),
('creation_date', models.DateTimeField(default=django.utils.timezone.now)),
('chat_room', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.chatroom')),
('user', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.professional')),
],
),
migrations.AddField(
model_name='chatroom',
name='users',
field=models.ManyToManyField(to='work_day.Professional'),
),
]
<file_sep># Generated by Django 3.1.2 on 2020-11-27 22:35
import datetime
from django.db import migrations, models
from django.utils.timezone import utc
class Migration(migrations.Migration):
dependencies = [
('work_day', '0006_auto_20201127_1732'),
]
operations = [
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 11, 28, 22, 35, 21, 35704, tzinfo=utc)),
),
]
<file_sep># Generated by Django 3.1.2 on 2020-11-27 02:18
import datetime
from django.db import migrations, models
import django.db.models.deletion
from django.utils.timezone import utc
import django.utils.timezone
class Migration(migrations.Migration):
dependencies = [
('work_day', '0002_auto_20201126_1916'),
]
operations = [
migrations.CreateModel(
name='Curriculum',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('owner', models.OneToOneField(on_delete=django.db.models.deletion.CASCADE, to='work_day.professional')),
],
),
migrations.AddField(
model_name='profession',
name='description',
field=models.CharField(default='', max_length=100),
),
migrations.CreateModel(
name='JobOffer',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('creation_date', models.DateTimeField(default=django.utils.timezone.now)),
('user', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.professional')),
],
),
migrations.CreateModel(
name='Job',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('description', models.TextField(default='', max_length=500)),
('start_date', models.DateTimeField(default=django.utils.timezone.now)),
('finish_date', models.DateTimeField(default=datetime.datetime(2020, 11, 28, 2, 18, 59, 358602, tzinfo=utc))),
('cv', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.curriculum')),
('profession', models.OneToOneField(on_delete=django.db.models.deletion.CASCADE, to='work_day.profession')),
],
),
migrations.CreateModel(
name='Employment',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('description', models.TextField(default='', max_length=200)),
('offer', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.joboffer')),
('profession', models.OneToOneField(on_delete=django.db.models.deletion.CASCADE, to='work_day.profession')),
],
),
]
<file_sep># Generated by Django 3.1.2 on 2020-12-11 21:58
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('work_day', '0023_auto_20201209_1207'),
]
operations = [
migrations.AlterModelOptions(
name='message',
options={'ordering': ['creation_date']},
),
]
<file_sep># Generated by Django 3.1.2 on 2020-12-09 00:18
import datetime
from django.db import migrations, models
from django.utils.timezone import utc
class Migration(migrations.Migration):
dependencies = [
('work_day', '0020_auto_20201208_1836'),
]
operations = [
migrations.AddField(
model_name='professional',
name='id_image',
field=models.ImageField(blank=True, upload_to=''),
),
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 12, 10, 0, 18, 58, 834065, tzinfo=utc)),
),
]
<file_sep># Generated by Django 3.1.2 on 2020-12-02 01:30
import datetime
from django.db import migrations, models
from django.utils.timezone import utc
class Migration(migrations.Migration):
dependencies = [
('work_day', '0014_auto_20201201_1940'),
]
operations = [
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 12, 3, 1, 30, 52, 942806, tzinfo=utc)),
),
]
<file_sep>import datetime
from django.test import (
TestCase,
Client
)
from django.urls import reverse
from django.utils import timezone
from work_day.models import *
class BaseTest(TestCase):
def setUp(self):
self.login_url = reverse('login')
self.register_url = reverse('register')
user = User.objects.create_user(
username='testUser', password='<PASSWORD>'
)
self.country = Country.objects.create(name='Peru')
self.city = City.objects.create(country=self.country, name='Arequipa')
self.professional = Professional.objects.create(
user=user, city=self.city, phone='999999999', id_number='11111111',
)
cv = Curriculum.objects.create(owner=self.professional)
return super().setUp()
class LoginTest(BaseTest):
def test_can_access_page(self):
response = self.client.get(self.login_url)
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'users/login.html')
def test_successful_login(self):
response = self.client.post(
self.login_url,
{'username': 'testUser', 'password': '<PASSWORD>'},
follow=True
)
self.assertEqual(response.status_code, 200)
self.assertTrue(response.context['user'].is_authenticated)
self.assertRedirects(response, reverse('home'))
def test_cant_login_with_invalid_username(self):
response = self.client.post(
self.login_url,
{'username': '', 'password': '<PASSWORD>'},
follow=True
)
self.assertFalse(response.context['user'].is_authenticated)
class RegisterTest(BaseTest):
def test_can_access_page(self):
response = self.client.get(self.register_url)
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'users/register.html')
class ViewsTest(BaseTest):
def test_index_view_doesnt_require_login(self):
response = self.client.get(reverse('index'), follow=True)
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'index.html')
def test_home_view_without_login(self):
response = self.client.get(reverse('home'), follow=True)
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'users/login.html')
def test_home_view_with_login(self):
self.client.login(username='testUser', password='<PASSWORD>')
response = self.client.get(reverse('home'), follow=True)
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'home.html')
def test_view_user_profile_without_login(self):
response = self.client.get(reverse('user_profile'), follow=True)
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'users/login.html')
def test_view_own_user_profile_with_login(self):
self.client.login(username='testUser', password='<PASSWORD>')
response = self.client.get(reverse('user_profile'), follow=True)
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'users/profile.html')
def test_view_professionals_without_login(self):
response = self.client.get(reverse('professionals'), follow=True)
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'users/login.html')
def test_view_professionals_with_login(self):
self.client.login(username='testUser', password='<PASSWORD>')
response = self.client.get(reverse('professionals'), follow=True)
print(response.context['professionals'][0])
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'professionals.html')
self.assertEqual(len(response.context['professionals']), 1)
def test_view_my_posts_receive_offers_list(self):
self.client.login(username='testUser', password='<PASSWORD>')
response = self.client.get(reverse('my_posts'), follow=True)
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'my_posts.html')
self.assertEqual(len(response.context['offers']), 0)<file_sep># Generated by Django 3.1.2 on 2020-12-07 14:25
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('work_day', '0016_auto_20201205_1839'),
('work_day', '0017_auto_20201206_1810'),
]
operations = [
]
<file_sep>import django_filters
from django_filters import CharFilter
from .models import *
class ProfessionalFilter(django_filters.FilterSet):
user__first_name = CharFilter(lookup_expr='icontains')
user__last_name = CharFilter(lookup_expr='icontains')
class Meta:
model = Professional
fields = ['city', 'professions']
<file_sep># Generated by Django 3.1.2 on 2020-11-27 22:15
import datetime
from django.db import migrations, models
import django.db.models.deletion
import django.utils.timezone
from django.utils.timezone import utc
import parler.models
class Migration(migrations.Migration):
dependencies = [
('work_day', '0003_auto_20201126_2118'),
]
operations = [
migrations.CreateModel(
name='City',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=50)),
],
bases=(parler.models.TranslatableModelMixin, models.Model),
),
migrations.CreateModel(
name='Country',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=40)),
('creation_date', models.DateTimeField(default=django.utils.timezone.now)),
],
bases=(parler.models.TranslatableModelMixin, models.Model),
),
migrations.RemoveField(
model_name='professional',
name='ruc',
),
migrations.AddField(
model_name='chatroom',
name='status',
field=models.BooleanField(default=True),
),
migrations.AddField(
model_name='curriculum',
name='contract_price',
field=models.IntegerField(default=0),
),
migrations.AddField(
model_name='curriculum',
name='score',
field=models.IntegerField(default=5),
),
migrations.AddField(
model_name='employment',
name='reward',
field=models.FloatField(default=0),
),
migrations.AddField(
model_name='employment',
name='status',
field=models.BooleanField(default=True),
),
migrations.AddField(
model_name='joboffer',
name='description',
field=models.CharField(default='', max_length=100),
),
migrations.AddField(
model_name='joboffer',
name='status',
field=models.BooleanField(default=True),
),
migrations.AddField(
model_name='professional',
name='id_number',
field=models.CharField(default='', max_length=11),
),
migrations.AddField(
model_name='professional',
name='phone',
field=models.CharField(default='', max_length=9),
),
migrations.AddField(
model_name='professional',
name='professions',
field=models.ManyToManyField(to='work_day.Profession'),
),
migrations.AddField(
model_name='professional',
name='status',
field=models.BooleanField(default=True),
),
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 11, 28, 22, 15, 29, 539038, tzinfo=utc)),
),
migrations.CreateModel(
name='School',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=200)),
('city', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.city')),
],
bases=(parler.models.TranslatableModelMixin, models.Model),
),
migrations.AddField(
model_name='city',
name='country',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.country'),
),
migrations.AddField(
model_name='joboffer',
name='city',
field=models.ForeignKey(default='', on_delete=django.db.models.deletion.CASCADE, to='work_day.city'),
),
migrations.AddField(
model_name='professional',
name='city',
field=models.ForeignKey(default='', on_delete=django.db.models.deletion.CASCADE, to='work_day.city'),
),
]
<file_sep># Generated by Django 3.1.2 on 2020-12-19 22:09
import datetime
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('work_day', '0025_auto_20201219_1616'),
]
operations = [
migrations.AddField(
model_name='professional',
name='birthdate',
field=models.DateField(default=datetime.date.today, verbose_name='Birthdate'),
),
migrations.AlterField(
model_name='professiontranslation',
name='description',
field=models.CharField(default='', max_length=200, verbose_name='Description'),
),
migrations.AlterField(
model_name='study',
name='name',
field=models.CharField(default='', max_length=100, verbose_name='Name'),
),
]
<file_sep>## Instalación
- Clonar repositorio
- pip install -r requirements.txt
- Establecer env variables en .env (copiar de .env_default)
## Base de datos
####Configuración
- Ejecutar los siguientes comandos en la terminal:
sudo su postgres -c psql
CREATE USER username WITH PASSWORD '<PASSWORD>';
CREATE DATABASE workday_db OWNER username ENCODING 'UTF8' LC_COLLATE = 'en_US.UTF-8' LC_CTYPE = 'en_US.UTF-8';
GRANT ALL PRIVILEGES ON DATABASE workday_db TO username;
GRANT ALL PRIVILEGES ON ALL TABLES IN SCHEMA public TO username;
ALTER USER username CREATEDB;
ALTER ROLE username SUPERUSER;
<file_sep># Generated by Django 3.1.2 on 2020-12-19 16:16
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
import django.utils.timezone
class Migration(migrations.Migration):
dependencies = [
migrations.swappable_dependency(settings.AUTH_USER_MODEL),
('work_day', '0024_auto_20201218_2312'),
]
operations = [
migrations.AlterField(
model_name='chatroom',
name='creation_date',
field=models.DateTimeField(default=django.utils.timezone.now, verbose_name='Creation Date'),
),
migrations.AlterField(
model_name='chatroom',
name='status',
field=models.BooleanField(default=True, verbose_name='Status'),
),
migrations.AlterField(
model_name='chatroom',
name='users',
field=models.ManyToManyField(to='work_day.Professional', verbose_name='Users'),
),
migrations.AlterField(
model_name='city',
name='country',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='cities', to='work_day.country', verbose_name='Country'),
),
migrations.AlterField(
model_name='curriculum',
name='contract_price',
field=models.IntegerField(default=0, verbose_name='Contract price'),
),
migrations.AlterField(
model_name='curriculum',
name='owner',
field=models.OneToOneField(on_delete=django.db.models.deletion.CASCADE, to='work_day.professional', verbose_name='Owner'),
),
migrations.AlterField(
model_name='curriculum',
name='score',
field=models.IntegerField(default=5, verbose_name='Score'),
),
migrations.AlterField(
model_name='employment',
name='offer',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='employments', to='work_day.joboffer', verbose_name='Offer'),
),
migrations.AlterField(
model_name='employment',
name='profession',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.profession', verbose_name='Profession'),
),
migrations.AlterField(
model_name='employment',
name='reward',
field=models.FloatField(default=0, verbose_name='Reward'),
),
migrations.AlterField(
model_name='employment',
name='status',
field=models.BooleanField(default=True, verbose_name='Status'),
),
migrations.AlterField(
model_name='job',
name='description',
field=models.TextField(default='', max_length=500, verbose_name='Description'),
),
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=django.utils.timezone.now, verbose_name='Finish Date'),
),
migrations.AlterField(
model_name='job',
name='profession',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.profession', verbose_name='Profession'),
),
migrations.AlterField(
model_name='job',
name='start_date',
field=models.DateTimeField(default=django.utils.timezone.now, verbose_name='Start Date'),
),
migrations.AlterField(
model_name='joboffer',
name='city',
field=models.ForeignKey(default='', on_delete=django.db.models.deletion.CASCADE, related_name='job_offers', to='work_day.city', verbose_name='City'),
),
migrations.AlterField(
model_name='joboffer',
name='creation_date',
field=models.DateTimeField(default=django.utils.timezone.now, verbose_name='Creation Date'),
),
migrations.AlterField(
model_name='joboffer',
name='status',
field=models.BooleanField(default=True, verbose_name='Status'),
),
migrations.AlterField(
model_name='joboffer',
name='user',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='job_offers', to='work_day.professional', verbose_name='User'),
),
migrations.AlterField(
model_name='message',
name='chat_room',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.chatroom', verbose_name='Chat Room'),
),
migrations.AlterField(
model_name='message',
name='creation_date',
field=models.DateTimeField(default=django.utils.timezone.now, verbose_name='Creation Date'),
),
migrations.AlterField(
model_name='message',
name='message',
field=models.CharField(max_length=400, verbose_name='Message'),
),
migrations.AlterField(
model_name='message',
name='user',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.professional', verbose_name='User'),
),
migrations.AlterField(
model_name='professional',
name='city',
field=models.ForeignKey(default='', on_delete=django.db.models.deletion.CASCADE, related_name='professionals', to='work_day.city', verbose_name='City'),
),
migrations.AlterField(
model_name='professional',
name='creation_date',
field=models.DateTimeField(default=django.utils.timezone.now, verbose_name='Creation Date'),
),
migrations.AlterField(
model_name='professional',
name='id_image',
field=models.ImageField(blank=True, upload_to='', verbose_name='Id Image'),
),
migrations.AlterField(
model_name='professional',
name='id_number',
field=models.CharField(default='', max_length=11, verbose_name='Id Number'),
),
migrations.AlterField(
model_name='professional',
name='phone',
field=models.CharField(default='', max_length=9, verbose_name='Phone'),
),
migrations.AlterField(
model_name='professional',
name='professions',
field=models.ManyToManyField(to='work_day.Profession', verbose_name='Professions'),
),
migrations.AlterField(
model_name='professional',
name='profile_picture',
field=models.ImageField(blank=True, null=True, upload_to='', verbose_name='Profile Picture'),
),
migrations.AlterField(
model_name='professional',
name='status',
field=models.BooleanField(default=True, verbose_name='Status'),
),
migrations.AlterField(
model_name='professional',
name='user',
field=models.OneToOneField(on_delete=django.db.models.deletion.CASCADE, to=settings.AUTH_USER_MODEL, verbose_name='User'),
),
migrations.AlterField(
model_name='school',
name='city',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.city', verbose_name='City'),
),
migrations.AlterField(
model_name='study',
name='image',
field=models.ImageField(blank=True, upload_to='', verbose_name='Image'),
),
migrations.AlterField(
model_name='study',
name='name',
field=models.CharField(default='', max_length=50, verbose_name='Name'),
),
migrations.AlterField(
model_name='study',
name='profession',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.profession', verbose_name='Profession'),
),
migrations.AlterField(
model_name='study',
name='school',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.school', verbose_name='School'),
),
]
<file_sep>{% extends "format/base.html" %}
{% load static %}
{% load i18n %}
{% block title %}
Edit profile
{% endblock %}
{% block css %}
<link rel="stylesheet"
href="{% static 'lib/adminlte-3.0.4/plugins/bootstrap-colorpicker/css/bootstrap-colorpicker.min.css' %}">
<link rel="stylesheet" href="{% static 'lib/adminlte-3.0.4/plugins/select2/css/select2.min.css' %}">
<link rel="stylesheet"
href="{% static 'lib/adminlte-3.0.4/plugins/select2-bootstrap4-theme/select2-bootstrap4.min.css' %}">
<link rel="stylesheet"
href="{% static 'lib/adminlte-3.0.4/plugins/bootstrap4-duallistbox/bootstrap-duallistbox.min.css' %}">
<link rel="stylesheet" href="{% static 'lib/adminlte-3.0.4/plugins/bs-stepper/css/bs-stepper.min.css' %}">
<link rel="stylesheet" href="{% static 'lib/adminlte-3.0.4/plugins/dropzone/min/dropzone.min.css' %}">
<link rel="stylesheet" href="{% static 'lib/adminlte-3.0.4/plugins/fontawesome-free/css/fontawesome.css' %}">
{% endblock %}
{% block content %}
<section class="content-header">
<div class="container-fluid">
<div class="row">
<div class="col-lg-5">
<h1 class="m-0"><i class="fa fa-cog" aria-hidden="true"></i>{% trans "Profile settings" %} </h1>
</div><!-- /.col -->
</div><!-- /.row -->
<!-- /.container-fluid -->
</div>
</section>
<section class="content">
<div class="container">
<div class="row mb-2">
<div class="col">
<div class="card card-default">
<div class="card-header">
<h3 class="card-title">{% trans "Personal information settings" %}</h3>
</div>
<!-- /.card-header -->
<div class="card-body">
<form method="POST" enctype="multipart/form-data">
<label for="id_email">{% trans "Email address:" %}</label><br>
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text"><i class="fas fa-envelope"></i></span>
</div>
{{ user_form.email }}
</div>
<label for="id_first_name">{% trans "First Name:" %}</label><br>
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text"><i class="fas fa-user"></i></span>
</div>
{{ user_form.first_name }}
</div>
<label for="id_last_name">{% trans "Last Name:" %}</label><br>
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text "><i class="fas fa-user"></i></span>
</div>
{{ user_form.last_name }}
</div>
<label for="id_city">{% trans 'City:' %}</label>
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text"><i class="fas fa-city"></i></span>
</div>
{{ professional_form.city }}
</div>
<label for="id_phone">{% trans "Phone number:" %}</label>
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text"><i class="fas fa-phone"></i></span>
</div>
{{ professional_form.phone }}
</div>
<label for="id_id_number">{% trans "ID Number:" %}</label>
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text"><i class="fas fa-id-card"></i></span>
</div>
{{ professional_form.id_number }}
</div>
<label for="exampleInputFile">{% trans "ID Card:" %}</label>
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text"><i class="fas fa-upload"></i></span>
</div>
<div class="custom-file">
{{ professional_form.id_image }}
<label class="custom-file-label" for="exampleInputFile">{% trans "Choose file" %}</label>
</div>
</div>
<label for="exampleInputFile">{% trans "Profile picture:" %}</label>
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text"><i class="fas fa-upload"></i></span>
</div>
<div class="custom-file">
{{ professional_form.profile_picture }}
<label class="custom-file-label" for="exampleInputFile">{% trans "Choose file" %}</label>
</div>
</div>
{% csrf_token %}
<button type="submit">{% trans 'Register' %}</button>
</form>
</div>
<!-- /.card-body -->
<div class="card-footer">
</div>
</div>
</div>
</div>
</div>
</section>
{% endblock %}
{% block scripts %}
<script src="{% static 'lib/adminlte-3.0.4/plugins/select2/js/select2.full.min.js' %}"></script>
<script src="{% static 'lib/adminlte-3.0.4/plugins/bootstrap4-duallistbox/jquery.bootstrap-duallistbox.min.js' %} "></script>
<script src="{% static 'lib/adminlte-3.0.4/plugins/bs-custom-file-input/bs-custom-file-input.min.js' %} "></script>
<script>
$(function () {
//Initialize Select2 Elements
$('.select2').select2()
//Date range picker
$('#reservationdate').datetimepicker({
format: 'L'
});
})
</script>
<script>
$(function () {
bsCustomFileInput.init();
});
</script>
{% endblock %}<file_sep>from selenium.webdriver.common.by import By
class RegisterLocator:
EMAIL_INPUT = (By.XPATH, "//input[@id='id_email']")
USERNAME_INPUT = (By.XPATH, "//input[@id='id_username']")
PASSWORD_INPUT = (By.XPATH, "//input[@id='<PASSWORD>']")
REPEAT_PASSWORD_INPUT = (By.XPATH, "//input[@id='<PASSWORD>']")
FIRST_NAME_INPUT = (By.XPATH, "//input[@id='id_first_name']")
LAST_NAME_INPUT = (By.XPATH, "//input[@id='id_last_name']")
PROFILE_PICTURE_INPUT = (By.XPATH, "//input[@id='id_profile_picture']")
CITY_SELECT = (By.XPATH, "//select[@id='id_city']")
PHONE_INPUT = (By.XPATH, "//input[@id='id_phone']")
ID_NUMBER_INPUT = (By.XPATH, "//input[@id='id_id_number']")
ID_PHOTO_INPUT = (By.XPATH, "//input[@id='id_id_image']")
REGISTER_BUTTON = (By.XPATH, "//button[contains(text(),'Register')]")
LOGIN_LINK = (By.LINK_TEXT, "I already have a membership")
<file_sep># Generated by Django 3.1.2 on 2020-12-08 23:36
import datetime
from django.db import migrations, models
from django.utils.timezone import utc
class Migration(migrations.Migration):
dependencies = [
('work_day', '0019_auto_20201208_1404'),
]
operations = [
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 12, 9, 23, 36, 44, 340220, tzinfo=utc)),
),
]
<file_sep># Generated by Django 3.1.2 on 2020-12-09 01:43
import datetime
from django.db import migrations, models
from django.utils.timezone import utc
class Migration(migrations.Migration):
dependencies = [
('work_day', '0021_auto_20201208_1918'),
]
operations = [
migrations.AddField(
model_name='study',
name='image',
field=models.ImageField(blank=True, upload_to=''),
),
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 12, 10, 1, 43, 25, 149024, tzinfo=utc)),
),
]
<file_sep>from django import forms
from django.contrib.auth.forms import UserCreationForm
from django.contrib.auth.forms import (
PasswordResetForm,
SetPasswordForm,
)
from django.contrib.auth import password_validation
from django.forms import inlineformset_factory
from .models import *
class UserForm(UserCreationForm):
class Meta:
model = User
fields = [
'email',
'username',
'password1',
'<PASSWORD>',
'first_name',
'last_name',
]
labels = {
'email': 'Email address',
'username': 'Username',
'password1': '<PASSWORD>',
'password2': '<PASSWORD>',
'first_name': 'First Name',
'last_name': 'Last Name',
}
widgets = {
'email': forms.EmailInput(
attrs={'class': 'form-control', 'placeholder': 'Email'}),
'username': forms.TextInput(
attrs={'class': 'form-control', 'placeholder': 'Username'}),
'first_name': forms.TextInput(
attrs={'class': 'form-control', 'placeholder': 'First name'}),
'last_name': forms.TextInput(
attrs={'class': 'form-control', 'placeholder': 'Last name'}),
}
def __init__(self, *args, **kwargs):
super(UserForm, self).__init__(*args, **kwargs)
self.fields['password1'].widget = forms.PasswordInput(
attrs={'class': 'form-control',
'placeholder': 'Password'})
self.fields['password2'].widget = forms.PasswordInput(
attrs={'class': 'form-control',
'placeholder': '<PASSWORD>'})
class ProfessionalForm(forms.ModelForm):
class Meta:
model = Professional
fields = [
'city', 'phone', 'id_number', 'id_image',
'status', 'birthdate', 'profile_picture',
]
labels = {
'city': 'City',
'phone': 'Phone number',
'id_number': 'ID Number',
'status': 'Status',
'birthdate': 'Birthdate'
}
widgets = {
'city': forms.Select(
attrs={'class': 'form-control select2bs4',
'placeholder': 'City',
'style': 'height:100%;'}
),
'phone': forms.TextInput(
attrs={'class': 'form-control',
'placeholder': 'Phone number'}
),
'id_number': forms.TextInput(
attrs={'class': 'form-control',
'placeholder': 'Identification document'}
),
'status': forms.CheckboxInput(),
'birthdate': forms.DateInput(
attrs={'class': 'form-control datetimepicker-input'}),
'id_image': forms.FileInput(
attrs={'class': 'custom-file-input'}
),
'profile_picture': forms.FileInput(
attrs={'class': 'custom-file-input'}
),
}
class JobForm(forms.ModelForm):
class Meta:
model = Job
fields = [
'profession', 'description', 'start_date', 'finish_date'
]
labels = {
'profession': 'Profession',
'description': 'Description',
'start_date': 'Start Date',
'finish_date': 'Finish Date',
}
widgets = {
'profession': forms.Select(attrs={'class': 'form-control'}),
'description': forms.TextInput(attrs={'class': 'form-control'}),
'start_date': forms.DateTimeInput(attrs={'class': 'form-control'}),
'finish_date': forms.DateTimeInput(
attrs={'class': 'form-control'}
),
}
class StudyForm(forms.ModelForm):
class Meta:
model = Study
fields = [
'school', 'profession', 'name', 'image'
]
labels = {
'school': 'Institution',
'profession': 'Profession',
'name': 'Degree',
}
widgets = {
'school': forms.Select(attrs={'class': 'form-control'}),
'profession': forms.Select(attrs={'class': 'form-control'}),
'name': forms.TextInput(attrs={'class': 'form-control'}),
'image': forms.FileInput(
attrs={'class': 'custom-file-input'}
),
}
class JobOfferForm(forms.ModelForm):
class Meta:
model = JobOffer
fields = ['city', 'description', 'status']
labels = {
'city': 'City',
'description': 'Description',
'status': 'Status',
}
widgets = {
'city': forms.Select(attrs={'class': 'form-control'}),
'description': forms.TextInput(
attrs={'class': 'form-control',
'placeholder': 'Enter description'}
),
}
class EmploymentForm(forms.ModelForm):
class Meta:
model = Employment
fields = ['profession', 'description', 'reward', 'status']
labels = {
'profession': 'Profession',
'description': 'Description',
'reward': 'Reward',
'status': 'Status',
}
widgets = {
'profession': forms.Select(attrs={'class': 'form-control'}),
'description': forms.Textarea(
attrs={'class': 'form-control',
'placeholder': 'Enter description'}
),
'reward': forms.TextInput(
attrs={'class': 'form-control',
'type': 'number'}
),
}
EmploymentInlineFormSet = inlineformset_factory(
JobOffer, Employment, form=EmploymentForm, extra=2, can_delete=True
)
class EditUserForm(forms.ModelForm):
class Meta:
model = User
fields = (
'email',
'first_name',
'last_name',
)
labels = {
'email': 'Email address',
'first_name': '<NAME>',
'last_name': '<NAME>',
}
widgets = {
'email': forms.EmailInput(
attrs={'class': 'form-control', 'placeholder': 'Email'}),
'first_name': forms.TextInput(
attrs={'class': 'form-control', 'placeholder': 'First name'}),
'last_name': forms.TextInput(
attrs={'class': 'form-control', 'placeholder': 'Last name'}),
}
class UserPasswordResetForm(PasswordResetForm):
email = forms.EmailField(
label='',
widget=forms.EmailInput(
attrs={'class': 'form-control', 'placeholder': 'Email'}
)
)
class UserPasswordResetConfirmForm(SetPasswordForm):
new_password1 = forms.CharField(
label="New password",
widget=forms.PasswordInput(
attrs={'autocomplete': 'new-password',
'class': 'form-control'}
),
strip=False,
help_text=password_validation.password_validators_help_text_html(),
)
new_password2 = forms.CharField(
label="New password confirmation",
strip=False,
widget=forms.PasswordInput(
attrs={'autocomplete': 'new-password', 'class': 'form-control'}
),
)
<file_sep>asgiref==3.2.10
coverage==5.3.1
Django==3.1.2
django-environ==0.4.5
django-filter==2.4.0
django-parler==2.2
Pillow==8.0.1
psycopg2-binary==2.8.6
pytz==2020.1
selenium==3.141.0
sqlparse==0.4.1
urllib3==1.26.2
<file_sep>from django.shortcuts import render, redirect, get_object_or_404
from django.contrib.auth import authenticate
from django.contrib.auth import logout as do_logout
from django.contrib.auth import login as do_login
from django.contrib.auth.forms import AuthenticationForm
from django.http import HttpResponseForbidden, HttpResponse
from django.template import loader
from django.contrib.auth.decorators import login_required
from django.urls import reverse
from .forms import *
from .filters import ProfessionalFilter
def register(request):
user_form = UserForm()
professional_form = ProfessionalForm()
user_form.fields['username'].help_text = None
user_form.fields['password1'].help_text = None
user_form.fields['password2'].help_text = None
if request.method == "POST":
user_form = UserForm(data=request.POST)
professional_form = ProfessionalForm(
data=request.POST, files=request.FILES
)
if user_form.is_valid() and professional_form.is_valid():
user = user_form.save()
professional = professional_form.save(commit=False)
professional.user = user
professional.save()
Curriculum.objects.create(owner=professional)
return redirect('login')
return render(
request,
"users/register.html",
{
'user_form': user_form,
'professional_form': professional_form
}
)
def login(request):
form = AuthenticationForm()
if request.method == "POST":
form = AuthenticationForm(data=request.POST)
if form.is_valid():
username = form.cleaned_data['username']
password = form.cleaned_data['<PASSWORD>']
user = authenticate(username=username, password=password)
if user is not None:
do_login(request, user)
return redirect('/home')
template = loader.get_template('users/login.html')
context = {
'form': form
}
return HttpResponse(template.render(context, request))
@login_required(login_url='/login')
def logout(request):
do_logout(request)
return redirect('/')
@login_required(login_url='/login')
def home(request):
try:
offers = JobOffer.objects.filter(
employments__profession=
request.user.professional.professions.all()[0]
).distinct()
except IndexError:
professional = Professional.objects.get(user=request.user)
offers = JobOffer.objects.filter(
city=professional.city
)
template = loader.get_template('home.html')
context = {
'offers': offers
}
return HttpResponse(template.render(context, request))
@login_required(login_url='/login')
def messages(request):
return render(request, "messages.html")
@login_required(login_url='/login')
def user_profile(request, pk=None):
if pk:
current_user = User.objects.get(pk=pk)
else:
current_user = request.user
professional = Professional.objects.get(user=current_user)
professions = professional.professions.all()
cv = Curriculum.objects.get(owner=professional)
studies = cv.studies.all()
jobs = cv.jobs.all()
template = loader.get_template('users/profile.html')
context = {
'user': current_user,
'professional': professional,
'professions': professions,
'cv': cv,
'studies': studies,
'jobs': jobs,
}
return HttpResponse(template.render(context, request))
@login_required(login_url='/login')
def view_professionals(request):
professionals = Professional.objects.filter(
status=True)
template = loader.get_template('professionals.html')
professional_filter = ProfessionalFilter(
request.GET, queryset=professionals
)
professionals = professional_filter.qs
context = {
'professionals': professionals,
'filter': professional_filter,
}
return HttpResponse(template.render(context, request))
@login_required(login_url='/login')
def add_job(request, pk=None):
if pk:
job = get_object_or_404(Job, pk=pk)
if job.cv.owner.user != request.user:
return HttpResponseForbidden()
else:
cv = Curriculum.objects.get(owner=request.user.professional)
job = Job(cv=cv)
form = JobForm(data=request.POST or None, instance=job)
if request.method == 'POST':
if form.is_valid():
form.save()
return redirect('home')
return render(
request, "add_job.html", {'form': form}
)
@login_required(login_url='/login')
def add_study(request, pk=None):
if pk:
study = get_object_or_404(Study, pk=pk)
if study.cv.owner.user != request.user:
return HttpResponseForbidden()
else:
cv = Curriculum.objects.get(owner=request.user.professional)
study = Study(cv=cv)
form = StudyForm(
data=request.POST or None,
instance=study,
files=request.FILES
)
if request.method == 'POST':
if form.is_valid():
saved_study = form.save()
request.user.professional.professions.add(saved_study.profession)
return redirect('../user-profile/')
if pk:
return render(
request, "add_study.html", {'form': StudyForm(instance=study)}
)
else:
return render(request, "add_study.html", {'form': form})
def index(request):
return render(request, "index.html")
@login_required(login_url='/login')
def my_posts(request):
current_user = request.user
professional = Professional.objects.get(user=current_user)
offers = professional.job_offers.all()
template = loader.get_template('my_posts.html')
context = {
'offers': offers,
}
return HttpResponse(template.render(context, request))
@login_required(login_url='/login')
def create_job_offer(request, pk=None):
if pk:
offer = get_object_or_404(JobOffer, pk=pk)
if offer.user.user != request.user:
return HttpResponseForbidden()
else:
offer = JobOffer(user=request.user.professional)
offer_form = JobOfferForm(data=request.POST or None, instance=offer)
employment_formset = EmploymentInlineFormSet(
data=request.POST or None, instance=offer)
if request.method == 'POST':
if offer_form.is_valid():
offer.save()
if employment_formset.is_valid():
employment_formset.save()
return redirect(reverse('my_posts'))
return render(
request,
"create_offer.html",
{
'offer_form': offer_form,
'employment_formset': employment_formset
}
)
@login_required(login_url='/login')
def job_offer(request, offer_id=None):
offer_list = JobOffer.objects.all()
if offer_id:
current_offer = JobOffer.objects.get(pk=offer_id)
else:
current_offer = JobOffer.objects.all().first()
context = {
'offers': offer_list,
'offer': current_offer,
}
return render(request, 'offers.html', context)
@login_required(login_url='/login')
def edit_profile(request):
user_form = EditUserForm(
data=request.POST or None,
instance=request.user,
)
professional_form = ProfessionalForm(
data=request.POST or None,
instance=request.user.professional,
files=request.FILES
)
if request.method == 'POST':
if user_form.is_valid() and professional_form.is_valid():
user_form.save()
professional_form.save()
return redirect('home')
context = {
'user_form': user_form,
'professional_form': ProfessionalForm(
instance=request.user.professional),
}
return render(request, 'users/edit_profile.html', context)
<file_sep># Generated by Django 3.1.2 on 2020-12-20 14:33
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('work_day', '0024_auto_20201211_2158'),
('work_day', '0026_auto_20201219_2209'),
]
operations = [
]
<file_sep># Generated by Django 3.1.2 on 2020-11-29 01:23
import datetime
from django.db import migrations, models
from django.utils.timezone import utc
class Migration(migrations.Migration):
dependencies = [
('work_day', '0008_auto_20201128_1939'),
]
operations = [
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 11, 30, 1, 23, 35, 863683, tzinfo=utc)),
),
]
<file_sep># Generated by Django 3.1.2 on 2020-12-09 17:07
from django.db import migrations, models
import django.utils.timezone
class Migration(migrations.Migration):
dependencies = [
('work_day', '0022_auto_20201208_2043'),
]
operations = [
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=django.utils.timezone.now),
),
]
<file_sep>from selenium.webdriver.chrome.webdriver import WebDriver
from work_day.tests.locators.register_locator import RegisterLocator
class RegisterPage:
"""
"""
def __init__(self, driver: WebDriver):
self.driver = driver
def get_email_input(self):
return self.driver.find_element(*RegisterLocator.EMAIL_INPUT)
def get_username_input(self):
return self.driver.find_element(*RegisterLocator.USERNAME_INPUT)
def get_password_input(self):
return self.driver.find_element(*RegisterLocator.PASSWORD_INPUT)
def get_password2_input(self):
return self.driver.find_element(*RegisterLocator.REPEAT_PASSWORD_INPUT)
def get_firstname_input(self):
return self.driver.find_element(*RegisterLocator.FIRST_NAME_INPUT)
def get_lastname_input(self):
return self.driver.find_element(*RegisterLocator.LAST_NAME_INPUT)
def get_profile_picture_input(self):
return self.driver.find_element(*RegisterLocator.PROFILE_PICTURE_INPUT)
def get_city_select(self):
return self.driver.find_element(*RegisterLocator.CITY_SELECT)
def get_phone_input(self):
return self.driver.find_element(*RegisterLocator.PHONE_INPUT)
def get_id_number_input(self):
return self.driver.find_element(*RegisterLocator.ID_NUMBER_INPUT)
def get_id_photo_input(self):
return self.driver.find_element(*RegisterLocator.ID_PHOTO_INPUT)
def get_register_button(self):
return self.driver.find_element(*RegisterLocator.REGISTER_BUTTON)
def get_login_link(self):
return self.driver.find_element()
<file_sep># Generated by Django 3.1.2 on 2020-12-05 23:39
import datetime
from django.db import migrations, models
import django.db.models.deletion
from django.utils.timezone import utc
class Migration(migrations.Migration):
dependencies = [
('work_day', '0015_auto_20201202_0130'),
]
operations = [
migrations.AlterField(
model_name='employment',
name='profession',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.profession'),
),
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 12, 6, 23, 39, 36, 743002, tzinfo=utc)),
),
migrations.AlterField(
model_name='job',
name='profession',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='work_day.profession'),
),
]
<file_sep>from django.test import TestCase
from work_day.models import *
class BaseTest(TestCase):
def setUp(self) -> None:
self.user = User.objects.create_user(
username='UserTest', password='<PASSWORD>')
self.country = Country.objects.create(name='CountryTest')
self.city = City.objects.create(country=self.country, name='CityTest')
self.school = School.objects.create(name='SchoolTest', city=self.city)
self.profession = Profession.objects.create(
name='ProfessionTest', description='ProfessionDescription')
self.professional = Professional.objects.create(
user=self.user, city=self.city, phone='999999999',
id_number='11111111', birthdate=datetime.date.today()
)
self.curriculum = Curriculum.objects.create(
owner=self.professional, contract_price=100, score=5)
self.job = Job.objects.create(
cv=self.curriculum, profession=self.profession,
description='JobDescription'
)
self.study = Study.objects.create(
cv=self.curriculum, school=self.school,
profession=self.profession, name='StudyTest'
)
self.job_offer = JobOffer.objects.create(
user=self.professional, city=self.city, description='JobOfferTest',
)
self.employment = Employment.objects.create(
offer=self.job_offer, profession=self.profession,
description='EmploymentTest', reward=1000,
)
class CountryModelTests(BaseTest):
def setUp(self) -> None:
super().setUp()
def test_country_is_created_with_the_right_name(self):
self.assertEqual(self.country.name, 'CountryTest')
def test_str_function(self):
self.assertEqual(self.country.__str__(), 'CountryTest')
def test_cities_related_name_is_correct(self):
self.assertEqual(len(self.country.cities.all()), 1)
class CityModelTests(BaseTest):
def setUp(self) -> None:
super().setUp()
def test_city_is_created_with_the_right_name(self):
self.assertEqual(self.city.name, 'CityTest')
def test_str_function(self):
self.assertEqual(self.city.__str__(), 'CityTest')
class SchoolModelTest(BaseTest):
def setUp(self) -> None:
super().setUp()
def test_school_is_created_with_the_right_name(self):
self.assertEqual(self.school.name, 'SchoolTest')
def test_str_function(self):
self.assertEqual(self.school.__str__(), 'SchoolTest')
class ProfessionModelTest(BaseTest):
def setUp(self) -> None:
super().setUp()
def test_profession_is_created_with_the_right_attrs(self):
self.assertEqual(self.profession.name, 'ProfessionTest')
self.assertEqual(self.profession.description, 'ProfessionDescription')
def test_str_function(self):
self.assertEqual(self.profession.__str__(), 'ProfessionTest')
class ProfessionalModelTest(BaseTest):
def setUp(self) -> None:
super().setUp()
def test_professional_is_created_with_the_right_attrs(self):
self.assertEqual(self.professional.user, self.user)
self.assertEqual(self.professional.city, self.city)
self.assertEqual(self.professional.phone, '999999999')
self.assertEqual(self.professional.id_number, '11111111')
self.assertEqual(self.professional.birthdate, datetime.date.today())
def test_str_function(self):
self.assertEqual(self.professional.__str__(), 'UserTest')
def test_professional_is_created_with_a_correct_birthdate(self):
self.assertGreaterEqual(
self.professional.birthdate, datetime.date.today())
class CurriculumModelTest(BaseTest):
def setUp(self) -> None:
super().setUp()
def test_curriculum_is_created_with_the_right_attrs(self):
self.assertEqual(self.curriculum.owner, self.professional)
self.assertEqual(self.curriculum.contract_price, 100)
self.assertEqual(self.curriculum.score, 5)
def test_str_function(self):
self.assertEqual(self.curriculum.__str__(), 'UserTest-cv')
class JobModelTest(BaseTest):
def setUp(self) -> None:
super().setUp()
def test_job_is_created_with_the_right_attrs(self):
self.assertEqual(self.job.cv, self.curriculum)
self.assertEqual(self.job.profession, self.profession)
self.assertEqual(self.job.description, 'JobDescription')
class StudyModelTest(BaseTest):
def setUp(self) -> None:
super().setUp()
def test_study_is_created_with_the_right_attrs(self):
self.assertEqual(self.study.cv, self.curriculum)
self.assertEqual(self.study.profession, self.profession)
self.assertEqual(self.study.name, 'StudyTest')
self.assertEqual(self.study.school, self.school)
class JobOfferModelTest(BaseTest):
def setUp(self) -> None:
super().setUp()
def test_job_offer_is_created_with_the_right_attrs(self):
self.assertEqual(self.job_offer.user, self.professional)
self.assertEqual(self.job_offer.city, self.city)
self.assertEqual(self.job_offer.description, 'JobOfferTest')
def test_job_offer_has_related_employments(self):
self.assertEqual(
self.job_offer.employments.all().first(), self.employment)
<file_sep># Generated by Django 3.1.2 on 2020-11-28 19:39
import datetime
from django.db import migrations, models
from django.utils.timezone import utc
class Migration(migrations.Migration):
dependencies = [
('work_day', '0007_auto_20201127_1735'),
]
operations = [
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 11, 29, 19, 39, 52, 367215, tzinfo=utc)),
),
]
<file_sep># Generated by Django 3.1.2 on 2020-12-08 19:04
import datetime
from django.db import migrations, models
import django.db.models.deletion
from django.utils.timezone import utc
class Migration(migrations.Migration):
dependencies = [
('work_day', '0018_merge_20201207_1425'),
]
operations = [
migrations.AddField(
model_name='professional',
name='profile_picture',
field=models.ImageField(blank=True, null=True, upload_to=''),
),
migrations.AlterField(
model_name='city',
name='country',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='cities', to='work_day.country'),
),
migrations.AlterField(
model_name='employment',
name='offer',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='employments', to='work_day.joboffer'),
),
migrations.AlterField(
model_name='job',
name='cv',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='jobs', to='work_day.curriculum'),
),
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 12, 9, 19, 4, 37, 960536, tzinfo=utc)),
),
migrations.AlterField(
model_name='joboffer',
name='city',
field=models.ForeignKey(default='', on_delete=django.db.models.deletion.CASCADE, related_name='job_offers', to='work_day.city'),
),
migrations.AlterField(
model_name='joboffer',
name='user',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='job_offers', to='work_day.professional'),
),
migrations.AlterField(
model_name='professional',
name='city',
field=models.ForeignKey(default='', on_delete=django.db.models.deletion.CASCADE, related_name='professionals', to='work_day.city'),
),
migrations.AlterField(
model_name='study',
name='cv',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='studies', to='work_day.curriculum'),
),
]
<file_sep># Generated by Django 3.1.2 on 2020-11-27 22:29
import datetime
from django.db import migrations, models
from django.utils.timezone import utc
class Migration(migrations.Migration):
dependencies = [
('work_day', '0004_auto_20201127_1715'),
]
operations = [
migrations.AlterField(
model_name='job',
name='finish_date',
field=models.DateTimeField(default=datetime.datetime(2020, 11, 28, 22, 29, 6, 362692, tzinfo=utc)),
),
]
<file_sep># Generated by Django 3.1.2 on 2020-12-18 23:12
from django.db import migrations, models
import django.db.models.deletion
import django.utils.timezone
import parler.fields
import parler.models
class Migration(migrations.Migration):
dependencies = [
('work_day', '0023_auto_20201209_1207'),
]
operations = [
migrations.AlterModelOptions(
name='chatroom',
options={'verbose_name': 'Chat Room', 'verbose_name_plural': 'Chat Rooms'},
),
migrations.AlterModelOptions(
name='city',
options={'verbose_name': 'City', 'verbose_name_plural': 'Cities'},
),
migrations.AlterModelOptions(
name='country',
options={'verbose_name': 'Country', 'verbose_name_plural': 'Countries'},
),
migrations.AlterModelOptions(
name='curriculum',
options={'verbose_name': 'Curriculum', 'verbose_name_plural': 'Curriculums'},
),
migrations.AlterModelOptions(
name='employment',
options={'verbose_name': 'Employment', 'verbose_name_plural': 'Employments'},
),
migrations.AlterModelOptions(
name='job',
options={'verbose_name': 'Job', 'verbose_name_plural': 'Jobs'},
),
migrations.AlterModelOptions(
name='joboffer',
options={'verbose_name': 'Job Offer', 'verbose_name_plural': 'Job Offers'},
),
migrations.AlterModelOptions(
name='message',
options={'verbose_name': 'Message', 'verbose_name_plural': 'Messages'},
),
migrations.AlterModelOptions(
name='profession',
options={'verbose_name': 'Profession', 'verbose_name_plural': 'Professions'},
),
migrations.AlterModelOptions(
name='professional',
options={'verbose_name': 'Professional', 'verbose_name_plural': 'Professionals'},
),
migrations.AlterModelOptions(
name='school',
options={'verbose_name': 'School', 'verbose_name_plural': 'Schools'},
),
migrations.AlterModelOptions(
name='study',
options={'verbose_name': 'Study', 'verbose_name_plural': 'Studies'},
),
migrations.RemoveField(
model_name='city',
name='name',
),
migrations.RemoveField(
model_name='country',
name='name',
),
migrations.RemoveField(
model_name='profession',
name='description',
),
migrations.RemoveField(
model_name='profession',
name='name',
),
migrations.RemoveField(
model_name='school',
name='name',
),
migrations.AlterField(
model_name='country',
name='creation_date',
field=models.DateTimeField(default=django.utils.timezone.now, verbose_name='Creation Date'),
),
migrations.AlterField(
model_name='employment',
name='description',
field=models.TextField(default='', max_length=200, verbose_name='Description'),
),
migrations.AlterField(
model_name='joboffer',
name='description',
field=models.CharField(default='', max_length=100, verbose_name='Description'),
),
migrations.CreateModel(
name='SchoolTranslation',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('language_code', models.CharField(db_index=True, max_length=15, verbose_name='Language')),
('name', models.CharField(max_length=200, verbose_name='Name')),
('master', parler.fields.TranslationsForeignKey(editable=False, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='translations', to='work_day.school')),
],
options={
'verbose_name': 'School Translation',
'db_table': 'work_day_school_translation',
'db_tablespace': '',
'managed': True,
'default_permissions': (),
'unique_together': {('language_code', 'master')},
},
bases=(parler.models.TranslatedFieldsModelMixin, models.Model),
),
migrations.CreateModel(
name='ProfessionTranslation',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('language_code', models.CharField(db_index=True, max_length=15, verbose_name='Language')),
('name', models.CharField(max_length=50, verbose_name='Name')),
('description', models.CharField(default='', max_length=100, verbose_name='Description')),
('master', parler.fields.TranslationsForeignKey(editable=False, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='translations', to='work_day.profession')),
],
options={
'verbose_name': 'Profession Translation',
'db_table': 'work_day_profession_translation',
'db_tablespace': '',
'managed': True,
'default_permissions': (),
'unique_together': {('language_code', 'master')},
},
bases=(parler.models.TranslatedFieldsModelMixin, models.Model),
),
migrations.CreateModel(
name='CountryTranslation',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('language_code', models.CharField(db_index=True, max_length=15, verbose_name='Language')),
('name', models.CharField(max_length=40, verbose_name='Name')),
('master', parler.fields.TranslationsForeignKey(editable=False, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='translations', to='work_day.country')),
],
options={
'verbose_name': 'Country Translation',
'db_table': 'work_day_country_translation',
'db_tablespace': '',
'managed': True,
'default_permissions': (),
'unique_together': {('language_code', 'master')},
},
bases=(parler.models.TranslatedFieldsModelMixin, models.Model),
),
migrations.CreateModel(
name='CityTranslation',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('language_code', models.CharField(db_index=True, max_length=15, verbose_name='Language')),
('name', models.CharField(max_length=50, verbose_name='Name')),
('master', parler.fields.TranslationsForeignKey(editable=False, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='translations', to='work_day.city')),
],
options={
'verbose_name': 'City Translation',
'db_table': 'work_day_city_translation',
'db_tablespace': '',
'managed': True,
'default_permissions': (),
'unique_together': {('language_code', 'master')},
},
bases=(parler.models.TranslatedFieldsModelMixin, models.Model),
),
]
<file_sep>from time import sleep
from .base_test_case import SeleniumBaseTestCase
class LoginTest(SeleniumBaseTestCase):
def setUp(self) -> None:
super().setUp()
self.driver.get("http://127.0.0.1:8000/login/")
def test_verify_login_with_valid_inputs(self):
self.driver.find_element_by_xpath(
"//*[@id='id_username']").send_keys('nur1234')
self.driver.find_element_by_xpath(
"//input[@id='id_password']").send_keys('shamas5432')
self.driver.find_element_by_xpath(
"//button[contains(text(),'Sign In')]").click()
self.assertEqual(
self.driver.current_url, 'http://127.0.0.1:8000/home/'
)
def test_verify_login_with_invalid_inputs(self):
self.driver.find_element_by_xpath(
"//*[@id='id_username']").send_keys('<PASSWORD>')
self.driver.find_element_by_xpath(
"//input[@id='id_password']").send_keys('<PASSWORD>')
self.driver.find_element_by_xpath(
"//button[contains(text(),'Sign In')]").click()
self.assertEqual(
self.driver.current_url, 'http://127.0.0.1:8000/login/'
)
def test_verify_login_with_empty_inputs(self):
self.driver.find_element_by_xpath(
"//*[@id='id_username']").send_keys('')
self.driver.find_element_by_xpath(
"//input[@id='id_password']").send_keys('')
self.driver.find_element_by_xpath(
"//button[contains(text(),'Sign In')]").click()
self.assertEqual(
self.driver.current_url, 'http://127.0.0.1:8000/login/'
)
def test_verify_password_recover_link(self):
self.driver.find_element_by_xpath(
"/html/body/div/div/div[2]/p[2]/a").click()
self.assertEqual(
self.driver.current_url, 'http://127.0.0.1:8000/resetPassword/'
)
def test_verify_register_link(self):
self.driver.find_element_by_xpath(
"/html/body/div/div/div[2]/p[3]/a").click()
self.assertEqual(
self.driver.current_url, 'http://127.0.0.1:8000/register/'
)<file_sep>from django.contrib import admin
from parler.admin import TranslatableAdmin
from .models import *
# Register your models here.
@admin.register(Country)
class CountryAdmin(TranslatableAdmin):
list_display = ('name', 'creation_date')
@admin.register(City)
class CityAdmin(TranslatableAdmin):
list_display = ('name', 'country')
@admin.register(School)
class SchoolAdmin(TranslatableAdmin):
list_display = ('name', 'city')
@admin.register(Profession)
class ProfessionAdmin(TranslatableAdmin):
list_display = ('name', 'description')
@admin.register(Professional)
class ProfessionalAdmin(admin.ModelAdmin):
list_display = (
'user', 'city', 'birthdate', 'phone', 'id', 'status', 'creation_date'
)
@admin.register(Curriculum)
class CurriculumAdmin(admin.ModelAdmin):
list_display = ('owner', 'contract_price', 'score')
@admin.register(Job)
class JobAdmin(admin.ModelAdmin):
list_display = ('description', 'cv', 'profession', 'start_date', 'finish_date')
@admin.register(JobOffer)
class JobOfferAdmin(admin.ModelAdmin):
list_display = ('user', 'city', 'description', 'creation_date', 'status')
@admin.register(Employment)
class EmploymentAdmin(admin.ModelAdmin):
list_display = ('offer', 'profession', 'description', 'reward', 'status')
@admin.register(Study)
class StudyAdmin(admin.ModelAdmin):
list_display = ('cv', 'school', 'profession', 'name')
@admin.register(ChatRoom)
class ChatRoomAdmin(admin.ModelAdmin):
list_display = ('creation_date', 'status')
@admin.register(Message)
class MessageAdmin(admin.ModelAdmin):
list_display = ('chat_room', 'user', 'message', 'creation_date')
<file_sep>from time import sleep
from selenium.webdriver.common.by import By
from selenium.webdriver.support.select import Select
from selenium.webdriver.support.wait import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from .base_test_case import SeleniumBaseTestCase
from ..pages.register_page import RegisterPage
class RegisterTest(SeleniumBaseTestCase):
def setUp(self) -> None:
super().setUp()
self.page = RegisterPage(self.driver)
self.driver.get("http://127.0.0.1:8000/register/")
def fill_register_inputs(self):
self.page.get_email_input().send_keys('<EMAIL>')
self.page.get_username_input().send_keys('myTestUsername')
self.page.get_password_input().send_keys('<PASSWORD>')
self.page.get_password2_input().send_keys('<PASSWORD>')
self.page.get_firstname_input().send_keys('MyNameTest')
self.page.get_lastname_input().send_keys('LastNameTest')
self.page.get_profile_picture_input().send_keys(
'/home/jhose/Nueva Carpeta/profile_photo.jpg'
)
select_city = Select(self.page.get_city_select())
select_city.select_by_index(0)
self.page.get_phone_input().send_keys('999999999')
self.page.get_id_number_input().send_keys('12345678')
self.page.get_id_photo_input().send_keys(
'/home/jhose/Nueva Carpeta/id_card_photo.jpg'
)
sleep(3)
def test_register_with_valid_inputs(self):
self.fill_register_inputs()
self.page.get_register_button().click()
self.assertEqual(
self.driver.current_url, 'http://127.0.0.1:8000/login/'
)
def test_register_with_blank_inputs(self):
self.page.get_register_button().click()
email_username = WebDriverWait(self.driver, 3).until(
EC.element_to_be_clickable(
(By.CSS_SELECTOR,
"input.cell.small-21.form-text.required#edit-name[name='name']")
)
)
print(email_username.get_attribute("validationMessage"))
self.assertEqual(
self.driver.current_url, "http://127.0.0.1:8000/register/"
)
| 6f5eef02ce7d609ba217b2a44993a3e06f7b42c1 | [
"Markdown",
"Python",
"Text",
"HTML"
] | 36 | Python | JoseHumire/proyecto_cs | d0036c09be3687b3cbc7ae74a87cb6a3e2d0dd38 | e7e4eb415629e8e45a5635be175f02c3c10326dc | |
refs/heads/master | <repo_name>parz3val/connection_test_sdk<file_sep>/index.js
// Entrypoint in the package
const ipTest = require("./lib/network.js");
const speedTest = require("./lib/speedTest.js");
module.exports = {
ipTest,
speedTest,
};
<file_sep>/README.md
# Connection Test SDK
Package to monitor the call quality and network status for a specific browser/clients
Returns a hashmap`{}`after running a suite of tests
## Tests
1. IP test - returns IP and location
2. IP Fraud Score - returns details like VPN and proxies. Uses quality score api.
3. Speed test to the cloudflare cli
<file_sep>/lib/network.js
//const fetch = require("node-fetch");
require("dotenv").config();
class ipTest {
/**
* Function to detect the IP
*
* @returns {Object}
*/
async detectIP() {
// may be we need to hit our own server which will hit multiple server if one is busy
let resp = [];
await fetch("https://extreme-ip-lookup.com/json/")
.then((res) => res.json())
.then((json) => {
resp.push(json);
})
.catch((error) => {
console.log("Request failed:", error);
});
return resp;
}
async detectVPN(ip, qualtyIpApiKey) {
// may be we need to hit our own server which will hit multiple server if one is busy
let resp = [];
const qualityIPURL =
"https://ipqualityscore.com/api/json/ip/" +
qualtyIpApiKey +
"/" +
ip +
"?strictness=1&user_agent=Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.114 Safari/537.36&user_language=en-US";
await fetch(qualityIPURL, { method: "GET" })
.then((res) => res.json())
.then((json) => {
resp.push(json);
})
.catch((error) => {
console.log("Request failed:", error);
});
return resp;
}
}
const iptest = new ipTest();
module.exports = iptest;
<file_sep>/tests/ip.test.js
const ipTest = require("../lib/network.js");
//
// Test the location and IP getting module
test("testIPLocation", async () => {
resp = await ipTest.detectIP();
json = resp[0];
console.log(json);
code = json.countryCode;
expect(code).toBe("NP");
});
//
// Test the If user is on vpn
test("testIF on VPN", async () => {
resp = await ipTest.detectVPN("172.16.31.10",process.env.QUALITY_IP_API_KEY);
json = resp[0];
code = json.vpn;
console.log(json);
expect(code).toBe(false);
});
| bde4cb400e9ae52d585dc4208e017d49d138cb3c | [
"JavaScript",
"Markdown"
] | 4 | JavaScript | parz3val/connection_test_sdk | ca7e89dfd70dbf671c9a162ed1dfd41bd5650957 | bd121813709a3afc4b43f1212c91a3ed727b6796 | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Security.Principal;
using System.Diagnostics;
using System.Threading;
using System.IO;
using System.IO.Pipes;
namespace PipeConnectionClient
{
class Program
{
private static int numClients = 2;
public static void Main(string[] args)
{
if (args.Length > 0)
{
if (args[0] == "spawnclient")
{
NamedPipeClientStream myPipeClient = new NamedPipeClientStream(".", "mypipe", PipeDirection.InOut, PipeOptions.None, TokenImpersonationLevel.Impersonation);
Console.WriteLine("Connecting to the server...");
myPipeClient.Connect();
StreamString theStreamString = new StreamString(myPipeClient);
// A signature string was hard coded in PipeConnection Server Side,
// Before we do the action, we need to verify and validate the server
if (theStreamString.ReadString() == "Hello, Friend")
{
// Sending the name of the file which will be returned by the server later
theStreamString.WriteString("openthis.txt");
Console.Write(theStreamString.ReadString());
} // END IF
else
{
Console.WriteLine("Server not found.");
} // END ELSE
myPipeClient.Close();
Thread.Sleep(4000);
} // END IF
}
else
{
Console.WriteLine("Name pipe client stream with impersonatin example");
startClient();
} // END ELSE
} // END main()
// This method create pipe process for client
private static void startClient()
{
int count;
string currentProcessName = Environment.CommandLine;
Process[] pList = new Process[numClients];
Console.WriteLine("Spawning client processes...");
if (currentProcessName.Contains(Environment.CurrentDirectory))
{
currentProcessName = currentProcessName.Replace(Environment.CurrentDirectory, String.Empty);
} // END IF
currentProcessName = currentProcessName.Replace("\\", String.Empty);
currentProcessName = currentProcessName.Replace("\"", String.Empty);
for (count = 0; count < numClients; count++)
{
pList[count] = Process.Start(currentProcessName, "spawnclient");
} // END FOR
while (count > 0)
{
for (int pCount = 0; pCount < numClients; pCount++)
{
if (pList[pCount] != null)
{
if (pList[pCount].HasExited)
{
Console.WriteLine("Client process[{0} has exited.]", pList[pCount].Id);
pList[pCount] = null;
count--;
} // END IF
else
{
Thread.Sleep(250);
} // END ELSE
} // END IF
} // END FOR
} // END WHILE
Console.WriteLine("Client processes finished, exiting.");
Console.WriteLine("Enter any key to exit the program");
Console.ReadLine();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
using System.IO.Pipes;
using System.Threading;
// This is the same class we written in PipeConnection Server side.
// Reuse of codes
namespace PipeConnectionClient
{
class StreamString
{
private Stream ioStream;
private UnicodeEncoding streamUniEncoding;
public StreamString()
{
// empty
}
public StreamString(Stream inIoStream)
{
this.ioStream = inIoStream;
streamUniEncoding = new UnicodeEncoding();
}
public string ReadString()
{
int length;
length = ioStream.ReadByte() * 256;
length += ioStream.ReadByte();
byte[] inBuffer = new byte[length];
ioStream.Read(inBuffer, 0, length);
return streamUniEncoding.GetString(inBuffer);
} // END ReadString()
public int WriteString(string inOutputString)
{
byte[] outputBuffer = streamUniEncoding.GetBytes(inOutputString);
int length = outputBuffer.Length;
if (length > UInt16.MaxValue)
{
length = (int)UInt16.MaxValue;
} // END IF
ioStream.WriteByte((byte)(length/256));
ioStream.WriteByte((byte)(length & 255));
ioStream.Write(outputBuffer, 0, length);
ioStream.Flush();
return outputBuffer.Length + 2;
} // END WriteString()
}
}
| 564b12eb3cea4ef25a15f03e1203f6d94a503506 | [
"C#"
] | 2 | C# | martineng/PipeConnectionClient | feb2f11477881b8a09585319c3e4e25f06155563 | 2d58e6b9ebbc346cdb2c53a8b747cc696b4db659 | |
refs/heads/master | <repo_name>guillaume-haerinck/lolimac<file_sep>/front/src/app/shared/components/event-card-notification/event-card-notification.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { Router } from '@angular/router';
import { Event } from 'app/shared/models/event';
@Component({
selector: 'app-event-card-notification',
templateUrl: './event-card-notification.component.html',
styleUrls: ['./event-card-notification.component.scss']
})
export class EventCardNotificationComponent implements OnInit {
@Input() event: Event;
constructor(private m_router: Router) { }
ngOnInit() {
}
goTo(url: string) {
this.m_router.navigateByUrl(url);
}
}
<file_sep>/back/api/controllers/NotificationCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \models\Notification;
use \models\NotificationManager;
use \models\User;
use \models\UserManager;
use \models\Event;
use \models\EventManager;
use \controllers\scans\TokenAccess;
class NotificationCRUD {
public function add($data) {
$notificationManager = new NotificationManager();
foreach ($data as $key => $value) {
if ($key == 'id') continue;
$notification = new Notification([
"id_event" => $data['id'],
'type_edit' => $key
]);
$notificationManager->add($notification);
}
}
public function read() {
$token = new TokenAccess();
$id_user = $token->getId();
$userManager = new UserManager();
$user = $userManager->readById($id_user);
$notificationManager = new NotificationManager();
$notifications = $notificationManager->readAll($id_user);
if ($notifications) {
$eventManager = new EventManager();
foreach ($notifications as $key => $notification) {
$notifications[$key] = $notification->toArray();
$event = $eventManager->readById_noException($notification->getId_event());
//var_dump($event);
if ($event) {
$event = new Event($event);
$notifications[$key]["title"] = $event->getTitle();
$notifications[$key]["photo_url"] = $event->getPhoto_url();
}
else {
unset($notifications[$key]);
}
}
$userManager->updateNotificationDate($id_user);
$notifications = \array_values($notifications);
echo json_encode([
"events" => $notifications
]);
}
else {
json_encode(['message'=>"Il n'y a pas de notifications"]);
}
}
public function count() {
$token = new TokenAccess();
$id_user = $token->getId();
$userManager = new UserManager();
$user = $userManager->readById($id_user);
$notificationManager = new NotificationManager();
$notifications = $notificationManager->countNotifications($id_user);
echo json_encode(["notifications" => $notifications]);
}
}
<file_sep>/front/src/app/modules/events/event.service.ts
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
import { environment } from 'environments/environment';
import { removeEmptyProperties } from 'app/shared/utility/change-objects';
@Injectable({
providedIn: 'root'
})
export class EventService {
constructor(private m_http: HttpClient) {}
createEvent(form: Object): Observable<any> {
form = removeEmptyProperties(form);
const URL = `${environment.backend.serverUrl}events`;
return this.m_http.post<any>(URL, JSON.stringify(form));
}
getEventById(id: number): Observable<any> {
const URL = `${environment.backend.serverUrl}events/${id}`;
return this.m_http.get<any>(URL);
}
getEventList(from: number, limit: number): Observable<any[]> {
const URL = `${environment.backend.serverUrl}events?from=${from}&limit=${limit}`;
return this.m_http.get<any>(URL);
}
getIcsLink(id: number) {
const URL = `${environment.backend.serverUrl}events/export/${id}`;
return this.m_http.get<any>(URL);
}
search(terms: string): Observable<any[]> {
const URL = `${environment.backend.serverUrl}events/search/?q=${terms}`;
return this.m_http.get<any>(URL);
}
update(id: number, form: Object): Observable<any> {
form = removeEmptyProperties(form);
const URL = `${environment.backend.serverUrl}events/${id}`;
return this.m_http.patch<any>(URL, JSON.stringify(form));
}
join(id: number): Observable<any> {
const URL = `${environment.backend.serverUrl}events/${id}/join`;
return this.m_http.post<any>(URL, undefined);
}
leave(id: number): Observable<any> {
const URL = `${environment.backend.serverUrl}events/${id}/leave`;
return this.m_http.delete<any>(URL, undefined);
}
delete(id: number): Observable<any> {
const URL = `${environment.backend.serverUrl}events/${id}`;
return this.m_http.delete<any>(URL);
}
}
<file_sep>/back/api/controllers/IcsCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \controllers\scans\TokenAccess;
use \controllers\parser\IcsParser;
use \controllers\EventCRUD;
use \controllers\Link_events_placesCRUD;
use \models\Event;
use \models\Link_events_places;
use \models\Place;
use \models\EventManager;
use \models\UserManager;
class IcsCRUD {
public function readOne($dataIn) {
$encryptionMethod = "AES-256-CBC";
$secretHash = "25c6c7ff35b9979b151f<PASSWORD>cd13b0ff";
$iv = "6546445734219402";
$token = openssl_decrypt($dataIn, $encryptionMethod, $secretHash, $options=0, $iv);
$data = \explode('/', $token);
$data['id_user'] = $data[0];
$data['id_event'] = $data[1];
$eventCRUD = new EventCRUD();
$event = $eventCRUD->readOBJ(['id' => $data['id_event']]);
$IcsParser = new IcsParser();
if ($event && $event->getDate_start() && $event->getDate_end()) {
$IcsParser->openEvent();
$IcsParser->setSUMMARY($event->getTitle());
$IcsParser->setUID($event->getId());
$IcsParser->setStatus();
$IcsParser->setDate_start($event->getDate_start());
$IcsParser->setDate_end($event->getDate_end());
$IcsParser->setDate_timeStamp();
$link_events_placesCRUD = new Link_events_placesCRUD();
$place = $link_events_placesCRUD->readPlace_OBJ(['id_event' => $event->getId()]);
if ($place) {
$IcsParser->setLocation($place);
}
$IcsParser->closeEvent();
$IcsParser->closeCalendar();
$filename = urlencode($event->getTitle());
header('Content-type: text/calendar; charset=utf-8');
header("Content-Disposition: inline; filename=lolimac-{$filename}.ics");
echo $IcsParser->ics;
exit;
}
else {
throw new \Exception("Impossible d'accéder à l'événement", 401);
}
}
public function readAll($dataIn) {
$scanDataIn = new ScanDataIn();
$encryptionMethod = "AES-256-CBC";
$secretHash = "<PASSWORD>ff<PASSWORD>";
$iv = "6546445734219402";
$token = openssl_decrypt($dataIn, $encryptionMethod, $secretHash, $options=0, $iv);
$data['pseudo'] = $token;
$data = $scanDataIn->failleXSS($data);
$userManager = new UserManager();
$user = $userManager->readByPseudo($data['pseudo']);
if (!$user) {
throw new \Exception("Accès refusé", 401);
}
$eventManager = new EventManager();
$events = $eventManager->readAllValid();
$IcsParser = new IcsParser();
if ($events) {
$link_events_placesCRUD = new Link_events_placesCRUD();
foreach ($events as $key => $event) {
$IcsParser->openEvent();
$IcsParser->setSUMMARY($event->getTitle());
$IcsParser->setUID($event->getId());
$IcsParser->setStatus();
$IcsParser->setDate_start($event->getDate_start());
$IcsParser->setDate_end($event->getDate_end());
$IcsParser->setDate_timeStamp();
$place = $link_events_placesCRUD->readPlace_OBJ(['id_event' => $event->getId()]);
if ($place) {
$IcsParser->setLocation($place);
}
$IcsParser->closeEvent();
}
$IcsParser->closeCalendar();
header('Content-type: text/calendar; charset=utf-8');
header('Content-Disposition: inline; filename=lolimac-calendar.ics');
echo $IcsParser->ics;
exit;
}
}
}
<file_sep>/back/api/models/CommentManager.php
<?php
namespace models;
class CommentManager extends DBAccess {
public function add(Comment $comment) {
$q = $this->db->prepare("INSERT INTO comments
(`content`, `date_created`, `date_edited`, `id_user`, `id_post`)
VALUES (:content, NOW(), NOW(), :id_user, :id_post);");
$q->bindValue(':content', $comment->getContent());
$q->bindValue(':id_user', $comment->getId_user());
$q->bindValue(':id_post', $comment->getId_post());
$q->execute();
$comment->hydrate(['id' => $this->db->lastInsertId()]);
return $comment;
}
public function count() {
return $this->db->query("SELECT COUNT(*) FROM comments;")->fetchColumn();
}
public function readById($id) {
$q = $this->db->query("SELECT * FROM comments WHERE id = {$id}");
$comment = $q->fetch(\PDO::FETCH_ASSOC);
$commentObject = new Comment($comment);
if($commentObject) {
return $commentObject;
} else {
throw new \Exception("Le commentaire n'existe pas.", 400);
}
}
public function readByPost($id_post) {
$allComment = [];
$q = $this->db->query("SELECT * FROM comments
WHERE id_post = {$id_post};");
for($i = 0;$data = $q->fetch(\PDO::FETCH_ASSOC); $i++) {
$allComments[$i] = new Comment($data);
}
if(!empty($allComments)) {
return $allComments;
}
}
public function update(Comment $comment) {
$q = $this->db->prepare('UPDATE comments SET content = :content, date_edited = NOW(), id_user = :id_user , id_post = :id_post WHERE id = :id');
$q->bindValue(':id', $comment->getId());
$q->bindValue(':content', $comment->getContent());
$q->bindValue(':id_user', $comment->getId_user());
$q->bindValue(':id_post', $comment->getId_post());
$q->execute();
return $comment;
}
public function deleteById($id) {
$this->db->exec('DELETE FROM comments WHERE id = '.$id.';');
return TRUE;
}
public function deleteByIdPost($id_post) {
$this->db->exec('DELETE FROM comments WHERE id_post = '.$id_post.';');
return TRUE;
}
}
<file_sep>/back/api/controllers/Link_events_eventtypesCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \controllers\scans\TokenAccess;
use \controllers\scans\TokenCreater;
use \controllers\EventTypeCRUD;
use \models\Link_events_eventtypes;
use \models\Link_events_eventtypesManager;
class Link_events_eventtypesCRUD {
public function add($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id_event", "id_type"]);
$data = $scanDataIn->failleXSS($dataIn);
$link = new Link_events_eventtypes($data);
$linkManager = new Link_events_eventtypesManager();
if ($linkManager->readById_event_type($link->getId_event(), $link->getId_type()) === FALSE) {
$linkManager->add($link);
} else {
throw new \Exception('Lien déjà existant.');
}
return TRUE;
}
public function readType_ARRAY($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id_event"]);
$data = $scanDataIn->failleXSS($dataIn);
$linkManager = new Link_events_eventtypesManager();
$link_events_eventTypes = $linkManager->readById_event($data["id_event"]);
if ($link_events_eventTypes) {
$eventTypeCRUD = new EventTypeCRUD();
$eventType = $eventTypeCRUD->read_OBJ(["id" => $link_events_eventTypes->getId_type()]);
return $eventType->toArray();
}
}
/*
public function updateByIdEvent($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$scanDataIn->exists($data, ['id_event', 'id_type']);
$linkManager = new Link_events_eventtypesManager();
$link_events_eventTypes = $linkManager->readById_event($data["id_event"]);
$link_events_eventTypes = $linkManager->updateType($data["id_event"]);
}
*/
public function readByIdEvent_OBJ($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$scanDataIn->exists($data, ["id_event"]);
$linkManager = new Link_events_eventtypesManager();
$link_events_eventTypes = $linkManager->readById_event($data["id_event"]);
if($link_events_eventTypes) {
return $link_events_eventTypes;
} else {
throw new Exception("Le type n'existe pas.");
}
}
public function addIfNotExist($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$scanDataIn->exists($data, ['id_event', 'id_type']);
$linkManager = new Link_events_eventtypesManager();
$link_events_eventTypes = $linkManager->readById_event($data["id_event"]);
if ($link_events_eventTypes) {
$linkManager->updateType($link_events_eventTypes, $data['id_type']);
}
else {
$link_events_eventTypes = new Link_events_eventtypes(['id_event' => $data['id_event'], 'id_type' => $data['id_type']]);
$linkManager->add($link_events_eventTypes);
}
}
public function delete($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id_event", "id_type"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
$linkManager = new Link_events_eventtypesManager();
$linkManager->deleteById($data["id_event"], $data["id_type"]);
return TRUE;
}
public function deleteByIdEvent($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id_event"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
$linkManager = new Link_events_eventtypesManager();
$linkManager->deleteByIdEvent($data["id_event"]);
return TRUE;
}
}
<file_sep>/front/src/app/modules/search/search.component.ts
import { Component, OnInit } from '@angular/core';
import { FormBuilder, FormGroup } from '@angular/forms';
import { Observable, Subject } from 'rxjs';
import { debounceTime, distinctUntilChanged, switchMap } from 'rxjs/operators';
import { Event } from 'app/shared/models/event';
import { EventService } from '../events/event.service';
@Component({
selector: 'app-search',
templateUrl: './search.component.html',
styleUrls: ['./search.component.scss']
})
export class SearchComponent implements OnInit {
searchResults$: Observable<Event[]>;
searchForm: FormGroup;
private m_searchTerms = new Subject<string>();
constructor(private m_eventService: EventService,
private m_formBuilder: FormBuilder) {
this.searchForm = this.m_formBuilder.group({
terms: ['']
});
}
ngOnInit() {
this.searchResults$ = this.m_searchTerms.pipe(
// wait 300ms after each keystroke before considering the term
debounceTime(300),
// ignore new term if same as previous term
distinctUntilChanged(),
// switch to new search observable each time the term changes
switchMap((term: string) => this.m_eventService.search(term))
);
}
// Push a search term into the observable stream.
search(terms: string): void {
this.m_searchTerms.next(terms);
}
}
<file_sep>/back/api/models/Place.php
<?php
namespace models;
class Place {
protected $id,
$postcode = NULL,
$street = NULL,
$number = NULL,
$city = NULL,
$name = NULL;
public function __construct(array $data) {
$this->hydrate($data);
}
//Gère la récupération de données des attributs lorsqu'elle proviennent de la bdd
public function hydrate(array $data) {
foreach ($data as $key => $value) {
$method = 'set'.ucfirst($key);
if (method_exists($this, $method)) {
$this->$method($value);
}
}
}
public function toArray() {
$array = [
"id_place" => $this->getId(),
"postcode" => $this->getPostcode(),
"street" => $this->getStreet(),
"number" => $this->getNumber(),
"city" => $this->getCity(),
"name" => $this->getName()
];
return $array;
}
// Getters
public function getId() {
return $this->id;
}
public function getPostcode() {
return $this->postcode;
}
public function getStreet() {
return $this->street;
}
public function getNumber() {
return $this->number;
}
public function getCity() {
return $this->city;
}
public function getName() {
return $this->name;
}
//Assesseurs
//Attention le nom de méthode doit obligatoirement être composé de "set" suivi du nom de l'attribut correspondant, avec une majuscule, pour que la méthode hydrate soit fonctionnelle.
public function setId($id) {
$id = (int) $id;
if ($id > 0) {
$this->id = $id;
}
}
public function setPostcode($postcode) {
$postcode = (int) $postcode;
$this->postcode = $postcode;
}
public function setStreet($street) {
if (is_string($street) && strlen($street) <= 255) {
$this->street = $street;
}
}
public function setNumber($number) {
$number = (int) $number;
$this->number = $number;
}
public function setCity($city) {
if (is_string($city) && strlen($city) <= 63 ) {
$this->city = $city;
}
}
public function setName($name) {
if (is_string($name) && strlen($name) < 63 ) {
$this->name = $name;
}
}
}
<file_sep>/front/src/app/modules/visitor/visitor.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { LoginComponent } from './login/login.component';
import { VisitorRoutingModule } from './visitor-routing.module';
import { SharedModule } from 'app/shared/shared.module';
import { InvitationComponent } from './invitation/invitation.component';
import { InscriptionComponent } from './inscription/inscription.component';
import { ForgotComponent } from './forgot/forgot.component';
import { UserService } from './user.service';
@NgModule({
declarations: [
LoginComponent,
InvitationComponent,
InscriptionComponent,
ForgotComponent
],
imports: [
CommonModule,
VisitorRoutingModule,
SharedModule
],
providers: [
UserService
]
})
export class VisitorModule { }
<file_sep>/front/src/app/shared/components/event-card-small/event-card-small.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { Router } from '@angular/router';
import { Event } from 'app/shared/models/event';
@Component({
selector: 'app-event-card-small',
templateUrl: './event-card-small.component.html',
styleUrls: ['./event-card-small.component.scss']
})
export class EventCardSmallComponent implements OnInit {
@Input() event: Event;
constructor(private m_router: Router) { }
ngOnInit() {
}
goTo(url: string) {
this.m_router.navigateByUrl(url);
}
}
<file_sep>/back/api/Root.php
<?php
use \controllers\UserCRUD;
use \controllers\PlaceCRUD;
use \controllers\EventCRUD;
use \controllers\PostCRUD;
use \controllers\IcsCRUD;
use \controllers\CommentCRUD;
use \controllers\ModuleCRUD;
use \controllers\Link_events_users_modulesCRUD;
use \controllers\NotificationCRUD;
use \controllers\Link_events_placesCRUD;
use \controllers\scans\ScanDataIn;
use \controllers\scans\CutURL;
class Root {
protected $root;
function __construct() {
header('Access-Control-Allow-Origin: *');
header('Access-Control-Allow-Methods: POST, GET, DELETE, PUT, PATCH, OPTIONS, HEAD');
header('Access-Control-Allow-Headers: Content-Type, Origin, Accept, Authorization, Content-Length, X-Requested-With');
header('Content-Type: application/json; charset=UTF-8');
$CutURL = new CutURL($_SERVER["REQUEST_URI"]);
$this->root = $CutURL->getURL_cut();
$key = $this->root[0];
if (!$key) {
// Called if the user access /api without any other argument
echo json_encode(
[
"status" => "running",
"commit" => trim(shell_exec("git rev-parse HEAD"), "\n")
]
);
}
else {
$this->$key();
}
}
public function login() {
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$_POST = json_decode(file_get_contents("php://input"), TRUE);
$userCRUD = new UserCRUD();
$userCRUD->auth($_POST);
}
}
public function users() {
switch ($_SERVER['REQUEST_METHOD']) {
case 'GET':
$userCRUD = new UserCRUD();
$userCRUD->read($this->root);
break;
case 'POST':
$_POST = json_decode(file_get_contents("php://input"), TRUE);
$userCRUD = new UserCRUD();
$userCRUD->add($_POST);
break;
case 'PATCH':
$_PATCH = json_decode(file_get_contents("php://input"), TRUE);
$userCRUD = new UserCRUD();
$userCRUD->update($_PATCH);
break;
case 'DELETE':
$userCRUD = new UserCRUD();
$_DELETE['id'] = $this->root[1];
$userCRUD->delete($_DELETE);
break;
default:
break;
}
}
public function me() {
switch ($_SERVER['REQUEST_METHOD']) {
case 'GET':
if (isset($this->root[1]) && $this->root[1] == "notifications") {
$notificationsCRUD = new NotificationCRUD();
if (isset($this->root[2]) && $this->root[2] == "count") {
$notificationsCRUD->count();
}
else {
$notificationsCRUD->read();
}
}
break;
}
}
public function ics() {
if ($_SERVER['REQUEST_METHOD'] == 'GET') {
if (isset($this->root[1])) {
$icsCRUD = new IcsCRUD();
if ($this->root[1] == "all") {
if (isset($this->root[2])) {
$icsCRUD->readAll($this->root[2]);
}
}
else {
$icsCRUD->readOne($this->root[1]);
}
}
}
}
public function events() {
switch ($_SERVER['REQUEST_METHOD']) {
case 'GET':
$eventCRUD = new EventCRUD();
$_GET;
//print_r($this->root);
if (isset($this->root[1])) {
if ($this->root[1] == "export") {
if (isset($this->root[2])) {
$eventCRUD->generateSingleEventICS($this->root[2]);
} else {
$eventCRUD->generateAllEventICS();
}
}
elseif ($this->root[1] == "search") {
$_GET['query'] = $_GET['q'];
$eventCRUD->search($_GET);
}
else if (empty($this->root[2])) {
$_GET["id"] = $this->root[1];
// We read one specific event
$eventCRUD->read($_GET);
}
}
else {
$eventCRUD->readMultiple($_GET);
//$eventCRUD->readOffsetLimit($_GET);
}
break;
case 'POST':
$_POST = json_decode(file_get_contents("php://input"), TRUE);
if(isset($this->root[2])) {
if($this->root[2] == "posts") {
if(isset($this->root[4])) {
if($this->root[4] == "comments") {
$commentCRUD = new CommentCRUD();
$_POST["id_post"] = $this->root[3];
$commentCRUD->add($_POST, $this->root[1]);
}
} else {
$postCRUD = new PostCRUD();
$_POST["id_event"] = $this->root[1];
$postCRUD->add($_POST);
}
} else if ($this->root[2] == "join") {
$linkCRUD = new Link_events_users_modulesCRUD();
$linkCRUD->add($this->root[1], 2);
}
} else {
$eventCRUD = new EventCRUD();
$eventCRUD->add($_POST);
}
break;
case 'PATCH':
$_PUT = json_decode(file_get_contents("php://input"), TRUE);
if(isset($this->root[2])) {
if($this->root[2] == "posts" && isset($this->root[3])) {
if(isset($this->root[4])) {
if($this->root[4] == "comments" && isset($this->root[5])) {
$commentCRUD = new CommentCRUD();
$_PUT["id"] = $this->root[5];
$commentCRUD->update($_PUT);
}
} else {
$postCRUD = new PostCRUD();
$_PUT['id'] = $this->root[3];
$postCRUD->update($_PUT);
}
}
} else {
$idEvent = $this->root[1];
$_PUT["id"] = $idEvent;
$eventCRUD = new EventCRUD();
$eventCRUD->update($_PUT);
}
break;
case 'DELETE':
if(isset($this->root[2])) {
if(isset($this->root[2])) {
if ($this->root[2] == "leave") {
$linkCRUD = new Link_events_users_modulesCRUD();
$linkCRUD->delete($this->root[1]);
} else if ($this->root[2] == "posts" && isset($this->root[3])) {
if(isset($this->root[4])) {
if ($this->root[4] == "comments" && isset($this->root[5])) {
$commentCRUD = new CommentCRUD();
$_DELETE['id'] = $this->root[5];
$commentCRUD->delete($_DELETE);
}
} else {
$postCRUD = new PostCRUD();
$_DELETE['id'] = $this->root[3];
$postCRUD->delete($_DELETE);
}
}
}
} else {
$idEvent = $this->root[1];
$_DELETE["id"] = $idEvent;
$eventCRUD = new EventCRUD();
$eventCRUD->delete($_DELETE);
}
break;
default:
break;
}
}
public function place() {
switch ($_SERVER['REQUEST_METHOD']) {
case 'GET':
$placeCRUD = new PlaceCRUD();
$placeCRUD->read($this->root);
break;
case 'POST':
$_POST = json_decode(file_get_contents("php://input"), TRUE);
$placeCRUD = new PlaceCRUD();
$placeCRUD->add($_POST);
break;
case 'PUT':
$_PUT = json_decode(file_get_contents("php://input"), TRUE);
$placeCRUD = new PlaceCRUD();
$placeCRUD->update($_PUT);
break;
case 'DELETE':
$_DELETE = json_decode(file_get_contents("php://input"), TRUE);
$placeCRUD = new PlaceCRUD();
$placeCRUD->delete($_DELETE);
break;
default:
break;
}
}
public function post() {
switch ($_SERVER['REQUEST_METHOD']) {
case 'GET':
$postCRUD = new PostCRUD();
$postCRUD->read($this->root);
break;
case 'POST':
$_POST = json_decode(file_get_contents("php://input"), TRUE);
$postCRUD = new PostCRUD();
$postCRUD->add($_POST);
break;
case 'PUT':
$_PUT = json_decode(file_get_contents("php://input"), TRUE);
$postCRUD = new PostCRUD();
$postCRUD->update($_PUT);
break;
case 'DELETE':
$_DELETE = json_decode(file_get_contents("php://input"), TRUE);
$postCRUD = new PostCRUD();
$postCRUD->delete($_DELETE);
break;
default:
break;
}
}
public function comment() {
switch ($_SERVER['REQUEST_METHOD']) {
case 'GET':
$commentCRUD = new CommentCRUD();
$commentCRUD->read($this->root);
break;
case 'POST':
$_POST = json_decode(file_get_contents("php://input"), TRUE);
$commentCRUD = new CommentCRUD();
$commentCRUD->add($_POST);
break;
case 'PUT':
$_PUT = json_decode(file_get_contents("php://input"), TRUE);
$commentCRUD = new CommentCRUD();
$commentCRUD->update($_PUT);
break;
case 'DELETE':
$_DELETE = json_decode(file_get_contents("php://input"), TRUE);
$commentCRUD = new CommentCRUD();
$commentCRUD->delete($_DELETE);
break;
default:
break;
}
}
public function module() {
switch ($_SERVER['REQUEST_METHOD']) {
case 'GET':
$moduleCRUD = new ink_events_users_modulesCRUD();
$moduleCRUD->read($this->root);
break;
case 'POST':
$_POST = json_decode(file_get_contents("php://input"), TRUE);
$moduleCRUD = new ModuleCRUD();
$moduleCRUD->add($_POST);
break;
case 'PUT':
$_PUT = json_decode(file_get_contents("php://input"), TRUE);
$moduleCRUD = new ModuleCRUD();
$moduleCRUD->update($_PUT);
break;
case 'DELETE':
$_DELETE = json_decode(file_get_contents("php://input"), TRUE);
$moduleCRUD = new ModuleCRUD();
$moduleCRUD->delete($_DELETE);
break;
default:
break;
}
}
public function linkEUM() {
switch ($_SERVER['REQUEST_METHOD']) {
case 'POST':
$_POST = json_decode(file_get_contents("php://input"), TRUE);
$linkCRUD = new Link_events_users_modulesCRUD();
$linkCRUD->add($_POST);
break;
case 'DELETE':
$_DELETE = json_decode(file_get_contents("php://input"), TRUE);
$linkCRUD = new Link_events_users_modulesCRUD();
$linkCRUD->delete($_DELETE);
break;
default:
break;
}
}
}
<file_sep>/back/api/Config.php
<?php
class Config {
protected $jwtKey,
$serverName;
public function __construct() {
include "configVar.php";
$this->jwtKey = $secretKey;
$this->serverName = $ip;
}
public function getJwtKey() {
return $this->jwtKey;
}
public function getServerName() {
return $this->serverName;
}
}<file_sep>/back/api/models/User.php
<?php
namespace models;
use \controllers\scans\ScanDataIn;
class User {
protected $id,
$firstname,
$lastname,
$pseudo,
$pwd_hash,
$mail,
$phone,
$photo_url,
$status,
$year_promotion,
$date_notification_check;
public function __construct(array $data) {
$this->hydrate($data);
}
//Gère la récupération de données des attributs lorsqu'elle proviennent de la bdd
public function hydrate(array $data) {
foreach ($data as $key => $value) {
$method = 'set'.ucfirst($key);
if (method_exists($this, $method)) {
$this->$method($value);
}
}
}
public function toArray() {
$array = [
"id" => $this->getId(),
"firstmane" => $this->getFirstname(),
"lastname" => $this->getLastname(),
"pseudo" => $this->getPseudo(),
"photo_url" => $this->getPhoto_url(),
"status" => $this->getStatus()
];
return $array;
}
// Getters
public function getId() {
return $this->id;
}
public function getFirstname() {
return $this->firstname;
}
public function getLastname() {
return $this->lastname;
}
public function getPseudo() {
return $this->pseudo;
}
public function getPwd_hash() {
return $this->pwd_hash;
}
public function getMail() {
return $this->mail;
}
public function getPhone() {
return $this->phone;
}
public function getPhoto_url() {
return $this->photo_url;
}
public function getStatus() {
return $this->status;
}
public function getYear_promotion() {
return $this->year_promotion;
}
public function getDate_notification_check() {
return $this->date_notification_check;
}
//Assesseurs
//Attention le nom de méthode doit obligatoirement être composé de "set" suivi du nom de l'attribut correspondant, avec une majuscule, pour que la méthode hydrate soit fonctionnelle.
public function setId($id) {
$id = (int) $id;
if ($id > 0) {
$this->id = $id;
}
}
public function setFirstname($firstname) {
if (is_string($firstname) && strlen($firstname) <= 22) {
$this->firstname = $firstname;
}
}
public function setLastname($lastname) {
if (is_string($lastname) && strlen($lastname) <= 22) {
$this->lastname = $lastname;
}
}
public function setPseudo($pseudo) {
if (is_string($pseudo) && strlen($pseudo) <= 25) {
$this->pseudo = $pseudo;
}
}
public function setPwd_hash($pwd_hash) {
if (is_string($pwd_hash)) {
$this->pwd_hash = $pwd_hash;
}
}
public function setMail($mail) {
$mail = filter_var($mail, FILTER_VALIDATE_EMAIL);
if ($mail == TRUE) {
$this->mail = $mail;
}
else {
throw new \Exception("L'adresse email est invalide.");
}
}
public function setPhone($phone) {
if (is_string($phone) && preg_match('#^(\d\d\s){4}(\d\d)$#', $phone)) {
$this->phone = $phone;
}
}
public function setPhoto_url($photo_url) {
if (is_string($photo_url) && strlen($photo_url) <= 255) {
$this->photo_url = $photo_url;
}
}
public function setStatus($status) {
$status = (int) $status;
$this->status = $status;
}
public function setYear_promotion($year_promotion) {
$year_promotion = (int) $year_promotion;
if ($year_promotion > 0) {
$this->year_promotion = $year_promotion;
}
}
public function setDate_notification_check($date_notification) {
$scanDataIn = new ScanDataIn();
if (\is_null($date_notification) || $scanDataIn->validateDate($date_notification)) {
$this->date_notification_check = $date_notification;
}
else {
throw new \Exception("Veuilliez entrer une date de vérification de notifications valide.");
}
}
}
<file_sep>/front/src/app/modules/events/edit-event/edit-event.component.ts
import { Component, OnInit } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
import { Router, ActivatedRoute } from '@angular/router';
import { ResponsiveService } from 'app/core/services/responsive.service';
import { EventService } from '../event.service';
import { fillUndefinedProperties, removeEmptyProperties, removeUnchangedProperties, isEmpty } from 'app/shared/utility/change-objects';
import { MatDialog } from '@angular/material';
import { DialogComponent } from 'app/shared/components/dialog/dialog.component';
@Component({
selector: 'app-edit-event',
templateUrl: './edit-event.component.html',
styleUrls: ['./edit-event.component.scss']
})
export class EditEventComponent implements OnInit {
bMobile: boolean;
eventId: number;
eventForm: FormGroup;
oldEventData: Object;
minDate = new Date();
bEndDetail = false;
bEndDetailControl = true;
constructor(responsiveService: ResponsiveService,
private m_router: Router,
private m_formBuilder: FormBuilder,
route: ActivatedRoute,
private m_eventService: EventService,
private m_dialog: MatDialog) {
this.eventId = Number(route.snapshot.paramMap.get('id'));
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
this.eventForm = this.m_formBuilder.group({
title: ['', Validators.required],
photo_url: ['https://images.unsplash.com/photo-1541532713592-79a0317b6b77?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=634&q=80', Validators.required],
description: '',
date_start: '',
date_start_hour: '0',
date_start_minute: '0',
date_end: '',
date_end_offset: '0',
date_end_hour: '0',
place: this.m_formBuilder.group({
name: '',
street: '',
number: '',
city: ''
})
});
}
ngOnInit() {
this.m_eventService.getEventById(this.eventId).subscribe((result) => {
// TODO store result as oldform, and remove matching terms
result = fillUndefinedProperties(result);
this.oldEventData = result;
if (result.date_start != '') {
const dateStart = new Date(result.date_start);
this.eventForm.patchValue({
date_start: dateStart,
date_start_hour: dateStart.getHours().toString(),
date_start_minute: dateStart.getMinutes().toString(),
});
}
if (result.date_end != '') {
this.bEndDetail = true;
this.bEndDetailControl = false;
const dateEnd = new Date(result.date_end);
this.eventForm.patchValue({
date_end: dateEnd,
date_end_hour: dateEnd.getHours().toString(),
});
}
this.eventForm.patchValue({
title: result.title,
photo_url: result.photo_url,
description: result.description,
place: result.place
});
}, error => {
});
}
goTo(url: string) {
this.m_router.navigateByUrl(url);
}
submitForm(): void {
let form = this.eventForm.value;
if (form.date_start_hour && form.date_start_minute) {
if (form.date_start instanceof Date) {
form.date_start.setHours(form.date_start_hour, form.date_start_minute);
}
}
if (!this.bEndDetail) {
form.date_end = new Date(form.date_start);
if (form.date_end_offset === 24) {
form.date_end.setHours(23);
} else {
form.date_end.setHours(form.date_end.getHours() + Number(form.date_end_offset), 0);
}
} else {
if (form.date_end instanceof Date && form.date_end_hour instanceof Date) {
form.date_end.setHours(form.date_end_hour);
}
}
delete form.date_start_hour;
delete form.date_start_minute;
delete form.date_end_hour;
delete form.date_end_offset;
form = removeEmptyProperties(form);
form = removeUnchangedProperties(this.oldEventData, form);
if (!isEmpty(form)) {
this.m_eventService.update(this.eventId, form).subscribe(result => {
this.m_router.navigate(['/evenements/detail/' + this.eventId]);
}, error => {
});
} else {
this.m_router.navigate(['/evenements/detail/' + this.eventId]);
}
}
deleteEvent(): void {
const dialogRef = this.m_dialog.open(DialogComponent, {
width: "500px",
data: {title: `Suppression d'un évènement`, text: `En êtes-vous bien sûr ?`, bValBtn: true}
});
dialogRef.afterClosed().subscribe(result => {
if (result.validated) {
this.m_eventService.delete(this.eventId).subscribe(result => {
this.m_router.navigate(['/tableau-de-bord']);
}, error => {
});
}
});
}
onDateEndDropChange(event: any): void {
if (event === 'more') {
this.bEndDetail = true;
this.eventForm.patchValue({
date_end: this.eventForm.value.date_start,
date_end_hour: (this.eventForm.value.date_start.getHours() + 2 ).toString()
});
} else {
this.bEndDetail = false;
}
}
}
<file_sep>/back/api/controllers/Link_events_users_modulesCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \controllers\scans\TokenAccess;
use \controllers\scans\TokenCreater;
use \models\Link_events_users_modules;
use \models\Link_events_users_modulesManager;
use \models\UserManager;
class Link_events_users_modulesCRUD {
public function add($id_event, $id_module) {
$token = new TokenAccess();
$id_user = $token->getId();
$data["id_event"] = $id_event;
$data["id_module"] = $id_module;
$data["id_user"] = $id_user;
$link = new Link_events_users_modules($data);
$linkManager = new Link_events_users_modulesManager();
if ($linkManager->readById_event_user_module($link->getId_event(), $link->getId_user(), $link->getId_module()) === FALSE) {
$linkManager->add($link);
} else {
throw new \Exception('Lien déjà existant.');
}
echo \json_encode([
'message' => 'Event joined.'
]);
return TRUE;
}
public function readParticipation($id_event) {
$token = new TokenAccess();
$id_user = $token->getId();
$linkManager = new Link_events_users_modulesManager();
$link = $linkManager->readById_user_event($id_user, $id_event);
if ($link != NULL) {
return $link->getId_module();
} else {
return 0;
}
}
public function readParticipants($id_event) {
$linkManager = new Link_events_users_modulesManager();
$listParticipants = $linkManager->readById_event($id_event);
if ($listParticipants != NULL) {
foreach ($listParticipants as $key => $value) {
$userManager = new UserManager();
$user = $userManager->readById($key);
if ($user) {
$participant = $user->toArray();
$listParticipants[$key] = $participant;
$listParticipants[$key]['participation'] = $value;
}
else {
unset($listParticipants[$key]);
}
}
return array_values($listParticipants);
}
}
public function delete($id_event) {
$token = new TokenAccess();
$id_user = $token->getId();
$linkManager = new Link_events_users_modulesManager();
$linkManager->deleteById($id_event, $id_user);
echo \json_encode([
'message' => 'Event leaved.'
]);
return TRUE;
}
}
<file_sep>/front/src/app/shared/components/comment-list/comment-list.component.ts
import { Component, OnInit, Input, Output, EventEmitter } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
import { Comment } from 'app/shared/models/comment';
import { PostService } from 'app/modules/events/post.service';
import { AuthService } from 'app/core/services/auth.service';
import { Router } from '@angular/router';
import { UserService } from 'app/modules/visitor/user.service';
import { MatDialog } from '@angular/material';
import { DialogComponent } from '../dialog/dialog.component';
@Component({
selector: 'app-comment-list',
templateUrl: './comment-list.component.html',
styleUrls: ['./comment-list.component.scss']
})
export class CommentListComponent implements OnInit {
@Input() comments: Comment[];
@Input() eventId: number;
@Input() postId: number;
@Output() updated = new EventEmitter<any>();
commentForm: FormGroup;
bEdit = false;
userId: number;
userPhotoUrl: string;
commentIdToUpdate: number;
constructor(private m_postService: PostService,
private m_formBuilder: FormBuilder,
authService: AuthService,
private m_router: Router,
private m_userService: UserService,
private m_dialog: MatDialog) {
this.commentForm = this.m_formBuilder.group({
content: ['', Validators.required]
});
this.userId = authService.getUserId();
}
ngOnInit() {
this.m_userService.get(this.userId).subscribe(result => {
this.userPhotoUrl = result.photo_url;
});
}
submitCreateForm(): void {
this.m_postService.createComment(this.eventId, this.postId, this.commentForm.value).subscribe(result => {
this.updated.emit(undefined);
}, error => {
});
}
submitEditForm(): void {
this.m_postService.updateComment(this.eventId, this.postId, this.commentIdToUpdate, this.commentForm.value).subscribe(result => {
this.bEdit = false;
this.commentIdToUpdate = undefined;
this.updated.emit(undefined);
}, error => {
});
}
deleteComment(): void {
const dialogRef = this.m_dialog.open(DialogComponent, {
width: "500px",
data: {title: `Suppression d'un commentaire`, text: `En êtes-vous bien sûr ?`, bValBtn: true}
});
dialogRef.afterClosed().subscribe(result => {
if (result.validated) {
this.m_postService.deleteComment(this.eventId, this.postId, this.commentIdToUpdate).subscribe(result => {
this.bEdit = false;
this.commentIdToUpdate = undefined;
this.updated.emit(undefined);
}, error => {
});
}
});
}
patchForm(comment: Comment | undefined): void {
if (comment == undefined) {
this.commentForm.patchValue({
content: ''
});
} else {
this.commentForm.patchValue({
content: comment.content
});
}
}
goTo(url: string) {
this.m_router.navigateByUrl(url);
}
}
<file_sep>/front/src/app/modules/visitor/user.service.ts
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
import { environment } from 'environments/environment';
@Injectable({
providedIn: 'root'
})
export class UserService {
constructor(private m_http: HttpClient) { }
create(form: JSON): Observable<any> {
const URL = `${environment.backend.serverUrl}users`;
return this.m_http.post<any>(URL, JSON.stringify(form));
}
get(id: number): Observable<any> {
const URL = `${environment.backend.serverUrl}users/${id}`;
return this.m_http.get<any>(URL);
}
update(id: number, form: JSON): Observable<any> {
const URL = `${environment.backend.serverUrl}users/${id}`;
return this.m_http.patch<any>(URL, JSON.stringify(form));
}
delete(id: number): Observable<any> {
const URL = `${environment.backend.serverUrl}users/${id}`;
return this.m_http.delete<any>(URL);
}
}
<file_sep>/front/src/app/shared/models/comment.ts
import { User } from './user';
export interface Comment {
id?: number;
content?: string;
date_created?: Date;
date_edited?: Date;
id_user?: number;
id_post?: number;
user?: User;
}<file_sep>/front/src/app/core/services/snack-bar.service.ts
import { Injectable } from '@angular/core';
import { MatSnackBar } from '@angular/material';
@Injectable({
providedIn: 'root'
})
export class SnackBarService {
constructor(private m_snackBar: MatSnackBar) { }
open(message: string, action = "ok", timeout = 5000): void {
this.m_snackBar.open(message, action, {
duration: timeout
});
}
}
<file_sep>/back/api/controllers/EventTypeCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \models\EventType;
use \models\EventTypeManager;
class EventTypeCRUD {
public function add($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$scanDataIn->exists($dataIn, ['name']);
$eventType = new EventType($data);
$eventTypeManager = new EventTypeManager();
$eventType = $eventTypeManager->add($eventType);
return $eventType;
}
public function update($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$scanDataIn->exists($data, ["id_event"]);
$eventTypeManager = new EventTypeManager();
$eventType = $eventTypeManager->readById($data["id_event"]);
$eventType->hydrate($data);
$eventType = $eventTypeManager->update($eventType);
return $eventType;
}
public function read($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$scanDataIn->exists($data, ["id"]);
$eventTypeManager = new EventTypeManager();
$eventType = $eventTypeManager->readById($data["id"]);
echo json_encode(array("name" => $eventType->GetName()));
}
public function read_OBJ($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$scanDataIn->exists($data, ["id"]);
$eventTypeManager = new EventTypeManager();
$eventType = $eventTypeManager->readById($data["id"]);
return $eventType;
}
public function delete($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$eventTypeManager = new EventTypeManager();
$eventTypeManager->deleteById($data["id"]);
return TRUE;
}
}
<file_sep>/front/src/app/shared/components/event-card/event-card.component.ts
import { Component, OnInit, Input } from '@angular/core';
import { Router } from '@angular/router';
import { Event } from 'app/shared/models/event';
import { EventService } from 'app/modules/events/event.service';
@Component({
selector: 'app-event-card',
templateUrl: './event-card.component.html',
styleUrls: ['./event-card.component.scss']
})
export class EventCardComponent implements OnInit {
@Input() event: Event;
constructor(private m_router: Router,
private m_eventService: EventService) { }
ngOnInit() {
}
goTo(url: string) {
this.m_router.navigateByUrl(url);
}
joinEvent(id: number) {
this.m_eventService.join(id).subscribe(result => {
}, error => {
})
}
leaveEvent(id: number) {
this.m_eventService.leave(id).subscribe(result => {
}, error => {
})
}
}
<file_sep>/back/api/models/PlaceManager.php
<?php
namespace models;
class PlaceManager extends DBAccess {
public function add(Place $place) {
$q = $this->db->prepare("INSERT INTO places
(`postcode`, `street`, `number`, `city`, `name`)
VALUES (:postcode, :street, :number, :city, :name);");
$q->bindValue(':postcode', $place->getPostcode());
$q->bindValue(':street', $place->getStreet());
$q->bindValue(':number', $place->getNumber());
$q->bindValue(':city', $place->getCity());
$q->bindValue(':name', $place->getName());
$q->execute();
$place->hydrate(['id' => $this->db->lastInsertId()]);
return $place;
}
public function count() {
return $this->db->query('SELECT COUNT(*) FROM places;')->fetchColumn();
}
public function readById($id) {
$q = $this->db->prepare("SELECT * FROM places WHERE id = :id;");
$q->bindValue(':id', $id);
$q->execute();
$place = $q->fetch(\PDO::FETCH_ASSOC);
if($place) {
return new Place($place);
} else {
throw new \Exception("Le lieu de l'évenement n'existe pas.", 400);
}
}
public function readByName($name) {
$q = $this->db->prepare("SELECT * FROM places WHERE name = :name");
$q->bindValue(':name', $name);
//$q->execute([':name' => $name]);
$place = $q->fetch(\PDO::FETCH_ASSOC);
return ($place) ? new Place($place) : false;
}
public function readAll() {
$allPlaces = [];
$q = $this->db->query("SELECT * FROM places");
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allPlaces[$data['id']] = new Place($data);
}
return $allPlaces;
}
public function update(Place $place) {
$q = $this->db->prepare('UPDATE places SET postcode = :postcode, street = :street, number = :number, city = :city, name = :name WHERE id = :id');
$q->bindValue(':id', $place->getId());
$q->bindValue(':postcode', $place->getPostcode());
$q->bindValue(':street', $place->getStreet());
$q->bindValue(':number', $place->getNumber());
$q->bindValue(':city', $place->getCity());
$q->bindValue(':name', $place->getName());
$q->execute();
return $place;
}
public function deleteById(int $id) {
$q = $this->db->prepare("DELETE FROM places WHERE id = :id;");
$q->bindValue(':id', $id);
return $q->execute();
}
}
<file_sep>/front/src/app/app-routing.module.ts
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { IsSignedInGuard } from './core/guards/is-signed-in.guard';
const routes: Routes = [
{
path: '',
redirectTo: 'tableau-de-bord',
pathMatch: 'full'
},
{
path: 'tableau-de-bord',
loadChildren: './modules/dashboard/dashboard.module#DashboardModule',
canActivate: [IsSignedInGuard]
},
{
path: 'notifications',
loadChildren: './modules/notifications/notifications.module#NotificationsModule',
canActivate: [IsSignedInGuard]
},
{
path: 'evenements',
loadChildren: './modules/events/events.module#EventsModule',
canActivate: [IsSignedInGuard]
},
{
path: 'profil',
loadChildren: './modules/profile/profile.module#ProfileModule',
canActivate: [IsSignedInGuard]
},
{
path: 'recherche',
loadChildren: './modules/search/search.module#SearchModule',
canActivate: [IsSignedInGuard]
},
{
path: 'visiteur',
loadChildren: './modules/visitor/visitor.module#VisitorModule'
},
{
path: 'mentions-legales',
loadChildren: './modules/legals/legals.module#LegalsModule'
},
{
path: '404',
loadChildren: './modules/page-not-found/page-not-found.module#PageNotFoundModule'
},
{
path: '**',
redirectTo: '404',
pathMatch: 'full'
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
<file_sep>/back/api/controllers/scans/ScanDataIn.php
<?php
namespace controllers\scans;
// Ensemble de méthode visant à effectuer des vérification sur des données entrantes dans l'api.
class ScanDataIn {
public function exists(array $dataIn, array $champs) {
foreach ($champs as $champ) {
if(!isset($dataIn[$champ])) {
throw new \Exception('Champ ' . $champ . ' non inexistant.', 400);
}
}
}
function validateDate($date, $format = 'Y-m-d H:i:s')
{
$d = \DateTime::createFromFormat($format, $date);
return $d && $d->format($format) === $date;
}
public function orgenize(array $dataIn, $numberIn, array $keys) {
$data = [];
for ($i=0; $i < $numberIn; $i++) {
if(!isset($dataIn[$i])) {
throw new \Exception('Champ ' . $keys[$i] . ' manquant pour satisfaire la requête.', 400);
}
$data[$keys[$i]] = $dataIn[$i];
}
return $data;
}
public function explodeSearchQuery($query) {
$keywords = [
'full'=>[],
'words'=>[]
];
\preg_match_all('/"([^"]+)"/', $query, $matches);
$keywords['full'] = $matches[1];
$splits = \preg_split('/"([^"]+)"/', $query);
foreach ($splits as $key => $split) {
$sp = preg_split('/[\s,]+/', $split);
foreach ($sp as $key => $value) {
if ($value) {
\array_push($keywords['words'], $value);
}
}
}
return $keywords;
}
public function failleXSS(array $dataIn) {
foreach ($dataIn as $data) {
if(is_string($data)) {
$data = htmlspecialchars($data);
}
}
return $dataIn;
}
}
<file_sep>/back/api/models/EventManager.php
<?php
namespace models;
class EventManager extends DBAccess {
public function add(Event $event) {
$q = $this->db->prepare("INSERT INTO events (`title`, `photo_url`, `description`, `date_start`, `date_end`, `date_created`) VALUES (:title, :photo_url, :description, :date_start, :date_end, NOW());");
$q->bindValue(':title', $event->getTitle());
$q->bindValue(':photo_url', $event->getPhoto_url());
$q->bindValue(':description', $event->getDescription());
$q->bindValue(':date_start', $event->getDate_start());
$q->bindValue(':date_end', $event->getDate_end());
$q->execute();
$event->hydrate(['id' => $this->db->lastInsertId()]);
return $event;
}
public function count() {
return $this->db->query('SELECT COUNT(*) FROM events;')->fetchColumn();
}
public function readById_noException($id) {
$q = $this->db->prepare("SELECT * FROM events WHERE id = :id");
$q->bindValue(':id', $id);
$q->execute();
$event = $q->fetch(\PDO::FETCH_ASSOC);
return $event;
}
public function readById($id) {
$q = $this->db->prepare("SELECT * FROM events WHERE id = :id");
$q->bindValue(':id', $id);
$q->execute();
$event = $q->fetch(\PDO::FETCH_ASSOC);
if ($event) {
return new Event($event);
}
else {
throw new \Exception("Aucun événement ne correspond à l'id $id", 400);
}
}
public function readOffsetLimit(int $offset, int $limit) {
$allEvents = [];
$q = $this->db->prepare("SELECT * FROM events WHERE date_start >= DATE_SUB(NOW(), INTERVAL 1 MONTH) OR date_start IS NULL ORDER BY CASE WHEN date_start IS NULL THEN 1 ELSE 0 END, date_start LIMIT :limit OFFSET :offset");
$q->bindValue(":limit", (int) $limit, \PDO::PARAM_INT);
$q->bindValue(':offset', (int) $offset, \PDO::PARAM_INT);
$q->execute();
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allEvents[$data['id']] = new Event($data);
}
return $allEvents;
}
public function readAll() {
$allEvents = [];
$q = $this->db->query("SELECT * FROM events WHERE date_start IS NOT NULL AND date_start >= NOW() ORDER BY date_start ASC LIMIT 10;");
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allEvents[$data['id']] = new Event($data);
}
return $allEvents;
}
public function search($keywords) {
$allEvents = [];
$query = "SELECT * FROM events";
$i = 0;
foreach ($keywords as $key => $keyword) {
if ($i != 0) $query .= " AND";
else $query .= " WHERE";
$query .= " title LIKE '%$keyword%'";
$i++;
}
$query .= ";";
$q = $this->db->query($query);
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allEvents[$data['id']] = new Event($data);
}
return $allEvents;
}
public function readAllValid() {
$allEvents = [];
$q = $this->db->query("SELECT * FROM events WHERE date_start < date_end;");
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allEvents[$data['id']] = new Event($data);
}
return $allEvents;
}
public function update(Event $event) {
$q = $this->db->prepare('UPDATE events SET title = :title, photo_url = :photo_url, description = :description, date_start = :date_start, date_end = :date_end, date_created = :date_created WHERE id = :id');
$q->bindValue(':id', $event->getId());
$q->bindValue(':title', $event->getTitle());
$q->bindValue(':photo_url', $event->getPhoto_url());
$q->bindValue(':description', $event->getDescription());
$q->bindValue(':date_start', $event->getDate_start());
$q->bindValue(':date_end', $event->getDate_end());
$q->bindValue(':date_created', $event->getDate_created());
$q->execute();
return $event;
}
public function deleteById(int $id) {
$this->db->exec("DELETE FROM events WHERE id = {$id};");
return TRUE;
}
}
<file_sep>/front/src/app/modules/visitor/inscription/inscription.component.ts
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, Validators } from '@angular/forms';
import { Router } from '@angular/router';
import { PasswordMatch } from 'app/shared/validators/password-match';
import { UserService } from '../user.service';
import { HttpErrorResponse } from '@angular/common/http';
import { ImageUrl } from 'app/shared/validators/image-url';
import { ResponsiveService } from 'app/core/services/responsive.service';
@Component({
selector: 'app-inscription',
templateUrl: './inscription.component.html',
styleUrls: ['./inscription.component.scss']
})
export class InscriptionComponent implements OnInit {
inscriptionForm: FormGroup;
bInscriptionDone = false;
bMobile = true;
constructor(private m_formBuilder: FormBuilder,
private m_userService: UserService,
private m_router: Router,
responsiveService: ResponsiveService) {
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
this.inscriptionForm = this.m_formBuilder.group({
page1: this.m_formBuilder.group({
pseudo: ['', Validators.required],
mail: ['', Validators.compose([
Validators.required,
Validators.pattern(/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/)
])],
year_promotion: ['', Validators.required]
}),
page2: this.m_formBuilder.group({
phone: '',
firstname: ['', Validators.required],
lastname: ['', Validators.required],
photo_url: ['https://i2.wp.com/rouelibrenmaine.fr/wp-content/uploads/2018/10/empty-avatar.png', Validators.required]
}, {
validators: ImageUrl('photo_url')
}),
page3: this.m_formBuilder.group({
pwd: ['', Validators.compose([
Validators.required,
Validators.minLength(4)
])],
confirm_pwd: ['', Validators.compose([
Validators.required,
Validators.minLength(4)
])]
}, {
validators: PasswordMatch('pwd','<PASSWORD>')
})
});
}
ngOnInit() {}
submitForm(): void {
// Prepare form
const form = Object.assign({}, this.inscriptionForm.value.page1, this.inscriptionForm.value.page2, this.inscriptionForm.value.page3);
delete form.confirm_pwd;
// Send form
this.m_userService.create(form).subscribe(response => {
this.bInscriptionDone = true;
setTimeout(() => {
this.m_router.navigate(['/visiteur/login]']);
}, 2500);
}, (error: HttpErrorResponse) => {
// TODO handles error
});
}
goTo(url: string): void {
this.m_router.navigate([url]);
}
}
<file_sep>/back/api/controllers/scans/TokenCreater.php
<?php
namespace controllers\scans;
use \Config;
use \vendor\jwt\src\JWT;
class TokenCreater {
public function createToken($id) {
$config = new Config();
/* NOTE: mcrypt_create_iv function was DEPRECATED in PHP 7.1.0, and REMOVED in PHP 7.2.0.
$tokenId = base64_encode(mcrypt_create_iv(32));*/
$tokenId = base64_encode(random_bytes(32));
$issuedAt = time();
$notBefore = $issuedAt + 10;
$expire = $notBefore + 10800;
$serverName = $config->getServerName();
$data = [
'iat' => $issuedAt,
'jti' => $tokenId,
'iss' => $serverName,
'nbf' => $notBefore,
'exp' => $expire,
'data' => [
'userId' => $id
]
];
$jwt = JWT::encode($data, $config->getJwtKey(),'HS512');
$unencodedArray = [
'jwt' => $jwt,
'id_user' => $id
];
echo json_encode($unencodedArray);
}
}
<file_sep>/back/api/models/EventTypeManager.php
<?php
namespace models;
class EventTypeManager extends DBAccess {
public function add(EventType $eventType) {
$q = $this->db->prepare("INSERT INTO eventtypes
(`name`)
VALUES (:name);");
$q->bindValue(':name', $eventType->getName());
$q->execute();
$eventType->hydrate(['id' => $this->db->lastInsertId()]);
return $eventType;
}
public function readById($id) {
$q = $this->db->prepare("SELECT * FROM eventtypes WHERE id = :id;");
$q->bindValue(':id', $id);
$q->execute();
$eventType = $q->fetch(\PDO::FETCH_ASSOC);
//\var_dump($place);
$eventTypeObj = new EventType($eventType);
if($eventTypeObj) {
return $eventTypeObj;
} else {
throw new \Exception("Le type n'existe pas.", 400);
}
}
public function readByName($name) {
$q = $this->db->prepare("SELECT * FROM eventtypes WHERE name = :name");
$q->bindValue(':name', $name);
//$q->execute([':name' => $name]);
$eventType = $q->fetch(\PDO::FETCH_ASSOC);
return ($eventType) ? new EventType($eventType) : false;
}
public function readAll() {
$allEventTypes = [];
$q = $this->db->query("SELECT * FROM eventtypes");
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allEventTypes[$data['id']] = new EventType($data);
}
return $allEventTypes;
}
public function update(EventType $eventType) {
$q = $this->db->prepare('UPDATE eventtypes SET name = :name WHERE id = :id');
$q->bindValue(':name', $eventType->getName());
$q->execute();
return $eventType;
}
public function deleteById(int $id) {
$q = $this->db->prepare("DELETE FROM eventtypes WHERE id = :id;");
$q->bindValue(':id', $id);
return $q->execute();
}
}
<file_sep>/front/documentation/js/menu-wc.js
'use strict';
customElements.define('compodoc-menu', class extends HTMLElement {
constructor() {
super();
this.isNormalMode = this.getAttribute('mode') === 'normal';
}
connectedCallback() {
this.render(this.isNormalMode);
}
render(isNormalMode) {
let tp = lithtml.html(`
<nav>
<ul class="list">
<li class="title">
<a href="index.html" data-type="index-link">imac-resoi-front documentation</a>
</li>
<li class="divider"></li>
${ isNormalMode ? `<div id="book-search-input" role="search"><input type="text" placeholder="Type to search"></div>` : '' }
<li class="chapter">
<a data-type="chapter-link" href="index.html"><span class="icon ion-ios-home"></span>Getting started</a>
<ul class="links">
<li class="link">
<a href="overview.html" data-type="chapter-link">
<span class="icon ion-ios-keypad"></span>Overview
</a>
</li>
<li class="link">
<a href="index.html" data-type="chapter-link">
<span class="icon ion-ios-paper"></span>README
</a>
</li>
<li class="link">
<a href="license.html" data-type="chapter-link">
<span class="icon ion-ios-paper"></span>LICENSE
</a>
</li>
<li class="link">
<a href="dependencies.html" data-type="chapter-link">
<span class="icon ion-ios-list"></span>Dependencies
</a>
</li>
</ul>
</li>
<li class="chapter modules">
<a data-type="chapter-link" href="modules.html">
<div class="menu-toggler linked" data-toggle="collapse" ${ isNormalMode ?
'data-target="#modules-links"' : 'data-target="#xs-modules-links"' }>
<span class="icon ion-ios-archive"></span>
<span class="link-name">Modules</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
</a>
<ul class="links collapse" ${ isNormalMode ? 'id="modules-links"' : 'id="xs-modules-links"' }>
<li class="link">
<a href="modules/AppModule.html" data-type="entity-link">AppModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-AppModule-1baba09bc7b7a38c6798e3b55ffefd74"' : 'data-target="#xs-components-links-module-AppModule-1baba09bc7b7a38c6798e3b55ffefd74"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-AppModule-1baba09bc7b7a38c6798e3b55ffefd74"' :
'id="xs-components-links-module-AppModule-1baba09bc7b7a38c6798e3b55ffefd74"' }>
<li class="link">
<a href="components/AppComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">AppComponent</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/AppRoutingModule.html" data-type="entity-link">AppRoutingModule</a>
</li>
<li class="link">
<a href="modules/AppServerModule.html" data-type="entity-link">AppServerModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-AppServerModule-6f845fdce6d80526e11ce4cddb70e623"' : 'data-target="#xs-components-links-module-AppServerModule-6f845fdce6d80526e11ce4cddb70e623"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-AppServerModule-6f845fdce6d80526e11ce4cddb70e623"' :
'id="xs-components-links-module-AppServerModule-6f845fdce6d80526e11ce4cddb70e623"' }>
<li class="link">
<a href="components/AppComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">AppComponent</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/CoreMaterialModule.html" data-type="entity-link">CoreMaterialModule</a>
</li>
<li class="link">
<a href="modules/CoreModule.html" data-type="entity-link">CoreModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-CoreModule-019fda25406c3d315c97aa9cf0a552fe"' : 'data-target="#xs-components-links-module-CoreModule-019fda25406c3d315c97aa9cf0a552fe"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-CoreModule-019fda25406c3d315c97aa9cf0a552fe"' :
'id="xs-components-links-module-CoreModule-019fda25406c3d315c97aa9cf0a552fe"' }>
<li class="link">
<a href="components/BottomNavigationComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">BottomNavigationComponent</a>
</li>
<li class="link">
<a href="components/TopNavigationComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">TopNavigationComponent</a>
</li>
</ul>
</li>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#injectables-links-module-CoreModule-019fda25406c3d315c97aa9cf0a552fe"' : 'data-target="#xs-injectables-links-module-CoreModule-019fda25406c3d315c97aa9cf0a552fe"' }>
<span class="icon ion-md-arrow-round-down"></span>
<span>Injectables</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="injectables-links-module-CoreModule-019fda25406c3d315c97aa9cf0a552fe"' :
'id="xs-injectables-links-module-CoreModule-019fda25406c3d315c97aa9cf0a552fe"' }>
<li class="link">
<a href="injectables/AuthService.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules" }>AuthService</a>
</li>
<li class="link">
<a href="injectables/ResponsiveService.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules" }>ResponsiveService</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/CreateEventModule.html" data-type="entity-link">CreateEventModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-CreateEventModule-1dc31bbf58b03dbe752879b28960acc3"' : 'data-target="#xs-components-links-module-CreateEventModule-1dc31bbf58b03dbe752879b28960acc3"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-CreateEventModule-1dc31bbf58b03dbe752879b28960acc3"' :
'id="xs-components-links-module-CreateEventModule-1dc31bbf58b03dbe752879b28960acc3"' }>
<li class="link">
<a href="components/CreateEventComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">CreateEventComponent</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/CreateEventRoutingModule.html" data-type="entity-link">CreateEventRoutingModule</a>
</li>
<li class="link">
<a href="modules/DashboardModule.html" data-type="entity-link">DashboardModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-DashboardModule-eee20dbd623b64326757842e12126d70"' : 'data-target="#xs-components-links-module-DashboardModule-eee20dbd623b64326757842e12126d70"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-DashboardModule-eee20dbd623b64326757842e12126d70"' :
'id="xs-components-links-module-DashboardModule-eee20dbd623b64326757842e12126d70"' }>
<li class="link">
<a href="components/DashboardComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">DashboardComponent</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/DashboardRoutingModule.html" data-type="entity-link">DashboardRoutingModule</a>
</li>
<li class="link">
<a href="modules/EditEventModule.html" data-type="entity-link">EditEventModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-EditEventModule-6b834132e3de09ab0c237f559d6bb3db"' : 'data-target="#xs-components-links-module-EditEventModule-6b834132e3de09ab0c237f559d6bb3db"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-EditEventModule-6b834132e3de09ab0c237f559d6bb3db"' :
'id="xs-components-links-module-EditEventModule-6b834132e3de09ab0c237f559d6bb3db"' }>
<li class="link">
<a href="components/EditEventComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">EditEventComponent</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/EditEventRoutingModule.html" data-type="entity-link">EditEventRoutingModule</a>
</li>
<li class="link">
<a href="modules/EventDetailModule.html" data-type="entity-link">EventDetailModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-EventDetailModule-494bd5a76125960439a258868cb76a69"' : 'data-target="#xs-components-links-module-EventDetailModule-494bd5a76125960439a258868cb76a69"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-EventDetailModule-494bd5a76125960439a258868cb76a69"' :
'id="xs-components-links-module-EventDetailModule-494bd5a76125960439a258868cb76a69"' }>
<li class="link">
<a href="components/EventDetailComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">EventDetailComponent</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/EventDetailRoutingModule.html" data-type="entity-link">EventDetailRoutingModule</a>
</li>
<li class="link">
<a href="modules/EventsModule.html" data-type="entity-link">EventsModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#injectables-links-module-EventsModule-b1488a9ad3d6042e16afdec85bc68306"' : 'data-target="#xs-injectables-links-module-EventsModule-b1488a9ad3d6042e16afdec85bc68306"' }>
<span class="icon ion-md-arrow-round-down"></span>
<span>Injectables</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="injectables-links-module-EventsModule-b1488a9ad3d6042e16afdec85bc68306"' :
'id="xs-injectables-links-module-EventsModule-b1488a9ad3d6042e16afdec85bc68306"' }>
<li class="link">
<a href="injectables/EventService.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules" }>EventService</a>
</li>
<li class="link">
<a href="injectables/PostService.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules" }>PostService</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/EventsRoutingModule.html" data-type="entity-link">EventsRoutingModule</a>
</li>
<li class="link">
<a href="modules/LegalsModule.html" data-type="entity-link">LegalsModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-LegalsModule-3a7232d3d4fd893148edc73ce1c0b8ac"' : 'data-target="#xs-components-links-module-LegalsModule-3a7232d3d4fd893148edc73ce1c0b8ac"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-LegalsModule-3a7232d3d4fd893148edc73ce1c0b8ac"' :
'id="xs-components-links-module-LegalsModule-3a7232d3d4fd893148edc73ce1c0b8ac"' }>
<li class="link">
<a href="components/LegalsComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">LegalsComponent</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/LegalsRoutingModule.html" data-type="entity-link">LegalsRoutingModule</a>
</li>
<li class="link">
<a href="modules/NotificationsModule.html" data-type="entity-link">NotificationsModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-NotificationsModule-080eb549465e4180c88c86e652079db6"' : 'data-target="#xs-components-links-module-NotificationsModule-080eb549465e4180c88c86e652079db6"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-NotificationsModule-080eb549465e4180c88c86e652079db6"' :
'id="xs-components-links-module-NotificationsModule-080eb549465e4180c88c86e652079db6"' }>
<li class="link">
<a href="components/NotificationsComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">NotificationsComponent</a>
</li>
</ul>
</li>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#injectables-links-module-NotificationsModule-080eb549465e4180c88c86e652079db6"' : 'data-target="#xs-injectables-links-module-NotificationsModule-080eb549465e4180c88c86e652079db6"' }>
<span class="icon ion-md-arrow-round-down"></span>
<span>Injectables</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="injectables-links-module-NotificationsModule-080eb549465e4180c88c86e652079db6"' :
'id="xs-injectables-links-module-NotificationsModule-080eb549465e4180c88c86e652079db6"' }>
<li class="link">
<a href="injectables/NotificationsService.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules" }>NotificationsService</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/NotificationsRoutingModule.html" data-type="entity-link">NotificationsRoutingModule</a>
</li>
<li class="link">
<a href="modules/PageNotFoundModule.html" data-type="entity-link">PageNotFoundModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-PageNotFoundModule-0bc830147d4044f6d50a71f02bb3e477"' : 'data-target="#xs-components-links-module-PageNotFoundModule-0bc830147d4044f6d50a71f02bb3e477"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-PageNotFoundModule-0bc830147d4044f6d50a71f02bb3e477"' :
'id="xs-components-links-module-PageNotFoundModule-0bc830147d4044f6d50a71f02bb3e477"' }>
<li class="link">
<a href="components/PageNotFoundComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">PageNotFoundComponent</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/PageNotFoundRoutingModule.html" data-type="entity-link">PageNotFoundRoutingModule</a>
</li>
<li class="link">
<a href="modules/ProfileModule.html" data-type="entity-link">ProfileModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-ProfileModule-3a43e8cd5888c0a8cc903ffad7e09556"' : 'data-target="#xs-components-links-module-ProfileModule-3a43e8cd5888c0a8cc903ffad7e09556"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-ProfileModule-3a43e8cd5888c0a8cc903ffad7e09556"' :
'id="xs-components-links-module-ProfileModule-3a43e8cd5888c0a8cc903ffad7e09556"' }>
<li class="link">
<a href="components/ChangePasswordComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">ChangePasswordComponent</a>
</li>
<li class="link">
<a href="components/EditProfileComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">EditProfileComponent</a>
</li>
<li class="link">
<a href="components/ProfileComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">ProfileComponent</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/ProfileRoutingModule.html" data-type="entity-link">ProfileRoutingModule</a>
</li>
<li class="link">
<a href="modules/SearchModule.html" data-type="entity-link">SearchModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-SearchModule-f91f897b9a44ee7da0d12eae88e59247"' : 'data-target="#xs-components-links-module-SearchModule-f91f897b9a44ee7da0d12eae88e59247"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-SearchModule-f91f897b9a44ee7da0d12eae88e59247"' :
'id="xs-components-links-module-SearchModule-f91f897b9a44ee7da0d12eae88e59247"' }>
<li class="link">
<a href="components/SearchComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">SearchComponent</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/SearchRoutingModule.html" data-type="entity-link">SearchRoutingModule</a>
</li>
<li class="link">
<a href="modules/SharedMaterialModule.html" data-type="entity-link">SharedMaterialModule</a>
</li>
<li class="link">
<a href="modules/SharedModule.html" data-type="entity-link">SharedModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-SharedModule-d4a7898cfced2451f209e2a9c9529e5b"' : 'data-target="#xs-components-links-module-SharedModule-d4a7898cfced2451f209e2a9c9529e5b"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-SharedModule-d4a7898cfced2451f209e2a9c9529e5b"' :
'id="xs-components-links-module-SharedModule-d4a7898cfced2451f209e2a9c9529e5b"' }>
<li class="link">
<a href="components/CommentListComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">CommentListComponent</a>
</li>
<li class="link">
<a href="components/EventCardComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">EventCardComponent</a>
</li>
<li class="link">
<a href="components/PostListComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">PostListComponent</a>
</li>
<li class="link">
<a href="components/TopAppBarComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">TopAppBarComponent</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/VisitorModule.html" data-type="entity-link">VisitorModule</a>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#components-links-module-VisitorModule-44b744e02433e4a304802c512af792b7"' : 'data-target="#xs-components-links-module-VisitorModule-44b744e02433e4a304802c512af792b7"' }>
<span class="icon ion-md-cog"></span>
<span>Components</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="components-links-module-VisitorModule-44b744e02433e4a304802c512af792b7"' :
'id="xs-components-links-module-VisitorModule-44b744e02433e4a304802c512af792b7"' }>
<li class="link">
<a href="components/ForgotComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">ForgotComponent</a>
</li>
<li class="link">
<a href="components/InscriptionComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">InscriptionComponent</a>
</li>
<li class="link">
<a href="components/InvitationComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">InvitationComponent</a>
</li>
<li class="link">
<a href="components/LoginComponent.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules">LoginComponent</a>
</li>
</ul>
</li>
<li class="chapter inner">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ?
'data-target="#injectables-links-module-VisitorModule-44b744e02433e4a304802c512af792b7"' : 'data-target="#xs-injectables-links-module-VisitorModule-44b744e02433e4a304802c512af792b7"' }>
<span class="icon ion-md-arrow-round-down"></span>
<span>Injectables</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="injectables-links-module-VisitorModule-44b744e02433e4a304802c512af792b7"' :
'id="xs-injectables-links-module-VisitorModule-44b744e02433e4a304802c512af792b7"' }>
<li class="link">
<a href="injectables/UserService.html"
data-type="entity-link" data-context="sub-entity" data-context-id="modules" }>UserService</a>
</li>
</ul>
</li>
</li>
<li class="link">
<a href="modules/VisitorRoutingModule.html" data-type="entity-link">VisitorRoutingModule</a>
</li>
</ul>
</li>
<li class="chapter">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ? 'data-target="#injectables-links"' :
'data-target="#xs-injectables-links"' }>
<span class="icon ion-md-arrow-round-down"></span>
<span>Injectables</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="injectables-links"' : 'id="xs-injectables-links"' }>
<li class="link">
<a href="injectables/AuthService.html" data-type="entity-link">AuthService</a>
</li>
<li class="link">
<a href="injectables/EventService.html" data-type="entity-link">EventService</a>
</li>
<li class="link">
<a href="injectables/NotificationsService.html" data-type="entity-link">NotificationsService</a>
</li>
<li class="link">
<a href="injectables/NotificationsService-1.html" data-type="entity-link">NotificationsService</a>
</li>
<li class="link">
<a href="injectables/PostService.html" data-type="entity-link">PostService</a>
</li>
<li class="link">
<a href="injectables/ResponsiveService.html" data-type="entity-link">ResponsiveService</a>
</li>
<li class="link">
<a href="injectables/SnackBarService.html" data-type="entity-link">SnackBarService</a>
</li>
<li class="link">
<a href="injectables/UserService.html" data-type="entity-link">UserService</a>
</li>
</ul>
</li>
<li class="chapter">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ? 'data-target="#interceptors-links"' :
'data-target="#xs-interceptors-links"' }>
<span class="icon ion-ios-swap"></span>
<span>Interceptors</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="interceptors-links"' : 'id="xs-interceptors-links"' }>
<li class="link">
<a href="interceptors/AuthInterceptor.html" data-type="entity-link">AuthInterceptor</a>
</li>
<li class="link">
<a href="interceptors/ErrorInterceptor.html" data-type="entity-link">ErrorInterceptor</a>
</li>
</ul>
</li>
<li class="chapter">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ? 'data-target="#guards-links"' :
'data-target="#xs-guards-links"' }>
<span class="icon ion-ios-lock"></span>
<span>Guards</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="guards-links"' : 'id="xs-guards-links"' }>
<li class="link">
<a href="guards/IsSignedInGuard.html" data-type="entity-link">IsSignedInGuard</a>
</li>
</ul>
</li>
<li class="chapter">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ? 'data-target="#interfaces-links"' :
'data-target="#xs-interfaces-links"' }>
<span class="icon ion-md-information-circle-outline"></span>
<span>Interfaces</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? ' id="interfaces-links"' : 'id="xs-interfaces-links"' }>
<li class="link">
<a href="interfaces/Comment.html" data-type="entity-link">Comment</a>
</li>
<li class="link">
<a href="interfaces/Event.html" data-type="entity-link">Event</a>
</li>
<li class="link">
<a href="interfaces/Notification.html" data-type="entity-link">Notification</a>
</li>
<li class="link">
<a href="interfaces/Place.html" data-type="entity-link">Place</a>
</li>
<li class="link">
<a href="interfaces/Post.html" data-type="entity-link">Post</a>
</li>
<li class="link">
<a href="interfaces/User.html" data-type="entity-link">User</a>
</li>
</ul>
</li>
<li class="chapter">
<div class="simple menu-toggler" data-toggle="collapse" ${ isNormalMode ? 'data-target="#miscellaneous-links"'
: 'data-target="#xs-miscellaneous-links"' }>
<span class="icon ion-ios-cube"></span>
<span>Miscellaneous</span>
<span class="icon ion-ios-arrow-down"></span>
</div>
<ul class="links collapse" ${ isNormalMode ? 'id="miscellaneous-links"' : 'id="xs-miscellaneous-links"' }>
<li class="link">
<a href="miscellaneous/enumerations.html" data-type="entity-link">Enums</a>
</li>
<li class="link">
<a href="miscellaneous/functions.html" data-type="entity-link">Functions</a>
</li>
<li class="link">
<a href="miscellaneous/variables.html" data-type="entity-link">Variables</a>
</li>
</ul>
</li>
<li class="chapter">
<a data-type="chapter-link" href="coverage.html"><span class="icon ion-ios-stats"></span>Documentation coverage</a>
</li>
<li class="divider"></li>
<li class="copyright">
Documentation generated using <a href="https://compodoc.app/" target="_blank">
<img data-src="images/compodoc-vectorise.png" class="img-responsive" data-type="compodoc-logo">
</a>
</li>
</ul>
</nav>
`);
this.innerHTML = tp.strings;
}
});<file_sep>/back/api/controllers/UserCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \controllers\scans\TokenAccess;
use \controllers\scans\TokenCreater;
use \models\User;
use \models\UserManager;
class UserCRUD {
public function add($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["firstname", "lastname", "pseudo", "pwd", "mail", "photo_url", "year_promotion"]);
$data = $scanDataIn->failleXSS($dataIn);
$data["pwd_hash"] = password_hash($data["pwd"], PASSWORD_DEFAULT);
$user = new User($data);
$userManager = new UserManager();
if ($userManager->readByPseudo($user->getPseudo()) === FALSE) {
$userManager->add($user);
} else {
throw new \Exception('Pseudo déjà existant.', 400);
}
echo json_encode([
"message" => "User added.",
]);;
}
public function update($dataIn) {
// TODO: vérifier les droits
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
$token->acompteAccess($data["id"]);
$userManager = new UserManager();
if (isset($data['pwd'])) {
$data['pwd_hash'] = password_hash($data["pwd"], PASSWORD_DEFAULT);
unset($data['pwd']);
}
$user = $userManager->readById($data["id"]);
if($user) {
$user->hydrate($data);
$userManager->update($user);
} else {
throw new Exception("L'utilisateur n'existe pas.", 400);
}
echo json_encode([
"message" => "User updated.",
]);;
}
public function read($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$data = $scanDataIn->orgenize($data, 2, ["type", "id"]);
$userManager = new UserManager();
$user = $userManager->readById($data["id"]);
if($user) {
echo json_encode([
"firstname" => $user->GetFirstname(),
"lastname" => $user->GetLastname(),
"pseudo" => $user->GetPseudo(),
"photo_url" => $user->GetPhoto_url(),
"status" => $user->GetStatus(),
"phone" => $user->GetPhone(),
"mail" => $user->GetMail(),
"year_promotion" => $user->getYear_promotion()
]);
} else {
throw new Exception("L'utilisateur n'existe pas.", 400);
}
}
public function delete($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
if($token->acompteAccess($data["id"]) OR $token->adminAccess(1)) {
$userManager = new UserManager();
$userManager->deleteById($data["id"]);
echo json_encode([
"message" => "User deleted.",
]);;
}
}
public function auth($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["pseudo", "pwd"]);
$data = $scanDataIn->failleXSS($dataIn);
$userManager = new UserManager();
$user = $userManager->readByPseudo($data["pseudo"]);
if ($user == FALSE) {
throw new \Exception("Le nom d'utilisateur est incorrect.", 401);
} else {
if(password_verify($data["pwd"], $user->getPwd_hash())) {
$tokenCreater = new TokenCreater();
$tokenCreater->createToken($user->GetId());
} else {
throw new \Exception("Le mot de passe est incorrect.", 401);
}
}
}
}
<file_sep>/front/src/app/modules/events/event-detail/event-detail.component.ts
import { Component, OnInit, ViewChild, ElementRef } from '@angular/core';
import { ActivatedRoute, Router } from '@angular/router';
import { Event } from 'app/shared/models/event';
import { EventService } from '../event.service';
import { ResponsiveService } from 'app/core/services/responsive.service';
import { MatDialog } from '@angular/material';
import { DialogComponent } from 'app/shared/components/dialog/dialog.component';
@Component({
selector: 'app-event-detail',
templateUrl: './event-detail.component.html',
styleUrls: ['./event-detail.component.scss']
})
export class EventDetailComponent implements OnInit {
@ViewChild('place') place: ElementRef;
event: Event;
bMobile = true;
bNoHour = true;
eventId: number;
constructor(private m_eventService: EventService,
route: ActivatedRoute,
private m_router: Router,
responsiveService: ResponsiveService,
private m_dialog: MatDialog) {
this.eventId = Number(route.snapshot.paramMap.get('id'));
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
}
ngOnInit() {
this.m_eventService.getEventById(this.eventId).subscribe(result => {
this.event = result;
if (result.date_start != '') {
const date = new Date(result.date_start);
if (date.getHours() != 0) {
this.bNoHour = false;
};
}
}, error => {
});
}
goTo(url: string) {
this.m_router.navigateByUrl(url);
}
joinEvent() {
this.m_eventService.join(this.eventId).subscribe(result => {
}, error => {
})
}
leaveEvent() {
this.m_eventService.leave(this.eventId).subscribe(result => {
}, error => {
})
}
reloadPosts(): void {
this.m_eventService.getEventById(this.eventId).subscribe(result => {
this.event.posts = result.posts;
}, error => {
});
}
getIcsLink(): void {
this.m_eventService.getIcsLink(this.eventId).subscribe(result => {
const dialogRef = this.m_dialog.open(DialogComponent, {
width: "500px",
data: {title: `Export de l'évènement`, text: `Copiez ce lien dans votre application de calendrier: ${result.url} `}
});
}, error => {
});
}
scrollToPlace(): void {
const target = this.place.nativeElement;
target.scrollIntoView({behavior: 'smooth'});
}
log(text: string): void {
console.log(text);
}
}
<file_sep>/front/src/app/modules/profile/change-password/change-password.component.ts
import { Component, OnInit } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
import { Router } from '@angular/router';
import { AuthService } from 'app/core/services/auth.service';
import { UserService } from 'app/modules/visitor/user.service';
import { PasswordMatch } from 'app/shared/validators/password-match';
import { ResponsiveService } from 'app/core/services/responsive.service';
@Component({
selector: 'app-change-password',
templateUrl: './change-password.component.html',
styleUrls: ['./change-password.component.scss']
})
export class ChangePasswordComponent implements OnInit {
userForm: FormGroup;
bUpdated = false;
bMobile: boolean;
constructor(private m_router: Router,
private m_formBuilder: FormBuilder,
private m_userService: UserService,
private m_authService: AuthService,
responsiveService: ResponsiveService) {
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
this.userForm = this.m_formBuilder.group({
pwd: ['', Validators.compose([
Validators.required,
Validators.minLength(4)
])],
confirm_pwd: ['', Validators.compose([
Validators.required,
Validators.minLength(4)
])]
}, {
validators: PasswordMatch('pwd','confirm_pwd')
});
}
ngOnInit() {
}
submitForm(): void {
let form = this.userForm.value;
form.id = this.m_authService.getUserId();
delete form.confirm_pwd;
this.m_userService.update(this.m_authService.getUserId(), form).subscribe(result => {
this.m_router.navigate(['/profil']);
}, error => {
});
}
goTo(url: string): void {
this.m_router.navigate([url]);
}
}
<file_sep>/front/src/app/shared/directives/defer-load.directive.ts
import { Directive, ElementRef, EventEmitter, Output } from '@angular/core';
@Directive({
selector: '[appDeferLoad]'
})
export class DeferLoadDirective {
@Output() public discovered: EventEmitter<any> = new EventEmitter();
private m_intersectionObserver?: IntersectionObserver;
constructor(private m_element: ElementRef) { }
ngAfterViewInit() {
this.m_intersectionObserver = new IntersectionObserver(entries => {
this.checkForIntersection(entries);
}, {});
this.m_intersectionObserver.observe(<Element>(this.m_element.nativeElement));
}
private checkForIntersection = (entries: Array<IntersectionObserverEntry>) => {
entries.forEach((entry: IntersectionObserverEntry) => {
if (this.checkIfIntersecting(entry)) {
this.discovered.emit();
this.m_intersectionObserver.unobserve(<Element>(this.m_element.nativeElement));
this.m_intersectionObserver.disconnect();
}
});
}
private checkIfIntersecting(entry: IntersectionObserverEntry) {
return (<any>entry).isIntersecting && entry.target === this.m_element.nativeElement;
}
}
<file_sep>/back/api/controllers/PlaceCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \models\Place;
use \models\PlaceManager;
class PlaceCRUD {
public function add($dataIn) {
$scanDataIn = new ScanDataIn();
//$scanDataIn->exists($dataIn, ["postcode", "street", "number", "city", "name"]);
$data = $scanDataIn->failleXSS($dataIn);
$place = new Place($data);
$placeManager = new PlaceManager();
$place = $placeManager->add($place);
return $place;
}
public function update($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$placeManager = new PlaceManager();
$place = $placeManager->readById($data["id"]);
$place->hydrate($data);
$place = $placeManager->update($place);
return $place;
}
public function read($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$scanDataIn->exists($data, ["id"]);
$placeManager = new PlaceManager();
$place = $placeManager->readById($data["id"]);
echo json_encode(array("name" => $place->GetName(), "postcode" => $place->GetPostcode(), "street" => $place->GetStreet(), "number" => $place->GetNumber(), "city" => $place->GetCity()));
}
public function read_OBJ($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$scanDataIn->exists($data, ["id"]);
$placeManager = new PlaceManager();
$place = $placeManager->readById($data["id"]);
return $place;
}
public function delete($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$placeManager = new PlaceManager();
$placeManager->deleteById($data["id"]);
return TRUE;
}
}
<file_sep>/front/src/app/modules/visitor/invitation/invitation.component.ts
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, Validators } from '@angular/forms';
import { ResponsiveService } from 'app/core/services/responsive.service';
import { Router } from '@angular/router';
@Component({
selector: 'app-invitation',
templateUrl: './invitation.component.html',
styleUrls: ['./invitation.component.scss']
})
export class InvitationComponent implements OnInit {
invitationForm: FormGroup;
bMobile: boolean;
constructor(private m_formBuilder: FormBuilder,
private m_router: Router,
responsiveService: ResponsiveService) {
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
this.invitationForm = this.m_formBuilder.group({
email: ['', Validators.compose([
Validators.required,
Validators.email
])],
name: ['', Validators.compose([
Validators.required,
Validators.minLength(4)
])],
promotion: ['', Validators.required],
message: ['']
});
}
ngOnInit() {
}
goTo(url: string): void {
this.m_router.navigate([url]);
}
}
<file_sep>/back/api/models/Comment.php
<?php
namespace models;
class Comment {
protected $id,
$content,
$date_created,
$date_edited,
$id_user,
$id_post;
public function __construct(array $data) {
$this->hydrate($data);
}
public function hydrate(array $data) {
foreach ($data as $key => $value) {
$method = 'set'.ucfirst($key);
if (method_exists($this, $method)) {
$this->$method($value);
}
}
}
public function toArray() {
$array = [
"id" => $this->getId(),
"content" => $this->getContent(),
"date_created" => $this->getDate_created(),
"date_edited" => $this->getDate_edited(),
"id_user" => $this->getId_user(),
"id_post" => $this->getId_post()
];
return $array;
}
public function getId() {
return $this->id;
}
public function getContent() {
return $this->content;
}
public function getDate_created() {
return $this->date_created;
}
public function getDate_edited() {
return $this->date_edited;
}
public function getId_user() {
return $this->id_user;
}
public function getId_post() {
return $this->id_post;
}
public function setId($id) {
$id = (int) $id;
if ($id > 0) {
$this->id = $id;
}
}
public function setContent($content) {
if (is_string($content) && strlen($content) <= 5000) {
$this->content = $content;
}
}
public function setDate_created($date_created) {
if (is_string($date_created)) {
$this->date_created = $date_created;
}
}
public function setDate_edited($date_edited) {
if (is_string($date_edited)) {
$this->date_edited = $date_edited;
}
}
public function setId_user($id_user) {
$id_user = (int) $id_user;
if ($id_user > 0) {
$this->id_user = $id_user;
}
}
public function setId_post($id_post) {
$id_post = (int) $id_post;
if ($id_post > 0) {
$this->id_post = $id_post;
}
}
}<file_sep>/front/src/app/modules/profile/edit-profile/edit-profile.component.ts
import { Component, OnInit } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
import { Router } from '@angular/router';
import { ResponsiveService } from 'app/core/services/responsive.service';
import { UserService } from 'app/modules/visitor/user.service';
import { ImageUrl } from 'app/shared/validators/image-url';
import { AuthService } from 'app/core/services/auth.service';
import { fillUndefinedProperties } from 'app/shared/utility/change-objects';
import { MatDialog } from '@angular/material';
import { DialogComponent } from 'app/shared/components/dialog/dialog.component';
@Component({
selector: 'app-edit-profile',
templateUrl: './edit-profile.component.html',
styleUrls: ['./edit-profile.component.scss']
})
export class EditProfileComponent implements OnInit {
bMobile: boolean;
userForm: FormGroup;
bUserDeleted = false;
constructor(responsiveService: ResponsiveService,
private m_router: Router,
private m_formBuilder: FormBuilder,
private m_userService: UserService,
private m_authService: AuthService,
private m_dialog: MatDialog) {
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
this.userForm = this.m_formBuilder.group({
pseudo: ['', Validators.required],
mail: ['', Validators.compose([
Validators.required,
Validators.pattern(/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/)
])],
year_promotion: ['', Validators.required],
phone: '',
firstname: ['', Validators.required],
lastname: ['', Validators.required],
photo_url: ['', Validators.required]
}, {
validators: ImageUrl('photo_url')
});
}
ngOnInit() {
this.m_userService.get(this.m_authService.getUserId()).subscribe(result => {
result = fillUndefinedProperties(result);
this.userForm.patchValue({
pseudo: result.pseudo,
mail: result.mail,
year_promotion: result.year_promotion.toString(),
phone: result.phone,
firstname: result.firstname,
lastname: result.lastname,
photo_url: result.photo_url
});
}, error => {
});
}
submitForm(): void {
let form = this.userForm.value;
form.id = this.m_authService.getUserId();
this.m_userService.update(this.m_authService.getUserId(), form).subscribe(result => {
this.m_router.navigate(['/profil']);
}, error => {
});
}
deleteUser(): void {
const dialogRef = this.m_dialog.open(DialogComponent, {
width: "500px",
data: {title: `Suppression de votre compte`, text: `On est triste de vous voir partir ! En êtes-vous sûr de sûr ?`, bValBtn: true}
});
dialogRef.afterClosed().subscribe(result => {
if (result.validated) {
this.m_userService.delete(this.m_authService.getUserId()).subscribe(result => {
this.bUserDeleted = true;
this.m_authService.logout();
this.m_router.navigate(['/visiteur/login]']);
}, error => {
});
}
});
}
goTo(url: string): void {
this.m_router.navigate([url]);
}
}
<file_sep>/front/src/app/modules/visitor/login/login.component.ts
import { Component, OnInit } from '@angular/core';
import { Router } from '@angular/router';
import { FormGroup, FormBuilder, Validators } from '@angular/forms';
// custom
import { AuthService } from 'app/core/services/auth.service';
import { HttpErrorResponse } from '@angular/common/http';
@Component({
selector: 'app-login',
templateUrl: './login.component.html',
styleUrls: ['./login.component.scss']
})
export class LoginComponent implements OnInit {
loginForm: FormGroup;
bInvalid = false;
constructor(private m_formBuilder: FormBuilder,
private m_authService: AuthService,
private m_router: Router)
{
this.loginForm = this.m_formBuilder.group({
username: ['', Validators.required],
password: ['', Validators.required]
});
}
ngOnInit() {
if (this.m_authService.isLoggedIn()) {
this.m_router.navigate(['/tableau-de-bord']);
}
}
login(username: string, password: string): void {
this.m_authService.login(username, password)
.subscribe(response => {
this.m_router.navigate([this.m_authService.redirectUrl]);
}, (error: HttpErrorResponse) => {
// TODO display error with the form field
});
}
}
<file_sep>/back/api/models/Notification.php
<?php
namespace models;
use \controllers\scans\ScanDataIn;
class Notification {
protected $id,
$id_event,
$date_edit,
$type_edit;
public function __construct(array $data) {
$this->hydrate($data);
}
//Gère la récupération de données des attributs lorsqu'elle proviennent de la bdd
public function hydrate(array $data) {
foreach ($data as $key => $value) {
$method = 'set'.ucfirst($key);
if (method_exists($this, $method)) {
$this->$method($value);
}
}
}
public function toArray() {
$array = [
"id_event" => $this->getId_event(),
"date_edit" => $this->getDate_edit(),
"type_edit" => $this->getType_edit()
];
return $array;
}
// Getters
public function getId() {
return $this->id;
}
public function getId_event() {
return $this->id_event;
}
public function getDate_edit() {
return $this->date_edit;
}
public function getType_edit() {
return $this->type_edit;
}
//Assesseurs
//Attention le nom de méthode doit obligatoirement être composé de "set" suivi du nom de l'attribut correspondant, avec une majuscule, pour que la méthode hydrate soit fonctionnelle.
public function setId($id) {
$id = (int) $id;
if ($id > 0) {
$this->id = $id;
}
}
public function setId_event($id) {
$id = (int) $id;
if ($id > 0) {
$this->id_event = $id;
}
}
public function setType_edit($type) {
if (is_string($type) && strlen($type) <= 255) {
$this->type_edit = $type;
}
}
public function setDate_edit($date_edit) {
$scanDataIn = new ScanDataIn();
if (\is_null($date_edit) || $scanDataIn->validateDate($date_edit)) {
$this->date_edit = $date_edit;
}
else {
throw new \Exception("Date de modification invalide.", 400);
}
}
}
<file_sep>/front/src/app/modules/events/post.service.ts
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
import { environment } from 'environments/environment';
@Injectable({
providedIn: 'root'
})
export class PostService {
constructor(private m_http: HttpClient) { }
// POSTS
createPost(eventId: number, form: Object): Observable<any> {
const URL = `${environment.backend.serverUrl}events/${eventId}/posts`;
return this.m_http.post<any>(URL, JSON.stringify(form));
}
updatePost(eventId: number, postId: number, form: Object): Observable<any> {
const URL = `${environment.backend.serverUrl}events/${eventId}/posts/${postId}`;
return this.m_http.patch<any>(URL, JSON.stringify(form));
}
deletePost(eventId: number, postId: number): Observable<any> {
const URL = `${environment.backend.serverUrl}events/${eventId}/posts/${postId}`;
return this.m_http.delete<any>(URL);
}
// COMMENTS
createComment(eventId: number, postId: number, form: Object): Observable<any> {
const URL = `${environment.backend.serverUrl}events/${eventId}/posts/${postId}/comments`;
return this.m_http.post<any>(URL, JSON.stringify(form));
}
updateComment(eventId: number, postId: number, commentId: number, form: Object): Observable<any> {
const URL = `${environment.backend.serverUrl}events/${eventId}/posts/${postId}/comments/${commentId}`;
return this.m_http.patch<any>(URL, JSON.stringify(form));
}
deleteComment(eventId: number, postId: number, commentId: number): Observable<any> {
const URL = `${environment.backend.serverUrl}events/${eventId}/posts/${postId}/comments/${commentId}`;
return this.m_http.delete<any>(URL);
}
}
<file_sep>/front/src/app/shared/models/notification.ts
import { Event } from './event';
export interface Notification {
event?: Event[];
}
<file_sep>/back/bdd/structure/lolimac.sql
-- phpMyAdmin SQL Dump
-- version 4.8.4
-- https://www.phpmyadmin.net/
--
-- Hôte : 127.0.0.1:3306
-- Généré le : Dim 19 mai 2019 à 11:27
-- Version du serveur : 10.3.9-MariaDB
-- Version de PHP : 7.0.33
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET AUTOCOMMIT = 0;
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Base de données : `lolimac`
--
-- --------------------------------------------------------
--
-- Structure de la table `comments`
--
DROP TABLE IF EXISTS `comments`;
CREATE TABLE IF NOT EXISTS `comments` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`content` text NOT NULL,
`date_created` date NOT NULL,
`date_edited` date NOT NULL,
`id_post` int(11) NOT NULL,
`id_user` int(11) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Structure de la table `events`
--
DROP TABLE IF EXISTS `events`;
CREATE TABLE IF NOT EXISTS `events` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`title` varchar(255) NOT NULL,
`photo_url` text NOT NULL,
`description` text DEFAULT NULL,
`date_start` datetime DEFAULT NULL,
`date_end` datetime DEFAULT NULL,
`date_created` datetime NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Structure de la table `eventtypes`
--
DROP TABLE IF EXISTS `eventtypes`;
CREATE TABLE IF NOT EXISTS `eventtypes` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Structure de la table `link_events_eventtypes`
--
DROP TABLE IF EXISTS `link_events_eventtypes`;
CREATE TABLE IF NOT EXISTS `link_events_eventtypes` (
`id_event` int(11) NOT NULL,
`id_type` int(11) NOT NULL,
PRIMARY KEY (`id_event`,`id_type`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Structure de la table `link_events_places`
--
DROP TABLE IF EXISTS `link_events_places`;
CREATE TABLE IF NOT EXISTS `link_events_places` (
`id_event` int(11) NOT NULL,
`id_place` int(11) NOT NULL,
PRIMARY KEY (`id_event`,`id_place`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Structure de la table `link_events_users_modules`
--
DROP TABLE IF EXISTS `link_events_users_modules`;
CREATE TABLE IF NOT EXISTS `link_events_users_modules` (
`id_event` int(11) NOT NULL,
`id_user` int(11) NOT NULL,
`id_module` int(11) NOT NULL,
PRIMARY KEY (`id_event`,`id_user`,`id_module`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Structure de la table `modules`
--
DROP TABLE IF EXISTS `modules`;
CREATE TABLE IF NOT EXISTS `modules` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Structure de la table `notification_events`
--
DROP TABLE IF EXISTS `notification_events`;
CREATE TABLE IF NOT EXISTS `notification_events` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`id_event` int(11) NOT NULL,
`date_edit` datetime NOT NULL,
`type_edit` varchar(255) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Structure de la table `places`
--
DROP TABLE IF EXISTS `places`;
CREATE TABLE IF NOT EXISTS `places` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`postcode` int(11) DEFAULT NULL,
`street` varchar(255) DEFAULT NULL,
`number` int(11) DEFAULT NULL,
`city` varchar(255) DEFAULT NULL,
`name` varchar(255) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Structure de la table `posts`
--
DROP TABLE IF EXISTS `posts`;
CREATE TABLE IF NOT EXISTS `posts` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`title` varchar(255) NOT NULL,
`content` text NOT NULL,
`date_created` date NOT NULL,
`date_edited` date NOT NULL,
`id_event` int(11) NOT NULL,
`id_user` int(11) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Structure de la table `promotions`
--
DROP TABLE IF EXISTS `promotions`;
CREATE TABLE IF NOT EXISTS `promotions` (
`end_year` int(11) NOT NULL,
`nickname` varchar(100) NOT NULL,
PRIMARY KEY (`end_year`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Structure de la table `users`
--
DROP TABLE IF EXISTS `users`;
CREATE TABLE IF NOT EXISTS `users` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`firstname` varchar(22) NOT NULL,
`lastname` varchar(22) NOT NULL,
`pseudo` varchar(25) NOT NULL,
`mail` varchar(100) NOT NULL,
`phone` varchar(14) DEFAULT NULL,
`pwd_hash` varchar(255) NOT NULL,
`photo_url` varchar(255) NOT NULL,
`status` int(1) NOT NULL,
`year_promotion` int(11) NOT NULL,
`date_notification_check` datetime NOT NULL DEFAULT current_timestamp(),
PRIMARY KEY (`id`),
UNIQUE KEY `pseudo` (`pseudo`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
<file_sep>/back/api/models/Link_events_eventtypesManager.php
<?php
namespace models;
class Link_events_eventtypesManager extends DBAccess {
public function add(Link_events_eventtypes $link) {
$q = $this->db->prepare("INSERT INTO link_events_eventtypes
(`id_event`, `id_type`)
VALUES (:id_event, :id_type);");
$q->bindValue(':id_event', $link->getId_event());
$q->bindValue(':id_type', $link->getId_type());
$q->execute();
$link->hydrate(['id' => $this->db->lastInsertId()]);
return $link;
}
public function count() {
return $this->db->query("SELECT COUNT(*) FROM link_events_eventtypes;")->fetchColumn();
}
public function updateType(Link_events_eventtypes $link_events_eventtypes, int $new_id_type) {
$q = $this->db->prepare("UPDATE link_events_eventtypes SET id_event = :id_event, id_type = :new_id_type WHERE id_event = :id_event AND id_type = :id_type");
$q->bindValue(':id_event', $link_events_eventtypes->getId_event());
$q->bindValue(':id_type', $link_events_eventtypes->getId_type());
$q->bindValue(':new_id_type', $new_id_type);
$q->execute();
return $link_events_eventtypes;
}
public function readById_event($id_event) {
$q = $this->db->prepare("SELECT * FROM link_events_eventtypes WHERE id_event = :id_event LIMIT 1;");
$q->bindValue(':id_event', $id_event);
$q->execute();
$link = $q->fetch(\PDO::FETCH_ASSOC);
if ($link) {
return new Link_events_eventtypes($link);
}
else {
return NULL;
}
}
public function readById_type($id_type) {
$q = $this->db->query("SELECT * FROM link_events_eventtypes WHERE id_type = :id_type");
$q->bindValue(':id_type', $id_type);
$link = $q->fetch(\PDO::FETCH_ASSOC);
if ($link) {
return new Link_events_eventtypes($link);
}
else {
return NULL;
}
}
public function readById_event_type($id_event, $id_type) {
$q = $this->db->prepare('SELECT * FROM link_events_eventtypes WHERE id_event = :id_event AND id_type = :id_type;');
$q->bindValue(':id_event', $id_event);
$q->bindValue(':id_type', $id_type);
$q->execute();
$link = $q->fetch(\PDO::FETCH_ASSOC);
return $link ? True : False;
}
public function readAll() {
$allLink = [];
$q = $this->db->query('SELECT * FROM link_events_eventtypes');
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allLink[$data['id']] = new Link_events_eventtypes($data);
}
return $allLink;
}
public function deleteById($id_event, $id_type) {
$q = $this->db->prepare('DELETE FROM link_events_eventtypes WHERE id_event = :id_event AND id_type = :id_type;');
$q->bindValue(':id_event', $id_event);
$q->bindValue(':id_type', $id_type);
$q->execute();
return TRUE;
}
public function deleteByIdEvent($id_event) {
$q = $this->db->prepare('DELETE FROM link_events_eventtypes WHERE id_event = :id_event;');
$q->bindValue(':id_event', $id_event);
$q->execute();
return TRUE;
}
}
<file_sep>/front/src/app/shared/models/post.ts
import { Comment } from './comment';
import { User } from './user';
export interface Post {
id?: number;
title?: string;
content?: string;
date_created?: Date;
date_edited?: Date;
id_user?: number;
id_event?: number;
comments?: Comment[];
user?: User;
}<file_sep>/back/api/Autoloader.php
<?php
// Ce fichier gère la récupération des fichiers de class en fonction des besoins de la requète et en prenant en compte les namespaces (devant correspondre à l'architecture de fichier).
class Autoloader {
function __construct()
{
$this->autoload();
}
public function autoload() {
spl_autoload_register(function ($class_name) {
include_once str_replace("\\", "/", $class_name) . '.php';
});
}
}<file_sep>/front/src/app/shared/models/place.ts
export interface Place {
id_place?: number;
street?: string;
number?: number;
city?: number;
name?: string;
}<file_sep>/front/src/app/shared/models/event.ts
import { Place } from './place';
import { Post } from './post';
import { User } from './user';
export interface Event {
id_event?: number;
title?: string;
description?: string;
date_start?: Date;
date_end?: Date;
date_created?: Date;
photo_url?: string;
participation: number;
place?: Place;
posts?: Post[];
participants?: User[];
type_edit?: string;
}<file_sep>/front/src/app/core/interceptors/error.interceptor.ts
import { Injectable } from '@angular/core';
import { HttpRequest, HttpHandler, HttpEvent, HttpInterceptor } from '@angular/common/http';
import { Router } from '@angular/router';
// 3rd party
import { Observable, throwError } from 'rxjs';
import { catchError } from 'rxjs/operators';
// custom
import { AuthService } from '../services/auth.service';
import { SnackBarService } from '../services/snack-bar.service';
@Injectable({
providedIn: 'root',
})
export class ErrorInterceptor implements HttpInterceptor {
constructor(private m_authService: AuthService, private m_router: Router, private m_snackbar: SnackBarService) { }
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
return next.handle(request).pipe(catchError(err => {
console.error(err);
switch(err.status) {
case 0:
this.m_snackbar.open('[Erreur] Le serveur est déconnecté !', 'Oh, zut alors', 10000);
break;
case 400:
if (err.error.message) {
this.m_snackbar.open('[Erreur] ' + err.error.message, 'Oh, zut alors', 10000);
} else {
this.m_snackbar.open('[Erreur] La requête envoyée au serveur est invalide !', 'Oh, zut alors', 10000);
}
break;
case 401:
// logout if token expired
//this.m_authService.redirectUrl = location.origin; // FIXME wrong route ?
if (this.m_authService.isLoggedIn()) {
this.m_snackbar.open('Votre connexion a expirée', 'Très bien', 10000);
this.m_authService.logout();
this.m_router.navigate(['/visiteur/login']);
} else {
this.m_snackbar.open('Mot de passe ou pseudo incorrect !', 'Je retente', 10000);
}
break;
case 500:
this.m_snackbar.open('[Erreur] Problème interne coté serveur !', 'Oh, zut alors', 10000);
break;
case 504:
this.m_snackbar.open('[Erreur] Le serveur a mit trop de temps à répondre, veuillez réessayer plus tard !', 'Oh, zut alors', 10000);
break;
default:
if (err.message.search("Http failure during parsing") == 0) {
this.m_snackbar.open('[Erreur] La réponse formulée par le serveur est incorrecte !', 'Oh, zut alors', 10000);
}
break;
}
return throwError(err);
}));
}
}
<file_sep>/back/api/models/NotificationManager.php
<?php
namespace models;
class NotificationManager extends DBAccess {
public function readAll($id_user) {
$q = $this->db->prepare("SELECT MAX(notification_events.id) AS id, notification_events.id_event, MAX(notification_events.date_edit) AS date_edit, notification_events.type_edit FROM notification_events INNER JOIN link_events_users_modules ON link_events_users_modules.id_event = notification_events.id_event INNER JOIN users ON users.id = link_events_users_modules.id_user WHERE link_events_users_modules.id_user = :id_user AND notification_events.date_edit >= users.date_notification_check GROUP BY notification_events.id_event, notification_events.type_edit ;");
$q->bindValue(':id_user', $id_user);
$q->execute();
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allNotifications[$data['id']] = new Notification($data);
}
if(isset($allNotifications)) {
return $allNotifications;
} else {
return NULL;
}
}
public function add(Notification $notification){
$q = $this->db->prepare('INSERT INTO notification_events (id_event, type_edit, date_edit) VALUES (:id_event, :type_edit, NOW());');
$q->bindValue(':id_event', $notification->getId_event());
$q->bindValue(':type_edit', $notification->getType_edit());
$q->execute();
}
public function countNotifications($id_user) {
$q = $this->db->prepare("SELECT COUNT(DISTINCT notification_events.type_edit ) AS Count FROM notification_events INNER JOIN link_events_users_modules ON link_events_users_modules.id_event = notification_events.id_event INNER JOIN users ON users.id = link_events_users_modules.id_user WHERE link_events_users_modules.id_user = :id_user AND notification_events.date_edit >= users.date_notification_check;");
$q->bindValue(':id_user', $id_user);
$q->execute();
return $q->fetch(\PDO::FETCH_ASSOC)['Count'];
}
}
<file_sep>/front/src/app/modules/notifications/notifications.service.ts
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
import { environment } from 'environments/environment';
@Injectable({
providedIn: 'root'
})
export class NotificationsService {
constructor(private m_http: HttpClient) {}
getAll(): Observable<any> {
const URL = `${environment.backend.serverUrl}me/notifications`;
return this.m_http.get<any>(URL);
}
getCount(): Observable<any> {
const URL = `${environment.backend.serverUrl}me/notifications/count`;
return this.m_http.get<any>(URL);
}
}
<file_sep>/front/src/environments/environment.prod.ts
export const environment = {
production: true,
backend: {
serverUrl: 'https://www.lolimac.fr/api/'
}
};
<file_sep>/front/src/app/app.component.ts
import { Component } from '@angular/core';
import { SwUpdate, SwPush } from '@angular/service-worker';
import { Router, RouterEvent, NavigationEnd } from '@angular/router';
import { AuthService } from './core/services/auth.service';
import { ResponsiveService } from './core/services/responsive.service';
import { NotificationsService } from './modules/notifications/notifications.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
bMobile = true;
bVisitor = true;
notificationCount = 0;
constructor(updates: SwUpdate, push: SwPush,
authService: AuthService,
responsiveService: ResponsiveService,
router: Router,
notificationsService: NotificationsService)
{
push.messages.subscribe(message => {
console.info("New push message recieved !");
console.log(message);
});
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
router.events.subscribe((event: RouterEvent) => {
if (event instanceof NavigationEnd) {
if (event.url.search("visiteur") != -1 || event.url.search("404") != -1) {
this.bVisitor = true;
} else {
this.bVisitor = false;
notificationsService.getCount().subscribe(result => {
this.notificationCount = Number(result.notifications);
});
}
if (event.url.search("notifications") != -1) {
this.notificationCount = 0;
}
}
});
}
}
<file_sep>/back/api/controllers/EventCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \controllers\scans\TokenAccess;
use \controllers\scans\TokenCreater;
use \models\Event;
use \models\EventManager;
use \models\Link_events_places;
use \models\Link_events_eventtypes;
use \models\Place;
use \controllers\EventTypeCRUD;
use \controllers\NotificationCRUD;
use \controllers\Link_events_placesCRUD;
use \controllers\Link_events_eventtypesCRUD;
use \controllers\Link_events_users_modulesCRUD;
use \models\Link_events_users_modules;
use \models\Link_events_users_modulesManager;
use \models\User;
use \models\UserManager;
class EventCRUD {
public function add($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["title", "photo_url"]);
$data = $scanDataIn->failleXSS($dataIn);
unset($data['date_created']);
$event = new Event($data);
$eventManager = new EventManager();
$event = $eventManager->add($event);
$token = new TokenAccess();
$link = new Link_events_users_modules([
'id_event'=>$event->getId(),
'id_module'=>1,
'id_user'=>$token->getId()
]);
$linkManager = new Link_events_users_modulesManager();
$linkManager->add($link);
if (isset($data["place"])) {
$placeCRUD = new PlaceCRUD();
if(\is_array($data["place"])) {
$place = $placeCRUD->add($data["place"]);
}
else {
$place = $placeCRUD->read_OBJ(['id' => $data['place']]);
}
$link_events_placesCRUD = new Link_events_placesCRUD();
$link_events_placesCRUD->add(["id_event"=>$event->getId(), "id_place" => $place->getId()]);
}
if (isset($data['type'])) {
$link_events_eventtypesCRUD = new Link_events_eventtypesCRUD();
$eventTypeCRUD = new EventTypeCRUD();
if (is_numeric($data['type'])) {
$eventType = $eventTypeCRUD->read_OBJ(['id' => $data['type']]);
$link_events_eventtypesCRUD->add(["id_event"=>$event->getId(), "id_type" => $eventType->getId()]);
}
else {
$eventType = $eventTypeCRUD->add($data['type']);
$link_events_eventtypesCRUD->add(["id_event"=>$event->getId(), "id_type" => $eventType->getId()]);
}
}
echo \json_encode([
'message' => 'New event added.',
'id_user' => $token->getId(),
'id_event' => $event->getId()
]);
return TRUE;
}
public function update($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
unset($data['date_created']); // NOTE: prevents user from modifying creation date
$eventManager = new EventManager();
$event = $eventManager->readById($data["id"]);
$event->hydrate($data);
$eventManager->update($event);
$NotificationCRUD = new NotificationCRUD();
$NotificationCRUD->add($data);
if (isset($data["place"])) {
$placeCRUD = new PlaceCRUD();
if(\is_array($data["place"])) { // NOTE: On ajoute un nouvel endroit
$place = $placeCRUD->add($data["place"]);
}
else { // On lie à un autre endroit déjà existant
$place = $placeCRUD->read_OBJ(['id' => $data['place']]);
}
$link_events_placesCRUD = new Link_events_placesCRUD();
$link_events_places = $link_events_placesCRUD->deleteByIdEvent(['id_event'=>$event->getId()]);
$link_events_placesCRUD->add(["id_event"=>$event->getId(), "id_place" => $place->getId()]);
}
if (isset($data['type'])) {
$link_events_eventtypesCRUD = new Link_events_eventtypesCRUD();
$eventTypeCRUD = new EventTypeCRUD();
if (is_numeric($data['type'])) {
$eventType = $eventTypeCRUD->read_OBJ(['id' => $data['type']]);
$link_events_eventtypesCRUD->addIfNotExist(["id_event"=>$event->getId(), "id_type" => $eventType->getId()]);
}
else {
$eventType = $eventTypeCRUD->add($data['type']);
$link_events_eventtypesCRUD->addIfNotExist(["id_event"=>$event->getId(), "id_type" => $eventType->getId()]);
}
}
echo \json_encode([
'message' => 'Event updated.',
]);
}
public function readMultiple($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$eventManager = new EventManager();
if (isset($data["limit"])) {
if (empty($data["from"])) {
$data["from"] = "0";
}
$events = $eventManager->readOffsetLimit($data["from"], $data["limit"]);
}
else {
$events = $eventManager->readAll();
}
if($events) {
$participantManager = new Link_events_users_modulesCRUD();
$link_events_placesCRUD = new Link_events_placesCRUD();
$link_events_eventtypesCRUD = new Link_events_eventtypesCRUD();
foreach ($events as $key => $event) {
$events[$key] = $event->toArray();
$participation = $participantManager->readParticipation($event->getId());
$events[$key]['participation'] = $participation;
$place = $link_events_placesCRUD->readPlace_ARRAY(['id_event' => $events[$key]["id_event"]]);
$events[$key]['place'] = $place;
$type = $link_events_eventtypesCRUD->readType_ARRAY(['id_event' => $events[$key]["id_event"]]);
$events[$key]['type'] = $type;
}
echo json_encode(array_values($events));
} else {
echo \json_encode([]);
}
}
public function search($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$scanDataIn->exists($dataIn, ["query"]);
$full = [];
$parts = [];
$eventManager = new EventManager();
$keywords = $scanDataIn->explodeSearchQuery($data['query']);
$keywords = array_merge($keywords['full'], $keywords['words']);
if (!empty($keywords)) {
$events = $eventManager->search($keywords);
}
else $events = [];
if($events) {
$participantManager = new Link_events_users_modulesCRUD();
$link_events_placesCRUD = new Link_events_placesCRUD();
$link_events_eventtypesCRUD = new Link_events_eventtypesCRUD();
foreach ($events as $key => $event) {
$events[$key] = $event->toArray();
$participation = $participantManager->readParticipation($event->getId());
$events[$key]['participation'] = $participation;
$place = $link_events_placesCRUD->readPlace_ARRAY(['id_event' => $events[$key]["id_event"]]);
$events[$key]['place'] = $place;
$type = $link_events_eventtypesCRUD->readType_ARRAY(['id_event' => $events[$key]["id_event"]]);
$events[$key]['type'] = $type;
}
echo json_encode(array_values($events));
} else {
echo \json_encode([]);
}
}
public function generateSingleEventICS($id_event) {
$token = new TokenAccess();
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS(['id_event'=>$id_event]);
$eventManager = new EventManager();
$event = $eventManager->readById($data['id_event']);
if ($event) {
if ($event->getDate_start() && $event->getDate_end()) {
$encryptionMethod = "AES-256-CBC";
$secretHash = "<KEY>";
$iv = "6546445734219402";
$token = "{$token->getId()}/{$data['id_event']}";
$encrypted = openssl_encrypt($token, $encryptionMethod, $secretHash, $options=0, $iv);
$url = "https://www.lolimac.fr/";
echo \json_encode([
'url'=> "{$url}api/ics/{$encrypted}"
], $options = JSON_UNESCAPED_SLASHES);
}
else {
throw new \Exception("Impossible de générer le lien iCalendar, l'événement n'est pas totalement prêt", 400);
}
}
else {
throw new \Exception("Evenement non existant", 400);
}
}
public function generateAllEventICS() {
$token = new TokenAccess();
$scanDataIn = new ScanDataIn();
$eventManager = new EventManager();
$encryptionMethod = "AES-256-CBC";
$secretHash = "2<PASSWORD>";
$userManager = new UserManager();
$user = $userManager->readById($token->getId());
$iv = "6546445734219402";
$token = "{$user->getPseudo()}";
$encrypted = openssl_encrypt($token, $encryptionMethod, $secretHash, $options=0, $iv);
$url = "https://www.lolimac.fr/";
echo \json_encode([
'url'=>"{$url}lolimac-back/api/ics/all/{$encrypted}"
], $options = JSON_UNESCAPED_SLASHES);
}
public function read($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$eventManager = new EventManager();
$event = $eventManager->readById($data["id"]);
$event = $event->toArray();
$participantManager = new Link_events_users_modulesCRUD();
$participation = $participantManager->readParticipation($event["id_event"]);
$event['participation'] = $participation;
$listParticipants = $participantManager->readParticipants($event["id_event"]);
$event['participants'] = $listParticipants;
$link_events_placesCRUD = new Link_events_placesCRUD();
$link_events_eventtypesCRUD = new Link_events_eventtypesCRUD();
$place = $link_events_placesCRUD->readPlace_ARRAY(['id_event' => $event["id_event"]]);
$event['place'] = $place;
$type = $link_events_eventtypesCRUD->readType_ARRAY(['id_event' => $event["id_event"]]);
$event['type'] = $type;
$postCRUD = new PostCRUD();
$posts = $postCRUD->read($data["id"]);
$event['posts'] = $posts;
echo json_encode($event);
}
public function readOBJ($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$eventManager = new EventManager();
$event = $eventManager->readById($data["id"]);
return $event;
}
public function delete($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$eventManager = new EventManager();
$event = $eventManager->readById($data["id"]);
$link_events_placesCRUD = new Link_events_placesCRUD();
$link_events_eventtypesCRUD = new Link_events_eventtypesCRUD();
$link_events_placesCRUD->deleteByIdEvent(['id_event'=>$event->getId()]);
$link_events_placesCRUD->deleteByIdEvent(['id_event'=>$event->getId()]);
$eventManager->deleteById($event->getId());
echo json_encode(["message" => "Event deleted"]);
}
}
<file_sep>/back/api/index.php
<?php
require_once('Root.php');
require_once("Autoloader.php");
new Autoloader();
try {
$index = new Root();
}
catch(Exception $e) {
http_response_code($e->getCode());
echo json_encode([
"code" => $e->getCode(),
"message" => $e->getMessage()
] );
}
<file_sep>/front/documentation/components/InscriptionComponent.html
<!doctype html>
<html class="no-js" lang="">
<head>
<meta charset="utf-8">
<meta http-equiv="x-ua-compatible" content="ie=edge">
<title>imac-resoi-front documentation</title>
<meta name="description" content="">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="../images/favicon.ico">
<link rel="stylesheet" href="../styles/style.css">
</head>
<body>
<div class="navbar navbar-default navbar-fixed-top visible-xs">
<a href="../" class="navbar-brand">imac-resoi-front documentation</a>
<button type="button" class="btn btn-default btn-menu ion-ios-menu" id="btn-menu"></button>
</div>
<div class="xs-menu menu" id="mobile-menu">
<div id="book-search-input" role="search"><input type="text" placeholder="Type to search"></div> <compodoc-menu></compodoc-menu>
</div>
<div class="container-fluid main">
<div class="row main">
<div class="hidden-xs menu">
<compodoc-menu mode="normal"></compodoc-menu>
</div>
<!-- START CONTENT -->
<div class="content component">
<div class="content-data">
<ol class="breadcrumb">
<li>Components</li>
<li>InscriptionComponent</li>
</ol>
<ul class="nav nav-tabs" role="tablist">
<li class="active">
<a href="#info" role="tab" id="info-tab" data-toggle="tab" data-link="info">Info</a>
</li>
<li >
<a href="#source" role="tab" id="source-tab" data-toggle="tab" data-link="source">Source</a>
</li>
<li >
<a href="#templateData" role="tab" id="templateData-tab" data-toggle="tab" data-link="template">Template</a>
</li>
<li >
<a href="#styleData" role="tab" id="styleData-tab" data-toggle="tab" data-link="style">Styles</a>
</li>
<li >
<a href="#tree" role="tab" id="tree-tab" data-toggle="tab" data-link="dom-tree">DOM Tree</a>
</li>
</ul>
<div class="tab-content">
<div class="tab-pane fade active in" id="c-info"><p class="comment">
<h3>File</h3>
</p>
<p class="comment">
<code>src/app/modules/visitor/inscription/inscription.component.ts</code>
</p>
<p class="comment">
<h3>Implements</h3>
</p>
<p class="comment">
<code><a href="https://angular.io/api/core/OnInit" target="_blank" >OnInit</a></code>
</p>
<section>
<h3>Metadata</h3>
<table class="table table-sm table-hover">
<tbody>
<tr>
<td class="col-md-3">selector</td>
<td class="col-md-9"><code>app-inscription</code></td>
</tr>
<tr>
<td class="col-md-3">styleUrls</td>
<td class="col-md-9"><code>./inscription.component.scss</code></td>
</tr>
<tr>
<td class="col-md-3">templateUrl</td>
<td class="col-md-9"><code>./inscription.component.html</code></td>
</tr>
</tbody>
</table>
</section>
<section>
<h3 id="index">Index</h3>
<table class="table table-sm table-bordered index-table">
<tbody>
<tr>
<td class="col-md-4">
<h6><b>Properties</b></h6>
</td>
</tr>
<tr>
<td class="col-md-4">
<ul class="index-list">
<li>
<a href="#bInscriptionDone">bInscriptionDone</a>
</li>
<li>
<a href="#bMobile">bMobile</a>
</li>
<li>
<a href="#inscriptionForm">inscriptionForm</a>
</li>
</ul>
</td>
</tr>
<tr>
<td class="col-md-4">
<h6><b>Methods</b></h6>
</td>
</tr>
<tr>
<td class="col-md-4">
<ul class="index-list">
<li>
<a href="#goTo">goTo</a>
</li>
<li>
<a href="#ngOnInit">ngOnInit</a>
</li>
<li>
<a href="#submitForm">submitForm</a>
</li>
</ul>
</td>
</tr>
</tbody>
</table>
</section>
<section>
<h3 id="constructor">Constructor</h3>
<table class="table table-sm table-bordered">
<tbody>
<tr>
<td class="col-md-4">
<code>constructor(m_formBuilder: <a href="https://angular.io/api/forms/FormBuilder" target="_blank">FormBuilder</a>, m_userService: <a href="../injectables/UserService.html">UserService</a>, m_router: <a href="https://angular.io/api/router/Router" target="_blank">Router</a>, responsiveService: <a href="../injectables/ResponsiveService.html">ResponsiveService</a>)</code>
</td>
</tr>
<tr>
<td class="col-md-4">
<div class="io-line">Defined in <a href="" data-line="19" class="link-to-prism">src/app/modules/visitor/inscription/inscription.component.ts:19</a></div>
</td>
</tr>
<tr>
<td class="col-md-4">
<div>
<b>Parameters :</b>
<table class="params">
<thead>
<tr>
<td>Name</td>
<td>Type</td>
<td>Optional</td>
</tr>
</thead>
<tbody>
<tr>
<td>m_formBuilder</td>
<td>
<code><a href="https://angular.io/api/forms/FormBuilder" target="_blank" >FormBuilder</a></code>
</td>
<td>
No
</td>
</tr>
<tr>
<td>m_userService</td>
<td>
<code><a href="../injectables/UserService.html" target="_self" >UserService</a></code>
</td>
<td>
No
</td>
</tr>
<tr>
<td>m_router</td>
<td>
<code><a href="https://angular.io/api/router/Router" target="_blank" >Router</a></code>
</td>
<td>
No
</td>
</tr>
<tr>
<td>responsiveService</td>
<td>
<code><a href="../injectables/ResponsiveService.html" target="_self" >ResponsiveService</a></code>
</td>
<td>
No
</td>
</tr>
</tbody>
</table>
</div>
</td>
</tr>
</tbody>
</table>
</section>
<section>
<h3 id="methods">
Methods
</h3>
<table class="table table-sm table-bordered">
<tbody>
<tr>
<td class="col-md-4">
<a name="goTo"></a>
<span class="name">
<b>
goTo
</b>
<a href="#goTo"><span class="icon ion-ios-link"></span></a>
</span>
</td>
</tr>
<tr>
<td class="col-md-4">
<code>goTo(url: <a href="https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/string" target="_blank">string</a>)</code>
</td>
</tr>
<tr>
<td class="col-md-4">
<div class="io-line">Defined in <a href="" data-line="84"
class="link-to-prism">src/app/modules/visitor/inscription/inscription.component.ts:84</a></div>
</td>
</tr>
<tr>
<td class="col-md-4">
<div class="io-description">
<b>Parameters :</b>
<table class="params">
<thead>
<tr>
<td>Name</td>
<td>Type</td>
<td>Optional</td>
</tr>
</thead>
<tbody>
<tr>
<td>url</td>
<td>
<code><a href="https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/string" target="_blank" >string</a></code>
</td>
<td>
No
</td>
</tr>
</tbody>
</table>
</div>
<div>
</div>
<div class="io-description">
<b>Returns : </b> <code><a href="https://www.typescriptlang.org/docs/handbook/basic-types.html" target="_blank" >void</a></code>
</div>
<div class="io-description">
</div>
</td>
</tr>
</tbody>
</table>
<table class="table table-sm table-bordered">
<tbody>
<tr>
<td class="col-md-4">
<a name="ngOnInit"></a>
<span class="name">
<b>
ngOnInit
</b>
<a href="#ngOnInit"><span class="icon ion-ios-link"></span></a>
</span>
</td>
</tr>
<tr>
<td class="col-md-4">
<code>ngOnInit()</code>
</td>
</tr>
<tr>
<td class="col-md-4">
<div class="io-line">Defined in <a href="" data-line="65"
class="link-to-prism">src/app/modules/visitor/inscription/inscription.component.ts:65</a></div>
</td>
</tr>
<tr>
<td class="col-md-4">
<div class="io-description">
<b>Returns : </b> <code><a href="https://www.typescriptlang.org/docs/handbook/basic-types.html" target="_blank" >void</a></code>
</div>
</td>
</tr>
</tbody>
</table>
<table class="table table-sm table-bordered">
<tbody>
<tr>
<td class="col-md-4">
<a name="submitForm"></a>
<span class="name">
<b>
submitForm
</b>
<a href="#submitForm"><span class="icon ion-ios-link"></span></a>
</span>
</td>
</tr>
<tr>
<td class="col-md-4">
<code>submitForm()</code>
</td>
</tr>
<tr>
<td class="col-md-4">
<div class="io-line">Defined in <a href="" data-line="67"
class="link-to-prism">src/app/modules/visitor/inscription/inscription.component.ts:67</a></div>
</td>
</tr>
<tr>
<td class="col-md-4">
<div class="io-description">
<b>Returns : </b> <code><a href="https://www.typescriptlang.org/docs/handbook/basic-types.html" target="_blank" >void</a></code>
</div>
</td>
</tr>
</tbody>
</table>
</section>
<section>
<h3 id="inputs">
Properties
</h3>
<table class="table table-sm table-bordered">
<tbody>
<tr>
<td class="col-md-4">
<a name="bInscriptionDone"></a>
<span class="name">
<b>
bInscriptionDone</b>
<a href="#bInscriptionDone"><span class="icon ion-ios-link"></span></a>
</span>
</td>
</tr>
<tr>
<td class="col-md-4">
<i>Default value : </i><code>false</code>
</td>
</tr>
<tr>
<td class="col-md-4">
<div class="io-line">Defined in <a href="" data-line="18" class="link-to-prism">src/app/modules/visitor/inscription/inscription.component.ts:18</a></div>
</td>
</tr>
</tbody>
</table>
<table class="table table-sm table-bordered">
<tbody>
<tr>
<td class="col-md-4">
<a name="bMobile"></a>
<span class="name">
<b>
bMobile</b>
<a href="#bMobile"><span class="icon ion-ios-link"></span></a>
</span>
</td>
</tr>
<tr>
<td class="col-md-4">
<i>Default value : </i><code>true</code>
</td>
</tr>
<tr>
<td class="col-md-4">
<div class="io-line">Defined in <a href="" data-line="19" class="link-to-prism">src/app/modules/visitor/inscription/inscription.component.ts:19</a></div>
</td>
</tr>
</tbody>
</table>
<table class="table table-sm table-bordered">
<tbody>
<tr>
<td class="col-md-4">
<a name="inscriptionForm"></a>
<span class="name">
<b>
inscriptionForm</b>
<a href="#inscriptionForm"><span class="icon ion-ios-link"></span></a>
</span>
</td>
</tr>
<tr>
<td class="col-md-4">
<i>Type : </i> <code><a href="https://angular.io/api/forms/FormGroup" target="_blank" >FormGroup</a></code>
</td>
</tr>
<tr>
<td class="col-md-4">
<div class="io-line">Defined in <a href="" data-line="17" class="link-to-prism">src/app/modules/visitor/inscription/inscription.component.ts:17</a></div>
</td>
</tr>
</tbody>
</table>
</section>
</div>
<div class="tab-pane fade tab-source-code" id="c-source">
<pre class="line-numbers compodoc-sourcecode"><code class="language-typescript">import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, Validators } from '@angular/forms';
import { Router } from '@angular/router';
import { PasswordMatch } from 'app/shared/validators/password-match';
import { UserService } from '../user.service';
import { HttpErrorResponse } from '@angular/common/http';
import { ImageUrl } from 'app/shared/validators/image-url';
import { ResponsiveService } from 'app/core/services/responsive.service';
@Component({
selector: 'app-inscription',
templateUrl: './inscription.component.html',
styleUrls: ['./inscription.component.scss']
})
export class InscriptionComponent implements OnInit {
inscriptionForm: FormGroup;
bInscriptionDone = false;
bMobile = true;
constructor(private m_formBuilder: FormBuilder,
private m_userService: UserService,
private m_router: Router,
responsiveService: ResponsiveService) {
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
this.inscriptionForm = this.m_formBuilder.group({
page1: this.m_formBuilder.group({
pseudo: ['', Validators.required],
mail: ['', Validators.compose([
Validators.required,
Validators.pattern(/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/)
])],
year_promotion: ['', Validators.required]
}),
page2: this.m_formBuilder.group({
phone: '',
firstname: ['', Validators.required],
lastname: ['', Validators.required],
photo_url: ['https://i2.wp.com/rouelibrenmaine.fr/wp-content/uploads/2018/10/empty-avatar.png', Validators.required]
}, {
validators: ImageUrl('photo_url')
}),
page3: this.m_formBuilder.group({
pwd: ['', Validators.compose([
Validators.required,
Validators.minLength(4)
])],
confirm_pwd: ['', Validators.compose([
Validators.required,
Validators.minLength(4)
])]
}, {
validators: PasswordMatch('pwd','confirm_pwd')
})
});
}
ngOnInit() {}
submitForm(): void {
// Prepare form
const form = Object.assign({}, this.inscriptionForm.value.page1, this.inscriptionForm.value.page2, this.inscriptionForm.value.page3);
delete form.confirm_pwd;
// Send form
this.m_userService.create(form).subscribe(response => {
this.bInscriptionDone = true;
setTimeout(() => {
this.m_router.navigate(['/visiteur/login]']);
}, 2500);
}, (error: HttpErrorResponse) => {
// TODO handles error
});
}
goTo(url: string): void {
this.m_router.navigate([url]);
}
}
</code></pre>
</div>
<div class="tab-pane fade " id="c-templateData">
<pre class="line-numbers"><code class="language-html"><app-top-app-bar *ngIf="bMobile" [title]="'Inscription'" [bReturn]="true" (return)="goTo('/visiteur/login')">
</app-top-app-bar>
<div id="inscription-page" class="full-page">
<h2 *ngIf="!bMobile">Inscription sur Lolimac</h2>
<mat-horizontal-stepper [linear]="true" *ngIf="!bInscriptionDone; else success">
<mat-step label="Identifiants" [stepControl]="inscriptionForm.get('page1')">
<form [formGroup]="inscriptionForm.get('page1')">
<mat-form-field appearance="fill">
<mat-label>Pseudo</mat-label>
<input matInput placeholder="CrousMan" formControlName="pseudo" required>
</mat-form-field>
<mat-form-field appearance="fill">
<mat-label>Email</mat-label>
<input matInput placeholder="<EMAIL>" type="email" formControlName="mail" required>
<mat-error>Email invalide !</mat-error>
</mat-form-field>
<mat-form-field appearance="fill">
<mat-label>Promotion</mat-label>
<mat-select placeholder="Promotion" formControlName="year_promotion" required>
<mat-option value="2021">2021</mat-option>
<mat-option value="2020">2020</mat-option>
<mat-option value="2019">2019</mat-option>
<mat-option value="2018">2018</mat-option>
<mat-option value="2017">2017</mat-option>
<mat-option value="2016">2016</mat-option>
<mat-option value="autre">Autre</mat-option>
</mat-select>
<mat-error>Veuillez indiquer votre promotion</mat-error>
</mat-form-field>
<button mat-button matStepperNext [disabled]="!inscriptionForm.get('page1').valid">Suite</button>
</form>
</mat-step>
<mat-step label="Identité">
<form [formGroup]="inscriptionForm.get('page2')">
<div class="avatar">
<img src="{{ inscriptionForm.get('page2').value.photo_url }}">
</div>
<mat-form-field appearance="fill">
<mat-label>Avatar</mat-label>
<input matInput type="url" formControlName="photo_url">
<mat-error>Vous devez spécifier une url d'image</mat-error>
</mat-form-field>
<mat-form-field appearance="fill">
<mat-label>Prénom</mat-label>
<input matInput placeholder="George" formControlName="lastname" required>
</mat-form-field>
<mat-form-field appearance="fill">
<mat-label>Nom</mat-label>
<input matInput placeholder="Brassens" formControlName="firstname" required>
</mat-form-field>
<mat-form-field appearance="fill">
<mat-label>Téléphone (Optionnel) </mat-label>
<input matInput type="tel" formControlName="phone">
</mat-form-field>
<button mat-button matStepperNext [disabled]="!inscriptionForm.get('page2').valid">Suite</button>
</form>
</mat-step>
<mat-step label="Validation">
<form [formGroup]="inscriptionForm.get('page3')">
<mat-form-field appearance="fill">
<mat-label>Mot de passe</mat-label>
<input matInput type="password" formControlName="pwd" required>
</mat-form-field>
<mat-form-field appearance="fill">
<mat-label>Confirmation du mot de passe</mat-label>
<input matInput type="password" formControlName="confirm_pwd" required>
<mat-error>Les mots de passe ne sont pas équivalents</mat-error>
</mat-form-field>
<button mat-raised-button color="accent"
aria-label="S'inscrire sur lolimac"
(click)="submitForm()"
[disabled]="!inscriptionForm.valid">
S'inscrire
</button>
</form>
</mat-step>
</mat-horizontal-stepper>
<ng-template #success>
<div class="success">
<mat-icon class="success-icon">done</mat-icon>
<h3>Inscription validée !</h3>
<p>Vous allez être redirigé à la page de login pour vous authentifier</p>
</div>
</ng-template>
<a id="subscribe" routerLink="/visiteur/login" *ngIf="!bMobile">Changement d'avis ? Retour à la page login</a>
</div>
</code></pre>
</div>
<div class="tab-pane fade " id="c-styleData">
<p class="comment">
<code>./inscription.component.scss</code>
</p>
<pre class="line-numbers"><code class="language-scss">
#inscription-page {
align-content: stretch;
grid-gap: 10px;
mat-horizontal-stepper, .success {
border-radius: var(--border-radius);
align-self: center;
overflow: hidden;
background-color: unset;
}
.success {
display: grid;
padding: 24px;
grid-auto-flow: row;
justify-items: center;
text-align: center;
background-color: var(--color-primary-lighter);
.success-icon {
font-size: 55px;
background-color: var(--color-accent);
border-radius: 50px;
height: 70px;
width: 70px;
line-height: 70px;
display: flex;
justify-content: center;
}
}
form {
display: grid;
grid-auto-flow: row;
grid-gap: 5px;
button {
margin-top: 5px;
}
}
a {
text-align: center;
}
}
</code></pre>
</div>
<div class="tab-pane fade " id="c-tree">
<div id="tree-container"></div>
<div class="tree-legend">
<div class="title">
<b>Legend</b>
</div>
<div>
<div class="color htmlelement"></div><span>Html element</span>
</div>
<div>
<div class="color component"></div><span>Component</span>
</div>
<div>
<div class="color directive"></div><span>Html element with directive</span>
</div>
</div>
</div>
</div>
<script src="../js/libs/vis.min.js"></script>
<script src="../js/libs/htmlparser.js"></script>
<script src="../js/libs/deep-iterator.js"></script>
<script>
var COMPONENT_TEMPLATE = '<div><app-top-app-bar *ngIf="bMobile" [title]="\'Inscription\'" [bReturn]="true" (return)="goTo(\'/visiteur/login\')"></app-top-app-bar><div id="inscription-page" class="full-page"> <h2 *ngIf="!bMobile">Inscription sur Lolimac</h2> <mat-horizontal-stepper [linear]="true" *ngIf="!bInscriptionDone; else success"> <mat-step label="Identifiants" [stepControl]="inscriptionForm.get(\'page1\')"> <form [formGroup]="inscriptionForm.get(\'page1\')"> <mat-form-field appearance="fill"> <mat-label>Pseudo</mat-label> <input matInput placeholder="CrousMan" formControlName="pseudo" required> </mat-form-field> <mat-form-field appearance="fill"> <mat-label>Email</mat-label> <input matInput placeholder="<EMAIL>" type="email" formControlName="mail" required> <mat-error>Email invalide !</mat-error> </mat-form-field> <mat-form-field appearance="fill"> <mat-label>Promotion</mat-label> <mat-select placeholder="Promotion" formControlName="year_promotion" required> <mat-option value="2021">2021</mat-option> <mat-option value="2020">2020</mat-option> <mat-option value="2019">2019</mat-option> <mat-option value="2018">2018</mat-option> <mat-option value="2017">2017</mat-option> <mat-option value="2016">2016</mat-option> <mat-option value="autre">Autre</mat-option> </mat-select> <mat-error>Veuillez indiquer votre promotion</mat-error> </mat-form-field> <button mat-button matStepperNext [disabled]="!inscriptionForm.get(\'page1\').valid">Suite</button> </form> </mat-step> <mat-step label="Identité"> <form [formGroup]="inscriptionForm.get(\'page2\')"> <div class="avatar"> <img src="{{ inscriptionForm.get(\'page2\').value.photo_url }}"> </div> <mat-form-field appearance="fill"> <mat-label>Avatar</mat-label> <input matInput type="url" formControlName="photo_url"> <mat-error>Vous devez spécifier une url d\'image</mat-error> </mat-form-field> <mat-form-field appearance="fill"> <mat-label>Prénom</mat-label> <input matInput placeholder="George" formControlName="lastname" required> </mat-form-field> <mat-form-field appearance="fill"> <mat-label>Nom</mat-label> <input matInput placeholder="Brassens" formControlName="firstname" required> </mat-form-field> <mat-form-field appearance="fill"> <mat-label>Téléphone (Optionnel) </mat-label> <input matInput type="tel" formControlName="phone"> </mat-form-field> <button mat-button matStepperNext [disabled]="!inscriptionForm.get(\'page2\').valid">Suite</button> </form> </mat-step> <mat-step label="Validation"> <form [formGroup]="inscriptionForm.get(\'page3\')"> <mat-form-field appearance="fill"> <mat-label>Mot de passe</mat-label> <input matInput type="password" formControlName="pwd" required> </mat-form-field> <mat-form-field appearance="fill"> <mat-label>Confirmation du mot de passe</mat-label> <input matInput type="password" formControlName="confirm_pwd" required> <mat-error>Les mots de passe ne sont pas équivalents</mat-error> </mat-form-field> <button mat-raised-button color="accent" aria-label="S\'inscrire sur lolimac" (click)="submitForm()" [disabled]="!inscriptionForm.valid"> S\'inscrire </button> </form> </mat-step> </mat-horizontal-stepper> <ng-template #success> <div class="success"> <mat-icon class="success-icon">done</mat-icon> <h3>Inscription validée !</h3> <p>Vous allez être redirigé à la page de login pour vous authentifier</p> </div> </ng-template> <a id="subscribe" routerLink="/visiteur/login" *ngIf="!bMobile">Changement d\'avis ? Retour à la page login</a></div></div>'
var COMPONENTS = [{'name': 'AppComponent', 'selector': 'app-root'},{'name': 'BottomNavigationComponent', 'selector': 'app-bottom-navigation'},{'name': 'ChangePasswordComponent', 'selector': 'app-change-password'},{'name': 'CommentListComponent', 'selector': 'app-comment-list'},{'name': 'CreateEventComponent', 'selector': 'app-create-event'},{'name': 'DashboardComponent', 'selector': 'app-dashboard'},{'name': 'EditEventComponent', 'selector': 'app-edit-event'},{'name': 'EditProfileComponent', 'selector': 'app-edit-profile'},{'name': 'EventCardComponent', 'selector': 'app-event-card'},{'name': 'EventDetailComponent', 'selector': 'app-event-detail'},{'name': 'ForgotComponent', 'selector': 'app-forgot'},{'name': 'InscriptionComponent', 'selector': 'app-inscription'},{'name': 'InvitationComponent', 'selector': 'app-invitation'},{'name': 'LegalsComponent', 'selector': 'app-legals'},{'name': 'LoginComponent', 'selector': 'app-login'},{'name': 'NotificationsComponent', 'selector': 'app-notifications'},{'name': 'PageNotFoundComponent', 'selector': 'app-page-not-found'},{'name': 'PostListComponent', 'selector': 'app-post-list'},{'name': 'ProfileComponent', 'selector': 'app-profile'},{'name': 'SearchComponent', 'selector': 'app-search'},{'name': 'TopAppBarComponent', 'selector': 'app-top-app-bar'},{'name': 'TopNavigationComponent', 'selector': 'app-top-navigation'}];
var DIRECTIVES = [];
var ACTUAL_COMPONENT = {'name': 'InscriptionComponent'};
</script>
<script src="../js/tree.js"></script>
</div><div class="search-results">
<div class="has-results">
<h1 class="search-results-title"><span class='search-results-count'></span> result-matching "<span class='search-query'></span>"</h1>
<ul class="search-results-list"></ul>
</div>
<div class="no-results">
<h1 class="search-results-title">No results matching "<span class='search-query'></span>"</h1>
</div>
</div>
</div>
<!-- END CONTENT -->
</div>
</div>
<script>
var COMPODOC_CURRENT_PAGE_DEPTH = 1;
var COMPODOC_CURRENT_PAGE_CONTEXT = 'component';
var COMPODOC_CURRENT_PAGE_URL = 'InscriptionComponent.html';
</script>
<script src="../js/libs/custom-elements.min.js"></script>
<script src="../js/libs/lit-html.js"></script>
<!-- Required to polyfill modern browsers as code is ES5 for IE... -->
<script src="../js/libs/custom-elements-es5-adapter.js" charset="utf-8" defer></script>
<script src="../js/menu-wc.js" defer></script>
<script src="../js/libs/bootstrap-native.js"></script>
<script src="../js/libs/es6-shim.min.js"></script>
<script src="../js/libs/EventDispatcher.js"></script>
<script src="../js/libs/promise.min.js"></script>
<script src="../js/libs/zepto.min.js"></script>
<script src="../js/compodoc.js"></script>
<script src="../js/tabs.js"></script>
<script src="../js/menu.js"></script>
<script src="../js/libs/clipboard.min.js"></script>
<script src="../js/libs/prism.js"></script>
<script src="../js/sourceCode.js"></script>
<script src="../js/search/search.js"></script>
<script src="../js/search/lunr.min.js"></script>
<script src="../js/search/search-lunr.js"></script>
<script src="../js/search/search_index.js"></script>
<script src="../js/lazy-load-graphs.js"></script>
</body>
</html>
<file_sep>/back/api/models/Post.php
<?php
namespace models;
class Post {
protected $id,
$title,
$content,
$date_created,
$date_edited,
$id_user,
$id_event;
public function __construct(array $data) {
$this->hydrate($data);
}
//Gère la récupération de données des attributs lorsqu'elle proviennent de la bdd
public function hydrate(array $data) {
foreach ($data as $key => $value) {
$method = 'set'.ucfirst($key);
if (method_exists($this, $method)) {
$this->$method($value);
}
}
}
public function toArray() {
$array = [
"id" => $this->getId(),
"title" => $this->getTitle(),
"content" => $this->getContent(),
"date_created" => $this->getDate_created(),
"date_edited" => $this->getDate_edited(),
"id_user" => $this->getId_user(),
"id_event" => $this->getId_event()
];
return $array;
}
// Getters
public function getId() {
return $this->id;
}
public function getTitle() {
return $this->title;
}
public function getContent() {
return $this->content;
}
public function getDate_created() {
return $this->date_created;
}
public function getDate_edited() {
return $this->date_edited;
}
public function getId_user() {
return $this->id_user;
}
public function getId_event() {
return $this->id_event;
}
//Assesseurs
//Attention le nom de méthode doit obligatoirement être composé de "set" suivi du nom de l'attribut correspondant, avec une majuscule, pour que la méthode hydrate soit fonctionnelle.
public function setId($id) {
$id = (int) $id;
if ($id > 0) {
$this->id = $id;
}
}
public function setTitle($title) {
if (is_string($title) && strlen($title) <= 255) {
$this->title = $title;
}
}
public function setContent($content) {
if (is_string($content) && strlen($content) <= 5000) {
$this->content = $content;
}
}
public function setDate_created($date_created) {
if (is_string($date_created)) {
$this->date_created = $date_created;
}
}
public function setDate_edited($date_edited) {
if (is_string($date_edited)) {
$this->date_edited = $date_edited;
}
}
public function setId_user($id_user) {
$id_user = (int) $id_user;
if ($id_user > 0) {
$this->id_user = $id_user;
}
}
public function setId_event($id_event) {
$id_event = (int) $id_event;
if ($id_event > 0) {
$this->id_event = $id_event;
}
}
}<file_sep>/front/src/app/modules/dashboard/dashboard.component.ts
import { Component, OnInit } from '@angular/core';
import { ResponsiveService } from 'app/core/services/responsive.service';
import { Event } from 'app/shared/models/event';
import { EventService } from '../events/event.service';
import { AuthService } from 'app/core/services/auth.service';
import { isEmpty } from 'app/shared/utility/change-objects';
enum DashBoardState {
Error,
Empty,
Done,
Loading,
NoMore
}
@Component({
selector: 'app-dashboard',
templateUrl: './dashboard.component.html',
styleUrls: ['./dashboard.component.scss']
})
export class DashboardComponent implements OnInit {
events: Event[] = [];
bMobile = true;
dashBoardState = DashBoardState;
currentState = DashBoardState.Loading;
private m_offset = 0;
private m_sizeToGet = 5;
constructor(responsiveService: ResponsiveService, private m_eventService: EventService, private m_authService: AuthService) {
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
if (!this.bMobile) {
this.m_sizeToGet = 15;
}
}
ngOnInit() {
this.m_eventService.getEventList(this.m_offset, this.m_sizeToGet).subscribe(result => {
this.currentState = DashBoardState.Done;
this.events = result;
this.m_offset += this.m_sizeToGet;
}, error => {
this.currentState = DashBoardState.Error;
});
}
increaseEventList() {
this.currentState = DashBoardState.Loading;
this.m_eventService.getEventList(this.m_offset, this.m_sizeToGet).subscribe(result => {
if (isEmpty(result)) {
this.currentState = DashBoardState.NoMore;
} else {
this.events = this.events.concat(result);
this.m_offset += this.m_sizeToGet;
this.currentState = DashBoardState.Done;
}
}, error => {
this.currentState = DashBoardState.Error;
});
}
}
<file_sep>/front/src/app/shared/utility/change-objects.ts
export function removeEmptyProperties(obj: Object, parent?: Object, key?: string): Object {
Object.keys(obj).forEach(key => {
if (obj[key] instanceof Date) {
obj[key] = dateToPhp(obj[key]);
}
if (obj[key] && typeof obj[key] === 'object') {
removeEmptyProperties(obj[key], obj, key);
} else if (obj[key] === '' || obj[key] === undefined || obj[key] === null) {
delete obj[key];
}
});
if (isEmpty(obj)) {
delete parent[key];
} else {
return obj;
}
}
export function fillUndefinedProperties(obj: Object): Object {
Object.keys(obj).forEach(key => {
if (obj[key] && typeof obj[key] === 'object') {
fillUndefinedProperties(obj[key]);
} else if (obj[key] === null || obj[key] === undefined) {
obj[key] = '';
}
});
return obj;
}
export function removeUnchangedProperties(objOld: Object, objNew: Object): Object {
Object.keys(objNew).forEach(key => {
if (objOld[key] != undefined) {
if (objOld[key] === objNew[key]) {
delete objNew[key];
}
}
});
return objNew;
}
export function isEmpty(obj: Object): boolean {
if (obj instanceof Date) {
return false;
} else {
for (let key in obj) {
if (obj.hasOwnProperty(key)) {
return false;
}
}
}
return true;
}
function dateToPhp(date: Date): string {
if (!isNaN(date.getTime())) {
let month = date.getMonth() + 1;
return `${date.getFullYear()}-${month.toLocaleString(undefined, {minimumIntegerDigits: 2})}-${date.getDate().toLocaleString(undefined, {minimumIntegerDigits: 2})} ${date.getHours().toLocaleString(undefined, {minimumIntegerDigits: 2})}:${date.getMinutes().toLocaleString(undefined, {minimumIntegerDigits: 2})}:${date.getSeconds().toLocaleString(undefined, {minimumIntegerDigits: 2})}`;
} else {
return '';
}
}
<file_sep>/front/src/app/modules/notifications/notifications.component.ts
import { Component, OnInit } from '@angular/core';
import { Router } from '@angular/router';
import { Observable } from 'rxjs';
import { Notification } from 'app/shared/models/notification';
import { ResponsiveService } from 'app/core/services/responsive.service';
import { NotificationsService } from './notifications.service';
@Component({
selector: 'app-notifications',
templateUrl: './notifications.component.html',
styleUrls: ['./notifications.component.scss']
})
export class NotificationsComponent implements OnInit {
bMobile = true;
notification$: Observable<Notification>;
constructor(responsiveService: ResponsiveService,
private m_notificationService: NotificationsService,
private m_router: Router) {
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
this.notification$ = this.m_notificationService.getAll();
}
ngOnInit() {
}
goTo(url: string) {
this.m_router.navigateByUrl(url);
}
}
<file_sep>/front/src/app/core/services/auth.service.ts
import { Injectable, PLATFORM_ID, Inject } from '@angular/core';
import { HttpClient } from '@angular/common/http';
// 3rd party
import { Observable } from 'rxjs';
import { tap } from 'rxjs/operators';
// Custom
import { environment } from 'environments/environment';
import { isPlatformBrowser } from '@angular/common';
@Injectable({
providedIn: 'root'
})
export class AuthService {
redirectUrl = '/tableau-de-bord';
private m_bLoggedIn = false;
constructor(private m_http: HttpClient, @Inject(PLATFORM_ID) private m_platformId: Object) {
if (isPlatformBrowser(this.m_platformId)) {
if (localStorage.getItem('jwt')) {
this.m_bLoggedIn = true;
// TODO test token and renew it
}
}
}
login(username: string, password: string): Observable<any> {
const URL = `${environment.backend.serverUrl}login`;
return this.m_http.post<any>(URL, {"pseudo": username, "pwd": <PASSWORD>})
.pipe(
tap(
data => { // logged-in
if ((data.jwt) && (data.id_user)) {
if (isPlatformBrowser(this.m_platformId)) {
localStorage.setItem('jwt', data.jwt);
localStorage.setItem('userId', data.id_user);
this.m_bLoggedIn = true;
} else {
console.error('[Login] Impossible de stocker token coté serveur');
}
} else {
console.error('[Login] Mauvaise structure de la réponse');
}
},
error => { // bad credentials or internal error
console.error(error);
}
)
);
}
logout(): void {
localStorage.removeItem('jwt');
localStorage.removeItem('userId');
this.m_bLoggedIn = false;
}
isLoggedIn(): boolean {
return this.m_bLoggedIn;
}
getToken(): string {
return localStorage.getItem('jwt');
}
getUserId(): number {
return Number(localStorage.getItem('userId'));
}
}
<file_sep>/front/src/app/shared/shared.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { ReactiveFormsModule } from '@angular/forms';
import { LazyLoadImageModule } from 'ng-lazyload-image';
import { SharedMaterialModule } from './modules/shared-material.module';
import { TopAppBarComponent } from './components/top-app-bar/top-app-bar.component';
import { EventCardComponent } from './components/event-card/event-card.component';
import { PostListComponent } from './components/post-list/post-list.component';
import { CommentListComponent } from './components/comment-list/comment-list.component';
import { DialogComponent } from './components/dialog/dialog.component';
import { EventCardSmallComponent } from './components/event-card-small/event-card-small.component';
import { EventCardNotificationComponent } from './components/event-card-notification/event-card-notification.component';
import { DeferLoadDirective } from './directives/defer-load.directive';
@NgModule({
declarations: [
TopAppBarComponent,
EventCardComponent,
PostListComponent,
CommentListComponent,
DialogComponent,
EventCardSmallComponent,
EventCardNotificationComponent,
DeferLoadDirective
],
imports: [
CommonModule,
SharedMaterialModule,
ReactiveFormsModule,
LazyLoadImageModule
],
entryComponents: [DialogComponent],
exports: [
SharedMaterialModule,
ReactiveFormsModule,
LazyLoadImageModule,
TopAppBarComponent,
EventCardComponent,
PostListComponent,
CommentListComponent,
DialogComponent,
EventCardSmallComponent,
EventCardNotificationComponent,
DeferLoadDirective
]
})
export class SharedModule { }
<file_sep>/front/src/app/shared/validators/image-url.ts
import { FormGroup } from '@angular/forms';
export function ImageUrl(controlName: string) {
return (formGroup: FormGroup) => {
const url = formGroup.controls[controlName].value;
if (formGroup.controls[controlName].errors && !formGroup.controls[controlName].errors.invalidImg) {
// return if another validator has already found an error
return;
}
if (!url.match(/\.(jpeg|jpg|gif|png)$/)) {
formGroup.controls[controlName].setErrors({ invalidImg: true });
} else {
formGroup.controls[controlName].setErrors(null);
}
}
}
<file_sep>/front/src/app/core/core.module.ts
import { NgModule, Optional, SkipSelf } from '@angular/core';
import { CommonModule } from '@angular/common';
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http';
import { RouterModule } from '@angular/router';
import { MAT_DATE_LOCALE } from '@angular/material/core';
import { registerLocaleData } from '@angular/common';
import { LOCALE_ID } from '@angular/core';
import localeFr from '@angular/common/locales/fr';
import { BottomNavigationComponent } from './components/bottom-navigation/bottom-navigation.component';
import { TopNavigationComponent } from './components/top-navigation/top-navigation.component';
import { AuthService } from './services/auth.service';
import { AuthInterceptor } from './interceptors/auth.interceptor';
import { ErrorInterceptor } from './interceptors/error.interceptor';
import { CoreMaterialModule } from './modules/core-material.module';
import { ResponsiveService } from './services/responsive.service';
registerLocaleData(localeFr, 'fr');
@NgModule({
declarations: [
BottomNavigationComponent,
TopNavigationComponent
],
imports: [
CommonModule,
RouterModule,
CoreMaterialModule
],
providers: [
HttpClientModule,
// Custom
AuthService,
ResponsiveService,
{
provide: HTTP_INTERCEPTORS,
useClass: AuthInterceptor,
multi: true
},
{
provide: HTTP_INTERCEPTORS,
useClass: ErrorInterceptor,
multi: true
},
{
provide: MAT_DATE_LOCALE,
useValue: 'fr-FR'
},
{
provide: LOCALE_ID,
useValue: 'fr-FR'
}
],
exports: [
HttpClientModule,
BottomNavigationComponent,
TopNavigationComponent,
CoreMaterialModule
]
})
export class CoreModule {
constructor(@Optional() @SkipSelf() parentModule: CoreModule) {
if (parentModule) {
throw new Error('CoreModule is already loaded. Import only in AppModule');
}
}
}
<file_sep>/back/api/controllers/parser/IcsParser.php
<?php
namespace controllers\parser;
use \models\Place;
class IcsParser {
protected $proid = "Lolimac Events//Lolimac//FR";
public $ics;
public function __construct() {
$this->ics = "BEGIN:VCALENDAR\r
METHOD:REQUEST\r
VERSION:2.0\r
PRODID:-//{$this->proid}\r\n";
}
public function openEvent() {
$this->ics .= "BEGIN:VEVENT\r\n";
}
public function closeEvent() {
$this->ics .= "END:VEVENT\r\n";
}
public function setDate_timeStamp() {
$date = new \DateTime("now");
$this->ics .= "DTSTAMP:{$date->format('Ymd\THis\Z')}\r\n";
}
public function setDate_start($date) {
$date = new \DateTime($date);
$this->ics .= "DTSTART:{$date->format('Ymd\THis\Z')}\r\n";
}
public function setDate_end($date) {
$date = new \DateTime($date);
$this->ics .= "DTEND:{$date->format('Ymd\THis\Z')}\r\n";
}
public function setStatus() {
$this->ics .= "STATUS:CONFIRMED\r\n";
}
public function setLocation(Place $place) {
if ($place->getName()) {
$this->ics .= "LOCATION:{$place->getName()}";
if ($place->getCity()) {
$this->ics .= " {$place->getCity()}";
}
if ($place->getPostcode()) {
$this->ics .= " {$place->getPostcode()}";
}
if ($place->getNumber()) {
$this->ics .= " n°{$place->getNumber()}";
}
if ($place->getStreet()) {
$this->ics .= " {$place->getStreet()}";
}
$this->ics .= "\r\n";
}
}
public function setUID($id) {
$id = md5($id);
$this->ics .= "UID:$id\r\n";
}
public function setSUMMARY($name) {
$this->ics .= "SUMMARY:$name\r\n";
}
public function closeCalendar() {
$this->ics .= "END:VCALENDAR\r\n";
}
}
<file_sep>/back/api/models/Link_events_users_modulesManager.php
<?php
namespace models;
class Link_events_users_modulesManager extends DBAccess {
public function add(Link_events_users_modules $link) {
$q = $this->db->prepare("INSERT INTO link_events_users_modules
(`id_event`, `id_user`, `id_module`)
VALUES (:id_event, :id_user, :id_module);");
$q->bindValue(':id_event', $link->getId_event());
$q->bindValue(':id_user', $link->getId_user());
$q->bindValue(':id_module', $link->getId_module());
$q->execute();
$link->hydrate(['id' => $this->db->lastInsertId()]);
return $link;
}
public function count() {
return $this->db->query('SELECT COUNT(*) FROM link_events_users_modules;')->fetchColumn();
}
public function readById_event($id_event) {
$q = $this->db->query('SELECT * FROM link_events_users_modules WHERE id_event = '.$id_event);
for($i = 0; $data = $q->fetch(\PDO::FETCH_ASSOC); $i++) {
$allParticipants[$data["id_user"]] = $data["id_module"];
}
if(isset($allParticipants)) {
return $allParticipants;
} else {
return NULL;
}
}
public function readById_user_event($id_user, $id_event) {
$q = $this->db->query('SELECT * FROM link_events_users_modules WHERE id_user = '. $id_user .' AND id_event = '. $id_event);
$link = $q->fetch(\PDO::FETCH_ASSOC);
if ($link != NULL) {
return new Link_events_users_modules($link);
} else {
return NULL;
}
}
public function readById_user_module($id_user, $id_module) {
$q = $this->db->query('SELECT * FROM link_events_users_modules WHERE id_user = '. $id_user .' AND id_module = '. $id_module);
$link = $q->fetch(\PDO::FETCH_ASSOC);
return new Link_events_users_modules($link);
}
public function readById_event_user_module($id_event, $id_user, $id_module) {
$q = $this->db->prepare('SELECT * FROM link_events_users_modules WHERE id_event = :id_event AND id_user = :id_user AND id_module = :id_module;');
$q->bindValue(':id_event', $id_event);
$q->bindValue(':id_user', $id_user);
$q->bindValue(':id_module', $id_module);
$q->execute();
$link = $q->fetch(\PDO::FETCH_ASSOC);
return $link ? True : False;
}
public function readAll() {
$allLink = [];
$q = $this->db->query('SELECT * FROM link_events_users_modules');
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allLink[$data['id']] = new Link_events_users_modules($data);
}
return $allLink;
}
public function deleteById($id_event, $id_user) {
$q = $this->db->prepare('DELETE FROM link_events_users_modules WHERE id_event = :id_event AND id_user = :id_user');
$q->bindValue(':id_event', $id_event);
$q->bindValue(':id_user', $id_user);
$q->execute();
return TRUE;
}
}<file_sep>/front/src/app/shared/components/post-list/post-list.component.ts
import { Component, OnInit, Input, Output, EventEmitter } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
import { Post } from 'app/shared/models/post';
import { PostService } from 'app/modules/events/post.service';
import { AuthService } from 'app/core/services/auth.service';
import { MatDialog } from '@angular/material';
import { DialogComponent } from '../dialog/dialog.component';
enum PostState {
Read,
Create,
Update
}
@Component({
selector: 'app-post-list',
templateUrl: './post-list.component.html',
styleUrls: ['./post-list.component.scss']
})
export class PostListComponent implements OnInit {
@Input() posts: Post[];
@Input() eventId: number;
@Output() updated = new EventEmitter<any>();
postForm: FormGroup;
postState = PostState;
currentState = PostState.Read;
userId: number;
postIdToUpdate: number;
constructor(private m_postService: PostService,
private m_formBuilder: FormBuilder,
authService: AuthService,
private m_dialog: MatDialog) {
this.postForm = this.m_formBuilder.group({
title: ['', Validators.required],
content: ['', Validators.required]
});
this.userId = authService.getUserId();
}
ngOnInit() {
}
submitCreateForm(): void {
this.m_postService.createPost(this.eventId, this.postForm.value).subscribe(result => {
this.currentState = PostState.Read;
this.updated.emit(undefined);
}, error => {
});
}
submitEditForm(): void {
this.m_postService.updatePost(this.eventId, this.postIdToUpdate, this.postForm.value).subscribe(result => {
this.currentState = PostState.Read;
this.postIdToUpdate = undefined;
this.patchForm(undefined);
this.updated.emit(undefined);
}, error => {
});
}
deletePost(): void {
const dialogRef = this.m_dialog.open(DialogComponent, {
width: "500px",
data: {title: `Suppression d'un post`, text: `En êtes-vous bien sûr ?`, bValBtn: true}
});
dialogRef.afterClosed().subscribe(result => {
if (result.validated) {
this.m_postService.deletePost(this.eventId, this.postIdToUpdate).subscribe(result => {
this.currentState = PostState.Read;
this.postIdToUpdate = undefined;
this.updated.emit(undefined);
}, error => {
});
}
});
}
reloadComments(): void {
this.updated.emit(undefined);
}
patchForm(post: Post | undefined): void {
if (post == undefined) {
this.postForm.patchValue({
title: '',
content: ''
});
} else {
this.postForm.patchValue({
title: post.title,
content: post.content
});
}
}
}
<file_sep>/back/api/models/UserManager.php
<?php
namespace models;
class UserManager extends DBAccess {
public function add(User $user) {
$q = $this->db->prepare("INSERT INTO users
(`firstname`, `lastname`, `pseudo`, `mail`, `phone`, `pwd_hash`, `photo_url`, `status`, `year_promotion`)
VALUES (:firstname, :lastname, :pseudo, :mail, :phone, :pwd_hash, :photo_url, 3, :year_promotion);");
$q->bindValue(':firstname', $user->getFirstname());
$q->bindValue(':lastname', $user->getLastname());
$q->bindValue(':pseudo', $user->getPseudo());
$q->bindValue(':phone', $user->getPhone());
$q->bindValue(':mail', $user->getMail());
$q->bindValue(':pwd_hash', $user->getPwd_hash());
$q->bindValue(':photo_url', $user->getPhoto_url());
$q->bindValue(':year_promotion', $user->getYear_promotion());
$q->execute();
$user->hydrate(['id' => $this->db->lastInsertId()]);
return $user;
}
public function count() {
return $this->db->query('SELECT COUNT(*) FROM users;')->fetchColumn();
}
public function readById($id) {
$q = $this->db->query('SELECT * FROM users WHERE id = '.$id);
$user = $q->fetch(\PDO::FETCH_ASSOC);
if ($user) {
return new User($user);
}
return NULL;
}
public function readByPseudo($pseudo) {
$q = $this->db->prepare('SELECT * FROM users WHERE pseudo = :pseudo');
$q->execute([':pseudo' => $pseudo]);
$user = $q->fetch(\PDO::FETCH_ASSOC);
return ($user) ? new User($user) : false;
}
public function readAll() {
$allUsers = [];
$q = $this->db->query('SELECT * FROM users');
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allUsers[$data['id']] = new User($data);
}
return $allUsers;
}
public function update(User $user) {
$q = $this->db->prepare('UPDATE users SET firstname = :firstname, lastname = :lastname, pseudo = :pseudo, mail = :mail, phone = :phone, pwd_hash = :pwd_hash, photo_url = :photo_url, status = :status, year_promotion = :year_promotion WHERE id = :id');
$q->bindValue(':id', $user->getId());
$q->bindValue(':firstname', $user->getFirstname());
$q->bindValue(':lastname', $user->getLastname());
$q->bindValue(':pseudo', $user->getPseudo());
$q->bindValue(':mail', $user->getMail());
$q->bindValue(':phone', $user->getPhone());
$q->bindValue(':pwd_hash', $user->getPwd_hash());
$q->bindValue(':photo_url', $user->getPhoto_url());
$q->bindValue(':status', $user->getStatus());
$q->bindValue(':year_promotion', $user->getYear_promotion());
$q->execute();
return $user;
}
public function updateNotificationDate($id_user) {
$q = $this->db->prepare('UPDATE users SET date_notification_check = NOW() WHERE id = :id_user;');
$q->bindValue(':id_user', $id_user);
$q->execute();
}
public function deleteById($id) {
$this->db->exec("DELETE FROM users WHERE id = $id;");
return TRUE;
}
}
<file_sep>/back/api/vendor/jwt/src/BeforeValidException.php
<?php
namespace vendor\jwt\src;
class BeforeValidException extends \UnexpectedValueException
{
}
<file_sep>/back/api/controllers/CommentCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \models\Comment;
use \models\CommentManager;
use \models\PostManager;
use \controllers\scans\TokenAccess;
use \controllers\Link_events_users_modulesCRUD;
class CommentCRUD {
public function add($dataIn, $id_event) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["content", "id_post"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
$data['id_user'] = $token->getId();
$postManager = new PostManager();
$postManager->readById($data["id_post"]);
$participantManager = new Link_events_users_modulesCRUD();
$participation = $participantManager->readParticipation($id_event);
if($participation != 0) {
$comment = new Comment($data);
$commentManager = new CommentManager();
$commentManager->add($comment);
} else {
throw new \Exception('Vous n\'etes pas autorisé à publier sur cette event.', 400);
}
echo \json_encode([
'message' => 'Comment added.'
]);
return TRUE;
}
public function update($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$commentManager = new CommentManager();
$comment = $commentManager->readById($data["id"]);
$comment->hydrate($data);
$token = new TokenAccess();
$token->acompteAccess($comment->getId_user());
$commentManager->update($comment);
echo \json_encode([
'message' => 'Comment updated.'
]);
}
public function read($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$data = $scanDataIn->orgenize($data, 2 ,["user", "id"]);
$commentManager = new CommentManager();
$comment = $commentManager->readById($data["id"]);
echo json_encode(array("content" => $comment->GetContent(), "id_post" => $comment->GetId_post(), "id_user" => $comment->GetId_user(), "date_created" => $comment->GetDate_created(), "date_edited" => $comment->GetDate_edited()));
}
public function delete($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$commentManager = new CommentManager();
$comment = $commentManager->readById($data["id"]);
$comment->hydrate($data);
$token = new TokenAccess();
$token->acompteAccess($comment->getId_user());
$commentManager->deleteById($data["id"]);
return TRUE;
}
}<file_sep>/back/README.md
# IMAC site d'organisation de soirée - BackEnd
Actuellement, sont fixés comme limite de taille pour les différents contenus du site :
- contenu commentaires : 1000
- contenu posts : 10 000
- titre evenements/posts : 255
- description evenement : 5000
- nom et prénom des utilisateurs : 22
- pseudo : 25
- mail : 50
## Getting started
Besoin de définir 2 fichiers PHP (à mettre à jour en production):
`configVar.php` dans `api`
```
<?php
$secretKey = "<KEY>
$ip = "127.0.0.1";
```
`dbID.php` dans `api/models`
```
<?php
$dbHost = "localhost";
$dbTableName ="lolimac";
$dbUsername = "root";
$dbPassword = "<PASSWORD>";
```
Il faut aussi vérifier que `Mcrpyt` (déprécié) est bien installé
## Inspirations
- [REST errors](https://blog.restcase.com/rest-api-error-codes-101/) - Best practices gestion erreurs api rest
- [Open Api errors](https://github.com/OAI/OpenAPI-Specification/issues/1392) - Proposition d'erreurs standardisés OpenAPI
- [Best practices](https://medium.com/studioarmix/learn-restful-api-design-ideals-c5ec915a430f) - Conseils quant à la création des endpoints
- [Microsoft guidelines](https://docs.microsoft.com/fr-fr/azure/architecture/best-practices/api-design) - Autre conseils d'organisations REST
## Dépendences
- [JWT](https://jwt.io/) - Json Web Tokens
<file_sep>/back/api/models/Link_events_users_modules.php
<?php
namespace models;
class Link_events_users_modules {
protected $id_event,
$id_user,
$id_module;
public function __construct(array $data) {
$this->hydrate($data);
}
public function hydrate(array $data) {
foreach ($data as $key => $value) {
$method = 'set'.ucfirst($key);
if (method_exists($this, $method)) {
$this->$method($value);
}
}
}
public function getId_event() {
return $this->id_event;
}
public function getId_user() {
return $this->id_user;
}
public function getId_module() {
return $this->id_module;
}
public function setId_event($id_event) {
$id_event = (int) $id_event;
if ($id_event > 0) {
$this->id_event = $id_event;
}
}
public function setId_user($id_user) {
$id_user = (int) $id_user;
if ($id_user > 0) {
$this->id_user = $id_user;
}
}
public function setId_module($id_module) {
$id_module = (int) $id_module;
if ($id_module >= 0) {
$this->id_module = $id_module;
}
}
}<file_sep>/back/api/vendor/jwt/src/SignatureInvalidException.php
<?php
namespace vendor\jwt\src;
class SignatureInvalidException extends \UnexpectedValueException
{
}
<file_sep>/README.md
# Lolimac
Event creation website made in Angular (front folder). It interacts with a REST api made in PHP (back folder).
<file_sep>/front/src/app/modules/events/event-detail/event-detail.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { EventDetailComponent } from './event-detail.component';
import { SharedModule } from 'app/shared/shared.module';
import { EventDetailRoutingModule } from './event-detail-routing.module';
@NgModule({
declarations: [EventDetailComponent],
imports: [
CommonModule,
SharedModule,
EventDetailRoutingModule
]
})
export class EventDetailModule { }
<file_sep>/front/src/app/shared/components/top-app-bar/top-app-bar.component.ts
import { Component, OnInit, Input, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-top-app-bar',
templateUrl: './top-app-bar.component.html',
styleUrls: ['./top-app-bar.component.scss']
})
export class TopAppBarComponent implements OnInit {
@Input() title: String;
@Input() bReturn: Boolean;
@Output() return = new EventEmitter<any>()
constructor() { }
ngOnInit() {
}
onReturn(event: any): void {
this.return.emit(undefined);
}
}
<file_sep>/back/api/controllers/scans/TokenAccess.php
<?php
namespace controllers\scans;
use \Config;
use \vendor\jwt\src\JWT;
use \models\UserManager;
use \models\Link_events_users_modulesManager;
class TokenAccess {
protected $token;
public function __construct() {
$config = new Config();
$headers = getallheaders();
if($headers['Authorization']) {
list($jwt) = sscanf($headers['Authorization'], 'Bearer %s');
if ($jwt) {
$this->token = JWT::decode($jwt, $config->getJwtKey(), array('HS512'));
} else {
throw new \Exception('Absense d\'autorization', 401);
}
}
}
public function getId() {
return $this->token->data->userId;
}
public function adminAccess($level) {
$userManager = new UserManager();
$user = $userManager->readById($this->token->data->userId);
if($user->getStatus() <= $level) {
return TRUE;
} else {
throw new \Exception('Droits utilisateur insuffisants', 400);
}
}
public function acompteAccess($id) {
$userManager = new UserManager();
$user = $userManager->readById($this->token->data->userId);
if($user->getId() == $id OR $this->adminAccess(1)) {
return TRUE;
} else {
throw new \Exception('Droits d\'accès au compte restrint', 400);
}
}
public function moduleAccess($event_id, $module_id) {
$userManager = new UserManager();
$user = $userManager->readById($this->token->data->userId);
$linkManager = new Link_events_users_modulesManager();
if($linkManager->readById_event_user_module($event_id, $user->getId(), $module_id)) {
return TRUE;
} else {
return FALSE;
}
}
}
<file_sep>/back/api/controllers/ModuleCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \controllers\scans\TokenAccess;
use \controllers\scans\TokenCreater;
use \models\Module;
use \models\ModuleManager;
class ModuleCRUD {
public function add($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["name"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
if($token->adminAccess(1)) {
$module = new Module($data);
$moduleManager = new ModuleManager();
if ($moduleManager->readByName($module->getName()) === FALSE) {
$moduleManager->add($module);
} else {
throw new \Exception('Nom de module déjà existant.');
}
return TRUE;
}
}
public function update($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
$token->adminAccess(1);
$moduleManager = new ModuleManager();
$module = $moduleManager->readById($data["id"]);
$module->hydrate($data);
$moduleManager->update($module);
}
public function read($dataIn) {
$scanDataIn = new ScanDataIn();
$data = $scanDataIn->failleXSS($dataIn);
$data = $scanDataIn->orgenize($data, 2, ["type", "id"]);
$moduleManager = new ModuleManager();
$module = $moduleManager->readById($data["id"]);
echo json_encode(array("name" => $module->GetName()));
}
public function delete($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
$token->adminAccess(1);
$moduleManager = new ModuleManager();
$moduleManager->deleteById($data["id"]);
}
}<file_sep>/back/api/controllers/Link_events_placesCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \controllers\scans\TokenAccess;
use \controllers\scans\TokenCreater;
use \controllers\PlaceCRUD;
use \models\Link_events_places;
use \models\Link_events_placesManager;
class Link_events_placesCRUD {
public function add($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id_event", "id_place"]);
$data = $scanDataIn->failleXSS($dataIn);
$link = new Link_events_places($data);
$linkManager = new Link_events_placesManager();
if ($linkManager->readById_event_place($link->getId_event(), $link->getId_place()) === FALSE) {
$linkManager->add($link);
} else {
throw new \Exception('Lien déjà existant.');
}
return TRUE;
}
public function readPlace_ARRAY($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id_event"]);
$data = $scanDataIn->failleXSS($dataIn);
$linkManager = new Link_events_placesManager();
$link_events_places = $linkManager->readById_event($data["id_event"]);
if ($link_events_places) {
$placeCRUD = new PlaceCRUD();
$place = $placeCRUD->read_OBJ(["id" => $link_events_places->getId_place()]);
return $place->toArray();
}
}
public function readPlace_OBJ($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id_event"]);
$data = $scanDataIn->failleXSS($dataIn);
$linkManager = new Link_events_placesManager();
$link_events_places = $linkManager->readById_event($data["id_event"]);
if ($link_events_places) {
$placeCRUD = new PlaceCRUD();
$place = $placeCRUD->read_OBJ(["id" => $link_events_places->getId_place()]);
return $place;
}
}
public function update($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id_event", "id_place"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
$linkManager = new Link_events_placesManager();
$link = $linkManager->readById_event($data["id_event"]);
if ($link) {
$linkManager->updatePlace($link, $data["id_place"]);
}
else {
self::add($dataIn);
}
return TRUE;
}
public function delete($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id_event", "id_place"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
$linkManager = new Link_events_placesManager();
$linkManager->deleteById($data["id_event"], $data["id_place"]);
return TRUE;
}
public function deleteByIdEvent($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id_event"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
$linkManager = new Link_events_placesManager();
$linkManager->deleteByIdEvent($data["id_event"]);
return TRUE;
}
}
<file_sep>/front/src/app/shared/models/user.ts
export interface User {
firstname?: string;
lastname?: string;
pseudo?: string;
photo_url?: string;
mail?: string;
phone?: string;
year_promotion?: number;
status?: number;
participation?: number;
}<file_sep>/front/src/app/core/services/responsive.service.ts
import { Injectable } from '@angular/core';
import { BreakpointObserver, BreakpointState } from '@angular/cdk/layout';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class ResponsiveService {
constructor(private m_breakpointObserver: BreakpointObserver) {}
isMobile(): Observable<BreakpointState> {
return this.m_breakpointObserver.observe(['(min-width: 800px)']);
}
}
<file_sep>/back/bdd/seed/lolimac.sql
-- phpMyAdmin SQL Dump
-- version 4.8.5
-- https://www.phpmyadmin.net/
--
-- Host: localhost:3306
-- Generation Time: May 19, 2019 at 01:19 PM
-- Server version: 5.7.26-0ubuntu0.18.04.1
-- PHP Version: 7.2.17-0ubuntu0.18.04.1
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET AUTOCOMMIT = 0;
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Database: `lolimac`
--
-- --------------------------------------------------------
--
-- Table structure for table `comments`
--
CREATE TABLE `comments` (
`id` int(11) NOT NULL,
`content` text NOT NULL,
`date_created` date NOT NULL,
`date_edited` date NOT NULL,
`id_post` int(11) NOT NULL,
`id_user` int(11) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `comments`
--
INSERT INTO `comments` (`id`, `content`, `date_created`, `date_edited`, `id_post`, `id_user`) VALUES
(1, 'Voila j\'ai changé ce que je voulait dire', '2019-04-20', '2019-04-20', 2, 12),
(2, 'Voila j\'ai changé ce que je voulait dire', '2019-04-20', '2019-04-20', 2, 12),
(3, 'Voila j\'ai changé ce que je voulait dire', '2019-04-20', '2019-04-20', 2, 12);
-- --------------------------------------------------------
--
-- Table structure for table `events`
--
CREATE TABLE `events` (
`id` int(11) NOT NULL,
`title` varchar(255) NOT NULL,
`photo_url` text NOT NULL,
`description` text,
`date_start` datetime DEFAULT NULL,
`date_end` datetime DEFAULT NULL,
`date_created` datetime NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `events`
--
INSERT INTO `events` (`id`, `title`, `photo_url`, `description`, `date_start`, `date_end`, `date_created`) VALUES
(1, 'Titre AAAAAeeAAA 1 <3', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', 'c\'est le lol', NULL, '2018-12-12 00:00:00', '2019-04-27 00:00:00'),
(2, 'euh coucou', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', 'c\'est le lol', '2019-05-23 10:10:10', '2019-12-12 10:10:10', '2019-04-27 23:42:06'),
(3, 'Titre AAAAAeeAAA 1 <3', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', 'c\'est le lol', '2019-05-20 10:00:00', '2019-05-21 00:00:00', '2019-04-27 23:44:54'),
(4, 'There we glkji', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-16 15:19:07'),
(5, 'There we godfodlkji', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-16 15:20:03'),
(6, 'There we godfodlkiojji', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-16 15:22:23'),
(7, 'There we godfodlkuihiuiojji', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-16 15:23:02'),
(8, 'There we godfodlkuihiuopiiojji', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-16 15:24:01'),
(9, 'There we godfodlkuihiuopoopiiojji', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-16 15:25:35'),
(11, 'Name', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-17 19:01:42'),
(12, 'Les slips en folie', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, '2019-05-24 18:22:12', '2019-05-24 23:25:12', '2019-05-17 19:08:44'),
(13, 'Name', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-17 19:08:45'),
(14, 'Name', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-17 19:08:46'),
(15, 'Name', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-17 19:08:46'),
(16, 'Name', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-17 19:08:46'),
(17, 'Name', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-17 19:09:15'),
(18, 'Name', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-17 19:09:34'),
(19, 'Name', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-17 19:10:35'),
(20, 'Nameeee', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-18 19:34:32'),
(21, 'Nameeee', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-18 19:35:09'),
(22, 'Nameeee', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-18 21:21:12'),
(23, 'Nameeee', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-18 21:21:14'),
(24, 'Nameeee', 'https://images.unsplash.com/photo-1504564321107-4aa3efddb5bd?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=2134&q=80', NULL, NULL, NULL, '2019-05-18 21:21:14');
-- --------------------------------------------------------
--
-- Table structure for table `eventtypes`
--
CREATE TABLE `eventtypes` (
`id` int(11) NOT NULL,
`name` varchar(255) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `eventtypes`
--
INSERT INTO `eventtypes` (`id`, `name`) VALUES
(1, '<NAME>'),
(2, 'salu'),
(3, '<NAME>'),
(4, 'n\'importe iugyt fr quoi'),
(5, 'n\'importe iugyt fr quoi'),
(6, 'n\'importe iugyt fr quoi'),
(7, 'n\'importe iugyt fr quoi'),
(8, 'n\'importe iugyt fr quoi');
-- --------------------------------------------------------
--
-- Table structure for table `link_events_eventtypes`
--
CREATE TABLE `link_events_eventtypes` (
`id_event` int(11) NOT NULL,
`id_type` int(11) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `link_events_eventtypes`
--
INSERT INTO `link_events_eventtypes` (`id_event`, `id_type`) VALUES
(1, 2),
(12, 8),
(19, 1),
(21, 3);
-- --------------------------------------------------------
--
-- Table structure for table `link_events_places`
--
CREATE TABLE `link_events_places` (
`id_event` int(11) NOT NULL,
`id_place` int(11) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `link_events_places`
--
INSERT INTO `link_events_places` (`id_event`, `id_place`) VALUES
(3, 10),
(12, 19);
-- --------------------------------------------------------
--
-- Table structure for table `link_events_users_modules`
--
CREATE TABLE `link_events_users_modules` (
`id_event` int(11) NOT NULL,
`id_user` int(11) NOT NULL,
`id_module` int(11) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `link_events_users_modules`
--
INSERT INTO `link_events_users_modules` (`id_event`, `id_user`, `id_module`) VALUES
(1, 21, 2),
(1, 22, 2),
(12, 22, 1),
(13, 22, 1),
(14, 22, 1),
(15, 22, 1),
(16, 22, 1),
(17, 22, 1),
(18, 22, 1),
(19, 22, 1),
(21, 22, 1),
(22, 22, 1),
(23, 22, 1),
(24, 22, 1);
-- --------------------------------------------------------
--
-- Table structure for table `modules`
--
CREATE TABLE `modules` (
`id` int(11) NOT NULL,
`name` varchar(255) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Table structure for table `notification_events`
--
CREATE TABLE `notification_events` (
`id` int(11) NOT NULL,
`id_event` int(11) NOT NULL,
`date_edit` datetime NOT NULL,
`type_edit` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- Dumping data for table `notification_events`
--
INSERT INTO `notification_events` (`id`, `id_event`, `date_edit`, `type_edit`) VALUES
(8, 1, '2019-05-17 18:34:12', 'title'),
(9, 1, '2019-05-17 18:34:12', 'id'),
(10, 1, '2019-05-17 18:34:50', 'date_start'),
(11, 1, '2019-05-17 18:34:50', 'title'),
(12, 1, '2019-05-17 18:34:57', 'date_start'),
(13, 1, '2019-05-17 18:34:57', 'title'),
(14, 1, '2019-05-17 19:31:31', 'date_start'),
(15, 1, '2019-05-17 19:31:31', 'title'),
(16, 1, '2019-05-17 19:31:31', 'type'),
(17, 1, '2019-05-17 19:35:46', 'date_start'),
(18, 1, '2019-05-17 19:35:46', 'title'),
(19, 1, '2019-05-17 19:35:46', 'type'),
(20, 3, '2019-05-19 01:14:09', 'date_start'),
(21, 3, '2019-05-19 01:14:09', 'title'),
(22, 3, '2019-05-19 01:14:09', 'place'),
(23, 3, '2019-05-19 01:18:07', 'date_start'),
(24, 3, '2019-05-19 01:18:07', 'title'),
(25, 3, '2019-05-19 01:18:07', 'place'),
(26, 12, '2019-05-19 12:36:44', 'title'),
(27, 12, '2019-05-19 12:36:44', 'place'),
(28, 12, '2019-05-19 12:36:44', 'type'),
(29, 12, '2019-05-19 12:36:44', 'date_start'),
(30, 12, '2019-05-19 12:36:44', 'date_end'),
(31, 12, '2019-05-19 12:49:35', 'title'),
(32, 12, '2019-05-19 12:49:35', 'place'),
(33, 12, '2019-05-19 12:49:35', 'type'),
(34, 12, '2019-05-19 12:49:35', 'date_start'),
(35, 12, '2019-05-19 12:49:35', 'date_end'),
(36, 12, '2019-05-19 12:58:28', 'title'),
(37, 12, '2019-05-19 12:58:28', 'place'),
(38, 12, '2019-05-19 12:58:28', 'type'),
(39, 12, '2019-05-19 12:58:28', 'date_start'),
(40, 12, '2019-05-19 12:58:28', 'date_end'),
(41, 12, '2019-05-19 13:01:47', 'title'),
(42, 12, '2019-05-19 13:01:47', 'place'),
(43, 12, '2019-05-19 13:01:47', 'type'),
(44, 12, '2019-05-19 13:01:47', 'date_start'),
(45, 12, '2019-05-19 13:01:47', 'date_end'),
(46, 12, '2019-05-19 13:02:25', 'title'),
(47, 12, '2019-05-19 13:02:25', 'place'),
(48, 12, '2019-05-19 13:02:25', 'type'),
(49, 12, '2019-05-19 13:02:25', 'date_start'),
(50, 12, '2019-05-19 13:02:25', 'date_end'),
(51, 12, '2019-05-19 13:02:28', 'title'),
(52, 12, '2019-05-19 13:02:28', 'place'),
(53, 12, '2019-05-19 13:02:28', 'type'),
(54, 12, '2019-05-19 13:02:28', 'date_start'),
(55, 12, '2019-05-19 13:02:28', 'date_end'),
(56, 12, '2019-05-19 13:02:29', 'title'),
(57, 12, '2019-05-19 13:02:29', 'place'),
(58, 12, '2019-05-19 13:02:29', 'type'),
(59, 12, '2019-05-19 13:02:29', 'date_start'),
(60, 12, '2019-05-19 13:02:29', 'date_end'),
(61, 12, '2019-05-19 13:03:15', 'title'),
(62, 12, '2019-05-19 13:03:15', 'place'),
(63, 12, '2019-05-19 13:03:15', 'type'),
(64, 12, '2019-05-19 13:03:15', 'date_start'),
(65, 12, '2019-05-19 13:03:15', 'date_end'),
(66, 12, '2019-05-19 13:09:45', 'type_edit'),
(67, 12, '2019-05-19 13:10:00', 'type_edit'),
(68, 12, '2019-05-19 13:11:37', 'post'),
(69, 12, '2019-05-19 13:11:54', 'post'),
(70, 12, '2019-05-19 13:11:59', 'title'),
(71, 12, '2019-05-19 13:11:59', 'place'),
(72, 12, '2019-05-19 13:11:59', 'type'),
(73, 12, '2019-05-19 13:11:59', 'date_start'),
(74, 12, '2019-05-19 13:11:59', 'date_end');
-- --------------------------------------------------------
--
-- Table structure for table `places`
--
CREATE TABLE `places` (
`id` int(11) NOT NULL,
`postcode` int(11) DEFAULT NULL,
`street` varchar(255) DEFAULT NULL,
`number` int(11) DEFAULT NULL,
`city` varchar(255) DEFAULT NULL,
`name` varchar(255) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `places`
--
INSERT INTO `places` (`id`, `postcode`, `street`, `number`, `city`, `name`) VALUES
(1, NULL, NULL, NULL, NULL, 'lalaspokzpela'),
(2, NULL, NULL, NULL, NULL, 'lalaspookpokzpela'),
(3, NULL, NULL, NULL, NULL, 'lalaspookpoiojkzpela'),
(4, NULL, NULL, NULL, NULL, 'lalaspookpoiojkzpela'),
(5, NULL, NULL, NULL, NULL, 'lalaspookpoiojkzpela'),
(6, NULL, NULL, NULL, NULL, 'lalaspookpoiojkzpela'),
(7, NULL, NULL, NULL, NULL, 'lalaspookpoiojkzpela'),
(8, NULL, NULL, NULL, NULL, 'lalaspookpoiojkzpela'),
(9, 98247, 'Groove St', 2, '<NAME>', 'C\'est là '),
(10, 98247, 'Groove St', 2, '<NAME>', 'C\'est là '),
(11, NULL, NULL, NULL, 'ta mère', 'lol'),
(12, 84848, 'Lalalol', 4, 'ta mère', 'lol'),
(13, 84848, 'Lalalol', 4, 'ta mère', 'lol'),
(14, 84848, 'Lalalol', 4, 'ta mère', 'lol'),
(15, 84848, 'Lalalol', 4, 'ta mère', 'lol'),
(16, 84848, 'Lalalol', 4, 'ta mère', 'lol'),
(17, 84848, 'Lalalol', 4, 'ta mère', 'lol'),
(18, 84848, 'Lalalol', 4, 'ta mère', 'lol'),
(19, 84848, 'Lalalol', 4, 'ta mère', 'lol');
-- --------------------------------------------------------
--
-- Table structure for table `posts`
--
CREATE TABLE `posts` (
`id` int(11) NOT NULL,
`title` varchar(255) NOT NULL,
`content` text NOT NULL,
`date_created` date NOT NULL,
`date_edited` date NOT NULL,
`id_event` int(11) NOT NULL,
`id_user` int(11) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `posts`
--
INSERT INTO `posts` (`id`, `title`, `content`, `date_created`, `date_edited`, `id_event`, `id_user`) VALUES
(2, 'J\'adore ta grosse saucisse', 'Enforne moi la stp.', '2019-05-19', '2019-05-19', 12, 22),
(3, 'Coucou', 'a stp.', '2019-05-19', '2019-05-19', 12, 22),
(4, 'Coucou toa', 'a stp.', '2019-05-19', '2019-05-19', 12, 22),
(5, 'Coucou toeeea', 'a stp.', '2019-05-19', '2019-05-19', 12, 22);
-- --------------------------------------------------------
--
-- Table structure for table `promotions`
--
CREATE TABLE `promotions` (
`end_year` int(11) NOT NULL,
`nickname` varchar(100) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Table structure for table `users`
--
CREATE TABLE `users` (
`id` int(11) NOT NULL,
`firstname` varchar(22) NOT NULL,
`lastname` varchar(22) NOT NULL,
`pseudo` varchar(25) NOT NULL,
`mail` varchar(100) NOT NULL,
`phone` varchar(14) DEFAULT NULL,
`pwd_hash` varchar(255) NOT NULL,
`photo_url` varchar(255) NOT NULL,
`status` int(1) NOT NULL,
`year_promotion` int(11) NOT NULL,
`date_notification_check` datetime NOT NULL DEFAULT CURRENT_TIMESTAMP
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `users`
--
INSERT INTO `users` (`id`, `firstname`, `lastname`, `pseudo`, `mail`, `phone`, `pwd_hash`, `photo_url`, `status`, `year_promotion`, `date_notification_check`) VALUES
(21, 'liuiyiuyl', 'lien', 'nicolnt', '<EMAIL>', '06 06 06 06 06', '$2y$10$iQs9LjUg./unNub/8xPQv.6zq2UXEdUukvigN3FH..AWCKbBAQQre', 'nom_photo.jpg', 1, 2021, '2019-05-17 14:33:49'),
(22, 'nico', 'lien', 'nicol', '<EMAIL>', '06 06 06 06 06', '$2y$10$YYYVYKgDq9iE6.zbzOp6zeAaoTmOYBcDfWAUUZgtdtiGUp7fH.S4W', 'nom_photo.jpg', 3, 2021, '2019-05-19 13:13:41'),
(23, 'nico', 'lien', 'lalal', '<EMAIL>', '06 06 06 06 06', '$2y$10$mXn.IcSl3Kk5.64zx3y7LOCAlndBrNgzreyKGT1veOyMk1FyvGT5m', 'nom_photo.jpg', 3, 2021, '2019-05-18 15:07:16'),
(24, 'nico', 'lien', 'yoyo', '<EMAIL>', NULL, '$2y$10$o8qfiU00HfqDN7GdmjdccOtuWOhOhScqJmjn2O.1tny/SXAJm.6Dm', 'nom_photo.jpg', 3, 2021, '2019-05-18 15:07:40');
--
-- Indexes for dumped tables
--
--
-- Indexes for table `comments`
--
ALTER TABLE `comments`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `events`
--
ALTER TABLE `events`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `eventtypes`
--
ALTER TABLE `eventtypes`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `link_events_eventtypes`
--
ALTER TABLE `link_events_eventtypes`
ADD PRIMARY KEY (`id_event`,`id_type`);
--
-- Indexes for table `link_events_places`
--
ALTER TABLE `link_events_places`
ADD PRIMARY KEY (`id_event`,`id_place`);
--
-- Indexes for table `link_events_users_modules`
--
ALTER TABLE `link_events_users_modules`
ADD PRIMARY KEY (`id_event`,`id_user`,`id_module`);
--
-- Indexes for table `modules`
--
ALTER TABLE `modules`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `notification_events`
--
ALTER TABLE `notification_events`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `places`
--
ALTER TABLE `places`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `posts`
--
ALTER TABLE `posts`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `promotions`
--
ALTER TABLE `promotions`
ADD PRIMARY KEY (`end_year`);
--
-- Indexes for table `users`
--
ALTER TABLE `users`
ADD PRIMARY KEY (`id`),
ADD UNIQUE KEY `pseudo` (`pseudo`);
--
-- AUTO_INCREMENT for dumped tables
--
--
-- AUTO_INCREMENT for table `comments`
--
ALTER TABLE `comments`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=5;
--
-- AUTO_INCREMENT for table `events`
--
ALTER TABLE `events`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=25;
--
-- AUTO_INCREMENT for table `eventtypes`
--
ALTER TABLE `eventtypes`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=9;
--
-- AUTO_INCREMENT for table `modules`
--
ALTER TABLE `modules`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
--
-- AUTO_INCREMENT for table `notification_events`
--
ALTER TABLE `notification_events`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=75;
--
-- AUTO_INCREMENT for table `places`
--
ALTER TABLE `places`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=20;
--
-- AUTO_INCREMENT for table `posts`
--
ALTER TABLE `posts`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=6;
--
-- AUTO_INCREMENT for table `users`
--
ALTER TABLE `users`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=25;
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
<file_sep>/back/api/vendor/jwt/src/ExpiredException.php
<?php
namespace vendor\jwt\src;
class ExpiredException extends \UnexpectedValueException
{
}
<file_sep>/front/src/app/shared/directives/defer-load.directive.spec.ts
import { DeferLoadDirective } from './defer-load.directive';
describe('DeferLoadDirective', () => {
it('should create an instance', () => {
const directive = new DeferLoadDirective();
expect(directive).toBeTruthy();
});
});
<file_sep>/front/src/app/modules/events/events.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { EventsRoutingModule } from './events-routing.module';
import { EventService } from './event.service';
import { PostService } from './post.service';
@NgModule({
declarations: [],
imports: [
CommonModule,
EventsRoutingModule
],
providers: [
EventService,
PostService
]
})
export class EventsModule { }
<file_sep>/back/api/models/DBAccess.php
<?php
namespace models;
// Classe mère de tous les modèles, gérant l'identification d'accès à la base de donnée.
abstract class DBAccess {
protected $db;
public function __construct()
{
include "dbID.php"; // This file must not be staged
$db = new \PDO("mysql:host={$dbHost};dbname={$dbTableName}", $dbUsername, $dbPassword);
$this->db = $db;
}
}
<file_sep>/back/api/models/Module.php
<?php
namespace models;
class Module {
protected $id,
$name;
public function __construct(array $data) {
$this->hydrate($data);
}
public function hydrate(array $data) {
foreach ($data as $key => $value) {
$method = 'set'.ucfirst($key);
if (method_exists($this, $method)) {
$this->$method($value);
}
}
}
public function getId() {
return $this->id;
}
public function getName() {
return $this->name;
}
public function setId($id) {
$id = (int) $id;
if ($id > 0) {
$this->id = $id;
}
}
public function setName($name) {
if (is_string($name) && strlen($name) <= 255) {
$this->name = $name;
}
}
}<file_sep>/back/api/models/EventType.php
<?php
namespace models;
class EventType {
protected $id,
$name = NULL;
public function __construct(array $data) {
$this->hydrate($data);
}
public function hydrate(array $data) {
foreach ($data as $key => $value) {
$method = 'set'.ucfirst($key);
if (method_exists($this, $method)) {
$this->$method($value);
}
}
}
public function toArray() {
$array = [
"id_type" => $this->getId(),
"name" => $this->getName()
];
return $array;
}
// Getters
public function getId() {
return $this->id;
}
public function getName() {
return $this->name;
}
//Assesseurs
//Attention le nom de méthode doit obligatoirement être composé de "set" suivi du nom de l'attribut correspondant, avec une majuscule, pour que la méthode hydrate soit fonctionnelle.
public function setId($id) {
$id = (int) $id;
if ($id > 0) {
$this->id = $id;
}
}
public function setName($name) {
if (is_string($name) && strlen($name) < 63 ) {
$this->name = $name;
}
}
}
<file_sep>/front/src/app/modules/events/create-event/create-event.component.ts
import { Component, OnInit } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
import { ResponsiveService } from 'app/core/services/responsive.service';
import { EventService } from '../event.service';
import { HttpErrorResponse } from '@angular/common/http';
import { Router } from '@angular/router';
enum FormStep {
Page1,
Page2,
Page3,
Page4
};
@Component({
selector: 'app-create-event',
templateUrl: './create-event.component.html',
styleUrls: ['./create-event.component.scss']
})
export class CreateEventComponent implements OnInit {
bMobile = true;
eventForm: FormGroup;
currentStep = FormStep.Page1;
formStep = FormStep;
topBarTitle = "Création d'évènement";
minDate = new Date();
bEndDetail = false;
constructor(responsiveService: ResponsiveService,
private m_formBuilder: FormBuilder,
private m_eventService: EventService,
private m_router: Router)
{
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
this.eventForm = this.m_formBuilder.group({
title: ['', Validators.required],
photo_url: ['https://images.unsplash.com/photo-1541532713592-79a0317b6b77?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=634&q=80', Validators.required],
description: '',
date_start: '',
date_start_hour: '0',
date_start_minute: '0',
date_end: '',
date_end_offset: '',
date_end_hour: '0',
place: this.m_formBuilder.group({
name: '',
street: '',
number: '',
city: ''
})
});
}
ngOnInit() {
}
submitForm(): void {
let form = this.eventForm.value;
if (form.date_start != '') {
form.date_start.setHours(form.date_start_hour, form.date_start_minute);
form.date_end = new Date(form.date_start);
if (this.bEndDetail) {
form.date_end.setHours(form.date_end_hour);
} else {
if (form.date_end_offset != '') {
form.date_end.setHours(form.date_end.getHours() + Number(form.date_end_offset), 0);
} else {
form.date_end.setHours(0, 0);
}
}
delete form.date_start_hour;
delete form.date_start_minute;
delete form.date_end_offset;
}
// FIXME temp fix for api that refuses a place without name
if (form.place.street != '' || form.place.number != '' || form.place.city != '') {
if (form.place.name === '') {
form.place.name = ' ';
}
}
this.m_eventService.createEvent(form)
.subscribe(response => {
if (response.id_event) {
this.m_router.navigate([`/evenements/detail/${response.id_event}`]);
} else {
// this.m_router.navigate([`/tableau-de-bord`]);
console.error("Le back n'a pas indiqué l'ID de l'event créé");
}
}, (error: HttpErrorResponse) => {
console.error("error");
// TODO display error with the form field
});
}
previousStep(): void {
if (this.currentStep > 0) {
this.currentStep--;
}
}
updateTitle(): void {
switch(this.currentStep) {
case FormStep.Page1:
this.topBarTitle = "Création d'évènement";
break;
case FormStep.Page2:
this.topBarTitle = "Lieu et Date";
break;
case FormStep.Page3:
this.topBarTitle = "Précisions";
break;
case FormStep.Page4:
this.topBarTitle = "Adresse";
break;
}
}
onDateEndDropChange(event: any): void {
if (event === 'more') {
this.bEndDetail = true;
this.eventForm.patchValue({
date_end: this.eventForm.value.date_start
});
} else {
this.bEndDetail = false;
}
}
}
<file_sep>/front/src/app/modules/profile/profile.component.ts
import { Component, OnInit } from '@angular/core';
import { Router } from '@angular/router';
import { Observable } from 'rxjs';
import { ResponsiveService } from 'app/core/services/responsive.service';
import { AuthService } from 'app/core/services/auth.service';
import { UserService } from '../visitor/user.service';
import { EventService } from '../events/event.service';
import { User } from 'app/shared/models/user';
import { Event } from 'app/shared/models/event';
@Component({
selector: 'app-profile',
templateUrl: './profile.component.html',
styleUrls: ['./profile.component.scss']
})
export class ProfileComponent implements OnInit {
bMobile = true;
user$: Observable<User>;
events$: Observable<Event[]>
constructor(responsiveService: ResponsiveService,
private m_authService: AuthService,
private m_router: Router,
private m_userService: UserService,
private m_eventService: EventService) {
responsiveService.isMobile().subscribe(result => {
if (result.matches) {
this.bMobile = false;
} else {
this.bMobile = true;
}
});
this.user$ = this.m_userService.get(this.m_authService.getUserId());
this.events$ = this.m_eventService.getEventList(0, 20);
}
ngOnInit() {}
logout(): void {
this.m_authService.logout();
this.m_router.navigate(['/visiteur/login']);
}
goTo(url: string): void {
this.m_router.navigate([url]);
}
}
<file_sep>/back/api/models/PostManager.php
<?php
namespace models;
class PostManager extends DBAccess {
public function add(Post $post) {
$q = $this->db->prepare("INSERT INTO posts
(`title`, `content`, `date_created`, `date_edited`,`id_user` ,`id_event`)
VALUES (:title, :content, NOW(), NOW(), :id_user, :id_event);");
$q->bindValue(':title', $post->getTitle());
$q->bindValue(':content', $post->getContent());
$q->bindValue(':id_user', $post->getId_user());
$q->bindValue(':id_event', $post->getId_event());
$q->execute();
$post->hydrate(['id' => $this->db->lastInsertId()]);
return $post;
}
public function count() {
return $this->db->query('SELECT COUNT(*) FROM posts;')->fetchColumn();
}
public function readById($id) {
$q = $this->db->query('SELECT * FROM posts WHERE id = '.$id);
$post = $q->fetch(\PDO::FETCH_ASSOC);
if($post != NULL) {
return new Post($post);
} else {
throw new \Exception("La publication n'existe pas.", 400);
}
}
public function readByIdEvent($id_event) {
$q = $this->db->query('SELECT * FROM posts WHERE id_event = '.$id_event);
for($i = 0; $data = $q->fetch(\PDO::FETCH_ASSOC); $i++) {
$allPosts[$i] = new Post($data);
}
if (isset($allPosts)) {
return $allPosts;
}
else return NULL;
}
public function readByTitle($title) {
$q = $this->db->prepare('SELECT * FROM posts WHERE title = :title');
$q->execute([':title' => $title]);
$title = $q->fetch(\PDO::FETCH_ASSOC);
return ($title) ? new Post($title) : false;
}
public function readAll() {
$allPosts = [];
$q = $this->db->query('SELECT * FROM posts');
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allPosts[$data['id']] = new Post($data);
}
return $allPosts;
}
public function update(Post $post) {
$q = $this->db->prepare('UPDATE posts SET title = :title, content = :content, date_edited = NOW(), id_user = :id_user, id_event = :id_event WHERE id = :id');
$q->bindValue(':id', $post->getId());
$q->bindValue(':title', $post->getTitle());
$q->bindValue(':content', $post->getContent());
$q->bindValue(':id_user', $post->getId_user());
$q->bindValue(':id_event', $post->getId_event());
$q->execute();
return $post;
}
public function deleteById($id) {
$this->db->exec('DELETE FROM posts WHERE id = '.$id.';');
return TRUE;
}
}
<file_sep>/back/api/controllers/PostCRUD.php
<?php
namespace controllers;
use \controllers\scans\ScanDataIn;
use \models\Post;
use \models\PostManager;
use \models\CommentManager;
use \models\UserManager;
use \controllers\scans\TokenAccess;
use \controllers\Link_events_users_modulesCRUD;
use \controllers\NotificationCRUD;
class PostCRUD {
public function add($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["title", "content", "id_event"]);
$data = $scanDataIn->failleXSS($dataIn);
$token = new TokenAccess();
$data['id_user'] = $token->getId();
$post = new Post($data);
$participantManager = new Link_events_users_modulesCRUD();
$participation = $participantManager->readParticipation($post->getId_event());
if($participation != 0) {
$postManager = new PostManager();
$postManager->add($post);
} else {
throw new \Exception('Vous n\'etes pas autorisé à publier sur cette event.', 400);
}
$NotificationCRUD = new NotificationCRUD();
$NotificationCRUD->add([
'id' => $data['id_event'],
'post' => 'post'
]);
echo \json_encode([
'message' => 'Post added.'
]);
return TRUE;
}
public function update($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$postManager = new PostManager();
$post = $postManager->readById($data["id"]);
$post->hydrate($data);
$token = new TokenAccess();
$token->acompteAccess($post->getId_user());
$postManager->update($post);
echo \json_encode([
'message' => 'Post updated.'
]);
}
public function read($id_event) {
$postManager = new PostManager();
$posts = $postManager->readByIdEvent($id_event);
if($posts) {
$commentManager = new CommentManager();
$userManager = new UserManager();
foreach ($posts as $key => $post) {
$postArray = $post->toArray();
$comments = $commentManager->readByPost($post->getId());
if ($comments) {
foreach ($comments as $key2 => $comment) {
$commentInfos = $comment->toArray();
$userComment = $userManager->readById($comment->getId_user());
$userCommentInfos["id"] = $userComment->getId();
$userCommentInfos["firstname"] = $userComment->getFirstname();
$userCommentInfos["lastname"] = $userComment->getLastname();
$userCommentInfos["photo_url"] = $userComment->getPhoto_url();
$commentInfos["user"] = $userCommentInfos;
$comments[$key2] = $commentInfos;
}
$user = $userManager->readById($post->getId_user());
$userInfos["id"] = $user->getId();
$userInfos["firstname"] = $user->getFirstname();
$userInfos["lastname"] = $user->getLastname();
$userInfos["photo_url"] = $user->getPhoto_url();
$postArray["comments"] = $comments;
$postArray["user"] = $userInfos;
}
$posts[$key] = $postArray;
}
return $posts;
}
}
public function delete($dataIn) {
$scanDataIn = new ScanDataIn();
$scanDataIn->exists($dataIn, ["id"]);
$data = $scanDataIn->failleXSS($dataIn);
$postManager = new PostManager();
$post = $postManager->readById($data["id"]);
$post->hydrate($data);
$token = new TokenAccess();
$token->acompteAccess($post->getId_user());
$commentManager = new CommentManager();
$commentManager->deleteByIdPost($data["id"]);
$postManager->deleteById($data["id"]);
echo \json_encode([
'message' => 'Post deleted.'
]);
return TRUE;
}
}
<file_sep>/back/api/controllers/scans/CutURL.php
<?php
namespace controllers\scans;
class CutURL {
protected $URL_cut;
public function __construct($url) {
$url_cutted = parse_url($url, PHP_URL_PATH); // NOTE: Focus on the actual path of the URL, preventing errors with query arguments
$url_cutted = explode ("/" , $url_cutted);
while ($url_cutted[0] != "api") {
array_shift($url_cutted);
}
array_shift($url_cutted);
$this->URL_cut = $url_cutted;
}
public function getURL_cut() {
return $this->URL_cut;
}
}
<file_sep>/back/api/models/Link_events_placesManager.php
<?php
namespace models;
class Link_events_placesManager extends DBAccess {
public function add(Link_events_places $link) {
$q = $this->db->prepare("INSERT INTO link_events_places
(`id_event`, `id_place`)
VALUES (:id_event, :id_place);");
$q->bindValue(':id_event', $link->getId_event());
$q->bindValue(':id_place', $link->getId_place());
$q->execute();
return $link;
}
public function count() {
return $this->db->query("SELECT COUNT(*) FROM link_events_places;")->fetchColumn();
}
public function updatePlace(Link_events_places $link_events_places, int $new_id_place) {
$q = $this->db->prepare("UPDATE link_events_places SET id_event = :id_event, id_place = :new_id_place WHERE id_event = :id_event AND id_place = :id_place");
$q->bindValue(':id_event', $link_events_places->getId_event());
$q->bindValue(':id_place', $link_events_places->getId_place());
$q->bindValue(':new_id_place', $new_id_place);
$q->execute();
return $link_events_places;
}
public function readById_event($id_event) {
$q = $this->db->prepare("SELECT * FROM link_events_places WHERE id_event = :id_event LIMIT 1;");
$q->bindValue(':id_event', $id_event);
$q->execute();
$link = $q->fetch(\PDO::FETCH_ASSOC);
if ($link) {
return new Link_events_places($link);
}
else {
return NULL;
}
}
public function readById_place($id_place) {
$q = $this->db->query("SELECT * FROM link_events_places WHERE id_place = :id_place");
$q->bindValue(':id_place', $id_place);
$link = $q->fetch(\PDO::FETCH_ASSOC);
if ($link) {
return new Link_events_places($link);
}
else {
return NULL;
}
}
public function readById_event_place($id_event, $id_place) {
$q = $this->db->prepare('SELECT * FROM link_events_places WHERE id_event = :id_event AND id_place = :id_place;');
$q->bindValue(':id_event', $id_event);
$q->bindValue(':id_place', $id_place);
$q->execute();
$link = $q->fetch(\PDO::FETCH_ASSOC);
return $link ? True : False;
}
public function readAll() {
$allLink = [];
$q = $this->db->query('SELECT * FROM link_events_places');
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allLink[$data['id']] = new Link_events_places($data);
}
return $allLink;
}
public function deleteById($id_event, $id_place) {
$q = $this->db->prepare('DELETE FROM link_events_places WHERE id_event = :id_event AND id_place = :id_place;');
$q->bindValue(':id_event', $id_event);
$q->bindValue(':id_place', $id_place);
$q->execute();
return TRUE;
}
public function deleteByIdEvent($id_event) {
$q = $this->db->prepare('DELETE FROM link_events_places WHERE id_event = :id_event;');
$q->bindValue(':id_event', $id_event);
$q->execute();
return TRUE;
}
}
<file_sep>/back/api/models/Link_events_eventtypes.php
<?php
namespace models;
class Link_events_eventtypes {
protected $id_event,
$id_type;
public function __construct(array $data) {
$this->hydrate($data);
}
public function hydrate(array $data) {
foreach ($data as $key => $value) {
$method = 'set'.ucfirst($key);
if (method_exists($this, $method)) {
$this->$method($value);
}
}
}
public function toArray() {
$array = [
"id_event" => $this->getId_event(),
"id_type" => $this->getId_place()
];
return $array;
}
public function getId_event() {
return $this->id_event;
}
public function getId_type() {
return $this->id_type;
}
public function setId_event($id_event) {
if ($id_event > 0) {
$this->id_event = $id_event;
}
}
public function setId_type($id_type) {
if ($id_type > 0) {
$this->id_type = $id_type;
}
}
}
<file_sep>/back/api/models/ModuleManager.php
<?php
namespace models;
class ModuleManager extends DBAccess {
public function add(Module $module) {
$q = $this->db->prepare("INSERT INTO modules
(`name`)
VALUES (:name);");
$q->bindValue(':name', $module->getName());
$q->execute();
$module->hydrate(['id' => $this->db->lastInsertId()]);
return $module;
}
public function count() {
return $this->db->query('SELECT COUNT(*) FROM modules;')->fetchColumn();
}
public function readById($id) {
$q = $this->db->query('SELECT * FROM modules WHERE id = '.$id);
$module = $q->fetch(\PDO::FETCH_ASSOC);
$moduleObj = new Module($module);
if($moduleObj) {
return $moduleObj;
} else {
throw new \Exception("Le module n'existe pas.", 400);
}
}
public function readByName($name) {
$q = $this->db->prepare('SELECT * FROM modules WHERE name = :name');
$q->execute([':name' => $name]);
$module = $q->fetch(\PDO::FETCH_ASSOC);
return ($module) ? new Module($module) : false;
}
public function readAll() {
$allModules = [];
$q = $this->db->query('SELECT * FROM modules');
while ($data = $q->fetch(\PDO::FETCH_ASSOC)) {
$allModules[$data['id']] = new Module($data);
}
return $allModules;
}
public function update(Module $module) {
$q = $this->db->prepare('UPDATE modules SET name = :name WHERE id = :id');
$q->bindValue(':id', $module->getId());
$q->bindValue(':name', $module->getName());
$q->execute();
return $module;
}
public function deleteById($id) {
$this->db->exec('DELETE FROM modules WHERE id = '.$id.';');
return TRUE;
}
}
<file_sep>/back/api/models/Event.php
<?php
namespace models;
use \controllers\scans\ScanDataIn;
class Event {
protected $id,
$title,
$photo_url,
$description = NULL,
$date_start = NULL,
$date_end = NULL,
$date_created;
public function __construct(array $data) {
$this->hydrate($data);
}
public function toArray() {
$array = [
"id_event" => $this->getId(),
"title" => $this->getTitle(),
"description" => $this->getDescription(),
"date_start" => $this->getDate_start(),
"date_end" => $this->getDate_end(),
"date_created" => $this->getDate_created(),
"photo_url" => $this->getPhoto_url()
];
return $array;
}
public function __isset($property) {
return isset($this->$property);
}
//Gère la récupération de données des attributs lorsqu'elle proviennent de la bdd
public function hydrate(array $data) {
foreach ($data as $key => $value) {
$method = 'set'.ucfirst($key);
if (method_exists($this, $method)) {
$this->$method($value);
}
}
}
// Getters
public function getId() {
return $this->id;
}
public function getTitle() {
return $this->title;
}
public function getPhoto_url() {
return $this->photo_url;
}
public function getDescription() {
return $this->description;
}
public function getDate_start() {
return $this->date_start;
}
public function getDate_end() {
return $this->date_end;
}
public function getDate_created() {
return $this->date_created;
}
//Assesseurs
//Attention le nom de méthode doit obligatoirement être composé de "set" suivi du nom de l'attribut correspondant, avec une majuscule, pour que la méthode hydrate soit fonctionnelle.
public function setId(int $id) {
$id = (int) $id;
if ($id > 0) {
$this->id = $id;
}
}
public function setTitle($title) {
if (is_null($title)) {
throw new \Exception("Veuilliez entrer un titre");
}
if (is_string($title) && strlen($title) <= 255) {
$this->title = $title;
}
}
public function setPhoto_url($photo_url) {
if (is_null($photo_url)) {
throw new \Exception("Veuilliez indiquer une photo");
}
if (filter_var($photo_url, FILTER_VALIDATE_URL) && strlen($photo_url) <= 2083) {
$this->photo_url = $photo_url;
}
}
public function setDescription($description) {
if (is_string($description) && (strlen($description) <= 65535)) {
$this->description = $description;
}
}
public function setDate_start($date_start) {
$scanDataIn = new ScanDataIn();
if (\is_null($date_start) || $scanDataIn->validateDate($date_start)) {
$this->date_start = $date_start;
}
else {
throw new \Exception("Veuilliez entrer une date de début valide.", 400);
}
}
public function setDate_end($date_end) {
$scanDataIn = new ScanDataIn();
if (\is_null($date_end) || $scanDataIn->validateDate($date_end)) {
$this->date_end = $date_end;
}
else {
throw new \Exception("Veuilliez entrer une date de fin valide.", 400);
}
}
public function setDate_created($date_created) {
$scanDataIn = new ScanDataIn();
if (\is_null($date_created) || $scanDataIn->validateDate($date_created)) {
$this->date_created = $date_created;
}
else {
throw new \Exception("Veuilliez entrer une date de création valide.", 400);
}
}
}
<file_sep>/front/src/app/modules/events/events-routing.module.ts
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
const routes: Routes = [
{
path: 'creation',
loadChildren: './create-event/create-event.module#CreateEventModule'
},
{
path: 'detail/:id',
loadChildren: './event-detail/event-detail.module#EventDetailModule'
},
{
path: 'edition/:id',
loadChildren: './edit-event/edit-event.module#EditEventModule'
},
{
path: '404',
loadChildren: '../page-not-found/page-not-found.module#PageNotFoundModule'
},
{
path: '**',
redirectTo: '404',
pathMatch: 'full'
}
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class EventsRoutingModule { } | 0c9e9c5d7ab7b76f4598c2c169373323e8169e7f | [
"SQL",
"HTML",
"JavaScript",
"Markdown",
"PHP",
"TypeScript"
] | 97 | TypeScript | guillaume-haerinck/lolimac | 29a811c2d30777066ead07abfa1ca754cd6c44e4 | 6ba31ccc9602891678e177a454aa48ab9e8b4779 | |
refs/heads/master | <file_sep># Copyright 2020 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
import tensorflow as tf
from tensorflow_addons.utils import types
@tf.keras.utils.register_keras_serializable(package="Addons")
def snake(logits: types.TensorLike, frequency: types.Number = 1) -> tf.Tensor:
"""Snake activation to learn periodic functions.
https://arxiv.org/abs/2006.08195
Args:
logits: Input tensor.
frequency: A scalar, frequency of the periodic part.
Returns:
Tensor of the same type and shape as `logits`.
"""
logits = tf.convert_to_tensor(logits)
frequency = tf.cast(frequency, logits.dtype)
return logits + (1 - tf.cos(2 * frequency * logits)) / (2 * frequency)
| 0d01a3062e560de673b61211a0c2d680f6d32516 | [
"Python"
] | 1 | Python | omalleyt12/addons | e89d4f7f5fee633e06c387fe07f3e5a540a901bb | df8cad9f00997ddc19bac6e324d2aebfe27df4b4 | |
refs/heads/master | <file_sep>/* jshint esversion: 6, node: true */
'use strict';
const mongoose = require ('mongoose');
const Schema = mongoose.Schema;
const userSchema = new Schema({
userName: {
type: String,
required: true
},
name: {
type: String,
required: true
},
password: {
type: String,
required: true
},
email: {
type: String
},
summary: {
type: String
},
imageUrl: {
type: String
},
company: {
type: String
},
jobTitle: {
type: String
}
});
const User = mongoose.model('users', userSchema);
module.exports = User;
<file_sep>'use strict';
/* jshint esversion: 6, node: true */
const express = require('express');
const router = express.Router();
const bcrypt = require('bcrypt');
const saltRounds = 7;
const User = require('../models/User');
// routes
router.get('/', (req, res, next) => {
const user = req.session.currentUser;
if (user) {
res.render('home', {
user
});
return;
}
res.redirect('/login');
});
router.get('/login', (req, res, next) => {
const user = req.session.currentUser;
if (user) {
res.render('home', {
user
});
return;
}
res.render('authentication/login');
});
router.post('/login', (req, res, next) => {
const {
userName,
password
} = req.body;
if (userName === '' || password === '') {
return res.render('authentication/login', {
errorMsg: "username/password cannot be blank."
});
}
User.findOne({
userName
}, (err, user) => {
if (err || !user) {
return res.render('authentication/login', {
errorMsg: "username does not exist."
});
}
bcrypt.compare(password, user.password, (err, isValid) => {
if (err) return next(err);
if (!isValid) {
return res.render('authentication/login', {
errorMsg: "username/password combination is incorrect."
});
}
req.session.currentUser = {
_id: user._id,
userName,
name: user.name,
email: user.email
};
res.redirect('/');
});
});
});
router.get('/signup', (req, res, next) => {
const user = req.session.currentUser;
if (user) {
res.render('home', {
user
});
return;
}
res.render('authentication/signup');
});
router.post('/signup', (req, res, next) => {
const {
userName,
password,
name,
email
} = req.body;
if (userName === '' || password === '') {
return res.render('authentication/signup', {
errorMsg: "username/password cannot be blank."
});
}
User.findOne({
userName
}, (err, user) => {
if (err) return next(err);
if (user) return res.render('authentication/signup', {
errorMsg: "That username already exists."
});
bcrypt.hash(password, saltRounds, (err, hash) => {
if (err) return next(err);
const newUser = User({
userName,
password: <PASSWORD>,
name,
email
});
newUser.save((err, userDoc) => {
if (err) return next(err);
res.redirect('/login');
});
});
});
});
router.post('/logout', (req, res, next) => {
const user = req.session.currentUser;
if (user) {
req.session.destroy((err) => {
if (err) return next(err);
});
}
return res.redirect('/login');
});
module.exports = router;
| 3816bfaa14168ee9824a640208c9064ec22eb14b | [
"JavaScript"
] | 2 | JavaScript | bruscantini/lab-express-linkedin-clone | 010235028763c1c4e1d8ab318d9722c5a0fd8b63 | 40a0c4475dbf7bbbbaaed3fb5fd20bd0b5a3e184 | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using ToDerp.Data.Models;
using ToDerp.Data.Services;
namespace ToDerp.Areas.Api.Controllers
{
public class TodoController : Controller
{
private DerpRepository _repo;
public TodoController()
{
_repo = new DerpRepository(); // *very* poor man's IOC
}
//
// GET: /Api/Todo/
public JsonResult Index()
{
var items = _repo.AllItems().OrderBy(i => i.Completed).ThenByDescending(i => i.CreatedAt);
return Json(items.ToArray(), JsonRequestBehavior.AllowGet);
}
[HttpPost]
public JsonResult Item(TodoItem item)
{
_repo.Add(item);
return Json(item);
}
[HttpPut]
public JsonResult Item(int id, TodoItem item)
{
var dbItem = _repo.Single(id);
dbItem.Completed = item.Completed;
dbItem.TaskName = item.TaskName;
if (dbItem.Completed)
dbItem.CompletedAt = DateTime.Now;
else
dbItem.CompletedAt = null;
_repo.Save();
return Json(dbItem);
}
}
}
<file_sep>Basic Todo Application for CoffeeScript and Backbone demo
It's basically a crummy ASP.NET and Entity Framework (code first) backend to power an intro to CoffeeScript and Backbone demo. All the Backbone stuff happens in the app.coffee file.
<file_sep>using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using ToDerp.Data.Models;
namespace ToDerp.Data.Services
{
public class ToDerpContext : DbContext
{
public DbSet<TodoItem> TodoItems { get; set; }
}
public class DerpRepository : IDisposable
{
private ToDerpContext _context;
public DerpRepository()
{
_context = new ToDerpContext();
}
public IQueryable<TodoItem> AllItems()
{
return _context.TodoItems.AsQueryable();
}
public void Save()
{
_context.SaveChanges();
}
public void Add(TodoItem item)
{
_context.TodoItems.Add(item);
Save();
}
public void Dispose()
{
if (_context != null)
{
_context.Dispose();
}
}
public TodoItem Single(int itemId)
{
return AllItems().FirstOrDefault(i => i.TodoItemId == itemId);
}
}
}
<file_sep>(function() {
var parseSerializedDate;
parseSerializedDate = function(text) {
return new Date(parseInt(text.substr(6)));
};
Handlebars.registerHelper('prettydate', function(text) {
var ampm, d, hours, months;
if (text === void 0) {
return '';
}
if (text == null) {
return '';
}
d = parseSerializedDate(text);
hours = d.getHours() + 1;
ampm = "AM";
if (hours > 12) {
hours -= 12;
ampm = "PM";
}
months = ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'];
return "" + hours + ":" + (d.getMinutes()) + " " + ampm + " " + months[d.getMonth()] + " " + (d.getDate()) + ", " + (d.getFullYear());
});
}).call(this);
<file_sep>using System.Web;
using System.Web.Optimization;
namespace ToDerp
{
public class BundleConfig
{
// For more information on Bundling, visit http://go.microsoft.com/fwlink/?LinkId=254725
public static void RegisterBundles(BundleCollection bundles)
{
bundles.Add(new ScriptBundle("~/bundles/derp").Include(
"~/Scripts/lib/jquery-1.8.3.js",
"~/Scripts/lib/underscore.js",
"~/Scripts/lib/handlebars-1.0.rc.1.js",
"~/Scripts/lib/bootstrap.js",
"~/Scripts/lib/backbone.js",
"~/Scripts/extensions.js",
"~/Scripts/app.js"));
// Use the development version of Modernizr to develop with and learn from. Then, when you're
// ready for production, use the build tool at http://modernizr.com to pick only the tests you need.
bundles.Add(new ScriptBundle("~/bundles/modernizr").Include(
"~/Scripts/lib/modernizr-*"));
bundles.Add(new StyleBundle("~/bundles/style").Include(
"~/Content/bootstrap.css",
"~/Content/app.css"
));
}
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ToDerp.Data.Models
{
public class TodoItem
{
public TodoItem()
{
CreatedAt = DateTime.Now;
}
public int TodoItemId { get; set; }
public string TaskName { get; set; }
public bool Completed { get; set; }
public DateTime CreatedAt { get; set; }
public DateTime? CompletedAt { get; set; }
}
}
<file_sep>(function() {
var BaseView, Controller, FormView, TodoItem, TodoItems, TodoView, _ref,
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
TodoItem = (function(_super) {
__extends(TodoItem, _super);
function TodoItem() {
return TodoItem.__super__.constructor.apply(this, arguments);
}
TodoItem.prototype.urlRoot = '/api/Todo/Item';
TodoItem.prototype.idAttribute = 'TodoItemId';
return TodoItem;
})(Backbone.Model);
TodoItems = (function(_super) {
__extends(TodoItems, _super);
function TodoItems() {
return TodoItems.__super__.constructor.apply(this, arguments);
}
TodoItems.prototype.model = TodoItem;
TodoItems.prototype.url = '/api/Todo';
return TodoItems;
})(Backbone.Collection);
BaseView = (function(_super) {
__extends(BaseView, _super);
function BaseView() {
return BaseView.__super__.constructor.apply(this, arguments);
}
BaseView.prototype.parseForm = function() {
var inputs, parseFn,
_this = this;
inputs = this.$('input');
parseFn = function(obj, input) {
var inputName;
input = $(input);
inputName = input.attr('name');
obj[inputName] = input.is('[type=checkbox]') ? input.is(':checked') : input.val();
return obj;
};
return _.reduce(inputs, parseFn, {});
};
BaseView.prototype.createTemplate = function() {
var _ref;
this.template = (_ref = this.template) != null ? _ref : Handlebars.compile($(this.templateId).html());
return this.template;
};
BaseView.prototype.render = function() {
var tmpl;
tmpl = this.createTemplate();
this.$el.html(tmpl(this.model.toJSON()));
return this;
};
return BaseView;
})(Backbone.View);
FormView = (function(_super) {
__extends(FormView, _super);
function FormView() {
return FormView.__super__.constructor.apply(this, arguments);
}
FormView.prototype.el = '#create';
FormView.prototype.initialize = function() {
return this.reset();
};
FormView.prototype.events = {
'submit': 'formSubmitted'
};
FormView.prototype.reset = function() {
this.$('[name=TaskName]').val('');
return this.model = new TodoItem;
};
FormView.prototype.formSubmitted = function(ev) {
ev.preventDefault();
this.model.save(this.parseForm());
this.trigger('TodoItem:Created', this.model);
return this.reset();
};
return FormView;
})(BaseView);
TodoView = (function(_super) {
__extends(TodoView, _super);
function TodoView() {
return TodoView.__super__.constructor.apply(this, arguments);
}
TodoView.prototype.tagName = 'li';
TodoView.prototype.className = 'row';
TodoView.prototype.templateId = '#row-template';
TodoView.prototype.initialize = function() {
return this.model.on('change:Completed', this.remove, this);
};
TodoView.prototype.events = {
'change input[type=checkbox]': 'checkChanged'
};
TodoView.prototype.checkChanged = function() {
var formValues;
formValues = this.parseForm();
return this.model.save(formValues);
};
return TodoView;
})(BaseView);
Controller = (function() {
function Controller() {}
Controller.prototype.inProgressId = '#inprogress';
Controller.prototype.completeId = '#completed';
Controller.prototype.index = function() {
var coll, formView;
coll = new TodoItems;
coll.on('reset', this._renderItems, this);
formView = new FormView;
formView.on('TodoItem:Created', this._renderItem, this);
return coll.fetch();
};
Controller.prototype._renderItem = function(item) {
var inList, view;
view = new TodoView({
model: item
});
inList = item.get('Completed') ? this.completeId : this.inProgressId;
$(inList).append(view.render().el);
return item.once('sync', this._renderItem, this);
};
Controller.prototype._renderItems = function(coll) {
var item, _i, _len, _ref, _results;
_ref = coll.models;
_results = [];
for (_i = 0, _len = _ref.length; _i < _len; _i++) {
item = _ref[_i];
_results.push(this._renderItem(item));
}
return _results;
};
return Controller;
})();
jQuery(function() {
var c;
c = new Controller;
return c.index();
});
this.derp = (_ref = window.derp) != null ? _ref : {};
this.derp.TodoItem = TodoItem;
this.derp.TodoItems = TodoItems;
}).call(this);
| c424e53696420d2d25d9a1d603741295232c4630 | [
"Markdown",
"C#",
"JavaScript"
] | 7 | C# | swilliams/toderp | 19986a6c39cfb871b38546f4c83882532c914b07 | dfef66c2ca9acebd0a6b8da38785c7a95df15dd8 | |
refs/heads/master | <file_sep>var employees = JSON.parse(teamMembers);
for(var i=0; i<employees.length; i++)
{
document.getElementById('employees').innerHTML += `
<h2>${employees[i].name}</h2>
<h3>${employees[i].email}</h3>
<img alt ="sor<NAME>" src="${employees[i].image}"</h3>
<br><br>
`
}
<file_sep><!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<header>
<img id="logo" src="img/logo.png">
<img src="img/banner.png">
</header>
<nav>
<ul>
<li>Home</li>
<li><a href="team.html">Team</a></li>
</ul>
</nav>
<main>
<h1 id="first">Car insurance</h1>
<div id="second">
<h2>Great coverage begins with a custom quote</h2>
<form id="form">
<div>
<label>Your name:</label><br>
<input type="text" id="name">
</div>
<div>
<label>Age:</label><br>
<input type="text" id="age">
</div>
<div>
<label>Country:</label><br>
<select id="select">
<option value="Austria">Austria</option>
<option value="Hungary">Hungary</option>
<option value="Greece">Greece</option>
</select>
</div>
<div>
<label>Horsepower of your car:</label><br>
<input type="text" id="name">
</div>
</form>
<br>
<button id="btn">Calculate</button>
<p id="result"></p>
</div>
<div id="bottom">
<h3>Un-complicating car coverage</h3>
<p>Understanding your coverage options SHouldn't be so hard.</p>
<p>We'll guide you through available coverage types,</p>
<p>starting with who and what is covered</p>
</div>
</main>
<section>
<div id="flexbox">
<div class="square">
<h4>What comes standard</h4>
<br>
<p>✔The coverage you need</p>
<p>✔ Bodily Injury Liability</p>
<p>✔ Property Damage</p>
<br><br>
<h5>Benefits</h5>
<p>12-month rate guarantee | 24/7 customer service | Lifetime Repair Guarantee</p>
</div>
<div class="square">
<h4>Add-on protection & coverage</h4>
<br>
<p>✔ Better Car Replacement</p>
<p>✔ Towin & Labor</p>
<p>✔ Rental Car Coverage</p>
<br><br>
<h5>Earned Benefits</h5>
<p>Accident Forgiveness | New Car Replacement ™</p>
</div>
</div>
</section>
<footer>
<nav id=navFooter>
<ul>
<li>Home</li>
<li>Team</li>
</ul>
</nav>
<img src="img/logo-inv.png">
<h2><NAME> - CodeFactory 2020</h2>
</footer>
<script src="js/code review.js"></script>
</body>
</html><file_sep># CF-CR3-Alejandro
We were required to create a website that could calculate price insurances.
The layout is based on the picture provided by CodeFactory, using HTML and CSS.
The functions use the formula provided by CodeFactory, and are written in javascript.
<file_sep>var input = document.getElementsByTagName('input');
var result = document.getElementById('result');
var select = document.getElementById('select');
function calculateInsurance() {
var horsePower = input[2].value;
var age = input[1].value;
var name = input[0].value;
if (select.value == "Austria") {
result.innerHTML = name + ", your insurance costs " + Math.round((horsePower * 100 / age + 50)) + " eur";
}
else if (select.value == "Hungary") {
result.innerHTML = name + ", your insurance costs " + Math.round((horsePower * 120 / age + 50)) + " eur";
}
else {
result.innerHTML = name + ", your insurance costs " + Math.round((horsePower * 150 / (age + 3) + 50)) + " eur";
}
}
document.getElementById('btn').addEventListener('click', calculateInsurance, false); | 9fc6685b9a5b6976169bb87e5a14770d87f4e8da | [
"JavaScript",
"HTML",
"Markdown"
] | 4 | JavaScript | AlejandroRodriguez95/CF-CR3-Alejandro | 85794db17b46351efd269ec3c06e8d90087bfc47 | b46ff3da8d95a8386a184caeb2b671b5df650cc2 | |
refs/heads/master | <repo_name>crizon/SciHive<file_sep>/README.md
# SciHive
SciHive is a mobile application made for grade 6 to 7 students, an educational game app about science. This app is my thesis project so I made sure I'll be integrating as many technologies as possible. The full title of this thesis project is SCIHIVE: A Mobile Based E-Learning System with BITAP Algorithm in Science Subject of Grade 7 Basic Education Columban College, Inc., Olongapo City.
SciHive is a world about Science, the students can access lessons, games, activities and quizzes, RPG inspired navigation to make an immersive experience to students. Developed using HTML, CSS, JS, Cordova/Phonegap, PHP, MySQL.
Here are some of the key features / technologies in this app:
Lessons
Games
Quizzes
Motivational Quotes
Science Facts
Activities
Experiments
Videos
Score Rankings
Accounts Control (Admin/Teacher)
2D Visuals
3D Models
Text-to-Speech (Audio)
Virtual Reality (Goggle-less)
Chatbot (Artificial Intelligence)
Search Bar (Bitap Algorithm)
Barcode Scanner Login
Group Chat
<img src="https://i.ibb.co/DpwRxXV/Slide1.jpg" alt="Slide1" border="0">
<img src="https://i.ibb.co/m531XSF/Slide2.jpg" alt="Slide2" border="0">
<img src="https://i.ibb.co/JHqqczM/Slide3.jpg" alt="Slide3" border="0">
<img src="https://i.ibb.co/1qRTZqR/Slide4.jpg" alt="Slide4" border="0">
<img src="https://i.ibb.co/KWk5q8R/Slide5.jpg" alt="Slide5" border="0">
<file_sep>/SciHive Source Code (Server)/_ranks/quiz_4.php
<!DOCTYPE html>
<html style="background-color: black; height: 100%">
<head>
<meta name="viewport" content="initial-scale=1, width=device-width, viewport-fit=cover">
<title>SciHive</title>
<style>
html,body,button{height: 100%}
.hyperX{height: 100%}
</style>
<script src="vendor/jquery-1.11.1.min.js"></script>
<link rel="stylesheet" type="text/css" href="vendor/sweetalert2.css">
<link rel="stylesheet" type="text/css" href="vendor/animate.css">
<link href="vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="vendor/elusive-icons.css">
<link href="../vendor/fontawesome-free/css/all.min.css" rel="stylesheet" type="text/css">
<link rel="stylesheet" type="text/css" href="vendor/own.css">
<!-- TO DISABLE SELECTING -->
<style type="text/css">
*
{
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none; outline: none !important
}
input
{
-webkit-user-select: auto !important;
-khtml-user-select: auto !important;
-moz-user-select: auto !important;
-ms-user-select: auto !important;
user-select: auto !important;
}
img { border: none; }
button:focus {
border: none;
outline: none;
}
input:focus {
border: none;
outline: none;
}
</style>
<style type="text/css">
.bgDark{background-color: #000000; background-image: url("data:image/svg+xml,%3Csvg xmlns='http://www.w3.org/2000/svg' width='28' height='49' viewBox='0 0 28 49'%3E%3Cg fill-rule='evenodd'%3E%3Cg id='hexagons' fill='%2329261c' fill-opacity='0.4' fill-rule='nonzero'%3E%3Cpath d='M13.99 9.25l13 7.5v15l-13 7.5L1 31.75v-15l12.99-7.5zM3 17.9v12.7l10.99 6.34 11-6.35V17.9l-11-6.34L3 17.9zM0 15l12.98-7.5V0h-2v6.35L0 12.69v2.3zm0 18.5L12.98 41v8h-2v-6.85L0 35.81v-2.3zM15 0v7.5L27.99 15H28v-2.31h-.01L17 6.35V0h-2zm0 49v-8l12.99-7.5H28v2.31h-.01L17 42.15V49h-2z'/%3E%3C/g%3E%3C/g%3E%3C/svg%3E");}
.css-serial {
counter-reset: serial-number; /* Set the serial number counter to 0 */
}
.css-serial td:first-child:before {
counter-increment: serial-number; /* Increment the serial number counter */
content: counter(serial-number); /* Display the counter */
}
</style>
</head>
<body class="bgDark">
<div class="btn-warning" style="height: 55px; width: 100%;top: 0; right: 0;">
<button style="float: left" class="btn btn-warning btn-sm" onclick="location.assign('index.php')"> <h1> ◀ </h1> </button>
<center><h4>F M E Quiz</h4></center>
</div>
<br>
<div style="padding: 25px">
<table class="css-serial table table-sm table-hover" style="background-color: white">
<thead>
<tr style="background-color: pink; font-family: OJ">
<th><h3><center>Rank</center></h3></th>
<th><h3><center>Name</center></h3></th>
<th><h3><center>Score</center></h3></th>
</tr>
</thead>
<?php
include_once('../connector.php');
$game="quiz_4";
$kwandesu=$db->prepare("SELECT * FROM sci_scores WHERE score_game=:s_g ORDER BY sci_scores.score_actual DESC LIMIT 20");
$kwandesu->bindParam(':s_g',$game);
$kwandesu->execute();
foreach ($kwandesu as $scores) { ?>
<tr style="font-family: moon; font-size: 1.5em">
<td style="text-align: center;font-family: OJ !important"></td>
<td><center><?=$scores['score_student_Name']?></center></td>
<td><center><?=$scores['score_actual']?></center></td>
</tr>
<?php } ?>
</table>
</div>
<script crossorigin type="text/javascript" src="vendor/sweetalert2.js"></script>
<script src="vendor/jquery/jquery.min.js"></script>
<script src="vendor/bootstrap/js/bootstrap.bundle.min.js"></script>
</body>
</html>
<file_sep>/SciHive Source Code (Server)/_quiz/Quiz_5/score.php
<?php
require_once('../../connector.php');
if(!empty($_GET)){
$game_name = "quiz_5";
$score = $_GET["score"];
$nameX = $_GET["name"];
$IDX = $_GET["ID"];
$dateX = date('Y-m-d H:i:s');
$sql=$db->prepare("INSERT INTO sci_scores(score_game,score_actual,score_student_Name,score_student_ID,score_date_time)
VALUES('$game_name', '$score', '$nameX', '$IDX', '$dateX')");
$sql->execute();
}
header("location:../index.php");
?><file_sep>/SciHive Source Code (Server)/_chat/index.php
<?php
include_once('../connector.php');
session_start();
if(isset($_SESSION['account'])){
?>
<?php
$UserName = $_SESSION["welcomename"];
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>The Way</title>
<!-- <link rel="stylesheet" href=" vendor/jquery.mobile-1.4.5.min.css" /> -->
<script src=" vendor/jquery-1.11.1.min.js"></script>
<!-- <script src=" vendor/jquery.mobile-1.4.5.min.js"></script> -->
<link href=" vendor/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" type="text/css" href=" vendor/own.css">
<script src=" vendor/own.js"></script>
<link rel="stylesheet" type="text/css" href=" vendor/sweetalert.css">
<script src=" vendor/sweetalert.min.js"></script>
<script src="vendor/bootstrap-filestyle.min.js"></script>
<!-- TO DISABLE SELECTING -->
<style type="text/css">
*
{
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
input
{
-webkit-user-select: auto !important;
-khtml-user-select: auto !important;
-moz-user-select: auto !important;
-ms-user-select: auto !important;
user-select: auto !important;
}
img { border: none; }
button:focus {
border: none;
outline: none;
}
input:focus {
border: none;
outline: none;
}
</style>
</head>
<body style="background-color: beige">
<div class="btn-warning" style="height: 55px; width: 100%;top: 0; right: 0;">
<button style="float: left" class="btn btn-warning btn-sm" onclick="location.assign('../index.php')"> <h1> ◀ </h1></button>
<center><h3>Group Chat</h3></center>
</div>
<form action="process-register.php" method="post" enctype="multipart/form-data" style="background-color: #ffc335;border: 5px outset orange; padding: 10px;border-bottom-right-radius: 25px;border-bottom-left-radius: 25px;">
<table style="width: 100%; background-color: white; border: 1px solid orange">
<?php
require_once('../connector.php');
$stmt = $db->prepare("SELECT * FROM ( SELECT * FROM sci_convo ORDER BY convo_ID DESC LIMIT 20) sub ORDER BY convo_date_time ASC;");
$stmt->execute();
foreach($stmt as $studidents) {
?>
<tr>
<td>
<span style="background-color: black; color: white; margin-right: 5px; border-radius: 10px; padding: 1px"><?=$studidents['convo_user']?>: </span>
<?=$studidents['convo_content']?>
<img src="<?=$studidents['convo_loc']?>" width="100%">
</td>
</tr>
<?php } ?>
</table><br>
<center>
<div class="form-group">
<input type="text" name="xSentMessage" class="form-control" placeholder="Enter message here . . ." required="required" style="border: 1px solid orange; border-radius: 10px;height: 50px;" autocomplete="off" autofocus="autofocus">
<!-- <input type="file" name="fileToUpload" id="fileToUpload" class="filestyle" style="width: 100%;"> -->
<input type="text" name="xSender" class="form-control" required="required" hidden="hidden" value="<?php echo $UserName ?>">
</div>
<button style="width: 45%; color: white; font-size: 15pt; font-weight: bold; box-shadow: 3px 3px 5px 1px rgba(10,10,10,.7);" type="submit" name="submit" class="btn btn-success">➤</button>
</center>
</form>
<script src=" vendor/bootstrap.bundle.min.js"></script>
</body>
</html>
<?php
}else{
header('location:errorlogin.php');
}
?>
<file_sep>/SciHive Source Code (Client)/www/lessons/matter/gas.html
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1, width=device-width, viewport-fit=cover">
<title>Gas</title>
<style>
html,body{height: 100%}
.hyperX{height: 100%}
</style>
<script src="../../vendor/jquery-1.11.1.min.js"></script>
<link rel="stylesheet" type="text/css" href="../../vendor/sweetalert2.css">
<link rel="stylesheet" type="text/css" href="../../vendor/animate.css">
<link href="../../vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="../../vendor/elusive-icons.css">
<link href="../../vendor/fontawesome-free/css/all.min.css" rel="stylesheet" type="text/css">
<link rel="stylesheet" type="text/css" href="../../vendor/own.css">
<!-- TO DISABLE SELECTING -->
<style type="text/css">
*
{
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
input
{
-webkit-user-select: auto !important;
-khtml-user-select: auto !important;
-moz-user-select: auto !important;
-ms-user-select: auto !important;
user-select: auto !important;
}
img { border: none; }
button:focus {
border: none;
outline: none;
}
input:focus {
border: none;
outline: none;
}
</style>
<script type="text/javascript">
</script>
</head>
<body style="background-color: black; background-image: url(../../img/hexellence.png)">
<script type="text/javascript" src="../../cordova.js"></script>
<center>
<h4 style="background-color: #7714d1; padding: 5px; top:0 ; width: 100%; z-index: 1; position: fixed; color: white; background-image: url(../../img/hexellence.png);">Gas</h4>
<h4 onclick="balik()" class="fas fa-fw fa-chevron-left" style="z-index: 2; position: fixed; left: 5px; top: 10px; color: white"></h4>
</center>
<br>
<br>
<div style="text-align: justify; padding: 5%; margin: 2.5%; width: 95%; background-color: white;border-radius: 5px">
<div class="row" style="margin: 0">
<div id="tts">
<button onclick="read1()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>
</div>
<span style="color: rgba(0,0,0,0);">h</span>
<h3> Gas </h3>
</div>
<img src="gas/gas 1.jpg" width="100%" style="border: 5px outset orange"><br><br>
<p>
Gases are everywhere. You may have heard about the atmosphere. The atmosphere is an envelope of gases that surrounds the Earth. In <i>solids,</i> atoms and molecules are compact and close together. <i>Liquids</i> have atoms that are spread out a little more. The molecules in gases are really spread out, full of energy, and constantly moving around in random ways.
</p><br>
<p>
What is another <b>physical</b> characteristic of gases? Gases can fill a container of any size or shape. It doesn't matter how big the container is. The molecules spread out to fill the whole space equally. Think about a balloon. No matter what shape you make the balloon, it will be evenly filled with the gas molecules. Even if you make a balloon animal, the molecules are spread equally throughout the entire shape.
</p><br>
<p>
Liquids can only fill the bottom of a container, while gases can fill it entirely. The shape of liquids is very dependent on <b>gravity</b>, while less dense gases are light enough to have a more freedom to move.
</p><br>
<img src="gas/gas 2.jpg" width="100%" style="border: 5px outset orange"><br><br>
<div class="row" style="margin: 0">
<div id="tts2">
<button onclick="read2()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>
</div>
<span style="color: rgba(0,0,0,0);">h</span>
<h3> Gas or Vapor?</h3>
</div>
<p>
You might hear the term "<b>vapor</b>". Vapor and gas mean the same thing. The word vapor is used to describe gases that are usually liquids at room temperature. Good examples of these types of liquids include water and mercury. They get the vapor title when they are in a gaseous phase. You will probably hear the term “water vapor” which means water in a gas state. Compounds such as carbon dioxide are usually gases at room temperature. Scientists will rarely talk about carbon dioxide vapor.
</p><br>
<h3> Compressing Gases </h3>
<p>
Gases hold huge amounts of <b>energy</b> and their molecules are spread out as much as possible. When compared to solids or liquids, those spread out gaseous systems can be <b>compressed</b> with very little effort. Scientists and engineers use that physical trait all of the time. Combinations of increased pressure and decreased temperature force gases into containers that we use every day.
</p><br>
<img src="gas/gas 3.jpg" width="100%" style="border: 5px outset orange"><br><br>
<p>
You might have compressed air in a spray bottle or feel the carbon dioxide rush out of a can of soda. Those are both examples of gas forced into a smaller space at greater pressure. As soon as the gas is introduced to an environment with a lower pressure, it rushes out of the container. The gas molecules move from an area of high pressure to one of low pressure.</p><br>
<!--
<br><br>
<div id="carousel1" data-interval="2000" class="carousel slide" data-ride="carousel">
<div class="carousel-inner">
<div class="carousel-item active">
<img class="d-block w-100" src="liquid/L1.jpg" alt="First slide">
</div>
<div class="carousel-item">
<img class="d-block w-100" src="liquid/L2.jpg" alt="Second slide">
</div>
<div class="carousel-item">
<img class="d-block w-100" src="liquid/L3.jpg" alt="Third slide">
</div>
<div class="carousel-item">
<img class="d-block w-100" src="liquid/L4.jpg" alt="Fourth slide">
</div>
<div class="carousel-item">
<img class="d-block w-100" src="liquid/L5.jpg" alt="Fifth slide">
</div>
</div>
</div><br><br> -->
</div>
<script type="text/javascript">
//READ THE TEXT TO SPEECH
function read1(){
var line_1 = "Gases are everywhere. You may have heard about the atmosphere. The atmosphere is an envelope of gases that surrounds the Earth. In solids, atoms and molecules are compact and close together. Liquids have atoms that are spread out a little more. The molecules in gases are really spread out, full of energy, and constantly moving around in random ways. What is another physical characteristic of gases? Gases can fill a container of any size or shape. It doesn't matter how big the container is. The molecules spread out to fill the whole space equally. Think about a balloon. No matter what shape you make the balloon, it will be evenly filled with the gas molecules. Even if you make a balloon animal, the molecules are spread equally throughout the entire shape. Liquids can only fill the bottom of a container, while gases can fill it entirely. The shape of liquids is very dependent on gravity, while less dense gases are light enough to have a more freedom to move.";
TTS.speak({
text: line_1,
locale: 'en-US',
rate: 1
});
//
var codeBlock='<button onclick="stop1()" class="btn btn-secondary"><i class="fas fa-fw fa-volume-mute"></i></button>';
document.getElementById("tts").innerHTML = codeBlock;
}
function read2(){
var line_2 = "Gas or Vapor? You might hear the term vapor. Vapor and gas mean the same thing. The word vapor is used to describe gases that are usually liquids at room temperature. Good examples of these types of liquids include water and mercury. They get the vapor title when they are in a gaseous phase. You will probably hear the term “water vapor” which means water in a gas state. Compounds such as carbon dioxide are usually gases at room temperature. Scientists will rarely talk about carbon dioxide vapor. Compressing Gases. Gases hold huge amounts of energy and their molecules are spread out as much as possible. When compared to solids or liquids, those spread out gaseous systems can be compressed with very little effort. Scientists and engineers use that physical trait all of the time. Combinations of increased pressure and decreased temperature force gases into containers that we use every day. You might have compressed air in a spray bottle or feel the carbon dioxide rush out of a can of soda. Those are both examples of gas forced into a smaller space at greater pressure. As soon as the gas is introduced to an environment with a lower pressure, it rushes out of the container. The gas molecules move from an area of high pressure to one of low pressure.";
TTS.speak({
text: line_2,
locale: 'en-US',
rate: 1
});
//
var codeBlock2='<button onclick="stop2()" class="btn btn-secondary"><i class="fas fa-fw fa-volume-mute"></i></button>';
document.getElementById("tts2").innerHTML = codeBlock2;
}
//STOP THE TEXT TO SPEECH
function stop1(){
TTS.speak({text: ''});
//set the new icon to button
var codeBlock='<button onclick="read1()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>';
document.getElementById("tts").innerHTML = codeBlock;
}
function stop2(){
TTS.speak({text: ''});
//set the new icon to button
var codeBlock2='<button onclick="read2()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>';
document.getElementById("tts2").innerHTML = codeBlock2;
}
//STOP THE TEXT TO SPEECH AND GO BACK HISTORY using back button
document.addEventListener("deviceready", onDeviceReady, false);
function onDeviceReady() {
document.addEventListener("backbutton", function () {
TTS.speak({text: ''});
window.history.back();
}, false );
}
//STOP THE TEXT TO SPEECH AND GO BACK HISTORY using a link button
function balik(){
TTS.speak({text: ''});
window.history.back();
}
</script>
<script crossorigin type="text/javascript" src="../../vendor/sweetalert2.js"></script>
<script src="../../vendor/jquery/jquery.min.js"></script>
<script src="../../vendor/bootstrap/js/bootstrap.bundle.min.js"></script>
</body>
</html><file_sep>/SciHive Source Code (Server)/_quiz/Quiz_3/myjs.js
var scoreQ1 = 0;
function goodX(){
scoreQ1 = scoreQ1 + 1;
}
function scoreX(){
var scoreIs = "Score: " + scoreQ1;
}<file_sep>/SciHive Source Code (Server)/_quiz/index.php
<?php
session_start();
$UserName = $_SESSION["welcomename"];
$firstName = $_SESSION["welcomename"];
$ID_number = $_SESSION['Username'];
?>
<!DOCTYPE html>
<html style="background-color: black; height: 100%">
<head>
<meta name="viewport" content="initial-scale=1, width=device-width, viewport-fit=cover">
<title>SciHive</title>
<style>
html,body,button{height: 100%}
.hyperX{height: 100%}
</style>
<script src="vendor/jquery-1.11.1.min.js"></script>
<link rel="stylesheet" type="text/css" href="vendor/sweetalert2.css">
<link rel="stylesheet" type="text/css" href="vendor/animate.css">
<link href="vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="vendor/elusive-icons.css">
<link href="../vendor/fontawesome-free/css/all.min.css" rel="stylesheet" type="text/css">
<link rel="stylesheet" type="text/css" href="vendor/own.css">
<!-- TO DISABLE SELECTING -->
<style type="text/css">
*
{
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none; outline: none !important
}
input
{
-webkit-user-select: auto !important;
-khtml-user-select: auto !important;
-moz-user-select: auto !important;
-ms-user-select: auto !important;
user-select: auto !important;
}
img { border: none; }
button:focus {
border: none;
outline: none;
}
input:focus {
border: none;
outline: none;
}
a{text-decoration: none !important;}
.bgDark{background-color: #000000; background-image: url("data:image/svg+xml,%3Csvg xmlns='http://www.w3.org/2000/svg' width='28' height='49' viewBox='0 0 28 49'%3E%3Cg fill-rule='evenodd'%3E%3Cg id='hexagons' fill='%2329261c' fill-opacity='0.4' fill-rule='nonzero'%3E%3Cpath d='M13.99 9.25l13 7.5v15l-13 7.5L1 31.75v-15l12.99-7.5zM3 17.9v12.7l10.99 6.34 11-6.35V17.9l-11-6.34L3 17.9zM0 15l12.98-7.5V0h-2v6.35L0 12.69v2.3zm0 18.5L12.98 41v8h-2v-6.85L0 35.81v-2.3zM15 0v7.5L27.99 15H28v-2.31h-.01L17 6.35V0h-2zm0 49v-8l12.99-7.5H28v2.31h-.01L17 42.15V49h-2z'/%3E%3C/g%3E%3C/g%3E%3C/svg%3E");}
</style>
<script type="text/javascript">
var student_ID = "<?php echo $ID_number ?>";
localStorage.setItem("stud_ID", student_ID);
var student_name = "<?php echo $firstName ?>";
localStorage.setItem("stud_Name", student_name);
</script>
</head>
<body class="bgDark">
<div class="btn-warning" style="height: 55px; width: 100%;top: 0; right: 0;">
<button style="float: left" class="btn btn-warning btn-sm" onclick="location.assign('../index.php')"> <h1> ◀ </h1> </button>
<center><img src="quiz.png" width="50%" style="float: left"></center>
</div>
<br>
<center>
<h6 style="color: white">Choose a category: </h6><br>
<a href="Quiz_1/index.php">
<div class="btn-warning" style="width: 90%; padding: 20px">
<h2><i class="fas fa-fw fa-play-circle"> </i>Science</h2>
<center>
<img src="quiz_logo.png" width="25%">
</center>
</div>
</a>
<br>
<a href="Quiz_2/index.php">
<div class="btn-warning" style="width: 90%; padding: 20px">
<h2><i class="fas fa-fw fa-play-circle"> </i>Matter</h2>
<center>
<img src="quiz_logo.png" width="25%">
</center>
</div>
</a>
<br>
<a href="Quiz_3/index.php">
<div class="btn-warning" style="width: 90%; padding: 20px">
<h2><i class="fas fa-fw fa-play-circle"> </i>Living Things</h2>
<center>
<img src="quiz_logo.png" width="25%">
</center>
</div>
</a>
<br>
<a href="Quiz_4/index.php">
<div class="btn-warning" style="width: 90%; padding: 20px">
<h3><i class="fas fa-fw fa-play-circle"> </i>Force Motion Energy</h3>
<center>
<img src="quiz_logo.png" width="25%">
</center>
</div>
</a>
<br>
<a href="Quiz_5/index.php">
<div class="btn-warning" style="width: 90%; padding: 20px">
<h2><i class="fas fa-fw fa-play-circle"> </i>Earth and Space</h2>
<center>
<img src="quiz_logo.png" width="25%">
</center>
</div>
</a>
<br>
</center>
<script crossorigin type="text/javascript" src="vendor/sweetalert2.js"></script>
<script src="vendor/jquery/jquery.min.js"></script>
<script src="vendor/bootstrap/js/bootstrap.bundle.min.js"></script>
</body>
</html>
<file_sep>/SciHive Source Code (Client)/www/lessons/living_things/animals_cells.html
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1, width=device-width, viewport-fit=cover">
<title>Animal Cell</title>
<style>
html,body{height: 100%}
.hyperX{height: 100%}
</style>
<script src="../../vendor/jquery-1.11.1.min.js"></script>
<link rel="stylesheet" type="text/css" href="../../vendor/sweetalert2.css">
<link rel="stylesheet" type="text/css" href="../../vendor/animate.css">
<link href="../../vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="../../vendor/elusive-icons.css">
<link href="../../vendor/fontawesome-free/css/all.min.css" rel="stylesheet" type="text/css">
<link rel="stylesheet" type="text/css" href="../../vendor/own.css">
<!-- TO DISABLE SELECTING -->
<style type="text/css">
*
{
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
input
{
-webkit-user-select: auto !important;
-khtml-user-select: auto !important;
-moz-user-select: auto !important;
-ms-user-select: auto !important;
user-select: auto !important;
}
img { border: none; }
button:focus {
border: none;
outline: none;
}
input:focus {
border: none;
outline: none;
}
</style>
<script type="text/javascript">
</script>
</head>
<body style="background-color: black; background-image: url(../../img/hexellence.png)">
<script type="text/javascript" src="../../cordova.js"></script>
<center>
<h4 style="background-color: #7714d1; padding: 5px; top:0 ; width: 100%; z-index: 1; position: fixed; color: white; background-image: url(../../img/hexellence.png);">Animal Cell</h4>
<h4 onclick="balik()" class="fas fa-fw fa-chevron-left" style="z-index: 2; position: fixed; left: 5px; top: 10px; color: white"></h4>
</center>
<br>
<br>
<div style="text-align: justify; padding: 5%; margin: 2.5%; width: 95%; background-color: white;border-radius: 5px">
<div class="row" style="margin: 0">
<div id="tts">
<button onclick="read1()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>
</div>
<span style="color: rgba(0,0,0,0);">h</span>
<h3> Animal Cell</h3>
</div>
<script type="text/javascript" src="../../vendor/model-viewer.js"></script>
<center>
<model-viewer style="width: 100%; height: 300px" src="ANIMALS/animalcell.gltf" auto-rotate camera-controls background-color="black">
</model-viewer>
3D Model (Touch and Rotate)
</center>
<br>
<p>Animal cells are eukaryotic cells or cells with a membrane-bound nucleus. Unlike prokaryotic cells, DNA in animal cells is housed within the nucleus. In addition to having a nucleus, animal cells also contain other membrane-bound organelles, or tiny cellular structures, that carry out specific functions necessary for normal cellular operation. Organelles have a wide range of responsibilities that include everything from producing hormones and enzymes to providing energy for animal cells.<br><br>
<img src="ANIMALS/anicell1.jpg" width="100%"><br><br>
Plant and animal cells are similar in that they are both eukaryotic and have similar types of organelles. Plant cells tend to have more uniform sizes than animal cells.
</p><br>
<div class="row" style="margin: 0">
<div id="tts2">
<button onclick="read2()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>
</div>
<span style="color: rgba(0,0,0,0);">h</span>
<h3>Components of <br>
Animal Cells</h3>
</div>
<img src="ANIMALS/anicell2.jpg" width="100%" style="border: 5px outset orange"><br><br>
<p>The following are examples of structures and organelles that can be found in typical animal cells:
<br><br>
<li>Cell (Plasma) Membrane - thin, semi-permeable membrane that surrounds the cytoplasm of a cell, enclosing its contents.</li><br>
<li>Centrioles - cylindrical structures that organize the assembly of microtubules during cell division.</li><br>
<li>Cilia and flagella - specialized groupings of microtubules that protrude from some cells and aid in cellular locomotion.</li><br>
<li>Cytoplasm - gel-like substance within the cell.</li><br>
<li>Cytoskeleton - a network of fibers throughout the cell's cytoplasm that gives the cell support and helps to maintain its shape.</li><br>
<li>Endoplasmic Reticulum - an extensive network of membranes composed of both regions with ribosomes (rough ER) and regions without ribosomes (smooth ER).</li><br>
<li>Golgi Complex - also called the Golgi apparatus, this structure is responsible for manufacturing, storing and shipping certain cellular products.</li><br>
<li>Lysosomes - sacs of enzymes that digest cellular macromolecules such as nucleic acids.</li><br>
<li>Microtubules - hollow rods that function primarily to help support and shape the cell.</li><br>
<li>Mitochondria - cell components that generate energy for the cell and are the sites of cellular respiration.</li><br>
<li>Nucleus - membrane-bound structure that contains the cell's hereditary information.</li><br>
<li style="margin-left: 30px"><b>Nucleolus</b> - structure within the nucleus that helps in the synthesis of ribosomes.</li><br>
<li style="margin-left: 30px"><b>Nucleopore</b> - a tiny hole in the nuclear membrane that allows nucleic acids and proteins to move into and out of the nucleus.</li><br>
<li>Peroxisomes - enzyme containing structures that help to detoxify alcohol, form bile acid, and break down fats.</li><br>
<li>Ribosomes - consisting of RNA and proteins, ribosomes are responsible for protein assembly.</li><br>
</p><br>
<!-- <br><br>
<div id="carousel1" data-interval="2000" class="carousel slide" data-ride="carousel">
<div class="carousel-inner">
<div class="carousel-item active">
<img class="d-block w-100" src="liquid/L1.jpg" alt="First slide">
</div>
<div class="carousel-item">
<img class="d-block w-100" src="liquid/L2.jpg" alt="Second slide">
</div>
<div class="carousel-item">
<img class="d-block w-100" src="liquid/L3.jpg" alt="Third slide">
</div>
<div class="carousel-item">
<img class="d-block w-100" src="liquid/L4.jpg" alt="Fourth slide">
</div>
<div class="carousel-item">
<img class="d-block w-100" src="liquid/L5.jpg" alt="Fifth slide">
</div>
</div>
</div><br><br> -->
</div>
<script type="text/javascript">
//READ THE TEXT TO SPEECH
function read1(){
var line_1 = "Animal cells. Animal cells are eukaryotic cells or cells with a membrane-bound nucleus. Unlike prokaryotic cells, DNA in animal cells is housed within the nucleus. In addition to having a nucleus, animal cells also contain other membrane-bound organelles, or tiny cellular structures, that carry out specific functions necessary for normal cellular operation. Organelles have a wide range of responsibilities that include everything from producing hormones and enzymes to providing energy for animal cells.Plant and animal cells are similar in that they are both eukaryotic and have similar types of organelles. Plant cells tend to have more uniform sizes than animal cells.";
TTS.speak({
text: line_1,
locale: 'en-US',
rate: 1
});
//
var codeBlock='<button onclick="stop1()" class="btn btn-secondary"><i class="fas fa-fw fa-volume-mute"></i></button>';
document.getElementById("tts").innerHTML = codeBlock;
}
function read2(){
var line_2 = "Components of Animal Cell. The following are examples of structures and organelles that can be found in typical animal cells. Cell (Plasma) Membrane - thin, semi-permeable membrane that surrounds the cytoplasm of a cell, enclosing its contents. Centrioles - cylindrical structures that organize the assembly of microtubules during cell division. Cilia and flagella - specialized groupings of microtubules that protrude from some cells and aid in cellular locomotion. Cytoplasm - gel-like substance within the cell. Cytoskeleton - a network of fibers throughout the cell's cytoplasm that gives the cell support and helps to maintain its shape. Endoplasmic Reticulum - an extensive network of membranes composed of both regions with ribosomes and regions without ribosomes. Golgi Complex - also called the Golgi apparatus, this structure is responsible for manufacturing, storing and shipping certain cellular products. Lysosomes - sacs of enzymes that digest cellular macromolecules such as nucleic acids. Microtubules - hollow rods that function primarily to help support and shape the cell. Mitochondria - cell components that generate energy for the cell and are the sites of cellular respiration. Nucleus - membrane-bound structure that contains the cells hereditary information. Nucleolus - structure within the nucleus that helps in the synthesis of ribosomes. Nucleopore - a tiny hole in the nuclear membrane that allows nucleic acids and proteins to move into and out of the nucleus. Peroxisomes - enzyme containing structures that help to detoxify alcohol, form bile acid, and break down fats. Ribosomes - consisting of RNA and proteins, ribosomes are responsible for protein assembly.";
TTS.speak({
text: line_2,
locale: 'en-US',
rate: 1
});
//
var codeBlock2='<button onclick="stop2()" class="btn btn-secondary"><i class="fas fa-fw fa-volume-mute"></i></button>';
document.getElementById("tts2").innerHTML = codeBlock2;
}
//STOP THE TEXT TO SPEECH
function stop1(){
TTS.speak({text: ''});
//set the new icon to button
var codeBlock='<button onclick="read1()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>';
document.getElementById("tts").innerHTML = codeBlock;
}
function stop2(){
TTS.speak({text: ''});
//set the new icon to button
var codeBlock2='<button onclick="read2()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>';
document.getElementById("tts2").innerHTML = codeBlock2;
}
//STOP THE TEXT TO SPEECH AND GO BACK HISTORY using back button
document.addEventListener("deviceready", onDeviceReady, false);
function onDeviceReady() {
document.addEventListener("backbutton", function () {
TTS.speak({text: ''});
window.history.back();
}, false );
}
//STOP THE TEXT TO SPEECH AND GO BACK HISTORY using a link button
function balik(){
TTS.speak({text: ''});
window.history.back();
}
</script>
<script crossorigin type="text/javascript" src="../../vendor/sweetalert2.js"></script>
<script src="../../vendor/jquery/jquery.min.js"></script>
<script src="../../vendor/bootstrap/js/bootstrap.bundle.min.js"></script>
</body>
</html><file_sep>/SciHive Source Code (Server)/index.php
<?php
include_once('connector.php');
session_start();
if(isset($_SESSION['account'])){
?>
<?php
$UserName = $_SESSION["welcomename"];
$firstName = $_SESSION["welcomename"];
$ID_number = $_SESSION['Username'];
?>
<!DOCTYPE html>
<html >
<head>
<!-- Site made with Mobirise Website Builder v4.11.2, https://mobirise.com -->
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="generator" content="Mobirise v4.11.2, mobirise.com">
<meta name="viewport" content="width=device-width, initial-scale=1, minimum-scale=1">
<link rel="shortcut icon" href="assets/images/beebot-122x122.png" type="image/x-icon">
<meta name="description" content="">
<title>Home</title>
<link rel="stylesheet" href="assets/web/assets/mobirise-icons/mobirise-icons.css">
<link rel="stylesheet" href="assets/bootstrap/css/bootstrap.min.css">
<link rel="stylesheet" href="assets/bootstrap/css/bootstrap-grid.min.css">
<link rel="stylesheet" href="assets/bootstrap/css/bootstrap-reboot.min.css">
<link rel="stylesheet" href="assets/socicon/css/styles.css">
<link rel="stylesheet" href="assets/tether/tether.min.css">
<link rel="stylesheet" href="assets/dropdown/css/style.css">
<link rel="stylesheet" href="assets/theme/css/style.css">
<link href="assets/fonts/style.css" rel="stylesheet">
<link rel="preload" as="style" href="assets/mobirise/css/mbr-additional.css"><link rel="stylesheet" href="assets/mobirise/css/mbr-additional.css" type="text/css">
<style type="text/css">
.bgDark{background-color: #000000; background-image: url("data:image/svg+xml,%3Csvg xmlns='http://www.w3.org/2000/svg' width='28' height='49' viewBox='0 0 28 49'%3E%3Cg fill-rule='evenodd'%3E%3Cg id='hexagons' fill='%2329261c' fill-opacity='0.4' fill-rule='nonzero'%3E%3Cpath d='M13.99 9.25l13 7.5v15l-13 7.5L1 31.75v-15l12.99-7.5zM3 17.9v12.7l10.99 6.34 11-6.35V17.9l-11-6.34L3 17.9zM0 15l12.98-7.5V0h-2v6.35L0 12.69v2.3zm0 18.5L12.98 41v8h-2v-6.85L0 35.81v-2.3zM15 0v7.5L27.99 15H28v-2.31h-.01L17 6.35V0h-2zm0 49v-8l12.99-7.5H28v2.31h-.01L17 42.15V49h-2z'/%3E%3C/g%3E%3C/g%3E%3C/svg%3E");}
.icons{border-radius: 5px!important}
</style>
<script type="text/javascript">
var student_ID = "<?php echo $ID_number ?>";
localStorage.setItem("stud_ID", student_ID);
var student_name = "<?php echo $firstName ?>";
localStorage.setItem("stud_Name", student_name);
</script>
</head>
<body class="bgDark">
<br>
<!-- <section class="menu cid-rF1Sxo7O9m" once="menu" id="menu1-1">
<nav class="navbar navbar-expand beta-menu navbar-dropdown align-items-center navbar-fixed-top navbar-toggleable-sm">
<button class="navbar-toggler navbar-toggler-right" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation">
<div class="hamburger">
<span></span>
<span></span>
<span></span>
<span></span>
</div>
</button>
<div class="menu-logo">
<div class="navbar-brand">
<span class="navbar-logo">
<a href="#">
<img src="assets/images/beebot-122x122.png" alt="SciHive" title="" style="height: 3.8rem;">
</a>
</span>
<span class="navbar-caption-wrap"><a class="navbar-caption text-white display-5" href="#">
SciHive Online</a></span>
</div>
</div>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul class="navbar-nav nav-dropdown nav-right" data-app-modern-menu="true">
<li class="nav-item">
<a class="nav-link link text-white display-4" href="#">
<span class="mbri-user mbr-iconfont mbr-iconfont-btn"></span>
Logout
</a>
</li>
<li class="nav-item">
<a class="nav-link link text-white display-4" href="#">
<span class="mbri-home mbr-iconfont mbr-iconfont-btn"></span>Home</a>
</li>
<li class="nav-item">
<a class="nav-link link text-white display-4" href="#">
<span class="mbri-search mbr-iconfont mbr-iconfont-btn"></span>
About Us
</a>
</li>
</ul>
</div>
</nav>
</section> -->
<div class="container">
<div class="row" style="margin: 0 !important; padding: 0 !important">
<div class="col icons" style="margin: 5px; background-color: orange;">
<a href="_chat/index.php">
<img src="img/ico1.png" width="100%">
<pre style="margin: 0!important;padding: 0!important; background-color: orange !important"><center>Chat</center></pre>
</a>
</div>
<div class="col icons" style="margin: 5px; background-color: orange;">
<a href="_ranks/index.php">
<img src="img/ico2.png" width="100%">
<pre style="margin: 0!important;padding: 0!important; background-color: orange !important"><center>Scores</center></pre>
</a>
</div>
<div class="col icons" style="margin: 5px; background-color: orange;">
<a href="_quiz/index.php">
<img src="img/ico3.png" width="100%">
<pre style="margin: 0!important;padding: 0!important; background-color: orange !important"><center>Quiz</center></pre>
</a>
</div>
</div><br>
<div class="row" style="margin: 0 !important; padding: 0 !important">
<div class="col icons" style="margin: 5px; background-color: orange;">
<a href="_beebot/index.php">
<img src="img/ico4.png" width="100%">
<pre style="margin: 0!important;padding: 0!important; background-color: orange !important"><center>Chat Bot</center></pre>
</a>
</div>
<div class="col icons" style="margin: 5px; background-color: orange;">
<a href="_videos/index.php">
<img src="img/ico5.png" width="100%">
<pre style="margin: 0!important;padding: 0!important; background-color: orange !important"><center>Videos</center></pre>
</a>
</div>
<div class="col icons" style="margin: 5px; background-color: orange;">
<img src="img/ico6.png" width="100%">
<pre style="margin: 0!important;padding: 0!important; background-color: orange !important"><center>Web</center></pre>
</div>
</div>
</div>
<br>
<section class="engine"></section><section class="mbr-gallery gallery5 cid-rF1UNSstr8" id="gallery5-x">
<div class="container">
<h3 class="mbr-section-title align-center mbr-fonts-style display-2">
Hello <?php echo $UserName; ?>
</h3>
<h4 class="mbr-section-subtitle align-center pb-4 mbr-fonts-style display-5">
Many Fun Activities with SciHive.
</h4>
<a href="https://animalinyou.com/#test" target="_blank" style="color: black">
<img class="w-100" src="assets/images/background1.jpg">
The Animal in You
</a><br><br>
<a href="https://play.howstuffworks.com/quiz/which-element-are-you" target="_blank" style="color: black">
<img class="w-100" src="assets/images/background2.jpg">
Which Element are you?
</a><br><br>
<a href="https://youtu.be/xctlaTmJ_zQ" target="_blank" style="color: black">
<img class="w-100" src="assets/images/background3.jpg">
Science Experiments You Haven't Seen
</a><br><br>
<a href="https://science.sciencemag.org/" target="_blank" style="color: black">
<img class="w-100" src="assets/images/background4.jpg">
Science Magazines
</a><br><br>
</div>
</section>
<!-- FACEBOOK PAGE NAME -->
<?php
$fb_page = "https://www.facebook.com/SciHive-1682859158525543"
?>
<div id="fb-root"></div>
<script async defer crossorigin="anonymous" src="https://connect.facebook.net/en_US/sdk.js#xfbml=1&version=v4.0&appId=309155756670591&autoLogAppEvents=1"></script>
<div style="width: 100%; background-color: #eeeff1">
<center>
<div class="fb-page" data-href="<?php echo $fb_page ?>" data-tabs="timeline" data-width="" data-height="736" data-small-header="true" data-adapt-container-width="true" data-hide-cover="true" data-show-facepile="true"><blockquote cite="<?php echo $fb_page ?>" class="fb-xfbml-parse-ignore"><a href="<?php echo $fb_page ?>">SciHive</a></blockquote></div>
</center>
</div>
<section class="clients cid-rF1V27i0Sw" data-interval="false" id="clients-13" style="background-color: red !important">
<div class="container mb-5">
<div class="media-container-row">
<div class="col-12 align-center">
<h2 class="mbr-section-title pb-3 mbr-fonts-style display-2">
Special thanks to these
</h2>
<h3 class="mbr-section-subtitle mbr-light mbr-fonts-style display-5">
Awesome Companies
</h3>
</div>
</div>
</div>
<div class="container">
<div class="carousel slide" role="listbox" data-pause="true" data-keyboard="false" data-ride="carousel" data-interval="5000">
<div class="carousel-inner" data-visible="5">
<div class="carousel-item ">
<div class="media-container-row">
<div class="col-md-12">
<div class="wrap-img ">
<img src="assets/images/1.png" class="img-responsive clients-img">
</div>
</div>
</div>
</div><div class="carousel-item ">
<div class="media-container-row">
<div class="col-md-12">
<div class="wrap-img ">
<img src="assets/images/2.png" class="img-responsive clients-img">
</div>
</div>
</div>
</div><div class="carousel-item ">
<div class="media-container-row">
<div class="col-md-12">
<div class="wrap-img ">
<img src="assets/images/3.png" class="img-responsive clients-img">
</div>
</div>
</div>
</div><div class="carousel-item ">
<div class="media-container-row">
<div class="col-md-12">
<div class="wrap-img ">
<img src="assets/images/4.png" class="img-responsive clients-img">
</div>
</div>
</div>
</div><div class="carousel-item ">
<div class="media-container-row">
<div class="col-md-12">
<div class="wrap-img ">
<img src="assets/images/5.png" class="img-responsive clients-img">
</div>
</div>
</div>
</div></div>
<div class="carousel-controls">
<a data-app-prevent-settings="" class="carousel-control carousel-control-prev" role="button" data-slide="prev">
<span aria-hidden="true" class="mbri-left mbr-iconfont"></span>
<span class="sr-only">Previous</span>
</a>
<a data-app-prevent-settings="" class="carousel-control carousel-control-next" role="button" data-slide="next">
<span aria-hidden="true" class="mbri-right mbr-iconfont"></span>
<span class="sr-only">Next</span>
</a>
</div>
</div>
</div>
</section>
<section id="social-buttons2-12" >
<div class="container" style="background-color: orange !important">
<div class="media-container-row">
<div class="col-md-8 align-center">
<h4 class="pb-3 mbr-fonts-style display-2">
FOLLOW US!
</h4>
<div class="social-list pl-0 mb-0">
<a href="" target="_blank" style="color: black">
<span class="px-2 socicon-twitter socicon mbr-iconfont mbr-iconfont-social"></span>
</a>
<a href="https://www.facebook.com/SciHive-1682859158525543" target="_blank" style="color: black">
<span class="px-2 socicon-facebook socicon mbr-iconfont mbr-iconfont-social"></span>
</a>
<a href="" target="_blank" style="color: black">
<span class="px-2 socicon-instagram socicon mbr-iconfont mbr-iconfont-social"></span>
</a>
<a href="" target="_blank" style="color: black">
<span class="px-2 socicon-youtube socicon mbr-iconfont mbr-iconfont-social"></span>
</a>
<a href="" target="_blank" style="color: black">
<span class="px-2 socicon-github socicon mbr-iconfont mbr-iconfont-social"></span>
</a>
</div>
</div>
</div>
</div>
</section>
<script src="assets/web/assets/jquery/jquery.min.js"></script>
<script src="assets/popper/popper.min.js"></script>
<script src="assets/bootstrap/js/bootstrap.min.js"></script>
<script src="assets/smoothscroll/smooth-scroll.js"></script>
<script src="assets/tether/tether.min.js"></script>
<script src="assets/touchswipe/jquery.touch-swipe.min.js"></script>
<script src="assets/bootstrapcarouselswipe/bootstrap-carousel-swipe.js"></script>
<script src="assets/mbr-clients-slider/mbr-clients-slider.js"></script>
<script src="assets/sociallikes/social-likes.js"></script>
<script src="assets/dropdown/js/nav-dropdown.js"></script>
<script src="assets/dropdown/js/navbar-dropdown.js"></script>
<script src="assets/theme/js/script.js"></script>
</body>
</html>
<?php
}else{
header('location:errorlogin.php');
}
?>
<file_sep>/SciHive Source Code (Client)/www/games/candylandia/candy.js
/* Copyright (c) 2017 MIT 6.813/6.831 course staff, all rights reserved.
* Redistribution of original or derived work requires permission of course staff.
*/
/**
* This object represents a candy on the board. Candies have a row
* and a column, and a color
*/
var Candy = function(color, id)
{
////////////////////////////////////////////////
// Representation
//
// Two immutable properties
Object.defineProperty(this, 'color', {value: color, writable: false});
Object.defineProperty(this, 'id', {value: id, writable: false});
// Two mutable properties
this.row = null;
this.col = null;
////////////////////////////////////////////////
// Public methods
//
this.toString = function()
{
var name = this.color;
return name;
}
};
Candy.colors = [
'red',
'yellow',
'green',
'orange',
'blue',
'purple'
];
<file_sep>/SciHive Source Code (Server)/pamahalaan/process-student-register.php
<?php
require_once('../connector.php');
$s_ID = $_POST['s_ID'];
$s_fname = $_POST['s_fname'];
$s_lname = $_POST['s_lname'];
$s_sname = $_POST['s_sname'];
$s_bday = $_POST['s_bday'];
$s_gender = $_POST['s_gender'];
$s_uname = $_POST['s_uname'];
$s_password = $_POST['s_password'];
$s_section = $_POST['s_section'];
$s_teacher = $_POST['s_teacher'];
if(isset($_POST['submit'])){
$studenting=$db->prepare("INSERT INTO sci_students ( s_ID, s_fname, s_lname, s_sname, s_bday, s_gender, s_uname, s_password, s_section, s_teacher ) VALUES ( '$s_ID', '$s_fname', '$s_lname', '$s_sname', '$s_bday', '$s_gender', '$s_uname', '$s_password', '$s_section', '$s_teacher')");
$studenting->execute();
/* $xwxwxw = $db->prepare("UPDATE teachers SET Tnumofstud = Tnumofstud + 1");
$xwxwxw->execute();*/
header('location:registration-student-GOOD.php');
}else{
header('location:registration-BAD.php');
}
?>
<!-- may error tayo sa registration pag doble primary key, kailangan ierror handling exception ek ek --><file_sep>/SciHive Source Code (Client)/www/games/js/plugins/PictureWipe.js
//=============================================================================
// PictureWipe.js
//=============================================================================
/*:
* @plugindesc Transition effects for event pictures.
* @author <NAME>
*
* @help
*
* Plugin Command:
* PictureWipe 1 down in 60
*
* The above plugin command means that the "down" transition effect will be
* applied for displaying the picture #1, in 60 frames.
*
* The first argument specifies the picture ID.
*
* The second argument specifies the type of the effect.
* Can be selected from the following types:
* down - From the top to the bottom.
* up - From the bottom to the top.
* right - From the left to the right.
* left - From the right to the left.
* square - From the center to the edge with a square shape.
* circle - From the center to the edge with a circle shape.
* hblind - Horizontal blind effect.
* vblind - Vertical blind effect.
* grid - Grid effect.
*
* The third argument should be "in" to display or "out" to erase.
*
* The fourth argument specifies the transition time in frames.
*/
/*:ja
* @plugindesc ピクチャの切り替えエフェクトです。
* @author <NAME>
*
* @help
*
* プラグインコマンド:
* PictureWipe 1 down in 60
*
* 上記のプラグインコマンドは、ピクチャ1番を表示する際に「down」の切り替え
* エフェクトを60フレーム表示することを指定しています。
*
* 1つ目の引数には、ピクチャIDを指定します。
*
* 2つ目の引数には、エフェクトの種類を指定します。
* 以下の種類が選択可能です:
* down - 上から下へ。
* up - 下から上へ。
* right - 左から右へ。
* left - 右から左へ。
* square - 正方形で中央から端へ。
* circle - 円形で中央から端へ。
* hblind - 水平ブラインド効果。
* vblind - 垂直ブラインド効果。
* grid - グリッド効果。
*
* 3つ目の引数は、表示なら「in」、消去なら「out」とします。
*
* 4つめの引数は、トランジション時間をフレーム数で指定します。
*/
(function() {
var _Game_Interpreter_pluginCommand =
Game_Interpreter.prototype.pluginCommand;
Game_Interpreter.prototype.pluginCommand = function(command, args) {
_Game_Interpreter_pluginCommand.call(this, command, args);
if (command === 'PictureWipe') {
var pictureId = Number(args[0]);
var wipeType = String(args[1]) || 'down';
var wipeDirection = String(args[2]) || 'in';
var wipeMaxFrames = Number(args[3]) || 60;
var picture = $gameScreen.picture(pictureId);
if (picture) {
picture.wipeType = wipeType;
picture.wipeDirection = wipeDirection;
picture.wipeMaxFrames = wipeMaxFrames;
picture.wipeIndex = 0;
}
}
};
var _Game_Picture_update = Game_Picture.prototype.update;
Game_Picture.prototype.update = function() {
_Game_Picture_update.call(this);
this.updateWipe();
};
Game_Picture.prototype.updateWipe = function() {
if (this.wipeIndex < this.wipeMaxFrames) {
this.wipeIndex++;
}
};
var _Sprite_Picture_update = Sprite_Picture.prototype.update;
Sprite_Picture.prototype.update = function() {
_Sprite_Picture_update.call(this);
if (this.picture() && this.visible) {
this.updateWipe();
}
};
Sprite_Picture.prototype.updateWipe = function() {
var picture = this.picture();
if (picture.wipeIndex < picture.wipeMaxFrames) {
var source = ImageManager.loadPicture(this._pictureName);
if (source.isReady()) {
if (!this.bitmap || this.bitmap === source) {
this.bitmap = new Bitmap(source.width, source.height);
}
var density = 0;
if (picture.wipeDirection === 'in') {
density = picture.wipeIndex / picture.wipeMaxFrames;
} else if (picture.wipeDirection === 'out') {
density = 1 - picture.wipeIndex / picture.wipeMaxFrames;
}
this.bitmap.clear();
this.paintWipe(this.bitmap, picture.wipeType, density);
var context = this.bitmap.context;
context.save();
context.globalCompositeOperation = 'source-in';
context.drawImage(source.canvas, 0, 0);
context.restore();
}
} else if (picture.wipeDirection === 'in') {
this.bitmap = ImageManager.loadPicture(this._pictureName);
} else if (picture.wipeDirection === 'out') {
this.bitmap.clear();
}
};
Sprite_Picture.prototype.paintWipe = function(bitmap, type, density) {
var blindSize = 48;
var w = bitmap.width;
var h = bitmap.height;
var cx = w / 2;
var cy = h / 2;
var color = 'white';
var size, i, j;
switch (type) {
case 'down':
size = h * density;
bitmap.fillRect(0, 0, w, size, color);
break;
case 'up':
size = h * density;
bitmap.fillRect(0, h - size, w, size, color);
break;
case 'right':
size = w * density;
bitmap.fillRect(0, 0, size, h, color);
break;
case 'left':
size = w * density;
bitmap.fillRect(w - size, 0, size, h, color);
break;
case 'square':
size = Math.max(w, h) / 2 * density;
bitmap.fillRect(cx - size, cy - size, size * 2, size * 2, color);
break;
case 'circle':
size = Math.sqrt(w * w + h * h) / 2 * density;
bitmap.drawCircle(cx, cy, size, color);
break;
case 'hblind':
size = blindSize * density;
for (i = 0; i < h; i += blindSize) {
bitmap.fillRect(0, i, w, size, color);
}
break;
case 'vblind':
size = blindSize * density;
for (i = 0; i < w; i += blindSize) {
bitmap.fillRect(i, 0, size, h, color);
}
break;
case 'grid':
size = blindSize * density;
for (i = 0; i < h; i += blindSize) {
for (j = 0; j < w; j += blindSize) {
bitmap.fillRect(j, i, size, size, color);
}
}
break;
}
};
})();
<file_sep>/SciHive Source Code (Server)/pamahalaan/process-register.php
<?php
require_once('../connector.php');
$t_ID = $_POST['t_ID'];
$t_fname = $_POST['t_fname'];
$t_lname = $_POST['t_lname'];
$t_sname = $_POST['t_sname'];
$t_gender = $_POST['t_gender'];
$t_uname = $_POST['t_uname'];
$t_password = $_POST['t_password'];
if(isset($_POST['submit'])){
$teachering=$db->prepare("INSERT INTO sci_teachers ( t_ID, t_fname, t_lname, t_sname, t_gender, t_uname, t_password ) VALUES ( '$t_ID', '$t_fname', '$t_lname', '$t_sname', '$t_gender', '$t_uname', '$t_password' )");
$teachering->execute();
//$xwxwxw = $db->prepare("UPDATE dashboardesu SET TotalTeachers = TotalTeachers + 1");
//$xwxwxw->execute();
header('location:registration-GOOD.php');
}else{
header('location:registration-BAD.php');
}
?><file_sep>/SciHive Source Code (Server)/pamahalaan/process-student-update.php
<?php
require_once('../connector.php');
$s_ID = $_POST['s_ID'];
$s_fname = $_POST['s_fname'];
$s_lname = $_POST['s_lname'];
$s_sname = $_POST['s_sname'];
$s_bday = $_POST['s_bday'];
$s_gender = $_POST['s_gender'];
$s_uname = $_POST['s_uname'];
$s_password = $_POST['s_password'];
$s_section = $_POST['s_section'];
if(isset($_POST['submit'])){
$studenting=$db->prepare("UPDATE sci_students SET s_ID = '$s_ID', s_fname = '$s_fname', s_lname = '$s_lname', s_sname = '$s_sname', s_bday = '$s_bday', s_gender = '$s_gender', s_uname = '$s_uname', s_password = <PASSWORD>', s_section = '$s_section' WHERE s_ID = '$s_ID' ");
$studenting->execute();
header('location:teacherHUB.php');
}
?>
<!-- may error tayo sa registration pag doble primary key, kailangan ierror handling exception ek ek --><file_sep>/SciHive Source Code (Server)/vendor/own.js
function goodscorexss(){
var goodie = Math.floor(Math.random() * 11 );
var goodness;
if (goodie == 0) { goodness = "Nice!"}
else if (goodie == 1) { goodness = "Cool!"}
else if (goodie == 2) { goodness = "Correct!"}
else if (goodie == 3) { goodness = "Awesome!"}
else if (goodie == 4) { goodness = "Sweet!"}
else if (goodie == 5) { goodness = "Brilliant!"}
else if (goodie == 6) { goodness = "Excellent!"}
else if (goodie == 7) { goodness = "Good!"}
else if (goodie == 8) { goodness = "Yeah!"}
else if (goodie == 9) { goodness = "Okay!"}
else if (goodie == 10) { goodness = "Bright!"}
else goodness = "ewan";
swal({
title: goodness,
text: "Correct Answer",
imageUrl: "media/right.png",
showConfirmButton: false,
timer: 1200
})
}
function badscorexss(){
var badie = Math.floor(Math.random() * 6 );
var badness;
if (badie == 0) { badness = "Ooops!"}
else if (badie == 1) { badness = "Sorry!"}
else if (badie == 2) { badness = "Uh-oh!"}
else if (badie == 3) { badness = "Bad!"}
else if (badie == 4) { badness = "Wrong!"}
else if (badie == 5) { badness = "Miss!"}
else badness = "ewan";
swal({
title: badness,
text: "Incorrect Answer",
imageUrl: "media/wrong.png",
showConfirmButton: false,
timer: 1200
})
}
<file_sep>/SciHive Source Code (Server)/pamahalaan/teacherHUB.php
<?php
include_once('../connector.php');
session_start();
if(isset($_SESSION['teacher_account'])){
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Teachers Portal</title>
<!-- <link rel="stylesheet" href="../vendor/jquery.mobile-1.4.5.min.css" /> -->
<script src="../vendor/jquery-1.11.1.min.js"></script>
<link href="../vendor/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="../vendor/own.css">
<link rel="stylesheet" type="text/css" href="../vendor/sweetalert.css">
<link rel="stylesheet" type="text/css" href="../vendor/elusive-icons.css">
<!-- TO DISABLE SELECTING -->
<style type="text/css">
*
{
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
input
{
-webkit-user-select: auto !important;
-khtml-user-select: auto !important;
-moz-user-select: auto !important;
-ms-user-select: auto !important;
user-select: auto !important;
}
img { border: none; }
button:focus {
border: none;
outline: none;
}
input:focus {
border: none;
outline: none;
}
html {height: 100%};
body {height: 100%};
.skwa:hover {border: 5px solid pink}
.hoverer:hover {cursor: pointer;}
</style>
<style>
/* Paste this css to your style sheet file or under head tag */
/* This only works with JavaScript,
if it's not present, don't show loader */
.no-js #loader { display: none; }
.js #loader { display: block; position: absolute; left: 100px; top: 0; }
.se-pre-con {
position: fixed;
left: 0px;
top: 0px;
width: 100%;
height: 100%;
z-index: 9999;
background: url(../media/loader.gif) center no-repeat #fff;
}
</style>
<script type="text/javascript">
//paste this code under the head tag or in a separate js file.
// Wait for window load
$(window).load(function() {
// Animate loader off screen
$(".se-pre-con").fadeOut("slow");;
});
</script>
<script type="text/javascript">
function lalabas() {
swal({
title: "Logout ?",
text: "Are you sure you want to exit?",
type: "warning",
showCancelButton: true,
confirmButtonColor: "#ffc335",
confirmButtonText: "Yeah!!",
closeOnConfirm: false
},
function(){
window.location = "process-logout.php";
});
}
</script>
</head>
<body style="background-color: skyblue">
<div class="se-pre-con"></div>
<?php
$ohyeah = $_SESSION['xteacherID']; //gagamiting variable from session name ('WHERE' clause). . .
$gumanakaha=$db->prepare("SELECT * FROM sci_teachers WHERE t_ID=:xteacherID"); //the query
$gumanakaha->bindParam(':xteacherID',$ohyeah); //importateng ibind muna yung variables para magamit sa querying
$gumanakaha->execute(); //the go signal
$waw=$gumanakaha->fetch(PDO::FETCH_ASSOC); //importante to para ma output na yung laman ng database na tinawag sa query
?>
<nav class="navbar navbar-expand-lg navbar-dark" style="background-color: #ffc335; width: 100%; position: fixed; top: 0; left: 0">
<h3 class="navbar-brand" style="font-weight: bold"> <img src="beebot.png" width="25px"> SciHive Teachers Portal</h3>
<a class="hoverer" onclick="lalabas()" style="position: fixed;top: 15px; right: 10px; color: white; font-weight: bold;"> Logout <span style="transform: rotate(270deg);" class="el el-fw"></span></a>
</nav>
<br><br><br><br>
<div style="background-color: rgb(0,161,255); padding: 20px; border: 5px outset orange; border-radius: 25px; margin: 10px" >
<div class="row">
<div class="col-md-3">
<table>
<tr>
<td> <h6>Teacher: </h6> </td>
<td> <h5 style="color: rgba(0,0,0,0);">lol</h5> </td>
<td> <h5><?php echo $waw['t_fname'] . " " . $waw['t_lname'] . " " . $waw['t_sname'] ?> </h5> </td>
</tr>
<tr>
<td> <h6>ID number: </h6> </td>
<td> <h5 style="color: rgba(0,0,0,0);">lol</h5> </td>
<td> <h5><?php echo $waw['t_ID']?> </h5> </td>
</tr>
<!-- <tr>
<td> <h6>Class Name: </h6> </td>
<td> <h5 style="color: rgba(0,0,0,0);">lol</h5> </td>
<td> <h5><?php echo $waw['NameOfClass'] ?> </h5> </td>
</tr> -->
</table>
</div>
<!--
<a class="col-md-2 btn skwa" style="color: white; margin: 5px; background-color: orangered; border-radius: 25px; border: 5px ridge orangered">
<h1><?php echo $waw['Tnumofstud'] ?></h1>
<div style="bottom: 0; border-top: 1px solid white">
<h4><i class="el el-fw jellyrice" ></i> Students </h4>
</div>
</a>
<a class="col-md-2 btn skwa" style="color: white; margin: 5px; background-color: turquoise; border-radius: 25px; border: 5px ridge turquoise">
<h1><?php echo $waw['Tnumofonline'] ?></h1>
<div style="bottom: 0; border-top: 1px solid white">
<h4><i class="el el-fw jellyrice" ></i> Logins </h4>
</div>
</a>
<a class="col-md-2 btn skwa" style="color: white; margin: 5px; background-color: orange; border-radius: 25px; border: 5px ridge orange">
<h1><?php echo "0" ?></h1>
<div style="bottom: 0; border-top: 1px solid white">
<h4><i class="el el-fw jellyrice" ></i> Messages </h4>
</div>
</a>
<a class="col-md-2 btn skwa" style="color: white; margin: 5px; background-color: violet; border-radius: 25px; border: 5px ridge violet">
<h1><?php echo "0" ?></h1>
<div style="bottom: 0; border-top: 1px solid white">
<h4><i class="el el-fw jellyrice" ></i> Likes </h4>
</div>
</a> -->
</div>
</div>
<br>
<div style="background-color: rgb(0,161,255); padding: 10px; border: 5px outset orange; border-radius: 25px; margin: 10px" >
<h3 style="width: 100%">
<i class="el el-fw"></i> My Students <small>(By Section)</small>
<small>
<a href="#" id="yay" role="button" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false" class="el el-fw" style="color: white;font-size: 15pt"></a>
<div class="dropdown-menu" aria-labelledby="yay" style="padding: 5px; width: 30%">
<a class="btn btn-sm btn-success"><i class="el el-fw" style="color: white"></i></a> <?php echo " = " ?> Add New Student
<br><br>
<a class="btn btn-sm btn-warning"> <i class="el el-fw" style="color: white">  </i> </a> <?php echo " = " ?> Show Student's Scores
<br><br>
<a class="btn btn-sm btn-info"> <i class="el el-fw" style="color: white">  </i> </a> <?php echo " = " ?> Show Student's Information
<br><br>
<a class="btn btn-sm btn-secondary"> <i class="el el-fw" style="color: white">  </i> </a> <?php echo " = " ?> Refresh Window
<br><br>
<div class="row" style="margin: 0 !important; padding: 0 !important">
<div class="col">
<b>Progress Points </b> - how much progress does the student has done in the game, talking to people, visiting places and doing activities etc. In game they can use it as money etc.
</div>
<div class="col">
<img src="money.png" width="100%">
</div>
</div>
</div>
</small>
<button class="btn btn-success btn-sm" style="float: right;margin-bottom: 10px" data-toggle="modal" data-target="#signupmodal"><i class="el el-fw"></i></button>
<button onclick="location.reload()" class="btn btn-secondary btn-sm" style="float: right;margin-bottom: 10px; margin-right: 5px"><i class="el el-fw"></i></button>
</h3>
<table class="table table-sm table-hover" style="background-color: white">
<tr style="background-color: lightyellow">
<th>Student ID</th>
<th>Name</th>
<th>Gender</th>
<th>Section</th>
<th>Progress Points</th>
<th>Action</th>
</tr>
<?php
require_once('../connector.php');
$stmt = $db->prepare("SELECT * FROM sci_students WHERE s_teacher=:xteacherID ORDER BY s_section ASC");
$stmt->bindParam(':xteacherID',$ohyeah); //ginamit ko parin yung sa session para mafilter students
$stmt->execute();
foreach($stmt as $studidents) {
?>
<tr>
<td><?=$studidents['s_ID']?></td>
<td><?=$studidents['s_fname'] . " " . $studidents['s_lname'] . " " . $studidents['s_sname'] ?> </td>
<td> <?=$studidents['s_gender']?> </td>
<td> <?=$studidents['s_section']?> </td>
<td> <?=$studidents['s_GP']?> </td>
<td>
<a href="score_charts.php?id=<?=$studidents['s_ID']?>" class="btn btn-sm btn-warning" style="color: white"><i class="el el-fw">  </i> </a>
<a href="student-details.php?id=<?=$studidents['s_ID']?>" class="btn btn-sm btn-info"><i class="el el-fw">  </i> </a>
</td>
</tr>
<?php } ?>
</table>
</div>
<!-- student register modal ------------------------------------------------ -->
<div class="modal fade" id="signupmodal" tabindex="-1" role="dialog" arialabelledby="exampleModalLabel"
aria-hidden="true">
<div class="modal-dialog" role="document">
<form action="process-student-register.php" method="POST" style="background-color: #ffc335;border: 5px outset orange; padding: 10px; border-radius: 25px;">
<div class="modal-content" style="background-color: rgb(0,161,255);">
<br>
<center>
<h1 style="color: white; margin-top: 10px"> <i class="el el-fw"></i> Student Registration </h1>
</center>
<div class="modal-body" style="background-color: rgb(0,161,255);">
<br>
<div class="form-group">
<label for="s_ID">ID No.</label><input type="text" name="s_ID" id="s_ID" class="form-control" required="required" style="border: 1px solid orange; border-radius: 10px"/>
</div>
<div class="form-group">
<label for="s_fname">First Name</label><input type="text" name="s_fname" id="s_fname" class="form-control" required="required" style="border: 1px solid orange; border-radius: 10px"/>
</div>
<div class="form-group">
<label for="s_lname">Last Name</label><input type="text" name="s_lname" id="s_lname" class="form-control" required="required" style="border: 1px solid orange; border-radius: 10px"/>
</div>
<div class="form-group">
<label for="s_sname">Suffix Name</label><input type="text" name="s_sname" id="s_sname" class="form-control" style="border: 1px solid orange; border-radius: 10px"/>
</div>
<div class="form-group">
<label for="s_bday">Birthday</label><input type="date" name="s_bday" id="s_bday" class="form-control" required="required" style="border: 1px solid orange; border-radius: 10px"/>
</div>
<div class="form-group">
<label for="s_gender">Gender</label><select name="s_gender" id="s_gender" class="form-control" required="required" style="border: 1px solid orange; border-radius: 10px">
<option value="Male">Male</option>
<option value="Female">Female</option>
</select>
</div>
<div class="form-group">
<label for="s_uname">User Name</label><input type="text" name="s_uname" id="s_uname" class="form-control" required="required" style="border: 1px solid orange; border-radius: 10px"/>
</div>
<div class="form-group">
<label for="s_password">Password</label><input type="<PASSWORD>" name="s_password" id="s_password" class="form-control" required="required" style="border: 1px solid orange; border-radius: 10px"/>
</div>
<div class="form-group">
<label for="s_section">Section</label><input type="text" name="s_section" id="s_section" class="form-control" required="required" style="border: 1px solid orange; border-radius: 10px"/>
</div><br>
<div class="form-group" hidden="hidden">
<label for="s_teacher">Section</label><input type="text" name="s_teacher" id="s_teacher" class="form-control" required="required" value="<?php echo $ohyeah ?>" />
</div><br>
<center><div class="form-group">
<input type="checkbox" required="required" style="border: 2px solid orange;"> I agree to the <a href="" style="color: white"> <u> Terms and Data Use Policy </u> </a>.
</div></center>
<center>
<button style="width: 45%; color: white; font-size: 15pt; font-weight: bold; box-shadow: 3px 3px 5px 1px rgba(10,10,10,.7);" type="button" class="btn btn-danger" data-dismiss="modal">Cancel</button>
<button style="width: 45%; color: white; font-size: 15pt; font-weight: bold; box-shadow: 3px 3px 5px 1px rgba(10,10,10,.7);" type="submit" name="submit" class="btn btn-success" onclick="return validate();">Register</button>
</center>
</div>
</div>
</form>
</div>
</div>
<!-- <script src="../vendor/jquery.mobile-1.4.5.min.js"></script> -->
<script src="../vendor/bootstrap.bundle.min.js"></script>
<script src="../vendor/jquery.min.js"></script>
<script src="../vendor/sweetalert.min.js"></script>
<script src="../vendor/own.js"></script>
</body>
</html>
<?php
}else{
header('location:index.php');
}
?> <file_sep>/SciHive Source Code (Server)/login_process_1.php
<?php
require_once('connector.php');
$username = $_GET['uname'];
$sql=$db->prepare("SELECT * FROM sci_students WHERE s_ID=:uname");
$sql->bindParam(':uname',$username);
$sql->execute();
$wee=$sql->fetch(PDO::FETCH_ASSOC);
$starring = $wee['s_fname'];
$section = $wee['s_section'];
if($row=$sql->rowCount()<>0){
session_start();
$_SESSION['account']=true;
$_SESSION['welcomename'] = $starring; // FIRST NAME
$_SESSION['section'] = $section; // SECTION
$_SESSION['Username'] = $username; // ID NUMBER
//PANG UPDATE KO NG LAST LOGIN -----------------------------------------------------------------------------------------
$nowDate = date('Y-m-d H:i:s');
$lastLogin = $db->prepare("UPDATE sci_students SET s_lastlogin = :sNOW WHERE s_ID=:sID");
$lastLogin->bindParam(':sNOW',$nowDate);
$lastLogin->bindParam(':sID',$username);
$lastLogin->execute();
header('location:index.php');
}
else{
header('location:errorlogin.php');
}
// localhost/sciserver/login_process_1.php?uname=16330006
?>
<file_sep>/SciHive Source Code (Server)/pp_update.php
<?php
require_once('connector.php');
$userID = $_GET['uID'];
$userPP = $_GET['uPP'];
$waw = $db->prepare("UPDATE sci_students SET s_GP=:moneyX WHERE s_ID =:uID ");
$waw->bindParam(':uID',$userID);
$waw->bindParam(':moneyX',$userPP);
$waw->execute();
?>
<file_sep>/scihive.sql
-- phpMyAdmin SQL Dump
-- version 4.8.5
-- https://www.phpmyadmin.net/
--
-- Host: 127.0.0.1
-- Generation Time: Nov 06, 2019 at 02:23 PM
-- Server version: 10.1.38-MariaDB
-- PHP Version: 7.1.27
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET AUTOCOMMIT = 0;
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Database: `scihive`
--
-- --------------------------------------------------------
--
-- Table structure for table `sci_activity`
--
CREATE TABLE `sci_activity` (
`activity_ID` int(11) NOT NULL,
`activity_name` varchar(255) DEFAULT NULL,
`activity_likes` varchar(255) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
-- --------------------------------------------------------
--
-- Table structure for table `sci_convo`
--
CREATE TABLE `sci_convo` (
`convo_ID` int(11) NOT NULL,
`convo_name` varchar(255) DEFAULT NULL,
`convo_content` varchar(255) DEFAULT NULL,
`convo_user` varchar(255) DEFAULT NULL,
`convo_loc` varchar(255) DEFAULT NULL,
`convo_date_time` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
--
-- Dumping data for table `sci_convo`
--
INSERT INTO `sci_convo` (`convo_ID`, `convo_name`, `convo_content`, `convo_user`, `convo_loc`, `convo_date_time`) VALUES
(1, NULL, 'wehehehe', 'Crizon', '', '2019-10-17 14:33:09'),
(2, NULL, 'Hello', 'Crizon', NULL, '2019-10-17 14:37:00'),
(3, NULL, 'Ot', 'Crizon', 'uploads/71284904_2193102694129495_553667781770346496_n.jpg', '2019-10-17 14:38:12'),
(4, NULL, 'Ppoyek', 'Crizon', 'uploads/71284904_2193102694129495_553667781770346496_n.jpg', '2019-10-17 14:38:12'),
(5, NULL, 'Ppoyekhahaa', 'Crizon', 'uploads/71284904_2193102694129495_553667781770346496_n.jpg', '2019-10-17 14:38:12'),
(6, NULL, 'What', 'Crizon', NULL, '2019-10-17 14:38:43'),
(7, NULL, 'What the fuck', 'Crizon', NULL, '2019-10-17 14:38:57'),
(8, NULL, 'Daamn', 'Crizon', 'uploads/66834128_443106139870595_2399654247533641728_n.jpg', '2019-10-17 14:39:34'),
(9, NULL, 'anything wrong?', 'Crizon', NULL, '2019-10-17 14:40:21'),
(10, NULL, 'You are wrong', 'Frenole', NULL, '2019-10-17 14:41:12'),
(11, NULL, 'Anything?', 'Frenole', NULL, '2019-10-17 14:42:07'),
(12, NULL, 'I think were missing something', 'Frenole', NULL, '2019-10-17 14:42:30'),
(13, NULL, 'What could that be', 'Frenole', NULL, '2019-10-17 14:42:39'),
(14, NULL, 'Mga loko loko hahaha', 'Frenole', NULL, '2019-10-17 14:42:59'),
(15, NULL, 'Why am i talking tomy self?', 'Frenole', NULL, '2019-10-17 14:43:15'),
(16, NULL, 'ðŸ˜', 'Frenole', NULL, '2019-10-17 14:43:23'),
(17, NULL, 'Ihopethat emoji issupported', 'Frenole', NULL, '2019-10-17 14:43:38'),
(18, NULL, 'Yeahhahaha', 'Frenole', NULL, '2019-10-17 14:43:46'),
(19, NULL, 'Weee', 'Frenole', NULL, '2019-10-17 14:44:34'),
(20, NULL, 'Hello', 'Crizon', NULL, '2019-10-17 14:45:50'),
(21, NULL, 'Zup frenny', 'Crizon', NULL, '2019-10-17 14:46:01'),
(22, NULL, 'Hu', 'Crizon', NULL, '2019-10-17 14:47:30'),
(23, NULL, 'What the hell', 'Crizon', NULL, '2019-10-17 14:47:58'),
(24, NULL, 'Back', 'Crizon', NULL, '2019-10-17 14:49:00'),
(25, NULL, 'Simple chat app', 'Crizon', NULL, '2019-10-17 14:49:28'),
(26, NULL, 'Itsgreate tho', 'Crizon', NULL, '2019-10-17 14:49:35'),
(27, NULL, 'Yu', 'Crizon', NULL, '2019-10-17 14:50:04'),
(28, NULL, 'Yu', 'Crizon', NULL, '2019-10-17 14:50:28'),
(29, NULL, 'You think its cool', 'Crizon', NULL, '2019-10-17 14:55:14'),
(30, NULL, 'Well i guess', 'Crizon', NULL, '2019-10-17 14:55:21'),
(31, NULL, 'Helo', 'Crizon', NULL, '2019-10-17 15:26:55'),
(32, NULL, 'Potek', 'Crizon', NULL, '2019-10-17 15:27:01'),
(33, NULL, 'The more you type', 'Crizon', NULL, '2019-10-17 15:27:09'),
(34, NULL, 'Kusa naman pala aangat', 'Crizon', NULL, '2019-10-17 15:29:17'),
(35, NULL, 'Yow', 'Crizon', NULL, '2019-10-17 15:58:20'),
(36, NULL, 'Yo', 'Crizon', NULL, '2019-10-17 15:58:27'),
(37, NULL, 'Hello', 'Crizon', NULL, '2019-10-21 18:39:04'),
(38, NULL, 'Charf', 'Crizon', NULL, '2019-10-22 05:55:28'),
(39, NULL, 'Nakabuntis pinsan ko', 'Frenole', NULL, '2019-10-22 05:55:49'),
(40, NULL, 'Pota ka', 'Crizon', NULL, '2019-10-22 05:55:56');
-- --------------------------------------------------------
--
-- Table structure for table `sci_likes`
--
CREATE TABLE `sci_likes` (
`like_ID` int(11) NOT NULL,
`like_for` varchar(255) DEFAULT NULL,
`like_user` varchar(255) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
-- --------------------------------------------------------
--
-- Table structure for table `sci_scores`
--
CREATE TABLE `sci_scores` (
`score_ID` int(11) NOT NULL,
`score_game` varchar(255) DEFAULT NULL,
`score_actual` int(255) NOT NULL,
`score_student` varchar(255) DEFAULT NULL,
`score_date_time` date NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
--
-- Dumping data for table `sci_scores`
--
INSERT INTO `sci_scores` (`score_ID`, `score_game`, `score_actual`, `score_student`, `score_date_time`) VALUES
(1, 'quiz_1', 1, 'Crizon', '0000-00-00'),
(2, 'quiz_1', 3, 'CJ', '0000-00-00'),
(3, 'quiz_1', 3, 'Crzn', '0000-00-00'),
(4, 'quiz_1', 2, 'Lul', '0000-00-00'),
(5, 'quiz_1', 1, 'Toto', '0000-00-00'),
(6, 'quiz_1', 1, 'aako', '0000-00-00'),
(7, 'quiz_1', 3, 'RatBo', '0000-00-00'),
(8, 'quiz_1', 2, 'KingInadesu', '0000-00-00'),
(9, 'quiz_1', 3, 'Yawang', '0000-00-00'),
(10, 'quiz_1', 1, 'Jawa', '0000-00-00'),
(11, 'quiz_1', 1, 'Futa', '0000-00-00'),
(12, 'quiz_1', 2, '<NAME>', '0000-00-00'),
(13, 'quiz_1', 3, 'Damet', '0000-00-00'),
(14, 'quiz_1', 3, 'Jo', '0000-00-00'),
(15, 'quiz_1', 2, 'DIDI', '0000-00-00'),
(16, 'quiz_1', 1, 'FUTA', '0000-00-00'),
(17, 'quiz_1', 3, 'JAWA', '0000-00-00'),
(18, 'quiz_1', 1, 'awkko', '0000-00-00'),
(19, 'quiz_1', 3, 'aehmo', '0000-00-00'),
(20, 'quiz_1', 2, 'Dajkl', '0000-00-00'),
(37, 'quiz_1', 4, 'Waw', '0000-00-00'),
(38, 'quiz_1', 5, 'Chup', '0000-00-00'),
(39, 'quiz_1', 5, 'Buji', '0000-00-00');
-- --------------------------------------------------------
--
-- Table structure for table `sci_sections`
--
CREATE TABLE `sci_sections` (
`section_ID` int(11) NOT NULL,
`section_name` varchar(255) DEFAULT NULL,
`section_teacher` varchar(255) DEFAULT NULL,
`section_size` double DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
-- --------------------------------------------------------
--
-- Table structure for table `sci_students`
--
CREATE TABLE `sci_students` (
`s_ID` varchar(11) NOT NULL,
`s_fname` varchar(255) DEFAULT NULL,
`s_lname` varchar(255) DEFAULT NULL,
`s_sname` varchar(255) DEFAULT NULL,
`s_bday` date DEFAULT NULL,
`s_gender` varchar(255) DEFAULT NULL,
`s_uname` varchar(255) DEFAULT NULL,
`s_password` varchar(255) DEFAULT NULL,
`s_section` varchar(255) DEFAULT NULL,
`s_teacher` varchar(255) DEFAULT NULL,
`s_lastlogin` datetime DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
--
-- Dumping data for table `sci_students`
--
INSERT INTO `sci_students` (`s_ID`, `s_fname`, `s_lname`, `s_sname`, `s_bday`, `s_gender`, `s_uname`, `s_password`, `s_section`, `s_teacher`, `s_lastlogin`) VALUES
('14310792', 'Frenolie', 'Verses', 'III', '2019-11-06', 'FemaleX', 'FVNE', 'bbq', 'Flower Power', '911', NULL),
('16330006', 'Crizon', 'Parcia', 'Jr.', '1993-11-18', 'Male', 'crzn.io', 'crzn.io', '2', '911', '2019-11-06 13:08:01');
-- --------------------------------------------------------
--
-- Table structure for table `sci_teachers`
--
CREATE TABLE `sci_teachers` (
`t_ID` int(11) NOT NULL,
`t_fname` varchar(255) DEFAULT NULL,
`t_lname` varchar(255) DEFAULT NULL,
`t_sname` varchar(255) DEFAULT NULL,
`t_bday` date DEFAULT NULL,
`t_gender` varchar(255) DEFAULT NULL,
`t_uname` varchar(255) DEFAULT NULL,
`t_password` varchar(255) DEFAULT NULL,
`t_lastlogin` datetime DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
--
-- Dumping data for table `sci_teachers`
--
INSERT INTO `sci_teachers` (`t_ID`, `t_fname`, `t_lname`, `t_sname`, `t_bday`, `t_gender`, `t_uname`, `t_password`, `t_lastlogin`) VALUES
(911, 'John', 'Cena', 'Jr.', NULL, NULL, '[email protected]', 'xxx', '2019-11-06 14:18:28'),
(1234, 'qwer', 'qwer', 'qwer', NULL, 'Male', 'qwer', 'qwer', NULL),
(123456, 'qwert', 'qwer', 'qwer', NULL, 'Female', 'qwer', 'qwer', NULL);
--
-- Indexes for dumped tables
--
--
-- Indexes for table `sci_activity`
--
ALTER TABLE `sci_activity`
ADD PRIMARY KEY (`activity_ID`);
--
-- Indexes for table `sci_convo`
--
ALTER TABLE `sci_convo`
ADD PRIMARY KEY (`convo_ID`);
--
-- Indexes for table `sci_likes`
--
ALTER TABLE `sci_likes`
ADD PRIMARY KEY (`like_ID`);
--
-- Indexes for table `sci_scores`
--
ALTER TABLE `sci_scores`
ADD PRIMARY KEY (`score_ID`);
--
-- Indexes for table `sci_sections`
--
ALTER TABLE `sci_sections`
ADD PRIMARY KEY (`section_ID`);
--
-- Indexes for table `sci_students`
--
ALTER TABLE `sci_students`
ADD PRIMARY KEY (`s_ID`);
--
-- Indexes for table `sci_teachers`
--
ALTER TABLE `sci_teachers`
ADD PRIMARY KEY (`t_ID`);
--
-- AUTO_INCREMENT for dumped tables
--
--
-- AUTO_INCREMENT for table `sci_convo`
--
ALTER TABLE `sci_convo`
MODIFY `convo_ID` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=41;
--
-- AUTO_INCREMENT for table `sci_scores`
--
ALTER TABLE `sci_scores`
MODIFY `score_ID` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=40;
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
<file_sep>/SciHive Source Code (Client)/www/lessons/earth_space/lands.html
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1, width=device-width, viewport-fit=cover">
<title>Landforms</title>
<style>
html,body{height: 100%}
.hyperX{height: 100%}
</style>
<script src="../../vendor/jquery-1.11.1.min.js"></script>
<link rel="stylesheet" type="text/css" href="../../vendor/sweetalert2.css">
<link rel="stylesheet" type="text/css" href="../../vendor/animate.css">
<link href="../../vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="../../vendor/elusive-icons.css">
<link href="../../vendor/fontawesome-free/css/all.min.css" rel="stylesheet" type="text/css">
<link rel="stylesheet" type="text/css" href="../../vendor/own.css">
<!-- TO DISABLE SELECTING -->
<style type="text/css">
*
{
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
input
{
-webkit-user-select: auto !important;
-khtml-user-select: auto !important;
-moz-user-select: auto !important;
-ms-user-select: auto !important;
user-select: auto !important;
}
img { border: none; }
button:focus {
border: none;
outline: none;
}
input:focus {
border: none;
outline: none;
}
</style>
<script type="text/javascript">
</script>
</head>
<body style="background-color: black; background-image: url(../../img/hexellence.png)">
<script type="text/javascript" src="../../cordova.js"></script>
<center>
<h4 style="background-color: #7714d1; padding: 5px; top:0 ; width: 100%; z-index: 1; position: fixed; color: white; background-image: url(../../img/hexellence.png);">Landforms</h4>
<h4 onclick="balik()" class="fas fa-fw fa-chevron-left" style="z-index: 2; position: fixed; left: 5px; top: 10px; color: white"></h4>
</center>
<br>
<br>
<div style="text-align: justify; padding: 5%; margin: 2.5%; width: 95%; background-color: white;border-radius: 5px">
<div class="row" style="margin: 0">
<div id="tts">
<button onclick="read1()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>
</div>
<span style="color: rgba(0,0,0,0);">h</span>
<h3> Philippine Landforms </h3>
</div>
<script type="text/javascript" src="../../vendor/model-viewer.js"></script>
<center>
<model-viewer style="width: 100%; height: 300px" src="Philippines/land.gltf" auto-rotate camera-controls background-color="black">
</model-viewer>
3D Model (Touch and Rotate)
</center><br><br>
<img src="Philippines/land1.jpg" width="100%" style="border:3px outset orange">
<br><br>
<p>
A landform is a natural or artificial feature of the solid surface of the Earth or other planetary body. Landforms together make up a given terrain, and their arrangement in the landscape is known as topography.<br><br>
Philippines is a beautiful country because it has different land and water formations that shaped our country. Here are some land forms in the Philippines:<br><br>
<h5>Volcano (Bulkan)</h5>
<img src="Philippines/land2.jpg" width="100%" style="border:3px outset orange"><br><br>
A volcano is a mountain with a large hole at the top. There are active volcanoes and inactive volcanoes found in the Philippines. We have almost 200 volcanoes, but only 22 volcanoes are active. Mayon volcano is an example of an active volcano. It is located in Bicol province. It is one of the beautiful volcanoes because of its perfect cone. Another example is found in Talisay, Batangas. It is known as Taal Volcano. This is the smallest volcano in the world, and you will be amazed how beautiful it is.<br><br>
<h5>Hill (Burol)</h5>
<img src="Philippines/land3.jpg" width="100%" style="border:3px outset orange"><br><br>
A hill is a high mass of land less than a mountain. It is still a part of a mountain, but it is in the lower part. This land form is used by farmers to graze their cows, goats, and carabaos because of its abundant grass and plants. Chocolate Hill is the famous hill in the Philippines found in Bohol.<br><br>
<h5>Plain (Kapatagan)</h5>
<img src="Philippines/land4.jpg" width="100%" style="border:3px outset orange"><br><br>
A plain is a wide and flat land. Most developed cities in the Philippines are located in a plain land. It is also an ideal place for farming. The plain of Central Luzon is the largest plain in the country. It is also known as the rice granary of the Philippines.<br><br>
<h5>Mountain (Bundok)</h5>
<img src="Philippines/land5.jpg" width="100%" style="border:3px outset orange"><br><br>
Philippines is rich in mountains. Mt. Apo is the highest mountain in the Philippines, and it is located in Davao, Mindanao. The second highest mountain is Mt. Dulang-Dulang found in Bukidnon. The third highest mountain is Mt. Pulag in Benguet. There are four mountain ranges in our country: Sierra Madre Mountains are the longest mountain range; Zambales mountain range;Cordillera mountain range;and Caraballo mountain range.<br><br>
<h5>Plateau (Talampas)</h5>
<img src="Philippines/land6.jpg" width="100%" style="border:3px outset orange"><br><br>
A plateau is a wide plain on top of a hill or mountain. Many farmers lived here because it has a cool weather. It is also ideal for planting and growing vegetables and fruits. Tagaytay City and Baguio City are good examples of beautiful plateau in Philippines.<br><br>
<h5>Valley (Lambak)</h5>
<img src="Philippines/land7.jpg" width="100%" style="border:3px outset orange"><br><br>
A valley is a plain land between the foot of the hills or mountains. Cagayan valley is the largest valley in the country. La Trinidad valley in Benguet is used for planting vegetables and fruits. You can find here different vegetables and fruits.<br><br>
<h5>Cape (Tangos)</h5>
<img src="Philippines/land8.jpg" width="100%" style="border:3px outset orange"><br><br>
A cape is point of land running into the water. Some capes are used for docking sea vessels. Also, some constructions are built for good purposes. An example of a beautiful cape is in Pangasinan. It is called Cape Bolinao. A lighthouse was built there to guide sea travelers.<br><br>
</p><br><br>
<center>
<model-viewer style="width: 100%; height: 400px" src="blank.gltf" auto-rotate camera-controls background-image="Philippines/landX.jpg">
</model-viewer>
360° Photo (Touch and Rotate)
</center><br>
<center>
<a href="landVR.html" class="btn btn-danger"><i class="fas fa-vr-cardboard"></i> VR Landform</a>
</center>
</div>
<script type="text/javascript">
//READ THE TEXT TO SPEECH
function read1(){
var line_1 = "Landforms. The Landforms is an archipelago, or string of over 7,100 islands, in southeastern Asia between the South China Sea and the Pacific Ocean. The two largest islands, Luzon and Mindanao, make up for two-thirds of the total land area. Only about one third of the islands are inhabited. FAST FACTS. OFFICIAL NAME: Republic of the Landforms. FORM OF GOVERNMENT: Republic. CAPITAL: Manila. POPULATION: 105,893,381. OFFICIAL LANGUAGE: Filipino (based on Tagalog), English. MONEY: Philippine peso. AREA: About 115,831 square miles (300,000 square kilometers). PEOPLE & CULTURE. Filipinos are predominantly of Malay descent, frequently with Chinese and sometimes American or Spanish ancestry. Many Filipinos have Spanish names because of a 19th-century Spanish decree that required them to use Spanish surnames, or last names. Parents often name their children after the saint whose feast day was on the day of their birth. Elementary education in the Landforms starts at age seven, is required by law, and lasts for six years. Secondary education begins at age 13 and lasts for four years; undergraduate college instruction typically is four years. NATURE. The islands are home to many species of flowering plants and ferns, including hundreds of species of orchids. Tall grasses have replaced the forests, which have disappeared due to logging, mining, and development. The Landforms are inhabited by more than 200 species of mammals, including monkeys, squirrels, lemurs, mice, pangolins, chevrotains, mongooses, civet cats, and red and brown deer, among others. The binturong, or Asian bear cat, was once prominent, but now this furry mammal is vulnerable. The tamaraw, a species of small water buffalo found only on Mindoro, is critically endangered. Hundreds of species of birds live in the Landforms, either for all or part of the year, including peacocks, pheasants, doves, parrots, kingfishers, sunbirds, tailorbirds, weaverbirds, and hornbills. The endangered Philippine eagle, which eats monkeys, is barely surviving deforestation. GOVERNMENT. Filipinos elect their president. The president is the head of state and the commander-in-chief of the armed forces and serves a six-year term. The economy is based on agriculture, light industry, and services. The country produces bananas, rice, coconuts, corn, fish, mangos, pineapples, sugarcane, pork, and beef.";
TTS.speak({
text: line_1,
locale: 'en-US',
rate: 1
});
//
var codeBlock='<button onclick="stop1()" class="btn btn-secondary"><i class="fas fa-fw fa-volume-mute"></i></button>';
document.getElementById("tts").innerHTML = codeBlock;
}
//STOP THE TEXT TO SPEECH
function stop1(){
TTS.speak({text: ''});
//set the new icon to button
var codeBlock='<button onclick="read1()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>';
document.getElementById("tts").innerHTML = codeBlock;
}
//STOP THE TEXT TO SPEECH AND GO BACK HISTORY using back button
document.addEventListener("deviceready", onDeviceReady, false);
function onDeviceReady() {
document.addEventListener("backbutton", function () {
TTS.speak({text: ''});
window.history.back();
}, false );
}
//STOP THE TEXT TO SPEECH AND GO BACK HISTORY using a link button
function balik(){
TTS.speak({text: ''});
window.history.back();
}
</script>
<script crossorigin type="text/javascript" src="../../vendor/sweetalert2.js"></script>
<script src="../../vendor/jquery/jquery.min.js"></script>
<script src="../../vendor/bootstrap/js/bootstrap.bundle.min.js"></script>
</body>
</html><file_sep>/SciHive Source Code (Server)/pamahalaan/process-login.php
<?php
require_once('../connector.php');
$Tusername = $_POST['txtTemail'];
$Tpassword = $_POST['txtTpassword'];
$sql=$db->prepare("SELECT * FROM sci_teachers WHERE t_uname=:txtTemail AND t_password=:txtTpassword");
$sql->bindParam(':txtTemail',$Tusername);
$sql->bindParam(':txtTpassword',$Tpassword);
$sql->execute();
$wee=$sql->fetch(PDO::FETCH_ASSOC); /*ibabato ko kasi sa xteacher session yung teacher ID*/
if($row=$sql->rowCount()<>0){
session_start();
$_SESSION['teacher_account']=true;
$_SESSION['xteacherID']= $wee['t_ID']; /*galing sa table yung teacher ID*/
//PANG UPDATE KO NG LAST LOGIN -----------------------------------------------------------------------------------------
$tID = $wee['t_ID'];
$nowDate = date('Y-m-d H:i:s');
$lastLogin = $db->prepare("UPDATE sci_teachers SET t_lastlogin = :sNOW WHERE t_ID=:tID");
$lastLogin->bindParam(':sNOW',$nowDate);
$lastLogin->bindParam(':tID',$tID);
$lastLogin->execute();
header('location:teacherHUB.php');
}else{
header('location:login-BAD.php');
}
?>
<file_sep>/SciHive Source Code (Server)/_chat/process-register.php
<?php
require_once('../connector.php');
$sentMessage = $_POST['xSentMessage'];
$sender = $_POST['xSender'];
/*header('location:index.php');*/
$target_dir = "uploads/";
$target_file = $target_dir . basename($_FILES["fileToUpload"]["name"]);
$uploadOk = 1;
$imageFileType = strtolower(pathinfo($target_file,PATHINFO_EXTENSION));
// Check if image file is a actual image or fake image
if(isset($_POST["submit"])) {
$check = getimagesize($_FILES["fileToUpload"]["tmp_name"]);
if($check !== false) {
echo "File is an image - " . $check["mime"] . ".";
$uploadOk = 1;
} else {
echo "File is not an image.";
$uploadOk = 0;
$teachering=$db->prepare("INSERT INTO sci_convo(convo_user,convo_content,convo_date_time) VALUES(:Zsender,:ZsentMessage,now())");
$teachering->bindparam(':Zsender',$sender);
$teachering->bindparam(':ZsentMessage',$sentMessage);
$teachering->execute();
header('location:index.php');
}
}
if ($uploadOk == 0) {
echo "Sorry, your file was not uploaded.";
// if everything is ok, try to upload file
} else {
if (move_uploaded_file($_FILES["fileToUpload"]["tmp_name"], $target_file)) {
/*echo "The file ". basename( $_FILES["fileToUpload"]["name"]). " has been uploaded.";*/
$uploaded_file = "uploads/" . basename($_FILES["fileToUpload"]["name"]);
$teachering=$db->prepare("INSERT INTO sci_convo(convo_user,convo_content,convo_date_time,convo_loc) VALUES(:Zsender,:ZsentMessage,now(),:ZuploadedFile)");
$teachering->bindparam(':Zsender',$sender);
$teachering->bindparam(':ZsentMessage',$sentMessage);
$teachering->bindparam(':ZuploadedFile',$uploaded_file);
$teachering->execute();
header('location:index.php');
} else {
echo "Sorry, there was an error uploading your file.";
}
}
?><file_sep>/SciHive Source Code (Client)/www/games/candylandia/table.js
/* This script will construct a table and linked to index.html */
//DEFAULT_BOARD_SIZE = 8;
var img_array =[];
function lookupLetter(num) {
var letterArr = ["a","b","c","d","e","f","g","h"];
return letterArr[--num];
}
function colorTable() {
var tableInfo = "<div class='p-2 align-self-center' style='margin:-10px;'><table class='tableBorder'>";
for(var row = 0; row < DEFAULT_BOARD_SIZE; row++){
tableInfo += "<tr class='tableBorder'>";
for(var col = 0; col < DEFAULT_BOARD_SIZE; col++) {
var colName = lookupLetter(col+1);
var colorBack = board.getCandyAt(row, col);
var textColor = "white";
if( colorBack == "yellow") {
textColor = "#505050";
}
tableInfo += "<td class='tableBorder padCell text-center' style='background-color:" + colorBack +";color:"
+ textColor +"'>" + colName + (row+1) +"</td>";
// console.log(tableInfo);
// console.log(" ");
}
tableInfo += "</tr>";
// console.log(tableInfo);
}
tableInfo += "</table></div>";
return tableInfo;
}
// function load_img(imgToLoad) {
// var loaded = false;
// var counter = 0;
// for( var i=0; i<imgToLoad.length; i++){
// var img = new Image();
// console.log(imgToLoad.length);
// img.onload = function() {
// counter++;
// //console.log(imgToLoad[i]);
// console.log(counter);
// if(counter == imgToLoad.length){
// loaded = true;
// }
// }
// img.src = imgToLoad[i];
// console.log(img.src);
// img_array = img;
// }
// console.log(loaded);
// }
// function drawBoard() {
// load_img(['./graphics/blue-candy.png', './graphics/blue-special.png',
// './graphics/green-candy.png', './graphics/green-special.png',
// './graphics/orange-candy.png', './graphics/orange-special.png',
// './graphics/purple-candy.png', './graphics/purple-special.png',
// './graphics/red-candy.png', './graphics/red-special.png',
// './graphics/yellow-candy.png', './graphics/color-bomb.png']);
// var canvas = "<div class'p-2 align-self-center' style='margin:-10px;'> <canvas class='CanvasDraw'>";
// ctx = canvas.getContext('2d');
// for(var row = 0; row < DEFAULT_BOARD_SIZE; row++){
// for(var col = 0; col < DEFAULT_BOARD_SIZE; col++) {
// var colorBack = board.getCandyAt(row, col);
// console.log(colorBack);
// ctx.d
// }
// // console.log(tableInfo);
// }
// }
<file_sep>/SciHive Source Code (Client)/www/games/candylandia/README.md
# Candy-Crush
html javascript css candy crush game
<file_sep>/SciHive Source Code (Server)/pamahalaan/process-logout.php
<?php
require_once('../connector.php');
session_start();
session_destroy();
// $stmt = $db->prepare("UPDATE stats SET loggedin = loggedin - 1");
// $stmt->execute();
header('location:index.php');
?>
<file_sep>/SciHive Source Code (Client)/www/lessons/earth_space/solar.html
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1, width=device-width, viewport-fit=cover">
<title>Solar System</title>
<style>
html,body{height: 100%}
.hyperX{height: 100%}
</style>
<script src="../../vendor/jquery-1.11.1.min.js"></script>
<link rel="stylesheet" type="text/css" href="../../vendor/sweetalert2.css">
<link rel="stylesheet" type="text/css" href="../../vendor/animate.css">
<link href="../../vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="../../vendor/elusive-icons.css">
<link href="../../vendor/fontawesome-free/css/all.min.css" rel="stylesheet" type="text/css">
<link rel="stylesheet" type="text/css" href="../../vendor/own.css">
<!-- TO DISABLE SELECTING -->
<style type="text/css">
*
{
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
input
{
-webkit-user-select: auto !important;
-khtml-user-select: auto !important;
-moz-user-select: auto !important;
-ms-user-select: auto !important;
user-select: auto !important;
}
img { border: none; }
button:focus {
border: none;
outline: none;
}
input:focus {
border: none;
outline: none;
}
</style>
<script type="text/javascript">
</script>
</head>
<body style="background-color: black; background-image: url(../../img/hexellence.png)">
<script type="text/javascript" src="../../cordova.js"></script>
<center>
<h4 style="background-color: #7714d1; padding: 5px; top:0 ; width: 100%; z-index: 1; position: fixed; color: white; background-image: url(../../img/hexellence.png);">Solar System</h4>
<h4 onclick="balik()" class="fas fa-fw fa-chevron-left" style="z-index: 2; position: fixed; left: 5px; top: 10px; color: white"></h4>
</center>
<br>
<br>
<div style="text-align: justify; padding: 5%; margin: 2.5%; width: 95%; background-color: white;border-radius: 5px">
<div class="row" style="margin: 0">
<div id="tts">
<button onclick="read1()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>
</div>
<span style="color: rgba(0,0,0,0);">h</span>
<h3> Solar System</h3>
</div>
<script type="text/javascript" src="../../vendor/model-viewer.js"></script>
<center>
<model-viewer style="width: 100%; height: 300px" src="Earth/solar.glb" auto-rotate camera-controls background-image="Earth/bg2.jpg">
</model-viewer>
3D Model (Touch and Rotate)
</center>
<br>
<br>
<p>
The Solar System is the gravitationally bound system of the Sun and the objects that orbit it, either directly or indirectly. Of the objects that orbit the Sun directly, the largest are the eight planets, with the remainder being smaller objects, such as the five dwarf planets and small Solar System bodies.<br><br>
Known minor planets: 796,354 (as of 2019-08-27)<br><br>
Known planets: 8 (Mercury; Venus; Earth; Mars; Jupiter; Saturn; Uranus; Neptune)<br><br>
<li>The solar system includes the Sun and all the objects that orbit around it due to its gravity. This includes things such as planets, comets, asteroids, meteoroids and moons.</li><br>
<li>The Solar System formed around 4.6 billion years ago.</li><br>
<li>There are eight planets in the Solar System. The four inner planets are Mercury, Venus, Earth and Mars while the four outer planets are Jupiter, Saturn, Uranus and Neptune.</li><br>
<li>The inner planets (also known as terrestrial planets) are smaller and made mostly of rock and metal.</li><br>
<li>The outer planets (also known as gas giants) are much larger and made mostly of hydrogen, helium and other gases.</li><br>
<li>As of 2008, there are also five dwarf planets: Pluto, Ceres, Eris, Makemake & Haumea.</li><br>
<li>There is an asteroid belt which lies between the orbits or Mars and Jupiter, it features a large number of irregular shaped asteroids.</li><br>
<li>For thousands of years humans were unaware of the Solar System and believed that Earth was at the center of the Universe.</li><br>
<li>Astronomers such as Nicolaus Copernicus, Galileo Galilei, <NAME> and <NAME> helped develop a new model that explained the movement of the planets with the Sun at the center of the Solar System.</li><br>
<li>The Sun contains 99.86 percent of the Solar System's known mass, with Jupiter and Saturn making up making up most of the rest. The small inner planets which include Mercury, Venus, Earth and Mars make up a very small percentage of the Solar System's mass</li><br>.
<img src="Earth/solar1.jpg" width="100%" style="border: 5px outset orange; border-radius: 25px">
<br><br>
<img src="Earth/solar2.jpg" width="100%" style="border: 5px outset orange; border-radius: 25px">
<br><br>
<img src="Earth/solar3.jpg" width="100%" style="border: 5px outset orange; border-radius: 25px">
<br><br>
<img src="Earth/solar4.jpg" width="100%" style="border: 5px outset orange; border-radius: 25px">
<br><br>
<img src="Earth/solar5.jpg" width="100%" style="border: 5px outset orange; border-radius: 25px">
<br>
</p>
</div>
<script type="text/javascript">
//READ THE TEXT TO SPEECH
function read1(){
var line_1 = "Solar System. The Solar System is the gravitationally bound system of the Sun and the objects that orbit it, either directly or indirectly. Of the objects that orbit the Sun directly, the largest are the eight planets, with the remainder being smaller objects, such as the five dwarf planets and small Solar System bodies. Known minor planets: 796,354. Known planets: 8 Mercury; Venus; Earth; Mars; Jupiter; Saturn; Uranus; Neptune. The solar system includes the Sun and all the objects that orbit around it due to its gravity. This includes things such as planets, comets, asteroids, meteoroids and moons. The Solar System formed around 4.6 billion years ago. There are eight planets in the Solar System. The four inner planets are Mercury, Venus, Earth and Mars while the four outer planets are Jupiter, Saturn, Uranus and Neptune. The inner planets (also known as terrestrial planets) are smaller and made mostly of rock and metal. The outer planets (also known as gas giants) are much larger and made mostly of hydrogen, helium and other gases. As of 2008, there are also five dwarf planets: Pluto, Ceres, Eris, Makemake & Haumea. There is an asteroid belt which lies between the orbits or Mars and Jupiter, it features a large number of irregular shaped asteroids. For thousands of years humans were unaware of the Solar System and believed that Earth was at the center of the Universe. Astronomers such as Nicolaus Copernicus, Galileo Galilei, <NAME> and <NAME> helped develop a new model that explained the movement of the planets with the Sun at the center of the Solar System. The Sun contains 99.86 percent of the Solar System's known mass, with Jupiter and Saturn making up making up most of the rest. The small inner planets which include Mercury, Venus, Earth and Mars make up a very small percentage of the Solar System's mass.";
TTS.speak({
text: line_1,
locale: 'en-US',
rate: 1
});
//
var codeBlock='<button onclick="stop1()" class="btn btn-secondary"><i class="fas fa-fw fa-volume-mute"></i></button>';
document.getElementById("tts").innerHTML = codeBlock;
}
//STOP THE TEXT TO SPEECH
function stop1(){
TTS.speak({text: ''});
//set the new icon to button
var codeBlock='<button onclick="read1()" class="btn btn-secondary"><i class="fas fa-fw fa-play"></i></button>';
document.getElementById("tts").innerHTML = codeBlock;
}
//STOP THE TEXT TO SPEECH AND GO BACK HISTORY using back button
document.addEventListener("deviceready", onDeviceReady, false);
function onDeviceReady() {
document.addEventListener("backbutton", function () {
TTS.speak({text: ''});
window.history.back();
}, false );
}
//STOP THE TEXT TO SPEECH AND GO BACK HISTORY using a link button
function balik(){
TTS.speak({text: ''});
window.history.back();
}
</script>
<script crossorigin type="text/javascript" src="../../vendor/sweetalert2.js"></script>
<script src="../../vendor/jquery/jquery.min.js"></script>
<script src="../../vendor/bootstrap/js/bootstrap.bundle.min.js"></script>
</body>
</html> | a50164de720d2fcf0384c651a8e3e693f82db29f | [
"SQL",
"HTML",
"Markdown",
"JavaScript",
"PHP"
] | 26 | Markdown | crizon/SciHive | e1ee38de17739fe1d305844267ad95cbd18c87b3 | 755bf485dbaaf73ce0e9c617a65d195831ce8e83 | |
refs/heads/master | <repo_name>arvisKTU/IIP-project<file_sep>/IIP_Projektas/app/src/main/java/com/example/iip_projektas/Leaderboard.java
package com.example.iip_projektas;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.Context;
import android.database.Cursor;
import android.os.Bundle;
import android.os.AsyncTask;
import android.support.annotation.NonNull;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import android.widget.TextView;
import android.widget.Toast;
import org.json.JSONArray;
import org.json.JSONObject;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
class LeaderAdapter extends ArrayAdapter<Leader>
{
public LeaderAdapter(Context context, List<Leader> objects) {
super(context, R.layout.adapter_view_layout, objects);
}
@Override
public View getView(int position, View convertView, ViewGroup parent)
{
View v = convertView;
if(v==null)
{
LayoutInflater inflater = (LayoutInflater) getContext().getSystemService(Context.LAYOUT_INFLATER_SERVICE);
v = inflater.inflate(R.layout.adapter_view_layout,null);
}
TextView id = (TextView) v.findViewById(R.id.idView);
TextView username = (TextView) v.findViewById(R.id.usernameView);
TextView score = (TextView) v.findViewById(R.id.scoreView);
Leader leader = getItem(position);
id.setText(leader.getId());
username.setText(leader.getUsername());
score.setText(leader.getScore());
return v;
}
}
class Leader {
String id;
String username;
String score;
public Leader() {
}
public Leader(String id, String username, String score) {
this.id = id;
this.username = username;
this.score=score;
}
public String getId()
{
return id;
}
public void setId()
{
this.id=id;
}
public String getUsername()
{
return username;
}
public void setUsername()
{
this.username=username;
}
public String getScore()
{
return score;
}
public void setScore()
{
this.score=score;
}
}
public class Leaderboard extends Activity {
public LeaderAdapter adapter;
public ListView mListView;
LeaderboardDatabaseHandler dbhandler;
List<Leader> leaderList;
@Override
protected void onCreate(Bundle savedInstanceState) {
leaderList = new ArrayList<>();
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_leaderboard);
dbhandler = new LeaderboardDatabaseHandler(this);
mListView = (ListView)findViewById(R.id.leader_list) ;
//prepareContent();
String[] from = new String[] { "name", "score"};
int[] to = new int[] {R.id.listview_username, R.id.listview_score};
viewAll();
// deleteEntries();
}
public void viewAll()
{
int counter=1;
Cursor res = dbhandler.getEntries();
while(res.moveToNext()&& counter<=10)
{
Leader leader = new Leader(Integer.toString(counter),res.getString(1),res.getString(2));
leaderList.add(leader);
counter++;
}
adapter = new LeaderAdapter(this, leaderList);
mListView.setAdapter(adapter);
}
public void showEntries(String Message)
{
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setCancelable(true);
builder.setMessage(Message);
builder.show();
}
public void deleteEntries()
{
dbhandler.deleteEntries();
}
}
| 4d1d8b19f85fcc0b6784d12986d2ba13532f0719 | [
"Java"
] | 1 | Java | arvisKTU/IIP-project | 3a457364fb2ffec9c4d493999d3cf731ad3e5669 | 740eeb83c7a299a629f7315b53b1033c25388611 | |
refs/heads/master | <file_sep>package com.android.providers.downloads;
import static android.net.NetworkCapabilities.NET_CAPABILITY_NOT_METERED;
import static android.net.NetworkCapabilities.NET_CAPABILITY_NOT_ROAMING;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.when;
import android.app.job.JobParameters;
import android.content.Context;
import android.content.Intent;
import android.content.pm.PackageManager.NameNotFoundException;
import android.net.ConnectivityManager;
import android.net.Network;
import android.net.NetworkCapabilities;
import android.net.NetworkInfo;
import android.net.NetworkInfo.DetailedState;
import android.os.Bundle;
import org.mockito.invocation.InvocationOnMock;
import org.mockito.stubbing.Answer;
import java.io.IOException;
import java.net.URL;
import java.net.URLConnection;
import java.security.GeneralSecurityException;
import java.util.ArrayList;
import java.util.List;
import javax.net.ssl.SSLContext;
public class FakeSystemFacade implements SystemFacade {
long mTimeMillis = 0;
Integer mActiveNetworkType = ConnectivityManager.TYPE_WIFI;
boolean mIsRoaming = false;
boolean mIsMetered = false;
long mMaxBytesOverMobile = Long.MAX_VALUE;
long mRecommendedMaxBytesOverMobile = Long.MAX_VALUE;
List<Intent> mBroadcastsSent = new ArrayList<Intent>();
Bundle mLastBroadcastOptions;
boolean mCleartextTrafficPermitted = true;
private boolean mReturnActualTime = false;
private SSLContext mSSLContext = null;
public void setUp() {
mTimeMillis = 0;
mActiveNetworkType = ConnectivityManager.TYPE_WIFI;
mIsRoaming = false;
mIsMetered = false;
mMaxBytesOverMobile = Long.MAX_VALUE;
mRecommendedMaxBytesOverMobile = Long.MAX_VALUE;
mBroadcastsSent.clear();
mLastBroadcastOptions = null;
mReturnActualTime = false;
try {
mSSLContext = SSLContext.getDefault();
} catch (GeneralSecurityException e) {
throw new RuntimeException(e);
}
}
void incrementTimeMillis(long delta) {
mTimeMillis += delta;
}
@Override
public long currentTimeMillis() {
if (mReturnActualTime) {
return System.currentTimeMillis();
}
return mTimeMillis;
}
@Override
public Network getNetwork(JobParameters params) {
if (mActiveNetworkType == null) {
return null;
} else {
final Network network = mock(Network.class);
try {
when(network.openConnection(any())).then(new Answer<URLConnection>() {
@Override
public URLConnection answer(InvocationOnMock invocation) throws Throwable {
final URL url = (URL) invocation.getArguments()[0];
return url.openConnection();
}
});
} catch (IOException ignored) {
}
return network;
}
}
@Override
public NetworkCapabilities getNetworkCapabilities(Network network) {
if (mActiveNetworkType == null) {
return null;
} else {
final NetworkCapabilities.Builder builder = new NetworkCapabilities.Builder();
if (!mIsMetered) builder.addCapability(NET_CAPABILITY_NOT_METERED);
if (!mIsRoaming) builder.addCapability(NET_CAPABILITY_NOT_ROAMING);
return builder.build();
}
}
@Override
public long getMaxBytesOverMobile() {
return mMaxBytesOverMobile;
}
@Override
public long getRecommendedMaxBytesOverMobile() {
return mRecommendedMaxBytesOverMobile;
}
@Override
public void sendBroadcast(Intent intent) {
mBroadcastsSent.add(intent);
mLastBroadcastOptions = null;
}
@Override
public void sendBroadcast(Intent intent, String receiverPermission, Bundle options) {
mBroadcastsSent.add(intent);
mLastBroadcastOptions = options;
}
@Override
public boolean userOwnsPackage(int uid, String pckg) throws NameNotFoundException {
return true;
}
@Override
public boolean isCleartextTrafficPermitted(String packageName, String hostname) {
return mCleartextTrafficPermitted;
}
@Override
public SSLContext getSSLContextForPackage(Context context, String pckg) {
return mSSLContext;
}
public void setSSLContext(SSLContext context) {
mSSLContext = context;
}
public void setReturnActualTime(boolean flag) {
mReturnActualTime = flag;
}
}
<file_sep>/*
* Copyright (C) 2019 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android.providers.downloads;
import static android.provider.BaseColumns._ID;
import static android.provider.Downloads.Impl.ALL_DOWNLOADS_CONTENT_URI;
import static android.provider.Downloads.Impl.COLUMN_DESTINATION;
import static android.provider.Downloads.Impl.COLUMN_IS_VISIBLE_IN_DOWNLOADS_UI;
import static android.provider.Downloads.Impl.COLUMN_MEDIASTORE_URI;
import static android.provider.Downloads.Impl.COLUMN_MEDIA_SCANNED;
import static android.provider.Downloads.Impl.DESTINATION_EXTERNAL;
import static android.provider.Downloads.Impl.DESTINATION_FILE_URI;
import static android.provider.Downloads.Impl.DESTINATION_NON_DOWNLOADMANAGER_DOWNLOAD;
import static android.provider.Downloads.Impl.MEDIA_SCANNED;
import static android.provider.Downloads.Impl._DATA;
import static com.android.providers.downloads.Constants.MEDIA_SCAN_TRIGGER_JOB_ID;
import android.app.job.JobInfo;
import android.app.job.JobParameters;
import android.app.job.JobScheduler;
import android.app.job.JobService;
import android.content.ComponentName;
import android.content.ContentProviderClient;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.net.Uri;
import android.os.Environment;
import android.provider.Downloads;
import android.provider.MediaStore;
import android.util.Log;
import java.io.File;
/**
* Job to update MediaProvider with all the downloads and force mediascan on them.
*/
public class MediaScanTriggerJob extends JobService {
private volatile boolean mJobStopped;
@Override
public boolean onStartJob(JobParameters parameters) {
if (!Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
// External storage is not available yet, so defer this job to a later time.
jobFinished(parameters, true /* reschedule */);
return false;
}
Helpers.getAsyncHandler().post(() -> {
final String selection = _DATA + " IS NOT NULL"
+ " AND (" + COLUMN_IS_VISIBLE_IN_DOWNLOADS_UI + "=1"
+ " OR " + COLUMN_MEDIA_SCANNED + "=" + MEDIA_SCANNED + ")"
+ " AND (" + COLUMN_DESTINATION + "=" + DESTINATION_EXTERNAL
+ " OR " + COLUMN_DESTINATION + "=" + DESTINATION_FILE_URI
+ " OR " + COLUMN_DESTINATION + "=" + DESTINATION_NON_DOWNLOADMANAGER_DOWNLOAD
+ ")";
try (ContentProviderClient cpc
= getContentResolver().acquireContentProviderClient(Downloads.Impl.AUTHORITY);
ContentProviderClient mediaProviderClient
= getContentResolver().acquireContentProviderClient(MediaStore.AUTHORITY)) {
final DownloadProvider downloadProvider
= ((DownloadProvider) cpc.getLocalContentProvider());
try (Cursor cursor = downloadProvider.query(ALL_DOWNLOADS_CONTENT_URI,
null, selection, null, null)) {
final DownloadInfo.Reader reader
= new DownloadInfo.Reader(getContentResolver(), cursor);
final DownloadInfo info = new DownloadInfo(MediaScanTriggerJob.this);
while (cursor.moveToNext()) {
if (mJobStopped) {
return;
}
reader.updateFromDatabase(info);
// This indicates that this entry has been handled already (perhaps when
// this job ran earlier and got preempted), so skip.
if (info.mMediaStoreUri != null) {
continue;
}
final ContentValues mediaValues;
try {
mediaValues = downloadProvider.convertToMediaProviderValues(info);
} catch (IllegalArgumentException e) {
Log.e(Constants.TAG,
"Error getting media content values from " + info, e);
continue;
}
// Overriding size column value to 0 for forcing the mediascan
// later (to address http://b/138419471).
mediaValues.put(MediaStore.Files.FileColumns.SIZE, 0);
downloadProvider.updateMediaProvider(mediaProviderClient, mediaValues);
final Uri mediaStoreUri = Helpers.triggerMediaScan(mediaProviderClient,
new File(info.mFileName));
if (mediaStoreUri != null) {
final ContentValues downloadValues = new ContentValues();
downloadValues.put(COLUMN_MEDIASTORE_URI, mediaStoreUri.toString());
downloadProvider.update(ALL_DOWNLOADS_CONTENT_URI,
downloadValues, _ID + "=" + info.mId, null);
}
}
}
}
jobFinished(parameters, false /* reschedule */);
});
return true;
}
@Override
public boolean onStopJob(JobParameters parameters) {
mJobStopped = true;
return true;
}
public static void schedule(Context context) {
final JobScheduler scheduler = context.getSystemService(JobScheduler.class);
final JobInfo job = new JobInfo.Builder(MEDIA_SCAN_TRIGGER_JOB_ID,
new ComponentName(context, MediaScanTriggerJob.class))
.setRequiresCharging(true)
.setRequiresDeviceIdle(true)
.build();
scheduler.schedule(job);
}
}
<file_sep>/*
* Copyright (C) 2019 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android.providers.downloads;
import android.content.ContentUris;
import android.net.Uri;
import android.provider.MediaStore;
public class MediaStoreDownloadsHelper {
private static final String MEDIASTORE_DOWNLOAD_FILE_PREFIX = "msf:";
private static final String MEDIASTORE_DOWNLOAD_DIR_PREFIX = "msd:";
public static String getDocIdForMediaStoreDownload(long id, boolean isDir) {
return (isDir ? MEDIASTORE_DOWNLOAD_DIR_PREFIX : MEDIASTORE_DOWNLOAD_FILE_PREFIX) + id;
}
public static boolean isMediaStoreDownload(String docId) {
return docId != null && (docId.startsWith(MEDIASTORE_DOWNLOAD_FILE_PREFIX)
|| docId.startsWith(MEDIASTORE_DOWNLOAD_DIR_PREFIX));
}
public static long getMediaStoreId(String docId) {
return Long.parseLong(getMediaStoreIdString(docId));
}
public static String getMediaStoreIdString(String docId) {
final int index = docId.indexOf(":");
return docId.substring(index + 1);
}
public static boolean isMediaStoreDownloadDir(String docId) {
return docId != null && docId.startsWith(MEDIASTORE_DOWNLOAD_DIR_PREFIX);
}
/**
* The returned uri always appends external volume {@link MediaStore#VOLUME_EXTERNAL}.
* It doesn't consider the item is located on second volume. It can't be used to update
* or insert.
* @param docId the doc id
* @return external uri for query
*/
public static Uri getMediaStoreUriForQuery(String docId) {
return getMediaStoreUri(MediaStore.VOLUME_EXTERNAL, docId);
}
public static Uri getMediaStoreUri(String volume, String docId) {
return MediaStore.Downloads.getContentUri(volume, getMediaStoreId(docId));
}
}
<file_sep>/*
* Copyright (C) 2012 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android.providers.downloads;
import static android.app.DownloadManager.COLUMN_LOCAL_FILENAME;
import static android.app.DownloadManager.COLUMN_MEDIA_TYPE;
import static android.app.DownloadManager.COLUMN_URI;
import static android.provider.Downloads.Impl.ALL_DOWNLOADS_CONTENT_URI;
import static com.android.providers.downloads.Constants.TAG;
import android.app.DownloadManager;
import android.content.ActivityNotFoundException;
import android.content.ContentUris;
import android.content.Context;
import android.content.Intent;
import android.content.pm.PackageInstaller;
import android.content.pm.PackageManager;
import android.database.Cursor;
import android.net.Uri;
import android.os.Process;
import android.provider.DocumentsContract;
import android.provider.Downloads.Impl.RequestHeaders;
import android.provider.MediaStore;
import android.util.Log;
import java.io.File;
public class OpenHelper {
/**
* Build and start an {@link Intent} to view the download with given ID,
* handling subtleties around installing packages.
*/
public static boolean startViewIntent(Context context, long id, int intentFlags) {
final Intent intent = OpenHelper.buildViewIntent(context, id);
if (intent == null) {
Log.w(TAG, "No intent built for " + id);
return false;
}
intent.addFlags(intentFlags);
return startViewIntent(context, intent);
}
public static boolean startViewIntent(Context context, Intent intent) {
try {
context.startActivity(intent);
return true;
} catch (ActivityNotFoundException e) {
Log.w(TAG, "Failed to start " + intent + ": " + e);
return false;
}
}
public static Intent buildViewIntentForMediaStoreDownload(Context context,
Uri documentUri) {
final long mediaStoreId = MediaStoreDownloadsHelper.getMediaStoreId(
DocumentsContract.getDocumentId(documentUri));
final Uri queryUri = ContentUris.withAppendedId(
MediaStore.Downloads.EXTERNAL_CONTENT_URI, mediaStoreId);
try (Cursor cursor = context.getContentResolver().query(
queryUri, null, null, null)) {
if (cursor.moveToFirst()) {
final String mimeType = cursor.getString(
cursor.getColumnIndex(MediaStore.Downloads.MIME_TYPE));
final Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(documentUri, mimeType);
intent.setFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION
| Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
if ("application/vnd.android.package-archive".equals(mimeType)) {
// Also splice in details about where it came from
intent.putExtra(Intent.EXTRA_ORIGINATING_URI,
getCursorUri(cursor, MediaStore.Downloads.DOWNLOAD_URI));
intent.putExtra(Intent.EXTRA_REFERRER,
getCursorUri(cursor, MediaStore.Downloads.REFERER_URI));
final String ownerPackageName = getCursorString(cursor,
MediaStore.Downloads.OWNER_PACKAGE_NAME);
final int ownerUid = getPackageUid(context, ownerPackageName);
if (ownerUid > 0) {
intent.putExtra(Intent.EXTRA_ORIGINATING_UID, ownerUid);
}
}
return intent;
}
}
return null;
}
/**
* Build an {@link Intent} to view the download with given ID, handling
* subtleties around installing packages.
*/
private static Intent buildViewIntent(Context context, long id) {
final DownloadManager downManager = (DownloadManager) context.getSystemService(
Context.DOWNLOAD_SERVICE);
downManager.setAccessAllDownloads(true);
downManager.setAccessFilename(true);
final Cursor cursor = downManager.query(new DownloadManager.Query().setFilterById(id));
try {
if (!cursor.moveToFirst()) {
return null;
}
final File file = getCursorFile(cursor, COLUMN_LOCAL_FILENAME);
String mimeType = getCursorString(cursor, COLUMN_MEDIA_TYPE);
mimeType = DownloadDrmHelper.getOriginalMimeType(context, file, mimeType);
final Uri documentUri = DocumentsContract.buildDocumentUri(
Constants.STORAGE_AUTHORITY, String.valueOf(id));
final Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(documentUri, mimeType);
intent.setFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION
| Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
if ("application/vnd.android.package-archive".equals(mimeType)) {
// Also splice in details about where it came from
final Uri remoteUri = getCursorUri(cursor, COLUMN_URI);
intent.putExtra(Intent.EXTRA_ORIGINATING_URI, remoteUri);
intent.putExtra(Intent.EXTRA_REFERRER, getRefererUri(context, id));
intent.putExtra(Intent.EXTRA_ORIGINATING_UID, getOriginatingUid(context, id));
}
return intent;
} finally {
cursor.close();
}
}
private static Uri getRefererUri(Context context, long id) {
final Uri headersUri = Uri.withAppendedPath(
ContentUris.withAppendedId(ALL_DOWNLOADS_CONTENT_URI, id),
RequestHeaders.URI_SEGMENT);
final Cursor headers = context.getContentResolver()
.query(headersUri, null, null, null, null);
try {
while (headers.moveToNext()) {
final String header = getCursorString(headers, RequestHeaders.COLUMN_HEADER);
if ("Referer".equalsIgnoreCase(header)) {
return getCursorUri(headers, RequestHeaders.COLUMN_VALUE);
}
}
} finally {
headers.close();
}
return null;
}
private static int getOriginatingUid(Context context, long id) {
final Uri uri = ContentUris.withAppendedId(ALL_DOWNLOADS_CONTENT_URI, id);
final Cursor cursor = context.getContentResolver().query(uri, new String[]{Constants.UID},
null, null, null);
if (cursor != null) {
try {
if (cursor.moveToFirst()) {
final int uid = cursor.getInt(cursor.getColumnIndexOrThrow(Constants.UID));
if (uid != Process.myUid()) {
return uid;
}
}
} finally {
cursor.close();
}
}
return PackageInstaller.SessionParams.UID_UNKNOWN;
}
private static int getPackageUid(Context context, String packageName) {
if (packageName == null) {
return -1;
}
try {
return context.getPackageManager().getPackageUid(packageName, 0);
} catch (PackageManager.NameNotFoundException e) {
Log.e(TAG, "Couldn't get uid for " + packageName, e);
return -1;
}
}
private static String getCursorString(Cursor cursor, String column) {
return cursor.getString(cursor.getColumnIndexOrThrow(column));
}
private static Uri getCursorUri(Cursor cursor, String column) {
final String uriString = cursor.getString(cursor.getColumnIndexOrThrow(column));
return uriString == null ? null : Uri.parse(uriString);
}
private static File getCursorFile(Cursor cursor, String column) {
return new File(cursor.getString(cursor.getColumnIndexOrThrow(column)));
}
}
<file_sep>/*
* Copyright (C) 2013 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android.providers.downloads.ui;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.DialogFragment;
import android.app.DownloadManager;
import android.app.DownloadManager.Query;
import android.app.FragmentManager;
import android.content.ContentUris;
import android.content.Context;
import android.content.DialogInterface;
import android.content.Intent;
import android.database.Cursor;
import android.net.Uri;
import android.os.Bundle;
import android.provider.DocumentsContract;
import android.util.Log;
import android.widget.Toast;
import com.android.providers.downloads.Constants;
import com.android.providers.downloads.MediaStoreDownloadsHelper;
import com.android.providers.downloads.OpenHelper;
import com.android.providers.downloads.RawDocumentsHelper;
import libcore.io.IoUtils;
/**
* Intercept all download clicks to provide special behavior. For example,
* PackageInstaller really wants raw file paths.
*/
public class TrampolineActivity extends Activity {
private static final String TAG_PAUSED = "paused";
private static final String TAG_FAILED = "failed";
private static final String KEY_ID = "id";
private static final String KEY_REASON = "reason";
private static final String KEY_SIZE = "size";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Uri documentUri = getIntent().getData();
if (RawDocumentsHelper.isRawDocId(DocumentsContract.getDocumentId(documentUri))) {
if (!RawDocumentsHelper.startViewIntent(this, documentUri)) {
Toast.makeText(this, R.string.download_no_application_title, Toast.LENGTH_SHORT)
.show();
}
finish();
return;
}
if (MediaStoreDownloadsHelper.isMediaStoreDownload(
DocumentsContract.getDocumentId(documentUri))) {
final Intent intent = OpenHelper.buildViewIntentForMediaStoreDownload(
this, documentUri);
if (intent == null || !OpenHelper.startViewIntent(this, intent)) {
Toast.makeText(this, R.string.download_no_application_title, Toast.LENGTH_SHORT)
.show();
}
finish();
return;
}
final long id = ContentUris.parseId(documentUri);
final DownloadManager dm = (DownloadManager) getSystemService(Context.DOWNLOAD_SERVICE);
dm.setAccessAllDownloads(true);
final int status;
final int reason;
final long size;
final Cursor cursor = dm.query(new Query().setFilterById(id));
try {
if (cursor.moveToFirst()) {
status = cursor.getInt(cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_STATUS));
reason = cursor.getInt(cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_REASON));
size = cursor.getLong(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_TOTAL_SIZE_BYTES));
} else {
Toast.makeText(this, R.string.dialog_file_missing_body, Toast.LENGTH_SHORT).show();
finish();
return;
}
} finally {
IoUtils.closeQuietly(cursor);
}
Log.d(Constants.TAG, "Found " + id + " with status " + status + ", reason " + reason);
switch (status) {
case DownloadManager.STATUS_PENDING:
case DownloadManager.STATUS_RUNNING:
sendRunningDownloadClickedBroadcast(id);
finish();
break;
case DownloadManager.STATUS_PAUSED:
if (reason == DownloadManager.PAUSED_QUEUED_FOR_WIFI) {
PausedDialogFragment.show(getFragmentManager(), id, size);
} else {
sendRunningDownloadClickedBroadcast(id);
finish();
}
break;
case DownloadManager.STATUS_SUCCESSFUL:
if (!OpenHelper.startViewIntent(this, id, 0)) {
Toast.makeText(this, R.string.download_no_application_title, Toast.LENGTH_SHORT)
.show();
}
finish();
break;
case DownloadManager.STATUS_FAILED:
FailedDialogFragment.show(getFragmentManager(), id, reason);
break;
}
}
private void sendRunningDownloadClickedBroadcast(long id) {
final Intent intent = new Intent(Constants.ACTION_LIST);
intent.setPackage(Constants.PROVIDER_PACKAGE_NAME);
intent.putExtra(DownloadManager.EXTRA_NOTIFICATION_CLICK_DOWNLOAD_IDS, new long[] { id });
sendBroadcast(intent);
}
public static class PausedDialogFragment extends DialogFragment {
public static void show(FragmentManager fm, long id, long size) {
final PausedDialogFragment dialog = new PausedDialogFragment();
final Bundle args = new Bundle();
args.putLong(KEY_ID, id);
args.putLong(KEY_SIZE, size);
dialog.setArguments(args);
dialog.show(fm, TAG_PAUSED);
}
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
final Context context = getActivity();
final DownloadManager dm = (DownloadManager) context.getSystemService(
Context.DOWNLOAD_SERVICE);
dm.setAccessAllDownloads(true);
final long id = getArguments().getLong(KEY_ID);
final long size = getArguments().getLong(KEY_SIZE);
final AlertDialog.Builder builder = new AlertDialog.Builder(
context, android.R.style.Theme_DeviceDefault_Light_Dialog_Alert);
builder.setTitle(R.string.dialog_title_queued_body);
builder.setMessage(R.string.dialog_queued_body);
final Long maxSize = DownloadManager.getMaxBytesOverMobile(context);
if (maxSize != null && size > maxSize) {
// When we have a max size, we have no choice
builder.setPositiveButton(R.string.keep_queued_download, null);
} else {
// Give user the choice of starting now
builder.setPositiveButton(R.string.start_now_download,
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dm.forceDownload(id);
}
});
}
builder.setNegativeButton(
R.string.remove_download, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dm.remove(id);
}
});
return builder.create();
}
@Override
public void onDismiss(DialogInterface dialog) {
super.onDismiss(dialog);
final Activity activity = getActivity();
if (activity != null) {
activity.finish();
}
}
}
public static class FailedDialogFragment extends DialogFragment {
public static void show(FragmentManager fm, long id, int reason) {
final FailedDialogFragment dialog = new FailedDialogFragment();
final Bundle args = new Bundle();
args.putLong(KEY_ID, id);
args.putInt(KEY_REASON, reason);
dialog.setArguments(args);
dialog.show(fm, TAG_FAILED);
}
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
final Context context = getActivity();
final DownloadManager dm = (DownloadManager) context.getSystemService(
Context.DOWNLOAD_SERVICE);
dm.setAccessAllDownloads(true);
final long id = getArguments().getLong(KEY_ID);
final int reason = getArguments().getInt(KEY_REASON);
final AlertDialog.Builder builder = new AlertDialog.Builder(
context, android.R.style.Theme_DeviceDefault_Light_Dialog_Alert);
builder.setTitle(R.string.dialog_title_not_available);
switch (reason) {
case DownloadManager.ERROR_FILE_ALREADY_EXISTS:
builder.setMessage(R.string.dialog_file_already_exists);
break;
case DownloadManager.ERROR_INSUFFICIENT_SPACE:
builder.setMessage(R.string.dialog_insufficient_space_on_cache);
break;
case DownloadManager.ERROR_DEVICE_NOT_FOUND:
builder.setMessage(R.string.dialog_media_not_found);
break;
case DownloadManager.ERROR_CANNOT_RESUME:
builder.setMessage(R.string.dialog_cannot_resume);
break;
default:
builder.setMessage(R.string.dialog_failed_body);
}
builder.setNegativeButton(
R.string.delete_download, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dm.remove(id);
}
});
builder.setPositiveButton(
R.string.retry_download, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dm.restartDownload(id);
}
});
return builder.create();
}
@Override
public void onDismiss(DialogInterface dialog) {
super.onDismiss(dialog);
final Activity activity = getActivity();
if (activity != null) {
activity.finish();
}
}
}
}
<file_sep>/*
* Copyright (C) 2013 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android.providers.downloads;
import static com.android.providers.downloads.MediaStoreDownloadsHelper.getDocIdForMediaStoreDownload;
import static com.android.providers.downloads.MediaStoreDownloadsHelper.getMediaStoreIdString;
import static com.android.providers.downloads.MediaStoreDownloadsHelper.getMediaStoreUriForQuery;
import static com.android.providers.downloads.MediaStoreDownloadsHelper.isMediaStoreDownload;
import static com.android.providers.downloads.MediaStoreDownloadsHelper.isMediaStoreDownloadDir;
import android.annotation.NonNull;
import android.annotation.Nullable;
import android.app.DownloadManager;
import android.app.DownloadManager.Query;
import android.content.ContentResolver;
import android.content.ContentUris;
import android.content.Context;
import android.content.UriPermission;
import android.database.Cursor;
import android.database.MatrixCursor;
import android.database.MatrixCursor.RowBuilder;
import android.media.MediaFile;
import android.net.Uri;
import android.os.Binder;
import android.os.Bundle;
import android.os.CancellationSignal;
import android.os.Environment;
import android.os.FileObserver;
import android.os.FileUtils;
import android.os.ParcelFileDescriptor;
import android.provider.DocumentsContract;
import android.provider.DocumentsContract.Document;
import android.provider.DocumentsContract.Path;
import android.provider.DocumentsContract.Root;
import android.provider.Downloads;
import android.provider.MediaStore;
import android.provider.MediaStore.DownloadColumns;
import android.text.TextUtils;
import android.util.Log;
import android.util.Pair;
import com.android.internal.annotations.GuardedBy;
import com.android.internal.content.FileSystemProvider;
import libcore.io.IoUtils;
import java.io.File;
import java.io.FileNotFoundException;
import java.text.NumberFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Locale;
import java.util.Set;
/**
* Presents files located in {@link Environment#DIRECTORY_DOWNLOADS} and contents from
* {@link DownloadManager}. {@link DownloadManager} contents include active downloads and completed
* downloads added by other applications using
* {@link DownloadManager#addCompletedDownload(String, String, boolean, String, String, long, boolean, boolean, Uri, Uri)}
* .
*/
public class DownloadStorageProvider extends FileSystemProvider {
private static final String TAG = "DownloadStorageProvider";
private static final boolean DEBUG = false;
private static final String AUTHORITY = Constants.STORAGE_AUTHORITY;
private static final String DOC_ID_ROOT = Constants.STORAGE_ROOT_ID;
private static final String[] DEFAULT_ROOT_PROJECTION = new String[] {
Root.COLUMN_ROOT_ID, Root.COLUMN_FLAGS, Root.COLUMN_ICON,
Root.COLUMN_TITLE, Root.COLUMN_DOCUMENT_ID, Root.COLUMN_QUERY_ARGS
};
private static final String[] DEFAULT_DOCUMENT_PROJECTION = new String[] {
Document.COLUMN_DOCUMENT_ID, Document.COLUMN_MIME_TYPE, Document.COLUMN_DISPLAY_NAME,
Document.COLUMN_SUMMARY, Document.COLUMN_LAST_MODIFIED, Document.COLUMN_FLAGS,
Document.COLUMN_SIZE,
};
private DownloadManager mDm;
private static final int NO_LIMIT = -1;
@Override
public boolean onCreate() {
super.onCreate(DEFAULT_DOCUMENT_PROJECTION);
mDm = (DownloadManager) getContext().getSystemService(Context.DOWNLOAD_SERVICE);
mDm.setAccessAllDownloads(true);
mDm.setAccessFilename(true);
return true;
}
private static String[] resolveRootProjection(String[] projection) {
return projection != null ? projection : DEFAULT_ROOT_PROJECTION;
}
private static String[] resolveDocumentProjection(String[] projection) {
return projection != null ? projection : DEFAULT_DOCUMENT_PROJECTION;
}
private void copyNotificationUri(@NonNull MatrixCursor result, @NonNull Cursor cursor) {
final List<Uri> notifyUris = cursor.getNotificationUris();
if (notifyUris != null) {
result.setNotificationUris(getContext().getContentResolver(), notifyUris);
}
}
/**
* Called by {@link DownloadProvider} when deleting a row in the {@link DownloadManager}
* database.
*/
static void onDownloadProviderDelete(Context context, long id) {
final Uri uri = DocumentsContract.buildDocumentUri(AUTHORITY, Long.toString(id));
context.revokeUriPermission(uri, ~0);
}
static void onMediaProviderDownloadsDelete(Context context, long[] ids, String[] mimeTypes) {
for (int i = 0; i < ids.length; ++i) {
final boolean isDir = mimeTypes[i] == null;
final Uri uri = DocumentsContract.buildDocumentUri(AUTHORITY,
MediaStoreDownloadsHelper.getDocIdForMediaStoreDownload(ids[i], isDir));
context.revokeUriPermission(uri, ~0);
}
}
static void revokeAllMediaStoreUriPermissions(Context context) {
final List<UriPermission> uriPermissions =
context.getContentResolver().getOutgoingUriPermissions();
final int size = uriPermissions.size();
final StringBuilder sb = new StringBuilder("Revoking permissions for uris: ");
for (int i = 0; i < size; ++i) {
final Uri uri = uriPermissions.get(i).getUri();
if (AUTHORITY.equals(uri.getAuthority())
&& isMediaStoreDownload(DocumentsContract.getDocumentId(uri))) {
context.revokeUriPermission(uri, ~0);
sb.append(uri + ",");
}
}
Log.d(TAG, sb.toString());
}
@Override
public Cursor queryRoots(String[] projection) throws FileNotFoundException {
// It's possible that the folder does not exist on disk, so we will create the folder if
// that is the case. If user decides to delete the folder later, then it's OK to fail on
// subsequent queries.
getPublicDownloadsDirectory().mkdirs();
final MatrixCursor result = new MatrixCursor(resolveRootProjection(projection));
final RowBuilder row = result.newRow();
row.add(Root.COLUMN_ROOT_ID, DOC_ID_ROOT);
row.add(Root.COLUMN_FLAGS, Root.FLAG_LOCAL_ONLY | Root.FLAG_SUPPORTS_RECENTS
| Root.FLAG_SUPPORTS_CREATE | Root.FLAG_SUPPORTS_SEARCH);
row.add(Root.COLUMN_ICON, R.mipmap.ic_launcher_download);
row.add(Root.COLUMN_TITLE, getContext().getString(R.string.root_downloads));
row.add(Root.COLUMN_DOCUMENT_ID, DOC_ID_ROOT);
row.add(Root.COLUMN_QUERY_ARGS, SUPPORTED_QUERY_ARGS);
return result;
}
@Override
public Path findDocumentPath(@Nullable String parentDocId, String docId) throws FileNotFoundException {
// parentDocId is null if the client is asking for the path to the root of a doc tree.
// Don't share root information with those who shouldn't know it.
final String rootId = (parentDocId == null) ? DOC_ID_ROOT : null;
if (parentDocId == null) {
parentDocId = DOC_ID_ROOT;
}
final File parent = getFileForDocId(parentDocId);
final File doc = getFileForDocId(docId);
return new Path(rootId, findDocumentPath(parent, doc));
}
/**
* Calls on {@link FileSystemProvider#createDocument(String, String, String)}, and then creates
* a new database entry in {@link DownloadManager} if it is not a raw file and not a folder.
*/
@Override
public String createDocument(String parentDocId, String mimeType, String displayName)
throws FileNotFoundException {
// Delegate to real provider
final long token = Binder.clearCallingIdentity();
try {
String newDocumentId = super.createDocument(parentDocId, mimeType, displayName);
if (!Document.MIME_TYPE_DIR.equals(mimeType)
&& !RawDocumentsHelper.isRawDocId(parentDocId)
&& !isMediaStoreDownload(parentDocId)) {
File newFile = getFileForDocId(newDocumentId);
newDocumentId = Long.toString(mDm.addCompletedDownload(
newFile.getName(), newFile.getName(), true, mimeType,
newFile.getAbsolutePath(), 0L,
false, true));
}
return newDocumentId;
} finally {
Binder.restoreCallingIdentity(token);
}
}
@Override
public void deleteDocument(String docId) throws FileNotFoundException {
// Delegate to real provider
final long token = Binder.clearCallingIdentity();
try {
if (RawDocumentsHelper.isRawDocId(docId) || isMediaStoreDownload(docId)) {
super.deleteDocument(docId);
return;
}
if (mDm.remove(Long.parseLong(docId)) != 1) {
throw new IllegalStateException("Failed to delete " + docId);
}
} finally {
Binder.restoreCallingIdentity(token);
}
}
@Override
public String renameDocument(String docId, String displayName)
throws FileNotFoundException {
final long token = Binder.clearCallingIdentity();
try {
if (RawDocumentsHelper.isRawDocId(docId)
|| isMediaStoreDownloadDir(docId)) {
return super.renameDocument(docId, displayName);
}
displayName = FileUtils.buildValidFatFilename(displayName);
if (isMediaStoreDownload(docId)) {
return renameMediaStoreDownload(docId, displayName);
} else {
final long id = Long.parseLong(docId);
if (!mDm.rename(getContext(), id, displayName)) {
throw new IllegalStateException(
"Failed to rename to " + displayName + " in downloadsManager");
}
}
return null;
} finally {
Binder.restoreCallingIdentity(token);
}
}
@Override
public Cursor queryDocument(String docId, String[] projection) throws FileNotFoundException {
// Delegate to real provider
final long token = Binder.clearCallingIdentity();
Cursor cursor = null;
try {
if (RawDocumentsHelper.isRawDocId(docId)) {
return super.queryDocument(docId, projection);
}
final DownloadsCursor result = new DownloadsCursor(projection,
getContext().getContentResolver());
if (DOC_ID_ROOT.equals(docId)) {
includeDefaultDocument(result);
} else if (isMediaStoreDownload(docId)) {
cursor = getContext().getContentResolver().query(getMediaStoreUriForQuery(docId),
null, null, null);
copyNotificationUri(result, cursor);
if (cursor.moveToFirst()) {
includeDownloadFromMediaStore(result, cursor, null /* filePaths */,
false /* shouldExcludeMedia */);
}
} else {
cursor = mDm.query(new Query().setFilterById(Long.parseLong(docId)));
copyNotificationUri(result, cursor);
if (cursor.moveToFirst()) {
// We don't know if this queryDocument() call is from Downloads (manage)
// or Files. Safely assume it's Files.
includeDownloadFromCursor(result, cursor, null /* filePaths */,
null /* queryArgs */);
}
}
result.start();
return result;
} finally {
IoUtils.closeQuietly(cursor);
Binder.restoreCallingIdentity(token);
}
}
@Override
public Cursor queryChildDocuments(String parentDocId, String[] projection, String sortOrder)
throws FileNotFoundException {
return queryChildDocuments(parentDocId, projection, sortOrder, false);
}
@Override
public Cursor queryChildDocumentsForManage(
String parentDocId, String[] projection, String sortOrder)
throws FileNotFoundException {
return queryChildDocuments(parentDocId, projection, sortOrder, true);
}
private Cursor queryChildDocuments(String parentDocId, String[] projection,
String sortOrder, boolean manage) throws FileNotFoundException {
// Delegate to real provider
final long token = Binder.clearCallingIdentity();
Cursor cursor = null;
try {
if (RawDocumentsHelper.isRawDocId(parentDocId)) {
return super.queryChildDocuments(parentDocId, projection, sortOrder);
}
final DownloadsCursor result = new DownloadsCursor(projection,
getContext().getContentResolver());
final ArrayList<Uri> notificationUris = new ArrayList<>();
if (isMediaStoreDownloadDir(parentDocId)) {
includeDownloadsFromMediaStore(result, null /* queryArgs */,
null /* filePaths */, notificationUris,
getMediaStoreIdString(parentDocId), NO_LIMIT, manage);
} else {
assert (DOC_ID_ROOT.equals(parentDocId));
if (manage) {
cursor = mDm.query(
new DownloadManager.Query().setOnlyIncludeVisibleInDownloadsUi(true));
} else {
cursor = mDm.query(
new DownloadManager.Query().setOnlyIncludeVisibleInDownloadsUi(true)
.setFilterByStatus(DownloadManager.STATUS_SUCCESSFUL));
}
final Set<String> filePaths = new HashSet<>();
while (cursor.moveToNext()) {
includeDownloadFromCursor(result, cursor, filePaths, null /* queryArgs */);
}
notificationUris.add(cursor.getNotificationUri());
includeDownloadsFromMediaStore(result, null /* queryArgs */,
filePaths, notificationUris,
null /* parentId */, NO_LIMIT, manage);
includeFilesFromSharedStorage(result, filePaths, null);
}
result.setNotificationUris(getContext().getContentResolver(), notificationUris);
result.start();
return result;
} finally {
IoUtils.closeQuietly(cursor);
Binder.restoreCallingIdentity(token);
}
}
@Override
public Cursor queryRecentDocuments(String rootId, String[] projection,
@Nullable Bundle queryArgs, @Nullable CancellationSignal signal)
throws FileNotFoundException {
final DownloadsCursor result =
new DownloadsCursor(projection, getContext().getContentResolver());
// Delegate to real provider
final long token = Binder.clearCallingIdentity();
int limit = 12;
if (queryArgs != null) {
limit = queryArgs.getInt(ContentResolver.QUERY_ARG_LIMIT, -1);
if (limit < 0) {
// Use default value, and no QUERY_ARG* is honored.
limit = 12;
} else {
// We are honoring the QUERY_ARG_LIMIT.
Bundle extras = new Bundle();
result.setExtras(extras);
extras.putStringArray(ContentResolver.EXTRA_HONORED_ARGS, new String[]{
ContentResolver.QUERY_ARG_LIMIT
});
}
}
Cursor cursor = null;
final ArrayList<Uri> notificationUris = new ArrayList<>();
try {
cursor = mDm.query(new DownloadManager.Query().setOnlyIncludeVisibleInDownloadsUi(true)
.setFilterByStatus(DownloadManager.STATUS_SUCCESSFUL));
final Set<String> filePaths = new HashSet<>();
while (cursor.moveToNext() && result.getCount() < limit) {
final String mimeType = cursor.getString(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_MEDIA_TYPE));
final String uri = cursor.getString(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_MEDIAPROVIDER_URI));
// Skip images, videos and documents that have been inserted into the MediaStore so
// we don't duplicate them in the recent list. The audio root of
// MediaDocumentsProvider doesn't support recent, we add it into recent list.
if (mimeType == null || (MediaFile.isImageMimeType(mimeType)
|| MediaFile.isVideoMimeType(mimeType) || MediaFile.isDocumentMimeType(
mimeType)) && !TextUtils.isEmpty(uri)) {
continue;
}
includeDownloadFromCursor(result, cursor, filePaths,
null /* queryArgs */);
}
notificationUris.add(cursor.getNotificationUri());
// Skip media files that have been inserted into the MediaStore so we
// don't duplicate them in the recent list.
final Bundle args = new Bundle();
args.putBoolean(DocumentsContract.QUERY_ARG_EXCLUDE_MEDIA, true);
includeDownloadsFromMediaStore(result, args, filePaths,
notificationUris, null /* parentId */, (limit - result.getCount()),
false /* includePending */);
} finally {
IoUtils.closeQuietly(cursor);
Binder.restoreCallingIdentity(token);
}
result.setNotificationUris(getContext().getContentResolver(), notificationUris);
result.start();
return result;
}
@Override
public Cursor querySearchDocuments(String rootId, String[] projection, Bundle queryArgs)
throws FileNotFoundException {
final DownloadsCursor result =
new DownloadsCursor(projection, getContext().getContentResolver());
final ArrayList<Uri> notificationUris = new ArrayList<>();
// Delegate to real provider
final long token = Binder.clearCallingIdentity();
Cursor cursor = null;
try {
cursor = mDm.query(new DownloadManager.Query().setOnlyIncludeVisibleInDownloadsUi(true)
.setFilterByString(DocumentsContract.getSearchDocumentsQuery(queryArgs)));
final Set<String> filePaths = new HashSet<>();
while (cursor.moveToNext()) {
includeDownloadFromCursor(result, cursor, filePaths, queryArgs);
}
notificationUris.add(cursor.getNotificationUri());
includeDownloadsFromMediaStore(result, queryArgs, filePaths,
notificationUris, null /* parentId */, NO_LIMIT, true /* includePending */);
includeSearchFilesFromSharedStorage(result, projection, filePaths, queryArgs);
} finally {
IoUtils.closeQuietly(cursor);
Binder.restoreCallingIdentity(token);
}
final String[] handledQueryArgs = DocumentsContract.getHandledQueryArguments(queryArgs);
if (handledQueryArgs.length > 0) {
final Bundle extras = new Bundle();
extras.putStringArray(ContentResolver.EXTRA_HONORED_ARGS, handledQueryArgs);
result.setExtras(extras);
}
result.setNotificationUris(getContext().getContentResolver(), notificationUris);
result.start();
return result;
}
private void includeSearchFilesFromSharedStorage(DownloadsCursor result,
String[] projection, Set<String> filePaths,
Bundle queryArgs) throws FileNotFoundException {
final File downloadDir = getPublicDownloadsDirectory();
try (Cursor rawFilesCursor = super.querySearchDocuments(downloadDir,
projection, filePaths, queryArgs)) {
final boolean shouldExcludeMedia = queryArgs.getBoolean(
DocumentsContract.QUERY_ARG_EXCLUDE_MEDIA, false /* defaultValue */);
while (rawFilesCursor.moveToNext()) {
final String mimeType = rawFilesCursor.getString(
rawFilesCursor.getColumnIndexOrThrow(Document.COLUMN_MIME_TYPE));
// When the value of shouldExcludeMedia is true, don't add media files into
// the result to avoid duplicated files. MediaScanner will scan the files
// into MediaStore. If the behavior is changed, we need to add the files back.
if (!shouldExcludeMedia || !isMediaMimeType(mimeType)) {
String docId = rawFilesCursor.getString(
rawFilesCursor.getColumnIndexOrThrow(Document.COLUMN_DOCUMENT_ID));
File rawFile = getFileForDocId(docId);
includeFileFromSharedStorage(result, rawFile);
}
}
}
}
@Override
public String getDocumentType(String docId) throws FileNotFoundException {
// Delegate to real provider
final long token = Binder.clearCallingIdentity();
try {
if (RawDocumentsHelper.isRawDocId(docId)) {
return super.getDocumentType(docId);
}
final ContentResolver resolver = getContext().getContentResolver();
final Uri contentUri;
if (isMediaStoreDownload(docId)) {
contentUri = getMediaStoreUriForQuery(docId);
} else {
final long id = Long.parseLong(docId);
contentUri = mDm.getDownloadUri(id);
}
return resolver.getType(contentUri);
} finally {
Binder.restoreCallingIdentity(token);
}
}
@Override
public ParcelFileDescriptor openDocument(String docId, String mode, CancellationSignal signal)
throws FileNotFoundException {
// Delegate to real provider
final long token = Binder.clearCallingIdentity();
try {
if (RawDocumentsHelper.isRawDocId(docId)) {
return super.openDocument(docId, mode, signal);
}
final ContentResolver resolver = getContext().getContentResolver();
final Uri contentUri;
if (isMediaStoreDownload(docId)) {
contentUri = getMediaStoreUriForQuery(docId);
} else {
final long id = Long.parseLong(docId);
contentUri = mDm.getDownloadUri(id);
}
return resolver.openFileDescriptor(contentUri, mode, signal);
} finally {
Binder.restoreCallingIdentity(token);
}
}
@Override
protected File getFileForDocId(String docId, boolean visible) throws FileNotFoundException {
if (RawDocumentsHelper.isRawDocId(docId)) {
return new File(RawDocumentsHelper.getAbsoluteFilePath(docId));
}
if (isMediaStoreDownload(docId)) {
return getFileForMediaStoreDownload(docId);
}
if (DOC_ID_ROOT.equals(docId)) {
return getPublicDownloadsDirectory();
}
final long token = Binder.clearCallingIdentity();
Cursor cursor = null;
String localFilePath = null;
try {
cursor = mDm.query(new Query().setFilterById(Long.parseLong(docId)));
if (cursor.moveToFirst()) {
localFilePath = cursor.getString(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_LOCAL_FILENAME));
}
} finally {
IoUtils.closeQuietly(cursor);
Binder.restoreCallingIdentity(token);
}
if (localFilePath == null) {
throw new IllegalStateException("File has no filepath. Could not be found.");
}
return new File(localFilePath);
}
@Override
protected String getDocIdForFile(File file) throws FileNotFoundException {
return RawDocumentsHelper.getDocIdForFile(file);
}
@Override
protected Uri buildNotificationUri(String docId) {
return DocumentsContract.buildChildDocumentsUri(AUTHORITY, docId);
}
private static boolean isMediaMimeType(String mimeType) {
return MediaFile.isImageMimeType(mimeType) || MediaFile.isVideoMimeType(mimeType)
|| MediaFile.isAudioMimeType(mimeType) || MediaFile.isDocumentMimeType(mimeType);
}
private void includeDefaultDocument(MatrixCursor result) {
final RowBuilder row = result.newRow();
row.add(Document.COLUMN_DOCUMENT_ID, DOC_ID_ROOT);
// We have the same display name as our root :)
row.add(Document.COLUMN_DISPLAY_NAME,
getContext().getString(R.string.root_downloads));
row.add(Document.COLUMN_MIME_TYPE, Document.MIME_TYPE_DIR);
row.add(Document.COLUMN_FLAGS,
Document.FLAG_DIR_PREFERS_LAST_MODIFIED | Document.FLAG_DIR_SUPPORTS_CREATE);
}
/**
* Adds the entry from the cursor to the result only if the entry is valid. That is,
* if the file exists in the file system.
*/
private void includeDownloadFromCursor(MatrixCursor result, Cursor cursor,
Set<String> filePaths, Bundle queryArgs) {
final long id = cursor.getLong(cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_ID));
final String docId = String.valueOf(id);
final String displayName = cursor.getString(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_TITLE));
String summary = cursor.getString(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_DESCRIPTION));
String mimeType = cursor.getString(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_MEDIA_TYPE));
if (mimeType == null) {
// Provide fake MIME type so it's openable
mimeType = "vnd.android.document/file";
}
if (queryArgs != null) {
final boolean shouldExcludeMedia = queryArgs.getBoolean(
DocumentsContract.QUERY_ARG_EXCLUDE_MEDIA, false /* defaultValue */);
if (shouldExcludeMedia) {
final String uri = cursor.getString(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_MEDIAPROVIDER_URI));
// Skip media files that have been inserted into the MediaStore so we
// don't duplicate them in the search list.
if (isMediaMimeType(mimeType) && !TextUtils.isEmpty(uri)) {
return;
}
}
}
// size could be -1 which indicates that download hasn't started.
final long size = cursor.getLong(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_TOTAL_SIZE_BYTES));
String localFilePath = cursor.getString(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_LOCAL_FILENAME));
int extraFlags = Document.FLAG_PARTIAL;
final int status = cursor.getInt(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_STATUS));
switch (status) {
case DownloadManager.STATUS_SUCCESSFUL:
// Verify that the document still exists in external storage. This is necessary
// because files can be deleted from the file system without their entry being
// removed from DownloadsManager.
if (localFilePath == null || !new File(localFilePath).exists()) {
return;
}
extraFlags = Document.FLAG_SUPPORTS_RENAME; // only successful is non-partial
break;
case DownloadManager.STATUS_PAUSED:
summary = getContext().getString(R.string.download_queued);
break;
case DownloadManager.STATUS_PENDING:
summary = getContext().getString(R.string.download_queued);
break;
case DownloadManager.STATUS_RUNNING:
final long progress = cursor.getLong(cursor.getColumnIndexOrThrow(
DownloadManager.COLUMN_BYTES_DOWNLOADED_SO_FAR));
if (size > 0) {
String percent =
NumberFormat.getPercentInstance().format((double) progress / size);
summary = getContext().getString(R.string.download_running_percent, percent);
} else {
summary = getContext().getString(R.string.download_running);
}
break;
case DownloadManager.STATUS_FAILED:
default:
summary = getContext().getString(R.string.download_error);
break;
}
final long lastModified = cursor.getLong(
cursor.getColumnIndexOrThrow(DownloadManager.COLUMN_LAST_MODIFIED_TIMESTAMP));
if (!DocumentsContract.matchSearchQueryArguments(queryArgs, displayName, mimeType,
lastModified, size)) {
return;
}
includeDownload(result, docId, displayName, summary, size, mimeType,
lastModified, extraFlags, status == DownloadManager.STATUS_RUNNING);
if (filePaths != null && localFilePath != null) {
filePaths.add(localFilePath);
}
}
private void includeDownload(MatrixCursor result,
String docId, String displayName, String summary, long size,
String mimeType, long lastModifiedMs, int extraFlags, boolean isPending) {
int flags = Document.FLAG_SUPPORTS_DELETE | Document.FLAG_SUPPORTS_WRITE | extraFlags;
if (mimeType.startsWith("image/")) {
flags |= Document.FLAG_SUPPORTS_THUMBNAIL;
}
if (typeSupportsMetadata(mimeType)) {
flags |= Document.FLAG_SUPPORTS_METADATA;
}
final RowBuilder row = result.newRow();
row.add(Document.COLUMN_DOCUMENT_ID, docId);
row.add(Document.COLUMN_DISPLAY_NAME, displayName);
row.add(Document.COLUMN_SUMMARY, summary);
row.add(Document.COLUMN_SIZE, size == -1 ? null : size);
row.add(Document.COLUMN_MIME_TYPE, mimeType);
row.add(Document.COLUMN_FLAGS, flags);
// Incomplete downloads get a null timestamp. This prevents thrashy UI when a bunch of
// active downloads get sorted by mod time.
if (!isPending) {
row.add(Document.COLUMN_LAST_MODIFIED, lastModifiedMs);
}
}
/**
* Takes all the top-level files from the Downloads directory and adds them to the result.
*
* @param result cursor containing all documents to be returned by queryChildDocuments or
* queryChildDocumentsForManage.
* @param downloadedFilePaths The absolute file paths of all the files in the result Cursor.
* @param searchString query used to filter out unwanted results.
*/
private void includeFilesFromSharedStorage(DownloadsCursor result,
Set<String> downloadedFilePaths, @Nullable String searchString)
throws FileNotFoundException {
final File downloadsDir = getPublicDownloadsDirectory();
// Add every file from the Downloads directory to the result cursor. Ignore files that
// were in the supplied downloaded file paths.
for (File file : FileUtils.listFilesOrEmpty(downloadsDir)) {
boolean inResultsAlready = downloadedFilePaths.contains(file.getAbsolutePath());
boolean containsQuery = searchString == null || file.getName().contains(
searchString);
if (!inResultsAlready && containsQuery) {
includeFileFromSharedStorage(result, file);
}
}
}
/**
* Adds a file to the result cursor. It uses a combination of {@code #RAW_PREFIX} and its
* absolute file path for its id. Directories are not to be included.
*
* @param result cursor containing all documents to be returned by queryChildDocuments or
* queryChildDocumentsForManage.
* @param file file to be included in the result cursor.
*/
private void includeFileFromSharedStorage(MatrixCursor result, File file)
throws FileNotFoundException {
includeFile(result, null, file);
}
private static File getPublicDownloadsDirectory() {
return Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOWNLOADS);
}
private String renameMediaStoreDownload(String docId, String displayName) {
final File before = getFileForMediaStoreDownload(docId);
final File after = new File(before.getParentFile(), displayName);
if (after.exists()) {
throw new IllegalStateException("Already exists " + after);
}
if (!before.renameTo(after)) {
throw new IllegalStateException("Failed to rename from " + before + " to " + after);
}
final String noMedia = ".nomedia";
// Scan the file to update the database
// For file, check whether the file is renamed to .nomedia. If yes, to scan the parent
// directory to update all files in the directory. We don't consider the case of renaming
// .nomedia file. We don't show .nomedia file.
if (!after.isDirectory() && displayName.toLowerCase(Locale.ROOT).endsWith(noMedia)) {
final Uri newUri = MediaStore.scanFile(getContext().getContentResolver(),
after.getParentFile());
// the file will not show in the list, return the parent docId to avoid not finding
// the detail for the file.
return getDocIdForMediaStoreDownloadUri(newUri, true /* isDir */);
}
// update the database for the old file
MediaStore.scanFile(getContext().getContentResolver(), before);
// Update tne database for the new file and get the new uri
final Uri newUri = MediaStore.scanFile(getContext().getContentResolver(), after);
return getDocIdForMediaStoreDownloadUri(newUri, after.isDirectory());
}
private static String getDocIdForMediaStoreDownloadUri(Uri uri, boolean isDir) {
if (uri != null) {
return getDocIdForMediaStoreDownload(Long.parseLong(uri.getLastPathSegment()), isDir);
}
return null;
}
private File getFileForMediaStoreDownload(String docId) {
final Uri mediaStoreUri = getMediaStoreUriForQuery(docId);
final long token = Binder.clearCallingIdentity();
try (Cursor cursor = queryForSingleItem(mediaStoreUri,
new String[] { DownloadColumns.DATA }, null, null, null)) {
final String filePath = cursor.getString(0);
if (filePath == null) {
throw new IllegalStateException("Missing _data for " + mediaStoreUri);
}
return new File(filePath);
} catch (FileNotFoundException e) {
throw new IllegalStateException(e);
} finally {
Binder.restoreCallingIdentity(token);
}
}
private Pair<String, String> getRelativePathAndDisplayNameForDownload(long id) {
final Uri mediaStoreUri = ContentUris.withAppendedId(
MediaStore.Downloads.getContentUri(MediaStore.VOLUME_EXTERNAL), id);
final long token = Binder.clearCallingIdentity();
try (Cursor cursor = queryForSingleItem(mediaStoreUri,
new String[] { DownloadColumns.RELATIVE_PATH, DownloadColumns.DISPLAY_NAME },
null, null, null)) {
final String relativePath = cursor.getString(0);
final String displayName = cursor.getString(1);
if (relativePath == null || displayName == null) {
throw new IllegalStateException(
"relative_path and _display_name should not be null for " + mediaStoreUri);
}
return Pair.create(relativePath, displayName);
} catch (FileNotFoundException e) {
throw new IllegalStateException(e);
} finally {
Binder.restoreCallingIdentity(token);
}
}
/**
* Copied from MediaProvider.java
*
* Query the given {@link Uri}, expecting only a single item to be found.
*
* @throws FileNotFoundException if no items were found, or multiple items
* were found, or there was trouble reading the data.
*/
private Cursor queryForSingleItem(Uri uri, String[] projection,
String selection, String[] selectionArgs, CancellationSignal signal)
throws FileNotFoundException {
final Cursor c = getContext().getContentResolver().query(uri, projection,
ContentResolver.createSqlQueryBundle(selection, selectionArgs, null), signal);
if (c == null) {
throw new FileNotFoundException("Missing cursor for " + uri);
} else if (c.getCount() < 1) {
IoUtils.closeQuietly(c);
throw new FileNotFoundException("No item at " + uri);
} else if (c.getCount() > 1) {
IoUtils.closeQuietly(c);
throw new FileNotFoundException("Multiple items at " + uri);
}
if (c.moveToFirst()) {
return c;
} else {
IoUtils.closeQuietly(c);
throw new FileNotFoundException("Failed to read row from " + uri);
}
}
private void includeDownloadsFromMediaStore(@NonNull MatrixCursor result,
@Nullable Bundle queryArgs,
@Nullable Set<String> filePaths, @NonNull ArrayList<Uri> notificationUris,
@Nullable String parentId, int limit, boolean includePending) {
if (limit == 0) {
return;
}
final long token = Binder.clearCallingIdentity();
final Uri uriInner = MediaStore.Downloads.getContentUri(MediaStore.VOLUME_EXTERNAL);
final Bundle queryArgsInner = new Bundle();
final Pair<String, String[]> selectionPair = buildSearchSelection(
queryArgs, filePaths, parentId);
queryArgsInner.putString(ContentResolver.QUERY_ARG_SQL_SELECTION,
selectionPair.first);
queryArgsInner.putStringArray(ContentResolver.QUERY_ARG_SQL_SELECTION_ARGS,
selectionPair.second);
if (limit != NO_LIMIT) {
queryArgsInner.putInt(ContentResolver.QUERY_ARG_LIMIT, limit);
}
if (includePending) {
queryArgsInner.putInt(MediaStore.QUERY_ARG_MATCH_PENDING, MediaStore.MATCH_INCLUDE);
}
try (Cursor cursor = getContext().getContentResolver().query(uriInner,
null, queryArgsInner, null)) {
final boolean shouldExcludeMedia = queryArgs != null && queryArgs.getBoolean(
DocumentsContract.QUERY_ARG_EXCLUDE_MEDIA, false /* defaultValue */);
while (cursor.moveToNext()) {
includeDownloadFromMediaStore(result, cursor, filePaths, shouldExcludeMedia);
}
notificationUris.add(MediaStore.Files.getContentUri(MediaStore.VOLUME_EXTERNAL));
notificationUris.add(MediaStore.Downloads.getContentUri(MediaStore.VOLUME_EXTERNAL));
} finally {
Binder.restoreCallingIdentity(token);
}
}
private void includeDownloadFromMediaStore(@NonNull MatrixCursor result,
@NonNull Cursor mediaCursor, @Nullable Set<String> filePaths,
boolean shouldExcludeMedia) {
final String mimeType = getMimeType(mediaCursor);
// Image, Audio and Video are excluded from buildSearchSelection in querySearchDocuments
// and queryRecentDocuments. Only exclude document type here for both cases.
if (shouldExcludeMedia && MediaFile.isDocumentMimeType(mimeType)) {
return;
}
final boolean isDir = Document.MIME_TYPE_DIR.equals(mimeType);
final String docId = getDocIdForMediaStoreDownload(
mediaCursor.getLong(mediaCursor.getColumnIndex(DownloadColumns._ID)), isDir);
final String displayName = mediaCursor.getString(
mediaCursor.getColumnIndex(DownloadColumns.DISPLAY_NAME));
final long size = mediaCursor.getLong(
mediaCursor.getColumnIndex(DownloadColumns.SIZE));
final long lastModifiedMs = mediaCursor.getLong(
mediaCursor.getColumnIndex(DownloadColumns.DATE_MODIFIED)) * 1000;
final boolean isPending = mediaCursor.getInt(
mediaCursor.getColumnIndex(DownloadColumns.IS_PENDING)) == 1;
int extraFlags = isPending ? Document.FLAG_PARTIAL : 0;
if (Document.MIME_TYPE_DIR.equals(mimeType)) {
extraFlags |= Document.FLAG_DIR_SUPPORTS_CREATE;
}
if (!isPending) {
extraFlags |= Document.FLAG_SUPPORTS_RENAME;
}
includeDownload(result, docId, displayName, null /* description */, size, mimeType,
lastModifiedMs, extraFlags, isPending);
if (filePaths != null) {
filePaths.add(mediaCursor.getString(
mediaCursor.getColumnIndex(DownloadColumns.DATA)));
}
}
private String getMimeType(@NonNull Cursor mediaCursor) {
final String mimeType = mediaCursor.getString(
mediaCursor.getColumnIndex(DownloadColumns.MIME_TYPE));
if (mimeType == null) {
return Document.MIME_TYPE_DIR;
}
return mimeType;
}
// Copied from MediaDocumentsProvider with some tweaks
private Pair<String, String[]> buildSearchSelection(@Nullable Bundle queryArgs,
@Nullable Set<String> filePaths, @Nullable String parentId) {
final StringBuilder selection = new StringBuilder();
final ArrayList<String> selectionArgs = new ArrayList<>();
if (parentId == null && filePaths != null && filePaths.size() > 0) {
if (selection.length() > 0) {
selection.append(" AND ");
}
selection.append(DownloadColumns.DATA + " NOT IN (");
selection.append(TextUtils.join(",", Collections.nCopies(filePaths.size(), "?")));
selection.append(")");
selectionArgs.addAll(filePaths);
}
if (parentId != null) {
if (selection.length() > 0) {
selection.append(" AND ");
}
selection.append(DownloadColumns.RELATIVE_PATH + "=?");
final Pair<String, String> data = getRelativePathAndDisplayNameForDownload(
Long.parseLong(parentId));
selectionArgs.add(data.first + data.second + "/");
} else {
if (selection.length() > 0) {
selection.append(" AND ");
}
selection.append(DownloadColumns.RELATIVE_PATH + "=?");
selectionArgs.add(Environment.DIRECTORY_DOWNLOADS + "/");
}
if (queryArgs != null) {
final boolean shouldExcludeMedia = queryArgs.getBoolean(
DocumentsContract.QUERY_ARG_EXCLUDE_MEDIA, false /* defaultValue */);
if (shouldExcludeMedia) {
if (selection.length() > 0) {
selection.append(" AND ");
}
selection.append(DownloadColumns.MIME_TYPE + " NOT LIKE ?");
selectionArgs.add("image/%");
selection.append(" AND ");
selection.append(DownloadColumns.MIME_TYPE + " NOT LIKE ?");
selectionArgs.add("audio/%");
selection.append(" AND ");
selection.append(DownloadColumns.MIME_TYPE + " NOT LIKE ?");
selectionArgs.add("video/%");
}
final String displayName = queryArgs.getString(
DocumentsContract.QUERY_ARG_DISPLAY_NAME);
if (!TextUtils.isEmpty(displayName)) {
if (selection.length() > 0) {
selection.append(" AND ");
}
selection.append(DownloadColumns.DISPLAY_NAME + " LIKE ?");
selectionArgs.add("%" + displayName + "%");
}
final long lastModifiedAfter = queryArgs.getLong(
DocumentsContract.QUERY_ARG_LAST_MODIFIED_AFTER, -1 /* defaultValue */);
if (lastModifiedAfter != -1) {
if (selection.length() > 0) {
selection.append(" AND ");
}
selection.append(DownloadColumns.DATE_MODIFIED
+ " > " + lastModifiedAfter / 1000);
}
final long fileSizeOver = queryArgs.getLong(
DocumentsContract.QUERY_ARG_FILE_SIZE_OVER, -1 /* defaultValue */);
if (fileSizeOver != -1) {
if (selection.length() > 0) {
selection.append(" AND ");
}
selection.append(DownloadColumns.SIZE + " > " + fileSizeOver);
}
final String[] mimeTypes = queryArgs.getStringArray(
DocumentsContract.QUERY_ARG_MIME_TYPES);
if (mimeTypes != null && mimeTypes.length > 0) {
if (selection.length() > 0) {
selection.append(" AND ");
}
selection.append("(");
final List<String> tempSelectionArgs = new ArrayList<>();
final StringBuilder tempSelection = new StringBuilder();
List<String> wildcardMimeTypeList = new ArrayList<>();
for (int i = 0; i < mimeTypes.length; ++i) {
final String mimeType = mimeTypes[i];
if (!TextUtils.isEmpty(mimeType) && mimeType.endsWith("/*")) {
wildcardMimeTypeList.add(mimeType);
continue;
}
if (tempSelectionArgs.size() > 0) {
tempSelection.append(",");
}
tempSelection.append("?");
tempSelectionArgs.add(mimeType);
}
for (int i = 0; i < wildcardMimeTypeList.size(); i++) {
selection.append(DownloadColumns.MIME_TYPE + " LIKE ?")
.append((i != wildcardMimeTypeList.size() - 1) ? " OR " : "");
final String mimeType = wildcardMimeTypeList.get(i);
selectionArgs.add(mimeType.substring(0, mimeType.length() - 1) + "%");
}
if (tempSelectionArgs.size() > 0) {
if (wildcardMimeTypeList.size() > 0) {
selection.append(" OR ");
}
selection.append(DownloadColumns.MIME_TYPE + " IN (")
.append(tempSelection.toString())
.append(")");
selectionArgs.addAll(tempSelectionArgs);
}
selection.append(")");
}
}
return new Pair<>(selection.toString(), selectionArgs.toArray(new String[0]));
}
/**
* A MatrixCursor that spins up a file observer when the first instance is
* started ({@link #start()}, and stops the file observer when the last instance
* closed ({@link #close()}. When file changes are observed, a content change
* notification is sent on the Downloads content URI.
*
* <p>This is necessary as other processes, like ExternalStorageProvider,
* can access and modify files directly (without sending operations
* through DownloadStorageProvider).
*
* <p>Without this, contents accessible by one a Downloads cursor instance
* (like the Downloads root in Files app) can become state.
*/
private static final class DownloadsCursor extends MatrixCursor {
private static final Object mLock = new Object();
@GuardedBy("mLock")
private static int mOpenCursorCount = 0;
@GuardedBy("mLock")
private static @Nullable ContentChangedRelay mFileWatcher;
private final ContentResolver mResolver;
DownloadsCursor(String[] projection, ContentResolver resolver) {
super(resolveDocumentProjection(projection));
mResolver = resolver;
}
void start() {
synchronized (mLock) {
if (mOpenCursorCount++ == 0) {
mFileWatcher = new ContentChangedRelay(mResolver,
Arrays.asList(getPublicDownloadsDirectory()));
mFileWatcher.startWatching();
}
}
}
@Override
public void close() {
super.close();
synchronized (mLock) {
if (--mOpenCursorCount == 0) {
mFileWatcher.stopWatching();
mFileWatcher = null;
}
}
}
}
/**
* A file observer that notifies on the Downloads content URI(s) when
* files change on disk.
*/
private static class ContentChangedRelay extends FileObserver {
private static final int NOTIFY_EVENTS = ATTRIB | CLOSE_WRITE | MOVED_FROM | MOVED_TO
| CREATE | DELETE | DELETE_SELF | MOVE_SELF;
private File[] mDownloadDirs;
private final ContentResolver mResolver;
public ContentChangedRelay(ContentResolver resolver, List<File> downloadDirs) {
super(downloadDirs, NOTIFY_EVENTS);
mDownloadDirs = downloadDirs.toArray(new File[0]);
mResolver = resolver;
}
@Override
public void startWatching() {
super.startWatching();
if (DEBUG) Log.d(TAG, "Started watching for file changes in: "
+ Arrays.toString(mDownloadDirs));
}
@Override
public void stopWatching() {
super.stopWatching();
if (DEBUG) Log.d(TAG, "Stopped watching for file changes in: "
+ Arrays.toString(mDownloadDirs));
}
@Override
public void onEvent(int event, String path) {
if ((event & NOTIFY_EVENTS) != 0) {
if (DEBUG) Log.v(TAG, "Change detected at path: " + path);
mResolver.notifyChange(Downloads.Impl.ALL_DOWNLOADS_CONTENT_URI, null, false);
mResolver.notifyChange(Downloads.Impl.CONTENT_URI, null, false);
}
}
}
}
| 15e62d9d72a752f6c9ba462fc790273f948edeca | [
"Java"
] | 6 | Java | aosp-mirror/platform_packages_providers_downloadprovider | ef2e80fc732c3fca488b02ba8ba8b1ff870f77c7 | 3fd500194f2075c85f918327468470203251e79e | |
refs/heads/master | <file_sep>import { Component, OnInit } from '@angular/core';
import { CognitoAuth } from 'amazon-cognito-auth-js';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
title = 'app';
auth: any;
constructor() {
//
}
ngOnInit() {
this.auth = this.initCognitoSDK();
const curUrl = window.location.href;
console.log('curUrl: ', curUrl);
this.auth.parseCognitoWebResponse(curUrl);
}
initCognitoSDK() {
const authData = {
ClientId: '11b65vi93c758vp1lf6eda3715', // Your client id here
AppWebDomain: 'uat-univers.auth.ap-south-1.amazoncognito.com', // Exclude the "https://" part.
TokenScopesArray: ['openid'], // like ['openid','email','phone']...
RedirectUriSignIn: 'http://localhost:4200',
UserPoolId: 'ap-south-1_b3KpbRme1',
RedirectUriSignOut: 'http://localhost:4200',
IdentityProvider: '', // e.g. 'Facebook',
AdvancedSecurityDataCollectionFlag: false
};
const auth = new CognitoAuth(authData);
auth.userhandler = {
onSuccess: (result) => {
alert('Sign in success: ' + auth.getCurrentUser());
this.showSignedIn(result);
},
onFailure: (err) => {
alert('Error!');
}
};
auth.useCodeGrantFlow();
return auth;
}
getSession() {
this.auth.getSession();
}
showSignedIn(session) {
console.log('Session: ', session);
}
}
| 995927ef23f85bbed63487aad0787384ac4dd961 | [
"TypeScript"
] | 1 | TypeScript | daundkarmangesh1109/aws-cognito-auth-test | 1772e7ab57b7f32cdc3b93e41a10146dcf8fadd3 | e4a934157a19b5a881ea2014e8e2d6fa71095fc1 | |
refs/heads/master | <repo_name>shuheiktgw/benchmark_fast_jsonapi<file_sep>/spec/serializers/performance_with_json_api_adapter_spec.rb
require 'rails_helper'
describe 'Serializers Performance with json_api adapter' do
before(:all) do
ActiveModel::Serializer.config.adapter = :json_api
class AmsCompanySerializer < ActiveModel::Serializer
attributes :name, :updated_at, :created_at
has_many :ams_employees
end
class AmsEmployeeSerializer < ActiveModel::Serializer
attributes :name, :position, :age, :updated_at, :created_at
belongs_to :ams_company
end
class FjaCompanySerializer
include FastJsonapi::ObjectSerializer
attributes :name, :updated_at, :created_at
has_many :fja_employees
end
class FjaEmployeeSerializer
include FastJsonapi::ObjectSerializer
attributes :name, :position, :age, :updated_at, :created_at
belongs_to :fja_company
end
end
before(:all) { GC.disable }
after(:all) { GC.enable }
before do
ams_companies = create_list(:ams_company, company_count)
ams_companies.each do |ams_company|
create_list(:ams_employee, employee_count, ams_company: ams_company)
end
fja_companies = create_list(:fja_company, company_count)
fja_companies.each do |fja_company|
create_list(:fja_employee, employee_count, fja_company: fja_company)
end
end
shared_examples 'serialize record' do
it 'should serialize record' do
puts "#{ company_count } companies"
puts "#{ employee_count } employees"
fja_total_time = 0
ams_total_time = 0
100.times do
fja_total_time += Benchmark.measure { FjaCompanySerializer.new(FjaCompany.preload(:fja_employees).all, include: [:fja_employees]).serialized_json }.real * 1000
ams_total_time += Benchmark.measure { ActiveModelSerializers::SerializableResource.new(AmsCompany.preload(:ams_employees).all, include: [:ams_employees]).to_json }.real * 1000
end
print 'FastJsonapi: '
puts fja_total_time / 100.0
print 'ActiveModel::Serializer: '
puts ams_total_time / 100.0
end
end
context '1 companies' do
let(:company_count) { 1 }
context '1 employees' do
let(:employee_count) { 1 }
it_behaves_like 'serialize record'
end
context '25 employees' do
let(:employee_count) { 25 }
it_behaves_like 'serialize record'
end
context '250 employees' do
let(:employee_count) { 250 }
it_behaves_like 'serialize record'
end
end
context '25 companies' do
let(:company_count) { 25 }
context '1 employees' do
let(:employee_count) { 1 }
it_behaves_like 'serialize record'
end
context '25 employees' do
let(:employee_count) { 25 }
it_behaves_like 'serialize record'
end
context '250 employees' do
let(:employee_count) { 250 }
it_behaves_like 'serialize record'
end
end
end
<file_sep>/app/models/ams_company.rb
class AmsCompany < ApplicationRecord
has_many :ams_employees
end
<file_sep>/spec/factories/ams_company.rb
FactoryGirl.define do
factory :ams_company, class: AmsCompany do
name 'Gooogle'
end
end
<file_sep>/app/models/ams_employee.rb
class AmsEmployee < ApplicationRecord
belongs_to :ams_company
end
<file_sep>/app/models/fja_company.rb
class FjaCompany < ApplicationRecord
has_many :fja_employees
end
<file_sep>/README.md
# Benchmark for Netflix/fast_jsonapi
This is a sample Rails application to benchmark [Netflix/fast_jsonapi](https://github.com/Netflix/fast_jsonapi) in a real Rails environment.
# How it works?
I created `Company has many employees` relationship with `AmsCompany.rb` and `AmsEmployee.rb` to test `ActiveModel::Serializer`, and `FjaCompany.rb` and `FjaEmployee.rb` to test `FastJsonapi`.
Also, for `ActiveModel::Serializer` I used two adapters, `default adapter` and `json_api adapter`. `json_api adapter` formats the result in the similar form to Json:API.
All the benchmarks are taken in `spec/serializers/performance_with_json_api_adapter_spec.rb` and `performance_with_default_adapter_spec.rb`.
# Results
### `FastJsonapi` (FJA) against`ActiveModel::Serializer` (AMS) with `json_api adapter`
| | Serializer | 1 company | 25 companies |
|:-----------:|:------------:|:------------|:------------|
| 1 employee | AMS | 32.995999999911874 | 60.81799999992654 |
| 1 employee | FJA | 19.072999999934837 | 8.621999999832042 |
| 25 employees | AMS | 35.11500000013257 | 577.1009999998569 |
| 25 employees | FJA | 6.498000000192405 | 85.53800000026968 |
| 250 employees | AMS | 246.90600000030827 | 5089.174999999614 |
| 250 employees | FJA | 32.62899999981528 | 754.1630000000623 |
### `FastJsonapi` against`ActiveModel::Serializer` with `default adapter`
| | Serializer | 1 company | 25 companies |
|:-----------:|:------------:|:------------|:------------|
| 1 employee | AMS | 15.873000000283355 | 24.73100000042905 |
| 1 employee | FJA | 16.28900000014255 | 8.800000000519503 |
| 25 employees | AMS | 7.133000000067113 | 134.71600000048056 |
| 25 employees | FJA | 6.07899999977235 | 84.16800000031799 |
| 250 employees | AMS | 52.87399999997433 | 855.4990000002363 |
| 250 employees | FJA | 31.502000000728003 | 1261.395999999877 |
The results above show two things.
1. `FastJsonapi` is about 4 - 7 times faster than`ActiveModel::Serializer` with `json_api adapter`.
2. `FastJsonapi` is about 1.5 times faster than`ActiveModel::Serializer` with `default adapter`.
# How to take a benchmark?
Install dependencies first.
```
$ bundle install
```
Then create tables.
```
$ bundle ex rake db:create
$ bundle ex rake db:migrate
$ bundle ex rake db:migrate RIALS_ENV=test
```
Finally run spec.
```
$ bundle ex rspec
```
<file_sep>/app/models/fja_employee.rb
class FjaEmployee < ApplicationRecord
belongs_to :fja_company
end
<file_sep>/spec/factories/ams_employee.rb
FactoryGirl.define do
sequence :name do |n|
"Employee #{n}"
end
factory :ams_employee, class: AmsEmployee do
name
age 25
position 'engineer'
end
end
<file_sep>/db/migrate/20180313035401_create_fja_employees.rb
class CreateFjaEmployees < ActiveRecord::Migration[5.1]
def change
create_table :fja_employees do |t|
t.references :fja_company
t.string :name
t.string :position
t.integer :age
t.timestamps
end
end
end
<file_sep>/spec/serializers/profiler_spec.rb
require 'rails_helper'
require 'profiler'
require 'rblineprof'
require 'rblineprof-report'
describe 'Serializers Performance with default adapter' do
before(:all) do
class AmsCompanySerializer < ActiveModel::Serializer
attributes :name, :updated_at, :created_at
has_many :ams_employees
end
class AmsEmployeeSerializer < ActiveModel::Serializer
attributes :name, :position, :age, :updated_at, :created_at
belongs_to :ams_company
end
class FjaCompanySerializer
include FastJsonapi::ObjectSerializer
attributes :name, :updated_at, :created_at
has_many :fja_employees
end
class FjaEmployeeSerializer
include FastJsonapi::ObjectSerializer
attributes :name, :position, :age, :updated_at, :created_at
belongs_to :fja_company
end
end
before(:all) { GC.disable }
after(:all) { GC.enable }
before do
ams_companies = create_list(:ams_company, company_count)
ams_companies.each do |ams_company|
create_list(:ams_employee, employee_count, ams_company: ams_company)
end
fja_companies = create_list(:fja_company, company_count)
fja_companies.each do |fja_company|
create_list(:fja_employee, employee_count, fja_company: fja_company)
end
end
shared_examples 'serialize record' do
it 'should serialize record' do
puts '--------------------------------------------------------------------------------------------------------'
puts
puts
puts 'FastJsonapi'
puts (Benchmark.measure { FjaCompanySerializer.new(FjaCompany.preload(:fja_employees).all, include: [:fja_employees]).serialized_json }.real * 1000).to_s + ' ms'
puts
puts
puts '--------------------------------------------------------------------------------------------------------'
puts
puts
puts 'ActiveModelSerializers with default adaptor'
ActiveModel::Serializer.config.adapter = :attributes
puts (Benchmark.measure { ActiveModelSerializers::SerializableResource.new(AmsCompany.preload(:ams_employees).all, include: [:ams_employees]).to_json }.real * 1000).to_s + ' ms'
puts
puts
puts '--------------------------------------------------------------------------------------------------------'
puts
puts
puts 'ActiveModelSerializers with json_api adaptor'
ActiveModel::Serializer.config.adapter = :json_api
puts (Benchmark.measure { ActiveModelSerializers::SerializableResource.new(AmsCompany.preload(:ams_employees).all, include: [:ams_employees]).to_json }.real * 1000).to_s + ' ms'
end
end
shared_examples 'serialize record with profiler' do
it 'should serialize record' do
puts
puts
puts '--------------------------------------------------------------------------------------------------------'
puts
puts
puts 'FastJsonapi'
Profiler__::start_profile
FjaCompanySerializer.new(FjaCompany.preload(:fja_employees).all, include: [:fja_employees]).serialized_json
Profiler__::print_profile(STDERR)
Profiler__::stop_profile
puts
puts
puts '--------------------------------------------------------------------------------------------------------'
puts
puts
puts 'ActiveModelSerializers with default adaptor'
ActiveModel::Serializer.config.adapter = :attributes
Profiler__::start_profile
ActiveModelSerializers::SerializableResource.new(AmsCompany.preload(:ams_employees).all).to_json
Profiler__::print_profile(STDERR)
Profiler__::stop_profile
puts
puts
puts '--------------------------------------------------------------------------------------------------------'
puts
puts
puts 'ActiveModelSerializers with json_api adaptor'
ActiveModel::Serializer.config.adapter = :json_api
Profiler__::start_profile
ActiveModelSerializers::SerializableResource.new(AmsCompany.preload(:ams_employees).all).to_json
Profiler__::print_profile(STDERR)
Profiler__::stop_profile
end
end
shared_examples 'serialize record with line profiler' do
it 'should serialize record' do
puts
puts
puts '--------------------------------------------------------------------------------------------------------'
puts
puts
puts 'FastJsonapi'
fja_profile = lineprof(/./) do
FjaCompanySerializer.new(FjaCompany.preload(:fja_employees).all, include: [:fja_employees]).serialized_json
end
LineProf.report(fja_profile)
puts
puts
puts '--------------------------------------------------------------------------------------------------------'
puts
puts
puts 'ActiveModelSerializers with default adaptor'
ActiveModel::Serializer.config.adapter = :attributes
asm_profile_default = lineprof(/./) do
ActiveModelSerializers::SerializableResource.new(AmsCompany.preload(:ams_employees).all).to_json
end
LineProf.report(asm_profile_default)
puts
puts
puts '--------------------------------------------------------------------------------------------------------'
puts
puts
puts 'ActiveModelSerializers with json_api adaptor'
ActiveModel::Serializer.config.adapter = :json_api
asm_profile_json = lineprof(/./) do
ActiveModelSerializers::SerializableResource.new(AmsCompany.preload(:ams_employees).all).to_json
end
LineProf.report(asm_profile_json)
end
end
context '1 companies' do
let(:company_count) { 1 }
context '250 employees' do
let(:employee_count) { 250 }
it_behaves_like 'serialize record'
it_behaves_like 'serialize record with profiler'
it_behaves_like 'serialize record with line profiler'
end
end
end
<file_sep>/spec/factories/fja_employee.rb
FactoryGirl.define do
factory :fja_employee, class: FjaEmployee do
name
age 25
position 'engineer'
end
end
<file_sep>/spec/factories/fja_company.rb
FactoryGirl.define do
factory :fja_company, class: FjaCompany do
name 'Gooogle'
end
end
| 27d453b2b4da1bcabdd9ca2fb4c43733fe41509f | [
"Markdown",
"Ruby"
] | 12 | Ruby | shuheiktgw/benchmark_fast_jsonapi | 6274b799c31efa6de82400c3dc128ac88db57ae6 | 0e09234f1ea6c974af7317d395c590a3d86e012a | |
refs/heads/master | <repo_name>SciEco/IntelliEco<file_sep>/Server/RequestProcess.cpp
#include <string>
#include <string.h>
#include <fstream>
#include <iostream>
#include <windows.h>
#include <list>
#include "json.hpp"
using namespace std;
using namespace nlohmann;
int execute(const string & cmd)
{
DWORD dwExitCode = -1;
STARTUPINFO si;
PROCESS_INFORMATION pi;
ZeroMemory( &si, sizeof(si) );
si.cb = sizeof(si);
ZeroMemory( &pi, sizeof(pi) );
char cmd_[100] = {'\0'};
strcpy(cmd_, cmd.c_str());
// Start the child process.
if( !CreateProcess( NULL, // an exe file.
cmd_, // parameter for your exe file.
NULL, // Process handle not inheritable.
NULL, // Thread handle not inheritable.
FALSE, // Set handle inheritance to FALSE.
0, // No creation flags.
NULL, // Use parent's environment block.
NULL, // Use parent's starting directory.
&si, // Pointer to STARTUPINFO structure.
&pi ) // Pointer to PROCESS_INFORMATION structure.
)
{
MessageBox(NULL, "CreateProcess failed.", "ERROR",NULL );
}
// Wait until child process exits.
WaitForSingleObject( pi.hProcess, INFINITE );
GetExitCodeProcess(pi.hProcess, & dwExitCode);
// Close process and thread handles.
CloseHandle( pi.hProcess );
CloseHandle( pi.hThread );
return dwExitCode;
}
list<string> listFiles(const string & dir)
{
HANDLE hFind;
WIN32_FIND_DATA findData;
LARGE_INTEGER size;
char dir_[100] = {'\0'};
strcpy(dir_, dir.c_str());
strcat(dir_, "\\*.*");
hFind = FindFirstFile(dir_, &findData);
if (hFind == INVALID_HANDLE_VALUE)
{
//cout << "Failed to find first file!\n";
return list<string>();
}
list<string> filenames;
do
{
// 忽略"."和".."两个结果
if (strcmp(findData.cFileName, ".") == 0 || strcmp(findData.cFileName, "..") == 0) continue;
if (findData.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY) continue; // 是否是目录
filenames.emplace_back(findData.cFileName);
} while (FindNextFile(hFind, &findData));
return filenames;
}
string getExtName(const string & filename)
{
string extName;
auto pos = filename.find_last_of('.');
if (pos == string::npos) return string();
return filename.substr(pos + 1);
}
const string RootDir = "C:\\IntelliEco";
const string RecordDir = "C:\\IntelliEco\\record";
int main(int argc, char ** argv)
{
ifstream usersStream(RootDir + "\\users.json");
json users;
usersStream >> users;
string uuidDir = argv[1];
ifstream requestStream(uuidDir + string("\\request.json"));
json request;
requestStream >> request;
ofstream responseStream(uuidDir + string("\\response.json"));
json response;
string username, authority;
request["username"].get_to(username);
if (users.find(username) != users.end())
{
if (users[username]["password"] == request["password"])
{
users[username]["authority"].get_to(authority);
response["authority"] = authority;
cout << authority << ' ' << username << " logged in. \n";
string requestType;
request["request"].get_to(requestType);
if (requestType == "login");
if (requestType == "upload")
{
cout << username << " has uploaded a data sample. \n";
long long int time = 0;
double longtitude = 0, latitude = 0;
string imageFilename, newFilename;
request["time"].get_to(time);
request["longtitude"].get_to(longtitude);
request["latitude"].get_to(latitude);
request["imageFilename"].get_to(imageFilename);
int mothCount = execute("MothCount.exe " + string(uuidDir) + "\\" + imageFilename);
newFilename = to_string(time) + '_' + username;
json record;
record["uploader"] = username;
record["time"] = time;
record["longtitude"] = longtitude;
record["latitude"] = latitude;
record["mothCount"] = mothCount;
record["imageFilename"] = newFilename + ".jpg";
ofstream recordStream(RecordDir + "\\" + newFilename + ".json");
recordStream << record;
system(("move " + (uuidDir + "\\" + imageFilename) + ' ' + (RecordDir + "\\" + newFilename + ".jpg")).c_str());
response["mothCount"] = mothCount;
}
if (requestType == "refresh")
{
cout << username << " requested records. \n";
list<string> filenames = listFiles(RecordDir);
response["records"] = json::array();
for (string & filename : filenames)
{
string extName = getExtName(filename);
if (extName == "json")
{
json record;
ifstream recordStream(RecordDir + "\\" + filename);
recordStream >> record;
string uploader;
long long int time = 0;
double longtitude = 0, latitude = 0;
int mothCount = 0;
record["uploader"].get_to(uploader);
record["time"].get_to(time);
record["longtitude"].get_to(longtitude);
record["latitude"].get_to(latitude);
record["mothCount"].get_to(mothCount);
response["records"].push_back(json::object({
{"uploader", uploader},
{"time", time},
{"longtitude", longtitude},
{"latitude", latitude},
{"mothCount", mothCount}
}));
}
}
}
}
else
{
response["authority"] = "unauthorised";
cout << username << " unauthorised. \n";
}
}
else
{
response["authority"] = "unauthorised";
cout << username << " not found. \n";
}
responseStream << response.dump(4);
responseStream.close();
return 0;
}
<file_sep>/Server/README.md
## 服务端部署
### 概述
智慧生态项目的服务端(下称“服务端”)基于Windows Server,使用FTP协议实现json文件的传输,配合以相关程序对json文件进行的分析,实现对客户端请求的识别、处理和回应。
服务端包括以下模块:
1. FTP模块
2. 请求识别与处理模块
3. 记录模块
### 部署
#### 文件结构
本项目的文件结构如下:
- 根目录(手动创建,路径不允许包括空格)
- 请求识别与处理模块(手动部署)
- users.json(保存个人用户信息)
- buffer文件夹(自动生成)
- 多个以请求的uuid命名的文件夹,文件夹下临时存放具体的请求文件(自动生成)
- record文件夹(自动生成)
- 多对记录文件,每一对包括两个文件名相同的jpg和json文件(自动生成)
每一部分的部署将在下文中具体介绍。
#### FTP模块
本项目的FTP模块可以通过Windows Server原生的FTP功能实现,也可以使用其他FTP工具实现。无论使用哪一种方式,都需要将FTP设为被动模式,并开放一定数量的端口供文件传输使用。FTP的用户只需设定一个,不限制登陆数量,并允许其拥有buffer文件夹的读写权限。该用户应与Android客户端内硬编码的FTP用户一致。注意!该FTP用户可以看作任意一个客户端与服务端联系的通用通行证,与users.json内记录的个人用户并非同一个概念,且不存在联系。
#### 请求识别与处理模块
该模块包括以下程序/动态链接库文件,需要在编译后复制到根目录下:
- RequestScan.py
- RequestProcess.exe
- MothCount.exe
- concrt140.dll
- libgcc_s_seh-1.dll
- libstdc++-6.dll
- libwinpthread-1.dll
- msvcp140.dll
- opencv_world410.dll
- vcruntime140.dll
##### 请求识别模块(RequestScan.py)
该脚本是服务端的入口程序,会不间断地扫描buffer文件夹内出现的新请求,将请求传递给RequestProcess.exe进行进一步处理。处理完成后,该脚本会自动清理buffer内残留的临时文件。
另外,该脚本以Python3编写,如果服务器不存在Python3运行环境,则需要提前安装。具体参见Python官网。
##### 请求处理模块(RequestProcess.exe)
收到请求后,该程序会对请求进行分类处理。所有请求可分为下列三类:
- login(登陆请求)
- upload(上传请求)
- refresh(查看记录请求)
对于每一类请求,该程序都会读取请求信息并生成回应文件,等待客户端下载。其中upload请求涉及图像分析,该程序会进一步调用MothCount.exe获取结果,将结果保存进回应文件返回给客户端并在record内保留记录。
#### 记录模块
记录模块包括个人用户记录users.json和数据记录record文件夹。
users.json内记录了所有个人用户的用户名、密码和权限。目前,各种权限之间没有差异。可以通过后台登陆服务器并参照已有的记录修改users.json中的内容来完成用户的注册和注销等操作。
record文件夹记录所有用户上传的监测数据,不需要手动创建,服务端开始运行后会自动生成。每一个upload请求都会在record文件夹中留下两个文件名相同(文件名包括上传用户和上传时间)的jpg和json文件。其中jpg文件是用户上传的原始监测数据,json文件中则记录了该数据的上传用户、时间、经纬度和MothCount.exe对该数据的分析结果。Android客户端的refresh请求只能获取json文件中的信息,若需要查看原始监测数据图片,则需要通过后台登陆服务器。
### 运行与停止
部署完成后,执行RequestScan.py即可让服务端开始运行。运行中的任何时候,使用Ctrl + C终止RequestScan.py的运行窗口即可停止服务端。
<file_sep>/MothCount/MothCount_Thermal/MothCount-Thermal-CutImage/main.cpp
//
// main.cpp
// MothCount-Thermal
//
// Created by william on 2019/7/30.
// Copyright © 2019 william. All rights reserved.
//
#include <iostream>
#include <opencv2/opencv.hpp>
//#include "CutPicture.hpp"
#include "CutPicture_Gray.hpp"
using namespace std;
using namespace cv;
int main(int argc, const char * argv[]) {
//Read an image
//freopen("/Users/william/out.txt", "w", stdout);
string s = "/Users/william/Documents/20190703-“智慧生态”小课题/software/GitRepo/IntelliEco/MothCount/moth/ThermalSrc/Gray/IR_019";
string append;
cin >> append;
s+=append;
append = ".jpg";
s+=append;
Mat image = imread(s);
//BGR at default...
if (image.empty()) {
cerr << "No image input!" << endl;
return -2;
}
testImage("shabby src", image);
//Portait to horizontal
if (image.cols < image.rows)
{
transpose(image, image);
flip(image, image, 1);
}
//image lossy compression
while (image.cols >= 2000)
{
pyrDown(image, image, Size(image.cols / 2, image.rows / 2));
}
// FindBiggest(image, image);
//line(image, <#Point pt1#>, <#Point pt2#>, <#const Scalar &color#>)
// Mat line(image);
//
long beginTime = clock();
CutImage(image, image);
long endTime = clock();
cerr << (endTime - beginTime)/1000 << "ms" << endl;
// int areaSum = col * row;
// auto get = [&image](int x, int y) { return image.at<uchar>(y, x); };
// auto get_TripleChannel = [&image](int x,int y) { return image.at<Vec3b>(y, x); };
// cvtColor(image, image, CV_BGR2GRAY);
// Canny(image, line, 65, 95);
// cout << int(get_TripleChannel(21,21)[0]) << " " << int(get_TripleChannel(21,21)[1]) << " " << int(get_TripleChannel(21,21)[2]) << endl;
// testImage("shabby", image, true);
// testImage("shibby", line);
testImage("shabby res", image);
return 0;
}
<file_sep>/MothCount/MatToJson/DrawContour.hpp
//
// main.cpp
// DrawContour
//
// Created by william on 2019/7/6.
// Copyright © 2019 W-Hsu. All rights reserved.
//
#include <opencv2/imgproc.hpp>
#include <opencv2/highgui.hpp>
#include <iostream> //our best loved header
#include <vector> //No matter
#include <cmath> //abs()
using namespace cv;
using namespace std;
const int LinePerEdge = 2;
//Not defining global variables
void testImage(const string WindowName, Mat TestingImage, bool DestroyOrNot = true)
{
//Show an image on window "winname", then destroy the window when pressing a key.
namedWindow(WindowName); //Create an window
imshow(WindowName, TestingImage); //Show the image on the window
waitKey(); //Waiting for pressing a key
if (DestroyOrNot)
destroyWindow(WindowName); //Destroy the window
}
void FindBiggest(Mat& src, Mat& dst) //dst stores the countour lines
{
Mat dst_middle;
Canny( src, dst_middle, 100,150,5); //Edge detection using Canny:
//Dilate : The "Canny" function draws a rather thin line.
//So dilate the lines to allow the "findContours" function to find it.
//Much time to DE this BUG
Mat element = getStructuringElement(MORPH_RECT, Size(3, 3), Point(1, 1));
dilate(dst_middle, dst_middle, element);
//Get and store the edge of the "white" board:
vector<vector<Point> > contours;
vector<Vec4i> hierarchy; //The containers
findContours(dst_middle, contours, hierarchy, RETR_CCOMP, CHAIN_APPROX_SIMPLE); //Get the edges
//Find the biggest area then output it into dst:
vector<vector<Point> > polyContours(contours.size());
int maxArea = 0;
for (int index = 0; index < contours.size(); index++)
{
if (contourArea(contours[index]) > contourArea(contours[maxArea]))
maxArea = index;
approxPolyDP(contours[index], polyContours[index], 10, true);
//cout << contourArea(contours[index]) << endl;
}
//cout << endl << contourArea(contours[maxArea]) << endl; //Debugging command : print the size of the max area
dst = Mat::zeros(src.size(), CV_8UC3);
drawContours(dst, polyContours, maxArea, Scalar(0,0,255/*rand() & 255, rand() & 255, rand() & 255*/), 2);
}
void DetectAndDrawLines(Mat& LineImage, Mat& DstImage)
{
//To Detect lines:
vector<Vec2f> lines; //Container which will hold the results of the 1st-time detection
HoughLines(LineImage, lines, 1, CV_PI/180, 150,0 ,0); //Runs the actual detection
//USAGE : dst1: Source image; lines: container of line's parameter(rho,theta); 1: precision of rho; CV/PI/180: precision of theta(rad).
//To draw lines:
int upCount=0, downCount=0, leftCount=0, rightCount=0;
////cout << LineImage.cols << " " << LineImage.rows << "\n" << endl;
//Go through all the lines
for( size_t i = 0; i < lines.size(); i++ )
{
float rho = lines[i][0], theta = lines[i][1];
//"const int LinePerEdge" lines each edge:
//Controls the maximum number of lines on each edge.
if (rho < 0)
{
if (rightCount >= LinePerEdge)
continue;
else
{
rightCount++;
//cout << "right" << endl;
}
}
else if (rho >= 500)
{
if (downCount >= LinePerEdge)
continue;
else
{
downCount++;
//cout << "down" << endl;
}
}
else
{
if (theta >= 0.70)
{
if (upCount >= LinePerEdge)
continue;
else
{
upCount++;
//cout << "up" << endl;
}
}
else
{
if (leftCount >= LinePerEdge)
continue;
else
{
leftCount++;
//cout << "left" << endl;
}
}
}
Point pt1, pt2; //Using pt1 and pt2 as terminals to draw a segment.
if (theta < 0.001 && theta > -0.001) //If the line is close to vertical
{
//double k = -1 * cos(theta)/sin(theta), b = rho/sin(theta);
double n = -1 * rho/sin(theta) / tan(theta);
pt1.x = n;
pt2.x = n;
pt1.y = 20;
pt2.y = LineImage.rows - 20;
}
else
{
double k = -1 * cos(theta)/sin(theta), b = rho/sin(theta);
//double m = -1 * tan(theta), n = -1 * rho/sin(theta) / tan(theta);
for (int x0=0 ; x0<LineImage.cols ; x0++)
{
double y0 = k * x0 + b;
if (y0 < LineImage.rows && y0 > 0)
{
pt1.x = x0 + 40 * sin(theta);
if (k>0)
pt1.y = y0 + 40 * abs(cos(theta));
else
pt1.y = y0 - 40 * abs(cos(theta));
break;
}
}
for (int x0 = pt1.x + 1 ; x0<LineImage.cols ; x0++)
{
double y0 = k * x0 + b;
pt2.x = x0 - 40 * sin(theta);
if (k>0)
pt2.y = y0 - 40 * abs(cos(theta));
else
pt2.y = y0 + 40 * abs(cos(theta));
if (y0 > LineImage.rows || y0 < 0)
break;
}
}
line(DstImage, pt1, pt2, 255, 5, LINE_8, 0);
}
}
void GetCountour(Mat& InputImage)
{
//Define variables
Mat SourceImg, LineImg;
Mat& _SourceImg = SourceImg;
Mat& _LineImg = LineImg;
//Load an image:
SourceImg = InputImage;
FindBiggest(_SourceImg, _LineImg);
//IMPORTANT!! Must convert Polypic to GRAYSCALE to (draw lines by using Houghlines)
//Takes me much time to DE this BUG
cvtColor(LineImg, LineImg, COLOR_BGR2GRAY);
DetectAndDrawLines(_LineImg, InputImage);
}
<file_sep>/Android/FTPTest/app/src/main/java/cc/hurrypeng/ftptest/MainActivity.java
package cc.hurrypeng.ftptest;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.content.FileProvider;
import android.app.ProgressDialog;
import android.content.Context;
import android.content.Intent;
import android.content.SharedPreferences;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.net.Uri;
import android.os.AsyncTask;
import android.os.Build;
import android.os.Bundle;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPReply;
import android.provider.MediaStore;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import com.google.gson.Gson;
public class MainActivity extends AppCompatActivity {
SharedPreferences sp;
SharedPreferences.Editor spEdit;
LocationManager locationManager;
EditText editTextServer;
EditText editTextUsername;
EditText editTextPassword;
Button buttonSelectFile;
ProgressDialog progressDialog;
final int REQUEST_TAKE_PHOTO = 1;
Uri imageUri;
String server;
String username;
String password;
String imageFilePath;
String imageFilename;
Gson gson;
private class FtpTask extends AsyncTask<String, String, String> {
private String jsonPath;
private boolean success = false;
@Override
protected void onPreExecute() {
super.onPreExecute();
progressDialog.setTitle("Packing Data Sample");
progressDialog.show();
try {
long time = System.currentTimeMillis();
Location location = locationManager.getLastKnownLocation(LocationManager.GPS_PROVIDER);
double longtitude = location.getLongitude();
double latitude = location.getLatitude();
DataSample dataSample = new DataSample(username, time, longtitude, latitude, imageFilename);
String json = gson.toJson(dataSample);
jsonPath = getExternalCacheDir() + "/DataSample.json";
File jsonFile = new File(jsonPath);
if (jsonFile.exists()) jsonFile.delete();
jsonFile.createNewFile();
FileOutputStream fileOutputStream = new FileOutputStream(jsonFile);
fileOutputStream.write(json.getBytes());
}
catch (SecurityException e)
{
e.printStackTrace();
}
catch (Exception e)
{
e.printStackTrace();
}
progressDialog.setTitle("Uploading Data Sample");
}
@Override
protected String doInBackground(String... strings) {
new Thread(new Runnable() {
@Override
public void run() {
FTPClient ftp = new FTPClient();
try {
int reply;
ftp.connect(server);
// After connection attempt, you should check the reply code to verify
// success.
reply = ftp.getReplyCode();
if (!FTPReply.isPositiveCompletion(reply)) {
ftp.disconnect();
Log.e("TAG", "FTP server refused connection.");
}
success = ftp.login(username, password);
ftp.enterLocalPassiveMode();
ftp.setRemoteVerificationEnabled(false);
ftp.makeDirectory("/users/" + username);
ftp.changeWorkingDirectory("/users/" + username);
ftp.setFileType(FTP.BINARY_FILE_TYPE);
ftp.storeFile("DataSample.json", new FileInputStream(jsonPath));
ftp.storeFile(imageFilename, new FileInputStream(imageFilePath));
ftp.makeDirectory("/users/" + username + "/uploadfin");
//ftp.retrieveFile("/6.jpeg",new FileOutputStream("/storage/emulated/0/Android/data/cc.hurrypeng.ftptest/files/6.jpg"));
// ... // transfer files
//ftp.logout();
} catch (IOException e) {
e.printStackTrace();
}
}
}).run();
return null;
}
@Override
protected void onPostExecute(String s) {
progressDialog.setTitle("Server Processing");
progressDialog.dismiss();
if (!success) Toast.makeText(MainActivity.this, "FTP operation failed! ", Toast.LENGTH_LONG).show();
else Toast.makeText(MainActivity.this, "FTP upload successful", Toast.LENGTH_LONG).show();
}
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
sp = getSharedPreferences("ftp", MODE_PRIVATE);
spEdit = sp.edit();
server = sp.getString("server", "");
username = sp.getString("username", "");
password = sp.getString("password", "");
editTextServer = findViewById(R.id.EditTextServer);
editTextUsername = findViewById(R.id.EditTextUsername);
editTextPassword = findViewById(R.id.EditTextPassword);
buttonSelectFile = findViewById(R.id.ButtonSelectFile);
editTextServer.setText(server);
editTextUsername.setText(username);
editTextPassword.setText(password);
progressDialog = new ProgressDialog(MainActivity.this);
progressDialog.setMessage("Loading...");
progressDialog.setCancelable(false);
gson = new Gson();
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
if (savedInstanceState != null)
{
editTextServer.setText(savedInstanceState.getString("server"));
editTextUsername.setText(savedInstanceState.getString("username"));
editTextPassword.setText(savedInstanceState.getString("password"));
}
buttonSelectFile.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
server = editTextServer.getText().toString();
username = editTextUsername.getText().toString();
password = editTextPassword.getText().toString();
spEdit.putString("server", server);
spEdit.putString("username", username);
spEdit.putString("password", <PASSWORD>);
spEdit.apply();
/*
Intent intent = new Intent(Intent.ACTION_PICK, android.provider.MediaStore.Images.Media.EXTERNAL_CONTENT_URI);
intent.addCategory(Intent.CATEGORY_OPENABLE);
startActivityForResult(intent, REQUEST_CHOOSEFILE);
*/
imageFilePath = getExternalCacheDir() + "/cache.jpg";
imageFilename = imageFilePath.substring(imageFilePath.lastIndexOf('/') + 1, imageFilePath.length());
File photo = new File(imageFilePath);
try {
if (photo.exists()) photo.delete();
photo.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
if (Build.VERSION.SDK_INT >= 24) {
imageUri = FileProvider.getUriForFile(MainActivity.this,
"cc.hurrypeng.ftptest.fileprovider", photo);
} else {
imageUri = Uri.fromFile(photo);
}
Intent intent = new Intent("android.media.action.IMAGE_CAPTURE");
intent.putExtra(MediaStore.EXTRA_OUTPUT, imageUri);
startActivityForResult(intent, REQUEST_TAKE_PHOTO);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
switch (requestCode) {
case REQUEST_TAKE_PHOTO: {
new FtpTask().execute();
}
}
}
}
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString("server", editTextServer.getText().toString());
outState.putString("username", editTextUsername.getText().toString());
outState.putString("password", editTextPassword.getText().toString());
}
}
<file_sep>/MothCount/BranchCutter/BranchCutter.cpp
// [OBSOLETED]
// It's successful, but just TOO SLOW.
#include "opencv2/imgproc.hpp"
#include "opencv2/highgui.hpp"
#include <iostream>
#include <deque>
#include <list>
#include <queue>
#include <math.h>
using namespace std;
using namespace cv;
const string window_capture_name = "Video Capture";
bool Counted[2001][2001] = { false };
bool Checked[2001][2001] = { false };
long long int Repetition = 0;
int dist2(Point & p, Point & q)
{
return (p.x - q.x)*(p.x - q.x) + (p.y - q.y)*(p.y - q.y);
}
int taxicab(Point & p, Point & q)
{
return abs(p.x - q.x) + abs(p.y - q.y);
}
class Block
{
public:
Block();
void add(Point p);
int area() const;
Point getCog();
int getR2();
int areaPerR();
void fill(Mat & mat);
deque<Point> pixels;
bool white;
Point cog;
int r2;
};
Block::Block()
{
r2 = 0;
cog = Point(-1, -1);
}
void Block::add(Point p)
{
pixels.push_back(p);
}
int Block::area() const
{
return pixels.size();
}
Point Block::getCog()
{
if (cog != Point(-1, -1)) return cog;
int xSum = 0, ySum = 0;
for (Point & p : pixels) xSum += p.x, ySum += p.y;
cog.x = xSum / max(1, area()), cog.y = ySum / max(1, area());
return cog;
}
int Block::getR2()
{
if (r2) return r2;
getCog();
for (Point & p : pixels) r2 = max(r2, dist2(p, cog));
return r2;
}
int Block::areaPerR()
{
return 100 * area() / max(1, getR2());
// Single moth APR falls between 100 and 200
}
void Block::fill(Mat & mat)
{
for (Point & p : pixels) mat.at<uchar>(p.y, p.x) = white ? 0 : 255;
return;
}
int main(int argc, char* argv[])
{
namedWindow(window_capture_name, WINDOW_AUTOSIZE);
// Trackbars to set thresholds for HSV values
Mat image, imageHSV, imageThreshold, imageErode;
image = imread(R"(C:\Users\haora\Documents\visual studio 2015\Projects\IntelliEco\moth\moth1.jpg)", IMREAD_COLOR); // Read the file
if (image.empty()) // Check for invalid input
{
cout << "Could not open or find the image" << std::endl;
cin.get();
cin.get();
return -1;
}
// Compress
while (image.cols >= 2000)
{
pyrDown(image, image, Size(image.cols / 2, image.rows / 2));
}
// Calculate basic params
int col = image.cols, row = image.rows;
int areaSum = col * row;
auto get = [&imageErode](int x, int y) {return imageErode.at<uchar>(y, x); };
// Convert to HSV colourspace
cvtColor(image, imageHSV, COLOR_BGR2HSV);
// Threshold
int hSum1 = 0, sSum1 = 0, vSum1 = 0;
int area1 = 0;
auto get1 = [&imageHSV](int x, int y) {return imageHSV.at<uchar>(y, x); };
for (int i = 0; i < col * 3; i += 3) for (int j = 0; j < row; j++)
{
if (get1(i, j) >= 20 && get1(i, j) <= 50)
{
area1++;
hSum1 += get1(i, j);
sSum1 += get1(i + 1, j);
vSum1 += get1(i + 2, j);
}
}
int hMean = hSum1 / area1;
inRange(imageHSV, Scalar(hMean - 5, 19, 70), Scalar(hMean + 10, 255, 255), imageThreshold);
auto get2 = [&imageThreshold](int x, int y) -> uchar & {return imageThreshold.at<uchar>(y, x); };
auto bfs = [get2, col, row](int x, int y, int tax = 2000)
{
if (Checked[x][y]) return Block();
Block block;
block.white = (get2(x, y) == 255 ? true : false);
queue<Point> q;
q.emplace(x, y);
while (!q.empty())
{
Point p = q.front();
Repetition++;
q.pop();
if (Counted[p.x][p.y]) continue;
Counted[p.x][p.y] = Checked[p.x][p.y] = true;
block.add(p);
if (p.x - 1 >= 0 && taxicab(Point(x, y), Point(p.x - 1, p.y)) <= tax) if (get2(p.x - 1, p.y) == get2(x, y)) q.emplace(p.x - 1, p.y);
if (p.x + 1 < col && taxicab(Point(x, y), Point(p.x + 1, p.y)) <= tax) if (get2(p.x + 1, p.y) == get2(x, y)) q.emplace(p.x + 1, p.y);
if (p.y - 1 >= 0 && taxicab(Point(x, y), Point(p.x, p.y - 1)) <= tax) if (get2(p.x, p.y - 1) == get2(x, y)) q.emplace(p.x, p.y - 1);
if (p.y + 1 < row && taxicab(Point(x, y), Point(p.x, p.y + 1)) <= tax) if (get2(p.x, p.y + 1) == get2(x, y)) q.emplace(p.x, p.y + 1);
}
return block;
};
list<Block> blocks;
for (int i = 0; i < col; i++) for (int j = 0; j < row; j++)
{
memset(Counted, 0, sizeof(Counted));
Block block = bfs(i, j, 20);
//block.areaPerR();
if (block.areaPerR() <= 64)
{
block.fill(imageThreshold);
}
//if (block.area()) blocks.emplace_back(block);
}
//for (Block & block : blocks) cout << (block.white ? "white" : "black") << "\tarea: " << block.area() << "\tCoG: " << block.getCog().x << ' ' << block.getCog().y << "\tradius: " << block.getR() << "\tAPR: " << block.areaPerR() << '\n';
for (Block & block : blocks)
{
if (block.areaPerR() <= 64)
{
block.fill(imageThreshold);
}
}
imshow(window_capture_name, imageThreshold);
waitKey();
return 0;
}
<file_sep>/MothCount/MothCount_Thermal/MothCount_Thermal.cpp
#include <opencv2/core.hpp>
#include <opencv2/imgcodecs.hpp>
#include <opencv2/imgproc.hpp>
#include <opencv2/highgui.hpp>
#include <iostream>
#include <list>
#include <queue>
#include <deque>
#include "MothCount-Thermal-CutImage/CutPicture_Gray.hpp"
using namespace cv;
using namespace std;
bool Counted[2001][2001] = { false };
int dist(Point & p, Point & q)
{
return sqrt((p.x - q.x)*(p.x - q.x) + (p.y - q.y)*(p.y - q.y));
}
class Block
{
public:
Block();
void add(Point p);
long area() const;
Point getCog();
int getR();
int getAPR2();
void erase(Mat & mat);
deque<Point> pixels;
bool white;
Point cog;
int r;
};
Block::Block()
{
r = 0;
cog = Point(-1, -1);
}
void Block::add(Point p)
{
pixels.push_back(p);
}
long Block::area() const
{
return pixels.size();
}
Point Block::getCog()
{
if (cog != Point(-1, -1)) return cog;
int xSum = 0, ySum = 0;
for (Point & p : pixels)
xSum += p.x, ySum += p.y;
cog.x = xSum / area(), cog.y = ySum / area();
return cog;
}
int Block::getR()
{
if (r) return r;
getCog();
for (Point & p : pixels)
r = max(r, dist(p, cog));
return r;
}
int Block::getAPR2()
{
return 100 * area() / max(1, getR()) / max(1, getR());
// Single moth APR2 falls between 100 and 200
}
void Block::erase(Mat & mat)
{
for (Point & p : pixels)
mat.at<uchar>(p.y, p.x) = white ? 0 : 255;
return;
}
bool operator<(const Block & a, const Block & b)
{
return a.area() < b.area();
}
//string Filenames[8] = { "IR_01920.jpg", "IR_01921.jpg", "IR_01922.jpg", "IR_01926.jpg", "IR_01931.jpg", "IR_01938.jpg", "IR_01941.jpg", "IR_01944.jpg" };
int main(int argc, char ** argv)
{
string filename;
if (argc > 1) filename = argv[1];
else
{
cout << "Enter the path of the image to be tested: ";
getline(cin, filename);
}
Mat image;
image = imread(filename, IMREAD_GRAYSCALE); // Read the file
if (image.empty()) // Check for invalid input
{
//cout << "Could not open or find the image" << std::endl;
//cin.get();
//cin.get();
return -2;
}
//testImage("Source", image);
// Compress
while (image.cols >= 2000)
{
pyrDown(image, image, Size(image.cols / 2, image.rows / 2));
}
CutImage(image, image);
//testImage("cut", image);
// If oriented potrait, then transpose it landscape
if (image.cols < image.rows)
{
transpose(image, image);
flip(image, image, 1);
}
// Calculate basic params
int col = image.cols, row = image.rows;
int areaSum = col * row;
auto get = [&image](int x, int y) {return image.at<uchar>(y, x); };
// Threshold
//int greySum = 0;
//for (int i = 0; i < col; i++) for (int j = 0; j < row; j++) greySum += get(i, j);
//int greyMean = greySum / areaSum;
//threshold(image, image, greyMean * 0.75, 255, 0);
/*
cvtColor(image, image, COLOR_BGR2HSV);
{
Mat temp(image.rows, image.cols, CV_8UC1);
for (int i = 0; i < image.cols; i++) for (int j = 0; j < image.rows; j++)
{
temp.at<uchar>(j, i) = get(3 * i, j);
}
image = temp;
}
testImage("de-colour", image);
*/
// Adaptive Threshold
adaptiveThreshold(image, image, 255, ADAPTIVE_THRESH_GAUSSIAN_C, THRESH_BINARY, 55, 16);
//testImage("Thresholded", image);
// BFS algorithm
auto bfs = [get, col, row](int x, int y)
{
if (Counted[x][y])
return Block();
Block block;
block.white = (get(x, y) == 255 ? true : false);
queue<Point> q;
q.emplace(x, y);
while (!q.empty())
{
Point p = q.front();
q.pop();
if (Counted[p.x][p.y]) continue;
Counted[p.x][p.y] = true;
block.add(p);
if (p.x - 1 >= 0) if (get(p.x - 1, p.y) == get(x, y)) q.emplace(p.x - 1, p.y);
if (p.x + 1 < col) if (get(p.x + 1, p.y) == get(x, y)) q.emplace(p.x + 1, p.y);
if (p.y - 1 >= 0) if (get(p.x, p.y - 1) == get(x, y)) q.emplace(p.x, p.y - 1);
if (p.y + 1 < row) if (get(p.x, p.y + 1) == get(x, y)) q.emplace(p.x, p.y + 1);
}
return block;
};
// Block search lambda
list<Block> block_list;
auto blockSearch = [&block_list, bfs, &col, &row]()
{
block_list.clear();
memset(Counted, 0, sizeof(Counted));
for (int i = 0; i < col; i++) for (int j = 0; j < row; j++)
{
Block block = bfs(i, j);
if (block.area() != 0)
block_list.emplace_back(block);
}
};
// Print initial block info
//blockSearch();
//block_list.sort();
//for (Block & block : block_list)
// cout << (block.white ? "white" : "black") << "\tarea: " << block.area() << "\tCoG: " << block.getCog().x << ' ' << block.getCog().y << "\tradius: " << block.getR() << "\tAPR2: " << block.getAPR2() << '\n';
// Dilate
Mat element = getStructuringElement(MORPH_RECT, Size(3, 3), Point(1, 1));
dilate(image, image, element);
//testImage("Dilated", image);
//GetCountour(_image);
// Block search 1
blockSearch();
// Erase all tiny blocks and small long black blocks
for (Block & block : block_list)
{
if ((block.area() <= 50)
|| (block.area() <= 7000 && block.getAPR2() <= 50 && !block.white))
{
block.erase(image);
}
}
//testImage("Erased", image);
// Erode
//element = getStructuringElement(MORPH_RECT, Size(5, 5), Point(3, 3));
//erode(image, image, element);
//testImage("Eroded", image);
// Block search 2
blockSearch();
// Erase all tiny blocks and small white blocks
for (Block & block : block_list)
{
if ((block.area() <= 50)
|| (block.area() <= 7000 && block.white))
{
block.erase(image);
}
}
//testImage("Erased", image);
// Block search 3
blockSearch();
// Print block info
//block_list.sort();
//for (Block & block : block_list)
// cout << (block.white ? "white" : "black") << "\tarea: " << block.area() << "\tCoG: " << block.getCog().x << ' ' << block.getCog().y << "\tradius: " << block.getR() << "\tAPR2: " << block.getAPR2() << '\n';
//See if the board exists
long maximumWhite = 0;
for (Block & block : block_list)
{
if (maximumWhite < block.area() && block.white)
maximumWhite = block.area();
}
if (maximumWhite < 50000) return -1;
/*
// Calculate boardArea
int boardArea = 0;
for (Block & block : block_list)
{
if ((block.area() < 7000 && !block.white) || (block.area() >= 7000 && block.white))
boardArea += block.area();
} // TODO: replace 7000 with another variable depending on areaSum
// Calculate singleMothArea
int singleMothArea = boardArea * 3.00e-3;
int minMothArea = singleMothArea / 2;
*/
// 632.61 per moth with board area being 427280
// moth/board = 1.60e-3
// //cout << mothArea / 65.0 << ' ' << boardArea << '\n';
int mothArea = 0, approxMothCount = 0;
for (Block & block : block_list)
if (!block.white && block.area() < 7000) mothArea += block.area(), approxMothCount++;
int singleMothArea = mothArea / approxMothCount;
//cout << "board area: " << boardArea << '\n';
//cout << "single moth area: " << singleMothArea << '\n';
//cout << "total moth area: " << mothArea << '\n';
int mothCount = 0;
for (Block & block : block_list)
if (!block.white && block.area() >= singleMothArea / 2) mothCount++;
cout << mothCount << " moth(s) counted. ";
cin.get();
cin.get();
//testImage("Result", image); // Show our image inside it.
return mothCount;
}
<file_sep>/Android/IntelliEco/app/src/main/java/cc/hurrypeng/intellieco/RecordAdapter.java
package cc.hurrypeng.intellieco;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.view.animation.LinearInterpolator;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.RecyclerView;
import java.text.DecimalFormat;
import java.text.SimpleDateFormat;
import java.util.Collection;
import java.util.Collections;
import java.util.Date;
import java.util.List;
class RecordAdapter extends RecyclerView.Adapter {
private List<DataPack.Record> records;
static class ViewHolder extends RecyclerView.ViewHolder {
TextView textViewMothCount;
TextView textViewRecordInfo;
public ViewHolder(@NonNull View itemView) {
super(itemView);
textViewMothCount = itemView.findViewById(R.id.TextViewMothCount);
textViewRecordInfo = itemView.findViewById(R.id.TextViewRecordInfo);
}
}
public RecordAdapter(List<DataPack.Record> records) {
super();
this.records = records;
Collections.reverse(this.records);
}
@NonNull
@Override
public RecyclerView.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.record_item, parent, false);
ViewHolder holder = new ViewHolder(view);
return holder;
}
@Override
public void onBindViewHolder(@NonNull RecyclerView.ViewHolder holder, int position) {
ViewHolder holder1 = (ViewHolder) holder;
DataPack.Record record = records.get(position);
SimpleDateFormat format=new SimpleDateFormat("yyyy.MM.dd HH:mm:ss");
Date date = new Date(record.time);
String timeString = format.format(date);
DecimalFormat df = new DecimalFormat("#.0000");
if (record.mothCount >= 0) {
holder1.textViewMothCount.setTextColor(0x8A000000);
holder1.textViewMothCount.setText(String.valueOf(record.mothCount));
}
else {
holder1.textViewMothCount.setTextColor(0xFFFF0000);
holder1.textViewMothCount.setText("!");
}
holder1.textViewRecordInfo.setText("Uploader: " + record.uploader + "\n" +
"Time: " + timeString + '\n' +
"Location: " + df.format(record.longtitude) + ", " + df.format(record.latitude));
}
@Override
public int getItemCount() {
return records.size();
}
}
<file_sep>/Android/IntelliEco/app/src/main/java/cc/hurrypeng/intellieco/FTPTask.java
package cc.hurrypeng.intellieco;
import android.os.Handler;
import android.os.Message;
import androidx.annotation.NonNull;
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPReply;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.UUID;
class FTPTask extends Thread {
final static int RESPONSE_RECV = 1;
protected File response;
protected ArrayList<File> sends;
protected Handler handler;
protected String server;
protected String ftpUsername;
protected String ftpPassword;
protected String uuid;
FTPTask(File response, File... send) {
super();
this.response = response;
sends = new ArrayList<File>();
for (File file : send) sends.add(file);
handler = new FTPTaskHandler();
server = "ie.hurrypeng.cc";
ftpUsername = "IE_User";
ftpPassword = "<PASSWORD>";
uuid = UUID.randomUUID().toString().replaceAll("-", "");
}
void execute() {
onPreExecute();
start();
}
void onPreExecute() {
}
@Override
public void run() {
FTPClient ftp = new FTPClient();
try {
int reply;
ftp.connect(server);
// After connection attempt, you should check the reply code to verify
// success.
reply = ftp.getReplyCode();
if (!FTPReply.isPositiveCompletion(reply)) {
ftp.disconnect();
return;
}
if(!ftp.login(ftpUsername, ftpPassword)) {
return;
}
ftp.enterLocalPassiveMode();
ftp.setRemoteVerificationEnabled(false);
ftp.makeDirectory(uuid);
ftp.changeWorkingDirectory(uuid);
ftp.setFileType(FTP.BINARY_FILE_TYPE);
for (File file : sends) {
String filename = file.getName();
ftp.storeFile(filename, new FileInputStream(file));
}
ftp.makeDirectory("request");
// Wait for response
while (true)
{
if (ftp.removeDirectory("response")) break;
try {
Thread.sleep(1000);
}
catch (Exception e) {
e.printStackTrace();
}
}
if (response.exists()) response.delete();
response.createNewFile();
FileOutputStream responseStream = new FileOutputStream(response);
ftp.retrieveFile("response.json", responseStream);
responseStream.close();
ftp.makeDirectory("receive");
ftp.disconnect();
Message message = new Message();
message.what = RESPONSE_RECV;
handler.sendMessage(message);
} catch (IOException e) {
e.printStackTrace();
}
}
class FTPTaskHandler extends Handler {
@Override
public void handleMessage(@NonNull Message msg) {
super.handleMessage(msg);
switch (msg.what) {
case FTPTask.RESPONSE_RECV: {
onPostExecute();
break;
}
}
}
}
void onPostExecute() {
}
}
<file_sep>/MothCount/ThresholdTest/ThresholdTest.cpp
#include "opencv2/imgproc.hpp"
#include "opencv2/highgui.hpp"
#include <iostream>
using namespace std;
using namespace cv;
const int max_value_H = 360 / 2;
const int max_value = 255;
const String window_capture_name = "Video Capture";
const String window_detection_name = "Object Detection";
int low_H = 0, low_S = 0, low_V = 0;
int high_H = max_value_H, high_S = max_value, high_V = max_value;
Mat frame, frame_HSV, frame_threshold;
static void on_low_H_thresh_trackbar(int, void *)
{
low_H = min(high_H - 1, low_H);
setTrackbarPos("Low H", window_detection_name, low_H);
inRange(frame_HSV, Scalar(low_H, low_S, low_V), Scalar(high_H, high_S, high_V), frame_threshold);
imshow(window_capture_name, frame_threshold);
imshow(window_detection_name, frame);
}
static void on_high_H_thresh_trackbar(int, void *)
{
high_H = max(high_H, low_H + 1);
setTrackbarPos("High H", window_detection_name, high_H);
inRange(frame_HSV, Scalar(low_H, low_S, low_V), Scalar(high_H, high_S, high_V), frame_threshold);
imshow(window_capture_name, frame_threshold);
imshow(window_detection_name, frame);
}
static void on_low_S_thresh_trackbar(int, void *)
{
low_S = min(high_S - 1, low_S);
setTrackbarPos("Low S", window_detection_name, low_S);
inRange(frame_HSV, Scalar(low_H, low_S, low_V), Scalar(high_H, high_S, high_V), frame_threshold);
imshow(window_capture_name, frame_threshold);
imshow(window_detection_name, frame);
}
static void on_high_S_thresh_trackbar(int, void *)
{
high_S = max(high_S, low_S + 1);
setTrackbarPos("High S", window_detection_name, high_S);
inRange(frame_HSV, Scalar(low_H, low_S, low_V), Scalar(high_H, high_S, high_V), frame_threshold);
imshow(window_capture_name, frame_threshold);
imshow(window_detection_name, frame);
}
static void on_low_V_thresh_trackbar(int, void *)
{
low_V = min(high_V - 1, low_V);
setTrackbarPos("Low V", window_detection_name, low_V);
inRange(frame_HSV, Scalar(low_H, low_S, low_V), Scalar(high_H, high_S, high_V), frame_threshold);
imshow(window_capture_name, frame_threshold);
imshow(window_detection_name, frame);
}
static void on_high_V_thresh_trackbar(int, void *)
{
high_V = max(high_V, low_V + 1);
setTrackbarPos("High V", window_detection_name, high_V);
inRange(frame_HSV, Scalar(low_H, low_S, low_V), Scalar(high_H, high_S, high_V), frame_threshold);
imshow(window_capture_name, frame_threshold);
imshow(window_detection_name, frame);
}
int main(int argc, char* argv[])
{
namedWindow(window_capture_name);
namedWindow(window_detection_name, WINDOW_AUTOSIZE);
// Trackbars to set thresholds for HSV values
createTrackbar("Low H", window_detection_name, &low_H, max_value_H, on_low_H_thresh_trackbar);
createTrackbar("High H", window_detection_name, &high_H, max_value_H, on_high_H_thresh_trackbar);
createTrackbar("Low S", window_detection_name, &low_S, max_value, on_low_S_thresh_trackbar);
createTrackbar("High S", window_detection_name, &high_S, max_value, on_high_S_thresh_trackbar);
createTrackbar("Low V", window_detection_name, &low_V, max_value, on_low_V_thresh_trackbar);
createTrackbar("High V", window_detection_name, &high_V, max_value, on_high_V_thresh_trackbar);
frame = imread(R"(C:\Users\haora\Documents\visual studio 2015\Projects\IntelliEco\moth\moth5.jpg)", IMREAD_COLOR); // Read the file
pyrDown(frame, frame, Size(frame.cols / 2, frame.rows / 2));
pyrDown(frame, frame, Size(frame.cols / 2, frame.rows / 2));
// Convert from BGR to HSV colorspace
cvtColor(frame, frame_HSV, COLOR_BGR2HSV);
// Detect the object based on HSV Range Values
// Show the frames
int hSum = 0, hSum1 = 0, sSum = 0, sSum1 = 0, vSum = 0, vSum1 = 0;
int col = frame_HSV.cols, row = frame_HSV.rows;
int areaSum = col * row, area1 = 0;
auto get = [](int x, int y) {return frame_HSV.at<uchar>(y, x); };
for (int i = 0; i < col * 3; i += 3) for (int j = 0; j < row; j++)
{
// if (j == 0) cout << (int)get(i, j) << ' ' << (int)get(i + 1, j) << ' ' << (int)get(i + 2, j) << '\n';
if (get(i, j) >= 20 && get(i, j) <= 50)
{
area1++;
hSum1 += get(i, j);
sSum1 += get(i + 1, j);
vSum1 += get(i + 2, j);
}
hSum += get(i, j);
sSum += get(i + 1, j);
vSum += get(i + 2, j);
}
cout << hSum / areaSum << ' ' << sSum / areaSum << ' ' << vSum / areaSum << '\n';
cout << hSum1 / area1 << ' ' << sSum1 / area1 << ' ' << vSum1 / area1 << '\n';
waitKey();
return 0;
}
<file_sep>/Android/FTPTest/app/src/main/java/cc/hurrypeng/ftptest/DataSample.java
package cc.hurrypeng.ftptest;
class DataSample {
public String username;
public long time;
double longtitude;
double latitude;
public String imageFilename;
public DataSample()
{
username = null;
time = 0;
longtitude = latitude = 0.0;
imageFilename = null;
}
public DataSample(String username, long time, double longtitude, double latitude, String imageFilename)
{
this.username = username;
this.time = time;
this.longtitude = longtitude;
this.latitude = latitude;
this.imageFilename = imageFilename;
}
}
<file_sep>/Android/IntelliEco/app/src/main/java/cc/hurrypeng/intellieco/RecordActivity.java
package cc.hurrypeng.intellieco;
import android.app.AlertDialog;
import android.app.ProgressDialog;
import android.content.Context;
import android.content.Intent;
import android.content.SharedPreferences;
import android.location.Location;
import android.location.LocationManager;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import com.google.android.material.floatingactionbutton.FloatingActionButton;
import com.google.android.material.snackbar.Snackbar;
import com.google.gson.Gson;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import androidx.appcompat.widget.Toolbar;
import androidx.core.content.FileProvider;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import android.provider.MediaStore;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class RecordActivity extends AppCompatActivity {
ProgressDialog progressDialog;
Toolbar toolbar;
RecyclerView recyclerView;
FloatingActionButton fab;
String username;
String password;
File request;
File response;
File sampleImage;
SharedPreferences sp;
SharedPreferences.Editor spEdit;
long lastWarning;
LocationManager locationManager;
final int REQUEST_TAKE_PHOTO = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_record);
fab = findViewById(R.id.fab);
progressDialog = new ProgressDialog(this);
progressDialog.setTitle("Loading");
progressDialog.setCancelable(false);
toolbar = findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
recyclerView = findViewById(R.id.RecyclerViewRecords);
username = getIntent().getStringExtra("username");
password = getIntent().getStringExtra("<PASSWORD>");
request = new File(getExternalCacheDir() + "/request.json");
response = new File(getExternalCacheDir() + "/response.json");
sampleImage = new File(getExternalCacheDir() + "/cache.jpg");
sp = getSharedPreferences("warning", MODE_PRIVATE);
spEdit = sp.edit();
lastWarning = sp.getLong("lastWarning", 0);
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
try {
if (sampleImage.exists()) sampleImage.delete();
sampleImage.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
Uri imageUri;
if (Build.VERSION.SDK_INT >= 24) {
imageUri = FileProvider.getUriForFile(RecordActivity.this,
"cc.hurrypeng.intellieco.fileprovider", sampleImage);
} else {
imageUri = Uri.fromFile(sampleImage);
}
Intent intent = new Intent("android.media.action.IMAGE_CAPTURE");
intent.putExtra(MediaStore.EXTRA_OUTPUT, imageUri);
startActivityForResult(intent, REQUEST_TAKE_PHOTO);
}
});
toolbar.setOnMenuItemClickListener(new Toolbar.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem item) {
switch (item.getItemId()) {
case R.id.ActionRefresh: {
refresh();
break;
}
}
return false;
}
});
LinearLayoutManager layoutManager = new LinearLayoutManager(this);
recyclerView.setLayoutManager(layoutManager);
refresh();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu_record, menu);
return super.onCreateOptionsMenu(menu);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
switch (requestCode) {
case REQUEST_TAKE_PHOTO: {
progressDialog.setMessage("Uploading");
progressDialog.show();
try {
if (request.exists()) request.delete();
request.createNewFile();
DataPack.UploadRequest uploadRequest = new DataPack.UploadRequest();
uploadRequest.username = username;
uploadRequest.password = <PASSWORD>;
uploadRequest.request = "upload";
uploadRequest.time = System.currentTimeMillis();
Location location = locationManager.getLastKnownLocation(LocationManager.GPS_PROVIDER);
uploadRequest.longtitude = location.getLongitude();
uploadRequest.latitude = location.getLatitude();
uploadRequest.imageFilename = "cache.jpg";
FileOutputStream requestStream = new FileOutputStream(request);
requestStream.write(new Gson().toJson(uploadRequest).getBytes());
requestStream.close();
new FTPTask(response, request, sampleImage) {
@Override
void onPostExecute() {
super.onPostExecute();
progressDialog.dismiss();
try {
String responseContent = Util.getContent(response);
if (responseContent.equals("")) {
Snackbar.make(fab, "FTP connection failed! ", Snackbar.LENGTH_LONG).show();
}
DataPack.Response generalResponse = new Gson().fromJson(responseContent, DataPack.Response.class);
if (generalResponse.authority.equals("unauthorised")) Snackbar.make(fab, "Unauthorised! ", Snackbar.LENGTH_LONG).show();
else {
DataPack.UploadResponse uploadResponse = new Gson().fromJson(responseContent, DataPack.UploadResponse.class);
refresh();
if (uploadResponse.mothCount >= 0) {
AlertDialog.Builder dialog = new AlertDialog.Builder(RecordActivity.this);
dialog.setTitle("Analyse Result");
dialog.setMessage(uploadResponse.mothCount + " moth(s) have been detected. ");
dialog.setCancelable(true);
dialog.show();
}
}
}
catch (IOException e) {
e.printStackTrace();
}
}
}.execute();
}
catch (SecurityException e) {
e.printStackTrace();
}
catch (Exception e) {
e.printStackTrace();
}
break;
}
}
}
}
void refresh() {
progressDialog.setMessage("Refreshing Record");
progressDialog.show();
try {
if (request.exists()) request.delete();
request.createNewFile();
final DataPack.RefreshRequest refreshRequest = new DataPack.RefreshRequest();
refreshRequest.username = username;
refreshRequest.password = <PASSWORD>;
refreshRequest.request = "refresh";
FileOutputStream requestStream = new FileOutputStream(request);
requestStream.write(new Gson().toJson(refreshRequest).getBytes());
requestStream.close();
new FTPTask(response, request) {
@Override
void onPostExecute() {
super.onPostExecute();
progressDialog.dismiss();
try {
String responseContent = Util.getContent(response);
if (responseContent.equals("")) {
Snackbar.make(fab, "FTP connection failed! ", Snackbar.LENGTH_LONG).show();
}
DataPack.Response generalResponse = new Gson().fromJson(responseContent, DataPack.Response.class);
if (generalResponse.authority.equals("unauthorised")) Snackbar.make(fab, "Unauthorised! ", Snackbar.LENGTH_LONG).show();
else
{
DataPack.RefreshResponse refreshResponse = new Gson().fromJson(responseContent, DataPack.RefreshResponse.class);
RecordAdapter adapter = new RecordAdapter(refreshResponse.records);
recyclerView.setAdapter(adapter);
for (DataPack.Record record : refreshResponse.records)
{
if (record.mothCount < 0 && record.time > lastWarning)
{
AlertDialog.Builder dialog = new AlertDialog.Builder(RecordActivity.this);
dialog.setTitle("Warning");
dialog.setMessage("An exceptional record has been detected. It could be a bad sample, or a moth outburst. Please check it out on the server. ");
dialog.setCancelable(true);
dialog.show();
break;
}
}
lastWarning = System.currentTimeMillis();
spEdit.putLong("lastWarning", lastWarning);
spEdit.apply();
}
}
catch (IOException e) {
e.printStackTrace();
}
}
}.execute();
}
catch (SecurityException e) {
e.printStackTrace();
}
catch (Exception e) {
e.printStackTrace();
}
}
}
<file_sep>/Server/RequestScan.py
import os
import time
import subprocess
rootDir = "C:\\IntelliEco"
bufferDir = "C:\\IntelliEco\\buffer"
while True:
list = os.listdir(bufferDir)
for i in range(0, len(list)):
uuid = list[i]
uuidDir = bufferDir + "\\" + uuid;
requestDir = uuidDir + "\\request"
responseDir = uuidDir + "\\response"
receiveDir = uuidDir + "\\receive"
if os.path.exists(requestDir):
print(f"A request has been posted by {uuid}.")
os.rmdir(requestDir)
if os.path.exists(responseDir):
os.rmdir(responseDir)
subprocess.call(rootDir + "\\RequestProcess.exe " + uuidDir)
os.remove(uuidDir + "\\request.json")
os.mkdir(responseDir)
if os.path.exists(receiveDir):
print(f"A response has been fetched by {uuid}.")
os.rmdir(receiveDir)
os.remove(uuidDir + "\\response.json")
os.rmdir(uuidDir)
time.sleep(0.1)
<file_sep>/MothCount/MatToJson/MatToJson.cpp
#include <opencv2/core.hpp>
#include <opencv2/imgcodecs.hpp>
#include <opencv2/imgproc.hpp>
#include <opencv2/highgui.hpp>
#include <iostream>
#include <fstream>
#include <list>
#include <queue>
#include <deque>
#include "DrawContour.hpp"
#include "json.hpp"
using namespace cv;
using namespace nlohmann;
using namespace std;
bool Counted[2001][2001] = { false };
int dist(Point & p, Point & q)
{
return sqrt((p.x - q.x)*(p.x - q.x) + (p.y - q.y)*(p.y - q.y));
}
class Block
{
public:
Block();
void add(Point p);
long area() const;
Point getCog();
int getR();
int getAPR2();
void erase(Mat & mat);
deque<Point> pixels;
bool white;
Point cog;
int r;
};
Block::Block()
{
r = 0;
cog = Point(-1, -1);
}
void Block::add(Point p)
{
pixels.push_back(p);
}
long Block::area() const //
{
return pixels.size();
}
Point Block::getCog()
{
if (cog != Point(-1, -1)) return cog;
int xSum = 0, ySum = 0;
for (Point & p : pixels)
xSum += p.x, ySum += p.y;
cog.x = xSum / area(), cog.y = ySum / area();
return cog;
}
int Block::getR()
{
if (r) return r;
getCog();
for (Point & p : pixels)
r = max(r, dist(p, cog));
return r;
}
int Block::getAPR2()
{
return 100 * area() / max(1, getR()) / max(1, getR());
// Single moth APR2 falls between 100 and 200
}
void Block::erase(Mat & mat)
{
for (Point & p : pixels)
mat.at<uchar>(p.y, p.x) = white ? 0 : 255;
return;
}
bool operator<(const Block & a, const Block & b)
{
return a.area() < b.area();
}
int main(int argc, char ** argv)
{
Mat image;
Mat &_image = image;
image = imread(R"(C:\Users\haora\Documents\Visual Studio 2015\Projects\IntelliEco\moth\moth1.2.jpg)", IMREAD_GRAYSCALE); // Read the file
// Calculate basic params
int col = image.cols, row = image.rows;
int areaSum = col * row;
auto get = [&image](int x, int y) -> uchar & {return image.at<uchar>(y, x); };
for (int i = 0; i < col; i++) for (int j = 0; j < row; j++) get(i, j) = get(i, j) >= 128 ? 255 : 0;
// BFS algorithm
auto bfs = [get, col, row](int x, int y)
{
if (Counted[x][y])
return Block();
Block block;
block.white = (get(x, y) == 255 ? true : false);
queue<Point> q;
q.emplace(x, y);
while (!q.empty())
{
Point p = q.front();
q.pop();
if (Counted[p.x][p.y]) continue;
Counted[p.x][p.y] = true;
block.add(p);
if (p.x - 1 >= 0) if (get(p.x - 1, p.y) == get(x, y)) q.emplace(p.x - 1, p.y);
if (p.x + 1 < col) if (get(p.x + 1, p.y) == get(x, y)) q.emplace(p.x + 1, p.y);
if (p.y - 1 >= 0) if (get(p.x, p.y - 1) == get(x, y)) q.emplace(p.x, p.y - 1);
if (p.y + 1 < row) if (get(p.x, p.y + 1) == get(x, y)) q.emplace(p.x, p.y + 1);
}
return block;
};
// Block search lambda
list<Block> block_list;
auto blockSearch = [&block_list, bfs, &col, &row]()
{
block_list.clear();
memset(Counted, 0, sizeof(Counted));
for (int i = 0; i < col; i++) for (int j = 0; j < row; j++)
{
Block block = bfs(i, j);
if (block.area() != 0)
block_list.emplace_back(block);
}
};
ofstream ofs("bitmap.json");
json js;
js["col"] = col;
js["row"] = row;
for (int i = 0; i < col; i++) for (int j = 0; j < row; j++) js["pixels"].push_back(get(i, j));
ofs << js;
ofs.close();
// Block search
blockSearch();
testImage("Window", image);
// Print block info
block_list.sort();
for (Block & block : block_list) if (block.area() >= 10)
cout << (block.white ? "white" : "black") << "\tarea: " << block.area() << "\tCoG: " << block.getCog().x << ' ' << block.getCog().y << "\tradius: " << block.getR() << "\tAPR2: " << block.getAPR2() << '\n';
system("pause");
return 0;
}
<file_sep>/MothCount/MothCount_Thermal/MothCount-Thermal-CutImage/CutPicture_Gray.hpp
#include <opencv2/highgui.hpp>
#include <list> //No matter
#include <cmath> //abs()
// A milestone! No Mount. Bullshxt added!
void testImage(const std::string WindowName,\
cv::Mat TestingImage,\
bool DestroyOrNot = true)
{
//Show an image on window "winname", then destroy the window when pressing a key.
cv::namedWindow(WindowName); //Create an window
cv::imshow(WindowName, TestingImage); //Show the image on the window
cv::waitKey(); //Waiting for pressing a key
if (DestroyOrNot)
cv::destroyWindow(WindowName); //Destroy the window
}
void SingleCut_col(cv::Mat & ToBeCut,\
cv::Mat & Destination,\
int thresholdCol_mono=50)
{
//testImage("shabby 0", ToBeCut);
std::list<int>memcol;
int col=ToBeCut.cols, row=ToBeCut.rows;
for (int i_cols = 0 ; i_cols < col-5 ; i_cols+=4)
{
double avg_b=0, avg_g=0, avg_r=0;
//double avg_b_next=0, avg_g_next=0, avg_r_next=0;
for (int i_rows = 0 ; i_rows < row ; i_rows++)
{
avg_b += ToBeCut.at<uchar>(i_rows, i_cols);
avg_b -= ToBeCut.at<uchar>(i_rows, i_cols+4);
}
avg_b = std::abs(avg_b / row);
//std::cerr << avg_b << " " << avg_g << " " << avg_r << std::endl;
if (avg_b >= thresholdCol_mono)
{
memcol.push_back(i_cols);
//std::cerr << avg_b << "\t" << avg_g << "\t" << avg_r << "\tcolumn:" << i_cols << std::endl;
//cv::line(ToBeCut, cv::Point(i_cols,0), cv::Point(i_cols,row), cvScalar(255,255,255), 5, cv::LINE_8, 0);
//i_cols += 4;
}
else;
}
// testImage("shabby", ToBeCut);
//See if 0 or "col"/"row" is included
if (memcol.size()>=2)
{
if (memcol.front() == 0)
memcol.pop_front();
if (memcol.back() == col)
memcol.pop_back();
}
//See if found two egdes
cv::Rect m_select = cv::Rect(memcol.front(), 0, memcol.back()-memcol.front(), row);
//testImage("shabby 0", ToBeCut);
Destination = ToBeCut(m_select);
// testImage("shabby", Destination);
// std::cerr << std::endl;
}
void SingleCut_row(cv::Mat & ToBeCut,\
cv::Mat & Destination,\
int thresholdRow_mono=50)
{
std::list<int>memrow;
int col=ToBeCut.cols, row=ToBeCut.rows;
for (int i_rows = 0 ; i_rows < row-5 ; i_rows+=4)
{
double avg_b=0;
//double avg_b_next=0, avg_g_next=0, avg_r_next=0;
for (int i_cols = 0 ; i_cols < col ; i_cols++)
{
avg_b += ToBeCut.at<uchar>(i_rows, i_cols);
avg_b -= ToBeCut.at<uchar>(i_rows, i_cols+4);
}
avg_b = std::abs(avg_b / col);
//std::cerr << avg_b << " " << avg_g << " " << avg_r << std::endl;
if (avg_b >= thresholdRow_mono)
{
memrow.push_back(i_rows);
//std::cerr << avg_b << "\t" << avg_g << "\t" << avg_r << "\trow:" << i_rows << std::endl;
//cv::line(ToBeCut, cv::Point(i_rows,0), cv::Point(i_rows,row), cvScalar(255,255,255), 5, cv::LINE_8, 0);
}
else;
}
if (memrow.size()>=2)
{
if (memrow.front() == 0)
memrow.pop_front();
if (memrow.back() == row)
memrow.pop_back();
}
if (memrow.empty())
{
memrow.push_back(0);
memrow.push_back(row);
}
else if (memrow.size() == 1)
{
memrow.pop_back();
memrow.push_back(0);
memrow.push_back(row);
}
cv::Rect m_select = cv::Rect(0, memrow.front(), col, memrow.back()-memrow.front());
Destination = ToBeCut(m_select);
}
void CutImage(const cv::Mat & Source,\
cv::Mat & Destination)
{
Destination = Source;
//testImage("Shabby src", Source);
// Define controlling variables
bool colContinue = true, rowContinue = true;
int CutCount_Row=0, CutCount_Col=0;
// Basic metadata of the image
int col = Destination.cols, row = Destination.rows;
// Base Threshold definition
int monRow=20, monCol=10;
// Hold the result of last cutting
// To recover when a ~~failed~~ cut occurs
cv::Mat Lastime(Destination);
// Do the cutting job
// Stop when cut to 3/4 size
// Cut ROWs
while ((Destination.rows >= row * 3.0 / 4)\
&&\
(rowContinue)\
&&\
(CutCount_Row <= 200))
{
// Do a single cutting in rows
// When no cutting is done, reduce the threshold
SingleCut_row(Destination, Destination, monRow);
if (Lastime.rows == Destination.rows)
{
monRow--;
}
else;
// testImage("rows", Destination);
// To detect a bad cut
if (Destination.rows <= row * 0.83)
{
Destination = Lastime;
rowContinue = false;
monRow = 255;
}
// Update Last time
Lastime = Destination;
CutCount_Row++;
}
// Column pre-conditioning
cv::Rect m_select = cv::Rect(Destination.cols*0.20, 0, Destination.cols*0.60, Destination.rows);
Destination = Destination(m_select);
Lastime = Destination;
// testImage("Shabby Middle", Destination);
while (Destination.cols >= col * 0.5\
&&\
colContinue\
&&\
(CutCount_Col <= 70))
{
// Do a single cutting in rows
// When no cutting is done, reduce the threshold
SingleCut_col(Destination, Destination, monCol);
if (Lastime.cols == Destination.cols)
{
monCol++;
}
else;
// To Detect a bad cut
if (Destination.cols <= col * 0.30)
{
Destination = Lastime;
colContinue = false;
monCol = 255;
}
// Update Last time
Lastime = Destination;
CutCount_Col++;
}
//std::cerr << "CutTimes:" << CutCount_Row << " " << CutCount_Col << " ";
}
| 6275887f9270f3eb4dc8172b8cc0e7917189fdfd | [
"Markdown",
"Java",
"Python",
"C++"
] | 15 | C++ | SciEco/IntelliEco | 5b4f1e04e09058b71f97f8d17910854eaa3b4d07 | e2218aadb6a33a5d8fa88fb338105248392f3eee | |
refs/heads/master | <repo_name>heuberg/ImageViewer<file_sep>/app/src/main/java/com/github/clerfayt/imageviewer/ImageActivity.java
package com.github.clerfayt.imageviewer;
import android.app.Activity;
import android.content.ContentResolver;
import android.content.Context;
import android.content.Intent;
import android.database.Cursor;
import android.graphics.Bitmap;
import android.graphics.drawable.BitmapDrawable;
import android.net.Uri;
import android.os.Bundle;
import android.provider.MediaStore;
import android.util.DisplayMetrics;
import android.view.MotionEvent;
import android.view.View;
import android.widget.ImageView;
import android.widget.Toast;
import com.github.clerfayt.imageviewer.utils.DecodeUtils;
import java.io.File;
public class ImageActivity extends Activity {
private ImageViewTouch imageView;
@Override
protected void onStart() {
super.onStart();
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_image);
this.imageView = (ImageViewTouch)findViewById(R.id.imageView);
Intent receivedIntent = getIntent();
String receivedAction = receivedIntent.getAction();
System.out.println(receivedAction);
if(receivedAction.equals(Intent.ACTION_VIEW)) {
Uri uri = receivedIntent.getData();
final DisplayMetrics metrics = getResources().getDisplayMetrics();
int size = (int) (Math.min(metrics.widthPixels, metrics.heightPixels) / 0.55);
Bitmap bitmap = DecodeUtils.decode(this, uri, size, size);
this.imageView.setImageBitmap(bitmap, null, -1, -1);
this.imageView.setOnLongClickListener(new View.OnLongClickListener() {
@Override
public boolean onLongClick(View v) {
Toast.makeText(ImageActivity.this, getString(R.string.app_name) + " " + BuildConfig.VERSION_NAME + " for my Tolino. ST 2017-07", Toast.LENGTH_LONG).show();
return false;
}
});
}
}
@Override
protected void onStop() {
super.onStop();
this.imageView.setImageBitmap(null);
}
}
| 8ad26cf7443c49adf521f59fced5256856eac247 | [
"Java"
] | 1 | Java | heuberg/ImageViewer | 512e697db6b293c3112799b617ffc7576f57f366 | c647ec8e27e333531c95d8d44f9e223bb6cdfc5b | |
refs/heads/master | <repo_name>ytyaru0/GitHub.Upload.Http.Response.201703301257<file_sep>/web/http/Response.py
#!python3
#encoding:utf-8
import json
import time
from urllib.parse import urlparse
import re
from PIL import Image
from io import BytesIO
class Response(object):
def __init__(self):
self.Headers = Response.Headers()
def Get(self, r, sleep_time=2, is_show=True):
if is_show:
print('Request---------------------')
print(r.url)
print(r.request.headers)
print(r.request.data)
print('Response---------------------')
print("HTTP Status Code: {0} {1}".format(r.status_code, r.reason))
print(r.text)
time.sleep(sleep_time)
r.raise_for_status()
self.headers.ContentType.Split(r)
if 'application/json' == self.headers.ContentType.mime_type:
return r.json()
elif ('image/gif' == self.headers.ContentType.mime_type or
'image/jpeg' == self.headers.ContentType.mime_type or
'image/png' == self.headers.ContentType.mime_type
):
return Image.open(BytesIO(r.content))
# elif r.request.stream:
# return r.raw
else:
return r.text
class Headers:
def __init__(self):
self.ContentType = Response.Headers.ContentType()
self.Link = Response.Headers.Link()
class Link:
def __init__(self):
pass
def Get(self, r, rel='next'):
if None is r.links:
return None
if 'next' == rel or 'prev' == rel or 'first' == rel or 'last' == rel:
return r.links[rel]['url']
def Next(self, r):
return self.__get_page(r, 'next')
def Prev(self, r):
return self.__get_page(r, 'prev')
def First(self, r):
return self.__get_page(r, 'first')
def Last(self, r):
return self.__get_page(r, 'last')
def __get_page(self, r, rel='next'):
if None is r:
return None
print(r.links)
if rel in r.links.keys():
url = urlparse(r.links[rel]['url'])
print('page=' + url.query['page'])
return url.query['page']
else:
return None
class ContentType:
def __init__(self):
self.__re_charset = re.compile(r'charset=', re.IGNORECASE)
self.mime_type = None # 例: application/json
self.char_set = None # 例: utf8
# トップレベルタイプ名/サブタイプ名 [;パラメータ]
# トップレベルタイプ名/[ツリー.]サブタイプ名[+サフィックス] [;パラメータ1] [;パラメータ2] ...
self.top_level_type = None
self.sub_type = None
self.suffix = None
self.parameters = None
def Split(self, r):
self.mime_type = None
self.char_set = None
self.top_level_type = None
self.sub_type = None
self.suffix = None
self.parameters = None
if not('Content-Type' in r.headers) or (None is r.headers['Content-Type']) or ('' == r.headers['Content-Type']):
pass
else:
content_types = r.headers['Content-Type'].split(';')
self.__mime_type = content_types[0]
if 1 < len(content_types):
parameters = content_types[1:]
self.__parameters = []
for p in parameters:
key, value = p.split('=')
self.__parameters.append({key.strip(): value.strip()})
if None is not self.__mime_type:
self.__mime_type = self.__mime_type.strip()
self.__top_level_type, self.__sub_type = self.__mime_type.split('/')
if self.__sub_type.endswith('+'):
self.__suffix = self.__sub_type.split('+')[1]
if None is not self.__char_set:
# 'charset='に一致するならcharsetに設定する
for key in self.__parameters.keys():
if 'charset' == key.lower():
self.__char_set = self.__parameters[key]
# if self.__re_charset.match(self.__parameters):
# self.__char_set = re.sub(self.__re_charset, '', self.__parameters).strip()
print('MimeType: {0}'.format(self.__mime_type))
print('CharSet: {0}'.format(self.__char_set))
print('MimeType: {0}'.format(self.__top_level_type))
print('CharSet: {0}'.format(self.__sub_type))
print('MimeType: {0}'.format(self.__suffix))
print('CharSet: {0}'.format(self.__parameters))
| 1d0913f0a018aeb49ba8570f59ede74c945456b2 | [
"Python"
] | 1 | Python | ytyaru0/GitHub.Upload.Http.Response.201703301257 | 3926ad3779e8be4a50ce536b69cef50a7eb3e640 | 91fe42ca93af35e6128fc78cd5bfbf7e7662663a | |
refs/heads/master | <file_sep>// Copyright (c) 2012 <NAME>
// MIT License - http://opensource.org/licenses/mit-license.php
/**
* Support for handling 64-bit int numbers in Javascript (node.js)
*
* JS Numbers are IEEE-754 binary double-precision floats, which limits the
* range of values that can be represented with integer precision to:
*
* 2^^53 <= N <= 2^53
*
* Int64 objects wrap a node Buffer that holds the 8-bytes of int64 data. These
* objects operate directly on the buffer which means that if they are created
* using an existing buffer then setting the value will modify the Buffer, and
* vice-versa.
*
* Internal Representation
*
* The internal buffer format is Big Endian. I.e. the most-significant byte is
* at buffer[0], the least-significant at buffer[7]. For the purposes of
* converting to/from JS native numbers, the value is assumed to be a signed
* integer stored in 2's complement form.
*
* For details about IEEE-754 see:
* http://en.wikipedia.org/wiki/Double_precision_floating-point_format
*/
// For to/from DecimalString
const POW2_24 = Math.pow(2, 24)
const POW2_31 = Math.pow(2, 31)
const POW2_32 = Math.pow(2, 32)
const POW10_11 = Math.pow(10, 11)
// Useful masks and values for bit twiddling
// const MASK31 = 0x7fffffff
// const VAL31 = 0x80000000
// const MASK32 = 0xffffffff
const VAL32 = 0x100000000
// Map for converting hex octets to strings
const _HEX: string[] = []
for (let i = 0; i < 256; i++) {
_HEX[i] = (i > 0xF ? '' : '0') + i.toString(16)
}
//
// Int64
//
/**
* Constructor accepts any of the following argument types, it no longer supports Browserify:
*
* new Int64(buffer[, offset=0]) - Existing Buffer with byte offset
* new Int64(Uint8Array[, offset=0]) - Existing Uint8Array with a byte offset
* new Int64(string) - Hex string (throws if n is outside int64 range)
* new Int64(number) - Number (throws if n is outside int64 range)
* new Int64(hi, lo) - Raw bits as two 32-bit values
*/
export default class Int64 {
// Max integer value that JS can accurately represent
static MAX_INT = Math.pow(2, 53)
// Min integer value that JS can accurately represent
static MIN_INT = -Math.pow(2, 53)
public buffer: Buffer
public offset: number
constructor()
constructor(a1: string|number)
constructor(a1: number, a2?: number)
constructor(a1: Buffer, offset?: number)
constructor(a1?: Buffer|string|number, a2: number = 0) {
if (a1 instanceof Buffer) {
this.buffer = a1
this.offset = a2
} else {
this.buffer = this.buffer || Buffer.alloc(8)
this.offset = 0
this.setValue.apply(this, arguments)
}
}
/**
* Do in-place 2's compliment. See
* http://en.wikipedia.org/wiki/Two's_complement
*/
private _2scomp(): void {
const b = this.buffer
const o = this.offset
let carry = 1
for (let i = o + 7; i >= o; i--) {
let v = (b[i] ^ 0xff) + carry
b[i] = v & 0xff
carry = v >> 8
}
}
/**
* Set the value. Takes any of the following arguments:
*
* setValue(string) - A hexidecimal string
* setValue(number) - Number (throws if n is outside int64 range)
* setValue(hi, lo) - Raw bits as two 32-bit values
*/
setValue(hi: string): void
setValue(hi: number): void
setValue(hi: number, lo: number): void
setValue(hi: string|number, lo?: number): void {
let negate = false
if (arguments.length === 1) {
if (typeof hi === 'number') {
// Simplify bitfield retrieval by using abs() value. We restore sign
// later
negate = hi < 0
hi = Math.abs(hi)
lo = hi % VAL32
hi = hi / VAL32
if (hi > VAL32) { throw new RangeError(hi + ' is outside Int64 range') }
hi = hi | 0
} else if (typeof hi === 'string') {
hi = (hi + '').replace(/^0x/, '')
const loStr = hi.substr(-8)
hi = hi.length > 8 ? hi.substr(0, hi.length - 8) : ''
hi = parseInt(hi, 16) as number
lo = parseInt(loStr, 16)
} else {
throw new Error(hi + ' must be a Number or String')
}
}
// Tell ts that we've converted hi and lo to nums
lo = lo as number
hi = hi as number
// Technically we should throw if hi or lo is outside int32 range here, but
// it's not worth the effort. Anything past the 32'nd bit is ignored.
// Copy bytes to buffer
const b = this.buffer
const o = this.offset
for (let i = 7; i >= 0; i--) {
b[o + i] = lo & 0xff
lo = i === 4 ? hi : lo >>> 8
}
// Restore sign of passed argument
if (negate) { this._2scomp() }
}
/**
* Convert to a native JS number.
*
* WARNING: Do not expect this value to be accurate to integer precision for
* large (positive or negative) numbers!
*
* @param allowImprecise If true, no check is performed to verify the
* returned value is accurate to integer precision. If false, imprecise
* numbers (very large positive or negative numbers) will be forced to +/-
* Infinity.
*/
toNumber(allowImprecise: boolean = false): number {
const b = this.buffer
const o = this.offset
// Running sum of octets, doing a 2's complement
const negate = b[o] & 0x80
let x = 0
let carry = 1
for (let i = 7, m = 1; i >= 0; i--, m *= 256) {
let v = b[o + i]
// 2's complement for negative numbers
if (negate) {
v = (v ^ 0xff) + carry
carry = v >> 8
v = v & 0xff
}
x += v * m
}
// Return Infinity if we've lost integer precision
if (!allowImprecise && x >= Int64.MAX_INT) {
return negate ? -Infinity : Infinity
}
return negate ? -x : x
}
/**
* Alias toNumber()
* Convert to a JS Number. Returns +/-Infinity for values that can't be
* represented to integer precision.
*/
valueOf(): number {
return this.toNumber(false)
}
/**
* Return string value
*
* @param radix Just like Number#toString()'s radix
*/
toString(radix: number = 10) {
return this.valueOf().toString(radix)
}
/**
* Return a string showing the buffer octets, with MSB on the left.
*
* @param sep separator string. default is '' (empty string)
*/
toOctetString(sep: string = '') {
const out = new Array(8)
const b = this.buffer
const o = this.offset
for (let i = 0; i < 8; i++) {
out[i] = _HEX[b[o + i]]
}
return out.join(sep)
}
/**
* Returns the int64's 8 bytes in a buffer.
*
* @param {bool} [rawBuffer=false] If no offset and this is true, return the internal buffer. Should only be used if
* you're discarding the Int64 afterwards, as it breaks encapsulation.
*/
toBuffer(rawBuffer: boolean = false) {
if (rawBuffer && this.offset === 0) { return this.buffer }
let out = Buffer.alloc(8)
this.buffer.copy(out, 0, this.offset, this.offset + 8)
return out
}
/**
* Copy 8 bytes of int64 into target buffer at target offset.
*
* @param {Buffer} targetBuffer Buffer to copy into.
* @param {number} [targetOffset=0] Offset into target buffer.
*/
copy(targetBuffer: Buffer, targetOffset: number = 0) {
this.buffer.copy(targetBuffer, targetOffset, this.offset, this.offset + 8)
}
/**
* Returns a number indicating whether this comes before or after or is the
* same as the other in sort order.
*
* @param {Int64} other Other Int64 to compare.
*/
compare(other: Int64): number {
// If sign bits differ ...
// tslint:disable-next-line
if ((this.buffer[this.offset] & 0x80) != (other.buffer[other.offset] & 0x80)) {
return other.buffer[other.offset] - this.buffer[this.offset]
}
// otherwise, compare bytes lexicographically
for (let i = 0; i < 8; i++) {
if (this.buffer[this.offset + i] !== other.buffer[other.offset + i]) {
return this.buffer[this.offset + i] - other.buffer[other.offset + i]
}
}
return 0
}
/**
* Returns a boolean indicating if this integer is equal to other.
*
* @param {Int64} other Other Int64 to compare.
*/
equals(other: Int64) {
return this.compare(other) === 0
}
/**
* Pretty output in console.log
*/
inspect(): string {
return `[Int64 value: ${this} octets: ${this.toOctetString(' ')}]`
}
// TODO: Test
// Factored in from node-thrift
toDecimalString(): string {
let b = this.buffer
let o = this.offset
if ((!b[o] && !(b[o + 1] & 0xe0)) ||
(!~b[o] && !~(b[o + 1] & 0xe0))) {
// The magnitude is small enough.
return this.toString()
} else {
let negative = b[o] & 0x80
if (negative) {
// 2's complement
let incremented = false
let buffer = Buffer.alloc(8)
for (let i = 7; i >= 0; --i) {
buffer[i] = (~b[o + i] + (incremented ? 0 : 1)) & 0xff
incremented = incremented || !!b[o + i]
}
b = buffer
}
let high2 = b[o + 1] + (b[o] << 8)
// Lesser 11 digits with exceeding values but is under 53 bits capacity.
let low = b[o + 7] + (b[o + 6] << 8) + (b[o + 5] << 16)
+ b[o + 4] * POW2_24 // Bit shift renders 32th bit as sign, so use multiplication
+ (b[o + 3] + (b[o + 2] << 8)) * POW2_32 + high2 * 74976710656 // The literal is 2^48 % 10^11
// 12th digit and greater.
let high = Math.floor(low / POW10_11) + high2 * 2814 // The literal is 2^48 / 10^11
// Make it exactly 11 with leading zeros.
const lowStr = ('00000000000' + String(low % POW10_11)).slice(-11)
return (negative ? '-' : '') + String(high) + lowStr
}
}
// TODO: Test
static fromDecimalString(text: string): Int64 {
let negative = text.charAt(0) === '-'
if (text.length < (negative ? 17 : 16)) {
// The magnitude is smaller than 2^53.
return new Int64(+text)
} else if (text.length > (negative ? 20 : 19)) {
throw new RangeError('Too many digits for Int64: ' + text)
} else {
// Most significant (up to 5) digits
let high5 = +text.slice(negative ? 1 : 0, -15)
let low = +text.slice(-15) + high5 * 2764472320 // The literal is 10^15 % 2^32
let high = Math.floor(low / POW2_32) + high5 * 232830 // The literal is 10^15 / 2^&32
low = low % POW2_32
if (high >= POW2_31 &&
!(negative && high === POW2_31 && low === 0) // Allow minimum Int64
) {
throw new RangeError('The magnitude is too large for Int64.')
}
if (negative) {
// 2's complement
high = ~high
if (low === 0) {
high = (high + 1) & 0xffffffff
} else {
low = ~low + 1
}
high = 0x80000000 | high
}
return new Int64(high, low)
}
}
}
<file_sep>import assert = require('assert')
import Int64 from './index'
import {Test} from 'nodeunit'
export function setUp(done: Function) {
done()
}
export function testBufferToString(test: Test) {
let int = new Int64(0xfffaffff, 0xfffff700)
test.equal(
int.toBuffer().toString('hex'),
'fffafffffffff700',
'Buffer to string conversion',
)
test.done()
}
export function testBufferCopy(test: Test) {
let src = new Int64(0xfffaffff, 0xfffff700)
let dst = Buffer.alloc(8)
src.copy(dst)
test.deepEqual(
dst,
Buffer.from([0xff, 0xfa, 0xff, 0xff, 0xff, 0xff, 0xf7, 0x00]),
'Copy to buffer',
)
test.done()
}
export function testValueRepresentation(test: Test) {
let args = [
[0], '0000000000000000', 0,
[1], '0000000000000001', 1,
[-1], 'ffffffffffffffff', -1,
[1e18], '0de0b6b3a7640000', 1e18,
['0001234500654321'], '0001234500654321', 0x1234500654321,
['0ff1234500654321'], '0ff1234500654321', 0xff1234500654300, // Imprecise!
[0xff12345, 0x654321], '0ff1234500654321', 0xff1234500654300, // Imprecise!
[0xfffaffff, 0xfffff700], 'fffafffffffff700', -0x5000000000900,
[0xafffffff, 0xfffff700], 'affffffffffff700', -0x5000000000000800, // Imprecise!
['0x0000123450654321'], '0000123450654321', 0x123450654321,
['0xFFFFFFFFFFFFFFFF'], 'ffffffffffffffff', -1,
]
// Test constructor argments
for (let i = 0; i < args.length; i += 3) {
let a = args[i]
const octets = args[i + 1]
const num = args[i + 2]
// Create instance
let x: Int64
if (a instanceof Array) {
if (a.length === 1) {
x = new Int64(a[0])
} else if (a.length === 2) {
a = a as number[]
x = new Int64(a[0], a[1])
} else {
throw new Error("Invalid arg count")
}
} else {
x = new Int64(a)
}
test.equal(x.toOctetString(), octets, 'Constuctor with ' + args.join(', '))
test.equal(x.toNumber(true), num)
}
test.done()
}
export function testBufferOffsets(test: Test) {
let sourceBuffer = Buffer.alloc(16)
sourceBuffer.writeUInt32BE(0xfffaffff, 2)
sourceBuffer.writeUInt32BE(0xfffff700, 6)
let int = new Int64(sourceBuffer, 2)
assert.equal(
int.toBuffer().toString('hex'), 'fffafffffffff700',
'Construct from offset',
)
let targetBuffer = Buffer.alloc(16)
int.copy(targetBuffer, 4)
assert.equal(
targetBuffer.slice(4, 12).toString('hex'), 'fffafffffffff700',
'Copy to offset',
)
test.done()
}
export function testInstanceOf(test: Test) {
let x = new Int64()
assert(x instanceof Int64, 'Variable is not instance of Int64')
let y = {}
assert(!(y instanceof Int64), 'Object is an instance of Int64')
test.done()
}
export function testCompare(test: Test) {
let intMin = new Int64(2147483648, 0)
let intMinPlusOne = new Int64(2147483648, 1)
let zero = new Int64(0, 0)
let intMaxMinusOne = new Int64(2147483647, 4294967294)
let intMax = new Int64(2147483647, 4294967295)
assert(intMin.compare(intMinPlusOne) < 0, "INT64_MIN is not less than INT64_MIN+1")
assert(intMin.compare(zero) < 0, "INT64_MIN is not less than 0")
assert(intMax.compare(new Int64(intMin.compare(zero))) > 0, "INT64_MIN is not less than INT64_MAX")
assert(intMax.compare(intMaxMinusOne) > 0, "INT64_MAX is not greater than INT64_MAX-1")
assert(intMax.compare(zero) > 0, "INT64_MAX is not greater than 0")
assert(intMax.compare(intMin) > 0, "INT64_MAX is not greater than INT_MIN")
test.done()
}
export function testEquals(test: Test) {
let intMin = new Int64(2147483648, 0)
let zero = new Int64(0, 0)
let intMax = new Int64(2147483647, 4294967295)
assert(intMin.equals(intMin), "INT64_MIN !== INT64_MIN")
assert(intMax.equals(intMax), "INT64_MAX !== INT64_MAX")
assert(zero.equals(zero), "0 !== 0")
assert(!intMin.equals(zero), "INT64_MIN === 0")
assert(!intMin.equals(intMax), "INT64_MIN === INT64_MAX")
assert(!intMax.equals(zero), "INT64_MAX === 0")
assert(!intMax.equals(intMin), "INT64_MAX === INT64_MIN")
test.done()
}
| 5fbe5d89562547f85bfdc8bfc9c37777e0bd4145 | [
"TypeScript"
] | 2 | TypeScript | estk/nint64 | 5199bfe36e8a9792ed3622a35c6d07e4c604652a | 0c976f1166bb7b6f0c7cbe6e682ce7c0e5ddd4c7 | |
refs/heads/master | <file_sep>"""This function takes users interests as inputs and outputs a list of
ranked vacation destinations with top interests for each destination.
To rank destinations, the app uses data that has been collected from Twitter
and stored in MySQL. user_interests is a assumed to be a string
consisting of interests separated by commas. The output is a 2D list of
strings. The left column has destinations and the right column has
the top three interests for that destination."""
# Packages
import re
import time
import pandas as pd
import pymysql as mdb
from sqlalchemy import create_engine
# Files in this directory
from mysql_login_info import sql_username, sql_password
import preprocessing
def get_rankings(user_interests):
if user_interests == '':
return []
destinations=[]
df_destinations=pd.read_csv('vacation_destinations.txt', header=None, names=['Destination'])
destinations = sorted(df_destinations['Destination'].values.tolist())
# Connect to the local mySQL server and open the vacation database.
engine = create_engine('mysql+mysqldb://'+sql_username+':'+sql_password+'@127.0.0.1:3306/vacation', echo=False)
# Parse user input, stem it, and make regexes.
user_input = user_interests
def stem(s):
return preprocessing.preprocess_pipeline(s, return_as_str=True, do_remove_stopwords=True, do_clean_html=False)
interests_regex={}
max_interests = 10
for interest in user_input.split(',')[:max_interests]:
if interest == '':
continue
# Remove any characters that that aren't alphanumeric.
interest = re.sub(r'[^\w]+', '', interest)
if False:
# Insert MySQL regex for spaces (this isn't working yet). Once
# this is working, edit the re.sub line above
# so that it doesn't remove spaces.
interests_regex[interest.strip()]= re.sub(' ',r'[[:space:]]',stem(interest))
interests_regex[interest.strip()]= stem(interest)
interests=interests_regex.keys()
print len(interests), 'interests'
print interests_regex
# Put the destinations in a dataframe to use in the next step.
df_destinations = pd.DataFrame({'Destination':destinations}).set_index('Destination')
df_destinations.head()
# Count the number of tweets for each destination for each interest.
destinations_interests = df_destinations.copy()
db = mdb.connect(user=sql_username, host="localhost", passwd=sql_password, db="vacation", charset='utf8')
interests_processed = 0
for interest in interests:
sql_query = ("SELECT Destination, COUNT(*) AS " + interest +
" FROM timeline_tweets_stemmed_unique WHERE Tweet REGEXP '[[:<:]]" +
interests_regex[interest] + "[[:>:]]' GROUP BY Destination")
with db:
cur = db.cursor()
cur.execute(sql_query)
query_results = cur.fetchall()
query_results = map(list, zip(*[list(i) for i in query_results]))
if len(query_results) == 0:
interests_processed += 1
print interests_processed, 'interests processed'
continue
df_timeline = pd.DataFrame({'Destination': query_results[0], interest: query_results[1]}).set_index('Destination')
destinations_interests = destinations_interests.join(df_timeline, how='outer').fillna(0)
interests_processed += 1
print interests_processed, 'interests processed'
# To normalize rows, change normalize_rows to True.
normalize_rows = False
if normalize_rows:
# Count the number of timeline tweets for each destination.
db = mdb.connect(user=sql_username, host="localhost", passwd=sql_password, db="vacation", charset='utf8')
sql_query = ("SELECT Destination, COUNT(*)" +
" FROM timeline_tweets_stemmed_unique GROUP BY Destination")
with db:
cur = db.cursor()
cur.execute(sql_query)
query_results = cur.fetchall()
query_results = map(list, zip(*[list(i) for i in query_results]))
df_tweets_per_destination = pd.DataFrame({'Destination': query_results[0],
'Tweets': query_results[1]}).set_index('Destination')
destinations_interests_rows_normalized = destinations_interests.join(df_tweets_per_destination, how='outer').fillna(0)
for interest in interests:
destinations_interests_rows_normalized[interest] = (
destinations_interests_rows_normalized[interest].astype(float) /
destinations_interests_rows_normalized['Tweets'].astype(float))
destinations_interests_rows_normalized = destinations_interests_rows_normalized.drop('Tweets', axis=1)
else:
destinations_interests_rows_normalized = destinations_interests
# Normalize each interest column.
def normalize(x):
if x.std() > 0.01:
return (x-x.mean())/x.std()
else:
return x-x.mean()
destinations_interests_scores = destinations_interests_rows_normalized.copy()
destinations_interests_scores = destinations_interests_rows_normalized.apply(normalize)
# Rank the destinations and then
# store the top three interests for each destination in a dataframe.
destination_col = destinations_interests_scores.sum(axis=1).sort(ascending=False, inplace=False).index.values.tolist()
interest_col = []
for destination in destination_col:
interest_list=[]
# Rank the interests.
for interest in destinations_interests_scores.loc[destination].sort(ascending=False, inplace=False).index.values.tolist():
# Only include interests with associated tweets.
if destinations_interests.loc[[destination]][interest][0]>0:
interest_list.append(interest)
# Only use the top 3 interests.
interest_col.append(', '.join(interest_list[:3]))
df_interest_destination = pd.DataFrame({'Interest': interest_col, 'Destination': destination_col})
# Remove New York (The algorithm doens't work very well for
# New York because it is both a city and a state).
remove_new_york = True
if remove_new_york:
df_interest_destination = df_interest_destination[df_interest_destination.Destination != 'New York']
# Reorder the columns.
df_interest_destination = df_interest_destination[['Destination', 'Interest']]
# Drop destinations without any interests.
df_interest_destination=df_interest_destination[df_interest_destination['Interest'] != '']
df_interest_destination.head()
# Put the top interest for each destination in a list format.
interest_col = df_interest_destination['Interest'].values.tolist()
destination_col = df_interest_destination['Destination'].values.tolist()
interest_destination = []
length = len(interest_col)
for i in range(length):
interest_destination.append([destination_col[i],interest_col[i]])
# Return the results as a 2D list.
return interest_destination
<file_sep>"""This will stem all of the tweets in the timeline_tweets table and
put them in the timeline_tweets_stemmed table."""
import pandas as pd
from sqlalchemy import create_engine
from sqlalchemy_utils import database_exists, create_database
import time
import preprocessing
from mysql_login_info import sql_username, sql_password
import datetime
start_time = time.clock()
# connect to my local mySQL server and open the vacation database
engine = create_engine('mysql+mysqldb://'+sql_username+':'+sql_password+'@127.0.0.1:3306/vacation', echo=False)
chunk_size = 10000
i = 0
while True:
# Pull the timeline data out of the mySQL server
# and put it in a pandas dataframe.
sql_query = 'SELECT * FROM timeline_tweets ORDER BY `Tweet ID` LIMIT ' + str(chunk_size) + ' OFFSET ' + str(i*chunk_size)
df_timeline = pd.read_sql_query(sql_query,engine)
if len(df_timeline.index)<1:
break
# Stem the Tweet column
def stem(s):
return preprocessing.preprocess_pipeline(s, return_as_str=True, do_remove_stopwords=True, do_clean_html=False)
df_timeline['Tweet']=df_timeline['Tweet'].str.decode('utf-8').str.encode('ascii', errors='ignore').apply(stem)
# Save the data on my mySQL server
df_timeline.to_sql('timeline_tweets_stemmed', engine, if_exists='append', index=False)
i += 1
print datetime.datetime.today().__str__()
print 'chunk size:', chunk_size
print 'chunks processed:', i
end_time = time.clock()
print 'runtime:', (end_time - start_time) / 60, 'minutes'
<file_sep>"""Run this file every 15 minutes until all timeline data is pulled
from Twitter. The process will take about about 12 hours."""
from twython import Twython
import pandas as pd
import re
import matplotlib as plt
from sqlalchemy import create_engine
from sqlalchemy_utils import database_exists, create_database
import datetime
from mysql_login_info import sql_username, sql_password
from twitter_keys import APP_KEY, APP_SECRET, OAUTH_TOKEN, OAUTH_TOKEN_SECRET
# Print log file entry
print ''
print datetime.datetime.today().__str__()
# Twitter authentication
twitter = Twython(APP_KEY, APP_SECRET,
OAUTH_TOKEN, OAUTH_TOKEN_SECRET)
# connect to my local mySQL server and open the vacation database
engine = create_engine('mysql+mysqldb://'+sql_username+':'+sql_password+'@127.0.0.1:3306/vacation', echo=False)
# Pull the vacation tweet data out of the mySQL server
# and put in in the original dataframe.
sql_query = 'SELECT * FROM vacation_tweets_w_name'
df_both = pd.read_sql_query(sql_query,engine).sort('Tweet ID')
print len(df_both.index), 'total user timelines to process'
df_both.head(2)
# Get the chron progress from the dataframe
sql_query = 'SELECT * FROM chron_progress'
df_progress = pd.read_sql_query(sql_query,engine)
progress = df_progress['Progress'][0]
# Filter out the vacation tweet data that has already been processed
df_both = df_both[df_both['Tweet ID']>progress].sort('Tweet ID')
print len(df_both.index), 'user timelines left to process'
df_both.head(2)
# Get the first 85 users' timelines
# count=200 is the maximum allowed amount
# 180 of such requests are allowed per 15 minutes
timelines = []
length = len(df_both['Screen Name'].values)
users_skipped = 0
user_timelines_to_query = 85
queries_per_timeline = 2
for i in range(user_timelines_to_query):
if i >= length:
break
screen_name = df_both.iloc[i]['Screen Name']
try:
next_timeline = twitter.get_user_timeline(screen_name=screen_name, count=200)
except Exception as e:
print 'exception at query', i
print e
#break
print 'skipping user', screen_name
users_skipped += 1
continue
current_user_timeline=[next_timeline]
for j in range(1, queries_per_timeline):
if len(current_user_timeline[-1])<200:
break
max_id=min([status['id'] for status in current_user_timeline[-1]])-1
try:
next_timeline = twitter.get_user_timeline(screen_name=screen_name, count=200, max_id=max_id)
except:
break
current_user_timeline.append(next_timeline)
timelines.append(current_user_timeline)
progress = df_both.iloc[i]['Tweet ID']
print i+1-users_skipped, 'user timelines processed'
print users_skipped, 'user timelines skipped'
print len(df_both.index) - i - 1, 'user timelines left to process'
# Put the timeline tweets in a dataframe
time_timeline = []
time_texts = []
time_ids = []
time_times = []
time_destinations = []
time_names = []
time_screen_names = []
for i in range(len(timelines)):
screen_name = df_both.iloc[i]['Screen Name']
destination = df_both.iloc[i]['Destination']
for user_timeline in timelines[i]:
for tweet in user_timeline:
time_timeline.append(screen_name)
time_texts.append(tweet['text'])
time_ids.append(tweet['id'])
time_times.append(tweet['created_at'])
time_destinations.append(destination)
time_names.append(tweet['user']['name'])
time_screen_names.append(tweet['user']['screen_name'])
df_timeline = pd.DataFrame({'Timeline': time_timeline,
'Tweet': time_texts,
'Tweet ID': time_ids,
'Time': time_times,
'Destination': time_destinations,
'Full Name': time_names,
'Screen Name': time_screen_names
})
# Change the character encoding to utf-8.
# MySQL will complain otherwise.
for col in df_timeline.columns.values:
if df_timeline[col].dtype=='object':
df_timeline[col]=df_timeline[col].str.encode('utf-8', errors='ignore')
# Save the timelines to my mySQL server
df_timeline.to_sql('timeline_tweets', engine, if_exists='append', index=False)
# put the progress in a dataframe
df_progress = pd.DataFrame({'Progress':[progress]})
# copy the progress dataframe to the MySQL database
df_progress.to_sql('chron_progress', engine, if_exists='replace', index=False)
# Print the number of tweets processed
print len(df_timeline.index), 'tweets processed'
# Print the time remaining, assuming
# 85 timelines collected 3 times every hour
print '{0:.1f} hours remain'.format(len(df_both.index) * 1.0 / 85 / 3)
<file_sep>"""This dummy version of get_rankings imports pre-computed rankings
from the MySQL server and is used for testing purposes."""
# packages
import re
import time
import pandas as pd
import pymysql as mdb
from sqlalchemy import create_engine
# files in this directory
from mysql_login_info import sql_username, sql_password
import preprocessing
def get_rankings_dummy():
# Connect to my local mySQL server and open the vacation database
engine = create_engine('mysql+mysqldb://'+sql_username+':'+sql_password+'@127.0.0.1:3306/vacation', echo=False)
# Pull the pre-computed rankings out of MySQL
sql_query = 'SELECT * FROM interest_destination_dummy'
df_interest_destination = pd.read_sql_query(sql_query,engine)
# Put the pre-computed rankings in table format
interest_col = df_interest_destination['Interest'].values.tolist()
destination_col = df_interest_destination['Destination'].values.tolist()
interest_destination = []
length = len(interest_col)
for i in range(length):
interest_destination.append([destination_col[i],interest_col[i]])
return interest_destination
<file_sep>"""This is the 'main' part of my app. Flask uses it generate the
HTML on my website."""
# packages
from flask import render_template, request
from flask import Flask
import time
# files in this directory
import get_rankings
import get_rankings_dummy
app = Flask(__name__)
@app.route('/')
@app.route('/index')
@app.route('/input')
def cities_input():
return render_template("input.html")
@app.route('/output')
def cities_output():
interests = request.args.get('ID') # pull interests from the input page
start = time.time()
# If the user inputs the string 'cached_results', then the app will return
# cached results for boats, surfing, kayaking, and swimming. This is for
# testing and demonstration purposes only.
if interests == 'cached_results':
interests = 'boats, surfing, kayaking, swimming'
rankings = get_rankings_dummy.get_rankings_dummy()
else:
rankings = get_rankings.get_rankings(interests)
end = time.time()
print 'runtime', end-start, 'seconds'
length = min(20,len(rankings))
return render_template("output.html", rankings = rankings, interests=interests, length=length, runtime=end-start)
@app.route('/slides')
def slides():
return render_template("slides.html")
if __name__ == "__main__":
app.run(host='0.0.0.0', port=5000)
<file_sep>"""This initializes the chron_progress table in my MySQL database
to zero so that I can then use timeline_chron.py to begin pulling Twitter
users' Timelines from Twitter every 15 minutes. Each time that
timeline_chron.py runs, it will update the chron_progress table in MySQL."""
from twython import Twython
import pandas as pd
import re
import matplotlib as plt
from sqlalchemy import create_engine
from sqlalchemy_utils import database_exists, create_database
import datetime
from mysql_login_info import sql_username, sql_password
from twitter_keys import APP_KEY, APP_SECRET, OAUTH_TOKEN, OAUTH_TOKEN_SECRET
# connect to my local mySQL server and open the vacation database
engine = create_engine('mysql+mysqldb://'+sql_username+':'+sql_password+'@127.0.0.1:3306/vacation', echo=False)
# initialize progress to zero
df_progress = pd.DataFrame({'Progress':[0]})
# copy the progress to the database
df_progress.to_sql('chron_progress', engine, if_exists='replace', index=False)
<file_sep>Vacation Finder
by <NAME>
This web app uses Twitter data to recommend vacation destinations based on the user's interests and can be found at http://www.vacationfinder.xyz. It comes preloaded with 40 destinations and the Twitter timelines of Twitter users who have tweeted about going to these destinations on vacation. When the user of the app inputs interests, the app searches the stored Twitter timelines for those interests. The current data set contains about one million tweets.
Scripts in the data_collection folder were used to gather the Twitter data and store it in a MySQL database.
Files in the webapp folder are used to run the app.
The app uses Python, MySQL, Flask, and Bootstrap.
<file_sep>"""This will create a timeline_tweets_stemmed_unique table in MySQL that contains
all unique tweets from the timeline_tweets_stemmed table."""
import pandas as pd
import re
import matplotlib as plt
from sqlalchemy import create_engine
from sqlalchemy_utils import database_exists, create_database
import numpy as np
import time
from mysql_login_info import sql_username, sql_password
import preprocessing
# connect to my local mySQL server and open the vacation database
engine = create_engine('mysql+mysqldb://'+sql_username+':'+sql_password+'@127.0.0.1:3306/vacation', echo=False)
# Pull the timeline data out of the mySQL server
# and put in in the original dataframe.
sql_query = 'SELECT * FROM timeline_tweets_stemmed GROUP BY `Tweet ID`, Destination'
df_timeline = pd.read_sql_query(sql_query,engine)
print len(df_timeline.index), 'rows'
df_timeline.head(2)
# Save the data on my mySQL server
if False:
chunk_size = 10000
chunk = 0
while True:
df_chunk = df_timeline.iloc[chunk*chunk_size:(chunk + 1)*chunk_size]
if len(df_chunk.index) < 1:
break
df_chunk.to_sql('timeline_tweets_stemmed_unique', engine, if_exists='append', index=False)
chunk += 1
print chunk, 'chunks completed'
print 'task completed'
| 78b6f013d6f2214b2c38890bc365d9bbf6eb5d9e | [
"Python",
"Text"
] | 8 | Python | ktaliaferro/VacationFinder | 594c802d7d250aece3919028f31863b54e1bfc75 | 062e25c45ec2e2e3d808a0d39c060bdc67167789 | |
refs/heads/master | <repo_name>baldurk/rdoc_serialise_test<file_sep>/main.cpp
#include "serialiser.h"
#include "test_types.h"
#include "xml_codec.h"
#include <time.h>
const char *GetChunkName(uint32_t i)
{
// dummy function
return "main()";
}
struct struct1_pure_pod
{
int x[10000];
};
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, struct1_pure_pod &el)
{
ser.Serialise("el.x", el.x);
}
INSTANTIATE_SERIALISE_TYPE(struct1_pure_pod);
void perftests()
{
struct1_pure_pod foo = { };
double sum = 0.0;
const uint64_t runs = 10;
WriteSerialiser *ser = new WriteSerialiser(new StreamWriter(10000*10000*4 + 1024));
for(uint64_t run=0; run < runs; run++)
{
ser->GetWriter()->Rewind();
PerformanceTimer timer;
for(uint64_t i=0; i < 10000; i++)
ser->Serialise("foo", foo);
double d = timer.GetMilliseconds();
sum += d;
printf("time %u: %f\n", (uint32_t)run, d);
}
printf("avg: %f\n", sum / double(runs));
}
#ifdef WIN32
#include <direct.h>
#define getcwd _getcwd
#endif
#ifndef MAX_PATH
#define MAX_PATH 260
#endif
void real_world_test_read();
void real_world_test_write();
void real_world_test_xml2rdc();
void real_world_test_read2();
void streamio_test();
int main(int argc, char **argv)
{
char workdir[MAX_PATH+1] = {};
getcwd(workdir, MAX_PATH);
srand(time(NULL));
printf("Working Dir: %s\n\n", workdir);
printf("%d args:\n", argc);
for(int i=0; i < argc; i++)
printf("[%d]: %s\n", i, argv[i]);
WriteSerialiser *write = new WriteSerialiser(new StreamWriter(StreamWriter::DefaultScratchSize));
ResourceManager resourcemanager;
write->SetUserData(&resourcemanager);
size_t bufferSize = 200*1024;
int array_of_data[5] = { 0, 1, 2, 3, 4 };
const char *exts[] = { "VK_foo", "VK_bar" };
VulkanHandleThingy vulkan; vulkan.handle = 1818;
ID3DInterface *d3d; d3d = (ID3DInterface *)&write;
{
simpleStruct a;
complexStruct b;
b.vectorArray.resize(10);
podStruct c;
byte *buffer = new byte[bufferSize];
memset(buffer, 0x54, bufferSize);
b.numExts = ARRAY_COUNT(exts);
b.exts = exts;
b.cstring = (char *)"blah blah !";
const int *const_array_ptr = array_of_data;
const int *const_array_ptr2 = array_of_data;
int *array_ptr = array_of_data;
const float cfloat = 3.141f;
ResourceId d;
WriteSerialiser &ser = *write;
VulkanHandleThingy multivulkan[8];
for(int i=0; i < 8; i++) multivulkan[i].handle = rand();
SCOPED_SERIALISE_CONTEXT(5, 205*1024);
SERIALISE_ELEMENT(a);
SERIALISE_ELEMENT(b);
SERIALISE_ELEMENT(c);
SERIALISE_ELEMENT(d);
SERIALISE_ELEMENT_ARRAY(buffer, bufferSize);
SERIALISE_ELEMENT_ARRAY(const_array_ptr, FIXED_COUNT(5));
SERIALISE_ELEMENT_ARRAY(const_array_ptr2, FIXED_COUNT(5));
SERIALISE_ELEMENT_ARRAY(array_ptr, FIXED_COUNT(5));
SERIALISE_ELEMENT(cfloat);
SERIALISE_ELEMENT(vulkan);
SERIALISE_ELEMENT(d3d);
SERIALISE_ELEMENT(multivulkan);
SAFE_DELETE_ARRAY(buffer);
}
StructReadSerialiser *read = new StructReadSerialiser(new StreamReader(write->GetWriter()->GetData(), write->GetWriter()->GetOffset()));
read->ConfigureStructuredExport(&GetChunkName, false);
read->SetUserData(&resourcemanager);
{
simpleStruct a;
complexStruct b;
podStruct c;
ResourceId d;
byte *buffer = (byte *)0xbaadf00d;
const int *const_array_ptr = NULL;
const int *const_array_ptr2 = NULL;
int *array_ptr = (int *)0xbadf00d;
float cfloat = 0.0f;
VulkanHandleThingy multivulkan[8];
a.Empty();
b.Empty();
c.Empty();
d.innerSecretValue = 0;
StructReadSerialiser &ser = *read;
uint32_t chunk = read->BeginChunk(0);
SERIALISE_ELEMENT(a);
SERIALISE_ELEMENT(b);
SERIALISE_ELEMENT(c);
SERIALISE_ELEMENT(d);
SERIALISE_ELEMENT_ARRAY(buffer, bufferSize);
SERIALISE_ELEMENT_ARRAY(const_array_ptr, FIXED_COUNT(5));
SERIALISE_ELEMENT_ARRAY(const_array_ptr2, FIXED_COUNT(5));
SERIALISE_ELEMENT_ARRAY(array_ptr, FIXED_COUNT(5));
SERIALISE_ELEMENT(cfloat);
SERIALISE_ELEMENT(vulkan);
SERIALISE_ELEMENT(d3d);
SERIALISE_ELEMENT(multivulkan);
read->EndChunk();
}
std::string xml = Structured2XML(read->GetSerialisedChunks());
//puts("xml:\n\n");
//puts(xml.c_str());
delete write;
delete read;
real_world_test_write();
real_world_test_read();
real_world_test_xml2rdc();
real_world_test_read2();
streamio_test();
return 0;
}<file_sep>/stringize.h
/******************************************************************************
* The MIT License (MIT)
*
* Copyright (c) 2016 <NAME>
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
******************************************************************************/
#pragma once
#include <string>
#include <type_traits>
template <typename T>
std::string DoStringize(const T &el);
template <typename T, bool is_pointer = std::is_pointer<T>::value>
struct StringConverter
{
static std::string Do(const T &el)
{
return DoStringize<T>(el);
}
};
template <typename T>
struct StringConverter<T, true>
{
static std::string Do(const T &el)
{
return DoStringize<void *>(el);
}
};
template <typename T>
std::string ToStr(const T &el)
{
return StringConverter<T>::Do(el);
}
// helper macros for common enum switch
#define BEGIN_ENUM_STRINGIZE() \
switch(el) { default: break;
// stringize the parameter
#define STRINGIZE_ENUM(a) \
case a: return #a;
#define STRINGIZE_ENUM_NAMED(value, str) \
case value: return str;
#define END_ENUM_STRINGIZE(type) \
} return StringFormat::Fmt(#type "<%u>", el);
// helper macros for common bitfield check-and-append
#define BEGIN_BITFIELD_STRINGIZE() \
std::string ret;
#define STRINGIZE_BITFIELD_NAMED(b) \
if(el == b) return #b;
#define STRINGIZE_BITFIELD(b, str) \
if(el == b) return str;
#define STRINGIZE_BITFIELD_BIT(b) \
if(el & b) ret += " | " #b;
#define STRINGIZE_BITFIELD_BIT_NAMED(b, str) \
if(el & b) ret += " | " str;
#define END_BITFIELD_STRINGIZE() \
if(!ret.empty()) { ret = ret.substr(3); } return ret;
template <typename T>
class GetSerialiseTypeName
{
public:
static const char *Name();
};
#define SERIALISE_ENUM_TYPE(type) \
template <> \
const char *GetSerialiseTypeName<type>::Name() \
{ \
return #type; \
}
<file_sep>/test_types.h
#pragma once
#include "serialiser.h"
#include <map>
using std::vector;
using std::map;
struct ResourceId
{
ResourceId() : innerSecretValue(cur++) {}
bool operator < (const ResourceId &o) const
{
return innerSecretValue < o.innerSecretValue;
}
uint64_t innerSecretValue;
static uint64_t cur;
};
DECLARE_SERIALISE_TYPE(ResourceId);
struct VulkanHandleThingy
{
uint64_t handle;
};
DECLARE_SERIALISE_TYPE(VulkanHandleThingy);
struct ID3DInterface;
DECLARE_SERIALISE_TYPE(ID3DInterface*);
struct ResourceManager
{
std::map<ResourceId, ID3DInterface*> d3d;
std::map<ResourceId, VulkanHandleThingy> vk;
};
struct podStruct
{
int x, y, width, height;
podStruct() : x(5), y(5), width(10), height(20) {}
void Empty()
{
x = y = width = height = 0;
}
};
DECLARE_SERIALISE_TYPE(podStruct);
enum blah
{
blah_foo,
blah_bar,
blah_qux,
};
struct simpleStruct
{
int foo;
float bar;
string hello;
uint32_t really_a_blah;
podStruct secret;
simpleStruct() : foo(5), bar(2.3f), hello("hello"), really_a_blah(blah_qux) {}
void Empty()
{
foo = 0;
bar = 0.0f;
hello = "";
really_a_blah = 999;
secret.Empty();
}
};
DECLARE_SERIALISE_TYPE(simpleStruct);
struct complexStruct
{
simpleStruct basicArray[5];
vector<simpleStruct> vectorArray;
simpleStruct secret;
uint32_t numExts;
const char **exts;
char *cstring;
complexStruct() : numExts(0), exts(NULL), cstring(NULL) {}
void Empty()
{
for(size_t i=0; i < ARRAY_COUNT(basicArray); i++)
basicArray[i].Empty();
vectorArray.clear();
secret.Empty();
numExts = 0;
exts = NULL;
}
};
DECLARE_SERIALISE_TYPE(complexStruct);
DECLARE_DESERIALISE_TYPE(complexStruct);
<file_sep>/json_convert.cpp
#include "json_convert.h"
#include "common.h"
#include "string_utils.h"
#include "structured_data.h"
#include "miniz.h"
#include "picojson.h"
static std::string EncodeString(std::string str)
{
size_t i = 0;
do
{
i = str.find_first_of("\"\\\r\n\t", i);
if(i == std::string::npos)
break;
if(str[i] == '\n') str[i] = 'n';
if(str[i] == '\r') str[i] = 'r';
if(str[i] == '\t') str[i] = 't';
str.insert(str.begin()+i, '\\');i += 2;
} while(true);
return '"' + str + '"';
}
static std::string EncodeType(const SerialisedType &type)
{
const char *typeName = "unknown";
switch(type.basetype)
{
case SerialisedType::eChunk:
typeName = "chunk";
break;
case SerialisedType::eStruct:
typeName = "struct";
break;
case SerialisedType::eArray:
typeName = "array";
break;
case SerialisedType::eNull:
typeName = "null";
break;
case SerialisedType::eBuffer:
typeName = "buffer";
break;
case SerialisedType::eString:
typeName = "string";
break;
case SerialisedType::eEnum:
typeName = "enum";
break;
case SerialisedType::eUnsignedInteger:
typeName = "uint";
break;
case SerialisedType::eSignedInteger:
typeName = "sint";
break;
case SerialisedType::eFloat:
typeName = "float";
break;
case SerialisedType::eBoolean:
typeName = "bool";
break;
case SerialisedType::eCharacter:
typeName = "char";
break;
}
std::string name = (type.name == NULL || type.name[0] == '\0') ? typeName : type.name;
const char *nullable = (type.flags & SerialisedType::Nullable) ? "true" : "false";
return StringFormat::Fmt("{ \"baseType\": \"%s\", \"nullable\": %s, \"name\": %s, \"width\": %u }", typeName, nullable, EncodeString(name).c_str(), type.byteSize);
}
static std::string DumpObject(size_t indent, bool isArray, const SerialisedObject &obj, bool humanReadable)
{
const bool machineReadable = !humanReadable;
int conciseIndentOffset = (humanReadable ? 0 : 1);
const std::string ind(indent, '\t');
const std::string indVal(indent + conciseIndentOffset, '\t');
std::string ret;
for(size_t i=0; i < obj.data.children.size(); i++)
{
const SerialisedObject &child = *obj.data.children[i];
if(isArray)
ret += ind;
else
ret += ind + EncodeString(child.name) + ": ";
bool customString = (child.type.flags & SerialisedType::HasCustomString);
if(machineReadable)
{
ret += "{\n";
ret += indVal + "\"type\": " + EncodeType(child.type) + ",\n";
ret += indVal + "\"name\": " + EncodeString(child.name) + ",\n";
if(customString)
ret += indVal + "\"string\": " + EncodeString(child.data.str) + ",\n";
ret += indVal + "\"value\": ";
customString = false;
}
if(child.type.flags & SerialisedType::Hidden)
{
ret += "\"hidden object\"";
}
else if(child.type.basetype == SerialisedType::eEnum)
{
ret += "{ "
"\"__enum\": " + EncodeString(child.data.str) + ", "
"\"value\": " + StringFormat::Fmt("%" PRIu64, child.data.basic.u) +
" }";
}
else if(customString)
{
ret += EncodeString(child.data.str);
}
else
{
SerialisedType::BasicType t = child.type.basetype;
switch(child.type.basetype)
{
case SerialisedType::eChunk:
RDCASSERT("Should not have a chunk object!");
break;
case SerialisedType::eNull:
{
ret += humanReadable ? StringFormat::Fmt("/* %s */ null", child.type.name) : "null";
break;
}
case SerialisedType::eStruct:
{
ret += humanReadable ? StringFormat::Fmt("/* %s, %" PRIu64 " bytes */ {\n", child.type.name, child.type.byteSize) : "[\n";
ret += DumpObject(indent + 1 + conciseIndentOffset, machineReadable, child, humanReadable);
ret += indVal + (humanReadable ? "}" : "]");
break;
}
case SerialisedType::eArray:
{
ret += "[\n";
ret += DumpObject(indent + 1 + conciseIndentOffset, true, child, humanReadable);
ret += indVal + "]";
break;
}
case SerialisedType::eBuffer:
{
ret += "{ "
"\"buffername\": \"" + GetBufferName(child.data.basic.u) + "\", "
"\"length\": " + StringFormat::Fmt("%" PRIu64, child.type.byteSize) +
" }";
break;
}
case SerialisedType::eString:
ret += "\"" + child.data.str + "\"";
break;
case SerialisedType::eEnum:
RDCASSERT("enums should be handled above!");
break;
case SerialisedType::eUnsignedInteger:
ret += StringFormat::Fmt("%" PRIu64, child.data.basic.u);
break;
case SerialisedType::eSignedInteger:
ret += StringFormat::Fmt("%" PRId64, child.data.basic.i);
break;
case SerialisedType::eFloat:
ret += StringFormat::Fmt("%f", child.data.basic.d);
break;
case SerialisedType::eBoolean:
ret += (child.data.basic.b ? "true" : "false");
break;
case SerialisedType::eCharacter:
ret += "\"" + std::string(1, child.data.basic.c) + "\"";
break;
}
}
if(machineReadable)
{
ret += "\n" + ind + "}";
}
if(i+1 < obj.data.children.size())
ret += ",\n";
}
ret += "\n";
return ret;
}
std::string SerialisedFile2JSON(SerialisedFile file, bool humanReadable)
{
const bool machineReadable = !humanReadable;
std::string ret;
ret += humanReadable ? "{\n" : "[\n";
for(size_t i=0; i < file.chunks.size(); i++)
{
SerialisedObject *obj = file.chunks[i];
RDCASSERT(obj->type.basetype == SerialisedType::eChunk);
if(machineReadable)
{
ret += "\t{\n";
ret += "\t\t\"name\": " + EncodeString(obj->name) + ",\n";
}
else
{
ret += "\t" + EncodeString(obj->name) + ": {\n";
}
if(obj->chunkData)
{
ret += "\t\t\"__metadata\": {\n";
if(obj->chunkData->threadID)
ret += StringFormat::Fmt("\t\t\t\"threadID\": %u,\n", obj->chunkData->threadID);
if(obj->chunkData->timestamp)
ret += StringFormat::Fmt("\t\t\t\"timestamp\": %llu,\n", obj->chunkData->timestamp);
if(obj->chunkData->duration)
ret += StringFormat::Fmt("\t\t\t\"duration\": %llu,\n", obj->chunkData->duration);
if(obj->chunkData->callstack[0])
ret += "\t\t\t\"callstack\": \"...\",\n";
ret += StringFormat::Fmt("\t\t\t\"chunkID\": %u\n", obj->chunkData->chunkID);
ret += "\t\t},\n";
}
if(obj->data.children.size() == 1 && obj->data.children[0]->type.flags & SerialisedType::OpaqueChunk)
{
ret += "\t\t\"opaque\": { ";
ret += "\"buffername\": \"" + GetBufferName(obj->data.children[0]->data.basic.u) + "\", ";
ret += "\"length\": " + StringFormat::Fmt("%" PRIu64, obj->data.children[0]->type.byteSize);
ret += " }";
ret += "\n\t}";
}
else
{
if(machineReadable)
ret += "\t\t\"contents\": [\n";
ret += DumpObject(humanReadable ? 2 : 3, machineReadable, *obj, humanReadable);
ret += humanReadable ? StringFormat::Fmt("\t} /* %" PRIu64 " bytes */", obj->type.byteSize) : "\t\t]";
if(machineReadable)
ret += "\n\t}";
}
if(i+1 < file.chunks.size()) ret += ",";
ret += "\n";
}
ret += humanReadable ? "}\n" : "]\n";
return ret;
}
bool has(picojson::object o, const std::string &key)
{
return o.find(key) != o.end();
}
#include "serialiser.h"
std::vector<SerialisedBuffer> *bufList = NULL;
struct jsonisedStruct
{
picojson::value *val;
};
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, jsonisedStruct &el)
{
using namespace picojson;
RDCASSERT(el.val->is<array>());
array &structMembers = el.val->get<array>();
for(size_t i = 0; i < structMembers.size(); i++)
ser.Serialise("", structMembers[i]);
}
INSTANTIATE_SERIALISE_TYPE(jsonisedStruct);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, picojson::value &el)
{
using namespace picojson;
std::vector<SerialisedBuffer> &bufs = *bufList;
RDCASSERT(el.is<object>());
object &baseValue = el.get<object>();
RDCASSERT(has(baseValue, "type"));
RDCASSERT(has(baseValue, "value"));
picojson::value &t = baseValue["type"];
picojson::value &v = baseValue["value"];
RDCASSERT(t.is<object>());
object &typeData = t.get<object>();
RDCASSERT(has(typeData, "baseType"));
RDCASSERT(has(typeData, "nullable"));
RDCASSERT(has(typeData, "width"));
picojson::value &baseType = typeData["baseType"];
picojson::value &nullable = typeData["nullable"];
picojson::value &width = typeData["width"];
RDCASSERT(baseType.is<string>());
RDCASSERT(nullable.is<bool>());
RDCASSERT(width.is<int64_t>());
string &baseTypeName = baseType.get<string>();
bool isNullable = nullable.get<bool>();
int64_t byteSize = width.get<int64_t>();
if(baseTypeName == "chunk")
{
RDCERR("Did not expect chunk type");
}
else if(baseTypeName == "struct")
{
// structs are represented as arrays in json to preserve order
if(isNullable)
{
// indirect through a struct so it is serialised as nullable
jsonisedStruct s = { &v };
jsonisedStruct *ptr = &s;
ser.SerialiseNullable("", ptr);
}
else
{
RDCASSERT(v.is<array>());
array &structMembers = v.get<array>();
for(size_t i = 0; i < structMembers.size(); i++)
ser.Serialise("", structMembers[i]);
}
}
else if(baseTypeName == "array")
{
RDCASSERT(v.is<array>());
array &arrayMembers = v.get<array>();
ser.Serialise("", arrayMembers);
}
else if(baseTypeName == "null")
{
// any type will do since we're writing a marker that it's NULL
float *foo = NULL;
ser.SerialiseNullable("", foo);
}
else if(baseTypeName == "buffer")
{
RDCASSERT(v.is<object>());
object &bufferDetails = v.get<object>();
RDCASSERT(has(bufferDetails, "buffername"));
size_t fn = (size_t)strtoimax(bufferDetails["buffername"].get<string>().c_str(), NULL, 10);
if(fn < bufs.size())
{
byte *data = bufs[fn].first;
uint64_t size = bufs[fn].second;
ser.Serialise("", data, size);
}
}
else if(baseTypeName == "string")
{
RDCASSERT(v.is<string>());
string s = v.get<string>();
if(isNullable)
{
string *ptr = &s;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", s);
}
}
else if(baseTypeName == "enum")
{
RDCASSERT(v.is<object>());
object &enumdata = v.get<object>();
RDCASSERT(has(enumdata, "value"));
v = enumdata["value"];
RDCASSERT(v.is<int64_t>());
uint32_t e = (uint32_t)v.get<int64_t>();
if(isNullable)
{
uint32_t *ptr = &e;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", e);
}
}
else if(baseTypeName == "uint")
{
RDCASSERT(v.is<int64_t>());
uint64_t u = (uint64_t)v.get<int64_t>();
if(byteSize == 1)
{
uint8_t u1 = (uint8_t)u;
if(isNullable)
{
uint8_t *ptr = &u1;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", u1);
}
}
else if(byteSize == 2)
{
uint16_t u2 = (uint16_t)u;
if(isNullable)
{
uint16_t *ptr = &u2;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", u2);
}
}
else if(byteSize == 4)
{
uint32_t u4 = (uint32_t)u;
if(isNullable)
{
uint32_t *ptr = &u4;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", u4);
}
}
else if(byteSize == 8)
{
if(isNullable)
{
uint64_t *ptr = &u;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", u);
}
}
else
{
RDCERR("Unexpected byteSize for %s: %d", baseTypeName.c_str(), (int)byteSize);
}
}
else if(baseTypeName == "sint")
{
RDCASSERT(v.is<int64_t>());
int64_t i = v.get<int64_t>();
if(byteSize == 1)
{
int8_t i1 = (int8_t)i;
if(isNullable)
{
int8_t *ptr = &i1;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", i1);
}
}
else if(byteSize == 2)
{
int16_t i2 = (int16_t)i;
if(isNullable)
{
int16_t *ptr = &i2;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", i2);
}
}
else if(byteSize == 4)
{
int32_t i4 = (int32_t)i;
if(isNullable)
{
int32_t *ptr = &i4;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", i4);
}
}
else if(byteSize == 8)
{
if(isNullable)
{
int64_t *ptr = &i;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", i);
}
}
else
{
RDCERR("Unexpected byteSize for %s: %d", baseTypeName.c_str(), (int)byteSize);
}
}
else if(baseTypeName == "bool")
{
RDCASSERT(v.is<bool>());
bool b = v.get<bool>();
if(isNullable)
{
bool *ptr = &b;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", b);
}
}
else if(baseTypeName == "float")
{
RDCASSERT(v.is<double>());
double d = v.get<double>();
if(byteSize == 4)
{
float f = (float)d;
if(isNullable)
{
float *ptr = &f;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", f);
}
}
else if(byteSize == 8)
{
if(isNullable)
{
double *ptr = &d;
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", d);
}
}
else
{
RDCERR("Unexpected byteSize for %s: %d", baseTypeName.c_str(), (int)byteSize);
}
}
else if(baseTypeName == "char")
{
RDCASSERT(v.is<string>());
string s = v.get<string>();
if(byteSize == 1)
{
if(isNullable)
{
char *ptr = &s[0];
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", s[0]);
}
}
else if(byteSize == 2)
{
wstring w = L"-"; // convert from s
if(isNullable)
{
wchar_t *ptr = &w[0];
ser.SerialiseNullable("", ptr);
}
else
{
ser.Serialise("", w[0]);
}
}
else
{
RDCERR("Unexpected byteSize for %s: %d", baseTypeName.c_str(), (int)byteSize);
}
}
}
INSTANTIATE_SERIALISE_TYPE(picojson::value);
<file_sep>/Makefile
GCC=gcc
GPP=g++
CLANG=clang++
CFLAGS=-g -O0
CPP_FILES=common.cpp main.cpp serialiser.cpp string_utils.cpp test_types.cpp xml_codec.cpp real.cpp streamio.cpp lz4io.cpp zstdio.cpp
H_FILES=common.h custom_assert.h serialiser.h string_utils.h test_types.h xml_codec.h structured_data.h streamio.h lz4io.h zstdio.h
EXT=lz4/lz4.o miniz.o zstd/common/entropy_common.o zstd/common/error_private.o zstd/common/fse_decompress.o zstd/common/pool.o zstd/common/threading.o zstd/common/xxhash.o zstd/common/zstd_common.o zstd/compress/fse_compress.o zstd/compress/huf_compress.o zstd/compress/zstdmt_compress.o zstd/compress/zstd_compress.o zstd/decompress/huf_decompress.o zstd/decompress/zstd_decompress.o pugixml.o
.PHONY: all
all: gpp_bin clang_bin
pugixml.o: pugixml.cpp
$(GPP) -c pugixml.cpp -o pugixml.o
%.o : %.c
$(GCC) -c $< -o $@
gpp_bin: $(CPP_FILES) $(H_FILES) $(EXT)
$(GPP) -std=c++0x $(CFLAGS) $(CPP_FILES) -Wno-attributes -Wno-narrowing $(EXT) -o gpp_bin
cp gpp_bin /tmp
clang_bin: $(CPP_FILES) $(H_FILES) libext.a
$(CLANG) -std=c++0x $(CFLAGS) $(CPP_FILES) $(EXT) -o clang_bin
cp clang_bin /tmp
<file_sep>/xml_codec.cpp
#include "xml_codec.h"
#include "pugixml.hpp"
#include "miniz.h"
static const char *typeNames[] = {
"chunk",
"struct",
"array",
"null",
"buffer",
"string",
"enum",
"uint",
"int",
"float",
"bool",
"char",
};
template<typename inttype>
std::string GetBufferName(inttype i)
{
return StringFormat::Fmt("%06u", (uint32_t)i);
}
struct xml_string_writer: pugi::xml_writer
{
std::string str;
void write(const void* data, size_t size)
{
str.append((const char *)data, size);
}
};
static void Obj2XML(pugi::xml_node &parent, StructuredObject &child)
{
pugi::xml_node obj = parent.append_child(typeNames[child.type.basetype]);
obj.append_attribute("name") = child.name.c_str();
if(!child.type.name.empty())
obj.append_attribute("typename") = child.type.name.c_str();
if(child.type.basetype == SerialisedType::eUnsignedInteger ||
child.type.basetype == SerialisedType::eSignedInteger ||
child.type.basetype == SerialisedType::eFloat)
{
obj.append_attribute("width") = child.type.byteSize;
}
if(child.type.flags & SerialisedType::Hidden)
obj.append_attribute("hidden") = true;
if(child.type.flags & SerialisedType::Nullable)
obj.append_attribute("nullable") = true;
if(child.type.basetype == SerialisedType::eChunk)
{
RDCFATAL("Nested chunks!");
}
else if(child.type.basetype == SerialisedType::eNull)
{
// redundant
obj.remove_attribute("nullable");
}
else if(child.type.basetype == SerialisedType::eStruct || child.type.basetype == SerialisedType::eArray)
{
if(child.type.basetype == SerialisedType::eArray && !child.data.children.empty())
obj.remove_attribute("typename");
for(size_t o=0; o < child.data.children.size(); o++)
{
Obj2XML(obj, *child.data.children[o]);
if(child.type.basetype == SerialisedType::eArray)
obj.last_child().remove_attribute("name");
}
}
else if(child.type.basetype == SerialisedType::eBuffer)
{
obj.append_attribute("byteLength") = child.type.byteSize;
obj.text() = child.data.basic.u;
}
else
{
if(child.type.flags & SerialisedType::HasCustomString)
{
obj.append_attribute("string") = child.data.str.c_str();
}
switch(child.type.basetype)
{
case SerialisedType::eEnum:
case SerialisedType::eUnsignedInteger:
obj.text() = child.data.basic.u;
break;
case SerialisedType::eSignedInteger:
obj.text() = child.data.basic.i;
break;
case SerialisedType::eString:
obj.text() = child.data.str.c_str();
break;
case SerialisedType::eFloat:
obj.text() = child.data.basic.d;
break;
case SerialisedType::eBoolean:
obj.text() = child.data.basic.b;
break;
case SerialisedType::eCharacter:
{
char str[2] = { child.data.basic.c, '\0' };
obj.text().set(str);
break;
}
default:
RDCERR("Unexpected case");
}
}
}
std::string Structured2XML(const StructuredChunkList &chunks)
{
pugi::xml_document doc;
pugi::xml_node xRoot = doc.append_child("rdc");
for(size_t c=0; c < chunks.size(); c++)
{
pugi::xml_node xChunk = xRoot.append_child("chunk");
StructuredChunk *chunk = chunks[c];
xChunk.append_attribute("id") = chunk->metadata.chunkID;
xChunk.append_attribute("name") = chunk->name.c_str();
if(chunk->metadata.threadID)
xChunk.append_attribute("threadID") = chunk->metadata.threadID;
if(chunk->metadata.timestamp)
xChunk.append_attribute("timestamp") = chunk->metadata.timestamp;
if(chunk->metadata.duration)
xChunk.append_attribute("duration") = chunk->metadata.duration;
if(chunk->metadata.callstack[0])
{
pugi::xml_node stack = xChunk.append_child("callstack");
for(size_t i=0; i < ARRAY_COUNT(chunk->metadata.callstack); i++)
{
if(chunk->metadata.callstack[i] == 0)
break;
stack.append_child("address").text() = chunk->metadata.callstack[i];
}
}
if(chunk->metadata.flags & SerialisedChunkMetaData::OpaqueChunk)
{
xChunk.append_attribute("opaque") = true;
RDCASSERT(!chunk->data.children.empty());
pugi::xml_node opaque = xChunk.append_child("buffer");
opaque.append_attribute("byteLength") = chunk->data.children[0]->type.byteSize;
opaque.text() = chunk->data.children[0]->data.basic.u;
}
else
{
for(size_t o=0; o < chunk->data.children.size(); o++)
Obj2XML(xChunk, *chunk->data.children[o]);
}
}
xml_string_writer writer;
doc.save(writer);
return writer.str;
}
StructuredObject *XML2Obj(pugi::xml_node &obj)
{
StructuredObject *ret = new StructuredObject(obj.attribute("name").as_string(), obj.attribute("typename").as_string());
std::string name = obj.name();
for(size_t i = 0; i < ARRAY_COUNT(typeNames); i++)
{
if(name == typeNames[i])
{
ret->type.basetype = (SerialisedType::BasicType)i;
break;
}
}
if(ret->type.basetype == SerialisedType::eUnsignedInteger ||
ret->type.basetype == SerialisedType::eSignedInteger ||
ret->type.basetype == SerialisedType::eFloat)
{
ret->type.byteSize = obj.attribute("width").as_uint();
}
if(obj.attribute("hidden"))
ret->type.flags |= SerialisedType::Hidden;
if(obj.attribute("nullable"))
ret->type.flags |= SerialisedType::Nullable;
if(obj.attribute("typename"))
ret->type.name = obj.attribute("typename").as_string();
ret->name = obj.attribute("name").as_string();
if(ret->type.basetype == SerialisedType::eChunk)
{
RDCFATAL("Nested chunks!");
}
else if(ret->type.basetype == SerialisedType::eNull)
{
ret->type.flags |= SerialisedType::Nullable;
}
else if(ret->type.basetype == SerialisedType::eStruct || ret->type.basetype == SerialisedType::eArray)
{
for(pugi::xml_node child = obj.first_child(); child; child = child.next_sibling())
{
ret->data.children.push_back(XML2Obj(child));
if(ret->type.basetype == SerialisedType::eArray)
ret->data.children.back()->name = "$el";
}
if(ret->type.basetype == SerialisedType::eArray && !ret->data.children.empty())
ret->type.name = ret->data.children.back()->type.name;
}
else if(ret->type.basetype == SerialisedType::eBuffer)
{
ret->type.byteSize = obj.attribute("byteLength").as_ullong();
ret->data.basic.u = obj.text().as_uint();
}
else
{
if(obj.attribute("string"))
{
ret->type.flags |= SerialisedType::HasCustomString;
ret->data.str = obj.attribute("string").as_string();
}
switch(ret->type.basetype)
{
case SerialisedType::eEnum:
case SerialisedType::eUnsignedInteger:
ret->data.basic.u = obj.text().as_ullong();
break;
case SerialisedType::eSignedInteger:
ret->data.basic.i = obj.text().as_llong();
break;
case SerialisedType::eString:
ret->data.str = obj.text().as_string();
break;
case SerialisedType::eFloat:
ret->data.basic.d = obj.text().as_double();
break;
case SerialisedType::eBoolean:
ret->data.basic.b = obj.text().as_bool();
break;
case SerialisedType::eCharacter:
ret->data.basic.c = obj.text().as_string()[0];
break;
default:
RDCERR("Unexpected case");
}
}
return ret;
}
StructuredChunkList XML2Structured(const std::string &xml)
{
pugi::xml_document doc;
doc.load_string(xml.c_str());
pugi::xml_node root = doc.child("rdc");
if(!root)
{
RDCERR("Malformed document, expected rdc node");
return StructuredChunkList();
}
StructuredChunkList ret;
for(pugi::xml_node xChunk = root.first_child(); xChunk; xChunk = xChunk.next_sibling())
{
StructuredChunk *chunk = new StructuredChunk(xChunk.attribute("name").as_string(), "Chunk");
chunk->metadata.chunkID = xChunk.attribute("id").as_uint();
if(xChunk.attribute("threadID"))
chunk->metadata.threadID = xChunk.attribute("threadID").as_uint();
if(xChunk.attribute("timestamp"))
chunk->metadata.timestamp = xChunk.attribute("timestamp").as_ullong();
if(xChunk.attribute("duration"))
chunk->metadata.duration = xChunk.attribute("duration").as_ullong();
pugi::xml_node callstack = xChunk.child("callstack");
if(callstack)
{
size_t i = 0;
for(pugi::xml_node address = callstack.first_child(); address; address = address.next_sibling())
{
chunk->metadata.callstack[i] = address.text().as_ullong();
i++;
}
}
if(xChunk.attribute("opaque"))
{
pugi::xml_node opaque = xChunk.child("buffer");
chunk->metadata.flags |= SerialisedChunkMetaData::OpaqueChunk;
chunk->data.children.push_back(new StructuredObject("Opaque chunk", "Byte Buffer"));
chunk->data.children[0]->type.basetype = SerialisedType::eBuffer;
chunk->data.children[0]->type.byteSize = opaque.attribute("byteLength").as_ullong();
chunk->data.children[0]->data.basic.u = opaque.text().as_ullong();
}
else
{
for(pugi::xml_node child = xChunk.first_child(); child; child = child.next_sibling())
chunk->data.children.push_back(XML2Obj(child));
}
ret.push_back(chunk);
}
return ret;
}
void Buffers2ZIP(const char *filename, const SerialisedBufferList &buffers)
{
mz_zip_archive zip;
memset(&zip, 0, sizeof(zip));
mz_zip_writer_init_file(&zip, filename, 0);
for(size_t i = 0; i < buffers.size(); i++)
mz_zip_writer_add_mem(&zip, GetBufferName(i).c_str(), buffers[i].first, buffers[i].second, MZ_BEST_COMPRESSION);
mz_zip_writer_finalize_archive(&zip);
mz_zip_writer_end(&zip);
}
SerialisedBufferList ZIP2Buffers(const char *filename)
{
SerialisedBufferList ret;
mz_zip_archive zip;
memset(&zip, 0, sizeof(zip));
mz_bool b = mz_zip_reader_init_file(&zip, "in.zip", 0);
if(b)
{
mz_uint numfiles = mz_zip_reader_get_num_files(&zip);
ret.resize(numfiles);
for(mz_uint i = 0; i < numfiles; i++)
{
mz_zip_archive_file_stat zstat;
mz_zip_reader_file_stat(&zip, i, &zstat);
int bufname = atoi(zstat.m_filename);
size_t sz = 0;
byte *buf = (byte *)mz_zip_reader_extract_to_heap(&zip, i, &sz, 0);
if(bufname < ret.size())
{
ret[bufname].first = buf;
ret[bufname].second = (uint64_t)sz;
}
}
}
mz_zip_reader_end(&zip);
return ret;
}
<file_sep>/serialiser.h
/******************************************************************************
* The MIT License (MIT)
*
* Copyright (c) 2015-2016 <NAME>
* Copyright (c) 2014 Crytek
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
******************************************************************************/
#pragma once
#include <list>
#include <set>
#include <string>
#include <vector>
//#include "api/replay/basic_types.h"
#include "common.h"
#include "structured_data.h"
#include "streamio.h"
#include "stringize.h"
//#include "os/os_specific.h"
//#include "replay/type_helpers.h"
using std::string;
/////////////////////////////////////////////////////////////////////////////
// main serialisation templated function that is specialised for any type
// that needs to be serialised. Implementations will either be a struct type,
// in which case they'll call Serialise() on each of their members, or
// they'll be some custom leaf type (most basic types are specialised in this
// header) which will call SerialiseValue().
template <class SerialiserType, class T>
void DoSerialise(SerialiserType &ser, T &el);
// function to deallocate anything from a serialise. Default impl
// does no deallocation of anything.
template <class T>
void Deserialise(const T &el)
{
}
#define DECLARE_SERIALISE_TYPE(type) \
template <class SerialiserType> \
void DoSerialise(SerialiserType &ser, type &el);
#define DECLARE_DESERIALISE_TYPE(type) \
template <> \
void Deserialise(const type &el);
// this is definitely the ugliest hack here, but necessary. We don't want to
// have all of our DoSerialise functions defined in global cross-project
// headers and compiled everywhere, we just want to define them in .cpp files.
// However because they are template specializations, if they're not
// instantiated in that same file then the compiler will skip them and not
// include them in the build, so elsewhere we'll get undefined symbol errors.
// This code forces them to be included in a global variable's constructor,
// because it can't optimise out the volatile int check. But in reality it
// will always be 0 so we spend a couple of instructions per type on load.
// It's obviously not ideal, but it's the best compromise.
#define INSTANTIATE_SERIALISE_TYPE(type) \
template <> \
const char *GetSerialiseTypeName<type>::Name() \
{ \
return #type; \
} \
namespace { struct CONCAT(Instantiator, __LINE__) \
{ \
CONCAT(Instantiator, __LINE__)() \
{ \
volatile int dummy = 0; type *el = NULL; \
WriteSerialiser *a = NULL; ReadSerialiser *b = NULL; StructReadSerialiser *c = NULL; \
void (*do_a)(decltype(*a) &, type &) = NULL; \
void (*do_b)(decltype(*b) &, type &) = NULL; \
void (*do_c)(decltype(*c) &, type &) = NULL; \
if(dummy) \
{ \
do_a = (decltype(do_a))(&DoSerialise); \
do_b = (decltype(do_b))(&DoSerialise); \
do_c = (decltype(do_c))(&DoSerialise); \
} \
if(dummy) \
{ \
do_a(*a, *el); \
do_b(*b, *el); \
do_c(*c, *el); \
} \
} \
} CONCAT(dummy, __LINE__); }
typedef const char *(*ChunkLookup)(uint32_t chunkType);
template <class SerialiserType>
class ScopedContext;
// holds the memory, length and type for a given chunk, so that it can be
// passed around and moved between owners before being serialised out
class Chunk
{
public:
~Chunk();
byte *GetData() { return m_Data; }
uint32_t GetLength() { return m_Length; }
uint32_t GetChunkType() { return m_ChunkType; }
bool IsAligned() { return m_AlignedData; }
bool IsTemporary() { return m_Temporary; }
#if !defined(RELEASE)
static uint64_t NumLiveChunks() { return m_LiveChunks; }
static uint64_t TotalMem() { return m_TotalMem; }
#else
static uint64_t NumLiveChunks() { return 0; }
static uint64_t TotalMem() { return 0; }
#endif
// grab current contents of the serialiser into this chunk
template<class SerialiserType>
Chunk(SerialiserType &ser, uint32_t chunkType, bool temporary)
{
m_Length = (uint32_t)ser.GetWriter()->GetOffset();
RDCASSERT(ser.GetWriter()->GetOffset() < 0xffffffff);
m_ChunkType = chunkType;
m_Temporary = temporary;
if(ser.HasAlignedData())
{
m_Data = AllocAlignedBuffer(m_Length);
m_AlignedData = true;
}
else
{
m_Data = new byte[m_Length];
m_AlignedData = false;
}
memcpy(m_Data, ser.GetWriter()->GetData(), m_Length);
ser.GetWriter()->Rewind();
}
private:
// no copy semantics
Chunk(const Chunk &);
Chunk &operator=(const Chunk &);
template <class SerialiserType>
friend class ScopedContext;
bool m_AlignedData;
bool m_Temporary;
uint32_t m_ChunkType;
uint32_t m_Length;
byte *m_Data;
#if !defined(RELEASE)
static int64_t m_LiveChunks, m_MaxChunks, m_TotalMem;
#endif
};
struct SerialiserFlags
{
enum Flags
{
None = 0,
AllocateMemory = 0x1,
};
};
enum ContainerError
{
eContError_None = 0,
eContError_FileIO,
eContError_Corrupt,
eContError_UnsupportedVersion,
};
enum SectionFlags
{
eSectionFlag_None = 0x0,
eSectionFlag_ASCIIStored = 0x1,
eSectionFlag_LZ4Compressed = 0x2,
eSectionFlag_ZstdCompressed = 0x4,
};
enum SectionType
{
eSectionType_Unknown = 0,
eSectionType_FrameCapture, // renderdoc/internal/framecapture
eSectionType_ResolveDatabase, // renderdoc/internal/resolvedb
eSectionType_FrameBookmarks, // renderdoc/ui/bookmarks
eSectionType_Notes, // renderdoc/ui/notes
eSectionType_Num,
};
// this class has a few functions. It can be used to serialise chunks - on writing it enforces
// that we only ever write a single chunk, then pull out the data into a Chunk class and erase
// the contents of the serialiser ready to serialise the next (see the RDCASSERT at the start
// of BeginChunk).
//
// We use this functionality for sending and receiving data across the network as well as saving
// out to the capture logfile format.
//
// It's also used on reading where it will contain the stream of chunks that were written out
// to the logfile on capture.
//
// When reading, the Serialiser allocates a window of memory and scans through the file by reading
// data into that window and moving along through the file. The window will expand to accomodate
// whichever is the biggest single element within a chunk that's read (so that you can always
// guarantee
// while reading that the element you're interested in is always in memory).
struct SerialiserType
{
enum Types
{
Writing,
Reading,
StructuredReading,
};
};
class RDOCFile
{
public:
// opens a file either to write into, or read from
RDOCFile(const char *path, SerialiserType::Types mode);
bool HasError();
ContainerError ErrorCode();
bool HasSection(SectionType type);
void *GetSection(SectionType type);
void *AddSection(SectionType type);
};
struct CompressedFileIO;
template<SerialiserType::Types sertype>
class Serialiser
{
public:
const bool IsReading() const { return sertype != SerialiserType::Writing; }
const bool IsWriting() const { return sertype == SerialiserType::Writing; }
const bool ExportStructure() const { return sertype == SerialiserType::StructuredReading && !m_InternalElement; }
enum ChunkFlags
{
ChunkIndexMask = 0x0000ffff,
ChunkCallstack = 0x00010000,
ChunkThreadID = 0x00020000,
ChunkDuration = 0x00040000,
ChunkTimestamp = 0x00080000,
};
enum
{
BufferAlignment = 64,
};
// version number of overall file format or chunk organisation. If the contents/meaning/order of
// chunks have changed this does not need to be bumped, there are version numbers within each
// API that interprets the stream that can be bumped.
static const uint64_t SERIALISE_VERSION = 0x00000032;
//////////////////////////////////////////
// Init and error handling
Serialiser(StreamWriter *writer);
Serialiser(StreamReader *reader);
~Serialiser();
StreamWriter *GetWriter() { return m_Write; }
StreamReader *GetReader() { return m_Read; }
bool HasError() { return m_HasError; }
ContainerError ErrorCode() { return m_ErrorCode; }
void SetChunkMetadataRecording(uint32_t flags);
SerialisedChunkMetaData &ChunkMetadata() { return m_ChunkMetadata; }
//////////////////////////////////////////
// Utility functions
void *GetUserData() { return m_pUserData; }
void SetUserData(void *userData) { m_pUserData = userData; }
bool HasAlignedData() { return m_AlignedData; }
// jumps to the byte after the current chunk, can be called any time after BeginChunk
void SkipCurrentChunk();
void InitCallstackResolver();
bool HasCallstacks() { return m_KnownSections[eSectionType_ResolveDatabase] != NULL; }
const StructuredChunkList &GetSerialisedChunks()
{
return m_StructuredData;
}
const SerialisedBufferList &GetSerialisedBuffers()
{
return m_StructuredDataBuffers;
}
void WriteSerialisedFile(Serialiser &fileser, SerialisedFile file);
//////////////////////////////////////////
// Public serialisation interface
void ConfigureStructuredExport(ChunkLookup lookup, bool includeBuffers)
{
RDCASSERT(lookup);
if(lookup)
{
m_ExportBuffers = includeBuffers;
m_ChunkLookup = lookup;
}
}
uint32_t BeginChunk(uint32_t chunkID, uint32_t byteLength = 0);
void EndChunk();
// Write a chunk to disk
void Insert(Chunk *el);
void FlushToDisk();
/////////////////////////////////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////
// Templated serialisation functions
// Users of the serialisation call Serialise() on the objects they want
// to serialise
// for structure type members or external use, main entry point
template <typename T>
void SerialiseStringify(const T el)
{
if(ExportStructure())
{
m_StructureStack.back()->data.str = ToStr(el);
m_StructureStack.back()->type.flags |= SerialisedType::HasCustomString;
}
}
template <class SerialiserType, typename T, bool isEnum = std::is_enum<T>::value>
struct SerialiseDispatch
{
static void Do(SerialiserType &ser, T &el)
{
DoSerialise(ser, el);
}
};
template <class SerialiserType, typename T>
struct SerialiseDispatch<SerialiserType, T, true>
{
static void Do(SerialiserType &ser, T &el)
{
RDCCOMPILE_ASSERT(sizeof(T) == sizeof(uint32_t), "enum isn't 32-bit");
ser.SerialiseValue(SerialisedType::eEnum, sizeof(T), el);
ser.SerialiseStringify(el);
}
};
// serialise an object (either loose, or a structure member).
template <class T>
Serialiser &Serialise(const char *name, T &el, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
if(ExportStructure())
{
if(m_StructureStack.empty())
{
RDCERR("Serialising object outside of chunk context! Call BeginChunk before any Serialise!");
return *this;
}
StructuredObject ¤t = *m_StructureStack.back();
current.data.basic.numChildren++;
current.data.children.push_back(new StructuredObject(name, GetSerialiseTypeName<T>::Name()));
m_StructureStack.push_back(current.data.children.back());
StructuredObject &obj = *m_StructureStack.back();
obj.type.byteSize = sizeof(T);
}
SerialiseDispatch<Serialiser, T>::Do(*this, el);
if(ExportStructure())
m_StructureStack.pop_back();
return *this;
}
// special function for serialising buffers
Serialiser &Serialise(const char *name, byte *&el, uint64_t &byteSize, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
uint64_t count = byteSize;
// silently handle NULL buffers
if(IsWriting() && el == NULL)
count = 0;
{
m_InternalElement = true;
DoSerialise(*this, count);
m_InternalElement = false;
}
if(IsReading())
byteSize = count;
if(ExportStructure())
{
if(m_StructureStack.empty())
{
RDCERR("Serialising object outside of chunk context! Call BeginChunk before any Serialise!");
return *this;
}
StructuredObject ¤t = *m_StructureStack.back();
current.data.basic.numChildren++;
current.data.children.push_back(new StructuredObject(name, "Byte Buffer"));
m_StructureStack.push_back(current.data.children.back());
StructuredObject &obj = *m_StructureStack.back();
obj.type.basetype = SerialisedType::eBuffer;
obj.type.byteSize = byteSize;
}
{
if(IsWriting())
{
// ensure byte alignment
m_Write->AlignTo<BufferAlignment>();
m_AlignedData = true;
m_Write->Write(el, byteSize);
}
else if(IsReading())
{
// ensure byte alignment
m_Read->AlignTo<BufferAlignment>();
if(flags & SerialiserFlags::AllocateMemory)
el = AllocAlignedBuffer(byteSize);
m_Read->Read(el, byteSize);
}
}
// if we want byte data associated with SerialisedObject somewhere,
// here's where we'd also copy byteSize bytes from el elsewhere.
if(ExportStructure())
{
if(m_ExportBuffers)
{
StructuredObject &obj = *m_StructureStack.back();
obj.data.basic.u = m_StructuredDataBuffers.size();
byte *alloc = new byte[byteSize];
memcpy(alloc, el, byteSize);
m_StructuredDataBuffers.push_back(std::make_pair(alloc, byteSize));
}
m_StructureStack.pop_back();
}
return *this;
}
Serialiser &Serialise(const char *name, byte *&el, uint32_t &byteSize, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
uint64_t upcastSize = byteSize;
Serialise(name, el, byteSize, flags);
if(IsReading())
byteSize = (uint32_t)upcastSize;
return *this;
}
Serialiser &Serialise(const char *name, void *&el, uint64_t &byteSize, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
return Serialise(name, (byte*&)el, byteSize, flags);
}
Serialiser &Serialise(const char *name, const void *&el, uint64_t &byteSize, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
return Serialise(name, (byte*&)el, byteSize, flags);
}
Serialiser &Serialise(const char *name, void *&el, uint32_t &byteSize, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
return Serialise(name, (byte*&)el, byteSize, flags);
}
Serialiser &Serialise(const char *name, const void *&el, uint32_t &byteSize, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
return Serialise(name, (byte*&)el, byteSize, flags);
}
// serialise a fixed array like foo[4];
// never needs to allocate, just needs to be iterated
template <class T, size_t N>
Serialiser &Serialise(const char *name, T (&el)[N], SerialiserFlags::Flags flags = SerialiserFlags::None)
{
// for consistency with other arrays, even though this is redundant, we serialise out and in the size
{
uint64_t count = N;
m_InternalElement = true;
DoSerialise(*this, count);
m_InternalElement = false;
}
if(ExportStructure())
{
if(m_StructureStack.empty())
{
RDCERR("Serialising object outside of chunk context! Call BeginChunk before any Serialise!");
return *this;
}
StructuredObject &parent = *m_StructureStack.back();
parent.data.basic.numChildren++;
parent.data.children.push_back(new StructuredObject(name, GetSerialiseTypeName<T>::Name()));
m_StructureStack.push_back(parent.data.children.back());
StructuredObject &arr = *m_StructureStack.back();
arr.type.basetype = SerialisedType::eArray;
arr.type.byteSize = N;
arr.data.basic.numChildren = (uint64_t)N;
arr.data.children.resize(N);
for(size_t i=0; i < N; i++)
{
arr.data.children[i] = new StructuredObject("$el", GetSerialiseTypeName<T>::Name());
m_StructureStack.push_back(arr.data.children[i]);
StructuredObject &obj = *m_StructureStack.back();
// default to struct. This will be overwritten if appropriate
obj.type.basetype = SerialisedType::eStruct;
obj.type.byteSize = sizeof(T);
SerialiseDispatch<Serialiser, T>::Do(*this, el[i]);
m_StructureStack.pop_back();
}
m_StructureStack.pop_back();
}
else
{
for(size_t i=0; i < N; i++)
SerialiseDispatch<Serialiser, T>::Do(*this, el[i]);
}
return *this;
}
// special function for serialising dynamically sized arrays
template<class T>
Serialiser &Serialise(const char *name, T *&el, uint64_t &arrayCount, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
uint64_t count = arrayCount;
// silently handle NULL arrays
if(IsWriting() && el == NULL)
count = 0;
{
m_InternalElement = true;
DoSerialise(*this, count);
m_InternalElement = false;
}
if(IsReading())
arrayCount = count;
if(ExportStructure())
{
if(m_StructureStack.empty())
{
RDCERR("Serialising object outside of chunk context! Call BeginChunk before any Serialise!");
return *this;
}
StructuredObject &parent = *m_StructureStack.back();
parent.data.basic.numChildren++;
parent.data.children.push_back(new StructuredObject(name, GetSerialiseTypeName<T>::Name()));
m_StructureStack.push_back(parent.data.children.back());
StructuredObject &arr = *m_StructureStack.back();
arr.type.basetype = SerialisedType::eArray;
arr.type.byteSize = arrayCount;
arr.data.basic.numChildren = arrayCount;
arr.data.children.resize(arrayCount);
if(IsReading() && (flags & SerialiserFlags::AllocateMemory))
{
if(arrayCount > 0)
el = new T[arrayCount];
else
el = NULL;
}
for(uint64_t i=0; el && i < arrayCount; i++)
{
arr.data.children[i] = new StructuredObject("$el", GetSerialiseTypeName<T>::Name());
m_StructureStack.push_back(arr.data.children[i]);
StructuredObject &obj = *m_StructureStack.back();
// default to struct. This will be overwritten if appropriate
obj.type.basetype = SerialisedType::eStruct;
obj.type.byteSize = sizeof(T);
SerialiseDispatch<Serialiser, T>::Do(*this, el[i]);
m_StructureStack.pop_back();
}
m_StructureStack.pop_back();
}
else
{
if(IsReading() && (flags & SerialiserFlags::AllocateMemory))
{
if(arrayCount > 0)
el = new T[arrayCount];
else
el = NULL;
}
for(size_t i=0; el && i < arrayCount; i++)
SerialiseDispatch<Serialiser, T>::Do(*this, el[i]);
}
return *this;
}
template<class T>
Serialiser &Serialise(const char *name, T *&el, uint32_t &arrayCount, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
uint64_t upcastCount = arrayCount;
Serialise(name, el, upcastCount, flags);
if(IsReading())
arrayCount = (uint32_t)upcastCount;
return *this;
}
// specialisations for container types
template <class U>
Serialiser &Serialise(const char *name, std::vector<U> &el, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
uint64_t size = (uint64_t)el.size();
{
m_InternalElement = true;
DoSerialise(*this, size);
m_InternalElement = false;
}
if(ExportStructure())
{
if(m_StructureStack.empty())
{
RDCERR("Serialising object outside of chunk context! Call BeginChunk before any Serialise!");
return *this;
}
StructuredObject &parent = *m_StructureStack.back();
parent.data.basic.numChildren++;
parent.data.children.push_back(new StructuredObject(name, GetSerialiseTypeName<U>::Name()));
m_StructureStack.push_back(parent.data.children.back());
StructuredObject &arr = *m_StructureStack.back();
arr.type.basetype = SerialisedType::eArray;
arr.type.byteSize = size;
arr.data.basic.numChildren = size;
arr.data.children.resize(size);
if(IsReading())
el.resize(size);
for(uint64_t i=0; i < size; i++)
{
arr.data.children[i] = new StructuredObject("$el", GetSerialiseTypeName<U>::Name());
m_StructureStack.push_back(arr.data.children[i]);
StructuredObject &obj = *m_StructureStack.back();
// default to struct. This will be overwritten if appropriate
obj.type.basetype = SerialisedType::eStruct;
obj.type.byteSize = sizeof(U);
SerialiseDispatch<Serialiser, U>::Do(*this, el[i]);
m_StructureStack.pop_back();
}
m_StructureStack.pop_back();
}
else
{
if(IsReading())
el.resize(size);
for(uint64_t i=0; i < size; i++)
SerialiseDispatch<Serialiser, U>::Do(*this, el[i]);
}
return *this;
}
template <class U>
Serialiser &Serialise(const char *name, std::list<U> &el, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
RDCFATAL("Not implemented");
return *this;
}
template <class U, class V>
Serialiser &Serialise(const char *name, std::pair<U, V> &el, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
RDCFATAL("Not implemented");
return *this;
}
// for const types, to cast away the const!
// caller is responsible for ensuring that on write, it's safe to write into
// this pointer on the other end
template<class T>
Serialiser &Serialise(const char *name, const T &el, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
return Serialise(name, (T&)el, flags);
}
// dynamic array variant
template<class T>
Serialiser &Serialise(const char *name, const T *&el, uint64_t &arrayCount, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
return Serialise(name, (T *&)el, arrayCount, flags);
}
template <class T>
Serialiser &SerialiseNullable(const char *name, T *&el, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
bool present = (el != NULL);
{
m_InternalElement = true;
DoSerialise(*this, present);
m_InternalElement = false;
}
if(ExportStructure())
{
if(m_StructureStack.empty())
{
RDCERR("Serialising object outside of chunk context! Call BeginChunk before any Serialise!");
return *this;
}
if(IsReading())
{
if(present)
el = new T;
else
el = NULL;
}
if(el)
{
Serialise(name, *el, flags);
StructuredObject &parent = *m_StructureStack.back();
StructuredObject &nullable = *parent.data.children.back();
nullable.type.flags |= SerialisedType::Nullable;
}
else
{
StructuredObject &parent = *m_StructureStack.back();
parent.data.basic.numChildren++;
parent.data.children.push_back(new StructuredObject(name, GetSerialiseTypeName<T>::Name()));
StructuredObject &nullable = *parent.data.children.back();
nullable.type.basetype = SerialisedType::eNull;
nullable.type.byteSize = 0;
nullable.type.flags |= SerialisedType::Nullable;
}
}
else
{
if(el)
Serialise(name, *el, flags);
}
return *this;
}
template <class T>
Serialiser &SerialiseNullable(const char *name, const T *&el, SerialiserFlags::Flags flags = SerialiserFlags::None)
{
return SerialiseNullable(name, (T *&)el, flags);
}
Serialiser &Aligned()
{
m_AlignedData = true;
return *this;
}
// these functions can be chained onto the end of a Serialise() call or macro to
// set additional properties or change things
Serialiser &Hidden()
{
if(ExportStructure() && !m_StructureStack.empty())
{
StructuredObject ¤t = *m_StructureStack.back();
if(!current.data.children.empty())
current.data.children.back()->type.flags |= SerialisedType::Hidden;
}
return *this;
}
Serialiser &Named(const char *name)
{
if(ExportStructure() && !m_StructureStack.empty())
{
StructuredObject ¤t = *m_StructureStack.back();
if(!current.data.children.empty())
current.data.children.back()->name = name;
}
return *this;
}
/////////////////////////////////////////////////////////////////////////////
// for basic/leaf types. Read/written just as byte soup, MUST be plain old data
template <class T>
void SerialiseValue(SerialisedType::BasicType type, size_t byteSize, T &el)
{
if(IsWriting())
{
m_Write->Write(el);
}
else if(IsReading())
{
m_Read->Read(el);
}
if(!ExportStructure())
return;
StructuredObject ¤t = *m_StructureStack.back();
current.type.basetype = type;
current.type.byteSize = byteSize;
switch(type)
{
case SerialisedType::eChunk:
case SerialisedType::eStruct:
case SerialisedType::eArray:
case SerialisedType::eBuffer:
case SerialisedType::eNull:
RDCFATAL("Cannot call SerialiseValue for type %d!", type);
break;
case SerialisedType::eString:
RDCFATAL("eString should be specialised!");
break;
case SerialisedType::eEnum:
case SerialisedType::eUnsignedInteger:
if(byteSize == 1)
current.data.basic.u = (uint64_t)(uint8_t)el;
else if(byteSize == 2)
current.data.basic.u = (uint64_t)(uint16_t)el;
else if(byteSize == 4)
current.data.basic.u = (uint64_t)(uint32_t)el;
else if(byteSize == 8)
current.data.basic.u = (uint64_t)el;
else
RDCFATAL("Unsupported unsigned integer byte width: %u", byteSize);
break;
case SerialisedType::eSignedInteger:
if(byteSize == 1)
current.data.basic.i = (int64_t)(int8_t)el;
else if(byteSize == 2)
current.data.basic.i = (int64_t)(int16_t)el;
else if(byteSize == 4)
current.data.basic.i = (int64_t)(int32_t)el;
else if(byteSize == 8)
current.data.basic.i = (int64_t)el;
else
RDCFATAL("Unsupported signed integer byte width: %u", byteSize);
break;
case SerialisedType::eFloat:
if(byteSize == 4)
current.data.basic.d = (double)(float)el;
else if(byteSize == 8)
current.data.basic.d = (double)el;
else
RDCFATAL("Unsupported floating point byte width: %u", byteSize);
break;
case SerialisedType::eBoolean:
// co-erce to boolean
current.data.basic.b = !!(el);
break;
case SerialisedType::eCharacter:
current.data.basic.c = (char)(el);
break;
}
}
// the only non POD basic type
void SerialiseValue(SerialisedType::BasicType type, size_t byteSize, std::string &el)
{
{
if(IsReading())
{
uint32_t len = 0;
m_Read->Read(len);
el.resize(len);
if(len > 0)
m_Read->Read(&el[0], len);
}
else
{
uint32_t len = (uint32_t)el.length();
m_Write->Write(len);
m_Write->Write(el.c_str(), len);
}
}
if(ExportStructure())
{
StructuredObject ¤t = *m_StructureStack.back();
current.type.basetype = type;
current.type.byteSize = byteSize;
current.data.str = el;
}
}
void SerialiseValue(SerialisedType::BasicType type, size_t byteSize, char *&el)
{
{
if(IsReading())
{
uint32_t len = 0;
m_Read->Read(len);
string str;
str.resize(len);
if(len > 0)
m_Read->Read(&str[0], len);
auto it = m_StringDB.insert(str);
el = (char *)it.first->c_str();
}
else
{
uint32_t len = (uint32_t)strlen(el);
m_Write->Write(len);
m_Write->Write(el, len);
}
}
if(ExportStructure())
{
StructuredObject ¤t = *m_StructureStack.back();
current.type.basetype = type;
current.type.byteSize = byteSize;
current.data.str = el;
}
}
void SerialiseValue(SerialisedType::BasicType type, size_t byteSize, const char *&el)
{
SerialiseValue(type, byteSize, (char *&)el);
}
private:
// no copies
Serialiser(const Serialiser &other);
static void CreateResolver(void *ths);
// clean out for before constructor and after destructor (and other times probably)
void Reset()
{
m_pUserData = NULL;
m_ExportBuffers = false;
m_InternalElement = false;
m_ChunkMetadata = SerialisedChunkMetaData();
m_SerVer = SERIALISE_VERSION;
RDCEraseEl(m_KnownSections);
m_HasError = false;
m_ErrorCode = eContError_None;
m_ChunkLookup = NULL;
m_AlignedData = false;
m_ChunkFlags = 0;
m_LastChunkLen = 0;
m_LastChunkOffset = 0;
}
uint64_t m_SerVer;
ContainerError m_ErrorCode;
bool m_HasError;
void *m_pUserData;
StreamWriter *m_Write;
StreamReader *m_Read;
size_t m_LastChunkLen;
uint64_t m_LastChunkOffset;
bool m_AlignedData;
uint64_t m_ChunkFixup;
// reading from file:
struct Section
{
Section()
: type(eSectionType_Unknown), flags(eSectionFlag_None), fileoffset(0), size(0), compressedReader(NULL)
{
}
string name;
SectionType type;
SectionFlags flags;
uint64_t fileoffset;
uint64_t size;
std::vector<byte> data; // some sections can be loaded entirely into memory
CompressedFileIO *compressedReader;
};
// this lists all sections in file order
std::vector<Section *> m_Sections;
// this lists known sections, some may be NULL
Section *m_KnownSections[eSectionType_Num];
// writing to file
std::vector<Chunk *> m_Chunks;
bool m_ExportBuffers;
bool m_InternalElement;
StructuredChunkList m_StructuredData;
SerialisedBufferList m_StructuredDataBuffers;
std::vector<StructuredObject *> m_StructureStack;
uint32_t m_ChunkFlags;
SerialisedChunkMetaData m_ChunkMetadata;
// a database of strings read from the file, useful when serialised structures
// expect a char* to return and point to static memory
std::set<string> m_StringDB;
ChunkLookup m_ChunkLookup;
};
typedef Serialiser<SerialiserType::Writing> WriteSerialiser;
typedef Serialiser<SerialiserType::Reading> ReadSerialiser;
typedef Serialiser<SerialiserType::StructuredReading> StructReadSerialiser;
#define BASIC_TYPE_SERIALISE(typeName, member, type, byteSize) \
template <class SerialiserType> \
void DoSerialise(SerialiserType &ser, typeName &el) \
{ \
ser.SerialiseValue(SerialisedType::type, byteSize, member); \
}
#define BASIC_TYPE_SERIALISE_STRINGIFY(typeName, member, type, byteSize) \
template <class SerialiserType> \
void DoSerialise(SerialiserType &ser, typeName &el) \
{ \
ser.SerialiseValue(SerialisedType::type, byteSize, member); \
ser.SerialiseStringify(el); \
}
BASIC_TYPE_SERIALISE(int64_t, el, eSignedInteger, 8);
BASIC_TYPE_SERIALISE(uint64_t, el, eUnsignedInteger, 8);
BASIC_TYPE_SERIALISE(int32_t, el, eSignedInteger, 4);
BASIC_TYPE_SERIALISE(uint32_t, el, eUnsignedInteger, 4);
BASIC_TYPE_SERIALISE(int16_t, el, eSignedInteger, 2);
BASIC_TYPE_SERIALISE(uint16_t, el, eUnsignedInteger, 2);
BASIC_TYPE_SERIALISE(int8_t, el, eSignedInteger, 1);
BASIC_TYPE_SERIALISE(uint8_t, el, eUnsignedInteger, 1);
BASIC_TYPE_SERIALISE(double, el, eFloat, 8);
BASIC_TYPE_SERIALISE(float, el, eFloat, 4);
BASIC_TYPE_SERIALISE(bool, el, eBoolean, 1);
BASIC_TYPE_SERIALISE(char, el, eCharacter, 1);
BASIC_TYPE_SERIALISE(string, el, eString, 0);
BASIC_TYPE_SERIALISE(char *, el, eString, 0);
BASIC_TYPE_SERIALISE(const char *, el, eString, 0);
template<class SerialiserType>
class ScopedContext
{
public:
ScopedContext(SerialiserType &s, uint32_t i, uint32_t byteLength = 0)
: m_Idx(i), m_Ser(s), m_Ended(false)
{
m_Ser.BeginChunk(i, byteLength);
}
~ScopedContext()
{
if(!m_Ended)
End();
}
Chunk *Get(bool temporary = false)
{
End();
return new Chunk(m_Ser, m_Idx, temporary);
}
private:
SerialiserType &m_Ser;
uint32_t m_Idx;
bool m_Ended;
void End()
{
RDCASSERT(!m_Ended);
m_Ser.EndChunk();
m_Ended = true;
}
};
template <class T>
struct ScopedDeserialise
{
ScopedDeserialise(const T &el) : m_El(el) {}
~ScopedDeserialise() { Deserialise(m_El); }
const T &m_El;
};
template<class T>
ScopedDeserialise<T> DeserialiseAutoMaker(const ReadSerialiser &ser, const T &el)
{
(void)ser;
return ScopedDeserialise<T>(el);
}
template<class T>
ScopedDeserialise<T> DeserialiseAutoMaker(const StructReadSerialiser &ser, const T &el)
{
(void)ser;
return ScopedDeserialise<T>(el);
}
template <class T>
struct ScopedDeserialiseArray
{
ScopedDeserialiseArray(T **el) : m_El(el) {}
~ScopedDeserialiseArray() { delete[] *m_El; }
T **m_El;
};
template <>
struct ScopedDeserialiseArray<void>
{
ScopedDeserialiseArray(void **el) : m_El(el) {}
~ScopedDeserialiseArray() { FreeAlignedBuffer((byte *)*m_El); }
void **m_El;
};
template <>
struct ScopedDeserialiseArray<const void>
{
ScopedDeserialiseArray(const void **el) : m_El(el) {}
~ScopedDeserialiseArray() { FreeAlignedBuffer((byte *)*m_El); }
const void **m_El;
};
template <>
struct ScopedDeserialiseArray<byte>
{
ScopedDeserialiseArray(byte **el) : m_El(el) {}
~ScopedDeserialiseArray() { FreeAlignedBuffer(*m_El); }
byte **m_El;
};
template<class T>
ScopedDeserialiseArray<T> DeserialiseArrayAutoMaker(const ReadSerialiser &ser, T **el)
{
(void)ser;
return ScopedDeserialiseArray<T>(el);
}
template<class T>
ScopedDeserialiseArray<T> DeserialiseArrayAutoMaker(const StructReadSerialiser &ser, T **el)
{
(void)ser;
return ScopedDeserialiseArray<T>(el);
}
template <class T>
struct ScopedDeserialiseNullable
{
ScopedDeserialiseNullable(T **el) : m_El(el) {}
~ScopedDeserialiseNullable() { if(*m_El != NULL) { Deserialise(**m_El); delete *m_El; } }
T **m_El;
};
template<class T>
ScopedDeserialiseNullable<T> DeserialiseNullableAutoMaker(const ReadSerialiser &ser, T **el)
{
(void)ser;
return ScopedDeserialiseNullable<T>(el);
}
template<class T>
ScopedDeserialiseNullable<T> DeserialiseNullableAutoMaker(const StructReadSerialiser &ser, T **el)
{
(void)ser;
return ScopedDeserialiseNullable<T>(el);
}
template <class T>
struct EmptyClass
{
};
template<class T>
EmptyClass<T> DeserialiseAutoMaker(const WriteSerialiser &ser, const T &el)
{
(void)ser;
(void)el;
return EmptyClass<T>();
}
template<class T>
EmptyClass<T> DeserialiseArrayAutoMaker(const WriteSerialiser &ser, T **el)
{
(void)ser;
(void)el;
return EmptyClass<T>();
}
template<class T>
EmptyClass<T> DeserialiseNullableAutoMaker(const WriteSerialiser &ser, T **el)
{
(void)ser;
(void)el;
return EmptyClass<T>();
}
template<class T>
T *ForceAllocate(const T *garbage)
{
return new T;
}
// can be overridden to change the name in the helper macros locally
#define GET_SERIALISER (ser)
#define SCOPED_SERIALISE_CONTEXT(...) ScopedContext<WriteSerialiser> scope(GET_SERIALISER, __VA_ARGS__);
// force the data to be aligned
#define SERIALISE_ALIGNED() GET_SERIALISER.Aligned();
// these helper macros are intended for use when serialising objects in chunks
#define SERIALISE_ELEMENT(obj) \
auto CONCAT(deserialise_, __LINE__) = DeserialiseAutoMaker(GET_SERIALISER, obj); \
GET_SERIALISER.Serialise(#obj, obj)
// helper macro for when an array has a constant size. Uses a dummy variable to serialise
#define FIXED_COUNT(count) \
(CONCAT(dummy_array_count, __LINE__) = count,CONCAT(dummy_array_count, __LINE__))
#define SERIALISE_ELEMENT_ARRAY(obj, count) \
uint64_t CONCAT(dummy_array_count, __LINE__) = 0; \
auto CONCAT(deserialise_, __LINE__) = DeserialiseArrayAutoMaker(GET_SERIALISER, &obj); \
GET_SERIALISER.Serialise(#obj, obj, count, SerialiserFlags::AllocateMemory)
#define SERIALISE_ELEMENT_OPT(obj) \
auto CONCAT(deserialise_, __LINE__) = DeserialiseNullableAutoMaker(GET_SERIALISER, &obj); \
GET_SERIALISER.SerialiseNullable(#obj, obj)
#define SERIALISE_ELEMENT_LOCAL(obj, inValue) \
decltype(inValue) obj; \
auto CONCAT(deserialise_, __LINE__) = DeserialiseAutoMaker(GET_SERIALISER, obj); \
if(GET_SERIALISER.IsWriting()) \
obj = (inValue); \
GET_SERIALISER.Serialise(#obj, obj)
// these macros are for use when implementing a DoSerialise function
#define SERIALISE_MEMBER(obj) \
ser.Serialise(#obj, el.obj)
#define SERIALISE_MEMBER_TYPED(type, obj) \
ser.template Serialise<type>(#obj, (type &)el.obj)
#define SERIALISE_MEMBER_ARRAY(arrayObj, countObj) \
ser.Serialise(#arrayObj, el.arrayObj, el.countObj, SerialiserFlags::AllocateMemory)
// a member that is a pointer and could be NULL, so needs a hidden 'present'
// flag serialised out
#define SERIALISE_MEMBER_OPT(obj) \
ser.SerialiseNullable(#obj, el.obj)
<file_sep>/common.h
/******************************************************************************
* The MIT License (MIT)
*
* Copyright (c) 2015-2016 <NAME>
* Copyright (c) 2014 Crytek
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
******************************************************************************/
#pragma once
#include <stddef.h>
#include <stdint.h>
#include <stdlib.h>
#include <stdarg.h>
//#include "globalconfig.h"
#include <string>
#include <string.h>
#ifdef WIN32
#define PRIu64 "llu"
#define PRId64 "lld"
#else
#include <cinttypes>
#endif
#ifdef WIN32
#define __PRETTY_FUNCTION_SIGNATURE__ __FUNCSIG__
#define va_copy(dst, src) dst = src
#else
#define __PRETTY_FUNCTION_SIGNATURE__ __PRETTY_FUNCTION__
#define _snprintf snprintf
#endif
namespace StringFormat
{
inline std::string Fmt(const char *format, ...)
{
va_list args;
va_start(args, format);
va_list args2;
va_copy(args2, args);
int size = vsnprintf(NULL, 0, format, args2);
char *buf = new char[size + 1];
vsnprintf(buf, size + 1, format, args);
buf[size] = 0;
va_end(args);
va_end(args2);
std::string ret = buf;
delete[] buf;
return ret;
}
};
#if defined(__LP64__) || defined(_WIN64) || defined(__x86_64__) || defined(_M_X64) || \
defined(__ia64) || defined(_M_IA64) || defined(__aarch64__) || defined(__powerpc64__)
#define RDC64BIT 1
#endif
enum
{
RenderDoc_FirstCaptureNetworkPort = 38920,
RenderDoc_LastCaptureNetworkPort = RenderDoc_FirstCaptureNetworkPort + 7,
RenderDoc_ReplayNetworkPort = 39920,
};
#define FORCE_ASSERTS
#define DEBUGBREAK_ON_ERROR_LOG
/////////////////////////////////////////////////
// Utility macros
#ifndef SAFE_DELETE
#define SAFE_DELETE(p) \
do \
{ \
if(p) \
{ \
delete(p); \
(p) = NULL; \
} \
} while((void)0, 0)
#endif
#ifndef SAFE_DELETE_ARRAY
#define SAFE_DELETE_ARRAY(p) \
do \
{ \
if(p) \
{ \
delete[](p); \
(p) = NULL; \
} \
} while((void)0, 0)
#endif
#ifndef SAFE_ADDREF
#define SAFE_ADDREF(p) \
do \
{ \
if(p) \
{ \
(p)->AddRef(); \
} \
} while((void)0, 0)
#endif
#ifndef SAFE_RELEASE
#define SAFE_RELEASE(p) \
do \
{ \
if(p) \
{ \
(p)->Release(); \
(p) = NULL; \
} \
} while((void)0, 0)
#define SAFE_RELEASE_NOCLEAR(p) \
do \
{ \
if(p) \
{ \
(p)->Release(); \
} \
} while((void)0, 0)
#endif
#ifndef ARRAY_COUNT
#define ARRAY_COUNT(arr) (sizeof(arr) / sizeof(arr[0]))
#endif
#define RANDF(mn, mx) ((float(rand()) / float(RAND_MAX)) * ((mx) - (mn)) + (mn))
#define STRINGIZE2(a) #a
#define STRINGIZE(a) STRINGIZE2(a)
#define CONCAT2(a, b) a##b
#define CONCAT(a, b) CONCAT2(a, b)
//#include "os/os_specific.h"
typedef uint8_t byte;
#define RDCEraseMem(a, b) memset(a, 0, b)
#define RDCEraseEl(a) memset(&a, 0, sizeof(a))
template <typename T>
T RDCCLAMP(const T &val, const T &mn, const T &mx)
{
return val < mn ? mn : (val > mx ? mx : val);
}
template <typename T>
T RDCMIN(const T &a, const T &b)
{
return a < b ? a : b;
}
template <typename T>
T RDCMAX(const T &a, const T &b)
{
return a > b ? a : b;
}
template <typename T>
inline T AlignUp4(T x)
{
return (x + 0x3) & (~0x3);
}
template <typename T>
inline T AlignUp16(T x)
{
return (x + 0xf) & (~0xf);
}
template <typename T>
inline T AlignUp(T x, T a)
{
return (x + (a - 1)) & (~(a - 1));
}
template <typename T, typename A>
inline T AlignUpPtr(T x, A a)
{
return (T)AlignUp<uintptr_t>((uintptr_t)x, (uintptr_t)a);
}
#define MAKE_FOURCC(a, b, c, d) \
(((uint32_t)(d) << 24) | ((uint32_t)(c) << 16) | ((uint32_t)(b) << 8) | (uint32_t)(a))
bool FindDiffRange(void *a, void *b, size_t bufSize, size_t &diffStart, size_t &diffEnd);
uint32_t CalcNumMips(int Width, int Height, int Depth);
byte *AllocAlignedBuffer(size_t size, size_t alignment = 64);
void FreeAlignedBuffer(byte *buf);
/////////////////////////////////////////////////
// Debugging features
#if WIN32
#define FORCE_CRASH() *((int *)NULL) = 0
#else
#define FORCE_CRASH() __builtin_trap()
#define __debugbreak()
#endif
#define RDCDUMP() \
do \
{ \
FORCE_CRASH(); \
} while((void)0, 0)
#if !defined(RELEASE) || defined(FORCE_DEBUGBREAK)
#define RDCBREAK() \
do \
{ \
__debugbreak(); \
} while((void)0, 0)
#else
#define RDCBREAK() \
do \
{ \
} while((void)0, 0)
#endif
#define RDCUNIMPLEMENTED(...) \
do \
{ \
rdclog(RDCLog_Warning, "Unimplemented: " __VA_ARGS__); \
RDCBREAK(); \
} while((void)0, 0)
//
// Logging
//
enum LogType
{
RDCLog_First = -1,
RDCLog_Debug,
RDCLog_Comment,
RDCLog_Warning,
RDCLog_Error,
RDCLog_Fatal,
RDCLog_NumTypes,
};
#if defined(STRIP_LOG)
#define RDCLOGFILE(fn) \
do \
{ \
} while((void)0, 0)
#define RDCLOGDELETE() \
do \
{ \
} while((void)0, 0)
#define RDCDEBUG(...) \
do \
{ \
} while((void)0, 0)
#define RDCLOG(...) \
do \
{ \
} while((void)0, 0)
#define RDCWARN(...) \
do \
{ \
} while((void)0, 0)
#define RDCERR(...) \
do \
{ \
} while((void)0, 0)
#define RDCFATAL(...) \
do \
{ \
RDCDUMP(); \
exit(0); \
} while((void)0, 0)
#define RDCDUMPMSG(message) \
do \
{ \
RDCDUMP(); \
exit(0); \
} while((void)0, 0)
#else
// perform any operations necessary to flush the log
void rdclog_flush();
// actual low-level print to log output streams defined (useful for if we need to print
// fatal error messages from within the more complex log function).
void rdclogprint_int(const char *str);
// printf() style main logger function
void rdclog_int(LogType type, const char *file, unsigned int line, const char *fmt, ...);
#define rdclog(type, ...) rdclog_int(type, __FILE__, __LINE__, __VA_ARGS__)
const char *rdclog_getfilename();
void rdclog_filename(const char *filename);
#define RDCLOGFILE(fn) rdclog_filename(fn)
#define RDCGETLOGFILE() rdclog_getfilename()
#if(!defined(RELEASE) || defined(FORCE_DEBUG_LOGS)) && !defined(STRIP_DEBUG_LOGS)
#define RDCDEBUG(...) rdclog(RDCLog_Debug, __VA_ARGS__)
#else
#define RDCDEBUG(...) \
do \
{ \
} while((void)0, 0)
#endif
#define RDCLOG(...) rdclog(RDCLog_Comment, __VA_ARGS__)
#define RDCWARN(...) rdclog(RDCLog_Warning, __VA_ARGS__)
#if defined(DEBUGBREAK_ON_ERROR_LOG)
#define RDCERR(...) \
do \
{ \
rdclog(RDCLog_Error, __VA_ARGS__); \
rdclog_flush(); \
RDCBREAK(); \
} while((void)0, 0)
#else
#define RDCERR(...) rdclog(RDCLog_Error, __VA_ARGS__)
#endif
#define RDCFATAL(...) \
do \
{ \
rdclog(RDCLog_Fatal, __VA_ARGS__); \
rdclog_flush(); \
RDCDUMP(); \
exit(0); \
} while((void)0, 0)
#define RDCDUMPMSG(message) \
do \
{ \
rdclogprint_int(message); \
rdclog_flush(); \
RDCDUMP(); \
exit(0); \
} while((void)0, 0)
#endif
//
// Assert
//
#if !defined(RELEASE) || defined(FORCE_ASSERTS)
void rdcassert(const char *msg, const char *file, unsigned int line, const char *func);
// this defines the root macro, RDCASSERTMSG(msg, cond, ...)
// where it will check cond, then print msg (if it's not "") and the values of all values passed via
// varargs.
// the other asserts are defined in terms of that
#include "custom_assert.h"
#else
#define RDCASSERTMSG(cond) \
do \
{ \
} while((void)0, 0)
#endif
#define RDCASSERT(...) RDCASSERTMSG("", __VA_ARGS__)
#define RDCASSERTEQUAL(a, b) RDCASSERTMSG("", a == b, a, b)
#define RDCASSERTNOTEQUAL(a, b) RDCASSERTMSG("", a != b, a, b)
//
// Compile asserts
//
#if defined(STRIP_COMPILE_ASSERTS)
#define RDCCOMPILE_ASSERT(condition, message) \
do \
{ \
} while((void)0, 0)
#else
#define RDCCOMPILE_ASSERT(condition, message) static_assert(condition, message)
#endif
#ifdef WIN32
#include <windows.h>
namespace Timing
{
inline double GetTickFrequency()
{
LARGE_INTEGER li;
QueryPerformanceFrequency(&li);
return double(li.QuadPart) / 1000.0;
}
inline uint64_t GetTick()
{
LARGE_INTEGER li;
QueryPerformanceCounter(&li);
return li.QuadPart;
}
};
#else
#include <time.h>
#include <unistd.h>
namespace Timing
{
inline double GetTickFrequency()
{
return 1000000.0;
}
inline uint64_t GetTick()
{
timespec ts;
clock_gettime(CLOCK_MONOTONIC, &ts);
return uint64_t(ts.tv_sec) * 1000000000ULL + uint32_t(ts.tv_nsec & 0xffffffff);
}
};
#endif
class PerformanceTimer
{
public:
PerformanceTimer() : m_CounterFrequency(Timing::GetTickFrequency()) { Restart(); }
double GetMilliseconds() const
{
return double(Timing::GetTick() - m_Start) / m_CounterFrequency;
}
void Restart() { m_Start = Timing::GetTick(); }
private:
double m_CounterFrequency;
uint64_t m_Start;
};
class ScopedTimer
{
public:
ScopedTimer(const char *file, unsigned int line, const char *fmt, ...)
{
m_File = file;
m_Line = line;
va_list args;
va_start(args, fmt);
char buf[1024];
buf[1023] = 0;
vsnprintf(buf, 1023, fmt, args);
m_Message = buf;
va_end(args);
}
~ScopedTimer()
{
rdclog_int(RDCLog_Comment, m_File, m_Line, "Timer %s - %.3lf ms", m_Message.c_str(),
m_Timer.GetMilliseconds());
}
private:
const char *m_File;
unsigned int m_Line;
std::string m_Message;
PerformanceTimer m_Timer;
};
#define SCOPED_TIMER(...) ScopedTimer CONCAT(timer, __LINE__)(__FILE__, __LINE__, __VA_ARGS__);
#include <vector>
inline std::vector<uint64_t> CollectCallstack_stub()
{
std::vector<uint64_t> ret;
ret.resize(5 + rand()%10);
for(size_t i=0; i < ret.size(); i++)
{
uint64_t addr = uint64_t(rand()&0x7fff) << 20;
addr |= rand()&0x7fff;
ret[i] = addr;
}
return ret;
}
inline uint64_t CollectTimestamp_stub()
{
static uint64_t time = 0x844674407ULL + ((rand()&0x7fff)<<8);
time += (rand()&0x7fff)<<6;
return time;
}
inline uint32_t CollectThreadID_stub()
{
static uint32_t id = 1000 + rand()&0xff;
return id;
}<file_sep>/streamio.h
/******************************************************************************
* The MIT License (MIT)
*
* Copyright (c) 2017 <NAME>
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
******************************************************************************/
#pragma once
#include <vector>
#include <stdio.h>
#include "common.h"
class StreamWriter;
class StreamReader;
class Compressor
{
public:
Compressor(StreamWriter *write)
: m_Write(write)
{
}
virtual ~Compressor() {}
virtual void Write(const void *data, uint64_t numBytes) = 0;
virtual void Finish() = 0;
protected:
StreamWriter *m_Write;
};
class Decompressor
{
public:
Decompressor(StreamReader *read)
: m_Read(read)
{
}
virtual ~Decompressor() {}
virtual void Recompress(Compressor *comp) = 0;
virtual void Read(void *data, uint64_t numBytes) = 0;
protected:
StreamReader *m_Read;
};
class StreamReader
{
public:
StreamReader(const void *buffer, size_t bufferSize);
StreamReader(const std::vector<byte> &buffer);
StreamReader(FILE *file, size_t fileSize);
StreamReader(FILE *file);
StreamReader(Decompressor *decompressor, size_t uncompressedSize);
~StreamReader();
void SetOffset(uint64_t offs);
inline uint64_t GetOffset()
{
return m_BufferHead - m_BufferBase + m_ReadOffset;
}
inline uint64_t GetSize()
{
return m_InputSize;
}
inline bool AtEnd() { return GetOffset() >= GetSize(); }
template<size_t alignment>
void AlignTo()
{
uint64_t offs = GetOffset();
uint64_t alignedOffs = AlignUp(offs, alignment);
uint64_t bytesToAlign = alignedOffs - offs;
if(bytesToAlign > 0)
Read(NULL, bytesToAlign);
}
void Read(void *data, size_t numBytes)
{
// if we're reading past the end, error, read nothing (no partial reads) and return
if(GetOffset() + numBytes > GetSize())
{
RDCERR("Reading off the end of the stream");
m_BufferHead = m_BufferBase + m_BufferSize;
return;
}
// if we're reading from an external source, reserve enough bytes to do the read
if(m_File || m_Decompressor)
{
// This preserves everything from min(m_BufferBase, m_BufferHead - 64) -> end of buffer
// which will still be in place relative to m_BufferHead.
// In other words - reservation will keep the aleady-read data that's after the head pointer,
// as well as up to 64 bytes *behind* the head if it exists.
if(numBytes > Available())
Reserve(numBytes);
}
// perform the actual copy
if(data)
memcpy(data, m_BufferHead, numBytes);
// advance head
m_BufferHead += numBytes;
}
void SkipBytes(size_t numBytes)
{
Read(NULL, numBytes);
}
// compile-time constant element to let the compiler inline the memcpy
template<typename T>
void Read(T &data)
{
Read(&data, sizeof(T));
}
private:
inline uint64_t Available()
{
return m_BufferSize - (m_BufferHead - m_BufferBase);
}
void Reserve(size_t numBytes);
void ReadFromExternal(uint64_t bufferOffs, size_t length);
// base of the buffer allocation
byte *m_BufferBase;
// where we are currently reading from in the buffer
byte *m_BufferHead;
// the size of the buffer (just a window if reading from external source)
uint64_t m_BufferSize;
// the total size of the total input. This is how many bytes you can read, regardless
// of how many bytes might actually be stored on the other side of the source (i.e.
// this is the uncompressed output size)
uint64_t m_InputSize;
// file pointer, if we're reading from a file
FILE *m_File;
// the decompressor, if reading from it
Decompressor *m_Decompressor;
// the offset in the file/decompressor that corresponds to the start of m_BufferBase
uint64_t m_ReadOffset;
};
class StreamWriter
{
public:
StreamWriter(uint64_t initialBufSize);
StreamWriter(FILE *file);
StreamWriter(Compressor *compressor);
static const int DefaultScratchSize = 32 * 1024;
~StreamWriter();
void SetOffset(uint64_t offs)
{
if(!m_File && !m_Compressor)
{
m_BufferHead = m_BufferBase + offs;
return;
}
RDCERR("Can't seek a file/compressor stream writer");
}
void Rewind()
{
if(!m_File && !m_Compressor)
{
m_BufferHead = m_BufferBase;
m_WriteSize = 0;
return;
}
RDCERR("Can't rewind a file/compressor stream writer");
}
uint64_t GetOffset() { return m_WriteSize; }
const byte *GetData() { return m_BufferBase; }
template<size_t alignment>
void AlignTo()
{
if(!m_File && !m_Compressor)
{
uint64_t offs = m_BufferHead - m_BufferBase;
uint64_t alignedOffs = AlignUp(offs, alignment);
uint64_t bytesToAlign = alignedOffs - offs;
RDCCOMPILE_ASSERT(alignment <= sizeof(empty), "Empty array is not large enough - increase size to support alignment");
if(bytesToAlign > 0)
Write(empty, bytesToAlign);
return;
}
RDCERR("Can't align a file/compressor stream writer");
}
void Write(const void *data, size_t numBytes)
{
m_WriteSize += numBytes;
if(!m_File && !m_Compressor)
{
// in-memory path
size_t endByte = numBytes + size_t(m_BufferHead - m_BufferBase);
// are we about to write outside the buffer? Resize it larger
if(m_BufferSize < endByte)
{
// reallocate to a conservative size, don't 'double and allocate'
while(m_BufferSize < endByte)
m_BufferSize += 128 * 1024;
byte *newBuf = AllocAlignedBuffer((size_t)m_BufferSize);
size_t curUsed = m_BufferHead - m_BufferBase;
memcpy(newBuf, m_BufferBase, curUsed);
FreeAlignedBuffer(m_BufferBase);
m_BufferBase = newBuf;
m_BufferHead = newBuf + curUsed;
}
// perform the actual copy
memcpy(m_BufferHead, data, numBytes);
// advance head
m_BufferHead += numBytes;
}
else if(m_Compressor)
{
m_Compressor->Write(data, numBytes);
}
else
{
RDCASSERT(m_File);
fwrite(data, 1, numBytes, m_File);
}
}
// compile-time constant amount of data to let the compiler inline the memcpy
template<typename T>
void Write(const T &data)
{
Write(&data, sizeof(data));
}
void Finish()
{
if(m_Compressor)
m_Compressor->Finish();
else if(m_File)
fflush(m_File);
}
private:
// used for aligned writes
static const byte empty[128];
// base of the buffer allocation if we're writing to a buffer
byte *m_BufferBase;
// where we are currently writing to in the buffer
byte *m_BufferHead;
// the size of the buffer
uint64_t m_BufferSize;
// the total size of the file/compressor (ie. how much data flushed through it)
uint64_t m_WriteSize;
// file pointer, if we're writing to a file
FILE *m_File;
// the compressor, if writing to it
Compressor *m_Compressor;
};<file_sep>/json_convert.h
#pragma once
#include <string>
#include <vector>
#include "common.h"
#include "serialiser.h"
#define PICOJSON_USE_INT64
#undef min
#undef max
#include "picojson.h"
struct SerialisedFile;
typedef std::pair<byte*,uint64_t> SerialisedBuffer;
std::string SerialisedFile2JSON(SerialisedFile file, bool concise = true);
void Buffers2ZIP(const char *filename, SerialisedFile file);
bool has(picojson::object o, const std::string &key);
extern std::vector<SerialisedBuffer> *bufList;
DECLARE_SERIALISE_TYPE(picojson::value);
<file_sep>/zstdio.cpp
/******************************************************************************
* The MIT License (MIT)
*
* Copyright (c) 2017 <NAME>
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
******************************************************************************/
#define ZSTD_STATIC_LINKING_ONLY
#include "zstdio.h"
static const size_t zstdBlockSize = 128 * 1024;
ZSTDCompressor::ZSTDCompressor(StreamWriter *write) : Compressor(write)
{
m_Page = AllocAlignedBuffer(zstdBlockSize);
m_CompressBuffer = AllocAlignedBuffer(ZSTD_CStreamOutSize());
m_PageOffset = 0;
m_Stream = ZSTD_createCStream();
}
ZSTDCompressor::~ZSTDCompressor()
{
ZSTD_freeCStream(m_Stream);
FreeAlignedBuffer(m_Page);
FreeAlignedBuffer(m_CompressBuffer);
}
void ZSTDCompressor::Write(const void *data, uint64_t numBytes)
{
// if we encountered a stream error this will be NULL
if(!m_CompressBuffer)
return;
if(numBytes == 0)
return;
// this is largely similar to LZ4Compressor, so check the comments there for more details.
// The only difference is that the lz4 streaming compression assumes a history of 64kb, where
// here we use a larger block size but no history must be maintained. This means we can
// potentially do less copying in the compress-from-source path
if(m_PageOffset + numBytes <= zstdBlockSize)
{
// simplest path, no page wrapping/spanning at all
memcpy(m_Page + m_PageOffset, data, numBytes);
m_PageOffset += numBytes;
}
else if(numBytes <= 2 * zstdBlockSize)
{
// simple path, do through partial copies that span pages and flush as necessary
const byte *src = (const byte *)data;
// copy whatever will fit on this page
{
uint64_t firstBytes = zstdBlockSize - m_PageOffset;
memcpy(m_Page + m_PageOffset, src, firstBytes);
m_PageOffset += firstBytes;
numBytes -= firstBytes;
src += firstBytes;
}
while(numBytes > 0)
{
// flush page
FlushPage();
// how many bytes can we copy in this page?
uint64_t partialBytes = RDCMIN(zstdBlockSize, numBytes);
memcpy(m_Page, src, partialBytes);
// advance the source pointer, dest offset, and remove the bytes we read
m_PageOffset += partialBytes;
numBytes -= partialBytes;
src += partialBytes;
}
}
else
{
// complex path, do a partial copy to finish a page then directly stream through zstd to the writer.
const byte *src = (const byte *)data;
// copy into the partial page
{
uint64_t firstBytes = zstdBlockSize - m_PageOffset;
memcpy(m_Page + m_PageOffset, src, firstBytes);
m_PageOffset += firstBytes;
numBytes -= firstBytes;
src += firstBytes;
// flush out to disk
FlushPage();
}
// while we still have at least one whole page remaining, compress & write directly without using the pages
while(numBytes > zstdBlockSize)
{
ZSTD_inBuffer in = {src, zstdBlockSize, 0};
ZSTD_outBuffer out = {m_CompressBuffer, ZSTD_CStreamOutSize(), 0};
CompressZSTDFrame(in, out);
// if there was an error, bail
if(!m_CompressBuffer)
return;
// a bit redundant to write this but it means we can read the entire frame without
// doing multiple reads
m_Write->Write((uint32_t)out.pos);
m_Write->Write(m_CompressBuffer, out.pos);
numBytes -= zstdBlockSize;
src += zstdBlockSize;
}
// write the rest into the page as we know it fits on a page
memcpy(m_Page + m_PageOffset, src, numBytes);
m_PageOffset += numBytes;
}
}
void ZSTDCompressor::Finish()
{
// This function just writes the current page and closes zstd. Since we assume all blocks are precisely 64kb in size
// only the last one can be smaller, so we only write a partial page when finishing.
// Calling Write() after Finish() is illegal
FlushPage();
}
void ZSTDCompressor::FlushPage()
{
// if we encountered a stream error this will be NULL
if(!m_CompressBuffer)
return;
ZSTD_inBuffer in = {m_Page, m_PageOffset, 0};
ZSTD_outBuffer out = {m_CompressBuffer, ZSTD_CStreamOutSize(), 0};
CompressZSTDFrame(in, out);
// if there was an error, bail
if(!m_CompressBuffer)
return;
// a bit redundant to write this but it means we can read the entire frame without
// doing multiple reads
m_Write->Write((uint32_t)out.pos);
m_Write->Write(m_CompressBuffer, out.pos);
// start writing to the start of the page again
m_PageOffset = 0;
}
void ZSTDCompressor::CompressZSTDFrame(ZSTD_inBuffer &in, ZSTD_outBuffer &out)
{
size_t err = ZSTD_initCStream(m_Stream, 7);
if(ZSTD_isError(err))
{
RDCERR("Error compressing: %s", ZSTD_getErrorName(err));
FreeAlignedBuffer(m_Page);
FreeAlignedBuffer(m_CompressBuffer);
m_Page = m_CompressBuffer = NULL;
return;
}
// keep calling compressStream until everything is consumed
while(in.pos < in.size)
{
size_t inpos = in.pos;
size_t outpos = out.pos;
size_t err = ZSTD_compressStream(m_Stream, &out, &in);
if(ZSTD_isError(err) || (inpos == in.pos && outpos == out.pos))
{
if(ZSTD_isError(err))
RDCERR("Error compressing: %s", ZSTD_getErrorName(err));
else
RDCERR("Error compressing, no progress made");
FreeAlignedBuffer(m_Page);
FreeAlignedBuffer(m_CompressBuffer);
m_Page = m_CompressBuffer = NULL;
return;
}
}
err = ZSTD_endStream(m_Stream, &out);
if(ZSTD_isError(err) || err != 0)
{
if(ZSTD_isError(err))
RDCERR("Error compressing: %s", ZSTD_getErrorName(err));
else
RDCERR("Error compressing, couldn't end stream");
FreeAlignedBuffer(m_Page);
FreeAlignedBuffer(m_CompressBuffer);
m_Page = m_CompressBuffer = NULL;
return;
}
}
ZSTDDecompressor::ZSTDDecompressor(StreamReader *read) : Decompressor(read)
{
m_Page = AllocAlignedBuffer(zstdBlockSize);
m_CompressBuffer = AllocAlignedBuffer(ZSTD_DStreamOutSize());
m_PageOffset = 0;
m_PageLength = 0;
m_Stream = ZSTD_createDStream();
}
ZSTDDecompressor::~ZSTDDecompressor()
{
ZSTD_freeDStream(m_Stream);
FreeAlignedBuffer(m_Page);
FreeAlignedBuffer(m_CompressBuffer);
}
void ZSTDDecompressor::Recompress(Compressor *comp)
{
while(!m_Read->AtEnd())
{
FillPage();
comp->Write(m_Page, m_PageLength);
}
comp->Finish();
}
void ZSTDDecompressor::Read(void *data, uint64_t numBytes)
{
// if we encountered a stream error this will be NULL
if(!m_CompressBuffer)
return;
if(numBytes == 0)
return;
// this is simpler than the ZstdWriter::Write() implementation.
// At any point, m_Page contains the current window with uncompressed bytes.
// If we can satisfy a read from it, then we just memcpy and increment m_PageOffset.
// When we wrap around, we do a partial memcpy from m_Page, then decompress more bytes.
// in future we could do an optimisation similar to Write() to reduce copying on large reads.
// if we already have all the data in-memory, just copy and return
uint64_t available = m_PageLength - m_PageOffset;
if(numBytes <= available)
{
memcpy(data, m_Page + m_PageOffset, numBytes);
m_PageOffset += numBytes;
return;
}
byte *dst = (byte *)data;
// copy what remains in m_Page
memcpy(dst, m_Page + m_PageOffset, available);
// adjust what needs to be copied
dst += available;
numBytes -= available;
while(numBytes > 0)
{
FillPage();
// if we can now satisfy the remainder of the read, do so and return
if(numBytes <= m_PageLength)
{
memcpy(dst, m_Page, numBytes);
m_PageOffset += numBytes;
return;
}
// otherwise copy this page in and continue
memcpy(dst, m_Page, m_PageLength);
dst += m_PageLength;
numBytes -= m_PageLength;
}
}
void ZSTDDecompressor::FillPage()
{
uint32_t compSize = 0;
m_Read->Read(compSize);
m_Read->Read(m_CompressBuffer, compSize);
size_t err = ZSTD_initDStream(m_Stream);
if(ZSTD_isError(err))
{
RDCERR("Error decompressing: %s", ZSTD_getErrorName(err));
FreeAlignedBuffer(m_Page);
FreeAlignedBuffer(m_CompressBuffer);
m_Page = m_CompressBuffer = NULL;
return;
}
ZSTD_inBuffer in = {m_CompressBuffer, compSize, 0};
ZSTD_outBuffer out = {m_Page, ZSTD_DStreamOutSize(), 0};
// keep calling compressStream until everything is consumed
while(in.pos < in.size)
{
size_t inpos = in.pos;
size_t outpos = out.pos;
size_t err = ZSTD_decompressStream(m_Stream, &out, &in);
if(ZSTD_isError(err) || (inpos == in.pos && outpos == out.pos))
{
if(ZSTD_isError(err))
RDCERR("Error decompressing: %s", ZSTD_getErrorName(err));
else
RDCERR("Error decompressing, no progress made");
FreeAlignedBuffer(m_Page);
FreeAlignedBuffer(m_CompressBuffer);
m_Page = m_CompressBuffer = NULL;
return;
}
}
m_PageOffset = 0;
m_PageLength = out.pos;
}
<file_sep>/lz4io.cpp
/******************************************************************************
* The MIT License (MIT)
*
* Copyright (c) 2017 <NAME>
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
******************************************************************************/
#include "lz4io.h"
static const size_t lz4BlockSize = 64 * 1024;
LZ4Compressor::LZ4Compressor(StreamWriter *write) : Compressor(write)
{
m_Page[0] = AllocAlignedBuffer(lz4BlockSize);
m_Page[1] = AllocAlignedBuffer(lz4BlockSize);
m_CompressBuffer = AllocAlignedBuffer(LZ4_COMPRESSBOUND(lz4BlockSize));
m_PageOffset = 0;
LZ4_resetStream(&m_LZ4Comp);
}
LZ4Compressor::~LZ4Compressor()
{
FreeAlignedBuffer(m_Page[0]);
FreeAlignedBuffer(m_Page[1]);
FreeAlignedBuffer(m_CompressBuffer);
}
void LZ4Compressor::Write(const void *data, uint64_t numBytes)
{
// if we encountered a stream error this will be NULL
if(!m_CompressBuffer)
return;
if(numBytes == 0)
return;
// The basic plan is:
// Write into page N incrementally until it is completely full. When full, flush it out to lz4 and swap pages.
// This keeps lz4 happy with 64kb of history each time it compresses.
// If we are writing some data the crosses the boundary between pages, we write the part that will fit on one page,
// flush & swap, write the rest into the next page.
//
// To reduce the amount of copying, if we are writing some data that is > 3 * page size, then we do something different:
// if the current page isn't empty (which is almost certain), follow the steps above to copy in the part that will fit.
// next loop over the remainder of the data, for as many whole-page sizes as we can, flushing each page directly from
// the source into lz4.
// when we get to the last < 2 page sizes, we go back to the normal copy and flush. The reason being we can't be using
// any of the input data as history since it may no longer be around, so we must copy and flush one whole page for
// history from our buffers and start on the next via copying to our own second page.
// So worst case we copy (page size - 1) bytes at the start, and (2 * page size - 1) at the end, but the rest is fed
// directly into lz4 from the input data.
if(m_PageOffset + numBytes <= lz4BlockSize)
{
// simplest path, no page wrapping/spanning at all
memcpy(m_Page[0] + m_PageOffset, data, numBytes);
m_PageOffset += numBytes;
}
else if(numBytes <= 3 * lz4BlockSize)
{
// simple path, do through partial copies that span pages and flush as necessary
const byte *src = (const byte *)data;
// copy whatever will fit on this page
{
uint64_t firstBytes = lz4BlockSize - m_PageOffset;
memcpy(m_Page[0] + m_PageOffset, src, firstBytes);
m_PageOffset += firstBytes;
numBytes -= firstBytes;
src += firstBytes;
}
while(numBytes > 0)
{
// flush and swap pages
FlushPage0();
// how many bytes can we copy in this page?
uint64_t partialBytes = RDCMIN(lz4BlockSize, numBytes);
memcpy(m_Page[0], src, partialBytes);
// advance the source pointer, dest offset, and remove the bytes we read
m_PageOffset += partialBytes;
numBytes -= partialBytes;
src += partialBytes;
}
}
else
{
// complex path, do a partial copy to finish a page then directly stream through lz4 to the writer.
const byte *src = (const byte *)data;
// copy into the partial page
{
uint64_t firstBytes = lz4BlockSize - m_PageOffset;
memcpy(m_Page[0] + m_PageOffset, src, firstBytes);
m_PageOffset += firstBytes;
numBytes -= firstBytes;
src += firstBytes;
// flush out to disk
FlushPage0();
}
// while we still have more than two pages remaining, compress & write directly without using the pages
while(numBytes > lz4BlockSize * 2)
{
// compress a block from src into m_CompressBuffer
int32_t compSize = LZ4_compress_fast_continue(&m_LZ4Comp, (const char *)src,
(char *)m_CompressBuffer, lz4BlockSize,
(int)LZ4_COMPRESSBOUND(lz4BlockSize), 1);
if(compSize < 0)
{
RDCERR("Error compressing: %i", compSize);
FreeAlignedBuffer(m_Page[0]);
FreeAlignedBuffer(m_Page[1]);
FreeAlignedBuffer(m_CompressBuffer);
m_Page[0] = m_Page[1] = m_CompressBuffer = NULL;
return;
}
m_Write->Write(compSize);
m_Write->Write(m_CompressBuffer, compSize);
numBytes -= lz4BlockSize;
src += lz4BlockSize;
}
// do a recursive call for the rest, which will go through the simple path above
Write(src, numBytes);
}
}
void LZ4Compressor::Finish()
{
// This function just writes the current page and closes lz4. Since we assume all blocks are precisely 64kb in size
// only the last one can be smaller, so we only write a partial page when finishing.
// Calling Write() after Finish() is illegal
FlushPage0();
}
void LZ4Compressor::FlushPage0()
{
// if we encountered a stream error this will be NULL
if(!m_CompressBuffer)
return;
// m_PageOffset is the amount written, usually equal to lz4BlockSize except the last block.
int32_t compSize = LZ4_compress_fast_continue(&m_LZ4Comp, (const char *)m_Page[0],
(char *)m_CompressBuffer, (int)m_PageOffset,
(int)LZ4_COMPRESSBOUND(lz4BlockSize), 1);
if(compSize < 0)
{
RDCERR("Error compressing: %i", compSize);
FreeAlignedBuffer(m_Page[0]);
FreeAlignedBuffer(m_Page[1]);
FreeAlignedBuffer(m_CompressBuffer);
m_Page[0] = m_Page[1] = m_CompressBuffer = NULL;
return;
}
m_Write->Write(compSize);
m_Write->Write(m_CompressBuffer, compSize);
// swap pages
std::swap(m_Page[0], m_Page[1]);
// start writing to the start of the page again
m_PageOffset = 0;
}
LZ4Decompressor::LZ4Decompressor(StreamReader *read) : Decompressor(read)
{
m_Page[0] = AllocAlignedBuffer(lz4BlockSize);
m_Page[1] = AllocAlignedBuffer(lz4BlockSize);
m_CompressBuffer = AllocAlignedBuffer(LZ4_COMPRESSBOUND(lz4BlockSize));
m_PageOffset = 0;
m_PageLength = 0;
LZ4_setStreamDecode(&m_LZ4Decomp, NULL, 0);
}
LZ4Decompressor::~LZ4Decompressor()
{
FreeAlignedBuffer(m_Page[0]);
FreeAlignedBuffer(m_Page[1]);
FreeAlignedBuffer(m_CompressBuffer);
}
void LZ4Decompressor::Recompress(Compressor *comp)
{
while(!m_Read->AtEnd())
{
FillPage0();
comp->Write(m_Page[0], m_PageLength);
}
comp->Finish();
}
void LZ4Decompressor::Read(void *data, uint64_t numBytes)
{
// if we encountered a stream error this will be NULL
if(!m_CompressBuffer)
return;
if(numBytes == 0)
return;
// this is simpler than the LZ4Writer::Write() implementation.
// At any point, m_Page[0] contains the current window with uncompressed bytes.
// If we can satisfy a read from it, then we just memcpy and increment m_PageOffset.
// When we wrap around, we do a partial memcpy from m_Page[0], then swap the pages and
// decompress some more bytes into m_Page[0]. Thus, m_Page[1] contains the history (if
// it exists)
// in future we could do an optimisation similar to Write() to reduce copying on large reads.
// if we already have all the data in-memory, just copy and return
uint64_t available = m_PageLength - m_PageOffset;
if(numBytes <= available)
{
memcpy(data, m_Page[0] + m_PageOffset, numBytes);
m_PageOffset += numBytes;
return;
}
byte *dst = (byte *)data;
// copy what remains in m_Page[0]
memcpy(dst, m_Page[0] + m_PageOffset, available);
// adjust what needs to be copied
dst += available;
numBytes -= available;
while(numBytes > 0)
{
FillPage0();
// if we can now satisfy the remainder of the read, do so and return
if(numBytes <= m_PageLength)
{
memcpy(dst, m_Page[0], numBytes);
m_PageOffset += numBytes;
return;
}
// otherwise copy this page in and continue
memcpy(dst, m_Page[0], m_PageLength);
dst += m_PageLength;
numBytes -= m_PageLength;
}
}
void LZ4Decompressor::FillPage0()
{
// swap pages
std::swap(m_Page[0], m_Page[1]);
int32_t compSize = 0;
m_Read->Read(compSize);
m_Read->Read(m_CompressBuffer, compSize);
int32_t decompSize = LZ4_decompress_safe_continue(
&m_LZ4Decomp, (const char *)m_CompressBuffer, (char *)m_Page[0], compSize, lz4BlockSize);
if(decompSize < 0)
{
RDCERR("Error decompressing: %i", decompSize);
FreeAlignedBuffer(m_Page[0]);
FreeAlignedBuffer(m_Page[1]);
FreeAlignedBuffer(m_CompressBuffer);
m_Page[0] = m_Page[1] = m_CompressBuffer = NULL;
return;
}
m_PageOffset = 0;
m_PageLength = decompSize;
}
<file_sep>/structured_data.h
/******************************************************************************
* The MIT License (MIT)
*
* Copyright (c) 2016 <NAME>
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
******************************************************************************/
#pragma once
#include <stdint.h>
#include <string>
#include <vector>
struct SerialisedType
{
enum BasicType
{
eChunk,
eStruct,
eArray,
eNull,
eBuffer,
eString,
eEnum,
eUnsignedInteger,
eSignedInteger,
eFloat,
eBoolean,
eCharacter,
};
enum
{
HasCustomString = 0x1,
Hidden = 0x2,
Nullable = 0x4,
};
SerialisedType(const char *n) : name(n), basetype(eStruct), flags(0), byteSize(0) {}
std::string name;
BasicType basetype;
uint32_t flags;
uint64_t byteSize;
private:
// don't copy
SerialisedType(const SerialisedType &);
};
struct SerialisedChunkMetaData
{
SerialisedChunkMetaData()
{
chunkID = 0;
threadID = 0;
flags = 0;
duration = 0;
timestamp = 0;
RDCEraseEl(callstack);
}
enum
{
OpaqueChunk = 0x1,
};
uint32_t chunkID;
uint32_t threadID;
uint64_t flags;
uint64_t duration;
uint64_t timestamp;
uint64_t callstack[32];
};
struct StructuredObject
{
StructuredObject(const char *n, const char *t)
: type(t)
{
name = n;
data.basic.u = 0;
}
~StructuredObject()
{
for(size_t i=0; i < data.children.size(); i++)
delete data.children[i];
data.children.clear();
}
std::string name;
SerialisedType type;
struct
{
union
{
uint64_t u;
int64_t i;
double d;
bool b;
char c;
// mostly here just for debugging
uint64_t numChildren;
} basic;
std::string str;
std::vector<StructuredObject*> children;
} data;
private:
// don't copy
StructuredObject(const StructuredObject &);
};
struct StructuredChunk : StructuredObject
{
StructuredChunk(const char *n, const char *t) : StructuredObject(n, t)
{
type.basetype = SerialisedType::eChunk;
}
~StructuredChunk() { }
SerialisedChunkMetaData metadata;
};
typedef std::pair<byte *,uint64_t> SerialisedBuffer;
typedef std::vector<StructuredChunk *> StructuredChunkList;
typedef std::vector<SerialisedBuffer> SerialisedBufferList;
struct SerialisedFile
{
SerialisedFile(const StructuredChunkList &c, const SerialisedBufferList &d)
: chunks(c)
, data(d)
{
}
const StructuredChunkList &chunks;
const SerialisedBufferList &data;
};
<file_sep>/real.cpp
#include "serialiser.h"
#include "xml_codec.h"
// for ResourceId stuff already written
#include "test_types.h"
/////////////////////////////////////////////////////////////////
// minimal inlined structs/enums from dx headers
#pragma region headers
#include "official/vulkan.h"
typedef unsigned short WORD;
typedef int INT;
typedef signed int INT32;
typedef unsigned int UINT;
typedef unsigned int UINT32;
typedef long LONG;
typedef unsigned long ULONG;
typedef unsigned long DWORD;
typedef size_t SIZE_T;
typedef LONG HRESULT;
#define SUCCEEDED(hr) (((HRESULT)(hr)) >= 0)
#define FAILED(hr) (((HRESULT)(hr)) < 0)
#define S_OK ((HRESULT)0L)
struct ID3D11ClassLinkage;
struct ID3D11RenderTargetView;
struct ID3D11InputLayout;
struct ID3D11VertexShader;
#define D3D11_SDK_VERSION ( 7 )
typedef
enum D3D_DRIVER_TYPE
{
D3D_DRIVER_TYPE_UNKNOWN = 0,
D3D_DRIVER_TYPE_HARDWARE = ( D3D_DRIVER_TYPE_UNKNOWN + 1 ) ,
D3D_DRIVER_TYPE_REFERENCE = ( D3D_DRIVER_TYPE_HARDWARE + 1 ) ,
D3D_DRIVER_TYPE_NULL = ( D3D_DRIVER_TYPE_REFERENCE + 1 ) ,
D3D_DRIVER_TYPE_SOFTWARE = ( D3D_DRIVER_TYPE_NULL + 1 ) ,
D3D_DRIVER_TYPE_WARP = ( D3D_DRIVER_TYPE_SOFTWARE + 1 )
} D3D_DRIVER_TYPE;
typedef
enum D3D_FEATURE_LEVEL
{
D3D_FEATURE_LEVEL_9_1 = 0x9100,
D3D_FEATURE_LEVEL_9_2 = 0x9200,
D3D_FEATURE_LEVEL_9_3 = 0x9300,
D3D_FEATURE_LEVEL_10_0 = 0xa000,
D3D_FEATURE_LEVEL_10_1 = 0xa100,
D3D_FEATURE_LEVEL_11_0 = 0xb000,
D3D_FEATURE_LEVEL_11_1 = 0xb100,
D3D_FEATURE_LEVEL_12_0 = 0xc000,
D3D_FEATURE_LEVEL_12_1 = 0xc100
} D3D_FEATURE_LEVEL;
typedef
enum D3D11_INPUT_CLASSIFICATION
{
D3D11_INPUT_PER_VERTEX_DATA = 0,
D3D11_INPUT_PER_INSTANCE_DATA = 1
} D3D11_INPUT_CLASSIFICATION;
typedef enum DXGI_FORMAT
{
DXGI_FORMAT_UNKNOWN = 0,
DXGI_FORMAT_R32G32B32A32_TYPELESS = 1,
DXGI_FORMAT_R32G32B32A32_FLOAT = 2,
DXGI_FORMAT_R32G32B32A32_UINT = 3,
DXGI_FORMAT_R32G32B32A32_SINT = 4,
DXGI_FORMAT_R32G32B32_TYPELESS = 5,
DXGI_FORMAT_R32G32B32_FLOAT = 6,
DXGI_FORMAT_R32G32B32_UINT = 7,
DXGI_FORMAT_R32G32B32_SINT = 8,
DXGI_FORMAT_R16G16B16A16_TYPELESS = 9,
DXGI_FORMAT_R16G16B16A16_FLOAT = 10,
DXGI_FORMAT_R16G16B16A16_UNORM = 11,
DXGI_FORMAT_R16G16B16A16_UINT = 12,
DXGI_FORMAT_R16G16B16A16_SNORM = 13,
DXGI_FORMAT_R16G16B16A16_SINT = 14,
DXGI_FORMAT_R32G32_TYPELESS = 15,
DXGI_FORMAT_R32G32_FLOAT = 16,
DXGI_FORMAT_R32G32_UINT = 17,
DXGI_FORMAT_R32G32_SINT = 18,
DXGI_FORMAT_R32G8X24_TYPELESS = 19,
DXGI_FORMAT_D32_FLOAT_S8X24_UINT = 20,
DXGI_FORMAT_R32_FLOAT_X8X24_TYPELESS = 21,
DXGI_FORMAT_X32_TYPELESS_G8X24_UINT = 22,
DXGI_FORMAT_R10G10B10A2_TYPELESS = 23,
DXGI_FORMAT_R10G10B10A2_UNORM = 24,
DXGI_FORMAT_R10G10B10A2_UINT = 25,
DXGI_FORMAT_R11G11B10_FLOAT = 26,
DXGI_FORMAT_R8G8B8A8_TYPELESS = 27,
DXGI_FORMAT_R8G8B8A8_UNORM = 28,
DXGI_FORMAT_R8G8B8A8_UNORM_SRGB = 29,
DXGI_FORMAT_R8G8B8A8_UINT = 30,
DXGI_FORMAT_R8G8B8A8_SNORM = 31,
DXGI_FORMAT_R8G8B8A8_SINT = 32,
DXGI_FORMAT_R16G16_TYPELESS = 33,
DXGI_FORMAT_R16G16_FLOAT = 34,
DXGI_FORMAT_R16G16_UNORM = 35,
DXGI_FORMAT_R16G16_UINT = 36,
DXGI_FORMAT_R16G16_SNORM = 37,
DXGI_FORMAT_R16G16_SINT = 38,
DXGI_FORMAT_R32_TYPELESS = 39,
DXGI_FORMAT_D32_FLOAT = 40,
DXGI_FORMAT_R32_FLOAT = 41,
DXGI_FORMAT_R32_UINT = 42,
DXGI_FORMAT_R32_SINT = 43,
DXGI_FORMAT_R24G8_TYPELESS = 44,
DXGI_FORMAT_D24_UNORM_S8_UINT = 45,
DXGI_FORMAT_R24_UNORM_X8_TYPELESS = 46,
DXGI_FORMAT_X24_TYPELESS_G8_UINT = 47,
DXGI_FORMAT_R8G8_TYPELESS = 48,
DXGI_FORMAT_R8G8_UNORM = 49,
DXGI_FORMAT_R8G8_UINT = 50,
DXGI_FORMAT_R8G8_SNORM = 51,
DXGI_FORMAT_R8G8_SINT = 52,
DXGI_FORMAT_R16_TYPELESS = 53,
DXGI_FORMAT_R16_FLOAT = 54,
DXGI_FORMAT_D16_UNORM = 55,
DXGI_FORMAT_R16_UNORM = 56,
DXGI_FORMAT_R16_UINT = 57,
DXGI_FORMAT_R16_SNORM = 58,
DXGI_FORMAT_R16_SINT = 59,
DXGI_FORMAT_R8_TYPELESS = 60,
DXGI_FORMAT_R8_UNORM = 61,
DXGI_FORMAT_R8_UINT = 62,
DXGI_FORMAT_R8_SNORM = 63,
DXGI_FORMAT_R8_SINT = 64,
DXGI_FORMAT_A8_UNORM = 65,
DXGI_FORMAT_R1_UNORM = 66,
DXGI_FORMAT_R9G9B9E5_SHAREDEXP = 67,
DXGI_FORMAT_R8G8_B8G8_UNORM = 68,
DXGI_FORMAT_G8R8_G8B8_UNORM = 69,
DXGI_FORMAT_BC1_TYPELESS = 70,
DXGI_FORMAT_BC1_UNORM = 71,
DXGI_FORMAT_BC1_UNORM_SRGB = 72,
DXGI_FORMAT_BC2_TYPELESS = 73,
DXGI_FORMAT_BC2_UNORM = 74,
DXGI_FORMAT_BC2_UNORM_SRGB = 75,
DXGI_FORMAT_BC3_TYPELESS = 76,
DXGI_FORMAT_BC3_UNORM = 77,
DXGI_FORMAT_BC3_UNORM_SRGB = 78,
DXGI_FORMAT_BC4_TYPELESS = 79,
DXGI_FORMAT_BC4_UNORM = 80,
DXGI_FORMAT_BC4_SNORM = 81,
DXGI_FORMAT_BC5_TYPELESS = 82,
DXGI_FORMAT_BC5_UNORM = 83,
DXGI_FORMAT_BC5_SNORM = 84,
DXGI_FORMAT_B5G6R5_UNORM = 85,
DXGI_FORMAT_B5G5R5A1_UNORM = 86,
DXGI_FORMAT_B8G8R8A8_UNORM = 87,
DXGI_FORMAT_B8G8R8X8_UNORM = 88,
DXGI_FORMAT_R10G10B10_XR_BIAS_A2_UNORM = 89,
DXGI_FORMAT_B8G8R8A8_TYPELESS = 90,
DXGI_FORMAT_B8G8R8A8_UNORM_SRGB = 91,
DXGI_FORMAT_B8G8R8X8_TYPELESS = 92,
DXGI_FORMAT_B8G8R8X8_UNORM_SRGB = 93,
DXGI_FORMAT_BC6H_TYPELESS = 94,
DXGI_FORMAT_BC6H_UF16 = 95,
DXGI_FORMAT_BC6H_SF16 = 96,
DXGI_FORMAT_BC7_TYPELESS = 97,
DXGI_FORMAT_BC7_UNORM = 98,
DXGI_FORMAT_BC7_UNORM_SRGB = 99,
DXGI_FORMAT_AYUV = 100,
DXGI_FORMAT_Y410 = 101,
DXGI_FORMAT_Y416 = 102,
DXGI_FORMAT_NV12 = 103,
DXGI_FORMAT_P010 = 104,
DXGI_FORMAT_P016 = 105,
DXGI_FORMAT_420_OPAQUE = 106,
DXGI_FORMAT_YUY2 = 107,
DXGI_FORMAT_Y210 = 108,
DXGI_FORMAT_Y216 = 109,
DXGI_FORMAT_NV11 = 110,
DXGI_FORMAT_AI44 = 111,
DXGI_FORMAT_IA44 = 112,
DXGI_FORMAT_P8 = 113,
DXGI_FORMAT_A8P8 = 114,
DXGI_FORMAT_B4G4R4A4_UNORM = 115,
DXGI_FORMAT_P208 = 130,
DXGI_FORMAT_V208 = 131,
DXGI_FORMAT_V408 = 132,
DXGI_FORMAT_FORCE_UINT = 0xffffffff
} DXGI_FORMAT;
typedef struct D3D11_INPUT_ELEMENT_DESC
{
const char * SemanticName;
UINT SemanticIndex;
DXGI_FORMAT Format;
UINT InputSlot;
UINT AlignedByteOffset;
D3D11_INPUT_CLASSIFICATION InputSlotClass;
UINT InstanceDataStepRate;
} D3D11_INPUT_ELEMENT_DESC;
typedef struct D3D11_BOX
{
UINT left;
UINT top;
UINT front;
UINT right;
UINT bottom;
UINT back;
} D3D11_BOX;
#pragma endregion headers
/////////////////////////////////////////////////////////////////
// d3d11_common.cpp / .h
#pragma region d3d11_common.cpp
SERIALISE_ENUM_TYPE(D3D_DRIVER_TYPE);
template <>
string ToStr(const D3D_DRIVER_TYPE &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(D3D_DRIVER_TYPE_UNKNOWN);
STRINGIZE_ENUM(D3D_DRIVER_TYPE_HARDWARE);
STRINGIZE_ENUM(D3D_DRIVER_TYPE_REFERENCE);
STRINGIZE_ENUM(D3D_DRIVER_TYPE_NULL);
STRINGIZE_ENUM(D3D_DRIVER_TYPE_SOFTWARE);
STRINGIZE_ENUM(D3D_DRIVER_TYPE_WARP);
END_ENUM_STRINGIZE(D3D_DRIVER_TYPE);
}
SERIALISE_ENUM_TYPE(D3D_FEATURE_LEVEL);
template <>
string DoStringize(const D3D_FEATURE_LEVEL &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(D3D_FEATURE_LEVEL_9_1);
STRINGIZE_ENUM(D3D_FEATURE_LEVEL_9_2);
STRINGIZE_ENUM(D3D_FEATURE_LEVEL_9_3);
STRINGIZE_ENUM(D3D_FEATURE_LEVEL_10_0);
STRINGIZE_ENUM(D3D_FEATURE_LEVEL_10_1);
STRINGIZE_ENUM(D3D_FEATURE_LEVEL_11_0);
STRINGIZE_ENUM(D3D_FEATURE_LEVEL_11_1);
STRINGIZE_ENUM(D3D_FEATURE_LEVEL_12_0);
STRINGIZE_ENUM(D3D_FEATURE_LEVEL_12_1);
END_ENUM_STRINGIZE(D3D_FEATURE_LEVEL);
}
SERIALISE_ENUM_TYPE(D3D11_INPUT_CLASSIFICATION);
template <>
string DoStringize(const D3D11_INPUT_CLASSIFICATION &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(D3D11_INPUT_PER_VERTEX_DATA)
STRINGIZE_ENUM(D3D11_INPUT_PER_INSTANCE_DATA)
END_ENUM_STRINGIZE(D3D11_INPUT_CLASSIFICATION);
}
SERIALISE_ENUM_TYPE(DXGI_FORMAT);
template <>
string DoStringize(const DXGI_FORMAT &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(DXGI_FORMAT_UNKNOWN)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32B32A32_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32B32A32_FLOAT)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32B32A32_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32B32A32_SINT)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32B32_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32B32_FLOAT)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32B32_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32B32_SINT)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16B16A16_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16B16A16_FLOAT)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16B16A16_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16B16A16_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16B16A16_SNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16B16A16_SINT)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32_FLOAT)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R32G32_SINT)
STRINGIZE_ENUM(DXGI_FORMAT_R32G8X24_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_D32_FLOAT_S8X24_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R32_FLOAT_X8X24_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_X32_TYPELESS_G8X24_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R10G10B10A2_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_R10G10B10A2_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R10G10B10A2_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R11G11B10_FLOAT)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8B8A8_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8B8A8_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8B8A8_UNORM_SRGB)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8B8A8_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8B8A8_SNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8B8A8_SINT)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16_FLOAT)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16_SNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R16G16_SINT)
STRINGIZE_ENUM(DXGI_FORMAT_R32_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_D32_FLOAT)
STRINGIZE_ENUM(DXGI_FORMAT_R32_FLOAT)
STRINGIZE_ENUM(DXGI_FORMAT_R32_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R32_SINT)
STRINGIZE_ENUM(DXGI_FORMAT_R24G8_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_D24_UNORM_S8_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R24_UNORM_X8_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_X24_TYPELESS_G8_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8_SNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8_SINT)
STRINGIZE_ENUM(DXGI_FORMAT_R16_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_R16_FLOAT)
STRINGIZE_ENUM(DXGI_FORMAT_D16_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R16_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R16_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R16_SNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R16_SINT)
STRINGIZE_ENUM(DXGI_FORMAT_R8_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_R8_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R8_UINT)
STRINGIZE_ENUM(DXGI_FORMAT_R8_SNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R8_SINT)
STRINGIZE_ENUM(DXGI_FORMAT_A8_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R1_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R9G9B9E5_SHAREDEXP)
STRINGIZE_ENUM(DXGI_FORMAT_R8G8_B8G8_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_G8R8_G8B8_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_BC1_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_BC1_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_BC1_UNORM_SRGB)
STRINGIZE_ENUM(DXGI_FORMAT_BC2_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_BC2_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_BC2_UNORM_SRGB)
STRINGIZE_ENUM(DXGI_FORMAT_BC3_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_BC3_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_BC3_UNORM_SRGB)
STRINGIZE_ENUM(DXGI_FORMAT_BC4_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_BC4_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_BC4_SNORM)
STRINGIZE_ENUM(DXGI_FORMAT_BC5_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_BC5_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_BC5_SNORM)
STRINGIZE_ENUM(DXGI_FORMAT_B5G6R5_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_B5G5R5A1_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_B8G8R8A8_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_B8G8R8X8_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_R10G10B10_XR_BIAS_A2_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_B8G8R8A8_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_B8G8R8A8_UNORM_SRGB)
STRINGIZE_ENUM(DXGI_FORMAT_B8G8R8X8_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_B8G8R8X8_UNORM_SRGB)
STRINGIZE_ENUM(DXGI_FORMAT_BC6H_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_BC6H_UF16)
STRINGIZE_ENUM(DXGI_FORMAT_BC6H_SF16)
STRINGIZE_ENUM(DXGI_FORMAT_BC7_TYPELESS)
STRINGIZE_ENUM(DXGI_FORMAT_BC7_UNORM)
STRINGIZE_ENUM(DXGI_FORMAT_BC7_UNORM_SRGB)
// D3D11.1 formats
STRINGIZE_ENUM(DXGI_FORMAT_AYUV)
STRINGIZE_ENUM(DXGI_FORMAT_Y410)
STRINGIZE_ENUM(DXGI_FORMAT_Y416)
STRINGIZE_ENUM(DXGI_FORMAT_NV12)
STRINGIZE_ENUM(DXGI_FORMAT_P010)
STRINGIZE_ENUM(DXGI_FORMAT_P016)
STRINGIZE_ENUM(DXGI_FORMAT_420_OPAQUE)
STRINGIZE_ENUM(DXGI_FORMAT_YUY2)
STRINGIZE_ENUM(DXGI_FORMAT_Y210)
STRINGIZE_ENUM(DXGI_FORMAT_Y216)
STRINGIZE_ENUM(DXGI_FORMAT_NV11)
STRINGIZE_ENUM(DXGI_FORMAT_AI44)
STRINGIZE_ENUM(DXGI_FORMAT_IA44)
STRINGIZE_ENUM(DXGI_FORMAT_P8)
STRINGIZE_ENUM(DXGI_FORMAT_A8P8)
STRINGIZE_ENUM(DXGI_FORMAT_B4G4R4A4_UNORM)
// D3D11.2 formats
STRINGIZE_ENUM(DXGI_FORMAT_P208)
STRINGIZE_ENUM(DXGI_FORMAT_V208)
STRINGIZE_ENUM(DXGI_FORMAT_V408)
END_ENUM_STRINGIZE(DXGI_FORMAT);
}
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, D3D11_INPUT_ELEMENT_DESC &el)
{
SERIALISE_MEMBER(SemanticName);
SERIALISE_MEMBER(SemanticIndex);
SERIALISE_MEMBER(Format);
SERIALISE_MEMBER(InputSlot);
SERIALISE_MEMBER(AlignedByteOffset);
SERIALISE_MEMBER(InputSlotClass);
SERIALISE_MEMBER(InstanceDataStepRate);
}
INSTANTIATE_SERIALISE_TYPE(D3D11_INPUT_ELEMENT_DESC);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, D3D11_BOX &el)
{
SERIALISE_MEMBER(left);
SERIALISE_MEMBER(top);
SERIALISE_MEMBER(front);
SERIALISE_MEMBER(right);
SERIALISE_MEMBER(bottom);
SERIALISE_MEMBER(back);
}
INSTANTIATE_SERIALISE_TYPE(D3D11_BOX);
#pragma endregion
/////////////////////////////////////////////////////////////////
// core.cpp / .h
#pragma region core.cpp
enum ReplayCreateStatus
{
eReplayCreate_Success = 0,
eReplayCreate_UnknownError,
eReplayCreate_InternalError,
eReplayCreate_NetworkIOFailed,
eReplayCreate_FileIOFailed,
eReplayCreate_FileIncompatibleVersion,
eReplayCreate_FileCorrupted,
eReplayCreate_APIUnsupported,
eReplayCreate_APIInitFailed,
eReplayCreate_APIIncompatibleVersion,
eReplayCreate_APIHardwareUnsupported,
};
enum LogState
{
READING = 0,
EXECUTING,
WRITING,
WRITING_IDLE,
WRITING_CAPFRAME,
};
enum RDCDriver
{
RDC_Unknown = 0,
RDC_D3D11 = 1,
RDC_OpenGL = 2,
RDC_Mantle = 3,
RDC_D3D12 = 4,
RDC_D3D10 = 5,
RDC_D3D9 = 6,
RDC_Image = 7,
RDC_Vulkan = 8,
RDC_Custom = 100000,
RDC_Custom0 = RDC_Custom,
RDC_Custom1,
RDC_Custom2,
RDC_Custom3,
RDC_Custom4,
RDC_Custom5,
RDC_Custom6,
RDC_Custom7,
RDC_Custom8,
RDC_Custom9,
};
SERIALISE_ENUM_TYPE(RDCDriver);
template <>
string DoStringize(const RDCDriver &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM_NAMED(RDC_Unknown, "Unknown");
STRINGIZE_ENUM_NAMED(RDC_D3D11, "D3D11");
STRINGIZE_ENUM_NAMED(RDC_OpenGL, "OpenGL");
STRINGIZE_ENUM_NAMED(RDC_Mantle, "Mantle");
STRINGIZE_ENUM_NAMED(RDC_D3D12, "D3D12");
STRINGIZE_ENUM_NAMED(RDC_D3D10, "D3D10");
STRINGIZE_ENUM_NAMED(RDC_D3D9, "D3D9");
STRINGIZE_ENUM_NAMED(RDC_Image, "Image");
STRINGIZE_ENUM_NAMED(RDC_Vulkan, "Vulkan");
END_ENUM_STRINGIZE(RDCDriver);
}
struct DebugMessage
{
uint32_t eventID;
uint32_t messageID;
string description;
};
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, DebugMessage &el)
{
SERIALISE_MEMBER(eventID);
SERIALISE_MEMBER(messageID);
SERIALISE_MEMBER(description);
}
INSTANTIATE_SERIALISE_TYPE(DebugMessage);
enum ChunkType
{
// 0 is reserved as a 'null' chunk that is only for debug
CREATE_PARAMS = 1,
THUMBNAIL_DATA,
DRIVER_INIT_PARAMS,
INITIAL_CONTENTS,
FIRST_CHUNK_ID = 128,
CREATE_INPUT_LAYOUT,
CREATE_VERTEX_SHADER,
DO_VULKAN_STUFF,
DEBUG_MESSAGES,
CLEAR_RTV,
};
struct RDCInitParams
{
virtual ~RDCInitParams() {}
virtual void Serialise(WriteSerialiser &ser) = 0;
virtual void Serialise(ReadSerialiser &ser) = 0;
virtual void Serialise(StructReadSerialiser &ser) = 0;
virtual ReplayCreateStatus GetStatus() = 0;
};
// utility macro for derived classes to be able to just make a templated Serialise() function
#define INIT_PARAMS_SERIALISE() \
template<class SerialiserType> \
void DoSerialise(SerialiserType &ser); \
virtual void Serialise(WriteSerialiser &ser) { return DoSerialise(ser); } \
virtual void Serialise(ReadSerialiser &ser) { return DoSerialise(ser); } \
virtual void Serialise(StructReadSerialiser &ser) { return DoSerialise(ser); }
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, RDCInitParams &el)
{
// go through overloaded virtual interface
el.Serialise(ser);
}
INSTANTIATE_SERIALISE_TYPE(RDCInitParams);
WriteSerialiser *OpenWriteSerialiser(uint32_t frameNum, RDCInitParams ¶ms, byte *thpixels,
size_t thlen, uint32_t thwidth, uint32_t thheight)
{
#if defined(RELEASE)
const bool debugSerialiser = false;
#else
const bool debugSerialiser = true;
#endif
string m_CurrentLogFile = "test.rdc";
RDCDriver m_CurrentDriver = RDC_Mantle;
string m_CurrentDriverName = "Mantle";
FILE *file = fopen(m_CurrentLogFile.c_str(), "wb");
WriteSerialiser *fileSerialiser = new WriteSerialiser(new StreamWriter(file));
WriteSerialiser *chunkSerialiser = new WriteSerialiser(new StreamWriter(StreamWriter::DefaultScratchSize));
WriteSerialiser &ser = *chunkSerialiser;
{
SCOPED_SERIALISE_CONTEXT(THUMBNAIL_DATA);
SERIALISE_ELEMENT_LOCAL(HasThumbnail, thpixels != NULL && thwidth > 0 && thheight > 0);
if(HasThumbnail)
{
SERIALISE_ELEMENT(thwidth).Named("Thumbnail Width");
SERIALISE_ELEMENT(thheight).Named("Thumbnail Height");
SERIALISE_ELEMENT_ARRAY(thpixels, thlen).Named("Thumbnail Pixels");
}
SERIALISE_ALIGNED();
fileSerialiser->Insert(scope.Get(true));
}
{
SCOPED_SERIALISE_CONTEXT(CREATE_PARAMS);
SERIALISE_ELEMENT(m_CurrentDriver).Named("Driver Type");
SERIALISE_ELEMENT(m_CurrentDriverName).Named("Driver Name");
SERIALISE_ELEMENT(params).Named("Driver Params");
SERIALISE_ALIGNED();
fileSerialiser->Insert(scope.Get(true));
}
SAFE_DELETE(chunkSerialiser);
return fileSerialiser;
}
ReplayCreateStatus FillInitParams(const char *logFile, RDCDriver &driverType, string &driverName, RDCInitParams *params)
{
FILE *f = fopen(logFile, "rb");
StreamReader read(f);
ReadSerialiser ser(&read);
if(ser.HasError())
return eReplayCreate_FileIOFailed;
{
int chunkType = ser.BeginChunk(0);
if(chunkType != THUMBNAIL_DATA)
{
RDCERR("Malformed logfile '%s', first chunk isn't thumbnail data", logFile);
return eReplayCreate_FileCorrupted;
}
ser.SkipCurrentChunk();
ser.EndChunk();
}
{
int chunkType = ser.BeginChunk(0);
if(chunkType != CREATE_PARAMS)
{
RDCERR("Malformed logfile '%s', second chunk isn't create params", logFile);
return eReplayCreate_FileCorrupted;
}
SERIALISE_ELEMENT(driverType).Named("Driver Type");
SERIALISE_ELEMENT(driverName).Named("Driver Name");
if(params)
SERIALISE_ELEMENT(*params).Named("Driver Params");
}
// we can just throw away the serialiser, don't need to care about closing/popping contexts
return eReplayCreate_Success;
}
#pragma endregion
/////////////////////////////////////////////////////////////////
// vk_common.cpp
#pragma region vk_common.cpp
#define SERIALISE_RESOURCE_AS_ID(VulkanType) \
template <class SerialiserType> \
void DoSerialise(SerialiserType &ser, VulkanType &el) \
{ \
ResourceId id; \
\
if(ser.IsWriting()) \
{ \
id = ResourceId(); \
} \
\
DoSerialise(ser, id); \
\
if(ser.IsReading()) \
{ \
el = (VulkanType)id.innerSecretValue; \
} \
} \
INSTANTIATE_SERIALISE_TYPE(VulkanType);
SERIALISE_RESOURCE_AS_ID(VkRenderPass);
SERIALISE_RESOURCE_AS_ID(VkPipelineLayout);
SERIALISE_RESOURCE_AS_ID(VkPipeline);
SERIALISE_RESOURCE_AS_ID(VkImageView);
SERIALISE_RESOURCE_AS_ID(VkSampler);
SERIALISE_RESOURCE_AS_ID(VkBuffer);
SERIALISE_RESOURCE_AS_ID(VkBufferView);
SERIALISE_RESOURCE_AS_ID(VkShaderModule);
SERIALISE_RESOURCE_AS_ID(VkDescriptorSet);
SERIALISE_RESOURCE_AS_ID(VkDescriptorSetLayout);
enum VkFlagWithNoBits
{
FlagWithNoBits_Dummy_Bit = 1,
};
template <>
string DoStringize(const VkFlagWithNoBits &el)
{
if(el != 0)
return StringFormat::Fmt("Invalid bits set: %x", el);
return "";
}
SERIALISE_ENUM_TYPE(VkFlagWithNoBits);
template <>
string DoStringize(const VkShaderStageFlagBits &el)
{
BEGIN_BITFIELD_STRINGIZE()
{
STRINGIZE_BITFIELD_NAMED(VK_SHADER_STAGE_ALL_GRAPHICS);
STRINGIZE_BITFIELD_NAMED(VK_SHADER_STAGE_ALL);
STRINGIZE_BITFIELD_BIT(VK_SHADER_STAGE_VERTEX_BIT)
STRINGIZE_BITFIELD_BIT(VK_SHADER_STAGE_TESSELLATION_CONTROL_BIT)
STRINGIZE_BITFIELD_BIT(VK_SHADER_STAGE_TESSELLATION_EVALUATION_BIT)
STRINGIZE_BITFIELD_BIT(VK_SHADER_STAGE_GEOMETRY_BIT)
STRINGIZE_BITFIELD_BIT(VK_SHADER_STAGE_FRAGMENT_BIT)
STRINGIZE_BITFIELD_BIT(VK_SHADER_STAGE_COMPUTE_BIT)
}
END_BITFIELD_STRINGIZE();
}
SERIALISE_ENUM_TYPE(VkShaderStageFlagBits);
template <>
string DoStringize(const VkImageAspectFlagBits &el)
{
BEGIN_BITFIELD_STRINGIZE()
STRINGIZE_BITFIELD_BIT(VK_IMAGE_ASPECT_COLOR_BIT)
STRINGIZE_BITFIELD_BIT(VK_IMAGE_ASPECT_DEPTH_BIT)
STRINGIZE_BITFIELD_BIT(VK_IMAGE_ASPECT_STENCIL_BIT)
STRINGIZE_BITFIELD_BIT(VK_IMAGE_ASPECT_METADATA_BIT)
END_BITFIELD_STRINGIZE();
}
SERIALISE_ENUM_TYPE(VkImageAspectFlagBits);
template <>
string DoStringize(const VkStructureType &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_APPLICATION_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_INSTANCE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_DEVICE_QUEUE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_DEVICE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_SUBMIT_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_MEMORY_ALLOCATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_MAPPED_MEMORY_RANGE)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_FENCE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_BIND_SPARSE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_SEMAPHORE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_EVENT_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_QUERY_POOL_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_BUFFER_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_BUFFER_VIEW_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_IMAGE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_IMAGE_VIEW_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_SHADER_MODULE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_CACHE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_SHADER_STAGE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_VERTEX_INPUT_STATE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_INPUT_ASSEMBLY_STATE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_TESSELLATION_STATE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_VIEWPORT_STATE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_RASTERIZATION_STATE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_MULTISAMPLE_STATE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_DEPTH_STENCIL_STATE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_COLOR_BLEND_STATE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_DYNAMIC_STATE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_GRAPHICS_PIPELINE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_COMPUTE_PIPELINE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PIPELINE_LAYOUT_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_SAMPLER_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_DESCRIPTOR_SET_LAYOUT_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_DESCRIPTOR_POOL_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_DESCRIPTOR_SET_ALLOCATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_WRITE_DESCRIPTOR_SET)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_COPY_DESCRIPTOR_SET)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_FRAMEBUFFER_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_RENDER_PASS_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_COMMAND_POOL_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_COMMAND_BUFFER_ALLOCATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_COMMAND_BUFFER_BEGIN_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_RENDER_PASS_BEGIN_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_BUFFER_MEMORY_BARRIER)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_IMAGE_MEMORY_BARRIER)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_MEMORY_BARRIER)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_LOADER_INSTANCE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_LOADER_DEVICE_CREATE_INFO)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_SWAPCHAIN_CREATE_INFO_KHR)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_PRESENT_INFO_KHR)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_DISPLAY_MODE_CREATE_INFO_KHR)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_DISPLAY_SURFACE_CREATE_INFO_KHR)
STRINGIZE_ENUM(VK_STRUCTURE_TYPE_DISPLAY_PRESENT_INFO_KHR)
END_ENUM_STRINGIZE(VkStructureType);
}
SERIALISE_ENUM_TYPE(VkStructureType);
template <>
string DoStringize(const VkStencilOp &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM_NAMED(VK_STENCIL_OP_KEEP, "KEEP");
STRINGIZE_ENUM_NAMED(VK_STENCIL_OP_ZERO, "ZERO");
STRINGIZE_ENUM_NAMED(VK_STENCIL_OP_REPLACE, "REPLACE");
STRINGIZE_ENUM_NAMED(VK_STENCIL_OP_INCREMENT_AND_CLAMP, "INC_SAT");
STRINGIZE_ENUM_NAMED(VK_STENCIL_OP_DECREMENT_AND_CLAMP, "DEC_SAT");
STRINGIZE_ENUM_NAMED(VK_STENCIL_OP_INVERT, "INVERT");
STRINGIZE_ENUM_NAMED(VK_STENCIL_OP_INCREMENT_AND_WRAP, "INC_WRAP");
STRINGIZE_ENUM_NAMED(VK_STENCIL_OP_DECREMENT_AND_WRAP, "DEC_WRAP");
END_ENUM_STRINGIZE(VkStencilOp);
}
SERIALISE_ENUM_TYPE(VkStencilOp);
template <>
string DoStringize(const VkLogicOp &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_CLEAR, "CLEAR");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_AND, "AND");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_AND_REVERSE, "AND_REV");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_COPY, "COPY");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_AND_INVERTED, "AND_INV");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_NO_OP, "NOOP");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_XOR, "XOR");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_OR, "OR");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_NOR, "NOR");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_EQUIVALENT, "EQUIV");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_INVERT, "INVERT");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_OR_REVERSE, "OR_REV");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_COPY_INVERTED, "COPY_INV");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_OR_INVERTED, "OR_INV");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_NAND, "NAND");
STRINGIZE_ENUM_NAMED(VK_LOGIC_OP_SET, "SET");
END_ENUM_STRINGIZE(VkLogicOp);
}
SERIALISE_ENUM_TYPE(VkLogicOp);
template <>
string DoStringize(const VkCompareOp &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM_NAMED(VK_COMPARE_OP_NEVER, "NEVER");
STRINGIZE_ENUM_NAMED(VK_COMPARE_OP_LESS, "LESS");
STRINGIZE_ENUM_NAMED(VK_COMPARE_OP_EQUAL, "EQUAL");
STRINGIZE_ENUM_NAMED(VK_COMPARE_OP_LESS_OR_EQUAL, "LESS_EQUAL");
STRINGIZE_ENUM_NAMED(VK_COMPARE_OP_GREATER, "GREATER");
STRINGIZE_ENUM_NAMED(VK_COMPARE_OP_NOT_EQUAL, "NOT_EQUAL");
STRINGIZE_ENUM_NAMED(VK_COMPARE_OP_GREATER_OR_EQUAL, "GREATER_EQUAL");
STRINGIZE_ENUM_NAMED(VK_COMPARE_OP_ALWAYS, "ALWAYS");
END_ENUM_STRINGIZE(VkCompareOp);
}
SERIALISE_ENUM_TYPE(VkCompareOp);
template <>
string DoStringize(const VkVertexInputRate &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(VK_VERTEX_INPUT_RATE_VERTEX)
STRINGIZE_ENUM(VK_VERTEX_INPUT_RATE_INSTANCE)
END_ENUM_STRINGIZE(VkVertexInputRate);
}
SERIALISE_ENUM_TYPE(VkVertexInputRate);
template <>
string DoStringize(const VkPolygonMode &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(VK_POLYGON_MODE_FILL)
STRINGIZE_ENUM(VK_POLYGON_MODE_LINE)
STRINGIZE_ENUM(VK_POLYGON_MODE_POINT)
END_ENUM_STRINGIZE(VkPolygonMode);
}
SERIALISE_ENUM_TYPE(VkPolygonMode);
template <>
string DoStringize(const VkFrontFace &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(VK_FRONT_FACE_COUNTER_CLOCKWISE)
STRINGIZE_ENUM(VK_FRONT_FACE_CLOCKWISE)
END_ENUM_STRINGIZE(VkFrontFace);
}
SERIALISE_ENUM_TYPE(VkFrontFace);
template <>
string DoStringize(const VkBlendFactor &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_ZERO, "ZERO");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_ONE, "ONE");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_SRC_COLOR, "SRC_COLOR");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_ONE_MINUS_SRC_COLOR, "INV_SRC_COLOR");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_DST_COLOR, "DST_COLOR");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_ONE_MINUS_DST_COLOR, "INV_DST_COLOR");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_SRC_ALPHA, "SRC_ALPHA");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_ONE_MINUS_SRC_ALPHA, "INV_SRC_ALPHA");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_DST_ALPHA, "DST_ALPHA");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_ONE_MINUS_DST_ALPHA, "INV_DST_ALPHA");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_CONSTANT_COLOR, "CONST_COLOR");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_ONE_MINUS_CONSTANT_COLOR, "INV_CONST_COLOR");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_CONSTANT_ALPHA, "CONST_ALPHA");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_ONE_MINUS_CONSTANT_ALPHA, "INV_CONST_ALPHA");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_SRC_ALPHA_SATURATE, "SRC_ALPHA_SAT");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_SRC1_COLOR, "SRC1_COLOR");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_ONE_MINUS_SRC1_COLOR, "INV_SRC1_COLOR");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_SRC1_ALPHA, "SRC1_ALPHA");
STRINGIZE_ENUM_NAMED(VK_BLEND_FACTOR_ONE_MINUS_SRC1_ALPHA, "INV_SRC1_ALPHA");
END_ENUM_STRINGIZE(VkBlendFactor);
}
SERIALISE_ENUM_TYPE(VkBlendFactor);
template <>
string DoStringize(const VkBlendOp &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM_NAMED(VK_BLEND_OP_ADD, "ADD");
STRINGIZE_ENUM_NAMED(VK_BLEND_OP_SUBTRACT, "SUB");
STRINGIZE_ENUM_NAMED(VK_BLEND_OP_REVERSE_SUBTRACT, "REV_SUB");
STRINGIZE_ENUM_NAMED(VK_BLEND_OP_MIN, "MIN");
STRINGIZE_ENUM_NAMED(VK_BLEND_OP_MAX, "MAX");
END_ENUM_STRINGIZE(VkBlendOp);
}
SERIALISE_ENUM_TYPE(VkBlendOp);
template <>
string DoStringize(const VkPrimitiveTopology &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(VK_PRIMITIVE_TOPOLOGY_POINT_LIST)
STRINGIZE_ENUM(VK_PRIMITIVE_TOPOLOGY_LINE_LIST)
STRINGIZE_ENUM(VK_PRIMITIVE_TOPOLOGY_LINE_STRIP)
STRINGIZE_ENUM(VK_PRIMITIVE_TOPOLOGY_TRIANGLE_LIST)
STRINGIZE_ENUM(VK_PRIMITIVE_TOPOLOGY_TRIANGLE_STRIP)
STRINGIZE_ENUM(VK_PRIMITIVE_TOPOLOGY_TRIANGLE_FAN)
STRINGIZE_ENUM(VK_PRIMITIVE_TOPOLOGY_LINE_LIST_WITH_ADJACENCY)
STRINGIZE_ENUM(VK_PRIMITIVE_TOPOLOGY_LINE_STRIP_WITH_ADJACENCY)
STRINGIZE_ENUM(VK_PRIMITIVE_TOPOLOGY_TRIANGLE_LIST_WITH_ADJACENCY)
STRINGIZE_ENUM(VK_PRIMITIVE_TOPOLOGY_TRIANGLE_STRIP_WITH_ADJACENCY)
STRINGIZE_ENUM(VK_PRIMITIVE_TOPOLOGY_PATCH_LIST)
END_ENUM_STRINGIZE(VkPrimitiveTopology);
}
SERIALISE_ENUM_TYPE(VkPrimitiveTopology);
template <>
string DoStringize(const VkDescriptorType &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(VK_DESCRIPTOR_TYPE_SAMPLER)
STRINGIZE_ENUM(VK_DESCRIPTOR_TYPE_COMBINED_IMAGE_SAMPLER)
STRINGIZE_ENUM(VK_DESCRIPTOR_TYPE_SAMPLED_IMAGE)
STRINGIZE_ENUM(VK_DESCRIPTOR_TYPE_STORAGE_IMAGE)
STRINGIZE_ENUM(VK_DESCRIPTOR_TYPE_UNIFORM_TEXEL_BUFFER)
STRINGIZE_ENUM(VK_DESCRIPTOR_TYPE_STORAGE_TEXEL_BUFFER)
STRINGIZE_ENUM(VK_DESCRIPTOR_TYPE_UNIFORM_BUFFER)
STRINGIZE_ENUM(VK_DESCRIPTOR_TYPE_STORAGE_BUFFER)
STRINGIZE_ENUM(VK_DESCRIPTOR_TYPE_UNIFORM_BUFFER_DYNAMIC)
STRINGIZE_ENUM(VK_DESCRIPTOR_TYPE_STORAGE_BUFFER_DYNAMIC)
STRINGIZE_ENUM(VK_DESCRIPTOR_TYPE_INPUT_ATTACHMENT)
END_ENUM_STRINGIZE(VkDescriptorType);
}
SERIALISE_ENUM_TYPE(VkDescriptorType);
template <>
string DoStringize(const VkFormat &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(VK_FORMAT_UNDEFINED)
STRINGIZE_ENUM(VK_FORMAT_R4G4_UNORM_PACK8)
STRINGIZE_ENUM(VK_FORMAT_R4G4B4A4_UNORM_PACK16)
STRINGIZE_ENUM(VK_FORMAT_B4G4R4A4_UNORM_PACK16)
STRINGIZE_ENUM(VK_FORMAT_R5G6B5_UNORM_PACK16)
STRINGIZE_ENUM(VK_FORMAT_B5G6R5_UNORM_PACK16)
STRINGIZE_ENUM(VK_FORMAT_R5G5B5A1_UNORM_PACK16)
STRINGIZE_ENUM(VK_FORMAT_B5G5R5A1_UNORM_PACK16)
STRINGIZE_ENUM(VK_FORMAT_A1R5G5B5_UNORM_PACK16)
STRINGIZE_ENUM(VK_FORMAT_R8_UNORM)
STRINGIZE_ENUM(VK_FORMAT_R8_SNORM)
STRINGIZE_ENUM(VK_FORMAT_R8_USCALED)
STRINGIZE_ENUM(VK_FORMAT_R8_SSCALED)
STRINGIZE_ENUM(VK_FORMAT_R8_UINT)
STRINGIZE_ENUM(VK_FORMAT_R8_SINT)
STRINGIZE_ENUM(VK_FORMAT_R8_SRGB)
STRINGIZE_ENUM(VK_FORMAT_R8G8_UNORM)
STRINGIZE_ENUM(VK_FORMAT_R8G8_SNORM)
STRINGIZE_ENUM(VK_FORMAT_R8G8_USCALED)
STRINGIZE_ENUM(VK_FORMAT_R8G8_SSCALED)
STRINGIZE_ENUM(VK_FORMAT_R8G8_UINT)
STRINGIZE_ENUM(VK_FORMAT_R8G8_SINT)
STRINGIZE_ENUM(VK_FORMAT_R8G8_SRGB)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8_UNORM)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8_SNORM)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8_USCALED)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8_SSCALED)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8_UINT)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8_SINT)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8_SRGB)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8_UNORM)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8_SNORM)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8_USCALED)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8_SSCALED)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8_UINT)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8_SINT)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8_SRGB)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8A8_UNORM)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8A8_SNORM)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8A8_USCALED)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8A8_SSCALED)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8A8_UINT)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8A8_SINT)
STRINGIZE_ENUM(VK_FORMAT_R8G8B8A8_SRGB)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8A8_UNORM)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8A8_SNORM)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8A8_USCALED)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8A8_SSCALED)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8A8_UINT)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8A8_SINT)
STRINGIZE_ENUM(VK_FORMAT_B8G8R8A8_SRGB)
STRINGIZE_ENUM(VK_FORMAT_A8B8G8R8_UNORM_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A8B8G8R8_SNORM_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A8B8G8R8_USCALED_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A8B8G8R8_SSCALED_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A8B8G8R8_UINT_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A8B8G8R8_SINT_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A8B8G8R8_SRGB_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2R10G10B10_UNORM_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2R10G10B10_SNORM_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2R10G10B10_USCALED_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2R10G10B10_SSCALED_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2R10G10B10_UINT_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2R10G10B10_SINT_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2B10G10R10_UNORM_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2B10G10R10_SNORM_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2B10G10R10_USCALED_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2B10G10R10_SSCALED_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2B10G10R10_UINT_PACK32)
STRINGIZE_ENUM(VK_FORMAT_A2B10G10R10_SINT_PACK32)
STRINGIZE_ENUM(VK_FORMAT_R16_UNORM)
STRINGIZE_ENUM(VK_FORMAT_R16_SNORM)
STRINGIZE_ENUM(VK_FORMAT_R16_USCALED)
STRINGIZE_ENUM(VK_FORMAT_R16_SSCALED)
STRINGIZE_ENUM(VK_FORMAT_R16_UINT)
STRINGIZE_ENUM(VK_FORMAT_R16_SINT)
STRINGIZE_ENUM(VK_FORMAT_R16_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_R16G16_UNORM)
STRINGIZE_ENUM(VK_FORMAT_R16G16_SNORM)
STRINGIZE_ENUM(VK_FORMAT_R16G16_USCALED)
STRINGIZE_ENUM(VK_FORMAT_R16G16_SSCALED)
STRINGIZE_ENUM(VK_FORMAT_R16G16_UINT)
STRINGIZE_ENUM(VK_FORMAT_R16G16_SINT)
STRINGIZE_ENUM(VK_FORMAT_R16G16_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16_UNORM)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16_SNORM)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16_USCALED)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16_SSCALED)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16_UINT)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16_SINT)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16A16_UNORM)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16A16_SNORM)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16A16_USCALED)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16A16_SSCALED)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16A16_UINT)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16A16_SINT)
STRINGIZE_ENUM(VK_FORMAT_R16G16B16A16_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_R32_UINT)
STRINGIZE_ENUM(VK_FORMAT_R32_SINT)
STRINGIZE_ENUM(VK_FORMAT_R32_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_R32G32_UINT)
STRINGIZE_ENUM(VK_FORMAT_R32G32_SINT)
STRINGIZE_ENUM(VK_FORMAT_R32G32_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_R32G32B32_UINT)
STRINGIZE_ENUM(VK_FORMAT_R32G32B32_SINT)
STRINGIZE_ENUM(VK_FORMAT_R32G32B32_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_R32G32B32A32_UINT)
STRINGIZE_ENUM(VK_FORMAT_R32G32B32A32_SINT)
STRINGIZE_ENUM(VK_FORMAT_R32G32B32A32_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_R64_UINT)
STRINGIZE_ENUM(VK_FORMAT_R64_SINT)
STRINGIZE_ENUM(VK_FORMAT_R64_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_R64G64_UINT)
STRINGIZE_ENUM(VK_FORMAT_R64G64_SINT)
STRINGIZE_ENUM(VK_FORMAT_R64G64_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_R64G64B64_UINT)
STRINGIZE_ENUM(VK_FORMAT_R64G64B64_SINT)
STRINGIZE_ENUM(VK_FORMAT_R64G64B64_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_R64G64B64A64_UINT)
STRINGIZE_ENUM(VK_FORMAT_R64G64B64A64_SINT)
STRINGIZE_ENUM(VK_FORMAT_R64G64B64A64_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_B10G11R11_UFLOAT_PACK32)
STRINGIZE_ENUM(VK_FORMAT_E5B9G9R9_UFLOAT_PACK32)
STRINGIZE_ENUM(VK_FORMAT_D16_UNORM)
STRINGIZE_ENUM(VK_FORMAT_X8_D24_UNORM_PACK32)
STRINGIZE_ENUM(VK_FORMAT_D32_SFLOAT)
STRINGIZE_ENUM(VK_FORMAT_S8_UINT)
STRINGIZE_ENUM(VK_FORMAT_D16_UNORM_S8_UINT)
STRINGIZE_ENUM(VK_FORMAT_D24_UNORM_S8_UINT)
STRINGIZE_ENUM(VK_FORMAT_D32_SFLOAT_S8_UINT)
STRINGIZE_ENUM(VK_FORMAT_BC1_RGB_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC1_RGB_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC1_RGBA_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC1_RGBA_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC2_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC2_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC3_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC3_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC4_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC4_SNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC5_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC5_SNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC6H_UFLOAT_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC6H_SFLOAT_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC7_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_BC7_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ETC2_R8G8B8_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ETC2_R8G8B8_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ETC2_R8G8B8A1_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ETC2_R8G8B8A1_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ETC2_R8G8B8A8_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ETC2_R8G8B8A8_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_EAC_R11_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_EAC_R11_SNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_EAC_R11G11_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_EAC_R11G11_SNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_4x4_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_4x4_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_5x4_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_5x4_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_5x5_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_5x5_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_6x5_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_6x5_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_6x6_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_6x6_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_8x5_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_8x5_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_8x6_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_8x6_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_8x8_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_8x8_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_10x5_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_10x5_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_10x6_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_10x6_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_10x8_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_10x8_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_10x10_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_10x10_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_12x10_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_12x10_SRGB_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_12x12_UNORM_BLOCK)
STRINGIZE_ENUM(VK_FORMAT_ASTC_12x12_SRGB_BLOCK)
END_ENUM_STRINGIZE(VkFormat);
}
SERIALISE_ENUM_TYPE(VkFormat);
template <>
string DoStringize(const VkSampleCountFlagBits &el)
{
BEGIN_BITFIELD_STRINGIZE()
{
STRINGIZE_BITFIELD_BIT(VK_SAMPLE_COUNT_1_BIT)
STRINGIZE_BITFIELD_BIT(VK_SAMPLE_COUNT_2_BIT)
STRINGIZE_BITFIELD_BIT(VK_SAMPLE_COUNT_4_BIT)
STRINGIZE_BITFIELD_BIT(VK_SAMPLE_COUNT_8_BIT)
STRINGIZE_BITFIELD_BIT(VK_SAMPLE_COUNT_16_BIT)
STRINGIZE_BITFIELD_BIT(VK_SAMPLE_COUNT_32_BIT)
STRINGIZE_BITFIELD_BIT(VK_SAMPLE_COUNT_64_BIT)
}
END_BITFIELD_STRINGIZE();
}
SERIALISE_ENUM_TYPE(VkSampleCountFlagBits);
template <>
string DoStringize(const VkCullModeFlagBits &el)
{
BEGIN_BITFIELD_STRINGIZE()
{
// technically a bitfield but each combination has a particular meaning
STRINGIZE_BITFIELD_NAMED(VK_CULL_MODE_NONE)
STRINGIZE_BITFIELD(VK_CULL_MODE_FRONT_BIT, "VK_CULL_MODE_FRONT")
STRINGIZE_BITFIELD(VK_CULL_MODE_BACK_BIT, "VK_CULL_MODE_BACK")
STRINGIZE_BITFIELD_NAMED(VK_CULL_MODE_FRONT_AND_BACK)
}
END_BITFIELD_STRINGIZE();
}
SERIALISE_ENUM_TYPE(VkCullModeFlagBits);
template <>
string DoStringize(const VkPipelineCreateFlagBits &el)
{
BEGIN_BITFIELD_STRINGIZE()
{
STRINGIZE_BITFIELD_BIT(VK_PIPELINE_CREATE_DISABLE_OPTIMIZATION_BIT)
STRINGIZE_BITFIELD_BIT(VK_PIPELINE_CREATE_ALLOW_DERIVATIVES_BIT)
STRINGIZE_BITFIELD_BIT(VK_PIPELINE_CREATE_DERIVATIVE_BIT)
}
END_BITFIELD_STRINGIZE();
}
SERIALISE_ENUM_TYPE(VkPipelineCreateFlagBits);
template <>
string DoStringize(const VkDynamicState &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(VK_DYNAMIC_STATE_VIEWPORT)
STRINGIZE_ENUM(VK_DYNAMIC_STATE_SCISSOR)
STRINGIZE_ENUM(VK_DYNAMIC_STATE_LINE_WIDTH)
STRINGIZE_ENUM(VK_DYNAMIC_STATE_DEPTH_BIAS)
STRINGIZE_ENUM(VK_DYNAMIC_STATE_BLEND_CONSTANTS)
STRINGIZE_ENUM(VK_DYNAMIC_STATE_DEPTH_BOUNDS)
STRINGIZE_ENUM(VK_DYNAMIC_STATE_STENCIL_COMPARE_MASK)
STRINGIZE_ENUM(VK_DYNAMIC_STATE_STENCIL_WRITE_MASK)
STRINGIZE_ENUM(VK_DYNAMIC_STATE_STENCIL_REFERENCE)
END_ENUM_STRINGIZE(VkDynamicState);
}
SERIALISE_ENUM_TYPE(VkDynamicState);
template <>
string DoStringize(const VkImageLayout &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM_NAMED(VK_IMAGE_LAYOUT_UNDEFINED, "UNDEFINED");
STRINGIZE_ENUM_NAMED(VK_IMAGE_LAYOUT_GENERAL, "GENERAL");
STRINGIZE_ENUM_NAMED(VK_IMAGE_LAYOUT_COLOR_ATTACHMENT_OPTIMAL, "COLOR_ATTACHMENT_OPTIMAL");
STRINGIZE_ENUM_NAMED(VK_IMAGE_LAYOUT_DEPTH_STENCIL_ATTACHMENT_OPTIMAL, "DEPTH_STENCIL_ATTACHMENT_OPTIMAL");
STRINGIZE_ENUM_NAMED(VK_IMAGE_LAYOUT_DEPTH_STENCIL_READ_ONLY_OPTIMAL, "DEPTH_STENCIL_READ_ONLY_OPTIMAL");
STRINGIZE_ENUM_NAMED(VK_IMAGE_LAYOUT_SHADER_READ_ONLY_OPTIMAL, "SHADER_READ_ONLY_OPTIMAL");
STRINGIZE_ENUM_NAMED(VK_IMAGE_LAYOUT_TRANSFER_SRC_OPTIMAL, "TRANSFER_SRC_OPTIMAL");
STRINGIZE_ENUM_NAMED(VK_IMAGE_LAYOUT_TRANSFER_DST_OPTIMAL, "TRANSFER_DST_OPTIMAL");
STRINGIZE_ENUM_NAMED(VK_IMAGE_LAYOUT_PREINITIALIZED, "PREINITIALIZED");
STRINGIZE_ENUM_NAMED(VK_IMAGE_LAYOUT_PRESENT_SRC_KHR, "PRESENT_SRC_KHR");
END_ENUM_STRINGIZE(VkImageLayout);
}
SERIALISE_ENUM_TYPE(VkImageLayout);
template <class SerialiserType>
static void SerialiseNext(SerialiserType &ser, VkStructureType &sType, const void *&pNext)
{
SERIALISE_ELEMENT(sType);
// we don't support any extensions, so pNext must always be NULL
if(ser.IsReading())
pNext = NULL;
else
RDCASSERT(pNext == NULL);
}
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkExtent2D &el)
{
SERIALISE_MEMBER(width);
SERIALISE_MEMBER(height);
}
INSTANTIATE_SERIALISE_TYPE(VkExtent2D);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkExtent3D &el)
{
SERIALISE_MEMBER(width);
SERIALISE_MEMBER(height);
SERIALISE_MEMBER(depth);
}
INSTANTIATE_SERIALISE_TYPE(VkExtent3D);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkOffset2D &el)
{
SERIALISE_MEMBER(x);
SERIALISE_MEMBER(y);
}
INSTANTIATE_SERIALISE_TYPE(VkOffset2D);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkOffset3D &el)
{
SERIALISE_MEMBER(x);
SERIALISE_MEMBER(y);
SERIALISE_MEMBER(z);
}
INSTANTIATE_SERIALISE_TYPE(VkOffset3D);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkRect2D &el)
{
SERIALISE_MEMBER(extent);
SERIALISE_MEMBER(offset);
}
INSTANTIATE_SERIALISE_TYPE(VkRect2D);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkViewport &el)
{
SERIALISE_MEMBER(x);
SERIALISE_MEMBER(y);
SERIALISE_MEMBER(width);
SERIALISE_MEMBER(height);
SERIALISE_MEMBER(minDepth);
SERIALISE_MEMBER(maxDepth);
}
INSTANTIATE_SERIALISE_TYPE(VkViewport);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineLayoutCreateInfo &el)
{
RDCASSERT(ser.IsReading() || el.sType == VK_STRUCTURE_TYPE_PIPELINE_LAYOUT_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkFlagWithNoBits, flags);
// need to do this one by hand since it's just an array of objects that don't themselves have
// a Serialise overload
SERIALISE_MEMBER_ARRAY(pSetLayouts, setLayoutCount);
// skipped for sanity
// SERIALISE_MEMBER_ARRAY(pPushConstantRanges, pushConstantRangeCount);
el.pushConstantRangeCount = 0;
el.pPushConstantRanges = NULL;
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineLayoutCreateInfo);
template <>
void Deserialise(const VkPipelineLayoutCreateInfo &el)
{
RDCASSERT(el.pNext == NULL); // otherwise delete
delete[] el.pSetLayouts;
delete[] el.pPushConstantRanges;
}
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkVertexInputBindingDescription &el)
{
SERIALISE_MEMBER(binding);
SERIALISE_MEMBER(stride);
SERIALISE_MEMBER(inputRate);
}
INSTANTIATE_SERIALISE_TYPE(VkVertexInputBindingDescription);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkVertexInputAttributeDescription &el)
{
SERIALISE_MEMBER(location);
SERIALISE_MEMBER(binding);
SERIALISE_MEMBER(format);
SERIALISE_MEMBER(offset);
}
INSTANTIATE_SERIALISE_TYPE(VkVertexInputAttributeDescription);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineVertexInputStateCreateInfo &el)
{
RDCASSERT(ser.IsReading() || el.sType == VK_STRUCTURE_TYPE_PIPELINE_VERTEX_INPUT_STATE_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkFlagWithNoBits, flags);
SERIALISE_MEMBER_ARRAY(pVertexBindingDescriptions, vertexBindingDescriptionCount);
SERIALISE_MEMBER_ARRAY(pVertexAttributeDescriptions, vertexAttributeDescriptionCount);
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineVertexInputStateCreateInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineInputAssemblyStateCreateInfo &el)
{
RDCASSERT(ser.IsReading() ||
el.sType == VK_STRUCTURE_TYPE_PIPELINE_INPUT_ASSEMBLY_STATE_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkFlagWithNoBits, flags);
SERIALISE_MEMBER(topology);
SERIALISE_MEMBER(primitiveRestartEnable);
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineInputAssemblyStateCreateInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineTessellationStateCreateInfo &el)
{
RDCASSERT(ser.IsReading() ||
el.sType == VK_STRUCTURE_TYPE_PIPELINE_TESSELLATION_STATE_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkFlagWithNoBits, flags);
SERIALISE_MEMBER(patchControlPoints);
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineTessellationStateCreateInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineViewportStateCreateInfo &el)
{
RDCASSERT(ser.IsReading() || el.sType == VK_STRUCTURE_TYPE_PIPELINE_VIEWPORT_STATE_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkFlagWithNoBits, flags);
SERIALISE_MEMBER_ARRAY(pViewports, viewportCount);
SERIALISE_MEMBER_ARRAY(pScissors, scissorCount);
// need to serialise these separately afterwards, since viewport count can be
// positive even with a NULL array, if dynamic state is set
SERIALISE_MEMBER(viewportCount);
SERIALISE_MEMBER(scissorCount);
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineViewportStateCreateInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineRasterizationStateCreateInfo &el)
{
RDCASSERT(ser.IsReading() ||
el.sType == VK_STRUCTURE_TYPE_PIPELINE_RASTERIZATION_STATE_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkFlagWithNoBits, flags);
SERIALISE_MEMBER(depthClampEnable);
SERIALISE_MEMBER(rasterizerDiscardEnable);
SERIALISE_MEMBER(polygonMode);
SERIALISE_MEMBER(cullMode);
SERIALISE_MEMBER(frontFace);
SERIALISE_MEMBER(depthBiasEnable);
SERIALISE_MEMBER(depthBiasConstantFactor);
SERIALISE_MEMBER(depthBiasClamp);
SERIALISE_MEMBER(depthBiasSlopeFactor);
SERIALISE_MEMBER(lineWidth);
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineRasterizationStateCreateInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineMultisampleStateCreateInfo &el)
{
RDCASSERT(ser.IsReading() || el.sType == VK_STRUCTURE_TYPE_PIPELINE_MULTISAMPLE_STATE_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkFlagWithNoBits, flags);
SERIALISE_MEMBER(rasterizationSamples);
RDCASSERT(el.rasterizationSamples <= VK_SAMPLE_COUNT_32_BIT);
SERIALISE_MEMBER(sampleShadingEnable);
SERIALISE_MEMBER(minSampleShading);
SERIALISE_MEMBER_OPT(pSampleMask);
SERIALISE_MEMBER(alphaToCoverageEnable);
SERIALISE_MEMBER(alphaToOneEnable);
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineMultisampleStateCreateInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineColorBlendAttachmentState &el)
{
SERIALISE_MEMBER(blendEnable);
SERIALISE_MEMBER(srcColorBlendFactor);
SERIALISE_MEMBER(dstColorBlendFactor);
SERIALISE_MEMBER(colorBlendOp);
SERIALISE_MEMBER(srcAlphaBlendFactor);
SERIALISE_MEMBER(dstAlphaBlendFactor);
SERIALISE_MEMBER(alphaBlendOp);
SERIALISE_MEMBER(colorWriteMask);
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineColorBlendAttachmentState);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineColorBlendStateCreateInfo &el)
{
RDCASSERT(ser.IsReading() || el.sType == VK_STRUCTURE_TYPE_PIPELINE_COLOR_BLEND_STATE_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkFlagWithNoBits, flags);
SERIALISE_MEMBER(logicOpEnable);
SERIALISE_MEMBER(logicOp);
SERIALISE_MEMBER_ARRAY(pAttachments, attachmentCount);
SERIALISE_MEMBER(blendConstants);
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineColorBlendStateCreateInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkStencilOpState &el)
{
SERIALISE_MEMBER(failOp);
SERIALISE_MEMBER(passOp);
SERIALISE_MEMBER(depthFailOp);
SERIALISE_MEMBER(compareOp);
SERIALISE_MEMBER(compareMask);
SERIALISE_MEMBER(writeMask);
SERIALISE_MEMBER(reference);
}
INSTANTIATE_SERIALISE_TYPE(VkStencilOpState);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkImageSubresourceLayers &el)
{
SERIALISE_MEMBER_TYPED(VkImageAspectFlagBits, aspectMask);
SERIALISE_MEMBER(mipLevel);
SERIALISE_MEMBER(baseArrayLayer);
SERIALISE_MEMBER(layerCount);
}
INSTANTIATE_SERIALISE_TYPE(VkImageSubresourceLayers);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineShaderStageCreateInfo &el)
{
RDCASSERT(ser.IsReading() || el.sType == VK_STRUCTURE_TYPE_PIPELINE_SHADER_STAGE_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkFlagWithNoBits, flags);
SERIALISE_MEMBER(stage);
SERIALISE_MEMBER(module);
SERIALISE_MEMBER(pName);
// SERIALISE_MEMBER_OPT(pSpecializationInfo); // skipped for sanity
el.pSpecializationInfo = NULL;
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineShaderStageCreateInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineDepthStencilStateCreateInfo &el)
{
RDCASSERT(ser.IsReading() ||
el.sType == VK_STRUCTURE_TYPE_PIPELINE_DEPTH_STENCIL_STATE_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkFlagWithNoBits, flags);
SERIALISE_MEMBER(depthTestEnable);
SERIALISE_MEMBER(depthWriteEnable);
SERIALISE_MEMBER(depthCompareOp);
SERIALISE_MEMBER(depthBoundsTestEnable);
SERIALISE_MEMBER(stencilTestEnable);
SERIALISE_MEMBER(front);
SERIALISE_MEMBER(back);
SERIALISE_MEMBER(minDepthBounds);
SERIALISE_MEMBER(maxDepthBounds);
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineDepthStencilStateCreateInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkPipelineDynamicStateCreateInfo &el)
{
RDCASSERT(ser.IsReading() || el.sType == VK_STRUCTURE_TYPE_PIPELINE_DYNAMIC_STATE_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkFlagWithNoBits, flags);
SERIALISE_MEMBER_ARRAY(pDynamicStates, dynamicStateCount);
}
INSTANTIATE_SERIALISE_TYPE(VkPipelineDynamicStateCreateInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkGraphicsPipelineCreateInfo &el)
{
RDCASSERT(ser.IsReading() || el.sType == VK_STRUCTURE_TYPE_GRAPHICS_PIPELINE_CREATE_INFO);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER_TYPED(VkPipelineCreateFlagBits, flags);
SERIALISE_MEMBER(layout);
SERIALISE_MEMBER(renderPass);
SERIALISE_MEMBER(subpass);
SERIALISE_MEMBER(basePipelineHandle);
SERIALISE_MEMBER(basePipelineIndex);
SERIALISE_MEMBER_OPT(pVertexInputState);
SERIALISE_MEMBER_OPT(pInputAssemblyState);
SERIALISE_MEMBER_OPT(pTessellationState);
SERIALISE_MEMBER_OPT(pViewportState);
SERIALISE_MEMBER_OPT(pRasterizationState);
SERIALISE_MEMBER_OPT(pMultisampleState);
SERIALISE_MEMBER_OPT(pDepthStencilState);
SERIALISE_MEMBER_OPT(pColorBlendState);
SERIALISE_MEMBER_OPT(pDynamicState);
SERIALISE_MEMBER_ARRAY(pStages, stageCount);
}
INSTANTIATE_SERIALISE_TYPE(VkGraphicsPipelineCreateInfo);
template <>
void Deserialise(const VkGraphicsPipelineCreateInfo &el)
{
RDCASSERT(el.pNext == NULL); // otherwise delete
if(el.pVertexInputState)
{
RDCASSERT(el.pVertexInputState->pNext == NULL); // otherwise delete
delete[] el.pVertexInputState->pVertexBindingDescriptions;
delete[] el.pVertexInputState->pVertexAttributeDescriptions;
delete el.pVertexInputState;
}
if(el.pInputAssemblyState)
{
RDCASSERT(el.pInputAssemblyState->pNext == NULL); // otherwise delete
delete el.pInputAssemblyState;
}
if(el.pTessellationState)
{
RDCASSERT(el.pTessellationState->pNext == NULL); // otherwise delete
delete el.pTessellationState;
}
if(el.pViewportState)
{
RDCASSERT(el.pViewportState->pNext == NULL); // otherwise delete
if(el.pViewportState->pViewports)
delete[] el.pViewportState->pViewports;
if(el.pViewportState->pScissors)
delete[] el.pViewportState->pScissors;
delete el.pViewportState;
}
if(el.pRasterizationState)
{
RDCASSERT(el.pRasterizationState->pNext == NULL); // otherwise delete
delete el.pRasterizationState;
}
if(el.pMultisampleState)
{
RDCASSERT(el.pMultisampleState->pNext == NULL); // otherwise delete
delete el.pMultisampleState->pSampleMask;
delete el.pMultisampleState;
}
if(el.pDepthStencilState)
{
RDCASSERT(el.pDepthStencilState->pNext == NULL); // otherwise delete
delete el.pDepthStencilState;
}
if(el.pColorBlendState)
{
RDCASSERT(el.pColorBlendState->pNext == NULL); // otherwise delete
delete[] el.pColorBlendState->pAttachments;
delete el.pColorBlendState;
}
if(el.pDynamicState)
{
RDCASSERT(el.pDynamicState->pNext == NULL); // otherwise delete
if(el.pDynamicState->pDynamicStates)
delete[] el.pDynamicState->pDynamicStates;
delete el.pDynamicState;
}
for(uint32_t i = 0; i < el.stageCount; i++)
{
RDCASSERT(el.pStages[i].pNext == NULL); // otherwise delete
if(el.pStages[i].pSpecializationInfo)
{
delete[](byte *)(el.pStages[i].pSpecializationInfo->pData);
delete[] el.pStages[i].pSpecializationInfo->pMapEntries;
delete el.pStages[i].pSpecializationInfo;
}
}
delete[] el.pStages;
}
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkDescriptorImageInfo &el)
{
SERIALISE_MEMBER(sampler);
SERIALISE_MEMBER(imageView);
SERIALISE_MEMBER(imageLayout);
}
INSTANTIATE_SERIALISE_TYPE(VkDescriptorImageInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkDescriptorBufferInfo &el)
{
SERIALISE_MEMBER(buffer);
SERIALISE_MEMBER(offset);
SERIALISE_MEMBER(range);
}
INSTANTIATE_SERIALISE_TYPE(VkDescriptorBufferInfo);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkWriteDescriptorSet &el)
{
RDCASSERT(ser.IsReading() || el.sType == VK_STRUCTURE_TYPE_WRITE_DESCRIPTOR_SET);
SerialiseNext(ser, el.sType, el.pNext);
SERIALISE_MEMBER(dstSet);
SERIALISE_MEMBER(dstBinding);
SERIALISE_MEMBER(dstArrayElement);
SERIALISE_MEMBER(descriptorType);
if(ser.IsReading())
{
el.pImageInfo = NULL;
el.pBufferInfo = NULL;
el.pTexelBufferView = NULL;
}
// only serialise the array type used, the others are ignored
if(el.descriptorType == VK_DESCRIPTOR_TYPE_SAMPLER ||
el.descriptorType == VK_DESCRIPTOR_TYPE_COMBINED_IMAGE_SAMPLER ||
el.descriptorType == VK_DESCRIPTOR_TYPE_SAMPLED_IMAGE ||
el.descriptorType == VK_DESCRIPTOR_TYPE_STORAGE_IMAGE ||
el.descriptorType == VK_DESCRIPTOR_TYPE_INPUT_ATTACHMENT)
{
SERIALISE_MEMBER_ARRAY(pImageInfo, descriptorCount);
}
else if(el.descriptorType == VK_DESCRIPTOR_TYPE_UNIFORM_BUFFER ||
el.descriptorType == VK_DESCRIPTOR_TYPE_STORAGE_BUFFER ||
el.descriptorType == VK_DESCRIPTOR_TYPE_UNIFORM_BUFFER_DYNAMIC ||
el.descriptorType == VK_DESCRIPTOR_TYPE_STORAGE_BUFFER_DYNAMIC)
{
SERIALISE_MEMBER_ARRAY(pBufferInfo, descriptorCount);
}
else if(el.descriptorType == VK_DESCRIPTOR_TYPE_UNIFORM_TEXEL_BUFFER ||
el.descriptorType == VK_DESCRIPTOR_TYPE_STORAGE_TEXEL_BUFFER)
{
SERIALISE_MEMBER_ARRAY(pTexelBufferView, descriptorCount);
}
}
INSTANTIATE_SERIALISE_TYPE(VkWriteDescriptorSet);
template <>
void Deserialise(const VkWriteDescriptorSet &el)
{
RDCASSERT(el.pNext == NULL); // otherwise delete
delete[] el.pImageInfo;
delete[] el.pBufferInfo;
delete[] el.pTexelBufferView;
}
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VkImageBlit &el)
{
SERIALISE_MEMBER(srcSubresource);
SERIALISE_MEMBER(srcOffsets);
SERIALISE_MEMBER(dstSubresource);
SERIALISE_MEMBER(dstOffsets);
}
INSTANTIATE_SERIALISE_TYPE(VkImageBlit);
#pragma endregion
/////////////////////////////////////////////////////////////////
// d3d11_device.cpp / .h
#pragma region d3d11_device.cpp
// - WrappedID3D11DeviceContext::Serialise_DebugMessages
#define IMPLEMENT_FUNCTION_SERIALISED(ret, func, ...) \
ret func(__VA_ARGS__); \
template<class SerialiserType> \
bool CONCAT(Serialise_, func(SerialiserType &ser, __VA_ARGS__));
#define IMPLEMENT_FUNCTION_SERIALISED_NOARGS(ret, func) \
ret func(); \
template<class SerialiserType> \
bool CONCAT(Serialise_, func(SerialiserType &ser));
struct FakeD3DVulkanEtc
{
FakeD3DVulkanEtc() : m_Writer(NULL), m_Reader(NULL), m_State(WRITING) {}
WriteSerialiser *m_Writer;
StructReadSerialiser *m_Reader;
vector<Chunk *> m_Chunks;
LogState m_State;
IMPLEMENT_FUNCTION_SERIALISED(virtual HRESULT ,
CreateInputLayout, const D3D11_INPUT_ELEMENT_DESC *pInputElementDescs,
UINT NumElements,
const void *pShaderBytecodeWithInputSignature,
SIZE_T BytecodeLength,
ID3D11InputLayout **ppInputLayout);
IMPLEMENT_FUNCTION_SERIALISED(virtual HRESULT ,
CreateVertexShader, const void *pShaderBytecode, SIZE_T BytecodeLength,
ID3D11ClassLinkage *pClassLinkage,
ID3D11VertexShader **ppVertexShader);
IMPLEMENT_FUNCTION_SERIALISED(virtual HRESULT ,
ClearRenderTarget, ID3D11RenderTargetView *rtv, UINT foobar,
const float color[4],
D3D11_BOX *box);
IMPLEMENT_FUNCTION_SERIALISED(virtual HRESULT ,
DoSomeVulkanStuff, uint32_t blah, float foo,
VkGraphicsPipelineCreateInfo *pipeInfo,
VkWriteDescriptorSet *writeSet,
VkPipelineLayoutCreateInfo *layoutInfo);
vector<DebugMessage> GetDebugMessages()
{
DebugMessage foo;
foo.description = "bl <a> h";
foo.eventID = 1234;
foo.messageID = 42;
return vector<DebugMessage>(1, foo);
}
void ReadLog();
};
template <class SerialiserType>
bool FakeD3DVulkanEtc::Serialise_CreateInputLayout(SerialiserType &ser,
const D3D11_INPUT_ELEMENT_DESC *pInputElementDescs, UINT NumElements,
const void *pShaderBytecodeWithInputSignature, SIZE_T BytecodeLength,
ID3D11InputLayout **ppInputLayout)
{
SERIALISE_ELEMENT_ARRAY(pInputElementDescs, NumElements);
SERIALISE_ELEMENT_ARRAY(pShaderBytecodeWithInputSignature, BytecodeLength);
SERIALISE_ELEMENT_LOCAL(pLayout, ResourceId());
// pretend this is a drawcall, OK
vector<DebugMessage> msgs = GetDebugMessages();
SERIALISE_ELEMENT(msgs).Named("Debug Messages");
ID3D11InputLayout *ret = NULL;
if(m_State >= WRITING)
{
ret = *ppInputLayout;
}
else if(m_State == READING)
{
// do stuff!
}
return true;
}
HRESULT FakeD3DVulkanEtc::CreateInputLayout(const D3D11_INPUT_ELEMENT_DESC *pInputElementDescs,
UINT NumElements,
const void *pShaderBytecodeWithInputSignature,
SIZE_T BytecodeLength,
ID3D11InputLayout **ppInputLayout)
{
ID3D11InputLayout *real = NULL;
ID3D11InputLayout *wrapped = NULL;
HRESULT ret = S_OK;
if(SUCCEEDED(ret))
{
wrapped = real;
if(m_Writer)
{
WriteSerialiser &ser = *m_Writer;
SCOPED_SERIALISE_CONTEXT(CREATE_INPUT_LAYOUT);
Serialise_CreateInputLayout(ser, pInputElementDescs, NumElements,
pShaderBytecodeWithInputSignature, BytecodeLength, &wrapped);
// do stuff!
m_Chunks.push_back(scope.Get());
}
}
*ppInputLayout = wrapped;
return ret;
}
template<class SerialiserType>
bool FakeD3DVulkanEtc::Serialise_CreateVertexShader(SerialiserType &ser, const void *pShaderBytecode,
SIZE_T BytecodeLength,
ID3D11ClassLinkage *pClassLinkage,
ID3D11VertexShader **ppVertexShader)
{
SERIALISE_ELEMENT_ARRAY(pShaderBytecode, BytecodeLength);
SERIALISE_ELEMENT_LOCAL(pLinkage, ResourceId());
SERIALISE_ELEMENT_LOCAL(pShader, ResourceId());
if(m_State == READING)
{
// do stuff!
}
return true;
}
HRESULT FakeD3DVulkanEtc::CreateVertexShader(const void *pShaderBytecode, SIZE_T BytecodeLength,
ID3D11ClassLinkage *pClassLinkage,
ID3D11VertexShader **ppVertexShader)
{
ID3D11VertexShader *real = NULL;
ID3D11VertexShader *wrapped = NULL;
HRESULT ret = S_OK;
if(SUCCEEDED(ret))
{
wrapped = real;
if(m_Writer)
{
WriteSerialiser &ser = *m_Writer;
SCOPED_SERIALISE_CONTEXT(CREATE_VERTEX_SHADER);
Serialise_CreateVertexShader(ser, pShaderBytecode, BytecodeLength, pClassLinkage, &wrapped);
// do stuff!
m_Chunks.push_back(scope.Get());
}
else
{
// do stuff?
}
*ppVertexShader = wrapped;
}
return ret;
}
template<class SerialiserType>
bool FakeD3DVulkanEtc::Serialise_ClearRenderTarget(SerialiserType &ser, ID3D11RenderTargetView *rtv, UINT foobar, const float color[4], D3D11_BOX *box)
{
SERIALISE_ELEMENT_LOCAL(RTV, ResourceId());
SERIALISE_ELEMENT(foobar);
SERIALISE_ELEMENT_ARRAY(color, FIXED_COUNT(4));
SERIALISE_ELEMENT_OPT(box);
if(m_State == READING)
{
// do stuff!
}
return true;
}
HRESULT FakeD3DVulkanEtc::ClearRenderTarget(ID3D11RenderTargetView *rtv, UINT foobar, const float color[4], D3D11_BOX *box)
{
HRESULT ret = S_OK;
if(SUCCEEDED(ret))
{
if(m_Writer)
{
WriteSerialiser &ser = *m_Writer;
SCOPED_SERIALISE_CONTEXT(CLEAR_RTV);
Serialise_ClearRenderTarget(ser, rtv, foobar, color, box);
// do stuff!
m_Chunks.push_back(scope.Get());
}
else
{
// do stuff?
}
}
return ret;
}
template<class SerialiserType>
bool FakeD3DVulkanEtc::Serialise_DoSomeVulkanStuff(SerialiserType &ser, uint32_t blah, float foo,
VkGraphicsPipelineCreateInfo *pipeInfo,
VkWriteDescriptorSet *writeSet,
VkPipelineLayoutCreateInfo *layoutInfo)
{
SERIALISE_ELEMENT(blah);
SERIALISE_ELEMENT(foo);
SERIALISE_ELEMENT_OPT(pipeInfo);
SERIALISE_ELEMENT_OPT(writeSet);
SERIALISE_ELEMENT_OPT(layoutInfo);
return true;
}
HRESULT FakeD3DVulkanEtc::DoSomeVulkanStuff(uint32_t blah, float foo,
VkGraphicsPipelineCreateInfo *pipeInfo,
VkWriteDescriptorSet *writeSet,
VkPipelineLayoutCreateInfo *layoutInfo)
{
if(m_Writer)
{
WriteSerialiser &ser = *m_Writer;
SCOPED_SERIALISE_CONTEXT(DO_VULKAN_STUFF);
Serialise_DoSomeVulkanStuff(ser, blah, foo, pipeInfo, writeSet, layoutInfo);
// do stuff!
m_Chunks.push_back(scope.Get());
}
return S_OK;
}
void FakeD3DVulkanEtc::ReadLog()
{
StructReadSerialiser &ser = *m_Reader;
for(;;)
{
ChunkType context = (ChunkType)ser.BeginChunk(0);
if(context == CREATE_INPUT_LAYOUT)
Serialise_CreateInputLayout(ser, NULL, 0, NULL, 0, NULL);
else if(context == CREATE_VERTEX_SHADER)
Serialise_CreateVertexShader(ser, NULL, 0, NULL, NULL);
else if(context == DO_VULKAN_STUFF)
Serialise_DoSomeVulkanStuff(ser, 0, 0.0f, NULL, NULL, NULL);
else if(context == CLEAR_RTV)
Serialise_ClearRenderTarget(ser, NULL, 0, (float *)NULL, NULL);
else
ser.SkipCurrentChunk();
ser.EndChunk();
if(ser.GetReader()->AtEnd())
break;
}
}
struct D3D11InitParams : public RDCInitParams
{
D3D11InitParams()
{
SerialiseVersion = D3D11_SERIALISE_VERSION;
DriverType = D3D_DRIVER_TYPE_UNKNOWN;
Flags = 0;
SDKVersion = D3D11_SDK_VERSION;
NumFeatureLevels = 0;
RDCEraseEl(FeatureLevels);
}
INIT_PARAMS_SERIALISE();
D3D_DRIVER_TYPE DriverType;
UINT Flags;
UINT SDKVersion;
UINT NumFeatureLevels;
D3D_FEATURE_LEVEL FeatureLevels[16];
static const uint32_t D3D11_SERIALISE_VERSION = 0x0000009;
ReplayCreateStatus GetStatus() { return SerialiseVersion < D3D11_SERIALISE_VERSION ? eReplayCreate_APIIncompatibleVersion : eReplayCreate_Success; }
// version number internal to d3d11 stream
uint32_t SerialiseVersion;
};
template <class SerialiserType>
void D3D11InitParams::DoSerialise(SerialiserType &ser)
{
D3D11InitParams &el = *this;
SERIALISE_MEMBER(SerialiseVersion);
SERIALISE_MEMBER(DriverType);
SERIALISE_MEMBER(Flags);
SERIALISE_MEMBER(SDKVersion);
SERIALISE_MEMBER(NumFeatureLevels);
SERIALISE_MEMBER(FeatureLevels);
}
#pragma endregion
/////////////////////////////////////////////////////////////////
// test harness
#pragma region harness
const char *chunklookup(uint32_t idx)
{
if(idx == CREATE_PARAMS)
return "Create Params";
if(idx == THUMBNAIL_DATA)
return "Thumbnail Data";
if(idx == DRIVER_INIT_PARAMS)
return "Driver Init Params";
if(idx == INITIAL_CONTENTS)
return "Initial Contents";
if(idx == CREATE_INPUT_LAYOUT)
return "CreateInputLayout";
if(idx == CREATE_VERTEX_SHADER)
return "CreateVertexShader";
if(idx == DO_VULKAN_STUFF)
return "DoVulkanStuff";
if(idx == CLEAR_RTV)
return "ClearRenderTarget";
return "Unknown!";
}
void real_world_test_write()
{
// volatile just so the constants don't get folded in
volatile uint32_t frame = 34;
volatile size_t thlen = frame*frame;
byte *pixels = new byte[thlen];
volatile uint32_t w = frame + 5;
volatile uint32_t h = w - 10;
pixels[0] = 0x1;
pixels[1] = 0x2;
pixels[2] = 0x3;
pixels[3] = 0x2;
pixels[4] = 0x1;
D3D11InitParams init;
WriteSerialiser *fileWrite = OpenWriteSerialiser(frame, init, (byte *)pixels, thlen, w, h);
FakeD3DVulkanEtc etc;
etc.m_Writer = new WriteSerialiser(new StreamWriter(StreamWriter::DefaultScratchSize));
etc.m_State = WRITING_IDLE;
etc.m_Writer->SetChunkMetadataRecording(WriteSerialiser::ChunkThreadID | WriteSerialiser::ChunkTimestamp);
D3D11_INPUT_ELEMENT_DESC inputDesc;
inputDesc.SemanticName = "POSITION";
inputDesc.SemanticIndex = 0;
inputDesc.Format = DXGI_FORMAT_R32G32B32_FLOAT;
inputDesc.InputSlot = 0;
inputDesc.InputSlotClass = D3D11_INPUT_PER_VERTEX_DATA;
inputDesc.AlignedByteOffset = 0;
inputDesc.InstanceDataStepRate = 0;
ID3D11InputLayout *ilayout = NULL;
etc.CreateInputLayout(&inputDesc, 1, (const void *)pixels, thlen, &ilayout);
ID3D11VertexShader *vs = NULL;
etc.CreateVertexShader((const void *)pixels, thlen, NULL, &vs);
ID3D11RenderTargetView *rtv = (ID3D11RenderTargetView *)0xf00baa;
float magenta[] = { 1.0f, 0.0f, 1.0f, 0.5f };
etc.ClearRenderTarget(rtv, 1337, magenta, NULL);
{
// declare the pipeline creation info and all of its sub-structures
// these are modified as appropriate for each pipeline we create
VkPipelineShaderStageCreateInfo stages[2] = {
{VK_STRUCTURE_TYPE_PIPELINE_SHADER_STAGE_CREATE_INFO, NULL, 0, VK_SHADER_STAGE_VERTEX_BIT,
VK_NULL_HANDLE, "main", NULL},
{VK_STRUCTURE_TYPE_PIPELINE_SHADER_STAGE_CREATE_INFO, NULL, 0, VK_SHADER_STAGE_FRAGMENT_BIT,
VK_NULL_HANDLE, "main", NULL},
};
VkPipelineVertexInputStateCreateInfo vi = {
VK_STRUCTURE_TYPE_PIPELINE_VERTEX_INPUT_STATE_CREATE_INFO,
NULL,
0,
0,
NULL, // vertex bindings
0,
NULL, // vertex attributes
};
VkPipelineInputAssemblyStateCreateInfo ia = {
VK_STRUCTURE_TYPE_PIPELINE_INPUT_ASSEMBLY_STATE_CREATE_INFO,
NULL,
0,
VK_PRIMITIVE_TOPOLOGY_TRIANGLE_STRIP,
false,
};
VkRect2D scissor = {{0, 0}, {4096, 4096}};
VkPipelineViewportStateCreateInfo vp = {
VK_STRUCTURE_TYPE_PIPELINE_VIEWPORT_STATE_CREATE_INFO, NULL, 0, 1, NULL, 1, &scissor};
VkPipelineRasterizationStateCreateInfo rs = {
VK_STRUCTURE_TYPE_PIPELINE_RASTERIZATION_STATE_CREATE_INFO,
NULL,
0,
true,
false,
VK_POLYGON_MODE_FILL,
VK_CULL_MODE_NONE,
VK_FRONT_FACE_CLOCKWISE,
false,
0.0f,
0.0f,
0.0f,
1.0f,
};
VkPipelineMultisampleStateCreateInfo msaa = {
VK_STRUCTURE_TYPE_PIPELINE_MULTISAMPLE_STATE_CREATE_INFO,
NULL,
0,
VK_SAMPLE_COUNT_1_BIT,
false,
0.0f,
NULL,
false,
false,
};
VkPipelineDepthStencilStateCreateInfo ds = {
VK_STRUCTURE_TYPE_PIPELINE_DEPTH_STENCIL_STATE_CREATE_INFO,
NULL,
0,
false,
false,
VK_COMPARE_OP_ALWAYS,
false,
false,
{VK_STENCIL_OP_KEEP, VK_STENCIL_OP_KEEP, VK_STENCIL_OP_KEEP, VK_COMPARE_OP_ALWAYS, 0, 0, 0},
{VK_STENCIL_OP_KEEP, VK_STENCIL_OP_KEEP, VK_STENCIL_OP_KEEP, VK_COMPARE_OP_ALWAYS, 0, 0, 0},
0.0f,
1.0f,
};
VkPipelineColorBlendAttachmentState attState = {
false,
VK_BLEND_FACTOR_ONE,
VK_BLEND_FACTOR_ZERO,
VK_BLEND_OP_ADD,
VK_BLEND_FACTOR_ONE,
VK_BLEND_FACTOR_ZERO,
VK_BLEND_OP_ADD,
0xf,
};
VkPipelineColorBlendStateCreateInfo cb = {
VK_STRUCTURE_TYPE_PIPELINE_COLOR_BLEND_STATE_CREATE_INFO,
NULL,
0,
false,
VK_LOGIC_OP_NO_OP,
1,
&attState,
{1.0f, 1.0f, 1.0f, 1.0f}};
VkDynamicState dynstates[] = {VK_DYNAMIC_STATE_VIEWPORT, VK_DYNAMIC_STATE_SCISSOR, VK_DYNAMIC_STATE_LINE_WIDTH};
VkPipelineDynamicStateCreateInfo dyn = {
VK_STRUCTURE_TYPE_PIPELINE_DYNAMIC_STATE_CREATE_INFO,
NULL,
0,
ARRAY_COUNT(dynstates),
dynstates,
};
VkGraphicsPipelineCreateInfo pipeInfo = {
VK_STRUCTURE_TYPE_GRAPHICS_PIPELINE_CREATE_INFO,
NULL,
0,
2,
stages,
&vi,
&ia,
NULL, // tess
&vp,
&rs,
&msaa,
&ds,
&cb,
&dyn,
VK_NULL_HANDLE,
VK_NULL_HANDLE,
0, // sub pass
VK_NULL_HANDLE, // base pipeline handle
-1, // base pipeline index
};
VkDescriptorBufferInfo bufinfo = {};
bufinfo.offset = 1111;
VkWriteDescriptorSet textSetWrite =
{VK_STRUCTURE_TYPE_WRITE_DESCRIPTOR_SET, NULL, VkDescriptorSet(), 0, 0, 1,
VK_DESCRIPTOR_TYPE_UNIFORM_BUFFER_DYNAMIC, NULL, &bufinfo, NULL};
VkPipelineLayoutCreateInfo pipeLayoutInfo = {
VK_STRUCTURE_TYPE_PIPELINE_LAYOUT_CREATE_INFO,
NULL,
0,
4,
NULL,
0,
NULL, // push constant ranges
};
VkDescriptorSetLayout setLayouts[4];
pipeLayoutInfo.pSetLayouts = setLayouts;
etc.DoSomeVulkanStuff(32, 4.4f, &pipeInfo, &textSetWrite, &pipeLayoutInfo);
}
for(size_t i=0; i < etc.m_Chunks.size(); i++)
fileWrite->Insert(etc.m_Chunks[i]);
fileWrite->FlushToDisk();
delete fileWrite;
for(size_t i=0; i < etc.m_Chunks.size(); i++)
delete etc.m_Chunks[i];
delete etc.m_Writer;
delete[] pixels;
}
void real_world_test_read()
{
FILE *readfile = fopen("test.rdc", "rb");
FakeD3DVulkanEtc etc;
etc.m_Reader = new StructReadSerialiser(new StreamReader(readfile));
etc.m_Reader->ConfigureStructuredExport(chunklookup, true);
etc.m_State = READING;
etc.ReadLog();
std::string xml = Structured2XML(etc.m_Reader->GetSerialisedChunks());
//printf("result from real serialising:\n\n%s\n\n", xml.c_str());
FILE *f = fopen("out.xml", "w");
fwrite(xml.c_str(), 1, xml.size(), f);
fclose(f);
Buffers2ZIP("out.zip", etc.m_Reader->GetSerialisedBuffers());
f = fopen("test.xml", "w");
fwrite(xml.c_str(), 1, xml.size(), f);
fclose(f);
delete etc.m_Reader;
}
void real_world_test_xml2rdc()
{
FILE *f = fopen("in.xml", "r");
if(f)
{
vector<SerialisedBuffer> bufs;
fseek(f, 0, SEEK_END);
int len = ftell(f);
fseek(f, 0, SEEK_SET);
char *xml = new char[len+1];
fread(xml, 1, len, f);
xml[len] = 0;
fclose(f);
StructuredChunkList chunks = XML2Structured(xml);
SerialisedBufferList buffers = ZIP2Buffers("in.zip");
delete[] xml;
FILE *writefile = fopen("converted.rdc", "wb");
WriteSerialiser *fileSerialiser = new WriteSerialiser(new StreamWriter(writefile));
WriteSerialiser *chunkSerialiser = new WriteSerialiser(new StreamWriter(StreamWriter::DefaultScratchSize));
chunkSerialiser->WriteSerialisedFile(*fileSerialiser, SerialisedFile(chunks, buffers));
fileSerialiser->FlushToDisk();
delete chunkSerialiser;
delete fileSerialiser;
for(size_t i = 0; i < buffers.size(); i++)
free(buffers[i].first);
for(size_t i = 0; i < chunks.size(); i++)
delete chunks[i];
}
}
void real_world_test_read2()
{
FILE *f = fopen("converted.rdc", "r");
if(!f)
return;
FakeD3DVulkanEtc etc;
etc.m_Reader = new StructReadSerialiser(new StreamReader(f));
etc.m_Reader->ConfigureStructuredExport(chunklookup, true);
etc.m_State = READING;
etc.ReadLog();
std::string xml = Structured2XML(etc.m_Reader->GetSerialisedChunks());
f = fopen("converted.xml", "w");
fwrite(xml.c_str(), 1, xml.size(), f);
fclose(f);
delete etc.m_Reader;
}
#pragma endregion
/////////////////////////////////////////////////////////////////
<file_sep>/xml_codec.h
#pragma once
#include <string>
#include <vector>
#include "common.h"
#include "structured_data.h"
std::string Structured2XML(const StructuredChunkList &chunks);
StructuredChunkList XML2Structured(const std::string &xml);
void Buffers2ZIP(const char *filename, const SerialisedBufferList &buffers);
SerialisedBufferList ZIP2Buffers(const char *filename);
<file_sep>/test_types.cpp
#include "common.h"
#include "test_types.h"
uint64_t ResourceId::cur = 40;
template <>
string ToStr(const blah &el)
{
BEGIN_ENUM_STRINGIZE();
STRINGIZE_ENUM(blah_foo);
STRINGIZE_ENUM(blah_bar);
STRINGIZE_ENUM(blah_qux);
END_ENUM_STRINGIZE(blah);
}
SERIALISE_ENUM_TYPE(blah);
BASIC_TYPE_SERIALISE_STRINGIFY(ResourceId, el.innerSecretValue, eUnsignedInteger, 8);
INSTANTIATE_SERIALISE_TYPE(ResourceId);
template <>
string ToStr(const ResourceId &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "Secret_%" PRIu64, el.innerSecretValue);
return tostrBuf;
}
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, podStruct &el)
{
SERIALISE_MEMBER(x);
SERIALISE_MEMBER(y);
SERIALISE_MEMBER(width);
SERIALISE_MEMBER(height);
}
INSTANTIATE_SERIALISE_TYPE(podStruct);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, simpleStruct &el)
{
SERIALISE_MEMBER(foo);
SERIALISE_MEMBER(bar);
SERIALISE_MEMBER(secret).Hidden();
SERIALISE_MEMBER(hello);
SERIALISE_MEMBER_TYPED(blah, really_a_blah);
}
INSTANTIATE_SERIALISE_TYPE(simpleStruct);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, complexStruct &el)
{
SERIALISE_MEMBER(basicArray);
SERIALISE_MEMBER(secret).Hidden();
SERIALISE_MEMBER(vectorArray);
SERIALISE_MEMBER_ARRAY(exts, numExts);
SERIALISE_MEMBER(cstring).Named("C-style string");
}
template <>
void Deserialise(const complexStruct &el)
{
delete[] el.exts;
}
INSTANTIATE_SERIALISE_TYPE(complexStruct);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, VulkanHandleThingy &el)
{
ResourceManager *rm = (ResourceManager *)ser.GetUserData();
ResourceId id;
if(ser.IsWriting())
{
id = ResourceId(); // GetResID(el);
rm->vk.insert(std::make_pair(id, el));
}
DoSerialise(ser, id);
if(ser.IsReading())
{
el = rm->vk[id];
}
}
INSTANTIATE_SERIALISE_TYPE(VulkanHandleThingy);
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, ID3DInterface *&el)
{
ResourceManager *rm = (ResourceManager *)ser.GetUserData();
ResourceId id;
if(ser.IsWriting())
{
id = ResourceId(); // GetResID(el);
rm->d3d.insert(std::make_pair(id, el));
}
DoSerialise(ser, id);
if(ser.IsReading())
{
el = rm->d3d[id];
}
}
INSTANTIATE_SERIALISE_TYPE(ID3DInterface *);<file_sep>/serialiser.cpp
/******************************************************************************
* The MIT License (MIT)
*
* Copyright (c) 2015-2016 <NAME>
* Copyright (c) 2014 Crytek
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
******************************************************************************/
#include "serialiser.h"
#include <errno.h>
#include "lz4/lz4.h"
#include "string_utils.h"
#ifdef _MSC_VER
#pragma warning( \
disable : 4422) // warning C4422: 'snprintf' : too many arguments passed for format string
// false positive as VS is trying to parse renderdoc's custom format strings
#endif
#if !defined(RELEASE)
int64_t Chunk::m_LiveChunks = 0;
int64_t Chunk::m_TotalMem = 0;
int64_t Chunk::m_MaxChunks = 0;
#endif
static const uint32_t MAGIC_HEADER = MAKE_FOURCC('R', 'D', 'O', 'C');
// based on blockStreaming_doubleBuffer.c in lz4 examples
struct CompressedFileIO
{
// large block size
static const size_t BlockSize = 64 * 1024;
CompressedFileIO(FILE *f)
{
m_F = f;
LZ4_resetStream(&m_LZ4Comp);
LZ4_setStreamDecode(&m_LZ4Decomp, NULL, 0);
m_CompressedSize = m_UncompressedSize = 0;
m_PageIdx = m_PageOffset = 0;
m_PageData = 0;
m_CompressSize = LZ4_COMPRESSBOUND(BlockSize);
m_CompressBuf = new byte[m_CompressSize];
}
~CompressedFileIO() { SAFE_DELETE_ARRAY(m_CompressBuf); }
uint32_t GetCompressedSize() { return m_CompressedSize; }
uint32_t GetUncompressedSize() { return m_UncompressedSize; }
// write out some data - accumulate into the input pages, then
// when a page is full call Flush() to flush it out to disk
void Write(const void *data, size_t len)
{
if(data == NULL || len == 0)
return;
m_UncompressedSize += (uint32_t)len;
const byte *src = (const byte *)data;
size_t remainder = 0;
// loop continually, writing up to BlockSize out of what remains of data
do
{
remainder = 0;
// if we're about to copy more than the page, copy only
// what will fit, then copy the remainder after flushing
if(m_PageOffset + len > BlockSize)
{
remainder = len - (BlockSize - m_PageOffset);
len = BlockSize - m_PageOffset;
}
memcpy(m_InPages[m_PageIdx] + m_PageOffset, src, len);
m_PageOffset += len;
if(remainder > 0)
{
Flush(); // this will swap the input pages and reset the page offset
src += len;
len = remainder;
}
} while(remainder > 0);
}
// flush out the current page to disk
void Flush()
{
// m_PageOffset is the amount written, usually equal to BlockSize except the last block.
int32_t compSize = LZ4_compress_fast_continue(&m_LZ4Comp, (const char *)m_InPages[m_PageIdx],
(char *)m_CompressBuf, (int)m_PageOffset,
(int)m_CompressSize, 1);
if(compSize < 0)
{
RDCERR("Error compressing: %i", compSize);
return;
}
fwrite(&compSize, sizeof(compSize), 1, m_F);
fwrite(m_CompressBuf, 1, compSize, m_F);
m_CompressedSize += compSize + sizeof(int32_t);
m_PageOffset = 0;
m_PageIdx = 1 - m_PageIdx;
}
// Reset back to 0, only makes sense when reading as writing can't be undone
void Reset()
{
LZ4_setStreamDecode(&m_LZ4Decomp, NULL, 0);
m_CompressedSize = m_UncompressedSize = 0;
m_PageIdx = 0;
m_PageOffset = 0;
}
// read out some data - if the input page is empty we fill
// the next page with data from disk
void Read(byte *data, size_t len)
{
if(data == NULL || len == 0)
return;
m_UncompressedSize += (uint32_t)len;
// loop continually, writing up to BlockSize out of what remains of data
do
{
size_t readamount = len;
// if we're about to copy more than the page, copy only
// what will fit, then copy the remainder after refilling
if(readamount > m_PageData)
readamount = m_PageData;
if(readamount > 0)
{
memcpy(data, m_InPages[m_PageIdx] + m_PageOffset, readamount);
m_PageOffset += readamount;
m_PageData -= readamount;
data += readamount;
len -= readamount;
}
if(len > 0)
FillBuffer(); // this will swap the input pages and reset the page offset
} while(len > 0);
}
void FillBuffer()
{
int32_t compSize = 0;
fread(&compSize, sizeof(compSize), 1, m_F);
fread(m_CompressBuf, 1, compSize, m_F);
m_CompressedSize += compSize;
m_PageIdx = 1 - m_PageIdx;
int32_t decompSize = LZ4_decompress_safe_continue(
&m_LZ4Decomp, (const char *)m_CompressBuf, (char *)m_InPages[m_PageIdx], compSize, BlockSize);
if(decompSize < 0)
{
RDCERR("Error decompressing: %i", decompSize);
return;
}
m_PageOffset = 0;
m_PageData = decompSize;
}
static void Decompress(byte *destBuf, const byte *srcBuf, size_t len)
{
LZ4_streamDecode_t lz4;
LZ4_setStreamDecode(&lz4, NULL, 0);
const byte *srcBufEnd = srcBuf + len;
while(srcBuf + 4 < srcBufEnd)
{
const int32_t *compSize = (const int32_t *)srcBuf;
srcBuf = (const byte *)(compSize + 1);
if(srcBuf + *compSize > srcBufEnd)
break;
int32_t decompSize = LZ4_decompress_safe_continue(&lz4, (const char *)srcBuf, (char *)destBuf,
*compSize, BlockSize);
if(decompSize < 0)
return;
srcBuf += *compSize;
destBuf += decompSize;
}
}
LZ4_stream_t m_LZ4Comp;
LZ4_streamDecode_t m_LZ4Decomp;
FILE *m_F;
uint32_t m_CompressedSize, m_UncompressedSize;
byte m_InPages[2][BlockSize];
size_t m_PageIdx, m_PageOffset, m_PageData;
byte *m_CompressBuf;
size_t m_CompressSize;
};
Chunk::~Chunk()
{
if(m_AlignedData)
{
if(m_Data)
FreeAlignedBuffer(m_Data);
m_Data = NULL;
}
else
{
SAFE_DELETE_ARRAY(m_Data);
}
}
/*
-----------------------------
File format for version 0x31:
uint64_t MAGIC_HEADER;
uint64_t version = 0x00000031;
uint64_t filesize;
uint64_t resolveDBSize;
if(resolveDBSize > 0)
{
byte resolveDB[resolveDBSize];
byte paddingTo16Alignment[];
}
byte captureData[]; // remainder of the file
-----------------------------
File format for version 0x32:
uint64_t MAGIC_HEADER;
uint64_t version = 0x00000032;
1 or more sections:
Section
{
char isASCII = '\0' or 'A'; // indicates the section is ASCII or binary. ASCII allows for easy
appending by hand/script
if(isASCII == 'A')
{
// ASCII sections are discouraged for tools, but useful for hand-editing by just
// appending a simple text file
char newline = '\n';
char length[]; // length of just section data below, as decimal string
char newline = '\n';
char sectionType[]; // section type, see SectionType enum, as decimal string.
char newline = '\n';
char sectionName[]; // UTF-8 string name of section.
char newline = '\n';
// sectionName is an arbitrary string. If two sections exist with the
// exact same name, the last one to occur in the file will be taken, the
// others ignored.
byte sectiondata[ interpret(length) ]; // section data
}
else if(isASCII == '\0')
{
byte zero[3]; // pad out the above character with 0 bytes. Reserved for future use
uint32_t sectionFlags; // section flags - e.g. is compressed or not.
uint32_t sectionType; // section type enum, see SectionType. Could be eSectionType_Unknown
uint32_t sectionLength; // byte length of the actual section data
uint32_t sectionNameLength; // byte length of the string below (minimum 1, for null terminator)
char sectionName[sectionNameLength]; // UTF-8 string name of section, optional.
byte sectiondata[length]; // actual contents of the section
// note: compressed sections will contain the uncompressed length as a uint64_t
// before the compressed data.
}
};
// remainder of the file is tightly packed/unaligned section structures.
// The first section must always be the actual frame capture data in
// binary form
Section sections[];
*/
struct FileHeader
{
FileHeader()
{
magic = MAGIC_HEADER;
version = ReadSerialiser::SERIALISE_VERSION;
}
uint64_t magic;
uint64_t version;
};
struct BinarySectionHeader
{
// 0x0
byte isASCII;
// 0x0, 0x0, 0x0
byte zero[3];
// section flags - e.g. is compressed or not.
SectionFlags sectionFlags;
// section type enum, see SectionType. Could be eSectionType_Unknown
SectionType sectionType;
// byte length of the actual section data
uint32_t sectionLength;
// byte length of the string below (could be 0)
uint32_t sectionNameLength;
// actually sectionNameLength, but at least 1 for null terminator
char name[1];
// char name[sectionNameLength];
// byte data[sectionLength];
};
#define RETURNCORRUPT(...) \
{ \
RDCERR(__VA_ARGS__); \
m_ErrorCode = eContError_Corrupt; \
m_HasError = true; \
return; \
}
#if 0 // container file reading
template<>
void ReadSerialiser::Init(const char *path)
{
Reset();
m_Filename = path ? path : "";
FileHeader header;
{
m_ReadFileHandle = fopen(m_Filename.c_str(), "rb");
if(!m_ReadFileHandle)
{
RDCERR("Can't open capture file '%s' for read - errno %d", m_Filename.c_str(), errno);
m_ErrorCode = eContError_FileIO;
m_HasError = true;
return;
}
RDCDEBUG("Opened capture file for read");
fread(&header, 1, sizeof(FileHeader), m_ReadFileHandle);
if(header.magic != MAGIC_HEADER)
{
RDCWARN("Invalid capture file. Expected magic %08x, got %08x", MAGIC_HEADER,
(uint32_t)header.magic);
m_ErrorCode = eContError_Corrupt;
m_HasError = true;
fclose(m_ReadFileHandle);
m_ReadFileHandle = 0;
return;
}
m_SerVer = header.version;
if(header.version == 0x00000031) // backwards compatibility
{
uint64_t headerRemainder[2];
fread(&headerRemainder, 1, sizeof(headerRemainder), m_ReadFileHandle);
fseek(m_ReadFileHandle, 0, SEEK_END);
uint64_t realLength = ftell(m_ReadFileHandle);
if(headerRemainder[0] != realLength)
{
RDCERR("Truncated/overlong capture file. Expected length 0x016llx, got 0x016llx",
headerRemainder[0], realLength);
m_ErrorCode = eContError_Corrupt;
m_HasError = true;
fclose(m_ReadFileHandle);
m_ReadFileHandle = 0;
return;
}
fseek(m_ReadFileHandle, sizeof(FileHeader) + sizeof(headerRemainder), SEEK_SET);
size_t resolveDBSize = (size_t)headerRemainder[1];
if(resolveDBSize > 0)
{
Section *resolveDB = new Section();
resolveDB->type = eSectionType_ResolveDatabase;
resolveDB->flags = eSectionFlag_None;
resolveDB->fileoffset = sizeof(FileHeader) + sizeof(headerRemainder);
resolveDB->name = "renderdoc/internal/resolvedb";
resolveDB->data.resize(resolveDBSize);
// read resolve DB entirely into memory
fread(&resolveDB->data[0], 1, resolveDBSize, m_ReadFileHandle);
m_Sections.push_back(resolveDB);
m_KnownSections[eSectionType_ResolveDatabase] = resolveDB;
}
// seek to frame capture data
fseek(m_ReadFileHandle,
AlignUp16(sizeof(FileHeader) + sizeof(headerRemainder) + resolveDBSize),
SEEK_SET);
Section *frameCap = new Section();
frameCap->type = eSectionType_FrameCapture;
frameCap->flags = eSectionFlag_None;
frameCap->fileoffset = ftell(m_ReadFileHandle);
frameCap->name = "renderdoc/internal/framecapture";
frameCap->size = realLength - frameCap->fileoffset;
m_Sections.push_back(frameCap);
m_KnownSections[eSectionType_FrameCapture] = frameCap;
}
else if(header.version == SERIALISE_VERSION)
{
while(!feof(m_ReadFileHandle))
{
BinarySectionHeader sectionHeader = {0};
byte *reading = (byte *)§ionHeader;
fread(reading, 1, 1, m_ReadFileHandle);
reading++;
if(feof(m_ReadFileHandle))
break;
if(sectionHeader.isASCII == 'A')
{
// ASCII section
char c = 0;
fread(&c, 1, 1, m_ReadFileHandle);
if(c != '\n')
RETURNCORRUPT("Invalid ASCII data section '%hhx'", c);
if(feof(m_ReadFileHandle))
RETURNCORRUPT("Invalid truncated ASCII data section");
uint64_t length = 0;
c = '0';
while(!feof(m_ReadFileHandle) && c != '\n')
{
c = '0';
fread(&c, 1, 1, m_ReadFileHandle);
if(c == '\n')
break;
length *= 10;
length += int(c - '0');
}
if(feof(m_ReadFileHandle))
RETURNCORRUPT("Invalid truncated ASCII data section");
union
{
uint32_t u32;
SectionType t;
} type;
type.u32 = 0;
c = '0';
while(!feof(m_ReadFileHandle) && c != '\n')
{
c = '0';
fread(&c, 1, 1, m_ReadFileHandle);
if(c == '\n')
break;
type.u32 *= 10;
type.u32 += int(c - '0');
}
if(feof(m_ReadFileHandle))
RETURNCORRUPT("Invalid truncated ASCII data section");
string name;
c = 0;
while(!feof(m_ReadFileHandle) && c != '\n')
{
c = 0;
fread(&c, 1, 1, m_ReadFileHandle);
if(c == 0 || c == '\n')
break;
name.push_back(c);
}
if(feof(m_ReadFileHandle))
RETURNCORRUPT("Invalid truncated ASCII data section");
Section *sect = new Section();
sect->flags = eSectionFlag_ASCIIStored;
sect->type = type.t;
sect->name = name;
sect->size = length;
sect->data.resize((size_t)length);
sect->fileoffset = ftell(m_ReadFileHandle);
fread(§->data[0], 1, (size_t)length, m_ReadFileHandle);
if(sect->type != eSectionType_Unknown && sect->type < eSectionType_Num)
m_KnownSections[sect->type] = sect;
m_Sections.push_back(sect);
}
else if(sectionHeader.isASCII == 0x0)
{
fread(reading, 1, offsetof(BinarySectionHeader, name) - 1, m_ReadFileHandle);
Section *sect = new Section();
sect->flags = sectionHeader.sectionFlags;
sect->type = sectionHeader.sectionType;
sect->name.resize(sectionHeader.sectionNameLength - 1);
sect->size = sectionHeader.sectionLength;
fread(§->name[0], 1, sectionHeader.sectionNameLength - 1, m_ReadFileHandle);
char nullterm = 0;
fread(&nullterm, 1, 1, m_ReadFileHandle);
sect->fileoffset = ftell(m_ReadFileHandle);
if(sect->flags & eSectionFlag_LZ4Compressed)
{
sect->compressedReader = new CompressedFileIO(m_ReadFileHandle);
fread(§->size, 1, sizeof(uint64_t), m_ReadFileHandle);
sect->fileoffset += sizeof(uint64_t);
}
if(sect->type != eSectionType_Unknown && sect->type < eSectionType_Num)
m_KnownSections[sect->type] = sect;
m_Sections.push_back(sect);
// if section isn't frame capture data and is small enough, read it all into memory now,
// otherwise skip
if(sect->type != eSectionType_FrameCapture && sectionHeader.sectionLength < 4 * 1024 * 1024)
{
sect->data.resize(sectionHeader.sectionLength);
fread(§->data[0], 1, sectionHeader.sectionLength, m_ReadFileHandle);
}
else
{
fseek(m_ReadFileHandle, sectionHeader.sectionLength, SEEK_CUR);
}
}
else
{
RETURNCORRUPT("Unrecognised section type '%hhx'", sectionHeader.isASCII);
}
}
}
else
{
RDCERR(
"Capture file from wrong version. This program is logfile version %" PRIu64 ", file is logfile "
"version %" PRIu64,
SERIALISE_VERSION, header.version);
m_ErrorCode = eContError_UnsupportedVersion;
m_HasError = true;
fclose(m_ReadFileHandle);
m_ReadFileHandle = 0;
return;
}
if(m_KnownSections[eSectionType_FrameCapture] == NULL)
{
RDCERR("Capture file doesn't have a frame capture");
m_ErrorCode = eContError_Corrupt;
m_HasError = true;
fclose(m_ReadFileHandle);
m_ReadFileHandle = 0;
return;
}
m_BufferSize = m_KnownSections[eSectionType_FrameCapture]->size;
m_CurrentBufferSize = (size_t)RDCMIN(m_BufferSize, (uint64_t)64 * 1024);
m_BufferHead = m_Buffer = AllocAlignedBuffer(m_CurrentBufferSize);
m_ReadOffset = 0;
fseek(m_ReadFileHandle, m_KnownSections[eSectionType_FrameCapture]->fileoffset,
SEEK_SET);
// read initial buffer of data
ReadFromFile(0, m_CurrentBufferSize);
}
}
#endif
/////////////////////////////////////////////////////////////
// Read Serialiser functions
template<>
ReadSerialiser::Serialiser(StreamReader *reader)
{
// ensure binary sizes of enums
RDCCOMPILE_ASSERT(sizeof(SectionFlags) == sizeof(uint32_t), "Section flags not in uint32");
RDCCOMPILE_ASSERT(sizeof(SectionType) == sizeof(uint32_t), "Section type not in uint32");
RDCCOMPILE_ASSERT(offsetof(BinarySectionHeader, name) == sizeof(uint32_t) * 5,
"BinarySectionHeader size has changed or contains padding");
Reset();
m_Read = reader;
m_Write = NULL;
}
template<>
ReadSerialiser::~Serialiser()
{
for(auto it = m_StructuredData.begin(); it != m_StructuredData.end(); ++it)
delete *it;
m_StructuredData.clear();
for(auto it = m_StructuredDataBuffers.begin(); it != m_StructuredDataBuffers.end(); ++it)
delete[] it->first;
m_StructuredDataBuffers.clear();
for(size_t i = 0; i < m_Sections.size(); i++)
{
SAFE_DELETE(m_Sections[i]->compressedReader);
SAFE_DELETE(m_Sections[i]);
}
RDCASSERT(m_Chunks.empty());
}
template<>
uint32_t ReadSerialiser::BeginChunk(uint32_t, uint32_t)
{
uint32_t chunkID = 0;
m_ChunkMetadata = SerialisedChunkMetaData();
{
uint32_t c = 0;
m_Read->Read(c);
// chunk index 0 is not allowed in normal situations.
// allows us to indicate some control bytes
while(c == 0)
{
uint8_t controlByte;
m_Read->Read(controlByte);
if(controlByte == 0x0)
{
// padding
uint8_t padLength;
m_Read->Read(padLength);
// might have padded with these 5 control bytes,
// so a pad length of 0 IS VALID.
if(padLength > 0)
{
m_Read->SkipBytes(padLength);
}
}
else
{
RDCERR("Unexpected control byte: %x", (uint32_t)controlByte);
}
m_Read->Read(c);
}
chunkID = c & ChunkIndexMask;
/////////////////
m_ChunkMetadata.chunkID = chunkID;
if(c & ChunkCallstack)
{
size_t sz = sizeof(m_ChunkMetadata.callstack);
m_Read->Read(m_ChunkMetadata.callstack, sz);
}
if(c & ChunkThreadID)
m_Read->Read(m_ChunkMetadata.threadID);
if(c & ChunkDuration)
m_Read->Read(m_ChunkMetadata.duration);
if(c & ChunkTimestamp)
m_Read->Read(m_ChunkMetadata.timestamp);
uint32_t chunkSize = 0xbeebfeed;
m_Read->Read(chunkSize);
m_LastChunkLen = chunkSize;
m_LastChunkOffset = m_Read->GetOffset();
}
return chunkID;
}
template<>
void ReadSerialiser::SkipCurrentChunk()
{
uint64_t readBytes = m_Read->GetOffset() - m_LastChunkOffset;
if(readBytes > m_LastChunkLen)
{
RDCERR("Can't skip current chunk outside of {BeginChunk, EndChunk}");
return;
}
m_Read->SkipBytes(m_LastChunkLen - readBytes);
}
template<>
void ReadSerialiser::EndChunk()
{
// this will be a no-op if the last chunk length was accurate. If it was a
// conservative estimate of the length then we'll skip some padding bytes
SkipCurrentChunk();
}
/////////////////////////////////////////////////////////////
// Structured Read Serialiser functions
template<>
StructReadSerialiser::Serialiser(StreamReader *reader)
{
// ensure binary sizes of enums
RDCCOMPILE_ASSERT(sizeof(SectionFlags) == sizeof(uint32_t), "Section flags not in uint32");
RDCCOMPILE_ASSERT(sizeof(SectionType) == sizeof(uint32_t), "Section type not in uint32");
RDCCOMPILE_ASSERT(offsetof(BinarySectionHeader, name) == sizeof(uint32_t) * 5,
"BinarySectionHeader size has changed or contains padding");
Reset();
m_Read = reader;
m_Write = NULL;
}
template<>
StructReadSerialiser::~Serialiser()
{
for(auto it = m_StructuredData.begin(); it != m_StructuredData.end(); ++it)
delete *it;
m_StructuredData.clear();
for(auto it = m_StructuredDataBuffers.begin(); it != m_StructuredDataBuffers.end(); ++it)
delete[] it->first;
m_StructuredDataBuffers.clear();
for(size_t i = 0; i < m_Sections.size(); i++)
{
SAFE_DELETE(m_Sections[i]->compressedReader);
SAFE_DELETE(m_Sections[i]);
}
RDCASSERT(m_Chunks.empty());
}
template<>
uint32_t StructReadSerialiser::BeginChunk(uint32_t, uint32_t)
{
uint32_t chunkID = 0;
m_ChunkMetadata = SerialisedChunkMetaData();
{
uint32_t c = 0;
m_Read->Read(c);
// chunk index 0 is not allowed in normal situations.
// allows us to indicate some control bytes
while(c == 0)
{
uint8_t controlByte;
m_Read->Read(controlByte);
if(controlByte == 0x0)
{
// padding
uint8_t padLength;
m_Read->Read(padLength);
// might have padded with these 5 control bytes,
// so a pad length of 0 IS VALID.
if(padLength > 0)
{
m_Read->SkipBytes(padLength);
}
}
else
{
RDCERR("Unexpected control byte: %x", (uint32_t)controlByte);
}
m_Read->Read(c);
}
chunkID = c & ChunkIndexMask;
/////////////////
m_ChunkMetadata.chunkID = chunkID;
if(c & ChunkCallstack)
{
size_t sz = sizeof(m_ChunkMetadata.callstack);
m_Read->Read(m_ChunkMetadata.callstack, sz);
}
if(c & ChunkThreadID)
m_Read->Read(m_ChunkMetadata.threadID);
if(c & ChunkDuration)
m_Read->Read(m_ChunkMetadata.duration);
if(c & ChunkTimestamp)
m_Read->Read(m_ChunkMetadata.timestamp);
uint32_t chunkSize = 0xbeebfeed;
m_Read->Read(chunkSize);
m_LastChunkLen = chunkSize;
m_LastChunkOffset = m_Read->GetOffset();
}
if(ExportStructure())
{
const char *name = m_ChunkLookup ? m_ChunkLookup(chunkID) : NULL;
if(!name)
name = "<Unknown Chunk>";
StructuredChunk *chunk = new StructuredChunk(name, "Chunk");
chunk->metadata = m_ChunkMetadata;
m_StructuredData.push_back(chunk);
m_StructureStack.push_back(chunk);
m_InternalElement = false;
}
return chunkID;
}
template<>
void StructReadSerialiser::SkipCurrentChunk()
{
if(ExportStructure())
{
RDCASSERTMSG("Skipping chunk after we've begun serialising!", m_StructureStack.size() == 1, m_StructureStack.size());
StructuredObject ¤t = *m_StructureStack.back();
current.data.basic.numChildren++;
current.data.children.push_back(new StructuredObject("Opaque chunk", "Byte Buffer"));
StructuredObject &obj = *current.data.children.back();
obj.type.basetype = SerialisedType::eBuffer;
obj.type.byteSize = m_LastChunkLen;
if(m_StructureStack.size() == 1)
{
StructuredChunk *chunk = (StructuredChunk *)m_StructureStack.back();
chunk->metadata.flags |= SerialisedChunkMetaData::OpaqueChunk;
}
}
{
uint64_t readBytes = m_Read->GetOffset() - m_LastChunkOffset;
if(readBytes > m_LastChunkLen)
{
RDCERR("Can't skip current chunk outside of {BeginChunk, EndChunk}");
return;
}
if(readBytes > 0)
{
RDCWARN("Partially consumed bytes at SkipCurrentChunk - blob data will be truncated");
}
uint64_t chunkBytes = m_LastChunkLen - readBytes;
if(ExportStructure() && m_ExportBuffers)
{
StructuredObject ¤t = *m_StructureStack.back();
StructuredObject &obj = *current.data.children.back();
obj.data.basic.u = m_StructuredDataBuffers.size();
byte *alloc = new byte[chunkBytes];
m_Read->Read(alloc, chunkBytes);
m_StructuredDataBuffers.push_back(std::make_pair(alloc, chunkBytes));
}
else
{
m_Read->SkipBytes(chunkBytes);
}
}
}
template<>
void StructReadSerialiser::EndChunk()
{
if(ExportStructure())
{
RDCASSERTMSG("Object Stack is imbalanced!", m_StructureStack.size() <= 1, m_StructureStack.size());
if(!m_StructureStack.empty())
{
m_StructureStack.back()->type.byteSize = m_LastChunkLen;
m_StructureStack.pop_back();
}
}
// this will be a no-op if the last chunk length was accurate. If it was a
// conservative estimate of the length then we'll skip some padding bytes
uint64_t readBytes = m_Read->GetOffset() - m_LastChunkOffset;
m_Read->SkipBytes(m_LastChunkLen - readBytes);
}
template<>
WriteSerialiser::Serialiser(StreamWriter *writer)
{
Reset();
m_Write = writer;
m_Read = NULL;
}
template<>
WriteSerialiser::~Serialiser()
{
for(auto it = m_StructuredData.begin(); it != m_StructuredData.end(); ++it)
delete *it;
m_StructuredData.clear();
for(auto it = m_StructuredDataBuffers.begin(); it != m_StructuredDataBuffers.end(); ++it)
delete[] it->first;
m_StructuredDataBuffers.clear();
RDCASSERT(m_Sections.empty());
for(size_t i = 0; i < m_Chunks.size(); i++)
{
if(m_Chunks[i]->IsTemporary())
SAFE_DELETE(m_Chunks[i]);
}
m_Chunks.clear();
}
/////////////////////////////////////////////////////////////
// generic
template<>
void WriteSerialiser::Insert(Chunk *chunk)
{
m_Chunks.push_back(chunk);
}
template<>
void WriteSerialiser::FlushToDisk()
{
#if 0 // container file writing
if(m_Filename != "" && !m_HasError)
{
RDCDEBUG("writing capture files");
FILE *binFile = fopen(m_Filename.c_str(), "w+b");
if(!binFile)
{
RDCERR("Can't open capture file '%s' for write, errno %d", m_Filename.c_str(), errno);
m_ErrorCode = eContError_FileIO;
m_HasError = true;
return;
}
RDCDEBUG("Opened capture file for write");
FileHeader header; // automagically initialised with correct data
// write header
fwrite(&header, 1, sizeof(FileHeader), binFile);
static const byte padding[BufferAlignment] = {0};
uint64_t compressedSizeOffset = 0;
uint64_t uncompressedSizeOffset = 0;
// write frame capture section header
{
const char sectionName[] = "renderdoc/internal/framecapture";
BinarySectionHeader section = {0};
section.isASCII = 0; // redundant but explicit
section.sectionNameLength = sizeof(sectionName); // includes null terminator
section.sectionType = eSectionType_FrameCapture;
section.sectionFlags = eSectionFlag_LZ4Compressed;
section.sectionLength =
0; // will be fixed up later, to avoid having to compress everything into memory
compressedSizeOffset = ftell(binFile) + offsetof(BinarySectionHeader, sectionLength);
fwrite(§ion, 1, offsetof(BinarySectionHeader, name), binFile);
fwrite(sectionName, 1, sizeof(sectionName), binFile);
uint64_t len = 0; // will be fixed up later
uncompressedSizeOffset = ftell(binFile);
fwrite(&len, 1, sizeof(uint64_t), binFile);
}
CompressedFileIO fwriter(binFile);
// track offset so we can add padding. The padding is relative
// to the start of the decompressed buffer, so we start it from 0
uint64_t offs = 0;
uint64_t alignedoffs = 0;
// write frame capture contents
for(size_t i = 0; i < m_Chunks.size(); i++)
{
Chunk *chunk = m_Chunks[i];
alignedoffs = AlignUp(offs, (uint64_t)BufferAlignment);
if(offs != alignedoffs && chunk->IsAligned())
{
uint32_t chunkID = 0; // write a '0' chunk that indicates special behaviour
fwriter.Write(&chunkID, sizeof(chunkID));
offs += sizeof(chunkID);
uint8_t controlByte = 0; // control byte 0 indicates padding
fwriter.Write(&controlByte, sizeof(controlByte));
offs += sizeof(controlByte);
offs++; // we will have to write out a byte indicating how much padding exists, so add 1
alignedoffs = AlignUp(offs, (uint64_t)BufferAlignment);
RDCCOMPILE_ASSERT(BufferAlignment < 0x100,
"Buffer alignment must be less than 256"); // with a byte at most
// indicating how many bytes
// to pad,
// this is our maximal representable alignment
uint8_t padLength = (alignedoffs - offs) & 0xff;
fwriter.Write(&padLength, sizeof(padLength));
// we might have padded with the control bytes, so only write some bytes if we need to
if(padLength > 0)
{
fwriter.Write(padding, size_t(alignedoffs - offs));
offs += alignedoffs - offs;
}
}
fwriter.Write(chunk->GetData(), chunk->GetLength());
offs += chunk->GetLength();
if(chunk->IsTemporary())
SAFE_DELETE(chunk);
}
fwriter.Flush();
m_Chunks.clear();
// fixup section size
{
uint32_t compsize = 0;
uint64_t uncompsize = 0;
uint64_t curoffs = ftell(binFile);
fseek(binFile, compressedSizeOffset, SEEK_SET);
compsize = fwriter.GetCompressedSize();
fwrite(&compsize, 1, sizeof(compsize), binFile);
fseek(binFile, uncompressedSizeOffset, SEEK_SET);
uncompsize = fwriter.GetUncompressedSize();
fwrite(&uncompsize, 1, sizeof(uncompsize), binFile);
fseek(binFile, curoffs, SEEK_SET);
RDCLOG("Compressed frame capture data from %u to %u", fwriter.GetUncompressedSize(),
fwriter.GetCompressedSize());
}
fclose(binFile);
}
#endif
}
template<>
void WriteSerialiser::SetChunkMetadataRecording(uint32_t flags)
{
// cannot change this mid-chunk
RDCASSERT(m_Write->GetOffset() == 0);
m_ChunkFlags = flags;
}
template<>
uint32_t WriteSerialiser::BeginChunk(uint32_t chunkID, uint32_t byteLength)
{
{
// if writing, we should be writing into the start of the serialiser. A serialiser should
// only ever have one actively constructing chunk in it.
RDCASSERT(m_Write->GetOffset() == 0);
// chunk index needs to be valid
RDCASSERT(chunkID > 0);
{
uint32_t c = chunkID & ChunkIndexMask;
RDCASSERT(chunkID <= ChunkIndexMask);
c |= m_ChunkFlags;
m_ChunkMetadata.chunkID = chunkID;
/////////////////
m_Write->Write(c);
if(c & ChunkCallstack)
{
if(m_ChunkMetadata.callstack[0] == 0)
{
vector<uint64_t> cs = CollectCallstack_stub();
memcpy(m_ChunkMetadata.callstack, &cs[0], sizeof(uint64_t)*cs.size());
}
m_Write->Write(m_ChunkMetadata.callstack, sizeof(m_ChunkMetadata.callstack));
}
if(c & ChunkThreadID)
{
if(m_ChunkMetadata.threadID == 0)
m_ChunkMetadata.threadID = CollectThreadID_stub();
m_Write->Write(m_ChunkMetadata.threadID);
}
if(c & ChunkDuration)
m_Write->Write(m_ChunkMetadata.duration);
if(c & ChunkTimestamp)
{
if(m_ChunkMetadata.timestamp == 0)
m_ChunkMetadata.timestamp = CollectTimestamp_stub();
m_Write->Write(m_ChunkMetadata.timestamp);
}
if(byteLength > 0)
{
// write length, assuming it is an upper bound
m_ChunkFixup = 0;
m_Write->Write(byteLength);
m_LastChunkOffset = m_Write->GetOffset();
m_LastChunkLen = byteLength;
}
else
{
// length will be fixed up in EndChunk
uint32_t chunkSize = 0xbeebfeed;
m_ChunkFixup = m_Write->GetOffset();
m_Write->Write(chunkSize);
}
}
}
return chunkID;
}
template<>
void WriteSerialiser::EndChunk()
{
if(m_ChunkFixup != 0)
{
// fix up the chunk header
uint64_t chunkOffset = m_ChunkFixup;
m_ChunkFixup = 0;
uint64_t curOffset = m_Write->GetOffset();
RDCASSERT(curOffset > chunkOffset);
uint64_t chunkLength = (curOffset - chunkOffset) - sizeof(uint32_t);
RDCASSERT(chunkLength < 0xffffffff);
uint32_t chunklen = (uint32_t)chunkLength;
m_Write->SetOffset(chunkOffset);
m_Write->Write(chunklen);
m_Write->SetOffset(curOffset);
}
else if(m_Write->GetOffset() - m_LastChunkOffset < m_LastChunkLen)
{
uint64_t numPadBytes = m_LastChunkLen - (m_Write->GetOffset() - m_LastChunkOffset);
// need to write some padding bytes so that the length is accurate
for(uint64_t i=0; i < numPadBytes; i++)
{
byte padByte = 0xbb;
m_Write->Write(padByte);
}
}
m_ChunkMetadata = SerialisedChunkMetaData();
}
template<>
void WriteSerialiser::WriteSerialisedFile(WriteSerialiser &fileser, SerialisedFile file)
{
m_StructuredDataBuffers = file.data;
for(size_t i = 0; i < file.chunks.size(); i++)
{
const StructuredChunk &chunk = *file.chunks[i];
m_ChunkMetadata = chunk.metadata;
m_ChunkFlags = 0;
if(m_ChunkMetadata.callstack[0] != 0)
m_ChunkFlags |= ChunkCallstack;
if(m_ChunkMetadata.threadID != 0)
m_ChunkFlags |= ChunkThreadID;
if(m_ChunkMetadata.duration != 0)
m_ChunkFlags |= ChunkDuration;
if(m_ChunkMetadata.timestamp != 0)
m_ChunkFlags |= ChunkTimestamp;
BeginChunk(m_ChunkMetadata.chunkID);
if(chunk.metadata.flags & SerialisedChunkMetaData::OpaqueChunk)
{
RDCASSERT(chunk.data.children.size() == 1);
uint64_t bufID = chunk.data.children[0]->data.basic.u;
byte *ptr = m_StructuredDataBuffers[bufID].first;
uint64_t len = m_StructuredDataBuffers[bufID].second;
// the information about whether a chunk is aligned or not is
// lost and can't be easily retrieved. It is safer to just
// always align opaque chunks. The only downside is that this
// could mean unmodified logs won't be bitwise identical
m_AlignedData = true;
m_Write->Write(ptr, len);
}
else
{
for(size_t o = 0; o < chunk.data.children.size(); o++)
{
// note, we don't need names because we aren't exporting structured data
Serialise("", chunk.data.children[o]);
}
}
EndChunk();
fileser.Insert(new Chunk(*this, m_ChunkMetadata.chunkID, true));
}
// clear it again so we don't try and deallocate any of the memory,
// because this function doesn't own it.
m_StructuredDataBuffers.clear();
}
template <>
const char *GetSerialiseTypeName<StructuredObject *>::Name()
{
return "";
}
template <class SerialiserType>
void DoSerialise(SerialiserType &ser, StructuredObject *el)
{
if(el->type.flags & SerialisedType::Nullable)
{
bool present = el->type.basetype != SerialisedType::eNull;
ser.Serialise("", present);
}
const SerialisedBufferList &buffers = ser.GetSerialisedBuffers();
switch(el->type.basetype)
{
case SerialisedType::eChunk:
RDCERR("Unexpected chunk inside object!");
break;
case SerialisedType::eStruct:
for(size_t o = 0; o < el->data.children.size(); o++)
ser.Serialise("", el->data.children[o]);
break;
case SerialisedType::eArray:
ser.Serialise("", el->data.children);
break;
case SerialisedType::eNull:
// nothing to do, we serialised present flag above
RDCASSERT(el->type.flags & SerialisedType::Nullable);
break;
case SerialisedType::eBuffer:
{
uint64_t bufID = el->data.basic.u;
byte *buf = buffers[bufID].first;
uint64_t size = buffers[bufID].second;
ser.Serialise("", buf, size);
break;
}
case SerialisedType::eString:
ser.Serialise("", el->data.str);
break;
case SerialisedType::eEnum:
{
uint32_t e = (uint32_t)el->data.basic.u;
ser.Serialise("", e);
break;
}
case SerialisedType::eBoolean:
ser.Serialise("", el->data.basic.b);
break;
case SerialisedType::eCharacter:
ser.Serialise("", el->data.basic.c);
break;
case SerialisedType::eUnsignedInteger:
if(el->type.byteSize == 1)
{
uint8_t u = uint8_t(el->data.basic.u);
ser.Serialise("", u);
}
else if(el->type.byteSize == 2)
{
uint16_t u = uint16_t(el->data.basic.u);
ser.Serialise("", u);
}
else if(el->type.byteSize == 4)
{
uint32_t u = uint32_t(el->data.basic.u);
ser.Serialise("", u);
}
else if(el->type.byteSize == 8)
{
ser.Serialise("", el->data.basic.u);
}
else
{
RDCERR("Unexpeted integer size %u", el->type.byteSize);
}
break;
case SerialisedType::eSignedInteger:
if(el->type.byteSize == 1)
{
int8_t i = int8_t(el->data.basic.i);
ser.Serialise("", i);
}
else if(el->type.byteSize == 2)
{
int16_t i = int16_t(el->data.basic.i);
ser.Serialise("", i);
}
else if(el->type.byteSize == 4)
{
int32_t i = int32_t(el->data.basic.i);
ser.Serialise("", i);
}
else if(el->type.byteSize == 8)
{
ser.Serialise("", el->data.basic.i);
}
else
{
RDCERR("Unexpeted integer size %u", el->type.byteSize);
}
break;
case SerialisedType::eFloat:
if(el->type.byteSize == 4)
{
float f = float(el->data.basic.d);
ser.Serialise("", f);
}
else if(el->type.byteSize == 8)
{
ser.Serialise("", el->data.basic.d);
}
else
{
RDCERR("Unexpeted float size %u", el->type.byteSize);
}
break;
}
}
/////////////////////////////////////////////////////////////
// Basic types
#define BASIC_TYPE_SERIALISE_NAME(typeName) \
template <> \
const char *GetSerialiseTypeName<typeName>::Name() \
{ \
return #typeName; \
}
BASIC_TYPE_SERIALISE_NAME(int64_t);
BASIC_TYPE_SERIALISE_NAME(uint64_t);
BASIC_TYPE_SERIALISE_NAME(int32_t);
BASIC_TYPE_SERIALISE_NAME(uint32_t);
BASIC_TYPE_SERIALISE_NAME(int16_t);
BASIC_TYPE_SERIALISE_NAME(uint16_t);
BASIC_TYPE_SERIALISE_NAME(int8_t);
BASIC_TYPE_SERIALISE_NAME(uint8_t);
BASIC_TYPE_SERIALISE_NAME(double);
BASIC_TYPE_SERIALISE_NAME(float);
BASIC_TYPE_SERIALISE_NAME(bool);
BASIC_TYPE_SERIALISE_NAME(wchar_t);
BASIC_TYPE_SERIALISE_NAME(char);
BASIC_TYPE_SERIALISE_NAME(string);
BASIC_TYPE_SERIALISE_NAME(char *);
BASIC_TYPE_SERIALISE_NAME(const char *);
template <>
string DoStringize(void *const &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "0x%p", el);
return tostrBuf;
}
template <>
string DoStringize(const int64_t &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "%" PRId64, el);
return tostrBuf;
}
#if defined(RENDERDOC_PLATFORM_APPLE)
template <>
string DoStringize(const size_t &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "%" PRIu64, (uint64_t)el);
return tostrBuf;
}
#endif
template <>
string DoStringize(const uint64_t &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "%" PRIu64, el);
return tostrBuf;
}
template <>
string DoStringize(const uint32_t &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "%u", el);
return tostrBuf;
}
template <>
string DoStringize(const char &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "'%c'", el);
return tostrBuf;
}
template <>
string DoStringize(const wchar_t &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "'%lc'", el);
return tostrBuf;
}
template <>
string DoStringize(const byte &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "%u", (uint32_t)el);
return tostrBuf;
}
template <>
string DoStringize(const uint16_t &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "%04d", el);
return tostrBuf;
}
template <>
string DoStringize(const int &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "%d", el);
return tostrBuf;
}
template <>
string DoStringize(const short &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "%04d", el);
return tostrBuf;
}
template <>
string DoStringize(const float &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "%0.4f", el);
return tostrBuf;
}
template <>
string DoStringize(const double &el)
{
char tostrBuf[256] = {0};
_snprintf(tostrBuf, 255, "%0.4lf", el);
return tostrBuf;
}
template <>
string DoStringize(const bool &el)
{
if(el)
return "True";
return "False";
}
<file_sep>/streamio.cpp
/******************************************************************************
* The MIT License (MIT)
*
* Copyright (c) 2017 <NAME>
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
******************************************************************************/
#include "streamio.h"
static const uint64_t initialBufferSize = 64 * 1024;
const byte StreamWriter::empty[128] = {};
StreamReader::StreamReader(const void *buffer, size_t bufferSize)
{
m_InputSize = m_BufferSize = bufferSize;
m_BufferHead = m_BufferBase = AllocAlignedBuffer(m_BufferSize);
memcpy(m_BufferHead, buffer, m_BufferSize);
m_File = NULL;
m_Decompressor = NULL;
m_ReadOffset = 0;
}
StreamReader::StreamReader(const std::vector<byte> &buffer)
{
m_InputSize = m_BufferSize = buffer.size();
m_BufferHead = m_BufferBase = AllocAlignedBuffer(m_BufferSize);
memcpy(m_BufferHead, buffer.data(), m_BufferSize);
m_File = NULL;
m_Decompressor = NULL;
m_ReadOffset = 0;
}
StreamReader::StreamReader(FILE *file, size_t fileSize)
{
m_File = file;
m_Decompressor = NULL;
m_ReadOffset = 0;
m_InputSize = fileSize;
m_BufferSize = initialBufferSize;
m_BufferHead = m_BufferBase = AllocAlignedBuffer(m_BufferSize);
ReadFromExternal(0, m_BufferSize);
}
StreamReader::StreamReader(FILE *file)
{
m_File = file;
m_Decompressor = NULL;
m_ReadOffset = 0;
fseek(file, 0, SEEK_END);
m_InputSize = ftell(file);
fseek(file, 0, SEEK_SET);
m_BufferSize = initialBufferSize;
m_BufferHead = m_BufferBase = AllocAlignedBuffer(m_BufferSize);
ReadFromExternal(0, m_BufferSize);
}
StreamReader::StreamReader(Decompressor *decompressor, size_t uncompressedSize)
{
m_File = NULL;
m_Decompressor = decompressor;
m_ReadOffset = 0;
m_InputSize = uncompressedSize;
m_BufferSize = initialBufferSize;
m_BufferHead = m_BufferBase = AllocAlignedBuffer(m_BufferSize);
ReadFromExternal(0, RDCMIN(uncompressedSize, m_BufferSize));
}
StreamReader::~StreamReader()
{
FreeAlignedBuffer(m_BufferBase);
}
void StreamReader::SetOffset(uint64_t offs)
{
if(m_File || m_Decompressor)
{
RDCERR("File and decompress stream readers do not support seeking");
return;
}
m_BufferHead = m_BufferBase + offs;
}
void StreamReader::Reserve(size_t numBytes)
{
RDCASSERT(m_File || m_Decompressor);
// store old buffer and the read data, so we can move it into the new buffer
byte *oldBuffer = m_BufferBase;
// always keep at least a certain window behind what we read.
size_t backwardsWindow = RDCMIN((size_t)64, size_t(m_BufferHead - m_BufferBase));
byte *currentData = m_BufferHead - backwardsWindow;
size_t currentDataSize = m_BufferSize - (m_BufferHead - m_BufferBase) + backwardsWindow;
size_t BufferOffset = m_BufferHead - m_BufferBase;
// if we are reading more than our current buffer size, expand the buffer size
if(numBytes + backwardsWindow > m_BufferSize)
{
// very conservative resizing - don't do "double and add" - to avoid
// a 1GB buffer being read and needing to allocate 2GB. The cost is we
// will reallocate a bit more often
m_BufferSize = numBytes + backwardsWindow;
m_BufferBase = AllocAlignedBuffer(m_BufferSize);
}
// move the unread data into the buffer
memmove(m_BufferBase, currentData, currentDataSize);
if(BufferOffset > backwardsWindow)
{
m_ReadOffset += BufferOffset - backwardsWindow;
m_BufferHead = m_BufferBase + backwardsWindow;
}
else
{
m_BufferHead = m_BufferBase + BufferOffset;
}
// if there's anything left of the file to read in, do so now
ReadFromExternal(currentDataSize, RDCMIN(m_BufferSize - currentDataSize,
size_t(m_InputSize - m_ReadOffset - currentDataSize)));
if(oldBuffer != m_BufferBase)
FreeAlignedBuffer(oldBuffer);
}
void StreamReader::ReadFromExternal(uint64_t bufferOffs, size_t length)
{
if(m_Decompressor)
{
m_Decompressor->Read(m_BufferBase + bufferOffs, length);
}
else
{
RDCASSERT(m_File);
fread(m_BufferBase + bufferOffs, 1, length, m_File);
}
}
StreamWriter::StreamWriter(uint64_t initialBufSize)
{
m_BufferSize = initialBufSize;
m_BufferBase = m_BufferHead = AllocAlignedBuffer(initialBufSize);
m_WriteSize = 0;
m_File = NULL;
m_Compressor = NULL;
}
StreamWriter::StreamWriter(FILE *file)
{
m_BufferSize = 0;
m_BufferBase = m_BufferHead = NULL;
m_WriteSize = 0;
m_File = file;
m_Compressor = NULL;
}
StreamWriter::StreamWriter(Compressor *compressor)
{
m_BufferSize = 0;
m_BufferBase = m_BufferHead = NULL;
m_WriteSize = 0;
m_File = NULL;
m_Compressor = compressor;
}
StreamWriter::~StreamWriter()
{
FreeAlignedBuffer(m_BufferBase);
}
#include "lz4io.h"
#include "zstdio.h"
void streamio_test()
{
{
StreamWriter writer(512);
writer.Write<int>(512);
writer.Write<char>('c');
writer.Write<bool>(true);
writer.Write<float>(5.0f);
writer.AlignTo<64>();
writer.Write<double>(64.0);
unsigned char buffer[20] = {};
memset(buffer, 0xbb, sizeof(buffer));
writer.Write(buffer, sizeof(buffer));
RDCASSERT(writer.GetOffset() == 64 + 8 + 20);
writer.Finish();
StreamReader reader(writer.GetData(), (size_t)writer.GetOffset());
writer.Rewind();
RDCASSERT(writer.GetOffset() == 0);
int i = 0;
char c = '\0';
bool b = false;
float f = 0.0f;
double d = 0.0;
memset(buffer, 0, sizeof(buffer));
reader.Read(i);
reader.Read(c);
reader.Read(b);
reader.Read(f);
reader.AlignTo<64>();
reader.Read(d);
reader.Read(buffer, sizeof(buffer));
RDCASSERT(i == 512);
RDCASSERT(c == 'c');
RDCASSERT(b == true);
RDCASSERT(f == 5.0f);
RDCASSERT(d == 64.0);
for(size_t i=0; i < sizeof(buffer); i++)
RDCASSERT(buffer[i] == 0xbb);
}
{
// the actual destination buffer
StreamWriter compressedWriter(512);
// compressor into the buffer
LZ4Compressor lz4Compressor(&compressedWriter);
// writer into the compressor
StreamWriter lz4Writer(&lz4Compressor);
struct Dummy
{
int i[100];
float f;
double d;
char str[200];
bool b;
} dummy = {};
for(int i=0; i < 100; i++)
dummy.i[i] = i * 100;
strcpy(dummy.str, "Foo bar writer into the compressor// compressor into the bufferLZ4Compressor lz4Compressor");
dummy.b = true;
dummy.d = 3.1415926535;
dummy.f = 420.69f;
for(int i=0; i < 1000; i++)
lz4Writer.Write(dummy);
lz4Writer.Write<int>(0xb33b1337);
lz4Writer.Write<int>(0xb33b1337);
lz4Writer.Write<int>(0xb33b1337);
char *hugebuf = new char[1024*1024*128];
for(int i=0; i < 1024*1024; i++)
memset(hugebuf + i*128, (rand()>>8)&0xff, 128);
lz4Writer.Write(hugebuf, 1024*1024*128);
lz4Writer.Finish();
uint64_t uncomp = lz4Writer.GetOffset();
uint64_t comp = compressedWriter.GetOffset();
// reader of compressed data
StreamReader compressedReader(compressedWriter.GetData(), (size_t)compressedWriter.GetOffset());
// decompressor
LZ4Decompressor decompressor(&compressedReader);
StreamReader lz4Reader(&decompressor, uncomp);
for(int i=0; i < 1000; i++)
{
Dummy read = {};
lz4Reader.Read(read);
RDCASSERT(memcmp(&read, &dummy, sizeof(read)) == 0);
}
int marker = 0;
lz4Reader.Read(marker); RDCASSERT(marker == 0xb33b1337);
lz4Reader.Read(marker); RDCASSERT(marker == 0xb33b1337);
lz4Reader.Read(marker); RDCASSERT(marker == 0xb33b1337);
char *hugebuf2 = new char[1024*1024*128];
lz4Reader.Read(hugebuf2, 1024*1024*128);
RDCASSERT(memcmp(hugebuf, hugebuf2, 1024*1024*128) == 0);
StreamWriter recompressedWriter(512);
ZSTDCompressor zstdCompressor(&recompressedWriter);
StreamReader compressedReader2(compressedWriter.GetData(), (size_t)compressedWriter.GetOffset());
LZ4Decompressor decompressor2(&compressedReader2);
decompressor2.Recompress(&zstdCompressor);
uint64_t comp2 = recompressedWriter.GetOffset();
// reader of compressed data
StreamReader recompressedReader(recompressedWriter.GetData(), (size_t)recompressedWriter.GetOffset());
// decompressor
ZSTDDecompressor zstdDecompressor(&recompressedReader);
StreamReader zstdReader(&zstdDecompressor, uncomp);
for(int i=0; i < 1000; i++)
{
Dummy read = {};
zstdReader.Read(read);
RDCASSERT(memcmp(&read, &dummy, sizeof(read)) == 0);
}
zstdReader.Read(marker); RDCASSERT(marker == 0xb33b1337);
zstdReader.Read(marker); RDCASSERT(marker == 0xb33b1337);
zstdReader.Read(marker); RDCASSERT(marker == 0xb33b1337);
char *hugebuf3 = new char[1024*1024*128];
zstdReader.Read(hugebuf3, 1024*1024*128);
RDCASSERT(memcmp(hugebuf, hugebuf3, 1024*1024*128) == 0);
delete[] hugebuf;
delete[] hugebuf2;
delete[] hugebuf3;
}
} | c749a49fb2b5b5e078b41f56349df6c446e7270e | [
"Makefile",
"C++"
] | 18 | C++ | baldurk/rdoc_serialise_test | 4da5e3a03df3f956d3a3dedc6a3e94593120c66a | 361073feed1d912e783597ff73f31fbc08377a00 | |
refs/heads/master | <file_sep>from bot import db
class Enzyme(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(64), index=True, nullable=False)
catalogue_number = db.Column(db.String(64), index=True, unique=True, nullable=False)
quantity = db.Column(db.Integer, nullable=False)
def __repr__(self):
return f'<Enzyme {self.name}>'
<file_sep>from flask_wtf import FlaskForm
from wtforms import StringField, SubmitField
from wtforms.validators import DataRequired
class AddForm(FlaskForm):
name = StringField("Enzyme name", validators=[DataRequired()])
catalogue_number = StringField("Catalogue number", validators=[DataRequired()])
quantity = StringField("Quantity", validators=[DataRequired()])
submit = SubmitField("Submit")<file_sep>from flask import request, jsonify, render_template
from bot import app, db
from bot.functions import send_message
from bot.models import Enzyme
from bot.forms import AddForm
@app.route("/", methods=["GET", "POST"])
def index():
if request.method == "POST":
r = request.get_json()
chat_id = r["message"]["chat"]["id"]
message = r["message"]["text"]
input_data = message.split()
if len(input_data) == 2:
cat_id, amount = input_data
data = db.session.query(Enzyme).filter(Enzyme.catalogue_number==cat_id).first()
if data:
data.quantity -= int(amount)
db.session.commit()
send_message(chat_id, text=f"Current amount is {data.quantity}")
else:
send_message(chat_id, "Wrong cat_id")
else:
send_message(chat_id, "Wrong data format")
return jsonify(r)
return render_template("index.html")
@app.route("/info/")
def db_info():
all_data = db.session.query(Enzyme).all()
return render_template("db_info.html", all_data=all_data)
@app.route("/addition/", methods=["GET", "POST"])
def addition():
form = AddForm()
if request.method == "POST" and form.validate_on_submit():
name = form.name.data
catalogue_number = form.catalogue_number.data
quantity = form.quantity.data
enzyme = Enzyme(name=name, catalogue_number=catalogue_number, quantity=quantity)
db.session.add(enzyme)
db.session.commit()
return f"Enzyme {name} was successfully added"
else:
return render_template("addition.html", form=form)
<file_sep>import requests
from config import Config
URL = f"https://api.telegram.org/bot{Config.TOKEN}/"
def send_message(chat_id, text="blabla"):
url = URL + "sendMessage"
answer = {"chat_id": chat_id, "text": text}
r = requests.post(url, json=answer)
return r.json()
| 7aa82b598f207de1d138a28e477569ddb2cde944 | [
"Python"
] | 4 | Python | syntarch/TelegramBot_Flask | 3c1bd29487deff5746faa7f6979eba2a9d3afdd7 | 6334719e9a428c5796e402f85504ed55b638ec66 | |
refs/heads/master | <repo_name>ismael101/Card-Game-Java<file_sep>/src/com/ismael/Card.java
package com.ismael;
public class Card {
private String suits;
private int number;
//this is the card class that contains all the attributes of a card
Card(int num,String suit){
this.number = num;
this.suits = suit;
}
@Override
public String toString() {
String fullCard;
if (number!=11){
fullCard = number +" of "+ suits; }
else{
fullCard = "Ace of "+ suits;
}
return fullCard;}
//Qaalib helped me with this part of my code
//This retruns the number of the card
public int getNumber() {
return number;
}
//this returns the suit of the card if needed
public String getSuits() {
return suits;
}
//this allows me to check if to cards have the same suits
public boolean checksuits(Card cardsuits){
Boolean flag;
if(cardsuits.suits == this.suits){ flag = true; }
else{flag = false;}
return flag;}
}
<file_sep>/src/com/ismael/Computer.java
package com.ismael;
import java.util.ArrayList;
import java.util.Random;
public class Computer {
//i created a instance of the deck
Deck deck = new Deck();
ArrayList<Card> cpucards = deck.ComputerCard();
// this returns the card of a given position in the Arraylist
public Card getCard(int position) {
return cpucards.get(position);
}
//this was used in debugging tomsee all the cards of the computers deck and they were effected
public void showCards(){
int counter = 0;
for(Card i: cpucards){
System.out.println(counter+" # "+i);
counter = counter+1;}}
//this deletes a card after the after it is used in a round
public void deleteCard(int position){
cpucards.remove(position);
System.out.println("CPU CARD REMOVED");
}
}
| 52a87ba6481363267065801bd1913b5d12561955 | [
"Java"
] | 2 | Java | ismael101/Card-Game-Java | 1aeafcb63ede12a54992407f68d71aeeb03b1be9 | 4eb2f7f0b94bc3607669541b87b4c086b007bf28 | |
refs/heads/master | <repo_name>zhy1/ngAmap<file_sep>/ng-amap.js
"use strict";
var aMapDirective = [ "$parse", "ngAMapHelper", "$rootElement", function($parse, ngAMapHelper, $rootElement) {
return {
restrict: "E",
compile: function(element, attrs, transclude, ctrl) {
window.AMap || ngAMapHelper.injectCode($rootElement, void 0);
return {
pre: function(scope, element, attrs, ctrl) {
function SyncMapGeneratorContext() {
element.html("").append('<div id="' + mapId + '" style="' + elementStyles + '"></div>');
}
function AsyncMapImplements(firstLoadRunner) {
function eventHandler(event) {
if (event) {
var longitude = event.lnglat.getLng(), latitude = event.lnglat.getLat();
map.marker.setMap(map.map);
map.marker.setPosition([ longitude, latitude ]);
map.map.setCenter([ longitude, latitude ]);
if (scope) {
scope.amap = scope.amap || {};
scope.amap.marker = map.marker;
scope.amap.longitude = longitude;
scope.amap.latitude = latitude;
scope.amap.location = [ longitude, latitude ];
}
map.Geocoder.getAddress([ longitude, latitude ], function(status, result) {
if ("complete" === status && "OK" === result.info) {
var addressComponent = result.regeocode.addressComponent, formattedAddress = result.regeocode.formattedAddress, map = ngAMapHelper.getMap();
if (map[scope.$id]) {
var current = map[scope.$id];
current.map.setZoom(current.map.getZoom() + 2);
scope.$apply(function() {
if (scope) {
scope.amap = scope.amap || {};
scope.amap.zoom = current.map.getZoom();
scope.amap.addressComponent = addressComponent;
scope.amap.formattedAddress = formattedAddress;
scope.amap.address = formattedAddress;
scope.amap.district = addressComponent.district;
scope.amap.adcode = addressComponent.adcode;
scope.amap.city = addressComponent.province;
}
});
}
scope.amap && "function" == typeof scope.amap.whenMarkerMove && scope.amap.whenMarkerMove(status, result);
}
});
}
}
if (window.AMap && window.AMap.Map) {
firstLoadRunner && firstLoadRunner();
var mapConfig = {
resizeEnable: !0,
zoom: 5,
center: [ ngAMapHelper.getLongitude() || 121, ngAMapHelper.getLatitude() || 21 ]
}, map = {};
map.map = new window.AMap.Map(mapId, mapConfig);
map.placeSearch = new window.AMap.PlaceSearch({
lang: "zh_cn",
pageSize: 50,
pageIndex: 0,
map: map.map,
panel: ngAMapHelper.getOptions().CONSTANT.PANEL,
extensions: "all"
});
map.marker = new window.AMap.Marker({
position: map.map.getCenter(),
draggable: !0,
cursor: "move",
raiseOnDrag: !0,
map: map.map,
visible: !0
});
window.AMap.plugin([ ngAMapHelper.getOptions().CONSTANT.TOOL_BAR, ngAMapHelper.getOptions().CONSTANT.SCALE ], function() {
map.map.addControl(new window.AMap.ToolBar());
map.map.addControl(new window.AMap.Scale());
});
map.Geocoder = new window.AMap.Geocoder({
radius: 1e3,
extensions: "all"
});
map.marker.on("mouseup", eventHandler);
map.map.on("click", eventHandler);
ngAMapHelper.addInstance({
id: scope.$id,
map: map
});
return map;
}
}
scope.$on("$destroy", function() {
ngAMapHelper.setDestroyList(scope, "DESTROY SCOPE OF CONTROLLER BEFORE EXIT");
});
var elementStyles = attrs.style, mapId = "map" + scope.$id, mapGenerator = function() {
if (ngAMapHelper.getDestroyList(scope)) {
ngAMapHelper.setDestroyList(scope, void 0);
ngAMapHelper.setDestroyList(scope, void 0);
ngAMapHelper.removeById(scope.$id);
clearInterval(waitPollingWhile);
return "RunningOver";
}
if (window.AMap) {
SyncMapGeneratorContext();
AsyncMapImplements();
clearInterval(waitPollingWhile);
return "RunningOk";
}
return "RunningNoYet";
}, waitPollingWhile = setInterval(mapGenerator, 500);
},
post: function(scope, element, attrs, ctrl) {}
};
},
controller: function() {}
};
} ], aMapProvider = function() {
var $$options = {
protocol: "http:",
baseUrl: "//webapi.amap.com/maps",
version: 1.3,
key: "<KEY>",
plugin: "AMap.Autocomplete,AMap.PlaceSearch,AMap.Geocoder",
completed: "https://webapi.amap.com/maps?v=1.3&key=<KEY>&plugin=AMap.Autocomplete,AMap.PlaceSearch,AMap.Geocoder",
longitude: 121,
latitude: 21,
CONSTANT: {
PANEL: "panel",
SELECT: "select",
COMPLETE: "complete",
OK: "OK",
CLICK: "click",
MOUSEDOWN: "mouseup",
SCALE: "AMap.Scale",
TOOL_BAR: "AMap.ToolBar",
PLACE_SEARCH: "AMap.PlaceSearch"
}
}, $$exitsMapArray = {}, $$DestroyScopeArray = {};
this.setOptions = function(options) {
angular.extend($$options, options);
};
this.$get = [ "$rootElement", function($rootElement) {
return {
addInstance: function(newMapInstance) {
if ($$exitsMapArray[newMapInstance.id]) throw new Error("scope Error");
$$exitsMapArray[newMapInstance.id] = newMapInstance.map;
},
removeById: function(id) {
$$exitsMapArray[id] = void 0;
},
setOptions: function(options) {
$$options = angular.extend($$options, options);
},
getMap: function() {
return $$exitsMapArray;
},
getOptions: function() {
return $$options;
},
injectCode: function(element, callback) {
function getScript(url, callback) {
function scriptOnload(node, callback) {
node.addEventListener("load", callback, !1);
node.addEventListener("error", function() {
console.error("404 error:", node.src);
callback("error");
}, !1);
}
var node = document.createElement("script");
!function(callback) {
scriptOnload(node, function(SystemCallback) {
callback && "function" == typeof callback && callback();
});
}(callback);
node.async = !0;
node.src = url;
return head.insertBefore(node, head.firstChild);
}
function originPrepend() {
var link = '<link rel="stylesheet" href="http://cache.amap.com/lbs/static/main1119.css" />', scripts = '<script src="http://cache.amap.com/lbs/static/es5.min.js"></script>';
element.prepend(scripts + link);
}
var head = document.getElementsByTagName("head")[0], aMapUrl = $$options.protocol + $$options.baseUrl + "?v=" + $$options.version + "&key=" + $$options.key + "&plugin=" + $$options.plugin;
getScript(aMapUrl, function() {
originPrepend();
callback && callback();
});
},
setDestroyList: function(scope, data) {
$$DestroyScopeArray[scope.$id] = data;
},
getDestroyList: function(scope) {
return $$DestroyScopeArray[scope.$id];
},
getLongitude: function() {
return $$options.longitude;
},
getLatitude: function() {
return $$options.latitude;
}
};
} ];
}, aMapAutoComplete = [ "ngAMapHelper", function(ngAMapHelper) {
return {
restrict: "A",
compile: function(element, attrs, transclude, ctrl) {
return {
post: function(scope, element, attrs, ctrl) {
function SyncMapGeneratorContext() {
element[0].id = inputId;
}
function WaitFor() {
waitForSecond++;
if (MapArray[scope.$id]) {
SyncMapGeneratorContext();
var map = MapArray[scope.$id], auto = window.AMap.plugin("AMap.Autocomplete", function() {
var autoOptions = {
city: "",
input: inputId
};
map.autocomplete = new AMap.Autocomplete(autoOptions);
});
window.AMap.event.addListener(map.autocomplete, "select", function(event) {
var position = event.poi, address = event.poi.address, district = event.poi.district, adcode = event.poi.adcode;
if (scope) {
scope.amap = scope.amap || {};
scope.amap.zoom = map.map.getZoom();
scope.amap.map = map.map;
scope.amap.address = address;
scope.amap.district = district;
scope.amap.adcode = adcode;
}
if ("" === event.poi.location) return "city didn't have location";
var location = event.poi.location, I = event.poi.location.I, L = event.poi.location.L;
map.map.setCenter([ I, L ]);
map.marker.setPosition([ I, L ]);
map.map.setZoom(17);
scope.amap.I = I;
scope.amap.L = L;
scope.amap.location = location;
scope.amap.position = position;
scope.amap.whenSelect && "function" == typeof scope.amap.whenSelect && scope.amap.whenSelect();
});
MapArray[scope.$id].auto = auto;
clearInterval(waitPollingWhile);
}
if (waitForSecond > 10) {
clearInterval(waitPollingWhile);
return "running failed at 10 attempts";
}
}
var MapArray = ngAMapHelper.getMap(), inputId = "ipt" + scope.$id, waitForSecond = 0, waitPollingWhile = setInterval(WaitFor, 500);
}
};
},
controller: function() {}
};
} ];
angular.module("ngAMap", []).directive("aMap", aMapDirective).provider("ngAMapHelper", aMapProvider).directive("aMapAutoComplete", aMapAutoComplete);<file_sep>/README.md
example
local running.
git tag v1.0.0
git push origin --tags
bower register ngAmap https://github.com/zhy1/ngAmap
git push origin --delete tag v1.0.0
git push origin --delete tag origin | 2b08e9fba01f9ab231065adff2dc81ee7618dbe7 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | zhy1/ngAmap | a885497964b5c90c2bcf899b2ef25c4278471816 | 54bca781ad5a8263faae8600d8c8e2a22bcb5e4a | |
refs/heads/master | <file_sep>#!/usr/local/bin/python3
import cgi
import subprocess
print("content-type: text/html")
print()
my_input = cgi.FieldStorage()
command = my_input.getvalue("cmd")
output = subprocess.getoutput("sudo " +command)
print(output)
<file_sep># IIEC-Python
Task2 : Python program for the Voice Assistant.
It can execute Basic Linux commands.
<file_sep>import pyttsx3
import speech_recognition as sr
import webbrowser as wb
engine = pyttsx3.init()
rate = engine.getProperty('rate')
#print (rate)
engine.setProperty('rate', 200)
#volume = engine.getProperty('volume')
#print (volume)
#engine.setProperty('volume',1.0)
voices = engine.getProperty('voices')
engine.setProperty('voice', voices[1].id)
pyttsx3.speak("Hello Kajal")
print()
print("Welcome")
engine.runAndWait()
pyttsx3.speak("I am your voice assistant")
pyttsx3.speak("How can I help you...?")
while True:
print()
print("\t \t Tell me how can i help you... i am listening: ", end='')
r = sr.Recognizer()
with sr.Microphone() as source:
pyttsx3.speak('Tell me...')
audio = r.listen(source)
pyttsx3.speak('got it... please wait..!!')
ch = r.recognize_google(audio)
if("hello" in ch) or ("hi" in ch) and ("hey" in ch):
pyttsx3.speak("Hii Kajal , how are you ...?")
elif("fine" in ch) or ("what" in ch) and ("about" in ch):
pyttsx3.speak("i am good")
elif("tell" in ch) or ("about" in ch) and ("yourself" in ch):
pyttsx3.speak("i am your voice assistant, i can control your linux system")
elif("date" in ch):
wb.open("http://192.168.43.15/cgi-bin/kajal.py?x=date")
pyttsx3.speak("showing date")
elif("calendar" in ch):
wb.open("http://192.168.43.15/cgi-bin/kajal.py?x=cal")
pyttsx3.speak("showing calendar")
elif("IP" in ch )or ("ip address" in ch) or "address" in ch):
wb.open("http://192.168.43.15/cgi-bin/kajal.py?x=ifconfig")
pyttsx3.speak("showing IP address")
elif("amount" in ch) or ("disc" in ch) and ("space" in ch):
wb.open("http://192.168.43.15/cgi-bin/kajal.py?x=df%20-h")
pyttsx3.speak("showing available disc space")
elif("pip" in ch) or ("module" in ch) and ("list" in ch):
wb.open("http://192.168.43.15/cgi-bin/kajal.py?x=pip%20module%20list")
pyttsx3.speak("Here is the list of avaulable modules")
elif("user" in ch) or ("Kajal" in ch):
wb.open("http://192.168.43.15/cgi-bin/kajal.py?x=useradd%20Kajal")
pyttsx3.speak("Kajal user has been created")
elif("restrict" in ch) or ("K" in ch):
wb.open("http://192.168.43.15/cgi-bin/kajal.py?x=useradd%20-s%20/usr/bin/cal%20K")
pyttsx3.speak("K user has been created and restricted")
elif ("exit" in ch) or ("quit" in ch) or ("bye" in ch) or ("Stop" in ch):
pyttsx3.speak("Ok, see you soon, have a nice day..!!!")
break
else:
print("not understand, Say it again!!!") | 09657957ec5e6532c5778be4fa1c0040947526de | [
"Markdown",
"Python"
] | 3 | Python | Kajal5414/IIEC-Python | ce5416c3fd617038f20977683d109897971a01a2 | a3ee7b6bf0f80a47e5aa6a85dffc2cb69ee3f6bc | |
refs/heads/master | <file_sep>//Meteor.publish("users", function () {
// return Meteor.users.find({_id: this.userId()});
//});
Meteor.users.deny({
update: function() {
return true;
}
});<file_sep>ViewModel.addBinding("files", {
on: "change",
get(event, $elem) {
return $elem.prop("files");
}
});
<file_sep>// Class for binding nexuses
Nexus = class Nexus extends Base {
constructor(view, selector, binding, context = {}) {
// Ensure type of arguments
check(selector, Match.OneOf(String, Match.Where(_.isElement)));
check(binding, Match.OneOf(String, Binding));
check(context, Object);
// Possibly get binding
if (_.isString(binding))
binding = Binding.get(binding);
let is_detached = binding.option("detached"),
key;
// Call constructor of Base
super(view, binding.name);
// Possibly create nexuses list
if (!(this.view[ViewModel.nexusesKey] instanceof List))
this.view[ViewModel.nexusesKey] = new List;
// Static properties on nexus instance
defineProperties(this, {
// Element selector
selector: { value: selector },
// Calling context of bind
context: { value: context },
// Whether to run the set function when updating
setPrevented: { value: null, writable: true }
});
// Possibly add key to nexus
if (!is_detached && _.isArray(context.args) && _.isString(context.args[0])) {
key = context.args[0];
defineProperties(context, {
// Viewmodel key
key: { value: key }
});
}
// Static properties on context object
defineProperties(context, {
// Reference to view
view: { value: view },
// Reference to template instance
templateInstance: { value: templateInstance(view) },
// Method bound to instance
preventSet: { value: this.preventSet.bind(this) }
});
// Possibly ensure existence of a viewmodel
if (!is_detached && !(context.viewmodel instanceof ViewModel)) {
let vm = ViewModel.ensureViewmodel(view, key);
defineProperties(context, {
// Reference to viewmodel
viewmodel: { value: vm }
});
}
// Add binding resolved with context
defineProperties(this, {
// Binding definition
binding: { value: binding.definition(this.context) }
});
// Unbind element on view refreshed
this.onRefreshed(this.unbind);
// Unbind element on view destroyed
this.onDestroyed(this.unbind);
// Unbind element on computation invalidation
this.onInvalidate(() => this.unbind(true));
// Bind element on view ready
this.onReady(this.bind);
}
// Get viewmodel property
getProp() {
let vm = this.context.viewmodel,
has_prop = vm && !_.isUndefined(this.context.key) && _.isFunction(vm[this.context.key]);
return has_prop ? vm[this.context.key] : null;
}
// Whether the element is present in the document body
inBody() {
return document.body.contains(this.elem);
}
// Bind element
bind() {
let $elem = $(this.elem || this.selector);
if (!this.elem) {
// Save element on nexus
defineProperties(this, {
elem: { value: $elem[0] }
});
}
// Don't bind if element is no longer present
if (!this.inBody())
return false;
let binding = this.binding,
prop = this.getProp();
if (binding.init) {
// Ensure type of definition property
check(binding.init, Function);
let init_value = prop && prop();
// Run init function immediately
binding.init.call(this.context, $elem, init_value);
}
if (binding.on) {
// Ensure type of definition property
check(binding.on, String);
let listener = event => {
if (binding.get) {
// Ensure type of definition property
check(binding.get, Function);
let result = binding.get.call(this.context, event, $elem, prop);
// Call property if get returned a value other than undefined
if (prop && !_.isUndefined(result)) {
// Don't trigger set function from updating property
if (this.setPrevented !== false)
this.preventSet();
prop.call(this.context.viewmodel, result);
}
}
else if (prop) {
// Call property if get was omitted in the binding definition
prop.call(this.context.viewmodel, event, this.context.args, this.context.hash);
}
// Mark that we are exiting the update cycle
Tracker.afterFlush(this.preventSet.bind(this, null));
};
// Save listener for unbind
defineProperties(this, {
listener: { value: listener }
});
// Register event listener
$elem.on(binding.on, listener);
}
if (binding.set) {
// Ensure type of definition property
check(binding.set, Function);
// Wrap set function and add it to list of autoruns
this.autorun(comp => {
if (comp.firstRun) {
// Save computation for unbind
defineProperties(this, {
comp: { value: comp }
});
}
let new_value = prop && prop();
if (!this.setPrevented)
binding.set.call(this.context, $elem, new_value);
});
}
// Add to view list
this.view[ViewModel.nexusesKey].add(this);
// Add to global list
Nexus.add(this);
return true;
}
// Unbind element
unbind(do_unbind = this.view.isDestroyed || !this.inBody()) {
// Unbind elements that are no longer part of the DOM
if (do_unbind) {
let binding = this.binding,
prop = this.getProp();
// Possibly unregister event listener
if (this.listener)
$(this.elem).off(binding.on, this.listener);
// Possibly stop set autorun
if (this.comp)
this.comp.stop();
// Possibly run dispose function
if (binding.dispose) {
// Ensure type of definition property
check(binding.dispose, Function);
binding.dispose.call(this.context, prop);
}
// Remove from global list
Nexus.remove(this);
// Remove from view list
this.view[ViewModel.nexusesKey].remove(this);
}
return do_unbind;
}
// Change prevented state of nexus
preventSet(state = true) {
// Ensure type of argument
check(state, Match.OneOf(Boolean, null));
this.setPrevented = state;
}
};
// Decorate Nexus class with list methods operating on an internal list
List.decorate(Nexus);
<file_sep>ViewModel.addBinding("class", {
set($elem) {
let classes = this.hash;
// Keyword arguments must be present
if (_.isObject(classes)) {
// Possibly only use indicated keys
if (this.args.length)
classes = _.pick(classes, this.args);
_.each(classes, (value, class_name) => $elem.toggleClass(class_name, value || false));
}
}
}, {
// This binding doesn't need a viewmodel
detached: true
});
<file_sep>ViewModel.addBinding("key", {
on: "keyup",
get(event, $elem, prop) {
let use_hash = _.isNumber(_.isObject(this.hash) && this.hash.keyCode),
key_code = use_hash ? this.hash.keyCode : parseInt(this.args[1], 10);
if (event.which === key_code)
prop(event, this.args, this.hash);
}
});
<file_sep>Meteor.startup(function() {
$('body').attr('data-uk-observe', '1');
});<file_sep>ViewModel.addBinding("disabled", {
set($elem, new_value) {
$elem.prop("disabled", new_value);
}
});
<file_sep>// Class for reactive lists
List = class List extends Array {
constructor(...args) {
super(...args);
// Add dependency to list
defineProperties(this, {
dep: { value: new Tracker.Dependency }
});
}
// Reactively add an item
add(...items) {
this.push(...items);
this.dep.changed();
}
// Reactively remove an item
remove(...items) {
let result = false;
_.each(items, item => {
let index = this.indexOf(item),
is_found = !!~index;
if (is_found) {
this.splice(index, 1);
this.dep.changed();
result = true;
}
});
return result;
}
// Reactively get an array of matching items
find(test) {
this.dep.depend();
// Possibly remove items failing test
if (test) {
return _.filter(this, (...args) => {
if (_.isFunction(test))
return test(...args);
else if (_.isObject(args[0]) && _.isFunction(args[0].test))
return args[0].test(test);
});
}
return this.slice();
}
// Reactively get the first current item at index
findOne(test, index) {
if (_.isNumber(test))
index = test, test = null;
return this.find(test).slice(index || 0)[0] || null;
}
// Decorate an object with list methods operating on an internal list
static decorate(obj, reference_key) {
// Ensure type of arguments
check(obj, Match.OneOf(Object, Function));
check(reference_key, Match.Optional(String));
// Internal list
let list = new List;
// Property descriptors
let descriptor = {
add: { value: list.add.bind(list) },
remove: { value: list.remove.bind(list) },
find: { value: list.find.bind(list) },
findOne: { value: list.findOne.bind(list) }
};
// Possibly add a reference to the internal list
if (reference_key)
descriptor[reference_key] = { value: list };
// Add bound methods
defineProperties(obj, descriptor);
}
};
<file_sep>Template.ContactsList.viewmodel({
myName: function () {
if (Meteor.userId()) {
return Meteor.users.find({_id: Meteor.userId()}).fetch()._id;
}
},
addToTask: function () {
console.log("addToTask");
Session.set('addToTask', 'true');
},
showAddTask: function () {
return Session.get('addToTask');
},
onDestroyed: function () {
console.log("ondestroy");
},
onCreated: function () {
console.log("oncreated");
},
onRendered: function () {
var str = " <NAME> ";
var nameArr = s.words(str);
var nAl = nameArr.length;
var nextTLastW = s(nameArr[nAl -2]).trim().capitalize().value();
var lastWord = s(nameArr[nAl -1]).trim().capitalize().value();
console.log(nAl);
console.log(nextTLastW.charAt(0) + lastWord.charAt(0));
$('.popupthis').popup({
popup: '#popupthis',
on : 'click',
onVisible: function () {
console.log("onVisible");
},
exclusive: 'true'
});
}
});<file_sep>ViewModel.addBinding("value", function () {
let use_hash = _.isObject(this.hash),
throttle = use_hash && this.hash.throttle || parseInt(this.args[1], 10),
leading = use_hash && _.isBoolean(this.hash.leading) ? this.hash.leading : String(this.args[2]) === "true",
get = function (event, $elem, prop) {
this.preventSet();
prop($elem.val());
};
if (throttle)
get = _.throttle(get, throttle, { leading: leading });
return {
set($elem, new_value) {
$elem.val(new_value);
},
on: "cut paste keyup input change",
get: get
};
});
<file_sep>Template.Index.viewmodel({
//showCases: 'hello',
aCases: function () {
//$('.ui.modal').modal('show');
Session.set("cPanelContent", "CasesList")
},
aTasks: function () {
Session.set("cPanelContent", "TasksList")
},
aNotes: function () {
Session.set("cPanelContent", "NotesList")
},
aMsgs: function () {
Session.set("cPanelContent", "MsgsList")
},
aContacts: function () {
Session.set("cPanelContent", "ContactsList")
},
});
Template.CPanel.viewmodel({
aCases: function () {
var showCases = Session.get("cPanelContent");
if (showCases == 'CasesList'){
return 'CasesList'
}
return ''
},
aTasks: function () {
var aTasks = Session.get("cPanelContent");
if ( aTasks == 'TasksList'){
return 'TasklsList'
}
return ''
},
aNotes: function () {
var aNotes = Session.get("cPanelContent");
if ( aNotes == 'NotesList'){
return 'NotesList'
}
return ''
},
aMsgs: function () {
var aNotes = Session.get("cPanelContent");
if ( aNotes == 'MsgsList'){
return 'MsgsList'
}
return ''
},
aContacts: function () {
var aContacts = Session.get("cPanelContent");
if ( aContacts == 'ContactsList'){
return 'ContactsList'
}
return ''
},
});
<file_sep>ViewModel.addBinding("checked", {
set($elem, new_value) {
$elem.prop("checked", new_value);
},
on: "change",
get(event, $elem) {
return $elem.prop("checked");
}
});
<file_sep>ViewModel.addBinding("enterKey", {
on: "keyup",
get(event, $elem, prop) {
if (event.which === 13)
prop(event, this.args, this.hash);
}
});
<file_sep>AccountsTemplates.configureRoute('resetPwd', {
name: 'resetPwd',
path: '/reset-password',
template: 'signInAtForm',
layoutTemplate: 'defaultLayout',
layoutRegions: {
top: 'defaultHeader',
//footer: 'myFooter'
},
contentRegion: 'main'
});
AccountsTemplates.configureRoute('signIn', {
name: 'signin',
path: '/login',
template: 'signInAtForm',
layoutTemplate: 'defaultLayout',
layoutRegions: {
top: 'defaultHeader',
//footer: 'myFooter'
},
contentRegion: 'main'
});<file_sep>ViewModel.addBinding("radio", {
set($elem, new_value) {
if ($elem.val() === new_value)
$elem.prop("checked", true);
},
on: "change",
get(event, $elem) {
return $elem.val();
}
});
<file_sep>FlowRouter.route('/', {
triggersEnter: [AccountsTemplates.ensureSignedIn],
subscriptions: function() {
this.register('users', Meteor.subscribe('users'));
},
action: function() {
BlazeLayout.render("defaultLayout", {top: "defaultHeader", main: "Index"});
}
});
FlowRouter.route('/logout', {
action: function() {
Meteor.logout();
FlowRouter.go('/')
}
});
<file_sep>ViewModel.addBinding("focused", {
set($elem, new_value) {
if (new_value)
$elem.focus();
else
$elem.blur();
},
on: "focus blur",
get(event) {
return event.type === "focus";
}
});
<file_sep>/*
Private package utility functions
*/
// Use ES5 property definitions when available
defineProperties = function (obj, props) {
if (_.isFunction(Object.defineProperties))
Object.defineProperties(obj, props);
else
_.each(props, (prop, key) => obj[key] = prop.value);
};
// Get closest template instance for view
templateInstance = function (view) {
do if (view.template && view.name !== "(contentBlock)" && view.name !== "Template.__dynamic" && view.name !== "Template.__dynamicWithDataContext")
return view.templateInstance();
while (view = view.parentView);
return null;
};
// Get the current path, taking FlowRouter into account
// https://github.com/kadirahq/flow-router/issues/293
getPath = function () {
if (typeof FlowRouter !== "undefined")
return FlowRouter.current().path;
return location.pathname + location.search;
};
| 19dddd7336793bf123c9e92fe8461d2cb97c8961 | [
"JavaScript"
] | 18 | JavaScript | ahmadthd/caseManagementApp | a33730e321dbb2abee726ab43603dec93a8db3c7 | 214a1776ab73951e07581fc983b203d990e98d89 | |
refs/heads/master | <repo_name>yanhsiah/TheStream<file_sep>/Podfile
# Uncomment this line to define a global platform for your project
# platform :ios, '8.0'
# Uncomment this line if you're using Swift
# use_frameworks!
target 'TheStream' do
pod 'Realm'
pod 'AFNetworking', '~> 3.0'
pod 'SVPullToRefresh'
end
target 'TheStreamTests' do
pod 'Realm/Headers'
end
target 'TheStreamUITests' do
end
<file_sep>/README.md
# TheStream
Dependencies
---
* Web Service: https://github.com/AFNetworking/AFNetworking
* Data Model: https://realm.io
* Stream interaction: https://github.com/samvermette/SVPullToRefresh
| d6a9dd87e9c76f09d17e0250690c7f38b9d962fb | [
"Markdown",
"Ruby"
] | 2 | Ruby | yanhsiah/TheStream | f3be9fc4c47ed7b8f920999fcbd5e37ba275bfe4 | 54d4ce1f6aa97557830045cd7211409ef8bdd359 | |
refs/heads/master | <file_sep>#!/bin/bash
#This script allows to create a user <user> and access a linux instance without the use of a keypair. Created at launch
user=patrick
password=<PASSWORD>
echo $(curl -s http://169.254.169.254/latest/meta-data/public-hostname) | xargs sudo hostname
useradd -m $user
echo -e "$password\n$password" | passwd $user
usermod -aG sudo $user
usermod -aG wheel $user
#change this to yes --> /etc/ssh/sshd_config line PasswordAuthentication no
sed -i 's/PasswordAuthentication no/PasswordAuthentication yes/g' /etc/ssh/sshd_config
echo -e "/root/.ssh/id_rsa\n$password\n$password" | ssh-keygen -t rsa
cat /root/.ssh/id_rsa.pub >> /root/.ssh/authorized_keys
chmod 600 /root/.ssh/authorized_keys
/etc/init.d/ssh restart #debian systems
#/sbin/service sshd restart #rhel systems
wget -O /tmp/ssmagent.deb https://s3.amazonaws.com/ec2-downloads-windows/SSMAgent/latest/debian_amd64/amazon-ssm-agent.deb
dpkg -i /tmp/ssmagent.deb
<file_sep>This folder contains information on Ansible
<file_sep>
# [How to Inspect Instances for vulnerabilities]()
This markdown will take you through using AWS Inspector to scan EC2 instances for Common vulnerabilities and exposures (CVEs) and set up Amazon SNS notifications. This will have four subsections;
1. Install the Amazon Inspector agent by using EC2 Run Command.
2. Set up notifications using Amazon SNS to notify us of any findings.
3. Define an Amazon Inspector target and template to define what assessment to perform on our EC2 instance.
4. Schedule Amazon Inspector assessment runs to assess our EC2 instance on a regular interval.
##### Prerequisites
In order to scan instances for vulnerabilities, the instances should have the following;
- Correct tagging on the instances with ``Key: Patch Group`` and ``Value: (desired) Servers e.g Ubuntu Servers``
- EC2 role that enables the instances to communicate with Systems Manager
- SSM agent is installed in the instance
- Inspector agent is installed in the instance
##### Instance Tagging
In addition to the Name tagging, we require to have Patch Group tags for each instance we are going to scan. Refer to the image below.

##### IAM Role
The Iam role will have the following policies attached to it;
```
AmazonEC2RoleforSSM
AmazonSSMFullAccess
```
## 1. Install the Amazon Inspector.
Amazon Inspector can help you scan your EC2 instance using prebuilt rules packages, which are built and maintained by AWS. These prebuilt rules packages tell Amazon Inspector what to scan for on the EC2 instances you select. Amazon Inspector provides the following prebuilt packages
- Common Vulnerabilities and Exposures
- Security Best Practices
- CIS Operating System Security Configuration Benchmarks
- Runtime Behavior Analysis

We are going to make sure we keep our EC2 instances inspected for common vulnerabilities and exposures (CVEs). As a result, we will focus on how to use the CVE rules package and use our instance tags to identify the instances on which to run the CVE rules. If our EC2 instance is fully patched using Systems Manager, we should not have any findings with the CVE rules package. Regardless, as a best practice AWS recommends that we use Amazon Inspector as an additional layer for identifying any unexpected failures. This involves using Amazon CloudWatch to set up weekly Amazon Inspector scans, and configuring Amazon Inspector to notify of any findings through SNS topics. By acting on the notifications we receive, we can respond quickly to any CVEs on any of our EC2 instances to help ensure that malware using known CVEs does not affect our EC2 instances.
### Install the Amazon Inspector agent
#### (a) Using EC2 userdata to install both Inspector agent and SSM agent
Use the following text into the user data section before launching the instance:
##### ubuntu instance
```
#!/bin/bash
cd /tmp
wget https://s3.amazonaws.com/ec2-downloads-windows/SSMAgent/latest/debian_amd64/amazon-ssm-agent.deb
dpkg -i amazon-ssm-agent.deb
curl -O https://d1wk0tztpsntt1.cloudfront.net/linux/latest/install
chmod a+x /tmp/install
bash /tmp/install
```
##### Centos Instance
```
#!/bin/bash
cd /tmp
yum install -y https://s3.amazonaws.com/ec2-downloads-windows/SSMAgent/latest/linux_amd64/amazon-ssm-agent.rpm
curl -O https://d1wk0tztpsntt1.cloudfront.net/linux/latest/install
chmod a+x /tmp/install
bash /tmp/install
```
##### Amazon Linux
```
#!/bin/bash
cd /tmp
yum install -y https://s3.amazonaws.com/ec2-downloads-windows/SSMAgent/latest/linux_amd64/amazon-ssm-agent.rpm
curl -O https://d1wk0tztpsntt1.cloudfront.net/linux/latest/install
chmod a+x /tmp/install
bash /tmp/install
```
##### RHEL Instance
```
#!/bin/bash
cd /tmp
yum install -y https://s3.amazonaws.com/ec2-downloads-windows/SSMAgent/latest/linux_amd64/amazon-ssm-agent.rpm
curl -O https://d1wk0tztpsntt1.cloudfront.net/linux/latest/install
chmod a+x /tmp/install
bash /tmp/install
```
##### SUSE Linux Enterprise Server (SLES) 12
```
#!/bin/bash
cd /tmp
wget https://s3.amazonaws.com/ec2-downloads-windows/SSMAgent/latest/linux_amd64/amazon-ssm-agent.rpm
rpm --install amazon-ssm-agent.rpm
curl -O https://d1wk0tztpsntt1.cloudfront.net/linux/latest/install
chmod a+x /tmp/install
bash /tmp/install
```
#### (b)Installing Inspector Agent using EC2 SSM
To install the Amazon Inspector agent, you will use EC2 Run Command, which allows you to run any command on any of your EC2 instances that have the Systems Manager agent with an attached IAM role that allows access to Systems Manager.
1. Choose Run Command under Systems Manager Services in the navigation pane of the EC2 console. Then choose Run a command.

2. To install the Amazon Inspector agent, you will use an AWS managed and provided command document that downloads and installs the agent for you on the selected EC2 instance. Choose AmazonInspector-ManageAWSAgent. To choose the target EC2 instance where this command will be run, use the tag you previously assigned to your EC2 instance, Patch Group, with a value of Windows Servers. For this example, set the concurrent installations to 1 and tell Systems Manager to stop after 5 errors.

3. Retain the default values for all other settings on the Run a command page and choose Run. Back on the Run Command page, you can see if the command that installed the Amazon Inspector agent executed successfully on all selected EC2 instances.

### Set up notifications using Amazon SNS
Now that you have installed the Amazon Inspector agent, you will set up an SNS topic that will notify you of any findings after an Amazon Inspector run.
To set up an SNS topic:
1. In the AWS Management Console, choose Simple Notification Service under Messaging in the Services menu.
2. Choose Create topic, name your topic (only alphanumeric characters, hyphens, and underscores are allowed) and give it a display name to ensure you know what this topic does. Choose Create topic.

3. To allow Amazon Inspector to publish messages to your new topic, choose Other topic actions and choose Edit topic policy.
4. For Allow these users to publish messages to this topic and Allow these users to subscribe to this topic, choose Only these AWS users. Type the following ARN for the Region in which you are deploying the solution: ``arn:aws:iam::xxxxxxxxxxxxxxx:root. ``This is the ARN of Amazon Inspector itself.

5. To receive notifications from Amazon Inspector, subscribe to your new topic by choosing Create subscription and adding your email address. After confirming your subscription by clicking the link in the email, the topic should display your email address as a subscriber. Later, you will configure the Amazon Inspector template to publish to this topic.

### Define an Amazon Inspector target and template
Now that you have set up the notification topic by which Amazon Inspector can notify you of findings, you can create an Amazon Inspector target and template. A target defines which EC2 instances are in scope for Amazon Inspector. A template defines which packages to run, for how long, and on which target.
To create an Amazon Inspector target:
1. Navigate to the Amazon Inspector console and choose Get started.
2. For Amazon Inspector to be able to collect the necessary data from your EC2 instance, you must create an IAM service role for Amazon Inspector. We are going to use IAM service role which AWS has created for us.

3. Amazon Inspector also asks you to tag your EC2 instance and install the Amazon Inspector agent. You already performed these steps previously, so you can proceed by choosing Next. To define the Amazon Inspector target, choose the previously used Patch Group tag with the desired value. This is the same tag that you used to define the targets for patching. Then choose Next.

4. Now, define your Amazon Inspector template, and choose a name and the package you want to run. For this post, use the Common Vulnerabilities and Exposures package and choose the default duration of 1 hour. As you can see, the package has a version number, so always select the latest version of the rules package if multiple versions are available.

5. Configure Amazon Inspector to publish to your SNS topic when findings are reported. You can also choose to receive a notification of a started run, a finished run, or changes in the state of a run. For this demo, you want to receive notifications if there are any findings. To start, choose Assessment Templates from the Amazon Inspector console and choose your newly created Amazon Inspector assessment template. Choose the icon below SNS topics (see the following screenshot).

6. A pop-up appears in which you can choose the previously created topic and the events about which you want SNS to notify you (choose Finding reported).

### Schedule Amazon Inspector assessment runs]
The last step in using Amazon Inspector to assess for CVEs is to schedule the Amazon Inspector template to run using Amazon CloudWatch Events. This will make sure that Amazon Inspector assesses your EC2 instance on a regular basis. To do this, you need the Amazon Inspector template ARN, which you can find under Assessment templates in the Amazon Inspector console. CloudWatch Events can run your Amazon Inspector assessment at an interval you define using a Cron-based schedule. Cron is a well-known scheduling agent that is widely used on UNIX-like operating systems and uses the following syntax for CloudWatch Events.

All scheduled events use a UTC time zone, and the minimum precision for schedules is one minute. For more information about scheduling CloudWatch Events, see Schedule Expressions for Rules.
To create the CloudWatch Events rule:
1. Navigate to the CloudWatch console, choose Events, and choose Create rule.

2. On the next page, specify if you want to invoke your rule based on an event pattern or a schedule. For this task, you will select a schedule based on a Cron expression.
3. You can schedule the Amazon Inspector assessment any time you want using the Cron expression, or you can use the Cron expression I used in the following screenshot, which will run the Amazon Inspector assessment every Sunday at 00:00 A.M. GMT.

4. Choose Add target and choose Inspector assessment template from the drop-down menu. Paste the ARN of the Amazon Inspector template you previously created in the Amazon Inspector console in the Assessment template box and choose Create a new role for this specific resource. This new role is necessary so that CloudWatch Events has the necessary permissions to start the Amazon Inspector assessment. CloudWatch Events will automatically create the new role and grant the minimum set of permissions needed to run the Amazon Inspector assessment. To proceed, choose Configure details.

5. Next, give your rule a name and a description. I suggest using a name that describes what the rule does, as shown in the following screenshot.
6. Finish the wizard by choosing Create rule. The rule should appear in the Events – Rules section of the CloudWatch console.

7. To confirm your CloudWatch Events rule works, wait for the next time your CloudWatch Events rule is scheduled to run. For testing purposes, you can choose your CloudWatch Events rule and choose Edit to change the schedule to run it sooner than scheduled.

8. Now navigate to the Amazon Inspector console to confirm the launch of our first assessment run. The Start time column shows the time each assessment started and the Status column the status of our assessment. In the following screenshot, you can see Amazon Inspector is busy Collecting data from the selected assessment targets.

### Findings Report
This section shows the findings of the scanning



| bfed132b603d20c88e0836713ee7e297834a5ce6 | [
"Markdown",
"Shell"
] | 3 | Shell | patrickmukumbu/DevOps | 317cb3e1b4f24c2956d8e2e5b9324681dea77e0f | 10c4871c8fa8b21be751e68ab6ead20f7de5afac | |
refs/heads/master | <repo_name>t3patterson/explorations-knockoutjs<file_sep>/02-observable-arrays.md
##Obserbale Arrays
###Knockout in JS - Observable Arrays (@ source)
1. Put `ko.observable` on the data-type
2. Append observables to an array and pass array as argument to `ko.observable`
3. Establish bindings by passing the array as the vale of a name-spaced property to `ko.applyBindings`
```js
var Person = function(name){
//(1)
this.name = ko.observable(name)
}
var p1 = new Person('Travo'),
p2 = new Person('Roberto')
//(2)
var viewModel = {people: ko.observableArray([p1,p2])};
//(3)
ko.applyBindings(viewModel)
```
In HTML data can be accessed under `people()` namespace:
```
<h3>Records</h3>
<p data-bind="text: people().length"></p>
<ul data-bind="foreach: people">
<li data-bind="text: name"></li>
</ul>
```
Observables will you allow to change the value from the input:
```
<ul data-bind="foreach: people">
<li>
<span data-bind="text: name"></span>
<input type="text" data-bind="value: name"/>
</li>
</ul>
```
When you change the `input` with a `data-bind="value: «»"` , the `<span>` tag will change to match. This is because we passed the original value that generated the name to a *ko.observable*
Note: observables are *functions* that require `()`
```html
<p> Records <span data-bind="text: people().length"></span></p>
<p>First Person: <span data-bind="text: people()[0].name"></span>
```
**EXCEPT** for the 'right-most' property which is automatically 'unwrapped'
```html
<ul data-bind="foreach: people">
<li>
<p data-bind="text: name"></p>
<p data-bind="text: age"></p>
</li>
<ul>
```
(however you can use the `()`)
####Sometimes you might not need observables:
- Read Only
- One Time Binding
- Loading a list of objects
...
- Example of mix & match:
```js
var Person = function (_name,_age,_id){
this.id = _id;
this.name = ko.observable(_name);
this.age = ko.observable(_age);
}
```
##Debugging
####In the console
- **breakpoints** in `Sources` for determining the code's
+ call stack
+ scope variables
+
- **conditional breakpoints**
- a breakpoint to run if a function/event evaluates to t/f
- **Evaluate in Console**
- right click under `Sources` tab in chrome
- Use *Add to Watch* to watch certain variables
- **Workspaces**
- Setup workspaces in Settins
- **XHR Breakpoints**
##Inspecting Bindings
####In `.js`
```
viewModel = {
...
bindingInfo: ko.computed(function(){
return ko.toJSON(«ko-observable item/array»,null,2);
})
};
ko.applyBindings(viewModel);
```
####In `.html`
```
```
###Create DOM-node with bindings using custom `ko.addBindingHandlers` method
**The HTML**
```html
<div data-bind="dump: people"></div>
```
**The JS**
Note:
`domElement` --> DOM element
`valueAccessor()` *i.e. the context* --> people
`dump` --> the name of the bindingHandler
```js
ko.bindingHandlers.dump = {
init: function(domElement, valueAccessor, allBindingsAccessor,viewModel,bindingContext){
var context = valueAccessor();
var allBindings = allBindingsAccessor();
var pre = document.createElement('pre');
domElement.appendChild();
var dumpJSON = ko.computed({
read: function(){
ko.toJSON(context,null,2)
},
disposeWhenNodeIsRemoved: domElement
});
ko.applyBindingsToNode(
pre,
{text: dumpJSON}
)
}
}
```
##Misc-DEV Tips
####Iterate clean over an object
`for-in` loops also iterate over the prototype. to avoid this:
```
for (var prop in this){
if (this.hasOwnProperty(prop)){
//do x
}
}
```<file_sep>/app-sessions.js
window.onload = (function(){
//observables
// sessions() - observable-array
//
// sessions
// speaker
// imageName
// fullName
// timeSlot
// name
// room
// name
// level
// track
// trackname
// code
// title
// tagsFormatted
function Session(ops){
if ( ops.speaker ){
this.speaker = {
fullName : ops.speaker.fullName || "",
imageName : ops.speaker.imageName ||""
}
} else {
this.speaker = {
fullName:"",
imageName: ""
}
}
this.room = ops.room || ""
this.code = ops.code || ""
this.title = ops.title ||""
this.tagsFormatted = ops.tagsFormatted || "no tages"
this.level = ops.level || ""
if ( ops.track ){
this.track = {
name: ops.track.name || ""
}
} else {
ops.track = {
name: ""
}
}
if ( ops.timeSlot ){
this.timeSlot = {
name: ops.timeSlot.name || ""
}
} else {
ops.timeSlot = {
name: ""
}
}
this.createObservables()
}
Session.prototype.createObservables = function(){
for (var prop in this){
ko.observable(this[prop])
}
}
var user1 = {
speaker: {
fullName: "<NAME>",
imageName: "https://randomuser.me/api/portraits/men/0.jpg"
},
timeSlot: { name: "Saturday 10pm"},
room: {name: "Moon Room"},
level: "4",
track: {name: "Programming"},
code: "#9f8f9-e0a",
title: "How to be the man",
tagsFormatted: "Study, Work, Play"
}
var user2 = {
speaker: {
fullName: "<NAME>",
imageName: "https://randomuser.me/api/portraits/men/3.jpg"
},
timeSlot: { name: "Wednesday 4pm"},
room: {name: "Cloud Room"},
level: "2",
track: {name: "Intro to Everythgin"},
code: "#37829-e0a",
title: "Crawl before you walk",
tagsFormatted: "Intro, Start, Learn"
}
var evt = new Session(user1);
var evt = new Session(user2);
var viewModel = {
sessions: ko.observableArray([user1,user2])
}
ko.applyBindings(viewModel)
})();<file_sep>/01-simple-binding.md
##Simple Binding Example
####The HTML
```html
<input type="text" data-bind="value: person().name">
...
<tr>
<td data-bind="text: person().name"></td>
<td data-bind="text: person().age"></td>
</tr>
```
####The JS
```js
function Person(name, age){
this.name = ko.observable(name)
this.age = ko.observable(age)
}
var p1 = new Person("Travoo", 29);
var viewModel = {
person: ko.addObservable(p1)
}
viewModel
```
var p1 = {
name: "Travo",
age: 33
}
var viewModel = {
person: ko.observable(p1)
}
ko.applyBindings(viewModel);
###Knockout in HTML/View (Read Only Target)
uses `data-bind` attribute
```
<section data-bind="foreach: speakers">
<article>
<img data-bind="attr: {src: imageName}"/>
<p>
<span data-bind="text: firstName"></span>
<span data-bind="text: lastName"></span>
</p>
</article>
</section>
```
<file_sep>/README.md
#Knockout Notes
1. [The Basics](./00-the-basics.md)
2. [Simple Binding](./01-simple-binding.md)
3. [Observable Array](./02-observable-arrays.md)
4. [Inspecting Bindings](./03-inspect-bindings.md)
##Misc-DEV Tips / Things Learned
####Iterate clean over an object
`for-in` loops also iterate over the prototype. to avoid this:
```
for (var prop in this){
if (this.hasOwnProperty(prop)){
//do x
}
}
```<file_sep>/dump.js
//ko.bindingHandler.dump
ko.bindingHandlers.dump = {
init: function(ko_element, valueAccessor, allBindingsAccessor, viewModel, bindingContext ){
// ko_element - is the DOMelement that you're bound to
// valueAccessor - value your bound to
// allBindingsAccessor - other bindings on that particular element
// viewModel - whatever the page is bound to
// bindingContext - ???
var context = valueAccessor(); // the ko-observable we're referencing/bound to
var allBindings = allBindingsAccessor(); //ko-observable
var pre = document.createElement('pre');
ko_element.appendChild(pre); //appends to KO element
var dumpJSON = ko.computed({
read: function(){
return ko.toJSON(context,null,2);
},
disposeWhenNodeIsRemoved: ko_element
});
ko.applyBindingsToNode(
pre, {text: dumpJSON}
);
}
}
<file_sep>/03-inspect-bindings.md
##Inspect Bindings
###Debugging in the console
- **breakpoints** in `Sources` for determining the code's
+ call stack
+ scope variables
+
- **conditional breakpoints**
- a breakpoint to run if a function/event evaluates to t/f
- **Evaluate in Console**
- right click under `Sources` tab in chrome
- Use *Add to Watch* to watch certain variables
- **Workspaces**
- Setup workspaces in Settins
- **XHR Breakpoints**
### Using `ko.computed` in `.js` as a k
```
viewModel = {
...
bindingInfo: ko.computed(function(){
return ko.toJSON(«ko-observable item/array»,null,2);
})
};
ko.applyBindings(viewModel);
```
####In `.html`
```
<pre data-bind="text: bindingInfo">
```
###Create DOM-node with custom bindings using `ko.addBindingHandlers` method
**The HTML**
```html
<div data-bind="dump: people"></div>
```
**The JS**
Note:
`domElement` --> DOM element
`valueAccessor()` *i.e. the context* --> people
`dump` --> the name of the bindingHandler
```js
ko.bindingHandlers.dump = {
init: function(domElement, valueAccessor, allBindingsAccessor,viewModel,bindingContext){
var context = valueAccessor();
var allBindings = allBindingsAccessor();
var pre = document.createElement('pre');
domElement.appendChild();
var dumpJSON = ko.computed({
read: function(){
ko.toJSON(context,null,2)
},
disposeWhenNodeIsRemoved: domElement
});
ko.applyBindingsToNode(
pre,
{text: dumpJSON}
)
}
}
```
<file_sep>/00-the-basics.md
## The Basics
##Based on Observables
Observables are *critical* to knockout.
**3-Types**
1. Observable
2. Observable-Array
3. Computed
How it looks:
```
var name = ko.observable('Travis')
var age = ko.observable(32)
```
### 2Way Data Binding
```
*Event Happens* @ source ---> Target is notified
Source is notified <--- *Event Happens:* @ target
```
Notes
- [Simple Binding Example](./index0.html)
- []
###Knockout in JS - Observable Arrays (@ source)
1. Put `ko.observable` on the data-type
2. Append observables to an array and pass array as argument to `ko.observable`
3. Establish bindings by passing the array as the vale of a name-spaced property to `ko.applyBindings`
```js
var Person = function(name){
//(1)
this.name = ko.observable(name)
}
var p1 = new Person('Travo'),
p2 = new Person('Roberto')
//(2)
var viewModel = {people: ko.observableArray([p1,p2])};
//(3)
ko.applyBindings(viewModel)
```
In HTML data can be accessed under `people()` namespace:
```
<h3>Records</h3>
<p data-bind="text: people().length"></p>
<ul data-bind="foreach: people">
<li data-bind="text: name"></li>
</ul>
```
Observables will you allow to change the value from the input:
```
<ul data-bind="foreach: people">
<li>
<span data-bind="text: name"></span>
<input type="text" data-bind="value: name"/>
</li>
</ul>
```
When you change the `input` with a `data-bind="value: «»"` , the `<span>` tag will change to match. This is because we passed the original value that generated the name to a *ko.observable*
Note: observables are *functions* that require `()`
```html
<p> Records <span data-bind="text: people().length"></span></p>
<p>First Person: <span data-bind="text: people()[0].name"></span>
```
**EXCEPT** for the 'right-most' property which is automatically 'unwrapped'
```html
<ul data-bind="foreach: people">
<li>
<p data-bind="text: name"></p>
<p data-bind="text: age"></p>
</li>
<ul>
```
(however you can use the `()`)
####Sometimes you might not need observables:
- Read Only
- One Time Binding
- Loading a list of objects
...
- Example of mix & match:
```js
var Person = function (_name,_age,_id){
this.id = _id;
this.name = ko.observable(_name);
this.age = ko.observable(_age);
}
```
| 1bbf78b6d34a3001e09a8f7133cd21ae7758db73 | [
"Markdown",
"JavaScript"
] | 7 | Markdown | t3patterson/explorations-knockoutjs | fabbae4c8102ee37f071349cc6cb4667203ab9a6 | 2421bc19421ee6618d1e512230f6ed5caa68feda | |
refs/heads/master | <repo_name>Majo-pq/ConsoleApp5<file_sep>/Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp5
{
class Program
{
static void Main(string[] args)
{
Alumno a;
a = new Alumno();
Console.WriteLine("Fecha de nacimiento :");
a.FechaNacimiento = Console.ReadLine();
Console.WriteLine("Nombre:");
a.Nombre = Console.ReadLine();
Console.WriteLine("Matricula:");
a.Matricula = Console.ReadLine();
Console.WriteLine("Carrera que cursa:");
a.Carrera = Console.ReadLine();
Console.WriteLine(a.ToString());
Console.ReadKey();
}
}
}
<file_sep>/Persona.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp5
{
class Persona
{
private string fechaNacimiento;
private string nombre;
public string FechaNacimiento
{
get
{
return fechaNacimiento;
}
set
{
fechaNacimiento = value;
}
}
public string Nombre
{
get
{
return nombre;
}
set
{
nombre = value;
}
}
public Persona()
{
fechaNacimiento = "";
nombre = "";
}
public Persona(string fechaNacimiento, string nombre)
{
this.fechaNacimiento = fechaNacimiento;
this.nombre = nombre;
}
public override string ToString()
{
return "\nfechaNacimiento: " + FechaNacimiento + "nombre: " + Nombre;
}
}
}
| 379293170140c13954944ad29d1c737d456ec044 | [
"C#"
] | 2 | C# | Majo-pq/ConsoleApp5 | 3973bc2edac9edca0fddf1d0b833c89c5fac6261 | f91f73360c3b641e87405544c845b4259d39ebf0 | |
refs/heads/main | <repo_name>durdonadjalilova/captains-log-react<file_sep>/src/Components/Logs.js
import Log from "./Log"
const Logs = ({ logs }) => {
return (
<div className="Logs">
<section>
<table>
<thead>
<tr>
<th></th>
<th>Take me there</th>
<th>See this log</th>
</tr>
</thead>
<tbody>
{logs.map((log, index) => {
return <Log key={index} log={log} index={index} />;
})}
</tbody>
</table>
</section>
</div>
);
}
export default Logs<file_sep>/src/Components/LogDetails.js
import { useState, useEffect } from "react";
import { Link, useParams, useHistory, withRouter } from "react-router-dom";
import axios from "axios";
import { apiURL } from '../util/apiURL';
const API = apiURL();
function LogDetails(props) {
const { deleteLog } = props;
const [log, setLog] = useState([]);
let { index } = useParams();
let history = useHistory();
const fetchLog = async() =>{
try {
const response = await axios.get(`${API}/logs/${index}`);
// console.log(response.data);
setLog(response.data);
} catch (error) {
console.log(error)
}
}
useEffect(() => {
fetchLog()
}, []);
const handleDelete = () => {
deleteLog(index)
history.push('/logs')
};
return (
<article>
<h3>
{/* {log.mistakesWereMadeToday ? <span>😢</span> : <span>😃</span>} */}
{log.title}
</h3>
<h5>
<span>
{/* <a href={log.title}>{log.title}</a> */}
{log.title} - By {log.captainName}
</span>{" "}
</h5>
<h6>{log.post}</h6>
<p> {`Mistakes were made today: ${log.mistakesWereMadeToday}`}</p>
<p>Days since last crisis: {log.daysSinceLastCrisis}</p>
<div className="showNavigation">
<div>
{" "}
<Link to={`/logs`}>
<button>Back</button>
</Link>
</div>
<div>
{" "}
<Link to={`/logs/${index}/edit`}>
<button>Edit</button>
</Link>
</div>
<div>
{" "}
<button onClick={handleDelete}>Delete</button>
</div>
</div>
</article>
);
}
export default withRouter(LogDetails);
<file_sep>/src/Components/Log.js
import { Link } from "react-router-dom";
const Log = ({ log, index }) => {
return (
<tr>
<td>
{log.mistakesWereMadeToday ? (
<span>⭐️</span>
) : (
<span> </span>
)}
</td>
<td>
{/* <a href={log.title} target="_blank" rel="noreferrer"> */}
<Link to={`/logs/${index}`}>{log.title}</Link>
{/* </a> */}
</td>
<td>
<Link to={`/logs/${index}`}>✏️</Link>
</td>
</tr>
);
}
export default Log;
| 6c3b1d1601771c73c338b75b466e1199b66957f3 | [
"JavaScript"
] | 3 | JavaScript | durdonadjalilova/captains-log-react | 9004986221640f19c26e28767617dafcf48ee194 | 2ba1eb22c5a36630e35f37624223d75dad49181e | |
refs/heads/main | <file_sep>#!/usr/bin/env python
import json
from kafka import KafkaProducer
from flask import Flask, request
app = Flask(__name__)
producer = KafkaProducer(bootstrap_servers='kafka:29092')
# * Adds metadata such as Host/IP address, User-Agent to all generated events
# * Encodes it (default: encoding='utf-8', #errors='strict')
# * Produces event to Kafka
def log_to_kafka(topic, event):
event.update(request.headers)
#Adding remote header in case IP addresses outside of my environment make requests to our server
event.update({'remote_addr': request.remote_addr})
producer.send(topic, json.dumps(event).encode())
@app.route("/")
def default_response():
"""
* Default response if no specific API route is hit
"""
default_event = {'event_type': 'default'}
log_to_kafka('game_events', default_event)
return "This is the default response!\n"
@app.route("/purchase_sword")
def purchase_sword():
"""
* GET message here can either be a default purchase_sword or pass query params
* Both options get processed through via the log_to_kafka function while return back to the user the information of their purchase.
"""
#Data is passed via qury parameters, add the arguments to our JSON logged to Kafka
result = {}
for key, value in request.args.items():
result[key] = value
#Condition to check if params were specified in request
if len(result) == 0:
sword_event = {'event_type': 'purchase_sword'}
log_to_kafka('game_events', sword_event)
return "Sword Purchased\n"
else:
sword_event = {'event_type': 'purchase_sword'}
sword_event.update(result)
log_to_kafka('game_events', sword_event)
return "Sword Purchased: " + json.dumps(result) + "\n"
@app.route("/join_a_guild")
def join_a_guild():
"""
* GET message here can either be a default join_a_guild or pass query params
* Both options get processed through via the log_to_kafka function while return back to the user the information of their purchase.
"""
#Data is passed via qury parameters, add the arguments to our JSON logged to Kafka
result = {}
for key, value in request.args.items():
result[key] = value
#Condition to check if params were specified in request
if len(result) == 0:
join_a_guild_event = {'event_type': 'join_a_guild'}
log_to_kafka('game_events', join_a_guild_event)
return "Joined a Guild!\n"
else:
join_a_guild_event = {'event_type': 'join_a_guild'}
join_a_guild_event.update(result)
log_to_kafka('game_events', join_a_guild_event)
return "Joined a guild! " + json.dumps(result) + "\n"<file_sep># Understanding User Behavior
### 07/30/2020 - <NAME>
# Introduction
- You're a data scientist at a game development company
- Your latest mobile game has two events you're interested in tracking: `purchase a
sword` & `join guild`
- Each has metadata characterstic of such events (i.e., sword type, guild name,
etc)
Our goal here will be to simulate user interactions with our "mobile game" while tracking and processing events through the entire pipeline end to end. We will take advantage of a variety of tools to achieve each step of this pipeline which will be detailed below.
Below you will find a table of contents in essence for the various stages of this project.
## Preparing the Pipeline
To set up the infrastructure where we will pipe the data through, transform it, and ultimately land it in in a queriable structure, we will need various docker containers to help out.
The `docker-compose.yml` file is the config file that specifies all the details in order to spin up our pipeline. Please refer to this to understand the various containers that are being used. You will also find the setup of the ports that allow us to connect the containers together. Comments are included in the `docker-compose.yml` file.
## Building the Pipeline
`project3_final_report.ipynb` describes all the commands that are required to spin up the pipeline with all the necessary command line options. You will find details of each step, followed at the end by a runnable query engine setup with Presto. Here you will see some simple queries into HDFS.
## Other Files:
* `karthikrbabu-history.txt` includes the complete history of the commands I have run on my console. (It is un-altered!)
* `game_api.py` includes all the setup for our Flask server. This file defines what APIs are supported and handles the logging to our Kafka queue as requests come in.
* `write_events_stream.py` defines the start of our Spark streaming session. The code detailed here kicks off the Spark job, and will continue to listen and process events that stream in from Kafka. The business logic sits here for filtering events of different types and writing them to the respective file storage in HDFS.
* `write_hive_table.py` defines the Spark job that creates "phonebook" for Presto to read from HDFS using Hive as a meta store that points to the right location to query the data from HDFS. The Spark job here will use spark SQL to create external tables that we can use as a pointer and schema definition to then query into HDFS.
* ```scripts/*.sh``` to simulate user interactions with our "mobile app". These generate requests to our Flask server.
* ```basic_ab.sh```
* ```complex_ab_limit.sh```
* ```complex_ab_infinite.sh```
<file_sep>---
version: '2'
services:
# zookeeper container is used to manage our Apache environment, generally for a distributed system.
# Handles most of the config, registry, and fault tolerance setup
zookeeper:
image: confluentinc/cp-zookeeper:latest
environment:
ZOOKEEPER_CLIENT_PORT: 32181
ZOOKEEPER_TICK_TIME: 2000
#Here we expose various ports for other containers to connect to zookeeper mainly on port: 32181
expose:
- "2181"
- "2888"
- "32181"
- "3888"
extra_hosts:
- "moby:127.0.0.1"
#Kakfa is our distributed messaging queue that lets us produce messages into it and read messages out.
kafka:
image: confluentinc/cp-kafka:latest
depends_on:
- zookeeper
#We connect to zookeeper on port: 32181
environment:
KAFKA_BROKER_ID: 1
KAFKA_ZOOKEEPER_CONNECT: zookeeper:32181
KAFKA_ADVERTISED_LISTENERS: PLAINTEXT://kafka:29092
KAFKA_OFFSETS_TOPIC_REPLICATION_FACTOR: 1
#Expose these ports for us to write to and read from Kafka
expose:
- "9092"
- "29092"
extra_hosts:
- "moby:127.0.0.1"
#This is our HDFS container that allows for more persistent storage
cloudera:
image: midsw205/hadoop:0.0.2
hostname: cloudera
#We expose some ports for GUI look into our HDFS, and for the Hive metastore to peer into HDFS
expose:
- "8020" # nn
- "8888" # hue
- "9083" # hive thrift
- "10000" # hive jdbc
- "50070" # nn http
ports:
- "8888:8888"
extra_hosts:
- "moby:127.0.0.1"
#Spark container that gives us the framework to stream process and transform our data to then land it in HDFS
spark:
image: midsw205/spark-python:0.0.6
stdin_open: true
tty: true
#Add the volumes which lets us use and interact with local machine files
volumes:
- ~/w205:/w205
expose:
- "8890" #Added ports to open up a Jupyter Notebook based on my firewall rules
- "8888"
ports:
- "8889:8888" # 8888 conflicts with hue
- "8890" # Jupyter Notebook
- "8890:8890" # Jupyter Notebook
depends_on:
- cloudera
#Connecting the environment to our HDFS setup
environment:
HADOOP_NAMENODE: cloudera
HIVE_THRIFTSERVER: cloudera:9083
extra_hosts:
- "moby:127.0.0.1"
command: bash
#Pulling in Presto which is a query engine layer that lets us run queries on top of HDFS
presto:
image: midsw205/presto:0.0.1
hostname: presto
volumes:
- ~/w205:/w205
expose:
- "8080"
environment:
HIVE_THRIFTSERVER: cloudera:9083
extra_hosts:
- "moby:127.0.0.1"
#MIDS container that has many of the fundamental tools ready for us to use. Attaching volumes here as well
mids:
image: midsw205/base:0.1.9
stdin_open: true
tty: true
volumes:
- ~/w205:/w205
expose:
- "5000"
ports:
- "5000:5000"
extra_hosts:
- "moby:127.0.0.1"
<file_sep>#!/usr/bin/env python
"""Extract events from kafka and write them to hdfs
"""
import json
from pyspark.sql import SparkSession
from pyspark.sql.functions import udf, from_json
from pyspark.sql.types import StructType, StructField, StringType
def purchase_sword_event_schema():
"""
Defining the strucuture of the "purchase_events" store
|-- Accept: string (nullable = true)
|-- Host: string (nullable = true)
|-- User-Agent: string (nullable = true)
|-- event_type: string (nullable = true)
|-- metal: string (nullable = true)
|-- power_level: string (nullable = true)
|-- magical: string (nullable = true)
|-- remote_addr: string (nullable = true)
"""
return StructType([
StructField("Accept", StringType(), True),
StructField("Host", StringType(), True),
StructField("User_Agent", StringType(), True),
StructField("event_type", StringType(), True),
StructField('metal', StringType(), True),
StructField('power_level', StringType(), True),
StructField('magical', StringType(), True),
StructField("remote_addr", StringType(), True),
])
def join_a_guild_event_schema():
"""
Defining the strucuture of the "join_a_guild" store
|-- Accept: string (nullable = true)
|-- Host: string (nullable = true)
|-- User-Agent: string (nullable = true)
|-- event_type: string (nullable = true)
|-- timestamp: string (nullable = true)
|-- region: string (nullable = true)
|-- remote_addr: string (nullable = true)
"""
return StructType([
StructField("Accept", StringType(), True),
StructField("Host", StringType(), True),
StructField("User_Agent", StringType(), True),
StructField("event_type", StringType(), True),
StructField('region', StringType(), True),
StructField("remote_addr", StringType(), True),
])
@udf('boolean')
def is_sword_purchase(event_as_json):
"""udf for filtering purchase_sword events
"""
event = json.loads(event_as_json)
if event['event_type'] == 'purchase_sword':
return True
return False
@udf('boolean')
def is_join_a_guild(event_as_json):
"""udf for filtering join_a_guild events
"""
event = json.loads(event_as_json)
if event['event_type'] == 'join_a_guild':
return True
return False
def main():
"""main
"""
#Build the Spark Session
spark = SparkSession \
.builder \
.appName("ExtractEventsJob") \
.getOrCreate()
'''
Connect Spark to Kafka. Set it up in streaming mode to subscribe to the "game_events"
topic and read in messages.
'''
raw_events = spark \
.readStream \
.format("kafka") \
.option("kafka.bootstrap.servers", "kafka:29092") \
.option("subscribe", "game_events") \
.load()
'''
Apply our UDF to only filter for "purchase_sword" events.
In addition we add two columns, "raw_event" and "timestamp". In addition to all the
columns coming form the JSON message. We cast all columns to Strings
'''
sword_purchases = raw_events \
.filter(is_sword_purchase(raw_events.value.cast('string'))) \
.select(raw_events.value.cast('string').alias('raw_event'),
raw_events.timestamp.cast('string'),
from_json(raw_events.value.cast('string'),
purchase_sword_event_schema()).alias('json')) \
.select('raw_event', 'timestamp', 'json.*')
'''
Apply our UDF to only filter for "join_a_guild" events.
In addition we add two columns, "raw_event" and "timestamp". In addition to all the
columns coming form the JSON message. We cast all columns to Strings
'''
join_a_guild = raw_events \
.filter(is_join_a_guild(raw_events.value.cast('string'))) \
.select(raw_events.value.cast('string').alias('raw_event'),
raw_events.timestamp.cast('string'),
from_json(raw_events.value.cast('string'),
join_a_guild_event_schema()).alias('json')) \
.select('raw_event', 'timestamp', 'json.*')
'''
Below we setup two sinks, one for each type of event that we are filtering for. Here we indicate
all the configurations for how and where to write the data. We specife "parquet" as the format.
we indicate the directory under the /tmp/* folder in HDFS. Finally we set our trigger processing time
to 15 seconds, this means that our stream processing will run ever 15 seconds to pull whatever data
that has been put into Kafka and process it.
'''
sink1 = sword_purchases \
.writeStream \
.format("parquet") \
.option("checkpointLocation", "/tmp/checkpoints_for_purchase_events") \
.option("path", "/tmp/purchase_events") \
.trigger(processingTime="15 seconds") \
.start()
sink2 = join_a_guild \
.writeStream \
.format("parquet") \
.option("checkpointLocation", "/tmp/checkpoints_for_join_guild") \
.option("path", "/tmp/join_a_guild") \
.trigger(processingTime="15 seconds") \
.start()
'''
Using awaitTermination here we do not actually shut down the executor.
It will block until all tasks have completed execution after a shutdown request, or the timeout occurs,
or the current thread is interrupted, whichever happens first.
'''
spark.streams.awaitAnyTermination()
if __name__ == "__main__":
main()
if __name__ == "__main__":
main()
<file_sep>#!/usr/bin/env python
"""Extract events from kafka and write them to hdfs
"""
import json
from pyspark.sql import SparkSession, Row
# READ DIRECTLY FROM HDFS
def main():
"""main
"""
'''
Create a Spark job here to create an external table into our Hive metastore. We specify the schema
needed for the table with the reference name of "sword_purchases"
'''
spark = SparkSession \
.builder \
.appName("WriteHiveTables") \
.enableHiveSupport() \
.getOrCreate()
query_sword_purchases = """
create external table if not exists sword_purchases(
raw_event STRING, timestamp STRING, Accept STRING, Host STRING, User_Agent STRING,
event_type STRING, metal STRING, power_level STRING, magical STRING, remote_addr STRING)
stored as parquet
location '/tmp/purchase_events'
"""
spark.sql(query_sword_purchases)
'''
Create a Spark job here to create an external table into our Hive metastore. We specify the schema
needed for the table with the reference name of "guild_joins"
'''
query_guild_joins = """
create external table if not exists guild_joins(
raw_event STRING, timestamp STRING, Accept STRING, Host STRING, User_Agent STRING,
event_type STRING, region STRING, remote_addr STRING)
stored as parquet
location '/tmp/join_a_guild'
"""
spark.sql(query_guild_joins)
if __name__ == "__main__":
main()
<file_sep>#!/bin/sh
# We can set a max threshold for the loop to run and stop for testing purposes. This has to come from the command line whatever value we pass for the limit
# i.e. sh complex_ab_limit.sh 7
x=1
while [ $((x)) -le $1 ]
do
#Based on odd or even we vary the data requests that we make
if [ $((x%2)) -eq 0 ]:
#Use Apache Bench to fire requests to Flask Server
then
docker-compose exec mids ab -n 10 -H "Host: user1.att.com" 'http://localhost:5000/purchase_sword?metal=copper&power_level=50&magical=True'
docker-compose exec mids ab -n 10 -H "Host: user2.comcast.com" 'http://localhost:5000/join_a_guild?region=cali'
else
docker-compose exec mids ab -n 10 -H "Host: user1.att.com" 'http://localhost:5000/purchase_sword?metal=gold&power_level=100&magical=False'
docker-compose exec mids ab -n 10 -H "Host: user2.comcast.com" 'http://localhost:5000/join_a_guild?region=ny'
fi
#Fire loop once every 5 seconds
sleep 5
#Increment counter for the data variation and for when to stop iteration
x=$(( $x + 1 ))
done<file_sep>#!/bin/sh
# To simulate streaming, we have an infinite loop to request data.
x=1
while true
do
#Based on odd or even we vary the data requests that we make
if [ $((x%2)) -eq 0 ]:
#Use Apache Bench to fire requests to Flask Server
then
docker-compose exec mids ab -n 10 -H "Host: user1.att.com" 'http://localhost:5000/purchase_sword?metal=copper&power_level=50&magical=True'
docker-compose exec mids ab -n 10 -H "Host: user2.comcast.com" 'http://localhost:5000/join_a_guild?region=cali'
else
#Use Apache Bench to fire requests
docker-compose exec mids ab -n 10 -H "Host: user1.att.com" 'http://localhost:5000/purchase_sword?metal=gold&power_level=100&magical=False'
docker-compose exec mids ab -n 10 -H "Host: user2.comcast.com" 'http://localhost:5000/join_a_guild?region=ny'
fi
#Fire loop once every 10 seconds
sleep 10
#Increment counter for the data variation
x=$(( $x + 1 ))
done<file_sep>#!/bin/sh
#Use Apache Bench to fire sample basic requests to Flask Server
docker-compose exec mids ab -n 10 -H "Host: user1.comcast.com" 'http://localhost:5000/'
docker-compose exec mids ab -n 10 -H "Host: user1.comcast.com" 'http://localhost:5000/purchase_sword'
docker-compose exec mids ab -n 10 -H "Host: user2.att.com" 'http://localhost:5000/'
docker-compose exec mids ab -n 10 -H "Host: user2.att.com" 'http://localhost:5000/purchase_sword'
docker-compose exec mids ab -n 10 -H "Host: user1.att.com" 'http://localhost:5000/join_a_guild'
docker-compose exec mids ab -n 10 -H "Host: user2.att.com" 'http://localhost:5000/join_a_guild'
| abf1f429a5285db0562a68aadd587d053122e152 | [
"Markdown",
"Python",
"YAML",
"Shell"
] | 8 | Python | karthikrbabu/tracking-user-behavior | 8e32ea59c1e51c1acfce63f2013a315a6e21acf7 | 0401d3715cfb06c1d05086b77928bb8a16d3d47f | |
refs/heads/master | <file_sep>//
// adicionarCell.swift
// iRemedy
//
// Created by <NAME> on 13/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
<file_sep>//
// SobreACompraTableViewCell.swift
// iRemedy
//
// Created by <NAME> on 17/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
class SobreACompraTableViewCell: UITableViewCell {
private var dataDeValidadeSelected: Date = Date()
override func awakeFromNib() {
super.awakeFromNib()
// Initialization code
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
// Configure the view for the selected state
}
@IBOutlet weak var dataDeValidadeDatePicker: UIDatePicker!
@IBAction func dataDeValidadeDatePicker(_ sender: Any) {
dataDeValidadeSelected = dataDeValidadeDatePicker.date
}
@IBOutlet weak var precoTextField: UITextField!
@IBAction func precoTextField(_ sender: Any) {
}
public func getDataDeValidadeSelected() -> Date{
return dataDeValidadeSelected
}
public func getPrecoSelected() -> String{
if let preco = precoTextField.text{
return preco
}
return ""
}
}
<file_sep>//
// Remedio.swift
// iRemedy
//
// Created by <NAME> on 09/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
class Remedio{
internal init() {
nome = ""
photo = nil
dosagem = ""
comprimidos = ""
horario = nil
frequencia = nil
contraindicacao = ""
dataDeValidade = nil
preco = ""
sintomas = ""
medida = ""
hasPhoto = false
}
var nome : String
var photo: UIImage?
var dosagem: String
var comprimidos: String
var horario: Date?
var frequencia: Date?
var contraindicacao: String
var dataDeValidade: Date?
var preco: String
var sintomas: String
var medida: String
var hasPhoto: Bool
}
<file_sep>//
// HorarioTableViewCell.swift
// iRemedy
//
// Created by <NAME> on 17/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
class PeriodoTableViewCell: UITableViewCell {
private var horarioSelected: Date = Date()
private var frequenciaSelected: Date = Date()
@IBOutlet weak var horarioPickerView: UIDatePicker!
@IBOutlet weak var frequenciaDatePicker: UIDatePicker!
@IBAction func frequenciaDatePicker(_ sender: Any) {
frequenciaSelected = frequenciaDatePicker.date
}
@IBAction func horarioPicker(_ sender: Any) {
horarioSelected = horarioPickerView.date
}
public func getFrequenciaSelected() -> Date{
return frequenciaSelected
}
public func getHorarioSelected() -> Date{
return horarioSelected
}
override func awakeFromNib() {
super.awakeFromNib()
}
func periodoToString(){
let formatter = DateFormatter()
// initially set the format based on your datepicker date / server String
formatter.dateFormat = "yyyy-MM-dd HH:mm:ss"
let myString = formatter.string(from: frequenciaSelected) // string purpose I add here
// convert your string to date
let yourDate = formatter.date(from: myString)
//then again set the date format whhich type of output you need
formatter.dateFormat = "HH:mm"
// again convert your date to string
let myStringafd = formatter.string(from: yourDate!)
print(myStringafd)
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
// Configure the view for the selected state
}
}
<file_sep>//
// CaixaDeRemediosViewController.swift
// iRemedy
//
// Created by <NAME> on 27/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
class CaixaDeRemediosViewController: UIViewController, UITableViewDelegate, UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return Model.instance.allRemedios.count
}
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "RemedioTableViewCell", for: indexPath) as! RemedioTableViewCell
let remedio = Model.instance.allRemedios[indexPath.row]
let dosagemText = " " + remedio.dosagem + " " + remedio.medida
cell.nomeDoRemedioLabel.text = remedio.nome
cell.contraindicacoesLabel.text = cell.contraindicacoesLabel.text! + " " + remedio.contraindicacao
cell.precoPagoLabel.text = cell.precoPagoLabel.text! + " " + remedio.preco
cell.contraindicacoesLabel.text = cell.contraindicacoesLabel.text! + " " + remedio.contraindicacao
cell.dosagemLabel.text = cell.dosagemLabel.text! + dosagemText
cell.sintomasLabel.text = cell.sintomasLabel.text! + " " + remedio.sintomas
if remedio.hasPhoto{
cell.photoImageView.image = remedio.photo
}
if let dataDeValidade = remedio.dataDeValidade {
cell.dataDeValidadeLabel.text = " " + Model.instance.dateToHHmmString(date: dataDeValidade)
}
return cell
}
override func viewDidLoad() {
super.viewDidLoad()
}
override func viewDidAppear(_ animated: Bool) {
tableView.reloadData()
}
override func viewWillAppear(_ animated: Bool) {
}
@IBOutlet weak var tableView: UITableView!
}
<file_sep>//
// AdicionarViewController.swift
// iRemedy
//
// Created by <NAME> on 09/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
import UserNotifications
class AdicionarViewController: UIViewController, UIImagePickerControllerDelegate, UINavigationControllerDelegate, UITableViewDelegate, UITableViewDataSource{
var appDelegate = UIApplication.shared.delegate as? AppDelegate
var cell0: NameTableViewCell = NameTableViewCell()
var cell1: DosagemTableViewCell = DosagemTableViewCell()
var cell2: PeriodoTableViewCell = PeriodoTableViewCell()
var cell3: SobreACompraTableViewCell = SobreACompraTableViewCell()
var cell4: ObservacoesTableViewCell = ObservacoesTableViewCell()
var nameTextField = UITextField()
var cellIsOpened: [Bool] = []
var keyboardIsShown: Bool = false
var medidaValue: String = "mg"
var dosagemValue: String = ""
var comprimidosValue: Int = 0
var frequenciaValue: Date = Date()
var horarioValue: Date = Date()
var dataDeValidadeValue: Date = Date()
var precoValue: String = ""
var sintomasValue: String = ""
var contraindicacaoValue: String = ""
var userSettedPhoto: Bool = false
var photoButton: UIButton = UIButton.init()
@IBOutlet weak var bottomConstraint: NSLayoutConstraint!
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
if cellIsOpened[section] == true && section != 0{
return 2;
}else{
return 1;
}
}
func numberOfSections(in tableView: UITableView) -> Int {
return 5
}
@IBAction func adicionarRemedioButton(_ sender: Any) {
let remedio: Remedio = Remedio()
if let nome = cell0.nameTextField.text{
remedio.nome = nome
if userSettedPhoto == true{
let cell = cell0
remedio.photo = cell.cameraButton.imageView?.image
remedio.hasPhoto = true
}else{
remedio.photo = nil
}
if cellIsOpened[1] == true{
let cell = cell1
if let dosagem = cell.dosagemTextField.text{
dosagemValue = dosagem
}
remedio.dosagem = dosagemValue
remedio.comprimidos = String(cell.comprimidosStepper.value)
remedio.medida = cell.getMedidaEscolhida()
}
if cellIsOpened[2] == true{
let cell = cell2
remedio.frequencia = cell.getFrequenciaSelected()
remedio.horario = cell.getHorarioSelected()
cell.periodoToString()
let homeRemedio = HomeRemedio(nome: remedio.nome, photo: remedio.photo, comprimidos: remedio.comprimidos, dosagem: remedio.dosagem, horario: remedio.horario, frequencia: remedio.frequencia)
print(homeRemedio.dateToSeconds(date: remedio.frequencia!))
homeRemedio.setHomeRemedio()
Model.instance.savesHomeRemedioData()
}
if cellIsOpened[3] == true{
let cell = cell3
remedio.dataDeValidade = cell.getDataDeValidadeSelected()
remedio.preco = cell.precoTextField.text!
}
if cellIsOpened[4] == true{
let cell = cell4
remedio.sintomas = cell.sintomasTextField.text!
remedio.contraindicacao = cell.contraindicacaoTextField.text!
}
Model.instance.allRemedios.append(remedio)
Model.instance.savesRemedioData()
}
}
private func callFillDetails(){
print("fill the details")
}
@IBAction func cameraButton(_ sender: Any) {
if UIImagePickerController.isSourceTypeAvailable(.camera) {
let imagePicker = UIImagePickerController()
imagePicker.delegate = self
imagePicker.sourceType = .camera;
imagePicker.allowsEditing = false
self.present(imagePicker, animated: true, completion: nil)
}
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
if indexPath.section == 0{
let cell = tableView.dequeueReusableCell(withIdentifier: "nameCell", for: indexPath) as! NameTableViewCell
cell.transform = CGAffineTransform(rotationAngle: (-.pi))
cell.nameTextField.text = nil
cell0 = cell
return cell
}
if cellIsOpened[indexPath.section] == true{
if indexPath.row == 0{
if indexPath.section == 1 && indexPath.row == 0 {
let cell = tableView.dequeueReusableCell(withIdentifier: "DosagemTableViewCell", for: indexPath) as! DosagemTableViewCell
cell.transform = CGAffineTransform(rotationAngle: (-.pi))
cell1 = cell
return cell
}
if indexPath.section == 2 && indexPath.row == 0{
let cell = tableView.dequeueReusableCell(withIdentifier: "PeriodoTableViewCell", for: indexPath) as! PeriodoTableViewCell
cell.transform = CGAffineTransform(rotationAngle: (-.pi))
cell2 = cell
return cell
}
if indexPath.section == 3 && indexPath.row == 0{
let cell = tableView.dequeueReusableCell(withIdentifier: "SobreACompraTableViewCell", for: indexPath) as! SobreACompraTableViewCell
cell.transform = CGAffineTransform(rotationAngle: (-.pi))
cell3 = cell
return cell
}
if indexPath.section == 4 && indexPath.row == 0{
let cell = tableView.dequeueReusableCell(withIdentifier: "ObservacoesTableViewCell", for: indexPath) as! ObservacoesTableViewCell
cell.transform = CGAffineTransform(rotationAngle: (-.pi))
cell4 = cell
return cell
}
}
}
let cell = tableView.dequeueReusableCell(withIdentifier: "expansibleCell", for: indexPath) as! TableViewCell
let newImage = UIImage(named: "expansible\(indexPath.section)")
cell.backgroundImageView?.image = newImage
cell.backgroundImageView?.contentMode = .topLeft
cell.backgroundImageView?.clipsToBounds = true
cell.transform = CGAffineTransform(rotationAngle: (-.pi))
return cell
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
if indexPath.section == 0{
return 260
}
if cellIsOpened[indexPath.section] == true{
if indexPath.row == 0{
if indexPath.section == 1 || indexPath.section == 2{
return 123
}
if indexPath.section == 3{
return 203
}
if indexPath.section == 4{
return 214
}
}else{
return 80;
}
} else if indexPath.row == 0{
return 80
}
return 0;
}
func tableView(_ tableView: UITableView, heightForHeaderInSection section: Int) -> CGFloat {
return CGFloat.leastNonzeroMagnitude
}
func tableView(_ tableView: UITableView, heightForFooterInSection section: Int) -> CGFloat {
return CGFloat.leastNonzeroMagnitude
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath){
if keyboardIsShown {
view.endEditing(true)
keyboardIsShown = false
return
}
if indexPath.section != 0{
cellIsOpened[indexPath.section] = !cellIsOpened[indexPath.section]
let section = IndexSet.init(integer: indexPath.section)
tableView.reloadSections(section, with: .none)
}
}
@IBOutlet weak var tableView: UITableView!
func imagePickerController(_ picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [UIImagePickerController.InfoKey : Any]) {
let image = info[UIImagePickerController.InfoKey.originalImage] as! UIImage
cell0.cameraButton.setImage(image, for: .normal)
userSettedPhoto = true
dismiss(animated:true, completion: nil)
}
public func callHomeViewController(){
let vc = storyboard?.instantiateViewController(withIdentifier: "homeViewController")
present(vc!, animated: true)
self.navigationController?.pushViewController(vc!, animated: true)
}
private func checkIfHasUso(dosagem: String, comprimidos: String, horario: String, durante: String) -> Bool{
if !(dosagem.isEmpty && comprimidos.isEmpty && horario.isEmpty && durante.isEmpty){
return true
}
return false
}
override func viewDidLoad(){
super.viewDidLoad()
tableView.transform = CGAffineTransform(rotationAngle: (-.pi))
cellIsOpened.append(true)
for _ in 0...4{
cellIsOpened.append(false)
}
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillDisappear), name: UIResponder.keyboardWillHideNotification, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillAppear), name: UIResponder.keyboardWillShowNotification, object: nil)
}
@objc func keyboardWillAppear(notification: NSNotification) {
guard let keyboardRect = (notification.userInfo?[UIResponder.keyboardFrameEndUserInfoKey] as? NSValue)?.cgRectValue else{
return
}
keyboardIsShown = true
bottomConstraint.constant = keyboardRect.height + 45
view.layoutIfNeeded()
print(keyboardIsShown)
}
@objc func keyboardWillDisappear() {
keyboardIsShown = false
bottomConstraint.constant = 45
view.layoutIfNeeded()
print(keyboardIsShown)
}
override func viewWillDisappear(_ animated: Bool) {
super.viewWillDisappear(animated)
NotificationCenter.default.removeObserver(self)
}
func textFieldShouldReturn(_ textField: UITextField) -> Bool {
view.endEditing(true)
return false
}
}
<file_sep>//
// ViewController.swift
// iRemedy
//
// Created by <NAME> on 08/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
import UserNotifications
class ViewController: UIViewController, UICollectionViewDataSource, UICollectionViewDelegate {
let notifications = ["Local Notification",
"Local Notification with Action",
"Local Notification with Content",
"Push Notification with APNs",
"Push Notification with Firebase",
"Push Notification with Content"]
var appDelegate = UIApplication.shared.delegate as? AppDelegate
@IBOutlet weak var homeCollectionView: UICollectionView!
let DOSAGEM_SIZE_IN_LABELS = 3;
let USO_SIZE_IN_LABELS = 2;
let DETALHES_SIZE_IN_LABELS = 3;
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return Model.instance.homeRemedios.count
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let homeRemedio = Model.instance.homeRemedios[indexPath.row]
let cell = homeCollectionView.dequeueReusableCell(withReuseIdentifier: "homeCell", for: indexPath) as! HomeCollectionViewCell
cell.horarioLabel.text = homeRemedio.dateToHHmmString(date: homeRemedio.horario!)
cell.nameLabel.text = homeRemedio.nome
cell.quantidadeLabel.text = homeRemedio.comprimidos
if homeRemedio.hasPhoto() == true{
cell.remImageView.image = homeRemedio.photo
}else{
let image = UIImage(named: "Icon.png")
cell.remImageView.image = image
}
return cell
}
func generatesArrayOfExistingData(remedio: Remedio) -> [String]{
var numberOfProperValues: Int = 0;
var arrayOfExistingData: [String] = []
if !(remedio.dosagem == "") {
arrayOfExistingData.append(remedio.dosagem)
arrayOfExistingData.append(remedio.comprimidos)
numberOfProperValues += DOSAGEM_SIZE_IN_LABELS
}
if !(remedio.contraindicacao == ""){
arrayOfExistingData.append(remedio.contraindicacao);
numberOfProperValues += DETALHES_SIZE_IN_LABELS;
}
if numberOfProperValues != 0{
return arrayOfExistingData
}else{
return []
}
}
override func viewDidLoad() {
super.viewDidLoad()
homeCollectionView.delegate = self
homeCollectionView.delegate = self
Model.instance.homeRemediosFromUserDefaults()
Model.instance.allRemediosFromUserDefaults()
}
override func viewWillAppear(_ animated: Bool) {
homeCollectionView.reloadData()
}
override func viewDidAppear(_ animated: Bool) {
}
}
<file_sep>//
// Model.swift
// iRemedy
//
// Created by <NAME> on 09/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
class Model{
private init(){}
public static var instance = Model()
public var allRemedios: [Remedio] = []
public var homeRemedios: [HomeRemedio] = []
public func savesHomeRemedioData(){
var nomeArray: [String?] = []
var photoArray: [String] = []
var dosagemArray: [String?] = []
var comprimidosArray: [String?] = []
var horarioArray: [String] = []
var frequenciaArray: [String] = []
for remedio in homeRemedios{
nomeArray.append(remedio.nome)
dosagemArray.append(remedio.dosagem)
comprimidosArray.append(remedio.comprimidos)
if remedio.photo == nil{
photoArray.append("")
}else{
photoArray.append(convertImageToBase64(image: remedio.photo!))
}
if remedio.frequencia == nil{
frequenciaArray.append("")
}else{
frequenciaArray.append(remedio.dateToHHmmString(date: remedio.frequencia!))
}
if remedio.horario == nil{
horarioArray.append("")
}else{
horarioArray.append(remedio.dateToHHmmString(date: remedio.horario!))
}
}
let defaults = UserDefaults.standard
defaults.set(nomeArray, forKey: "homeRemedioNomeArray")
defaults.set(dosagemArray, forKey: "homeRemedioDosagemArray")
defaults.set(comprimidosArray, forKey: "homeRemedioComprimidosArray")
defaults.set(horarioArray, forKey: "homeRemedioHorarioArray")
defaults.set(frequenciaArray, forKey: "homeRemedioFrequenciaArray")
defaults.set(photoArray, forKey: "homeRemedioPhotoArray")
}
public func homeRemediosFromUserDefaults(){
let defaults = UserDefaults.standard
let nomeArray = defaults.stringArray(forKey: "homeRemedioNomeArray") ?? [String]()
let dosagemArray = defaults.stringArray(forKey: "homeRemedioDosagemArray") ?? [String]()
let comprimidosArray = defaults.stringArray(forKey: "homeRemedioComprimidosArray") ?? [String]()
let horarioArray = defaults.stringArray(forKey: "homeRemedioHorarioArray") ?? [String]()
let frequenciaArray = defaults.stringArray(forKey: "homeRemedioFrequenciaArray") ?? [String]()
let photosArray = defaults.stringArray(forKey: "homeRemedioPhotoArray") ?? [String]()
if nomeArray.count > 0{
for i in 0..<nomeArray.count{
let image = convertBase64ToImage(imageString: photosArray[0])
let remedio: HomeRemedio = HomeRemedio(nome: nomeArray[i], photo: image, comprimidos: comprimidosArray[i], dosagem: dosagemArray[i], horario: stringToDate(string: horarioArray[i]), frequencia: stringToDate(string: frequenciaArray[i]))
Model.instance.homeRemedios.append(remedio)
}
}
}
public func savesRemedioData(){
var nomeArray: [String] = []
var photoArray: [String] = []
var dosagemArray: [String] = []
var comprimidosArray: [String] = []
var horarioArray: [String] = []
var frequenciaArray: [String] = []
var contraindicacaoArray: [String] = []
var dataDeValidade: [String] = []
var precoArray: [String] = []
var sintomasArray: [String] = []
var medidaArray: [String] = []
var hasPhotoArray: [Bool] = []
for remedio in allRemedios{
nomeArray.append(remedio.nome)
dosagemArray.append(remedio.dosagem)
comprimidosArray.append(remedio.comprimidos)
contraindicacaoArray.append(remedio.contraindicacao)
precoArray.append(remedio.preco)
sintomasArray.append(remedio.sintomas)
medidaArray.append(remedio.medida)
hasPhotoArray.append(remedio.hasPhoto)
if remedio.horario == nil{
horarioArray.append("")
}else{
horarioArray.append(dateToHHmmString(date: remedio.horario!))
}
if remedio.frequencia == nil{
frequenciaArray.append("")
}else{
frequenciaArray.append(dateToHHmmString(date: remedio.frequencia!))
}
if remedio.photo == nil{
photoArray.append("")
}else{
photoArray.append(convertImageToBase64(image: remedio.photo!))
}
}
let defaults = UserDefaults.standard
defaults.set(nomeArray, forKey: "allRemedioNomeArray")
defaults.set(dosagemArray, forKey: "allRemedioDosagemArray")
defaults.set(comprimidosArray, forKey: "allRemedioComprimidosArray")
defaults.set(horarioArray, forKey: "allRemedioHorarioArray")
defaults.set(frequenciaArray, forKey: "allRemedioFrequenciaArray")
defaults.set(contraindicacaoArray, forKey: "allRemedioContraindicacaoArray")
defaults.set(precoArray, forKey: "allRemedioPrecoArray")
defaults.set(sintomasArray, forKey: "allRemedioSintomasArray")
defaults.set(medidaArray, forKey: "allRemedioMedidaArray")
defaults.set(hasPhotoArray, forKey: "allRemedioHasPhotoArray")
defaults.set(photoArray, forKey: "homeRemedioPhotoArray")
}
public func allRemediosFromUserDefaults(){
let defaults = UserDefaults.standard
let nomeArray = defaults.stringArray(forKey: "allRemedioNomeArray") ?? [String]()
let dosagemArray = defaults.stringArray(forKey: "allRemedioDosagemArray") ?? [String]()
let comprimidosArray = defaults.stringArray(forKey: "allRemedioComprimidosArray") ?? [String]()
let horarioArray = defaults.stringArray(forKey: "allRemedioHorarioArray") ?? [String]()
let frequenciaArray = defaults.stringArray(forKey: "allRemedioFrequenciaArray") ?? [String]()
let contraindicacaoArray = defaults.stringArray(forKey: "allRemedioContraindicacaoArray") ?? [String]()
let precoArray = defaults.stringArray(forKey: "allRemedioPrecoArray") ?? [String]()
let sintomasArray = defaults.stringArray(forKey: "allRemedioSintomasArray") ?? [String]()
let medidaArray = defaults.stringArray(forKey: "allRemedioMedidaArray") ?? [String]()
let hasPhotoArray: [Bool] = defaults.array(forKey: "allRemedioHasPhotoArray") as? [Bool] ?? [Bool]()
let photosArray = defaults.stringArray(forKey: "homeRemedioPhotoArray") ?? [String]()
for i in 0..<nomeArray.count{
let remedio: Remedio = Remedio()
remedio.comprimidos = comprimidosArray[i]
remedio.nome = nomeArray[i]
remedio.dosagem = dosagemArray[i]
remedio.horario = stringToDate(string: horarioArray[i])
remedio.frequencia = stringToDate(string: frequenciaArray[i])
remedio.contraindicacao = contraindicacaoArray[i]
remedio.preco = precoArray[i]
remedio.sintomas = sintomasArray[i]
remedio.medida = medidaArray[i]
remedio.hasPhoto = hasPhotoArray[i]
remedio.photo = convertBase64ToImage(imageString: photosArray[i])
allRemedios.append(remedio)
}
}
public func stringToDate(string: String) -> Date?{
if string == ""{
return nil
}
let formatter = DateFormatter()
formatter.dateFormat = "HH:mm"
let yourDate = formatter.date(from: string)
return yourDate!
}
func dateToHHmmString(date: Date) -> String{
let formatter = DateFormatter()
formatter.dateFormat = "yyyy-MM-dd HH:mm:ss"
let myString = formatter.string(from: date)
let yourDate = formatter.date(from: myString)
formatter.dateFormat = "HH:mm"
let myStringafd = formatter.string(from: yourDate!)
return myStringafd
}
func convertImageToBase64(image: UIImage?) -> String {
if image == nil{
return ""
}
let imageData = image!.pngData()!
return imageData.base64EncodedString(options: Data.Base64EncodingOptions.lineLength64Characters)
}
func convertBase64ToImage(imageString: String) -> UIImage? {
if imageString == ""{
return nil
}
let imageData = Data(base64Encoded: imageString, options: Data.Base64DecodingOptions.ignoreUnknownCharacters)!
return UIImage(data: imageData)!
}
}
<file_sep>//
// TableViewCell.swift
// iRemedy
//
// Created by <NAME> on 13/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
class TableViewCell: UITableViewCell {
override func awakeFromNib() {
super.awakeFromNib()
// Initialization code
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
// Configure the view for the selected state
}
@IBOutlet weak var backgroundImageView: UIImageView!
}
<file_sep>//
// HomeCollectionViewCell.swift
// iRemedy
//
// Created by <NAME> on 09/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
class HomeCollectionViewCell: UICollectionViewCell {
@IBOutlet weak var remImageView: UIImageView!
@IBOutlet weak var nameLabel: UILabel!
@IBOutlet weak var quantidadeLabel: UILabel!
@IBOutlet weak var horarioLabel: UILabel!
}
<file_sep>//
// NameTableViewCell.swift
// iRemedy
//
// Created by <NAME> on 13/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
class NameTableViewCell: UITableViewCell {
override func awakeFromNib() {
super.awakeFromNib()
// Initialization code
nameQuestionTitle.text = "Nome do remédio"
nameQuestionSubtilte.text = "(maior nome escrito na caixa)"
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
// Configure the view for the selected state
}
@IBOutlet weak var cameraButton: UIButton!
@IBOutlet weak var nameTextField: UITextField!
@IBOutlet weak var nameQuestionSubtilte: UILabel!
@IBOutlet weak var nameQuestionTitle: UILabel!
}
<file_sep>//
// ObservacoesTableViewCell.swift
// iRemedy
//
// Created by <NAME> on 17/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
class ObservacoesTableViewCell: UITableViewCell {
private var sintomasSelected: String = ""
private var contraindicacaoSelected: String = ""
override func awakeFromNib() {
super.awakeFromNib()
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
// Configure the view for the selected state
}
@IBOutlet weak var sintomasTextField: UITextField!
@IBOutlet weak var contraindicacaoTextField: UITextField!
@IBAction func contraindicacaoTextField(_ sender: Any) {
print("entrou no contra")
if let chosenText = contraindicacaoTextField.text{
contraindicacaoSelected = chosenText
}else{
contraindicacaoSelected = ""
}
}
@IBAction func sintomasTextField(_ sender: Any) {
print("entrou no sint")
if let chosenText = sintomasTextField.text{
sintomasSelected = chosenText
}else{
sintomasSelected = ""
}
}
public func getSintomasSelected() -> String{
return sintomasSelected
}
public func getContraindicacoesSelected() -> String{
return contraindicacaoSelected
}
}
<file_sep>//
// File.swift
// iRemedy
//
// Created by <NAME> on 09/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
<file_sep>//
// DosagemTableViewCell.swift
// iRemedy
//
// Created by <NAME> on 14/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
class DosagemTableViewCell: UITableViewCell, UIPickerViewDataSource, UIPickerViewDelegate {
@IBOutlet weak var dosagemTextField: UITextField!
@IBOutlet weak var pickerView: UIPickerView!
@IBOutlet weak var comprimidosStepper: UIStepper!
@IBOutlet weak var numeroDeComprimidosLabel: UILabel!
let medidasOptions: [String] = ["mg", "g", "µg"]
static var medidasSelected: String = ""
static var dosagemSelected: String = ""
static var comprimidosSelected: Int = 0
func numberOfComponents(in pickerView: UIPickerView) -> Int {
return 1
}
func pickerView(_ pickerView: UIPickerView, numberOfRowsInComponent component: Int) -> Int {
return medidasOptions.count
}
func pickerView(_ pickerView: UIPickerView, titleForRow row: Int, forComponent component: Int) -> String? {
return medidasOptions[row]
}
func pickerView(_ pickerView: UIPickerView, didSelectRow row: Int, inComponent component: Int) {
DosagemTableViewCell.medidasSelected = medidasOptions[row]
}
public func getMedidaEscolhida() -> String{
return DosagemTableViewCell.medidasSelected
}
@IBAction func dosagemTextField(_ sender: Any) {
print("entrou no dosagem")
if let dosagem = dosagemTextField.text{
DosagemTableViewCell.dosagemSelected = dosagem
}
}
override func awakeFromNib() {
super.awakeFromNib()
// Initialization code
pickerView.delegate = self
pickerView.dataSource = self
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
}
@IBAction func stepperPressed(_ sender: Any) {
DosagemTableViewCell.comprimidosSelected = Int(comprimidosStepper.value)
numeroDeComprimidosLabel.text = String(DosagemTableViewCell.comprimidosSelected)
}
}
<file_sep>//
// remedios.swift
// iRemedy
//
// Created by <NAME> on 12/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
class HomeRemedio{
var appDelegate = UIApplication.shared.delegate as? AppDelegate
internal init(nome: String?, photo: UIImage?, comprimidos: String?, dosagem: String?, horario: Date?, frequencia: Date?) {
self.nome = nome
self.photo = photo
self.dosagem = dosagem
self.comprimidos = comprimidos
self.horario = horario
self.frequencia = frequencia
}
var nome: String?
var photo: UIImage?
var dosagem: String?
var comprimidos: String?
var horario: Date?
var frequencia: Date?
public func hasPhoto() -> Bool{
if photo == nil{
return false
}
return true
}
func dateToHHmmString(date: Date) -> String{
let formatter = DateFormatter()
// initially set the format based on your datepicker date / server String
formatter.dateFormat = "yyyy-MM-dd HH:mm:ss"
let myString = formatter.string(from: date) // string purpose I add here
// convert your string to date
let yourDate = formatter.date(from: myString)
//then again set the date format whhich type of output you need
formatter.dateFormat = "HH:mm"
// again convert your date to string
let myStringafd = formatter.string(from: yourDate!)
return myStringafd
}
public func dateToSeconds(date: Date) -> Int{
let formatter = DateFormatter()
// initially set the format based on your datepicker date / server String
formatter.dateFormat = "yyyy-MM-dd HH:mm:ss"
let myString = formatter.string(from: date) // string purpose I add here
// convert your string to date
let yourDate = formatter.date(from: myString)
//then again set the date format whhich type of output you need
formatter.dateFormat = "mm"
// again convert your date to string
let myStringafd = formatter.string(from: yourDate!)
let asInt = Int(myStringafd)! * 60 + hoursToSeconds(date: date)
return asInt
}
private func hoursToSeconds(date: Date) -> Int{
let formatter = DateFormatter()
// initially set the format based on your datepicker date / server String
formatter.dateFormat = "yyyy-MM-dd HH:mm:ss"
let myString = formatter.string(from: date) // string purpose I add here
// convert your string to date
let yourDate = formatter.date(from: myString)
//then again set the date format whhich type of output you need
formatter.dateFormat = "HH"
// again convert your date to string
let myStringafd = formatter.string(from: yourDate!)
let asInt = Int(myStringafd)! * 3600
return asInt
}
public func setHomeRemedio(){
Model.instance.homeRemedios.append(self)
setNotifications(remedio: self)
}
private func setNotifications(remedio: HomeRemedio){
var notificationDate: Date = remedio.horario!
let notificationFrequencia: Double = Double(remedio.dateToSeconds(date: remedio.frequencia!))
let iterations: Int = Int((24*60*60)/Double(notificationFrequencia))
for i in 0...iterations{
if i < 10{
self.appDelegate?.scheduleNotification(remedio: remedio, notificationDate: notificationDate, iteration: i)
notificationDate = Date(timeInterval: notificationFrequencia, since: notificationDate)
}
}
}
}
<file_sep>//
// RemedioTableViewCell.swift
// iRemedy
//
// Created by <NAME> on 27/06/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
class RemedioTableViewCell: UITableViewCell {
@IBOutlet weak var nomeDoRemedioLabel: UILabel!
@IBOutlet weak var dosagemLabel: UILabel!
@IBOutlet weak var dataDeValidadeLabel: UILabel!
@IBOutlet weak var precoPagoLabel: UILabel!
@IBOutlet weak var contraindicacoesLabel: UILabel!
@IBOutlet weak var sintomasLabel: UILabel!
@IBOutlet weak var photoImageView: UIImageView!
override func awakeFromNib() {
super.awakeFromNib()
// Initialization code
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
// Configure the view for the selected state
}
}
| 8982756f86c5c5252affb94d1f0ad82c2de6bbc4 | [
"Swift"
] | 16 | Swift | joaoraffs/iMedicine | 875883b8ead6f4e419a2bd5426c36ca2af69450e | 79444ba4912f2b3116c81dc2c1e65502cadec706 | |
refs/heads/master | <repo_name>vedhashrees/generator-hapi-restful-version<file_sep>/generators/hapiApiService/index.js
const Path = require("path");
const config = require("konfig")({
path: Path.join(__dirname, "../config")
}).app;
const baseName = Path.basename(__dirname);
const Generator = require('yeoman-generator');
const { prompt } = require('inquirer');
const Mkdirp = require("mkdirp");
const chalk = require('chalk');
module.exports = class extends Generator {
constructor(args, opts) {
super(args, opts);
}
askFor() {
return this.prompt(config.q[baseName]).then((answers) => {
if (answers.apiPort === "0000") {
answers.apiPort = Math.floor(Math.random() * 10000 + 1);
}
Object.assign(this, answers);
});
}
createProjectWithVersionDir() {
this.destinationRoot(Path.join(this.appName, "v" + this.apiVersion));
if (this.fs.exists(this.destinationPath("server.js"))) {
console.log(chalk.red(`Already project ${chalk.cyan(this.appName)} has found in file system`));
process.exit(1);
}
}
copyProjectIndexes() {
this.fs.copyTpl(
this.templatePath("_package.json"),
this.destinationPath('package.json'),
{
appName: this.appName,
appVersion: this.apiVersion
}
);
this.fs.copyTpl(
this.templatePath("_npm-shrinkwrap.json"),
this.destinationPath('npm-shrinkwrap.json'),
{
appName: this.appName
}
);
this.fs.copy(
this.templatePath("_README.md"),
this.destinationPath('README.md')
);
this.fs.copyTpl(
this.templatePath("_server.js"),
this.destinationPath('server.js'),
{
apiPort: this.apiPort,
apiVersion: this.apiVersion
}
);
this.fs.copy(
this.templatePath('gulpfile.js'),
this.destinationPath('gulpfile.js')
);
this.fs.copy(
this.templatePath('.retireignore.json'),
this.destinationPath('.retireignore.json')
);
this.fs.copy(
this.templatePath('.editorconfig'),
this.destinationPath('.editorconfig')
);
this.fs.copy(
this.templatePath('LICENSE'),
this.destinationPath('LICENSE')
);
}
copyDocsDir() {
this.fs.copy(
this.templatePath('docs'),
this.destinationPath('docs')
);
}
createAppConfDirAndCopyAll() {
this.fs.copyTpl(
this.templatePath("config/app.json"),
this.destinationPath('config/app.json'),
{
apiPort: this.apiPort,
basePrefix: this.basePrefix,
apiVersion: this.apiVersion
}
);
}
createAppDefaultModuleAndCopyAll() {
const filesName = ["router", "validator", "controller", "model", "query"];
for (let file in filesName) {
this.fs.copyTpl(
this.templatePath(`app/modules/default/default-${filesName[file]}.js`),
this.destinationPath("app/modules/"+ this.defaultModule +"/"+ this.defaultModule + `-${filesName[file]}.js`),
{
defaultModule: this.defaultModule
}
);
}
}
createAppRegisterDirAndCopyAll() {
this.fs.copy(
this.templatePath("app/register/app-register.js"),
this.destinationPath('app/register/app-register.js')
);
this.fs.copyTpl(
this.templatePath("app/register/app-router.js"),
this.destinationPath('app/register/app-router.js'),
{
defaultModule: this.defaultModule
}
);
this.installDependencies();
}
}
<file_sep>/generators/hapiApiService/templates/gulpfile.js
const gulp = require("gulp");
const { spawn } = require('child_process');
const gutil = require('gulp-util');
gulp.task("default", () => {
var child = spawn('retire', [], {
shell: true,
cwd: process.cwd()
});
child.stdout.setEncoding('utf8');
child.stdout.on('data', function (data) {
gutil.log(data);
});
child.stderr.setEncoding('utf8');
child.stderr.on('data', function (data) {
gutil.log(gutil.colors.red(data));
gutil.beep();
});
});
gulp.task('retireWatch', ['default'], function (done) {
// Watch all javascript files and package.json
gulp.watch(['app/**/*.js', 'server.js', 'package.json'], ['default']);
});
<file_sep>/generators/hapiApiService/templates/app/modules/default/default-query.js
exports.<%= defaultModule %>Query = {
testQuery() {
return "Hello World";
}
}
<file_sep>/README.md
# generator-hapi-restful-version
>This is a *yeoman generator* utility module to generate a sample hapi api service project.
#### Note: This is tailored for our project requirements.
## Installation
Install the following two packages globally as below.
```sh
$ npm install yo -g
$ npm install retire -g
$ npm install gulp -g
$ npm install generator-hapi-restful-version -g
```
## Usage:
1) To generate the skeleton of a Hapi REST API service node project , use the following command
```sh
$ yo hapi-restful-version:hapiApiService
```
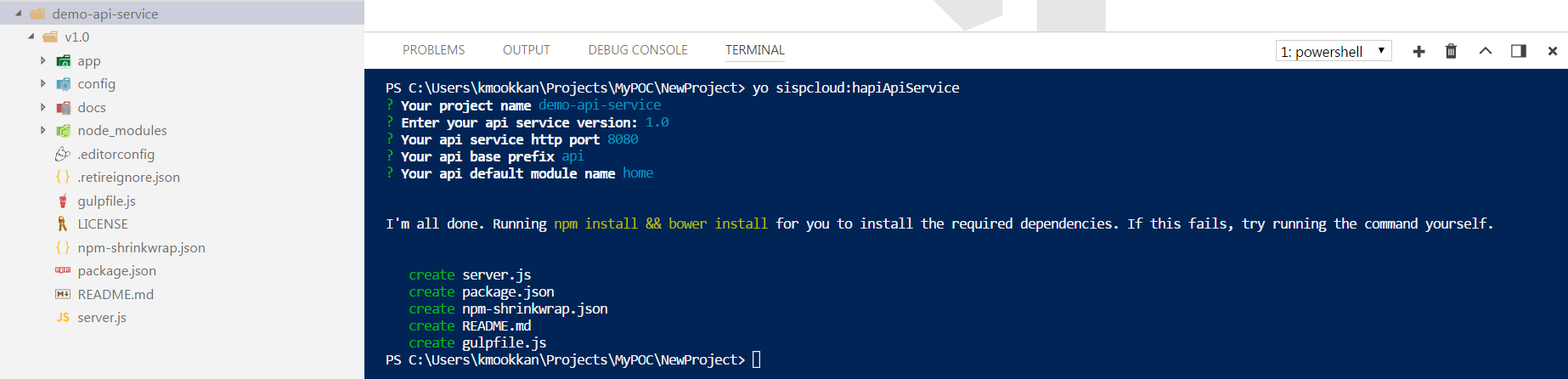
2) To generate a Hapi REST API service with a new version , use the following command
```sh
$ yo hapi-restful-version:hapiApiVersion
```
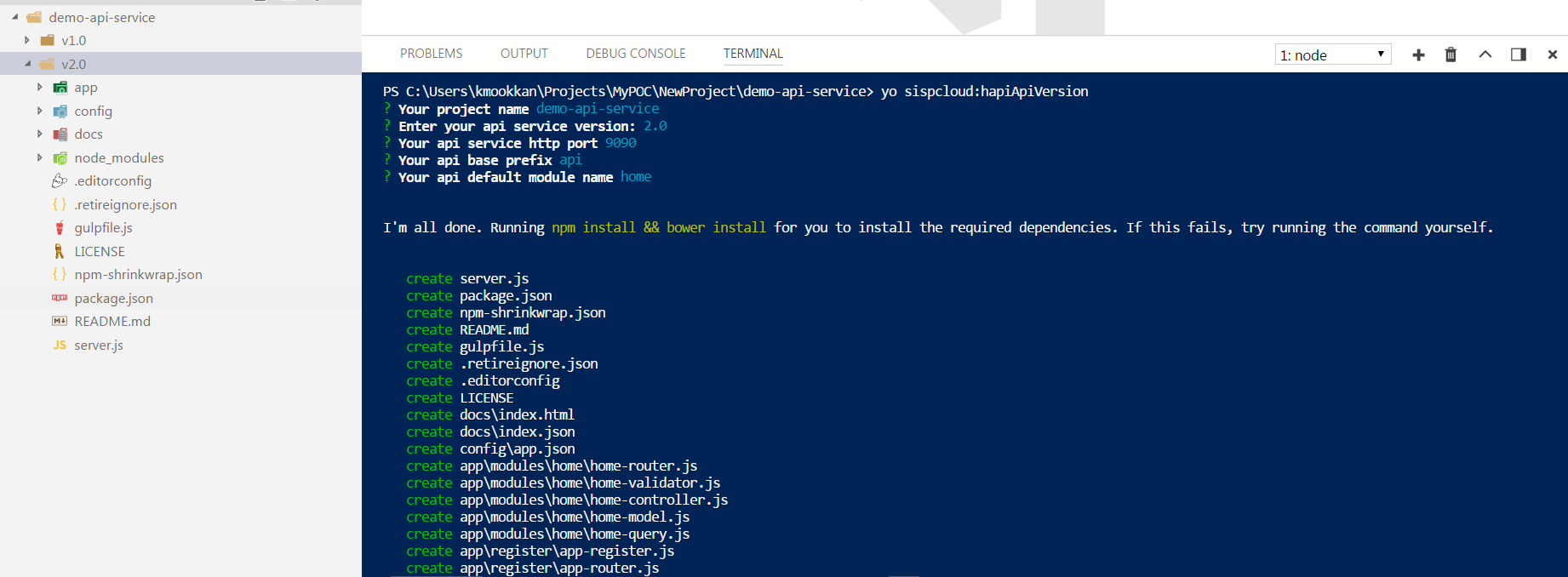
3) To generate a Hapi REST API service with a new module , use the following command
```sh
$ yo hapi-restful-version:hapiApiModule
```
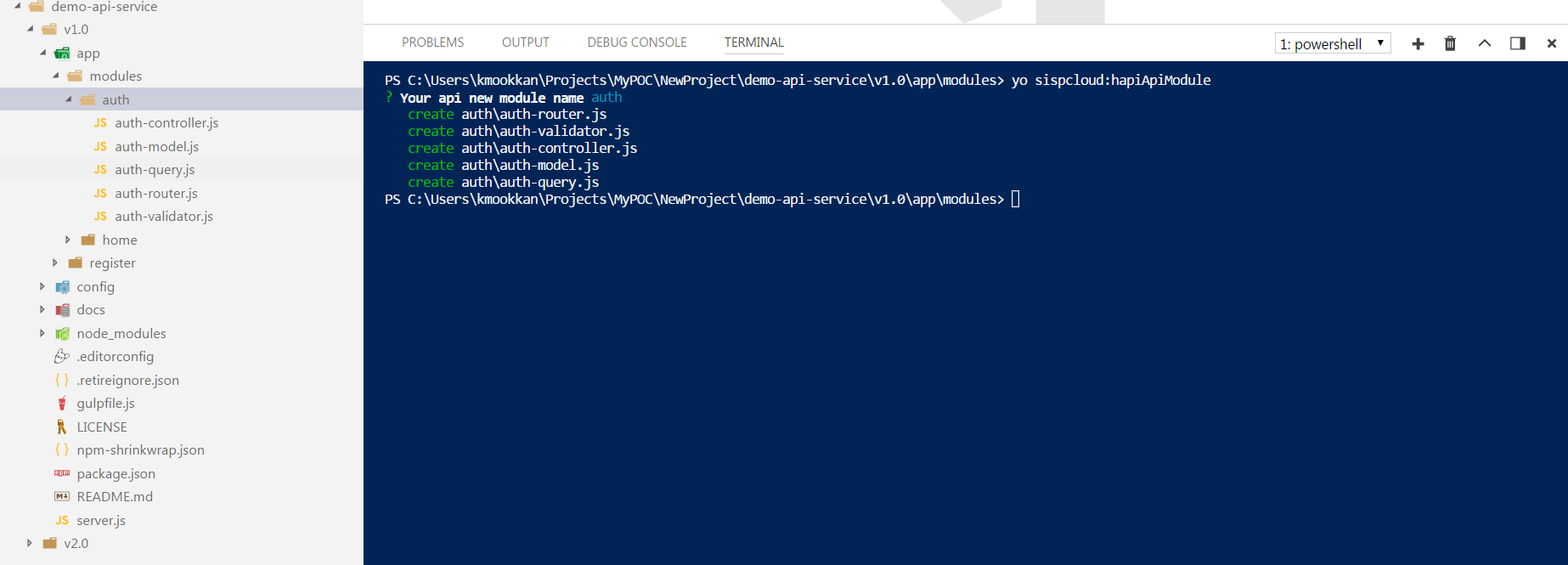
4) After the successful creation of new module `eg. auth` ,
goto `app-router.js` file in the folder path `app/register` and add the following line as below as highlighted in red in the screenshot.
```javascript
const {authRouter} = require(",,/modules/auth/auth-router");
```
Inside appRouter function add the following line.
```javascript
appRouter(server);
```
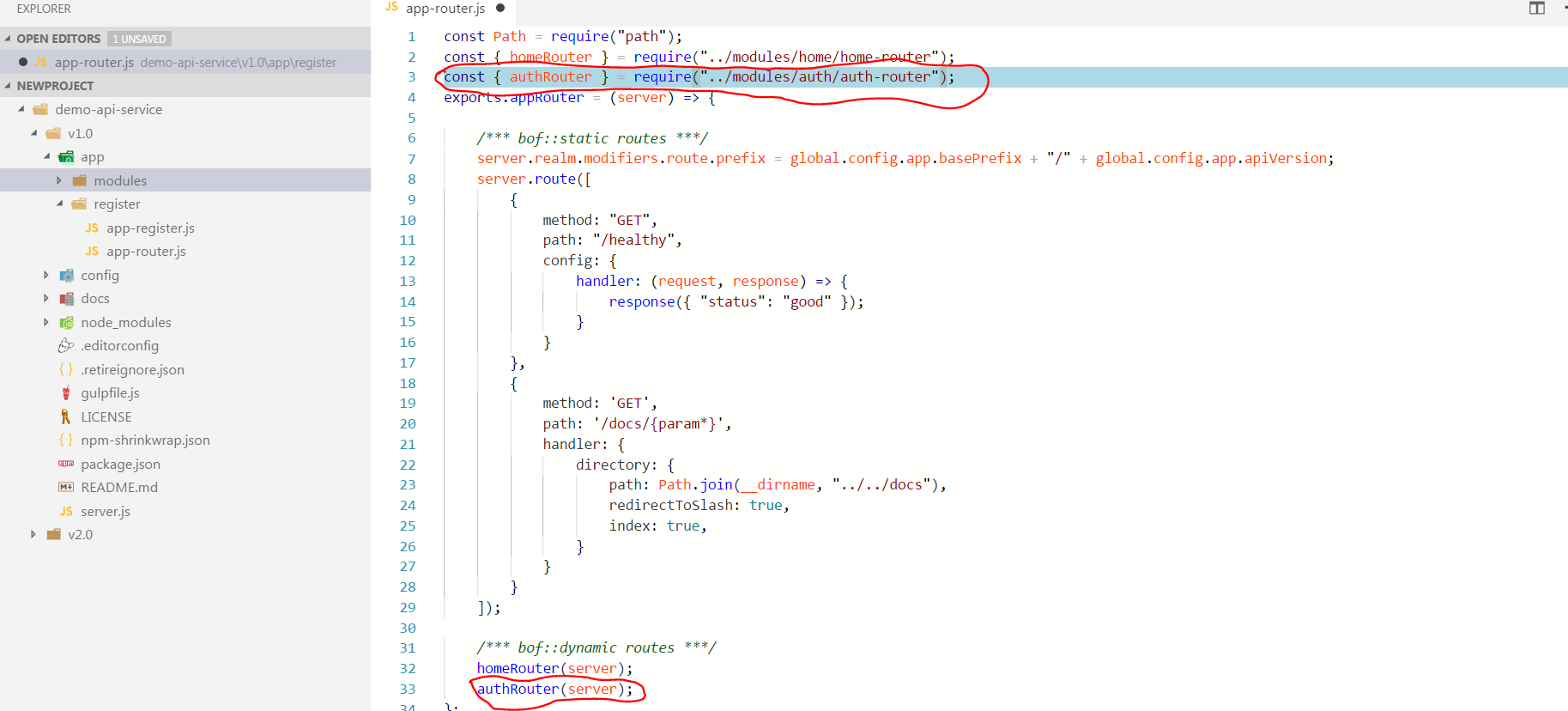
####Note: any project related config values can be initialized in `config\app.json`
To get the configuration values in the project , use as below
`eg . global.config.app.apiPort`
if the new config file is added in the name of user.json into config directory as `config\user.json`, then use as below `eg . global.config.user.name`
<file_sep>/generators/hapiApiModule/index.js
const Path = require("path");
const config = require("konfig")({
path: Path.join(__dirname, "../config")
}).app;
const baseName = Path.basename(__dirname);
const Generator = require('yeoman-generator');
const { prompt } = require('inquirer');
const Mkdirp = require("mkdirp");
const chalk = require('chalk');
module.exports = class extends Generator {
constructor(args, opts) {
super(args, opts);
}
askFor() {
return this.prompt(config.q[baseName]).then((answers) => {
if (answers.apiPort === "0000") {
answers.apiPort = Math.floor(Math.random() * 10000 + 1);
}
Object.assign(this, answers);
});
}
createProjectWithVersionDir() {
this.destinationRoot();
}
createAppDefaultModuleAndCopyAll() {
if (this.fs.exists(this.destinationPath(this.defaultModule + "/" + this.defaultModule + `-router.js`))) {
console.log(chalk.red(`Already api ${chalk.cyan(this.defaultModule)} module has found in file system.`));
process.exit(1);
}
const filesName = ["router", "validator", "controller", "model", "query"];
for (let file in filesName) {
this.fs.copyTpl(
this.templatePath(`app/modules/default/default-${filesName[file]}.js`),
this.destinationPath(this.defaultModule +"/"+ this.defaultModule + `-${filesName[file]}.js`),
{
defaultModule: this.defaultModule
}
);
}
}
}
<file_sep>/generators/hapiApiVersion/templates/app/modules/default/default-model.js
const { <%= defaultModule %>Query } = require("./<%= defaultModule %>-query");
exports.<%= defaultModule %>Model = {
testModel() {
return <%= defaultModule %>Query.testQuery();
}
};
<file_sep>/generators/hapiApiModule/templates/app/modules/default/default-router.js
exports.<%= defaultModule %>Router = (server) => {
const { <%= defaultModule %>Controller } = require("./<%= defaultModule %>-controller");
const { <%= defaultModule %>Validator } = require("./<%= defaultModule %>-validator");
// Prefix grouping specific route
server.realm.modifiers.route.prefix = global.config.app.basePrefix + "/" + global.config.app.apiVersion + "/<%= defaultModule %>";
server.route([
{
method: "GET",
path: "/",
config: {
handler: <%= defaultModule %>Controller.test
}
}
]);
};
<file_sep>/generators/hapiApiModule/templates/app/modules/default/default-validator.js
exports.<%= defaultModule %>Validator = {
}
<file_sep>/generators/hapiApiService/templates/app/modules/default/default-controller.js
const { <%= defaultModule %>Model } = require("./<%= defaultModule %>-model");
class <%= defaultModule %>Controller {
constructor() {
}
test(request, response) {
response("Hello Home Controller v1.0" + <%= defaultModule %>Model.testModel());
}
}
exports.<%= defaultModule %>Controller = new <%= defaultModule %>Controller();
| 69aab46e6204eea674cfdb463ee8a9650a737ced | [
"JavaScript",
"Markdown"
] | 9 | JavaScript | vedhashrees/generator-hapi-restful-version | 9072152a23a507f1c0f7e7aa6fa575078d0585d4 | 2e28e7816449ff393cd2173ceb89ff2398b9a7b4 | |
refs/heads/master | <file_sep>app.controller('ShowController', ['$scope', '$http', '$routeParams', '$location', function($scope, $http, $routeParams, $location) {
console.log("Show controller.");
$http.get('http://localhost:8080/api/swords/' + $routeParams.id).then(function(response) { // SHOW
$scope.sword = response.data;
console.log($scope.sword);
}, function(response) {
console.log("Invalid URL");
});
}]);
<file_sep>app.controller('NewController', ["$scope", '$http', '$location', function($scope, $http, $location){
console.log("New controller");
$scope.addSword = function(sword){
var sword = {
swordName: $scope.sword.swordName,
swordOwner: $scope.sword.swordOwner
}
$http.post('http://localhost:8080/api/swords/', sword).then(function(response) { // NEW
console.log("Sword added.");
$location.path( "/" );
}, function(response) {
console.log("Invalid URL");
});
}
}]);
<file_sep>app.controller('HomeController', ['$scope', '$http', function($scope, $http){
console.log("Home controller.");
$http.get('http://localhost:8080/api/swords/').then(function(response) { // INDEX
$scope.swords = response.data;
}, function(response) {
console.log("Invalid URL");
});
}]);
$scope.deleteSword = function(sword) { // DESTROY
console.log("Deleting sword.");
$http.delete('http://localhost:8080/api/swords/' + sword._id).then(function(response){
console.log("Sword deleted.");
$route.reload();
}, function(response) {
console.log("Failed to reload page.");
});
};
| b86f7063004f877302a4bb349385acd5e96fa369 | [
"JavaScript"
] | 3 | JavaScript | kimschles/angular-express-testapp | b37623420f9d7db152bc8927222762030e7b5959 | e59c7d344d724fde990d74b13c3149fb2e8e077b | |
refs/heads/main | <repo_name>jyoung9154/ITbank<file_sep>/Project/src/main/java/com/project/proto/dao/Dao.java
package com.project.proto.dao;
import java.util.List;
import org.apache.ibatis.session.SqlSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
@Repository
public class Dao {
@Autowired
private SqlSession sqlSession;
/*전체 사원 수*/
public List<Dao> selectMember() {
return sqlSession.selectList("selectList");
}
}
<file_sep>/Project/src/main/java/com/project/proto/command/Main.java
package com.project.proto.command;
import org.springframework.ui.Model;
import com.project.proto.dao.Dao;
public class Main implements Command {
@Override
public void execute(Model model,Dao dao) {
// TODO Auto-generated method stub
model.addAttribute("member",dao.selectMember());
}
}
<file_sep>/README.md
# ITbank
팀 프로젝트 저장소
<file_sep>/Project/src/main/java/com/project/proto/MainController.java
package com.project.proto;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import com.project.proto.command.Command;
import com.project.proto.command.Main;
import com.project.proto.dao.Dao;
@Controller
public class MainController {
Command comm;
@Autowired
Dao dao;
@RequestMapping(value = "/")
public String main(Model model) {
comm = new Main();
comm.execute(model,dao);
return "main";
}
}
| e0f4e2c5eebc2939fed65b4a8b8a91951e5156d2 | [
"Markdown",
"Java"
] | 4 | Java | jyoung9154/ITbank | 6aa100398892a8c3c67770ca659d040b0cf54565 | de900420beaefa320d30b6f745c7c85b4f506bd4 | |
refs/heads/master | <file_sep>use notify;
use notify::{RecommendedWatcher, Watcher, RecursiveMode, op};
use notify::DebouncedEvent::*;
use std::sync::mpsc::channel;
use std::time::Duration;
use std::net::UdpSocket;
use std::net;
use std::env;
use std::path::PathBuf;
use std::process::exit;
pub fn listen_and_broadcast(socket: &UdpSocket, bind_addr: &net::SocketAddrV4) {
println!("It's stream time");
if let Err(e) = watch(socket, bind_addr) {
println!("error: {:?}", e)
}
}
fn watch(socket: &UdpSocket, bind_addr: &net::SocketAddrV4) -> notify::Result<()> {
let (tx, rx) = channel();
let mut watcher: RecommendedWatcher = try!(Watcher::new(tx, Duration::from_secs(2)));
let path: PathBuf = env::current_dir().expect("Current directory is invalid for watching");
try!(watcher.watch(path.as_path(), RecursiveMode::Recursive));
loop {
match rx.recv() {
Ok(event) => {
let data = data(event);
println!("sending data to {}: {:?}", &bind_addr, &data);
socket.send_to(data.as_bytes(), bind_addr).expect("couldn't send message");
},
Err(e) => println!("watch error: {:?}", e)
}
}
}
fn data(event: notify::DebouncedEvent) -> String {
match event {
NoticeWrite(x) => data_format(op::WRITE, x),
NoticeRemove(x) => data_format(op::REMOVE, x),
Create(x) => data_format(op::CREATE, x),
Write(x) => data_format(op::WRITE, x),
Chmod(x) => data_format(op::CHMOD, x),
Remove(x) => data_format(op::REMOVE, x),
Rename(_, x) => data_format(op::RENAME, x),
Rescan => exit(1),
Error(_, _) => exit(1)
}
}
fn data_format(event: notify::Op, path: PathBuf) -> String {
format!("{:?}|{}", event, path.display())
}
<file_sep>[package]
name = "ven"
description = "Virtual Event Network, to recreate file system events from a host machine onto a virtual machine."
version = "0.1.0"
authors = ["<NAME>"]
[dependencies]
docopt = "0.8.1"
serde = "1.0.16"
serde_derive = "1.0.16"
notify = "4.0.0"
<file_sep>#![feature(slice_patterns)]
#[macro_use]
extern crate serde_derive;
extern crate docopt;
extern crate notify;
mod actor;
mod stream;
use docopt::Docopt;
use std::process::exit;
use std::str::FromStr;
use std::net::UdpSocket;
use std::net;
static USAGE: &'static str = "
Usage:
ven [--stream | --actor] -b <bind_address>
ven [-s | -a]
ven [-s | -a] -b <bind_address>
ven (-h | --help)
ven (-v | --version)
Flags:
-s, --stream Stream mode to stream OS events to a bound connection [default]
-a, --actor Actor mode for reading events form the port and triggering events
-h, --help Prints help information
-v, --version Prints version information
Options:
-b <bind_address> The address and port you want to send to and listen from [default: 127.0.0.1:34254]
-r <broadcast_address> A host socket to send from if broadcasting and receiving from the same machine. Not normally required [default: 127.0.0.1:45243]
";
#[derive(Debug, Deserialize)]
struct Args {
flag_s: bool,
flag_a: bool,
flag_v: bool,
flag_b: Option<String>,
flag_r: Option<String>
}
fn addr_parser(maybe_addr: Option<String>) -> net::SocketAddrV4 {
match net::SocketAddrV4::from_str(&maybe_addr.unwrap()) {
Ok(x) => x,
Err(e) => {
println!("Could not parse the provided address as a valid IPv4: {}", e);
exit(1)
}
}
}
fn main() {
let args: Args = Docopt::new(USAGE)
.and_then(|d| d.deserialize())
.unwrap_or_else(|e| e.exit());
let bind_addr = addr_parser(args.flag_b);
let broadcast_addr = addr_parser(args.flag_r);
if args.flag_v {
println!("{} {}", env!("CARGO_PKG_NAME"), env!("CARGO_PKG_VERSION"));
exit(0);
} else if args.flag_a {
let socket = UdpSocket::bind(bind_addr).expect("couldn't bind to address");
actor::listen_and_mimic(&socket);
} else {
let socket = UdpSocket::bind(broadcast_addr).expect("couldn't bind to address");
stream::listen_and_broadcast(&socket, &bind_addr);
};
}
<file_sep>ven - A virtual event network
=====
<img src="https://raw.githubusercontent.com/brianp/ven/master/ven.png" alt="A Venn Diagram containing nothing at all" align="right" />
## We're here to recreate file system events from a host machine onto a virtual machine. Quickly.
Ever ran a vm with a shared directory? Ever made a change to a file on the host
machine, expecting a fs event to trigger in the VM?
Then *ven* is for you.
(I'm looking at you vagrant shared directory and guard | grunt | gulp | watcher systems.)
## Setup
### It takes two
The program runs in one of two modes. Stream, or Actor. And requires you to run each
mode in a differnt place.
#### Stream
The stream mode binds to a local udp port (broadcast address) and sits around
listening for OS events. When it receives one it broadcasts the event to the
bind address. That's it. Listen, and yell.
#### Actor
The actor hangs around bound to the bind address just waiting for a juicy
event to come in. When it gets one, it tries its hardest to mimic it.
Recreating a local fs event on a different system.
### Setup the Stream on the system you'd like to capture events _from_
`ven --stream`
### Setup the Actor on the system you'd like to to trigger events _on_
`ven --actor`
Now sit back and watch it play out
## Usage Options
```shell
Usage:
ven [--stream | --actor] -b <bind_address>
ven [-s | -a]
ven [-s | -a] -b <bind_address>
ven (-h | --help)
ven (-v | --version)
Flags:
-s, --stream Stream mode to stream OS events to a bound connection [default]
-a, --actor Actor mode for reading events form the port and triggering events
-h, --help Prints help information
-v, --version Prints version information
Options:
-b <bind_address> The address and port you want to send to and listen from [default: 127.0.0.1:34254]
-r <broadcast_address> A host socket to send from if broadcasting and receiving from the same machine. Not normally required [default: 127.0.0.1:45243]
```
## Copyright
Copyright (c) 2017-2018 <NAME>. See [LICENSE](https://github.com/brianp/ven/blob/master/LICENSE) for further details.
<file_sep>use std::net::UdpSocket;
use std::path::PathBuf;
use std::process::{Command, exit};
use notify::op;
use notify;
pub fn listen_and_mimic(socket: &UdpSocket) {
println!("listening started, ready to accept");
let mut buf = [0; 64000];
println!("Reading data");
loop {
let result = socket.recv_from(&mut buf);
drop(socket);
match result {
Ok((amt, src)) => {
let data = Vec::from(&buf[..amt]);
let string = String::from_utf8_lossy(&data);
println!("received data from {}: {}", src, &string);
execute(string.into_owned());
},
Err(err) => panic!("Read error: {}", err)
}
}
}
fn execute(data: String) {
let vec: Vec<&str> = data.split("|").collect();
let file: PathBuf = PathBuf::from(vec[1]);
let file_str: &str = file.to_str().expect("Couldn't parse file path");
match vec[0] {
"CREATE" => {
Command::new("sed")
.args(&["-n", "", "-i", file_str])
.spawn()
.expect("sed command failed to start");
},
&_ => {
Command::new("touch")
.args(&["-r", file_str, file_str])
.spawn()
.expect("touch command failed to start");
}
}
}
| f996017b5b3bb598eae346357a24e34054292abb | [
"TOML",
"Rust",
"Markdown"
] | 5 | Rust | brianp/ven | e1b20124443d6f26c91ba2c2834d4c28c74ced4f | 03bd594f9a225d776b0c8cbe08f5d03914d6e262 | |
refs/heads/master | <repo_name>CodeLegacy/RestuarantReviewer<file_sep>/seeds.js
var mongoose = require("mongoose")
var Blog = require("./models/blogs"),
Comment = require("./models/comment"),
data = [
{
title:"France",
image: "https://encrypted-tbn0.gstatic.com/images?q=tbn%3AANd9GcSIe6Xu8hGwEmaP4fb3HKwCSPFZhUF-9uTHwx6_pu6YDmjMboON",
body:"Not of France"
},{
title: "India",
image: "https://encrypted-tbn0.gstatic.com/images?q=tbn%3AANd9GcTbWts0RTdJiP2p_Vv59V6x7LcvtG5wFC-LfXY8HKA0BgyrtVzx",
body: "Not of India"
},{
title: "Italy",
image: "https://images.livemint.com/img/2019/09/23/600x338/hotels-kZnG--621x414@LiveMint_1569216860852.jpg",
body: "Not of Italy"
}
]
function seedDB(){
Blog.remove({}, function (err) {
if (err) {
console.log(err)
}
console.log("removed hotels")
data.forEach(function (seed) {
Blog.create(seed, function (err, hotel) {
if (err) {
console.log(err)
} else {
console.log("added a hotel")
}
})
})
})
}
module.exports = seedDB<file_sep>/app.js
var express = require("express")
app =express()
bodyParser=require("body-parser")
expressSanitizer=require("express-sanitizer")
mongoose=require("mongoose")
methodOverride=require("method-override")
passport = require("passport")
LocalStrategy = require("passport-local")
Blog = require("./models/blogs")
User = require("./models/user")
Comment = require("./models/comment")
seedDB = require("./seeds")
seedDB()
//mongoose.connect("mongodb://localhost/restaurant2", { useNewUrlParser: true,useUnifiedTopology:true })
//mongoose.connect("mongodb + srv://Samay:@cluster<EMAIL>/test?retryWrites=true&w=majority", { useNewUrlParser: true, useUnifiedTopology: true })
//const MongoClient = require('mongodb').MongoClient;
//const uri = "mongodb+srv://Samay:<EMAIL>/test?retryWrites=true&w=majority";
const uri = process.env.DATABASEURL
mongoose.connect(uri,{
useNewUrlParser: true, useUnifiedTopology: true
})
// const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true });
// client.connect(err => {
// const collection = client.db("test").collection("devices");
// // perform actions on the collection object
// client.close();
// })
mongoose.connection.on("connected",() => {
console.log("Mongoose is connected!!!!!")
})
app.set("view engine","ejs")
app.use(express.static("public"))
app.use(bodyParser.urlencoded({extended: true}))
app.use(expressSanitizer())
app.use(methodOverride("_method"))
app.use(require("express-session")({
secret: "Just like that",
resave: false,
saveUninitialized: false
}))
app.use(passport.initialize())
app.use(passport.session())
passport.use(new LocalStrategy(User.authenticate()))
passport.serializeUser(User.serializeUser())
passport.deserializeUser(User.deserializeUser())
app.use(function (req, res, next) {
res.locals.currentUser = req.user
next()
})
app.get("/",function(req,res){
Blog.find({},function(err,blogs){
if(err){
console.log("ERROR!")
}
else{
res.render("home",{blogs:blogs,currentUser:req.user})
}
})
})
app.get("/blogs",function(req,res){
if(req.query.search){
const regex= new RegExp(escapeRegex(req.query.search),'gi')
Blog.find({title: regex}, function (err, blogs) {
if (err) {
console.log("ERROR!!")
}
else {
res.render("index", { blogs: blogs,currentUser:req.user })
}
})
}else{
Blog.find({},function(err,blogs){
if(err){
console.log("ERROR!!")
}
else{
res.render("index",{blogs:blogs})
}
})
}}
)
app.get("/blogs/register", function (req, res) {
Blog.find({}, function (err, blogs) {
if (err) {
console.log("ERROR!!")
} else {
res.render("register")
}
})
})
app.post("/blogs/register",function(req,res){
var newUser = new User({username:req.body.username})
User.register(newUser,req.body.password, function(err,user){
if(err){
console.log(err)
return res.render("register")
}
passport.authenticate("local")(req,res,function(){
res.redirect("/")
})
})
})
app.get("/blogs/login", function (req, res) {
Blog.find({}, function (err, blogs) {
if (err) {
console.log("ERROR!!")
}
else {
res.render("login", { blogs: blogs })
}
})
})
app.post("/blogs/login", passport.authenticate("local",
{
successRedirect:"/",
failureRedirect:"/blogs/login"
}),function(req,res){
})
app.get("/blogs/new",function(req,res){
res.render("new")
})
app.post("/blogs",function(req,res){
req.body.blog.body = req.sanitize(req.body.blog.body)
Blog.create(req.body.blog,function(err,newBlog){
if(err)
{
res.render("new")
}
else{
res.redirect("/blogs")
}
})
})
app.get("/blogs/:id",function(req,res){
Blog.findById(req.params.id).populate("comments").exec(function(err,foundBlog){
if(err)
{
res.redirect("/blogs")
}
else
{
// console.log(foundBlog )
res.render("show",{blog:foundBlog})
}
})
})
app.get("/blogs/:id/edit",function(req,res){
Blog.findById(req.params.id,function(err,foundBlog){
if(err)
{
res.redirect("/blogs")
}
else
{
res.render("edit",{blog:foundBlog})
}
})
})
app.put("/blogs/:id",function(req,res){
req.body.blog.body = req.sanitize(req.body.blog.body)
Blog.findByIdAndUpdate(req.params.id,req.body.blog,function(err,updatedBlog){
if(err)
{
res.redirect("/blogs")
}
else{
res.redirect("/blogs/"+req.params.id)
}
})
})
app.delete("/blogs/:id",function(req,res)
{
Blog.findByIdAndRemove(req.params.id,function(err){
if(err){
res.redirect("/blogs")
}
else
{
res.redirect("/blogs")
}
})
})
app.get("/logout",function(req,res){
req.logout()
res.redirect("/")
})
//COMMENT ROUTES
app.get("/blogs/:id/comments/new",function(req,res){
Blog.findById(req.params.id,function(err,blog){
if(err)
console.log(err)
else{
res.render("comments/new",{blog:blog})
}
})
})
app.post("/blogs/:id/comments",function(req,res){
Blog.findById(req.params.id, function (err, blog) {
if (err)
{console.log(err)
res.redirect("/blogs")
}
else {
Comment.create(req.body.comment,function(err,comment){
if(err){
console.log(err)
}
else{
blog.comments.push(comment)
blog.save();
res.redirect('/blogs/'+blog._id)
}
})
}
})
})
function escapeRegex(text){
return text.replace(/[-[\]{}()*+?.,\\^$|#\s]/g,"\\s&")
}
app.listen(process.env.PORT,process.env.IP,function(){
console.log("Server is Running")
})<file_sep>/README.md
# RestaurantReviewer
<p>This project focuses on the development of restaurant reviewer , a website where one can search for hotels and can give or read the reviews given also about the menu , timing of the restuarant and location of the restaurant.</p>
<h2>WebPage Link: <a href="https://agile-everglades-67068.herokuapp.com">Go to Site</a></h2>
<h1>Prerequirements:</h1>
<ul>
<li>nodejs</li>
<li>npm</li>
<li>mongo</li>
</ul>
<h2>Packages to be installed </h2>
<ul>
<li>passport</li>
<li>express</li>
<li>mongoose</li>
<li>body-parser</li>
<li>ejs</li>
</ul>
<h3>To login as admin, type your username as "Admin"</h3>
| 808e186f586457356f63a3cdd32c8bfd3bda4200 | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | CodeLegacy/RestuarantReviewer | 5fc86113d48ddccac80f21dedbf3def9c029d107 | 769ea5026eff32f666231f54b228c611f5d1b078 | |
refs/heads/master | <file_sep># daily-bible-reader
for reading a part of Bible in every day's mass.
<file_sep>import datetime
import sys
import requests
import re
from bs4 import BeautifulSoup
def today_string():
today = datetime.date.today()
if today.month > 9:
today_string_out = str(today.month)
else:
today_string_out = "0"+str(today.month)
if today.day > 9:
today_string_out = today_string_out + str(today.day)
else:
today_string_out = today_string_out + "0" + str(today.day)
year_string = str(today.year)
today_string_out = today_string_out + year_string[2] + year_string[3]
return today_string_out
def print_today_bible(daystring):
bible_reading_url='http://www.usccb.org/bible/readings/' + daystring + '.cfm'
r = requests.get(bible_reading_url)
html = r.text
soup = BeautifulSoup(html, "html.parser")
for each in soup.find_all(class_="bibleReadingsWrapper"):
if len(str(each.parent))>50:
out_text = re.sub(r'</em>|<em>|</br>|<strong>|</strong>|<div>|</div>|<h4>|</h4>|</a>|<br/>|<div¥ class=¥"bibleReadingsWrapper¥">|<div class=¥"¥">', " ", str(each.text))
out_text2 = re.sub(r'<br>', ' ', out_text)
print(str(out_text2))
else:
return "TWO"
return "OK"
print (datetime.date.today())
rtn = print_today_bible(today_string())
if rtn=="TWO": # for the feast of Assumption etc ?
print("Vigil Mass:")
print_today_bible(today_string()+"-vigil")
print("Day Mass:")
print_today_bible(today_string()+"-day-mass")
| 6d5c2edde54675e130bded122db82d086f78d101 | [
"Markdown",
"Python"
] | 2 | Markdown | jst223/daily-bible-reader | 88924d0777226e7c9a8a1e224b62ab5a52dd0667 | 12fb560a767f397f9f639ccec4a400e5946c0b80 | |
refs/heads/master | <file_sep>class Patient
attr_accessor :name, :appointments
def initialize(name)
@name =name
@appointments = []
end
def add_appointment(appointment)
@appointments << appointment
appointment.patient = self
end
def doctors
@appointments.collect{|appointment| appointment.doctor
}
end
end
| 404b2afceac5ebce65272777d39224ec9dfcea17 | [
"Ruby"
] | 1 | Ruby | illusioninlost/ruby-objects-has-many-through-lab-v-000 | 6ef7c66ffbc8d65b86c783a3b6bdf7293f863364 | 9097c0f0292d7be3e627a6d5862c67f87f28911d | |
refs/heads/master | <file_sep>/*
* Copyright 2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jboss.fuse.mvnd.logging.smart;
import java.util.List;
import java.util.Map;
import org.apache.maven.cli.logging.Slf4jLogger;
import org.apache.maven.eventspy.AbstractEventSpy;
import org.apache.maven.execution.ExecutionEvent;
import org.apache.maven.plugin.MojoExecution;
import org.apache.maven.project.MavenProject;
import org.jboss.fuse.mvnd.common.Message;
import org.jline.utils.AttributedString;
import org.slf4j.MDC;
import static org.jboss.fuse.mvnd.logging.smart.ProjectBuildLogAppender.KEY_PROJECT_ID;
public abstract class AbstractLoggingSpy extends AbstractEventSpy {
private static AbstractLoggingSpy instance;
public static AbstractLoggingSpy instance() {
if (instance == null) {
if ("mvns".equals(System.getProperty("mvnd.logging", "mvn"))) {
instance = new MavenLoggingSpy();
} else {
instance = new AbstractLoggingSpy() {
};
}
}
return instance;
}
public static void instance(AbstractLoggingSpy instance) {
AbstractLoggingSpy.instance = instance;
}
protected Map<String, ProjectBuild> projects;
protected List<Message.BuildMessage> events;
@Override
public synchronized void init(Context context) throws Exception {
}
@Override
public synchronized void close() throws Exception {
projects = null;
}
@Override
public void onEvent(Object event) throws Exception {
if (event instanceof ExecutionEvent) {
ExecutionEvent executionEvent = (ExecutionEvent) event;
switch (executionEvent.getType()) {
case SessionStarted:
notifySessionStart(executionEvent);
break;
case SessionEnded:
notifySessionFinish(executionEvent);
break;
case ProjectStarted:
notifyProjectBuildStart(executionEvent);
break;
case ProjectSucceeded:
case ProjectFailed:
case ProjectSkipped:
notifyProjectBuildFinish(executionEvent);
break;
case MojoStarted:
notifyMojoExecutionStart(executionEvent);
break;
case MojoSucceeded:
case MojoSkipped:
case MojoFailed:
notifyMojoExecutionFinish(executionEvent);
break;
default:
break;
}
}
}
protected void notifySessionStart(ExecutionEvent event) {
}
protected void notifySessionFinish(ExecutionEvent event) {
}
protected void notifyProjectBuildStart(ExecutionEvent event) {
onStartProject(getProjectId(event), getProjectDisplay(event));
}
protected void notifyProjectBuildFinish(ExecutionEvent event) throws Exception {
onStopProject(getProjectId(event), getProjectDisplay(event));
}
protected void notifyMojoExecutionStart(ExecutionEvent event) {
onStartMojo(getProjectId(event), getProjectDisplay(event));
}
protected void notifyMojoExecutionFinish(ExecutionEvent event) {
onStopMojo(getProjectId(event), getProjectDisplay(event));
}
protected void onStartProject(String projectId, String display) {
MDC.put(KEY_PROJECT_ID, projectId);
}
protected void onStopProject(String projectId, String display) {
MDC.remove(KEY_PROJECT_ID);
}
protected void onStartMojo(String projectId, String display) {
Slf4jLogger.setCurrentProject(projectId);
MDC.put(KEY_PROJECT_ID, projectId);
}
protected void onStopMojo(String projectId, String display) {
MDC.put(KEY_PROJECT_ID, projectId);
}
protected void onProjectLog(String projectId, String message) {
MDC.put(KEY_PROJECT_ID, projectId);
}
private String getProjectId(ExecutionEvent event) {
return event.getProject().getArtifactId();
}
private String getProjectDisplay(ExecutionEvent event) {
String projectId = getProjectId(event);
String disp = event.getMojoExecution() != null
? ":" + projectId + ":" + event.getMojoExecution().toString()
: ":" + projectId;
return disp;
}
public void append(String projectId, String event) {
String msg = event.endsWith("\n") ? event.substring(0, event.length() - 1) : event;
onProjectLog(projectId, msg);
}
protected static class ProjectBuild {
MavenProject project;
volatile MojoExecution execution;
@Override
public String toString() {
MojoExecution e = execution;
return e != null ? ":" + project.getArtifactId() + ":" + e.toString() : ":" + project.getArtifactId();
}
public AttributedString toDisplay() {
return new AttributedString(toString());
}
public String projectId() {
return project.getArtifactId();
}
}
}
<file_sep># Changelog
## [Unreleased](https://github.com/mvndaemon/mvnd/tree/HEAD)
[Full Changelog](https://github.com/mvndaemon/mvnd/compare/0.0.6...HEAD)
**Closed issues:**
- The output of modules being built in parallel is interleaved [\#78](https://github.com/mvndaemon/mvnd/issues/78)
- Show test output while running [\#77](https://github.com/mvndaemon/mvnd/issues/77)
- Explain project better in README [\#75](https://github.com/mvndaemon/mvnd/issues/75)
**Merged pull requests:**
- Honor the -X / --debug / --quiet arguments on the command line [\#90](https://github.com/mvndaemon/mvnd/pull/90) ([gnodet](https://github.com/gnodet))
- Fix display [\#88](https://github.com/mvndaemon/mvnd/pull/88) ([gnodet](https://github.com/gnodet))
- Use Visual Studio 2019 pre-installed on Windows CI workers to save some [\#84](https://github.com/mvndaemon/mvnd/pull/84) ([ppalaga](https://github.com/ppalaga))
- Use a maven proxy for integration tests to speed them up [\#83](https://github.com/mvndaemon/mvnd/pull/83) ([gnodet](https://github.com/gnodet))
- Improvements [\#81](https://github.com/mvndaemon/mvnd/pull/81) ([gnodet](https://github.com/gnodet))
- Replace the jpm library with the jdk ProcessHandle interface, \#36 [\#80](https://github.com/mvndaemon/mvnd/pull/80) ([gnodet](https://github.com/gnodet))
- Provide smarter output on the client, fixes \#77 [\#79](https://github.com/mvndaemon/mvnd/pull/79) ([gnodet](https://github.com/gnodet))
- Explain project better in README \#75 [\#76](https://github.com/mvndaemon/mvnd/pull/76) ([ppalaga](https://github.com/ppalaga))
## [0.0.6](https://github.com/mvndaemon/mvnd/tree/0.0.6) (2020-09-29)
[Full Changelog](https://github.com/mvndaemon/mvnd/compare/0.0.5...0.0.6)
**Closed issues:**
- CachingProjectBuilder ignored [\#72](https://github.com/mvndaemon/mvnd/issues/72)
- Goals of mvnd [\#71](https://github.com/mvndaemon/mvnd/issues/71)
- Keep a changelog file [\#64](https://github.com/mvndaemon/mvnd/issues/64)
**Merged pull requests:**
- Wait for the deamon to become idle before rebuilding in UpgradesInBom… [\#74](https://github.com/mvndaemon/mvnd/pull/74) ([ppalaga](https://github.com/ppalaga))
- CachingProjectBuilder ignored [\#73](https://github.com/mvndaemon/mvnd/pull/73) ([ppalaga](https://github.com/ppalaga))
- Added a changelog automatic update gh action [\#70](https://github.com/mvndaemon/mvnd/pull/70) ([oscerd](https://github.com/oscerd))
- Fixup publishing new versions via sdkman vendor API \#67 [\#69](https://github.com/mvndaemon/mvnd/pull/69) ([ppalaga](https://github.com/ppalaga))
## [0.0.5](https://github.com/mvndaemon/mvnd/tree/0.0.5) (2020-09-17)
[Full Changelog](https://github.com/mvndaemon/mvnd/compare/0.0.4...0.0.5)
**Closed issues:**
- Publish new versions via sdkman vendor API [\#67](https://github.com/mvndaemon/mvnd/issues/67)
- Cannot re-use daemon with sdkman java 8.0.265.hs-adpt [\#65](https://github.com/mvndaemon/mvnd/issues/65)
- List mvnd on sdkman.io [\#48](https://github.com/mvndaemon/mvnd/issues/48)
**Merged pull requests:**
- Publish new versions via sdkman vendor API [\#68](https://github.com/mvndaemon/mvnd/pull/68) ([ppalaga](https://github.com/ppalaga))
- Cannot re-use daemon with sdkman java 8.0.265.hs-adpt [\#66](https://github.com/mvndaemon/mvnd/pull/66) ([ppalaga](https://github.com/ppalaga))
- Upgrade to GraalVM 20.2.0 [\#62](https://github.com/mvndaemon/mvnd/pull/62) ([ppalaga](https://github.com/ppalaga))
## [0.0.4](https://github.com/mvndaemon/mvnd/tree/0.0.4) (2020-08-20)
[Full Changelog](https://github.com/mvndaemon/mvnd/compare/0.0.3...0.0.4)
**Closed issues:**
- Question: when running mvnd, it looks like regular mvn is still running [\#60](https://github.com/mvndaemon/mvnd/issues/60)
- Question: is there a way to limit the concurrency? [\#59](https://github.com/mvndaemon/mvnd/issues/59)
**Merged pull requests:**
- Allow \<mvnd.builder.rule\> entries to be separated by whitespace [\#61](https://github.com/mvndaemon/mvnd/pull/61) ([ppalaga](https://github.com/ppalaga))
## [0.0.3](https://github.com/mvndaemon/mvnd/tree/0.0.3) (2020-08-15)
[Full Changelog](https://github.com/mvndaemon/mvnd/compare/0.0.2...0.0.3)
**Closed issues:**
- Require Java 8+ instead of Java 11+ at runtime [\#56](https://github.com/mvndaemon/mvnd/issues/56)
- Using MAVEN\_HOME may clash with other tools [\#53](https://github.com/mvndaemon/mvnd/issues/53)
- Could not notify CliPluginRealmCache [\#49](https://github.com/mvndaemon/mvnd/issues/49)
**Merged pull requests:**
- Use amd64 arch label also on Mac [\#58](https://github.com/mvndaemon/mvnd/pull/58) ([ppalaga](https://github.com/ppalaga))
## [0.0.2](https://github.com/mvndaemon/mvnd/tree/0.0.2) (2020-08-14)
[Full Changelog](https://github.com/mvndaemon/mvnd/compare/0.0.1...0.0.2)
**Closed issues:**
- differences between `mvn clean install` and `mvnd clean install` [\#25](https://github.com/mvndaemon/mvnd/issues/25)
**Merged pull requests:**
- Fix \#56 Require Java 8+ instead of Java 11+ at runtime [\#57](https://github.com/mvndaemon/mvnd/pull/57) ([ppalaga](https://github.com/ppalaga))
- Include native clients in platform specific distros [\#55](https://github.com/mvndaemon/mvnd/pull/55) ([ppalaga](https://github.com/ppalaga))
- Fix \#53 Using MAVEN\_HOME may clash with other tools [\#54](https://github.com/mvndaemon/mvnd/pull/54) ([ppalaga](https://github.com/ppalaga))
- Add curl -L flag to cope with redirects [\#51](https://github.com/mvndaemon/mvnd/pull/51) ([fvaleri](https://github.com/fvaleri))
- Fix \#49 Could not notify CliPluginRealmCache [\#50](https://github.com/mvndaemon/mvnd/pull/50) ([ppalaga](https://github.com/ppalaga))
## [0.0.1](https://github.com/mvndaemon/mvnd/tree/0.0.1) (2020-07-30)
[Full Changelog](https://github.com/mvndaemon/mvnd/compare/844f3ddd7f4278b2ba097d817def4c3b46d574e7...0.0.1)
**Closed issues:**
- Running maven daemon fails on windows [\#35](https://github.com/mvndaemon/mvnd/issues/35)
- Add some integration tests [\#23](https://github.com/mvndaemon/mvnd/issues/23)
- Class loader clash during Quarkus augmentation [\#22](https://github.com/mvndaemon/mvnd/issues/22)
- Allow to plug a custom rule resolver [\#19](https://github.com/mvndaemon/mvnd/issues/19)
- Chaotic output if the console's height is less then the number of threads [\#17](https://github.com/mvndaemon/mvnd/issues/17)
- Concurrency issues while handling log events in the daemon [\#16](https://github.com/mvndaemon/mvnd/issues/16)
- Do not display exceptions stack trace when trying to stop already stopped daemons [\#15](https://github.com/mvndaemon/mvnd/issues/15)
- Concurrent access to the local repository [\#14](https://github.com/mvndaemon/mvnd/issues/14)
- Support for hidden dependencies [\#12](https://github.com/mvndaemon/mvnd/issues/12)
- mvnd should fail cleanly with unknown CLI options [\#11](https://github.com/mvndaemon/mvnd/issues/11)
- Properly configure logging in the client [\#10](https://github.com/mvndaemon/mvnd/issues/10)
- mvnd does not pick the BoM from the source tree [\#9](https://github.com/mvndaemon/mvnd/issues/9)
- Add a correct NOTICE file [\#8](https://github.com/mvndaemon/mvnd/issues/8)
- Do not require the mvn in PATH to come from mvnd dist [\#7](https://github.com/mvndaemon/mvnd/issues/7)
- Provide an option to kill/stop the daemon [\#6](https://github.com/mvndaemon/mvnd/issues/6)
- Options / system properties are not reset in the daemon after a build [\#5](https://github.com/mvndaemon/mvnd/issues/5)
- Add a LICENSE file [\#4](https://github.com/mvndaemon/mvnd/issues/4)
- The mvn output appears all at once at the very end [\#3](https://github.com/mvndaemon/mvnd/issues/3)
- mvnd -version does not work [\#2](https://github.com/mvndaemon/mvnd/issues/2)
- mvnd fails if there is no .mvn/ dir in the user home [\#42](https://github.com/mvndaemon/mvnd/issues/42)
- Cannot clean on Windows as long as mvnd keeps a plugin from the tree loaded [\#40](https://github.com/mvndaemon/mvnd/issues/40)
- Maven mojo change ignored [\#33](https://github.com/mvndaemon/mvnd/issues/33)
**Merged pull requests:**
- Fix \#42 mvnd fails if there is no .mvn/ dir in the user home [\#46](https://github.com/mvndaemon/mvnd/pull/46) ([ppalaga](https://github.com/ppalaga))
- Fix \#40 Cannot clean on Windows as long as mvnd keeps a plugin from t… [\#45](https://github.com/mvndaemon/mvnd/pull/45) ([ppalaga](https://github.com/ppalaga))
- Add code formatter plugins [\#44](https://github.com/mvndaemon/mvnd/pull/44) ([ppalaga](https://github.com/ppalaga))
\* *This Changelog was automatically generated by [github_changelog_generator](https://github.com/github-changelog-generator/github-changelog-generator)*
| c08ff2bb33d3d59969a9aa9d5c7a67b20078106f | [
"Markdown",
"Java"
] | 2 | Java | zulkar/mvnd | 68fcf3d7333a7c9ddcd873a5a73f52b1cbe1e2d8 | 786cb426d188413da68028155eccd4c1f0343cbb | |
refs/heads/dev | <file_sep>
const types = {
SINGLE: 0,
};
const collection = {
ding: {
src: "ding.mp3",
type: types.SINGLE,
label: "Ding",
}
};
export { collection };<file_sep>
import '../scss/index.scss';
import Vue from 'vue';
import PingApp from '../components/PingApp.vue';
const app = new Vue( {
el: '#app',
components: {
PingApp,
},
} );
<file_sep># Ping!
[](https://opensource.org/licenses/MIT)
[](https://david-dm.org/nandenjin/ping)
[](https://david-dm.org/nandenjin/ping?type=dev)
[](https://travis-ci.org/nandenjin/ping)
### Play with Sounds.
| 44245af646d21a6b89a8e67fb1f58a0f2470c33a | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | nandenjin/ping | d1e376c20b1cec8c3ae481d26b6855a43008b191 | 841f9acc7394b0fe7beb3cb1bc7914eece7a8644 | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace JasChess {
public partial class Pensando : Form {
DateTime inicio;
public Pensando() {
InitializeComponent();
this.inicio = System.DateTime.Now;
}
int estado = 0;
private void timer1_Tick(object sender, EventArgs e) {
if(estado == 0) {
Opacity -= 0.07;
}
else {
Opacity += 0.07;
}
estado = Opacity == 0 || Opacity == 1 ? 1 - estado : estado;
}
//public int tempo = 0;
private void timer2_Tick(object sender, EventArgs e) {
Busca.x = DateTime.Now.Subtract(inicio).TotalSeconds;
}
}
}
<file_sep># JasChess
Xadrez didático desenvolvido durante projeto de Iniciação Científica
<file_sep>using System;
using System.Drawing;
using System.Windows.Forms;
using static JasChess.Jogo;
using static JasChess.Pecas;
using static JasChess.Tabuleiro;
namespace JasChess {
public partial class GUI : Form {
public GUI() {
InitializeComponent();
}
private void pictureBox_MouseEnter(object sender, EventArgs e) {
PictureBox c = sender as PictureBox;
c.Image = Properties.Resources.selecao;
c.Cursor = c.BackgroundImage != null || c.BackColor == proxV ? Cursors.Hand : Cursors.Arrow;
int ind;
for(ind = 0; ind < 64; ind++)
if(casa[ind] == (PictureBox)sender) {
if(casa[ind].BackgroundImage != null)
tsFoco.Text = Nome(ind);
break;
}
}
private void pictureBox_MouseLeave(object sender, EventArgs e) {
PictureBox c = sender as PictureBox;
c.Image = null;
tsFoco.Text = null;
}
private void tsJNH_Click(object sender, EventArgs e) {
NovoJogo(false);
tsVez.Text = "Vez das: Brancas";
}
private void tsNJH_Click(object sender, EventArgs e) {
NovoJogo(true);
tsVez.Text = "Vez das: Brancas";
}
private void tsSobre_Click(object sender, EventArgs e) {
new Sobre().ShowDialog();
}
public static Color atual = Color.Gold;
public static Color proxV = Color.PaleGreen;
public static Color proxO = Color.IndianRed;
public static Color antO = Color.LightSkyBlue;
public static Color antD = Color.PowderBlue;
public static int lino;
public static int colo;
public static int lind;
public static int cold;
public static bool flag = false, temSom = true;
private void tocarSonsToolStripMenuItem_Click(object sender, EventArgs e) {
tocarSonsToolStripMenuItem.Checked = !tocarSonsToolStripMenuItem.Checked;
temSom = tocarSonsToolStripMenuItem.Checked;
}
private void GUI_Load(object sender, EventArgs e) {
}
private void pictureBox_Click(object sender, EventArgs e) {
int ind;
int lin = -1;
int col = -1;
for(ind = 0; ind < 64; ind++) {
if(casa[ind] == (PictureBox)sender) {
lin = lind = ind / 8 + 2;
col = cold = ind % 8 + 2;
break;
}
}
if(!TemCoisa(lin, col) && !flag)
ResetCor();
else if(flag && (casa[ind].BackColor == proxO || casa[ind].BackColor == proxV)) {
Jogar();
tsVez.Text = "Vez das: " + new string[] { "Brancas", "Pretas" }[jogador];
}
else if(TemCoisa(lin, col) && Cor(lin, col) == jogador) {
ResetCor();
casa[ind].BackColor = atual;
lino = ind / 8 + 2;
colo = ind % 8 + 2;
flag = true;
MostrarPosicoes(lin, col);
}
else ResetCor();
}
}
}
<file_sep>using System.Drawing;
using static JasChess.Properties.Resources;
using static JasChess.Tabuleiro;
namespace JasChess {
public class Pecas {
public const int PEAO_BRANCO = 10;
public const int BISPO_BRANCO = 20;
public const int CAVALO_BRANCO = 30;
public const int TORRE_BRANCA = 40;
public const int DAMA_BRANCA = 50;
public const int REI_BRANCO = 60;
public const int PEAO_PRETO = 11;
public const int BISPO_PRETO = 21;
public const int CAVALO_PRETO = 31;
public const int TORRE_PRETA = 41;
public const int DAMA_PRETA = 51;
public const int REI_PRETO = 61;
public const int PEAO = 1;
public const int BISPO = 2;
public const int CAVALO = 3;
public const int TORRE = 4;
public const int DAMA = 5;
public const int REI = 6;
public const int BRANCA = 0;
public const int PRETA = 1;
public static int[] rei = new int[2];
public static Image[,] peca = { { _10, _20, _30, _40, _50, _60 }, { _11, _21, _31, _41, _51, _61 } };
public static int[,] ATAQUES_PEAO = new int[68, 6];
public static int[,] ATAQUES_BISPO = new int[92, 28];
public static int[,] ATAQUES_CAVALO = new int[92, 8];
public static int[,] ATAQUES_TORRE = new int[92, 28];
public static int Cor(int peca) {
return peca % 10;
}
public static int Cor(int lin, int col) {
return tabuleiro[lin, col] % 10;
}
public static int Tipo(int lin, int col) {
return tabuleiro[lin, col] / 10;
}
public static int Tipo(int peca) {
return peca / 10;
}
public static int Quantidade() {
int q = 0;
for(int i = 2; i < 9; i++)
for(int j = 2; j < 9; j++)
if(TemCoisa(i, j)) q++;
return q;
}
public static int Quantidade(int cor) {
int q = 0;
for(int i = 2; i < 9; i++)
for(int j = 2; j < 9; j++)
if(TemCoisa(i, j) && Cor(i,j) == cor) q++;
return q;
}
public static bool TemPar(int peca) {
bool achou = false;
for(int i = 2; i < 10; i++)
for(int j = 2; j < 10; j++)
if(tabuleiro[i,j] == peca)
if(achou) return true;
else achou = true;
return false;
}
public static string Nome(int pos) {
pos = (pos / 8+2) * 12 + (pos % 8+2);
string[] tipo = { "Peão", "Bispo", "Cavalo", "Torre", "Rainha", "Rei" };
string[] cor = { "branco", "preto", "branca", "preta"};
if(TemCoisa(pos / 12, pos % 12) && ÉValida(pos / 12, pos % 12)) {
int a = Tipo(pos / 12, pos % 12) - 1;
int b = a == TORRE-1 || a == DAMA -1? Cor(pos / 12, pos % 12) + 2 : Cor(pos / 12, pos % 12);
return tipo[a] + " " + cor[b];
} else
return null;
}
public static void Promover(int jog) {
int lin = 7 * jog + 2;
for(int col = 2; col < 10; col++) {
if(Tipo(lin, col) == PEAO) {
if(!Jogo.humano && Cor(lin,col) == PRETA) {
tabuleiro[lin, col] = DAMA_PRETA;
casa[(lin - 2) * 8 + col - 2].BackgroundImage = peca[PRETA, DAMA - 1];
} else {
Promocao p = new Promocao();
p.ShowDialog();
tabuleiro[lin, col] = (p.nova + 1) * 10 + jog;
casa[(lin - 2) * 8 + col - 2].BackgroundImage = peca[jog, p.nova];
}
if(GUI.temSom) Jogo.sons[1].Play();
}
}
}
public static void AtribuirValores() {
int lin, col;
int[] bispo = { -1, 1 };
int[,] cavalo = { { -1, 1 }, { 2, -2 } };
int[] torreA = { -1, 0, 1, 0 };
int[] torreB = { 0, 1, 0, -1 };
for(int cont = 0, x = 26, y = 0; cont < 92; cont++, x++, y = 0) {
// CAVALO
for(int i = 0; i <= 1; i++)
for(int j = 0; j <= 1; j++)
for(int k = 0; k <= 1; k++) {
lin = cavalo[i, j] + x / 12;
col = cavalo[1 - i, k] + x % 12;
ATAQUES_CAVALO[cont, y++] = lin * 12 + col;
}
y = 0;
// TORRE
for(int i = 1; i < 8; i++)
for(int j = 0; j < 4; j++, y++) {
lin = (i * torreA[j]) + x / 12;
col = (i * torreB[j]) + x % 12;
if((lin * 12 + col > -1) && (lin * 12 + col < 144))
ATAQUES_TORRE[cont, y] = lin * 12 + col;
}
y = 0;
// BISPO
for(int k = 1; k < 8; k++)
for(int i = 0; i <= 1; i++)
for(int j = 0; j <= 1; j++, y++) {
lin = (k * bispo[i]) + x / 12;
col = (k * bispo[j]) + x % 12;
if((lin * 12 + col > -1) && (lin * 12 + col < 144))
ATAQUES_BISPO[cont, y] = lin * 12 + col;
}
}
// PEÃO
for(int cont = 0, x = 38, y = 0; cont < 68; cont++, x++, y = 0)
for(int i = -1; i < 2; i += 2)
for(int j = -1; j < 2; j++, y++) {
lin = i + x / 12;
col = j + x % 12;
ATAQUES_PEAO[cont, y] = lin * 12 + col;
}
}
}
}<file_sep>using System.Media;
using System.Windows.Forms;
using static JasChess.GUI;
using static JasChess.Movimentos;
using static JasChess.Pecas;
using static JasChess.Tabuleiro;
using static JasChess.Busca;
using static JasChess.Properties.Resources;
namespace JasChess {
class Jogo {
public const int XEQUE = -1;
public const int XEQUE_MATE = 0;
public const int REI_AFOGADO = 1;
public const int EMPATE = 2;
private static string[] sentenca = { "Xeque-mate", "Rei afogado", "Empate" };
private static string[] resultado = { "vitória das brancas", "vitória das pretas", "não houve vencedor" };
public static SoundPlayer[] sons = { new SoundPlayer(move), new SoundPlayer(promocao),
new SoundPlayer(xeque), new SoundPlayer(empate),
new SoundPlayer(uder), new SoundPlayer(uvi)};
private static int situacao = 3;
private static int vencedor = -1;
public static int jogador = 0;
public static bool humano = true;
public static void NovoJogo(bool human) {
IniciarTabuleiro();
jogador = 0;
humano = human;
gui.tsTempo.Visible = !humano;
if(temSom) sons[1].Play();
MessageBox.Show("Tudo pronto! As brancas começam.", "JasChess", MessageBoxButtons.OK, MessageBoxIcon.None);
}
public static void Jogar() {
FacaMovimento(lino, colo, lind, cold, true);
Promover(jogador);
lino = colo = -1;
flag = false;
jogador = 1 - jogador;
if(FimJogo(ref jogador, ref situacao, ref vencedor))
Finaliza();
else {
if(situacao == XEQUE) {
int a = (rei[jogador] / 12 - 2) * 8;
int b = a + (rei[jogador] % 12) - 2;
casa[b].BackColor = proxO;
if(temSom) sons[2].Play();
MessageBox.Show("Xeque!", "JasChess", MessageBoxButtons.OK, MessageBoxIcon.None);
}
if(jogador == PRETA && !humano) {
ComputadorJoga();
lino = colo = -1;
flag = false;
jogador = 1 - jogador;
if(FimJogo(ref jogador, ref situacao, ref vencedor))
Finaliza();
else {
if(situacao == XEQUE) {
int a = (rei[jogador] / 12 - 2) * 8;
int b = a + (rei[jogador] % 12) - 2;
casa[b].BackColor = proxO;
if(temSom) sons[2].Play();
MessageBox.Show("Xeque!", "JasChess", MessageBoxButtons.OK, MessageBoxIcon.None);
}
}
}
}
}
public static void MostrarPosicoes(int lin, int col) {
switch(Tipo(lin, col)) {
case PEAO:
for(int i = 0; i < 64; i++) {
int nil = i / 8 + 2;
int loc = i % 8 + 2;
if(MoverPeao(lin * 12 + col, nil * 12 + loc, jogador)) {
casa[i].BackColor = TemCoisa(nil, loc) ? proxO : proxV;
}
}
break;
case BISPO:
for(int i = 0; i < 64; i++) {
int nil = i / 8 + 2;
int loc = i % 8 + 2;
if(MoverBispo(lin * 12 + col, nil * 12 + loc, jogador)) {
casa[i].BackColor = TemCoisa(nil, loc) ? proxO : proxV;
}
}
break;
case CAVALO:
for(int i = 0; i < 64; i++) {
int nil = i / 8 + 2;
int loc = i % 8 + 2;
if(MoverCavalo(lin * 12 + col, nil * 12 + loc, jogador)) {
casa[i].BackColor = TemCoisa(nil, loc) ? proxO : proxV;
}
}
break;
case TORRE:
for(int i = 0; i < 64; i++) {
int nil = i / 8 + 2;
int loc = i % 8 + 2;
if(MoverTorre(lin * 12 + col, nil * 12 + loc, jogador)) {
casa[i].BackColor = TemCoisa(nil, loc) ? proxO : proxV;
}
}
break;
case DAMA:
for(int i = 0; i < 64; i++) {
int nil = i / 8 + 2;
int loc = i % 8 + 2;
if(MoverDama(lin * 12 + col, nil * 12 + loc, jogador)) {
casa[i].BackColor = TemCoisa(nil, loc) ? proxO : proxV;
}
}
break;
case REI:
for(int i = 0; i < 64; i++) {
int nil = i / 8 + 2;
int loc = i % 8 + 2;
if(MoverRei(lin * 12 + col, nil * 12 + loc, jogador)) {
casa[i].BackColor = TemCoisa(nil, loc) ? proxO : proxV;
}
}
break;
}
}
public static bool FimJogo(ref int jog, ref int sit, ref int venc) {
if(Xeque(jog)) {
if(!ExisteMovimentosValidos(jog)) {
sit = XEQUE_MATE;
venc = 1 - jog;
return true;
} else {
sit = XEQUE;
return false;
}
} else if(!ExisteMovimentosValidos(jog)) {
sit = REI_AFOGADO;
venc = 2;
return true;
} else if(Quantidade() == 2) {
sit = venc = EMPATE;
return true;
}
sit = 3;
return false;
}
static void Finaliza() {
if(temSom)
switch(situacao) {
case XEQUE_MATE:
int a = (rei[jogador] / 12 - 2) * 8;
int b = a + (rei[jogador] % 12) - 2;
casa[b].BackColor = proxO;
sons[5 - vencedor].Play();
break;
case REI_AFOGADO:
case EMPATE:
sons[3].Play();
break;
}
string msg = string.Format("{0}, {1}.", sentenca[situacao], resultado[vencedor]);
MessageBox.Show(msg, "\nFim de Jogo.", MessageBoxButtons.OK, MessageBoxIcon.None);
DialogResult d = MessageBox.Show("Iniciar nova partida?", "JasChess", MessageBoxButtons.YesNo, MessageBoxIcon.Question);
if(d == DialogResult.Yes)
NovoJogo(humano);
else
for(int i = 0; i < 64; casa[i++].Enabled = false) ;
}
}
}<file_sep>using static JasChess.Pecas;
using static JasChess.Tabuleiro;
namespace JasChess {
class Movimentos {
public static void FacaMovimento(int lin_o, int col_o, int lin_d, int col_d, bool real) {
if(Tipo(lin_o, col_o) == REI)
rei[Jogo.jogador] = lin_d * 12 + col_d;
tabuleiro[lin_d, col_d] = tabuleiro[lin_o, col_o];
tabuleiro[lin_o, col_o] = CASA_VAZIA;
if(real) {
ResetCor();
casa[(lin_o - 2) * 8 + col_o - 2].BackColor = GUI.antO;
casa[(lin_d - 2) * 8 + col_d - 2].BackColor = GUI.antD;
casa[(lin_d - 2) * 8 + col_d - 2].BackgroundImage = casa[(lin_o - 2) * 8 + col_o - 2].BackgroundImage;
casa[(lin_o - 2) * 8 + col_o - 2].BackgroundImage = null;
if(GUI.temSom) Jogo.sons[0].Play();
}
}
public static bool PeaoAtaca(int pos_o, int pos_d, int jogador) {
// VERIFICA SE O PEÃO DE jogador SE MOVE DE pos_o PARA pos_d
// *j <- -1, PARA O JOGADOR 0}
// *j <- 1, PARA O JOGADOR 1}
for(int i = 0, j = (2 * jogador - 1); i < 6; i++)
if(pos_d == ATAQUES_PEAO[pos_o - 38, i])
return (pos_o / 12 + j == pos_d / 12);
return false;
}
public static bool BispoAtaca(int pos_o, int pos_d, int max) {
int casa;
max = 4 * max - 1;
// VERIFICA SE O "BISPO" SE MOVE DE pos_o PARA pos_d
for(int i = 0; i <= max; i++) {
if(pos_d == ATAQUES_BISPO[pos_o - 26, i]) {
// VERIFICA SE HÁ ALGUMA PEÇA NO CAMINHO OU SE CHEGOU NO FIM DO TABULEIRO
for(int j = i % 4; j < i; j += 4) {
casa = ATAQUES_BISPO[pos_o - 26, j];
if(TemCoisa(casa / 12, casa % 12))
return false;
}
return true;
}
}
return false;
}
public static bool CavaloAtaca(int pos_o, int pos_d) {
for(int i = 0; i < 8; i++)
if(pos_d == ATAQUES_CAVALO[pos_o - 26, i])
return true;
return false;
}
public static bool TorreAtaca(int pos_o, int pos_d, int max) {
int casa;
max = 4 * max;
// VERIFICA SE A "TORRE" SE MOVE DE pos_o PARA pos_d
for(int i = 0; i < max; i++) {
if(pos_d == ATAQUES_TORRE[pos_o - 26, i]) {
// VERIFICA SE HÁ ALGUMA PEÇA NO CAMINHO OU SE CHEGOU NO FIM DO TABULEIRO
for(int j = i % 4; j < i; j += 4) {
casa = ATAQUES_TORRE[pos_o - 26, j];
if(TemCoisa(casa / 12, casa % 12))
return false;
}
return true;
}
}
return false;
}
public static bool Xeque(int jogador) {
// VERIFICA SE O REI DE jogador ESTÁ EM XEQUE
int x, y, oponente = 1 - jogador;
// ATAQUE POR CAVALO
for(int i = 0; i < 8; i++) {
x = ATAQUES_CAVALO[rei[jogador] - 26, i] / 12;
y = ATAQUES_CAVALO[rei[jogador] - 26, i] % 12;
if(Tipo(x, y) == CAVALO && Cor(x, y) == oponente)
return true;
}
// ATAQUE POR TORRE, REI OU RAINHA (EM LINHA RETA)
for(int i = 0; i < 4; i++) {
for(int j = i; j < 28; j += 4) {
x = (ATAQUES_TORRE[rei[jogador] - 26, j] / 12);
y = (ATAQUES_TORRE[rei[jogador] - 26, j] % 12);
if(i == j && tabuleiro[x, y] == 60 + oponente)
return true;
switch(Tipo(x, y)) {
case TORRE:
case DAMA:
if(TorreAtaca((x * 12 + y), rei[jogador], 7) && Cor(x, y) == oponente)
return true;
break;
}
}
}
// ATAQUE POR BISPO, REI OU RAINHA (EM DIAGONAL)
for(int i = 0; i < 4; i++)
for(int j = i; j < 28; j += 4) {
x = (ATAQUES_BISPO[rei[jogador] - 26, j] / 12);
y = (ATAQUES_BISPO[rei[jogador] - 26, j] % 12);
if(i == j && tabuleiro[x, y] == 60 + oponente)
return true;
switch(Tipo(x, y)) {
case BISPO:
case DAMA:
if(BispoAtaca((x * 12 + y), rei[jogador], 7) && Cor(x, y) == oponente)
return true;
break;
}
}
// ATAQUE POR PEÃO
for(int i = -1; i < 2; i += 2) {
x = (rei[jogador] / 12) + (2 * jogador - 1);
y = (rei[jogador] % 12) + i;
if(tabuleiro[x, y] == 10 + oponente)
return true;
}
return false;
}
public static bool ExisteMovimentosValidos(int jogador) {
// VERIFICA SE O jogador PODE FAZER ALGUM L<NAME>
int origem, destino;
for(int lin = 2; lin < 10; lin++)
for(int col = 2; col < 10; col++)
if(TemCoisa(lin, col) && (Cor(lin, col) == jogador)) {
origem = lin * 12 + col;
switch(Tipo(lin, col)) {
case PEAO:
for(int i = 0; i < 3; i++) {
destino = ATAQUES_PEAO[origem - 38, 3 * jogador + i];
if(MoverPeao(origem, destino, jogador))
return true;
}
if((lin == 2 && Cor(lin, col) == PRETA) || (lin == 9 && Cor(lin, col) == BRANCA)) {
destino = lin * 12 + col + (24 * (2 * jogador - 1));
if(MoverPeao(origem, destino, jogador))
return true;
}
break;
case CAVALO:
for(int i = 0; i < 8; i++) {
destino = ATAQUES_CAVALO[origem - 26, i];
if(MoverCavalo(origem, destino, jogador))
return true;
}
break;
case BISPO:
case DAMA:
for(int i = 0; i < 28; i++) {
destino = ATAQUES_BISPO[origem - 26, i];
if(MoverBispo(origem, destino, jogador))
return true;
}
if(Tipo(lin, col) == DAMA) goto case TORRE;
break;
case TORRE:
for(int i = 0; i < 28; i++) {
destino = ATAQUES_TORRE[origem - 26, i];
if(MoverTorre(origem, destino, jogador))
return true;
}
break;
case REI:
for(int i = -1; i < 2; i++) {
for(int j = -1; j < 2; j++) {
destino = (lin + i) * 12 + col + j;
if(ÉValida(lin + i, col + j))
if(MoverRei(origem, destino, jogador))
return true;
}
}
break;
}
}
return false;
}
public static bool Ilegal(int pos_o, int pos_d, int jogador) {
// EXECUTA O MOVIMENTO, VERIFICA SE O REI ESTARÁ EM XEQUE, DESFAZ A JOGADA
// RETORNA VERDADEIRO SE O REI FICARÁ EM XEQUE
int lin_o = pos_o / 12;
int col_o = pos_o % 12;
int lin_d = pos_d / 12;
int col_d = pos_d % 12;
int aux = tabuleiro[lin_d, col_d];
FacaMovimento(lin_o, col_o, lin_d, col_d, false);
bool ret = Xeque(jogador);
tabuleiro[lin_o, col_o] = tabuleiro[lin_d, col_d];
tabuleiro[lin_d, col_d] = aux;
return ret;
}
public static bool MoverPeao(int pos_o, int pos_d, int jogador) {
int lin_o = pos_o / 12;
int col_o = pos_o % 12;
int lin_d = pos_d / 12;
int col_d = pos_d % 12;
bool valido = !Ilegal(pos_o, pos_d, jogador) && (ÉValida(lin_d, col_d));
if(Tipo(lin_o, col_o) == PEAO)
// VERIFICA SE ESTÁ NA PRIMEIRA FILA
if((lin_o == (5 * (1 - jogador) + 3)) && (col_o == col_d)) {
int i = (2 * jogador - 1);
// UMA OU DUAS CASAS À FRENTE
for(int j = 1; j < 3; j++)
if((lin_o + i * j) == lin_d && j == 1)
return !TemCoisa(lin_d, col_d) && valido;
else if((lin_o + i * j) == lin_d && j == 2)
return !TemCoisa(lin_d, col_d) && valido && !TemCoisa(lin_d - i, col_d);
} else {
if(PeaoAtaca(pos_o, pos_d, jogador))
if(col_o == col_d)
// MOVIMENTO SIMPLES(PARA FRENTE)
return (!TemCoisa(lin_d, col_d)) && valido;
else
// MOVIMENTO DE CAPTURA (NA DIAGONAL)
return ((Cor(lin_d, col_d) != jogador) && TemCoisa(lin_d, col_d)) && valido;
}
return false;
}
public static bool MoverBispo(int pos_o, int pos_d, int jogador) {
int lin_o = pos_o / 12;
int col_o = pos_o % 12;
int lin_d = pos_d / 12;
int col_d = pos_d % 12;
// VERIFICA SE É BISPO OU RAINHA
if((Tipo(lin_o, col_o) == BISPO) || (Tipo(lin_o, col_o) == DAMA)) {
// VALIDAÇÃO DO MOVIMENTO
if(BispoAtaca(pos_o, pos_d, 7))
if((tabuleiro[lin_d, col_d] == CASA_VAZIA) || ((Cor(lin_d, col_d) != jogador) && (tabuleiro[lin_d, col_d] != CASA_NULA)))
return !Ilegal(pos_o, pos_d, jogador);
}
return false;
}
public static bool MoverCavalo(int pos_o, int pos_d, int jogador) {
int lin_o = pos_o / 12;
int col_o = pos_o % 12;
int lin_d = pos_d / 12;
int col_d = pos_d % 12;
// VERIFICA SE É CAVALO
if(Tipo(lin_o, col_o) == CAVALO) {
// VALIDAÇÃO DO MOVIMENTO
if(CavaloAtaca(pos_o, pos_d))
if((tabuleiro[lin_d, col_d] == CASA_VAZIA) || ((Cor(lin_d, col_d) != jogador) && (tabuleiro[lin_d, col_d] != CASA_NULA)))
return !Ilegal(pos_o, pos_d, jogador);
}
return false;
}
public static bool MoverTorre(int pos_o, int pos_d, int jogador) {
int lin_o = pos_o / 12;
int col_o = pos_o % 12;
int lin_d = pos_d / 12;
int col_d = pos_d % 12;
// VERIFICA SE É TORRE OU RAINHA
if((Tipo(lin_o, col_o) == TORRE) || (Tipo(lin_o, col_o) == DAMA)) {
// VALIDAÇÃO DO MOVIMENTO
if(TorreAtaca(pos_o, pos_d, 7))
if((tabuleiro[lin_d, col_d] == CASA_VAZIA) || ((Cor(lin_d, col_d) != jogador) && (tabuleiro[lin_d, col_d] != CASA_NULA)))
return !Ilegal(pos_o, pos_d, jogador);
}
return false;
}
public static bool MoverDama(int pos_o, int pos_d, int jogador) {
int lin_o = pos_o / 12;
int col_o = pos_o % 12;
int lin_d = pos_d / 12;
int col_d = pos_d % 12;
// VERIFICA SE É RAINHA
if(Tipo(lin_o, col_o) == DAMA)
if(((lin_o == lin_d) && (col_o != col_d)) || ((lin_o != lin_d) && (col_o == col_d)))
// MOVIMENTO EM LINHA RETA
return MoverTorre(pos_o, pos_d, jogador);
else
//MOVIMENTO EM DIAGONAL
return MoverBispo(pos_o, pos_d, jogador);
return false;
}
public static bool MoverRei(int pos_o, int pos_d, int jogador) {
int lin_o = pos_o / 12;
int col_o = pos_o % 12;
int lin_d = pos_d / 12;
int col_d = pos_d % 12;
if(!ÉValida(lin_d, col_d)) return false;
bool valido = false;
// VERIFICA SE É REI
if(Tipo(lin_o, col_o) == REI)
// PODE SE MOVIMENTAR
if(((lin_o == lin_d) && (col_o != col_d)) || ((lin_o != lin_d) && (col_o == col_d)))
// - EM LINHA RETA?
valido = TorreAtaca(pos_o, pos_d, 1);
else
// - EM DIAGONAL?
valido = BispoAtaca(pos_o, pos_d, 1);
if(valido) {
// VERIFICA SE O REI FICARÁ EM XEQUE
rei[jogador] = pos_d;
valido = !Ilegal(pos_o, pos_d, jogador);
valido &= !TemCoisa(lin_d, col_d) || ((Cor(lin_d, col_d) != jogador) && (ÉValida(lin_d, col_d)));
rei[jogador] = pos_o;
return valido;
}
return false;
}
}
}<file_sep>using System.Resources;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Runtime.InteropServices;
// General Information about an assembly is controlled through the following
// set of attributes. Change these attribute values to modify the information
// associated with an assembly.
[assembly: AssemblyTitle("JasChess")]
[assembly: AssemblyDescription("JasChess é um jogo de xadrez didático desenvolvido durante um trabalho de Iniciação Científica, com a lógica de movimentação baseada em pré-indicações para onde uma peça poderia se mover a partir de tal posição. A Inteligência Artificial presente está baseada na Busca em Profundidade, integrando os algoritmos Minimax e Poda Alfa-Beta com implementação na linguagem Microsoft Visual C#, sendo desenvolvida na versão 4.5.2 do Microsoft .NET Framework. {0}{0}Seus algoritmos foram inteiramente escritos por Josué Fonseca, sob a orientação do Me. <NAME>, com ajuda intelectual de <NAME> e do Dr. <NAME>. A interface gráfica foi programada pelo autor, sendo as peças desenhadas por <NAME>.")]
[assembly: AssemblyConfiguration("")]
[assembly: AssemblyCompany("IFSP - Campus Cubatão")]
[assembly: AssemblyProduct("JasChess")]
[assembly: AssemblyCopyright("© 2018 Josué Fonseca")]
[assembly: AssemblyTrademark("")]
[assembly: AssemblyCulture("")]
// Setting ComVisible to false makes the types in this assembly not visible
// to COM components. If you need to access a type in this assembly from
// COM, set the ComVisible attribute to true on that type.
[assembly: ComVisible(false)]
// The following GUID is for the ID of the typelib if this project is exposed to COM
[assembly: Guid("ea557216-784e-444b-8e60-7d2f34072d91")]
// Version information for an assembly consists of the following four values:
//
// Major Version
// Minor Version
// Build Number
// Revision
//
// You can specify all the values or you can default the Build and Revision Numbers
// by using the '*' as shown below:
// [assembly: AssemblyVersion("1.0.*")]
[assembly: AssemblyVersion("2.1.1.0")]
[assembly: AssemblyFileVersion("2.0.0.0")]
[assembly: NeutralResourcesLanguage("pt-BR")]
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using static JasChess.Pecas;
using static JasChess.Movimentos;
using static JasChess.Tabuleiro;
namespace JasChess {
class Heuristica {
public static int Avaliacao() {
int pontuacao = 0;
for(int lin = 2; lin < 10; lin++)
for(int col = 2; col < 10; col++)
if(TemCoisa(lin, col))
pontuacao += ValorDe(tabuleiro[lin, col], lin);
return pontuacao;
}
private static int ValorDe(int peca, int lin) {
int valor = 0;
switch(Tipo(peca)) {
case PEAO:
valor = ((Cor(peca) == PRETA && lin >= 5) || (Cor(peca) == BRANCA && lin <= 6)) ? 125 : 100;
valor = ((Cor(peca) == PRETA && lin == 9) || (Cor(peca) == BRANCA && lin == 2)) ? 500 : valor;
valor += int.Parse(Math.Pow(2, (Cor(peca) == PRETA ? lin - 2 : 9 - lin)).ToString());
break;
case BISPO:
case CAVALO:
valor = TemPar(peca) ? 325 : 300;
break;
case TORRE:
valor = TemPar(peca) ? 425 : 400;
break;
case DAMA:
valor = TemPar(peca) ? 900 : 500;
break;
case REI:
valor = Xeque(Cor(peca)) ? -2000 : 0;
break;
}
return Cor(peca) == BRANCA ? -valor : valor;
}
}
}<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace JasChess {
public partial class Promocao : Form {
public Promocao() {
InitializeComponent();
}
private int _nova;
public int nova {
get { return _nova; }
set { _nova = value; }
}
private void btn_Click(object sender, EventArgs e) {
Button b = sender as Button;
nova = b.TabIndex;
this.Close();
}
}
}<file_sep>[LocalizedFileNames]
tada.wav=@%windir%\system32\mmres.dll,-710
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using static JasChess.Program;
using static JasChess.Pecas;
namespace JasChess {
class Tabuleiro {
public const int CASA_VAZIA = 0;
public const int CASA_NULA = -1;
public static GUI gui = new GUI();
public static int[,] tabuleiro = new int[12, 12];
public static PictureBox[] casa = new PictureBox[64];
public static bool TemCoisa(int lin, int col) {
return tabuleiro[lin, col] != CASA_VAZIA;
}
public static bool ÉValida(int lin, int col) {
return tabuleiro[lin, col] != CASA_NULA;
}
public static void IniciarTabuleiro() {
int i = 0;
foreach(Control c in gui.Controls) {
if(c is PictureBox) {
casa[i] = c as PictureBox;
casa[i].Enabled = true;
casa[i++].BackgroundImage = null;
}
}
ResetCor();
// MOLDURA
for(int lin = 0; lin < 2; lin++)
for(int col = 0; col < 12; col++)
tabuleiro[lin, col] = CASA_NULA;
//--------
// PEÇAS PRETAS (COMPUTADOR)
{
tabuleiro[2, 0] = CASA_NULA;
tabuleiro[2, 1] = CASA_NULA;
tabuleiro[2, 2] = TORRE_PRETA; casa[0].BackgroundImage = peca[PRETA, TORRE - 1];
tabuleiro[2, 3] = CAVALO_PRETO; casa[1].BackgroundImage = peca[PRETA, CAVALO - 1];
tabuleiro[2, 4] = BISPO_PRETO; casa[2].BackgroundImage = peca[PRETA, BISPO - 1];
tabuleiro[2, 5] = DAMA_PRETA; casa[3].BackgroundImage = peca[PRETA, DAMA - 1];
tabuleiro[2, 6] = REI_PRETO; casa[4].BackgroundImage = peca[PRETA, REI - 1];
tabuleiro[2, 7] = BISPO_PRETO; casa[5].BackgroundImage = peca[PRETA, BISPO - 1];
tabuleiro[2, 8] = CAVALO_PRETO; casa[6].BackgroundImage = peca[PRETA, CAVALO - 1];
tabuleiro[2, 9] = TORRE_PRETA; casa[7].BackgroundImage = peca[PRETA, TORRE - 1];
tabuleiro[2, 10] = CASA_NULA;
tabuleiro[2, 11] = CASA_NULA;
tabuleiro[3, 0] = CASA_NULA;
tabuleiro[3, 1] = CASA_NULA;
for(int col = 2; col < 10; tabuleiro[3, col] = PEAO_PRETO, casa[col + 6].BackgroundImage = peca[PRETA, PEAO - 1], col++) ;
tabuleiro[3, 10] = CASA_NULA;
tabuleiro[3, 11] = CASA_NULA;
rei[1] = 30;
}
//--------
// MEIO DO TABULEIRO
for(int lin = 4; lin < 8; lin++) {
for(int col = 0; col < 2; tabuleiro[lin, col] = CASA_NULA, col++) ;
for(int col = 2; col < 10; tabuleiro[lin, col] = CASA_VAZIA, col++) ;
for(int col = 10; col < 12; tabuleiro[lin, col] = CASA_NULA, col++) ;
}
//--------
// <NAME> (<NAME>)
{
tabuleiro[8, 0] = CASA_NULA;
tabuleiro[8, 1] = CASA_NULA;
for(int col = 2; col < 10; tabuleiro[8, col] = PEAO_BRANCO, casa[col + 46].BackgroundImage = peca[BRANCA, PEAO - 1], col++) ;
tabuleiro[8, 10] = CASA_NULA;
tabuleiro[8, 11] = CASA_NULA;
tabuleiro[9, 0] = CASA_NULA;
tabuleiro[9, 1] = CASA_NULA;
tabuleiro[9, 2] = TORRE_BRANCA; casa[56].BackgroundImage = peca[BRANCA, TORRE - 1];
tabuleiro[9, 3] = CAVALO_BRANCO; casa[57].BackgroundImage = peca[BRANCA, CAVALO - 1];
tabuleiro[9, 4] = BISPO_BRANCO; casa[58].BackgroundImage = peca[BRANCA, BISPO - 1];
tabuleiro[9, 5] = DAMA_BRANCA; casa[59].BackgroundImage = peca[BRANCA, DAMA - 1];
tabuleiro[9, 6] = REI_BRANCO; casa[60].BackgroundImage = peca[BRANCA, REI - 1];
tabuleiro[9, 7] = BISPO_BRANCO; casa[61].BackgroundImage = peca[BRANCA, BISPO - 1];
tabuleiro[9, 8] = CAVALO_BRANCO; casa[62].BackgroundImage = peca[BRANCA, CAVALO - 1];
tabuleiro[9, 9] = TORRE_BRANCA; casa[63].BackgroundImage = peca[BRANCA, TORRE - 1];
tabuleiro[9, 10] = CASA_NULA;
tabuleiro[9, 11] = CASA_NULA;
rei[0] = 114;
}
// MOLDURA
for(int lin = 10; lin < 12; lin++)
for(int col = 0; col < 12; col++)
tabuleiro[lin, col] = CASA_NULA;
//--------
}
public static void ResetCor() {
for(int i = 0; i < 64; i++)
casa[i].BackColor = (i % 8 % 2 == 1) && (i / 8 % 2 == 0) ||
(i % 8 % 2 == 0) && (i / 8 % 2 == 1) ?
System.Drawing.Color.SaddleBrown : System.Drawing.Color.SandyBrown;
}
}
} | 705866128137d4b475ad7fd0f8e286391153d5b2 | [
"Markdown",
"C#",
"INI"
] | 11 | C# | josuefonseca/JasChess | 7e72a760c5a6f9280bd9f8162f79b951c7b78501 | a60830210fd2c69f48497a554809c2fc125dcdde | |
refs/heads/master | <repo_name>oldy777/phpstan-src<file_sep>/tests/PHPStan/Rules/Exceptions/data/unthrown-exception.php
<?php
namespace UnthrownException;
class Foo
{
public function doFoo(): void
{
try {
$foo = 1;
} catch (\Throwable $e) {
// pass
}
}
public function doBar(): void
{
try {
$foo = 1;
} catch (\Exception $e) {
// pass
}
}
/** @throws \InvalidArgumentException */
public function throwIae(): void
{
}
public function doBaz(): void
{
try {
$this->throwIae();
} catch (\InvalidArgumentException $e) {
} catch (\Exception $e) {
// dead
} catch (\Throwable $e) {
// not dead
}
}
public function doLorem(): void
{
try {
$this->throwIae();
} catch (\RuntimeException $e) {
// dead
} catch (\Throwable $e) {
}
}
public function doIpsum(): void
{
try {
$this->throwIae();
} catch (\Throwable $e) {
}
}
public function doDolor(): void
{
try {
throw new \InvalidArgumentException();
} catch (\InvalidArgumentException $e) {
} catch (\Throwable $e) {
}
}
public function doSit(): void
{
try {
try {
\ThrowPoints\Helpers\maybeThrows();
} catch (\InvalidArgumentException $e) {
}
} catch (\InvalidArgumentException $e) {
}
}
/**
* @throws \InvalidArgumentException
* @throws \DomainException
*/
public function doAmet()
{
}
public function doAmet1()
{
try {
$this->doAmet();
} catch (\InvalidArgumentException $e) {
} catch (\DomainException $e) {
} catch (\Throwable $e) {
// not dead
}
}
public function doAmet2()
{
try {
throw new \InvalidArgumentException();
} catch (\InvalidArgumentException $e) {
} catch (\DomainException $e) {
// dead
} catch (\Throwable $e) {
// dead
}
}
public function doConsecteur()
{
try {
if (false) {
} elseif ($this->doAmet()) {
}
} catch (\InvalidArgumentException $e) {
}
}
}
class InlineThrows
{
public function doFoo()
{
try {
/** @throws \InvalidArgumentException */
echo 1;
} catch (\InvalidArgumentException $e) {
}
}
public function doBar()
{
try {
/** @throws \InvalidArgumentException */
$i = 1;
} catch (\InvalidArgumentException $e) {
}
}
}
class TestDateTime
{
public function doFoo(): void
{
try {
new \DateTime();
} catch (\Exception $e) {
}
}
public function doBar(): void
{
try {
new \DateTime('now');
} catch (\Exception $e) {
}
}
public function doBaz(string $s): void
{
try {
new \DateTime($s);
} catch (\Exception $e) {
}
}
/**
* @phpstan-param 'now'|class-string $s
*/
public function doSuperBaz(string $s): void
{
try {
new \DateTime($s);
} catch (\Exception $e) {
}
}
}
class TestDateInterval
{
public function doFoo(): void
{
try {
new \DateInterval('invalid format');
} catch (\Exception $e) {
}
}
public function doBar(): void
{
try {
new \DateInterval('P10D');
} catch (\Exception $e) {
}
}
public function doBaz(string $s): void
{
try {
new \DateInterval($s);
} catch (\Exception $e) {
}
}
/**
* @phpstan-param 'P10D'|class-string $s
*/
public function doSuperBaz(string $s): void
{
try {
new \DateInterval($s);
} catch (\Exception $e) {
}
}
}
class TestIntdiv
{
public function doFoo(): void
{
try {
intdiv(1, 1);
intdiv(1, -1);
} catch (\ArithmeticError $e) {
}
try {
intdiv(PHP_INT_MIN, -1);
} catch (\ArithmeticError $e) {
}
try {
intdiv(1, 0);
} catch (\ArithmeticError $e) {
}
}
public function doBar(int $int): void
{
try {
intdiv($int, 1);
} catch (\ArithmeticError $e) {
}
try {
intdiv($int, -1);
} catch (\ArithmeticError $e) {
}
}
public function doBaz(int $int): void
{
try {
intdiv(1, $int);
} catch (\ArithmeticError $e) {
}
try {
intdiv(PHP_INT_MIN, $int);
} catch (\ArithmeticError $e) {
}
}
}
class TestSimpleXMLElement
{
public function doFoo(): void
{
try {
new \SimpleXMLElement('<?xml version="1.0" encoding="UTF-8"?><root></root>');
} catch (\Exception $e) {
}
}
public function doBar(): void
{
try {
new \SimpleXMLElement('foo');
} catch (\Exception $e) {
}
}
public function doBaz(string $string): void
{
try {
new \SimpleXMLElement($string);
} catch (\Exception $e) {
}
}
}
<file_sep>/tests/PHPStan/Rules/Api/ApiRuleHelperTest.php
<?php declare(strict_types = 1);
namespace PHPStan\Rules\Api;
use PHPUnit\Framework\TestCase;
class ApiRuleHelperTest extends TestCase
{
public function dataIsInPhpStanNamespace(): array
{
return [
[
null,
false,
],
[
'PHPStan',
true,
],
[
'PhpStan',
true,
],
[
'PHPStan\\Foo',
true,
],
[
'PhpStan\\Foo',
true,
],
[
'App\\Foo',
false,
],
[
'PHPStanWorkshop',
false,
],
[
'PHPStanWorkshop\\',
false,
],
[
'PHPStan\\PhpDocParser\\',
false,
],
];
}
/**
* @dataProvider dataIsInPhpStanNamespace
* @param string|null $namespace
* @param bool $expected
*/
public function testIsInPhpStanNamespace(?string $namespace, bool $expected): void
{
$rule = new ApiRuleHelper();
$this->assertSame($expected, $rule->isInPhpStanNamespace($namespace));
}
}
<file_sep>/src/Rules/Api/ApiClassImplementsRule.php
<?php declare(strict_types = 1);
namespace PHPStan\Rules\Api;
use PhpParser\Node;
use PhpParser\Node\Stmt\Class_;
use PHPStan\Analyser\Scope;
use PHPStan\Analyser\TypeSpecifierAwareExtension;
use PHPStan\Command\ErrorFormatter\ErrorFormatter;
use PHPStan\PhpDoc\TypeNodeResolverAwareExtension;
use PHPStan\PhpDoc\TypeNodeResolverExtension;
use PHPStan\Reflection\BrokerAwareExtension;
use PHPStan\Reflection\ClassMemberReflection;
use PHPStan\Reflection\MethodReflection;
use PHPStan\Reflection\MethodsClassReflectionExtension;
use PHPStan\Reflection\ParameterReflection;
use PHPStan\Reflection\ParametersAcceptor;
use PHPStan\Reflection\PropertiesClassReflectionExtension;
use PHPStan\Reflection\PropertyReflection;
use PHPStan\Reflection\ReflectionProvider;
use PHPStan\Rules\Constants\AlwaysUsedClassConstantsExtension;
use PHPStan\Rules\Exceptions\ExceptionTypeResolver;
use PHPStan\Rules\Properties\ReadWritePropertiesExtension;
use PHPStan\Rules\Rule;
use PHPStan\Rules\RuleError;
use PHPStan\Rules\RuleErrorBuilder;
use PHPStan\Type\DynamicFunctionReturnTypeExtension;
use PHPStan\Type\DynamicFunctionThrowTypeExtension;
use PHPStan\Type\DynamicMethodReturnTypeExtension;
use PHPStan\Type\DynamicMethodThrowTypeExtension;
use PHPStan\Type\DynamicStaticMethodReturnTypeExtension;
use PHPStan\Type\DynamicStaticMethodThrowTypeExtension;
use PHPStan\Type\FunctionTypeSpecifyingExtension;
use PHPStan\Type\MethodTypeSpecifyingExtension;
use PHPStan\Type\OperatorTypeSpecifyingExtension;
use PHPStan\Type\StaticMethodTypeSpecifyingExtension;
/**
* @implements Rule<Class_>
*/
class ApiClassImplementsRule implements Rule
{
public const INTERFACES = [
PropertiesClassReflectionExtension::class,
PropertyReflection::class,
MethodsClassReflectionExtension::class,
MethodReflection::class,
ParametersAcceptor::class,
ParameterReflection::class,
ClassMemberReflection::class,
DynamicMethodReturnTypeExtension::class,
DynamicFunctionReturnTypeExtension::class,
DynamicStaticMethodReturnTypeExtension::class,
DynamicMethodThrowTypeExtension::class,
DynamicFunctionThrowTypeExtension::class,
DynamicStaticMethodThrowTypeExtension::class,
OperatorTypeSpecifyingExtension::class,
MethodTypeSpecifyingExtension::class,
FunctionTypeSpecifyingExtension::class,
StaticMethodTypeSpecifyingExtension::class,
ErrorFormatter::class,
Rule::class,
TypeSpecifierAwareExtension::class,
BrokerAwareExtension::class,
AlwaysUsedClassConstantsExtension::class,
ReadWritePropertiesExtension::class,
ExceptionTypeResolver::class,
TypeNodeResolverExtension::class,
TypeNodeResolverAwareExtension::class,
];
private ApiRuleHelper $apiRuleHelper;
private ReflectionProvider $reflectionProvider;
public function __construct(
ApiRuleHelper $apiRuleHelper,
ReflectionProvider $reflectionProvider
)
{
$this->apiRuleHelper = $apiRuleHelper;
$this->reflectionProvider = $reflectionProvider;
}
public function getNodeType(): string
{
return Class_::class;
}
public function processNode(Node $node, Scope $scope): array
{
if ($this->apiRuleHelper->isInPhpStanNamespace($scope->getNamespace())) {
return [];
}
$errors = [];
foreach ($node->implements as $implements) {
$errors = array_merge($errors, $this->checkName($implements));
}
return $errors;
}
/**
* @param Node\Name $name
* @return RuleError[]
*/
private function checkName(Node\Name $name): array
{
$implementedClassName = (string) $name;
if (!$this->reflectionProvider->hasClass($implementedClassName)) {
return [];
}
$implementedClassReflection = $this->reflectionProvider->getClass($implementedClassName);
if (!$this->apiRuleHelper->isInPhpStanNamespace($implementedClassReflection->getName())) {
return [];
}
if (in_array($implementedClassReflection->getName(), self::INTERFACES, true)) {
return [];
}
$ruleError = RuleErrorBuilder::message(sprintf(
'Implementing %s is not covered by backward compatibility promise. The interface might change in a minor PHPStan version.',
$implementedClassReflection->getDisplayName()
))->tip(sprintf(
"If you think it should be covered by backward compatibility promise, open a discussion:\n %s\n\n See also:\n https://phpstan.org/developing-extensions/backward-compatibility-promise",
'https://github.com/phpstan/phpstan/discussions'
))->build();
return [$ruleError];
}
}
<file_sep>/src/Rules/Api/ApiRuleHelper.php
<?php declare(strict_types = 1);
namespace PHPStan\Rules\Api;
class ApiRuleHelper
{
public function isInPhpStanNamespace(?string $namespace): bool
{
if ($namespace === null) {
return false;
}
if (strtolower($namespace) === 'phpstan') {
return true;
}
if (strpos($namespace, 'PHPStan\\PhpDocParser\\') === 0) {
return false;
}
return stripos($namespace, 'PHPStan\\') === 0;
}
}
| 24406542cacf4d593ec301b045c85385ea720330 | [
"PHP"
] | 4 | PHP | oldy777/phpstan-src | de00c86689b4cb8f865aa9e811a7712b69fff9d3 | 642142dcca009124f6d889981543f679032e62e4 | |
refs/heads/master | <file_sep># -*- coding: utf-8 -*-
import numpy as np
from os import listdir
from sklearn.svm import SVC
def im2vector(filname):#对数据进行扁平化处理
returnvect=np.zeros((32,32))
file=open(filname)
linestr=file.readlines()
index=0
for i in linestr:
words=i.strip()
words=list(words)
returnvect[index,:]=words[0:32]
index+=1
returnvect=returnvect.flatten()
return returnvect
def handwritingclasstest():
hwlabels=[]
trainingflilelist=listdir('trainingdigits')
m=len(trainingflilelist)
trainningmat=np.zeros((m,1024))
for i in range(m):
filenamestr=trainingflilelist[i]
classnumber=int(filenamestr.split('_')[0])
hwlabels.append(classnumber)
trainningmat[i,:]=im2vector('trainingDigits/'+filenamestr)
clf=SVC(C=200)
clf.fit(trainningmat,hwlabels)
testfilelist=listdir('testDigits')
errorcount=0
mtest=len(testfilelist)
for i in range(mtest):
filenamestr=testfilelist[i]
classnumber=int(filenamestr.split('_')[0])
vectorundertest=im2vector('testDigits/'+filenamestr)
classifiterresult=clf.predict(vectorundertest.reshape(1,-1))
print("分类返回结果为%d\t真实结果为%d" % (classifiterresult, classnumber))
if classifiterresult!=classnumber:
errorcount+=1
print('共预测错误%d个数\n错误率为%f%%'%(errorcount,errorcount/mtest*100))
if __name__=="__main__":
# filename='D:/程序/python/dl/trainingDigits/0_0.txt'
# im2vector(filename)
handwritingclasstest()<file_sep># ML
常用机器学习算法,原理及实战
<file_sep>import matplotlib.pyplot as plt
import numpy as np
import random
def loaddataset(filename):
datamat = []
labelmat =[]
fr = open(filename)
for line in fr.readlines():
linearr = line.strip().split('\t')
datamat.append([float(linearr[0]),float(linearr[1])])
labelmat.append(float(linearr[2]))
return datamat,labelmat
def selectjrand(i,m):
j=i
while(j==i):
j=int(random.uniform(0,m))
return j
def clipalpha(aj,h,l):
if aj>h:
aj=h
if l > aj:
aj=l
return aj
def smosimple(datamatin,classlabels,c,toler,maxiter):
datamatrix = np.mat(datamatin)
labelmat = np.mat(classlabels).transpose()
b=0
m,n=np.shape(datamatrix)
alphas = np.mat(np.zeros((m,1)))
iter_num = 0
while (iter_num < maxiter):
alphapairschanged = 0
for i in range(m):
fxi = float(np.multiply(alphas,labelmat).T * (datamatrix * datamatrix[i,:].T))+b
ei=fxi - float(labelmat[i])
if ((labelmat[i]*ei < -toler) and (alphas[i] <c)) or ((labelmat[i] * ei >toler) and (alphas[i] > 0)):
j = selectjrand(i,m)
fxj= float(np.multiply(alphas,labelmat).T * (datamatrix * datamatrix[j,:].T))+b
ej=fxj - float(labelmat[j])
alphaiold = alphas[i].copy()
alphajold = alphas[j].copy()
if (labelmat[i] != labelmat[j]):
l= max(0,alphas[j]-alphas[i])
h= min(c,c+alphas[j] - alphas[i])
else :
l = max(0,alphas[j] + alphas[i] -c)
h = min(c, alphas[j] + alphas[i])
if l == h :
print('l==h')
continue
eta = 2.0 * datamatrix[i,:]*datamatrix[j,:].T - datamatrix[i,:] * datamatrix[i,:].T - datamatrix[j,:] * datamatrix[j,:].T
if eta >=0:
print('eta>=0')
continue
alphas[j] -=labelmat[j] * (ei - ej)/eta
alphas[j] = clipalpha(alphas[j],h,l)
if (abs(alphas[j] - alphajold) < 0.00001):
print('alpha_ja too small')
continue
alphas[i] +=labelmat[j] * labelmat[i] * (alphajold - alphas[j])
b1 = b - ei -labelmat[i]*(alphas[i]-alphaiold)*datamatrix[i,:]*datamatrix[i,:].T - labelmat[j]*(alphas[j]-alphajold)*datamatrix[i,:]*datamatrix[j,:].T
b2 = b - ei -labelmat[i]*(alphas[i]-alphaiold)*datamatrix[i,:]*datamatrix[j,:].T - labelmat[j]*(alphas[j]-alphajold)*datamatrix[j,:]*datamatrix[j,:].T
if (0 < alphas[i]) and (c > alphas[i]):
b=b1
elif (0 < alphas[j]) and (c > alphas[j]):
b=b2
else:
b=(b1+b2)/2.0
alphapairschanged +=1
print('the %d iteration,sampel:%d,aplpha optimize num:%d'%(iter_num,i,alphapairschanged))
if (alphapairschanged == 0):
iter_num +=1
else:
iter_num = 0
print('iteration num : %d:'% iter_num)
return b,alphas
def showclassifer(datamat,labelmat,w,b):
data_plus=[]
data_minus = []
for i in range(len(datamat)):
if labelmat[i]>0:
data_plus.append(datamat[i])
data_minus.append(datamat[i])
data_plus_np = np.array(data_plus)
data_minus_np = np.array(data_minus)
plt.scatter(np.transpose(data_plus_np)[0],np.transpose(data_plus_np)[1])
plt.scatter(np.transpose(data_minus_np)[0],np.transpose(data_minus_np)[1])
x1 = max (datamat)[0]
x2 = min(datamat)[0]
a1,a2 = w
b = float(b)
a1= float(a1[0])
a2 = float(a2[0])
y1,y2 = (-b-a1*x1)/a2,(-b-a1*x2)/a2
plt.plot([x1,x2],[y1,y2])
for i ,alpha in enumerate(alphas):
if abs(alpha) > 0:
x,y= datamat[i]
plt.scatter([x],[y],s=150,c='none',alpha=0.7,linewidths=1.5,edgecolors='red')
plt.show()
def get_w(datamat,labelmat,alphas):
alphas,datamat,labelmat=np.array(alphas),np.array(datamat),np.array(labelmat)
w = np.dot((np.tile(labelmat.reshape(1,-1).T,(1,2))*datamat).T,alphas)
return w.tolist()
if __name__=='__main__':
datamat,labelmat = loaddataset('testSet.txt')
b,alphas = smosimple(datamat,labelmat,0.6,0.001,40)
w = get_w(datamat,labelmat,alphas)
showclassifer(datamat,labelmat,w,b)
<file_sep># -*- coding: utf-8 -*-
"""
Spyder Editor
This is a temporary script file.
"""
import matplotlib.pyplot as plt
import numpy as np
import random
import time
class optstruct:
def __init__(self,datamatin,classlabels,c,toler):
self.x = datamatin
self.labelmat = classlabels
self.c = c
self.tol = toler
self.b = 0
self.m = np.shape(datamatin)[0]
self.alphas = np.mat(np.zeros((self.m , 1)))
self.ecache = np.mat(np.zeros((self.m,2)))
def loaddataset(filename):
datamat = []
labelmat =[]
fr = open(filename)
for line in fr.readlines():
linearr = line.strip().split('\t')
datamat.append([float(linearr[0]),float(linearr[1])])
labelmat.append(float(linearr[2]))
return datamat,labelmat
def calcek(os,k):
fxk = float(np.multiply(os.alphas,os.labelmat).T * (os.x * os.x[k,:].T))+os.b
ek = fxk - float(os.labelmat[k])
return ek
def selectjrand(i,m):
j=i
while(j==i):
j=int(random.uniform(0,m))
return j
def selectj(i,os,ei):
maxk = -1
maxdeltae=0
ej=0
os.ecache[i] = [1,ei]
validecachelist = np.nonzero(os.ecache[:,0].A)[0]
if (len(validecachelist))>1:
for k in validecachelist:
if k == i:
continue
ek = calcek(os,k)
deltae = abs(ei - ek)
if (deltae > maxdeltae):
maxk = k
maxdeltae = deltae
ej = ek
return maxk , ej
else:
j=selectjrand(i,os.m)
ej = calcek(os,j)
return j,ej
def updataek(os,k):
ek = calcek(os,k)
os.ecache[k] = [1,ek]
def clipalpha(aj,h,l):
if aj>h:
aj=h
if l > aj:
aj=l
return aj
def innerl(i,os):
ei = calcek(os,i)
if ((os.labelmat[i]*ei < -os.tol) and (os.alphas[i] < os.c)) or ((os.labelmat[i]*ei > -os.tol) and (os.alphas[i] > 0)):
j,ej = selectj(i,os,ei)
alphaiold = os.alphas[i].copy()
alphajold = os.alphas[j].copy()
if (os.labelmat[i]) != os.labelmat[j]:
l = max(0,os.alphas[j] + os.alphas[i])
h = min(os.c ,os.c+os.alphas[j] - os.alphas[i])
else:
l = max(0,os.alphas[j] + os.alphas[i] - os.c)
h = min(os.c,os.alphas[j]+os.alphas[i])
if l == h:
print('l==h')
return 0
eta = 2.0 * os.x[i,:] * os.x[j,:].T - os.x[i,:] * os.x[i,:].T - os.x[j,:] * os.x[j,:].T
if eta >= 0:
print("eta>=0")
return 0
#步骤4:更新alpha_j
os.alphas[j] -= os.labelmat[j] * (ei - ej)/eta
#步骤5:修剪alpha_j
os.alphas[j] = clipalpha(os.alphas[j],h,l)
#更新Ej至误差缓存
updataek(os, j)
if (abs(os.alphas[j] - alphajold) < 0.00001):
print("alpha_j变化太小")
return 0
os.alphas[i] += os.labelmat[j] * os.labelmat[i] * (alphaiold - os.alphas[j])
updataek(os,i)
b1 = os.b - ei - os.labelmat[i] * (os.alphas[i] - alphaiold) * os.x[i,:] * os.x[i,:].T- os.labelmat[j]*(os.alphas[j]-alphajold)*os.x[i,:]*os.x[j,:].T
b2 = os.b - ej - os.labelmat[i] * (os.alphas[i] - alphaiold) * os.x[i,:] * os.x[j,:].T- os.labelmat[j]*(os.alphas[j]-alphajold)*os.x[j,:]*os.x[j,:].T
if (0 < os.alphas[i]) and (os.c > os.alphas[i]):
os.b =b1
elif (0 < os.alphas[j]) and (os.c > os.alphas[j]):
os.b = b2
else:
os.b = (b1 + b2)/2.0
return 1
else:
return 0
def smop(datamatin,classlabels,c,toler,maxiter):
os = optstruct(np.mat(datamatin),np.mat(classlabels).transpose(),c,toler)
iter = 0
entireset = True
alphapairschanged = 0
while (iter < maxiter) and ((alphapairschanged > 0) or (entireset)):
alphapairschanged= 0
if entireset:
for i in range(os.m):
alphapairschanged += innerl(i,os)
print('all sample:the %d iteration,sampel:%d,aplpha optimize num:%d'%(iter,i,alphapairschanged))
iter +=1
else:
nonboundis = np.nonzero((os.alphas.A >0) * (os.alphas.A < c))[0]
for i in nonboundis:
alphapairschanged +=innerl(i,os)
print('nonboundis:the %d iteration,sampel:%d,aplpha optimize num:%d'%(iter,i,alphapairschanged))
iter +=1
if entireset:
entireset = False
elif (alphapairschanged == 0):
entireset = True
print('iteration num : %d'%iter)
return os.b,os.alphas
def showclassifer(datamat,labelmat,w,b):
data_plus=[]
data_minus = []
for i in range(len(datamat)):
if labelmat[i]>0:
data_plus.append(datamat[i])
else:
data_minus.append(datamat[i])
data_plus_np = np.array(data_plus)
data_minus_np = np.array(data_minus)
plt.scatter(np.transpose(data_plus_np)[0],np.transpose(data_plus_np)[1])
plt.scatter(np.transpose(data_minus_np)[0],np.transpose(data_minus_np)[1])
x1 = max (datamat)[0]
x2 = min(datamat)[0]
a1,a2 = w
b = float(b)
a1= float(a1[0])
a2 = float(a2[0])
y1,y2 = (-b-a1*x1)/a2,(-b-a1*x2)/a2
plt.plot([x1,x2],[y1,y2])
for i ,alpha in enumerate(alphas):
if abs(alpha) > 0:
x,y= datamat[i]
plt.scatter([x],[y],s=150,c='none',alpha=0.7,linewidths=1.5,edgecolors='red')
plt.ylim(-20,20)
plt.show()
def get_w(alphas,dataarr,classlabels):
x = np.mat(dataarr)
labelmat = np.mat(classlabels).transpose()
m,n = np.shape(x)
w = np.zeros((n,1))
for i in range(m):
w +=np.multiply(alphas[i] * labelmat[i],x[i,:].T)
return w
if __name__=='__main__':
datamat,labelmat = loaddataset('testSet.txt')
start = time.time()
b,alphas = smop(datamat,labelmat,0.6,0.001,40)
w = get_w(alphas,datamat,labelmat)
showclassifer(datamat,labelmat,w,b)
end = time.time()
print('times is:%d'%(end-start) ) | e2e267f5dab2464a29e8ec914a21e0917a1e38ad | [
"Markdown",
"Python"
] | 4 | Python | whudddddd/ML | 32aeb755c00825b669f9e3e5c5ab8a48f27175d7 | 773e972be65b80e9f2ae0e3d9a7650336ed925fa | |
refs/heads/main | <file_sep>/*jslint maxparams: 4, maxdepth: 4, maxstatements: 20, maxcomplexity: 8, esversion: 6 */
(function ( window ) {
const
SHIP_DIRECTION_RIGHT = 'right',
SHIP_DIRECTION_LEFT = 'left',
SHIP_DIRECTION_UP = 'up',
SHIP_DIRECTION_DOWN = 'down',
BULLETS = [],
ALIENS = [],
MIN_SHIP_SPEED_X = 0,
MAX_SHIP_SPEED_X = 5,
MIN_SHIP_SPEED_Y = 0,
MAX_SHIP_SPEED_Y = 3,
BULLET_SPEED = 4,
// The current speed is multiplied by this number. Probably just keep the value of one, seems pretty good.
SHIP_MOVE_X_BASE = 1,
SHIP_MOVE_Y_BASE = 1,
MIN_STARS = 30,
MAX_STARS = 40,
ODDS_OF_MAKING_A_NEW_STAR = 0.1
;
let
lastTimeStamp = 0,
lastDirectionRequest,
currentShipDirectionHorizontal = SHIP_DIRECTION_RIGHT,
currentShipDirectionVertical,
currentShipSpeedHorizontal = 0,
currentShipSpeedVertical = 0,
furthestLeftSoFar = 0,
furthestRightSoFar = 0,
starFieldRight = 0,
starFieldLeft = 0,
cont,
dbg,
ship,
star_field
;
const
document = window.document,
LAND_1 = '\u25A9',
SHIP_FACING_RIGHT = '\u1405',
// ALIEN_SHIP_FACING_UP = '\u1403',
ALIEN_SHIP_FACING_UP = '\u16DF',
ALIEN_BEAM_START = '\u16D8',
ALIEN_BEAM_START_B = '\u16D9',
ALIEN_SHIP_RAY_1 = '\u15d1',
ALIEN_SHIP_RAY_2 = '\u16e3',
ALIEN_SHIP_WITH_PERSON = '\u16E4',
PERSON_A = '\uC6C3',
PERSON_B = '\uD83D\uDEB6\uFE0E',
INTERESTING = [
'\u005e',
'\u02c4',
'\u02c6',
'\u02f0',
'\u0311',
'\u1403'
],
createElement = ( tag, classes = [] ) => {
const elem = document.createElement( tag );
const ca = Array.isArray( classes ) ? classes : classes.split( ' ' );
ca
.forEach(
( c ) => {
elem.classList.add( c );
}
)
;
return elem;
},
createDiv = ( classes = [] ) => {
return createElement( 'div', classes );
},
getRandomIntInclusive = ( min, max ) => {
min = Math.ceil( min );
max = Math.floor( max );
return Math.floor( Math.random() * (max - min + 1) ) + min; //The maximum is inclusive and the minimum is inclusive
},
getContainerBox = () => {
return cont.getClientRects()[ 0 ];
},
getShipBox = () => {
return ship.getClientRects()[ 0 ];
},
fireGun = () => {
const
cb = getContainerBox(),
sb = getShipBox()
;
// debugger;
BULLETS
.push(
{
dir: currentShipDirectionHorizontal,
x: SHIP_DIRECTION_RIGHT === currentShipDirectionHorizontal ? sb.right : sb.left,
y: sb.top,
}
)
;
},
handleKeyPress = ( evt ) => {
switch ( evt.code ) {
case 'ArrowRight':
lastDirectionRequest = SHIP_DIRECTION_RIGHT;
break;
case 'ArrowLeft':
lastDirectionRequest = SHIP_DIRECTION_LEFT;
break;
case 'ArrowUp':
lastDirectionRequest = SHIP_DIRECTION_UP;
break;
case 'ArrowDown':
lastDirectionRequest = SHIP_DIRECTION_DOWN;
break;
case 'Space':
fireGun();
break;
}
},
maybeMoveStars = () => {
if ( 0 === currentShipSpeedHorizontal ) {
return;
}
const m = (SHIP_DIRECTION_LEFT === currentShipDirectionHorizontal ? +1 : -1) * currentShipSpeedHorizontal;
star_field
.childNodes
.forEach(
( n ) => {
const ol = parseInt( n.style.left, 10 );
n.style.left = ol + m + 'px';
}
)
;
},
clamp = ( min, val, max ) => {
return Math.max( min, Math.min( val, max ) );
},
maybeMoveShip = () => {
const
curLeft = parseInt( ship.style.left, 10 ),
curTop = parseInt( ship.style.top, 10 ),
shipBox = getShipBox(),
contBox = getContainerBox()
;
ship.classList.toggle( SHIP_DIRECTION_LEFT, currentShipDirectionHorizontal === SHIP_DIRECTION_LEFT );
ship.classList.toggle( SHIP_DIRECTION_RIGHT, currentShipDirectionHorizontal === SHIP_DIRECTION_RIGHT );
let
newLeft = curLeft,
newTop = curTop
;
switch ( currentShipDirectionHorizontal ) {
case SHIP_DIRECTION_RIGHT:
newLeft = curLeft + (SHIP_MOVE_X_BASE * currentShipSpeedHorizontal);
break;
case SHIP_DIRECTION_LEFT:
newLeft = curLeft - (SHIP_MOVE_X_BASE * currentShipSpeedHorizontal);
break;
}
switch ( currentShipDirectionVertical ) {
case SHIP_DIRECTION_DOWN:
newTop = curTop + (SHIP_MOVE_Y_BASE * currentShipSpeedVertical);
break;
case SHIP_DIRECTION_UP:
newTop = curTop - (SHIP_MOVE_Y_BASE * currentShipSpeedVertical);
break;
}
newTop = clamp( 0, newTop, contBox.height - shipBox.height );
newLeft = clamp( 0, newLeft, (contBox.width / 2) );
ship.style.left = newLeft + 'px';
ship.style.top = newTop + 'px';
},
setRandomStarPosition = ( star, box ) => {
if ( !box ) {
box = getContainerBox();
}
const l = getRandomIntInclusive( box.left, box.right );
const t = getRandomIntInclusive( box.top, box.bottom );
star.style.left = l + 'px';
star.style.top = t + 'px';
},
maybeDrawNewStars = () => {
const maybeMoveBy = currentShipSpeedHorizontal * SHIP_MOVE_X_BASE;
let maybeMakeNewStar = false;
let leftOrRight = 'neither';
switch ( currentShipDirectionHorizontal ) {
case SHIP_DIRECTION_RIGHT:
starFieldLeft -= maybeMoveBy;
starFieldRight += maybeMoveBy;
if ( starFieldRight > furthestRightSoFar ) {
maybeMakeNewStar = true;
furthestRightSoFar = starFieldRight;
leftOrRight = SHIP_DIRECTION_RIGHT;
}
break;
case SHIP_DIRECTION_LEFT:
starFieldLeft += maybeMoveBy;
starFieldRight -= maybeMoveBy;
if ( starFieldLeft > furthestLeftSoFar ) {
maybeMakeNewStar = true;
furthestLeftSoFar = starFieldLeft;
leftOrRight = SHIP_DIRECTION_LEFT;
}
break;
}
if ( maybeMakeNewStar ) {
const shouldMakeNewStar = Math.random() < ODDS_OF_MAKING_A_NEW_STAR;
if ( shouldMakeNewStar ) {
const star = createDiv();
const contBox = getContainerBox();
const box = {
top: contBox.top,
bottom: contBox.bottom,
};
if ( SHIP_DIRECTION_LEFT === leftOrRight ) {
box.left = 0 - furthestLeftSoFar;
box.right = 0 - furthestLeftSoFar + maybeMoveBy;
} else {
box.left = furthestRightSoFar - maybeMoveBy;
box.right = furthestRightSoFar;
}
setRandomStarPosition( star, box );
star_field.appendChild( star );
}
}
},
maybeHandleShipDirection = () => {
if ( !lastDirectionRequest ) {
return;
}
const
ld = lastDirectionRequest
;
lastDirectionRequest = null;
switch ( ld ) {
case SHIP_DIRECTION_RIGHT:
case SHIP_DIRECTION_LEFT:
if ( ld === currentShipDirectionHorizontal ) {
if ( currentShipSpeedHorizontal < MAX_SHIP_SPEED_X ) {
currentShipSpeedHorizontal++;
}
return;
}
if ( currentShipSpeedHorizontal > MIN_SHIP_SPEED_X ) {
currentShipSpeedHorizontal--;
return;
}
currentShipDirectionHorizontal = ld;
currentShipSpeedHorizontal = 1;
break;
case SHIP_DIRECTION_DOWN:
case SHIP_DIRECTION_UP:
if ( ld === currentShipDirectionVertical ) {
if ( currentShipSpeedVertical < MAX_SHIP_SPEED_Y ) {
currentShipSpeedVertical++;
}
return;
}
if ( currentShipSpeedVertical > MIN_SHIP_SPEED_Y ) {
currentShipSpeedVertical--;
return;
}
currentShipDirectionVertical = ld;
currentShipSpeedVertical = 1;
break;
}
},
getOrMakeBulletElement = ( bullet ) => {
if ( bullet.hasOwnProperty( 'obj' ) ) {
return bullet.obj;
}
const
obj = createDiv( 'bullet' )
;
obj.style.left = bullet.x + 'px';
obj.style.top = bullet.y + 'px';
obj.appendChild( document.createTextNode( '-' ) );
cont.appendChild( obj );
bullet.obj = obj;
return obj;
},
maybeDrawBullets = () => {
const cb = getContainerBox();
BULLETS
.forEach(
( bullet, idx ) => {
const obj = getOrMakeBulletElement( bullet );
const
oldLeft = parseInt( obj.style.left, 10 ),
m = SHIP_DIRECTION_LEFT === bullet.dir ? -1 : 1,
newLeft = oldLeft + (m * BULLET_SPEED),
oob = (SHIP_DIRECTION_LEFT === bullet.dir && newLeft <= cb.left)
||
(SHIP_DIRECTION_RIGHT === bullet.dir && newLeft >= cb.right)
;
if ( oob ) {
BULLETS.splice( idx, 1 );
obj.remove();
} else {
obj.style.left = newLeft + 'px';
}
}
)
;
},
maybeDrawAliens = () => {
if ( !ALIENS.length ) {
const alien = createDiv( 'alien' );
alien.appendChild( document.createTextNode( ALIEN_SHIP_FACING_UP ) );
cont.appendChild( alien );
ALIENS.push( alien );
const person_a = createDiv( 'person' );
person_a.appendChild( document.createTextNode( PERSON_A ) );
cont.appendChild( person_a );
ALIENS.push( person_a );
const person_b = createDiv( 'person' );
person_b.appendChild( document.createTextNode( PERSON_B ) );
cont.appendChild( person_b );
ALIENS.push( person_b );
}
},
logDebug = () => {
dbg.querySelector( '[data-debug~=direction]' ).innerText = currentShipDirectionHorizontal;
dbg.querySelector( '[data-debug~="h-speed"]' ).innerText = currentShipSpeedHorizontal;
dbg.querySelector( '[data-debug~="v-speed"]' ).innerText = currentShipSpeedVertical;
dbg.querySelector( '[data-debug~="star-field-left"]' ).innerText = starFieldLeft;
dbg.querySelector( '[data-debug~="star-field-right"]' ).innerText = starFieldRight;
},
mainEventLoop = ( ts ) => {
lastTimeStamp = ts;
maybeHandleShipDirection();
logDebug();
maybeMoveShip();
maybeMoveStars();
maybeDrawNewStars();
maybeDrawBullets();
// maybeDrawAliens();
window.requestAnimationFrame( mainEventLoop );
},
createShip = () => {
ship = createDiv( [ 'ship', SHIP_DIRECTION_RIGHT ] );
ship.appendChild( document.createTextNode( SHIP_FACING_RIGHT ) );
ship.style.left = '0';
ship.style.top = '50px';
return ship;
},
createLand = () => {
const MAX_LAND_X = 50;
let last_land_y = 1;
const land_c = createDiv( 'land-container' );
for ( let i = 0; i < MAX_LAND_X; i++ ) {
const land_v_stripe = createDiv( 'land-v-stripe' );
for ( let j = 0; j < 40; j++ ) {
const c = createDiv();
land_v_stripe.appendChild( c );
}
land_c.appendChild( land_v_stripe );
}
return land_c;
},
createStarField = () => {
star_field = createDiv( 'star-field' );
const newStarCount = getRandomIntInclusive( MIN_STARS, MAX_STARS );
for ( let i = 0; i < newStarCount; i++ ) {
const star = createDiv();
setRandomStarPosition( star );
star_field.appendChild( star );
}
furthestRightSoFar = getContainerBox().width;
starFieldRight = furthestRightSoFar;
return star_field;
},
createEverything = () => {
cont = createDiv( 'container' );
document.body.appendChild( cont );
cont.appendChild( createShip() );
cont.appendChild( createStarField() );
cont.appendChild( createLand() );
},
setupListeners = () => {
document.addEventListener( 'keydown', handleKeyPress );
},
setupMainEventLoop = () => {
window.requestAnimationFrame( mainEventLoop );
},
setupDebugger = () => {
dbg = createDiv( 'debugger' );
[ 'Direction', 'H Speed', 'V Speed', 'Star Field Left', 'Star Field Right' ]
.forEach(
( i ) => {
const
c = createDiv(),
h = createDiv( 'header' ),
d = createDiv()
;
h.appendChild( document.createTextNode( i ) );
d.setAttribute( 'data-debug', i.toLocaleLowerCase()
.replace( /\s/g, '-' ) );
c.appendChild( h );
c.appendChild( d );
dbg.appendChild( c );
}
)
;
document.body.appendChild( dbg );
},
run = () => {
createEverything();
setupListeners();
setupMainEventLoop();
setupDebugger();
},
init = () => {
run();
}
;
init();
}
)( window );
| 002a882c86f14823ec44d205ec38c6e1ea241e72 | [
"JavaScript"
] | 1 | JavaScript | cjhaas/space | 6c22df3dae7eabbaa920696cdf82f281cbe10070 | de304916d8bcfaf8c00b46f4639845e353df7e15 | |
refs/heads/master | <repo_name>notnaa/counter-yii<file_sep>/basic/models/Group.php
<?php
namespace app\models;
use Yii;
/**
* This is the model class for table "group".
*
* @property integer $group_id
* @property string $group_name
* @property integer $kafedra
* @property integer $created_at
* @property integer $updated_at
*
* @property Kafedra $kafedra0
* @property User[] $users
*/
class Group extends \yii\db\ActiveRecord
{
/**
* @inheritdoc
*/
public static function tableName()
{
return 'group';
}
/**
* @inheritdoc
*/
public function rules()
{
return [
[['group_name', 'kafedra', 'created_at', 'updated_at'], 'required'],
[['kafedra', 'created_at', 'updated_at'], 'integer'],
[['group_name'], 'string', 'max' => 255],
[['kafedra'], 'unique'],
[['kafedra'], 'exist', 'skipOnError' => true, 'targetClass' => Kafedra::className(), 'targetAttribute' => ['kafedra' => 'kafedra_id']],
];
}
/**
* @inheritdoc
*/
public function attributeLabels()
{
return [
'group_id' => 'Group ID',
'group_name' => 'Group Name',
'kafedra' => 'Kafedra',
'created_at' => 'Created At',
'updated_at' => 'Updated At',
];
}
/**
* @return \yii\db\ActiveQuery
*/
public function getKafedra0()
{
return $this->hasOne(Kafedra::className(), ['kafedra_id' => 'kafedra']);
}
/**
* @return \yii\db\ActiveQuery
*/
public function getUsers()
{
return $this->hasMany(User::className(), ['group' => 'group_id']);
}
}
<file_sep>/counter_yii.sql
-- phpMyAdmin SQL Dump
-- version 4.6.5.2
-- https://www.phpmyadmin.net/
--
-- Хост: 127.0.0.1:3306
-- Время создания: Авг 03 2017 г., 14:56
-- Версия сервера: 5.7.16
-- Версия PHP: 5.6.29
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- База данных: `counter_yii`
--
-- --------------------------------------------------------
--
-- Структура таблицы `auth_assignment`
--
CREATE TABLE `auth_assignment` (
`item_name` varchar(64) COLLATE utf8_unicode_ci NOT NULL,
`user_id` int(64) NOT NULL,
`created_at` int(11) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
--
-- Дамп данных таблицы `auth_assignment`
--
INSERT INTO `auth_assignment` (`item_name`, `user_id`, `created_at`) VALUES
('admin', 2, 1497138271),
('student', 6, 1497138284),
('teacher', 3, 1497138279);
-- --------------------------------------------------------
--
-- Структура таблицы `auth_item`
--
CREATE TABLE `auth_item` (
`name` varchar(64) COLLATE utf8_unicode_ci NOT NULL,
`type` smallint(6) NOT NULL,
`description` text COLLATE utf8_unicode_ci,
`rule_name` varchar(64) COLLATE utf8_unicode_ci DEFAULT NULL,
`data` blob,
`created_at` int(11) DEFAULT NULL,
`updated_at` int(11) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
--
-- Дамп данных таблицы `auth_item`
--
INSERT INTO `auth_item` (`name`, `type`, `description`, `rule_name`, `data`, `created_at`, `updated_at`) VALUES
('/admin/*', 2, NULL, NULL, NULL, 1497136134, 1497136134),
('/admin/group/*', 2, NULL, NULL, NULL, 1497136176, 1497136176),
('/admin/test/delete', 2, NULL, NULL, NULL, 1497199967, 1497199967),
('/admin/test/index', 2, NULL, NULL, NULL, 1497199833, 1497199833),
('/admin/test/view', 2, NULL, NULL, NULL, 1497136310, 1497136310),
('/admin/users/*', 2, NULL, NULL, NULL, 1497137185, 1497137185),
('/admin/users/update', 2, NULL, NULL, NULL, 1497137204, 1497137204),
('/admin/users/view', 2, NULL, NULL, NULL, 1497137240, 1497137240),
('/rbac/*', 2, NULL, NULL, NULL, 1497136117, 1497136117),
('/site/*', 2, NULL, NULL, NULL, 1497136112, 1497136112),
('admin', 1, 'Главный администратор', NULL, NULL, 1497138110, 1497138110),
('adminAccess', 2, 'Доступ к административным разделам.', NULL, NULL, 1497137698, 1497137698),
('authorizeAccess', 2, 'Доступ к данным аккаунта и результату тестирования', NULL, NULL, 1497137529, 1497138048),
('moderatorAccess', 2, 'Доступ к всем результатам тестирования. Доступ к добавлению, удалению, просмотру и обновлению информации о группах.', NULL, NULL, 1497137381, 1497199971),
('student', 1, 'Обучающийся авторизованный пользователь', NULL, NULL, 1497138213, 1497138213),
('teacher', 1, 'Преподаватель', NULL, NULL, 1497138173, 1497138173);
-- --------------------------------------------------------
--
-- Структура таблицы `auth_item_child`
--
CREATE TABLE `auth_item_child` (
`parent` varchar(64) COLLATE utf8_unicode_ci NOT NULL,
`child` varchar(64) COLLATE utf8_unicode_ci NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
--
-- Дамп данных таблицы `auth_item_child`
--
INSERT INTO `auth_item_child` (`parent`, `child`) VALUES
('adminAccess', '/admin/*'),
('moderatorAccess', '/admin/group/*'),
('moderatorAccess', '/admin/test/delete'),
('moderatorAccess', '/admin/test/index'),
('authorizeAccess', '/admin/test/view'),
('moderatorAccess', '/admin/test/view'),
('authorizeAccess', '/admin/users/update'),
('authorizeAccess', '/admin/users/view'),
('moderatorAccess', '/admin/users/view'),
('adminAccess', '/rbac/*'),
('admin', 'adminAccess'),
('student', 'authorizeAccess'),
('teacher', 'moderatorAccess');
-- --------------------------------------------------------
--
-- Структура таблицы `auth_rule`
--
CREATE TABLE `auth_rule` (
`name` varchar(64) COLLATE utf8_unicode_ci NOT NULL,
`data` blob,
`created_at` int(11) DEFAULT NULL,
`updated_at` int(11) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
-- --------------------------------------------------------
--
-- Структура таблицы `group`
--
CREATE TABLE `group` (
`group_id` int(11) NOT NULL,
`group_name` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`kafedra` int(11) NOT NULL,
`created_at` int(11) NOT NULL,
`updated_at` int(11) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
--
-- Дамп данных таблицы `group`
--
INSERT INTO `group` (`group_id`, `group_name`, `kafedra`, `created_at`, `updated_at`) VALUES
(1, '122', 1, 11111, 11111);
-- --------------------------------------------------------
--
-- Структура таблицы `kafedra`
--
CREATE TABLE `kafedra` (
`kafedra_id` int(11) NOT NULL,
`name` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`created_at` int(11) NOT NULL,
`updated_at` int(11) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
--
-- Дамп данных таблицы `kafedra`
--
INSERT INTO `kafedra` (`kafedra_id`, `name`, `created_at`, `updated_at`) VALUES
(1, 'test', 11111, 11111);
-- --------------------------------------------------------
--
-- Структура таблицы `menu`
--
CREATE TABLE `menu` (
`id` int(11) NOT NULL,
`name` varchar(128) NOT NULL,
`parent` int(11) DEFAULT NULL,
`route` varchar(255) DEFAULT NULL,
`order` int(11) DEFAULT NULL,
`data` blob
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Структура таблицы `migration`
--
CREATE TABLE `migration` (
`version` varchar(180) NOT NULL,
`apply_time` int(11) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- Дамп данных таблицы `migration`
--
INSERT INTO `migration` (`version`, `apply_time`) VALUES
('m000000_000000_base', 1496867517),
('m140506_102106_rbac_init', 1497133215),
('m140602_111327_create_menu_table', 1497133175),
('m160312_050000_create_user', 1497133175),
('m170608_143514_create_user_table', 1496935077),
('m170609_090307_create_test', 1496999745),
('m170610_211053_create_kafedra', 1497129401),
('m170610_211811_create_group', 1497129852);
-- --------------------------------------------------------
--
-- Структура таблицы `test`
--
CREATE TABLE `test` (
`test_id` int(11) NOT NULL,
`user_id` int(11) NOT NULL,
`results` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`created_at` int(11) NOT NULL,
`updated_at` int(11) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
--
-- Дамп данных таблицы `test`
--
INSERT INTO `test` (`test_id`, `user_id`, `results`, `created_at`, `updated_at`) VALUES
(2, 2, '5', 11111, 11111);
-- --------------------------------------------------------
--
-- Структура таблицы `user`
--
CREATE TABLE `user` (
`user_id` int(11) NOT NULL,
`username` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`group` int(11) NOT NULL,
`name` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`lastname` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`thirdname` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`auth_key` varchar(32) COLLATE utf8_unicode_ci NOT NULL,
`password_hash` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`password_reset_token` varchar(255) COLLATE utf8_unicode_ci DEFAULT NULL,
`email` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`status` smallint(6) NOT NULL DEFAULT '10',
`created_at` int(11) NOT NULL,
`updated_at` int(11) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
--
-- Дамп данных таблицы `user`
--
INSERT INTO `user` (`user_id`, `username`, `group`, `name`, `lastname`, `thirdname`, `auth_key`, `password_hash`, `password_reset_token`, `email`, `status`, `created_at`, `updated_at`) VALUES
(2, 'admin', 1, 'Anton', 'Drogin', 'Romanovich', '1o2-J_YxXvsOgXe-v0u4mj7BYPWhUJsw', '$2y$13$FPN0NGOcUCdHfizyS3xYw.fKU8OJ8rXednM8.B213la3XrLWP9ON.', NULL, '<EMAIL>', 10, 1497130828, 1497130828),
(3, 'astaf', 1, 'Иван', 'Астафьев', 'Сергеевич', 'Pz4iP30SzQlm_H1nWYlg2tONTC4gHGv_', '$2y$13$E4cnrkqMSyrdQUe6ucp0c.U7UyeJzgbAZyp.kPCUuQw/0Hd..ie0C', 'YPNouY0F0xwMjul5xvSffB1-OlEHXzfh_1497566030', '<EMAIL>', 10, 1497134862, 1497566030),
(6, 'zemlyak', 1, 'Сергей', 'Земляков', 'Рустамович', 'TYRNN1kDG8AqEMZq5h_CfBHe6rFgfjah', '$2y$13$IOJGa/C2e0SQmu/y/aPS7u2L3bK3scQFkooL5SOonMKkm3zt/YGea', NULL, '<EMAIL>', 10, 1497135589, 1497135589);
--
-- Индексы сохранённых таблиц
--
--
-- Индексы таблицы `auth_assignment`
--
ALTER TABLE `auth_assignment`
ADD PRIMARY KEY (`item_name`,`user_id`),
ADD KEY `user_id` (`user_id`);
--
-- Индексы таблицы `auth_item`
--
ALTER TABLE `auth_item`
ADD PRIMARY KEY (`name`),
ADD KEY `rule_name` (`rule_name`),
ADD KEY `idx-auth_item-type` (`type`);
--
-- Индексы таблицы `auth_item_child`
--
ALTER TABLE `auth_item_child`
ADD PRIMARY KEY (`parent`,`child`),
ADD KEY `child` (`child`);
--
-- Индексы таблицы `auth_rule`
--
ALTER TABLE `auth_rule`
ADD PRIMARY KEY (`name`);
--
-- Индексы таблицы `group`
--
ALTER TABLE `group`
ADD PRIMARY KEY (`group_id`),
ADD UNIQUE KEY `kafedra` (`kafedra`);
--
-- Индексы таблицы `kafedra`
--
ALTER TABLE `kafedra`
ADD PRIMARY KEY (`kafedra_id`);
--
-- Индексы таблицы `menu`
--
ALTER TABLE `menu`
ADD PRIMARY KEY (`id`),
ADD KEY `parent` (`parent`);
--
-- Индексы таблицы `migration`
--
ALTER TABLE `migration`
ADD PRIMARY KEY (`version`);
--
-- Индексы таблицы `test`
--
ALTER TABLE `test`
ADD PRIMARY KEY (`test_id`),
ADD KEY `test_ibfk_1` (`user_id`);
--
-- Индексы таблицы `user`
--
ALTER TABLE `user`
ADD PRIMARY KEY (`user_id`),
ADD UNIQUE KEY `email` (`email`),
ADD UNIQUE KEY `password_reset_token` (`<PASSWORD>token`),
ADD KEY `group` (`group`);
--
-- AUTO_INCREMENT для сохранённых таблиц
--
--
-- AUTO_INCREMENT для таблицы `group`
--
ALTER TABLE `group`
MODIFY `group_id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=2;
--
-- AUTO_INCREMENT для таблицы `kafedra`
--
ALTER TABLE `kafedra`
MODIFY `kafedra_id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=2;
--
-- AUTO_INCREMENT для таблицы `menu`
--
ALTER TABLE `menu`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
--
-- AUTO_INCREMENT для таблицы `test`
--
ALTER TABLE `test`
MODIFY `test_id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=3;
--
-- AUTO_INCREMENT для таблицы `user`
--
ALTER TABLE `user`
MODIFY `user_id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=7;
--
-- Ограничения внешнего ключа сохраненных таблиц
--
--
-- Ограничения внешнего ключа таблицы `auth_assignment`
--
ALTER TABLE `auth_assignment`
ADD CONSTRAINT `auth_assignment_ibfk_1` FOREIGN KEY (`item_name`) REFERENCES `auth_item` (`name`) ON DELETE CASCADE ON UPDATE CASCADE,
ADD CONSTRAINT `auth_assignment_ibfk_2` FOREIGN KEY (`user_id`) REFERENCES `user` (`user_id`);
--
-- Ограничения внешнего ключа таблицы `auth_item`
--
ALTER TABLE `auth_item`
ADD CONSTRAINT `auth_item_ibfk_1` FOREIGN KEY (`rule_name`) REFERENCES `auth_rule` (`name`) ON DELETE SET NULL ON UPDATE CASCADE;
--
-- Ограничения внешнего ключа таблицы `auth_item_child`
--
ALTER TABLE `auth_item_child`
ADD CONSTRAINT `auth_item_child_ibfk_1` FOREIGN KEY (`parent`) REFERENCES `auth_item` (`name`) ON DELETE CASCADE ON UPDATE CASCADE,
ADD CONSTRAINT `auth_item_child_ibfk_2` FOREIGN KEY (`child`) REFERENCES `auth_item` (`name`) ON DELETE CASCADE ON UPDATE CASCADE;
--
-- Ограничения внешнего ключа таблицы `group`
--
ALTER TABLE `group`
ADD CONSTRAINT `group_ibfk_1` FOREIGN KEY (`kafedra`) REFERENCES `kafedra` (`kafedra_id`);
--
-- Ограничения внешнего ключа таблицы `menu`
--
ALTER TABLE `menu`
ADD CONSTRAINT `menu_ibfk_1` FOREIGN KEY (`parent`) REFERENCES `menu` (`id`) ON DELETE SET NULL ON UPDATE CASCADE;
--
-- Ограничения внешнего ключа таблицы `test`
--
ALTER TABLE `test`
ADD CONSTRAINT `test_ibfk_1` FOREIGN KEY (`user_id`) REFERENCES `user` (`user_id`);
--
-- Ограничения внешнего ключа таблицы `user`
--
ALTER TABLE `user`
ADD CONSTRAINT `user_ibfk_1` FOREIGN KEY (`group`) REFERENCES `group` (`group_id`);
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
<file_sep>/basic/views/site/signup.php
<?php
use yii\helpers\Html;
use yii\bootstrap\ActiveForm;
$this->title = 'Регистрация нового пользователя';
$this->params['breadcrumbs'][] = $this->title;
?>
<div class="site-signup">
<h1><?= Html::encode($this->title) ?></h1>
<div class="row">
<div class="col-md-8">
<?php $form = ActiveForm::begin(['id' => 'form-signup']); ?>
<?= $form->field($model, 'lastname')->label('Фамилия'); ?>
<?= $form->field($model, 'name')->label('Имя'); ?>
<?= $form->field($model, 'thirdname')->label('Отчество'); ?>
<?= $form->field($model, 'group')->label('Номер группы')->dropDownList([
'0' => 'У-134',
'1' => 'У-133',
'2' => 'А-155',
]);
?>
<?= $form->field($model, 'username')->label('Логин'); ?>
<?= $form->field($model, 'email')->label('Email'); ?>
<?= $form->field($model, 'password')->passwordInput()->label('Пароль'); ?>
<div class="form-group">
<?= Html::submitButton('Зарегистрироваться', ['class' => 'btn btn-primary', 'name' => 'signup-button']) ?>
</div>
<?php ActiveForm::end(); ?>
</div>
</div>
</div><file_sep>/basic/web/js/counter.js
var i = 0; // Временный костыль для запрета множественного одновременного запуска функции onStart
var status="none"; // пока никак не задействована. Думаю хранить там состояние установки: работает или нет (следовательно, если один из статусов принят, то она уже включалась хотя бы раз)
var num; // Переменная показаний счетчика. Пришлось как глобальную объявить, чтобы у функций был к ней доступ
function onStart(){ // Основная функция, которая запускает установку (можно сказать, это main)
document.addEventListener('DOMContentLoaded', onDomReady); // нужно подгружать до функции onDomReady
document.addEventListener('DOMContentLoaded', timerPanel); // нужно подгружать до функции timerPanel
count(); // Запуск счетчика
randomInteger(); // Запуск рандома для показаний температуры/влажности
onDomReady(); // Вывод данных температуры/влажности. В функции описал почему данный этап разбит на две функции
timerPanel(); // Запуск и вывод таймера
}
function count(){ // Функция счетчика (Codepen)
// num = parseInt(Math.random() * 100000, 10);
num = 0;
setTimeout(function(){
$('.cou-item').find('ul').each(function(i, el){
var val = pad(num, 5, 0).split("");
var $el = $(this);
$el.removeClass();
$el.addClass('goto-' + val[i]);
})
}, 1000);
setTimeout(function(){
counter();
}, 0)
}
function pad(n, width, z) { // Функция счетчика (Codepen)
z = z || '0';
n = n + '';
return n.length >= width ? n : new Array(width - n.length + 1).join(z) + n;
}
function counter() { // Функция счетчика (Codepen)
setInterval(function(){
$('.cou-item').find('ul').each(function(i, el){
num += 1;
var val = pad(num, 5, 0).split("");
var $el = $(this);
$el.removeClass();
$el.addClass('goto-' + val[i]);
})
}, 250);
}
// custom
// генерирует целое значение в нужном диапозоне
function randomInteger(min, max) {
var rand = min - 0.5 + Math.random() * (max - min + 1)
rand = Math.round(rand);
return rand;
}
// выводит значение в нужное место через заданный промежуток времени. Есть смысл дописать brake или придумать другой стопор функции (она зациклена)
function onDomReady(place) {
var place = document.getElementById('temperature'); // к примеру, температура. В идеале нужно передавать в функцию id нужного элемента для изменения
(function iterate(i) {
value = randomInteger(15, 25); // лучше передавать эти данные в функцию с помощью доп. параметра
timeout = randomInteger(1000, 2000); // рандомная задержка между выводом значения 1000...2000 мсек
place.innerHTML = value;
// setTimeout(function() { iterate(i + 1); }, timeout);
})(0);
}
function timerPanel(){ // Функция создания и вывода таймера
var hour = 0;
var minute = 0;
var second = 0;
var milsecond = 0;
var hour_zero = '0';
var minute_zero = '0';
var second_zero = '0';
var milsecond_zero = '0';
// var place = document.getElementById('timer');
var place_hour = document.getElementById('hour');
var place_minute = document.getElementById('minute');
var place_second = document.getElementById('second');
var place_milsecond = document.getElementById('milsecond');
(function iterate(i) {
milsecond++;
if(milsecond == 100){
milsecond = 0;
second++;
}
if(second == 60){
second = 0;
minute++;
}
if(minute == 60){
minute = 0;
hour++;
}
if(milsecond > 9){
milsecond_zero = '';
}else{
milsecond_zero = '0';
}
if(second > 9){
second_zero = '';
}else{
second_zero = '0';
}
if(minute > 9){
minute_zero = '';
}else{
minute_zero = '0';
}
if(hour > 9){
hour_zero = '';
}else{
hour_zero = '0';
}
// console.log(hour+' | '+minute+' | '+second+' | '+milsecond);
place_hour.innerHTML = hour_zero+hour;
place_minute.innerHTML = minute_zero+minute;
place_second.innerHTML = second_zero+second;
place_milsecond.innerHTML = milsecond_zero+milsecond;
// place.innerHTML = hour_zero+hour+':'+minute_zero+minute+':'+second_zero+second+':'+milsecond_zero+milsecond;
setTimeout(function() { iterate(i + 1); }, 10);
})(0);
}<file_sep>/basic/models/SignupForm.php
<?php
namespace app\models;
use Yii;
use yii\base\Model;
/**
* Signup form
*/
class SignupForm extends Model
{
public $username;
public $email;
public $group;
public $name;
public $lastname;
public $thirdname;
public $password;
/**
* @inheritdoc
*/
public function rules()
{
return [
['username', 'trim'],
['username', 'required', 'message' => 'Поле не должно быть пустым'],
['username', 'unique', 'targetClass' => '\app\models\User', 'message' => 'Логин занят. Попробуйте другой.'],
['username', 'string', 'max' => 32, 'message' => 'Логин слишком длинный'],
['email', 'trim'],
['email', 'required', 'message' => 'Поле не должно быть пустым'],
['email', 'email'],
['email', 'string', 'max' => 45],
['email', 'unique', 'targetClass' => '\app\models\User', 'message' => 'Данный Email был зарегистрирован ранее.'],
['group', 'trim'],
['group', 'required', 'message' => 'Поле не должно быть пустым'],
['group', 'string'],
['name', 'trim'],
['name', 'required', 'message' => 'Поле не должно быть пустым'],
['name', 'string', 'max' => 32],
['lastname', 'trim'],
['lastname', 'required', 'message' => 'Поле не должно быть пустым'],
['lastname', 'string', 'max' => 32],
['thirdname', 'trim'],
['thirdname', 'required', 'message' => 'Поле не должно быть пустым'],
['thirdname', 'string', 'max' => 32],
['password', 'required', 'message' => 'Поле не должно быть пустым'],
['password', 'string', 'min' => 6, 'message' => 'Пароль должен содержать не менее 6 символов'],
];
}
/**
* Signs user up.
*
* @return User|null the saved model or null if saving fails
*/
public function signup()
{
if (!$this->validate()) {
return null;
}
$user = new User();
$user->username = $this->username;
$user->email = $this->email;
$user->group = $this->group;
$user->name = $this->name;
$user->lastname = $this->lastname;
$user->thirdname = $this->thirdname;
$user->setPassword($<PASSWORD>);
$user->generateAuthKey();
return $user->save() ? $user : null;
}
}<file_sep>/basic/views/site/index.php
<?php
/* @var $this yii\web\View */
$this->title = 'Тренажер поверки газового счетчика';
$this->params['breadcrumbs'][] = $this->title;
?>
<div class="container">
<div class="col-md-4 gas-meter">
<div class="odometer">
<ul class="digits">
<li class="digit" style="border-left: 0px solid #1c3c50 !important;">
<span class="cou-item cou-item-anim">
<ul>
<li>0</li>
<li>0</li>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
<li>5</li>
<li>6</li>
<li>7</li>
<li>8</li>
<li>9</li>
<li>0</li>
</ul>
</span>
</li>
<li class="digit">
<span class="cou-item">
<ul>
<li>0</li>
<li>0</li>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
<li>5</li>
<li>6</li>
<li>7</li>
<li>8</li>
<li>9</li>
<li>0</li>
</ul>
</span>
</li>
<li class="digit">
<span class="cou-item">
<ul>
<li>0</li>
<li>0</li>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
<li>5</li>
<li>6</li>
<li>7</li>
<li>8</li>
<li>9</li>
<li>0</li>
</ul>
</span>
</li>
<li class="digit">
<span class="cou-item">
<ul>
<li>0</li>
<li>0</li>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
<li>5</li>
<li>6</li>
<li>7</li>
<li>8</li>
<li>9</li>
<li>0</li>
</ul>
</span>
</li>
<li class="digit">
<span class="cou-item">
<ul>
<li>0</li>
<li>0</li>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
<li>5</li>
<li>6</li>
<li>7</li>
<li>8</li>
<li>9</li>
<li>0</li>
</ul>
</span>
</li>
</ul>
</div>
</div>
<div class="col-md-8">
<div class="scheme-device">
<div class="popup" onclick="myFunction()">
<img class="rotate" src="img/circle-animate.png">
<span class="popuptext" id="myPopup">A Simple Popup!</span>
</div>
</div>
</div>
<div class="col-md-8 on-start">
<button class="start" id="start">Запуск</button>
</div>
<div class="col-md-8 panel">
<div class="panel-use">
<div class="indication-1">
<span id="temperature">
0
</span>
</div>
<div class="indication-2">
<span id="chastota">
0
</span>
</div>
<div class="indication-3">
<span id="davlenie">
2206
</span>
</div>
<div class="indication-4">
<span id="timer">
<span id="hour">00</span>:<span id="minute">00</span>:<span id="second">00</span>:<span id="milsecond">00</span>
</span>
</div>
<div class="indication-5">
<span id="zadvijka">
49.7
</span>
</div>
<div class="indication-6">
<span id="vakuum">
24.07
</span>
</div>
</div>
</div>
</div>
<!--<script>-->
<!-- // When the user clicks on div, open the popup-->
<!-- // function myFunction() {-->
<!-- // var popup = document.getElementById("myPopup");-->
<!-- // popup.classList.toggle("show");-->
<!-- // }-->
<!--</script>-->
<?php
$script = <<< JS
document.getElementById('start').onclick = function() {
if (i == 0){
onStart();
i++;
}
}
JS;
$this->registerJs($script, yii\web\View::POS_END);
?>
<!-- var i = 0;-->
<!-- if (i == 0){-->
<!-- var script = document.createElement('script');-->
<!-- script.src = "js/counter.js";-->
<!-- document.getElementsByTagName('head')[0].appendChild(script);-->
<!-- }-->
<!-- i++;-->
<!-- onStart();-->
<!-- }--> | e11abf8f1f7d6c307882afab165657c5a2c6a309 | [
"JavaScript",
"SQL",
"PHP"
] | 6 | PHP | notnaa/counter-yii | 9d21bdf8ad30cd75fcfb12b171fe213a704cafd6 | 8f4cb97647202856c6f5a2ce7cea56c87ed5b0e8 | |
refs/heads/master | <repo_name>taiki45/mikutter_sad_happy<file_sep>/README.md
# mikutter_sad_happy.rb
みくったは深夜のつらぽよから救う
## About
つくってみました.
>深夜、皆必然的に…のついたツイートしてしまうし全自動で♡に変換するようにして欲しい
http://twitter.com/morisoba_nzr/status/313336279972593665
<file_sep>/mikutter_sad_happy.rb
# -*- coding: utf-8 -*-
Plugin.create :mikutter_sad_happy do
texts = ["にゃー♡", "にゃんにゃん♡"]
filter_gui_postbox_post do |box|
if (0..6).cover? Time.now.hour
buff = ::Plugin.create(:gtk).widgetof(box).widget_post.buffer
if rand(20) == 0
buff.text = Array.new(rand(1..6)) { texts.sample }.join
else
buff.text = buff.text.gsub(/…|\./u, "♡")
end
end
[box]
end
end
| 3d9f59d72406e3c4a6195e1dc187efa6972ecf7a | [
"Markdown",
"Ruby"
] | 2 | Markdown | taiki45/mikutter_sad_happy | 31e4364625e561126675f28001cc688c4c452776 | bf2bd19bfc580cfc50b2a58dc51b541fd2f9cc14 | |
refs/heads/master | <file_sep>const path = require('path');
const glob = require('glob');
const resolverFileGlob = '**/*.js';
const CWD = process.cwd();
const slash_REG = /\/$/;
/*
* PageMapper will require all the files in a folder and its sub folders and let you use `findPage` to access them
* This makes it easy to setup a single route to manage all your pages.
* You can also setup a default 404 page here
*/
module.exports = class PageMapper {
constructor(uri, ignore = []) {
const resolverFiles = path.join(uri, resolverFileGlob);
const jsFiles = glob.sync(resolverFiles, { ignore: ignore }) || [];
this.modules = jsFiles.reduce((a, f) => {
const convertedURL = convertToUrl(f, uri);
a[convertedURL] = require(path.join(CWD, f));
if (convertedURL.includes('404')) this._404 = a[convertedURL];
return a;
}, {});
}
findPage(url) {
if (this._root && url === '/') url = '/' + this._root;
const module = this.modules[url.replace(slash_REG, '')];
if (typeof module !== 'function' && typeof module !== 'object') return this._404 || noop;
return this.modules[url.replace(slash_REG, '')];
}
set pageNotFount(url) {
this._404 = this.modules[url.replace(slash_REG, '')];
}
set root(url) {
this._root = url;
}
};
function convertToUrl(str, uri) {
return str
.replace(uri, '') // remove folder path
.replace('index.js', '') // do not use index.js. insterad use folder
.replace('.js', '') //js extension
.replace(slash_REG, ''); // trailing slash
}
function noop() {
return {};
}
<file_sep>/*
* Cache
* This is inteded to increase performance
* Using caching can make perfance for serving the website close to the performance of using static files
* The one caveot is the first time you render a page after the server being started it will not be optomized yet
* The cache is stored in memory
*/
// TODO look into alowing cache to be stored and managed outside of memory
exports.memoize = fn => {
return variadic.bind(
this,
fn,
new Cache()
);
};
/*
* https://en.wikipedia.org/wiki/Variadic_function
* This just means it is expeting multiple arguments, but it will work with 0 and 1
*/
function variadic(fn, cache) {
const args = Array.prototype.slice.call(arguments, 2);
const cacheKey = JSON.stringify(args);
let computedValue = cache.get(cacheKey);
if (typeof computedValue === 'undefined') {
computedValue = fn.apply(this, args);
cache.set(cacheKey, computedValue);
}
return computedValue;
}
// Basic in memory cache class
class Cache {
constructor() {
this.cache = Object.create(null);
}
has(key) {
return (key in this.cache);
}
get(key) {
return this.cache[key];
}
set(key, value) {
this.cache[key] = value;
}
}
<file_sep>const customElements = require('./src/customElements');
const PageMapper = require('./src/PageMapper');
const fileHandler = require('./src/fileHandler');
const { set } = require('./src/config');
const { css, html } = require('./src/template-literal-tags');
const HTMLElementExtended = require('./src/HTMLElementExtended');
const Page = require('./src/Page');
module.exports = {
customElements,
PageMapper,
html,
css,
setConfig: set,
fileHandler,
HTMLElementExtended,
Page
};
<file_sep>module.exports = {
css: basic,
html: basic
};
// this just builds the remplate literal
// Basically this will enable colors in your editor
function basic(strings, ...expressionValues) {
let finalString = '';
let i = 0;
let length = strings.length;
for(; i < length; i++) {
if (i > 0) finalString += expressionValues[i - 1];
finalString += strings[i];
}
return finalString;
}
<file_sep>module.exports = class HTMLElementExtended {
constructor() {
this.style = {};
}
appendChild() {}
attachShadow() {
this.shadowRoot = new ShadowRoot();
}
hasAttribute() {}
getAttribute() {}
setAttribute() {}
removeAttribute() {}
addEventListener() {}
removeEventListener() {}
getAttribute() { return ''; }
hasAttribute() {}
setAttribute() {}
// override methods
html() { return ''; }
css() { return ''; }
externalCSS() { return ''; }
// client methods
// do not override
render() {}
cloneTemplate() {}
// building methods
getTemplateElementAsIIFE(name) {
return `(function(){
var t=document.createElement('template');
t.setAttribute('id','${name}');
t.innerHTML=\`
<style>
${this.css()}
</style>
<render-block>
${this.html()}
</render-block>
\`;
document.body.insertAdjacentElement('beforeend', t);
}());`;
}
getClassAsString(name) {
let cstr = this.constructor.toString();
// remove class declaration
cstr = cstr.slice(cstr.indexOf('{') + 1, cstr.lastIndexOf('}'));
return `
customElements.define("${name}", class extends HTMLElement {
${cstr}
render() {
const renderBlock = document.querySelector('render-block');
if (!renderBlock) throw Error('Could not find <render-block>');
renderBlock.innerHTML = this.html();
}
cloneTemplate() {
var template = document.getElementById('${name}');
var templateContent = template.content;
var shadowRoot = this.shadowRoot ? this.shadowRoot : this.attachShadow({mode: 'open'});
shadowRoot.appendChild(templateContent.cloneNode(true));
}
});
`;
}
};
class ShadowRoot {
innerHTML() {}
}
<file_sep># Attention: project moved and renamed
This project has been moved under [webformula](https://github.com/webformula) and renamed to [pax-core](https://github.com/webformula/pax-core)
<file_sep>// Global Configuration
const config = {
/*
* This will memoize certain methods to prevent unnecessary processing
* This is essantially equal to static file performance after the first request
*/
memoize: true,
/*
* default: true
* user built in service worker to manage cache
*/
serviceWorker: true
};
exports.get = (name) => name !== undefined ? config[name] : config;
exports.set = (params = {}) => {
Object.keys(config).forEach(key => {
if (params[key] !== undefined) config[key] = params[key];
});
};
<file_sep>const config = require('./config');
const { memoize } = require('./cache');
const components = {};
module.exports = {
define(name, constructor) {
if (components[name]) throw Error(`component "${name}" has already been registered. Please change the components name`);
components[name] = constructor;
},
getStaticExternalCSS() {
if (this.memoize) {
if (!this.getStaticExternalCSSMemoized) this.getStaticExternalCSSMemoized = memoize(_getStaticExternalCSS);
return this.getStaticExternalCSSMemoized();
}
return _getStaticExternalCSS();
},
getStaticFile() {
if (this.memoize) {
if (!this.getStaticFileMemoized) this.getStaticFileMemoized = memoize(_getStaticFile);
return this.getStaticFileMemoized();
}
return _getStaticFile();
}
};
function _getStaticExternalCSS() {
return Object
.keys(components)
.map(key => new components[key]().externalCSS())
.join('\n');
}
function _getStaticFile() {
return `
document.addEventListener("DOMContentLoaded", function (event) {
${Object
.keys(components)
.map(key => {
const comp = new components[key]();
return [
comp.getTemplateElementAsIIFE(key),
comp.getClassAsString(key)
].join('\n');
})
.join('\n')}
});
`;
}
<file_sep>module.exports = () => `
var _cacheName = 'wcn-cache';
self.addEventListener('install', function (event) {
event.waitUntil(
caches.open(_cacheName).then(function (cache) {
return cache.addAll(
[
// TODO get list of html css and javascript
// can use page mapper and then parse links and scripts
]
);
})
);
});
self.addEventListener('activate', function (event) {
event.waitUntil(
caches.keys().then(function (cacheNames) {
return Promise.all(
cacheNames.filter(function (cacheName) {
return cacheName === _cacheName;
}).map(function (cacheName) {
return caches.delete(cacheName);
})
);
})
);
});
self.addEventListener('fetch', function (event) {
event.respondWith(
caches.open(_cacheName).then(function (cache) {
return cache.match(event.request).then(function (response) {
return response || fetch(event.request).then(function (response) {
if (!event.request.url.includes('load-service-worker.js')) cache.put(event.request, response.clone());
return response;
}).catch(function() {
return caches.match('/offline.html');
}).catch(function() {
return new Response("<h2>No page loaded</h2><p>You are currently offline and the page is not cahced, Please check you internet and reload to access this page.</p>", {
headers: {'Content-Type': 'text/html'}
});
});
});
}).catch(function (e) {
throw e;
})
);
});
`;
<file_sep>const components = require('../componentRegistry');
const files = {
main_js: require('./main.js'),
serviceWorkerLoader_js: require('./service-worker-loader'),
serviceWorker_js: require('./service-worker'),
components_js: components.staticFile,
components_css: components.staticComponentCSS
};
const reg = /-([a-z])/g;
function toCamelCase(str) {
return str.replace(reg, g => g[1].toUpperCase());
}
module.exports = params => {
const cameled = toCamelCase(params.fileName.replace('.js', '_js').replace('.css', '_css'));
if (!files[cameled]) throw Error('file not found');
return {
content: files[cameled](params),
mime: getMime(params.fileName)
};
};
function getMime(file) {
if (file.includes('.js')) return 'text/javascript';
if (file.includes('.css')) return 'text/css';
if (file.includes('.html')) return 'text/html';
return 'text/plain';
}
exports.getJavascript = () => {
}
<file_sep>module.exports = params => `
window.html = function (strings, ...expressionValues) {
let finalString = '';
let i = 0;
let length = strings.length;
for(; i < length; i++) {
if (i > 0) finalString += expressionValues[i - 1];
finalString += strings[i];
}
return finalString;
};
window.htmlSafe = window.html;
window.css = window.html;
`;
| 7dd1f7ea41726448b74b4a720d9e38cc2aea3568 | [
"JavaScript",
"Markdown"
] | 11 | JavaScript | B-3PO/web-components-node | bdfb8783f3996d10a77bd8fab9febbf71aac346c | 117246f9e25f21093e2e97de50dc4cb05cd2ab2f | |
refs/heads/main | <repo_name>suhaib079/city-explorer<file_sep>/server.js
'use strict';
const express = require('express');
const server = express();
const dot = require('dotenv');
dot.config();
const pg = require('pg')
// const client = new pg.Client(process.env.DATABASE_URL)
const client = new pg.Client({ connectionString: process.env.DATABASE_URL, ssl: { rejectUnauthorized: false } });
client.connect();
const cors = require('cors');
server.use(cors());
const port = process.env.PORT || 4000;
const axios = require('axios')
const superAgent = require('superagent')
// Routes
server.get('/location', locHandler)
server.get('/weather', wetherHandler)
server.get('/parks', parksHandler)
server.get('/movies', moviesHandler)
server.get('/yelp', yelpHandler)
server.get('*', errHandler)
// Handler Functions
// http://localhost:3500/location?city=amman
function locHandler(req, res) {
const cityName = req.query.city
let key = process.env.LOCATION_KEY;
let url = `https://api.locationiq.com/v1/autocomplete.php?key=${key}&q=${cityName}`;
let SQL = `SELECT * FROM locations;`;
client.query(SQL)
.then(result => {
if (result.rows.filter(value => value.search_query === `${cityName}`).length > 0) {
let SQL = `SELECT * FROM locations WHERE search_query='${cityName}';`
client.query(SQL)
.then(result => {
let x = new LocationFromDB(cityName, result.rows[0])
res.send(x)
})
} else {
console.log("Adding To DataBase");
superAgent.get(url)
.then(result => {
let x = new Location(cityName, result.body[0])
let cityname = x.search_query;
let format = x.formatted_query;
let lati = x.latitude;
let long = x.longitude;
let SQL = `INSERT INTO locations VALUES ($1,$2,$3,$4) RETURNING *;`;
let safeValues = [cityname,format,lati,long];
client.query(SQL,safeValues)
.then(()=>{
res.send(x)
})
})
}
})
}
// http://localhost:3500/weather?search_query=amman&formatted_query=Amman%2C%20Amman%2C%2011181%2C%20Jordan&latitude=31.9515694&longitude=35.9239625&page=1
function wetherHandler(req, res) {
const cityName = req.query.search_query;
let key = process.env.WEATHER_KEY;
let url = `https://api.weatherbit.io/v2.0/forecast/daily?city=${cityName}&key=${key}`;
superAgent.get(url)
.then(wethData => {
let wethObjArr = wethData.body.data.map(value => {
return new Weather(value)
})
res.send(wethObjArr);
})
}
// http://localhost:3500/parks?search_query=amman&formatted_query=Amman%2C%20Amman%2C%2011181%2C%20Jordan&latitude=31.9515694&longitude=35.9239625&page=1
function parksHandler(req, res) {
const cityName = req.query.search_query;
let key = process.env.PARKS_KEY;
let url = `https://developer.nps.gov/api/v1/parks?q=${cityName}&api_key=${key}`
superAgent.get(url)
.then(parksData => {
let parksObjArr = parksData.body.data.map(value => {
return new Parks(value)
})
res.send(parksObjArr);
})
}
// http://localhost:2000/movies?search_query=london&formatted_query=London%2C%20London%2C%20England%2C%20SW1A%202DX%2C%20United%20Kingdom&latitude=51.50732190000000&longitude=51.50732190000000&page=1
function moviesHandler(req, res) {
const cityName = req.query.search_query;
let key = process.env.MOVIES_KEY;
let url = `https://api.themoviedb.org/3/search/movie?api_key=${key}&query=${cityName}`
superAgent.get(url)
.then(moviesData => {
let moviesObjArr = moviesData.body.results.map(value => {
return new Movies(value)
})
res.send(moviesObjArr)
})
}
// http://localhost:2000/yelp?search_query=seattle&formatted_query=Seattle%2C%20Seattle%2C%20Washington%2C%2098104%2C%20USA&latitude=47.6038321&longitude=-122.3300624&page=1
function yelpHandler(req, res) {
let cityName = req.query.search_query;
let page = req.query.page;
const limitNum = 5;
const start = ((page - 1) * limitNum + 1)
let key = process.env.YELP_KEY;
let url = `https://api.yelp.com/v3/businesses/search?location=${cityName}&limit=${limitNum}&offset=${start}`
let yelpRest = axios.create({
baseURL: "https://api.yelp.com/v3/",
headers: {
Authorization: `Bearer ${key}`,
"Content-type": "application/json",
},
})
yelpRest(url)
.then(({ data }) => {
let yelpObjArr = data.businesses.map(value => {
return new Yelp(value)
})
res.send(yelpObjArr)
})
}
function errHandler(req, res) {
let errObj = {
status: 500,
responseText: "Sorry, something went wrong"
}
res.send(errObj);
}
// Constructors
function Location(cityName, locData) {
this.search_query = cityName;
this.formatted_query = locData.display_name;
this.latitude = locData.lat;
this.longitude = locData.lon;
}
function LocationFromDB(city, data) {
this.search_query = city;
this.formatted_query = data.formatted_query;
this.latitude = data.latitude;
this.longitude = data.longitude;
}
function Weather(wetherData) {
this.forecast = wetherData.weather.description;
this.time = new Date(wetherData.datetime).toString().slice(0, 15);;
}
function Parks(parksData) {
this.name = parksData.fullName;
this.address = `${parksData.addresses[0].line1}" "${parksData.addresses[0].city}" "${parksData.addresses[0].stateCode}" "${parksData.addresses[0].postalCode}`;
this.fee = parksData.entranceFees[0].cost;
this.description = parksData.description;
this.url = parksData.url;
}
function Movies(movieData) {
this.title = movieData.title;
this.overview = movieData.overview;
this.average_votes = movieData.vote_average;
this.image_url = `https://image.tmdb.org/t/p/w500${movieData.poster_path}`;
this.popularity = movieData.popularity;
this.released_on = movieData.release_date;
}
function Yelp(yelpData) {
this.name = yelpData.name;
this.image_url = yelpData.image_url;
this.price = yelpData.price;
this.rating = yelpData.rating;
this.url = yelpData.url;
}
server.listen(port, () => {
console.log(`Server is listening on Port ${port}`)
}) | 884f9ae94d7ef932798357ace8c9aa2de5ef820b | [
"JavaScript"
] | 1 | JavaScript | suhaib079/city-explorer | 42f52fe50d0a02110b87f9a88b57498f18acbb61 | c3c26ef401c5110a64fd90d8341d3a741d475453 | |
refs/heads/master | <repo_name>shieldfy/is-serverless<file_sep>/lib/is-azure-function.js
'use strict'
module.exports = !!(
process.env.AzureWebJobsStorage ||
false
)
<file_sep>/test/isAzurefunction.js
'use strict'
var assert = require('assert')
//------- Azure --------
process.env.AzureWebJobsStorage = 'Value'
var isServerless = require('../')
assert(isServerless.result)
assert.equal(isServerless.whichOne,'Azure')<file_sep>/lib/is-cloudflare-worker.js
'use strict'
module.exports = !!(
process.env.CLOUDFLARE_ZONE_ID ||
false
)
<file_sep>/README.md
# is-serverless
Detection library for serverless environment, currently supports ([AWS Lambda](https://aws.amazon.com/lambda/), [GCP cloud functions](https://cloud.google.com/functions/), [Azure functions](https://azure.microsoft.com/en-in/services/functions/), [Cloudflare workers](https://workers.cloudflare.com))
## Inspiration
This package inspired by `is-lambda` package by [@watson](https://github.com/watson)
## Installation
```
npm install is-serverless
```
## Usage
```js
var isServerless = require('is-serverless')
if (isServerless.result) {
console.log('The code is running on a serverless environment : ' + isServerless.whichOne)
}
```
## Contribution
Feel free to fork, commit and submit pull request if you find a bug, or you want to add support to a new environment. Contributions are very welcome.
## License
MIT<file_sep>/lib/index.js
'use strict'
module.exports = {
'isAWSLambda' : require('./is-aws-lambda'),
'isGCPCloudfunction' : require('./is-gcp-cloudfunction.js'),
'isAzureFunction' : require('./is-azure-function.js'),
'isCloudflareWorker' : require('./is-cloudflare-worker.js'),
'isVercel' : require('./is-vercel.js')
}<file_sep>/lib/is-aws-lambda.js
'use strict'
var isLambda = require('is-lambda')
module.exports = isLambda;<file_sep>/test/isLambda.js
'use strict'
var assert = require('assert')
//------ AWS -----------
process.env.AWS_EXECUTION_ENV = 'AWS_Lambda_nodejs6.10'
process.env.LAMBDA_TASK_ROOT = '/var/task'
var isServerless = require('../')
assert(isServerless.result)
assert.equal(isServerless.whichOne,'AWS') | 313cb2215f5039f0aec9fe086cb9a7cc8bfb9c5d | [
"JavaScript",
"Markdown"
] | 7 | JavaScript | shieldfy/is-serverless | f170b6a7c9db4dfb774c094ee8db5e7c1f0dd311 | 634965cedc1c5a3ae83bb6230f2735acf8b97150 | |
refs/heads/main | <repo_name>zhang-zhoujie-optim/install-vnc<file_sep>/vnc.sh
#!/bin/bash
echo "Installing vnc..."
sudo apt update
sudo apt install -y xfce4 xfce4-goodies
sudo apt install -y tightvncserver
vncserver
vncserver -kill :1
mv ~/.vnc/xstartup ~/.vnc/xstartup.bak
mkdir -p ~/.vnc/
touch ~/.vnc/xstartup
cat <<EOT >> ~/.vnc/xstartup
#!/bin/bash
xrdb \$HOME/.Xresources
startxfce4 &
EOT
chmod +x ~/.vnc/xstartup
touch [email protected]
cat <<EOT >> ./[email protected]
[Unit]
Description=Start TightVNC server at startup
After=syslog.target network.target
[Service]
Type=forking
User=$USER
Group=$USER
WorkingDirectory=$HOME
PIDFile=$HOME/.vnc/%H:%i.pid
ExecStartPre=-/usr/bin/vncserver -kill :%i > /dev/null 2>&1
ExecStart=/usr/bin/vncserver -depth 24 -geometry 1280x800 -localhost :%i
ExecStop=/usr/bin/vncserver -kill :%i
[Install]
WantedBy=multi-user.target
EOT
sudo mv ./[email protected] /etc/systemd/system/[email protected]
sudo systemctl daemon-reload
sudo systemctl enable [email protected]
sudo systemctl start vncserver@1
<file_sep>/README.md
# install-vnc
This script installs VNC for a linux server.
For more information, see [this guide](https://www.digitalocean.com/community/tutorials/how-to-install-and-configure-vnc-on-ubuntu-20-04).
## How to use
```bash
chmod +x vnc.sh
./vnc.sh
```
During installation, you may be prompted to choose a default display manager for Xfce. Select either one and press `ENTER`.
Then you’ll be prompted to enter and verify a password to access your machine remotely:
```bash
You will require a password to access your desktops.
Password:
Verify:
# The password must be between six and eight characters long. Passwords more than 8 characters will be truncated automatically.
Would you like to enter a view-only password (y/n)?
# n
```
| 7b2f88ba3f6f2a61b3c071b5fa638401e15b8f7d | [
"Markdown",
"Shell"
] | 2 | Shell | zhang-zhoujie-optim/install-vnc | c38940b2e4395def4c80a9a1aac0dcb34b97c857 | ae70f63ea198d8ccbf417c6bf71c94a9845d61b3 | |
refs/heads/master | <file_sep>animals = {"sugar glider"=>"Australia","aye-aye"=> "Madagascar","red-footed tortoise"=>"Panama","kangaroo"=> "Australia","tomato frog"=>"Madagascar","koala"=>"Australia"}
require 'pry'
class Hash
def keys_of(*arguments)
value_returned = []
arguments.each do |arg|
self.each {|key,value| value_returned << key unless value != arg}
end
value_returned
end
end
| dd6e662a6cd6af272eb6dcd6f4ec68e29692903e | [
"Ruby"
] | 1 | Ruby | cstatro/keys-of-hash-nyc-web-062419 | 78e8d766165b022251df4dd5e3644204e3ea2a34 | 51f155496d06da8cc960fce34a25783c5a52c15a | |
refs/heads/master | <file_sep><?php
$time = microtime();
$time = explode(' ', $time);
$time = $time[1] + $time[0];
$finish = $time;
$total_time = round(($finish - $start), 4);
//echo '<p style="text-align: center; color: black;">Page generated in '.$total_time.' seconds.'."</p>\n";
$link->close();
?><file_sep><?php
header('Content-type: text/javascript');
include("../includes/vars.php");
?>
var DEFAULT_ID = 0;
var POSTERS_PER_ROW = 7;
var titleStorage = new Array();
var selectedMov = 0;
var processingMovie = false;
// Local database ----------------------------------------------------------
var db;
// Type Constants
var NOT_TMDB = 0;
var YES_TMDB = 1;
// Status Constants
var NOT_WATCHED = 0;
var WATCHED = 1;
db = openDatabase('Shelf', '1.0', 'Shelf', 5*1024*1024);
if (db)
db.transaction(function(transaction) {
// Creat tables in the database
transaction.executeSql(
'CREATE TABLE IF NOT EXISTS movies ' +
' (id INTEGER NOT NULL PRIMARY KEY AUTOINCREMENT, ' +
' type INTEGER NOT NULL, ' + // 0 = not TMDb 1 = TMDb
' status INTEGER NOT NULL, ' + // <0 = not watched >1 = watched
' TMDb INTEGER NOT NULL, ' +
' overview TEXT NOT NULL, ' +
' release TEXT NOT NULL, ' +
' image TEXT NOT NULL, ' +
' url TEXT NOT NULL, ' +
' runtime INTEGER NOT NULL, ' +
' title TEXT NOT NULL );'
, []);
});
// Get from database
function updateMovies() {
var search =
"<div class=\"listItem\">" +
"<input type=\"text\" id=\"searchBox\" class=\"searchBox, search\" onChange=\"search(this.value);\" placeholder=\"Search for Movies Titles you want to add to your list.\" />" +
"<a type=\"text\" id=\"searchButton\" class=\"searchButton\">Search</a>" +
"<div id=\"searchResults\"></div>" +
"</div>";
var notList = "Not";
var seenList = "Seen";
// Write Movie to list of movies
function writeMovie(row) {
if (row.type == NOT_TMDB)
listItem =
'<div class="listItem" id="listItem' + row.id + '">' +
'<h3 class="itemContents, movieTitle">' + row.title + '</h3>' +
'<div class="itemControls">' +
'<button class="itemControls" onClick="seeMovie(' + row.id + ');">Mark Watched</button>' +
'<button class="itemControls" onClick="removeItem(' + row.id + ');">Remove</button>' +
'</div>' +
'</div>';
else {
var homepage = "", time = "", date = "", watch = "", image = "";
// Check there is a url to link to
if (row.url !== "")
homepage = '<span class="movieSite"><a target="TOP" href="' + row.url + '">Movie Website</a></span>';
// Check there is a time to show
if (row.runtime !== "")
time = '<span class="movieRuntime">Runs for: <span id="movieRuntime">' + row.runtime + '</span> min</span>';
// Check there is a realse date to show
if (row.release !== "")
date = '<span>Released On: <span id="movieRelease_date">' + row.release + '</span></span>';
// Watched on not watched
if (row.status == WATCHED)
watch = '<button class="itemControls" onClick="unseeMovie(' + row.id + ');">Unwatch</button>';
else
watch = '<button class="itemControls" onClick="seeMovie(' + row.id + ');">Mark Watched</button>';
// Check there is a Image on record
if (row.image == "")
image = '<img class="movieImage" src="http://graphicport.net/mySoftware/images/no_image.gif"/>';
else
image = '<img class="movieImage" src="' + row.image + '"/>';
listItem =
'<div class="listItem" id="listItem' + row.id + '">' +
'<div class="movieImage">' +
image +
'</div>' +
'<div class="movieInfo">' +
'<h3 class="itemContents, movieTitle">' + row.title + '</h3>' +
'<p id="movieOverview" class="movieOverview, movieDescription">' + row.overview + '</p>' +
'<div class="movieControlsAnd">' +
'<div class="movieData">' +
date +
time +
homepage +
'</div>' +
'<div class="movieControls">' +
watch +
'<button class="itemControls" onClick="removeItem(' + row.id + ');">Remove</button>' +
'</div>' +
'</div>' +
'</div>' +
'</div>';
}
return listItem;
}
// Write List to screen
function writeList(status) {
db.transaction(function(transaction) {
transaction.executeSql('SELECT * FROM movies WHERE status = ?',[status], function (trx, result) {
var list = "";
var section = "";
// If needed write Seen movies header
if (status == WATCHED)
section = "Watched Movies";
else
section = "Movies Not Yet Watched";
for (var i=0; i < result.rows.length; i++) {
list = writeMovie(result.rows.item(i)) + list;
}
if (result.rows.length >= 1) {
list =
'<div class="listItem">' +
'<h2 class="itemContents, movieTitle">' + section + '</h2>' +
'</div>' + list;
}
if (status == WATCHED)
seenList = list;
else {
if (processingMovie) {
list =
'<div class="listItem">' +
'<h2 class="itemContents, movieTitle">Currently adding \'' + titleStorage[selectedMov] + '\' to your list. It just takes a few seconds, cause we have to talk to the blokes a TMDb again.</h2>' +
'</div>' + list;
processingMovie = false;
}
notList = list;
}
// Show help statment if no movies in list
if ((notList + seenList) == "")
notList =
'<div class="listItem">' +
'<h2 class="itemContents, movieTitle">Type a Movie Title in the Box above, to start your Movie list.</h2>' +
'</div>';
$('#movieList').html(search + notList + seenList);
$("#searchBox").focus();
}, errorHandler);
});
}
writeList(NOT_WATCHED);
// Watched movies
writeList(WATCHED);
}
updateMovies();
// Fill tables in the databasevar
function addMovie(type, tmdbId) {
var status = NOT_WATCHED, runtime = 0;
var overview = "", release = "", image = "", homepage = "";
processingMovie = true;
selectedMov = tmdbId;
title = titleStorage[tmdbId];
updateMovies();
if (type == YES_TMDB) {
// JSON AJAX request
var url = "http://api.themoviedb.org/2.1/Movie.getInfo/en/json/<?php echo($tmdb_api_key);?>/" + encodeURIComponent(tmdbId) + "?callback=?";
$.ajax(url, {
crossDomain:true,
dataType: "jsonp",
success:function(data,text,xhqr){
$("#tmdbSearch").html("");
if (data[0] !== "Nothing found.") {
$.each(data, function(i, item) {
var count = 0;
runtime = item.runtime;
overview = item.overview;
release = item.released;
$.each(item.posters, function(i, item) {
if (count++ == 0)
image = item.image.url;
});
homepage = item.homepage;
title = item.name;
});
db.transaction(function(transaction) {
transaction.executeSql('INSERT INTO movies (type, status, TMDb, overview, release, image, url, runtime, title) VALUES (?, ?, ?, ?, ?, ?, ?, ?, ?);',
[YES_TMDB, status, tmdbId, overview, release, image, homepage, runtime, title]);
});
updateMovies();
}
},
error:function (xhr, ajaxOptions, thrownError){
console.log(xhr.status);
}
})
} else {
db.transaction(function(transaction) {
transaction.executeSql('INSERT INTO movies (type, status, TMDb, overview, release, image, url, runtime, title) VALUES (?, ?, ?, ?, ?, ?, ?, ?, ?);',
[NOT_TMDB, status, tmdbId, overview, release, image, homepage, runtime, title]);
});
updateMovies();
}
return false; // Stop page refresh
}
function clearTable() {
db.transaction(function(transaction) {
transaction.executeSql('DELETE FROM movies', [], function(){
updateMovies();
}, errorHandler);
});
}
function removeItem(id) {
db.transaction(function(transaction) {
transaction.executeSql('DELETE FROM movies WHERE id = ?', [id], function(){
updateMovies();
}, errorHandler);
});
}
function errorHandler(transaction, error) {
alert('Oops. Error was '+error.message+' (Code '+error.code+')');
return false;
}
function unseeMovie(id) {
db.transaction(function(transaction) {
transaction.executeSql('UPDATE movies SET status = ? WHERE id = ?', [NOT_WATCHED, id], function(){
updateMovies();
}, errorHandler);
});
}
function seeMovie(id) {
db.transaction(function(transaction) {
transaction.executeSql('UPDATE movies SET status = ? WHERE id = ?', [WATCHED, id], function(){
updateMovies();
}, errorHandler);
});
}
// TMDb talk ----------------------------------------------------------
var req = new Array();
function search(title) {
var posterCount = 0;
var recentDiv = "";
var arrLast = new Array(); // Array of ids with current row in it
var highPoster = 0;
for(var i = 0; i < req.length; i++)
req[i].abort();
$("#searchResults").html("<div id=\"homemadeSearch\"></div><div id=\"tmdbSearch\"></div>"); // Clear list
function addChoice(type, title, tmdbId, img) {
if (type == NOT_TMDB) {
$("#homemadeSearch").append("<div id=\"searchResult\" onClick=\"addMovie(" + type + ", " + tmdbId + ");\" class=\"search\">Just '" + title + "'</div>");
} else {
var HEIGHT_DIF = 22; // Difference in height bettween components and row
if ((posterCount % POSTERS_PER_ROW) == 0) {
divId = "posterRow" + (posterCount / POSTERS_PER_ROW);
arrLast = new Array();
$("#posterSearch").append("<div class=\"posterRow\" id=\"" + divId + "\"></div>");
recentDiv = divId;
highPoster = 0;
}
var posterId = "poster" + posterCount;
var rowId = "#" + recentDiv;
$(rowId).append(
"<div class=\"posterItem\" id=\"" + posterId + "\" onClick=\"addMovie(" + type + ", " + tmdbId + ");\">" +
"<img class=\"movieImage posterItem\" src=\"" + img + "\"/>" +
"<a class=\"posterItem\">" + title + "</a>" +
"</div>");
arrLast.push(posterId);
if ($("#" + posterId).height() < highPoster)
$("#" + posterId).height(highPoster);
if ((highPoster + HEIGHT_DIF) < ($(rowId).height())) {
highPoster = $("#" + posterId).height(); // (Most recent poster = the one above) + extra distace for format
for(var i = 0; i < arrLast.length; i++)
$("#" + arrLast[i]).height(highPoster);
}
posterCount++;
}
titleStorage[tmdbId] = title;
}
if (title.length < 1) // Check something is there
return;
if (!(/\S/.test(title))) // Check something is there
return;
addChoice(NOT_TMDB, title, DEFAULT_ID, "");
$("#tmdbSearch").html("<h6 class=\"tmdbSearch\">Yeah Mate, we're just looking through the disks at TMDb to give you more suggestions. Give us a minute</h6>");
// JSON AJAX request
var url = "http://api.themoviedb.org/2.1/Movie.search/en/json/<?php echo($tmdb_api_key);?>/" + encodeURIComponent(title.replace(" ","+")) + "?callback=?";
var request;
req.push(
request = $.ajax(url, {
crossDomain:true,
dataType: "jsonp",
success:function(data,text,xhqr){
$("#tmdbSearch").html("<div id=\"posterSearch\" class=\"posterSearch\"></div>");
if (data[0] !== "Nothing found.")
$.each(data, function(i, item) {
var image = "http://graphicport.net/mySoftware/images/no_image.gif";
// Get Image
var count = 0;
$.each(item.posters, function(i, item) {
if (count++ == 0)
image = item.image.url;
});
addChoice(YES_TMDB, item.name, item.id, image);
});
},
error:function (xhr, ajaxOptions, thrownError){
console.log(xhr.status);
$("#tmdbSearch").html("");
}
})
);
request.fail(function(jqXHR, textStatus) {
$("#tmdbSearch").html("<h6 class=\"tmdbSearch\">Request failed: " + textStatus + "</h6>");
});
return false; // Stop page refresh
}<file_sep><?php
include("includes/vars.php");
include($phpheader);
?>
<!DOCTYPE html>
<html manifest="movies.appcache">
<head>
<title>Movies | List the movies you want to see.</title>
<?php include($head);?>
<link rel="stylesheet" type="text/css" href="<?php echo($indexCss); ?>" />
<script type="text/javascript" src="<?php echo($jsIndex); ?>"></script>
</head>
<body id="body">
<?php include($header);?>
<div class="content" id="content">
<div id="movieList" class="movieList">
<div class="listItem"><h3 class="itemContents">Javascript Problems? Try reloading. Otherwise we recomend <a href="http://www.google.com/chrome">Google Chrome</a></h3></div>
</div>
<div class="listControls">
<button onClick="clearTable();">Remove All Movies</button>
</div>
</div>
<?php include($footer);?>
<script type="text/javascript" src="http://lab.kaiserapps.com/backfun/js/backfunmin.js"></script>
<script>
// the Backfun class takes (centerDivId,BackgroundColor) as its arguements
var back = new Backfun("body", "#ffffff", true, "black");
// add the mouse spawn point
back.addMouse();
// the start function sets off the animation
back.start();
</script>
</body>
</html>
<?php include($phpfooter);?><file_sep><?php
header('Content-type: text/css');
include("../includes/vars.php");
?>
/* Generic all pages style */
body {
overflow-y: scroll;
}
html, body{
width: 100%;
margin: -5px 0 0 0;
padding: 5px 0;
color: <?php echo($color[4]);?>;
}
html {
height: 100%;
}
.mainContainer {
width: 860px;
margin: 10px auto;
border: 1px solid <?php echo($color[4]);?>;
border-radius: 25px;
text-align: center;
padding: 20px;
background-color: white;
box-shadow: 5px 5px 20px #CCC;
}
header {
width: inherit;
font-size: 10pt;
}
header h1 {
font-family: Calibri;
line-height: 50px;
font-size: 89px;
margin: 30px;
color: <?php echo($color[4]);?>;
}
.center {
text-align: center;
}
footer {
text-align: center;
color: silver;
margin-top: 20px;
}
h2 {
color: <?php echo($color[4]);?>;
text-align: left;
font-family: Arial;
}
.content {
text-align: left;
}<file_sep><?php
// Vars for app.
$incFolder = "includes/";
$cssFolder = "css/";
$jsFolder = "js/";
// Include folder vars
$phpheader = $incFolder . "phpheader.php";
$head = $incFolder . "head.php";
$header = $incFolder . "header.php";
$footer = $incFolder . "footer.php";
$phpfooter = $incFolder . "phpfooter.php";
$mainCss = $cssFolder . "style_css.php"; // Main Project CSS file
$indexCss = $cssFolder . "app_css.php";
$jsIndex = $jsFolder . "app_js.php";
$tmdb_api_key = "<KEY>";
// CSS Color vars
$color[0] = "#022859";
$color[1] = "#45648C";
$color[2] = "#7C8EA6";
$color[3] = "#CCCCCC";
$color[4] = "#383838";
?><file_sep><?php
header('Content-type: text/css');
include("../includes/vars.php");
?>
.movieList {
border: 1px solid <?php echo($color[4]);?>;
border-radius: 15px;
background-color: <?php echo($color[1]);?>;
}
.listItem {
border: 1px solid <?php echo($color[4]);?>;
border-radius: 10px;
padding: 10px;
margin: 10px;
background-color: white;
}
h3 {
color: <?php echo($color[4]);?>;
font-family: Arial;
line-height: 10%;
}
.search {
color: <?php echo($color[4]);?>;
font-family: Arial;
border: 1px solid black;
border-radius: 5px;
padding: 5px;
margin: 5px;
}
div.search:hover {
color: white;
background-color: <?php echo($color[2]);?>;
cursor: pointer;
}
form {
margin: 0;
}
.itemControls {
text-align: right;
}
.movieTitle {
text-align: left;
}
div.movieImage {
float: left;
}
img.movieImage {
width: 92px;
height: 138px;
border-radius: 5px;
}
div.movieInfo {
margin-left: 100px;
}
p.movieDescription {
font-family: Times New Roman;
text-align: left;
min-height: 60px;
}
div.movieControlsAnd {
margin-bottom: 0;
color: gray;
font-family: Arial;
}
span.movieRuntime {
margin-left: 20px;
}
span.movieSite {
margin-left: 20px;
}
div.movieControls {
text-align: right;
}
div.movieData {
width: 500px;
float: left;
}
h2 {
color: <?php echo($color[4]);?>;
margin: 0;
}
div.listControls {
margin: 10px;
}
h6.tmdbSearch {
margin: 5px;
padding: 5px;
font-family: Arial;
font-size: 20px;
}
input.search {
width: 727px;
}
a.searchButton {
padding: 5px;
margin: 5px;
border-radius: 5px;
font-family: Arial;
font-size: 14px;
color: white;
cursor: pointer;
text-decoration: none;
border: 1px solid <?php echo($color[4]);?>;
background-color: <?php echo($color[1]);?>;
}
div.posterItem {
width: 100px;
padding: 2px;
padding-top: 6px;
font-family: Arial;
border-radius: 5px;
font-size: 10px;
text-align: center;
display: inline-table;
margin: 4px;
border: 1px solid <?php echo($color[4]);?>;
}
div.posterItem:hover {
color: white;
cursor: pointer;
background-color: <?php echo($color[1]);?>;
}
div.posterSearch {
color: <?php echo($color[4]);?>;
font-family: Arial;
margin: 5px;
}
div.posterRow {
padding: 1px;
}
/* Bubles */
#canvas{
position: absolute;
z-index: -5;
height: 100%;
width: 100%;
padding: 0;
margin: -10px 0;
}
<file_sep><meta name="description" content="HTML5 Web App that stores movies you want to see." />
<meta name="keywords" content="Movies, Movie List, Movies I want to see, Movie, Film, TV, Television, Cinema" />
<meta name="author" content="<NAME>" />
<?php
// determine if working local or server side
if($_SERVER['SERVER_NAME'] == "localhost") { ?>
<base href="http://localhost/v/movies/" target="_blank" />
<?php
} else { ?>
<base href="http://movies.vicary.tk/" target="_blank" />
<?php
}?>
<link rel="shortcut icon" href="favicon.ico" />
<link rel="stylesheet" type="text/css" href="<?php echo($mainCss); ?>" />
<?php // Get APIs ?>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js" type="text/javascript"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jqueryui/1.8.18/jquery-ui.min.js" type="text/javascript"></script><file_sep><?php
session_start();
// determine if working local or server side
if($_SERVER['SERVER_NAME'] == "localhost") {
$mysql_host = "localhost";
$mysql_database = "movies";
$mysql_user = "root";
$mysql_password = "<PASSWORD>";
} else {
$mysql_host = "mysql17.000webhost.com";
$mysql_database = "a5390394_movies";
$mysql_user = "a5390394_movies";
$mysql_password = "<PASSWORD>";
}
// this block connects to the mysql server and opens my database
$link = new mysqli($mysql_host, $mysql_user, $mysql_password, $mysql_database);
if ($link->connect_error){
die('Could not connect ('.$link->connect_errno.')'.$link->connect_error);
}
// Debug
$time = microtime();
$time = explode(' ', $time);
$time = $time[1] + $time[0];
$start = $time;
?><file_sep>Movies I want to see
====================
By <NAME>
Mainly to use client side storage. | c78a2db6e99cc320fd4d427ef4372e675f21100c | [
"Markdown",
"PHP"
] | 9 | PHP | willem-h/movies | ab2e3164c91b4b586c9d004474dc815ffb51e6f9 | 23bf04645fd1eb9696deae4ac5f8a3fa15667851 | |
refs/heads/main | <repo_name>nourelhouda95/midad<file_sep>/SQL_CODE/midad.sql
-- phpMyAdmin SQL Dump
-- version 5.1.1
-- https://www.phpmyadmin.net/
--
-- Host: 127.0.0.1
-- Generation Time: Aug 12, 2021 at 11:08 PM
-- Server version: 10.4.20-MariaDB
-- PHP Version: 8.0.8
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Database: `midad`
--
-- --------------------------------------------------------
--
-- Table structure for table `attestation`
--
CREATE TABLE `attestation` (
`id` int(11) NOT NULL,
`id_group` int(11) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
-- --------------------------------------------------------
--
-- Table structure for table `bon`
--
CREATE TABLE `bon` (
`id` int(11) NOT NULL,
`date_creation` text NOT NULL,
`versement` text NOT NULL,
`rest_a_paye` text NOT NULL,
`id_user` varchar(5) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
-- --------------------------------------------------------
--
-- Table structure for table `etudiant_group`
--
CREATE TABLE `etudiant_group` (
`id_etudiant` varchar(5) NOT NULL,
`id_group` int(11) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
-- --------------------------------------------------------
--
-- Table structure for table `formation`
--
CREATE TABLE `formation` (
`id` int(11) NOT NULL,
`titre_formation` text NOT NULL,
`prix_formation` text NOT NULL,
`date_debut` text NOT NULL,
`volume_hor` varchar(3) NOT NULL,
`date_fin` text NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
-- --------------------------------------------------------
--
-- Table structure for table `groupe`
--
CREATE TABLE `groupe` (
`id` int(11) NOT NULL,
`id_formation` int(11) NOT NULL,
`id_formateur` varchar(5) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
-- --------------------------------------------------------
--
-- Table structure for table `inscription`
--
CREATE TABLE `inscription` (
`id` int(11) NOT NULL,
`date_insc` text NOT NULL,
`date_deb` text NOT NULL,
`id_formation` int(11) DEFAULT NULL,
`id_user` varchar(5) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
-- --------------------------------------------------------
--
-- Table structure for table `utilisateur`
--
CREATE TABLE `utilisateur` (
`id` varchar(5) NOT NULL,
`nom` text NOT NULL,
`prenom` text NOT NULL,
`numero_tel` text NOT NULL,
`email` text NOT NULL,
`password` longtext NOT NULL,
`type` int(11) NOT NULL,
`remise` int(11) DEFAULT NULL,
`cause_remise` mediumtext DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Indexes for dumped tables
--
--
-- Indexes for table `attestation`
--
ALTER TABLE `attestation`
ADD PRIMARY KEY (`id`),
ADD KEY `fk_group_attestation` (`id_group`);
--
-- Indexes for table `bon`
--
ALTER TABLE `bon`
ADD PRIMARY KEY (`id`),
ADD KEY `fk_user_bon` (`id_user`);
--
-- Indexes for table `etudiant_group`
--
ALTER TABLE `etudiant_group`
ADD PRIMARY KEY (`id_etudiant`,`id_group`),
ADD KEY `fk_groupe` (`id_group`);
--
-- Indexes for table `formation`
--
ALTER TABLE `formation`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `groupe`
--
ALTER TABLE `groupe`
ADD PRIMARY KEY (`id`),
ADD KEY `fk_formation_group` (`id_formation`),
ADD KEY `fk_formateur_group` (`id_formateur`);
--
-- Indexes for table `inscription`
--
ALTER TABLE `inscription`
ADD PRIMARY KEY (`id`),
ADD KEY `fk_formation` (`id_formation`),
ADD KEY `fk_user` (`id_user`);
--
-- Indexes for table `utilisateur`
--
ALTER TABLE `utilisateur`
ADD PRIMARY KEY (`id`);
--
-- AUTO_INCREMENT for dumped tables
--
--
-- AUTO_INCREMENT for table `bon`
--
ALTER TABLE `bon`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
--
-- AUTO_INCREMENT for table `formation`
--
ALTER TABLE `formation`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
--
-- AUTO_INCREMENT for table `groupe`
--
ALTER TABLE `groupe`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
--
-- AUTO_INCREMENT for table `inscription`
--
ALTER TABLE `inscription`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
--
-- Constraints for dumped tables
--
--
-- Constraints for table `attestation`
--
ALTER TABLE `attestation`
ADD CONSTRAINT `fk_group_attestation` FOREIGN KEY (`id_group`) REFERENCES `groupe` (`id`);
--
-- Constraints for table `bon`
--
ALTER TABLE `bon`
ADD CONSTRAINT `fk_user_bon` FOREIGN KEY (`id_user`) REFERENCES `utilisateur` (`id`);
--
-- Constraints for table `etudiant_group`
--
ALTER TABLE `etudiant_group`
ADD CONSTRAINT `fk_etudiant_group` FOREIGN KEY (`id_etudiant`) REFERENCES `utilisateur` (`id`) ON DELETE CASCADE,
ADD CONSTRAINT `fk_groupe` FOREIGN KEY (`id_group`) REFERENCES `groupe` (`id`) ON DELETE CASCADE;
--
-- Constraints for table `groupe`
--
ALTER TABLE `groupe`
ADD CONSTRAINT `fk_formateur_group` FOREIGN KEY (`id_formateur`) REFERENCES `utilisateur` (`id`) ON DELETE CASCADE,
ADD CONSTRAINT `fk_formation_group` FOREIGN KEY (`id_formation`) REFERENCES `formation` (`id`) ON DELETE CASCADE;
--
-- Constraints for table `inscription`
--
ALTER TABLE `inscription`
ADD CONSTRAINT `fk_formation` FOREIGN KEY (`id_formation`) REFERENCES `formation` (`id`) ON DELETE SET NULL ON UPDATE CASCADE,
ADD CONSTRAINT `fk_user` FOREIGN KEY (`id_user`) REFERENCES `utilisateur` (`id`) ON DELETE CASCADE ON UPDATE CASCADE;
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
| 80f68b141bf1d7174c33e3356c0135ccc3cb8598 | [
"SQL"
] | 1 | SQL | nourelhouda95/midad | 3c16bbc377ee462e132ca98bd7ca62b929da8298 | da3d8f05289efd3ff4352e47b49f36f3e4f623db | |
refs/heads/master | <file_sep>package com.example.mostpopularapi;
import android.database.Cursor;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.design.widget.FloatingActionButton;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.Toolbar;
import android.view.MenuItem;
import android.widget.ImageView;
import android.widget.TextView;
import com.squareup.picasso.Picasso;
public class FavoriteArticle extends AppCompatActivity {
private TextView articleText;
private TextView conten;
private TextView link;
private long id;
private DBHelper db;
private Cursor cursor;
private FloatingActionButton fab;
private ImageView imageView;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_article);
fab = findViewById(R.id.btn_favorites);
fab.hide();
db = new DBHelper(this, 1);
id = Import.id;
articleText = findViewById(R.id.articleText);
conten = findViewById(R.id.contentTextArticle);
link = findViewById(R.id.link);
imageView = findViewById(R.id.iv);
cursor = db.viewArticleId(id);
cursor.moveToFirst();
articleText.setText(cursor.getString(cursor.getColumnIndex("title")));
String content;
content = "" +
"keywords: " + cursor.getString(cursor.getColumnIndex("keywords")) +
"\ncount type: " + cursor.getString(cursor.getColumnIndex("count_type")) +
"\ncolumn: " + cursor.getString(cursor.getColumnIndex("colum")) +
"\nsection: " + cursor.getString(cursor.getColumnIndex("section")) +
"\nbyline: " + cursor.getString(cursor.getColumnIndex("byline")) +
"\nabstract: " + cursor.getString(cursor.getColumnIndex("abstract")) +
"\npublished date: " + cursor.getString(cursor.getColumnIndex("published_date")) +
"\nupdated: " + cursor.getString(cursor.getColumnIndex("updated"));
conten.setText(content);
Picasso.get().load(cursor.getString(cursor.getColumnIndex("url_image"))).into(imageView);
link.setText("Link: " + cursor.getString(cursor.getColumnIndex("url")));
Toolbar toolbar = findViewById(R.id.toolbarArticle);
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayShowTitleEnabled(false);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
if (id == android.R.id.home) {
finish();
}
return super.onOptionsItemSelected(item);
}
}
<file_sep>package com.example.mostpopularapi;
import android.database.Cursor;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.MenuItem;
import android.support.v7.widget.Toolbar;
public class FavoritesActivity extends AppCompatActivity {
private RecyclerView favoritesArticle;
private FavoritesAdapter favoritesAdapter;
private Cursor cursor;
private DBHelper db;
private int countItems;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.favorites_activity);
db = new DBHelper(this, 1);
cursor = db.viewArticle();
countItems = db.viewKol();
favoritesArticle = findViewById(R.id.rv_fav);
LinearLayoutManager layoutManager = new LinearLayoutManager(this);
favoritesArticle.setLayoutManager(layoutManager);
favoritesAdapter = new FavoritesAdapter( countItems,this, cursor);
favoritesArticle.setAdapter(favoritesAdapter);
Toolbar toolbar = findViewById(R.id.toolbarFav);
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayShowTitleEnabled(false);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
if (id == android.R.id.home) {
finish();
}
return super.onOptionsItemSelected(item);
}
}
<file_sep>package com.example.mostpopularapi;
import java.util.List;
class Import {
static Result ob;
static Long id;
}
<file_sep>package com.example.mostpopularapi;
import android.content.Context;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentPagerAdapter;
public class ViewPagerAdapter extends FragmentPagerAdapter {
final int NumbOfTabs = 3;
private String Titles[] = new String[] { "Emailed", "Shared", "Viewed" };
private Context context;
public ViewPagerAdapter(FragmentManager fm, Context context) {
super(fm);
this.context = context;
}
@Override
public Fragment getItem(int position) {
if (position == 0) {
EmailedFragment tab = new EmailedFragment();
return tab;
}
if (position == 1) {
SharedFragment tab = new SharedFragment();
return tab;
}
else {
ViewedFragment tab = new ViewedFragment();
return tab;
}
}
@Override
public CharSequence getPageTitle(int position) {
return Titles[position];
}
@Override
public int getCount() {
return NumbOfTabs;
}
}<file_sep>package com.example.mostpopularapi;
import android.content.Context;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import android.widget.Toast;
import java.util.List;
import static android.support.constraint.Constraints.TAG;
public class ArticlesAdapter extends RecyclerView.Adapter<ArticlesAdapter.ArticlesViewHolder>{
private Context parent;
private List<Result> mArticles;
private Result articles;
public ArticlesAdapter(Context parent, List<Result> dataList){
this.parent = parent;
mArticles = dataList;
}
@NonNull
@Override
public ArticlesViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
Context context = viewGroup.getContext();
LayoutInflater inflater = LayoutInflater.from(context);
View v = inflater.inflate(R.layout.item, viewGroup, false);
ArticlesViewHolder viewHolder = new ArticlesViewHolder(v);
return viewHolder;
}
@Override
public void onBindViewHolder(@NonNull ArticlesViewHolder articlesViewHolder, int position) {
articles = mArticles.get(position);
articlesViewHolder.listItemView.setText(articles.getTitle());
}
@Override
public int getItemCount() {
return mArticles.size();
}
class ArticlesViewHolder extends RecyclerView.ViewHolder {
TextView listItemView;
public ArticlesViewHolder(@NonNull View itemView) {
super(itemView);
listItemView = itemView.findViewById(R.id.text_title);
itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int positionIndex = getAdapterPosition();
Result impID = mArticles.get(positionIndex);
Intent intent = new Intent(parent, ArticlesActivity.class);
Import.ob = impID;
parent.startActivity(intent);
}
});
}
}
}
<file_sep>package com.example.mostpopularapi;
import android.os.Bundle;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.support.v4.app.Fragment;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Toast;
import com.example.mostpopularapi.retrofit.API;
import com.example.mostpopularapi.retrofit.RetroArticle;
import java.util.List;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
public class SharedFragment extends Fragment {
private RecyclerView sharedArticle;
private ArticlesAdapter sharedAdapter;
private List<Result> sharedList;
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
final View v = inflater.inflate(R.layout.fragment_articles, container, false);
if(InternetConnection.checkConnection(v.getContext())){
API api = RetroArticle.getRetrofitIntance().create(API.class);
Call<Example> call = api.getSharedJSON();
call.enqueue(new Callback<Example>() {
@Override
public void onResponse(Call<Example> call, Response<Example> response) {
sharedList = response.body().getResults();
sharedArticle = v.findViewById(R.id.rv_articles);
LinearLayoutManager layoutManager = new LinearLayoutManager(v.getContext());
sharedArticle.setLayoutManager(layoutManager);
sharedAdapter = new ArticlesAdapter(v.getContext(), sharedList);
sharedArticle.setAdapter(sharedAdapter);
}
@Override
public void onFailure(Call<Example> call, Throwable t) {
Toast.makeText(v.getContext(), t.getMessage(), Toast.LENGTH_SHORT).show();
}
});
}
else{
Toast.makeText(v.getContext(),"No Internet Connection", Toast.LENGTH_SHORT).show();
}
return v;
}
}
| b94b596f69ed5162a279c6c8e976120b23b4ecce | [
"Java"
] | 6 | Java | DanyilBohdan/MostPopularNYT | 0b94079f02c1c18aa565f6724b37c0a0c802ed31 | 5e6253c86e1f631f4de3c8d0588b6742ea27ed03 | |
refs/heads/main | <repo_name>bpeskett/flashcards<file_sep>/src/Layout/Study/Study.js
import React, { useEffect, useState } from "react";
import { useHistory } from "react-router";
import NotEnoughCards from "../Cards/NotEnoughCards";
export default function Study({ deck, cards }) {
const history = useHistory();
const [cardNumber, setCardNumber] = useState(0); //used to decide which card to display from cards array
const enoughCards = cards.length > 2; //boolean used to determine if there are enough cards to study (there must be at least 3)
const [cardSide, setCardSide] = useState("front"); //front or back. always start with front.
const [nextButtonDisplay, setNextButtonDisplay] = useState(""); //next button is hidden when cardSide === 'front'
const [shuffledCards, setShuffledCards] = useState([...cards]);
const [card, setCard] = useState(cards[0]); //current card object to be displayed
const [cardClass, setCardClass] = useState("text-dark bg-light");
//on first render, shuffle the cards
useEffect(() => {
setShuffledCards(shuffle(cards))
// eslint-disable-next-line react-hooks/exhaustive-deps
},[])
//when cards are shuffled, set first card to first shuffled card
useEffect(() => {
setCard(shuffledCards[0]);
},[shuffledCards])
//function to make a copy of the cards array and shuffle it
function shuffle(cards) {
const cardsToShuffle = [...cards];
for (let i = cardsToShuffle.length - 1; i > 0; i--){
const j = Math.floor(Math.random() * (i + 1));
[cardsToShuffle[i], cardsToShuffle[j]] = [cardsToShuffle[j], cardsToShuffle[i]];
}
return cardsToShuffle
}
function nextHandler() {
//handles clicking next
if (cardNumber + 1 < cards.length) {
//what to do if the current card if not the last card
setNextButtonDisplay(""); //hides the next button
setCardSide("front"); //switches the next card to front
setCard(shuffledCards[cardNumber + 1]); //updates the 'card _ of _' number
setCardNumber(cardNumber + 1); //moves to the next card
setCardClass("text-dark bg-light");
} else {
//what to do if the deck is finished
const message =
"Restart cards? \n \n Clisck 'cancel' to return to the home page."; //displays message
if (window.confirm(message)) {
//if message is confirmed, reset the deck
setCardSide("front");
setCardNumber(0);
setShuffledCards(shuffle(cards));
setCard(shuffledCards[0]);
setNextButtonDisplay("");
setCardClass("text-dark bg-light");
} else {
//if pushed cancel, go home.
history.push("/");
}
}
}
function flipHandler() {
//handles flip button
if (cardSide === "back") {
//if previous state of the card was the backside...
setCardSide("front"); //switch to front
setCardClass("text-dark bg-light");
setNextButtonDisplay(""); //hide the next button
} else {
//if previous state of the card was the frontside...
setCardSide("back"); //switch to backside
setCardClass("text-white bg-dark");
setNextButtonDisplay(
//display the next button
<button onClick={nextHandler} className="btn btn-primary mx-2">
Next
<span className="oi oi-chevron-right ml-2" />
</button>
);
}
}
const progressbarStyles = {
display: 'flex',
height: '.5rem',
overflow: 'hidden',
margin: '-1px'
}
return enoughCards ? (
<>
<h2>Study: {deck.name}</h2>
<div
className={`card mb-3 ${cardClass}`}
style={{ maxWidth: "40rem", minHeight: "14rem" }}
>
<div style = {progressbarStyles}>
<div
className="progress-bar bg-success"
role="progressbar"
style={{width: `${(((cardNumber) / (cards.length )) + (1 / cards.length) ) * 100}%`}}
aria-valuenow={(((cardNumber) / (cards.length )) + (1 / cards.length) ) * 100}
aria-valuemin="0"
aria-valuemax="100"
>
</div>
</div>
<div className="card-header">{`Card ${cardNumber + 1} of ${
cards.length
}`}
</div>
<div className="card-body">
<p className="card-text">{card[cardSide]} </p>
</div>
</div>
<button onClick={flipHandler} className="btn btn-secondary">
<span className="oi oi-loop-circular mr-2" />
Flip
</button>
{/* next button ony displays when the back of a card is showing */}
{nextButtonDisplay}
</>
) : (
/* display NotEnoughCards component if the deck has less than 3 cards */
<NotEnoughCards deck={deck} />
);
}
<file_sep>/src/Layout/Cards/CardCard.js
import React from "react";
import { Link } from "react-router-dom";
import { deleteCard } from "../../utils/api";
//individual card componant displaying both sides and edit/ delete buttons (not what is shown when studying)
export default function CardCard({ card, deckUpdated }) {
//trunkate card text if longer than 150 characters.
let trunkatedFront = card.front;
let trunkatedBack = card.back;
if (card.front.length > 150) {
trunkatedFront = card.front.slice(0, 150) + "...";
}
if (card.back.length > 150) {
trunkatedBack = card.back.slice(0, 150) + "...";
}
//confirm deletion than go to the deck view and refresh
const deleteHandler = () => {
const message =
"Delete this card? \n \n You will not be able to recover it.";
if (window.confirm(message)) {
deleteCard(card.id).then(() => {
deckUpdated();
});
}
};
return (
<>
<div className="my-3 col col-12 col-md-6 ">
{/*front of card*/}
<div
className="card text-dark bg-light mb-1"
style={{ minHeight: "11rem" }}
>
<div className="card-header">Front</div>
<div className="card-body ">
<p className="card-text ">{trunkatedFront} </p>
</div>
</div>
{/*back of card*/}
<div className="card text-white bg-dark" style={{ minHeight: "11rem" }}>
<div className="card-header">Back</div>
<div className="card-body">
<p className="card-text">{trunkatedBack} </p>
</div>
</div>
<div className="col mt-2">
{/*delete button*/}
<button
to="/"
className="btn btn-danger mx-2 float-right"
onClick={deleteHandler}
>
<span className="oi oi-trash ml-1" />
</button>
{/*edit button*/}
<Link
to={`/decks/${card.deckId}/cards/${card.id}/edit`}
className="btn btn-secondary float-right"
>
<span className="oi oi-pencil mr-2" />
Edit
</Link>
</div>
</div>
</>
);
}
<file_sep>/src/Layout/Decks/DeckList.js
import React, { useEffect, useState } from "react";
import DeckCard from "./DeckCard";
//list of all the decks (as shown on the home page)
export default function DeckList({ deckList, deckView, deckListUpdated }) {
const [listAllDecks, setListAllDecks] = useState("");
//map the deckList into DeckCard components wrapped in bootstrap columns.
useEffect(() => {
setListAllDecks(
deckList.map((deck, index) => {
return (
<div key={index} className="col col-12 col-lg-6 col-xl-4">
<DeckCard deck={deck} deckView={deckView} deckListUpdated={deckListUpdated} />
</div>
);
})
);
// eslint-disable-next-line react-hooks/exhaustive-deps
}, [deckList, deckView]);
//render list of decks wrapped in a bootstrap row
return (
<>
<h2>Decks</h2>
<div className="row">{listAllDecks}</div>
</>
);
}
<file_sep>/src/Layout/Decks/DeckForm.js
import React, { useEffect, useState } from "react";
import { Link, useHistory } from "react-router-dom";
import { createDeck, updateDeck } from "../../utils/api";
import SubmitAlert from "../Common/SubmitAlert";
//form for creating and editing decks
//props: deck being edited (if editing) formType: (edit or create) depending on how the form is being used
export default function DeckForm({ deck, formType, deckUpdated }) {
//name and description of the deck (being created or edited)
const [name, setName] = useState("");
const [description, setDescription] = useState("");
const [alertType, setAlertType] = useState("");
const history = useHistory();
//if editing a deck, set the state variables to the name and description of the deck being edited
useEffect(() => {
if (formType === "Edit") {
setName(deck.name);
setDescription(deck.description);
}
}, [deck, formType]);
//submit handler
const handleSubmit = (event) => {
event.preventDefault();
setAlertType("warning");
//create a new deck object using form values
const newDeck = { name: name, description: description };
//update the or create the new deck
if (formType === "Edit") {
const updatedDeck = { ...newDeck, id: deck.id };
updateDeck(updatedDeck).then((result) => {
history.push(`/decks/${result.id}/success`);
deckUpdated();
})
.catch(() => {
setAlertType("danger");
});
} else {
createDeck(newDeck).then((result) => {
history.push(`/decks/${result.id}/success`);
})
.catch(() => {
setAlertType("danger");
});
}
};
return (
<>
<h2>{formType} Deck</h2>
<form onSubmit={handleSubmit}>
{/*name input*/}
<div className="form-group">
<label htmlFor="name">Name</label>
<input
className="form-control"
id="name"
type="text"
name="name"
onChange={(event) => {
setName(event.target.value);
}}
value={name}
maxLength="50"
/>
</div>
{/*description input*/}
<div className="form-group">
<label htmlFor="description">Description</label>
<textarea
className="form-control"
id="description"
rows="3"
name="description"
onChange={(event) => {
setDescription(event.target.value);
}}
value={description}
></textarea>
</div>
{/*cancel button*/}
<Link className="btn btn-secondary mx-1 mb-3" to="/">
<span className="oi oi-circle-x mr-2" />
Cancel
</Link>
{/*submit button*/}
<button className="btn btn-primary mx-1 mb-3" type="submit">
<span className="oi oi-circle-check mr-2" />
Submit
</button>
<SubmitAlert form="deck" alertType={alertType} />
</form>
</>
);
}
<file_sep>/src/Layout/Common/Loading.js
import React from "react";
//spinning wheel and "Loading..." displays on pages while waiting for api fetch
export default function Loading() {
return (
<div>
<span className="spinner-border float-left mt-1 mr-3"></span>{" "}
<h2 className="mt-1">Loading...</h2>
</div>
);
}
<file_sep>/README.md

#
# Flashcard-o-matic
Thinkful student project built using React and Bootstrap. Users can create decks and cards, then study them as flashcards. Both decks and cards can be edited or deleted. Additional cards can be added to a deck.
#
## SKILLS USED
* React
* Javascript
* HTML / JSX
* CSS
* Bootstrap
* React Router
* React Hooks (useState, useEffect)
#
## FEATURES BEYOND THE SCOPE OF THE PROJECT
I went beyond the scope of the project and added the following features
* Fully responsive
* Flashcards shuffle when studying
* shuffle algorithm uses a runtime of O(n)
* Error handling that gives feedback to user with alerts for
* Saving...
* Loading...
* Your `[deck, card]` is saved!
* Unable to save your `[deck, card]`.
* Status bar showing percentage of cards a user has studied
* Truncation for long names and descriptions
#
## FUTURE FEATURES
Some features that I would like to impliment in the future
* Get server up and running
* Grading. Track right/ wrong answers while studying
* Add different types of decks / cards (multiple choice, etc)
* Double sided deck option
* Update favicon
#
## SCREENSHOTS
### Home Page:
The home page displays a card for each deck as well as a button to create a new deck.
`path = '/'`

### Study:
Study a deck. Flip to reveal the back of a card.
`path = '/decks/:deckId/study'`

### Deck View:
Viewing a deck reveals the deck's full description, all it's associated cards and options to study, edit, or delete the deck, and add to, edit or delete the cards.
`path = '/decks/:deckId'`
<file_sep>/src/Layout/Decks/DeckCard.js
import React from "react";
import { Link, useHistory } from "react-router-dom";
import { deleteDeck } from "../../utils/api";
//individual deck card as seen on home page and '/decks/:deckId' route (deck view)
//deck prop passes the deck object. the deckView prop (boolean) tells if this component is being used in the deck view route or not ('/decks/:deckId')
export default function DeckCard({ deck, deckView, deckListUpdated }) {
const history = useHistory();
//trunkate the deck's description if being displayed on home screen and if the description is long
let trunkatedDescription = deck.description;
if (!deckView & (deck.description.length > 115)) {
trunkatedDescription = deck.description.slice(0, 115) + "...";
}
// some buttons should only be displayed in some cases
const deckViewButton = deckView ? (
""
) : (
<Link to={`/decks/${deck.id}`} className="btn btn-sm mb-1 btn-secondary mx-1">
<span className="oi oi-eye mr-2" />
View
</Link>
);
const deckEditButton = deckView ? (
<Link
to={`/decks/${deck.id}/edit`}
className="btn btn-sm mb-1 btn-secondary mx-1 float-right"
>
<span className="oi oi-pencil mr-2" />
Edit
</Link>
) : (
""
);
//delete deck, go to root directory and refresh
const deleteHandler = () => {
const message =
"Delete this deck? \n \n You will not be able to recover it.";
if (window.confirm(message)) {
deleteDeck(deck.id).then(() => {
history.push("/");
!deckView && deckListUpdated();
});
}
};
//card count should not be plural if there is only one card
const cardCount =
deck.cards.length === 1 ? `1 Card` : `${deck.cards.length} cards`;
return (
<>
<div className="card mb-3">
<div className="card-header bg-dark text-white pb-0">
{/* number of cards in the deck */}
<span className="float-right badge bg-success text-white ml-3">
{cardCount}
</span>
{/* name of the deck */}
<h5 className="card-title text-truncate pb-2 my-0">{deck.name}</h5>
</div>
<div className="card-body row">
<div className="col col-12">
{/* deck description */}
<p className="" style={{ minHeight: "5rem" }}>
{trunkatedDescription}{" "}
</p>
</div>
<div className="col">
{/* view button */}
{deckViewButton}
{/* study button */}
<Link to={`/decks/${deck.id}/study`} className="btn btn-sm mb-1 btn-primary mx-1">
<span className="oi oi-book mr-2" />
Study
</Link>
{/* delete button */}
<button
className="btn btn-sm mb-1 btn-danger mx-1 float-right"
onClick={deleteHandler}
>
<span className="oi oi-trash ml-1" />
</button>
{/* edit button */}
{deckEditButton}
</div>
</div>
</div>
</>
);
}
<file_sep>/src/Layout/Decks/Deck.js
import React from "react";
import DeckCard from "./DeckCard";
import CardList from "../Cards/CardList";
// 'decks/:deckId' route. display the deck details and a list of cards.
export default function Deck({ deck, cards, deckUpdated }) {
return (
<>
<DeckCard deck={deck} deckView={true} />
<CardList deck={deck} cards={cards} deckUpdated={deckUpdated} />
</>
);
}
<file_sep>/src/Layout/Cards/NotEnoughCards.js
import React from "react";
import { Link } from "react-router-dom";
import CardList from "./CardList";
//component displays when trying to study a deck with less than 3 cards
export default function NotEnoughCards({ deck }) {
const notEnoughMessage =
deck.cards.length === 1 ? "is 1 card" : `are ${deck.cards.length} cards`;
return (
<>
<div className="alert alert-warning" role="alert">
Not enough cards. You need at least 3 cards to study. There{" "}
{notEnoughMessage} in this deck.
</div>
<h2>Study: {deck.name}</h2>
<br />
<Link
className="btn btn-primary mb-3 btn"
to={`/decks/${deck.id}/cards/new`}
>
<span className="oi oi-plus pr-2" />
Add Cards
</Link>
<CardList deck={deck} cards={deck.cards} />
</>
);
}
<file_sep>/src/Layout/Cards/CardList.js
import React, { useEffect, useState } from "react";
import { Link, useLocation, useParams } from "react-router-dom";
import CardCard from "./CardCard";
//list all given cards. used on '/decks/:deckId' route
export default function CardList({ deck, cards, deckUpdated }) {
const deckId = useParams().deckId;
const addCardsBtn = (
<Link
to={`/decks/${deckId}/cards/new`}
className="btn btn-sm mb-3 btn-success mx-1 float-right"
>
<span className="oi oi-plus mr-2" />
Add Cards
</Link>
);
const [listAllCards, setListAllCards] = useState("");
const [cardHeadDisplay, setCardHeadDisplay] = useState("");
const [addCardsBtnDisplay, setAddCardBtnDisplay] = useState(addCardsBtn);
const location = useLocation().pathname;
const currentPage = location.substring(location.lastIndexOf('/') + 1)
useEffect(() => {
if (currentPage === "new" || currentPage === "edit" || currentPage === "study"){
setAddCardBtnDisplay("");
}
},[currentPage])
//map cards into list of Card components
useEffect(() => {
setListAllCards(
cards.map((card, index) => {
return <CardCard key={index} card={card} deckUpdated={deckUpdated} />;
})
);
//only display "Cards" headline if there is at least one card in the deck
cards.length > 0 && setCardHeadDisplay(<h3 className="mb-3">Cards</h3>);
// eslint-disable-next-line react-hooks/exhaustive-deps
}, [cards]);
return (
<>
{addCardsBtnDisplay}
{cardHeadDisplay}
<div className="row">{listAllCards}</div>
</>
);
}
<file_sep>/src/Layout/index.js
import React from "react";
import { Route, Switch } from "react-router";
import Header from "./Header";
import Home from "./Home/Home";
import Decks from "./Decks/Decks";
import NotFound from "./NotFound";
import BreadCrumbs from "./Common/BreadCrumbs";
import DeckForm from "./Decks/DeckForm";
function Layout() {
//bread crumbs for for new deck bread crumbs
const newCrumbList = [{ name: "Create Deck", path: `/decks/new` }];
return (
<div>
<Header />
<div className="container pb-3">
<Switch>
<Route exact={true} path="/">
{" "}
{/* route for root directory */}
<Home />
</Route>
<Route exact={true} path="/decks/new">
{" "}
{/* route for new deck */}
<BreadCrumbs crumbs={newCrumbList} />
<DeckForm formType={"Create"} />
</Route>
<Route path="/decks/:deckId">
{" "}
{/* route for any page that contains deckId */}
<Decks />
</Route>
<Route>
{" "}
{/* route for unknown pages */}
<NotFound />
</Route>
</Switch>
</div>
</div>
);
}
export default Layout;
| 24a9bdfe84da9462a969e3b55894dcfc9ac87ada | [
"JavaScript",
"Markdown"
] | 11 | JavaScript | bpeskett/flashcards | d8e90a95c6d01d0ba03752ecc5b78f3cdd17c172 | 5d0a07325e889a8efebd2b05d81b5cf17555cbab | |
refs/heads/master | <file_sep>source 'https://rubygems.org'
gem 'rails', '3.2.12'
gem 'toto'
group :production do
gem "pg"
end
group :development, :test do
gem "sqlite3"
end
| ef9d5812e4f5c86997b57bb50cfb41c170720a81 | [
"Ruby"
] | 1 | Ruby | NickG22293/dorothy | a057fb5e6a70f0a84df1491d84646df02206c5f3 | 1c6d864eae49f0e138c302284546d18cd9103493 | |
refs/heads/master | <file_sep>using datalogic_ce_sync;
using System;
using System.Collections.Generic;
using System.IO;
namespace USBLAN_Sample
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("~~~ Datalogic USB/LAN API Test App ~~~");
Console.WriteLine("1) Start Process");
Console.WriteLine("2) Find Files");
Console.WriteLine("3) Find Directories");
Console.WriteLine("4) Create Directory");
Console.WriteLine("5) Push File To Device");
Console.WriteLine("6) Pull File From Device");
Console.WriteLine("7) Set File Date and Time");
Console.WriteLine("8) Delete File");
Console.WriteLine("Enter) Exit");
Console.WriteLine("\nChoose test...");
string command;
while ((command = Console.ReadLine()) != "")
{
try
{
Console.WriteLine("Starting test {0}...", command);
switch (command)
{
case "1": TestStartProcess(); break;
case "2": TestFindFiles(); break;
case "3": TestFindDirectories(); break;
case "4": TestCreateDirectory(); break;
case "5": TestPushFileToDevice(); break;
case "6": TestPullFileFromDevice(); break;
case "7": TestSetFileDateTime(); break;
case "8": TestDeleteFile(); break;
default: Console.WriteLine("Invalid input."); break;
}
}
catch (Exception e)
{
Console.WriteLine(e);
}
Console.WriteLine("Done");
}
}
private static void TestStartProcess()
{
bool result = USBLAN.StartProcess("/Windows/ctlpnl.exe", "/Windows/Audio.cpl");
Console.WriteLine("response: " + result);
}
private static void TestFindFiles()
{
IEnumerable<SimpleFileInfo> files = USBLAN.FindFiles("/Windows/", "DeviceImage.pn*");
if (files != null)
{
int count = 0;
Console.WriteLine("Files: ");
foreach (var file in files)
{
Console.WriteLine(++count + ") " + file.Name + " : " + file.Length);
Console.WriteLine("\t" + file.FullName + " : " + file.Extension);
}
}
else
Console.WriteLine("returned null");
}
private static void TestFindDirectories()
{
IEnumerable<SimpleFileInfo> dirs = USBLAN.FindDirectories("/Windows/", "*ro*");
if (dirs != null)
{
Console.WriteLine("Dirs: ");
int count = 0;
foreach (var dir in dirs)
Console.WriteLine(++count + ") " + dir.Name);
}
else
Console.WriteLine("returned null");
}
private static void TestCreateDirectory()
{
bool result = USBLAN.CreateDirectory("/My Documents/testdir");
Console.WriteLine("response: " + result);
}
private static void TestPushFileToDevice()
{
bool result = USBLAN.PushFileToDevice("C:/Users/mchew/Documents/IT_71796/big.jpg", "/Temp/big.jpg", false);
Console.WriteLine("response: " + result);
}
private static void TestPullFileFromDevice()
{
bool result = USBLAN.PullFileFromDevice("/Temp/big.jpg", "C:/Users/mchew/Documents/IT_71796/pull.jpg", false);
Console.WriteLine("response: " + result);
}
private static void TestSetFileDateTime()
{
DateTime dt = new DateTime(1995, 10, 25, 3, 57, 0, 050);
Console.WriteLine(dt.ToFileTimeUtc());
bool result = USBLAN.SetFileDateTime("Temp/push.txt", dt);
Console.WriteLine("response: " + result);
}
private static void TestDeleteFile()
{
bool result = USBLAN.DeleteFile("/Temp/push.txt");
Console.WriteLine("response: " + result);
}
private static bool CmpInfo(FileInfo fi, SimpleFileInfo sfi)
{
return fi.FullName == sfi.FullName
&& fi.Name == sfi.Name
&& fi.Length == sfi.Length
&& fi.LastWriteTime == sfi.LastWriteTime
&& fi.Extension == sfi.Extension
&& fi.DirectoryName == sfi.DirectoryName
;
}
}
}
| ad578d9c129fd6f2623bf5496c7754be61533c0e | [
"C#"
] | 1 | C# | datalogic/USBLAN_Sample | 16569c6de816829141888433d5ed0b06e88adb1e | c4666a40371f3b67c80e5d433803d89fa1196843 | |
refs/heads/master | <file_sep>######### Band should initialize with a name and hometown
######### Band should have a Band#name and Band#hometown and a band should be able to change it's name
##### Band should have a method Band.all that returns all the instances of Band
##### Band should have a method Band#play_in_venue that takes a venue and date as a string as arguments and associates the band to that venue
##### Band should have a method Band#concerts should return an array of all that band's concerts
##### Band should have a method Band#venues that returns an array of all the venues the band has concerts in
# Band should have a method Band.all_introductions that puts out a message of "Hello, we are {insert band name here} and we're from {insert hometown here}" for each band
class Band
attr_accessor :name
attr_reader :hometown
@@all = []
def initialize(name, hometown)
@name = name
@hometown = hometown
@@all << self
end
def self.all
@@all
end
def play_in_venue(venue, date)
# takes a venue and date as a string as arguments and associates the band to that venue
if Concert.all.any? {|concert| concert.venue == venue && concert.date == date}
puts "ya booked!"
else
Concert.new(date, self, venue)
end
end
def concerts
# return an array of all that band's concerts
Concert.all.select do |concert|
concert.band == self
end
end
def venues
# returns an array of all the venues the band has concerts in
self.concerts.map do |concert|
concert.venue
end
end
# Band should have a method Band.all_introductions that puts out a message of "Hello, we are {insert band name here} and we're from {insert hometown here}" for each band
def self.all_introductions
all.each do |band|
puts "Hello, we are #{band.name} and we're from #{band.hometown}"
end
end
end
<file_sep>### Concert should have a Concert.all method which returns all the instances of Concert
#### Concert should initialize with a date, band, and venue
#### Concert should have methods Concert#band and Concert#venue that return the band and venue associated to the Concert
#### Concert should have a method Concert#hometown_show? that returns true if the concert is in the band's hometown
class Concert
attr_accessor :date, :band, :venue
@@all = []
def initialize(date, band, venue)
@date = date
@band = band
@venue = venue
@@all << self
end
def self.all
@@all
end
def hometown_show?
# returns true if the concert is in the band's hometown
self.venue.city == self.band.hometown
end
def introduction
if self.hometown_show?
puts "Hello, we are #{band.name} and we're happy to be home in #{venue.city}!"
else
puts "Hello, we are #{band.name} and we're happy to be in #{venue.city}."
end
end
end
<file_sep>require 'pry'
require_relative './band.rb'
require_relative './concert.rb'
require_relative './venue.rb'
green_day = Band.new("Green Day", "Sanduski, OH")
paramore = Band.new("Paramore", "Shrug... Canada?")
band = Band.new("the band", "prolly like georgia")
aboot_playhouse = Venue.new("Aboot Playhouse", "Shrug... Canada?")
msg = Venue.new("Madison Square Garden", "NYC")
paramore_show_ca = Concert.new("03/24/18", paramore, aboot_playhouse)
green_day_msg = Concert.new("02/24/18", green_day, msg)
band_msg = Concert.new("03/24/76", band, msg)
binding.pry
hi = 'hello'
<file_sep>##### Venue should initialize with a title and city
##### Venue should have a method Venue.all method which returns all the instances of Venue
##### Venue should have a method Venue#concerts that lists all the concerts that have ever been performed in that venue
##### Venue should have a method Venue#bands that lists all the bands that have ever played in that venue
class Venue
attr_reader :title, :city
@@all = []
def initialize(title, city)
@title = title
@city = city
@@all << self
end
def self.all
@@all
end
def concerts
# lists all the concerts that have ever been performed in that venue
concerts = Concert.all.select do |concert|
concert.venue == self
end
concerts
end
def bands
# lists all the bands that have ever played in that venue
self.concerts.map do |concert|
concert.band
end
end
end
| 1e3f914d5c33af0e2f8d37e1fa79c277a28de0e2 | [
"Ruby"
] | 4 | Ruby | SaigeXSaige/practice-oo-associations | 74ef1c4d61fc57702ab22ebfb6a06b1a0ceacce5 | a66658f9bee4fcaf32b9d92e5ff0f2d7b6665887 | |
refs/heads/master | <repo_name>phanvantan73/roll-system<file_sep>/database/seeds/UserTableSeeder.php
<?php
use Illuminate\Database\Seeder;
use App\User;
use App\Subject;
class UserTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
$faker = Faker\Factory::create();
factory(User::class)->create([
'email' => '<EMAIL>',
'name' => 'Admin',
'password' => bcrypt('<PASSWORD>'),
'is_admin' => 1,
]);
for ($i = 0; $i <= 10; $i++) {
$mssv = "$i$i$i$i$i$i$i";
$user = User::create([
'name' => $faker->name,
'email' => $mssv . '@sv.com.vn',
'password' => bcrypt('<PASSWORD>'),
]);
$user->student()->create([
'name' => $user->name,
'mssv' => $mssv,
'class' => ($i > 0 && $i < 10 ? '1' . $i . 'T1' : '11T4'),
]);
}
Subject::create([
'name' => 'Môn học 1',
'start_time' => '07:00:00',
'end_time' => '12:00:00',
'learn_time' => 1,
]);
Subject::create([
'name' => 'Môn học 2',
'start_time' => '13:00:00',
'end_time' => '17:00:00',
'learn_time' => 1,
]);
Subject::create([
'name' => 'Môn học 3',
'start_time' => '07:00:00',
'end_time' => '12:00:00',
'learn_time' => 2,
]);
Subject::create([
'name' => '<NAME>',
'start_time' => '13:00:00',
'end_time' => '17:00:00',
'learn_time' => 2,
]);
Subject::create([
'name' => '<NAME>',
'start_time' => '07:00:00',
'end_time' => '12:00:00',
'learn_time' => 3,
]);
Subject::create([
'name' => '<NAME>',
'start_time' => '13:00:00',
'end_time' => '17:00:00',
'learn_time' => 3,
]);
Subject::create([
'name' => '<NAME>',
'start_time' => '07:00:00',
'end_time' => '12:00:00',
'learn_time' => 4,
]);
Subject::create([
'name' => '<NAME>',
'start_time' => '13:00:00',
'end_time' => '17:00:00',
'learn_time' => 4,
]);
Subject::create([
'name' => '<NAME>',
'start_time' => '07:00:00',
'end_time' => '12:00:00',
'learn_time' => 5,
]);
Subject::create([
'name' => '<NAME>',
'start_time' => '13:00:00',
'end_time' => '17:00:00',
'learn_time' => 5,
]);
}
}
<file_sep>/app/Student.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
use App\Subject;
use App\User;
class Student extends Model
{
protected $fillable = [
'mssv',
'name',
'class',
'user_id',
];
public function subjects()
{
return $this->belongsToMany(Subject::class)->withPivot('status')->withTimestamps();
}
public function user()
{
return $this->belongsTo(User::class);
}
public static function getStudentFromMssv($mssv)
{
return Student::where('mssv', $mssv)->first();
}
public function checkRollcallByDay($day, $subject_id)
{
return $this->subjects()->whereDate('student_subject.created_at', $day)
->where('student_subject.subject_id', $subject_id)
->exists();
}
}
<file_sep>/app/Http/Controllers/Admin/AdminController.php
<?php
namespace App\Http\Controllers\Admin;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
use App\Student;
use App\Subject;
use App\User;
use Carbon\Carbon;
use File;
use Auth;
use DB;
class AdminController extends Controller
{
public function index()
{
return view('admin.index');
}
public function getAll()
{
$requestDay = Carbon::now();
$day = $requestDay->dayOfWeek;
$requestDay = $requestDay->format('d-m-Y');
$path = public_path('file/' . Carbon::now()->toDateString() . '.txt');
$data = [];
$errors = [];
if (File::exists($path)) {
$contents = File::get($path);
$contents = explode(PHP_EOL, $contents);
$subjectsInDay = Subject::where('learn_time', $day)->get();
for ($i = 0; $i < count($contents) - 1; $i++) {
$content = explode(',', $contents[$i]);
$student = Student::getStudentFromMssv($content[0]);
if ($student) {
$rollTime = $content[1];
$data[$i] = [
'student' => $student,
'rollTime' => $rollTime,
];
foreach ($subjectsInDay as $key => $subject) {
$check = DB::table('student_subject')->where('student_id', $student->id)->where('subject_id', $subject->id)
->whereDate('created_at', Carbon::today()->toDateString())->oldest()->first();
if ($rollTime >= $subject->start_time && $rollTime <= $subject->end_time ) {
if (!$check) {
$student->subjects()->attach($subject->id, [
'created_at' => Carbon::today()->toDateString() . ' ' . $rollTime,
'status' => 1,
]);
} else {
if ($rollTime < Carbon::create($check->created_at)->format('H:i:s')) {
DB::table('student_subject')->delete($check->id);
$student->subjects()->attach($subject->id, [
'created_at' => Carbon::today()->toDateString() . ' ' . $rollTime,
'status' => 1,
]);
}
}
}
}
}
}
} else {
$errors['error'] = 'Camera chưa cập nhật kết quả!';
}
return view('sumary', compact('requestDay', 'data', 'errors'));
}
public function addStudent(Request $request)
{
$name = $request->name;
$mssv = $request->mssv;
$user = User::create([
'name' => $name,
'email' => $mssv . '@sv.com.vn',
'password' => bcrypt('<PASSWORD>'),
]);
$student = $user->student()->create([
'name' => $name,
'mssv' => $mssv,
'class' => '13T1',
]);
$user = $user->load('student');
return response()->json([
'success' => true,
'user' => $user,
]);
}
public function getAllStudent(Request $request)
{
$users = User::where('id', '!=', Auth::user()->id)->get();
return view('admin.student', compact('users'));
}
}
<file_sep>/readme.md
Sau khi đã clone project từ github, làm theo những bước sau:<br>
+ Mở terminal và chạy lệnh "composer install" hoặc "composer update"<br>
+ Tạo file .env bằng cách copy file .env.example và đổi tên.<br>
+ Vào file .env chỉnh sửa claij cấu hình database như sau:<br>
+ DB_DATABASE = tên cơ sở dữ liệu<br>
+ DB_USERNAME = tên đăng nhập mySql<br>
+ DB_PASSWORD = <PASSWORD><br>
+ Tiếp đó mở terminal và chạy lệnh "php artisan key:generate".<br>
+ Tiếp theo nhập vào dòng lệnh "php artisan migrate" để migration cơ sở dữ liệu.<br>
+ Sau đó chạy tiếp "php artisan db:seed" để seed dữ liệu.<br>
+ Cuối cùng chạy lệnh "php artisan make:auth" để tạo authentication.<br>
Sau khi hoàn thành các bước trên, chạy lệnh "php artisan serve" rồi truy cập vào địa chỉ<br>
http://127.0.0.1:8000 để xem kết quả.<br>
<file_sep>/app/Http/Controllers/StudentController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Student;
use App\Subject;
use Carbon\Carbon;
use File;
use Auth;
class StudentController extends Controller
{
public function getResult(Request $request)
{
$student = Auth::user()->student;
$subjects = Subject::pluck('name', 'id')->toArray();
$month = Carbon::today()->format('m');
$year = Carbon::today()->format('Y');
$date = Carbon::today()->format('d');
$defaultSubjectId = Subject::orderBy('created_at')->first()->id;
$defaultSubjectName = Subject::orderBy('created_at')->first()->name;
$defaultDay = $year . '-' . $month. '-' . $date;
$data = $this->getData($student, $defaultSubjectId, $defaultDay);
$defaultDay = Carbon::create($defaultDay)->format('d-m-Y');
if ($request->ajax()) {
$defaultSubjectId = $request->subject_id;
$defaultDay = $request->day;
$data = $this->getData($student, $defaultSubjectId, $defaultDay);
return response()->json([
'html' => count($data) ? view('table', compact('data'))->render() : [],
'message' => 'successfully',
]);
}
return view('result', compact('data', 'student', 'subjects', 'defaultDay', 'defaultSubjectName'));
}
public function getData($student, $subjectId, $day)
{
$data = [];
foreach ($student->subjects as $subject) {
if ($subject->pivot->subject_id == $subjectId && $subject->pivot->created_at->format('Y-m-d') == $day) {
array_push($data, $subject);
}
}
return $data;
}
}
| c8543c7c8124325b681af5f4b581ff1bd1933f1c | [
"Markdown",
"PHP"
] | 5 | PHP | phanvantan73/roll-system | dcc9bfda3098a272567a146e6c253ffa28fc0950 | 12e761688216d31926928a0b0fa413428d8f8e0d | |
refs/heads/master | <repo_name>baharestani/shokunin.10xdev<file_sep>/10xDev.Tests/PresenterTests.cs
using System;
using Shouldly;
using Xunit;
namespace _10xDev.Tests
{
public class PresenterTests
{
[Fact]
public void Get10XDev_throws_exception_for_more_than_one_results()
{
var results = new[]
{
new[] {"name1", "name2"},
new[] {"name2", "name1"}
};
var presenter = new Presenter();
Should.Throw<InvalidOperationException>(() => presenter.Get10XDeveloper(results));
}
[Fact]
public void Get10XDev_announce_firstName_if_only_one_result_found()
{
var results = new[]
{
new[]
{
"Winner", "Other"
}
};
var presenter = new Presenter();
presenter.Get10XDeveloper(results).ShouldBe("The 10X Developer is Winner");
}
[Fact]
public void GetLeaderBoard_throws_exception_for_more_than_one_results()
{
var results = new[]
{
new[] {"name1", "name2"},
new[] {"name2", "name1"}
};
var presenter = new Presenter();
Should.Throw<InvalidOperationException>(() => presenter.GetLeaderBoard(results));
}
[Fact]
public void GetLeaderBoard_should_announce_all_names_if_single_result()
{
var results = new[]
{
new[] {"Name1", "Name2", "Name3"},
};
var presenter = new Presenter();
var leaderBoard = presenter.GetLeaderBoard(results);
leaderBoard.ShouldBe("Leader board:\n" +
"1. Name1\n" +
"2. Name2\n" +
"3. Name3\n"
);
}
}
}
<file_sep>/10xDev/RulesProvider.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text.RegularExpressions;
using _10xDev.Rules;
namespace _10xDev
{
public class RulesProvider : IRuleProvider
{
private readonly IFactsRepository _factsRepository;
public RulesProvider(IFactsRepository factsRepository)
{
_factsRepository = factsRepository;
}
public IEnumerable<ISortingRule> GetRules()
{
var facts = _factsRepository.GetFacts();
var ruleTypes = CollectAllRuleTypes();
return ruleTypes.SelectMany(ruleType =>
Regex.Matches(facts, ruleType.GetCustomAttribute<RuleAttribute>().Pattern)
.Select(match => ActivateRule(ruleType, match)));
}
private static ISortingRule ActivateRule(Type ruleType, Match regexMatch)
{
const int groupMatchingWhole = 1;
var args = regexMatch.Groups.Skip(groupMatchingWhole).Select(g => (object) g.Value).ToArray();
return (ISortingRule) Activator.CreateInstance(ruleType, args);
}
private static IEnumerable<Type> CollectAllRuleTypes()
{
return Assembly.GetCallingAssembly()
.GetExportedTypes()
.Where(t => Attribute.IsDefined(t, typeof(RuleAttribute)));
}
}
}
<file_sep>/10xDev/TextFileFactsRepository.cs
using System.IO;
namespace _10xDev
{
public class TextFileFactsRepository : IFactsRepository
{
private readonly string _path;
public TextFileFactsRepository(string path)
{
_path = path;
}
public string GetFacts()
{
return File.ReadAllText(_path);
}
}
}
<file_sep>/10xDev/IFactsRepository.cs
namespace _10xDev
{
public interface IFactsRepository
{
string GetFacts();
}
}
<file_sep>/README.md
# August Challenge: Find the 10x Developer
A solution to ThoughtWorks Australia shokunin coding challenge
## Background
Jessie, Evan, John, Sarah and Matt are all engineers in the same delivery team (note: any resemblance to actual TWers, living or dead, is purely coincidental)... and each of them has a different level of coding skill to the other. This means it possible to order them from best to... "least best". Importantly, the best of them is the mythical 10x Developer!!!
## Here's what we know
- Jessie is not the best developer
- Evan is not the worst developer
- John is not the best developer or the worst developer
- Sarah is a better developer than Evan
- Matt is not directly below or above John as a developer
- John is not directly below or above Evan as a developer
## Challenge
You need to write a solution to compute these answers:
1. Who is the 10x developer on the team?
1. What is the correct ordering of the members of the team according to their coding skills?
## Prerequisite
.NET Core 2.2 ([download](https://dotnet.microsoft.com/download))
## Usage
Define the facts as English sentences in `facts.txt` file.
### Mac OS, Linux
- Run the application: `./go`
- Run the tests: `./go test`
### Other OS
- Run the application: `dotnet run -p 10xDev`
- Run the tests: `dotnet test`
<file_sep>/go
#!/usr/bin/env sh
if [ $# = 0 ]; then
dotnet run -p 10xDev
fi
if [ "$1" = "test" ]; then
dotnet test 10xDev.Tests
fi
<file_sep>/10xDev/PermutationService.cs
using System.Collections.Generic;
using System.Linq;
namespace _10xDev
{
public static class PermutationService
{
public static IEnumerable<IEnumerable<string>> GenerateFor(string[] items)
{
if (items.Length == 1)
yield return items;
foreach (var item in items)
{
var otherItems = items.Except(new[] {item});
foreach (var permutation in GenerateFor(otherItems.ToArray()))
{
yield return permutation.Prepend(item);
}
}
}
}
}
<file_sep>/10xDev/Rules/RuleAttribute.cs
using System;
namespace _10xDev.Rules
{
public class RuleAttribute : Attribute
{
public string Pattern { get; }
public RuleAttribute(string pattern)
{
Pattern = pattern;
}
}
}
<file_sep>/10xDev.Tests/SortServiceTests.cs
using System.Collections.Generic;
using System.Linq;
using _10xDev.Rules;
using Shouldly;
using Xunit;
namespace _10xDev.Tests
{
public class SortServiceTests
{
private readonly string[] _names = {"Jessie", "Evan", "John", "Sarah", "Matt"};
private readonly IEnumerable<IEnumerable<string>> _possibleResults;
public SortServiceTests()
{
var sortService = new SortService(new RulesProvider(new TextFileFactsRepository("facts.txt")));
_possibleResults = sortService.GetPossibleSorts(_names);
}
[Fact]
public void Sorted_names_should_always_contain_all_of_them()
{
_possibleResults.ShouldAllBe(p => p.Count() == _names.Length);
_possibleResults.ShouldAllBe(p=> p.Contains("Jessie"));
_possibleResults.ShouldAllBe(p=> p.Contains("Evan"));
_possibleResults.ShouldAllBe(p=> p.Contains("John"));
_possibleResults.ShouldAllBe(p=> p.Contains("Sarah"));
_possibleResults.ShouldAllBe(p=> p.Contains("Matt"));
}
[Fact]
public void Jessie_is_not_the_best_developer()
{
_possibleResults.ShouldNotContain(p => p.First() == "Jessie");
}
[Fact]
public void Evan_is_not_the_worst_developer()
{
_possibleResults.ShouldNotContain(p => p.Last() == "Evan");
}
[Fact]
public void John_is_not_the_best_developer_or_the_worst_developer()
{
_possibleResults.ShouldNotContain(p => p.Last() == "John");
_possibleResults.ShouldNotContain(p => p.First() == "John");
}
[Fact]
public void Sarah_is_a_better_developer_than_Evan()
{
_possibleResults.ShouldAllBe(result =>
result.IndexOf("Sarah") < result.IndexOf("Evan")
);
}
[Fact]
public void Matt_is_not_directly_below_or_above_John_as_a_developer()
{
_possibleResults.ShouldNotContain(result => result.Stringify().Contains("Matt,John"));
_possibleResults.ShouldNotContain(result => result.Stringify().Contains("John,Matt"));
}
[Fact]
public void John_is_not_directly_below_or_above_Evan_as_a_developer()
{
_possibleResults.ShouldNotContain(result => result.Stringify().Contains("John,Evan"));
_possibleResults.ShouldNotContain(result => result.Stringify().Contains("Evan,John"));
}
[Fact]
public void When_all_rules_apply_there_will_be_one_result()
{
_possibleResults.Count().ShouldBe(1);
}
}
}
<file_sep>/10xDev/Rules/NotTheWorstRule.cs
using System.Collections.Generic;
using System.Linq;
namespace _10xDev.Rules
{
[Rule(@"\s*(.+) is not.*the worst")]
public class NotTheWorstRule : ISortingRule
{
private readonly string _name;
public NotTheWorstRule(string name) => _name = name;
public bool Matches(IEnumerable<string> names) => names.Last() != _name;
}
}
<file_sep>/10xDev/Presenter.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace _10xDev
{
public class Presenter
{
public string Get10XDeveloper(IEnumerable<string>[] results)
{
var result = EnsureSingleResult(results);
return $"The 10X Developer is {result[0]}";
}
public string GetLeaderBoard(IEnumerable<string>[] results)
{
var result = EnsureSingleResult(results);
var leaderBoardBuilder = new StringBuilder();
leaderBoardBuilder.AppendLine("Leader board:");
for (var i = 0; i < result.Length; i++)
{
leaderBoardBuilder.AppendLine($"{i + 1}. {result[i]}");
}
return leaderBoardBuilder.ToString();
}
private static string[] EnsureSingleResult(IEnumerable<string>[] results)
{
return results.Length == 1 ?
results.Single().ToArray() :
throw new InvalidOperationException("More than one possible ordering! You many not have enough rules.");
}
}
}
<file_sep>/10xDev/Extensions.cs
using System;
using System.Collections.Generic;
using System.Linq;
namespace _10xDev
{
public static class Extensions
{
public static int IndexOf<T>(this IEnumerable<T> @this, T element)
{
return Array.IndexOf(@this.ToArray(), element);
}
public static string Stringify(this IEnumerable<string> @this)
{
return string.Join(',', @this);
}
}
}
<file_sep>/10xDev/IRuleProvider.cs
using System.Collections.Generic;
using _10xDev.Rules;
namespace _10xDev
{
public interface IRuleProvider
{
IEnumerable<ISortingRule> GetRules();
}
}
<file_sep>/10xDev.Tests/PermutationServiceTests.cs
using System;
using System.Collections;
using System.Linq;
using Shouldly;
using Xunit;
namespace _10xDev.Tests
{
public class PermutationServiceTests
{
[Fact]
public void GeneratesPermutationsForGivenNames()
{
var items = new[] {"a", "b", "c"};
var permutations = PermutationService.GenerateFor(items).ToArray();
permutations.Length.ShouldBe(6);
permutations.ShouldContain(p => p.SequenceEqual(new[] {"a", "b", "c"}));
permutations.ShouldContain(p => p.SequenceEqual(new[] {"b", "a", "c"}));
permutations.ShouldContain(p => p.SequenceEqual(new[] {"c", "a", "b"}));
permutations.ShouldContain(p => p.SequenceEqual(new[] {"a", "c", "b"}));
permutations.ShouldContain(p => p.SequenceEqual(new[] {"b", "c", "a"}));
permutations.ShouldContain(p => p.SequenceEqual(new[] {"c", "b", "a"}));
}
[Fact]
public void GenerateNoResultsWithNoErrorsForEmptyInput()
{
var items = new string[0];
var permutations = PermutationService.GenerateFor(items).ToArray();
permutations.Length.ShouldBe(0);
}
}
}
<file_sep>/10xDev/Rules/ISortingRule.cs
using System.Collections.Generic;
namespace _10xDev.Rules
{
public interface ISortingRule
{
bool Matches(IEnumerable<string> names);
}
}
<file_sep>/10xDev/Rules/NotNextToRule.cs
using System;
using System.Collections.Generic;
namespace _10xDev.Rules
{
[Rule(@"\s*(.+) is not directly below or above (.+) as a developer")]
public class NotNextToRule : ISortingRule
{
private readonly string _name1;
private readonly string _name2;
public NotNextToRule(string name1, string name2)
{
_name1 = name1;
_name2 = name2;
}
public bool Matches(IEnumerable<string> names) => Math.Abs(names.IndexOf(_name1) - names.IndexOf(_name2)) > 1;
}
}
<file_sep>/10xDev/Rules/BetterRule.cs
using System.Collections.Generic;
using System.Linq;
namespace _10xDev.Rules
{
[Rule(@"\s*(.+) is a better developer than (.+)")]
public class BetterRule : ISortingRule
{
private readonly string _better;
private readonly string _worse;
public BetterRule(string better, string worse)
{
_better = better;
_worse = worse;
}
public bool Matches(IEnumerable<string> names)
{
var namesArray = names.ToArray();
return namesArray.IndexOf(_better) < namesArray.IndexOf(_worse);
}
}
}
<file_sep>/10xDev/Program.cs
using System;
using System.Linq;
namespace _10xDev
{
class Program
{
static void Main(string[] args)
{
try
{
string[] names = {"Jessie", "Evan", "John", "Sarah", "Matt"};
var sortService = new SortService(new RulesProvider(new TextFileFactsRepository("facts.txt")));
var presenter = new Presenter();
var results = sortService.GetPossibleSorts(names).ToArray();
Console.WriteLine(presenter.Get10XDeveloper(results));
Console.WriteLine(presenter.GetLeaderBoard(results));
}
catch (Exception e)
{
Console.ForegroundColor = ConsoleColor.Red;
Console.WriteLine(e.Message);
Console.ResetColor();
}
}
}
}
<file_sep>/10xDev/SortService.cs
using System.Collections.Generic;
using System.Linq;
using _10xDev.Rules;
namespace _10xDev
{
public class SortService
{
private readonly IRuleProvider _ruleProvider;
public SortService(IRuleProvider ruleProvider)
{
_ruleProvider = ruleProvider;
}
public IEnumerable<IEnumerable<string>> GetPossibleSorts(string[] names)
{
var permutations = PermutationService.GenerateFor(names);
var rules = _ruleProvider.GetRules();
return permutations.Where(p => rules.All(r => r.Matches(p)));
}
}
}
| 9e54f677b859371531f66290f8f042e7809ae9da | [
"Markdown",
"C#",
"Shell"
] | 19 | C# | baharestani/shokunin.10xdev | c3de9b279757779f9875b94b613c9a033f879f61 | bb2b20679d6eacd7304ebafe28be3d08370941e3 | |
refs/heads/master | <file_sep>let packageName = "mp3-compress";
let fs = require('fire-fs');
let path = require('fire-path');
let Electron = require('electron');
let fs_extra = require('fs-extra');
let lameJs = Editor.require('packages://' + packageName + '/node_modules/lamejs');
let co = Editor.require('packages://' + packageName + '/node_modules/co');
let child_process = require('child_process');
let mp3item = Editor.require('packages://' + packageName + '/panel/item/mp3item.js');
// 同步执行exec
child_process.execPromise = function (cmd, options, callback) {
return new Promise(function (resolve, reject) {
child_process.exec(cmd, options, function (err, stdout, stderr) {
// console.log("执行完毕!");
if (err) {
console.log(err);
callback && callback(stderr);
reject(err);
return;
}
resolve();
})
});
};
Editor.Panel.extend({
style: fs.readFileSync(Editor.url('packages://' + packageName + '/panel/index.css', 'utf8')) + "",
template: fs.readFileSync(Editor.url('packages://' + packageName + '/panel/index.html', 'utf8')) + "",
$: {
logTextArea: '#logTextArea',
},
ready() {
let logCtrl = this.$logTextArea;
let logListScrollToBottom = function () {
setTimeout(function () {
logCtrl.scrollTop = logCtrl.scrollHeight;
}, 10);
};
mp3item.init();
window.plugin = new window.Vue({
el: this.shadowRoot,
created() {
this._getLamePath();
this.onBtnClickGetProjectMusic();
},
init() {
},
data: {
logView: "",
mp3Path: null,
lameFilePath: null,// lame程序路径
mp3Array: [
{uuid: "88a33018-0323-4c25-9b0c-c54f147f5dd8"},
],
},
methods: {
_addLog(str) {
let time = new Date();
// this.logView = "[" + time.toLocaleString() + "]: " + str + "\n" + this.logView;
this.logView += "[" + time.toLocaleString() + "]: " + str + "\n";
logListScrollToBottom();
},
onBtnClickCompressAll() {
console.log("压缩整个项目音频文件");
this._compressMp3(this.mp3Array);
},
// 检索项目中的声音文件mp3类型
onBtnClickGetProjectMusic() {
this.mp3Array = [];
Editor.assetdb.queryAssets('db://assets/**\/*', 'audio-clip', function (err, results) {
results.forEach(function (result) {
let ext = path.extname(result.path);
if (ext === '.mp3') {
this.mp3Array.push(result);
}
}.bind(this));
}.bind(this));
},
onItemCompress(data) {
console.log("onItemCompress");
// 进行压缩
this._compressMp3([data]);
},
_getLamePath() {
let lamePath = null;
let lameBasePath = path.join(Editor.projectInfo.path, "packages/mp3-compress/file");
let runPlatform = cc.sys.os;
if (runPlatform === "Windows") {
lamePath = path.join(lameBasePath, 'lame.exe');
} else if (runPlatform === "OS X") {
// 添加执行权限
lamePath = path.join(lameBasePath, 'lame');
let cmd = "chmod u+x " + lamePath;
child_process.exec(cmd, null, function (err) {
if(err){
console.log(err);
}
//console.log("添加执行权限成功");
}.bind(this));
}
return lamePath;
},
_getTempMp3Dir() {
let userPath = Electron.remote.app.getPath('userData');
let tempMp3Dir = path.join(userPath, "/mp3Compress");// 临时目录
return tempMp3Dir;
},
_compressMp3(fileDataArray) {
// 设置lame路径
let lamePath = this._getLamePath();
if (!fs.existsSync(lamePath)) {
this._addLog("文件不存在: " + lamePath);
return;
}
console.log("压缩");
co(function* () {
// 处理要压缩的音频文件
for (let i = 0; i < fileDataArray.length; i++) {
let voiceFile = fileDataArray[i].path;
let voiceFileUrl = fileDataArray[i].url;
if (!fs.existsSync(voiceFile)) {
this._addLog("声音文件不存在: " + voiceFile);
return;
}
if (path.extname(voiceFile) === ".mp3") {
// 检测临时缓存目录
let tempMp3Dir = this._getTempMp3Dir();// 临时目录
if (!fs.existsSync(tempMp3Dir)) {
fs.mkdirSync(tempMp3Dir);
}
console.log("mp3目录: " + tempMp3Dir);
let dir = path.dirname(voiceFile);
let arr = voiceFile.split('.');
let fileName = arr[0].substr(dir.length + 1, arr[0].length - dir.length);
let tempMp3Path = path.join(tempMp3Dir, 'temp_' + fileName + '.mp3');
// 压缩mp3
let cmd = `${lamePath} -V 0 -q 0 -b 45 -B 80 --abr 64 "${voiceFile}" "${tempMp3Path}"`;
console.log("------------------------------exec began" + i);
yield child_process.execPromise(cmd, null, function (err) {
this._addLog("出现错误: \n" + err);
}.bind(this));
console.log("------------------------------exec end" + i);
// 临时文件重命名
let newNamePath = path.join(tempMp3Dir, fileName + '.mp3');
fs.renameSync(tempMp3Path, newNamePath);
this._addLog(`压缩成功: ${voiceFileUrl} `);
let fullFileName = fileName + '.mp3';
let url = voiceFileUrl.substr(0, voiceFileUrl.length - fullFileName.length - 1);
// 导入到项目原位置
Editor.assetdb.import([newNamePath], url,
function (err, results) {
console.log("11111111111111111");
results.forEach(function (result) {
console.log(result.path);
// result.uuid
// result.parentUuid
// result.url
// result.path
// result.type
});
}.bind(this));
} else {
console.log("不支持的文件类型:" + voiceFile);
}
}
// TODO 删除临时目录的文件
// let dir = this._getTempMp3Dir();// 临时目录
// if (fs.existsSync(dir)) {
// fs.unlinkSync(dir);// 删除临时文件
// }
this._addLog("处理完毕!");
}.bind(this));
},
onBtnCompress() {
},
dropFile(event) {
event.preventDefault();
let files = event.dataTransfer.files;
if (files.length > 0) {
let file = files[0].path;
console.log(file);
this.mp3Path = file;
} else {
console.log("no file");
}
},
drag(event) {
event.preventDefault();
event.stopPropagation();
// console.log("dragOver");
},
}
});
},
messages: {
'mp3-compress:hello'(event, target) {
console.log("刷新文件列表");
// 检测变动的文件里面是否包含mp3
let b = false;
for (let i = 0; i < target.length; i++) {
let ext = require('fire-path').extname(target[i].path || target[i].destPath);
if (ext === '.mp3') {
b = true;
break;
}
}
if (b) {
window.plugin.onBtnClickGetProjectMusic();
} else {
console.log("未发现音频文件,无需刷新:");
}
}
}
});<file_sep>var FS = require("fire-fs");
Editor.Panel.extend({
style: FS.readFileSync(Editor.url('packages://creator-chat-room/panel/index.css', 'utf8')) + "",
template: FS.readFileSync(Editor.url('packages://creator-chat-room/panel/index.html', 'utf8')) + "",
$: {},
ready() {
window.plugin = new window.Vue({
el: this.shadowRoot,
created() {
},
init() {
},
data: {
word: "test word",
},
methods: {
_checkFir(url) {
let xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && ((xhr.status >= 200 && xhr.status < 400))) {
let text = xhr.responseText;
let result = JSON.parse(text);
console.log(result);
}
};
xhr.open('GET', url, true);
xhr.send();
},
onTest() {
console.log("test");
let url = "https://download.fir.im/game";
this._checkFir(url);
return;
let wildDog = Editor.require("packages://creator-chat-room/node_modules/wilddog");
let config = {
syncURL: "https://wild-hare-4781.wilddogio.com" //输入节点 URL
};
wildDog.initializeApp(config);
let ref = wildDog.sync().ref();
ref.on('value', function (snapshot) {
let str = snapshot.val();
this.word = str;
console.log(str);
}.bind(this));
},
},
});
},
messages: {
'creator-chat-room:hello'(event) {
}
}
});<file_sep># 插件说明
在保证mp3音频文件质量的前提下,对mp3文件进行瘦身,减小mp3文件体积的大小
## 其他说明
项目中使用了lame
- npm地址: https://www.npmjs.com/package/node-lame
- sourceforge地址: http://lame.sourceforge.net/
## 插件使用
- 打开快捷键: Cmd/Ctrl + m
- 目前插件只会对mp3进行压缩,所以建议项目使用mp3类型音频文件
## 使用动态图

## 压缩效果
- [压缩前文件 9.79k](../../doc/mp3-Compress/testcase.mp3)
- [压缩前文件 4.94k](../../doc/mp3-Compress/testcase-compress.mp3)
## 帮助
在mac平台下,执行lame需要运行权限,插件会自动授予权限,如果插件提示了权限问题,需要再次检查下lame的权限

<file_sep>cc.Class({
extends: cc.Component,
editor: CC_EDITOR && {
inspector: "packages://foobar/inspector.js",
},
properties: {
foo: 'Foo',
bar: 'Bar'
}
});
<file_sep>module.exports={
title:'mp3-compress',
};<file_sep>module.exports={
title:'音频压缩',
};<file_sep># 项目简介
目前包含的免费插件有:
[热更新插件](packages/hot-update-tools/README.md)
[Bugly插件](packages/plugin-bugly/README.md)
[4399原创平台SDK插件](packages/plugin-4399-web-js-sdk/README.md)
[Cocos-Creator-Inspector](CocosCreatorInspector/README.md)
[Excel工具](packages/excel-killer/README.md)
[贝塞尔编辑工具](packages/bezier/README.md)
[音频压缩工具](packages/mp3-compress/README.md)
## 2款收费插件,有需求的支持下呗
- 字体瘦身
- 购买链接: http://store.cocos.com/stuff/show/178910.html
- BMFont位图字体生成工具
- 购买链接: http://store.cocos.com/stuff/show/178913.html
- 帮助文档: [点击](doc/bitmap-font/README.md)
# 福利代码
项目中的代码是个人开发沉淀,希望对你有所帮助!
- [Observer.js](assets/core/Observer.js)
- [ObserverMgr.js](assets/core/ObserverMgr.js)
# 支持一下呗

| b485eba9840fd00b920c515f85a23e8a2499e924 | [
"JavaScript",
"Markdown"
] | 7 | JavaScript | Lucassssss/CocosCreatorPlugins | 130cb3fac58c5bd69d49b42dba7b706904d2dd79 | beaba58a9cd9c28bcdba5660da8c033cade39d54 | |
refs/heads/master | <repo_name>nidhhoggr/GPRS-googleVoice<file_sep>/classes/GPRS_SMS_API.php
<?php
/**
* GPRS_SMS_API
*
* This class has some loose coupling with PhpSerial, a class for interfacing
* a device connected to a computer. the purpose of this class is to interface
* SMS functionality of the connected GPRS shield.
*
* @abstract
* @package
* @version $id$
* @copyright
* @author <NAME> <<EMAIL>>
* @license
*/
abstract class GPRS_SMS_API
{
protected $sms_ids = array(1,2,3,4,5);
protected $sms_last_index = 1;
/**
* __construct
*
* @param PhpSerial $serial
* @param mixed $device
* @param int $baud
* @access protected
* @return void
*/
function __construct(PhpSerial $serial, $device, $baud = 9600)
{
$serial->deviceSet($device);
$serial->confBaudRate($baud);
$serial->deviceOpen();
$this->serial = $serial;
$this->init();
}
/**
* boot_load
*
* @access public
* @return void
*/
function boot_load()
{
$this->serial->sendMessage("AT\r",1);
}
/**
* setSmsLastIndex
*
* @param mixed $sms_last_index
* @access public
* @return void
*/
public function setSmsLastIndex($sms_last_index)
{
$this->sms_last_index = $sms_last_index;
}
/**
* setSmsIds
*
* @param array $sms_ids
* @access public
* @return void
*/
public function setSmsIds($sms_ids = array())
{
$this->sms_ids = $sms_ids;
}
/**
* getSerial
*
* @access public
* @return PhpSerial $serial
*/
public function getSerial()
{
return $this->serial;
}
/**
* _getSmsIds
*
* @access protected
* @return array $sms_ids
*/
protected function _getSmsIds()
{
return $this->sms_ids;
}
/**
* _getSmsLastIndex
*
* @access protected
* @return int $sms_last_index
*/
protected function _getSmsLastIndex()
{
return $this->sms_last_index;
}
/**
* listAllIncomingSMSBySmsIds
*
* @access public
* @return void
*/
public function listAllIncomingSMSBySmsIds()
{
$this->serial->sendMessage("AT+CMGF=1\r",1);
$sms_ids = $this->_getSmsIds();
foreach($sms_ids as $sms_id)
{
$this->lastCmd = "AT+CMGR=$sms_id\r";
$this->setial->readPort();
$this->serial->sendMessage($this->lastCmd,1);
$this->_readSms($this->serial->readPort());
}
}
/**
* listAllIncomingSMSFromLastIndex
*
* @param mixed $sms_processor
* @access public
* @return void
*/
public function listAllIncomingSMSFromLastIndex($sms_processor)
{
$this->serial->sendMessage("AT+CMGF=1\r",1);
$sms_id = $this->_getSmsLastIndex();
do
{
$this->lastCmd = "AT+CMGR=$sms_id\r";
$this->serial->sendMessage($this->lastCmd,1);
$sms = $this->_readSms($sms_id, $this->serial->readPort());
if($sms)
{
$this->_saveSms($sms);
}
$sms_processor($sms);
$sms_id++;
}
while($sms);
}
/**
* _saveSms
*
* @param mixed array $sms
* @abstract
* @access protected
* @return void
*/
abstract protected function _saveSms($sms);
/**
* _readSms
*
* @param mixed $sms_id
* @param mixed $deviceBuffer
* @access private
* @return void
*/
private function _readSms($sms_id, $deviceBuffer)
{
//the SMS query should be the last command
if(!strstr($deviceBuffer, $this->lastCmd))
{
return FALSE;
}
$replacable = array(
$this->lastCmd,
"+CMGR: ",
"OK"
);
foreach($replacable as $to_replace)
{
$deviceBuffer = str_replace($to_replace,'',$deviceBuffer);
}
$trimmed_message = trim($deviceBuffer);
if(empty($trimmed_message))
{
return FALSE;
}
else
{
$contents = explode("\r\n", $trimmed_message);
$info = explode(',', $contents[0]);
$sms_info_keys = $this->_getSmsInfoKeys();
foreach($info as $info_key=>$info_val)
{
$key_name = $sms_info_keys[$info_key];
if($key_name !== "omit")
{
$info[$key_name] = str_replace('"','',$info_val);
}
unset($info[$info_key]);
}
$info['index'] = $sms_id;
$sms['info'] = $info;
if(empty($contents[1]))
{
return FALSE;
}
$sms['msg'] = $contents[1];
return $sms;
}
}
/**
* _getSmsInfoKeys
*
* @access protected
* @return array $sms_info_keys
*/
protected function _getSmsInfoKeys()
{
/**
array(5) {
[0]=>
string(10) ""REC READ""
[1]=>
string(14) ""+13214277445""
[2]=>
string(2) """"
[3]=>
string(9) ""14/12/06"
[4]=>
string(12) "16:46:18-20""
**/
return array(
'status',
'recipient_phone_number',
'omit',
'date',
'time'
);
}
}
<file_sep>/classes/SMSForwarder.php
<?php
/**
* SMSForwarder
*
* This class is not only a concrete implementation of an SMSProcessor
* but it also acts to decouple database persietnce from abstracted functionality
* in the GPRS_SMS_API. The main purpose of this class is to play Google Tranlsate
* MP3's of the lastest text message recieved and them as processed.
*
* @uses GPRS_SMS_API
* @uses SMSProcessor
* @package
* @version $id$
* @copyright
* @author <NAME> <<EMAIL>>
* @license
*/
class SMSForwarder extends GPRS_SMS_API implements SMSProcessor
{
const AUDIO_DIR = 'audio';
const GOOGLE_TRANSLATE_API_CALL = 'http://translate.google.com/translate_tts?ie=UTF-8&tl=en&q=';
/**
* init
*
* @access public
* @return void
*/
public function init()
{
R::setup('mysql:host=localhost;dbname=gprs','root','');
}
/**
* _saveSms
*
* @param array $sms
* @access protected
* @return void
*/
protected function _saveSms($sms = array())
{
$sms_bean = R::dispense('sms');
$sms_info = $sms['info'];
$sms_bean->index = $sms_info['index'];
$sms_bean->status = $sms_info['status'];
$sms_bean->recipient_phone_number = $sms_info['recipient_phone_number'];
$sms_bean->date = $sms_info['date'];
$sms_bean->time = $sms_info['time'];
$sms_bean->msg = $sms['msg'];
$sms_bean->is_sent = FALSE;
$id = R::store($sms_bean);
}
/**
* processIncomingSMS
*
* @access public
* @return void
*/
public function processIncomingSMS()
{
$sendableSms = R::find('sms', ' is_sent = 0');
foreach($sendableSms as $sms_to_send)
{
$message = urlencode($sms_to_send->msg);
$audio_file = self::AUDIO_DIR . "/output-{$sms_to_send->id}.mp3";
shell_exec("wget -q -U Mozilla -O {$audio_file} '".self::GOOGLE_TRANSLATE_API_CALL."{$message}'");
if(file_exists($audio_file))
{
exec("mplayer " . $audio_file);
exec("rm -rf $audio_file");
$sms_to_send->is_sent = TRUE;
R::store($sms_to_send);
}
}
}
/**
* setSmsLastIndex
*
* @access public
* @return void
*/
public function setSmsLastIndex()
{
$lastSms = R::findOne('sms', ' ORDER BY id DESC LIMIT 1');
$smsLastIndex = $lastSms->index + 1;
parent::setSmsLastIndex($smsLastIndex);
}
}
<file_sep>/autoload.php
<?php
include('vendor/autoload.php');
function myload($class)
{
$libClass = 'libs/'.$class.'.php';
$classClass = 'classes/'.$class.'.php';
if (is_file($libClass))
{
require_once ($libClass);
}
else if(is_file($classClass))
{
require_once ($classClass);
}
}
spl_autoload_register("myload");
<file_sep>/README.md
# GPRS-Google Voice
>Author: <NAME> | Version: 0.1
The purpose of the repository is to house multiple libraries and useful scripts related to interfacing a GPRS shield and Google voice.
###SMSForwarder
this is a script that connects to a GRPS shield through USB serial port. The script checks for any new SMS text messages and saves them to a database. to process the SMS records the script utilizes the google translate API to download an MP3 containing the message in the SMS record and plays the record using Mplayer. After the record is played the record is updated as is_sent(boolean).
#####TODO
* add functionality to store phone number and keep a record of a name associated to the phone number
* In the MP3 for the text message the voice will provide who texted you what such as "Bob said [contents of sms message]" when the phone number is associated to a user name bob
* When a SMS message is recieved an SMS message will be sent asking for a name if the number doesn't already have an associated name
##### Installation
* Create a database named GPRS and configure the database username and password. Thats it!
```sh
mysql> CREATE DATABASE gprs;
```
* You need to install mapler to play the MP3's
```sh
$ sudo apt-get install mplayey
```
##### Usage
Plugin in your device with USB serial. The default baud rate is 9600.If another baud rate is desired you will have to explicity configure in the SMSForwarder instantion with a thrid parameter on the gprs script.
1) Send a text message to your Device
2) probe the shield for the new SMS message
```sh
$ ./gprs -d /dev/ttyACMO
```
3) probe the shield again. This time an MP3 will be played containing the latest SMS text message
```sh
$ ./gprs -d /dev/ttyACMO
```
###GoogleVoice
##### Usage
```sh
$ ./googleVoice
```
#####LINKS
* GPRS Shield Documentation
http://www.seeedstudio.com/wiki/GPRS_Shield_V1.0
* SIM900 AT Commands Manual
http://www.seeedstudio.com/wiki/images/a/a8/SIM900_AT_Command_Manual_V1.03.pdf
* Youtube videos coming soon
<file_sep>/googleVoice
#!/usr/bin/php
<?php
$options = getopt('u:p:n:m:f:h::');
extract($options);
//$p = "<PASSWORD>";
if(isset($h) || !isset($u) || !isset($p) || !isset($n) || (!isset($m) && !isset($f)))
{
echo "usage: ./googleVoice -u [google voice email] -p [google voice password] -n [recipient phone number or from phone number for calls] -m [message] -f [to phone number for calls only]";
}
else
{
include('autoload.php');
$gv = new GoogleVoice($u, $p);
if(isset($m)) {
$gv->sendSMS($n, $m);
} else if(isset($f)) {
$gv->callNumber($n, $f);
}
}
<file_sep>/gprs
#!/usr/bin/php
<?php
$options = getopt('d:');
if(!isset($options['d']))
{
Throw new Exception('Must specify a device');
}
else if(!file_exists($options['d']))
{
Throw new Exception('No such device: ' . $options['d']);
}
require_once('autoload.php');
$serial = new PhpSerial;
$smsF = new SMSForwarder($serial, $options['d']);
$smsF->processIncomingSMS();
$smsF->boot_load();
$smsF->setSmsLastIndex();
$smsF->listAllIncomingSMSFromLastIndex(function($sms) {
var_dump($sms);
});
<file_sep>/classes/SMSProcessor.php
<?php
/**
* SMSProcessor
*
* @package
* @version $id$
* @author <NAME> <<EMAIL>>
* @license
*/
interface SMSProcessor
{
/**
* init
*
* this funtion is called in the constructor of the super class
*
* @access public
* @return void
*/
function init();
/**
* processIncomingSMS
*
* This is why the child class is implementing me.
*
* @access public
* @return void
*/
function processIncomingSMS();
}
| 783c6b729c51bc04aabd5c580f80b7225079c97c | [
"Markdown",
"PHP"
] | 7 | PHP | nidhhoggr/GPRS-googleVoice | 20f4cdb6baf8f798e91d1d90413d8142f679d966 | 91510c1ad9bdda18010ea00b9589c5f050548038 | |
refs/heads/master | <file_sep>using AssessmentTest03.Domain;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using System;
namespace AssessmentTest03
{
[TestClass]
public class Level1EfTests
{
[TestMethod]
public void add_parties_and_make_queries()
{
EfMethods.RecreateDatabase(); // To student: comment this line when the database is created
EfMethods.ClearDatabase();
var lib = new PoliticalParty
{
Name = "Liberalerna",
AbbreviatedName = "L",
Founded = new DateTime(1934, 8, 5),
Members = 15390,
};
var miljo = new PoliticalParty
{
Name = "<NAME>",
AbbreviatedName = "MP",
Founded = new DateTime(1981, 9, 20),
Members = 10719,
};
var soc = new PoliticalParty
{
Name = "<NAME>",
AbbreviatedName = "S",
Founded = new DateTime(1889, 4, 23),
Members = 89010,
};
EfMethods.AddParty(miljo);
EfMethods.AddParty(lib);
EfMethods.AddParty(soc);
string[] result = EfMethods.GetAllAbbreviatedNamesOrderedByFoundedDate();
string[] expected = new[] { "S", "L", "MP" };
CollectionAssert.AreEqual(expected, result);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace AssessmentTest03
{
public class CSharpMethods
{
public static int SumEdges(int[] arr, bool add10000)
{
var eka = arr.First();
var vika = arr.Last();
int tonni = 10000;
if (add10000)
{
return eka + vika + tonni;
}
return eka + vika;
}
}
}
<file_sep>using Microsoft.VisualStudio.TestTools.UnitTesting;
namespace AssessmentTest03
{
[TestClass]
public class Level1CSharpTest
{
/*
Create the method "SumEdges" so the following tests will pass.
The method should add the first and last number in the array
If add10000 is true then the method should add 10000 to the result
(you don't need to take care of edge cases like null or arrays with 0 or 1 elements)
*/
[TestMethod]
public void test1()
{
var arr = new[] { 4, 11, 15 };
bool add10000 = false;
int expected = 19; // 4+15
int result = CSharpMethods.SumEdges(arr, add10000);
Assert.AreEqual(expected, result);
}
[TestMethod]
public void test2()
{
var arr = new[] { 4, 11, 15 };
bool add10000 = true;
int expected = 10019; // 4+15+10000
int result = CSharpMethods.SumEdges(arr, add10000);
Assert.AreEqual(expected, result);
}
[TestMethod]
public void test3()
{
var arr = new[] { 6, 2 };
bool add10000 = false;
int expected = 8; // 6+2
int result = CSharpMethods.SumEdges(arr, add10000);
Assert.AreEqual(expected, result);
}
[TestMethod]
public void test4()
{
var arr = new[] { 6, 2 };
bool add10000 = true;
int expected = 10008; // 6+2+10000
int result = CSharpMethods.SumEdges(arr, add10000);
Assert.AreEqual(expected, result);
}
[TestMethod]
public void test5()
{
var arr = new[] { 100, 200, 500, 77 };
bool add10000 = false;
int expected = 177; // 100+77
int result = CSharpMethods.SumEdges(arr, add10000);
Assert.AreEqual(expected, result);
}
[TestMethod]
public void test6()
{
var arr = new[] { 100, 200, 500, 77 };
bool add10000 = true;
int expected = 10177; // 100+77+10000
int result = CSharpMethods.SumEdges(arr, add10000);
Assert.AreEqual(expected, result);
}
}
}
<file_sep>using AssessmentTest03.Domain;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace AssessmentTest03
{
public class EfMethods
{
internal static void AddParty(PoliticalParty party)
{
var context = new PoliticalContext();
context.PoliticalParties.Add(party);
context.SaveChanges();
}
internal static string[] GetAllAbbreviatedNamesOrderedByFoundedDate()
{
var context = new PoliticalContext();
return context.PoliticalParties.OrderBy(p => p.Founded).Select(c => c.AbbreviatedName).ToArray();
}
internal static void RecreateDatabase()
{
var context = new PoliticalContext();
context.Database.EnsureDeleted();
context.Database.EnsureCreated();
}
internal static void ClearDatabase()
{
var context = new PoliticalContext();
context.RemoveRange(context.PoliticalParties);
context.SaveChanges();
}
}
}
<file_sep>USE PoliticalParties
select * from person
-- (1) Remove all rows from Person, Country, PoliticalParties
DELETE FROM Person;
DELETE FROM Country;
DELETE FROM PoliticalParties
SET IDENTITY_INSERT Person ON
INSERT INTO Person (Id, FirstName, LastName)
VALUES (5000, 'Jonas', 'Sjöstedt')
INSERT INTO Person (Id, FirstName, LastName)
VALUES (5001, 'Ebba', '<NAME>')
INSERT INTO Person (Id, FirstName, LastName)
VALUES (5002, 'Ulf', 'Kristersson')
-- (2) Add three people (Jonas, Ebba, Ulf)
SET IDENTITY_INSERT Person OFF
SET IDENTITY_INSERT PoliticalParties ON
insert into PoliticalParties (Id, Name) values (5000, 'Väntsterpartiet')
insert into PoliticalParties (Id, Name) values (5001, 'Kristdemokraterna')
insert into PoliticalParties (Id, Name) values (5002, 'Moderaterna')
-- (3) Add three political parties
SET IDENTITY_INSERT PoliticalParties OFF
select * from Person
select Person.Id, Name, FirstName, LastName from PoliticalParties join Person on PoliticalParties.Id = Person.Id
-- (4) Write a "select" which gives info about the political parties
-- Verify that you get exactly the same result as in SQL-output.png
<file_sep>using Microsoft.EntityFrameworkCore;
namespace AssessmentTest03.Domain
{
public class PoliticalContext : DbContext
{
public DbSet<PoliticalParty> PoliticalParties { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
if (!optionsBuilder.IsConfigured)
optionsBuilder.UseSqlServer("Server = (localdb)\\mssqllocaldb; Database = PoliticalParties; Trusted_Connection = True; ");
}
}
}
<file_sep>using System;
namespace AssessmentTest03.Domain
{
public class PoliticalParty
{
public int Id { get; set; }
public string Name { get; set; }
public DateTime? Founded { get; set; }
public int? Members { get; set; }
public string AbbreviatedName { get; set; }
public Person Chairman { get; set; }
public Country Country { get; set; }
}
}
| 868bff040aaed8e6916429ca8ffcb11036cd89c6 | [
"C#",
"SQL"
] | 7 | C# | johnur/AssessmentTest03 | 5c1b578af9e7e453973c0143c2af3cbec6f76bb1 | eb6060b676191dc5a2182fefc433ed927287085d | |
refs/heads/master | <file_sep>let run: game.LedSprite = null
let char: game.LedSprite = null
basic.forever(function () {
run = game.createSprite(randint(0, 4), randint(0, 4))
char = game.createSprite(2, 2)
while (true) {
if (char.isTouching(run)) {
run.change(LedSpriteProperty.X, randint(0, 4))
run.change(LedSpriteProperty.Y, randint(0, 4))
}
if (input.acceleration(Dimension.Y) > 0) {
char.change(LedSpriteProperty.Y, 1)
basic.pause(100)
}
if (input.acceleration(Dimension.Y) < 0) {
char.change(LedSpriteProperty.Y, -1)
basic.pause(100)
}
if (input.acceleration(Dimension.X) < 0) {
char.change(LedSpriteProperty.X, -1)
basic.pause(100)
}
if (input.acceleration(Dimension.X) > 0) {
char.change(LedSpriteProperty.X, 1)
basic.pause(100)
}
}
})
| a282dd377073b35818614be56059a95bb75974b3 | [
"TypeScript"
] | 1 | TypeScript | umtozl/yakalamaca | 2c8fd3ab76944a7face8c3aa5b8bc47db37beb99 | 5e897802ceaccbda1eb7697df9467bd6b3f4b13c | |
refs/heads/master | <repo_name>bouhenic/MysqlPython<file_sep>/BddMysql/Sammysql.py
import pymysql
class Bdd:
def __init__(self, host, port, user, password, db):
self.host = host
self.port = port
self.user = user
self.password = <PASSWORD>
self.db = db
def Lastselect(self):
with connection.cursor() as cursor:
sql = "SELECT * FROM `TEMPERATURE` ORDER BY ID DESC LIMIT 1"
try:
cursor.execute(sql)
result = cursor.fetchall()
for row in result:
print(str(row[0]) + " " + str(row[1]) + " " + str(row[2]))
except:
print("Oops! Something wrong")
connection.commit()
def selectById(self,Id):
with connection.cursor() as cursor:
sql = f"SELECT * FROM `TEMPERATURE` WHERE ID={Id} LIMIT 1"
try:
cursor.execute(sql)
result = cursor.fetchall()
for row in result:
print(str(row[0]) + " " + str(row[1]) + " " + str(row[2]))
except:
print("Oops! Something wrong")
connection.commit()
def insert(self,temp):
with connection.cursor() as cursor:
sql = f"INSERT INTO temperature(TEMP) VALUES({temp})"
try:
cursor.execute(sql)
except:
print("Oops! Something wrong")
connection.commit()
def update(self,temp,Id):
with connection.cursor() as cursor:
sql = f"UPDATE TEMPERATURE SET TEMP = {temp} WHERE id = {Id}"
try:
cursor.execute(sql)
except:
print("Oops! Something wrong")
connection.commit()
def delete(self,Id):
with connection.cursor() as cursor:
sql = f"DELETE FROM TEMPERATURE WHERE id={Id}"
try:
cursor.execute(sql)
except:
print("Oops! Something wrong")
connection.commit()
bdd = Bdd('localhost', 8889, 'root', 'root', 'METEO')
connection = pymysql.connect(
host=bdd.host,
port=bdd.port,
user=bdd.user,
password=<PASSWORD>,
db=bdd.db
)
bdd.insert(27)
bdd.delete(1008)
bdd.Lastselect()
bdd.selectById(1006)
connection.close() | 5f73d72faa990a1f6eaae5b93ef2de5c8075ced6 | [
"Python"
] | 1 | Python | bouhenic/MysqlPython | 43e1f438ce316f68bf31f29af4f41e271330c2c8 | 4ab031d3cb6424a08f239f3977ab6f9030cb7ebc | |
refs/heads/master | <file_sep>module Gry
class CLI
def initialize(argv)
@argv = argv
end
def run(writer)
opt = Option.new(@argv)
if opt.version
rubocop_version, = *Open3.capture3('rubocop', '--verbose-version')
writer.puts "gry #{VERSION} (RuboCop #{rubocop_version.chomp})"
return
end
cops = opt.args.empty? ? RubocopAdapter.configurable_cops : opt.args
if opt.fast
cops.reject!{|cop| cop == 'Style/AlignHash'}
end
pilot_study = Gry::PilotStudy.new(cops, process: opt.process)
bills = pilot_study.analyze
congress = Congress.new(
max_count: opt.max_count,
min_difference: opt.min_difference,
metrics_percentile: opt.metrics_percentile,
)
laws = bills.map do |cop_name, bill|
congress.discuss(cop_name, bill)
end
fmt = Formatter.new(display_disabled_cops: opt.display_disabled_cops)
writer.puts fmt.format(laws)
end
end
end
<file_sep>appraise "rubocop-head" do
gem "rubocop", github: 'bbatsov/rubocop'
end
appraise "rubocop-0.47.0" do
gem "rubocop", "0.47.0"
end
| fa47efeee67fdf753f4b41b8589a73aa0d29d789 | [
"Ruby"
] | 2 | Ruby | koic/gry | f739203d28a75be663536b9a467709c03c703860 | c2addc9232aecf64e4e31a3ab9be16de76a67355 | |
refs/heads/master | <repo_name>nayeemzen/koin-merchant-android<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/checkout/CheckoutPresenter.java
package com.sendkoin.merchant.ui.checkout;
import com.annimon.stream.Stream;
import com.google.gson.Gson;
import com.pushtorefresh.storio.sqlite.operations.delete.DeleteResult;
import com.sendkoin.api.InitiateDynamicTransactionRequest;
import com.sendkoin.api.InitiateDynamicTransactionResponse;
import com.sendkoin.api.QrCode;
import com.sendkoin.api.SaleItem;
import com.sendkoin.events.TransactionProcessedEvent;
import com.sendkoin.merchant.data.onboarding.MerchantDataStore;
import com.sendkoin.merchant.data.transactions.SaleItemDataStore;
import com.sendkoin.merchant.data.transactions.TransactionService;
import com.sendkoin.sql.entities.MerchantEntity;
import org.greenrobot.eventbus.EventBus;
import org.greenrobot.eventbus.Subscribe;
import org.greenrobot.eventbus.ThreadMode;
import java.util.List;
import javax.inject.Inject;
import okhttp3.WebSocket;
import rx.Observer;
import rx.Subscription;
import rx.android.schedulers.AndroidSchedulers;
import rx.schedulers.Schedulers;
import rx.subscriptions.CompositeSubscription;
import timber.log.Timber;
import static com.sendkoin.events.TransactionProcessedEvent.Status.SUCCESSFUL;
class CheckoutPresenter implements CheckoutContract.Presenter {
private static final String TAG = CheckoutPresenter.class.getSimpleName();
private final CheckoutContract.View view;
private final SaleItemDataStore saleItemDataStore;
private MerchantDataStore merchantDataStore;
private final TransactionService transactionService;
private WebSocket webSocket;
private EventBus eventBus;
private Gson gson;
private final CompositeSubscription compositeSubscription = new CompositeSubscription();
private String transactionToken;
@Inject
CheckoutPresenter(CheckoutContract.View view,
SaleItemDataStore saleItemDataStore,
MerchantDataStore merchantDataStore,
TransactionService transactionService,
WebSocket webSocket,
EventBus eventBus,
Gson gson) {
this.view = view;
this.saleItemDataStore = saleItemDataStore;
this.merchantDataStore = merchantDataStore;
this.transactionService = transactionService;
this.webSocket = webSocket;
this.eventBus = eventBus;
this.gson = gson;
}
@Override
public void subscribe() {
eventBus.register(this);
loadMerchant();
loadCurrentSale();
}
@Override
public void loadMerchant() {
Subscription subscription = merchantDataStore.getOnly()
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(merchant -> {
MerchantEntity merchantEntity = merchant.get();
view.showInstruction(merchantEntity.getStoreName());
});
compositeSubscription.add(subscription);
}
@Override
public void unsubscribe() {
// Defined status codes - https://tools.ietf.org/html/rfc6455#section-7.4.
eventBus.unregister(this);
webSocket.close(1001, "Presenter::unsubscribe called");
compositeSubscription.clear();
}
@Override
public void loadCurrentSale() {
Subscription subscription = saleItemDataStore.getAllItems()
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.doOnNext(items -> view.showCurrentSaleAmount(Stream.of(items)
.mapToInt(item -> item.getPrice().intValue() * item.getQuantity().intValue())
.sum()))
.flatMap(items -> transactionService.initiateTransaction(
new InitiateDynamicTransactionRequest.Builder()
.sale_items(Stream.of(items).map(SaleItemDataStore::toWire).toList())
.build())
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread()))
.subscribe(new Observer<InitiateDynamicTransactionResponse>() {
@Override
public void onCompleted() {
}
@Override
public void onError(Throwable e) {
Timber.e(e, "Error in checkout");
}
@Override
public void onNext(InitiateDynamicTransactionResponse initiateTransactionResponse) {
transactionToken = initiateTransactionResponse.transaction_token;
String qrCodeJson = gson.toJson(initiateTransactionResponse.qr_code, QrCode.class);
view.showPaymentQrCode(qrCodeJson);
}
});
compositeSubscription.add(subscription);
}
@Subscribe(threadMode = ThreadMode.MAIN)
@Override
public void onTransactionProcessed(TransactionProcessedEvent event) {
Timber.d("Received event! [TransactionProcessedEvent=%s]", event);
if (event.transaction_token.equals(transactionToken)) {
view.showCheckoutProcessing();
if (event.status != SUCCESSFUL) {
view.showFailedCheckout(event.status, event.failure_reason);
return;
}
Subscription subscription = saleItemDataStore.removeAllItems()
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(new Observer<DeleteResult>() {
@Override
public void onCompleted() {
}
@Override
public void onError(Throwable e) {
Timber.e(e);
}
@Override
public void onNext(DeleteResult deleteResult) {
view.showSuccessfulCheckout();
}
});
compositeSubscription.add(subscription);
}
}
static final class SaleSummary {
final List<SaleItem> saleItems;
final String merchantName;
SaleSummary(List<SaleItem> saleItems, String merchantName) {
this.saleItems = saleItems;
this.merchantName = merchantName;
}
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/orders/list/OrderListContract.java
package com.sendkoin.merchant.ui.orders.list;
import com.sendkoin.merchant.ui.BasePresenter;
import com.sendkoin.sql.entities.KoinOrderEntity;
import java.util.List;
public interface OrderListContract {
interface View {
void showPendingOrders(List<KoinOrderEntity> orderEntities);
void showOrderDetails(KoinOrderEntity order);
}
interface Presenter extends BasePresenter {
void loadPendingOrdersFromLocal();
void loadNewPendingOrdersFromServer();
}
}
<file_sep>/app/src/main/sql/merchant.sql
CREATE TABLE Merchant (
id INTEGER NOT NULL PRIMARY KEY,
store_name TEXT NOT NULL,
store_location TEXT NOT NULL,
store_type TEXT NOT NULL,
phone_number TEXT NULL DEFAULT NULL,
email_address TEXT NULL DEFAULT NULL
);<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/orders/list/OrderListFragment.java
package com.sendkoin.merchant.ui.orders.list;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.v4.app.Fragment;
import android.support.v7.app.ActionBar;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import com.sendkoin.merchant.KoinMerchantApp;
import com.sendkoin.merchant.R;
import com.sendkoin.merchant.data.orders.OrderDataStore;
import com.sendkoin.merchant.ui.MainActivity;
import com.sendkoin.merchant.ui.orders.details.OrderDetailsFragment;
import com.sendkoin.merchant.ui.utils.DividerItemDecoration;
import com.sendkoin.sql.entities.KoinOrderEntity;
import java.util.List;
import javax.inject.Inject;
import butterknife.BindView;
import butterknife.ButterKnife;
import butterknife.Unbinder;
import static android.support.v7.widget.LinearLayoutManager.VERTICAL;
public class OrderListFragment extends Fragment implements OrderListContract.View {
@BindView(R.id.rv_transactions_pending_review) RecyclerView recyclerView;
@BindView(R.id.tv_empty_activity) TextView emptyTextView;
@Inject OrderListContract.Presenter presenter;
private Unbinder unbinder;
private PendingOrdersListAdapter recyclerViewAdapter;
@Nullable
@Override
public View onCreateView(LayoutInflater inflater,
@Nullable ViewGroup container,
@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
View view = inflater.inflate(R.layout.order_list_fragment, container, false);
unbinder = ButterKnife.bind(this, view);
setHasOptionsMenu(true);
return view;
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
ActionBar supportActionBar = ((MainActivity) getActivity()).getSupportActionBar();
supportActionBar.setTitle("Sales");
supportActionBar.show();
setUpDagger();
setUpRecyclerView();
}
@Override
public void showPendingOrders(List<KoinOrderEntity> pendingOrders) {
if (pendingOrders.isEmpty()) {
recyclerView.setVisibility(View.GONE);
emptyTextView.setVisibility(View.VISIBLE);
} else {
emptyTextView.setVisibility(View.GONE);
recyclerView.setVisibility(View.VISIBLE);
recyclerViewAdapter.setPendingOrdersList(pendingOrders);
}
}
private void setUpRecyclerView() {
LinearLayoutManager layoutManager = new LinearLayoutManager(getActivity(), VERTICAL, false);
recyclerView.addItemDecoration(new DividerItemDecoration(getActivity()));
recyclerView.setLayoutManager(layoutManager);
recyclerView.setHasFixedSize(true);
recyclerViewAdapter = new PendingOrdersListAdapter(this);
recyclerView.setAdapter(recyclerViewAdapter);
}
@Override
public void onResume() {
super.onResume();
presenter.subscribe();
}
@Override
public void onPause() {
super.onPause();
presenter.unsubscribe();
}
@Override
public void onDestroyView() {
unbinder.unbind();
super.onDestroyView();
}
private void setUpDagger() {
DaggerOrderListComponent.builder()
.orderListModule(new OrderListModule(this))
.netComponent(((KoinMerchantApp) getActivity()
.getApplicationContext())
.getNetComponent())
.build()
.inject(this);
}
@Override
public void showOrderDetails(KoinOrderEntity order) {
Bundle bundle = new Bundle();
bundle.putLong("order_details.local_id", order.getLocalId());
bundle.putByteArray("order_details.order", OrderDataStore.toWire(order).encode());
OrderDetailsFragment orderDetailsFragment = new OrderDetailsFragment();
orderDetailsFragment.setArguments(bundle);
getActivity().getSupportFragmentManager()
.beginTransaction()
.replace(R.id.fragment_placeholder, orderDetailsFragment)
.addToBackStack(null)
.commit();
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/transactions/review/TransactionReviewPresenter.java
package com.sendkoin.merchant.ui.transactions.review;
import com.annimon.stream.Stream;
import com.pushtorefresh.storio.sqlite.operations.delete.DeleteResult;
import com.sendkoin.merchant.data.transactions.SaleItemDataStore;
import com.sendkoin.sql.entities.SaleItemEntity;
import java.util.List;
import javax.inject.Inject;
import rx.Observer;
import rx.Subscription;
import rx.android.schedulers.AndroidSchedulers;
import rx.schedulers.Schedulers;
import rx.subscriptions.CompositeSubscription;
import timber.log.Timber;
import static com.annimon.stream.Collectors.summingInt;
class TransactionReviewPresenter implements TransactionReviewContract.Presenter {
private static final String TAG = TransactionReviewPresenter.class.getSimpleName();
private final SaleItemDataStore saleItemDataStore;
private TransactionReviewContract.View view;
private CompositeSubscription compositeSubscription = new CompositeSubscription();
@Inject
TransactionReviewPresenter(TransactionReviewContract.View view,
SaleItemDataStore saleItemDataStore) {
this.view = view;
this.saleItemDataStore = saleItemDataStore;
}
@Override
public void subscribe() {
loadItems();
}
@Override
public void loadItems() {
Subscription subscription = saleItemDataStore.getAllItems()
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(new Observer<List<SaleItemEntity>>() {
@Override
public void onCompleted() {
Timber.d("Load items complete!");
}
@Override
public void onError(Throwable e) {
Timber.e(e, "Load items failed!");
}
@Override
public void onNext(List<SaleItemEntity> saleItems) {
// TODO(Zen): Fix duplicated and inefficient summing logic in
// TransactionReviewPresenter and CurrentSalePresenter.
Integer total = Stream.of(saleItems).collect(
summingInt(i -> i.getPrice().intValue() * i.getQuantity().intValue()));
view.showCurrentSaleAmount(total);
view.showNumItems(saleItems.size());
view.showTransactionReviewItems(saleItems);
}
});
compositeSubscription.add(subscription);
}
@Override
public void deleteItem(SaleItemEntity saleItem) {
Subscription subscription = saleItemDataStore.removeItem(saleItem.getId())
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(new Observer<DeleteResult>() {
@Override
public void onCompleted() {
Timber.d("Delete item [id=%s] complete!", saleItem.getId());
}
@Override
public void onError(Throwable e) {
Timber.d("Delete item [id=%s] failed!", saleItem.getId());
}
@Override
public void onNext(DeleteResult deleteResult) {
view.showItemDeleted(saleItem.getTitle());
}
});
compositeSubscription.add(subscription);
}
@Override
public void unsubscribe() {
compositeSubscription.clear();
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/data/onboarding/MerchantService.java
package com.sendkoin.merchant.data.onboarding;
import com.sendkoin.api.Merchant;
import com.sendkoin.api.OnboardMerchantRequest;
import com.sendkoin.api.OnboardMerchantResponse;
import retrofit2.http.Body;
import retrofit2.http.GET;
import retrofit2.http.POST;
import rx.Observable;
public interface MerchantService {
@POST("merchant/onboard")
Observable<OnboardMerchantResponse> onboardMerchant(
@Body OnboardMerchantRequest onboardMerchantRequest);
@GET("merchant")
Observable<Merchant> getMerchant();
}
<file_sep>/app/src/test/java/com/sendkoin/merchant/ui/currentsale/CurrentSaleTestComponent.java
package com.sendkoin.merchant.ui.currentsale;
import com.sendkoin.merchant.KoinTestComponent;
import com.sendkoin.merchant.ui.CustomScope;
import dagger.Component;
@CustomScope
@Component(dependencies = KoinTestComponent.class, modules = CurrentSaleModule.class)
public interface CurrentSaleTestComponent {
void inject(CurrentSaleTest currentSaleTest);
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/transactions/history/TransactionHistoryModule.java
package com.sendkoin.merchant.ui.transactions.history;
import com.sendkoin.merchant.data.transactions.TransactionDataStore;
import com.sendkoin.merchant.data.transactions.TransactionRepository;
import com.sendkoin.merchant.data.transactions.TransactionService;
import com.sendkoin.merchant.ui.CustomScope;
import dagger.Module;
import dagger.Provides;
import retrofit2.Retrofit;
@Module
public class TransactionHistoryModule {
private TransactionHistoryContract.View view;
public TransactionHistoryModule(TransactionHistoryContract.View view) {
this.view = view;
}
@Provides
@CustomScope
public TransactionHistoryContract.View provideTransactionHistoryView() {
return view;
}
@Provides
@CustomScope
public TransactionService transactionService(Retrofit retrofit) {
return retrofit.create(TransactionService.class);
}
@Provides
@CustomScope
public TransactionRepository provideTransactionRepository(
TransactionService transactionService, TransactionDataStore transactionDataStore) {
return new TransactionRepository(transactionDataStore, transactionService);
}
@Provides
@CustomScope
public TransactionHistoryContract.Presenter provideTransactionHistoryPresenter(
TransactionHistoryContract.View view,
TransactionRepository transactionRepository) {
return new TransactionHistoryPresenter(view, transactionRepository);
}
}
<file_sep>/app/src/main/sql/transaction.sql
CREATE TABLE KoinTransaction (
transaction_token TEXT PRIMARY KEY NOT NULL,
amount INTEGER NOT NULL,
created_at INTEGER NOT NULL,
state TEXT NOT NULL
);<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/orders/details/OrderDetailsComponent.java
package com.sendkoin.merchant.ui.orders.details;
import com.sendkoin.merchant.dagger.component.NetComponent;
import com.sendkoin.merchant.ui.CustomScope;
import dagger.Component;
@CustomScope
@Component(dependencies = NetComponent.class, modules = OrderDetailsModule.class)
public interface OrderDetailsComponent {
void inject(OrderDetailsFragment orderDetailsFragment);
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/transactions/review/TransactionReviewContract.java
package com.sendkoin.merchant.ui.transactions.review;
import com.sendkoin.merchant.ui.BasePresenter;
import com.sendkoin.sql.entities.SaleItemEntity;
import java.util.List;
public class TransactionReviewContract {
public interface View {
void showCurrentSaleAmount(int currentSaleAmount);
void showTransactionReviewItems(List<SaleItemEntity> items);
void showItemDeleted(String itemName);
void showNumItems(int numItems);
}
interface Presenter extends BasePresenter {
void loadItems();
void deleteItem(SaleItemEntity item);
}
}
<file_sep>/app/src/main/sql/order_items.sql
CREATE TABLE OrderItem (
id INTEGER NOT NULL PRIMARY KEY,
order_id INTEGER NOT NULL,
order_item_id INTEGER NOT NULL,
name TEXT NOT NULL,
price INTEGER NOT NULL,
quantity INTEGER NOT NULL,
customer_notes TEXT NULL DEFAULT NULL,
sale_type TEXT NOT NULL,
created_at INTEGER NOT NULL,
updated_at INTEGER NOT NULL
);<file_sep>/app/src/main/java/com/sendkoin/merchant/data/notifications/RegisterDeviceService.java
package com.sendkoin.merchant.data.notifications;
import com.sendkoin.api.RegisterDeviceRequest;
import com.sendkoin.api.RegisterDeviceResponse;
import retrofit2.http.Body;
import retrofit2.http.POST;
import rx.Observable;
public interface RegisterDeviceService {
@POST("register-device/merchant/firebase")
Observable<RegisterDeviceResponse> registerDevice(@Body RegisterDeviceRequest request);
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/onboarding/OnboardingPresenter.java
package com.sendkoin.merchant.ui.onboarding;
import com.sendkoin.api.Merchant;
import com.sendkoin.api.OnboardMerchantRequest;
import com.sendkoin.merchant.data.onboarding.MerchantDataStore;
import com.sendkoin.merchant.data.onboarding.MerchantService;
import javax.inject.Inject;
import rx.Observer;
import rx.Subscription;
import rx.android.schedulers.AndroidSchedulers;
import rx.schedulers.Schedulers;
import rx.subscriptions.CompositeSubscription;
import timber.log.Timber;
class OnboardingPresenter implements OnboardingContract.Presenter {
private static final String TAG = OnboardingPresenter.class.getSimpleName();
private OnboardingContract.View view;
private MerchantService merchantService;
private MerchantDataStore merchantDataStore;
private CompositeSubscription compositeSubscription = new CompositeSubscription();
@Inject
public OnboardingPresenter(OnboardingContract.View view,
MerchantService merchantService,
MerchantDataStore merchantDataStore) {
this.view = view;
this.merchantService = merchantService;
this.merchantDataStore = merchantDataStore;
}
@Override
public void subscribe() {
}
@Override
public void unsubscribe() {
compositeSubscription.clear();
}
@Override
public void saveOnboardingData(Merchant merchant) {
view.showResultLoading();
OnboardMerchantRequest onboardMerchantRequest = new OnboardMerchantRequest.Builder()
.store_name(merchant.store_name)
.store_location(merchant.store_location)
.store_type(merchant.store_type)
.build();
Subscription subscription = merchantService.onboardMerchant(onboardMerchantRequest)
.subscribeOn(Schedulers.io())
.flatMap(onboardMerchantResponse ->
merchantDataStore.createOrUpdate(onboardMerchantResponse.merchant))
.observeOn(AndroidSchedulers.mainThread())
.subscribe(new Observer<Merchant>() {
@Override
public void onCompleted() {
}
@Override
public void onError(Throwable e) {
Timber.d(e, "Error saving onboarding to server");
view.showOnboardingFailure();
}
@Override
public void onNext(Merchant merchant1) {
view.showOnboardingSuccess();
}
});
compositeSubscription.add(subscription);
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/checkout/CheckoutComponent.java
package com.sendkoin.merchant.ui.checkout;
import com.sendkoin.merchant.dagger.component.NetComponent;
import com.sendkoin.merchant.ui.CustomScope;
import dagger.Component;
@CustomScope
@Component(dependencies = NetComponent.class, modules = CheckoutModule.class)
public interface CheckoutComponent {
void inject(CheckoutFragment fragment);
}
<file_sep>/app/src/main/sql/saleitem.sql
CREATE TABLE SaleItem (
id INTEGER NOT NULL PRIMARY KEY AUTOINCREMENT,
title TEXT NOT NULL,
price INTEGER NOT NULL,
quantity INTEGER NOT NULL,
sale_type TEXT NULL DEFAULT NULL,
);<file_sep>/app/src/main/java/com/sendkoin/merchant/KoinFirebaseMessagingService.java
package com.sendkoin.merchant;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.content.Context;
import android.content.Intent;
import android.support.v4.app.NotificationCompat;
import android.support.v4.content.ContextCompat;
import com.google.firebase.messaging.FirebaseMessagingService;
import com.google.firebase.messaging.RemoteMessage;
import com.sendkoin.merchant.data.notifications.DaggerKoinFirebaseComponent;
import com.sendkoin.merchant.data.notifications.KoinFirebaseModule;
import com.sendkoin.merchant.data.notifications.PushDataProcessor;
import com.sendkoin.merchant.ui.MainActivity;
import javax.inject.Inject;
public class KoinFirebaseMessagingService extends FirebaseMessagingService {
@Inject PushDataProcessor pushDataProcessor;
@Override
public void onCreate() {
setUpDagger();
}
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
String description = remoteMessage.getData().get("description");
String data = remoteMessage.getData().get("data");
String type = remoteMessage.getData().get("type");
pushDataProcessor.process(type, data);
showNotification(description);
}
private void setUpDagger() {
DaggerKoinFirebaseComponent.builder()
.netComponent(((KoinMerchantApp) getApplicationContext()).getNetComponent())
.koinFirebaseModule(new KoinFirebaseModule())
.build()
.inject(this);
}
@Override
public void onDeletedMessages() {
super.onDeletedMessages();
}
private void showNotification(String description) {
Intent intent = new Intent(this, MainActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0 /* Request code */, intent,
PendingIntent.FLAG_ONE_SHOT);
NotificationCompat.InboxStyle inboxStyle = new NotificationCompat.InboxStyle()
.setBigContentTitle("Koin Merchant")
.setSummaryText(description);
NotificationCompat.Builder notificationBuilder = new NotificationCompat.Builder(getApplicationContext())
.setSmallIcon(R.drawable.ic_add_tag)
.setColor(ContextCompat.getColor(getApplicationContext(), R.color.colorPrimary))
.setContentTitle("Koin Merchant")
.setContentText(description)
.setAutoCancel(true)
.setLights(ContextCompat.getColor(getApplicationContext(), R.color.colorPrimary), 100, 1900)
.setPriority(NotificationCompat.PRIORITY_HIGH)
.setDefaults(NotificationCompat.DEFAULT_SOUND | NotificationCompat.DEFAULT_VIBRATE)
.setStyle(inboxStyle);
NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
notificationManager.notify(0 /* ID of notification */, notificationBuilder.build());
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/transactions/history/TransactionHistoryContract.java
package com.sendkoin.merchant.ui.transactions.history;
import com.sendkoin.api.Transaction;
import com.sendkoin.merchant.ui.BasePresenter;
import java.util.List;
public interface TransactionHistoryContract {
interface View {
void showTransactions(List<Transaction> transactions);
void addTransactions(List<Transaction> transactions);
}
interface Presenter extends BasePresenter {
void loadNewTransactionsFromServer();
void loadTransactionsFromLocal();
void loadOldTransactionsFromServer();
}
}
<file_sep>/app/src/test/java/com/sendkoin/merchant/ui/currentsale/CurrentSaleTest.java
package com.sendkoin.merchant.ui.currentsale;
import com.sendkoin.api.SaleItem;
import com.sendkoin.merchant.DaggerKoinTestComponent;
import com.sendkoin.merchant.KoinTestModule;
import com.sendkoin.merchant.RxSchedulersOverrideRule;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.robolectric.RobolectricTestRunner;
import org.robolectric.annotation.Config;
import javax.inject.Inject;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.times;
import static org.mockito.Mockito.verify;
@RunWith(RobolectricTestRunner.class)
@Config(manifest="src/main/AndroidManifest.xml")
public class CurrentSaleTest {
@Inject CurrentSaleContract.View currentSaleView;
@Inject CurrentSaleContract.Presenter currentSalePresenter;
@Rule public RxSchedulersOverrideRule rxSchedulersOverrideRule = new RxSchedulersOverrideRule();
@Before
public void setUp() {
DaggerCurrentSaleTestComponent.builder()
.koinTestComponent(DaggerKoinTestComponent.builder()
.koinTestModule(new KoinTestModule())
.build())
.currentSaleModule(new CurrentSaleModule(mock(CurrentSaleFragment.class)))
.build()
.inject(this);
}
@Test
public void quickSaleItemsLoadedInCurrentSaleView() throws Exception {
currentSalePresenter.loadItems();
currentSalePresenter.addItem(new SaleItem.Builder()
.sale_type(SaleItem.SaleType.QUICK_SALE)
.name("Coffee")
.price(300)
.quantity(2)
.build());
currentSalePresenter.addItem(new SaleItem.Builder()
.sale_type(SaleItem.SaleType.QUICK_SALE)
.name("Red Velvet Cake")
.price(500)
.quantity(1)
.build());
verify(currentSaleView).showTotalAmount(1100);
}
@Test
public void quickSaleNumPadUpdatesView() throws Exception {
// Add item with price 750, quantity 10.
currentSalePresenter.numPadClicked("7", true, false);
currentSalePresenter.numPadClicked("5", true, false);
currentSalePresenter.numPadClicked("0", true, false);
currentSalePresenter.numPadClicked("0", false, true);
verify(currentSaleView).showPrice("750");
verify(currentSaleView).showQuantity("10");
// Remove the price and quantity.
currentSalePresenter.numPadClicked("<", true, false);
currentSalePresenter.numPadClicked("<", true, false);
currentSalePresenter.numPadClicked("<", true, false);
verify(currentSaleView, times(2)).showPrice("75");
verify(currentSaleView, times(2)).showPrice("7");
verify(currentSaleView).showPrice("");
currentSalePresenter.numPadClicked("<", false, true);
currentSalePresenter.numPadClicked("<", false, true);
currentSalePresenter.numPadClicked("<", false, true);
verify(currentSaleView).showQuantity("1");
verify(currentSaleView).showQuantity("");
// Add item with price 5 and quantity 5.
currentSalePresenter.numPadClicked("5", true, false);
currentSalePresenter.numPadClicked("5", false, true);
verify(currentSaleView).showPrice("5");
verify(currentSaleView).showQuantity("5");
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/home/summary/SummaryContact.java
package com.sendkoin.merchant.ui.home.summary;
import com.sendkoin.merchant.ui.BasePresenter;
public interface SummaryContact {
interface View {
}
interface Presenter extends BasePresenter {
}
}
<file_sep>/app/src/main/sql/order.sql
CREATE TABLE KoinOrder (
local_id INTEGER NOT NULL PRIMARY KEY AUTOINCREMENT,
order_id INTEGER NOT NULL,
created_at INTEGER NOT NULL,
updated_at INTEGER NOT NULL,
transaction_token TEXT NOT NULL,
amount INTEGER NOT NULL,
state TEXT NOT NULL
);<file_sep>/app/src/main/java/com/sendkoin/merchant/data/orders/OrderDataStore.java
package com.sendkoin.merchant.data.orders;
import com.annimon.stream.Stream;
import com.pushtorefresh.storio.sqlite.StorIOSQLite;
import com.pushtorefresh.storio.sqlite.operations.delete.DeleteResult;
import com.pushtorefresh.storio.sqlite.operations.put.PutResults;
import com.pushtorefresh.storio.sqlite.queries.DeleteQuery;
import com.pushtorefresh.storio.sqlite.queries.Query;
import com.sendkoin.api.Order;
import com.sendkoin.api.OrderState;
import com.sendkoin.sql.entities.KoinOrderEntity;
import com.sendkoin.sql.entities.OrderItemEntity;
import com.sendkoin.sql.tables.KoinOrderTable;
import com.sendkoin.sql.tables.OrderItemTable;
import java.util.List;
import rx.Observable;
public class OrderDataStore {
private final StorIOSQLite storIOSQLite;
public OrderDataStore(StorIOSQLite storIOSQLite) {
this.storIOSQLite = storIOSQLite;
}
public Observable<PutResults<KoinOrderEntity>> createOrUpdate(List<Order> orders) {
return storIOSQLite.put()
.objects(Stream.of(orders)
.map(OrderDataStore::fromWire)
.toList())
.withPutResolver(new KoinOrderEntityCustomPutResolver())
.prepare()
.asRxObservable();
}
public Observable<List<KoinOrderEntity>> getAllOrders() {
return storIOSQLite.get()
.listOfObjects(KoinOrderEntity.class)
.withQuery(Query.builder()
.table(KoinOrderTable.TABLE)
.orderBy(KoinOrderTable.COLUMN_UPDATED_AT + " DESC")
.build())
.prepare()
.asRxObservable();
}
public Observable<List<OrderItemEntity>> getOrderDetails(long orderId) {
return storIOSQLite.get()
.listOfObjects(OrderItemEntity.class)
.withQuery(Query.builder()
.table(OrderItemTable.TABLE)
.where(OrderItemTable.COLUMN_ORDER_ID + " = ?")
.whereArgs(orderId)
.build())
.prepare()
.asRxObservable();
}
public static KoinOrderEntity fromWire(Order order) {
return new KoinOrderEntity(
null,
order.order_id,
order.created_at,
order.updated_at,
order.transaction.token,
order.transaction.amount.longValue(),
order.state.name());
}
public Observable<KoinOrderEntity> getLatestOrder() {
return storIOSQLite.get()
.object(KoinOrderEntity.class)
.withQuery(Query.builder()
.table(KoinOrderTable.TABLE)
.limit(1)
.orderBy(KoinOrderTable.COLUMN_UPDATED_AT + " DESC")
.build())
.prepare()
.asRxObservable();
}
public Observable<DeleteResult> deleteOrder(long localOrderId) {
return storIOSQLite.delete()
.byQuery(DeleteQuery.builder()
.table(KoinOrderTable.TABLE)
.where(KoinOrderTable.COLUMN_LOCAL_ID + " = ?")
.whereArgs(localOrderId)
.build())
.prepare()
.asRxObservable();
}
public static Order toWire(KoinOrderEntity orderEntity) {
return new Order.Builder()
.order_id(orderEntity.getOrderId())
.state(OrderState.valueOf(orderEntity.getState()))
.created_at(orderEntity.getCreatedAt())
.updated_at(orderEntity.getUpdatedAt())
.build();
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/transactions/history/TransactionHistoryPresenter.java
package com.sendkoin.merchant.ui.transactions.history;
import com.annimon.stream.Stream;
import com.pushtorefresh.storio.sqlite.operations.put.PutResults;
import com.sendkoin.api.ListTransactionsResponse;
import com.sendkoin.api.QueryParameters;
import com.sendkoin.api.TransactionState;
import com.sendkoin.merchant.data.transactions.TransactionDataStore;
import com.sendkoin.merchant.data.transactions.TransactionRepository;
import com.sendkoin.sql.entities.KoinTransactionEntity;
import java.util.List;
import javax.inject.Inject;
import rx.Observer;
import rx.Subscription;
import rx.subscriptions.CompositeSubscription;
import timber.log.Timber;
import static com.sendkoin.api.QueryParameters.Order.ASCENDING;
import static com.sendkoin.api.QueryParameters.Order.DESCENDING;
class TransactionHistoryPresenter implements TransactionHistoryContract.Presenter {
private final CompositeSubscription compositeSubscription = new CompositeSubscription();
private final TransactionHistoryContract.View view;
private TransactionRepository transactionRepository;
@Inject
TransactionHistoryPresenter(TransactionHistoryContract.View view,
TransactionRepository transactionRepository) {
this.view = view;
this.transactionRepository = transactionRepository;
}
/**
* Do these steps in parallel.
* 1. Load all transactions from the local database.
* 2. Load new transactions from the server and insert into the database.
*/
@Override
public void subscribe() {
loadTransactionsFromLocal();
loadNewTransactionsFromServer();
}
@Override
public void loadTransactionsFromLocal() {
Subscription subscription = transactionRepository.loadTransactionsFromLocal()
.subscribe(new Observer<List<KoinTransactionEntity>>() {
@Override
public void onCompleted() {
Timber.d("Completed loadTransactionsFromLocal().");
}
@Override
public void onError(Throwable e) {
Timber.e(e);
}
@Override
public void onNext(List<KoinTransactionEntity> transactionEntities) {
Timber.d("Loaded %d orderEntity entities.", transactionEntities.size());
view.showTransactions(Stream.of(transactionEntities)
.map(TransactionDataStore::toWire)
.toList());
}
});
compositeSubscription.add(subscription);
}
@Override
public void loadNewTransactionsFromServer() {
Subscription subscription = transactionRepository.loadNewTransactionsFromServer(
new QueryParameters.Builder()
.order(ASCENDING)
.transaction_state(TransactionState.PROCESSING)
.build())
.subscribe(new Observer<PutResults<KoinTransactionEntity>>() {
@Override
public void onCompleted() {
Timber.d("loadNewPendingOrdersFromServer complete.");
}
@Override
public void onError(Throwable e) {
Timber.e(e);
}
@Override
public void onNext(PutResults<KoinTransactionEntity> putResults) {
Timber.d(
"Fetched from server and locally saved %d transactions, updated %d transactions",
putResults.numberOfInserts(),
putResults.numberOfUpdates());
}
});
compositeSubscription.add(subscription);
}
@Override
public void loadOldTransactionsFromServer() {
Subscription subscription = transactionRepository.loadOldTransactionsFromServer(
new QueryParameters.Builder()
.order(DESCENDING)
.transaction_state(TransactionState.PROCESSING)
.build())
.subscribe(new Observer<ListTransactionsResponse>() {
@Override
public void onCompleted() {
Timber.d("loadNewPendingOrdersFromServer complete.");
}
@Override
public void onError(Throwable e) {
Timber.e(e);
}
@Override
public void onNext(ListTransactionsResponse listTransactionsResponse) {
Timber.d("Fetched %d old transactions from server.",
listTransactionsResponse.transactions.size());
view.addTransactions(listTransactionsResponse.transactions);
}
});
compositeSubscription.add(subscription);
}
@Override
public void unsubscribe() {
compositeSubscription.clear();
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/orders/list/OrderListPresenter.java
package com.sendkoin.merchant.ui.orders.list;
import com.pushtorefresh.storio.sqlite.operations.put.PutResults;
import com.sendkoin.api.QueryParameters;
import com.sendkoin.merchant.data.orders.OrderRepository;
import com.sendkoin.sql.entities.KoinOrderEntity;
import java.util.List;
import javax.inject.Inject;
import rx.Observer;
import rx.Subscription;
import rx.subscriptions.CompositeSubscription;
import timber.log.Timber;
import static com.sendkoin.api.OrderState.ORDER_PROCESSING;
import static com.sendkoin.api.QueryParameters.Order.ASCENDING;
public class OrderListPresenter implements OrderListContract.Presenter {
private final OrderListContract.View view;
private final CompositeSubscription compositeSubscription = new CompositeSubscription();
private final OrderRepository orderRepository;
@Inject
public OrderListPresenter(OrderListContract.View view, OrderRepository orderRepository) {
this.view = view;
this.orderRepository = orderRepository;
}
@Override
public void subscribe() {
loadNewPendingOrdersFromServer();
loadPendingOrdersFromLocal();
}
@Override
public void loadPendingOrdersFromLocal() {
Subscription subscription = orderRepository.loadOrdersFromLocal()
.subscribe(new Observer<List<KoinOrderEntity>>() {
@Override
public void onCompleted() {
Timber.d("Completed loadPendingOrdersFromLocal().");
}
@Override
public void onError(Throwable e) {
Timber.e(e);
}
@Override
public void onNext(List<KoinOrderEntity> orderEntities) {
Timber.d("Loaded %d order entities.", orderEntities.size());
view.showPendingOrders(orderEntities);
}
});
compositeSubscription.add(subscription);
}
@Override
public void loadNewPendingOrdersFromServer() {
Subscription subscription = orderRepository.loadNewOrdersFromServer(new QueryParameters.Builder()
.order(ASCENDING)
.order_state(ORDER_PROCESSING)
.build())
.subscribe(new Observer<PutResults<KoinOrderEntity>>() {
@Override
public void onCompleted() {
Timber.d("loadNewPendingOrdersFromServer complete.");
}
@Override
public void onError(Throwable e) {
Timber.e(e);
}
@Override
public void onNext(PutResults<KoinOrderEntity> putResults) {
Timber.d(
"Fetched new orders from server and locally saved %d orders, updated %d orders",
putResults.numberOfInserts(),
putResults.numberOfUpdates());
}
});
compositeSubscription.add(subscription);
}
@Override
public void unsubscribe() {
compositeSubscription.clear();
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/onboarding/OnboardingContract.java
package com.sendkoin.merchant.ui.onboarding;
import com.sendkoin.api.Merchant;
import com.sendkoin.merchant.ui.BasePresenter;
public interface OnboardingContract {
interface View {
void onBackPressed();
boolean canGoBack();
void showOnboardingSuccess();
void showOnboardingFailure();
void showResultLoading();
}
interface Presenter extends BasePresenter {
void saveOnboardingData(Merchant merchant);
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/currentsale/CurrentSaleModule.java
package com.sendkoin.merchant.ui.currentsale;
import com.sendkoin.merchant.data.transactions.SaleItemDataStore;
import com.sendkoin.merchant.ui.CustomScope;
import com.sendkoin.merchant.ui.currentsale.CurrentSaleContract.Presenter;
import com.sendkoin.merchant.ui.currentsale.CurrentSaleContract.View;
import dagger.Module;
import dagger.Provides;
@Module
public class CurrentSaleModule {
private final View view;
public CurrentSaleModule(View view) {
this.view = view;
}
@Provides
@CustomScope
View provideCurrentSaleView() {
return view;
}
@Provides
@CustomScope
Presenter provideCurrentSalePresenter(View view,
SaleItemDataStore saleItemDataStore) {
return new CurrentSalePresenter(view, saleItemDataStore);
}
}
<file_sep>/app/src/test/java/com/sendkoin/merchant/ui/transactions/review/TransactionReviewTest.java
package com.sendkoin.merchant.ui.transactions.review;
import com.annimon.stream.Stream;
import com.google.common.collect.ImmutableList;
import com.sendkoin.api.SaleItem;
import com.sendkoin.merchant.DaggerKoinTestComponent;
import com.sendkoin.merchant.KoinTestModule;
import com.sendkoin.merchant.RxSchedulersOverrideRule;
import com.sendkoin.merchant.data.transactions.SaleItemDataStore;
import com.sendkoin.merchant.ui.currentsale.CurrentSaleContract;
import com.sendkoin.merchant.ui.currentsale.CurrentSaleFragment;
import com.sendkoin.merchant.ui.currentsale.CurrentSaleModule;
import com.sendkoin.sql.entities.SaleItemEntity;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.ArgumentCaptor;
import org.mockito.Captor;
import org.mockito.MockitoAnnotations;
import org.robolectric.RobolectricTestRunner;
import org.robolectric.annotation.Config;
import java.util.List;
import javax.inject.Inject;
import static com.sendkoin.api.SaleItem.SaleType.QUICK_SALE;
import static org.assertj.core.api.Java6Assertions.assertThat;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.times;
import static org.mockito.Mockito.verify;
@RunWith(RobolectricTestRunner.class)
@Config(manifest = "src/main/AndroidManifest.xml")
public class TransactionReviewTest {
@Captor ArgumentCaptor<List<SaleItemEntity>> argumentCaptor;
@Inject CurrentSaleContract.Presenter currentSalePresenter;
@Inject TransactionReviewContract.View transactionReviewView;
@Inject TransactionReviewContract.Presenter transactionReviewPresenter;
@Rule public RxSchedulersOverrideRule rxSchedulersOverrideRule = new RxSchedulersOverrideRule();
@Before
public void setUp() {
DaggerTransactionReviewTestComponent.builder()
.koinTestComponent(DaggerKoinTestComponent.builder()
.koinTestModule(new KoinTestModule())
.build())
.transactionReviewModule(new TransactionReviewModule(mock(TransactionReviewFragment.class)))
.currentSaleModule(new CurrentSaleModule(mock(CurrentSaleFragment.class)))
.build()
.inject(this);
MockitoAnnotations.initMocks(this);
}
@Test
public void transactionReviewDisplaysItemsAndSummary() throws Exception {
SaleItem milkShake = new SaleItem.Builder()
.name("MilkShake")
.price(200)
.quantity(4)
.sale_type(QUICK_SALE)
.build();
SaleItem cheeseBurger = new SaleItem.Builder()
.name("<NAME>")
.price(500)
.quantity(4)
.sale_type(QUICK_SALE)
.build();
transactionReviewPresenter.loadItems();
currentSalePresenter.addItem(milkShake);
currentSalePresenter.addItem(cheeseBurger);
verify(transactionReviewView).showCurrentSaleAmount(2800);
verify(transactionReviewView).showNumItems(2);
verify(transactionReviewView, times(3)).showTransactionReviewItems(argumentCaptor.capture());
List<SaleItemEntity> saleItemEntities = argumentCaptor.getValue();
assertThat(Stream.of(saleItemEntities).map(SaleItemDataStore::toWire).toList())
.isEqualTo(ImmutableList.of(milkShake, cheeseBurger));
}
@Test
public void removingItemsUpdatesSummary() throws Exception {
SaleItem milkShake = new SaleItem.Builder()
.name("MilkShake")
.price(200)
.quantity(4)
.sale_type(QUICK_SALE)
.build();
SaleItem cheeseBurger = new SaleItem.Builder()
.name("<NAME>")
.price(500)
.quantity(4)
.sale_type(QUICK_SALE)
.build();
transactionReviewPresenter.loadItems();
verify(transactionReviewView).showNumItems(0);
verify(transactionReviewView).showCurrentSaleAmount(0);
currentSalePresenter.addItem(milkShake);
verify(transactionReviewView).showCurrentSaleAmount(800);
verify(transactionReviewView).showNumItems(1);
currentSalePresenter.addItem(cheeseBurger);
verify(transactionReviewView).showNumItems(2);
verify(transactionReviewView).showCurrentSaleAmount(2800);
verify(transactionReviewView, times(3)).showTransactionReviewItems(argumentCaptor.capture());
List<SaleItemEntity> saleItemEntities = argumentCaptor.getValue();
assertThat(Stream.of(saleItemEntities).map(SaleItemDataStore::toWire).toList())
.isEqualTo(ImmutableList.of(milkShake, cheeseBurger));
transactionReviewPresenter.deleteItem(saleItemEntities.get(0));
verify(transactionReviewView, times(2)).showNumItems(1);
verify(transactionReviewView).showCurrentSaleAmount(2000);
verify(transactionReviewView, times(4)).showTransactionReviewItems(argumentCaptor.capture());
saleItemEntities = argumentCaptor.getValue();
assertThat(Stream.of(saleItemEntities).map(SaleItemDataStore::toWire).toList())
.isEqualTo(ImmutableList.of(cheeseBurger));
transactionReviewPresenter.deleteItem(saleItemEntities.get(0));
verify(transactionReviewView, times(2)).showNumItems(0);
verify(transactionReviewView, times(2)).showCurrentSaleAmount(0);
verify(transactionReviewView, times(5)).showTransactionReviewItems(argumentCaptor.capture());
saleItemEntities = argumentCaptor.getValue();
assertThat(Stream.of(saleItemEntities).map(SaleItemDataStore::toWire).toList())
.isEqualTo(ImmutableList.of());
}
}<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/transactions/review/TransactionReviewModule.java
package com.sendkoin.merchant.ui.transactions.review;
import com.sendkoin.merchant.data.transactions.SaleItemDataStore;
import com.sendkoin.merchant.ui.CustomScope;
import dagger.Module;
import dagger.Provides;
@Module
public class TransactionReviewModule {
private final TransactionReviewContract.View view;
public TransactionReviewModule(TransactionReviewContract.View view) {
this.view = view;
}
@Provides
@CustomScope
TransactionReviewContract.View providesTransactionReviewScreenView() {
return view;
}
@Provides
@CustomScope
TransactionReviewContract.Presenter providesTransactionReviewScreenPresenter(TransactionReviewContract.View view,
SaleItemDataStore saleItemDataStore) {
return new TransactionReviewPresenter(view, saleItemDataStore);
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/login/LoginFragment.java
package com.sendkoin.merchant.ui.login;
import android.content.Intent;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentTransaction;
import android.text.TextUtils;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import com.facebook.CallbackManager;
import com.facebook.FacebookCallback;
import com.facebook.FacebookException;
import com.facebook.login.LoginManager;
import com.facebook.login.LoginResult;
import com.sendkoin.api.Merchant;
import com.sendkoin.merchant.KoinMerchantApp;
import com.sendkoin.merchant.R;
import com.sendkoin.merchant.ui.MainActivity;
import com.sendkoin.merchant.ui.orders.list.OrderListFragment;
import com.sendkoin.merchant.ui.login.LoginContract.Presenter;
import com.sendkoin.merchant.ui.onboarding.OnboardingFragment;
import java.util.Collections;
import javax.inject.Inject;
import butterknife.ButterKnife;
import butterknife.OnClick;
import butterknife.Unbinder;
import cn.pedant.SweetAlert.SweetAlertDialog;
import timber.log.Timber;
import static android.support.v4.content.ContextCompat.getColor;
public class LoginFragment extends Fragment implements LoginContract.View {
private static final String TAG = LoginFragment.class.getSimpleName();
@Inject Presenter presenter;
private Unbinder unbinder;
private CallbackManager callbackManager;
private SweetAlertDialog pDialog;
@Nullable
@Override
public View onCreateView(LayoutInflater inflater,
@Nullable ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.login_fragment, container, false);
unbinder = ButterKnife.bind(this, view);
initFacebookLoginCallback();
return view;
}
private void initFacebookLoginCallback() {
callbackManager = CallbackManager.Factory.create();
LoginManager.getInstance().registerCallback(callbackManager,
new FacebookCallback<LoginResult>() {
@Override
public void onSuccess(LoginResult loginResult) {
presenter.loginWithFacebook(loginResult.getAccessToken().getToken());
}
@Override
public void onCancel() {
Timber.d("Facebook login cancelled");
}
@Override
public void onError(FacebookException e) {
Timber.e(e, "Facebook login error!");
}
});
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
((MainActivity) getActivity()).getSupportActionBar().hide();
setUpDagger();
}
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
callbackManager.onActivityResult(requestCode, resultCode, data);
}
@OnClick(R.id.btn_login_sms)
void loginWithSms() {
presenter.loginWithSms();
}
@OnClick(R.id.btn_login_fb)
void loginWithFacebook() {
LoginManager.getInstance().logInWithReadPermissions(this, Collections.singletonList("email"));
}
@Override
public void onResume() {
super.onResume();
presenter.subscribe();
}
@Override
public void onPause() {
super.onPause();
presenter.unsubscribe();
}
@Override
public void navigateHome(Merchant merchant) {
FragmentTransaction fragmentTransaction = getFragmentManager().beginTransaction();
if (merchant != null && isOnboardingComplete(merchant)) {
fragmentTransaction.replace(R.id.fragment_placeholder, new OrderListFragment());
} else {
fragmentTransaction.replace(
R.id.fragment_placeholder, new OnboardingFragment(), OnboardingFragment.TAG);
}
fragmentTransaction.commit();
}
private boolean isOnboardingComplete(Merchant merchant) {
return !TextUtils.isEmpty(merchant.store_name)
&& !TextUtils.isEmpty(merchant.store_location)
&& !TextUtils.isEmpty(merchant.store_type);
}
@Override
public void showLoginLoading() {
pDialog = new SweetAlertDialog(getContext(), SweetAlertDialog.PROGRESS_TYPE);
pDialog.getProgressHelper().setBarColor(getColor(getContext(), R.color.colorAccent));
pDialog.setTitleText(getString(R.string.login_loading_message));
pDialog.setCancelable(false);
pDialog.show();
}
@Override
public void showLoginSuccessful() {
pDialog.dismissWithAnimation();
}
@Override
public void showLoginFailed(Throwable e) {
pDialog.setTitleText("Login failed");
pDialog.changeAlertType(SweetAlertDialog.ERROR_TYPE);
}
@Override
public void onDestroyView() {
super.onDestroyView();
unbinder.unbind();
}
private void setUpDagger() {
DaggerLoginScreenComponent.builder()
.netComponent(((KoinMerchantApp) getActivity()
.getApplicationContext())
.getNetComponent())
.loginScreenModule(new LoginScreenModule(this))
.build()
.inject(this);
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ContextProvider.java
package com.sendkoin.merchant;
import android.content.Context;
interface ContextProvider {
Context getActivityContext();
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/data/transactions/TransactionRepository.java
package com.sendkoin.merchant.data.transactions;
import com.pushtorefresh.storio.sqlite.operations.delete.DeleteResult;
import com.pushtorefresh.storio.sqlite.operations.put.PutResults;
import com.sendkoin.api.ListTransactionsRequest;
import com.sendkoin.api.ListTransactionsResponse;
import com.sendkoin.api.QueryParameters;
import com.sendkoin.api.Transaction;
import com.sendkoin.sql.entities.KoinTransactionEntity;
import java.util.List;
import javax.inject.Inject;
import rx.Observable;
import rx.android.schedulers.AndroidSchedulers;
import rx.schedulers.Schedulers;
public class TransactionRepository {
private static final int MAX_TRANSACTIONS = 3000;
private final TransactionDataStore transactionDataStore;
private final TransactionService transactionService;
private Integer pageNumber = 1;
@Inject
public TransactionRepository(TransactionDataStore transactionDataStore,
TransactionService transactionService) {
this.transactionDataStore = transactionDataStore;
this.transactionService = transactionService;
}
/**
* 1. Load transactions with a limit of MAX_TRANSACTIONS + 1. We fetch 1 more than
* MAX_TRANSACTIONS to see if we have more than our limit in the database.
* <p>
* 2. If the size of our results is greater than MAX_TRANSACTIONS,
* we delete (MAX_TRANSACTIONS / 2) old transactions from the local database.
*/
public Observable<List<KoinTransactionEntity>> loadTransactionsFromLocal() {
return transactionDataStore.getAllTransactions(MAX_TRANSACTIONS + 1)
.subscribeOn(Schedulers.io())
.map(transactionEntities -> {
if (transactionEntities.size() > MAX_TRANSACTIONS) {
Long olderThan = transactionEntities.get(MAX_TRANSACTIONS / 2).getCreatedAt();
deleteTransactionsOlderThan(olderThan);
}
return transactionEntities;
})
.observeOn(AndroidSchedulers.mainThread());
}
private Observable<DeleteResult> deleteTransactionsOlderThan(Long olderThan) {
return transactionDataStore.deleteTransactionsOlderThan(olderThan)
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread());
}
/**
* Load the latest unseen transactions from the server and save to the local database.
* It needs to fetch in ASCENDING order in case it doesn't finish loading from the server and
* then getLatestTransaction() will return the latest and the missing results won't be fetched.
* <p>
* 1. Fetch latest orderEntity from the local database.
* 2. Query server for all transactions newer than that.
* 3. Save results returned by server in the local database.
*
* @param queryParameters
*/
public Observable<PutResults<KoinTransactionEntity>> loadNewTransactionsFromServer(
QueryParameters queryParameters) {
return transactionDataStore.getLatestTransaction()
.subscribeOn(Schedulers.io())
.take(1)
.flatMap(latestTransactionEntity -> {
long updatesAfter =
latestTransactionEntity != null ? latestTransactionEntity.getCreatedAt() : 0;
ListTransactionsRequest listTransactionsRequest = new ListTransactionsRequest.Builder()
.query_parameters(queryParameters.newBuilder().updates_after(updatesAfter).build())
.build();
return recursivelyLoadTransactionsFromServer(listTransactionsRequest, 1);
})
.flatMap(transactionDataStore::createOrUpdate)
.observeOn(AndroidSchedulers.mainThread());
}
public Observable<ListTransactionsResponse> loadOldTransactionsFromServer(
QueryParameters queryParameters) {
return transactionDataStore.getEarliestTransaction()
.subscribeOn(Schedulers.io())
.take(1)
.flatMap(earliestTransactionEntity -> {
long updatesBefore =
earliestTransactionEntity != null ? earliestTransactionEntity.getCreatedAt() : 0;
ListTransactionsRequest listTransactionsRequest = new ListTransactionsRequest.Builder()
.query_parameters(queryParameters.newBuilder().updates_before(updatesBefore).build())
.build();
return transactionService.listTransactions(listTransactionsRequest, pageNumber++);
})
.observeOn(AndroidSchedulers.mainThread());
}
/**
* Fetches the transactions from the server and if `has_next_page` is true, recursively is called
* again until `has_next_page` is false.
* <p>
* Using `concatWith` to combine all the results of the
* recursive calls into one observable.
* <p>
* ConcatMap ensures that there is no interleaving between mutliple observables from the multiple
* recursive calls.
*
* @param listTransactionsRequest The request defining our server query.
* @param page The page number of the results returned by the server.
* @return An observable consisting of the combined transactions from the pages.
*/
private Observable<List<Transaction>> recursivelyLoadTransactionsFromServer(
ListTransactionsRequest listTransactionsRequest, int page) {
return transactionService.listTransactions(listTransactionsRequest, page)
.subscribeOn(Schedulers.io())
.concatMap(listTransactionsResponse -> {
if (listTransactionsResponse.has_next_page) {
return Observable.just(listTransactionsResponse.transactions).concatWith(
recursivelyLoadTransactionsFromServer(listTransactionsRequest, page + 1));
} else {
return Observable.just(listTransactionsResponse.transactions);
}
});
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/MainActivity.java
package com.sendkoin.merchant.ui;
import android.content.Context;
import android.os.Bundle;
import android.support.design.widget.NavigationView;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentTransaction;
import android.support.v4.view.GravityCompat;
import android.support.v4.widget.DrawerLayout;
import android.support.v7.app.ActionBarDrawerToggle;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.Toolbar;
import android.view.MenuItem;
import android.view.View;
import android.widget.TextView;
import android.widget.Toast;
import com.sendkoin.merchant.KoinMerchantApp;
import com.sendkoin.merchant.R;
import com.sendkoin.merchant.dagger.component.NetComponent;
import com.sendkoin.merchant.data.authentication.SessionManager;
import com.sendkoin.merchant.ui.home.HomeFragment;
import com.sendkoin.merchant.ui.login.LoginFragment;
import com.sendkoin.merchant.ui.onboarding.OnboardingFragment;
import com.sendkoin.merchant.ui.transactions.history.TransactionHistoryFragment;
import butterknife.BindView;
import butterknife.ButterKnife;
import rx.Subscription;
import rx.android.schedulers.AndroidSchedulers;
import rx.schedulers.Schedulers;
import rx.subscriptions.CompositeSubscription;
import uk.co.chrisjenx.calligraphy.CalligraphyContextWrapper;
public class MainActivity extends AppCompatActivity {
@BindView(R.id.toolbar) Toolbar toolbar;
@BindView(R.id.drawer_layout) DrawerLayout drawer;
@BindView(R.id.nav_view) NavigationView navigationView;
private ActionBarDrawerToggle drawerToggle;
private CompositeSubscription compositeSubscription = new CompositeSubscription();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.bind(this);
setUpToolbar();
if (savedInstanceState == null) {
navigationView.setNavigationItemSelectedListener(this::onNavigationItemSelected);
loadMerchant();
String sessionToken = sessionManager().getSessionToken();
Fragment fragment = sessionToken != null ? new HomeFragment() : new LoginFragment();
getSupportFragmentManager()
.beginTransaction()
.replace(R.id.fragment_placeholder, fragment)
.commit();
}
}
private void loadMerchant() {
Subscription subscription = getNetComponent().merchantDataStore().getOnly()
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(merchant -> {
View headerView = navigationView.getHeaderView(0);
TextView merchantName = (TextView) headerView.findViewById(R.id.tv_drawer_merchant_name);
TextView merchantAddress = (TextView) headerView.findViewById(R.id.tv_drawer_merchant_location);
merchantName.setText(merchant.get().getStoreName());
merchantAddress.setText(merchant.get().getStoreLocation());
});
compositeSubscription.add(subscription);
}
@Override
protected void onDestroy() {
super.onDestroy();
compositeSubscription.unsubscribe();
}
private SessionManager sessionManager() {
return getNetComponent().sessionManager();
}
private NetComponent getNetComponent() {
return ((KoinMerchantApp) getApplication()).getNetComponent();
}
@Override
protected void attachBaseContext(Context newBase) {
super.attachBaseContext(CalligraphyContextWrapper.wrap(newBase));
}
/* TODO(Zen): Implement. */
private void setUpToolbar() {
setSupportActionBar(toolbar);
drawerToggle = new ActionBarDrawerToggle(this, drawer, toolbar,
R.string.navigation_drawer_open, R.string.navigation_drawer_close);
drawer.addDrawerListener(drawerToggle);
drawerToggle.syncState();
}
/* Auto-generated. TODO(Zen): Implement. */
public void onBackPressed() {
if (drawer.isDrawerOpen(GravityCompat.START)) {
drawer.closeDrawer(GravityCompat.START);
}
// Delegate back presses for onboarding fragment to the fragment's back press handler in order
// to allow it to navigate to a previous view in the view pager. If the back button is pressed
// when at the first view of the view pager, then call super.onBackPressed().
OnboardingFragment onboardingFragment =
(OnboardingFragment) getSupportFragmentManager().findFragmentByTag(OnboardingFragment.TAG);
if (onboardingFragment != null) {
if (onboardingFragment.canGoBack()) {
onboardingFragment.onBackPressed();
} else {
super.onBackPressed();
}
} else {
super.onBackPressed();
}
}
public boolean onNavigationItemSelected(MenuItem item) {
// Handle navigation view item clicks here.
int id = item.getItemId();
Fragment targetFragment = new HomeFragment();
if (id == R.id.nav_history) {
targetFragment = new TransactionHistoryFragment();
} else if (id == R.id.nav_profile) {
Toast.makeText(this, "Unsupported operation", Toast.LENGTH_SHORT).show();
} else if (id == R.id.nav_share) {
Toast.makeText(this, "Unsupported operation", Toast.LENGTH_SHORT).show();
}
FragmentTransaction fragmentTransaction = getSupportFragmentManager()
.beginTransaction()
.replace(R.id.fragment_placeholder, targetFragment)
.addToBackStack(null);
drawer.closeDrawer(GravityCompat.START);
fragmentTransaction.commit();
return true;
}
public ActionBarDrawerToggle getDrawerToggle() {
return drawerToggle;
}
public void navigateToLoginScreen() {
getSupportFragmentManager()
.beginTransaction()
.replace(R.id.fragment_placeholder, new LoginFragment())
.commit();
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/data/sql/KoinSQLiteOpenHelper.java
package com.sendkoin.merchant.data.sql;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import com.sendkoin.sql.tables.KoinTransactionTable;
import com.sendkoin.sql.tables.MerchantTable;
import com.sendkoin.sql.tables.OrderItemTable;
import com.sendkoin.sql.tables.KoinOrderTable;
import com.sendkoin.sql.tables.SaleItemTable;
public class KoinSQLiteOpenHelper extends SQLiteOpenHelper {
public static final int VERSION = 6;
public static final String DATABASE_NAME = "koin_merchant_db";
public KoinSQLiteOpenHelper(Context context) {
super(context, DATABASE_NAME, null, VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(MerchantTable.getCreateTableQuery());
db.execSQL(SaleItemTable.getCreateTableQuery());
db.execSQL(KoinTransactionTable.getCreateTableQuery());
db.execSQL(KoinOrderTable.getCreateTableQuery());
db.execSQL(OrderItemTable.getCreateTableQuery());
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/orders/details/OrderDetailsListAdapter.java
package com.sendkoin.merchant.ui.orders.details;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import com.sendkoin.api.SaleItem;
import com.sendkoin.merchant.R;
import java.util.List;
import butterknife.BindView;
import butterknife.ButterKnife;
class OrderDetailsListAdapter extends RecyclerView.Adapter<RecyclerView.ViewHolder> {
public static final String DEFAULT_ITEM_NAME = "One Time Payment";
private List<SaleItem> saleItems;
public void setOrderDetailsList(List<SaleItem> saleItems) {
this.saleItems = saleItems;
notifyDataSetChanged();
}
@Override
public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
LayoutInflater layoutInflater = LayoutInflater.from(parent.getContext());
View inflatedView = layoutInflater.inflate(R.layout.order_details_list_item, parent, false);
return new OrderDetailsViewHolder(inflatedView);
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) {
SaleItem saleItem = saleItems.get(position);
((OrderDetailsViewHolder) holder).itemName.setText(saleItem.name != null
? saleItem.name
: DEFAULT_ITEM_NAME);
((OrderDetailsViewHolder) holder).quantity.setText(String.valueOf(saleItem.quantity));
if (saleItem.customer_notes != null) {
((OrderDetailsViewHolder) holder).customerNotesTitle.setVisibility(View.VISIBLE);
((OrderDetailsViewHolder) holder).customerNotesTitle.setText(R.string.customer_notes_title);
((OrderDetailsViewHolder) holder).customerNotes.setVisibility(View.VISIBLE);
((OrderDetailsViewHolder) holder).customerNotes.setText(saleItem.customer_notes);
}
}
@Override
public int getItemCount() {
return saleItems != null ? saleItems.size() : 0;
}
class OrderDetailsViewHolder extends RecyclerView.ViewHolder {
@BindView(R.id.tv_order_details_item_name) TextView itemName;
@BindView(R.id.tv_order_details_quantity) TextView quantity;
@BindView(R.id.tv_order_details_customer_notes) TextView customerNotes;
@BindView(R.id.tv_order_details_customer_notes_title) TextView customerNotesTitle;
OrderDetailsViewHolder(View itemView) {
super(itemView);
ButterKnife.bind(this, itemView);
}
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/transactions/verify/VerifyOrderModule.java
package com.sendkoin.merchant.ui.transactions.verify;
import com.sendkoin.merchant.data.orders.OrderService;
import com.sendkoin.merchant.ui.CustomScope;
import com.sendkoin.merchant.ui.transactions.verify.VerifyOrderContract.Presenter;
import dagger.Module;
import dagger.Provides;
import retrofit2.Retrofit;
@Module
public class VerifyOrderModule {
private final VerifyOrderContract.View view;
public VerifyOrderModule(VerifyOrderContract.View view) {
this.view = view;
}
@Provides
@CustomScope
VerifyOrderContract.View provideVerifyTransactionView() {
return view;
}
@Provides
@CustomScope
public OrderService orderService(Retrofit retrofit) {
return retrofit.create(OrderService.class);
}
@Provides
@CustomScope
Presenter provideVerifyTransactionPresenter(VerifyOrderContract.View view,
OrderService orderService) {
return new VerifyOrderPreseneter(view, orderService);
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/onboarding/OnboardingComponent.java
package com.sendkoin.merchant.ui.onboarding;
import com.sendkoin.merchant.dagger.component.NetComponent;
import com.sendkoin.merchant.ui.CustomScope;
import dagger.Component;
@CustomScope
@Component(dependencies = NetComponent.class, modules = OnboardingModule.class)
public interface OnboardingComponent {
void inject(OnboardingFragment onboardingFragment);
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/transactions/verify/VerifyOrderContract.java
package com.sendkoin.merchant.ui.transactions.verify;
import com.sendkoin.api.PickUpOrderResponse;
import com.sendkoin.api.Transaction;
import com.sendkoin.merchant.ui.BasePresenter;
public interface VerifyOrderContract {
interface View {
void showSuccessful(PickUpOrderResponse pickUpOrderResponse);
void showPreviouslyVerified(Transaction transaction);
void showFailed(Transaction transaction);
void startCamera();
void stopCamera();
}
interface Presenter extends BasePresenter {
void verifyOrder(String transactionToken);
}
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/transactions/history/TransactionHistoryComponent.java
package com.sendkoin.merchant.ui.transactions.history;
import com.sendkoin.merchant.dagger.component.NetComponent;
import com.sendkoin.merchant.ui.CustomScope;
import dagger.Component;
@Component(dependencies = NetComponent.class, modules = TransactionHistoryModule.class)
@CustomScope
public interface TransactionHistoryComponent {
void inject(TransactionHistoryFragment transactionHistoryFragment);
}
<file_sep>/app/src/main/java/com/sendkoin/merchant/ui/currentsale/CurrentSaleComponent.java
package com.sendkoin.merchant.ui.currentsale;
import com.sendkoin.merchant.dagger.component.NetComponent;
import com.sendkoin.merchant.ui.CustomScope;
import dagger.Component;
@CustomScope
@Component(dependencies = NetComponent.class, modules = CurrentSaleModule.class)
public interface CurrentSaleComponent {
void inject(CurrentSaleFragment fragment);
}
| 7b7fb931db72c7fa7088938f61c38e33d72ef344 | [
"Java",
"SQL"
] | 39 | Java | nayeemzen/koin-merchant-android | 17541ce27a1186591eae3a1c0db607791bbf0016 | 8ba79d37aa5aa3490020cd6200580bfe14f5c27f | |
refs/heads/master | <repo_name>richmengsix/Tweather<file_sep>/js/GoogleAPI.js
/**
* @author SHarmony
*/
var geocoder;
var map;
var mc;
var mcOptions;
function initialize() {
geocoder = new google.maps.Geocoder();
var mapOptions = {
center: new google.maps.LatLng(40.807979,-73.963137),
zoom: 18,
mapTypeId: google.maps.MapTypeId.SATELLITE
};
map = new google.maps.Map(document.getElementById("map_canvas"),
mapOptions);
var markers=new Array();
//Adding points
markers[0]=pining(40.806979,-73.964137,"Columbia University","<h4>Columbia University</h4><h5>- Columbia University</h4><a id='stata-get' href='#' onclick=''>Get Inside Direction</a>",'http://maps.google.com/mapfiles/ms/icons/green-dot.png');
markers[1]=pining(40.907979,-73.863137,"Columbia University","<h4>Columbia University</h4><h5>- Columbia University</h4><a id='stata-get' href='#' onclick=''>Get Inside Direction</a>",'http://maps.google.com/mapfiles/ms/icons/green-dot.png');
markers[2]=pining(40.927979,-73.893137,"Columbia University","<h4>Columbia University</h4><h5>- Columbia University</h4><a id='stata-get' href='#' onclick=''>Get Inside Direction</a>",'http://maps.google.com/mapfiles/ms/icons/green-dot.png');
markers[3]=pining(40.807989,-73.963137,"Columbia University","<h4>Columbia University</h4><h5>- Columbia University</h4><a id='stata-get' href='#' onclick=''>Get Inside Direction</a>",'http://maps.google.com/mapfiles/ms/icons/green-dot.png');
markers[4]=pining(40.907999,-73.863137,"Columbia University","<h4>Columbia University</h4><h5>- Columbia University</h4><a id='stata-get' href='#' onclick=''>Get Inside Direction</a>",'http://maps.google.com/mapfiles/ms/icons/green-dot.png');
markers[5]=pining(40.927969,-73.893137,"Columbia University","<h4>Columbia University</h4><h5>- Columbia University</h4><a id='stata-get' href='#' onclick=''>Get Inside Direction</a>",'http://maps.google.com/mapfiles/ms/icons/green-dot.png');
//set style options for marker clusters (these are the default styles)
mcOptions = {gridSize: 30, maxZoom: 15,
styles: [{
height: 64,
url: "img/sunny64.png",
width: 64
},
{
height: 64,
url: "img/sunny64.png",
width: 64
},
{
height: 64,
url: "img/cloudy64.png",
width: 64
},
{
height: 64,
url: "img/snowy64.png",
width: 64
},
{
height: 64,
url: "img/rainy64.png",
width: 64
}]};
mc = new MarkerClusterer(map,markers,mcOptions);
}
function codeAddress() {
var address = document.getElementById("Google-address").value;
geocoder.geocode( { 'address': address}, function(results, status) {
if (status == google.maps.GeocoderStatus.OK) {
map.setCenter(results[0].geometry.location);
var marker = new google.maps.Marker({
map: map,
position: results[0].geometry.location
});
// alert('(lng,lat): '+ [results[0].geometry.location.lng(),results[0].geometry.location.lat()]);
getNowCoordinate(address,[results[0].geometry.location.lng(),results[0].geometry.location.lat()]);
$("#current-addr").html("Current Address: "+address);
} else {
alert("Geocode was not successful for the following reason: " + status);
}
});
}
function refresh(pinary)
{
var iconPath;
var iconSmallPath;
var content;
var CurrentMarkers = new Array();
for(var i=0;i<pinary.length;i++){
iconPath=getWeatherPic(pinary[i].weather);
iconSmallPath=getWeatherSmallPic(pinary[i].weather);
content="<div class='mapitem'>"+
"<div class='mapitem-left'>"+
"<p class='mapitem-title'>"+pinary[i].name+"</p>"+
"<img class='mapitem-line' src='img/line22.png'/>"+
"<p class='mapitem-tweet'>"+pinary[i].tweet+"</p>"+
"<p class='mapitem-time'>"+pinary[i].nowtime+"</p>"+
"</div>"+
"<div class='mapitem-right'>"+
"<img class='mapitem-img' src='"+iconPath+"' />"+
"</div>"+
"</div>";
CurrentMarkers[i]=pining(pinary[i].lat,pinary[i].lng,pinary[i].description,content,iconSmallPath);
}
mc.clearMarkers();
mc.addMarkers(CurrentMarkers,false);
}
function getWeatherPic(weather){
var sunny="img/sunny.png";
var cloudy="img/cloudy.png";
var rainy="img/rainy.png";
var snowy="img/snow.png";
var defaut="img/clearmoon.png";
if(weather=="sunny") return sunny;
else if (weather=="cloudy") return cloudy;
else if (weather=="rainy") return rainy;
else if (weather=="snowy") return snowy;
else return defaut;
}
function getWeatherSmallPic(weather){
var sunny="img/sunny64.png";
var cloudy="img/cloudy64.png";
var rainy="img/rainy64.png";
var snowy="img/snow64.png";
var defaut="img/sunny_night64.png";
if(weather=="sunny") return sunny;
else if (weather=="cloudy") return cloudy;
else if (weather=="rainy") return rainy;
else if (weather=="snowy") return snowy;
else return defaut;
}
function pining(lat,lng,description,contentString,iconPath)
{
var myLatlng = new google.maps.LatLng(lat,lng);
/* contentS="<div class='mapitem'>"+
"<div class='mapitem-left'>"+
"<p class='mapitem-title'>Columbia University</p>"+
"<img class='mapitem-line' src='img/line22.png'/>"+
"<p class='mapitem-tweet'>Wow! It's sunny outside... Finally!</p>"+
"<p class='mapitem-time'>-30 minutes ago</p>"+
"</div>"+
"<div class='mapitem-right'>"+
"<img class='mapitem-img' src='img/sunny.png' />"+
"</div>"+
"</div>";
*/
var infowindow = new google.maps.InfoWindow({
content: contentString
});
var marker = new google.maps.Marker({
position: myLatlng,
map: map,
flat: true,
title: description,
});
iconFile = iconPath;
marker.setIcon(iconFile);
// The function after click
google.maps.event.addListener(marker, 'click', function() {
infowindow.open(map,marker);
});
return marker;
}<file_sep>/js/weather.js
/**
* @author SHarmony
*/
//Node object
$(document).ready(function(){
getJASON();
$('#refresh-button').click(function(){
refresh(WeatherNodes);
});
});
function getJASON(){
$.getJSON("temp.json", function(json) {
console.log(json); // this will show the info it in firebug console
$.each(json.results, function(i, item) {
var created_at = item.created_at;
var user = item.from_user;
var lat = item.geo.coordinates[0];
var lng = item.geo.coordinates[1];
var tweet = item.text;
var weather = item.weather;
addWeatherNode(user,lat,lng,user,tweet,created_at,weather);
$('<p>').text(tweet).appendTo('body');
});
});
}
function WeatherNode(name,lat,lng,description,tweet,nowtime,weather){
this.name=name;
this.lat=lat;
this.lng=lng;
this.description=description;
this.tweet=tweet;
this.nowtime=nowtime;
this.weather=weather;
}
var WeatherNodes=new Array();
WeatherNodes[0]=new WeatherNode("Columbia University",40.807979,-73.963137,"Columbia University","Wow! It's sunny outside... Finally!",getTimeAgo("Sat, 09 Feb 2013 03:55:38 +0000"),"sunny");
WeatherNodes[1]=new WeatherNode("Time Square",40.756035,-73.986676,"Time Square","Cloudy!! It's so bad!",getTimeAgo("Sat, 09 Feb 2013 04:55:38 +0000"),"snowy");
function addWeatherNode(name,lat,lng,description,tweet,nowtime,weather){
var length = WeatherNodes.length;
WeatherNodes[length] = new WeatherNode(name,lat,lng,name,tweet,getTimeAgo(nowtime),weather);
alert(WeatherNodes.length);
}
function getTimeAgo(timestatement){
var date = new Date();
var second = date.getSeconds();
var hour = date.getHours();
var minute = date.getMinutes();
//Sat, 09 Feb 2013 03:55:38 +0000
var TweetHour = timestatement.split(" ")[4].split(":")[0];
var TweetMinute = timestatement.split(" ")[4].split(":")[1];
var TweetSecond = timestatement.split(" ")[4].split(":")[2];
var OffsetHour = hour-(TweetHour-5);
var OffsetMinute = minute-TweetMinute;
//modify cross day
if (OffsetHour<0) OffsetHour=OffsetHour+24;
//modify cross hour
if (OffsetHour>0 && OffsetMinute<0){
OffsetHour--;
OffsetMinute=OffsetMinute+60;
}
//Output
if (OffsetHour==0) return " - "+OffsetMinute+" min ago";
else return " - "+OffsetHour+" hr and "+OffsetMinute+" min ago";
}
function loadScript() {
var script = document.createElement("script");
script.type = "text/javascript";
script.src = "http://maps.google.com/maps/api/js?key=<KEY>&sensor=false&callback=initialize";
document.body.appendChild(script);
}
window.onload = loadScript;
| 6ff22ae8293fe21e1f6f56ca051f5f55355d76a1 | [
"JavaScript"
] | 2 | JavaScript | richmengsix/Tweather | 9d0a1434c6f09acf56b7d18cb39aaf22c2ccbfb7 | 1d4a066889cb4a966d6009499129655c8c6b0229 | |
refs/heads/master | <repo_name>ds147000/pannelVr<file_sep>/src/run.js
var VR = pannellum.viewer('panorama', {
"type": "equirectangular",
"panorama": "./src/images/alma.jpg",
});
function hotspot(hotSpotDiv, args) {
hotSpotDiv.classList.add('custom-tooltip');
var div = document.createElement('div');
div.innerHTML = args[0];
hotSpotDiv.appendChild(div);
div.style.marginLeft = -(div.scrollWidth - hotSpotDiv.offsetWidth) / 2 + 'px';
div.style.marginTop = -div.scrollHeight + 80 + 'px';
div.addEventListener('click', function() {
alert('报修内容是' + args[1])
})
}
//创建热点
function addHot(pitch, yaw, r) {
VR.addHotSpot({
"pitch": pitch,
"yaw": yaw,
"cssClass": "custom-hotspot",
"createTooltipFunc": hotspot,
"createTooltipArgs": ["保修点", r]
})
}
var clickTime = 0
//绑定事件
VR.on('mousedown', function (e) {
clickTime = new Date().getTime()
})
VR.on('mouseup', function (e) {
if (new Date().getTime() - clickTime < 150) {
var r = prompt('请输入保修内容')
if (r) {
var hotData = VR.mouseEventToCoords(e)
addHot(hotData[0], hotData[1], r)
}
}
}) | 266471f0d2f7e825aeb7e349b559890d0702fcc4 | [
"JavaScript"
] | 1 | JavaScript | ds147000/pannelVr | 746f64d493709a6827f6738244292990713ee635 | 878e3f7d7d3cb28829690fdf3124fbe1c0fd23e8 | |
refs/heads/main | <repo_name>Sedriskor/chat<file_sep>/chat_3/chat_split.py
def read_file(filename): #read file
lines =[]
with open(filename, 'r', encoding='utf-8-sig') as f:
for line in f:
lines.append(line.strip())#strip()去掉換行
return lines
def chat_split(lines): #split chat
new =[]
for line in lines:
line_split = line.split(' ', 1)#這邊切成兩部分(只切第一個空格)就好:['13:32Allen', '請問分公司代號是 9432 嗎']
time = line_split[0][:5]
name = line_split[0][5:]
chat = line_split[1]
new.append(time + ' ' + name + ' ' + chat)
return new
def write_file(filename, new): #output file
with open(filename, 'w', encoding='utf-8') as f:
for line in new:
f.write(line + '\n')
def main():
lines = read_file('3.txt')
new = chat_split(lines)
write_file('3_split.txt', new)
main() | 286bbd5a6734c3d07446b03850e474a731855eb2 | [
"Python"
] | 1 | Python | Sedriskor/chat | 3c9f6e7707f84b1855a1e5bc7bfbc6461099d7d8 | 60e028dc658b5406785794b59b4e4c347d5d1348 | |
refs/heads/master | <file_sep>import numpy as np
import urllib.request
import json
import csv
def parseData(fname):
for l in urllib.request.urlopen(fname):
yield eval(l)
def one_hot_year(y, max_year, min_year):
ohv = np.zeros(max_year-min_year) # zero vector represents max_year
if y - min_year == 0:
return ohv
else:
ohv[y-min_year-1] = 1
return ohv
def main():
data = list(parseData("http://cseweb.ucsd.edu/classes/fa19/cse258-a/data/beer_50000.json"))
prepared_data = {}
feature_list = ['beer/ABV', 'review/text', 'review/timeStruct', 'review/appearance']
target = 'review/overall'
# process first feature
ABV = [d[feature_list[0]] for d in data]
print('ABV shape:', np.shape(ABV))
# prepared_data[feature_list[0]] = ABV
# process second feature
review_length = [len(d[feature_list[1]]) for d in data]
print('review length:', np.shape(review_length))
# prepared_data[feature_list[1]] = review_length
# process third feature
years = [d[feature_list[2]]['year'] for d in data]
min_year = np.min(years)
max_year = np.max(years)
ohvs = [one_hot_year(y, max_year, min_year) for y in years]
print('ohvs shape:', np.shape(ohvs))
# prepared_data['review/ohv_year'] = ohvs
# process fourth feature
appearance = [d[feature_list[3]] for d in data]
print('appearance shape:', np.shape(appearance))
# prepared_data[feature_list[3]] = appearance
# process target
y = [d['review/overall'] for d in data]
print('y shape:', np.shape(y))
Xy = [[a,b,c,d,e] for a,b,c,d,e in zip(ABV, review_length, ohvs, appearance, y)]
with open('prepared_data.csv', 'w') as f:
# write_string = ''
# for entry in ['ABV', 'review_length', 'ohv_years', 'appearance', 'y']:
# write_string = write_string + entry + ', '
# f.write(write_string + '\n')
for row in Xy:
write_string = ''
for entry in row:
# print(np.shape(entry))
if len(np.shape(entry))>0:
for e in entry:
write_string = write_string + str(e) + ', '
else:
write_string = write_string + str(entry) + ', '
f.write(write_string[:-2] + '\n')
# with open('prepared_data.json', 'w') as f:
# json.dump(prepared_data, f)
if __name__ == '__main__':
main()
<file_sep>import numpy as np
import matplotlib.pyplot as plt
import torch
from torch.autograd import Variable
from torch.utils.data import TensorDataset
from torch.utils.data import DataLoader
# define class for linear regression
class linearRegression(torch.nn.Module):
def __init__(self, inputSize, outputSize):
super(linearRegression, self).__init__()
self.linear = torch.nn.Linear(inputSize, outputSize)
def forward(self, x):
out = self.linear(x)
return out
# define train test split function
def ttss(X,y,ratio_train):
full_data = np.hstack([X,y])
np.random.shuffle(full_data)
train = full_data[:round(np.shape(full_data)[0]*ratio_train),:]
test = full_data[round(np.shape(full_data)[0]*ratio_train):,:]
return train[:,:-1], test[:,:-1], np.expand_dims(train[:,-1], axis=1), np.expand_dims(test[:,-1], axis=1) # Xtrain, Xtest, ytrain, ytest
# read in data from prepared_data.csv
with open('prepared_data.csv') as f:
Xy = np.loadtxt(f, delimiter=',')
inputs = Xy[:,[1]].astype(np.float32) # pick one column from data
targets = np.expand_dims(Xy[:,-1], axis=1).astype(np.float32) # last column is target
train_ratio = .8
inputDim = np.shape(inputs)[1]
outputDim = 1
learningRate = 0.000001
epochs = 10
X_train, X_test, y_train, y_test = ttss(inputs, targets, train_ratio)
print(np.shape(X_train), np.shape(X_test), np.shape(y_train), np.shape(y_test))
# inputs = torch.from_numpy(Xy[:,:-2])
# targets = torch.from_numpy(Xy[:,-1])
# dataset = TensorDataset(inputs, targets)
# batch_size = 3
# train_loader = DataLoader(dataset, batch_size=batch_size, shuffle=True)
# instantate the model
model = linearRegression(inputDim, outputDim)
# For GPU
if torch.cuda.is_available():
model.cuda()
# define MSE as the learning rule
criterion = torch.nn.MSELoss()
optimizer = torch.optim.SGD(model.parameters(), lr=learningRate)
# start training
for epoch in range(epochs):
# converting inputs and labels to torch variables
if torch.cuda.is_available():
inputs = Variable(torch.from_numpy(X_train).cuda())
labels = Variable(torch.from_numpy(y_train).cuda())
else:
inputs = Variable(torch.from_numpy(X_train))
labels = Variable(torch.from_numpy(y_train))
# clear gradient buffers
optimizer.zero_grad()
# get output from the model, given the inputs
outputs = model(inputs)
# calculate MSE loss using criterion
loss = criterion(outputs, labels)
# calculate gradients of the loss function with respect to the parameters
loss.backward()
#update the parameters
optimizer.step()
print('epoch {}, loss {}'.format(epoch, loss.item()))
for layer in model.children():
if isinstance(layer, torch.nn.Linear):
print('bias: ',layer.state_dict()['bias'] ,' weight(s): ', layer.state_dict()['weight'])
with torch.no_grad(): # we don't need gradients in the testing phase
if torch.cuda.is_available():
predicted = model(Variable(torch.from_numpy(X_test).cuda())).cpu().data.numpy()
else:
predicted = model(Variable(torch.from_numpy(X_test))).data.numpy()
print(predicted)
plt.clf()
plt.plot(X_train, y_train, 'go', label='True data', alpha=0.5)
plt.plot(X_test, predicted, '--', label='Predictions', alpha=0.5)
plt.legend(loc='best')
plt.show() | 275a9cf175ea6d3bd99ff3dbf9922882426cb993 | [
"Python"
] | 2 | Python | nolan4/PyTorch-Linear-Regression | b6ddaa22a1a8f6f92952a58507319d837c7d1786 | 444f4b3d352fe847edac3d4366935fde569eecaf | |
refs/heads/master | <repo_name>zhangchch/exercise7<file_sep>/lib/quantified_group.js
module.exports = function (str) {
var arr = str.split(/\s/);
arr = arr.filter((item) => item);
if(arr.length != 8) {
return false;
}
arr.forEach((item) => {
let regExp = /Ox[A-Fa-f0-9]{2}/;
if(!regExp.test(item)) {
return false;
}
});
return true;
}<file_sep>/lib/capture.js
module.exports = function (str) {
let regExpNum = /.*\bx=([1-9][0-9]*)\b.*/;
if(Array.isArray(regExpNum.exec(str))) {
return regExpNum.exec(str)[1];
}
return null;
} | 50033cc77dd2fde482400158270b57abfd3388cc | [
"JavaScript"
] | 2 | JavaScript | zhangchch/exercise7 | 80955a0499308d4ba26bdd633613d562c8d4ee04 | 0b84f0e3cb5de2fb1799b3e008e21b1c00fc3a5c | |
refs/heads/master | <repo_name>Zain517/java-s_FianalProject<file_sep>/finalproject/WEB-INF/classes/neworder.java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
import java.sql.*;
import javax.swing.*;
public class neworder extends HttpServlet {
//Process the HTTP Get request
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
HttpSession session=request.getSession(false);
if(session==null)
{RequestDispatcher rd=request.getRequestDispatcher("login.html");
rd.forward(request, response);}
String sid=(String)session.getAttribute("type");
System.out.println(sid);
if( sid.equals("0"))
{RequestDispatcher rd=request.getRequestDispatcher("login.html");
rd.forward(request, response);}
response.setContentType("text/html");
// get PrintWriter object
PrintWriter out = response.getWriter();
//cvalidate.......
out.println("<html>");
out.println("<head>");
out.println("<title> Add Order</title>");
out.println("<script language=\"JavaScript\" type=\"text/javaScript\"> ");
out.println("function cvalidate(){if ( document.cf.rid.value == \"\" ){");
out.println("var errors=\"Customer's ID is empty!!\";");
out.println("document.getElementById(\"rid\").innerHTML=errors; document.cf.rid.focus();");
out.println("return false;}");
out.println("if ( document.cf.fn.value == \"\" ){");
out.println("var errors=\"First name is empty!!\";");
out.println("document.getElementById(\"fn\").innerHTML=errors;");
out.println("document.cf.fn.focus();return false;}");
out.println("if ( document.cf.ln.value == \"\" ) {var errors=\"Last name is empty!!\";");
out.println("document.getElementById(\"ln\").innerHTML=errors;");
out.println("document.cf.ln.focus();return false;}");
out.println("return true;}");
//validate......
out.println("function validate(){if ( document.f.id.value == \"\" ){");
out.println("var errors=\"Order's ID is empty!!\"; ");
out.println("document.getElementById(\"ID\").innerHTML=errors;");
out.println("document.f.id.focus();return false; } ");
out.println("if ( document.f.na.value == \"\" ){var errors=\"Customer's name is empty!!\";document.getElementById(\"name\").innerHTML=errors;");
out.println("document.f.na.focus();return false; }");
out.println("if ( document.f.sq.value == \"\" ){var errors=\"Quantity is empty!!\";");
out.println("document.getElementById(\"sq\").innerHTML=errors;");
out.println("document.f.sq.focus();return false;} ");
out.println("var mail=document.forms['f']['sq'].value;");
out.println("if(mail>=99 ){var errors=\"Too much Suit(s) Quantity [1-99].....!!\";document.getElementById(\"co\").innerHTML=errors;document.f.sq.focus();return false; }");
out.println("if ( document.f.co.value == \"\" ){var errors=\"Color-Desc is empty!!\";document.getElementById(\"co\").innerHTML=errors; document.f.co.focus();return false;}");
out.println("if(mail<='0' ){var errors=\"In_Valid Suit(s) Quantity[1-99].....!!\";document.getElementById(\"co\").innerHTML=errors;document.f.sq.focus();return false; }");
out.println("if ( document.f.dt.value == \"\" ){var errors=\"Design-type is empty!!\";document.getElementById(\"dt\").innerHTML=errors;document.f.dt.focus();return false;}");
out.println("if((document.f.subDate.value) > (document.f.deliDate.value)) ");
out.println(" {var errors=\"Submission Date always less than delivery date!!\";document.getElementById(\"date\").innerHTML=errors;return false;}");
out.println("return true;}");
//letternumber.....
out.println("function letternumber(event){ var keychar;keychar = event.key; if (((\".+-0123456789\").indexOf(keychar) > -1)) return true; if (event.keyCode === 13){ event.preventDefault();document.getElementById(\"order\").click();}else{ alert(\" Should only Numerics.....!!\"); return false; }}");
//nameVelidation....
out.println("function nameVelidation(event) { var keychar; keychar = event.key; if (((\"abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ \").indexOf(keychar) > -1)) return true; if (event.keyCode === 13){ event.preventDefault();document.getElementById(\"order\").click();} else{alert(\"Special & numeric charaters not Allowed...!!\"); return false; } }");
out.println("</script> ");
//ScriptEnd.....
out.println("<style>");
out.println(".button{");
out.println("background-color:blue;");
out.println(" border:2px solid #white;");
out.println("opacity:0.80;");
out.println(" border-radius:1px;");
out.println("width:105px;");
out.println("height:35px;");
out.println("color:white;");
out.println("transition:0.5s;");
out.println("transition:0.5s;}");
out.println("</style>");
out.println("</head> <body>");
//Header End Body Start from here....
//
out.println("<div>"); //main div
out.println("<div style=\" float:right;\"> "); //right div
out.println("<fieldset style=\"width:65px; margin-right:200px;\"> ");
out.println("<legend><h1><b><i>( New Order's Details ) </i></b></h1></legend> ");
out.println("<form name=\"f\" action=\"generatereceipt\" method=\"post\" onsubmit=\"return validate()\" > ");
out.println("<input style=\"width:350px;padding:10px;\" type=\"text\" placeholder=\"New Order ID \" name=\"id\" onKeyPress=\"return letternumber(event)\"> <div id=\"ID\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<input style=\"width:350px;padding:10px;\" type=\"text\" placeholder=\"Customer Name \" name=\"na\" onKeyPress=\"return nameVelidation(event)\"> <div id=\"name\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<input style=\"width:350px;padding:10px;\" type=\"text\" placeholder=\"Suit(s) Quantity \" name=\"sq\" onKeyPress=\"return letternumber(event)\"> <div id=\"sq\" style=\"color:red\"></div>");
out.println("<br>");
//out.println("<input style=\"width:350px;padding:10px;\" type=\"text\" placeholder=\"Colors-Description \" name=\"co\" > <div id=\"co\" style=\"color:red\"></div>");
out.println("<textarea name=\"co\" rows=\"3\" cols=\"48\" placeholder=\"Colors- Description......\"></textarea> <div id=\"co\" style=\"color:red\"></div> ");
out.println("<br>");
// out.println("<input style=\"width:350px;padding:10px;\" type=\"text\" placeholder=\"Design-type /Style \" name=\"dt\"> <div id=\"dt\" style=\"color:red\"></div>");
out.println("<textarea name=\"dt\" rows=\"3\" cols=\"48\" placeholder=\"Design-Type......\"></textarea> <div id=\"dt\" style=\"color:red\"></div> ");
out.println("<br>");
out.println("<strong> Submit Date:        Delivery Date:</strong><br> ");
out.println("<input type=\"date\" name=\"subDate\" value=\"2020-01-01\">    ");
out.println("<input type=\"date\" name=\"deliDate\" value=\"2020-01-07\"> ");
out.println("<div id=\"date\" style=\"color:red\"></div> ");
out.println("<br><br>");
out.println("<textarea name=\"t\" rows=\"5\" cols=\"48\" placeholder=\" Description......\"></textarea> ");
out.println("<br><br>");
out.println("<input style=float:right; class=\"button\" type=\"submit\" id=\"order\" value=\"Add Order\" /> ");
out.println("</form> </fieldset> </div> ");
//right div end
out.println("<div style=\"float:left;\">"); //left div start
out.println("<fieldset style=\"width:65px; margin-left:100px;\"> ");
out.println("<legend><h1><b><i>( Customer's Details ) </i></b></h1></legend> ");
out.println("<form name=\"cf\" action=\"customersearch\" method=\"post\" onsubmit=\"return cvalidate()\" > ");
out.println("<input style=\"width:350px;padding:10px;\" type=\"text\" placeholder=\"Registered Customer ID \" name=\"rid\" onKeyPress=\"return letternumber(event)\"> ");
out.println("<div id=\"rid\" style=\"color:red\"></div> ");
out.println("<br>");
out.println("<input style=\"width:350px;padding:10px;\" type=\"text\" placeholder=\"<NAME> \" name=\"fn\" onKeyPress=\"return nameVelidation(event)\"> <div id=\"fn\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<input style=\"width:350px;padding:10px;\" type=\"text\" placeholder=\"<NAME> \" name=\"ln\" onKeyPress=\"return nameVelidation(event)\"> <div id=\"ln\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<input style=float:right; class=\"button\" type=\"submit\" id=\"search\" value=\"Search \" /> ");
out.println("</form> </fieldset> <br>");
out.println("<div>"); //left down div
//out.println("<fieldset style=\"width:350px; height:250px; margin-left:100px;\"> ");
String s=(String)request.getAttribute("status");
///////Search Result
if(s.equals("1")){
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
int id=(Integer)request.getAttribute("cid");
String fn=(String)request.getAttribute("fn");
String ln=(String)request.getAttribute("ln");
String mb=(String)request.getAttribute("mb");
String add=(String)request.getAttribute("add");
double kl=(Double)request.getAttribute("kl");
double al=(Double)request.getAttribute("al");
double sh=(Double)request.getAttribute("sh");
double ch=(Double)request.getAttribute("ch");
double co=(Double)request.getAttribute("co");
double fi=(Double)request.getAttribute("fi");
double tl=(Double)request.getAttribute("tl");
double bo=(Double)request.getAttribute("bo");
String po=(String)request.getAttribute("po");
String da=(String)request.getAttribute("da");
out.println("<h1 style=\"color:green;\">Record found...</h1>");
out.println("<strong>Customer ID:</strong> " +id+" ");out.println("<br>");
out.println("<strong>First Name:</strong> " +fn+" ");out.println("<br>");
out.println("<strong>Last Name:</strong> " +ln+" ");out.println("<br>");
out.println("<strong>Mobile#:</strong> " +mb+" ");out.println("<br>");
out.println("<strong>Address:</strong> " +add+" ");out.println("<br>");
out.println("<strong>Kameez Length:</strong> " +kl+ " inches ");out.println("<br>");
out.println("<strong>Arm Length:</strong> " +al+ " inches ");out.println("<br>");
out.println("<strong>Shoulder:</strong> " +sh+ " inches ");out.println("<br>");
out.println("<strong>Chest:</strong> " +ch+ " inches ");out.println("<br>");
out.println("<strong>Collar:</strong> " +co+ " inches ");out.println("<br>");
out.println("<strong>Fitting:</strong> " +fi+ " inches ");out.println("<br>");
out.println("<strong>Trouser Length:</strong> " +tl+ " inches ");out.println("<br>");
out.println("<strong>Narrow Bottom:</strong> " +bo+ " inches ");out.println("<br>");
out.println("<strong>Pockects:</strong> " +po+" ");out.println("<br>");
out.println("<strong>Daman Style:</strong> " +da+" ");out.println("<br>");
out.println("<br>");
out.println("<form name=\"uf\" action=\"edit\" method=\"post\" > ");
out.println("<input style=float:right; class=\"button\" type=submit value=update>");
out.println("</form>");
out.println("<form name=\"uf\" action=\"remove\" method=\"post\" > ");
out.println("<input style=float:right; class=\"button\" type=submit value=Delete>");
out.println("</form>");
}
//////Search Invalid
if(s.equals("0"))
{
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
String n=(String)request.getAttribute("error");
out.println("<h1 style=\"color:red;\"> Record not found.. </h1><br>");
out.println("<form name=\"uf\" action=\"newcustomer\" method=\"post\" > ");
out.println("<input style=float:right;width:150px; class=\"button\" type=submit value=\"Add new Customer\">");
out.println("</form>");
}
////Record Update
if(s.equals("true")){
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
out.println("<h1 style=\"color:green;\"> Record Updated...</h1><br>");
}
////not Update Record
if(s.equals("false")){
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
out.println("<h1 style=\"color:red;\"> Updation Error... </h1><br>");
}
////Delete
if(s.equals("delete")){
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
out.println("<h1 style=\"color:green;\"> Record Deleted...</h1><br>");
}
///Not Delete Record
if(s.equals("not delete")){
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
out.println("<h1 style=\"color:red;\"> Deletion Error... </h1><br>");
}
////already present
if(s.equals("found")){
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
out.println("<h1 style=\"color:red;\"> Already in Record...</h1><br>");
out.println("<strong> Customer ID already taken, Please change it...</strong><br>");
}
///inserted
if(s.equals("added")){
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
out.println("<h1 style=\"color:green;\"> Insert Successfully... </h1><br>");
}
///not Inserted
if(s.equals("not added")){
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
out.println("<h1 style=\"color:red;\"> Insertion Error ... </h1><br>");
}
////order founded....
if(s.equals("order found")){
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
out.println("<h1 style=\"color:green;\"> Order Already Present... </h1><br>");
out.println("<strong> Order ID already taken, Please change it...</strong><br>");
}
///Order not Inserted
if(s.equals("order not added")){
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
out.println("<h1 style=\"color:red;\"> Order Insertion Error ... </h1><br>");
}
//invalid order ID input by jOptionPane.....
if(s.equals("order false")){
out.println("<fieldset style=\"width:350px; margin-left:100px;\"> ");
out.println("<h1 style=\"color:red;\"> Invalid Order ID ... </h1><br>");
out.println("<strong> Please enter Valid Order ID...</strong><br>");
}
out.println("</fieldset> </div> "); //left down div
out.println("</div>"); //left div
out.println("</div>"); //main div
out.println("</body></html>");
}
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request,response);
}
}
<file_sep>/finalproject/WEB-INF/classes/update.java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
import java.sql.*;
public class update extends HttpServlet{
public void doPost(HttpServletRequest request,HttpServletResponse response) throws IOException
{
HttpSession session=request.getSession(false);
if(session==null)
{response.sendRedirect("login.html");}
else{
String id=(String)session.getAttribute("c_searchId");
//String fname=(String)request.getAttribute("c_searchname");
System.out.println(id);
response.setContentType("text/html");
PrintWriter out=response.getWriter();
// Cookie c[]=request.getCookies();
// //for(int i=0;i<c.length;i++){
// String fname=c[0].getName();
// String id=c[0].getValue();
try{
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://127.0.0.1/darzikhana";
Connection con=DriverManager.getConnection(url,"root","zain4744");
Statement st=con.createStatement();
String query="Select * from customerdetails where customerId="+id+" ";
ResultSet rs = st.executeQuery( query );
if(rs.next()){
//out.println("<h1>Record found</h1>");
int cid = rs.getInt("customerId");
String fn = rs.getString("firstName");
String ln = rs.getString("lastName");
String mb = rs.getString("mobileNumber");
String add = rs.getString("address");
// System.out.println(add);
double kl = rs.getDouble("kameezLength");
double al = rs.getDouble("armLength");
double sh = rs.getDouble("shoulder");
double ch = rs.getDouble("chest");
double co = rs.getDouble("collar");
double fi = rs.getDouble("fitting");
double tl = rs.getDouble("trouserLength");
double bo = rs.getDouble("bottomOpenning");
String po = rs.getString("pocket");
String da = rs.getString("daman");
System.out.println(po);
// out.println("Welcome " + name+"<br>Your password is "+password+"<br>");
//}
out.println("<html> <head> <title> Update Customer</title>");
out.println("<script language=\"JavaScript\" type=\"text/javaScript\"> ");
out.println("function validate(){ ");
out.println("if ( document.f.mb.value == \"\" ) {var errors=\"Mobile no. is empty!!\"; document.getElementById(\"mobile\").innerHTML=errors;document.f.mb.focus(); return false; } ");
out.println("var mail=document.forms['f']['mb'].value;");
out.println("if(mail.length<11 || mail.length>15 ) { var errors=\"In_Valid (minimum 11 digits).....!!\";document.getElementById(\"mobile\").innerHTML=errors;document.f.mb.focus();return false; }");
out.println("if ( document.f.add.value == \"\" ){ var errors=\"Address is empty!!\"; document.getElementById(\"address\").innerHTML=errors;document.f.add.focus(); return false;}");
out.println("if ( document.f.kl.value == \"\" ) { var errors=\"kameez-Length is Empty...!!\";document.getElementById(\"kl\").innerHTML=errors;document.f.kl.focus();return false; }");
out.println("if ( document.f.al.value == \"\" ){var errors=\"Arm-Length is Empty...!!\"; document.getElementById(\"al\").innerHTML=errors;document.f.al.focus(); return false; }");
out.println("if ( document.f.sh.value == \"\" ){var errors=\"Shoulder-Length is Empty...!!\";document.getElementById(\"sh\").innerHTML=errors;document.f.sh.focus();return false; }");
out.println("if ( document.f.ch.value == \"\" ){var errors=\"Chest-Length is Empty...!!\";document.getElementById(\"ch\").innerHTML=errors;document.f.ch.focus();return false;}");
out.println("if ( document.f.co.value == \"\" ){var errors=\"Collar-Length is Empty...!!\";document.getElementById(\"co\").innerHTML=errors;document.f.co.focus(); return false; }");
out.println("if ( document.f.fi.value == \"\" ) { var errors=\"Fitting-Length is Empty...!!\";document.getElementById(\"fi\").innerHTML=errors;document.f.fi.focus();return false;}");
out.println("if ( document.f.tl.value == \"\" ){var errors=\"Trouser-Length is Empty...!!\"; document.getElementById(\"tl\").innerHTML=errors;document.f.tl.focus(); return false;}");
out.println("if ( document.f.bo.value == \"\" ){ var errors=\"Bottom-Opening-Length is Empty...!!\";document.getElementById(\"bo\").innerHTML=errors;document.f.bo.focus(); return false;}");
out.println("if ( document.f.po.value == \"\" ){var errors=\"Number of Pockets are Empty...!!\";document.getElementById(\"po\").innerHTML=errors;document.f.po.focus(); return false; }");
out.println("return true;}");
//letternumber.....
out.println("function letternumber(event){ var keychar;keychar = event.key; if (((\".+-0123456789\").indexOf(keychar) > -1)) return true; if (event.keyCode === 13){ event.preventDefault();document.getElementById(\"order\").click();}else{ alert(\" Should only Numerics.....!!\"); return false; }}");
//nameVelidation....
out.println("function nameVelidation(event) { var keychar; keychar = event.key; if (((\"abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ \").indexOf(keychar) > -1)) return true; if (event.keyCode === 13){ event.preventDefault();document.getElementById(\"order\").click();} else{alert(\"Special & numeric charaters not Allowed...!!\"); return false; } }");
out.println("</script> ");
//ScriptEnd.....
out.println("<style>");
out.println(".button{");
out.println("background-color:blue;");
out.println(" border:2px solid #white;");
out.println("opacity:0.80;");
out.println(" border-radius:1px;");
out.println("width:150px;");
out.println("height:35px;");
out.println("color:white;");
out.println("transition:0.5s;");
out.println("transition:0.5s;}");
out.println("</style>");
out.println("</head> <body>");
//Header End Body Start from here....
out.println("<div > <fieldset style=\"width:480px; margin-left:500px;\"> ");
out.println("<form name=\"f\" action=\"finalupdate\" method=\"post\" onsubmit=\"return validate()\" > ");
out.println("<h1><b>Update Customer's Details </b></h1><br>");
out.println("<strong>Mobile# : </strong><input style=\"width:350px;padding:10px;\" type=\"text\" placeholder=\"Mobile Number \" value=" + mb+" name=\"mb\" onKeyPress=\"return letternumber(event)\"><div id=\"mobile\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<strong>Address : </strong><input style=\"width:350px;padding:10px;\" type=\"text\" placeholder=\"Address \" value=\" " + add+ " \" name=\"add\"><div id=\"address\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<h1><b>Customer's Measurements<sup> (inches)</sup></b></h1> ");
out.println("<br>");
out.println("<strong>Kameez Length : </strong><input style=\"width:150px;padding:10px;\" type=\"text\" placeholder=\"Kameez-Length \" value=" + kl+" name=\"kl\" onKeyPress=\"return letternumber(event)\"> <div id=\"kl\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<strong>Arm Length : </strong><input style=\"width:150px;padding:10px;\" type=\"text\" placeholder=\"Arm-Length \" value=" + al+" name=\"al\" onKeyPress=\"return letternumber(event)\"> <div id=\"al\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<strong>Shoulder : </strong><input style=\"width:150px;padding:10px;\" type=\"text\" placeholder=\"Shoulder \" value=" + sh+" name=\"sh\" onKeyPress=\"return letternumber(event)\"> <div id=\"sh\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<strong>Chest : </strong><input style=\"width:150px;padding:10px;\" type=\"text\" placeholder=\"Chest \" value=" +ch+" name=\"ch\" onKeyPress=\"return letternumber(event)\"> <div id=\"ch\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<strong>Collar : </strong><input style=\"width:150px;padding:10px;\" type=\"text\" placeholder=\"Collar \" value=" + co+" name=\"co\" onKeyPress=\"return letternumber(event)\"> <div id=\"co\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<strong>Fitting : </strong><input style=\"width:150px;padding:10px;\" type=\"text\" placeholder=\"Fitting \" value=" + fi+" name=\"fi\" onKeyPress=\"return letternumber(event)\"> <div id=\"fi\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<strong>Trouser Length : </strong><input style=\"width:150px;padding:10px;\" type=\"text\" placeholder=\"Trouser-Length \" value=" + tl+" name=\"tl\" onKeyPress=\"return letternumber(event)\"> <div id=\"tl\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<strong>Bottom Openning : </strong><input style=\"width:150px;padding:10px;\" type=\"text\" placeholder=\"Bottom-Opening \" value=" + bo+" name=\"bo\" onKeyPress=\"return letternumber(event)\"> <div id=\"bo\" style=\"color:red\"></div>");
out.println("<br>");
out.println("<strong>Pockets : </strong><input style=\"width:150px;padding:10px;\" type=\"text\" placeholder=\"Pockets \" value=\" "+ po+" \" name=\"po\" > <div id=\"po\" style=\"color:red\"></div>");
out.println("<br><strong> Daman : </strong>");
out.println("<select name=Daman : ><option value=\"square\">Square</option> <option value=\"circle\">Circular</option></select>");
out.println("<br>");
out.println("<input style=float:right; class=\"button\" type=\"submit\" id=\"order\" value=\"Update Customer\" /> ");
out.println("</form>");
out.println("</fieldset></div>");
// String s=(String)request.getAttribute("status");
out.println(" </div></body> </html> ");
}
else{
//out.println("else");
// out.println(i);
String err="No record found........";
}
//out.println("</body></html>");
st.close();
con.close();
}catch(Exception e){
out.println(" ");
}
}
}
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request,response);
}
}<file_sep>/finalproject/WEB-INF/classes/Search.java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
import java.sql.*;
public class Search extends HttpServlet {
//Process the HTTP Get request
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
PrintWriter out = response.getWriter();
String u_name=request.getParameter("n");
String pwd=request.getParameter("p");
System.out.println(u_name);
try{
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://127.0.0.1/darzikhana";
Connection con=DriverManager.getConnection(url,"root","zain4744");
Statement st=con.createStatement();
String query="Select * from login where username='"+u_name+"' AND password='"+pwd+"' ";
ResultSet rs = st.executeQuery( query );
if(rs.next()){
String name = rs.getString("username");
String pass = rs.getString("password");
String type = rs.getString("type");
HttpSession session = request.getSession(true);
session.setAttribute("username", name);
session.setAttribute("password", <PASSWORD>);
session.setAttribute("type", type);
if(type.equals("1"))
{
RequestDispatcher rd=request.getRequestDispatcher("/home");
rd.forward(request, response);
}
if(type.equals("0"))
{
RequestDispatcher rd=request.getRequestDispatcher("extra.html");
rd.forward(request, response);
}
}
else{
out.println(" <html> <head> <title> login</title> <script language=\"JavaScript\" type=\"text/javaScript\"> function validate() ");
out.println(" { if ( document.f.n.value == \"\" ){var errors=\"Username is empty!!\"; document.getElementById(\"name\").innerHTML=errors; document.f.n.focus();return false; } ");
out.println(" if(document.f.p.value == \"\") {var errors=\"password is empty!!\"; document.getElementById(\"p\").innerHTML=errors; document.f.p.focus();return false;} ");
out.println(" return true;} ");
out.println(" function fun(){alert(\"Username Password Error\");}");
out.println("</script><style>");
out.println(".button{");
out.println("background-color:blue;");
out.println(" border:2px solid #white;");
out.println("opacity:0.80;");
out.println(" border-radius:1px;");
out.println("width:105px;");
out.println("height:35px;");
out.println("color:white;");
out.println("transition:0.5s;");
out.println("transition:0.5s;}");
out.println("</style> </head> ");
out.println(" <body onload=\"fun()\"> ");
out.println(" <fieldset style=\"width:440px; margin-left:500px;\"> ");
out.println(" <h1><b>Welcome the Darzi-Khana</b></h1> <br> ");
out.println(" <form name=\"f\" action=\"Search\" method=\"post\" onsubmit=\"return validate()\" > <br>");
out.println(" <input style=\"width:350px;padding:10px;\" type=\"text\" placeholder=\"Username \" name=\"n\"> ");
out.println("<div id=\"name\" style=\"color:red\"></div> <br> ");
out.println(" <input type=\"password\" style=padding:10px; placeholder=\"Password\" name=\"p\"><div id=\"p\" style=\"color:red\"></div> ");
out.println(" <input style=float:right; class=\"button\" type=\"submit\" value=\"Next\" /> ");
out.println(" </form> </fieldset> ");
out.println(" </body> ");
out.println(" </html>");
}
st.close();
con.close();
}catch(Exception e){
out.println(" ");
}
}
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request,response);}
}
<file_sep>/finalproject/WEB-INF/classes/logout.java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
import java.sql.*;
import javax.swing.*;
public class logout extends HttpServlet{
public void doPost(HttpServletRequest request,HttpServletResponse response) throws IOException
{
HttpSession session=request.getSession(false);
if(session==null)
{response.sendRedirect("login.html");}
else{
response.setContentType("text/html");
PrintWriter out=response.getWriter();
session.invalidate();
response.sendRedirect("login.html");
}
}
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request,response);
}
} | 7e8c0ea43b1ef979d54638ac2d56daa2f6163b72 | [
"Java"
] | 4 | Java | Zain517/java-s_FianalProject | e1e05fbc3c84523e6fe9d60759bd050eb563cdd9 | 3acfc0e09909a4c3b9fb88be25cb3e7ae22bc151 | |
refs/heads/master | <file_sep>import Vue from "vue";
import Vuex from "vuex";
Vue.use(Vuex);
export default new Vuex.Store({
state: {
userName: "<NAME>",
userAge: 70,
friendsList: ["<NAME>", "<NAME>", "Case 250"]
},
mutations: {
changeUserAge(state, data){
state.userAge = data;
}
},
actions: {
updateLater: function(context){
setTimeout(function(){ context.commit("changeUserAge", 50)}, 5000)
}
},
getters:{
lousdUserName: function(state){
return state.userName.toUpperCase();
}
},
modules: {}
});
| 066f7170e2bb4c6083807143c7138775aafee3b7 | [
"JavaScript"
] | 1 | JavaScript | nighatm/Assignment13BGrid | 4744645c4317e671343b8df5015449b7a50ea1a2 | 555a5493d18f8685875ee6c865de8bb0f28c112b | |
refs/heads/master | <file_sep># This is a sample Python script to work on how to open a file.
from pathlib import Path
'''
****************************************************
First Task: OPEN, READ, ASSIGN
Step 1: open text file
Step 2: assign the data to list.
Step 3: decode the given list
Step 4: assign the decoded list to a new_list
#***************************************************
'''
# assign data to "text"
dataFolder = Path(r'C:\Users\ncssa\PycharmProjects\dreamWork\sample-1.txt')
text = dataFolder.read_text()
# split string to a list
list1 = text.rsplit(" ")
print("list to decode: ", list1)
# this is the decoder function
def decoder(work):
number = 0 # int variable to store converted numbers - temporary
list2 = [] # This is a new list to hold the decoded numbers
for item in work:
number += int(item)
list2.append(number)
return list2
# call the decoder function
decoded_list1 = decoder(list1)
print("decoded list: ", decoded_list1)
'''
****************************************************
Second Task: CONVERT, CREATE NEW TEXT FILE
Step 1: convert list to String
Step 2: create new Text File
Step 3: save the string to the new Text File
#***************************************************
'''
f = open("final-1.txt", "a+") # create/open a new txt file for the final result
# write the decoded_list_1 in the text file.
for i in decoded_list1:
f.write("%s " % i)
f.close()
'''
****************************************************
Third Task: TEST the script
Step 1: write unittest
Step 2: run unittest
https://github.com/NorbertSapi/unitTestDreamWork.git
****************************************************
'''
# unittest is up and running for the decoder.
'''
****************************************************
FUTURE Task:
- use decorator to reduce time and save memory.
- open and loop through multiple files
- for line sys.stdin:
****************************************************
'''<file_sep># DREAM BIG
UNITTEST:
https://github.com/NorbertSapi/unitTestDreamWork.git
Study Project while I am doing the research:
https://github.com/NorbertSapi/try_for_loop.git
https://github.com/NorbertSapi/open_data_from_text.git
https://github.com/NorbertSapi/dream.git
First Task: OPEN, READ, ASSIGN
Step 1: open text file
Step 2: assign the data to list.
Step 3: decode the given list
Step 4: assign the decoded list to a new_list
Second Task: CONVERT, CREATE NEW TEXT FILE
Step 1: convert list to String
Step 2: create new Text File
Step 3: save the string to the new Text File
Third Task: TEST the script
Step 1: open multiple files
Step 2: run test
https://github.com/NorbertSapi/unitTestDreamWork.git
FUTURE Task:
- use decorator to reduce time and save memory.
- open and loop through multiple files
- for line sys.stdin:
- extract online data (this could be the next project)
| 2e30b119bf963244f48add6a26a6df9931c9a3c0 | [
"Markdown",
"Python"
] | 2 | Python | NorbertSapi/dreamWork | b96a6c568e0b7684ff565cd83c5dfb830855950c | 1d7e0f809a104b4038a8ae3d0cab71d920543da6 | |
refs/heads/master | <repo_name>tonyfruzza/AwsSsmAgentMonitoringPoC<file_sep>/README.md
## SSM Agent Monitor
Triggers a CloudWatch Alarm when a managed instance is not capable of receiving SSM commands and generating an CloudWatch Metric within the allotted threshold of time.
### Configuration
1. Set `alarm_sns_arn` within conifg-common.yml for where the alarm should be directed.
2. Duplicate the example CloudWatch alarm in the resources section to match your managed instances. One for each instance.
Your IAM role assigned to your managed instance should include at least these managed policies:
* AmazonSSMManagedInstanceCore
* CloudWatchAgentServerPolicy
### Management
This serverless framework is designed to be launched by runway. Create a module directory with a .sls folder extension, add the module to your runway.yml list. The example configuration files use -common.yml extensions, if you'd rather launch in a different environment you'll need to change those to match your desired environment name.
### Deployment
AWS infrastructure managed by [Serverles Framework](https://github.com/serverless/serverless) follow the install guidelines for your OS and AWS CLI. This example comes with a autoscaling group which launches an instance to be monitored. There are parameter values that must be configured to launch this within your VPC within `deploy.sh`. Configure your AWS credentials and then execute the provided `./deploy.sh` after performing the Configuration step to set your SNS topic.
<file_sep>/deploy.sh
#!/bin/sh
REGION=us-west-2
sls deploy --stage common --region $REGION
<file_sep>/lambda/ssm-run-doc.rb
require 'aws-sdk-ssm'
def lambda_handler(event:, context:)
ssm = Aws::SSM::Client.new(region: ENV['AWS_REGION'])
# Get list of instances that are checking into SSM
managed_instances = []
next_token = nil
loop do
dii = ssm.describe_instance_information({next_token: next_token}).to_h
managed_instances += dii[:instance_information_list].map{|i| i[:instance_id]}
break unless next_token = dii[:next_token]
next_token = dii[:next_token]
end
puts "Targeting #{managed_instances}"
managed_instances.each do |mi|
ssm.send_command({
targets: [{key: 'InstanceIds', values: managed_instances}],
document_name: 'AWS-RunPowerShellScript',
parameters: {
commands: ["aws --region #{ENV['AWS_REGION']} cloudwatch put-metric-data --metric-name heart-beat --namespace mi-health --value 1 --dimensions InstanceID=#{mi}"]
},
timeout_seconds: 300
})
end
end
| f709be17930b6f3dc0d29404fc4b2ce316348b0e | [
"Markdown",
"Ruby",
"Shell"
] | 3 | Markdown | tonyfruzza/AwsSsmAgentMonitoringPoC | b6f0b33fb47072341c01a48460b08e722d480dfe | 6affc575a4f21b728fd8a847bee75a5170347d22 | |
refs/heads/master | <repo_name>xj0707/tigergame<file_sep>/.svn/pristine/07/077b9c6eab368804a18c9523f493fade2980d3e1.svn-base
<?php
namespace application\admin\controller;
use think\Db;
class GameReportsController extends CommonController{
//玩家报表
public function index(){
if(!$this->come_where['parent'][1]){
$this->error('无商户无玩家信息!!');
}
$where['g.parent']=$this->come_where['parent'];
// $players=Db::name('gameReports')->alias('g')->join('na_users u','g.parent=u.uid')
// ->field('g.gameName gg,g.wct gwct,g.totalBetCount gtbc,g.avgRTP ga,g.totalPays gtp,g.totalBets gtb,g.totalPlayers gt,g.updatedAt gu,u.suffix us,u.username uu')
// ->where($where)->paginate(20);
$merchantIds=session('tiger_parentId');
$gameReports=array();
foreach ($merchantIds as $key=>$parent){
$totalBetCount=Db::name('playerReports')->where('parent',$parent)->sum('totalBetCount');//投注次数
if(!$totalBetCount){
unset($gameReports[$key]);
continue;
}
$totalPays=Db::name('playerReports')->where('parent',$parent)->sum('totalPays');//中奖金额
$totalBets=Db::name('playerReports')->where('parent',$parent)->sum('totalBets');//投注金额
$totalusers=Db::name('playerReports')->where('parent',$parent)->count();//玩家总数
$avgRTP='0.00';
if($totalBets){
$avgRTP=round($totalPays/$totalBets,2);//RTP;
}
$merch=Db::name('users')->field('username,nickname')->where('uid',$parent)->find();
$gameReports[$key]['gameName']='塔罗之谜';
$gameReports[$key]['totalBets']=sprintf("%.2f", $totalBets);
$gameReports[$key]['totalPays']=sprintf("%.2f", $totalPays);
$gameReports[$key]['totalusers']=$totalusers;
$gameReports[$key]['totalBetCount']=$totalBetCount;
$gameReports[$key]['avgRTP']=$avgRTP;
$gameReports[$key]['merch']=$merch['nickname'];
}
$role=session('tiger_role');
switch ($role){
case 1://平台管理
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 2://顶级代理
$data1=Db::name('users')->where('msn','0')->where('levelIndex','<>','0')->select();
$this->assign('data1',$data1);
break;
case 3://线路商
$id=session('tiger_uid');
$users=Db::name('users')->field('uid')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','-1')->where('levelindex','like',"%$uid%")->select();
$data2=Db::name('users')->where('msn','<>','-1')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 4://次级代理
$id=session('tiger_uid');
$users=Db::name('users')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','0')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
break;
case 5://商户
break;
case 6://超管
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$data3=Db::name('users')->where('msn','0')->where('levelindex','<>','0')->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
$this->assign('data3',$data3);
break;
}
$this->assign('role',$role);
$this->assign('players',$gameReports);
return $this->fetch();
}
/**
* 线路商查询
*/
public function xl(){
$id=input('get.id');
if($id==-1){
$this->error('请选择线路商!!!');
}
$info=Db::name('users')->field('uid')->where('id',$id)->find();
$role=session('tiger_role');
if($info){
$uid=$info['uid'];
$user_ids=Db::name('users')->field('uid')->where('levelindex','like',"%$uid%")->select();
$gameReports=array();
foreach ($user_ids as $key=>$user_id){
$parent=$user_id['uid'];
$totalBetCount=Db::name('playerReports')->where('parent',$parent)->sum('totalBetCount');//投注次数
$totalPays=Db::name('playerReports')->where('parent',$parent)->sum('totalPays');//中奖金额
$totalBets=Db::name('playerReports')->where('parent',$parent)->sum('totalBets');//投注金额
$totalusers=Db::name('playerReports')->where('parent',$parent)->count();//玩家总数
$avgRTP=0;
if($totalBets){
$avgRTP=round($totalPays/$totalBets,2);//RTP;
}
$merch=Db::name('users')->field('username,nickname')->where('uid',$parent)->find();
$gameReports[$key]['gameName']='塔罗之谜';
$gameReports[$key]['totalBets']=sprintf("%.2f", $totalBets);
$gameReports[$key]['totalPays']=sprintf("%.2f", $totalPays);
$gameReports[$key]['totalusers']=$totalusers;
$gameReports[$key]['totalBetCount']=$totalBetCount;
$gameReports[$key]['avgRTP']=$avgRTP;
$gameReports[$key]['merch']=$merch['nickname'];
}
//
// $players=Db::name('gameReports')->alias('g')->join('na_users u','g.parent=u.uid')
// ->field('g.gameName gg,g.wct gwct,g.totalBetCount gtbc,g.avgRTP ga,g.totalPays gtp,g.totalBets gtb,g.totalPlayers gt,g.updatedAt gu,u.suffix us,u.username uu')
// ->where('g.parent','in',$guanli)->paginate(20);
switch ($role){
case 1://平台管理
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 2://顶级代理
$data1=Db::name('users')->where('msn','0')->where('levelIndex','<>','0')->select();
$this->assign('data1',$data1);
break;
case 3://线路商
$id=session('tiger_uid');
$users=Db::name('users')->field('uid')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','-1')->where('levelindex','like',"%$uid%")->select();
$data2=Db::name('users')->where('msn','<>','-1')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 4://次级代理
$id=session('tiger_uid');
$users=Db::name('users')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','0')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
break;
case 5://商户
break;
case 6://超管
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$data3=Db::name('users')->where('msn','0')->where('levelindex','<>','0')->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
$this->assign('data3',$data3);
break;
}
$this->assign('role',$role);
$this->assign('players',$gameReports);
return $this->fetch('index');
}
}
/**
* 商户查询
*/
public function sh(){
$id=input('get.id');
if($id==-1){
$this->error('请选择商户!!!');
}
$info=Db::name('users')->field('uid')->where('id',$id)->find();
$role=session('tiger_role');
if($info){
$parent=$info['uid'];
$totalBetCount=Db::name('playerReports')->where('parent',$parent)->sum('totalBetCount');//投注次数
$totalPays=Db::name('playerReports')->where('parent',$parent)->sum('totalPays');//中奖金额
$totalBets=Db::name('playerReports')->where('parent',$parent)->sum('totalBets');//投注金额
$totalusers=Db::name('playerReports')->where('parent',$parent)->count();//玩家总数
$avgRTP=0;
if($totalBets){
$avgRTP=round($totalPays/$totalBets,2);//RTP;
}
$merch=Db::name('users')->field('username,nickname')->where('uid',$parent)->find();
$gameReports[0]['gameName']='塔罗之谜';
$gameReports[0]['totalBets']=sprintf("%.2f", $totalBets);
$gameReports[0]['totalPays']=sprintf("%.2f", $totalPays);
$gameReports[0]['totalusers']=$totalusers;
$gameReports[0]['totalBetCount']=$totalBetCount;
$gameReports[0]['avgRTP']=$avgRTP;
$gameReports[0]['merch']=$merch['nickname'];
// $players=Db::name('gameReports')->alias('g')->join('na_users u','g.parent=u.uid')
// ->field('g.gameName gg,g.wct gwct,g.totalBetCount gtbc,g.avgRTP ga,g.totalPays gtp,g.totalBets gtb,g.totalPlayers gt,g.updatedAt gu,u.suffix us,u.username uu')
// ->where('g.parent',$uid)->paginate(20);
switch ($role){
case 1://平台管理
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 2://顶级代理
$data1=Db::name('users')->where('msn','0')->where('levelIndex','<>','0')->select();
$this->assign('data1',$data1);
break;
case 3://线路商
$id=session('tiger_uid');
$users=Db::name('users')->field('uid')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','-1')->where('levelindex','like',"%$uid%")->select();
$data2=Db::name('users')->where('msn','<>','-1')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 4://次级代理
$id=session('tiger_uid');
$users=Db::name('users')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','0')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
break;
case 5://商户
break;
case 6://超管
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$data3=Db::name('users')->where('msn','0')->where('levelindex','<>','0')->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
$this->assign('data3',$data3);
break;
}
$this->assign('role',$role);
$this->assign('players',$gameReports);
return $this->fetch('index');
}
}
/**
* 代理商查询
*/
public function dl(){
$id=input('get.id');
if($id==-1){
$this->error('请选择代理!!!');
}
$info=Db::name('users')->field('uid')->where('id',$id)->find();
$role=session('tiger_role');
if($info){
$uid=$info['uid'];
$user_ids=Db::name('users')->field('uid')->where('levelindex','like',"%$uid%")->select();
$gameReports=array();
foreach ($user_ids as $key=>$user_id){
$parent=$user_id['uid'];
$totalBetCount=Db::name('playerReports')->where('parent',$parent)->sum('totalBetCount');//投注次数
$totalPays=Db::name('playerReports')->where('parent',$parent)->sum('totalPays');//中奖金额
$totalBets=Db::name('playerReports')->where('parent',$parent)->sum('totalBets');//投注金额
$totalusers=Db::name('playerReports')->where('parent',$parent)->count();//玩家总数
$avgRTP=0;
if($totalBets){
$avgRTP=round($totalPays/$totalBets,2);//RTP;
}
$merch=Db::name('users')->field('username,nickname')->where('uid',$parent)->find();
$gameReports[$key]['gameName']='塔罗之谜';
$gameReports[$key]['totalBets']=sprintf("%.2f", $totalBets);
$gameReports[$key]['totalPays']=sprintf("%.2f", $totalPays);
$gameReports[$key]['totalusers']=$totalusers;
$gameReports[$key]['totalBetCount']=$totalBetCount;
$gameReports[$key]['avgRTP']=$avgRTP;
$gameReports[$key]['merch']=$merch['nickname'];
}
// $players=Db::name('gameReports')->alias('g')->join('na_users u','g.parent=u.uid')
// ->field('g.gameName gg,g.wct gwct,g.totalBetCount gtbc,g.avgRTP ga,g.totalPays gtp,g.totalBets gtb,g.totalPlayers gt,g.updatedAt gu,u.suffix us,u.username uu')
// ->where('g.parent','in',$guanli)->paginate(20);
switch ($role){
case 1://平台管理
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 2://顶级代理
$data1=Db::name('users')->where('msn','0')->where('levelIndex','<>','0')->select();
$this->assign('data1',$data1);
break;
case 3://线路商
$id=session('tiger_uid');
$users=Db::name('users')->field('uid')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','-1')->where('levelindex','like',"%$uid%")->select();
$data2=Db::name('users')->where('msn','<>','-1')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 4://次级代理
$id=session('tiger_uid');
$users=Db::name('users')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','0')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
break;
case 5://商户
break;
case 6://超管
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$data3=Db::name('users')->where('msn','0')->where('levelindex','<>','0')->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
$this->assign('data3',$data3);
break;
}
$this->assign('role',$role);
$this->assign('players',$gameReports);
return $this->fetch('index');
}
}
/**
*游戏名称查询
*/
public function likeGamename(){
$gamename=input('post.gamename');
$role=session('tiger_role');
$where['g.parent']=$this->come_where['parent'];
$players=Db::name('gameReports')->alias('g')->join('na_users u','g.parent=u.uid')
->field('g.gameName gg,g.wct gwct,g.totalBetCount gtbc,g.avgRTP ga,g.totalPays gtp,g.totalBets gtb,g.totalPlayers gt,g.updatedAt gu,u.suffix us,u.username uu')
->where('g.gameName','like',"%$gamename%")->where($where)->paginate(20);
switch ($role){
case 1://平台管理
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 2://顶级代理
$data1=Db::name('users')->where('msn','0')->where('levelIndex','<>','0')->select();
$this->assign('data1',$data1);
break;
case 3://线路商
$id=session('tiger_uid');
$users=Db::name('users')->field('uid')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','-1')->where('levelindex','like',"%$uid%")->select();
$data2=Db::name('users')->where('msn','<>','-1')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 4://次级代理
$id=session('tiger_uid');
$users=Db::name('users')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','0')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
break;
case 5://商户
break;
case 6://超管
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$data3=Db::name('users')->where('msn','0')->where('levelindex','<>','0')->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
$this->assign('data3',$data3);
break;
}
$this->assign('role',$role);
$this->assign('players',$players);
return $this->fetch('index');
}
}<file_sep>/.svn/pristine/64/646ae46212594d32ea35028012e7e7077f6e990e.svn-base
<?php
namespace application\admin\controller;
use think\Db;
use think\Session;
class GameSetController extends CommonController{
//
public function index(){
$userIds=session('tiger_parentId');
if(count($userIds)){
$userinfos=Db::name('users')->where('uid','in',$userIds)->where('msn','<>',-1)->paginate(20);
$this->assign('userinfos',$userinfos);
return $this->fetch();
}else{
$this->error('无任何商户信息!');
}
}
//线数线注设置
public function xsxz(){
//$this->error('暂未开通');
$uid=input('uid');
$info=Db::name('users')->where('uid',$uid)->find();
//初始线注
$xzinit=[
['v1'=>0.01,'istrue'=>0],['v1'=>0.25,'istrue'=>0],['v1'=>0.50,'istrue'=>0],['v1'=>1.0,'istrue'=>0],
['v1'=>1.25,'istrue'=>0],['v1'=>2.50,'istrue'=>0],['v1'=>3.75,'istrue'=>0],['v1'=>5.0,'istrue'=>0],
['v1'=>10.0,'istrue'=>0],['v1'=>20.0,'istrue'=>0],['v1'=>25.0,'istrue'=>0],['v1'=>50.0,'istrue'=>0],
['v1'=>100.0,'istrue'=>0],['v1'=>125.0,'istrue'=>0],['v1'=>200.0,'istrue'=>0]
];
$xzinfo=explode(',',$info['betcfg']);
foreach ($xzinit as $key=>$xz){
if(in_array($xz['v1'],$xzinfo)){
$xzinit[$key]['istrue']=1;
}
}
$this->assign('nickname',$info['nickname']);
$this->assign('xzinit',$xzinit);
$this->assign('xs',$info['linecfg']);
$this->assign('uid',$uid);
return $this->fetch();
}
//线数请求
public function doxs(){
$uid=input('uid');
$xs=input('xs');
$typeId=input('typeId');
if($typeId==40001){
$url=config('xsxz40001');
}elseif($typeId==40002){
$url=config('xsxz40002');
}
$data=[
'uid'=>$uid,
'linecfg'=>$xs
];
if($xs>25 || $xs<0){
$this->error('线数应该在0-25之间');
}
$jsonstr=json_encode($data);
$ch=curl_init();
curl_setopt($ch,CURLOPT_URL,$url);
curl_setopt($ch,CURLOPT_HEADER,0);
curl_setopt($ch,CURLOPT_RETURNTRANSFER,1);
curl_setopt($ch,CURLOPT_POST,true);
curl_setopt($ch,CURLOPT_POSTFIELDS,$jsonstr);
// 设置HTTPS支持
date_default_timezone_set('PRC');
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 2);
$output=curl_exec($ch);
curl_close($ch);
if($output){
$obj=json_decode($output);
if($obj->code==0) {
return json(['code'=>1,'message'=>'操作成功!']);
}else{
return json(['code'=>-1,'message'=>'操作失败!'.$obj->err]);
}
}else{
return json(['code'=>-1,'message'=>'请求失败!']);
}
}
//线注请求修改
public function doxz(){
$uid=input('uid');
$xzstr=input('xz');
$typeId=input('typeId');
if($typeId==40001){
$url=config('xsxz40001');
}elseif($typeId==40002){
$url=config('xsxz40002');
}
$data=[
'uid'=>$uid,
'betcfg'=>$xzstr
];
$jsonstr=json_encode($data);
$ch=curl_init();
curl_setopt($ch,CURLOPT_URL,$url);
curl_setopt($ch,CURLOPT_HEADER,0);
curl_setopt($ch,CURLOPT_RETURNTRANSFER,1);
curl_setopt($ch,CURLOPT_POST,true);
curl_setopt($ch,CURLOPT_POSTFIELDS,$jsonstr);
// 设置HTTPS支持
date_default_timezone_set('PRC');
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 2);
$output=curl_exec($ch);
curl_close($ch);
//var_dump($output);
if($output){
$obj=json_decode($output);
if($obj->code==0) {
return json(['code'=>1,'message'=>'操作成功!']);
}else{
return json(['code'=>-1,'message'=>'操作失败!'.$obj->err]);
}
}else{
return json(['code'=>-1,'message'=>'请求失败!']);
}
}
}<file_sep>/.svn/pristine/51/5171a67e0d4ea250482f8235a87fb618ff2074cc.svn-base
/*
Navicat MySQL Data Transfer
Source Server : localhost_3306
Source Server Version : 50617
Source Host : localhost:3306
Source Database : na_tigergame
Target Server Type : MYSQL
Target Server Version : 50617
File Encoding : 65001
Date: 2017-09-30 03:18:23
*/
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- Table structure for na_total_reports
-- ----------------------------
DROP TABLE IF EXISTS `na_total_reports`;
CREATE TABLE `na_total_reports` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`gameUserId` varchar(36) DEFAULT NULL,
`username` varchar(50) DEFAULT NULL,
`nickname` varchar(10) DEFAULT NULL,
`balance` decimal(20,2) DEFAULT NULL,
`parentId` varchar(36) DEFAULT NULL,
`betCount` varchar(255) DEFAULT NULL,
`winlose` decimal(20,2) DEFAULT NULL,
`totalBet` varchar(20) DEFAULT NULL,
`wcr` decimal(20,2) DEFAULT NULL,
`ret` decimal(20,2) DEFAULT NULL,
`agentBalance` decimal(20,2) DEFAULT NULL,
`agentRate` varchar(5) DEFAULT NULL,
`giveback` decimal(20,2) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of na_total_reports
-- ----------------------------
<file_sep>/.svn/pristine/a3/a38bdbf4b3eaecb665f8d09061ef6681bb19ef18.svn-base
<?php
namespace application\admin\controller;
use think\Db;
class PlayerReportsBController extends CommonController{
//选择游戏
public function typeSelect(){
return $this->fetch();
}
//玩家报表
public function index(){
//$typeid=input('typeid');
if(!$this->come_where['parent'][1]){
$this->error('无商户无玩家信息!!');
}
$where['p.parent']=$this->come_where['parent'];
$players=Db::name('playerReportsB')->alias('p')->join('na_users u','p.parent=u.uid')
->field('p.username pu,p.wct pwct,p.msn pm,p.balance pb,p.waterflow pw,p.totalBetCount ptbc,p.avgRTP pa,p.totalPays ptp,p.totalBets ptb,u.suffix us,u.username uu,p.pid,u.nickname uni')
->where($where)->paginate(20);
$role=session('tiger_role');
switch ($role){
case 1://平台管理
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 2://顶级代理
$data1=Db::name('users')->where('msn','0')->where('levelIndex','<>','0')->select();
$this->assign('data1',$data1);
break;
case 3://线路商
$id=session('tiger_uid');
$users=Db::name('users')->field('uid')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','-1')->where('levelindex','like',"%$uid%")->select();
$data2=Db::name('users')->where('msn','<>','-1')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 4://次级代理
$id=session('tiger_uid');
$users=Db::name('users')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','0')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
break;
case 5://商户
break;
case 6://超管
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$data3=Db::name('users')->where('msn','0')->where('levelindex','<>','0')->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
$this->assign('data3',$data3);
break;
}
// if($typeid){
// if($typeid==40001){
// $typename='塔罗之谜';
// $this->assign('typename',$typename);
// }
// }
$this->assign('typeId',40002);
$this->assign('role',$role);
$this->assign('players',$players);
return $this->fetch();
}
/**
* 线路商查询
*/
public function xl(){
$id=input('get.id');
if($id==-1){
$this->error('请选择线路商!!!');
}
$info=Db::name('users')->field('uid')->where('id',$id)->find();
$role=session('tiger_role');
if($info){
$uid=$info['uid'];
$user_ids=Db::name('users')->field('uid')->where('levelindex','like',"%$uid%")->select();
$guanli=[$uid];
foreach ($user_ids as $user_id){
$guanli[]=$user_id['uid'];
}
$players=Db::name('playerReportsB')->alias('p')->join('na_users u','p.parent=u.uid')
->field('p.username pu,p.wct pwct,p.msn pm,p.balance pb,p.waterflow pw,p.totalBetCount ptbc,p.avgRTP pa,p.totalPays ptp,p.totalBets ptb,u.suffix us,u.username uu,p.pid,u.nickname uni')
->where('p.parent','in',$guanli)->paginate(20,false,['query' => request()->param()]);
switch ($role){
case 1://平台管理
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 2://顶级代理
$data1=Db::name('users')->where('msn','0')->where('levelIndex','<>','0')->select();
$this->assign('data1',$data1);
break;
case 3://线路商
$id=session('tiger_uid');
$users=Db::name('users')->field('uid')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','-1')->where('levelindex','like',"%$uid%")->select();
$data2=Db::name('users')->where('msn','<>','-1')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 4://次级代理
$id=session('tiger_uid');
$users=Db::name('users')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','0')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
break;
case 5://商户
break;
case 6://超管
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$data3=Db::name('users')->where('msn','0')->where('levelindex','<>','0')->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
$this->assign('data3',$data3);
break;
}
$this->assign('typeId',40002);
$this->assign('role',$role);
$this->assign('players',$players);
return $this->fetch('index');
}
}
/**
* 商户查询
*/
public function sh(){
$id=input('get.id');
if($id==-1){
$this->error('请选择商户!!!');
}
$info=Db::name('users')->field('uid')->where('id',$id)->find();
$role=session('tiger_role');
if($info){
$uid=$info['uid'];
$players=Db::name('playerReportsB')->alias('p')->join('na_users u','p.parent=u.uid')
->field('p.username pu,p.wct pwct,p.msn pm,p.balance pb,p.waterflow pw,p.totalBetCount ptbc,p.avgRTP pa,p.totalPays ptp,p.totalBets ptb,u.suffix us,u.username uu,p.pid,u.nickname uni')
->where('p.parent',$uid)->paginate(20,false,['query' => request()->param()]);
switch ($role){
case 1://平台管理
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 2://顶级代理
$data1=Db::name('users')->where('msn','0')->where('levelIndex','<>','0')->select();
$this->assign('data1',$data1);
break;
case 3://线路商
$id=session('tiger_uid');
$users=Db::name('users')->field('uid')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','-1')->where('levelindex','like',"%$uid%")->select();
$data2=Db::name('users')->where('msn','<>','-1')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 4://次级代理
$id=session('tiger_uid');
$users=Db::name('users')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','0')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
break;
case 5://商户
break;
case 6://超管
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$data3=Db::name('users')->where('msn','0')->where('levelindex','<>','0')->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
$this->assign('data3',$data3);
break;
}
$this->assign('typeId',40002);
$this->assign('role',$role);
$this->assign('players',$players);
return $this->fetch('index');
}
}
/**
* 代理商查询
*/
public function dl(){
$id=input('get.id');
if($id==-1){
$this->error('请选择代理!!!');
}
$info=Db::name('users')->field('uid')->where('id',$id)->find();
$role=session('tiger_role');
if($info){
$uid=$info['uid'];
$user_ids=Db::name('users')->field('uid')->where('levelindex','like',"%$uid%")->select();
$guanli=[$uid];
foreach ($user_ids as $user_id){
$guanli[]=$user_id['uid'];
}
$players=Db::name('playerReportsB')->alias('p')->join('na_users u','p.parent=u.uid')
->field('p.username pu,p.wct pwct,p.msn pm,p.balance pb,p.waterflow pw,p.totalBetCount ptbc,p.avgRTP pa,p.totalPays ptp,p.totalBets ptb,u.suffix us,u.username uu,p.pid,u.nickname uni')
->where('p.parent','in',$guanli)->paginate(20,false,['query' => request()->param()]);
switch ($role){
case 1://平台管理
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 2://顶级代理
$data1=Db::name('users')->where('msn','0')->where('levelIndex','<>','0')->select();
$this->assign('data1',$data1);
break;
case 3://线路商
$id=session('tiger_uid');
$users=Db::name('users')->field('uid')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','-1')->where('levelindex','like',"%$uid%")->select();
$data2=Db::name('users')->where('msn','<>','-1')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 4://次级代理
$id=session('tiger_uid');
$users=Db::name('users')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','0')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
break;
case 5://商户
break;
case 6://超管
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$data3=Db::name('users')->where('msn','0')->where('levelindex','<>','0')->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
$this->assign('data3',$data3);
break;
}
$this->assign('typeId',40002);
$this->assign('role',$role);
$this->assign('players',$players);
return $this->fetch('index');
}
}
/**
*玩家用户名查询
*/
public function likeUsername(){
$username=input('post.username');
$role=session('tiger_role');
$where['p.parent']=$this->come_where['parent'];
$players=Db::name('playerReportsB')->alias('p')->join('na_users u','p.parent=u.uid')
->field('p.username pu,p.wct pwct,p.msn pm,p.balance pb,p.waterflow pw,p.totalBetCount ptbc,p.avgRTP pa,p.totalPays ptp,p.totalBets ptb,u.suffix us,u.username uu,p.pid,u.nickname uni')
->where('p.username','like',"%$username%")->where($where)->paginate(20);
switch ($role){
case 1://平台管理
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 2://顶级代理
$data1=Db::name('users')->where('msn','0')->where('levelIndex','<>','0')->select();
$this->assign('data1',$data1);
break;
case 3://线路商
$id=session('tiger_uid');
$users=Db::name('users')->field('uid')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','-1')->where('levelindex','like',"%$uid%")->select();
$data2=Db::name('users')->where('msn','<>','-1')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
break;
case 4://次级代理
$id=session('tiger_uid');
$users=Db::name('users')->where('id',$id)->find();
$uid=$users['uid'];
$data1=Db::name('users')->where('msn','0')->where('levelindex','like',"%$uid%")->select();
$this->assign('data1',$data1);
break;
case 5://商户
break;
case 6://超管
$data1=Db::name('users')->where('msn','-1')->where('suffix','<>','Platform')->select();
$data2=Db::name('users')->where("msn !='-1' and msn != '0'")->select();
$data3=Db::name('users')->where('msn','0')->where('levelindex','<>','0')->select();
$this->assign('data1',$data1);
$this->assign('data2',$data2);
$this->assign('data3',$data3);
break;
}
$this->assign('typeId',40002);
$this->assign('role',$role);
$this->assign('players',$players);
return $this->fetch('index');
}
}<file_sep>/application/admin/controller/IndexController.php
<?php
/**
*
* @file Index.php
* @date 2016-8-23 16:03:10
* @author <NAME><<EMAIL>>
* @version SVN:$Id:$
*/
namespace application\admin\controller;
class IndexController extends CommonController{
/**
* 后台首页
*/
public function index(){
$role=session('tiger_role');
$msg='';
switch ($role){
case 1:
$msg='当前身份:管理员';
break;
case 2:
$msg='当前身份:代理管理员';
break;
case 3:
$msg='当前身份:线路商';
break;
case 4:
$msg='当前身份:代理';
break;
case 5:
$msg='当前身份:商户';
break;
case 6:
$msg='当前身份:超管';
break;
}
$this->assign('msg',$msg);
return $this->fetch();
}
}<file_sep>/sql/na_tigergame.sql
/*
Navicat MySQL Data Transfer
Source Server : localhost_3306
Source Server Version : 50617
Source Host : localhost:3306
Source Database : na_tigergame
Target Server Type : MYSQL
Target Server Version : 50617
File Encoding : 65001
Date: 2017-09-01 11:45:57
*/
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- Table structure for na_admin
-- ----------------------------
DROP TABLE IF EXISTS `na_admin`;
CREATE TABLE `na_admin` (
`id` mediumint(6) unsigned NOT NULL AUTO_INCREMENT,
`username` varchar(20) NOT NULL DEFAULT '',
`password` varchar(32) NOT NULL DEFAULT '',
`encrypt` varchar(6) NOT NULL DEFAULT '',
`lastloginip` int(10) NOT NULL DEFAULT '0',
`lastlogintime` int(10) unsigned NOT NULL DEFAULT '0',
`email` varchar(40) NOT NULL DEFAULT '',
`mobile` varchar(11) NOT NULL DEFAULT '',
`realname` varchar(50) NOT NULL DEFAULT '',
`openid` varchar(255) NOT NULL DEFAULT '',
`status` tinyint(1) NOT NULL DEFAULT '1' COMMENT '是否有效(2:无效,1:有效)',
`updatetime` int(10) NOT NULL DEFAULT '0',
`uid` varchar(50) DEFAULT NULL COMMENT '平台管理员的uid ',
PRIMARY KEY (`id`),
KEY `username` (`username`),
KEY `status` (`status`)
) ENGINE=InnoDB AUTO_INCREMENT=2 DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of na_admin
-- ----------------------------
INSERT INTO `na_admin` VALUES ('1', 'admin', '21232f297a57a5a743894a0e4a801fc3', '', '2130706433', '1504233919', '<EMAIL>', '13076050636', '小肖', '', '1', '1502875535', null);
-- ----------------------------
-- Table structure for na_admin_group
-- ----------------------------
DROP TABLE IF EXISTS `na_admin_group`;
CREATE TABLE `na_admin_group` (
`id` tinyint(3) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(50) NOT NULL,
`description` text,
`rules` varchar(500) NOT NULL DEFAULT '' COMMENT '用户组拥有的规则id,多个规则 , 隔开',
`listorder` smallint(5) unsigned NOT NULL DEFAULT '0',
`updatetime` int(11) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `listorder` (`listorder`)
) ENGINE=InnoDB AUTO_INCREMENT=6 DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of na_admin_group
-- ----------------------------
INSERT INTO `na_admin_group` VALUES ('1', '系统首页', '拥有系统首页模块', '31', '0', '1503288249');
INSERT INTO `na_admin_group` VALUES ('4', '商户管理', '拥有商户管理权限', '48,50,51,55,56,57,58,52', '0', '1503539486');
INSERT INTO `na_admin_group` VALUES ('5', '报表统计', '拥有报表统计模块查看', '49,53,59,60,61,62,54,63,64,65,66', '0', '1503539502');
-- ----------------------------
-- Table structure for na_admin_group_access
-- ----------------------------
DROP TABLE IF EXISTS `na_admin_group_access`;
CREATE TABLE `na_admin_group_access` (
`uid` int(10) unsigned NOT NULL COMMENT '用户id',
`group_id` mediumint(8) unsigned NOT NULL COMMENT '用户组id',
UNIQUE KEY `uid_group_id` (`uid`,`group_id`),
KEY `uid` (`uid`),
KEY `group_id` (`group_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of na_admin_group_access
-- ----------------------------
INSERT INTO `na_admin_group_access` VALUES ('20', '1');
INSERT INTO `na_admin_group_access` VALUES ('21', '1');
INSERT INTO `na_admin_group_access` VALUES ('22', '1');
INSERT INTO `na_admin_group_access` VALUES ('23', '1');
INSERT INTO `na_admin_group_access` VALUES ('24', '1');
INSERT INTO `na_admin_group_access` VALUES ('25', '1');
INSERT INTO `na_admin_group_access` VALUES ('26', '1');
INSERT INTO `na_admin_group_access` VALUES ('20', '4');
INSERT INTO `na_admin_group_access` VALUES ('21', '4');
INSERT INTO `na_admin_group_access` VALUES ('22', '4');
INSERT INTO `na_admin_group_access` VALUES ('23', '4');
INSERT INTO `na_admin_group_access` VALUES ('24', '4');
INSERT INTO `na_admin_group_access` VALUES ('25', '4');
INSERT INTO `na_admin_group_access` VALUES ('26', '4');
INSERT INTO `na_admin_group_access` VALUES ('20', '5');
INSERT INTO `na_admin_group_access` VALUES ('21', '5');
INSERT INTO `na_admin_group_access` VALUES ('22', '5');
INSERT INTO `na_admin_group_access` VALUES ('23', '5');
INSERT INTO `na_admin_group_access` VALUES ('24', '5');
INSERT INTO `na_admin_group_access` VALUES ('25', '5');
INSERT INTO `na_admin_group_access` VALUES ('26', '5');
-- ----------------------------
-- Table structure for na_admin_log
-- ----------------------------
DROP TABLE IF EXISTS `na_admin_log`;
CREATE TABLE `na_admin_log` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`m` varchar(15) NOT NULL,
`c` varchar(20) NOT NULL,
`a` varchar(20) NOT NULL,
`querystring` varchar(255) NOT NULL,
`userid` mediumint(8) unsigned NOT NULL DEFAULT '0',
`username` varchar(20) NOT NULL,
`ip` int(10) NOT NULL,
`time` datetime NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=32 DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of na_admin_log
-- ----------------------------
INSERT INTO `na_admin_log` VALUES ('1', 'admin', 'Menu', 'del', '?id=45', '1', 'admin', '2130706433', '2017-08-15 16:25:18');
INSERT INTO `na_admin_log` VALUES ('2', 'admin', 'Group', 'index', '', '1', 'admin', '2130706433', '2017-08-15 16:27:00');
INSERT INTO `na_admin_log` VALUES ('3', 'admin', 'Admin', 'public_edit_info', '', '1', 'admin', '2130706433', '2017-08-15 16:27:01');
INSERT INTO `na_admin_log` VALUES ('4', 'admin', 'Group', 'index', '', '1', 'admin', '2130706433', '2017-08-15 16:30:54');
INSERT INTO `na_admin_log` VALUES ('5', 'admin', 'Admin', 'public_edit_info', '', '1', 'admin', '2130706433', '2017-08-15 16:30:56');
INSERT INTO `na_admin_log` VALUES ('6', 'admin', 'Admin', 'index', '', '1', 'admin', '2130706433', '2017-08-15 16:30:57');
INSERT INTO `na_admin_log` VALUES ('7', 'admin', 'Menu', 'info', '', '1', 'admin', '2130706433', '2017-08-15 16:31:36');
INSERT INTO `na_admin_log` VALUES ('8', 'admin', 'Menu', 'add', '', '1', 'admin', '2130706433', '2017-08-15 16:32:53');
INSERT INTO `na_admin_log` VALUES ('9', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-15 16:34:22');
INSERT INTO `na_admin_log` VALUES ('10', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-15 16:34:37');
INSERT INTO `na_admin_log` VALUES ('11', 'admin', 'Player', 'favicon.ico', '', '1', 'admin', '2130706433', '2017-08-15 16:34:37');
INSERT INTO `na_admin_log` VALUES ('12', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-15 16:35:09');
INSERT INTO `na_admin_log` VALUES ('13', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-15 16:35:24');
INSERT INTO `na_admin_log` VALUES ('14', 'admin', 'Index', 'favicon.ico', '', '1', 'admin', '2130706433', '2017-08-16 10:32:17');
INSERT INTO `na_admin_log` VALUES ('15', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-16 11:33:24');
INSERT INTO `na_admin_log` VALUES ('16', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-16 11:33:32');
INSERT INTO `na_admin_log` VALUES ('17', 'admin', 'Player', 'favicon.ico', '', '1', 'admin', '2130706433', '2017-08-16 11:33:32');
INSERT INTO `na_admin_log` VALUES ('18', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-16 11:33:51');
INSERT INTO `na_admin_log` VALUES ('19', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-16 11:35:31');
INSERT INTO `na_admin_log` VALUES ('20', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-16 11:35:46');
INSERT INTO `na_admin_log` VALUES ('21', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-16 11:36:11');
INSERT INTO `na_admin_log` VALUES ('22', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-16 11:37:30');
INSERT INTO `na_admin_log` VALUES ('23', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-16 11:38:54');
INSERT INTO `na_admin_log` VALUES ('24', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-16 11:46:38');
INSERT INTO `na_admin_log` VALUES ('25', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-16 12:15:30');
INSERT INTO `na_admin_log` VALUES ('26', 'admin', 'Player', 'index', '', '1', 'admin', '2130706433', '2017-08-16 12:19:14');
INSERT INTO `na_admin_log` VALUES ('27', 'admin', 'Menu', 'favicon.ico', '', '1', 'admin', '2130706433', '2017-08-16 12:20:02');
INSERT INTO `na_admin_log` VALUES ('28', 'admin', 'Menu', 'info', '', '1', 'admin', '2130706433', '2017-08-16 12:20:13');
INSERT INTO `na_admin_log` VALUES ('29', 'admin', 'Menu', 'add', '', '1', 'admin', '2130706433', '2017-08-16 12:21:20');
INSERT INTO `na_admin_log` VALUES ('30', 'admin', 'GameReports', 'index', '', '1', 'admin', '2130706433', '2017-08-16 14:41:41');
INSERT INTO `na_admin_log` VALUES ('31', 'admin', 'Log', 'favicon.ico', '', '1', 'admin', '2130706433', '2017-08-16 14:43:51');
-- ----------------------------
-- Table structure for na_game_reports
-- ----------------------------
DROP TABLE IF EXISTS `na_game_reports`;
CREATE TABLE `na_game_reports` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`gameName` varchar(50) DEFAULT NULL COMMENT '游戏名称',
`updatedAt` int(11) DEFAULT NULL COMMENT '更新时间',
`totalPays` float DEFAULT NULL COMMENT '中奖金额',
`totalBets` float DEFAULT NULL COMMENT '投注金额',
`totalBetCount` int(11) DEFAULT NULL COMMENT '投注次数',
`totalPlayers` int(11) DEFAULT NULL COMMENT '投注用户数',
`avgRTP` float DEFAULT NULL,
`parent` varchar(36) DEFAULT NULL COMMENT '对应商户的uid',
`wct` float(10,2) DEFAULT NULL COMMENT '洗马量',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of na_game_reports
-- ----------------------------
-- ----------------------------
-- Table structure for na_menu
-- ----------------------------
DROP TABLE IF EXISTS `na_menu`;
CREATE TABLE `na_menu` (
`id` smallint(6) unsigned NOT NULL AUTO_INCREMENT,
`name` char(40) NOT NULL DEFAULT '',
`parentid` smallint(6) DEFAULT '0',
`icon` varchar(20) NOT NULL DEFAULT '',
`c` varchar(20) DEFAULT NULL,
`a` varchar(20) DEFAULT NULL,
`data` varchar(50) NOT NULL DEFAULT '',
`tip` varchar(255) NOT NULL DEFAULT '' COMMENT '提示',
`group` varchar(50) DEFAULT '' COMMENT '分组',
`listorder` smallint(6) unsigned NOT NULL DEFAULT '999',
`display` tinyint(1) NOT NULL DEFAULT '1' COMMENT '是否显示(1:显示,2:不显示)',
`updatetime` int(11) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `listorder` (`listorder`),
KEY `parentid` (`parentid`)
) ENGINE=InnoDB AUTO_INCREMENT=67 DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of na_menu
-- ----------------------------
INSERT INTO `na_menu` VALUES ('1', '管理设置', '0', 'fa-users', 'Admin', 'admin', '', '', '', '1', '1', '1476175413');
INSERT INTO `na_menu` VALUES ('2', '管理员管理', '1', '', 'Admin', 'index', '', '', '', '0', '1', '1476175413');
INSERT INTO `na_menu` VALUES ('3', '详情', '2', '', 'Admin', 'info', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('4', '添加', '2', '', 'Admin', 'add', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('5', '修改', '2', '', 'Admin', 'edit', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('6', '删除', '2', '', 'Admin', 'del', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('7', '权限管理', '1', '', 'Group', 'index', '', '', '', '0', '1', '1488781190');
INSERT INTO `na_menu` VALUES ('8', '详情', '7', '', 'Group', 'info', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('9', '添加', '7', '', 'Group', 'add', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('10', '修改', '7', '', 'Group', 'edit', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('11', '删除', '7', '', 'Group', 'del', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('12', '菜单管理', '18', '', 'Menu', 'index', '', '', '', '0', '1', '1476175413');
INSERT INTO `na_menu` VALUES ('13', '查看', '12', '', 'Menu', 'info', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('14', '添加', '12', '', 'Menu', 'add', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('15', '修改', '12', '', 'Menu', 'edit', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('16', '删除', '12', '', 'Menu', 'del', '', '', '', '0', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('18', '系统设置', '0', 'fa-cogs', 'Menu', 'index', '', '', '', '2', '1', '1476175413');
INSERT INTO `na_menu` VALUES ('22', '权限设置', '2', '', 'Group', 'rule', '', '', '', '999', '2', '1476175413');
INSERT INTO `na_menu` VALUES ('23', '个人设置', '1', '', 'Admin', 'public_edit_info', '', '', '', '999', '2', '1502865964');
INSERT INTO `na_menu` VALUES ('27', '日志管理', '18', '', 'Log', 'index', '', '', '', '999', '2', '1502865919');
INSERT INTO `na_menu` VALUES ('31', '系统首页', '0', 'fa-bank', 'Index', 'index', '', '', '', '0', '1', '1476175413');
INSERT INTO `na_menu` VALUES ('48', '商户管理', '0', 'fa-user-plus', 'Merchant', 'index', '', '', '', '999', '1', '1502867010');
INSERT INTO `na_menu` VALUES ('49', '报表统计', '0', 'fa-area-chart', 'GameReports', 'index', '', '', '', '999', '1', '1502866845');
INSERT INTO `na_menu` VALUES ('50', '商户信息', '48', '', 'Merchant', 'index', '', '', '', '999', '2', '1502874043');
INSERT INTO `na_menu` VALUES ('51', '玩家信息', '48', '', 'Player', 'index', '', '', '', '999', '1', '1502867129');
INSERT INTO `na_menu` VALUES ('52', '额度记录', '48', '', 'Limit', 'index', '', '', '', '999', '2', '1502874053');
INSERT INTO `na_menu` VALUES ('53', '玩家报表', '49', '', 'PlayerReports', 'index', '', '', '', '999', '1', '1502866923');
INSERT INTO `na_menu` VALUES ('54', '游戏报表', '49', '', 'GameReports', 'index', '', '', '', '999', '1', null);
INSERT INTO `na_menu` VALUES ('55', '线路商查询', '51', '', 'Player', 'xl', '', '', '', '999', '2', '1503374844');
INSERT INTO `na_menu` VALUES ('56', '商户查询', '51', '', 'Player', 'sh', '', '', '', '999', '2', '1503374863');
INSERT INTO `na_menu` VALUES ('57', '玩家用户名查询', '51', '', 'Player', 'likeUsername', '', '', '', '999', '2', '1503374878');
INSERT INTO `na_menu` VALUES ('58', '代理商查询', '51', '', 'Player', 'dl', '', '', '', '999', '2', '1503374893');
INSERT INTO `na_menu` VALUES ('59', '线路商查询', '53', '', 'PlayerReports', 'xl', '', '', '', '999', '2', null);
INSERT INTO `na_menu` VALUES ('60', '商户查询', '53', '', 'PlayerReports', 'sh', '', '', '', '999', '2', null);
INSERT INTO `na_menu` VALUES ('61', '玩家用户名查询', '53', '', 'PlayerReports', 'likeUsername', '', '', '', '999', '2', null);
INSERT INTO `na_menu` VALUES ('62', '代理商查询', '53', '', 'PlayerReports', 'dl', '', '', '', '999', '2', null);
INSERT INTO `na_menu` VALUES ('63', '线路商查询', '54', '', 'GameReports', 'xl', '', '', '', '999', '2', null);
INSERT INTO `na_menu` VALUES ('64', '商户查询', '54', '', 'GameReports', 'sh', '', '', '', '999', '2', null);
INSERT INTO `na_menu` VALUES ('65', '代理商查询', '54', '', 'GameReports', 'dl', '', '', '', '999', '2', null);
INSERT INTO `na_menu` VALUES ('66', '游戏名称查询', '54', '', 'GameReports', 'likeGamename', '', '', '', '999', '2', null);
-- ----------------------------
-- Table structure for na_merchants
-- ----------------------------
DROP TABLE IF EXISTS `na_merchants`;
CREATE TABLE `na_merchants` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`username` varchar(255) DEFAULT NULL,
`msn` varchar(255) DEFAULT NULL,
`displayName` varchar(255) DEFAULT NULL,
`userId` int(11) DEFAULT NULL,
`userStatus` varchar(255) DEFAULT NULL,
`createdAt` datetime DEFAULT NULL,
`updatedAt` datetime DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of na_merchants
-- ----------------------------
-- ----------------------------
-- Table structure for na_players
-- ----------------------------
DROP TABLE IF EXISTS `na_players`;
CREATE TABLE `na_players` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT COMMENT '主键自增长的ID',
`uid` varchar(36) DEFAULT NULL COMMENT '玩家的uid',
`parent` varchar(36) DEFAULT NULL COMMENT '所属商户的uid',
`username` varchar(50) DEFAULT NULL COMMENT '玩家账户',
`nickname` varchar(10) DEFAULT NULL COMMENT '玩家昵称',
`suffix` varchar(20) DEFAULT NULL COMMENT '玩家前缀',
`msn` varchar(5) DEFAULT NULL COMMENT '线路号',
`createdAt` datetime DEFAULT NULL COMMENT '创建时间',
`balance` float DEFAULT NULL COMMENT '玩家余额',
`waterflow` float DEFAULT NULL COMMENT '玩家流水总额',
`content` text COMMENT 'josn格式的数据',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of na_players
-- ----------------------------
-- ----------------------------
-- Table structure for na_player_reports
-- ----------------------------
DROP TABLE IF EXISTS `na_player_reports`;
CREATE TABLE `na_player_reports` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`username` varchar(50) DEFAULT NULL COMMENT '玩家账户',
`totalBetCount` int(11) DEFAULT NULL COMMENT '投注次数',
`avgRTP` float DEFAULT NULL,
`totalPays` float DEFAULT NULL COMMENT '中奖金额',
`totalBets` float DEFAULT NULL COMMENT '投注金额',
`balance` float DEFAULT NULL COMMENT '玩家余额',
`waterflow` float DEFAULT NULL COMMENT '流水总额',
`msn` varchar(5) DEFAULT NULL,
`parent` varchar(36) DEFAULT NULL COMMENT '对应商户的uid',
`wct` float(10,2) DEFAULT NULL COMMENT '洗马量',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of na_player_reports
-- ----------------------------
-- ----------------------------
-- Table structure for na_users
-- ----------------------------
DROP TABLE IF EXISTS `na_users`;
CREATE TABLE `na_users` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`uid` varchar(36) DEFAULT NULL COMMENT '管理员,线路商,商户,代理,的ID',
`parent` varchar(36) DEFAULT NULL COMMENT '上级用户uid',
`ancestor` varchar(36) DEFAULT NULL COMMENT '根用户uid',
`username` varchar(50) DEFAULT NULL COMMENT '用户账号',
`suffix` varchar(10) DEFAULT NULL COMMENT '当前用户前缀',
`msn` varchar(5) DEFAULT NULL COMMENT '当前用户线路号',
`content` text COMMENT 'json格式数据',
`createdAt` int(11) DEFAULT NULL COMMENT '创建时间',
`updatedAt` int(11) DEFAULT NULL COMMENT '更新时间',
`levelindex` varchar(1800) DEFAULT NULL COMMENT '层级关系所属',
`wcr` float(10,2) DEFAULT NULL COMMENT '洗马比',
`ptr` float(10,2) DEFAULT NULL COMMENT '成数',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of na_users
-- ----------------------------
<file_sep>/.svn/pristine/c9/c93b5b5423500e90eb869aec2c7390599da81320.svn-base
<?php
namespace application\admin\controller;
use think\Controller;
use think\Db;
class ApiController extends Controller{
//写入管理员数据
public function addAdminInfo(){
header("Access-Control-Allow-Origin:*");
$info=file_get_contents('php://input');
$data=json_decode($info);
$datainfo['uid']=$data->uid;
$datainfo['parent']=$data->parent;
$datainfo['ancestor']=$data->ancestor;
$datainfo['username']=$data->username;
$datainfo['nickname']=$data->nickname;
$datainfo['suffix']=$data->suffix;
$datainfo['msn']=$data->msn;
$datainfo['content']=$data->content;
$datainfo['createdAt']=date("Y-m-d H:i:s");
$datainfo['updatedAt']=date("Y-m-d H:i:s");
$datainfo['levelindex']=$data->levelindex;
$datainfo['wcr']=$data->wcr;
$datainfo['ptr']=$data->ptr;
$datainfo['linecfg']=$data->linecfg;
$datainfo['betcfg']=$data->betcfg;
if(!$datainfo['uid'] || !$datainfo['suffix'] ){
return json(['code'=>0,'message'=>'数据不完整']);
}
$uinfo=Db::name('users')->where('uid',$data->uid)->find();
if($uinfo){
unset($datainfo['createdAt']);
$res=Db::name('users')->where('id',$uinfo['id'])->update($datainfo);
if($res){
return json(['code'=>1,'message'=>'更新成功']);
}else{
return json(['code'=>0,'message'=>'更新失败']);
}
}else{
$res=Db::name('users')->insert($datainfo);
if($res){
$adminId = Db::name('users')->getLastInsID();
$groups=Db::name('adminGroup')->field('id')->select();
foreach($groups as $v) {
Db::name('adminGroupAccess')->insert(['uid' => $adminId, 'group_id' => $v['id']]);//生成权限
}
return json(['code'=>1,'message'=>'插入成功']);
}else{
return json(['code'=>0,'message'=>'插入失败']);
}
}
}
//写入玩家数据
public function addPlayers(){
header("Access-Control-Allow-Origin:*");
$info=file_get_contents('php://input');
$data=json_decode($info);
$playerinfo['uid']=$data->uid;
$playerinfo['parent']=$data->parent;
$playerinfo['username']=$data->username;
$playerinfo['nickname']=$data->nickname;
$playerinfo['suffix']=$data->suffix;
$playerinfo['msn']=$data->msn;
$playerinfo['createdAt']=date("Y-m-d H:i:s",time());
$playerinfo['balance']=$data->balance;
$playerinfo['waterflow']=$data->waterflow;
$playerinfo['content']=$data->content;
if(!$playerinfo['uid'] || !$playerinfo['parent'] || !$playerinfo['username']){
return json(['code'=>0,'message'=>'数据不完整']);
}
$uinfo=Db::name('players')->where('uid',$data->uid)->find();
if($uinfo){
$res=Db::name('players')->where('id',$uinfo['id'])->update($playerinfo);
if($res){
return json(['code'=>1,'message'=>'操作成功']);
}else{
return json(['code'=>0,'message'=>'操作失败']);
}
}else{
$res=Db::name('players')->insert($playerinfo);
if($res){
return json(['code'=>1,'message'=>'操作成功']);
}else{
return json(['code'=>0,'message'=>'操作失败']);
}
}
}
//写入游戏报表数据
public function addGameReports(){
header("Access-Control-Allow-Origin:*");
$info=file_get_contents('php://input');
$data=json_decode($info);
$playerinfo['gameName']=$data->gameName;
$playerinfo['updatedAt']=$data->updatedAt;
$playerinfo['totalPays']=$data->totalPays;
$playerinfo['totalBets']=$data->totalBets;
$playerinfo['totalBetCount']=$data->totalBetCount;
$playerinfo['totalPlayers']=$data->totalPlayers;
$playerinfo['avgRTP']=$data->avgRTP;
$playerinfo['parent']=$data->parent;
$playerinfo['wct']=$data->wct;
if(!$playerinfo['gameName'] || !$playerinfo['parent']){
return json(['code'=>0,'message'=>'数据不完整']);
}
$res=Db::name('gameReports')->insert($playerinfo);
if($res){
return json(['code'=>1,'message'=>'操作成功']);
}else{
return json(['code'=>0,'message'=>'操作失败']);
}
}
//写入玩家报表数据之塔罗之谜
public function addPlayerReports(){
header("Access-Control-Allow-Origin:*");
$info=file_get_contents('php://input');
$data=json_decode($info);
$playerinfo['pid']=$data->pid;
$playerinfo['username']=$data->username;
$playerinfo['totalBetCount']=$data->totalBetCount;
$playerinfo['avgRTP']=$data->avgRTP;
$playerinfo['totalPays']=$data->totalPays;
$playerinfo['totalBets']=$data->totalBets;
$playerinfo['balance']=$data->balance;
$playerinfo['waterflow']=$data->waterflow;
$playerinfo['msn']=$data->msn;
$playerinfo['parent']=$data->parent;
$playerinfo['wct']=$data->wct;
if(!$playerinfo['username'] || !$playerinfo['parent'] || !$playerinfo['pid']){
return json(['code'=>0,'message'=>'数据不完整']);
}
$prinfo=Db::name('playerReports')->where('pid',$playerinfo['pid'])->find();
if($prinfo){//更新p
$res=Db::name('playerReports')->where('id',$prinfo['id'])->update($playerinfo);
if($res){
return json(['code'=>1,'message'=>'更新成功']);
}else{
return json(['code'=>0,'message'=>'更新失败']);
}
}else{
$res=Db::name('playerReports')->insert($playerinfo);
if($res){
return json(['code'=>1,'message'=>'操作成功']);
}else{
return json(['code'=>0,'message'=>'操作失败']);
}
}
}
//写入玩家报表数据之塔罗之谜
public function addPlayerReportsB(){
header("Access-Control-Allow-Origin:*");
$info=file_get_contents('php://input');
$data=json_decode($info);
$playerinfo['pid']=$data->pid;
$playerinfo['username']=$data->username;
$playerinfo['totalBetCount']=$data->totalBetCount;
$playerinfo['avgRTP']=$data->avgRTP;
$playerinfo['totalPays']=$data->totalPays;
$playerinfo['totalBets']=$data->totalBets;
$playerinfo['balance']=$data->balance;
$playerinfo['waterflow']=$data->waterflow;
$playerinfo['msn']=$data->msn;
$playerinfo['parent']=$data->parent;
$playerinfo['wct']=$data->wct;
if(!$playerinfo['username'] || !$playerinfo['parent'] || !$playerinfo['pid']){
return json(['code'=>0,'message'=>'数据不完整']);
}
$prinfo=Db::name('playerReportsB')->where('pid',$playerinfo['pid'])->find();
if($prinfo){//更新p
$res=Db::name('playerReportsB')->where('id',$prinfo['id'])->update($playerinfo);
if($res){
return json(['code'=>1,'message'=>'更新成功']);
}else{
return json(['code'=>0,'message'=>'更新失败']);
}
}else{
$res=Db::name('playerReportsB')->insert($playerinfo);
if($res){
return json(['code'=>1,'message'=>'操作成功']);
}else{
return json(['code'=>0,'message'=>'操作失败']);
}
}
}
//写入玩家总记录数
public function totalReports(){
header("Access-Control-Allow-Origin:*");
$info=file_get_contents('php://input');
$data=json_decode($info);
$playerinfo['gameUserId']=$data->gameUserId;
$playerinfo['username']=$data->username;
$playerinfo['nickname']=$data->nickname;
$playerinfo['balance']=$data->balance;
$playerinfo['parentId']=$data->parentId;
$playerinfo['betCount']=$data->betCount;
$playerinfo['winlose']=$data->winlose;
$playerinfo['totalBet']=$data->totalBet;
$playerinfo['wcr']=$data->wcr;
$playerinfo['ret']=$data->ret;
$playerinfo['agentBalance']=$data->agentBalance;
$playerinfo['agentRate']=$data->agentRate;
$playerinfo['giveback']=$data->giveback;
if(!$playerinfo['gameUserId'] || !$playerinfo['parentId'] ){
return json(['code'=>0,'message'=>'数据不完整']);
}
$prinfo=Db::name('totalReports')->where('gameUserId',$playerinfo['gameUserId'])->find();
if($prinfo){//更新p
$res=Db::name('totalReports')->where('id',$prinfo['id'])->update($playerinfo);
if($res){
return json(['code'=>1,'message'=>'更新成功']);
}else{
return json(['code'=>0,'message'=>'更新失败']);
}
}else{
$res=Db::name('totalReports')->insert($playerinfo);
if($res){
return json(['code'=>1,'message'=>'操作成功']);
}else{
return json(['code'=>0,'message'=>'操作失败']);
}
}
}
//写入商户数据
//新接口验证身份信息 返回登录URL ---NA平台
public function checkLoginAccess(){
header("Access-Control-Allow-Origin:*");
$info=file_get_contents('php://input');
$data=json_decode($info);
$uid=$data->id;
$timestamp=$data->timestamp;
$sign=$data->sign;
if(!$uid || !$timestamp || !$sign){
return json(['code'=>1,'msg'=>'参数不完整!']);
}
$str='id'.$uid.'timestamp'.$timestamp;
$str=urlencode($str);
$gameKey=config('tigerkey');//定义老虎机的key
$truesign=$gameKey.$str.$gameKey;
$truesign=hash('sha256',$truesign);
if($truesign!=$sign){
$jsonarr=[
'code'=>1,
'msg'=>'签名验证失败!',
];
return json($jsonarr);
}
//用uid去查找这个商户信息存在不?
$info=Db::name('users')->where('uid',$uid)->find();
if(!$info){
$jsonarr=[
'code'=>1,
'msg'=>'信息没有同步,后台没有检测到商户,请联系管理员!',
];
return json($jsonarr);
}
//取出msn /suffix;
$msn=$info['msn'];
$suffix=$info['suffix'];
$levelIndex=$info['levelindex'];
$guanli=[];
$time=time();
//定义身份
if($levelIndex==0 && $suffix=='Platform'){//管理员
//找出所有的线路商或者商户
$guanliinfos=Db::name('users')->field('uid')->where('levelindex','like','%01%')->where('msn','<>',0)->where('suffix','<>','Platform')->select();
foreach ($guanliinfos as $guanliinfo){
$guanli[]=$guanliinfo['uid'];
}
}
if($levelIndex==0 && $msn==0){//代理管理员 没有玩家的
$dailiinfos=Db::name('users')->field('uid')->where('levelindex','like','%01%')->where('msn',0)->select();
foreach ($dailiinfos as $dailiinfo){
$guanli[]=$dailiinfo['uid'];
}
}
if($msn==-1 && $levelIndex!=0){//值为null就是线路商
$xianlus=Db::name('users')->field('uid')->where('levelindex','like',"%$uid%")->select();
foreach ($xianlus as $xianlu){
$guanli[]=$xianlu['uid'];
}
}
if($msn==0 && $levelIndex!=0 ){//代理
$guanli[]=$uid;
$dailiinfos=Db::name('users')->field('uid')->where('levelindex','like',"%$uid%")->select();
foreach ($dailiinfos as $dailiinfo){
$guanli[]=$dailiinfo['uid'];
}
}
if($levelIndex!=0 && $msn!=0 && $msn !=-1){//商户
$guanli[]=$uid;
}
if(!count($guanli)){
$jsonarr=[
'code'=>1,
'msg'=>'无商户无玩家信息',
];
return json($jsonarr);
}
//生成URL
$data=[
'uid'=>$info['id'],
'time'=>$time
];
$token=serialize($data);
$token = encry_code($token);//加密
$jsonarr=[
'code'=>0,
'msg'=>'success',
'url'=>'http://'.config('naurl').'/admin/login/apilogin/token/'.$token.'.html'
];
return json($jsonarr);
}
//线路
public function treeXianLu($uid){
$xinlus=Db::name('users')->where('parent',$uid)->select();
static $uids=array();
foreach ($xinlus as $xinlu){
if($xinlu['msn']){
$uids[]=$xinlu['uid'];
}else{
$this->treeXianLu($xinlu['uid']);
}
}
return $uids;
}
//代理
public function treeDaiLi(){
}
} | dbef0c956b571c4e863b9c7bd3a922c74d979e8a | [
"SQL",
"PHP"
] | 7 | PHP | xj0707/tigergame | 4efface5b2626d6446e99d25be779f9939cc4ac1 | 9814f1a0c8578d34478461b90f39551b97df483c | |
refs/heads/master | <file_sep>// import preact
import { h, render, Component } from 'preact';
// import stylesheets for ipad & button
import style from './style';
import style_iphone from '../button/style_iphone';
//import undersground stylesheet
import undergroundStyle from './styleUnderground';
// import jquery for API calls
import $ from 'jquery';
// import the Button component
import Button from '../button';
export default class Iphone extends Component {
//var Iphone = React.createClass({
// a constructor with initial set states
constructor(props){
super(props);
// temperature state
this.state.temp = "";
// button display state
//this.setState({ display: true });
}
// a call to fetch weather data via wunderground for London
componentDidMount(){
this.fetchWeatherDataLondon();
//open hourly forecast nav
document.getElementById("hourlyNav").style.width = "414px";
//close daily forecast nav
document.getElementById("dailyNav").style.width = "0px";
this.fetchAllLines();
}
//FETCH UNDERGROUND DATA
fetchAllLines = () => {
//fetch all underground lines
var linesUrl = "https://api.tfl.gov.uk/line/mode/tube,overground,dlr,tflrail/status?detail=true&app_id=bd4e5952&app_key=c132ec0ed9e2a5a7d767bf0b8c393ea5"
$.ajax({
url: linesUrl,
dataType: "json",
success: this.parseLineStatus,
error : function(req, err){ console.log('API call failed ' + err); }
});
}
//FETCH DAILY AND HOURLY FORECAST DATA
// a call to fetch weather data via wunderground for London
fetchWeatherDataLondon = () => {
// API URL with a structure of : http://api.wunderground.com/api/key/feature/q/country-code/city.json
//Change the background image
document.getElementById("backgroundImage").style.backgroundImage = "url('../../assets/backgrounds/London.jpg')";
//change Underground icon visibility
document.getElementById("train").style.visibility = "visible";
//fetch daily forecast
this.fetchDailyForecastLondon();
this.fetchHourlyForecastLondon();
this.closeNav();
var url = "http://api.wunderground.com/api/b5f4673cdd4aa31e/conditions/q/UK/London.json";
$.ajax({
url: url,
dataType: "jsonp",
success : this.parseResponse,
error : function(req, err){ console.log('API call failed ' + err); }
});
// once the data grabbed, hide the button
//this.setState({ display: false });
}
//4 day forecast London
fetchDailyForecastLondon = () => {
var dailyForecast = "http://api.wunderground.com/api/b5f4673cdd4aa31e/forecast10day/q/UK/London.json";
$.ajax({
url: dailyForecast,
dataType: "jsonp",
success : this.parseDailyForecast,
error : function(req, err){ console.log('API call failed ' + err); }
});
}
// hourly forecast London
fetchHourlyForecastLondon = () => {
var hourlyForecast = "http://api.wunderground.com/api/b5f4673cdd4aa31e/hourly/q/UK/London.json";
$.ajax({
url: hourlyForecast,
dataType: "jsonp",
success : this.parseHourlyForecast,
error : function(req, err){ console.log('API call failed ' + err); }
});
}
// a call to fetch weather data via wunderground for New York
fetchWeatherDataNY = () => {
// API URL with a structure of : http://api.wunderground.com/api/key/feature/q/country-code/city.json
//Change the background image
document.getElementById("backgroundImage").style.backgroundImage = "url('../../assets/backgrounds/NY.jpg')";
//change Underground icon visibility
document.getElementById("train").style.visibility = "hidden";
//fetch daily forecast
this.fetchDailyForecastNY();
this.closeNav();
var url = "http://api.wunderground.com/api/b5f4673cdd4aa31e/conditions/q/US/NewYork.json";
$.ajax({
url: url,
dataType: "jsonp",
success : this.parseResponse,
error : function(req, err){ console.log('API call failed ' + err); }
});
// once the data grabbed, hide the button
//this.setState({ display: false });
}
fetchDailyForecastNY = () => {
var dailyForecast = "http://api.wunderground.com/api/b5f4673cdd4aa31e/forecast10day/q/US/NewYork.json";
//console.log(dailyForecast);
$.ajax({
url: dailyForecast,
dataType: "jsonp",
success : this.parseDailyForecast,
error : function(req, err){ console.log('API call failed ' + err); }
});
}
// a call to fetch weather data via wunderground for Singapore
fetchWeatherDataSingapore = () => {
// API URL with a structure of : http://api.wunderground.com/api/key/feature/q/country-code/city.json
//Change the background image
document.getElementById("backgroundImage").style.backgroundImage = "url('../../assets/backgrounds/Singapore.jpg')";
//change Underground icon visibility
document.getElementById("train").style.visibility = "hidden";
//fetch daily forecast
this.fetchDailyForecastSingapore();
this.closeNav();
var url = "http://api.wunderground.com/api/b5f4673cdd4aa31e/conditions/q/SG/Singapore.json";
$.ajax({
url: url,
dataType: "jsonp",
success : this.parseResponse,
error : function(req, err){ console.log('API call failed ' + err); }
});
// once the data grabbed, hide the button
//this.setState({ display: false });
}
fetchDailyForecastSingapore = () => {
var dailyForecast = "http://api.wunderground.com/api/b5f4673cdd4aa31e/forecast10day/q/SG/Singapore.json";
//console.log(dailyForecast);
$.ajax({
url: dailyForecast,
dataType: "jsonp",
success : this.parseDailyForecast,
error : function(req, err){ console.log('API call failed ' + err); }
});
}
// a call to fetch weather data via wunderground for Paris
fetchWeatherDataParis = () => {
// API URL with a structure of : http://api.wunderground.com/api/key/feature/q/country-code/city.json
//Change the background image
document.getElementById("backgroundImage").style.backgroundImage = "url('../../assets/backgrounds/Paris.jpg')";
//change Underground icon visibility
document.getElementById("train").style.visibility = "hidden";
//fetch daily forecast
this.fetchDailyForecastParis();
this.closeNav();
var url = "http://api.wunderground.com/api/b5f4673cdd4aa31e/conditions/q/FR/Paris.json";
$.ajax({
url: url,
dataType: "jsonp",
success : this.parseResponse,
error : function(req, err){ console.log('API call failed ' + err); }
});
// once the data grabbed, hide the button
//this.setState({ display: false });
}
fetchDailyForecastParis = () => {
var dailyForecast = "http://api.wunderground.com/api/b5f4673cdd4aa31e/forecast10day/q/FR/Paris.json";
//console.log(dailyForecast);
$.ajax({
url: dailyForecast,
dataType: "jsonp",
success : this.parseDailyForecast,
error : function(req, err){ console.log('API call failed ' + err); }
});
}
// a call to fetch weather data via wunderground for Rome
fetchWeatherDataRome = () => {
// API URL with a structure of : http://api.wunderground.com/api/key/feature/q/country-code/city.json
//Change the background image
document.getElementById("backgroundImage").style.backgroundImage = "url('../../assets/backgrounds/Rome.jpg')";
//change Underground icon visibility
document.getElementById("train").style.visibility = "hidden";
//fetch daily forecast
this.fetchDailyForecastRome();
this.closeNav();
var url = "http://api.wunderground.com/api/b5f4673cdd4aa31e/conditions/q/IT/Rome.json";
$.ajax({
url: url,
dataType: "jsonp",
success : this.parseResponse,
error : function(req, err){ console.log('API call failed ' + err); }
});
// once the data grabbed, hide the button
//this.setState({ display: false });
}
fetchDailyForecastRome = () => {
var dailyForecast = "http://api.wunderground.com/api/b5f4673cdd4aa31e/forecast10day/q/IT/Rome.json";
//console.log(dailyForecast);
$.ajax({
url: dailyForecast,
dataType: "jsonp",
success : this.parseDailyForecast,
error : function(req, err){ console.log('API call failed ' + err); }
});
}
//SIDE NAVIGATIONAL BAR COMPONENTS MANAGEMENT
//Open the city side navigation bar
openNav = () => {
document.getElementById("myNav").style.width = "415px";
}
//Close city the side navigation bar
closeNav = () => {
document.getElementById("myNav").style.width = "0px";
}
//Open the underground side navigation bar
openNav2 = () => {
document.getElementById("myNav2").style.width = "415px";
}
//Close underground the side navigation bar
closeNav2 = () => {
document.getElementById("myNav2").style.width = "0px";
}
//Show hour forecast div section
hourlyNav = () => {
document.getElementById("hourlyNav").style.width = "415px";
document.getElementById("dailyNav").style.width = "0px";
}
//Show days forecast div section
dailyNav = () => {
document.getElementById("hourlyNav").style.width = "0px";
document.getElementById("dailyNav").style.width = "415px";
}
//RENDER COMPONENT OF APPLICATION
render(){
// check if temperature data is fetched, if so add the sign styling to the page
const tempStyles = this.state.temp ? `${style.temperature} ${style.filled}` : style.temperature;
// display all weather data
return (
<div id="backgroundImage" class={ style.container }>
{/* Container for the side bar menu icon */}
<div class={ style.iconMenuContainer }>
<img src="../../assets/weather-icon/menu64px.png" class={ style.menu } onClick={ this.openNav }> </img>
</div>
{/* Container for the side bar menu icon */}
<div class={ style.iconMenuContainer2 }>
<img id="train" src="../../assets/weather-icon/Tfl2.png" class={ style.Tfl } onClick={ this.openNav2 }> </img>
</div>
{/* Side navigation bar for cities */}
<div id="myNav" class={ style.sideNav }>
<span class={ style.close } onClick={ this.closeNav }> × </span>
{/* Cities list container */}
<div class={ style.citiesContainer }>
<span class={ style.cities } onClick={ this.fetchWeatherDataLondon }> London </span>
<span class={ style.cities } onClick={ this.fetchWeatherDataNY }> New York </span>
<span class={ style.cities } onClick={ this.fetchWeatherDataParis }> Paris </span>
<span class={ style.cities } onClick={ this.fetchWeatherDataRome }> Rome </span>
<span class={ style.cities } onClick={ this.fetchWeatherDataSingapore }> Singapore </span>
</div>
</div>
{/* Side navigation bar for underground */}
<div id="myNav2" class={ style.sideNav2 }>
<span class={ style.close2 } onClick={ this.closeNav2 }> × </span>
{/* Underground list container */}
<div class={ undergroundStyle.underground }>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.Bakerloo }> {this.state.line1} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status1} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.Central }> {this.state.line2} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status2} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.Circle }> {this.state.line3} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status3} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.District }> {this.state.line4} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status4} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.DLR }> {this.state.line5} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status5} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.Hammersmith }> {this.state.line6} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status6} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.Jubilee }> {this.state.line7} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status7} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.Overground }> {this.state.line8} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status8} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.Metropolitan }> {this.state.line9} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status9} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.Northern }> {this.state.line10} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status10} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.Picadilly }> {this.state.line11} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status11} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.TfL }> {this.state.line12} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status12} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.Victoria }> {this.state.line13} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status13} </span>
</div>
<div class={ undergroundStyle.undergroundLinesStatusContainer } >
<span class={ undergroundStyle.WaterlooCity }> {this.state.line14} </span>
<span class={ undergroundStyle.undergroundStatus }> {this.state.status14} </span>
</div>
</div>
</div>
{/* Rendering the conditions information in the screen */}
<div class={ style.header }>
<div class={ style.city }> { this.state.locate } </div>
<div class={ style.conditions }> { this.state.cond } </div>
<span class={ tempStyles }> { this.state.temp } </span>
</div>
<div class={ style.details }> </div>
{/*
<div class={ style_iphone.container }>
{ this.state.display ? <Button class={ style_iphone.button } clickFunction={ this.fetchWeatherData }/ > : null }
</div>
*/}
{/* Daily and hourly buttons rendering */}
<div class={ style.buttonContainer }>
<button id="hourButton" class={ style.buttonStyle1 } onClick={ this.hourlyNav }> Hourly </button>
<button id="dayButton" class={ style.buttonStyle2 } onClick={ this.dailyNav }> Daily </button>
</div>
{/* Daily and hourly forecast container */}
<div class={ style.hourlyDailyContainer }>
{/* Hourly forecast display */}
<div id="hourlyNav" class={ style.hourlyForecastContainer }>
{/* printing data from api hour 1 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour1 } </span>
</div>
<div class={ style.hourlyCondition }>
<span> <img src={ this.state.img1 } class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp1 } </span>
</div>
</div>
{/* printing data from api hour 2 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour2 } </span>
</div>
<div class={ style.hourlyCondition }>
<span> <img src={this.state.img2} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp2 } </span>
</div>
</div>
{/* printing data from api hour 3 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour3 } </span>
</div>
<div class={ style.hourlyCondition }>
<span> <img src={this.state.img3} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp3 } </span>
</div>
</div>
{/* printing data from api hour 4 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour4 } </span>
</div>
<div class={ style.hourlyCondition }>
<span> <img src={this.state.img4} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp4 } </span>
</div>
</div>
{/* printing data from api hour 5 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour5 } </span>
</div>
<div class={ style.hourlyCondition }>
<span> <img src={this.state.img5} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp5 } </span>
</div>
</div>
{/* printing data from api hour 6 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour6 } </span>
</div>
<div class={ style.hourlyCondition }>
<span> <img src={this.state.img6} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp6 } </span>
</div>
</div>
{/* printing data from api hour 7 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour7 } </span>
</div>
<div class={ style.hourlyCondition }>
<span> <img src={this.state.img7} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp7 } </span>
</div>
</div>
{/* printing data from api hour 8 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour8 } </span>
</div>
<div class={ style.hourlyCondition }>
<span> <img src={this.state.img8} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp8 } </span>
</div>
</div>
{/* printing data from api hour 9 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour9 } </span>
</div>
<div class={ style.hourlyCondition }>
<span> <img src={this.state.img9} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp9 } </span>
</div>
</div>
{/* printing data from api hour 10 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour10 } </span>
</div>
<div class={ style.hourlyCondition }>
<span> <img src={this.state.img10} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp10 } </span>
</div>
</div>
{/* printing data from api hour 11 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour11 } </span>
</div>
<div class={ style.hourlyCondition }>
<span> <img src={this.state.img11} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp11 } </span>
</div>
</div>
{/* printing data from api hour 12 */}
<div class={ style.dayContainer }>
<div class={ style.hour }>
<span class={ style.textStyle }> { this.state.hour12 } </span>
</div>
<div class={ style.hourlyCondition }>
<span><img src={this.state.img12} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.hourlyTemp }>
<span> { this.state.temp12 } </span>
</div>
</div>
</div>
{/* Daily forecast display */}
<div id="dailyNav" class={ style.dailyForecastContainer }>
{/* printing data from api day 1 */}
<div class={ style.dayContainer }>
<div class={ style.day }>
<span class={ style.textStyle}> { this.state.Day1} </span>
</div>
<div class={ style.dayCondition }>
<span> <img src={ this.state.img1 } class={ style.conditionIcon } /> </span>
</div>
<div class={ style.dayHighTemp }>
<span> { this.state.dayHigh1 } </span>
</div>
<div class={ style.dayLowTemp }>
<span> { this.state.dayLow1 } </span>
</div>
</div>
{/* printing data from api day 2 */}
<div class={ style.dayContainer }>
<div class={ style.day }>
<span class={ style.textStyle }> { this.state.Day2 } </span>
</div>
<div class={ style.dayCondition }>
<span> <img src={ this.state.img2 } class={ style.conditionIcon } /> </span>
</div>
<div class={ style.dayHighTemp }>
<span> { this.state.dayHigh2 } </span>
</div>
<div class={ style.dayLowTemp }>
<span> { this.state.dayLow2 } </span>
</div>
</div>
{/* printing data from api day 3 */}
<div class={ style.dayContainer }>
<div class={ style.day }>
<span class={ style.textStyle }> { this.state.Day3 } </span>
</div>
<div class={ style.dayCondition }>
<span> <img src={ this.state.img3 } class={ style.conditionIcon } /> </span>
</div>
<div class={ style.dayHighTemp }>
<span> { this.state.dayHigh3 } </span>
</div>
<div class={ style.dayLowTemp }>
<span> { this.state.dayLow3 } </span>
</div>
</div>
{/* printing data from api day 4 */}
<div class={ style.dayContainer }>
<div class={ style.day }>
<span class={ style.textStyle }> { this.state.Day4 } </span>
</div>
<div class={ style.dayCondition }>
<span> <img src={ this.state.img4 } class={ style.conditionIcon } /> </span>
</div>
<div class={ style.dayHighTemp }>
<span> { this.state.dayHigh4 } </span>
</div>
<div class={ style.dayLowTemp }>
<span> { this.state.dayLow4 } </span>
</div>
</div>
{/* printing data from api day 5 */}
<div class={ style.dayContainer }>
<div class={ style.day }>
<span class={ style.textStyle }> { this.state.Day5 } </span>
</div>
<div class={ style.dayCondition }>
<span> <img src={ this.state.img5 } class={ style.conditionIcon } /> </span>
</div>
<div class={ style.dayHighTemp }>
<span> { this.state.dayHigh5 } </span>
</div>
<div class={ style.dayLowTemp }>
<span> { this.state.dayLow5 } </span>
</div>
</div>
{/* printing data from api day 6 */}
<div class={ style.dayContainer }>
<div class={ style.day }>
<span class={ style.textStyle }> { this.state.Day6 } </span>
</div>
<div class={ style.dayCondition }>
<span> <img src={ this.state.img6 } class={ style.conditionIcon } /></span>
</div>
<div class={ style.dayHighTemp }>
<span> { this.state.dayHigh6 } </span>
</div>
<div class={ style.dayLowTemp }>
<span> { this.state.dayLow6 } </span>
</div>
</div>
{/* printing data from api day 7 */}
<div class={ style.dayContainer }>
<div class={ style.day }>
<span class={ style.textStyle }> { this.state.Day7 } </span>
</div>
<div class={ style.dayCondition }>
<span> <img src={this.state.img7} class={ style.conditionIcon } /> </span>
</div>
<div class={ style.dayHighTemp }>
<span> { this.state.dayHigh7 } </span>
</div>
<div class={ style.dayLowTemp }>
<span> { this.state.dayLow7 } </span>
</div>
</div>
</div>
</div>
</div>
);
}
parseResponse = (parsed_json) => {
var location = parsed_json['current_observation']['display_location']['city'];
var temp_c = Math.round(parsed_json['current_observation']['temp_c']);
var conditions = parsed_json['current_observation']['weather'];
// set states for fields so they could be rendered later on
this.setState({
locate: location,
temp: temp_c,
cond : conditions
});
}
//getting data from api - highest, lowest and conditions, 4 day forecast, working on the icons
parseDailyForecast = (parsed_json) => {
var count = 1;
var arrDay = new Array();
var arrHigh = new Array();
var arrLow = new Array();
var arrConditions = new Array();
var arrImg = new Array();
//using count var to get the data from subtrees 0 to 3
for(var i = 1 ; i < 8 ; i++) {
//saving data
var day = parsed_json['forecast']['simpleforecast']['forecastday'][count]['date']['weekday'];
var dayHighTemp = parsed_json['forecast']['simpleforecast']['forecastday'][count]['high']['celsius'];
var dayLowTemp = parsed_json['forecast']['simpleforecast']['forecastday'][count]['low']['celsius'];
var conditions = parsed_json['forecast']['simpleforecast']['forecastday'][count]['conditions'];
var img = parsed_json['forecast']['simpleforecast']['forecastday'][count]['icon'];
//pushing data onto the arrays
arrDay.push(day);
arrHigh.push(dayHighTemp);
arrLow.push(dayLowTemp);
arrConditions.push(conditions);
arrImg.push(img);
count++;
}
this.setState({
Day1: arrDay[0],
Day2: arrDay[1],
Day3: arrDay[2],
Day4: arrDay[3],
Day5: arrDay[4],
Day6: arrDay[5],
Day7: arrDay[6],
dayHigh1: arrHigh[0],
dayHigh2: arrHigh[1],
dayHigh3: arrHigh[2],
dayHigh4: arrHigh[3],
dayHigh5: arrHigh[4],
dayHigh6: arrHigh[5],
dayHigh7: arrHigh[6],
dayLow1: arrLow[0],
dayLow2: arrLow[1],
dayLow3: arrLow[2],
dayLow4: arrLow[3],
dayLow5: arrLow[4],
dayLow6: arrLow[5],
dayLow7: arrLow[6],
day1Conditions: arrConditions[0],
day2Conditions: arrConditions[1],
day3Conditions: arrConditions[2],
day4Conditions: arrConditions[3],
day5Conditions: arrConditions[4],
day6Conditions: arrConditions[5],
day7Conditions: arrConditions[6],
img1 : "https://icons.wxug.com/i/c/i/"+arrImg[0]+".gif",
img2 : "https://icons.wxug.com/i/c/i/"+arrImg[1]+".gif",
img3 : "https://icons.wxug.com/i/c/i/"+arrImg[2]+".gif",
img4 : "https://icons.wxug.com/i/c/i/"+arrImg[3]+".gif",
img5 : "https://icons.wxug.com/i/c/i/"+arrImg[4]+".gif",
img6 : "https://icons.wxug.com/i/c/i/"+arrImg[5]+".gif",
img7 : "https://icons.wxug.com/i/c/i/"+arrImg[6]+".gif",
});
}
// parse hourly forecast
parseHourlyForecast = (parsed_json) => {
var count = 0;
var arrHour = new Array();
var arrTemp = new Array();
var arrConditions = new Array();
var arrImg = new Array();
for(var i = 0 ; i < 12 ; i++)
{
var Hour = parsed_json['hourly_forecast'][count]['FCTTIME']['civil'];
var Temp = parsed_json['hourly_forecast'][count]['temp']['metric'];
var Conditions = parsed_json['hourly_forecast'][count]['condition'];
var img = parsed_json['hourly_forecast'][count]['icon'];
//pushing fetched data into the array
arrHour.push(Hour);
arrTemp.push(Temp);
arrConditions.push(Conditions);
arrImg.push(img);
count++;
}
this.setState({
hour1: arrHour[0],
hour2: arrHour[1],
hour3: arrHour[2],
hour4: arrHour[3],
hour5: arrHour[4],
hour6: arrHour[5],
hour7: arrHour[6],
hour8: arrHour[7],
hour9: arrHour[8],
hour10: arrHour[9],
hour11: arrHour[10],
hour12: arrHour[11],
temp1: arrTemp[0],
temp2: arrTemp[1],
temp3: arrTemp[2],
temp4: arrTemp[3],
temp5: arrTemp[4],
temp6: arrTemp[5],
temp7: arrTemp[6],
temp8: arrTemp[7],
temp9: arrTemp[8],
temp10: arrTemp[9],
temp11: arrTemp[10],
temp12: arrTemp[11],
condition1: arrConditions[0],
condition2: arrConditions[1],
condition3: arrConditions[2],
condition4: arrConditions[3],
condition5: arrConditions[4],
condition6: arrConditions[5],
condition7: arrConditions[6],
condition8: arrConditions[7],
condition9: arrConditions[8],
condition10: arrConditions[9],
condition11: arrConditions[10],
condition12: arrConditions[11],
img1 : "https://icons.wxug.com/i/c/i/"+arrImg[0]+".gif",
img2 : "https://icons.wxug.com/i/c/i/"+arrImg[1]+".gif",
img3 : "https://icons.wxug.com/i/c/i/"+arrImg[2]+".gif",
img4 : "https://icons.wxug.com/i/c/i/"+arrImg[3]+".gif",
img5 : "https://icons.wxug.com/i/c/i/"+arrImg[4]+".gif",
img6 : "https://icons.wxug.com/i/c/i/"+arrImg[5]+".gif",
img7 : "https://icons.wxug.com/i/c/i/"+arrImg[6]+".gif",
img8 : "https://icons.wxug.com/i/c/i/"+arrImg[7]+".gif",
img9 : "https://icons.wxug.com/i/c/i/"+arrImg[8]+".gif",
img10 : "https://icons.wxug.com/i/c/i/"+arrImg[9]+".gif",
img11 : "https://icons.wxug.com/i/c/i/"+arrImg[10]+".gif",
img12 : "https://icons.wxug.com/i/c/i/"+arrImg[11]+".gif"
});
}
parseLineStatus = (parsed_json) => {
var count = 0;
var arrLinesName = new Array();
var arrLinesStatus = new Array();
for(var i = 0 ; i < 14 ; i++)
{
var name = parsed_json[count]['name'];
var status = parsed_json[count]['lineStatuses'][0]['statusSeverityDescription'];
//pushing fetched data into array
arrLinesName.push(name);
arrLinesStatus.push(status);
count++;
console.log(name + "" + status);
}
this.setState({
line1: arrLinesName[0],
line2: arrLinesName[1],
line3: arrLinesName[2],
line4: arrLinesName[3],
line5: arrLinesName[4],
line6: arrLinesName[5],
line7: arrLinesName[6],
line8: arrLinesName[7],
line9: arrLinesName[8],
line10: arrLinesName[9],
line11: arrLinesName[10],
line12: arrLinesName[11],
line13: arrLinesName[12],
line14: arrLinesName[13],
status1: arrLinesStatus[0],
status2: arrLinesStatus[1],
status3: arrLinesStatus[2],
status4: arrLinesStatus[3],
status5: arrLinesStatus[4],
status6: arrLinesStatus[5],
status7: arrLinesStatus[6],
status8: arrLinesStatus[7],
status9: arrLinesStatus[8],
status10: arrLinesStatus[9],
status11: arrLinesStatus[10],
status12: arrLinesStatus[11],
status13: arrLinesStatus[12],
status14: arrLinesStatus[13]
});
}
};
| 7a6d78b2a3b0924dad8648b2e671885d59c1383d | [
"JavaScript"
] | 1 | JavaScript | dewicz/GUI | 7f5b1be581983b5fec51286f824c439253ab5879 | ddc58eb4ec8dcdfd5f6ca1b5c7fa1ef97282c5a5 | |
refs/heads/master | <file_sep>if [[ $- != *i* ]] ; then
# Shell is non-interactive. Be done now!
return
fi
#USER config
export USER_SHORTPATH=true
#enable bash completion
[ -f /etc/profile.d/bash-completion ] && source /etc/profile.d/bash-completion
# make less more friendly for non-text input files, see lesspipe(1)
[ -x /usr/bin/lesspipe ] && eval "$(lesspipe)"
# Shell variables
export PAGER=less
export EDITOR=vim
export PATH=$PATH:$HOME/bin:$HOME/node_modules/.bin:/usr/local/sbin
export LESS='-R'
export HISTCONTROL=ignoredups
export HISTSIZE=5000
export HISTFILESIZE=5000
export HISTIGNORE="&:ls:ll:la:l.:pwd:exit:clear"
if [ -d /opt/local/bin ]; then
PATH="/opt/local/bin:$PATH"
fi
if [ -d /usr/lib/cw ] ; then
PATH="/usr/lib/cw:$PATH"
fi
complete -cf sudo # Tab complete for sudo
## shopt options
shopt -s cdspell # This will correct minor spelling errors in a cd command.
shopt -s histappend # Append to history rather than overwrite
shopt -s checkwinsize # Check window after each command
shopt -s dotglob # files beginning with . to be returned in the results of path-name expansion.
shopt -s extglob # use extended globbing
## set options
set -o noclobber # prevent overwriting files with cat
set -o ignoreeof # stops ctrl+d from logging me out
# Set appropriate ls alias
case $(uname -s) in
Darwin|FreeBSD)
alias ls="ls -hFG"
;;
Linux)
alias ls="ls --color=always -hF"
;;
NetBSD|OpenBSD)
alias ls="ls -hF"
;;
esac
alias rm="rm -i"
alias mv="mv -i"
alias cp="cp -i"
alias ll="ls -hl"
alias cd..="cd .."
alias mkdir='mkdir -p -v'
alias df='df -h'
alias du='du -h -c'
alias lsd="ls -hdlf */"
alias ":q"="exit"
alias s="ls | GREP_COLOR='1;34' grep --color '.*@'"
alias l="ls"
#PS1='\h:\W \u\$ '
# Make bash check its window size after a process completes
##############################################################################
# Color variables
##############################################################################
txtblk='\e[0;30m' # Black - Regular
txtred='\e[0;31m' # Red
txtgrn='\e[0;32m' # Green
txtylw='\e[0;33m' # Yellow
txtblu='\e[0;34m' # Blue
txtpur='\e[0;35m' # Purple
txtcyn='\e[0;36m' # Cyan
txtwht='\e[0;37m' # White
bldblk='\e[1;30m' # Black - Bold
bldred='\e[1;31m' # Red
bldgrn='\e[1;32m' # Green
bldylw='\e[1;33m' # Yellow
bldblu='\e[1;34m' # Blue
bldpur='\e[1;35m' # Purple
bldcyn='\e[1;36m' # Cyan
bldwht='\e[1;37m' # White
unkblk='\e[4;30m' # Black - Underline
undred='\e[4;31m' # Red
undgrn='\e[4;32m' # Green
undylw='\e[4;33m' # Yellow
undblu='\e[4;34m' # Blue
undpur='\e[4;35m' # Purple
undcyn='\e[4;36m' # Cyan
undwht='\e[4;37m' # White
bakblk='\e[40m' # Black - Background
bakred='\e[41m' # Red
badgrn='\e[42m' # Green
bakylw='\e[43m' # Yellow
bakblu='\e[44m' # Blue
bakpur='\e[45m' # Purple
bakcyn='\e[46m' # Cyan
bakwht='\e[47m' # White
txtrst='\e[0m' # Text Reset
##############################################################################
short_path() {
echo "$PWD" | sed "s|$HOME|~|g" | sed 's|/\(...\)[^/]*|/\1|g'
}
if [ $(id -u) -eq 0 ]; then # you are root, set red colour prompt
export PS1="[\[$txtred\]\u\[$txtylw\]@\[$txtrst\]\h] \[$txtgrn\]\W\[$txtrst\]# "
else
if $USER_SHORTPATH; then
pth='$(eval "short_path")'
else
pth=$PWD
fi
export PS1="\[$txtcyn\]\D{%m-%d} \A \[$bldcyn\]$pth $ \[$txtrst\]"
#shopt -s extdebug; trap "tput sgr0" DEBUG
export SUDO_PS1="[\[$txtred\]\u\[$txtylw\]@\[$txtrst\]\h] \[$txtgrn\]\W\[$txtrst\]# "
export LSCOLORS="fxgxcxdxbxegedabagacad"
fi
export GREP_OPTIONS=--color=auto
export LESS_TERMCAP_mb=$'\E[01;31m'
export LESS_TERMCAP_md=$'\E[01;31m'
export LESS_TERMCAP_me=$'\E[0m'
export LESS_TERMCAP_se=$'\E[0m'
export LESS_TERMCAP_so=$'\E[01;44;33m'
export LESS_TERMCAP_ue=$'\E[0m'
export LESS_TERMCAP_us=$'\E[01;32m'
export CLICOLOR=1
##############################################################################
# Functions
##############################################################################
# Delete line from known_hosts
# courtesy of rpetre from reddit
ssh-del() {
sed -i -e ${1}d ~/.ssh/known_hosts
}
psgrep() {
if [ ! -z $1 ] ; then
echo "Grepping for processes matching $1..."
ps aux | grep $1 | grep -v grep
else
echo "!! Need name to grep for"
fi
}
alias searchjobs=psgrep
alias numbersum="paste -s -d+ - | bc"
# clock - a little clock that appeares in the terminal window.
# Usage: clock.
clock () {
while true;do clear;echo "===========";date +"%r";echo "===========";sleep 1;done
}
# showip - show the current IP address if connected to the internet.
# Usage: showip.
showip () {
lynx -dump -hiddenlinks=ignore -nolist http://checkip.dyndns.org:8245/ | awk '{ print $4 }' | sed '/^$/d; s/^[ ]*//g; s/[ ]*$//g'
}
##################
#extract files eg: ex tarball.tar#
##################
ex () {
if [ -f $1 ] ; then
case $1 in
*.tar.bz2) tar xjf $1 ;;
*.tar.gz) tar xzf $1 ;;
*.bz2) bunzip2 $1 ;;
*.rar) rar x $1 ;;
*.gz) gunzip $1 ;;
*.tar) tar xf $1 ;;
*.tbz2) tar xjf $1 ;;
*.tgz) tar xzf $1 ;;
*.zip) unzip $1 ;;
*.Z) uncompress $1 ;;
*.7z) 7z x $1 ;;
*) echo "'$1' can not be extracted via extract()" ;;
esac
else
echo "'$1' is not a valid file"
fi
}
# map the given function to the inputs
function map {
if [ $# -le 1 ]; then
return
else
local f=$1
local x=$2
shift 2
local xs=$@
$f $x
map "$f" $xs
fi
}
#log all history always
promptFunc() {
# right before prompting for the next command, save the previous
# command in a file.
echo "$(date +%Y-%m-%d--%H-%M-%S) $(hostname) $PWD $(history 1)" >> ~/.full_history
}
PROMPT_COMMAND=promptFunc
function histgrep {
cat ~/.full_history | grep "$@" | tail
}
# Add bash completion for ssh: it tries to complete the host to which you
# want to connect from the list of the ones contained in ~/.ssh/known_hosts
__ssh_known_hosts() {
if [[ -f ~/.ssh/known_hosts ]]; then
cut -d " " -f1 ~/.ssh/known_hosts | cut -d "," -f1
fi
}
_ssh() {
local cur known_hosts
COMPREPLY=()
cur="${COMP_WORDS[COMP_CWORD]}"
known_hosts="$(__ssh_known_hosts)"
if [[ ! ${cur} == -* ]] ; then
COMPREPLY=( $(compgen -W "${known_hosts}" -- ${cur}) )
return 0
fi
}
complete -o bashdefault -o default -o nospace -F _ssh ssh 2>/dev/null \
|| complete -o default -o nospace -F _ssh ssh
if [ "$TERM" = "screen" ] && [ "$HAS_256_COLORS" = "yes" ]
then
export TERM=screen-256color
fi
#name the current tab
function nametab {
export PROMPT_COMMAND="echo -ne '\033]0;$@\007'"
}
alias nt=nametab
function git-vimerge {
vim -p $(git --no-pager diff --name-status --diff-filter=U | awk 'BEGIN {x=""} {x=x" "$2;} END {print x}')
}
function activate {
tses=`tmux display-message -p "#S"`
if [ $tses ]; then
echo "now tmux'n"
else
echo "starting tmux..."
tmux new -s first
fi
}
source ~/.${USER}.bashrc
activate
export NVM_DIR="/home/whaikung/.nvm"
[ -s "$NVM_DIR/nvm.sh" ] && . "$NVM_DIR/nvm.sh" # This loads nvm
| 5e549664b866b6ac0fd5c2b8a4b168b2c17a4472 | [
"Shell"
] | 1 | Shell | singlewhai/dotfiles | 362566316fbdbd7e59cef63948990755d0efd71c | dca8f5cab27e2b0057bccd67152263d386142468 | |
refs/heads/master | <repo_name>ValentinAlexandrovGeorgiev/SeekForGeek<file_sep>/javascript/setup/geek/profile.js
$(document).ready(function(){
var currentUser = Parse.User.current();
$('#username').append(currentUser.get('username'));
$('#emailToChange').append(currentUser.get('email'));
$('#emailInput').val(currentUser.get('email'));
$('#changePassword').click(function(){
$('.change').fadeToggle(700);
});
$('#changeEmail').click(function(){
$('#emailToChange').hide();
$('#changeEmail').hide();
$('#emailInput').show();
$('#saveEmail').show();
});
$('#saveEmail').click(function(){
var email = $('#emailInput').val();
currentUser.set('email', email);
currentUser.save()
.then(function(){
$('#emailToChange').show().empty().append(currentUser.get('email'));
$('#changeEmail').show();
$('#emailInput').hide();
$('#saveEmail').hide();
});
});
});
<file_sep>/javascript/setup/home.js
(function(){
$(".navbarTemplate").css("display", "none");
}());
$(document).ready(function () {
$('#loginInRegister').click(loginInRegister);
$('#registerInLogin').click(registerInLogin);
$('#btnForgotPass').click(registerInLogin);
$('#registerButton').click(registerForm);
$('#register-customer').click(registerFormCustomer);
$('#register-geek').click(registerFormGeek);
$("#register-conf-pass").keyup(validateRegisterPasswords);
$('#modal-register').on('shown.bs.modal',function(){
$('#register-email').focus();
});
});
function loginInRegister() {
$("#closeRegister").trigger("click");
}
function registerInLogin() {
$("#closeLogIn").trigger("click");
}
function registerForm() {
var type;
if ($('#register-hire').is(':checked')) {
type = 'customer';
}
if ($('#register-work').is(':checked')) {
type = 'geek';
}
var registerData = {
email: $('#register-email').val(),
username: $('#register-username').val(),
password: $('#register-<PASSWORD>').val(),
type: type
};
if (validateRegisterForm(registerData)) {
var User = new Parse.User();
User.set("username", registerData.username);
User.set("email", registerData.email);
User.set("password", <PASSWORD>Data.<PASSWORD>);
User.set("type", registerData.type);
User.signUp(null, {
success: function (user) {
alert(user + " is registered successfully");
$(location).attr('href','/#/geek/profile');
location.reload();
},
error: function (user, error) {
//TODO div with error
alert(user + " ERROR: " + error);
console.log(error);
}
})
}
}
function validateRegisterForm(registerData) {
var patternForEmail = /^\w+@[a-zA-Z_]+?\.[a-zA-Z]{2,3}$/;
var confirmPassword = $("#register-conf-pass");
var emailForm = $('#email-form');
var usernameForm = $('#username-form');
var passwordForm = $('#password-form');
var typeForm = $('#type-form');
if (!registerData.email.match(patternForEmail)) {
if (emailForm.hasClass('has-success')) {
emailForm.removeClass('has-success');
}
emailForm.addClass('has-error');
return false;
} else {
if (emailForm.hasClass('has-error')) {
emailForm.removeClass('has-error');
}
emailForm.addClass('has-success');
}
if (registerData.username.length < 6) {
if (usernameForm.hasClass('has-success')) {
usernameForm.removeClass('has-success');
}
usernameForm.addClass('has-error');
return false
} else {
if (usernameForm.hasClass('has-error')) {
usernameForm.removeClass('has-error');
}
usernameForm.addClass('has-success');
}
if (registerData.password.length < 6) {
if (passwordForm.hasClass('has-success')) {
passwordForm.removeClass('has-success');
}
passwordForm.addClass('has-error');
return false;
} else {
if (passwordForm.hasClass('has-error')) {
passwordForm.removeClass('has-error');
}
passwordForm.addClass('has-success');
}
if (registerData.type != 'customer' && registerData.type != 'geek') {
if (typeForm.hasClass('has-success')) {
typeForm.removeClass('has-success');
}
typeForm.addClass('has-error');
return false;
} else {
if (typeForm.hasClass('has-error')) {
typeForm.removeClass('has-error');
}
typeForm.addClass('has-success');
}
return true;
}
function registerFormCustomer() {
$("#register-button").trigger("click");
$("#register-hire").prop("checked", true);
}
function registerFormGeek() {
$("#register-button").trigger("click");
$("#register-work").prop("checked", true);
}
function validateRegisterPasswords() {
var password = $("#register-pass").val();
var confirmPassword = $("#register-conf-pass").val();
var confPassForm = $("#conf-pass-form");
if (password === confirmPassword) {
if (confPassForm.hasClass('has-error')) {
confPassForm.removeClass('has-error');
}
confPassForm.addClass('has-success');
return true;
} else {
if (confPassForm.hasClass('has-success')) {
confPassForm.removeClass('has-success');
}
confPassForm.addClass('has-error');
return false;
}
} | e5e8d23de1dc0b92874775c1c199bc105b6c87b9 | [
"JavaScript"
] | 2 | JavaScript | ValentinAlexandrovGeorgiev/SeekForGeek | d0a52c949fb515f4a48d983a568f48c4b6589b73 | 37262a22bd483981b5e5c214d698879010441c1c | |
refs/heads/master | <file_sep>import React from 'react';
import { Card, Row, Col, Icon, Switch, Modal, Button,AutoComplete, Input,Popover,Form, Select, message, DatePicker, Radio, Upload, InputNumber, Slider } from 'antd';
import './projects.less';
const Option = AutoComplete.Option;
const OptGroup = AutoComplete.OptGroup;
const FormItem = Form.Item;
const RadioButton = Radio.Button;
const RadioGroup = Radio.Group;
const { TextArea } = Input;
export default class projectFoundComponent extends React.Component {
render() {
const gridStyle = {
width: '25%',
textAlign: 'center',
};
return (
<div className="project_contentbox">
<div className="project_checkbox">
<div className="project_checktiitle" style={{marginBottom:'30px'}}>项目信息</div>
<Row gutter={8} style={{ marginTop: '20px' }}>
<Col span={24}>
<Demo6App />
</Col>
</Row>
</div>
</div >
)
}
}
class Demo6 extends React.Component {
handleSubmit = (e) => {
e.preventDefault();
this.props.form.validateFields((err, values) => {
if (!err) {
console.log('Received values of form: ', values);
}
});
}
normFile = (e) => {
console.log('Upload event:', e);
if (Array.isArray(e)) {
return e;
}
return e && e.fileList;
}
render() {
const { getFieldDecorator } = this.props.form;
const formItemLayout = {
labelCol: { span: 6 },
wrapperCol: { span: 14 },
};
return (
<Form onSubmit={this.handleSubmit}>
<FormItem
label="项目名称"
{...formItemLayout}
>
{getFieldDecorator('projectname', {
rules: [
{ required: true, message: '请输入项目名称!' },
],
})(
<Input placeholder="项目名称" />
)}
</FormItem>
<FormItem
{...formItemLayout}
label="场景分类"
>
{getFieldDecorator('select', {
rules: [
{ required: true, message: '请选择场景分类!' },
],
})(
<Select placeholder="场景分类">
<Option value="china">场景分类一</Option>
<Option value="use">场景分类二</Option>
</Select>
)}
</FormItem>
<FormItem
{...formItemLayout}
label="项目图片"
>
<div className="dropbox">
{getFieldDecorator('dragger', {
valuePropName: 'fileList',
getValueFromEvent: this.normFile,
})(
<Upload.Dragger name="files" action="/upload.do">
<p className="ant-upload-drag-icon">
<Icon type="inbox" />
</p>
<p className="ant-upload-text">上传图片</p>
<p className="ant-upload-hint"></p>
</Upload.Dragger>
)}
</div>
</FormItem>
<FormItem
{...formItemLayout}
label="项目文件"
extra="支持扩展名zip"
>
{getFieldDecorator('upload', {
valuePropName: 'fileList',
getValueFromEvent: this.normFile,
})(
<Upload name="logo" action="/upload.do" listType="picture">
<Button>
<Icon type="upload" /> 上传文件
</Button>
</Upload>
)}
</FormItem>
<FormItem
label="项目描述"
{...formItemLayout}
>
{getFieldDecorator('projectdepict', {
rules: [
{ required: true, message: '请输入项目名称!' },
],
})(
<AutoComplete
style={{ width: 368, height: 70 }}
onSearch={this.handleSearch}
placeholder="请输入"
>
<TextArea onKeyPress={this.handleKeyPress} style={{ height: 70 }} />
</AutoComplete>
)}
</FormItem>
<FormItem
wrapperCol={{ span: 12, offset: 6 }}
>
<div className="project_fbuttomboxs">
<Button style={{marginRight:'20px'}}>取消</Button>
<Button type="primary" htmlType="submit">提交</Button>
</div>
</FormItem>
</Form>
);
}
}
const Demo6App = Form.create()(Demo6);
<file_sep>import React from 'react';
import { Card, Row, Col, Icon, Switch, Modal, Button,AutoComplete, Input,Popover } from 'antd';
import './projects.less';
import CardDemo from './projectcheck'
import FoundComponent from './projectfound'
const imgsrc = require('../../assets/img/pone.png');
const imgsrcone = require('../../assets/img/logo.png');
const imgsrcproject = require('../../assets/img/projectone.png');
const imgsrcprojecttwo = require('../../assets/img/projecttwo.png');
const imgsrcprojectthree = require('../../assets/img/projectthree.png');
const imgsrcprojecttwos = require('../../assets/img/projecttwos.png');
const Option = AutoComplete.Option;
const OptGroup = AutoComplete.OptGroup;
const { TextArea } = Input;
const text = <span className="popovertopbox">余杭区海创科技中心建模项目余杭区海创科技中心建模项目</span>;
export default class ProjectsCardComponent extends React.Component {
constructor(props) {
super(props);
this.onClickButtonReview = this.onClickButtonReview.bind(this);
this.onClickButtonReviews = this.onClickButtonReviews.bind(this);
this.state = {
isShowProjectDetail : false
};
this.state = {
isShowProjectDetails : false
};
}
onClickButtonReviews(){
this.setState({
isShowProjectDetails : true
});
}
onClickButtonReview(){
this.setState({
isShowProjectDetail : true
});
}
render() {
if(this.state.isShowProjectDetail == true){
return(
<CardDemo/>
)
}
if(this.state.isShowProjectDetails == true){
return(
<FoundComponent/>
)
}
return (
<div className="project_contentbox">
<Row gutter={8} style={{ marginTop: '20px' }}>
<Col span={4}>
<Card bodyStyle={{ padding: 0 }} noHovering>
<div className="addcard" onClick={this.onClickButtonReviews}>增加项目</div>
</Card>
</Col>
<Col span={4}>
<Card bodyStyle={{ padding: 0 }} noHovering>
<Popover placement="topLeft" title={text} className="popoverboxmid">
<Button className="popoverbox">余杭区海创科技中心建模项目余杭区海创科技中心建模项目</Button>
</Popover>
<div style={{position:'relative'}}>
<img style={{ display: 'block' }} alt="example" width="100%" src={imgsrc} />
<div style={{ padding: '3px 0px' }} className="projectmenage">
<Button className="popoverlook" onClick={this.onClickButtonReview}>查看</Button>
<Button className="popoverlook" onClick={this.onClickButtonReview}>修改</Button>
<span onClick={() => {
Modal.confirm({
title: '您确定要删除该项目吗?',
content: '删除后,该项目信息及模型将被清除',
onOk() {
console.log('OK');
},
onCancel() {
console.log('Cancel');
},
});
}} className="projectlook" >
删除
</span>
</div>
</div>
<div className="project_contentime">添加于 2017-10-09 17:09</div>
</Card>
</Col>
</Row>
</div>
);
}
}
<file_sep>import React from 'react';
import { Card, Row, Col, Icon, Switch, Modal, Button,AutoComplete, Input,Popover } from 'antd';
const imgsrc = require('../../assets/img/pone.png');
const imgsrcone = require('../../assets/img/logo.png');
const Option = AutoComplete.Option;
const OptGroup = AutoComplete.OptGroup;
const { TextArea } = Input;
const text = <span>余杭区海创科技中心建模项目余杭区海创科技中心建模项目</span>;
class CardDemo extends React.Component {
render() {
const gridStyle = {
width: '25%',
textAlign: 'center',
};
return (
<div style={{height:'100%'}}>
<div className="projectleftbox">
<div className="project_leftmid">
<div className="project_logo">
<img style={{ display: 'block' }} alt="example" src={imgsrcone} />
</div>
<ul className="project_leftmenage">
<li><a href=""><img src="" alt=""/><span>用户管理</span></a></li>
<li><a href=""><img src="" alt=""/><span>项目管理</span></a></li>
<li><a href=""><img src="" alt=""/><span>分类管理</span></a></li>
</ul>
</div>
</div>
<div className="project_topbox">
<h5>智慧消防管理后台</h5>
<div className="project_topboxright">
<Icon type="user" style={{paddingRight:'8px'}}/>登录
</div>
</div>
<div className="project_contentbox">
<div className="project_contenttitle"><em></em><span>项目管理</span></div>
<div className="project_contentsearch">
<Col span={8}>
<div className="certain-category-search-wrapper" style={{ width: 250,marginTop:15,marginLeft:15 }}>
<AutoComplete
className="certain-category-search"
dropdownClassName="certain-category-search-dropdown"
dropdownMatchSelectWidth={false}
dropdownStyle={{ width: 300 }}
size="large"
style={{ width: '100%' }}
placeholder="请输入项目名称"
optionLabelProp="value"
>
<Input suffix={<Icon type="search" className="certain-category-icon" />} />
</AutoComplete>
</div>
</Col>
</div>
<Row gutter={8} style={{ marginTop: '20px' }}>
<Col span={4}>
<Card bodyStyle={{ padding: 0 }} noHovering>
<Popover placement="topLeft" title={text} className="popoverboxmid">
<Button className="popoverbox">余杭区海创科技中心建模项目余杭区海创科技中心建模项目</Button>
</Popover>
<div style={{position:'relative'}}>
<img style={{ display: 'block' }} alt="example" width="100%" src={imgsrc} />
<div style={{ padding: '10px 0px' }} className="projectmenage">
<a href="#/projectcheck"><span className="projectlook" >查看</span></a>
<span className="projectlook" >编辑</span>
<span onClick={() => {
Modal.confirm({
title: '您确定要删除该项目吗?',
content: '删除后,该项目信息及模型将被清除',
onOk() {
console.log('OK');
},
onCancel() {
console.log('Cancel');
},
});
}} className="projectlook" >
删除
</span>
</div>
</div>
<div className="project_contentime">添加于 2017-10-09 17:09</div>
</Card>
</Col>
<Col span={4}>
<Card bodyStyle={{ padding: 0 }} noHovering>
<Popover placement="topLeft" title={text} className="popoverboxmid">
<Button className="popoverbox">余杭区海创科技中心建模项目余杭区海创科技中心建模项目</Button>
</Popover>
<div style={{position:'relative'}}>
<img style={{ display: 'block' }} alt="example" width="100%" src={imgsrc} />
<div style={{ padding: '10px 0px' }} className="projectmenage">
<span className="projectlook" >查看</span>
<span className="projectlook" >编辑</span>
<span onClick={() => {
Modal.confirm({
title: '您确定要删除该项目吗?',
content: '删除后,该项目信息及模型将被清除',
onOk() {
console.log('OK');
},
onCancel() {
console.log('Cancel');
},
});
}} className="projectlook" >
删除
</span>
</div>
</div>
<div className="project_contentime">添加于 2017-10-09 17:09</div>
</Card>
</Col>
<Col span={4}>
<Card bodyStyle={{ padding: 0 }} noHovering>
<Popover placement="topLeft" title={text} className="popoverboxmid">
<Button className="popoverbox">余杭区海创科技中心建模项目余杭区海创科技中心建模项目</Button>
</Popover>
<div style={{position:'relative'}}>
<img style={{ display: 'block' }} alt="example" width="100%" src={imgsrc} />
<div style={{ padding: '10px 0px' }} className="projectmenage">
<span className="projectlook" >查看</span>
<span className="projectlook" >编辑</span>
<span onClick={() => {
Modal.confirm({
title: '您确定要删除该项目吗?',
content: '删除后,该项目信息及模型将被清除',
onOk() {
console.log('OK');
},
onCancel() {
console.log('Cancel');
},
});
}} className="projectlook" >
删除
</span>
</div>
</div>
<div className="project_contentime">添加于 2017-10-09 17:09</div>
</Card>
</Col>
<Col span={4}>
<Card bodyStyle={{ padding: 0 }} noHovering>
<Popover placement="topLeft" title={text} className="popoverboxmid">
<Button className="popoverbox">余杭区海创科技中心建模项目余杭区海创科技中心建模项目</Button>
</Popover>
<div style={{position:'relative'}}>
<img style={{ display: 'block' }} alt="example" width="100%" src={imgsrc} />
<div style={{ padding: '10px 0px' }} className="projectmenage">
<span className="projectlook" >查看</span>
<span className="projectlook" >编辑</span>
<span onClick={() => {
Modal.confirm({
title: '您确定要删除该项目吗?',
content: '删除后,该项目信息及模型将被清除',
onOk() {
console.log('OK');
},
onCancel() {
console.log('Cancel');
},
});
}} className="projectlook" >
删除
</span>
</div>
</div>
<div className="project_contentime">添加于 2017-10-09 17:09</div>
</Card>
</Col>
<Col span={4}>
<Card bodyStyle={{ padding: 0 }} noHovering>
<Popover placement="topLeft" title={text} className="popoverboxmid">
<Button className="popoverbox">余杭区海创科技中心建模项目余杭区海创科技中心建模项目</Button>
</Popover>
<div style={{position:'relative'}}>
<img style={{ display: 'block' }} alt="example" width="100%" src={imgsrc} />
<div style={{ padding: '10px 0px' }} className="projectmenage">
<span className="projectlook" >查看</span>
<span className="projectlook" >编辑</span>
<span onClick={() => {
Modal.confirm({
title: '您确定要删除该项目吗?',
content: '删除后,该项目信息及模型将被清除',
onOk() {
console.log('OK');
},
onCancel() {
console.log('Cancel');
},
});
}} className="projectlook" >
删除
</span>
</div>
</div>
<div className="project_contentime">添加于 2017-10-09 17:09</div>
</Card>
</Col>
<Col span={4}>
<Card bodyStyle={{ padding: 0 }} noHovering>
<Popover placement="topLeft" title={text} className="popoverboxmid">
<Button className="popoverbox">余杭区海创科技中心建模项目余杭区海创科技中心建模项目</Button>
</Popover>
<div style={{position:'relative'}}>
<img style={{ display: 'block' }} alt="example" width="100%" src={imgsrc} />
<div style={{ padding: '10px 0px' }} className="projectmenage">
<span className="projectlook" >查看</span>
<span className="projectlook" >编辑</span>
<span onClick={() => {
Modal.confirm({
title: '您确定要删除该项目吗?',
content: '删除后,该项目信息及模型将被清除',
onOk() {
console.log('OK');
},
onCancel() {
console.log('Cancel');
},
});
}} className="projectlook" >
删除
</span>
</div>
</div>
<div className="project_contentime">添加于 2017-10-09 17:09</div>
</Card>
</Col>
</Row>
</div>
</div>
)
}
}
export default CardDemo;
<file_sep>import React from 'react';
import {Col, Icon,AutoComplete, Input,Popover } from 'antd';
import './projects.less';
export default class ProjectsSearchComponent extends React.Component {
constructor(props) {
super(props);
}
render() {
return(
<div className="project_contentsearch">
<Col span={8}>
<div className="certain-category-search-wrapper" style={{ width: 250,marginTop:15,marginLeft:15 }}>
<AutoComplete
className="certain-category-search"
dropdownClassName="certain-category-search-dropdown"
dropdownMatchSelectWidth={false}
dropdownStyle={{ width: 300 }}
size="large"
style={{ width: '100%' }}
placeholder="请输入项目名称"
optionLabelProp="value"
>
<Input suffix={<Icon type="search" className="certain-category-icon" />} />
</AutoComplete>
</div>
</Col>
</div>
);
}
}
<file_sep>import React from 'react';
import { Card, Row, Col, Icon, Switch, Modal, Button,AutoComplete, Input,Popover } from 'antd';
const imgsrc = require('../../assets/img/pone.png');
const imgsrcone = require('../../assets/img/logo.png');
const Option = AutoComplete.Option;
const OptGroup = AutoComplete.OptGroup;
class CardDemo extends React.Component {
render() {
const gridStyle = {
width: '25%',
textAlign: 'center',
};
return (
<div style={{height:'100%'}}>
<div className="projectleftbox">
<div className="project_leftmid">
<div className="project_logo">
<img style={{ display: 'block' }} alt="example" src={imgsrcone} />
</div>
<ul className="project_leftmenage">
<li><a href=""><img src="" alt=""/><span>用户管理</span></a></li>
<li><a href=""><img src="" alt=""/><span>项目管理</span></a></li>
<li><a href=""><img src="" alt=""/><span>分类管理</span></a></li>
</ul>
</div>
</div>
<div className="project_topbox">
<h5>智慧消防管理后台</h5>
<div className="project_topboxright">
<Icon type="user" style={{paddingRight:'8px'}}/>登录
</div>
</div>
<div className="project_contentbox">
<div className="project_checkbox">
<div className="project_checktiitle">项目信息</div>
</div>
</div>
</div>
)
}
}
export default CardDemo;
<file_sep>import React from 'react';
export default class MainApp extends React.Component {
constructor(props) {
super(props);
this.isLogin = this.isLogin.bind(this);
}
isLogin() {
return false;
}
render() {
return (
<div>
{
this.isLogin() == true ?
<h1 >yyyyy</h1> :
<h1 >nononon</h1>
}
</div>
);
}
}
<file_sep>import React from 'react';
import LoginPage from '../01_Login/Login/login'
import ProjectsPage from '../08_Projects/projects'
export default class MainApp extends React.Component {
constructor(props) {
super(props);
this.isLogin = this.isLogin.bind(this);
this.state = {
isLogin : false
};
}
componentDidMount() {
this.loginWithRequest();
}
loginWithRequest() {
//TODO: 请求登录接口
if(true) {
this.setState({
isLogin : true
});
} else {
console.log('Please confirm the password!!!');
}
}
isLogin() {
return this.state.isLogin;
}
render() {
return (
<div>
{/*<LoginPage>*/}
{/*</LoginPage>*/}
{/*<ProjectsPage > */}
{/*{this.props.children}*/}
{/*</ProjectsPage>*/}
{/*this.isLogin() == true ?*/}
{/*<ProjectsPage ></ProjectsPage>*/}
{/*:*/}
{/*<LoginPage ></LoginPage>*/}
</div>
);
}
}
<file_sep># 更新日志
## 1.0.0
`2017-09-27`
- 功能
- 完成 [#1](https://github.com/huzzbuzz/bear-admin/issues/1) :菜单布局在顶部时,可配置固定
- 完成 [#1](https://github.com/huzzbuzz/bear-admin/issues/1) :升级 dva.js 至 2.x
- 其他
- 优化代码
<file_sep>import React from 'react';
import { connect } from 'dva';
import { withRouter } from 'dva/router';
import { Spin } from 'antd';
import LayoutLeft from './Layout/Left/Header';
import LayoutTop from './Layout/Left/Layout';
import './App.less';
import './projects.less';
import '../07_ThirdParty/projectone.less';
import Compont from '../07_ThirdParty/projectone';
class App extends React.Component {
render() {
const { app } = this.props;
const { menuMode, fakeGlobal } = app;
return (
<div>
<Spin size="large" spinning={fakeGlobal}>
{
<Compont {...this.props} ></Compont>
}
</Spin>
</div>
);
}
}
export default withRouter(connect(({ app }) => ({ app }))(App));
<file_sep>import React from 'react';
import LayoutDemo1 from './Layout';
// import { Card, Row, Col, Form, Icon, Input, Button, Checkbox, Select, AutoComplete, Tooltip, Cascader } from 'antd';
// const FormItem = Form.Item;
import { Layout } from 'antd';
const { Header, Footer, Sider, Content } = Layout;
export default class LoginPage extends React.Component {
constructor(props) {
super(props);
}
render() {
return (
<div>
<LayoutDemo1/>
</div>
);
}
}
<file_sep>import React from 'react';
import {Layout}from 'antd';
import {row,column }from 'antd';
import { Card, Row, Col, Icon, Switch, Modal, Button,AutoComplete, Input,Popover } from 'antd';
import './projects.less';
import CardDemo from './projectcheck'
import FoundComponent from './projectfound'
import LeftComponent from './projectsLeftComponent'
import SearchComponent from './projectsSearchComponent'
import CardComponent from './projectsCardComponent'
import HeaderComponent from './projectsHeaderComponent'
const { Content ,Sider}=Layout;
const imgsrc = require('../../assets/img/pone.png');
const imgsrcone = require('../../assets/img/logo.png');
const imgsrcproject = require('../../assets/img/projectone.png');
const imgsrcprojecttwo = require('../../assets/img/projecttwo.png');
const imgsrcprojectthree = require('../../assets/img/projectthree.png');
const imgsrcprojecttwos = require('../../assets/img/projecttwos.png');
const Option = AutoComplete.Option;
const OptGroup = AutoComplete.OptGroup;
const { TextArea } = Input;
const text = <span className="popovertopbox">余杭区海创科技中心建模项目余杭区海创科技中心建模项目</span>;
export default class ProjectsPage extends React.Component {
constructor(props) {
super(props);
this.onClickButtonReview = this.onClickButtonReview.bind(this);
// this.createCards = this.createCards.bind(this);
this.state = {
isShowProjectDetail : false
};
this.dataSource = {
card1 : "card1",
card2 : "card2"
}
}
onClickButtonReview(){
this.setState({
isShowProjectDetail : true
});
}
// createCards() {
//
// return (
// <CardComponent />
// );
// this.dataSource && this.dataSource.map(() => {
// return (
// <CardComponent />
// );
// });
render() {
return (
<div>
<Layout>
<Sider>
<LeftComponent />
</Sider>
<Layout>
<HeaderComponent/>
CardComponent
<Content>
<SearchComponent/>
<CardComponent/>
</Content>
{/*<SearchComponent />*/}
{/*{*/}
{/*this.createCards()*/}
{/*}*/}
</Layout>
</Layout>
</div>
);
}
}
<file_sep>import React from 'react';
import {Link} from 'dva/router';
import { Card, Row, Col, Icon, Switch, Modal, Button,AutoComplete, Input,Popover } from 'antd';
import './projects.less';
import CardDemo from './projectcheck'
const imgsrc = require('../../assets/img/pone.png');
const imgsrcone = require('../../assets/img/logo.png');
const imgsrcproject = require('../../assets/img/projectone.png');
const imgsrcprojecttwo = require('../../assets/img/projecttwo.png');
const imgsrcprojectthree = require('../../assets/img/projectthree.png');
const imgsrcprojecttwos = require('../../assets/img/projecttwos.png');
export default class ProjectsLeftComponent extends React.Component {
constructor(props) {
super(props);
}
render() {
return (
<div className="projectleftbox">
<div className="project_leftmid">
<div className="project_logo">
<img style={{ display: 'block' }} alt="example" src={imgsrcone} />
</div>
<ul className="project_leftmenage">
<li>
<Link to="/register">
<img style={{ display: 'block',margin:'0 auto' }} alt="example" src={imgsrcproject} />
<span>用户管理</span>
</Link>
</li>
<li>
<Link to="/projectcheck">
<img style={{ display: 'block',margin:'0 auto' }} alt="example" src={imgsrcprojecttwos} />
<span>项目管理</span>
</Link>ss
</li>
<li>
<a href="">
<img style={{ display: 'block',margin:'0 auto' }} alt="example" src={imgsrcprojectthree} />
<span>分类管理</span>
</a>
</li>
</ul>
</div>
</div>
)
}
}
<file_sep>import React from 'react';
import { Link } from 'dva/router';
import {history} from 'dva/router'
import log from '../../assets/img/001.png';
import { Layout } from 'antd';
import reqwest from 'reqwest';
// import Demo2 from './Demo2';
import './Layout.less';
import { Card, Row, Col, Form, Icon, Input, Button, Checkbox, } from 'antd';
import './Layout.less';
const FormItem = Form.Item;
const { Header, Footer, Sider, Content } = Layout;
class LayoutDemo extends React.Component {
render() {
const head_footer = {
background: '-webkit-linear-gradient(left,#272E3E,#4E5462); ',
color: '#fff'
}
// const sider = {
// background: ' #252B39',
// color: '#fff',
// lineHeight: '120px'
// }
const content ={
// background: 'rgba(16, 142, 233, 1)',
color: '#fff',
// minHeight: '120px',
// lineHeight: '120px'
}
return (
<div style={{ textAlign: 'center' }}>
<Layout className={"layout"}>
<Sider className="sider" ><img src={log} alt="brand"/></Sider>
<Layout className={"layout2"}>
<Header style={head_footer}>智慧消防管理后台
{/*<label className={"con"} > <Icon className={"circle"} type="user"/><a href="">登录</a></label>*/}
</Header>
<Content style={content} className="content">
<Card className="card" noHovering title="登录框">
<Demo2Form />
</Card>
</Content>
{/*<Footer style={Object.assign(head_footer, footer)}>Footer</Footer>*/}
</Layout>
</Layout>
</div>
)
}
}
export default LayoutDemo;
class Demo2 extends React.Component {
constructor(props) {
super(props);
this.state = {};
}
isOk = () => {
// let data = {
// acctId : "qiujun",
//
// acctPassword : <PASSWORD>
// };
// const context =this;}
}
handleSubmit = (e) => {
e.preventDefault();
this.props.form.validateFields((err, values) => {
if (!err) {
reqwest({
url: 'login',
method: 'POST',
contentType:"application/json; charset=UTF-8",
data: JSON.stringify({
"acctId": values.userName,
"acctPassword" : <PASSWORD>
}
),
type: 'json',
}).then((data)=>{
if(data.message =="success")
{
alert("欢迎"+values.userName+"!!!");
window.location.href="#/app";
// this.context.router.push('/ProjectsPage');
}
else{
alert("账号或者密码错误!!!");
window.location.href="#/"
}
});
}
});
}
render() {
const { getFieldDecorator } = this.props.form;
return (
<div id="components-form-demo-normal-login">
<Form onSubmit={this.handleSubmit} className="login-form">
<FormItem>
{getFieldDecorator('userName', {
rules: [{ required: true, message: 'Please input your username!' }],
})(
<Input size ="large" prefix={<Icon type="user" style={{ fontSize: 13 }} />} placeholder="Username" />
)}
</FormItem>
<FormItem>
{getFieldDecorator('password', {
rules: [{ required: true, message: 'Please input your Password!' }],
})(
<Input size="large" prefix={<Icon type="lock" style={{ fontSize: 13 }} />} type="password" placeholder="<PASSWORD>" />
)}
</FormItem>
<FormItem>
{/*{getFieldDecorator('remember', {*/}
{/*valuePropName: 'checked',*/}
{/*initialValue: true,*/}
{/*})(*/}
{/*<Checkbox>Remember me</Checkbox>*/}
{/*)}*/}
{/*<a className="login-form-forgot" href="">Forgot password</a>*/}
{/*<Link to="/ProjectsPage"> <Button type="primary" htmlType="submit" className="login-form-button">*/}
{/*Log in*/}
{/*</Button>*/}
{/*</Link>*/}
<Button size="large" type="primary" htmlType="submit" className="login-form-button">
Log in
</Button>
{/*Or <Link to="/register">register now!</Link>*/}
</FormItem>
</Form>
</div>
);
}
}
const Demo2Form = Form.create()(Demo2);
<file_sep>import React from 'react';
import { connect } from 'dva';
import { withRouter } from 'dva/router';
import MainApp from './mainApp'
class FireControlApp extends React.Component {
render() {
return (
<div>
<MainApp >
</MainApp>
</div>
);
}
}
export default withRouter(connect(({ app }) => ({ app }))(FireControlApp));
| de697ecac96d802decf9eaf700994a5342f1df92 | [
"JavaScript",
"Markdown"
] | 14 | JavaScript | HanTengfeifei/react | 7fbdaef859a8e9f8b5db0a7ab39df3be332ef75c | e615431f908e109050e0a33a6c67ac145577225c | |
refs/heads/master | <repo_name>Toansmile/gos<file_sep>/gos.go
/*
Go ↔︎ SQL, tool for mapping between Go structs and SQL records. NOT AN ORM, and
should be used instead of an ORM. Expressly designed to let you WRITE PLAIN SQL.
Example usage:
type External struct {
Id string `db:"id"`
Name string `db:"name"`
}
type Internal struct {
Id string `db:"id"`
Name string `db:"name"`
}
// Step 1: generate query.
var result []External
query := fmt.Sprintf(`select %v from (
select
external.*,
internal as internal
from
external
cross join internal
) as _
`, gos.Cols(result))
// Resulting query (formatted here for readability):
// select
// "id",
// "name",
// ("internal")."id" as "internal.id",
// ("internal")."name" as "internal.name"
// from (
// ...
// ) as _
// Step 2: use query.
err := gos.Query(ctx, conn, &result, query, nil)
fmt.Printf("%#v\n", result)
Struct rules
When decoding a row into a struct, Gos observes the following rules.
1. Columns are matched to public struct fields whose `db` tag exactly matches
the column name. Private fields or fields without `db` are completely
ignored. Example:
type Result struct {
A string `db:"a"`
B string // ignored: no `db` tag
c string // ignored: private
}
2. Fields of embedded structs are treated as part of the enclosing struct. For
example, these two definitions are completely equivalent:
type Result struct {
A string `db:"a"`
Embedded
}
type Embedded struct {
B string `db:"b"`
}
type Result struct {
A string `db:"a"`
B string `db:"b"`
}
3. Fields of nested non-embedded structs are matched with columns whose aliases
look like `"outer_field.inner_field.innermost_field"` with arbitrary nesting.
Example:
-- Query:
select
'one' as "outer_val",
'two' as "inner.inner_val";
// Go types:
type Outer struct {
OuterVal string `db:"outer_val"`
Inner Inner `db:"inner"`
}
type Inner struct {
InnerVal string `db:"inner_val"`
}
4. If every column from a nested struct is null or missing, the entire nested
struct is considered null. If the field is not nilable (struct, not pointer
to struct), this will produce an error. Otherwise, the field is left nil and
not allocated. This convention is extremely useful for outer joins, where
nested records are often null. Example:
-- Query:
select
'one' as "outer_val",
null as "inner.inner_val";
// Go types:
type Outer struct {
OuterVal string `db:"outer_val"`
Inner *Inner `db:"inner"`
}
type Inner struct {
InnerVal string `db:"inner_val"`
}
// Output:
Outer{OuterVal: "one", Inner: nil}
Differences from sqlx
Gos is very similar to https://github.com/jmoiron/sqlx. Key differences:
* Supports null records in outer joins, as nested struct pointers
* Selects fields explicitly without relying on `select *`
* Much simpler API, does not wrap `database/sql`
* Explicit field-column mapping, no hidden renaming
* No special utilities for named parameters
* Depends only on the standard library (the `go.mod` dependencies are test-only)
Notes on array support
Gos doesn't specially support SQL arrays. Generally speaking, SQL arrays are
usable only for primitive types such as numbers or strings. Some databases, such
as Postgres, have their own implementations of multi-dimensional arrays, which
are non-standard and have so many quirks and limitations that it's more
practical to just use JSON. Arrays of primitives are already supported in
adapters such as "github.com/lib/pq", which are orthogonal to Gos and used in
combination with it.
*/
package gos
import (
"context"
"database/sql"
"errors"
"fmt"
"reflect"
"strings"
"time"
)
/*
Main API of this library. Scans columns into the destination, which may be one
of:
* single scalar
* slice of scalars
* single struct
* slice of structs
If the destination is a non-slice, there must be exactly one row. Less or more
will result in an error. If the destination is a struct, this will decode
columns into struct fields, following the rules outlined above in the package
overview.
The `select` part of the query must be generated by calling `Cols(dest)`. See
the example below.
*/
func Query(ctx context.Context, conn Queryer, dest interface{}, query string, args []interface{}) error {
rval := reflect.ValueOf(dest)
if !isNonNilPointer(rval) {
return ErrInvalidDest.because(fmt.Errorf(`destination must be a non-nil pointer, received %#v`, dest))
}
rtype := derefRtype(rval.Type())
if rtype.Kind() == reflect.Slice {
elemRtype := derefRtype(rtype.Elem())
if elemRtype.Kind() == reflect.Struct && !isScannableRtype(elemRtype) {
return queryStructs(ctx, conn, rval, query, args)
}
return queryScalars(ctx, conn, rval, query, args)
}
if rtype.Kind() == reflect.Struct && !isScannableRtype(rtype) {
return queryStruct(ctx, conn, rval, query, args)
}
return queryScalar(ctx, conn, dest, query, args)
}
/*
Takes a struct or struct slice and generates a string for inclusion into
`select`. Allowed inputs: struct, struct pointer, slice of structs, slice of
struct pointers, pointer to slice. Any other input causes a panic.
Should be used in conjunction with `Query()`. See the examples below.
*/
func Cols(dest interface{}) string {
rtype := derefRtype(reflect.TypeOf(dest))
if rtype.Kind() == reflect.Slice {
rtype = derefRtype(rtype.Elem())
}
idents := structTypeSqlIdents(rtype)
return sqlIdent{idents: idents}.selectString()
}
/*
Use these blank errors to detect error type:
if errors.Is(err, gos.ErrNoRows) {
// Handle specific error.
}
Note that errors returned by Gos can't be compared via `==` because they may
include additional details about the circumstances. Use `errors.Is`.
*/
var (
ErrNoRows Err = Err{Code: ErrCodeNoRows, Cause: sql.ErrNoRows}
ErrMultipleRows Err = Err{Code: ErrCodeMultipleRows, Cause: errors.New(`expected one row, got multiple`)}
ErrInvalidDest Err = Err{Code: ErrCodeInvalidDest, Cause: errors.New(`invalid destination type`)}
ErrNoColDest Err = Err{Code: ErrCodeNoColDest, Cause: errors.New(`column has no matching destination`)}
ErrRedundantCol Err = Err{Code: ErrCodeRedundantCol, Cause: errors.New(`redundant column occurrence`)}
ErrNull Err = Err{Code: ErrCodeNull, Cause: errors.New(`null column for non-nilable field`)}
ErrScan Err = Err{Code: ErrCodeScan, Cause: errors.New(`error while scanning into field`)}
)
// Describes a Gos error.
type Err struct {
Code ErrCode
While string
Cause error
}
// Implement `error`.
func (self Err) Error() string {
if self == (Err{}) {
return ""
}
msg := `SQL error`
if self.Code != ErrCodeUnknown {
msg += fmt.Sprintf(` %s`, self.Code)
}
if self.While != "" {
msg += fmt.Sprintf(` while %v`, self.While)
}
if self.Cause != nil {
msg += `: ` + self.Cause.Error()
}
return msg
}
// Implement a hidden interface in "errors".
func (self Err) Is(other error) bool {
if self.Cause != nil && errors.Is(self.Cause, other) {
return true
}
err, ok := other.(Err)
return ok && err.Code == self.Code
}
// Implement a hidden interface in "errors".
func (self Err) Unwrap() error {
return self.Cause
}
func (self Err) while(while string) Err {
self.While = while
return self
}
func (self Err) because(cause error) Err {
self.Cause = cause
return self
}
/*
Describes the specific error type. You probably shouldn't use this directly;
instead, use `errors.Is` with the various `Err` variables.
*/
type ErrCode byte
const (
ErrCodeUnknown ErrCode = iota
ErrCodeNoRows
ErrCodeMultipleRows
ErrCodeInvalidDest
ErrCodeNoColDest
ErrCodeRedundantCol
ErrCodeNull
ErrCodeScan
)
// Implement `fmt.Stringer`.
func (self ErrCode) String() string {
switch self {
case ErrCodeNoRows:
return "ErrNoRows"
case ErrCodeMultipleRows:
return "ErrMultipleRows"
case ErrCodeInvalidDest:
return "ErrInvalidDest"
case ErrCodeNoColDest:
return "ErrNoColDest"
case ErrCodeRedundantCol:
return "ErrRedundantCol"
case ErrCodeNull:
return "ErrNull"
case ErrCodeScan:
return "ErrScan"
default:
return ""
}
}
/*
Database connection passed to `Query()`. Satisfied by `*sql.DB`, `*sql.Tx`, may
be satisfied by application-custom types.
*/
type Queryer interface {
QueryContext(context.Context, string, ...interface{}) (*sql.Rows, error)
}
/* Internal */
const expectedStructDepth = 8
type tDestSpec struct {
colNames []string
colRtypes map[string]reflect.Type
typeSpec tTypeSpec
}
type tTypeSpec struct {
rtype reflect.Type
fieldSpecs []tFieldSpec
}
type tFieldSpec struct {
parentFieldSpec *tFieldSpec
typeSpec tTypeSpec
fieldIndex int
fieldPath []int // Relative to root struct.
colName string
uniqColAlias string
colIndex int // Must be initialized to -1.
sfield reflect.StructField
}
type tDecodeState struct {
colPtrs []interface{}
}
/*
The destination must be a pointer to a non-scannable struct.
*/
func queryStruct(ctx context.Context, conn Queryer, rval reflect.Value, query string, args []interface{}) error {
rows, err := conn.QueryContext(ctx, query, args...)
if err != nil {
return Err{While: `querying rows`, Cause: err}
}
defer rows.Close()
spec, err := prepareDestSpec(rows, rval.Type())
if err != nil {
return err
}
state, err := prepareDecodeState(rows, spec)
if err != nil {
return err
}
if !rows.Next() {
return ErrNoRows.while(`preparing row`)
}
err = rows.Scan(state.colPtrs...)
if err != nil {
return Err{While: `scanning row`, Cause: err}
}
err = traverseDecode(rval, spec, state, &spec.typeSpec, nil)
if err != nil {
return err
}
if rows.Next() {
return ErrMultipleRows.while(`verifying row count`)
}
return nil
}
/*
The destination must be a pointer to a slice of non-scannable structs or
pointers to those structs.
*/
func queryStructs(ctx context.Context, conn Queryer, rval reflect.Value, query string, args []interface{}) error {
elemRtype := derefRtype(rval.Type()).Elem()
rows, err := conn.QueryContext(ctx, query, args...)
if err != nil {
return Err{While: `querying rows`, Cause: err}
}
defer rows.Close()
spec, err := prepareDestSpec(rows, reflect.PtrTo(elemRtype))
if err != nil {
return err
}
sliceRval := derefAllocRval(rval)
// When the slice is nil, this leaves it as nil.
sliceRval.SetLen(0)
for rows.Next() {
state, err := prepareDecodeState(rows, spec)
if err != nil {
return err
}
err = rows.Scan(state.colPtrs...)
if err != nil {
return Err{While: `scanning row`, Cause: err}
}
elemPtrRval := reflect.New(elemRtype)
err = traverseDecode(elemPtrRval, spec, state, &spec.typeSpec, nil)
if err != nil {
return err
}
sliceRval.Set(reflect.Append(sliceRval, elemPtrRval.Elem()))
}
return nil
}
func queryScalar(ctx context.Context, conn Queryer, dest interface{}, query string, args []interface{}) error {
rows, err := conn.QueryContext(ctx, query, args...)
if err != nil {
return Err{While: `querying rows`, Cause: err}
}
defer rows.Close()
if !rows.Next() {
return ErrNoRows.while(`preparing row`)
}
err = rows.Scan(dest)
if err != nil {
return Err{While: `scanning row`, Cause: err}
}
if rows.Next() {
return ErrMultipleRows.while(`verifying row count`)
}
return nil
}
/*
The destination must be a pointer to a slice of scannables or primitives.
*/
func queryScalars(ctx context.Context, conn Queryer, rval reflect.Value, query string, args []interface{}) error {
elemRtype := derefRtype(rval.Type()).Elem()
rows, err := conn.QueryContext(ctx, query, args...)
if err != nil {
return Err{While: `querying rows`, Cause: err}
}
defer rows.Close()
sliceRval := derefAllocRval(rval)
// When the slice is nil, this leaves it as nil.
sliceRval.SetLen(0)
for rows.Next() {
elemPtrRval := reflect.New(elemRtype)
err = rows.Scan(elemPtrRval.Interface())
if err != nil {
return Err{While: `scanning row`, Cause: err}
}
sliceRval.Set(reflect.Append(sliceRval, elemPtrRval.Elem()))
}
return nil
}
func prepareDestSpec(rows *sql.Rows, rtype reflect.Type) (*tDestSpec, error) {
if rtype == nil || rtype.Kind() != reflect.Ptr || derefRtype(rtype).Kind() != reflect.Struct {
return nil, Err{
Code: ErrCodeInvalidDest,
While: `preparing destination spec`,
Cause: fmt.Errorf(`expected destination type to be a struct pointer, got %q`, rtype),
}
}
colNames, err := rows.Columns()
if err != nil {
return nil, Err{While: `getting columns`, Cause: err}
}
spec := &tDestSpec{
typeSpec: tTypeSpec{rtype: rtype},
colNames: colNames,
colRtypes: map[string]reflect.Type{},
}
colPath := make([]string, 0, expectedStructDepth)
fieldPath := make([]int, 0, expectedStructDepth)
err = traverseMakeSpec(spec, &spec.typeSpec, nil, colPath, fieldPath)
if err != nil {
return nil, err
}
for _, colName := range colNames {
if spec.colRtypes[colName] == nil {
return nil, Err{
Code: ErrCodeNoColDest,
While: `preparing destination spec`,
Cause: fmt.Errorf(`column %q doesn't have a matching destination in type %q`, colName, rtype),
}
}
}
return spec, nil
}
func prepareDecodeState(rows *sql.Rows, spec *tDestSpec) (*tDecodeState, error) {
colPtrs := make([]interface{}, 0, len(spec.colNames))
for _, colName := range spec.colNames {
if spec.colRtypes[colName] == nil {
return nil, Err{
Code: ErrCodeNoColDest,
While: `preparing decode state`,
Cause: fmt.Errorf(`column %q doesn't have a matching destination in type %q`,
colName, spec.typeSpec.rtype),
}
}
colPtrs = append(colPtrs, reflect.New(reflect.PtrTo(spec.colRtypes[colName])).Interface())
}
return &tDecodeState{colPtrs: colPtrs}, nil
}
func traverseMakeSpec(spec *tDestSpec, typeSpec *tTypeSpec, parentFieldSpec *tFieldSpec, colPath []string, fieldPath []int) error {
rtypeElem := derefRtype(typeSpec.rtype)
typeSpec.fieldSpecs = make([]tFieldSpec, rtypeElem.NumField())
for i := 0; i < rtypeElem.NumField(); i++ {
sfield := rtypeElem.Field(i)
fieldRtype := derefRtype(sfield.Type)
fieldPath := append(fieldPath, i)
fieldSpec := &typeSpec.fieldSpecs[i]
*fieldSpec = tFieldSpec{
parentFieldSpec: parentFieldSpec,
typeSpec: tTypeSpec{rtype: sfield.Type},
fieldIndex: i,
fieldPath: copyIntSlice(fieldPath),
colIndex: -1,
sfield: sfield,
}
if !isStructFieldPublic(sfield) {
continue
}
if sfield.Anonymous && fieldRtype.Kind() == reflect.Struct {
err := traverseMakeSpec(spec, &fieldSpec.typeSpec, fieldSpec, colPath, fieldPath)
if err != nil {
return err
}
continue
}
fieldSpec.colName = structFieldColumnName(sfield)
if fieldSpec.colName == "" {
continue
}
colPath := append(colPath, fieldSpec.colName)
fieldSpec.uniqColAlias = strings.Join(colPath, ".")
fieldSpec.colIndex = stringIndex(spec.colNames, fieldSpec.uniqColAlias)
if spec.colRtypes[fieldSpec.uniqColAlias] != nil {
return Err{
Code: ErrCodeRedundantCol,
While: `preparing destination spec`,
Cause: fmt.Errorf(`redundant occurrence of column %q`, fieldSpec.uniqColAlias),
}
}
spec.colRtypes[fieldSpec.uniqColAlias] = sfield.Type
if fieldRtype.Kind() == reflect.Struct && !isScannableRtype(fieldRtype) {
err := traverseMakeSpec(spec, &fieldSpec.typeSpec, fieldSpec, colPath, fieldPath)
if err != nil {
return err
}
}
}
return nil
}
func traverseDecode(rootRval reflect.Value, spec *tDestSpec, state *tDecodeState, typeSpec *tTypeSpec, fieldSpec *tFieldSpec) error {
everyColValueIsNil := true
for i := range typeSpec.fieldSpecs {
fieldSpec := &typeSpec.fieldSpecs[i]
sfield := fieldSpec.sfield
fieldRtype := derefRtype(sfield.Type)
if !isStructFieldPublic(sfield) {
continue
}
if sfield.Anonymous && fieldRtype.Kind() == reflect.Struct {
err := traverseDecode(rootRval, spec, state, &fieldSpec.typeSpec, fieldSpec)
if err != nil {
return err
}
continue
}
if fieldSpec.colName == "" {
continue
}
if fieldRtype.Kind() == reflect.Struct && !isScannableRtype(fieldRtype) {
err := traverseDecode(rootRval, spec, state, &fieldSpec.typeSpec, fieldSpec)
if err != nil {
return err
}
continue
}
if !(fieldSpec.colIndex >= 0) {
continue
}
colRval := reflect.ValueOf(state.colPtrs[fieldSpec.colIndex]).Elem()
if !colRval.IsNil() {
everyColValueIsNil = false
}
}
isNested := fieldSpec != nil
if everyColValueIsNil && isNested && isNilableOrHasNilableNonRootAncestor(fieldSpec) {
return nil
}
for _, fieldSpec := range typeSpec.fieldSpecs {
if !(fieldSpec.colIndex >= 0) {
continue
}
sfield := fieldSpec.sfield
colRval := reflect.ValueOf(state.colPtrs[fieldSpec.colIndex]).Elem()
if colRval.IsNil() {
if isNilableRkind(sfield.Type.Kind()) {
continue
}
fieldRval := derefAllocStructRvalAt(rootRval, fieldSpec.fieldPath)
scanner, ok := fieldRval.Addr().Interface().(sql.Scanner)
if ok {
err := scanner.Scan(nil)
if err != nil {
return Err{Code: ErrCodeScan, While: `scanning into field`, Cause: err}
}
continue
}
return Err{
Code: ErrCodeNull,
While: `decoding into struct`,
Cause: fmt.Errorf(`type %q at field %q of struct %q is not nilable, but corresponding column %q was null`,
sfield.Type, sfield.Name, typeSpec.rtype, fieldSpec.uniqColAlias),
}
}
fieldRval := derefAllocStructRvalAt(rootRval, fieldSpec.fieldPath)
fieldRval.Set(colRval.Elem())
}
return nil
}
func stringIndex(strs []string, str string) int {
for i := range strs {
if strs[i] == str {
return i
}
}
return -1
}
var timeRtype = reflect.TypeOf(time.Time{})
var sqlScannerRtype = reflect.TypeOf((*sql.Scanner)(nil)).Elem()
func isScannableRtype(rtype reflect.Type) bool {
return rtype != nil &&
(rtype == timeRtype || reflect.PtrTo(rtype).Implements(sqlScannerRtype))
}
func isNonNilPointer(rval reflect.Value) bool {
return rval.IsValid() && rval.Kind() == reflect.Ptr && !rval.IsNil()
}
func copyIntSlice(vals []int) []int {
out := make([]int, len(vals), len(vals))
copy(out, vals)
return out
}
func isNilableOrHasNilableNonRootAncestor(fieldSpec *tFieldSpec) bool {
for fieldSpec != nil {
if isNilableRkind(fieldSpec.typeSpec.rtype.Kind()) {
return true
}
fieldSpec = fieldSpec.parentFieldSpec
}
return false
}
func structTypeSqlIdents(structRtype reflect.Type) []sqlIdent {
var idents []sqlIdent
traverseStructFields(structRtype, func(sfield reflect.StructField) {
colName := structFieldColumnName(sfield)
if colName == "" {
return
}
fieldRtype := derefRtype(sfield.Type)
if fieldRtype.Kind() == reflect.Struct && !isScannableRtype(fieldRtype) {
idents = append(idents, sqlIdent{
name: colName,
idents: structTypeSqlIdents(fieldRtype),
})
return
}
idents = append(idents, sqlIdent{name: colName})
return
})
return idents
}
type sqlIdent struct {
name string
idents []sqlIdent
}
func (self sqlIdent) selectString() string {
return bytesToString(self.appendSelect(nil, nil))
}
func (self sqlIdent) appendSelect(buf []byte, path []string) []byte {
if len(self.idents) == 0 {
if self.name == "" {
return buf
}
if len(buf) > 0 {
buf = append(buf, ", "...)
}
if len(path) == 0 {
buf = appendIdentAlias(buf, path, self.name)
} else {
buf = appendIdentPath(buf, path, self.name)
buf = append(buf, " as "...)
buf = appendIdentAlias(buf, path, self.name)
}
return buf
}
if self.name != "" {
path = append(path, self.name)
}
for _, ident := range self.idents {
buf = ident.appendSelect(buf, path)
}
return buf
}
func appendIdentPath(buf []byte, path []string, ident string) []byte {
for i, name := range path {
if i == 0 {
buf = append(buf, '(', '"')
buf = append(buf, name...)
buf = append(buf, '"', ')')
} else {
buf = append(buf, '"')
buf = append(buf, name...)
buf = append(buf, '"')
}
buf = append(buf, '.')
}
buf = append(buf, '"')
buf = append(buf, ident...)
buf = append(buf, '"')
return buf
}
func appendIdentAlias(buf []byte, path []string, ident string) []byte {
buf = append(buf, '"')
for _, name := range path {
buf = append(buf, name...)
buf = append(buf, '.')
}
buf = append(buf, ident...)
buf = append(buf, '"')
return buf
}
func bytesToString(bytes []byte) string { return string(bytes) }
func stringToBytes(input string) []byte { return []byte(input) }
func traverseStructFields(rtype reflect.Type, fun func(sfield reflect.StructField)) {
rtype = derefRtype(rtype)
if rtype == nil || rtype.Kind() != reflect.Struct {
panic(fmt.Errorf("expected a struct type, got a %q", rtype))
}
for i := 0; i < rtype.NumField(); i++ {
sfield := rtype.Field(i)
if !isStructFieldPublic(sfield) {
continue
}
/**
If this is an embedded struct, traverse its fields as if they're in the
parent struct.
*/
if sfield.Anonymous && derefRtype(sfield.Type).Kind() == reflect.Struct {
traverseStructFields(sfield.Type, fun)
continue
}
fun(sfield)
}
}
func derefRtype(rtype reflect.Type) reflect.Type {
for rtype != nil && rtype.Kind() == reflect.Ptr {
rtype = rtype.Elem()
}
return rtype
}
/*
Derefs the provided value until it's no longer a pointer, allocating as
necessary. Returns a non-pointer value. The input value must be settable or a
non-nil pointer, otherwise this causes a panic.
*/
func derefAllocRval(rval reflect.Value) reflect.Value {
for rval.Kind() == reflect.Ptr {
if rval.IsNil() {
rval.Set(reflect.New(rval.Type().Elem()))
}
rval = rval.Elem()
}
return rval
}
/*
Finds or allocates an rval at the given struct field path, returning the
resulting reflect value. If the starting value is settable, the resulting value
should also be settable. Ditto if the starting value is a non-nil pointer and
the path is not empty.
Assumes that every type on the path, starting with the root, is a struct type or
an arbitrarily nested struct pointer type. Panics if the assumption doesn't
hold.
*/
func derefAllocStructRvalAt(rval reflect.Value, path []int) reflect.Value {
for _, i := range path {
rval = derefAllocRval(rval)
rval = rval.Field(i)
}
return rval
}
func isStructFieldPublic(sfield reflect.StructField) bool {
return sfield.PkgPath == ""
}
func structFieldColumnName(sfield reflect.StructField) string {
tag := sfield.Tag.Get("db")
if tag == "-" {
return ""
}
return tag
}
func isNilableRkind(kind reflect.Kind) bool {
switch kind {
case reflect.Chan, reflect.Func, reflect.Interface, reflect.Map, reflect.Ptr, reflect.Slice:
return true
default:
return false
}
}
<file_sep>/gos_test.go
package gos
import (
"context"
"database/sql"
"errors"
"fmt"
"os"
"os/user"
"reflect"
"strings"
"testing"
"time"
"unsafe"
_ "github.com/lib/pq"
)
var testCtx = context.Background()
var testTx *sql.Tx
const testDbName = `gos_test_db`
func TestMain(m *testing.M) {
os.Exit(runTestMain(m))
}
// This is a separate function to allow `defer` before `os.Exit`.
func runTestMain(m *testing.M) int {
connParams := []string{
`host=localhost`,
`sslmode=disable`,
`dbname=gos_test_db`,
}
/**
Try using the current OS user as the Postgres user. Works on MacOS when
Postgres is installed via Homebrew. Might fail in other configurations.
*/
usr, err := user.Current()
if err != nil {
panic(err)
}
connParams = append(connParams, `user=`+usr.Username)
/**
Create a test database and drop it at the end. Note that two concurrent
instances of this test would conflict; we could create databases with random
names to allow multiple instances of the test, but that seems like decadent
overkill.
*/
_ = dropDb(connParams, testDbName)
err = createDb(connParams, testDbName)
if err != nil {
panic(err)
}
defer dropDb(connParams, testDbName)
testConnParams := append(connParams, `dbname=`+testDbName)
db, err := sql.Open("postgres", strings.Join(testConnParams, ` `))
if err != nil {
panic(err)
}
defer db.Close()
/**
Use a single DB transaction for testing, rolling it back at the end, to avoid
storing test-generated garbage even if database deletion fails.
*/
testTx, err = db.Begin()
if err != nil {
panic(fmt.Errorf("failed to start DB transaction: %+v", err))
}
defer testTx.Rollback()
return m.Run()
}
func TestScalarBasic(t *testing.T) {
var result string
query := `select 'blah'`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := "blah"
if expected != result {
t.Fatalf(`expected %q, got %q`, expected, result)
}
}
func TestScalarNonNullable(t *testing.T) {
var result string
query := `select null`
err := Query(testCtx, testTx, &result, query, nil)
/**
Why this doesn't inspect the error: the error comes from `database/sql`;
there's no programmatic API to detect its type. We return an `ErrNull` in
some other scenarios.
*/
if err == nil {
t.Fatalf(`expected scanning null into non-nullable scalar to produce an error`)
}
}
func TestScalarNullable(t *testing.T) {
var result *string
query := `select 'blah'`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := "blah"
if expected != *result {
t.Fatalf(`expected %q, got %q`, expected, *result)
}
query = `select null`
err = Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
if result != nil {
t.Fatalf(`expected selecting null to produce nil, got %q`, *result)
}
}
func TestScalarsBasic(t *testing.T) {
var results []string
query := `select * from (values ('one'), ('two'), ('three')) as _`
err := Query(testCtx, testTx, &results, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := []string{"one", "two", "three"}
if !reflect.DeepEqual(expected, results) {
t.Fatalf(`expected %#v, got %#v`, expected, results)
}
}
func TestScalarsNonNullable(t *testing.T) {
var results []string
query := `select * from (values ('one'), (null), ('three')) as _`
err := Query(testCtx, testTx, &results, query, nil)
/**
Why this doesn't inspect the error: the error comes from `database/sql`;
there's no programmatic API to detect its type. We return an `ErrNull` in
some other scenarios.
*/
if err == nil {
t.Fatalf(`expected scanning null into non-nullable scalar to produce an error`)
}
}
func TestScalarsNullable(t *testing.T) {
var results []*string
query := `select * from (values ('one'), (null), ('three')) as _`
err := Query(testCtx, testTx, &results, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := []*string{strptr("one"), nil, strptr("three")}
if !reflect.DeepEqual(expected, results) {
t.Fatalf(`expected %#v, got %#v`, expected, results)
}
}
// Verify that we treat `time.Time` as an atomic scannable rather than a struct.
func TestScalarTime(t *testing.T) {
var result time.Time
query := `select '0001-01-01'::timestamp`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := timeMustParse(`0001-01-01T00:00:00Z`)
if expected.UnixNano() != result.UnixNano() {
t.Fatalf(`expected %v, got %v`, expected, result)
}
}
// Verify that we treat `[]time.Time` as atomic scannables rather than structs.
func TestScalarsTime(t *testing.T) {
var results []time.Time
query := `select * from (values ('0001-01-01'::timestamp), ('0002-01-01'::timestamp)) as _`
err := Query(testCtx, testTx, &results, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := []int64{
timeMustParse(`0001-01-01T00:00:00Z`).UnixNano(),
timeMustParse(`0002-01-01T00:00:00Z`).UnixNano(),
}
received := []int64{
results[0].UnixNano(),
results[1].UnixNano(),
}
if !reflect.DeepEqual(expected, received) {
t.Fatalf(`expected %#v, got %#v`, expected, received)
}
}
func TestScalarScannable(t *testing.T) {
var result ScannableString
query := `select 'blah'`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := "blah_scanned"
received := string(result)
if expected != received {
t.Fatalf(`expected %q, got %q`, expected, received)
}
}
func TestScalarsScannable(t *testing.T) {
var results []ScannableString
query := `select * from (values ('one'), ('two'), ('three')) as _`
err := Query(testCtx, testTx, &results, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := []string{"one_scanned", "two_scanned", "three_scanned"}
received := *(*[]string)(unsafe.Pointer(&results))
if !reflect.DeepEqual(expected, received) {
t.Fatalf(`expected %#v, got %#v`, expected, received)
}
}
func TestStructScannable(t *testing.T) {
var result ScannableStruct
query := `select 'blah'`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := ScannableStruct{Value: "blah_scanned"}
if expected != result {
t.Fatalf(`expected %q, got %q`, expected, result)
}
}
func TestStructsScannable(t *testing.T) {
var results []ScannableStruct
query := `select * from (values ('one'), ('two'), ('three')) as _`
err := Query(testCtx, testTx, &results, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := []ScannableStruct{{"one_scanned"}, {"two_scanned"}, {"three_scanned"}}
if !reflect.DeepEqual(expected, results) {
t.Fatalf(`expected %#v, got %#v`, expected, results)
}
}
func TestStructWithBasicTypes(t *testing.T) {
var result struct {
Int32 int32 `db:"int32"`
Int64 int64 `db:"int64"`
Float32 float32 `db:"float32"`
Float64 float64 `db:"float64"`
String string `db:"string"`
Bool bool `db:"bool"`
Time time.Time `db:"time"`
Scan ScannableString `db:"scan"`
}
query := `
select
1 :: int4 as int32,
2 :: int8 as int64,
3 :: float4 as float32,
4 :: float8 as float64,
'5' :: text as string,
true :: bool as bool,
current_timestamp :: timestamp as time,
'scan' :: text as scan
`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
tFieldEq(t, "Int32", result.Int32, int32(1))
tFieldEq(t, "Int64", result.Int64, int64(2))
tFieldEq(t, "Float32", result.Float32, float32(3))
tFieldEq(t, "Float64", result.Float64, float64(4))
tFieldEq(t, "String", result.String, "5")
tFieldEq(t, "Bool", result.Bool, true)
if result.Time.IsZero() {
t.Fatalf(`expected time to be non-zero`)
}
tFieldEq(t, "Scan", result.Scan, ScannableString("scan_scanned"))
}
func TestStructFieldNaming(t *testing.T) {
var result struct {
One string `db:"one"`
Two *string `db:"six"`
Three **string `db:"seven"`
Four string `db:"-"`
Five string
}
two := "2"
three := "3"
three_ := &three
result.One = "1"
result.Two = &two
result.Three = &three_
result.Four = "4"
result.Five = "5"
query := `
select
'one' as one,
'two' as six,
'three' as seven
`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
tFieldEq(t, "One", result.One, "one")
tFieldEq(t, "Two", *result.Two, "two")
tFieldEq(t, "Three", **result.Three, "three")
tFieldEq(t, "Four", result.Four, "4")
tFieldEq(t, "Five", result.Five, "5")
}
func TestStructNoRows(t *testing.T) {
var result struct{}
query := `select where false`
err := Query(testCtx, testTx, &result, query, nil)
if !errors.Is(err, ErrNoRows) {
t.Fatalf(`expected error ErrNoRows, got %+v`, err)
}
}
func TestStructMultipleRows(t *testing.T) {
var result struct {
Val string `db:"val"`
}
query := `select * from (values ('one'), ('two')) as vals (val)`
err := Query(testCtx, testTx, &result, query, nil)
if !errors.Is(err, ErrMultipleRows) {
t.Fatalf(`expected error ErrMultipleRows, got %+v`, err)
}
}
func TestInvalidDest(t *testing.T) {
err := Query(testCtx, testTx, nil, `select`, nil)
if !errors.Is(err, ErrInvalidDest) {
t.Fatalf(`expected error ErrInvalidDest, got %+v`, err)
}
err = Query(testCtx, testTx, "str", `select`, nil)
if !errors.Is(err, ErrInvalidDest) {
t.Fatalf(`expected error ErrInvalidDest, got %+v`, err)
}
err = Query(testCtx, testTx, struct{}{}, `select`, nil)
if !errors.Is(err, ErrInvalidDest) {
t.Fatalf(`expected error ErrInvalidDest, got %+v`, err)
}
err = Query(testCtx, testTx, []struct{}{}, `select`, nil)
if !errors.Is(err, ErrInvalidDest) {
t.Fatalf(`expected error ErrInvalidDest, got %+v`, err)
}
}
func TestStructFieldNullability(t *testing.T) {
type Result struct {
NonNilable string `db:"non_nilable"`
Nilable *string `db:"nilable"`
}
var result Result
query := `
select
'one' as non_nilable,
null as nilable
`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := Result{NonNilable: "one", Nilable: nil}
if expected != result {
t.Fatalf("expected %#v, got %#v", expected, result)
}
}
func TestStructs(t *testing.T) {
type Result struct {
One string `db:"one"`
Two int64 `db:"two"`
}
var results []Result
query := `select * from (values ('one', 10), ('two', 20)) as vals (one, two)`
err := Query(testCtx, testTx, &results, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := []Result{{"one", 10}, {"two", 20}}
if !reflect.DeepEqual(expected, results) {
t.Fatalf(`expected %#v, got %#v`, expected, results)
}
}
func TestStructMissingColDest(t *testing.T) {
var result struct {
One string `db:"one"`
}
{
query := `select 'one' as one, 'two' as two`
err := Query(testCtx, testTx, &result, query, nil)
if !errors.Is(err, ErrNoColDest) {
t.Fatalf(`expected error ErrNoColDest, got %+v`, err)
}
}
{
query := `select 'one' as one, null as two`
err := Query(testCtx, testTx, &result, query, nil)
if !errors.Is(err, ErrNoColDest) {
t.Fatalf(`expected error ErrNoColDest, got %+v`, err)
}
}
}
func TestScalarsEmptyResult(t *testing.T) {
results := []string{"one", "two", "three"}
query := `select where false`
err := Query(testCtx, testTx, &results, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := []string{}
if !reflect.DeepEqual(expected, results) {
t.Fatalf(`expected %#v, got %#v`, expected, results)
}
}
func TestStructsEmptyResult(t *testing.T) {
results := []struct{}{{}, {}, {}}
query := `select where false`
err := Query(testCtx, testTx, &results, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := []struct{}{}
if !reflect.DeepEqual(expected, results) {
t.Fatalf(`expected %#v, got %#v`, expected, results)
}
}
func TestStructNestedNotNullNotNilable(t *testing.T) {
type Nested struct {
Val string `db:"val"`
}
type Nesting struct {
Val string `db:"val"`
Nested Nested `db:"nested"`
}
var result Nesting
query := `
select
'one' as "val",
'two' as "nested.val"
`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := Nesting{Val: "one", Nested: Nested{Val: "two"}}
if !reflect.DeepEqual(expected, result) {
t.Fatalf(`expected %#v, got %#v`, expected, result)
}
}
func TestStructNestedNotNullNilableStruct(t *testing.T) {
type Nested struct {
Val string `db:"val"`
}
type Nesting struct {
Val string `db:"val"`
Nested *Nested `db:"nested"`
}
var result Nesting
query := `
select
'one' as "val",
'two' as "nested.val"
`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := Nesting{Val: "one", Nested: &Nested{Val: "two"}}
if !reflect.DeepEqual(expected, result) {
t.Fatalf(`expected %#v, got %#v`, expected, result)
}
}
func TestStructNestedNotNullNilableField(t *testing.T) {
type Nested struct {
Val *string `db:"val"`
}
type Nesting struct {
Val string `db:"val"`
Nested Nested `db:"nested"`
}
var result Nesting
query := `
select
'one' as "val",
'two' as "nested.val"
`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := Nesting{Val: "one", Nested: Nested{Val: strptr("two")}}
if !reflect.DeepEqual(expected, result) {
t.Fatalf(`expected %#v, got %#v`, expected, result)
}
}
func TestStructNestedNotNullNilableBoth(t *testing.T) {
type Nested struct {
Val *string `db:"val"`
}
type Nesting struct {
Val string `db:"val"`
Nested *Nested `db:"nested"`
}
var result Nesting
query := `
select
'one' as "val",
'two' as "nested.val"
`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := Nesting{Val: "one", Nested: &Nested{Val: strptr("two")}}
if !reflect.DeepEqual(expected, result) {
t.Fatalf(`expected %#v, got %#v`, expected, result)
}
}
func TestStructNestedNullNotNilable(t *testing.T) {
type Nested struct {
Val string `db:"val"`
}
type Nesting struct {
Val string `db:"val"`
Nested Nested `db:"nested"`
}
var result Nesting
query := `
select
'one' as "val",
null as "nested.val"
`
err := Query(testCtx, testTx, &result, query, nil)
if !errors.Is(err, ErrNull) {
t.Fatalf(`expected error ErrNull, got %+v`, err)
}
}
// This also tests for on-demand allocation: if all fields of the inner struct
// are nil, the struct is not allocated.
func TestStructNestedNullNilableStruct(t *testing.T) {
type Nested struct {
Val string `db:"val"`
}
type Nesting struct {
Val string `db:"val"`
Nested *Nested `db:"nested"`
}
var result Nesting
query := `
select
'one' as "val",
null as "nested.val"
`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := Nesting{Val: "one", Nested: nil}
if !reflect.DeepEqual(expected, result) {
t.Fatalf(`expected %#v, got %#v`, expected, result)
}
}
func TestStructNestedNullNilableField(t *testing.T) {
type Nested struct {
Val *string `db:"val"`
}
type Nesting struct {
Val string `db:"val"`
Nested Nested `db:"nested"`
}
var result Nesting
query := `
select
'one' as "val",
null as "nested.val"
`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := Nesting{Val: "one", Nested: Nested{Val: nil}}
if !reflect.DeepEqual(expected, result) {
t.Fatalf(`expected %#v, got %#v`, expected, result)
}
}
// This also tests for on-demand allocation: if all fields of the inner struct
// are nil, the struct is not allocated.
func TestStructNestedNullNilableBoth(t *testing.T) {
type Nested struct {
Val *string `db:"val"`
}
type Nesting struct {
Val string `db:"val"`
Nested *Nested `db:"nested"`
}
var result Nesting
query := `
select
'one' as "val",
null as "nested.val"
`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := Nesting{Val: "one", Nested: nil}
if !reflect.DeepEqual(expected, result) {
t.Fatalf(`expected %#v, got %#v`, expected, result)
}
}
func TestStructNestedPartiallyNull(t *testing.T) {
type Nested struct {
One *string `db:"one"`
Two *string `db:"two"`
}
type Nesting struct {
Nested *Nested `db:"nested"`
Three string `db:"three"`
}
var result Nesting
query := `
select
'one' as "nested.one",
'three' as "three"
`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := Nesting{Nested: &Nested{One: strptr("one")}, Three: "three"}
if !reflect.DeepEqual(expected, result) {
t.Fatalf(`expected %#v, got %#v`, expected, result)
}
}
/*
Fields without a matching source column must be left untouched. If they have
non-zero values, the existing values must be preserved.
*/
func TestStructMissingColSrc(t *testing.T) {
type Result struct {
One string `db:"one"`
Two string `db:"two"`
Three *string `db:"-"`
Four *Result
}
result := Result{Two: "two", Three: strptr("three"), Four: &Result{One: "four"}}
query := `select 'one' as one`
err := Query(testCtx, testTx, &result, query, nil)
if err != nil {
t.Fatalf("%+v", err)
}
expected := Result{One: "one", Two: "two", Three: strptr("three"), Four: &Result{One: "four"}}
if !reflect.DeepEqual(expected, result) {
t.Fatalf(`expected %#v, got %#v`, expected, result)
}
}
func createDb(connParams []string, dbName string) error {
return withPostgresDb(connParams, func(db *sql.DB) error {
_, err := db.Exec(`create database ` + dbName)
return err
})
}
func dropDb(connParams []string, dbName string) error {
return withPostgresDb(connParams, func(db *sql.DB) error {
_, err := db.Exec(`drop database ` + dbName)
return err
})
}
func withPostgresDb(connParams []string, fun func(db *sql.DB) error) error {
connParams = append(connParams, `dbname=postgres`)
db, err := sql.Open("postgres", strings.Join(connParams, ` `))
if err != nil {
return err
}
err = fun(db)
if err != nil {
return err
}
return db.Close()
}
func tFieldEq(t *testing.T, fieldName string, left interface{}, right interface{}) {
if !reflect.DeepEqual(left, right) {
t.Fatalf(`mismatch in field %q: %#v vs. %#v`, fieldName, left, right)
}
}
func tExec(t *testing.T, query string) {
_, err := testTx.Exec(query)
if err != nil {
t.Fatalf("%+v", err)
}
}
type ScannableString string
func (self *ScannableString) Scan(input interface{}) error {
*self = ScannableString(input.(string) + "_scanned")
return nil
}
func strptr(str string) *string { return &str }
func timeMustParse(str string) time.Time {
out, err := time.Parse(time.RFC3339, str)
if err != nil {
panic(err)
}
return out
}
type ScannableStruct struct {
Value string
}
func (self *ScannableStruct) Scan(input interface{}) error {
switch input := input.(type) {
case nil:
return nil
case string:
self.Value = input + "_scanned"
return nil
case []byte:
self.Value = string(input) + "_scanned"
return nil
default:
return fmt.Errorf("unrecognized input for type %T: type %T, value %v", self, input, input)
}
}
<file_sep>/go.mod
module github.com/mitranim/gos
go 1.13
// All dependencies are test-only.
require (
github.com/davecgh/go-spew v1.1.1
github.com/lib/pq v1.3.0
)
<file_sep>/readme.md
## Overview
**Go** ↔︎ **S**QL: tool for mapping between Go and SQL. Features:
* Decodes SQL records into Go structs
* Supports nested records/structs
* Supports nilable nested records/structs in outer joins
* Helps to generate queries from structs
**This is not an ORM**, and should be used **instead** of an ORM. This tool is expressly designed to let you **write plain SQL**.
See the full documentation at https://godoc.org/github.com/mitranim/gos.
## Differences from [jmoiron/sqlx](https://github.com/jmoiron/sqlx)
Gos is very similar to [jmoiron/sqlx](https://github.com/jmoiron/sqlx). Key differences:
* Supports null records in outer joins, as nested struct pointers
* Selects fields explicitly without relying on `select *`
* Much simpler API, does not wrap `database/sql`
* Explicit field-column mapping, no hidden renaming
* No special utilities for named parameters
* Depends only on the standard library (the `go.mod` dependencies are test-only)
## Usage Example
See the full documentation at https://godoc.org/github.com/mitranim/gos.
```go
type External struct {
Id string `db:"id"`
Name string `db:"name"`
}
type Internal struct {
Id string `db:"id"`
Name string `db:"name"`
}
// Step 1: generate query.
var result []External
query := fmt.Sprintf(`select %v from (
select
external.*,
internal as internal
from
external
cross join internal
) as _
`, gos.Cols(result))
/*
Resulting query (formatted here for readability):
select
"id",
"name",
("internal")."id" as "internal.id",
("internal")."name" as "internal.name"
from (
...
) as _
*/
// Step 2: use query.
err := gos.Query(ctx, conn, &result, query, nil)
fmt.Printf("%#v\n", result)
```
## Features Under Consideration
1. Utilities for insert and update queries.
The current API helps generate select queries from structs, but not insert or update queries. This needs to be rectified.
2. Short column aliases.
Like many similar libraries, when selecting fields for nested records, Gos relies on column aliases like `"column_name.column_name"`. With enough nesting, they can become too long. At the time of writing, Postgres 12 has an identifier length limit of 63 and will _silently truncate_ the remainder, causing queries to fail. One solution is shorter aliases, such as `"1.2.3"` from struct field indexes. We still want to support long alises for manually-written queries, which means the library would have to support _both_ alias types, which could potentially cause collisions. Unclear what's the best approach.
3. `select *`
Currently Gos requires you to generate queries with the help of `gos.Cols()`. It would be nice to just pass `select *` and have Gos automatically wrap the query into another `select`, listing the fields. Unfortunately not every query can be wrapped, but this can be provided as an additional function, alongside `Query()`.
4. Streaming API.
An API for scanning rows one-by-one, like `database/sql.Rows.Scan()` but for structs. This would allow streaming and might be useful when processing a very large amount of rows. Scanning into structs involves reflecting on the type and generating a spec, and this must be done only once; the API would have to be designed to make it seamless for the user. One option is to wrap `sql.Rows` into an object that provides `.Next()` and `.Scan()`, generates the output spec on the first call to `.Scan()` and stores it, and on subsequent calls ensures that the same destination type was provided.
## Contributing
Issues and pull requests are welcome! The development setup is simple:
```sh
git clone https://github.com/mitranim/gos
cd gos
go test
```
Tests currently require a local instance of Postgres on the default port. They create a separate "database", make no persistent changes, and drop it at the end.
## License
https://en.wikipedia.org/wiki/WTFPL
## Misc
I'm receptive to suggestions. If this library _almost_ satisfies you but needs changes, open an issue or chat me up. Contacts: https://mitranim.com/#contacts
| b392676f15c8b5b25a9822c290e1a287cbf4afa7 | [
"Markdown",
"Go Module",
"Go"
] | 4 | Go | Toansmile/gos | c048fd10939daac847b7df5ee7b06801dab66e75 | e9f2cf2f45df6df3acf3d145cef5a9112ec9cab4 | |
refs/heads/master | <repo_name>userrails/GrapeApi<file_sep>/app/api/api.rb
require 'grape-swagger'
class API < Grape::API
prefix 'api'
version 'v1', using: :path
mount Employee::Data
add_swagger_documentation
# add_swagger_documentation :format => :json,
# :api_version => 'v1',
# :base_path => "http://localhost:3000/api/",
# :hide_documentation_path => true
end<file_sep>/app/api/employee/data.rb
module Employee
class Data < Grape::API
resource :employee_data do
desc "list of the employee data"
get do
EmpData.all
end
#curl http://localhost:3000/api/v1/employee_data.json
desc "create a new employee data"
## This takes care of parameter validation
params do
requires :name, type: String
requires :address, type: String
requires :age, type: Integer
end
## This takes care of creating employee
post do
EmpData.create!({
name: params[:name],
address: params[:address],
age: params[:age]
})
end
#curl http://localhost:3000/api/v1/employee_data.json -d "name=jay;address=delhi;age=25"
## delete an employee
params do
requires :id, type: String
end
delete ':id' do
EmpData.find(params[:id]).destroy!
end
# curl -X DELETE http://localhost:3000/api/v1/employee_data/5.json
## update the employee records
params do
requires :id, type:String
requires :name, type:String
requires :address, type:String
requires :age, type:Integer
end
put ':id' do
EmpData.find(params[:id]).update!({
address: params[:address],
name: params[:name],
age: params[:age]
})
end
end
end
end | 42cf479fcadc2158d8d3e879d792c9dbb5f24e60 | [
"Ruby"
] | 2 | Ruby | userrails/GrapeApi | 1ee55ce250acde228487f5480253f13ab407c49a | 36e957a8bdf236fce6d5f9c097c2fe2110445e70 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.