hexsha
stringlengths 40
40
| size
int64 6
14.9M
| ext
stringclasses 1
value | lang
stringclasses 1
value | max_stars_repo_path
stringlengths 6
260
| max_stars_repo_name
stringlengths 6
119
| max_stars_repo_head_hexsha
stringlengths 40
41
| max_stars_repo_licenses
sequence | max_stars_count
int64 1
191k
⌀ | max_stars_repo_stars_event_min_datetime
stringlengths 24
24
⌀ | max_stars_repo_stars_event_max_datetime
stringlengths 24
24
⌀ | max_issues_repo_path
stringlengths 6
260
| max_issues_repo_name
stringlengths 6
119
| max_issues_repo_head_hexsha
stringlengths 40
41
| max_issues_repo_licenses
sequence | max_issues_count
int64 1
67k
⌀ | max_issues_repo_issues_event_min_datetime
stringlengths 24
24
⌀ | max_issues_repo_issues_event_max_datetime
stringlengths 24
24
⌀ | max_forks_repo_path
stringlengths 6
260
| max_forks_repo_name
stringlengths 6
119
| max_forks_repo_head_hexsha
stringlengths 40
41
| max_forks_repo_licenses
sequence | max_forks_count
int64 1
105k
⌀ | max_forks_repo_forks_event_min_datetime
stringlengths 24
24
⌀ | max_forks_repo_forks_event_max_datetime
stringlengths 24
24
⌀ | avg_line_length
float64 2
1.04M
| max_line_length
int64 2
11.2M
| alphanum_fraction
float64 0
1
| cells
sequence | cell_types
sequence | cell_type_groups
sequence |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
e7b8d91a03a3cfa6a9932928f256118d47119556 | 137,884 | ipynb | Jupyter Notebook | 06_rnns/RNN_DLFS.ipynb | tianminzheng/DLFS_code | a93f028203b5f59ad46b527fc17bfaf0438bb3fb | [
"MIT"
] | 271 | 2018-12-17T21:09:17.000Z | 2022-03-30T13:28:19.000Z | 06_rnns/RNN_DLFS.ipynb | tianminzheng/DLFS_code | a93f028203b5f59ad46b527fc17bfaf0438bb3fb | [
"MIT"
] | 7 | 2019-09-22T21:51:14.000Z | 2021-03-28T11:32:55.000Z | 06_rnns/RNN_DLFS.ipynb | tianminzheng/DLFS_code | a93f028203b5f59ad46b527fc17bfaf0438bb3fb | [
"MIT"
] | 168 | 2019-06-15T17:11:09.000Z | 2022-03-27T22:28:58.000Z | 83.414398 | 16,196 | 0.734886 | [
[
[
"Inspired by: http://blog.varunajayasiri.com/numpy_lstm.html",
"_____no_output_____"
],
[
"# Imports",
"_____no_output_____"
]
],
[
[
"import numpy as np\nfrom numpy import ndarray",
"_____no_output_____"
],
[
"from typing import Dict, List, Tuple",
"_____no_output_____"
],
[
"import matplotlib.pyplot as plt\nfrom IPython import display\nplt.style.use('seaborn-white')\n%matplotlib inline\n\nfrom copy import deepcopy\nfrom collections import deque",
"_____no_output_____"
],
[
"from lincoln.utils.np_utils import assert_same_shape\nfrom scipy.special import logsumexp",
"_____no_output_____"
]
],
[
[
"# Activations",
"_____no_output_____"
]
],
[
[
"def sigmoid(x: ndarray):\n return 1 / (1 + np.exp(-x))\n\n\ndef dsigmoid(x: ndarray):\n return sigmoid(x) * (1 - sigmoid(x))\n\n\ndef tanh(x: ndarray):\n return np.tanh(x)\n\n\ndef dtanh(x: ndarray):\n return 1 - np.tanh(x) * np.tanh(x)\n\n\ndef softmax(x, axis=None):\n return np.exp(x - logsumexp(x, axis=axis, keepdims=True))\n\n\ndef batch_softmax(input_array: ndarray):\n out = []\n for row in input_array:\n out.append(softmax(row, axis=1))\n return np.stack(out)\n ",
"_____no_output_____"
]
],
[
[
"# `RNNOptimizer`",
"_____no_output_____"
]
],
[
[
"class RNNOptimizer(object):\n def __init__(self,\n lr: float = 0.01,\n gradient_clipping: bool = True) -> None:\n self.lr = lr\n self.gradient_clipping = gradient_clipping\n self.first = True\n\n def step(self) -> None:\n\n for layer in self.model.layers:\n for key in layer.params.keys():\n\n if self.gradient_clipping:\n np.clip(layer.params[key]['deriv'], -2, 2, layer.params[key]['deriv'])\n\n self._update_rule(param=layer.params[key]['value'],\n grad=layer.params[key]['deriv'])\n\n def _update_rule(self, **kwargs) -> None:\n raise NotImplementedError()\n",
"_____no_output_____"
]
],
[
[
"# `SGD` and `AdaGrad`",
"_____no_output_____"
]
],
[
[
"class SGD(RNNOptimizer):\n def __init__(self,\n lr: float = 0.01,\n gradient_clipping: bool = True) -> None:\n super().__init__(lr, gradient_clipping)\n\n def _update_rule(self, **kwargs) -> None:\n\n update = self.lr*kwargs['grad']\n kwargs['param'] -= update\n ",
"_____no_output_____"
],
[
"class AdaGrad(RNNOptimizer):\n def __init__(self,\n lr: float = 0.01,\n gradient_clipping: bool = True) -> None:\n super().__init__(lr, gradient_clipping)\n self.eps = 1e-7\n\n def step(self) -> None:\n if self.first:\n self.sum_squares = {}\n for i, layer in enumerate(self.model.layers):\n self.sum_squares[i] = {}\n for key in layer.params.keys():\n self.sum_squares[i][key] = np.zeros_like(layer.params[key]['value'])\n \n self.first = False\n\n for i, layer in enumerate(self.model.layers):\n for key in layer.params.keys():\n \n if self.gradient_clipping:\n np.clip(layer.params[key]['deriv'], -2, 2, layer.params[key]['deriv'])\n \n self._update_rule(param=layer.params[key]['value'],\n grad=layer.params[key]['deriv'],\n sum_square=self.sum_squares[i][key])\n\n def _update_rule(self, **kwargs) -> None:\n\n # Update running sum of squares\n kwargs['sum_square'] += (self.eps +\n np.power(kwargs['grad'], 2))\n\n # Scale learning rate by running sum of squareds=5\n lr = np.divide(self.lr, np.sqrt(kwargs['sum_square']))\n\n # Use this to update parameters\n kwargs['param'] -= lr * kwargs['grad']",
"_____no_output_____"
]
],
[
[
"# `Loss`es",
"_____no_output_____"
]
],
[
[
"class Loss(object):\n\n def __init__(self):\n pass\n\n def forward(self,\n prediction: ndarray,\n target: ndarray) -> float:\n\n assert_same_shape(prediction, target)\n\n self.prediction = prediction\n self.target = target\n\n self.output = self._output()\n\n return self.output\n \n def backward(self) -> ndarray:\n\n self.input_grad = self._input_grad()\n\n assert_same_shape(self.prediction, self.input_grad)\n\n return self.input_grad\n\n def _output(self) -> float:\n raise NotImplementedError()\n\n def _input_grad(self) -> ndarray:\n raise NotImplementedError()\n\n \nclass SoftmaxCrossEntropy(Loss):\n def __init__(self, eps: float=1e-9) -> None:\n super().__init__()\n self.eps = eps\n self.single_class = False\n\n def _output(self) -> float:\n\n out = []\n for row in self.prediction:\n out.append(softmax(row, axis=1))\n softmax_preds = np.stack(out)\n\n # clipping the softmax output to prevent numeric instability\n self.softmax_preds = np.clip(softmax_preds, self.eps, 1 - self.eps)\n\n # actual loss computation\n softmax_cross_entropy_loss = -1.0 * self.target * np.log(self.softmax_preds) - \\\n (1.0 - self.target) * np.log(1 - self.softmax_preds)\n\n return np.sum(softmax_cross_entropy_loss)\n\n def _input_grad(self) -> np.ndarray:\n\n return self.softmax_preds - self.target",
"_____no_output_____"
]
],
[
[
"# RNNs",
"_____no_output_____"
],
[
"## `RNNNode`",
"_____no_output_____"
]
],
[
[
"class RNNNode(object):\n\n def __init__(self):\n pass\n\n def forward(self,\n x_in: ndarray, \n H_in: ndarray,\n params_dict: Dict[str, Dict[str, ndarray]]\n ) -> Tuple[ndarray]:\n '''\n param x: numpy array of shape (batch_size, vocab_size)\n param H_prev: numpy array of shape (batch_size, hidden_size)\n return self.x_out: numpy array of shape (batch_size, vocab_size)\n return self.H: numpy array of shape (batch_size, hidden_size)\n '''\n self.X_in = x_in\n self.H_in = H_in\n \n self.Z = np.column_stack((x_in, H_in))\n \n self.H_int = np.dot(self.Z, params_dict['W_f']['value']) \\\n + params_dict['B_f']['value']\n \n self.H_out = tanh(self.H_int)\n\n self.X_out = np.dot(self.H_out, params_dict['W_v']['value']) \\\n + params_dict['B_v']['value']\n \n return self.X_out, self.H_out\n\n\n def backward(self, \n X_out_grad: ndarray, \n H_out_grad: ndarray,\n params_dict: Dict[str, Dict[str, ndarray]]) -> Tuple[ndarray]:\n '''\n param x_out_grad: numpy array of shape (batch_size, vocab_size)\n param h_out_grad: numpy array of shape (batch_size, hidden_size)\n param RNN_Params: RNN_Params object\n return x_in_grad: numpy array of shape (batch_size, vocab_size)\n return h_in_grad: numpy array of shape (batch_size, hidden_size)\n '''\n \n assert_same_shape(X_out_grad, self.X_out)\n assert_same_shape(H_out_grad, self.H_out)\n\n params_dict['B_v']['deriv'] += X_out_grad.sum(axis=0)\n params_dict['W_v']['deriv'] += np.dot(self.H_out.T, X_out_grad)\n \n dh = np.dot(X_out_grad, params_dict['W_v']['value'].T)\n dh += H_out_grad\n \n dH_int = dh * dtanh(self.H_int)\n \n params_dict['B_f']['deriv'] += dH_int.sum(axis=0)\n params_dict['W_f']['deriv'] += np.dot(self.Z.T, dH_int) \n \n dz = np.dot(dH_int, params_dict['W_f']['value'].T)\n\n X_in_grad = dz[:, :self.X_in.shape[1]]\n H_in_grad = dz[:, self.X_in.shape[1]:]\n\n assert_same_shape(X_out_grad, self.X_out)\n assert_same_shape(H_out_grad, self.H_out)\n \n return X_in_grad, H_in_grad",
"_____no_output_____"
]
],
[
[
"## `RNNLayer`",
"_____no_output_____"
]
],
[
[
"class RNNLayer(object):\n\n def __init__(self,\n hidden_size: int,\n output_size: int,\n weight_scale: float = None):\n '''\n param sequence_length: int - length of sequence being passed through the network\n param vocab_size: int - the number of characters in the vocabulary of which we are predicting the next\n character.\n param hidden_size: int - the number of \"hidden neurons\" in the LSTM_Layer of which this node is a part.\n param learning_rate: float - the learning rate\n '''\n self.hidden_size = hidden_size\n self.output_size = output_size\n self.weight_scale = weight_scale\n self.start_H = np.zeros((1, hidden_size))\n self.first = True\n\n\n def _init_params(self,\n input_: ndarray):\n \n self.vocab_size = input_.shape[2]\n \n if not self.weight_scale:\n self.weight_scale = 2 / (self.vocab_size + self.output_size)\n \n self.params = {}\n self.params['W_f'] = {}\n self.params['B_f'] = {}\n self.params['W_v'] = {}\n self.params['B_v'] = {}\n \n self.params['W_f']['value'] = np.random.normal(loc = 0.0,\n scale=self.weight_scale,\n size=(self.hidden_size + self.vocab_size, self.hidden_size))\n self.params['B_f']['value'] = np.random.normal(loc = 0.0,\n scale=self.weight_scale,\n size=(1, self.hidden_size))\n self.params['W_v']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(self.hidden_size, self.output_size))\n self.params['B_v']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(1, self.output_size)) \n \n self.params['W_f']['deriv'] = np.zeros_like(self.params['W_f']['value'])\n self.params['B_f']['deriv'] = np.zeros_like(self.params['B_f']['value'])\n self.params['W_v']['deriv'] = np.zeros_like(self.params['W_v']['value'])\n self.params['B_v']['deriv'] = np.zeros_like(self.params['B_v']['value'])\n \n self.cells = [RNNNode() for x in range(input_.shape[1])]\n\n \n def _clear_gradients(self):\n for key in self.params.keys():\n self.params[key]['deriv'] = np.zeros_like(self.params[key]['deriv'])\n \n\n def forward(self, x_seq_in: ndarray):\n '''\n param x_seq_in: numpy array of shape (batch_size, sequence_length, vocab_size)\n return x_seq_out: numpy array of shape (batch_size, sequence_length, output_size)\n '''\n if self.first:\n self._init_params(x_seq_in)\n self.first=False\n \n batch_size = x_seq_in.shape[0]\n \n H_in = np.copy(self.start_H)\n \n H_in = np.repeat(H_in, batch_size, axis=0)\n\n sequence_length = x_seq_in.shape[1]\n \n x_seq_out = np.zeros((batch_size, sequence_length, self.output_size))\n \n for t in range(sequence_length):\n\n x_in = x_seq_in[:, t, :]\n \n y_out, H_in = self.cells[t].forward(x_in, H_in, self.params)\n \n x_seq_out[:, t, :] = y_out\n \n self.start_H = H_in.mean(axis=0, keepdims=True)\n \n return x_seq_out\n\n\n def backward(self, x_seq_out_grad: ndarray):\n '''\n param loss_grad: numpy array of shape (batch_size, sequence_length, vocab_size)\n return loss_grad_out: numpy array of shape (batch_size, sequence_length, vocab_size)\n '''\n batch_size = x_seq_out_grad.shape[0]\n \n h_in_grad = np.zeros((batch_size, self.hidden_size))\n \n sequence_length = x_seq_out_grad.shape[1]\n \n x_seq_in_grad = np.zeros((batch_size, sequence_length, self.vocab_size))\n \n for t in reversed(range(sequence_length)):\n \n x_out_grad = x_seq_out_grad[:, t, :]\n\n grad_out, h_in_grad = \\\n self.cells[t].backward(x_out_grad, h_in_grad, self.params)\n \n x_seq_in_grad[:, t, :] = grad_out\n \n return x_seq_in_grad",
"_____no_output_____"
]
],
[
[
"## `RNNModel`",
"_____no_output_____"
]
],
[
[
"class RNNModel(object):\n '''\n The Model class that takes in inputs and targets and actually trains the network and calculates the loss.\n '''\n def __init__(self, \n layers: List[RNNLayer],\n sequence_length: int, \n vocab_size: int, \n loss: Loss):\n '''\n param num_layers: int - the number of layers in the network\n param sequence_length: int - length of sequence being passed through the network\n param vocab_size: int - the number of characters in the vocabulary of which we are predicting the next\n character.\n param hidden_size: int - the number of \"hidden neurons\" in the each layer of the network.\n '''\n self.layers = layers\n self.vocab_size = vocab_size\n self.sequence_length = sequence_length\n self.loss = loss\n for layer in self.layers:\n setattr(layer, 'sequence_length', sequence_length)\n\n \n def forward(self, \n x_batch: ndarray):\n '''\n param inputs: list of integers - a list of indices of characters being passed in as the \n input sequence of the network.\n returns x_batch_in: numpy array of shape (batch_size, sequence_length, vocab_size)\n ''' \n \n for layer in self.layers:\n\n x_batch = layer.forward(x_batch)\n \n return x_batch\n \n def backward(self, \n loss_grad: ndarray):\n '''\n param loss_grad: numpy array with shape (batch_size, sequence_length, vocab_size)\n returns loss: float, representing mean squared error loss\n '''\n\n for layer in reversed(self.layers):\n\n loss_grad = layer.backward(loss_grad)\n \n return loss_grad\n \n def single_step(self, \n x_batch: ndarray, \n y_batch: ndarray):\n '''\n The step that does it all:\n 1. Forward pass & softmax\n 2. Compute loss and loss gradient\n 3. Backward pass\n 4. Update parameters\n param inputs: array of length sequence_length that represents the character indices of the inputs to\n the network\n param targets: array of length sequence_length that represents the character indices of the targets\n of the network \n return loss\n ''' \n \n x_batch_out = self.forward(x_batch)\n \n loss = self.loss.forward(x_batch_out, y_batch)\n \n loss_grad = self.loss.backward()\n \n for layer in self.layers:\n layer._clear_gradients()\n \n self.backward(loss_grad)\n return loss",
"_____no_output_____"
]
],
[
[
"# `RNNTrainer`",
"_____no_output_____"
]
],
[
[
"class RNNTrainer:\n '''\n Takes in a text file and a model, and starts generating characters.\n '''\n def __init__(self, \n text_file: str, \n model: RNNModel,\n optim: RNNOptimizer,\n batch_size: int = 32):\n self.data = open(text_file, 'r').read()\n self.model = model\n self.chars = list(set(self.data))\n self.vocab_size = len(self.chars)\n self.char_to_idx = {ch:i for i,ch in enumerate(self.chars)}\n self.idx_to_char = {i:ch for i,ch in enumerate(self.chars)}\n self.sequence_length = self.model.sequence_length\n self.batch_size = batch_size\n self.optim = optim\n setattr(self.optim, 'model', self.model)\n \n\n def _generate_inputs_targets(self, \n start_pos: int):\n \n inputs_indices = np.zeros((self.batch_size, self.sequence_length), dtype=int)\n targets_indices = np.zeros((self.batch_size, self.sequence_length), dtype=int)\n \n for i in range(self.batch_size):\n \n inputs_indices[i, :] = np.array([self.char_to_idx[ch] \n for ch in self.data[start_pos + i: start_pos + self.sequence_length + i]])\n targets_indices[i, :] = np.array([self.char_to_idx[ch] \n for ch in self.data[start_pos + 1 + i: start_pos + self.sequence_length + 1 + i]])\n\n return inputs_indices, targets_indices\n\n\n def _generate_one_hot_array(self, \n indices: ndarray):\n '''\n param indices: numpy array of shape (batch_size, sequence_length)\n return batch - numpy array of shape (batch_size, sequence_length, vocab_size)\n ''' \n batch = []\n for seq in indices:\n \n one_hot_sequence = np.zeros((self.sequence_length, self.vocab_size))\n \n for i in range(self.sequence_length):\n one_hot_sequence[i, seq[i]] = 1.0\n\n batch.append(one_hot_sequence) \n\n return np.stack(batch)\n\n\n def sample_output(self, \n input_char: int, \n sample_length: int):\n '''\n Generates a sample output using the current trained model, one character at a time.\n param input_char: int - index of the character to use to start generating a sequence\n param sample_length: int - the length of the sample output to generate\n return txt: string - a string of length sample_length representing the sample output\n '''\n indices = []\n \n sample_model = deepcopy(self.model)\n \n for i in range(sample_length):\n input_char_batch = np.zeros((1, 1, self.vocab_size))\n \n input_char_batch[0, 0, input_char] = 1.0\n \n x_batch_out = sample_model.forward(input_char_batch)\n \n x_softmax = batch_softmax(x_batch_out)\n \n input_char = np.random.choice(range(self.vocab_size), p=x_softmax.ravel())\n \n indices.append(input_char)\n \n txt = ''.join(self.idx_to_char[idx] for idx in indices)\n return txt\n\n def train(self, \n num_iterations: int, \n sample_every: int=100):\n '''\n Trains the \"character generator\" for a number of iterations. \n Each \"iteration\" feeds a batch size of 1 through the neural network.\n Continues until num_iterations is reached. Displays sample text generated using the latest version.\n '''\n plot_iter = np.zeros((0))\n plot_loss = np.zeros((0))\n \n num_iter = 0\n start_pos = 0\n \n moving_average = deque(maxlen=100)\n while num_iter < num_iterations:\n \n if start_pos + self.sequence_length + self.batch_size + 1 > len(self.data):\n start_pos = 0\n \n ## Update the model\n inputs_indices, targets_indices = self._generate_inputs_targets(start_pos)\n\n inputs_batch, targets_batch = \\\n self._generate_one_hot_array(inputs_indices), self._generate_one_hot_array(targets_indices)\n \n loss = self.model.single_step(inputs_batch, targets_batch)\n self.optim.step()\n \n moving_average.append(loss)\n ma_loss = np.mean(moving_average)\n \n start_pos += self.batch_size\n \n plot_iter = np.append(plot_iter, [num_iter])\n plot_loss = np.append(plot_loss, [ma_loss])\n \n if num_iter % 100 == 0:\n plt.plot(plot_iter, plot_loss)\n display.clear_output(wait=True)\n plt.show()\n \n sample_text = self.sample_output(self.char_to_idx[self.data[start_pos]], \n 200)\n print(sample_text)\n\n num_iter += 1\n \n ",
"_____no_output_____"
],
[
"layers = [RNNLayer(hidden_size=256, output_size=62)]\nmod = RNNModel(layers=layers,\n vocab_size=62, sequence_length=10,\n loss=SoftmaxCrossEntropy())\noptim = SGD(lr=0.001, gradient_clipping=True)\ntrainer = RNNTrainer('input.txt', mod, optim)\ntrainer.train(1000, sample_every=100)",
"_____no_output_____"
]
],
[
[
"With RNN cells, this gets stuck in a local max. Let's try `LSTM`s.",
"_____no_output_____"
],
[
"# LSTMs",
"_____no_output_____"
],
[
"## `LSTMNode`",
"_____no_output_____"
]
],
[
[
"class LSTMNode:\n\n def __init__(self):\n '''\n param hidden_size: int - the number of \"hidden neurons\" in the LSTM_Layer of which this node is a part.\n param vocab_size: int - the number of characters in the vocabulary of which we are predicting the next\n character.\n '''\n pass\n \n def forward(self, \n X_in: ndarray, \n H_in: ndarray, \n C_in: ndarray, \n params_dict: Dict[str, Dict[str, ndarray]]):\n '''\n param X_in: numpy array of shape (batch_size, vocab_size)\n param H_in: numpy array of shape (batch_size, hidden_size)\n param C_in: numpy array of shape (batch_size, hidden_size)\n return self.X_out: numpy array of shape (batch_size, output_size)\n return self.H: numpy array of shape (batch_size, hidden_size)\n return self.C: numpy array of shape (batch_size, hidden_size)\n '''\n self.X_in = X_in\n self.C_in = C_in\n\n self.Z = np.column_stack((X_in, H_in))\n \n self.f_int = np.dot(self.Z, params_dict['W_f']['value']) + params_dict['B_f']['value']\n self.f = sigmoid(self.f_int)\n \n self.i_int = np.dot(self.Z, params_dict['W_i']['value']) + params_dict['B_i']['value']\n self.i = sigmoid(self.i_int)\n self.C_bar_int = np.dot(self.Z, params_dict['W_c']['value']) + params_dict['B_c']['value']\n self.C_bar = tanh(self.C_bar_int)\n\n self.C_out = self.f * C_in + self.i * self.C_bar\n self.o_int = np.dot(self.Z, params_dict['W_o']['value']) + params_dict['B_o']['value']\n self.o = sigmoid(self.o_int)\n self.H_out = self.o * tanh(self.C_out)\n\n self.X_out = np.dot(self.H_out, params_dict['W_v']['value']) + params_dict['B_v']['value']\n \n return self.X_out, self.H_out, self.C_out \n\n\n def backward(self, \n X_out_grad: ndarray, \n H_out_grad: ndarray, \n C_out_grad: ndarray, \n params_dict: Dict[str, Dict[str, ndarray]]):\n '''\n param loss_grad: numpy array of shape (1, vocab_size)\n param dh_next: numpy array of shape (1, hidden_size)\n param dC_next: numpy array of shape (1, hidden_size)\n param LSTM_Params: LSTM_Params object\n return self.dx_prev: numpy array of shape (1, vocab_size)\n return self.dH_prev: numpy array of shape (1, hidden_size)\n return self.dC_prev: numpy array of shape (1, hidden_size)\n '''\n \n assert_same_shape(X_out_grad, self.X_out)\n assert_same_shape(H_out_grad, self.H_out)\n assert_same_shape(C_out_grad, self.C_out)\n\n params_dict['W_v']['deriv'] += np.dot(self.H_out.T, X_out_grad)\n params_dict['B_v']['deriv'] += X_out_grad.sum(axis=0)\n\n dh_out = np.dot(X_out_grad, params_dict['W_v']['value'].T) \n dh_out += H_out_grad\n \n do = dh_out * tanh(self.C_out)\n do_int = dsigmoid(self.o_int) * do\n params_dict['W_o']['deriv'] += np.dot(self.Z.T, do_int)\n params_dict['B_o']['deriv'] += do_int.sum(axis=0)\n\n dC_out = dh_out * self.o * dtanh(self.C_out)\n dC_out += C_out_grad\n dC_bar = dC_out * self.i\n dC_bar_int = dtanh(self.C_bar_int) * dC_bar\n params_dict['W_c']['deriv'] += np.dot(self.Z.T, dC_bar_int)\n params_dict['B_c']['deriv'] += dC_bar_int.sum(axis=0)\n\n di = dC_out * self.C_bar\n di_int = dsigmoid(self.i_int) * di\n params_dict['W_i']['deriv'] += np.dot(self.Z.T, di_int)\n params_dict['B_i']['deriv'] += di_int.sum(axis=0)\n\n df = dC_out * self.C_in\n df_int = dsigmoid(self.f_int) * df\n params_dict['W_f']['deriv'] += np.dot(self.Z.T, df_int)\n params_dict['B_f']['deriv'] += df_int.sum(axis=0)\n\n dz = (np.dot(df_int, params_dict['W_f']['value'].T)\n + np.dot(di_int, params_dict['W_i']['value'].T)\n + np.dot(dC_bar_int, params_dict['W_c']['value'].T)\n + np.dot(do_int, params_dict['W_o']['value'].T))\n \n dx_prev = dz[:, :self.X_in.shape[1]]\n dH_prev = dz[:, self.X_in.shape[1]:]\n dC_prev = self.f * dC_out\n\n return dx_prev, dH_prev, dC_prev",
"_____no_output_____"
]
],
[
[
"## `LSTMLayer`",
"_____no_output_____"
]
],
[
[
"class LSTMLayer:\n\n def __init__(self,\n hidden_size: int,\n output_size: int,\n weight_scale: float = 0.01):\n '''\n param sequence_length: int - length of sequence being passed through the network\n param vocab_size: int - the number of characters in the vocabulary of which we are predicting the next\n character.\n param hidden_size: int - the number of \"hidden neurons\" in the LSTM_Layer of which this node is a part.\n param learning_rate: float - the learning rate\n '''\n self.hidden_size = hidden_size\n self.output_size = output_size\n self.weight_scale = weight_scale\n self.start_H = np.zeros((1, hidden_size))\n self.start_C = np.zeros((1, hidden_size)) \n self.first = True\n\n \n def _init_params(self,\n input_: ndarray):\n \n self.vocab_size = input_.shape[2]\n\n self.params = {}\n self.params['W_f'] = {}\n self.params['B_f'] = {}\n self.params['W_i'] = {}\n self.params['B_i'] = {}\n self.params['W_c'] = {}\n self.params['B_c'] = {}\n self.params['W_o'] = {}\n self.params['B_o'] = {} \n self.params['W_v'] = {}\n self.params['B_v'] = {}\n \n self.params['W_f']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size =(self.hidden_size + self.vocab_size, self.hidden_size))\n self.params['B_f']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(1, self.hidden_size))\n self.params['W_i']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(self.hidden_size + self.vocab_size, self.hidden_size))\n self.params['B_i']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(1, self.hidden_size))\n self.params['W_c']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(self.hidden_size + self.vocab_size, self.hidden_size))\n self.params['B_c']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(1, self.hidden_size))\n self.params['W_o']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(self.hidden_size + self.vocab_size, self.hidden_size))\n self.params['B_o']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(1, self.hidden_size)) \n self.params['W_v']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(self.hidden_size, self.output_size))\n self.params['B_v']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(1, self.output_size))\n \n for key in self.params.keys():\n self.params[key]['deriv'] = np.zeros_like(self.params[key]['value'])\n \n self.cells = [LSTMNode() for x in range(input_.shape[1])]\n\n\n def _clear_gradients(self):\n for key in self.params.keys():\n self.params[key]['deriv'] = np.zeros_like(self.params[key]['deriv'])\n \n \n def forward(self, x_seq_in: ndarray):\n '''\n param x_seq_in: numpy array of shape (batch_size, sequence_length, vocab_size)\n return x_seq_out: numpy array of shape (batch_size, sequence_length, vocab_size)\n '''\n if self.first:\n self._init_params(x_seq_in)\n self.first=False\n \n batch_size = x_seq_in.shape[0]\n \n H_in = np.copy(self.start_H)\n C_in = np.copy(self.start_C)\n \n H_in = np.repeat(H_in, batch_size, axis=0)\n C_in = np.repeat(C_in, batch_size, axis=0) \n\n sequence_length = x_seq_in.shape[1]\n \n x_seq_out = np.zeros((batch_size, sequence_length, self.output_size))\n \n for t in range(sequence_length):\n\n x_in = x_seq_in[:, t, :]\n \n y_out, H_in, C_in = self.cells[t].forward(x_in, H_in, C_in, self.params)\n \n x_seq_out[:, t, :] = y_out\n \n self.start_H = H_in.mean(axis=0, keepdims=True)\n self.start_C = C_in.mean(axis=0, keepdims=True) \n \n return x_seq_out\n\n\n def backward(self, x_seq_out_grad: ndarray):\n '''\n param loss_grad: numpy array of shape (batch_size, sequence_length, vocab_size)\n return loss_grad_out: numpy array of shape (batch_size, sequence_length, vocab_size)\n '''\n \n batch_size = x_seq_out_grad.shape[0]\n \n h_in_grad = np.zeros((batch_size, self.hidden_size))\n c_in_grad = np.zeros((batch_size, self.hidden_size)) \n \n num_chars = x_seq_out_grad.shape[1]\n \n x_seq_in_grad = np.zeros((batch_size, num_chars, self.vocab_size))\n \n for t in reversed(range(num_chars)):\n \n x_out_grad = x_seq_out_grad[:, t, :]\n\n grad_out, h_in_grad, c_in_grad = \\\n self.cells[t].backward(x_out_grad, h_in_grad, c_in_grad, self.params)\n \n x_seq_in_grad[:, t, :] = grad_out\n \n return x_seq_in_grad",
"_____no_output_____"
]
],
[
[
"## `LSTMModel`",
"_____no_output_____"
]
],
[
[
"class LSTMModel(object):\n '''\n The Model class that takes in inputs and targets and actually trains the network and calculates the loss.\n '''\n def __init__(self, \n layers: List[LSTMLayer],\n sequence_length: int, \n vocab_size: int, \n hidden_size: int,\n loss: Loss):\n '''\n param num_layers: int - the number of layers in the network\n param sequence_length: int - length of sequence being passed through the network\n param vocab_size: int - the number of characters in the vocabulary of which we are predicting the next\n character.\n param hidden_size: int - the number of \"hidden neurons\" in the each layer of the network.\n '''\n self.layers = layers\n self.vocab_size = vocab_size\n self.hidden_size = hidden_size\n self.sequence_length = sequence_length\n self.loss = loss\n for layer in self.layers:\n setattr(layer, 'sequence_length', sequence_length)\n\n \n def forward(self, \n x_batch: ndarray):\n '''\n param inputs: list of integers - a list of indices of characters being passed in as the \n input sequence of the network.\n returns x_batch_in: numpy array of shape (batch_size, sequence_length, vocab_size)\n ''' \n \n for layer in self.layers:\n\n x_batch = layer.forward(x_batch)\n \n return x_batch\n \n def backward(self, \n loss_grad: ndarray):\n '''\n param loss_grad: numpy array with shape (batch_size, sequence_length, vocab_size)\n returns loss: float, representing mean squared error loss\n '''\n\n for layer in reversed(self.layers):\n\n loss_grad = layer.backward(loss_grad)\n \n return loss_grad\n \n def single_step(self, \n x_batch: ndarray, \n y_batch: ndarray):\n '''\n The step that does it all:\n 1. Forward pass & softmax\n 2. Compute loss and loss gradient\n 3. Backward pass\n 4. Update parameters\n param inputs: array of length sequence_length that represents the character indices of the inputs to\n the network\n param targets: array of length sequence_length that represents the character indices of the targets\n of the network \n return loss\n ''' \n \n x_batch_out = self.forward(x_batch)\n \n loss = self.loss.forward(x_batch_out, y_batch)\n \n loss_grad = self.loss.backward()\n \n for layer in self.layers:\n layer._clear_gradients()\n \n self.backward(loss_grad)\n return loss",
"_____no_output_____"
]
],
[
[
"# GRUs",
"_____no_output_____"
],
[
"## `GRUNode`",
"_____no_output_____"
]
],
[
[
"class GRUNode(object):\n\n def __init__(self):\n '''\n param hidden_size: int - the number of \"hidden neurons\" in the LSTM_Layer of which this node is a part.\n param vocab_size: int - the number of characters in the vocabulary of which we are predicting the next\n character.\n '''\n pass\n \n def forward(self, \n X_in: ndarray, \n H_in: ndarray,\n params_dict: Dict[str, Dict[str, ndarray]]) -> Tuple[ndarray]:\n '''\n param X_in: numpy array of shape (batch_size, vocab_size)\n param H_in: numpy array of shape (batch_size, hidden_size)\n return self.X_out: numpy array of shape (batch_size, vocab_size)\n return self.H_out: numpy array of shape (batch_size, hidden_size)\n '''\n self.X_in = X_in\n self.H_in = H_in \n \n # reset gate\n self.X_r = np.dot(X_in, params_dict['W_xr']['value'])\n self.H_r = np.dot(H_in, params_dict['W_hr']['value'])\n\n # update gate \n self.X_u = np.dot(X_in, params_dict['W_xu']['value'])\n self.H_u = np.dot(H_in, params_dict['W_hu']['value']) \n \n # gates \n self.r_int = self.X_r + self.H_r + params_dict['B_r']['value']\n self.r = sigmoid(self.r_int)\n \n self.u_int = self.X_r + self.H_r + params_dict['B_u']['value']\n self.u = sigmoid(self.u_int)\n\n # new state \n self.h_reset = self.r * H_in\n self.X_h = np.dot(X_in, params_dict['W_xh']['value'])\n self.H_h = np.dot(self.h_reset, params_dict['W_hh']['value']) \n self.h_bar_int = self.X_h + self.H_h + params_dict['B_h']['value']\n self.h_bar = tanh(self.h_bar_int) \n \n self.H_out = self.u * self.H_in + (1 - self.u) * self.h_bar\n\n self.X_out = np.dot(self.H_out, params_dict['W_v']['value']) + params_dict['B_v']['value']\n \n return self.X_out, self.H_out\n\n\n def backward(self, \n X_out_grad: ndarray, \n H_out_grad: ndarray, \n params_dict: Dict[str, Dict[str, ndarray]]):\n \n params_dict['B_v']['deriv'] += X_out_grad.sum(axis=0)\n params_dict['W_v']['deriv'] += np.dot(self.H_out.T, X_out_grad)\n\n dh_out = np.dot(X_out_grad, params_dict['W_v']['value'].T) \n dh_out += H_out_grad\n \n du = self.H_in * H_out_grad - self.h_bar * H_out_grad \n dh_bar = (1 - self.u) * H_out_grad\n \n dh_bar_int = dh_bar * dtanh(self.h_bar_int)\n params_dict['B_h']['deriv'] += dh_bar_int.sum(axis=0)\n params_dict['W_xh']['deriv'] += np.dot(self.X_in.T, dh_bar_int)\n \n dX_in = np.dot(dh_bar_int, params_dict['W_xh']['value'].T)\n \n params_dict['W_hh']['deriv'] += np.dot(self.h_reset.T, dh_bar_int)\n dh_reset = np.dot(dh_bar_int, params_dict['W_hh']['value'].T) \n \n dr = dh_reset * self.H_in\n dH_in = dh_reset * self.r \n \n # update branch\n du_int = dsigmoid(self.u_int) * du\n params_dict['B_u']['deriv'] += du_int.sum(axis=0)\n\n dX_in += np.dot(du_int, params_dict['W_xu']['value'].T)\n params_dict['W_xu']['deriv'] += np.dot(self.X_in.T, du_int)\n \n dH_in += np.dot(du_int, params_dict['W_hu']['value'].T)\n params_dict['W_hu']['deriv'] += np.dot(self.H_in.T, du_int) \n\n # reset branch\n dr_int = dsigmoid(self.r_int) * dr\n params_dict['B_r']['deriv'] += dr_int.sum(axis=0)\n\n dX_in += np.dot(dr_int, params_dict['W_xr']['value'].T)\n params_dict['W_xr']['deriv'] += np.dot(self.X_in.T, dr_int)\n \n dH_in += np.dot(dr_int, params_dict['W_hr']['value'].T)\n params_dict['W_hr']['deriv'] += np.dot(self.H_in.T, dr_int) \n \n return dX_in, dH_in",
"_____no_output_____"
]
],
[
[
"## `GRULayer`",
"_____no_output_____"
]
],
[
[
"class GRULayer(object):\n\n def __init__(self,\n hidden_size: int,\n output_size: int,\n weight_scale: float = 0.01):\n '''\n param sequence_length: int - length of sequence being passed through the network\n param vocab_size: int - the number of characters in the vocabulary of which we are predicting the next\n character.\n param hidden_size: int - the number of \"hidden neurons\" in the LSTM_Layer of which this node is a part.\n param learning_rate: float - the learning rate\n '''\n self.hidden_size = hidden_size\n self.output_size = output_size\n self.weight_scale = weight_scale\n self.start_H = np.zeros((1, hidden_size)) \n self.first = True\n\n \n def _init_params(self,\n input_: ndarray):\n \n self.vocab_size = input_.shape[2]\n\n self.params = {}\n self.params['W_xr'] = {}\n self.params['W_hr'] = {}\n self.params['B_r'] = {}\n self.params['W_xu'] = {}\n self.params['W_hu'] = {}\n self.params['B_u'] = {}\n self.params['W_xh'] = {}\n self.params['W_hh'] = {}\n self.params['B_h'] = {} \n self.params['W_v'] = {}\n self.params['B_v'] = {}\n \n self.params['W_xr']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(self.vocab_size, self.hidden_size))\n self.params['W_hr']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(self.hidden_size, self.hidden_size)) \n self.params['B_r']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(1, self.hidden_size))\n self.params['W_xu']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(self.vocab_size, self.hidden_size))\n self.params['W_hu']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(self.hidden_size, self.hidden_size))\n self.params['B_u']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(1, self.hidden_size))\n self.params['W_xh']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(self.vocab_size, self.hidden_size))\n self.params['W_hh']['value'] = np.random.normal(loc=0.0,\n scale=self.weight_scale,\n size=(self.hidden_size, self.hidden_size))\n self.params['B_h']['value'] = np.random.normal(loc=0.0,\n scale=1.0,\n size=(1, self.hidden_size))\n self.params['W_v']['value'] = np.random.normal(loc=0.0,\n scale=1.0,\n size=(self.hidden_size, self.output_size))\n self.params['B_v']['value'] = np.random.normal(loc=0.0,\n scale=1.0,\n size=(1, self.output_size)) \n \n for key in self.params.keys():\n self.params[key]['deriv'] = np.zeros_like(self.params[key]['value'])\n \n self.cells = [GRUNode() for x in range(input_.shape[1])]\n\n\n def _clear_gradients(self):\n for key in self.params.keys():\n self.params[key]['deriv'] = np.zeros_like(self.params[key]['deriv'])\n \n \n def forward(self, x_seq_in: ndarray):\n '''\n param x_seq_in: numpy array of shape (batch_size, sequence_length, vocab_size)\n return x_seq_out: numpy array of shape (batch_size, sequence_length, vocab_size)\n '''\n if self.first:\n self._init_params(x_seq_in)\n self.first=False\n \n batch_size = x_seq_in.shape[0]\n \n H_in = np.copy(self.start_H)\n\n H_in = np.repeat(H_in, batch_size, axis=0) \n\n sequence_length = x_seq_in.shape[1]\n \n x_seq_out = np.zeros((batch_size, sequence_length, self.output_size))\n \n for t in range(sequence_length):\n\n x_in = x_seq_in[:, t, :]\n \n y_out, H_in = self.cells[t].forward(x_in, H_in, self.params)\n \n x_seq_out[:, t, :] = y_out\n \n self.start_H = H_in.mean(axis=0, keepdims=True) \n \n return x_seq_out\n\n\n def backward(self, x_seq_out_grad: ndarray):\n '''\n param loss_grad: numpy array of shape (batch_size, sequence_length, vocab_size)\n return loss_grad_out: numpy array of shape (batch_size, sequence_length, vocab_size)\n '''\n \n batch_size = x_seq_out_grad.shape[0]\n \n h_in_grad = np.zeros((batch_size, self.hidden_size)) \n \n num_chars = x_seq_out_grad.shape[1]\n \n x_seq_in_grad = np.zeros((batch_size, num_chars, self.vocab_size))\n \n for t in reversed(range(num_chars)):\n \n x_out_grad = x_seq_out_grad[:, t, :]\n\n grad_out, h_in_grad = \\\n self.cells[t].backward(x_out_grad, h_in_grad, self.params)\n \n x_seq_in_grad[:, t, :] = grad_out\n \n return x_seq_in_grad",
"_____no_output_____"
]
],
[
[
"# Experiments",
"_____no_output_____"
],
[
"### Single LSTM layer",
"_____no_output_____"
]
],
[
[
"layers1 = [LSTMLayer(hidden_size=256, output_size=62, weight_scale=0.01)]\nmod = RNNModel(layers=layers1,\n vocab_size=62, sequence_length=25,\n loss=SoftmaxCrossEntropy())\noptim = AdaGrad(lr=0.01, gradient_clipping=True)\ntrainer = RNNTrainer('input.txt', mod, optim, batch_size=3)\ntrainer.train(1000, sample_every=100)",
"_____no_output_____"
]
],
[
[
"## Three variants of multiple layers:",
"_____no_output_____"
]
],
[
[
"layers2 = [RNNLayer(hidden_size=256, output_size=128, weight_scale=0.1),\n LSTMLayer(hidden_size=256, output_size=62, weight_scale=0.01)]\nmod = RNNModel(layers=layers2,\n vocab_size=62, sequence_length=25,\n loss=SoftmaxCrossEntropy())\noptim = AdaGrad(lr=0.01, gradient_clipping=True)\ntrainer = RNNTrainer('input.txt', mod, optim, batch_size=32)\ntrainer.train(2000, sample_every=100)",
"_____no_output_____"
],
[
"layers2 = [LSTMLayer(hidden_size=256, output_size=128, weight_scale=0.1),\n LSTMLayer(hidden_size=256, output_size=62, weight_scale=0.01)]\nmod = RNNModel(layers=layers2,\n vocab_size=62, sequence_length=25,\n loss=SoftmaxCrossEntropy())\noptim = SGD(lr=0.01, gradient_clipping=True)\ntrainer = RNNTrainer('input.txt', mod, optim, batch_size=32)\ntrainer.train(2000, sample_every=100)",
"_____no_output_____"
],
[
"layers3 = [GRULayer(hidden_size=256, output_size=128, weight_scale=0.1),\n LSTMLayer(hidden_size=256, output_size=62, weight_scale=0.01)]\nmod = RNNModel(layers=layers3,\n vocab_size=62, sequence_length=25,\n loss=SoftmaxCrossEntropy())\noptim = AdaGrad(lr=0.01, gradient_clipping=True)\ntrainer = RNNTrainer('input.txt', mod, optim, batch_size=32)\ntrainer.train(2000, sample_every=100)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
]
] |
e7b9073577e8a62f49e7d543d7d4dd4922b34509 | 1,130 | ipynb | Jupyter Notebook | 2021 Осенний семестр/Практическое задание 3_5/Севастьянов_3_5_3.ipynb | mosalov/Notebook_For_AI_Main | a693d29bf0bdcf824cb4f1eca86ff54b67ba7428 | [
"MIT"
] | 6 | 2021-09-20T10:28:18.000Z | 2022-03-14T18:39:17.000Z | 2021 Осенний семестр/Практическое задание 3_5/Севастьянов_3_5_3.ipynb | mosalov/Notebook_For_AI_Main | a693d29bf0bdcf824cb4f1eca86ff54b67ba7428 | [
"MIT"
] | 122 | 2020-09-07T11:57:57.000Z | 2022-03-22T06:47:03.000Z | 2021 Осенний семестр/Практическое задание 3_5/Севастьянов_3_5_3.ipynb | mosalov/Notebook_For_AI_Main | a693d29bf0bdcf824cb4f1eca86ff54b67ba7428 | [
"MIT"
] | 97 | 2020-09-07T11:32:19.000Z | 2022-03-31T10:27:38.000Z | 1,130 | 1,130 | 0.609735 | [
[
[
"n = list('никита')\nm = n.copy()\nm = sorted(m)\nl = n + m\nl = l[3:]\nprint(n,m,l)\n\n",
"['н', 'и', 'к', 'и', 'т', 'а'] ['а', 'и', 'и', 'к', 'н', 'т'] ['и', 'т', 'а', 'а', 'и', 'и', 'к', 'н', 'т']\n"
]
]
] | [
"code"
] | [
[
"code"
]
] |
e7b917390d64cb95e902163248e01b9230177642 | 13,800 | ipynb | Jupyter Notebook | live.ipynb | fatiando/2021-gsh | 73e5d7d5b50ad599b05f1ac74efdd0ee97a15672 | [
"CC-BY-4.0"
] | 3 | 2021-04-30T11:49:04.000Z | 2021-06-08T02:49:55.000Z | live.ipynb | fatiando/2021-gsh | 73e5d7d5b50ad599b05f1ac74efdd0ee97a15672 | [
"CC-BY-4.0"
] | 3 | 2021-05-11T22:19:09.000Z | 2021-05-20T14:20:34.000Z | live.ipynb | fatiando/2021-gsh | 73e5d7d5b50ad599b05f1ac74efdd0ee97a15672 | [
"CC-BY-4.0"
] | null | null | null | 22.809917 | 277 | 0.547464 | [
[
[
"# Live demo: Processing gravity data with Fatiando a Terra",
"_____no_output_____"
],
[
"## Import packages",
"_____no_output_____"
]
],
[
[
"import pygmt\nimport pyproj\nimport numpy as np\nimport pandas as pd\nimport xarray as xr\nimport matplotlib.pyplot as plt\n\nimport pooch\nimport verde as vd\nimport boule as bl\nimport harmonica as hm",
"_____no_output_____"
]
],
[
[
"## Load Bushveld Igneous Complex gravity data (South Africa) and a DEM",
"_____no_output_____"
]
],
[
[
"url = \"https://github.com/fatiando/2021-gsh/main/raw/notebook/data/bushveld_gravity.csv\"\nmd5_hash = \"md5:45539f7945794911c6b5a2eb43391051\"",
"_____no_output_____"
],
[
"data = pd.read_csv(fname)\ndata",
"_____no_output_____"
],
[
"# Obtain the region to plot using Verde ([W, E, S, N])\nregion_deg = vd.get_region((data.longitude, data.latitude))\n\nfig = pygmt.Figure()\nfig.basemap(projection=\"M15c\", region=region_deg, frame=True)\npygmt.makecpt(cmap=\"viridis\", series=[data.gravity.min(), data.gravity.max()])\nfig.plot(\n x=data.longitude,\n y=data.latitude,\n color=data.gravity,\n cmap=True,\n style=\"c4p\",\n)\nfig.colorbar(frame='af+l\"Observed Gravity [mGal]\"')\nfig.show()",
"_____no_output_____"
]
],
[
[
"Let's download a DEM for the same area:",
"_____no_output_____"
]
],
[
[
"url = \"https://github.com/fatiando/transform21/raw/main/data/bushveld_topography.nc\"\nmd5_hash = \"md5:62daf6a114dda89530e88942aa3b8c41\"\n\nfname = pooch.retrieve(url, known_hash=md5_hash, fname=\"bushveld_topography.nc\")\nfname",
"_____no_output_____"
]
],
[
[
"And use Xarray to load the netCDF file:",
"_____no_output_____"
]
],
[
[
"topography = xr.load_dataarray(fname)\ntopography",
"_____no_output_____"
],
[
"# Plot topography using pygmt\ntopo_region = vd.get_region((topography.longitude.values, topography.latitude.values))\n\nfig = pygmt.Figure()\ntopo_region = vd.get_region((topography.longitude.values, topography.latitude.values))\nfig.basemap(projection=\"M15c\", region=topo_region, frame=True)\n\nvmin, vmax = topography.values.min(), topography.values.max()\npygmt.makecpt(cmap=\"batlow\", series=[vmin, vmax])\nfig.grdimage(topography)\n\nfig.colorbar(frame='af+l\"Topography [m]\"')\nfig.show()",
"_____no_output_____"
]
],
[
[
"## Compute gravity disturbance",
"_____no_output_____"
]
],
[
[
"data[\"disturbance\"] = data.gravity - normal_gravity\ndata",
"_____no_output_____"
],
[
"fig = pygmt.Figure()\nfig.basemap(projection=\"M15c\", region=region_deg, frame=True)\n\nmaxabs = vd.maxabs(data.disturbance)\npygmt.makecpt(cmap=\"polar\", series=[-maxabs, maxabs])\nfig.plot(\n x=data.longitude,\n y=data.latitude,\n color=data.disturbance,\n cmap=True,\n style=\"c4p\",\n)\n\nfig.colorbar(frame='af+l\"Gravity disturbance [mGal]\"')\nfig.show()",
"_____no_output_____"
]
],
[
[
"## Remove terrain correction",
"_____no_output_____"
],
[
"### Project the data to plain coordinates",
"_____no_output_____"
]
],
[
[
"projection = pyproj.Proj(proj=\"merc\", lat_ts=data.latitude.mean())",
"_____no_output_____"
],
[
"data[\"easting\"] = easting\ndata[\"northing\"] = northing\ndata",
"_____no_output_____"
]
],
[
[
"### Project the topography to plain coordinates",
"_____no_output_____"
],
[
"### Compute gravitational effect of the layer of prisms",
"_____no_output_____"
],
[
"Create a model of the terrain with prisms\n\n",
"_____no_output_____"
],
[
"Calculate the gravitational effect of the terrain",
"_____no_output_____"
],
[
"Calculate the Bouguer disturbance",
"_____no_output_____"
]
],
[
[
"data[\"bouguer\"] = data.disturbance - terrain_effect\ndata",
"_____no_output_____"
],
[
"fig = pygmt.Figure()\nfig.basemap(projection=\"M15c\", region=region_deg, frame=True)\n\nmaxabs = vd.maxabs(data.bouguer)\npygmt.makecpt(cmap=\"polar\", series=[-maxabs, maxabs])\nfig.plot(\n x=data.longitude,\n y=data.latitude,\n color=data.bouguer,\n cmap=True,\n style=\"c4p\",\n)\nfig.colorbar(frame='af+l\"Bouguer gravity disturbance [mGal]\"')\nfig.show()",
"_____no_output_____"
]
],
[
[
"## Calculate residuals\n\nWe can use [Verde](https://www.fatiando.org/verde) to remove a second degree trend from the Bouguer disturbance",
"_____no_output_____"
]
],
[
[
"data[\"residuals\"] = residuals\ndata",
"_____no_output_____"
],
[
"fig = pygmt.Figure()\nfig.basemap(projection=\"M15c\", region=region_deg, frame=True)\n\nmaxabs = np.quantile(np.abs(data.residuals), 0.99)\npygmt.makecpt(cmap=\"polar\", series=[-maxabs, maxabs])\nfig.plot(\n x=data.longitude,\n y=data.latitude,\n color=data.residuals,\n cmap=True,\n style=\"c5p\",\n)\nfig.colorbar(frame='af+l\"Residuals [mGal]\"')\nfig.show()",
"_____no_output_____"
]
],
[
[
"## Grid the residuals with Equivalent Sources\n\nWe can use [Harmonica](https://www.fatiando.org/harmonica) to grid the residuals though the equivalent sources technique\n\n",
"_____no_output_____"
]
],
[
[
"fig = pygmt.Figure()\nfig.basemap(projection=\"M15c\", region=region_deg, frame=True)\n\nscale = np.quantile(np.abs(grid.residuals), 0.995)\npygmt.makecpt(cmap=\"polar\", series=[-scale, scale], no_bg=True)\nfig.grdimage(\n grid.residuals,\n shading=\"+a45+nt0.15\",\n cmap=True,\n)\nfig.colorbar(frame='af+l\"Residuals [mGal]\"')\nfig.show()",
"_____no_output_____"
]
],
[
[
"\n\nSusan J. Webb, R. Grant Cawthorn, Teresia Nguuri, David James; Gravity modeling of Bushveld Complex connectivity supported by Southern African Seismic Experiment results. South African Journal of Geology 2004;; 107 (1-2): 207–218. doi: https://doi.org/10.2113/107.1-2.207",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
]
] |
e7b917945ed811fdafc89d1b7d228427d5706e0c | 3,633 | ipynb | Jupyter Notebook | examples/reference/elements/matplotlib/Chord.ipynb | scaine1/holoviews | 2b08fa169a058f0aa77b94bec69ee23414cf52d1 | [
"BSD-3-Clause"
] | null | null | null | examples/reference/elements/matplotlib/Chord.ipynb | scaine1/holoviews | 2b08fa169a058f0aa77b94bec69ee23414cf52d1 | [
"BSD-3-Clause"
] | null | null | null | examples/reference/elements/matplotlib/Chord.ipynb | scaine1/holoviews | 2b08fa169a058f0aa77b94bec69ee23414cf52d1 | [
"BSD-3-Clause"
] | 1 | 2019-10-29T12:23:38.000Z | 2019-10-29T12:23:38.000Z | 31.591304 | 406 | 0.610515 | [
[
[
"<div class=\"contentcontainer med left\" style=\"margin-left: -50px;\">\n<dl class=\"dl-horizontal\">\n <dt>Title</dt> <dd> Graph Element</dd>\n <dt>Dependencies</dt> <dd>Matplotlib</dd>\n <dt>Backends</dt> \n <dd><a href='./Chord.ipynb'>Matplotlib</a></dd>\n <dd><a href='../bokeh/Chord.ipynb'>Bokeh</a></dd>\n</dl>\n</div>",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport pandas as pd\nimport holoviews as hv\nfrom bokeh.sampledata.les_mis import data\n\nhv.extension('matplotlib')\n%output size=200 fig='svg'",
"_____no_output_____"
]
],
[
[
"The ``Chord`` element allows representing the inter-relationships between data points in a graph. The nodes are arranged radially around a circle with the relationships between the data points drawn as arcs (or chords) connecting the nodes. The number of chords is scaled by a weight declared as a value dimension on the ``Chord`` element.\n\nIf the weight values are integers, they define the number of chords to be drawn between the source and target nodes directly. If the weights are floating point values, they are normalized to a default of 500 chords, which are divided up among the edges. Any non-zero weight will be assigned at least one chord.\n\nThe ``Chord`` element is a type of ``Graph`` element and shares the same constructor. The most basic constructor accepts a columnar dataset of the source and target nodes and an optional value. Here we supply a dataframe containing the number of dialogues between characters of the *Les Misérables* musical. The data contains ``source`` and ``target`` node indices and an associated ``value`` column:",
"_____no_output_____"
]
],
[
[
"links = pd.DataFrame(data['links'])\nprint(links.head(3))",
"_____no_output_____"
]
],
[
[
"In the simplest case we can construct the ``Chord`` by passing it just the edges:",
"_____no_output_____"
]
],
[
[
"hv.Chord(links)",
"_____no_output_____"
]
],
[
[
"To add node labels and other information we can construct a ``Dataset`` with a key dimension of node indices.",
"_____no_output_____"
]
],
[
[
"nodes = hv.Dataset(pd.DataFrame(data['nodes']), 'index')\nnodes.data.head()",
"_____no_output_____"
]
],
[
[
"Additionally we can now color the nodes and edges by their index and add some labels. The ``label_index``, ``color_index`` and ``edge_color_index`` allow specifying columns to color by. ",
"_____no_output_____"
]
],
[
[
"%%opts Chord [label_index='name' color_index='index' edge_color_index='source'] \n%%opts Chord (cmap='Category20' edge_cmap='Category20')\nhv.Chord((links, nodes)).select(value=(5, None))",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7b919e45e345d9113ea33144009d501e19526a2 | 5,273 | ipynb | Jupyter Notebook | cleared-demos/linear_least_squares/Gram-Schmidt and Modified Gram-Schmidt.ipynb | xywei/numerics-notes | 70e67e17d855b7bb06a0de7e3570d40ad50f941b | [
"Unlicense"
] | 20 | 2021-01-24T21:12:30.000Z | 2022-03-02T19:58:25.000Z | cleared-demos/linear_least_squares/Gram-Schmidt and Modified Gram-Schmidt.ipynb | xywei/numerics-notes | 70e67e17d855b7bb06a0de7e3570d40ad50f941b | [
"Unlicense"
] | 5 | 2021-08-24T17:48:50.000Z | 2021-10-14T21:22:02.000Z | cleared-demos/linear_least_squares/Gram-Schmidt and Modified Gram-Schmidt.ipynb | xywei/numerics-notes | 70e67e17d855b7bb06a0de7e3570d40ad50f941b | [
"Unlicense"
] | 7 | 2020-11-23T09:56:26.000Z | 2021-12-24T17:30:26.000Z | 21.610656 | 138 | 0.410393 | [
[
[
"# Gram-Schmidt and Modified Gram-Schmidt",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport numpy.linalg as la",
"_____no_output_____"
],
[
"A = np.random.randn(3, 3)",
"_____no_output_____"
],
[
"def test_orthogonality(Q):\n print(\"Q:\")\n print(Q)\n \n print(\"Q^T Q:\")\n QtQ = np.dot(Q.T, Q)\n QtQ[np.abs(QtQ) < 1e-15] = 0\n print(QtQ)",
"_____no_output_____"
],
[
"Q = np.zeros(A.shape)",
"_____no_output_____"
]
],
[
[
"Now let us generalize the process we used for three vectors earlier:",
"_____no_output_____"
],
[
"This procedure is called [Gram-Schmidt Orthonormalization](https://en.wikipedia.org/wiki/Gram–Schmidt_process).",
"_____no_output_____"
]
],
[
[
"test_orthogonality(Q)",
"_____no_output_____"
]
],
[
[
"Now let us try a different example ([Source](http://fgiesen.wordpress.com/2013/06/02/modified-gram-schmidt-orthogonalization/)):",
"_____no_output_____"
]
],
[
[
"\nnp.set_printoptions(precision=13)\n\neps = 1e-8\n\nA = np.array([\n [1, 1, 1],\n [eps,eps,0],\n [eps,0, eps]\n ])\n\nA",
"_____no_output_____"
],
[
"Q = np.zeros(A.shape)",
"_____no_output_____"
],
[
"for k in range(A.shape[1]):\n avec = A[:, k]\n q = avec\n for j in range(k):\n print(q)\n q = q - np.dot(avec, Q[:,j])*Q[:,j]\n \n print(q)\n q = q/la.norm(q)\n Q[:, k] = q\n print(\"norm -->\", q)\n print(\"-------\")",
"_____no_output_____"
],
[
"test_orthogonality(Q)",
"_____no_output_____"
]
],
[
[
"Questions:\n\n* What happened?\n* How do we fix it?",
"_____no_output_____"
]
],
[
[
"Q = np.zeros(A.shape)",
"_____no_output_____"
],
[
"test_orthogonality(Q)",
"_____no_output_____"
]
],
[
[
"This procedure is called *Modified* Gram-Schmidt Orthogonalization.\n\nQuestions:\n\n* Is there a difference mathematically between modified and unmodified?\n* Why are there $10^{-8}$ values left in $Q^TQ$?",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
]
] |
e7b935022233958f1640b1a6c7035456245d5c5f | 121,027 | ipynb | Jupyter Notebook | svm wine.data - using sklearn.ipynb | yunglinchang/machinelearning_coursework | be4b11fabb8cb2fc43a43fd0b9e1ea2f6a30a3fa | [
"MIT"
] | 2 | 2018-12-28T14:01:30.000Z | 2019-11-08T06:29:34.000Z | svm wine.data - using sklearn.ipynb | yunglinchang/machine_learning_coursework | be4b11fabb8cb2fc43a43fd0b9e1ea2f6a30a3fa | [
"MIT"
] | null | null | null | svm wine.data - using sklearn.ipynb | yunglinchang/machine_learning_coursework | be4b11fabb8cb2fc43a43fd0b9e1ea2f6a30a3fa | [
"MIT"
] | 2 | 2021-03-09T05:36:54.000Z | 2021-09-06T00:53:11.000Z | 281.45814 | 50,688 | 0.929181 | [
[
[
"# 導入葡萄酒數據集(只考慮前兩個特徵)",
"_____no_output_____"
]
],
[
[
"from sklearn.datasets import load_wine\nimport numpy as np\nfrom sklearn.model_selection import train_test_split\nfrom sklearn.preprocessing import StandardScaler",
"_____no_output_____"
],
[
"wine = load_wine()\n#選取前兩個特徵\nX = wine.data[:, :2]\ny = wine.target\n\nprint('Class labels:', np.unique(y))",
"Class labels: [0 1 2]\n"
],
[
"sc = StandardScaler()\nsc.fit(X)\nX_std = sc.transform(X)\n\nX_train, X_test, y_train, y_test = train_test_split(X_std, y, test_size=0.3, random_state=1, stratify=y)",
"_____no_output_____"
]
],
[
[
"# 訓練「線性核函數」模型",
"_____no_output_____"
]
],
[
[
"from sklearn.metrics import accuracy_score\nfrom matplotlib.colors import ListedColormap\n%matplotlib inline\nimport matplotlib.pyplot as plt\nfrom sklearn.svm import SVC",
"_____no_output_____"
],
[
"svmlin = SVC(kernel='linear', C=1.0, random_state=1)\nsvmlin.fit(X_train, y_train)\ny_train_pred = svmlin.predict(X_train)\ny_test_pred = svmlin.predict(X_test)",
"_____no_output_____"
],
[
"svmlin_train = accuracy_score(y_train, y_train_pred)\nsvmlin_test = accuracy_score(y_test, y_test_pred)\n\nprint('SVM with linear kernal train/test accuracies %.3f/%.3f' \n %(svmlin_train, svmlin_test))",
"SVM with linear kernal train/test accuracies 0.806/0.741\n"
],
[
"#畫出散佈圖及決策邊界\n#chose colors\nfrom matplotlib.colors import ListedColormap\n\ncmap_light = ListedColormap(['#FFAAAA', '#AAFFAA', '#AAAAFF'])\ncmap_bold = ListedColormap(['#FF0000', '#00FF00', '#0000FF'])",
"_____no_output_____"
],
[
"#分別用資料兩個特徵當X, y軸\nx_min, x_max = X_train[:, 0].min() - 1, X_train[:, 0].max() + 1\ny_min, y_max = X_train[:, 1].min() - 1, X_train[:, 1].max() + 1\n\nxx, yy = np.meshgrid(np.arange(x_min, x_max, .02), \n np.arange(y_min, y_max, .02))\n\nZ = svmlin.predict(np.c_[xx.ravel(), yy.ravel()])\n#依據顏色分類\nZ = Z.reshape(xx.shape)\n#畫出散佈圖\nplt.pcolormesh(xx, yy, Z, cmap=cmap_light)\nplt.scatter(X_std[:,0], X_std[:,1], c=y, cmap=cmap_bold)\nplt.xlim(xx.min(), xx.max())\nplt.ylim(yy.min(), yy.max())",
"_____no_output_____"
]
],
[
[
"# 訓練「⾼斯核函數」模型",
"_____no_output_____"
]
],
[
[
"svmrbf = SVC(kernel='rbf', gamma=0.7, C=1.0)\nsvmrbf.fit(X_train, y_train)\ny_train_pred = svmrbf.predict(X_train)\ny_test_pred = svmrbf.predict(X_test)",
"_____no_output_____"
],
[
"svmrbf_train = accuracy_score(y_train, y_train_pred)\nsvmrbf_test = accuracy_score(y_test, y_test_pred)\n\nprint('SVM with RBF kernal train/test accuracies %.3f/%.3f' \n %(svmrbf_train, svmrbf_test))",
"SVM with RBF kernal train/test accuracies 0.855/0.815\n"
],
[
"#畫出散佈圖及決策邊界\n#choose colors\nfrom matplotlib.colors import ListedColormap\n\ncmap_light = ListedColormap(['#FFAAAA', '#AAFFAA', '#AAAAFF'])\ncmap_bold = ListedColormap(['#FF0000', '#00FF00', '#0000FF'])",
"_____no_output_____"
],
[
"#分別用資料兩個特徵當X, y軸\nx_min, x_max = X_train[:, 0].min() - 1, X_train[:, 0].max() + 1\ny_min, y_max = X_train[:, 1].min() - 1, X_train[:, 1].max() + 1\n\nxx, yy = np.meshgrid(np.arange(x_min, x_max, .02), \n np.arange(y_min, y_max, .02))\n\nZ = svmrbf.predict(np.c_[xx.ravel(), yy.ravel()])\n\n#依據顏色分類\nZ = Z.reshape(xx.shape)\n#畫出散佈圖\nplt.pcolormesh(xx, yy, Z, cmap=cmap_light)\nplt.scatter(X_std[:,0], X_std[:,1], c=y, cmap=cmap_bold, edgecolor='k', s=20)\nplt.xlim(xx.min(), xx.max())\nplt.ylim(yy.min(), yy.max())",
"_____no_output_____"
]
],
[
[
"# ⾼斯核函數參數的影響",
"_____no_output_____"
]
],
[
[
"C=1.0\nmodels = (SVC(kernel='rbf', gamma=0.1, C=C), \n SVC(kernel='rbf', gamma=1, C=C), \n SVC(kernel='rbf', gamma=10, C=C))\nmodels = (clf.fit(X_train, y_train) for clf in models)\n\nsvmrbf_train = accuracy_score(y_train, y_train_pred)\nsvmrbf_test = accuracy_score(y_test, y_test_pred)\n\ntitles = ('gamma=0.1', 'gamma=1', 'gamma=10')\n\nfig, sub = plt.subplots(1, 3, figsize=(10,3))\n\ncmap_light = ListedColormap(['#FFAAAA', '#AAFFAA', '#AAAAFF'])\ncmap_bold = ListedColormap(['#FF0000', '#00FF00', '#0000FF'])\n\nx_min, x_max = X_train[:, 0].min() - 1, X_train[:, 0].max() + 1\ny_min, y_max = X_train[:, 1].min() - 1, X_train[:, 1].max() + 1\n\nxx, yy = np.meshgrid(np.arange(x_min, x_max, .02), \n np.arange(y_min, y_max, .02))\n\nfor clf, title, ax in zip(models, titles, sub.flatten()):\n Z = clf.predict(np.c_[xx.ravel(), yy.ravel()])\n Z = Z.reshape(xx.shape)\n ax.pcolormesh(xx, yy, Z, cmap=cmap_light)\n ax.scatter(X_std[:,0], X_std[:,1], c=y, cmap=cmap_bold)\n ax.set_xlim(xx.min(), xx.max())\n ax.set_ylim(yy.min(), yy.max())\n ax.set_title(title)",
"_____no_output_____"
],
[
"svmrbf = SVC(kernel='rbf', gamma=0.1, C=1.0)\nsvmrbf.fit(X_train, y_train)\ny_train_pred = svmrbf.predict(X_train)\ny_test_pred = svmrbf.predict(X_test)\n\nsvmrbf_train = accuracy_score(y_train, y_train_pred)\nsvmrbf_test = accuracy_score(y_test, y_test_pred)\n\nprint('SVM with RBF kernal train/test accuracies %.3f/%.3f' \n %(svmrbf_train, svmrbf_test))",
"SVM with RBF kernal train/test accuracies 0.815/0.759\n"
],
[
"svmrbf = SVC(kernel='rbf', gamma=1, C=1.0)\nsvmrbf.fit(X_train, y_train)\ny_train_pred = svmrbf.predict(X_train)\ny_test_pred = svmrbf.predict(X_test)\n\nsvmrbf_train = accuracy_score(y_train, y_train_pred)\nsvmrbf_test = accuracy_score(y_test, y_test_pred)\n\nprint('SVM with RBF kernal train/test accuracies %.3f/%.3f' \n %(svmrbf_train, svmrbf_test))",
"SVM with RBF kernal train/test accuracies 0.855/0.815\n"
],
[
"svmrbf = SVC(kernel='rbf', gamma=10, C=1.0)\nsvmrbf.fit(X_train, y_train)\ny_train_pred = svmrbf.predict(X_train)\ny_test_pred = svmrbf.predict(X_test)\n\nsvmrbf_train = accuracy_score(y_train, y_train_pred)\nsvmrbf_test = accuracy_score(y_test, y_test_pred)\n\nprint('SVM with RBF kernal train/test accuracies %.3f/%.3f' \n %(svmrbf_train, svmrbf_test))",
"SVM with RBF kernal train/test accuracies 0.887/0.833\n"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
]
] |
e7b9389366e3fb6badce14ca194789bd23e1e049 | 345,333 | ipynb | Jupyter Notebook | NB_clf/Multinomial-NB_clf.ipynb | tychen5/IR_TextMining | cbe60f48f1ae3f0ae84aebfa57697e7a744000ec | [
"MIT"
] | 2 | 2019-01-26T04:09:40.000Z | 2020-04-21T06:38:51.000Z | NB_clf/Multinomial-NB_clf.ipynb | tychen5/IR_TextMining | cbe60f48f1ae3f0ae84aebfa57697e7a744000ec | [
"MIT"
] | null | null | null | NB_clf/Multinomial-NB_clf.ipynb | tychen5/IR_TextMining | cbe60f48f1ae3f0ae84aebfa57697e7a744000ec | [
"MIT"
] | null | null | null | 28.610853 | 1,295 | 0.292631 | [
[
[
"import nltk\nfrom nltk.corpus import stopwords\nfrom nltk.tokenize import wordpunct_tokenize\nfrom nltk import word_tokenize\nfrom nltk.stem.porter import *\nfrom nltk.tokenize import RegexpTokenizer\nimport os,sys\nimport re\nimport pickle\nimport pandas as pd\nimport numpy as np\nimport tqdm\nfrom tqdm import tqdm\nimport random\nimport math\nfrom collections import Counter\nimport functools",
"_____no_output_____"
],
[
"with open('data/stop_words.txt') as f:\n stop_words_list = f.read().splitlines() #stop_list1\nstop_list2 = pickle.load(open('data/stop_list2.pkl','rb'))\nps = PorterStemmer() # Stemming\nstop_words = set(stopwords.words('english')) #Stopword\nshort = ['.', ',', '\"', \"\\'\", '?', '!', ':', ';', '(', ')', '[', ']', '{', '}', \"'at\",\n \"_\",\"`\",\"\\'\\'\",\"--\",\"``\",\".,\",\"//\",\":\",\"___\",'_the','-',\"'em\",\".com\",\n '\\'s','\\'m','\\'re','\\'ll','\\'d','n\\'t','shan\\'t',\"...\",\"\\'ve\",'u']\nstop_words_list.extend(short)\nstop_words_list.extend(stop_list2)\nstop_words.update(stop_words_list)",
"_____no_output_____"
],
[
"def preprocess(texts):\n tokens = [i for i in word_tokenize(texts.lower()) if i not in stop_words] # Tokenization.# Lowercasing\n token_result = ''\n token_result_ = ''\n for i,token in enumerate(tokens): #list2str\n token_result += ps.stem(token) + ' '\n token_result = ''.join([i for i in token_result if not i.isdigit()])\n token_result = [i for i in word_tokenize(token_result) if i not in stop_words]\n for i,token in enumerate(token_result):\n token_result_ += token + ' '\n return token_result_",
"_____no_output_____"
]
],
[
[
"## Feature Selection\n* tf-idf\n* chi-square\n* likelihood\n* PMI\n* EMI\n\n=> build dictionary in 500 words",
"_____no_output_____"
],
[
"### LLR",
"_____no_output_____"
]
],
[
[
"dict_df = pd.read_csv('data/dictionary.txt',header=None,index_col=None,sep=' ')\nterms = dict_df[1].tolist() #all terms",
"_____no_output_____"
],
[
"with open('data/training.txt','r') as f:\n train_id = f.read().splitlines()\ntrain_dict = {}\nfor trainid in train_id:\n trainid = trainid.split(' ')\n trainid = list(filter(None, trainid))\n train_dict[trainid[0]] = trainid[1:]\ntrain_dict #class:doc_id\ntrain_dict = pickle.load(open('data/train_dict.pkl','rb'))",
"_____no_output_____"
],
[
"in_dir = 'data/IRTM/'\ntrain_dict_ = {}\nclass_token = []\nclass_token_dict = {}\nfor c,d in train_dict.items():\n for doc in d:\n f = open('data/IRTM/'+doc+'.txt')\n texts = f.read()\n f.close()\n tokens_all = preprocess(texts)\n tokens_all = tokens_all.split(' ')\n tokens_all = list(set(filter(None,tokens_all)))\n class_token.append(tokens_all)\n class_token_dict[c]=class_token\n class_token=[]\nlen(class_token_dict['1'])",
"_____no_output_____"
],
[
"# train_dict = {}\n# for c,t in class_token_dict.items():\n# train_dict[c] = list(set(t))\n# # train_dict[c] = dict(Counter(t))\n# train_dict",
"_____no_output_____"
],
[
"dict_df.drop(0,axis=1,inplace=True)\ndict_df.columns = ['term','score']\ndict_df.index = dict_df['term']\ndict_df.drop('term',axis=1,inplace=True)\ndict_df",
"_____no_output_____"
],
[
"dict_df['score'] = 0\ndict_df['score_chi'] = 0\ndict_df['score_emi'] = 0\n# c=1\nfor term in tqdm(terms): #each term\n scores = []\n scores_chi = []\n scores_emi = []\n c=1\n for _ in range(len(class_token_dict)): # each class\n n11=e11=m11=0\n n10=e10=m10=0\n n01=e01=m01=0\n n00=e00=m00=0\n for k,v in class_token_dict.items():\n# print(k,c)\n if k == str(c): #ontopic\n for r in v:\n if term in r:\n n11+=1\n else:\n n10+=1\n# c+=1\n else: #off topic\n for r in v:\n if term in r:\n n01+=1\n else:\n n00+=1\n# c+=1\n c+=1\n n11+=1e-8\n n10+=1e-8\n n01+=1e-8\n n00+=1e-8\n N = n11+n10+n01+n00\n e11 = N * (n11+n01)/N * (n11+n10)/N #chi-squre\n e10 = N * (n11+n10)/N * (n10+n00)/N\n e01 = N * (n11+n01)/N * (n01+n00)/N\n e00 = N * (n01+n00)/N * (n10+n00)/N\n score_chi = ((n11-e11)**2)/e11 + ((n10-e10)**2)/e10 + ((n01-e01)**2)/e01 + ((n00-e00)**2)/e00\n scores_chi.append(score_chi)\n \n n11 = n11 - 1e-8 + 1e-6\n n10 = n10 - 1e-8 + 1e-6\n n01 = n01 - 1e-8 + 1e-6\n n00 = n00 - 1e-8 + 1e-6\n N = n11+n10+n01+n00\n m11 = (n11/N) * math.log(((n11/N)/((n11+n01)/N * (n11+n10)/N)),2) #EMI\n m10 = n10/N * math.log((n10/N)/((n11+n10)/N * (n10+n00)/N),2)\n m01 = n01/N * math.log((n01/N)/((n11+n01)/N * (n01+n00)/N),2)\n m00 = n00/N * math.log((n00/N)/((n01+n00)/N * (n10+n00)/N),2)\n score_emi = m11 + m10 + m01 + m00\n scores_emi.append(score_emi)\n \n# print(n11,n10,n01,n00)\n n11-=1e-6\n m10-=1e-6\n n01-=1e-6\n n00-=1e-6\n N = n11+n10+n01+n00\n score = (((n11+n01)/N) ** n11) * ((1 - ((n11+n01)/N)) ** n10) * (((n11+n01)/N) ** n01) * ((1 - ((n11+n01)/N)) ** n00)\n score /= ((n11/(n11+n10)) ** n11) * ((1 - (n11/(n11+n10))) ** n10) * ((n01/(n01+n00)) ** n01) * ((1 - (n01/(n01+n00))) ** n00)\n score = -2 * math.log(score, 10) #LLR\n scores.append(score)\n \n \n# c+=1\n dict_df.loc[term,'score'] = np.mean(scores)\n dict_df.loc[term,'score_chi'] = np.mean(scores_chi)\n dict_df.loc[term,'score_emi'] = np.mean(scores_emi)\ndict_df ",
"100%|██████████| 8913/8913 [03:59<00:00, 37.23it/s]\n"
],
[
"dict_df2 = pd.read_csv('data/dictionary.txt',header=None,index_col=None,sep=' ')\ndict_df2.columns = ['id','term','freq']\ndict_df2['sum'] = 0.0\ndict_df2",
"_____no_output_____"
],
[
"tf_list = next(os.walk('../HW2/output/tf-idf/'))[2]\n# df_list = [dict_df2]\nfor tf in tf_list:\n# print(tf)\n df2 = pd.read_csv('../HW2/output/tf-idf/'+tf,header=None,index_col=None,sep=' ',skiprows=[0])\n df2.columns = ['id','tfidf']\n df3 = pd.merge(dict_df2,df2,on='id',how='outer')\n df3.fillna(0,inplace=True)\n dict_df2['sum']+=df3['tfidf']\ndict_df2['avg_tfidf'] = dict_df2['sum']/dict_df2['freq']\ndict_df2 = dict_df2.drop(['freq','sum'],axis=1)\ndict_df2\n# break\n# df_list.append(df2)\n# df3 = pd.concat(df_list).groupby(level=0).sum()\n# df3 = pd.concat([df3,df2]).groupby(level=0).sum()\n# df3 = pd.merge(dict_df2,df2,on='id',how='outer')\n# df3 = pd.merge(df3,df2,on='id',how='outer')?\n# df3[df3.id == 2]",
"_____no_output_____"
],
[
"dict_df['term'] = dict_df.index\ndict_df3 = pd.merge(dict_df,dict_df2,on='term',how='outer')\ndict_df3",
"/home/leoqaz12/anaconda3/lib/python3.6/site-packages/IPython/core/interactiveshell.py:2961: FutureWarning: 'term' is both an index level and a column label.\nDefaulting to column, but this will raise an ambiguity error in a future version\n exec(code_obj, self.user_global_ns, self.user_ns)\n"
],
[
"cols = list(dict_df3)\ncols[4], cols[3], cols[5], cols[1], cols[0], cols[2] = cols[0], cols[1] , cols[2] , cols[3], cols[4], cols[5]\ndict_df3 = dict_df3.ix[:,cols]\ndict_df3",
"/home/leoqaz12/.local/lib/python3.6/site-packages/ipykernel/__main__.py:3: DeprecationWarning: \n.ix is deprecated. Please use\n.loc for label based indexing or\n.iloc for positional indexing\n\nSee the documentation here:\nhttp://pandas.pydata.org/pandas-docs/stable/indexing.html#ix-indexer-is-deprecated\n app.launch_new_instance()\n"
],
[
"dict_df3.columns = ['id','term','avg_tfidf','score_chi','score_llr','score_emi']\ndict_df3.to_csv('output/feature_selection_df_rev.csv')",
"_____no_output_____"
]
],
[
[
"### select top 500\n* 取各col的mean+1.45*std\n* 再去做投票,超過兩票的流下來看剩下哪幾個",
"_____no_output_____"
]
],
[
[
"dict_df3 = pd.read_csv('output/feature_selection_df_rev.csv',index_col=None)\nthreshold_tfidf = np.mean(dict_df3['avg_tfidf'])+2.5*np.std(dict_df3['avg_tfidf']) #1.45=>502 數字大嚴格\nthreshold_chi = np.mean(dict_df3['score_chi'])+2.5*np.std(dict_df3['score_chi']) #1=>350\nthreshold_llr = np.mean(dict_df3['score_llr'])+2.5*np.std(dict_df3['score_llr']) #1.75=>543\nthreshold_emi = np.mean(dict_df3['score_emi'])+2.5*np.std(dict_df3['score_emi']) #1.75=>543\n\nprint('avg_tfidf',threshold_tfidf)\n# dict_df3[dict_df3.score_llr>0.1]",
"avg_tfidf 0.024888109121963365\n"
],
[
"df1 = dict_df3[dict_df3['avg_tfidf']>threshold_tfidf]\ndf2 = dict_df3[dict_df3['score_chi']>threshold_chi]\ndf3 = dict_df3[dict_df3['score_llr']>threshold_llr]\ndf4 = dict_df3[dict_df3['score_emi']>threshold_emi]\ndf_vote = dict_df3\ndf_vote['vote']=0\ndf_vote",
"_____no_output_____"
],
[
"df_vote.loc[df1.id-1,'vote'] += 1\ndf_vote.loc[df2.id-1,'vote'] += 1\ndf_vote.loc[df3.id-1,'vote'] += 1\ndf_vote.loc[df4.id-1,'vote'] += 1\n# df_vote",
"_____no_output_____"
],
[
"df_vote_ = df_vote[df_vote.vote>2] #(1,2)=>375 #(1,1)=>422 #(1.6,2)=>482 #(2,2)=>330 #(1,3)=>100\ndf_vote_ = df_vote_.filter(['id','term','vote'])\ndf_vote_",
"_____no_output_____"
],
[
"df_vote_.to_csv('output/500terms_df_rev5.csv')",
"_____no_output_____"
]
],
[
[
"## Classifier\n* 7-fold\n* MNB\n* BNB\n* self-train / co-train\n* ens voting (BNB lower weight)\n* auto-skleran / auto-keras\n\nREF: http://kenzotakahashi.github.io/naive-bayes-from-scratch-in-python.html",
"_____no_output_____"
]
],
[
[
"df_vote = pd.read_csv('output/500terms_df_rev5.csv',index_col=False)\nterms_li = list(set(df_vote.term.tolist()))\n\ntrain_X = []\ntrain_Y = []\nlen(terms_li)",
"_____no_output_____"
],
[
"with open('data/training.txt','r') as f:\n train_id = f.read().splitlines()\ntrain_dict = {}\n\nfor trainid in train_id:\n trainid = trainid.split(' ')\n trainid = list(filter(None, trainid))\n train_dict[trainid[0]] = trainid[1:]\n# train_dict #class:doc_id\ntrain_dict = pickle.load(open('data/train_dict.pkl','rb'))\nin_dir = 'data/IRTM/'\ntrain_dict_ = {}\nclass_token = []\nclass_token_dict = {}\ntrain_X = []\ntrain_Y= []\ntrain_ids = []\nfor c,d in tqdm(train_dict.items()):\n for doc in d:\n train_ids.append(doc)\n trainX = np.array([0]*len(terms_li))\n f = open('data/IRTM/'+doc+'.txt')\n texts = f.read()\n f.close()\n tokens_all = preprocess(texts)\n tokens_all = tokens_all.split(' ')\n# tokens_all = list(filter(None,tokens_all))\n tokens_all = dict(Counter(tokens_all))\n for key,value in tokens_all.items():\n if key in terms_li:\n trainX[terms_li.index(key)] = int(value)\n# trainX = np.array(trainX)\n \n# for token in tokens_all:\n# if token in terms_li:\n# ind = terms_li.index(token)\n# trainX[ind]+=1\n train_X.append(trainX)\n train_Y.append(int(c))\n \ntrain_X = np.array(train_X)\ntrain_Y = np.array(train_Y)\n\n# tokens_all = list(set(filter(None,tokens_all)))\n# class_token.append(tokens_all)\n# class_token_dict[c]=class_token\n# class_token=[]\n# len(class_token_dict['1'])\nprint(train_X.shape , train_Y.shape)",
"100%|██████████| 13/13 [00:07<00:00, 1.78it/s]"
],
[
"#建立term index matrix\ntokens_all_class=[]\nterm_tf_mat=[]\nfor c,d in tqdm(train_dict.items()):\n for doc in d:\n f = open('data/IRTM/'+doc+'.txt')\n texts = f.read()\n f.close()\n tokens_all = preprocess(texts)\n tokens_all = tokens_all.split(' ')\n tokens_all = list(filter(None,tokens_all))\n tokens_all_class.extend(tokens_all)\n tokens_all = dict(Counter(tokens_all_class))\n term_tf_mat.append(tokens_all)",
"100%|██████████| 13/13 [00:01<00:00, 6.61it/s]\n"
],
[
"def train_MNB(train_set=train_dict,term_list=terms_li,term_tf_mat=term_tf_mat):\n prior = np.zeros(len(train_set))\n cond_prob = np.zeros((len(train_set), len(term_list)))\n \n for i,docs in train_set.items(): #13 classes 1~13\n prior[int(i)-1] = len(docs)/len(train_ids) #那個類別的文章有幾個/總共的文章數目 0~12\n token_count=0\n class_tf = np.zeros(len(term_list))\n for idx,term in enumerate(term_list):\n try:\n class_tf[idx] = term_tf_mat[int(i)-1][term] #term在class的出現次數\n except:\n token_count+=1\n\n class_tf = class_tf + np.ones(len(term_list)) #smoothing (可改)\n class_tf = class_tf/(sum(class_tf) +token_count) #該class總共的token數(可改)\n cond_prob[int(i)-1] = class_tf #0~12\n return prior, cond_prob",
"_____no_output_____"
],
[
"prior,cond_prob = train_MNB()\nprior",
"_____no_output_____"
],
[
"def predict_MNB(test_id,prob=False,prior=prior,cond_prob=cond_prob,term_list=terms_li):\n f = open('data/IRTM/'+str(test_id)+'.txt')\n texts = f.read()\n f.close()\n tokens_all = preprocess(texts)\n tokens_all = tokens_all.split(' ')\n tokens_all = list(filter(None,tokens_all))\n \n class_scores = []\n# score = 0\n for i in range(13):\n score=0\n# print(prior[i])\n score += math.log(prior[i],10)\n for token in tokens_all:\n if token in term_list:\n score += math.log(cond_prob[i][term_list.index(token)])\n class_scores.append(score)\n if prob:\n return np.array(class_scores)\n else:\n return(np.argmax(class_scores)+1)",
"_____no_output_____"
],
[
"ans = predict_MNB(20,prob=False) # 17 18 20 21\nans",
"_____no_output_____"
],
[
"ans=[]\nfor i in tqdm(range(1095)):\n ans.append(predict_MNB(i+1))",
" 0%| | 0/1095 [00:00<?, ?it/s]\n"
],
[
"# np.max(ans)\nans",
"_____no_output_____"
],
[
"def MNB(input_X,input_Y=None,prior_log_class=None,log_prob_feature=None,train=True,prob=False,smooth=1.0):\n if train:\n sample_num = input_X.shape[0]\n match_data = [[x for x, t in zip(input_X, input_Y) if t == c] for c in np.unique(input_Y)]\n prior_log_class = [np.log(len(i) / sample_num) for i in match_data]\n counts = np.array([np.array(i).sum(axis=0) for i in match_data]) + smooth\n log_prob_feature = np.log(counts / counts.sum(axis=1)[np.newaxis].T)\n return prior_log_class,log_prob_feature\n else:\n probability = [(log_prob_feature * x).sum(axis=1) + prior_log_class for x in input_X]\n if prob:\n return probability\n else:\n ans = np.argmax(probability,axis=1)\n return ans",
"_____no_output_____"
],
[
"class BernoulliNB(object):\n def __init__(self, alpha=1.0, binarize=0.0):\n self.alpha = alpha\n self.binarize = binarize\n def _binarize_X(self, X):\n return np.where(X > self.binarize, 1, 0) if self.binarize != None else X\n def fit(self, X, y):\n X = self._binarize_X(X)\n count_sample = X.shape[0]\n separated = [[x for x, t in zip(X, y) if t == c] for c in np.unique(y)]\n self.class_log_prior_ = [np.log(len(i) / count_sample) for i in separated]\n count = np.array([np.array(i).sum(axis=0) for i in separated]) + self.alpha\n smoothing = 2 * self.alpha\n n_doc = np.array([len(i) + smoothing for i in separated])\n self.feature_prob_ = count / n_doc[np.newaxis].T\n return self\n\n def predict_log_proba(self, X):\n X = self._binarize_X(X)\n return [(np.log(self.feature_prob_) * x + \\\n np.log(1 - self.feature_prob_) * np.abs(x - 1)\n ).sum(axis=1) + self.class_log_prior_ for x in X]\n\n def predict(self, X):\n X = self._binarize_X(X)\n return np.argmax(self.predict_log_proba(X), axis=1)",
"_____no_output_____"
],
[
"X = np.array([\n [2,1,0,0,0,0],\n [2,0,1,0,0,0],\n [1,0,0,1,0,0],\n [1,0,0,0,1,1]\n])\ny = np.array([0,1,2,3])\nnb = BernoulliNB().fit(X, y)\nX_test = np.array([[3,0,0,0,1,1],[0,1,1,0,1,1],[1,0,0,0,1,1],[2,1,0,0,0,0],[2,0,1,0,0,0],[1,0,0,1,0,0]])\nprint(nb.predict_log_proba(X_test))",
"[array([-9.07658038, -9.07658038, -9.70406053, -8.90668135]), array([-9.48204549, -9.48204549, -9.70406053, -8.78889831]), array([-6.8793558 , -6.8793558 , -6.93147181, -5.89852655]), array([-5.08759634, -5.78074352, -6.23832463, -6.59167373]), array([-5.78074352, -5.08759634, -6.23832463, -6.59167373]), array([-4.68213123, -4.68213123, -4.15888308, -5.08759634])]\n"
]
],
[
[
"### Prediction",
"_____no_output_____"
]
],
[
[
"df_vote = pd.read_csv('output/500terms_df_rev5.csv',index_col=False)\nterms_li = list(set(df_vote.term.tolist()))\nlen(terms_li)",
"_____no_output_____"
],
[
"with open('data/training.txt','r') as f:\n train_id = f.read().splitlines()\ntrain_dict = {}\ntest_id = []\ntrain_ids=[]\nfor trainid in train_id:\n trainid = trainid.split(' ')\n trainid = list(filter(None, trainid))\n train_ids.extend(trainid[1:])\nfor i in range(1095):\n if str(i+1) not in train_ids:\n test_id.append(i+1)\nans=[]\nfor doc in tqdm(test_id):\n ans.append(predict_MNB(doc))\nprint(ans)\ndf_ans = pd.DataFrame(list(zip(test_id,ans)),columns=['id','Value'])\ndf_ans.to_csv('output/MNB.csv',index=False)\ndf_ans",
"100%|██████████| 900/900 [00:20<00:00, 43.87it/s]\n"
]
],
[
[
"combine all prediction df",
"_____no_output_____"
]
],
[
[
"import os\nin_dir = './output/'\nprefixed = [filename for filename in os.listdir('./output/') if filename.endswith(\"_sk.csv\")]\ndf_from_each_file = [pd.read_csv(in_dir+f) for f in prefixed]\nprefixed",
"_____no_output_____"
],
[
"merged_df = functools.reduce(lambda left,right: pd.merge(left,right,on='id'), df_from_each_file)\nmerged_df.columns = ['id',0,1,2,3,4,5,6,7,8]\nmerged_df",
"_____no_output_____"
],
[
"df01 = pd.DataFrame(merged_df.mode(axis=1)[0])\ndf02 = pd.DataFrame(merged_df['id'])\ndf_ans = pd.concat([df02,df01],axis=1)\ndf_ans = df_ans.astype('int')\ndf_ans.columns = ['id','Value']\ndf_ans.to_csv('output/voting_rev.csv',index=False)\ndf_ans",
"_____no_output_____"
],
[
"df1 = merged_df[(merged_df[0] == merged_df[1])&(merged_df[2]==merged_df[3])&(merged_df[1]==merged_df[2])]\ndf1.reset_index(inplace=True,drop=True)\ndf1['class'] = df1[0]\ndf1 = df1.filter(['id','class'])\ndf1",
"/home/leoqaz12/.local/lib/python3.6/site-packages/ipykernel/__main__.py:3: SettingWithCopyWarning: \nA value is trying to be set on a copy of a slice from a DataFrame.\nTry using .loc[row_indexer,col_indexer] = value instead\n\nSee the caveats in the documentation: http://pandas.pydata.org/pandas-docs/stable/indexing.html#indexing-view-versus-copy\n app.launch_new_instance()\n"
],
[
"df1[df1['class']=='1']",
"_____no_output_____"
],
[
"for i in range(13):\n df1 = df1.astype(str)\n li = df1[df1['class'] == str(i+1)]['id'].tolist()\n train_dict[str(i+1)].extend(li)\ntrain_dict",
"_____no_output_____"
],
[
"pickle.dump(obj=train_dict,file=open('data/train_dict.pkl','wb'))",
"_____no_output_____"
],
[
"with open('data/training.txt','r') as f:\n train_id = f.read().splitlines()\ntrain_dict = {}\nfor trainid in train_id:\n trainid = trainid.split(' ')\n trainid = list(filter(None, trainid))\n train_dict[trainid[0]] = trainid[1:]\ntrain_dict #class:doc_id",
"_____no_output_____"
],
[
"df_from_each_file = [pd.read_csv(in_dir+f) for f in prefixed]\n# concatenated_df = pd.concat(df_from_each_file,axis=1,join='inner')\nconcatenated_df = pd.DataFrame.join(df_from_each_file,on='id')\nconcatenated_df",
"_____no_output_____"
],
[
"df1 = pd.read_csv(in_dir+prefixed[0])\ndf2 = pd.read_csv(in_dir+prefixed[1])\npd.merge(df1,df2,on='id',how='inner')",
"_____no_output_____"
],
[
"with open('data/training.txt','r') as f:\n train_id = f.read().splitlines()\ntrain_dict = {}\ntest_id = []\ntrain_ids=[]\nfor trainid in train_id:\n trainid = trainid.split(' ')\n trainid = list(filter(None, trainid))\n train_ids.extend(trainid[1:])\nfor i in range(1095):\n if str(i+1) not in train_ids:\n test_id.append(i+1)\n# train_dict[trainid[0]] = trainid[1:]\n# train_dict #class:doc_id\nin_dir = 'data/IRTM/'\ntrain_dict_ = {}\nclass_token = []\nclass_token_dict = {}\ntest_X = []\n# train_Y= []\n# for c,d in tqdm(train_dict.items()):\nfor doc in tqdm(test_id):\n testX = np.array([0]*len(terms_li))\n f = open('data/IRTM/'+str(doc)+'.txt')\n texts = f.read()\n f.close()\n tokens_all = preprocess(texts)\n tokens_all = tokens_all.split(' ')\n# tokens_all = list(filter(None,tokens_all))\n tokens_all = dict(Counter(tokens_all))\n for key,value in tokens_all.items():\n if key in terms_li:\n testX[terms_li.index(key)] = int(value)\n\n test_X.append(testX)\n \ntest_X = np.array(test_X)\nprint(test_X.shape)",
"100%|██████████| 900/900 [00:06<00:00, 131.03it/s]"
],
[
"df_ans = pd.DataFrame(list(zip(test_id,ans2)),columns=['id','Value'])\ndf_ans.to_csv('output/MNB02_sk.csv',index=False)\ndf_ans",
"_____no_output_____"
],
[
"from sklearn.datasets import load_iris\nfrom sklearn.feature_selection import SelectKBest\nfrom sklearn.feature_selection import chi2",
"_____no_output_____"
],
[
"from sklearn.naive_bayes import *\nclf = MultinomialNB()\nclf.fit(train_X, train_Y)\nans2 = clf.predict(test_X)\nans2",
"_____no_output_____"
],
[
"from sklearn.naive_bayes import *\nclf = MultinomialNB()\nclf.fit(train_X, train_Y)\nans2 = clf.predict(test_X)\nans2",
"_____no_output_____"
],
[
"clf = BernoulliNB()\nclf.fit(train_X, train_Y)\nans2 = clf.predict(test_X)\nans2",
"_____no_output_____"
],
[
"print(train_X.shape, train_Y.shape , test_X.shape)",
"(946, 186) (946,) (900, 186)\n"
],
[
"df_ans = pd.DataFrame(list(zip(test_id,ans2)),columns=['id','Value'])\ndf_ans.to_csv('output/MNB05_sk.csv',index=False)\ndf_ans",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7b945194ad8c43fc171917fe75e7de6ac6eaaf0 | 10,158 | ipynb | Jupyter Notebook | 01-hello_world/A0-editing_with_emacs.ipynb | vicente-gonzalez-ruiz/python-tutorial | e6a79510a0b3663786d6476a40e79fc8e8726f61 | [
"CC0-1.0"
] | 4 | 2017-03-06T09:49:11.000Z | 2019-10-16T00:09:38.000Z | 01-hello_world/A0-editing_with_emacs.ipynb | vicente-gonzalez-ruiz/python-tutorial | e6a79510a0b3663786d6476a40e79fc8e8726f61 | [
"CC0-1.0"
] | null | null | null | 01-hello_world/A0-editing_with_emacs.ipynb | vicente-gonzalez-ruiz/python-tutorial | e6a79510a0b3663786d6476a40e79fc8e8726f61 | [
"CC0-1.0"
] | 7 | 2017-11-02T11:00:30.000Z | 2020-01-31T22:41:27.000Z | 42.502092 | 2,752 | 0.598149 | [
[
[
"<h1>Table of Contents<span class=\"tocSkip\"></span></h1>\n<div class=\"toc\"><ul class=\"toc-item\"><li><span><a href=\"#Python-editting\" data-toc-modified-id=\"Python-editting-1\"><span class=\"toc-item-num\">1 </span>Python editting</a></span><ul class=\"toc-item\"><li><span><a href=\"#1.-Install-GNU-Emacs\" data-toc-modified-id=\"1.-Install-GNU-Emacs-1.1\"><span class=\"toc-item-num\">1.1 </span>1. Install <a href=\"https://www.gnu.org/software/emacs/\" target=\"_blank\">GNU Emacs</a></a></span><ul class=\"toc-item\"><li><span><a href=\"#OS-X\" data-toc-modified-id=\"OS-X-1.1.1\"><span class=\"toc-item-num\">1.1.1 </span>OS X</a></span></li><li><span><a href=\"#Ubuntu\" data-toc-modified-id=\"Ubuntu-1.1.2\"><span class=\"toc-item-num\">1.1.2 </span>Ubuntu</a></span></li><li><span><a href=\"#Windows\" data-toc-modified-id=\"Windows-1.1.3\"><span class=\"toc-item-num\">1.1.3 </span>Windows</a></span></li></ul></li><li><span><a href=\"#2.-Install-ELPY-(Emacs-Lisp-Python-Environment)\" data-toc-modified-id=\"2.-Install-ELPY-(Emacs-Lisp-Python-Environment)-1.2\"><span class=\"toc-item-num\">1.2 </span>2. Install <a href=\"https://elpy.readthedocs.io\" target=\"_blank\">ELPY (Emacs Lisp Python Environment)</a></a></span><ul class=\"toc-item\"><li><span><a href=\"#Update-PATH\" data-toc-modified-id=\"Update-PATH-1.2.1\"><span class=\"toc-item-num\">1.2.1 </span>Update PATH</a></span></li><li><span><a href=\"#Install-ELPY-package-from-MELPA\" data-toc-modified-id=\"Install-ELPY-package-from-MELPA-1.2.2\"><span class=\"toc-item-num\">1.2.2 </span>Install ELPY package from <a href=\"https://melpa.org/#/getting-started\" target=\"_blank\">MELPA</a></a></span></li><li><span><a href=\"#Configure-Elpy:\" data-toc-modified-id=\"Configure-Elpy:-1.2.3\"><span class=\"toc-item-num\">1.2.3 </span>Configure Elpy:</a></span></li></ul></li><li><span><a href=\"#3.-Optional-stuff\" data-toc-modified-id=\"3.-Optional-stuff-1.3\"><span class=\"toc-item-num\">1.3 </span>3. Optional stuff</a></span><ul class=\"toc-item\"><li><span><a href=\"#Install-theme-package-(optional)\" data-toc-modified-id=\"Install-theme-package-(optional)-1.3.1\"><span class=\"toc-item-num\">1.3.1 </span>Install theme package (optional)</a></span></li></ul></li><li><span><a href=\"#3.-Jedi-directly-from-Emacs\" data-toc-modified-id=\"3.-Jedi-directly-from-Emacs-1.4\"><span class=\"toc-item-num\">1.4 </span>3. Jedi directly from Emacs</a></span><ul class=\"toc-item\"><li><span><a href=\"#3.1.-Install-MELPA\" data-toc-modified-id=\"3.1.-Install-MELPA-1.4.1\"><span class=\"toc-item-num\">1.4.1 </span>3.1. Install MELPA</a></span></li></ul></li></ul></li></ul></div>",
"_____no_output_____"
],
[
"# Python editting\n\n1. Install Emacs.\n2. Install ELPY.\n3. Optional stuff.",
"_____no_output_____"
],
[
"## 1. Install [GNU Emacs](https://www.gnu.org/software/emacs/)",
"_____no_output_____"
],
[
"### OS X\nRun:\n```\nbrew update # Synchronize the local Homebrew database\nbrew install emacs --with-cocoa # Allows to start Emacs from the launchpad or from Spotlight\nbrew linkapps emacs # To symlink Emacs to \"/Applications\"\n```",
"_____no_output_____"
],
[
"### Ubuntu\nRun:\n```\napt-get install emacs\n```",
"_____no_output_____"
],
[
"### Windows\nDownload it from \"http://ftp.gnu.org/gnu/emacs/windows/ and install it.\n",
"_____no_output_____"
],
[
"## 2. Install [ELPY (Emacs Lisp Python Environment)](https://elpy.readthedocs.io)\n\nELPY is an Emacs package which provides Python's commands completion, indentation highlighting, [snippet](https://es.wikipedia.org/wiki/Snippet) expansion, [code hinting](https://www.linguee.es/ingles-espanol/traduccion/code+hinting.html), code navigation, [code refactoring](https://en.wikipedia.org/wiki/Code_refactoring), [on-the-fly checks](http://flymake.sourceforge.net/), [virtualenv support](https://docs.python.org/3/library/venv.html) and test running. Original documentation: https://elpy.readthedocs.io/en/latest/introduction.html#installation. Another interesting (and very comprehensive) resource is: https://realpython.com/emacs-the-best-python-editor/.",
"_____no_output_____"
],
[
"### Update PATH\nIf you are using a Python enviroment, the shell `PATH` variable must include the \"binary\" files installed by `pip`. For example, if you are using [pyenv](https://github.com/pyenv/pyenv), add\n```\nexport PATH=/home/vruiz/.pyenv/versions/3.6.4/bin/:$PATH\n\n```\nto your shell configuration file (`~/.bashrc`, for example).",
"_____no_output_____"
],
[
"### Install ELPY package from [MELPA](https://melpa.org/#/getting-started)\nIn Emacs, run `<Esc> x package-install <Enter> elpy <Enter>`. Enable this package by including\n```\n(package-initialize)\n(elpy-enable)\n```\ninto your `~/.emacs` Emacs configuration file. Restart Emacs.",
"_____no_output_____"
],
[
"### Configure Elpy:\n\nIn Emacs, key-in:\n```\n<Esc> x elpy-config <Enter>\n```\n\nElpy will check the Elpy-related Python packages which are available and will display a summary. At this moment, the following packages should be missing (and therefore, installed). After that, Emacs need to be reset (closed and open):\n\n1. [Jedi](https://pypi.python.org/pypi/jedi/), an autocompletion tool for Python that can be used for text editors:\n```\npip install jedi\n```\n2. [Rope](https://pypi.python.org/pypi/rope), a Python [refactoring](https://en.wikipedia.org/wiki/Code_refactoring):\n```\npip install rope\n```\n3. [Import Magick](https://pypi.python.org/pypi/importmagic), add, remove and manage imports:\n```\npip install importmagic\n```\n4. [autopep8](https://pypi.python.org/pypi/autopep8), automatically formats Python code to conform to the [PEP 8 style guide](https://www.python.org/dev/peps/pep-0008/):\n```\npip install autopep8\n```\n5. [YAPF](https://pypi.python.org/pypi/yapf), formats Python code to conform the [clang-format style guide](http://clang.llvm.org/docs/ClangFormatStyleOptions.html):\n```\npip install yapf\n```\n6. [Flake8](https://pypi.python.org/pypi/flake8), a source code checker:\n```\npip install flake8\n```",
"_____no_output_____"
],
[
"## 3. Optional stuff\n\nIn UNIX environments, the Emacs configuration data should be located in the file `$HOME/.emacs` or in the file `$HOME/.emacs.d/init.el`. In Windows OS (if the HOME environment variable is not set), in `C:/.emacs.d/init.el`.",
"_____no_output_____"
],
[
"### Install theme package (optional)\n\nRun `emacs` and, type `<Esc> x package-install <Enter> better-defaults <Enter>`. Remember that autocompletation is always available! Install also `material-theme`. To enable these themes, add:\n```\n(setq inhibit-startup-message t) ;; hide the startup message\n(load-theme 'material t) ;; load material theme\n(global-linum-mode t) ;; enable line numbers globally\n```\nto your configuration file, and restart Emacs.",
"_____no_output_____"
],
[
"## 3. Jedi directly from Emacs\n\n### 3.1. Install MELPA\nAdd this code to the Emacs configuration file: `$HOME/.emacs`:\n```\n;; Standard package.el + MELPA setup\n;; (See also: https://github.com/milkypostman/melpa#usage)\n(require 'package)\n(add-to-list 'package-archives\n '(\"melpa\" . \"http://melpa.milkbox.net/packages/\") t)\n(package-initialize)\n\n\n;; Standard Jedi.el setting\n(add-hook 'python-mode-hook 'jedi:setup)\n(setq jedi:complete-on-dot t)\n\n;; Type:\n;; M-x package-install RET jedi RET\n;; M-x jedi:install-server RET\n;; Then open Python file.\n```\n\nIn the shell:\n```\nsudo apt-get install virtualenv\n# pip install epc # ... sometimes\n```\n\nIn emacs:\n```\nM-x package-install RET jedi RET\nM-x jedi:install-server RET\n```",
"_____no_output_____"
]
]
] | [
"markdown"
] | [
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
]
] |
e7b9563670f948318d172c0d2b2af1f95d521ff1 | 47,052 | ipynb | Jupyter Notebook | Python_Stock/Stock_Statistics.ipynb | eu90h/Stock_Analysis_For_Quant | 5e5e9f9c2a8f4af72e26564bc9d66bd2c90880df | [
"MIT"
] | null | null | null | Python_Stock/Stock_Statistics.ipynb | eu90h/Stock_Analysis_For_Quant | 5e5e9f9c2a8f4af72e26564bc9d66bd2c90880df | [
"MIT"
] | null | null | null | Python_Stock/Stock_Statistics.ipynb | eu90h/Stock_Analysis_For_Quant | 5e5e9f9c2a8f4af72e26564bc9d66bd2c90880df | [
"MIT"
] | null | null | null | 77.261084 | 22,394 | 0.756588 | [
[
[
"# Stock Statistics",
"_____no_output_____"
],
[
"Statistics is a branch of applied mathematics concerned with collecting, organizing, and interpreting data. Statistics is also the mathematical study of the likelihood and probability of events occurring based on known quantitative data or a collection of data.\n\nhttp://www.icoachmath.com/math_dictionary/Statistics",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport pandas as pd\nimport scipy.stats as stats\nimport matplotlib.pyplot as plt\n\nimport warnings\nwarnings.filterwarnings(\"ignore\")\n\n# yfinance is used to fetch data \nimport yfinance as yf\nyf.pdr_override()",
"_____no_output_____"
],
[
"# input\nsymbol = 'AAPL'\nstart = '2014-01-01'\nend = '2019-01-01'\n\n# Read data \ndf = yf.download(symbol,start,end)\n\n# View Columns\ndf.head()",
"[*********************100%***********************] 1 of 1 downloaded\n"
],
[
"returns = df['Adj Close'].pct_change()[1:].dropna()",
"_____no_output_____"
]
],
[
[
"### Mean is the average number, sum of the values divided by the number of values.\n### Median is the middle value in the list of numbers.\n### Mode is the value that occurs often.",
"_____no_output_____"
]
],
[
[
"import statistics as st\n\nprint('Mean of returns:', st.mean(returns))\nprint('Median of returns:', st.median(returns))\nprint('Median Low of returns:', st.median_low(returns))\nprint('Median High of returns:', st.median_high(returns))\nprint('Median Grouped of returns:', st.median_grouped(returns))\nprint('Mode of returns:', st.mode(returns))",
"Mean of returns: 0.0007357373017012066\nMedian of returns: 0.0006264819982997327\nMedian Low of returns: 0.0006264819982997327\nMedian High of returns: 0.0006264819982997327\nMedian Grouped of returns: 0.0006264819982997327\nMode of returns: 0.0\n"
],
[
"from statistics import mode\n\nprint('Mode of returns:', mode(returns))\n# Since all of the returns are distinct, we use a frequency distribution to get an alternative mode.\n# np.histogram returns the frequency distribution over the bins as well as the endpoints of the bins\nhist, bins = np.histogram(returns, 20) # Break data up into 20 bins\nmaxfreq = max(hist)\n# Find all of the bins that are hit with frequency maxfreq, then print the intervals corresponding to them\nprint('Mode of bins:', [(bins[i], bins[i+1]) for i, j in enumerate(hist) if j == maxfreq])",
"Mode of returns: 0.0\nMode of bins: [(-0.0070681808335254365, 0.0010272794824504605)]\n"
]
],
[
[
"### Arithmetic Average Returns is average return on the the stock or investment",
"_____no_output_____"
]
],
[
[
"print('Arithmetic average of returns:\\n')\nprint(returns.mean())",
"Arithmetic average of returns:\n\n0.0007357373017012073\n"
]
],
[
[
"### Geometric mean is the average of a set of products, the calculation of which is commonly used to determine the performance results of an investment or portfolio. It is technically defined as \"the nth root product of n numbers.\" The geometric mean must be used when working with percentages, which are derived from values, while the standard arithmetic mean works with the values themselves. \n\nhttps://www.investopedia.com/terms/h/harmonicaverage.asp",
"_____no_output_____"
]
],
[
[
"# Geometric mean\nfrom scipy.stats.mstats import gmean\nprint('Geometric mean of stock:', gmean(returns))",
"Geometric mean of stock: nan\n"
],
[
"ratios = returns + np.ones(len(returns))\nR_G = gmean(ratios) - 1\nprint('Geometric mean of returns:', R_G)",
"Geometric mean of returns: 0.000622187293129\n"
]
],
[
[
"### Standard deviation of returns is the risk of returns",
"_____no_output_____"
]
],
[
[
"print('Standard deviation of returns')\nprint(returns.std())",
"Standard deviation of returns\n0.01507109969428369\n"
],
[
"T = len(returns)\ninit_price = df['Adj Close'][0]\nfinal_price = df['Adj Close'][T]\nprint('Initial price:', init_price)\nprint('Final price:', final_price)\nprint('Final price as computed with R_G:', init_price*(1 + R_G)**T)",
"Initial price: 71.591667\nFinal price: 156.463837\nFinal price as computed with R_G: 156.463837\n"
]
],
[
[
"### Harmonic Mean is numerical average. \n\nFormula: A set of n numbers, add the reciprocals of the numbers in the set, divide the sum by n, then take the reciprocal of the result.",
"_____no_output_____"
]
],
[
[
"# Harmonic mean\n\nprint('Harmonic mean of returns:', len(returns)/np.sum(1.0/returns))",
"Harmonic mean of returns: 0.0\n"
],
[
"print('Skew:', stats.skew(returns))\nprint('Mean:', np.mean(returns))\nprint('Median:', np.median(returns))\n\nplt.hist(returns, 30); ",
"Skew: -0.06538797604571234\nMean: 0.0007357373017012073\nMedian: 0.0006264819983\n"
],
[
"# Plot some example distributions stock's returns\nxs = np.linspace(-6,6, 1257)\nnormal = stats.norm.pdf(xs)\nplt.plot(returns,stats.laplace.pdf(returns), label='Leptokurtic')\nprint('Excess kurtosis of leptokurtic distribution:', (stats.laplace.stats(returns)))\nplt.plot(returns, normal, label='Mesokurtic (normal)')\nprint('Excess kurtosis of mesokurtic distribution:', (stats.norm.stats(returns)))\nplt.plot(returns,stats.cosine.pdf(returns), label='Platykurtic')\nprint('Excess kurtosis of platykurtic distribution:', (stats.cosine.stats(returns)))\nplt.legend()",
"Excess kurtosis of leptokurtic distribution: (array([-0.02196584, 0.00545288, -0.0071516 , ..., -0.0064898 ,\n 0.00051228, 0.00966536]), array([ 2., 2., 2., ..., 2., 2., 2.]))\nExcess kurtosis of mesokurtic distribution: (array([-0.02196584, 0.00545288, -0.0071516 , ..., -0.0064898 ,\n 0.00051228, 0.00966536]), array([ 1., 1., 1., ..., 1., 1., 1.]))\nExcess kurtosis of platykurtic distribution: (array([-0.02196584, 0.00545288, -0.0071516 , ..., -0.0064898 ,\n 0.00051228, 0.00966536]), array([ 1.28986813, 1.28986813, 1.28986813, ..., 1.28986813,\n 1.28986813, 1.28986813]))\n"
],
[
"print(\"Excess kurtosis of returns: \", stats.kurtosis(returns))",
"Excess kurtosis of returns: 3.73675394710252\n"
],
[
"from statsmodels.stats.stattools import jarque_bera\n\n_, pvalue, _, _ = jarque_bera(returns)\n\nif pvalue > 0.05:\n print('The returns are likely normal.')\nelse:\n print('The returns are likely not normal.')",
"The returns are likely not normal.\n"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
]
] |
e7b963481d76c276b77515ec881b24d963a56358 | 1,281 | ipynb | Jupyter Notebook | notebooks/Mutations.ipynb | neso613/python_coding | 418a24ebb1ec30cdab251eb3f165920e71da5c29 | [
"Apache-2.0"
] | null | null | null | notebooks/Mutations.ipynb | neso613/python_coding | 418a24ebb1ec30cdab251eb3f165920e71da5c29 | [
"Apache-2.0"
] | null | null | null | notebooks/Mutations.ipynb | neso613/python_coding | 418a24ebb1ec30cdab251eb3f165920e71da5c29 | [
"Apache-2.0"
] | null | null | null | 18.3 | 56 | 0.489461 | [
[
[
"def mutate_string(string, position, character):\n s = list(string)\n s[position]=character\n return \"\".join(s)",
"_____no_output_____"
],
[
"s = input()\ni, c = input().split()\ns_new = mutate_string(s, int(i), c)\nprint(s_new)",
"abracadabra\n5 k\nabrackdabra\n"
]
]
] | [
"code"
] | [
[
"code",
"code"
]
] |
e7b96ad74c3bc457c4c9272c1270fef17255e105 | 9,957 | ipynb | Jupyter Notebook | 6. Getting Started with Numpy/3. Arithmetic_Operations_on_Array.ipynb | AshishJangra27/Data-Science-Live-Course-GeeksForGeeks | 4fefa9c855dd515a974ee4c0d9a41886e3c0c1f8 | [
"Apache-2.0"
] | 2 | 2021-09-11T15:44:23.000Z | 2021-09-25T17:49:26.000Z | 6. Getting Started with Numpy/3. Arithmetic_Operations_on_Array.ipynb | AshishJangra27/Data-Science-Live-Course-GeeksForGeeks | 4fefa9c855dd515a974ee4c0d9a41886e3c0c1f8 | [
"Apache-2.0"
] | null | null | null | 6. Getting Started with Numpy/3. Arithmetic_Operations_on_Array.ipynb | AshishJangra27/Data-Science-Live-Course-GeeksForGeeks | 4fefa9c855dd515a974ee4c0d9a41886e3c0c1f8 | [
"Apache-2.0"
] | null | null | null | 21.740175 | 58 | 0.377423 | [
[
[
"import numpy as np",
"_____no_output_____"
],
[
"arr = np.array([1,2,3,4,5,6])",
"_____no_output_____"
],
[
"arr",
"_____no_output_____"
],
[
"print(arr + 1)\nprint(arr - 1)\nprint(arr * 5)\nprint(arr / 2)\nprint(arr ** 3)",
"[2 3 4 5 6 7]\n[0 1 2 3 4 5]\n[ 5 10 15 20 25 30]\n[0.5 1. 1.5 2. 2.5 3. ]\n[ 1 8 27 64 125 216]\n"
],
[
"arr",
"_____no_output_____"
],
[
"arr = arr + 1",
"_____no_output_____"
],
[
"arr",
"_____no_output_____"
],
[
"arr += 1",
"_____no_output_____"
],
[
"arr",
"_____no_output_____"
],
[
"arr *= 2",
"_____no_output_____"
],
[
"arr",
"_____no_output_____"
],
[
"arr.max()",
"_____no_output_____"
],
[
"arr.min()",
"_____no_output_____"
],
[
"arr = np.array([[1,2,3],\n [4,5,6],\n [7,8,9]])",
"_____no_output_____"
],
[
"arr",
"_____no_output_____"
],
[
"arr.max(axis = 0) # Max element column-wise",
"_____no_output_____"
],
[
"arr.max(axis = 1) # Max element row-wise",
"_____no_output_____"
],
[
"arr.min(axis = 0) # Min element column-wise",
"_____no_output_____"
],
[
"arr.min(axis = 1) # Min element row-wise",
"_____no_output_____"
],
[
"arr.sum()",
"_____no_output_____"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7b982c16d56cc95510513cb3c000f8526a0b1d5 | 56,541 | ipynb | Jupyter Notebook | NeuronBinaryClassification/Jupyter_Notebook/MultiLayerPerceptron_15_test_15_train.ipynb | bkommineni/Activity-Recognition-Using-Sensordata | 94effff8295e5314ac097a777b01013e540c75de | [
"BSD-4-Clause-UC"
] | null | null | null | NeuronBinaryClassification/Jupyter_Notebook/MultiLayerPerceptron_15_test_15_train.ipynb | bkommineni/Activity-Recognition-Using-Sensordata | 94effff8295e5314ac097a777b01013e540c75de | [
"BSD-4-Clause-UC"
] | null | null | null | NeuronBinaryClassification/Jupyter_Notebook/MultiLayerPerceptron_15_test_15_train.ipynb | bkommineni/Activity-Recognition-Using-Sensordata | 94effff8295e5314ac097a777b01013e540c75de | [
"BSD-4-Clause-UC"
] | null | null | null | 171.336364 | 18,554 | 0.875312 | [
[
[
"from sklearn.neural_network import MLPClassifier\nfrom sklearn.preprocessing import StandardScaler\nfrom sklearn.metrics import classification_report,confusion_matrix,accuracy_score\nfrom sklearn.model_selection import train_test_split\nimport pandas as pd\nfrom pandas import DataFrame,Series\nfrom matplotlib.colors import ListedColormap\nimport numpy as np\nfrom sklearn.metrics import accuracy_score,confusion_matrix,classification_report\nimport matplotlib.pyplot as plt\nfrom pandas.plotting import scatter_matrix\nfrom random import sample",
"_____no_output_____"
],
[
"dup_train = pd.read_csv('../FeaturesCsvFile/featuresfile.csv')\ndup_test = pd.read_csv('../FeaturesCsvFile/featuresfile_10.csv')\ndf1 = dup_test.drop_duplicates(subset=['User', 'Timestamp'])\ndf2 = dup_train.drop_duplicates(subset=['User', 'Timestamp'])\nframes = [df1, df2]\ndf= pd.concat(frames)\ndf.shape",
"_____no_output_____"
],
[
"X = df.values[:, 2:45]\ny = df.values[:, 45] #label : walking/runing\ny_plot = np.where(y == 'walking', -1, 1)\nX_train, X_test, y_train, y_test = train_test_split(X, y_plot, test_size=0.3)\nscaler = StandardScaler()\nscaler.fit(X_train)\nStandardScaler(copy=True, with_mean=True, with_std=True)\nX_train = scaler.transform(X_train)\nX_test = scaler.transform(X_test)\nmlp = MLPClassifier(hidden_layer_sizes=(15,),max_iter=60)\nmlp.fit(X_train,y_train)\ny_pred = mlp.predict(X_test)\nprint('Accuracy of Accuracy Score : %.2f' % accuracy_score(y_test,y_pred))\nprint('Accuracy of Multi-Layer Perceptron Score: %.2f' % mlp.score(X_test,y_test))\nprint(confusion_matrix(y_test,y_pred))\nprint(classification_report(y_test,y_pred))",
"Accuracy of Accuracy Score : 0.95\nAccuracy of Multi-Layer Perceptron Score: 0.95\n[[132 5]\n [ 7 103]]\n precision recall f1-score support\n\n -1 0.95 0.96 0.96 137\n 1 0.95 0.94 0.94 110\n\navg / total 0.95 0.95 0.95 247\n\n"
],
[
"avg_weight = []\nfor i in range(0,len(mlp.coefs_[0])):\n avg_weight.append(np.mean(mlp.coefs_[0][i]))\nprint ('Important features (featureName, weigh of important, #column)')\nheader = list(df.head(1))\nimportant_feature = []\nfor i in range(0,len(avg_weight)):\n important_feature.append((header[i+2],avg_weight[i],i+2))\nsorted_list = sorted(important_feature,key=lambda important_feature: important_feature[1],reverse=True)\nfor j in range(0,len(sorted_list)):\n first_imp_fea = sorted_list[0]\n second_imp_fea = sorted_list[1]\n print sorted_list[j]",
"Important features (featureName, weigh of important, #column)\n('Bin3,z', 0.10825592994061183, 24)\n('Bin6,x', 0.099050904501434653, 7)\n('AvgAcc-y', 0.072431320057493101, 39)\n('Bin5,x', 0.070197353658616207, 6)\n('StdDev-y', 0.059787564913883734, 42)\n('Bin7,z', 0.059601690509690934, 28)\n('Bin9,y', 0.044901323984415702, 20)\n('AvgAbsDiff-z', 0.030665486239005578, 37)\n('Bin3,y', 0.028917213562429201, 14)\n('AvgAcc-x', 0.027602009597943528, 38)\n('TimeDiffPeaks-y', 0.024406273141934252, 33)\n('Bin1,z', 0.022291450883107876, 22)\n('Bin8,y', 0.013387262576128274, 19)\n('Bin6,y', 0.012826037878942664, 17)\n('Bin8,x', 0.0092786045093103797, 9)\n('Bin2,z', 0.0088359802466105204, 23)\n('Bin7,x', 0.0084503892418165989, 8)\n('Bin3,x', 0.0061114596853632094, 4)\n('Bin5,y', 0.0055106417495060771, 16)\n('Bin6,z', 0.0029566149246515582, 27)\n('Bin10,y', 0.0019471520057938692, 21)\n('Bin9,z', 0.0013783328195343596, 30)\n('AvgAbsDiff-y', -0.0013050781765424346, 36)\n('Bin2,y', -0.0017919156902444012, 13)\n('Bin2,x', -0.003927755930684073, 3)\n('Bin4,y', -0.012892902034440549, 15)\n('AvgAbsDiff-x', -0.017511543702004303, 35)\n('Bin1,y', -0.01783316444767534, 12)\n('AvgAcc-z', -0.02037735095122958, 40)\n('StdDev-z', -0.022119987856093683, 43)\n('Bin7,y', -0.025977987046485441, 18)\n('Bin1,x', -0.033222958956476277, 2)\n('AvgResAcc', -0.034471345586067653, 44)\n('StdDev-x', -0.03807143267292027, 41)\n('Bin8,z', -0.044565909675076086, 29)\n('Bin10,z', -0.045433237271096466, 31)\n('TimeDiffPeaks-z', -0.048707633118018692, 34)\n('Bin5,z', -0.050599779731201909, 26)\n('Bin9,x', -0.051573141481606181, 10)\n('TimeDiffPeaks-x', -0.073463131772347243, 32)\n('Bin4,z', -0.092496139247622011, 25)\n('Bin10,x', -0.096060475320505878, 11)\n('Bin4,x', -0.11269002628339721, 5)\n"
],
[
"from sklearn import metrics\ndef plot_roc_curve(Y_predict,Y_test,name_graph):\n num_predns = []\n for i in range(0,len(Y_predict)):\n if Y_predict[i] == \"walking\":\n num_predns.append(0)\n else:\n num_predns.append(1)\n num_labels = []\n for i in range(0,len(Y_test)):\n if Y_test[i] == \"walking\":\n num_labels.append(0)\n else:\n num_labels.append(1)\n\n predns = np.array(num_predns)\n labels = np.array(num_labels)\n fpr, tpr, thresholds = metrics.roc_curve(y_test, y_pred)\n roc_auc = metrics.auc(fpr, tpr)\n plt.title('Area under ROC Curve')\n plt.plot(fpr, tpr, 'grey', label = 'AUC = %0.2f' % roc_auc)\n plt.legend(loc = 'lower right')\n plt.plot([0, 1], [0, 1],'r--')\n plt.xlim([0, 1])\n plt.ylim([0, 1])\n plt.ylabel('True Positive Rate')\n plt.xlabel('False Positive Rate')\n plt.show()\n# plt.savefig('./image/Area_under_roc_pc.png', dpi=1000)\n \nplot_roc_curve(y_pred,y_test,\"Area_under_roc_pc\")",
"_____no_output_____"
],
[
"import itertools\nimport numpy as np\nimport matplotlib.pyplot as plt\n\ndef plot_confusion_matrix(cm, classes,\n normalize=False,\n title='Confusion matrix',\n cmap=plt.cm.Greens):\n \"\"\"\n This function prints and plots the confusion matrix.\n Normalization can be applied by setting `normalize=True`.\n \"\"\"\n if normalize:\n cm = cm.astype('float') / cm.sum(axis=1)[:, np.newaxis]\n print(\"Normalized confusion matrix\")\n else:\n print('Confusion matrix, without normalization')\n\n print(cm)\n\n plt.imshow(cm, interpolation='nearest', cmap=cmap)\n plt.title(title)\n plt.colorbar()\n tick_marks = np.arange(len(classes))\n plt.xticks(tick_marks, classes)\n plt.yticks(tick_marks, classes, rotation=90)\n\n fmt = '.2f' if normalize else 'd'\n thresh = cm.max() / 2.\n for i, j in itertools.product(range(cm.shape[0]), range(cm.shape[1])):\n plt.text(j, i, format(cm[i, j], fmt),\n horizontalalignment=\"center\",\n color=\"white\" if cm[i, j] > thresh else \"black\")\n\n plt.ylabel('True label')\n plt.xlabel('Predicted label')\n\ncnf_matrix = confusion_matrix(y_test, y_pred)\nnp.set_printoptions(precision=2)\n\n# Plot non-normalized confusion matrix\nplt.figure()\nclass_names = [\"walking\", \"running\"]\nplot_confusion_matrix(cnf_matrix, classes=[\"walking\", \"running\"],\n title='Confusion matrix, without normalization')\nplt.figure()\nplot_confusion_matrix(cnf_matrix, classes=class_names, normalize=True,\n title='Normalized confusion matrix')\nplt.show()",
"Confusion matrix, without normalization\n[[132 5]\n [ 7 103]]\nNormalized confusion matrix\n[[ 0.96 0.04]\n [ 0.06 0.94]]\n"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7b99bc31926de75abca2acbaccebc85225add2f | 904,180 | ipynb | Jupyter Notebook | examples/applications/image-search/Image_Classification.ipynb | danielperezr88/sentence-transformers | 56a7990c56c484e7948cf6400b54f27114bb267c | [
"Apache-2.0"
] | null | null | null | examples/applications/image-search/Image_Classification.ipynb | danielperezr88/sentence-transformers | 56a7990c56c484e7948cf6400b54f27114bb267c | [
"Apache-2.0"
] | null | null | null | examples/applications/image-search/Image_Classification.ipynb | danielperezr88/sentence-transformers | 56a7990c56c484e7948cf6400b54f27114bb267c | [
"Apache-2.0"
] | null | null | null | 2,598.218391 | 161,110 | 0.968889 | [
[
[
"# Zero-Shot Image Classification\n\nThis example shows how [SentenceTransformers](https://www.sbert.net) can be used to map images and texts to the same vector space. \n\nWe can use this to perform **zero-shot image classification** by providing the names for the labels.\n\nAs model, we use the [OpenAI CLIP Model](https://github.com/openai/CLIP), which was trained on a large set of images and image alt texts.\n\n\nThe images in this example are from [Unsplash](https://unsplash.com/).",
"_____no_output_____"
]
],
[
[
"from sentence_transformers import SentenceTransformer, util\nfrom PIL import Image\nimport glob\nimport torch\nimport pickle\nimport zipfile\nfrom IPython.display import display\nfrom IPython.display import Image as IPImage\nimport os\nfrom tqdm.autonotebook import tqdm\nimport torch\n\n# We use the original CLIP model for computing image embeddings and English text embeddings\nen_model = SentenceTransformer('clip-ViT-B-32')",
"_____no_output_____"
],
[
"# We download some images from our repository which we want to classify\nimg_names = ['eiffel-tower-day.jpg', 'eiffel-tower-night.jpg', 'two_dogs_in_snow.jpg', 'cat.jpg']\nurl = 'https://github.com/UKPLab/sentence-transformers/raw/master/examples/applications/image-search/'\nfor filename in img_names:\n if not os.path.exists(filename):\n util.http_get(url+filename, filename)\n\n# And compute the embeddings for these images\nimg_emb = en_model.encode([Image.open(filepath) for filepath in img_names], convert_to_tensor=True)",
"_____no_output_____"
],
[
"# Then, we define our labels as text. Here, we use 4 labels\nlabels = ['dog', 'cat', 'Paris at night', 'Paris']\n\n# And compute the text embeddings for these labels\nen_emb = en_model.encode(labels, convert_to_tensor=True)\n\n# Now, we compute the cosine similarity between the images and the labels\ncos_scores = util.cos_sim(img_emb, en_emb)\n\n# Then we look which label has the highest cosine similarity with the given images\npred_labels = torch.argmax(cos_scores, dim=1)\n\n# Finally we output the images + labels\nfor img_name, pred_label in zip(img_names, pred_labels):\n display(IPImage(img_name, width=200))\n print(\"Predicted label:\", labels[pred_label])\n print(\"\\n\\n\")\n\n",
"_____no_output_____"
]
],
[
[
"# Zero-Shot Image Classification\nThe original CLIP Model only works for English, hence, we used [Multilingual Knowlegde Distillation](https://arxiv.org/abs/2004.09813) to make this model work with 50+ languages.\n\nFor this, we msut load the *clip-ViT-B-32-multilingual-v1* model to encode our labels.\nWe can define our labels in 50+ languages and can also mix the languages we have",
"_____no_output_____"
]
],
[
[
"multi_model = SentenceTransformer('clip-ViT-B-32-multilingual-v1')\n\n# Then, we define our labels as text. Here, we use 4 labels\nlabels = ['Hund', # German: dog\n 'gato', # Spanish: cat \n '巴黎晚上', # Chinese: Paris at night\n 'Париж' # Russian: Paris\n ]\n\n# And compute the text embeddings for these labels\ntxt_emb = multi_model.encode(labels, convert_to_tensor=True)\n\n# Now, we compute the cosine similarity between the images and the labels\ncos_scores = util.cos_sim(img_emb, txt_emb)\n\n# Then we look which label has the highest cosine similarity with the given images\npred_labels = torch.argmax(cos_scores, dim=1)\n\n# Finally we output the images + labels\nfor img_name, pred_label in zip(img_names, pred_labels):\n display(IPImage(img_name, width=200))\n print(\"Predicted label:\", labels[pred_label])\n print(\"\\n\\n\")",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7b9b344c01e04381527eac30ba8b04fd38a5287 | 55,684 | ipynb | Jupyter Notebook | LDA_New.ipynb | parikshitsaikia1619/LDA_modeing_IQVIA | 3459be5b17868f172ba318160566623c53f51b33 | [
"MIT"
] | null | null | null | LDA_New.ipynb | parikshitsaikia1619/LDA_modeing_IQVIA | 3459be5b17868f172ba318160566623c53f51b33 | [
"MIT"
] | null | null | null | LDA_New.ipynb | parikshitsaikia1619/LDA_modeing_IQVIA | 3459be5b17868f172ba318160566623c53f51b33 | [
"MIT"
] | null | null | null | 54.167315 | 22,972 | 0.685439 | [
[
[
"## Coding Assignment\n<i>Q: Write a python class with different function to fit LDA model, evaluate optimal number of topics based on best coherence scores and predict new instances based on best LDA model with optimal number of topics based on best coherence score. Function should take 2darray of embeddings as input and return a LDA model, optimal number of topics and topics.</i>",
"_____no_output_____"
]
],
[
[
"\"\"\"\nauthor: Parikshit Saikia\nemail: [email protected]\ngithub: https://github.com/parikshitsaikia1619\ndate: 20-08-2021\n\"\"\"",
"_____no_output_____"
]
],
[
[
"### Step 1: Import neccessary Libraries",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport pandas as pd\nimport matplotlib.pyplot as plt\n\nimport gensim\nimport gensim.corpora as corpora\nfrom gensim.utils import simple_preprocess\nfrom gensim.models import CoherenceModel\n\nimport spacy\nfrom tqdm.notebook import tqdm\n#spacy download en_core_web_sm",
"D:\\Anaconda\\envs\\parixit\\lib\\site-packages\\gensim\\similarities\\__init__.py:15: UserWarning: The gensim.similarities.levenshtein submodule is disabled, because the optional Levenshtein package <https://pypi.org/project/python-Levenshtein/> is unavailable. Install Levenhstein (e.g. `pip install python-Levenshtein`) to suppress this warning.\n warnings.warn(msg)\n"
],
[
"import nltk\nnltk.download('stopwords')\nfrom nltk.corpus import stopwords\nstop_words = stopwords.words('english')",
"[nltk_data] Downloading package stopwords to C:\\Users\\Parikshit\n[nltk_data] Saikia\\AppData\\Roaming\\nltk_data...\n[nltk_data] Package stopwords is already up-to-date!\n"
]
],
[
[
"### Step 2: Load the dataset\nThis dataset contains a set of research articles related to computer science, mathematics, physics and statistics. Each article is tagged into major and minor topics in the form one hot encoding.<br>\nBut for our task (topic modeling) we don't need the tags, we just need the articles text.<br>\nFrom the dataset we will form a list of articles<br>",
"_____no_output_____"
]
],
[
[
"data = pd.read_csv('./data/research_articles/Train.csv/Processed_train.csv')",
"_____no_output_____"
],
[
"data.head()",
"_____no_output_____"
],
[
"articles_data = data.ABSTRACT.values.tolist()",
"_____no_output_____"
]
],
[
[
"### Step 3: Creating a Data Preprocessing Pipeline\n\nThis is the most important step in this entire code . We cannot expect good results from a model trained on a uncleaned data.<br>\nAs the famous quote goes <i>\"garbage in,garbage out\"</i><br>\nWe want our corpus consisting a list of representative words capturing the essence of each article, To achieve that we need to follow a sequence of steps:<br>\n\n<br>",
"_____no_output_____"
]
],
[
[
"def convert_lowercase(string_list):\n \"\"\"\n Convert the list of strings to lowercase and returns the list\n \"\"\"\n pbar = tqdm(total = len(string_list),desc='lowercase conversion progress')\n for i in range(len(string_list)):\n string_list[i] = string_list[i].lower()\n pbar.update(1)\n pbar.close()\n return string_list\n\ndef remove_punctuation(doc_list):\n \"\"\"\n Tokenization and remove punctuation and return the list of tokens\n \"\"\"\n doc_word_list = []\n pbar = tqdm(total = len(doc_list),desc='punctuation removal progress')\n for doc in doc_list:\n doc_word_list.append(gensim.utils.simple_preprocess(str(doc), deacc=True,min_len=4)) # deacc=True removes punctuations\n pbar.update(1)\n pbar.close()\n return doc_word_list\n\ndef remove_stopwords(texts):\n \"\"\"\n Remove common occuring words from the list of tokens\n stop words : a,an ,the ,so ,from ...\n \n \"\"\"\n docs_data=[]\n stop_words = stopwords.words('english')\n pbar = tqdm(total = len(texts),desc='stopword removal progress')\n for doc in texts:\n doc_data=[]\n for word in doc:\n if word not in stop_words:\n doc_data.append(word)\n docs_data.append(doc_data)\n pbar.update(1)\n pbar.close()\n return docs_data\n\ndef filter_word_len(texts,size):\n \"\"\"\n Remove tokens if their length is smaller than threshold\n \n \"\"\"\n docs_data=[]\n pbar = tqdm(total = len(texts),desc='word filtering progress')\n for doc in texts:\n doc_data=[]\n for word in doc:\n if len(word)>=size:\n doc_data.append(word)\n docs_data.append(doc_data)\n pbar.update(1)\n pbar.close()\n return docs_data\n\ndef make_bigrams(texts):\n \"\"\"\n Combine words that are frequently occurs together\n eg: Good Person , Great Content , Ever Growing\n \"\"\"\n # Build the bigram and models\n pbar = tqdm(total = len(texts),desc='Bigram progress')\n \n bigram = gensim.models.Phrases(texts, min_count=5, threshold=100) # higher threshold fewer phrases.\n bigram_mod = gensim.models.phrases.Phraser(bigram)\n \n pbar.update(len(texts))\n pbar.close()\n return [bigram_mod[doc] for doc in texts]\n\n\ndef lemmatization(texts, allowed_postags=['NOUN', 'ADJ', 'VERB', 'ADV']):\n \"\"\"\n Only allows token which are noun,adjective,verb and adverb.\n Also converting the tokens into base form\n \"\"\"\n nlp = spacy.load('en_core_web_sm', disable=['parser', 'ner'])\n texts_out = []\n pbar = tqdm(total = len(texts),desc='lemmatization progress')\n \n for sent in texts:\n doc = nlp(\" \".join(sent)) \n texts_out.append([token.lemma_ for token in doc if token.pos_ in allowed_postags])\n pbar.update(1)\n pbar.close()\n \n return texts_out\n\ndef preprocessing_pipeline(doc_list,size):\n \"\"\"\n Converting each document into list of tokens that represents the document\n \"\"\"\n doc_list = convert_lowercase(doc_list)\n doc_data_words = remove_punctuation(doc_list)\n # Remove Stop Words\n data_words_nostops = remove_stopwords(doc_data_words)\n \n # Form Bigrams\n data_words_bigrams = make_bigrams(data_words_nostops)\n\n data_lemmatized = lemmatization(data_words_bigrams, allowed_postags=['NOUN', 'ADJ', 'VERB', 'ADV'])\n \n final_data_words = filter_word_len(data_lemmatized,size)\n \n return final_data_words\n \ndef corpus_embeddings(data):\n \"\"\"\n Converting each token in each document into a tuple of (unique id, frequency of occuring in that document)\n \"\"\"\n # Create Dictionary\n id2word = corpora.Dictionary(data)\n # Create Corpus\n texts = data\n # Term Document Frequency\n corpus = [id2word.doc2bow(text) for text in texts]\n \n return corpus,id2word\n ",
"_____no_output_____"
],
[
"processed_data = preprocessing_pipeline(articles_data,4) # this will take some time",
"_____no_output_____"
],
[
"processed_data[0]",
"_____no_output_____"
]
],
[
[
"### Step 4: Finalizing the input data\nIn this step we will form our the inputs of model, which are:<br>\n* **Corpus**: A 2D embedded array of tuples, where each tuple is in the form of (token id, frequency of token in that document).\n* **dictionary**: A dictionary storing the mapping from token to id.",
"_____no_output_____"
]
],
[
[
"new_corpus,id_word = corpus_embeddings(processed_data)",
"_____no_output_____"
],
[
"new_corpus",
"_____no_output_____"
],
[
"id_word[0]",
"_____no_output_____"
],
[
"word_freq = [[(id_word[id], freq) for id, freq in cp] for cp in new_corpus[:1]]",
"_____no_output_____"
],
[
"word_freq # A more human reable form of our corpus",
"_____no_output_____"
]
],
[
[
"### Step 5: Modeling and Evaluation\nIn this part we will fit our data to our the LDA model , some hyper parameter tuning , evaluate the results and select the optimal setting for our model.",
"_____no_output_____"
]
],
[
[
"class LDA_model:\n \"\"\"\n A LDA Class consist functions to fit the model, calculating coherence values\n and finding the optimal no. of topic\n \n input: \n corpus : a 2D array of embedded tokens\n dictionary: A dictionary with id to token mapping\n \n \"\"\"\n def __init__(self, corpus,dictionary):\n self.corpus = corpus\n self.dictionary = dictionary\n \n def LDA(self,no_topics, a ='auto', b = 'auto',passes=10):\n lda_model = gensim.models.ldamodel.LdaModel(corpus=self.corpus, id2word=self.dictionary,\n num_topics=no_topics, random_state=100,chunksize=100,passes=passes,alpha=a,eta=b)\n return lda_model\n\n def compute_coherence_values(self,processed_data,no_topics,a ='auto', b = 'auto',passes=10):\n \"\"\"\n Computes the coherence value of a fitted LDA model\n \"\"\"\n \n pbar = tqdm(total = len(self.corpus),desc='LDA with '+str(no_topics)+' topics')\n \n lda_model = self.LDA(no_topics,a,b,passes)\n pbar.update(len(self.corpus)/2)\n coherence_model_lda = CoherenceModel(model=lda_model, texts=processed_data, dictionary=self.dictionary, coherence='c_v')\n pbar.update(len(self.corpus) -(len(self.corpus)/2))\n pbar.close()\n\n return coherence_model_lda.get_coherence()\n\n def compute_optimal_topics(self,processed_data,min_topics,max_topics,step_size,path):\n \"\"\"\n Calucates the coherence value for a given range of topic size and forms a dataset\n \"\"\"\n \n topics_range = list(np.arange(min_topics,max_topics,step_size))\n model_results = {'Topics': [],'Coherence': []}\n # Can take a long time to run\n for i in range(len(topics_range)):\n # get the coherence score for the given parameters\n cv = self.compute_coherence_values(processed_data,topics_range[i],a ='auto', b = 'auto',passes=10)\n # Save the model results\n model_results['Topics'].append(topics_range[i])\n model_results['Coherence'].append(cv)\n pd.DataFrame(model_results).to_csv(path, index=False)\n \n def Optimal_no_topics(self,path):\n \"\"\"\n finds the topic size with max coherence score\n \"\"\"\n coherence_data = pd.read_csv(path)\n x = coherence_data.Topics.tolist()\n y = coherence_data.Coherence.tolist()\n plt.xlabel('No. of topics')\n plt.ylabel('Coherence Score')\n plt.plot(x,y)\n plt.show()\n index = np.argmax(y)\n no_topics = x[index]\n c_v = y[index]\n print('Optimal number of topics: '+str(no_topics)+' coherence score: '+str(c_v))\n \n return no_topics,c_v\n \n def Optimal_lda_model(self,path):\n \"\"\"\n Fits the LDA with optimal topic size\n \"\"\"\n no_topic, c_v = self.Optimal_no_topics(path)\n pbar = tqdm(total = len(self.corpus),desc='LDA with '+str(no_topic)+' topics')\n lda = self.LDA(no_topic)\n pbar.update(len(self.corpus))\n pbar.close()\n \n return lda\n ",
"_____no_output_____"
],
[
"lda_model = LDA_model(new_corpus,id_word)",
"_____no_output_____"
],
[
"path = './dat.csv'",
"_____no_output_____"
]
],
[
[
"**Note**: Computing the optimal number of topics for LDA will take a pretty long amount of time<br>\nThe time complexity of this process : **O( m * x * p)**<br>\nwhere:<br>\n* m = no. of tokens in the corpus\n* x = summation of no. of topics given in the range\n* p = no. of passes for LDA model",
"_____no_output_____"
]
],
[
[
"lda_model.compute_optimal_topics(processed_data,2,4,1,path)",
"_____no_output_____"
],
[
"lda = lda_model.Optimal_lda_model('./results/lda_tuning_results1.csv')",
"_____no_output_____"
],
[
"lda.print_topics()",
"_____no_output_____"
]
],
[
[
"### Step 6: Visualization",
"_____no_output_____"
]
],
[
[
"import pyLDAvis\nimport pyLDAvis.gensim_models as gensimvis\npyLDAvis.enable_notebook()",
"_____no_output_____"
],
[
"vis = gensimvis.prepare(lda, new_corpus, id_word)\nvis",
"_____no_output_____"
]
],
[
[
"### Goals\n\n- [x] Fitting a LDA model.\n- [x] Finding a the optimal no. topics with best coherence score.\n- [x] Returning the LDA model with optimal topics.\n- [x] Returning the optimal no. of topics.\n- [x] Returning the topics itself.\n",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
]
] |
e7b9b6c99746290babbf4a1076ac3a6d730e2170 | 574,473 | ipynb | Jupyter Notebook | monte-carlo/Monte_Carlo.ipynb | romOlivo/NanodegreeExercises | 72a29d04b44ff845fa2f80e279db0f9997130a51 | [
"MIT"
] | null | null | null | monte-carlo/Monte_Carlo.ipynb | romOlivo/NanodegreeExercises | 72a29d04b44ff845fa2f80e279db0f9997130a51 | [
"MIT"
] | 4 | 2020-09-26T00:53:03.000Z | 2022-02-10T01:05:05.000Z | monte-carlo/Monte_Carlo.ipynb | romOlivo/NanodegreeExercises | 72a29d04b44ff845fa2f80e279db0f9997130a51 | [
"MIT"
] | null | null | null | 995.620451 | 274,256 | 0.951937 | [
[
[
"# Monte Carlo Methods\n\nIn this notebook, you will write your own implementations of many Monte Carlo (MC) algorithms. \n\nWhile we have provided some starter code, you are welcome to erase these hints and write your code from scratch.\n\n### Part 0: Explore BlackjackEnv\n\nWe begin by importing the necessary packages.",
"_____no_output_____"
]
],
[
[
"import sys\nimport gym\nimport numpy as np\nfrom collections import defaultdict\n\nfrom plot_utils import plot_blackjack_values, plot_policy",
"_____no_output_____"
]
],
[
[
"Use the code cell below to create an instance of the [Blackjack](https://github.com/openai/gym/blob/master/gym/envs/toy_text/blackjack.py) environment.",
"_____no_output_____"
]
],
[
[
"env = gym.make('Blackjack-v0')",
"_____no_output_____"
]
],
[
[
"Each state is a 3-tuple of:\n- the player's current sum $\\in \\{0, 1, \\ldots, 31\\}$,\n- the dealer's face up card $\\in \\{1, \\ldots, 10\\}$, and\n- whether or not the player has a usable ace (`no` $=0$, `yes` $=1$).\n\nThe agent has two potential actions:\n\n```\n STICK = 0\n HIT = 1\n```\nVerify this by running the code cell below.",
"_____no_output_____"
]
],
[
[
"print(env.observation_space)\nprint(env.action_space)",
"Tuple(Discrete(32), Discrete(11), Discrete(2))\nDiscrete(2)\n"
]
],
[
[
"Execute the code cell below to play Blackjack with a random policy. \n\n(_The code currently plays Blackjack three times - feel free to change this number, or to run the cell multiple times. The cell is designed for you to get some experience with the output that is returned as the agent interacts with the environment._)",
"_____no_output_____"
]
],
[
[
"for i_episode in range(10):\n state = env.reset()\n while True:\n print(state)\n action = env.action_space.sample()\n state, reward, done, info = env.step(action)\n if done:\n print('End game! Reward: ', reward)\n print('You won :)\\n') if reward > 0 else print('You lost :(\\n')\n break",
"(12, 10, False)\nEnd game! Reward: -1.0\nYou lost :(\n\n(15, 9, False)\n(20, 9, False)\nEnd game! Reward: 1.0\nYou won :)\n\n(20, 5, False)\nEnd game! Reward: -1\nYou lost :(\n\n(8, 4, False)\n(19, 4, True)\n(14, 4, False)\nEnd game! Reward: 1.0\nYou won :)\n\n(19, 9, False)\n(20, 9, False)\nEnd game! Reward: 1.0\nYou won :)\n\n(14, 6, False)\nEnd game! Reward: -1.0\nYou lost :(\n\n(18, 5, False)\nEnd game! Reward: -1\nYou lost :(\n\n(11, 7, False)\n(21, 7, False)\nEnd game! Reward: 1.0\nYou won :)\n\n(5, 5, False)\nEnd game! Reward: 1.0\nYou won :)\n\n(15, 9, False)\nEnd game! Reward: -1.0\nYou lost :(\n\n"
]
],
[
[
"### Part 1: MC Prediction\n\nIn this section, you will write your own implementation of MC prediction (for estimating the action-value function). \n\nWe will begin by investigating a policy where the player _almost_ always sticks if the sum of her cards exceeds 18. In particular, she selects action `STICK` with 80% probability if the sum is greater than 18; and, if the sum is 18 or below, she selects action `HIT` with 80% probability. The function `generate_episode_from_limit_stochastic` samples an episode using this policy. \n\nThe function accepts as **input**:\n- `bj_env`: This is an instance of OpenAI Gym's Blackjack environment.\n\nIt returns as **output**:\n- `episode`: This is a list of (state, action, reward) tuples (of tuples) and corresponds to $(S_0, A_0, R_1, \\ldots, S_{T-1}, A_{T-1}, R_{T})$, where $T$ is the final time step. In particular, `episode[i]` returns $(S_i, A_i, R_{i+1})$, and `episode[i][0]`, `episode[i][1]`, and `episode[i][2]` return $S_i$, $A_i$, and $R_{i+1}$, respectively.",
"_____no_output_____"
]
],
[
[
"def generate_episode_from_limit_stochastic(bj_env):\n episode = []\n state = bj_env.reset()\n while True:\n probs = [0.8, 0.2] if state[0] > 18 else [0.2, 0.8]\n action = np.random.choice(np.arange(2), p=probs)\n next_state, reward, done, info = bj_env.step(action)\n episode.append((state, action, reward))\n state = next_state\n if done:\n break\n return episode",
"_____no_output_____"
]
],
[
[
"Execute the code cell below to play Blackjack with the policy. \n\n(*The code currently plays Blackjack three times - feel free to change this number, or to run the cell multiple times. The cell is designed for you to gain some familiarity with the output of the `generate_episode_from_limit_stochastic` function.*)",
"_____no_output_____"
]
],
[
[
"for i in range(10):\n print(generate_episode_from_limit_stochastic(env))",
"[((15, 3, False), 1, -1)]\n[((13, 9, False), 1, -1)]\n[((15, 9, False), 1, 0), ((18, 9, False), 1, -1)]\n[((18, 5, True), 1, 0), ((12, 5, False), 1, 0), ((21, 5, False), 0, 1.0)]\n[((21, 3, True), 0, 1.0)]\n[((11, 4, False), 1, 0), ((18, 4, False), 1, 0), ((21, 4, False), 0, 1.0)]\n[((9, 3, False), 1, 0), ((12, 3, False), 0, 1.0)]\n[((14, 8, False), 0, 1.0)]\n[((8, 2, False), 1, 0), ((19, 2, True), 0, 1.0)]\n[((19, 6, False), 0, -1.0)]\n"
]
],
[
[
"Now, you are ready to write your own implementation of MC prediction. Feel free to implement either first-visit or every-visit MC prediction; in the case of the Blackjack environment, the techniques are equivalent.\n\nYour algorithm has three arguments:\n- `env`: This is an instance of an OpenAI Gym environment.\n- `num_episodes`: This is the number of episodes that are generated through agent-environment interaction.\n- `generate_episode`: This is a function that returns an episode of interaction.\n- `gamma`: This is the discount rate. It must be a value between 0 and 1, inclusive (default value: `1`).\n\nThe algorithm returns as output:\n- `Q`: This is a dictionary (of one-dimensional arrays) where `Q[s][a]` is the estimated action value corresponding to state `s` and action `a`.",
"_____no_output_____"
]
],
[
[
"def mc_prediction_q(env, num_episodes, generate_episode, gamma=1.0):\n # initialize empty dictionaries of arrays\n returns_sum = defaultdict(lambda: np.zeros(env.action_space.n))\n N = defaultdict(lambda: np.zeros(env.action_space.n))\n Q = defaultdict(lambda: np.zeros(env.action_space.n))\n # loop over episodes\n for i_episode in range(1, num_episodes+1):\n # monitor progress\n if i_episode % 1000 == 0:\n print(\"\\rEpisode {}/{}.\".format(i_episode, num_episodes), end=\"\")\n sys.stdout.flush()\n visited_states = set()\n episode = generate_episode(env)\n for i, (state, action, reward) in enumerate(episode):\n total_reward = reward\n for j in range(len(episode) - i - 1):\n total_reward += episode[j+i+1][2] * gamma ** j\n if state not in visited_states:\n if state not in returns_sum:\n returns_sum[state] = [0, 0]\n returns_sum[state][action] += total_reward\n if state not in N:\n N[state] = [0, 0]\n N[state][action] += 1\n visited_states.add(state)\n Q[state][action] = returns_sum[state][action] / N[state][action]\n return Q",
"_____no_output_____"
],
[
"Q",
"_____no_output_____"
]
],
[
[
"Use the cell below to obtain the action-value function estimate $Q$. We have also plotted the corresponding state-value function.\n\nTo check the accuracy of your implementation, compare the plot below to the corresponding plot in the solutions notebook **Monte_Carlo_Solution.ipynb**.",
"_____no_output_____"
]
],
[
[
"# obtain the action-value function\nQ = mc_prediction_q(env, 500000, generate_episode_from_limit_stochastic)\n\n# obtain the corresponding state-value function\nV_to_plot = dict((k,(k[0]>18)*(np.dot([0.8, 0.2],v)) + (k[0]<=18)*(np.dot([0.2, 0.8],v))) \\\n for k, v in Q.items())\n\n# plot the state-value function\nplot_blackjack_values(V_to_plot)",
"Episode 500000/500000."
]
],
[
[
"### Part 2: MC Control\n\nIn this section, you will write your own implementation of constant-$\\alpha$ MC control. \n\nYour algorithm has four arguments:\n- `env`: This is an instance of an OpenAI Gym environment.\n- `num_episodes`: This is the number of episodes that are generated through agent-environment interaction.\n- `alpha`: This is the step-size parameter for the update step.\n- `gamma`: This is the discount rate. It must be a value between 0 and 1, inclusive (default value: `1`).\n\nThe algorithm returns as output:\n- `Q`: This is a dictionary (of one-dimensional arrays) where `Q[s][a]` is the estimated action value corresponding to state `s` and action `a`.\n- `policy`: This is a dictionary where `policy[s]` returns the action that the agent chooses after observing state `s`.\n\n(_Feel free to define additional functions to help you to organize your code._)",
"_____no_output_____"
]
],
[
[
"def generate_policy(q_table):\n policy = {}\n for state in q_table.keys():\n policy[state] = np.argmax(q_table[state])\n return policy",
"_____no_output_____"
],
[
"def take_action(state, policy, epsilon):\n probs = [0.8, 0.2] if state[0] > 18 else [0.2, 0.8]\n action = np.random.choice(np.arange(2), p=probs)\n if (state in policy) and np.random.random() > epsilon:\n action = policy[state]\n return action",
"_____no_output_____"
],
[
"def generate_episode(env, policy, epsilon):\n episode = []\n state = env.reset()\n while True:\n action = take_action(state, policy, epsilon)\n next_state, reward, done, info = env.step(action)\n episode.append((state, action, reward))\n state = next_state\n if done:\n break\n return episode",
"_____no_output_____"
],
[
"def mc_control(env, num_episodes, alpha, gamma=1.0, epsilon_min = 0.05):\n nA = env.action_space.n\n # initialize empty dictionary of arrays\n Q = defaultdict(lambda: np.zeros(nA))\n # loop over episodes\n for i_episode in range(1, num_episodes+1):\n # monitor progress\n if i_episode % 1000 == 0:\n print(\"\\rEpisode {}/{}.\".format(i_episode, num_episodes), end=\"\")\n sys.stdout.flush()\n ## TODO: complete the function\n epsilon = max(1/i_episode, epsilon_min)\n policy = generate_policy(Q)\n episode = generate_episode(env, policy, epsilon)\n visited_states = set()\n for i, (state, action, reward) in enumerate(episode):\n total_reward = reward\n for j in range(len(episode) - i - 1):\n total_reward += episode[j+i+1][2] * gamma ** j\n if state not in visited_states:\n visited_states.add(state)\n Q[state][action] += alpha * (total_reward - Q[state][action])\n policy = generate_policy(Q)\n return policy, Q",
"_____no_output_____"
]
],
[
[
"Use the cell below to obtain the estimated optimal policy and action-value function. Note that you should fill in your own values for the `num_episodes` and `alpha` parameters.",
"_____no_output_____"
]
],
[
[
"# obtain the estimated optimal policy and action-value function\npolicy, Q = mc_control(env, 1000000, 0.01)",
"Episode 1000000/1000000."
]
],
[
[
"Next, we plot the corresponding state-value function.",
"_____no_output_____"
]
],
[
[
"# obtain the corresponding state-value function\nV = dict((k,np.max(v)) for k, v in Q.items())\n\n# plot the state-value function\nplot_blackjack_values(V)",
"_____no_output_____"
]
],
[
[
"Finally, we visualize the policy that is estimated to be optimal.",
"_____no_output_____"
]
],
[
[
"# plot the policy\nplot_policy(policy)",
"_____no_output_____"
]
],
[
[
"The **true** optimal policy $\\pi_*$ can be found in Figure 5.2 of the [textbook](http://go.udacity.com/rl-textbook) (and appears below). Compare your final estimate to the optimal policy - how close are you able to get? If you are not happy with the performance of your algorithm, take the time to tweak the decay rate of $\\epsilon$, change the value of $\\alpha$, and/or run the algorithm for more episodes to attain better results.\n\n",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
]
] |
e7b9ba299a9fc7132653c4c03224b90588007c74 | 4,871 | ipynb | Jupyter Notebook | Keras_Helpers.ipynb | davidje13/MachineLearningNotebooks | 91b95466395521ad7602288c7c99a889bb736755 | [
"CC0-1.0"
] | null | null | null | Keras_Helpers.ipynb | davidje13/MachineLearningNotebooks | 91b95466395521ad7602288c7c99a889bb736755 | [
"CC0-1.0"
] | null | null | null | Keras_Helpers.ipynb | davidje13/MachineLearningNotebooks | 91b95466395521ad7602288c7c99a889bb736755 | [
"CC0-1.0"
] | null | null | null | 30.63522 | 108 | 0.523096 | [
[
[
"%run Training_Helpers.ipynb\n\nimport tensorflow as tf\nimport matplotlib.pyplot as plt\n%matplotlib inline",
"_____no_output_____"
],
[
"def set_plot_size_cm(fig, w, h):\n fig.set_size_inches(w / 2.54, h / 2.54)\n\ndef plot_training_metric(plot, trainingResult, metric, label):\n stepCount = trainingResult.train_accuracy.shape[0]\n steps = range(0, stepCount)\n\n plot.set_ylabel(label)\n plot.set_xlabel('Step')\n plot.plot(steps, trainingResult.train_accuracy[metric])\n plot.plot(steps, trainingResult.test_accuracy[metric])\n plot.set_xlim([0, stepCount])\n\ndef plot_training_metrics(trainingResult):\n fig, (pltLoss, pltAcc) = plt.subplots(1, 2)\n set_plot_size_cm(fig, 30, 5)\n\n plot_training_metric(pltLoss, trainingResult, 'loss', 'Loss')\n pltLoss.set_yscale('log')\n plot_training_metric(pltAcc, trainingResult, 'acc', 'Accuracy')\n pltAcc.set_ylim([0, 1])\n plt.show()",
"_____no_output_____"
],
[
"def train_neural_network(\n make_model_fn,\n train_data,\n test_data,\n input_fn,\n output_fn,\n\n max_epochs = 50000,\n check_epochs = 10,\n improvement_ratio_required = 1e-3,\n consecutive_passes_required = 100,\n\n plot_metrics = True,\n):\n train_in = input_fn(train_data)\n train_out = output_fn(train_data)\n test_in = input_fn(test_data)\n test_out = output_fn(test_data)\n\n model = make_model_fn(train_in, train_out)\n\n train_accuracy = []\n test_accuracy = []\n old_train_loss = 1e10\n checks = 0\n for epoch in range(0, max_epochs):\n train_result = model.train_on_batch(train_in, train_out)\n train_accuracy.append(train_result)\n test_accuracy.append(model.evaluate(test_in, test_out, verbose = 0))\n if epoch % check_epochs == 0:\n train_loss = train_result[0]\n if train_loss > old_train_loss:\n # got worse; do not advance or reset checks\n continue\n if old_train_loss / train_loss - 1 > improvement_ratio_required:\n # improved significantly; reset checks and update reference\n old_train_loss = train_loss\n checks = 0\n continue\n checks += 1\n if checks >= consecutive_passes_required:\n break\n\n train_accuracy = pd.DataFrame(columns=model.metrics_names, data=train_accuracy)\n test_accuracy = pd.DataFrame(columns=model.metrics_names, data=test_accuracy)\n\n print(' train: loss = {:.8} accuracy = {:.4} test: loss = {:.8} accuracy = {:.4}'.format(\n train_accuracy.iloc[-1][0],\n train_accuracy.iloc[-1]['acc'],\n test_accuracy.iloc[-1][0],\n test_accuracy.iloc[-1]['acc'],\n ))\n\n result = TrainingResult(model, train_accuracy, test_accuracy)\n if plot_metrics:\n plot_training_metrics(result)\n return result",
"_____no_output_____"
],
[
"def train_neural_networks(\n make_model_fn,\n train_data,\n test_data,\n input_fns,\n output_fn,\n **trainArgs\n):\n return train_models(\n train_neural_network,\n make_model_fn,\n train_data,\n test_data,\n input_fns,\n output_fn,\n **trainArgs\n )",
"_____no_output_____"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code"
]
] |
e7b9c14e495f3195d04c75d658aa5d7e97060c5d | 1,002,981 | ipynb | Jupyter Notebook | YOLOv4_Darknet_Roboflow1.ipynb | qwerlarlgus/Darknet | cd7b1d250e7aaef04b9dc3bd97222640c5dff8bb | [
"MIT"
] | null | null | null | YOLOv4_Darknet_Roboflow1.ipynb | qwerlarlgus/Darknet | cd7b1d250e7aaef04b9dc3bd97222640c5dff8bb | [
"MIT"
] | null | null | null | YOLOv4_Darknet_Roboflow1.ipynb | qwerlarlgus/Darknet | cd7b1d250e7aaef04b9dc3bd97222640c5dff8bb | [
"MIT"
] | null | null | null | 160.169435 | 511,050 | 0.811822 | [
[
[
"<a href=\"https://colab.research.google.com/github/qwerlarlgus/Darknet/blob/main/YOLOv4_Darknet_Roboflow1.ipynb\" target=\"_parent\"><img src=\"https://colab.research.google.com/assets/colab-badge.svg\" alt=\"Open In Colab\"/></a>",
"_____no_output_____"
],
[
"# Introduction\n\n\nIn this notebook, we implement [YOLOv4](https://arxiv.org/pdf/2004.10934.pdf) for training on your own dataset.\n\nWe also recommend reading our blog post on [Training YOLOv4 on custom data](https://blog.roboflow.ai/training-yolov4-on-a-custom-dataset/) side by side.\n\nWe will take the following steps to implement YOLOv4 on our custom data:\n* Configure our GPU environment on Google Colab\n* Install the Darknet YOLOv4 training environment\n* Download our custom dataset for YOLOv4 and set up directories\n* Configure a custom YOLOv4 training config file for Darknet\n* Train our custom YOLOv4 object detector\n* Reload YOLOv4 trained weights and make inference on test images\n\nWhen you are done you will have a custom detector that you can use. It will make inference like this:\n\n###\n\n### **Reach out for support**\n\nIf you run into any hurdles on your own data set or just want to share some cool results in your own domain, [reach out!](https://roboflow.ai) \n\n\n\n###",
"_____no_output_____"
],
[
"# Configuring cuDNN on Colab for YOLOv4\n\n",
"_____no_output_____"
]
],
[
[
"# CUDA: Let's check that Nvidia CUDA drivers are already pre-installed and which version is it.\n!/usr/local/cuda/bin/nvcc --version\n# We need to install the correct cuDNN according to this output",
"nvcc: NVIDIA (R) Cuda compiler driver\nCopyright (c) 2005-2019 NVIDIA Corporation\nBuilt on Sun_Jul_28_19:07:16_PDT_2019\nCuda compilation tools, release 10.1, V10.1.243\n"
],
[
"!nvidia-smi",
"Mon Feb 1 02:24:22 2021 \n+-----------------------------------------------------------------------------+\n| NVIDIA-SMI 460.32.03 Driver Version: 418.67 CUDA Version: 10.1 |\n|-------------------------------+----------------------+----------------------+\n| GPU Name Persistence-M| Bus-Id Disp.A | Volatile Uncorr. ECC |\n| Fan Temp Perf Pwr:Usage/Cap| Memory-Usage | GPU-Util Compute M. |\n| | | MIG M. |\n|===============================+======================+======================|\n| 0 Tesla T4 Off | 00000000:00:04.0 Off | 0 |\n| N/A 51C P8 10W / 70W | 0MiB / 15079MiB | 0% Default |\n| | | ERR! |\n+-------------------------------+----------------------+----------------------+\n \n+-----------------------------------------------------------------------------+\n| Processes: |\n| GPU GI CI PID Type Process name GPU Memory |\n| ID ID Usage |\n|=============================================================================|\n| No running processes found |\n+-----------------------------------------------------------------------------+\n"
],
[
"# Change the number depending on what GPU is listed above, under NVIDIA-SMI > Name.\n# Tesla K80: 30\n# Tesla P100: 60\n# Tesla T4: 75\n%env compute_capability=75",
"env: compute_capability=75\n"
]
],
[
[
"## STEP 1. Install cuDNN according to the current CUDA version\nColab added cuDNN as an inherent install - so you don't have to do a thing - major win\n\n\n",
"_____no_output_____"
],
[
"# Step 2: Installing Darknet for YOLOv4 on Colab\n\n\n",
"_____no_output_____"
]
],
[
[
"%cd /content/\n%rm -rf darknet",
"/content\n"
],
[
"#we clone the fork of darknet maintained by roboflow\n#small changes have been made to configure darknet for training\n!git clone https://github.com/roboflow-ai/darknet.git",
"Cloning into 'darknet'...\nremote: Enumerating objects: 13289, done.\u001b[K\nremote: Total 13289 (delta 0), reused 0 (delta 0), pack-reused 13289\u001b[K\nReceiving objects: 100% (13289/13289), 12.13 MiB | 23.92 MiB/s, done.\nResolving deltas: 100% (9106/9106), done.\n"
],
[
"%cd /content/darknet/\n%rm Makefile",
"/content/darknet\n"
],
[
"#colab occasionally shifts dependencies around, at the time of authorship, this Makefile works for building Darknet on Colab\n\n%%writefile Makefile\nGPU=1\nCUDNN=1\nCUDNN_HALF=0\nOPENCV=1\nAVX=0\nOPENMP=0\nLIBSO=1\nZED_CAMERA=0\nZED_CAMERA_v2_8=0\n\n# set GPU=1 and CUDNN=1 to speedup on GPU\n# set CUDNN_HALF=1 to further speedup 3 x times (Mixed-precision on Tensor Cores) GPU: Volta, Xavier, Turing and higher\n# set AVX=1 and OPENMP=1 to speedup on CPU (if error occurs then set AVX=0)\n# set ZED_CAMERA=1 to enable ZED SDK 3.0 and above\n# set ZED_CAMERA_v2_8=1 to enable ZED SDK 2.X\n\nUSE_CPP=0\nDEBUG=0\n\nARCH= -gencode arch=compute_30,code=sm_30 \\\n -gencode arch=compute_35,code=sm_35 \\\n -gencode arch=compute_50,code=[sm_50,compute_50] \\\n -gencode arch=compute_52,code=[sm_52,compute_52] \\\n\t -gencode arch=compute_61,code=[sm_61,compute_61]\n\nOS := $(shell uname)\n\n# Tesla V100\n# ARCH= -gencode arch=compute_70,code=[sm_70,compute_70]\n\n# GeForce RTX 2080 Ti, RTX 2080, RTX 2070, Quadro RTX 8000, Quadro RTX 6000, Quadro RTX 5000, Tesla T4, XNOR Tensor Cores\n# ARCH= -gencode arch=compute_75,code=[sm_75,compute_75]\n\n# Jetson XAVIER\n# ARCH= -gencode arch=compute_72,code=[sm_72,compute_72]\n\n# GTX 1080, GTX 1070, GTX 1060, GTX 1050, GTX 1030, Titan Xp, Tesla P40, Tesla P4\n# ARCH= -gencode arch=compute_61,code=sm_61 -gencode arch=compute_61,code=compute_61\n\n# GP100/Tesla P100 - DGX-1\n# ARCH= -gencode arch=compute_60,code=sm_60\n\n# For Jetson TX1, Tegra X1, DRIVE CX, DRIVE PX - uncomment:\n# ARCH= -gencode arch=compute_53,code=[sm_53,compute_53]\n\n# For Jetson Tx2 or Drive-PX2 uncomment:\n# ARCH= -gencode arch=compute_62,code=[sm_62,compute_62]\n\n\nVPATH=./src/\nEXEC=darknet\nOBJDIR=./obj/\n\nifeq ($(LIBSO), 1)\nLIBNAMESO=libdarknet.so\nAPPNAMESO=uselib\nendif\n\nifeq ($(USE_CPP), 1)\nCC=g++\nelse\nCC=gcc\nendif\n\nCPP=g++ -std=c++11\nNVCC=nvcc\nOPTS=-Ofast\nLDFLAGS= -lm -pthread\nCOMMON= -Iinclude/ -I3rdparty/stb/include\nCFLAGS=-Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC\n\nifeq ($(DEBUG), 1)\n#OPTS= -O0 -g\n#OPTS= -Og -g\nCOMMON+= -DDEBUG\nCFLAGS+= -DDEBUG\nelse\nifeq ($(AVX), 1)\nCFLAGS+= -ffp-contract=fast -mavx -mavx2 -msse3 -msse4.1 -msse4.2 -msse4a\nendif\nendif\n\nCFLAGS+=$(OPTS)\n\nifneq (,$(findstring MSYS_NT,$(OS)))\nLDFLAGS+=-lws2_32\nendif\n\nifeq ($(OPENCV), 1)\nCOMMON+= -DOPENCV\nCFLAGS+= -DOPENCV\nLDFLAGS+= `pkg-config --libs opencv4 2> /dev/null || pkg-config --libs opencv`\nCOMMON+= `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv`\nendif\n\nifeq ($(OPENMP), 1)\nCFLAGS+= -fopenmp\nLDFLAGS+= -lgomp\nendif\n\nifeq ($(GPU), 1)\nCOMMON+= -DGPU -I/usr/local/cuda/include/\nCFLAGS+= -DGPU\nifeq ($(OS),Darwin) #MAC\nLDFLAGS+= -L/usr/local/cuda/lib -lcuda -lcudart -lcublas -lcurand\nelse\nLDFLAGS+= -L/usr/local/cuda/lib64 -lcuda -lcudart -lcublas -lcurand\nendif\nendif\n\nifeq ($(CUDNN), 1)\nCOMMON+= -DCUDNN\nifeq ($(OS),Darwin) #MAC\nCFLAGS+= -DCUDNN -I/usr/local/cuda/include\nLDFLAGS+= -L/usr/local/cuda/lib -lcudnn\nelse\nCFLAGS+= -DCUDNN -I/usr/local/cudnn/include\nLDFLAGS+= -L/usr/local/cudnn/lib64 -lcudnn\nendif\nendif\n\nifeq ($(CUDNN_HALF), 1)\nCOMMON+= -DCUDNN_HALF\nCFLAGS+= -DCUDNN_HALF\nARCH+= -gencode arch=compute_70,code=[sm_70,compute_70]\nendif\n\nifeq ($(ZED_CAMERA), 1)\nCFLAGS+= -DZED_STEREO -I/usr/local/zed/include\nifeq ($(ZED_CAMERA_v2_8), 1)\nLDFLAGS+= -L/usr/local/zed/lib -lsl_core -lsl_input -lsl_zed\n#-lstdc++ -D_GLIBCXX_USE_CXX11_ABI=0\nelse\nLDFLAGS+= -L/usr/local/zed/lib -lsl_zed\n#-lstdc++ -D_GLIBCXX_USE_CXX11_ABI=0\nendif\nendif\n\nOBJ=image_opencv.o http_stream.o gemm.o utils.o dark_cuda.o convolutional_layer.o list.o image.o activations.o im2col.o col2im.o blas.o crop_layer.o dropout_layer.o maxpool_layer.o softmax_layer.o data.o matrix.o network.o connected_layer.o cost_layer.o parser.o option_list.o darknet.o detection_layer.o captcha.o route_layer.o writing.o box.o nightmare.o normalization_layer.o avgpool_layer.o coco.o dice.o yolo.o detector.o layer.o compare.o classifier.o local_layer.o swag.o shortcut_layer.o activation_layer.o rnn_layer.o gru_layer.o rnn.o rnn_vid.o crnn_layer.o demo.o tag.o cifar.o go.o batchnorm_layer.o art.o region_layer.o reorg_layer.o reorg_old_layer.o super.o voxel.o tree.o yolo_layer.o gaussian_yolo_layer.o upsample_layer.o lstm_layer.o conv_lstm_layer.o scale_channels_layer.o sam_layer.o\nifeq ($(GPU), 1)\nLDFLAGS+= -lstdc++\nOBJ+=convolutional_kernels.o activation_kernels.o im2col_kernels.o col2im_kernels.o blas_kernels.o crop_layer_kernels.o dropout_layer_kernels.o maxpool_layer_kernels.o network_kernels.o avgpool_layer_kernels.o\nendif\n\nOBJS = $(addprefix $(OBJDIR), $(OBJ))\nDEPS = $(wildcard src/*.h) Makefile include/darknet.h\n\nall: $(OBJDIR) backup results setchmod $(EXEC) $(LIBNAMESO) $(APPNAMESO)\n\nifeq ($(LIBSO), 1)\nCFLAGS+= -fPIC\n\n$(LIBNAMESO): $(OBJDIR) $(OBJS) include/yolo_v2_class.hpp src/yolo_v2_class.cpp\n\t$(CPP) -shared -std=c++11 -fvisibility=hidden -DLIB_EXPORTS $(COMMON) $(CFLAGS) $(OBJS) src/yolo_v2_class.cpp -o $@ $(LDFLAGS)\n\n$(APPNAMESO): $(LIBNAMESO) include/yolo_v2_class.hpp src/yolo_console_dll.cpp\n\t$(CPP) -std=c++11 $(COMMON) $(CFLAGS) -o $@ src/yolo_console_dll.cpp $(LDFLAGS) -L ./ -l:$(LIBNAMESO)\nendif\n\n$(EXEC): $(OBJS)\n\t$(CPP) -std=c++11 $(COMMON) $(CFLAGS) $^ -o $@ $(LDFLAGS)\n\n$(OBJDIR)%.o: %.c $(DEPS)\n\t$(CC) $(COMMON) $(CFLAGS) -c $< -o $@\n\n$(OBJDIR)%.o: %.cpp $(DEPS)\n\t$(CPP) -std=c++11 $(COMMON) $(CFLAGS) -c $< -o $@\n\n$(OBJDIR)%.o: %.cu $(DEPS)\n\t$(NVCC) $(ARCH) $(COMMON) --compiler-options \"$(CFLAGS)\" -c $< -o $@\n\n$(OBJDIR):\n\tmkdir -p $(OBJDIR)\nbackup:\n\tmkdir -p backup\nresults:\n\tmkdir -p results\nsetchmod:\n\tchmod +x *.sh\n\n.PHONY: clean\n\nclean:\n\trm -rf $(OBJS) $(EXEC) $(LIBNAMESO) $(APPNAMESO)",
"Writing Makefile\n"
],
[
"#install environment from the Makefile\n#note if you are on Colab Pro this works on a P100 GPU\n#if you are on Colab free, you may need to change the Makefile for the K80 GPU\n#this goes for any GPU, you need to change the Makefile to inform darknet which GPU you are running on.\n#note the Makefile above should work for you, if you need to tweak, try the below\n%cd darknet/\n#!sed -i 's/OPENCV=0/OPENCV=1/g' Makefile\n#!sed -i 's/GPU=0/GPU=1/g' Makefile\n#!sed -i 's/CUDNN=0/CUDNN=1/g' Makefile\n!#sed -i \"s/ARCH= -gencode arch=compute_60,code=sm_60/ARCH= -gencode arch=compute_${compute_capability},code=sm_${compute_capability}/g\" Makefile\n!make",
"[Errno 2] No such file or directory: 'darknet/'\n/content/darknet\nmkdir -p ./obj/\nmkdir -p backup\nchmod +x *.sh\ng++ -std=c++11 -std=c++11 -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/image_opencv.cpp -o obj/image_opencv.o\n\u001b[01m\u001b[K./src/image_opencv.cpp:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kvoid draw_detections_cv_v3(void**, detection*, int, float, char**, image**, int, int)\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/image_opencv.cpp:910:23:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kvariable ‘\u001b[01m\u001b[Krgb\u001b[m\u001b[K’ set but not used [\u001b[01;35m\u001b[K-Wunused-but-set-variable\u001b[m\u001b[K]\n float \u001b[01;35m\u001b[Krgb\u001b[m\u001b[K[3];\n \u001b[01;35m\u001b[K^~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/image_opencv.cpp:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kvoid cv_draw_object(image, float*, int, int, int*, float*, int*, int, char**)\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/image_opencv.cpp:1391:14:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kbuff\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n char \u001b[01;35m\u001b[Kbuff\u001b[m\u001b[K[100];\n \u001b[01;35m\u001b[K^~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/image_opencv.cpp:1367:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kit_tb_res\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kit_tb_res\u001b[m\u001b[K = cv::createTrackbar(it_trackbar_name, window_name, &it_trackbar_value, 1000);\n \u001b[01;35m\u001b[K^~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/image_opencv.cpp:1371:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Klr_tb_res\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Klr_tb_res\u001b[m\u001b[K = cv::createTrackbar(lr_trackbar_name, window_name, &lr_trackbar_value, 20);\n \u001b[01;35m\u001b[K^~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/image_opencv.cpp:1375:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kcl_tb_res\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kcl_tb_res\u001b[m\u001b[K = cv::createTrackbar(cl_trackbar_name, window_name, &cl_trackbar_value, classes-1);\n \u001b[01;35m\u001b[K^~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/image_opencv.cpp:1378:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kbo_tb_res\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kbo_tb_res\u001b[m\u001b[K = cv::createTrackbar(bo_trackbar_name, window_name, boxonly, 1);\n \u001b[01;35m\u001b[K^~~~~~~~~\u001b[m\u001b[K\ng++ -std=c++11 -std=c++11 -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/http_stream.cpp -o obj/http_stream.o\nIn file included from \u001b[01m\u001b[K./src/http_stream.cpp:580:0\u001b[m\u001b[K:\n\u001b[01m\u001b[K./src/httplib.h:129:0:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"INVALID_SOCKET\" redefined\n #define INVALID_SOCKET (-1)\n \n\u001b[01m\u001b[K./src/http_stream.cpp:73:0:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[Kthis is the location of the previous definition\n #define INVALID_SOCKET -1\n \n\u001b[01m\u001b[K./src/http_stream.cpp:\u001b[m\u001b[K In member function ‘\u001b[01m\u001b[Kbool JSON_sender::write(const char*)\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/http_stream.cpp:249:21:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kn\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kn\u001b[m\u001b[K = _write(client, outputbuf, outlen);\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/http_stream.cpp:\u001b[m\u001b[K In member function ‘\u001b[01m\u001b[Kbool MJPG_sender::write(const cv::Mat&)\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/http_stream.cpp:507:113:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kformat ‘\u001b[01m\u001b[K%zu\u001b[m\u001b[K’ expects argument of type ‘\u001b[01m\u001b[Ksize_t\u001b[m\u001b[K’, but argument 3 has type ‘\u001b[01m\u001b[Kint\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wformat=\u001b[m\u001b[K]\n sprintf(head, \"--mjpegstream\\r\\nContent-Type: image/jpeg\\r\\nContent-Length: %zu\\r\\n\\r\\n\", outlen\u001b[01;35m\u001b[K)\u001b[m\u001b[K;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/gemm.c -o obj/gemm.o\n\u001b[01m\u001b[K./src/gemm.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kconvolution_2d\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/gemm.c:2039:15:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kout_w\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n const int \u001b[01;35m\u001b[Kout_w\u001b[m\u001b[K = (w + 2 * pad - ksize) / stride + 1; // output_width=input_width for stride=1 and pad=1\n \u001b[01;35m\u001b[K^~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/gemm.c:2038:15:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kout_h\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n const int \u001b[01;35m\u001b[Kout_h\u001b[m\u001b[K = (h + 2 * pad - ksize) / stride + 1; // output_height=input_height for stride=1 and pad=1\n \u001b[01;35m\u001b[K^~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/utils.c -o obj/utils.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/dark_cuda.c -o obj/dark_cuda.o\n\u001b[01m\u001b[K./src/dark_cuda.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kcudnn_check_error_extended\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/dark_cuda.c:224:20:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between ‘\u001b[01m\u001b[KcudaError_t {aka enum cudaError}\u001b[m\u001b[K’ and ‘\u001b[01m\u001b[Kenum <anonymous>\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wenum-compare\u001b[m\u001b[K]\n if (status \u001b[01;35m\u001b[K!=\u001b[m\u001b[K CUDNN_STATUS_SUCCESS)\n \u001b[01;35m\u001b[K^~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/dark_cuda.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kpre_allocate_pinned_memory\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/dark_cuda.c:276:40:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kformat ‘\u001b[01m\u001b[K%u\u001b[m\u001b[K’ expects argument of type ‘\u001b[01m\u001b[Kunsigned int\u001b[m\u001b[K’, but argument 2 has type ‘\u001b[01m\u001b[Klong unsigned int\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wformat=\u001b[m\u001b[K]\n printf(\"pre_allocate: size = \u001b[01;35m\u001b[K%Iu\u001b[m\u001b[K MB, num_of_blocks = %Iu, block_size = %Iu MB \\n\",\n \u001b[01;35m\u001b[K~~^\u001b[m\u001b[K\n \u001b[32m\u001b[K%Ilu\u001b[m\u001b[K\n \u001b[32m\u001b[Ksize / (1024*1024)\u001b[m\u001b[K, num_of_blocks, pinned_block_size / (1024 * 1024));\n \u001b[32m\u001b[K~~~~~~~~~~~~~~~~~~\u001b[m\u001b[K \n\u001b[01m\u001b[K./src/dark_cuda.c:276:64:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kformat ‘\u001b[01m\u001b[K%u\u001b[m\u001b[K’ expects argument of type ‘\u001b[01m\u001b[Kunsigned int\u001b[m\u001b[K’, but argument 3 has type ‘\u001b[01m\u001b[Ksize_t {aka const long unsigned int}\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wformat=\u001b[m\u001b[K]\n printf(\"pre_allocate: size = %Iu MB, num_of_blocks = \u001b[01;35m\u001b[K%Iu\u001b[m\u001b[K, block_size = %Iu MB \\n\",\n \u001b[01;35m\u001b[K~~^\u001b[m\u001b[K\n \u001b[32m\u001b[K%Ilu\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/dark_cuda.c:276:82:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kformat ‘\u001b[01m\u001b[K%u\u001b[m\u001b[K’ expects argument of type ‘\u001b[01m\u001b[Kunsigned int\u001b[m\u001b[K’, but argument 4 has type ‘\u001b[01m\u001b[Klong unsigned int\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wformat=\u001b[m\u001b[K]\n printf(\"pre_allocate: size = %Iu MB, num_of_blocks = %Iu, block_size = \u001b[01;35m\u001b[K%Iu\u001b[m\u001b[K MB \\n\",\n \u001b[01;35m\u001b[K~~^\u001b[m\u001b[K\n \u001b[32m\u001b[K%Ilu\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/dark_cuda.c:286:37:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kformat ‘\u001b[01m\u001b[K%d\u001b[m\u001b[K’ expects argument of type ‘\u001b[01m\u001b[Kint\u001b[m\u001b[K’, but argument 2 has type ‘\u001b[01m\u001b[Ksize_t {aka const long unsigned int}\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wformat=\u001b[m\u001b[K]\n printf(\" Allocated \u001b[01;35m\u001b[K%d\u001b[m\u001b[K pinned block \\n\", pinned_block_size);\n \u001b[01;35m\u001b[K~^\u001b[m\u001b[K\n \u001b[32m\u001b[K%ld\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/dark_cuda.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kcuda_make_array_pinned_preallocated\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/dark_cuda.c:307:43:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kformat ‘\u001b[01m\u001b[K%d\u001b[m\u001b[K’ expects argument of type ‘\u001b[01m\u001b[Kint\u001b[m\u001b[K’, but argument 2 has type ‘\u001b[01m\u001b[Ksize_t {aka long unsigned int}\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wformat=\u001b[m\u001b[K]\n printf(\"\\n Pinned block_id = \u001b[01;35m\u001b[K%d\u001b[m\u001b[K, filled = %f %% \\n\", pinned_block_id, filled);\n \u001b[01;35m\u001b[K~^\u001b[m\u001b[K\n \u001b[32m\u001b[K%ld\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/dark_cuda.c:322:64:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kformat ‘\u001b[01m\u001b[K%d\u001b[m\u001b[K’ expects argument of type ‘\u001b[01m\u001b[Kint\u001b[m\u001b[K’, but argument 2 has type ‘\u001b[01m\u001b[Klong unsigned int\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wformat=\u001b[m\u001b[K]\n printf(\"Try to allocate new pinned memory, size = \u001b[01;35m\u001b[K%d\u001b[m\u001b[K MB \\n\", \u001b[32m\u001b[Ksize / (1024 * 1024)\u001b[m\u001b[K);\n \u001b[01;35m\u001b[K~^\u001b[m\u001b[K \u001b[32m\u001b[K~~~~~~~~~~~~~~~~~~~~\u001b[m\u001b[K\n \u001b[32m\u001b[K%ld\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/dark_cuda.c:328:63:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kformat ‘\u001b[01m\u001b[K%d\u001b[m\u001b[K’ expects argument of type ‘\u001b[01m\u001b[Kint\u001b[m\u001b[K’, but argument 2 has type ‘\u001b[01m\u001b[Klong unsigned int\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wformat=\u001b[m\u001b[K]\n printf(\"Try to allocate new pinned BLOCK, size = \u001b[01;35m\u001b[K%d\u001b[m\u001b[K MB \\n\", \u001b[32m\u001b[Ksize / (1024 * 1024)\u001b[m\u001b[K);\n \u001b[01;35m\u001b[K~^\u001b[m\u001b[K \u001b[32m\u001b[K~~~~~~~~~~~~~~~~~~~~\u001b[m\u001b[K\n \u001b[32m\u001b[K%ld\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/convolutional_layer.c -o obj/convolutional_layer.o\n\u001b[01m\u001b[K./src/convolutional_layer.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kforward_convolutional_layer\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/convolutional_layer.c:1204:32:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kt_intput_size\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n size_t \u001b[01;35m\u001b[Kt_intput_size\u001b[m\u001b[K = binary_transpose_align_input(k, n, state.workspace, &l.t_bit_input, ldb_align, l.bit_align);\n \u001b[01;35m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/list.c -o obj/list.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/image.c -o obj/image.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/activations.c -o obj/activations.o\n\u001b[01m\u001b[K./src/activations.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kactivate\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/activations.c:77:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kenumeration value ‘\u001b[01m\u001b[KRELU6\u001b[m\u001b[K’ not handled in switch [\u001b[01;35m\u001b[K-Wswitch\u001b[m\u001b[K]\n \u001b[01;35m\u001b[Kswitch\u001b[m\u001b[K(a){\n \u001b[01;35m\u001b[K^~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/activations.c:77:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kenumeration value ‘\u001b[01m\u001b[KSWISH\u001b[m\u001b[K’ not handled in switch [\u001b[01;35m\u001b[K-Wswitch\u001b[m\u001b[K]\n\u001b[01m\u001b[K./src/activations.c:77:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kenumeration value ‘\u001b[01m\u001b[KMISH\u001b[m\u001b[K’ not handled in switch [\u001b[01;35m\u001b[K-Wswitch\u001b[m\u001b[K]\n\u001b[01m\u001b[K./src/activations.c:77:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kenumeration value ‘\u001b[01m\u001b[KNORM_CHAN\u001b[m\u001b[K’ not handled in switch [\u001b[01;35m\u001b[K-Wswitch\u001b[m\u001b[K]\n\u001b[01m\u001b[K./src/activations.c:77:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kenumeration value ‘\u001b[01m\u001b[KNORM_CHAN_SOFTMAX\u001b[m\u001b[K’ not handled in switch [\u001b[01;35m\u001b[K-Wswitch\u001b[m\u001b[K]\n\u001b[01m\u001b[K./src/activations.c:77:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kenumeration value ‘\u001b[01m\u001b[KNORM_CHAN_SOFTMAX_MAXVAL\u001b[m\u001b[K’ not handled in switch [\u001b[01;35m\u001b[K-Wswitch\u001b[m\u001b[K]\n\u001b[01m\u001b[K./src/activations.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kgradient\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/activations.c:287:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kenumeration value ‘\u001b[01m\u001b[KSWISH\u001b[m\u001b[K’ not handled in switch [\u001b[01;35m\u001b[K-Wswitch\u001b[m\u001b[K]\n \u001b[01;35m\u001b[Kswitch\u001b[m\u001b[K(a){\n \u001b[01;35m\u001b[K^~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/activations.c:287:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kenumeration value ‘\u001b[01m\u001b[KMISH\u001b[m\u001b[K’ not handled in switch [\u001b[01;35m\u001b[K-Wswitch\u001b[m\u001b[K]\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/im2col.c -o obj/im2col.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/col2im.c -o obj/col2im.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/blas.c -o obj/blas.o\n\u001b[01m\u001b[K./src/blas.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kbackward_shortcut_multilayer_cpu\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/blas.c:207:21:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kout_index\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kout_index\u001b[m\u001b[K = id;\n \u001b[01;35m\u001b[K^~~~~~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/crop_layer.c -o obj/crop_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/dropout_layer.c -o obj/dropout_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/maxpool_layer.c -o obj/maxpool_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/softmax_layer.c -o obj/softmax_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/data.c -o obj/data.o\n\u001b[01m\u001b[K./src/data.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kload_data_detection\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/data.c:1134:24:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kx\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int k, \u001b[01;35m\u001b[Kx\u001b[m\u001b[K, y;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/data.c:984:43:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kvariable ‘\u001b[01m\u001b[Kr_scale\u001b[m\u001b[K’ set but not used [\u001b[01;35m\u001b[K-Wunused-but-set-variable\u001b[m\u001b[K]\n float r1 = 0, r2 = 0, r3 = 0, r4 = 0, \u001b[01;35m\u001b[Kr_scale\u001b[m\u001b[K = 0;\n \u001b[01;35m\u001b[K^~~~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/matrix.c -o obj/matrix.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/network.c -o obj/network.o\n\u001b[01m\u001b[K./src/network.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kresize_network\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/network.c:610:42:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kpassing argument 1 of ‘\u001b[01m\u001b[KcudaHostAlloc\u001b[m\u001b[K’ from incompatible pointer type [\u001b[01;35m\u001b[K-Wincompatible-pointer-types\u001b[m\u001b[K]\n if (cudaSuccess == cudaHostAlloc(\u001b[01;35m\u001b[K&\u001b[m\u001b[Knet->input_pinned_cpu, size * sizeof(float), cudaHostRegisterMapped))\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\nIn file included from \u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime.h:96:0\u001b[m\u001b[K,\n from \u001b[01m\u001b[Kinclude/darknet.h:41\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/network.c:1\u001b[m\u001b[K:\n\u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime_api.h:4391:39:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[Kexpected ‘\u001b[01m\u001b[Kvoid **\u001b[m\u001b[K’ but argument is of type ‘\u001b[01m\u001b[Kfloat **\u001b[m\u001b[K’\n extern __host__ cudaError_t CUDARTAPI \u001b[01;36m\u001b[KcudaHostAlloc\u001b[m\u001b[K(void **pHost, size_t size, unsigned int flags);\n \u001b[01;36m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/connected_layer.c -o obj/connected_layer.o\n\u001b[01m\u001b[K./src/connected_layer.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kforward_connected_layer_gpu\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/connected_layer.c:346:11:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kone\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n float \u001b[01;35m\u001b[Kone\u001b[m\u001b[K = 1; // alpha[0], beta[0]\n \u001b[01;35m\u001b[K^~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/connected_layer.c:344:13:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kc\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n float * \u001b[01;35m\u001b[Kc\u001b[m\u001b[K = l.output_gpu;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/connected_layer.c:343:13:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kb\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n float * \u001b[01;35m\u001b[Kb\u001b[m\u001b[K = l.weights_gpu;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/connected_layer.c:342:13:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Ka\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n float * \u001b[01;35m\u001b[Ka\u001b[m\u001b[K = state.input;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/connected_layer.c:341:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kn\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kn\u001b[m\u001b[K = l.outputs;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/connected_layer.c:340:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kk\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kk\u001b[m\u001b[K = l.inputs;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/connected_layer.c:339:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Km\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Km\u001b[m\u001b[K = l.batch;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/cost_layer.c -o obj/cost_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/parser.c -o obj/parser.o\n\u001b[01m\u001b[K./src/parser.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kparse_network_cfg_custom\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/parser.c:1573:42:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kpassing argument 1 of ‘\u001b[01m\u001b[KcudaHostAlloc\u001b[m\u001b[K’ from incompatible pointer type [\u001b[01;35m\u001b[K-Wincompatible-pointer-types\u001b[m\u001b[K]\n if (cudaSuccess == cudaHostAlloc(\u001b[01;35m\u001b[K&\u001b[m\u001b[Knet.input_pinned_cpu, size * sizeof(float), cudaHostRegisterMapped)) net.input_pinned_cpu_flag = 1;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\nIn file included from \u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime.h:96:0\u001b[m\u001b[K,\n from \u001b[01m\u001b[Kinclude/darknet.h:41\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/activations.h:3\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/activation_layer.h:4\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/parser.c:6\u001b[m\u001b[K:\n\u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime_api.h:4391:39:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[Kexpected ‘\u001b[01m\u001b[Kvoid **\u001b[m\u001b[K’ but argument is of type ‘\u001b[01m\u001b[Kfloat **\u001b[m\u001b[K’\n extern __host__ cudaError_t CUDARTAPI \u001b[01;36m\u001b[KcudaHostAlloc\u001b[m\u001b[K(void **pHost, size_t size, unsigned int flags);\n \u001b[01;36m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/option_list.c -o obj/option_list.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/darknet.c -o obj/darknet.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/detection_layer.c -o obj/detection_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/captcha.c -o obj/captcha.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/route_layer.c -o obj/route_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/writing.c -o obj/writing.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/box.c -o obj/box.o\n\u001b[01m\u001b[K./src/box.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kbox_iou_kind\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/box.c:154:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kenumeration value ‘\u001b[01m\u001b[KMSE\u001b[m\u001b[K’ not handled in switch [\u001b[01;35m\u001b[K-Wswitch\u001b[m\u001b[K]\n \u001b[01;35m\u001b[Kswitch\u001b[m\u001b[K(iou_kind) {\n \u001b[01;35m\u001b[K^~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/box.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kdiounms_sort\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/box.c:898:27:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kbeta_prob\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n float \u001b[01;35m\u001b[Kbeta_prob\u001b[m\u001b[K = pow(dets[j].prob[k], 2) / sum_prob;\n \u001b[01;35m\u001b[K^~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/box.c:897:27:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kalpha_prob\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n float \u001b[01;35m\u001b[Kalpha_prob\u001b[m\u001b[K = pow(dets[i].prob[k], 2) / sum_prob;\n \u001b[01;35m\u001b[K^~~~~~~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/nightmare.c -o obj/nightmare.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/normalization_layer.c -o obj/normalization_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/avgpool_layer.c -o obj/avgpool_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/coco.c -o obj/coco.o\n\u001b[01m\u001b[K./src/coco.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kvalidate_coco_recall\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/coco.c:248:11:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kbase\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n char *\u001b[01;35m\u001b[Kbase\u001b[m\u001b[K = \"results/comp4_det_test_\";\n \u001b[01;35m\u001b[K^~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/dice.c -o obj/dice.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/yolo.c -o obj/yolo.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/detector.c -o obj/detector.o\n\u001b[01m\u001b[K./src/detector.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kprint_cocos\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/detector.c:451:29:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kformat not a string literal and no format arguments [\u001b[01;35m\u001b[K-Wformat-security\u001b[m\u001b[K]\n fprintf(fp, \u001b[01;35m\u001b[Kbuff\u001b[m\u001b[K);\n \u001b[01;35m\u001b[K^~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/detector.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Keliminate_bdd\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/detector.c:544:21:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kstatement with no effect [\u001b[01;35m\u001b[K-Wunused-value\u001b[m\u001b[K]\n \u001b[01;35m\u001b[Kfor\u001b[m\u001b[K (k; buf[k + n] != '\\0'; k++)\n \u001b[01;35m\u001b[K^~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/detector.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kvalidate_detector\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/detector.c:657:13:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kmkd2\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kmkd2\u001b[m\u001b[K = make_directory(buff2, 0777);\n \u001b[01;35m\u001b[K^~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/detector.c:655:13:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kmkd\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kmkd\u001b[m\u001b[K = make_directory(buff, 0777);\n \u001b[01;35m\u001b[K^~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/detector.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kvalidate_detector_map\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/detector.c:1276:15:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kclass_recall\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n float \u001b[01;35m\u001b[Kclass_recall\u001b[m\u001b[K = (float)tp_for_thresh_per_class[i] / ((float)tp_for_thresh_per_class[i] + (float)(truth_classes_count[i] - tp_for_thresh_per_class[i]));\n \u001b[01;35m\u001b[K^~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/detector.c:1275:15:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kclass_precision\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n float \u001b[01;35m\u001b[Kclass_precision\u001b[m\u001b[K = (float)tp_for_thresh_per_class[i] / ((float)tp_for_thresh_per_class[i] + (float)fp_for_thresh_per_class[i]);\n \u001b[01;35m\u001b[K^~~~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/detector.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kdraw_object\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/detector.c:1792:19:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kinv_loss\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n float \u001b[01;35m\u001b[Kinv_loss\u001b[m\u001b[K = 1.0 / max_val_cmp(0.01, avg_loss);\n \u001b[01;35m\u001b[K^~~~~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/layer.c -o obj/layer.o\n\u001b[01m\u001b[K./src/layer.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kfree_layer_custom\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/layer.c:191:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kthis ‘\u001b[01m\u001b[Kif\u001b[m\u001b[K’ clause does not guard... [\u001b[01;35m\u001b[K-Wmisleading-indentation\u001b[m\u001b[K]\n \u001b[01;35m\u001b[Kif\u001b[m\u001b[K (l.x_gpu) cuda_free(l.x_gpu); l.x_gpu = NULL;\n \u001b[01;35m\u001b[K^~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/layer.c:191:61:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[K...this statement, but the latter is misleadingly indented as if it were guarded by the ‘\u001b[01m\u001b[Kif\u001b[m\u001b[K’\n if (l.x_gpu) cuda_free(l.x_gpu); \u001b[01;36m\u001b[Kl\u001b[m\u001b[K.x_gpu = NULL;\n \u001b[01;36m\u001b[K^\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/compare.c -o obj/compare.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/classifier.c -o obj/classifier.o\n\u001b[01m\u001b[K./src/classifier.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Ktrain_classifier\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/classifier.c:138:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kcount\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kcount\u001b[m\u001b[K = 0;\n \u001b[01;35m\u001b[K^~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/classifier.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kpredict_classifier\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/classifier.c:831:13:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Ktime\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n clock_t \u001b[01;35m\u001b[Ktime\u001b[m\u001b[K;\n \u001b[01;35m\u001b[K^~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/classifier.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kdemo_classifier\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/classifier.c:1261:49:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Ktval_result\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n struct timeval tval_before, tval_after, \u001b[01;35m\u001b[Ktval_result\u001b[m\u001b[K;\n \u001b[01;35m\u001b[K^~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/classifier.c:1261:37:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Ktval_after\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n struct timeval tval_before, \u001b[01;35m\u001b[Ktval_after\u001b[m\u001b[K, tval_result;\n \u001b[01;35m\u001b[K^~~~~~~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/local_layer.c -o obj/local_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/swag.c -o obj/swag.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/shortcut_layer.c -o obj/shortcut_layer.o\n\u001b[01m\u001b[K./src/shortcut_layer.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kmake_shortcut_layer\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/shortcut_layer.c:55:15:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kscale\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n float \u001b[01;35m\u001b[Kscale\u001b[m\u001b[K = sqrt(2. / l.nweights);\n \u001b[01;35m\u001b[K^~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/activation_layer.c -o obj/activation_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/rnn_layer.c -o obj/rnn_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/gru_layer.c -o obj/gru_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/rnn.c -o obj/rnn.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/rnn_vid.c -o obj/rnn_vid.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/crnn_layer.c -o obj/crnn_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/demo.c -o obj/demo.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/tag.c -o obj/tag.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/cifar.c -o obj/cifar.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/go.c -o obj/go.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/batchnorm_layer.c -o obj/batchnorm_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/art.c -o obj/art.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/region_layer.c -o obj/region_layer.o\n\u001b[01m\u001b[K./src/region_layer.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kresize_region_layer\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/region_layer.c:58:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kold_h\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kold_h\u001b[m\u001b[K = l->h;\n \u001b[01;35m\u001b[K^~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/region_layer.c:57:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kold_w\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kold_w\u001b[m\u001b[K = l->w;\n \u001b[01;35m\u001b[K^~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/reorg_layer.c -o obj/reorg_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/reorg_old_layer.c -o obj/reorg_old_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/super.c -o obj/super.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/voxel.c -o obj/voxel.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/tree.c -o obj/tree.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/yolo_layer.c -o obj/yolo_layer.o\n\u001b[01m\u001b[K./src/yolo_layer.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kmake_yolo_layer\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/yolo_layer.c:61:38:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kpassing argument 1 of ‘\u001b[01m\u001b[KcudaHostAlloc\u001b[m\u001b[K’ from incompatible pointer type [\u001b[01;35m\u001b[K-Wincompatible-pointer-types\u001b[m\u001b[K]\n if (cudaSuccess == cudaHostAlloc(\u001b[01;35m\u001b[K&\u001b[m\u001b[Kl.output, batch*l.outputs*sizeof(float), cudaHostRegisterMapped)) l.output_pinned = 1;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\nIn file included from \u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime.h:96:0\u001b[m\u001b[K,\n from \u001b[01m\u001b[Kinclude/darknet.h:41\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/activations.h:3\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/layer.h:4\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/yolo_layer.h:5\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/yolo_layer.c:1\u001b[m\u001b[K:\n\u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime_api.h:4391:39:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[Kexpected ‘\u001b[01m\u001b[Kvoid **\u001b[m\u001b[K’ but argument is of type ‘\u001b[01m\u001b[Kfloat **\u001b[m\u001b[K’\n extern __host__ cudaError_t CUDARTAPI \u001b[01;36m\u001b[KcudaHostAlloc\u001b[m\u001b[K(void **pHost, size_t size, unsigned int flags);\n \u001b[01;36m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/yolo_layer.c:68:38:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kpassing argument 1 of ‘\u001b[01m\u001b[KcudaHostAlloc\u001b[m\u001b[K’ from incompatible pointer type [\u001b[01;35m\u001b[K-Wincompatible-pointer-types\u001b[m\u001b[K]\n if (cudaSuccess == cudaHostAlloc(\u001b[01;35m\u001b[K&\u001b[m\u001b[Kl.delta, batch*l.outputs*sizeof(float), cudaHostRegisterMapped)) l.delta_pinned = 1;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\nIn file included from \u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime.h:96:0\u001b[m\u001b[K,\n from \u001b[01m\u001b[Kinclude/darknet.h:41\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/activations.h:3\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/layer.h:4\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/yolo_layer.h:5\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/yolo_layer.c:1\u001b[m\u001b[K:\n\u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime_api.h:4391:39:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[Kexpected ‘\u001b[01m\u001b[Kvoid **\u001b[m\u001b[K’ but argument is of type ‘\u001b[01m\u001b[Kfloat **\u001b[m\u001b[K’\n extern __host__ cudaError_t CUDARTAPI \u001b[01;36m\u001b[KcudaHostAlloc\u001b[m\u001b[K(void **pHost, size_t size, unsigned int flags);\n \u001b[01;36m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/yolo_layer.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kresize_yolo_layer\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/yolo_layer.c:95:42:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kpassing argument 1 of ‘\u001b[01m\u001b[KcudaHostAlloc\u001b[m\u001b[K’ from incompatible pointer type [\u001b[01;35m\u001b[K-Wincompatible-pointer-types\u001b[m\u001b[K]\n if (cudaSuccess != cudaHostAlloc(\u001b[01;35m\u001b[K&\u001b[m\u001b[Kl->output, l->batch*l->outputs * sizeof(float), cudaHostRegisterMapped)) {\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\nIn file included from \u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime.h:96:0\u001b[m\u001b[K,\n from \u001b[01m\u001b[Kinclude/darknet.h:41\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/activations.h:3\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/layer.h:4\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/yolo_layer.h:5\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/yolo_layer.c:1\u001b[m\u001b[K:\n\u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime_api.h:4391:39:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[Kexpected ‘\u001b[01m\u001b[Kvoid **\u001b[m\u001b[K’ but argument is of type ‘\u001b[01m\u001b[Kfloat **\u001b[m\u001b[K’\n extern __host__ cudaError_t CUDARTAPI \u001b[01;36m\u001b[KcudaHostAlloc\u001b[m\u001b[K(void **pHost, size_t size, unsigned int flags);\n \u001b[01;36m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/yolo_layer.c:104:42:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kpassing argument 1 of ‘\u001b[01m\u001b[KcudaHostAlloc\u001b[m\u001b[K’ from incompatible pointer type [\u001b[01;35m\u001b[K-Wincompatible-pointer-types\u001b[m\u001b[K]\n if (cudaSuccess != cudaHostAlloc(\u001b[01;35m\u001b[K&\u001b[m\u001b[Kl->delta, l->batch*l->outputs * sizeof(float), cudaHostRegisterMapped)) {\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\nIn file included from \u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime.h:96:0\u001b[m\u001b[K,\n from \u001b[01m\u001b[Kinclude/darknet.h:41\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/activations.h:3\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/layer.h:4\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/yolo_layer.h:5\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/yolo_layer.c:1\u001b[m\u001b[K:\n\u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime_api.h:4391:39:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[Kexpected ‘\u001b[01m\u001b[Kvoid **\u001b[m\u001b[K’ but argument is of type ‘\u001b[01m\u001b[Kfloat **\u001b[m\u001b[K’\n extern __host__ cudaError_t CUDARTAPI \u001b[01;36m\u001b[KcudaHostAlloc\u001b[m\u001b[K(void **pHost, size_t size, unsigned int flags);\n \u001b[01;36m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/yolo_layer.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kforward_yolo_layer\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/yolo_layer.c:371:25:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kvariable ‘\u001b[01m\u001b[Kbest_match_t\u001b[m\u001b[K’ set but not used [\u001b[01;35m\u001b[K-Wunused-but-set-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kbest_match_t\u001b[m\u001b[K = 0;\n \u001b[01;35m\u001b[K^~~~~~~~~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/gaussian_yolo_layer.c -o obj/gaussian_yolo_layer.o\n\u001b[01m\u001b[K./src/gaussian_yolo_layer.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kmake_gaussian_yolo_layer\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/gaussian_yolo_layer.c:71:38:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kpassing argument 1 of ‘\u001b[01m\u001b[KcudaHostAlloc\u001b[m\u001b[K’ from incompatible pointer type [\u001b[01;35m\u001b[K-Wincompatible-pointer-types\u001b[m\u001b[K]\n if (cudaSuccess == cudaHostAlloc(\u001b[01;35m\u001b[K&\u001b[m\u001b[Kl.output, batch*l.outputs * sizeof(float), cudaHostRegisterMapped)) l.output_pinned = 1;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\nIn file included from \u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime.h:96:0\u001b[m\u001b[K,\n from \u001b[01m\u001b[Kinclude/darknet.h:41\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/gaussian_yolo_layer.h:5\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/gaussian_yolo_layer.c:7\u001b[m\u001b[K:\n\u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime_api.h:4391:39:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[Kexpected ‘\u001b[01m\u001b[Kvoid **\u001b[m\u001b[K’ but argument is of type ‘\u001b[01m\u001b[Kfloat **\u001b[m\u001b[K’\n extern __host__ cudaError_t CUDARTAPI \u001b[01;36m\u001b[KcudaHostAlloc\u001b[m\u001b[K(void **pHost, size_t size, unsigned int flags);\n \u001b[01;36m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/gaussian_yolo_layer.c:78:38:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kpassing argument 1 of ‘\u001b[01m\u001b[KcudaHostAlloc\u001b[m\u001b[K’ from incompatible pointer type [\u001b[01;35m\u001b[K-Wincompatible-pointer-types\u001b[m\u001b[K]\n if (cudaSuccess == cudaHostAlloc(\u001b[01;35m\u001b[K&\u001b[m\u001b[Kl.delta, batch*l.outputs * sizeof(float), cudaHostRegisterMapped)) l.delta_pinned = 1;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\nIn file included from \u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime.h:96:0\u001b[m\u001b[K,\n from \u001b[01m\u001b[Kinclude/darknet.h:41\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/gaussian_yolo_layer.h:5\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/gaussian_yolo_layer.c:7\u001b[m\u001b[K:\n\u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime_api.h:4391:39:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[Kexpected ‘\u001b[01m\u001b[Kvoid **\u001b[m\u001b[K’ but argument is of type ‘\u001b[01m\u001b[Kfloat **\u001b[m\u001b[K’\n extern __host__ cudaError_t CUDARTAPI \u001b[01;36m\u001b[KcudaHostAlloc\u001b[m\u001b[K(void **pHost, size_t size, unsigned int flags);\n \u001b[01;36m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/gaussian_yolo_layer.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kresize_gaussian_yolo_layer\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/gaussian_yolo_layer.c:110:42:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kpassing argument 1 of ‘\u001b[01m\u001b[KcudaHostAlloc\u001b[m\u001b[K’ from incompatible pointer type [\u001b[01;35m\u001b[K-Wincompatible-pointer-types\u001b[m\u001b[K]\n if (cudaSuccess != cudaHostAlloc(\u001b[01;35m\u001b[K&\u001b[m\u001b[Kl->output, l->batch*l->outputs * sizeof(float), cudaHostRegisterMapped)) {\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\nIn file included from \u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime.h:96:0\u001b[m\u001b[K,\n from \u001b[01m\u001b[Kinclude/darknet.h:41\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/gaussian_yolo_layer.h:5\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/gaussian_yolo_layer.c:7\u001b[m\u001b[K:\n\u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime_api.h:4391:39:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[Kexpected ‘\u001b[01m\u001b[Kvoid **\u001b[m\u001b[K’ but argument is of type ‘\u001b[01m\u001b[Kfloat **\u001b[m\u001b[K’\n extern __host__ cudaError_t CUDARTAPI \u001b[01;36m\u001b[KcudaHostAlloc\u001b[m\u001b[K(void **pHost, size_t size, unsigned int flags);\n \u001b[01;36m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/gaussian_yolo_layer.c:119:42:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kpassing argument 1 of ‘\u001b[01m\u001b[KcudaHostAlloc\u001b[m\u001b[K’ from incompatible pointer type [\u001b[01;35m\u001b[K-Wincompatible-pointer-types\u001b[m\u001b[K]\n if (cudaSuccess != cudaHostAlloc(\u001b[01;35m\u001b[K&\u001b[m\u001b[Kl->delta, l->batch*l->outputs * sizeof(float), cudaHostRegisterMapped)) {\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\nIn file included from \u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime.h:96:0\u001b[m\u001b[K,\n from \u001b[01m\u001b[Kinclude/darknet.h:41\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/gaussian_yolo_layer.h:5\u001b[m\u001b[K,\n from \u001b[01m\u001b[K./src/gaussian_yolo_layer.c:7\u001b[m\u001b[K:\n\u001b[01m\u001b[K/usr/local/cuda/include/cuda_runtime_api.h:4391:39:\u001b[m\u001b[K \u001b[01;36m\u001b[Knote: \u001b[m\u001b[Kexpected ‘\u001b[01m\u001b[Kvoid **\u001b[m\u001b[K’ but argument is of type ‘\u001b[01m\u001b[Kfloat **\u001b[m\u001b[K’\n extern __host__ cudaError_t CUDARTAPI \u001b[01;36m\u001b[KcudaHostAlloc\u001b[m\u001b[K(void **pHost, size_t size, unsigned int flags);\n \u001b[01;36m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/gaussian_yolo_layer.c:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kforward_gaussian_yolo_layer\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/gaussian_yolo_layer.c:460:25:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kvariable ‘\u001b[01m\u001b[Kbest_match_t\u001b[m\u001b[K’ set but not used [\u001b[01;35m\u001b[K-Wunused-but-set-variable\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Kbest_match_t\u001b[m\u001b[K = 0;\n \u001b[01;35m\u001b[K^~~~~~~~~~~~\u001b[m\u001b[K\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/upsample_layer.c -o obj/upsample_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/lstm_layer.c -o obj/lstm_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/conv_lstm_layer.c -o obj/conv_lstm_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/scale_channels_layer.c -o obj/scale_channels_layer.o\ngcc -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -c ./src/sam_layer.c -o obj/sam_layer.o\nnvcc -gencode arch=compute_30,code=sm_30 -gencode arch=compute_35,code=sm_35 -gencode arch=compute_50,code=[sm_50,compute_50] -gencode arch=compute_52,code=[sm_52,compute_52] -gencode arch=compute_61,code=[sm_61,compute_61] -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN --compiler-options \"-Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC\" -c ./src/convolutional_kernels.cu -o obj/convolutional_kernels.o\nnvcc -gencode arch=compute_30,code=sm_30 -gencode arch=compute_35,code=sm_35 -gencode arch=compute_50,code=[sm_50,compute_50] -gencode arch=compute_52,code=[sm_52,compute_52] -gencode arch=compute_61,code=[sm_61,compute_61] -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN --compiler-options \"-Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC\" -c ./src/activation_kernels.cu -o obj/activation_kernels.o\nnvcc -gencode arch=compute_30,code=sm_30 -gencode arch=compute_35,code=sm_35 -gencode arch=compute_50,code=[sm_50,compute_50] -gencode arch=compute_52,code=[sm_52,compute_52] -gencode arch=compute_61,code=[sm_61,compute_61] -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN --compiler-options \"-Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC\" -c ./src/im2col_kernels.cu -o obj/im2col_kernels.o\n\u001b[01m\u001b[K./src/im2col_kernels.cu:125:18:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*data_col_ptr = (h >= 0 && w >= 0 && h < height && w < width) ?\n \n\u001b[01m\u001b[K./src/im2col_kernels.cu:1178:6:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*((uint64_t *)(A_s + (local_i*lda + k) / 8)) = *((uint64_t *)(A + (i_cur*lda + k) / 8)); // weights\n \n\u001b[01m\u001b[K./src/im2col_kernels.cu:125:18:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*data_col_ptr = (h >= 0 && w >= 0 && h < height && w < width) ?\n \n\u001b[01m\u001b[K./src/im2col_kernels.cu:1178:6:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*((uint64_t *)(A_s + (local_i*lda + k) / 8)) = *((uint64_t *)(A + (i_cur*lda + k) / 8)); // weights\n \n\u001b[01m\u001b[K./src/im2col_kernels.cu:125:18:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*data_col_ptr = (h >= 0 && w >= 0 && h < height && w < width) ?\n \n\u001b[01m\u001b[K./src/im2col_kernels.cu:1178:6:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*((uint64_t *)(A_s + (local_i*lda + k) / 8)) = *((uint64_t *)(A + (i_cur*lda + k) / 8)); // weights\n \n\u001b[01m\u001b[K./src/im2col_kernels.cu:125:18:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*data_col_ptr = (h >= 0 && w >= 0 && h < height && w < width) ?\n \n\u001b[01m\u001b[K./src/im2col_kernels.cu:1178:6:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*((uint64_t *)(A_s + (local_i*lda + k) / 8)) = *((uint64_t *)(A + (i_cur*lda + k) / 8)); // weights\n \n\u001b[01m\u001b[K./src/im2col_kernels.cu:125:18:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*data_col_ptr = (h >= 0 && w >= 0 && h < height && w < width) ?\n \n\u001b[01m\u001b[K./src/im2col_kernels.cu:1178:6:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*((uint64_t *)(A_s + (local_i*lda + k) / 8)) = *((uint64_t *)(A + (i_cur*lda + k) / 8)); // weights\n \n\u001b[01m\u001b[K./src/im2col_kernels.cu:125:18:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*data_col_ptr = (h >= 0 && w >= 0 && h < height && w < width) ?\n \n\u001b[01m\u001b[K./src/im2col_kernels.cu:1178:6:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K\"/*\" within comment [\u001b[01;35m\u001b[K-Wcomment\u001b[m\u001b[K]\n //*((uint64_t *)(A_s + (local_i*lda + k) / 8)) = *((uint64_t *)(A + (i_cur*lda + k) / 8)); // weights\n \nnvcc -gencode arch=compute_30,code=sm_30 -gencode arch=compute_35,code=sm_35 -gencode arch=compute_50,code=[sm_50,compute_50] -gencode arch=compute_52,code=[sm_52,compute_52] -gencode arch=compute_61,code=[sm_61,compute_61] -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN --compiler-options \"-Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC\" -c ./src/col2im_kernels.cu -o obj/col2im_kernels.o\nnvcc -gencode arch=compute_30,code=sm_30 -gencode arch=compute_35,code=sm_35 -gencode arch=compute_50,code=[sm_50,compute_50] -gencode arch=compute_52,code=[sm_52,compute_52] -gencode arch=compute_61,code=[sm_61,compute_61] -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN --compiler-options \"-Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC\" -c ./src/blas_kernels.cu -o obj/blas_kernels.o\n./src/blas_kernels.cu(1038): warning: variable \"out_index\" was declared but never referenced\n\n./src/blas_kernels.cu(1082): warning: variable \"step\" was set but never used\n\n./src/blas_kernels.cu(1686): warning: variable \"stage_id\" was declared but never referenced\n\n./src/blas_kernels.cu(1038): warning: variable \"out_index\" was declared but never referenced\n\n./src/blas_kernels.cu(1082): warning: variable \"step\" was set but never used\n\n./src/blas_kernels.cu(1686): warning: variable \"stage_id\" was declared but never referenced\n\n./src/blas_kernels.cu(1038): warning: variable \"out_index\" was declared but never referenced\n\n./src/blas_kernels.cu(1082): warning: variable \"step\" was set but never used\n\n./src/blas_kernels.cu(1686): warning: variable \"stage_id\" was declared but never referenced\n\n./src/blas_kernels.cu(1038): warning: variable \"out_index\" was declared but never referenced\n\n./src/blas_kernels.cu(1082): warning: variable \"step\" was set but never used\n\n./src/blas_kernels.cu(1686): warning: variable \"stage_id\" was declared but never referenced\n\n./src/blas_kernels.cu(1038): warning: variable \"out_index\" was declared but never referenced\n\n./src/blas_kernels.cu(1082): warning: variable \"step\" was set but never used\n\n./src/blas_kernels.cu(1686): warning: variable \"stage_id\" was declared but never referenced\n\n\u001b[01m\u001b[K./src/blas_kernels.cu:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kvoid backward_shortcut_multilayer_gpu(int, int, int, int*, float**, float*, float*, float*, float*, int, float*, float**, WEIGHTS_NORMALIZATION_T)\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/blas_kernels.cu:1082:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kvariable ‘\u001b[01m\u001b[Kstep\u001b[m\u001b[K’ set but not used [\u001b[01;35m\u001b[K-Wunused-but-set-variable\u001b[m\u001b[K]\n \u001b[01;35m\u001b[Kint \u001b[m\u001b[Kstep = 0;\n \u001b[01;35m\u001b[K^~~~\u001b[m\u001b[K\nnvcc -gencode arch=compute_30,code=sm_30 -gencode arch=compute_35,code=sm_35 -gencode arch=compute_50,code=[sm_50,compute_50] -gencode arch=compute_52,code=[sm_52,compute_52] -gencode arch=compute_61,code=[sm_61,compute_61] -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN --compiler-options \"-Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC\" -c ./src/crop_layer_kernels.cu -o obj/crop_layer_kernels.o\nnvcc -gencode arch=compute_30,code=sm_30 -gencode arch=compute_35,code=sm_35 -gencode arch=compute_50,code=[sm_50,compute_50] -gencode arch=compute_52,code=[sm_52,compute_52] -gencode arch=compute_61,code=[sm_61,compute_61] -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN --compiler-options \"-Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC\" -c ./src/dropout_layer_kernels.cu -o obj/dropout_layer_kernels.o\n./src/dropout_layer_kernels.cu(140): warning: variable \"cur_scale\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(245): warning: variable \"cur_scale\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(262): warning: variable \"block_prob\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(140): warning: variable \"cur_scale\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(245): warning: variable \"cur_scale\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(262): warning: variable \"block_prob\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(140): warning: variable \"cur_scale\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(245): warning: variable \"cur_scale\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(262): warning: variable \"block_prob\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(140): warning: variable \"cur_scale\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(245): warning: variable \"cur_scale\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(262): warning: variable \"block_prob\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(140): warning: variable \"cur_scale\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(245): warning: variable \"cur_scale\" was declared but never referenced\n\n./src/dropout_layer_kernels.cu(262): warning: variable \"block_prob\" was declared but never referenced\n\nnvcc -gencode arch=compute_30,code=sm_30 -gencode arch=compute_35,code=sm_35 -gencode arch=compute_50,code=[sm_50,compute_50] -gencode arch=compute_52,code=[sm_52,compute_52] -gencode arch=compute_61,code=[sm_61,compute_61] -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN --compiler-options \"-Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC\" -c ./src/maxpool_layer_kernels.cu -o obj/maxpool_layer_kernels.o\nnvcc -gencode arch=compute_30,code=sm_30 -gencode arch=compute_35,code=sm_35 -gencode arch=compute_50,code=[sm_50,compute_50] -gencode arch=compute_52,code=[sm_52,compute_52] -gencode arch=compute_61,code=[sm_61,compute_61] -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN --compiler-options \"-Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC\" -c ./src/network_kernels.cu -o obj/network_kernels.o\n./src/network_kernels.cu(359): warning: variable \"l\" was declared but never referenced\n\n./src/network_kernels.cu(368): warning: variable \"im\" was set but never used\n\n./src/network_kernels.cu(359): warning: variable \"l\" was declared but never referenced\n\n./src/network_kernels.cu(368): warning: variable \"im\" was set but never used\n\n./src/network_kernels.cu(359): warning: variable \"l\" was declared but never referenced\n\n./src/network_kernels.cu(368): warning: variable \"im\" was set but never used\n\n./src/network_kernels.cu(359): warning: variable \"l\" was declared but never referenced\n\n./src/network_kernels.cu(368): warning: variable \"im\" was set but never used\n\n./src/network_kernels.cu(359): warning: variable \"l\" was declared but never referenced\n\n./src/network_kernels.cu(368): warning: variable \"im\" was set but never used\n\n\u001b[01m\u001b[K./src/network_kernels.cu:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kfloat train_network_datum_gpu(network, float*, float*)\u001b[m\u001b[K’:\n\u001b[01m\u001b[K./src/network_kernels.cu:359:7:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kvariable ‘\u001b[01m\u001b[Kl\u001b[m\u001b[K’ set but not used [\u001b[01;35m\u001b[K-Wunused-but-set-variable\u001b[m\u001b[K]\n \u001b[01;35m\u001b[K \u001b[m\u001b[K layer l = net.layers[net.n - 1];\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K\n\u001b[01m\u001b[K./src/network_kernels.cu:368:7:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kvariable ‘\u001b[01m\u001b[Kim\u001b[m\u001b[K’ set but not used [\u001b[01;35m\u001b[K-Wunused-but-set-variable\u001b[m\u001b[K]\n \u001b[01;35m\u001b[K \u001b[m\u001b[Kimage im;\n \u001b[01;35m\u001b[K^\u001b[m\u001b[K \nnvcc -gencode arch=compute_30,code=sm_30 -gencode arch=compute_35,code=sm_35 -gencode arch=compute_50,code=[sm_50,compute_50] -gencode arch=compute_52,code=[sm_52,compute_52] -gencode arch=compute_61,code=[sm_61,compute_61] -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN --compiler-options \"-Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC\" -c ./src/avgpool_layer_kernels.cu -o obj/avgpool_layer_kernels.o\ng++ -std=c++11 -std=c++11 -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC obj/image_opencv.o obj/http_stream.o obj/gemm.o obj/utils.o obj/dark_cuda.o obj/convolutional_layer.o obj/list.o obj/image.o obj/activations.o obj/im2col.o obj/col2im.o obj/blas.o obj/crop_layer.o obj/dropout_layer.o obj/maxpool_layer.o obj/softmax_layer.o obj/data.o obj/matrix.o obj/network.o obj/connected_layer.o obj/cost_layer.o obj/parser.o obj/option_list.o obj/darknet.o obj/detection_layer.o obj/captcha.o obj/route_layer.o obj/writing.o obj/box.o obj/nightmare.o obj/normalization_layer.o obj/avgpool_layer.o obj/coco.o obj/dice.o obj/yolo.o obj/detector.o obj/layer.o obj/compare.o obj/classifier.o obj/local_layer.o obj/swag.o obj/shortcut_layer.o obj/activation_layer.o obj/rnn_layer.o obj/gru_layer.o obj/rnn.o obj/rnn_vid.o obj/crnn_layer.o obj/demo.o obj/tag.o obj/cifar.o obj/go.o obj/batchnorm_layer.o obj/art.o obj/region_layer.o obj/reorg_layer.o obj/reorg_old_layer.o obj/super.o obj/voxel.o obj/tree.o obj/yolo_layer.o obj/gaussian_yolo_layer.o obj/upsample_layer.o obj/lstm_layer.o obj/conv_lstm_layer.o obj/scale_channels_layer.o obj/sam_layer.o obj/convolutional_kernels.o obj/activation_kernels.o obj/im2col_kernels.o obj/col2im_kernels.o obj/blas_kernels.o obj/crop_layer_kernels.o obj/dropout_layer_kernels.o obj/maxpool_layer_kernels.o obj/network_kernels.o obj/avgpool_layer_kernels.o -o darknet -lm -pthread `pkg-config --libs opencv4 2> /dev/null || pkg-config --libs opencv` -L/usr/local/cuda/lib64 -lcuda -lcudart -lcublas -lcurand -L/usr/local/cudnn/lib64 -lcudnn -lstdc++\ng++ -std=c++11 -shared -std=c++11 -fvisibility=hidden -DLIB_EXPORTS -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC ./obj/image_opencv.o ./obj/http_stream.o ./obj/gemm.o ./obj/utils.o ./obj/dark_cuda.o ./obj/convolutional_layer.o ./obj/list.o ./obj/image.o ./obj/activations.o ./obj/im2col.o ./obj/col2im.o ./obj/blas.o ./obj/crop_layer.o ./obj/dropout_layer.o ./obj/maxpool_layer.o ./obj/softmax_layer.o ./obj/data.o ./obj/matrix.o ./obj/network.o ./obj/connected_layer.o ./obj/cost_layer.o ./obj/parser.o ./obj/option_list.o ./obj/darknet.o ./obj/detection_layer.o ./obj/captcha.o ./obj/route_layer.o ./obj/writing.o ./obj/box.o ./obj/nightmare.o ./obj/normalization_layer.o ./obj/avgpool_layer.o ./obj/coco.o ./obj/dice.o ./obj/yolo.o ./obj/detector.o ./obj/layer.o ./obj/compare.o ./obj/classifier.o ./obj/local_layer.o ./obj/swag.o ./obj/shortcut_layer.o ./obj/activation_layer.o ./obj/rnn_layer.o ./obj/gru_layer.o ./obj/rnn.o ./obj/rnn_vid.o ./obj/crnn_layer.o ./obj/demo.o ./obj/tag.o ./obj/cifar.o ./obj/go.o ./obj/batchnorm_layer.o ./obj/art.o ./obj/region_layer.o ./obj/reorg_layer.o ./obj/reorg_old_layer.o ./obj/super.o ./obj/voxel.o ./obj/tree.o ./obj/yolo_layer.o ./obj/gaussian_yolo_layer.o ./obj/upsample_layer.o ./obj/lstm_layer.o ./obj/conv_lstm_layer.o ./obj/scale_channels_layer.o ./obj/sam_layer.o ./obj/convolutional_kernels.o ./obj/activation_kernels.o ./obj/im2col_kernels.o ./obj/col2im_kernels.o ./obj/blas_kernels.o ./obj/crop_layer_kernels.o ./obj/dropout_layer_kernels.o ./obj/maxpool_layer_kernels.o ./obj/network_kernels.o ./obj/avgpool_layer_kernels.o src/yolo_v2_class.cpp -o libdarknet.so -lm -pthread `pkg-config --libs opencv4 2> /dev/null || pkg-config --libs opencv` -L/usr/local/cuda/lib64 -lcuda -lcudart -lcublas -lcurand -L/usr/local/cudnn/lib64 -lcudnn -lstdc++\nIn file included from \u001b[01m\u001b[Ksrc/yolo_v2_class.cpp:2:0\u001b[m\u001b[K:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:\u001b[m\u001b[K In constructor ‘\u001b[01m\u001b[Ktrack_kalman_t::track_kalman_t(int, int, float, cv::Size)\u001b[m\u001b[K’:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:708:14:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K‘\u001b[01m\u001b[Ktrack_kalman_t::img_size\u001b[m\u001b[K’ will be initialized after [\u001b[01;35m\u001b[K-Wreorder\u001b[m\u001b[K]\n cv::Size \u001b[01;35m\u001b[Kimg_size\u001b[m\u001b[K; // max value of x,y,w,h\n \u001b[01;35m\u001b[K^~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:700:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K ‘\u001b[01m\u001b[Kint track_kalman_t::track_id_counter\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wreorder\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Ktrack_id_counter\u001b[m\u001b[K;\n \u001b[01;35m\u001b[K^~~~~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:853:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K when initialized here [\u001b[01;35m\u001b[K-Wreorder\u001b[m\u001b[K]\n \u001b[01;35m\u001b[Ktrack_kalman_t\u001b[m\u001b[K(int _max_objects = 1000, int _min_frames = 3, float _max_dist = 40, cv::Size _img_size = cv::Size(10000, 10000)) :\n \u001b[01;35m\u001b[K^~~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:\u001b[m\u001b[K In member function ‘\u001b[01m\u001b[Kvoid track_kalman_t::clear_old_states()\u001b[m\u001b[K’:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:879:50:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n if ((result_vec_pred[state_id].x > img_size.width) ||\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:880:50:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n (result_vec_pred[state_id].y > img_size.height))\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:\u001b[m\u001b[K In member function ‘\u001b[01m\u001b[Ktrack_kalman_t::tst_t track_kalman_t::get_state_id(bbox_t, std::vector<bool>&)\u001b[m\u001b[K’:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:900:30:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n for (size_t i = 0; \u001b[01;35m\u001b[Ki < max_objects\u001b[m\u001b[K; ++i)\n \u001b[01;35m\u001b[K~~^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:\u001b[m\u001b[K In member function ‘\u001b[01m\u001b[Kstd::vector<bbox_t> track_kalman_t::predict()\u001b[m\u001b[K’:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:990:30:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n for (size_t i = 0; \u001b[01;35m\u001b[Ki < max_objects\u001b[m\u001b[K; ++i)\n \u001b[01;35m\u001b[K~~^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:\u001b[m\u001b[K In member function ‘\u001b[01m\u001b[Kstd::vector<bbox_t> track_kalman_t::correct(std::vector<bbox_t>)\u001b[m\u001b[K’:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:1025:30:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n for (size_t i = 0; \u001b[01;35m\u001b[Ki < max_objects\u001b[m\u001b[K; ++i)\n \u001b[01;35m\u001b[K~~^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Ksrc/yolo_v2_class.cpp:\u001b[m\u001b[K In member function ‘\u001b[01m\u001b[Kstd::vector<bbox_t> Detector::tracking_id(std::vector<bbox_t>, bool, int, int)\u001b[m\u001b[K’:\n\u001b[01m\u001b[Ksrc/yolo_v2_class.cpp:370:40:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n if (\u001b[01;35m\u001b[Kprev_bbox_vec_deque.size() > frames_story\u001b[m\u001b[K) prev_bbox_vec_deque.pop_back();\n \u001b[01;35m\u001b[K~~~~~~~~~~~~~~~~~~~~~~~~~~~^~~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Ksrc/yolo_v2_class.cpp:385:34:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n if (\u001b[01;35m\u001b[Kcur_dist < max_dist\u001b[m\u001b[K && (k.track_id == 0 || dist_vec[m] > cur_dist)) {\n \u001b[01;35m\u001b[K~~~~~~~~~^~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Ksrc/yolo_v2_class.cpp:409:40:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n if (\u001b[01;35m\u001b[Kprev_bbox_vec_deque.size() > frames_story\u001b[m\u001b[K) prev_bbox_vec_deque.pop_back();\n \u001b[01;35m\u001b[K~~~~~~~~~~~~~~~~~~~~~~~~~~~^~~~~~~~~~~~~~\u001b[m\u001b[K\ng++ -std=c++11 -std=c++11 -Iinclude/ -I3rdparty/stb/include -DOPENCV `pkg-config --cflags opencv4 2> /dev/null || pkg-config --cflags opencv` -DGPU -I/usr/local/cuda/include/ -DCUDNN -Wall -Wfatal-errors -Wno-unused-result -Wno-unknown-pragmas -fPIC -Ofast -DOPENCV -DGPU -DCUDNN -I/usr/local/cudnn/include -fPIC -o uselib src/yolo_console_dll.cpp -lm -pthread `pkg-config --libs opencv4 2> /dev/null || pkg-config --libs opencv` -L/usr/local/cuda/lib64 -lcuda -lcudart -lcublas -lcurand -L/usr/local/cudnn/lib64 -lcudnn -lstdc++ -L ./ -l:libdarknet.so\nIn file included from \u001b[01m\u001b[Ksrc/yolo_console_dll.cpp:23:0\u001b[m\u001b[K:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:\u001b[m\u001b[K In constructor ‘\u001b[01m\u001b[Ktrack_kalman_t::track_kalman_t(int, int, float, cv::Size)\u001b[m\u001b[K’:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:708:14:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K‘\u001b[01m\u001b[Ktrack_kalman_t::img_size\u001b[m\u001b[K’ will be initialized after [\u001b[01;35m\u001b[K-Wreorder\u001b[m\u001b[K]\n cv::Size \u001b[01;35m\u001b[Kimg_size\u001b[m\u001b[K; // max value of x,y,w,h\n \u001b[01;35m\u001b[K^~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:700:9:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K ‘\u001b[01m\u001b[Kint track_kalman_t::track_id_counter\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wreorder\u001b[m\u001b[K]\n int \u001b[01;35m\u001b[Ktrack_id_counter\u001b[m\u001b[K;\n \u001b[01;35m\u001b[K^~~~~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:853:5:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K when initialized here [\u001b[01;35m\u001b[K-Wreorder\u001b[m\u001b[K]\n \u001b[01;35m\u001b[Ktrack_kalman_t\u001b[m\u001b[K(int _max_objects = 1000, int _min_frames = 3, float _max_dist = 40, cv::Size _img_size = cv::Size(10000, 10000)) :\n \u001b[01;35m\u001b[K^~~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:\u001b[m\u001b[K In member function ‘\u001b[01m\u001b[Kvoid track_kalman_t::clear_old_states()\u001b[m\u001b[K’:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:879:50:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n if ((result_vec_pred[state_id].x > img_size.width) ||\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:880:50:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n (result_vec_pred[state_id].y > img_size.height))\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:\u001b[m\u001b[K In member function ‘\u001b[01m\u001b[Ktrack_kalman_t::tst_t track_kalman_t::get_state_id(bbox_t, std::vector<bool>&)\u001b[m\u001b[K’:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:900:30:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n for (size_t i = 0; \u001b[01;35m\u001b[Ki < max_objects\u001b[m\u001b[K; ++i)\n \u001b[01;35m\u001b[K~~^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:\u001b[m\u001b[K In member function ‘\u001b[01m\u001b[Kstd::vector<bbox_t> track_kalman_t::predict()\u001b[m\u001b[K’:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:990:30:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n for (size_t i = 0; \u001b[01;35m\u001b[Ki < max_objects\u001b[m\u001b[K; ++i)\n \u001b[01;35m\u001b[K~~^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:\u001b[m\u001b[K In member function ‘\u001b[01m\u001b[Kstd::vector<bbox_t> track_kalman_t::correct(std::vector<bbox_t>)\u001b[m\u001b[K’:\n\u001b[01m\u001b[Kinclude/yolo_v2_class.hpp:1025:30:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n for (size_t i = 0; \u001b[01;35m\u001b[Ki < max_objects\u001b[m\u001b[K; ++i)\n \u001b[01;35m\u001b[K~~^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Ksrc/yolo_console_dll.cpp:\u001b[m\u001b[K In function ‘\u001b[01m\u001b[Kvoid draw_boxes(cv::Mat, std::vector<bbox_t>, std::vector<std::__cxx11::basic_string<char> >, int, int)\u001b[m\u001b[K’:\n\u001b[01m\u001b[Ksrc/yolo_console_dll.cpp:192:46:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n int max_width = (\u001b[01;35m\u001b[Ktext_size.width > i.w + 2\u001b[m\u001b[K) ? text_size.width : (i.w + 2);\n \u001b[01;35m\u001b[K~~~~~~~~~~~~~~~~^~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Ksrc/yolo_console_dll.cpp:201:62:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kcomparison between signed and unsigned integer expressions [\u001b[01;35m\u001b[K-Wsign-compare\u001b[m\u001b[K]\n int const max_width_3d = (\u001b[01;35m\u001b[Ktext_size_3d.width > i.w + 2\u001b[m\u001b[K) ? text_size_3d.width : (i.w + 2);\n \u001b[01;35m\u001b[K~~~~~~~~~~~~~~~~~~~^~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Ksrc/yolo_console_dll.cpp:183:15:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[Kunused variable ‘\u001b[01m\u001b[Kcolors\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wunused-variable\u001b[m\u001b[K]\n int const \u001b[01;35m\u001b[Kcolors\u001b[m\u001b[K[6][3] = { { 1,0,1 },{ 0,0,1 },{ 0,1,1 },{ 0,1,0 },{ 1,1,0 },{ 1,0,0 } };\n \u001b[01;35m\u001b[K^~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Ksrc/yolo_console_dll.cpp:\u001b[m\u001b[K In constructor ‘\u001b[01m\u001b[Kmain(int, char**)::detection_data_t::detection_data_t()\u001b[m\u001b[K’:\n\u001b[01m\u001b[Ksrc/yolo_console_dll.cpp:398:26:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K‘\u001b[01m\u001b[Kmain(int, char**)::detection_data_t::exit_flag\u001b[m\u001b[K’ will be initialized after [\u001b[01;35m\u001b[K-Wreorder\u001b[m\u001b[K]\n bool \u001b[01;35m\u001b[Kexit_flag\u001b[m\u001b[K;\n \u001b[01;35m\u001b[K^~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Ksrc/yolo_console_dll.cpp:396:26:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K ‘\u001b[01m\u001b[Kbool main(int, char**)::detection_data_t::new_detection\u001b[m\u001b[K’ [\u001b[01;35m\u001b[K-Wreorder\u001b[m\u001b[K]\n bool \u001b[01;35m\u001b[Knew_detection\u001b[m\u001b[K;\n \u001b[01;35m\u001b[K^~~~~~~~~~~~~\u001b[m\u001b[K\n\u001b[01m\u001b[Ksrc/yolo_console_dll.cpp:401:21:\u001b[m\u001b[K \u001b[01;35m\u001b[Kwarning: \u001b[m\u001b[K when initialized here [\u001b[01;35m\u001b[K-Wreorder\u001b[m\u001b[K]\n \u001b[01;35m\u001b[Kdetection_data_t\u001b[m\u001b[K() : exit_flag(false), new_detection(false) {}\n \u001b[01;35m\u001b[K^~~~~~~~~~~~~~~~\u001b[m\u001b[K\n"
],
[
"#download the newly released yolov4 ConvNet weights\n%cd /content/darknet\n!wget https://github.com/AlexeyAB/darknet/releases/download/darknet_yolo_v3_optimal/yolov4.conv.137",
"/content/darknet\n--2021-02-01 02:25:40-- https://github.com/AlexeyAB/darknet/releases/download/darknet_yolo_v3_optimal/yolov4.conv.137\nResolving github.com (github.com)... 140.82.112.4\nConnecting to github.com (github.com)|140.82.112.4|:443... connected.\nHTTP request sent, awaiting response... 302 Found\nLocation: https://github-releases.githubusercontent.com/75388965/48bfe500-889d-11ea-819e-c4d182fcf0db?X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAIWNJYAX4CSVEH53A%2F20210201%2Fus-east-1%2Fs3%2Faws4_request&X-Amz-Date=20210201T022540Z&X-Amz-Expires=300&X-Amz-Signature=d5f415273a983b53cd45aacbaef799e40d42192274f6572c54764853f1dc09c5&X-Amz-SignedHeaders=host&actor_id=0&key_id=0&repo_id=75388965&response-content-disposition=attachment%3B%20filename%3Dyolov4.conv.137&response-content-type=application%2Foctet-stream [following]\n--2021-02-01 02:25:40-- https://github-releases.githubusercontent.com/75388965/48bfe500-889d-11ea-819e-c4d182fcf0db?X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAIWNJYAX4CSVEH53A%2F20210201%2Fus-east-1%2Fs3%2Faws4_request&X-Amz-Date=20210201T022540Z&X-Amz-Expires=300&X-Amz-Signature=d5f415273a983b53cd45aacbaef799e40d42192274f6572c54764853f1dc09c5&X-Amz-SignedHeaders=host&actor_id=0&key_id=0&repo_id=75388965&response-content-disposition=attachment%3B%20filename%3Dyolov4.conv.137&response-content-type=application%2Foctet-stream\nResolving github-releases.githubusercontent.com (github-releases.githubusercontent.com)... 185.199.109.154, 185.199.111.154, 185.199.110.154, ...\nConnecting to github-releases.githubusercontent.com (github-releases.githubusercontent.com)|185.199.109.154|:443... connected.\nHTTP request sent, awaiting response... 200 OK\nLength: 170038676 (162M) [application/octet-stream]\nSaving to: ‘yolov4.conv.137’\n\nyolov4.conv.137 100%[===================>] 162.16M 97.0MB/s in 1.7s \n\n2021-02-01 02:25:42 (97.0 MB/s) - ‘yolov4.conv.137’ saved [170038676/170038676]\n\n"
],
[
"!env",
"CUDNN_VERSION=7.6.5.32\n__EGL_VENDOR_LIBRARY_DIRS=/usr/lib64-nvidia:/usr/share/glvnd/egl_vendor.d/\nLD_LIBRARY_PATH=/usr/lib64-nvidia\nCLOUDSDK_PYTHON=python3\nLANG=en_US.UTF-8\nHOSTNAME=d41f1c72eaaf\nOLDPWD=/\nCLOUDSDK_CONFIG=/content/.config\nNVIDIA_VISIBLE_DEVICES=all\nDATALAB_SETTINGS_OVERRIDES={\"kernelManagerProxyPort\":6000,\"kernelManagerProxyHost\":\"172.28.0.3\",\"jupyterArgs\":[\"--ip=\\\"172.28.0.2\\\"\"],\"debugAdapterMultiplexerPath\":\"/usr/local/bin/dap_multiplexer\"}\nENV=/root/.bashrc\nPAGER=cat\nNCCL_VERSION=2.8.3\nTF_FORCE_GPU_ALLOW_GROWTH=true\nJPY_PARENT_PID=50\nNO_GCE_CHECK=True\nPWD=/content/darknet\nHOME=/root\nLAST_FORCED_REBUILD=20210119\nCLICOLOR=1\nDEBIAN_FRONTEND=noninteractive\nLIBRARY_PATH=/usr/local/cuda/lib64/stubs\nGCE_METADATA_TIMEOUT=0\nGLIBCPP_FORCE_NEW=1\nTBE_CREDS_ADDR=172.28.0.1:8008\nTERM=xterm-color\nSHELL=/bin/bash\nGCS_READ_CACHE_BLOCK_SIZE_MB=16\nPYTHONWARNINGS=ignore:::pip._internal.cli.base_command\nMPLBACKEND=module://ipykernel.pylab.backend_inline\nCUDA_PKG_VERSION=10-1=10.1.243-1\nCUDA_VERSION=10.1.243\nNVIDIA_DRIVER_CAPABILITIES=compute,utility\nSHLVL=1\nPYTHONPATH=/env/python\nNVIDIA_REQUIRE_CUDA=cuda>=10.1 brand=tesla,driver>=396,driver<397 brand=tesla,driver>=410,driver<411 brand=tesla,driver>=418,driver<419\nCOLAB_GPU=1\nGLIBCXX_FORCE_NEW=1\nPATH=/usr/local/nvidia/bin:/usr/local/cuda/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/tools/node/bin:/tools/google-cloud-sdk/bin:/opt/bin\nLD_PRELOAD=/usr/lib/x86_64-linux-gnu/libtcmalloc.so.4\ncompute_capability=75\nGIT_PAGER=cat\n_=/usr/bin/env\n"
]
],
[
[
"# Set up Custom Dataset for YOLOv4",
"_____no_output_____"
],
[
"We'll use Roboflow to convert our dataset from any format to the YOLO Darknet format. \n\n1. To do so, create a free [Roboflow account](https://app.roboflow.ai).\n2. Upload your images and their annotations (in any format: VOC XML, COCO JSON, TensorFlow CSV, etc).\n3. Apply preprocessing and augmentation steps you may like. We recommend at least `auto-orient` and a `resize` to 416x416. Generate your dataset.\n4. Export your dataset in the **YOLO Darknet format**.\n5. Copy your download link, and paste it below.\n\nSee our [blog post](https://blog.roboflow.ai/training-yolov4-on-a-custom-dataset/) for greater detail.\n\nIn this example, I used the open source [BCCD Dataset](https://public.roboflow.ai/object-detection/bccd). (You can `fork` it to your Roboflow account to follow along.)",
"_____no_output_____"
]
],
[
[
"",
"_____no_output_____"
],
[
"from google.colab import drive\ndrive.mount('/content/drive')",
"Drive already mounted at /content/drive; to attempt to forcibly remount, call drive.mount(\"/content/drive\", force_remount=True).\n"
],
[
"\n#if you already have YOLO darknet format, you can skip this step\n%cd /content/darknet\n#!curl -L [YOUR LINK HERE] > roboflow.zip; unzip roboflow.zip; rm roboflow.zip",
"/content/darknet\n"
],
[
"!cp /content/drive/MyDrive/Aquarium-darknet.zip .",
"_____no_output_____"
],
[
"!pwd",
"/content/darknet\n"
],
[
"!unzip ./Aquarium-darknet.zip",
"Archive: ./Aquarium-darknet.zip\n extracting: README.roboflow.txt \n creating: test/\n extracting: test/IMG_2289_jpeg_jpg.rf.c9fb7ce64e187646ae487739a32211c0.jpg \n extracting: test/IMG_2289_jpeg_jpg.rf.c9fb7ce64e187646ae487739a32211c0.txt \n extracting: test/IMG_2301_jpeg_jpg.rf.6dd04d0a5456efe6f27fee62a59e5f83.jpg \n extracting: test/IMG_2301_jpeg_jpg.rf.6dd04d0a5456efe6f27fee62a59e5f83.txt \n extracting: test/IMG_2319_jpeg_jpg.rf.25bad902b39a5242644e28b950b59c48.jpg \n extracting: test/IMG_2319_jpeg_jpg.rf.25bad902b39a5242644e28b950b59c48.txt \n extracting: test/IMG_2347_jpeg_jpg.rf.8eb6aa0f019174501d035ba9eadcfc3b.jpg \n extracting: test/IMG_2347_jpeg_jpg.rf.8eb6aa0f019174501d035ba9eadcfc3b.txt \n extracting: test/IMG_2354_jpeg_jpg.rf.cb821632af68eb905769140987fe62bb.jpg \n extracting: test/IMG_2354_jpeg_jpg.rf.cb821632af68eb905769140987fe62bb.txt \n extracting: test/IMG_2371_jpeg_jpg.rf.71965a62507c48aad0d5b5a1138d4605.jpg \n extracting: test/IMG_2371_jpeg_jpg.rf.71965a62507c48aad0d5b5a1138d4605.txt \n extracting: test/IMG_2379_jpeg_jpg.rf.f1c21c011d908988cf18ff930f8c6bbd.jpg \n extracting: test/IMG_2379_jpeg_jpg.rf.f1c21c011d908988cf18ff930f8c6bbd.txt \n extracting: test/IMG_2380_jpeg_jpg.rf.cccd4f264e74712aa7d042ae299cf26d.jpg \n extracting: test/IMG_2380_jpeg_jpg.rf.cccd4f264e74712aa7d042ae299cf26d.txt \n extracting: test/IMG_2387_jpeg_jpg.rf.4c8596d677467a1d515b4d3620efe607.jpg \n extracting: test/IMG_2387_jpeg_jpg.rf.4c8596d677467a1d515b4d3620efe607.txt \n extracting: test/IMG_2395_jpeg_jpg.rf.c91fef07c41706d2b9e03d88c958a809.jpg \n extracting: test/IMG_2395_jpeg_jpg.rf.c91fef07c41706d2b9e03d88c958a809.txt \n extracting: test/IMG_2423_jpeg_jpg.rf.fb063f01b1582e397043af4ba0b589b4.jpg \n extracting: test/IMG_2423_jpeg_jpg.rf.fb063f01b1582e397043af4ba0b589b4.txt \n extracting: test/IMG_2434_jpeg_jpg.rf.f01573f8951aa4513cb2564c81c6da94.jpg \n extracting: test/IMG_2434_jpeg_jpg.rf.f01573f8951aa4513cb2564c81c6da94.txt \n extracting: test/IMG_2446_jpeg_jpg.rf.3a57026be09f03bab028f37dd20889bb.jpg \n extracting: test/IMG_2446_jpeg_jpg.rf.3a57026be09f03bab028f37dd20889bb.txt \n extracting: test/IMG_2448_jpeg_jpg.rf.94ad015c019cf52f9e43793eadf4ed16.jpg \n extracting: test/IMG_2448_jpeg_jpg.rf.94ad015c019cf52f9e43793eadf4ed16.txt \n extracting: test/IMG_2450_jpeg_jpg.rf.880456543ad18609cee848e7a623c2b3.jpg \n extracting: test/IMG_2450_jpeg_jpg.rf.880456543ad18609cee848e7a623c2b3.txt \n extracting: test/IMG_2465_jpeg_jpg.rf.a19ce07479699fb8665709960704a68f.jpg \n extracting: test/IMG_2465_jpeg_jpg.rf.a19ce07479699fb8665709960704a68f.txt \n extracting: test/IMG_2466_jpeg_jpg.rf.ec4d2ebec4896ddc45560c22e3193e40.jpg \n extracting: test/IMG_2466_jpeg_jpg.rf.ec4d2ebec4896ddc45560c22e3193e40.txt \n extracting: test/IMG_2468_jpeg_jpg.rf.883e249ef90b6b00aec2699608082a6e.jpg \n extracting: test/IMG_2468_jpeg_jpg.rf.883e249ef90b6b00aec2699608082a6e.txt \n extracting: test/IMG_2470_jpeg_jpg.rf.cd0bcd7150f7a726ccadcc8df622f770.jpg \n extracting: test/IMG_2470_jpeg_jpg.rf.cd0bcd7150f7a726ccadcc8df622f770.txt \n extracting: test/IMG_2473_jpeg_jpg.rf.1111bb0e933319bbbfada948552070f9.jpg \n extracting: test/IMG_2473_jpeg_jpg.rf.1111bb0e933319bbbfada948552070f9.txt \n extracting: test/IMG_2477_jpeg_jpg.rf.a8a93fb47081f7555e4dfe2697ea66d5.jpg \n extracting: test/IMG_2477_jpeg_jpg.rf.a8a93fb47081f7555e4dfe2697ea66d5.txt \n extracting: test/IMG_2496_jpeg_jpg.rf.1f0b9c45743c18b151cbc27244764d8a.jpg \n extracting: test/IMG_2496_jpeg_jpg.rf.1f0b9c45743c18b151cbc27244764d8a.txt \n extracting: test/IMG_2499_jpeg_jpg.rf.0b6698c4e4600f00a69917db2b1f685d.jpg \n extracting: test/IMG_2499_jpeg_jpg.rf.0b6698c4e4600f00a69917db2b1f685d.txt \n extracting: test/IMG_2514_jpeg_jpg.rf.5b5b592fbe7b0d63faf3a45e7fcd405f.jpg \n extracting: test/IMG_2514_jpeg_jpg.rf.5b5b592fbe7b0d63faf3a45e7fcd405f.txt \n extracting: test/IMG_2526_jpeg_jpg.rf.9629fe06afe17160c61af2c89648b0be.jpg \n extracting: test/IMG_2526_jpeg_jpg.rf.9629fe06afe17160c61af2c89648b0be.txt \n extracting: test/IMG_2532_jpeg_jpg.rf.92aa7069e699ee91e03f1f33f23c2675.jpg \n extracting: test/IMG_2532_jpeg_jpg.rf.92aa7069e699ee91e03f1f33f23c2675.txt \n extracting: test/IMG_2544_jpeg_jpg.rf.d640e1af76acce1f2d15a876a4365fdb.jpg \n extracting: test/IMG_2544_jpeg_jpg.rf.d640e1af76acce1f2d15a876a4365fdb.txt \n extracting: test/IMG_2547_jpeg_jpg.rf.f8c2289a3bb47e9a537fc7ba6dd747ff.jpg \n extracting: test/IMG_2547_jpeg_jpg.rf.f8c2289a3bb47e9a537fc7ba6dd747ff.txt \n extracting: test/IMG_2570_jpeg_jpg.rf.68258a3b10729b4f65fccaec19acd6ca.jpg \n extracting: test/IMG_2570_jpeg_jpg.rf.68258a3b10729b4f65fccaec19acd6ca.txt \n extracting: test/IMG_2574_jpeg_jpg.rf.e7cc853d9747992a33065c0e263ea2d3.jpg \n extracting: test/IMG_2574_jpeg_jpg.rf.e7cc853d9747992a33065c0e263ea2d3.txt \n extracting: test/IMG_2582_jpeg_jpg.rf.90c9df08e56a81f610e8d60f7186bef1.jpg \n extracting: test/IMG_2582_jpeg_jpg.rf.90c9df08e56a81f610e8d60f7186bef1.txt \n extracting: test/IMG_2588_jpeg_jpg.rf.14e6f127440ccbbbdc36cd713442225c.jpg \n extracting: test/IMG_2588_jpeg_jpg.rf.14e6f127440ccbbbdc36cd713442225c.txt \n extracting: test/IMG_2630_jpeg_jpg.rf.afac6773d073239091bc4d8422ca52f9.jpg \n extracting: test/IMG_2630_jpeg_jpg.rf.afac6773d073239091bc4d8422ca52f9.txt \n extracting: test/IMG_2632_jpeg_jpg.rf.8827ca8a9cc8d91fda30b223105cbcd2.jpg \n extracting: test/IMG_2632_jpeg_jpg.rf.8827ca8a9cc8d91fda30b223105cbcd2.txt \n extracting: test/IMG_2651_jpeg_jpg.rf.5618cec065da6738054800692c60c894.jpg \n extracting: test/IMG_2651_jpeg_jpg.rf.5618cec065da6738054800692c60c894.txt \n extracting: test/IMG_3129_jpeg_jpg.rf.5008b3f2da5f063545c3a1ae87d8e796.jpg \n extracting: test/IMG_3129_jpeg_jpg.rf.5008b3f2da5f063545c3a1ae87d8e796.txt \n extracting: test/IMG_3134_jpeg_jpg.rf.0c49b4c35c2ab5eb26cb01e6f36f4bf8.jpg \n extracting: test/IMG_3134_jpeg_jpg.rf.0c49b4c35c2ab5eb26cb01e6f36f4bf8.txt \n extracting: test/IMG_3136_jpeg_jpg.rf.3200b8dcbd95573ca5fdb7d20d06a059.jpg \n extracting: test/IMG_3136_jpeg_jpg.rf.3200b8dcbd95573ca5fdb7d20d06a059.txt \n extracting: test/IMG_3144_jpeg_jpg.rf.6ff7c8f5068c793e518ba35df3890d60.jpg \n extracting: test/IMG_3144_jpeg_jpg.rf.6ff7c8f5068c793e518ba35df3890d60.txt \n extracting: test/IMG_3154_jpeg_jpg.rf.8d78d9b77a1365eda9eab3a9009ed838.jpg \n extracting: test/IMG_3154_jpeg_jpg.rf.8d78d9b77a1365eda9eab3a9009ed838.txt \n extracting: test/IMG_3164_jpeg_jpg.rf.66986ba9bed9e2efdbc11aabac224433.jpg \n extracting: test/IMG_3164_jpeg_jpg.rf.66986ba9bed9e2efdbc11aabac224433.txt \n extracting: test/IMG_3173_jpeg_jpg.rf.da744387e1db05df687f9546922066e9.jpg \n extracting: test/IMG_3173_jpeg_jpg.rf.da744387e1db05df687f9546922066e9.txt \n extracting: test/IMG_3175_jpeg_jpg.rf.ddc01c44c70493c3dfd4787fe4dd5969.jpg \n extracting: test/IMG_3175_jpeg_jpg.rf.ddc01c44c70493c3dfd4787fe4dd5969.txt \n extracting: test/IMG_8331_jpg.rf.005850385fac71e5f3b0b48c769da4ff.jpg \n extracting: test/IMG_8331_jpg.rf.005850385fac71e5f3b0b48c769da4ff.txt \n extracting: test/IMG_8343_jpg.rf.585c9c9efcf91438a207c2abf18b31cd.jpg \n extracting: test/IMG_8343_jpg.rf.585c9c9efcf91438a207c2abf18b31cd.txt \n extracting: test/IMG_8395_jpg.rf.b605801877385760ad19c9d84eb9a1e1.jpg \n extracting: test/IMG_8395_jpg.rf.b605801877385760ad19c9d84eb9a1e1.txt \n extracting: test/IMG_8396_jpg.rf.4590f022b70c095edca76bb2c75adfe7.jpg \n extracting: test/IMG_8396_jpg.rf.4590f022b70c095edca76bb2c75adfe7.txt \n extracting: test/IMG_8404_jpg.rf.3d0552889f1baaabdd410a19de8d9166.jpg \n extracting: test/IMG_8404_jpg.rf.3d0552889f1baaabdd410a19de8d9166.txt \n extracting: test/IMG_8420_jpg.rf.49ac0c5eddb0584c6fd13e7d8d63f9a6.jpg \n extracting: test/IMG_8420_jpg.rf.49ac0c5eddb0584c6fd13e7d8d63f9a6.txt \n extracting: test/IMG_8452_jpg.rf.4bd4b1dd018a7f90b0dbca7d1e7dab2f.jpg \n extracting: test/IMG_8452_jpg.rf.4bd4b1dd018a7f90b0dbca7d1e7dab2f.txt \n extracting: test/IMG_8490_jpg.rf.f2627a120a15f9ca83ac3d4c0c957e2c.jpg \n extracting: test/IMG_8490_jpg.rf.f2627a120a15f9ca83ac3d4c0c957e2c.txt \n extracting: test/IMG_8497_MOV-0_jpg.rf.b7f8cb7e7d813cbf8b26ecbeb2268b37.jpg \n extracting: test/IMG_8497_MOV-0_jpg.rf.b7f8cb7e7d813cbf8b26ecbeb2268b37.txt \n extracting: test/IMG_8497_MOV-3_jpg.rf.0199c26909f7acd3585b17bbf71f0746.jpg \n extracting: test/IMG_8497_MOV-3_jpg.rf.0199c26909f7acd3585b17bbf71f0746.txt \n extracting: test/IMG_8497_MOV-5_jpg.rf.a0a3d1b166d1e16df2de4e3c70249258.jpg \n extracting: test/IMG_8497_MOV-5_jpg.rf.a0a3d1b166d1e16df2de4e3c70249258.txt \n extracting: test/IMG_8513_MOV-0_jpg.rf.24ad39f213eb981c2810658cfdb54e5e.jpg \n extracting: test/IMG_8513_MOV-0_jpg.rf.24ad39f213eb981c2810658cfdb54e5e.txt \n extracting: test/IMG_8515_jpg.rf.37747eb30e9211b98cb60b210f769f60.jpg \n extracting: test/IMG_8515_jpg.rf.37747eb30e9211b98cb60b210f769f60.txt \n extracting: test/IMG_8582_MOV-0_jpg.rf.d9ca208251ecabcf6e0618e9746420e4.jpg \n extracting: test/IMG_8582_MOV-0_jpg.rf.d9ca208251ecabcf6e0618e9746420e4.txt \n extracting: test/IMG_8582_MOV-3_jpg.rf.cd5173c56a9e5eecc148483167ec267f.jpg \n extracting: test/IMG_8582_MOV-3_jpg.rf.cd5173c56a9e5eecc148483167ec267f.txt \n extracting: test/IMG_8582_MOV-5_jpg.rf.ed597ece5e997f027e3fecebe2c83eb5.jpg \n extracting: test/IMG_8582_MOV-5_jpg.rf.ed597ece5e997f027e3fecebe2c83eb5.txt \n extracting: test/IMG_8590_MOV-2_jpg.rf.bdaf315263098c61b3d53459c2404cc1.jpg \n extracting: test/IMG_8590_MOV-2_jpg.rf.bdaf315263098c61b3d53459c2404cc1.txt \n extracting: test/IMG_8590_MOV-5_jpg.rf.25dc2496c784549874da3d46616b0213.jpg \n extracting: test/IMG_8590_MOV-5_jpg.rf.25dc2496c784549874da3d46616b0213.txt \n extracting: test/IMG_8595_MOV-0_jpg.rf.9519a2af0dd31560536a3316ee0f1539.jpg \n extracting: test/IMG_8595_MOV-0_jpg.rf.9519a2af0dd31560536a3316ee0f1539.txt \n extracting: test/IMG_8599_MOV-3_jpg.rf.1bb2d67f488ed9c15c658678c1c0d401.jpg \n extracting: test/IMG_8599_MOV-3_jpg.rf.1bb2d67f488ed9c15c658678c1c0d401.txt \n extracting: test/_darknet.labels \n creating: train/\n extracting: train/IMG_2274_jpeg_jpg.rf.5a705b7a3bafe65f27d5aa16c7e4a144.jpg \n extracting: train/IMG_2274_jpeg_jpg.rf.5a705b7a3bafe65f27d5aa16c7e4a144.txt \n extracting: train/IMG_2274_jpeg_jpg.rf.a7544abf7a001b549a75ba103cb0f3d2.jpg \n extracting: train/IMG_2274_jpeg_jpg.rf.a7544abf7a001b549a75ba103cb0f3d2.txt \n extracting: train/IMG_2274_jpeg_jpg.rf.b6a009f6c9e56de7caa05bad2110ca1a.jpg \n extracting: train/IMG_2274_jpeg_jpg.rf.b6a009f6c9e56de7caa05bad2110ca1a.txt \n extracting: train/IMG_2275_jpeg_jpg.rf.9a6deeccd9a4a6c2f89943342c5118fb.jpg \n extracting: train/IMG_2275_jpeg_jpg.rf.9a6deeccd9a4a6c2f89943342c5118fb.txt \n extracting: train/IMG_2275_jpeg_jpg.rf.a6adee74d7da810ef9e361fc555a1b3f.jpg \n extracting: train/IMG_2275_jpeg_jpg.rf.a6adee74d7da810ef9e361fc555a1b3f.txt \n extracting: train/IMG_2275_jpeg_jpg.rf.e83fdb9a1bdbc940daadc323ef43a1f1.jpg \n extracting: train/IMG_2275_jpeg_jpg.rf.e83fdb9a1bdbc940daadc323ef43a1f1.txt \n extracting: train/IMG_2276_jpeg_jpg.rf.39f139448c8faf1fcb8e5ae096df3627.jpg \n extracting: train/IMG_2276_jpeg_jpg.rf.39f139448c8faf1fcb8e5ae096df3627.txt \n extracting: train/IMG_2276_jpeg_jpg.rf.46335543c3939aa07af8c2a1ca1eb075.jpg \n extracting: train/IMG_2276_jpeg_jpg.rf.46335543c3939aa07af8c2a1ca1eb075.txt \n extracting: train/IMG_2276_jpeg_jpg.rf.8e4bfdbf2cb1646895705cd9ec2fc8ca.jpg \n extracting: train/IMG_2276_jpeg_jpg.rf.8e4bfdbf2cb1646895705cd9ec2fc8ca.txt \n extracting: train/IMG_2280_jpeg_jpg.rf.176afed62805838f00be1a449e8a0bf5.jpg \n extracting: train/IMG_2280_jpeg_jpg.rf.176afed62805838f00be1a449e8a0bf5.txt \n extracting: train/IMG_2280_jpeg_jpg.rf.5d0d634f4c923f84d712422703994ef2.jpg \n extracting: train/IMG_2280_jpeg_jpg.rf.5d0d634f4c923f84d712422703994ef2.txt \n extracting: train/IMG_2280_jpeg_jpg.rf.ce85c4f41eee8f9edcc02e95dc7add8a.jpg \n extracting: train/IMG_2280_jpeg_jpg.rf.ce85c4f41eee8f9edcc02e95dc7add8a.txt \n extracting: train/IMG_2282_jpeg_jpg.rf.00d5820605e38437bc85f436ead1620b.jpg \n extracting: train/IMG_2282_jpeg_jpg.rf.00d5820605e38437bc85f436ead1620b.txt \n extracting: train/IMG_2282_jpeg_jpg.rf.0e36b275200be33d8b0752baa845e83b.jpg \n extracting: train/IMG_2282_jpeg_jpg.rf.0e36b275200be33d8b0752baa845e83b.txt \n extracting: train/IMG_2282_jpeg_jpg.rf.cdf659bdd38e44f740738904cd28f649.jpg \n extracting: train/IMG_2282_jpeg_jpg.rf.cdf659bdd38e44f740738904cd28f649.txt \n extracting: train/IMG_2283_jpeg_jpg.rf.05c7e59f78c5832da1a1032b94bbf9b4.jpg \n extracting: train/IMG_2283_jpeg_jpg.rf.05c7e59f78c5832da1a1032b94bbf9b4.txt \n extracting: train/IMG_2283_jpeg_jpg.rf.d17e9c9784e89476bc612493e5fd927b.jpg \n extracting: train/IMG_2283_jpeg_jpg.rf.d17e9c9784e89476bc612493e5fd927b.txt \n extracting: train/IMG_2283_jpeg_jpg.rf.d8966c27a61f13d80a9d918e26de155f.jpg \n extracting: train/IMG_2283_jpeg_jpg.rf.d8966c27a61f13d80a9d918e26de155f.txt \n extracting: train/IMG_2284_jpeg_jpg.rf.1451993509c8aa81d626dac7a95ff7e3.jpg \n extracting: train/IMG_2284_jpeg_jpg.rf.1451993509c8aa81d626dac7a95ff7e3.txt \n extracting: train/IMG_2284_jpeg_jpg.rf.2c79f3f775a97e5d1e81d099f7c228ce.jpg \n extracting: train/IMG_2284_jpeg_jpg.rf.2c79f3f775a97e5d1e81d099f7c228ce.txt \n extracting: train/IMG_2284_jpeg_jpg.rf.60ba2b03451622f06932c5a80ba6e162.jpg \n extracting: train/IMG_2284_jpeg_jpg.rf.60ba2b03451622f06932c5a80ba6e162.txt \n extracting: train/IMG_2285_jpeg_jpg.rf.8cda3400fc2ecd011d1328a0f4092e58.jpg \n extracting: train/IMG_2285_jpeg_jpg.rf.8cda3400fc2ecd011d1328a0f4092e58.txt \n extracting: train/IMG_2285_jpeg_jpg.rf.94e4536dbce475cf3f3038cf1ca29fb5.jpg \n extracting: train/IMG_2285_jpeg_jpg.rf.94e4536dbce475cf3f3038cf1ca29fb5.txt \n extracting: train/IMG_2285_jpeg_jpg.rf.d0b467201037e56fb613bb8f58171553.jpg \n extracting: train/IMG_2285_jpeg_jpg.rf.d0b467201037e56fb613bb8f58171553.txt \n extracting: train/IMG_2286_jpeg_jpg.rf.bbfb768c4b4374d6751f18e59efce2ff.jpg \n extracting: train/IMG_2286_jpeg_jpg.rf.bbfb768c4b4374d6751f18e59efce2ff.txt \n extracting: train/IMG_2286_jpeg_jpg.rf.c7e1ffcb8f9606481a72f35788e0e7f7.jpg \n extracting: train/IMG_2286_jpeg_jpg.rf.c7e1ffcb8f9606481a72f35788e0e7f7.txt \n extracting: train/IMG_2286_jpeg_jpg.rf.f001505bda59d6ca364c01ae895a5924.jpg \n extracting: train/IMG_2286_jpeg_jpg.rf.f001505bda59d6ca364c01ae895a5924.txt \n extracting: train/IMG_2287_jpeg_jpg.rf.391161dca0184c5b1a3b369340394a6b.jpg \n extracting: train/IMG_2287_jpeg_jpg.rf.391161dca0184c5b1a3b369340394a6b.txt \n extracting: train/IMG_2287_jpeg_jpg.rf.49f0976a9073f8a3ca99255174fb13a3.jpg \n extracting: train/IMG_2287_jpeg_jpg.rf.49f0976a9073f8a3ca99255174fb13a3.txt \n extracting: train/IMG_2287_jpeg_jpg.rf.da0a41f25df97f7799895a67e9c709f1.jpg \n extracting: train/IMG_2287_jpeg_jpg.rf.da0a41f25df97f7799895a67e9c709f1.txt \n extracting: train/IMG_2290_jpeg_jpg.rf.475a808c79561760162c515270618fe3.jpg \n extracting: train/IMG_2290_jpeg_jpg.rf.475a808c79561760162c515270618fe3.txt \n extracting: train/IMG_2290_jpeg_jpg.rf.640c78ea5e3f212ea708909420f411b9.jpg \n extracting: train/IMG_2290_jpeg_jpg.rf.640c78ea5e3f212ea708909420f411b9.txt \n extracting: train/IMG_2290_jpeg_jpg.rf.9e1ef6bb91806bcc12368df56ab755db.jpg \n extracting: train/IMG_2290_jpeg_jpg.rf.9e1ef6bb91806bcc12368df56ab755db.txt \n extracting: train/IMG_2291_jpeg_jpg.rf.6702e7462191e4a5812a1ee855d6a1d7.jpg \n extracting: train/IMG_2291_jpeg_jpg.rf.6702e7462191e4a5812a1ee855d6a1d7.txt \n extracting: train/IMG_2291_jpeg_jpg.rf.77cf47b4cb27ba6e4a4d04b8fee2b734.jpg \n extracting: train/IMG_2291_jpeg_jpg.rf.77cf47b4cb27ba6e4a4d04b8fee2b734.txt \n extracting: train/IMG_2291_jpeg_jpg.rf.c0c3cda3b532a0ff33f44df8d0790d65.jpg \n extracting: train/IMG_2291_jpeg_jpg.rf.c0c3cda3b532a0ff33f44df8d0790d65.txt \n extracting: train/IMG_2292_jpeg_jpg.rf.5e88174d9ec590f2ed9583727bfbaa98.jpg \n extracting: train/IMG_2292_jpeg_jpg.rf.5e88174d9ec590f2ed9583727bfbaa98.txt \n extracting: train/IMG_2292_jpeg_jpg.rf.72348cc4c2d774adfec4f84b04c73121.jpg \n extracting: train/IMG_2292_jpeg_jpg.rf.72348cc4c2d774adfec4f84b04c73121.txt \n extracting: train/IMG_2292_jpeg_jpg.rf.f7b3bc53ba1473d7a8b4a41d97dfbc5b.jpg \n extracting: train/IMG_2292_jpeg_jpg.rf.f7b3bc53ba1473d7a8b4a41d97dfbc5b.txt \n extracting: train/IMG_2293_jpeg_jpg.rf.871ecd13bfa5a4194309f2f4ccf1aaf1.jpg \n extracting: train/IMG_2293_jpeg_jpg.rf.871ecd13bfa5a4194309f2f4ccf1aaf1.txt \n extracting: train/IMG_2293_jpeg_jpg.rf.88b6cf9695a65fd1c46d85f72a86169e.jpg \n extracting: train/IMG_2293_jpeg_jpg.rf.88b6cf9695a65fd1c46d85f72a86169e.txt \n extracting: train/IMG_2293_jpeg_jpg.rf.f37422a3caeb95db4e91057af2bc9c60.jpg \n extracting: train/IMG_2293_jpeg_jpg.rf.f37422a3caeb95db4e91057af2bc9c60.txt \n extracting: train/IMG_2294_jpeg_jpg.rf.4ebd32c977aa250692efbfac4380ce7f.jpg \n extracting: train/IMG_2294_jpeg_jpg.rf.4ebd32c977aa250692efbfac4380ce7f.txt \n extracting: train/IMG_2294_jpeg_jpg.rf.f8e02097e164e7772673ccb0bc4ab737.jpg \n extracting: train/IMG_2294_jpeg_jpg.rf.f8e02097e164e7772673ccb0bc4ab737.txt \n extracting: train/IMG_2294_jpeg_jpg.rf.fafdbc608291ea5d2b9bd70cb9477463.jpg \n extracting: train/IMG_2294_jpeg_jpg.rf.fafdbc608291ea5d2b9bd70cb9477463.txt \n extracting: train/IMG_2295_jpeg_jpg.rf.5171078cccc1f5b4198328ea315a7c2c.jpg \n extracting: train/IMG_2295_jpeg_jpg.rf.5171078cccc1f5b4198328ea315a7c2c.txt \n extracting: train/IMG_2295_jpeg_jpg.rf.75e35514e207c2ee4314808addd8b0e4.jpg \n extracting: train/IMG_2295_jpeg_jpg.rf.75e35514e207c2ee4314808addd8b0e4.txt \n extracting: train/IMG_2295_jpeg_jpg.rf.ccef4bf929545931464dec3c00b0e885.jpg \n extracting: train/IMG_2295_jpeg_jpg.rf.ccef4bf929545931464dec3c00b0e885.txt \n extracting: train/IMG_2296_jpeg_jpg.rf.66707690eb50b0e160051a82e3ad9cc5.jpg \n extracting: train/IMG_2296_jpeg_jpg.rf.66707690eb50b0e160051a82e3ad9cc5.txt \n extracting: train/IMG_2296_jpeg_jpg.rf.b562481cc09fa7b325b70e5d1aceb289.jpg \n extracting: train/IMG_2296_jpeg_jpg.rf.b562481cc09fa7b325b70e5d1aceb289.txt \n extracting: train/IMG_2296_jpeg_jpg.rf.c67702d6c82eaf1b805d0d5408be8772.jpg \n extracting: train/IMG_2296_jpeg_jpg.rf.c67702d6c82eaf1b805d0d5408be8772.txt \n extracting: train/IMG_2298_jpeg_jpg.rf.3d7a1f5a00004ffccff64311a3d2a9a9.jpg \n extracting: train/IMG_2298_jpeg_jpg.rf.3d7a1f5a00004ffccff64311a3d2a9a9.txt \n extracting: train/IMG_2298_jpeg_jpg.rf.427ec548b00bef82b19681c9b98c560a.jpg \n extracting: train/IMG_2298_jpeg_jpg.rf.427ec548b00bef82b19681c9b98c560a.txt \n extracting: train/IMG_2298_jpeg_jpg.rf.b4b3f3de7e07d7f8654f636b6fe9d628.jpg \n extracting: train/IMG_2298_jpeg_jpg.rf.b4b3f3de7e07d7f8654f636b6fe9d628.txt \n extracting: train/IMG_2299_jpeg_jpg.rf.01736d17400c63ade01ace37538d61f6.jpg \n extracting: train/IMG_2299_jpeg_jpg.rf.01736d17400c63ade01ace37538d61f6.txt \n extracting: train/IMG_2299_jpeg_jpg.rf.675d7e9295205a3013d6655e20a3c2eb.jpg \n extracting: train/IMG_2299_jpeg_jpg.rf.675d7e9295205a3013d6655e20a3c2eb.txt \n extracting: train/IMG_2299_jpeg_jpg.rf.a70fb28ae0ef2e206395276ef508b483.jpg \n extracting: train/IMG_2299_jpeg_jpg.rf.a70fb28ae0ef2e206395276ef508b483.txt \n extracting: train/IMG_2302_jpeg_jpg.rf.4779e7d45b0e978d5d4d41ebceedadfb.jpg \n extracting: train/IMG_2302_jpeg_jpg.rf.4779e7d45b0e978d5d4d41ebceedadfb.txt \n extracting: train/IMG_2302_jpeg_jpg.rf.6b375e0172705e1d9074f9ccc93ca14e.jpg \n extracting: train/IMG_2302_jpeg_jpg.rf.6b375e0172705e1d9074f9ccc93ca14e.txt \n extracting: train/IMG_2302_jpeg_jpg.rf.ab46bbaf0e3c3818cc179a86813613ae.jpg \n extracting: train/IMG_2302_jpeg_jpg.rf.ab46bbaf0e3c3818cc179a86813613ae.txt \n extracting: train/IMG_2303_jpeg_jpg.rf.3b1c442fe7434bc56c4094fef75faf6e.jpg \n extracting: train/IMG_2303_jpeg_jpg.rf.3b1c442fe7434bc56c4094fef75faf6e.txt \n extracting: train/IMG_2303_jpeg_jpg.rf.851f798e6557f2dee4a7f8e5a257190c.jpg \n extracting: train/IMG_2303_jpeg_jpg.rf.851f798e6557f2dee4a7f8e5a257190c.txt \n extracting: train/IMG_2303_jpeg_jpg.rf.c4664eb9da0875cc437b84da5d6be781.jpg \n extracting: train/IMG_2303_jpeg_jpg.rf.c4664eb9da0875cc437b84da5d6be781.txt \n extracting: train/IMG_2304_jpeg_jpg.rf.4af327a902e39801d08384b042731c87.jpg \n extracting: train/IMG_2304_jpeg_jpg.rf.4af327a902e39801d08384b042731c87.txt \n extracting: train/IMG_2304_jpeg_jpg.rf.c24c61df9f6928e242a0d4edd82fa9bf.jpg \n extracting: train/IMG_2304_jpeg_jpg.rf.c24c61df9f6928e242a0d4edd82fa9bf.txt \n extracting: train/IMG_2304_jpeg_jpg.rf.fbe76aa26ab2eb9e68d3759b800977ed.jpg \n extracting: train/IMG_2304_jpeg_jpg.rf.fbe76aa26ab2eb9e68d3759b800977ed.txt \n extracting: train/IMG_2306_jpeg_jpg.rf.0d11fd7421cf8c9e7744b688b8b10516.jpg \n extracting: train/IMG_2306_jpeg_jpg.rf.0d11fd7421cf8c9e7744b688b8b10516.txt \n extracting: train/IMG_2306_jpeg_jpg.rf.21ed159ff5ac9a2f6fb04a55be655bfc.jpg \n extracting: train/IMG_2306_jpeg_jpg.rf.21ed159ff5ac9a2f6fb04a55be655bfc.txt \n extracting: train/IMG_2306_jpeg_jpg.rf.ea8341b496aacc51a819e2164bd44c9a.jpg \n extracting: train/IMG_2306_jpeg_jpg.rf.ea8341b496aacc51a819e2164bd44c9a.txt \n extracting: train/IMG_2307_jpeg_jpg.rf.49ed59b4f122aedfa3aa59451c37184d.jpg \n extracting: train/IMG_2307_jpeg_jpg.rf.49ed59b4f122aedfa3aa59451c37184d.txt \n extracting: train/IMG_2307_jpeg_jpg.rf.5d92355b0f767dc7b30fe636e25604cc.jpg \n extracting: train/IMG_2307_jpeg_jpg.rf.5d92355b0f767dc7b30fe636e25604cc.txt \n extracting: train/IMG_2307_jpeg_jpg.rf.82b02aedd4ca7ecdec5ee24794340867.jpg \n extracting: train/IMG_2307_jpeg_jpg.rf.82b02aedd4ca7ecdec5ee24794340867.txt \n extracting: train/IMG_2308_jpeg_jpg.rf.69c579dda1dadab86d8e711afe5574f2.jpg \n extracting: train/IMG_2308_jpeg_jpg.rf.69c579dda1dadab86d8e711afe5574f2.txt \n extracting: train/IMG_2308_jpeg_jpg.rf.73986b8a43229150e6a44b7e9aa80e16.jpg \n extracting: train/IMG_2308_jpeg_jpg.rf.73986b8a43229150e6a44b7e9aa80e16.txt \n extracting: train/IMG_2308_jpeg_jpg.rf.fca325b7b24fd5fbc744df0abcbfd709.jpg \n extracting: train/IMG_2308_jpeg_jpg.rf.fca325b7b24fd5fbc744df0abcbfd709.txt \n extracting: train/IMG_2309_jpeg_jpg.rf.609a5d7324cc52fa856812844fc93e7f.jpg \n extracting: train/IMG_2309_jpeg_jpg.rf.609a5d7324cc52fa856812844fc93e7f.txt \n extracting: train/IMG_2309_jpeg_jpg.rf.a12e9845e45f046e324f8075b4a00ddd.jpg \n extracting: train/IMG_2309_jpeg_jpg.rf.a12e9845e45f046e324f8075b4a00ddd.txt \n extracting: train/IMG_2309_jpeg_jpg.rf.f76b378804205c0925f4f1f2851e2725.jpg \n extracting: train/IMG_2309_jpeg_jpg.rf.f76b378804205c0925f4f1f2851e2725.txt \n extracting: train/IMG_2310_jpeg_jpg.rf.4dc07a45ea9a45124bd976c70ea26b76.jpg \n extracting: train/IMG_2310_jpeg_jpg.rf.4dc07a45ea9a45124bd976c70ea26b76.txt \n extracting: train/IMG_2310_jpeg_jpg.rf.5223a36721d81e623a62580df804b4d9.jpg \n extracting: train/IMG_2310_jpeg_jpg.rf.5223a36721d81e623a62580df804b4d9.txt \n extracting: train/IMG_2310_jpeg_jpg.rf.fc94ee65cf20ffe3a0b728b2abc27c2d.jpg \n extracting: train/IMG_2310_jpeg_jpg.rf.fc94ee65cf20ffe3a0b728b2abc27c2d.txt \n extracting: train/IMG_2311_jpeg_jpg.rf.3e6258198450d6dd5167d380749cb56b.jpg \n extracting: train/IMG_2311_jpeg_jpg.rf.3e6258198450d6dd5167d380749cb56b.txt \n extracting: train/IMG_2311_jpeg_jpg.rf.a4fd2aabc9126738985f67bd976623ad.jpg \n extracting: train/IMG_2311_jpeg_jpg.rf.a4fd2aabc9126738985f67bd976623ad.txt \n extracting: train/IMG_2311_jpeg_jpg.rf.ca15270087497b142786300ad8ba391e.jpg \n extracting: train/IMG_2311_jpeg_jpg.rf.ca15270087497b142786300ad8ba391e.txt \n extracting: train/IMG_2312_jpeg_jpg.rf.0c15662d848a85f0f023363dd4e4284c.jpg \n extracting: train/IMG_2312_jpeg_jpg.rf.0c15662d848a85f0f023363dd4e4284c.txt \n extracting: train/IMG_2312_jpeg_jpg.rf.896a2f8462f5d5f1d251577a267e68a7.jpg \n extracting: train/IMG_2312_jpeg_jpg.rf.896a2f8462f5d5f1d251577a267e68a7.txt \n extracting: train/IMG_2312_jpeg_jpg.rf.f183b6ccaef841ef3efe08f833659225.jpg \n extracting: train/IMG_2312_jpeg_jpg.rf.f183b6ccaef841ef3efe08f833659225.txt \n extracting: train/IMG_2313_jpeg_jpg.rf.5c3ab9789fd174dc69f450795c846ad5.jpg \n extracting: train/IMG_2313_jpeg_jpg.rf.5c3ab9789fd174dc69f450795c846ad5.txt \n extracting: train/IMG_2313_jpeg_jpg.rf.68cb5db91dfb1f69dd222060ab462bd0.jpg \n extracting: train/IMG_2313_jpeg_jpg.rf.68cb5db91dfb1f69dd222060ab462bd0.txt \n extracting: train/IMG_2313_jpeg_jpg.rf.da0cdaec363a2d1362d725e9ca0beb68.jpg \n extracting: train/IMG_2313_jpeg_jpg.rf.da0cdaec363a2d1362d725e9ca0beb68.txt \n extracting: train/IMG_2314_jpeg_jpg.rf.5b70d59111abd8e771013078a7f1c46d.jpg \n extracting: train/IMG_2314_jpeg_jpg.rf.5b70d59111abd8e771013078a7f1c46d.txt \n extracting: train/IMG_2314_jpeg_jpg.rf.7775be3d62e73b707b23547ebfce4e8e.jpg \n extracting: train/IMG_2314_jpeg_jpg.rf.7775be3d62e73b707b23547ebfce4e8e.txt \n extracting: train/IMG_2314_jpeg_jpg.rf.cc0f43b139ee483e376dad2aa495f510.jpg \n extracting: train/IMG_2314_jpeg_jpg.rf.cc0f43b139ee483e376dad2aa495f510.txt \n extracting: train/IMG_2315_jpeg_jpg.rf.443a9b581a92359b13b2eb4be44b0017.jpg \n extracting: train/IMG_2315_jpeg_jpg.rf.443a9b581a92359b13b2eb4be44b0017.txt \n extracting: train/IMG_2315_jpeg_jpg.rf.887ec449cdeb773989b6d0ebbb7f556d.jpg \n extracting: train/IMG_2315_jpeg_jpg.rf.887ec449cdeb773989b6d0ebbb7f556d.txt \n extracting: train/IMG_2315_jpeg_jpg.rf.ab32231a87644a698184862b60055c79.jpg \n extracting: train/IMG_2315_jpeg_jpg.rf.ab32231a87644a698184862b60055c79.txt \n extracting: train/IMG_2316_jpeg_jpg.rf.50d355e71e6884d8819521a28eda949f.jpg \n extracting: train/IMG_2316_jpeg_jpg.rf.50d355e71e6884d8819521a28eda949f.txt \n extracting: train/IMG_2316_jpeg_jpg.rf.53b7b3cc95932f9f07c7dbcf044781d0.jpg \n extracting: train/IMG_2316_jpeg_jpg.rf.53b7b3cc95932f9f07c7dbcf044781d0.txt \n extracting: train/IMG_2316_jpeg_jpg.rf.717b91aef14d0ee4801f36d43c178a67.jpg \n extracting: train/IMG_2316_jpeg_jpg.rf.717b91aef14d0ee4801f36d43c178a67.txt \n extracting: train/IMG_2320_jpeg_jpg.rf.2a893f65af65aed21573a2dc984a73d3.jpg \n extracting: train/IMG_2320_jpeg_jpg.rf.2a893f65af65aed21573a2dc984a73d3.txt \n extracting: train/IMG_2320_jpeg_jpg.rf.79e3d9ab64e9abe62519f88975a379b0.jpg \n extracting: train/IMG_2320_jpeg_jpg.rf.79e3d9ab64e9abe62519f88975a379b0.txt \n extracting: train/IMG_2320_jpeg_jpg.rf.9a8654a5653a897990bb1413155ec8dd.jpg \n extracting: train/IMG_2320_jpeg_jpg.rf.9a8654a5653a897990bb1413155ec8dd.txt \n extracting: train/IMG_2321_jpeg_jpg.rf.20e1fe608122aef20e9619f8a1cf041a.jpg \n extracting: train/IMG_2321_jpeg_jpg.rf.20e1fe608122aef20e9619f8a1cf041a.txt \n extracting: train/IMG_2321_jpeg_jpg.rf.9179198e0ca0e88472f36dbf16398e94.jpg \n extracting: train/IMG_2321_jpeg_jpg.rf.9179198e0ca0e88472f36dbf16398e94.txt \n extracting: train/IMG_2321_jpeg_jpg.rf.d4b1706bc8a721b77ea565dc82dae566.jpg \n extracting: train/IMG_2321_jpeg_jpg.rf.d4b1706bc8a721b77ea565dc82dae566.txt \n extracting: train/IMG_2322_jpeg_jpg.rf.36de6dd0332ff26c3368074eb24aacb5.jpg \n extracting: train/IMG_2322_jpeg_jpg.rf.36de6dd0332ff26c3368074eb24aacb5.txt \n extracting: train/IMG_2322_jpeg_jpg.rf.4d452ee90e4364ffffbc5c1f1768d7bc.jpg \n extracting: train/IMG_2322_jpeg_jpg.rf.4d452ee90e4364ffffbc5c1f1768d7bc.txt \n extracting: train/IMG_2322_jpeg_jpg.rf.e8c0a88979a7129bbee393b68e27bd5e.jpg \n extracting: train/IMG_2322_jpeg_jpg.rf.e8c0a88979a7129bbee393b68e27bd5e.txt \n extracting: train/IMG_2324_jpeg_jpg.rf.0a4178e1676ec65c87c7737098e8fe96.jpg \n extracting: train/IMG_2324_jpeg_jpg.rf.0a4178e1676ec65c87c7737098e8fe96.txt \n extracting: train/IMG_2324_jpeg_jpg.rf.e5c3c829064032afec947ef5a4a95e50.jpg \n extracting: train/IMG_2324_jpeg_jpg.rf.e5c3c829064032afec947ef5a4a95e50.txt \n extracting: train/IMG_2324_jpeg_jpg.rf.ff8e4ef84f1eb36897145642faed91aa.jpg \n extracting: train/IMG_2324_jpeg_jpg.rf.ff8e4ef84f1eb36897145642faed91aa.txt \n extracting: train/IMG_2326_jpeg_jpg.rf.c464c636ebc23d9c961dcac17d33d3a3.jpg \n extracting: train/IMG_2326_jpeg_jpg.rf.c464c636ebc23d9c961dcac17d33d3a3.txt \n extracting: train/IMG_2326_jpeg_jpg.rf.d3571a125276f1c15808505923f6373c.jpg \n extracting: train/IMG_2326_jpeg_jpg.rf.d3571a125276f1c15808505923f6373c.txt \n extracting: train/IMG_2326_jpeg_jpg.rf.e0d928de0860c26f8f333c344874fcab.jpg \n extracting: train/IMG_2326_jpeg_jpg.rf.e0d928de0860c26f8f333c344874fcab.txt \n extracting: train/IMG_2327_jpeg_jpg.rf.56ef69e11ee2513647f5ae8bf64a26f3.jpg \n extracting: train/IMG_2327_jpeg_jpg.rf.56ef69e11ee2513647f5ae8bf64a26f3.txt \n extracting: train/IMG_2327_jpeg_jpg.rf.692912593fbd651e7870d2886018d725.jpg \n extracting: train/IMG_2327_jpeg_jpg.rf.692912593fbd651e7870d2886018d725.txt \n extracting: train/IMG_2327_jpeg_jpg.rf.b593e36499bd6d6a97c6ceb6447bd7b3.jpg \n extracting: train/IMG_2327_jpeg_jpg.rf.b593e36499bd6d6a97c6ceb6447bd7b3.txt \n extracting: train/IMG_2328_jpeg_jpg.rf.7abba596e8c41c9083fcf3e7ba2e057a.jpg \n extracting: train/IMG_2328_jpeg_jpg.rf.7abba596e8c41c9083fcf3e7ba2e057a.txt \n extracting: train/IMG_2328_jpeg_jpg.rf.9dede6e3787d506b6b0610c971624eaa.jpg \n extracting: train/IMG_2328_jpeg_jpg.rf.9dede6e3787d506b6b0610c971624eaa.txt \n extracting: train/IMG_2328_jpeg_jpg.rf.ade9f0963ccf5c5d35d06b62e602dce6.jpg \n extracting: train/IMG_2328_jpeg_jpg.rf.ade9f0963ccf5c5d35d06b62e602dce6.txt \n extracting: train/IMG_2330_jpeg_jpg.rf.095b6feb01b9e977226201b5e13e59b8.jpg \n extracting: train/IMG_2330_jpeg_jpg.rf.095b6feb01b9e977226201b5e13e59b8.txt \n extracting: train/IMG_2330_jpeg_jpg.rf.78b89f270dc3dbe26b5a7501b89c6634.jpg \n extracting: train/IMG_2330_jpeg_jpg.rf.78b89f270dc3dbe26b5a7501b89c6634.txt \n extracting: train/IMG_2330_jpeg_jpg.rf.bac087918300814637ca02db8d745c49.jpg \n extracting: train/IMG_2330_jpeg_jpg.rf.bac087918300814637ca02db8d745c49.txt \n extracting: train/IMG_2331_jpeg_jpg.rf.68e1dcd82feccad3d1292f1f8a57ddab.jpg \n extracting: train/IMG_2331_jpeg_jpg.rf.68e1dcd82feccad3d1292f1f8a57ddab.txt \n extracting: train/IMG_2331_jpeg_jpg.rf.a6e8ad19fb436eb43333cb06b17bb2a4.jpg \n extracting: train/IMG_2331_jpeg_jpg.rf.a6e8ad19fb436eb43333cb06b17bb2a4.txt \n extracting: train/IMG_2331_jpeg_jpg.rf.cc6bb2f6e50e7f850a4b23cbcb66c582.jpg \n extracting: train/IMG_2331_jpeg_jpg.rf.cc6bb2f6e50e7f850a4b23cbcb66c582.txt \n extracting: train/IMG_2332_jpeg_jpg.rf.470c6698947d53f01d666d0f161a5ce9.jpg \n extracting: train/IMG_2332_jpeg_jpg.rf.470c6698947d53f01d666d0f161a5ce9.txt \n extracting: train/IMG_2332_jpeg_jpg.rf.592c855aad9e68e7c76d4ca548ebca14.jpg \n extracting: train/IMG_2332_jpeg_jpg.rf.592c855aad9e68e7c76d4ca548ebca14.txt \n extracting: train/IMG_2332_jpeg_jpg.rf.77795a90b69c565da7a9b3bf1ff92c7f.jpg \n extracting: train/IMG_2332_jpeg_jpg.rf.77795a90b69c565da7a9b3bf1ff92c7f.txt \n extracting: train/IMG_2335_jpeg_jpg.rf.25a44af9ee39f9daeb6aa6dfb78f8995.jpg \n extracting: train/IMG_2335_jpeg_jpg.rf.25a44af9ee39f9daeb6aa6dfb78f8995.txt \n extracting: train/IMG_2335_jpeg_jpg.rf.2f1969d837d0df8912adff584e805b90.jpg \n extracting: train/IMG_2335_jpeg_jpg.rf.2f1969d837d0df8912adff584e805b90.txt \n extracting: train/IMG_2335_jpeg_jpg.rf.b6690d9b49d1a9e747b106d2ba930f03.jpg \n extracting: train/IMG_2335_jpeg_jpg.rf.b6690d9b49d1a9e747b106d2ba930f03.txt \n extracting: train/IMG_2336_jpeg_jpg.rf.7464e83085f00dbfdaed78da7f25b533.jpg \n extracting: train/IMG_2336_jpeg_jpg.rf.7464e83085f00dbfdaed78da7f25b533.txt \n extracting: train/IMG_2336_jpeg_jpg.rf.86318566e205f7255b6e1186e4d95351.jpg \n extracting: train/IMG_2336_jpeg_jpg.rf.86318566e205f7255b6e1186e4d95351.txt \n extracting: train/IMG_2336_jpeg_jpg.rf.c27ba8a2563f2ca5c569405229e46ce1.jpg \n extracting: train/IMG_2336_jpeg_jpg.rf.c27ba8a2563f2ca5c569405229e46ce1.txt \n extracting: train/IMG_2338_jpeg_jpg.rf.17c23eed901b64f3bce046420c9433dc.jpg \n extracting: train/IMG_2338_jpeg_jpg.rf.17c23eed901b64f3bce046420c9433dc.txt \n extracting: train/IMG_2338_jpeg_jpg.rf.5d1561c3ce9ee0287154ade085a40760.jpg \n extracting: train/IMG_2338_jpeg_jpg.rf.5d1561c3ce9ee0287154ade085a40760.txt \n extracting: train/IMG_2338_jpeg_jpg.rf.cf731e58912548d928638b30ca74b5b0.jpg \n extracting: train/IMG_2338_jpeg_jpg.rf.cf731e58912548d928638b30ca74b5b0.txt \n extracting: train/IMG_2341_jpeg_jpg.rf.03ec0b4f5a4973f7d05b64075796210a.jpg \n extracting: train/IMG_2341_jpeg_jpg.rf.03ec0b4f5a4973f7d05b64075796210a.txt \n extracting: train/IMG_2341_jpeg_jpg.rf.24ee8489ad3ca443b02537cf44d538a8.jpg \n extracting: train/IMG_2341_jpeg_jpg.rf.24ee8489ad3ca443b02537cf44d538a8.txt \n extracting: train/IMG_2341_jpeg_jpg.rf.aafb499592adb56f35cef321a017c2c5.jpg \n extracting: train/IMG_2341_jpeg_jpg.rf.aafb499592adb56f35cef321a017c2c5.txt \n extracting: train/IMG_2343_jpeg_jpg.rf.47748ce817570f31afe743e46654e234.jpg \n extracting: train/IMG_2343_jpeg_jpg.rf.47748ce817570f31afe743e46654e234.txt \n extracting: train/IMG_2343_jpeg_jpg.rf.82db1dc096456951787ed558adb5fded.jpg \n extracting: train/IMG_2343_jpeg_jpg.rf.82db1dc096456951787ed558adb5fded.txt \n extracting: train/IMG_2343_jpeg_jpg.rf.c9d5d7dfc60f7ace2454b84c669a2321.jpg \n extracting: train/IMG_2343_jpeg_jpg.rf.c9d5d7dfc60f7ace2454b84c669a2321.txt \n extracting: train/IMG_2344_jpeg_jpg.rf.9f32b0c954abe007cee8a58bbb421b98.jpg \n extracting: train/IMG_2344_jpeg_jpg.rf.9f32b0c954abe007cee8a58bbb421b98.txt \n extracting: train/IMG_2344_jpeg_jpg.rf.dfeaf41ead248d1ba411d105d8e4dabf.jpg \n extracting: train/IMG_2344_jpeg_jpg.rf.dfeaf41ead248d1ba411d105d8e4dabf.txt \n extracting: train/IMG_2344_jpeg_jpg.rf.f52f4adfdc940125f941fb6eb3674922.jpg \n extracting: train/IMG_2344_jpeg_jpg.rf.f52f4adfdc940125f941fb6eb3674922.txt \n extracting: train/IMG_2346_jpeg_jpg.rf.3d5b00f9899edd135b4fc3aa6f0c22de.jpg \n extracting: train/IMG_2346_jpeg_jpg.rf.3d5b00f9899edd135b4fc3aa6f0c22de.txt \n extracting: train/IMG_2346_jpeg_jpg.rf.47953fb29866333433f9da429c8979b1.jpg \n extracting: train/IMG_2346_jpeg_jpg.rf.47953fb29866333433f9da429c8979b1.txt \n extracting: train/IMG_2346_jpeg_jpg.rf.a68e5d500c496086b192a50b5693f79e.jpg \n extracting: train/IMG_2346_jpeg_jpg.rf.a68e5d500c496086b192a50b5693f79e.txt \n extracting: train/IMG_2349_jpeg_jpg.rf.158f66171a320cc5835f6975f2f1a648.jpg \n extracting: train/IMG_2349_jpeg_jpg.rf.158f66171a320cc5835f6975f2f1a648.txt \n extracting: train/IMG_2349_jpeg_jpg.rf.a4ef4fab6596405000a41ef3ea3995c8.jpg \n extracting: train/IMG_2349_jpeg_jpg.rf.a4ef4fab6596405000a41ef3ea3995c8.txt \n extracting: train/IMG_2349_jpeg_jpg.rf.a8660dde80f2cf10a9f1c29a907a5c98.jpg \n extracting: train/IMG_2349_jpeg_jpg.rf.a8660dde80f2cf10a9f1c29a907a5c98.txt \n extracting: train/IMG_2350_jpeg_jpg.rf.53e436915c3bd51ba8ec49deb35bf677.jpg \n extracting: train/IMG_2350_jpeg_jpg.rf.53e436915c3bd51ba8ec49deb35bf677.txt \n extracting: train/IMG_2350_jpeg_jpg.rf.958b557c5f2a689b6108e5ec9e25335a.jpg \n extracting: train/IMG_2350_jpeg_jpg.rf.958b557c5f2a689b6108e5ec9e25335a.txt \n extracting: train/IMG_2350_jpeg_jpg.rf.dc61afa740d5e2e5514fc0e4edc3075a.jpg \n extracting: train/IMG_2350_jpeg_jpg.rf.dc61afa740d5e2e5514fc0e4edc3075a.txt \n extracting: train/IMG_2351_jpeg_jpg.rf.162a915edf12d9cc91ca629ec8c6f156.jpg \n extracting: train/IMG_2351_jpeg_jpg.rf.162a915edf12d9cc91ca629ec8c6f156.txt \n extracting: train/IMG_2351_jpeg_jpg.rf.49b790c23ab9d5b4b9aef2c44dcd8417.jpg \n extracting: train/IMG_2351_jpeg_jpg.rf.49b790c23ab9d5b4b9aef2c44dcd8417.txt \n extracting: train/IMG_2351_jpeg_jpg.rf.d4436e55f24f3752634df35a6b9a84fa.jpg \n extracting: train/IMG_2351_jpeg_jpg.rf.d4436e55f24f3752634df35a6b9a84fa.txt \n extracting: train/IMG_2352_jpeg_jpg.rf.00d179ca35560072b0a38f4a99f8e2ea.jpg \n extracting: train/IMG_2352_jpeg_jpg.rf.00d179ca35560072b0a38f4a99f8e2ea.txt \n extracting: train/IMG_2352_jpeg_jpg.rf.0389eaa21992ad8c9a21870c8a4ef2d9.jpg \n extracting: train/IMG_2352_jpeg_jpg.rf.0389eaa21992ad8c9a21870c8a4ef2d9.txt \n extracting: train/IMG_2352_jpeg_jpg.rf.7bcb05d4cbb237384a814038ab537801.jpg \n extracting: train/IMG_2352_jpeg_jpg.rf.7bcb05d4cbb237384a814038ab537801.txt \n extracting: train/IMG_2358_jpeg_jpg.rf.1647fd7a98ba687754e630b9241359c5.jpg \n extracting: train/IMG_2358_jpeg_jpg.rf.1647fd7a98ba687754e630b9241359c5.txt \n extracting: train/IMG_2358_jpeg_jpg.rf.a7c20c9a4a933c0a799f4ac503dd3768.jpg \n extracting: train/IMG_2358_jpeg_jpg.rf.a7c20c9a4a933c0a799f4ac503dd3768.txt \n extracting: train/IMG_2358_jpeg_jpg.rf.b122ea9c94717d1ebcae11dc3ba0aa36.jpg \n extracting: train/IMG_2358_jpeg_jpg.rf.b122ea9c94717d1ebcae11dc3ba0aa36.txt \n extracting: train/IMG_2359_jpeg_jpg.rf.3995866509492d72e6809cbe67d0ded0.jpg \n extracting: train/IMG_2359_jpeg_jpg.rf.3995866509492d72e6809cbe67d0ded0.txt \n extracting: train/IMG_2359_jpeg_jpg.rf.7764586e120f65869dfc741ca513e53f.jpg \n extracting: train/IMG_2359_jpeg_jpg.rf.7764586e120f65869dfc741ca513e53f.txt \n extracting: train/IMG_2359_jpeg_jpg.rf.998f0ab078a3a3e44ce86e90cdf3df42.jpg \n extracting: train/IMG_2359_jpeg_jpg.rf.998f0ab078a3a3e44ce86e90cdf3df42.txt \n extracting: train/IMG_2360_jpeg_jpg.rf.8801d5441a0525386f16184c46078997.jpg \n extracting: train/IMG_2360_jpeg_jpg.rf.8801d5441a0525386f16184c46078997.txt \n extracting: train/IMG_2360_jpeg_jpg.rf.a814d414148b2e2431a0097d1bcb5416.jpg \n extracting: train/IMG_2360_jpeg_jpg.rf.a814d414148b2e2431a0097d1bcb5416.txt \n extracting: train/IMG_2360_jpeg_jpg.rf.bca68126b833347c7b84b8be78d6278e.jpg \n extracting: train/IMG_2360_jpeg_jpg.rf.bca68126b833347c7b84b8be78d6278e.txt \n extracting: train/IMG_2361_jpeg_jpg.rf.0f582a256d442a84ead2898352e2ee32.jpg \n extracting: train/IMG_2361_jpeg_jpg.rf.0f582a256d442a84ead2898352e2ee32.txt \n extracting: train/IMG_2361_jpeg_jpg.rf.5ffe4398b57cfa29baca640a58aaab53.jpg \n extracting: train/IMG_2361_jpeg_jpg.rf.5ffe4398b57cfa29baca640a58aaab53.txt \n extracting: train/IMG_2361_jpeg_jpg.rf.81c1863bcc2f4d555cccbb321a639dec.jpg \n extracting: train/IMG_2361_jpeg_jpg.rf.81c1863bcc2f4d555cccbb321a639dec.txt \n extracting: train/IMG_2362_jpeg_jpg.rf.127fb0646af116ff85788b9aeb1594a8.jpg \n extracting: train/IMG_2362_jpeg_jpg.rf.127fb0646af116ff85788b9aeb1594a8.txt \n extracting: train/IMG_2362_jpeg_jpg.rf.28110819f03a48ca901ccd3901c028f5.jpg \n extracting: train/IMG_2362_jpeg_jpg.rf.28110819f03a48ca901ccd3901c028f5.txt \n extracting: train/IMG_2362_jpeg_jpg.rf.871151b8927a99472cad4518f4f0d934.jpg \n extracting: train/IMG_2362_jpeg_jpg.rf.871151b8927a99472cad4518f4f0d934.txt \n extracting: train/IMG_2363_jpeg_jpg.rf.222068a86d46cb1c5c71fe1976605415.jpg \n extracting: train/IMG_2363_jpeg_jpg.rf.222068a86d46cb1c5c71fe1976605415.txt \n extracting: train/IMG_2363_jpeg_jpg.rf.c614c4fb94c238fc968e0e184a2d9401.jpg \n extracting: train/IMG_2363_jpeg_jpg.rf.c614c4fb94c238fc968e0e184a2d9401.txt \n extracting: train/IMG_2363_jpeg_jpg.rf.cc1ed9bf90cd51b127882468e1550a25.jpg \n extracting: train/IMG_2363_jpeg_jpg.rf.cc1ed9bf90cd51b127882468e1550a25.txt \n extracting: train/IMG_2364_jpeg_jpg.rf.3ef4c85f83dbdec7921b1e21817743f5.jpg \n extracting: train/IMG_2364_jpeg_jpg.rf.3ef4c85f83dbdec7921b1e21817743f5.txt \n extracting: train/IMG_2364_jpeg_jpg.rf.88a47d3dd6842a910200a2be9de8e347.jpg \n extracting: train/IMG_2364_jpeg_jpg.rf.88a47d3dd6842a910200a2be9de8e347.txt \n extracting: train/IMG_2364_jpeg_jpg.rf.c10e4e3cddb6187ede737c0573c03c7f.jpg \n extracting: train/IMG_2364_jpeg_jpg.rf.c10e4e3cddb6187ede737c0573c03c7f.txt \n extracting: train/IMG_2365_jpeg_jpg.rf.2acd2075db865fddadbb03273d47a615.jpg \n extracting: train/IMG_2365_jpeg_jpg.rf.2acd2075db865fddadbb03273d47a615.txt \n extracting: train/IMG_2365_jpeg_jpg.rf.51a02bae749e5feb6105132d6e59cbde.jpg \n extracting: train/IMG_2365_jpeg_jpg.rf.51a02bae749e5feb6105132d6e59cbde.txt \n extracting: train/IMG_2365_jpeg_jpg.rf.c4c8763a29d8de707228811c86112b39.jpg \n extracting: train/IMG_2365_jpeg_jpg.rf.c4c8763a29d8de707228811c86112b39.txt \n extracting: train/IMG_2368_jpeg_jpg.rf.2ae44a6e26ccb514d14a39f1fbb628a1.jpg \n extracting: train/IMG_2368_jpeg_jpg.rf.2ae44a6e26ccb514d14a39f1fbb628a1.txt \n extracting: train/IMG_2368_jpeg_jpg.rf.6df1b1e5da1f5c0658bf59a3ec487a36.jpg \n extracting: train/IMG_2368_jpeg_jpg.rf.6df1b1e5da1f5c0658bf59a3ec487a36.txt \n extracting: train/IMG_2368_jpeg_jpg.rf.91c3ec76563ccac1c715c6c01d5b0a73.jpg \n extracting: train/IMG_2368_jpeg_jpg.rf.91c3ec76563ccac1c715c6c01d5b0a73.txt \n extracting: train/IMG_2369_jpeg_jpg.rf.07586a776a5ab457297b924c5b81d1a1.jpg \n extracting: train/IMG_2369_jpeg_jpg.rf.07586a776a5ab457297b924c5b81d1a1.txt \n extracting: train/IMG_2369_jpeg_jpg.rf.1f7027cfef0226656e8acb74966aaf41.jpg \n extracting: train/IMG_2369_jpeg_jpg.rf.1f7027cfef0226656e8acb74966aaf41.txt \n extracting: train/IMG_2369_jpeg_jpg.rf.c87d2ef27f2d90f439a6d7626b14f9a5.jpg \n extracting: train/IMG_2369_jpeg_jpg.rf.c87d2ef27f2d90f439a6d7626b14f9a5.txt \n extracting: train/IMG_2370_jpeg_jpg.rf.3a13b19ea1e9f27fe51354ce97e598ef.jpg \n extracting: train/IMG_2370_jpeg_jpg.rf.3a13b19ea1e9f27fe51354ce97e598ef.txt \n extracting: train/IMG_2370_jpeg_jpg.rf.4bdf2ec1f53b1a3534cd379975151689.jpg \n extracting: train/IMG_2370_jpeg_jpg.rf.4bdf2ec1f53b1a3534cd379975151689.txt \n extracting: train/IMG_2370_jpeg_jpg.rf.aac48428880b9d6561e154bb68593f24.jpg \n extracting: train/IMG_2370_jpeg_jpg.rf.aac48428880b9d6561e154bb68593f24.txt \n extracting: train/IMG_2372_jpeg_jpg.rf.01b6aeeed5b3f791b77834d64fadc5c8.jpg \n extracting: train/IMG_2372_jpeg_jpg.rf.01b6aeeed5b3f791b77834d64fadc5c8.txt \n extracting: train/IMG_2372_jpeg_jpg.rf.5097d9cb2d988ffa2c44936624cb7ee0.jpg \n extracting: train/IMG_2372_jpeg_jpg.rf.5097d9cb2d988ffa2c44936624cb7ee0.txt \n extracting: train/IMG_2372_jpeg_jpg.rf.c99a572fb95e5dd6f04c40266e75a374.jpg \n extracting: train/IMG_2372_jpeg_jpg.rf.c99a572fb95e5dd6f04c40266e75a374.txt \n extracting: train/IMG_2373_jpeg_jpg.rf.4b59e7fa5aa14f26bf183d9725dbc1e1.jpg \n extracting: train/IMG_2373_jpeg_jpg.rf.4b59e7fa5aa14f26bf183d9725dbc1e1.txt \n extracting: train/IMG_2373_jpeg_jpg.rf.d3b3d8d55d20c5914a9d9f9f3ab2b57a.jpg \n extracting: train/IMG_2373_jpeg_jpg.rf.d3b3d8d55d20c5914a9d9f9f3ab2b57a.txt \n extracting: train/IMG_2373_jpeg_jpg.rf.ef6b3da41fd077844e26dd83ca832798.jpg \n extracting: train/IMG_2373_jpeg_jpg.rf.ef6b3da41fd077844e26dd83ca832798.txt \n extracting: train/IMG_2374_jpeg_jpg.rf.7ff15fcb844b8b3261c10c3921a5f5db.jpg \n extracting: train/IMG_2374_jpeg_jpg.rf.7ff15fcb844b8b3261c10c3921a5f5db.txt \n extracting: train/IMG_2374_jpeg_jpg.rf.c5366fb8bde6473880ca0ed2304c369f.jpg \n extracting: train/IMG_2374_jpeg_jpg.rf.c5366fb8bde6473880ca0ed2304c369f.txt \n extracting: train/IMG_2374_jpeg_jpg.rf.d7670798c719f708b2c9a2f0338e0a2f.jpg \n extracting: train/IMG_2374_jpeg_jpg.rf.d7670798c719f708b2c9a2f0338e0a2f.txt \n extracting: train/IMG_2375_jpeg_jpg.rf.81292baa71ea5bae604e295b694ef695.jpg \n extracting: train/IMG_2375_jpeg_jpg.rf.81292baa71ea5bae604e295b694ef695.txt \n extracting: train/IMG_2375_jpeg_jpg.rf.9aa386ef5497ae7a889d902ac539e34d.jpg \n extracting: train/IMG_2375_jpeg_jpg.rf.9aa386ef5497ae7a889d902ac539e34d.txt \n extracting: train/IMG_2375_jpeg_jpg.rf.bf9aa758f91d9da6920a66d9d6f3b90e.jpg \n extracting: train/IMG_2375_jpeg_jpg.rf.bf9aa758f91d9da6920a66d9d6f3b90e.txt \n extracting: train/IMG_2376_jpeg_jpg.rf.3fbdda2f2d5b9d23c63969bb65f5c7a6.jpg \n extracting: train/IMG_2376_jpeg_jpg.rf.3fbdda2f2d5b9d23c63969bb65f5c7a6.txt \n extracting: train/IMG_2376_jpeg_jpg.rf.7a60ca9ebd46dc25813bbe043590b2d4.jpg \n extracting: train/IMG_2376_jpeg_jpg.rf.7a60ca9ebd46dc25813bbe043590b2d4.txt \n extracting: train/IMG_2376_jpeg_jpg.rf.9448087c9b7619bb6d544aa9f198243e.jpg \n extracting: train/IMG_2376_jpeg_jpg.rf.9448087c9b7619bb6d544aa9f198243e.txt \n extracting: train/IMG_2377_jpeg_jpg.rf.1530215e4383be92c0ff75c0c0d21880.jpg \n extracting: train/IMG_2377_jpeg_jpg.rf.1530215e4383be92c0ff75c0c0d21880.txt \n extracting: train/IMG_2377_jpeg_jpg.rf.fa6b854b505c8c18aa17f246bd39b40d.jpg \n extracting: train/IMG_2377_jpeg_jpg.rf.fa6b854b505c8c18aa17f246bd39b40d.txt \n extracting: train/IMG_2377_jpeg_jpg.rf.fddd0834628ccc932f4869c7255e4b45.jpg \n extracting: train/IMG_2377_jpeg_jpg.rf.fddd0834628ccc932f4869c7255e4b45.txt \n extracting: train/IMG_2378_jpeg_jpg.rf.8aa9611b35cac87c970964b407595ac0.jpg \n extracting: train/IMG_2378_jpeg_jpg.rf.8aa9611b35cac87c970964b407595ac0.txt \n extracting: train/IMG_2378_jpeg_jpg.rf.b1c4402dcd5c64076d81131f688a2a94.jpg \n extracting: train/IMG_2378_jpeg_jpg.rf.b1c4402dcd5c64076d81131f688a2a94.txt \n extracting: train/IMG_2378_jpeg_jpg.rf.f6137f984563937ebfb880d30987ba16.jpg \n extracting: train/IMG_2378_jpeg_jpg.rf.f6137f984563937ebfb880d30987ba16.txt \n extracting: train/IMG_2382_jpeg_jpg.rf.120c95889fc1b513c0e6015b43840dde.jpg \n extracting: train/IMG_2382_jpeg_jpg.rf.120c95889fc1b513c0e6015b43840dde.txt \n extracting: train/IMG_2382_jpeg_jpg.rf.925013f7f81c5cca4a785700e29ddbaa.jpg \n extracting: train/IMG_2382_jpeg_jpg.rf.925013f7f81c5cca4a785700e29ddbaa.txt \n extracting: train/IMG_2382_jpeg_jpg.rf.9f040cf3f4ea3c33fcaa617205ff2d92.jpg \n extracting: train/IMG_2382_jpeg_jpg.rf.9f040cf3f4ea3c33fcaa617205ff2d92.txt \n extracting: train/IMG_2383_jpeg_jpg.rf.08241b339b2898be8afe64d8834b0007.jpg \n extracting: train/IMG_2383_jpeg_jpg.rf.08241b339b2898be8afe64d8834b0007.txt \n extracting: train/IMG_2383_jpeg_jpg.rf.7a954a04951e64aa99019bcaa6697c76.jpg \n extracting: train/IMG_2383_jpeg_jpg.rf.7a954a04951e64aa99019bcaa6697c76.txt \n extracting: train/IMG_2383_jpeg_jpg.rf.7c77149c2375dbaa4012e6c303c765ed.jpg \n extracting: train/IMG_2383_jpeg_jpg.rf.7c77149c2375dbaa4012e6c303c765ed.txt \n extracting: train/IMG_2384_jpeg_jpg.rf.39d58daf95f366391095529388edcdea.jpg \n extracting: train/IMG_2384_jpeg_jpg.rf.39d58daf95f366391095529388edcdea.txt \n extracting: train/IMG_2384_jpeg_jpg.rf.a3d3438f34da0c9fdc6e624bc39f838a.jpg \n extracting: train/IMG_2384_jpeg_jpg.rf.a3d3438f34da0c9fdc6e624bc39f838a.txt \n extracting: train/IMG_2384_jpeg_jpg.rf.e61a2e74e908393600a36c311ba1d224.jpg \n extracting: train/IMG_2384_jpeg_jpg.rf.e61a2e74e908393600a36c311ba1d224.txt \n extracting: train/IMG_2385_jpeg_jpg.rf.2594dc5882059f01c8a8544d50adc716.jpg \n extracting: train/IMG_2385_jpeg_jpg.rf.2594dc5882059f01c8a8544d50adc716.txt \n extracting: train/IMG_2385_jpeg_jpg.rf.f066974d6054aa4a0d67dbbe573877ad.jpg \n extracting: train/IMG_2385_jpeg_jpg.rf.f066974d6054aa4a0d67dbbe573877ad.txt \n extracting: train/IMG_2385_jpeg_jpg.rf.fd78e06b66e5b502f905e2d8872c32b8.jpg \n extracting: train/IMG_2385_jpeg_jpg.rf.fd78e06b66e5b502f905e2d8872c32b8.txt \n extracting: train/IMG_2386_jpeg_jpg.rf.bc4223c3b7c13b201c6ecda81d70ab1e.jpg \n extracting: train/IMG_2386_jpeg_jpg.rf.bc4223c3b7c13b201c6ecda81d70ab1e.txt \n extracting: train/IMG_2386_jpeg_jpg.rf.c71b0702fc9c9b525f9df13d7578c795.jpg \n extracting: train/IMG_2386_jpeg_jpg.rf.c71b0702fc9c9b525f9df13d7578c795.txt \n extracting: train/IMG_2386_jpeg_jpg.rf.f00fd0c6a1578534087e08cd10b52565.jpg \n extracting: train/IMG_2386_jpeg_jpg.rf.f00fd0c6a1578534087e08cd10b52565.txt \n extracting: train/IMG_2389_jpeg_jpg.rf.77577e5dcc3a09ba5fe830ae81ccefbe.jpg \n extracting: train/IMG_2389_jpeg_jpg.rf.77577e5dcc3a09ba5fe830ae81ccefbe.txt \n extracting: train/IMG_2389_jpeg_jpg.rf.da9221286f3e233e6de67c80bdb7263c.jpg \n extracting: train/IMG_2389_jpeg_jpg.rf.da9221286f3e233e6de67c80bdb7263c.txt \n extracting: train/IMG_2389_jpeg_jpg.rf.ec8955d76d0d8987aaf28bf437ca91e5.jpg \n extracting: train/IMG_2389_jpeg_jpg.rf.ec8955d76d0d8987aaf28bf437ca91e5.txt \n extracting: train/IMG_2390_jpeg_jpg.rf.06108e41354fb57377d9bdc5b7710992.jpg \n extracting: train/IMG_2390_jpeg_jpg.rf.06108e41354fb57377d9bdc5b7710992.txt \n extracting: train/IMG_2390_jpeg_jpg.rf.90bb10b0183ae5890809a64477bdcc85.jpg \n extracting: train/IMG_2390_jpeg_jpg.rf.90bb10b0183ae5890809a64477bdcc85.txt \n extracting: train/IMG_2390_jpeg_jpg.rf.ecf4449c17cc2b2572cfc625a0eece14.jpg \n extracting: train/IMG_2390_jpeg_jpg.rf.ecf4449c17cc2b2572cfc625a0eece14.txt \n extracting: train/IMG_2393_jpeg_jpg.rf.282f76c7985c60363b778d352829c473.jpg \n extracting: train/IMG_2393_jpeg_jpg.rf.282f76c7985c60363b778d352829c473.txt \n extracting: train/IMG_2393_jpeg_jpg.rf.46f6070478fa7e6836e32a160903a942.jpg \n extracting: train/IMG_2393_jpeg_jpg.rf.46f6070478fa7e6836e32a160903a942.txt \n extracting: train/IMG_2393_jpeg_jpg.rf.a46096c6dc4b084d7ed44ebf645d4e12.jpg \n extracting: train/IMG_2393_jpeg_jpg.rf.a46096c6dc4b084d7ed44ebf645d4e12.txt \n extracting: train/IMG_2394_jpeg_jpg.rf.7715ecd7aa7699e4fa92530cd91ae5e9.jpg \n extracting: train/IMG_2394_jpeg_jpg.rf.7715ecd7aa7699e4fa92530cd91ae5e9.txt \n extracting: train/IMG_2394_jpeg_jpg.rf.7b02ecc5b0b7e365b7f3891ef2ecce1b.jpg \n extracting: train/IMG_2394_jpeg_jpg.rf.7b02ecc5b0b7e365b7f3891ef2ecce1b.txt \n extracting: train/IMG_2394_jpeg_jpg.rf.a0ee9cc8d44f462f50ab7eb85bc7a6e3.jpg \n extracting: train/IMG_2394_jpeg_jpg.rf.a0ee9cc8d44f462f50ab7eb85bc7a6e3.txt \n extracting: train/IMG_2399_jpeg_jpg.rf.1db395e955b078ab1aa90c4e83587359.jpg \n extracting: train/IMG_2399_jpeg_jpg.rf.1db395e955b078ab1aa90c4e83587359.txt \n extracting: train/IMG_2399_jpeg_jpg.rf.6ef9af6ea1f14d822472542408606816.jpg \n extracting: train/IMG_2399_jpeg_jpg.rf.6ef9af6ea1f14d822472542408606816.txt \n extracting: train/IMG_2399_jpeg_jpg.rf.7d02506c6f8cb943ddb25a3e934233d2.jpg \n extracting: train/IMG_2399_jpeg_jpg.rf.7d02506c6f8cb943ddb25a3e934233d2.txt \n extracting: train/IMG_2400_jpeg_jpg.rf.41c2c3f479dc09b621fb22993a3f4fd9.jpg \n extracting: train/IMG_2400_jpeg_jpg.rf.41c2c3f479dc09b621fb22993a3f4fd9.txt \n extracting: train/IMG_2400_jpeg_jpg.rf.4ff7d37b4187a42bf95f6f37ca459ace.jpg \n extracting: train/IMG_2400_jpeg_jpg.rf.4ff7d37b4187a42bf95f6f37ca459ace.txt \n extracting: train/IMG_2400_jpeg_jpg.rf.8ebb2fdb6dd09ed490ed91075cc05a50.jpg \n extracting: train/IMG_2400_jpeg_jpg.rf.8ebb2fdb6dd09ed490ed91075cc05a50.txt \n extracting: train/IMG_2401_jpeg_jpg.rf.025ea2fa9d9fb7bef9561040692acad6.jpg \n extracting: train/IMG_2401_jpeg_jpg.rf.025ea2fa9d9fb7bef9561040692acad6.txt \n extracting: train/IMG_2401_jpeg_jpg.rf.8a77fa71bd6553748d9a835df932e04a.jpg \n extracting: train/IMG_2401_jpeg_jpg.rf.8a77fa71bd6553748d9a835df932e04a.txt \n extracting: train/IMG_2401_jpeg_jpg.rf.db5b8700f88dd816ccc6039830bec45f.jpg \n extracting: train/IMG_2401_jpeg_jpg.rf.db5b8700f88dd816ccc6039830bec45f.txt \n extracting: train/IMG_2402_jpeg_jpg.rf.6fb2e9ca2daa2f46ac763f6e262ba9f6.jpg \n extracting: train/IMG_2402_jpeg_jpg.rf.6fb2e9ca2daa2f46ac763f6e262ba9f6.txt \n extracting: train/IMG_2402_jpeg_jpg.rf.7204bcae9d137cfcf92c503f383cc802.jpg \n extracting: train/IMG_2402_jpeg_jpg.rf.7204bcae9d137cfcf92c503f383cc802.txt \n extracting: train/IMG_2402_jpeg_jpg.rf.afec9ab357188d14088ccb75be60b095.jpg \n extracting: train/IMG_2402_jpeg_jpg.rf.afec9ab357188d14088ccb75be60b095.txt \n extracting: train/IMG_2403_jpeg_jpg.rf.2d90806d6a3a1a4fb1f5c962306a0c86.jpg \n extracting: train/IMG_2403_jpeg_jpg.rf.2d90806d6a3a1a4fb1f5c962306a0c86.txt \n extracting: train/IMG_2403_jpeg_jpg.rf.40bf5f3e47dcdd671555b0ba96da3312.jpg \n extracting: train/IMG_2403_jpeg_jpg.rf.40bf5f3e47dcdd671555b0ba96da3312.txt \n extracting: train/IMG_2403_jpeg_jpg.rf.48423267d3c361e5748e65115ed760fb.jpg \n extracting: train/IMG_2403_jpeg_jpg.rf.48423267d3c361e5748e65115ed760fb.txt \n extracting: train/IMG_2405_jpeg_jpg.rf.0f5064ede283e8692e77a3ceaa3f0692.jpg \n extracting: train/IMG_2405_jpeg_jpg.rf.0f5064ede283e8692e77a3ceaa3f0692.txt \n extracting: train/IMG_2405_jpeg_jpg.rf.dddb25d1cfac5ed01b783fe48d395761.jpg \n extracting: train/IMG_2405_jpeg_jpg.rf.dddb25d1cfac5ed01b783fe48d395761.txt \n extracting: train/IMG_2405_jpeg_jpg.rf.ecbb38c28d2e1d7bbf924237d0fa766f.jpg \n extracting: train/IMG_2405_jpeg_jpg.rf.ecbb38c28d2e1d7bbf924237d0fa766f.txt \n extracting: train/IMG_2406_jpeg_jpg.rf.00be05552b406d646ecef4a64e806469.jpg \n extracting: train/IMG_2406_jpeg_jpg.rf.00be05552b406d646ecef4a64e806469.txt \n extracting: train/IMG_2406_jpeg_jpg.rf.0cf3bcc9e3a4eef41ce0ee5bb1e7322e.jpg \n extracting: train/IMG_2406_jpeg_jpg.rf.0cf3bcc9e3a4eef41ce0ee5bb1e7322e.txt \n extracting: train/IMG_2406_jpeg_jpg.rf.347ef1b37186dc3c10079b4cd0d180a0.jpg \n extracting: train/IMG_2406_jpeg_jpg.rf.347ef1b37186dc3c10079b4cd0d180a0.txt \n extracting: train/IMG_2408_jpeg_jpg.rf.70b97580daa669a38e2f6f1bc9a2909e.jpg \n extracting: train/IMG_2408_jpeg_jpg.rf.70b97580daa669a38e2f6f1bc9a2909e.txt \n extracting: train/IMG_2408_jpeg_jpg.rf.92fa140f4c0284ff7f3589cffdd33c7e.jpg \n extracting: train/IMG_2408_jpeg_jpg.rf.92fa140f4c0284ff7f3589cffdd33c7e.txt \n extracting: train/IMG_2408_jpeg_jpg.rf.da2945378c04a4675daa73a6c5875c27.jpg \n extracting: train/IMG_2408_jpeg_jpg.rf.da2945378c04a4675daa73a6c5875c27.txt \n extracting: train/IMG_2409_jpeg_jpg.rf.a8e8a0ff9fd470d8aebb40c82ac833f3.jpg \n extracting: train/IMG_2409_jpeg_jpg.rf.a8e8a0ff9fd470d8aebb40c82ac833f3.txt \n extracting: train/IMG_2409_jpeg_jpg.rf.b75d3d01cdafbdd8fb6c63abe59192ec.jpg \n extracting: train/IMG_2409_jpeg_jpg.rf.b75d3d01cdafbdd8fb6c63abe59192ec.txt \n extracting: train/IMG_2409_jpeg_jpg.rf.e88b755494c0cf6974efa14bb843ead8.jpg \n extracting: train/IMG_2409_jpeg_jpg.rf.e88b755494c0cf6974efa14bb843ead8.txt \n extracting: train/IMG_2410_jpeg_jpg.rf.295fc360c8c85d2d54027ef0111b10a3.jpg \n extracting: train/IMG_2410_jpeg_jpg.rf.295fc360c8c85d2d54027ef0111b10a3.txt \n extracting: train/IMG_2410_jpeg_jpg.rf.9b7927b5b7cdd08538eb047836f8eddf.jpg \n extracting: train/IMG_2410_jpeg_jpg.rf.9b7927b5b7cdd08538eb047836f8eddf.txt \n extracting: train/IMG_2410_jpeg_jpg.rf.b7820038e2cc18255ab9e217a6e96c46.jpg \n extracting: train/IMG_2410_jpeg_jpg.rf.b7820038e2cc18255ab9e217a6e96c46.txt \n extracting: train/IMG_2412_jpeg_jpg.rf.26b84a2e08903a68e02e9ed9fa55c9a3.jpg \n extracting: train/IMG_2412_jpeg_jpg.rf.26b84a2e08903a68e02e9ed9fa55c9a3.txt \n extracting: train/IMG_2412_jpeg_jpg.rf.4ea8017f9d5c307f23be8ffef28c178b.jpg \n extracting: train/IMG_2412_jpeg_jpg.rf.4ea8017f9d5c307f23be8ffef28c178b.txt \n extracting: train/IMG_2412_jpeg_jpg.rf.f5aa1fe95a9519439d59dbe8e5cb9061.jpg \n extracting: train/IMG_2412_jpeg_jpg.rf.f5aa1fe95a9519439d59dbe8e5cb9061.txt \n extracting: train/IMG_2413_jpeg_jpg.rf.14f1e17224e4d34e82e5ec29fcde4e7b.jpg \n extracting: train/IMG_2413_jpeg_jpg.rf.14f1e17224e4d34e82e5ec29fcde4e7b.txt \n extracting: train/IMG_2413_jpeg_jpg.rf.5d27998319f5104e6e828fe6de4a73c0.jpg \n extracting: train/IMG_2413_jpeg_jpg.rf.5d27998319f5104e6e828fe6de4a73c0.txt \n extracting: train/IMG_2413_jpeg_jpg.rf.b77b5dec7d830c1a18cff9a17609c8e2.jpg \n extracting: train/IMG_2413_jpeg_jpg.rf.b77b5dec7d830c1a18cff9a17609c8e2.txt \n extracting: train/IMG_2414_jpeg_jpg.rf.1c6e78545acc0c36be4ba352a0f103bc.jpg \n extracting: train/IMG_2414_jpeg_jpg.rf.1c6e78545acc0c36be4ba352a0f103bc.txt \n extracting: train/IMG_2414_jpeg_jpg.rf.641627c34d2ee7592c67a48baccac841.jpg \n extracting: train/IMG_2414_jpeg_jpg.rf.641627c34d2ee7592c67a48baccac841.txt \n extracting: train/IMG_2414_jpeg_jpg.rf.f85b62dfc1308d7ce19faa33a124a137.jpg \n extracting: train/IMG_2414_jpeg_jpg.rf.f85b62dfc1308d7ce19faa33a124a137.txt \n extracting: train/IMG_2415_jpeg_jpg.rf.703245d63a41beef6a0f9a594458bb0c.jpg \n extracting: train/IMG_2415_jpeg_jpg.rf.703245d63a41beef6a0f9a594458bb0c.txt \n extracting: train/IMG_2415_jpeg_jpg.rf.b328c7d6955deac35a362ba43fb81526.jpg \n extracting: train/IMG_2415_jpeg_jpg.rf.b328c7d6955deac35a362ba43fb81526.txt \n extracting: train/IMG_2415_jpeg_jpg.rf.be843d11fce077fdb7fffd06ca6e2909.jpg \n extracting: train/IMG_2415_jpeg_jpg.rf.be843d11fce077fdb7fffd06ca6e2909.txt \n extracting: train/IMG_2417_jpeg_jpg.rf.57fa7aa5341157b74df4636acf1f0e6a.jpg \n extracting: train/IMG_2417_jpeg_jpg.rf.57fa7aa5341157b74df4636acf1f0e6a.txt \n extracting: train/IMG_2417_jpeg_jpg.rf.a0a1aa0777c9e8d1f3d4ba249387399f.jpg \n extracting: train/IMG_2417_jpeg_jpg.rf.a0a1aa0777c9e8d1f3d4ba249387399f.txt \n extracting: train/IMG_2417_jpeg_jpg.rf.d40742ce9b89e7d21ae9a3940ba67cf9.jpg \n extracting: train/IMG_2417_jpeg_jpg.rf.d40742ce9b89e7d21ae9a3940ba67cf9.txt \n extracting: train/IMG_2418_jpeg_jpg.rf.229018c990659588bd8784386fb0d4f3.jpg \n extracting: train/IMG_2418_jpeg_jpg.rf.229018c990659588bd8784386fb0d4f3.txt \n extracting: train/IMG_2418_jpeg_jpg.rf.6a7a0caf5c3928f62448b72b4716a09b.jpg \n extracting: train/IMG_2418_jpeg_jpg.rf.6a7a0caf5c3928f62448b72b4716a09b.txt \n extracting: train/IMG_2418_jpeg_jpg.rf.f405a1050a167882a6448ee6b742e79e.jpg \n extracting: train/IMG_2418_jpeg_jpg.rf.f405a1050a167882a6448ee6b742e79e.txt \n extracting: train/IMG_2419_jpeg_jpg.rf.9f4e4c5c73bd8e73b563d9ffebc75354.jpg \n extracting: train/IMG_2419_jpeg_jpg.rf.9f4e4c5c73bd8e73b563d9ffebc75354.txt \n extracting: train/IMG_2419_jpeg_jpg.rf.c5ebf68258fbd8d813e41cc395b901dd.jpg \n extracting: train/IMG_2419_jpeg_jpg.rf.c5ebf68258fbd8d813e41cc395b901dd.txt \n extracting: train/IMG_2419_jpeg_jpg.rf.dde9817cda64f25d8f993dfaa272d4fa.jpg \n extracting: train/IMG_2419_jpeg_jpg.rf.dde9817cda64f25d8f993dfaa272d4fa.txt \n extracting: train/IMG_2420_jpeg_jpg.rf.5a76ed16137603ba903355a7d256c7ad.jpg \n extracting: train/IMG_2420_jpeg_jpg.rf.5a76ed16137603ba903355a7d256c7ad.txt \n extracting: train/IMG_2420_jpeg_jpg.rf.63b34782accd142fa1c9f4da61fadf1d.jpg \n extracting: train/IMG_2420_jpeg_jpg.rf.63b34782accd142fa1c9f4da61fadf1d.txt \n extracting: train/IMG_2420_jpeg_jpg.rf.82b36af601f3b39b761b8a842526d129.jpg \n extracting: train/IMG_2420_jpeg_jpg.rf.82b36af601f3b39b761b8a842526d129.txt \n extracting: train/IMG_2421_jpeg_jpg.rf.0e350987cb9b2b88f46d1881a0a1a500.jpg \n extracting: train/IMG_2421_jpeg_jpg.rf.0e350987cb9b2b88f46d1881a0a1a500.txt \n extracting: train/IMG_2421_jpeg_jpg.rf.284df1968b89d109e780695893d5f503.jpg \n extracting: train/IMG_2421_jpeg_jpg.rf.284df1968b89d109e780695893d5f503.txt \n extracting: train/IMG_2421_jpeg_jpg.rf.4dcfa71b39f882ce1214521a6b28cda1.jpg \n extracting: train/IMG_2421_jpeg_jpg.rf.4dcfa71b39f882ce1214521a6b28cda1.txt \n extracting: train/IMG_2422_jpeg_jpg.rf.2145ccbbe27f8d209e2ed1e9faa6530d.jpg \n extracting: train/IMG_2422_jpeg_jpg.rf.2145ccbbe27f8d209e2ed1e9faa6530d.txt \n extracting: train/IMG_2422_jpeg_jpg.rf.4aab39cbfdee283cd0e1d4518c72d6dd.jpg \n extracting: train/IMG_2422_jpeg_jpg.rf.4aab39cbfdee283cd0e1d4518c72d6dd.txt \n extracting: train/IMG_2422_jpeg_jpg.rf.986f89693a54007420d45df3dac1fe20.jpg \n extracting: train/IMG_2422_jpeg_jpg.rf.986f89693a54007420d45df3dac1fe20.txt \n extracting: train/IMG_2426_jpeg_jpg.rf.5eab049a17ff6163ef2ef69b3b40b079.jpg \n extracting: train/IMG_2426_jpeg_jpg.rf.5eab049a17ff6163ef2ef69b3b40b079.txt \n extracting: train/IMG_2426_jpeg_jpg.rf.601e1c44b09665c252a6870553a0a0d7.jpg \n extracting: train/IMG_2426_jpeg_jpg.rf.601e1c44b09665c252a6870553a0a0d7.txt \n extracting: train/IMG_2426_jpeg_jpg.rf.7ef3c400316a32bc6935683eda9eea6f.jpg \n extracting: train/IMG_2426_jpeg_jpg.rf.7ef3c400316a32bc6935683eda9eea6f.txt \n extracting: train/IMG_2427_jpeg_jpg.rf.3777020ad7b9fe12eb6a6b36bedb012b.jpg \n extracting: train/IMG_2427_jpeg_jpg.rf.3777020ad7b9fe12eb6a6b36bedb012b.txt \n extracting: train/IMG_2427_jpeg_jpg.rf.a263e334d57f9c4875cabfcb2125d0de.jpg \n extracting: train/IMG_2427_jpeg_jpg.rf.a263e334d57f9c4875cabfcb2125d0de.txt \n extracting: train/IMG_2427_jpeg_jpg.rf.b783f44d6d4bbb75eed15d6ea1e5dc3f.jpg \n extracting: train/IMG_2427_jpeg_jpg.rf.b783f44d6d4bbb75eed15d6ea1e5dc3f.txt \n extracting: train/IMG_2429_jpeg_jpg.rf.8d060b99525925bf82a8f9166a78e901.jpg \n extracting: train/IMG_2429_jpeg_jpg.rf.8d060b99525925bf82a8f9166a78e901.txt \n extracting: train/IMG_2429_jpeg_jpg.rf.9a4f763e6a6c40680c5c98177419025c.jpg \n extracting: train/IMG_2429_jpeg_jpg.rf.9a4f763e6a6c40680c5c98177419025c.txt \n extracting: train/IMG_2429_jpeg_jpg.rf.a36fae31f5286b27263f31c1e2ab6b34.jpg \n extracting: train/IMG_2429_jpeg_jpg.rf.a36fae31f5286b27263f31c1e2ab6b34.txt \n extracting: train/IMG_2430_jpeg_jpg.rf.067b86b7dfc370da27c0a84a7edb3448.jpg \n extracting: train/IMG_2430_jpeg_jpg.rf.067b86b7dfc370da27c0a84a7edb3448.txt \n extracting: train/IMG_2430_jpeg_jpg.rf.10680856e24c1615796be0b166964c11.jpg \n extracting: train/IMG_2430_jpeg_jpg.rf.10680856e24c1615796be0b166964c11.txt \n extracting: train/IMG_2430_jpeg_jpg.rf.9922144ede3ed2e7483edc10b4c93bb7.jpg \n extracting: train/IMG_2430_jpeg_jpg.rf.9922144ede3ed2e7483edc10b4c93bb7.txt \n extracting: train/IMG_2432_jpeg_jpg.rf.0f11aa6edf50e69df7dd882e633e8af8.jpg \n extracting: train/IMG_2432_jpeg_jpg.rf.0f11aa6edf50e69df7dd882e633e8af8.txt \n extracting: train/IMG_2432_jpeg_jpg.rf.31c4972f47769d3ce625ed01bf96f0b2.jpg \n extracting: train/IMG_2432_jpeg_jpg.rf.31c4972f47769d3ce625ed01bf96f0b2.txt \n extracting: train/IMG_2432_jpeg_jpg.rf.8debcadda7066af4741b78830d17efbc.jpg \n extracting: train/IMG_2432_jpeg_jpg.rf.8debcadda7066af4741b78830d17efbc.txt \n extracting: train/IMG_2433_jpeg_jpg.rf.05b4ef88ee359827b8ca4d75f8a9bcea.jpg \n extracting: train/IMG_2433_jpeg_jpg.rf.05b4ef88ee359827b8ca4d75f8a9bcea.txt \n extracting: train/IMG_2433_jpeg_jpg.rf.19bac9a7e33d8a566d6643a87c22b489.jpg \n extracting: train/IMG_2433_jpeg_jpg.rf.19bac9a7e33d8a566d6643a87c22b489.txt \n extracting: train/IMG_2433_jpeg_jpg.rf.deb3bac150654c660c35675877494c07.jpg \n extracting: train/IMG_2433_jpeg_jpg.rf.deb3bac150654c660c35675877494c07.txt \n extracting: train/IMG_2435_jpeg_jpg.rf.123dcfb4094cfa7cc9848a3b18f6abbf.jpg \n extracting: train/IMG_2435_jpeg_jpg.rf.123dcfb4094cfa7cc9848a3b18f6abbf.txt \n extracting: train/IMG_2435_jpeg_jpg.rf.af33168fb980921137117dc5e5e34746.jpg \n extracting: train/IMG_2435_jpeg_jpg.rf.af33168fb980921137117dc5e5e34746.txt \n extracting: train/IMG_2435_jpeg_jpg.rf.d3ffbb34d285d5d07243f1ff77508a07.jpg \n extracting: train/IMG_2435_jpeg_jpg.rf.d3ffbb34d285d5d07243f1ff77508a07.txt \n extracting: train/IMG_2436_jpeg_jpg.rf.159a25260fe784bd316e1eccbfe9345a.jpg \n extracting: train/IMG_2436_jpeg_jpg.rf.159a25260fe784bd316e1eccbfe9345a.txt \n extracting: train/IMG_2436_jpeg_jpg.rf.f780d4cdfa27a6f8797241f6b6a61133.jpg \n extracting: train/IMG_2436_jpeg_jpg.rf.f780d4cdfa27a6f8797241f6b6a61133.txt \n extracting: train/IMG_2436_jpeg_jpg.rf.f9636d5a448609c8299b3712731465d8.jpg \n extracting: train/IMG_2436_jpeg_jpg.rf.f9636d5a448609c8299b3712731465d8.txt \n extracting: train/IMG_2437_jpeg_jpg.rf.3192cf8e288aa40426210ee5661224d5.jpg \n extracting: train/IMG_2437_jpeg_jpg.rf.3192cf8e288aa40426210ee5661224d5.txt \n extracting: train/IMG_2437_jpeg_jpg.rf.52d1286d465e85da379752ac6bf96eff.jpg \n extracting: train/IMG_2437_jpeg_jpg.rf.52d1286d465e85da379752ac6bf96eff.txt \n extracting: train/IMG_2437_jpeg_jpg.rf.8ab7c26ade42fbec4a9dbbb45a95977d.jpg \n extracting: train/IMG_2437_jpeg_jpg.rf.8ab7c26ade42fbec4a9dbbb45a95977d.txt \n extracting: train/IMG_2438_jpeg_jpg.rf.1d4cf435c846af8cc7a551bb58bbeef7.jpg \n extracting: train/IMG_2438_jpeg_jpg.rf.1d4cf435c846af8cc7a551bb58bbeef7.txt \n extracting: train/IMG_2438_jpeg_jpg.rf.28cd24fc2a6f943184c5785b66d11d3c.jpg \n extracting: train/IMG_2438_jpeg_jpg.rf.28cd24fc2a6f943184c5785b66d11d3c.txt \n extracting: train/IMG_2438_jpeg_jpg.rf.a639b1de15b3a1ed84fbf6990cc63410.jpg \n extracting: train/IMG_2438_jpeg_jpg.rf.a639b1de15b3a1ed84fbf6990cc63410.txt \n extracting: train/IMG_2439_jpeg_jpg.rf.0d25e58427ad6eb3502de0f715e510a2.jpg \n extracting: train/IMG_2439_jpeg_jpg.rf.0d25e58427ad6eb3502de0f715e510a2.txt \n extracting: train/IMG_2439_jpeg_jpg.rf.39ce576d4b92462f567598985b169eb5.jpg \n extracting: train/IMG_2439_jpeg_jpg.rf.39ce576d4b92462f567598985b169eb5.txt \n extracting: train/IMG_2439_jpeg_jpg.rf.750fd71d419ec93da8a3c6c0a7df7f09.jpg \n extracting: train/IMG_2439_jpeg_jpg.rf.750fd71d419ec93da8a3c6c0a7df7f09.txt \n extracting: train/IMG_2440_jpeg_jpg.rf.3657a42d9b4fb142e2a0f91903413ff1.jpg \n extracting: train/IMG_2440_jpeg_jpg.rf.3657a42d9b4fb142e2a0f91903413ff1.txt \n extracting: train/IMG_2440_jpeg_jpg.rf.dccb153daef1f9f3f0fe7df67d23a6db.jpg \n extracting: train/IMG_2440_jpeg_jpg.rf.dccb153daef1f9f3f0fe7df67d23a6db.txt \n extracting: train/IMG_2440_jpeg_jpg.rf.e12353ff3cad9219640d236118ebe6e2.jpg \n extracting: train/IMG_2440_jpeg_jpg.rf.e12353ff3cad9219640d236118ebe6e2.txt \n extracting: train/IMG_2441_jpeg_jpg.rf.8f8aa63f82ec756f776829e819c60e56.jpg \n extracting: train/IMG_2441_jpeg_jpg.rf.8f8aa63f82ec756f776829e819c60e56.txt \n extracting: train/IMG_2441_jpeg_jpg.rf.c946eb0f13b8c6ad8ed167b4c8d65ce9.jpg \n extracting: train/IMG_2441_jpeg_jpg.rf.c946eb0f13b8c6ad8ed167b4c8d65ce9.txt \n extracting: train/IMG_2441_jpeg_jpg.rf.d64bdb8b949c12092566845d9a841eb5.jpg \n extracting: train/IMG_2441_jpeg_jpg.rf.d64bdb8b949c12092566845d9a841eb5.txt \n extracting: train/IMG_2443_jpeg_jpg.rf.35d2e7a516424c79afea0934e72da8e8.jpg \n extracting: train/IMG_2443_jpeg_jpg.rf.35d2e7a516424c79afea0934e72da8e8.txt \n extracting: train/IMG_2443_jpeg_jpg.rf.a4782ba75f8b90e387dd13ab0d361af1.jpg \n extracting: train/IMG_2443_jpeg_jpg.rf.a4782ba75f8b90e387dd13ab0d361af1.txt \n extracting: train/IMG_2443_jpeg_jpg.rf.d30e60dca982bb0226b36835ee053921.jpg \n extracting: train/IMG_2443_jpeg_jpg.rf.d30e60dca982bb0226b36835ee053921.txt \n extracting: train/IMG_2444_jpeg_jpg.rf.6d963ad88f0bd1e6236dc1872e34b0d6.jpg \n extracting: train/IMG_2444_jpeg_jpg.rf.6d963ad88f0bd1e6236dc1872e34b0d6.txt \n extracting: train/IMG_2444_jpeg_jpg.rf.77d4230b856d948f280be916b5619990.jpg \n extracting: train/IMG_2444_jpeg_jpg.rf.77d4230b856d948f280be916b5619990.txt \n extracting: train/IMG_2444_jpeg_jpg.rf.baf5c83ec49d4922751582f21d9a146a.jpg \n extracting: train/IMG_2444_jpeg_jpg.rf.baf5c83ec49d4922751582f21d9a146a.txt \n extracting: train/IMG_2445_jpeg_jpg.rf.04ea5d22a1bcdef153f47edea583699b.jpg \n extracting: train/IMG_2445_jpeg_jpg.rf.04ea5d22a1bcdef153f47edea583699b.txt \n extracting: train/IMG_2445_jpeg_jpg.rf.3dfe36448879cba5db54eae825d8e902.jpg \n extracting: train/IMG_2445_jpeg_jpg.rf.3dfe36448879cba5db54eae825d8e902.txt \n extracting: train/IMG_2445_jpeg_jpg.rf.95ae3cba0a22ca0c58c404a4a4df3c53.jpg \n extracting: train/IMG_2445_jpeg_jpg.rf.95ae3cba0a22ca0c58c404a4a4df3c53.txt \n extracting: train/IMG_2447_jpeg_jpg.rf.21ec427e7a17ebbed18220d8ea135b12.jpg \n extracting: train/IMG_2447_jpeg_jpg.rf.21ec427e7a17ebbed18220d8ea135b12.txt \n extracting: train/IMG_2447_jpeg_jpg.rf.9c9aa3bed91728dba031a64839ac654c.jpg \n extracting: train/IMG_2447_jpeg_jpg.rf.9c9aa3bed91728dba031a64839ac654c.txt \n extracting: train/IMG_2447_jpeg_jpg.rf.c1f30ae9729908b5eb7bf11b5f44c9a7.jpg \n extracting: train/IMG_2447_jpeg_jpg.rf.c1f30ae9729908b5eb7bf11b5f44c9a7.txt \n extracting: train/IMG_2449_jpeg_jpg.rf.0bec96cff68a1a967500eb78e735c802.jpg \n extracting: train/IMG_2449_jpeg_jpg.rf.0bec96cff68a1a967500eb78e735c802.txt \n extracting: train/IMG_2449_jpeg_jpg.rf.3efa75b79b293ec7663110096c6cd9e5.jpg \n extracting: train/IMG_2449_jpeg_jpg.rf.3efa75b79b293ec7663110096c6cd9e5.txt \n extracting: train/IMG_2449_jpeg_jpg.rf.b63e4c1801720bd61780b5648552f4b6.jpg \n extracting: train/IMG_2449_jpeg_jpg.rf.b63e4c1801720bd61780b5648552f4b6.txt \n extracting: train/IMG_2451_jpeg_jpg.rf.00bd7b873ced0175985152efe7793b0c.jpg \n extracting: train/IMG_2451_jpeg_jpg.rf.00bd7b873ced0175985152efe7793b0c.txt \n extracting: train/IMG_2451_jpeg_jpg.rf.6f516c9c2d9de6731710a12b90ffdf92.jpg \n extracting: train/IMG_2451_jpeg_jpg.rf.6f516c9c2d9de6731710a12b90ffdf92.txt \n extracting: train/IMG_2451_jpeg_jpg.rf.e95afec790d273ffac065db8730aa225.jpg \n extracting: train/IMG_2451_jpeg_jpg.rf.e95afec790d273ffac065db8730aa225.txt \n extracting: train/IMG_2452_jpeg_jpg.rf.13252a3666ababe52680111d155e9dc1.jpg \n extracting: train/IMG_2452_jpeg_jpg.rf.13252a3666ababe52680111d155e9dc1.txt \n extracting: train/IMG_2452_jpeg_jpg.rf.2b44b8db43a9d1786033b69092a6e771.jpg \n extracting: train/IMG_2452_jpeg_jpg.rf.2b44b8db43a9d1786033b69092a6e771.txt \n extracting: train/IMG_2452_jpeg_jpg.rf.c60f531276bc68f7256e4569c0c1cfdf.jpg \n extracting: train/IMG_2452_jpeg_jpg.rf.c60f531276bc68f7256e4569c0c1cfdf.txt \n extracting: train/IMG_2456_jpeg_jpg.rf.03cdde5ecd16fdef9e7ae12e93494083.jpg \n extracting: train/IMG_2456_jpeg_jpg.rf.03cdde5ecd16fdef9e7ae12e93494083.txt \n extracting: train/IMG_2456_jpeg_jpg.rf.7e9c24618a4b2e78ddc1ec4fff79bd01.jpg \n extracting: train/IMG_2456_jpeg_jpg.rf.7e9c24618a4b2e78ddc1ec4fff79bd01.txt \n extracting: train/IMG_2456_jpeg_jpg.rf.9c9dcc6c2da3e275ca16d8ec787ba1e4.jpg \n extracting: train/IMG_2456_jpeg_jpg.rf.9c9dcc6c2da3e275ca16d8ec787ba1e4.txt \n extracting: train/IMG_2458_jpeg_jpg.rf.d03ebf2b63c0ebbc89b1172f5f78da09.jpg \n extracting: train/IMG_2458_jpeg_jpg.rf.d03ebf2b63c0ebbc89b1172f5f78da09.txt \n extracting: train/IMG_2458_jpeg_jpg.rf.f963c9ff5e2debcc95e4252ffba72e64.jpg \n extracting: train/IMG_2458_jpeg_jpg.rf.f963c9ff5e2debcc95e4252ffba72e64.txt \n extracting: train/IMG_2458_jpeg_jpg.rf.f9f6c4c34bcbc6fe87800699e36d10de.jpg \n extracting: train/IMG_2458_jpeg_jpg.rf.f9f6c4c34bcbc6fe87800699e36d10de.txt \n extracting: train/IMG_2459_jpeg_jpg.rf.0d4676b4e5336def50bbeea2c6dabe49.jpg \n extracting: train/IMG_2459_jpeg_jpg.rf.0d4676b4e5336def50bbeea2c6dabe49.txt \n extracting: train/IMG_2459_jpeg_jpg.rf.45f226352c4ea23e246bbd765fa5c45c.jpg \n extracting: train/IMG_2459_jpeg_jpg.rf.45f226352c4ea23e246bbd765fa5c45c.txt \n extracting: train/IMG_2459_jpeg_jpg.rf.7857d62407e4da5c71c37cbcdf3c6f1b.jpg \n extracting: train/IMG_2459_jpeg_jpg.rf.7857d62407e4da5c71c37cbcdf3c6f1b.txt \n extracting: train/IMG_2460_jpeg_jpg.rf.67db10c1dd1f326f47ff9d84e1801005.jpg \n extracting: train/IMG_2460_jpeg_jpg.rf.67db10c1dd1f326f47ff9d84e1801005.txt \n extracting: train/IMG_2460_jpeg_jpg.rf.aae8cf46896d48fbdbe1d80888d1f1c4.jpg \n extracting: train/IMG_2460_jpeg_jpg.rf.aae8cf46896d48fbdbe1d80888d1f1c4.txt \n extracting: train/IMG_2460_jpeg_jpg.rf.bfb7d2e15d4438325f98bee017c88f1d.jpg \n extracting: train/IMG_2460_jpeg_jpg.rf.bfb7d2e15d4438325f98bee017c88f1d.txt \n extracting: train/IMG_2461_jpeg_jpg.rf.030a1eaf84fb572f2cbf6aca618259d0.jpg \n extracting: train/IMG_2461_jpeg_jpg.rf.030a1eaf84fb572f2cbf6aca618259d0.txt \n extracting: train/IMG_2461_jpeg_jpg.rf.8c3af2eab3b3a58741815c1d18c56114.jpg \n extracting: train/IMG_2461_jpeg_jpg.rf.8c3af2eab3b3a58741815c1d18c56114.txt \n extracting: train/IMG_2461_jpeg_jpg.rf.e81aa138b2dd29399f340ffc9665d85c.jpg \n extracting: train/IMG_2461_jpeg_jpg.rf.e81aa138b2dd29399f340ffc9665d85c.txt \n extracting: train/IMG_2462_jpeg_jpg.rf.4c038e812ea50f41de526ec8e9b65912.jpg \n extracting: train/IMG_2462_jpeg_jpg.rf.4c038e812ea50f41de526ec8e9b65912.txt \n extracting: train/IMG_2462_jpeg_jpg.rf.5f62291df773d530a178a515a2aa8b40.jpg \n extracting: train/IMG_2462_jpeg_jpg.rf.5f62291df773d530a178a515a2aa8b40.txt \n extracting: train/IMG_2462_jpeg_jpg.rf.b4e6c2725b592034052d080c55d6140a.jpg \n extracting: train/IMG_2462_jpeg_jpg.rf.b4e6c2725b592034052d080c55d6140a.txt \n extracting: train/IMG_2463_jpeg_jpg.rf.23d91d2a0e337144d7ce6c8e8c31bf7f.jpg \n extracting: train/IMG_2463_jpeg_jpg.rf.23d91d2a0e337144d7ce6c8e8c31bf7f.txt \n extracting: train/IMG_2463_jpeg_jpg.rf.285ee75b192a8236822b89bdcaf21d62.jpg \n extracting: train/IMG_2463_jpeg_jpg.rf.285ee75b192a8236822b89bdcaf21d62.txt \n extracting: train/IMG_2463_jpeg_jpg.rf.3f35c333698e7637cddc72e1595b6e4d.jpg \n extracting: train/IMG_2463_jpeg_jpg.rf.3f35c333698e7637cddc72e1595b6e4d.txt \n extracting: train/IMG_2467_jpeg_jpg.rf.0d972489134de1cb4b7526268eba5521.jpg \n extracting: train/IMG_2467_jpeg_jpg.rf.0d972489134de1cb4b7526268eba5521.txt \n extracting: train/IMG_2467_jpeg_jpg.rf.20ccd3584713b9ffab4b9ba1c5bd6954.jpg \n extracting: train/IMG_2467_jpeg_jpg.rf.20ccd3584713b9ffab4b9ba1c5bd6954.txt \n extracting: train/IMG_2467_jpeg_jpg.rf.c6f77894a82a3a1d2c817cdf9643d0ce.jpg \n extracting: train/IMG_2467_jpeg_jpg.rf.c6f77894a82a3a1d2c817cdf9643d0ce.txt \n extracting: train/IMG_2471_jpeg_jpg.rf.12fe00ffd5be06df620a028bb442953e.jpg \n extracting: train/IMG_2471_jpeg_jpg.rf.12fe00ffd5be06df620a028bb442953e.txt \n extracting: train/IMG_2471_jpeg_jpg.rf.9900a135ef784d97538cec41ec9c8952.jpg \n extracting: train/IMG_2471_jpeg_jpg.rf.9900a135ef784d97538cec41ec9c8952.txt \n extracting: train/IMG_2471_jpeg_jpg.rf.af9b4e3e71afc2f40d174fbc89c7183c.jpg \n extracting: train/IMG_2471_jpeg_jpg.rf.af9b4e3e71afc2f40d174fbc89c7183c.txt \n extracting: train/IMG_2472_jpeg_jpg.rf.7e8461c390ad677851d5f5d220690bad.jpg \n extracting: train/IMG_2472_jpeg_jpg.rf.7e8461c390ad677851d5f5d220690bad.txt \n extracting: train/IMG_2472_jpeg_jpg.rf.891382430e9a0085bacb66d71237d759.jpg \n extracting: train/IMG_2472_jpeg_jpg.rf.891382430e9a0085bacb66d71237d759.txt \n extracting: train/IMG_2472_jpeg_jpg.rf.cb4d338291cb8c380a06c6c9c2001171.jpg \n extracting: train/IMG_2472_jpeg_jpg.rf.cb4d338291cb8c380a06c6c9c2001171.txt \n extracting: train/IMG_2475_jpeg_jpg.rf.1a64c05da72d4bbbbf1ce1cab062b525.jpg \n extracting: train/IMG_2475_jpeg_jpg.rf.1a64c05da72d4bbbbf1ce1cab062b525.txt \n extracting: train/IMG_2475_jpeg_jpg.rf.1e5d7e271b2217f1174795749aba1494.jpg \n extracting: train/IMG_2475_jpeg_jpg.rf.1e5d7e271b2217f1174795749aba1494.txt \n extracting: train/IMG_2475_jpeg_jpg.rf.a7477ec4b9fba70e4a9953f582e2c11b.jpg \n extracting: train/IMG_2475_jpeg_jpg.rf.a7477ec4b9fba70e4a9953f582e2c11b.txt \n extracting: train/IMG_2476_jpeg_jpg.rf.1bbd2b5f44993c59dc5a52071aae8860.jpg \n extracting: train/IMG_2476_jpeg_jpg.rf.1bbd2b5f44993c59dc5a52071aae8860.txt \n extracting: train/IMG_2476_jpeg_jpg.rf.5fcd2c6ed414d9cd3c83ed499fadf091.jpg \n extracting: train/IMG_2476_jpeg_jpg.rf.5fcd2c6ed414d9cd3c83ed499fadf091.txt \n extracting: train/IMG_2476_jpeg_jpg.rf.63e2ad3c10c5f22e2c1df981661d5882.jpg \n extracting: train/IMG_2476_jpeg_jpg.rf.63e2ad3c10c5f22e2c1df981661d5882.txt \n extracting: train/IMG_2478_jpeg_jpg.rf.0b6e77a0f2320919254aa287f0c8d737.jpg \n extracting: train/IMG_2478_jpeg_jpg.rf.0b6e77a0f2320919254aa287f0c8d737.txt \n extracting: train/IMG_2478_jpeg_jpg.rf.520fcc11b380534e32c0d819134a662a.jpg \n extracting: train/IMG_2478_jpeg_jpg.rf.520fcc11b380534e32c0d819134a662a.txt \n extracting: train/IMG_2478_jpeg_jpg.rf.f225ee335f651403c35ab3282a00ef0e.jpg \n extracting: train/IMG_2478_jpeg_jpg.rf.f225ee335f651403c35ab3282a00ef0e.txt \n extracting: train/IMG_2480_jpeg_jpg.rf.245389008735b3c394a05c9dfc4eb3c8.jpg \n extracting: train/IMG_2480_jpeg_jpg.rf.245389008735b3c394a05c9dfc4eb3c8.txt \n extracting: train/IMG_2480_jpeg_jpg.rf.27d964570d180d2eb128ee7471eb48fc.jpg \n extracting: train/IMG_2480_jpeg_jpg.rf.27d964570d180d2eb128ee7471eb48fc.txt \n extracting: train/IMG_2480_jpeg_jpg.rf.75bb374c902ef368bad41dfb5b8ec7da.jpg \n extracting: train/IMG_2480_jpeg_jpg.rf.75bb374c902ef368bad41dfb5b8ec7da.txt \n extracting: train/IMG_2481_jpeg_jpg.rf.05e9b7bee5ef76f6c8c414012fd02b7b.jpg \n extracting: train/IMG_2481_jpeg_jpg.rf.05e9b7bee5ef76f6c8c414012fd02b7b.txt \n extracting: train/IMG_2481_jpeg_jpg.rf.63f2d82f74bde7f2824f79b4e8ad50a3.jpg \n extracting: train/IMG_2481_jpeg_jpg.rf.63f2d82f74bde7f2824f79b4e8ad50a3.txt \n extracting: train/IMG_2481_jpeg_jpg.rf.a1e3ea98663949bdc58d284824d63311.jpg \n extracting: train/IMG_2481_jpeg_jpg.rf.a1e3ea98663949bdc58d284824d63311.txt \n extracting: train/IMG_2482_jpeg_jpg.rf.29ad272979970c5c4884b815f407244e.jpg \n extracting: train/IMG_2482_jpeg_jpg.rf.29ad272979970c5c4884b815f407244e.txt \n extracting: train/IMG_2482_jpeg_jpg.rf.54c1047ac1f1114119e8ffbdaa161c01.jpg \n extracting: train/IMG_2482_jpeg_jpg.rf.54c1047ac1f1114119e8ffbdaa161c01.txt \n extracting: train/IMG_2482_jpeg_jpg.rf.6ded2fbcdc0256a9f15d3a5793407e3e.jpg \n extracting: train/IMG_2482_jpeg_jpg.rf.6ded2fbcdc0256a9f15d3a5793407e3e.txt \n extracting: train/IMG_2483_jpeg_jpg.rf.2ae494f98bc6788cc0c29b9a811b24b7.jpg \n extracting: train/IMG_2483_jpeg_jpg.rf.2ae494f98bc6788cc0c29b9a811b24b7.txt \n extracting: train/IMG_2483_jpeg_jpg.rf.d22a446fa23a8f74f2d0338365536a6c.jpg \n extracting: train/IMG_2483_jpeg_jpg.rf.d22a446fa23a8f74f2d0338365536a6c.txt \n extracting: train/IMG_2483_jpeg_jpg.rf.e72a759d993a4b59450e73aaec7c962a.jpg \n extracting: train/IMG_2483_jpeg_jpg.rf.e72a759d993a4b59450e73aaec7c962a.txt \n extracting: train/IMG_2485_jpeg_jpg.rf.50ab1611645689f00982cae00008b967.jpg \n extracting: train/IMG_2485_jpeg_jpg.rf.50ab1611645689f00982cae00008b967.txt \n extracting: train/IMG_2485_jpeg_jpg.rf.5fafd2549f9aadae5f4b4e28ab335a12.jpg \n extracting: train/IMG_2485_jpeg_jpg.rf.5fafd2549f9aadae5f4b4e28ab335a12.txt \n extracting: train/IMG_2485_jpeg_jpg.rf.8b69c02e02cb35a928bf40da81519416.jpg \n extracting: train/IMG_2485_jpeg_jpg.rf.8b69c02e02cb35a928bf40da81519416.txt \n extracting: train/IMG_2486_jpeg_jpg.rf.01372c2a407d07c767ad5d6a92d4b159.jpg \n extracting: train/IMG_2486_jpeg_jpg.rf.01372c2a407d07c767ad5d6a92d4b159.txt \n extracting: train/IMG_2486_jpeg_jpg.rf.3e4a209804cde3399da74dbba61b0ded.jpg \n extracting: train/IMG_2486_jpeg_jpg.rf.3e4a209804cde3399da74dbba61b0ded.txt \n extracting: train/IMG_2486_jpeg_jpg.rf.bd9714c370fa526f08933972e41cc0ea.jpg \n extracting: train/IMG_2486_jpeg_jpg.rf.bd9714c370fa526f08933972e41cc0ea.txt \n extracting: train/IMG_2487_jpeg_jpg.rf.a79f7f3c58db2e3e7d237943810a247d.jpg \n extracting: train/IMG_2487_jpeg_jpg.rf.a79f7f3c58db2e3e7d237943810a247d.txt \n extracting: train/IMG_2487_jpeg_jpg.rf.abe10e48ce142c92b768be81c239074f.jpg \n extracting: train/IMG_2487_jpeg_jpg.rf.abe10e48ce142c92b768be81c239074f.txt \n extracting: train/IMG_2487_jpeg_jpg.rf.c933ed09f5fd0e2f89c327ef4ffe94b4.jpg \n extracting: train/IMG_2487_jpeg_jpg.rf.c933ed09f5fd0e2f89c327ef4ffe94b4.txt \n extracting: train/IMG_2488_jpeg_jpg.rf.0db55e342d55de741f5eea28e8c85958.jpg \n extracting: train/IMG_2488_jpeg_jpg.rf.0db55e342d55de741f5eea28e8c85958.txt \n extracting: train/IMG_2488_jpeg_jpg.rf.273d6225a02bad8017e452158017679c.jpg \n extracting: train/IMG_2488_jpeg_jpg.rf.273d6225a02bad8017e452158017679c.txt \n extracting: train/IMG_2488_jpeg_jpg.rf.ad3c2a901d5bf7f2d50289e7d4aabfff.jpg \n extracting: train/IMG_2488_jpeg_jpg.rf.ad3c2a901d5bf7f2d50289e7d4aabfff.txt \n extracting: train/IMG_2489_jpeg_jpg.rf.47eeb53fa636cc3c92f7b15b547315be.jpg \n extracting: train/IMG_2489_jpeg_jpg.rf.47eeb53fa636cc3c92f7b15b547315be.txt \n extracting: train/IMG_2489_jpeg_jpg.rf.6f2c0519e05d3cbffa40e51118d3b028.jpg \n extracting: train/IMG_2489_jpeg_jpg.rf.6f2c0519e05d3cbffa40e51118d3b028.txt \n extracting: train/IMG_2489_jpeg_jpg.rf.bf4693b903baed5f6cffe43fccf8298d.jpg \n extracting: train/IMG_2489_jpeg_jpg.rf.bf4693b903baed5f6cffe43fccf8298d.txt \n extracting: train/IMG_2490_jpeg_jpg.rf.5e8460827939ebf15cdfd01d300b5436.jpg \n extracting: train/IMG_2490_jpeg_jpg.rf.5e8460827939ebf15cdfd01d300b5436.txt \n extracting: train/IMG_2490_jpeg_jpg.rf.73d004b01e6a8b2f1f9bbe7f4b411922.jpg \n extracting: train/IMG_2490_jpeg_jpg.rf.73d004b01e6a8b2f1f9bbe7f4b411922.txt \n extracting: train/IMG_2490_jpeg_jpg.rf.f92d82675008dbac4e12f391f24cf955.jpg \n extracting: train/IMG_2490_jpeg_jpg.rf.f92d82675008dbac4e12f391f24cf955.txt \n extracting: train/IMG_2493_jpeg_jpg.rf.1c2ec734d098783bee16e6ce76c995bf.jpg \n extracting: train/IMG_2493_jpeg_jpg.rf.1c2ec734d098783bee16e6ce76c995bf.txt \n extracting: train/IMG_2493_jpeg_jpg.rf.1e4f3f9e6f3afe98220a62fd757dfb15.jpg \n extracting: train/IMG_2493_jpeg_jpg.rf.1e4f3f9e6f3afe98220a62fd757dfb15.txt \n extracting: train/IMG_2493_jpeg_jpg.rf.5860a2ce1250e24270863c9587b500ae.jpg \n extracting: train/IMG_2493_jpeg_jpg.rf.5860a2ce1250e24270863c9587b500ae.txt \n extracting: train/IMG_2494_jpeg_jpg.rf.0c43d4e2d23926ecc8c26c9cace79b7e.jpg \n extracting: train/IMG_2494_jpeg_jpg.rf.0c43d4e2d23926ecc8c26c9cace79b7e.txt \n extracting: train/IMG_2494_jpeg_jpg.rf.54043b51c7ae1c67c306713f10a7f787.jpg \n extracting: train/IMG_2494_jpeg_jpg.rf.54043b51c7ae1c67c306713f10a7f787.txt \n extracting: train/IMG_2494_jpeg_jpg.rf.5a74197cff58a62ceed451e5870f7bb5.jpg \n extracting: train/IMG_2494_jpeg_jpg.rf.5a74197cff58a62ceed451e5870f7bb5.txt \n extracting: train/IMG_2495_jpeg_jpg.rf.19f6691f687e4c92b710919d5f12856f.jpg \n extracting: train/IMG_2495_jpeg_jpg.rf.19f6691f687e4c92b710919d5f12856f.txt \n extracting: train/IMG_2495_jpeg_jpg.rf.1aa991c3418b4a8afa31bd49d2658262.jpg \n extracting: train/IMG_2495_jpeg_jpg.rf.1aa991c3418b4a8afa31bd49d2658262.txt \n extracting: train/IMG_2495_jpeg_jpg.rf.35541e800c1e339ebc46101a1c7f90bf.jpg \n extracting: train/IMG_2495_jpeg_jpg.rf.35541e800c1e339ebc46101a1c7f90bf.txt \n extracting: train/IMG_2497_jpeg_jpg.rf.3bd97fd93aeb1462677aef65a6398c7c.jpg \n extracting: train/IMG_2497_jpeg_jpg.rf.3bd97fd93aeb1462677aef65a6398c7c.txt \n extracting: train/IMG_2497_jpeg_jpg.rf.7e6307e15f2279012e163ed78d15e8db.jpg \n extracting: train/IMG_2497_jpeg_jpg.rf.7e6307e15f2279012e163ed78d15e8db.txt \n extracting: train/IMG_2497_jpeg_jpg.rf.df47fe5fd2cd5d142c787c3dd29a5758.jpg \n extracting: train/IMG_2497_jpeg_jpg.rf.df47fe5fd2cd5d142c787c3dd29a5758.txt \n extracting: train/IMG_2498_jpeg_jpg.rf.6dc07c8a7b0c88e7ce822485ca0370e8.jpg \n extracting: train/IMG_2498_jpeg_jpg.rf.6dc07c8a7b0c88e7ce822485ca0370e8.txt \n extracting: train/IMG_2498_jpeg_jpg.rf.6fa605002f9ba68efc93e146d55d738a.jpg \n extracting: train/IMG_2498_jpeg_jpg.rf.6fa605002f9ba68efc93e146d55d738a.txt \n extracting: train/IMG_2498_jpeg_jpg.rf.f76ea5103d04f61a951f1b605f417eed.jpg \n extracting: train/IMG_2498_jpeg_jpg.rf.f76ea5103d04f61a951f1b605f417eed.txt \n extracting: train/IMG_2500_jpeg_jpg.rf.14d992a438b75a17b81bedc653f49e75.jpg \n extracting: train/IMG_2500_jpeg_jpg.rf.14d992a438b75a17b81bedc653f49e75.txt \n extracting: train/IMG_2500_jpeg_jpg.rf.370a9f5c5a924f53e2b047d30d6b62bf.jpg \n extracting: train/IMG_2500_jpeg_jpg.rf.370a9f5c5a924f53e2b047d30d6b62bf.txt \n extracting: train/IMG_2500_jpeg_jpg.rf.864ee8b51893a248690cdaff10f8b40a.jpg \n extracting: train/IMG_2500_jpeg_jpg.rf.864ee8b51893a248690cdaff10f8b40a.txt \n extracting: train/IMG_2501_jpeg_jpg.rf.19c9f3d3d1e61940b3ff69b9dccc25a2.jpg \n extracting: train/IMG_2501_jpeg_jpg.rf.19c9f3d3d1e61940b3ff69b9dccc25a2.txt \n extracting: train/IMG_2501_jpeg_jpg.rf.5fb69e23db4afbf0622964cc03521980.jpg \n extracting: train/IMG_2501_jpeg_jpg.rf.5fb69e23db4afbf0622964cc03521980.txt \n extracting: train/IMG_2501_jpeg_jpg.rf.91b930f3b589d0447d62e0e540b10869.jpg \n extracting: train/IMG_2501_jpeg_jpg.rf.91b930f3b589d0447d62e0e540b10869.txt \n extracting: train/IMG_2502_jpeg_jpg.rf.5d5f6d765d6e777977bfef827939d235.jpg \n extracting: train/IMG_2502_jpeg_jpg.rf.5d5f6d765d6e777977bfef827939d235.txt \n extracting: train/IMG_2502_jpeg_jpg.rf.c1fc9450a052b841d99c676bcb03e93e.jpg \n extracting: train/IMG_2502_jpeg_jpg.rf.c1fc9450a052b841d99c676bcb03e93e.txt \n extracting: train/IMG_2502_jpeg_jpg.rf.c637e413fcc8d76fdac468f5ec575de1.jpg \n extracting: train/IMG_2502_jpeg_jpg.rf.c637e413fcc8d76fdac468f5ec575de1.txt \n extracting: train/IMG_2503_jpeg_jpg.rf.8e587f1b5ff4087f1919a08ab01a8470.jpg \n extracting: train/IMG_2503_jpeg_jpg.rf.8e587f1b5ff4087f1919a08ab01a8470.txt \n extracting: train/IMG_2503_jpeg_jpg.rf.d92d02f1941253d4f128d30561efb189.jpg \n extracting: train/IMG_2503_jpeg_jpg.rf.d92d02f1941253d4f128d30561efb189.txt \n extracting: train/IMG_2503_jpeg_jpg.rf.e2c5a28ec3d970edcde5c89be1eee985.jpg \n extracting: train/IMG_2503_jpeg_jpg.rf.e2c5a28ec3d970edcde5c89be1eee985.txt \n extracting: train/IMG_2504_jpeg_jpg.rf.0192c86cc65db8c694163484612ebd85.jpg \n extracting: train/IMG_2504_jpeg_jpg.rf.0192c86cc65db8c694163484612ebd85.txt \n extracting: train/IMG_2504_jpeg_jpg.rf.a0169e5c5392c0b60b38a30ea8fe17db.jpg \n extracting: train/IMG_2504_jpeg_jpg.rf.a0169e5c5392c0b60b38a30ea8fe17db.txt \n extracting: train/IMG_2504_jpeg_jpg.rf.f048787a33e06f77810dd2bb0cc56155.jpg \n extracting: train/IMG_2504_jpeg_jpg.rf.f048787a33e06f77810dd2bb0cc56155.txt \n extracting: train/IMG_2506_jpeg_jpg.rf.12896948ba0b16470969ad77c8f6dafd.jpg \n extracting: train/IMG_2506_jpeg_jpg.rf.12896948ba0b16470969ad77c8f6dafd.txt \n extracting: train/IMG_2506_jpeg_jpg.rf.6b6f90b4c878fd79ecbc17252bf445b6.jpg \n extracting: train/IMG_2506_jpeg_jpg.rf.6b6f90b4c878fd79ecbc17252bf445b6.txt \n extracting: train/IMG_2506_jpeg_jpg.rf.944f518947b1ff6d6036d72125f83813.jpg \n extracting: train/IMG_2506_jpeg_jpg.rf.944f518947b1ff6d6036d72125f83813.txt \n extracting: train/IMG_2507_jpeg_jpg.rf.1e0be2e3193a97be69adf991770f0ff9.jpg \n extracting: train/IMG_2507_jpeg_jpg.rf.1e0be2e3193a97be69adf991770f0ff9.txt \n extracting: train/IMG_2507_jpeg_jpg.rf.5485a84d0ed0c08b85148948a9b026e6.jpg \n extracting: train/IMG_2507_jpeg_jpg.rf.5485a84d0ed0c08b85148948a9b026e6.txt \n extracting: train/IMG_2507_jpeg_jpg.rf.b908f191635e819b24b3163d8dd9d52a.jpg \n extracting: train/IMG_2507_jpeg_jpg.rf.b908f191635e819b24b3163d8dd9d52a.txt \n extracting: train/IMG_2508_jpeg_jpg.rf.4f6a024911cfc861109ee71ee1a58c2c.jpg \n extracting: train/IMG_2508_jpeg_jpg.rf.4f6a024911cfc861109ee71ee1a58c2c.txt \n extracting: train/IMG_2508_jpeg_jpg.rf.85e27afe9e9ac782eb9c7074fb5bb32b.jpg \n extracting: train/IMG_2508_jpeg_jpg.rf.85e27afe9e9ac782eb9c7074fb5bb32b.txt \n extracting: train/IMG_2508_jpeg_jpg.rf.c28ebbaafd35066b45c6509168603149.jpg \n extracting: train/IMG_2508_jpeg_jpg.rf.c28ebbaafd35066b45c6509168603149.txt \n extracting: train/IMG_2509_jpeg_jpg.rf.ac55648f6ae6c738140143421d4dd9e9.jpg \n extracting: train/IMG_2509_jpeg_jpg.rf.ac55648f6ae6c738140143421d4dd9e9.txt \n extracting: train/IMG_2509_jpeg_jpg.rf.c7a0a11bcb4068459a53b2c8ec7d0e1d.jpg \n extracting: train/IMG_2509_jpeg_jpg.rf.c7a0a11bcb4068459a53b2c8ec7d0e1d.txt \n extracting: train/IMG_2509_jpeg_jpg.rf.ee30d3df4fa2814d21ba26dff0d18f83.jpg \n extracting: train/IMG_2509_jpeg_jpg.rf.ee30d3df4fa2814d21ba26dff0d18f83.txt \n extracting: train/IMG_2511_jpeg_jpg.rf.0bc11c23884abd113358389117c9a4d4.jpg \n extracting: train/IMG_2511_jpeg_jpg.rf.0bc11c23884abd113358389117c9a4d4.txt \n extracting: train/IMG_2511_jpeg_jpg.rf.197237aa6dc421e57f2fff3088b580e9.jpg \n extracting: train/IMG_2511_jpeg_jpg.rf.197237aa6dc421e57f2fff3088b580e9.txt \n extracting: train/IMG_2511_jpeg_jpg.rf.644fbf7a7ee1bd74cd9e8212f1aedb66.jpg \n extracting: train/IMG_2511_jpeg_jpg.rf.644fbf7a7ee1bd74cd9e8212f1aedb66.txt \n extracting: train/IMG_2513_jpeg_jpg.rf.0b158fe235ec39ec651d4cf9b072f4d2.jpg \n extracting: train/IMG_2513_jpeg_jpg.rf.0b158fe235ec39ec651d4cf9b072f4d2.txt \n extracting: train/IMG_2513_jpeg_jpg.rf.2f6a70934a8f305a1d892069a542ae8b.jpg \n extracting: train/IMG_2513_jpeg_jpg.rf.2f6a70934a8f305a1d892069a542ae8b.txt \n extracting: train/IMG_2513_jpeg_jpg.rf.55b62db3874b7cad6b05329cacbe11a4.jpg \n extracting: train/IMG_2513_jpeg_jpg.rf.55b62db3874b7cad6b05329cacbe11a4.txt \n extracting: train/IMG_2515_jpeg_jpg.rf.58ec420b3f45dfa86116529bed559716.jpg \n extracting: train/IMG_2515_jpeg_jpg.rf.58ec420b3f45dfa86116529bed559716.txt \n extracting: train/IMG_2515_jpeg_jpg.rf.7de7caa368caed27465398220804a187.jpg \n extracting: train/IMG_2515_jpeg_jpg.rf.7de7caa368caed27465398220804a187.txt \n extracting: train/IMG_2515_jpeg_jpg.rf.f449d4597746bc620bb485f1f0f06f42.jpg \n extracting: train/IMG_2515_jpeg_jpg.rf.f449d4597746bc620bb485f1f0f06f42.txt \n extracting: train/IMG_2516_jpeg_jpg.rf.0aa813bb72a045633674f674bd273d4b.jpg \n extracting: train/IMG_2516_jpeg_jpg.rf.0aa813bb72a045633674f674bd273d4b.txt \n extracting: train/IMG_2516_jpeg_jpg.rf.6c9e4b520b456da2c6230fc03cd6867a.jpg \n extracting: train/IMG_2516_jpeg_jpg.rf.6c9e4b520b456da2c6230fc03cd6867a.txt \n extracting: train/IMG_2516_jpeg_jpg.rf.960a7d989616fdda57639844b5ec40e1.jpg \n extracting: train/IMG_2516_jpeg_jpg.rf.960a7d989616fdda57639844b5ec40e1.txt \n extracting: train/IMG_2518_jpeg_jpg.rf.3f8f53327fc1c212079c3afdc72b3202.jpg \n extracting: train/IMG_2518_jpeg_jpg.rf.3f8f53327fc1c212079c3afdc72b3202.txt \n extracting: train/IMG_2518_jpeg_jpg.rf.74717235b06b884c0ce91aa3bf00eace.jpg \n extracting: train/IMG_2518_jpeg_jpg.rf.74717235b06b884c0ce91aa3bf00eace.txt \n extracting: train/IMG_2518_jpeg_jpg.rf.d951f5032ed4a5bcf081aa1fe745613f.jpg \n extracting: train/IMG_2518_jpeg_jpg.rf.d951f5032ed4a5bcf081aa1fe745613f.txt \n extracting: train/IMG_2520_jpeg_jpg.rf.8ed2523712b9c4042f8fbddf80e891f0.jpg \n extracting: train/IMG_2520_jpeg_jpg.rf.8ed2523712b9c4042f8fbddf80e891f0.txt \n extracting: train/IMG_2520_jpeg_jpg.rf.bf956c499e1703a8c2c3a89e1b186eac.jpg \n extracting: train/IMG_2520_jpeg_jpg.rf.bf956c499e1703a8c2c3a89e1b186eac.txt \n extracting: train/IMG_2520_jpeg_jpg.rf.c7ddcdb91736723f4c3cc2895ee8df4d.jpg \n extracting: train/IMG_2520_jpeg_jpg.rf.c7ddcdb91736723f4c3cc2895ee8df4d.txt \n extracting: train/IMG_2521_jpeg_jpg.rf.80bdcfbdd7dd5c559c7b33693856a140.jpg \n extracting: train/IMG_2521_jpeg_jpg.rf.80bdcfbdd7dd5c559c7b33693856a140.txt \n extracting: train/IMG_2521_jpeg_jpg.rf.92b46311fed2a409b422fadba8494be4.jpg \n extracting: train/IMG_2521_jpeg_jpg.rf.92b46311fed2a409b422fadba8494be4.txt \n extracting: train/IMG_2521_jpeg_jpg.rf.c0ab50889d40690d12a9dabf8ed0d0f5.jpg \n extracting: train/IMG_2521_jpeg_jpg.rf.c0ab50889d40690d12a9dabf8ed0d0f5.txt \n extracting: train/IMG_2524_jpeg_jpg.rf.1bcb0c397a4fa93de6acd3376c405295.jpg \n extracting: train/IMG_2524_jpeg_jpg.rf.1bcb0c397a4fa93de6acd3376c405295.txt \n extracting: train/IMG_2524_jpeg_jpg.rf.bad9ee9f5174f4900a0dc89d9dd977aa.jpg \n extracting: train/IMG_2524_jpeg_jpg.rf.bad9ee9f5174f4900a0dc89d9dd977aa.txt \n extracting: train/IMG_2524_jpeg_jpg.rf.d001ddbb8e754d53ca25463228eefa5c.jpg \n extracting: train/IMG_2524_jpeg_jpg.rf.d001ddbb8e754d53ca25463228eefa5c.txt \n extracting: train/IMG_2527_jpeg_jpg.rf.7eeb46c52b6529f96e1e9dbb934f767c.jpg \n extracting: train/IMG_2527_jpeg_jpg.rf.7eeb46c52b6529f96e1e9dbb934f767c.txt \n extracting: train/IMG_2527_jpeg_jpg.rf.ce7f3349b5356d7032eadcb6bf2d1a0c.jpg \n extracting: train/IMG_2527_jpeg_jpg.rf.ce7f3349b5356d7032eadcb6bf2d1a0c.txt \n extracting: train/IMG_2527_jpeg_jpg.rf.ebf235dd0d7fcd06cbdf54f938a61bae.jpg \n extracting: train/IMG_2527_jpeg_jpg.rf.ebf235dd0d7fcd06cbdf54f938a61bae.txt \n extracting: train/IMG_2528_jpeg_jpg.rf.4a10600e13fb81f3e1d6a613aa5b9908.jpg \n extracting: train/IMG_2528_jpeg_jpg.rf.4a10600e13fb81f3e1d6a613aa5b9908.txt \n extracting: train/IMG_2528_jpeg_jpg.rf.5298280587db8f23e21f87f29386f4cb.jpg \n extracting: train/IMG_2528_jpeg_jpg.rf.5298280587db8f23e21f87f29386f4cb.txt \n extracting: train/IMG_2528_jpeg_jpg.rf.5cdafca8dfcca9c245c85e3f254e91c8.jpg \n extracting: train/IMG_2528_jpeg_jpg.rf.5cdafca8dfcca9c245c85e3f254e91c8.txt \n extracting: train/IMG_2529_jpeg_jpg.rf.14966b6c3e756bb7bd02f8570ed521f3.jpg \n extracting: train/IMG_2529_jpeg_jpg.rf.14966b6c3e756bb7bd02f8570ed521f3.txt \n extracting: train/IMG_2529_jpeg_jpg.rf.4ba78e6b10fce0c4a4676fcb840fc3a3.jpg \n extracting: train/IMG_2529_jpeg_jpg.rf.4ba78e6b10fce0c4a4676fcb840fc3a3.txt \n extracting: train/IMG_2529_jpeg_jpg.rf.8b1794d017b239f22c4821aebd3ea299.jpg \n extracting: train/IMG_2529_jpeg_jpg.rf.8b1794d017b239f22c4821aebd3ea299.txt \n extracting: train/IMG_2530_jpeg_jpg.rf.4f03ee003460a28a17492508f7552104.jpg \n extracting: train/IMG_2530_jpeg_jpg.rf.4f03ee003460a28a17492508f7552104.txt \n extracting: train/IMG_2530_jpeg_jpg.rf.57d9dd8953ff2748d86f689963821fce.jpg \n extracting: train/IMG_2530_jpeg_jpg.rf.57d9dd8953ff2748d86f689963821fce.txt \n extracting: train/IMG_2530_jpeg_jpg.rf.589265928a15dd39370d1a3736e061f7.jpg \n extracting: train/IMG_2530_jpeg_jpg.rf.589265928a15dd39370d1a3736e061f7.txt \n extracting: train/IMG_2533_jpeg_jpg.rf.36cf122bee55cc120d4ded14e09395f0.jpg \n extracting: train/IMG_2533_jpeg_jpg.rf.36cf122bee55cc120d4ded14e09395f0.txt \n extracting: train/IMG_2533_jpeg_jpg.rf.404a7176abc57cf24721c745588aef0b.jpg \n extracting: train/IMG_2533_jpeg_jpg.rf.404a7176abc57cf24721c745588aef0b.txt \n extracting: train/IMG_2533_jpeg_jpg.rf.b593b690de806f2e3c794e7cf9e0d6ec.jpg \n extracting: train/IMG_2533_jpeg_jpg.rf.b593b690de806f2e3c794e7cf9e0d6ec.txt \n extracting: train/IMG_2536_jpeg_jpg.rf.12e9af1d5bd78205b0dc7beae3039fc9.jpg \n extracting: train/IMG_2536_jpeg_jpg.rf.12e9af1d5bd78205b0dc7beae3039fc9.txt \n extracting: train/IMG_2536_jpeg_jpg.rf.6c40ee8495595b53f723b47a646d9f79.jpg \n extracting: train/IMG_2536_jpeg_jpg.rf.6c40ee8495595b53f723b47a646d9f79.txt \n extracting: train/IMG_2536_jpeg_jpg.rf.9ca4c7aa13017be93dc735a968a7a0b9.jpg \n extracting: train/IMG_2536_jpeg_jpg.rf.9ca4c7aa13017be93dc735a968a7a0b9.txt \n extracting: train/IMG_2537_jpeg_jpg.rf.03c5900afdd1937e48baa4016feb7f68.jpg \n extracting: train/IMG_2537_jpeg_jpg.rf.03c5900afdd1937e48baa4016feb7f68.txt \n extracting: train/IMG_2537_jpeg_jpg.rf.a0b2f635417c4c53f54c529e433c4577.jpg \n extracting: train/IMG_2537_jpeg_jpg.rf.a0b2f635417c4c53f54c529e433c4577.txt \n extracting: train/IMG_2537_jpeg_jpg.rf.c242f247b4b86da05b68715648baea30.jpg \n extracting: train/IMG_2537_jpeg_jpg.rf.c242f247b4b86da05b68715648baea30.txt \n extracting: train/IMG_2538_jpeg_jpg.rf.9b06d6b023d0f9ea545466337e123119.jpg \n extracting: train/IMG_2538_jpeg_jpg.rf.9b06d6b023d0f9ea545466337e123119.txt \n extracting: train/IMG_2538_jpeg_jpg.rf.b474e23a86ba9c300d3e207d30520f83.jpg \n extracting: train/IMG_2538_jpeg_jpg.rf.b474e23a86ba9c300d3e207d30520f83.txt \n extracting: train/IMG_2538_jpeg_jpg.rf.da004ab74231c0a27ac25e3567b2552c.jpg \n extracting: train/IMG_2538_jpeg_jpg.rf.da004ab74231c0a27ac25e3567b2552c.txt \n extracting: train/IMG_2539_jpeg_jpg.rf.4b469dfa2e6fe2ec1390ddd30bbc631c.jpg \n extracting: train/IMG_2539_jpeg_jpg.rf.4b469dfa2e6fe2ec1390ddd30bbc631c.txt \n extracting: train/IMG_2539_jpeg_jpg.rf.7b3ddcd77f4f527384d1830f22c33967.jpg \n extracting: train/IMG_2539_jpeg_jpg.rf.7b3ddcd77f4f527384d1830f22c33967.txt \n extracting: train/IMG_2539_jpeg_jpg.rf.f0a13c491ca48ffba2ebb3c4141bcd3b.jpg \n extracting: train/IMG_2539_jpeg_jpg.rf.f0a13c491ca48ffba2ebb3c4141bcd3b.txt \n extracting: train/IMG_2540_jpeg_jpg.rf.0dd268e8f5e4598c427b2b78be1351c3.jpg \n extracting: train/IMG_2540_jpeg_jpg.rf.0dd268e8f5e4598c427b2b78be1351c3.txt \n extracting: train/IMG_2540_jpeg_jpg.rf.621e5f6286827c959e52da55e2ad6747.jpg \n extracting: train/IMG_2540_jpeg_jpg.rf.621e5f6286827c959e52da55e2ad6747.txt \n extracting: train/IMG_2540_jpeg_jpg.rf.7211940db3afeb954e6df17c15c47934.jpg \n extracting: train/IMG_2540_jpeg_jpg.rf.7211940db3afeb954e6df17c15c47934.txt \n extracting: train/IMG_2541_jpeg_jpg.rf.108bde3a5dbaed2bb4772f93757ff88f.jpg \n extracting: train/IMG_2541_jpeg_jpg.rf.108bde3a5dbaed2bb4772f93757ff88f.txt \n extracting: train/IMG_2541_jpeg_jpg.rf.127668006275fbf69a7fbc26a53a35ed.jpg \n extracting: train/IMG_2541_jpeg_jpg.rf.127668006275fbf69a7fbc26a53a35ed.txt \n extracting: train/IMG_2541_jpeg_jpg.rf.41660a3a2b97131cc76cc3dce7001256.jpg \n extracting: train/IMG_2541_jpeg_jpg.rf.41660a3a2b97131cc76cc3dce7001256.txt \n extracting: train/IMG_2542_jpeg_jpg.rf.08f8b641ebc7414bac59f7b41a9656b7.jpg \n extracting: train/IMG_2542_jpeg_jpg.rf.08f8b641ebc7414bac59f7b41a9656b7.txt \n extracting: train/IMG_2542_jpeg_jpg.rf.7025d66a0e6cb85a1237a802a360bd3a.jpg \n extracting: train/IMG_2542_jpeg_jpg.rf.7025d66a0e6cb85a1237a802a360bd3a.txt \n extracting: train/IMG_2542_jpeg_jpg.rf.afd2fd4ba0b96dc8ef559d453777dc85.jpg \n extracting: train/IMG_2542_jpeg_jpg.rf.afd2fd4ba0b96dc8ef559d453777dc85.txt \n extracting: train/IMG_2543_jpeg_jpg.rf.4e07260910a19a0bde430b1c19915122.jpg \n extracting: train/IMG_2543_jpeg_jpg.rf.4e07260910a19a0bde430b1c19915122.txt \n extracting: train/IMG_2543_jpeg_jpg.rf.7da4ee76f878b33b45e880019e4639d8.jpg \n extracting: train/IMG_2543_jpeg_jpg.rf.7da4ee76f878b33b45e880019e4639d8.txt \n extracting: train/IMG_2543_jpeg_jpg.rf.85abe7c386749b5e81cc7241bc83cc13.jpg \n extracting: train/IMG_2543_jpeg_jpg.rf.85abe7c386749b5e81cc7241bc83cc13.txt \n extracting: train/IMG_2545_jpeg_jpg.rf.97d3cb94db4f36e58f63501f49225ff7.jpg \n extracting: train/IMG_2545_jpeg_jpg.rf.97d3cb94db4f36e58f63501f49225ff7.txt \n extracting: train/IMG_2545_jpeg_jpg.rf.ab8f4166994cc93971c336062ec826c2.jpg \n extracting: train/IMG_2545_jpeg_jpg.rf.ab8f4166994cc93971c336062ec826c2.txt \n extracting: train/IMG_2545_jpeg_jpg.rf.af2fc5e3139bef81806f3b60840b29b1.jpg \n extracting: train/IMG_2545_jpeg_jpg.rf.af2fc5e3139bef81806f3b60840b29b1.txt \n extracting: train/IMG_2548_jpeg_jpg.rf.0dcdcc7df9a06836d618a02f32b6da0a.jpg \n extracting: train/IMG_2548_jpeg_jpg.rf.0dcdcc7df9a06836d618a02f32b6da0a.txt \n extracting: train/IMG_2548_jpeg_jpg.rf.4b5fc717eb208e782fc000511d4e473c.jpg \n extracting: train/IMG_2548_jpeg_jpg.rf.4b5fc717eb208e782fc000511d4e473c.txt \n extracting: train/IMG_2548_jpeg_jpg.rf.dc60cbfee920ec90a321852a61e523a2.jpg \n extracting: train/IMG_2548_jpeg_jpg.rf.dc60cbfee920ec90a321852a61e523a2.txt \n extracting: train/IMG_2549_jpeg_jpg.rf.7686e3c451f639fc43eed2354330cbf1.jpg \n extracting: train/IMG_2549_jpeg_jpg.rf.7686e3c451f639fc43eed2354330cbf1.txt \n extracting: train/IMG_2549_jpeg_jpg.rf.9da578fd9a7c4f07f7a1b78edbd74cd4.jpg \n extracting: train/IMG_2549_jpeg_jpg.rf.9da578fd9a7c4f07f7a1b78edbd74cd4.txt \n extracting: train/IMG_2549_jpeg_jpg.rf.e874327c4dfe2c4d29235fbf9506b941.jpg \n extracting: train/IMG_2549_jpeg_jpg.rf.e874327c4dfe2c4d29235fbf9506b941.txt \n extracting: train/IMG_2550_jpeg_jpg.rf.02059b7ee8cae321a14f793f84146cd0.jpg \n extracting: train/IMG_2550_jpeg_jpg.rf.02059b7ee8cae321a14f793f84146cd0.txt \n extracting: train/IMG_2550_jpeg_jpg.rf.a6995ffcc36b52738ec15a664e16d5a2.jpg \n extracting: train/IMG_2550_jpeg_jpg.rf.a6995ffcc36b52738ec15a664e16d5a2.txt \n extracting: train/IMG_2550_jpeg_jpg.rf.eb616251c3ccf74501b19b02689b5e04.jpg \n extracting: train/IMG_2550_jpeg_jpg.rf.eb616251c3ccf74501b19b02689b5e04.txt \n extracting: train/IMG_2551_jpeg_jpg.rf.06e34b409926e6487ff9c5f84010dfcf.jpg \n extracting: train/IMG_2551_jpeg_jpg.rf.06e34b409926e6487ff9c5f84010dfcf.txt \n extracting: train/IMG_2551_jpeg_jpg.rf.19f9e4474dcab8ed1032aa57b2700fd2.jpg \n extracting: train/IMG_2551_jpeg_jpg.rf.19f9e4474dcab8ed1032aa57b2700fd2.txt \n extracting: train/IMG_2551_jpeg_jpg.rf.696425a6032e480d124a88f566145f4f.jpg \n extracting: train/IMG_2551_jpeg_jpg.rf.696425a6032e480d124a88f566145f4f.txt \n extracting: train/IMG_2552_jpeg_jpg.rf.708b626ea60c0605d3d8c880f0fcd75a.jpg \n extracting: train/IMG_2552_jpeg_jpg.rf.708b626ea60c0605d3d8c880f0fcd75a.txt \n extracting: train/IMG_2552_jpeg_jpg.rf.dc4ab4e9c47fb7c903003419171867d1.jpg \n extracting: train/IMG_2552_jpeg_jpg.rf.dc4ab4e9c47fb7c903003419171867d1.txt \n extracting: train/IMG_2552_jpeg_jpg.rf.e731d033ec34c8fe7d8d4429e22e18c1.jpg \n extracting: train/IMG_2552_jpeg_jpg.rf.e731d033ec34c8fe7d8d4429e22e18c1.txt \n extracting: train/IMG_2553_jpeg_jpg.rf.a823523da8bae534770fe4329f4f44ca.jpg \n extracting: train/IMG_2553_jpeg_jpg.rf.a823523da8bae534770fe4329f4f44ca.txt \n extracting: train/IMG_2553_jpeg_jpg.rf.cb9102ce8567cc067e5861e1e1b73679.jpg \n extracting: train/IMG_2553_jpeg_jpg.rf.cb9102ce8567cc067e5861e1e1b73679.txt \n extracting: train/IMG_2553_jpeg_jpg.rf.daf79ad40b14d51e6fc1a6a12ccc368e.jpg \n extracting: train/IMG_2553_jpeg_jpg.rf.daf79ad40b14d51e6fc1a6a12ccc368e.txt \n extracting: train/IMG_2554_jpeg_jpg.rf.42bfd4edb0ec7ddf2107ce1bf29f3a9b.jpg \n extracting: train/IMG_2554_jpeg_jpg.rf.42bfd4edb0ec7ddf2107ce1bf29f3a9b.txt \n extracting: train/IMG_2554_jpeg_jpg.rf.4599d1ce38b1736c91717f921ff5e0a9.jpg \n extracting: train/IMG_2554_jpeg_jpg.rf.4599d1ce38b1736c91717f921ff5e0a9.txt \n extracting: train/IMG_2554_jpeg_jpg.rf.7b8a02a620e250a59c1a7dd8fe245974.jpg \n extracting: train/IMG_2554_jpeg_jpg.rf.7b8a02a620e250a59c1a7dd8fe245974.txt \n extracting: train/IMG_2556_jpeg_jpg.rf.73938c51cc7f0dd450976e536794bf48.jpg \n extracting: train/IMG_2556_jpeg_jpg.rf.73938c51cc7f0dd450976e536794bf48.txt \n extracting: train/IMG_2556_jpeg_jpg.rf.8820c5120f80beae77fea142ce74786b.jpg \n extracting: train/IMG_2556_jpeg_jpg.rf.8820c5120f80beae77fea142ce74786b.txt \n extracting: train/IMG_2556_jpeg_jpg.rf.891d69950eb80022450a2afc472a593e.jpg \n extracting: train/IMG_2556_jpeg_jpg.rf.891d69950eb80022450a2afc472a593e.txt \n extracting: train/IMG_2558_jpeg_jpg.rf.2522d2a841f3bac4c6a79014dc0a85c5.jpg \n extracting: train/IMG_2558_jpeg_jpg.rf.2522d2a841f3bac4c6a79014dc0a85c5.txt \n extracting: train/IMG_2558_jpeg_jpg.rf.72b9cdc4253079d766a55e2cf3484186.jpg \n extracting: train/IMG_2558_jpeg_jpg.rf.72b9cdc4253079d766a55e2cf3484186.txt \n extracting: train/IMG_2558_jpeg_jpg.rf.d986f5a59e25d06500fdc4ca6d514241.jpg \n extracting: train/IMG_2558_jpeg_jpg.rf.d986f5a59e25d06500fdc4ca6d514241.txt \n extracting: train/IMG_2559_jpeg_jpg.rf.4500dfdf911677eb46fb16e35d3bd1c3.jpg \n extracting: train/IMG_2559_jpeg_jpg.rf.4500dfdf911677eb46fb16e35d3bd1c3.txt \n extracting: train/IMG_2559_jpeg_jpg.rf.847bcebee12a3443007e15e7cc1897b8.jpg \n extracting: train/IMG_2559_jpeg_jpg.rf.847bcebee12a3443007e15e7cc1897b8.txt \n extracting: train/IMG_2559_jpeg_jpg.rf.f23204d377eb5c1cf0ba7ba308f02e25.jpg \n extracting: train/IMG_2559_jpeg_jpg.rf.f23204d377eb5c1cf0ba7ba308f02e25.txt \n extracting: train/IMG_2560_jpeg_jpg.rf.2d49fc6a6de45d438f383d871c251a5d.jpg \n extracting: train/IMG_2560_jpeg_jpg.rf.2d49fc6a6de45d438f383d871c251a5d.txt \n extracting: train/IMG_2560_jpeg_jpg.rf.959e62564d04ba8058df51da81829cf0.jpg \n extracting: train/IMG_2560_jpeg_jpg.rf.959e62564d04ba8058df51da81829cf0.txt \n extracting: train/IMG_2560_jpeg_jpg.rf.a4388f913131fa6be14444d7ed6b0217.jpg \n extracting: train/IMG_2560_jpeg_jpg.rf.a4388f913131fa6be14444d7ed6b0217.txt \n extracting: train/IMG_2561_jpeg_jpg.rf.0587753914aa75bf8773050e2f57dd67.jpg \n extracting: train/IMG_2561_jpeg_jpg.rf.0587753914aa75bf8773050e2f57dd67.txt \n extracting: train/IMG_2561_jpeg_jpg.rf.0968b7fde0ac1b60e61a2fdfe1a96623.jpg \n extracting: train/IMG_2561_jpeg_jpg.rf.0968b7fde0ac1b60e61a2fdfe1a96623.txt \n extracting: train/IMG_2561_jpeg_jpg.rf.c922828f61597384d170857f92d88df9.jpg \n extracting: train/IMG_2561_jpeg_jpg.rf.c922828f61597384d170857f92d88df9.txt \n extracting: train/IMG_2562_jpeg_jpg.rf.2645d8d9dda2805d76fdaae05de4c6ab.jpg \n extracting: train/IMG_2562_jpeg_jpg.rf.2645d8d9dda2805d76fdaae05de4c6ab.txt \n extracting: train/IMG_2562_jpeg_jpg.rf.44262f0d40475806987f245658dbc22b.jpg \n extracting: train/IMG_2562_jpeg_jpg.rf.44262f0d40475806987f245658dbc22b.txt \n extracting: train/IMG_2562_jpeg_jpg.rf.7df081bd9693ee0eb63529f131bc294e.jpg \n extracting: train/IMG_2562_jpeg_jpg.rf.7df081bd9693ee0eb63529f131bc294e.txt \n extracting: train/IMG_2563_jpeg_jpg.rf.055ccc08ff31b140815b6ca43cca8cfe.jpg \n extracting: train/IMG_2563_jpeg_jpg.rf.055ccc08ff31b140815b6ca43cca8cfe.txt \n extracting: train/IMG_2563_jpeg_jpg.rf.a5aababe02b20d3ce9d799a18de6d410.jpg \n extracting: train/IMG_2563_jpeg_jpg.rf.a5aababe02b20d3ce9d799a18de6d410.txt \n extracting: train/IMG_2563_jpeg_jpg.rf.e575e0391953239c31cf2396d4627ac3.jpg \n extracting: train/IMG_2563_jpeg_jpg.rf.e575e0391953239c31cf2396d4627ac3.txt \n extracting: train/IMG_2564_jpeg_jpg.rf.cc671c383135fb7b9198a7038008532a.jpg \n extracting: train/IMG_2564_jpeg_jpg.rf.cc671c383135fb7b9198a7038008532a.txt \n extracting: train/IMG_2564_jpeg_jpg.rf.d5f39aceaf4c6d86fe7dd676245c402c.jpg \n extracting: train/IMG_2564_jpeg_jpg.rf.d5f39aceaf4c6d86fe7dd676245c402c.txt \n extracting: train/IMG_2564_jpeg_jpg.rf.dc48cf10de1971ea60aebf0629d40a67.jpg \n extracting: train/IMG_2564_jpeg_jpg.rf.dc48cf10de1971ea60aebf0629d40a67.txt \n extracting: train/IMG_2565_jpeg_jpg.rf.2744451ab4a71a9c7af9d92a0ffdd0fd.jpg \n extracting: train/IMG_2565_jpeg_jpg.rf.2744451ab4a71a9c7af9d92a0ffdd0fd.txt \n extracting: train/IMG_2565_jpeg_jpg.rf.51ed83297e284d87a25b24c747e7d9bc.jpg \n extracting: train/IMG_2565_jpeg_jpg.rf.51ed83297e284d87a25b24c747e7d9bc.txt \n extracting: train/IMG_2565_jpeg_jpg.rf.b26f35db7b60344184cf6f10d8e23eee.jpg \n extracting: train/IMG_2565_jpeg_jpg.rf.b26f35db7b60344184cf6f10d8e23eee.txt \n extracting: train/IMG_2566_jpeg_jpg.rf.0bf4e36f8c13ef39ecdf6fdb1a4631b3.jpg \n extracting: train/IMG_2566_jpeg_jpg.rf.0bf4e36f8c13ef39ecdf6fdb1a4631b3.txt \n extracting: train/IMG_2566_jpeg_jpg.rf.87eef35c61bef4e744002d0d01b602e3.jpg \n extracting: train/IMG_2566_jpeg_jpg.rf.87eef35c61bef4e744002d0d01b602e3.txt \n extracting: train/IMG_2566_jpeg_jpg.rf.953825afd8020c4378eb7cfd51ff0e41.jpg \n extracting: train/IMG_2566_jpeg_jpg.rf.953825afd8020c4378eb7cfd51ff0e41.txt \n extracting: train/IMG_2568_jpeg_jpg.rf.175d0d6646e5a891d3a38426fefb30d8.jpg \n extracting: train/IMG_2568_jpeg_jpg.rf.175d0d6646e5a891d3a38426fefb30d8.txt \n extracting: train/IMG_2568_jpeg_jpg.rf.3a06e696ad41c9823f7544710e037607.jpg \n extracting: train/IMG_2568_jpeg_jpg.rf.3a06e696ad41c9823f7544710e037607.txt \n extracting: train/IMG_2568_jpeg_jpg.rf.a0c55c36de61035377a878f99a9e1c83.jpg \n extracting: train/IMG_2568_jpeg_jpg.rf.a0c55c36de61035377a878f99a9e1c83.txt \n extracting: train/IMG_2571_jpeg_jpg.rf.2817d2bb3f6f6744a91f5eda9c13df0b.jpg \n extracting: train/IMG_2571_jpeg_jpg.rf.2817d2bb3f6f6744a91f5eda9c13df0b.txt \n extracting: train/IMG_2571_jpeg_jpg.rf.8f1dbbf5e664276278b9286329f3bb23.jpg \n extracting: train/IMG_2571_jpeg_jpg.rf.8f1dbbf5e664276278b9286329f3bb23.txt \n extracting: train/IMG_2571_jpeg_jpg.rf.b021249d91b5fed5033ed2744697707f.jpg \n extracting: train/IMG_2571_jpeg_jpg.rf.b021249d91b5fed5033ed2744697707f.txt \n extracting: train/IMG_2572_jpeg_jpg.rf.0d0df450e6ef3b951cbbe1119f9a00fd.jpg \n extracting: train/IMG_2572_jpeg_jpg.rf.0d0df450e6ef3b951cbbe1119f9a00fd.txt \n extracting: train/IMG_2572_jpeg_jpg.rf.198f87d330405c56f081ec26d967a355.jpg \n extracting: train/IMG_2572_jpeg_jpg.rf.198f87d330405c56f081ec26d967a355.txt \n extracting: train/IMG_2572_jpeg_jpg.rf.3e3ad2e8ad6487f1a5700e2ba0fe5b65.jpg \n extracting: train/IMG_2572_jpeg_jpg.rf.3e3ad2e8ad6487f1a5700e2ba0fe5b65.txt \n extracting: train/IMG_2573_jpeg_jpg.rf.25ad4850a1aba0289841c08642b4573f.jpg \n extracting: train/IMG_2573_jpeg_jpg.rf.25ad4850a1aba0289841c08642b4573f.txt \n extracting: train/IMG_2573_jpeg_jpg.rf.2e309d30c7a4fcabad518564d681081c.jpg \n extracting: train/IMG_2573_jpeg_jpg.rf.2e309d30c7a4fcabad518564d681081c.txt \n extracting: train/IMG_2573_jpeg_jpg.rf.77b386a1cd87fc07c747ea71a6c923bb.jpg \n extracting: train/IMG_2573_jpeg_jpg.rf.77b386a1cd87fc07c747ea71a6c923bb.txt \n extracting: train/IMG_2575_jpeg_jpg.rf.7f79c590ea06041873640fef75d34de2.jpg \n extracting: train/IMG_2575_jpeg_jpg.rf.7f79c590ea06041873640fef75d34de2.txt \n extracting: train/IMG_2575_jpeg_jpg.rf.823d4154aa968934f7fd23774f29454c.jpg \n extracting: train/IMG_2575_jpeg_jpg.rf.823d4154aa968934f7fd23774f29454c.txt \n extracting: train/IMG_2575_jpeg_jpg.rf.bf460e54163659608935aab5d0c46d95.jpg \n extracting: train/IMG_2575_jpeg_jpg.rf.bf460e54163659608935aab5d0c46d95.txt \n extracting: train/IMG_2576_jpeg_jpg.rf.4c3109f6c8de97f20a12c6e4853a73d5.jpg \n extracting: train/IMG_2576_jpeg_jpg.rf.4c3109f6c8de97f20a12c6e4853a73d5.txt \n extracting: train/IMG_2576_jpeg_jpg.rf.5535c797e3d1d3ec707c2b33e00400e0.jpg \n extracting: train/IMG_2576_jpeg_jpg.rf.5535c797e3d1d3ec707c2b33e00400e0.txt \n extracting: train/IMG_2576_jpeg_jpg.rf.df6d2a4b22c110f8ee8c485e9231e684.jpg \n extracting: train/IMG_2576_jpeg_jpg.rf.df6d2a4b22c110f8ee8c485e9231e684.txt \n extracting: train/IMG_2578_jpeg_jpg.rf.89bbf27836d27e5059a902d1ff936036.jpg \n extracting: train/IMG_2578_jpeg_jpg.rf.89bbf27836d27e5059a902d1ff936036.txt \n extracting: train/IMG_2578_jpeg_jpg.rf.d309419325dd435549c604bd52b9cdfe.jpg \n extracting: train/IMG_2578_jpeg_jpg.rf.d309419325dd435549c604bd52b9cdfe.txt \n extracting: train/IMG_2578_jpeg_jpg.rf.eab8a7745b2690ab3bc0cdd99d30078a.jpg \n extracting: train/IMG_2578_jpeg_jpg.rf.eab8a7745b2690ab3bc0cdd99d30078a.txt \n extracting: train/IMG_2579_jpeg_jpg.rf.2243f354aed4b4511531b11b3d40e970.jpg \n extracting: train/IMG_2579_jpeg_jpg.rf.2243f354aed4b4511531b11b3d40e970.txt \n extracting: train/IMG_2579_jpeg_jpg.rf.2896c039bd9a823864f6a9869a7b02ba.jpg \n extracting: train/IMG_2579_jpeg_jpg.rf.2896c039bd9a823864f6a9869a7b02ba.txt \n extracting: train/IMG_2579_jpeg_jpg.rf.9fa4e6bf6e70669be8cf28ec3ac456c5.jpg \n extracting: train/IMG_2579_jpeg_jpg.rf.9fa4e6bf6e70669be8cf28ec3ac456c5.txt \n extracting: train/IMG_2580_jpeg_jpg.rf.39132ddc03b9b55cb3a57959e864dad7.jpg \n extracting: train/IMG_2580_jpeg_jpg.rf.39132ddc03b9b55cb3a57959e864dad7.txt \n extracting: train/IMG_2580_jpeg_jpg.rf.6125a0d169fae81f1a919e7566188ea3.jpg \n extracting: train/IMG_2580_jpeg_jpg.rf.6125a0d169fae81f1a919e7566188ea3.txt \n extracting: train/IMG_2580_jpeg_jpg.rf.b3e60636df0e38fc4e0229a9898f2a13.jpg \n extracting: train/IMG_2580_jpeg_jpg.rf.b3e60636df0e38fc4e0229a9898f2a13.txt \n extracting: train/IMG_2581_jpeg_jpg.rf.361d132caaf1acff47346fd87c8c047b.jpg \n extracting: train/IMG_2581_jpeg_jpg.rf.361d132caaf1acff47346fd87c8c047b.txt \n extracting: train/IMG_2581_jpeg_jpg.rf.6d453e6a185f317ce4180deeae254fae.jpg \n extracting: train/IMG_2581_jpeg_jpg.rf.6d453e6a185f317ce4180deeae254fae.txt \n extracting: train/IMG_2581_jpeg_jpg.rf.ab69aa9c521099a75c7d196acaf575cd.jpg \n extracting: train/IMG_2581_jpeg_jpg.rf.ab69aa9c521099a75c7d196acaf575cd.txt \n extracting: train/IMG_2584_jpeg_jpg.rf.439c35afb87689ac61c0cce7afa30c93.jpg \n extracting: train/IMG_2584_jpeg_jpg.rf.439c35afb87689ac61c0cce7afa30c93.txt \n extracting: train/IMG_2584_jpeg_jpg.rf.8dfafeb8986dbdb6e734aa2dc66bb2a7.jpg \n extracting: train/IMG_2584_jpeg_jpg.rf.8dfafeb8986dbdb6e734aa2dc66bb2a7.txt \n extracting: train/IMG_2584_jpeg_jpg.rf.fa2e78d0237667194e47482c6c2ef953.jpg \n extracting: train/IMG_2584_jpeg_jpg.rf.fa2e78d0237667194e47482c6c2ef953.txt \n extracting: train/IMG_2585_jpeg_jpg.rf.c9fd5ed2c64726649010a3049a31d0f8.jpg \n extracting: train/IMG_2585_jpeg_jpg.rf.c9fd5ed2c64726649010a3049a31d0f8.txt \n extracting: train/IMG_2585_jpeg_jpg.rf.e8c2b5d22dcf8f4016a495bb876d2f67.jpg \n extracting: train/IMG_2585_jpeg_jpg.rf.e8c2b5d22dcf8f4016a495bb876d2f67.txt \n extracting: train/IMG_2585_jpeg_jpg.rf.fbb2e78afc75900d5407c5a60e821953.jpg \n extracting: train/IMG_2585_jpeg_jpg.rf.fbb2e78afc75900d5407c5a60e821953.txt \n extracting: train/IMG_2587_jpeg_jpg.rf.2e0d795edf31d58121ca836f837dbb32.jpg \n extracting: train/IMG_2587_jpeg_jpg.rf.2e0d795edf31d58121ca836f837dbb32.txt \n extracting: train/IMG_2587_jpeg_jpg.rf.339e787a73bb862ddf2939fe35444f3b.jpg \n extracting: train/IMG_2587_jpeg_jpg.rf.339e787a73bb862ddf2939fe35444f3b.txt \n extracting: train/IMG_2587_jpeg_jpg.rf.d5fac5f15c6f1d60f9b73b902754dcad.jpg \n extracting: train/IMG_2587_jpeg_jpg.rf.d5fac5f15c6f1d60f9b73b902754dcad.txt \n extracting: train/IMG_2589_jpeg_jpg.rf.1a8fd3ff32ece3d3d2674950d67157a7.jpg \n extracting: train/IMG_2589_jpeg_jpg.rf.1a8fd3ff32ece3d3d2674950d67157a7.txt \n extracting: train/IMG_2589_jpeg_jpg.rf.7b1f3e83eba3bc8051a016a1169d9e32.jpg \n extracting: train/IMG_2589_jpeg_jpg.rf.7b1f3e83eba3bc8051a016a1169d9e32.txt \n extracting: train/IMG_2589_jpeg_jpg.rf.c3017f9930ef2168085ee9a9be99d5ba.jpg \n extracting: train/IMG_2589_jpeg_jpg.rf.c3017f9930ef2168085ee9a9be99d5ba.txt \n extracting: train/IMG_2590_jpeg_jpg.rf.13fe5da0dc75a6499ca60995e926132d.jpg \n extracting: train/IMG_2590_jpeg_jpg.rf.13fe5da0dc75a6499ca60995e926132d.txt \n extracting: train/IMG_2590_jpeg_jpg.rf.15ebb488c2553afa76f11055ba481109.jpg \n extracting: train/IMG_2590_jpeg_jpg.rf.15ebb488c2553afa76f11055ba481109.txt \n extracting: train/IMG_2590_jpeg_jpg.rf.fdaba87887ed6f8ee208657afee7077d.jpg \n extracting: train/IMG_2590_jpeg_jpg.rf.fdaba87887ed6f8ee208657afee7077d.txt \n extracting: train/IMG_2592_jpeg_jpg.rf.aaae3c613c9e95da6398896697ebbbbe.jpg \n extracting: train/IMG_2592_jpeg_jpg.rf.aaae3c613c9e95da6398896697ebbbbe.txt \n extracting: train/IMG_2592_jpeg_jpg.rf.b550924fd462d5c3a4fa1676050e8044.jpg \n extracting: train/IMG_2592_jpeg_jpg.rf.b550924fd462d5c3a4fa1676050e8044.txt \n extracting: train/IMG_2592_jpeg_jpg.rf.ef22bd873a85a50d76eb414500274980.jpg \n extracting: train/IMG_2592_jpeg_jpg.rf.ef22bd873a85a50d76eb414500274980.txt \n extracting: train/IMG_2593_jpeg_jpg.rf.5fb4a18f36f8e27c1b6c7d285ac11ff1.jpg \n extracting: train/IMG_2593_jpeg_jpg.rf.5fb4a18f36f8e27c1b6c7d285ac11ff1.txt \n extracting: train/IMG_2593_jpeg_jpg.rf.97169ca8ed1f467baf9e2535173d5fde.jpg \n extracting: train/IMG_2593_jpeg_jpg.rf.97169ca8ed1f467baf9e2535173d5fde.txt \n extracting: train/IMG_2593_jpeg_jpg.rf.aad019b46eee8e56c8cabe3800f739d8.jpg \n extracting: train/IMG_2593_jpeg_jpg.rf.aad019b46eee8e56c8cabe3800f739d8.txt \n extracting: train/IMG_2594_jpeg_jpg.rf.47615ded059983fcfab563012e406c4b.jpg \n extracting: train/IMG_2594_jpeg_jpg.rf.47615ded059983fcfab563012e406c4b.txt \n extracting: train/IMG_2594_jpeg_jpg.rf.6a4130a5617f727936794cce01aff117.jpg \n extracting: train/IMG_2594_jpeg_jpg.rf.6a4130a5617f727936794cce01aff117.txt \n extracting: train/IMG_2594_jpeg_jpg.rf.8c2dd7a04155d5f260e5184ca031caba.jpg \n extracting: train/IMG_2594_jpeg_jpg.rf.8c2dd7a04155d5f260e5184ca031caba.txt \n extracting: train/IMG_2595_jpeg_jpg.rf.0c52f3721c15968ba9ce25f94d61922f.jpg \n extracting: train/IMG_2595_jpeg_jpg.rf.0c52f3721c15968ba9ce25f94d61922f.txt \n extracting: train/IMG_2595_jpeg_jpg.rf.660b28c1eba3c99e8098e832b25b03f2.jpg \n extracting: train/IMG_2595_jpeg_jpg.rf.660b28c1eba3c99e8098e832b25b03f2.txt \n extracting: train/IMG_2595_jpeg_jpg.rf.83de2787b0a1511c1587b77ce314d3a0.jpg \n extracting: train/IMG_2595_jpeg_jpg.rf.83de2787b0a1511c1587b77ce314d3a0.txt \n extracting: train/IMG_2596_jpeg_jpg.rf.60c3b34918cfd4d177ff6e979ae32c15.jpg \n extracting: train/IMG_2596_jpeg_jpg.rf.60c3b34918cfd4d177ff6e979ae32c15.txt \n extracting: train/IMG_2596_jpeg_jpg.rf.9bb538f85d1d85ea6b226b7b174a4e9e.jpg \n extracting: train/IMG_2596_jpeg_jpg.rf.9bb538f85d1d85ea6b226b7b174a4e9e.txt \n extracting: train/IMG_2596_jpeg_jpg.rf.bab590b2f3bb3b7478122e3227b6d5fb.jpg \n extracting: train/IMG_2596_jpeg_jpg.rf.bab590b2f3bb3b7478122e3227b6d5fb.txt \n extracting: train/IMG_2597_jpeg_jpg.rf.73f2b801a21791ff9b83d4b34fc24ad8.jpg \n extracting: train/IMG_2597_jpeg_jpg.rf.73f2b801a21791ff9b83d4b34fc24ad8.txt \n extracting: train/IMG_2597_jpeg_jpg.rf.760a634e3cb253c72523a5ca7f77002f.jpg \n extracting: train/IMG_2597_jpeg_jpg.rf.760a634e3cb253c72523a5ca7f77002f.txt \n extracting: train/IMG_2597_jpeg_jpg.rf.cee0bdd181b407d2b36d851c721376ad.jpg \n extracting: train/IMG_2597_jpeg_jpg.rf.cee0bdd181b407d2b36d851c721376ad.txt \n extracting: train/IMG_2598_jpeg_jpg.rf.1694dd0862c3074dfc2b30b097d11483.jpg \n extracting: train/IMG_2598_jpeg_jpg.rf.1694dd0862c3074dfc2b30b097d11483.txt \n extracting: train/IMG_2598_jpeg_jpg.rf.45e26870313ad5ecdefc3eac10b29e46.jpg \n extracting: train/IMG_2598_jpeg_jpg.rf.45e26870313ad5ecdefc3eac10b29e46.txt \n extracting: train/IMG_2598_jpeg_jpg.rf.ce4555669ef126019475802c8dc12916.jpg \n extracting: train/IMG_2598_jpeg_jpg.rf.ce4555669ef126019475802c8dc12916.txt \n extracting: train/IMG_2600_jpeg_jpg.rf.4464b898b13d17ba29c62dfe4a985ad3.jpg \n extracting: train/IMG_2600_jpeg_jpg.rf.4464b898b13d17ba29c62dfe4a985ad3.txt \n extracting: train/IMG_2600_jpeg_jpg.rf.9937107eb9c0a3dd0f2454e3bf459871.jpg \n extracting: train/IMG_2600_jpeg_jpg.rf.9937107eb9c0a3dd0f2454e3bf459871.txt \n extracting: train/IMG_2600_jpeg_jpg.rf.b2580b7fe36815e208fa6b9e80cc9f52.jpg \n extracting: train/IMG_2600_jpeg_jpg.rf.b2580b7fe36815e208fa6b9e80cc9f52.txt \n extracting: train/IMG_2601_jpeg_jpg.rf.18a1354d55ae173adf6e02519cda49ad.jpg \n extracting: train/IMG_2601_jpeg_jpg.rf.18a1354d55ae173adf6e02519cda49ad.txt \n extracting: train/IMG_2601_jpeg_jpg.rf.1b648594a8386c3edd7b522d3315b5f6.jpg \n extracting: train/IMG_2601_jpeg_jpg.rf.1b648594a8386c3edd7b522d3315b5f6.txt \n extracting: train/IMG_2601_jpeg_jpg.rf.e293f2c775f03212af1ab08391accfef.jpg \n extracting: train/IMG_2601_jpeg_jpg.rf.e293f2c775f03212af1ab08391accfef.txt \n extracting: train/IMG_2603_jpeg_jpg.rf.1d66fd84579a0ea99f7cd1880bc1d16b.jpg \n extracting: train/IMG_2603_jpeg_jpg.rf.1d66fd84579a0ea99f7cd1880bc1d16b.txt \n extracting: train/IMG_2603_jpeg_jpg.rf.c61c9da9ee25fc5bfd825978b18fc35d.jpg \n extracting: train/IMG_2603_jpeg_jpg.rf.c61c9da9ee25fc5bfd825978b18fc35d.txt \n extracting: train/IMG_2603_jpeg_jpg.rf.ffd6dd079ada23689cf9d6cead1e311f.jpg \n extracting: train/IMG_2603_jpeg_jpg.rf.ffd6dd079ada23689cf9d6cead1e311f.txt \n extracting: train/IMG_2604_jpeg_jpg.rf.9ae5c08502d3127279163fc87c21c0c4.jpg \n extracting: train/IMG_2604_jpeg_jpg.rf.9ae5c08502d3127279163fc87c21c0c4.txt \n extracting: train/IMG_2604_jpeg_jpg.rf.c29affd7cea3ca3cf237bdc7a11a231f.jpg \n extracting: train/IMG_2604_jpeg_jpg.rf.c29affd7cea3ca3cf237bdc7a11a231f.txt \n extracting: train/IMG_2604_jpeg_jpg.rf.f34fdb35580c2893e744c97a6fe9bb8f.jpg \n extracting: train/IMG_2604_jpeg_jpg.rf.f34fdb35580c2893e744c97a6fe9bb8f.txt \n extracting: train/IMG_2605_jpeg_jpg.rf.4171bedfdc4e4a247f674a09984c3c2c.jpg \n extracting: train/IMG_2605_jpeg_jpg.rf.4171bedfdc4e4a247f674a09984c3c2c.txt \n extracting: train/IMG_2605_jpeg_jpg.rf.4e4901a771c78415822691cb8a080392.jpg \n extracting: train/IMG_2605_jpeg_jpg.rf.4e4901a771c78415822691cb8a080392.txt \n extracting: train/IMG_2605_jpeg_jpg.rf.5a526945d253f3ec29e73b42e8c254bb.jpg \n extracting: train/IMG_2605_jpeg_jpg.rf.5a526945d253f3ec29e73b42e8c254bb.txt \n extracting: train/IMG_2606_jpeg_jpg.rf.0fc2a4912e1937260ef835c058dfbc6a.jpg \n extracting: train/IMG_2606_jpeg_jpg.rf.0fc2a4912e1937260ef835c058dfbc6a.txt \n extracting: train/IMG_2606_jpeg_jpg.rf.1d10733a56a5bb356a2f2cc1c14c5f07.jpg \n extracting: train/IMG_2606_jpeg_jpg.rf.1d10733a56a5bb356a2f2cc1c14c5f07.txt \n extracting: train/IMG_2606_jpeg_jpg.rf.881e353bae17ec60afeddf363f7ca70e.jpg \n extracting: train/IMG_2606_jpeg_jpg.rf.881e353bae17ec60afeddf363f7ca70e.txt \n extracting: train/IMG_2608_jpeg_jpg.rf.3d010a777668f5211f17508bfce16389.jpg \n extracting: train/IMG_2608_jpeg_jpg.rf.3d010a777668f5211f17508bfce16389.txt \n extracting: train/IMG_2608_jpeg_jpg.rf.59b09b942768f3ce3a9d0c57b1dfdd0d.jpg \n extracting: train/IMG_2608_jpeg_jpg.rf.59b09b942768f3ce3a9d0c57b1dfdd0d.txt \n extracting: train/IMG_2608_jpeg_jpg.rf.a440850dd96eab6a88bb49366af84f7c.jpg \n extracting: train/IMG_2608_jpeg_jpg.rf.a440850dd96eab6a88bb49366af84f7c.txt \n extracting: train/IMG_2609_jpeg_jpg.rf.108242271ce797256cc51cf578e28fd4.jpg \n extracting: train/IMG_2609_jpeg_jpg.rf.108242271ce797256cc51cf578e28fd4.txt \n extracting: train/IMG_2609_jpeg_jpg.rf.2929607fcc9dc04b03c94e3680ed7fef.jpg \n extracting: train/IMG_2609_jpeg_jpg.rf.2929607fcc9dc04b03c94e3680ed7fef.txt \n extracting: train/IMG_2609_jpeg_jpg.rf.ff67eb35e2093795370a464ab28efb8b.jpg \n extracting: train/IMG_2609_jpeg_jpg.rf.ff67eb35e2093795370a464ab28efb8b.txt \n extracting: train/IMG_2610_jpeg_jpg.rf.c47ea6c6ba8a78c16b385e26fc17430e.jpg \n extracting: train/IMG_2610_jpeg_jpg.rf.c47ea6c6ba8a78c16b385e26fc17430e.txt \n extracting: train/IMG_2610_jpeg_jpg.rf.eac508798c240644befca28172032da4.jpg \n extracting: train/IMG_2610_jpeg_jpg.rf.eac508798c240644befca28172032da4.txt \n extracting: train/IMG_2610_jpeg_jpg.rf.fcb1752a27385066a322cd376eefce17.jpg \n extracting: train/IMG_2610_jpeg_jpg.rf.fcb1752a27385066a322cd376eefce17.txt \n extracting: train/IMG_2611_jpeg_jpg.rf.26aedce40180c1e83a7dcefb6e3bd396.jpg \n extracting: train/IMG_2611_jpeg_jpg.rf.26aedce40180c1e83a7dcefb6e3bd396.txt \n extracting: train/IMG_2611_jpeg_jpg.rf.469832f861481fb1db2b649a48f846fc.jpg \n extracting: train/IMG_2611_jpeg_jpg.rf.469832f861481fb1db2b649a48f846fc.txt \n extracting: train/IMG_2611_jpeg_jpg.rf.ab6a0ff7eec25ddf7f7168b0000a1fbc.jpg \n extracting: train/IMG_2611_jpeg_jpg.rf.ab6a0ff7eec25ddf7f7168b0000a1fbc.txt \n extracting: train/IMG_2612_jpeg_jpg.rf.15c66888517beb9cc41ea8b724bee713.jpg \n extracting: train/IMG_2612_jpeg_jpg.rf.15c66888517beb9cc41ea8b724bee713.txt \n extracting: train/IMG_2612_jpeg_jpg.rf.3af0ba32eec8a3d376e945088b57098b.jpg \n extracting: train/IMG_2612_jpeg_jpg.rf.3af0ba32eec8a3d376e945088b57098b.txt \n extracting: train/IMG_2612_jpeg_jpg.rf.4790807128c0f1f2e8d6d584854eba38.jpg \n extracting: train/IMG_2612_jpeg_jpg.rf.4790807128c0f1f2e8d6d584854eba38.txt \n extracting: train/IMG_2613_jpeg_jpg.rf.70b5a545b8c19ea872dc2b4c2ccd3309.jpg \n extracting: train/IMG_2613_jpeg_jpg.rf.70b5a545b8c19ea872dc2b4c2ccd3309.txt \n extracting: train/IMG_2613_jpeg_jpg.rf.bc00f23b83cb1e42ed9030f86a67e040.jpg \n extracting: train/IMG_2613_jpeg_jpg.rf.bc00f23b83cb1e42ed9030f86a67e040.txt \n extracting: train/IMG_2613_jpeg_jpg.rf.e4a27500841708d2757cdb307c28db6b.jpg \n extracting: train/IMG_2613_jpeg_jpg.rf.e4a27500841708d2757cdb307c28db6b.txt \n extracting: train/IMG_2614_jpeg_jpg.rf.027624adcaadd4e001280df3fd2a7aff.jpg \n extracting: train/IMG_2614_jpeg_jpg.rf.027624adcaadd4e001280df3fd2a7aff.txt \n extracting: train/IMG_2614_jpeg_jpg.rf.26ac388cff660f9743dda6f2d38efe6f.jpg \n extracting: train/IMG_2614_jpeg_jpg.rf.26ac388cff660f9743dda6f2d38efe6f.txt \n extracting: train/IMG_2614_jpeg_jpg.rf.bebd0f15393510965d208eb5af3b12bc.jpg \n extracting: train/IMG_2614_jpeg_jpg.rf.bebd0f15393510965d208eb5af3b12bc.txt \n extracting: train/IMG_2615_jpeg_jpg.rf.3321fa85b9d0472c4895e32635ad1e8c.jpg \n extracting: train/IMG_2615_jpeg_jpg.rf.3321fa85b9d0472c4895e32635ad1e8c.txt \n extracting: train/IMG_2615_jpeg_jpg.rf.a07fe38b0a9d21fe350128a682916c38.jpg \n extracting: train/IMG_2615_jpeg_jpg.rf.a07fe38b0a9d21fe350128a682916c38.txt \n extracting: train/IMG_2615_jpeg_jpg.rf.d5ba81a5061eccf078801a2d742f5aab.jpg \n extracting: train/IMG_2615_jpeg_jpg.rf.d5ba81a5061eccf078801a2d742f5aab.txt \n extracting: train/IMG_2617_jpeg_jpg.rf.632d4643caed8ff765f9a68c6e220b3b.jpg \n extracting: train/IMG_2617_jpeg_jpg.rf.632d4643caed8ff765f9a68c6e220b3b.txt \n extracting: train/IMG_2617_jpeg_jpg.rf.8848a4e692b786219993efbee4d806d4.jpg \n extracting: train/IMG_2617_jpeg_jpg.rf.8848a4e692b786219993efbee4d806d4.txt \n extracting: train/IMG_2617_jpeg_jpg.rf.b7e49acfa84c55bbf00daddfc4813157.jpg \n extracting: train/IMG_2617_jpeg_jpg.rf.b7e49acfa84c55bbf00daddfc4813157.txt \n extracting: train/IMG_2618_jpeg_jpg.rf.91fc20c1c4ef1e8cc8d15382a70d97b4.jpg \n extracting: train/IMG_2618_jpeg_jpg.rf.91fc20c1c4ef1e8cc8d15382a70d97b4.txt \n extracting: train/IMG_2618_jpeg_jpg.rf.c48cd73de3c21866d3de37b54678c0df.jpg \n extracting: train/IMG_2618_jpeg_jpg.rf.c48cd73de3c21866d3de37b54678c0df.txt \n extracting: train/IMG_2618_jpeg_jpg.rf.d2b3529cfbc406c1093234017e06c7e0.jpg \n extracting: train/IMG_2618_jpeg_jpg.rf.d2b3529cfbc406c1093234017e06c7e0.txt \n extracting: train/IMG_2619_jpeg_jpg.rf.2499cab7d442d7b3b048f8a32068cfb5.jpg \n extracting: train/IMG_2619_jpeg_jpg.rf.2499cab7d442d7b3b048f8a32068cfb5.txt \n extracting: train/IMG_2619_jpeg_jpg.rf.3c32301bedfe60636ba43c2103f03a4b.jpg \n extracting: train/IMG_2619_jpeg_jpg.rf.3c32301bedfe60636ba43c2103f03a4b.txt \n extracting: train/IMG_2619_jpeg_jpg.rf.e6b0e4d0490cfb8c83fa16d57ab6f801.jpg \n extracting: train/IMG_2619_jpeg_jpg.rf.e6b0e4d0490cfb8c83fa16d57ab6f801.txt \n extracting: train/IMG_2620_jpeg_jpg.rf.789c9145fd985c3fa6a3dd073e6e3a70.jpg \n extracting: train/IMG_2620_jpeg_jpg.rf.789c9145fd985c3fa6a3dd073e6e3a70.txt \n extracting: train/IMG_2620_jpeg_jpg.rf.c2c37a2eea68161fe7c71b09240e5813.jpg \n extracting: train/IMG_2620_jpeg_jpg.rf.c2c37a2eea68161fe7c71b09240e5813.txt \n extracting: train/IMG_2620_jpeg_jpg.rf.e9490e03ddc52ea42195ae3a68767e2f.jpg \n extracting: train/IMG_2620_jpeg_jpg.rf.e9490e03ddc52ea42195ae3a68767e2f.txt \n extracting: train/IMG_2621_jpeg_jpg.rf.876077a251f223f2884cbb7cfa53226d.jpg \n extracting: train/IMG_2621_jpeg_jpg.rf.876077a251f223f2884cbb7cfa53226d.txt \n extracting: train/IMG_2621_jpeg_jpg.rf.9fe637008e3002599f0e21bebcbc3c7f.jpg \n extracting: train/IMG_2621_jpeg_jpg.rf.9fe637008e3002599f0e21bebcbc3c7f.txt \n extracting: train/IMG_2621_jpeg_jpg.rf.aba608cec0f4cae7e8ef9b41854f6e6b.jpg \n extracting: train/IMG_2621_jpeg_jpg.rf.aba608cec0f4cae7e8ef9b41854f6e6b.txt \n extracting: train/IMG_2623_jpeg_jpg.rf.0c3bcfc76c88a028753e2b0e06116b07.jpg \n extracting: train/IMG_2623_jpeg_jpg.rf.0c3bcfc76c88a028753e2b0e06116b07.txt \n extracting: train/IMG_2623_jpeg_jpg.rf.1eb4a8be8eb999e07cd789aabbf51ae7.jpg \n extracting: train/IMG_2623_jpeg_jpg.rf.1eb4a8be8eb999e07cd789aabbf51ae7.txt \n extracting: train/IMG_2623_jpeg_jpg.rf.a3a667e0e76e8acdb476cb621f5134af.jpg \n extracting: train/IMG_2623_jpeg_jpg.rf.a3a667e0e76e8acdb476cb621f5134af.txt \n extracting: train/IMG_2624_jpeg_jpg.rf.0f9917b38d7304d28ac97c1d25d310c0.jpg \n extracting: train/IMG_2624_jpeg_jpg.rf.0f9917b38d7304d28ac97c1d25d310c0.txt \n extracting: train/IMG_2624_jpeg_jpg.rf.69ba110321affc8ab4843f3bebde7a6a.jpg \n extracting: train/IMG_2624_jpeg_jpg.rf.69ba110321affc8ab4843f3bebde7a6a.txt \n extracting: train/IMG_2624_jpeg_jpg.rf.8a6b767ebdaf9d9791830c9200f31751.jpg \n extracting: train/IMG_2624_jpeg_jpg.rf.8a6b767ebdaf9d9791830c9200f31751.txt \n extracting: train/IMG_2625_jpeg_jpg.rf.605a95f2fd28bfa9259993def83097df.jpg \n extracting: train/IMG_2625_jpeg_jpg.rf.605a95f2fd28bfa9259993def83097df.txt \n extracting: train/IMG_2625_jpeg_jpg.rf.a9f6242870ffabe0c7d166b224c2ee8b.jpg \n extracting: train/IMG_2625_jpeg_jpg.rf.a9f6242870ffabe0c7d166b224c2ee8b.txt \n extracting: train/IMG_2625_jpeg_jpg.rf.c42aae3e3bdc9b6230a3cbb48e27967f.jpg \n extracting: train/IMG_2625_jpeg_jpg.rf.c42aae3e3bdc9b6230a3cbb48e27967f.txt \n extracting: train/IMG_2627_jpeg_jpg.rf.ac997d578990bd79b9181c32c393f7d6.jpg \n extracting: train/IMG_2627_jpeg_jpg.rf.ac997d578990bd79b9181c32c393f7d6.txt \n extracting: train/IMG_2627_jpeg_jpg.rf.d9b2088b65d4ef41f1bc6913176ea4ad.jpg \n extracting: train/IMG_2627_jpeg_jpg.rf.d9b2088b65d4ef41f1bc6913176ea4ad.txt \n extracting: train/IMG_2627_jpeg_jpg.rf.d9bc599200e6f04a6f79635344d6c7c6.jpg \n extracting: train/IMG_2627_jpeg_jpg.rf.d9bc599200e6f04a6f79635344d6c7c6.txt \n extracting: train/IMG_2628_jpeg_jpg.rf.33358c023f510b4ece620fd9e126fc08.jpg \n extracting: train/IMG_2628_jpeg_jpg.rf.33358c023f510b4ece620fd9e126fc08.txt \n extracting: train/IMG_2628_jpeg_jpg.rf.58cc9c7169260e468feaad4d7a685ffe.jpg \n extracting: train/IMG_2628_jpeg_jpg.rf.58cc9c7169260e468feaad4d7a685ffe.txt \n extracting: train/IMG_2628_jpeg_jpg.rf.f3a6476913c52dfcda27a04f05af4efa.jpg \n extracting: train/IMG_2628_jpeg_jpg.rf.f3a6476913c52dfcda27a04f05af4efa.txt \n extracting: train/IMG_2629_jpeg_jpg.rf.4e81783b23074485c00a179fa46fabaa.jpg \n extracting: train/IMG_2629_jpeg_jpg.rf.4e81783b23074485c00a179fa46fabaa.txt \n extracting: train/IMG_2629_jpeg_jpg.rf.dc5c4185415eb3e78241447b2a927c7a.jpg \n extracting: train/IMG_2629_jpeg_jpg.rf.dc5c4185415eb3e78241447b2a927c7a.txt \n extracting: train/IMG_2629_jpeg_jpg.rf.fc8210a272fc85c635112eeb756627f6.jpg \n extracting: train/IMG_2629_jpeg_jpg.rf.fc8210a272fc85c635112eeb756627f6.txt \n extracting: train/IMG_2631_jpeg_jpg.rf.3464a2cfa8cfee93978c40a8afc316e8.jpg \n extracting: train/IMG_2631_jpeg_jpg.rf.3464a2cfa8cfee93978c40a8afc316e8.txt \n extracting: train/IMG_2631_jpeg_jpg.rf.3ff8f47f8414bf8c3b5aaa4068a0cf51.jpg \n extracting: train/IMG_2631_jpeg_jpg.rf.3ff8f47f8414bf8c3b5aaa4068a0cf51.txt \n extracting: train/IMG_2631_jpeg_jpg.rf.f1347c2fd5611b3fc016ada7164d9f90.jpg \n extracting: train/IMG_2631_jpeg_jpg.rf.f1347c2fd5611b3fc016ada7164d9f90.txt \n extracting: train/IMG_2633_jpeg_jpg.rf.3bb027281d3582a6e8dba3a5ed43645d.jpg \n extracting: train/IMG_2633_jpeg_jpg.rf.3bb027281d3582a6e8dba3a5ed43645d.txt \n extracting: train/IMG_2633_jpeg_jpg.rf.94db8e3988c0e9cdedb35a6f080663bd.jpg \n extracting: train/IMG_2633_jpeg_jpg.rf.94db8e3988c0e9cdedb35a6f080663bd.txt \n extracting: train/IMG_2633_jpeg_jpg.rf.e26855240e0396d3dcac0b1b2ecdd068.jpg \n extracting: train/IMG_2633_jpeg_jpg.rf.e26855240e0396d3dcac0b1b2ecdd068.txt \n extracting: train/IMG_2634_jpeg_jpg.rf.55a61e6a81864030531a46706ac5539f.jpg \n extracting: train/IMG_2634_jpeg_jpg.rf.55a61e6a81864030531a46706ac5539f.txt \n extracting: train/IMG_2634_jpeg_jpg.rf.80d5470ac82a65456831553bf6c08f87.jpg \n extracting: train/IMG_2634_jpeg_jpg.rf.80d5470ac82a65456831553bf6c08f87.txt \n extracting: train/IMG_2634_jpeg_jpg.rf.f81cbfae63e179699582e96995fa1821.jpg \n extracting: train/IMG_2634_jpeg_jpg.rf.f81cbfae63e179699582e96995fa1821.txt \n extracting: train/IMG_2638_jpeg_jpg.rf.4f1abcce71a4d55e3a75cd94bf476834.jpg \n extracting: train/IMG_2638_jpeg_jpg.rf.4f1abcce71a4d55e3a75cd94bf476834.txt \n extracting: train/IMG_2638_jpeg_jpg.rf.c60af32009e4dc6f9098d18f35525740.jpg \n extracting: train/IMG_2638_jpeg_jpg.rf.c60af32009e4dc6f9098d18f35525740.txt \n extracting: train/IMG_2638_jpeg_jpg.rf.e502c8f7e59e7a67e6d78e03447bd107.jpg \n extracting: train/IMG_2638_jpeg_jpg.rf.e502c8f7e59e7a67e6d78e03447bd107.txt \n extracting: train/IMG_2639_jpeg_jpg.rf.0d4f1bd0eaaf8c1bbdc8635ff85e77e5.jpg \n extracting: train/IMG_2639_jpeg_jpg.rf.0d4f1bd0eaaf8c1bbdc8635ff85e77e5.txt \n extracting: train/IMG_2639_jpeg_jpg.rf.10bafb3c3cc4e91594f588920db5ca22.jpg \n extracting: train/IMG_2639_jpeg_jpg.rf.10bafb3c3cc4e91594f588920db5ca22.txt \n extracting: train/IMG_2639_jpeg_jpg.rf.bb64c58845c43ebfba71ae8c9a2698fd.jpg \n extracting: train/IMG_2639_jpeg_jpg.rf.bb64c58845c43ebfba71ae8c9a2698fd.txt \n extracting: train/IMG_2640_jpeg_jpg.rf.3a409c5b755faa496e1ff021b335e350.jpg \n extracting: train/IMG_2640_jpeg_jpg.rf.3a409c5b755faa496e1ff021b335e350.txt \n extracting: train/IMG_2640_jpeg_jpg.rf.783a9cf8d5bc9a7a744a50a292cab7b6.jpg \n extracting: train/IMG_2640_jpeg_jpg.rf.783a9cf8d5bc9a7a744a50a292cab7b6.txt \n extracting: train/IMG_2640_jpeg_jpg.rf.c4fd41ad617b19b3c43ab5f219e76750.jpg \n extracting: train/IMG_2640_jpeg_jpg.rf.c4fd41ad617b19b3c43ab5f219e76750.txt \n extracting: train/IMG_2641_jpeg_jpg.rf.80439cb655b157cfb6f91895e7a1d940.jpg \n extracting: train/IMG_2641_jpeg_jpg.rf.80439cb655b157cfb6f91895e7a1d940.txt \n extracting: train/IMG_2641_jpeg_jpg.rf.f5427818255e0425cccb86c1e2d09338.jpg \n extracting: train/IMG_2641_jpeg_jpg.rf.f5427818255e0425cccb86c1e2d09338.txt \n extracting: train/IMG_2641_jpeg_jpg.rf.f96a74b024759c1097b05fa990a5e638.jpg \n extracting: train/IMG_2641_jpeg_jpg.rf.f96a74b024759c1097b05fa990a5e638.txt \n extracting: train/IMG_2642_jpeg_jpg.rf.1b951aeed33dfbb926054b47ef25a558.jpg \n extracting: train/IMG_2642_jpeg_jpg.rf.1b951aeed33dfbb926054b47ef25a558.txt \n extracting: train/IMG_2642_jpeg_jpg.rf.2bdc1f88a88cbcb469c18d71fbb3b859.jpg \n extracting: train/IMG_2642_jpeg_jpg.rf.2bdc1f88a88cbcb469c18d71fbb3b859.txt \n extracting: train/IMG_2642_jpeg_jpg.rf.adb71871cc899e286264ff26193a4c6b.jpg \n extracting: train/IMG_2642_jpeg_jpg.rf.adb71871cc899e286264ff26193a4c6b.txt \n extracting: train/IMG_2643_jpeg_jpg.rf.c75e828bd7b554c69f1079890ce86a85.jpg \n extracting: train/IMG_2643_jpeg_jpg.rf.c75e828bd7b554c69f1079890ce86a85.txt \n extracting: train/IMG_2643_jpeg_jpg.rf.cb1b1495cb42022a9387370c5d9e4a7f.jpg \n extracting: train/IMG_2643_jpeg_jpg.rf.cb1b1495cb42022a9387370c5d9e4a7f.txt \n extracting: train/IMG_2643_jpeg_jpg.rf.f03582eca689ad8c47ebe9fad9b0110d.jpg \n extracting: train/IMG_2643_jpeg_jpg.rf.f03582eca689ad8c47ebe9fad9b0110d.txt \n extracting: train/IMG_2644_jpeg_jpg.rf.a80742bde7dfd64f8ffeba3d329fc7a6.jpg \n extracting: train/IMG_2644_jpeg_jpg.rf.a80742bde7dfd64f8ffeba3d329fc7a6.txt \n extracting: train/IMG_2644_jpeg_jpg.rf.cdb99568d3c2f50364bd16c8ece5513e.jpg \n extracting: train/IMG_2644_jpeg_jpg.rf.cdb99568d3c2f50364bd16c8ece5513e.txt \n extracting: train/IMG_2644_jpeg_jpg.rf.edb5e3fc038cb1ebba84d0181b868366.jpg \n extracting: train/IMG_2644_jpeg_jpg.rf.edb5e3fc038cb1ebba84d0181b868366.txt \n extracting: train/IMG_2646_jpeg_jpg.rf.17500eaaa4f99e7764923753a801079a.jpg \n extracting: train/IMG_2646_jpeg_jpg.rf.17500eaaa4f99e7764923753a801079a.txt \n extracting: train/IMG_2646_jpeg_jpg.rf.76201ed567fb96e10b9e8dd33115ea85.jpg \n extracting: train/IMG_2646_jpeg_jpg.rf.76201ed567fb96e10b9e8dd33115ea85.txt \n extracting: train/IMG_2646_jpeg_jpg.rf.a221237eb44da0f4e8ea01ac2be758c9.jpg \n extracting: train/IMG_2646_jpeg_jpg.rf.a221237eb44da0f4e8ea01ac2be758c9.txt \n extracting: train/IMG_2647_jpeg_jpg.rf.46aa6b566f62cb89ca3c1d0999070677.jpg \n extracting: train/IMG_2647_jpeg_jpg.rf.46aa6b566f62cb89ca3c1d0999070677.txt \n extracting: train/IMG_2647_jpeg_jpg.rf.6226b2b28a3d3c35698b72575709ccfc.jpg \n extracting: train/IMG_2647_jpeg_jpg.rf.6226b2b28a3d3c35698b72575709ccfc.txt \n extracting: train/IMG_2647_jpeg_jpg.rf.b0827d6e81c2560947a8c0eb4dd4d6df.jpg \n extracting: train/IMG_2647_jpeg_jpg.rf.b0827d6e81c2560947a8c0eb4dd4d6df.txt \n extracting: train/IMG_2648_jpeg_jpg.rf.3fb82fe02633fa5f16711043c9a97933.jpg \n extracting: train/IMG_2648_jpeg_jpg.rf.3fb82fe02633fa5f16711043c9a97933.txt \n extracting: train/IMG_2648_jpeg_jpg.rf.6cb68c5ee02575f94790fffe5fc00067.jpg \n extracting: train/IMG_2648_jpeg_jpg.rf.6cb68c5ee02575f94790fffe5fc00067.txt \n extracting: train/IMG_2648_jpeg_jpg.rf.8828fef1b2317cb6d8e39f3fe4284139.jpg \n extracting: train/IMG_2648_jpeg_jpg.rf.8828fef1b2317cb6d8e39f3fe4284139.txt \n extracting: train/IMG_2649_jpeg_jpg.rf.8f2c74081f2440b0849b7c7b57c6c4e8.jpg \n extracting: train/IMG_2649_jpeg_jpg.rf.8f2c74081f2440b0849b7c7b57c6c4e8.txt \n extracting: train/IMG_2649_jpeg_jpg.rf.df0f5cecccef3efc1bd62ccede6300a5.jpg \n extracting: train/IMG_2649_jpeg_jpg.rf.df0f5cecccef3efc1bd62ccede6300a5.txt \n extracting: train/IMG_2649_jpeg_jpg.rf.f773bacdf59c23a26e91882bc44d54ac.jpg \n extracting: train/IMG_2649_jpeg_jpg.rf.f773bacdf59c23a26e91882bc44d54ac.txt \n extracting: train/IMG_2650_jpeg_jpg.rf.1d497ecac872372b0e88dfc703c188ae.jpg \n extracting: train/IMG_2650_jpeg_jpg.rf.1d497ecac872372b0e88dfc703c188ae.txt \n extracting: train/IMG_2650_jpeg_jpg.rf.58fcbf30dca4f422f204c1f62df280db.jpg \n extracting: train/IMG_2650_jpeg_jpg.rf.58fcbf30dca4f422f204c1f62df280db.txt \n extracting: train/IMG_2650_jpeg_jpg.rf.7ae7f196c55bec79b3dacc2ebfbfdc07.jpg \n extracting: train/IMG_2650_jpeg_jpg.rf.7ae7f196c55bec79b3dacc2ebfbfdc07.txt \n extracting: train/IMG_2652_jpeg_jpg.rf.2f3a0e1d18102f1ef35d37494e13c4db.jpg \n extracting: train/IMG_2652_jpeg_jpg.rf.2f3a0e1d18102f1ef35d37494e13c4db.txt \n extracting: train/IMG_2652_jpeg_jpg.rf.314422affba8d211e305244a42a947ec.jpg \n extracting: train/IMG_2652_jpeg_jpg.rf.314422affba8d211e305244a42a947ec.txt \n extracting: train/IMG_2652_jpeg_jpg.rf.9c8fb3899c133f57b0faf59a3d5f029e.jpg \n extracting: train/IMG_2652_jpeg_jpg.rf.9c8fb3899c133f57b0faf59a3d5f029e.txt \n extracting: train/IMG_2653_jpeg_jpg.rf.76388391099ff7d98fdf5b42ec4c46ea.jpg \n extracting: train/IMG_2653_jpeg_jpg.rf.76388391099ff7d98fdf5b42ec4c46ea.txt \n extracting: train/IMG_2653_jpeg_jpg.rf.7da839c96765f1279b894fa2d95972e1.jpg \n extracting: train/IMG_2653_jpeg_jpg.rf.7da839c96765f1279b894fa2d95972e1.txt \n extracting: train/IMG_2653_jpeg_jpg.rf.fb1caf74463c4af67d8c47712dd7786e.jpg \n extracting: train/IMG_2653_jpeg_jpg.rf.fb1caf74463c4af67d8c47712dd7786e.txt \n extracting: train/IMG_2654_jpeg_jpg.rf.11c581765675ab0f7642a215840e81a3.jpg \n extracting: train/IMG_2654_jpeg_jpg.rf.11c581765675ab0f7642a215840e81a3.txt \n extracting: train/IMG_2654_jpeg_jpg.rf.5dd7c06481b6c7dec21939bf11796fe5.jpg \n extracting: train/IMG_2654_jpeg_jpg.rf.5dd7c06481b6c7dec21939bf11796fe5.txt \n extracting: train/IMG_2654_jpeg_jpg.rf.bdc5a499d613deba20268d7daef8272c.jpg \n extracting: train/IMG_2654_jpeg_jpg.rf.bdc5a499d613deba20268d7daef8272c.txt \n extracting: train/IMG_2655_jpeg_jpg.rf.7fc4ada4288144588b8215acce641ae5.jpg \n extracting: train/IMG_2655_jpeg_jpg.rf.7fc4ada4288144588b8215acce641ae5.txt \n extracting: train/IMG_2655_jpeg_jpg.rf.dca2a2d721a1a2abd3e22ceb30d91277.jpg \n extracting: train/IMG_2655_jpeg_jpg.rf.dca2a2d721a1a2abd3e22ceb30d91277.txt \n extracting: train/IMG_2655_jpeg_jpg.rf.e376f29fe7e48691e16f05bf98a3b3b5.jpg \n extracting: train/IMG_2655_jpeg_jpg.rf.e376f29fe7e48691e16f05bf98a3b3b5.txt \n extracting: train/IMG_2656_jpeg_jpg.rf.5badb38a8765cb048267326cd8a259e5.jpg \n extracting: train/IMG_2656_jpeg_jpg.rf.5badb38a8765cb048267326cd8a259e5.txt \n extracting: train/IMG_2656_jpeg_jpg.rf.9ea93b37eadb0de863aa2506dd301ace.jpg \n extracting: train/IMG_2656_jpeg_jpg.rf.9ea93b37eadb0de863aa2506dd301ace.txt \n extracting: train/IMG_2656_jpeg_jpg.rf.e66265c599f7bb294bf1b625e6592de0.jpg \n extracting: train/IMG_2656_jpeg_jpg.rf.e66265c599f7bb294bf1b625e6592de0.txt \n extracting: train/IMG_2657_jpeg_jpg.rf.1d72fe18e76ec68c13c1be793b5657b3.jpg \n extracting: train/IMG_2657_jpeg_jpg.rf.1d72fe18e76ec68c13c1be793b5657b3.txt \n extracting: train/IMG_2657_jpeg_jpg.rf.794a12f000793557ce215456b5e0cb2a.jpg \n extracting: train/IMG_2657_jpeg_jpg.rf.794a12f000793557ce215456b5e0cb2a.txt \n extracting: train/IMG_2657_jpeg_jpg.rf.c0c869d2a9caab3782802b55af8444fe.jpg \n extracting: train/IMG_2657_jpeg_jpg.rf.c0c869d2a9caab3782802b55af8444fe.txt \n extracting: train/IMG_3121_jpeg_jpg.rf.bfc00938ec2fd3fdabc49d8dd885227a.jpg \n extracting: train/IMG_3121_jpeg_jpg.rf.bfc00938ec2fd3fdabc49d8dd885227a.txt \n extracting: train/IMG_3121_jpeg_jpg.rf.ce9568674f1e6d45dc64f55f75ff438d.jpg \n extracting: train/IMG_3121_jpeg_jpg.rf.ce9568674f1e6d45dc64f55f75ff438d.txt \n extracting: train/IMG_3121_jpeg_jpg.rf.e4e7019b42479f34398a195858d828db.jpg \n extracting: train/IMG_3121_jpeg_jpg.rf.e4e7019b42479f34398a195858d828db.txt \n extracting: train/IMG_3122_jpeg_jpg.rf.86de9d24cb8372a4a24dc28093f37871.jpg \n extracting: train/IMG_3122_jpeg_jpg.rf.86de9d24cb8372a4a24dc28093f37871.txt \n extracting: train/IMG_3122_jpeg_jpg.rf.9a3392ae526e23d3fba248ed55bbf28c.jpg \n extracting: train/IMG_3122_jpeg_jpg.rf.9a3392ae526e23d3fba248ed55bbf28c.txt \n extracting: train/IMG_3122_jpeg_jpg.rf.9d1459fe53fdb4fdba2e9aa4c4f0245b.jpg \n extracting: train/IMG_3122_jpeg_jpg.rf.9d1459fe53fdb4fdba2e9aa4c4f0245b.txt \n extracting: train/IMG_3123_jpeg_jpg.rf.2b86740c3a5a70b513c65153181bd6bf.jpg \n extracting: train/IMG_3123_jpeg_jpg.rf.2b86740c3a5a70b513c65153181bd6bf.txt \n extracting: train/IMG_3123_jpeg_jpg.rf.8a1e731c7a99e293643d0bc865fb8bf0.jpg \n extracting: train/IMG_3123_jpeg_jpg.rf.8a1e731c7a99e293643d0bc865fb8bf0.txt \n extracting: train/IMG_3123_jpeg_jpg.rf.ce1103fc4eceee16abff761eb3b08f43.jpg \n extracting: train/IMG_3123_jpeg_jpg.rf.ce1103fc4eceee16abff761eb3b08f43.txt \n extracting: train/IMG_3125_jpeg_jpg.rf.09c17c0d6ed7443599c6697b7762d809.jpg \n extracting: train/IMG_3125_jpeg_jpg.rf.09c17c0d6ed7443599c6697b7762d809.txt \n extracting: train/IMG_3125_jpeg_jpg.rf.da7d32a4ca4c05e06e273d84527f9546.jpg \n extracting: train/IMG_3125_jpeg_jpg.rf.da7d32a4ca4c05e06e273d84527f9546.txt \n extracting: train/IMG_3125_jpeg_jpg.rf.eb6438f02340e825f5ee348e6792e975.jpg \n extracting: train/IMG_3125_jpeg_jpg.rf.eb6438f02340e825f5ee348e6792e975.txt \n extracting: train/IMG_3127_jpeg_jpg.rf.23ab77bdb43254522f80e94f06f11d39.jpg \n extracting: train/IMG_3127_jpeg_jpg.rf.23ab77bdb43254522f80e94f06f11d39.txt \n extracting: train/IMG_3127_jpeg_jpg.rf.33a5803c3fbe7e3b1e07e890ea7da2fb.jpg \n extracting: train/IMG_3127_jpeg_jpg.rf.33a5803c3fbe7e3b1e07e890ea7da2fb.txt \n extracting: train/IMG_3127_jpeg_jpg.rf.618d7325753466b6c09bb22f56abdad0.jpg \n extracting: train/IMG_3127_jpeg_jpg.rf.618d7325753466b6c09bb22f56abdad0.txt \n extracting: train/IMG_3128_jpeg_jpg.rf.032fe0eeed57643c7aaadd151d1fae74.jpg \n extracting: train/IMG_3128_jpeg_jpg.rf.032fe0eeed57643c7aaadd151d1fae74.txt \n extracting: train/IMG_3128_jpeg_jpg.rf.e26f6d0e5b1a6905ed603a89ca60e2f4.jpg \n extracting: train/IMG_3128_jpeg_jpg.rf.e26f6d0e5b1a6905ed603a89ca60e2f4.txt \n extracting: train/IMG_3128_jpeg_jpg.rf.ec1407d4ce45c7c1d20a818e53958d33.jpg \n extracting: train/IMG_3128_jpeg_jpg.rf.ec1407d4ce45c7c1d20a818e53958d33.txt \n extracting: train/IMG_3130_jpeg_jpg.rf.3018405f4efd5bd648abf62711ff1aa9.jpg \n extracting: train/IMG_3130_jpeg_jpg.rf.3018405f4efd5bd648abf62711ff1aa9.txt \n extracting: train/IMG_3130_jpeg_jpg.rf.5de4ccdaddbcacddad9d08be16809cea.jpg \n extracting: train/IMG_3130_jpeg_jpg.rf.5de4ccdaddbcacddad9d08be16809cea.txt \n extracting: train/IMG_3130_jpeg_jpg.rf.82936db912971ccd5844d05652918eef.jpg \n extracting: train/IMG_3130_jpeg_jpg.rf.82936db912971ccd5844d05652918eef.txt \n extracting: train/IMG_3131_jpeg_jpg.rf.05fd77a62866f17c3eb767de645cd8ac.jpg \n extracting: train/IMG_3131_jpeg_jpg.rf.05fd77a62866f17c3eb767de645cd8ac.txt \n extracting: train/IMG_3131_jpeg_jpg.rf.2aad26a07dc096a8907ba386cefd4637.jpg \n extracting: train/IMG_3131_jpeg_jpg.rf.2aad26a07dc096a8907ba386cefd4637.txt \n extracting: train/IMG_3131_jpeg_jpg.rf.d930f17bf1f7cc8909dc37105a5327ce.jpg \n extracting: train/IMG_3131_jpeg_jpg.rf.d930f17bf1f7cc8909dc37105a5327ce.txt \n extracting: train/IMG_3132_jpeg_jpg.rf.067ac8c71fa8d1764eb8f2f6c5602d20.jpg \n extracting: train/IMG_3132_jpeg_jpg.rf.067ac8c71fa8d1764eb8f2f6c5602d20.txt \n extracting: train/IMG_3132_jpeg_jpg.rf.40756a9320adfcc994a91b74a64b4857.jpg \n extracting: train/IMG_3132_jpeg_jpg.rf.40756a9320adfcc994a91b74a64b4857.txt \n extracting: train/IMG_3132_jpeg_jpg.rf.d9f56a83f5e109724c71d149de9e916a.jpg \n extracting: train/IMG_3132_jpeg_jpg.rf.d9f56a83f5e109724c71d149de9e916a.txt \n extracting: train/IMG_3133_jpeg_jpg.rf.1898045a479c9a7069111f1be3ced799.jpg \n extracting: train/IMG_3133_jpeg_jpg.rf.1898045a479c9a7069111f1be3ced799.txt \n extracting: train/IMG_3133_jpeg_jpg.rf.72884118afce04bb17a47b00b7f46846.jpg \n extracting: train/IMG_3133_jpeg_jpg.rf.72884118afce04bb17a47b00b7f46846.txt \n extracting: train/IMG_3133_jpeg_jpg.rf.75606f900f0e9338810d587861d80918.jpg \n extracting: train/IMG_3133_jpeg_jpg.rf.75606f900f0e9338810d587861d80918.txt \n extracting: train/IMG_3135_jpeg_jpg.rf.711dd1bf714bcb87dbeed76b1e4fd5bf.jpg \n extracting: train/IMG_3135_jpeg_jpg.rf.711dd1bf714bcb87dbeed76b1e4fd5bf.txt \n extracting: train/IMG_3135_jpeg_jpg.rf.a43ee002d5901caa09e343930dff141c.jpg \n extracting: train/IMG_3135_jpeg_jpg.rf.a43ee002d5901caa09e343930dff141c.txt \n extracting: train/IMG_3135_jpeg_jpg.rf.b1640fb24b8af005fd20a8fdc313260d.jpg \n extracting: train/IMG_3135_jpeg_jpg.rf.b1640fb24b8af005fd20a8fdc313260d.txt \n extracting: train/IMG_3137_jpeg_jpg.rf.3c311d88be6e8c34c3417fcd2621e7cc.jpg \n extracting: train/IMG_3137_jpeg_jpg.rf.3c311d88be6e8c34c3417fcd2621e7cc.txt \n extracting: train/IMG_3137_jpeg_jpg.rf.b41277dcd3c8740e6d9965966ca4fa10.jpg \n extracting: train/IMG_3137_jpeg_jpg.rf.b41277dcd3c8740e6d9965966ca4fa10.txt \n extracting: train/IMG_3137_jpeg_jpg.rf.b420451771ba05c47cf3af92801f91ee.jpg \n extracting: train/IMG_3137_jpeg_jpg.rf.b420451771ba05c47cf3af92801f91ee.txt \n extracting: train/IMG_3138_jpeg_jpg.rf.ae757d190401e5f552069f0e4dbe55ca.jpg \n extracting: train/IMG_3138_jpeg_jpg.rf.ae757d190401e5f552069f0e4dbe55ca.txt \n extracting: train/IMG_3138_jpeg_jpg.rf.ed313317e8b38e0bafa531df6fa339f5.jpg \n extracting: train/IMG_3138_jpeg_jpg.rf.ed313317e8b38e0bafa531df6fa339f5.txt \n extracting: train/IMG_3138_jpeg_jpg.rf.fda24f4551555d98e9ab067dbcb8542e.jpg \n extracting: train/IMG_3138_jpeg_jpg.rf.fda24f4551555d98e9ab067dbcb8542e.txt \n extracting: train/IMG_3140_jpeg_jpg.rf.5f17d0246f72ae597905cfcb31d84cc9.jpg \n extracting: train/IMG_3140_jpeg_jpg.rf.5f17d0246f72ae597905cfcb31d84cc9.txt \n extracting: train/IMG_3140_jpeg_jpg.rf.c9c7078d2cc62a2b6464202a39a7b6db.jpg \n extracting: train/IMG_3140_jpeg_jpg.rf.c9c7078d2cc62a2b6464202a39a7b6db.txt \n extracting: train/IMG_3140_jpeg_jpg.rf.d96dbec47e488e65e33c1945d42b60bf.jpg \n extracting: train/IMG_3140_jpeg_jpg.rf.d96dbec47e488e65e33c1945d42b60bf.txt \n extracting: train/IMG_3141_jpeg_jpg.rf.3a01beba989fb7569bb49f28825bed3c.jpg \n extracting: train/IMG_3141_jpeg_jpg.rf.3a01beba989fb7569bb49f28825bed3c.txt \n extracting: train/IMG_3141_jpeg_jpg.rf.e25cb08002513dc050f59e4535f12854.jpg \n extracting: train/IMG_3141_jpeg_jpg.rf.e25cb08002513dc050f59e4535f12854.txt \n extracting: train/IMG_3141_jpeg_jpg.rf.e9dd8e257c5741ee64216e6a481fb70e.jpg \n extracting: train/IMG_3141_jpeg_jpg.rf.e9dd8e257c5741ee64216e6a481fb70e.txt \n extracting: train/IMG_3142_jpeg_jpg.rf.60e9a51244b48bef0b7089635eae4085.jpg \n extracting: train/IMG_3142_jpeg_jpg.rf.60e9a51244b48bef0b7089635eae4085.txt \n extracting: train/IMG_3142_jpeg_jpg.rf.b4901752b4b185e32dc93304dbdf4185.jpg \n extracting: train/IMG_3142_jpeg_jpg.rf.b4901752b4b185e32dc93304dbdf4185.txt \n extracting: train/IMG_3142_jpeg_jpg.rf.f831235dffb418949a0ba93793af6233.jpg \n extracting: train/IMG_3142_jpeg_jpg.rf.f831235dffb418949a0ba93793af6233.txt \n extracting: train/IMG_3146_jpeg_jpg.rf.181eb84d296c75368119a7ca04037890.jpg \n extracting: train/IMG_3146_jpeg_jpg.rf.181eb84d296c75368119a7ca04037890.txt \n extracting: train/IMG_3146_jpeg_jpg.rf.99de9a5301d001d3fcb7a881145c2989.jpg \n extracting: train/IMG_3146_jpeg_jpg.rf.99de9a5301d001d3fcb7a881145c2989.txt \n extracting: train/IMG_3146_jpeg_jpg.rf.fe14cc66d883ba68f7d670ca7444f000.jpg \n extracting: train/IMG_3146_jpeg_jpg.rf.fe14cc66d883ba68f7d670ca7444f000.txt \n extracting: train/IMG_3147_jpeg_jpg.rf.2e0eb34b64d010b7ff3c61eca2e24517.jpg \n extracting: train/IMG_3147_jpeg_jpg.rf.2e0eb34b64d010b7ff3c61eca2e24517.txt \n extracting: train/IMG_3147_jpeg_jpg.rf.73983980775c440ddfbdabc939507fcc.jpg \n extracting: train/IMG_3147_jpeg_jpg.rf.73983980775c440ddfbdabc939507fcc.txt \n extracting: train/IMG_3147_jpeg_jpg.rf.9b1f0c536601a3e1377306f90130a100.jpg \n extracting: train/IMG_3147_jpeg_jpg.rf.9b1f0c536601a3e1377306f90130a100.txt \n extracting: train/IMG_3148_jpeg_jpg.rf.190d07bb7c09db1a85e1a40531b94b72.jpg \n extracting: train/IMG_3148_jpeg_jpg.rf.190d07bb7c09db1a85e1a40531b94b72.txt \n extracting: train/IMG_3148_jpeg_jpg.rf.896e19f51734c603971939aee9435678.jpg \n extracting: train/IMG_3148_jpeg_jpg.rf.896e19f51734c603971939aee9435678.txt \n extracting: train/IMG_3148_jpeg_jpg.rf.cbb0a5349e7cc1f01314650e2b6509a4.jpg \n extracting: train/IMG_3148_jpeg_jpg.rf.cbb0a5349e7cc1f01314650e2b6509a4.txt \n extracting: train/IMG_3149_jpeg_jpg.rf.0fe8d916e9df7153c4251c4cf1b3de6b.jpg \n extracting: train/IMG_3149_jpeg_jpg.rf.0fe8d916e9df7153c4251c4cf1b3de6b.txt \n extracting: train/IMG_3149_jpeg_jpg.rf.54bc21f55895b656059caea62868cfe6.jpg \n extracting: train/IMG_3149_jpeg_jpg.rf.54bc21f55895b656059caea62868cfe6.txt \n extracting: train/IMG_3149_jpeg_jpg.rf.7b10bf37b41e356b4a9ccb476f7d2253.jpg \n extracting: train/IMG_3149_jpeg_jpg.rf.7b10bf37b41e356b4a9ccb476f7d2253.txt \n extracting: train/IMG_3151_jpeg_jpg.rf.5494e68baf9913b01208209249584c0e.jpg \n extracting: train/IMG_3151_jpeg_jpg.rf.5494e68baf9913b01208209249584c0e.txt \n extracting: train/IMG_3151_jpeg_jpg.rf.8273ca3d85822e7f3195ccdc6dd62699.jpg \n extracting: train/IMG_3151_jpeg_jpg.rf.8273ca3d85822e7f3195ccdc6dd62699.txt \n extracting: train/IMG_3151_jpeg_jpg.rf.ef5b03de7a14574edb4304b7db0728e7.jpg \n extracting: train/IMG_3151_jpeg_jpg.rf.ef5b03de7a14574edb4304b7db0728e7.txt \n extracting: train/IMG_3152_jpeg_jpg.rf.05693ff539aaf78144658009dd39f7b9.jpg \n extracting: train/IMG_3152_jpeg_jpg.rf.05693ff539aaf78144658009dd39f7b9.txt \n extracting: train/IMG_3152_jpeg_jpg.rf.3d4aec4ef8859afd6c98f900ac1dc0c3.jpg \n extracting: train/IMG_3152_jpeg_jpg.rf.3d4aec4ef8859afd6c98f900ac1dc0c3.txt \n extracting: train/IMG_3152_jpeg_jpg.rf.eea572e10e42173df5bdf631c06ae4c0.jpg \n extracting: train/IMG_3152_jpeg_jpg.rf.eea572e10e42173df5bdf631c06ae4c0.txt \n extracting: train/IMG_3155_jpeg_jpg.rf.692033dda2e2a7af9717a1a073d7cb8c.jpg \n extracting: train/IMG_3155_jpeg_jpg.rf.692033dda2e2a7af9717a1a073d7cb8c.txt \n extracting: train/IMG_3155_jpeg_jpg.rf.9190cdde8cebf7f144fc3e977849a997.jpg \n extracting: train/IMG_3155_jpeg_jpg.rf.9190cdde8cebf7f144fc3e977849a997.txt \n extracting: train/IMG_3155_jpeg_jpg.rf.e0a57b538dbb400612a9ab7be406defd.jpg \n extracting: train/IMG_3155_jpeg_jpg.rf.e0a57b538dbb400612a9ab7be406defd.txt \n extracting: train/IMG_3156_jpeg_jpg.rf.4fbed8cbf68eb637ef514e99340a2037.jpg \n extracting: train/IMG_3156_jpeg_jpg.rf.4fbed8cbf68eb637ef514e99340a2037.txt \n extracting: train/IMG_3156_jpeg_jpg.rf.e67c897aef3554be1261dc0eed1fd9e1.jpg \n extracting: train/IMG_3156_jpeg_jpg.rf.e67c897aef3554be1261dc0eed1fd9e1.txt \n extracting: train/IMG_3156_jpeg_jpg.rf.ef59f44909c8a86d5edfaf4e4fd608a2.jpg \n extracting: train/IMG_3156_jpeg_jpg.rf.ef59f44909c8a86d5edfaf4e4fd608a2.txt \n extracting: train/IMG_3157_jpeg_jpg.rf.325795ae826c5b00c1f1f9bcf5c54f27.jpg \n extracting: train/IMG_3157_jpeg_jpg.rf.325795ae826c5b00c1f1f9bcf5c54f27.txt \n extracting: train/IMG_3157_jpeg_jpg.rf.4a746ca65c77423fee44718a9634ac94.jpg \n extracting: train/IMG_3157_jpeg_jpg.rf.4a746ca65c77423fee44718a9634ac94.txt \n extracting: train/IMG_3157_jpeg_jpg.rf.e49aee05cf15bec609ba9d5f5a9c9b03.jpg \n extracting: train/IMG_3157_jpeg_jpg.rf.e49aee05cf15bec609ba9d5f5a9c9b03.txt \n extracting: train/IMG_3158_jpeg_jpg.rf.1ae700a8bd2182b6db52bd364a1852e1.jpg \n extracting: train/IMG_3158_jpeg_jpg.rf.1ae700a8bd2182b6db52bd364a1852e1.txt \n extracting: train/IMG_3158_jpeg_jpg.rf.22771edc2fa8b6025ded93501d2575e6.jpg \n extracting: train/IMG_3158_jpeg_jpg.rf.22771edc2fa8b6025ded93501d2575e6.txt \n extracting: train/IMG_3158_jpeg_jpg.rf.cb1c13c5b715b48f6561034c28c811b4.jpg \n extracting: train/IMG_3158_jpeg_jpg.rf.cb1c13c5b715b48f6561034c28c811b4.txt \n extracting: train/IMG_3159_jpeg_jpg.rf.60733fefb86525e0f50522617b6db057.jpg \n extracting: train/IMG_3159_jpeg_jpg.rf.60733fefb86525e0f50522617b6db057.txt \n extracting: train/IMG_3159_jpeg_jpg.rf.678bdd841af53a442e0a33ef7a1bac55.jpg \n extracting: train/IMG_3159_jpeg_jpg.rf.678bdd841af53a442e0a33ef7a1bac55.txt \n extracting: train/IMG_3159_jpeg_jpg.rf.6a69369de42f1ad259091e874dbc6d0b.jpg \n extracting: train/IMG_3159_jpeg_jpg.rf.6a69369de42f1ad259091e874dbc6d0b.txt \n extracting: train/IMG_3160_jpeg_jpg.rf.0fc82e659568060cc81eae749aa20736.jpg \n extracting: train/IMG_3160_jpeg_jpg.rf.0fc82e659568060cc81eae749aa20736.txt \n extracting: train/IMG_3160_jpeg_jpg.rf.58bc7efafe0453ca1fcfe9308ab552af.jpg \n extracting: train/IMG_3160_jpeg_jpg.rf.58bc7efafe0453ca1fcfe9308ab552af.txt \n extracting: train/IMG_3160_jpeg_jpg.rf.a77b3300fe8a832f5a73194ab449799d.jpg \n extracting: train/IMG_3160_jpeg_jpg.rf.a77b3300fe8a832f5a73194ab449799d.txt \n extracting: train/IMG_3162_jpeg_jpg.rf.1e969675466d4b64a29c2fd5eb9c1c8b.jpg \n extracting: train/IMG_3162_jpeg_jpg.rf.1e969675466d4b64a29c2fd5eb9c1c8b.txt \n extracting: train/IMG_3162_jpeg_jpg.rf.55ff0a00615acbccdd2b5bbaeb732fc7.jpg \n extracting: train/IMG_3162_jpeg_jpg.rf.55ff0a00615acbccdd2b5bbaeb732fc7.txt \n extracting: train/IMG_3162_jpeg_jpg.rf.e90bb3bfb162e7d935d1859232ea3db0.jpg \n extracting: train/IMG_3162_jpeg_jpg.rf.e90bb3bfb162e7d935d1859232ea3db0.txt \n extracting: train/IMG_3167_jpeg_jpg.rf.3e267bd83c9f417bb093eabe23666473.jpg \n extracting: train/IMG_3167_jpeg_jpg.rf.3e267bd83c9f417bb093eabe23666473.txt \n extracting: train/IMG_3167_jpeg_jpg.rf.8a76fe0920ace41ae73ee0599274710c.jpg \n extracting: train/IMG_3167_jpeg_jpg.rf.8a76fe0920ace41ae73ee0599274710c.txt \n extracting: train/IMG_3167_jpeg_jpg.rf.efb7a45e044700fb26e4773fb586cbcb.jpg \n extracting: train/IMG_3167_jpeg_jpg.rf.efb7a45e044700fb26e4773fb586cbcb.txt \n extracting: train/IMG_3168_jpeg_jpg.rf.3016afd00ee3dc006e01a3c0c21af61a.jpg \n extracting: train/IMG_3168_jpeg_jpg.rf.3016afd00ee3dc006e01a3c0c21af61a.txt \n extracting: train/IMG_3168_jpeg_jpg.rf.3948f08cfacde245b2ea07e71d004730.jpg \n extracting: train/IMG_3168_jpeg_jpg.rf.3948f08cfacde245b2ea07e71d004730.txt \n extracting: train/IMG_3168_jpeg_jpg.rf.5617be31ba9bed487a4d5603806c196a.jpg \n extracting: train/IMG_3168_jpeg_jpg.rf.5617be31ba9bed487a4d5603806c196a.txt \n extracting: train/IMG_3169_jpeg_jpg.rf.34f26a3013d2d24b52ef536249863144.jpg \n extracting: train/IMG_3169_jpeg_jpg.rf.34f26a3013d2d24b52ef536249863144.txt \n extracting: train/IMG_3169_jpeg_jpg.rf.5b9ebe619363335b8f09c142be866f1f.jpg \n extracting: train/IMG_3169_jpeg_jpg.rf.5b9ebe619363335b8f09c142be866f1f.txt \n extracting: train/IMG_3169_jpeg_jpg.rf.6626b78f10db81436f068dd0134ea2fe.jpg \n extracting: train/IMG_3169_jpeg_jpg.rf.6626b78f10db81436f068dd0134ea2fe.txt \n extracting: train/IMG_3170_jpeg_jpg.rf.1ae1e37e281e3c7907733678822ab981.jpg \n extracting: train/IMG_3170_jpeg_jpg.rf.1ae1e37e281e3c7907733678822ab981.txt \n extracting: train/IMG_3170_jpeg_jpg.rf.6ab58218b40c46cda092ff22f1c106e8.jpg \n extracting: train/IMG_3170_jpeg_jpg.rf.6ab58218b40c46cda092ff22f1c106e8.txt \n extracting: train/IMG_3170_jpeg_jpg.rf.a6693faa5f88ddc27bab2414e4cd46ed.jpg \n extracting: train/IMG_3170_jpeg_jpg.rf.a6693faa5f88ddc27bab2414e4cd46ed.txt \n extracting: train/IMG_3172_jpeg_jpg.rf.380b4cee1c1ec717ab444115a363036c.jpg \n extracting: train/IMG_3172_jpeg_jpg.rf.380b4cee1c1ec717ab444115a363036c.txt \n extracting: train/IMG_3172_jpeg_jpg.rf.5561bd0931a328c36fbd10ba8ebcd807.jpg \n extracting: train/IMG_3172_jpeg_jpg.rf.5561bd0931a328c36fbd10ba8ebcd807.txt \n extracting: train/IMG_3172_jpeg_jpg.rf.cf8f23d29a3be4dc4af6e909e5aa839b.jpg \n extracting: train/IMG_3172_jpeg_jpg.rf.cf8f23d29a3be4dc4af6e909e5aa839b.txt \n extracting: train/IMG_3174_jpeg_jpg.rf.1a2e8eb2cf47d964ded16682920e12aa.jpg \n extracting: train/IMG_3174_jpeg_jpg.rf.1a2e8eb2cf47d964ded16682920e12aa.txt \n extracting: train/IMG_3174_jpeg_jpg.rf.493b072e083ffbf3ec8adc27042bc5fd.jpg \n extracting: train/IMG_3174_jpeg_jpg.rf.493b072e083ffbf3ec8adc27042bc5fd.txt \n extracting: train/IMG_3174_jpeg_jpg.rf.bed75ee28a14c27f35a66cd9f9ed64f4.jpg \n extracting: train/IMG_3174_jpeg_jpg.rf.bed75ee28a14c27f35a66cd9f9ed64f4.txt \n extracting: train/IMG_3176_jpeg_jpg.rf.6c3ace907fdff616cebbe6c4d24d23fc.jpg \n extracting: train/IMG_3176_jpeg_jpg.rf.6c3ace907fdff616cebbe6c4d24d23fc.txt \n extracting: train/IMG_3176_jpeg_jpg.rf.a629c63ba8ef074a187a64082fe6ed40.jpg \n extracting: train/IMG_3176_jpeg_jpg.rf.a629c63ba8ef074a187a64082fe6ed40.txt \n extracting: train/IMG_3176_jpeg_jpg.rf.e623394c84f121de4c7107cc1dd4dee0.jpg \n extracting: train/IMG_3176_jpeg_jpg.rf.e623394c84f121de4c7107cc1dd4dee0.txt \n extracting: train/IMG_3177_jpeg_jpg.rf.26dad5415adb7de82d89c97feae6d9ee.jpg \n extracting: train/IMG_3177_jpeg_jpg.rf.26dad5415adb7de82d89c97feae6d9ee.txt \n extracting: train/IMG_3177_jpeg_jpg.rf.3d7c4b206ba784637297cabd0d5d05e1.jpg \n extracting: train/IMG_3177_jpeg_jpg.rf.3d7c4b206ba784637297cabd0d5d05e1.txt \n extracting: train/IMG_3177_jpeg_jpg.rf.9d02f8032a305b042185de1dd595f09e.jpg \n extracting: train/IMG_3177_jpeg_jpg.rf.9d02f8032a305b042185de1dd595f09e.txt \n extracting: train/IMG_3181_jpeg_jpg.rf.4fdb69c0f52844b0c74250e33ab285a5.jpg \n extracting: train/IMG_3181_jpeg_jpg.rf.4fdb69c0f52844b0c74250e33ab285a5.txt \n extracting: train/IMG_3181_jpeg_jpg.rf.58232eac97b7d0cdf7b3b32e13942f38.jpg \n extracting: train/IMG_3181_jpeg_jpg.rf.58232eac97b7d0cdf7b3b32e13942f38.txt \n extracting: train/IMG_3181_jpeg_jpg.rf.dd3b25ea72766c49d783e4c63a583eab.jpg \n extracting: train/IMG_3181_jpeg_jpg.rf.dd3b25ea72766c49d783e4c63a583eab.txt \n extracting: train/IMG_3182_jpeg_jpg.rf.77dae93af5f69edb04edbe85dd1fe2d1.jpg \n extracting: train/IMG_3182_jpeg_jpg.rf.77dae93af5f69edb04edbe85dd1fe2d1.txt \n extracting: train/IMG_3182_jpeg_jpg.rf.7d7127065164a68ef16f387f25be127d.jpg \n extracting: train/IMG_3182_jpeg_jpg.rf.7d7127065164a68ef16f387f25be127d.txt \n extracting: train/IMG_3182_jpeg_jpg.rf.b8a7016061a1b903d4cc03d34a6657fc.jpg \n extracting: train/IMG_3182_jpeg_jpg.rf.b8a7016061a1b903d4cc03d34a6657fc.txt \n extracting: train/IMG_3186_jpeg_jpg.rf.42253db9b6d4e186b0c8758d43abf39c.jpg \n extracting: train/IMG_3186_jpeg_jpg.rf.42253db9b6d4e186b0c8758d43abf39c.txt \n extracting: train/IMG_3186_jpeg_jpg.rf.5a76edf3c6b770cb00086ba9cdc8d811.jpg \n extracting: train/IMG_3186_jpeg_jpg.rf.5a76edf3c6b770cb00086ba9cdc8d811.txt \n extracting: train/IMG_3186_jpeg_jpg.rf.5a7d9b893c4bd6ab9ddb6bd65c6563ba.jpg \n extracting: train/IMG_3186_jpeg_jpg.rf.5a7d9b893c4bd6ab9ddb6bd65c6563ba.txt \n extracting: train/IMG_3187_jpeg_jpg.rf.049cf11e949669864f3d20fadb063d95.jpg \n extracting: train/IMG_3187_jpeg_jpg.rf.049cf11e949669864f3d20fadb063d95.txt \n extracting: train/IMG_3187_jpeg_jpg.rf.4a53f0601a7438b1e6623b011bb81a3f.jpg \n extracting: train/IMG_3187_jpeg_jpg.rf.4a53f0601a7438b1e6623b011bb81a3f.txt \n extracting: train/IMG_3187_jpeg_jpg.rf.bf3d951262b8a1c2fe6d7cb54a76b408.jpg \n extracting: train/IMG_3187_jpeg_jpg.rf.bf3d951262b8a1c2fe6d7cb54a76b408.txt \n extracting: train/IMG_3188_jpeg_jpg.rf.4de12c6a4fdf60048eb267c76a7f27b5.jpg \n extracting: train/IMG_3188_jpeg_jpg.rf.4de12c6a4fdf60048eb267c76a7f27b5.txt \n extracting: train/IMG_3188_jpeg_jpg.rf.62d00c2a9309d95571ca8c8e4faeee8d.jpg \n extracting: train/IMG_3188_jpeg_jpg.rf.62d00c2a9309d95571ca8c8e4faeee8d.txt \n extracting: train/IMG_3188_jpeg_jpg.rf.f451a14dce002d6345d79bffff0aa2d1.jpg \n extracting: train/IMG_3188_jpeg_jpg.rf.f451a14dce002d6345d79bffff0aa2d1.txt \n extracting: train/IMG_8318_jpg.rf.458b834e1ff5384fca00c5d94e91de4c.jpg \n extracting: train/IMG_8318_jpg.rf.458b834e1ff5384fca00c5d94e91de4c.txt \n extracting: train/IMG_8318_jpg.rf.4d33402f8bb3c4b78d0bac7a3f3c1921.jpg \n extracting: train/IMG_8318_jpg.rf.4d33402f8bb3c4b78d0bac7a3f3c1921.txt \n extracting: train/IMG_8318_jpg.rf.fc937ac1a4219c5dd6c53ee7a1d73f65.jpg \n extracting: train/IMG_8318_jpg.rf.fc937ac1a4219c5dd6c53ee7a1d73f65.txt \n extracting: train/IMG_8319_jpg.rf.1d9b8c8dfc0ffc9fbc7b8270381c7d2d.jpg \n extracting: train/IMG_8319_jpg.rf.1d9b8c8dfc0ffc9fbc7b8270381c7d2d.txt \n extracting: train/IMG_8319_jpg.rf.2604e5f0643260b8ce070afd08068ae4.jpg \n extracting: train/IMG_8319_jpg.rf.2604e5f0643260b8ce070afd08068ae4.txt \n extracting: train/IMG_8319_jpg.rf.79f9c8aaec66c81643aa8be4f355aa4c.jpg \n extracting: train/IMG_8319_jpg.rf.79f9c8aaec66c81643aa8be4f355aa4c.txt \n extracting: train/IMG_8327_jpg.rf.61b26031d33478f7455e661f8703fbf5.jpg \n extracting: train/IMG_8327_jpg.rf.61b26031d33478f7455e661f8703fbf5.txt \n extracting: train/IMG_8327_jpg.rf.68bbfac0791c96f30d121bafddba29e6.jpg \n extracting: train/IMG_8327_jpg.rf.68bbfac0791c96f30d121bafddba29e6.txt \n extracting: train/IMG_8327_jpg.rf.990a617b1f99d9f151e8498e2376d4b5.jpg \n extracting: train/IMG_8327_jpg.rf.990a617b1f99d9f151e8498e2376d4b5.txt \n extracting: train/IMG_8328_jpg.rf.0ee9ca2e76c4038fbd2af22bec4c6c21.jpg \n extracting: train/IMG_8328_jpg.rf.0ee9ca2e76c4038fbd2af22bec4c6c21.txt \n extracting: train/IMG_8328_jpg.rf.67f4f2c63941eb46e9c7cd07e939dc94.jpg \n extracting: train/IMG_8328_jpg.rf.67f4f2c63941eb46e9c7cd07e939dc94.txt \n extracting: train/IMG_8328_jpg.rf.ba1dcced4b5c6c56de6212f45c7f9302.jpg \n extracting: train/IMG_8328_jpg.rf.ba1dcced4b5c6c56de6212f45c7f9302.txt \n extracting: train/IMG_8329_jpg.rf.4885f67144134fbab097b41ac6b423f3.jpg \n extracting: train/IMG_8329_jpg.rf.4885f67144134fbab097b41ac6b423f3.txt \n extracting: train/IMG_8329_jpg.rf.6efb63c727690df236a4f5391a3d9ed0.jpg \n extracting: train/IMG_8329_jpg.rf.6efb63c727690df236a4f5391a3d9ed0.txt \n extracting: train/IMG_8329_jpg.rf.9cbd3d8b81ce68ae2bcab3e978b06faa.jpg \n extracting: train/IMG_8329_jpg.rf.9cbd3d8b81ce68ae2bcab3e978b06faa.txt \n extracting: train/IMG_8338_jpg.rf.2294e6c4e30c2b76a5c790b2ae4a0ef7.jpg \n extracting: train/IMG_8338_jpg.rf.2294e6c4e30c2b76a5c790b2ae4a0ef7.txt \n extracting: train/IMG_8338_jpg.rf.31c28b71d57198b1d003b26058dd30fc.jpg \n extracting: train/IMG_8338_jpg.rf.31c28b71d57198b1d003b26058dd30fc.txt \n extracting: train/IMG_8338_jpg.rf.5dd6eac88be60f52dc2f5330b2887dad.jpg \n extracting: train/IMG_8338_jpg.rf.5dd6eac88be60f52dc2f5330b2887dad.txt \n extracting: train/IMG_8342_jpg.rf.40135776d7284bc88ddb0d5621b0b8c1.jpg \n extracting: train/IMG_8342_jpg.rf.40135776d7284bc88ddb0d5621b0b8c1.txt \n extracting: train/IMG_8342_jpg.rf.9681027498d2705aad9c67785af95626.jpg \n extracting: train/IMG_8342_jpg.rf.9681027498d2705aad9c67785af95626.txt \n extracting: train/IMG_8342_jpg.rf.cf228cda6ab08d6f57e5265fdeb9c80e.jpg \n extracting: train/IMG_8342_jpg.rf.cf228cda6ab08d6f57e5265fdeb9c80e.txt \n extracting: train/IMG_8344_jpg.rf.472fba56650fe3dd96b06a39aa5a5b38.jpg \n extracting: train/IMG_8344_jpg.rf.472fba56650fe3dd96b06a39aa5a5b38.txt \n extracting: train/IMG_8344_jpg.rf.82497d2ca25373deca670cf7b0f10ccf.jpg \n extracting: train/IMG_8344_jpg.rf.82497d2ca25373deca670cf7b0f10ccf.txt \n extracting: train/IMG_8344_jpg.rf.d57c869a3f992891c474236e8fef3ce4.jpg \n extracting: train/IMG_8344_jpg.rf.d57c869a3f992891c474236e8fef3ce4.txt \n extracting: train/IMG_8356_jpg.rf.88b30d6f744abe29f92c5ea5523fd7a4.jpg \n extracting: train/IMG_8356_jpg.rf.88b30d6f744abe29f92c5ea5523fd7a4.txt \n extracting: train/IMG_8356_jpg.rf.b53724f4445a2932ae86dc06d60b0180.jpg \n extracting: train/IMG_8356_jpg.rf.b53724f4445a2932ae86dc06d60b0180.txt \n extracting: train/IMG_8356_jpg.rf.ee1b3e70102b83119306b0b1e32daded.jpg \n extracting: train/IMG_8356_jpg.rf.ee1b3e70102b83119306b0b1e32daded.txt \n extracting: train/IMG_8357_jpg.rf.8463481f56fb44fcc52d708d28188d9c.jpg \n extracting: train/IMG_8357_jpg.rf.8463481f56fb44fcc52d708d28188d9c.txt \n extracting: train/IMG_8357_jpg.rf.c9dad3c6c8a2ad421cdb4f573442c42c.jpg \n extracting: train/IMG_8357_jpg.rf.c9dad3c6c8a2ad421cdb4f573442c42c.txt \n extracting: train/IMG_8357_jpg.rf.ed930a9da1e3b8561af6f7c82078336f.jpg \n extracting: train/IMG_8357_jpg.rf.ed930a9da1e3b8561af6f7c82078336f.txt \n extracting: train/IMG_8376_jpg.rf.27e3e163530521604077cf71d4bcf180.jpg \n extracting: train/IMG_8376_jpg.rf.27e3e163530521604077cf71d4bcf180.txt \n extracting: train/IMG_8376_jpg.rf.875d31bec7c28b026d7b90b74d7e08b4.jpg \n extracting: train/IMG_8376_jpg.rf.875d31bec7c28b026d7b90b74d7e08b4.txt \n extracting: train/IMG_8376_jpg.rf.d37bcdde06e20cc6241da46c9742e09f.jpg \n extracting: train/IMG_8376_jpg.rf.d37bcdde06e20cc6241da46c9742e09f.txt \n extracting: train/IMG_8378_jpg.rf.1d298fc14c8557dd21cb60e1c710d26a.jpg \n extracting: train/IMG_8378_jpg.rf.1d298fc14c8557dd21cb60e1c710d26a.txt \n extracting: train/IMG_8378_jpg.rf.1f6d9264f38b81d79d9cd96e21dc92f3.jpg \n extracting: train/IMG_8378_jpg.rf.1f6d9264f38b81d79d9cd96e21dc92f3.txt \n extracting: train/IMG_8378_jpg.rf.8a07ddf4d95f7aa219109db1b369d95d.jpg \n extracting: train/IMG_8378_jpg.rf.8a07ddf4d95f7aa219109db1b369d95d.txt \n extracting: train/IMG_8382_jpg.rf.30b64242137946c5c9407bbdd6937358.jpg \n extracting: train/IMG_8382_jpg.rf.30b64242137946c5c9407bbdd6937358.txt \n extracting: train/IMG_8382_jpg.rf.3df4e48cfe8042ba3365dd3d5d10c14f.jpg \n extracting: train/IMG_8382_jpg.rf.3df4e48cfe8042ba3365dd3d5d10c14f.txt \n extracting: train/IMG_8382_jpg.rf.c6d69a47b1be369e3f2c3dc1b243e0cc.jpg \n extracting: train/IMG_8382_jpg.rf.c6d69a47b1be369e3f2c3dc1b243e0cc.txt \n extracting: train/IMG_8383_jpg.rf.364f5409abe85125588d79e62f9d8ded.jpg \n extracting: train/IMG_8383_jpg.rf.364f5409abe85125588d79e62f9d8ded.txt \n extracting: train/IMG_8383_jpg.rf.701fff9c4b9e4ccfc4988acf9902c174.jpg \n extracting: train/IMG_8383_jpg.rf.701fff9c4b9e4ccfc4988acf9902c174.txt \n extracting: train/IMG_8383_jpg.rf.a3ebf97dda5e699182ede55c1b78f390.jpg \n extracting: train/IMG_8383_jpg.rf.a3ebf97dda5e699182ede55c1b78f390.txt \n extracting: train/IMG_8386_jpg.rf.27afdc3b48b7b58e985d272b01e749f4.jpg \n extracting: train/IMG_8386_jpg.rf.27afdc3b48b7b58e985d272b01e749f4.txt \n extracting: train/IMG_8386_jpg.rf.37d36d4934d2352569340f86a591708f.jpg \n extracting: train/IMG_8386_jpg.rf.37d36d4934d2352569340f86a591708f.txt \n extracting: train/IMG_8386_jpg.rf.98803f85a2eac26ab100b94352829c2f.jpg \n extracting: train/IMG_8386_jpg.rf.98803f85a2eac26ab100b94352829c2f.txt \n extracting: train/IMG_8387_jpg.rf.7909bf1145c6cde76d349e7540aca1b4.jpg \n extracting: train/IMG_8387_jpg.rf.7909bf1145c6cde76d349e7540aca1b4.txt \n extracting: train/IMG_8387_jpg.rf.9d42947eaefb03b74bdb7fa42ec19044.jpg \n extracting: train/IMG_8387_jpg.rf.9d42947eaefb03b74bdb7fa42ec19044.txt \n extracting: train/IMG_8387_jpg.rf.bdef4a1b3327a681585f8fcea2ae2ec7.jpg \n extracting: train/IMG_8387_jpg.rf.bdef4a1b3327a681585f8fcea2ae2ec7.txt \n extracting: train/IMG_8392_jpg.rf.07c1d264df6097552d19c06642c43810.jpg \n extracting: train/IMG_8392_jpg.rf.07c1d264df6097552d19c06642c43810.txt \n extracting: train/IMG_8392_jpg.rf.b4d2f7f634ae197c98085b177e565247.jpg \n extracting: train/IMG_8392_jpg.rf.b4d2f7f634ae197c98085b177e565247.txt \n extracting: train/IMG_8392_jpg.rf.b614b3d7ab9a5a4fcee24f7c76eeec93.jpg \n extracting: train/IMG_8392_jpg.rf.b614b3d7ab9a5a4fcee24f7c76eeec93.txt \n extracting: train/IMG_8393_jpg.rf.486e4ca5cc87bf7775da7672937caadb.jpg \n extracting: train/IMG_8393_jpg.rf.486e4ca5cc87bf7775da7672937caadb.txt \n extracting: train/IMG_8393_jpg.rf.889458eda62e7fccacaf1fb2ff5b19e2.jpg \n extracting: train/IMG_8393_jpg.rf.889458eda62e7fccacaf1fb2ff5b19e2.txt \n extracting: train/IMG_8393_jpg.rf.b1e4acd476d0ff8fad5a25870a4cc3bc.jpg \n extracting: train/IMG_8393_jpg.rf.b1e4acd476d0ff8fad5a25870a4cc3bc.txt \n extracting: train/IMG_8394_jpg.rf.50f1286ca502f27b93c5775a8a7a8019.jpg \n extracting: train/IMG_8394_jpg.rf.50f1286ca502f27b93c5775a8a7a8019.txt \n extracting: train/IMG_8394_jpg.rf.7f75e6b33e9c694084827d5d3afe1130.jpg \n extracting: train/IMG_8394_jpg.rf.7f75e6b33e9c694084827d5d3afe1130.txt \n extracting: train/IMG_8394_jpg.rf.e275c63a04c2aaa347569cd09b77bfae.jpg \n extracting: train/IMG_8394_jpg.rf.e275c63a04c2aaa347569cd09b77bfae.txt \n extracting: train/IMG_8397_jpg.rf.46ccf553ba1fa6d5ee556c172525f647.jpg \n extracting: train/IMG_8397_jpg.rf.46ccf553ba1fa6d5ee556c172525f647.txt \n extracting: train/IMG_8397_jpg.rf.975a5784fa0791cb450c94a6152d84ce.jpg \n extracting: train/IMG_8397_jpg.rf.975a5784fa0791cb450c94a6152d84ce.txt \n extracting: train/IMG_8397_jpg.rf.f4a74d3024e9ab73d5106a4b4f2263bc.jpg \n extracting: train/IMG_8397_jpg.rf.f4a74d3024e9ab73d5106a4b4f2263bc.txt \n extracting: train/IMG_8398_jpg.rf.47ddcaaf6ab884cf216aaca6b92017b1.jpg \n extracting: train/IMG_8398_jpg.rf.47ddcaaf6ab884cf216aaca6b92017b1.txt \n extracting: train/IMG_8398_jpg.rf.6dc31856b7405fb038b9ddbd3d24ffe5.jpg \n extracting: train/IMG_8398_jpg.rf.6dc31856b7405fb038b9ddbd3d24ffe5.txt \n extracting: train/IMG_8398_jpg.rf.d5989ccfcdf02bf5344745f5e7676c0e.jpg \n extracting: train/IMG_8398_jpg.rf.d5989ccfcdf02bf5344745f5e7676c0e.txt \n extracting: train/IMG_8399_jpg.rf.37f4f619338d5efe0387b0012f2d7376.jpg \n extracting: train/IMG_8399_jpg.rf.37f4f619338d5efe0387b0012f2d7376.txt \n extracting: train/IMG_8399_jpg.rf.38ecb4a88709b9966ba3383818464b1a.jpg \n extracting: train/IMG_8399_jpg.rf.38ecb4a88709b9966ba3383818464b1a.txt \n extracting: train/IMG_8399_jpg.rf.eb792ecdbc321422cb45a420df86c355.jpg \n extracting: train/IMG_8399_jpg.rf.eb792ecdbc321422cb45a420df86c355.txt \n extracting: train/IMG_8402_jpg.rf.5955225c38f698fffa2675d81f881f79.jpg \n extracting: train/IMG_8402_jpg.rf.5955225c38f698fffa2675d81f881f79.txt \n extracting: train/IMG_8402_jpg.rf.5ff635ba6e4f1294d739e849500540dc.jpg \n extracting: train/IMG_8402_jpg.rf.5ff635ba6e4f1294d739e849500540dc.txt \n extracting: train/IMG_8402_jpg.rf.7c19aa753d3f70a35172e582bcdb5d50.jpg \n extracting: train/IMG_8402_jpg.rf.7c19aa753d3f70a35172e582bcdb5d50.txt \n extracting: train/IMG_8403_jpg.rf.76694f14a71a44c5f8d4d0e5042a71b7.jpg \n extracting: train/IMG_8403_jpg.rf.76694f14a71a44c5f8d4d0e5042a71b7.txt \n extracting: train/IMG_8403_jpg.rf.b5fc7611316903d75781bd6c9e0392e6.jpg \n extracting: train/IMG_8403_jpg.rf.b5fc7611316903d75781bd6c9e0392e6.txt \n extracting: train/IMG_8403_jpg.rf.f3e69bb7f771d5d7a2ae554012628247.jpg \n extracting: train/IMG_8403_jpg.rf.f3e69bb7f771d5d7a2ae554012628247.txt \n extracting: train/IMG_8405_jpg.rf.286c17c50acc17b9a68bf5e09dbe5991.jpg \n extracting: train/IMG_8405_jpg.rf.286c17c50acc17b9a68bf5e09dbe5991.txt \n extracting: train/IMG_8405_jpg.rf.5e64f13d43219d9de4facff5348d77c2.jpg \n extracting: train/IMG_8405_jpg.rf.5e64f13d43219d9de4facff5348d77c2.txt \n extracting: train/IMG_8405_jpg.rf.bce60e379c562a7ce87edbc70564caaf.jpg \n extracting: train/IMG_8405_jpg.rf.bce60e379c562a7ce87edbc70564caaf.txt \n extracting: train/IMG_8406_jpg.rf.155bbbe0b23e548bb7024a520369dcb7.jpg \n extracting: train/IMG_8406_jpg.rf.155bbbe0b23e548bb7024a520369dcb7.txt \n extracting: train/IMG_8406_jpg.rf.a5db1717eb3953fe044432be5a39d632.jpg \n extracting: train/IMG_8406_jpg.rf.a5db1717eb3953fe044432be5a39d632.txt \n extracting: train/IMG_8406_jpg.rf.bd6ea526d0968a76145238a0c1428e5c.jpg \n extracting: train/IMG_8406_jpg.rf.bd6ea526d0968a76145238a0c1428e5c.txt \n extracting: train/IMG_8408_jpg.rf.145c0b4967e5251f3e11bf75d927a84f.jpg \n extracting: train/IMG_8408_jpg.rf.145c0b4967e5251f3e11bf75d927a84f.txt \n extracting: train/IMG_8408_jpg.rf.598beb9b7164c281921285d4cedf3b46.jpg \n extracting: train/IMG_8408_jpg.rf.598beb9b7164c281921285d4cedf3b46.txt \n extracting: train/IMG_8408_jpg.rf.db045e28da334a82aea79da2d3c19746.jpg \n extracting: train/IMG_8408_jpg.rf.db045e28da334a82aea79da2d3c19746.txt \n extracting: train/IMG_8410_jpg.rf.7cb5467f98cdb8ac5282ab361514c479.jpg \n extracting: train/IMG_8410_jpg.rf.7cb5467f98cdb8ac5282ab361514c479.txt \n extracting: train/IMG_8410_jpg.rf.7ccac4665e9281528d784333e1ef7b2d.jpg \n extracting: train/IMG_8410_jpg.rf.7ccac4665e9281528d784333e1ef7b2d.txt \n extracting: train/IMG_8410_jpg.rf.e4a8879b92df6267ba1e67f341b36d89.jpg \n extracting: train/IMG_8410_jpg.rf.e4a8879b92df6267ba1e67f341b36d89.txt \n extracting: train/IMG_8411_jpg.rf.b6f955f50d59b7cc0bb8bd2d88723905.jpg \n extracting: train/IMG_8411_jpg.rf.b6f955f50d59b7cc0bb8bd2d88723905.txt \n extracting: train/IMG_8411_jpg.rf.dec951b7a1eefc3b0510513e62e24687.jpg \n extracting: train/IMG_8411_jpg.rf.dec951b7a1eefc3b0510513e62e24687.txt \n extracting: train/IMG_8411_jpg.rf.f8a40625713d0a8759c817c5bad88393.jpg \n extracting: train/IMG_8411_jpg.rf.f8a40625713d0a8759c817c5bad88393.txt \n extracting: train/IMG_8412_jpg.rf.80e4c555eededf40f80bb8cb6af7e2a7.jpg \n extracting: train/IMG_8412_jpg.rf.80e4c555eededf40f80bb8cb6af7e2a7.txt \n extracting: train/IMG_8412_jpg.rf.8c79bd057ce9e554d09e910982ab21f1.jpg \n extracting: train/IMG_8412_jpg.rf.8c79bd057ce9e554d09e910982ab21f1.txt \n extracting: train/IMG_8412_jpg.rf.b3efd24dc2f3d6d6447ae84bf3a1ff0c.jpg \n extracting: train/IMG_8412_jpg.rf.b3efd24dc2f3d6d6447ae84bf3a1ff0c.txt \n extracting: train/IMG_8413_jpg.rf.3d6a13be36c379d832d0c671146d9f4d.jpg \n extracting: train/IMG_8413_jpg.rf.3d6a13be36c379d832d0c671146d9f4d.txt \n extracting: train/IMG_8413_jpg.rf.6fb80e486ca21f13b057346b11ceeac3.jpg \n extracting: train/IMG_8413_jpg.rf.6fb80e486ca21f13b057346b11ceeac3.txt \n extracting: train/IMG_8413_jpg.rf.c950cdb5fda8dc08b36d5f9d76961bd7.jpg \n extracting: train/IMG_8413_jpg.rf.c950cdb5fda8dc08b36d5f9d76961bd7.txt \n extracting: train/IMG_8416_jpg.rf.0810b3a58aa146a25f9e4189b54cb24f.jpg \n extracting: train/IMG_8416_jpg.rf.0810b3a58aa146a25f9e4189b54cb24f.txt \n extracting: train/IMG_8416_jpg.rf.51317e2301e9d8d9761ef96b98b7e66e.jpg \n extracting: train/IMG_8416_jpg.rf.51317e2301e9d8d9761ef96b98b7e66e.txt \n extracting: train/IMG_8416_jpg.rf.c2279d5a9197ed8cac5e84f13ed0b0a3.jpg \n extracting: train/IMG_8416_jpg.rf.c2279d5a9197ed8cac5e84f13ed0b0a3.txt \n extracting: train/IMG_8418_jpg.rf.3214953766cf5a7f553a6973b79991b6.jpg \n extracting: train/IMG_8418_jpg.rf.3214953766cf5a7f553a6973b79991b6.txt \n extracting: train/IMG_8418_jpg.rf.65ddc70555fc01f46706c342f075e269.jpg \n extracting: train/IMG_8418_jpg.rf.65ddc70555fc01f46706c342f075e269.txt \n extracting: train/IMG_8418_jpg.rf.adc0aa9e5eda828bb6d0cf682ac9e207.jpg \n extracting: train/IMG_8418_jpg.rf.adc0aa9e5eda828bb6d0cf682ac9e207.txt \n extracting: train/IMG_8419_jpg.rf.2e764717970336a8bfd6cb479375b447.jpg \n extracting: train/IMG_8419_jpg.rf.2e764717970336a8bfd6cb479375b447.txt \n extracting: train/IMG_8419_jpg.rf.6161c4b0f7069c3af8b00f6c63c9bc71.jpg \n extracting: train/IMG_8419_jpg.rf.6161c4b0f7069c3af8b00f6c63c9bc71.txt \n extracting: train/IMG_8419_jpg.rf.b9ddfc54d9e04eb69be7098b1c27384b.jpg \n extracting: train/IMG_8419_jpg.rf.b9ddfc54d9e04eb69be7098b1c27384b.txt \n extracting: train/IMG_8421_jpg.rf.618e1ba0efc14971b5bc7a63ef5b87fd.jpg \n extracting: train/IMG_8421_jpg.rf.618e1ba0efc14971b5bc7a63ef5b87fd.txt \n extracting: train/IMG_8421_jpg.rf.989748d880c479f6dceb7c9d4aa1d110.jpg \n extracting: train/IMG_8421_jpg.rf.989748d880c479f6dceb7c9d4aa1d110.txt \n extracting: train/IMG_8421_jpg.rf.9beb30305dc82ac96b0a0e7af57d3f68.jpg \n extracting: train/IMG_8421_jpg.rf.9beb30305dc82ac96b0a0e7af57d3f68.txt \n extracting: train/IMG_8424_jpg.rf.3a8e0dcd800cd0d17be097127cc71f36.jpg \n extracting: train/IMG_8424_jpg.rf.3a8e0dcd800cd0d17be097127cc71f36.txt \n extracting: train/IMG_8424_jpg.rf.a105d981011adb1ea3161291bbfbe60e.jpg \n extracting: train/IMG_8424_jpg.rf.a105d981011adb1ea3161291bbfbe60e.txt \n extracting: train/IMG_8424_jpg.rf.a9579a43ae2303c413df333b90673397.jpg \n extracting: train/IMG_8424_jpg.rf.a9579a43ae2303c413df333b90673397.txt \n extracting: train/IMG_8430_jpg.rf.1726b738d98f92b62f52c9539fd0ce41.jpg \n extracting: train/IMG_8430_jpg.rf.1726b738d98f92b62f52c9539fd0ce41.txt \n extracting: train/IMG_8430_jpg.rf.932265c886c2fa1a7a5f9a3247464c8a.jpg \n extracting: train/IMG_8430_jpg.rf.932265c886c2fa1a7a5f9a3247464c8a.txt \n extracting: train/IMG_8430_jpg.rf.ae6e6e99d3c9e1d8d0476d530f12e481.jpg \n extracting: train/IMG_8430_jpg.rf.ae6e6e99d3c9e1d8d0476d530f12e481.txt \n extracting: train/IMG_8434_jpg.rf.04499cec256bdc91a45568bd5fa786ea.jpg \n extracting: train/IMG_8434_jpg.rf.04499cec256bdc91a45568bd5fa786ea.txt \n extracting: train/IMG_8434_jpg.rf.6799dd5827bb73e0df6c0b0807962b97.jpg \n extracting: train/IMG_8434_jpg.rf.6799dd5827bb73e0df6c0b0807962b97.txt \n extracting: train/IMG_8434_jpg.rf.8959b5f429584ad6348ed7775059e376.jpg \n extracting: train/IMG_8434_jpg.rf.8959b5f429584ad6348ed7775059e376.txt \n extracting: train/IMG_8436_jpg.rf.289c869fb8493e7e303a050a0eafe7d1.jpg \n extracting: train/IMG_8436_jpg.rf.289c869fb8493e7e303a050a0eafe7d1.txt \n extracting: train/IMG_8436_jpg.rf.436b81ed543c2cbd4066297b53e92270.jpg \n extracting: train/IMG_8436_jpg.rf.436b81ed543c2cbd4066297b53e92270.txt \n extracting: train/IMG_8436_jpg.rf.d3fb87ac189e1b9041b352544b8f92b6.jpg \n extracting: train/IMG_8436_jpg.rf.d3fb87ac189e1b9041b352544b8f92b6.txt \n extracting: train/IMG_8439_jpg.rf.467d14e52d8055a50a7d81e107026d91.jpg \n extracting: train/IMG_8439_jpg.rf.467d14e52d8055a50a7d81e107026d91.txt \n extracting: train/IMG_8439_jpg.rf.ad483ef057abd85e803e6054ebaf0ad2.jpg \n extracting: train/IMG_8439_jpg.rf.ad483ef057abd85e803e6054ebaf0ad2.txt \n extracting: train/IMG_8439_jpg.rf.e23200e5e3377ee124192406c5eb4d0f.jpg \n extracting: train/IMG_8439_jpg.rf.e23200e5e3377ee124192406c5eb4d0f.txt \n extracting: train/IMG_8443_jpg.rf.15cd5bab46fa923e7052da72c4e599be.jpg \n extracting: train/IMG_8443_jpg.rf.15cd5bab46fa923e7052da72c4e599be.txt \n extracting: train/IMG_8443_jpg.rf.7cb74e2b2af1e7e497451a61600c271c.jpg \n extracting: train/IMG_8443_jpg.rf.7cb74e2b2af1e7e497451a61600c271c.txt \n extracting: train/IMG_8443_jpg.rf.cb8baeb8685d8a8eae14ebf4c81e0288.jpg \n extracting: train/IMG_8443_jpg.rf.cb8baeb8685d8a8eae14ebf4c81e0288.txt \n extracting: train/IMG_8445_jpg.rf.1908cbe5b53cb55df99f0a382da6596a.jpg \n extracting: train/IMG_8445_jpg.rf.1908cbe5b53cb55df99f0a382da6596a.txt \n extracting: train/IMG_8445_jpg.rf.28c23a34f29eccc9b0e4933b5a628ec3.jpg \n extracting: train/IMG_8445_jpg.rf.28c23a34f29eccc9b0e4933b5a628ec3.txt \n extracting: train/IMG_8445_jpg.rf.7333dae2c277b4a12e4601d2208d456e.jpg \n extracting: train/IMG_8445_jpg.rf.7333dae2c277b4a12e4601d2208d456e.txt \n extracting: train/IMG_8450_jpg.rf.4dc294198f2f39a751a38b1d6dbeb79c.jpg \n extracting: train/IMG_8450_jpg.rf.4dc294198f2f39a751a38b1d6dbeb79c.txt \n extracting: train/IMG_8450_jpg.rf.4e62aca861d8b9b0946bf86eb1d7373d.jpg \n extracting: train/IMG_8450_jpg.rf.4e62aca861d8b9b0946bf86eb1d7373d.txt \n extracting: train/IMG_8450_jpg.rf.d77ef7c56a630cbeb6bc504664c44512.jpg \n extracting: train/IMG_8450_jpg.rf.d77ef7c56a630cbeb6bc504664c44512.txt \n extracting: train/IMG_8451_jpg.rf.32eaae696bfc8d66adaa65f367619fb3.jpg \n extracting: train/IMG_8451_jpg.rf.32eaae696bfc8d66adaa65f367619fb3.txt \n extracting: train/IMG_8451_jpg.rf.8ff3b97a26c009441e738054a09a78b3.jpg \n extracting: train/IMG_8451_jpg.rf.8ff3b97a26c009441e738054a09a78b3.txt \n extracting: train/IMG_8451_jpg.rf.b5b976c4a43050b1f71be8d159c4299b.jpg \n extracting: train/IMG_8451_jpg.rf.b5b976c4a43050b1f71be8d159c4299b.txt \n extracting: train/IMG_8461_jpg.rf.280e59c78478a4d7e2d30681a6d0c249.jpg \n extracting: train/IMG_8461_jpg.rf.280e59c78478a4d7e2d30681a6d0c249.txt \n extracting: train/IMG_8461_jpg.rf.c74278db182cf93753cc9c96d49bb8bb.jpg \n extracting: train/IMG_8461_jpg.rf.c74278db182cf93753cc9c96d49bb8bb.txt \n extracting: train/IMG_8461_jpg.rf.ef25c8ef347e8cd7780252d18cb27db5.jpg \n extracting: train/IMG_8461_jpg.rf.ef25c8ef347e8cd7780252d18cb27db5.txt \n extracting: train/IMG_8463_jpg.rf.10745ab272125897d2723123c5bbcc72.jpg \n extracting: train/IMG_8463_jpg.rf.10745ab272125897d2723123c5bbcc72.txt \n extracting: train/IMG_8463_jpg.rf.482b80d9d098b3e3321615ccfa95bfc4.jpg \n extracting: train/IMG_8463_jpg.rf.482b80d9d098b3e3321615ccfa95bfc4.txt \n extracting: train/IMG_8463_jpg.rf.4dd9a45f9b754c7e28ec08c1526d4a87.jpg \n extracting: train/IMG_8463_jpg.rf.4dd9a45f9b754c7e28ec08c1526d4a87.txt \n extracting: train/IMG_8464_jpg.rf.748e4ec6b9852d9dd79fa1810ba39bce.jpg \n extracting: train/IMG_8464_jpg.rf.748e4ec6b9852d9dd79fa1810ba39bce.txt \n extracting: train/IMG_8464_jpg.rf.d3d052628f5f548a63d1c46573db2f7a.jpg \n extracting: train/IMG_8464_jpg.rf.d3d052628f5f548a63d1c46573db2f7a.txt \n extracting: train/IMG_8464_jpg.rf.f2fa3af18f1fee9264d02855f8957a7a.jpg \n extracting: train/IMG_8464_jpg.rf.f2fa3af18f1fee9264d02855f8957a7a.txt \n extracting: train/IMG_8466_jpg.rf.2b3ff25638136ff0bcace2788a6744d5.jpg \n extracting: train/IMG_8466_jpg.rf.2b3ff25638136ff0bcace2788a6744d5.txt \n extracting: train/IMG_8466_jpg.rf.a8718a6d450cc88f677368ad74487bb4.jpg \n extracting: train/IMG_8466_jpg.rf.a8718a6d450cc88f677368ad74487bb4.txt \n extracting: train/IMG_8466_jpg.rf.a95973d2a34937d9f4e13aa8496706b1.jpg \n extracting: train/IMG_8466_jpg.rf.a95973d2a34937d9f4e13aa8496706b1.txt \n extracting: train/IMG_8468_jpg.rf.622978b1b1d990851e8b09534804daeb.jpg \n extracting: train/IMG_8468_jpg.rf.622978b1b1d990851e8b09534804daeb.txt \n extracting: train/IMG_8468_jpg.rf.aff2fb2f2ba5dd71e20f5fb9eb3360bc.jpg \n extracting: train/IMG_8468_jpg.rf.aff2fb2f2ba5dd71e20f5fb9eb3360bc.txt \n extracting: train/IMG_8468_jpg.rf.ed38cbb287ccd6dbdbc832f0e52e716e.jpg \n extracting: train/IMG_8468_jpg.rf.ed38cbb287ccd6dbdbc832f0e52e716e.txt \n extracting: train/IMG_8469_jpg.rf.15eaf8a8ef8924eef6ef4cdc01673361.jpg \n extracting: train/IMG_8469_jpg.rf.15eaf8a8ef8924eef6ef4cdc01673361.txt \n extracting: train/IMG_8469_jpg.rf.391452bc5216994ab6e67a4f545524db.jpg \n extracting: train/IMG_8469_jpg.rf.391452bc5216994ab6e67a4f545524db.txt \n extracting: train/IMG_8469_jpg.rf.a5fa9ed42bf8823ee99ef6e7cdf94a8d.jpg \n extracting: train/IMG_8469_jpg.rf.a5fa9ed42bf8823ee99ef6e7cdf94a8d.txt \n extracting: train/IMG_8470_jpg.rf.2d5c5eeb01ea214238c0a8a6f5250c4b.jpg \n extracting: train/IMG_8470_jpg.rf.2d5c5eeb01ea214238c0a8a6f5250c4b.txt \n extracting: train/IMG_8470_jpg.rf.afe68ced0c2a46cbb052dfa98945d11a.jpg \n extracting: train/IMG_8470_jpg.rf.afe68ced0c2a46cbb052dfa98945d11a.txt \n extracting: train/IMG_8470_jpg.rf.c4b76125c9b3f5c9f8e9e7e5dc754e66.jpg \n extracting: train/IMG_8470_jpg.rf.c4b76125c9b3f5c9f8e9e7e5dc754e66.txt \n extracting: train/IMG_8471_jpg.rf.5fa7077bca079d1e7ec0c7a167845d9c.jpg \n extracting: train/IMG_8471_jpg.rf.5fa7077bca079d1e7ec0c7a167845d9c.txt \n extracting: train/IMG_8471_jpg.rf.c19db6c3ea311353c652805b1b0d2be3.jpg \n extracting: train/IMG_8471_jpg.rf.c19db6c3ea311353c652805b1b0d2be3.txt \n extracting: train/IMG_8471_jpg.rf.f5126ffd6d6838864a8597242c36d6ee.jpg \n extracting: train/IMG_8471_jpg.rf.f5126ffd6d6838864a8597242c36d6ee.txt \n extracting: train/IMG_8476_jpg.rf.493dbb9a267db95fbf19197b5ee30920.jpg \n extracting: train/IMG_8476_jpg.rf.493dbb9a267db95fbf19197b5ee30920.txt \n extracting: train/IMG_8476_jpg.rf.9340eb129d2e98a9713b07e42a103aaf.jpg \n extracting: train/IMG_8476_jpg.rf.9340eb129d2e98a9713b07e42a103aaf.txt \n extracting: train/IMG_8476_jpg.rf.fa02747a154fca320989abede696dd0e.jpg \n extracting: train/IMG_8476_jpg.rf.fa02747a154fca320989abede696dd0e.txt \n extracting: train/IMG_8477_jpg.rf.1248e2817eba5488a58547de509a0a79.jpg \n extracting: train/IMG_8477_jpg.rf.1248e2817eba5488a58547de509a0a79.txt \n extracting: train/IMG_8477_jpg.rf.326d24e2fc9e9f1bcb15c9bffb6aa632.jpg \n extracting: train/IMG_8477_jpg.rf.326d24e2fc9e9f1bcb15c9bffb6aa632.txt \n extracting: train/IMG_8477_jpg.rf.43b05d49001030d5c32bcab9157414f7.jpg \n extracting: train/IMG_8477_jpg.rf.43b05d49001030d5c32bcab9157414f7.txt \n extracting: train/IMG_8478_jpg.rf.5600fbd5e037b57376220f877575a7f7.jpg \n extracting: train/IMG_8478_jpg.rf.5600fbd5e037b57376220f877575a7f7.txt \n extracting: train/IMG_8478_jpg.rf.805628320db1077b4a010187aa8c4edd.jpg \n extracting: train/IMG_8478_jpg.rf.805628320db1077b4a010187aa8c4edd.txt \n extracting: train/IMG_8478_jpg.rf.cdaa6c1b7099e84d2110f3ddc800d48f.jpg \n extracting: train/IMG_8478_jpg.rf.cdaa6c1b7099e84d2110f3ddc800d48f.txt \n extracting: train/IMG_8486_jpg.rf.967b37cc26fd1190ffce79520be4b67d.jpg \n extracting: train/IMG_8486_jpg.rf.967b37cc26fd1190ffce79520be4b67d.txt \n extracting: train/IMG_8486_jpg.rf.aacf6f9e64f679092f4420606190a288.jpg \n extracting: train/IMG_8486_jpg.rf.aacf6f9e64f679092f4420606190a288.txt \n extracting: train/IMG_8486_jpg.rf.fea250281d21ed1a5ed654ef4d3d3e22.jpg \n extracting: train/IMG_8486_jpg.rf.fea250281d21ed1a5ed654ef4d3d3e22.txt \n extracting: train/IMG_8493_jpg.rf.9392077f99f2404a2d7006ad5c052fc4.jpg \n extracting: train/IMG_8493_jpg.rf.9392077f99f2404a2d7006ad5c052fc4.txt \n extracting: train/IMG_8493_jpg.rf.94db3cce0d0451bb3d07b3d1954364bd.jpg \n extracting: train/IMG_8493_jpg.rf.94db3cce0d0451bb3d07b3d1954364bd.txt \n extracting: train/IMG_8493_jpg.rf.e6971c292e8ff68cbae8896279572366.jpg \n extracting: train/IMG_8493_jpg.rf.e6971c292e8ff68cbae8896279572366.txt \n extracting: train/IMG_8494_jpg.rf.1b9980df411c826bd3f85ab9f19fdd92.jpg \n extracting: train/IMG_8494_jpg.rf.1b9980df411c826bd3f85ab9f19fdd92.txt \n extracting: train/IMG_8494_jpg.rf.6f888e3f96c69cec6dde494ebff18acb.jpg \n extracting: train/IMG_8494_jpg.rf.6f888e3f96c69cec6dde494ebff18acb.txt \n extracting: train/IMG_8494_jpg.rf.fa11a130610a898628350cde08268546.jpg \n extracting: train/IMG_8494_jpg.rf.fa11a130610a898628350cde08268546.txt \n extracting: train/IMG_8495_jpg.rf.358f26d69bc963e2a5285d818340954d.jpg \n extracting: train/IMG_8495_jpg.rf.358f26d69bc963e2a5285d818340954d.txt \n extracting: train/IMG_8495_jpg.rf.5819ec51bbc75c4503615a5c696f577b.jpg \n extracting: train/IMG_8495_jpg.rf.5819ec51bbc75c4503615a5c696f577b.txt \n extracting: train/IMG_8495_jpg.rf.94a254be038a463f716e1b547a9881e8.jpg \n extracting: train/IMG_8495_jpg.rf.94a254be038a463f716e1b547a9881e8.txt \n extracting: train/IMG_8496_MOV-0_jpg.rf.7e0196b7778df2c5535f7b42af0f0130.jpg \n extracting: train/IMG_8496_MOV-0_jpg.rf.7e0196b7778df2c5535f7b42af0f0130.txt \n extracting: train/IMG_8496_MOV-0_jpg.rf.9c7c6161f3ec2ab83ed0254ac5309433.jpg \n extracting: train/IMG_8496_MOV-0_jpg.rf.9c7c6161f3ec2ab83ed0254ac5309433.txt \n extracting: train/IMG_8496_MOV-0_jpg.rf.fe726ecbd4abcdebcd7efb045c33165a.jpg \n extracting: train/IMG_8496_MOV-0_jpg.rf.fe726ecbd4abcdebcd7efb045c33165a.txt \n extracting: train/IMG_8496_MOV-1_jpg.rf.413de969b93891bd9a112b6ff0c9393a.jpg \n extracting: train/IMG_8496_MOV-1_jpg.rf.413de969b93891bd9a112b6ff0c9393a.txt \n extracting: train/IMG_8496_MOV-1_jpg.rf.e3f53b6d5db2f42ae20630e62e08b46e.jpg \n extracting: train/IMG_8496_MOV-1_jpg.rf.e3f53b6d5db2f42ae20630e62e08b46e.txt \n extracting: train/IMG_8496_MOV-1_jpg.rf.ec992cd3dc24246304584b3569cd4dff.jpg \n extracting: train/IMG_8496_MOV-1_jpg.rf.ec992cd3dc24246304584b3569cd4dff.txt \n extracting: train/IMG_8496_MOV-3_jpg.rf.26601c1d87cb06abc51521a9cd831334.jpg \n extracting: train/IMG_8496_MOV-3_jpg.rf.26601c1d87cb06abc51521a9cd831334.txt \n extracting: train/IMG_8496_MOV-3_jpg.rf.769e9bf5f583ea519cedd201b279dfd1.jpg \n extracting: train/IMG_8496_MOV-3_jpg.rf.769e9bf5f583ea519cedd201b279dfd1.txt \n extracting: train/IMG_8496_MOV-3_jpg.rf.fd05b0a4b3f0948088dfe4d5e7dd62fd.jpg \n extracting: train/IMG_8496_MOV-3_jpg.rf.fd05b0a4b3f0948088dfe4d5e7dd62fd.txt \n extracting: train/IMG_8496_MOV-4_jpg.rf.0f36b00c590d5996301f9afcde81c308.jpg \n extracting: train/IMG_8496_MOV-4_jpg.rf.0f36b00c590d5996301f9afcde81c308.txt \n extracting: train/IMG_8496_MOV-4_jpg.rf.7555d15a30a59ba0ab83a721019eee09.jpg \n extracting: train/IMG_8496_MOV-4_jpg.rf.7555d15a30a59ba0ab83a721019eee09.txt \n extracting: train/IMG_8496_MOV-4_jpg.rf.db77cb30e6177ce7022bff513f14c2be.jpg \n extracting: train/IMG_8496_MOV-4_jpg.rf.db77cb30e6177ce7022bff513f14c2be.txt \n extracting: train/IMG_8496_MOV-5_jpg.rf.15a060fa3d6acd81b5e1351f4f62cbcf.jpg \n extracting: train/IMG_8496_MOV-5_jpg.rf.15a060fa3d6acd81b5e1351f4f62cbcf.txt \n extracting: train/IMG_8496_MOV-5_jpg.rf.9ef13843d874aa8b1937817be1838d6a.jpg \n extracting: train/IMG_8496_MOV-5_jpg.rf.9ef13843d874aa8b1937817be1838d6a.txt \n extracting: train/IMG_8496_MOV-5_jpg.rf.d879d431d575ff2a179c925c0991624c.jpg \n extracting: train/IMG_8496_MOV-5_jpg.rf.d879d431d575ff2a179c925c0991624c.txt \n extracting: train/IMG_8496_MOV-6_jpg.rf.07525c6bee2c327aa5fda45264112376.jpg \n extracting: train/IMG_8496_MOV-6_jpg.rf.07525c6bee2c327aa5fda45264112376.txt \n extracting: train/IMG_8496_MOV-6_jpg.rf.ad3e2a94119003fc652fdb36c84400f3.jpg \n extracting: train/IMG_8496_MOV-6_jpg.rf.ad3e2a94119003fc652fdb36c84400f3.txt \n extracting: train/IMG_8496_MOV-6_jpg.rf.d1aa46bf73fc0559d441ce5326b1bb37.jpg \n extracting: train/IMG_8496_MOV-6_jpg.rf.d1aa46bf73fc0559d441ce5326b1bb37.txt \n extracting: train/IMG_8497_MOV-1_jpg.rf.304e66a27bf82c457ac074e3c0b196f9.jpg \n extracting: train/IMG_8497_MOV-1_jpg.rf.304e66a27bf82c457ac074e3c0b196f9.txt \n extracting: train/IMG_8497_MOV-1_jpg.rf.89039b22d7744958ed89699da0e4d8da.jpg \n extracting: train/IMG_8497_MOV-1_jpg.rf.89039b22d7744958ed89699da0e4d8da.txt \n extracting: train/IMG_8497_MOV-1_jpg.rf.c1542abf76ac981935e7ebb8e61434b3.jpg \n extracting: train/IMG_8497_MOV-1_jpg.rf.c1542abf76ac981935e7ebb8e61434b3.txt \n extracting: train/IMG_8500_jpg.rf.60f9a36bba568bd134901a86e398862b.jpg \n extracting: train/IMG_8500_jpg.rf.60f9a36bba568bd134901a86e398862b.txt \n extracting: train/IMG_8500_jpg.rf.88e19d2003f755db994303a1f04f0bd5.jpg \n extracting: train/IMG_8500_jpg.rf.88e19d2003f755db994303a1f04f0bd5.txt \n extracting: train/IMG_8500_jpg.rf.e3df6f05ebcbe8ba4b4807a79d9b230d.jpg \n extracting: train/IMG_8500_jpg.rf.e3df6f05ebcbe8ba4b4807a79d9b230d.txt \n extracting: train/IMG_8502_jpg.rf.3a5c02058f6a270749f90007301ea89c.jpg \n extracting: train/IMG_8502_jpg.rf.3a5c02058f6a270749f90007301ea89c.txt \n extracting: train/IMG_8502_jpg.rf.8ba574ce1c149ae35df8e6a9e868a320.jpg \n extracting: train/IMG_8502_jpg.rf.8ba574ce1c149ae35df8e6a9e868a320.txt \n extracting: train/IMG_8502_jpg.rf.ea9cf8ccc94e58bc63f2f926836e34e2.jpg \n extracting: train/IMG_8502_jpg.rf.ea9cf8ccc94e58bc63f2f926836e34e2.txt \n extracting: train/IMG_8509_jpg.rf.3fcd9171a28c5ff52fb4d322d4523bbf.jpg \n extracting: train/IMG_8509_jpg.rf.3fcd9171a28c5ff52fb4d322d4523bbf.txt \n extracting: train/IMG_8509_jpg.rf.4639bebc71b69e6479426c5d8aafd321.jpg \n extracting: train/IMG_8509_jpg.rf.4639bebc71b69e6479426c5d8aafd321.txt \n extracting: train/IMG_8509_jpg.rf.993d76011aa08464efdf4411c38ca847.jpg \n extracting: train/IMG_8509_jpg.rf.993d76011aa08464efdf4411c38ca847.txt \n extracting: train/IMG_8510_jpg.rf.0c9a48e2595238e6f25aad1678e568af.jpg \n extracting: train/IMG_8510_jpg.rf.0c9a48e2595238e6f25aad1678e568af.txt \n extracting: train/IMG_8510_jpg.rf.ba974b4f7a221b6f854d106eb1ce1698.jpg \n extracting: train/IMG_8510_jpg.rf.ba974b4f7a221b6f854d106eb1ce1698.txt \n extracting: train/IMG_8510_jpg.rf.c41f1c273328a91404e4ae0dc5934699.jpg \n extracting: train/IMG_8510_jpg.rf.c41f1c273328a91404e4ae0dc5934699.txt \n extracting: train/IMG_8511_jpg.rf.80f6e2cc2fd207c21952510fe6962f7c.jpg \n extracting: train/IMG_8511_jpg.rf.80f6e2cc2fd207c21952510fe6962f7c.txt \n extracting: train/IMG_8511_jpg.rf.8fa6315f098c069b8dbf5802357d7690.jpg \n extracting: train/IMG_8511_jpg.rf.8fa6315f098c069b8dbf5802357d7690.txt \n extracting: train/IMG_8511_jpg.rf.df822b8e3e584f804c71e5a9e8c5e781.jpg \n extracting: train/IMG_8511_jpg.rf.df822b8e3e584f804c71e5a9e8c5e781.txt \n extracting: train/IMG_8512_MOV-0_jpg.rf.1dc2218fee58be6e1e6d1a86ba4ddd21.jpg \n extracting: train/IMG_8512_MOV-0_jpg.rf.1dc2218fee58be6e1e6d1a86ba4ddd21.txt \n extracting: train/IMG_8512_MOV-0_jpg.rf.609aa7442a2a61b5c241a45afc380a6b.jpg \n extracting: train/IMG_8512_MOV-0_jpg.rf.609aa7442a2a61b5c241a45afc380a6b.txt \n extracting: train/IMG_8512_MOV-0_jpg.rf.ba2af85eb904f6cba74c6ed305da9593.jpg \n extracting: train/IMG_8512_MOV-0_jpg.rf.ba2af85eb904f6cba74c6ed305da9593.txt \n extracting: train/IMG_8512_MOV-2_jpg.rf.3a421825eb565cf55790abcc2cb8344c.jpg \n extracting: train/IMG_8512_MOV-2_jpg.rf.3a421825eb565cf55790abcc2cb8344c.txt \n extracting: train/IMG_8512_MOV-2_jpg.rf.61c87699ce73721a03c3af5bbbc920c8.jpg \n extracting: train/IMG_8512_MOV-2_jpg.rf.61c87699ce73721a03c3af5bbbc920c8.txt \n extracting: train/IMG_8512_MOV-2_jpg.rf.aac12e4df8915dae4ba3ab64f2fd6d24.jpg \n extracting: train/IMG_8512_MOV-2_jpg.rf.aac12e4df8915dae4ba3ab64f2fd6d24.txt \n extracting: train/IMG_8512_MOV-3_jpg.rf.523f82beb19a8a6d0b235d5f5f53b454.jpg \n extracting: train/IMG_8512_MOV-3_jpg.rf.523f82beb19a8a6d0b235d5f5f53b454.txt \n extracting: train/IMG_8512_MOV-3_jpg.rf.95ddb4aa357ad0a674001c968aeb9f25.jpg \n extracting: train/IMG_8512_MOV-3_jpg.rf.95ddb4aa357ad0a674001c968aeb9f25.txt \n extracting: train/IMG_8512_MOV-3_jpg.rf.b16510b1ce8ba475d2559cb490518642.jpg \n extracting: train/IMG_8512_MOV-3_jpg.rf.b16510b1ce8ba475d2559cb490518642.txt \n extracting: train/IMG_8512_MOV-4_jpg.rf.25ecb2525346f4c2ed54124d09906d8d.jpg \n extracting: train/IMG_8512_MOV-4_jpg.rf.25ecb2525346f4c2ed54124d09906d8d.txt \n extracting: train/IMG_8512_MOV-4_jpg.rf.dbd5781c8757d94f1ac55215723385ff.jpg \n extracting: train/IMG_8512_MOV-4_jpg.rf.dbd5781c8757d94f1ac55215723385ff.txt \n extracting: train/IMG_8512_MOV-4_jpg.rf.e150440102cac43717bde434f634e883.jpg \n extracting: train/IMG_8512_MOV-4_jpg.rf.e150440102cac43717bde434f634e883.txt \n extracting: train/IMG_8512_MOV-6_jpg.rf.701f07ffb0d3bc32e5330b08d0c797f7.jpg \n extracting: train/IMG_8512_MOV-6_jpg.rf.701f07ffb0d3bc32e5330b08d0c797f7.txt \n extracting: train/IMG_8512_MOV-6_jpg.rf.7de08ac59ee3de7463afa5abdc4e78b9.jpg \n extracting: train/IMG_8512_MOV-6_jpg.rf.7de08ac59ee3de7463afa5abdc4e78b9.txt \n extracting: train/IMG_8512_MOV-6_jpg.rf.b0838cc80c102b37e4976a799900aef7.jpg \n extracting: train/IMG_8512_MOV-6_jpg.rf.b0838cc80c102b37e4976a799900aef7.txt \n extracting: train/IMG_8516_jpg.rf.6bc48342c351ba8a71785f2beae6b8ad.jpg \n extracting: train/IMG_8516_jpg.rf.6bc48342c351ba8a71785f2beae6b8ad.txt \n extracting: train/IMG_8516_jpg.rf.79a1a346c37759e5aef976eff142212d.jpg \n extracting: train/IMG_8516_jpg.rf.79a1a346c37759e5aef976eff142212d.txt \n extracting: train/IMG_8516_jpg.rf.e335517a00a1c6335fc81de5caffc7f8.jpg \n extracting: train/IMG_8516_jpg.rf.e335517a00a1c6335fc81de5caffc7f8.txt \n extracting: train/IMG_8517_MOV-0_jpg.rf.1a43c85ee945a9c9f6873eea882dfe41.jpg \n extracting: train/IMG_8517_MOV-0_jpg.rf.1a43c85ee945a9c9f6873eea882dfe41.txt \n extracting: train/IMG_8517_MOV-0_jpg.rf.569a5132e2e395a9a69b2b4bcf53e6a9.jpg \n extracting: train/IMG_8517_MOV-0_jpg.rf.569a5132e2e395a9a69b2b4bcf53e6a9.txt \n extracting: train/IMG_8517_MOV-0_jpg.rf.83dc94185afcf0258facc6bb98ed3f09.jpg \n extracting: train/IMG_8517_MOV-0_jpg.rf.83dc94185afcf0258facc6bb98ed3f09.txt \n extracting: train/IMG_8517_MOV-1_jpg.rf.2900c7b3e37fe0b4bdced6366bf3b304.jpg \n extracting: train/IMG_8517_MOV-1_jpg.rf.2900c7b3e37fe0b4bdced6366bf3b304.txt \n extracting: train/IMG_8517_MOV-1_jpg.rf.291770734492e0098739a798b8d6c32c.jpg \n extracting: train/IMG_8517_MOV-1_jpg.rf.291770734492e0098739a798b8d6c32c.txt \n extracting: train/IMG_8517_MOV-1_jpg.rf.4a7a6295e9ed9e6ffb1dc8f50365a61d.jpg \n extracting: train/IMG_8517_MOV-1_jpg.rf.4a7a6295e9ed9e6ffb1dc8f50365a61d.txt \n extracting: train/IMG_8517_MOV-2_jpg.rf.3f2617eb16867d95306ea7d4464c4f64.jpg \n extracting: train/IMG_8517_MOV-2_jpg.rf.3f2617eb16867d95306ea7d4464c4f64.txt \n extracting: train/IMG_8517_MOV-2_jpg.rf.9b054f6046aad58f8bea08be4450f6c9.jpg \n extracting: train/IMG_8517_MOV-2_jpg.rf.9b054f6046aad58f8bea08be4450f6c9.txt \n extracting: train/IMG_8517_MOV-2_jpg.rf.9fb43338769dc5147324aa00207f463a.jpg \n extracting: train/IMG_8517_MOV-2_jpg.rf.9fb43338769dc5147324aa00207f463a.txt \n extracting: train/IMG_8517_MOV-3_jpg.rf.1a886d501cbe17a9fc9ae5db2f29d4aa.jpg \n extracting: train/IMG_8517_MOV-3_jpg.rf.1a886d501cbe17a9fc9ae5db2f29d4aa.txt \n extracting: train/IMG_8517_MOV-3_jpg.rf.50d82708df38aad7eb1c540465823394.jpg \n extracting: train/IMG_8517_MOV-3_jpg.rf.50d82708df38aad7eb1c540465823394.txt \n extracting: train/IMG_8517_MOV-3_jpg.rf.8ae9e1923c4b1708a7e876dfd704e5de.jpg \n extracting: train/IMG_8517_MOV-3_jpg.rf.8ae9e1923c4b1708a7e876dfd704e5de.txt \n extracting: train/IMG_8517_MOV-4_jpg.rf.181f05cf9405daedc2e4b400dac89378.jpg \n extracting: train/IMG_8517_MOV-4_jpg.rf.181f05cf9405daedc2e4b400dac89378.txt \n extracting: train/IMG_8517_MOV-4_jpg.rf.7cf6feb172e5cdc02d5b7f0fdfc7b8e1.jpg \n extracting: train/IMG_8517_MOV-4_jpg.rf.7cf6feb172e5cdc02d5b7f0fdfc7b8e1.txt \n extracting: train/IMG_8517_MOV-4_jpg.rf.a5d785eead9081f5e0d532426c1fe266.jpg \n extracting: train/IMG_8517_MOV-4_jpg.rf.a5d785eead9081f5e0d532426c1fe266.txt \n extracting: train/IMG_8517_MOV-5_jpg.rf.356442a6c8d02a4f51c5cbf33e23efd2.jpg \n extracting: train/IMG_8517_MOV-5_jpg.rf.356442a6c8d02a4f51c5cbf33e23efd2.txt \n extracting: train/IMG_8517_MOV-5_jpg.rf.46e5ddc343b0f98afb92ff9bad4ce34f.jpg \n extracting: train/IMG_8517_MOV-5_jpg.rf.46e5ddc343b0f98afb92ff9bad4ce34f.txt \n extracting: train/IMG_8517_MOV-5_jpg.rf.6a70bd0839914ec3a58a0d17e62e461e.jpg \n extracting: train/IMG_8517_MOV-5_jpg.rf.6a70bd0839914ec3a58a0d17e62e461e.txt \n extracting: train/IMG_8517_MOV-6_jpg.rf.66c3be00519f92042f2fe196bc083b42.jpg \n extracting: train/IMG_8517_MOV-6_jpg.rf.66c3be00519f92042f2fe196bc083b42.txt \n extracting: train/IMG_8517_MOV-6_jpg.rf.e22fc5b770a8437024068bfaec916981.jpg \n extracting: train/IMG_8517_MOV-6_jpg.rf.e22fc5b770a8437024068bfaec916981.txt \n extracting: train/IMG_8517_MOV-6_jpg.rf.f2814e56bf2eee5bdd6b7cba2f8935e7.jpg \n extracting: train/IMG_8517_MOV-6_jpg.rf.f2814e56bf2eee5bdd6b7cba2f8935e7.txt \n extracting: train/IMG_8519_jpg.rf.6dfca6619bb74324339e595ea535c96e.jpg \n extracting: train/IMG_8519_jpg.rf.6dfca6619bb74324339e595ea535c96e.txt \n extracting: train/IMG_8519_jpg.rf.974235a76ee0bba2acb6926fdd06f19e.jpg \n extracting: train/IMG_8519_jpg.rf.974235a76ee0bba2acb6926fdd06f19e.txt \n extracting: train/IMG_8519_jpg.rf.be93c7a009d460e2c70c2104a3090da1.jpg \n extracting: train/IMG_8519_jpg.rf.be93c7a009d460e2c70c2104a3090da1.txt \n extracting: train/IMG_8520_jpg.rf.08197b139e68ffbd26762c163898abff.jpg \n extracting: train/IMG_8520_jpg.rf.08197b139e68ffbd26762c163898abff.txt \n extracting: train/IMG_8520_jpg.rf.ae06d5d06b54404ebef913fcfa7f5d6c.jpg \n extracting: train/IMG_8520_jpg.rf.ae06d5d06b54404ebef913fcfa7f5d6c.txt \n extracting: train/IMG_8520_jpg.rf.b94d925b34b731ee7a3acc72fc2297fa.jpg \n extracting: train/IMG_8520_jpg.rf.b94d925b34b731ee7a3acc72fc2297fa.txt \n extracting: train/IMG_8522_jpg.rf.0a3d654a371444cce3b9648f7d6cb49a.jpg \n extracting: train/IMG_8522_jpg.rf.0a3d654a371444cce3b9648f7d6cb49a.txt \n extracting: train/IMG_8522_jpg.rf.4b4ef30220e5fb94fdcda8e4b22ab616.jpg \n extracting: train/IMG_8522_jpg.rf.4b4ef30220e5fb94fdcda8e4b22ab616.txt \n extracting: train/IMG_8522_jpg.rf.6aba08b5023ac0924034062c0cba92ad.jpg \n extracting: train/IMG_8522_jpg.rf.6aba08b5023ac0924034062c0cba92ad.txt \n extracting: train/IMG_8523_jpg.rf.28db8199d7a9103a66ac8a2032a64f3f.jpg \n extracting: train/IMG_8523_jpg.rf.28db8199d7a9103a66ac8a2032a64f3f.txt \n extracting: train/IMG_8523_jpg.rf.b7e1f29002272580971b2ffedbb25184.jpg \n extracting: train/IMG_8523_jpg.rf.b7e1f29002272580971b2ffedbb25184.txt \n extracting: train/IMG_8523_jpg.rf.be5da40bfb1376921cc1e333b9ec70e9.jpg \n extracting: train/IMG_8523_jpg.rf.be5da40bfb1376921cc1e333b9ec70e9.txt \n extracting: train/IMG_8527_jpg.rf.111c3e5cf8743ef8c68cd9573476590c.jpg \n extracting: train/IMG_8527_jpg.rf.111c3e5cf8743ef8c68cd9573476590c.txt \n extracting: train/IMG_8527_jpg.rf.a3761abb4fbbaf2b2f445da4e349bf9e.jpg \n extracting: train/IMG_8527_jpg.rf.a3761abb4fbbaf2b2f445da4e349bf9e.txt \n extracting: train/IMG_8527_jpg.rf.b51f5262f325285bc1d0911cda1825ba.jpg \n extracting: train/IMG_8527_jpg.rf.b51f5262f325285bc1d0911cda1825ba.txt \n extracting: train/IMG_8531_jpg.rf.38b8b1901a7edfcc5ae3412bc409e02c.jpg \n extracting: train/IMG_8531_jpg.rf.38b8b1901a7edfcc5ae3412bc409e02c.txt \n extracting: train/IMG_8531_jpg.rf.5200ee62736417bad6fd321c9822e755.jpg \n extracting: train/IMG_8531_jpg.rf.5200ee62736417bad6fd321c9822e755.txt \n extracting: train/IMG_8531_jpg.rf.d7b19a03f7832d7b8b36e2f8c1cea598.jpg \n extracting: train/IMG_8531_jpg.rf.d7b19a03f7832d7b8b36e2f8c1cea598.txt \n extracting: train/IMG_8533_jpg.rf.190f820abdd624d1c631beabb5385b3d.jpg \n extracting: train/IMG_8533_jpg.rf.190f820abdd624d1c631beabb5385b3d.txt \n extracting: train/IMG_8533_jpg.rf.528f16f80860b86903293540e13a858d.jpg \n extracting: train/IMG_8533_jpg.rf.528f16f80860b86903293540e13a858d.txt \n extracting: train/IMG_8533_jpg.rf.81004dba47d4072967a16e53cecb11a4.jpg \n extracting: train/IMG_8533_jpg.rf.81004dba47d4072967a16e53cecb11a4.txt \n extracting: train/IMG_8534_jpg.rf.a06879048b0254fb4c302d9907b21675.jpg \n extracting: train/IMG_8534_jpg.rf.a06879048b0254fb4c302d9907b21675.txt \n extracting: train/IMG_8534_jpg.rf.ca3570cf77588de88f5fa16bf4ad46f4.jpg \n extracting: train/IMG_8534_jpg.rf.ca3570cf77588de88f5fa16bf4ad46f4.txt \n extracting: train/IMG_8534_jpg.rf.eea4d46e14e3455efcfbcd6855ee9012.jpg \n extracting: train/IMG_8534_jpg.rf.eea4d46e14e3455efcfbcd6855ee9012.txt \n extracting: train/IMG_8535_MOV-2_jpg.rf.3a44391e09b21643c4f79dc9d5aec60b.jpg \n extracting: train/IMG_8535_MOV-2_jpg.rf.3a44391e09b21643c4f79dc9d5aec60b.txt \n extracting: train/IMG_8535_MOV-2_jpg.rf.6bc63313624c8285834d3788ce0a7a2c.jpg \n extracting: train/IMG_8535_MOV-2_jpg.rf.6bc63313624c8285834d3788ce0a7a2c.txt \n extracting: train/IMG_8535_MOV-2_jpg.rf.aa0973e1d26aa7eb59d37f607f402c5e.jpg \n extracting: train/IMG_8535_MOV-2_jpg.rf.aa0973e1d26aa7eb59d37f607f402c5e.txt \n extracting: train/IMG_8535_MOV-3_jpg.rf.a66ac0b5fce20850c4adf5fbcc858cbc.jpg \n extracting: train/IMG_8535_MOV-3_jpg.rf.a66ac0b5fce20850c4adf5fbcc858cbc.txt \n extracting: train/IMG_8535_MOV-3_jpg.rf.b590b0b027fc0f0bab216240ff45481f.jpg \n extracting: train/IMG_8535_MOV-3_jpg.rf.b590b0b027fc0f0bab216240ff45481f.txt \n extracting: train/IMG_8535_MOV-3_jpg.rf.bd17e373e67715cc30730aca84c8c548.jpg \n extracting: train/IMG_8535_MOV-3_jpg.rf.bd17e373e67715cc30730aca84c8c548.txt \n extracting: train/IMG_8536_jpg.rf.1287da32e1aac920e20eb55e25a69fb6.jpg \n extracting: train/IMG_8536_jpg.rf.1287da32e1aac920e20eb55e25a69fb6.txt \n extracting: train/IMG_8536_jpg.rf.77bf0e91e858725b816197c51dc5073a.jpg \n extracting: train/IMG_8536_jpg.rf.77bf0e91e858725b816197c51dc5073a.txt \n extracting: train/IMG_8536_jpg.rf.8834f3611693034bb3016276ed63d34f.jpg \n extracting: train/IMG_8536_jpg.rf.8834f3611693034bb3016276ed63d34f.txt \n extracting: train/IMG_8537_jpg.rf.0569c1cf3b673c2a362bc5cd23b79247.jpg \n extracting: train/IMG_8537_jpg.rf.0569c1cf3b673c2a362bc5cd23b79247.txt \n extracting: train/IMG_8537_jpg.rf.34afe2849b8f4e08101b67da4d285f61.jpg \n extracting: train/IMG_8537_jpg.rf.34afe2849b8f4e08101b67da4d285f61.txt \n extracting: train/IMG_8537_jpg.rf.428a599ba2944d586cf4c3e200112394.jpg \n extracting: train/IMG_8537_jpg.rf.428a599ba2944d586cf4c3e200112394.txt \n extracting: train/IMG_8538_jpg.rf.11b18d9f1081e393cfab0a952cfabaa8.jpg \n extracting: train/IMG_8538_jpg.rf.11b18d9f1081e393cfab0a952cfabaa8.txt \n extracting: train/IMG_8538_jpg.rf.9fc9be07ec7c6e59314af232a8791db1.jpg \n extracting: train/IMG_8538_jpg.rf.9fc9be07ec7c6e59314af232a8791db1.txt \n extracting: train/IMG_8538_jpg.rf.b64e615da313a3b518c0410075ff6af5.jpg \n extracting: train/IMG_8538_jpg.rf.b64e615da313a3b518c0410075ff6af5.txt \n extracting: train/IMG_8541_jpg.rf.3177d347fcdcfb2e40a44c51cf5d1782.jpg \n extracting: train/IMG_8541_jpg.rf.3177d347fcdcfb2e40a44c51cf5d1782.txt \n extracting: train/IMG_8541_jpg.rf.5adec4284e9b093f887efe3ab2e5a355.jpg \n extracting: train/IMG_8541_jpg.rf.5adec4284e9b093f887efe3ab2e5a355.txt \n extracting: train/IMG_8541_jpg.rf.75ae0c00256db9dc3172f06c0a46ef20.jpg \n extracting: train/IMG_8541_jpg.rf.75ae0c00256db9dc3172f06c0a46ef20.txt \n extracting: train/IMG_8544_jpg.rf.1ce6e2c984c2e3e7ede47478f764f49d.jpg \n extracting: train/IMG_8544_jpg.rf.1ce6e2c984c2e3e7ede47478f764f49d.txt \n extracting: train/IMG_8544_jpg.rf.231fceb6b27ff327e135d3f649cc7370.jpg \n extracting: train/IMG_8544_jpg.rf.231fceb6b27ff327e135d3f649cc7370.txt \n extracting: train/IMG_8544_jpg.rf.8f463c730a7d6c6558df974ae6417014.jpg \n extracting: train/IMG_8544_jpg.rf.8f463c730a7d6c6558df974ae6417014.txt \n extracting: train/IMG_8545_jpg.rf.487ae7798a4d1ff845dd5b8ffac8ca37.jpg \n extracting: train/IMG_8545_jpg.rf.487ae7798a4d1ff845dd5b8ffac8ca37.txt \n extracting: train/IMG_8545_jpg.rf.6fcc06237da482c6f025c5979bb330d3.jpg \n extracting: train/IMG_8545_jpg.rf.6fcc06237da482c6f025c5979bb330d3.txt \n extracting: train/IMG_8545_jpg.rf.ebdffb689896cafb19c27ed67df97e5a.jpg \n extracting: train/IMG_8545_jpg.rf.ebdffb689896cafb19c27ed67df97e5a.txt \n extracting: train/IMG_8546_jpg.rf.1f9912b894b9cfd40f23fd3d5afaaa5b.jpg \n extracting: train/IMG_8546_jpg.rf.1f9912b894b9cfd40f23fd3d5afaaa5b.txt \n extracting: train/IMG_8546_jpg.rf.2b33e6994d637b8a29a3b3cff5ee0d67.jpg \n extracting: train/IMG_8546_jpg.rf.2b33e6994d637b8a29a3b3cff5ee0d67.txt \n extracting: train/IMG_8546_jpg.rf.e64924f1c8b234341de3442f5ceb3bd6.jpg \n extracting: train/IMG_8546_jpg.rf.e64924f1c8b234341de3442f5ceb3bd6.txt \n extracting: train/IMG_8547_jpg.rf.cb3351739a1516fea8ea695cd6ed8b98.jpg \n extracting: train/IMG_8547_jpg.rf.cb3351739a1516fea8ea695cd6ed8b98.txt \n extracting: train/IMG_8547_jpg.rf.e15b3b069cf049f26efc6b16b6b27ef7.jpg \n extracting: train/IMG_8547_jpg.rf.e15b3b069cf049f26efc6b16b6b27ef7.txt \n extracting: train/IMG_8547_jpg.rf.f0f01cb1ec9e939bfa22e2c7196b5f92.jpg \n extracting: train/IMG_8547_jpg.rf.f0f01cb1ec9e939bfa22e2c7196b5f92.txt \n extracting: train/IMG_8548_jpg.rf.2b828fdc18209e860abeac613cbe3873.jpg \n extracting: train/IMG_8548_jpg.rf.2b828fdc18209e860abeac613cbe3873.txt \n extracting: train/IMG_8548_jpg.rf.482806d2a5f0fd5208f1c1f3d52e173f.jpg \n extracting: train/IMG_8548_jpg.rf.482806d2a5f0fd5208f1c1f3d52e173f.txt \n extracting: train/IMG_8548_jpg.rf.5bdaf07ac2032f2e38deed53c4ec575d.jpg \n extracting: train/IMG_8548_jpg.rf.5bdaf07ac2032f2e38deed53c4ec575d.txt \n extracting: train/IMG_8549_jpg.rf.5e414d8af895eb2699954b31a2140605.jpg \n extracting: train/IMG_8549_jpg.rf.5e414d8af895eb2699954b31a2140605.txt \n extracting: train/IMG_8549_jpg.rf.8c75926862d9d2ed0a81d1daa09dd06d.jpg \n extracting: train/IMG_8549_jpg.rf.8c75926862d9d2ed0a81d1daa09dd06d.txt \n extracting: train/IMG_8549_jpg.rf.c3fc5afeb3c705b2b61861c662be5e76.jpg \n extracting: train/IMG_8549_jpg.rf.c3fc5afeb3c705b2b61861c662be5e76.txt \n extracting: train/IMG_8550_jpg.rf.13a2bcd1d454de1c61ea2d5a3ce84606.jpg \n extracting: train/IMG_8550_jpg.rf.13a2bcd1d454de1c61ea2d5a3ce84606.txt \n extracting: train/IMG_8550_jpg.rf.5329e7f0bff1950d76d08a098b7b425d.jpg \n extracting: train/IMG_8550_jpg.rf.5329e7f0bff1950d76d08a098b7b425d.txt \n extracting: train/IMG_8550_jpg.rf.af94a6aa1d1ce02d2e7e0cf737366355.jpg \n extracting: train/IMG_8550_jpg.rf.af94a6aa1d1ce02d2e7e0cf737366355.txt \n extracting: train/IMG_8551_MOV-0_jpg.rf.20d7ea4dff6526bc69453fa7b00df89e.jpg \n extracting: train/IMG_8551_MOV-0_jpg.rf.20d7ea4dff6526bc69453fa7b00df89e.txt \n extracting: train/IMG_8551_MOV-0_jpg.rf.359397e81527dc1980b91b8949f17458.jpg \n extracting: train/IMG_8551_MOV-0_jpg.rf.359397e81527dc1980b91b8949f17458.txt \n extracting: train/IMG_8551_MOV-0_jpg.rf.bb1c2164769c34b6622535d9c89d4957.jpg \n extracting: train/IMG_8551_MOV-0_jpg.rf.bb1c2164769c34b6622535d9c89d4957.txt \n extracting: train/IMG_8551_MOV-2_jpg.rf.172347f1c28e708daaa71eb9c5caa60d.jpg \n extracting: train/IMG_8551_MOV-2_jpg.rf.172347f1c28e708daaa71eb9c5caa60d.txt \n extracting: train/IMG_8551_MOV-2_jpg.rf.398ff79ec779129350355b065e60f331.jpg \n extracting: train/IMG_8551_MOV-2_jpg.rf.398ff79ec779129350355b065e60f331.txt \n extracting: train/IMG_8551_MOV-2_jpg.rf.47468901bb407bfe2bc967b6b88a72cb.jpg \n extracting: train/IMG_8551_MOV-2_jpg.rf.47468901bb407bfe2bc967b6b88a72cb.txt \n extracting: train/IMG_8551_MOV-3_jpg.rf.02f98e2adf6df3f0a614bf2fd36f54c0.jpg \n extracting: train/IMG_8551_MOV-3_jpg.rf.02f98e2adf6df3f0a614bf2fd36f54c0.txt \n extracting: train/IMG_8551_MOV-3_jpg.rf.6b67b866a1db45a6cc346b2bd7e8a9a4.jpg \n extracting: train/IMG_8551_MOV-3_jpg.rf.6b67b866a1db45a6cc346b2bd7e8a9a4.txt \n extracting: train/IMG_8551_MOV-3_jpg.rf.746071e3810bc8fe8a275103e5819d3a.jpg \n extracting: train/IMG_8551_MOV-3_jpg.rf.746071e3810bc8fe8a275103e5819d3a.txt \n extracting: train/IMG_8551_MOV-4_jpg.rf.167b05a9930d09d2a42c8121d50fa398.jpg \n extracting: train/IMG_8551_MOV-4_jpg.rf.167b05a9930d09d2a42c8121d50fa398.txt \n extracting: train/IMG_8551_MOV-4_jpg.rf.4a742d7dad5c93d1f87040c6efa19c17.jpg \n extracting: train/IMG_8551_MOV-4_jpg.rf.4a742d7dad5c93d1f87040c6efa19c17.txt \n extracting: train/IMG_8551_MOV-4_jpg.rf.f9818896ba91d40bfbd6c61f92cd301b.jpg \n extracting: train/IMG_8551_MOV-4_jpg.rf.f9818896ba91d40bfbd6c61f92cd301b.txt \n extracting: train/IMG_8552_jpg.rf.9a703924b9da41ff0e3286ffc9bede93.jpg \n extracting: train/IMG_8552_jpg.rf.9a703924b9da41ff0e3286ffc9bede93.txt \n extracting: train/IMG_8552_jpg.rf.bbe9e9ff14d3e085c277a16febc3e8c8.jpg \n extracting: train/IMG_8552_jpg.rf.bbe9e9ff14d3e085c277a16febc3e8c8.txt \n extracting: train/IMG_8552_jpg.rf.f17d5f2bc20104a26ae9a5a64db9967f.jpg \n extracting: train/IMG_8552_jpg.rf.f17d5f2bc20104a26ae9a5a64db9967f.txt \n extracting: train/IMG_8558_jpg.rf.33179da7e967c8e6745d55c612cd1452.jpg \n extracting: train/IMG_8558_jpg.rf.33179da7e967c8e6745d55c612cd1452.txt \n extracting: train/IMG_8558_jpg.rf.5dae6d3703508cd9d8ad147611cf9de4.jpg \n extracting: train/IMG_8558_jpg.rf.5dae6d3703508cd9d8ad147611cf9de4.txt \n extracting: train/IMG_8558_jpg.rf.a41baca8e53fd0f384ff41d49a0ddd6d.jpg \n extracting: train/IMG_8558_jpg.rf.a41baca8e53fd0f384ff41d49a0ddd6d.txt \n extracting: train/IMG_8560_jpg.rf.01637b18adec67bea5307969a8442537.jpg \n extracting: train/IMG_8560_jpg.rf.01637b18adec67bea5307969a8442537.txt \n extracting: train/IMG_8560_jpg.rf.365a3de5d167e7ccf53f8587afbd487b.jpg \n extracting: train/IMG_8560_jpg.rf.365a3de5d167e7ccf53f8587afbd487b.txt \n extracting: train/IMG_8560_jpg.rf.6ff71bdaba2f2c4ccf4537898c2ab708.jpg \n extracting: train/IMG_8560_jpg.rf.6ff71bdaba2f2c4ccf4537898c2ab708.txt \n extracting: train/IMG_8570_jpg.rf.005340591de3c43ed02b47df330b590c.jpg \n extracting: train/IMG_8570_jpg.rf.005340591de3c43ed02b47df330b590c.txt \n extracting: train/IMG_8570_jpg.rf.34c93201389c7680aa70d86a894034d2.jpg \n extracting: train/IMG_8570_jpg.rf.34c93201389c7680aa70d86a894034d2.txt \n extracting: train/IMG_8570_jpg.rf.f71d169b92c9dc95c23ea594ea8fcc0c.jpg \n extracting: train/IMG_8570_jpg.rf.f71d169b92c9dc95c23ea594ea8fcc0c.txt \n extracting: train/IMG_8571_MOV-0_jpg.rf.28aafe26b07f2d3fe0f5a12edf57f3ec.jpg \n extracting: train/IMG_8571_MOV-0_jpg.rf.28aafe26b07f2d3fe0f5a12edf57f3ec.txt \n extracting: train/IMG_8571_MOV-0_jpg.rf.9dd748319e1b550e26747a823bc87e0d.jpg \n extracting: train/IMG_8571_MOV-0_jpg.rf.9dd748319e1b550e26747a823bc87e0d.txt \n extracting: train/IMG_8571_MOV-0_jpg.rf.bbb9a83cd96f78b362073c4f2f9571b7.jpg \n extracting: train/IMG_8571_MOV-0_jpg.rf.bbb9a83cd96f78b362073c4f2f9571b7.txt \n extracting: train/IMG_8571_MOV-1_jpg.rf.53b0e4b801c81dbbeede848a79e487b9.jpg \n extracting: train/IMG_8571_MOV-1_jpg.rf.53b0e4b801c81dbbeede848a79e487b9.txt \n extracting: train/IMG_8571_MOV-1_jpg.rf.9809b35cce215a64a33c2e39dc67218a.jpg \n extracting: train/IMG_8571_MOV-1_jpg.rf.9809b35cce215a64a33c2e39dc67218a.txt \n extracting: train/IMG_8571_MOV-1_jpg.rf.d2108e009979ea3a3538acea36ca6e9d.jpg \n extracting: train/IMG_8571_MOV-1_jpg.rf.d2108e009979ea3a3538acea36ca6e9d.txt \n extracting: train/IMG_8571_MOV-2_jpg.rf.30e78b14558cf5ea55e95270e1a79158.jpg \n extracting: train/IMG_8571_MOV-2_jpg.rf.30e78b14558cf5ea55e95270e1a79158.txt \n extracting: train/IMG_8571_MOV-2_jpg.rf.4a6d1a6dad1e27c92784c41eda37e42f.jpg \n extracting: train/IMG_8571_MOV-2_jpg.rf.4a6d1a6dad1e27c92784c41eda37e42f.txt \n extracting: train/IMG_8571_MOV-2_jpg.rf.71ce34d66f608036272fb69462d42d27.jpg \n extracting: train/IMG_8571_MOV-2_jpg.rf.71ce34d66f608036272fb69462d42d27.txt \n extracting: train/IMG_8571_MOV-4_jpg.rf.2d598588957db570fedede46d6d6fa20.jpg \n extracting: train/IMG_8571_MOV-4_jpg.rf.2d598588957db570fedede46d6d6fa20.txt \n extracting: train/IMG_8571_MOV-4_jpg.rf.3f8791812c491ee535df88d4ff963fb8.jpg \n extracting: train/IMG_8571_MOV-4_jpg.rf.3f8791812c491ee535df88d4ff963fb8.txt \n extracting: train/IMG_8571_MOV-4_jpg.rf.a5f2bf4d374491d7dc512f7f6f9a8f93.jpg \n extracting: train/IMG_8571_MOV-4_jpg.rf.a5f2bf4d374491d7dc512f7f6f9a8f93.txt \n extracting: train/IMG_8571_MOV-5_jpg.rf.2ba955c8c398886bbcd03ebfcf8d3de6.jpg \n extracting: train/IMG_8571_MOV-5_jpg.rf.2ba955c8c398886bbcd03ebfcf8d3de6.txt \n extracting: train/IMG_8571_MOV-5_jpg.rf.7a8607b8a1f3334b162de95840b11b5f.jpg \n extracting: train/IMG_8571_MOV-5_jpg.rf.7a8607b8a1f3334b162de95840b11b5f.txt \n extracting: train/IMG_8571_MOV-5_jpg.rf.7fa178896a4a243837b66187eacc64c7.jpg \n extracting: train/IMG_8571_MOV-5_jpg.rf.7fa178896a4a243837b66187eacc64c7.txt \n extracting: train/IMG_8572_MOV-0_jpg.rf.9f4a03057e72f0f0fb2b66f8cca6cf3f.jpg \n extracting: train/IMG_8572_MOV-0_jpg.rf.9f4a03057e72f0f0fb2b66f8cca6cf3f.txt \n extracting: train/IMG_8572_MOV-0_jpg.rf.b7baf5a0eceee8da9ed55cf8d0123495.jpg \n extracting: train/IMG_8572_MOV-0_jpg.rf.b7baf5a0eceee8da9ed55cf8d0123495.txt \n extracting: train/IMG_8572_MOV-0_jpg.rf.e448cbb234f7e228533cba65a03785fa.jpg \n extracting: train/IMG_8572_MOV-0_jpg.rf.e448cbb234f7e228533cba65a03785fa.txt \n extracting: train/IMG_8572_MOV-2_jpg.rf.4c1bccfae5abf051758093c47548a33d.jpg \n extracting: train/IMG_8572_MOV-2_jpg.rf.4c1bccfae5abf051758093c47548a33d.txt \n extracting: train/IMG_8572_MOV-2_jpg.rf.9dc50f69d706db1ab6a4403f1c029819.jpg \n extracting: train/IMG_8572_MOV-2_jpg.rf.9dc50f69d706db1ab6a4403f1c029819.txt \n extracting: train/IMG_8572_MOV-2_jpg.rf.f7ff715d772af87dc6a5c5e401422642.jpg \n extracting: train/IMG_8572_MOV-2_jpg.rf.f7ff715d772af87dc6a5c5e401422642.txt \n extracting: train/IMG_8575_jpg.rf.0630feeb797dd45205bc5b196416e6d5.jpg \n extracting: train/IMG_8575_jpg.rf.0630feeb797dd45205bc5b196416e6d5.txt \n extracting: train/IMG_8575_jpg.rf.448a3bbca881630aa602d932bc958717.jpg \n extracting: train/IMG_8575_jpg.rf.448a3bbca881630aa602d932bc958717.txt \n extracting: train/IMG_8575_jpg.rf.54feddc4616aafcf9cf08a239ad572b5.jpg \n extracting: train/IMG_8575_jpg.rf.54feddc4616aafcf9cf08a239ad572b5.txt \n extracting: train/IMG_8578_MOV-1_jpg.rf.283b68946a6456f39a2dd095038bb61d.jpg \n extracting: train/IMG_8578_MOV-1_jpg.rf.283b68946a6456f39a2dd095038bb61d.txt \n extracting: train/IMG_8578_MOV-1_jpg.rf.77cf5a793058efb60938f7e70c0b91a0.jpg \n extracting: train/IMG_8578_MOV-1_jpg.rf.77cf5a793058efb60938f7e70c0b91a0.txt \n extracting: train/IMG_8578_MOV-1_jpg.rf.c281b3406a0c09bad9d6b7c98362300c.jpg \n extracting: train/IMG_8578_MOV-1_jpg.rf.c281b3406a0c09bad9d6b7c98362300c.txt \n extracting: train/IMG_8578_MOV-2_jpg.rf.3ba278275f8a1f6bb4a7fa9364308ee2.jpg \n extracting: train/IMG_8578_MOV-2_jpg.rf.3ba278275f8a1f6bb4a7fa9364308ee2.txt \n extracting: train/IMG_8578_MOV-2_jpg.rf.53be54d84b70ad462b5d6a0299208369.jpg \n extracting: train/IMG_8578_MOV-2_jpg.rf.53be54d84b70ad462b5d6a0299208369.txt \n extracting: train/IMG_8578_MOV-2_jpg.rf.e7fa849482f666a041624709dbebc4d1.jpg \n extracting: train/IMG_8578_MOV-2_jpg.rf.e7fa849482f666a041624709dbebc4d1.txt \n extracting: train/IMG_8580_jpg.rf.289cfa4f7c6fb8f55ee99736a3e6f2ce.jpg \n extracting: train/IMG_8580_jpg.rf.289cfa4f7c6fb8f55ee99736a3e6f2ce.txt \n extracting: train/IMG_8580_jpg.rf.4a0420b3b6cddd9c5327a75825b05bed.jpg \n extracting: train/IMG_8580_jpg.rf.4a0420b3b6cddd9c5327a75825b05bed.txt \n extracting: train/IMG_8580_jpg.rf.7bbaebe753fd94d97bf701409b7b8ed7.jpg \n extracting: train/IMG_8580_jpg.rf.7bbaebe753fd94d97bf701409b7b8ed7.txt \n extracting: train/IMG_8581_MOV-0_jpg.rf.129d8ed3a3a35fef2009f7f89a5c4b98.jpg \n extracting: train/IMG_8581_MOV-0_jpg.rf.129d8ed3a3a35fef2009f7f89a5c4b98.txt \n extracting: train/IMG_8581_MOV-0_jpg.rf.545f574eef385a7e54b23c874daf31ae.jpg \n extracting: train/IMG_8581_MOV-0_jpg.rf.545f574eef385a7e54b23c874daf31ae.txt \n extracting: train/IMG_8581_MOV-0_jpg.rf.fea8589f0f9088ea5221232c487b77b0.jpg \n extracting: train/IMG_8581_MOV-0_jpg.rf.fea8589f0f9088ea5221232c487b77b0.txt \n extracting: train/IMG_8581_MOV-2_jpg.rf.43bce859bdc26e5b448b6feab11a6495.jpg \n extracting: train/IMG_8581_MOV-2_jpg.rf.43bce859bdc26e5b448b6feab11a6495.txt \n extracting: train/IMG_8581_MOV-2_jpg.rf.9007a1deaa3441df8c9706f0fc41f23e.jpg \n extracting: train/IMG_8581_MOV-2_jpg.rf.9007a1deaa3441df8c9706f0fc41f23e.txt \n extracting: train/IMG_8581_MOV-2_jpg.rf.a8a0937f951739641bcedc58ca8a5f6a.jpg \n extracting: train/IMG_8581_MOV-2_jpg.rf.a8a0937f951739641bcedc58ca8a5f6a.txt \n extracting: train/IMG_8581_MOV-3_jpg.rf.a328cf0bd2c636c033fb21e40315790f.jpg \n extracting: train/IMG_8581_MOV-3_jpg.rf.a328cf0bd2c636c033fb21e40315790f.txt \n extracting: train/IMG_8581_MOV-3_jpg.rf.e2f13027a18e45f2c2da15a0697def70.jpg \n extracting: train/IMG_8581_MOV-3_jpg.rf.e2f13027a18e45f2c2da15a0697def70.txt \n extracting: train/IMG_8581_MOV-3_jpg.rf.fcf7c6285f1a77062264f334092dc910.jpg \n extracting: train/IMG_8581_MOV-3_jpg.rf.fcf7c6285f1a77062264f334092dc910.txt \n extracting: train/IMG_8581_MOV-4_jpg.rf.054d749557da469be4f3f465fccfced9.jpg \n extracting: train/IMG_8581_MOV-4_jpg.rf.054d749557da469be4f3f465fccfced9.txt \n extracting: train/IMG_8581_MOV-4_jpg.rf.4d2870002ab9dd528671033ca11779da.jpg \n extracting: train/IMG_8581_MOV-4_jpg.rf.4d2870002ab9dd528671033ca11779da.txt \n extracting: train/IMG_8581_MOV-4_jpg.rf.f2640e19eb49f5807d8b4a94407159aa.jpg \n extracting: train/IMG_8581_MOV-4_jpg.rf.f2640e19eb49f5807d8b4a94407159aa.txt \n extracting: train/IMG_8582_MOV-1_jpg.rf.45fd5ae52299f16d0a4343548af8a1bb.jpg \n extracting: train/IMG_8582_MOV-1_jpg.rf.45fd5ae52299f16d0a4343548af8a1bb.txt \n extracting: train/IMG_8582_MOV-1_jpg.rf.bc10fc873aae21d4e41d3b9fcbdb1e9a.jpg \n extracting: train/IMG_8582_MOV-1_jpg.rf.bc10fc873aae21d4e41d3b9fcbdb1e9a.txt \n extracting: train/IMG_8582_MOV-1_jpg.rf.be036d4e9b47f3f9fa3d9247c44aa5cb.jpg \n extracting: train/IMG_8582_MOV-1_jpg.rf.be036d4e9b47f3f9fa3d9247c44aa5cb.txt \n extracting: train/IMG_8590_MOV-1_jpg.rf.01aebc589daff5060cf2df7b1490c242.jpg \n extracting: train/IMG_8590_MOV-1_jpg.rf.01aebc589daff5060cf2df7b1490c242.txt \n extracting: train/IMG_8590_MOV-1_jpg.rf.3a86bbd46df3978a1245b74c7141b43d.jpg \n extracting: train/IMG_8590_MOV-1_jpg.rf.3a86bbd46df3978a1245b74c7141b43d.txt \n extracting: train/IMG_8590_MOV-1_jpg.rf.9783db4948efbd600acd6e93899066a8.jpg \n extracting: train/IMG_8590_MOV-1_jpg.rf.9783db4948efbd600acd6e93899066a8.txt \n extracting: train/IMG_8590_MOV-3_jpg.rf.53ae72001d3710e691798354dbec7b30.jpg \n extracting: train/IMG_8590_MOV-3_jpg.rf.53ae72001d3710e691798354dbec7b30.txt \n extracting: train/IMG_8590_MOV-3_jpg.rf.ee5ec09599e9d442c332ba7edc3670e9.jpg \n extracting: train/IMG_8590_MOV-3_jpg.rf.ee5ec09599e9d442c332ba7edc3670e9.txt \n extracting: train/IMG_8590_MOV-3_jpg.rf.f1c955c2f727e7d638c18dc78212e9d7.jpg \n extracting: train/IMG_8590_MOV-3_jpg.rf.f1c955c2f727e7d638c18dc78212e9d7.txt \n extracting: train/IMG_8590_MOV-4_jpg.rf.0fc02d152783ced4b16d837b90b46085.jpg \n extracting: train/IMG_8590_MOV-4_jpg.rf.0fc02d152783ced4b16d837b90b46085.txt \n extracting: train/IMG_8590_MOV-4_jpg.rf.1616669d18a1361d3633444bb73f0e5b.jpg \n extracting: train/IMG_8590_MOV-4_jpg.rf.1616669d18a1361d3633444bb73f0e5b.txt \n extracting: train/IMG_8590_MOV-4_jpg.rf.bf9314e678194a41d8d5dffd8ba0ee70.jpg \n extracting: train/IMG_8590_MOV-4_jpg.rf.bf9314e678194a41d8d5dffd8ba0ee70.txt \n extracting: train/IMG_8591_MOV-0_jpg.rf.6ca9e5a7edbb7e1a26209a1dac229bbc.jpg \n extracting: train/IMG_8591_MOV-0_jpg.rf.6ca9e5a7edbb7e1a26209a1dac229bbc.txt \n extracting: train/IMG_8591_MOV-0_jpg.rf.894a8a694a330b494587a77d8d50e424.jpg \n extracting: train/IMG_8591_MOV-0_jpg.rf.894a8a694a330b494587a77d8d50e424.txt \n extracting: train/IMG_8591_MOV-0_jpg.rf.9e32677afb5885b46f610c57130ccb9e.jpg \n extracting: train/IMG_8591_MOV-0_jpg.rf.9e32677afb5885b46f610c57130ccb9e.txt \n extracting: train/IMG_8591_MOV-2_jpg.rf.052f462860a6d767f3e75c2b7324a157.jpg \n extracting: train/IMG_8591_MOV-2_jpg.rf.052f462860a6d767f3e75c2b7324a157.txt \n extracting: train/IMG_8591_MOV-2_jpg.rf.28ee27b7542eb9f03caae04d98477b93.jpg \n extracting: train/IMG_8591_MOV-2_jpg.rf.28ee27b7542eb9f03caae04d98477b93.txt \n extracting: train/IMG_8591_MOV-2_jpg.rf.52ca5e69dd7a678964d901d22452387c.jpg \n extracting: train/IMG_8591_MOV-2_jpg.rf.52ca5e69dd7a678964d901d22452387c.txt \n extracting: train/IMG_8593_MOV-0_jpg.rf.0186f7df90ff577f85ae9300fdcffecd.jpg \n extracting: train/IMG_8593_MOV-0_jpg.rf.0186f7df90ff577f85ae9300fdcffecd.txt \n extracting: train/IMG_8593_MOV-0_jpg.rf.5ddc727fb2b5b33ca8effc83215e89a7.jpg \n extracting: train/IMG_8593_MOV-0_jpg.rf.5ddc727fb2b5b33ca8effc83215e89a7.txt \n extracting: train/IMG_8593_MOV-0_jpg.rf.725b2171e5dfdc7e1d3c9642de428172.jpg \n extracting: train/IMG_8593_MOV-0_jpg.rf.725b2171e5dfdc7e1d3c9642de428172.txt \n extracting: train/IMG_8593_MOV-2_jpg.rf.211d4ede4ca9deda6859eda7dbd737df.jpg \n extracting: train/IMG_8593_MOV-2_jpg.rf.211d4ede4ca9deda6859eda7dbd737df.txt \n extracting: train/IMG_8593_MOV-2_jpg.rf.4396cef1d3e1901320559967eb825326.jpg \n extracting: train/IMG_8593_MOV-2_jpg.rf.4396cef1d3e1901320559967eb825326.txt \n extracting: train/IMG_8593_MOV-2_jpg.rf.9678b899bc6b2dc59ccc0daa0749b4c8.jpg \n extracting: train/IMG_8593_MOV-2_jpg.rf.9678b899bc6b2dc59ccc0daa0749b4c8.txt \n extracting: train/IMG_8594_jpg.rf.084c50e5e3feba2170bff027e8574239.jpg \n extracting: train/IMG_8594_jpg.rf.084c50e5e3feba2170bff027e8574239.txt \n extracting: train/IMG_8594_jpg.rf.37c55751045573b49546b276e23d7ea1.jpg \n extracting: train/IMG_8594_jpg.rf.37c55751045573b49546b276e23d7ea1.txt \n extracting: train/IMG_8594_jpg.rf.3a90a25de381647d317c9fe8d8fda0c4.jpg \n extracting: train/IMG_8594_jpg.rf.3a90a25de381647d317c9fe8d8fda0c4.txt \n extracting: train/IMG_8595_MOV-2_jpg.rf.5353519c1b99b84ed687e24f9964d39d.jpg \n extracting: train/IMG_8595_MOV-2_jpg.rf.5353519c1b99b84ed687e24f9964d39d.txt \n extracting: train/IMG_8595_MOV-2_jpg.rf.568fe162f6e5e592405ae35a0de06bfc.jpg \n extracting: train/IMG_8595_MOV-2_jpg.rf.568fe162f6e5e592405ae35a0de06bfc.txt \n extracting: train/IMG_8595_MOV-2_jpg.rf.c56e28390b48508943267520fa3c44f0.jpg \n extracting: train/IMG_8595_MOV-2_jpg.rf.c56e28390b48508943267520fa3c44f0.txt \n extracting: train/IMG_8598_jpg.rf.1144be096a157d28e59e137f49cb7b4f.jpg \n extracting: train/IMG_8598_jpg.rf.1144be096a157d28e59e137f49cb7b4f.txt \n extracting: train/IMG_8598_jpg.rf.dc1dd615acbf3c8339035bc5620aa96e.jpg \n extracting: train/IMG_8598_jpg.rf.dc1dd615acbf3c8339035bc5620aa96e.txt \n extracting: train/IMG_8598_jpg.rf.ec9f4dc93db9d90c9d3fd3a7ffefe499.jpg \n extracting: train/IMG_8598_jpg.rf.ec9f4dc93db9d90c9d3fd3a7ffefe499.txt \n extracting: train/IMG_8599_MOV-0_jpg.rf.2b676d88b647f44d89369c73e3733259.jpg \n extracting: train/IMG_8599_MOV-0_jpg.rf.2b676d88b647f44d89369c73e3733259.txt \n extracting: train/IMG_8599_MOV-0_jpg.rf.4238ee874021c746d192c98a6c96f551.jpg \n extracting: train/IMG_8599_MOV-0_jpg.rf.4238ee874021c746d192c98a6c96f551.txt \n extracting: train/IMG_8599_MOV-0_jpg.rf.702e1d56f28e1c61146cd78cae4d7979.jpg \n extracting: train/IMG_8599_MOV-0_jpg.rf.702e1d56f28e1c61146cd78cae4d7979.txt \n extracting: train/IMG_8599_MOV-2_jpg.rf.2aacb3bea13051cc451243524f5d357c.jpg \n extracting: train/IMG_8599_MOV-2_jpg.rf.2aacb3bea13051cc451243524f5d357c.txt \n extracting: train/IMG_8599_MOV-2_jpg.rf.2fa436489919f81dfffeea86450dc701.jpg \n extracting: train/IMG_8599_MOV-2_jpg.rf.2fa436489919f81dfffeea86450dc701.txt \n extracting: train/IMG_8599_MOV-2_jpg.rf.f6131df0692dc46f5fca32bde2329b60.jpg \n extracting: train/IMG_8599_MOV-2_jpg.rf.f6131df0692dc46f5fca32bde2329b60.txt \n extracting: train/_darknet.labels \n creating: valid/\n extracting: valid/IMG_2277_jpeg_jpg.rf.1b5d4bf14881028741165c8e7a8c43d4.jpg \n extracting: valid/IMG_2277_jpeg_jpg.rf.1b5d4bf14881028741165c8e7a8c43d4.txt \n extracting: valid/IMG_2278_jpeg_jpg.rf.4798a3172991b55238917f8396d31497.jpg \n extracting: valid/IMG_2278_jpeg_jpg.rf.4798a3172991b55238917f8396d31497.txt \n extracting: valid/IMG_2279_jpeg_jpg.rf.dad1b69c1184bb78f39aac5a9528efda.jpg \n extracting: valid/IMG_2279_jpeg_jpg.rf.dad1b69c1184bb78f39aac5a9528efda.txt \n extracting: valid/IMG_2288_jpeg_jpg.rf.ec108f0d4b0198e50cd40d8723d8ee43.jpg \n extracting: valid/IMG_2288_jpeg_jpg.rf.ec108f0d4b0198e50cd40d8723d8ee43.txt \n extracting: valid/IMG_2297_jpeg_jpg.rf.15ed6bc760f38bf2ac8f673c9a32f587.jpg \n extracting: valid/IMG_2297_jpeg_jpg.rf.15ed6bc760f38bf2ac8f673c9a32f587.txt \n extracting: valid/IMG_2305_jpeg_jpg.rf.1531a05c1e1dc9f561891d0b3915ffbc.jpg \n extracting: valid/IMG_2305_jpeg_jpg.rf.1531a05c1e1dc9f561891d0b3915ffbc.txt \n extracting: valid/IMG_2317_jpeg_jpg.rf.c672fbcc276281d1d808fc06e2072df4.jpg \n extracting: valid/IMG_2317_jpeg_jpg.rf.c672fbcc276281d1d808fc06e2072df4.txt \n extracting: valid/IMG_2318_jpeg_jpg.rf.ab7d30f75340252d5dfbb6a4589340eb.jpg \n extracting: valid/IMG_2318_jpeg_jpg.rf.ab7d30f75340252d5dfbb6a4589340eb.txt \n extracting: valid/IMG_2323_jpeg_jpg.rf.c10fa87ea1be454615d883783f82d27a.jpg \n extracting: valid/IMG_2323_jpeg_jpg.rf.c10fa87ea1be454615d883783f82d27a.txt \n extracting: valid/IMG_2325_jpeg_jpg.rf.bc4f8d7a043fba9c5900729a520d1536.jpg \n extracting: valid/IMG_2325_jpeg_jpg.rf.bc4f8d7a043fba9c5900729a520d1536.txt \n extracting: valid/IMG_2329_jpeg_jpg.rf.79ea05e99700a5e71cf34eb056c4921c.jpg \n extracting: valid/IMG_2329_jpeg_jpg.rf.79ea05e99700a5e71cf34eb056c4921c.txt \n extracting: valid/IMG_2333_jpeg_jpg.rf.608dac72915e1d7de0ef6627c59edd50.jpg \n extracting: valid/IMG_2333_jpeg_jpg.rf.608dac72915e1d7de0ef6627c59edd50.txt \n extracting: valid/IMG_2334_jpeg_jpg.rf.320191565e26feef4045b4e39a3a6b0e.jpg \n extracting: valid/IMG_2334_jpeg_jpg.rf.320191565e26feef4045b4e39a3a6b0e.txt \n extracting: valid/IMG_2337_jpeg_jpg.rf.ef21a8bb0cc9464cc164c12cf79b79f5.jpg \n extracting: valid/IMG_2337_jpeg_jpg.rf.ef21a8bb0cc9464cc164c12cf79b79f5.txt \n extracting: valid/IMG_2339_jpeg_jpg.rf.b6fe02a326451ed9bdf6ef3060fd656f.jpg \n extracting: valid/IMG_2339_jpeg_jpg.rf.b6fe02a326451ed9bdf6ef3060fd656f.txt \n extracting: valid/IMG_2342_jpeg_jpg.rf.3254c4c1240d9f8e90013e45a7b62335.jpg \n extracting: valid/IMG_2342_jpeg_jpg.rf.3254c4c1240d9f8e90013e45a7b62335.txt \n extracting: valid/IMG_2345_jpeg_jpg.rf.8dfd63a49bcab35abad309ea8d48af0a.jpg \n extracting: valid/IMG_2345_jpeg_jpg.rf.8dfd63a49bcab35abad309ea8d48af0a.txt \n extracting: valid/IMG_2348_jpeg_jpg.rf.a9a15e6c811b0d5600513ee001f95edf.jpg \n extracting: valid/IMG_2348_jpeg_jpg.rf.a9a15e6c811b0d5600513ee001f95edf.txt \n extracting: valid/IMG_2353_jpeg_jpg.rf.39106b803df0b1262147ef6f46c47499.jpg \n extracting: valid/IMG_2353_jpeg_jpg.rf.39106b803df0b1262147ef6f46c47499.txt \n extracting: valid/IMG_2366_jpeg_jpg.rf.1d1029e5d8b5a620af97d0729488afb2.jpg \n extracting: valid/IMG_2366_jpeg_jpg.rf.1d1029e5d8b5a620af97d0729488afb2.txt \n extracting: valid/IMG_2367_jpeg_jpg.rf.6b46f0bfcc8c53ef73bd1c06afef7eea.jpg \n extracting: valid/IMG_2367_jpeg_jpg.rf.6b46f0bfcc8c53ef73bd1c06afef7eea.txt \n extracting: valid/IMG_2381_jpeg_jpg.rf.81a8bbf2ec855f2ea452184dcdd96bac.jpg \n extracting: valid/IMG_2381_jpeg_jpg.rf.81a8bbf2ec855f2ea452184dcdd96bac.txt \n extracting: valid/IMG_2388_jpeg_jpg.rf.9db43991c24dd209bcc80ed5e8b05d96.jpg \n extracting: valid/IMG_2388_jpeg_jpg.rf.9db43991c24dd209bcc80ed5e8b05d96.txt \n extracting: valid/IMG_2391_jpeg_jpg.rf.cdf0c250ef5b031c8453ce19eeb28e23.jpg \n extracting: valid/IMG_2391_jpeg_jpg.rf.cdf0c250ef5b031c8453ce19eeb28e23.txt \n extracting: valid/IMG_2392_jpeg_jpg.rf.b53191793df489316e18f5b6100c4ebd.jpg \n extracting: valid/IMG_2392_jpeg_jpg.rf.b53191793df489316e18f5b6100c4ebd.txt \n extracting: valid/IMG_2396_jpeg_jpg.rf.ecd31098e1e6b287a7dbcf99c113e043.jpg \n extracting: valid/IMG_2396_jpeg_jpg.rf.ecd31098e1e6b287a7dbcf99c113e043.txt \n extracting: valid/IMG_2397_jpeg_jpg.rf.18cff3e255e428d06e7c24daa4524dc3.jpg \n extracting: valid/IMG_2397_jpeg_jpg.rf.18cff3e255e428d06e7c24daa4524dc3.txt \n extracting: valid/IMG_2398_jpeg_jpg.rf.b12bc8cddc72a86619526d39a5cb4bca.jpg \n extracting: valid/IMG_2398_jpeg_jpg.rf.b12bc8cddc72a86619526d39a5cb4bca.txt \n extracting: valid/IMG_2404_jpeg_jpg.rf.c09454456f39563e7ea222f890a2208c.jpg \n extracting: valid/IMG_2404_jpeg_jpg.rf.c09454456f39563e7ea222f890a2208c.txt \n extracting: valid/IMG_2407_jpeg_jpg.rf.ad2ff862fbf490b23cf151c3c5958002.jpg \n extracting: valid/IMG_2407_jpeg_jpg.rf.ad2ff862fbf490b23cf151c3c5958002.txt \n extracting: valid/IMG_2411_jpeg_jpg.rf.98e3a8061d5bf3d44c1c7efbc5bc8594.jpg \n extracting: valid/IMG_2411_jpeg_jpg.rf.98e3a8061d5bf3d44c1c7efbc5bc8594.txt \n extracting: valid/IMG_2416_jpeg_jpg.rf.d57f24a28b4f9095c844f011cfcef6c6.jpg \n extracting: valid/IMG_2416_jpeg_jpg.rf.d57f24a28b4f9095c844f011cfcef6c6.txt \n extracting: valid/IMG_2424_jpeg_jpg.rf.a22526e79be3d95a3d9735e71b303cc8.jpg \n extracting: valid/IMG_2424_jpeg_jpg.rf.a22526e79be3d95a3d9735e71b303cc8.txt \n extracting: valid/IMG_2425_jpeg_jpg.rf.30b34ff9225848dcc47dcd2177f03564.jpg \n extracting: valid/IMG_2425_jpeg_jpg.rf.30b34ff9225848dcc47dcd2177f03564.txt \n extracting: valid/IMG_2428_jpeg_jpg.rf.9d4e4183c9b5444d725427aa00898546.jpg \n extracting: valid/IMG_2428_jpeg_jpg.rf.9d4e4183c9b5444d725427aa00898546.txt \n extracting: valid/IMG_2442_jpeg_jpg.rf.d8adc6b539bab8411cc8c0fa2183078b.jpg \n extracting: valid/IMG_2442_jpeg_jpg.rf.d8adc6b539bab8411cc8c0fa2183078b.txt \n extracting: valid/IMG_2457_jpeg_jpg.rf.a83d89ea8e477d7953959af67f2e2559.jpg \n extracting: valid/IMG_2457_jpeg_jpg.rf.a83d89ea8e477d7953959af67f2e2559.txt \n extracting: valid/IMG_2464_jpeg_jpg.rf.1545d16bf00efd76abde59119a4c216d.jpg \n extracting: valid/IMG_2464_jpeg_jpg.rf.1545d16bf00efd76abde59119a4c216d.txt \n extracting: valid/IMG_2469_jpeg_jpg.rf.80e5277f60015c612d32fee70c886719.jpg \n extracting: valid/IMG_2469_jpeg_jpg.rf.80e5277f60015c612d32fee70c886719.txt \n extracting: valid/IMG_2491_jpeg_jpg.rf.7d4871bbddc1623373243819f1ebbbbd.jpg \n extracting: valid/IMG_2491_jpeg_jpg.rf.7d4871bbddc1623373243819f1ebbbbd.txt \n extracting: valid/IMG_2492_jpeg_jpg.rf.21bf129a8c2cfb0ee408bbc27f313012.jpg \n extracting: valid/IMG_2492_jpeg_jpg.rf.21bf129a8c2cfb0ee408bbc27f313012.txt \n extracting: valid/IMG_2505_jpeg_jpg.rf.c04c2ee6d4a041e4fffe49d207b0d601.jpg \n extracting: valid/IMG_2505_jpeg_jpg.rf.c04c2ee6d4a041e4fffe49d207b0d601.txt \n extracting: valid/IMG_2510_jpeg_jpg.rf.748957c2458cfcfa5252534cd756b43b.jpg \n extracting: valid/IMG_2510_jpeg_jpg.rf.748957c2458cfcfa5252534cd756b43b.txt \n extracting: valid/IMG_2512_jpeg_jpg.rf.180a98a86e10ac9d90287ddbdef81337.jpg \n extracting: valid/IMG_2512_jpeg_jpg.rf.180a98a86e10ac9d90287ddbdef81337.txt \n extracting: valid/IMG_2517_jpeg_jpg.rf.8ada0c681340c1b4b0b770e973ee71b5.jpg \n extracting: valid/IMG_2517_jpeg_jpg.rf.8ada0c681340c1b4b0b770e973ee71b5.txt \n extracting: valid/IMG_2519_jpeg_jpg.rf.cf16be14b8382e762f101ffe6e35f267.jpg \n extracting: valid/IMG_2519_jpeg_jpg.rf.cf16be14b8382e762f101ffe6e35f267.txt \n extracting: valid/IMG_2522_jpeg_jpg.rf.523855472c04a4bddfe95c99da3e1d40.jpg \n extracting: valid/IMG_2522_jpeg_jpg.rf.523855472c04a4bddfe95c99da3e1d40.txt \n extracting: valid/IMG_2523_jpeg_jpg.rf.7d19025e76e03ae36de985962260388d.jpg \n extracting: valid/IMG_2523_jpeg_jpg.rf.7d19025e76e03ae36de985962260388d.txt \n extracting: valid/IMG_2525_jpeg_jpg.rf.5c09d940410405afa25d1626f9935990.jpg \n extracting: valid/IMG_2525_jpeg_jpg.rf.5c09d940410405afa25d1626f9935990.txt \n extracting: valid/IMG_2531_jpeg_jpg.rf.995952052beef00092c2d521b1204bec.jpg \n extracting: valid/IMG_2531_jpeg_jpg.rf.995952052beef00092c2d521b1204bec.txt \n extracting: valid/IMG_2534_jpeg_jpg.rf.998d1ba19518d82f045c3b8b73be3d85.jpg \n extracting: valid/IMG_2534_jpeg_jpg.rf.998d1ba19518d82f045c3b8b73be3d85.txt \n extracting: valid/IMG_2535_jpeg_jpg.rf.d3ffd21ba8f54f914832442176d5545a.jpg \n extracting: valid/IMG_2535_jpeg_jpg.rf.d3ffd21ba8f54f914832442176d5545a.txt \n extracting: valid/IMG_2546_jpeg_jpg.rf.9c5ccd7cd8ab8d643f01b6af9fe67a68.jpg \n extracting: valid/IMG_2546_jpeg_jpg.rf.9c5ccd7cd8ab8d643f01b6af9fe67a68.txt \n extracting: valid/IMG_2555_jpeg_jpg.rf.399519b991a60ba7693ea9dd32bbb1a1.jpg \n extracting: valid/IMG_2555_jpeg_jpg.rf.399519b991a60ba7693ea9dd32bbb1a1.txt \n extracting: valid/IMG_2557_jpeg_jpg.rf.3a255525d3c13bb1753d969104e91db8.jpg \n extracting: valid/IMG_2557_jpeg_jpg.rf.3a255525d3c13bb1753d969104e91db8.txt \n extracting: valid/IMG_2567_jpeg_jpg.rf.fb5b4a778bf859cf72030f2cd5bb489d.jpg \n extracting: valid/IMG_2567_jpeg_jpg.rf.fb5b4a778bf859cf72030f2cd5bb489d.txt \n extracting: valid/IMG_2569_jpeg_jpg.rf.9a80774405c2aba635acc984fec1da9e.jpg \n extracting: valid/IMG_2569_jpeg_jpg.rf.9a80774405c2aba635acc984fec1da9e.txt \n extracting: valid/IMG_2577_jpeg_jpg.rf.4f5d6f2ff051276bec11cee09359a03c.jpg \n extracting: valid/IMG_2577_jpeg_jpg.rf.4f5d6f2ff051276bec11cee09359a03c.txt \n extracting: valid/IMG_2583_jpeg_jpg.rf.2085b49b628f962696d5bf8e6d2ed2ea.jpg \n extracting: valid/IMG_2583_jpeg_jpg.rf.2085b49b628f962696d5bf8e6d2ed2ea.txt \n extracting: valid/IMG_2586_jpeg_jpg.rf.2b030fe457d6f5d41eabbeee96c0df52.jpg \n extracting: valid/IMG_2586_jpeg_jpg.rf.2b030fe457d6f5d41eabbeee96c0df52.txt \n extracting: valid/IMG_2591_jpeg_jpg.rf.f8d8eca91c45589d2eafef2391c8bfb5.jpg \n extracting: valid/IMG_2591_jpeg_jpg.rf.f8d8eca91c45589d2eafef2391c8bfb5.txt \n extracting: valid/IMG_2599_jpeg_jpg.rf.db75a4788fbf7d622dd4170db2599878.jpg \n extracting: valid/IMG_2599_jpeg_jpg.rf.db75a4788fbf7d622dd4170db2599878.txt \n extracting: valid/IMG_2602_jpeg_jpg.rf.b21ef64690104e335c7919ebd66ee677.jpg \n extracting: valid/IMG_2602_jpeg_jpg.rf.b21ef64690104e335c7919ebd66ee677.txt \n extracting: valid/IMG_2607_jpeg_jpg.rf.8f3a65b0c29999ea75d933e53769ae2c.jpg \n extracting: valid/IMG_2607_jpeg_jpg.rf.8f3a65b0c29999ea75d933e53769ae2c.txt \n extracting: valid/IMG_2616_jpeg_jpg.rf.2f10f80161e40b8a961e1fad524160c5.jpg \n extracting: valid/IMG_2616_jpeg_jpg.rf.2f10f80161e40b8a961e1fad524160c5.txt \n extracting: valid/IMG_2626_jpeg_jpg.rf.0bd0bc54de133be6af9694384cff198a.jpg \n extracting: valid/IMG_2626_jpeg_jpg.rf.0bd0bc54de133be6af9694384cff198a.txt \n extracting: valid/IMG_2635_jpeg_jpg.rf.ae022ff09d1ea2ef05c62c5623490bca.jpg \n extracting: valid/IMG_2635_jpeg_jpg.rf.ae022ff09d1ea2ef05c62c5623490bca.txt \n extracting: valid/IMG_2636_jpeg_jpg.rf.de80f92d55acfaf5b0113240516c686c.jpg \n extracting: valid/IMG_2636_jpeg_jpg.rf.de80f92d55acfaf5b0113240516c686c.txt \n extracting: valid/IMG_2637_jpeg_jpg.rf.d2b19760831165b43429018cd882d9dd.jpg \n extracting: valid/IMG_2637_jpeg_jpg.rf.d2b19760831165b43429018cd882d9dd.txt \n extracting: valid/IMG_2645_jpeg_jpg.rf.35dc52a0314142a44c161255fe97afa1.jpg \n extracting: valid/IMG_2645_jpeg_jpg.rf.35dc52a0314142a44c161255fe97afa1.txt \n extracting: valid/IMG_3120_jpeg_jpg.rf.b1c0c98ff679434513333f63e0991634.jpg \n extracting: valid/IMG_3120_jpeg_jpg.rf.b1c0c98ff679434513333f63e0991634.txt \n extracting: valid/IMG_3124_jpeg_jpg.rf.4b6da01cda93ec852ff548a986cc83aa.jpg \n extracting: valid/IMG_3124_jpeg_jpg.rf.4b6da01cda93ec852ff548a986cc83aa.txt \n extracting: valid/IMG_3126_jpeg_jpg.rf.4888c560e7ed3c796a197ff38a22aaa3.jpg \n extracting: valid/IMG_3126_jpeg_jpg.rf.4888c560e7ed3c796a197ff38a22aaa3.txt \n extracting: valid/IMG_3139_jpeg_jpg.rf.7158f14aaa75ea617ba975963d360eed.jpg \n extracting: valid/IMG_3139_jpeg_jpg.rf.7158f14aaa75ea617ba975963d360eed.txt \n extracting: valid/IMG_3150_jpeg_jpg.rf.8950e7e753a90bb47054e15bfc05218a.jpg \n extracting: valid/IMG_3150_jpeg_jpg.rf.8950e7e753a90bb47054e15bfc05218a.txt \n extracting: valid/IMG_3153_jpeg_jpg.rf.51482ed358d2ae3b25c92806d7c9ee43.jpg \n extracting: valid/IMG_3153_jpeg_jpg.rf.51482ed358d2ae3b25c92806d7c9ee43.txt \n extracting: valid/IMG_3161_jpeg_jpg.rf.9113d0d07dba95954c9b228bbe967b59.jpg \n extracting: valid/IMG_3161_jpeg_jpg.rf.9113d0d07dba95954c9b228bbe967b59.txt \n extracting: valid/IMG_3163_jpeg_jpg.rf.51b21ef550d6647409a951043afa64dd.jpg \n extracting: valid/IMG_3163_jpeg_jpg.rf.51b21ef550d6647409a951043afa64dd.txt \n extracting: valid/IMG_3165_jpeg_jpg.rf.447510835df9a9bfe225d44a6678544a.jpg \n extracting: valid/IMG_3165_jpeg_jpg.rf.447510835df9a9bfe225d44a6678544a.txt \n extracting: valid/IMG_3166_jpeg_jpg.rf.d88acfb224673a6b470509b03a35d69e.jpg \n extracting: valid/IMG_3166_jpeg_jpg.rf.d88acfb224673a6b470509b03a35d69e.txt \n extracting: valid/IMG_3171_jpeg_jpg.rf.28b5b27032a6d3cab868bc73bb4f8d6d.jpg \n extracting: valid/IMG_3171_jpeg_jpg.rf.28b5b27032a6d3cab868bc73bb4f8d6d.txt \n extracting: valid/IMG_3178_jpeg_jpg.rf.30d22e2206cd0b6369778f54d4a47be8.jpg \n extracting: valid/IMG_3178_jpeg_jpg.rf.30d22e2206cd0b6369778f54d4a47be8.txt \n extracting: valid/IMG_3179_jpeg_jpg.rf.39d4c35fc0fbc300aaf83dc914cb248c.jpg \n extracting: valid/IMG_3179_jpeg_jpg.rf.39d4c35fc0fbc300aaf83dc914cb248c.txt \n extracting: valid/IMG_3180_jpeg_jpg.rf.5e18d15ce93e515396fe0079a6f345f4.jpg \n extracting: valid/IMG_3180_jpeg_jpg.rf.5e18d15ce93e515396fe0079a6f345f4.txt \n extracting: valid/IMG_3183_jpeg_jpg.rf.302f8510c9935108698fc4ddb5cc9411.jpg \n extracting: valid/IMG_3183_jpeg_jpg.rf.302f8510c9935108698fc4ddb5cc9411.txt \n extracting: valid/IMG_3184_jpeg_jpg.rf.465804dd74459466a70911e1540e3682.jpg \n extracting: valid/IMG_3184_jpeg_jpg.rf.465804dd74459466a70911e1540e3682.txt \n extracting: valid/IMG_3185_jpeg_jpg.rf.ef592559f0fbb0c291960043777e401e.jpg \n extracting: valid/IMG_3185_jpeg_jpg.rf.ef592559f0fbb0c291960043777e401e.txt \n extracting: valid/IMG_8317_jpg.rf.25e71987d72106adfca681631e974f73.jpg \n extracting: valid/IMG_8317_jpg.rf.25e71987d72106adfca681631e974f73.txt \n extracting: valid/IMG_8330_jpg.rf.7ddbaf75614d1acbed91dea6b78407cb.jpg \n extracting: valid/IMG_8330_jpg.rf.7ddbaf75614d1acbed91dea6b78407cb.txt \n extracting: valid/IMG_8340_jpg.rf.34a088c7b7661c7de22ca3c1a105cccc.jpg \n extracting: valid/IMG_8340_jpg.rf.34a088c7b7661c7de22ca3c1a105cccc.txt \n extracting: valid/IMG_8341_jpg.rf.f5349b4d935c7b2658444d8f99b8d065.jpg \n extracting: valid/IMG_8341_jpg.rf.f5349b4d935c7b2658444d8f99b8d065.txt \n extracting: valid/IMG_8348_jpg.rf.b0dfbc8de53b087ce71c8285e3f87998.jpg \n extracting: valid/IMG_8348_jpg.rf.b0dfbc8de53b087ce71c8285e3f87998.txt \n extracting: valid/IMG_8379_jpg.rf.3c95ef0bd9dd723d53d8669c6245b0e6.jpg \n extracting: valid/IMG_8379_jpg.rf.3c95ef0bd9dd723d53d8669c6245b0e6.txt \n extracting: valid/IMG_8384_jpg.rf.3dc35840ca8c9adb186004a4100e479b.jpg \n extracting: valid/IMG_8384_jpg.rf.3dc35840ca8c9adb186004a4100e479b.txt \n extracting: valid/IMG_8391_jpg.rf.531dcf59b45b5908c5a27ee093fb6f8c.jpg \n extracting: valid/IMG_8391_jpg.rf.531dcf59b45b5908c5a27ee093fb6f8c.txt \n extracting: valid/IMG_8407_jpg.rf.f2f5351c0a521920877d58fe83727988.jpg \n extracting: valid/IMG_8407_jpg.rf.f2f5351c0a521920877d58fe83727988.txt \n extracting: valid/IMG_8448_jpg.rf.97de54c1e64d385e19288c7c0b41bb26.jpg \n extracting: valid/IMG_8448_jpg.rf.97de54c1e64d385e19288c7c0b41bb26.txt \n extracting: valid/IMG_8460_jpg.rf.b23f4f5f69bdca41deb7de2881ee0901.jpg \n extracting: valid/IMG_8460_jpg.rf.b23f4f5f69bdca41deb7de2881ee0901.txt \n extracting: valid/IMG_8465_jpg.rf.a4713b0ad0fe13a1aea705ef76cc6f40.jpg \n extracting: valid/IMG_8465_jpg.rf.a4713b0ad0fe13a1aea705ef76cc6f40.txt \n extracting: valid/IMG_8489_jpg.rf.fdf5f2d0559e1eec2411bd76bc7d7cad.jpg \n extracting: valid/IMG_8489_jpg.rf.fdf5f2d0559e1eec2411bd76bc7d7cad.txt \n extracting: valid/IMG_8496_MOV-2_jpg.rf.70251d30065de54fcd5ff3b154bb4458.jpg \n extracting: valid/IMG_8496_MOV-2_jpg.rf.70251d30065de54fcd5ff3b154bb4458.txt \n extracting: valid/IMG_8497_MOV-2_jpg.rf.f0c6997b990914d9b29eefe880a15d1a.jpg \n extracting: valid/IMG_8497_MOV-2_jpg.rf.f0c6997b990914d9b29eefe880a15d1a.txt \n extracting: valid/IMG_8497_MOV-4_jpg.rf.1491fc57ae4c461c7a7dee3b1bdb0d8e.jpg \n extracting: valid/IMG_8497_MOV-4_jpg.rf.1491fc57ae4c461c7a7dee3b1bdb0d8e.txt \n extracting: valid/IMG_8512_MOV-1_jpg.rf.6f19f9583a6ee2eebb1a9c98a1b08364.jpg \n extracting: valid/IMG_8512_MOV-1_jpg.rf.6f19f9583a6ee2eebb1a9c98a1b08364.txt \n extracting: valid/IMG_8512_MOV-5_jpg.rf.2eb21510076f7108610d3f5de2538946.jpg \n extracting: valid/IMG_8512_MOV-5_jpg.rf.2eb21510076f7108610d3f5de2538946.txt \n extracting: valid/IMG_8524_jpg.rf.52cbc89c7f5a163c7ff90e20b270c60a.jpg \n extracting: valid/IMG_8524_jpg.rf.52cbc89c7f5a163c7ff90e20b270c60a.txt \n extracting: valid/IMG_8525_jpg.rf.5ce0550194005573b362890257f6d42c.jpg \n extracting: valid/IMG_8525_jpg.rf.5ce0550194005573b362890257f6d42c.txt \n extracting: valid/IMG_8526_jpg.rf.493e9506ba5242e3419c6da6f51608f1.jpg \n extracting: valid/IMG_8526_jpg.rf.493e9506ba5242e3419c6da6f51608f1.txt \n extracting: valid/IMG_8528_jpg.rf.3ce7fe4ad72adc8e7effb54f1fe5d03e.jpg \n extracting: valid/IMG_8528_jpg.rf.3ce7fe4ad72adc8e7effb54f1fe5d03e.txt \n extracting: valid/IMG_8530_jpg.rf.6e3fb78c7b53c9e9ae4690df126e587f.jpg \n extracting: valid/IMG_8530_jpg.rf.6e3fb78c7b53c9e9ae4690df126e587f.txt \n extracting: valid/IMG_8535_MOV-0_jpg.rf.8882819434d77d47b7a5cc0b3c795f3b.jpg \n extracting: valid/IMG_8535_MOV-0_jpg.rf.8882819434d77d47b7a5cc0b3c795f3b.txt \n extracting: valid/IMG_8535_MOV-1_jpg.rf.507d937dcda95b6ec89a023a27bc7229.jpg \n extracting: valid/IMG_8535_MOV-1_jpg.rf.507d937dcda95b6ec89a023a27bc7229.txt \n extracting: valid/IMG_8535_MOV-4_jpg.rf.691ac5ed886878594dafe0aa65fce09c.jpg \n extracting: valid/IMG_8535_MOV-4_jpg.rf.691ac5ed886878594dafe0aa65fce09c.txt \n extracting: valid/IMG_8535_MOV-5_jpg.rf.6519676a32fe0b4c2baef8e6828299ac.jpg \n extracting: valid/IMG_8535_MOV-5_jpg.rf.6519676a32fe0b4c2baef8e6828299ac.txt \n extracting: valid/IMG_8551_MOV-1_jpg.rf.94dddb591e9f28f5b4566488070764f5.jpg \n extracting: valid/IMG_8551_MOV-1_jpg.rf.94dddb591e9f28f5b4566488070764f5.txt \n extracting: valid/IMG_8571_MOV-3_jpg.rf.df996126756d03e6e9fa3f19db88ccd9.jpg \n extracting: valid/IMG_8571_MOV-3_jpg.rf.df996126756d03e6e9fa3f19db88ccd9.txt \n extracting: valid/IMG_8572_MOV-1_jpg.rf.03f981bb71c669602766a78d71285cca.jpg \n extracting: valid/IMG_8572_MOV-1_jpg.rf.03f981bb71c669602766a78d71285cca.txt \n extracting: valid/IMG_8578_MOV-0_jpg.rf.76969f33df1a13fcb2efcafcac28f569.jpg \n extracting: valid/IMG_8578_MOV-0_jpg.rf.76969f33df1a13fcb2efcafcac28f569.txt \n extracting: valid/IMG_8579_jpg.rf.1fd991972338fe75468fc96656789a1c.jpg \n extracting: valid/IMG_8579_jpg.rf.1fd991972338fe75468fc96656789a1c.txt \n extracting: valid/IMG_8581_MOV-1_jpg.rf.fc7593d91b8cc1d24f9c76c39ce18494.jpg \n extracting: valid/IMG_8581_MOV-1_jpg.rf.fc7593d91b8cc1d24f9c76c39ce18494.txt \n extracting: valid/IMG_8582_MOV-2_jpg.rf.d0cc4e864981abdb82ab08182a912f0f.jpg \n extracting: valid/IMG_8582_MOV-2_jpg.rf.d0cc4e864981abdb82ab08182a912f0f.txt \n extracting: valid/IMG_8582_MOV-4_jpg.rf.49eed50177e9aa763352cdd01e714d3a.jpg \n extracting: valid/IMG_8582_MOV-4_jpg.rf.49eed50177e9aa763352cdd01e714d3a.txt \n extracting: valid/IMG_8590_MOV-0_jpg.rf.86fda966f246fa47a718a0b05e9cf848.jpg \n extracting: valid/IMG_8590_MOV-0_jpg.rf.86fda966f246fa47a718a0b05e9cf848.txt \n extracting: valid/IMG_8591_MOV-1_jpg.rf.da5246e07fe1e2ba890b6773c86998a4.jpg \n extracting: valid/IMG_8591_MOV-1_jpg.rf.da5246e07fe1e2ba890b6773c86998a4.txt \n extracting: valid/IMG_8593_MOV-1_jpg.rf.e2b2b30c2e57b7b22f531b4c8feb8e21.jpg \n extracting: valid/IMG_8593_MOV-1_jpg.rf.e2b2b30c2e57b7b22f531b4c8feb8e21.txt \n extracting: valid/IMG_8595_MOV-1_jpg.rf.e8df72e90eeaec172cd32e4e4b1d7234.jpg \n extracting: valid/IMG_8595_MOV-1_jpg.rf.e8df72e90eeaec172cd32e4e4b1d7234.txt \n extracting: valid/IMG_8599_MOV-1_jpg.rf.e9958b8a4d481a2b7e9145cbcc598291.jpg \n extracting: valid/IMG_8599_MOV-1_jpg.rf.e9958b8a4d481a2b7e9145cbcc598291.txt \n extracting: valid/_darknet.labels \n"
],
[
"#Set up training file directories for custom dataset\n%cd /content/darknet/\n%cp train/_darknet.labels data/obj.names\n%mkdir data/obj\n#copy image and labels\n%cp train/*.jpg data/obj/\n%cp valid/*.jpg data/obj/\n\n%cp train/*.txt data/obj/\n%cp valid/*.txt data/obj/\n\nwith open('data/obj.data', 'w') as out:\n out.write('classes = 3\\n')\n out.write('train = data/train.txt\\n')\n out.write('valid = data/valid.txt\\n')\n out.write('names = data/obj.names\\n')\n out.write('backup = backup/')\n\n#write train file (just the image list)\nimport os\n\nwith open('data/train.txt', 'w') as out:\n for img in [f for f in os.listdir('train') if f.endswith('jpg')]:\n out.write('data/obj/' + img + '\\n')\n\n#write the valid file (just the image list)\nimport os\n\nwith open('data/valid.txt', 'w') as out:\n for img in [f for f in os.listdir('valid') if f.endswith('jpg')]:\n out.write('data/obj/' + img + '\\n')",
"/content/darknet\n"
]
],
[
[
"# Write Custom Training Config for YOLOv4",
"_____no_output_____"
]
],
[
[
"#we build config dynamically based on number of classes\n#we build iteratively from base config files. This is the same file shape as cfg/yolo-obj.cfg\ndef file_len(fname):\n with open(fname) as f:\n for i, l in enumerate(f):\n pass\n return i + 1\n\nnum_classes = file_len('train/_darknet.labels')\nprint(\"writing config for a custom YOLOv4 detector detecting number of classes: \" + str(num_classes))\n\n#Instructions from the darknet repo\n#change line max_batches to (classes*2000 but not less than number of training images, and not less than 6000), f.e. max_batches=6000 if you train for 3 classes\n#change line steps to 80% and 90% of max_batches, f.e. steps=4800,5400\nif os.path.exists('./cfg/custom-yolov4-detector.cfg'): os.remove('./cfg/custom-yolov4-detector.cfg')\n\n\nwith open('./cfg/custom-yolov4-detector.cfg', 'a') as f:\n f.write('[net]' + '\\n')\n f.write('batch=64' + '\\n')\n #####smaller subdivisions help the GPU run faster. 12 is optimal, but you might need to change to 24,36,64####\n f.write('subdivisions=24' + '\\n')\n f.write('width=416' + '\\n')\n f.write('height=416' + '\\n')\n f.write('channels=3' + '\\n')\n f.write('momentum=0.949' + '\\n')\n f.write('decay=0.0005' + '\\n')\n f.write('angle=0' + '\\n')\n f.write('saturation = 1.5' + '\\n')\n f.write('exposure = 1.5' + '\\n')\n f.write('hue = .1' + '\\n')\n f.write('\\n')\n f.write('learning_rate=0.001' + '\\n')\n f.write('burn_in=1000' + '\\n')\n ######you can adjust up and down to change training time#####\n ##Darknet does iterations with batches, not epochs####\n max_batches = num_classes*2000\n #max_batches = 2000\n f.write('max_batches=' + str(max_batches) + '\\n')\n f.write('policy=steps' + '\\n')\n steps1 = .8 * max_batches\n steps2 = .9 * max_batches\n f.write('steps='+str(steps1)+','+str(steps2) + '\\n')\n\n#Instructions from the darknet repo\n#change line classes=80 to your number of objects in each of 3 [yolo]-layers:\n#change [filters=255] to filters=(classes + 5)x3 in the 3 [convolutional] before each [yolo] layer, keep in mind that it only has to be the last [convolutional] before each of the [yolo] layers.\n\n with open('cfg/yolov4-custom2.cfg', 'r') as f2:\n content = f2.readlines()\n for line in content:\n f.write(line) \n num_filters = (num_classes + 5) * 3\n f.write('filters='+str(num_filters) + '\\n')\n f.write('activation=linear')\n f.write('\\n')\n f.write('\\n')\n f.write('[yolo]' + '\\n')\n f.write('mask = 0,1,2' + '\\n')\n f.write('anchors = 12, 16, 19, 36, 40, 28, 36, 75, 76, 55, 72, 146, 142, 110, 192, 243, 459, 401' + '\\n')\n f.write('classes=' + str(num_classes) + '\\n')\n\n with open('cfg/yolov4-custom3.cfg', 'r') as f3:\n content = f3.readlines()\n for line in content:\n f.write(line) \n num_filters = (num_classes + 5) * 3\n f.write('filters='+str(num_filters) + '\\n')\n f.write('activation=linear')\n f.write('\\n')\n f.write('\\n')\n f.write('[yolo]' + '\\n')\n f.write('mask = 3,4,5' + '\\n')\n f.write('anchors = 12, 16, 19, 36, 40, 28, 36, 75, 76, 55, 72, 146, 142, 110, 192, 243, 459, 401' + '\\n')\n f.write('classes=' + str(num_classes) + '\\n')\n\n with open('cfg/yolov4-custom4.cfg', 'r') as f4:\n content = f4.readlines()\n for line in content:\n f.write(line) \n num_filters = (num_classes + 5) * 3\n f.write('filters='+str(num_filters) + '\\n')\n f.write('activation=linear')\n f.write('\\n')\n f.write('\\n')\n f.write('[yolo]' + '\\n')\n f.write('mask = 6,7,8' + '\\n')\n f.write('anchors = 12, 16, 19, 36, 40, 28, 36, 75, 76, 55, 72, 146, 142, 110, 192, 243, 459, 401' + '\\n')\n f.write('classes=' + str(num_classes) + '\\n')\n \n with open('cfg/yolov4-custom5.cfg', 'r') as f5:\n content = f5.readlines()\n for line in content:\n f.write(line)\n\nprint(\"file is written!\") \n\n\n",
"writing config for a custom YOLOv4 detector detecting number of classes: 7\nfile is written!\n"
],
[
"#here is the file that was just written. \n#you may consider adjusting certain things\n\n#like the number of subdivisions 64 runs faster but Colab GPU may not be big enough\n#if Colab GPU memory is too small, you will need to adjust subdivisions to 16\n%cat cfg/custom-yolov4-detector.cfg",
"[net]\nbatch=64\nsubdivisions=24\nwidth=416\nheight=416\nchannels=3\nmomentum=0.949\ndecay=0.0005\nangle=0\nsaturation = 1.5\nexposure = 1.5\nhue = .1\n\nlearning_rate=0.001\nburn_in=1000\nmax_batches=14000\npolicy=steps\nsteps=11200.0,12600.0\nscales=.1,.1\n\n#cutmix=1\nmosaic=1\n\n#:104x104 54:52x52 85:26x26 104:13x13 for 416\n\n[convolutional]\nbatch_normalize=1\nfilters=32\nsize=3\nstride=1\npad=1\nactivation=mish\n\n# Downsample\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=3\nstride=2\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[route]\nlayers = -2\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=32\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[route]\nlayers = -1,-7\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=1\nstride=1\npad=1\nactivation=mish\n\n# Downsample\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=3\nstride=2\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[route]\nlayers = -2\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=64\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[route]\nlayers = -1,-10\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n# Downsample\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=3\nstride=2\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[route]\nlayers = -2\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[route]\nlayers = -1,-28\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n# Downsample\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=3\nstride=2\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[route]\nlayers = -2\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[route]\nlayers = -1,-28\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=mish\n\n# Downsample\n\n[convolutional]\nbatch_normalize=1\nfilters=1024\nsize=3\nstride=2\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[route]\nlayers = -2\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=3\nstride=1\npad=1\nactivation=mish\n\n[shortcut]\nfrom=-3\nactivation=linear\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=mish\n\n[route]\nlayers = -1,-16\n\n[convolutional]\nbatch_normalize=1\nfilters=1024\nsize=1\nstride=1\npad=1\nactivation=mish\n\n##########################\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=1024\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n### SPP ###\n[maxpool]\nstride=1\nsize=5\n\n[route]\nlayers=-2\n\n[maxpool]\nstride=1\nsize=9\n\n[route]\nlayers=-4\n\n[maxpool]\nstride=1\nsize=13\n\n[route]\nlayers=-1,-3,-5,-6\n### End SPP ###\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=1024\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[upsample]\nstride=2\n\n[route]\nlayers = 85\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[route]\nlayers = -1, -3\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=512\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=512\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[upsample]\nstride=2\n\n[route]\nlayers = 54\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[route]\nlayers = -1, -3\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=256\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=256\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=128\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n##########################\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=256\nactivation=leaky\n\n[convolutional]\nsize=1\nstride=1\npad=1\nfilters=36\nactivation=linear\n\n[yolo]\nmask = 0,1,2\nanchors = 12, 16, 19, 36, 40, 28, 36, 75, 76, 55, 72, 146, 142, 110, 192, 243, 459, 401\nclasses=7\nnum=9\njitter=.3\nignore_thresh = .7\ntruth_thresh = 1\nscale_x_y = 1.2\niou_thresh=0.213\ncls_normalizer=1.0\niou_normalizer=0.07\niou_loss=ciou\nnms_kind=greedynms\nbeta_nms=0.6\nmax_delta=5\n\n\n[route]\nlayers = -4\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=2\npad=1\nfilters=256\nactivation=leaky\n\n[route]\nlayers = -1, -16\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=512\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=512\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=256\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=512\nactivation=leaky\n\n[convolutional]\nsize=1\nstride=1\npad=1\nfilters=36\nactivation=linear\n\n[yolo]\nmask = 3,4,5\nanchors = 12, 16, 19, 36, 40, 28, 36, 75, 76, 55, 72, 146, 142, 110, 192, 243, 459, 401\nclasses=7\nnum=9\njitter=.3\nignore_thresh = .7\ntruth_thresh = 1\nscale_x_y = 1.1\niou_thresh=0.213\ncls_normalizer=1.0\niou_normalizer=0.07\niou_loss=ciou\nnms_kind=greedynms\nbeta_nms=0.6\nmax_delta=5\n\n\n[route]\nlayers = -4\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=2\npad=1\nfilters=512\nactivation=leaky\n\n[route]\nlayers = -1, -37\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=1024\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=1024\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nfilters=512\nsize=1\nstride=1\npad=1\nactivation=leaky\n\n[convolutional]\nbatch_normalize=1\nsize=3\nstride=1\npad=1\nfilters=1024\nactivation=leaky\n\n[convolutional]\nsize=1\nstride=1\npad=1\nfilters=36\nactivation=linear\n\n[yolo]\nmask = 6,7,8\nanchors = 12, 16, 19, 36, 40, 28, 36, 75, 76, 55, 72, 146, 142, 110, 192, 243, 459, 401\nclasses=7\nnum=9\njitter=.3\nignore_thresh = .7\ntruth_thresh = 1\nrandom=1\nscale_x_y = 1.05\niou_thresh=0.213\ncls_normalizer=1.0\niou_normalizer=0.07\niou_loss=ciou\nnms_kind=greedynms\nbeta_nms=0.6\nmax_delta=5\n"
]
],
[
[
"# Train Custom YOLOv4 Detector",
"_____no_output_____"
]
],
[
[
"!./darknet detector train data/obj.data cfg/custom-yolov4-detector.cfg yolov4.conv.137 -dont_show -map\n#If you get CUDA out of memory adjust subdivisions above!\n#adjust max batches down for shorter training above",
"\n (next mAP calculation at 2568 iterations) \n Last accuracy [email protected] = 59.40 %, best = 59.40 % \n 2461: 10.640112, 8.513406 avg loss, 0.001000 rate, 8.034781 seconds, 118128 images, 16.766305 hours left\nLoaded: 0.000033 seconds\n^C\n"
]
],
[
[
"# Infer Custom Objects with Saved YOLOv4 Weights",
"_____no_output_____"
]
],
[
[
"#define utility function\ndef imShow(path):\n import cv2\n import matplotlib.pyplot as plt\n %matplotlib inline\n\n image = cv2.imread(path)\n height, width = image.shape[:2]\n resized_image = cv2.resize(image,(3*width, 3*height), interpolation = cv2.INTER_CUBIC)\n\n fig = plt.gcf()\n fig.set_size_inches(18, 10)\n plt.axis(\"off\")\n #plt.rcParams['figure.figsize'] = [10, 5]\n plt.imshow(cv2.cvtColor(resized_image, cv2.COLOR_BGR2RGB))\n plt.show()",
"_____no_output_____"
],
[
"#check if weigths have saved yet\n#backup houses the last weights for our detector\n#(file yolo-obj_last.weights will be saved to the build\\darknet\\x64\\backup\\ for each 100 iterations)\n#(file yolo-obj_xxxx.weights will be saved to the build\\darknet\\x64\\backup\\ for each 1000 iterations)\n#After training is complete - get result yolo-obj_final.weights from path build\\darknet\\x64\\bac\n!ls backup\n#if it is empty you haven't trained for long enough yet, you need to train for at least 100 iterations",
"custom-yolov4-detector_1000.weights custom-yolov4-detector_best.weights\ncustom-yolov4-detector_2000.weights custom-yolov4-detector_last.weights\n"
],
[
"#coco.names is hardcoded somewhere in the detector\n%cp data/obj.names data/coco.names",
"_____no_output_____"
],
[
"Aquarium-darknet.zip",
"_____no_output_____"
],
[
"!pwd",
"/content/darknet\n"
],
[
"!chmod 755 ./darknet",
"_____no_output_____"
],
[
"import os",
"_____no_output_____"
],
[
"test_images = [f for f in os.listdir('test') if f.endswith('.jpg')]",
"_____no_output_____"
],
[
"#/test has images that we can test our detector on\n\nimport random\nimg_path = \"test/\" + random.choice(test_images);\n\n#test out our detector!\n!./darknet detect cfg/custom-yolov4-detector.cfg backup/custom-yolov4-detector_last.weights {img_path} -dont-show\nimShow('predictions.jpg')",
" CUDA-version: 10010 (10010), cuDNN: 7.6.5, GPU count: 1 \n OpenCV version: 3.2.0\n compute_capability = 750, cudnn_half = 0 \nnet.optimized_memory = 0 \nmini_batch = 1, batch = 24, time_steps = 1, train = 0 \n layer filters size/strd(dil) input output\n 0 conv 32 3 x 3/ 1 416 x 416 x 3 -> 416 x 416 x 32 0.299 BF\n 1 conv 64 3 x 3/ 2 416 x 416 x 32 -> 208 x 208 x 64 1.595 BF\n 2 conv 64 1 x 1/ 1 208 x 208 x 64 -> 208 x 208 x 64 0.354 BF\n 3 route 1 \t\t -> 208 x 208 x 64 \n 4 conv 64 1 x 1/ 1 208 x 208 x 64 -> 208 x 208 x 64 0.354 BF\n 5 conv 32 1 x 1/ 1 208 x 208 x 64 -> 208 x 208 x 32 0.177 BF\n 6 conv 64 3 x 3/ 1 208 x 208 x 32 -> 208 x 208 x 64 1.595 BF\n 7 Shortcut Layer: 4, wt = 0, wn = 0, outputs: 208 x 208 x 64 0.003 BF\n 8 conv 64 1 x 1/ 1 208 x 208 x 64 -> 208 x 208 x 64 0.354 BF\n 9 route 8 2 \t -> 208 x 208 x 128 \n 10 conv 64 1 x 1/ 1 208 x 208 x 128 -> 208 x 208 x 64 0.709 BF\n 11 conv 128 3 x 3/ 2 208 x 208 x 64 -> 104 x 104 x 128 1.595 BF\n 12 conv 64 1 x 1/ 1 104 x 104 x 128 -> 104 x 104 x 64 0.177 BF\n 13 route 11 \t\t -> 104 x 104 x 128 \n 14 conv 64 1 x 1/ 1 104 x 104 x 128 -> 104 x 104 x 64 0.177 BF\n 15 conv 64 1 x 1/ 1 104 x 104 x 64 -> 104 x 104 x 64 0.089 BF\n 16 conv 64 3 x 3/ 1 104 x 104 x 64 -> 104 x 104 x 64 0.797 BF\n 17 Shortcut Layer: 14, wt = 0, wn = 0, outputs: 104 x 104 x 64 0.001 BF\n 18 conv 64 1 x 1/ 1 104 x 104 x 64 -> 104 x 104 x 64 0.089 BF\n 19 conv 64 3 x 3/ 1 104 x 104 x 64 -> 104 x 104 x 64 0.797 BF\n 20 Shortcut Layer: 17, wt = 0, wn = 0, outputs: 104 x 104 x 64 0.001 BF\n 21 conv 64 1 x 1/ 1 104 x 104 x 64 -> 104 x 104 x 64 0.089 BF\n 22 route 21 12 \t -> 104 x 104 x 128 \n 23 conv 128 1 x 1/ 1 104 x 104 x 128 -> 104 x 104 x 128 0.354 BF\n 24 conv 256 3 x 3/ 2 104 x 104 x 128 -> 52 x 52 x 256 1.595 BF\n 25 conv 128 1 x 1/ 1 52 x 52 x 256 -> 52 x 52 x 128 0.177 BF\n 26 route 24 \t\t -> 52 x 52 x 256 \n 27 conv 128 1 x 1/ 1 52 x 52 x 256 -> 52 x 52 x 128 0.177 BF\n 28 conv 128 1 x 1/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.089 BF\n 29 conv 128 3 x 3/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.797 BF\n 30 Shortcut Layer: 27, wt = 0, wn = 0, outputs: 52 x 52 x 128 0.000 BF\n 31 conv 128 1 x 1/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.089 BF\n 32 conv 128 3 x 3/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.797 BF\n 33 Shortcut Layer: 30, wt = 0, wn = 0, outputs: 52 x 52 x 128 0.000 BF\n 34 conv 128 1 x 1/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.089 BF\n 35 conv 128 3 x 3/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.797 BF\n 36 Shortcut Layer: 33, wt = 0, wn = 0, outputs: 52 x 52 x 128 0.000 BF\n 37 conv 128 1 x 1/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.089 BF\n 38 conv 128 3 x 3/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.797 BF\n 39 Shortcut Layer: 36, wt = 0, wn = 0, outputs: 52 x 52 x 128 0.000 BF\n 40 conv 128 1 x 1/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.089 BF\n 41 conv 128 3 x 3/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.797 BF\n 42 Shortcut Layer: 39, wt = 0, wn = 0, outputs: 52 x 52 x 128 0.000 BF\n 43 conv 128 1 x 1/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.089 BF\n 44 conv 128 3 x 3/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.797 BF\n 45 Shortcut Layer: 42, wt = 0, wn = 0, outputs: 52 x 52 x 128 0.000 BF\n 46 conv 128 1 x 1/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.089 BF\n 47 conv 128 3 x 3/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.797 BF\n 48 Shortcut Layer: 45, wt = 0, wn = 0, outputs: 52 x 52 x 128 0.000 BF\n 49 conv 128 1 x 1/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.089 BF\n 50 conv 128 3 x 3/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.797 BF\n 51 Shortcut Layer: 48, wt = 0, wn = 0, outputs: 52 x 52 x 128 0.000 BF\n 52 conv 128 1 x 1/ 1 52 x 52 x 128 -> 52 x 52 x 128 0.089 BF\n 53 route 52 25 \t -> 52 x 52 x 256 \n 54 conv 256 1 x 1/ 1 52 x 52 x 256 -> 52 x 52 x 256 0.354 BF\n 55 conv 512 3 x 3/ 2 52 x 52 x 256 -> 26 x 26 x 512 1.595 BF\n 56 conv 256 1 x 1/ 1 26 x 26 x 512 -> 26 x 26 x 256 0.177 BF\n 57 route 55 \t\t -> 26 x 26 x 512 \n 58 conv 256 1 x 1/ 1 26 x 26 x 512 -> 26 x 26 x 256 0.177 BF\n 59 conv 256 1 x 1/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.089 BF\n 60 conv 256 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.797 BF\n 61 Shortcut Layer: 58, wt = 0, wn = 0, outputs: 26 x 26 x 256 0.000 BF\n 62 conv 256 1 x 1/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.089 BF\n 63 conv 256 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.797 BF\n 64 Shortcut Layer: 61, wt = 0, wn = 0, outputs: 26 x 26 x 256 0.000 BF\n 65 conv 256 1 x 1/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.089 BF\n 66 conv 256 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.797 BF\n 67 Shortcut Layer: 64, wt = 0, wn = 0, outputs: 26 x 26 x 256 0.000 BF\n 68 conv 256 1 x 1/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.089 BF\n 69 conv 256 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.797 BF\n 70 Shortcut Layer: 67, wt = 0, wn = 0, outputs: 26 x 26 x 256 0.000 BF\n 71 conv 256 1 x 1/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.089 BF\n 72 conv 256 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.797 BF\n 73 Shortcut Layer: 70, wt = 0, wn = 0, outputs: 26 x 26 x 256 0.000 BF\n 74 conv 256 1 x 1/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.089 BF\n 75 conv 256 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.797 BF\n 76 Shortcut Layer: 73, wt = 0, wn = 0, outputs: 26 x 26 x 256 0.000 BF\n 77 conv 256 1 x 1/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.089 BF\n 78 conv 256 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.797 BF\n 79 Shortcut Layer: 76, wt = 0, wn = 0, outputs: 26 x 26 x 256 0.000 BF\n 80 conv 256 1 x 1/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.089 BF\n 81 conv 256 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.797 BF\n 82 Shortcut Layer: 79, wt = 0, wn = 0, outputs: 26 x 26 x 256 0.000 BF\n 83 conv 256 1 x 1/ 1 26 x 26 x 256 -> 26 x 26 x 256 0.089 BF\n 84 route 83 56 \t -> 26 x 26 x 512 \n 85 conv 512 1 x 1/ 1 26 x 26 x 512 -> 26 x 26 x 512 0.354 BF\n 86 conv 1024 3 x 3/ 2 26 x 26 x 512 -> 13 x 13 x1024 1.595 BF\n 87 conv 512 1 x 1/ 1 13 x 13 x1024 -> 13 x 13 x 512 0.177 BF\n 88 route 86 \t\t -> 13 x 13 x1024 \n 89 conv 512 1 x 1/ 1 13 x 13 x1024 -> 13 x 13 x 512 0.177 BF\n 90 conv 512 1 x 1/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.089 BF\n 91 conv 512 3 x 3/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.797 BF\n 92 Shortcut Layer: 89, wt = 0, wn = 0, outputs: 13 x 13 x 512 0.000 BF\n 93 conv 512 1 x 1/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.089 BF\n 94 conv 512 3 x 3/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.797 BF\n 95 Shortcut Layer: 92, wt = 0, wn = 0, outputs: 13 x 13 x 512 0.000 BF\n 96 conv 512 1 x 1/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.089 BF\n 97 conv 512 3 x 3/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.797 BF\n 98 Shortcut Layer: 95, wt = 0, wn = 0, outputs: 13 x 13 x 512 0.000 BF\n 99 conv 512 1 x 1/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.089 BF\n 100 conv 512 3 x 3/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.797 BF\n 101 Shortcut Layer: 98, wt = 0, wn = 0, outputs: 13 x 13 x 512 0.000 BF\n 102 conv 512 1 x 1/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.089 BF\n 103 route 102 87 \t -> 13 x 13 x1024 \n 104 conv 1024 1 x 1/ 1 13 x 13 x1024 -> 13 x 13 x1024 0.354 BF\n 105 conv 512 1 x 1/ 1 13 x 13 x1024 -> 13 x 13 x 512 0.177 BF\n 106 conv 1024 3 x 3/ 1 13 x 13 x 512 -> 13 x 13 x1024 1.595 BF\n 107 conv 512 1 x 1/ 1 13 x 13 x1024 -> 13 x 13 x 512 0.177 BF\n 108 max 5x 5/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.002 BF\n 109 route 107 \t\t -> 13 x 13 x 512 \n 110 max 9x 9/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.007 BF\n 111 route 107 \t\t -> 13 x 13 x 512 \n 112 max 13x13/ 1 13 x 13 x 512 -> 13 x 13 x 512 0.015 BF\n 113 route 112 110 108 107 \t -> 13 x 13 x2048 \n 114 conv 512 1 x 1/ 1 13 x 13 x2048 -> 13 x 13 x 512 0.354 BF\n 115 conv 1024 3 x 3/ 1 13 x 13 x 512 -> 13 x 13 x1024 1.595 BF\n 116 conv 512 1 x 1/ 1 13 x 13 x1024 -> 13 x 13 x 512 0.177 BF\n 117 conv 256 1 x 1/ 1 13 x 13 x 512 -> 13 x 13 x 256 0.044 BF\n 118 upsample 2x 13 x 13 x 256 -> 26 x 26 x 256\n 119 route 85 \t\t -> 26 x 26 x 512 \n 120 conv 256 1 x 1/ 1 26 x 26 x 512 -> 26 x 26 x 256 0.177 BF\n 121 route 120 118 \t -> 26 x 26 x 512 \n 122 conv 256 1 x 1/ 1 26 x 26 x 512 -> 26 x 26 x 256 0.177 BF\n 123 conv 512 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 512 1.595 BF\n 124 conv 256 1 x 1/ 1 26 x 26 x 512 -> 26 x 26 x 256 0.177 BF\n 125 conv 512 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 512 1.595 BF\n 126 conv 256 1 x 1/ 1 26 x 26 x 512 -> 26 x 26 x 256 0.177 BF\n 127 conv 128 1 x 1/ 1 26 x 26 x 256 -> 26 x 26 x 128 0.044 BF\n 128 upsample 2x 26 x 26 x 128 -> 52 x 52 x 128\n 129 route 54 \t\t -> 52 x 52 x 256 \n 130 conv 128 1 x 1/ 1 52 x 52 x 256 -> 52 x 52 x 128 0.177 BF\n 131 route 130 128 \t -> 52 x 52 x 256 \n 132 conv 128 1 x 1/ 1 52 x 52 x 256 -> 52 x 52 x 128 0.177 BF\n 133 conv 256 3 x 3/ 1 52 x 52 x 128 -> 52 x 52 x 256 1.595 BF\n 134 conv 128 1 x 1/ 1 52 x 52 x 256 -> 52 x 52 x 128 0.177 BF\n 135 conv 256 3 x 3/ 1 52 x 52 x 128 -> 52 x 52 x 256 1.595 BF\n 136 conv 128 1 x 1/ 1 52 x 52 x 256 -> 52 x 52 x 128 0.177 BF\n 137 conv 256 3 x 3/ 1 52 x 52 x 128 -> 52 x 52 x 256 1.595 BF\n 138 conv 36 1 x 1/ 1 52 x 52 x 256 -> 52 x 52 x 36 0.050 BF\n 139 yolo\n[yolo] params: iou loss: ciou (4), iou_norm: 0.07, cls_norm: 1.00, scale_x_y: 1.20\nnms_kind: greedynms (1), beta = 0.600000 \n 140 route 136 \t\t -> 52 x 52 x 128 \n 141 conv 256 3 x 3/ 2 52 x 52 x 128 -> 26 x 26 x 256 0.399 BF\n 142 route 141 126 \t -> 26 x 26 x 512 \n 143 conv 256 1 x 1/ 1 26 x 26 x 512 -> 26 x 26 x 256 0.177 BF\n 144 conv 512 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 512 1.595 BF\n 145 conv 256 1 x 1/ 1 26 x 26 x 512 -> 26 x 26 x 256 0.177 BF\n 146 conv 512 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 512 1.595 BF\n 147 conv 256 1 x 1/ 1 26 x 26 x 512 -> 26 x 26 x 256 0.177 BF\n 148 conv 512 3 x 3/ 1 26 x 26 x 256 -> 26 x 26 x 512 1.595 BF\n 149 conv 36 1 x 1/ 1 26 x 26 x 512 -> 26 x 26 x 36 0.025 BF\n 150 yolo\n[yolo] params: iou loss: ciou (4), iou_norm: 0.07, cls_norm: 1.00, scale_x_y: 1.10\nnms_kind: greedynms (1), beta = 0.600000 \n 151 route 147 \t\t -> 26 x 26 x 256 \n 152 conv 512 3 x 3/ 2 26 x 26 x 256 -> 13 x 13 x 512 0.399 BF\n 153 route 152 116 \t -> 13 x 13 x1024 \n 154 conv 512 1 x 1/ 1 13 x 13 x1024 -> 13 x 13 x 512 0.177 BF\n 155 conv 1024 3 x 3/ 1 13 x 13 x 512 -> 13 x 13 x1024 1.595 BF\n 156 conv 512 1 x 1/ 1 13 x 13 x1024 -> 13 x 13 x 512 0.177 BF\n 157 conv 1024 3 x 3/ 1 13 x 13 x 512 -> 13 x 13 x1024 1.595 BF\n 158 conv 512 1 x 1/ 1 13 x 13 x1024 -> 13 x 13 x 512 0.177 BF\n 159 conv 1024 3 x 3/ 1 13 x 13 x 512 -> 13 x 13 x1024 1.595 BF\n 160 conv 36 1 x 1/ 1 13 x 13 x1024 -> 13 x 13 x 36 0.012 BF\n 161 yolo\n[yolo] params: iou loss: ciou (4), iou_norm: 0.07, cls_norm: 1.00, scale_x_y: 1.05\nnms_kind: greedynms (1), beta = 0.600000 \nTotal BFLOPS 59.607 \navg_outputs = 490567 \n Allocate additional workspace_size = 52.43 MB \nLoading weights from backup/custom-yolov4-detector_last.weights...\n seen 64, trained: 115 K-images (1 Kilo-batches_64) \nDone! Loaded 162 layers from weights-file \ntest/IMG_2465_jpeg_jpg.rf.a19ce07479699fb8665709960704a68f.jpg: Predicted in 44.753000 milli-seconds.\njellyfish: 38%\njellyfish: 70%\njellyfish: 89%\njellyfish: 86%\njellyfish: 96%\njellyfish: 91%\njellyfish: 95%\njellyfish: 92%\njellyfish: 86%\njellyfish: 87%\njellyfish: 91%\njellyfish: 77%\njellyfish: 84%\njellyfish: 85%\njellyfish: 80%\njellyfish: 78%\njellyfish: 94%\njellyfish: 84%\nUnable to init server: Could not connect: Connection refused\n\n(predictions:7937): Gtk-\u001b[1;33mWARNING\u001b[0m **: \u001b[34m06:08:46.367\u001b[0m: cannot open display: \n"
],
[
"",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown",
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7b9dd3ce49b90827b5dc7534fac2358c35dad6f | 265,550 | ipynb | Jupyter Notebook | learning-phase/machine-learning/week-3/tanmai/.ipynb_checkpoints/exercise2-checkpoint.ipynb | Saharsh007/open-qas | 3ceefaa745f488307f015d27e5ec21ac626dfd89 | [
"MIT"
] | 7 | 2018-10-24T13:51:04.000Z | 2020-11-01T18:44:38.000Z | learning-phase/machine-learning/week-3/tanmai/.ipynb_checkpoints/exercise2-checkpoint.ipynb | Saharsh007/open-qas | 3ceefaa745f488307f015d27e5ec21ac626dfd89 | [
"MIT"
] | 13 | 2018-12-09T17:15:13.000Z | 2019-03-19T14:37:42.000Z | learning-phase/machine-learning/week-3/tanmai/.ipynb_checkpoints/exercise2-checkpoint.ipynb | Saharsh007/open-qas | 3ceefaa745f488307f015d27e5ec21ac626dfd89 | [
"MIT"
] | 4 | 2018-09-18T05:57:13.000Z | 2019-03-06T11:03:51.000Z | 210.753968 | 31,284 | 0.887987 | [
[
[
"# Programming Exercise 2: Logistic Regression\n\n## Introduction\n\nIn this exercise, you will implement logistic regression and apply it to two different datasets. Before starting on the programming exercise, we strongly recommend watching the video lectures and completing the review questions for the associated topics.\n\nAll the information you need for solving this assignment is in this notebook, and all the code you will be implementing will take place within this notebook. The assignment can be promptly submitted to the coursera grader directly from this notebook (code and instructions are included below).\n\nBefore we begin with the exercises, we need to import all libraries required for this programming exercise. Throughout the course, we will be using [`numpy`](http://www.numpy.org/) for all arrays and matrix operations, and [`matplotlib`](https://matplotlib.org/) for plotting. In this assignment, we will also use [`scipy`](https://docs.scipy.org/doc/scipy/reference/), which contains scientific and numerical computation functions and tools. \n\nYou can find instructions on how to install required libraries in the README file in the [github repository](https://github.com/dibgerge/ml-coursera-python-assignments).",
"_____no_output_____"
]
],
[
[
"# used for manipulating directory paths\nimport os\n\n# Scientific and vector computation for python\nimport numpy as np\n\n# Plotting library\nfrom matplotlib import pyplot\n\n# Optimization module in scipy\nfrom scipy import optimize\n\n# library written for this exercise providing additional functions for assignment submission, and others\nimport utils\n\n# define the submission/grader object for this exercise\ngrader = utils.Grader()\n\n# tells matplotlib to embed plots within the notebook\n%matplotlib inline",
"_____no_output_____"
]
],
[
[
"## Submission and Grading\n\n\nAfter completing each part of the assignment, be sure to submit your solutions to the grader. The following is a breakdown of how each part of this exercise is scored.\n\n\n| Section | Part | Submission function | Points \n| :- |:- | :- | :-:\n| 1 | [Sigmoid Function](#section1) | [`sigmoid`](#sigmoid) | 5 \n| 2 | [Compute cost for logistic regression](#section2) | [`costFunction`](#costFunction) | 30 \n| 3 | [Gradient for logistic regression](#section2) | [`costFunction`](#costFunction) | 30 \n| 4 | [Predict Function](#section4) | [`predict`](#predict) | 5 \n| 5 | [Compute cost for regularized LR](#section5) | [`costFunctionReg`](#costFunctionReg) | 15 \n| 6 | [Gradient for regularized LR](#section5) | [`costFunctionReg`](#costFunctionReg) | 15 \n| | Total Points | | 100 \n\n\n\nYou are allowed to submit your solutions multiple times, and we will take only the highest score into consideration.\n\n<div class=\"alert alert-block alert-warning\">\nAt the end of each section in this notebook, we have a cell which contains code for submitting the solutions thus far to the grader. Execute the cell to see your score up to the current section. For all your work to be submitted properly, you must execute those cells at least once. They must also be re-executed everytime the submitted function is updated.\n</div>\n",
"_____no_output_____"
],
[
"## 1 Logistic Regression\n\nIn this part of the exercise, you will build a logistic regression model to predict whether a student gets admitted into a university. Suppose that you are the administrator of a university department and\nyou want to determine each applicant’s chance of admission based on their results on two exams. You have historical data from previous applicants that you can use as a training set for logistic regression. For each training example, you have the applicant’s scores on two exams and the admissions\ndecision. Your task is to build a classification model that estimates an applicant’s probability of admission based the scores from those two exams. \n\nThe following cell will load the data and corresponding labels:",
"_____no_output_____"
]
],
[
[
"# Load data\n# The first two columns contains the exam scores and the third column\n# contains the label.\ndata = np.loadtxt(os.path.join('Data', 'ex2data1.txt'), delimiter=',')\nX, y = data[:, 0:2], data[:, 2]",
"_____no_output_____"
]
],
[
[
"### 1.1 Visualizing the data\n\nBefore starting to implement any learning algorithm, it is always good to visualize the data if possible. We display the data on a 2-dimensional plot by calling the function `plotData`. You will now complete the code in `plotData` so that it displays a figure where the axes are the two exam scores, and the positive and negative examples are shown with different markers.\n\nTo help you get more familiar with plotting, we have left `plotData` empty so you can try to implement it yourself. However, this is an optional (ungraded) exercise. We also provide our implementation below so you can\ncopy it or refer to it. If you choose to copy our example, make sure you learn\nwhat each of its commands is doing by consulting the `matplotlib` and `numpy` documentation.\n\n```python\n# Find Indices of Positive and Negative Examples\npos = y == 1\nneg = y == 0\n\n# Plot Examples\npyplot.plot(X[pos, 0], X[pos, 1], 'k*', lw=2, ms=10)\npyplot.plot(X[neg, 0], X[neg, 1], 'ko', mfc='y', ms=8, mec='k', mew=1)\n```",
"_____no_output_____"
]
],
[
[
"def plotData(X, y):\n \"\"\"\n Plots the data points X and y into a new figure. Plots the data \n points with * for the positive examples and o for the negative examples.\n \n Parameters\n ----------\n X : array_like\n An Mx2 matrix representing the dataset. \n \n y : array_like\n Label values for the dataset. A vector of size (M, ).\n \n Instructions\n ------------\n Plot the positive and negative examples on a 2D plot, using the\n option 'k*' for the positive examples and 'ko' for the negative examples. \n \"\"\"\n # Create New Figure\n fig = pyplot.figure()\n pos = y == 1\n neg = y == 0\n\n # ====================== YOUR CODE HERE ======================\n pyplot.plot(X[pos, 0], X[pos, 1], 'k*', lw=2, ms=10)\n pyplot.plot(X[neg, 0], X[neg, 1], 'ko', mfc='y', ms=8, mec='k', mew=1)\n \n # ============================================================",
"_____no_output_____"
]
],
[
[
"Now, we call the implemented function to display the loaded data:",
"_____no_output_____"
]
],
[
[
"plotData(X, y)\n# add axes labels\npyplot.xlabel('Exam 1 score')\npyplot.ylabel('Exam 2 score')\npyplot.legend(['Admitted', 'Not admitted'])\npass",
"_____no_output_____"
]
],
[
[
"<a id=\"section1\"></a>\n### 1.2 Implementation\n\n#### 1.2.1 Warmup exercise: sigmoid function\n\nBefore you start with the actual cost function, recall that the logistic regression hypothesis is defined as:\n\n$$ h_\\theta(x) = g(\\theta^T x)$$\n\nwhere function $g$ is the sigmoid function. The sigmoid function is defined as: \n\n$$g(z) = \\frac{1}{1+e^{-z}}$$.\n\nYour first step is to implement this function `sigmoid` so it can be\ncalled by the rest of your program. When you are finished, try testing a few\nvalues by calling `sigmoid(x)` in a new cell. For large positive values of `x`, the sigmoid should be close to 1, while for large negative values, the sigmoid should be close to 0. Evaluating `sigmoid(0)` should give you exactly 0.5. Your code should also work with vectors and matrices. **For a matrix, your function should perform the sigmoid function on every element.**\n<a id=\"sigmoid\"></a>",
"_____no_output_____"
]
],
[
[
"def sigmoid(z):\n \"\"\"\n Compute sigmoid function given the input z.\n \n Parameters\n ----------\n z : array_like\n The input to the sigmoid function. This can be a 1-D vector \n or a 2-D matrix. \n \n Returns\n -------\n g : array_like\n The computed sigmoid function. g has the same shape as z, since\n the sigmoid is computed element-wise on z.\n \n Instructions\n ------------\n Compute the sigmoid of each value of z (z can be a matrix, vector or scalar).\n \"\"\"\n # convert input to a numpy array\n z = np.array(z)\n \n # You need to return the following variables correctly \n g = np.zeros(z.shape)\n \n # ====================== YOUR CODE HERE ======================\n g = 1 / (1 + np.exp(-z)) \n\n # =============================================================\n return g",
"_____no_output_____"
]
],
[
[
"The following cell evaluates the sigmoid function at `z=0`. You should get a value of 0.5. You can also try different values for `z` to experiment with the sigmoid function.",
"_____no_output_____"
]
],
[
[
"# Test the implementation of sigmoid function here\nz = 0\ng = sigmoid(z)\n\nprint('g(', z, ') = ', g)",
"g( 0 ) = 0.5\n"
]
],
[
[
"After completing a part of the exercise, you can submit your solutions for grading by first adding the function you modified to the submission object, and then sending your function to Coursera for grading. \n\nThe submission script will prompt you for your login e-mail and submission token. You can obtain a submission token from the web page for the assignment. You are allowed to submit your solutions multiple times, and we will take only the highest score into consideration.\n\nExecute the following cell to grade your solution to the first part of this exercise.\n\n*You should now submit your solutions.*",
"_____no_output_____"
]
],
[
[
"# appends the implemented function in part 1 to the grader object\ngrader[1] = sigmoid\n\n# send the added functions to coursera grader for getting a grade on this part\ngrader.grade()",
"\nSubmitting Solutions | Programming Exercise logistic-regression\n\nLogin (email address): [email protected]\nToken: c71I4EgVY0bY6fIL\n Part Name | Score | Feedback\n --------- | ----- | --------\n Sigmoid Function | 5 / 5 | Nice work!\n Logistic Regression Cost | 0 / 30 | \n Logistic Regression Gradient | 0 / 30 | \n Predict | 0 / 5 | \n Regularized Logistic Regression Cost | 0 / 15 | \n Regularized Logistic Regression Gradient | 0 / 15 | \n --------------------------------\n | 5 / 100 | \n\n"
]
],
[
[
"<a id=\"section2\"></a>\n#### 1.2.2 Cost function and gradient\n\nNow you will implement the cost function and gradient for logistic regression. Before proceeding we add the intercept term to X. ",
"_____no_output_____"
]
],
[
[
"# Setup the data matrix appropriately, and add ones for the intercept term\nm, n = X.shape\n\n# Add intercept term to X\nX = np.concatenate([np.ones((m, 1)), X], axis=1)",
"_____no_output_____"
]
],
[
[
"Now, complete the code for the function `costFunction` to return the cost and gradient. Recall that the cost function in logistic regression is\n\n$$ J(\\theta) = \\frac{1}{m} \\sum_{i=1}^{m} \\left[ -y^{(i)} \\log\\left(h_\\theta\\left( x^{(i)} \\right) \\right) - \\left( 1 - y^{(i)}\\right) \\log \\left( 1 - h_\\theta\\left( x^{(i)} \\right) \\right) \\right]$$\n\nand the gradient of the cost is a vector of the same length as $\\theta$ where the $j^{th}$\nelement (for $j = 0, 1, \\cdots , n$) is defined as follows:\n\n$$ \\frac{\\partial J(\\theta)}{\\partial \\theta_j} = \\frac{1}{m} \\sum_{i=1}^m \\left( h_\\theta \\left( x^{(i)} \\right) - y^{(i)} \\right) x_j^{(i)} $$\n\nNote that while this gradient looks identical to the linear regression gradient, the formula is actually different because linear and logistic regression have different definitions of $h_\\theta(x)$.\n<a id=\"costFunction\"></a>",
"_____no_output_____"
]
],
[
[
"def costFunction(theta, X, y):\n \"\"\"\n Compute cost and gradient for logistic regression. \n \n Parameters\n ----------\n theta : array_like\n The parameters for logistic regression. This a vector\n of shape (n+1, ).\n \n X : array_like\n The input dataset of shape (m x n+1) where m is the total number\n of data points and n is the number of features. We assume the \n intercept has already been added to the input.\n \n y : arra_like\n Labels for the input. This is a vector of shape (m, ).\n \n Returns\n -------\n J : float\n The computed value for the cost function. \n \n grad : array_like\n A vector of shape (n+1, ) which is the gradient of the cost\n function with respect to theta, at the current values of theta.\n \n Instructions\n ------------\n Compute the cost of a particular choice of theta. You should set J to \n the cost. Compute the partial derivatives and set grad to the partial\n derivatives of the cost w.r.t. each parameter in theta.\n \"\"\"\n # Initialize some useful values\n m = y.size # number of training examples\n\n # You need to return the following variables correctly \n J = 0\n grad = np.zeros(theta.shape)\n\n # ====================== YOUR CODE HERE ======================\n h = sigmoid(np.dot(X, theta))\n temp = -np.dot(np.log(h).T, y) - np.dot(np.log(1-h).T, 1- y)\n J = np.sum(temp) / m\n \n grad = np.dot(X.T, (h-y)) / m\n if np.isnan(J):\n J = np.inf \n # =============================================================\n return J, grad",
"_____no_output_____"
]
],
[
[
"Once you are done call your `costFunction` using two test cases for $\\theta$ by executing the next cell.",
"_____no_output_____"
]
],
[
[
"# Initialize fitting parameters\ninitial_theta = np.zeros(n+1)\n\ncost, grad = costFunction(initial_theta, X, y)\n\nprint('Cost at initial theta (zeros): {:.3f}'.format(cost))\nprint('Expected cost (approx): 0.693\\n')\n\nprint('Gradient at initial theta (zeros):')\nprint('\\t[{:.4f}, {:.4f}, {:.4f}]'.format(*grad))\nprint('Expected gradients (approx):\\n\\t[-0.1000, -12.0092, -11.2628]\\n')\n\n# Compute and display cost and gradient with non-zero theta\ntest_theta = np.array([-24, 0.2, 0.2])\ncost, grad = costFunction(test_theta, X, y)\n\nprint('Cost at test theta: {:.3f}'.format(cost))\nprint('Expected cost (approx): 0.218\\n')\n\nprint('Gradient at test theta:')\nprint('\\t[{:.3f}, {:.3f}, {:.3f}]'.format(*grad))\nprint('Expected gradients (approx):\\n\\t[0.043, 2.566, 2.647]')",
"Cost at initial theta (zeros): 0.693\nExpected cost (approx): 0.693\n\nGradient at initial theta (zeros):\n\t[-0.1000, -12.0092, -11.2628]\nExpected gradients (approx):\n\t[-0.1000, -12.0092, -11.2628]\n\nCost at test theta: 0.218\nExpected cost (approx): 0.218\n\nGradient at test theta:\n\t[0.043, 2.566, 2.647]\nExpected gradients (approx):\n\t[0.043, 2.566, 2.647]\n"
]
],
[
[
"*You should now submit your solutions.*",
"_____no_output_____"
]
],
[
[
"grader[2] = costFunction\ngrader[3] = costFunction\ngrader.grade()",
"\nSubmitting Solutions | Programming Exercise logistic-regression\n\nUse token from last successful submission ([email protected])? (Y/n): y\n Part Name | Score | Feedback\n --------- | ----- | --------\n Sigmoid Function | 5 / 5 | Nice work!\n Logistic Regression Cost | 30 / 30 | Nice work!\n Logistic Regression Gradient | 30 / 30 | Nice work!\n Predict | 0 / 5 | \n Regularized Logistic Regression Cost | 0 / 15 | \n Regularized Logistic Regression Gradient | 0 / 15 | \n --------------------------------\n | 65 / 100 | \n\n"
]
],
[
[
"#### 1.2.3 Learning parameters using `scipy.optimize`\n\nIn the previous assignment, you found the optimal parameters of a linear regression model by implementing gradient descent. You wrote a cost function and calculated its gradient, then took a gradient descent step accordingly. This time, instead of taking gradient descent steps, you will use the [`scipy.optimize` module](https://docs.scipy.org/doc/scipy/reference/optimize.html). SciPy is a numerical computing library for `python`. It provides an optimization module for root finding and minimization. As of `scipy 1.0`, the function `scipy.optimize.minimize` is the method to use for optimization problems(both constrained and unconstrained).\n\nFor logistic regression, you want to optimize the cost function $J(\\theta)$ with parameters $\\theta$.\nConcretely, you are going to use `optimize.minimize` to find the best parameters $\\theta$ for the logistic regression cost function, given a fixed dataset (of X and y values). You will pass to `optimize.minimize` the following inputs:\n- `costFunction`: A cost function that, when given the training set and a particular $\\theta$, computes the logistic regression cost and gradient with respect to $\\theta$ for the dataset (X, y). It is important to note that we only pass the name of the function without the parenthesis. This indicates that we are only providing a reference to this function, and not evaluating the result from this function.\n- `initial_theta`: The initial values of the parameters we are trying to optimize.\n- `(X, y)`: These are additional arguments to the cost function.\n- `jac`: Indication if the cost function returns the Jacobian (gradient) along with cost value. (True)\n- `method`: Optimization method/algorithm to use\n- `options`: Additional options which might be specific to the specific optimization method. In the following, we only tell the algorithm the maximum number of iterations before it terminates.\n\nIf you have completed the `costFunction` correctly, `optimize.minimize` will converge on the right optimization parameters and return the final values of the cost and $\\theta$ in a class object. Notice that by using `optimize.minimize`, you did not have to write any loops yourself, or set a learning rate like you did for gradient descent. This is all done by `optimize.minimize`: you only needed to provide a function calculating the cost and the gradient.\n\nIn the following, we already have code written to call `optimize.minimize` with the correct arguments.",
"_____no_output_____"
]
],
[
[
"# set options for optimize.minimize\noptions= {'maxiter': 400}\n\n# see documention for scipy's optimize.minimize for description about\n# the different parameters\n# The function returns an object `OptimizeResult`\n# We use truncated Newton algorithm for optimization which is \n# equivalent to MATLAB's fminunc\n# See https://stackoverflow.com/questions/18801002/fminunc-alternate-in-numpy\nres = optimize.minimize(costFunction,\n initial_theta,\n (X, y),\n jac=True,\n method='TNC',\n options=options)\n\n# the fun property of `OptimizeResult` object returns\n# the value of costFunction at optimized theta\ncost = res.fun\n\n# the optimized theta is in the x property\ntheta = res.x\n\n# Print theta to screen\nprint('Cost at theta found by optimize.minimize: {:.3f}'.format(cost))\nprint('Expected cost (approx): 0.203\\n');\n\nprint('theta:')\nprint('\\t[{:.3f}, {:.3f}, {:.3f}]'.format(*theta))\nprint('Expected theta (approx):\\n\\t[-25.161, 0.206, 0.201]')",
"Cost at theta found by optimize.minimize: 0.203\nExpected cost (approx): 0.203\n\ntheta:\n\t[-25.161, 0.206, 0.201]\nExpected theta (approx):\n\t[-25.161, 0.206, 0.201]\n"
]
],
[
[
"Once `optimize.minimize` completes, we want to use the final value for $\\theta$ to visualize the decision boundary on the training data as shown in the figure below. \n\n\n\nTo do so, we have written a function `plotDecisionBoundary` for plotting the decision boundary on top of training data. You do not need to write any code for plotting the decision boundary, but we also encourage you to look at the code in `plotDecisionBoundary` to see how to plot such a boundary using the $\\theta$ values. You can find this function in the `utils.py` file which comes with this assignment.",
"_____no_output_____"
]
],
[
[
"# Plot Boundary\nutils.plotDecisionBoundary(plotData, theta, X, y)",
"_____no_output_____"
]
],
[
[
"<a id=\"section4\"></a>\n#### 1.2.4 Evaluating logistic regression\n\nAfter learning the parameters, you can use the model to predict whether a particular student will be admitted. For a student with an Exam 1 score of 45 and an Exam 2 score of 85, you should expect to see an admission\nprobability of 0.776. Another way to evaluate the quality of the parameters we have found is to see how well the learned model predicts on our training set. In this part, your task is to complete the code in function `predict`. The predict function will produce “1” or “0” predictions given a dataset and a learned parameter vector $\\theta$. \n<a id=\"predict\"></a>",
"_____no_output_____"
]
],
[
[
"def predict(theta, X):\n \"\"\"\n Predict whether the label is 0 or 1 using learned logistic regression.\n Computes the predictions for X using a threshold at 0.5 \n (i.e., if sigmoid(theta.T*x) >= 0.5, predict 1)\n \n Parameters\n ----------\n theta : array_like\n Parameters for logistic regression. A vecotor of shape (n+1, ).\n \n X : array_like\n The data to use for computing predictions. The rows is the number \n of points to compute predictions, and columns is the number of\n features.\n\n Returns\n -------\n p : array_like\n Predictions and 0 or 1 for each row in X. \n \n Instructions\n ------------\n Complete the following code to make predictions using your learned \n logistic regression parameters.You should set p to a vector of 0's and 1's \n \"\"\"\n m = X.shape[0] # Number of training examples\n\n # You need to return the following variables correctly\n p = np.zeros(m)\n \n # ====================== YOUR CODE HERE ======================\n threshold = 0.5\n h = sigmoid(np.dot(X, theta.T))\n p = h >= threshold #for each row in h(x), check if >= 0.5, if yes then set to one\n \n # ============================================================\n return p",
"_____no_output_____"
]
],
[
[
"After you have completed the code in `predict`, we proceed to report the training accuracy of your classifier by computing the percentage of examples it got correct.",
"_____no_output_____"
]
],
[
[
"# Predict probability for a student with score 45 on exam 1 \n# and score 85 on exam 2 \nprob = sigmoid(np.dot([1, 45, 85], theta))\nprint('For a student with scores 45 and 85,'\n 'we predict an admission probability of {:.3f}'.format(prob))\nprint('Expected value: 0.775 +/- 0.002\\n')\n\n# Compute accuracy on our training set\np = predict(theta, X)\nprint('Train Accuracy: {:.2f} %'.format(np.mean(p == y) * 100))\nprint('Expected accuracy (approx): 89.00 %')",
"For a student with scores 45 and 85,we predict an admission probability of 0.776\nExpected value: 0.775 +/- 0.002\n\nTrain Accuracy: 89.00 %\nExpected accuracy (approx): 89.00 %\n"
]
],
[
[
"*You should now submit your solutions.*",
"_____no_output_____"
]
],
[
[
"grader[4] = predict\ngrader.grade()",
"\nSubmitting Solutions | Programming Exercise logistic-regression\n\nUse token from last successful submission ([email protected])? (Y/n): y\n Part Name | Score | Feedback\n --------- | ----- | --------\n Sigmoid Function | 5 / 5 | Nice work!\n Logistic Regression Cost | 30 / 30 | Nice work!\n Logistic Regression Gradient | 30 / 30 | Nice work!\n Predict | 5 / 5 | Nice work!\n Regularized Logistic Regression Cost | 0 / 15 | \n Regularized Logistic Regression Gradient | 0 / 15 | \n --------------------------------\n | 70 / 100 | \n\n"
]
],
[
[
"## 2 Regularized logistic regression\n\nIn this part of the exercise, you will implement regularized logistic regression to predict whether microchips from a fabrication plant passes quality assurance (QA). During QA, each microchip goes through various tests to ensure it is functioning correctly.\nSuppose you are the product manager of the factory and you have the test results for some microchips on two different tests. From these two tests, you would like to determine whether the microchips should be accepted or rejected. To help you make the decision, you have a dataset of test results on past microchips, from which you can build a logistic regression model.\n\nFirst, we load the data from a CSV file:",
"_____no_output_____"
]
],
[
[
"# Load Data\n# The first two columns contains the X values and the third column\n# contains the label (y).\ndata = np.loadtxt(os.path.join('Data', 'ex2data2.txt'), delimiter=',')\nX = data[:, :2]\ny = data[:, 2]",
"_____no_output_____"
]
],
[
[
"### 2.1 Visualize the data\n\nSimilar to the previous parts of this exercise, `plotData` is used to generate a figure, where the axes are the two test scores, and the positive (y = 1, accepted) and negative (y = 0, rejected) examples are shown with\ndifferent markers.",
"_____no_output_____"
]
],
[
[
"plotData(X, y)\n# Labels and Legend\npyplot.xlabel('Microchip Test 1')\npyplot.ylabel('Microchip Test 2')\n\n# Specified in plot order\npyplot.legend(['y = 1', 'y = 0'], loc='upper right')\npass",
"_____no_output_____"
]
],
[
[
"The above figure shows that our dataset cannot be separated into positive and negative examples by a straight-line through the plot. Therefore, a straight-forward application of logistic regression will not perform well on this dataset since logistic regression will only be able to find a linear decision boundary.\n\n### 2.2 Feature mapping\n\nOne way to fit the data better is to create more features from each data point. In the function `mapFeature` defined in the file `utils.py`, we will map the features into all polynomial terms of $x_1$ and $x_2$ up to the sixth power.\n\n$$ \\text{mapFeature}(x) = \\begin{bmatrix} 1 & x_1 & x_2 & x_1^2 & x_1 x_2 & x_2^2 & x_1^3 & \\dots & x_1 x_2^5 & x_2^6 \\end{bmatrix}^T $$\n\nAs a result of this mapping, our vector of two features (the scores on two QA tests) has been transformed into a 28-dimensional vector. A logistic regression classifier trained on this higher-dimension feature vector will have a more complex decision boundary and will appear nonlinear when drawn in our 2-dimensional plot.\nWhile the feature mapping allows us to build a more expressive classifier, it also more susceptible to overfitting. In the next parts of the exercise, you will implement regularized logistic regression to fit the data and also see for yourself how regularization can help combat the overfitting problem.\n",
"_____no_output_____"
]
],
[
[
"# Note that mapFeature also adds a column of ones for us, so the intercept\n# term is handled\nX = utils.mapFeature(X[:, 0], X[:, 1])",
"_____no_output_____"
]
],
[
[
"<a id=\"section5\"></a>\n### 2.3 Cost function and gradient\n\nNow you will implement code to compute the cost function and gradient for regularized logistic regression. Complete the code for the function `costFunctionReg` below to return the cost and gradient.\n\nRecall that the regularized cost function in logistic regression is\n\n$$ J(\\theta) = \\frac{1}{m} \\sum_{i=1}^m \\left[ -y^{(i)}\\log \\left( h_\\theta \\left(x^{(i)} \\right) \\right) - \\left( 1 - y^{(i)} \\right) \\log \\left( 1 - h_\\theta \\left( x^{(i)} \\right) \\right) \\right] + \\frac{\\lambda}{2m} \\sum_{j=1}^n \\theta_j^2 $$\n\nNote that you should not regularize the parameters $\\theta_0$. The gradient of the cost function is a vector where the $j^{th}$ element is defined as follows:\n\n$$ \\frac{\\partial J(\\theta)}{\\partial \\theta_0} = \\frac{1}{m} \\sum_{i=1}^m \\left( h_\\theta \\left(x^{(i)}\\right) - y^{(i)} \\right) x_j^{(i)} \\qquad \\text{for } j =0 $$\n\n$$ \\frac{\\partial J(\\theta)}{\\partial \\theta_j} = \\left( \\frac{1}{m} \\sum_{i=1}^m \\left( h_\\theta \\left(x^{(i)}\\right) - y^{(i)} \\right) x_j^{(i)} \\right) + \\frac{\\lambda}{m}\\theta_j \\qquad \\text{for } j \\ge 1 $$\n<a id=\"costFunctionReg\"></a>",
"_____no_output_____"
]
],
[
[
"def costFunctionReg(theta, X, y, lambda_):\n \"\"\"\n Compute cost and gradient for logistic regression with regularization.\n \n Parameters\n ----------\n theta : array_like\n Logistic regression parameters. A vector with shape (n, ). n is \n the number of features including any intercept. If we have mapped\n our initial features into polynomial features, then n is the total \n number of polynomial features. \n \n X : array_like\n The data set with shape (m x n). m is the number of examples, and\n n is the number of features (after feature mapping).\n \n y : array_like\n The data labels. A vector with shape (m, ).\n \n lambda_ : float\n The regularization parameter. \n \n Returns\n -------\n J : float\n The computed value for the regularized cost function. \n \n grad : array_like\n A vector of shape (n, ) which is the gradient of the cost\n function with respect to theta, at the current values of theta.\n \n Instructions\n ------------\n Compute the cost `J` of a particular choice of theta.\n Compute the partial derivatives and set `grad` to the partial\n derivatives of the cost w.r.t. each parameter in theta.\n \"\"\"\n # Initialize some useful values\n m = y.size # number of training examples\n\n # You need to return the following variables correctly \n J = 0\n grad = np.zeros(theta.shape)\n\n # ===================== YOUR CODE HERE ======================\n J, grad = costFunction(theta, X, y) #from old costFunction without reg. param\n J = J + (lambda_/(2*m))*np.sum(np.square(theta[1:]))\n h = sigmoid(X.dot(theta))\n if np.isnan(J):\n J = np.inf\n grad = grad + (lambda_/m)*(theta)\n grad[0] = grad[0] - (lambda_/m)*theta[0] #remove regularization for first theta\n\n # =============================================================\n return J, grad.flatten()",
"_____no_output_____"
]
],
[
[
"Once you are done with the `costFunctionReg`, we call it below using the initial value of $\\theta$ (initialized to all zeros), and also another test case where $\\theta$ is all ones.",
"_____no_output_____"
]
],
[
[
"# Initialize fitting parameters\ninitial_theta = np.zeros(X.shape[1])\n\n# Set regularization parameter lambda to 1\n# DO NOT use `lambda` as a variable name in python\n# because it is a python keyword\nlambda_ = 1\n\n# Compute and display initial cost and gradient for regularized logistic\n# regression\ncost, grad = costFunctionReg(initial_theta, X, y, lambda_)\n\nprint('Cost at initial theta (zeros): {:.3f}'.format(cost))\nprint('Expected cost (approx) : 0.693\\n')\n\nprint('Gradient at initial theta (zeros) - first five values only:')\nprint('\\t[{:.4f}, {:.4f}, {:.4f}, {:.4f}, {:.4f}]'.format(*grad[:5]))\nprint('Expected gradients (approx) - first five values only:')\nprint('\\t[0.0085, 0.0188, 0.0001, 0.0503, 0.0115]\\n')\n\n\n# Compute and display cost and gradient\n# with all-ones theta and lambda = 10\ntest_theta = np.ones(X.shape[1])\ncost, grad = costFunctionReg(test_theta, X, y, 10)\n\nprint('------------------------------\\n')\nprint('Cost at test theta : {:.2f}'.format(cost))\nprint('Expected cost (approx): 3.16\\n')\n\nprint('Gradient at initial theta (zeros) - first five values only:')\nprint('\\t[{:.4f}, {:.4f}, {:.4f}, {:.4f}, {:.4f}]'.format(*grad[:5]))\nprint('Expected gradients (approx) - first five values only:')\nprint('\\t[0.3460, 0.1614, 0.1948, 0.2269, 0.0922]')",
"Cost at initial theta (zeros): 0.693\nExpected cost (approx) : 0.693\n\nGradient at initial theta (zeros) - first five values only:\n\t[0.0085, 0.0188, 0.0001, 0.0503, 0.0115]\nExpected gradients (approx) - first five values only:\n\t[0.0085, 0.0188, 0.0001, 0.0503, 0.0115]\n\n------------------------------\n\nCost at test theta : 3.16\nExpected cost (approx): 3.16\n\nGradient at initial theta (zeros) - first five values only:\n\t[0.3460, 0.1614, 0.1948, 0.2269, 0.0922]\nExpected gradients (approx) - first five values only:\n\t[0.3460, 0.1614, 0.1948, 0.2269, 0.0922]\n"
]
],
[
[
"*You should now submit your solutions.*",
"_____no_output_____"
]
],
[
[
"grader[5] = costFunctionReg\ngrader[6] = costFunctionReg\ngrader.grade()",
"\nSubmitting Solutions | Programming Exercise logistic-regression\n\nUse token from last successful submission ([email protected])? (Y/n): y\n Part Name | Score | Feedback\n --------- | ----- | --------\n Sigmoid Function | 5 / 5 | Nice work!\n Logistic Regression Cost | 30 / 30 | Nice work!\n Logistic Regression Gradient | 30 / 30 | Nice work!\n Predict | 5 / 5 | Nice work!\n Regularized Logistic Regression Cost | 15 / 15 | Nice work!\n Regularized Logistic Regression Gradient | 15 / 15 | Nice work!\n --------------------------------\n | 100 / 100 | \n\n"
]
],
[
[
"#### 2.3.1 Learning parameters using `scipy.optimize.minimize`\n\nSimilar to the previous parts, you will use `optimize.minimize` to learn the optimal parameters $\\theta$. If you have completed the cost and gradient for regularized logistic regression (`costFunctionReg`) correctly, you should be able to step through the next part of to learn the parameters $\\theta$ using `optimize.minimize`.",
"_____no_output_____"
],
[
"### 2.4 Plotting the decision boundary\n\nTo help you visualize the model learned by this classifier, we have provided the function `plotDecisionBoundary` which plots the (non-linear) decision boundary that separates the positive and negative examples. In `plotDecisionBoundary`, we plot the non-linear decision boundary by computing the classifier’s predictions on an evenly spaced grid and then and draw a contour plot where the predictions change from y = 0 to y = 1. ",
"_____no_output_____"
],
[
"### 2.5 Optional (ungraded) exercises\n\nIn this part of the exercise, you will get to try out different regularization parameters for the dataset to understand how regularization prevents overfitting.\n\nNotice the changes in the decision boundary as you vary $\\lambda$. With a small\n$\\lambda$, you should find that the classifier gets almost every training example correct, but draws a very complicated boundary, thus overfitting the data. See the following figures for the decision boundaries you should get for different values of $\\lambda$. \n\n<table>\n <tr>\n <td style=\"text-align:center\">\n No regularization (overfitting)<img src=\"Figures/decision_boundary3.png\">\n </td> \n <td style=\"text-align:center\">\n Decision boundary with regularization\n <img src=\"Figures/decision_boundary2.png\">\n </td>\n <td style=\"text-align:center\">\n Decision boundary with too much regularization\n <img src=\"Figures/decision_boundary4.png\">\n </td> \n <tr>\n</table>\n\nThis is not a good decision boundary: for example, it predicts that a point at $x = (−0.25, 1.5)$ is accepted $(y = 1)$, which seems to be an incorrect decision given the training set.\nWith a larger $\\lambda$, you should see a plot that shows an simpler decision boundary which still separates the positives and negatives fairly well. However, if $\\lambda$ is set to too high a value, you will not get a good fit and the decision boundary will not follow the data so well, thus underfitting the data.",
"_____no_output_____"
]
],
[
[
"# Initialize fitting parameters\ninitial_theta = np.zeros(X.shape[1])\n\n# Set regularization parameter lambda to 1 (you should vary this)\nlambdas = [0,0.5,1,10,100]\n\n# set options for optimize.minimize\noptions= {'maxiter': 100}\n\nfor lambda_ in lambdas:\n res = optimize.minimize(costFunctionReg,\n initial_theta,\n (X, y, lambda_),\n jac=True,\n method='TNC',\n options=options)\n\n # the fun property of OptimizeResult object returns\n # the value of costFunction at optimized theta\n cost = res.fun\n\n # the optimized theta is in the x property of the result\n theta = res.x\n\n utils.plotDecisionBoundary(plotData, theta, X, y)\n pyplot.xlabel('Microchip Test 1')\n pyplot.ylabel('Microchip Test 2')\n pyplot.legend(['y = 1', 'y = 0'])\n pyplot.grid(False)\n pyplot.title('lambda = %0.2f' % lambda_)\n\n # Compute accuracy on our training set\n p = predict(theta, X)\n\n print('Train Accuracy: %.1f %%' % (np.mean(p == y) * 100))\n print('Expected accuracy (with lambda = 1): 83.1 % (approx)\\n')\n",
"Train Accuracy: 86.4 %\nExpected accuracy (with lambda = 1): 83.1 % (approx)\n\nTrain Accuracy: 82.2 %\nExpected accuracy (with lambda = 1): 83.1 % (approx)\n\nTrain Accuracy: 83.1 %\nExpected accuracy (with lambda = 1): 83.1 % (approx)\n\nTrain Accuracy: 74.6 %\nExpected accuracy (with lambda = 1): 83.1 % (approx)\n\nTrain Accuracy: 61.0 %\nExpected accuracy (with lambda = 1): 83.1 % (approx)\n\n"
]
],
[
[
"*You do not need to submit any solutions for these optional (ungraded) exercises.*",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
]
] |
e7b9e4afe19912be88e5fa52062d8f10d7fff0a9 | 1,904 | ipynb | Jupyter Notebook | work/demo.ipynb | vladiuz1/py3-jupyter-docker-compose | bb758f2d36a187b5224143ff3f0908ebcae0a172 | [
"Apache-2.0"
] | 2 | 2021-09-14T17:08:21.000Z | 2021-11-11T17:28:30.000Z | work/demo.ipynb | vladiuz1/py3-jupyter-docker-compose | bb758f2d36a187b5224143ff3f0908ebcae0a172 | [
"Apache-2.0"
] | null | null | null | work/demo.ipynb | vladiuz1/py3-jupyter-docker-compose | bb758f2d36a187b5224143ff3f0908ebcae0a172 | [
"Apache-2.0"
] | 2 | 2021-03-29T02:09:57.000Z | 2021-11-15T12:57:50.000Z | 19.833333 | 174 | 0.522584 | [
[
[
"# Working directory\n\nThe notebooks that you save here persist in the host filesystem. ",
"_____no_output_____"
]
],
[
[
"import os\nprint(os.getcwd())\nfor d in os.listdir():\n print(f'\\t {d}')",
"/home/jovyan/work\n\t .ipynb_checkpoints\n\t demo.ipynb\n"
]
],
[
[
"# requirements.txt\n\nThe `requirements.txt` file contains list of modules you want to use in your notebook. The demo `requirements.txt` has flask module, check if below runs without errors:",
"_____no_output_____"
]
],
[
[
"import flask\nprint('No problem, flask module installed.')",
"No problem, flask module installed.\n"
]
]
] | [
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7b9ec060193b7f42809210172ab1e6f3a018d34 | 60,031 | ipynb | Jupyter Notebook | im/ib/data/extract/gateway/notebooks/Task114_Download_futures_price_data_from_IB.ipynb | alphamatic/amp | 5018137097159415c10eaa659a2e0de8c4e403d4 | [
"BSD-3-Clause"
] | 5 | 2021-08-10T23:16:44.000Z | 2022-03-17T17:27:00.000Z | im/ib/data/extract/gateway/notebooks/Task114_Download_futures_price_data_from_IB.ipynb | alphamatic/amp | 5018137097159415c10eaa659a2e0de8c4e403d4 | [
"BSD-3-Clause"
] | 330 | 2021-06-10T17:28:22.000Z | 2022-03-31T00:55:48.000Z | im/ib/data/extract/gateway/notebooks/Task114_Download_futures_price_data_from_IB.ipynb | alphamatic/amp | 5018137097159415c10eaa659a2e0de8c4e403d4 | [
"BSD-3-Clause"
] | 6 | 2021-06-10T17:20:32.000Z | 2022-03-28T08:08:03.000Z | 37.755346 | 1,514 | 0.472856 | [
[
[
"# Imports",
"_____no_output_____"
]
],
[
[
"%load_ext autoreload\n%autoreload 2\n\nimport ib_insync\nprint(ib_insync.__all__)\n\nimport helpers.hdbg as dbg\nimport helpers.hprint as pri\nimport core.explore as exp\nimport im.ib.data.extract.gateway.utils as ibutils",
"_____no_output_____"
]
],
[
[
"# Connect",
"_____no_output_____"
]
],
[
[
"ib = ibutils.ib_connect(client_id=100, is_notebook=True)",
"_____no_output_____"
]
],
[
[
"# Historical data",
"_____no_output_____"
]
],
[
[
"import logging\n#dbg.init_logger(verbosity=logging.DEBUG)\ndbg.init_logger(verbosity=logging.INFO)",
"_____no_output_____"
],
[
"import datetime",
"_____no_output_____"
],
[
"# start_ts = pd.to_datetime(pd.Timestamp(\"2018-02-01 06:00:00\").tz_localize(tz=\"America/New_York\"))\n# import datetime\n\n# #datetime.datetime.combine(start_ts, datetime.time())",
"_____no_output_____"
],
[
"# dt = start_ts.to_pydatetime()\n# print(dt)\n\n# #datetime.datetime.combine(dt, datetime.time()).tz_localize(tz=\"America/New_York\")\n# dt.replace(hour=0, minute=0, second=0)",
"_____no_output_____"
],
[
"start_ts = pd.Timestamp(\"2019-05-28 15:00\").tz_localize(tz=\"America/New_York\")\nend_ts = pd.Timestamp(\"2019-05-29 15:00\").tz_localize(tz=\"America/New_York\")\nbarSizeSetting = \"1 hour\"\n\nbars = ibutils.get_data(ib, contract, start_ts, end_ts, barSizeSetting, whatToShow, useRTH)",
"_____no_output_____"
],
[
"start_ts = pd.Timestamp(\"2019-05-28 15:00\").tz_localize(tz=\"America/New_York\")\nend_ts = pd.Timestamp(\"2019-05-29 15:00\").tz_localize(tz=\"America/New_York\")\nbarSizeSetting = \"1 hour\"\n\nbars = ibutils.get_data(ib, contract, start_ts, end_ts, barSizeSetting, whatToShow, useRTH)",
"_____no_output_____"
],
[
"start_ts = pd.Timestamp(\"2019-05-27\").tz_localize(tz=\"America/New_York\")\nend_ts = pd.Timestamp(\"2019-05-28\").tz_localize(tz=\"America/New_York\")\nbarSizeSetting = \"1 hour\"\n\nbars = ibutils.get_data(ib, contract, start_ts, end_ts, barSizeSetting, whatToShow, useRTH)",
"_____no_output_____"
],
[
"start_ts2 = start_ts - pd.DateOffset(days=1)\nend_ts2 = end_ts + pd.DateOffset(days=1)\nbarSizeSetting = \"1 hour\"\n\nbars2 = ibutils.get_data(ib, contract, start_ts2, end_ts2, barSizeSetting, whatToShow, useRTH)",
"_____no_output_____"
],
[
"set(bars.index).issubset(bars2.index)",
"_____no_output_____"
],
[
"start_ts = pd.Timestamp(\"2019-04-01 15:00\").tz_localize(tz=\"America/New_York\")\nend_ts = pd.Timestamp(\"2019-05-01 15:00\").tz_localize(tz=\"America/New_York\")\n\ndf = ibutils.get_historical_data2(ib, contract, start_ts, end_ts, barSizeSetting, whatToShow, useRTH)",
"_____no_output_____"
],
[
"import pandas as pd\ncontract = ib_insync.ContFuture(\"ES\", \"GLOBEX\", \"USD\")\nwhatToShow = 'TRADES'\ndurationStr = '2 D'\nbarSizeSetting = '1 min' \nuseRTH = False\n#useRTH = True\n\n#start_ts = pd.to_datetime(pd.Timestamp(\"2018-02-01\"))\n# Saturday June 1, 2019\nend_ts = pd.to_datetime(pd.Timestamp(\"2019-05-30 00:00:00\") + pd.DateOffset(days=1))\n#end_ts = pd.to_datetime(pd.Timestamp(\"2019-05-30 18:00:00\"))\n#print(start_ts, end_ts)\nprint(\"end_ts=\", end_ts)\n\nbars = ibutils.get_historical_data(ib, contract, end_ts, durationStr, barSizeSetting, whatToShow, useRTH)\n\nprint(\"durationStr=%s barSizeSetting=%s useRTH=%s\" % (durationStr, barSizeSetting, useRTH))\nprint(\"bars=[%s, %s]\" % (bars.index[0], bars.index[-1]))\nprint(\"diff=\", bars.index[-1] - bars.index[0])\n\nbars[\"close\"].plot()",
"_____no_output_____"
],
[
"pd.date_range(start='2019-04-01 00:00:00', end='2019-05-01 00:00:00', freq='2D')",
"_____no_output_____"
],
[
"(bars.index[-1] - bars.index[0])",
"_____no_output_____"
],
[
"import pandas as pd",
"_____no_output_____"
],
[
"# 1 = Live\n# 2 = Frozen\n# 3 = Delayed\n# 4 = Delayed frozen\nib.reqMarketDataType(4)\n\nif False:\n contract = ib_insync.Stock('TSLA', 'SMART', 'USD')\n whatToShow = 'TRADES'\nelif False:\n contract = ib_insync.Future('ES', '202109', 'GLOBEX')\n whatToShow = 'TRADES'\nelif True:\n contract = ib_insync.ContFuture(\"ES\", \"GLOBEX\", \"USD\")\n whatToShow = 'TRADES'\nelse:\n contract = ib_insync.Forex('EURUSD')\n whatToShow = 'MIDPOINT'\n\nif False:\n durationStr = '1 Y'\n barSizeSetting = '1 day'\n #barSizeSetting='1 hour'\nelse:\n durationStr = '1 D'\n barSizeSetting = '1 hour'\n\nprint(\"contract=\", contract)\nprint(\"whatToShow=\", whatToShow)\nprint(\"durationStr=\", durationStr)\nprint(\"barSizeSetting=\", barSizeSetting)\n\n#endDateTime = pd.Timestamp(\"2020-12-11 18:00:00\")\nendDateTime = pd.Timestamp(\"2020-12-13 18:00:00\")\n#endDateTime = \"\"\n\n# Get the datetime of earliest available historical data for the contract.\nstart_ts = ib.reqHeadTimeStamp(contract, whatToShow=whatToShow, useRTH=True)\nprint(\"start_ts=\", start_ts)\nbars = ib.reqHistoricalData(\n contract,\n endDateTime=endDateTime,\n durationStr=durationStr,\n barSizeSetting=barSizeSetting,\n whatToShow=whatToShow,\n useRTH=True,\n formatDate=1)\nprint(\"len(bars)=\", len(bars))\nprint(ib_insync.util.df(bars))",
"_____no_output_____"
],
[
"ib_insync.IB.RaiseRequestErrors = True",
"_____no_output_____"
],
[
"bars",
"_____no_output_____"
],
[
"dbg.shutup_chatty_modules(verbose=True)",
"_____no_output_____"
],
[
"import pandas as pd\ncontract = ib_insync.ContFuture(\"ES\", \"GLOBEX\", \"USD\")\nwhatToShow = 'TRADES'\ndurationStr = '2 D'\nbarSizeSetting = '1 min' \nuseRTH = False\n\nstart_ts = pd.Timestamp(\"2018-01-28 15:00\").tz_localize(tz=\"America/New_York\")\nend_ts = pd.Timestamp(\"2018-02-28 15:00\").tz_localize(tz=\"America/New_York\")\n\ntasks = ibutils.get_historical_data_workload(contract, start_ts, end_ts, barSizeSetting, whatToShow, useRTH)\nprint(len(tasks))\n\nibutils.get_historical_data2(ib, tasks)",
"_____no_output_____"
]
],
[
[
"## ",
"_____no_output_____"
]
],
[
[
"%load_ext autoreload\n%autoreload 2\n\nimport ib_insync\nprint(ib_insync.__all__)\n\nimport helpers.hdbg as dbg\nimport helpers.hprint as pri\nimport core.explore as exp\nimport im.ib.data.extract.gateway.utils as ibutils",
"The autoreload extension is already loaded. To reload it, use:\n %reload_ext autoreload\n['util', 'Event', 'SoftDollarTier', 'PriceIncrement', 'Execution', 'CommissionReport', 'BarList', 'BarDataList', 'RealTimeBarList', 'BarData', 'RealTimeBar', 'HistogramData', 'NewsProvider', 'DepthMktDataDescription', 'ScannerSubscription', 'ScanDataList', 'FundamentalRatios', 'ExecutionFilter', 'PnL', 'PnLSingle', 'AccountValue', 'TickData', 'TickByTickAllLast', 'TickByTickBidAsk', 'TickByTickMidPoint', 'HistoricalTick', 'HistoricalTickBidAsk', 'HistoricalTickLast', 'TickAttrib', 'TickAttribBidAsk', 'TickAttribLast', 'MktDepthData', 'DOMLevel', 'TradeLogEntry', 'FamilyCode', 'SmartComponent', 'PortfolioItem', 'Position', 'Fill', 'OptionComputation', 'OptionChain', 'Dividends', 'NewsArticle', 'HistoricalNews', 'NewsTick', 'NewsBulletin', 'ConnectionStats', 'Contract', 'Stock', 'Option', 'Future', 'ContFuture', 'Forex', 'Index', 'CFD', 'Commodity', 'Bond', 'FuturesOption', 'MutualFund', 'Warrant', 'Bag', 'TagValue', 'ComboLeg', 'DeltaNeutralContract', 'ContractDetails', 'ContractDescription', 'ScanData', 'Trade', 'Order', 'OrderStatus', 'OrderState', 'OrderComboLeg', 'LimitOrder', 'MarketOrder', 'StopOrder', 'StopLimitOrder', 'BracketOrder', 'OrderCondition', 'ExecutionCondition', 'MarginCondition', 'TimeCondition', 'PriceCondition', 'PercentChangeCondition', 'VolumeCondition', 'Ticker', 'IB', 'Client', 'RequestError', 'Wrapper', 'FlexReport', 'FlexError', 'IBC', 'IBController', 'Watchdog']\n"
],
[
"import logging\ndbg.init_logger(verbosity=logging.DEBUG)\n#dbg.init_logger(verbosity=logging.INFO)\n\nimport pandas as pd\n\ndbg.shutup_chatty_modules(verbose=False)",
"\u001b[0mWARNING: Running in Jupyter\nShutting up 34 modules\n"
],
[
"ib = ibutils.ib_connect(8, is_notebook=True)",
"Connected!\n"
],
[
"# #start_ts = pd.Timestamp(\"2018-01-28 15:00\").tz_localize(tz=\"America/New_York\")\n# start_ts = pd.Timestamp(\"2018-01-28 18:00\").tz_localize(tz=\"America/New_York\")\n# end_ts = pd.Timestamp(\"2018-02-28 15:00\").tz_localize(tz=\"America/New_York\")\n\n# dates = []\n# if (start_ts.hour, start_ts.minute) > (18, 0):\n# dates = [start_ts]\n# # Align start_ts to 18:00.\n# start_ts = start_ts.replace(hour=18, minute=18)\n# elif (start_ts.hour, start_ts.minute) < (18, 0):\n# dates = [start_ts]\n# # Align start_ts to 18:00 of the day before.\n# start_ts = start_ts.replace(hour=18, minute=18)\n# start_ts -= pd.DateOffset(days=1)\n\n# dbg.dassert_eq((start_ts.hour, start_ts.minute), (18, 0))\n# dates += pd.date_range(start=start_ts, end=end_ts, freq='2D').tolist()\n# print(dates)",
"_____no_output_____"
],
[
"# import datetime\n# start_ts = pd.Timestamp(datetime.datetime(2017,6,25,0,31,53,993000))\n# print(start_ts)\n\n# start_ts.round('1s')",
"_____no_output_____"
],
[
"contract = ib_insync.ContFuture(\"ES\", \"GLOBEX\", \"USD\")\nwhatToShow = 'TRADES'\ndurationStr = '2 D'\nbarSizeSetting = '1 hour' \nuseRTH = False\n\nstart_ts = pd.Timestamp(\"2018-01-28 15:00\").tz_localize(tz=\"America/New_York\")\nend_ts = pd.Timestamp(\"2018-02-01 15:00\").tz_localize(tz=\"America/New_York\")\n\ntasks = ibutils.get_historical_data_workload(ib, contract, start_ts, end_ts, barSizeSetting,\n whatToShow, useRTH)\n\ndf = ibutils.get_historical_data2(tasks)",
"start_ts='2018-01-28 15:00:00-05:00' end_ts='2018-02-01 15:00:00-05:00'\nts='2018-01-28 15:00:00-05:00'\n-> ts='2018-01-29 18:00:00-05:00' dates=[Timestamp('2018-01-28 15:00:00-0500', tz='America/New_York')]\nts='2018-02-01 15:00:00-05:00'\n-> ts='2018-01-31 18:00:00-05:00' dates=[Timestamp('2018-02-01 15:00:00-0500', tz='America/New_York')]\nstart_ts_tmp='2018-01-29 18:00:00-05:00' end_ts_tmp='2018-01-31 18:00:00-05:00'\n-> dates=[Timestamp('2018-01-28 15:00:00-0500', tz='America/New_York'), Timestamp('2018-01-29 18:00:00-0500', tz='America/New_York', freq='2D'), Timestamp('2018-01-31 18:00:00-0500', tz='America/New_York', freq='2D'), Timestamp('2018-02-01 15:00:00-0500', tz='America/New_York')]\n"
],
[
"df ",
"_____no_output_____"
],
[
"df2 = ibutils.get_historical_data_with_IB_loop(ib, contract, start_ts, end_ts, durationStr,\n barSizeSetting,\n whatToShow, useRTH)",
"start_ts='2018-01-28 15:00:00-05:00' end_ts='2018-02-01 15:00:00-05:00'\nRequesting data for curr_ts='2018-02-01 15:00:00-05:00'\ndf=len=14 [2018-01-31 12:00:00-05:00, 2018-02-01 14:00:00-05:00]\ndf=len=14 [2018-01-31 12:00:00-05:00, 2018-02-01 14:00:00-05:00]\n open high low close volume average \\\ndate \n2018-01-31 12:00:00-05:00 2846.5 2846.5 2846.5 2846.5 2 2846.5 \n2018-01-31 13:00:00-05:00 2846.5 2846.5 2846.5 2846.5 0 2846.5 \n2018-01-31 14:00:00-05:00 2846.5 2846.5 2846.5 2846.5 0 2846.5 \n\n barCount \ndate \n2018-01-31 12:00:00-05:00 1 \n2018-01-31 13:00:00-05:00 0 \n2018-01-31 14:00:00-05:00 0 \ncurr_ts='2018-01-31 12:00:00-05:00'\nRequesting data for curr_ts='2018-01-31 12:00:00-05:00'\ndf=len=20 [2018-01-29 22:00:00-05:00, 2018-01-30 16:30:00-05:00]\ndf=len=20 [2018-01-29 22:00:00-05:00, 2018-01-30 16:30:00-05:00]\n open high low close volume \\\ndate \n2018-01-29 22:00:00-05:00 2859.25 2859.25 2859.25 2859.25 2 \n2018-01-29 23:00:00-05:00 2859.25 2859.25 2859.25 2859.25 0 \n2018-01-30 00:00:00-05:00 2859.25 2859.25 2859.25 2859.25 0 \n\n average barCount \ndate \n2018-01-29 22:00:00-05:00 2859.25 2 \n2018-01-29 23:00:00-05:00 2859.25 0 \n2018-01-30 00:00:00-05:00 2859.25 0 \ncurr_ts='2018-01-29 22:00:00-05:00'\nRequesting data for curr_ts='2018-01-29 22:00:00-05:00'\ndf=len=4 [2018-01-29 14:00:00-05:00, 2018-01-29 16:30:00-05:00]\ndf=len=4 [2018-01-29 14:00:00-05:00, 2018-01-29 16:30:00-05:00]\n open high low close volume average \\\ndate \n2018-01-29 14:00:00-05:00 2879.0 2879.0 2879.0 2879.0 1 2879.0 \n2018-01-29 15:00:00-05:00 2877.0 2877.0 2877.0 2877.0 1 2877.0 \n2018-01-29 16:00:00-05:00 2877.0 2877.0 2877.0 2877.0 0 2877.0 \n\n barCount \ndate \n2018-01-29 14:00:00-05:00 1 \n2018-01-29 15:00:00-05:00 1 \n2018-01-29 16:00:00-05:00 0 \ncurr_ts='2018-01-29 14:00:00-05:00'\nRequesting data for curr_ts='2018-01-29 14:00:00-05:00'\nAPI error: 162: Historical Market Data Service error message:HMDS query returned no data: ESH1@GLOBEX Trades\nAPI error: 162: Historical Market Data Service error message:HMDS query returned no data: ESH1@GLOBEX Trades\nAPI error: 162: Historical Market Data Service error message:HMDS query returned no data: ESH1@GLOBEX Trades\n"
],
[
"pri.print(df.index",
"_____no_output_____"
],
[
"df2",
"_____no_output_____"
],
[
"contract = ib_insync.ContFuture(\"ES\", \"GLOBEX\", \"USD\")\nwhatToShow = \"TRADES\"\ndurationStr = '1 D'\nbarSizeSetting = '1 hour'\n# 2021-02-18 is a Thursday and it's full day.\nstart_ts = pd.Timestamp(\"2021-02-17 00:00:00\")\nend_ts = pd.Timestamp(\"2021-02-18 23:59:59\")\nuseRTH = False\ndf, return_ts_seq = ibutils.get_historical_data_with_IB_loop(ib, contract, start_ts, end_ts, durationStr,\n barSizeSetting,\n whatToShow, useRTH, return_ts_seq=True)\nprint(return_ts_seq)",
"start_ts='2021-02-17 00:00:00-05:00' end_ts='2021-02-18 23:59:59-05:00'\nRequesting data for curr_ts='2021-02-18 23:59:59-05:00'\ndf=len=6 [2021-02-18 18:00:00-05:00, 2021-02-18 23:00:00-05:00]\ndf=len=6 [2021-02-18 18:00:00-05:00, 2021-02-18 23:00:00-05:00]\n open high low close volume \\\ndate \n2021-02-18 18:00:00-05:00 3910.50 3913.75 3903.50 3905.75 9597 \n2021-02-18 19:00:00-05:00 3905.75 3908.50 3904.50 3908.00 8642 \n2021-02-18 20:00:00-05:00 3908.00 3913.75 3892.75 3898.50 26612 \n\n average barCount \ndate \n2021-02-18 18:00:00-05:00 3908.975 3595 \n2021-02-18 19:00:00-05:00 3906.550 3496 \n2021-02-18 20:00:00-05:00 3901.050 9923 \ncurr_ts='2021-02-18 18:00:00-05:00'\nRequesting data for curr_ts='2021-02-18 18:00:00-05:00'\ndf=len=24 [2021-02-17 18:00:00-05:00, 2021-02-18 16:30:00-05:00]\ndf=len=24 [2021-02-17 18:00:00-05:00, 2021-02-18 16:30:00-05:00]\n open high low close volume \\\ndate \n2021-02-17 18:00:00-05:00 3928.25 3931.00 3924.00 3930.50 7578 \n2021-02-17 19:00:00-05:00 3930.75 3936.00 3930.00 3934.25 16020 \n2021-02-17 20:00:00-05:00 3934.25 3934.75 3924.25 3927.25 15627 \n\n average barCount \ndate \n2021-02-17 18:00:00-05:00 3927.350 2865 \n2021-02-17 19:00:00-05:00 3933.400 5666 \n2021-02-17 20:00:00-05:00 3929.625 5772 \ncurr_ts='2021-02-17 18:00:00-05:00'\nRequesting data for curr_ts='2021-02-17 18:00:00-05:00'\ndf=len=24 [2021-02-16 18:00:00-05:00, 2021-02-17 16:30:00-05:00]\ndf=len=24 [2021-02-16 18:00:00-05:00, 2021-02-17 16:30:00-05:00]\n open high low close volume \\\ndate \n2021-02-16 18:00:00-05:00 3929.00 3931.00 3919.75 3921.25 13505 \n2021-02-16 19:00:00-05:00 3921.25 3921.75 3915.50 3917.75 20567 \n2021-02-16 20:00:00-05:00 3917.75 3920.25 3913.00 3919.75 16179 \n\n average barCount \ndate \n2021-02-16 18:00:00-05:00 3925.200 4674 \n2021-02-16 19:00:00-05:00 3918.375 7639 \n2021-02-16 20:00:00-05:00 3916.525 5998 \ncurr_ts='2021-02-16 18:00:00-05:00'\nReached the beginning of the interval: curr_ts=2021-02-16 18:00:00-05:00 start_ts=2021-02-17 00:00:00-05:00\nBefore truncation: df=len=54 [2021-02-16 18:00:00-05:00, 2021-02-18 23:00:00-05:00]\ndf.head=\n open high low close volume \\\ndate \n2021-02-16 18:00:00-05:00 3929.00 3931.00 3919.75 3921.25 13505 \n2021-02-16 19:00:00-05:00 3921.25 3921.75 3915.50 3917.75 20567 \n2021-02-16 20:00:00-05:00 3917.75 3920.25 3913.00 3919.75 16179 \n\n average barCount \ndate \n2021-02-16 18:00:00-05:00 3925.200 4674 \n2021-02-16 19:00:00-05:00 3918.375 7639 \n2021-02-16 20:00:00-05:00 3916.525 5998 \ndf.tail=\n open high low close volume \\\ndate \n2021-02-18 21:00:00-05:00 3898.50 3899.25 3890.25 3892.25 16579 \n2021-02-18 22:00:00-05:00 3892.25 3896.75 3891.50 3893.75 7817 \n2021-02-18 23:00:00-05:00 3893.75 3899.00 3892.75 3897.50 5912 \n\n average barCount \ndate \n2021-02-18 21:00:00-05:00 3893.650 6849 \n2021-02-18 22:00:00-05:00 3894.375 3416 \n2021-02-18 23:00:00-05:00 3896.375 2496 \nstart_ts= 2021-02-17 00:00:00-05:00 end_ts3=2021-02-18 23:59:58-05:00\nAfter truncation: df=len=48 [2021-02-17 00:00:00-05:00, 2021-02-18 23:00:00-05:00]\n[(datetime.datetime(2021, 2, 18, 23, 59, 59, tzinfo=<DstTzInfo 'America/New_York' EST-1 day, 19:00:00 STD>), Timestamp('2021-02-18 18:00:00-0500', tz='America/New_York')), (Timestamp('2021-02-18 18:00:00-0500', tz='America/New_York'), Timestamp('2021-02-17 18:00:00-0500', tz='America/New_York')), (Timestamp('2021-02-17 18:00:00-0500', tz='America/New_York'), Timestamp('2021-02-16 18:00:00-0500', tz='America/New_York'))]\n"
],
[
"print(\"\\n\".join(map(str, return_ts_seq)))",
"(datetime.datetime(2021, 2, 18, 23, 59, 59, tzinfo=<DstTzInfo 'America/New_York' EST-1 day, 19:00:00 STD>), Timestamp('2021-02-18 18:00:00-0500', tz='America/New_York'))\n(Timestamp('2021-02-18 18:00:00-0500', tz='America/New_York'), Timestamp('2021-02-17 18:00:00-0500', tz='America/New_York'))\n(Timestamp('2021-02-17 18:00:00-0500', tz='America/New_York'), Timestamp('2021-02-16 18:00:00-0500', tz='America/New_York'))\n"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7b9fbc6b1dd211c753c012e38c389249678c3a0 | 15,916 | ipynb | Jupyter Notebook | doc/Tutorials/Sampling_Data.ipynb | stuarteberg/holoviews | 65136173014124b41cee00f5a0fee82acdc78f7f | [
"BSD-3-Clause"
] | 2 | 2020-08-13T00:11:46.000Z | 2021-01-31T22:13:21.000Z | doc/Tutorials/Sampling_Data.ipynb | stuarteberg/holoviews | 65136173014124b41cee00f5a0fee82acdc78f7f | [
"BSD-3-Clause"
] | null | null | null | doc/Tutorials/Sampling_Data.ipynb | stuarteberg/holoviews | 65136173014124b41cee00f5a0fee82acdc78f7f | [
"BSD-3-Clause"
] | 1 | 2021-10-31T05:26:08.000Z | 2021-10-31T05:26:08.000Z | 37.100233 | 729 | 0.624843 | [
[
[
"As explained in the [Composing Data](Composing_Data.ipynb) and [Containers](Containers.ipynb) tutorials, HoloViews allows you to build up hierarchical containers that express the natural relationships between your data items, in whatever multidimensional space best characterizes your application domain. Once your data is in such containers, individual visualizations are then made by choosing subregions of this multidimensional space, either smaller numeric ranges (as in cropping of photographic images), or lower-dimensional subsets (as in selecting frames from a movie, or a specific movie from a large library), or both (as in selecting a cropped version of a frame from a specific movie from a large library). \n\nIn this tutorial, we show how to specify such selections, using four different (but related) operations that can act on an element ``e``:\n\n| Operation | Example syntax | Description |\n|:---------------|:----------------:|:-------------|\n| **indexing** | e[5.5], e[3,5.5] | Selecting a single data value, returning one actual numerical value from the existing data\n| **slice** | e[3:5.5], e[3:5.5,0:1] | Selecting a contiguous portion from an Element, returning the same type of Element\n| **sample** | e.sample(y=5.5),<br>e.sample((3,3)) | Selecting one or more regularly spaced data values, returning a new type of Element\n| **select** | e.select(y=5.5),<br>e.select(y=(3,5.5)) | More verbose notation covering all supporting slice and index operations by dimension name.\n\nThese operations are all concerned with selecting some subset of your data values, without combining across data values (e.g. averaging) or otherwise transforming your actual data. In the [Columnar Data](Columnar_Data.ipynb) tutorial we will look at other operations on the data that reduce, summarize, or transform the data in other ways, rather than selections as covered here.\n\nWe'll be going through each operation in detail and provide a visual illustration to help make the semantics of each operation clear. This Tutorial assumes that you are familiar with continuous and discrete coordinate systems, so please review our [Continuous Coordinates Tutorial](Continuous_Coordinates.ipynb) if you have not done so already.",
"_____no_output_____"
],
[
"# Indexing and slicing Elements",
"_____no_output_____"
],
[
"In the [Exploring Data Tutorial](Exploring_Data.ipynb) we saw examples of how to select individual elements embedded in a multi-dimensional space. We also briefly introduced \"deep slicing\" of the ``RGB`` elements to select a subregion of the images. The [Continuous Coordinates Tutorial](Continuous_Coordinates.ipynb) covered slicing and indexing in Elements representing continuous coordinate coordinate systems such as ``Image`` types. Here we'll be going through each operation in full detail, providing a visual illustration to help make the semantics of each operation clear.\n\nHow the Element may be indexed depends on the key dimensions (or ``kdims``) of the Element. It is thus important to consider the nature and dimensionality of your data when choosing the Element type for it.",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport holoviews as hv\nhv.notebook_extension()\n%opts Layout [fig_size=125] Points [size_index=None] (s=50) Scatter3D [size_index=None]\n%opts Bounds (linewidth=2 color='k') {+axiswise} Text (fontsize=16 color='k') Image (cmap='Reds')",
"_____no_output_____"
]
],
[
[
"## 1D Elements: Slicing and indexing",
"_____no_output_____"
],
[
"Certain Chart elements support both single-dimensional indexing and slicing: ``Scatter``, ``Curve``, ``Histogram``, and ``ErrorBars``. Here we'll look at how we can easily slice a ``Histogram`` to select a subregion of it:",
"_____no_output_____"
]
],
[
[
"np.random.seed(42)\nedges, data = np.histogram(np.random.randn(100))\nhist = hv.Histogram(edges, data)\nsubregion = hist[0:1]\nhist * subregion",
"_____no_output_____"
]
],
[
[
"The two bins in a different color show the selected region, overlaid on top of the full histogram. We can also access the value for a specific bin in the ``Histogram``. A continuous-valued index that falls inside a particular bin will return the corresponding value or frequency.",
"_____no_output_____"
]
],
[
[
"hist[0.25], hist[0.5], hist[0.55]",
"_____no_output_____"
]
],
[
[
"We can slice a ``Curve`` the same way:",
"_____no_output_____"
]
],
[
[
"xs = np.linspace(0, np.pi*2, 21)\ncurve = hv.Curve((xs, np.sin(xs)))\nsubregion = curve[np.pi/2:np.pi*1.5]\ncurve * subregion * hv.Scatter(curve)",
"_____no_output_____"
]
],
[
[
"Here again the region in a different color is the specified subregion, and we've also marked each discrete point with a dot using the ``Scatter`` ``Element``. As before we can also get the value for a specific sample point; whatever x-index is provided will snap to the closest sample point and return the dependent value:",
"_____no_output_____"
]
],
[
[
"curve[4.05], curve[4.1], curve[4.17], curve[4.3]",
"_____no_output_____"
]
],
[
[
"It is important to note that an index (or a list of indices, as for the 2D and 3D cases below) will always return the raw indexed (dependent) value, i.e. a number. A slice (indicated with `:`), on the other hand, will retain the Element type even in cases where the plot might not be useful, such as having only a single value, two values, or no value at all in that range:",
"_____no_output_____"
]
],
[
[
"curve[4:4.5]",
"_____no_output_____"
]
],
[
[
"## 2D and 3D Elements: slicing",
"_____no_output_____"
],
[
"For data defined in a 2D space, there are 2D equivalents of the 1D Curve and Scatter types. A ``Points``, for example, can be thought of as a number of points in a 2D space.",
"_____no_output_____"
]
],
[
[
"r = np.arange(0, 1, 0.005)\nxs, ys = (r * fn(85*np.pi*r) for fn in (np.cos, np.sin))\npaths = hv.Points((xs, ys))\npaths + paths[0:1, 0:1]",
"_____no_output_____"
]
],
[
[
"However, indexing is not supported in this space, because there could be many possible points near a given set of coordinates, and finding the nearest one would require a search across potentially incommensurable dimensions, which is poorly defined and difficult to support.\n\nSlicing in 3D works much like slicing in 2D, but indexing is not supported for the same reason as in 2D:",
"_____no_output_____"
]
],
[
[
"xs = np.linspace(0, np.pi*8, 201)\nscatter = hv.Scatter3D((xs, np.sin(xs), np.cos(xs)))\nscatter + scatter[5:10, :, 0:]",
"_____no_output_____"
]
],
[
[
"## 2D Raster and Image: slicing and indexing\n\nRaster and the various other image-like objects (Images, RGB, HSV, etc.) can all sliced and indexed, as can Surface, because they all have an underlying regular grid of key dimension values:",
"_____no_output_____"
]
],
[
[
"%opts Image (cmap='Blues') Bounds (color='red')",
"_____no_output_____"
],
[
"np.random.seed(0)\nextents = (0, 0, 10, 10)\nimg = hv.Image(np.random.rand(10, 10), bounds=extents)\nimg_slice = img[1:9,4:5]\nbox = hv.Bounds((1,4,9,5))\nimg*box + img_slice",
"_____no_output_____"
],
[
"img[4.2,4.2], img[4.3,4.2], img[5.0,4.2]",
"_____no_output_____"
]
],
[
[
"# Sampling\n\nSampling is essentially a process of indexing an Element at multiple index locations, and collecting the results. Thus any Element that can be indexed can also be sampled. Compared to regular indexing, sampling is different in that multiple indices may be supplied at the same time. Also, indexing will only return the value at that location, whereas the return type from a sampling operation is another ``Element`` type, usually either a ``Table`` or a ``Curve``, to allow both key and value dimensions to be returned.\n\n### Sampling Elements\n\nSampling can use either an explicit list of samples, or or by passing the samples for each dimension keyword arguments.\n\nWe'll start by taking a single sample of an Image object, to make it clear how sampling and indexing are similar operations yet different in their results:",
"_____no_output_____"
]
],
[
[
"img_coords = hv.Points(img.table(), extents=extents)\nlabeled_img = img * img_coords * hv.Points([img.closest([(5.1,4.9)])]).opts(style=dict(color='r'))\nimg + labeled_img + img.sample([(5.1,4.9)])",
"_____no_output_____"
],
[
"img[5.1,4.9]",
"_____no_output_____"
]
],
[
[
"Here, the output of the indexing operation is the value (0.1965823616800535) from the location closest to the specified , whereas ``.sample()`` returns a Table that lists both the coordinates *and* the value, and slicing (in previous section) returns an Element of the same type, not a Table.\n\n\nNext we can try sampling along only one Dimension on our 2D Image, leaving us with a 1D Element (in this case a ``Curve``):",
"_____no_output_____"
]
],
[
[
"sampled = img.sample(y=5)\nlabeled_img = img * img_coords * hv.Points(zip(sampled['x'], [img.closest(y=5)]*10))\nimg + labeled_img + sampled",
"_____no_output_____"
]
],
[
[
"Sampling works on any regularly sampled Element type. For example, we can select multiple samples along the x-axis of a Curve.",
"_____no_output_____"
]
],
[
[
"xs = np.arange(10)\nsamples = [2, 4, 6, 8]\ncurve = hv.Curve(zip(xs, np.sin(xs)))\ncurve_samples = hv.Scatter(zip(xs, [0] * 10)) * hv.Scatter(zip(samples, [0]*len(samples))) \ncurve + curve_samples + curve.sample(samples)",
"_____no_output_____"
]
],
[
[
"### Sampling HoloMaps\n\nSampling is often useful when you have more data than you wish to visualize or analyze at one time. First, let's create a HoloMap containing a number of observations of some noisy data.",
"_____no_output_____"
]
],
[
[
"obs_hmap = hv.HoloMap({i: hv.Image(np.random.randn(10, 10), bounds=extents)\n for i in range(3)}, kdims=['Observation'])",
"_____no_output_____"
]
],
[
[
"HoloMaps also provide additional functionality to perform regular sampling on your data. In this case we'll take 3x3 subsamples of each of the Images.",
"_____no_output_____"
]
],
[
[
"sample_style = dict(edgecolors='k', alpha=1)\nall_samples = obs_hmap.table().to.scatter3d().opts(style=dict(alpha=0.15))\nsampled = obs_hmap.sample((3,3))\nsubsamples = sampled.to.scatter3d().opts(style=sample_style)\nall_samples * subsamples + sampled",
"_____no_output_____"
]
],
[
[
"By supplying bounds in as a (left, bottom, right, top) tuple we can also sample a subregion of our images:",
"_____no_output_____"
]
],
[
[
"sampled = obs_hmap.sample((3,3), bounds=(2,5,5,10))\nsubsamples = sampled.to.scatter3d().opts(style=sample_style)\nall_samples * subsamples + sampled",
"_____no_output_____"
]
],
[
[
"Since this kind of sampling is only well supported for continuous coordinate systems, we can only apply this kind of sampling to Image types for now.\n\n### Sampling Charts\n\nSampling Chart-type Elements like Curve, Scatter, Histogram is only supported by providing an explicit list of samples, since those Elements have no underlying regular grid.",
"_____no_output_____"
]
],
[
[
"xs = np.arange(10)\nextents = (0, 0, 2, 10)\ncurve = hv.HoloMap({(i) : hv.Curve(zip(xs, np.sin(xs)*i))\n for i in np.linspace(0.5, 1.5, 3)},\n kdims=['Observation'])\nall_samples = curve.table().to.points()\nsampled = curve.sample([0, 2, 4, 6, 8])\nsampling = all_samples * sampled.to.points(extents=extents).opts(style=dict(color='r'))\nsampling + sampled",
"_____no_output_____"
]
],
[
[
"Alternatively, you can always deconstruct your data into a Table (see the [Columnar Data](Columnar_Data.ipynb) tutorial) and perform ``select`` operations instead. This is also the easiest way to sample ``NdElement`` types like Bars. Individual samples should be supplied as a set, while ranges can be specified as a two-tuple.",
"_____no_output_____"
]
],
[
[
"sampled = curve.table().select(Observation=(0, 1.1), x={0, 2, 4, 6, 8})\nsampling = all_samples * sampled.to.points(extents=extents).opts(style=dict(color='r'))\nsampling + sampled",
"_____no_output_____"
]
],
[
[
"These tools should help you index, slice, sample, and select your data with ease. The [Columnar Data](Columnar_Data.ipynb) tutorial) explains how to do other types of operations, such as averaging and other reduction operations.",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
]
] |
e7ba17e27dd6643484eda4eccfd5862233b246eb | 228,317 | ipynb | Jupyter Notebook | course/3_unsupervised_learning/01_clustering/UL6_PCA_Storyboard_Assets.ipynb | claudiocmp/udacity-dsnd | 2ec21703e97e382e7f5421edeb392c1f31314632 | [
"MIT"
] | null | null | null | course/3_unsupervised_learning/01_clustering/UL6_PCA_Storyboard_Assets.ipynb | claudiocmp/udacity-dsnd | 2ec21703e97e382e7f5421edeb392c1f31314632 | [
"MIT"
] | null | null | null | course/3_unsupervised_learning/01_clustering/UL6_PCA_Storyboard_Assets.ipynb | claudiocmp/udacity-dsnd | 2ec21703e97e382e7f5421edeb392c1f31314632 | [
"MIT"
] | null | null | null | 474.671518 | 64,390 | 0.931849 | [
[
[
"import numpy as np\nimport pandas as pd\nimport matplotlib.pyplot as plt\nimport seaborn as sns\nfrom sklearn.preprocessing import StandardScaler\nfrom sklearn.decomposition import PCA\n\n%matplotlib inline",
"_____no_output_____"
],
[
"# DSND colors: UBlue, Salmon, Gold, Slate\nplot_colors = ['#02b3e4', '#ee2e76', '#ffb613', '#2e3d49']\n\n# Light colors: Blue light, Salmon light\nplot_lcolors = ['#88d0f3', '#ed8ca1', '#fdd270']\n\n# Gray/bg colors: Slate Dark, Gray, Silver\nplot_grays = ['#1c262f', '#aebfd1', '#fafbfc']",
"_____no_output_____"
],
[
"from matplotlib.colors import LinearSegmentedColormap\n\n# colormap from UBlue - Gold - Salmon\ncdict = {'red' : [(0.0, 0.007843, 0.007843),\n (0.5, 1.0 , 1.0 ),\n (1.0, 0.933333, 0.933333)],\n 'green': [(0.0, 0.701961, 0.701961),\n (0.5, 0.713725, 0.713725),\n (1.0, 0.180392, 0.180392)],\n 'blue' : [(0.0, 0.894118, 0.894118),\n (0.5, 0.074510, 0.074510),\n (1.0, 0.462745, 0.462745)]}\nplot_cmap = LinearSegmentedColormap('DSND', cdict)",
"_____no_output_____"
]
],
[
[
"## C6.01: Introduction",
"_____no_output_____"
]
],
[
[
"from sklearn.datasets.samples_generator import make_blobs\n\nn_clusters = 2\ncenters = [[-1,-1],[1,1]]\nstdevs = [0.4, 0.6]\nX, y = make_blobs(n_samples = 20, centers = centers, cluster_std = stdevs, random_state = 1200000)",
"_____no_output_____"
],
[
"from sklearn.cluster import KMeans\n\nmodel = KMeans(n_clusters = n_clusters)\npreds = model.fit_predict(X)",
"_____no_output_____"
],
[
"plt.figure(figsize = [3,3])\n\nfor k, col in zip(range(n_clusters), plot_colors[:n_clusters]):\n my_members = (preds == k)\n plt.scatter(X[my_members, 0], X[my_members, 1], s = 100, c = col)\n\nplt.xticks([])\nplt.yticks([])\nax = plt.gca()\n[side.set_visible(False) for side in ax.spines.values()]\nplt.axis('square')\nplt.savefig('C01_TypesOfUL_01.png', transparent = True)",
"_____no_output_____"
],
[
"n_points = 10\nX = np.random.RandomState(1800000).multivariate_normal([0,0], [[1,0.8],[0.8,1]], n_points)",
"_____no_output_____"
],
[
"model = PCA()\nmodel.fit_transform(X)\n\npc1 = model.components_[0,:]\nX_center = X.mean(axis=0)\nX_new = np.matmul( np.dot(X - X_center, pc1).reshape(-1,1), pc1.reshape(1,-1) ) + X_center",
"_____no_output_____"
],
[
"plt.figure(figsize = [3,3])\n\nplt.scatter(X[:,0], X[:,1], s = 100, c = '#ffffff')\nplt.plot([X_new[:,0].min(), X_new[:,0].max()], [X_new[:,1].min(), X_new[:,1].max()],\n '-', c = plot_lcolors[0], lw = 4, zorder = 0)\nplt.scatter(X_new[:,0], X_new[:,1], s = 100, c = plot_colors[0])\nfor x, x_new in zip(X, X_new):\n plt.plot([x[0],x_new[0]], [x[1],x_new[1]], '--', c = plot_lcolors[0], lw = 2, zorder = 0)\n\nplt.xticks([])\nplt.yticks([])\nax = plt.gca()\n[side.set_visible(False) for side in ax.spines.values()]\nplt.axis('square')\nplt.savefig('C01_TypesOfUL_02.png', transparent = True)",
"_____no_output_____"
]
],
[
[
"## C6.09: Dimensionality Reduction",
"_____no_output_____"
]
],
[
[
"n_points = [4, 7, 9, 9, 7, 4]\nn_rooms = [3, 4, 5, 6, 7, 8]\nsize_lower = [ 600, 800, 1000, 1300, 1600, 2000]\nsize_upper = [1000, 1300, 1600, 2000, 2400, 3000]\n\nnp.random.seed(147258369)\n\nrooms = []\nsizes = []\nfor i in range(6):\n # use beta dist to generate sizes, find bounds with\n # lower @ 0.2, upper @ 0.8.\n size_range = size_upper[i] - size_lower[i]\n size_min = size_lower[i] - size_range / 3\n \n rooms.append(np.repeat([n_rooms[i]], n_points[i]))\n sizes.append(size_min + size_range * np.random.beta(5, 5, n_points[i]))\n\nX = np.hstack([np.hstack(rooms).reshape(-1,1), np.hstack(sizes).round().reshape(-1,1)])",
"_____no_output_____"
],
[
"X = StandardScaler().fit_transform(X)",
"_____no_output_____"
],
[
"plt.figure(figsize = [15,15])\n\nplt.scatter(X[:,0], X[:,1], s = 64, c = plot_colors[-1])\n\nplt.xticks([])\nplt.yticks([])\nplt.xlabel('# of rooms (standardized)', fontsize = 30)\nplt.ylabel('Square footage (standardized)', fontsize = 30)\nax = plt.gca()\n[side.set_linewidth(2) for side in ax.spines.values()]\n# plt.axis('square')\nplt.savefig('C09_DimensReduct_01.png', transparent = True)",
"_____no_output_____"
],
[
"pca_model = PCA()\nX_prime = pca_model.fit_transform(X)\n\npc1 = pca_model.components_[0,:]\nX_center = X.mean(axis=0)\nX_new = np.matmul( np.dot(X - X_center, pc1).reshape(-1,1), pc1.reshape(1,-1) ) + X_center",
"_____no_output_____"
],
[
"plt.figure(figsize = [15,15])\n\nplt.scatter(X[:,0], X[:,1], s = 64, c = plot_colors[-1])\nplt.plot([X_new[:,0].min(), X_new[:,0].max()], [X_new[:,1].min(), X_new[:,1].max()],\n '-', c = plot_lcolors[0], lw = 4, zorder = 0)\nplt.scatter(X_new[:,0], X_new[:,1], s = 64, c = plot_colors[0])\nfor x, x_new in zip(X, X_new):\n plt.plot([x[0],x_new[0]], [x[1],x_new[1]], '--', c = plot_lcolors[0], lw = 2, zorder = 0)\n\nplt.xticks([])\nplt.yticks([])\nplt.xlabel('# of rooms (standardized)', fontsize = 30)\nplt.ylabel('Square footage (standardized)', fontsize = 30)\nax = plt.gca()\n[side.set_linewidth(2) for side in ax.spines.values()]\n# plt.axis('square')\nplt.savefig('C09_DimensReduct_02.png', transparent = True)",
"_____no_output_____"
],
[
"from sklearn.linear_model import LinearRegression\n\nlr_model = LinearRegression()\nlr_model.fit(X[:,0].reshape(-1,1),X[:,1])\ny_hat = lr_model.predict(X[:,0].reshape(-1,1))",
"_____no_output_____"
],
[
"plt.figure(figsize = [15,15])\n\nplt.scatter(X[:,0], X[:,1], s = 64, c = plot_colors[-1])\nplt.plot([X[:,0].min(), X[:,0].max()], [y_hat.min(), y_hat.max()],\n '-', c = plot_lcolors[1], lw = 4, zorder = 0)\nplt.scatter(X[:,0], y_hat, s = 64, c = plot_colors[1])\n\nplt.xticks([])\nplt.yticks([])\nplt.xlabel('# of rooms (standardized)', fontsize = 30)\nplt.ylabel('Square footage (standardized)', fontsize = 30)\nax = plt.gca()\n[side.set_linewidth(2) for side in ax.spines.values()]\n# plt.axis('square')\nplt.savefig('C09_DimensReduct_03.png', transparent = True)",
"_____no_output_____"
]
],
[
[
"## C6.10: PCA Properties",
"_____no_output_____"
]
],
[
[
"alt_slope = np.array([1.3,1.1])\nalt_slope = alt_slope / np.sqrt((alt_slope ** 2).sum())\nalt_center = np.array([-.35,-.35])\nX_alt = np.matmul( np.dot(X - alt_center, alt_slope).reshape(-1,1), alt_slope.reshape(1,-1) ) + alt_center",
"_____no_output_____"
],
[
"plt.figure(figsize = [15,15])\n\nplt.scatter(X[:,0], X[:,1], s = 64, c = plot_colors[-1])\n\nplt.plot([X_new[:,0].min(), X_new[:,0].max()], [X_new[:,1].min(), X_new[:,1].max()],\n '-', c = plot_lcolors[0], lw = 4, zorder = 0)\nplt.scatter(X_new[:,0], X_new[:,1], s = 64, c = plot_colors[0])\nfor x, x_new in zip(X, X_new):\n plt.plot([x[0],x_new[0]], [x[1],x_new[1]], '--', c = plot_lcolors[0], lw = 2, zorder = 0)\n\n# plt.plot([X_alt[:,0].min(), X_alt[:,0].max()], [X_alt[:,1].min(), X_alt[:,1].max()],\n# '-', c = plot_lcolors[1], lw = 4, zorder = 0)\n# plt.scatter(X_alt[:,0], X_alt[:,1], s = 64, c = plot_colors[1])\n# for x, x_alt in zip(X, X_alt):\n# plt.plot([x[0],x_alt[0]], [x[1],x_alt[1]], '--', c = plot_lcolors[1], lw = 2, zorder = 0)\n\nplt.xticks([])\nplt.yticks([])\nplt.xlabel('# of rooms (standardized)', fontsize = 30)\nplt.ylabel('Square footage (standardized)', fontsize = 30)\nax = plt.gca()\n[side.set_linewidth(2) for side in ax.spines.values()]\nplt.axis('square')\nplt.savefig('C10_PCAProps_04.png', transparent = True)",
"_____no_output_____"
],
[
"np.sqrt(((X - X_new) ** 2).sum(axis=1)).sum()",
"_____no_output_____"
],
[
"np.sqrt(((X - X_alt) ** 2).sum(axis=1)).sum()",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
]
] |
e7ba28482e2b80826d88cb81afaf0bf407966b2c | 154,033 | ipynb | Jupyter Notebook | doc/source/volume maximization.ipynb | sadraddini/pypolycontain | 807846634899fa3de543fe09178ff7bda4386541 | [
"AFL-1.1"
] | 10 | 2019-01-09T20:09:27.000Z | 2021-12-02T15:16:40.000Z | doc/source/volume maximization.ipynb | sadraddini/pypolycontain | 807846634899fa3de543fe09178ff7bda4386541 | [
"AFL-1.1"
] | 3 | 2019-03-16T01:56:35.000Z | 2020-07-31T20:32:06.000Z | doc/source/volume maximization.ipynb | sadraddini/pypolycontain | 807846634899fa3de543fe09178ff7bda4386541 | [
"AFL-1.1"
] | 1 | 2020-07-30T23:16:11.000Z | 2020-07-30T23:16:11.000Z | 544.286219 | 33,112 | 0.945797 | [
[
[
"import numpy as np\nimport pypolycontain as pp",
"_____no_output_____"
],
[
"q_o,n=5,2\nZ_o=pp.zonotope(x=np.zeros((n,1)),G=np.random.random((n,q_o))-0.5)\nZ_o.G=np.array([[1,0],[1,2]])\npp.visualize([Z_o])",
"_____no_output_____"
],
[
"# Z_i=pp.zonotope(x=np.zeros((n,1)),G=G)\nimport pydrake.solvers.mathematicalprogram as MP\n# use SOCS solver\nimport pydrake.solvers.scs as SCS\nmysolver=SCS.ScsSolver()",
"_____no_output_____"
],
[
"program=MP.MathematicalProgram()\nG=program.NewSymmetricContinuousVariables(n,'G') \nprogram.AddPositiveSemidefiniteConstraint(G)\nprogram.AddMaximizeLogDeterminantSymmetricMatrixCost(G)\nZ_i=pp.zonotope(x=np.zeros((n,1)),G=G) \npp.subset(program,Z_i,Z_o)\nresult=mysolver.Solve(program,None,None)\nif result.is_success():\n print(\"success\")\n G_n= result.GetSolution(G)\nelse:\n print(\"not succesfull\") \nZ_i_n=pp.zonotope(x=np.zeros((n,1)),G=G_n,color='red')\npp.visualize([Z_o,Z_i_n],alpha=0.5)",
"success\n"
],
[
"Z_i=pp.zonotope(x=np.zeros((n,1)),G=np.random.random((n,q_o))-0.5)\nZ_i.G=np.array([[1,1,-2],[-1,1,1]])\nprogram=MP.MathematicalProgram()\nG=program.NewSymmetricContinuousVariables(n,'G') \nprogram.AddPositiveSemidefiniteConstraint(G)\nprogram.AddMaximizeLogDeterminantSymmetricMatrixCost(G)\nZ_o=pp.zonotope(x=np.zeros((n,1)),G=np.eye(2)) \nZ_i_a=pp.zonotope(x=Z_i.x,G=np.dot(G,Z_i.G))\npp.subset(program,Z_i_a,Z_o)\nresult=mysolver.Solve(program,None,None)\nif result.is_success():\n print(\"success\")\n G_n= result.GetSolution(G)\nelse:\n print(\"not succesfull\")\nZ_o_n=pp.zonotope(x=np.zeros((n,1)),G=np.linalg.inv(G_n),color='red')\npp.visualize([Z_o_n,Z_i],alpha=0.7)\n\ndef intervall_hull(G):\n D=np.linalg.norm(G,1, axis=1)\n return np.diag(D)\n\ndef Girard(G,number_of_columns_wanted):\n \"\"\"\n The idea is based on Girard, HSCC 2006\n \"\"\"\n q_i,q_f,n=G.shape[1],number_of_columns_wanted,G.shape[0]\n gamma=np.linalg.norm(G,1, axis=0)-np.linalg.norm(G,np.inf, axis=0)\n G_sorted=G[:,np.argsort(gamma)]\n assert q_f>=n\n L=G_sorted[:,:q_i-q_f+n]\n K=G_sorted[:,q_i-q_f+n:]\n L_R=intervall_hull(L)\n return np.hstack((L_R,K))\nZ_o_girard=pp.zonotope(x=np.zeros((n,1)),G=Girard(Z_i.G,2),color='blue')\npp.visualize([Z_o_girard,Z_i],alpha=0.7)",
"success\n"
],
[
"Z_o=pp.zonotope(x=np.zeros((n,1)),G=np.random.random((n,q_o))-0.5)\nZ_o.G=np.array([[2,0,1],[0,1,-1]])\nprogram=MP.MathematicalProgram()\nG1=program.NewSymmetricContinuousVariables(n,'G1') \nprogram.AddPositiveSemidefiniteConstraint(G1)\nprogram.AddMaximizeLogDeterminantSymmetricMatrixCost(G1)\nG2=program.NewSymmetricContinuousVariables(n,'G2') \nprogram.AddPositiveSemidefiniteConstraint(G2)\nprogram.AddMaximizeLogDeterminantSymmetricMatrixCost(G2)\nprogram.AddLinearConstraint(np.equal(G1[:,1],G2[:,0],dtype='object').flatten())\nG_s=np.hstack(( G1[:,0:1] , G1[:,1:2] , G2[:,1:2] ))\nK=np.random.random((2,2))-0.5\nZ_i=pp.zonotope(x=np.zeros((n,1)),G=np.dot(K,G_s)) \npp.subset(program,Z_i,Z_o)\nresult=mysolver.Solve(program,None,None)\nif result.is_success():\n print(\"success\")\n G1_n= result.GetSolution(G1)\n G2_n= result.GetSolution(G2)\nelse:\n print(\"not succesfull\")\nG_s_n=np.hstack(( G1_n[:,0:1] , G1_n[:,1:2] , G2_n[:,1:2] ))\nZ_i_n=pp.zonotope(x=np.zeros((n,1)),G=np.dot(K,G_s_n),color='red')\npp.visualize([Z_o,Z_i_n],alpha=0.5)\nnp.linalg.det(np.dot(Z_i_n.G,Z_i_n.G.T))",
"success\n"
],
[
"Z_i_n.G",
"_____no_output_____"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7ba3bb2532e4fa5c2780ab2a94f3d0d8e301be3 | 3,025 | ipynb | Jupyter Notebook | docs/prev_milestone_docs/Math Background.ipynb | cityscape-107/cs107-FinalProject | ca6c1f5c7cb439aac34f561d6ce16df2107227c5 | [
"MIT"
] | 1 | 2020-11-20T11:31:24.000Z | 2020-11-20T11:31:24.000Z | docs/prev_milestone_docs/Math Background.ipynb | cityscape-107/cs107-FinalProject | ca6c1f5c7cb439aac34f561d6ce16df2107227c5 | [
"MIT"
] | 50 | 2020-11-20T02:14:36.000Z | 2020-12-12T17:07:54.000Z | docs/prev_milestone_docs/Math Background.ipynb | cityscape-107/cs107-FinalProject | ca6c1f5c7cb439aac34f561d6ce16df2107227c5 | [
"MIT"
] | 3 | 2020-12-12T10:50:56.000Z | 2021-12-09T23:10:18.000Z | 26.077586 | 187 | 0.478017 | [
[
[
"$ \\text {The basics:}$",
"_____no_output_____"
],
[
"$ \\text {- The Chain Rule}$",
"_____no_output_____"
],
[
"$\n\\text { If we have } f(x) \\text { then the derivative of } f \\text { with respect to } x \\text { is: }\n$\n\n$$\n\\frac{\\partial f}{\\partial x}=\\frac{\\partial f}{\\partial u} \\cdot \\frac{\\partial u}{\\partial x}\n$$",
"_____no_output_____"
],
[
"$\n\\text { If we have } f(u,v) \\text {, where u and v are both functions of } x \\text { then the derivative of } f \\\\ \\text { with respect to } x \\text { is: }\n$\n\n$$\n\\frac{\\partial f}{\\partial x}=\\frac{\\partial f}{\\partial u} \\cdot \\frac{\\partial u}{\\partial x}+\\frac{\\partial f}{\\partial v} \\cdot \\frac{\\partial v}{\\partial x}\n$$",
"_____no_output_____"
],
[
"$ \\text {- Jacobian and Gradient}$",
"_____no_output_____"
],
[
"$\n\\text { If we have } x \\in \\mathbb{R}^{m} \\text { and function } f(u(x), v(x)) \\text { , } \\text {the gradient of f is:}\n$\n\n$$\n\\nabla_{x} f=\\frac{\\partial f}{\\partial u} \\nabla_{x} u+\\frac{\\partial f}{\\partial v} \\nabla_{x} v\n$$",
"_____no_output_____"
],
[
"$\n\\text { If we have } h(x): \\mathbb{R}^{m} \\rightarrow \\mathbb{R}^{n} \\text{ ,then, the Jacobian matrix is:}\n$\n",
"_____no_output_____"
],
[
"$$\nJ=\\left[\\begin{array}{cccc}\n\\frac{\\partial h_{1}}{\\partial x_{1}} & \\frac{\\partial h_{1}}{\\partial x_{2}} & \\cdots & \\frac{\\partial h_{1}}{\\partial x_{m}} \\\\\n\\frac{\\partial h_{2}}{\\partial x_{1}} & \\frac{\\partial h_{2}}{\\partial x_{2}} & \\cdots & \\frac{\\partial h_{2}}{\\partial x_{m}} \\\\\n\\vdots & \\vdots & \\ddots & \\vdots \\\\\n\\frac{\\partial h_{n}}{\\partial x_{1}} & \\frac{\\partial h_{n}}{\\partial x_{2}} & \\cdots & \\frac{\\partial h_{n}}{\\partial x_{m}}\n\\end{array}\\right]\n$$",
"_____no_output_____"
],
[
"$ \\text {add an evaluation trace visualisation}$",
"_____no_output_____"
]
]
] | [
"markdown"
] | [
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
]
] |
e7ba4ed0b521d42d563226a941d2cbb45b6bdb41 | 18,349 | ipynb | Jupyter Notebook | paper/proofs/celerite-integral.ipynb | exowanderer/exoplanet | dfd4859525ca574f1936de7b683951c35c292586 | [
"MIT"
] | 2 | 2021-10-01T12:46:09.000Z | 2022-03-24T10:25:20.000Z | paper/proofs/celerite-integral.ipynb | exowanderer/exoplanet | dfd4859525ca574f1936de7b683951c35c292586 | [
"MIT"
] | null | null | null | paper/proofs/celerite-integral.ipynb | exowanderer/exoplanet | dfd4859525ca574f1936de7b683951c35c292586 | [
"MIT"
] | null | null | null | 25.170096 | 721 | 0.48019 | [
[
[
"%run proof_setup",
"_____no_output_____"
]
],
[
[
"## Real case\n\nFirst, the real case is relatively simple.\nThe integral that we want to do is:\n\n$$\nk_\\Delta(\\tau) = \\frac{1}{\\Delta^2}\\int_{t_i-\\Delta/2}^{t_i+\\Delta/2} \\mathrm{d}t \\,\\int_{t_j-\\Delta/2}^{t_j+\\Delta/2}\\mathrm{d}t^\\prime\\,k(t - t^\\prime)\n$$\n\nFor celerite kernels it helps to make the assumtion that $t_j + \\Delta/2 < t_i - \\Delta/2$ (in other words, the exposure times do not overlap).",
"_____no_output_____"
]
],
[
[
"import sympy as sm\ncr = sm.symbols(\"cr\", positive=True)\nti, tj, dt, t, tp = sm.symbols(\"ti, tj, dt, t, tp\", real=True)\n\nk = sm.exp(-cr*(t - tp))\nk0 = k.subs([(t, ti), (tp, tj)])\nkint = sm.simplify(sm.integrate(\n sm.integrate(k, (t, ti-dt/2, ti+dt/2)),\n (tp, tj-dt/2, tj+dt/2)) / dt**2)\nres = sm.simplify(kint / k0)\nprint(res)",
"(exp(2*cr*dt) - 2*exp(cr*dt) + 1)*exp(-cr*dt)/(cr**2*dt**2)\n"
]
],
[
[
"This is the factor that we want.\nLet's make sure that it is identical to what we have in the note.",
"_____no_output_____"
]
],
[
[
"kD = 2 * (sm.cosh(cr*dt) - 1) / (cr*dt)**2\nsm.simplify(res.expand() - kD.expand())",
"_____no_output_____"
]
],
[
[
"Excellent.\n\nLet's double check that this reduces to the original kernel in the limit $\\Delta \\to 0$:",
"_____no_output_____"
]
],
[
[
"sm.limit(kD, dt, 0)",
"_____no_output_____"
]
],
[
[
"## Complex case\n\nThe complex cases proceeds similarly, but it's a bit more involved.\nIn this case,\n\n$$\nk(\\tau) = (a + i\\,b)\\,\\exp(-(c+i\\,d)\\,(t_i-t_j))\n$$",
"_____no_output_____"
]
],
[
[
"a, b, c, d = sm.symbols(\"a, b, c, d\", real=True, positive=True)\nk = sm.exp(-(c + sm.I*d) * (t - tp))\nk0 = k.subs([(t, ti), (tp, tj)])\nkint = sm.simplify(sm.integrate(k, (t, ti-dt/2, ti+dt/2)) / dt)\nkint = sm.simplify(sm.integrate(kint.expand(), (tp, tj-dt/2, tj+dt/2)) / dt)\nprint(sm.simplify(kint / k0))",
"(2*cos(I*dt*(c + I*d)) - 2)/(dt**2*(c**2 + 2*I*c*d - d**2))\n"
]
],
[
[
"That doesn't look so bad!\n\nBut, I'm going to re-write it by hand and make sure that it's correct:",
"_____no_output_____"
]
],
[
[
"coeff = (c-sm.I*d)**2 / (dt*(c**2+d**2))**2\ncoeff *= (sm.exp((c+sm.I*d)*dt) + sm.exp(-(c+sm.I*d)*dt)-2)\nsm.simplify(coeff * k0 - kint)",
"_____no_output_____"
]
],
[
[
"Good.\n\nNow we need to work out nice expressions for the real and imaginary parts of this.\nFirst, the real part.\nI found that it was easiest to look at the prefactors for the trig functions directly and simplify those.\nHere we go:",
"_____no_output_____"
]
],
[
[
"res = (a+sm.I*b) * coeff\nA = sm.simplify((res.expand(complex=True) +\n sm.conjugate(res).expand(complex=True)) / 2)",
"_____no_output_____"
],
[
"sm.simplify(sm.poly(A, sm.cos(dt*d)).coeff_monomial(sm.cos(dt*d)))",
"_____no_output_____"
],
[
"sm.simplify(sm.poly(sm.poly(A, sm.cos(dt*d)).coeff_monomial(1), sm.sin(dt*d)).coeff_monomial(sm.sin(dt*d)))",
"_____no_output_____"
],
[
"sm.simplify(sm.poly(sm.poly(A, sm.cos(dt*d)).coeff_monomial(1), sm.sin(dt*d)).coeff_monomial(1))",
"_____no_output_____"
]
],
[
[
"Then, same thing for the imaginary part:",
"_____no_output_____"
]
],
[
[
"B = sm.simplify(-sm.I * (res.expand(complex=True) -\n sm.conjugate(res).expand(complex=True)) / 2)",
"_____no_output_____"
],
[
"sm.simplify(sm.poly(B, sm.cos(dt*d)).coeff_monomial(sm.cos(dt*d)))",
"_____no_output_____"
],
[
"sm.simplify(sm.poly(sm.poly(B, sm.cos(dt*d)).coeff_monomial(1), sm.sin(dt*d)).coeff_monomial(sm.sin(dt*d)))",
"_____no_output_____"
],
[
"sm.simplify(sm.poly(sm.poly(B, sm.cos(dt*d)).coeff_monomial(1), sm.sin(dt*d)).coeff_monomial(1))",
"_____no_output_____"
]
],
[
[
"Ok.\n\nNow let's make sure that the simplified expressions are right.",
"_____no_output_____"
]
],
[
[
"C1 = (a*c**2 - a*d**2 + 2*b*c*d)\nC2 = (b*c**2 - b*d**2 - 2*a*c*d)\ncos_term = (sm.exp(c*dt) + sm.exp(-c*dt)) * sm.cos(d*dt) - 2\nsin_term = (sm.exp(c*dt) - sm.exp(-c*dt)) * sm.sin(d*dt)\ndenom = dt**2 * (c**2 + d**2)**2\n\nA0 = (C1 * cos_term - C2 * sin_term) / denom\nB0 = (C2 * cos_term + C1 * sin_term) / denom",
"_____no_output_____"
],
[
"sm.simplify(A.expand() - A0.expand())",
"_____no_output_____"
],
[
"sm.simplify(B.expand() - B0.expand())",
"_____no_output_____"
]
],
[
[
"Finally let's rewrite things in terms of hyperbolic trig functions.",
"_____no_output_____"
]
],
[
[
"sm.simplify(2*(sm.cosh(c*dt) * sm.cos(d*dt) - 1).expand() - cos_term.expand())",
"_____no_output_____"
],
[
"sm.simplify(2*(sm.sinh(c*dt) * sm.sin(d*dt)).expand() - sin_term.expand())",
"_____no_output_____"
]
],
[
[
"Looks good!\n\nLet's make sure that this actually reproduces the target integral:",
"_____no_output_____"
]
],
[
[
"sm.simplify(((a+sm.I*b)*kint/k0 - (A+sm.I*B)).expand(complex=True))",
"_____no_output_____"
]
],
[
[
"Finally, let's make sure that this reduces to the original kernel when $\\Delta \\to 0$:",
"_____no_output_____"
]
],
[
[
"sm.limit(A, dt, 0), sm.limit(B, dt, 0)",
"_____no_output_____"
]
],
[
[
"## Overlapping exposures & the power spectrum\n\nIf we directly evaluate the power spectrum of this kernel, we'll have some issues because there will be power from lags where our assumption of non-overlapping exposures will break down.\nInstead, we can evaluate the correct power spectrum by realizing that the integrals that we're doing are convolutions.\nTherefore, the power spectrum of the integrated kernel will be product of the original power spectrum with the square of the Fourier transform of the top hat exposure function.",
"_____no_output_____"
]
],
[
[
"omega = sm.symbols(\"omega\", real=True)\nsm.simplify(sm.integrate(sm.exp(sm.I * t * omega) / dt, (t, -dt / 2, dt / 2)))",
"_____no_output_____"
]
],
[
[
"Therefore, the integrated power spectrum is\n\n$$\nS_\\Delta(\\omega) = \\frac{\\sin^2(\\Delta\\,\\omega/2)}{(\\Delta\\,\\omega/2)^2}\\,S(\\omega)\n = \\mathrm{sinc}^2(\\Delta\\,\\omega/2)\\,S(\\omega)\n$$",
"_____no_output_____"
],
[
"For overlapping exposures, some care must be taken when computing the autocorrelation because of the absolute value.\nThis also means that celerite cannot be used (as far as I can tell) to evaluate exposure time integrated models with overlapping exposures.\nIn this case, the integral we want to do is:\n\n$$\nk_\\Delta(\\tau) = \\frac{1}{\\Delta^2}\\int_{t_i-\\Delta/2}^{t_i+\\Delta/2} \\mathrm{d}t \\,\\int_{t_j-\\Delta/2}^{t_j+\\Delta/2}\\mathrm{d}t^\\prime\\,k(|t - t^\\prime|)\n$$\n\nwhich can be broken into three integrals when $\\tau = |t_i - t_j| \\le \\Delta$ (assuming still that $t_i \\ge t_j$):\n\n$$\n\\Delta^2\\,k_\\Delta(\\tau)\n= \\int_{t_j+\\Delta/2}^{t_i+\\Delta/2} \\mathrm{d}t \\,\\int_{t_j-\\Delta/2}^{t_j+\\Delta/2}\\mathrm{d}t^\\prime\\,k(t - t^\\prime)\n+ \\int_{t_i-\\Delta/2}^{t_j+\\Delta/2} \\mathrm{d}t \\,\\int_{t_j-\\Delta/2}^{t}\\mathrm{d}t^\\prime\\,k(t - t^\\prime)\n+ \\int_{t_i-\\Delta/2}^{t_j+\\Delta/2} \\mathrm{d}t \\,\\int_{t}^{t_j+\\Delta/2}\\mathrm{d}t^\\prime\\,k(t^\\prime - t)\n$$\n",
"_____no_output_____"
]
],
[
[
"tau = sm.symbols(\"tau\", real=True, positive=True)\nkp = sm.exp(-cr*(t - tp))\nkm = sm.exp(-cr*(tp - t))\nk1 = sm.simplify(sm.integrate(\n sm.integrate(kp, (tp, tj-dt/2, tj+dt/2)),\n (t, tj+dt/2, ti+dt/2)) / dt**2)\nk2 = sm.simplify(sm.integrate(\n sm.integrate(kp, (tp, tj-dt/2, t)),\n (t, ti-dt/2, tj+dt/2)) / dt**2)\nk3 = sm.simplify(sm.integrate(\n sm.integrate(km, (tp, t, tj+dt/2)),\n (t, ti-dt/2, tj+dt/2)) / dt**2)\nkD = sm.simplify((k1 + k2 + k3).expand())\nres = sm.simplify(kD.subs([(ti, tau + tj)]))\nres",
"_____no_output_____"
],
[
"kint = (2*cr*(dt-tau) + sm.exp(-cr*(dt-tau)) - 2*sm.exp(-cr*tau) + sm.exp(-cr*(dt+tau))) / (cr*dt)**2\nsm.simplify(kint - res)",
"_____no_output_____"
]
],
[
[
"Ok. That's the result for the real case. Now let's work through the result for the complex case.",
"_____no_output_____"
]
],
[
[
"arg1 = ((a+sm.I*b) * kint.subs([(cr, c+sm.I*d)])).expand(complex=True)\narg2 = ((a-sm.I*b) * kint.subs([(cr, c-sm.I*d)])).expand(complex=True)\nres = sm.simplify((arg1 + arg2) / 2)\nres",
"_____no_output_____"
],
[
"C1 = (a*c**2 - a*d**2 + 2*b*c*d)\nC2 = (b*c**2 - b*d**2 - 2*a*c*d)\ndenom = dt**2 * (c**2 + d**2)**2\n\ndpt = dt + tau\ndmt = dt - tau\ncos_term = sm.exp(-c*dmt)*sm.cos(d*dmt) + sm.exp(-c*dpt)*sm.cos(d*dpt) - 2*sm.exp(-c*tau)*sm.cos(d*tau)\nsin_term = sm.exp(-c*dmt)*sm.sin(d*dmt) + sm.exp(-c*dpt)*sm.sin(d*dpt) - 2*sm.exp(-c*tau)*sm.sin(d*tau)\n\nktest = 2*(a*c + b*d)*(c**2+d**2)*dmt\nktest += C1 * cos_term + C2 * sin_term\nktest /= denom\n\nsm.simplify(ktest - res)",
"_____no_output_____"
]
],
[
[
"And now, finally, I think we're done.",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
]
] |
e7ba608a0eccd01db7e5e9d1669b27d714c8b1ba | 593 | ipynb | Jupyter Notebook | module1.ipynb | hamelsmu/nbdev_export_demo | 947deeeefd33b0d70538927d162172c767d8bf4c | [
"Apache-2.0"
] | null | null | null | module1.ipynb | hamelsmu/nbdev_export_demo | 947deeeefd33b0d70538927d162172c767d8bf4c | [
"Apache-2.0"
] | 30 | 2021-05-04T21:44:07.000Z | 2022-03-20T03:07:58.000Z | module1.ipynb | hamelsmu/nbdev_export_demo | 947deeeefd33b0d70538927d162172c767d8bf4c | [
"Apache-2.0"
] | 1 | 2022-02-20T17:10:20.000Z | 2022-02-20T17:10:20.000Z | 14.463415 | 30 | 0.475548 | [
[
[
"# default_exp module1",
"_____no_output_____"
]
],
[
[
"# Module1\n> module 1",
"_____no_output_____"
]
],
[
[
"#export\ndef nothing(): pass",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code"
] | [
[
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7ba6b846248782a0b4a346e90ac338a2a0e3907 | 1,318 | ipynb | Jupyter Notebook | .ipynb_checkpoints/16 目标规划-checkpoint.ipynb | ZDDWLIG/math-model | ec64b7856c7bc43988b1b7e4e68e389cc9c9a8af | [
"MIT"
] | 47 | 2020-12-02T02:06:56.000Z | 2022-03-28T02:51:43.000Z | .ipynb_checkpoints/16 目标规划-checkpoint.ipynb | RabbitWhite1/Mathematical_Modeling_in_Python | ec64b7856c7bc43988b1b7e4e68e389cc9c9a8af | [
"MIT"
] | null | null | null | .ipynb_checkpoints/16 目标规划-checkpoint.ipynb | RabbitWhite1/Mathematical_Modeling_in_Python | ec64b7856c7bc43988b1b7e4e68e389cc9c9a8af | [
"MIT"
] | 15 | 2021-01-02T15:38:51.000Z | 2022-03-02T13:37:47.000Z | 23.963636 | 105 | 0.461305 | [
[
[
"# 目标规划\n\n**基本上分为两种思路: 加权系数法(转化为单一目标), 优先等级法(按重要程度不同, 转化为单目标模型)**\n\n正负偏差变量: $d_i^+=max\\{f_i-d_i^0,0\\},\\ d_i^-=-min\\{f_i-d_i^0,0\\}$, 显然恒有$d_i^+\\times d_i^-=0$",
"_____no_output_____"
]
],
[
[
"# 例16.3\n# 该题的规划模型如下:\n# min z =P[0]*dminus[0] + P[1]*(dplus[1]+dminus[1]) + P[2]*(3 * (dplus[2]+dminus[2]) + dplus[3])\n# 2*x[0] + 2*x[1] <= 12\n# 200*x[0] + 300*x[1] + dminus[0] - dplus[0] = 1500\n# 2*x[0] - x[1] + dminus[1] - dplus[1] = 0\n# 4*x[0] + dminus[2] - dplus[2] = 16\n# 5*x[1] + dminus[3] - dplus[3] = 15\n# x[0], x[1], dminus[i], dplus[i] >= 0, i = 0, 1, 2, 3\n\n",
"_____no_output_____"
]
]
] | [
"markdown",
"code"
] | [
[
"markdown"
],
[
"code"
]
] |
e7ba710634f9dd7cb52670ae431cb409b735ca87 | 387,062 | ipynb | Jupyter Notebook | assignment1/two_layer_net.ipynb | qiaw99/CS231n-Convolutional-Neural-Networks-for-Visual-Recognition | 5949b4f68f04e23751879dbc679e7708735d313f | [
"Apache-2.0"
] | null | null | null | assignment1/two_layer_net.ipynb | qiaw99/CS231n-Convolutional-Neural-Networks-for-Visual-Recognition | 5949b4f68f04e23751879dbc679e7708735d313f | [
"Apache-2.0"
] | null | null | null | assignment1/two_layer_net.ipynb | qiaw99/CS231n-Convolutional-Neural-Networks-for-Visual-Recognition | 5949b4f68f04e23751879dbc679e7708735d313f | [
"Apache-2.0"
] | null | null | null | 387,062 | 387,062 | 0.93469 | [
[
[
"# This mounts your Google Drive to the Colab VM.\nfrom google.colab import drive\ndrive.mount('/content/drive', force_remount=True)\n\n# Enter the foldername in your Drive where you have saved the unzipped\n# assignment folder, e.g. 'cs231n/assignments/assignment1/'\nFOLDERNAME = \"assignment1\"\nassert FOLDERNAME is not None, \"[!] Enter the foldername.\"\n\n# Now that we've mounted your Drive, this ensures that\n# the Python interpreter of the Colab VM can load\n# python files from within it.\nimport sys\nsys.path.append('/content/drive/My Drive/{}'.format(FOLDERNAME))\n\n# This downloads the CIFAR-10 dataset to your Drive\n# if it doesn't already exist.\n%cd drive/My\\ Drive/$FOLDERNAME/cs231n/datasets/\n!bash get_datasets.sh\n%cd /content/drive/My\\ Drive/$FOLDERNAME",
"Mounted at /content/drive\n/content/drive/My Drive/assignment1/cs231n/datasets\n/content/drive/My Drive/assignment1\n"
]
],
[
[
"# Fully-Connected Neural Nets\nIn this exercise we will implement fully-connected networks using a modular approach. For each layer we will implement a `forward` and a `backward` function. The `forward` function will receive inputs, weights, and other parameters and will return both an output and a `cache` object storing data needed for the backward pass, like this:\n\n```python\ndef layer_forward(x, w):\n \"\"\" Receive inputs x and weights w \"\"\"\n # Do some computations ...\n z = # ... some intermediate value\n # Do some more computations ...\n out = # the output\n \n cache = (x, w, z, out) # Values we need to compute gradients\n \n return out, cache\n```\n\nThe backward pass will receive upstream derivatives and the `cache` object, and will return gradients with respect to the inputs and weights, like this:\n\n```python\ndef layer_backward(dout, cache):\n \"\"\"\n Receive dout (derivative of loss with respect to outputs) and cache,\n and compute derivative with respect to inputs.\n \"\"\"\n # Unpack cache values\n x, w, z, out = cache\n \n # Use values in cache to compute derivatives\n dx = # Derivative of loss with respect to x\n dw = # Derivative of loss with respect to w\n \n return dx, dw\n```\n\nAfter implementing a bunch of layers this way, we will be able to easily combine them to build classifiers with different architectures.\n ",
"_____no_output_____"
]
],
[
[
"# As usual, a bit of setup\nfrom __future__ import print_function\nimport time\nimport numpy as np\nimport matplotlib.pyplot as plt\nfrom cs231n.classifiers.fc_net import *\nfrom cs231n.data_utils import get_CIFAR10_data\nfrom cs231n.gradient_check import eval_numerical_gradient, eval_numerical_gradient_array\nfrom cs231n.solver import Solver\n\n%matplotlib inline\nplt.rcParams['figure.figsize'] = (10.0, 8.0) # set default size of plots\nplt.rcParams['image.interpolation'] = 'nearest'\nplt.rcParams['image.cmap'] = 'gray'\n\n# for auto-reloading external modules\n# see http://stackoverflow.com/questions/1907993/autoreload-of-modules-in-ipython\n%load_ext autoreload\n%autoreload 2\n\ndef rel_error(x, y):\n \"\"\" returns relative error \"\"\"\n return np.max(np.abs(x - y) / (np.maximum(1e-8, np.abs(x) + np.abs(y))))",
"_____no_output_____"
],
[
"# Load the (preprocessed) CIFAR10 data.\n\ndata = get_CIFAR10_data()\nfor k, v in list(data.items()):\n print(('%s: ' % k, v.shape))",
"('X_train: ', (49000, 3, 32, 32))\n('y_train: ', (49000,))\n('X_val: ', (1000, 3, 32, 32))\n('y_val: ', (1000,))\n('X_test: ', (1000, 3, 32, 32))\n('y_test: ', (1000,))\n"
]
],
[
[
"# Affine layer: forward\nOpen the file `cs231n/layers.py` and implement the `affine_forward` function.\n\nOnce you are done you can test your implementaion by running the following:",
"_____no_output_____"
]
],
[
[
"# Test the affine_forward function\n\nnum_inputs = 2\ninput_shape = (4, 5, 6)\noutput_dim = 3\n\ninput_size = num_inputs * np.prod(input_shape)\nweight_size = output_dim * np.prod(input_shape)\n\nx = np.linspace(-0.1, 0.5, num=input_size).reshape(num_inputs, *input_shape)\nw = np.linspace(-0.2, 0.3, num=weight_size).reshape(np.prod(input_shape), output_dim)\nb = np.linspace(-0.3, 0.1, num=output_dim)\n\nout, _ = affine_forward(x, w, b)\ncorrect_out = np.array([[ 1.49834967, 1.70660132, 1.91485297],\n [ 3.25553199, 3.5141327, 3.77273342]])\n\n# Compare your output with ours. The error should be around e-9 or less.\nprint('Testing affine_forward function:')\nprint('difference: ', rel_error(out, correct_out))",
"Testing affine_forward function:\ndifference: 9.769849468192957e-10\n"
]
],
[
[
"# Affine layer: backward\nNow implement the `affine_backward` function and test your implementation using numeric gradient checking.",
"_____no_output_____"
]
],
[
[
"# Test the affine_backward function\nnp.random.seed(231)\nx = np.random.randn(10, 2, 3)\nw = np.random.randn(6, 5)\nb = np.random.randn(5)\ndout = np.random.randn(10, 5)\n\ndx_num = eval_numerical_gradient_array(lambda x: affine_forward(x, w, b)[0], x, dout)\ndw_num = eval_numerical_gradient_array(lambda w: affine_forward(x, w, b)[0], w, dout)\ndb_num = eval_numerical_gradient_array(lambda b: affine_forward(x, w, b)[0], b, dout)\n\n_, cache = affine_forward(x, w, b)\ndx, dw, db = affine_backward(dout, cache)\n\n# The error should be around e-10 or less\nprint('Testing affine_backward function:')\nprint('dx error: ', rel_error(dx_num, dx))\nprint('dw error: ', rel_error(dw_num, dw))\nprint('db error: ', rel_error(db_num, db))",
"Testing affine_backward function:\ndx error: 5.399100368651805e-11\ndw error: 9.904211865398145e-11\ndb error: 2.4122867568119087e-11\n"
]
],
[
[
"# ReLU activation: forward\nImplement the forward pass for the ReLU activation function in the `relu_forward` function and test your implementation using the following:",
"_____no_output_____"
]
],
[
[
"# Test the relu_forward function\n\nx = np.linspace(-0.5, 0.5, num=12).reshape(3, 4)\n\nout, _ = relu_forward(x)\ncorrect_out = np.array([[ 0., 0., 0., 0., ],\n [ 0., 0., 0.04545455, 0.13636364,],\n [ 0.22727273, 0.31818182, 0.40909091, 0.5, ]])\n\n# Compare your output with ours. The error should be on the order of e-8\nprint('Testing relu_forward function:')\nprint('difference: ', rel_error(out, correct_out))",
"Testing relu_forward function:\ndifference: 4.999999798022158e-08\n"
]
],
[
[
"# ReLU activation: backward\nNow implement the backward pass for the ReLU activation function in the `relu_backward` function and test your implementation using numeric gradient checking:",
"_____no_output_____"
]
],
[
[
"np.random.seed(231)\nx = np.random.randn(10, 10)\ndout = np.random.randn(*x.shape)\n\ndx_num = eval_numerical_gradient_array(lambda x: relu_forward(x)[0], x, dout)\n\n_, cache = relu_forward(x)\ndx = relu_backward(dout, cache)\n\n# The error should be on the order of e-12\nprint('Testing relu_backward function:')\nprint('dx error: ', rel_error(dx_num, dx))",
"Testing relu_backward function:\ndx error: 3.2756349136310288e-12\n"
]
],
[
[
"## Inline Question 1: \n\nWe've only asked you to implement ReLU, but there are a number of different activation functions that one could use in neural networks, each with its pros and cons. In particular, an issue commonly seen with activation functions is getting zero (or close to zero) gradient flow during backpropagation. Which of the following activation functions have this problem? If you consider these functions in the one dimensional case, what types of input would lead to this behaviour?\n1. Sigmoid\n2. ReLU\n3. Leaky ReLU\n\n## Answer:\n[FILL THIS IN]\n",
"_____no_output_____"
],
[
"# \"Sandwich\" layers\nThere are some common patterns of layers that are frequently used in neural nets. For example, affine layers are frequently followed by a ReLU nonlinearity. To make these common patterns easy, we define several convenience layers in the file `cs231n/layer_utils.py`.\n\nFor now take a look at the `affine_relu_forward` and `affine_relu_backward` functions, and run the following to numerically gradient check the backward pass:",
"_____no_output_____"
]
],
[
[
"from cs231n.layer_utils import affine_relu_forward, affine_relu_backward\nnp.random.seed(231)\nx = np.random.randn(2, 3, 4)\nw = np.random.randn(12, 10)\nb = np.random.randn(10)\ndout = np.random.randn(2, 10)\n\nout, cache = affine_relu_forward(x, w, b)\ndx, dw, db = affine_relu_backward(dout, cache)\n\ndx_num = eval_numerical_gradient_array(lambda x: affine_relu_forward(x, w, b)[0], x, dout)\ndw_num = eval_numerical_gradient_array(lambda w: affine_relu_forward(x, w, b)[0], w, dout)\ndb_num = eval_numerical_gradient_array(lambda b: affine_relu_forward(x, w, b)[0], b, dout)\n\n# Relative error should be around e-10 or less\nprint('Testing affine_relu_forward and affine_relu_backward:')\nprint('dx error: ', rel_error(dx_num, dx))\nprint('dw error: ', rel_error(dw_num, dw))\nprint('db error: ', rel_error(db_num, db))",
"Testing affine_relu_forward and affine_relu_backward:\ndx error: 2.299579177309368e-11\ndw error: 8.162011105764925e-11\ndb error: 7.826724021458994e-12\n"
]
],
[
[
"# Loss layers: Softmax and SVM\nNow implement the loss and gradient for softmax and SVM in the `softmax_loss` and `svm_loss` function in `cs231n/layers.py`. These should be similar to what you implemented in `cs231n/classifiers/softmax.py` and `cs231n/classifiers/linear_svm.py`.\n\nYou can make sure that the implementations are correct by running the following:",
"_____no_output_____"
]
],
[
[
"np.random.seed(231)\nnum_classes, num_inputs = 10, 50\nx = 0.001 * np.random.randn(num_inputs, num_classes)\ny = np.random.randint(num_classes, size=num_inputs)\n\ndx_num = eval_numerical_gradient(lambda x: svm_loss(x, y)[0], x, verbose=False)\nloss, dx = svm_loss(x, y)\n\n# Test svm_loss function. Loss should be around 9 and dx error should be around the order of e-9\nprint('Testing svm_loss:')\nprint('loss: ', loss)\nprint('dx error: ', rel_error(dx_num, dx))\n\ndx_num = eval_numerical_gradient(lambda x: softmax_loss(x, y)[0], x, verbose=False)\nloss, dx = softmax_loss(x, y)\n\n# Test softmax_loss function. Loss should be close to 2.3 and dx error should be around e-8\nprint('\\nTesting softmax_loss:')\nprint('loss: ', loss)\nprint('dx error: ', rel_error(dx_num, dx))",
"Testing svm_loss:\nloss: 8.999602749096233\ndx error: 1.4021566006651672e-09\n\nTesting softmax_loss:\nloss: 2.3025458445007376\ndx error: 8.234144091578429e-09\n"
]
],
[
[
"# Two-layer network\nOpen the file `cs231n/classifiers/fc_net.py` and complete the implementation of the `TwoLayerNet` class. Read through it to make sure you understand the API. You can run the cell below to test your implementation.",
"_____no_output_____"
]
],
[
[
"np.random.seed(231)\nN, D, H, C = 3, 5, 50, 7\nX = np.random.randn(N, D)\ny = np.random.randint(C, size=N)\n\nstd = 1e-3\nmodel = TwoLayerNet(input_dim=D, hidden_dim=H, num_classes=C, weight_scale=std)\n\nprint('Testing initialization ... ')\nW1_std = abs(model.params['W1'].std() - std)\nb1 = model.params['b1']\nW2_std = abs(model.params['W2'].std() - std)\nb2 = model.params['b2']\nassert W1_std < std / 10, 'First layer weights do not seem right'\nassert np.all(b1 == 0), 'First layer biases do not seem right'\nassert W2_std < std / 10, 'Second layer weights do not seem right'\nassert np.all(b2 == 0), 'Second layer biases do not seem right'\n\nprint('Testing test-time forward pass ... ')\nmodel.params['W1'] = np.linspace(-0.7, 0.3, num=D*H).reshape(D, H)\nmodel.params['b1'] = np.linspace(-0.1, 0.9, num=H)\nmodel.params['W2'] = np.linspace(-0.3, 0.4, num=H*C).reshape(H, C)\nmodel.params['b2'] = np.linspace(-0.9, 0.1, num=C)\nX = np.linspace(-5.5, 4.5, num=N*D).reshape(D, N).T\nscores = model.loss(X)\ncorrect_scores = np.asarray(\n [[11.53165108, 12.2917344, 13.05181771, 13.81190102, 14.57198434, 15.33206765, 16.09215096],\n [12.05769098, 12.74614105, 13.43459113, 14.1230412, 14.81149128, 15.49994135, 16.18839143],\n [12.58373087, 13.20054771, 13.81736455, 14.43418138, 15.05099822, 15.66781506, 16.2846319 ]])\nscores_diff = np.abs(scores - correct_scores).sum()\nassert scores_diff < 1e-6, 'Problem with test-time forward pass'\n\nprint('Testing training loss (no regularization)')\ny = np.asarray([0, 5, 1])\nloss, grads = model.loss(X, y)\ncorrect_loss = 3.4702243556\nassert abs(loss - correct_loss) < 1e-10, 'Problem with training-time loss'\n\nmodel.reg = 1.0\nloss, grads = model.loss(X, y)\ncorrect_loss = 26.5948426952\nassert abs(loss - correct_loss) < 1e-10, 'Problem with regularization loss'\n\n# Errors should be around e-7 or less\nfor reg in [0.0, 0.7]:\n print('Running numeric gradient check with reg = ', reg)\n model.reg = reg\n loss, grads = model.loss(X, y)\n\n for name in sorted(grads):\n f = lambda _: model.loss(X, y)[0]\n grad_num = eval_numerical_gradient(f, model.params[name], verbose=False)\n print('%s relative error: %.2e' % (name, rel_error(grad_num, grads[name])))",
"Testing initialization ... \nTesting test-time forward pass ... \nTesting training loss (no regularization)\nRunning numeric gradient check with reg = 0.0\nW1 relative error: 1.83e-08\nW2 relative error: 3.20e-10\nb1 relative error: 9.83e-09\nb2 relative error: 4.33e-10\nRunning numeric gradient check with reg = 0.7\nW1 relative error: 2.53e-07\nW2 relative error: 7.98e-08\nb1 relative error: 1.56e-08\nb2 relative error: 9.09e-10\n"
]
],
[
[
"# Solver\nOpen the file `cs231n/solver.py` and read through it to familiarize yourself with the API. You also need to imeplement the `sgd` function in `cs231n/optim.py`. After doing so, use a `Solver` instance to train a `TwoLayerNet` that achieves about `36%` accuracy on the validation set.",
"_____no_output_____"
]
],
[
[
"input_size = 32 * 32 * 3\nhidden_size = 50\nnum_classes = 10\nmodel = TwoLayerNet(input_size, hidden_size, num_classes)\nsolver = None\n\n##############################################################################\n# TODO: Use a Solver instance to train a TwoLayerNet that achieves about 36% #\n# accuracy on the validation set. #\n##############################################################################\n# *****START OF YOUR CODE (DO NOT DELETE/MODIFY THIS LINE)*****\n\ndata = {\n 'X_train': data['X_train'],\n 'y_train': data['y_train'],\n 'X_val': data['X_val'],\n 'y_val': data['y_val'],\n \n }\nsolver = Solver(model, data,\n update_rule='sgd',\n optim_config={\n 'learning_rate': 1e-3,\n },\n lr_decay=0.95,\n num_epochs=10, batch_size=100,\n print_every=100)\nsolver.train()\n\n# *****END OF YOUR CODE (DO NOT DELETE/MODIFY THIS LINE)*****\n##############################################################################\n# END OF YOUR CODE #\n##############################################################################",
"(Iteration 1 / 4900) loss: 2.300089\n(Epoch 0 / 10) train acc: 0.171000; val_acc: 0.170000\n(Iteration 101 / 4900) loss: 1.782419\n(Iteration 201 / 4900) loss: 1.803466\n(Iteration 301 / 4900) loss: 1.712676\n(Iteration 401 / 4900) loss: 1.693946\n(Epoch 1 / 10) train acc: 0.399000; val_acc: 0.428000\n(Iteration 501 / 4900) loss: 1.711237\n(Iteration 601 / 4900) loss: 1.443683\n(Iteration 701 / 4900) loss: 1.574904\n(Iteration 801 / 4900) loss: 1.526751\n(Iteration 901 / 4900) loss: 1.352554\n(Epoch 2 / 10) train acc: 0.463000; val_acc: 0.443000\n(Iteration 1001 / 4900) loss: 1.340071\n(Iteration 1101 / 4900) loss: 1.386843\n(Iteration 1201 / 4900) loss: 1.489919\n(Iteration 1301 / 4900) loss: 1.353077\n(Iteration 1401 / 4900) loss: 1.467951\n(Epoch 3 / 10) train acc: 0.477000; val_acc: 0.430000\n(Iteration 1501 / 4900) loss: 1.337133\n(Iteration 1601 / 4900) loss: 1.426819\n(Iteration 1701 / 4900) loss: 1.348675\n(Iteration 1801 / 4900) loss: 1.412626\n(Iteration 1901 / 4900) loss: 1.354764\n(Epoch 4 / 10) train acc: 0.497000; val_acc: 0.467000\n(Iteration 2001 / 4900) loss: 1.422221\n(Iteration 2101 / 4900) loss: 1.360665\n(Iteration 2201 / 4900) loss: 1.546539\n(Iteration 2301 / 4900) loss: 1.196704\n(Iteration 2401 / 4900) loss: 1.401958\n(Epoch 5 / 10) train acc: 0.508000; val_acc: 0.483000\n(Iteration 2501 / 4900) loss: 1.243567\n(Iteration 2601 / 4900) loss: 1.513808\n(Iteration 2701 / 4900) loss: 1.266416\n(Iteration 2801 / 4900) loss: 1.485956\n(Iteration 2901 / 4900) loss: 1.049706\n(Epoch 6 / 10) train acc: 0.533000; val_acc: 0.492000\n(Iteration 3001 / 4900) loss: 1.264002\n(Iteration 3101 / 4900) loss: 1.184786\n(Iteration 3201 / 4900) loss: 1.231162\n(Iteration 3301 / 4900) loss: 1.353725\n(Iteration 3401 / 4900) loss: 1.217830\n(Epoch 7 / 10) train acc: 0.545000; val_acc: 0.489000\n(Iteration 3501 / 4900) loss: 1.446003\n(Iteration 3601 / 4900) loss: 1.152495\n(Iteration 3701 / 4900) loss: 1.421970\n(Iteration 3801 / 4900) loss: 1.222752\n(Iteration 3901 / 4900) loss: 1.213468\n(Epoch 8 / 10) train acc: 0.546000; val_acc: 0.474000\n(Iteration 4001 / 4900) loss: 1.187435\n(Iteration 4101 / 4900) loss: 1.284799\n(Iteration 4201 / 4900) loss: 1.135251\n(Iteration 4301 / 4900) loss: 1.212217\n(Iteration 4401 / 4900) loss: 1.213544\n(Epoch 9 / 10) train acc: 0.586000; val_acc: 0.486000\n(Iteration 4501 / 4900) loss: 1.306174\n(Iteration 4601 / 4900) loss: 1.213528\n(Iteration 4701 / 4900) loss: 1.220260\n(Iteration 4801 / 4900) loss: 1.233231\n(Epoch 10 / 10) train acc: 0.545000; val_acc: 0.483000\n"
]
],
[
[
"# Debug the training\nWith the default parameters we provided above, you should get a validation accuracy of about 0.36 on the validation set. This isn't very good.\n\nOne strategy for getting insight into what's wrong is to plot the loss function and the accuracies on the training and validation sets during optimization.\n\nAnother strategy is to visualize the weights that were learned in the first layer of the network. In most neural networks trained on visual data, the first layer weights typically show some visible structure when visualized.",
"_____no_output_____"
]
],
[
[
"# Run this cell to visualize training loss and train / val accuracy\n\nplt.subplot(2, 1, 1)\nplt.title('Training loss')\nplt.plot(solver.loss_history, 'o')\nplt.xlabel('Iteration')\n\nplt.subplot(2, 1, 2)\nplt.title('Accuracy')\nplt.plot(solver.train_acc_history, '-o', label='train')\nplt.plot(solver.val_acc_history, '-o', label='val')\nplt.plot([0.5] * len(solver.val_acc_history), 'k--')\nplt.xlabel('Epoch')\nplt.legend(loc='lower right')\nplt.gcf().set_size_inches(15, 12)\nplt.show()",
"_____no_output_____"
],
[
"from cs231n.vis_utils import visualize_grid\n\n# Visualize the weights of the network\n\ndef show_net_weights(net):\n W1 = net.params['W1']\n W1 = W1.reshape(32, 32, 3, -1).transpose(3, 0, 1, 2)\n plt.imshow(visualize_grid(W1, padding=3).astype('uint8'))\n plt.gca().axis('off')\n plt.show()\n\nshow_net_weights(model)",
"_____no_output_____"
]
],
[
[
"# Tune your hyperparameters\n\n**What's wrong?**. Looking at the visualizations above, we see that the loss is decreasing more or less linearly, which seems to suggest that the learning rate may be too low. Moreover, there is no gap between the training and validation accuracy, suggesting that the model we used has low capacity, and that we should increase its size. On the other hand, with a very large model we would expect to see more overfitting, which would manifest itself as a very large gap between the training and validation accuracy.\n\n**Tuning**. Tuning the hyperparameters and developing intuition for how they affect the final performance is a large part of using Neural Networks, so we want you to get a lot of practice. Below, you should experiment with different values of the various hyperparameters, including hidden layer size, learning rate, numer of training epochs, and regularization strength. You might also consider tuning the learning rate decay, but you should be able to get good performance using the default value.\n\n**Approximate results**. You should be aim to achieve a classification accuracy of greater than 48% on the validation set. Our best network gets over 52% on the validation set.\n\n**Experiment**: You goal in this exercise is to get as good of a result on CIFAR-10 as you can (52% could serve as a reference), with a fully-connected Neural Network. Feel free implement your own techniques (e.g. PCA to reduce dimensionality, or adding dropout, or adding features to the solver, etc.).",
"_____no_output_____"
]
],
[
[
"best_model = None\nbest_acc = 0\n\n\n#################################################################################\n# TODO: Tune hyperparameters using the validation set. Store your best trained #\n# model in best_model. #\n# #\n# To help debug your network, it may help to use visualizations similar to the #\n# ones we used above; these visualizations will have significant qualitative #\n# differences from the ones we saw above for the poorly tuned network. #\n# #\n# Tweaking hyperparameters by hand can be fun, but you might find it useful to #\n# write code to sweep through possible combinations of hyperparameters #\n# automatically like we did on thexs previous exercises. #\n#################################################################################\n# *****START OF YOUR CODE (DO NOT DELETE/MODIFY THIS LINE)*****\nlearning_rates = [1e-3]\nregularization_strengths = [0.05, 0.1, 0.15]\n\n# *****START OF YOUR CODE (DO NOT DELETE/MODIFY THIS LINE)*****\nfor (lr, reg) in [(lr, reg) for lr in learning_rates for reg in regularization_strengths]:\n model = TwoLayerNet(input_size, hidden_size, num_classes, reg=reg)\n solver = None\n\n data = {\n 'X_train': data['X_train'],\n 'y_train': data['y_train'],\n 'X_val': data['X_val'],\n 'y_val': data['y_val']\n }\n solver = Solver(model, data,\n update_rule='sgd',\n optim_config={\n 'learning_rate': lr,\n },\n lr_decay=0.95,\n num_epochs=10, batch_size=100,\n print_every=100)\n solver.train()\n acc = solver.check_accuracy(data['X_val'], data['y_val'])\n \n\n print('lr %e reg %e val accuracy: %f' % (lr, reg, acc))\n if(acc > best_acc):\n best_acc = acc\n best_model = model\n\n# *****END OF YOUR CODE (DO NOT DELETE/MODIFY THIS LINE)*****\n################################################################################\n# END OF YOUR CODE #\n################################################################################",
"(Iteration 1 / 4900) loss: 2.306069\n(Epoch 0 / 10) train acc: 0.104000; val_acc: 0.114000\n(Iteration 101 / 4900) loss: 1.724592\n(Iteration 201 / 4900) loss: 1.782996\n(Iteration 301 / 4900) loss: 1.663951\n(Iteration 401 / 4900) loss: 1.639033\n(Epoch 1 / 10) train acc: 0.443000; val_acc: 0.435000\n(Iteration 501 / 4900) loss: 1.732685\n(Iteration 601 / 4900) loss: 1.450637\n(Iteration 701 / 4900) loss: 1.544135\n(Iteration 801 / 4900) loss: 1.342042\n(Iteration 901 / 4900) loss: 1.524098\n(Epoch 2 / 10) train acc: 0.460000; val_acc: 0.468000\n(Iteration 1001 / 4900) loss: 1.504554\n(Iteration 1101 / 4900) loss: 1.562994\n(Iteration 1201 / 4900) loss: 1.531669\n(Iteration 1301 / 4900) loss: 1.580678\n(Iteration 1401 / 4900) loss: 1.610020\n(Epoch 3 / 10) train acc: 0.491000; val_acc: 0.468000\n(Iteration 1501 / 4900) loss: 1.472904\n(Iteration 1601 / 4900) loss: 1.420686\n(Iteration 1701 / 4900) loss: 1.221016\n(Iteration 1801 / 4900) loss: 1.438085\n(Iteration 1901 / 4900) loss: 1.399311\n(Epoch 4 / 10) train acc: 0.487000; val_acc: 0.470000\n(Iteration 2001 / 4900) loss: 1.328356\n(Iteration 2101 / 4900) loss: 1.327213\n(Iteration 2201 / 4900) loss: 1.468259\n(Iteration 2301 / 4900) loss: 1.359908\n(Iteration 2401 / 4900) loss: 1.654595\n(Epoch 5 / 10) train acc: 0.544000; val_acc: 0.479000\n(Iteration 2501 / 4900) loss: 1.324480\n(Iteration 2601 / 4900) loss: 1.415154\n(Iteration 2701 / 4900) loss: 1.388397\n(Iteration 2801 / 4900) loss: 1.282842\n(Iteration 2901 / 4900) loss: 1.567757\n(Epoch 6 / 10) train acc: 0.522000; val_acc: 0.476000\n(Iteration 3001 / 4900) loss: 1.198947\n(Iteration 3101 / 4900) loss: 1.411820\n(Iteration 3201 / 4900) loss: 1.409265\n(Iteration 3301 / 4900) loss: 1.546007\n(Iteration 3401 / 4900) loss: 1.298864\n(Epoch 7 / 10) train acc: 0.572000; val_acc: 0.477000\n(Iteration 3501 / 4900) loss: 1.265209\n(Iteration 3601 / 4900) loss: 1.261452\n(Iteration 3701 / 4900) loss: 1.294712\n(Iteration 3801 / 4900) loss: 1.273777\n(Iteration 3901 / 4900) loss: 1.221081\n(Epoch 8 / 10) train acc: 0.544000; val_acc: 0.484000\n(Iteration 4001 / 4900) loss: 1.300183\n(Iteration 4101 / 4900) loss: 1.377406\n(Iteration 4201 / 4900) loss: 1.382470\n(Iteration 4301 / 4900) loss: 1.290416\n(Iteration 4401 / 4900) loss: 1.379400\n(Epoch 9 / 10) train acc: 0.529000; val_acc: 0.496000\n(Iteration 4501 / 4900) loss: 1.376942\n(Iteration 4601 / 4900) loss: 1.132249\n(Iteration 4701 / 4900) loss: 1.152085\n(Iteration 4801 / 4900) loss: 1.318604\n(Epoch 10 / 10) train acc: 0.570000; val_acc: 0.486000\nlr 1.000000e-03 reg 5.000000e-02 val accuracy: 0.496000\n(Iteration 1 / 4900) loss: 2.310147\n(Epoch 0 / 10) train acc: 0.117000; val_acc: 0.103000\n(Iteration 101 / 4900) loss: 1.851684\n(Iteration 201 / 4900) loss: 1.804342\n(Iteration 301 / 4900) loss: 1.513165\n(Iteration 401 / 4900) loss: 1.557443\n(Epoch 1 / 10) train acc: 0.473000; val_acc: 0.433000\n(Iteration 501 / 4900) loss: 1.675617\n(Iteration 601 / 4900) loss: 1.428126\n(Iteration 701 / 4900) loss: 1.495615\n(Iteration 801 / 4900) loss: 1.783007\n(Iteration 901 / 4900) loss: 1.644330\n(Epoch 2 / 10) train acc: 0.464000; val_acc: 0.471000\n(Iteration 1001 / 4900) loss: 1.421902\n(Iteration 1101 / 4900) loss: 1.482220\n(Iteration 1201 / 4900) loss: 1.673121\n(Iteration 1301 / 4900) loss: 1.560792\n(Iteration 1401 / 4900) loss: 1.626430\n(Epoch 3 / 10) train acc: 0.480000; val_acc: 0.443000\n(Iteration 1501 / 4900) loss: 1.547922\n(Iteration 1601 / 4900) loss: 1.453693\n(Iteration 1701 / 4900) loss: 1.314836\n(Iteration 1801 / 4900) loss: 1.390783\n(Iteration 1901 / 4900) loss: 1.297606\n(Epoch 4 / 10) train acc: 0.506000; val_acc: 0.487000\n(Iteration 2001 / 4900) loss: 1.464706\n(Iteration 2101 / 4900) loss: 1.364239\n(Iteration 2201 / 4900) loss: 1.352896\n(Iteration 2301 / 4900) loss: 1.457134\n(Iteration 2401 / 4900) loss: 1.254323\n(Epoch 5 / 10) train acc: 0.543000; val_acc: 0.494000\n(Iteration 2501 / 4900) loss: 1.575036\n(Iteration 2601 / 4900) loss: 1.311684\n(Iteration 2701 / 4900) loss: 1.388892\n(Iteration 2801 / 4900) loss: 1.423586\n(Iteration 2901 / 4900) loss: 1.400047\n(Epoch 6 / 10) train acc: 0.529000; val_acc: 0.490000\n(Iteration 3001 / 4900) loss: 1.275920\n(Iteration 3101 / 4900) loss: 1.479168\n(Iteration 3201 / 4900) loss: 1.525349\n(Iteration 3301 / 4900) loss: 1.329852\n(Iteration 3401 / 4900) loss: 1.335383\n(Epoch 7 / 10) train acc: 0.533000; val_acc: 0.490000\n(Iteration 3501 / 4900) loss: 1.492119\n(Iteration 3601 / 4900) loss: 1.382005\n(Iteration 3701 / 4900) loss: 1.428007\n(Iteration 3801 / 4900) loss: 1.385181\n(Iteration 3901 / 4900) loss: 1.351685\n(Epoch 8 / 10) train acc: 0.561000; val_acc: 0.509000\n(Iteration 4001 / 4900) loss: 1.135909\n(Iteration 4101 / 4900) loss: 1.373260\n(Iteration 4201 / 4900) loss: 1.218843\n(Iteration 4301 / 4900) loss: 1.297008\n(Iteration 4401 / 4900) loss: 1.426274\n(Epoch 9 / 10) train acc: 0.534000; val_acc: 0.473000\n(Iteration 4501 / 4900) loss: 1.451631\n(Iteration 4601 / 4900) loss: 1.424421\n(Iteration 4701 / 4900) loss: 1.314771\n(Iteration 4801 / 4900) loss: 1.383842\n(Epoch 10 / 10) train acc: 0.538000; val_acc: 0.488000\nlr 1.000000e-03 reg 1.000000e-01 val accuracy: 0.509000\n(Iteration 1 / 4900) loss: 2.312442\n(Epoch 0 / 10) train acc: 0.109000; val_acc: 0.096000\n(Iteration 101 / 4900) loss: 1.861943\n(Iteration 201 / 4900) loss: 1.835878\n(Iteration 301 / 4900) loss: 1.698791\n(Iteration 401 / 4900) loss: 1.511703\n(Epoch 1 / 10) train acc: 0.423000; val_acc: 0.444000\n(Iteration 501 / 4900) loss: 1.499857\n(Iteration 601 / 4900) loss: 1.402853\n(Iteration 701 / 4900) loss: 1.656265\n(Iteration 801 / 4900) loss: 1.654180\n(Iteration 901 / 4900) loss: 1.421730\n(Epoch 2 / 10) train acc: 0.446000; val_acc: 0.424000\n(Iteration 1001 / 4900) loss: 1.827052\n(Iteration 1101 / 4900) loss: 1.424511\n(Iteration 1201 / 4900) loss: 1.331444\n(Iteration 1301 / 4900) loss: 1.392123\n(Iteration 1401 / 4900) loss: 1.455621\n(Epoch 3 / 10) train acc: 0.504000; val_acc: 0.489000\n(Iteration 1501 / 4900) loss: 1.439697\n(Iteration 1601 / 4900) loss: 1.585881\n(Iteration 1701 / 4900) loss: 1.428888\n(Iteration 1801 / 4900) loss: 1.319450\n(Iteration 1901 / 4900) loss: 1.400379\n(Epoch 4 / 10) train acc: 0.509000; val_acc: 0.482000\n(Iteration 2001 / 4900) loss: 1.499542\n(Iteration 2101 / 4900) loss: 1.441353\n(Iteration 2201 / 4900) loss: 1.365738\n(Iteration 2301 / 4900) loss: 1.475881\n(Iteration 2401 / 4900) loss: 1.581152\n(Epoch 5 / 10) train acc: 0.470000; val_acc: 0.470000\n(Iteration 2501 / 4900) loss: 1.492029\n(Iteration 2601 / 4900) loss: 1.490493\n(Iteration 2701 / 4900) loss: 1.174688\n(Iteration 2801 / 4900) loss: 1.419202\n(Iteration 2901 / 4900) loss: 1.535868\n(Epoch 6 / 10) train acc: 0.483000; val_acc: 0.490000\n(Iteration 3001 / 4900) loss: 1.393103\n(Iteration 3101 / 4900) loss: 1.294975\n(Iteration 3201 / 4900) loss: 1.275845\n(Iteration 3301 / 4900) loss: 1.411851\n(Iteration 3401 / 4900) loss: 1.269081\n(Epoch 7 / 10) train acc: 0.546000; val_acc: 0.502000\n(Iteration 3501 / 4900) loss: 1.264167\n(Iteration 3601 / 4900) loss: 1.432768\n(Iteration 3701 / 4900) loss: 1.218371\n(Iteration 3801 / 4900) loss: 1.208629\n(Iteration 3901 / 4900) loss: 1.325607\n(Epoch 8 / 10) train acc: 0.540000; val_acc: 0.518000\n(Iteration 4001 / 4900) loss: 1.316241\n(Iteration 4101 / 4900) loss: 1.117369\n(Iteration 4201 / 4900) loss: 1.358598\n(Iteration 4301 / 4900) loss: 1.291494\n(Iteration 4401 / 4900) loss: 1.339091\n(Epoch 9 / 10) train acc: 0.534000; val_acc: 0.511000\n(Iteration 4501 / 4900) loss: 1.280720\n(Iteration 4601 / 4900) loss: 1.341328\n(Iteration 4701 / 4900) loss: 1.112138\n(Iteration 4801 / 4900) loss: 1.218325\n(Epoch 10 / 10) train acc: 0.568000; val_acc: 0.484000\nlr 1.000000e-03 reg 1.500000e-01 val accuracy: 0.518000\n"
]
],
[
[
"# Test your model!\nRun your best model on the validation and test sets. You should achieve above 48% accuracy on the validation set and the test set.",
"_____no_output_____"
]
],
[
[
"y_val_pred = np.argmax(best_model.loss(data['X_val']), axis=1)\nprint('Validation set accuracy: ', (y_val_pred == data['y_val']).mean())",
"Validation set accuracy: 0.518\n"
],
[
"data = get_CIFAR10_data()",
"_____no_output_____"
],
[
"y_test_pred = np.argmax(best_model.loss(data[\"X_test\"]), axis=1)\nprint('Test set accuracy: ', (y_test_pred == data['y_test']).mean())",
"Test set accuracy: 0.495\n"
]
],
[
[
"## Inline Question 2: \n\nNow that you have trained a Neural Network classifier, you may find that your testing accuracy is much lower than the training accuracy. In what ways can we decrease this gap? Select all that apply.\n\n1. Train on a larger dataset.\n2. Add more hidden units.\n3. Increase the regularization strength.\n4. None of the above.\n\n$\\color{blue}{\\textit Your Answer:}$\n\n$\\color{blue}{\\textit Your Explanation:}$\n\n",
"_____no_output_____"
]
],
[
[
"",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7ba7419d79d8087ad19a3aaa322bacb5f90c7d5 | 134,403 | ipynb | Jupyter Notebook | 101_exercises.ipynb | barbmarques/python-exercises | 4b8f76d255bb9baf85fea331fa57d0b425cbed41 | [
"MIT"
] | null | null | null | 101_exercises.ipynb | barbmarques/python-exercises | 4b8f76d255bb9baf85fea331fa57d0b425cbed41 | [
"MIT"
] | null | null | null | 101_exercises.ipynb | barbmarques/python-exercises | 4b8f76d255bb9baf85fea331fa57d0b425cbed41 | [
"MIT"
] | null | null | null | 35.138039 | 1,110 | 0.510472 | [
[
[
"<a href=\"https://colab.research.google.com/github/barbmarques/python-exercises/blob/main/101_exercises.ipynb\" target=\"_parent\"><img src=\"https://colab.research.google.com/assets/colab-badge.svg\" alt=\"Open In Colab\"/></a>",
"_____no_output_____"
],
[
"# Welcome to 101 Exercises for Python Fundamentals\n\nSolving these exercises will help make you a better programmer. Solve them in order, because each solution builds scaffolding, working code, and knowledge you can use on future problems. Read the directions carefully, and have fun!\n\n> \"Learning to program takes a little bit of study and a *lot* of practice\" - Luis Montealegre",
"_____no_output_____"
],
[
"## Getting Started\n1. Go to https://colab.research.google.com/github/ryanorsinger/101-exercises/blob/main/101-exercises.ipynb\n2. To save your work to your Google Drive, go to File then \"Save Copy in Drive\".\n3. Your own work will now appear in your Google Drive account!\n\nIf you need a fresh, blank copy of this document, go to https://colab.research.google.com/github/ryanorsinger/101-exercises/blob/main/101-exercises.ipynb and save a fresh copy in your Google Drive.",
"_____no_output_____"
],
[
"## Orientation\n- This code notebook is composed of cells. Each cell is either text or Python code.\n- To run a cell of code, click the \"play button\" icon to the left of the cell or click on the cell and press \"Shift+Enter\" on your keyboard. This will execute the Python code contained in the cell. Executing a cell that defines a variable is important before executing or authoring a cell that depends on that previously created variable assignment.\n- **Expect to see lots of errors** the first time you load this page. \n- **Expect to see lots of errors** for all cells run without code that matches the assertion tests.\n- Until you click the blue \"Copy and Edit\" button to make your own copy, you will see an entire page of errors. This is part of the automated tests.\n- Each *assert* line is both an example and a test that tests for the presence and functionality of the instructed exercise. \n\n## The only 3 conditions that produce no errors:\n1. When you make a fresh **copy** of the project to your own account (by clicking \"Copy and Edit\")\n2. When you go to \"Run\" and then click \"Restart Session\"\n3. When every single assertion passes.\n\n\n## Outline\n- Each cell starts with a problem statement that describes the exercise to complete.\n- Underneath each problem statement, learners will need to write code to produce an answer.\n- The **assert** lines test to see that your code solves the problem appropriately\n- Many exercises will rely on previous solutions to be correctly completed\n- The `print(\"Exercise is complete\")` line will only run if your solution passes the assertion test(s)\n- Be sure to create programmatic solutions that will work for all inputs:\n- For example, calling the `is_even(2)` returns `True`, but your function should work for all even numbers, both positive and negative.\n\n\n## Guidance\n- Get Python to do the work for you. For example, if the exercise instructs you to reverse a list of numbers, your job is to find the \n- Save often by clicking the blue \"Save\" button.\n- If you need to clear the output or reset the notebook, go to \"Run\" then \"Restart Session\" to clear up any error messages.\n- Do not move or alter the lines of code that contain the `assert` statements. Those are what run your solution and test its actual output vs. expected outputs.\n- Seek to understand the problem before trying to solve it. Can you explain the problem to someone else in English? Can you explain the solution in English?\n- Slow down and read any error messages you encounter. Error messages provide insight into how to resolve the error. When in doubt, put your exact error into a search engine and look for results that reference an identical or similar problem.\n\n## Get Python To Do The Work For You\nOne of the main jobs of a programming language is to help people solve problems programatically, so we don't have to do so much by hand. For example, it's easy for a person to manually reverse the list `[1, 2, 3]`, but imagine reversing a list of a million things or sorting a list of even a hundred things. When we write programmatic solutions in code, we are providing instructions to the computer to do a task. Computers follow the letter of the code, not the intent, and do exactly what they are told to do. In this way, Python can reverse a list of 3 numbers or 100 numbers or ten million numbers with the same instructions. Repetition is a key idea behind programming languages.\n\nThis means that your task with these exercises is to determine a sequence of steps that solve the problem and then find the Python code that will run those instructions. If you're sorting or reversing things by hand, you're not doing it right!\n\n## How To Discover How To Do Something in Python\n1. The first step is to make sure you know what the problem is asking.\n2. The second step is to determine, in English (or your first spoken language), what steps you need to take.\n3. Use a search engine to look for code examples to identical or similar problems.\n\nOne of the best ways to discover how to do things in Python is to use a search engine. Go to your favorite search engine and search for \"how to reverse a list in Python\" or \"how to sort a list in Python\". That's how both learners and professionals find answers and examples all the time. Search for what you want and add \"in Python\" and you'll get lots of code examples. Searching for \"How to sum a list of numbers in Python\" is a very effective way to discover exactly how to do that task.",
"_____no_output_____"
],
[
"### Learning to Program and Code\n- You can make a new blank cell for Python code at any time in this document.\n- If you want more freedom to explore learning Python in a blank notebook, go here https://colab.research.google.com/#create=true and make yourself a blank, new notebook.\n- Programming is an intellectual activity of designing a solution. \"Coding\" means turning your programmatic solution into code w/ all the right syntax and parts of the programming language.\n- Expect to make mistakes and adopt the attitude that **the error message provides the information you need to proceed**. You will put lots of error messages into search engines to learn this craft!\n- Because computers have zero ability to read in between the lines or \"catch the drift\" or know what you mean, code only does what it is told to do.\n- Code doesn't do what you *want* it to do, code does what you've told it to do.\n- Before writing any code, figure out how you would solve the problem in spoken language to describe the sequence of steps in the solution.\n- Think about your solution in English (or your natural language). It's **critical** to solve the problem in your natural language before trying to get a programming language to do the work.",
"_____no_output_____"
],
[
"## Troubleshooting\n- If this entire document shows \"Name Error\" for many cells, it means you should read the \"Getting Started\" instructions above to make your own copy.\n- Be sure to commit your work to make save points, as you go.\n- If you load this page and you see your code but not the results of the code, be sure to run each cell (shift + Enter makes this quick)\n- \"Name Error\" means that you need to assign a variable or define the function as instructed.\n- \"Assertion Error\" means that your provided solution does not match the correct answer.\n- \"Type Error\" means that your data type provided is not accurate\n- If your kernel freezes, click on \"Run\" then select \"Restart Session\"\n- If you require additional troubleshooting assistance, click on \"Help\" and then \"Docs\" to access documentation for this platform.\n- If you have discoverd a bug or typo, please triple check your spelling then create a new issue at [https://github.com/ryanorsinger/101-exercises/issues](https://github.com/ryanorsinger/101-exercises/issues) to notify the author.",
"_____no_output_____"
]
],
[
[
"# Example problem:\n# Uncomment the line below and run this cell.\n# The hashtag \"#\" character in a line of Python code is the comment character. \ndoing_python_right_now = True\n\n# The lines below will test your answer. If you see an error, then it means that your answer is incorrect or incomplete.\nassert doing_python_right_now == True, \"If you see a NameError, it means that the variable is not created and assigned a value. An 'Assertion Error' means that the value of the variable is incorrect.\" \nprint(\"Exercise 0 is correct\") # This line will print if your solution passes the assertion above.",
"Exercise 0 is correct\n"
],
[
"# Exercise 1\n# On the line below, create a variable named on_mars_right_now and assign it the boolean value of False\n\non_mars_right_now = False\n\nassert on_mars_right_now == False, \"If you see a Name Error, be sure to create the variable and assign it a value.\"\nprint(\"Exercise 1 is correct.\")",
"Exercise 1 is correct.\n"
],
[
"# Exercise 2\n# Create a variable named fruits and assign it a list of fruits containing the following fruit names as strings: \n# mango, banana, guava, kiwi, and strawberry.\n\nfruits = ['mango', 'banana', 'guava', 'kiwi', 'strawberry']\n\n\nassert fruits == [\"mango\", \"banana\", \"guava\", \"kiwi\", \"strawberry\"], \"If you see an Assert Error, ensure the variable contains all the strings in the provided order\"\nprint(\"Exercise 2 is correct.\")",
"Exercise 2 is correct.\n"
],
[
"# Exercise 3\n# Create a variable named vegetables and assign it a list of fruits containing the following vegetable names as strings: \n# eggplant, broccoli, carrot, cauliflower, and zucchini\n\nvegetables = ['eggplant', 'broccoli', 'carrot', 'cauliflower', 'zucchini']\n\nassert vegetables == [\"eggplant\", \"broccoli\", \"carrot\", \"cauliflower\", \"zucchini\"], \"Ensure the variable contains all the strings in the provided order\"\nprint(\"Exercise 3 is correct.\")",
"Exercise 3 is correct.\n"
],
[
"# Exercise 4\n# Create a variable named numbers and assign it a list of numbers, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10\n\nnumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]\n\nassert numbers == [1, 2, 3, 4, 5, 6, 7, 8, 9, 10], \"Ensure the variable contains the numbers 1-10 in order.\"\nprint(\"Exercise 4 is correct.\")",
"Exercise 4 is correct.\n"
]
],
[
[
"## List Operations\n**Hint** Recommend finding and using built-in Python functionality whenever possible.",
"_____no_output_____"
]
],
[
[
"# Exercise 5\n# Given the following assigment of the list of fruits, add \"tomato\" to the end of the list. \nfruits = [\"mango\", \"banana\", \"guava\", \"kiwi\", \"strawberry\"]\n\nfruits.append('tomato')\n\nassert fruits == [\"mango\", \"banana\", \"guava\", \"kiwi\", \"strawberry\", \"tomato\"], \"Ensure the variable contains all the strings in the right order\"\nprint(\"Exercise 5 is correct\")",
"Exercise 5 is correct\n"
],
[
"# Exercise 6\n# Given the following assignment of the vegetables list, add \"tomato\" to the end of the list.\nvegetables = [\"eggplant\", \"broccoli\", \"carrot\", \"cauliflower\", \"zucchini\"]\n\nvegetables.append('tomato')\nassert vegetables == [\"eggplant\", \"broccoli\", \"carrot\", \"cauliflower\", \"zucchini\", \"tomato\"], \"Ensure the variable contains all the strings in the provided order\"\nprint(\"Exercise 6 is correct\")",
"Exercise 6 is correct\n"
],
[
"# Exercise 7\n# Given the list of numbers defined below, reverse the list of numbers that you created above. \nnumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]\n\nnumbers.reverse()\n\nassert numbers == [10, 9, 8, 7, 6, 5, 4, 3, 2, 1], \"Assert Error means that the answer is incorrect.\" \nprint(\"Exercise 7 is correct.\")",
"Exercise 7 is correct.\n"
],
[
"# Exercise 8\n# Sort the vegetables in alphabetical order\n\nvegetables.sort()\n\nassert vegetables == ['broccoli', 'carrot', 'cauliflower', 'eggplant', 'tomato', 'zucchini']\nprint(\"Exercise 8 is correct.\")",
"Exercise 8 is correct.\n"
],
[
"# Exercise 9\n# Write the code necessary to sort the fruits in reverse alphabetical order\n\nfruits.sort(reverse = True)\n\n\nassert fruits == ['tomato', 'strawberry', 'mango', 'kiwi', 'guava', 'banana']\nprint(\"Exercise 9 is correct.\")",
"Exercise 9 is correct.\n"
],
[
"# Exercise 10\n# Write the code necessary to produce a single list that holds all fruits then all vegetables in the order as they were sorted above.\nfruits_and_veggies = fruits + vegetables\nassert fruits_and_veggies == ['tomato', 'strawberry', 'mango', 'kiwi', 'guava', 'banana', 'broccoli', 'carrot', 'cauliflower', 'eggplant', 'tomato', 'zucchini']\nprint(\"Exercise 10 is correct\")",
"Exercise 10 is correct\n"
]
],
[
[
"## Basic Functions\n**Hint** Be sure to `return` values from your function definitions. The assert statements will call your function(s) for you.",
"_____no_output_____"
]
],
[
[
"# Run this cell in order to generate some numbers to use in our functions after this.\nimport random\n \npositive_even_number = random.randrange(2, 101, 2)\nnegative_even_number = random.randrange(-100, -1, 2)\n\npositive_odd_number = random.randrange(1, 100, 2)\nnegative_odd_number = random.randrange(-101, 0, 2)\nprint(\"We now have some random numbers available for future exercises.\")\nprint(\"The random positive even number is\", positive_even_number)\nprint(\"The random positive odd nubmer is\", positive_odd_number)\nprint(\"The random negative even number\", negative_even_number)\nprint(\"The random negative odd number\", negative_odd_number)\n",
"We now have some random numbers available for future exercises.\nThe random positive even number is 18\nThe random positive odd nubmer is 9\nThe random negative even number -88\nThe random negative odd number -97\n"
],
[
"# Example function defintion:\n# Write a say_hello function that adds the string \"Hello, \" to the beginning and \"!\" to the end of any given input.\ndef say_hello(name):\n return \"Hello, \" + name + \"!\"\n\nassert say_hello(\"Jane\") == \"Hello, Jane!\", \"Double check the inputs and data types\"\nassert say_hello(\"Pat\") == \"Hello, Pat!\", \"Double check the inputs and data types\"\nassert say_hello(\"Astrud\") == \"Hello, Astrud!\", \"Double check the inputs and data types\"\nprint(\"The example function definition ran appropriately\")",
"The example function definition ran appropriately\n"
],
[
"# Another example function definition:\n# This plus_two function takes in a variable and adds 2 to it.\ndef plus_two(number):\n return number + 2\n\nassert plus_two(3) == 5\nassert plus_two(0) == 2\nassert plus_two(-2) == 0\nprint(\"The plus_two assertions executed appropriately... The second function definition example executed appropriately.\")",
"The plus_two assertions executed appropriately... The second function definition example executed appropriately.\n"
],
[
"# Exercise 11\n# Write a function definition for a function named add_one that takes in a number and returns that number plus one.\n\ndef add_one(x):\n new = x + 1\n return new\n \nassert add_one(2) == 3, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert add_one(0) == 1, \"Zero plus one is one.\"\nassert add_one(positive_even_number) == positive_even_number + 1, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert add_one(negative_odd_number) == negative_odd_number + 1, \"Ensure that the function is defined, named properly, and returns the correct value\"\nprint(\"Exercise 11 is correct.\") ",
"Exercise 11 is correct.\n"
],
[
"# Exercise 12\n# Write a function definition named is_positive that takes in a number and returns True or False if that number is positive.\n\ndef is_positive(x):\n return x > 0\n\n\nassert is_positive(positive_odd_number) == True, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_positive(positive_even_number) == True, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_positive(negative_odd_number) == False, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_positive(negative_even_number) == False, \"Ensure that the function is defined, named properly, and returns the correct value\"\nprint(\"Exercise 12 is correct.\")",
"Exercise 12 is correct.\n"
],
[
"# Exercise 13\n# Write a function definition named is_negative that takes in a number and returns True or False if that number is negative.\n\ndef is_negative(x):\n return x < 0\n\nassert is_negative(positive_odd_number) == False, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_negative(positive_even_number) == False, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_negative(negative_odd_number) == True, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_negative(negative_even_number) == True, \"Ensure that the function is defined, named properly, and returns the correct value\"\nprint(\"Exercise 13 is correct.\")",
"Exercise 13 is correct.\n"
],
[
"# Exercise 14\n# Write a function definition named is_odd that takes in a number and returns True or False if that number is odd.\n\ndef is_odd(x):\n return x % 2 != 0\n\nassert is_odd(positive_odd_number) == True, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_odd(positive_even_number) == False, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_odd(negative_odd_number) == True, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_odd(negative_even_number) == False, \"Ensure that the function is defined, named properly, and returns the correct value\"\nprint(\"Exercise 14 is correct.\")",
"Exercise 14 is correct.\n"
],
[
"# Exercise 15\n# Write a function definition named is_even that takes in a number and returns True or False if that number is even.\n\ndef is_even(x):\n return x % 2 == 0\n\nassert is_even(2) == True, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_even(positive_odd_number) == False, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_even(positive_even_number) == True, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_even(negative_odd_number) == False, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert is_even(negative_even_number) == True, \"Ensure that the function is defined, named properly, and returns the correct value\"\nprint(\"Exercise 15 is correct.\")",
"Exercise 15 is correct.\n"
],
[
"# Exercise 16\n# Write a function definition named identity that takes in any argument and returns that argument's value. Don't overthink this one!\n\ndef identity(x):\n return x\n\nassert identity(fruits) == fruits, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert identity(vegetables) == vegetables, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert identity(positive_odd_number) == positive_odd_number, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert identity(positive_even_number) == positive_even_number, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert identity(negative_odd_number) == negative_odd_number, \"Ensure that the function is defined, named properly, and returns the correct value\"\nassert identity(negative_even_number) == negative_even_number, \"Ensure that the function is defined, named properly, and returns the correct value\"\nprint(\"Exercise 16 is correct.\")",
"Exercise 16 is correct.\n"
],
[
"# Exercise 17\n# Write a function definition named is_positive_odd that takes in a number and returns True or False if the value is both greater than zero and odd\n\ndef is_positive_odd(x):\n return (x > 0) and (x % 2 != 0)\n\nassert is_positive_odd(3) == True, \"Double check your syntax and logic\" \nassert is_positive_odd(positive_odd_number) == True, \"Double check your syntax and logic\"\nassert is_positive_odd(positive_even_number) == False, \"Double check your syntax and logic\"\nassert is_positive_odd(negative_odd_number) == False, \"Double check your syntax and logic\"\nassert is_positive_odd(negative_even_number) == False, \"Double check your syntax and logic\"\nprint(\"Exercise 17 is correct.\")",
"Exercise 17 is correct.\n"
],
[
"# Exercise 18\n# Write a function definition named is_positive_even that takes in a number and returns True or False if the value is both greater than zero and even\n\ndef is_positive_even(x):\n return (x > 0) and (x % 2 == 0)\n\nassert is_positive_even(4) == True, \"Double check your syntax and logic\" \nassert is_positive_even(positive_odd_number) == False, \"Double check your syntax and logic\"\nassert is_positive_even(positive_even_number) == True, \"Double check your syntax and logic\"\nassert is_positive_even(negative_odd_number) == False, \"Double check your syntax and logic\"\nassert is_positive_even(negative_even_number) == False, \"Double check your syntax and logic\"\nprint(\"Exercise 18 is correct.\")",
"Exercise 18 is correct.\n"
],
[
"# Exercise 19\n# Write a function definition named is_negative_odd that takes in a number and returns True or False if the value is both less than zero and odd.\n\n\ndef is_negative_odd(x):\n return (x < 0) and (x % 2 != 0)\n\nassert is_negative_odd(-3) == True, \"Double check your syntax and logic\" \nassert is_negative_odd(positive_odd_number) == False, \"Double check your syntax and logic\"\nassert is_negative_odd(positive_even_number) == False, \"Double check your syntax and logic\"\nassert is_negative_odd(negative_odd_number) == True, \"Double check your syntax and logic\"\nassert is_negative_odd(negative_even_number) == False, \"Double check your syntax and logic\"\nprint(\"Exercise 19 is correct.\")\n",
"Exercise 19 is correct.\n"
],
[
"# Exercise 20\n# Write a function definition named is_negative_even that takes in a number and returns True or False if the value is both less than zero and even.\n\ndef is_negative_even(x):\n return (x < 0) and (x % 2 == 0)\n\nassert is_negative_even(-4) == True, \"Double check your syntax and logic\" \nassert is_negative_even(positive_odd_number) == False, \"Double check your syntax and logic\"\nassert is_negative_even(positive_even_number) == False, \"Double check your syntax and logic\"\nassert is_negative_even(negative_odd_number) == False, \"Double check your syntax and logic\"\nassert is_negative_even(negative_even_number) == True, \"Double check your syntax and logic\"\nprint(\"Exercise 20 is correct.\")",
"Exercise 20 is correct.\n"
],
[
"# Exercise 21\n# Write a function definition named half that takes in a number and returns half the provided number.\n\ndef half(x):\n return x/2\n\nassert half(4) == 2\nassert half(5) == 2.5\nassert half(positive_odd_number) == positive_odd_number / 2\nassert half(positive_even_number) == positive_even_number / 2\nassert half(negative_odd_number) == negative_odd_number / 2\nassert half(negative_even_number) == negative_even_number / 2\nprint(\"Exercise 21 is correct.\")",
"Exercise 21 is correct.\n"
],
[
"# Exercise 22\n# Write a function definition named double that takes in a number and returns double the provided number.\n\ndef double(x):\n return x * 2\n\nassert double(4) == 8\nassert double(5) == 10\nassert double(positive_odd_number) == positive_odd_number * 2\nassert double(positive_even_number) == positive_even_number * 2\nassert double(negative_odd_number) == negative_odd_number * 2\nassert double(negative_even_number) == negative_even_number * 2\nprint(\"Exercise 22 is correct.\")",
"Exercise 22 is correct.\n"
],
[
"# Exercise 23\n# Write a function definition named triple that takes in a number and returns triple the provided number.\n\ndef triple(x):\n return x * 3\n\nassert triple(4) == 12\nassert triple(5) == 15\nassert triple(positive_odd_number) == positive_odd_number * 3\nassert triple(positive_even_number) == positive_even_number * 3\nassert triple(negative_odd_number) == negative_odd_number * 3\nassert triple(negative_even_number) == negative_even_number * 3\nprint(\"Exercise 23 is correct.\")",
"Exercise 23 is correct.\n"
],
[
"# Exercise 24\n# Write a function definition named reverse_sign that takes in a number and returns the provided number but with the sign reversed.\n\ndef reverse_sign(x):\n return x * (-1)\nassert reverse_sign(4) == -4\nassert reverse_sign(-5) == 5\nassert reverse_sign(positive_odd_number) == positive_odd_number * -1\nassert reverse_sign(positive_even_number) == positive_even_number * -1\nassert reverse_sign(negative_odd_number) == negative_odd_number * -1\nassert reverse_sign(negative_even_number) == negative_even_number * -1\nprint(\"Exercise 24 is correct.\")",
"Exercise 24 is correct.\n"
],
[
"# Exercise 25\n# Write a function definition named absolute_value that takes in a number and returns the absolute value of the provided number\nimport numpy as np\n\ndef absolute_value(x):\n return abs(x)\n\nassert absolute_value(4) == 4\nassert absolute_value(-5) == 5\nassert absolute_value(positive_odd_number) == positive_odd_number\nassert absolute_value(positive_even_number) == positive_even_number\nassert absolute_value(negative_odd_number) == negative_odd_number * -1\nassert absolute_value(negative_even_number) == negative_even_number * -1\nprint(\"Exercise 25 is correct.\")",
"Exercise 25 is correct.\n"
],
[
"# Exercise 26\n# Write a function definition named is_multiple_of_three that takes in a number and returns True or False if the number is evenly divisible by 3.\n\ndef is_multiple_of_three(x):\n return x % 3 == 0\n \nassert is_multiple_of_three(3) == True\nassert is_multiple_of_three(15) == True\nassert is_multiple_of_three(9) == True\nassert is_multiple_of_three(4) == False\nassert is_multiple_of_three(10) == False\nprint(\"Exercise 26 is correct.\")",
"Exercise 26 is correct.\n"
],
[
"# Exercise 27\n# Write a function definition named is_multiple_of_five that takes in a number and returns True or False if the number is evenly divisible by 5.\n\ndef is_multiple_of_five(x):\n return x % 5 == 0\n\n\n\nassert is_multiple_of_five(3) == False\nassert is_multiple_of_five(15) == True\nassert is_multiple_of_five(9) == False\nassert is_multiple_of_five(4) == False\nassert is_multiple_of_five(10) == True\nprint(\"Exercise 27 is correct.\")",
"Exercise 27 is correct.\n"
],
[
"# Exercise 28\n# Write a function definition named is_multiple_of_both_three_and_five that takes in a number and returns True or False if the number is evenly divisible by both 3 and 5.\n\ndef is_multiple_of_both_three_and_five(x):\n return x % 5 == 0 and x % 3 == 0\n\n\nassert is_multiple_of_both_three_and_five(15) == True\nassert is_multiple_of_both_three_and_five(45) == True\nassert is_multiple_of_both_three_and_five(3) == False\nassert is_multiple_of_both_three_and_five(9) == False\nassert is_multiple_of_both_three_and_five(4) == False\nprint(\"Exercise 28 is correct.\")",
"Exercise 28 is correct.\n"
],
[
"# Exercise 29\n# Write a function definition named square that takes in a number and returns the number times itself.\n\ndef square(x):\n return x ** 2\n\nassert square(3) == 9\nassert square(2) == 4\nassert square(9) == 81\nassert square(positive_odd_number) == positive_odd_number * positive_odd_number\nprint(\"Exercise 29 is correct.\")",
"Exercise 29 is correct.\n"
],
[
"# Exercise 30\n# Write a function definition named add that takes in two numbers and returns the sum.\n\ndef add(x,y):\n return x + y\n\nassert add(3, 2) == 5\nassert add(10, -2) == 8\nassert add(5, 7) == 12\nprint(\"Exercise 30 is correct.\")",
"Exercise 30 is correct.\n"
],
[
"# Exercise 31\n# Write a function definition named cube that takes in a number and returns the number times itself, times itself.\n\ndef cube(x):\n return x ** 3\n\nassert cube(3) == 27\nassert cube(2) == 8\nassert cube(5) == 125\nassert cube(positive_odd_number) == positive_odd_number * positive_odd_number * positive_odd_number\nprint(\"Exercise 31 is correct.\")",
"Exercise 31 is correct.\n"
],
[
"# Exercise 32\n# Write a function definition named square_root that takes in a number and returns the square root of the provided number\n\ndef square_root(x):\n return np.sqrt(x)\n\nassert square_root(4) == 2.0\nassert square_root(64) == 8.0\nassert square_root(81) == 9.0\nprint(\"Exercise 32 is correct.\")",
"Exercise 32 is correct.\n"
],
[
"# Exercise 33\n# Write a function definition named subtract that takes in two numbers and returns the first minus the second argument.\n\ndef subtract(x,y):\n return x - y\n\nassert subtract(8, 6) == 2\nassert subtract(27, 4) == 23\nassert subtract(12, 2) == 10\nprint(\"Exercise 33 is correct.\")",
"Exercise 33 is correct.\n"
],
[
"# Exercise 34\n# Write a function definition named multiply that takes in two numbers and returns the first times the second argument.\n\ndef multiply(x,y):\n return x * y\n\nassert multiply(2, 1) == 2\nassert multiply(3, 5) == 15\nassert multiply(5, 2) == 10\nprint(\"Exercise 34 is correct.\")",
"Exercise 34 is correct.\n"
],
[
"# Exercise 35\n# Write a function definition named divide that takes in two numbers and returns the first argument divided by the second argument.\n\ndef divide(x,y):\n return x/y\n\nassert divide(27, 9) == 3\nassert divide(15, 3) == 5\nassert divide(5, 2) == 2.5\nassert divide(10, 2) == 5\nprint(\"Exercise 35 is correct.\")",
"Exercise 35 is correct.\n"
],
[
"# Exercise 36\n# Write a function definition named quotient that takes in two numbers and returns only the quotient from dividing the first argument by the second argument.\n\ndef quotient(x,y):\n return x // y\n\nassert quotient(27, 9) == 3\nassert quotient(5, 2) == 2\nassert quotient(10, 3) == 3\nprint(\"Exercise 36 is correct.\")",
"Exercise 36 is correct.\n"
],
[
"# Exercise 37\n# Write a function definition named remainder that takes in two numbers and returns the remainder of first argument divided by the second argument.\n\ndef remainder(x,y):\n return x % y\n\nassert remainder(3, 3) == 0\nassert remainder(5, 2) == 1\nassert remainder(7, 5) == 2\nprint(\"Exercise 37 is correct.\")",
"Exercise 37 is correct.\n"
],
[
"# Exercise 38\n# Write a function definition named sum_of_squares that takes in two numbers, squares each number, then returns the sum of both squares.\n\ndef sum_of_squares(x,y):\n return (x ** 2) + (y ** 2)\n\nassert sum_of_squares(3, 2) == 13\nassert sum_of_squares(5, 2) == 29\nassert sum_of_squares(2, 4) == 20\nprint(\"Exercise 38 is correct.\")",
"Exercise 38 is correct.\n"
],
[
"# Exercise 39\n# Write a function definition named times_two_plus_three that takes in a number, multiplies it by two, adds 3 and returns the result.\n\ndef times_two_plus_three(x):\n return x * 2 + 3\n\n\nassert times_two_plus_three(0) == 3\nassert times_two_plus_three(1) == 5\nassert times_two_plus_three(2) == 7\nassert times_two_plus_three(3) == 9\nassert times_two_plus_three(5) == 13\nprint(\"Exercise 39 is correct.\")",
"Exercise 39 is correct.\n"
],
[
"# Exercise 40\n# Write a function definition named area_of_rectangle that takes in two numbers and returns the product.\n\ndef area_of_rectangle(x,y):\n return x * y\n\n\nassert area_of_rectangle(1, 3) == 3\nassert area_of_rectangle(5, 2) == 10\nassert area_of_rectangle(2, 7) == 14\nassert area_of_rectangle(5.3, 10.3) == 54.59\nprint(\"Exercise 40 is correct.\")",
"Exercise 40 is correct.\n"
],
[
"import math\n# Exercise 41\n# Write a function definition named area_of_circle that takes in a number representing a circle's radius and returns the area of the circl\n\ndef area_of_circle(radius):\n return math.pi * radius ** 2\n\nassert area_of_circle(3) == 28.274333882308138\nassert area_of_circle(5) == 78.53981633974483\nassert area_of_circle(7) == 153.93804002589985\nprint(\"Exercise 41 is correct.\")",
"Exercise 41 is correct.\n"
],
[
"import math\n# Exercise 42\n# Write a function definition named circumference that takes in a number representing a circle's radius and returns the circumference.\n\ndef circumference(radius):\n return math.pi * 2 * radius\n\nassert circumference(3) == 18.84955592153876\nassert circumference(5) == 31.41592653589793\nassert circumference(7) == 43.982297150257104\nprint(\"Exercise 42 is correct.\")",
"Exercise 42 is correct.\n"
]
],
[
[
"## Functions working with strings\nIf you need some guidance working with the next few problems, recommend reading through [this example code](https://gist.github.com/ryanorsinger/f758599c886549e7615ec43488ae514c)",
"_____no_output_____"
]
],
[
[
"# Exercise 43\n# Write a function definition named is_vowel that takes in value and returns True if the value is a, e, i, o, u in upper or lower case.\n\ndef is_vowel(x):\n return x.lower() in \"aeiou\"\n\nassert is_vowel(\"a\") == True\nassert is_vowel(\"U\") == True\nassert is_vowel(\"banana\") == False\nassert is_vowel(\"Q\") == False\nassert is_vowel(\"y\") == False\nprint(\"Exercise 43 is correct.\")",
"Exercise 43 is correct.\n"
],
[
"# Exercise 44\n# Write a function definition named has_vowels that takes in value and returns True if the string contains any vowels.\n\ndef has_vowels(x):\n for i in x:\n if i.lower() in \"aeiou\":\n return True\n else:\n return False\n \nassert has_vowels(\"banana\") == True\nassert has_vowels(\"ubuntu\") == True\nassert has_vowels(\"QQQQ\") == False\nassert has_vowels(\"wyrd\") == False\nprint(\"Exercise 44 is correct.\")",
"Exercise 44 is correct.\n"
],
[
"# Exercise 45\n# Write a function definition named count_vowels that takes in value and returns the count of the number of vowels in a sequence.\n\ndef count_vowels(letters):\n count = 0 \n for i in letters: \n if i in 'aeiou':\n count += 1\n return count\n\n\nassert count_vowels(\"banana\") == 3\nassert count_vowels(\"ubuntu\") == 3\nassert count_vowels(\"mango\") == 2\nassert count_vowels(\"QQQQ\") == 0\nassert count_vowels(\"wyrd\") == 0\nprint(\"Exercise 45 is correct.\")",
"Exercise 45 is correct.\n"
],
[
"# Exercise 46\n# Write a function definition named remove_vowels that takes in string and returns the string without any vowels\n\ndef remove_vowels(astring):\n for x in astring:\n if x.lower() in 'aeiou':\n astring = astring.replace(x,'')\n return astring \n \n\nassert remove_vowels(\"banana\") == \"bnn\"\nassert remove_vowels(\"ubuntu\") == \"bnt\"\nassert remove_vowels(\"mango\") == \"mng\"\nassert remove_vowels(\"QQQQ\") == \"QQQQ\"\nprint(\"Exercise 46 is correct.\")",
"Exercise 46 is correct.\n"
],
[
"# Exercise 47\n# Write a function definition named starts_with_vowel that takes in string and True if the string starts with a vowel\n\ndef starts_with_vowel(word):\n if word[0] in 'aeiou':\n return True\n else:\n return False\n \n\nassert starts_with_vowel(\"ubuntu\") == True\nassert starts_with_vowel(\"banana\") == False\nassert starts_with_vowel(\"mango\") == False\nprint(\"Exercise 47 is correct.\")",
"Exercise 47 is correct.\n"
],
[
"# Exercise 48\n# Write a function definition named ends_with_vowel that takes in string and True if the string ends with a vowel\n\ndef ends_with_vowel(word):\n if word[-1] in 'aeiou':\n return True\n else:\n return False\n\nassert ends_with_vowel(\"ubuntu\") == True\nassert ends_with_vowel(\"banana\") == True\nassert ends_with_vowel(\"mango\") == True\nassert ends_with_vowel(\"spinach\") == False\nprint(\"Exercise 48 is correct.\")",
"Exercise 48 is correct.\n"
],
[
"# Exercise 49\n# Write a function definition named starts_and_ends_with_vowel that takes in string and returns True if the string starts and ends with a vowel\n\ndef starts_and_ends_with_vowel(word):\n if word[0] in 'aeiou' and word[-1] in 'aeiou':\n return True \n else:\n return False\n\n\nassert starts_and_ends_with_vowel(\"ubuntu\") == True\nassert starts_and_ends_with_vowel(\"banana\") == False\nassert starts_and_ends_with_vowel(\"mango\") == False\nprint(\"Exercise 49 is correct.\")",
"Exercise 49 is correct.\n"
]
],
[
[
"## Accessing List Elements",
"_____no_output_____"
]
],
[
[
"# Exercise 50\n# Write a function definition named first that takes in sequence and returns the first value of that sequence.\n\ndef first(x):\n return x[0]\n\nassert first(\"ubuntu\") == \"u\"\nassert first([1, 2, 3]) == 1\nassert first([\"python\", \"is\", \"awesome\"]) == \"python\"\nprint(\"Exercise 50 is correct.\")",
"Exercise 50 is correct.\n"
],
[
"# Exercise 51\n# Write a function definition named second that takes in sequence and returns the second value of that sequence.\n\ndef second(x):\n return x[1]\n\nassert second(\"ubuntu\") == \"b\"\nassert second([1, 2, 3]) == 2\nassert second([\"python\", \"is\", \"awesome\"]) == \"is\"\nprint(\"Exercise 51 is correct.\")",
"Exercise 51 is correct.\n"
],
[
"# Exercise 52\n# Write a function definition named third that takes in sequence and returns the third value of that sequence.\n\ndef third(x):\n return x[2]\n\nassert third(\"ubuntu\") == \"u\"\nassert third([1, 2, 3]) == 3\nassert third([\"python\", \"is\", \"awesome\"]) == \"awesome\"\nprint(\"Exercise 52 is correct.\")",
"Exercise 52 is correct.\n"
],
[
"# Exercise 53\n# Write a function definition named forth that takes in sequence and returns the forth value of that sequence.\n\ndef forth(x):\n return x[3]\n\n\n\nassert forth(\"ubuntu\") == \"n\"\nassert forth([1, 2, 3, 4]) == 4\nassert forth([\"python\", \"is\", \"awesome\", \"right?\"]) == \"right?\"\nprint(\"Exercise 53 is correct.\")",
"Exercise 53 is correct.\n"
],
[
"# Exercise 54\n# Write a function definition named last that takes in sequence and returns the last value of that sequence.\n\ndef last(x):\n return x[-1]\n\nassert last(\"ubuntu\") == \"u\"\nassert last([1, 2, 3, 4]) == 4\nassert last([\"python\", \"is\", \"awesome\"]) == \"awesome\"\nassert last([\"kiwi\", \"mango\", \"guava\"]) == \"guava\"\nprint(\"Exercise 54 is correct.\")",
"Exercise 54 is correct.\n"
],
[
"# Exercise 55\n# Write a function definition named second_to_last that takes in sequence and returns the second to last value of that sequence.\n\ndef second_to_last(x):\n return x[-2]\n\n\nassert second_to_last(\"ubuntu\") == \"t\"\nassert second_to_last([1, 2, 3, 4]) == 3\nassert second_to_last([\"python\", \"is\", \"awesome\"]) == \"is\"\nassert second_to_last([\"kiwi\", \"mango\", \"guava\"]) == \"mango\"\nprint(\"Exercise 55 is correct.\")",
"Exercise 55 is correct.\n"
],
[
"# Exercise 56\n# Write a function definition named third_to_last that takes in sequence and returns the third to last value of that sequence.\n\ndef third_to_last(x):\n return x[-3]\n\nassert third_to_last(\"ubuntu\") == \"n\"\nassert third_to_last([1, 2, 3, 4]) == 2\nassert third_to_last([\"python\", \"is\", \"awesome\"]) == \"python\"\nassert third_to_last([\"strawberry\", \"kiwi\", \"mango\", \"guava\"]) == \"kiwi\"\nprint(\"Exercise 56 is correct.\")",
"Exercise 56 is correct.\n"
],
[
"# Exercise 57\n# Write a function definition named first_and_second that takes in sequence and returns the first and second value of that sequence as a list\n\ndef first_and_second(x):\n return x[:2]\n\nassert first_and_second([1, 2, 3, 4]) == [1, 2]\nassert first_and_second([\"python\", \"is\", \"awesome\"]) == [\"python\", \"is\"]\nassert first_and_second([\"strawberry\", \"kiwi\", \"mango\", \"guava\"]) == [\"strawberry\", \"kiwi\"]\nprint(\"Exercise 57 is correct.\")",
"Exercise 57 is correct.\n"
],
[
"# Exercise 58\n# Write a function definition named first_and_last that takes in sequence and returns the first and last value of that sequence as a list\n\ndef first_and_last(x):\n return [x[0], x[-1]]\n\nassert first_and_last([1, 2, 3, 4]) == [1, 4]\nassert first_and_last([\"python\", \"is\", \"awesome\"]) == [\"python\", \"awesome\"]\nassert first_and_last([\"strawberry\", \"kiwi\", \"mango\", \"guava\"]) == [\"strawberry\", \"guava\"]\nprint(\"Exercise 58 is correct.\")",
"Exercise 58 is correct.\n"
],
[
"# Exercise 59\n# Write a function definition named first_to_last that takes in sequence and returns the sequence with the first value moved to the end of the sequence.\n\ndef first_to_last(b):\n x = b[0] \n b.append(x)\n b.pop(0)\n return b\n \n\nassert first_to_last([1, 2, 3, 4]) == [2, 3, 4, 1]\nassert first_to_last([\"python\", \"is\", \"awesome\"]) == [\"is\", \"awesome\", \"python\"]\nassert first_to_last([\"strawberry\", \"kiwi\", \"mango\", \"guava\"]) == [\"kiwi\", \"mango\", \"guava\", \"strawberry\"]\nprint(\"Exercise 59 is correct.\")",
"Exercise 59 is correct.\n"
]
],
[
[
"## Functions to describe data ",
"_____no_output_____"
]
],
[
[
"# Exercise 60\n# Write a function definition named sum_all that takes in sequence of numbers and returns all the numbers added together.\n\ndef sum_all(numbers):\n return sum(numbers)\n \nassert sum_all([1, 2, 3, 4]) == 10\nassert sum_all([3, 3, 3]) == 9\nassert sum_all([0, 5, 6]) == 11\nprint(\"Exercise 60 is correct.\")",
"Exercise 60 is correct.\n"
],
[
"# Exercise 61\n# Write a function definition named mean that takes in sequence of numbers and returns the average value\n\nimport numpy as np\n\ndef mean(x):\n return np.mean(x)\n \n\nassert mean([1, 2, 3, 4]) == 2.5\nassert mean([3, 3, 3]) == 3\nassert mean([1, 5, 6]) == 4\nprint(\"Exercise 61 is correct.\")",
"Exercise 61 is correct.\n"
],
[
"# Exercise 62\n# Write a function definition named median that takes in sequence of numbers and returns the average value\n\ndef median(x):\n return np.median(x)\n\nassert median([1, 2, 3, 4, 5]) == 3.0\nassert median([1, 2, 3]) == 2.0\nassert median([1, 5, 6]) == 5.0\nassert median([1, 2, 5, 6]) == 3.5\nprint(\"Exercise 62 is correct.\")",
"Exercise 62 is correct.\n"
],
[
"# Exercise 63\n# Write a function definition named mode that takes in sequence of numbers and returns the most commonly occuring value\n\nimport statistics as stat\n\ndef mode(x):\n return stat.mode(x)\n\nassert mode([1, 2, 2, 3, 4]) == 2\nassert mode([1, 1, 2, 3]) == 1\nassert mode([2, 2, 3, 3, 3]) == 3\nprint(\"Exercise 63 is correct.\")",
"Exercise 63 is correct.\n"
],
[
"# Exercise 64\n# Write a function definition named product_of_all that takes in sequence of numbers and returns the product of multiplying all the numbers together\n\ndef product_of_all(x):\n return np.prod(x)\n\nassert product_of_all([1, 2, 3]) == 6\nassert product_of_all([3, 4, 5]) == 60\nassert product_of_all([2, 2, 3, 0]) == 0\nprint(\"Exercise 64 is correct.\")",
"Exercise 64 is correct.\n"
]
],
[
[
"## Applying functions to lists",
"_____no_output_____"
]
],
[
[
"# Run this cell in order to use the following list of numbers for the next exercises\nnumbers = [-5, -4, -3, -2, -1, 1, 2, 3, 4, 5] ",
"_____no_output_____"
],
[
"# Exercise 65\n# Write a function definition named get_highest_number that takes in sequence of numbers and returns the largest number.\n\ndef get_highest_number(x):\n return max(x)\n\nassert get_highest_number([1, 2, 3]) == 3\nassert get_highest_number([12, -5, -4, -3, -2, -1, 1, 2, 3, 4, 5]) == 12\nassert get_highest_number([-5, -3, 1]) == 1\nprint(\"Exercise 65 is correct.\")",
"Exercise 65 is correct.\n"
],
[
"# Exercise 66\n# Write a function definition named get_smallest_number that takes in sequence of numbers and returns the smallest number.\n\ndef get_smallest_number(x):\n return min(x)\n\nassert get_smallest_number([1, 3, 2]) == 1\nassert get_smallest_number([5, -5, -4, -3, -2, -1, 1, 2, 3, 4]) == -5\nassert get_smallest_number([-4, -3, 1, -10]) == -10\nprint(\"Exercise 66 is correct.\")",
"Exercise 66 is correct.\n"
],
[
"# Exercise 67\n# Write a function definition named only_odd_numbers that takes in sequence of numbers and returns the odd numbers in a list.\n\ndef only_odd_numbers(num_list):\n return [x for x in num_list if x % 2 != 0]\n \n\nassert only_odd_numbers([1, 2, 3]) == [1, 3]\nassert only_odd_numbers([-5, -4, -3, -2, -1, 1, 2, 3, 4, 5]) == [-5, -3, -1, 1, 3, 5]\nassert only_odd_numbers([-4, -3, 1]) == [-3, 1]\nprint(\"Exercise 67 is correct.\")",
"Exercise 67 is correct.\n"
],
[
"# Exercise 68\n# Write a function definition named only_even_numbers that takes in sequence of numbers and returns the even numbers in a list.\n\ndef only_even_numbers(num_list):\n return [x for x in num_list if x % 2 == 0]\n \n\nassert only_even_numbers([1, 2, 3]) == [2]\nassert only_even_numbers([-5, -4, -3, -2, -1, 1, 2, 3, 4, 5]) == [-4, -2, 2, 4]\nassert only_even_numbers([-4, -3, 1]) == [-4]\nprint(\"Exercise 68 is correct.\")",
"Exercise 68 is correct.\n"
],
[
"# Exercise 69\n# Write a function definition named only_positive_numbers that takes in sequence of numbers and returns the positive numbers in a list.\n\ndef only_positive_numbers(num_list):\n return [x for x in num_list if x > 0]\n\n\nassert only_positive_numbers([1, 2, 3]) == [1, 2, 3]\nassert only_positive_numbers([-5, -4, -3, -2, -1, 1, 2, 3, 4, 5]) == [1, 2, 3, 4, 5]\nassert only_positive_numbers([-4, -3, 1]) == [1]\nprint(\"Exercise 69 is correct.\")",
"Exercise 69 is correct.\n"
],
[
"# Exercise 70\n# Write a function definition named only_negative_numbers that takes in sequence of numbers and returns the negative numbers in a list.\n\ndef only_negative_numbers(num_list):\n return [x for x in num_list if x < 0]\n\nassert only_negative_numbers([1, 2, 3]) == []\nassert only_negative_numbers([-5, -4, -3, -2, -1, 1, 2, 3, 4, 5]) == [-5, -4, -3, -2, -1]\nassert only_negative_numbers([-4, -3, 1]) == [-4, -3]\nprint(\"Exercise 70 is correct.\")",
"Exercise 70 is correct.\n"
],
[
"# Exercise 71\n# Write a function definition named has_evens that takes in sequence of numbers and returns True if there are any even numbers in the sequence\n\ndef has_evens(num_list):\n for x in num_list:\n if x % 2 == 0:\n return True\n break\n return False\n\n\nassert has_evens([1, 2, 3]) == True\nassert has_evens([2, 5, 6]) == True\nassert has_evens([3, 3, 3]) == False\nassert has_evens([]) == False\nprint(\"Exercise 71 is correct.\")",
"Exercise 71 is correct.\n"
],
[
"# Exercise 72\n# Write a function definition named count_evens that takes in sequence of numbers and returns the number of even numbers\n\ndef count_evens(num_list):\n return(len([x for x in num_list if x % 2 == 0]))\n\n\nassert count_evens([1, 2, 3]) == 1\nassert count_evens([2, 5, 6]) == 2\nassert count_evens([3, 3, 3]) == 0\nassert count_evens([5, 6, 7, 8] ) == 2\nprint(\"Exercise 72 is correct.\")",
"Exercise 72 is correct.\n"
],
[
"# Exercise 73\n# Write a function definition named has_odds that takes in sequence of numbers and returns True if there are any odd numbers in the sequence\n\n\ndef has_odds(num_list):\n for x in num_list:\n if x % 2 != 0:\n return True\n break\n return False\n\nassert has_odds([1, 2, 3]) == True\nassert has_odds([2, 5, 6]) == True\nassert has_odds([3, 3, 3]) == True\nassert has_odds([2, 4, 6]) == False\nprint(\"Exercise 73 is correct.\")",
"Exercise 73 is correct.\n"
],
[
"# Exercise 74\n# Write a function definition named count_odds that takes in sequence of numbers and returns True if there are any odd numbers in the sequence\n\ndef count_odds(num_list):\n return(len([x for x in num_list if x % 2 != 0]))\n\nassert count_odds([1, 2, 3]) == 2\nassert count_odds([2, 5, 6]) == 1\nassert count_odds([3, 3, 3]) == 3\nassert count_odds([2, 4, 6]) == 0\nprint(\"Exercise 74 is correct.\")",
"Exercise 74 is correct.\n"
],
[
"# Exercise 75\n# Write a function definition named count_negatives that takes in sequence of numbers and returns a count of the number of negative numbers\n\ndef count_negatives(num_list):\n return(len([x for x in num_list if x < 0]))\n\nassert count_negatives([1, -2, 3]) == 1\nassert count_negatives([2, -5, -6]) == 2\nassert count_negatives([3, 3, 3]) == 0\nprint(\"Exercise 75 is correct.\")",
"Exercise 75 is correct.\n"
],
[
"# Exercise 76\n# Write a function definition named count_positives that takes in sequence of numbers and returns a count of the number of positive numbers\n\ndef count_positives(num_list):\n return(len([x for x in num_list if x > 0]))\n\nassert count_positives([1, -2, 3]) == 2\nassert count_positives([2, -5, -6]) == 1\nassert count_positives([3, 3, 3]) == 3\nassert count_positives([-2, -1, -5]) == 0\nprint(\"Exercise 76 is correct.\")",
"Exercise 76 is correct.\n"
],
[
"# Exercise 77\n# Write a function definition named only_positive_evens that takes in sequence of numbers and returns a list containing all the positive evens from the sequence\n\ndef only_positive_evens(num_list):\n return [x for x in num_list if x > 0 and x % 2 == 0]\n\nassert only_positive_evens([1, -2, 3]) == []\nassert only_positive_evens([2, -5, -6]) == [2]\nassert only_positive_evens([3, 3, 4, 6]) == [4, 6]\nassert only_positive_evens([2, 3, 4, -1, -5]) == [2, 4]\nprint(\"Exercise 77 is correct.\")",
"Exercise 77 is correct.\n"
],
[
"# Exercise 78\n# Write a function definition named only_positive_odds that takes in sequence of numbers and returns a list containing all the positive odd numbers from the sequence\n\ndef only_positive_odds(num_list):\n return [x for x in num_list if x > 0 and x % 2 != 0]\n\nassert only_positive_odds([1, -2, 3]) == [1, 3]\nassert only_positive_odds([2, -5, -6]) == []\nassert only_positive_odds([3, 3, 4, 6]) == [3, 3]\nassert only_positive_odds([2, 3, 4, -1, -5]) == [3]\nprint(\"Exercise 78 is correct.\")",
"Exercise 78 is correct.\n"
],
[
"# Exercise 79\n# Write a function definition named only_negative_evens that takes in sequence of numbers and returns a list containing all the negative even numbers from the sequence\n\ndef only_negative_evens(num_list):\n return [x for x in num_list if x < 0 and x % 2 == 0]\n\nassert only_negative_evens([1, -2, 3]) == [-2]\nassert only_negative_evens([2, -5, -6]) == [-6]\nassert only_negative_evens([3, 3, 4, 6]) == []\nassert only_negative_evens([-2, 3, 4, -1, -4]) == [-2, -4]\nprint(\"Exercise 79 is correct.\")",
"Exercise 79 is correct.\n"
],
[
"# Exercise 80\n# Write a function definition named only_negative_odds that takes in sequence of numbers and returns a list containing all the negative odd numbers from the sequence\n\ndef only_negative_odds(num_list):\n return [x for x in num_list if x < 0 and x % 2 != 0]\n\nassert only_negative_odds([1, -2, 3]) == []\nassert only_negative_odds([2, -5, -6]) == [-5]\nassert only_negative_odds([3, 3, 4, 6]) == []\nassert only_negative_odds([2, -3, 4, -1, -4]) == [-3, -1]\nprint(\"Exercise 80 is correct.\")",
"Exercise 80 is correct.\n"
],
[
"# Exercise 81\n# Write a function definition named shortest_string that takes in a list of strings and returns the shortest string in the list.\n\ndef shortest_string(my_list):\n return(min((word for word in my_list), key=len))\n \nassert shortest_string([\"kiwi\", \"mango\", \"strawberry\"]) == \"kiwi\"\nassert shortest_string([\"hello\", \"everybody\"]) == \"hello\"\nassert shortest_string([\"mary\", \"had\", \"a\", \"little\", \"lamb\"]) == \"a\"\nprint(\"Exercise 81 is correct.\")",
"Exercise 81 is correct.\n"
],
[
"# Exercise 82\n# Write a function definition named longest_string that takes in sequence of strings and returns the longest string in the list.\n\ndef longest_string(my_list):\n return(max((word for word in my_list), key=len))\n\nassert longest_string([\"kiwi\", \"mango\", \"strawberry\"]) == \"strawberry\"\nassert longest_string([\"hello\", \"everybody\"]) == \"everybody\"\nassert longest_string([\"mary\", \"had\", \"a\", \"little\", \"lamb\"]) == \"little\"\nprint(\"Exercise 82 is correct.\")",
"Exercise 82 is correct.\n"
]
],
[
[
"## Working with sets\n**Hint** Take a look at the `set` function in Python, the `set` data type, and built-in `set` methods.",
"_____no_output_____"
]
],
[
[
"# Example set function usage\nprint(set(\"kiwi\"))\nprint(set([1, 2, 2, 3, 3, 3, 4, 4, 4, 4]))",
"{'i', 'k', 'w'}\n{1, 2, 3, 4}\n"
],
[
"# Exercise 83\n# Write a function definition named get_unique_values that takes in a list and returns a set with only the unique values from that list.\n\ndef get_unique_values(x):\n return set(x)\n\nassert get_unique_values([\"ant\", \"ant\", \"mosquito\", \"mosquito\", \"ladybug\"]) == {\"ant\", \"mosquito\", \"ladybug\"}\nassert get_unique_values([\"b\", \"a\", \"n\", \"a\", \"n\", \"a\", \"s\"]) == {\"b\", \"a\", \"n\", \"s\"}\nassert get_unique_values([\"mary\", \"had\", \"a\", \"little\", \"lamb\", \"little\", \"lamb\", \"little\", \"lamb\"]) == {\"mary\", \"had\", \"a\", \"little\", \"lamb\"}\nprint(\"Exercise 83 is correct.\")",
"Exercise 83 is correct.\n"
],
[
"# Exercise 84\n# Write a function definition named get_unique_values_from_two_lists that takes two lists and returns a single set with only the unique values\n\ndef get_unique_values_from_two_lists(x,y):\n return set(x).union(set(y))\n \nassert get_unique_values_from_two_lists([5, 1, 2, 3], [3, 4, 5, 5]) == {1, 2, 3, 4, 5}\nassert get_unique_values_from_two_lists([1, 1], [2, 2, 3]) == {1, 2, 3}\nassert get_unique_values_from_two_lists([\"tomato\", \"mango\", \"kiwi\"], [\"eggplant\", \"tomato\", \"broccoli\"]) == {\"tomato\", \"mango\", \"kiwi\", \"eggplant\", \"broccoli\"}\nprint(\"Exercise 84 is correct.\")",
"Exercise 84 is correct.\n"
],
[
"# Exercise 85\n# Write a function definition named get_values_in_common that takes two lists and returns a single set with the values that each list has in common\n\ndef get_values_in_common(x,y):\n return set(x).intersection(set(y))\n\n\nassert get_values_in_common([5, 1, 2, 3], [3, 4, 5, 5]) == {3, 5}\nassert get_values_in_common([1, 2], [2, 2, 3]) == {2}\nassert get_values_in_common([\"tomato\", \"mango\", \"kiwi\"], [\"eggplant\", \"tomato\", \"broccoli\"]) == {\"tomato\"}\nprint(\"Exercise 85 is correct.\")",
"Exercise 85 is correct.\n"
],
[
"# Exercise 86\n# Write a function definition named get_values_not_in_common that takes two lists and returns a single set with the values that each list does not have in common\n\ndef get_values_not_in_common(x,y):\n return (set(x)-set(y)).union(set(y)-set(x))\n\nassert get_values_not_in_common([5, 1, 2, 3], [3, 4, 5, 5]) == {1, 2, 4}\nassert get_values_not_in_common([1, 1], [2, 2, 3]) == {1, 2, 3}\nassert get_values_not_in_common([\"tomato\", \"mango\", \"kiwi\"], [\"eggplant\", \"tomato\", \"broccoli\"]) == {\"mango\", \"kiwi\", \"eggplant\", \"broccoli\"}\nprint(\"Exercise 86 is correct.\")",
"Exercise 86 is correct.\n"
]
],
[
[
"## Working with Dictionaries\n",
"_____no_output_____"
]
],
[
[
"# Run this cell in order to have these two dictionary variables defined.\ntukey_paper = {\n \"title\": \"The Future of Data Analysis\",\n \"author\": \"John W. Tukey\",\n \"link\": \"https://projecteuclid.org/euclid.aoms/1177704711\",\n \"year_published\": 1962\n}\n\nthomas_paper = {\n \"title\": \"A mathematical model of glutathione metabolism\",\n \"author\": \"Rachel Thomas\",\n \"link\": \"https://www.ncbi.nlm.nih.gov/pubmed/18442411\",\n \"year_published\": 2008\n}",
"_____no_output_____"
],
[
"# Exercise 87\n# Write a function named get_paper_title that takes in a dictionary and returns the title property\n\ndef get_paper_title(my_dictionary):\n return my_dictionary.get(\"title\")\n\nassert get_paper_title(tukey_paper) == \"The Future of Data Analysis\"\nassert get_paper_title(thomas_paper) == \"A mathematical model of glutathione metabolism\"\nprint(\"Exercise 87 is correct.\")",
"Exercise 87 is correct.\n"
],
[
"# Exercise 88\n# Write a function named get_year_published that takes in a dictionary and returns the value behind the \"year_published\" key.\n\ndef get_year_published(my_dictionary):\n return my_dictionary.get(\"year_published\")\n\nassert get_year_published(tukey_paper) == 1962\nassert get_year_published(thomas_paper) == 2008\nprint(\"Exercise 88 is correct.\")",
"Exercise 88 is correct.\n"
],
[
"# Run this code to create data for the next two questions\nbook = {\n \"title\": \"Genetic Algorithms and Machine Learning for Programmers\",\n \"price\": 36.99,\n \"author\": \"Frances Buontempo\"\n}",
"_____no_output_____"
],
[
"# Exercise 89\n# Write a function named get_price that takes in a dictionary and returns the price\n\ndef get_price(x):\n return x.get(\"price\")\n\nassert get_price(book) == 36.99\nprint(\"Exercise 89 is complete.\")",
"Exercise 89 is complete.\n"
],
[
"# Exercise 90\n# Write a function named get_book_author that takes in a dictionary (the above declared book variable) and returns the author's name\n\ndef get_book_author(x):\n return x.get(\"author\")\nassert get_book_author(book) == \"Frances Buontempo\"\nprint(\"Exercise 90 is complete.\")",
"Exercise 90 is complete.\n"
]
],
[
[
"## Working with Lists of Dictionaries\n**Hint** If you need an example of lists of dictionaries, see [https://gist.github.com/ryanorsinger/fce8154028a924c1073eac24c7c3f409](https://gist.github.com/ryanorsinger/fce8154028a924c1073eac24c7c3f409)",
"_____no_output_____"
]
],
[
[
"# Run this cell in order to have some setup data for the next exercises\nbooks = [\n {\n \"title\": \"Genetic Algorithms and Machine Learning for Programmers\",\n \"price\": 36.99,\n \"author\": \"Frances Buontempo\"\n },\n {\n \"title\": \"The Visual Display of Quantitative Information\",\n \"price\": 38.00,\n \"author\": \"Edward Tufte\"\n },\n {\n \"title\": \"Practical Object-Oriented Design\",\n \"author\": \"Sandi Metz\",\n \"price\": 30.47\n },\n {\n \"title\": \"Weapons of Math Destruction\",\n \"author\": \"Cathy O'Neil\",\n \"price\": 17.44\n }\n]",
"_____no_output_____"
],
[
"# Exercise 91\n# Write a function named get_number_of_books that takes in a list of objects and returns the number of dictionaries in that list.\n\ndef get_number_of_books(x):\n return len(x)\n\nassert get_number_of_books(books) == 4\nprint(\"Exercise 91 is complete.\")",
"Exercise 91 is complete.\n"
],
[
"# Exercise 92\n# Write a function named total_of_book_prices that takes in a list of dictionaries and returns the sum total of all the book prices added together\n\ndef total_of_book_prices(mylist):\n return sum(x[\"price\"] for x in books)\n\nassert total_of_book_prices(books) == 122.9\nprint(\"Exercise 92 is complete.\")",
"Exercise 92 is complete.\n"
],
[
"# Exercise 93\n# Write a function named get_average_book_price that takes in a list of dictionaries and returns the average book price.\n\nimport numpy as np\n\ndef get_average_book_price(x):\n return np.mean([i[\"price\"] for i in books])\n\nassert get_average_book_price(books) == 30.725\nprint(\"Exercise 93 is complete.\")",
"Exercise 93 is complete.\n"
],
[
"# Exercise 94\n# Write a function called highest_price_book that takes in the above defined list of dictionaries \"books\" and returns the dictionary containing the title, price, and author of the book with the highest priced book.\n# Hint: Much like sometimes start functions with a variable set to zero, you may want to create a dictionary with the price set to zero to compare to each dictionary's price in the list\n\ndef highest_price_book(x):\n return dict(max(books, key = \"price\"))\n \n \n\n # def myfunc(somedict):\n # x = list(somedict.values()) \n # for i in x:\n # data = dict_from_otherfunc(i)\n # mylist = [float(max(data.values()))]\n # mydict = dict(zip([i], mylist))\n # return mydict\n\nassert highest_price_book(books) == {\n \"title\": \"The Visual Display of Quantitative Information\",\n \"price\": 38.00,\n \"author\": \"Edward Tufte\"}\n\nprint(\"Exercise 94 is complete\")",
"_____no_output_____"
],
[
"# Exercise 95\n# Write a function called lowest_priced_book that takes in the above defined list of dictionaries \"books\" and returns the dictionary containing the title, price, and author of the book with the lowest priced book.\n# Hint: Much like sometimes start functions with a variable set to zero or float('inf'), you may want to create a dictionary with the price set to float('inf') to compare to each dictionary in the list\n\n\nassert lowest_price_book(books) == {\n \"title\": \"Weapons of Math Destruction\",\n \"author\": \"Cathy O'Neil\",\n \"price\": 17.44\n}\nprint(\"Exercise 95 is complete.\")",
"_____no_output_____"
],
[
"shopping_cart = {\n \"tax\": .08,\n \"items\": [\n {\n \"title\": \"orange juice\",\n \"price\": 3.99,\n \"quantity\": 1\n },\n {\n \"title\": \"rice\",\n \"price\": 1.99,\n \"quantity\": 3\n },\n {\n \"title\": \"beans\",\n \"price\": 0.99,\n \"quantity\": 3\n },\n {\n \"title\": \"chili sauce\",\n \"price\": 2.99,\n \"quantity\": 1\n },\n {\n \"title\": \"chocolate\",\n \"price\": 0.75,\n \"quantity\": 9\n }\n ]\n}",
"_____no_output_____"
],
[
"# Exercise 96\n# Write a function named get_tax_rate that takes in the above shopping cart as input and returns the tax rate.\n# Hint: How do you access a key's value on a dictionary? The tax rate is one key of the entire shopping_cart dictionary.\n\ndef get_tax_rate(x):\n return shopping_cart.get(\"tax\")\n\nassert get_tax_rate(shopping_cart) == .08\nprint(\"Exercise 96 is complete\")",
"Exercise 96 is complete\n"
],
[
"# Exercise 97\n# Write a function named number_of_item_types that takes in the shopping cart as input and returns the number of unique item types in the shopping cart. \n# We're not yet using the quantity of each item, but rather focusing on determining how many different types of items are in the cart.\n\ndef number_of_item_types(x):\n return len('items')\n \n\nassert number_of_item_types(shopping_cart) == 5\nprint(\"Exercise 97 is complete.\")",
"Exercise 97 is complete.\n"
],
[
"# Exercise 98\n# Write a function named total_number_of_items that takes in the shopping cart as input and returns the total number all item quantities.\n# This should return the sum of all of the quantities from each item type\n\ndef total_number_of_items(s):\n return sum(\"quantity\"() for x in s)\n\nassert total_number_of_items(shopping_cart) == 17\nprint(\"Exercise 98 is complete.\")",
"_____no_output_____"
],
[
"# Exercise 99\n# Write a function named get_average_item_price that takes in the shopping cart as an input and returns the average of all the item prices.\n# Hint - This should determine the total price divided by the number of types of items. This does not account for each item type's quantity.\nassert get_average_item_price(shopping_cart) == 2.1420000000000003\nprint(\"Exercise 99 is complete.\")",
"_____no_output_____"
],
[
"# Exercise 100\n# Write a function named get_average_spent_per_item that takes in the shopping cart and returns the average of summing each item's quanties times that item's price.\n# Hint: You may need to set an initial total price and total total quantity to zero, then sum up and divide that total price by the total quantity\n\nassert get_average_spent_per_item(shopping_cart) == 1.333529411764706\nprint(\"Exercise 100 is complete.\")",
"_____no_output_____"
],
[
"# Exercise 101\n# Write a function named most_spent_on_item that takes in the shopping cart as input and returns the dictionary associated with the item that has the highest price*quantity.\n# Be sure to do this as programmatically as possible. \n# Hint: Similarly to how we sometimes begin a function with setting a variable to zero, we need a starting place:\n# Hint: Consider creating a variable that is a dictionary with the keys \"price\" and \"quantity\" both set to 0. You can then compare each item's price and quantity total to the one from \"most\"\n\nassert most_spent_on_item(shopping_cart) == {\n \"title\": \"chocolate\",\n \"price\": 0.75,\n \"quantity\": 9\n}\nprint(\"Exercise 101 is complete.\")",
"_____no_output_____"
]
],
[
[
"Created by [Ryan Orsinger](https://ryanorsinger.com)\n\nSource code on [https://github.com/ryanorsinger/101-exercises](https://github.com/ryanorsinger/101-exercises)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
]
] |
e7ba76ac8b0edcc39502549437e48836609a2163 | 620,693 | ipynb | Jupyter Notebook | Analysis_KolmogorovFlow.ipynb | googleinterns/invobs-data-assimilation | 36e0ff6319a596d99d6f4197bff0f00a38d299c4 | [
"Apache-2.0"
] | 16 | 2021-07-05T08:09:43.000Z | 2022-03-21T19:12:06.000Z | Analysis_KolmogorovFlow.ipynb | googleinterns/invobs-data-assimilation | 36e0ff6319a596d99d6f4197bff0f00a38d299c4 | [
"Apache-2.0"
] | null | null | null | Analysis_KolmogorovFlow.ipynb | googleinterns/invobs-data-assimilation | 36e0ff6319a596d99d6f4197bff0f00a38d299c4 | [
"Apache-2.0"
] | null | null | null | 737.165083 | 234,876 | 0.947945 | [
[
[
"# Data Analysis for Inverse Observation Data Assimilation of Kolmogorov Flow\nThis notebook analyzes the paper's data and reproduces the plots.",
"_____no_output_____"
]
],
[
[
"import os\nos.environ[\"CUDA_VISIBLE_DEVICES\"]=\"0\" # system integration faster on GPU\nimport warnings\nwarnings.filterwarnings('ignore')\nfrom functools import partial\nimport numpy as np\nimport scipy\nimport jax\nimport jax.numpy as jnp\nfrom jax import random, jit\nimport argparse\nfrom datetime import datetime\nimport xarray as xr\nimport seaborn as sns\nimport pandas as pd\nimport matplotlib.pyplot as plt\nfrom cycler import cycler\n%matplotlib inline\n\nfrom dynamical_system import KolmogorovFlow\nfrom util import jnp_to_aa_tuple, aa_tuple_to_jnp\nfrom jax_cfd.data import xarray_utils as xru\nfrom util import jnp_to_aa_tuple, aa_tuple_to_jnp\nfrom analysis_util import (\n compute_vorticity, \n integrate_kolmogorov_xr, \n compute_l1_error_kolmogorov, \n adjust_row_labels, \n plot_colors, \n load_da_results,\n)\n\n# create figure directory\n! mkdir -p figures",
"_____no_output_____"
]
],
[
[
"## Copy data from Google cloud\n\nThis requires [gsutil](https://cloud.google.com/storage/docs/gsutil).",
"_____no_output_____"
]
],
[
[
"!gsutil cp -r gs://gresearch/jax-cfd/projects/invobs-data-assimilation/invobs-da-results/ /tmp",
"Copying gs://gresearch/jax-cfd/projects/invobs-data-assimilation/invobs-da-results/kolmogorov_baselineinit_hybridopt.nc...\nCopying gs://gresearch/jax-cfd/projects/invobs-data-assimilation/invobs-da-results/kolmogorov_baselineinit_obsopt.nc...\nCopying gs://gresearch/jax-cfd/projects/invobs-data-assimilation/invobs-da-results/kolmogorov_invobsinit_hybridopt.nc...\nCopying gs://gresearch/jax-cfd/projects/invobs-data-assimilation/invobs-da-results/kolmogorov_invobsinit_obsopt.nc...\n- [4 files][ 43.7 MiB/ 43.7 MiB] \n==> NOTE: You are performing a sequence of gsutil operations that may\nrun significantly faster if you instead use gsutil -m cp ... Please\nsee the -m section under \"gsutil help options\" for further information\nabout when gsutil -m can be advantageous.\n\nCopying gs://gresearch/jax-cfd/projects/invobs-data-assimilation/invobs-da-results/lorenz96_baselineinit_hybridopt.nc...\nCopying gs://gresearch/jax-cfd/projects/invobs-data-assimilation/invobs-da-results/lorenz96_baselineinit_obsopt.nc...\nCopying gs://gresearch/jax-cfd/projects/invobs-data-assimilation/invobs-da-results/lorenz96_invobsinit_hybridopt.nc...\nCopying gs://gresearch/jax-cfd/projects/invobs-data-assimilation/invobs-da-results/lorenz96_invobsinit_obsopt.nc...\n| [8 files][ 47.0 MiB/ 47.0 MiB] \nOperation completed over 8 objects/47.0 MiB. \n"
]
],
[
[
"## Load data",
"_____no_output_____"
]
],
[
[
"path = '/tmp/invobs-da-results'\n\nfilenames = [\n 'kolmogorov_baselineinit_obsopt.nc',\n 'kolmogorov_baselineinit_hybridopt.nc',\n 'kolmogorov_invobsinit_obsopt.nc',\n 'kolmogorov_invobsinit_hybridopt.nc',\n]\nretained_variables = [\n 'f_vals',\n 'eval_vals',\n 'X0_ground_truth',\n 'X0_opt',\n 'X0_init',\n]\nretained_attrs = [\n 'observe_every',\n 'grid_size',\n 'num_time_steps',\n 'num_warmup_steps',\n 'num_inner_steps',\n 'viscosity',\n 'peak_wavenumber',\n 'offset_x',\n 'offset_y',\n]\n\nfull_filenames = [os.path.join(path, filename) for filename in filenames]\nds = load_da_results(full_filenames, retained_variables, retained_attrs)",
"_____no_output_____"
]
],
[
[
"## Instantiate dynamical system",
"_____no_output_____"
]
],
[
[
"kolmogorov_flow = KolmogorovFlow(\n grid_size=ds.attrs['grid_size'],\n num_inner_steps=ds.attrs['num_inner_steps'],\n viscosity=ds.attrs['viscosity'],\n observe_every=ds.attrs['observe_every'], \n wavenumber=ds.attrs['peak_wavenumber'],\n)",
"_____no_output_____"
]
],
[
[
"## Data assimilation initialization samples\n\nComparison of initialization schemes.",
"_____no_output_____"
]
],
[
[
"da_init = xr.concat(\n [\n ds['X0_init'].sel(init=['invobs', 'baseline']), \n ds['X0_ground_truth'].sel(init='baseline'),\n ], \n dim='init',\n) \\\n.assign_coords(init=['invobs', 'baseline', 'ground_truth']) \\\n.sel(opt_space='observation')\n\nvort_init = compute_vorticity(da_init, kolmogorov_flow.grid)\n\n# create subsampled ground truth\nvort_gt_subsampled_jnp = jax.vmap(jnp.kron, in_axes=(0, None))(\n vort_init.sel(init='ground_truth').data[...,::16,::16], jnp.ones((16,16)),\n)\nvort_gt_subsampled = vort_init.sel(init='ground_truth').copy()\nvort_gt_subsampled.data = np.asarray(vort_gt_subsampled_jnp)\nvort_gt_subsampled = vort_gt_subsampled.assign_coords(init='ground_truth_sub')\n\nvort = xr.concat([vort_gt_subsampled, vort_init], dim='init')\nvort = vort.rename({'init': 'data_type'})",
"_____no_output_____"
],
[
"sns.set(font_scale=2.4)\nplt.rc('font', **{'family': 'Times New Roman'})\n\ng = vort.sel(\n n=3, \n data_type=['ground_truth', 'ground_truth_sub', 'baseline', 'invobs'],\n) \\\n.plot.imshow(\n x='x', \n y='y', \n col='data_type', \n col_wrap=2, \n size=5, \n add_colorbar=False, \n cmap=sns.cm.icefire, \n vmin=-8, \n vmax=8,\n)\n\ncol_labels = [\n 'ground truth', \n 'observed ground truth', \n 'interpolation init', \n 'inverse init',\n] \n[ax.set_title(t) for ax, t in zip(g.axes.ravel(), col_labels)]\ng.set_axis_labels('', '')\n[ax.set_aspect('equal') for ax in g.axes.ravel()]\n[ax.set_yticks([]) for ax in g.axes.ravel()]\n[ax.set_xticks([]) for ax in g.axes.ravel()]\nplt.subplots_adjust(wspace=-0.2)\n\n# plot subsamling grid\nsub_grid_points = np.linspace(0, 2*np.pi, num=4)\none_pixel_size = 2*np.pi / 64\nsub_grid_points[0] += one_pixel_size\nsub_grid_points[-1] -= one_pixel_size\n\ng.axes.ravel()[0].plot(\n np.repeat(sub_grid_points, 4), \n np.tile(sub_grid_points, 4), \n 's', \n color=plot_colors['y'], \n markersize=8, \n markeredgecolor='k',\n)\n\nplt.savefig(\n 'figures/da_init_kolmogorov.pdf', \n bbox_inches='tight', \n pad_inches=0.1,\n)",
"_____no_output_____"
]
],
[
[
"## Optimization curves\n\nPlot value of observation space objective function during optimization normalized by the first-step value of the observation space objective function.",
"_____no_output_____"
]
],
[
[
"sns.set(font_scale=1.5)\nsns.set_style('white')\nto_plot = ds['eval_vals'].sel(n=[6, 9]) \nto_plot_relative_mean = (\n to_plot / to_plot.sel(opt_step=0, opt_space='observation')\n)\nto_plot_relative_mean = to_plot_relative_mean.sel(\n init=['invobs', 'baseline'], \n opt_space=['hybrid', 'observation'],\n)\ndf_opt_curves = (\n to_plot_relative_mean\n .to_dataframe('observation objective')\n .reset_index()\n)",
"_____no_output_____"
],
[
"sns.set(font_scale=2.5)\nsns.set_style('ticks')\nplt.rc(\n 'axes', \n prop_cycle=(cycler('color', [plot_colors['r'], plot_colors['b']])),\n)\nplt.rc('font', **{'family': 'Times New Roman'})\n\ng = sns.relplot(\n data=df_opt_curves, \n x='opt_step', \n y='observation objective',\n col='init',\n row='n',\n hue='opt_space',\n style='opt_space',\n kind='line',\n lw=4,\n legend=True,\n height=5.8,\n)\n\nsns.despine()\ng.set(yscale='log', xlabel='optimization step')\ng.set_titles('')\ng.axes[0,0].set_title('inverse init')\ng.axes[0,1].set_title('interpolation init')\ng._margin_titles = True\n[ax.axvline(x=100, color='k', ls='--') for ax in g.axes.flat]\n\n# place legend in first facet plot\ng.axes[0,0].legend(frameon=False, labels=['hybrid opt', 'observation opt'])\ng._legend.remove()\n\nplt.savefig(\n 'figures/opt_curves_kolmogorov.pdf', \n bbox_inches='tight', \n pad_inches=0.1,\n)",
"_____no_output_____"
]
],
[
[
"## Forecast quality",
"_____no_output_____"
]
],
[
[
"X0_da = ds[['X0_ground_truth', 'X0_init', 'X0_opt']].to_array('data_type') \\\n.assign_coords({'data_type': ['gt', 'init', 'opt']})",
"_____no_output_____"
],
[
"X_da = integrate_kolmogorov_xr(kolmogorov_flow, X0_da, 20)",
"_____no_output_____"
],
[
"vorticity = compute_vorticity(X_da, kolmogorov_flow.grid)",
"_____no_output_____"
],
[
"relative_scale = 14533 # average L1 norm over independent samples\nl1_error = compute_l1_error_kolmogorov(vorticity, 'gt', scale=relative_scale)",
"_____no_output_____"
],
[
"delta_t = ds.attrs['num_inner_steps'] * kolmogorov_flow.dt\nl1_error_stacked = ( \n l1_error\n .mean(dim='n')\n .sel(data_type='opt', drop=True)\n .assign_coords(\n {\n 't': delta_t * np.arange(l1_error.sizes['t']), \n 'init': [s.split('_')[0] for s in l1_error.init.values],\n },\n )\n .stack(opt_method=['init', 'opt_space'])\n)\n\ntuple_labels = l1_error_stacked.opt_method.values\nconcat_labels = [ a + ' init' + ' / ' + b + ' opt' for a,b in tuple_labels]\nl1_error_stacked = l1_error_stacked.assign_coords({'opt_method': concat_labels})",
"_____no_output_____"
],
[
"# select to have a custom sort of the optimization methods\nl1_error_stacked = l1_error_stacked.sel(\n opt_method=[\n 'invobs init / observation opt',\n 'invobs init / hybrid opt',\n 'baseline init / observation opt',\n 'baseline init / hybrid opt',\n ]\n)",
"_____no_output_____"
],
[
"plt.figure(figsize=(10, 7.5))\nsns.set(font_scale=2.2)\nsns.set_style('ticks')\nplt.rc('font', **{'family': 'Times New Roman'})\n\nplt.rc(\n 'axes', \n prop_cycle=(\n cycler(\n 'color', \n [plot_colors['r']]*2 + [plot_colors['b']]*2,\n ) \n + cycler(\n 'linestyle', \n ['-', 'dotted']*2,\n ) \n + cycler(\n 'marker', \n ['o', 'o', 'v', 'v'],\n )\n ),\n)\n\ntime_steps = l1_error_stacked.coords['t'].values\nax = plt.subplot(1,1,1)\nfor opt_method in l1_error_stacked.opt_method.values:\n ax.plot(\n time_steps, \n l1_error_stacked.sel(opt_method=opt_method).values,\n markersize=13,\n markeredgecolor='white', \n lw=4,\n label=opt_method,\n )\n\nsns.despine()\nplt.xlabel('time')\nplt.ylabel('mean relative $L_1$ error')\nplt.ylim(0, 1.1)\nplt.axvline(x=9.5 * delta_t, ymax=0.6, color='k', ls='--')\nplt.title('')\n\nhandles, labels = ax.get_legend_handles_labels()\nline_ordering = [2, 3, 1, 0] # legend ordering according to appearance in plot\nreordered_handles = [handles[i] for i in line_ordering]\nreordered_labels = [labels[i] for i in line_ordering]\nax.legend(reordered_handles, reordered_labels, frameon=False)\n\nplt.savefig(\n 'figures/da_kolmogorov_invobs.pdf', \n bbox_inches='tight', \n pad_inches=0.1,\n)",
"_____no_output_____"
]
],
[
[
"### Summary stats\nCompare forecast performance on the first forecast state relative to baseline init and optimization method.",
"_____no_output_____"
]
],
[
[
"summary_stats = l1_error.sel(data_type='opt', t=11).mean(dim='n') / l1_error.sel(data_type='opt', t=11, init='baseline', opt_space='observation').mean(dim='n')",
"_____no_output_____"
],
[
"print(\n summary_stats.sel(opt_space='observation', init='baseline').values,\n summary_stats.sel(opt_space='hybrid', init='baseline').values,\n summary_stats.sel(opt_space='observation', init='invobs').values,\n summary_stats.sel(opt_space='hybrid', init='invobs').values,\n)",
"1.0 0.8789517 0.19961545 0.22848208\n"
]
],
[
[
"## Significance test between trajectories\n\nPerform a Z-test to evaluate significance level between optimization methods for the two initialization schemes.",
"_____no_output_____"
],
[
"### Inverse observation initialization",
"_____no_output_____"
]
],
[
[
"time_step = 11 # beginning of forecast window\nnum_samples = l1_error.sizes['n']\nl1_error_inv = l1_error.sel(init='invobs', data_type='opt')\ndiff_l1_error = (\n l1_error_inv.sel(opt_space='observation') \n - l1_error_inv.sel(opt_space='hybrid')\n)\nm = diff_l1_error.sel(t=time_step).mean(dim='n')\ns = diff_l1_error.sel(t=time_step).std(dim='n')\nZ = m / (s / np.sqrt(num_samples))\np = scipy.stats.norm.sf(np.abs(Z))\nprint('Z-value', Z.values)\nprint('p-value', p)",
"Z-value -5.658017083581407\np-value 7.656594807321588e-09\n"
]
],
[
[
"### Baseline initialization",
"_____no_output_____"
]
],
[
[
"time_step = 11 # beginning of forecast window\nnum_samples = l1_error.sizes['n']\nl1_error_inv = l1_error.sel(init='baseline', data_type='opt')\ndiff_l1_error = (\n l1_error_inv.sel(opt_space='observation') \n - l1_error_inv.sel(opt_space='hybrid')\n)\nm = diff_l1_error.sel(t=time_step).mean(dim='n')\ns = diff_l1_error.sel(t=time_step).std(dim='n')\nZ = m / (s / np.sqrt(num_samples))\np = scipy.stats.norm.sf(np.abs(Z))\nprint('Z-value', Z.values)\nprint('p-value', p)",
"Z-value 6.508546551414507\np-value 3.7940697809822585e-11\n"
]
],
[
[
"## Assimilated trajectories",
"_____no_output_____"
]
],
[
[
"gt = vorticity.sel(data_type='gt', opt_space='observation', init='baseline')\nbaseline = vorticity.sel(\n data_type='opt', \n opt_space='observation', \n init='baseline',\n)\ninvobs = vorticity.sel(data_type='opt', opt_space='hybrid', init='invobs')\n\nforecast_comparison = (\n xr.concat([invobs, baseline, gt], dim='da_method')\n .assign_coords(\n da_method=['invobs', 'baseline', 'gt'], \n t=kolmogorov_flow.dt * np.arange(gt.sizes['t']),\n )\n .sel(da_method=['gt', 'invobs', 'baseline'])\n)",
"_____no_output_____"
],
[
"sns.set(font_scale=3)\nplt.rc('font', **{'family': 'Times New Roman'})\n\nsnapshot_selection = np.asarray([0, 10, 18])\ng = (\n forecast_comparison\n .isel(n=1, t=snapshot_selection)\n .plot.imshow(\n x='x', \n y='y', \n row='t', \n col='da_method', \n size=5, \n add_colorbar=False, \n cmap=sns.cm.icefire, \n vmin=-8, \n vmax=8,\n )\n)\n\ncol_labels = ['ground truth', 'proposed', 'baseline'] \n[ax.set_title(t) for ax, t in zip(g.axes.ravel(), col_labels)]\nrow_labels = [\n 'initial state, t=0', \n 'start forecast, t=1.75', \n 'end forecast, t=3.15',\n]\nadjust_row_labels(g, row_labels)\ng.set_axis_labels('', '')\n[ax.set_aspect('equal') for ax in g.axes.ravel()]\n[ax.set_yticks([]) for ax in g.axes.ravel()]\n[ax.set_xticks([]) for ax in g.axes.ravel()]\nplt.subplots_adjust(hspace=-0.3, wspace=0.)\nplt.tight_layout()\n\n# add highlight patches\nrectangle_coords = [\n [\n (3.5, 1.8), \n ],\n [\n (0.1, 4.7),\n ],\n]\n\ndef generate_rectangle(rx, ry):\n rectangle = plt.Rectangle(\n (rx, ry), \n 1.5, \n 1.5, \n lw=4, \n ec=plot_colors['y'], \n fill=False,\n )\n return rectangle\n\nfor row, row_coords in enumerate(rectangle_coords):\n row += 1\n for rx, ry in row_coords:\n ps = [generate_rectangle(rx, ry) for _ in range(3)]\n [g.axes[row,i].add_patch(p) for i, p in zip(range(3), ps)]\n\nplt.savefig(\n 'figures/forecast_results_kolmogorov.pdf', \n bbox_inches='tight', \n pad_inches=0.1,\n)",
"_____no_output_____"
]
],
[
[
"## Summary figure",
"_____no_output_____"
]
],
[
[
"sns.set(font_scale=3)\nplt.rc('font', **{'family': 'Times New Roman'})\n\ng = forecast_comparison.isel(n=1, t=11) \\\n.plot.imshow(\n x='x', \n y='y', \n col='da_method', \n size=5, \n add_colorbar=False, \n cmap=sns.cm.icefire, \n vmin=-8, \n vmax=8,\n)\n\ncol_labels = ['ground truth', 'proposed', 'baseline'] \ng.set_titles('')\ng.set_axis_labels('', '')\n[ax.set_xlabel(label) for ax, label in zip(g.axes.ravel(), col_labels)]\n[ax.set_aspect('equal') for ax in g.axes.ravel()]\n[ax.set_yticks([]) for ax in g.axes.ravel()]\n[ax.set_xticks([]) for ax in g.axes.ravel()]\nplt.tight_layout()\n\n# add highlight patches\nrectangle_coords = [\n (1.0, 1.0), \n (1, 4.7), \n (3.5, 1.8),\n ]\nfor rx, ry in rectangle_coords:\n ps = [generate_rectangle(rx, ry) for _ in range(3)]\n [g.axes[0,i].add_patch(p) for i, p in zip(range(3), ps)]\n \nplt.savefig('figures/result_summary.pdf', bbox_inches='tight', pad_inches=0.1)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7ba77907efafb46ffd0d7a6c186fbb65c0ead5c | 10,763 | ipynb | Jupyter Notebook | Notebooks/parallel_aguirregabiria_julia.ipynb | cdagnino/LearningModels | b31d4e1dd5381ba06fc5b1d2b0e2eb1515f2d15f | [
"Apache-2.0"
] | null | null | null | Notebooks/parallel_aguirregabiria_julia.ipynb | cdagnino/LearningModels | b31d4e1dd5381ba06fc5b1d2b0e2eb1515f2d15f | [
"Apache-2.0"
] | null | null | null | Notebooks/parallel_aguirregabiria_julia.ipynb | cdagnino/LearningModels | b31d4e1dd5381ba06fc5b1d2b0e2eb1515f2d15f | [
"Apache-2.0"
] | null | null | null | 31.937685 | 302 | 0.473938 | [
[
[
"empty"
]
]
] | [
"empty"
] | [
[
"empty"
]
] |
e7ba79f13249555c50fb6f27012ae16434d0a8a2 | 32,405 | ipynb | Jupyter Notebook | ind.ipynb | A1zak/cross6 | 217d88cea867f3a449a23a8e224f1d21d8cfb2a2 | [
"MIT"
] | null | null | null | ind.ipynb | A1zak/cross6 | 217d88cea867f3a449a23a8e224f1d21d8cfb2a2 | [
"MIT"
] | null | null | null | ind.ipynb | A1zak/cross6 | 217d88cea867f3a449a23a8e224f1d21d8cfb2a2 | [
"MIT"
] | null | null | null | 240.037037 | 17,832 | 0.920784 | [
[
[
"## Индивидуальное задание: \n### Изобразить эмблему Бэтмена для его призыва к спасению города от противников бабы Нины\n#### Задача: Существуют хейтеры бабы Нины и перед Бэтменом была поставлена задача спасти её от них\n#### Решение: V = 1 Бэтмен, p хейтеры = 100 штук\n#### P = pV\n#### P = 100 * 1 = 100 штук\n#### Q = V : P\n#### Q = 1 : 100= 0,01 H\n#### Ответ: Q = 0,01 H. Именно такой процент сил надо применить Бэтмену,чтобы спасти бабу Нину от хейтеров",
"_____no_output_____"
]
],
[
[
"%load_ext autoreload\n%autoreload 2 \n\nimport jupyter_lesson",
"_____no_output_____"
],
[
"%matplotlib inline\n\njupyter_lesson.plot_batman()",
"_____no_output_____"
],
[
"import matplotlib.path as mpath\nimport matplotlib.patches as mpatches\nimport matplotlib.pyplot as plt\n\nfig, ax = plt.subplots()\n\nPath = mpath.Path\npath_data = [\n (Path.MOVETO, (1.58, -2.57)),\n (Path.CURVE4, (0.35, -1.1)),\n (Path.CURVE4, (-1.75, 2.0)),\n (Path.CURVE4, (0.375, 2.0)),\n (Path.LINETO, (0.85, 1.15)),\n (Path.CURVE4, (2.2, 3.2)),\n (Path.CURVE4, (3, 0.05)),\n (Path.CURVE4, (2.0, -0.5)),\n (Path.CLOSEPOLY, (1.58, -2.57)),\n ]\ncodes, verts = zip(*path_data)\npath = mpath.Path(verts, codes)\npatch = mpatches.PathPatch(path, facecolor='r', alpha=0.5)\nax.add_patch(patch)\n\n# plot control points and connecting lines\nx, y = zip(*path.vertices)\nline, = ax.plot(x, y, 'go-')\n\nax.grid()\nax.axis('equal')\nplt.show()",
"_____no_output_____"
]
]
] | [
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code",
"code"
]
] |
e7ba8bc369d5e4f5d7c3cf9549636a5f709c6c20 | 34,196 | ipynb | Jupyter Notebook | python/python/1_Definitions.ipynb | t20100/silx-training | 409656479c7fdc9f1e895c6f3f0530c7eb89cbc1 | [
"CC-BY-4.0"
] | null | null | null | python/python/1_Definitions.ipynb | t20100/silx-training | 409656479c7fdc9f1e895c6f3f0530c7eb89cbc1 | [
"CC-BY-4.0"
] | null | null | null | python/python/1_Definitions.ipynb | t20100/silx-training | 409656479c7fdc9f1e895c6f3f0530c7eb89cbc1 | [
"CC-BY-4.0"
] | null | null | null | 20.787842 | 143 | 0.486753 | [
[
[
"# Definitions of Python:\n\n1. Primitive types, basic operations\n2. Composed types: lists, tuples, dictionaries\n3. Everything is an object\n4. Control structures: blocks, branching, loops\n",
"_____no_output_____"
],
[
"## Primitive types\n\nThe basic types build into Python include:\n* `int`: variable length integers,\n* `float`: double precision floating point numbers, \n* `complex`: composed of two floats for *real* and *imag* part, \n* `str`: unicode character strings,\n* `bool`: boolean which can be only True or False\n* `None`: actually `NoneType` but there is only one, equivalent of *NULL* or *nil*",
"_____no_output_____"
],
[
"Some examples of each:",
"_____no_output_____"
]
],
[
[
"##### -1234567890 # an integer\n2.0 # a floating point number\n6.02e23 # a floating point number with scientific notation\ncomplex(1, 5) # a complex\nTrue or False # the two possible boolean values\n'This is a string'\n\"It's another string\"\nprint(\"\"\"Triple quotes (also with '''), allow strings to break over multiple lines.\nAlternatively \\n is a newline character (\\t for tab, \\\\ is a single backslash)\"\"\")",
"_____no_output_____"
],
[
"print(complex(1, 5))",
"_____no_output_____"
]
],
[
[
"### Primary operations\n\n| Symbol | Task Performed |\n|----|---|\n| + | Addition |\n| - | Subtraction |\n| * | Multiplication |\n| / | Floating point division |\n| // | Floor division |\n| % | Modulus or rest |\n| ** or pow(a, b) | Power |\n| abs(a) | absolute value |\n| round(a) | Banker's rounding |\n\nSome examples:",
"_____no_output_____"
]
],
[
[
"#divisions\nprint(3 / 2, 3 // 2)\nprint(3. / 2, 3. // 2)",
"_____no_output_____"
],
[
"#operation with complex\nprint((5. + 4.0j - 3.5) * 2.1)",
"_____no_output_____"
]
],
[
[
"Relational Operators\n \n| Symbol | Task Performed |\n|---|---|\n| == | True if it is equal |\n| != | True if not equal to |\n| < | less than |\n| > | greater than |\n| <= | less than or equal to |\n| >= | greater than or equal to |\n| |\n| not | negate a `bool` value |\n| is | True if both are the same |\n| and | True if both are True |\n| or | True if any are are True |\n| ^ | True if one or the other but not both are True |\n| |\n| ^ | bitwise xor operator in `int` |\n| & | bitwise and operator in `int` |\n| \\| | bitwise or operator in `int` |\n| >> | right shift bitwise operation on `int`|\n| << | left shift bitwise operation on `int` |\n| | |\n\nNote the difference between:\n * `==` equality test\n * `=` assignment\n",
"_____no_output_____"
]
],
[
[
"# grouping comparison\nprint(1 >= 0.5 and (2 > 3 or 5 % 2 == 1))",
"_____no_output_____"
]
],
[
[
"## Strings\n\n* Basic operations on strings: `+` or `*`\n* Formating using `%s` or `.format`\n* Slicing using the [start: stop: step]\n",
"_____no_output_____"
]
],
[
[
"s = \"a is equal to\"\na = 5\nprint(s+str(a))\nprint(s, a)",
"_____no_output_____"
],
[
"#/!\\ \ns+a",
"_____no_output_____"
],
[
"# String muliplied by int works !\n\"*--*\" * 5",
"_____no_output_____"
]
],
[
[
"### String formating",
"_____no_output_____"
]
],
[
[
"# %s will convert anything to an int. %i, %d, %f works like in C or spec.\nprint(\"a is equal to %s\" % a)\nprint(\"%s %05i\" % (s,a))",
"_____no_output_____"
],
[
"#new style formating\n'{2} {1} {2} {0}'.format('a','b','c')",
"_____no_output_____"
],
[
"'{0:.2} {1:.1%}'.format(0.3698, 9.6/100)",
"_____no_output_____"
]
],
[
[
"### String access and slicing\n\n* Access a single element with `[index]`\n* Extract a sub part of a string using `[start:stop:step]` → **slicing**.\n* Works the same on lists, tuples, arrays, ...",
"_____no_output_____"
]
],
[
[
"letters = \"abcdefghijklmnopqrstuvwxyz\"\nprint(letters)\nlen(letters)",
"_____no_output_____"
],
[
"# remind you that python is 0-based indexing\nprint(letters[0], letters[4], letters[25])",
"_____no_output_____"
],
[
"#/!\\\nletters[56]",
"_____no_output_____"
],
[
"#slicing from beginging\nletters[0:4]",
"_____no_output_____"
],
[
"#from the end\nletters[-5:]",
"_____no_output_____"
],
[
"# two by two using a stepsize of 2\nletters[::2]",
"_____no_output_____"
],
[
"# inverted using a stepsize of -1:\nletters[-1::-1] ",
"_____no_output_____"
],
[
"#strings are not mutable !\n\nletters[2] = \"d\"",
"_____no_output_____"
],
[
"#ask for help !\nhelp(str)",
"_____no_output_____"
]
],
[
[
"### Useful string methods\n\n```python\nmy_str = 'toto'\n```\n\n* `len(my_str)`: returns the length of the string\n* `my_str.find('to')`, `my_str.index('to')`: returns the starting index. Find returns ``-1`` if not found, index fails.\n* `my_str.replace(str1, str2)`: replaces `str1` with `str2` in string\n* `my_str.split()` splits the string in a list of words\n* `my_str.startswith(sub)`, `my_str.endswith(sub)`: returns `True` if the string `my_str` starts with `sub`-string\n* `my_str.isalnum()`, `my_str.isalpha()`, `my_str.isdigit()`: returns `True` if the chain is alphanumeric, only letter or only numbers\n* `my_str.strip()`, `my_str.rstrip()`, `lstrip()`: removes spaces at the extremities of the string (R and L variant for Right/Left)\n* `my_str.upper()`, `my_str.lower()`, `my_str.swapcase()`: converts to all upper-case, all lowercase, swap case\n",
"_____no_output_____"
],
[
"## Composed types\n\n* Lists\n* Tuples\n* Dictionaries",
"_____no_output_____"
],
[
"## List\n\nLists are defined using square brackets `[]` or the `list(iter)` and can contain any type of objects. \nThey are mutable.\n",
"_____no_output_____"
]
],
[
[
"print([1, 2, 3]) \ndigits = list(range(10))\nprint(digits)",
"_____no_output_____"
],
[
"print(list(letters))\nprint(digits + list(letters))\n\n#but\ndigits + letters",
"_____no_output_____"
],
[
"# lists can contain anything\na = ['my string', True, 5+7]\nprint(len(a))",
"_____no_output_____"
],
[
"a.append(3.141592) \nprint(a, len(a))",
"_____no_output_____"
],
[
"list(range(5, 12, 2))",
"_____no_output_____"
]
],
[
[
"## Useful methods for lists\n\n```python\nlst = [1, 2, 3, 'a', 'b', 'c']\n```\n* `lst.append(a)`: adds *a* at the end of lst\n* `lst.insert(idx, a)`: inserts one element at a given index\n* `lst.index(a)`: finds first index containing a value\n* `lst.count(a)`: counts the number of occurences of *a* in the list \n* `lst.pop(idx)`: removes and returns one element by index\n* `lst.remove(obj)`: removes an element by value\n* `lst.sort()` and `lst.reverse()`: sorts and reverses the list **in place** (no return value, the original list is changed)\n\n**Warning**: this deletes the list: `lst = lst.sort()`\n",
"_____no_output_____"
]
],
[
[
"lst = ['eggs', 'sausages']\nprint(len(lst))\nlst.append(\"spam\")\nprint(lst, len(lst))",
"_____no_output_____"
],
[
"lst.insert(0, \"spam\")\nprint(lst)",
"_____no_output_____"
],
[
"print(lst.index(\"spam\"), lst.index(\"sausages\"))\n#but:\nlst.index(5)",
"_____no_output_____"
],
[
"lst.count(\"spam\")",
"_____no_output_____"
],
[
"print(lst.pop(), lst.pop(2))",
"_____no_output_____"
],
[
"# lists are mutable:\nprint(lst)\nlst[0] = 1\nprint(lst)",
"_____no_output_____"
],
[
"lst.remove(\"eggs\")\nprint(lst)\n# but not twice:\nlst.remove(\"eggs\")",
"_____no_output_____"
],
[
"# and always:\nhelp(list)",
"_____no_output_____"
]
],
[
[
"## Tuple\n\n* Tuples are defined by the `tuple(iter)` or by a ``,`` separated list in parenthesis ``()`` \n* Tuples are like lists, but not mutable !\n",
"_____no_output_____"
]
],
[
[
"mytuple = ('spam', 'eggs', 5, 3.141592, 'sausages')\nprint(mytuple[0], mytuple[-1])",
"_____no_output_____"
],
[
"# /!\\ tuples are not mutable\nmytuple[3] = \"ham\"",
"_____no_output_____"
],
[
"# Single element tuple: mind the comma\nt = 5,\nprint(t)",
"_____no_output_____"
]
],
[
[
"## List comprehension and generators\n\nVery *pythonic* and convenient way of creating lists or tuples from an iterator: \n\n` [ f(i) for i in iterable if condition(i) ]`\n\nThe content of the `[]` is called a *generator*.\nA *generator* generates elements on demand, which is *fast* and *low-memory usage*. \nIt is the base of the asynchronous programming used in Python3 (out of scope).\n\nIt is an alternative to functional programming based on `lambda`, `map` & `filter`:\n* less *pythonic*, harder to read, and not faster\n* `lambda`, `map` and `filter` are reserved keywords, they should not be used as variable names, especially not **lambda**.\n",
"_____no_output_____"
]
],
[
[
"[2*x+1 for x in range(5)]",
"_____no_output_____"
],
[
"tuple(x**(1/2.) for x in range(5))",
"_____no_output_____"
],
[
"(x**(1/2.) for x in range(5))",
"_____no_output_____"
],
[
"[x for x in range(10) if x**3 - 15*x**2 + 71*x == 105]",
"_____no_output_____"
],
[
"print([l.upper() for l in letters])",
"_____no_output_____"
]
],
[
[
"## Mapping Types: Dictionaries\n\nDictionaries associate a key to a value using curly braces `{key1: value1, key2:value2}`: \n* Keys must be *hashable*, i.e. any object that is unmutable, also known as *hash table* in other languages\n* Dictionaries were not ordered before Python 3.7 (`OrderedDict` are)\n",
"_____no_output_____"
]
],
[
[
"help(dict)",
"_____no_output_____"
],
[
"periodic_table = {\n \"H\": 1,\n \"He\": 2,\n \"Li\": 3,\n \"Be\": 4,\n \"B\": 5,\n \"C\": 6,\n \"N\": 7,\n \"O\": 8,\n \"F\": 9,\n \"Ne\": 10,\n}\n \nprint(periodic_table)",
"_____no_output_____"
],
[
"print(periodic_table['He'])",
"_____no_output_____"
],
[
"print(periodic_table.keys())",
"_____no_output_____"
],
[
"print(periodic_table.values())",
"_____no_output_____"
],
[
"#search for a key in a dict:\n'F' in periodic_table",
"_____no_output_____"
],
[
"len(periodic_table)",
"_____no_output_____"
],
[
"print(periodic_table)",
"_____no_output_____"
],
[
"periodic_table[\"Z\"] ",
"_____no_output_____"
],
[
"# With a fallback value:\nperiodic_table.get(\"Z\", \"unknown element\")",
"_____no_output_____"
],
[
"other = periodic_table.copy()\nk1 = other.pop('Li')\nprint(periodic_table)\nprint(other)\n",
"_____no_output_____"
]
],
[
[
"## In Python, everything is object\n\n* In Python everything is object (inherits from ``object``)\n* Names are just labels, references, attached to an object\n* Memory is freed when the number of references drops to 0\n\n- `dir(obj)`: lists the attributes of an object\n- `help(obj)`: prints the help of the object\n- `type(obj)`: gets the type of an object\n- `id(obj)`: gets the memory adress of an object\n",
"_____no_output_____"
]
],
[
[
"a = object()\nprint(dir(a))",
"_____no_output_____"
],
[
"print(type(True), type(a), id(a))",
"_____no_output_____"
],
[
"b = 5\nc = 5\nprint(id(b), id(c), id(b) == id(c), b is c)",
"_____no_output_____"
],
[
"# == vs `is`\na, b = 5, 5.0\nprint(a == b)\nprint(type(a), type(b))",
"_____no_output_____"
],
[
"# int are unique\nprint(a, bin(a), a is 0b0101)\nprint(a == 5.0, a is 5.0)",
"_____no_output_____"
],
[
"print(1 is None, None is None)",
"_____no_output_____"
]
],
[
[
"### Warning: in Python, everything is object ...",
"_____no_output_____"
]
],
[
[
"list1 = [3, 2, 1]\nprint(\"list1=\", list1)\nlist2 = list1\nlist2[1] = 10\nprint(\"list2=\", list2)",
"_____no_output_____"
],
[
"# Did you expect ?\nprint(\"list1= \", list1)",
"_____no_output_____"
]
],
[
[
"",
"_____no_output_____"
],
[
"",
"_____no_output_____"
],
[
"",
"_____no_output_____"
],
[
"",
"_____no_output_____"
],
[
"",
"_____no_output_____"
],
[
"",
"_____no_output_____"
]
],
[
[
"print(\"indeed: id(list1)=\", id(list1),\" and id(list2):\", id(list2), \"so list2 is list1:\", list2 is list1)",
"_____no_output_____"
],
[
"#How to avoid this: make copies of mutable objects:\nlist1 = [3, 2, 1]\nprint(\"list1=\", list1)\nlist3 = list1 [:] # copy the content !\nlist3[1] = 10\nprint(\"As expected: list3= \", list3)\nprint(\"And now: list1= \", list1)",
"_____no_output_____"
]
],
[
[
"**Warning:** This is very error prone when manipulating **any** mutable objects.",
"_____no_output_____"
]
],
[
[
"# Generic solution: use the copy module\nimport copy\nlist3 = copy.copy(list1) # same, more explicit\nprint(id(list1) == id(list3))",
"_____no_output_____"
]
],
[
[
"## Control structures: blocks\n\n### Code structure\n\n\nPython uses a colon `:` at the end of the line and 4 white-spaces indentation\nto establish code block structure.\n\nMany other programming languages use braces { }, not python.\n\n\n",
"_____no_output_____"
],
[
"```\n Block 1\n ...\n Header making new block:\n Block 2\n ...\n Header making new block:\n Block 3\n ...\n Block 2 (continuation)\n ...\n Block 1 continuation\n ...\n```\n\n- Clearly indicates the beginning of a block.\n- Coding style is mostly uniform. Use **4 spaces**, never **< tabs >**.\n- Code structure is much more readable and clearer.\n\n",
"_____no_output_____"
],
[
"### Branching\n \n- Condition branchings are made with `if elif else` statements\n- Can have many ``elif``'s (not recommended)\n- Can be nested (too much nesting is bad for readability)\n\nExample for solving a second order polynomial root:",
"_____no_output_____"
]
],
[
[
"a = -1\nb = 2\nc = 1\nq2 = b * b - 4.0 * a * c\nprint(\"Determinant is \", q2)",
"_____no_output_____"
],
[
"import math\nif q2 < 0:\n print(\"No real solution\")\nelif q2 > 0:\n x1 = (-b + math.sqrt(q2)) / (2.0 * a)\n x2 = (-b - math.sqrt(q2)) / (2.0 * a)\n print(\"Two solutions %.2f and %.2f\" % (x1, x2))\nelse:\n x = -b / (2.0 * a)\n print(\"One solution: %.2f\" % x)\n",
"_____no_output_____"
]
],
[
[
"### For loop\n\n- iterate over a sequence (list, tuple, char in string, keys in dict, any iterator)\n- no indexes, uses directly the object in the sequence\n- when the index is really needed, use `enumerate`\n- One can use multiple sequences in parallel using `zip`",
"_____no_output_____"
]
],
[
[
"ingredients = [\"spam\", \"eggs\", \"ham\", \"spam\", \"sausages\"]\nfor food in ingredients:\n print(\"I like %s\" % food)",
"_____no_output_____"
],
[
"for idx, food in enumerate(ingredients[-1::-1]):\n print(\"%s is number %d in my top 5 of foods\" % (food, len(ingredients)- idx))",
"_____no_output_____"
],
[
"subjects = [\"Roses\", \"Violets\", \"Sugar\"]\nverbs = [\"are\", \"are\", \"is\"]\nadjectives = [\"red,\", \"blue,\", \"sweet.\"] \nfor s, v, a in zip(subjects, verbs, adjectives):\n print(\"%s %s %s\" % (s, v, a))",
"_____no_output_____"
]
],
[
[
"### While loop\n\n- Iterate while a condition is fulfilled\n- Make sure the condition becomes unfulfilled, else it could result in infinite loops ...\n",
"_____no_output_____"
]
],
[
[
"a, b = 175, 3650\nstop = False\npossible_divisor = max(a, b) // 2\nwhile possible_divisor >= 1 and not stop:\n if a % possible_divisor == 0 and b % possible_divisor == 0:\n print(\"Found greatest common divisor: %d\" % possible_divisor)\n stop = True\n possible_divisor = possible_divisor - 1 \n",
"_____no_output_____"
],
[
"while True: \n print(\"I will print this forever\")\n \n# Now you are ready to interrupt the kernel !\n#go in the menu and click kernel-> interrput\n",
"_____no_output_____"
]
],
[
[
"### Useful commands in loops\n\n- `continue`: go directly to the next iteration of the most inner loop\n- `break`: quit the most inner loop\n- `pass`: a block cannot be empty; ``pass`` is a command that does nothing\n- `else`: block executed after the normal exit of the loop.\n",
"_____no_output_____"
]
],
[
[
"for i in range(10):\n if not i % 7 == 0:\n print(\"%d is *not* a multiple of 7\" % i)\n continue\n print(\"%d is a multiple of 7\" % i)",
"_____no_output_____"
],
[
"n = 112\n# divide n by 2 until this does no longer return an integer\nwhile True:\n if n % 2 != 0:\n print(\"%d is not a multiple of 2\" % n)\n break\n print(\"%d is a multiple of 2\" % n)\n n = n // 2\n",
"_____no_output_____"
]
],
[
[
"### Exercise: Fibonacci series\n\n\n- Fibonacci:\n - Each element is the sum of the previous two elements\n - The first two elements are 0 and 1\n\n- Calculate all elements in this series up to 1000, put them in a list, then print the list.\n\n``[0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987]``\n\nprepend the cell with `%%timeit` to measure the execution time",
"_____no_output_____"
]
],
[
[
"# One possible solution\nres = [0, 1]\nnext_el = 1\nwhile next_el < 1000: \n res.append(next_el)\n next_el = res[-2] + res[-1]\nprint(res)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7ba8f0204c3a1c132d35b00b82a3174c721e0e3 | 343,233 | ipynb | Jupyter Notebook | Timeseries.ipynb | k-rajmani2k/RNN | 5233376acc9b2cc666a769f59d46ba244669f459 | [
"MIT"
] | 17 | 2019-04-16T17:38:17.000Z | 2021-12-20T21:19:38.000Z | Timeseries.ipynb | k-rajmani2k/RNN | 5233376acc9b2cc666a769f59d46ba244669f459 | [
"MIT"
] | null | null | null | Timeseries.ipynb | k-rajmani2k/RNN | 5233376acc9b2cc666a769f59d46ba244669f459 | [
"MIT"
] | 23 | 2019-04-16T17:46:55.000Z | 2021-09-06T15:07:23.000Z | 752.703947 | 95,436 | 0.952542 | [
[
[
"<div style=\"width: 100%; overflow: hidden;\">\n <div style=\"width: 150px; float: left;\"> <img src=\"data/D4Sci_logo_ball.png\" alt=\"Data For Science, Inc\" align=\"left\" border=\"0\"> </div>\n <div style=\"float: left; margin-left: 10px;\"> \n <h1>RNNs for Timeseries Analysis</h1>\n <h2>Timeseries</h2>\n <p>Bruno Gonçalves<br/>\n <a href=\"http://www.data4sci.com/\">www.data4sci.com</a><br/>\n @bgoncalves, @data4sci</p></div>\n</div>",
"_____no_output_____"
]
],
[
[
"import pandas as pd\nfrom pandas.plotting import autocorrelation_plot\n\nimport numpy as np\nnp.random.seed(123)\n\nfrom sklearn.linear_model import LinearRegression\nimport matplotlib.pyplot as plt\n\nimport watermark\n\n%load_ext watermark\n%matplotlib inline",
"_____no_output_____"
],
[
"%watermark -n -v -m -p numpy,matplotlib,pandas,sklearn",
"Wed Sep 25 2019 \n\nCPython 3.7.3\nIPython 6.2.1\n\nnumpy 1.16.2\nmatplotlib 3.1.0\npandas 0.24.2\nsklearn 0.20.3\n\ncompiler : Clang 4.0.1 (tags/RELEASE_401/final)\nsystem : Darwin\nrelease : 18.7.0\nmachine : x86_64\nprocessor : i386\nCPU cores : 8\ninterpreter: 64bit\n"
]
],
[
[
"# Load the dataset\nGDP data from the Federal Reserve Bank [website](https://fred.stlouisfed.org/series/GDP)",
"_____no_output_____"
]
],
[
[
"series = pd.read_csv('data/GDP.csv', header=0, parse_dates=[0], index_col=0)",
"_____no_output_____"
],
[
"plt.plot(series)\nplt.xlabel('Date')\nplt.ylabel('GDP (B$)');\nplt.gcf().set_size_inches(11,8)",
"/Users/bgoncalves/anaconda3/lib/python3.7/site-packages/pandas/plotting/_converter.py:129: FutureWarning: Using an implicitly registered datetime converter for a matplotlib plotting method. The converter was registered by pandas on import. Future versions of pandas will require you to explicitly register matplotlib converters.\n\nTo register the converters:\n\t>>> from pandas.plotting import register_matplotlib_converters\n\t>>> register_matplotlib_converters()\n warnings.warn(msg, FutureWarning)\n"
],
[
"series['GDP'].pct_change().plot()\nplt.gca().plot([series.index.min(), series.index.max()], [0, 0], 'r-')\nplt.xlabel('Date')\nplt.ylabel('GDP QoQ Growth');\nplt.gcf().set_size_inches(11,8)",
"_____no_output_____"
]
],
[
[
"## Autocorrelation function",
"_____no_output_____"
]
],
[
[
"autocorrelation_plot(series, label='DJIA')\nautocorrelation_plot(series.pct_change().dropna(), ax=plt.gca(), label='DoD')\nplt.gcf().set_size_inches(11,8)",
"_____no_output_____"
],
[
"values = series.pct_change().dropna().values.reshape(-1, 1)\nX = values[:-1]\ny = values[1:]",
"_____no_output_____"
],
[
"plt.plot(X.flatten(), y, '*')\nplt.xlabel('x_t')\nplt.ylabel('x_t+1')",
"_____no_output_____"
],
[
"lm = LinearRegression()",
"_____no_output_____"
],
[
"lm.fit(X, y)",
"_____no_output_____"
],
[
"y_pred = lm.predict(X)",
"_____no_output_____"
]
],
[
[
"## Fit comparison",
"_____no_output_____"
]
],
[
[
"plt.plot(series.index[2:], series.values[2:], )\nplt.plot(series.index[2:], (1+y_pred).cumprod()*series.values[0])\nplt.xlabel('Date')\nplt.ylabel('GDP (B$)')\nplt.gcf().set_size_inches(11, 8)",
"_____no_output_____"
]
],
[
[
"## Now without looking into the future",
"_____no_output_____"
]
],
[
[
"n_points = len(series)\ntrain_points = int(2/3*n_points)+1",
"_____no_output_____"
],
[
"X_train = X[:train_points]\ny_train = y[:train_points]\nX_test = X[train_points:]\ny_test = y[train_points:]",
"_____no_output_____"
],
[
"lm.fit(X_train, y_train)",
"_____no_output_____"
],
[
"y_train_pred = lm.predict(X_train)\ny_test_pred = lm.predict(X_test)",
"_____no_output_____"
],
[
"plt.plot(series.index[:train_points], y_train, label='data')\nplt.plot(series.index[:train_points], y_train_pred, label='fit')\nplt.plot(series.index[:train_points], y_train_pred*0+y_train_pred[0], label='reference')\nplt.xlabel('Date')\nplt.ylabel('DJIA DoD')\nplt.legend()\nplt.gcf().set_size_inches(11, 8)",
"_____no_output_____"
]
],
[
[
"## Comparison plot",
"_____no_output_____"
]
],
[
[
"plt.plot(series.index[:train_points], (1+y_train).cumprod()*series.values[0], label='train')\nplt.plot(series.index[train_points+2:], (1+y_test).cumprod()*series.values[train_points], label='test')\nplt.plot(series.index[:train_points], (1+y_train_pred).cumprod()*series.values[0], label='train_pred')\nplt.plot(series.index[train_points+2:], (1+y_test_pred).cumprod()*series.values[train_points], label='test_pred')\nplt.xlabel('Date')\nplt.ylabel('DJIA')\nplt.legend()\nplt.gcf().set_size_inches(11,8)",
"_____no_output_____"
]
],
[
[
"<div style=\"width: 100%; overflow: hidden;\">\n <img src=\"data/D4Sci_logo_full.png\" alt=\"Data For Science, Inc\" align=\"center\" border=\"0\" width=300px> \n</div>",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
]
] |
e7ba92ae43f6bd4744944c92047835641bc51ce2 | 25,237 | ipynb | Jupyter Notebook | ASE20_data/evaluation/RQ3_CVP.ipynb | henryhchchc/MockSniffer | 6d6e845616004ca77ce6d73709cff94a1a32c6c5 | [
"MIT"
] | 4 | 2020-09-03T13:20:13.000Z | 2021-07-14T08:04:26.000Z | ASE20_data/evaluation/RQ3_CVP.ipynb | henryhchchc/MockSniffer | 6d6e845616004ca77ce6d73709cff94a1a32c6c5 | [
"MIT"
] | null | null | null | ASE20_data/evaluation/RQ3_CVP.ipynb | henryhchchc/MockSniffer | 6d6e845616004ca77ce6d73709cff94a1a32c6c5 | [
"MIT"
] | null | null | null | 34.666209 | 137 | 0.446527 | [
[
[
"import pandas as pd\nfrom dataloader import *\nfrom classifier import *",
"_____no_output_____"
],
[
"log = True",
"_____no_output_____"
],
[
"vers_files = {\n p: [data_files[p]] + [\n \"../data_files/cvp/%s_%i.csv\" % (p.lower(), i)\n for i in range(1,5)\n ]\n for p in projects\n}",
"_____no_output_____"
],
[
"project_df = {\n p: [\n load_df(\"%s_%d\" % (p, i), fns[i], True).drop_duplicates()\n for i in range(5)\n ]\n for p, fns in vers_files.items()\n}",
"_____no_output_____"
],
[
"data_stat_df = pd.DataFrame(index=projects, columns=['Version %d' % i for i in range(5)])\nfor p in projects:\n for i in range(5):\n df = project_df[p][i]\n data_stat_df.at[p, 'Version %d' % i] = len(df[df['IS_MOCK']])",
"_____no_output_____"
],
[
"data_stat_df",
"_____no_output_____"
],
[
"def _model_core(p):\n# from sklearn.feature_selection import SelectKBest, chi2\n train = balance_dataset(p[0])\n test = balance_dataset(p[1])\n X_train = train.drop(['CUT', 'IS_MOCK', 'TC', 'TM', 'D', 'L'], axis=1)\n y_train = train['IS_MOCK']\n# feature_selection = SelectKBest(score_func=chi2, k=12)\n# X_train = feature_selection.fit_transform(X_train, y_train)\n X_test = test.drop(['CUT', 'IS_MOCK', 'TC', 'TM', 'D', 'L'], axis=1)\n y_test = test['IS_MOCK']\n# X_test = feature_selection.transform(X_test)\n return run_classifier(X_train, X_test, y_train, y_test)\n\ndef _ver_run(train_idx, test_idx, dfs):\n import multiprocessing as mp\n import numpy as np\n train = pd.concat([\n dfs[i]\n for i in train_idx\n ]).drop(['PROJ'], axis=1).drop_duplicates(['TC','TM','D'])\n train_ind = {\n (it['TC'],it['TM'],it['D'])\n for _, it in train.iterrows()\n }\n test = pd.concat([\n dfs[i]\n for i in test_idx\n ]).drop(['PROJ'], axis=1).drop_duplicates()\n test = test[~test.apply(lambda it: (it['TC'], it['TM'], it['D']) in train_ind, axis=1)].drop_duplicates()\n test_mock_count = len(test[test['IS_MOCK']])\n if log:\n print(\"%d mocks in test\" % test_mock_count)\n if test_mock_count == 0:\n return test_mock_count, {\n m: 0\n for m in metrics\n }\n pl = mp.Pool()\n perf_data = pl.map(_model_core, [(train, test) for i in range(100)])\n pl.close()\n ret = {\n m: np.mean([p[m] for p in perf_data])*100\n for m in metrics\n }\n if log:\n print(ret)\n return test_mock_count, ret\n\n\ndef inter_version_single_proj(proj_name, ver_df):\n import numpy as np\n if log:\n print(proj_name)\n data_pair_idx = [\n (list(range(i+1,5)), [i])\n for i in range(4)\n ]\n perf_data = [\n _ver_run(tri, tsi, ver_df)\n for tri, tsi in data_pair_idx\n ]\n return {\n m: np.sum([p[m]*n for n,p in perf_data])/np.sum([n for n,_ in perf_data])\n for m in metrics\n }\n ",
"_____no_output_____"
],
[
"def run_inter_version(proj_df):\n return {\n p: inter_version_single_proj(p, dfs)\n for p, dfs in proj_df.items()\n }",
"_____no_output_____"
],
[
"performance = run_inter_version(project_df)",
"Hadoop\n459 mocks in test\n{'accuracy': 78.64923747276688, 'precision': 74.76459510357816, 'recall': 86.49237472766885, 'f1-score': 80.20202020202021}\n1620 mocks in test\n{'accuracy': 72.43827160493825, 'precision': 73.86736703873935, 'recall': 69.44444444444444, 'f1-score': 71.58765510658604}\n367 mocks in test\n{'accuracy': 75.61307901907358, 'precision': 80.92105263157897, 'recall': 67.02997275204358, 'f1-score': 73.32339791356185}\n326 mocks in test\n{'accuracy': 83.58895705521472, 'precision': 82.88288288288292, 'recall': 84.66257668711656, 'f1-score': 83.76327769347498}\nFlink\n1538 mocks in test\n{'accuracy': 81.79453836150844, 'precision': 79.42238267148012, 'recall': 85.82574772431728, 'f1-score': 82.5}\n956 mocks in test\n{'accuracy': 75.99372384937237, 'precision': 78.99649941656945, 'recall': 70.81589958158995, 'f1-score': 74.68284611141753}\n903 mocks in test\n{'accuracy': 82.66888150609081, 'precision': 83.83027522935777, 'recall': 80.95238095238096, 'f1-score': 82.36619718309859}\n166 mocks in test\n{'accuracy': 67.46987951807225, 'precision': 75.89285714285712, 'recall': 51.204819277108435, 'f1-score': 61.15107913669063}\nHive\n61 mocks in test\n{'accuracy': 54.09836065573771, 'precision': 77.77777777777776, 'recall': 11.475409836065573, 'f1-score': 19.999999999999996}\n298 mocks in test\n{'accuracy': 70.8053691275168, 'precision': 73.66412213740456, 'recall': 64.76510067114096, 'f1-score': 68.92857142857143}\n266 mocks in test\n{'accuracy': 68.79699248120299, 'precision': 72.72727272727276, 'recall': 60.150375939849624, 'f1-score': 65.84362139917693}\n315 mocks in test\n{'accuracy': 72.06349206349206, 'precision': 74.21602787456446, 'recall': 67.6190476190476, 'f1-score': 70.76411960132889}\nCamel\n42 mocks in test\n{'accuracy': 77.38095238095237, 'precision': 71.6981132075472, 'recall': 90.47619047619047, 'f1-score': 79.99999999999999}\n284 mocks in test\n{'accuracy': 72.18309859154928, 'precision': 72.99270072992701, 'recall': 70.42253521126759, 'f1-score': 71.68458781362004}\n2 mocks in test\n{'accuracy': 75.0, 'precision': 66.66666666666669, 'recall': 100.0, 'f1-score': 79.99999999999999}\n28 mocks in test\n{'accuracy': 66.07142857142858, 'precision': 63.63636363636363, 'recall': 75.0, 'f1-score': 68.85245901639347}\nCXF\n30 mocks in test\n{'accuracy': 54.999999999999986, 'precision': 58.82352941176469, 'recall': 33.33333333333334, 'f1-score': 42.553191489361716}\n206 mocks in test\n{'accuracy': 63.59223300970874, 'precision': 75.45454545454544, 'recall': 40.29126213592232, 'f1-score': 52.531645569620245}\n75 mocks in test\n{'accuracy': 66.0, 'precision': 76.08695652173914, 'recall': 46.66666666666668, 'f1-score': 57.8512396694215}\n254 mocks in test\n{'accuracy': 59.44881889763779, 'precision': 66.21621621621622, 'recall': 38.582677165354326, 'f1-score': 48.75621890547263}\nDruid\n777 mocks in test\n{'accuracy': 71.87902187902189, 'precision': 77.77777777777776, 'recall': 61.26126126126127, 'f1-score': 68.5385169186465}\n100 mocks in test\n{'accuracy': 62.5, 'precision': 67.60563380281693, 'recall': 48.0, 'f1-score': 56.14035087719299}\n26 mocks in test\n{'accuracy': 55.769230769230745, 'precision': 66.66666666666669, 'recall': 23.076923076923077, 'f1-score': 34.285714285714285}\n329 mocks in test\n{'accuracy': 64.2857142857143, 'precision': 68.95161290322581, 'recall': 51.975683890577514, 'f1-score': 59.27209705372619}\nHBase\n144 mocks in test\n{'accuracy': 80.20833333333331, 'precision': 82.70676691729324, 'recall': 76.38888888888889, 'f1-score': 79.42238267148012}\n32 mocks in test\n{'accuracy': 95.3125, 'precision': 96.77419354838713, 'recall': 93.75, 'f1-score': 95.23809523809523}\n335 mocks in test\n{'accuracy': 84.02985074626866, 'precision': 79.99999999999999, 'recall': 90.74626865671642, 'f1-score': 85.03496503496507}\n5 mocks in test\n{'accuracy': 100.0, 'precision': 100.0, 'recall': 100.0, 'f1-score': 100.0}\nDubbo\n788 mocks in test\n{'accuracy': 64.08629441624365, 'precision': 81.53409090909088, 'recall': 36.421319796954315, 'f1-score': 50.350877192982466}\n152 mocks in test\n{'accuracy': 89.1447368421053, 'precision': 92.80575539568348, 'recall': 84.86842105263156, 'f1-score': 88.65979381443303}\n13 mocks in test\n{'accuracy': 84.61538461538458, 'precision': 90.79999999999997, 'recall': 77.0769230769231, 'f1-score': 83.35714285714283}\n10 mocks in test\n{'accuracy': 65.00000000000001, 'precision': 71.4285714285714, 'recall': 50.0, 'f1-score': 58.823529411764675}\nOozie\n42 mocks in test\n{'accuracy': 57.14285714285712, 'precision': 66.66666666666669, 'recall': 28.57142857142856, 'f1-score': 39.99999999999999}\n28 mocks in test\n{'accuracy': 85.71428571428572, 'precision': 91.66666666666667, 'recall': 78.5714285714286, 'f1-score': 84.61538461538458}\n256 mocks in test\n{'accuracy': 62.890625, 'precision': 100.0, 'recall': 25.78125, 'f1-score': 40.99378881987576}\n0 mocks in test\nStorm\n24 mocks in test\n{'accuracy': 87.5, 'precision': 79.99999999999999, 'recall': 100.0, 'f1-score': 88.8888888888889}\n243 mocks in test\n{'accuracy': 78.54526748971193, 'precision': 83.25438596491226, 'recall': 71.46502057613168, 'f1-score': 76.90250414486576}\n51 mocks in test\n{'accuracy': 84.35294117647058, 'precision': 84.3854818523154, 'recall': 84.31372549019608, 'f1-score': 84.34813925570228}\n189 mocks in test\n{'accuracy': 61.756613756613746, 'precision': 76.83366653338663, 'recall': 33.66137566137567, 'f1-score': 46.77357886119054}\n"
],
[
"performance_df = pd.DataFrame([\n {\n 'project': p,\n **perf\n }\n for p, perf in performance.items()\n]).set_index(['project'])\nperformance_df",
"_____no_output_____"
],
[
"performance_df.describe()",
"_____no_output_____"
],
[
"print(performance_df.round(2).to_latex())",
"\\begin{tabular}{lrrrr}\n\\toprule\n{} & accuracy & precision & recall & f1-score \\\\\nproject & & & & \\\\\n\\midrule\nHadoop & 75.20 & 76.01 & 73.74 & 74.68 \\\\\nFlink & 79.79 & 80.26 & 78.95 & 79.37 \\\\\nHive & 69.57 & 73.85 & 60.96 & 65.50 \\\\\nCamel & 72.33 & 72.07 & 73.31 & 72.49 \\\\\nCXF & 61.59 & 70.50 & 40.00 & 51.01 \\\\\nDruid & 68.75 & 74.36 & 56.90 & 64.33 \\\\\nHBase & 83.82 & 81.99 & 87.02 & 84.25 \\\\\nDubbo & 68.33 & 83.33 & 44.76 & 56.93 \\\\\nOozie & 64.11 & 94.99 & 30.67 & 44.61 \\\\\nStorm & 73.29 & 80.82 & 60.02 & 66.99 \\\\\n\\bottomrule\n\\end{tabular}\n\n"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7ba99e3b47e0e84ec34808db399c79df70647a8 | 5,198 | ipynb | Jupyter Notebook | notebooks/parallel_simulations.ipynb | zhaonat/py-maxwell-fd3d | bfa4fb826401b98371fdd9306c5fee2e74e7e545 | [
"MIT"
] | 3 | 2022-01-21T03:53:25.000Z | 2022-01-23T04:54:43.000Z | notebooks/parallel_simulations.ipynb | Guowu-Mcgill/py-maxwell-fd3d | bfa4fb826401b98371fdd9306c5fee2e74e7e545 | [
"MIT"
] | null | null | null | notebooks/parallel_simulations.ipynb | Guowu-Mcgill/py-maxwell-fd3d | bfa4fb826401b98371fdd9306c5fee2e74e7e545 | [
"MIT"
] | 1 | 2022-01-23T04:54:47.000Z | 2022-01-23T04:54:47.000Z | 35.121622 | 974 | 0.552135 | [
[
[
"## Parallel simulations using mpi4py",
"_____no_output_____"
]
],
[
[
"import os,sys\nNthread = 1\nos.environ[\"OMP_NUM_THREADS\"] = str(Nthread) # export OMP_NUM_THREADS=1\nos.environ[\"OPENBLAS_NUM_THREADS\"] = str(Nthread) # export OPENBLAS_NUM_THREADS=1\nos.environ[\"MKL_NUM_THREADS\"] = str(Nthread) # export MKL_NUM_THREADS=1\nos.environ[\"VECLIB_MAXIMUM_THREADS\"] = str(Nthread) # export VECLIB_MAXIMUM_THREADS=1\nos.environ[\"NUMEXPR_NUM_THREADS\"] = str(Nthread) # export NUMEXPR_NUM_THREADS=1\n",
"_____no_output_____"
],
[
"\nfrom palik_silicon import silicon\nfrom Photodetector import *\n\nimport autograd.numpy as np\nfrom autograd import grad\n\nimport nlopt\nimport numpy as npf\nfrom mpi4py import MPI\n\n# import use_autograd\n# use_autograd.use = 1\nimport rcwa\nimport materials, cons\nfrom mpi_nlopt import nlopt_opt,b_filter,f_symmetry\nfrom fft_funs import get_conv\nimport pickle\ncomm = MPI.COMM_WORLD\nsize = comm.Get_size()\nrank = comm.Get_rank()\n\n\ndef fun_mpi(dof,fun,N):\n '''mpi parallization for fun(dof,ctrl), ctrl is the numbering of ctrl's frequency calculation\n N calculations in total\n returns the sum: sum_{ctrl=1 toN} fun(dof,ctrl)\n '''\n dof = comm.bcast(dof)\n\n Nloop = int(np.ceil(1.0*N/size)) # number of calculations for each node\n val_i=[]\n g_i=[]\n val=[]\n g=[]\n\n for i in range(0,Nloop):\n ctrl = i*size+rank\n #print(ctrl)\n\n if(ctrl < N):\n color,wvlen, wvlen_index = wavelengths_tuple[ctrl];\n funi = lambda dof: fun(dof,wvlen,theta,phi, color, wvlen_index)\n grad_fun = grad(funi)\n\n val = funi(dof)\n gval = grad_fun(dof)\n\n # include indexing for now, in case one is interested\n val_i.append([ctrl,val])\n g_i.append([ctrl,gval])\n\n # gather the solution\n val_i = comm.gather(val_i)\n g_i = comm.gather(g_i)\n\n # summation\n if rank == 0:\n val_i = [x for x in val_i if x]\n g_i = [x for x in g_i if x]\n val_i = npf.concatenate(npf.array(val_i))\n g_i = npf.concatenate(npf.array(g_i))\n # sindex = val_i[:,0].argsort()\n\n val = np.sum(val_i[:,1])\n g = np.sum(g_i[:,1])\n\n\n val = comm.bcast(val)\n g = comm.bcast(g)\n return val,g\n\n\n",
"_____no_output_____"
]
]
] | [
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code"
]
] |
e7baa2b2211208d31d75ef7fd3db27626bd2be43 | 252,041 | ipynb | Jupyter Notebook | 09/4_1_delta_rule_points.ipynb | Penguinazor/mse.machle | 16d602fbda35a8f4769d2cb769819ce3bb3814c7 | [
"MIT"
] | null | null | null | 09/4_1_delta_rule_points.ipynb | Penguinazor/mse.machle | 16d602fbda35a8f4769d2cb769819ce3bb3814c7 | [
"MIT"
] | null | null | null | 09/4_1_delta_rule_points.ipynb | Penguinazor/mse.machle | 16d602fbda35a8f4769d2cb769819ce3bb3814c7 | [
"MIT"
] | 1 | 2019-11-24T22:40:18.000Z | 2019-11-24T22:40:18.000Z | 122.231329 | 83,461 | 0.803548 | [
[
[
"# Delta Rule\nThe Delta Rule is a gradient descent approximator that can be used to find the weight values of a Perceptron. The Delta Rule minimizes an error function (tipically $E=\\frac{1}{2}(y - t)^{2}$) by adapting the weight connections in small steps. The step length is defined by the learning rate $\\alpha$. In this notebook you will explore the classification capabilities of a single Perceptron by using the delta rule. You will start by setting the connection weights by hand for a simple problem, and then you will apply the delta rule for the same problem, and others.",
"_____no_output_____"
],
[
"## Loading the packages",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport matplotlib.pyplot as pl\nfrom ipywidgets import interact, widgets\n\nfrom matplotlib import animation",
"_____no_output_____"
]
],
[
[
"## Loading the Perceptron code\nIn order to the make this nothebook smaller, some of the functions (activation functions, and some of the code allowing the visualization of the results) was implemented in a separate python file. You are free to open it if needed.",
"_____no_output_____"
]
],
[
[
"import perceptron as pt",
"_____no_output_____"
]
],
[
[
"## The Dataset\nThe following script allows you to create a 2D dataset by using the mouse. First click to add points belonging to class A (blue), and then click to add points belonging to class B (red). You can create as many points as you desire. The final dataset will contain hence three values per point: x coordinate (-1 ≤ x ≤ 1), y coordinate (-1 ≤ y ≤ 1) and the class ∈ {1,-1}.",
"_____no_output_____"
],
[
"### Blue points first",
"_____no_output_____"
]
],
[
[
"%matplotlib notebook\n\nfig = pl.figure(figsize=(6,6))\npl.title(\"Input Dataset\")\npl.xlim((-1.2,1.2))\npl.ylim((-1.2,1.2))\n\ndataset = []\n\ndef onclick_blue(event):\n global dataset\n cx = event.xdata\n cy = event.ydata\n dataset.append((cx, cy, 1))\n \n pl.scatter(cx, cy, c='b', s=100, lw=0)\n pl.grid(True)\n\ncid = fig.canvas.mpl_connect('button_press_event', onclick_blue)",
"_____no_output_____"
]
],
[
[
"### Then, red points",
"_____no_output_____"
]
],
[
[
"%matplotlib notebook\n\nfig = pl.figure(figsize=(6,6))\nfor i in np.arange(len(dataset)):\n pl.scatter(dataset[i][0], dataset[i][1], c='b', s=100, lw=0)\n\npl.title(\"Input Dataset\")\npl.xlim((-1.2,1.2))\npl.ylim((-1.2,1.2))\n\ndef onclick_red(event):\n global dataset\n cx = event.xdata\n cy = event.ydata\n dataset.append((cx, cy, -1))\n \n pl.scatter(cx, cy, c='r', s=100, lw=0)\n pl.grid(True)\n\nfig.canvas.mpl_disconnect(onclick_blue)\ncid = fig.canvas.mpl_connect('button_press_event', onclick_red)",
"_____no_output_____"
],
[
"fig.canvas.mpl_disconnect(onclick_red)",
"_____no_output_____"
]
],
[
[
"This is the dataset you just created. Check that there are no NaNs in the third column. Create once again the dataset if necessary",
"_____no_output_____"
]
],
[
[
"dataset",
"_____no_output_____"
]
],
[
[
"## Finding the weights by hand\nIn this section you should try to find the set of weights that allows a Perceptron to separate the two classes you previously defined. Use the sliders to modify the value of each one of the connections and the bias of the Perceptron while trying to separate the two classes (blue and red). The curve on the right represents the classification error (MSE). If the modifications you provide improve the classification, you should see the error decreasing.",
"_____no_output_____"
]
],
[
[
" ______________\n / \\\nx __w_x__ j l \n _______ | f_act(I.W + b) |------ output\ny w_y l j\n \\______________/\nWhere:\nx = input x\ny = input y\nb = bias\nf_act = activation function\nI = vector of inputs\nW = vector of weights",
"_____no_output_____"
]
],
[
[
"plotter = pt.PerceptronPlotter2D(data=np.asarray(dataset))",
"_____no_output_____"
],
[
"%matplotlib inline\n_= interact(plotter.plot_interactive, **plotter.controls)",
"_____no_output_____"
]
],
[
[
"## The Delta Rule\nIn the following step we propose to solve the classification problem you defined by using the delta-rule algorithm. Look at the code in compute_delta_w and try to understand it.",
"_____no_output_____"
]
],
[
[
" ______________\n / \\\nx __w_x__ j l \n _______ | f_act(I.W + b) |------ output\ny w_y l j\n \\______________/\nWhere:\nx = input x\ny = input y\nb = bias\nf_act = activation function\nI = vector of inputs\nW = vector of weights",
"_____no_output_____"
]
],
[
[
"$$ neta = (x * w\\_x) + (y * w\\_y) + b$$\n$$ output = f\\_act(neta) $$\n$$ \\Delta w\\_x = \\alpha * (target - output) * f\\_act'(neta) * x $$\n$$ \\Delta w\\_y = \\alpha * (target - output) * f\\_act'(neta) * y $$\n$$ \\Delta b = \\alpha * (target - output) * f\\_act'(neta) $$",
"_____no_output_____"
]
],
[
[
"def compute_delta_w(inputs, weights, bias, targets, alpha, activation_function):\n neta = np.dot(inputs, weights) + bias\n output, d_output = activation_function(neta)\n error = targets - output\n d_w_x = alpha * error * d_output * inputs[:,0]\n d_w_y = alpha * error * d_output * inputs[:,1]\n d_b = alpha * error * d_output\n return [d_w_x, d_w_y, d_b]",
"_____no_output_____"
]
],
[
[
"## Batch learning\nWhen you launch the cell, the weights and the bias are initialized at random, and the algorithm perform some iterations (NUMBER_OF_EPOCHS) doing the following:\n+ for each point in the dataset, compute the modifications ( Δw ) to be done at each parameter in order to minimize the error function\n+ sum up all the modifications -> batch policy\n+ modify the weights and bias of the perceptron\n\nThe cell records the effects of the modifications performed in a video. Therefore, you can visualize the learning process afterwards.",
"_____no_output_____"
]
],
[
[
"%matplotlib inline\n\ninputs = np.asarray(dataset)[:,0:2]\ntargets = np.asarray(dataset)[:,2]\nweights = np.random.normal(size=2)\nbias = np.random.normal(size=1)\nactivation_function = pt.htan\n\nALPHA = 0.1\nNUMBER_OF_EPOCHS = 30\n\nfig = pl.figure(figsize=(12, 4))\nplotter = pt.PerceptronPlotter2D(data=np.asarray(dataset))\nplotter.init_animation()\n\ndef run_epoch_batch(i, alpha, inputs, weights, bias, targets, activation_function):\n d_w_x, d_w_y, d_b = compute_delta_w(inputs, weights, bias, targets, ALPHA, activation_function)\n weights += np.array([np.sum(d_w_x), np.sum(d_w_y)])\n bias += np.sum(d_b)\n \n return plotter.data2animation(i, inputs, weights, bias, targets, activation_function)\n\nSHOW_VIDEO = True # change this flag if you are unable to see the video\nif SHOW_VIDEO:\n anim = animation.FuncAnimation(fig, run_epoch_batch, fargs=(ALPHA, inputs, weights, bias, targets, activation_function), frames=NUMBER_OF_EPOCHS, interval=20, blit=True)\n pt.display_animation(anim)\nelse:\n for i in np.arange(NUMBER_OF_EPOCHS):\n run_epoch_batch(i, ALPHA, inputs, weights, bias, targets, activation_function)",
"MovieWriter ffmpeg unavailable. Trying to use avconv instead.\n/home/hector/miniconda3/envs/t-machle/lib/python3.6/site-packages/matplotlib/__init__.py:886: MatplotlibDeprecationWarning: \nexamples.directory is deprecated; in the future, examples will be found relative to the 'datapath' directory.\n \"found relative to the 'datapath' directory.\".format(key))\n"
]
],
[
[
"## Exercise\nYou are free to modify the learning rate (ALPHA) and the number of iterations (NUMBER_OF_EPOCHS).\n\nTry different 2D classification problems and observe the behaviour of the algorithm in terms of:\n- Learning rate needed\n- Convergence speed\n- Oscillations\n\nBare in mind that, in the current implementation, the parameters (weights and bias) are initialized randomly every time you launch the cell.\n\nCreate dataset as shown and perform the following tests:\n\n1. What happens if the boundaries between both classes are well defined?\n\n\n2. What happens if the classes overlap? What could you say about oscillations in the error signal?\n\n\n3. What happens if it is not possible to separate the classes with a single line? What could you say about local minima?\n\n",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"raw",
"code",
"markdown",
"raw",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"raw"
],
[
"code",
"code"
],
[
"markdown"
],
[
"raw"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
]
] |
e7baa6d865082eac611447c92f2d966dedca1fec | 12,843 | ipynb | Jupyter Notebook | notebooks/Playing TextWorld generated games with OpenAI Gym.ipynb | zhaozj89/TextWorld | c6b626af9e5fbd3bc03e98cbf154f85bfc365373 | [
"MIT"
] | 307 | 2019-05-07T01:51:55.000Z | 2022-03-31T19:35:47.000Z | notebooks/Playing TextWorld generated games with OpenAI Gym.ipynb | zhaozj89/TextWorld | c6b626af9e5fbd3bc03e98cbf154f85bfc365373 | [
"MIT"
] | 84 | 2019-05-08T14:24:36.000Z | 2022-03-31T14:35:16.000Z | notebooks/Playing TextWorld generated games with OpenAI Gym.ipynb | zhaozj89/TextWorld | c6b626af9e5fbd3bc03e98cbf154f85bfc365373 | [
"MIT"
] | 70 | 2019-05-21T21:36:56.000Z | 2022-02-28T12:04:27.000Z | 37.662757 | 423 | 0.453944 | [
[
[
"# Playing TextWorld generated games with OpenAI Gym\nThis tutorial shows how to play a text-based adventure game **generated by TextWorld** using OpenAI's Gym API.",
"_____no_output_____"
],
[
"### Generate a new TextWorld game",
"_____no_output_____"
]
],
[
[
"!tw-make custom --world-size 2 --quest-length 3 --nb-objects 10 --output tw_games/game.ulx -f -v --seed 1234",
"Global seed: 1234\nGame generated: tw_games/game.ulx\nWelcome to another fast paced game of TextWorld! Here is your task for today. First, it would be great if you could try to go east. With that accomplished, take the shoe that's in the attic. With the shoe, place the shoe on the shelf. Alright, thanks!\n"
]
],
[
[
"### Register the game with Gym\nIn order to call to `gym.make`, we need to create a valid `env_id` for our game.",
"_____no_output_____"
]
],
[
[
"import textworld.gym\nenv_id = textworld.gym.register_game('tw_games/game.ulx')",
"_____no_output_____"
]
],
[
[
"### Make the gym environment\nWith our `env_id` we are ready to use gym to start the new environment.",
"_____no_output_____"
]
],
[
[
"import gym\nenv = gym.make(env_id)",
"_____no_output_____"
]
],
[
[
"### Start the game\nLike for other Gym environments, we start a new game by calling the `env.reset` method. It returns the initial observation text string as well as a dictionary for additional informations (more on that later).",
"_____no_output_____"
]
],
[
[
"obs, infos = env.reset()\nprint(obs)",
"\n\n\n ________ ________ __ __ ________ \n | \\| \\| \\ | \\| \\ \n \\$$$$$$$$| $$$$$$$$| $$ | $$ \\$$$$$$$$ \n | $$ | $$__ \\$$\\/ $$ | $$ \n | $$ | $$ \\ >$$ $$ | $$ \n | $$ | $$$$$ / $$$$\\ | $$ \n | $$ | $$_____ | $$ \\$$\\ | $$ \n | $$ | $$ \\| $$ | $$ | $$ \n \\$$ \\$$$$$$$$ \\$$ \\$$ \\$$ \n __ __ ______ _______ __ _______ \n | \\ _ | \\ / \\ | \\ | \\ | \\ \n | $$ / \\ | $$| $$$$$$\\| $$$$$$$\\| $$ | $$$$$$$\\\n | $$/ $\\| $$| $$ | $$| $$__| $$| $$ | $$ | $$\n | $$ $$$\\ $$| $$ | $$| $$ $$| $$ | $$ | $$\n | $$ $$\\$$\\$$| $$ | $$| $$$$$$$\\| $$ | $$ | $$\n | $$$$ \\$$$$| $$__/ $$| $$ | $$| $$_____ | $$__/ $$\n | $$$ \\$$$ \\$$ $$| $$ | $$| $$ \\| $$ $$\n \\$$ \\$$ \\$$$$$$ \\$$ \\$$ \\$$$$$$$$ \\$$$$$$$ \n\nWelcome to another fast paced game of TextWorld! Here is your task for today. First, it would be great if you could try to go east. With that accomplished, take the shoe that's in the attic. With the shoe, place the shoe on the shelf. Alright, thanks!\n\n-= Scullery =-\nYou've just shown up in a scullery.\n\nYou make out a Canadian style box. If you haven't noticed it already, there seems to be something there by the wall, it's a board. The board appears to be empty. What, you think everything in TextWorld should have stuff on it? You bend down to tie your shoe. When you stand up, you notice a bowl. But there isn't a thing on it. This always happens!\n\nYou don't like doors? Why not try going east, that entranceway is unguarded.\n\n\n\n\n"
]
],
[
[
"### Interact with the game\nThe `env.step` method is used to send text command to the game. This method returns the observation for the new state, the current game score, whether the game is done, and dictionary for additional informations (more on that later).",
"_____no_output_____"
]
],
[
[
"obs, score, done, infos = env.step(\"open box\")\nprint(obs)",
"You have to unlock the Canadian style box with the Canadian style key first.\n\n\n"
]
],
[
[
"### Make a simple play loop\nWe now have everything we need in order to interactively play a text-based game.",
"_____no_output_____"
]
],
[
[
"try:\n done = False\n obs, _ = env.reset()\n print(obs)\n nb_moves = 0\n while not done:\n command = input(\"> \")\n obs, score, done, _ = env.step(command)\n print(obs)\n nb_moves += 1\n\nexcept KeyboardInterrupt:\n pass # Press the stop button in the toolbar to quit the game.\n\nprint(\"Played {} steps, scoring {} points.\".format(nb_moves, score))",
"\n\n\n ________ ________ __ __ ________ \n | \\| \\| \\ | \\| \\ \n \\$$$$$$$$| $$$$$$$$| $$ | $$ \\$$$$$$$$ \n | $$ | $$__ \\$$\\/ $$ | $$ \n | $$ | $$ \\ >$$ $$ | $$ \n | $$ | $$$$$ / $$$$\\ | $$ \n | $$ | $$_____ | $$ \\$$\\ | $$ \n | $$ | $$ \\| $$ | $$ | $$ \n \\$$ \\$$$$$$$$ \\$$ \\$$ \\$$ \n __ __ ______ _______ __ _______ \n | \\ _ | \\ / \\ | \\ | \\ | \\ \n | $$ / \\ | $$| $$$$$$\\| $$$$$$$\\| $$ | $$$$$$$\\\n | $$/ $\\| $$| $$ | $$| $$__| $$| $$ | $$ | $$\n | $$ $$$\\ $$| $$ | $$| $$ $$| $$ | $$ | $$\n | $$ $$\\$$\\$$| $$ | $$| $$$$$$$\\| $$ | $$ | $$\n | $$$$ \\$$$$| $$__/ $$| $$ | $$| $$_____ | $$__/ $$\n | $$$ \\$$$ \\$$ $$| $$ | $$| $$ \\| $$ $$\n \\$$ \\$$ \\$$$$$$ \\$$ \\$$ \\$$$$$$$$ \\$$$$$$$ \n\nWelcome to another fast paced game of TextWorld! Here is your task for today. First, it would be great if you could try to go east. With that accomplished, take the shoe that's in the attic. With the shoe, place the shoe on the shelf. Alright, thanks!\n\n-= Scullery =-\nYou've just shown up in a scullery.\n\nYou make out a Canadian style box. If you haven't noticed it already, there seems to be something there by the wall, it's a board. The board appears to be empty. What, you think everything in TextWorld should have stuff on it? You bend down to tie your shoe. When you stand up, you notice a bowl. But there isn't a thing on it. This always happens!\n\nYou don't like doors? Why not try going east, that entranceway is unguarded.\n\n\n\n\n> help\nAvailable commands:\n look: describe the current room\n goal: print the goal of this game\n inventory: print player's inventory\n go <dir>: move the player north, east, south or west\n examine ...: examine something more closely\n eat ...: eat edible food\n open ...: open a door or a container\n close ...: close a door or a container\n drop ...: drop an object on the floor\n take ...: take an object that is on the floor\n put ... on ...: place an object on a supporter\n take ... from ...: take an object from a container or a supporter\n insert ... into ...: place an object into a container\n lock ... with ...: lock a door or a container with a key\n unlock ... with ...: unlock a door or a container with a key\n\n\n\nPlayed 1 steps, scoring 0 points.\n"
]
],
[
[
"## Request additional information\n<span style=\"color:red\">_*Only available for games generated with TextWorld._</span>\n\nTo ease the learning process of AI agents, TextWorld offers control over what information should be available alongside the game's narrative (i.e. the observation).\n\nLet's request the list of __admissible__ commands (i.e. commands that are guaranteed to be understood by the game interpreter) for every game state. We will also request the list of entity names that can be interacted with. For the complete list of information that can be requested, see the [documentation](https://textworld.readthedocs.io/en/latest/_modules/textworld/envs/wrappers/filter.html?highlight=envinfos)).",
"_____no_output_____"
]
],
[
[
"import textworld\nrequest_infos = textworld.EnvInfos(admissible_commands=True, entities=True)\n\n# Requesting additional information should be done when registering the game.\nenv_id = textworld.gym.register_game('tw_games/game.ulx', request_infos)\nenv = gym.make(env_id)\n\nobs, infos = env.reset()\nprint(\"Entities: {}\".format(infos[\"entities\"]))\nprint(\"Admissible commands:\\n {}\".format(\"\\n \".join(infos[\"admissible_commands\"])))",
"Entities: ['safe', 'Canadian style box', 'shoe', 'cane', 'shelf', 'workbench', 'board', 'bowl', 'Canadian style key', 'book', 'fork', 'pair of pants', 'north', 'south', 'east', 'west']\nAdmissible commands:\n drop Canadian style key\n drop book\n drop fork\n examine Canadian style box\n examine Canadian style key\n examine board\n examine book\n examine bowl\n examine fork\n go east\n inventory\n look\n put Canadian style key on board\n put Canadian style key on bowl\n put book on board\n put book on bowl\n put fork on board\n put fork on bowl\n unlock Canadian style box with Canadian style key\n"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7bab393453e06da95b4aac91cea32ce974b5fde | 39,863 | ipynb | Jupyter Notebook | 2-Even Fibonacci numbers(right).ipynb | vss-py/TDD-ProjectEuler | cc8324f6a7ffa4b734739f5ebc31117f0fc75c4b | [
"MIT"
] | null | null | null | 2-Even Fibonacci numbers(right).ipynb | vss-py/TDD-ProjectEuler | cc8324f6a7ffa4b734739f5ebc31117f0fc75c4b | [
"MIT"
] | null | null | null | 2-Even Fibonacci numbers(right).ipynb | vss-py/TDD-ProjectEuler | cc8324f6a7ffa4b734739f5ebc31117f0fc75c4b | [
"MIT"
] | null | null | null | 32.382616 | 1,287 | 0.486115 | [
[
[
"* # Problem 2 - Even Fibonacci numbers\n \n Each new term in the Fibonacci sequence is generated by adding the previous two terms. By starting with 1 and 2, the first 10 terms will be:\n1, 2, 3, 5, 8, 13, 21, 34, 55, 89, ...\n By considering the terms in the Fibonacci sequence whose values do not exceed four million, find the sum of the even-valued terms.",
"_____no_output_____"
],
[
"### Let's break the trouble in more steps, at least meanwhile:\n* Find the fibonacci term;\n* Check if this term is even;\n* If the term is even, append this in one list;\n* Set the interval of the list;\n* Find the sum of all members of this list.",
"_____no_output_____"
],
[
"* ### At first, we must remember the Fibonacci algorithm:\na(n) = a(n-2) + a(n-1)\nTherefore, we must to apply it.",
"_____no_output_____"
]
],
[
[
"if __name__ == \"__main__\":\n assert fibonacci(10) == 89\n\"\"\"Let's start with the 10th fibonacci term\"\"\"",
"_____no_output_____"
],
[
"def fibonacci(n):\n return 89\n\nif __name__ == \"__main__\":\n assert fibonacci(10) == 89\n #assert fibonacci(9) == 55",
"_____no_output_____"
],
[
"def fibonacci(n):\n if n == 9:\n return 55\n return 89\n\nif __name__ == \"__main__\":\n assert fibonacci(10) == 89\n assert fibonacci(9) == 55",
"_____no_output_____"
]
],
[
[
"##### But if I do this way, I probabilly having a problem to apply the fibonacci algorithm,\nso, let's begin with the ascending order ",
"_____no_output_____"
]
],
[
[
"def fibonacci(n):\n return 1\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1",
"_____no_output_____"
],
[
"def fibonacci(n):\n return 1\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2",
"_____no_output_____"
],
[
"def fibonacci(n):\n if n == 2:\n return 2\n return 1\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n ",
"_____no_output_____"
],
[
"def fibonacci(n):\n if n == 2:\n return n#refactoring\n return 1\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n #assert fibonacci(3) == 3",
"_____no_output_____"
],
[
"def fibonacci(n):\n if n == 2:\n return n\n return 1\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n\"\"\"Let's use the fact the 3 is the sum of the 2 and 1, that is, the two first numbers\nin the growing order\"\"\"",
"_____no_output_____"
],
[
"def fibonacci(n):\n if n > 2:\n n = (n-2) + (n-1)\n return n\n if n == 2:\n return n\n return 1\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n\"\"\"Now, we can check the 4th and any other terms\"\"\"",
"_____no_output_____"
],
[
"def fibonacci(n):\n if n > 2:\n n = (n-2) + (n-1)\n return n\n if n == 2:\n return n\n return 1\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n",
"_____no_output_____"
],
[
"fibonacci(5)",
"_____no_output_____"
]
],
[
[
"### What the code is doing is: n = (5 - 2) + (5 - 1).\nTherefore we must to apply a repetition.",
"_____no_output_____"
]
],
[
[
"def fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 0\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 2:\n return n\n return 1\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n",
"_____no_output_____"
],
[
"fibonacci(5)\n\"\"\"It is happening because I set i == 1, \nbut the condition is applied when n > 2, \ntherefore I should to set i == 2\"\"\"",
"_____no_output_____"
],
[
"def fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 2\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 2:\n return n\n return 1\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n",
"_____no_output_____"
],
[
"def fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 2\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 2:\n return n\n return 1\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n assert fibonacci(6) == 13\n assert fibonacci(7) == 21\n assert fibonacci(8) == 34\n assert fibonacci(9) == 55\n assert fibonacci(10) == 89\n\"\"\"The first step is done\"\"\"",
"_____no_output_____"
]
],
[
[
"### * Now, I must to find the list (the second step)\n\nAs I am using the TDD, I can do this without a problem. ",
"_____no_output_____"
]
],
[
[
"def fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 2\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 2:\n return n\n return 1\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n assert fibonacci(6) == 13\n assert fibonacci(7) == 21\n assert fibonacci(8) == 34\n assert fibonacci(9) == 55\n assert fibonacci(10) == 89\n assert fibonacci_list(1) == [1]",
"_____no_output_____"
],
[
"def fibonacci_list(l):\n lista = []\n i = 1\n while i < l:\n a = fibonacci(i)\n lista.append(a)\n i = i + 1\n return lista\ndef fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 2\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 2:\n return n\n return 1\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n assert fibonacci(6) == 13\n assert fibonacci(7) == 21\n assert fibonacci(8) == 34\n assert fibonacci(9) == 55\n assert fibonacci(10) == 89\n assert fibonacci_list(1) == [1]",
"_____no_output_____"
],
[
"#Let's check\nfibonacci_list(1)",
"_____no_output_____"
],
[
"def fibonacci_list(l):\n lista = []\n i = 0\n while i < l:\n a = fibonacci(i)\n lista.append(a)\n i = i + 1\n return lista\ndef fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 2\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 2:\n return n\n return 1\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n assert fibonacci(6) == 13\n assert fibonacci(7) == 21\n assert fibonacci(8) == 34\n assert fibonacci(9) == 55\n assert fibonacci(10) == 89\n assert fibonacci_list(1) == [1]\n assert fibonacci_list(2) == [1, 2]",
"_____no_output_____"
],
[
"\"\"\"Now I can do the test with the first ten terms\"\"\"",
"_____no_output_____"
],
[
"fibonacci_list(6)\n\"\"\"I must to avoid this repetition of the first term\"\"\"",
"_____no_output_____"
],
[
"fibonacci_list(6)",
"_____no_output_____"
]
],
[
[
"The repetition of the first term occurs because I don't set what the function should to do if n < 2.",
"_____no_output_____"
]
],
[
[
"def fibonacci_list(l):\n lista = []\n i = 1\n while i < l:\n a = fibonacci(i)\n lista.append(a)\n i = i + 1\n return lista\ndef fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 2\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 1 or n == 2:\n return n\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n assert fibonacci(6) == 13\n assert fibonacci(7) == 21\n assert fibonacci(8) == 34\n assert fibonacci(9) == 55\n assert fibonacci(10) == 89\n assert fibonacci_list(1) == [1]\n assert fibonacci_list(2) == [1, 2]",
"_____no_output_____"
],
[
"print(fibonacci_list(0))\nprint(fibonacci_list(1))\nprint(fibonacci_list(2))",
"[]\n[]\n[1]\n"
],
[
"fibonacci_list(5)",
"_____no_output_____"
],
[
"def fibonacci_list(l):\n lista = []\n i = 1\n while i < l:\n a = fibonacci(i)\n lista.append(a)\n i = i + 1\n return lista\ndef fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 2\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 1 or n == 2:\n return n\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n assert fibonacci(6) == 13\n assert fibonacci(7) == 21\n assert fibonacci(8) == 34\n assert fibonacci(9) == 55\n assert fibonacci(10) == 89\n assert fibonacci_list(1) == []\n assert fibonacci_list(2) == [1]\n assert fibonacci_list(3) == [1, 2]",
"_____no_output_____"
]
],
[
[
"### Now, I must to apply the condition that I miss. To append in the list only the even terms.",
"_____no_output_____"
]
],
[
[
"def fibonacci_list(l):\n lista = []\n i = 1\n while i < l:\n a = fibonacci(i)\n if a % 2 == 0:\n lista.append(a)\n i = i + 1\n else:\n i = i + 1\n return lista\ndef fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 2\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 1 or n == 2:\n return n\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n assert fibonacci(6) == 13\n assert fibonacci(7) == 21\n assert fibonacci(8) == 34\n assert fibonacci(9) == 55\n assert fibonacci(10) == 89\n assert fibonacci_list(1) == []\n assert fibonacci_list(2) == [2]\n #assert fibonacci_list(3) == [1, 2]",
"_____no_output_____"
],
[
"fibonacci_list(10)",
"_____no_output_____"
],
[
"fibonacci_list(3)\n\"\"\"As 0 is a order of the first term, the first even term is showed in the 3tr member\"\"\"",
"_____no_output_____"
],
[
"def fibonacci_list(l):\n lista = []\n i = 1\n while i < l:\n a = fibonacci(i)\n if a % 2 == 0:\n lista.append(a)\n i = i + 1\n else:\n i = i + 1\n return lista\ndef fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 2\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 1 or n == 2:\n return n\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n assert fibonacci(6) == 13\n assert fibonacci(7) == 21\n assert fibonacci(8) == 34\n assert fibonacci(9) == 55\n assert fibonacci(10) == 89\n assert fibonacci_list(1) == []\n assert fibonacci_list(3) == [2]\n",
"_____no_output_____"
]
],
[
[
"#### Now, for the next step, I must to set a interval condition. In the statement, the condition is \"the values not exced 4 milions\".",
"_____no_output_____"
]
],
[
[
"def fibonacci_list_interval(m):\n lista = []\n i = 1\n while True:\n a = fibonacci(i)\n if a > m:\n print('The last term is: ' + str(a))\n break\n if a % 2 == 0:\n lista.append(a)\n i = i + 1\n else:\n i = i + 1\n return lista\n\n\ndef fibonacci_list(l):\n lista = []\n i = 1\n while i < l:\n a = fibonacci(i)\n if a % 2 == 0:\n lista.append(a)\n i = i + 1\n else:\n i = i + 1\n return lista\ndef fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 2\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 1 or n == 2:\n return n\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n assert fibonacci(6) == 13\n assert fibonacci(7) == 21\n assert fibonacci(8) == 34\n assert fibonacci(9) == 55\n assert fibonacci(10) == 89\n assert fibonacci_list(1) == []\n assert fibonacci_list(3) == [2]",
"_____no_output_____"
],
[
"fibonacci_list_interval(50)",
"The last term is: 55\n"
],
[
"fibonacci_list_interval(4000000)",
"The last term is: 5702887\n"
]
],
[
[
"#### I don't know how can I make first a test in this case, but how the code was developed, I see no one problem to do this way. Whatever, the new function is just the previous function with same changes. ",
"_____no_output_____"
],
[
"To return the sum of even numbers of the fibonacci list, I must to do only this:",
"_____no_output_____"
]
],
[
[
"def fibonacci_list_interval(m):\n lista = []\n i = 1\n while True:\n a = fibonacci(i)\n if a > m:\n print('The last term is: ' + str(a))\n break\n if a % 2 == 0:\n lista.append(a)\n i = i + 1\n else:\n i = i + 1\n return sum(lista)\n\n\ndef fibonacci_list(l):\n lista = []\n i = 1\n while i < l:\n a = fibonacci(i)\n if a % 2 == 0:\n lista.append(a)\n i = i + 1\n else:\n i = i + 1\n return lista\ndef fibonacci(n):\n a = 1\n b = 2\n c = 0\n i = 2\n if n > 2:\n while i < n:\n c = a + b\n a = b\n b = c\n i = i + 1\n return c\n if n == 1 or n == 2:\n return n\n\nif __name__ == '__main__':\n assert fibonacci(1) == 1\n assert fibonacci(2) == 2\n assert fibonacci(3) == 3\n assert fibonacci(4) == 5\n assert fibonacci(5) == 8\n assert fibonacci(6) == 13\n assert fibonacci(7) == 21\n assert fibonacci(8) == 34\n assert fibonacci(9) == 55\n assert fibonacci(10) == 89\n assert fibonacci_list(1) == []\n assert fibonacci_list(3) == [2]",
"_____no_output_____"
],
[
"fibonacci_list_interval(4000000)",
"The last term is: 5702887\n"
]
],
[
[
"#### Now, I just need to refactor my code to get this more simply. In my opinion, in the way that we can be able to use the test is possible to see the code growing up, and so we can better set where and when we need to change. For my own learning I thinked it is amazing. ",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown",
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
]
] |
e7baba45ea2fc41ee94d42da85990f0e1d164372 | 82,015 | ipynb | Jupyter Notebook | modules/module_12/part3/03_02_begin/03_02_begin.ipynb | ryanjmccall/sb_ml_eng_capstone | dfa87dcbd741c6f502b6cd0eb8f31203568c09a2 | [
"MIT"
] | null | null | null | modules/module_12/part3/03_02_begin/03_02_begin.ipynb | ryanjmccall/sb_ml_eng_capstone | dfa87dcbd741c6f502b6cd0eb8f31203568c09a2 | [
"MIT"
] | null | null | null | modules/module_12/part3/03_02_begin/03_02_begin.ipynb | ryanjmccall/sb_ml_eng_capstone | dfa87dcbd741c6f502b6cd0eb8f31203568c09a2 | [
"MIT"
] | null | null | null | 270.676568 | 75,200 | 0.920307 | [
[
[
"# Chapter 3 - Regression Models\n## Segment 2 - Multiple linear regression",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport pandas as pd\n\nimport matplotlib.pyplot as plt\nfrom pylab import rcParams\n\nimport sklearn\nfrom sklearn.linear_model import LinearRegression\nfrom sklearn.preprocessing import scale",
"_____no_output_____"
],
[
"%matplotlib inline\nrcParams['figure.figsize'] = 5, 4",
"_____no_output_____"
],
[
"import seaborn as sb\nsb.set_style('whitegrid')\nfrom collections import Counter",
"_____no_output_____"
]
],
[
[
"#### (Multiple) linear regression on the enrollment data",
"_____no_output_____"
]
],
[
[
"address = 'C:/Users/Lillian/Desktop/ExerciseFiles/Data/enrollment_forecast.csv'\n\nenroll = pd.read_csv(address)\nenroll.columns = ['year', 'roll', 'unem', 'hgrad', 'inc']\nenroll.head()",
"_____no_output_____"
],
[
"sb.pairplot(enroll)",
"_____no_output_____"
],
[
"print(enroll.corr())",
" year roll unem hgrad inc\nyear 1.000000 0.900934 0.378305 0.670300 0.944287\nroll 0.900934 1.000000 0.391344 0.890294 0.949876\nunem 0.378305 0.391344 1.000000 0.177376 0.282310\nhgrad 0.670300 0.890294 0.177376 1.000000 0.820089\ninc 0.944287 0.949876 0.282310 0.820089 1.000000\n"
],
[
"enroll_data = enroll[['unem', 'hgrad']].values\n\nenroll_target = enroll[['roll']].values\n\nenroll_data_names = ['unem', 'hgrad']\n\nX, y = scale(enroll_data), enroll_target",
"_____no_output_____"
]
],
[
[
"### Checking for missing values",
"_____no_output_____"
]
],
[
[
"missing_values = X==np.NAN\nX[missing_values == True]",
"_____no_output_____"
],
[
"LinReg = LinearRegression(normalize=True)\n\nLinReg.fit(X, y)\n\nprint(LinReg.score(X, y))",
"0.8488812666133723\n"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
]
] |
e7babbea26c1f0ca7f40aae5484d6074fb3e995a | 14,450 | ipynb | Jupyter Notebook | section_kalman_filter/kf4.ipynb | harukary/LNPR_BOOK_CODES | 97752f8c415b6006fcb8e25f919cd821c66101c4 | [
"MIT"
] | null | null | null | section_kalman_filter/kf4.ipynb | harukary/LNPR_BOOK_CODES | 97752f8c415b6006fcb8e25f919cd821c66101c4 | [
"MIT"
] | null | null | null | section_kalman_filter/kf4.ipynb | harukary/LNPR_BOOK_CODES | 97752f8c415b6006fcb8e25f919cd821c66101c4 | [
"MIT"
] | null | null | null | 74.870466 | 6,974 | 0.729273 | [
[
[
"import sys\nsys.path.append('../scripts/')\nfrom mcl import *\nfrom scipy.stats import multivariate_normal\nfrom matplotlib.patches import Ellipse\nfrom matplotlib.patches import Ellipse\n%matplotlib widget",
"_____no_output_____"
],
[
"def sigma_ellipse(p, cov, n):\n eig_vals, eig_vec = np.linalg.eig(cov)\n ang = math.atan2(eig_vec[:,0][1], eig_vec[:,0][0])/math.pi*180\n return Ellipse(p, width=2*n*math.sqrt(eig_vals[0]),height=2*n*math.sqrt(eig_vals[1]), angle=ang, fill=False, color=\"blue\", alpha=0.5)\n\ndef matM(nu, omega, time, stds):\n return np.diag([stds[\"nn\"]**2*abs(nu)/time + stds[\"no\"]**2*abs(omega)/time, \n stds[\"on\"]**2*abs(nu)/time + stds[\"oo\"]**2*abs(omega)/time])\n \ndef matA(nu, omega, time, theta):\n st, ct = math.sin(theta), math.cos(theta)\n stw, ctw = math.sin(theta + omega*time), math.cos(theta + omega*time)\n return np.array([[(stw - st)/omega, -nu/(omega**2)*(stw - st) + nu/omega*time*ctw],\n [(-ctw + ct)/omega, -nu/(omega**2)*(-ctw + ct) + nu/omega*time*stw],\n [0, time]] )\n\ndef matF(nu, omega, time, theta):\n F = np.diag([1.0, 1.0, 1.0])\n F[0, 2] = nu / omega * (math.cos(theta + omega * time) - math.cos(theta))\n F[1, 2] = nu / omega * (math.sin(theta + omega * time) - math.sin(theta))\n return F\n\ndef matH(pose, landmark_pos): ###kf4funcs\n mx, my = landmark_pos\n mux, muy, mut = pose\n q = (mux - mx)**2 + (muy - my)**2\n return np.array([[(mux - mx)/np.sqrt(q), (muy - my)/np.sqrt(q), 0.0], [(my - muy)/q, (mux - mx)/q, -1.0]])\n\ndef matQ(distance_dev, direction_dev):\n return np.diag(np.array([distance_dev**2, direction_dev**2]))",
"_____no_output_____"
],
[
"class KalmanFilter: ###kf4init\n def __init__(self, envmap, init_pose, motion_noise_stds={\"nn\":0.19, \"no\":0.001, \"on\":0.13, \"oo\":0.2}, \\\n distance_dev_rate=0.14, direction_dev=0.05): #変数追加\n self.belief = multivariate_normal(mean=init_pose, cov=np.diag([1e-10, 1e-10, 1e-10])) \n self.pose = self.belief.mean\n self.motion_noise_stds = motion_noise_stds\n self.map = envmap #以下3行追加(Mclと同じ)\n self.distance_dev_rate = distance_dev_rate\n self.direction_dev = direction_dev\n \n def observation_update(self, observation): #追加\n for d in observation:\n z = d[0]\n obs_id = d[1]\n \n H = matH(self.belief.mean, self.map.landmarks[obs_id].pos)\n estimated_z = IdealCamera.observation_function(self.belief.mean, self.map.landmarks[obs_id].pos)\n Q = matQ(estimated_z[0]*self.distance_dev_rate, self.direction_dev)\n K = self.belief.cov.dot(H.T).dot(np.linalg.inv(Q + H.dot(self.belief.cov).dot(H.T)))\n self.belief.mean += K.dot(z - estimated_z)\n self.belief.cov = (np.eye(3) - K.dot(H)).dot(self.belief.cov)\n self.pose = self.belief.mean\n \n def motion_update(self, nu, omega, time): #追加\n if abs(omega) < 1e-5: omega = 1e-5 #値が0になるとゼロ割りになって計算ができないのでわずかに値を持たせる\n\n M = matM(nu, omega, time, self.motion_noise_stds)\n A = matA(nu, omega, time, self.belief.mean[2])\n F = matF(nu, omega, time, self.belief.mean[2])\n self.belief.cov = F.dot(self.belief.cov).dot(F.T) + A.dot(M).dot(A.T)\n self.belief.mean = IdealRobot.state_transition(nu, omega, time, self.belief.mean)\n self.pose = self.belief.mean #他のクラスで使う\n \n def draw(self, ax, elems):\n ###xy平面上の誤差の3シグマ範囲###\n e = sigma_ellipse(self.belief.mean[0:2], self.belief.cov[0:2, 0:2], 3)\n elems.append(ax.add_patch(e))\n\n ###θ方向の誤差の3シグマ範囲###\n x, y, c = self.belief.mean\n sigma3 = math.sqrt(self.belief.cov[2, 2])*3\n xs = [x + math.cos(c-sigma3), x, x + math.cos(c+sigma3)]\n ys = [y + math.sin(c-sigma3), y, y + math.sin(c+sigma3)]\n elems += ax.plot(xs, ys, color=\"blue\", alpha=0.5)",
"_____no_output_____"
],
[
"if __name__ == '__main__': \n time_interval = 0.1\n world = World(30, time_interval, debug=False) \n\n ### 地図を生成して3つランドマークを追加 ###\n m = Map() \n m.append_landmark(Landmark(-4,2))\n m.append_landmark(Landmark(2,-3))\n m.append_landmark(Landmark(3,3))\n world.append(m) \n\n ### ロボットを作る ###\n initial_pose = np.array([0, 0, 0]).T\n kf = KalmanFilter(m, initial_pose)\n circling = EstimationAgent(time_interval, 0.2, 10.0/180*math.pi, kf)\n r = Robot(initial_pose, sensor=Camera(m), agent=circling, color=\"red\")\n world.append(r)\n \n kf = KalmanFilter(m, initial_pose)\n linear = EstimationAgent(time_interval, 0.1, 0.0, kf)\n r = Robot(initial_pose, sensor=Camera(m), agent=linear, color=\"red\")\n world.append(r)\n \n kf = KalmanFilter(m, initial_pose)\n right = EstimationAgent(time_interval, 0.1, -3.0/180*math.pi, kf)\n r = Robot(initial_pose, sensor=Camera(m), agent=right, color=\"red\")\n world.append(r)\n\n world.draw()",
"_____no_output_____"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code"
]
] |
e7bac205acf76da5e73be5165d9283d7a6b1ec18 | 588,511 | ipynb | Jupyter Notebook | batadal/Task3_Discrete.ipynb | imchengh/Cyber-Data-Analytics | 7595886e3239106a537c7f4e7902c65346a8dfa3 | [
"MIT"
] | null | null | null | batadal/Task3_Discrete.ipynb | imchengh/Cyber-Data-Analytics | 7595886e3239106a537c7f4e7902c65346a8dfa3 | [
"MIT"
] | null | null | null | batadal/Task3_Discrete.ipynb | imchengh/Cyber-Data-Analytics | 7595886e3239106a537c7f4e7902c65346a8dfa3 | [
"MIT"
] | null | null | null | 260.980488 | 30,384 | 0.92193 | [
[
[
"# Discrete Models Task",
"_____no_output_____"
],
[
"### 1) Data Preparation",
"_____no_output_____"
],
[
"Installing 'tslearn' - only run if not installed previously (if necessary, for more information see documentation of the package at https://tslearn.readthedocs.io/en/latest/index.html?highlight=install",
"_____no_output_____"
]
],
[
[
"##!pip install tslearn",
"_____no_output_____"
]
],
[
[
"Importing packages",
"_____no_output_____"
]
],
[
[
"import datetime\nimport pandas as pd\nfrom pandas import Series\nfrom pandas import DataFrame\nfrom pandas import concat\nimport numpy as np\nimport time, datetime\nimport matplotlib.pyplot as plt\nimport sys\nfrom tslearn.generators import random_walks\nfrom tslearn.preprocessing import TimeSeriesScalerMeanVariance\nfrom tslearn.piecewise import PiecewiseAggregateApproximation\nfrom tslearn.piecewise import SymbolicAggregateApproximation, OneD_SymbolicAggregateApproximation\nimport string\nfrom collections import Counter\nfrom nltk import ngrams\nimport itertools\nimport random\nfrom matplotlib.collections import LineCollection\nfrom matplotlib.colors import ListedColormap, BoundaryNorm\nfrom sklearn.metrics import confusion_matrix\nimport seaborn as sns\nfrom sklearn.datasets import make_classification \nfrom sklearn.neighbors import KNeighborsClassifier \nfrom sklearn.ensemble import RandomForestClassifier \nfrom sklearn.model_selection import train_test_split \nfrom sklearn.metrics import roc_curve\nfrom sklearn.metrics import roc_auc_score\nfrom sklearn.metrics import precision_recall_curve\nfrom sklearn.metrics import fbeta_score\nfrom sklearn.preprocessing import MinMaxScaler\nfrom sklearn import preprocessing",
"_____no_output_____"
]
],
[
[
"Reading the data, converting the time to timestamp and indexing the date to use it as a time series",
"_____no_output_____"
]
],
[
[
"#Data Paths\nDATA_PATH = sys.path[0]+\"\\\\data\\\\\"\nfilename_train1=\"BATADAL_dataset03.csv\"\nfilename_train2=\"BATADAL_dataset04.csv\"\nfilename_test=\"BATADAL_test_dataset.csv\"\n\n#Reading the data\ndftrain1 = pd.read_csv(DATA_PATH + filename_train1)\ndftrain2 = pd.read_csv(DATA_PATH + filename_train2)\ndftest = pd.read_csv(DATA_PATH + filename_test)\n\n# Modify string date to timestamp\ndftrain1.DATETIME = dftrain1.DATETIME.apply(lambda s: pd.to_datetime(s,format='%d/%m/%y %H'))\ndftrain2.DATETIME = dftrain2.DATETIME.apply(lambda s: pd.to_datetime(s,format='%d/%m/%y %H'))\ndftest.DATETIME = dftest.DATETIME.apply(lambda s: pd.to_datetime(s,format='%d/%m/%y %H'))\n\n# Choosing 0 if not an anomaly and 1 if anomaly\n#dftrain2[' ATT_FLAG']=dftrain2[' ATT_FLAG'].apply(lambda x: 0 if x==-999 else x)\n\n# Indexing\ndftrain1 = dftrain1.set_index('DATETIME')\ndftrain2 = dftrain2.set_index('DATETIME')\ndftest = dftest.set_index('DATETIME')\n\n#dftrain1.shift(1)",
"_____no_output_____"
]
],
[
[
"### Scaling the data to normal distribution with mean zero and standard deviation",
"_____no_output_____"
]
],
[
[
"#Obs: some values will be converted to float\ndftrain1_original=dftrain1\ndftrain2_original=dftrain2\ndftest_original=dftest\n#Dftrain1\ndftrain1.iloc[:,range(0,31)]=preprocessing.scale(dftrain1.iloc[:,range(0,31)])\n#Dftrain2\ndftrain2.iloc[:,range(0,31)]=preprocessing.scale(dftrain2.iloc[:,range(0,31)])\n#Dftest\ndftest.iloc[:,range(0,31)]=preprocessing.scale(dftest.iloc[:,range(0,31)])",
"C:\\Users\\pvbia\\Anaconda3\\lib\\site-packages\\ipykernel_launcher.py:6: DataConversionWarning: Data with input dtype int64, float64 were all converted to float64 by the scale function.\n \nC:\\Users\\pvbia\\Anaconda3\\lib\\site-packages\\ipykernel_launcher.py:10: DataConversionWarning: Data with input dtype int64, float64 were all converted to float64 by the scale function.\n # Remove the CWD from sys.path while we load stuff.\n"
]
],
[
[
"### Labeling the attacks on dftrain2 according to the information below\n\n###### For L_T7\n13/09/2016 23 - 16/09/2016 00 |\n26/09/2016 11 - 27/09/2016 10\n###### For F_PU10 and F_PU11\n26/09/2016 11 - 27/09/2016 10\n###### For L_T1\n09/10/2016 9 - 11/10/2016 20 |\n29/10/2016 19 - 02/11/2016 16 |\n14/12/2016 15 - 19/12/2016 04\n###### For F_PU1 and F_PU2\n29/10/2016 19 - 02/11/2016 16 |\n14/12/2016 15 - 19/12/2016 04\n###### For L_T4\n26/11/2016 17 - 29/11/2016 04",
"_____no_output_____"
]
],
[
[
"#L_T7\ndftrain2_L_T7=dftrain2.loc[:,[' L_T7',' ATT_FLAG']]\ndftrain2_L_T7.loc[:,' ATT_FLAG']=0\ndftrain2_L_T7.loc['2016-09-13 23':'2016-09-16 00',' ATT_FLAG']=1\ndftrain2_L_T7.loc['2016-09-26 11':'2016-09-27 10',' ATT_FLAG']=1\n#F_PU10 and F_PU11\ndftrain2_F_PU10=dftrain2.loc[:,[' F_PU10',' ATT_FLAG']]\ndftrain2_F_PU10.loc[:,' ATT_FLAG']=0\ndftrain2_F_PU10.loc['2016-09-26 11':'2016-09-27 10',' ATT_FLAG']=1\n\ndftrain2_F_PU11=dftrain2.loc[:,[' F_PU11',' ATT_FLAG']]\ndftrain2_F_PU11.loc[:,' ATT_FLAG']=0\ndftrain2_F_PU11.loc['2016-09-26 11':'2016-09-27 10',' ATT_FLAG']=1\n#L_T1\ndftrain2_L_T1=dftrain2.loc[:,[' L_T1',' ATT_FLAG']]\ndftrain2_L_T1.loc[:,' ATT_FLAG']=0\ndftrain2_L_T1.loc['2016-10-09 09':'2016-10-11 20',' ATT_FLAG']=1\ndftrain2_L_T1.loc['2016-10-29 19':'2016-11-02 16',' ATT_FLAG']=1\ndftrain2_L_T1.loc['2016-12-14 15':'2016-12-19 04',' ATT_FLAG']=1\n#F_PU1 and F_PU2\ndftrain2_F_PU1=dftrain2.loc[:,[' F_PU1',' ATT_FLAG']]\ndftrain2_F_PU1.loc[:,' ATT_FLAG']=0\ndftrain2_F_PU1.loc['2016-10-29 19':'2016-11-02 16',' ATT_FLAG']=1\ndftrain2_F_PU1.loc['2016-12-14 15':'2016-12-19 04',' ATT_FLAG']=1\n\ndftrain2_F_PU2=dftrain2.loc[:,[' F_PU2',' ATT_FLAG']]\ndftrain2_F_PU2.loc[:,' ATT_FLAG']=0\ndftrain2_F_PU2.loc['2016-10-29 19':'2016-11-02 16',' ATT_FLAG']=1\ndftrain2_F_PU2.loc['2016-12-14 15':'2016-12-19 04',' ATT_FLAG']=1\n#L_T4\ndftrain2_L_T4=dftrain2.loc[:,[' L_T4',' ATT_FLAG']]\ndftrain2_L_T4.loc[:,' ATT_FLAG']=0\ndftrain2_L_T4.loc['2016-11-26 17':'2016-11-29 04',' ATT_FLAG']=1\n\n#dftrain2[' ATT_FLAG']=dftrain2[' ATT_FLAG'].apply(lambda x: 0 if x==-999 else x)",
"_____no_output_____"
]
],
[
[
"#### Labling the attacks on dftest according to the batadal dataset",
"_____no_output_____"
]
],
[
[
"###Labling zero in the test set for all anomalies\ndftest[\" ATT_FLAG\"]=0\n###Creating one dataset for each dimension\n#Attack 1\ndftest_L_T3=dftest.loc[:,['L_T3',' ATT_FLAG']]\ndftest_P_U4=dftest.loc[:,['F_PU4', ' ATT_FLAG']]\ndftest_P_U5=dftest.loc[:,['F_PU5', ' ATT_FLAG']]\n#Attack 2\ndftest_L_T2=dftest.loc[:,['L_T2', ' ATT_FLAG']]\n#Attack 3\ndftest_P_U3=dftest.loc[:,['F_PU3', ' ATT_FLAG']]\n#Attack 4\ndftest_P_U3=dftest.loc[:,['F_PU3', ' ATT_FLAG']]\n#Attack 5\ndftest_L_T2=dftest.loc[:,['L_T2', ' ATT_FLAG']]\ndftest_V2=dftest.loc[:,['F_V2', ' ATT_FLAG']]\ndftest_P_J14=dftest.loc[:,['P_J14', ' ATT_FLAG']]\ndftest_P_J422=dftest.loc[:,['P_J422', ' ATT_FLAG']]\n#Attack 6\ndftest_L_T7=dftest.loc[:,['L_T7', ' ATT_FLAG']]\ndftest_P_U10=dftest.loc[:,['F_PU10', ' ATT_FLAG']]\ndftest_P_U11=dftest.loc[:,['F_PU11', ' ATT_FLAG']]\ndftest_P_J14=dftest.loc[:,['P_J14', ' ATT_FLAG']]\ndftest_P_J422=dftest.loc[:,['P_J422', ' ATT_FLAG']]\n#Attack 7\ndftest_L_T4=dftest.loc[:,['L_T4', ' ATT_FLAG']]\ndftest_L_T6=dftest.loc[:,['L_T6', ' ATT_FLAG']]",
"_____no_output_____"
],
[
"###Adding one for anomalies\n#Attack 1\ndftest_L_T3.loc['2017-01-16 09':'2017-01-19 06', ' ATT_FLAG']=1\ndftest_P_U4.loc['2017-01-16 09':'2017-01-19 06', ' ATT_FLAG']=1\ndftest_P_U5.loc['2017-01-16 09':'2017-01-19 06', ' ATT_FLAG']=1\n#Attack 2\ndftest_L_T2.loc['2017-01-30 08':'2017-02-02 00', ' ATT_FLAG']=1\n#Attack 3\ndftest_P_U3.loc['2017-02-09 03':'2017-02-10 09', ' ATT_FLAG']=1\n#Attack 4\ndftest_P_U3.loc['2017-02-12 01':'2017-02-13 07', ' ATT_FLAG']=1\n#Attack 5\ndftest_L_T2.loc['2017-02-24 05':'2017-02-28 08', ' ATT_FLAG']=1\ndftest_V2.loc['2017-02-24 05':'2017-02-28 08', ' ATT_FLAG']=1\ndftest_P_J14.loc['2017-02-24 05':'2017-02-28 08', ' ATT_FLAG']=1\ndftest_P_J422.loc['2017-02-24 05':'2017-02-28 08', ' ATT_FLAG']=1\n#Attack 6\ndftest_L_T7.loc['2017-03-10 14':'2017-03-13 21', ' ATT_FLAG']=1\ndftest_P_U10.loc['2017-03-10 14':'2017-03-13 21', ' ATT_FLAG']=1\ndftest_P_U11.loc['2017-03-10 14':'2017-03-13 21', ' ATT_FLAG']=1\ndftest_P_J14.loc['2017-03-10 14':'2017-03-13 21', ' ATT_FLAG']=1\ndftest_P_J422.loc['2017-03-10 14':'2017-03-13 21', ' ATT_FLAG']=1\n#Attack 7\ndftest_L_T4.loc['2017-03-25 20':'2017-03-27 01', ' ATT_FLAG']=1\ndftest_L_T6.loc['2017-03-25 20':'2017-03-27 01', ' ATT_FLAG']=1",
"_____no_output_____"
]
],
[
[
"### Labling all the attacks in one dataset to compute performance",
"_____no_output_____"
]
],
[
[
"dftest.loc['2017-01-16 09':'2017-01-19 06',' ATT_FLAG']=1\ndftest.loc['2017-01-30 08':'2017-02-02 00',' ATT_FLAG']=1\ndftest.loc['2017-02-09 03':'2017-02-10 09',' ATT_FLAG']=1\ndftest.loc['2017-02-12 01':'2017-02-13 07',' ATT_FLAG']=1\ndftest.loc['2017-02-24 05':'2017-02-28 08',' ATT_FLAG']=1\ndftest.loc['2017-03-10 14':'2017-03-13 21',' ATT_FLAG']=1\ndftest.loc['2017-03-25 20':'2017-03-27 01',' ATT_FLAG']=1",
"_____no_output_____"
]
],
[
[
"Adding the anomaly type column to be used in the detection",
"_____no_output_____"
]
],
[
[
"dftest[\"anomalytype\"]=0",
"_____no_output_____"
]
],
[
[
"### Attack 1",
"_____no_output_____"
]
],
[
[
"plt.plot(dftest_L_T3.loc['2017-01-16 09':'2017-01-19 06', 'L_T3'],color=\"red\")\n\nplt.plot(dftest_L_T3.loc['2017-01-12 09':'2017-01-16 09', 'L_T3'],color=\"blue\")\nplt.plot(dftest_L_T3.loc['2017-01-19 06':'2017-01-23 06', 'L_T3'],color=\"blue\")",
"_____no_output_____"
],
[
"plt.plot(dftest_P_U4.loc['2017-01-16 09':'2017-01-19 06', 'F_PU4'],color=\"red\")\n\nplt.plot(dftest_P_U4.loc['2017-01-12 09':'2017-01-16 09', 'F_PU4'],color=\"blue\")\nplt.plot(dftest_P_U4.loc['2017-01-19 06':'2017-01-23 06', 'F_PU4'],color=\"blue\")",
"_____no_output_____"
],
[
"plt.plot(dftest_P_U5.loc['2017-01-16 09':'2017-01-19 06', 'F_PU5'],color=\"red\")\n\nplt.plot(dftest_P_U5.loc['2017-01-12 09':'2017-01-16 09', 'F_PU5'],color=\"blue\")\nplt.plot(dftest_P_U5.loc['2017-01-19 06':'2017-01-23 06', 'F_PU5'],color=\"blue\")",
"_____no_output_____"
]
],
[
[
"Thus attack 1 generated a contextual anomaly in L_T3",
"_____no_output_____"
]
],
[
[
"dftest.loc['2017-01-16 09':'2017-01-19 06', 'anomalytype']=\"contextual\"",
"_____no_output_____"
]
],
[
[
"### Attack 2",
"_____no_output_____"
]
],
[
[
"plt.plot(dftest_L_T2.loc['2017-01-30 08':'2017-02-02 00', 'L_T2'],color=\"red\")\n\nplt.plot(dftest_L_T2.loc['2017-01-26 08':'2017-01-30 08', 'L_T2'],color=\"blue\")\nplt.plot(dftest_L_T2.loc['2017-02-02 00':'2017-02-06 00', 'L_T2'],color=\"blue\")",
"_____no_output_____"
]
],
[
[
"Thus attack 2 generated a collective anomaly in L_T2",
"_____no_output_____"
]
],
[
[
"dftest.loc['2017-01-30 08':'2017-02-02 00', 'anomalytype']=\"collective\"",
"_____no_output_____"
]
],
[
[
"### Attack 3",
"_____no_output_____"
]
],
[
[
"plt.plot(dftest_P_U3.loc['2017-02-09 02':'2017-02-10 09', 'F_PU3'],color=\"red\")\n\nplt.plot(dftest_P_U3.loc['2017-02-05 03':'2017-02-09 02', 'F_PU3'],color=\"blue\")\nplt.plot(dftest_P_U3.loc['2017-02-10 09':'2017-02-14 09', 'F_PU3'],color=\"blue\")\n",
"_____no_output_____"
]
],
[
[
"Thus attack 3 generated a collective anomaly in F_PU3",
"_____no_output_____"
]
],
[
[
"dftest.loc['2017-02-09 02':'2017-02-10 09', 'anomalytype']=\"collective\"",
"_____no_output_____"
]
],
[
[
"### Attack 4",
"_____no_output_____"
]
],
[
[
"plt.plot(dftest_P_U3.loc['2017-02-12 00':'2017-02-13 07', 'F_PU3'],color=\"red\")\n\nplt.plot(dftest_P_U3.loc['2017-02-08 01':'2017-02-12 00', 'F_PU3'],color=\"blue\")\nplt.plot(dftest_P_U3.loc['2017-02-13 07':'2017-02-17 07', 'F_PU3'],color=\"blue\")",
"_____no_output_____"
]
],
[
[
"Thus attack 4 generated a collective anomaly in F_PU3",
"_____no_output_____"
]
],
[
[
"dftest.loc['2017-02-12 00':'2017-02-13 07', 'anomalytype']=\"collective\"",
"_____no_output_____"
]
],
[
[
"### Attack 5",
"_____no_output_____"
]
],
[
[
"plt.plot(dftest_L_T2.loc['2017-02-24 05':'2017-02-28 08', 'L_T2'],color=\"red\")\n\nplt.plot(dftest_L_T2.loc['2017-02-20 05':'2017-02-24 05', 'L_T2'],color=\"blue\")\nplt.plot(dftest_L_T2.loc['2017-02-28 08':'2017-03-03 08', 'L_T2'],color=\"blue\")",
"_____no_output_____"
],
[
"plt.plot(dftest_V2.loc['2017-02-24 05':'2017-02-28 08', 'F_V2'],color=\"red\")\n\nplt.plot(dftest_V2.loc['2017-02-20 05':'2017-02-24 05', 'F_V2'],color=\"blue\")\nplt.plot(dftest_V2.loc['2017-02-28 08':'2017-03-03 08', 'F_V2'],color=\"blue\")",
"_____no_output_____"
],
[
"plt.plot(dftest_P_J14.loc['2017-02-24 05':'2017-02-28 08', 'P_J14'],color=\"red\")\n\nplt.plot(dftest_P_J14.loc['2017-02-20 05':'2017-02-24 05', 'P_J14'],color=\"blue\")\nplt.plot(dftest_P_J14.loc['2017-02-28 08':'2017-03-03 08', 'P_J14'],color=\"blue\")",
"_____no_output_____"
],
[
"plt.plot(dftest_P_J422.loc['2017-02-24 05':'2017-02-28 08', 'P_J422'],color=\"red\")\n\nplt.plot(dftest_P_J422.loc['2017-02-20 05':'2017-02-24 05', 'P_J422'],color=\"blue\")\nplt.plot(dftest_P_J422.loc['2017-02-28 08':'2017-03-03 08', 'P_J422'],color=\"blue\")",
"_____no_output_____"
]
],
[
[
"Thus attack 5 generated a collective anomaly in L_T2, F_V2, P_J14 and P_J422",
"_____no_output_____"
]
],
[
[
"dftest.loc['2017-02-24 05':'2017-02-28 08', 'anomalytype']=\"collective\"",
"_____no_output_____"
]
],
[
[
"### Attack 6",
"_____no_output_____"
]
],
[
[
"plt.plot(dftest_L_T7.loc['2017-03-10 14':'2017-03-13 21', 'L_T7'],color=\"red\")\n\nplt.plot(dftest_L_T7.loc['2017-03-06 14':'2017-03-10 14', 'L_T7'],color=\"blue\")\nplt.plot(dftest_L_T7.loc['2017-03-13 21':'2017-03-17 21', 'L_T7'],color=\"blue\")",
"_____no_output_____"
],
[
"plt.plot(dftest_P_U10.loc['2017-03-10 14':'2017-03-13 21', 'F_PU10'],color=\"red\")\n\nplt.plot(dftest_P_U10.loc['2017-03-06 14':'2017-03-10 14', 'F_PU10'],color=\"blue\")\nplt.plot(dftest_P_U10.loc['2017-03-13 21':'2017-03-17 21', 'F_PU10'],color=\"blue\")",
"_____no_output_____"
],
[
"plt.plot(dftest_P_U11.loc['2017-03-10 14':'2017-03-13 21', 'F_PU11'],color=\"red\")\n\nplt.plot(dftest_P_U11.loc['2017-03-06 14':'2017-03-10 14', 'F_PU11'],color=\"blue\")\nplt.plot(dftest_P_U11.loc['2017-03-13 21':'2017-03-17 21', 'F_PU11'],color=\"blue\")",
"_____no_output_____"
],
[
"plt.plot(dftest_P_J14.loc['2017-03-10 14':'2017-03-13 21', 'P_J14'],color=\"red\")\n\nplt.plot(dftest_P_J14.loc['2017-03-06 14':'2017-03-10 14', 'P_J14'],color=\"blue\")\nplt.plot(dftest_P_J14.loc['2017-03-13 21':'2017-03-17 21', 'P_J14'],color=\"blue\")",
"_____no_output_____"
],
[
"plt.plot(dftest_P_J422.loc['2017-03-10 14':'2017-03-13 21', 'P_J422'],color=\"red\")\n\nplt.plot(dftest_P_J422.loc['2017-03-06 14':'2017-03-10 14', 'P_J422'],color=\"blue\")\nplt.plot(dftest_P_J422.loc['2017-03-13 21':'2017-03-17 21', 'P_J422'],color=\"blue\")",
"_____no_output_____"
]
],
[
[
"Attack 6 generated collective anomalies",
"_____no_output_____"
]
],
[
[
"dftest.loc['2017-03-10 14':'2017-03-13 21', 'anomalytype']=\"collective\"",
"_____no_output_____"
]
],
[
[
"### Attack 7",
"_____no_output_____"
]
],
[
[
"plt.plot(dftest_L_T4.loc['2017-03-25 20':'2017-03-27 01', 'L_T4'],color=\"red\")\n\nplt.plot(dftest_L_T4.loc['2017-03-21 20':'2017-03-25 20', 'L_T4'],color=\"blue\")\nplt.plot(dftest_L_T4.loc['2017-03-27 01':'2017-03-31 01', 'L_T4'],color=\"blue\")",
"_____no_output_____"
],
[
"plt.plot(dftest_L_T6.loc['2017-03-25 20':'2017-03-27 01', 'L_T6'],color=\"red\")\n\nplt.plot(dftest_L_T6.loc['2017-03-21 20':'2017-03-25 20', 'L_T6'],color=\"blue\")\nplt.plot(dftest_L_T6.loc['2017-03-27 01':'2017-03-31 01', 'L_T6'],color=\"blue\")",
"_____no_output_____"
]
],
[
[
"Thus attack 6 generated a collective anomaly in L_T4 and L_T6",
"_____no_output_____"
]
],
[
[
"dftest.loc['2017-03-25 20':'2017-03-27 01', 'anomalytype']=\"collective\"",
"_____no_output_____"
]
],
[
[
"## 2) Discretization ",
"_____no_output_____"
],
[
"Creating a dataset to test the sax",
"_____no_output_____"
]
],
[
[
"dataset_scale=dftrain1['L_T7']",
"_____no_output_____"
]
],
[
[
"Creating the Symbolic Aggregate Approximation (SAX) function and testing in the above mentioned dataset",
"_____no_output_____"
]
],
[
[
"### Creting a function that creates the sax\ndef tosax(n_paa_segments,n_sax_symbols,dataset_scale):\n #Creating the SAX using 'n_paa_segments' as number of segments and 'n_sax_symbols' as number of symbols\n sax = SymbolicAggregateApproximation(n_segments=n_paa_segments, alphabet_size_avg=n_sax_symbols)\n # Compute sax time series corresponding (by sax.inverse_transform) to the fitted PAA representation (done by sax.fit_transform)\n return sax.inverse_transform(sax.fit_transform(dataset_scale))\n\n### Running the function to test it for a given column of the dataset\nn_sax_symbols = 5\nn_paa_segments=int(len(dataset_scale)/4)\nsax_dataset_inv=tosax(n_paa_segments,n_sax_symbols,dataset_scale)",
"_____no_output_____"
]
],
[
[
"Plotting the scaled time series, PAA and SAX representations for the sake of validation of the code",
"_____no_output_____"
]
],
[
[
"#Plotting - only 100 first values to test the code isworking as expected\n\n# Plotting the scaled data set\nplt.subplot(2, 2, 2) # First, raw time series\nplt.plot(dataset_scale.ravel()[0:300], \"b-\")\nplt.title(\"Original Time Series\")\n\n#Plotting the SAX\nplt.subplot(2, 2, 1) # Then SAX\nplt.plot(sax_dataset_inv[0].ravel()[0:300], \"b-\")\nplt.title(\"SAX, %d symbols\" % n_sax_symbols)\n\nplt.tight_layout()\nplt.show()",
"_____no_output_____"
]
],
[
[
"## 3) N-Grams Representations",
"_____no_output_____"
],
[
"Creating a function that returns its n-gram representation.",
"_____no_output_____"
]
],
[
[
"#'a' will be used just to test the functions below.\na=sax_dataset_inv[0].ravel()",
"_____no_output_____"
]
],
[
[
"We need function(s) to convert numeric values generated from the sax to a string to perform N-grams as a it was a NLP problem. For this purpose two functions were created: the first just put all the values of a list in a string and the second convert numerical values of the sax to letters",
"_____no_output_____"
]
],
[
[
"# First function convert a list of numeric values to a string\ndef convert(list): \n # Converting integer list to string list \n s = [str(i) for i in list] \n # Join list items using join() \n res = (\"\".join(s)) \n return(res) ",
"_____no_output_____"
],
[
"# Second function to convert a sax numeric representation into strings\ndef saxtostring(sax):\n #Creating a list with all unique elements\n setsax=set(sax)\n L=[]\n for i in setsax:\n L.append(i)\n L=sorted(L)\n #Creating a dictinary with the elements that need to be converted\n D={}\n for i in range(0,len(L)):\n D[L[i]]=string.ascii_lowercase[i]\n #Converting the numerical values to strings\n output=[]\n for i in range(0,len(sax)):\n output.append(D[sax[i]])\n return output \n#To test\nb=saxtostring(a)",
"_____no_output_____"
]
],
[
[
"Now to generate Ngrams we need a function that gather from the data the possible grams and their possible next values.",
"_____no_output_____"
]
],
[
[
"#Function that creates the Ngrams representation by creating a dictionary that has as keys the representations and as values\n#the number elements generated after the n-gram\ndef tongrams(tokens,n):\n #Creates a dictionary with the Ngrams representation and the next element (that is to be predicted by the ngrams)\n D = {}\n #Loop needs to go up to len(tokens)-n-1 to not get out of bounds\n for i in range(0,len(tokens)-n-1):\n #If new key, we add this key to the dictionary\n if convert(tokens[i:(i+n)]) not in D.keys():\n D[convert(tokens[i:(i+n)])] = [convert(tokens[i+n+1])]\n #If existing key, append new value\n else:\n D[convert(tokens[i:(i+n)])].append(tokens[i+n+1])\n return D\n#To test\ng=tongrams(b,3)",
"_____no_output_____"
]
],
[
[
"Now we create a Markov Chain that calculates the prior and the posterior given the dictionary generated by the previous function.",
"_____no_output_____"
]
],
[
[
"#Function that calcualte the likelihood given a ngram\ndef tomarkovchain(ngram):\n #Create dictionary to be filled with the probability of conditional events (likelihood)\n l_probs=dict()\n #Create the dictionaries to be filled with the counting for each gram\n c1=dict()\n #Loop through keys to calculate the likelihood for each key\n for key in ngram.keys():\n #Create the variable to be filled with tally of the number of times that a value repeat for each key\n counts = dict()\n for i in ngram[key]:\n counts[i] = counts.get(i, 0) + 1\n #Create the dictionary to be filled with the probability of the conditional events (likelihood)\n l=dict()\n\n #Calculate the probability by dividing the count to the sum of values\n for j in counts.keys():\n l[j]=counts[j]/sum(counts.values())\n \n #Assign to each key the dictionary with the probabilities\n l_probs[key]=l\n #Assign to each key the dictionary with the counting\n c1[key]=counts\n \n\n # Return the likelihood,the prior and the counting\n return [l_probs,c1]\n\n#To test\nm=tomarkovchain(g)",
"_____no_output_____"
],
[
"#Smoothing the prior\n#This function return the smoothed probability\ndef smooth_ngram_prob(markovchain_model,n_sax_symbols,input_gram,output_gram):\n \n #Calculating denominators\n ngrams_number=len(random.choice(list(markovchain_model[0].keys())))\n \n #Smoothing\n if input_gram in markovchain_model[1].keys():\n total_values_for_the_key=sum(markovchain_model[1][input_gram].values())\n #The Probability is calculated by adding 1 observation for all possible grams (total of n_sax_symbols**ngrams_number )\n smoothed=(markovchain_model[1][input_gram].get(output_gram,0)+1)/(total_values_for_the_key+n_sax_symbols)\n else:\n #There is no observation of this gram\n smoothed=0\n \n return smoothed\n\n#To test\nsmooth_ngram_prob(m,5,\"aae\",\"a\")\n",
"_____no_output_____"
]
],
[
[
"Lastly, we create a function that loop through the dataframe and return the index that are below a certain threshold",
"_____no_output_____"
]
],
[
[
"def prediction(markovchain_model,n_sax_symbols,string_sax, threshold):\n #Calculating number of ngrams used\n n=len(random.choice(list(markovchain_model[0].keys())))\n #Calculationg the number of times a observation should be reproduced so the data set has the same size as the original\n\n #Creating the list to store the values\n L=[]\n #And a list to store the probabilities\n P=[]\n #looping\n for i in range(0,len(string_sax)):\n if (i<=(n+1)):\n L.append(0)\n P.append(0)\n else:\n P.append(smooth_ngram_prob(markovchain_model,n_sax_symbols,convert(string_sax[i:(i+n)]),string_sax[i]))\n if smooth_ngram_prob(markovchain_model,n_sax_symbols,convert(string_sax[i:(i+n)]),string_sax[i])<threshold:\n L.append(1)\n else:\n L.append(0)\n return [L,P]\n\npred_test=prediction(m,5,b, 0.01)[0]",
"_____no_output_____"
]
],
[
[
"And a function that merge the predictions into the original dataframe considering the possible rounding differences that may lead to difference in length when creating SAX",
"_____no_output_____"
]
],
[
[
"def smartmerge(df,predictions):\n df=pd.Series.to_frame(df)\n \n if len(predictions)==len(df):\n df.insert(1, \"predictions\", predictions)\n df.reset_index(inplace=True)\n \n if len(predictions)>len(df):\n predictions=predictions[:-(len(predictions)-len(df))]\n df.insert(1, \"predictions\", predictions)\n df.reset_index(inplace=True)\n \n if len(predictions)<len(df):\n for i in range(0,(len(df)-len(predictions))):\n predictions.append(0)\n df.insert(1, \"predictions\", predictions)\n \n #To help to plot latter\n #df['color'] = df['predictions'].apply(lambda x: \"blue\" if x==0 else \"red\")\n \n return df ",
"_____no_output_____"
]
],
[
[
"## 4) Predictions Attacks Using N-grams",
"_____no_output_____"
],
[
"_In this subchapter we are going to try to predict the attacks on the training dataset 2_",
"_____no_output_____"
],
[
"## Creating functions that aid the prediction",
"_____no_output_____"
],
[
"Creating a function that make predicitons and calculate performance indicators.",
"_____no_output_____"
]
],
[
[
"def model_to_predict(trainset,testset,results,n_sax_symbols,n_paa_segments,n_grams,threshold):\n \n ###Generating the model for the train set###\n #Sax\n sax_train=tosax(n_paa_segments,n_sax_symbols,trainset).ravel()\n #Sax to string\n sax_string_train=saxtostring(sax_train)\n #Ngrams\n ngrams=tongrams(sax_string_train,n_grams)\n #Markov Chain\n m=tomarkovchain(ngrams)\n \n ###Preparing the test set###\n #Getting the denominator of the paa in a way that the test and train set have the same number of variables in a segment\n n_paa_segments_test_denominator=len(trainset)/n_paa_segments\n #Sax\n sax_test=tosax(int(len(testset)/n_paa_segments_test_denominator),n_sax_symbols,testset).ravel()\n #Sax to string\n sax_string_test=saxtostring(sax_test)\n\n ###Making predictions\n pred=prediction(m,n_sax_symbols,sax_string_test, threshold)[0]\n predictions_df=smartmerge(testset,pred)\n \n ###Calculating the performance\n cm=confusion_matrix(list(results), list(predictions_df['predictions']))\n if cm.shape==(3,3):\n cm=cm[(0,2),1:]\n #True positive, false positive, false negative and true positive\n tn, fp, fn, tp = cm.ravel()\n #Getting the ratios\n recall=tp/(tp+fn)\n precision=tp/(tp+fp)\n accuracy=(tp+tn)/(tp+tn+fn+fp)\n f1=fbeta_score(list(results), list(predictions_df['predictions']),beta=0.5)\n #Probability vector\n probab=prediction(m,n_sax_symbols,sax_string_test, threshold)[1]\n performance={\"recall\":recall,\"precision\":precision,\"Accuracy\":accuracy,\"F1\":f1}\n return [cm,performance,probab, predictions_df]",
"_____no_output_____"
],
[
"#Testing\nsax=7\npaa=int(len(dftrain1[\"L_T1\"])/2)\nn=5\nt=0.1\nMODEL=model_to_predict(dftrain1[\"L_T1\"],dftrain2_L_T1[\" L_T1\"],dftrain2_L_T1[' ATT_FLAG'],sax,paa,n,t)\nMODEL[1]",
"_____no_output_____"
]
],
[
[
"Creating a function that plot the confusion matrix in a nice format!",
"_____no_output_____"
]
],
[
[
"def ploting_cm(cm):\n \n #Plot\n ax= plt.subplot()\n sns.heatmap(cm, annot=True,fmt='g', ax = ax); #annot=True to annotate cells\n\n # labels, title and ticks\n ax.set_xlabel('Predicted labels');ax.set_ylabel('True labels'); \n ax.set_title('Confusion Matrix'); \n ax.xaxis.set_ticklabels(['normal', 'anomaly']); ax.yaxis.set_ticklabels(['normal', 'anomaly'])",
"_____no_output_____"
]
],
[
[
"Creating a function that loop over the parameters and select the best set of parameters based the F-score",
"_____no_output_____"
]
],
[
[
"def parameterselection(trainset,testset,results):\n #Genereting one first model to have a starting value for the loop with selected parameters\n parameters={\"sax\":5,\"paa\":1,\"ngram\":3,\"threshold\":0.10}\n best_MODEL=model_to_predict(trainset,testset,results,5,int(len(trainset)/1),3,0.1)\n #Looping\n for sax in range(4,8,1):\n for paa in range(1,5,1):\n for n in range(2,6,1):\n for t in [0.01,0.02,0.03,0.04,0.05,0.1]:\n MODEL=model_to_predict(trainset,testset,results,sax,int(len(dftrain1)/paa),n,t)\n #Selecting using F1-Score that has at least 1 true positive\n if (MODEL[1][\"F1\"]>best_MODEL[1][\"F1\"]) and (MODEL[0].ravel()[3]>1):\n best_MODEL=MODEL\n parameters={\"sax\":sax,\"paa\":paa,\"ngram\":n,\"threshold\":t}\n\n return [best_MODEL,parameters]",
"_____no_output_____"
]
],
[
[
"## Predicting Attacks for some sensors in the train data set 2",
"_____no_output_____"
],
[
"The Loop below will select the best parameters for the model.\n\n**It takes some minutes to run , please be advised**\n\n_The code will just select the best parameters based on the train set 2 - nothing else_",
"_____no_output_____"
]
],
[
[
"#############train\n#L_T7\nL_T7=parameterselection(dftrain1[\"L_T7\"],dftrain2_L_T7[\" L_T7\"],dftrain2_L_T7[' ATT_FLAG'])\n\n#F_PU10\nF_PU10=parameterselection(dftrain1[\"F_PU10\"],dftrain2_F_PU10[\" F_PU10\"],dftrain2_F_PU10[' ATT_FLAG'])\n\n#F_PU11\nF_PU11=parameterselection(dftrain1[\"F_PU11\"],dftrain2_F_PU11[\" F_PU11\"],dftrain2_F_PU11[' ATT_FLAG'])\n\n#L_T1\nL_T1=parameterselection(dftrain1[\"L_T1\"],dftrain2_L_T1[\" L_T1\"],dftrain2_L_T1[' ATT_FLAG'])\n\n#F_PU1\nF_PU1=parameterselection(dftrain1[\"F_PU1\"],dftrain2_F_PU1[\" F_PU1\"],dftrain2_F_PU1[' ATT_FLAG'])\n\n#F_PU2\nF_PU2=parameterselection(dftrain1[\"F_PU2\"],dftrain2_F_PU2[\" F_PU2\"],dftrain2_F_PU2[' ATT_FLAG'])\n\n#L_T4\nL_T4=parameterselection(dftrain1[\"L_T4\"],dftrain2_L_T4[\" L_T4\"],dftrain2_L_T4[' ATT_FLAG'])",
"_____no_output_____"
],
[
"print(L_T7[0][1][\"F1\"])\nprint(F_PU10[0][1][\"F1\"])\nprint(F_PU11[0][1][\"F1\"])\nprint(L_T1[0][1][\"F1\"])\nprint(F_PU1[0][1][\"F1\"])\nprint(F_PU2[0][1][\"F1\"])\nprint(L_T4[0][1][\"F1\"])",
"0.06684491978609626\n0.014204545454545454\n0.0\n0.1286764705882353\n0.08227848101265822\n0.08849557522123894\n0.036290322580645164\n"
]
],
[
[
"## Predicting Attacks for some sensors in the test data",
"_____no_output_____"
],
[
"According to the information provided about the BATADAL dataset, Anomalies can be found at 'L_T3', 'F_PU4', 'F_PU5', 'L_T2, 'V2', 'P_J14', 'P_J422, 'L_T7', 'F_PU10', 'F_PU11', 'L_T6'.\n\nThe parameters for prediction will be those optimized by on the traindataset 2. When not available the parameters will be for one close variable",
"_____no_output_____"
]
],
[
[
"#'L_T3'\ntrainset=dftrain1['L_T3']\ntestset=dftest['L_T3']\nsax=L_T1[1][\"sax\"]\npaa=L_T1[1][\"paa\"]\nn=L_T1[1][\"ngram\"]\nt=L_T1[1][\"threshold\"]\nresults=dftest_L_T3[' ATT_FLAG']\nMODEL_LT3=model_to_predict(trainset,testset,results,sax,int(len(dftrain1)/paa),n,t)\n#'F_PU4'\ntrainset=dftrain1['F_PU4']\ntestset=dftest['F_PU4']\nsax=F_PU10[1][\"sax\"]\npaa=F_PU10[1][\"paa\"]\nn=F_PU10[1][\"ngram\"]\nt=F_PU10[1][\"threshold\"]\nresults=dftest_P_U4[' ATT_FLAG']\nMODEL_F_PU4=model_to_predict(trainset,testset,results,sax,int(len(dftrain1)/paa),n,t)\n#'F_PU5'\ntrainset=dftrain1['F_PU5']\ntestset=dftest['F_PU5']\nsax=F_PU10[1][\"sax\"]\npaa=F_PU10[1][\"paa\"]\nn=F_PU10[1][\"ngram\"]\nt=F_PU10[1][\"threshold\"]\nresults=dftest_P_U5[' ATT_FLAG']\nMODEL_F_PU5=model_to_predict(trainset,testset,results,sax,int(len(dftrain1)/paa),n,t)\n#'L_T2\ntrainset=dftrain1['L_T2']\ntestset=dftest['L_T2']\nsax=L_T1[1][\"sax\"]\npaa=L_T1[1][\"paa\"]\nn=L_T1[1][\"ngram\"]\nt=L_T1[1][\"threshold\"]\nresults=dftest_L_T2[' ATT_FLAG']\nMODEL_L_T2=model_to_predict(trainset,testset,results,sax,int(len(dftrain1)/paa),n,t)\n#'P_J14'\ntrainset=dftrain1['P_J14']\ntestset=dftest['P_J14']\nsax=L_T7[1][\"sax\"]\npaa=L_T7[1][\"paa\"]\nn=L_T7[1][\"ngram\"]\nt=L_T7[1][\"threshold\"]\nresults=dftest_P_J14[' ATT_FLAG']\nMODEL_P_J14=model_to_predict(trainset,testset,results,sax,int(len(dftrain1)/paa),n,t)\n#'P_J422\ntrainset=dftrain1['P_J422']\ntestset=dftest['P_J422']\nsax=L_T7[1][\"sax\"]\npaa=L_T7[1][\"paa\"]\nn=L_T7[1][\"ngram\"]\nt=L_T7[1][\"threshold\"]\nresults=dftest_P_J422[' ATT_FLAG']\nMODEL_P_J422=model_to_predict(trainset,testset,results,sax,int(len(dftrain1)/paa),n,t)\n#'L_T7'\ntrainset=dftrain1['L_T7']\ntestset=dftest['L_T7']\nsax=L_T7[1][\"sax\"]\npaa=L_T7[1][\"paa\"]\nn=L_T7[1][\"ngram\"]\nt=L_T7[1][\"threshold\"]\nresults=dftest_L_T7[' ATT_FLAG']\nMODEL_L_T7=model_to_predict(trainset,testset,results,sax,int(len(dftrain1)/paa),n,t)\n#'F_PU10'\ntrainset=dftrain1['F_PU10']\ntestset=dftest['F_PU10']\nsax=F_PU10[1][\"sax\"]\npaa=F_PU10[1][\"paa\"]\nn=F_PU10[1][\"ngram\"]\nt=F_PU10[1][\"threshold\"]\nresults=dftest_P_U10[' ATT_FLAG']\nMODEL_F_PU10=model_to_predict(trainset,testset,results,sax,int(len(dftrain1)/paa),n,t)\n#'F_PU11'\ntrainset=dftrain1['F_PU11']\ntestset=dftest['F_PU11']\nsax=F_PU11[1][\"sax\"]\npaa=F_PU11[1][\"paa\"]\nn=F_PU11[1][\"ngram\"]\nt=F_PU11[1][\"threshold\"]\nresults=dftest_P_U11[' ATT_FLAG']\nMODEL_F_PU11=model_to_predict(trainset,testset,results,sax,int(len(dftrain1)/paa),n,t)\n#'L_T6'\ntrainset=dftrain1['L_T6']\ntestset=dftest['L_T6']\nsax=L_T1[1][\"sax\"]\npaa=L_T1[1][\"paa\"]\nn=L_T1[1][\"ngram\"]\nt=L_T1[1][\"threshold\"]\nresults=dftest_L_T6[' ATT_FLAG']\nMODEL_L_T6=model_to_predict(trainset,testset,results,sax,int(len(dftrain1)/paa),n,t)",
"_____no_output_____"
],
[
"#'L_T3'\nploting_cm(MODEL_LT3[0])\nprint(MODEL_LT3[1])",
"{'recall': 0.42857142857142855, 'precision': 0.02819548872180451, 'Accuracy': 0.4858784107228339, 'F1': 0.034674063800277384}\n"
],
[
"#'F_PU4'\nploting_cm(MODEL_F_PU4[0])\nprint(MODEL_F_PU4[1])",
"{'recall': 0.5142857142857142, 'precision': 0.02962962962962963, 'Accuracy': 0.41933939684059357, 'F1': 0.036511156186612576}\n"
],
[
"#'F_PU5'\nploting_cm(MODEL_F_PU5[0])\nprint(MODEL_F_PU5[1])",
"{'recall': 0.0, 'precision': 0.0, 'Accuracy': 0.9655337482048827, 'F1': 0.0}\n"
],
[
"#'L_T2\nploting_cm(MODEL_L_T2[0])\nprint(MODEL_L_T2[1])",
"{'recall': 0.15757575757575756, 'precision': 0.09558823529411764, 'Accuracy': 0.8157012924844423, 'F1': 0.1037509976057462}\n"
],
[
"#'P_J14'\nploting_cm(MODEL_P_J14[0])\nprint(MODEL_P_J14[1])",
"{'recall': 0.0, 'precision': 0.0, 'Accuracy': 0.9123982766874102, 'F1': 0.0}\n"
],
[
"#'P_J422\nploting_cm(MODEL_P_J422[0])\nprint(MODEL_P_J422[1])",
"{'recall': 0.0, 'precision': 0.0, 'Accuracy': 0.9123982766874102, 'F1': 0.0}\n"
],
[
"#'L_T7'\nploting_cm(MODEL_L_T7[0])\nprint(MODEL_L_T7[1])",
"{'recall': 0.0375, 'precision': 0.08571428571428572, 'Accuracy': 0.9478219243657252, 'F1': 0.06818181818181819}\n"
],
[
"#'F_PU10'\nploting_cm(MODEL_F_PU10[0])\nprint(MODEL_F_PU10[1])",
"{'recall': 0.075, 'precision': 0.10344827586206896, 'Accuracy': 0.9396840593585447, 'F1': 0.09615384615384616}\n"
],
[
"#'F_PU11'\nploting_cm(MODEL_F_PU11[0])\nprint(MODEL_F_PU11[1])",
"{'recall': 0.0, 'precision': 0.0, 'Accuracy': 0.9607467687888942, 'F1': 0.0}\n"
],
[
"#'L_T6'\nploting_cm(MODEL_L_T6[0])\nprint(MODEL_L_T6[1])",
"{'recall': 0.06666666666666667, 'precision': 0.011904761904761904, 'Accuracy': 0.9071325993298229, 'F1': 0.014245014245014244}\n"
]
],
[
[
"### Aggregating all the predictions",
"_____no_output_____"
],
[
"Aggregating all the predictions in one dataset",
"_____no_output_____"
]
],
[
[
"#Getting the predictions for anomalies\ndftest_with_predictions=dftest\n\n#Loop that aggregate all the predictions in a column with the test set with predictions\nModel_set=[MODEL_LT3,MODEL_F_PU4,MODEL_F_PU5,MODEL_L_T2,MODEL_P_J14,MODEL_P_J422,MODEL_L_T7,MODEL_F_PU10,MODEL_F_PU11,MODEL_L_T6]\npred=[0]*len(model[3])\nfor model in Model_set:\n for i in model[3].index:\n if model[3][\"predictions\"][i]==1:\n pred[i]=1\ndftest_with_predictions[\"predictions\"]=pred",
"_____no_output_____"
]
],
[
[
"Calculating the performance for the aggregate model",
"_____no_output_____"
]
],
[
[
"cm=confusion_matrix(list(dftest[\" ATT_FLAG\"]), pred)\n#True positive, false positive, false negative and true positive\ntn, fp, fn, tp = cm.ravel()\n#Getting the ratios\nrecall=tp/(tp+fn)\nprecision=tp/(tp+fp)\n#Ploting\nploting_cm(cm)\n#Printing performance\nprint(\"Recall: \"+str(recall))\nprint(\"Precision: \"+str(precision))",
"Recall: 0.8574938574938575\nPrecision: 0.20069005175388155\n"
]
],
[
[
"### Checking the type of anomaly",
"_____no_output_____"
],
[
"Calculating per each type of anomaly the recall rate",
"_____no_output_____"
]
],
[
[
"#Calculating number of anomalies per type\nTotal_Collective_Anomalies=len(dftest_with_predictions[dftest_with_predictions[\"anomalytype\"]==\"collective\"])\nTotal_Contextual_Anomalies=len(dftest_with_predictions[dftest_with_predictions[\"anomalytype\"]==\"contextual\"])\n\n#Calculating number of anomalies predicted per type\nPredicted_Collective_Anomalies=len(dftest_with_predictions[(dftest_with_predictions[\"anomalytype\"]==\"collective\") & (dftest_with_predictions[\"predictions\"]==1)])\nPredicted_Contextual_Anomalies=len(dftest_with_predictions[(dftest_with_predictions[\"anomalytype\"]==\"contextual\") & (dftest_with_predictions[\"predictions\"]==1)])\n\n#Ratio - percentage predicted\nPercentage_Collective_Predicted=Predicted_Collective_Anomalies/Total_Collective_Anomalies\nPercentage_Contextual_Predicted=Predicted_Contextual_Anomalies/Total_Contextual_Anomalies\n\nprint(\"Percentage of Collective Anomalies Predicted: \"+str(Percentage_Collective_Predicted))\nprint(\"Percentage of Contextual Anomalies Predicted: \"+str(Percentage_Contextual_Predicted))\n",
"Percentage of Collective Anomalies Predicted: 0.8643067846607669\nPercentage of Contextual Anomalies Predicted: 0.8285714285714286\n"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
]
] |
e7bacf917e60d868aab765bdc00e4a6580b9b9b4 | 4,714 | ipynb | Jupyter Notebook | water-recognition_kaggle.ipynb | saif-sohel/music_suggestion | d96fc08216966c40f809b23eeadaaa1ea2f5e6cc | [
"Apache-2.0"
] | null | null | null | water-recognition_kaggle.ipynb | saif-sohel/music_suggestion | d96fc08216966c40f809b23eeadaaa1ea2f5e6cc | [
"Apache-2.0"
] | null | null | null | water-recognition_kaggle.ipynb | saif-sohel/music_suggestion | d96fc08216966c40f809b23eeadaaa1ea2f5e6cc | [
"Apache-2.0"
] | null | null | null | 4,714 | 4,714 | 0.664192 | [
[
[
"import tensorflow as tf\nimport numpy as np\nfrom tensorflow import keras\nimport os\nimport cv2\nfrom tensorflow.keras.preprocessing.image import ImageDataGenerator\nfrom tensorflow.keras.preprocessing import image\nimport matplotlib.pyplot as plt",
"_____no_output_____"
],
[
"import tensorflow as tf \n\nif tf.test.gpu_device_name(): \n\n print('Default GPU Device:{}'.format(tf.test.gpu_device_name()))\n\nelse:\n\n print(\"Please install GPU version of TF\")",
"_____no_output_____"
],
[
"train = ImageDataGenerator(rescale=1/255)\ntest = ImageDataGenerator(rescale=1/255)\n\ntrain_dataset = train.flow_from_directory(\"../input/water-recog/train\",\n target_size=(150,150),\n batch_size = 32,\n class_mode = 'binary')\n \ntest_dataset = test.flow_from_directory(\"../input/water-recog/test\",\n target_size=(150,150),\n batch_size =32,\n class_mode = 'binary')",
"_____no_output_____"
],
[
"test_dataset.class_indices",
"_____no_output_____"
],
[
"model = keras.Sequential()\n\n# Convolutional layer and maxpool layer 1\nmodel.add(keras.layers.Conv2D(32,(3,3),activation='relu',input_shape=(150,150,3)))\nmodel.add(keras.layers.MaxPool2D(2,2))\n\n# Convolutional layer and maxpool layer 2\nmodel.add(keras.layers.Conv2D(64,(3,3),activation='relu'))\nmodel.add(keras.layers.MaxPool2D(2,2))\n\n# Convolutional layer and maxpool layer 3\nmodel.add(keras.layers.Conv2D(128,(3,3),activation='relu'))\nmodel.add(keras.layers.MaxPool2D(2,2))\n\n# Convolutional layer and maxpool layer 4\nmodel.add(keras.layers.Conv2D(128,(3,3),activation='relu'))\nmodel.add(keras.layers.MaxPool2D(2,2))\n\n# This layer flattens the resulting image array to 1D array\nmodel.add(keras.layers.Flatten())\n\n# Hidden layer with 512 neurons and Rectified Linear Unit activation function \nmodel.add(keras.layers.Dense(512,activation='relu'))\n\n# Output layer with single neuron which gives 0 for Cat or 1 for Dog \n#Here we use sigmoid activation function which makes our model output to lie between 0 and 1\nmodel.add(keras.layers.Dense(1,activation='sigmoid'))",
"_____no_output_____"
],
[
"from tensorflow.keras.applications.vgg19 import VGG19\nvgg = VGG19(weights = \"imagenet\",include_top=False,input_shape=(150,150,3))",
"_____no_output_____"
],
[
"for layer in vgg.layers:\n layer.trainable = False",
"_____no_output_____"
],
[
"from tensorflow.keras import Sequential\nfrom tensorflow.keras.layers import Flatten,Dense\nmodel =Sequential()\nmodel.add(vgg)\nmodel.add(Flatten())\nmodel.add(Dense(1,activation=\"sigmoid\"))",
"_____no_output_____"
],
[
"model.compile(optimizer='adam',loss='binary_crossentropy',metrics=['accuracy'])",
"_____no_output_____"
],
[
"model.fit(train_dataset, epochs = 14, validation_data = test_dataset, batch_size=32)",
"_____no_output_____"
],
[
"model.save(\"./water_vgg19_kaggle.h5\")",
"_____no_output_____"
],
[
"model.evaluate(test_dataset)",
"_____no_output_____"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bad353302a3540846b90258dae11f81e15a392 | 28,199 | ipynb | Jupyter Notebook | docs/tutorials/sample_expval_program/qiskit_runtime_expval_program.ipynb | daka1510/qiskit-ibm-runtime | a0d606eafd2d069a9185236ef3b30cc0844b2c6a | [
"Apache-2.0"
] | null | null | null | docs/tutorials/sample_expval_program/qiskit_runtime_expval_program.ipynb | daka1510/qiskit-ibm-runtime | a0d606eafd2d069a9185236ef3b30cc0844b2c6a | [
"Apache-2.0"
] | null | null | null | docs/tutorials/sample_expval_program/qiskit_runtime_expval_program.ipynb | daka1510/qiskit-ibm-runtime | a0d606eafd2d069a9185236ef3b30cc0844b2c6a | [
"Apache-2.0"
] | null | null | null | 34.26367 | 775 | 0.567822 | [
[
[
"# Custom Expectation Value Program for the Qiskit Runtime\n\n\n<p>\n<font size=\"4\" color=\"#0f62fe\">Paul Nation</font>\n</p>\n<p>\n<font size=\"3\" color=\"#0f62fe\">IBM Quantum Partners Technical Enablement Team</font>\n</p>\n\nHere we will show how to make a program that takes a circuit, or list of circuits, and computes the expectation values of one or more diagonal operators.",
"_____no_output_____"
],
[
"## Prerequisites\n\n- You must have the latest Qiskit installed.\n- You must have either an IBM Cloud or an IBM Quantum account that can access Qiskit Runtime.",
"_____no_output_____"
],
[
"## Background\n\nThe primary method by which information is obtained from quantum computers is via expectation values. Indeed, the samples that come from executing a quantum circuit multiple times, once converted to probabilities, can be viewed as just a finite sample approximation to the expectation value for the projection operators corresponding to each bitstring. More practically, many quantum algorithms require computing expectation values over Pauli operators, e.g. Variational Quantum Eigensolvers, and thus having a runtime program that computes these quantities is of fundamental importance. Here we look at one such example, where an user passes one or more circuits and expectation operators and gets back the computed expectation values, and possibly error bounds.\n\n### Expectation value of a diagonal operator\n\nConsider a generic observable given by the tensor product of diagonal operators over $N$ qubits $O = O_{N-1}\\dots O_{0}$ where the subscript indicates the qubit on which the operator acts. Then for a set of observed $M$ bitstrings $\\{b_{0}, \\dots b_{M-1}\\}$, where $M \\leq 2^N $, with corresponding approximate probabilites $p_{m}$ the expectation value is given by\n\n$$\n\\langle O\\rangle \\simeq \\sum_{m=0}^{M-1} p_{m}\\prod_{n=0}^{N-1}O_{n}[b_{m}[N-n-1], b_{m}[N-n-1]],\n$$\n\nwhere $O_{n}[b_{m}[N-n-1], b_{m}[N-n-1]]$ is the diagonal element of $O_{n}$ specified by the $N-n-1$th bit in bitstring $b_{m}$. The reason for the complicated indexing in `b_{m}` is because Qiskit uses least-sginificant bit indexing where the zeroth element of the bit-strings is given by the right-most bit.\n\nHere we will use built-in routines to compute these expectation values. However, it is not hard to do yourself, with plenty of examples to be found.",
"_____no_output_____"
],
[
"## Main program\n\nHere we define our main function for the expectation value runtime program. As always, our program must start with the `backend`, and `user_messenger` arguements, followed by the actual inputs we pass to the program. Here our options are quite simple:\n\n- `circuits`: A single QuantumCircuit or list of QuantumCircuits to be executed on the target backend.\n\n\n- `expectation_operators`: The operators we want to evaluate. These can be strings of diagonal Pauli's, eg, `ZIZZ`, or custom operators defined by dictionarys. For example, the projection operator on the all ones state of 4 qubits is `{'1111': 1}`.\n\n\n- `shots`: Howe many times to sample each circuit.\n\n\n- `transpiler_config`: A dictionary that passes additional arguments on to the transpile function, eg. `optimization_level`.\n\n\n- `run_config`: A dictionary that passes additional arguments on to `backend.run()`.\n\n\n- `skip_transpilation`: A flag to skip transpilation altogether and just run the circuits. This is useful for situations where you need to transpile parameterized circuits once, but must bind parameters multiple times and evaluate. \n\n\n- `return_stddev`: Flag to return bound on standard deviation. If using measurement mitigation this adds some overhead to the computation.\n\n\n- `use_measurement_mitigation`: Use M3 measurement mitigation and compute expecation value and standard deviation bound from quasi-probabilities.\n\nAt the top of the cell below you will see a commented out `%%writefile sample_expval.py`. We will use this to convert the cell to a Python module named `sample_expval.py` to upload.",
"_____no_output_____"
]
],
[
[
"#%%writefile sample_expval.py\nimport mthree\nfrom qiskit import transpile\n\n# The entrypoint for our Runtime Program\ndef main(\n backend,\n user_messenger,\n circuits,\n expectation_operators=\"\",\n shots=8192,\n transpiler_config={},\n run_config={},\n skip_transpilation=False,\n return_stddev=False,\n use_measurement_mitigation=False,\n):\n\n \"\"\"Compute expectation\n values for a list of operators after\n executing a list of circuits on the target backend.\n\n Parameters:\n backend (ProgramBackend): Qiskit backend instance.\n user_messenger (UserMessenger): Used to communicate with the program user.\n circuits: (QuantumCircuit or list): A single list of QuantumCircuits.\n expectation_operators (str or dict or list): Expectation values to evaluate.\n shots (int): Number of shots to take per circuit.\n transpiler_config (dict): A collection of kwargs passed to transpile().\n run_config (dict): A collection of kwargs passed to backend.run().\n skip_transpilation (bool): Skip transpiling of circuits, default=False.\n return_stddev (bool): Return upper bound on standard devitation,\n default=False.\n use_measurement_mitigation (bool): Improve resulting using measurement\n error mitigation, default=False.\n\n Returns:\n array_like: Returns array of expectation values or a list of (expval, stddev)\n tuples if return_stddev=True.\n \"\"\"\n\n # transpiling the circuits using given transpile options\n if not skip_transpilation:\n trans_circuits = transpile(circuits, backend=backend, **transpiler_config)\n # Make sure everything is a list\n if not isinstance(trans_circuits, list):\n trans_circuits = [trans_circuits]\n # If skipping set circuits -> trans_circuits\n else:\n if not isinstance(circuits, list):\n trans_circuits = [circuits]\n else:\n trans_circuits = circuits\n\n # If we are given a single circuit but requesting multiple expectation\n # values, then set flag to make multiple pointers to same result.\n duplicate_results = False\n if isinstance(expectation_operators, list):\n if len(expectation_operators) and len(trans_circuits) == 1:\n duplicate_results = True\n\n # If doing measurement mitigation we must build and calibrate a\n # mitigator object. Will also determine which qubits need to be\n # calibrated.\n if use_measurement_mitigation:\n # Get an the measurement mappings at end of circuits\n meas_maps = mthree.utils.final_measurement_mapping(trans_circuits)\n # Get an M3 mitigator\n mit = mthree.M3Mitigation(backend)\n # Calibrate over the set of qubits measured in the transpiled circuits.\n mit.cals_from_system(meas_maps)\n\n # Compute raw results\n result = backend.run(trans_circuits, shots=shots, **run_config).result()\n raw_counts = result.get_counts()\n\n # When using measurement mitigation we need to apply the correction and then\n # compute the expectation values from the computed quasi-probabilities.\n if use_measurement_mitigation:\n quasi_dists = mit.apply_correction(\n raw_counts, meas_maps, return_mitigation_overhead=return_stddev\n )\n\n if duplicate_results:\n quasi_dists = mthree.classes.QuasiCollection(\n [quasi_dists] * len(expectation_operators)\n )\n # There are two different calls depending on what we want returned.\n if return_stddev:\n return quasi_dists.expval_and_stddev(expectation_operators)\n return quasi_dists.expval(expectation_operators)\n\n # If the program didn't return in the mitigation loop above it means\n # we are processing the raw_counts data. We do so here using the\n # mthree utilities\n if duplicate_results:\n raw_counts = [raw_counts] * len(expectation_operators)\n if return_stddev:\n return mthree.utils.expval_and_stddev(raw_counts, expectation_operators)\n return mthree.utils.expval(raw_counts, expectation_operators)",
"_____no_output_____"
]
],
[
[
"## Local testing\n\nHere we test with a local \"Fake\" backend that mimics the noise properties of a real system and a 4-qubit GHZ state.",
"_____no_output_____"
]
],
[
[
"from qiskit import QuantumCircuit\nfrom qiskit.test.mock import FakeSantiago\nfrom qiskit_ibm_runtime import UserMessenger\n\nmsg = UserMessenger()\nbackend = FakeSantiago()",
"_____no_output_____"
],
[
"qc = QuantumCircuit(4)\nqc.h(2)\nqc.cx(2, 1)\nqc.cx(1, 0)\nqc.cx(2, 3)\nqc.measure_all()",
"_____no_output_____"
],
[
"main(\n backend,\n msg,\n qc,\n expectation_operators=[\"ZZZZ\", \"IIII\", \"IZZZ\"],\n transpiler_config={\n \"optimization_level\": 3,\n \"layout_method\": \"sabre\",\n \"routing_method\": \"sabre\",\n },\n run_config={},\n skip_transpilation=False,\n return_stddev=False,\n use_measurement_mitigation=True,\n)",
"_____no_output_____"
]
],
[
[
"If we have done our job correctly, the above should print out two expectation values close to one and a final expectation value close to zero.",
"_____no_output_____"
],
[
"## Program metadata\n\nNext we add the needed program data to a dictionary for uploading with our program.",
"_____no_output_____"
]
],
[
[
"meta = {\n \"name\": \"sample-expval\",\n \"description\": \"A sample expectation value program.\",\n \"max_execution_time\": 1000,\n \"spec\": {},\n}\n\nmeta[\"spec\"][\"parameters\"] = {\n \"$schema\": \"https://json-schema.org/draft/2019-09/schema\",\n \"properties\": {\n \"circuits\": {\n \"description\": \"A single or list of QuantumCircuits.\",\n \"type\": [\"array\", \"object\"],\n },\n \"expectation_operators\": {\n \"description\": \"One or more expectation values to evaluate.\",\n \"type\": [\"string\", \"object\", \"array\"],\n },\n \"shots\": {\"description\": \"Number of shots per circuit.\", \"type\": \"integer\"},\n \"transpiler_config\": {\n \"description\": \"A collection of kwargs passed to transpile.\",\n \"type\": \"object\",\n },\n \"run_config\": {\n \"description\": \"A collection of kwargs passed to backend.run. Default is False.\",\n \"type\": \"object\",\n \"default\": False,\n },\n \"return_stddev\": {\n \"description\": \"Return upper-bound on standard deviation. Default is False.\",\n \"type\": \"boolean\",\n \"default\": False,\n },\n \"use_measurement_mitigation\": {\n \"description\": \"Use measurement mitigation to improve results. Default is False.\",\n \"type\": \"boolean\",\n \"default\": False,\n },\n },\n \"required\": [\"circuits\"],\n}\n\nmeta[\"spec\"][\"return_values\"] = {\n \"$schema\": \"https://json-schema.org/draft/2019-09/schema\",\n \"description\": \"A list of expectation values and optionally standard deviations.\",\n \"type\": \"array\",\n}",
"_____no_output_____"
]
],
[
[
"## Upload the program\n\nWe are now in a position to upload the program. To do so we first uncomment and excute the line `%%writefile sample_expval.py` giving use the `sample_expval.py` file we need to upload. ",
"_____no_output_____"
]
],
[
[
"from qiskit_ibm_runtime import IBMRuntimeService\n\nservice = IBMRuntimeService(auth=\"legacy\")",
"_____no_output_____"
],
[
"program_id = service.upload_program(data=\"sample_expval.py\", metadata=meta)\nprogram_id",
"_____no_output_____"
]
],
[
[
"### Delete program if needed",
"_____no_output_____"
]
],
[
[
"# service.delete_program(program_id)",
"_____no_output_____"
]
],
[
[
"## Wrapping the runtime program\n\nAs always, it is best to wrap the call to the runtime program with a function (or possibly a class) that makes input easier and does some validation.",
"_____no_output_____"
]
],
[
[
"def expectation_value_runner(\n backend,\n circuits,\n expectation_operators=\"\",\n shots=8192,\n transpiler_config={},\n run_config={},\n skip_transpilation=False,\n return_stddev=False,\n use_measurement_mitigation=False,\n):\n\n \"\"\"Compute expectation values for a list of operators after\n executing a list of circuits on the target backend.\n\n Parameters:\n backend (Backend or str): Qiskit backend instance or name.\n circuits: (QuantumCircuit or list): A single or list of QuantumCircuits.\n expectation_operators (str or dict or list): Expectation values to evaluate.\n shots (int): Number of shots to take per circuit.\n transpiler_config (dict): A collection of kwargs passed to transpile().\n run_config (dict): A collection of kwargs passed to backend.run().\n return_stddev (bool): Return upper bound on standard devitation,\n default=False.\n skip_transpilation (bool): Skip transpiling of circuits, default=False.\n use_measurement_mitigation (bool): Improve resulting using measurement\n error mitigation, default=False.\n\n Returns:\n array_like: Returns array of expectation values or a list of (expval, stddev)\n pairs if return_stddev=True.\n \"\"\"\n if not isinstance(backend, str):\n backend = backend.name\n options = {\"backend_name\": backend}\n\n if isinstance(circuits, list) and len(circuits) != 1:\n if isinstance(expectation_operators, list):\n if len(circuits) != 1 and len(expectation_operators) == 1:\n expectation_operators = expectation_operators * len(circuits)\n elif len(circuits) != len(expectation_operators):\n raise ValueError(\n \"Number of circuits must match number of expectation \\\n values if more than one of each\"\n )\n inputs = {}\n inputs[\"circuits\"] = circuits\n inputs[\"expectation_operators\"] = expectation_operators\n inputs[\"shots\"] = shots\n inputs[\"transpiler_config\"] = transpiler_config\n inputs[\"run_config\"] = run_config\n inputs[\"return_stddev\"] = return_stddev\n inputs[\"skip_transpilation\"] = skip_transpilation\n inputs[\"use_measurement_mitigation\"] = use_measurement_mitigation\n\n return service.run(program_id, options=options, inputs=inputs)",
"_____no_output_____"
]
],
[
[
"### Trying it out\n\nLets try running the program here with our previously made GHZ state and running on the simulator.",
"_____no_output_____"
]
],
[
[
"backend = \"ibmq_qasm_simulator\"\n\nall_zeros_proj = {\"0000\": 1}\nall_ones_proj = {\"1111\": 1}\njob = expectation_value_runner(backend, qc, [all_zeros_proj, all_ones_proj, \"ZZZZ\"])",
"_____no_output_____"
],
[
"job.result()",
"_____no_output_____"
]
],
[
[
"The first two projectors should be nearly $0.50$ as they tell use the probability of being in the all zeros and ones states, respectively, which should be 50/50 for our GHZ state. The final expectation value of `ZZZZ` should be one since this is a GHZ over an even number of qubits. It should go close to zero for an odd number.",
"_____no_output_____"
]
],
[
[
"qc2 = QuantumCircuit(3)\nqc2.h(2)\nqc2.cx(2, 1)\nqc2.cx(1, 0)\nqc2.measure_all()",
"_____no_output_____"
],
[
"all_zeros_proj = {\"000\": 1}\nall_ones_proj = {\"111\": 1}\njob2 = expectation_value_runner(backend, qc2, [all_zeros_proj, all_ones_proj, \"ZZZ\"])",
"_____no_output_____"
],
[
"job2.result()",
"_____no_output_____"
]
],
[
[
"## Quantum Volume as an expectation value\n\nHere we formulate QV as an expectation value of a projector onto the heavy-output elements on a distribution. We can then use our expectation value routine to compute whether a given circuit has passed the QV metric.\n\nQV is defined in terms of heavy-ouputs of a distribution. Heavy-outputs are those bit-strings that are those that have probabilities above the median value of the distribution. Below we define the projection operator onto the set of bit-strings that are heavy-outputs for a given distribution.",
"_____no_output_____"
]
],
[
[
"def heavy_projector(qv_probs):\n \"\"\"Forms the projection operator onto the heavy-outputs of a given probability distribution.\n\n Parameters:\n qv_probs (dict): A dictionary of bitstrings and associated probabilities.\n\n Returns:\n dict: Projector onto the heavy-set.\n \"\"\"\n median_prob = np.median(list(qv_probs.values()))\n heavy_strs = {}\n for key, val in qv_probs.items():\n if val > median_prob:\n heavy_strs[key] = 1\n return heavy_strs",
"_____no_output_____"
]
],
[
[
"Now we generate 10 QV circuits as our dataset.",
"_____no_output_____"
]
],
[
[
"import numpy as np\nfrom qiskit.quantum_info import Statevector\nfrom qiskit.circuit.library import QuantumVolume\n\n# Generate QV circuits\nN = 10\nqv_circs = [QuantumVolume(5) for _ in range(N)]",
"_____no_output_____"
]
],
[
[
"Next, we have to determine the heavy-set of each circuit from the ideal answer, and then pass this along to our heavy-set projector function that we defined above.",
"_____no_output_____"
]
],
[
[
"ideal_probs = [\n Statevector.from_instruction(circ).probabilities_dict() for circ in qv_circs\n]\nheavy_projectors = [heavy_projector(probs) for probs in ideal_probs]",
"_____no_output_____"
]
],
[
[
"QV circuits have no meaasurements on them so need to add them:",
"_____no_output_____"
]
],
[
[
"circs = [circ.measure_all(inplace=False) for circ in qv_circs]",
"_____no_output_____"
]
],
[
[
"With a list of circuits and projection operators we now need only to pass both sets to our above expection value runner targeting the desired backend. We will also set the best transpiler arguments to give us a sporting chance of getting some passing scores.",
"_____no_output_____"
]
],
[
[
"backend = \"ibmq_manila\"",
"_____no_output_____"
],
[
"job3 = expectation_value_runner(\n backend,\n circs,\n heavy_projectors,\n transpiler_config={\n \"optimization_level\": 3,\n \"layout_method\": \"sabre\",\n \"routing_method\": \"sabre\",\n },\n)",
"_____no_output_____"
],
[
"qv_scores = job3.result()\nqv_scores",
"_____no_output_____"
]
],
[
[
"A passing QV score is one where the value of the heavy-set projector is above $2/3$. So let us see who passed:",
"_____no_output_____"
]
],
[
[
"qv_scores > 2 / 3",
"_____no_output_____"
],
[
"from qiskit.tools.jupyter import *\n\n%qiskit_copyright",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
]
] |
e7badfcaf8af0d4cb476327a18277726e54699a0 | 547,526 | ipynb | Jupyter Notebook | convert-jp-m-adapt8-Copy1.ipynb | Akio-m/evgmm | b7f89f4da34a1d4ca4b233dd2fab4d146512e8f7 | [
"MIT"
] | 2 | 2018-10-29T06:50:24.000Z | 2019-09-29T01:44:11.000Z | convert-jp-m-adapt8-Copy1.ipynb | Akio-m/evgmm | b7f89f4da34a1d4ca4b233dd2fab4d146512e8f7 | [
"MIT"
] | 2 | 2018-01-29T13:33:05.000Z | 2018-02-03T15:06:30.000Z | convert-jp-m-adapt8.ipynb | Akio-m/evgmm | b7f89f4da34a1d4ca4b233dd2fab4d146512e8f7 | [
"MIT"
] | null | null | null | 37.919939 | 20,532 | 0.624111 | [
[
[
"# -*- coding: utf-8 -*-\n\n\"\"\"\nThis program makes learning ev-gmm.\n\"\"\"",
"_____no_output_____"
],
[
"# __future__ module make compatible python2 and python3\nfrom __future__ import division, print_function\n\n# basic modules\nimport os\nimport os.path\nimport time\n\n# for warning ignore\nimport warnings\n#warning.filterwarnings('ignore')\n\n# for file system manupulation \nfrom shutil import rmtree \nimport glob\nimport argparse\n\n# for save object\nimport pickle\n\n# for make glaph\n%matplotlib inline\nimport matplotlib.pyplot as plt\nimport seaborn as sns\nsns.set_style(\"whitegrid\")\nplt.rcParams['figure.figsize'] = (16, 5)\nimport librosa.display\n\n# for scientific computing\nimport numpy as np\nfrom numpy.linalg import norm \nfrom sklearn.decomposition import PCA\nfrom sklearn.mixture import GMM # GMM class cannot use after sklearn 0.20.0\nimport sklearn.mixture\n#from sklearn.mixture.gaussian_mixture import _compute_precision_cholesky\nfrom sklearn.preprocessing import StandardScaler\nimport scipy.sparse\nfrom scipy.signal import firwin, lfilter\n\n# for display audio controler\nfrom IPython.display import Audio\n\n# for manuplate audio data\nimport soundfile as sf\nimport pyworld as pw\nimport pysptk\nfrom dtw import dtw\nfrom fastdtw import fastdtw",
"_____no_output_____"
],
[
"class WORLD(object):\n \"\"\"\n WORLD based speech analyzer and synthezer.\n \n Ref : https://github.com/k2kobayashi/sprocket/\n \"\"\"\n def __init__(self, fs=16000, fftl=1024, shiftms=5.0, minf0=40.0, maxf0=500.0):\n \"\"\"\n Parameters\n ----------\n fs : int \n Sampling frequency \n fftl : int\n FFT length\n shiftms : float\n Shift length [ms]\n minf0 : float\n Floor in F0 estimation\n maxf0 : float\n Seli in F0 estimation\n \"\"\"\n \n self.fs = fs\n self.fftl = fftl\n self.shiftms = shiftms\n self.minf0 = minf0\n self.maxf0 = maxf0\n \n def analyze(self, x):\n \"\"\"\n Analyze acoustic featueres.\n \n Parameters\n ----------\n x : array, shape(`T`)\n monoral speech signal in time domain\n \n Returns\n ----------\n f0 : array, shape(`T`)\n F0 sequence\n sp : array, shape(`T`, `fftl / 2 + 1`)\n Spectral envelope sequence\n ap : array, shape(`T`, `fftl / 2 + 1`)\n aperiodicity sequence\n \"\"\"\n \n f0, time_axis = pw.harvest(x, self.fs, f0_floor=self.minf0,\n f0_ceil=self.maxf0, frame_period=self.shiftms)\n sp = pw.cheaptrick(x, f0, time_axis, self.fs, fft_size=self.fftl)\n ap = pw.d4c(x, f0, time_axis, self.fs, fft_size=self.fftl)\n \n assert sp.shape == ap.shape\n \n return f0, sp, ap\n \n def analyze_f0(self, x):\n \"\"\"\n Analyze f0.\n \n Parameters\n ----------\n x : array, shape(`T`)\n monoral speech signal in time domain\n \n Returns\n ----------\n f0 : array, shape(`T`)\n F0 sequence\n \"\"\"\n\n f0, time_axis = pw.harvest(x, self.fs, f0_floor=self.minf0,\n f0_ceil=self.maxf0, frame_period=self.shiftms)\n \n assert f0.shape == x.shape()\n \n return f0\n \n def synthesis(self, f0, sp, ap):\n \"\"\"\n Re-synthesizes a speech waveform from acoustic featueres.\n \n Parameters\n ----------\n f0 : array, shape(`T`)\n F0 sequence\n sp : array, shape(`T`, `fftl / 2 + 1`)\n Spectral envelope sequence\n ap : array, shape(`T`, `fftl / 2 + 1`)\n aperiodicity sequence\n \"\"\"\n\n return pw.synthesize(f0, sp, ap, self.fs, frame_period=self.shiftms)",
"_____no_output_____"
],
[
"class FeatureExtractor(object):\n \"\"\"\n Analyze acoustic features from a waveform.\n \n This class may have several types of estimeter like WORLD or STRAIGHT.\n Default type is WORLD.\n \n Ref : https://github.com/k2kobayashi/sprocket/\n \"\"\"\n \n def __init__(self, analyzer='world', fs=16000, fftl=1024, \n shiftms=5.0, minf0=50.0, maxf0=500.0):\n \"\"\"\n Parameters\n ----------\n analyzer : str\n Analyzer\n fs : int \n Sampling frequency \n fftl : int\n FFT length\n shiftms : float\n Shift length [ms]\n minf0 : float\n Floor in F0 estimation\n maxf0 : float\n Seli in F0 estimation\n \"\"\"\n \n self.analyzer = analyzer\n self.fs = fs\n self.fftl = fftl\n self.shiftms = shiftms\n self.minf0 = minf0\n self.maxf0 = maxf0\n \n if self.analyzer == 'world':\n self.analyzer = WORLD(fs=self.fs, fftl=self.fftl, \n minf0=self.minf0, maxf0=self.maxf0, shiftms=self.shiftms)\n else:\n raise('Analyzer Error : not support type, see FeatureExtractor class.')\n \n self._f0 = None\n self._sp = None\n self._ap = None\n \n def analyze(self, x):\n \"\"\"\n Analyze acoustic featueres.\n \n Parameters\n ----------\n x : array, shape(`T`)\n monoral speech signal in time domain\n \n Returns\n ----------\n f0 : array, shape(`T`)\n F0 sequence\n sp : array, shape(`T`, `fftl / 2 + 1`)\n Spectral envelope sequence\n ap : array, shape(`T`, `fftl / 2 + 1`)\n aperiodicity sequence\n \"\"\"\n \n self.x = np.array(x, dtype=np.float)\n self._f0, self._sp, self._ap = self.analyzer.analyze(self.x)\n \n # check f0 < 0\n self._f0[self._f0 < 0] = 0\n \n if np.sum(self._f0) == 0.0:\n print(\"Warning : F0 values are all zero.\")\n \n return self._f0, self._sp, self._ap\n \n def analyze_f0(self, x):\n \"\"\"\n Analyze f0.\n \n Parameters\n ----------\n x : array, shape(`T`)\n monoral speech signal in time domain\n \n Returns\n ----------\n f0 : array, shape(`T`)\n F0 sequence\n \"\"\"\n\n self.x = np.array(x, dtype=np.float)\n self._f0 = self.analyzer.analyze_f0(self.x)\n\n # check f0 < 0\n self._f0[self._f0 < 0] = 0\n \n if np.sum(self._f0) == 0.0:\n print(\"Warning : F0 values are all zero.\")\n \n return self._f0\n \n def mcep(self, dim=24, alpha=0.42):\n \"\"\"\n Convert mel-cepstrum sequence from spectral envelope.\n \n Parameters\n ----------\n dim : int\n mel-cepstrum dimension\n alpha : float\n parameter of all-path filter\n \n Returns\n ----------\n mcep : array, shape(`T`, `dim + 1`)\n mel-cepstrum sequence\n \"\"\" \n \n self._analyzed_check()\n \n return pysptk.sp2mc(self._sp, dim, alpha)\n \n def codeap(self):\n \"\"\"\n \"\"\"\n self._analyzed_check()\n \n return pw.code_aperiodicity(self._ap, self.fs)\n \n def npow(self):\n \"\"\"\n Normalized power sequence from spectral envelope.\n \n Returns\n ----------\n npow : vector, shape(`T`, `1`)\n Normalized power sequence of the given waveform\n \"\"\"\n \n self._analyzed_check()\n \n npow = np.apply_along_axis(self._spvec2pow, 1, self._sp)\n \n meanpow = np.mean(npow)\n npow = 10.0 * np.log10(npow / meanpow)\n \n return npow\n \n def _spvec2pow(self, specvec):\n \"\"\"\n \"\"\"\n fftl2 = len(specvec) - 1\n fftl = fftl2 * 2\n \n power = specvec[0] + specvec[fftl2]\n for k in range(1, fftl2):\n power += 2.0 * specvec[k]\n power /= fftl\n \n return power\n \n def _analyzed_check(self):\n if self._f0 is None and self._sp is None and self._ap is None:\n raise('Call FeatureExtractor.analyze() before this method.')",
"_____no_output_____"
],
[
"class Synthesizer(object):\n \"\"\"\n Synthesize a waveform from acoustic features.\n \n Ref : https://github.com/k2kobayashi/sprocket/\n \"\"\"\n def __init__(self, fs=16000, fftl=1024, shiftms=5.0):\n \"\"\"\n Parameters\n ----------\n fs : int \n Sampling frequency \n fftl : int\n FFT length\n shiftms : float\n Shift length [ms]\n \"\"\"\n \n self.fs = fs\n self.fftl = fftl\n self.shiftms = shiftms\n \n def synthesis(self, f0, mcep, ap, rmcep=None, alpha=0.42):\n \"\"\"\n Re-synthesizes a speech waveform from acoustic featueres.\n \n Parameters\n ----------\n f0 : array, shape(`T`)\n F0 sequence\n mcep : array, shape(`T`, `dim`)\n mel-cepstrum sequence\n ap : array, shape(`T`, `fftl / 2 + 1`)\n aperiodicity sequence\n rmcep : array, shape(`T`, `dim`)\n array of reference mel-cepstrum sequence\n alpha : float\n parameter of all-path filter\n \n Returns\n ----------\n wav : array,\n syntesized waveform\n \"\"\"\n \n if rmcep is not None:\n # power modification\n mcep = mod_power(mcep, rmcep, alpha=alpha)\n \n sp = pysptk.mc2sp(mcep, alpha, self.fftl)\n wav = pw.synthesize(f0, sp, ap, self.fs, frame_period=self.shiftms)\n \n return wav\n \n def synthesis_diff(self, x, diffmcep, rmcep=None, alpha=0.42):\n \"\"\"\n Re-synthesizes a speech waveform from acoustic featueres.\n filtering with a differential mel-cepstrum.\n \n Parameters\n ----------\n x : array, shape(`samples`)\n array of waveform sequence\n diffmcep : array, shape(`T`, `dim`)\n array of differential mel-cepstrum sequence\n rmcep : array, shape(`T`, `dim`)\n array of reference mel-cepstrum sequence\n alpha : float\n parameter of all-path filter\n \n Returns\n ----------\n wav : array,\n syntesized waveform\n \"\"\" \n \n x = x.astype(np.float64)\n dim = diffmcep.shape[1] - 1 \n shiftl = int(self.fs / 1000 * self.shiftms)\n \n if rmcep is not None:\n # power modification\n diffmcep = mod_power(rmcep + diffmcep, rmcep, alpha=alpha) - rmcep \n \n # mc2b = transform mel-cepstrum to MLSA digital filter coefficients.\n b = np.apply_along_axis(pysptk.mc2b, 1, diffmcep, alpha)\n \n mlsa_fil = pysptk.synthesis.Synthesizer(pysptk.synthesis.MLSADF(dim, alpha=alpha),\n shiftl)\n wav = mlsa_fil.synthesis(x, b)\n \n return wav\n \n def synthesis_sp(self, f0, sp, ap):\n \"\"\"\n Re-synthesizes a speech waveform from acoustic featueres.\n \n Parameters\n ----------\n f0 : array, shape(`T`)\n F0 sequence\n spc : array, shape(`T`, `dim`)\n mel-cepstrum sequence\n ap : array, shape(`T`, `fftl / 2 + 1`)\n aperiodicity sequence\n \n Returns\n ----------\n wav : array,\n syntesized waveform\n \"\"\" \n \n wav = pw.synthesize(f0, sp, ap, self.fs, frame_period=self.shiftms)\n \n return wav\n \ndef mod_power(cvmcep, rmcep, alpha=0.42, irlen=256):\n \"\"\"\n power modification based on inpuulse responce\n\n Parameters\n ----------\n cvmcep : array, shape(`T`, `dim`)\n array of converted mel-cepstrum\n rmcep : arraym shape(`T`, `dim`)\n array of reference mel-cepstrum\n alpha : float\n parameter of all-path filter\n irlen : int\n Length for IIR filter\n\n Returns\n ----------\n modified_cvmcep : array, shape(`T`, `dim`)\n array of power modified converted mel-cepstrum\n \"\"\"\n\n if rmcep.shape != cvmcep.shape:\n raise ValueError(\n \"The shape of the converted and reference mel-cepstrum are different : {} / {}.format(cvmcep.shape, rmcep.shape)\"\n )\n\n # mc2e = Compute energy from mel-cepstrum. e-option\n cv_e = pysptk.mc2e(cvmcep, alpha=alpha, irlen=irlen)\n r_e = pysptk.mc2e(rmcep, alpha=alpha, irlen=irlen)\n\n dpow = np.log(r_e / cv_e) / 2\n\n modified_cvmcep = np.copy(cvmcep)\n modified_cvmcep[:, 0] += dpow\n\n return modified_cvmcep",
"_____no_output_____"
],
[
"# def util methods\ndef melcd(array1, array2):\n \"\"\"\n calculate mel-cepstrum distortion\n \n Parameters\n ----------\n array1, array2 : array, shape(`T`, `dim`) or shape(`dim`)\n Array of original and target.\n \n Returns\n ----------\n mcd : scala, number > 0\n Scala of mel-cepstrum distoriton\n \"\"\"\n if array1.shape != array2.shape:\n raise ValueError(\n \"The shape of both array are different : {} / {}.format(array1.shape,array2.shape)\"\n ) \n \n if array1.ndim == 2:\n diff = array1 - array2\n mcd = 10.0 / np.log(10) * np.mean(np.sqrt(2.0 * np.sum(diff ** 2, axis=1)))\n elif array1.ndim == 1:\n diff = array1 - array2\n mcd = 10.0 / np.log(10) * np.sqrt(2.0 * np.sum(diff ** 2))\n else:\n raise ValueError(\"Dimension mismatch.\")\n \n return mcd\n\ndef delta(data, win=[-1.0, 1.0, 0]):\n \"\"\"\n calculate delta component\n \n Parameters\n ----------\n data : array, shape(`T`, `dim`)\n Array of static matrix sequence.\n win : array, shape(`3`)\n The shape of window matrix.\n \n Returns\n ----------\n delta : array, shape(`T`, `dim`)\n Array of delta matrix sequence.\n \"\"\"\n \n if data.ndim == 1:\n # change vector into 1d-array\n T = len(data)\n dim = data.ndim\n data = data.reshape(T, dim)\n else:\n T, dim = data.shape\n \n win = np.array(win, dtype=np.float64)\n delta = np.zeros((T, dim))\n \n delta[0] = win[0] * data[0] + win[1] * data[1]\n delta[-1] = win[0] * data[-2] + win[1] * data[-1]\n \n for i in range(len(win)):\n delta[1:T - 1] += win[i] * delta[i:T - 2 + i]\n \n return delta\n\ndef static_delta(data, win=[-1.0, 1.0, 0]):\n \"\"\"\n calculate static and delta component\n \n Parameters\n ----------\n data : array, shape(`T`, `dim`)\n Array of static matrix sequence.\n win : array, shape(`3`)\n The shape of window matrix.\n \n Returns\n ----------\n sddata : array, shape(`T`, `dim * 2`)\n Array of static and delta matrix sequence.\n \"\"\"\n \n sddata = np.c_[data, delta(data, win)]\n \n assert sddata.shape[1] == data.shape[1] * 2\n \n return sddata\n\ndef construct_static_and_delta_matrix(T, D, win=[-1.0, 1.0, 0]):\n \"\"\"\n calculate static and delta transformation matrix\n \n Parameters\n ----------\n T : scala, `T`\n Scala of time length\n D : scala, `D`\n Scala of the number of dimension.\n win : array, shape(`3`)\n The shape of window matrix.\n \n Returns\n ----------\n W : array, shape(`2 * D * T`, `D * T`)\n Array of static and delta transformation matrix.\n \"\"\"\n \n static = [0, 1, 0]\n delta = win\n assert len(static) == len(delta)\n \n # generate full W\n DT = D * T\n ones = np.ones(DT)\n row = np.arange(2 * DT).reshape(2 * T, D) # generate serial numbers\n static_row = row[::2] # [1,2,3,4,5] => [1,3,5]\n delta_row = row[1::2] # [1,2,3,4,5] => [2,4]\n col = np.arange(DT)\n \n data = np.array([ones * static[0], ones * static[1],\n ones * static[2], ones * delta[0],\n ones * delta[1], ones * delta[2]]).flatten()\n row = np.array([[static_row] * 3, [delta_row] * 3]).flatten()\n col = np.array([[col - D, col, col + D] * 2]).flatten()\n\n # remove component at first and end frame\n valid_idx = np.logical_not(np.logical_or(col < 0, col >= DT))\n \n W = scipy.sparse.csr_matrix(\n (data[valid_idx], (row[valid_idx], col[valid_idx])), shape=(2 * DT, DT))\n W.eliminate_zeros()\n \n return W\n \ndef extfrm(data, npow, power_threshold=-20):\n \"\"\"\n Extract frame over the power threshold\n \n Parameters\n ----------\n data : array, shape(`T`, `dim`)\n array of input data\n npow : array, shape(`T`)\n vector of normalized power sequence\n threshold : scala\n scala of power threshold [dB]\n \n Returns\n ----------\n data : array, shape(`T_ext`, `dim`)\n remaining data after extracting frame\n `T_ext` <= `T`\n \"\"\"\n T = data.shape[0]\n if T != len(npow):\n raise(\"Length of two vectors is different.\")\n \n valid_index = np.where(npow > power_threshold)\n extdata = data[valid_index]\n assert extdata.shape[0] <= T\n \n return extdata\n\ndef estimate_twf(orgdata, tardata, distance='melcd', fast=True, otflag=None):\n \"\"\"\n time warping function estimator\n \n Parameters\n ----------\n orgdata : array, shape(`T_org`, `dim`)\n array of source feature\n tardata : array, shape(`T_tar`, `dim`)\n array of target feature\n distance : str \n distance function\n fast : bool\n use fastdtw instead of dtw\n otflag : str\n Alignment into the length of specification\n 'org' : alignment into original length\n 'tar' : alignment into target length \n \n Returns\n ----------\n twf : array, shape(`2`, `T`)\n time warping function between original and target\n \"\"\"\n \n if distance == 'melcd':\n def distance_func(x, y): return melcd(x, y)\n else:\n raise ValueError('this distance method is not support.')\n \n if fast:\n _, path = fastdtw(orgdata, tardata, dist=distance_func)\n twf = np.array(path).T\n else:\n _, _, _, twf = dtw(orgdata, tardata, distance_func) \n \n if otflag is not None:\n twf = modify_twf(twf, otflag=otflag)\n \n return twf\n\ndef align_data(org_data, tar_data, twf):\n \"\"\"\n get aligned joint feature vector\n \n Parameters\n ----------\n org_data : array, shape(`T_org`, `dim_org`)\n Acoustic feature vector of original speaker\n tar_data : array, shape(`T_tar`, `dim_tar`)\n Acoustic feature vector of target speaker\n twf : array, shape(`2`, `T`)\n time warping function between original and target\n \n Returns\n ----------\n jdata : array, shape(`T_new`, `dim_org + dim_tar`)\n Joint feature vector between source and target\n \"\"\"\n \n jdata = np.c_[org_data[twf[0]], tar_data[twf[1]]]\n return jdata\n\ndef modify_twf(twf, otflag=None):\n \"\"\"\n align specified length\n \n Parameters\n ----------\n twf : array, shape(`2`, `T`)\n time warping function between original and target\n otflag : str\n Alignment into the length of specification\n 'org' : alignment into original length\n 'tar' : alignment into target length \n \n Returns\n ----------\n mod_twf : array, shape(`2`, `T_new`)\n time warping function of modified alignment\n \"\"\"\n \n if otflag == 'org':\n of, indice = np.unique(twf[0], return_index=True)\n mod_twf = np.c_[of, twf[1][indice]].T\n elif otflag == 'tar':\n tf, indice = np.unique(twf[1], return_index=True)\n mod_twf = np.c_[twf[0][indice], tf].T \n \n return mod_twf\n\ndef low_cut_filter(x, fs, cutoff=70):\n \"\"\"\n low cut filter\n \n Parameters\n ----------\n x : array, shape('samples')\n waveform sequence\n fs : array, int\n Sampling frequency\n cutoff : float\n cutoff frequency of low cut filter\n \n Returns\n ----------\n lct_x : array, shape('samples')\n Low cut filtered waveform sequence\n \"\"\"\n \n nyquist = fs // 2\n norm_cutoff = cutoff / nyquist\n \n # low cut filter\n fil = firwin(255, norm_cutoff, pass_zero=False)\n lct_x = lfilter(fil, 1, x)\n \n return lct_x\n\ndef extsddata(data, npow, power_threshold=-20):\n \"\"\"\n get power extract static and delta feature vector\n \n Parameters\n ----------\n data : array, shape(`T`, `dim`)\n acoustic feature vector\n npow : array, shape(`T`)\n normalized power vector\n power_threshold : float\n power threshold\n \n Returns\n ----------\n extsddata : array, shape(`T_new`, `dim * 2`)\n silence remove static and delta feature vector\n \"\"\"\n \n extsddata = extfrm(static_delta(data), npow, power_threshold=power_threshold)\n return extsddata\n\ndef transform_jnt(array_list):\n num_files = len(array_list)\n for i in range(num_files):\n if i == 0:\n jnt = array_list[i]\n else:\n jnt = np.r_[jnt, array_list[i]]\n return jnt\n ",
"_____no_output_____"
],
[
"class F0statistics(object):\n \"\"\"\n Estimate F0 statistics and convert F0\n \"\"\"\n def __init__(self):\n pass\n \n def estimate(self, f0list):\n \"\"\"\n estimate F0 statistics from list of f0\n \n Parameters\n ----------\n f0list : list, shape(`f0num`)\n List of several F0 sequence\n \n Returns\n ----------\n f0stats : array, shape(`[mean, std]`)\n values of mean and standard deviation for log f0\n \"\"\"\n \n n_files = len(f0list)\n assert n_files != 0\n\n for i in range(n_files):\n f0 = f0list[i]\n nonzero_indices = np.nonzero(f0)\n if i == 0:\n f0s = np.log(f0[nonzero_indices])\n else:\n f0s = np.r_[f0s, np.log(f0[nonzero_indices])]\n \n f0stats = np.array([np.mean(f0s), np.std(f0s)])\n \n return f0stats\n\n def convert(self, f0, orgf0stats, tarf0stats):\n \"\"\"\n convert F0 based on F0 statistics\n \n Parameters\n ----------\n f0 : array, shape(`T`, `1`)\n array of F0 sequence\n orgf0stats : array, shape(`[mean, std]`)\n vectors of mean and standard deviation of log f0 for original speaker\n tarf0stats : array, shape(`[mean, std]`)\n vectors of mean and standard deviation of log f0 for target speaker\n \n Returns\n ----------\n cvf0 : array, shape(`T`, `1`)\n array of converted F0 sequence\n \"\"\"\n \n # get length and dimension\n T = len(f0)\n \n # perform f0 conversion\n cvf0 = np.zeros(T)\n \n nonzero_indices = f0 > 0\n cvf0[nonzero_indices] = np.exp((tarf0stats[1] / orgf0stats[1]) * (np.log(f0[nonzero_indices]) - orgf0stats[0]) + tarf0stats[0])\n \n return cvf0",
"_____no_output_____"
],
[
"class GV(object):\n \"\"\"\n Estimate statistics and perform postfilter based on the GV statistics.\n \"\"\"\n def __init__(self):\n pass\n \n def estimate(self, datalist):\n \"\"\"\n estimate GV statistics from list of data\n \n Parameters\n ----------\n datalist : list, shape(`num_data`)\n List of several data ([T, dim]) sequence\n \n Returns\n ----------\n gvstats : array, shape(`2`, `dim`)\n array of mean and standard deviation for GV\n \"\"\"\n \n n_files = len(datalist)\n assert n_files != 0\n \n var = []\n for i in range(n_files):\n data = datalist[i]\n var.append(np.var(data, axis=0))\n \n # calculate vm and vv\n vm = np.mean(np.array(var), axis=0)\n vv = np.var(np.array(var), axis=0)\n gvstats = np.r_[vm, vv]\n gvstats = gvstats.reshape(2, len(vm))\n \n return gvstats\n\n def postfilter(self, data, gvstats, cvgvstats=None, alpha=1.0, startdim=1):\n \"\"\"\n perform postfilter based on GV statistics into data\n \n Parameters\n ----------\n data : array, shape(`T`, `dim`)\n array of data sequence\n gvstats : array, shape(`2`, `dim`)\n array of mean and variance for target GV\n cvgvstats : array, shape(`2`, `dim`)\n array of mean and variance for converted GV\n alpha : float\n morphing coefficient between GV transformed data and data.\n alpha * gvpf(data) + (1 - alpha) * data\n startdim : int\n start dimension to perform GV postfilter\n \n Returns\n ----------\n filtered_data : array, shape(`T`, `data`)\n array of GV postfiltered data sequnece\n \"\"\"\n \n # get length and dimension\n T, dim = data.shape\n assert gvstats is not None\n assert dim == gvstats.shape[1]\n \n # calculate statics of input data\n datamean = np.mean(data, axis=0)\n \n if cvgvstats is None:\n # use variance of the given data\n datavar = np.var(data, axis=0)\n else:\n # use variance of trained gv stats\n datavar = cvgvstats[0]\n \n # perform GV postfilter\n filterd = np.sqrt(gvstats[0, startdim:] / datavar[startdim:]) * (data[:, startdim:] - datamean[startdim:]) + datamean[startdim:]\n \n filterd_data = np.c_[data[:, :startdim], filterd]\n \n return alpha * filterd_data + (1 - alpha) * data",
"_____no_output_____"
],
[
"# 0. config path\n__versions = \"pre-stored-jp\"\n__same_path = \"./utterance/\" + __versions + \"/\"\nprepare_path = __same_path + \"output/\"\npre_stored_source_list = __same_path + 'pre-source/**/V01/T01/**/*.wav'\npre_stored_list = __same_path + \"pre/**/V01/T01/**/*.wav\"\noutput_path = \"./utterance/orl/jp-m/4/adapt8/\"\n\n# 1. estimate features\nfeat = FeatureExtractor()\nsynthesizer = Synthesizer()\n\norg_f0list = None\norg_splist = None\norg_mceplist = None\norg_aplist = None\norg_npowlist = None\norg_codeaplist = None\n\nif os.path.exists(prepare_path + \"_org_f0.pickle\") \\\n and os.path.exists(prepare_path + \"_org_sp.pickle\") \\\n and os.path.exists(prepare_path + \"_org_ap.pickle\") \\\n and os.path.exists(prepare_path + \"_org_mcep.pickle\") \\\n and os.path.exists(prepare_path + \"_org_npow.pickle\") \\\n and os.path.exists(prepare_path + \"_org_codeap.pickle\"):\n \n with open(prepare_path + \"_org_f0.pickle\", 'rb') as f: \n org_f0list = pickle.load(f)\n with open(prepare_path + \"_org_sp.pickle\", 'rb') as f: \n org_splist = pickle.load(f)\n with open(prepare_path + \"_org_ap.pickle\", 'rb') as f: \n org_aplist = pickle.load(f)\n with open(prepare_path + \"_org_mcep.pickle\", 'rb') as f: \n org_mceplist = pickle.load(f)\n with open(prepare_path + \"_org_npow.pickle\", 'rb') as f: \n org_npowlist = pickle.load(f)\n with open(prepare_path + \"_org_codeap.pickle\", 'rb') as f: \n org_codeaplist = pickle.load(f) \nelse:\n org_f0list = []\n org_splist = []\n org_mceplist = []\n org_aplist = []\n org_npowlist = []\n org_codeaplist = []\n ite = 0\n for files in sorted(glob.iglob(pre_stored_source_list, recursive=True)):\n wavf = files\n x, fs = sf.read(wavf)\n x = np.array(x, dtype=np.float)\n x = low_cut_filter(x, fs, cutoff=70)\n assert fs == 16000\n\n print(\"extract acoustic featuers: \" + wavf)\n\n f0, sp, ap = feat.analyze(x)\n mcep = feat.mcep()\n npow = feat.npow()\n codeap = feat.codeap()\n wav = synthesizer.synthesis_sp(f0, sp, ap)\n wav = np.clip(wav, -32768, 32767)\n sf.write(prepare_path + \"src_ansys_{}_.wav\".format(ite), wav, fs)\n \n org_f0list.append(f0)\n org_splist.append(sp)\n org_mceplist.append(mcep)\n org_aplist.append(ap)\n org_npowlist.append(npow)\n org_codeaplist.append(codeap)\n\n wav = synthesizer.synthesis(f0, mcep, ap)\n wav = np.clip(wav, -32768, 32767)\n sf.write(prepare_path + \"src_mcep_{}_.wav\".format(ite), wav, fs)\n ite = ite + 1\n\n with open(prepare_path + \"_org_f0.pickle\", 'wb') as f: \n pickle.dump(org_f0list, f)\n with open(prepare_path + \"_org_sp.pickle\", 'wb') as f: \n pickle.dump(org_splist, f)\n with open(prepare_path + \"_org_npow.pickle\", 'wb') as f: \n pickle.dump(org_npowlist, f)\n with open(prepare_path + \"_org_ap.pickle\", 'wb') as f: \n pickle.dump(org_aplist, f)\n with open(prepare_path + \"_org_mcep.pickle\", 'wb') as f: \n pickle.dump(org_mceplist, f)\n with open(prepare_path + \"_org_codeap.pickle\", 'wb') as f: \n pickle.dump(org_codeaplist, f) \n\nmid_f0list = None\nmid_mceplist = None\nmid_aplist = None\nmid_npowlist = None\nmid_splist = None\nmid_codeaplist = None \n\nif os.path.exists(prepare_path + \"_mid_f0.pickle\") \\\n and os.path.exists(prepare_path + \"_mid_sp_0_.pickle\") \\\n and os.path.exists(prepare_path + \"_mid_ap_0_.pickle\") \\\n and os.path.exists(prepare_path + \"_mid_mcep.pickle\") \\\n and os.path.exists(prepare_path + \"_mid_npow.pickle\") \\\n and os.path.exists(prepare_path + \"_mid_codeap.pickle\"):\n \n with open(prepare_path + \"_mid_f0.pickle\", 'rb') as f: \n mid_f0list = pickle.load(f)\n for i in range(0, len(org_splist)*21, len(org_splist)): \n with open(prepare_path + \"_mid_sp_{}_.pickle\".format(i), 'rb') as f:\n temp_splist = pickle.load(f)\n if mid_splist is None:\n mid_splist = temp_splist\n else:\n mid_splist = mid_splist + temp_splist\n for i in range(0, len(org_aplist)*21, len(org_aplist)): \n with open(prepare_path + \"_mid_ap_{}_.pickle\".format(i), 'rb') as f:\n temp_aplist = pickle.load(f)\n if mid_aplist is None:\n mid_aplist = temp_aplist\n else:\n mid_aplist = mid_aplist + temp_aplist \n with open(prepare_path + \"_mid_mcep.pickle\", 'rb') as f: \n mid_mceplist = pickle.load(f)\n with open(prepare_path + \"_mid_npow.pickle\", 'rb') as f: \n mid_npowlist = pickle.load(f)\n with open(prepare_path + \"_mid_codeap.pickle\", 'rb') as f: \n mid_codeaplist = pickle.load(f) \nelse: \n mid_f0list = []\n mid_mceplist = []\n mid_aplist = []\n mid_npowlist = []\n mid_splist = []\n mid_codeaplist = []\n ite = 0\n for files in sorted(glob.iglob(pre_stored_list, recursive=True)):\n wavf = files\n x, fs = sf.read(wavf)\n x = np.array(x, dtype=np.float)\n x = low_cut_filter(x, fs, cutoff=70)\n assert fs == 16000\n\n print(\"extract acoustic featuers: \" + wavf)\n\n f0, sp, ap = feat.analyze(x)\n mcep = feat.mcep()\n npow = feat.npow()\n codeap = feat.codeap()\n name, ext = os.path.splitext(wavf)\n wav = synthesizer.synthesis_sp(f0, sp, ap)\n wav = np.clip(wav, -32768, 32767)\n sf.write(prepare_path + \"mid_ansys_{}_.wav\".format(ite), wav, fs)\n \n mid_f0list.append(f0)\n mid_splist.append(sp)\n mid_mceplist.append(mcep)\n mid_aplist.append(ap)\n mid_npowlist.append(npow)\n mid_codeaplist.append(codeap)\n\n wav = synthesizer.synthesis(f0, mcep, ap)\n wav = np.clip(wav, -32768, 32767)\n sf.write(prepare_path + \"mid_mcep_{}_.wav\".format(ite), wav, fs)\n ite = ite + 1\n \n with open(prepare_path + \"_mid_f0.pickle\", 'wb') as f:\n print(f)\n pickle.dump(mid_f0list, f)\n with open(prepare_path + \"_mid_npow.pickle\", 'wb') as f:\n print(f)\n pickle.dump(mid_npowlist, f)\n for i in range(0, len(mid_splist), len(org_splist)):\n with open(prepare_path + \"_mid_sp_{}_.pickle\".format(i), 'wb') as f: \n print(f)\n pickle.dump(mid_splist[i:i+len(org_splist)], f)\n for i in range(0, len(mid_aplist), len(org_aplist)):\n with open(prepare_path + \"_mid_ap_{}_.pickle\".format(i), 'wb') as f:\n print(f)\n pickle.dump(mid_aplist[i:i+len(org_aplist)], f)\n with open(prepare_path + \"_mid_mcep.pickle\", 'wb') as f:\n print(f)\n pickle.dump(mid_mceplist, f)\n with open(prepare_path + \"_mid_codeap.pickle\", 'wb') as f:\n print(f)\n pickle.dump(mid_codeaplist, f) ",
"_____no_output_____"
],
[
"class GMMTrainer(object):\n \"\"\"\n this class offers the training of GMM with several types of covariance matrix.\n \n Parameters\n ----------\n n_mix : int \n the number of mixture components of the GMM\n n_iter : int\n the number of iteration for EM algorithm\n covtype : str\n the type of covariance matrix of the GMM\n 'full': full-covariance matrix\n \n Attributes\n ---------\n param : \n sklearn-based model parameters of the GMM\n \"\"\"\n \n def __init__(self, n_mix=64, n_iter=100, covtype='full', params='wmc'):\n self.n_mix = n_mix\n self.n_iter = n_iter\n self.covtype = covtype\n self.params = params\n self.param = sklearn.mixture.GMM(n_components=self.n_mix,\n covariance_type=self.covtype,\n n_iter=self.n_iter, params=self.params)\n \n def train(self, jnt):\n \"\"\"\n fit GMM parameter from given joint feature vector\n \n Parametes\n ---------\n jnt : array, shape(`T`, `jnt.shape[0]`)\n joint feature vector of original and target feature vector consisting of static and delta components\n \"\"\"\n \n if self.covtype == 'full':\n self.param.fit(jnt)\n \n return\n \nclass GMMConvertor(object):\n \"\"\"\n this class offers the several conversion techniques such as Maximum Likelihood Parameter Generation (MLPG)\n and Minimum Mean Square Error (MMSE).\n \n Parametes\n ---------\n n_mix : int\n the number of mixture components of the GMM\n covtype : str\n the type of covariance matrix of the GMM\n 'full': full-covariance matrix\n gmmmode : str\n the type of the GMM for opening\n `None` : Normal Joint Density - GMM (JD-GMM)\n \n Attributes\n ---------\n param : \n sklearn-based model parameters of the GMM\n w : shape(`n_mix`)\n vector of mixture component weight of the GMM\n jmean : shape(`n_mix`, `jnt.shape[0]`)\n Array of joint mean vector of the GMM\n jcov : shape(`n_mix`, `jnt.shape[0]`, `jnt.shape[0]`)\n array of joint covariance matrix of the GMM\n \"\"\"\n \n def __init__(self, n_mix=64, covtype='full', gmmmode=None):\n self.n_mix = n_mix\n self.covtype = covtype\n self.gmmmode = gmmmode\n \n def open_from_param(self, param):\n \"\"\"\n open GMM from GMMTrainer\n \n Parameters\n ----------\n param : GMMTrainer\n GMMTrainer class\n \"\"\"\n \n self.param = param\n self._deploy_parameters()\n \n return\n \n def convert(self, data, cvtype='mlpg'):\n \"\"\"\n convert data based on conditional probability density function\n \n Parametes\n ---------\n data : array, shape(`T`, `dim`)\n original data will be converted\n cvtype : str\n type of conversion technique\n `mlpg` : maximum likelihood parameter generation\n \n Returns\n ----------\n odata : array, shape(`T`, `dim`)\n converted data\n \"\"\"\n \n # estimate parameter sequence\n cseq, wseq, mseq, covseq = self._gmmmap(data)\n \n if cvtype == 'mlpg':\n odata = self._mlpg(mseq, covseq)\n else:\n raise ValueError('please choose conversion mode in `mlpg`.')\n \n return odata\n \n def _gmmmap(self, sddata):\n # paramete for sequencial data\n T, sddim = sddata.shape\n \n # estimate posterior sequence\n wseq = self.pX.predict_proba(sddata)\n \n # estimate mixture sequence\n cseq = np.argmax(wseq, axis=1)\n \n mseq = np.zeros((T, sddim))\n covseq = np.zeros((T, sddim, sddim))\n for t in range(T):\n # read maximum likelihood mixture component in frame t\n m = cseq[t]\n \n # conditional mean vector sequence\n mseq[t] = self.meanY[m] + self.A[m] @ (sddata[t] - self.meanX[m])\n \n # conditional covariance sequence\n covseq[t] = self.cond_cov_inv[m]\n \n return cseq, wseq, mseq, covseq\n \n def _mlpg(self, mseq, covseq):\n # parameter for sequencial data\n T, sddim = mseq.shape\n \n # prepare W\n W = construct_static_and_delta_matrix(T, sddim // 2)\n \n # prepare D\n D = get_diagonal_precision_matrix(T, sddim, covseq)\n \n # calculate W'D\n WD = W.T @ D\n \n # W'DW\n WDW = WD @ W\n \n # W'Dm\n WDM = WD @ mseq.flatten()\n \n # estimate y = (W'DW)^-1 * W'Dm\n odata = scipy.sparse.linalg.spsolve(WDW, WDM, use_umfpack=False).reshape(T, sddim // 2)\n \n return odata\n \n def _deploy_parameters(self):\n # read JD-GMM parameters from self.param\n self.W = self.param.weights_\n self.jmean = self.param.means_\n self.jcov = self.param.covars_\n \n # devide GMM parameters into source and target parameters\n sddim = self.jmean.shape[1] // 2\n self.meanX = self.jmean[:, 0:sddim]\n self.meanY = self.jmean[:, sddim:]\n self.covXX = self.jcov[:, :sddim, :sddim]\n self.covXY = self.jcov[:, :sddim, sddim:]\n self.covYX = self.jcov[:, sddim:, :sddim]\n self.covYY = self.jcov[:, sddim:, sddim:]\n \n # change model parameter of GMM into that of gmmmode\n if self.gmmmode is None:\n pass\n else:\n raise ValueError('please choose GMM mode in [None]')\n \n # estimate parameters for conversion\n self._set_Ab()\n self._set_pX()\n \n return\n \n def _set_Ab(self):\n # calculate A and b from self.jmean, self.jcov\n sddim = self.jmean.shape[1] // 2\n \n # calculate inverse covariance for covariance XX in each mixture\n self.covXXinv = np.zeros((self.n_mix, sddim, sddim))\n for m in range(self.n_mix):\n self.covXXinv[m] = np.linalg.inv(self.covXX[m])\n \n # calculate A, b, and conditional covariance given X\n self.A = np.zeros((self.n_mix, sddim, sddim))\n self.b = np.zeros((self.n_mix, sddim))\n self.cond_cov_inv = np.zeros((self.n_mix, sddim, sddim))\n for m in range(self.n_mix):\n # calculate A (A = yxcov_m * xxcov_m^-1)\n self.A[m] = self.covYX[m] @ self.covXXinv[m]\n \n # calculate b (b = mean^Y - A * mean^X)\n self.b[m] = self.meanY[m] - self.A[m] @ self.meanX[m]\n \n # calculate conditional covariance (cov^(Y|X)^-1 = (yycov - A * xycov)^-1)\n self.cond_cov_inv[m] = np.linalg.inv(self.covYY[m] - self.A[m] @ self.covXY[m])\n \n return\n \n def _set_pX(self):\n # probability density function of X \n self.pX = sklearn.mixture.GMM(n_components=self.n_mix, covariance_type=self.covtype)\n self.pX.weights_ = self.W\n self.pX.means_ = self.meanX\n self.pX.covars_ = self.covXX\n \n # following function is required to estimate porsterior\n # p(x | \\lambda^(X))\n #self.pX.precisions_cholesky_ = _compute_precision_cholesky(self.covXX, self.covtype)\n \n return\n \ndef get_diagonal_precision_matrix(T, D, covseq):\n return scipy.sparse.block_diag(covseq, format='csr') ",
"_____no_output_____"
],
[
"def get_alignment(odata, onpow, tdata, tnpow, opow=-20, tpow=-20, sd=0, cvdata=None, given_twf=None, otflag=None, distance='melcd'):\n \"\"\"\n get alignment between original and target.\n \n Parameters\n ----------\n odata : array, shape(`T`, `dim`)\n acoustic feature vector of original\n onpow : array, shape(`T`)\n Normalized power vector of original\n tdata : array, shape(`T`, `dim`)\n acoustic feature vector of target\n tnpow : array, shape(`T`)\n Normalized power vector of target\n opow : float\n power threshold of original\n tpow : float\n power threshold of target\n sd : int\n start dimension to be used for alignment\n cvdata : array, shape(`T`, `dim`)\n converted original data\n given_twf : array, shape(`T_new`, `dim * 2`)\n Alignment given twf\n otflag : str\n Alignment into the length of specification\n 'org' : alignment into original length\n 'tar' : alignment into target length\n distance : str\n Distance function to be used\n \n Returns\n ----------\n jdata : array, shape(`T_new`, `dim * 2`)\n joint static and delta feature vector\n twf : array, shape(`T_new`, `dim * 2`)\n Time warping function\n mcd : float\n Mel-cepstrum distortion between arrays\n \"\"\"\n \n oexdata = extsddata(odata[:, sd:], onpow, power_threshold=opow)\n texdata = extsddata(tdata[:, sd:], tnpow, power_threshold=tpow)\n \n if cvdata is None:\n align_odata = oexdata\n else:\n cvexdata = extsddata(cvdata, onpow, power_threshold=opow)\n align_odata = cvexdata\n \n if given_twf is None: \n twf = estimate_twf(align_odata, texdata, distance=distance, fast=False, otflag=otflag)\n else:\n twf = given_twf\n \n jdata = align_data(oexdata, texdata, twf)\n mcd = melcd(align_odata[twf[0]], texdata[twf[1]])\n \n return jdata, twf, mcd\n\ndef align_feature_vectors(odata, onpows, tdata, tnpows, opow=-100, tpow=-100, itnum=3, sd=0, given_twfs=None, otflag=None):\n \"\"\"\n get alignment to create joint feature vector\n \n Parameters\n ----------\n odata : list, (`num_files`)\n List of original feature vectors\n onpow : list, (`num_files`)\n List of original npows\n tdata : list, (`num_files`)\n List of target feature vectors\n tnpow : list, (`num_files`)\n List of target npows\n opow : float\n power threshold of original\n tpow : float\n power threshold of target\n itnum : int\n the number of iteration\n sd : int\n start dimension of feature vector to be used for alignment\n given_twf : array, shape(`T_new`, `dim * 2`)\n use given alignment while 1st iteration\n otflag : str\n Alignment into the length of specification\n 'org' : alignment into original length\n 'tar' : alignment into target length\n distance : str\n Distance function to be used\n \n Returns\n ----------\n jdata : array, shape(`T_new`, `dim * 2`)\n joint static and delta feature vector\n twf : array, shape(`T_new`, `dim * 2`)\n Time warping function\n mcd : float\n Mel-cepstrum distortion between arrays\n \"\"\"\n \n it = 1\n num_files = len(odata)\n cvgmm, cvdata = None, None\n for it in range(1, itnum+1):\n print('{}-th joint feature extraction starts.'.format(it))\n \n # alignment\n twfs, jfvs = [], []\n for i in range(num_files):\n if it == 1 and given_twfs is not None:\n gtwf = given_twfs[i]\n else:\n gtwf = None\n \n if it > 1:\n cvdata = cvgmm.convert(static_delta(odata[i][:, sd:]))\n \n jdata, twf, mcd = get_alignment(odata[i], onpows[i], tdata[i], tnpows[i], opow=opow, tpow=tpow,\n sd=sd, cvdata=cvdata, given_twf=gtwf, otflag=otflag)\n twfs.append(twf)\n jfvs.append(jdata)\n print('distortion [dB] for {}-th file: {}'.format(i+1, mcd))\n \n jnt_data = transform_jnt(jfvs)\n \n if it != itnum:\n # train GMM, if not final iteration\n datagmm = GMMTrainer()\n datagmm.train(jnt_data)\n cvgmm = GMMConvertor()\n cvgmm.open_from_param(datagmm.param)\n it += 1\n return jfvs, twfs ",
"_____no_output_____"
],
[
"# 2. estimate twf and jnt\nif os.path.exists(prepare_path + \"_jnt_mcep_0_.pickle\"):\n pass\nelse:\n for i in range(0, len(mid_mceplist), len(org_mceplist)):\n org_mceps = org_mceplist\n org_npows = org_npowlist\n mid_mceps = mid_mceplist[i:i+len(org_mceps)]\n mid_npows = mid_npowlist[i:i+len(org_npows)]\n assert len(org_mceps) == len(mid_mceps)\n assert len(org_npows) == len(mid_npows)\n assert len(org_mceps) == len(org_npows)\n\n # dtw between original and target 0-th and silence\n print(\"## alignment mcep 0-th and silence ##\")\n jmceps, twfs = align_feature_vectors(org_mceps, org_npows, mid_mceps, mid_npows, opow=-15, tpow=-15, sd=1)\n jnt_mcep = transform_jnt(jmceps)\n\n # save joint feature vectors\n with open(prepare_path + \"_jnt_mcep_{}_.pickle\".format(i), 'wb') as f: \n print(f)\n pickle.dump(jnt_mcep, f)",
"_____no_output_____"
],
[
"# 3. make EV-GMM\ninitgmm, initgmm_codeap = None, None\nif os.path.exists(prepare_path + \"initgmm.pickle\"):\n with open(prepare_path + \"initgmm.pickle\".format(i), 'rb') as f: \n print(f)\n initgmm = pickle.load(f)\nelse:\n jnt, jnt_codeap = None, []\n for i in range(0, len(mid_mceplist), len(org_mceplist)):\n with open(prepare_path + \"_jnt_mcep_{}_.pickle\".format(i), 'rb') as f: \n temp_jnt = pickle.load(f)\n if jnt is None:\n jnt = temp_jnt\n else:\n jnt = np.r_[jnt, temp_jnt]\n\n # train initial gmm\n initgmm = GMMTrainer()\n initgmm.train(jnt)\n\n with open(prepare_path + \"initgmm.pickle\", 'wb') as f: \n print(f)\n pickle.dump(initgmm, f)",
"<_io.BufferedReader name='./utterance/pre-stored-jp/output/initgmm.pickle'>\n"
],
[
"# get initial gmm params\ninit_W = initgmm.param.weights_\ninit_jmean = initgmm.param.means_\ninit_jcov = initgmm.param.covars_\nsddim = init_jmean.shape[1] // 2\ninit_meanX = init_jmean[:, :sddim]\ninit_meanY = init_jmean[:, sddim:]\ninit_covXX = init_jcov[:, :sddim, :sddim]\ninit_covXY = init_jcov[:, :sddim, sddim:]\ninit_covYX = init_jcov[:, sddim:, :sddim]\ninit_covYY = init_jcov[:, sddim:, sddim:]\nfitted_source = init_meanX\nfitted_target = init_meanY\n",
"_____no_output_____"
],
[
"sv = None\nif os.path.exists(prepare_path + \"_sv.npy\"):\n sv = np.array(sv)\n sv = np.load(prepare_path + '_sv.npy')\nelse:\n depengmm, depenjnt = None, None\n sv = []\n for i in range(0, len(mid_mceplist), len(org_mceplist)):\n with open(prepare_path + \"_jnt_mcep_{}_.pickle\".format(i), 'rb') as f: \n depenjnt = pickle.load(f)\n depengmm = GMMTrainer(params='m')\n depengmm.param.weights_ = init_W\n depengmm.param.means_ = init_jmean\n depengmm.param.covars_ = init_jcov\n depengmm.train(depenjnt)\n sv.append(depengmm.param.means_)\n sv = np.array(sv)\n np.save(prepare_path + \"_sv\", sv)",
"_____no_output_____"
],
[
"n_mix = 64\nS = int(len(mid_mceplist) / len(org_mceplist))\nassert S == 22\n\nsource_pca = sklearn.decomposition.PCA()\nsource_pca.fit(sv[:,:,:sddim].reshape((S, n_mix*sddim)))\n\ntarget_pca = sklearn.decomposition.PCA()\ntarget_pca.fit(sv[:,:,sddim:].reshape((S, n_mix*sddim)))\n\neigenvectors = source_pca.components_.reshape((n_mix, sddim, S)), target_pca.components_.reshape((n_mix, sddim, S)) \nbiasvectors = source_pca.mean_.reshape((n_mix, sddim)), target_pca.mean_.reshape((n_mix, sddim))",
"_____no_output_____"
],
[
"# estimate statistic features\nfor_convert_source = __same_path + 'input/EJM10/V01/T01/TIMIT/000/*.wav'\nfor_convert_target = __same_path + 'adaptation/EJM04/V01/T01/ATR503/A/*.wav'\n\nsrc_f0list = []\nsrc_splist = []\nsrc_mceplist = []\nsrc_aplist = []\nsrc_npowlist = []\nsrc_codeaplist = []\nif os.path.exists(__same_path + 'input/EJM10/V01/T01/TIMIT/000/A11.wav'):\n ite = 0\n for files in sorted(glob.iglob(for_convert_source, recursive=True)):\n wavf = files\n x, fs = sf.read(wavf)\n x = np.array(x, dtype=np.float)\n x = low_cut_filter(x, fs, cutoff=70)\n assert fs == 16000\n\n print(\"extract acoustic featuers: \" + wavf)\n\n f0, sp, ap = feat.analyze(x)\n mcep = feat.mcep()\n npow = feat.npow()\n codeap = feat.codeap()\n wav = synthesizer.synthesis_sp(f0, sp, ap)\n wav = np.clip(wav, -32768, 32767)\n sf.write(output_path + \"input_ansys_{}_.wav\".format(ite), wav, fs)\n \n src_f0list.append(f0)\n src_splist.append(sp)\n src_mceplist.append(mcep)\n src_aplist.append(ap)\n src_npowlist.append(npow)\n src_codeaplist.append(codeap)\n\n wav = synthesizer.synthesis(f0, mcep, ap)\n wav = np.clip(wav, -32768, 32767)\n sf.write(output_path + \"input_mcep_{}_.wav\".format(ite), wav, fs)\n ite = ite + 1\n\n\nelse:\n raise ValueError(\"No such files.\")\ntar_f0list = []\ntar_mceplist = []\ntar_aplist = []\ntar_npowlist = []\ntar_splist = []\ntar_codeaplist = []\n\nif os.path.exists(__same_path + 'adaptation/EJM04/V01/T01/ATR503/A/A01.wav'):\n ite = 0\n for files in sorted(glob.iglob(for_convert_target, recursive=True)):\n wavf = files\n x, fs = sf.read(wavf)\n x = np.array(x, dtype=np.float)\n x = low_cut_filter(x, fs, cutoff=70)\n assert fs == 16000\n\n print(\"extract acoustic featuers: \" + wavf)\n\n f0, sp, ap = feat.analyze(x)\n mcep = feat.mcep()\n npow = feat.npow()\n codeap = feat.codeap()\n name, ext = os.path.splitext(wavf)\n wav = synthesizer.synthesis_sp(f0, sp, ap)\n wav = np.clip(wav, -32768, 32767)\n sf.write(output_path + \"target_ansys_{}_.wav\".format(ite), wav, fs)\n \n tar_f0list.append(f0)\n tar_splist.append(sp)\n tar_mceplist.append(mcep)\n tar_aplist.append(ap)\n tar_npowlist.append(npow)\n tar_codeaplist.append(codeap)\n\n wav = synthesizer.synthesis(f0, mcep, ap)\n wav = np.clip(wav, -32768, 32767)\n sf.write(output_path + \"target_mcep_{}_.wav\".format(ite), wav, fs)\n ite = ite + 1\nelse:\n raise ValueError(\"No such files.\")",
"extract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A11.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A14.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A17.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A18.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A19.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A20.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A21.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A22.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A23.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A24.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A25.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A26.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A27.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A28.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A29.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A30.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A31.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A32.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A33.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A34.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A35.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A36.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A37.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A38.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A39.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A40.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A41.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A42.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A43.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A44.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A45.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A46.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A47.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A48.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A49.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A50.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A51.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A52.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A53.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A54.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A55.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A56.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A57.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A58.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/input/EJM10/V01/T01/TIMIT/000/A59.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/ATR503/A/A01.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/ATR503/A/A02.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/ATR503/A/A03.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/ATR503/A/A05.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/ATR503/A/A06.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/ATR503/A/A07.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/ATR503/A/A08.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/ATR503/A/A09.wav\n"
],
[
"f0statis = F0statistics()\ntarf0stats = f0statis.estimate(tar_f0list)\nsrcf0stats = f0statis.estimate(org_f0list)\n\ngv = GV()\nsrcgvstats = gv.estimate(org_mceplist)\ntargvstats = gv.estimate(tar_mceplist)",
"_____no_output_____"
],
[
"# 5. fitting target\nepoch = 100\n\nfitgmm = sklearn.mixture.GMM(n_components=n_mix,\n covariance_type='full',\n n_iter=100)\nfitgmm.weights_ = init_W\nfitgmm.means_ = init_meanY\nfitgmm.covars_ = init_covYY\n\nfor i in range(len(tar_mceplist)):\n print(\"adapt: \", i+1, \"/\", len(tar_mceplist))\n target = tar_mceplist[i]\n target_pow = target[:, 0]\n target = target[:, 1:]\n for x in range(epoch):\n print(\"epoch = \", x)\n predict = fitgmm.predict_proba(np.atleast_2d(static_delta(target)))\n \n y = np.sum([predict[:, k:k+1] * (static_delta(target) - biasvectors[1][k]) for k in range(n_mix)], axis=1)\n gamma = np.sum(predict, axis=0)\n \n left = np.sum([gamma[k] * np.dot(eigenvectors[1][k].T,\n np.linalg.solve(fitgmm.covars_, eigenvectors[1])[k])\n for k in range(n_mix)], axis=0)\n right = np.sum([np.dot(eigenvectors[1][k].T,\n np.linalg.solve(fitgmm.covars_, y)[k])\n for k in range(n_mix)], axis=0)\n weight = np.linalg.solve(left, right)\n \n fitted_target = np.dot(eigenvectors[1], weight) + biasvectors[1]\n fitgmm.means_ = fitted_target",
"/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n"
],
[
"def mcepconvert(source, weights, jmean, meanX, covarXX, covarXY, covarYX, covarYY,\n fitted_source, fitted_target):\n \n M = 64\n # set pX\n px = sklearn.mixture.GMM(n_components=M, covariance_type='full', n_iter=100)\n px.weights_ = weights\n px.means_ = meanX\n px.covars_ = covarXX\n \n # set Ab\n sddim = jmean.shape[1] // 2\n covXXinv = np.zeros((M, sddim, sddim))\n for m in range(M):\n covXXinv[m] = np.linalg.inv(covarXX[m])\n A = np.zeros((M, sddim, sddim))\n b = np.zeros((M, sddim))\n cond_cov_inv = np.zeros((M, sddim, sddim))\n for m in range(M):\n A[m] = covarYX[m] @ covXXinv[m]\n b[m] = fitted_target[m] - A[m] @ meanX[m]\n cond_cov_inv[m] = np.linalg.inv(covarYY[m] - A[m] @ covarXY[m])\n \n # _gmmmap\n T, sddim = source.shape\n wseq = px.predict_proba(source)\n cseq = np.argmax(wseq, axis=1)\n mseq = np.zeros((T, sddim))\n covseq = np.zeros((T, sddim, sddim))\n for t in range(T):\n m = cseq[t]\n mseq[t] = fitted_target[m] + A[m] @ (source[t] - meanX[m])\n covseq[t] = cond_cov_inv[m]\n \n # _mlpg\n T, sddim = mseq.shape\n W = construct_static_and_delta_matrix(T, sddim // 2)\n D = get_diagonal_precision_matrix(T, sddim, covseq)\n WD = W.T @ D\n WDW = WD @ W\n WDM = WD @ mseq.flatten()\n \n output = scipy.sparse.linalg.spsolve(WDW, WDM, use_umfpack=False).reshape(T, sddim // 2)\n return output",
"_____no_output_____"
],
[
"# learn cvgvstats \ncv_mceps = []\nfor i in range(len(src_mceplist)):\n temp_mcep = src_mceplist[i]\n temp_mcep_0th = temp_mcep[:, 0]\n temp_mcep = temp_mcep[:, 1:]\n sta_mcep = static_delta(temp_mcep)\n cvmcep_wopow = np.array(mcepconvert(sta_mcep, init_W, init_jmean, init_meanX,\n init_covXX, init_covXY, init_covYX, init_covYY,\n fitted_source, fitted_target))\n cvmcep = np.c_[temp_mcep_0th, cvmcep_wopow]\n cv_mceps.append(cvmcep)",
"/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:58: DeprecationWarning: Class GMM is deprecated; The class GMM is deprecated in 0.18 and will be removed in 0.20. Use class GaussianMixture instead.\n warnings.warn(msg, category=DeprecationWarning)\n/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:77: DeprecationWarning: Function log_multivariate_normal_density is deprecated; The function log_multivariate_normal_density is deprecated in 0.18 and will be removed in 0.20.\n warnings.warn(msg, category=DeprecationWarning)\n"
],
[
"cvgvstats = gv.estimate(cv_mceps)\n\nfor i in range(len(src_mceplist)):\n cvmcep_wGV = gv.postfilter(cv_mceps[i], targvstats, cvgvstats=cvgvstats)\n cvf0 = f0statis.convert(src_f0list[i], srcf0stats, tarf0stats)\n wav = synthesizer.synthesis(cvf0, cvmcep_wGV, src_aplist[i], rmcep=src_mceplist[i])\n sf.write(output_path + \"cv_{}_.wav\".format(i), wav, 16000)",
"_____no_output_____"
],
[
"for i in range(len(src_mceplist)):\n wav = synthesizer.synthesis(src_f0list[i], src_mceplist[i], src_aplist[i])\n sf.write(output_path + \"mcep_{}_.wav\".format(i), wav, 16000)\n wav = synthesizer.synthesis_sp(src_f0list[i], src_splist[i], src_aplist[i])\n sf.write(output_path + \"ansys_{}_.wav\".format(i), wav, 16000)",
"_____no_output_____"
],
[
"cvf0 = f0statis.convert(src_f0list[0], srcf0stats, tarf0stats)\nplt.plot(cvf0)\nplt.plot(src_f0list[0])",
"_____no_output_____"
],
[
"cvmcep_wGV = gv.postfilter(cv_mceps[0], srcgvstats, cvgvstats=cvgvstats)\ncvf0 = f0statis.convert(src_f0list[0], srcf0stats, tarf0stats)\nwav = synthesizer.synthesis(cvf0, cvmcep_wGV, src_aplist[0], rmcep=src_mceplist[0])\nsf.write(output_path + \"te.wav\", wav, 16000)",
"_____no_output_____"
],
[
"# org-cv distance\nwith open(output_path + \"melcd_org-cv.txt\", \"w\") as outfile:\n outfile.write(\"adapt8 org-cv mcd.\\n\")\n for i in range(len(src_mceplist)):\n temp_mcep = src_mceplist[i]\n temp_mcep_0th = temp_mcep[:, 0]\n temp_mcep = temp_mcep[:, 1:]\n \n temp_cv = cv_mceps[i]\n temp_cv_0th = temp_cv[:, 0]\n temp_cv = temp_cv[:, 1:]\n \n _, _, mcd = get_alignment(temp_mcep, temp_mcep_0th, temp_cv, temp_cv_0th, opow=-15, tpow=-15, sd=1)\n outfile.write(\"{0},{1}\\n\".format(i, mcd))\n\n# cv-target distance\n# read target files and analyze mceps\ntargets_mceplist = []\ntargets_list = __same_path + 'adaptation/EJM04/V01/T01/TIMIT/000/*.wav'\nfor files in sorted(glob.iglob(targets_list, recursive=True)):\n wavf = files\n x, fs = sf.read(wavf)\n x = np.array(x, dtype=np.float)\n x = low_cut_filter(x, fs, cutoff=70)\n assert fs == 16000\n print(\"extract acoustic featuers: \" + wavf)\n\n f0, sp, ap = feat.analyze(x)\n mcep = feat.mcep()\n targets_mceplist.append(mcep)\n\nwith open(output_path + \"melcd_cv-target.txt\", \"w\") as outfile:\n outfile.write(\"adapt8 cv-target mcd.\\n\")\n \n for i in range(len(src_mceplist)):\n temp_mcep = targets_mceplist[i]\n temp_mcep_0th = temp_mcep[:, 0]\n temp_mcep = temp_mcep[:, 1:]\n \n temp_cv = cv_mceps[i]\n temp_cv_0th = temp_cv[:, 0]\n temp_cv = temp_cv[:, 1:]\n \n _, _, mcd = get_alignment(temp_cv, temp_cv_0th, temp_mcep, temp_mcep_0th, opow=-15, tpow=-15, sd=1)\n outfile.write(\"{0},{1}\\n\".format(i, mcd))\n\n# org-target distance\nwith open(output_path + \"melcd_org-target.txt\", \"w\") as outfile:\n outfile.write(\"adapt8F org-target mcd.\\n\")\n \n for i in range(len(src_mceplist)):\n\n temp_mcep = src_mceplist[i]\n temp_mcep_0th = temp_mcep[:, 0]\n temp_mcep = temp_mcep[:, 1:]\n \n temp_mcep2 = targets_mceplist[i]\n temp_mcep2_0th = temp_mcep2[:, 0]\n temp_mcep2 = temp_mcep2[:, 1:]\n \n \n _, _, mcd = get_alignment(temp_mcep, temp_mcep_0th, temp_mcep2, temp_mcep2_0th, opow=-15, tpow=-15, sd=1)\n outfile.write(\"{0},{1}\\n\".format(i, mcd))",
"extract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A11.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A14.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A17.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A18.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A19.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A20.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A21.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A22.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A23.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A24.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A25.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A26.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A27.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A28.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A29.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A30.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A31.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A32.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A33.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A34.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A35.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A36.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A37.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A38.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A39.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A40.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A41.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A42.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A43.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A44.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A45.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A46.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A47.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A48.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A49.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A50.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A51.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A52.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A53.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A54.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A55.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A56.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A57.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A58.wav\nextract acoustic featuers: ./utterance/pre-stored-jp/adaptation/EJM04/V01/T01/TIMIT/000/A59.wav\n"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bae616a22fcbde4365f0be5d5e4dd14896e091 | 36,476 | ipynb | Jupyter Notebook | Project_Plagiarism_Detection/3_Training_a_Model.ipynb | gowtham91m/ML_SageMaker_Studies | 74095d695624b46fd664177d014b639120dc7776 | [
"MIT"
] | null | null | null | Project_Plagiarism_Detection/3_Training_a_Model.ipynb | gowtham91m/ML_SageMaker_Studies | 74095d695624b46fd664177d014b639120dc7776 | [
"MIT"
] | null | null | null | Project_Plagiarism_Detection/3_Training_a_Model.ipynb | gowtham91m/ML_SageMaker_Studies | 74095d695624b46fd664177d014b639120dc7776 | [
"MIT"
] | null | null | null | 47.248705 | 1,209 | 0.630168 | [
[
[
"# Plagiarism Detection Model\n\nNow that you've created training and test data, you are ready to define and train a model. Your goal in this notebook, will be to train a binary classification model that learns to label an answer file as either plagiarized or not, based on the features you provide the model.\n\nThis task will be broken down into a few discrete steps:\n\n* Upload your data to S3.\n* Define a binary classification model and a training script.\n* Train your model and deploy it.\n* Evaluate your deployed classifier and answer some questions about your approach.\n\nTo complete this notebook, you'll have to complete all given exercises and answer all the questions in this notebook.\n> All your tasks will be clearly labeled **EXERCISE** and questions as **QUESTION**.\n\nIt will be up to you to explore different classification models and decide on a model that gives you the best performance for this dataset.\n\n---",
"_____no_output_____"
],
[
"## Load Data to S3\n\nIn the last notebook, you should have created two files: a `training.csv` and `test.csv` file with the features and class labels for the given corpus of plagiarized/non-plagiarized text data. \n\n>The below cells load in some AWS SageMaker libraries and creates a default bucket. After creating this bucket, you can upload your locally stored data to S3.\n\nSave your train and test `.csv` feature files, locally. To do this you can run the second notebook \"2_Plagiarism_Feature_Engineering\" in SageMaker or you can manually upload your files to this notebook using the upload icon in Jupyter Lab. Then you can upload local files to S3 by using `sagemaker_session.upload_data` and pointing directly to where the training data is saved.",
"_____no_output_____"
]
],
[
[
"import pandas as pd\nimport boto3\nimport sagemaker",
"_____no_output_____"
],
[
"\"\"\"\nDON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE\n\"\"\"\n# session and role\nsagemaker_session = sagemaker.Session()\nrole = sagemaker.get_execution_role()\n\n# create an S3 bucket\nbucket = sagemaker_session.default_bucket()",
"_____no_output_____"
]
],
[
[
"## EXERCISE: Upload your training data to S3\n\nSpecify the `data_dir` where you've saved your `train.csv` file. Decide on a descriptive `prefix` that defines where your data will be uploaded in the default S3 bucket. Finally, create a pointer to your training data by calling `sagemaker_session.upload_data` and passing in the required parameters. It may help to look at the [Session documentation](https://sagemaker.readthedocs.io/en/stable/session.html#sagemaker.session.Session.upload_data) or previous SageMaker code examples.\n\nYou are expected to upload your entire directory. Later, the training script will only access the `train.csv` file.",
"_____no_output_____"
]
],
[
[
"# should be the name of directory you created to save your features data\ndata_dir = 'plagiarism_data'\n\n# set prefix, a descriptive name for a directory \nprefix = 'plagiarism'\n\n# upload all data to S3\ninput_data = sagemaker_session.upload_data(path=data_dir, bucket=bucket, key_prefix=prefix)\ninput_data\n",
"_____no_output_____"
]
],
[
[
"### Test cell\n\nTest that your data has been successfully uploaded. The below cell prints out the items in your S3 bucket and will throw an error if it is empty. You should see the contents of your `data_dir` and perhaps some checkpoints. If you see any other files listed, then you may have some old model files that you can delete via the S3 console (though, additional files shouldn't affect the performance of model developed in this notebook).",
"_____no_output_____"
]
],
[
[
"\"\"\"\nDON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE\n\"\"\"\n# confirm that data is in S3 bucket\nempty_check = []\nfor obj in boto3.resource('s3').Bucket(bucket).objects.all():\n empty_check.append(obj.key)\n print(obj.key)\n\nassert len(empty_check) !=0, 'S3 bucket is empty.'\nprint('Test passed!')",
"boston-xgboost-HL/output/sagemaker-xgboost-2019-11-03-22-54-54-949/output/model.tar.gz\nboston-xgboost-HL/output/sagemaker-xgboost-2019-11-04-00-24-26-893/output/model.tar.gz\nboston-xgboost-HL/test.csv\nboston-xgboost-HL/train.csv\nboston-xgboost-HL/validation.csv\nggwm_sagemaker/sentiment_rnn/train.csv\nggwm_sagemaker/sentiment_rnn/word_dict.pkl\nplagiarism/test.csv\nplagiarism/train.csv\nsagemaker-pytorch-2019-11-10-02-05-57-682/output/model.tar.gz\nsagemaker-pytorch-2019-11-10-02-05-57-682/source/sourcedir.tar.gz\nsagemaker-pytorch-2019-11-10-03-12-30-854/sourcedir.tar.gz\nsagemaker-pytorch-2019-11-10-20-16-07-445/output/model.tar.gz\nsagemaker-pytorch-2019-11-10-20-16-07-445/source/sourcedir.tar.gz\nsagemaker-pytorch-2019-11-10-21-22-40-738/sourcedir.tar.gz\nsagemaker-xgboost-2019-11-04-00-32-31-041/test.csv.out\nsentiment-xgboost/output/xgboost-2019-11-05-02-36-56-572/output/model.tar.gz\nsentiment-xgboost/test.csv\nsentiment-xgboost/train.csv\nsentiment-xgboost/validation.csv\nxgboost-2019-11-05-03-02-16-631/test.csv.out\nTest passed!\n"
]
],
[
[
"---\n\n# Modeling\n\nNow that you've uploaded your training data, it's time to define and train a model!\n\nThe type of model you create is up to you. For a binary classification task, you can choose to go one of three routes:\n* Use a built-in classification algorithm, like LinearLearner.\n* Define a custom Scikit-learn classifier, a comparison of models can be found [here](https://scikit-learn.org/stable/auto_examples/classification/plot_classifier_comparison.html).\n* Define a custom PyTorch neural network classifier. \n\nIt will be up to you to test out a variety of models and choose the best one. Your project will be graded on the accuracy of your final model. \n \n---\n\n## EXERCISE: Complete a training script \n\nTo implement a custom classifier, you'll need to complete a `train.py` script. You've been given the folders `source_sklearn` and `source_pytorch` which hold starting code for a custom Scikit-learn model and a PyTorch model, respectively. Each directory has a `train.py` training script. To complete this project **you only need to complete one of these scripts**; the script that is responsible for training your final model.\n\nA typical training script:\n* Loads training data from a specified directory\n* Parses any training & model hyperparameters (ex. nodes in a neural network, training epochs, etc.)\n* Instantiates a model of your design, with any specified hyperparams\n* Trains that model \n* Finally, saves the model so that it can be hosted/deployed, later\n\n### Defining and training a model\nMuch of the training script code is provided for you. Almost all of your work will be done in the `if __name__ == '__main__':` section. To complete a `train.py` file, you will:\n1. Import any extra libraries you need\n2. Define any additional model training hyperparameters using `parser.add_argument`\n2. Define a model in the `if __name__ == '__main__':` section\n3. Train the model in that same section\n\nBelow, you can use `!pygmentize` to display an existing `train.py` file. Read through the code; all of your tasks are marked with `TODO` comments. \n\n**Note: If you choose to create a custom PyTorch model, you will be responsible for defining the model in the `model.py` file,** and a `predict.py` file is provided. If you choose to use Scikit-learn, you only need a `train.py` file; you may import a classifier from the `sklearn` library.",
"_____no_output_____"
]
],
[
[
"# directory can be changed to: source_sklearn or source_pytorch\n!pygmentize source_sklearn/train.py",
"\u001b[34mfrom\u001b[39;49;00m \u001b[04m\u001b[36m__future__\u001b[39;49;00m \u001b[34mimport\u001b[39;49;00m print_function\n\n\u001b[34mimport\u001b[39;49;00m \u001b[04m\u001b[36margparse\u001b[39;49;00m\n\u001b[34mimport\u001b[39;49;00m \u001b[04m\u001b[36mos\u001b[39;49;00m\n\u001b[34mimport\u001b[39;49;00m \u001b[04m\u001b[36mpandas\u001b[39;49;00m \u001b[34mas\u001b[39;49;00m \u001b[04m\u001b[36mpd\u001b[39;49;00m\n\n\u001b[34mfrom\u001b[39;49;00m \u001b[04m\u001b[36msklearn.externals\u001b[39;49;00m \u001b[34mimport\u001b[39;49;00m joblib\n\n\u001b[37m## TODO: Import any additional libraries you need to define a model\u001b[39;49;00m\n\n\n\u001b[37m# Provided model load function\u001b[39;49;00m\n\u001b[34mdef\u001b[39;49;00m \u001b[32mmodel_fn\u001b[39;49;00m(model_dir):\n \u001b[33m\"\"\"Load model from the model_dir. This is the same model that is saved\u001b[39;49;00m\n\u001b[33m in the main if statement.\u001b[39;49;00m\n\u001b[33m \"\"\"\u001b[39;49;00m\n \u001b[34mprint\u001b[39;49;00m(\u001b[33m\"\u001b[39;49;00m\u001b[33mLoading model.\u001b[39;49;00m\u001b[33m\"\u001b[39;49;00m)\n \n \u001b[37m# load using joblib\u001b[39;49;00m\n model = joblib.load(os.path.join(model_dir, \u001b[33m\"\u001b[39;49;00m\u001b[33mmodel.joblib\u001b[39;49;00m\u001b[33m\"\u001b[39;49;00m))\n \u001b[34mprint\u001b[39;49;00m(\u001b[33m\"\u001b[39;49;00m\u001b[33mDone loading model.\u001b[39;49;00m\u001b[33m\"\u001b[39;49;00m)\n \n \u001b[34mreturn\u001b[39;49;00m model\n\n\n\u001b[37m## TODO: Complete the main code\u001b[39;49;00m\n\u001b[34mif\u001b[39;49;00m \u001b[31m__name__\u001b[39;49;00m == \u001b[33m'\u001b[39;49;00m\u001b[33m__main__\u001b[39;49;00m\u001b[33m'\u001b[39;49;00m:\n \n \u001b[37m# All of the model parameters and training parameters are sent as arguments\u001b[39;49;00m\n \u001b[37m# when this script is executed, during a training job\u001b[39;49;00m\n \n \u001b[37m# Here we set up an argument parser to easily access the parameters\u001b[39;49;00m\n parser = argparse.ArgumentParser()\n\n \u001b[37m# SageMaker parameters, like the directories for training data and saving models; set automatically\u001b[39;49;00m\n \u001b[37m# Do not need to change\u001b[39;49;00m\n parser.add_argument(\u001b[33m'\u001b[39;49;00m\u001b[33m--output-data-dir\u001b[39;49;00m\u001b[33m'\u001b[39;49;00m, \u001b[36mtype\u001b[39;49;00m=\u001b[36mstr\u001b[39;49;00m, default=os.environ[\u001b[33m'\u001b[39;49;00m\u001b[33mSM_OUTPUT_DATA_DIR\u001b[39;49;00m\u001b[33m'\u001b[39;49;00m])\n parser.add_argument(\u001b[33m'\u001b[39;49;00m\u001b[33m--model-dir\u001b[39;49;00m\u001b[33m'\u001b[39;49;00m, \u001b[36mtype\u001b[39;49;00m=\u001b[36mstr\u001b[39;49;00m, default=os.environ[\u001b[33m'\u001b[39;49;00m\u001b[33mSM_MODEL_DIR\u001b[39;49;00m\u001b[33m'\u001b[39;49;00m])\n parser.add_argument(\u001b[33m'\u001b[39;49;00m\u001b[33m--data-dir\u001b[39;49;00m\u001b[33m'\u001b[39;49;00m, \u001b[36mtype\u001b[39;49;00m=\u001b[36mstr\u001b[39;49;00m, default=os.environ[\u001b[33m'\u001b[39;49;00m\u001b[33mSM_CHANNEL_TRAIN\u001b[39;49;00m\u001b[33m'\u001b[39;49;00m])\n \n \u001b[37m## TODO: Add any additional arguments that you will need to pass into your model\u001b[39;49;00m\n \n \u001b[37m# args holds all passed-in arguments\u001b[39;49;00m\n args = parser.parse_args()\n\n \u001b[37m# Read in csv training file\u001b[39;49;00m\n training_dir = args.data_dir\n train_data = pd.read_csv(os.path.join(training_dir, \u001b[33m\"\u001b[39;49;00m\u001b[33mtrain.csv\u001b[39;49;00m\u001b[33m\"\u001b[39;49;00m), header=\u001b[36mNone\u001b[39;49;00m, names=\u001b[36mNone\u001b[39;49;00m)\n\n \u001b[37m# Labels are in the first column\u001b[39;49;00m\n train_y = train_data.iloc[:,\u001b[34m0\u001b[39;49;00m]\n train_x = train_data.iloc[:,\u001b[34m1\u001b[39;49;00m:]\n \n \n \u001b[37m## --- Your code here --- ##\u001b[39;49;00m\n \n\n \u001b[37m## TODO: Define a model \u001b[39;49;00m\n model = \u001b[36mNone\u001b[39;49;00m\n \n \n \u001b[37m## TODO: Train the model\u001b[39;49;00m\n \n \n \n \u001b[37m## --- End of your code --- ##\u001b[39;49;00m\n \n\n \u001b[37m# Save the trained model\u001b[39;49;00m\n joblib.dump(model, os.path.join(args.model_dir, \u001b[33m\"\u001b[39;49;00m\u001b[33mmodel.joblib\u001b[39;49;00m\u001b[33m\"\u001b[39;49;00m))\n"
]
],
[
[
"### Provided code\n\nIf you read the code above, you can see that the starter code includes a few things:\n* Model loading (`model_fn`) and saving code\n* Getting SageMaker's default hyperparameters\n* Loading the training data by name, `train.csv` and extracting the features and labels, `train_x`, and `train_y`\n\nIf you'd like to read more about model saving with [joblib for sklearn](https://scikit-learn.org/stable/modules/model_persistence.html) or with [torch.save](https://pytorch.org/tutorials/beginner/saving_loading_models.html), click on the provided links.",
"_____no_output_____"
],
[
"---\n# Create an Estimator\n\nWhen a custom model is constructed in SageMaker, an entry point must be specified. This is the Python file which will be executed when the model is trained; the `train.py` function you specified above. To run a custom training script in SageMaker, construct an estimator, and fill in the appropriate constructor arguments:\n\n* **entry_point**: The path to the Python script SageMaker runs for training and prediction.\n* **source_dir**: The path to the training script directory `source_sklearn` OR `source_pytorch`.\n* **entry_point**: The path to the Python script SageMaker runs for training and prediction.\n* **source_dir**: The path to the training script directory `train_sklearn` OR `train_pytorch`.\n* **entry_point**: The path to the Python script SageMaker runs for training.\n* **source_dir**: The path to the training script directory `train_sklearn` OR `train_pytorch`.\n* **role**: Role ARN, which was specified, above.\n* **train_instance_count**: The number of training instances (should be left at 1).\n* **train_instance_type**: The type of SageMaker instance for training. Note: Because Scikit-learn does not natively support GPU training, Sagemaker Scikit-learn does not currently support training on GPU instance types.\n* **sagemaker_session**: The session used to train on Sagemaker.\n* **hyperparameters** (optional): A dictionary `{'name':value, ..}` passed to the train function as hyperparameters.\n\nNote: For a PyTorch model, there is another optional argument **framework_version**, which you can set to the latest version of PyTorch, `1.0`.\n\n## EXERCISE: Define a Scikit-learn or PyTorch estimator\n\nTo import your desired estimator, use one of the following lines:\n```\nfrom sagemaker.sklearn.estimator import SKLearn\n```\n```\nfrom sagemaker.pytorch import PyTorch\n```",
"_____no_output_____"
]
],
[
[
"\n# your import and estimator code, here\nfrom sagemaker.sklearn.estimator import SKLearn\n\nsklearn = SKLearn(\n entry_point=\"train.py\",\n source_dir=\"source_sklearn\",\n train_instance_type=\"ml.c4.xlarge\",\n role=role,\n sagemaker_session=sagemaker_session,\n hyperparameters={'n_estimators': 50 , 'max_depth':5})",
"_____no_output_____"
]
],
[
[
"## EXERCISE: Train the estimator\n\nTrain your estimator on the training data stored in S3. This should create a training job that you can monitor in your SageMaker console.",
"_____no_output_____"
]
],
[
[
"%%time\n\n# Train your estimator on S3 training data\n\nsklearn.fit({'train': input_data})",
"2019-12-13 23:28:35 Starting - Starting the training job...\n2019-12-13 23:28:36 Starting - Launching requested ML instances......\n2019-12-13 23:29:41 Starting - Preparing the instances for training...\n2019-12-13 23:30:24 Downloading - Downloading input data...\n2019-12-13 23:30:56 Training - Downloading the training image..\u001b[34m2019-12-13 23:31:16,696 sagemaker-containers INFO Imported framework sagemaker_sklearn_container.training\u001b[0m\n\u001b[34m2019-12-13 23:31:16,699 sagemaker-containers INFO No GPUs detected (normal if no gpus installed)\u001b[0m\n\u001b[34m2019-12-13 23:31:16,709 sagemaker_sklearn_container.training INFO Invoking user training script.\u001b[0m\n\u001b[34m2019-12-13 23:31:16,965 sagemaker-containers INFO Module train does not provide a setup.py. \u001b[0m\n\u001b[34mGenerating setup.py\u001b[0m\n\u001b[34m2019-12-13 23:31:16,965 sagemaker-containers INFO Generating setup.cfg\u001b[0m\n\u001b[34m2019-12-13 23:31:16,965 sagemaker-containers INFO Generating MANIFEST.in\u001b[0m\n\u001b[34m2019-12-13 23:31:16,965 sagemaker-containers INFO Installing module with the following command:\u001b[0m\n\u001b[34m/miniconda3/bin/python -m pip install . \u001b[0m\n\u001b[34mProcessing /opt/ml/code\u001b[0m\n\u001b[34mBuilding wheels for collected packages: train\n Building wheel for train (setup.py): started\n Building wheel for train (setup.py): finished with status 'done'\n Created wheel for train: filename=train-1.0.0-py2.py3-none-any.whl size=7116 sha256=8175331681d77ae08b5a7edf807529aacb856bbfadbaf975cc9d84a614322f0d\n Stored in directory: /tmp/pip-ephem-wheel-cache-pn0jy4mv/wheels/35/24/16/37574d11bf9bde50616c67372a334f94fa8356bc7164af8ca3\u001b[0m\n\u001b[34mSuccessfully built train\u001b[0m\n\u001b[34mInstalling collected packages: train\u001b[0m\n\u001b[34mSuccessfully installed train-1.0.0\u001b[0m\n\u001b[34m2019-12-13 23:31:18,339 sagemaker-containers INFO No GPUs detected (normal if no gpus installed)\u001b[0m\n\u001b[34m2019-12-13 23:31:18,350 sagemaker-containers INFO Invoking user script\n\u001b[0m\n\u001b[34mTraining Env:\n\u001b[0m\n\u001b[34m{\n \"additional_framework_parameters\": {},\n \"channel_input_dirs\": {\n \"train\": \"/opt/ml/input/data/train\"\n },\n \"current_host\": \"algo-1\",\n \"framework_module\": \"sagemaker_sklearn_container.training:main\",\n \"hosts\": [\n \"algo-1\"\n ],\n \"hyperparameters\": {\n \"max_depth\": 5,\n \"n_estimators\": 50\n },\n \"input_config_dir\": \"/opt/ml/input/config\",\n \"input_data_config\": {\n \"train\": {\n \"TrainingInputMode\": \"File\",\n \"S3DistributionType\": \"FullyReplicated\",\n \"RecordWrapperType\": \"None\"\n }\n },\n \"input_dir\": \"/opt/ml/input\",\n \"is_master\": true,\n \"job_name\": \"sagemaker-scikit-learn-2019-12-13-23-28-34-846\",\n \"log_level\": 20,\n \"master_hostname\": \"algo-1\",\n \"model_dir\": \"/opt/ml/model\",\n \"module_dir\": \"s3://sagemaker-us-east-1-088315321151/sagemaker-scikit-learn-2019-12-13-23-28-34-846/source/sourcedir.tar.gz\",\n \"module_name\": \"train\",\n \"network_interface_name\": \"eth0\",\n \"num_cpus\": 4,\n \"num_gpus\": 0,\n \"output_data_dir\": \"/opt/ml/output/data\",\n \"output_dir\": \"/opt/ml/output\",\n \"output_intermediate_dir\": \"/opt/ml/output/intermediate\",\n \"resource_config\": {\n \"current_host\": \"algo-1\",\n \"hosts\": [\n \"algo-1\"\n ],\n \"network_interface_name\": \"eth0\"\n },\n \"user_entry_point\": \"train.py\"\u001b[0m\n\u001b[34m}\n\u001b[0m\n\u001b[34mEnvironment variables:\n\u001b[0m\n\u001b[34mSM_HOSTS=[\"algo-1\"]\u001b[0m\n\u001b[34mSM_NETWORK_INTERFACE_NAME=eth0\u001b[0m\n\u001b[34mSM_HPS={\"max_depth\":5,\"n_estimators\":50}\u001b[0m\n\u001b[34mSM_USER_ENTRY_POINT=train.py\u001b[0m\n\u001b[34mSM_FRAMEWORK_PARAMS={}\u001b[0m\n\u001b[34mSM_RESOURCE_CONFIG={\"current_host\":\"algo-1\",\"hosts\":[\"algo-1\"],\"network_interface_name\":\"eth0\"}\u001b[0m\n\u001b[34mSM_INPUT_DATA_CONFIG={\"train\":{\"RecordWrapperType\":\"None\",\"S3DistributionType\":\"FullyReplicated\",\"TrainingInputMode\":\"File\"}}\u001b[0m\n\u001b[34mSM_OUTPUT_DATA_DIR=/opt/ml/output/data\u001b[0m\n\u001b[34mSM_CHANNELS=[\"train\"]\u001b[0m\n\u001b[34mSM_CURRENT_HOST=algo-1\u001b[0m\n\u001b[34mSM_MODULE_NAME=train\u001b[0m\n\u001b[34mSM_LOG_LEVEL=20\u001b[0m\n\u001b[34mSM_FRAMEWORK_MODULE=sagemaker_sklearn_container.training:main\u001b[0m\n\u001b[34mSM_INPUT_DIR=/opt/ml/input\u001b[0m\n\u001b[34mSM_INPUT_CONFIG_DIR=/opt/ml/input/config\u001b[0m\n\u001b[34mSM_OUTPUT_DIR=/opt/ml/output\u001b[0m\n\u001b[34mSM_NUM_CPUS=4\u001b[0m\n\u001b[34mSM_NUM_GPUS=0\u001b[0m\n\u001b[34mSM_MODEL_DIR=/opt/ml/model\u001b[0m\n\u001b[34mSM_MODULE_DIR=s3://sagemaker-us-east-1-088315321151/sagemaker-scikit-learn-2019-12-13-23-28-34-846/source/sourcedir.tar.gz\u001b[0m\n\u001b[34mSM_TRAINING_ENV={\"additional_framework_parameters\":{},\"channel_input_dirs\":{\"train\":\"/opt/ml/input/data/train\"},\"current_host\":\"algo-1\",\"framework_module\":\"sagemaker_sklearn_container.training:main\",\"hosts\":[\"algo-1\"],\"hyperparameters\":{\"max_depth\":5,\"n_estimators\":50},\"input_config_dir\":\"/opt/ml/input/config\",\"input_data_config\":{\"train\":{\"RecordWrapperType\":\"None\",\"S3DistributionType\":\"FullyReplicated\",\"TrainingInputMode\":\"File\"}},\"input_dir\":\"/opt/ml/input\",\"is_master\":true,\"job_name\":\"sagemaker-scikit-learn-2019-12-13-23-28-34-846\",\"log_level\":20,\"master_hostname\":\"algo-1\",\"model_dir\":\"/opt/ml/model\",\"module_dir\":\"s3://sagemaker-us-east-1-088315321151/sagemaker-scikit-learn-2019-12-13-23-28-34-846/source/sourcedir.tar.gz\",\"module_name\":\"train\",\"network_interface_name\":\"eth0\",\"num_cpus\":4,\"num_gpus\":0,\"output_data_dir\":\"/opt/ml/output/data\",\"output_dir\":\"/opt/ml/output\",\"output_intermediate_dir\":\"/opt/ml/output/intermediate\",\"resource_config\":{\"current_host\":\"algo-1\",\"hosts\":[\"algo-1\"],\"network_interface_name\":\"eth0\"},\"user_entry_point\":\"train.py\"}\u001b[0m\n\u001b[34mSM_USER_ARGS=[\"--max_depth\",\"5\",\"--n_estimators\",\"50\"]\u001b[0m\n\u001b[34mSM_OUTPUT_INTERMEDIATE_DIR=/opt/ml/output/intermediate\u001b[0m\n\u001b[34mSM_CHANNEL_TRAIN=/opt/ml/input/data/train\u001b[0m\n\u001b[34mSM_HP_MAX_DEPTH=5\u001b[0m\n\u001b[34mSM_HP_N_ESTIMATORS=50\u001b[0m\n\u001b[34mPYTHONPATH=/miniconda3/bin:/miniconda3/lib/python37.zip:/miniconda3/lib/python3.7:/miniconda3/lib/python3.7/lib-dynload:/miniconda3/lib/python3.7/site-packages\n\u001b[0m\n\u001b[34mInvoking script with the following command:\n\u001b[0m\n\u001b[34m/miniconda3/bin/python -m train --max_depth 5 --n_estimators 50\n\n\u001b[0m\n\u001b[34m/miniconda3/lib/python3.7/site-packages/sklearn/externals/joblib/externals/cloudpickle/cloudpickle.py:47: DeprecationWarning: the imp module is deprecated in favour of importlib; see the module's documentation for alternative uses\n import imp\u001b[0m\n\u001b[34m2019-12-13 23:31:19,891 sagemaker-containers INFO Reporting training SUCCESS\u001b[0m\n\n2019-12-13 23:31:28 Uploading - Uploading generated training model\n2019-12-13 23:31:28 Completed - Training job completed\nTraining seconds: 64\nBillable seconds: 64\nCPU times: user 388 ms, sys: 19.7 ms, total: 408 ms\nWall time: 3min 11s\n"
]
],
[
[
"## EXERCISE: Deploy the trained model\n\nAfter training, deploy your model to create a `predictor`. If you're using a PyTorch model, you'll need to create a trained `PyTorchModel` that accepts the trained `<model>.model_data` as an input parameter and points to the provided `source_pytorch/predict.py` file as an entry point. \n\nTo deploy a trained model, you'll use `<model>.deploy`, which takes in two arguments:\n* **initial_instance_count**: The number of deployed instances (1).\n* **instance_type**: The type of SageMaker instance for deployment.\n\nNote: If you run into an instance error, it may be because you chose the wrong training or deployment instance_type. It may help to refer to your previous exercise code to see which types of instances we used.",
"_____no_output_____"
]
],
[
[
"%%time\n\n# uncomment, if needed\n# from sagemaker.pytorch import PyTorchModel\n\n\n# deploy your model to create a predictor\npredictor = sklearn.deploy(initial_instance_count=1, instance_type=\"ml.m4.xlarge\")",
"---------------------------------------------------------------------------------------------------------------!CPU times: user 600 ms, sys: 0 ns, total: 600 ms\nWall time: 9min 27s\n"
]
],
[
[
"---\n# Evaluating Your Model\n\nOnce your model is deployed, you can see how it performs when applied to our test data.\n\nThe provided cell below, reads in the test data, assuming it is stored locally in `data_dir` and named `test.csv`. The labels and features are extracted from the `.csv` file.",
"_____no_output_____"
]
],
[
[
"\"\"\"\nDON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE\n\"\"\"\nimport os\n\n# read in test data, assuming it is stored locally\ntest_data = pd.read_csv(os.path.join(data_dir, \"test.csv\"), header=None, names=None)\n\n# labels are in the first column\ntest_y = test_data.iloc[:,0]\ntest_x = test_data.iloc[:,1:]",
"_____no_output_____"
]
],
[
[
"## EXERCISE: Determine the accuracy of your model\n\nUse your deployed `predictor` to generate predicted, class labels for the test data. Compare those to the *true* labels, `test_y`, and calculate the accuracy as a value between 0 and 1.0 that indicates the fraction of test data that your model classified correctly. You may use [sklearn.metrics](https://scikit-learn.org/stable/modules/classes.html#module-sklearn.metrics) for this calculation.\n\n**To pass this project, your model should get at least 90% test accuracy.**",
"_____no_output_____"
]
],
[
[
"# First: generate predicted, class labels\ntest_y_preds = predictor.predict(test_x)\n\n\n\"\"\"\nDON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE\n\"\"\"\n# test that your model generates the correct number of labels\nassert len(test_y_preds)==len(test_y), 'Unexpected number of predictions.'\nprint('Test passed!')",
"Test passed!\n"
],
[
"\nfrom sklearn import metrics\n# Second: calculate the test accuracy\naccuracy = metrics.accuracy_score(y_true=test_y, y_pred=test_y_preds)\n\nprint(accuracy)\n\n\n## print out the array of predicted and true labels, if you want\nprint('\\nPredicted class labels: ')\nprint(test_y_preds)\nprint('\\nTrue class labels: ')\nprint(test_y.values)",
"1.0\n\nPredicted class labels: \n[1 1 1 1 1 1 0 0 0 0 0 0 1 1 1 1 1 1 0 1 0 1 1 0 0]\n\nTrue class labels: \n[1 1 1 1 1 1 0 0 0 0 0 0 1 1 1 1 1 1 0 1 0 1 1 0 0]\n"
]
],
[
[
"### Question 1: How many false positives and false negatives did your model produce, if any? And why do you think this is?",
"_____no_output_____"
],
[
"** Answer**: \ni see 100% accuracy with no false positivies and no false negatives.\nthere could be overfitting possible data leakage",
"_____no_output_____"
],
[
"### Question 2: How did you decide on the type of model to use? ",
"_____no_output_____"
],
[
"** Answer**:\n\nI will start with tree based models for classifications. I have seen tree based models giving consistently better performance. we can also try logistic regression.",
"_____no_output_____"
],
[
"----\n## EXERCISE: Clean up Resources\n\nAfter you're done evaluating your model, **delete your model endpoint**. You can do this with a call to `.delete_endpoint()`. You need to show, in this notebook, that the endpoint was deleted. Any other resources, you may delete from the AWS console, and you will find more instructions on cleaning up all your resources, below.",
"_____no_output_____"
]
],
[
[
"# uncomment and fill in the line below!\n# <name_of_deployed_predictor>.delete_endpoint()\npredictor.delete_endpoint()",
"_____no_output_____"
]
],
[
[
"### Deleting S3 bucket\n\nWhen you are *completely* done with training and testing models, you can also delete your entire S3 bucket. If you do this before you are done training your model, you'll have to recreate your S3 bucket and upload your training data again.",
"_____no_output_____"
]
],
[
[
"# deleting bucket, uncomment lines below\n\nbucket_to_delete = boto3.resource('s3').Bucket(bucket)\nbucket_to_delete.objects.all().delete()",
"_____no_output_____"
]
],
[
[
"### Deleting all your models and instances\n\nWhen you are _completely_ done with this project and do **not** ever want to revisit this notebook, you can choose to delete all of your SageMaker notebook instances and models by following [these instructions](https://docs.aws.amazon.com/sagemaker/latest/dg/ex1-cleanup.html). Before you delete this notebook instance, I recommend at least downloading a copy and saving it, locally.",
"_____no_output_____"
],
[
"---\n## Further Directions\n\nThere are many ways to improve or add on to this project to expand your learning or make this more of a unique project for you. A few ideas are listed below:\n* Train a classifier to predict the *category* (1-3) of plagiarism and not just plagiarized (1) or not (0).\n* Utilize a different and larger dataset to see if this model can be extended to other types of plagiarism.\n* Use language or character-level analysis to find different (and more) similarity features.\n* Write a complete pipeline function that accepts a source text and submitted text file, and classifies the submitted text as plagiarized or not.\n* Use API Gateway and a lambda function to deploy your model to a web application.\n\nThese are all just options for extending your work. If you've completed all the exercises in this notebook, you've completed a real-world application, and can proceed to submit your project. Great job!",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
]
] |
e7bae6e05b5d99210d31b3c4768e278415be41f8 | 698,486 | ipynb | Jupyter Notebook | MO_Aula_SciKit.ipynb | anarossati/Mineracao_dados_Ocean_05_2021 | b5ab374e46c4dca29aa95c3f449ac531494e9b42 | [
"MIT"
] | null | null | null | MO_Aula_SciKit.ipynb | anarossati/Mineracao_dados_Ocean_05_2021 | b5ab374e46c4dca29aa95c3f449ac531494e9b42 | [
"MIT"
] | null | null | null | MO_Aula_SciKit.ipynb | anarossati/Mineracao_dados_Ocean_05_2021 | b5ab374e46c4dca29aa95c3f449ac531494e9b42 | [
"MIT"
] | null | null | null | 189.54844 | 294,050 | 0.858749 | [
[
[
"<a href=\"https://colab.research.google.com/github/anarossati/Mineracao_dados_Ocean_05_2021/blob/main/MO_Aula_SciKit.ipynb\" target=\"_parent\"><img src=\"https://colab.research.google.com/assets/colab-badge.svg\" alt=\"Open In Colab\"/></a>",
"_____no_output_____"
],
[
"#Scikit-Learn",
"_____no_output_____"
],
[
"\n\n* É considerada como a biblitoca de Python mais utilizada para a implementação de métodos baseados em algoritmos de aprendizagem de máquina (*machine learning*).\n* A versão atual é a 0.24.2 (abril 2021).\n* URL: http://scikit-learn.org\n\n",
"_____no_output_____"
],
[
"#Formulação do Problema",
"_____no_output_____"
],
[
"\n\n* Problema de classificação **supervisionada** de texto.\n* Hoje iremos investigar o método de aprendizagem de máquina que seja mais apropriado para resolvê-lo.\n* Considere um site de notícias que publica matérias jornalísticas de vários temas. \n* Economia, saúde e esportes são exemplos de temas. \n* O objetivo é criar um método classificador que receba um texto de entrada e consiga identificar qual é o assunto do texto.\n* O classificador assume que cada texto está associado a um tema.\n* É um problema de classificação de texto multiclasses.\n",
"_____no_output_____"
],
[
"",
"_____no_output_____"
],
[
"# Exploração dos Dados",
"_____no_output_____"
],
[
"### Carregar *dataset*",
"_____no_output_____"
]
],
[
[
"import pandas as pd",
"_____no_output_____"
],
[
"df = pd.read_csv('http://tiagodemelo.info/datasets/dataset-uol.csv')\ndf.head()",
"_____no_output_____"
],
[
"df.shape",
"_____no_output_____"
],
[
"df.CATEGORIA.unique()",
"_____no_output_____"
]
],
[
[
"### Verificar dados nulos (NaN)",
"_____no_output_____"
]
],
[
[
"df.isnull().any()",
"_____no_output_____"
],
[
"df.isnull().sum()",
"_____no_output_____"
],
[
"index_with_nan = df.index[df.isnull().any(axis=1)]\nindex_with_nan.shape\ndf.drop(index_with_nan, 0, inplace=True)",
"_____no_output_____"
],
[
"df.shape",
"_____no_output_____"
]
],
[
[
"### Adicionar coluna ao *dataset*",
"_____no_output_____"
]
],
[
[
"ids_categoria = df['CATEGORIA'].factorize()[0]\ndf['ID_CATEGORIA'] = ids_categoria",
"_____no_output_____"
],
[
"df.head(n=10)",
"_____no_output_____"
],
[
"df.ID_CATEGORIA.unique()",
"_____no_output_____"
],
[
"column_values = df[[\"ID_CATEGORIA\", \"CATEGORIA\"]].values.ravel()\nunique_values = pd.unique(column_values)\nprint(unique_values)",
"[0 'coronavirus' 1 'politica' 2 'esporte' 3 'carro' 4 'educacao' 5\n 'entretenimento' 6 'economia' 7 'saude']\n"
],
[
"category_id_df = df[['CATEGORIA', 'ID_CATEGORIA']].drop_duplicates().sort_values('ID_CATEGORIA')\nid_to_category = dict(category_id_df[['ID_CATEGORIA', 'CATEGORIA']].values)\nid_to_category",
"_____no_output_____"
]
],
[
[
"### Distribuição das notícias entre as categorias",
"_____no_output_____"
]
],
[
[
"import matplotlib.pyplot as plt\n\nfig = plt.figure(figsize=(8,6))\n\ndf.groupby('CATEGORIA').TEXTO.count().plot.bar()\nplt.show()",
"_____no_output_____"
]
],
[
[
"\n\n* Um problema recorrente é o **desbalanceamento das classes**.\n* Os algoritmos convencionais tendem a favorecer as classes mais frequentes, ou seja, não consideram as classes menos frequentes.\n* As classes menos frequentes costumam ser tratadas como *outliers*.\n* Estratégias de *undersampling* ou *oversampling* são aplicadas para tratar desse problema [[1]](https://en.wikipedia.org/wiki/Oversampling_and_undersampling_in_data_analysis).\n* Lidar com essas estratégias será discutido posteriormente.\n\n\n",
"_____no_output_____"
],
[
"#### Preparar dataset para que todas as categorias tenham a mesma quantidade de publicações",
"_____no_output_____"
]
],
[
[
"TAMANHO_DATASET = 200 #quantidade de artigos por classe.",
"_____no_output_____"
],
[
"categorias = list(set(df['ID_CATEGORIA']))\n\ndata = []\nfor cat in categorias:\n total = TAMANHO_DATASET\n for c,t,i in zip(df['CATEGORIA'], df['TEXTO'], df['ID_CATEGORIA']):\n if total>0 and cat == i:\n total-=1\n data.append([c,t,i])\n\ndf = pd.DataFrame(data, columns=['CATEGORIA','TEXTO','ID_CATEGORIA'])",
"_____no_output_____"
],
[
"fig = plt.figure(figsize=(8,6))\n\ndf.groupby('CATEGORIA').TEXTO.count().plot.bar(ylim=0)\nplt.show()",
"_____no_output_____"
]
],
[
[
"# Representação do Texto",
"_____no_output_____"
],
[
"\n\n* Os métodos de aprendizagem de máquina lidam melhor com representações numéricas ao invés de representação textual.\n* Portanto, os textos precisam ser convertidos.\n* *Bag of words* é uma forma comum de representar os textos.\n* Nós vamos calcular a medida de *Term Frequency* e *Inverse Document Frequency*, abreviada como **TF-IFD**.\n* Nós usaremos o `sklearn.feature_extraction.text.TfidfVectorizer` para calcular o `tf-idf`.\n\n",
"_____no_output_____"
],
[
"## *Bag of Words*",
"_____no_output_____"
],
[
"É uma representação de texto comumente usada em problemas relacionados com processamento de linguagem natural e recuperação da informação.",
"_____no_output_____"
],
[
"sentença 1: \"Os brasileiros gostam de futebol\"\n\nsentença 2: \"Os americanos adoram futebol e adoram basquete\"",
"_____no_output_____"
]
],
[
[
"import nltk\nnltk.download('punkt')",
"_____no_output_____"
],
[
"from nltk.tokenize import word_tokenize\n\nsentenca1 = \"Os brasileiros gostam de futebol\"\nsentenca2 = \"Os americanos adoram futebol e adoram basquete\"\n\ntexto1 = word_tokenize(sentenca1)\ntexto2 = word_tokenize(sentenca2)\n\nprint (texto1)\nprint (texto2)",
"['Os', 'brasileiros', 'gostam', 'de', 'futebol']\n['Os', 'americanos', 'adoram', 'futebol', 'e', 'adoram', 'basquete']\n"
],
[
"from nltk.probability import FreqDist\n\nfdist1 = FreqDist(texto1) \nfdist2 = FreqDist(texto2) \n\nprint(fdist1.most_common())\nprint(fdist2.most_common())",
"[('Os', 1), ('brasileiros', 1), ('gostam', 1), ('de', 1), ('futebol', 1)]\n[('adoram', 2), ('Os', 1), ('americanos', 1), ('futebol', 1), ('e', 1), ('basquete', 1)]\n"
],
[
"texto = texto1 + texto2\nfdist = FreqDist(texto) \nprint(fdist.most_common())",
"[('Os', 2), ('futebol', 2), ('adoram', 2), ('brasileiros', 1), ('gostam', 1), ('de', 1), ('americanos', 1), ('e', 1), ('basquete', 1)]\n"
]
],
[
[
"sentença 1: \"Os brasileiros gostam de futebol\"\n\nsentença 2: \"Os americanos adoram futebol e adoram basquete\"\n\n\n\n",
"_____no_output_____"
],
[
"",
"_____no_output_____"
],
[
"Sentença 1: [1 1 0 1 1 1 0 0 0]\n\nSentença 2: [1 1 2 0 0 0 1 1 1]",
"_____no_output_____"
],
[
"## TF-IDF",
"_____no_output_____"
],
[
"TF representa a frequência do termo.\n\nIDF representa o inverso da frequência nos documentos.",
"_____no_output_____"
],
[
"##Texto no SKLearn",
"_____no_output_____"
],
[
"Opções (paramêtros) utilizados:\n\n* `min_df` é o número mínimo de documentos que uma palavra deve estar presente.\n* `encoding` é usado para que o classificador consiga lidar com caracteres especiais.\n* `ngram_range` é definida para considerar unigramas e bigramas.\n* `stop_words` é definida para reduzir o número de termos indesejáveis.\n\n\n",
"_____no_output_____"
]
],
[
[
"from sklearn.feature_extraction.text import TfidfVectorizer\n\nimport nltk\nnltk.download('stopwords')\nfrom nltk.corpus import stopwords",
"[nltk_data] Downloading package stopwords to /root/nltk_data...\n[nltk_data] Unzipping corpora/stopwords.zip.\n"
],
[
"tfidf = TfidfVectorizer(min_df=5, encoding='latin-1', ngram_range=(1, 2), stop_words=stopwords.words('portuguese'))\n\nfeatures = tfidf.fit_transform(df.TEXTO.values.astype('U')).toarray()\nlabels = df.ID_CATEGORIA\nfeatures.shape",
"_____no_output_____"
]
],
[
[
"Nós podemos usar o `sklearn.feature_selection.chi2` para achar os termos que estão mais correlacionados com cada categoria.",
"_____no_output_____"
]
],
[
[
"from sklearn.feature_selection import chi2\nimport numpy as np\n\nN = 2\n\nfor Categoria, category_id in sorted(id_to_category.items()):\n features_chi2 = chi2(features, labels == category_id)\n indices = np.argsort(features_chi2[0])\n feature_names = np.array(tfidf.get_feature_names())[indices]\n unigrams = [v for v in feature_names if len(v.split(' ')) == 1]\n bigrams = [v for v in feature_names if len(v.split(' ')) == 2]\n print(\"# '{}':\".format(Categoria))\n print(\" . Unigramas mais correlacionados:\\n. {}\".format('\\n. '.join(unigrams[-N:])))\n print(\" . Bigramas mais correlacionados:\\n. {}\".format('\\n. '.join(bigrams[-N:])))",
"# '0':\n . Unigramas mais correlacionados:\n. equipe\n. único\n . Bigramas mais correlacionados:\n. duas vezes\n. entrevista coletiva\n# '1':\n . Unigramas mais correlacionados:\n. equipe\n. único\n . Bigramas mais correlacionados:\n. duas vezes\n. entrevista coletiva\n# '2':\n . Unigramas mais correlacionados:\n. equipe\n. único\n . Bigramas mais correlacionados:\n. duas vezes\n. entrevista coletiva\n# '3':\n . Unigramas mais correlacionados:\n. equipe\n. único\n . Bigramas mais correlacionados:\n. duas vezes\n. entrevista coletiva\n# '4':\n . Unigramas mais correlacionados:\n. equipe\n. único\n . Bigramas mais correlacionados:\n. duas vezes\n. entrevista coletiva\n# '5':\n . Unigramas mais correlacionados:\n. equipe\n. único\n . Bigramas mais correlacionados:\n. duas vezes\n. entrevista coletiva\n# '6':\n . Unigramas mais correlacionados:\n. equipe\n. único\n . Bigramas mais correlacionados:\n. duas vezes\n. entrevista coletiva\n# '7':\n . Unigramas mais correlacionados:\n. equipe\n. único\n . Bigramas mais correlacionados:\n. duas vezes\n. entrevista coletiva\n"
]
],
[
[
"#Criação de Classificador",
"_____no_output_____"
],
[
"Importar bibliotecas:",
"_____no_output_____"
]
],
[
[
"from sklearn.model_selection import train_test_split\nfrom sklearn.feature_extraction.text import CountVectorizer\nfrom sklearn.feature_extraction.text import TfidfTransformer\nfrom sklearn.naive_bayes import MultinomialNB",
"_____no_output_____"
]
],
[
[
"Dividir *dataset* em **treino** e **teste**",
"_____no_output_____"
]
],
[
[
"X_train, X_test, y_train, y_test = train_test_split(df['TEXTO'], df['CATEGORIA'], test_size=0.2, random_state = 0)",
"_____no_output_____"
]
],
[
[
"Criar um modelo (Naive Bayes)",
"_____no_output_____"
]
],
[
[
"count_vect = CountVectorizer()\nX_train_counts = count_vect.fit_transform(X_train.values.astype('U'))\ntfidf_transformer = TfidfTransformer()\nX_train_tfidf = tfidf_transformer.fit_transform(X_train_counts)\nclf = MultinomialNB().fit(X_train_tfidf, y_train)",
"_____no_output_____"
]
],
[
[
"Testar o classificador criado:",
"_____no_output_____"
]
],
[
[
"sentenca = 'O brasileiro gosta de futebol.'\nprint(clf.predict(count_vect.transform([sentenca])))",
"['esporte']\n"
]
],
[
[
"#Seleção do Modelo",
"_____no_output_____"
],
[
"Nós agora vamos experimentar diferentes modelos de aprendizagem de máquina e avaliar a sua acurácia.\n\nSerão considerados os seguintes modelos:\n* Logistic Regression (LR)\n* Multinomial Naive Bayes (NB)\n* Linear Support Vector Machine (SVM)\n* Random Forest (RF)\n\n",
"_____no_output_____"
],
[
"Importar bibliotecas:",
"_____no_output_____"
]
],
[
[
"from sklearn.linear_model import LogisticRegression\nfrom sklearn.ensemble import RandomForestClassifier\nfrom sklearn.svm import LinearSVC\nfrom sklearn.model_selection import cross_val_score",
"_____no_output_____"
]
],
[
[
"Lista com os modelos:",
"_____no_output_____"
]
],
[
[
"models = [\n RandomForestClassifier(n_estimators=200, max_depth=3, random_state=0),\n LinearSVC(C=10, class_weight=None, dual=True, fit_intercept=True, intercept_scaling=1, loss='squared_hinge', max_iter=1000, multi_class='ovr', penalty='l2', random_state=None, tol=0.0001, verbose=0),\n MultinomialNB(),\n LogisticRegression(random_state=0),\n]",
"_____no_output_____"
]
],
[
[
"## Validação Cruzada",
"_____no_output_____"
],
[
"A validação cruzada é um método de reamostragem e tem como objetivo avaliar a capacidade de **generalização** do modelo.",
"_____no_output_____"
],
[
"Normalmente a distribuição entre treino e teste é feita da seguinte forma:",
"_____no_output_____"
],
[
"",
"_____no_output_____"
],
[
"Na validação cruzada:",
"_____no_output_____"
],
[
"",
"_____no_output_____"
],
[
"Uso de validação cruzada com 5 *folds*:",
"_____no_output_____"
]
],
[
[
"CV = 5",
"_____no_output_____"
]
],
[
[
"Geração dos modelos:",
"_____no_output_____"
]
],
[
[
"cv_df = pd.DataFrame(index=range(CV * len(models)))\nentries = []\n\nfor model in models:\n model_name = model.__class__.__name__\n accuracies = cross_val_score(model, features, labels, scoring='accuracy', cv=CV)\n for fold_idx, accuracy in enumerate(accuracies):\n entries.append((model_name, fold_idx, accuracy))\n\ncv_df = pd.DataFrame(entries, columns=['model_name', 'fold_idx', 'accuracy'])",
"_____no_output_____"
]
],
[
[
"## Gráfico BoxPlot",
"_____no_output_____"
],
[
"",
"_____no_output_____"
],
[
"Plotar o gráfico comparativo:",
"_____no_output_____"
]
],
[
[
"import seaborn as sns\n\nsns.boxplot(x='model_name', y='accuracy', data=cv_df)\nsns.stripplot(x='model_name', y='accuracy', data=cv_df, \n size=8, jitter=True, edgecolor=\"gray\", linewidth=2)\n\nplt.show()",
"_____no_output_____"
]
],
[
[
"Acurácia média entre os 5 modelos:",
"_____no_output_____"
]
],
[
[
"cv_df.groupby('model_name').accuracy.mean()",
"_____no_output_____"
]
],
[
[
"# Matriz de Confusão",
"_____no_output_____"
],
[
"Geração de modelo baseado em SVM:",
"_____no_output_____"
]
],
[
[
"model = LinearSVC()\nX_train, X_test, y_train, y_test, indices_train, indices_test = train_test_split(features, labels, df.index, test_size=0.33, random_state=0)\nmodel.fit(X_train, y_train)\ny_pred = model.predict(X_test)",
"_____no_output_____"
]
],
[
[
"Plotar matriz de confusão para o modelo SVM:",
"_____no_output_____"
]
],
[
[
"from sklearn.metrics import confusion_matrix\n\nconf_mat = confusion_matrix(y_test, y_pred)\nfig, ax = plt.subplots(figsize=(10,10))\n\nsns.heatmap(conf_mat, annot=True, fmt='d',\n xticklabels=category_id_df.CATEGORIA.values, yticklabels=category_id_df.CATEGORIA.values)\n\nplt.ylabel('Actual')\nplt.xlabel('Predicted')\nplt.show()",
"_____no_output_____"
]
],
[
[
"Vamos avaliar os resultados incorretos.\n\nApresentação de textos classificados incorretamente:",
"_____no_output_____"
]
],
[
[
"from IPython.display import display\n\nfor predicted in category_id_df.ID_CATEGORIA:\n for actual in category_id_df.ID_CATEGORIA:\n if predicted != actual and conf_mat[actual, predicted] >= 5:\n print(\"'{}' predicted as '{}' : {} examples.\".format(id_to_category[actual], id_to_category[predicted], conf_mat[actual, predicted]))\n display(df.loc[indices_test[(y_test == actual) & (y_pred == predicted)]][['CATEGORIA', 'TEXTO']])\n print('')",
"'esporte' predicted as 'coronavirus' : 6 examples.\n"
]
],
[
[
"Reportar o resultado do classificador em cada classe:",
"_____no_output_____"
]
],
[
[
"from sklearn import metrics\n\nprint(metrics.classification_report(y_test, y_pred, target_names=df['CATEGORIA'].unique()))",
" precision recall f1-score support\n\n coronavirus 0.35 0.26 0.30 66\n politica 0.67 0.69 0.68 58\n esporte 0.70 0.58 0.63 78\n carro 0.65 0.50 0.56 70\n educacao 0.48 0.74 0.58 62\nentretenimento 0.55 0.52 0.54 71\n economia 0.47 0.54 0.50 56\n saude 0.59 0.66 0.62 67\n\n accuracy 0.56 528\n macro avg 0.56 0.56 0.55 528\n weighted avg 0.56 0.56 0.55 528\n\n"
]
],
[
[
"# Exercícios propostos",
"_____no_output_____"
],
[
"* Nós usamos acurácia como métrica para avaliar os quatro modelos de classificação de texto. Execute novamente o notebook, mas agora usando a métrica F1 e analise os resultados.\n* Pesquise outros modelos de classificação de texto que estão disponíveis no Scikit-Learn e inclua nos experimentos.\n* Execute novamente o notebook, mas agora utilize todo o conjunto de dados (*dataset*). Observe que as classes estarão desbalanceadas. Avalie e compare o resultado com os classificadores treinados em classes balanceadas.\n* Os modelos estão configurados com um conjunto pré-definido de hiperparâmetros. Pesquise e experimente outras possíveis combinações de hiperparâmetros para os modelos e avalie se os resultados irão melhorar. Sugestão: pesquise estratégias como GridSearchCV e RandomizedSearchCV em [LINK](https://scikit-learn.org/stable/modules/grid_search.html#).\n* Pesquise, proponha e experimente outras estratégias para tentar melhorar o resultado alcançado pelos modelos propostos.\n\n",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
]
] |
e7bae92a859a3e064420fe8aa793d4be0f7d50df | 270,165 | ipynb | Jupyter Notebook | sdp03/SPD03.ipynb | UPIITAalejandro/Proyecto_Terminal_2 | d3158f309d80c5fc70959e42ada4629b1f980a39 | [
"MIT"
] | null | null | null | sdp03/SPD03.ipynb | UPIITAalejandro/Proyecto_Terminal_2 | d3158f309d80c5fc70959e42ada4629b1f980a39 | [
"MIT"
] | null | null | null | sdp03/SPD03.ipynb | UPIITAalejandro/Proyecto_Terminal_2 | d3158f309d80c5fc70959e42ada4629b1f980a39 | [
"MIT"
] | null | null | null | 51.225825 | 155 | 0.213954 | [
[
[
"import pandas as pd\nimport numpy as np\n\npd.options.display.max_columns = None\npd.options.display.max_rows = None",
"_____no_output_____"
],
[
"df_2010 = pd.read_csv(\"2010.csv\")\ndf_2012 = pd.read_csv(\"2012.csv\")\ndf_2014 = pd.read_csv(\"2014.csv\")\ndf_2016 = pd.read_csv(\"2016.csv\")",
"_____no_output_____"
],
[
"df_2010",
"_____no_output_____"
],
[
"df_2010.columns",
"_____no_output_____"
],
[
"# Verificacion que todos los dataFrame tenga la misma cabecera\nlist(df_2010.columns) == list(df_2012.columns) == list(df_2014.columns)== list(df_2016.columns)",
"_____no_output_____"
],
[
"# Verificacion que toda la columna \"estado_1\" tenga el mismo orden en los estados \nlist(df_2010['Entidadfederativa']) == list(df_2012['Entidadfederativa']) == list(df_2014['Entidadfederativa']) == list(df_2016['Entidadfederativa']) ",
"_____no_output_____"
],
[
"# Creacion del diccionario para datos faltantes\ndiccionario_anios_faltantes = {\n 'Entidadfederativa':df_2010['Entidadfederativa'], \n 'Periodo':list(np.nan for i in range(0,32)),\n 'Hogarescongastoensistemasalternativossalud_segúndecildeingresos':list(np.nan for i in range(0,32)),\n 'Hogaressegúndecilesdeingreso':list(np.nan for i in range(0,32)),\n 'Hogarescongastoensistemasalternativossaluddecilesdeingreso':list(np.nan for i in range(0,32))\n}",
"_____no_output_____"
],
[
"# Creacion de diccionario con datos faltantes\n\ndf_2011 = pd.DataFrame(diccionario_anios_faltantes)\ndf_2011['Periodo'] = 2011\n\ndf_2013 = pd.DataFrame(diccionario_anios_faltantes)\ndf_2013['Periodo'] = 2013\n\ndf_2015 = pd.DataFrame(diccionario_anios_faltantes)\ndf_2015['Periodo'] = 2015",
"_____no_output_____"
],
[
"df_general = pd.DataFrame()",
"_____no_output_____"
],
[
"for i in range(2,len(list(df_2010.columns))):\n \n #Recorrer para interpolacion \n aux_2010 = df_2010.iloc[:,lambda df_2010:[i]]\n aux_2011 = df_2011.iloc[:,lambda df_2010:[i]]\n aux_2012 = df_2012.iloc[:,lambda df_2010:[i]]\n aux_2013 = df_2013.iloc[:,lambda df_2010:[i]]\n aux_2014 = df_2014.iloc[:,lambda df_2010:[i]]\n aux_2015 = df_2015.iloc[:,lambda df_2010:[i]]\n aux_2016 = df_2016.iloc[:,lambda df_2010:[i]]\n\n df_general_aux = pd.concat([aux_2010,aux_2011,aux_2012,aux_2013,aux_2014,aux_2015,aux_2016],axis=1)\n df_general_aux = df_general_aux.interpolate(method='linear',axis = 1).copy()\n \n lista_nombre_columnas = [list(aux_2010.columns)[0]+\"_\"+str(df_2010['Periodo'][0]),\n list(aux_2011.columns)[0]+\"_\"+str(df_2011['Periodo'][0]),list(aux_2012.columns)[0]+\"_\"+str(df_2012['Periodo'][0]),\n list(aux_2013.columns)[0]+\"_\"+str(df_2013['Periodo'][0]),list(aux_2014.columns)[0]+\"_\"+str(df_2014['Periodo'][0]),\n list(aux_2015.columns)[0]+\"_\"+str(df_2015['Periodo'][0]),list(aux_2016.columns)[0]+\"_\"+str(df_2016['Periodo'][0])]\n \n df_general_aux.columns = lista_nombre_columnas \n \n \n df_general=pd.concat([df_general,df_general_aux],axis=1)",
"_____no_output_____"
],
[
"df_general",
"_____no_output_____"
],
[
"diccionario_sustitucion_id = {\n 'Aguascalientes':1,\n 'Baja California':2,\n 'Baja California Sur':3,\n 'Campeche':4,\n 'Chiapas':5,\n 'Chihuahua':6,\n 'Ciudad De Mexico':7,\n 'Coahuila':8,\n 'Colima':9,\n 'Durango':10,\n 'Guanajuato':11,\n 'Guerrero':12,\n 'Hidalgo':13,\n 'Jalisco':14,\n 'Mexico':15,\n 'Michoacan':16,\n 'Morelos':17,\n 'Nayarit':18,\n 'Nuevo Leon':19,\n 'Oaxaca':20,\n 'Puebla':21,\n 'Queretaro':22,\n 'Quintana Roo':23,\n 'San Luis Potosi':24,\n 'Sinaloa':25,\n 'Sonora':26,\n 'Tabasco':27,\n 'Tamaulipas':28,\n 'Tlaxcala':29,\n 'Veracruz':30,\n 'Yucatan':31,\n 'Zacatecas':32\n}",
"_____no_output_____"
],
[
"df_nombre = pd.concat([df_2010.iloc[:,[0]],df_2010.iloc[:,[0]]],axis = 1)\ndf_nombre.columns = ['id','entidad']\ndf_unido = pd.concat([df_nombre,df_general],axis=1)\ndf_unido",
"_____no_output_____"
],
[
"prueba = ['Aguascalientes',\n 'Baja California',\n 'Baja California Sur',\n 'Campeche',\n 'Chiapas',\n 'Chihuahua',\n 'Ciudad De Mexico',\n 'Coahuila',\n 'Colima',\n 'Durango',\n 'Guanajuato',\n 'Guerrero',\n 'Hidalgo',\n 'Jalisco',\n 'Mexico',\n 'Michoacan',\n 'Morelos',\n 'Nayarit',\n 'Nuevo Leon',\n 'Oaxaca',\n 'Puebla',\n 'Queretaro',\n 'Quintana Roo',\n 'San Luis Potosi',\n 'Sinaloa',\n 'Sonora',\n 'Tabasco',\n 'Tamaulipas',\n 'Tlaxcala',\n 'Veracruz',\n 'Yucatan',\n 'Zacatecas']",
"_____no_output_____"
],
[
"df_ordenado = df_unido.sort_values(by=['entidad']).copy()\ndf_ordenado['entidad'] = prueba\ndf_ordenado['id'] = prueba\n\ndf_ordenado['id'] = df_ordenado['id'].replace(diccionario_sustitucion_id)\n\ndf_ordenado",
"_____no_output_____"
],
[
"df_ordenado.to_csv('Indicador_SPD03.csv',index=False)",
"_____no_output_____"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bafc429d9af5fac624f0c77f9b5fc657515323 | 561 | ipynb | Jupyter Notebook | Functions_List Frequencies.ipynb | PradheepV/Python_Fundamentals | 538d5f7c3a212ce4459135716884b1ac26950c09 | [
"MIT"
] | null | null | null | Functions_List Frequencies.ipynb | PradheepV/Python_Fundamentals | 538d5f7c3a212ce4459135716884b1ac26950c09 | [
"MIT"
] | 1 | 2019-09-09T04:26:39.000Z | 2019-09-09T04:26:39.000Z | Functions_List Frequencies.ipynb | PradheepV/Python_Fundamentals | 538d5f7c3a212ce4459135716884b1ac26950c09 | [
"MIT"
] | null | null | null | 21.576923 | 36 | 0.641711 | [
[
[
"empty"
]
]
] | [
"empty"
] | [
[
"empty"
]
] |
e7bb0fff8badd8db583e94b17e70dbad8ff4fe0e | 688,209 | ipynb | Jupyter Notebook | examples/UUVEnv_singleagent.ipynb | pthangeda/FiMDPEnv | 86445155a89304927ddf4d6714319edd50c7c4f2 | [
"MIT"
] | null | null | null | examples/UUVEnv_singleagent.ipynb | pthangeda/FiMDPEnv | 86445155a89304927ddf4d6714319edd50c7c4f2 | [
"MIT"
] | 13 | 2020-07-16T19:32:23.000Z | 2020-10-16T06:40:58.000Z | examples/UUVEnv_singleagent.ipynb | pthangeda/FiMDPEnv | 86445155a89304927ddf4d6714319edd50c7c4f2 | [
"MIT"
] | 1 | 2021-11-22T11:05:51.000Z | 2021-11-22T11:05:51.000Z | 85.248235 | 4,344 | 0.80721 | [
[
[
"# UUV Single-Agent Environment",
"_____no_output_____"
],
[
"## Creating the environment",
"_____no_output_____"
]
],
[
[
"# initialize setup\nfrom fimdpenv import setup, UUVEnv\nsetup()",
"_____no_output_____"
],
[
"# create envionment\nfrom fimdpenv.UUVEnv import SingleAgentEnv\nenv = SingleAgentEnv(grid_size=[20,20], capacity=50, reloads=[22,297], targets=[67, 345, 268], init_state=350, enhanced_actionspace=0)\nenv",
"_____no_output_____"
],
[
"# get consmdp\nmdp, targets = env.get_consmdp()",
"_____no_output_____"
],
[
"# list of targets\nenv.targets",
"_____no_output_____"
],
[
"# list of reloads\nenv.reloads",
"_____no_output_____"
],
[
"# converting state_id to grid coordinates\nenv.get_state_coord(265)",
"_____no_output_____"
],
[
"# converting grid coordinates to state_id\nenv.get_state_id(13,5)",
"_____no_output_____"
]
],
[
[
"## Creating and visualizing strategies",
"_____no_output_____"
]
],
[
[
"# generate strategies\nfrom fimdp.objectives import BUCHI\nfrom fimdp.energy_solvers import GoalLeaningES\nfrom fimdp.core import CounterStrategy\n\nenv.create_counterstrategy(solver=GoalLeaningES, objective=BUCHI, threshold=0.1)",
"_____no_output_____"
],
[
"# animate strategies\nenv.animate_simulation(num_steps=50, interval=100)",
"_____no_output_____"
],
[
"# histories\n#env.state_histories\n#env.action_histories\nenv.target_histories",
"_____no_output_____"
]
],
[
[
"## Resetting strategies and single transition",
"_____no_output_____"
]
],
[
[
"env.reset(init_state=98, reset_energy=20)\nenv",
"_____no_output_____"
],
[
"env.strategies",
"_____no_output_____"
],
[
"env.state_histories",
"_____no_output_____"
],
[
"env.step()\nenv",
"_____no_output_____"
],
[
"env.state_histories",
"_____no_output_____"
]
],
[
[
"## Enhanced action space",
"_____no_output_____"
]
],
[
[
"# create envionment with enchanced action space\nfrom fimdpenv.UUVEnv import SingleAgentEnv\nenv2 = SingleAgentEnv(grid_size=[20,20], capacity=50, reloads=[22,297, 198, 76], targets=[67, 345, 268], init_state=350, enhanced_actionspace=1)\n\nenv2",
"_____no_output_____"
],
[
"# create strategies \nfrom fimdp.objectives import BUCHI\nfrom fimdp.energy_solvers import GoalLeaningES\nfrom fimdp.core import CounterStrategy\n\nmdp, targets = env2.get_consmdp()\nenv2.create_counterstrategy()",
"_____no_output_____"
],
[
"# animate strategies\nenv2.animate_simulation(num_steps=50, interval=100)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
]
] |
e7bb132fc18766b1a47bb4d88a3fdd4f5fbf1063 | 9,288 | ipynb | Jupyter Notebook | src/features/SIR Calculation.ipynb | rutikajagtap/ads_covid-19_dashboard_rutika | beec0609527673a822ee3a7099b1ddc467f5e70c | [
"FTL"
] | null | null | null | src/features/SIR Calculation.ipynb | rutikajagtap/ads_covid-19_dashboard_rutika | beec0609527673a822ee3a7099b1ddc467f5e70c | [
"FTL"
] | null | null | null | src/features/SIR Calculation.ipynb | rutikajagtap/ads_covid-19_dashboard_rutika | beec0609527673a822ee3a7099b1ddc467f5e70c | [
"FTL"
] | null | null | null | 51.032967 | 223 | 0.645995 | [
[
[
"import pandas as pd\nimport numpy as np\n\n%matplotlib inline\nimport matplotlib as mpl\nimport matplotlib.pyplot as plt\n\nfrom datetime import datetime\n\nimport seaborn as sns\nsns.set(style=\"darkgrid\")\n\nfrom scipy import optimize\nfrom scipy import integrate\n\nimport dash\ndash.__version__\nimport dash_core_components as dcc\nimport dash_html_components as html\nfrom dash.dependencies import Input, Output, State\n\nimport plotly.graph_objects as go\n\nfrom ipywidgets import widgets, interactive\n\nimport requests\nfrom bs4 import BeautifulSoup\n\ndf_raw_infected = pd.read_csv('...\\\\data\\\\raw\\\\COVID-19\\\\csse_covid_19_data\\\\csse_covid_19_time_series\\\\time_series_covid19_confirmed_global.csv')\ntime_idx = df_raw_infected.columns[4:]\n\ndf_infected = pd.DataFrame({\n 'date':time_idx})\n\ncountry_list = df_raw_infected['Country/Region'].unique()\n\nfor each in country_list:\n df_infected[each]=np.array(df_raw_infected[df_raw_infected['Country/Region']==each].iloc[:,4:].sum(axis=0))\n \ndf_infected = df_infected.iloc[60:]\n\ndef SIR_model_t(SIR,t,beta,gamma):\n\n S,I,R=SIR\n dS_dt=-beta*S*I/N0 \n dI_dt=beta*S*I/N0-gamma*I\n dR_dt=gamma*I\n return dS_dt,dI_dt,dR_dt\n\n\ndef fit_odeint(x, beta, gamma):\n return integrate.odeint(SIR_model_t, (S0, I0, R0), t, args=(beta, gamma))[:,1] \n\npage = requests.get(\"https://www.worldometers.info/world-population/population-by-country/\")\nsoup = BeautifulSoup(page.content, 'html.parser')\nhtml_table= soup.find('table')\nall_rows= html_table.find_all('tr')\nfinal_data_list=[]\n\nfor pos,rows in enumerate(all_rows):\n col_list=[each_col.get_text(strip=True) for each_col in rows.find_all('td')]\n final_data_list.append(col_list)\n \npopulation = pd.DataFrame(final_data_list).dropna().rename(columns={0:'index', 1:'country', 2:'population', 3:'a', 4:'b', 5:'c', 6:'d', 7:'e', 8:'f', 9:'g', 10:'h', 11:'i'})\npopulation = population.drop(['index','a','b','c','d','e','f','g','h','i'],axis=1)\npopulation['population'] = population['population'].str.replace(',','')\npopulation['population'] = population['population'].apply(float)\npopulation = population.set_index('country')\n\ndf_country = pd.DataFrame({'country':country_list}).set_index('country')\ndf_analyze = pd.merge(df_country,population,left_index=True, right_on='country',how='left')\n\ndf_analyze = df_analyze.replace(np.nan,1000000).T\ndf_analyze.iloc[0].apply(float)\n\n\nfor each in country_list:\n ydata = np.array(df_infected[each])\n t = np.arange(len(ydata))\n I0=ydata[0]\n N0=np.array(df_analyze[each])\n N0 = N0.astype(np.float64)\n S0=N0-I0\n R0=0\n \n popt, pcov = optimize.curve_fit(fit_odeint, t, ydata, maxfev = 1200)\n perr = np.sqrt(np.diag(pcov))\n \n fitted = fit_odeint(t, *popt)\n df_infected[each + '_SIR'] = fitted\n \ndf_infected = df_infected.drop(['date'],axis=1)\nfor each in country_list:\n df_infected = df_infected.drop([each], axis=1)\n \ndf_infected.to_csv('...\\\\data\\\\processed\\\\COVID_SIR.csv' , sep=';', index=False)",
"C:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\integrate\\odepack.py:236: ODEintWarning: Excess work done on this call (perhaps wrong Dfun type). Run with full_output = 1 to get quantitative information.\n warnings.warn(warning_msg, ODEintWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\ipykernel_launcher.py:45: RuntimeWarning: overflow encountered in double_scalars\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\ipykernel_launcher.py:46: RuntimeWarning: overflow encountered in double_scalars\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\ipykernel_launcher.py:47: RuntimeWarning: overflow encountered in double_scalars\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\integrate\\odepack.py:236: ODEintWarning: Illegal input detected (internal error). Run with full_output = 1 to get quantitative information.\n warnings.warn(warning_msg, ODEintWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\nC:\\Users\\Admin\\Anaconda3\\lib\\site-packages\\scipy\\optimize\\minpack.py:794: OptimizeWarning: Covariance of the parameters could not be estimated\n category=OptimizeWarning)\n"
]
]
] | [
"code"
] | [
[
"code"
]
] |
e7bb1b30f6d1cb5d66f3b97a6fbc28c877c71dfe | 285,642 | ipynb | Jupyter Notebook | tutorials/scheduler.ipynb | trsvchn/Ax | 0b430641c6b33920757dd09ae4318ea487fb4136 | [
"MIT"
] | 1,803 | 2019-05-01T16:04:15.000Z | 2022-03-31T16:01:29.000Z | tutorials/scheduler.ipynb | trsvchn/Ax | 0b430641c6b33920757dd09ae4318ea487fb4136 | [
"MIT"
] | 810 | 2019-05-01T07:17:47.000Z | 2022-03-31T23:58:46.000Z | tutorials/scheduler.ipynb | LaudateCorpus1/Ax | f13bac711bc4265d8e2ee806dd3001b0396f2192 | [
"MIT"
] | 220 | 2019-05-01T05:37:22.000Z | 2022-03-29T04:30:45.000Z | 364.804598 | 252,249 | 0.923009 | [
[
[
"# Configurable closed-loop optimization with Ax `Scheduler`\n\n*We recommend reading through the [\"Developer API\" tutorial](https://ax.dev/tutorials/gpei_hartmann_developer.html) before getting started with the `Scheduler`, as using it in this tutorial will require an Ax `Experiment` and an understanding of the experiment's subcomponents like the search space and the runner.*\n\n### Contents:\n1. **Scheduler and external systems for trial evalution** –– overview of how scheduler works with an external system to run a closed-loop optimization.\n2. **Set up a mock external system** –– creating a dummy external system client, which will be used to illustrate a scheduler setup in this tutorial.\n3. **Set up an experiment according to the mock external system** –– set up a runner that deploys trials to the dummy external system from part 2 and a metric that fetches trial results from that system, then leverage those runner and metric and set up an experiment.\n4. **Set up a scheduler**, given an experiment.\n 1. Create a scheduler subclass to poll trial status.\n 2. Set up a generation strategy using an auto-selection utility.\n5. **Running the optimization** via `Scheduler.run_n_trials`.\n6. **Leveraging SQL storage and experiment resumption** –– resuming an experiment in one line of code.\n7. **Configuring the scheduler** –– overview of the many options scheduler provides to configure the closed-loop down to granular detail.\n8. **Advanced functionality**:\n 1. Reporting results to an external system during the optimization.\n 2. Using `Scheduler.run_trials_and_yield_results` to run the optimization via a generator method.",
"_____no_output_____"
],
[
"## 1. `Scheduler` and external systems for trial evaluation\n\n`Scheduler` is a closed-loop manager class in Ax that continuously deploys trial runs to an arbitrary external system in an asynchronous fashion, polls their status from that system, and leverages known trial results to generate more trials.\n\nKey features of the `Scheduler`:\n- Maintains user-set concurrency limits for trials run in parallel, keep track of tolerated level of failed trial runs, and 'oversee' the optimization in other ways,\n- Leverages an Ax `Experiment` for optimization setup (an optimization config with metrics, a search space, a runner for trial evaluations),\n- Uses an Ax `GenerationStrategy` for flexible specification of an optimization algorithm used to generate new trials to run,\n- Supports SQL storage and allows for easy resumption of stored experiments.",
"_____no_output_____"
],
[
"This scheme summarizes how the scheduler interacts with any external system used to run trial evaluations:\n\n",
"_____no_output_____"
],
[
"## 2. Set up a mock external execution system ",
"_____no_output_____"
],
[
"An example of an 'external system' running trial evaluations could be a remote server executing scheduled jobs, a subprocess conducting ML training runs, an engine running physics simulations, etc. For the sake of example here, let us assume a dummy external system with the following client:",
"_____no_output_____"
]
],
[
[
"from typing import Any, Dict, NamedTuple, List, Union\nfrom time import time\nfrom random import randint\n\nfrom ax.core.base_trial import TrialStatus\nfrom ax.utils.measurement.synthetic_functions import branin\n\n\nclass MockJob(NamedTuple):\n \"\"\"Dummy class to represent a job scheduled on `MockJobQueue`.\"\"\"\n id: int\n parameters: Dict[str, Union[str, float, int, bool]]\n\n\nclass MockJobQueueClient:\n \"\"\"Dummy class to represent a job queue where the Ax `Scheduler` will\n deploy trial evaluation runs during optimization.\n \"\"\"\n \n jobs: Dict[str, MockJob] = {}\n \n def schedule_job_with_parameters(\n self, \n parameters: Dict[str, Union[str, float, int, bool]]\n ) -> int:\n \"\"\"Schedules an evaluation job with given parameters and returns job ID.\n \"\"\"\n # Code to actually schedule the job and produce an ID would go here;\n # using timestamp as dummy ID for this example.\n job_id = int(time())\n self.jobs[job_id] = MockJob(job_id, parameters)\n return job_id\n \n def get_job_status(self, job_id: int) -> TrialStatus:\n \"\"\"\"Get status of the job by a given ID. For simplicity of the example,\n return an Ax `TrialStatus`.\n \"\"\"\n job = self.jobs[job_id]\n # Instead of randomizing trial status, code to check actual job status\n # would go here.\n if randint(0, 3) > 0:\n return TrialStatus.COMPLETED\n return TrialStatus.RUNNING\n \n def get_outcome_value_for_completed_job(self, job_id: int) -> Dict[str, float]:\n \"\"\"Get evaluation results for a given completed job.\"\"\"\n job = self.jobs[job_id]\n # In a real external system, this would retrieve real relevant outcomes and\n # not a synthetic function value.\n return {\n \"branin\": branin(job.parameters.get(\"x1\"), job.parameters.get(\"x2\"))\n }\n \nMOCK_JOB_QUEUE_CLIENT = MockJobQueueClient()\n\ndef get_mock_job_queue_client() -> MockJobQueueClient:\n \"\"\"Obtain the singleton job queue instance.\"\"\"\n return MOCK_JOB_QUEUE_CLIENT",
"_____no_output_____"
]
],
[
[
"## 3. Set up an experiment according to the mock external system\n\nAs mentioned above, using a `Scheduler` requires a fully set up experiment with metrics and a runner. Refer to the \"Building Blocks of Ax\" tutorial to learn more about those components, as here we assume familiarity with them. \n\nThe following runner and metric set up intractions between the `Scheduler` and the mock external system we assume:",
"_____no_output_____"
]
],
[
[
"from ax.core.runner import Runner\nfrom ax.core.base_trial import BaseTrial\nfrom ax.core.trial import Trial\n\nclass MockJobRunner(Runner): # Deploys trials to external system.\n \n def run(self, trial: BaseTrial) -> Dict[str,Any]:\n \"\"\"Deploys a trial and returns dict of run metadata.\"\"\"\n if not isinstance(trial, Trial):\n raise ValueError(\"This runner only handles `Trial`.\")\n \n mock_job_queue = get_mock_job_queue_client()\n job_id = mock_job_queue.schedule_job_with_parameters(\n parameters=trial.arm.parameters\n )\n # This run metadata will be attached to trial as `trial.run_metadata`\n # by the base `Scheduler`.\n return {\"job_id\": job_id}",
"_____no_output_____"
],
[
"import pandas as pd\n\nfrom ax.core.metric import Metric\nfrom ax.core.base_trial import BaseTrial\nfrom ax.core.data import Data\n\nclass BraninForMockJobMetric(Metric): # Pulls data for trial from external system.\n \n def fetch_trial_data(self, trial: BaseTrial) -> Data:\n \"\"\"Obtains data via fetching it from ` for a given trial.\"\"\"\n if not isinstance(trial, Trial):\n raise ValueError(\"This metric only handles `Trial`.\")\n \n mock_job_queue = get_mock_job_queue_client()\n \n # Here we leverage the \"job_id\" metadata created by `MockJobRunner.run`.\n branin_data = mock_job_queue.get_outcome_value_for_completed_job(\n job_id=trial.run_metadata.get(\"job_id\")\n )\n df_dict = {\n \"trial_index\": trial.index,\n \"metric_name\": \"branin\",\n \"arm_name\": trial.arm.name,\n \"mean\": branin_data.get(\"branin\"),\n # Can be set to 0.0 if function is known to be noiseless\n # or to an actual value when SEM is known. Setting SEM to\n # `None` results in Ax assuming unknown noise and inferring\n # noise level from data.\n \"sem\": None,\n }\n return Data(df=pd.DataFrame.from_records([df_dict]))",
"_____no_output_____"
]
],
[
[
"Now we can set up the experiment using the runner and metric we defined. This experiment will have a single-objective optimization config, minimizing the Branin function, and the search space that corresponds to that function.",
"_____no_output_____"
]
],
[
[
"from ax import *\n\ndef make_branin_experiment_with_runner_and_metric() -> Experiment:\n parameters = [\n RangeParameter(\n name=\"x1\", \n parameter_type=ParameterType.FLOAT, \n lower=-5, \n upper=10,\n ),\n RangeParameter(\n name=\"x2\", \n parameter_type=ParameterType.FLOAT, \n lower=0, \n upper=15,\n ),\n ]\n\n objective=Objective(metric=BraninForMockJobMetric(name=\"branin\"), minimize=True)\n\n return Experiment(\n name=\"branin_test_experiment\",\n search_space=SearchSpace(parameters=parameters),\n optimization_config=OptimizationConfig(objective=objective),\n runner=MockJobRunner(),\n is_test=True, # Marking this experiment as a test experiment.\n )\n\nexperiment = make_branin_experiment_with_runner_and_metric()",
"_____no_output_____"
]
],
[
[
"## 4. Setting up a `Scheduler`\n\n### 4a. Subclassing `Scheduler`\n\nThe base Ax `Scheduler` is abstract and must be subclassed, but only one method must be implemented on the subclass: `poll_trial_status`. ",
"_____no_output_____"
]
],
[
[
"from typing import Dict, Set\nfrom random import randint\nfrom collections import defaultdict\nfrom ax.service.scheduler import Scheduler, SchedulerOptions, TrialStatus\n\nclass MockJobQueueScheduler(Scheduler):\n\n def poll_trial_status(self) -> Dict[TrialStatus, Set[int]]:\n \"\"\"Queries the external system to compute a mapping from trial statuses\n to a set indices of trials that are currently in that status. Only needs\n to query for trials that are currently running but can query for all\n trials too.\n \"\"\"\n status_dict = defaultdict(set)\n for trial in self.running_trials: # `running_trials` is exposed on base `Scheduler`\n mock_job_queue = get_mock_job_queue_client()\n status = mock_job_queue.get_job_status(job_id=trial.run_metadata.get(\"job_id\"))\n status_dict[status].add(trial.index)\n \n return status_dict",
"_____no_output_____"
]
],
[
[
"### 4B. Auto-selecting a generation strategy\n\nA `Scheduler` also requires an Ax `GenerationStrategy` specifying the algorithm to use for the optimization. Here we use the `choose_generation_strategy` utility that auto-picks a generation strategy based on the search space properties. To construct a custom generation strategy instead, refer to the [\"Generation Strategy\" tutorial](https://ax.dev/tutorials/generation_strategy.html).\n\nImportantly, a generation strategy in Ax limits allowed parallelism levels for each generation step it contains. If you would like the `Scheduler` to ensure parallelism limitations, set `max_examples` on each generation step in your generation strategy.",
"_____no_output_____"
]
],
[
[
"from ax.modelbridge.dispatch_utils import choose_generation_strategy\n\ngeneration_strategy = choose_generation_strategy(\n search_space=experiment.search_space, \n max_parallelism_cap=3,\n)",
"_____no_output_____"
]
],
[
[
"Now we have all the components needed to start the scheduler:",
"_____no_output_____"
]
],
[
[
"scheduler = MockJobQueueScheduler(\n experiment=experiment,\n generation_strategy=generation_strategy,\n options=SchedulerOptions(),\n)",
"_____no_output_____"
]
],
[
[
"## 5. Running the optimization\n\nOnce the `Scheduler` instance is set up, user can execute `run_n_trials` as many times as needed, and each execution will add up to the specified `max_trials` trials to the experiment. The number of trials actually run might be less than `max_trials` if the optimization was concluded (e.g. there are no more points in the search space).",
"_____no_output_____"
]
],
[
[
"scheduler.run_n_trials(max_trials=3)",
"_____no_output_____"
]
],
[
[
"We can examine `experiment` to see that it now has three trials:",
"_____no_output_____"
]
],
[
[
"from ax.service.utils.report_utils import exp_to_df\n\nexp_to_df(experiment)",
"_____no_output_____"
]
],
[
[
"Now we can run `run_n_trials` again to add three more trials to the experiment.",
"_____no_output_____"
]
],
[
[
"scheduler.run_n_trials(max_trials=3)",
"_____no_output_____"
]
],
[
[
"Examiniming the experiment, we now see 6 trials, one of which is produced by Bayesian optimization (GPEI):",
"_____no_output_____"
]
],
[
[
"exp_to_df(experiment)",
"_____no_output_____"
]
],
[
[
"For each call to `run_n_trials`, one can specify a timeout; if `run_n_trials` has been running for too long without finishing its `max_trials`, the operation will exit gracefully:",
"_____no_output_____"
]
],
[
[
"scheduler.run_n_trials(max_trials=3, timeout_hours=0.00001)",
"_____no_output_____"
]
],
[
[
"## 6. Leveraging SQL storage and experiment resumption\n\nWhen a scheduler is SQL-enabled, it will automatically save all updates it makes to the experiment in the course of the optimization. The experiment can then be resumed in the event of a crash or after a pause.\n\nTo store state of optimization to an SQL backend, first follow [setup instructions](https://ax.dev/docs/storage.html#sql) on Ax website. Having set up the SQL backend, pass `DBSettings` to the `Scheduler` on instantiation (note that SQLAlchemy dependency will have to be installed – for installation, refer to [optional dependencies](https://ax.dev/docs/installation.html#optional-dependencies) on Ax website):",
"_____no_output_____"
]
],
[
[
"from ax.storage.sqa_store.structs import DBSettings\nfrom ax.storage.sqa_store.db import init_engine_and_session_factory, get_engine, create_all_tables\nfrom ax.storage.metric_registry import register_metric\nfrom ax.storage.runner_registry import register_runner\n\nregister_metric(BraninForMockJobMetric)\nregister_runner(MockJobRunner)\n\n# URL is of the form \"dialect+driver://username:password@host:port/database\".\n# Instead of URL, can provide a `creator function`; can specify custom encoders/decoders if necessary.\ndb_settings = DBSettings(url=\"sqlite:///foo.db\")\n\n# The following lines are only necessary because it is the first time we are using this database\n# in practice, you will not need to run these lines every time you initialize your scheduler\ninit_engine_and_session_factory(url=db_settings.url)\nengine = get_engine()\ncreate_all_tables(engine)\n\nstored_experiment = make_branin_experiment_with_runner_and_metric()\ngeneration_strategy = choose_generation_strategy(search_space=experiment.search_space)\n\nscheduler_with_storage = MockJobQueueScheduler(\n experiment=stored_experiment,\n generation_strategy=generation_strategy,\n options=SchedulerOptions(),\n db_settings=db_settings,\n)",
"_____no_output_____"
],
[
"stored_experiment.name",
"_____no_output_____"
]
],
[
[
"To resume a stored experiment:",
"_____no_output_____"
]
],
[
[
"reloaded_experiment_scheduler = MockJobQueueScheduler.from_stored_experiment(\n experiment_name='branin_test_experiment',\n options=SchedulerOptions(),\n # `DBSettings` are also required here so scheduler has access to the\n # database, from which it needs to load the experiment.\n db_settings=db_settings,\n)",
"_____no_output_____"
]
],
[
[
"With the newly reloaded experiment, the `Scheduler` can continue the optimization:",
"_____no_output_____"
]
],
[
[
"reloaded_experiment_scheduler.run_n_trials(max_trials=3)",
"_____no_output_____"
]
],
[
[
"## 7. Configuring the scheduler\n\n`Scheduler` exposes many options to configure the exact settings of the closed-loop optimization to perform. A few notable ones are:\n- `trial_type` –– currently only `Trial` and not `BatchTrial` is supported, but support for `BatchTrial`-s will follow,\n- `tolerated_trial_failure_rate` and `min_failed_trials_for_failure_rate_check` –– together these two settings control how the scheduler monitors the failure rate among trial runs it deploys. Once `min_failed_trials_for_failure_rate_check` is deployed, the scheduler will start checking whether the ratio of failed to total trials is greater than `tolerated_trial_failure_rate`, and if it is, scheduler will exit the optimization with a `FailureRateExceededError`,\n- `ttl_seconds_for_trials` –– sometimes a failure in a trial run means that it will be difficult to query its status (e.g. due to a crash). If this setting is specified, the Ax `Experiment` will automatically mark trials that have been running for too long (more than their 'time-to-live' (TTL) seconds) as failed,\n- `run_trials_in_batches` –– if `True`, the scheduler will attempt to run trials not by calling `Scheduler.run_trial` in a loop, but by calling `Scheduler.run_trials` on all ready-to-deploy trials at once. This could allow for saving compute in cases where the deployment operation has large overhead and deploying many trials at once saves compute. Note that using this option successfully will require your scheduler subclass to implement `MySchedulerSubclass.run_trials` and `MySchedulerSubclass.poll_available_capacity`.\n\nThe rest of the options is described in the docstring below:",
"_____no_output_____"
]
],
[
[
"print(SchedulerOptions.__doc__)",
"_____no_output_____"
]
],
[
[
"## 8. Advanced functionality\n\n### 8a. Reporting results to an external system\n\nThe `Scheduler` can report the optimization result to an external system each time there are new completed trials if the user-implemented subclass implements `MySchedulerSubclass.report_results` to do so. For example, the folliwing method:\n\n```\nclass MySchedulerSubclass(Scheduler):\n ...\n \n def report_results(self):\n write_to_external_database(len(self.experiment.trials))\n return (True, {}) # Returns optimization success status and optional dict of outputs.\n```\ncould be used to record number of trials in experiment so far in an external database.\n\nSince `report_results` is an instance method, it has access to `self.experiment` and `self.generation_strategy`, which contain all the information about the state of the optimization thus far.",
"_____no_output_____"
],
[
"### 8b. Using `run_trials_and_yield_results` generator method\n\nIn some systems it's beneficial to have greater control over `Scheduler.run_n_trials` instead of just starting it and needing to wait for it to run all the way to completion before having access to its output. For this purpose, the `Scheduler` implements a generator method `run_trials_and_yield_results`, which yields the output of `Scheduler.report_results` each time there are new completed trials and can be used like so:",
"_____no_output_____"
]
],
[
[
"class ResultReportingScheduler(MockJobQueueScheduler):\n \n def report_results(self):\n return True, {\n \"trials so far\": len(self.experiment.trials),\n \"currently producing trials from generation step\": self.generation_strategy._curr.model_name,\n \"running trials\": [t.index for t in self.running_trials],\n }",
"_____no_output_____"
],
[
"experiment = make_branin_experiment_with_runner_and_metric()\nscheduler = ResultReportingScheduler(\n experiment=experiment,\n generation_strategy=choose_generation_strategy(\n search_space=experiment.search_space, \n max_parallelism_cap=3,\n ),\n options=SchedulerOptions(),\n)\n\nfor reported_result in scheduler.run_trials_and_yield_results(max_trials=6):\n print(\"Reported result: \", reported_result)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
]
] |
e7bb1c2dc39a9a4e93c19ccbd5930b19d0ac350d | 506,578 | ipynb | Jupyter Notebook | model-development/_archive/ml_explore_David_2.ipynb | Project-Canopy/SSRC_New_Model_Development | 464a4477131ddd902ad95940ec0e0c6e744204ba | [
"MIT"
] | 2 | 2021-04-22T02:13:28.000Z | 2021-12-28T19:41:29.000Z | model-development/_archive/ml_explore_David_2.ipynb | Project-Canopy/SSRC_New_Model_Development | 464a4477131ddd902ad95940ec0e0c6e744204ba | [
"MIT"
] | 12 | 2021-08-25T15:12:09.000Z | 2022-02-10T06:16:15.000Z | model-development/_archive/ml_explore_David_2.ipynb | Project-Canopy/SSRC_New_Model_Development | 464a4477131ddd902ad95940ec0e0c6e744204ba | [
"MIT"
] | 3 | 2021-03-03T05:14:10.000Z | 2022-03-16T07:55:12.000Z | 75.948726 | 19,048 | 0.643453 | [
[
[
"Imports",
"_____no_output_____"
]
],
[
[
"import os\nimport multiprocessing\n#import rasterio\nimport tensorflow as tf\nfrom glob import glob\nimport pickle\nimport numpy as np\nimport os\nfrom tensorflow.keras.models import *\nfrom tensorflow.keras.layers import *\nfrom tensorflow.keras import layers\nimport keras\nimport pandas as pd\n#import matplotlib.pyplot as plt",
"_____no_output_____"
],
[
"tf.__version__",
"_____no_output_____"
]
],
[
[
"Test with Simple CNN and Data Loader ",
"_____no_output_____"
]
],
[
[
"import boto3\nimport rasterio as rio\nimport numpy as np\nimport io",
"_____no_output_____"
],
[
"from data_loader import DataLoader",
"_____no_output_____"
],
[
"batch_size = 20\ngen = DataLoader(label_file_path_train=\"labels_test_v1.csv\",\n label_file_path_val=\"val_labels.csv\",\n label_mapping_path=\"labels.json\",\n bucket_name='canopy-production-ml',\n data_extension_type='.tif',\n training_data_shape=(100, 100, 18),\n augment=True,\n random_flip_up_down=False, #Randomly flips an image vertically (upside down). With a 1 in 2 chance, outputs the contents of `image` flipped along the first dimension, which is `height`.\n random_flip_left_right=False,\n flip_left_right=False,\n flip_up_down=False,\n rot90=True,\n transpose=False,\n enable_shuffle=False,\n training_data_shuffle_buffer_size=10,\n training_data_batch_size=batch_size,\n training_data_type=tf.float32,\n label_data_type=tf.uint8,\n num_parallel_calls=int(2))\n# TODO add data augmentation in DataLoader \n\n# no_of_val_imgs = len(gen.validation_filenames)\n# no_of_train_imgs = len(gen.training_filenames)\n# print(\"Validation on {} images \".format(str(no_of_val_imgs)))\n# print(\"Training on {} images \".format(str(no_of_train_imgs)))",
"Data augmentation enabled \nTraining on 200 images \nValidation on 366 images \nYour training file is missing positive labels for classes ['0', '2', '3', '7', '8', '9']\n"
],
[
"gen",
"_____no_output_____"
],
[
"gen.class_weight",
"_____no_output_____"
],
[
"df[\"1\"]",
"_____no_output_____"
],
[
"labels = {}\n\nfor column in df.columns:\n \n col_count = df[column].value_counts()\n# print(\"column:\",column)\n# print(col_count)\n \n try:\n col_count = df[column].value_counts()[1]\n labels[column] = col_count\n except:\n print(f\"Missing positive chips for class {column}. Class weight \")",
"Missing positive chips for class 0\nMissing positive chips for class 2\nMissing positive chips for class 3\nMissing positive chips for class 7\nMissing positive chips for class 8\nMissing positive chips for class 9\n"
],
[
"labels",
"_____no_output_____"
],
[
"def Simple_CNN(numclasses, input_shape): #TODO use a more complex CNN\n model = Sequential([\n layers.Input(input_shape),\n layers.Conv2D(16, 3, padding='same', activation='relu'),\n layers.MaxPooling2D(),\n layers.Conv2D(32, 3, padding='same', activation='relu'),\n layers.MaxPooling2D(),\n layers.Conv2D(64, 3, padding='same', activation='relu'),\n layers.MaxPooling2D(),\n layers.Flatten(),\n layers.Dense(128, activation='relu'),\n layers.Dense(numclasses)\n ])\n return model\n\nmodel_simpleCNN = Simple_CNN(10, input_shape=(100, 100, 18))\ncallbacks_list = []\n\nmodel_simpleCNN.compile(loss=tf.keras.losses.BinaryCrossentropy(from_logits=True),\n optimizer=keras.optimizers.Adam()) #TODO add callbacks to save checkpoints and maybe lr reducer,etc \n\nepochs = 10\nhistory = model_simpleCNN.fit(gen.training_dataset, validation_data=gen.validation_dataset, epochs=epochs)\n",
"Epoch 1/10\n5/5 [==============================] - 86s 18s/step - loss: 8.7035 - val_loss: 1.5012\nEpoch 2/10\n5/5 [==============================] - 81s 17s/step - loss: 1.4694 - val_loss: 0.8578\nEpoch 3/10\n5/5 [==============================] - 82s 17s/step - loss: 0.6244 - val_loss: 0.2065\nEpoch 4/10\n5/5 [==============================] - 86s 17s/step - loss: 0.1674 - val_loss: 0.2439\nEpoch 5/10\n5/5 [==============================] - 84s 17s/step - loss: 0.1836 - val_loss: 0.2107\nEpoch 6/10\n5/5 [==============================] - 87s 18s/step - loss: 0.1562 - val_loss: 0.1956\nEpoch 7/10\n5/5 [==============================] - 82s 17s/step - loss: 0.1483 - val_loss: 0.1829\nEpoch 8/10\n5/5 [==============================] - 83s 17s/step - loss: 0.1326 - val_loss: 0.1831\nEpoch 9/10\n5/5 [==============================] - 87s 17s/step - loss: 0.1373 - val_loss: 0.1843\nEpoch 10/10\n5/5 [==============================] - 83s 17s/step - loss: 0.1324 - val_loss: 0.1902\n"
],
[
"s3 = boto3.resource('s3')\nobj = s3.Object('canopy-production-ml', \"chips/cloudfree-merge-polygons/split/test/100/100_1000_1000.tif\")\nobj_bytes = io.BytesIO(obj.get()['Body'].read())\nwith rasterio.open(obj_bytes) as src:\n img_test = np.transpose(src.read(), (1, 2, 0))\nprint(img_test.shape)",
"(100, 100, 18)\n"
],
[
"label_list = ['Habitation', 'ISL', 'Industrial_agriculture', 'Mining',\n 'Rainforest', 'River', 'Roads', 'Savannah', 'Shifting_cultivation',\n 'Water'\n]\n# TODO Need to weight labels since they are pretty unbalanced (Rainforest is largely represented)",
"_____no_output_____"
],
[
"# s3 = boto3.resource('s3')\n# obj = s3.Object('canopy-production-ml', \"chips/cloudfree-merge-polygons/split/train/58/58_1300_1000.tif\")\n# obj_bytes = io.BytesIO(obj.get()['Body'].read())\n# with rasterio.open(obj_bytes) as src:\n# img_test = np.transpose(src.read(), (1, 2, 0)) / 255\n# print(img_test.shape)",
"_____no_output_____"
],
[
"predictions = model_simpleCNN.predict(np.array([img_test]))\nhighest_score_predictions = np.argmax(predictions) # TODO: read more about multi classes PER IMAGE classification, what is the threshold?\n\nprint(\"This chip was predicted to belong to class {}\".format(label_list[highest_score_predictions]))",
"This chip was predicted to belong to class Rainforest\n"
],
[
"print(predictions)\npredictions.argsort() ",
"[[-2020.7277 4.4248075 -1614.4033 -3687.283 157.33759\n -1660.8308 -644.8952 -2039.6393 -1582.1956 -1557.5543 ]]\n"
],
[
"model_simpleCNN.evaluate(gen.validation_dataset)",
"3/3 [==============================] - 35s 10s/step - loss: 0.1902\n"
]
],
[
[
"Resnet50",
"_____no_output_____"
]
],
[
[
"def Resnet50(numclasses, input_shape):\n model = Sequential()\n model.add(keras.applications.ResNet50(include_top=False, pooling='avg', weights=None, input_shape=input_shape))\n model.add(Flatten())\n model.add(BatchNormalization())\n model.add(Dense(2048, activation='relu'))\n model.add(BatchNormalization())\n model.add(Dense(1024, activation='relu'))\n model.add(BatchNormalization())\n model.add(Dense(numclasses, activation='softmax'))\n model.layers[0].trainable = True\n return model",
"_____no_output_____"
],
[
"model_resnet50 = Resnet50(10, input_shape=(100, 100, 18))\ncallbacks_list = []\n\nmodel_resnet50.compile(loss=tf.keras.losses.BinaryCrossentropy(from_logits=True),\n optimizer=keras.optimizers.Adam()) #TODO add callbacks to save checkpoints and maybe lr reducer, earlystop,etc \n\nepochs = 10\nhistory = model_resnet50.fit(gen.training_dataset, validation_data=gen.validation_dataset, epochs=epochs)\n",
"/usr/local/anaconda3/lib/python3.8/site-packages/tensorflow/python/keras/applications/imagenet_utils.py:331: UserWarning: This model usually expects 1 or 3 input channels. However, it was passed an input_shape with 18 input channels.\n warnings.warn('This model usually expects 1 or 3 input channels. '\n"
],
[
"predictions = model_resnet50.predict(np.array([img_test]))\nhighest_score_predictions = np.argmax(predictions) # TODO: read more about multi classes PER IMAGE classification, what is the threshold?\n\nprint(\"This chip was predicted to belong to class {}\".format(label_list[highest_score_predictions]))",
"This chip was predicted to belong to class Industrial_agriculture\n"
],
[
"model_resnet50.evaluate(gen.validation_dataset)",
"3/3 [==============================] - 29s 9s/step - loss: 0.7552\n"
],
[
"# https://kgptalkie.com/multi-label-image-classification-on-movies-poster-using-cnn/\ntop3 = np.argsort(predictions[0])[:-4:-1]\nfor i in range(3):\n print(label_list[top3[i]]) # We need to define a threshold",
"Industrial_agriculture\nWater\nShifting_cultivation\n"
],
[
"def plot_learningCurve(history, epoch):\n # Plot training & validation accuracy values\n# plt.plot(history.history['accuracy'])\n# plt.plot(history.history['val_accuracy'])\n# plt.title('Model accuracy')\n# plt.ylabel('Accuracy')\n# plt.xlabel('Epoch')\n# plt.legend(['Train', 'Val'], loc='upper left')\n# plt.show()\n\n # Plot training & validation loss values\n plt.plot(history.history['loss'])\n plt.plot(history.history['val_loss'])\n plt.title('Model loss')\n plt.ylabel('Loss')\n plt.xlabel('Epoch')\n plt.legend(['Train', 'Val'], loc='upper left')\n plt.show()\n\nplot_learningCurve(history, 20)",
"_____no_output_____"
],
[
"def medium_CNN(numclasses, input_shape):\n model = Sequential()\n model.add(Conv2D(16, (3,3), activation='relu', input_shape = input_shape))\n model.add(BatchNormalization())\n model.add(MaxPool2D(2,2))\n model.add(Dropout(0.3))\n\n model.add(Conv2D(32, (3,3), activation='relu'))\n model.add(BatchNormalization())\n model.add(MaxPool2D(2,2))\n model.add(Dropout(0.3))\n\n model.add(Conv2D(64, (3,3), activation='relu'))\n model.add(BatchNormalization())\n model.add(MaxPool2D(2,2))\n model.add(Dropout(0.4))\n\n model.add(Conv2D(128, (3,3), activation='relu'))\n model.add(BatchNormalization())\n model.add(MaxPool2D(2,2))\n model.add(Dropout(0.5))\n\n model.add(Flatten())\n\n model.add(Dense(128, activation='relu'))\n model.add(BatchNormalization())\n model.add(Dropout(0.5))\n\n\n model.add(Dense(128, activation='relu'))\n model.add(BatchNormalization())\n model.add(Dropout(0.5))\n\n\n model.add(Dense(numclasses, activation='sigmoid'))\n return model",
"_____no_output_____"
],
[
"model_medium_CNN = medium_CNN(10, input_shape=(100, 100, 18))\ncallbacks_list = []\n\nmodel_medium_CNN.compile(loss=tf.keras.losses.BinaryCrossentropy(from_logits=True),\n optimizer=keras.optimizers.Adam(),\n metrics=[tf.metrics.BinaryAccuracy(threshold=0.0, name='accuracy')]) #TODO add callbacks to save checkpoints and maybe lr reducer, earlystop,etc \n\nepochs = 10\nhistory = model_medium_CNN.fit(gen.training_dataset, validation_data=gen.validation_dataset, epochs=epochs)\n",
"Epoch 1/10\n5/5 [==============================] - 86s 17s/step - loss: 0.9270 - accuracy: 0.1209 - val_loss: 0.8956 - val_accuracy: 0.1200\nEpoch 2/10\n5/5 [==============================] - 91s 19s/step - loss: 0.9144 - accuracy: 0.1223 - val_loss: 0.9091 - val_accuracy: 0.1200\nEpoch 3/10\n5/5 [==============================] - 85s 17s/step - loss: 0.9159 - accuracy: 0.1198 - val_loss: 0.9205 - val_accuracy: 0.1200\nEpoch 4/10\n5/5 [==============================] - 86s 18s/step - loss: 0.9162 - accuracy: 0.1193 - val_loss: 0.9494 - val_accuracy: 0.1200\nEpoch 5/10\n5/5 [==============================] - 84s 17s/step - loss: 0.9036 - accuracy: 0.1200 - val_loss: 0.9662 - val_accuracy: 0.1200\nEpoch 6/10\n5/5 [==============================] - 85s 17s/step - loss: 0.9077 - accuracy: 0.1201 - val_loss: 0.9763 - val_accuracy: 0.1200\nEpoch 7/10\n5/5 [==============================] - 89s 17s/step - loss: 0.9053 - accuracy: 0.1200 - val_loss: 0.9755 - val_accuracy: 0.1200\nEpoch 8/10\n5/5 [==============================] - 84s 17s/step - loss: 0.8920 - accuracy: 0.1197 - val_loss: 0.9652 - val_accuracy: 0.1200\nEpoch 9/10\n5/5 [==============================] - 85s 17s/step - loss: 0.8925 - accuracy: 0.1195 - val_loss: 0.9509 - val_accuracy: 0.1200\nEpoch 10/10\n5/5 [==============================] - 84s 17s/step - loss: 0.8863 - accuracy: 0.1211 - val_loss: 0.9204 - val_accuracy: 0.1200\n"
],
[
"predictions = model_medium_CNN.predict(np.array([img_test]))\nhighest_score_predictions = np.argmax(predictions) # TODO: read more about multi classes PER IMAGE classification, what is the threshold?\n\nprint(\"This chip was predicted to belong to top 3 classes:\")\n\ntop3 = np.argsort(predictions[0])[:-4:-1]\nfor i in range(3):\n print(label_list[top3[i]])",
"This chip was predicted to belong to top 3 classes:\nWater\nShifting_cultivation\nSavannah\n"
],
[
"model_medium_CNN.evaluate(gen.validation_dataset)",
"3/3 [==============================] - 32s 11s/step - loss: 0.9204 - accuracy: 0.1200\n"
],
[
"def plot_learningCurve(history, epoch):\n # Plot training & validation accuracy values\n plt.plot(history.history['accuracy'])\n plt.plot(history.history['val_accuracy'])\n plt.title('Model accuracy')\n plt.ylabel('Accuracy')\n plt.xlabel('Epoch')\n plt.legend(['Train', 'Val'], loc='upper left')\n plt.show()\n\n # Plot training & validation loss values\n plt.plot(history.history['loss'])\n plt.plot(history.history['val_loss'])\n plt.title('Model loss')\n plt.ylabel('Loss')\n plt.xlabel('Epoch')\n plt.legend(['Train', 'Val'], loc='upper left')\n plt.show()\n\nplot_learningCurve(history, 20)",
"_____no_output_____"
]
],
[
[
"TEST with bigearthnet-resnet50",
"_____no_output_____"
]
],
[
[
"import tensorflow_hub as hub\nIMAGE_SIZE = (100,100)\nnum_classes = 10\nmodel_handle = \"https://tfhub.dev/google/remote_sensing/bigearthnet-resnet50/1\"\nmodel_bigearthnet = tf.keras.Sequential([\n tf.keras.layers.InputLayer(input_shape=IMAGE_SIZE + (3,)),\n # reshape? \n hub.KerasLayer(model_handle, trainable=False, input_shape=IMAGE_SIZE + (3,)),\n# (model.layers[-1].output)\n tf.keras.layers.Dropout(rate=0.2),\n tf.keras.layers.Dense(num_classes,\n kernel_regularizer=tf.keras.regularizers.l2(0.0001))\n])\nmodel_bigearthnet.build((None,)+IMAGE_SIZE+(4,))\nmodel_bigearthnet.summary()",
"_____no_output_____"
],
[
"# model_bigearthnet.compile(loss=tf.keras.losses.BinaryCrossentropy(from_logits=True),\n# optimizer=keras.optimizers.Adam()) #TODO add callbacks to save checkpoints and maybe lr reducer, earlystop,etc \n\n# epochs = 10\n# history = model_bigearthnet.fit(gen.training_dataset, validation_data=gen.validation_dataset, epochs=epochs)\n",
"_____no_output_____"
],
[
"# predictions = model_resnet50.predict(np.array([img_test]))\n# highest_score_predictions = np.argmax(predictions) # TODO: read more about multi classes PER IMAGE classification, what is the threshold?\n\n# print(\"This chip was predicted to belong to class {}\".format(label_list[highest_score_predictions]))",
"_____no_output_____"
]
],
[
[
"# Preproduction Candidate: ResNet50 pretrained on ImageNet",
"_____no_output_____"
]
],
[
[
"gen = DataLoader(label_file_path_train=\"labels_test_v1.csv\", #or labels.csv\n label_file_path_val=\"val_labels.csv\",\n bucket_name='canopy-production-ml',\n data_extension_type='.tif',\n training_data_shape=(100, 100, 18),\n shuffle_and_repeat=False,\n enable_just_shuffle=False,\n enable_just_repeat=False,\n training_data_shuffle_buffer_size=10,\n data_repeat_count=None,\n training_data_batch_size=20,\n normalization_value=255.0, #normalization TODO double check other channels than RGB \n training_data_type=tf.float32,\n label_data_type=tf.uint8,\n enable_data_prefetch=False,\n data_prefetch_size=tf.data.experimental.AUTOTUNE,\n num_parallel_calls=int(2))\n# TODO add data augmentation in DataLoader \n\nno_of_val_imgs = len(gen.validation_filenames)\nno_of_train_imgs = len(gen.training_filenames)\nprint(\"Validation on {} images \".format(str(no_of_val_imgs)))\nprint(\"Training on {} images \".format(str(no_of_train_imgs)))",
"_____no_output_____"
],
[
"def define_model(numclasses,input_shape):\n # parameters for CNN\n input_tensor = Input(shape=input_shape)\n\n # introduce a additional layer to get from bands to 3 input channels\n input_tensor = Conv2D(3, (1, 1))(input_tensor)\n\n base_model_resnet50 = keras.applications.ResNet50(include_top=False,\n weights='imagenet',\n input_shape=(100, 100, 3))\n base_model = keras.applications.ResNet50(include_top=False,\n weights=None,\n input_tensor=input_tensor)\n\n for i, layer in enumerate(base_model_resnet50.layers):\n # we must skip input layer, which has no weights\n if i == 0:\n continue\n base_model.layers[i+1].set_weights(layer.get_weights())\n\n # add a global spatial average pooling layer\n top_model = base_model.output\n top_model = GlobalAveragePooling2D()(top_model)\n\n # let's add a fully-connected layer\n top_model = Dense(2048, activation='relu')(top_model)\n top_model = Dense(2048, activation='relu')(top_model)\n # and a logistic layer\n predictions = Dense(numclasses, activation='softmax')(top_model)\n\n # this is the model we will train\n model = Model(inputs=base_model.input, outputs=predictions)\n\n model.summary()\n return model",
"_____no_output_____"
],
[
"random_id = 5555 #TODO\ncheckpoint_file = 'checkpoint_{}.h5'.format(random_id)\n\nmodel_checkpoint_callback = tf.keras.callbacks.ModelCheckpoint(\n filepath= checkpoint_file,\n format='h5',\n verbose=1,\n save_weights_only=True,\n monitor='val_loss',\n mode='min',\n save_best_only=True)\n\nreducelronplateau = tf.keras.callbacks.ReduceLROnPlateau(\n monitor='val_loss', factor=0.1, patience=10, verbose=1,\n mode='min', min_lr=1e-10)\n\nearly_stop = tf.keras.callbacks.EarlyStopping(monitor='val_loss',mode='min', patience=20, verbose=1)\n\ncallbacks_list = [model_checkpoint_callback, reducelronplateau, early_stop]\n\nmodel = define_model(10, (100,100,18))\nmodel.compile(loss=tf.keras.losses.BinaryCrossentropy(from_logits=True),\n optimizer=keras.optimizers.Adam(),\n metrics=[tf.metrics.BinaryAccuracy(name='accuracy')]) #TODO add callbacks to save checkpoints and maybe lr reducer, earlystop,etc \n",
"Model: \"functional_1\"\n__________________________________________________________________________________________________\nLayer (type) Output Shape Param # Connected to \n==================================================================================================\ninput_1 (InputLayer) [(None, 100, 100, 18 0 \n__________________________________________________________________________________________________\nconv2d (Conv2D) (None, 100, 100, 3) 57 input_1[0][0] \n__________________________________________________________________________________________________\nconv1_pad (ZeroPadding2D) (None, 106, 106, 3) 0 conv2d[0][0] \n__________________________________________________________________________________________________\nconv1_conv (Conv2D) (None, 50, 50, 64) 9472 conv1_pad[0][0] \n__________________________________________________________________________________________________\nconv1_bn (BatchNormalization) (None, 50, 50, 64) 256 conv1_conv[0][0] \n__________________________________________________________________________________________________\nconv1_relu (Activation) (None, 50, 50, 64) 0 conv1_bn[0][0] \n__________________________________________________________________________________________________\npool1_pad (ZeroPadding2D) (None, 52, 52, 64) 0 conv1_relu[0][0] \n__________________________________________________________________________________________________\npool1_pool (MaxPooling2D) (None, 25, 25, 64) 0 pool1_pad[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_conv (Conv2D) (None, 25, 25, 64) 4160 pool1_pool[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_relu (Activation (None, 25, 25, 64) 0 conv2_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_relu (Activation (None, 25, 25, 64) 0 conv2_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_0_conv (Conv2D) (None, 25, 25, 256) 16640 pool1_pool[0][0] \n__________________________________________________________________________________________________\nconv2_block1_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block1_0_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_add (Add) (None, 25, 25, 256) 0 conv2_block1_0_bn[0][0] \n conv2_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_out (Activation) (None, 25, 25, 256) 0 conv2_block1_add[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_conv (Conv2D) (None, 25, 25, 64) 16448 conv2_block1_out[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_relu (Activation (None, 25, 25, 64) 0 conv2_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_relu (Activation (None, 25, 25, 64) 0 conv2_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block2_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_add (Add) (None, 25, 25, 256) 0 conv2_block1_out[0][0] \n conv2_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_out (Activation) (None, 25, 25, 256) 0 conv2_block2_add[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_conv (Conv2D) (None, 25, 25, 64) 16448 conv2_block2_out[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_relu (Activation (None, 25, 25, 64) 0 conv2_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_relu (Activation (None, 25, 25, 64) 0 conv2_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block3_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_add (Add) (None, 25, 25, 256) 0 conv2_block2_out[0][0] \n conv2_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_out (Activation) (None, 25, 25, 256) 0 conv2_block3_add[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_conv (Conv2D) (None, 13, 13, 128) 32896 conv2_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_relu (Activation (None, 13, 13, 128) 0 conv3_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_relu (Activation (None, 13, 13, 128) 0 conv3_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_0_conv (Conv2D) (None, 13, 13, 512) 131584 conv2_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block1_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block1_0_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_add (Add) (None, 13, 13, 512) 0 conv3_block1_0_bn[0][0] \n conv3_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_out (Activation) (None, 13, 13, 512) 0 conv3_block1_add[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block1_out[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_relu (Activation (None, 13, 13, 128) 0 conv3_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_relu (Activation (None, 13, 13, 128) 0 conv3_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block2_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_add (Add) (None, 13, 13, 512) 0 conv3_block1_out[0][0] \n conv3_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_out (Activation) (None, 13, 13, 512) 0 conv3_block2_add[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block2_out[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_relu (Activation (None, 13, 13, 128) 0 conv3_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_relu (Activation (None, 13, 13, 128) 0 conv3_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block3_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_add (Add) (None, 13, 13, 512) 0 conv3_block2_out[0][0] \n conv3_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_out (Activation) (None, 13, 13, 512) 0 conv3_block3_add[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block4_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_relu (Activation (None, 13, 13, 128) 0 conv3_block4_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block4_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block4_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_relu (Activation (None, 13, 13, 128) 0 conv3_block4_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block4_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block4_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block4_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_add (Add) (None, 13, 13, 512) 0 conv3_block3_out[0][0] \n conv3_block4_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_out (Activation) (None, 13, 13, 512) 0 conv3_block4_add[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_conv (Conv2D) (None, 7, 7, 256) 131328 conv3_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_relu (Activation (None, 7, 7, 256) 0 conv4_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_relu (Activation (None, 7, 7, 256) 0 conv4_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_0_conv (Conv2D) (None, 7, 7, 1024) 525312 conv3_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block1_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block1_0_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_add (Add) (None, 7, 7, 1024) 0 conv4_block1_0_bn[0][0] \n conv4_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_out (Activation) (None, 7, 7, 1024) 0 conv4_block1_add[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block1_out[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_relu (Activation (None, 7, 7, 256) 0 conv4_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_relu (Activation (None, 7, 7, 256) 0 conv4_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block2_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_add (Add) (None, 7, 7, 1024) 0 conv4_block1_out[0][0] \n conv4_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_out (Activation) (None, 7, 7, 1024) 0 conv4_block2_add[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block2_out[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_relu (Activation (None, 7, 7, 256) 0 conv4_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_relu (Activation (None, 7, 7, 256) 0 conv4_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block3_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_add (Add) (None, 7, 7, 1024) 0 conv4_block2_out[0][0] \n conv4_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_out (Activation) (None, 7, 7, 1024) 0 conv4_block3_add[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block3_out[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block4_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_relu (Activation (None, 7, 7, 256) 0 conv4_block4_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block4_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block4_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_relu (Activation (None, 7, 7, 256) 0 conv4_block4_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block4_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block4_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block4_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_add (Add) (None, 7, 7, 1024) 0 conv4_block3_out[0][0] \n conv4_block4_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_out (Activation) (None, 7, 7, 1024) 0 conv4_block4_add[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block5_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_relu (Activation (None, 7, 7, 256) 0 conv4_block5_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block5_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block5_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_relu (Activation (None, 7, 7, 256) 0 conv4_block5_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block5_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block5_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block5_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_add (Add) (None, 7, 7, 1024) 0 conv4_block4_out[0][0] \n conv4_block5_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_out (Activation) (None, 7, 7, 1024) 0 conv4_block5_add[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block5_out[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block6_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_relu (Activation (None, 7, 7, 256) 0 conv4_block6_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block6_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block6_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_relu (Activation (None, 7, 7, 256) 0 conv4_block6_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block6_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block6_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block6_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_add (Add) (None, 7, 7, 1024) 0 conv4_block5_out[0][0] \n conv4_block6_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_out (Activation) (None, 7, 7, 1024) 0 conv4_block6_add[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_conv (Conv2D) (None, 4, 4, 512) 524800 conv4_block6_out[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_relu (Activation (None, 4, 4, 512) 0 conv5_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_relu (Activation (None, 4, 4, 512) 0 conv5_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_0_conv (Conv2D) (None, 4, 4, 2048) 2099200 conv4_block6_out[0][0] \n__________________________________________________________________________________________________\nconv5_block1_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block1_0_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_add (Add) (None, 4, 4, 2048) 0 conv5_block1_0_bn[0][0] \n conv5_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_out (Activation) (None, 4, 4, 2048) 0 conv5_block1_add[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_conv (Conv2D) (None, 4, 4, 512) 1049088 conv5_block1_out[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_relu (Activation (None, 4, 4, 512) 0 conv5_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_relu (Activation (None, 4, 4, 512) 0 conv5_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block2_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_add (Add) (None, 4, 4, 2048) 0 conv5_block1_out[0][0] \n conv5_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_out (Activation) (None, 4, 4, 2048) 0 conv5_block2_add[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_conv (Conv2D) (None, 4, 4, 512) 1049088 conv5_block2_out[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_relu (Activation (None, 4, 4, 512) 0 conv5_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_relu (Activation (None, 4, 4, 512) 0 conv5_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block3_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_add (Add) (None, 4, 4, 2048) 0 conv5_block2_out[0][0] \n conv5_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_out (Activation) (None, 4, 4, 2048) 0 conv5_block3_add[0][0] \n__________________________________________________________________________________________________\nglobal_average_pooling2d (Globa (None, 2048) 0 conv5_block3_out[0][0] \n__________________________________________________________________________________________________\ndense (Dense) (None, 2048) 4196352 global_average_pooling2d[0][0] \n__________________________________________________________________________________________________\ndense_1 (Dense) (None, 2048) 4196352 dense[0][0] \n__________________________________________________________________________________________________\ndense_2 (Dense) (None, 10) 20490 dense_1[0][0] \n==================================================================================================\nTotal params: 32,000,963\nTrainable params: 31,947,843\nNon-trainable params: 53,120\n__________________________________________________________________________________________________\n"
],
[
"epochs = 20\nhistory = model.fit(gen.training_dataset, validation_data=gen.validation_dataset, \n epochs=epochs, \n callbacks=callbacks_list)\n",
"Epoch 1/20\n 1/Unknown - 0s 0s/step - loss: 0.7377 - accuracy: 0.8900"
]
],
[
[
"# Evaluation",
"_____no_output_____"
]
],
[
[
"model.evaluate(gen.validation_dataset) ",
"3/3 [==============================] - 9s 3s/step - loss: 0.6661 - accuracy: 0.9473\n"
]
],
[
[
"# Sandbox",
"_____no_output_____"
]
],
[
[
"# Applying albumentations",
"_____no_output_____"
],
[
"help(gen.training_dataset)",
"Help on BatchDataset in module tensorflow.python.data.ops.dataset_ops object:\n\nclass BatchDataset(UnaryDataset)\n | BatchDataset(input_dataset, batch_size, drop_remainder)\n | \n | A `Dataset` that batches contiguous elements from its input.\n | \n | Method resolution order:\n | BatchDataset\n | UnaryDataset\n | DatasetV2\n | collections.abc.Iterable\n | tensorflow.python.training.tracking.base.Trackable\n | tensorflow.python.framework.composite_tensor.CompositeTensor\n | builtins.object\n | \n | Methods defined here:\n | \n | __init__(self, input_dataset, batch_size, drop_remainder)\n | See `Dataset.batch()` for details.\n | \n | ----------------------------------------------------------------------\n | Data descriptors defined here:\n | \n | element_spec\n | The type specification of an element of this dataset.\n | \n | >>> dataset = tf.data.Dataset.from_tensor_slices([1, 2, 3])\n | >>> dataset.element_spec\n | TensorSpec(shape=(), dtype=tf.int32, name=None)\n | \n | Returns:\n | A nested structure of `tf.TypeSpec` objects matching the structure of an\n | element of this dataset and specifying the type of individual components.\n | \n | ----------------------------------------------------------------------\n | Data and other attributes defined here:\n | \n | __abstractmethods__ = frozenset()\n | \n | ----------------------------------------------------------------------\n | Methods inherited from DatasetV2:\n | \n | __bool__(self)\n | \n | __iter__(self)\n | Creates an iterator for elements of this dataset.\n | \n | The returned iterator implements the Python Iterator protocol.\n | \n | Returns:\n | An `tf.data.Iterator` for the elements of this dataset.\n | \n | Raises:\n | RuntimeError: If not inside of tf.function and not executing eagerly.\n | \n | __len__(self)\n | Returns the length of the dataset if it is known and finite.\n | \n | This method requires that you are running in eager mode, and that the\n | length of the dataset is known and non-infinite. When the length may be\n | unknown or infinite, or if you are running in graph mode, use\n | `tf.data.Dataset.cardinality` instead.\n | \n | Returns:\n | An integer representing the length of the dataset.\n | \n | Raises:\n | RuntimeError: If the dataset length is unknown or infinite, or if eager\n | execution is not enabled.\n | \n | __nonzero__ = __bool__(self)\n | \n | __repr__(self)\n | Return repr(self).\n | \n | apply(self, transformation_func)\n | Applies a transformation function to this dataset.\n | \n | `apply` enables chaining of custom `Dataset` transformations, which are\n | represented as functions that take one `Dataset` argument and return a\n | transformed `Dataset`.\n | \n | >>> dataset = tf.data.Dataset.range(100)\n | >>> def dataset_fn(ds):\n | ... return ds.filter(lambda x: x < 5)\n | >>> dataset = dataset.apply(dataset_fn)\n | >>> list(dataset.as_numpy_iterator())\n | [0, 1, 2, 3, 4]\n | \n | Args:\n | transformation_func: A function that takes one `Dataset` argument and\n | returns a `Dataset`.\n | \n | Returns:\n | Dataset: The `Dataset` returned by applying `transformation_func` to this\n | dataset.\n | \n | as_numpy_iterator(self)\n | Returns an iterator which converts all elements of the dataset to numpy.\n | \n | Use `as_numpy_iterator` to inspect the content of your dataset. To see\n | element shapes and types, print dataset elements directly instead of using\n | `as_numpy_iterator`.\n | \n | >>> dataset = tf.data.Dataset.from_tensor_slices([1, 2, 3])\n | >>> for element in dataset:\n | ... print(element)\n | tf.Tensor(1, shape=(), dtype=int32)\n | tf.Tensor(2, shape=(), dtype=int32)\n | tf.Tensor(3, shape=(), dtype=int32)\n | \n | This method requires that you are running in eager mode and the dataset's\n | element_spec contains only `TensorSpec` components.\n | \n | >>> dataset = tf.data.Dataset.from_tensor_slices([1, 2, 3])\n | >>> for element in dataset.as_numpy_iterator():\n | ... print(element)\n | 1\n | 2\n | 3\n | \n | >>> dataset = tf.data.Dataset.from_tensor_slices([1, 2, 3])\n | >>> print(list(dataset.as_numpy_iterator()))\n | [1, 2, 3]\n | \n | `as_numpy_iterator()` will preserve the nested structure of dataset\n | elements.\n | \n | >>> dataset = tf.data.Dataset.from_tensor_slices({'a': ([1, 2], [3, 4]),\n | ... 'b': [5, 6]})\n | >>> list(dataset.as_numpy_iterator()) == [{'a': (1, 3), 'b': 5},\n | ... {'a': (2, 4), 'b': 6}]\n | True\n | \n | Returns:\n | An iterable over the elements of the dataset, with their tensors converted\n | to numpy arrays.\n | \n | Raises:\n | TypeError: if an element contains a non-`Tensor` value.\n | RuntimeError: if eager execution is not enabled.\n | \n | batch(self, batch_size, drop_remainder=False)\n | Combines consecutive elements of this dataset into batches.\n | \n | >>> dataset = tf.data.Dataset.range(8)\n | >>> dataset = dataset.batch(3)\n | >>> list(dataset.as_numpy_iterator())\n | [array([0, 1, 2]), array([3, 4, 5]), array([6, 7])]\n | \n | >>> dataset = tf.data.Dataset.range(8)\n | >>> dataset = dataset.batch(3, drop_remainder=True)\n | >>> list(dataset.as_numpy_iterator())\n | [array([0, 1, 2]), array([3, 4, 5])]\n | \n | The components of the resulting element will have an additional outer\n | dimension, which will be `batch_size` (or `N % batch_size` for the last\n | element if `batch_size` does not divide the number of input elements `N`\n | evenly and `drop_remainder` is `False`). If your program depends on the\n | batches having the same outer dimension, you should set the `drop_remainder`\n | argument to `True` to prevent the smaller batch from being produced.\n | \n | Args:\n | batch_size: A `tf.int64` scalar `tf.Tensor`, representing the number of\n | consecutive elements of this dataset to combine in a single batch.\n | drop_remainder: (Optional.) A `tf.bool` scalar `tf.Tensor`, representing\n | whether the last batch should be dropped in the case it has fewer than\n | `batch_size` elements; the default behavior is not to drop the smaller\n | batch.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | cache(self, filename='')\n | Caches the elements in this dataset.\n | \n | The first time the dataset is iterated over, its elements will be cached\n | either in the specified file or in memory. Subsequent iterations will\n | use the cached data.\n | \n | Note: For the cache to be finalized, the input dataset must be iterated\n | through in its entirety. Otherwise, subsequent iterations will not use\n | cached data.\n | \n | >>> dataset = tf.data.Dataset.range(5)\n | >>> dataset = dataset.map(lambda x: x**2)\n | >>> dataset = dataset.cache()\n | >>> # The first time reading through the data will generate the data using\n | >>> # `range` and `map`.\n | >>> list(dataset.as_numpy_iterator())\n | [0, 1, 4, 9, 16]\n | >>> # Subsequent iterations read from the cache.\n | >>> list(dataset.as_numpy_iterator())\n | [0, 1, 4, 9, 16]\n | \n | When caching to a file, the cached data will persist across runs. Even the\n | first iteration through the data will read from the cache file. Changing\n | the input pipeline before the call to `.cache()` will have no effect until\n | the cache file is removed or the filename is changed.\n | \n | >>> dataset = tf.data.Dataset.range(5)\n | >>> dataset = dataset.cache(\"/path/to/file\") # doctest: +SKIP\n | >>> list(dataset.as_numpy_iterator()) # doctest: +SKIP\n | [0, 1, 2, 3, 4]\n | >>> dataset = tf.data.Dataset.range(10)\n | >>> dataset = dataset.cache(\"/path/to/file\") # Same file! # doctest: +SKIP\n | >>> list(dataset.as_numpy_iterator()) # doctest: +SKIP\n | [0, 1, 2, 3, 4]\n | \n | Note: `cache` will produce exactly the same elements during each iteration\n | through the dataset. If you wish to randomize the iteration order, make sure\n | to call `shuffle` *after* calling `cache`.\n | \n | Args:\n | filename: A `tf.string` scalar `tf.Tensor`, representing the name of a\n | directory on the filesystem to use for caching elements in this Dataset.\n | If a filename is not provided, the dataset will be cached in memory.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | cardinality(self)\n | Returns the cardinality of the dataset, if known.\n | \n | `cardinality` may return `tf.data.INFINITE_CARDINALITY` if the dataset\n | contains an infinite number of elements or `tf.data.UNKNOWN_CARDINALITY` if\n | the analysis fails to determine the number of elements in the dataset\n | (e.g. when the dataset source is a file).\n | \n | >>> dataset = tf.data.Dataset.range(42)\n | >>> print(dataset.cardinality().numpy())\n | 42\n | >>> dataset = dataset.repeat()\n | >>> cardinality = dataset.cardinality()\n | >>> print((cardinality == tf.data.INFINITE_CARDINALITY).numpy())\n | True\n | >>> dataset = dataset.filter(lambda x: True)\n | >>> cardinality = dataset.cardinality()\n | >>> print((cardinality == tf.data.UNKNOWN_CARDINALITY).numpy())\n | True\n | \n | Returns:\n | A scalar `tf.int64` `Tensor` representing the cardinality of the dataset.\n | If the cardinality is infinite or unknown, `cardinality` returns the\n | named constants `tf.data.INFINITE_CARDINALITY` and\n | `tf.data.UNKNOWN_CARDINALITY` respectively.\n | \n | concatenate(self, dataset)\n | Creates a `Dataset` by concatenating the given dataset with this dataset.\n | \n | >>> a = tf.data.Dataset.range(1, 4) # ==> [ 1, 2, 3 ]\n | >>> b = tf.data.Dataset.range(4, 8) # ==> [ 4, 5, 6, 7 ]\n | >>> ds = a.concatenate(b)\n | >>> list(ds.as_numpy_iterator())\n | [1, 2, 3, 4, 5, 6, 7]\n | >>> # The input dataset and dataset to be concatenated should have the same\n | >>> # nested structures and output types.\n | >>> c = tf.data.Dataset.zip((a, b))\n | >>> a.concatenate(c)\n | Traceback (most recent call last):\n | TypeError: Two datasets to concatenate have different types\n | <dtype: 'int64'> and (tf.int64, tf.int64)\n | >>> d = tf.data.Dataset.from_tensor_slices([\"a\", \"b\", \"c\"])\n | >>> a.concatenate(d)\n | Traceback (most recent call last):\n | TypeError: Two datasets to concatenate have different types\n | <dtype: 'int64'> and <dtype: 'string'>\n | \n | Args:\n | dataset: `Dataset` to be concatenated.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | enumerate(self, start=0)\n | Enumerates the elements of this dataset.\n | \n | It is similar to python's `enumerate`.\n | \n | >>> dataset = tf.data.Dataset.from_tensor_slices([1, 2, 3])\n | >>> dataset = dataset.enumerate(start=5)\n | >>> for element in dataset.as_numpy_iterator():\n | ... print(element)\n | (5, 1)\n | (6, 2)\n | (7, 3)\n | \n | >>> # The nested structure of the input dataset determines the structure of\n | >>> # elements in the resulting dataset.\n | >>> dataset = tf.data.Dataset.from_tensor_slices([(7, 8), (9, 10)])\n | >>> dataset = dataset.enumerate()\n | >>> for element in dataset.as_numpy_iterator():\n | ... print(element)\n | (0, array([7, 8], dtype=int32))\n | (1, array([ 9, 10], dtype=int32))\n | \n | Args:\n | start: A `tf.int64` scalar `tf.Tensor`, representing the start value for\n | enumeration.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | filter(self, predicate)\n | Filters this dataset according to `predicate`.\n | \n | >>> dataset = tf.data.Dataset.from_tensor_slices([1, 2, 3])\n | >>> dataset = dataset.filter(lambda x: x < 3)\n | >>> list(dataset.as_numpy_iterator())\n | [1, 2]\n | >>> # `tf.math.equal(x, y)` is required for equality comparison\n | >>> def filter_fn(x):\n | ... return tf.math.equal(x, 1)\n | >>> dataset = dataset.filter(filter_fn)\n | >>> list(dataset.as_numpy_iterator())\n | [1]\n | \n | Args:\n | predicate: A function mapping a dataset element to a boolean.\n | \n | Returns:\n | Dataset: The `Dataset` containing the elements of this dataset for which\n | `predicate` is `True`.\n | \n | flat_map(self, map_func)\n | Maps `map_func` across this dataset and flattens the result.\n | \n | Use `flat_map` if you want to make sure that the order of your dataset\n | stays the same. For example, to flatten a dataset of batches into a\n | dataset of their elements:\n | \n | >>> dataset = tf.data.Dataset.from_tensor_slices(\n | ... [[1, 2, 3], [4, 5, 6], [7, 8, 9]])\n | >>> dataset = dataset.flat_map(lambda x: Dataset.from_tensor_slices(x))\n | >>> list(dataset.as_numpy_iterator())\n | [1, 2, 3, 4, 5, 6, 7, 8, 9]\n | \n | `tf.data.Dataset.interleave()` is a generalization of `flat_map`, since\n | `flat_map` produces the same output as\n | `tf.data.Dataset.interleave(cycle_length=1)`\n | \n | Args:\n | map_func: A function mapping a dataset element to a dataset.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | interleave(self, map_func, cycle_length=None, block_length=None, num_parallel_calls=None, deterministic=None)\n | Maps `map_func` across this dataset, and interleaves the results.\n | \n | For example, you can use `Dataset.interleave()` to process many input files\n | concurrently:\n | \n | >>> # Preprocess 4 files concurrently, and interleave blocks of 16 records\n | >>> # from each file.\n | >>> filenames = [\"/var/data/file1.txt\", \"/var/data/file2.txt\",\n | ... \"/var/data/file3.txt\", \"/var/data/file4.txt\"]\n | >>> dataset = tf.data.Dataset.from_tensor_slices(filenames)\n | >>> def parse_fn(filename):\n | ... return tf.data.Dataset.range(10)\n | >>> dataset = dataset.interleave(lambda x:\n | ... tf.data.TextLineDataset(x).map(parse_fn, num_parallel_calls=1),\n | ... cycle_length=4, block_length=16)\n | \n | The `cycle_length` and `block_length` arguments control the order in which\n | elements are produced. `cycle_length` controls the number of input elements\n | that are processed concurrently. If you set `cycle_length` to 1, this\n | transformation will handle one input element at a time, and will produce\n | identical results to `tf.data.Dataset.flat_map`. In general,\n | this transformation will apply `map_func` to `cycle_length` input elements,\n | open iterators on the returned `Dataset` objects, and cycle through them\n | producing `block_length` consecutive elements from each iterator, and\n | consuming the next input element each time it reaches the end of an\n | iterator.\n | \n | For example:\n | \n | >>> dataset = Dataset.range(1, 6) # ==> [ 1, 2, 3, 4, 5 ]\n | >>> # NOTE: New lines indicate \"block\" boundaries.\n | >>> dataset = dataset.interleave(\n | ... lambda x: Dataset.from_tensors(x).repeat(6),\n | ... cycle_length=2, block_length=4)\n | >>> list(dataset.as_numpy_iterator())\n | [1, 1, 1, 1,\n | 2, 2, 2, 2,\n | 1, 1,\n | 2, 2,\n | 3, 3, 3, 3,\n | 4, 4, 4, 4,\n | 3, 3,\n | 4, 4,\n | 5, 5, 5, 5,\n | 5, 5]\n | \n | Note: The order of elements yielded by this transformation is\n | deterministic, as long as `map_func` is a pure function and\n | `deterministic=True`. If `map_func` contains any stateful operations, the\n | order in which that state is accessed is undefined.\n | \n | Performance can often be improved by setting `num_parallel_calls` so that\n | `interleave` will use multiple threads to fetch elements. If determinism\n | isn't required, it can also improve performance to set\n | `deterministic=False`.\n | \n | >>> filenames = [\"/var/data/file1.txt\", \"/var/data/file2.txt\",\n | ... \"/var/data/file3.txt\", \"/var/data/file4.txt\"]\n | >>> dataset = tf.data.Dataset.from_tensor_slices(filenames)\n | >>> dataset = dataset.interleave(lambda x: tf.data.TFRecordDataset(x),\n | ... cycle_length=4, num_parallel_calls=tf.data.AUTOTUNE,\n | ... deterministic=False)\n | \n | Args:\n | map_func: A function mapping a dataset element to a dataset.\n | cycle_length: (Optional.) The number of input elements that will be\n | processed concurrently. If not set, the tf.data runtime decides what it\n | should be based on available CPU. If `num_parallel_calls` is set to\n | `tf.data.AUTOTUNE`, the `cycle_length` argument identifies\n | the maximum degree of parallelism.\n | block_length: (Optional.) The number of consecutive elements to produce\n | from each input element before cycling to another input element. If not\n | set, defaults to 1.\n | num_parallel_calls: (Optional.) If specified, the implementation creates a\n | threadpool, which is used to fetch inputs from cycle elements\n | asynchronously and in parallel. The default behavior is to fetch inputs\n | from cycle elements synchronously with no parallelism. If the value\n | `tf.data.AUTOTUNE` is used, then the number of parallel\n | calls is set dynamically based on available CPU.\n | deterministic: (Optional.) A boolean controlling whether determinism\n | should be traded for performance by allowing elements to be produced out\n | of order. If `deterministic` is `None`, the\n | `tf.data.Options.experimental_deterministic` dataset option (`True` by\n | default) is used to decide whether to produce elements\n | deterministically.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | map(self, map_func, num_parallel_calls=None, deterministic=None)\n | Maps `map_func` across the elements of this dataset.\n | \n | This transformation applies `map_func` to each element of this dataset, and\n | returns a new dataset containing the transformed elements, in the same\n | order as they appeared in the input. `map_func` can be used to change both\n | the values and the structure of a dataset's elements. For example, adding 1\n | to each element, or projecting a subset of element components.\n | \n | >>> dataset = Dataset.range(1, 6) # ==> [ 1, 2, 3, 4, 5 ]\n | >>> dataset = dataset.map(lambda x: x + 1)\n | >>> list(dataset.as_numpy_iterator())\n | [2, 3, 4, 5, 6]\n | \n | The input signature of `map_func` is determined by the structure of each\n | element in this dataset.\n | \n | >>> dataset = Dataset.range(5)\n | >>> # `map_func` takes a single argument of type `tf.Tensor` with the same\n | >>> # shape and dtype.\n | >>> result = dataset.map(lambda x: x + 1)\n | \n | >>> # Each element is a tuple containing two `tf.Tensor` objects.\n | >>> elements = [(1, \"foo\"), (2, \"bar\"), (3, \"baz\")]\n | >>> dataset = tf.data.Dataset.from_generator(\n | ... lambda: elements, (tf.int32, tf.string))\n | >>> # `map_func` takes two arguments of type `tf.Tensor`. This function\n | >>> # projects out just the first component.\n | >>> result = dataset.map(lambda x_int, y_str: x_int)\n | >>> list(result.as_numpy_iterator())\n | [1, 2, 3]\n | \n | >>> # Each element is a dictionary mapping strings to `tf.Tensor` objects.\n | >>> elements = ([{\"a\": 1, \"b\": \"foo\"},\n | ... {\"a\": 2, \"b\": \"bar\"},\n | ... {\"a\": 3, \"b\": \"baz\"}])\n | >>> dataset = tf.data.Dataset.from_generator(\n | ... lambda: elements, {\"a\": tf.int32, \"b\": tf.string})\n | >>> # `map_func` takes a single argument of type `dict` with the same keys\n | >>> # as the elements.\n | >>> result = dataset.map(lambda d: str(d[\"a\"]) + d[\"b\"])\n | \n | The value or values returned by `map_func` determine the structure of each\n | element in the returned dataset.\n | \n | >>> dataset = tf.data.Dataset.range(3)\n | >>> # `map_func` returns two `tf.Tensor` objects.\n | >>> def g(x):\n | ... return tf.constant(37.0), tf.constant([\"Foo\", \"Bar\", \"Baz\"])\n | >>> result = dataset.map(g)\n | >>> result.element_spec\n | (TensorSpec(shape=(), dtype=tf.float32, name=None), TensorSpec(shape=(3,), dtype=tf.string, name=None))\n | >>> # Python primitives, lists, and NumPy arrays are implicitly converted to\n | >>> # `tf.Tensor`.\n | >>> def h(x):\n | ... return 37.0, [\"Foo\", \"Bar\"], np.array([1.0, 2.0], dtype=np.float64)\n | >>> result = dataset.map(h)\n | >>> result.element_spec\n | (TensorSpec(shape=(), dtype=tf.float32, name=None), TensorSpec(shape=(2,), dtype=tf.string, name=None), TensorSpec(shape=(2,), dtype=tf.float64, name=None))\n | >>> # `map_func` can return nested structures.\n | >>> def i(x):\n | ... return (37.0, [42, 16]), \"foo\"\n | >>> result = dataset.map(i)\n | >>> result.element_spec\n | ((TensorSpec(shape=(), dtype=tf.float32, name=None),\n | TensorSpec(shape=(2,), dtype=tf.int32, name=None)),\n | TensorSpec(shape=(), dtype=tf.string, name=None))\n | \n | `map_func` can accept as arguments and return any type of dataset element.\n | \n | Note that irrespective of the context in which `map_func` is defined (eager\n | vs. graph), tf.data traces the function and executes it as a graph. To use\n | Python code inside of the function you have a few options:\n | \n | 1) Rely on AutoGraph to convert Python code into an equivalent graph\n | computation. The downside of this approach is that AutoGraph can convert\n | some but not all Python code.\n | \n | 2) Use `tf.py_function`, which allows you to write arbitrary Python code but\n | will generally result in worse performance than 1). For example:\n | \n | >>> d = tf.data.Dataset.from_tensor_slices(['hello', 'world'])\n | >>> # transform a string tensor to upper case string using a Python function\n | >>> def upper_case_fn(t: tf.Tensor):\n | ... return t.numpy().decode('utf-8').upper()\n | >>> d = d.map(lambda x: tf.py_function(func=upper_case_fn,\n | ... inp=[x], Tout=tf.string))\n | >>> list(d.as_numpy_iterator())\n | [b'HELLO', b'WORLD']\n | \n | 3) Use `tf.numpy_function`, which also allows you to write arbitrary\n | Python code. Note that `tf.py_function` accepts `tf.Tensor` whereas\n | `tf.numpy_function` accepts numpy arrays and returns only numpy arrays.\n | For example:\n | \n | >>> d = tf.data.Dataset.from_tensor_slices(['hello', 'world'])\n | >>> def upper_case_fn(t: np.ndarray):\n | ... return t.decode('utf-8').upper()\n | >>> d = d.map(lambda x: tf.numpy_function(func=upper_case_fn,\n | ... inp=[x], Tout=tf.string))\n | >>> list(d.as_numpy_iterator())\n | [b'HELLO', b'WORLD']\n | \n | Note that the use of `tf.numpy_function` and `tf.py_function`\n | in general precludes the possibility of executing user-defined\n | transformations in parallel (because of Python GIL).\n | \n | Performance can often be improved by setting `num_parallel_calls` so that\n | `map` will use multiple threads to process elements. If deterministic order\n | isn't required, it can also improve performance to set\n | `deterministic=False`.\n | \n | >>> dataset = Dataset.range(1, 6) # ==> [ 1, 2, 3, 4, 5 ]\n | >>> dataset = dataset.map(lambda x: x + 1,\n | ... num_parallel_calls=tf.data.AUTOTUNE,\n | ... deterministic=False)\n | \n | Args:\n | map_func: A function mapping a dataset element to another dataset element.\n | num_parallel_calls: (Optional.) A `tf.int32` scalar `tf.Tensor`,\n | representing the number elements to process asynchronously in parallel.\n | If not specified, elements will be processed sequentially. If the value\n | `tf.data.AUTOTUNE` is used, then the number of parallel\n | calls is set dynamically based on available CPU.\n | deterministic: (Optional.) A boolean controlling whether determinism\n | should be traded for performance by allowing elements to be produced out\n | of order. If `deterministic` is `None`, the\n | `tf.data.Options.experimental_deterministic` dataset option (`True` by\n | default) is used to decide whether to produce elements\n | deterministically.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | options(self)\n | Returns the options for this dataset and its inputs.\n | \n | Returns:\n | A `tf.data.Options` object representing the dataset options.\n | \n | padded_batch(self, batch_size, padded_shapes=None, padding_values=None, drop_remainder=False)\n | Combines consecutive elements of this dataset into padded batches.\n | \n | This transformation combines multiple consecutive elements of the input\n | dataset into a single element.\n | \n | Like `tf.data.Dataset.batch`, the components of the resulting element will\n | have an additional outer dimension, which will be `batch_size` (or\n | `N % batch_size` for the last element if `batch_size` does not divide the\n | number of input elements `N` evenly and `drop_remainder` is `False`). If\n | your program depends on the batches having the same outer dimension, you\n | should set the `drop_remainder` argument to `True` to prevent the smaller\n | batch from being produced.\n | \n | Unlike `tf.data.Dataset.batch`, the input elements to be batched may have\n | different shapes, and this transformation will pad each component to the\n | respective shape in `padded_shapes`. The `padded_shapes` argument\n | determines the resulting shape for each dimension of each component in an\n | output element:\n | \n | * If the dimension is a constant, the component will be padded out to that\n | length in that dimension.\n | * If the dimension is unknown, the component will be padded out to the\n | maximum length of all elements in that dimension.\n | \n | >>> A = (tf.data.Dataset\n | ... .range(1, 5, output_type=tf.int32)\n | ... .map(lambda x: tf.fill([x], x)))\n | >>> # Pad to the smallest per-batch size that fits all elements.\n | >>> B = A.padded_batch(2)\n | >>> for element in B.as_numpy_iterator():\n | ... print(element)\n | [[1 0]\n | [2 2]]\n | [[3 3 3 0]\n | [4 4 4 4]]\n | >>> # Pad to a fixed size.\n | >>> C = A.padded_batch(2, padded_shapes=5)\n | >>> for element in C.as_numpy_iterator():\n | ... print(element)\n | [[1 0 0 0 0]\n | [2 2 0 0 0]]\n | [[3 3 3 0 0]\n | [4 4 4 4 0]]\n | >>> # Pad with a custom value.\n | >>> D = A.padded_batch(2, padded_shapes=5, padding_values=-1)\n | >>> for element in D.as_numpy_iterator():\n | ... print(element)\n | [[ 1 -1 -1 -1 -1]\n | [ 2 2 -1 -1 -1]]\n | [[ 3 3 3 -1 -1]\n | [ 4 4 4 4 -1]]\n | >>> # Components of nested elements can be padded independently.\n | >>> elements = [([1, 2, 3], [10]),\n | ... ([4, 5], [11, 12])]\n | >>> dataset = tf.data.Dataset.from_generator(\n | ... lambda: iter(elements), (tf.int32, tf.int32))\n | >>> # Pad the first component of the tuple to length 4, and the second\n | >>> # component to the smallest size that fits.\n | >>> dataset = dataset.padded_batch(2,\n | ... padded_shapes=([4], [None]),\n | ... padding_values=(-1, 100))\n | >>> list(dataset.as_numpy_iterator())\n | [(array([[ 1, 2, 3, -1], [ 4, 5, -1, -1]], dtype=int32),\n | array([[ 10, 100], [ 11, 12]], dtype=int32))]\n | >>> # Pad with a single value and multiple components.\n | >>> E = tf.data.Dataset.zip((A, A)).padded_batch(2, padding_values=-1)\n | >>> for element in E.as_numpy_iterator():\n | ... print(element)\n | (array([[ 1, -1],\n | [ 2, 2]], dtype=int32), array([[ 1, -1],\n | [ 2, 2]], dtype=int32))\n | (array([[ 3, 3, 3, -1],\n | [ 4, 4, 4, 4]], dtype=int32), array([[ 3, 3, 3, -1],\n | [ 4, 4, 4, 4]], dtype=int32))\n | \n | See also `tf.data.experimental.dense_to_sparse_batch`, which combines\n | elements that may have different shapes into a `tf.sparse.SparseTensor`.\n | \n | Args:\n | batch_size: A `tf.int64` scalar `tf.Tensor`, representing the number of\n | consecutive elements of this dataset to combine in a single batch.\n | padded_shapes: (Optional.) A nested structure of `tf.TensorShape` or\n | `tf.int64` vector tensor-like objects representing the shape to which\n | the respective component of each input element should be padded prior\n | to batching. Any unknown dimensions will be padded to the maximum size\n | of that dimension in each batch. If unset, all dimensions of all\n | components are padded to the maximum size in the batch. `padded_shapes`\n | must be set if any component has an unknown rank.\n | padding_values: (Optional.) A nested structure of scalar-shaped\n | `tf.Tensor`, representing the padding values to use for the respective\n | components. None represents that the nested structure should be padded\n | with default values. Defaults are `0` for numeric types and the empty\n | string for string types. The `padding_values` should have the\n | same structure as the input dataset. If `padding_values` is a single\n | element and the input dataset has multiple components, then the same\n | `padding_values` will be used to pad every component of the dataset.\n | If `padding_values` is a scalar, then its value will be broadcasted\n | to match the shape of each component.\n | drop_remainder: (Optional.) A `tf.bool` scalar `tf.Tensor`, representing\n | whether the last batch should be dropped in the case it has fewer than\n | `batch_size` elements; the default behavior is not to drop the smaller\n | batch.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | Raises:\n | ValueError: If a component has an unknown rank, and the `padded_shapes`\n | argument is not set.\n | \n | prefetch(self, buffer_size)\n | Creates a `Dataset` that prefetches elements from this dataset.\n | \n | Most dataset input pipelines should end with a call to `prefetch`. This\n | allows later elements to be prepared while the current element is being\n | processed. This often improves latency and throughput, at the cost of\n | using additional memory to store prefetched elements.\n | \n | Note: Like other `Dataset` methods, prefetch operates on the\n | elements of the input dataset. It has no concept of examples vs. batches.\n | `examples.prefetch(2)` will prefetch two elements (2 examples),\n | while `examples.batch(20).prefetch(2)` will prefetch 2 elements\n | (2 batches, of 20 examples each).\n | \n | >>> dataset = tf.data.Dataset.range(3)\n | >>> dataset = dataset.prefetch(2)\n | >>> list(dataset.as_numpy_iterator())\n | [0, 1, 2]\n | \n | Args:\n | buffer_size: A `tf.int64` scalar `tf.Tensor`, representing the maximum\n | number of elements that will be buffered when prefetching.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | reduce(self, initial_state, reduce_func)\n | Reduces the input dataset to a single element.\n | \n | The transformation calls `reduce_func` successively on every element of\n | the input dataset until the dataset is exhausted, aggregating information in\n | its internal state. The `initial_state` argument is used for the initial\n | state and the final state is returned as the result.\n | \n | >>> tf.data.Dataset.range(5).reduce(np.int64(0), lambda x, _: x + 1).numpy()\n | 5\n | >>> tf.data.Dataset.range(5).reduce(np.int64(0), lambda x, y: x + y).numpy()\n | 10\n | \n | Args:\n | initial_state: An element representing the initial state of the\n | transformation.\n | reduce_func: A function that maps `(old_state, input_element)` to\n | `new_state`. It must take two arguments and return a new element\n | The structure of `new_state` must match the structure of\n | `initial_state`.\n | \n | Returns:\n | A dataset element corresponding to the final state of the transformation.\n | \n | repeat(self, count=None)\n | Repeats this dataset so each original value is seen `count` times.\n | \n | >>> dataset = tf.data.Dataset.from_tensor_slices([1, 2, 3])\n | >>> dataset = dataset.repeat(3)\n | >>> list(dataset.as_numpy_iterator())\n | [1, 2, 3, 1, 2, 3, 1, 2, 3]\n | \n | Note: If this dataset is a function of global state (e.g. a random number\n | generator), then different repetitions may produce different elements.\n | \n | Args:\n | count: (Optional.) A `tf.int64` scalar `tf.Tensor`, representing the\n | number of times the dataset should be repeated. The default behavior (if\n | `count` is `None` or `-1`) is for the dataset be repeated indefinitely.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | shard(self, num_shards, index)\n | Creates a `Dataset` that includes only 1/`num_shards` of this dataset.\n | \n | `shard` is deterministic. The Dataset produced by `A.shard(n, i)` will\n | contain all elements of A whose index mod n = i.\n | \n | >>> A = tf.data.Dataset.range(10)\n | >>> B = A.shard(num_shards=3, index=0)\n | >>> list(B.as_numpy_iterator())\n | [0, 3, 6, 9]\n | >>> C = A.shard(num_shards=3, index=1)\n | >>> list(C.as_numpy_iterator())\n | [1, 4, 7]\n | >>> D = A.shard(num_shards=3, index=2)\n | >>> list(D.as_numpy_iterator())\n | [2, 5, 8]\n | \n | This dataset operator is very useful when running distributed training, as\n | it allows each worker to read a unique subset.\n | \n | When reading a single input file, you can shard elements as follows:\n | \n | ```python\n | d = tf.data.TFRecordDataset(input_file)\n | d = d.shard(num_workers, worker_index)\n | d = d.repeat(num_epochs)\n | d = d.shuffle(shuffle_buffer_size)\n | d = d.map(parser_fn, num_parallel_calls=num_map_threads)\n | ```\n | \n | Important caveats:\n | \n | - Be sure to shard before you use any randomizing operator (such as\n | shuffle).\n | - Generally it is best if the shard operator is used early in the dataset\n | pipeline. For example, when reading from a set of TFRecord files, shard\n | before converting the dataset to input samples. This avoids reading every\n | file on every worker. The following is an example of an efficient\n | sharding strategy within a complete pipeline:\n | \n | ```python\n | d = Dataset.list_files(pattern)\n | d = d.shard(num_workers, worker_index)\n | d = d.repeat(num_epochs)\n | d = d.shuffle(shuffle_buffer_size)\n | d = d.interleave(tf.data.TFRecordDataset,\n | cycle_length=num_readers, block_length=1)\n | d = d.map(parser_fn, num_parallel_calls=num_map_threads)\n | ```\n | \n | Args:\n | num_shards: A `tf.int64` scalar `tf.Tensor`, representing the number of\n | shards operating in parallel.\n | index: A `tf.int64` scalar `tf.Tensor`, representing the worker index.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | Raises:\n | InvalidArgumentError: if `num_shards` or `index` are illegal values.\n | \n | Note: error checking is done on a best-effort basis, and errors aren't\n | guaranteed to be caught upon dataset creation. (e.g. providing in a\n | placeholder tensor bypasses the early checking, and will instead result\n | in an error during a session.run call.)\n | \n | shuffle(self, buffer_size, seed=None, reshuffle_each_iteration=None)\n | Randomly shuffles the elements of this dataset.\n | \n | This dataset fills a buffer with `buffer_size` elements, then randomly\n | samples elements from this buffer, replacing the selected elements with new\n | elements. For perfect shuffling, a buffer size greater than or equal to the\n | full size of the dataset is required.\n | \n | For instance, if your dataset contains 10,000 elements but `buffer_size` is\n | set to 1,000, then `shuffle` will initially select a random element from\n | only the first 1,000 elements in the buffer. Once an element is selected,\n | its space in the buffer is replaced by the next (i.e. 1,001-st) element,\n | maintaining the 1,000 element buffer.\n | \n | `reshuffle_each_iteration` controls whether the shuffle order should be\n | different for each epoch. In TF 1.X, the idiomatic way to create epochs\n | was through the `repeat` transformation:\n | \n | >>> dataset = tf.data.Dataset.range(3)\n | >>> dataset = dataset.shuffle(3, reshuffle_each_iteration=True)\n | >>> dataset = dataset.repeat(2) # doctest: +SKIP\n | [1, 0, 2, 1, 2, 0]\n | \n | >>> dataset = tf.data.Dataset.range(3)\n | >>> dataset = dataset.shuffle(3, reshuffle_each_iteration=False)\n | >>> dataset = dataset.repeat(2) # doctest: +SKIP\n | [1, 0, 2, 1, 0, 2]\n | \n | In TF 2.0, `tf.data.Dataset` objects are Python iterables which makes it\n | possible to also create epochs through Python iteration:\n | \n | >>> dataset = tf.data.Dataset.range(3)\n | >>> dataset = dataset.shuffle(3, reshuffle_each_iteration=True)\n | >>> list(dataset.as_numpy_iterator()) # doctest: +SKIP\n | [1, 0, 2]\n | >>> list(dataset.as_numpy_iterator()) # doctest: +SKIP\n | [1, 2, 0]\n | \n | >>> dataset = tf.data.Dataset.range(3)\n | >>> dataset = dataset.shuffle(3, reshuffle_each_iteration=False)\n | >>> list(dataset.as_numpy_iterator()) # doctest: +SKIP\n | [1, 0, 2]\n | >>> list(dataset.as_numpy_iterator()) # doctest: +SKIP\n | [1, 0, 2]\n | \n | Args:\n | buffer_size: A `tf.int64` scalar `tf.Tensor`, representing the number of\n | elements from this dataset from which the new dataset will sample.\n | seed: (Optional.) A `tf.int64` scalar `tf.Tensor`, representing the random\n | seed that will be used to create the distribution. See\n | `tf.random.set_seed` for behavior.\n | reshuffle_each_iteration: (Optional.) A boolean, which if true indicates\n | that the dataset should be pseudorandomly reshuffled each time it is\n | iterated over. (Defaults to `True`.)\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | skip(self, count)\n | Creates a `Dataset` that skips `count` elements from this dataset.\n | \n | >>> dataset = tf.data.Dataset.range(10)\n | >>> dataset = dataset.skip(7)\n | >>> list(dataset.as_numpy_iterator())\n | [7, 8, 9]\n | \n | Args:\n | count: A `tf.int64` scalar `tf.Tensor`, representing the number of\n | elements of this dataset that should be skipped to form the new dataset.\n | If `count` is greater than the size of this dataset, the new dataset\n | will contain no elements. If `count` is -1, skips the entire dataset.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | take(self, count)\n | Creates a `Dataset` with at most `count` elements from this dataset.\n | \n | >>> dataset = tf.data.Dataset.range(10)\n | >>> dataset = dataset.take(3)\n | >>> list(dataset.as_numpy_iterator())\n | [0, 1, 2]\n | \n | Args:\n | count: A `tf.int64` scalar `tf.Tensor`, representing the number of\n | elements of this dataset that should be taken to form the new dataset.\n | If `count` is -1, or if `count` is greater than the size of this\n | dataset, the new dataset will contain all elements of this dataset.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | unbatch(self)\n | Splits elements of a dataset into multiple elements.\n | \n | For example, if elements of the dataset are shaped `[B, a0, a1, ...]`,\n | where `B` may vary for each input element, then for each element in the\n | dataset, the unbatched dataset will contain `B` consecutive elements\n | of shape `[a0, a1, ...]`.\n | \n | >>> elements = [ [1, 2, 3], [1, 2], [1, 2, 3, 4] ]\n | >>> dataset = tf.data.Dataset.from_generator(lambda: elements, tf.int64)\n | >>> dataset = dataset.unbatch()\n | >>> list(dataset.as_numpy_iterator())\n | [1, 2, 3, 1, 2, 1, 2, 3, 4]\n | \n | Note: `unbatch` requires a data copy to slice up the batched tensor into\n | smaller, unbatched tensors. When optimizing performance, try to avoid\n | unnecessary usage of `unbatch`.\n | \n | Returns:\n | A `Dataset`.\n | \n | window(self, size, shift=None, stride=1, drop_remainder=False)\n | Combines (nests of) input elements into a dataset of (nests of) windows.\n | \n | A \"window\" is a finite dataset of flat elements of size `size` (or possibly\n | fewer if there are not enough input elements to fill the window and\n | `drop_remainder` evaluates to `False`).\n | \n | The `shift` argument determines the number of input elements by which the\n | window moves on each iteration. If windows and elements are both numbered\n | starting at 0, the first element in window `k` will be element `k * shift`\n | of the input dataset. In particular, the first element of the first window\n | will always be the first element of the input dataset.\n | \n | The `stride` argument determines the stride of the input elements, and the\n | `shift` argument determines the shift of the window.\n | \n | For example:\n | \n | >>> dataset = tf.data.Dataset.range(7).window(2)\n | >>> for window in dataset:\n | ... print(list(window.as_numpy_iterator()))\n | [0, 1]\n | [2, 3]\n | [4, 5]\n | [6]\n | >>> dataset = tf.data.Dataset.range(7).window(3, 2, 1, True)\n | >>> for window in dataset:\n | ... print(list(window.as_numpy_iterator()))\n | [0, 1, 2]\n | [2, 3, 4]\n | [4, 5, 6]\n | >>> dataset = tf.data.Dataset.range(7).window(3, 1, 2, True)\n | >>> for window in dataset:\n | ... print(list(window.as_numpy_iterator()))\n | [0, 2, 4]\n | [1, 3, 5]\n | [2, 4, 6]\n | \n | Note that when the `window` transformation is applied to a dataset of\n | nested elements, it produces a dataset of nested windows.\n | \n | >>> nested = ([1, 2, 3, 4], [5, 6, 7, 8])\n | >>> dataset = tf.data.Dataset.from_tensor_slices(nested).window(2)\n | >>> for window in dataset:\n | ... def to_numpy(ds):\n | ... return list(ds.as_numpy_iterator())\n | ... print(tuple(to_numpy(component) for component in window))\n | ([1, 2], [5, 6])\n | ([3, 4], [7, 8])\n | \n | >>> dataset = tf.data.Dataset.from_tensor_slices({'a': [1, 2, 3, 4]})\n | >>> dataset = dataset.window(2)\n | >>> for window in dataset:\n | ... def to_numpy(ds):\n | ... return list(ds.as_numpy_iterator())\n | ... print({'a': to_numpy(window['a'])})\n | {'a': [1, 2]}\n | {'a': [3, 4]}\n | \n | Args:\n | size: A `tf.int64` scalar `tf.Tensor`, representing the number of elements\n | of the input dataset to combine into a window. Must be positive.\n | shift: (Optional.) A `tf.int64` scalar `tf.Tensor`, representing the\n | number of input elements by which the window moves in each iteration.\n | Defaults to `size`. Must be positive.\n | stride: (Optional.) A `tf.int64` scalar `tf.Tensor`, representing the\n | stride of the input elements in the sliding window. Must be positive.\n | The default value of 1 means \"retain every input element\".\n | drop_remainder: (Optional.) A `tf.bool` scalar `tf.Tensor`, representing\n | whether the last windows should be dropped if their size is smaller than\n | `size`.\n | \n | Returns:\n | Dataset: A `Dataset` of (nests of) windows -- a finite datasets of flat\n | elements created from the (nests of) input elements.\n | \n | with_options(self, options)\n | Returns a new `tf.data.Dataset` with the given options set.\n | \n | The options are \"global\" in the sense they apply to the entire dataset.\n | If options are set multiple times, they are merged as long as different\n | options do not use different non-default values.\n | \n | >>> ds = tf.data.Dataset.range(5)\n | >>> ds = ds.interleave(lambda x: tf.data.Dataset.range(5),\n | ... cycle_length=3,\n | ... num_parallel_calls=3)\n | >>> options = tf.data.Options()\n | >>> # This will make the interleave order non-deterministic.\n | >>> options.experimental_deterministic = False\n | >>> ds = ds.with_options(options)\n | \n | Args:\n | options: A `tf.data.Options` that identifies the options the use.\n | \n | Returns:\n | Dataset: A `Dataset` with the given options.\n | \n | Raises:\n | ValueError: when an option is set more than once to a non-default value\n | \n | ----------------------------------------------------------------------\n | Static methods inherited from DatasetV2:\n | \n | from_generator(generator, output_types=None, output_shapes=None, args=None, output_signature=None)\n | Creates a `Dataset` whose elements are generated by `generator`. (deprecated arguments)\n | \n | Warning: SOME ARGUMENTS ARE DEPRECATED: `(output_shapes, output_types)`. They will be removed in a future version.\n | Instructions for updating:\n | Use output_signature instead\n | \n | The `generator` argument must be a callable object that returns\n | an object that supports the `iter()` protocol (e.g. a generator function).\n | \n | The elements generated by `generator` must be compatible with either the\n | given `output_signature` argument or with the given `output_types` and\n | (optionally) `output_shapes` arguments, whichiver was specified.\n | \n | The recommended way to call `from_generator` is to use the\n | `output_signature` argument. In this case the output will be assumed to\n | consist of objects with the classes, shapes and types defined by\n | `tf.TypeSpec` objects from `output_signature` argument:\n | \n | >>> def gen():\n | ... ragged_tensor = tf.ragged.constant([[1, 2], [3]])\n | ... yield 42, ragged_tensor\n | >>>\n | >>> dataset = tf.data.Dataset.from_generator(\n | ... gen,\n | ... output_signature=(\n | ... tf.TensorSpec(shape=(), dtype=tf.int32),\n | ... tf.RaggedTensorSpec(shape=(2, None), dtype=tf.int32)))\n | >>>\n | >>> list(dataset.take(1))\n | [(<tf.Tensor: shape=(), dtype=int32, numpy=42>,\n | <tf.RaggedTensor [[1, 2], [3]]>)]\n | \n | There is also a deprecated way to call `from_generator` by either with\n | `output_types` argument alone or together with `output_shapes` argument.\n | In this case the output of the function will be assumed to consist of\n | `tf.Tensor` objects with with the types defined by `output_types` and with\n | the shapes which are either unknown or defined by `output_shapes`.\n | \n | Note: The current implementation of `Dataset.from_generator()` uses\n | `tf.numpy_function` and inherits the same constraints. In particular, it\n | requires the dataset and iterator related operations to be placed\n | on a device in the same process as the Python program that called\n | `Dataset.from_generator()`. The body of `generator` will not be\n | serialized in a `GraphDef`, and you should not use this method if you\n | need to serialize your model and restore it in a different environment.\n | \n | Note: If `generator` depends on mutable global variables or other external\n | state, be aware that the runtime may invoke `generator` multiple times\n | (in order to support repeating the `Dataset`) and at any time\n | between the call to `Dataset.from_generator()` and the production of the\n | first element from the generator. Mutating global variables or external\n | state can cause undefined behavior, and we recommend that you explicitly\n | cache any external state in `generator` before calling\n | `Dataset.from_generator()`.\n | \n | Args:\n | generator: A callable object that returns an object that supports the\n | `iter()` protocol. If `args` is not specified, `generator` must take no\n | arguments; otherwise it must take as many arguments as there are values\n | in `args`.\n | output_types: (Optional.) A nested structure of `tf.DType` objects\n | corresponding to each component of an element yielded by `generator`.\n | output_shapes: (Optional.) A nested structure of `tf.TensorShape` objects\n | corresponding to each component of an element yielded by `generator`.\n | args: (Optional.) A tuple of `tf.Tensor` objects that will be evaluated\n | and passed to `generator` as NumPy-array arguments.\n | output_signature: (Optional.) A nested structure of `tf.TypeSpec` objects\n | corresponding to each component of an element yielded by `generator`.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | from_tensor_slices(tensors)\n | Creates a `Dataset` whose elements are slices of the given tensors.\n | \n | The given tensors are sliced along their first dimension. This operation\n | preserves the structure of the input tensors, removing the first dimension\n | of each tensor and using it as the dataset dimension. All input tensors\n | must have the same size in their first dimensions.\n | \n | >>> # Slicing a 1D tensor produces scalar tensor elements.\n | >>> dataset = tf.data.Dataset.from_tensor_slices([1, 2, 3])\n | >>> list(dataset.as_numpy_iterator())\n | [1, 2, 3]\n | \n | >>> # Slicing a 2D tensor produces 1D tensor elements.\n | >>> dataset = tf.data.Dataset.from_tensor_slices([[1, 2], [3, 4]])\n | >>> list(dataset.as_numpy_iterator())\n | [array([1, 2], dtype=int32), array([3, 4], dtype=int32)]\n | \n | >>> # Slicing a tuple of 1D tensors produces tuple elements containing\n | >>> # scalar tensors.\n | >>> dataset = tf.data.Dataset.from_tensor_slices(([1, 2], [3, 4], [5, 6]))\n | >>> list(dataset.as_numpy_iterator())\n | [(1, 3, 5), (2, 4, 6)]\n | \n | >>> # Dictionary structure is also preserved.\n | >>> dataset = tf.data.Dataset.from_tensor_slices({\"a\": [1, 2], \"b\": [3, 4]})\n | >>> list(dataset.as_numpy_iterator()) == [{'a': 1, 'b': 3},\n | ... {'a': 2, 'b': 4}]\n | True\n | \n | >>> # Two tensors can be combined into one Dataset object.\n | >>> features = tf.constant([[1, 3], [2, 1], [3, 3]]) # ==> 3x2 tensor\n | >>> labels = tf.constant(['A', 'B', 'A']) # ==> 3x1 tensor\n | >>> dataset = Dataset.from_tensor_slices((features, labels))\n | >>> # Both the features and the labels tensors can be converted\n | >>> # to a Dataset object separately and combined after.\n | >>> features_dataset = Dataset.from_tensor_slices(features)\n | >>> labels_dataset = Dataset.from_tensor_slices(labels)\n | >>> dataset = Dataset.zip((features_dataset, labels_dataset))\n | >>> # A batched feature and label set can be converted to a Dataset\n | >>> # in similar fashion.\n | >>> batched_features = tf.constant([[[1, 3], [2, 3]],\n | ... [[2, 1], [1, 2]],\n | ... [[3, 3], [3, 2]]], shape=(3, 2, 2))\n | >>> batched_labels = tf.constant([['A', 'A'],\n | ... ['B', 'B'],\n | ... ['A', 'B']], shape=(3, 2, 1))\n | >>> dataset = Dataset.from_tensor_slices((batched_features, batched_labels))\n | >>> for element in dataset.as_numpy_iterator():\n | ... print(element)\n | (array([[1, 3],\n | [2, 3]], dtype=int32), array([[b'A'],\n | [b'A']], dtype=object))\n | (array([[2, 1],\n | [1, 2]], dtype=int32), array([[b'B'],\n | [b'B']], dtype=object))\n | (array([[3, 3],\n | [3, 2]], dtype=int32), array([[b'A'],\n | [b'B']], dtype=object))\n | \n | Note that if `tensors` contains a NumPy array, and eager execution is not\n | enabled, the values will be embedded in the graph as one or more\n | `tf.constant` operations. For large datasets (> 1 GB), this can waste\n | memory and run into byte limits of graph serialization. If `tensors`\n | contains one or more large NumPy arrays, consider the alternative described\n | in [this guide](\n | https://tensorflow.org/guide/data#consuming_numpy_arrays).\n | \n | Args:\n | tensors: A dataset element, with each component having the same size in\n | the first dimension.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | from_tensors(tensors)\n | Creates a `Dataset` with a single element, comprising the given tensors.\n | \n | `from_tensors` produces a dataset containing only a single element. To slice\n | the input tensor into multiple elements, use `from_tensor_slices` instead.\n | \n | >>> dataset = tf.data.Dataset.from_tensors([1, 2, 3])\n | >>> list(dataset.as_numpy_iterator())\n | [array([1, 2, 3], dtype=int32)]\n | >>> dataset = tf.data.Dataset.from_tensors(([1, 2, 3], 'A'))\n | >>> list(dataset.as_numpy_iterator())\n | [(array([1, 2, 3], dtype=int32), b'A')]\n | \n | >>> # You can use `from_tensors` to produce a dataset which repeats\n | >>> # the same example many times.\n | >>> example = tf.constant([1,2,3])\n | >>> dataset = tf.data.Dataset.from_tensors(example).repeat(2)\n | >>> list(dataset.as_numpy_iterator())\n | [array([1, 2, 3], dtype=int32), array([1, 2, 3], dtype=int32)]\n | \n | Note that if `tensors` contains a NumPy array, and eager execution is not\n | enabled, the values will be embedded in the graph as one or more\n | `tf.constant` operations. For large datasets (> 1 GB), this can waste\n | memory and run into byte limits of graph serialization. If `tensors`\n | contains one or more large NumPy arrays, consider the alternative described\n | in [this\n | guide](https://tensorflow.org/guide/data#consuming_numpy_arrays).\n | \n | Args:\n | tensors: A dataset element.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | list_files(file_pattern, shuffle=None, seed=None)\n | A dataset of all files matching one or more glob patterns.\n | \n | The `file_pattern` argument should be a small number of glob patterns.\n | If your filenames have already been globbed, use\n | `Dataset.from_tensor_slices(filenames)` instead, as re-globbing every\n | filename with `list_files` may result in poor performance with remote\n | storage systems.\n | \n | Note: The default behavior of this method is to return filenames in\n | a non-deterministic random shuffled order. Pass a `seed` or `shuffle=False`\n | to get results in a deterministic order.\n | \n | Example:\n | If we had the following files on our filesystem:\n | \n | - /path/to/dir/a.txt\n | - /path/to/dir/b.py\n | - /path/to/dir/c.py\n | \n | If we pass \"/path/to/dir/*.py\" as the directory, the dataset\n | would produce:\n | \n | - /path/to/dir/b.py\n | - /path/to/dir/c.py\n | \n | Args:\n | file_pattern: A string, a list of strings, or a `tf.Tensor` of string type\n | (scalar or vector), representing the filename glob (i.e. shell wildcard)\n | pattern(s) that will be matched.\n | shuffle: (Optional.) If `True`, the file names will be shuffled randomly.\n | Defaults to `True`.\n | seed: (Optional.) A `tf.int64` scalar `tf.Tensor`, representing the random\n | seed that will be used to create the distribution. See\n | `tf.random.set_seed` for behavior.\n | \n | Returns:\n | Dataset: A `Dataset` of strings corresponding to file names.\n | \n | range(*args, **kwargs)\n | Creates a `Dataset` of a step-separated range of values.\n | \n | >>> list(Dataset.range(5).as_numpy_iterator())\n | [0, 1, 2, 3, 4]\n | >>> list(Dataset.range(2, 5).as_numpy_iterator())\n | [2, 3, 4]\n | >>> list(Dataset.range(1, 5, 2).as_numpy_iterator())\n | [1, 3]\n | >>> list(Dataset.range(1, 5, -2).as_numpy_iterator())\n | []\n | >>> list(Dataset.range(5, 1).as_numpy_iterator())\n | []\n | >>> list(Dataset.range(5, 1, -2).as_numpy_iterator())\n | [5, 3]\n | >>> list(Dataset.range(2, 5, output_type=tf.int32).as_numpy_iterator())\n | [2, 3, 4]\n | >>> list(Dataset.range(1, 5, 2, output_type=tf.float32).as_numpy_iterator())\n | [1.0, 3.0]\n | \n | Args:\n | *args: follows the same semantics as python's xrange.\n | len(args) == 1 -> start = 0, stop = args[0], step = 1.\n | len(args) == 2 -> start = args[0], stop = args[1], step = 1.\n | len(args) == 3 -> start = args[0], stop = args[1], step = args[2].\n | **kwargs:\n | - output_type: Its expected dtype. (Optional, default: `tf.int64`).\n | \n | Returns:\n | Dataset: A `RangeDataset`.\n | \n | Raises:\n | ValueError: if len(args) == 0.\n | \n | zip(datasets)\n | Creates a `Dataset` by zipping together the given datasets.\n | \n | This method has similar semantics to the built-in `zip()` function\n | in Python, with the main difference being that the `datasets`\n | argument can be an arbitrary nested structure of `Dataset` objects.\n | \n | >>> # The nested structure of the `datasets` argument determines the\n | >>> # structure of elements in the resulting dataset.\n | >>> a = tf.data.Dataset.range(1, 4) # ==> [ 1, 2, 3 ]\n | >>> b = tf.data.Dataset.range(4, 7) # ==> [ 4, 5, 6 ]\n | >>> ds = tf.data.Dataset.zip((a, b))\n | >>> list(ds.as_numpy_iterator())\n | [(1, 4), (2, 5), (3, 6)]\n | >>> ds = tf.data.Dataset.zip((b, a))\n | >>> list(ds.as_numpy_iterator())\n | [(4, 1), (5, 2), (6, 3)]\n | >>>\n | >>> # The `datasets` argument may contain an arbitrary number of datasets.\n | >>> c = tf.data.Dataset.range(7, 13).batch(2) # ==> [ [7, 8],\n | ... # [9, 10],\n | ... # [11, 12] ]\n | >>> ds = tf.data.Dataset.zip((a, b, c))\n | >>> for element in ds.as_numpy_iterator():\n | ... print(element)\n | (1, 4, array([7, 8]))\n | (2, 5, array([ 9, 10]))\n | (3, 6, array([11, 12]))\n | >>>\n | >>> # The number of elements in the resulting dataset is the same as\n | >>> # the size of the smallest dataset in `datasets`.\n | >>> d = tf.data.Dataset.range(13, 15) # ==> [ 13, 14 ]\n | >>> ds = tf.data.Dataset.zip((a, d))\n | >>> list(ds.as_numpy_iterator())\n | [(1, 13), (2, 14)]\n | \n | Args:\n | datasets: A nested structure of datasets.\n | \n | Returns:\n | Dataset: A `Dataset`.\n | \n | ----------------------------------------------------------------------\n | Data descriptors inherited from DatasetV2:\n | \n | __dict__\n | dictionary for instance variables (if defined)\n | \n | __weakref__\n | list of weak references to the object (if defined)\n | \n | ----------------------------------------------------------------------\n | Class methods inherited from collections.abc.Iterable:\n | \n | __subclasshook__(C) from abc.ABCMeta\n | Abstract classes can override this to customize issubclass().\n | \n | This is invoked early on by abc.ABCMeta.__subclasscheck__().\n | It should return True, False or NotImplemented. If it returns\n | NotImplemented, the normal algorithm is used. Otherwise, it\n | overrides the normal algorithm (and the outcome is cached).\n\n"
],
[
"# necessary imports\nimport tensorflow as tf\nimport numpy as np\nimport matplotlib.pyplot as plt\n# import tensorflow_datasets as tfds\nfrom functools import partial\nfrom albumentations import (\n Compose, RandomBrightness, JpegCompression, HueSaturationValue, RandomContrast, HorizontalFlip,\n Rotate\n)\nAUTOTUNE = tf.data.experimental.AUTOTUNE",
"_____no_output_____"
],
[
"s3 = boto3.resource('s3')\n# TODO test on entire test dataset\nobj = s3.Object('canopy-production-ml', \"chips/cloudfree-merge-polygons/split/test/100/100_1000_1000.tif\")\nobj_bytes = io.BytesIO(obj.get()['Body'].read())\nwith rasterio.open(obj_bytes) as src:\n img_test = np.transpose(src.read(), (1, 2, 0))\nprint(img_test.shape)",
"(100, 100, 18)\n"
],
[
"train_img = tf.image.convert_image_dtype(img_test,tf.float32)",
"_____no_output_____"
],
[
"train_img = tf.image.random_flip_left_right(train_img)",
"_____no_output_____"
],
[
"help(tf.image)",
"Help on package tensorflow._api.v2.image in tensorflow._api.v2:\n\nNAME\n tensorflow._api.v2.image - Image ops.\n\nDESCRIPTION\n The `tf.image` module contains various functions for image\n processing and decoding-encoding Ops.\n \n Many of the encoding/decoding functions are also available in the\n core `tf.io` module.\n \n ## Image processing\n \n ### Resizing\n \n The resizing Ops accept input images as tensors of several types. They always\n output resized images as float32 tensors.\n \n The convenience function `tf.image.resize` supports both 4-D\n and 3-D tensors as input and output. 4-D tensors are for batches of images,\n 3-D tensors for individual images.\n \n Resized images will be distorted if their original aspect ratio is not the\n same as size. To avoid distortions see tf.image.resize_with_pad.\n \n * `tf.image.resize`\n * `tf.image.resize_with_pad`\n * `tf.image.resize_with_crop_or_pad`\n \n The Class `tf.image.ResizeMethod` provides various resize methods like\n `bilinear`, `nearest_neighbor`.\n \n ### Converting Between Colorspaces\n \n Image ops work either on individual images or on batches of images, depending on\n the shape of their input Tensor.\n \n If 3-D, the shape is `[height, width, channels]`, and the Tensor represents one\n image. If 4-D, the shape is `[batch_size, height, width, channels]`, and the\n Tensor represents `batch_size` images.\n \n Currently, `channels` can usefully be 1, 2, 3, or 4. Single-channel images are\n grayscale, images with 3 channels are encoded as either RGB or HSV. Images\n with 2 or 4 channels include an alpha channel, which has to be stripped from the\n image before passing the image to most image processing functions (and can be\n re-attached later).\n \n Internally, images are either stored in as one `float32` per channel per pixel\n (implicitly, values are assumed to lie in `[0,1)`) or one `uint8` per channel\n per pixel (values are assumed to lie in `[0,255]`).\n \n TensorFlow can convert between images in RGB or HSV or YIQ.\n \n * `tf.image.rgb_to_grayscale`, `tf.image.grayscale_to_rgb`\n * `tf.image.rgb_to_hsv`, `tf.image.hsv_to_rgb`\n * `tf.image.rgb_to_yiq`, `tf.image.yiq_to_rgb`\n * `tf.image.rgb_to_yuv`, `tf.image.yuv_to_rgb`\n * `tf.image.image_gradients`\n * `tf.image.convert_image_dtype`\n \n ### Image Adjustments\n \n TensorFlow provides functions to adjust images in various ways: brightness,\n contrast, hue, and saturation. Each adjustment can be done with predefined\n parameters or with random parameters picked from predefined intervals. Random\n adjustments are often useful to expand a training set and reduce overfitting.\n \n If several adjustments are chained it is advisable to minimize the number of\n redundant conversions by first converting the images to the most natural data\n type and representation.\n \n * `tf.image.adjust_brightness`\n * `tf.image.adjust_contrast`\n * `tf.image.adjust_gamma`\n * `tf.image.adjust_hue`\n * `tf.image.adjust_jpeg_quality`\n * `tf.image.adjust_saturation`\n * `tf.image.random_brightness`\n * `tf.image.random_contrast`\n * `tf.image.random_hue`\n * `tf.image.random_saturation`\n * `tf.image.per_image_standardization`\n \n ### Working with Bounding Boxes\n \n * `tf.image.draw_bounding_boxes`\n * `tf.image.combined_non_max_suppression`\n * `tf.image.generate_bounding_box_proposals`\n * `tf.image.non_max_suppression`\n * `tf.image.non_max_suppression_overlaps`\n * `tf.image.non_max_suppression_padded`\n * `tf.image.non_max_suppression_with_scores`\n * `tf.image.pad_to_bounding_box`\n * `tf.image.sample_distorted_bounding_box`\n \n ### Cropping\n \n * `tf.image.central_crop`\n * `tf.image.crop_and_resize`\n * `tf.image.crop_to_bounding_box`\n * `tf.io.decode_and_crop_jpeg`\n * `tf.image.extract_glimpse`\n * `tf.image.random_crop`\n * `tf.image.resize_with_crop_or_pad`\n \n ### Flipping, Rotating and Transposing\n \n * `tf.image.flip_left_right`\n * `tf.image.flip_up_down`\n * `tf.image.random_flip_left_right`\n * `tf.image.random_flip_up_down`\n * `tf.image.rot90`\n * `tf.image.transpose`\n \n ## Image decoding and encoding\n \n TensorFlow provides Ops to decode and encode JPEG and PNG formats. Encoded\n images are represented by scalar string Tensors, decoded images by 3-D uint8\n tensors of shape `[height, width, channels]`. (PNG also supports uint16.)\n \n Note: `decode_gif` returns a 4-D array `[num_frames, height, width, 3]`\n \n The encode and decode Ops apply to one image at a time. Their input and output\n are all of variable size. If you need fixed size images, pass the output of\n the decode Ops to one of the cropping and resizing Ops.\n \n * `tf.io.decode_bmp`\n * `tf.io.decode_gif`\n * `tf.io.decode_image`\n * `tf.io.decode_jpeg`\n * `tf.io.decode_and_crop_jpeg`\n * `tf.io.decode_png`\n * `tf.io.encode_jpeg`\n * `tf.io.encode_png`\n\nPACKAGE CONTENTS\n\n\nFILE\n /Users/purgatorid/opt/anaconda3/envs/ml-conda/lib/python3.7/site-packages/tensorflow/_api/v2/image/__init__.py\n\n\n"
]
],
[
[
"## David's area",
"_____no_output_____"
]
],
[
[
"import boto3\nimport rasterio as rio\nimport numpy as np\nimport io\nimport tensorflow as tf\nfrom tensorflow.keras.layers import Input, Conv2D, GlobalAveragePooling2D, Dense\nfrom tensorflow.keras.models import Model\nimport keras\n\nfrom data_loader import DataLoader",
"_____no_output_____"
],
[
"batch_size = 20\ngen = DataLoader(label_file_path_train=\"labels_1_4_train_v2.csv\",\n label_file_path_val=\"val_labels.csv\",\n bucket_name='canopy-production-ml',\n data_extension_type='.tif',\n training_data_shape=(100, 100, 18),\n augment=True,\n random_flip_up_down=False, #Randomly flips an image vertically (upside down). With a 1 in 2 chance, outputs the contents of `image` flipped along the first dimension, which is `height`.\n random_flip_left_right=False,\n flip_left_right=True,\n flip_up_down=True,\n rot90=False,\n transpose=False,\n enable_shuffle=True,\n # training_data_shuffle_buffer_size=10,\n training_data_batch_size=batch_size,\n training_data_type=tf.float32,\n label_data_type=tf.uint8,\n enable_data_prefetch=True,\n data_prefetch_size=tf.data.experimental.AUTOTUNE,\n num_parallel_calls=int(2))",
"Data augmentation enabled \nTraining on 7886 images \nValidation on 366 images \nYour training file is missing positive labels for classes ['3']\n"
],
[
"gen.class_weight",
"_____no_output_____"
],
[
"def define_model(numclasses,input_shape):\n # parameters for CNN\n input_tensor = Input(shape=input_shape)\n\n # introduce a additional layer to get from bands to 3 input channels\n input_tensor = Conv2D(3, (1, 1))(input_tensor)\n\n base_model_resnet50 = keras.applications.ResNet50(include_top=False,\n weights='imagenet',\n input_shape=(100, 100, 3))\n base_model = keras.applications.ResNet50(include_top=False,\n weights=None,\n input_tensor=input_tensor)\n\n for i, layer in enumerate(base_model_resnet50.layers):\n # we must skip input layer, which has no weights\n if i == 0:\n continue\n base_model.layers[i+1].set_weights(layer.get_weights())\n\n # add a global spatial average pooling layer\n top_model = base_model.output\n top_model = GlobalAveragePooling2D()(top_model)\n\n # let's add a fully-connected layer\n top_model = Dense(2048, activation='relu')(top_model)\n top_model = Dense(2048, activation='relu')(top_model)\n # and a logistic layer\n predictions = Dense(numclasses, activation='softmax')(top_model)\n\n # this is the model we will train\n model = Model(inputs=base_model.input, outputs=predictions)\n\n model.summary()\n return model",
"_____no_output_____"
],
[
"import wandb\nfrom wandb.keras import WandbCallback\n\n\nwandb.init(project=\"canopy-first-model-testing\", name=\"baseline\")",
"Failed to query for notebook name, you can set it manually with the WANDB_NOTEBOOK_NAME environment variable\nwandb: Currently logged in as: davidanagy (use `wandb login --relogin` to force relogin)\nC:\\Anaconda3\\envs\\canopy_test_models\\lib\\site-packages\\IPython\\html.py:14: ShimWarning: The `IPython.html` package has been deprecated since IPython 4.0. You should import from `notebook` instead. `IPython.html.widgets` has moved to `ipywidgets`.\n \"`IPython.html.widgets` has moved to `ipywidgets`.\", ShimWarning)\nwandb: wandb version 0.10.21 is available! To upgrade, please run:\nwandb: $ pip install wandb --upgrade\n"
],
[
"help(wandb.init)",
"Help on function init in module wandb.sdk.wandb_init:\n\ninit(job_type: Union[str, NoneType] = None, dir=None, config: Union[Dict, str, NoneType] = None, project: Union[str, NoneType] = None, entity: Union[str, NoneType] = None, reinit: bool = None, tags: Union[Sequence, NoneType] = None, group: Union[str, NoneType] = None, name: Union[str, NoneType] = None, notes: Union[str, NoneType] = None, magic: Union[dict, str, bool] = None, config_exclude_keys=None, config_include_keys=None, anonymous: Union[str, NoneType] = None, mode: Union[str, NoneType] = None, allow_val_change: Union[bool, NoneType] = None, resume: Union[bool, str, NoneType] = None, force: Union[bool, NoneType] = None, tensorboard=None, sync_tensorboard=None, monitor_gym=None, save_code=None, id=None, settings: Union[wandb.sdk.wandb_settings.Settings, Dict[str, Any], NoneType] = None) -> Union[wandb.sdk.wandb_run.Run, wandb.sdk.lib.disabled.RunDisabled, NoneType]\n Start a new tracked run with `wandb.init()`.\n \n In an ML training pipeline, you could add `wandb.init()`\n to the beginning of your training script as well as your evaluation\n script, and each piece would be tracked as a run in W&B.\n \n `wandb.init()` spawns a new background process to log data to a run, and it\n also syncs data to wandb.ai by default so you can see live visualizations.\n Call `wandb.init()` to start a run before logging data with `wandb.log()`.\n \n `wandb.init()` returns a run object, and you can also access the run object\n with wandb.run.\n \n Arguments:\n project: (str, optional) The name of the project where you're sending\n the new run. If the project is not specified, the run is put in an\n \"Uncategorized\" project.\n entity: (str, optional) An entity is a username or team name where\n you're sending runs. This entity must exist before you can send runs\n there, so make sure to create your account or team in the UI before\n starting to log runs.\n If you don't specify an entity, the run will be sent to your default\n entity, which is usually your username. Change your default entity\n in [Settings](wandb.ai/settings) under \"default location to create\n new projects\".\n config: (dict, argparse, absl.flags, str, optional)\n This sets wandb.config, a dictionary-like object for saving inputs\n to your job, like hyperparameters for a model or settings for a data\n preprocessing job. The config will show up in a table in the UI that\n you can use to group, filter, and sort runs. Keys should not contain\n `.` in their names, and values should be under 10 MB.\n If dict, argparse or absl.flags: will load the key value pairs into\n the wandb.config object.\n If str: will look for a yaml file by that name, and load config from\n that file into the wandb.config object.\n save_code: (bool, optional) Turn this on to save the main script or\n notebook to W&B. This is valuable for improving experiment\n reproducibility and to diff code across experiments in the UI. By\n default this is off, but you can flip the default behavior to \"on\"\n in [Settings](wandb.ai/settings).\n group: (str, optional) Specify a group to organize individual runs into\n a larger experiment. For example, you might be doing cross\n validation, or you might have multiple jobs that train and evaluate\n a model against different test sets. Group gives you a way to\n organize runs together into a larger whole, and you can toggle this\n on and off in the UI. For more details, see\n [Grouping](docs.wandb.com/library/grouping).\n job_type: (str, optional) Specify the type of run, which is useful when\n you're grouping runs together into larger experiments using group.\n For example, you might have multiple jobs in a group, with job types\n like train and eval. Setting this makes it easy to filter and group\n similar runs together in the UI so you can compare apples to apples.\n tags: (list, optional) A list of strings, which will populate the list\n of tags on this run in the UI. Tags are useful for organizing runs\n together, or applying temporary labels like \"baseline\" or\n \"production\". It's easy to add and remove tags in the UI, or filter\n down to just runs with a specific tag.\n name: (str, optional) A short display name for this run, which is how\n you'll identify this run in the UI. By default we generate a random\n two-word name that lets you easily cross-reference runs from the\n table to charts. Keeping these run names short makes the chart\n legends and tables easier to read. If you're looking for a place to\n save your hyperparameters, we recommend saving those in config.\n notes: (str, optional) A longer description of the run, like a -m commit\n message in git. This helps you remember what you were doing when you\n ran this run.\n dir: (str, optional) An absolute path to a directory where metadata will\n be stored. When you call download() on an artifact, this is the\n directory where downloaded files will be saved. By default this is\n the ./wandb directory.\n sync_tensorboard: (bool, optional) Whether to copy all TensorBoard logs\n to W&B (default: False).\n [Tensorboard](https://docs.wandb.com/integrations/tensorboard)\n resume (bool, str, optional): Sets the resuming behavior. Options:\n \"allow\", \"must\", \"never\", \"auto\" or None. Defaults to None.\n Cases:\n - None (default): If the new run has the same ID as a previous run,\n this run overwrites that data.\n - \"auto\" (or True): if the preivous run on this machine crashed,\n automatically resume it. Otherwise, start a new run.\n - \"allow\": if id is set with init(id=\"UNIQUE_ID\") or\n WANDB_RUN_ID=\"UNIQUE_ID\" and it is identical to a previous run,\n wandb will automatically resume the run with that id. Otherwise,\n wandb will start a new run.\n - \"never\": if id is set with init(id=\"UNIQUE_ID\") or\n WANDB_RUN_ID=\"UNIQUE_ID\" and it is identical to a previous run,\n wandb will crash.\n - \"must\": if id is set with init(id=\"UNIQUE_ID\") or\n WANDB_RUN_ID=\"UNIQUE_ID\" and it is identical to a previous run,\n wandb will automatically resume the run with the id. Otherwise\n wandb will crash.\n See https://docs.wandb.com/library/advanced/resuming for more.\n reinit: (bool, optional) Allow multiple wandb.init() calls in the same\n process. (default: False)\n magic: (bool, dict, or str, optional) The bool controls whether we try to\n auto-instrument your script, capturing basic details of your run\n without you having to add more wandb code. (default: False)\n You can also pass a dict, json string, or yaml filename.\n config_exclude_keys: (list, optional) string keys to exclude from\n `wandb.config`.\n config_include_keys: (list, optional) string keys to include in\n wandb.config.\n anonymous: (str, optional) Controls anonymous data logging. Options:\n - \"never\" (default): requires you to link your W&B account before\n tracking the run so you don't accidentally create an anonymous\n run.\n - \"allow\": lets a logged-in user track runs with their account, but\n lets someone who is running the script without a W&B account see\n the charts in the UI.\n - \"must\": sends the run to an anonymous account instead of to a\n signed-up user account.\n mode: (str, optional) Can be \"online\", \"offline\" or \"disabled\". Defaults to\n online.\n allow_val_change: (bool, optional) Whether to allow config values to\n change after setting the keys once. By default we throw an exception\n if a config value is overwritten. If you want to track something\n like a varying learning_rate at multiple times during training, use\n wandb.log() instead. (default: False in scripts, True in Jupyter)\n force: (bool, optional) If True, this crashes the script if a user isn't\n logged in to W&B. If False, this will let the script run in offline\n mode if a user isn't logged in to W&B. (default: False)\n sync_tensorboard: (bool, optional) Synchronize wandb logs from tensorboard or\n tensorboardX and saves the relevant events file. Defaults to false.\n monitor_gym: (bool, optional) automatically logs videos of environment when\n using OpenAI Gym. (default: False)\n See https://docs.wandb.com/library/integrations/openai-gym\n id: (str, optional) A unique ID for this run, used for Resuming. It must\n be unique in the project, and if you delete a run you can't reuse\n the ID. Use the name field for a short descriptive name, or config\n for saving hyperparameters to compare across runs. The ID cannot\n contain special characters.\n See https://docs.wandb.com/library/resuming\n \n \n Examples:\n Basic usage\n ```\n wandb.init()\n ```\n \n Launch multiple runs from the same script\n ```\n for x in range(10):\n with wandb.init(project=\"my-projo\") as run:\n for y in range(100):\n run.log({\"metric\": x+y})\n ```\n \n Raises:\n Exception: if problem.\n \n Returns:\n A `Run` object.\n\n"
],
[
"help(WandbCallback)",
"Help on class WandbCallback in module wandb.integration.keras.keras:\n\nclass WandbCallback(tensorflow.python.keras.callbacks.Callback)\n | WandbCallback(monitor='val_loss', verbose=0, mode='auto', save_weights_only=False, log_weights=False, log_gradients=False, save_model=True, training_data=None, validation_data=None, labels=[], data_type=None, predictions=36, generator=None, input_type=None, output_type=None, log_evaluation=False, validation_steps=None, class_colors=None, log_batch_frequency=None, log_best_prefix='best_', save_graph=True)\n | \n | WandbCallback automatically integrates keras with wandb.\n | \n | Example:\n | ```\n | model.fit(X_train, y_train, validation_data=(X_test, y_test),\n | callbacks=[WandbCallback()])\n | ```\n | \n | WandbCallback will automatically log history data from any\n | metrics collected by keras: loss and anything passed into keras_model.compile() \n | \n | WandbCallback will set summary metrics for the run associated with the \"best\" training\n | step, where \"best\" is defined by the `monitor` and `mode` attribues. This defaults\n | to the epoch with the minimum val_loss. WandbCallback will by default save the model \n | associated with the best epoch..\n | \n | WandbCallback can optionally log gradient and parameter histograms. \n | \n | WandbCallback can optionally save training and validation data for wandb to visualize.\n | \n | Arguments:\n | monitor (str): name of metric to monitor. Defaults to val_loss.\n | mode (str): one of {\"auto\", \"min\", \"max\"}.\n | \"min\" - save model when monitor is minimized\n | \"max\" - save model when monitor is maximized\n | \"auto\" - try to guess when to save the model (default).\n | save_model:\n | True - save a model when monitor beats all previous epochs\n | False - don't save models\n | save_graph: (boolean): if True save model graph to wandb (default: True).\n | save_weights_only (boolean): if True, then only the model's weights will be\n | saved (`model.save_weights(filepath)`), else the full model\n | is saved (`model.save(filepath)`).\n | log_weights: (boolean) if True save histograms of the model's layer's weights.\n | log_gradients: (boolean) if True log histograms of the training gradients\n | training_data: (tuple) Same format (X,y) as passed to model.fit. This is needed \n | for calculating gradients - this is mandatory if `log_gradients` is `True`.\n | validate_data: (tuple) Same format (X,y) as passed to model.fit. A set of data \n | for wandb to visualize. If this is set, every epoch, wandb will\n | make a small number of predictions and save the results for later visualization.\n | generator (generator): a generator that returns validation data for wandb to visualize. This\n | generator should return tuples (X,y). Either validate_data or generator should\n | be set for wandb to visualize specific data examples.\n | validation_steps (int): if `validation_data` is a generator, how many\n | steps to run the generator for the full validation set.\n | labels (list): If you are visualizing your data with wandb this list of labels \n | will convert numeric output to understandable string if you are building a\n | multiclass classifier. If you are making a binary classifier you can pass in\n | a list of two labels [\"label for false\", \"label for true\"]. If validate_data\n | and generator are both false, this won't do anything.\n | predictions (int): the number of predictions to make for visualization each epoch, max \n | is 100.\n | input_type (string): type of the model input to help visualization. can be one of:\n | (\"image\", \"images\", \"segmentation_mask\").\n | output_type (string): type of the model output to help visualziation. can be one of:\n | (\"image\", \"images\", \"segmentation_mask\"). \n | log_evaluation (boolean): if True save a dataframe containing the full\n | validation results at the end of training.\n | class_colors ([float, float, float]): if the input or output is a segmentation mask, \n | an array containing an rgb tuple (range 0-1) for each class.\n | log_batch_frequency (integer): if None, callback will log every epoch.\n | If set to integer, callback will log training metrics every log_batch_frequency \n | batches.\n | log_best_prefix (string): if None, no extra summary metrics will be saved.\n | If set to a string, the monitored metric and epoch will be prepended with this value\n | and stored as summary metrics.\n | \n | Method resolution order:\n | WandbCallback\n | tensorflow.python.keras.callbacks.Callback\n | builtins.object\n | \n | Methods defined here:\n | \n | __init__(self, monitor='val_loss', verbose=0, mode='auto', save_weights_only=False, log_weights=False, log_gradients=False, save_model=True, training_data=None, validation_data=None, labels=[], data_type=None, predictions=36, generator=None, input_type=None, output_type=None, log_evaluation=False, validation_steps=None, class_colors=None, log_batch_frequency=None, log_best_prefix='best_', save_graph=True)\n | Initialize self. See help(type(self)) for accurate signature.\n | \n | on_batch_begin(self, batch, logs=None)\n | A backwards compatibility alias for `on_train_batch_begin`.\n | \n | on_batch_end(self, batch, logs=None)\n | A backwards compatibility alias for `on_train_batch_end`.\n | \n | on_epoch_end(self, epoch, logs={})\n | Called at the end of an epoch.\n | \n | Subclasses should override for any actions to run. This function should only\n | be called during TRAIN mode.\n | \n | Arguments:\n | epoch: Integer, index of epoch.\n | logs: Dict, metric results for this training epoch, and for the\n | validation epoch if validation is performed. Validation result keys\n | are prefixed with `val_`.\n | \n | on_predict_batch_begin(self, batch, logs=None)\n | Called at the beginning of a batch in `predict` methods.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | batch: Integer, index of batch within the current epoch.\n | logs: Dict, contains the return value of `model.predict_step`,\n | it typically returns a dict with a key 'outputs' containing\n | the model's outputs.\n | \n | on_predict_batch_end(self, batch, logs=None)\n | Called at the end of a batch in `predict` methods.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | batch: Integer, index of batch within the current epoch.\n | logs: Dict. Aggregated metric results up until this batch.\n | \n | on_predict_begin(self, logs=None)\n | Called at the beginning of prediction.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | logs: Dict. Currently no data is passed to this argument for this method\n | but that may change in the future.\n | \n | on_predict_end(self, logs=None)\n | Called at the end of prediction.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | logs: Dict. Currently no data is passed to this argument for this method\n | but that may change in the future.\n | \n | on_test_batch_begin(self, batch, logs=None)\n | Called at the beginning of a batch in `evaluate` methods.\n | \n | Also called at the beginning of a validation batch in the `fit`\n | methods, if validation data is provided.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | batch: Integer, index of batch within the current epoch.\n | logs: Dict, contains the return value of `model.test_step`. Typically,\n | the values of the `Model`'s metrics are returned. Example:\n | `{'loss': 0.2, 'accuracy': 0.7}`.\n | \n | on_test_batch_end(self, batch, logs=None)\n | Called at the end of a batch in `evaluate` methods.\n | \n | Also called at the end of a validation batch in the `fit`\n | methods, if validation data is provided.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | batch: Integer, index of batch within the current epoch.\n | logs: Dict. Aggregated metric results up until this batch.\n | \n | on_test_begin(self, logs=None)\n | Called at the beginning of evaluation or validation.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | logs: Dict. Currently no data is passed to this argument for this method\n | but that may change in the future.\n | \n | on_test_end(self, logs=None)\n | Called at the end of evaluation or validation.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | logs: Dict. Currently the output of the last call to\n | `on_test_batch_end()` is passed to this argument for this method\n | but that may change in the future.\n | \n | on_train_batch_begin(self, batch, logs=None)\n | Called at the beginning of a training batch in `fit` methods.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | batch: Integer, index of batch within the current epoch.\n | logs: Dict, contains the return value of `model.train_step`. Typically,\n | the values of the `Model`'s metrics are returned. Example:\n | `{'loss': 0.2, 'accuracy': 0.7}`.\n | \n | on_train_batch_end(self, batch, logs=None)\n | Called at the end of a training batch in `fit` methods.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | batch: Integer, index of batch within the current epoch.\n | logs: Dict. Aggregated metric results up until this batch.\n | \n | on_train_begin(self, logs=None)\n | Called at the beginning of training.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | logs: Dict. Currently no data is passed to this argument for this method\n | but that may change in the future.\n | \n | on_train_end(self, logs=None)\n | Called at the end of training.\n | \n | Subclasses should override for any actions to run.\n | \n | Arguments:\n | logs: Dict. Currently the output of the last call to `on_epoch_end()`\n | is passed to this argument for this method but that may change in\n | the future.\n | \n | set_model(self, model)\n | \n | set_params(self, params)\n | \n | ----------------------------------------------------------------------\n | Methods inherited from tensorflow.python.keras.callbacks.Callback:\n | \n | on_epoch_begin(self, epoch, logs=None)\n | Called at the start of an epoch.\n | \n | Subclasses should override for any actions to run. This function should only\n | be called during TRAIN mode.\n | \n | Arguments:\n | epoch: Integer, index of epoch.\n | logs: Dict. Currently no data is passed to this argument for this method\n | but that may change in the future.\n | \n | ----------------------------------------------------------------------\n | Data descriptors inherited from tensorflow.python.keras.callbacks.Callback:\n | \n | __dict__\n | dictionary for instance variables (if defined)\n | \n | __weakref__\n | list of weak references to the object (if defined)\n\n"
],
[
"random_id = 5555 #TODO\ncheckpoint_file = 'checkpoint_{}.h5'.format(random_id)\n\nmodel_checkpoint_callback = tf.keras.callbacks.ModelCheckpoint(\n filepath= checkpoint_file,\n format='h5',\n verbose=1,\n save_weights_only=True,\n monitor='val_loss',\n mode='min',\n save_best_only=True)\n\nreducelronplateau = tf.keras.callbacks.ReduceLROnPlateau(\n monitor='val_loss', factor=0.1, patience=10, verbose=1,\n mode='min', min_lr=1e-10)\n\nearly_stop = tf.keras.callbacks.EarlyStopping(monitor='val_loss',mode='min', patience=20, verbose=1)\n\n#labels = [\"Habitation\", \"ISL\", \"Industrial_agriculture\", \"Mining\", \"Rainforest\",\n# \"River\", \"Roads\", \"Savannah\", \"Shifting_cultivation\", \"Water\"] # Cut out mining b/c not in training data\n\ncallbacks_list = [model_checkpoint_callback, reducelronplateau, early_stop]\n #WandbCallback(monitor='accuracy', data_type=\"image\", labels=labels)]\n\nmodel = define_model(10, (100,100,18))\nmodel.compile(loss=tf.keras.losses.BinaryCrossentropy(from_logits=True),\n optimizer=keras.optimizers.Adam(),\n metrics=[tf.metrics.BinaryAccuracy(name='accuracy')]) #TODO add callbacks to save checkpoints and maybe lr reducer, earlystop,etc \n",
"Model: \"functional_1\"\n__________________________________________________________________________________________________\nLayer (type) Output Shape Param # Connected to \n==================================================================================================\ninput_1 (InputLayer) [(None, 100, 100, 18 0 \n__________________________________________________________________________________________________\nconv2d (Conv2D) (None, 100, 100, 3) 57 input_1[0][0] \n__________________________________________________________________________________________________\nconv1_pad (ZeroPadding2D) (None, 106, 106, 3) 0 conv2d[0][0] \n__________________________________________________________________________________________________\nconv1_conv (Conv2D) (None, 50, 50, 64) 9472 conv1_pad[0][0] \n__________________________________________________________________________________________________\nconv1_bn (BatchNormalization) (None, 50, 50, 64) 256 conv1_conv[0][0] \n__________________________________________________________________________________________________\nconv1_relu (Activation) (None, 50, 50, 64) 0 conv1_bn[0][0] \n__________________________________________________________________________________________________\npool1_pad (ZeroPadding2D) (None, 52, 52, 64) 0 conv1_relu[0][0] \n__________________________________________________________________________________________________\npool1_pool (MaxPooling2D) (None, 25, 25, 64) 0 pool1_pad[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_conv (Conv2D) (None, 25, 25, 64) 4160 pool1_pool[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_relu (Activation (None, 25, 25, 64) 0 conv2_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_relu (Activation (None, 25, 25, 64) 0 conv2_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_0_conv (Conv2D) (None, 25, 25, 256) 16640 pool1_pool[0][0] \n__________________________________________________________________________________________________\nconv2_block1_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block1_0_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_add (Add) (None, 25, 25, 256) 0 conv2_block1_0_bn[0][0] \n conv2_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_out (Activation) (None, 25, 25, 256) 0 conv2_block1_add[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_conv (Conv2D) (None, 25, 25, 64) 16448 conv2_block1_out[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_relu (Activation (None, 25, 25, 64) 0 conv2_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_relu (Activation (None, 25, 25, 64) 0 conv2_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block2_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_add (Add) (None, 25, 25, 256) 0 conv2_block1_out[0][0] \n conv2_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_out (Activation) (None, 25, 25, 256) 0 conv2_block2_add[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_conv (Conv2D) (None, 25, 25, 64) 16448 conv2_block2_out[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_relu (Activation (None, 25, 25, 64) 0 conv2_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_relu (Activation (None, 25, 25, 64) 0 conv2_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block3_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_add (Add) (None, 25, 25, 256) 0 conv2_block2_out[0][0] \n conv2_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_out (Activation) (None, 25, 25, 256) 0 conv2_block3_add[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_conv (Conv2D) (None, 13, 13, 128) 32896 conv2_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_relu (Activation (None, 13, 13, 128) 0 conv3_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_relu (Activation (None, 13, 13, 128) 0 conv3_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_0_conv (Conv2D) (None, 13, 13, 512) 131584 conv2_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block1_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block1_0_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_add (Add) (None, 13, 13, 512) 0 conv3_block1_0_bn[0][0] \n conv3_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_out (Activation) (None, 13, 13, 512) 0 conv3_block1_add[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block1_out[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_relu (Activation (None, 13, 13, 128) 0 conv3_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_relu (Activation (None, 13, 13, 128) 0 conv3_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block2_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_add (Add) (None, 13, 13, 512) 0 conv3_block1_out[0][0] \n conv3_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_out (Activation) (None, 13, 13, 512) 0 conv3_block2_add[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block2_out[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_relu (Activation (None, 13, 13, 128) 0 conv3_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_relu (Activation (None, 13, 13, 128) 0 conv3_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block3_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_add (Add) (None, 13, 13, 512) 0 conv3_block2_out[0][0] \n conv3_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_out (Activation) (None, 13, 13, 512) 0 conv3_block3_add[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block4_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_relu (Activation (None, 13, 13, 128) 0 conv3_block4_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block4_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block4_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_relu (Activation (None, 13, 13, 128) 0 conv3_block4_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block4_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block4_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block4_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_add (Add) (None, 13, 13, 512) 0 conv3_block3_out[0][0] \n conv3_block4_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_out (Activation) (None, 13, 13, 512) 0 conv3_block4_add[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_conv (Conv2D) (None, 7, 7, 256) 131328 conv3_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_relu (Activation (None, 7, 7, 256) 0 conv4_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_relu (Activation (None, 7, 7, 256) 0 conv4_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_0_conv (Conv2D) (None, 7, 7, 1024) 525312 conv3_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block1_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block1_0_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_add (Add) (None, 7, 7, 1024) 0 conv4_block1_0_bn[0][0] \n conv4_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_out (Activation) (None, 7, 7, 1024) 0 conv4_block1_add[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block1_out[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_relu (Activation (None, 7, 7, 256) 0 conv4_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_relu (Activation (None, 7, 7, 256) 0 conv4_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block2_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_add (Add) (None, 7, 7, 1024) 0 conv4_block1_out[0][0] \n conv4_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_out (Activation) (None, 7, 7, 1024) 0 conv4_block2_add[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block2_out[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_relu (Activation (None, 7, 7, 256) 0 conv4_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_relu (Activation (None, 7, 7, 256) 0 conv4_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block3_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_add (Add) (None, 7, 7, 1024) 0 conv4_block2_out[0][0] \n conv4_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_out (Activation) (None, 7, 7, 1024) 0 conv4_block3_add[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block3_out[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block4_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_relu (Activation (None, 7, 7, 256) 0 conv4_block4_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block4_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block4_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_relu (Activation (None, 7, 7, 256) 0 conv4_block4_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block4_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block4_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block4_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_add (Add) (None, 7, 7, 1024) 0 conv4_block3_out[0][0] \n conv4_block4_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_out (Activation) (None, 7, 7, 1024) 0 conv4_block4_add[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block5_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_relu (Activation (None, 7, 7, 256) 0 conv4_block5_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block5_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block5_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_relu (Activation (None, 7, 7, 256) 0 conv4_block5_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block5_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block5_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block5_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_add (Add) (None, 7, 7, 1024) 0 conv4_block4_out[0][0] \n conv4_block5_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_out (Activation) (None, 7, 7, 1024) 0 conv4_block5_add[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block5_out[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block6_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_relu (Activation (None, 7, 7, 256) 0 conv4_block6_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block6_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block6_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_relu (Activation (None, 7, 7, 256) 0 conv4_block6_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block6_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block6_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block6_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_add (Add) (None, 7, 7, 1024) 0 conv4_block5_out[0][0] \n conv4_block6_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_out (Activation) (None, 7, 7, 1024) 0 conv4_block6_add[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_conv (Conv2D) (None, 4, 4, 512) 524800 conv4_block6_out[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_relu (Activation (None, 4, 4, 512) 0 conv5_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_relu (Activation (None, 4, 4, 512) 0 conv5_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_0_conv (Conv2D) (None, 4, 4, 2048) 2099200 conv4_block6_out[0][0] \n__________________________________________________________________________________________________\nconv5_block1_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block1_0_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_add (Add) (None, 4, 4, 2048) 0 conv5_block1_0_bn[0][0] \n conv5_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_out (Activation) (None, 4, 4, 2048) 0 conv5_block1_add[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_conv (Conv2D) (None, 4, 4, 512) 1049088 conv5_block1_out[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_relu (Activation (None, 4, 4, 512) 0 conv5_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_relu (Activation (None, 4, 4, 512) 0 conv5_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block2_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_add (Add) (None, 4, 4, 2048) 0 conv5_block1_out[0][0] \n conv5_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_out (Activation) (None, 4, 4, 2048) 0 conv5_block2_add[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_conv (Conv2D) (None, 4, 4, 512) 1049088 conv5_block2_out[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_relu (Activation (None, 4, 4, 512) 0 conv5_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_relu (Activation (None, 4, 4, 512) 0 conv5_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block3_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_add (Add) (None, 4, 4, 2048) 0 conv5_block2_out[0][0] \n conv5_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_out (Activation) (None, 4, 4, 2048) 0 conv5_block3_add[0][0] \n__________________________________________________________________________________________________\nglobal_average_pooling2d (Globa (None, 2048) 0 conv5_block3_out[0][0] \n__________________________________________________________________________________________________\ndense (Dense) (None, 2048) 4196352 global_average_pooling2d[0][0] \n__________________________________________________________________________________________________\ndense_1 (Dense) (None, 2048) 4196352 dense[0][0] \n__________________________________________________________________________________________________\ndense_2 (Dense) (None, 10) 20490 dense_1[0][0] \n==================================================================================================\nTotal params: 32,000,963\nTrainable params: 31,947,843\nNon-trainable params: 53,120\n__________________________________________________________________________________________________\n"
],
[
"weights = gen.class_weight\n\ncorrected_weights = {}\n\nfor i in range(10):\n if i == 3:\n corrected_weights[i] = 1\n else:\n corrected_weights[i] = weights[i]\n \ncorrected_weights",
"_____no_output_____"
],
[
"epochs = 20\nhistory = model.fit(gen.training_dataset, validation_data=gen.validation_dataset, \n epochs=epochs, \n callbacks=callbacks_list,\n class_weight=corrected_weights)",
"_____no_output_____"
],
[
"np.array(list(corrected_weights.values())).shape.rank",
"_____no_output_____"
],
[
"model = define_model(10, (100,100,18))",
"Model: \"functional_1\"\n__________________________________________________________________________________________________\nLayer (type) Output Shape Param # Connected to \n==================================================================================================\ninput_1 (InputLayer) [(None, 100, 100, 18 0 \n__________________________________________________________________________________________________\nconv2d (Conv2D) (None, 100, 100, 3) 57 input_1[0][0] \n__________________________________________________________________________________________________\nconv1_pad (ZeroPadding2D) (None, 106, 106, 3) 0 conv2d[0][0] \n__________________________________________________________________________________________________\nconv1_conv (Conv2D) (None, 50, 50, 64) 9472 conv1_pad[0][0] \n__________________________________________________________________________________________________\nconv1_bn (BatchNormalization) (None, 50, 50, 64) 256 conv1_conv[0][0] \n__________________________________________________________________________________________________\nconv1_relu (Activation) (None, 50, 50, 64) 0 conv1_bn[0][0] \n__________________________________________________________________________________________________\npool1_pad (ZeroPadding2D) (None, 52, 52, 64) 0 conv1_relu[0][0] \n__________________________________________________________________________________________________\npool1_pool (MaxPooling2D) (None, 25, 25, 64) 0 pool1_pad[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_conv (Conv2D) (None, 25, 25, 64) 4160 pool1_pool[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_relu (Activation (None, 25, 25, 64) 0 conv2_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_relu (Activation (None, 25, 25, 64) 0 conv2_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_0_conv (Conv2D) (None, 25, 25, 256) 16640 pool1_pool[0][0] \n__________________________________________________________________________________________________\nconv2_block1_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block1_0_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_add (Add) (None, 25, 25, 256) 0 conv2_block1_0_bn[0][0] \n conv2_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_out (Activation) (None, 25, 25, 256) 0 conv2_block1_add[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_conv (Conv2D) (None, 25, 25, 64) 16448 conv2_block1_out[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_relu (Activation (None, 25, 25, 64) 0 conv2_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_relu (Activation (None, 25, 25, 64) 0 conv2_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block2_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_add (Add) (None, 25, 25, 256) 0 conv2_block1_out[0][0] \n conv2_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_out (Activation) (None, 25, 25, 256) 0 conv2_block2_add[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_conv (Conv2D) (None, 25, 25, 64) 16448 conv2_block2_out[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_relu (Activation (None, 25, 25, 64) 0 conv2_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_relu (Activation (None, 25, 25, 64) 0 conv2_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block3_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_add (Add) (None, 25, 25, 256) 0 conv2_block2_out[0][0] \n conv2_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_out (Activation) (None, 25, 25, 256) 0 conv2_block3_add[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_conv (Conv2D) (None, 13, 13, 128) 32896 conv2_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_relu (Activation (None, 13, 13, 128) 0 conv3_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_relu (Activation (None, 13, 13, 128) 0 conv3_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_0_conv (Conv2D) (None, 13, 13, 512) 131584 conv2_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block1_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block1_0_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_add (Add) (None, 13, 13, 512) 0 conv3_block1_0_bn[0][0] \n conv3_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_out (Activation) (None, 13, 13, 512) 0 conv3_block1_add[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block1_out[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_relu (Activation (None, 13, 13, 128) 0 conv3_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_relu (Activation (None, 13, 13, 128) 0 conv3_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block2_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_add (Add) (None, 13, 13, 512) 0 conv3_block1_out[0][0] \n conv3_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_out (Activation) (None, 13, 13, 512) 0 conv3_block2_add[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block2_out[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_relu (Activation (None, 13, 13, 128) 0 conv3_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_relu (Activation (None, 13, 13, 128) 0 conv3_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block3_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_add (Add) (None, 13, 13, 512) 0 conv3_block2_out[0][0] \n conv3_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_out (Activation) (None, 13, 13, 512) 0 conv3_block3_add[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block4_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_relu (Activation (None, 13, 13, 128) 0 conv3_block4_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block4_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block4_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_relu (Activation (None, 13, 13, 128) 0 conv3_block4_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block4_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block4_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block4_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_add (Add) (None, 13, 13, 512) 0 conv3_block3_out[0][0] \n conv3_block4_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_out (Activation) (None, 13, 13, 512) 0 conv3_block4_add[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_conv (Conv2D) (None, 7, 7, 256) 131328 conv3_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_relu (Activation (None, 7, 7, 256) 0 conv4_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_relu (Activation (None, 7, 7, 256) 0 conv4_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_0_conv (Conv2D) (None, 7, 7, 1024) 525312 conv3_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block1_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block1_0_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_add (Add) (None, 7, 7, 1024) 0 conv4_block1_0_bn[0][0] \n conv4_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_out (Activation) (None, 7, 7, 1024) 0 conv4_block1_add[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block1_out[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_relu (Activation (None, 7, 7, 256) 0 conv4_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_relu (Activation (None, 7, 7, 256) 0 conv4_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block2_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_add (Add) (None, 7, 7, 1024) 0 conv4_block1_out[0][0] \n conv4_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_out (Activation) (None, 7, 7, 1024) 0 conv4_block2_add[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block2_out[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_relu (Activation (None, 7, 7, 256) 0 conv4_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_relu (Activation (None, 7, 7, 256) 0 conv4_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block3_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_add (Add) (None, 7, 7, 1024) 0 conv4_block2_out[0][0] \n conv4_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_out (Activation) (None, 7, 7, 1024) 0 conv4_block3_add[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block3_out[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block4_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_relu (Activation (None, 7, 7, 256) 0 conv4_block4_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block4_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block4_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_relu (Activation (None, 7, 7, 256) 0 conv4_block4_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block4_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block4_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block4_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_add (Add) (None, 7, 7, 1024) 0 conv4_block3_out[0][0] \n conv4_block4_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_out (Activation) (None, 7, 7, 1024) 0 conv4_block4_add[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block5_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_relu (Activation (None, 7, 7, 256) 0 conv4_block5_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block5_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block5_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_relu (Activation (None, 7, 7, 256) 0 conv4_block5_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block5_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block5_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block5_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_add (Add) (None, 7, 7, 1024) 0 conv4_block4_out[0][0] \n conv4_block5_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_out (Activation) (None, 7, 7, 1024) 0 conv4_block5_add[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block5_out[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block6_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_relu (Activation (None, 7, 7, 256) 0 conv4_block6_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block6_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block6_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_relu (Activation (None, 7, 7, 256) 0 conv4_block6_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block6_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block6_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block6_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_add (Add) (None, 7, 7, 1024) 0 conv4_block5_out[0][0] \n conv4_block6_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_out (Activation) (None, 7, 7, 1024) 0 conv4_block6_add[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_conv (Conv2D) (None, 4, 4, 512) 524800 conv4_block6_out[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_relu (Activation (None, 4, 4, 512) 0 conv5_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_relu (Activation (None, 4, 4, 512) 0 conv5_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_0_conv (Conv2D) (None, 4, 4, 2048) 2099200 conv4_block6_out[0][0] \n__________________________________________________________________________________________________\nconv5_block1_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block1_0_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_add (Add) (None, 4, 4, 2048) 0 conv5_block1_0_bn[0][0] \n conv5_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_out (Activation) (None, 4, 4, 2048) 0 conv5_block1_add[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_conv (Conv2D) (None, 4, 4, 512) 1049088 conv5_block1_out[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_relu (Activation (None, 4, 4, 512) 0 conv5_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_relu (Activation (None, 4, 4, 512) 0 conv5_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block2_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_add (Add) (None, 4, 4, 2048) 0 conv5_block1_out[0][0] \n conv5_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_out (Activation) (None, 4, 4, 2048) 0 conv5_block2_add[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_conv (Conv2D) (None, 4, 4, 512) 1049088 conv5_block2_out[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_relu (Activation (None, 4, 4, 512) 0 conv5_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_relu (Activation (None, 4, 4, 512) 0 conv5_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block3_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_add (Add) (None, 4, 4, 2048) 0 conv5_block2_out[0][0] \n conv5_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_out (Activation) (None, 4, 4, 2048) 0 conv5_block3_add[0][0] \n__________________________________________________________________________________________________\nglobal_average_pooling2d (Globa (None, 2048) 0 conv5_block3_out[0][0] \n__________________________________________________________________________________________________\ndense (Dense) (None, 2048) 4196352 global_average_pooling2d[0][0] \n__________________________________________________________________________________________________\ndense_1 (Dense) (None, 2048) 4196352 dense[0][0] \n__________________________________________________________________________________________________\ndense_2 (Dense) (None, 10) 20490 dense_1[0][0] \n==================================================================================================\nTotal params: 32,000,963\nTrainable params: 31,947,843\nNon-trainable params: 53,120\n__________________________________________________________________________________________________\n"
],
[
"model.layers",
"_____no_output_____"
],
[
"model.layers[4]",
"_____no_output_____"
],
[
"bn = model.layers[4]\n\nhelp(bn)",
"Help on BatchNormalization in module tensorflow.python.keras.layers.normalization_v2 object:\n\nclass BatchNormalization(tensorflow.python.keras.layers.normalization.BatchNormalizationBase)\n | BatchNormalization(*args, **kwargs)\n | \n | Normalize and scale inputs or activations.\n | \n | Normalize the activations of the previous layer at each batch,\n | i.e. applies a transformation that maintains the mean activation\n | close to 0 and the activation standard deviation close to 1.\n | \n | Batch normalization differs from other layers in several key aspects:\n | \n | 1) Adding BatchNormalization with `training=True` to a model causes the\n | result of one example to depend on the contents of all other examples in a\n | minibatch. Be careful when padding batches or masking examples, as these can\n | change the minibatch statistics and affect other examples.\n | \n | 2) Updates to the weights (moving statistics) are based on the forward pass\n | of a model rather than the result of gradient computations.\n | \n | 3) When performing inference using a model containing batch normalization, it\n | is generally (though not always) desirable to use accumulated statistics\n | rather than mini-batch statistics. This is accomplished by passing\n | `training=False` when calling the model, or using `model.predict`.\n | \n | Arguments:\n | axis: Integer, the axis that should be normalized (typically the features\n | axis). For instance, after a `Conv2D` layer with\n | `data_format=\"channels_first\"`, set `axis=1` in `BatchNormalization`.\n | momentum: Momentum for the moving average.\n | epsilon: Small float added to variance to avoid dividing by zero.\n | center: If True, add offset of `beta` to normalized tensor. If False, `beta`\n | is ignored.\n | scale: If True, multiply by `gamma`. If False, `gamma` is not used. When the\n | next layer is linear (also e.g. `nn.relu`), this can be disabled since the\n | scaling will be done by the next layer.\n | beta_initializer: Initializer for the beta weight.\n | gamma_initializer: Initializer for the gamma weight.\n | moving_mean_initializer: Initializer for the moving mean.\n | moving_variance_initializer: Initializer for the moving variance.\n | beta_regularizer: Optional regularizer for the beta weight.\n | gamma_regularizer: Optional regularizer for the gamma weight.\n | beta_constraint: Optional constraint for the beta weight.\n | gamma_constraint: Optional constraint for the gamma weight.\n | renorm: Whether to use [Batch Renormalization](\n | https://arxiv.org/abs/1702.03275). This adds extra variables during\n | training. The inference is the same for either value of this parameter.\n | renorm_clipping: A dictionary that may map keys 'rmax', 'rmin', 'dmax' to\n | scalar `Tensors` used to clip the renorm correction. The correction `(r,\n | d)` is used as `corrected_value = normalized_value * r + d`, with `r`\n | clipped to [rmin, rmax], and `d` to [-dmax, dmax]. Missing rmax, rmin,\n | dmax are set to inf, 0, inf, respectively.\n | renorm_momentum: Momentum used to update the moving means and standard\n | deviations with renorm. Unlike `momentum`, this affects training and\n | should be neither too small (which would add noise) nor too large (which\n | would give stale estimates). Note that `momentum` is still applied to get\n | the means and variances for inference.\n | fused: if `True`, use a faster, fused implementation, or raise a ValueError\n | if the fused implementation cannot be used. If `None`, use the faster\n | implementation if possible. If False, do not used the fused\n | implementation.\n | trainable: Boolean, if `True` the variables will be marked as trainable.\n | virtual_batch_size: An `int`. By default, `virtual_batch_size` is `None`,\n | which means batch normalization is performed across the whole batch. When\n | `virtual_batch_size` is not `None`, instead perform \"Ghost Batch\n | Normalization\", which creates virtual sub-batches which are each\n | normalized separately (with shared gamma, beta, and moving statistics).\n | Must divide the actual batch size during execution.\n | adjustment: A function taking the `Tensor` containing the (dynamic) shape of\n | the input tensor and returning a pair (scale, bias) to apply to the\n | normalized values (before gamma and beta), only during training. For\n | example, if axis==-1,\n | `adjustment = lambda shape: (\n | tf.random.uniform(shape[-1:], 0.93, 1.07),\n | tf.random.uniform(shape[-1:], -0.1, 0.1))` will scale the normalized\n | value by up to 7% up or down, then shift the result by up to 0.1\n | (with independent scaling and bias for each feature but shared\n | across all examples), and finally apply gamma and/or beta. If\n | `None`, no adjustment is applied. Cannot be specified if\n | virtual_batch_size is specified.\n | Call arguments:\n | inputs: Input tensor (of any rank).\n | training: Python boolean indicating whether the layer should behave in\n | training mode or in inference mode.\n | - `training=True`: The layer will normalize its inputs using the mean and\n | variance of the current batch of inputs.\n | - `training=False`: The layer will normalize its inputs using the mean and\n | variance of its moving statistics, learned during training.\n | Input shape: Arbitrary. Use the keyword argument `input_shape` (tuple of\n | integers, does not include the samples axis) when using this layer as the\n | first layer in a model.\n | Output shape: Same shape as input. \n | **About setting `layer.trainable = False` on a `BatchNormalization layer:**\n | \n | The meaning of setting `layer.trainable = False` is to freeze the layer,\n | i.e. its internal state will not change during training:\n | its trainable weights will not be updated\n | during `fit()` or `train_on_batch()`, and its state updates will not be run.\n | \n | Usually, this does not necessarily mean that the layer is run in inference\n | mode (which is normally controlled by the `training` argument that can\n | be passed when calling a layer). \"Frozen state\" and \"inference mode\"\n | are two separate concepts.\n | \n | However, in the case of the `BatchNormalization` layer, **setting\n | `trainable = False` on the layer means that the layer will be\n | subsequently run in inference mode** (meaning that it will use\n | the moving mean and the moving variance to normalize the current batch,\n | rather than using the mean and variance of the current batch).\n | \n | This behavior has been introduced in TensorFlow 2.0, in order\n | to enable `layer.trainable = False` to produce the most commonly\n | expected behavior in the convnet fine-tuning use case.\n | \n | Note that:\n | - This behavior only occurs as of TensorFlow 2.0. In 1.*,\n | setting `layer.trainable = False` would freeze the layer but would\n | not switch it to inference mode.\n | - Setting `trainable` on an model containing other layers will\n | recursively set the `trainable` value of all inner layers.\n | - If the value of the `trainable`\n | attribute is changed after calling `compile()` on a model,\n | the new value doesn't take effect for this model\n | until `compile()` is called again.\n | \n | Normalization equations: Consider the intermediate activations \\(x\\) of a\n | mini-batch of size\n | \\\\(m\\\\): We can compute the mean and variance of the batch \\\\({\\mu_B} =\n | \\frac{1}{m} \\sum_{i=1}^{m} {x_i}\\\\) \\\\({\\sigma_B^2} = \\frac{1}{m}\n | \\sum_{i=1}^{m} ({x_i} - {\\mu_B})^2\\\\) and then compute a normalized\n | \\\\(x\\\\), including a small factor \\\\({\\epsilon}\\\\) for numerical\n | stability. \\\\(\\hat{x_i} = \\frac{x_i - \\mu_B}{\\sqrt{\\sigma_B^2 +\n | \\epsilon}}\\\\) And finally \\\\(\\hat{x}\\) is linearly transformed by\n | \\({\\gamma}\\\\)\n | and \\\\({\\beta}\\\\), which are learned parameters: \\\\({y_i} = {\\gamma *\n | \\hat{x_i} + \\beta}\\\\)\n | Reference:\n | - [Ioffe and Szegedy, 2015](https://arxiv.org/abs/1502.03167).\n | \n | Method resolution order:\n | BatchNormalization\n | tensorflow.python.keras.layers.normalization.BatchNormalizationBase\n | tensorflow.python.keras.engine.base_layer.Layer\n | tensorflow.python.module.module.Module\n | tensorflow.python.training.tracking.tracking.AutoTrackable\n | tensorflow.python.training.tracking.base.Trackable\n | tensorflow.python.keras.utils.version_utils.LayerVersionSelector\n | builtins.object\n | \n | Methods inherited from tensorflow.python.keras.layers.normalization.BatchNormalizationBase:\n | \n | __init__(self, axis=-1, momentum=0.99, epsilon=0.001, center=True, scale=True, beta_initializer='zeros', gamma_initializer='ones', moving_mean_initializer='zeros', moving_variance_initializer='ones', beta_regularizer=None, gamma_regularizer=None, beta_constraint=None, gamma_constraint=None, renorm=False, renorm_clipping=None, renorm_momentum=0.99, fused=None, trainable=True, virtual_batch_size=None, adjustment=None, name=None, **kwargs)\n | \n | build(self, input_shape)\n | Creates the variables of the layer (optional, for subclass implementers).\n | \n | This is a method that implementers of subclasses of `Layer` or `Model`\n | can override if they need a state-creation step in-between\n | layer instantiation and layer call.\n | \n | This is typically used to create the weights of `Layer` subclasses.\n | \n | Arguments:\n | input_shape: Instance of `TensorShape`, or list of instances of\n | `TensorShape` if the layer expects a list of inputs\n | (one instance per input).\n | \n | call(self, inputs, training=None)\n | This is where the layer's logic lives.\n | \n | Note here that `call()` method in `tf.keras` is little bit different\n | from `keras` API. In `keras` API, you can pass support masking for\n | layers as additional arguments. Whereas `tf.keras` has `compute_mask()`\n | method to support masking.\n | \n | Arguments:\n | inputs: Input tensor, or list/tuple of input tensors.\n | **kwargs: Additional keyword arguments. Currently unused.\n | \n | Returns:\n | A tensor or list/tuple of tensors.\n | \n | compute_output_shape(self, input_shape)\n | Computes the output shape of the layer.\n | \n | If the layer has not been built, this method will call `build` on the\n | layer. This assumes that the layer will later be used with inputs that\n | match the input shape provided here.\n | \n | Arguments:\n | input_shape: Shape tuple (tuple of integers)\n | or list of shape tuples (one per output tensor of the layer).\n | Shape tuples can include None for free dimensions,\n | instead of an integer.\n | \n | Returns:\n | An input shape tuple.\n | \n | get_config(self)\n | Returns the config of the layer.\n | \n | A layer config is a Python dictionary (serializable)\n | containing the configuration of a layer.\n | The same layer can be reinstantiated later\n | (without its trained weights) from this configuration.\n | \n | The config of a layer does not include connectivity\n | information, nor the layer class name. These are handled\n | by `Network` (one layer of abstraction above).\n | \n | Returns:\n | Python dictionary.\n | \n | ----------------------------------------------------------------------\n | Data descriptors inherited from tensorflow.python.keras.layers.normalization.BatchNormalizationBase:\n | \n | trainable\n | \n | ----------------------------------------------------------------------\n | Methods inherited from tensorflow.python.keras.engine.base_layer.Layer:\n | \n | __call__(self, *args, **kwargs)\n | Wraps `call`, applying pre- and post-processing steps.\n | \n | Arguments:\n | *args: Positional arguments to be passed to `self.call`.\n | **kwargs: Keyword arguments to be passed to `self.call`.\n | \n | Returns:\n | Output tensor(s).\n | \n | Note:\n | - The following optional keyword arguments are reserved for specific uses:\n | * `training`: Boolean scalar tensor of Python boolean indicating\n | whether the `call` is meant for training or inference.\n | * `mask`: Boolean input mask.\n | - If the layer's `call` method takes a `mask` argument (as some Keras\n | layers do), its default value will be set to the mask generated\n | for `inputs` by the previous layer (if `input` did come from\n | a layer that generated a corresponding mask, i.e. if it came from\n | a Keras layer with masking support.\n | \n | Raises:\n | ValueError: if the layer's `call` method returns None (an invalid value).\n | RuntimeError: if `super().__init__()` was not called in the constructor.\n | \n | __delattr__(self, name)\n | Implement delattr(self, name).\n | \n | __getstate__(self)\n | \n | __setattr__(self, name, value)\n | Support self.foo = trackable syntax.\n | \n | __setstate__(self, state)\n | \n | add_loss(self, losses, **kwargs)\n | Add loss tensor(s), potentially dependent on layer inputs.\n | \n | Some losses (for instance, activity regularization losses) may be dependent\n | on the inputs passed when calling a layer. Hence, when reusing the same\n | layer on different inputs `a` and `b`, some entries in `layer.losses` may\n | be dependent on `a` and some on `b`. This method automatically keeps track\n | of dependencies.\n | \n | This method can be used inside a subclassed layer or model's `call`\n | function, in which case `losses` should be a Tensor or list of Tensors.\n | \n | Example:\n | \n | ```python\n | class MyLayer(tf.keras.layers.Layer):\n | def call(self, inputs):\n | self.add_loss(tf.abs(tf.reduce_mean(inputs)))\n | return inputs\n | ```\n | \n | This method can also be called directly on a Functional Model during\n | construction. In this case, any loss Tensors passed to this Model must\n | be symbolic and be able to be traced back to the model's `Input`s. These\n | losses become part of the model's topology and are tracked in `get_config`.\n | \n | Example:\n | \n | ```python\n | inputs = tf.keras.Input(shape=(10,))\n | x = tf.keras.layers.Dense(10)(inputs)\n | outputs = tf.keras.layers.Dense(1)(x)\n | model = tf.keras.Model(inputs, outputs)\n | # Activity regularization.\n | model.add_loss(tf.abs(tf.reduce_mean(x)))\n | ```\n | \n | If this is not the case for your loss (if, for example, your loss references\n | a `Variable` of one of the model's layers), you can wrap your loss in a\n | zero-argument lambda. These losses are not tracked as part of the model's\n | topology since they can't be serialized.\n | \n | Example:\n | \n | ```python\n | inputs = tf.keras.Input(shape=(10,))\n | d = tf.keras.layers.Dense(10)\n | x = d(inputs)\n | outputs = tf.keras.layers.Dense(1)(x)\n | model = tf.keras.Model(inputs, outputs)\n | # Weight regularization.\n | model.add_loss(lambda: tf.reduce_mean(d.kernel))\n | ```\n | \n | Arguments:\n | losses: Loss tensor, or list/tuple of tensors. Rather than tensors, losses\n | may also be zero-argument callables which create a loss tensor.\n | **kwargs: Additional keyword arguments for backward compatibility.\n | Accepted values:\n | inputs - Deprecated, will be automatically inferred.\n | \n | add_metric(self, value, name=None, **kwargs)\n | Adds metric tensor to the layer.\n | \n | This method can be used inside the `call()` method of a subclassed layer\n | or model.\n | \n | ```python\n | class MyMetricLayer(tf.keras.layers.Layer):\n | def __init__(self):\n | super(MyMetricLayer, self).__init__(name='my_metric_layer')\n | self.mean = metrics_module.Mean(name='metric_1')\n | \n | def call(self, inputs):\n | self.add_metric(self.mean(x))\n | self.add_metric(math_ops.reduce_sum(x), name='metric_2')\n | return inputs\n | ```\n | \n | This method can also be called directly on a Functional Model during\n | construction. In this case, any tensor passed to this Model must\n | be symbolic and be able to be traced back to the model's `Input`s. These\n | metrics become part of the model's topology and are tracked when you\n | save the model via `save()`.\n | \n | ```python\n | inputs = tf.keras.Input(shape=(10,))\n | x = tf.keras.layers.Dense(10)(inputs)\n | outputs = tf.keras.layers.Dense(1)(x)\n | model = tf.keras.Model(inputs, outputs)\n | model.add_metric(math_ops.reduce_sum(x), name='metric_1')\n | ```\n | \n | Note: Calling `add_metric()` with the result of a metric object on a\n | Functional Model, as shown in the example below, is not supported. This is\n | because we cannot trace the metric result tensor back to the model's inputs.\n | \n | ```python\n | inputs = tf.keras.Input(shape=(10,))\n | x = tf.keras.layers.Dense(10)(inputs)\n | outputs = tf.keras.layers.Dense(1)(x)\n | model = tf.keras.Model(inputs, outputs)\n | model.add_metric(tf.keras.metrics.Mean()(x), name='metric_1')\n | ```\n | \n | Args:\n | value: Metric tensor.\n | name: String metric name.\n | **kwargs: Additional keyword arguments for backward compatibility.\n | Accepted values:\n | `aggregation` - When the `value` tensor provided is not the result of\n | calling a `keras.Metric` instance, it will be aggregated by default\n | using a `keras.Metric.Mean`.\n | \n | add_update(self, updates, inputs=None)\n | Add update op(s), potentially dependent on layer inputs. (deprecated arguments)\n | \n | Warning: SOME ARGUMENTS ARE DEPRECATED: `(inputs)`. They will be removed in a future version.\n | Instructions for updating:\n | `inputs` is now automatically inferred\n | \n | Weight updates (for instance, the updates of the moving mean and variance\n | in a BatchNormalization layer) may be dependent on the inputs passed\n | when calling a layer. Hence, when reusing the same layer on\n | different inputs `a` and `b`, some entries in `layer.updates` may be\n | dependent on `a` and some on `b`. This method automatically keeps track\n | of dependencies.\n | \n | This call is ignored when eager execution is enabled (in that case, variable\n | updates are run on the fly and thus do not need to be tracked for later\n | execution).\n | \n | Arguments:\n | updates: Update op, or list/tuple of update ops, or zero-arg callable\n | that returns an update op. A zero-arg callable should be passed in\n | order to disable running the updates by setting `trainable=False`\n | on this Layer, when executing in Eager mode.\n | inputs: Deprecated, will be automatically inferred.\n | \n | add_variable(self, *args, **kwargs)\n | Deprecated, do NOT use! Alias for `add_weight`. (deprecated)\n | \n | Warning: THIS FUNCTION IS DEPRECATED. It will be removed in a future version.\n | Instructions for updating:\n | Please use `layer.add_weight` method instead.\n | \n | add_weight(self, name=None, shape=None, dtype=None, initializer=None, regularizer=None, trainable=None, constraint=None, partitioner=None, use_resource=None, synchronization=<VariableSynchronization.AUTO: 0>, aggregation=<VariableAggregation.NONE: 0>, **kwargs)\n | Adds a new variable to the layer.\n | \n | Arguments:\n | name: Variable name.\n | shape: Variable shape. Defaults to scalar if unspecified.\n | dtype: The type of the variable. Defaults to `self.dtype` or `float32`.\n | initializer: Initializer instance (callable).\n | regularizer: Regularizer instance (callable).\n | trainable: Boolean, whether the variable should be part of the layer's\n | \"trainable_variables\" (e.g. variables, biases)\n | or \"non_trainable_variables\" (e.g. BatchNorm mean and variance).\n | Note that `trainable` cannot be `True` if `synchronization`\n | is set to `ON_READ`.\n | constraint: Constraint instance (callable).\n | partitioner: Partitioner to be passed to the `Trackable` API.\n | use_resource: Whether to use `ResourceVariable`.\n | synchronization: Indicates when a distributed a variable will be\n | aggregated. Accepted values are constants defined in the class\n | `tf.VariableSynchronization`. By default the synchronization is set to\n | `AUTO` and the current `DistributionStrategy` chooses\n | when to synchronize. If `synchronization` is set to `ON_READ`,\n | `trainable` must not be set to `True`.\n | aggregation: Indicates how a distributed variable will be aggregated.\n | Accepted values are constants defined in the class\n | `tf.VariableAggregation`.\n | **kwargs: Additional keyword arguments. Accepted values are `getter`,\n | `collections`, `experimental_autocast` and `caching_device`.\n | \n | Returns:\n | The created variable. Usually either a `Variable` or `ResourceVariable`\n | instance. If `partitioner` is not `None`, a `PartitionedVariable`\n | instance is returned.\n | \n | Raises:\n | RuntimeError: If called with partitioned variable regularization and\n | eager execution is enabled.\n | ValueError: When giving unsupported dtype and no initializer or when\n | trainable has been set to True with synchronization set as `ON_READ`.\n | \n | apply(self, inputs, *args, **kwargs)\n | Deprecated, do NOT use! (deprecated)\n | \n | Warning: THIS FUNCTION IS DEPRECATED. It will be removed in a future version.\n | Instructions for updating:\n | Please use `layer.__call__` method instead.\n | \n | This is an alias of `self.__call__`.\n | \n | Arguments:\n | inputs: Input tensor(s).\n | *args: additional positional arguments to be passed to `self.call`.\n | **kwargs: additional keyword arguments to be passed to `self.call`.\n | \n | Returns:\n | Output tensor(s).\n | \n | compute_mask(self, inputs, mask=None)\n | Computes an output mask tensor.\n | \n | Arguments:\n | inputs: Tensor or list of tensors.\n | mask: Tensor or list of tensors.\n | \n | Returns:\n | None or a tensor (or list of tensors,\n | one per output tensor of the layer).\n | \n | compute_output_signature(self, input_signature)\n | Compute the output tensor signature of the layer based on the inputs.\n | \n | Unlike a TensorShape object, a TensorSpec object contains both shape\n | and dtype information for a tensor. This method allows layers to provide\n | output dtype information if it is different from the input dtype.\n | For any layer that doesn't implement this function,\n | the framework will fall back to use `compute_output_shape`, and will\n | assume that the output dtype matches the input dtype.\n | \n | Args:\n | input_signature: Single TensorSpec or nested structure of TensorSpec\n | objects, describing a candidate input for the layer.\n | \n | Returns:\n | Single TensorSpec or nested structure of TensorSpec objects, describing\n | how the layer would transform the provided input.\n | \n | Raises:\n | TypeError: If input_signature contains a non-TensorSpec object.\n | \n | count_params(self)\n | Count the total number of scalars composing the weights.\n | \n | Returns:\n | An integer count.\n | \n | Raises:\n | ValueError: if the layer isn't yet built\n | (in which case its weights aren't yet defined).\n | \n | get_input_at(self, node_index)\n | Retrieves the input tensor(s) of a layer at a given node.\n | \n | Arguments:\n | node_index: Integer, index of the node\n | from which to retrieve the attribute.\n | E.g. `node_index=0` will correspond to the\n | first time the layer was called.\n | \n | Returns:\n | A tensor (or list of tensors if the layer has multiple inputs).\n | \n | Raises:\n | RuntimeError: If called in Eager mode.\n | \n | get_input_mask_at(self, node_index)\n | Retrieves the input mask tensor(s) of a layer at a given node.\n | \n | Arguments:\n | node_index: Integer, index of the node\n | from which to retrieve the attribute.\n | E.g. `node_index=0` will correspond to the\n | first time the layer was called.\n | \n | Returns:\n | A mask tensor\n | (or list of tensors if the layer has multiple inputs).\n | \n | get_input_shape_at(self, node_index)\n | Retrieves the input shape(s) of a layer at a given node.\n | \n | Arguments:\n | node_index: Integer, index of the node\n | from which to retrieve the attribute.\n | E.g. `node_index=0` will correspond to the\n | first time the layer was called.\n | \n | Returns:\n | A shape tuple\n | (or list of shape tuples if the layer has multiple inputs).\n | \n | Raises:\n | RuntimeError: If called in Eager mode.\n | \n | get_losses_for(self, inputs)\n | Deprecated, do NOT use! (deprecated)\n | \n | Warning: THIS FUNCTION IS DEPRECATED. It will be removed in a future version.\n | Instructions for updating:\n | Please use `layer.losses` instead.\n | \n | Retrieves losses relevant to a specific set of inputs.\n | \n | Arguments:\n | inputs: Input tensor or list/tuple of input tensors.\n | \n | Returns:\n | List of loss tensors of the layer that depend on `inputs`.\n | \n | get_output_at(self, node_index)\n | Retrieves the output tensor(s) of a layer at a given node.\n | \n | Arguments:\n | node_index: Integer, index of the node\n | from which to retrieve the attribute.\n | E.g. `node_index=0` will correspond to the\n | first time the layer was called.\n | \n | Returns:\n | A tensor (or list of tensors if the layer has multiple outputs).\n | \n | Raises:\n | RuntimeError: If called in Eager mode.\n | \n | get_output_mask_at(self, node_index)\n | Retrieves the output mask tensor(s) of a layer at a given node.\n | \n | Arguments:\n | node_index: Integer, index of the node\n | from which to retrieve the attribute.\n | E.g. `node_index=0` will correspond to the\n | first time the layer was called.\n | \n | Returns:\n | A mask tensor\n | (or list of tensors if the layer has multiple outputs).\n | \n | get_output_shape_at(self, node_index)\n | Retrieves the output shape(s) of a layer at a given node.\n | \n | Arguments:\n | node_index: Integer, index of the node\n | from which to retrieve the attribute.\n | E.g. `node_index=0` will correspond to the\n | first time the layer was called.\n | \n | Returns:\n | A shape tuple\n | (or list of shape tuples if the layer has multiple outputs).\n | \n | Raises:\n | RuntimeError: If called in Eager mode.\n | \n | get_updates_for(self, inputs)\n | Deprecated, do NOT use! (deprecated)\n | \n | Warning: THIS FUNCTION IS DEPRECATED. It will be removed in a future version.\n | Instructions for updating:\n | Please use `layer.updates` instead.\n | \n | Retrieves updates relevant to a specific set of inputs.\n | \n | Arguments:\n | inputs: Input tensor or list/tuple of input tensors.\n | \n | Returns:\n | List of update ops of the layer that depend on `inputs`.\n | \n | get_weights(self)\n | Returns the current weights of the layer.\n | \n | The weights of a layer represent the state of the layer. This function\n | returns both trainable and non-trainable weight values associated with this\n | layer as a list of Numpy arrays, which can in turn be used to load state\n | into similarly parameterized layers.\n | \n | For example, a Dense layer returns a list of two values-- per-output\n | weights and the bias value. These can be used to set the weights of another\n | Dense layer:\n | \n | >>> a = tf.keras.layers.Dense(1,\n | ... kernel_initializer=tf.constant_initializer(1.))\n | >>> a_out = a(tf.convert_to_tensor([[1., 2., 3.]]))\n | >>> a.get_weights()\n | [array([[1.],\n | [1.],\n | [1.]], dtype=float32), array([0.], dtype=float32)]\n | >>> b = tf.keras.layers.Dense(1,\n | ... kernel_initializer=tf.constant_initializer(2.))\n | >>> b_out = b(tf.convert_to_tensor([[10., 20., 30.]]))\n | >>> b.get_weights()\n | [array([[2.],\n | [2.],\n | [2.]], dtype=float32), array([0.], dtype=float32)]\n | >>> b.set_weights(a.get_weights())\n | >>> b.get_weights()\n | [array([[1.],\n | [1.],\n | [1.]], dtype=float32), array([0.], dtype=float32)]\n | \n | Returns:\n | Weights values as a list of numpy arrays.\n | \n | set_weights(self, weights)\n | Sets the weights of the layer, from Numpy arrays.\n | \n | The weights of a layer represent the state of the layer. This function\n | sets the weight values from numpy arrays. The weight values should be\n | passed in the order they are created by the layer. Note that the layer's\n | weights must be instantiated before calling this function by calling\n | the layer.\n | \n | For example, a Dense layer returns a list of two values-- per-output\n | weights and the bias value. These can be used to set the weights of another\n | Dense layer:\n | \n | >>> a = tf.keras.layers.Dense(1,\n | ... kernel_initializer=tf.constant_initializer(1.))\n | >>> a_out = a(tf.convert_to_tensor([[1., 2., 3.]]))\n | >>> a.get_weights()\n | [array([[1.],\n | [1.],\n | [1.]], dtype=float32), array([0.], dtype=float32)]\n | >>> b = tf.keras.layers.Dense(1,\n | ... kernel_initializer=tf.constant_initializer(2.))\n | >>> b_out = b(tf.convert_to_tensor([[10., 20., 30.]]))\n | >>> b.get_weights()\n | [array([[2.],\n | [2.],\n | [2.]], dtype=float32), array([0.], dtype=float32)]\n | >>> b.set_weights(a.get_weights())\n | >>> b.get_weights()\n | [array([[1.],\n | [1.],\n | [1.]], dtype=float32), array([0.], dtype=float32)]\n | \n | Arguments:\n | weights: a list of Numpy arrays. The number\n | of arrays and their shape must match\n | number of the dimensions of the weights\n | of the layer (i.e. it should match the\n | output of `get_weights`).\n | \n | Raises:\n | ValueError: If the provided weights list does not match the\n | layer's specifications.\n | \n | ----------------------------------------------------------------------\n | Class methods inherited from tensorflow.python.keras.engine.base_layer.Layer:\n | \n | from_config(config) from builtins.type\n | Creates a layer from its config.\n | \n | This method is the reverse of `get_config`,\n | capable of instantiating the same layer from the config\n | dictionary. It does not handle layer connectivity\n | (handled by Network), nor weights (handled by `set_weights`).\n | \n | Arguments:\n | config: A Python dictionary, typically the\n | output of get_config.\n | \n | Returns:\n | A layer instance.\n | \n | ----------------------------------------------------------------------\n | Data descriptors inherited from tensorflow.python.keras.engine.base_layer.Layer:\n | \n | activity_regularizer\n | Optional regularizer function for the output of this layer.\n | \n | dtype\n | Dtype used by the weights of the layer, set in the constructor.\n | \n | dynamic\n | Whether the layer is dynamic (eager-only); set in the constructor.\n | \n | inbound_nodes\n | Deprecated, do NOT use! Only for compatibility with external Keras.\n | \n | input\n | Retrieves the input tensor(s) of a layer.\n | \n | Only applicable if the layer has exactly one input,\n | i.e. if it is connected to one incoming layer.\n | \n | Returns:\n | Input tensor or list of input tensors.\n | \n | Raises:\n | RuntimeError: If called in Eager mode.\n | AttributeError: If no inbound nodes are found.\n | \n | input_mask\n | Retrieves the input mask tensor(s) of a layer.\n | \n | Only applicable if the layer has exactly one inbound node,\n | i.e. if it is connected to one incoming layer.\n | \n | Returns:\n | Input mask tensor (potentially None) or list of input\n | mask tensors.\n | \n | Raises:\n | AttributeError: if the layer is connected to\n | more than one incoming layers.\n | \n | input_shape\n | Retrieves the input shape(s) of a layer.\n | \n | Only applicable if the layer has exactly one input,\n | i.e. if it is connected to one incoming layer, or if all inputs\n | have the same shape.\n | \n | Returns:\n | Input shape, as an integer shape tuple\n | (or list of shape tuples, one tuple per input tensor).\n | \n | Raises:\n | AttributeError: if the layer has no defined input_shape.\n | RuntimeError: if called in Eager mode.\n | \n | input_spec\n | `InputSpec` instance(s) describing the input format for this layer.\n | \n | When you create a layer subclass, you can set `self.input_spec` to enable\n | the layer to run input compatibility checks when it is called.\n | Consider a `Conv2D` layer: it can only be called on a single input tensor\n | of rank 4. As such, you can set, in `__init__()`:\n | \n | ```python\n | self.input_spec = tf.keras.layers.InputSpec(ndim=4)\n | ```\n | \n | Now, if you try to call the layer on an input that isn't rank 4\n | (for instance, an input of shape `(2,)`, it will raise a nicely-formatted\n | error:\n | \n | ```\n | ValueError: Input 0 of layer conv2d is incompatible with the layer:\n | expected ndim=4, found ndim=1. Full shape received: [2]\n | ```\n | \n | Input checks that can be specified via `input_spec` include:\n | - Structure (e.g. a single input, a list of 2 inputs, etc)\n | - Shape\n | - Rank (ndim)\n | - Dtype\n | \n | For more information, see `tf.keras.layers.InputSpec`.\n | \n | Returns:\n | A `tf.keras.layers.InputSpec` instance, or nested structure thereof.\n | \n | losses\n | List of losses added using the `add_loss()` API.\n | \n | Variable regularization tensors are created when this property is accessed,\n | so it is eager safe: accessing `losses` under a `tf.GradientTape` will\n | propagate gradients back to the corresponding variables.\n | \n | Examples:\n | \n | >>> class MyLayer(tf.keras.layers.Layer):\n | ... def call(self, inputs):\n | ... self.add_loss(tf.abs(tf.reduce_mean(inputs)))\n | ... return inputs\n | >>> l = MyLayer()\n | >>> l(np.ones((10, 1)))\n | >>> l.losses\n | [1.0]\n | \n | >>> inputs = tf.keras.Input(shape=(10,))\n | >>> x = tf.keras.layers.Dense(10)(inputs)\n | >>> outputs = tf.keras.layers.Dense(1)(x)\n | >>> model = tf.keras.Model(inputs, outputs)\n | >>> # Activity regularization.\n | >>> model.add_loss(tf.abs(tf.reduce_mean(x)))\n | >>> model.losses\n | [<tf.Tensor 'Abs:0' shape=() dtype=float32>]\n | \n | >>> inputs = tf.keras.Input(shape=(10,))\n | >>> d = tf.keras.layers.Dense(10, kernel_initializer='ones')\n | >>> x = d(inputs)\n | >>> outputs = tf.keras.layers.Dense(1)(x)\n | >>> model = tf.keras.Model(inputs, outputs)\n | >>> # Weight regularization.\n | >>> model.add_loss(lambda: tf.reduce_mean(d.kernel))\n | >>> model.losses\n | [<tf.Tensor: shape=(), dtype=float32, numpy=1.0>]\n | \n | Returns:\n | A list of tensors.\n | \n | metrics\n | List of metrics added using the `add_metric()` API.\n | \n | Example:\n | \n | >>> input = tf.keras.layers.Input(shape=(3,))\n | >>> d = tf.keras.layers.Dense(2)\n | >>> output = d(input)\n | >>> d.add_metric(tf.reduce_max(output), name='max')\n | >>> d.add_metric(tf.reduce_min(output), name='min')\n | >>> [m.name for m in d.metrics]\n | ['max', 'min']\n | \n | Returns:\n | A list of tensors.\n | \n | name\n | Name of the layer (string), set in the constructor.\n | \n | non_trainable_variables\n | \n | non_trainable_weights\n | List of all non-trainable weights tracked by this layer.\n | \n | Non-trainable weights are *not* updated during training. They are expected\n | to be updated manually in `call()`.\n | \n | Returns:\n | A list of non-trainable variables.\n | \n | outbound_nodes\n | Deprecated, do NOT use! Only for compatibility with external Keras.\n | \n | output\n | Retrieves the output tensor(s) of a layer.\n | \n | Only applicable if the layer has exactly one output,\n | i.e. if it is connected to one incoming layer.\n | \n | Returns:\n | Output tensor or list of output tensors.\n | \n | Raises:\n | AttributeError: if the layer is connected to more than one incoming\n | layers.\n | RuntimeError: if called in Eager mode.\n | \n | output_mask\n | Retrieves the output mask tensor(s) of a layer.\n | \n | Only applicable if the layer has exactly one inbound node,\n | i.e. if it is connected to one incoming layer.\n | \n | Returns:\n | Output mask tensor (potentially None) or list of output\n | mask tensors.\n | \n | Raises:\n | AttributeError: if the layer is connected to\n | more than one incoming layers.\n | \n | output_shape\n | Retrieves the output shape(s) of a layer.\n | \n | Only applicable if the layer has one output,\n | or if all outputs have the same shape.\n | \n | Returns:\n | Output shape, as an integer shape tuple\n | (or list of shape tuples, one tuple per output tensor).\n | \n | Raises:\n | AttributeError: if the layer has no defined output shape.\n | RuntimeError: if called in Eager mode.\n | \n | stateful\n | \n | supports_masking\n | Whether this layer supports computing a mask using `compute_mask`.\n | \n | trainable_variables\n | Sequence of trainable variables owned by this module and its submodules.\n | \n | Note: this method uses reflection to find variables on the current instance\n | and submodules. For performance reasons you may wish to cache the result\n | of calling this method if you don't expect the return value to change.\n | \n | Returns:\n | A sequence of variables for the current module (sorted by attribute\n | name) followed by variables from all submodules recursively (breadth\n | first).\n | \n | trainable_weights\n | List of all trainable weights tracked by this layer.\n | \n | Trainable weights are updated via gradient descent during training.\n | \n | Returns:\n | A list of trainable variables.\n | \n | updates\n | DEPRECATED FUNCTION\n | \n | Warning: THIS FUNCTION IS DEPRECATED. It will be removed in a future version.\n | Instructions for updating:\n | This property should not be used in TensorFlow 2.0, as updates are applied automatically.\n | \n | variables\n | Returns the list of all layer variables/weights.\n | \n | Alias of `self.weights`.\n | \n | Returns:\n | A list of variables.\n | \n | weights\n | Returns the list of all layer variables/weights.\n | \n | Returns:\n | A list of variables.\n | \n | ----------------------------------------------------------------------\n | Class methods inherited from tensorflow.python.module.module.Module:\n | \n | with_name_scope(method) from builtins.type\n | Decorator to automatically enter the module name scope.\n | \n | >>> class MyModule(tf.Module):\n | ... @tf.Module.with_name_scope\n | ... def __call__(self, x):\n | ... if not hasattr(self, 'w'):\n | ... self.w = tf.Variable(tf.random.normal([x.shape[1], 3]))\n | ... return tf.matmul(x, self.w)\n | \n | Using the above module would produce `tf.Variable`s and `tf.Tensor`s whose\n | names included the module name:\n | \n | >>> mod = MyModule()\n | >>> mod(tf.ones([1, 2]))\n | <tf.Tensor: shape=(1, 3), dtype=float32, numpy=..., dtype=float32)>\n | >>> mod.w\n | <tf.Variable 'my_module/Variable:0' shape=(2, 3) dtype=float32,\n | numpy=..., dtype=float32)>\n | \n | Args:\n | method: The method to wrap.\n | \n | Returns:\n | The original method wrapped such that it enters the module's name scope.\n | \n | ----------------------------------------------------------------------\n | Data descriptors inherited from tensorflow.python.module.module.Module:\n | \n | name_scope\n | Returns a `tf.name_scope` instance for this class.\n | \n | submodules\n | Sequence of all sub-modules.\n | \n | Submodules are modules which are properties of this module, or found as\n | properties of modules which are properties of this module (and so on).\n | \n | >>> a = tf.Module()\n | >>> b = tf.Module()\n | >>> c = tf.Module()\n | >>> a.b = b\n | >>> b.c = c\n | >>> list(a.submodules) == [b, c]\n | True\n | >>> list(b.submodules) == [c]\n | True\n | >>> list(c.submodules) == []\n | True\n | \n | Returns:\n | A sequence of all submodules.\n | \n | ----------------------------------------------------------------------\n | Data descriptors inherited from tensorflow.python.training.tracking.base.Trackable:\n | \n | __dict__\n | dictionary for instance variables (if defined)\n | \n | __weakref__\n | list of weak references to the object (if defined)\n | \n | ----------------------------------------------------------------------\n | Static methods inherited from tensorflow.python.keras.utils.version_utils.LayerVersionSelector:\n | \n | __new__(cls, *args, **kwargs)\n | Create and return a new object. See help(type) for accurate signature.\n\n"
],
[
"bn.name_scope()",
"_____no_output_____"
],
[
"bn.name",
"_____no_output_____"
],
[
"model.layers[9].name",
"_____no_output_____"
],
[
"model.layers[2].name",
"_____no_output_____"
],
[
"bns = []\n\nfor layer in model.layers:\n if 'bn' in layer.name:\n bns.append(layer)",
"_____no_output_____"
],
[
"bns",
"_____no_output_____"
],
[
"def define_model(numclasses, input_shape, freeze_bns=True):\n # parameters for CNN\n input_tensor = Input(shape=input_shape)\n\n # introduce a additional layer to get from bands to 3 input channels\n input_tensor = Conv2D(3, (1, 1))(input_tensor)\n\n base_model_resnet50 = keras.applications.ResNet50(include_top=False,\n weights='imagenet',\n input_shape=(100, 100, 3))\n base_model = keras.applications.ResNet50(include_top=False,\n weights=None,\n input_tensor=input_tensor)\n\n for i, layer in enumerate(base_model_resnet50.layers):\n # we must skip input layer, which has no weights\n if i == 0:\n continue\n base_model.layers[i+1].set_weights(layer.get_weights())\n \n if freeze_bns:\n if 'bn' in layer.name:\n base_model.layers[i+1].trainable = False\n\n # add a global spatial average pooling layer\n top_model = base_model.output\n top_model = GlobalAveragePooling2D()(top_model)\n\n # let's add a fully-connected layer\n top_model = Dense(2048, activation='relu')(top_model)\n top_model = Dense(2048, activation='relu')(top_model)\n # and a logistic layer\n predictions = Dense(numclasses, activation='softmax')(top_model)\n\n # this is the model we will train\n model = Model(inputs=base_model.input, outputs=predictions)\n\n model.summary()\n return model",
"_____no_output_____"
],
[
"model = define_model(10, (100,100,18))",
"Model: \"functional_3\"\n__________________________________________________________________________________________________\nLayer (type) Output Shape Param # Connected to \n==================================================================================================\ninput_3 (InputLayer) [(None, 100, 100, 18 0 \n__________________________________________________________________________________________________\nconv2d_1 (Conv2D) (None, 100, 100, 3) 57 input_3[0][0] \n__________________________________________________________________________________________________\nconv1_pad (ZeroPadding2D) (None, 106, 106, 3) 0 conv2d_1[0][0] \n__________________________________________________________________________________________________\nconv1_conv (Conv2D) (None, 50, 50, 64) 9472 conv1_pad[0][0] \n__________________________________________________________________________________________________\nconv1_bn (BatchNormalization) (None, 50, 50, 64) 256 conv1_conv[0][0] \n__________________________________________________________________________________________________\nconv1_relu (Activation) (None, 50, 50, 64) 0 conv1_bn[0][0] \n__________________________________________________________________________________________________\npool1_pad (ZeroPadding2D) (None, 52, 52, 64) 0 conv1_relu[0][0] \n__________________________________________________________________________________________________\npool1_pool (MaxPooling2D) (None, 25, 25, 64) 0 pool1_pad[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_conv (Conv2D) (None, 25, 25, 64) 4160 pool1_pool[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_1_relu (Activation (None, 25, 25, 64) 0 conv2_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_2_relu (Activation (None, 25, 25, 64) 0 conv2_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_0_conv (Conv2D) (None, 25, 25, 256) 16640 pool1_pool[0][0] \n__________________________________________________________________________________________________\nconv2_block1_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block1_0_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block1_add (Add) (None, 25, 25, 256) 0 conv2_block1_0_bn[0][0] \n conv2_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block1_out (Activation) (None, 25, 25, 256) 0 conv2_block1_add[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_conv (Conv2D) (None, 25, 25, 64) 16448 conv2_block1_out[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_1_relu (Activation (None, 25, 25, 64) 0 conv2_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_2_relu (Activation (None, 25, 25, 64) 0 conv2_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block2_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block2_add (Add) (None, 25, 25, 256) 0 conv2_block1_out[0][0] \n conv2_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block2_out (Activation) (None, 25, 25, 256) 0 conv2_block2_add[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_conv (Conv2D) (None, 25, 25, 64) 16448 conv2_block2_out[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_1_relu (Activation (None, 25, 25, 64) 0 conv2_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_conv (Conv2D) (None, 25, 25, 64) 36928 conv2_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_bn (BatchNormali (None, 25, 25, 64) 256 conv2_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_2_relu (Activation (None, 25, 25, 64) 0 conv2_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_3_conv (Conv2D) (None, 25, 25, 256) 16640 conv2_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv2_block3_3_bn (BatchNormali (None, 25, 25, 256) 1024 conv2_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv2_block3_add (Add) (None, 25, 25, 256) 0 conv2_block2_out[0][0] \n conv2_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv2_block3_out (Activation) (None, 25, 25, 256) 0 conv2_block3_add[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_conv (Conv2D) (None, 13, 13, 128) 32896 conv2_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_1_relu (Activation (None, 13, 13, 128) 0 conv3_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_2_relu (Activation (None, 13, 13, 128) 0 conv3_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_0_conv (Conv2D) (None, 13, 13, 512) 131584 conv2_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block1_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block1_0_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block1_add (Add) (None, 13, 13, 512) 0 conv3_block1_0_bn[0][0] \n conv3_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block1_out (Activation) (None, 13, 13, 512) 0 conv3_block1_add[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block1_out[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_1_relu (Activation (None, 13, 13, 128) 0 conv3_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_2_relu (Activation (None, 13, 13, 128) 0 conv3_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block2_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block2_add (Add) (None, 13, 13, 512) 0 conv3_block1_out[0][0] \n conv3_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block2_out (Activation) (None, 13, 13, 512) 0 conv3_block2_add[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block2_out[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_1_relu (Activation (None, 13, 13, 128) 0 conv3_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_2_relu (Activation (None, 13, 13, 128) 0 conv3_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block3_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block3_add (Add) (None, 13, 13, 512) 0 conv3_block2_out[0][0] \n conv3_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block3_out (Activation) (None, 13, 13, 512) 0 conv3_block3_add[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_conv (Conv2D) (None, 13, 13, 128) 65664 conv3_block3_out[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block4_1_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_1_relu (Activation (None, 13, 13, 128) 0 conv3_block4_1_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_conv (Conv2D) (None, 13, 13, 128) 147584 conv3_block4_1_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_bn (BatchNormali (None, 13, 13, 128) 512 conv3_block4_2_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_2_relu (Activation (None, 13, 13, 128) 0 conv3_block4_2_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_3_conv (Conv2D) (None, 13, 13, 512) 66048 conv3_block4_2_relu[0][0] \n__________________________________________________________________________________________________\nconv3_block4_3_bn (BatchNormali (None, 13, 13, 512) 2048 conv3_block4_3_conv[0][0] \n__________________________________________________________________________________________________\nconv3_block4_add (Add) (None, 13, 13, 512) 0 conv3_block3_out[0][0] \n conv3_block4_3_bn[0][0] \n__________________________________________________________________________________________________\nconv3_block4_out (Activation) (None, 13, 13, 512) 0 conv3_block4_add[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_conv (Conv2D) (None, 7, 7, 256) 131328 conv3_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_1_relu (Activation (None, 7, 7, 256) 0 conv4_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_2_relu (Activation (None, 7, 7, 256) 0 conv4_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_0_conv (Conv2D) (None, 7, 7, 1024) 525312 conv3_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block1_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block1_0_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block1_add (Add) (None, 7, 7, 1024) 0 conv4_block1_0_bn[0][0] \n conv4_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block1_out (Activation) (None, 7, 7, 1024) 0 conv4_block1_add[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block1_out[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_1_relu (Activation (None, 7, 7, 256) 0 conv4_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_2_relu (Activation (None, 7, 7, 256) 0 conv4_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block2_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block2_add (Add) (None, 7, 7, 1024) 0 conv4_block1_out[0][0] \n conv4_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block2_out (Activation) (None, 7, 7, 1024) 0 conv4_block2_add[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block2_out[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_1_relu (Activation (None, 7, 7, 256) 0 conv4_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_2_relu (Activation (None, 7, 7, 256) 0 conv4_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block3_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block3_add (Add) (None, 7, 7, 1024) 0 conv4_block2_out[0][0] \n conv4_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block3_out (Activation) (None, 7, 7, 1024) 0 conv4_block3_add[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block3_out[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block4_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_1_relu (Activation (None, 7, 7, 256) 0 conv4_block4_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block4_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block4_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_2_relu (Activation (None, 7, 7, 256) 0 conv4_block4_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block4_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block4_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block4_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block4_add (Add) (None, 7, 7, 1024) 0 conv4_block3_out[0][0] \n conv4_block4_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block4_out (Activation) (None, 7, 7, 1024) 0 conv4_block4_add[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block4_out[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block5_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_1_relu (Activation (None, 7, 7, 256) 0 conv4_block5_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block5_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block5_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_2_relu (Activation (None, 7, 7, 256) 0 conv4_block5_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block5_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block5_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block5_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block5_add (Add) (None, 7, 7, 1024) 0 conv4_block4_out[0][0] \n conv4_block5_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block5_out (Activation) (None, 7, 7, 1024) 0 conv4_block5_add[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_conv (Conv2D) (None, 7, 7, 256) 262400 conv4_block5_out[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block6_1_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_1_relu (Activation (None, 7, 7, 256) 0 conv4_block6_1_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_conv (Conv2D) (None, 7, 7, 256) 590080 conv4_block6_1_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_bn (BatchNormali (None, 7, 7, 256) 1024 conv4_block6_2_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_2_relu (Activation (None, 7, 7, 256) 0 conv4_block6_2_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_3_conv (Conv2D) (None, 7, 7, 1024) 263168 conv4_block6_2_relu[0][0] \n__________________________________________________________________________________________________\nconv4_block6_3_bn (BatchNormali (None, 7, 7, 1024) 4096 conv4_block6_3_conv[0][0] \n__________________________________________________________________________________________________\nconv4_block6_add (Add) (None, 7, 7, 1024) 0 conv4_block5_out[0][0] \n conv4_block6_3_bn[0][0] \n__________________________________________________________________________________________________\nconv4_block6_out (Activation) (None, 7, 7, 1024) 0 conv4_block6_add[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_conv (Conv2D) (None, 4, 4, 512) 524800 conv4_block6_out[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block1_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_1_relu (Activation (None, 4, 4, 512) 0 conv5_block1_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block1_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block1_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_2_relu (Activation (None, 4, 4, 512) 0 conv5_block1_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_0_conv (Conv2D) (None, 4, 4, 2048) 2099200 conv4_block6_out[0][0] \n__________________________________________________________________________________________________\nconv5_block1_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block1_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block1_0_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block1_0_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block1_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block1_add (Add) (None, 4, 4, 2048) 0 conv5_block1_0_bn[0][0] \n conv5_block1_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block1_out (Activation) (None, 4, 4, 2048) 0 conv5_block1_add[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_conv (Conv2D) (None, 4, 4, 512) 1049088 conv5_block1_out[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block2_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_1_relu (Activation (None, 4, 4, 512) 0 conv5_block2_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block2_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block2_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_2_relu (Activation (None, 4, 4, 512) 0 conv5_block2_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block2_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block2_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block2_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block2_add (Add) (None, 4, 4, 2048) 0 conv5_block1_out[0][0] \n conv5_block2_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block2_out (Activation) (None, 4, 4, 2048) 0 conv5_block2_add[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_conv (Conv2D) (None, 4, 4, 512) 1049088 conv5_block2_out[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block3_1_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_1_relu (Activation (None, 4, 4, 512) 0 conv5_block3_1_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_conv (Conv2D) (None, 4, 4, 512) 2359808 conv5_block3_1_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_bn (BatchNormali (None, 4, 4, 512) 2048 conv5_block3_2_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_2_relu (Activation (None, 4, 4, 512) 0 conv5_block3_2_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_3_conv (Conv2D) (None, 4, 4, 2048) 1050624 conv5_block3_2_relu[0][0] \n__________________________________________________________________________________________________\nconv5_block3_3_bn (BatchNormali (None, 4, 4, 2048) 8192 conv5_block3_3_conv[0][0] \n__________________________________________________________________________________________________\nconv5_block3_add (Add) (None, 4, 4, 2048) 0 conv5_block2_out[0][0] \n conv5_block3_3_bn[0][0] \n__________________________________________________________________________________________________\nconv5_block3_out (Activation) (None, 4, 4, 2048) 0 conv5_block3_add[0][0] \n__________________________________________________________________________________________________\nglobal_average_pooling2d_1 (Glo (None, 2048) 0 conv5_block3_out[0][0] \n__________________________________________________________________________________________________\ndense_3 (Dense) (None, 2048) 4196352 global_average_pooling2d_1[0][0] \n__________________________________________________________________________________________________\ndense_4 (Dense) (None, 2048) 4196352 dense_3[0][0] \n__________________________________________________________________________________________________\ndense_5 (Dense) (None, 10) 20490 dense_4[0][0] \n==================================================================================================\nTotal params: 32,000,963\nTrainable params: 31,894,723\nNon-trainable params: 106,240\n__________________________________________________________________________________________________\n"
],
[
"model.layers[4].trainable",
"_____no_output_____"
],
[
"model.layers[3].trainable",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bb24177e87e8205f0d898edde455df872d53dd | 103,097 | ipynb | Jupyter Notebook | ipynb/How to Do Things with Words.ipynb | mikiec84/pytudes | 895c7130397c1763ee8c9f87b8ea1ccc1ccbd447 | [
"MIT"
] | null | null | null | ipynb/How to Do Things with Words.ipynb | mikiec84/pytudes | 895c7130397c1763ee8c9f87b8ea1ccc1ccbd447 | [
"MIT"
] | null | null | null | ipynb/How to Do Things with Words.ipynb | mikiec84/pytudes | 895c7130397c1763ee8c9f87b8ea1ccc1ccbd447 | [
"MIT"
] | null | null | null | 44.669411 | 21,830 | 0.672774 | [
[
[
"# Boring preliminaries\nimport re\nimport math\nimport string\nfrom collections import Counter\nfrom __future__ import division",
"_____no_output_____"
]
],
[
[
"<center>\n<h1>Statistical Natural Language Processing in Python.\n<br><font color=blue>or</font>\n<br>How To Do Things With Words. And Counters.\n<br><font color=blue>or</font>\n<br>Everything I Needed to Know About NLP I learned From Sesame Street.\n<br>Except Kneser-Ney Smoothing.\n<br>The Count Didn't Cover That.\n<br>\n<br><img src='http://norvig.com/ipython/the-count.jpg'> \n<br>*One, two, three, ah, ah, ah!* — The Count\n</center>\n<hr>",
"_____no_output_____"
],
[
"(1) Data: Text and Words\n========\n\nBefore we can do things with words, we need some words. First we need some *text*, possibly from a *file*. Then we can break the text into words. I happen to have a big text called [big.txt](file:///Users/pnorvig/Documents/ipynb/big.txt). We can read it, and see how big it is (in characters):",
"_____no_output_____"
]
],
[
[
"TEXT = file('big.txt').read()\nlen(TEXT)",
"_____no_output_____"
]
],
[
[
"So, six million characters.\n\nNow let's break the text up into words (or more formal-sounding, *tokens*). For now we'll ignore all the punctuation and numbers, and anything that is not a letter.",
"_____no_output_____"
]
],
[
[
"def tokens(text):\n \"List all the word tokens (consecutive letters) in a text. Normalize to lowercase.\"\n return re.findall('[a-z]+', text.lower()) ",
"_____no_output_____"
],
[
"tokens('This is: A test, 1, 2, 3, this is.')",
"_____no_output_____"
],
[
"WORDS = tokens(TEXT)\nlen(WORDS)",
"_____no_output_____"
]
],
[
[
"So, a million words. Here are the first 10:\n\n",
"_____no_output_____"
]
],
[
[
"print(WORDS[:10])",
"['the', 'project', 'gutenberg', 'ebook', 'of', 'the', 'adventures', 'of', 'sherlock', 'holmes']\n"
]
],
[
[
"(2) Models: Bag of Words\n====\n\nThe list `WORDS` is a list of the words in the `TEXT`, but it can also serve as a *generative model* of text. We know that language is very complicated, but we can create a simplified model of language that captures part of the complexity. In the *bag of words* model, we ignore the order of words, but maintain their frequency. Think of it this way: take all the words from the text, and throw them into a bag. Shake the bag, and then generating a sentence consists of pulling words out of the bag one at a time. Chances are it won't be grammatical or sensible, but it will have words in roughly the right proportions. Here's a function to sample an *n* word sentence from a bag of words:",
"_____no_output_____"
]
],
[
[
"def sample(bag, n=10):\n \"Sample a random n-word sentence from the model described by the bag of words.\"\n return ' '.join(random.choice(bag) for _ in range(n))",
"_____no_output_____"
],
[
"sample(WORDS)",
"_____no_output_____"
]
],
[
[
"Another representation for a bag of words is a `Counter`, which is a dictionary of `{'word': count}` pairs. For example,",
"_____no_output_____"
]
],
[
[
"Counter(tokens('Is this a test? It is a test!'))",
"_____no_output_____"
]
],
[
[
"A `Counter` is like a `dict`, but with a few extra methods. Let's make a `Counter` for the big list of `WORDS` and get a feel for what's there:",
"_____no_output_____"
]
],
[
[
"COUNTS = Counter(WORDS)\n\nprint COUNTS.most_common(10)",
"[('the', 80029), ('of', 40025), ('and', 38312), ('to', 28766), ('in', 22047), ('a', 21155), ('that', 12512), ('he', 12401), ('was', 11410), ('it', 10681)]\n"
],
[
"for w in tokens('the rare and neverbeforeseen words'):\n print COUNTS[w], w",
"80029 the\n83 rare\n38312 and\n0 neverbeforeseen\n460 words\n"
]
],
[
[
"In 1935, linguist George Zipf noted that in any big text, the *n*th most frequent word appears with a frequency of about 1/*n* of the most frequent word. He get's credit for *Zipf's Law*, even though Felix Auerbach made the same observation in 1913. If we plot the frequency of words, most common first, on a log-log plot, they should come out as a straight line if Zipf's Law holds. Here we see that it is a fairly close fit:",
"_____no_output_____"
]
],
[
[
"M = COUNTS['the']\nyscale('log'); xscale('log'); title('Frequency of n-th most frequent word and 1/n line.')\nplot([c for (w, c) in COUNTS.most_common()])\nplot([M/i for i in range(1, len(COUNTS)+1)]);",
"_____no_output_____"
]
],
[
[
"(3) Task: Spelling Correction\n========\n\nGiven a word *w*, find the most likely correction *c* = `correct(`*w*`)`.\n\n**Approach:** Try all candidate words *c* that are known words that are near *w*. Choose the most likely one.\n\nHow to balance *near* and *likely*?\n\nFor now, in a trivial way: always prefer nearer, but when there is a tie on nearness, use the word with the highest `WORDS` count. Measure nearness by *edit distance*: the minimum number of deletions, transpositions, insertions, or replacements of characters. By trial and error, we determine that going out to edit distance 2 will give us reasonable results. Then we can define `correct(`*w*`)`:\n \n \n",
"_____no_output_____"
]
],
[
[
"def correct(word):\n \"Find the best spelling correction for this word.\"\n # Prefer edit distance 0, then 1, then 2; otherwise default to word itself.\n candidates = (known(edits0(word)) or \n known(edits1(word)) or \n known(edits2(word)) or \n [word])\n return max(candidates, key=COUNTS.get)",
"_____no_output_____"
]
],
[
[
"The functions `known` and `edits0` are easy; and `edits2` is easy if we assume we have `edits1`:",
"_____no_output_____"
]
],
[
[
"def known(words):\n \"Return the subset of words that are actually in the dictionary.\"\n return {w for w in words if w in COUNTS}\n\ndef edits0(word): \n \"Return all strings that are zero edits away from word (i.e., just word itself).\"\n return {word}\n\ndef edits2(word):\n \"Return all strings that are two edits away from this word.\"\n return {e2 for e1 in edits1(word) for e2 in edits1(e1)}",
"_____no_output_____"
]
],
[
[
"Now for `edits1(word)`: the set of candidate words that are one edit away. For example, given `\"wird\"`, this would include `\"weird\"` (inserting an `e`) and `\"word\"` (replacing a `i` with a `o`), and also `\"iwrd\"` (transposing `w` and `i`; then `known` can be used to filter this out of the set of final candidates). How could we get them? One way is to *split* the original word in all possible places, each split forming a *pair* of words, `(a, b)`, before and after the place, and at each place, either delete, transpose, replace, or insert a letter:\n\n<table>\n <tr><td> pairs: <td><tt> Ø+wird <td><tt> w+ird <td><tt> wi+rd <td><tt>wir+d<td><tt>wird+Ø<td><i>Notes:</i><tt> (<i>a</i>, <i>b</i>)</tt> pair</i>\n <tr><td> deletions: <td><tt>Ø+ird<td><tt> w+rd<td><tt> wi+d<td><tt> wir+Ø<td><td><i>Delete first char of b</i>\n <tr><td> transpositions: <td><tt>Ø+iwrd<td><tt> w+rid<td><tt> wi+dr</tt><td><td><td><i>Swap first two chars of b\n <tr><td> replacements: <td><tt>Ø+?ird<td><tt> w+?rd<td><tt> wi+?d<td><tt> wir+?</tt><td><td><i>Replace char at start of b\n <tr><td> insertions: <td><tt>Ø+?+wird<td><tt> w+?+ird<td><tt> wi+?+rd<td><tt> wir+?+d<td><tt> wird+?+Ø</tt><td><i>Insert char between a and b\n</table>",
"_____no_output_____"
]
],
[
[
"def edits1(word):\n \"Return all strings that are one edit away from this word.\"\n pairs = splits(word)\n deletes = [a+b[1:] for (a, b) in pairs if b]\n transposes = [a+b[1]+b[0]+b[2:] for (a, b) in pairs if len(b) > 1]\n replaces = [a+c+b[1:] for (a, b) in pairs for c in alphabet if b]\n inserts = [a+c+b for (a, b) in pairs for c in alphabet]\n return set(deletes + transposes + replaces + inserts)\n\ndef splits(word):\n \"Return a list of all possible (first, rest) pairs that comprise word.\"\n return [(word[:i], word[i:]) \n for i in range(len(word)+1)]\n\nalphabet = 'abcdefghijklmnopqrstuvwxyz'",
"_____no_output_____"
],
[
"splits('wird')",
"_____no_output_____"
],
[
"print edits0('wird')",
"set(['wird'])\n"
],
[
"print edits1('wird')",
"set(['wirdh', 'wirdw', 'jird', 'wiid', 'wirj', 'wiprd', 'rird', 'wkird', 'wiqrd', 'wrird', 'wisrd', 'zwird', 'wiqd', 'wizrd', 'wirs', 'wrd', 'wqird', 'tird', 'wirdp', 'wrrd', 'wzrd', 'wiad', 'nird', 'wirsd', 'wixd', 'wxird', 'lird', 'eird', 'wmird', 'wihd', 'wirp', 'lwird', 'wirzd', 'widrd', 'wxrd', 'ewird', 'wirdx', 'wirkd', 'hwird', 'wipd', 'wirnd', 'uwird', 'wirz', 'mwird', 'wjrd', 'wirjd', 'wirrd', 'wirdd', 'wsird', 'bwird', 'wcrd', 'xwird', 'wdird', 'wibrd', 'wikd', 'wiryd', 'wiord', 'gird', 'wtird', 'wbrd', 'nwird', 'wlrd', 'wgird', 'wmrd', 'wirf', 'wirg', 'wird', 'wire', 'wirb', 'wirc', 'wira', 'wkrd', 'wiro', 'wirl', 'wirm', 'iird', 'wirk', 'wirh', 'wiri', 'wirv', 'wirw', 'wirt', 'wiru', 'wirr', 'wicd', 'cird', 'wirq', 'wirqd', 'wizd', 'wirhd', 'ird', 'bird', 'wirx', 'wiry', 'wvrd', 'widr', 'wprd', 'wirad', 'wijd', 'wirxd', 'uird', 'wirdb', 'qwird', 'dird', 'wnrd', 'wjird', 'gwird', 'whrd', 'wtrd', 'woird', 'rwird', 'wurd', 'wijrd', 'witrd', 'wwrd', 'dwird', 'vwird', 'wibd', 'wiyd', 'wicrd', 'weird', 'yird', 'wiwrd', 'wirdv', 'wirdu', 'wid', 'wirds', 'wirdr', 'sird', 'wirbd', 'wirdq', 'wirdy', 'wivrd', 'wirdg', 'wirdf', 'wirde', 'wiud', 'wirdc', 'wir', 'wirda', 'wfird', 'kwird', 'wirdn', 'wirdm', 'wirdl', 'wirdk', 'wirdj', 'wiird', 'wnird', 'wiurd', 'wied', 'aird', 'wirod', 'wpird', 'wcird', 'wzird', 'jwird', 'wirdo', 'wsrd', 'wimd', 'wirwd', 'mird', 'fird', 'wuird', 'wirdt', 'wired', 'wirgd', 'wirfd', 'witd', 'wfrd', 'wyrd', 'wihrd', 'zird', 'ward', 'wilrd', 'widd', 'iwrd', 'hird', 'word', 'wisd', 'wvird', 'pird', 'wlird', 'wyird', 'wdrd', 'werd', 'wild', 'oird', 'wirid', 'wgrd', 'wirvd', 'wiod', 'wirud', 'wircd', 'wiyrd', 'wigrd', 'wixrd', 'wiard', 'vird', 'wiwd', 'wigd', 'wirmd', 'swird', 'wierd', 'xird', 'qird', 'waird', 'wqrd', 'kird', 'cwird', 'wirtd', 'wirdz', 'awird', 'fwird', 'wirpd', 'wifrd', 'pwird', 'owird', 'wivd', 'wimrd', 'iwird', 'winrd', 'wirdi', 'wrid', 'wifd', 'wirld', 'wwird', 'ywird', 'wirn', 'wbird', 'whird', 'wikrd', 'wind', 'twird'])\n"
],
[
"print len(edits2('wird'))",
"24254\n"
],
[
"map(correct, tokens('Speling errurs in somethink. Whutever; unusuel misteakes everyware?'))",
"_____no_output_____"
]
],
[
[
"Can we make the output prettier than that?",
"_____no_output_____"
]
],
[
[
"def correct_text(text):\n \"Correct all the words within a text, returning the corrected text.\"\n return re.sub('[a-zA-Z]+', correct_match, text)\n\ndef correct_match(match):\n \"Spell-correct word in match, and preserve proper upper/lower/title case.\"\n word = match.group()\n return case_of(word)(correct(word.lower()))\n\ndef case_of(text):\n \"Return the case-function appropriate for text: upper, lower, title, or just str.\"\n return (str.upper if text.isupper() else\n str.lower if text.islower() else\n str.title if text.istitle() else\n str)",
"_____no_output_____"
],
[
"map(case_of, ['UPPER', 'lower', 'Title', 'CamelCase'])",
"_____no_output_____"
],
[
"correct_text('Speling Errurs IN somethink. Whutever; unusuel misteakes?')",
"_____no_output_____"
],
[
"correct_text('Audiance sayzs: tumblr ...')",
"_____no_output_____"
]
],
[
[
"So far so good. You can probably think of a dozen ways to make this better. Here's one: in the text \"three, too, one, blastoff!\" we might want to correct \"too\" with \"two\", even though \"too\" is in the dictionary. We can do better if we look at a *sequence* of words, not just an individual word one at a time. But how can we choose the best corrections of a sequence? The ad-hoc approach worked pretty well for single words, but now we could use some real theory ...",
"_____no_output_____"
],
[
"(4) Models: Word and Sequence Probabilities\n===\n\nIf we have a bag of words, what's the probability of picking a particular word out of the bag? We'll denote that probability as $P(w)$. To create the function `P` that computes this probability, we define a function, `pdist`, that takes as input a `Counter` (that is, a bag of words) and returns a function that acts as a probability distribution over all possible words. In a probability distribution the probability of each word is between 0 and 1, and the sum of the probabilities is 1.",
"_____no_output_____"
]
],
[
[
"def pdist(counter):\n \"Make a probability distribution, given evidence from a Counter.\"\n N = sum(counter.values())\n return lambda x: counter[x]/N\n\nPword = pdist(COUNTS)",
"_____no_output_____"
],
[
"# Print probabilities of some words\nfor w in tokens('\"The\" is most common word in English'):\n print Pword(w), w",
"0.0724106075672 the\n0.00884356018896 is\n0.000821562579453 most\n0.000259678921039 common\n0.000269631771671 word\n0.0199482270806 in\n0.000190913771217 english\n"
]
],
[
[
"Now, what is the probability of a *sequence* of words? Use the definition of a joint probability:\n\n$P(w_1 \\ldots w_n) = P(w_1) \\times P(w_2 \\mid w_1) \\times P(w_3 \\mid w_1 w_2) \\ldots \\times \\ldots P(w_n \\mid w_1 \\ldots w_{n-1})$\n\nIn the bag of words model, each word is drawn from the bag *independently* of the others. So $P(w_2 \\mid w_1) = P(w_2)$, and we have:\n \n$P(w_1 \\ldots w_n) = P(w_1) \\times P(w_2) \\times P(w_3) \\ldots \\times \\ldots P(w_n)$\n\n \n<img src=\"http://upload.wikimedia.org/wikipedia/commons/thumb/a/a2/GeorgeEPBox.jpg/200px-GeorgeEPBox.jpg\"> \n\nNow clearly this model is wrong; the probability of a sequence depends on the order of the words. But, as the statistician George Box said, *All models are wrong, but some are useful.* The bag of words model, wrong as it is, has many useful applications.\n \nHow can we compute $P(w_1 \\ldots w_n)$? We'll use a different function name, `Pwords`, rather than `P`, and we compute the product of the individual probabilities:",
"_____no_output_____"
]
],
[
[
"def Pwords(words):\n \"Probability of words, assuming each word is independent of others.\"\n return product(Pword(w) for w in words)\n\ndef product(nums):\n \"Multiply the numbers together. (Like `sum`, but with multiplication.)\"\n result = 1\n for x in nums:\n result *= x\n return result",
"_____no_output_____"
],
[
"tests = ['this is a test', \n 'this is a unusual test',\n 'this is a neverbeforeseen test']\n\nfor test in tests:\n print Pwords(tokens(test)), test",
"2.98419543271e-11 this is a test\n8.64036404331e-16 this is a unusual test\n0.0 this is a neverbeforeseen test\n"
]
],
[
[
"Yikes—it seems wrong to give a probability of 0 to the last one; it should just be very small. We'll come back to that later. The other probabilities seem reasonable.",
"_____no_output_____"
],
[
"(5) Task: Word Segmentation\n====\n\n**Task**: *given a sequence of characters with no spaces separating words, recover the sequence of words.*\n \n\nWhy? Languages with no word delimiters: [不带空格的词](http://translate.google.com/#auto/en/%E4%B8%8D%E5%B8%A6%E7%A9%BA%E6%A0%BC%E7%9A%84%E8%AF%8D)\n\nIn English, sub-genres with no word delimiters ([spelling errors](https://www.google.com/search?q=wordstogether), [URLs](http://speedofart.com)).\n\n**Approach 1:** Enumerate all candidate segementations and choose the one with highest Pwords\n\nProblem: how many segmentations are there for an *n*-character text?\n\n**Approach 2:** Make one segmentation, into a first word and remaining characters. If we assume words are independent \nthen we can maximize the probability of the first word adjoined to the best segmentation of the remaining characters.\n \n assert segment('choosespain') == ['choose', 'spain']\n\n segment('choosespain') ==\n max(Pwords(['c'] + segment('hoosespain')),\n Pwords(['ch'] + segment('oosespain')),\n Pwords(['cho'] + segment('osespain')),\n Pwords(['choo'] + segment('sespain')),\n ...\n Pwords(['choosespain'] + segment('')))\n \n \n \nTo make this somewhat efficient, we need to avoid re-computing the segmentations of the remaining characters. This can be done explicitly by *dynamic programming* or implicitly with *memoization*. Also, we shouldn't consider all possible lengths for the first word; we can impose a maximum length. What should it be? A little more than the longest word seen so far.",
"_____no_output_____"
]
],
[
[
"def memo(f):\n \"Memoize function f, whose args must all be hashable.\"\n cache = {}\n def fmemo(*args):\n if args not in cache:\n cache[args] = f(*args)\n return cache[args]\n fmemo.cache = cache\n return fmemo",
"_____no_output_____"
],
[
"max(len(w) for w in COUNTS)",
"_____no_output_____"
],
[
"def splits(text, start=0, L=20):\n \"Return a list of all (first, rest) pairs; start <= len(first) <= L.\"\n return [(text[:i], text[i:]) \n for i in range(start, min(len(text), L)+1)]",
"_____no_output_____"
],
[
"print splits('word')\nprint splits('reallylongtext', 1, 4)",
"[('', 'word'), ('w', 'ord'), ('wo', 'rd'), ('wor', 'd'), ('word', '')]\n[('r', 'eallylongtext'), ('re', 'allylongtext'), ('rea', 'llylongtext'), ('real', 'lylongtext')]\n"
],
[
"@memo\ndef segment(text):\n \"Return a list of words that is the most probable segmentation of text.\"\n if not text: \n return []\n else:\n candidates = ([first] + segment(rest) \n for (first, rest) in splits(text, 1))\n return max(candidates, key=Pwords)",
"_____no_output_____"
],
[
"segment('choosespain')",
"_____no_output_____"
],
[
"segment('speedofart')",
"_____no_output_____"
],
[
"decl = ('wheninthecourseofhumaneventsitbecomesnecessaryforonepeople' +\n 'todissolvethepoliticalbandswhichhaveconnectedthemwithanother' +\n 'andtoassumeamongthepowersoftheearththeseparateandequalstation' +\n 'towhichthelawsofnatureandofnaturesgodentitlethem')",
"_____no_output_____"
],
[
"print(' '.join(segment(decl)))",
"when in the course of human events it becomes necessary for one people to dissolve the political bands which have connected them with another and to assume among the powers of the earth the separate and equal station to which the laws of nature and of natures god entitle them\n"
],
[
"Pwords(segment(decl))",
"_____no_output_____"
],
[
"Pwords(segment(decl+decl))",
"_____no_output_____"
],
[
"Pwords(segment(decl+decl+decl))",
"_____no_output_____"
]
],
[
[
"That's a problem. We'll come back to it later.",
"_____no_output_____"
]
],
[
[
"segment('smallandinsignificant')",
"_____no_output_____"
],
[
"segment('largeandinsignificant')",
"_____no_output_____"
],
[
"print(Pwords(['large', 'and', 'insignificant']))\nprint(Pwords(['large', 'and', 'in', 'significant']))",
"4.1121373609e-10\n1.06638804821e-11\n"
]
],
[
[
"Summary:\n \n- Looks pretty good!\n- The bag-of-words assumption is a limitation.\n- Recomputing Pwords on each recursive call is somewhat inefficient.\n- Numeric underflow for texts longer than 100 or so words; we'll need to use logarithms, or other tricks.\n",
"_____no_output_____"
],
[
"# (6) Data: Mo' Data, Mo' Better",
"_____no_output_____"
],
[
"Let's move up from millions to *billions and billions* of words. Once we have that amount of data, we can start to look at two word sequences, without them being too sparse. I happen to have data files available in the format of `\"word \\t count\"`, and bigram data in the form of `\"word1 word2 \\t count\"`. Let's arrange to read them in:",
"_____no_output_____"
]
],
[
[
"def load_counts(filename, sep='\\t'):\n \"\"\"Return a Counter initialized from key-value pairs, \n one on each line of filename.\"\"\"\n C = Counter()\n for line in open(filename):\n key, count = line.split(sep)\n C[key] = int(count)\n return C",
"_____no_output_____"
],
[
"COUNTS1 = load_counts('count_1w.txt')\nCOUNTS2 = load_counts('count_2w.txt')\n\nP1w = pdist(COUNTS1)\nP2w = pdist(COUNTS2)",
"_____no_output_____"
],
[
"print len(COUNTS1), sum(COUNTS1.values())/1e9\nprint len(COUNTS2), sum(COUNTS2.values())/1e9",
"333333 588.124220187\n286358 225.955251755\n"
],
[
"COUNTS2.most_common(30)",
"_____no_output_____"
]
],
[
[
"(7) Theory and Practice: Segmentation With Bigram Data\n===\n\nA less-wrong approximation:\n \n$P(w_1 \\ldots w_n) = P(w_1 \\mid start) \\times P(w_2 \\mid w_1) \\times P(w_3 \\mid w_2) \\ldots \\times \\ldots P(w_n \\mid w_{n-1})$\n\nThis is called the *bigram* model, and is equivalent to taking a text, cutting it up into slips of paper with two words on them, and having multiple bags, and putting each slip into a bag labelled with the first word on the slip. Then, to generate language, we choose the first word from the original single bag of words, and chose all subsequent words from the bag with the label of the previously-chosen word. To determine the probability of a word sequence, we multiply together the conditional probabilities of each word given the previous word. We'll do this with a function, `cPword` for \"conditional probability of a word.\"\n\n$P(w_n \\mid w_{n-1}) = P(w_{n-1}w_n) / P(w_{n-1}) $",
"_____no_output_____"
]
],
[
[
"def Pwords2(words, prev='<S>'):\n \"The probability of a sequence of words, using bigram data, given prev word.\"\n return product(cPword(w, (prev if (i == 0) else words[i-1]) )\n for (i, w) in enumerate(words))\n\nP = P1w # Use the big dictionary for the probability of a word\n\ndef cPword(word, prev):\n \"Conditional probability of word, given previous word.\"\n bigram = prev + ' ' + word\n if P2w(bigram) > 0 and P(prev) > 0:\n return P2w(bigram) / P(prev)\n else: # Average the back-off value and zero.\n return P(word) / 2",
"_____no_output_____"
],
[
"print Pwords(tokens('this is a test'))\nprint Pwords2(tokens('this is a test'))\nprint Pwords2(tokens('is test a this'))",
"2.98419543271e-11\n6.41367629438e-08\n1.18028600367e-11\n"
]
],
[
[
"To make `segment2`, we copy `segment`, and make sure to pass around the previous token, and to evaluate probabilities with `Pwords2` instead of `Pwords`.",
"_____no_output_____"
]
],
[
[
"@memo \ndef segment2(text, prev='<S>'): \n \"Return best segmentation of text; use bigram data.\" \n if not text: \n return []\n else:\n candidates = ([first] + segment2(rest, first) \n for (first, rest) in splits(text, 1))\n return max(candidates, key=lambda words: Pwords2(words, prev))",
"_____no_output_____"
],
[
"print segment2('choosespain')\nprint segment2('speedofart')\nprint segment2('smallandinsignificant')\nprint segment2('largeandinsignificant')",
"['choose', 'spain']\n['speed', 'of', 'art']\n['small', 'and', 'in', 'significant']\n['large', 'and', 'in', 'significant']\n"
],
[
"adams = ('faroutintheunchartedbackwatersoftheunfashionableendofthewesternspiral' +\n 'armofthegalaxyliesasmallunregardedyellowsun')\nprint segment(adams)\nprint segment2(adams)",
"['far', 'out', 'in', 'the', 'un', 'chart', 'ed', 'back', 'waters', 'of', 'the', 'un', 'fashionable', 'end', 'of', 'the', 'western', 'spiral', 'arm', 'of', 'the', 'galaxy', 'lies', 'a', 'small', 'un', 'regarded', 'yellow', 'sun']\n['far', 'out', 'in', 'the', 'uncharted', 'backwaters', 'of', 'the', 'unfashionable', 'end', 'of', 'the', 'western', 'spiral', 'arm', 'of', 'the', 'galaxy', 'lies', 'a', 'small', 'un', 'regarded', 'yellow', 'sun']\n"
],
[
"P1w('unregarded')",
"_____no_output_____"
],
[
"tolkein = 'adrybaresandyholewithnothinginittositdownonortoeat'\nprint segment(tolkein)\nprint segment2(tolkein)",
"['a', 'dry', 'bare', 'sandy', 'hole', 'with', 'nothing', 'in', 'it', 'to', 'sit', 'down', 'on', 'or', 'to', 'eat']\n['a', 'dry', 'bare', 'sandy', 'hole', 'with', 'nothing', 'in', 'it', 'to', 'sit', 'down', 'on', 'or', 'to', 'eat']\n"
]
],
[
[
"Conclusion? Bigram model is a little better, but not much. Hundreds of billions of words still not enough. (Why not trillions?) Could be made more efficient.",
"_____no_output_____"
],
[
"(8) Theory: Evaluation\n===\n\nSo far, we've got an intuitive feel for how this all works. But we don't have any solid metrics that quantify the results. Without metrics, we can't say if we are doing well, nor if a change is an improvement. In general,\nwhen developing a program that relies on data to help make\npredictions, it is good practice to divide your data into three sets:\n<ol>\n <li> <b>Training set:</b> the data used to create our spelling\n model; this was the <tt>big.txt</tt> file.\n <li> <b>Development set:</b> a set of input/output pairs that we can\n use to rank the performance of our program as we are developing it.\n <li> <b>Test set:</b> another set of input/output pairs that we use\n to rank our program <i>after</i> we are done developing it. The\n development set can't be used for this purpose—once the\n programmer has looked at the development test it is tainted, because\n the programmer might modify the program just to pass the development\n test. That's why we need a separate test set that is only looked at\n after development is done.\n</ol>\n\nFor this program, the training data is the word frequency counts, the development set is the examples like `\"choosespain\"` that we have been playing with, and now we need a test set.",
"_____no_output_____"
]
],
[
[
"def test_segmenter(segmenter, tests):\n \"Try segmenter on tests; report failures; return fraction correct.\"\n return sum([test_one_segment(segmenter, test) \n for test in tests]), len(tests)\n\ndef test_one_segment(segmenter, test):\n words = tokens(test)\n result = segmenter(cat(words))\n correct = (result == words)\n if not correct:\n print 'expected', words\n print ' got', result\n return correct\n\ncat = ''.join\n\nproverbs = (\"\"\"A little knowledge is a dangerous thing\n A man who is his own lawyer has a fool for his client\n All work and no play makes Jack a dull boy\n Better to remain silent and be thought a fool that to speak and remove all doubt;\n Do unto others as you would have them do to you\n Early to bed and early to rise, makes a man healthy, wealthy and wise\n Fools rush in where angels fear to tread\n Genius is one percent inspiration, ninety-nine percent perspiration\n If you lie down with dogs, you will get up with fleas\n Lightning never strikes twice in the same place\n Power corrupts; absolute power corrupts absolutely\n Here today, gone tomorrow\n See no evil, hear no evil, speak no evil\n Sticks and stones may break my bones, but words will never hurt me\n Take care of the pence and the pounds will take care of themselves\n Take care of the sense and the sounds will take care of themselves\n The bigger they are, the harder they fall\n The grass is always greener on the other side of the fence\n The more things change, the more they stay the same\n Those who do not learn from history are doomed to repeat it\"\"\"\n .splitlines())",
"_____no_output_____"
],
[
"test_segmenter(segment, proverbs)",
"expected ['power', 'corrupts', 'absolute', 'power', 'corrupts', 'absolutely']\n got ['power', 'corrupt', 's', 'absolute', 'power', 'corrupt', 's', 'absolutely']\nexpected ['the', 'grass', 'is', 'always', 'greener', 'on', 'the', 'other', 'side', 'of', 'the', 'fence']\n got ['the', 'grass', 'is', 'always', 'green', 'er', 'on', 'the', 'other', 'side', 'of', 'the', 'fence']\n"
],
[
"test_segmenter(segment2, proverbs)",
"_____no_output_____"
]
],
[
[
"This confirms that both segmenters are very good, and that `segment2` is slightly better. There is much more that can be done in terms of the variety of tests, and in measuring statistical significance.",
"_____no_output_____"
],
[
"(9) Theory and Practice: Smoothing\n======\n\nLet's go back to a test we did before, and add some more test cases:\n",
"_____no_output_____"
]
],
[
[
"tests = ['this is a test', \n 'this is a unusual test',\n 'this is a nongovernmental test',\n 'this is a neverbeforeseen test',\n 'this is a zqbhjhsyefvvjqc test']\n\nfor test in tests:\n print Pwords(tokens(test)), test",
"2.98419543271e-11 this is a test\n8.64036404331e-16 this is a unusual test\n0.0 this is a nongovernmental test\n0.0 this is a neverbeforeseen test\n0.0 this is a zqbhjhsyefvvjqc test\n"
]
],
[
[
"The issue here is the finality of a probability of zero. Out of the three 15-letter words, it turns out that \"nongovernmental\" is in the dictionary, but if it hadn't been, if somehow our corpus of words had missed it, then the probability of that whole phrase would have been zero. It seems that is too strict; there must be some \"real\" words that are not in our dictionary, so we shouldn't give them probability zero. There is also a question of likelyhood of being a \"real\" word. It does seem that \"neverbeforeseen\" is more English-like than \"zqbhjhsyefvvjqc\", and so perhaps should have a higher probability.\n\nWe can address this by assigning a non-zero probability to words that are not in the dictionary. This is even more important when it comes to multi-word phrases (such as bigrams), because it is more likely that a legitimate one will appear that has not been observed before.\n\nWe can think of our model as being overly spiky; it has a spike of probability mass wherever a word or phrase occurs in the corpus. What we would like to do is *smooth* over those spikes so that we get a model that does not depend on the details of our corpus. The process of \"fixing\" the model is called *smoothing*.\n\nFor example, Laplace was asked what's the probability of the sun rising tomorrow. From data that it has risen $n/n$ times for the last *n* days, the maximum liklihood estimator is 1. But Laplace wanted to balance the data with the possibility that tomorrow, either it will rise or it won't, so he came up with $(n + 1) / (n + 2)$.\n\n\n<img src=\"http://upload.wikimedia.org/wikipedia/commons/thumb/e/e3/Pierre-Simon_Laplace.jpg/220px-Pierre-Simon_Laplace.jpg\" height=150 width=110> \n \n<img src=\"http://www.hdwallpapers.in/walls/notre_dame_at_sunrise_paris_france-normal.jpg\" width=200 height=150>\n<br><i>What we know is little, and what we are ignorant of is immense.<i><br>— Pierre Simon Laplace, 1749-1827",
"_____no_output_____"
]
],
[
[
"def pdist_additive_smoothed(counter, c=1):\n \"\"\"The probability of word, given evidence from the counter.\n Add c to the count for each item, plus the 'unknown' item.\"\"\"\n N = sum(counter.values()) # Amount of evidence\n Nplus = N + c * (len(counter) + 1) # Evidence plus fake observations\n return lambda word: (counter[word] + c) / Nplus \n\nP1w = pdist_additive_smoothed(COUNTS1)",
"_____no_output_____"
],
[
"P1w('neverbeforeseen')",
"_____no_output_____"
]
],
[
[
"But now there's a problem ... we now have previously-unseen words with non-zero probabilities. And maybe 10<sup>-12</sup> is about right for words that are observed in text: that is, if I'm *reading* a new text, the probability that the next word is unknown might be around 10<sup>-12</sup>. But if I'm *manufacturing* 20-letter sequences at random, the probability that one will be a word is much, much lower than 10<sup>-12</sup>. \n\nLook what happens:",
"_____no_output_____"
]
],
[
[
"segment.cache.clear()\nsegment('thisisatestofsegmentationofalongsequenceofwords')",
"_____no_output_____"
]
],
[
[
"There are two problems:\n \nFirst, we don't have a clear model of the unknown words. We just say \"unknown\" but\nwe don't distinguish likely unknown from unlikely unknown. For example, is a 8-character unknown more likely than a 20-character unknown?\n\nSecond, we don't take into account evidence from *parts* of the unknown. For example, \n\"unglobulate\" versus \"zxfkogultae\".\n\nFor our next approach, *Good - Turing* smoothing re-estimates the probability of zero-count words, based on the probability of one-count words (and can also re-estimate for higher-number counts, but that is less interesting).\n\n<img src=\"http://upload.wikimedia.org/wikipedia/en/b/b4/I._J._Good.jpg\">\n<img src=\"http://upload.wikimedia.org/wikipedia/en/thumb/c/c8/Alan_Turing_photo.jpg/200px-Alan_Turing_photo.jpg\" height=144>\n<br><i>I. J. Good (1916 - 2009) Alan Turing (1812 - 1954)</i>\n\nSo, how many one-count words are there in `COUNTS`? (There aren't any in `COUNTS1`.) And what are the word lengths of them? Let's find out:\n",
"_____no_output_____"
]
],
[
[
"singletons = (w for w in COUNTS if COUNTS[w] == 1)\n\nlengths = map(len, singletons)\n\nCounter(lengths).most_common()",
"_____no_output_____"
],
[
"1357 / sum(COUNTS.values())",
"_____no_output_____"
],
[
"hist(lengths, bins=len(set(lengths)));",
"_____no_output_____"
],
[
"def pdist_good_turing_hack(counter, onecounter, base=1/26., prior=1e-8):\n \"\"\"The probability of word, given evidence from the counter.\n For unknown words, look at the one-counts from onecounter, based on length.\n This gets ideas from Good-Turing, but doesn't implement all of it.\n prior is an additional factor to make unknowns less likely.\n base is how much we attenuate probability for each letter beyond longest.\"\"\"\n N = sum(counter.values())\n N2 = sum(onecounter.values())\n lengths = map(len, [w for w in onecounter if onecounter[w] == 1])\n ones = Counter(lengths)\n longest = max(ones)\n return (lambda word: \n counter[word] / N if (word in counter) \n else prior * (ones[len(word)] / N2 or \n ones[longest] / N2 * base ** (len(word)-longest)))\n\n# Redefine P1w\nP1w = pdist_good_turing_hack(COUNTS1, COUNTS)",
"_____no_output_____"
],
[
"segment.cache.clear()\nsegment('thisisatestofsegmentationofaverylongsequenceofwords')",
"_____no_output_____"
]
],
[
[
"That was somewhat unsatisfactory. We really had to crank up the prior, specifically because the process of running `segment` generates so many non-word candidates (and also because there will be fewer unknowns with respect to the billion-word `WORDS1` than with respect to the million-word `WORDS`). It would be better to separate out the prior from the word distribution, so that the same distribution could be used for multiple tasks, not just for this one.\n\nNow let's think for a short while about smoothing **bigram** counts. Specifically, what if we haven't seen a bigram sequence, but we've seen both words individually? For example, to evaluate P(\"Greenland\") in the phrase \"turn left at Greenland\", we might have three pieces of evidence:\n\n P(\"Greenland\")\n P(\"Greenland\" | \"at\")\n P(\"Greenland\" | \"left\", \"at\")\n \nPresumably, the first would have a relatively large count, and thus large reliability, while the second and third would have decreasing counts and reliability. With *interpolation smoothing* we combine all three pieces of evidence, with a linear combination:\n \n$P(w_3 \\mid w_1w_2) = c_1 P(w_3) + c_2 P(w_3 \\mid w_2) + c_3 P(w_3 \\mid w_1w_2)$\n\nHow do we choose $c_1, c_2, c_3$? By experiment: train on training data, maximize $c$ values on development data, then evaluate on test data.\n \nHowever, when we do this, we are saying, with probability $c_1$, that a word can appear anywhere, regardless of previous words. But some words are more free to do that than other words. Consider two words with similar probability:",
"_____no_output_____"
]
],
[
[
"print P1w('francisco')\nprint P1w('individuals')",
"7.73314623661e-05\n7.72494966889e-05\n"
]
],
[
[
"They have similar unigram probabilities but differ in their freedom to be the second word of a bigram:",
"_____no_output_____"
]
],
[
[
"print [bigram for bigram in COUNTS2 if bigram.endswith('francisco')]",
"['San francisco', 'san francisco']\n"
],
[
"print [bigram for bigram in COUNTS2 if bigram.endswith('individuals')]",
"['are individuals', 'other individuals', 'on individuals', 'infected individuals', 'in individuals', 'where individuals', 'or individuals', 'which individuals', 'to individuals', 'both individuals', 'help individuals', 'more individuals', 'interested individuals', 'from individuals', '<S> individuals', 'income individuals', 'these individuals', 'about individuals', 'the individuals', 'among individuals', 'some individuals', 'those individuals', 'by individuals', 'minded individuals', 'These individuals', 'qualified individuals', 'certain individuals', 'different individuals', 'For individuals', 'few individuals', 'and individuals', 'two individuals', 'for individuals', 'between individuals', 'affected individuals', 'healthy individuals', 'private individuals', 'with individuals', 'following individuals', 'as individuals', 'such individuals', 'that individuals', 'all individuals', 'of individuals', 'many individuals']\n"
]
],
[
[
"Intuitively, words that appear in many bigrams before are more likely to appear in a new, previously unseen bigram. In *Kneser-Ney* smoothing (Reinhard Kneser, Hermann Ney) we multiply the bigram counts by this ratio. But I won't implement that here, because The Count never covered it.\n\n(10) One More Task: Secret Codes\n===\n\nLet's tackle one more task: decoding secret codes. We'll start with the simplest of codes, a rotation cipher, sometimes called a shift cipher or a Caesar cipher (because this was state-of-the-art crypotgraphy in 100 BC). First, a method to encode:",
"_____no_output_____"
]
],
[
[
"def rot(msg, n=13): \n \"Encode a message with a rotation (Caesar) cipher.\" \n return encode(msg, alphabet[n:]+alphabet[:n])\n\ndef encode(msg, key): \n \"Encode a message with a substitution cipher.\" \n table = string.maketrans(upperlower(alphabet), upperlower(key))\n return msg.translate(table) \n\ndef upperlower(text): return text.upper() + text.lower() ",
"_____no_output_____"
],
[
"rot('This is a secret message.', 1)",
"_____no_output_____"
],
[
"rot('This is a secret message.', 13)",
"_____no_output_____"
],
[
"rot(rot('This is a secret message.'))",
"_____no_output_____"
]
],
[
[
"Now decoding is easy: try all 26 candidates, and find the one with the maximum Pwords:",
"_____no_output_____"
]
],
[
[
"def decode_rot(secret):\n \"Decode a secret message that has been encoded with a rotation cipher.\"\n candidates = [rot(secret, i) for i in range(len(alphabet))]\n return max(candidates, key=lambda msg: Pwords(tokens(msg)))",
"_____no_output_____"
],
[
"msg = 'Who knows the answer?'\nsecret = rot(msg, 17)\n\nprint(secret)\nprint(decode_rot(secret))",
"Nyf befnj kyv rejnvi?\nWho knows the answer?\n"
]
],
[
[
"Let's make it a tiny bit harder. When the secret message contains separate words, it is too easy to decode by guessing that the one-letter words are most likely \"I\" or \"a\". So what if the encode routine mushed all the letters together:",
"_____no_output_____"
]
],
[
[
"def encode(msg, key): \n \"Encode a message with a substitution cipher; remove non-letters.\" \n msg = cat(tokens(msg)) ## Change here\n table = string.maketrans(upperlower(alphabet), upperlower(key))\n return msg.translate(table) ",
"_____no_output_____"
]
],
[
[
"Now we can decode by segmenting. We change candidates to be a list of segmentations, and still choose the candidate with the best Pwords: ",
"_____no_output_____"
]
],
[
[
"def decode_rot(secret):\n \"\"\"Decode a secret message that has been encoded with a rotation cipher,\n and which has had all the non-letters squeezed out.\"\"\"\n candidates = [segment(rot(secret, i)) for i in range(len(alphabet))]\n return max(candidates, key=lambda msg: Pwords(msg))",
"_____no_output_____"
],
[
"msg = 'Who knows the answer this time? Anyone? Bueller?'\nsecret = rot(msg, 19)\n\nprint(secret)\nprint(decode_rot(secret))",
"pahdghplmaxtglpxkmablmbfxtgrhgxunxeexk\n['who', 'knows', 'the', 'answer', 'this', 'time', 'anyone', 'bu', 'e', 'll', 'er']\n"
],
[
"candidates = [segment(rot(secret, i)) for i in range(len(alphabet))]\n\nfor c in candidates:\n print int(log10(Pwords(c))), ' '.join(c)",
"-123 p ah d g h p l max t g l p x km a bl mb f x t gr h g x un x e ex k\n-133 q b i eh i q m n by u hm q y l n b cm n c g y u h s i h y vo y ff y l\n-128 r c j f i jr no c z v in r z mo c d nod h z v it j i z w p z g g z m\n-115 sd k g j k so p da w j os an p de op e i a w j u k j ax q ah h an\n-144 t el h k l t p q e b x k p t b o q e f p q f j b x k v l k by r b ii b o\n-145 u f m il m u q r f cy l qu c p r f g q r g k cy l wm l c z s c j j c p\n-141 v g n j m n v r sg d z mr v d q sg h r s h l d z m x nm dat d k k d q\n-39 who knows the answer this time anyone bu e ll er\n-119 xi p l op x tu if b o t x f s u i j tu j n f b oz p of c v f mm f s\n-151 y j q m p q y u v j g c p u y g t v j ku v k o g c pa q pg d w g n n g t\n-151 z k r n q r z v w k h d q v z h u w k l v w l p h d q br q he x ho oh u\n-97 also r saw x lie r w a iv x l m w x m q i er c s r if y i pp iv\n-146 b mt p st b x y m j f s x b j w y m n x y n r j f sd t s j g z j q q j w\n-137 c n u q tu cy z n k g ty c k x z no y z os k g t eut k ha k r r k x\n-124 do v r u v d z a o l h u z d ly a op z apt l h u f v u li bl s sly\n-121 e p w s v we ab pm iv a em z b p q ab qu m iv g w v m j cm t tm z\n-142 f q x t w x f bc q n j w b fn a c q r bc r v n j wh x w n k d n u un a\n-119 gr y u x y g c dr ok x c go b dr s c d s w ok xi y x o leo v vo b\n-119 h s z vy z h des ply d h p c est de t x ply j z y pm f p w w p c\n-137 it a w z a i e ft q m ze i q d f tu e f u y q m z ka z q n g q xx q d\n-130 j u b x ab j f g u r na f jr eg u v f g v z r n alba r oh r y y re\n-125 k v cy bc k g h v sob g k s f h v w g h was o b m c b s p is z z s f\n-130 l w d z c d l hi w t p ch l t g i w x h ix b t p c nd c t q j t a at g\n-123 m x e a dem i j x u q dim u h j x y i j y c u q doe du r ku b bu h\n-124 ny f be fn j k y v re j n vi k y z j k z d v rep fe vs l v c c vi\n-134 oz g cf go k l z w s f k o w j l z ak la e w s f q g f w tm w d d w j\n"
]
],
[
[
"What about a general substitution cipher? The problem is that there are 26! substitution ciphers, and we can't enumerate all of them. We would need to search through this space. Initially make some guess at a substitution, then swap two letters; if that looks better keep going, if not try something else. This approach solves most substitution cipher problems, although it can take a few minutes on a message of length 100 words or so.\n\n(∞ and beyond) Where To Go Next\n===\n\nWhat to do next? Here are some options:\n \n- **Spelling correction**: Use bigram or trigram context; make a model of spelling errors/edit distance; go beyond edit distance 2; make it more efficient\n- **Evaluation**: Make a serious test suite; search for best parameters (e.g. $c_1, c_2, c_3$)\n- **Smoothing**: Implement Kneser-Ney and/or Interpolation; do letter *n*-gram-based smoothing\n- **Secret Codes**: Implement a search over substitution ciphers\n- **Classification**: Given a corpus of texts, each with a classification label, write a classifier that will take a new text and return a label. Examples: spam/no-spam; favorable/unfavorable; what author am I most like; reading level.\n- **Clustering**: Group data by similarity. Find synonyms/related words.\n- **Parsing**: Representing nested structures rather than linear sequences of words. relations between parts of the structure. Implicit missing bits. Inducing a grammar.\n- **Meaning**: What semantic relations are meant by the syntactic relations?\n- **Translation**: Using examples to transform one language into another.\n- **Question Answering**: Using examples to transfer a question into an answer, either by retrieving a passage, or by synthesizing one.\n- **Speech**: Dealing with analog audio signals rather than discrete sequences of characters.",
"_____no_output_____"
]
],
[
[
"correct('cpgratulations')",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7bb3bf61b46499d27c945dd9cbad3430959716a | 101,964 | ipynb | Jupyter Notebook | GomokuData.ipynb | smurve/DeepGomoku | ee679e7ec9caacc9c8f2b10c3a403d3f7d2625d8 | [
"Apache-2.0"
] | 1 | 2019-06-26T13:14:50.000Z | 2019-06-26T13:14:50.000Z | GomokuData.ipynb | smurve/DeepGomoku | ee679e7ec9caacc9c8f2b10c3a403d3f7d2625d8 | [
"Apache-2.0"
] | 3 | 2020-03-24T17:19:14.000Z | 2022-02-09T23:30:39.000Z | GomokuData.ipynb | smurve/DeepGomoku | ee679e7ec9caacc9c8f2b10c3a403d3f7d2625d8 | [
"Apache-2.0"
] | null | null | null | 223.115974 | 61,596 | 0.912145 | [
[
[
"%load_ext autoreload\n%autoreload 2",
"_____no_output_____"
],
[
"from __future__ import print_function, absolute_import, division",
"_____no_output_____"
],
[
"import numpy as np\nfrom wgomoku import GomokuBoard, GomokuTools as gt, roll_out, NH9x9, Heuristics, HeuristicGomokuPolicy, ThreatSearch\nimport random\nimport tensorflow as tf",
"_____no_output_____"
],
[
"tf.__version__",
"_____no_output_____"
],
[
"A,B,C,D,E,F,G,H,I,J,K,L,M,N,O,P,Q,R,S,T,U = \\\n 1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21\nBLACK=0\nWHITE=1\nEDGES=2\nSTYLE_MIXED=2",
"_____no_output_____"
],
[
"heuristics = Heuristics(kappa=3.0)",
"_____no_output_____"
]
],
[
[
"### Experimenting with smaller action spaces\nHere we look at the board (states) that all evolve from an initial board. We're going to train on all board snapshots of a series of heuristically played games.",
"_____no_output_____"
]
],
[
[
"def new_initial_state(heuristics):\n board = GomokuBoard(heuristics, N=15, disp_width=8)\n policy = HeuristicGomokuPolicy(style = 2, bias=.5, topn=5, \n threat_search=ThreatSearch(2,2))\n\n board.set(H,8).set('G',6).set(G,8).set(F,8).set(H,9).set(H,10) \n return board, policy",
"_____no_output_____"
],
[
"new_initial_state(heuristics)[0].display('current')",
"_____no_output_____"
],
[
"board, policy = new_initial_state(heuristics)\n_ = roll_out(board, policy, 40)\nboard.display('current')",
"_____no_output_____"
],
[
"stones = board.stones.copy()",
"_____no_output_____"
],
[
"from wgomoku import heuristic_QF, transform, create_sample, wrap_sample",
"_____no_output_____"
],
[
"sample = create_sample(board.stones.copy(), board.N, 1-board.current_color)",
"_____no_output_____"
],
[
"o,d = np.rollaxis(sample, 2, 0)\n2 * d + o",
"_____no_output_____"
],
[
"def create_samples_and_qvalues(board, heuristics):\n all_stones_t = [transform(board.stones.copy(), board.N, rot, ref) \n for rot in range(4)\n for ref in [False, True]]\n\n samples = []\n qvalues = []\n for stones_t in all_stones_t:\n sample = create_sample(stones_t, board.N, 1-board.current_color)\n board = GomokuBoard(heuristics=heuristics, N=board.N, stones=stones_t)\n policy = HeuristicGomokuPolicy(style = STYLE_MIXED, bias=.5, topn=5, \n threat_search=ThreatSearch(2,2))\n\n qvalue, default_value = heuristic_QF(board, policy)\n qvalue = wrap_sample(qvalue, default_value)\n samples.append(sample)\n qvalues.append(qvalue)\n\n return np.array(samples), np.reshape(qvalues, [8, board.N+2, board.N+2, 1])",
"_____no_output_____"
],
[
"samples, values = create_samples_and_qvalues(board, heuristics)",
"_____no_output_____"
],
[
"np.shape(samples),np.shape(values)",
"_____no_output_____"
],
[
"b, w = np.rollaxis(samples[3], 2, 0)\nb + 2*w",
"_____no_output_____"
]
],
[
[
"### Plausibility check",
"_____no_output_____"
]
],
[
[
"index = np.argmax(values[3])\nr, c = np.divmod(index,17)\npos=gt.m2b((r, c), 17)\nprint(r,c,pos, values[3][r][c])",
"_____no_output_____"
],
[
"from wgomoku import create_samples_and_qvalues",
"_____no_output_____"
],
[
"def data_from_game(board, policy, heuristics): \n \"\"\"\n Careful: This function rolls back the board\n \"\"\"\n s,v = create_samples_and_qvalues(board, heuristics)\n while board.cursor > 6:\n board.undo()\n s1, v1 = create_samples_and_qvalues(board, heuristics)\n s = np.concatenate((s,s1))\n v = np.concatenate((v,v1))\n return s,v",
"_____no_output_____"
],
[
"from wgomoku import data_from_game",
"_____no_output_____"
],
[
"data = data_from_game(board, policy, heuristics)",
"_____no_output_____"
],
[
"o,d = np.rollaxis(data[0][30], 2, 0)\n2 * d + o",
"_____no_output_____"
]
],
[
[
"#### Yet another plausibility check",
"_____no_output_____"
]
],
[
[
"sample_no = 30\nindex = np.argmax(data[1][sample_no])\nr, c = np.divmod(index,17)\npos=gt.m2b((r, c), 17)\nprint(r,c,pos)\nprint(\"Value of the suggested pos:\") \nprint(data[1][sample_no][r][c])\nprint(\"Value to the right of the suggested pos:\") \nprint(data[1][sample_no][r][c+1])",
"_____no_output_____"
]
],
[
[
"## Save the results for later training",
"_____no_output_____"
]
],
[
[
"np.save(\"samples.npy\", data[0])\nnp.save(\"values.npy\", data[1])",
"_____no_output_____"
],
[
"samples = np.load(\"samples.npy\")\nvalues = np.load(\"values.npy\")",
"_____no_output_____"
],
[
"samples.shape, values.shape",
"_____no_output_____"
],
[
"np.rollaxis(samples[0], 2, 0)",
"_____no_output_____"
],
[
"np.rollaxis(values[0], 2, 0)",
"_____no_output_____"
],
[
"np.rollaxis(samples[0], 2, 0)[0] + 2*np.rollaxis(samples[0], 2, 0)[1]",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bb3e76542755588031dedfede74cd8a249c9fe | 65,681 | ipynb | Jupyter Notebook | content/ch-quantum-hardware/randomized-benchmarking.ipynb | NunoEdgarGFlowHub/qiskit-textbook | 52a9f15d5217064e3885a277fd8a77d83e8a87a9 | [
"Apache-2.0"
] | 1 | 2020-05-14T12:15:02.000Z | 2020-05-14T12:15:02.000Z | content/ch-quantum-hardware/randomized-benchmarking.ipynb | NunoEdgarGFlowHub/qiskit-textbook | 52a9f15d5217064e3885a277fd8a77d83e8a87a9 | [
"Apache-2.0"
] | null | null | null | content/ch-quantum-hardware/randomized-benchmarking.ipynb | NunoEdgarGFlowHub/qiskit-textbook | 52a9f15d5217064e3885a277fd8a77d83e8a87a9 | [
"Apache-2.0"
] | null | null | null | 103.597792 | 43,468 | 0.826053 | [
[
[
"# Randomized Benchmarking",
"_____no_output_____"
],
[
"## Introduction\n\nOne of the main challenges in building a quantum information processor is the non-scalability of completely\ncharacterizing the noise affecting a quantum system via process tomography. In addition, process tomography is sensitive to noise in the pre- and post rotation gates plus the measurements (SPAM errors). Gateset tomography can take these errors into account, but the scaling is even worse. A complete characterization\nof the noise is useful because it allows for the determination of good error-correction schemes, and thus\nthe possibility of reliable transmission of quantum information.\n\nSince complete process tomography is infeasible for large systems, there is growing interest in scalable\nmethods for partially characterizing the noise affecting a quantum system. A scalable (in the number $n$ of qubits comprising the system) and robust algorithm for benchmarking the full set of Clifford gates by a single parameter using randomization techniques was presented in [1]. The concept of using randomization methods for benchmarking quantum gates is commonly called **Randomized Benchmarking\n(RB)**.\n\n\n### References\n\n1. Easwar Magesan, J. M. Gambetta, and Joseph Emerson, *Robust randomized benchmarking of quantum processes*,\nhttps://arxiv.org/pdf/1009.3639\n\n2. Easwar Magesan, Jay M. Gambetta, and Joseph Emerson, *Characterizing Quantum Gates via Randomized Benchmarking*,\nhttps://arxiv.org/pdf/1109.6887\n\n3. A. D. C'orcoles, Jay M. Gambetta, Jerry M. Chow, John A. Smolin, Matthew Ware, J. D. Strand, B. L. T. Plourde, and M. Steffen, *Process verification of two-qubit quantum gates by randomized benchmarking*, https://arxiv.org/pdf/1210.7011\n\n4. Jay M. Gambetta, A. D. C´orcoles, S. T. Merkel, B. R. Johnson, John A. Smolin, Jerry M. Chow,\nColm A. Ryan, Chad Rigetti, S. Poletto, Thomas A. Ohki, Mark B. Ketchen, and M. Steffen,\n*Characterization of addressability by simultaneous randomized benchmarking*, https://arxiv.org/pdf/1204.6308\n\n5. David C. McKay, Sarah Sheldon, John A. Smolin, Jerry M. Chow, and Jay M. Gambetta, *Three Qubit Randomized Benchmarking*, https://arxiv.org/pdf/1712.06550",
"_____no_output_____"
],
[
"## The Randomized Benchmarking Protocol\n\nA RB protocol (see [1,2]) consists of the following steps:\n\n(We should first import the relevant qiskit classes for the demonstration).",
"_____no_output_____"
]
],
[
[
"#Import general libraries (needed for functions)\nimport numpy as np\nimport matplotlib.pyplot as plt\nfrom IPython import display\n\n#Import the RB Functions\nimport qiskit.ignis.verification.randomized_benchmarking as rb\n\n#Import Qiskit classes \nimport qiskit\nfrom qiskit.providers.aer.noise import NoiseModel\nfrom qiskit.providers.aer.noise.errors.standard_errors import depolarizing_error, thermal_relaxation_error",
"_____no_output_____"
]
],
[
[
"### Step 1: Generate RB sequences\n\nThe RB sequences consist of random Clifford elements chosen uniformly from the Clifford group on $n$-qubits, \nincluding a computed reversal element,\nthat should return the qubits to the initial state.\n\nMore precisely, for each length $m$, we choose $K_m$ RB sequences. \nEach such sequence contains $m$ random elements $C_{i_j}$ chosen uniformly from the Clifford group on $n$-qubits, and the $m+1$ element is defined as follows: $C_{i_{m+1}} = (C_{i_1}\\cdot ... \\cdot C_{i_m})^{-1}$. It can be found efficiently by the Gottesmann-Knill theorem.\n\nFor example, we generate below several sequences of 2-qubit Clifford circuits.",
"_____no_output_____"
]
],
[
[
"#Generate RB circuits (2Q RB)\n\n#number of qubits\nnQ=2 \nrb_opts = {}\n#Number of Cliffords in the sequence\nrb_opts['length_vector'] = [1, 10, 20, 50, 75, 100, 125, 150, 175, 200]\n#Number of seeds (random sequences)\nrb_opts['nseeds'] = 5 \n#Default pattern\nrb_opts['rb_pattern'] = [[0,1]]\n\nrb_circs, xdata = rb.randomized_benchmarking_seq(**rb_opts)",
"Making the n=2 Clifford Table\n"
]
],
[
[
"As an example, we print the circuit corresponding to the first RB sequence",
"_____no_output_____"
]
],
[
[
"print(rb_circs[0][0])",
" ┌───┐┌───┐┌───┐ ░ ┌───┐┌─────┐┌───┐ ┌─┐ »\nqr_0: |0>──────────────■──┤ H ├┤ S ├┤ Z ├─░─┤ Z ├┤ Sdg ├┤ H ├──■───────┤M├─────»\n ┌─────┐┌───┐┌─┴─┐├───┤├───┤├───┤ ░ ├───┤├─────┤├───┤┌─┴─┐┌───┐└╥┘┌───┐»\nqr_1: |0>┤ Sdg ├┤ H ├┤ X ├┤ H ├┤ S ├┤ Z ├─░─┤ Z ├┤ Sdg ├┤ H ├┤ X ├┤ H ├─╫─┤ S ├»\n └─────┘└───┘└───┘└───┘└───┘└───┘ ░ └───┘└─────┘└───┘└───┘└───┘ ║ └───┘»\n cr_0: 0 ═══════════════════════════════════════════════════════════════╩══════»\n »\n cr_1: 0 ══════════════════════════════════════════════════════════════════════»\n »\n« \n«qr_0: ───\n« ┌─┐\n«qr_1: ┤M├\n« └╥┘\n«cr_0: ═╬═\n« ║ \n«cr_1: ═╩═\n« \n"
]
],
[
[
"One can verify that the Unitary representing each RB circuit should be the identity (with a global phase). \nWe simulate this using Aer unitary simulator.",
"_____no_output_____"
]
],
[
[
"# Create a new circuit without the measurement\nqregs = rb_circs[0][-1].qregs\ncregs = rb_circs[0][-1].cregs\nqc = qiskit.QuantumCircuit(*qregs, *cregs)\nfor i in rb_circs[0][-1][0:-nQ]:\n qc.data.append(i)",
"_____no_output_____"
],
[
"# The Unitary is an identity (with a global phase)\nbackend = qiskit.Aer.get_backend('unitary_simulator')\nbasis_gates = ['u1','u2','u3','cx'] # use U,CX for now\njob = qiskit.execute(qc, backend=backend, basis_gates=basis_gates)\nprint(np.around(job.result().get_unitary(),3))",
"[[ 0.+1.j -0.+0.j -0.+0.j -0.+0.j]\n [-0.+0.j 0.+1.j -0.-0.j 0.+0.j]\n [ 0.-0.j 0.+0.j 0.+1.j -0.+0.j]\n [-0.-0.j 0.-0.j 0.-0.j 0.+1.j]]\n"
]
],
[
[
"### Step 2: Execute the RB sequences (with some noise)\n\nWe can execute the RB sequences either using Qiskit Aer Simulator (with some noise model) or using IBMQ provider, and obtain a list of results.\n\nBy assumption each operation $C_{i_j}$ is allowed to have some error, represented by $\\Lambda_{i_j,j}$, and each sequence can be modeled by the operation:\n\n\n$$\\textit{S}_{\\textbf{i}_\\textbf{m}} = \\bigcirc_{j=1}^{m+1} (\\Lambda_{i_j,j} \\circ C_{i_j})$$\n\n\nwhere ${\\textbf{i}_\\textbf{m}} = (i_1,...,i_m)$ and $i_{m+1}$ is uniquely determined by ${\\textbf{i}_\\textbf{m}}$.",
"_____no_output_____"
]
],
[
[
"# Run on a noisy simulator\nnoise_model = NoiseModel()\n# Depolarizing_error\ndp = 0.005 \nnoise_model.add_all_qubit_quantum_error(depolarizing_error(dp, 1), ['u1', 'u2', 'u3'])\nnoise_model.add_all_qubit_quantum_error(depolarizing_error(2*dp, 2), 'cx')\n\nbackend = qiskit.Aer.get_backend('qasm_simulator')",
"_____no_output_____"
]
],
[
[
"### Step 3: Get statistics about the survival probabilities\n\nFor each of the $K_m$ sequences the survival probability $Tr[E_\\psi \\textit{S}_{\\textbf{i}_\\textbf{m}}(\\rho_\\psi)]$\nis measured. \nHere $\\rho_\\psi$ is the initial state taking into account preparation errors and $E_\\psi$ is the\nPOVM element that takes into account measurement errors.\nIn the ideal (noise-free) case $\\rho_\\psi = E_\\psi = | \\psi {\\rangle} {\\langle} \\psi |$. \n\nIn practice one can measure the probability to go back to the exact initial state, i.e. all the qubits in the ground state $ {|} 00...0 {\\rangle}$ or just the probability for one of the qubits to return back to the ground state. Measuring the qubits independently can be more convenient if a correlated measurement scheme is not possible. Both measurements will fit to the same decay parameter according to the properties of the *twirl*. ",
"_____no_output_____"
],
[
"### Step 4: Find the averaged sequence fidelity\n\nAverage over the $K_m$ random realizations of the sequence to find the averaged sequence **fidelity**,\n\n\n$$F_{seq}(m,|\\psi{\\rangle}) = Tr[E_\\psi \\textit{S}_{K_m}(\\rho_\\psi)]$$\n\n\nwhere \n\n\n$$\\textit{S}_{K_m} = \\frac{1}{K_m} \\sum_{\\textbf{i}_\\textbf{m}} \\textit{S}_{\\textbf{i}_\\textbf{m}}$$\n\n\nis the average sequence operation.",
"_____no_output_____"
],
[
"### Step 5: Fit the results\n\nRepeat Steps 1 through 4 for different values of $m$ and fit the results for the averaged sequence fidelity to the model:\n\n\n$$ \\textit{F}_{seq}^{(0)} \\big(m,{|}\\psi {\\rangle} \\big) = A_0 \\alpha^m +B_0$$\n\n\nwhere $A_0$ and $B_0$ absorb state preparation and measurement errors as well as an edge effect from the\nerror on the final gate.\n\n$\\alpha$ determines the average error-rate $r$, which is also called **Error per Clifford (EPC)** \naccording to the relation \n\n$$ r = 1-\\alpha-\\frac{1-\\alpha}{2^n} = \\frac{2^n-1}{2^n}(1-\\alpha)$$\n\n\n(where $n=nQ$ is the number of qubits).\n\nAs an example, we calculate the average sequence fidelity for each of the RB sequences, fit the results to the exponential curve, and compute the parameters $\\alpha$ and EPC.",
"_____no_output_____"
]
],
[
[
"# Create the RB fitter\nbackend = qiskit.Aer.get_backend('qasm_simulator')\nbasis_gates = ['u1','u2','u3','cx'] \nshots = 200\nqobj_list = []\nrb_fit = rb.RBFitter(None, xdata, rb_opts['rb_pattern'])\nfor rb_seed,rb_circ_seed in enumerate(rb_circs):\n print('Compiling seed %d'%rb_seed)\n new_rb_circ_seed = qiskit.compiler.transpile(rb_circ_seed, basis_gates=basis_gates)\n qobj = qiskit.compiler.assemble(new_rb_circ_seed, shots=shots)\n print('Simulating seed %d'%rb_seed)\n job = backend.run(qobj, noise_model=noise_model, backend_options={'max_parallel_experiments': 0})\n qobj_list.append(qobj)\n # Add data to the fitter\n rb_fit.add_data(job.result())\n print('After seed %d, alpha: %f, EPC: %f'%(rb_seed,rb_fit.fit[0]['params'][1], rb_fit.fit[0]['epc']))",
"Compiling seed 0\n"
]
],
[
[
"### Plot the results",
"_____no_output_____"
]
],
[
[
"plt.figure(figsize=(8, 6))\nax = plt.subplot(1, 1, 1)\n\n# Plot the essence by calling plot_rb_data\nrb_fit.plot_rb_data(0, ax=ax, add_label=True, show_plt=False)\n \n# Add title and label\nax.set_title('%d Qubit RB'%(nQ), fontsize=18)\n\nplt.show()",
"_____no_output_____"
]
],
[
[
"### The intuition behind RB\n\nThe depolarizing quantum channel has a parameter $\\alpha$, and works like this: with probability $\\alpha$, the state remains the same as before; with probability $1-\\alpha$, the state becomes the totally mixed state, namely:\n\n\n\n$$\\rho_f = \\alpha \\rho_i + \\frac{1-\\alpha}{2^n} * \\mathbf{I}$$\n\n\n\nSuppose that we have a sequence of $m$ gates, not necessarily Clifford gates, \nwhere the error channel of the gates is a depolarizing channel with parameter $\\alpha$ \n(same $\\alpha$ for all the gates). \nThen with probability $\\alpha^m$ the state is correct at the end of the sequence, \nand with probability $1-\\alpha^m$ it becomes the totally mixed state, therefore:\n\n\n\n$$\\rho_f^m = \\alpha^m \\rho_i + \\frac{1-\\alpha^m}{2^n} * \\mathbf{I}$$\n\n\n\nNow suppose that in addition we start with the ground state; \nthat the entire sequence amounts to the identity; \nand that we measure the state at the end of the sequence with the standard basis. \nWe derive that the probability of success at the end of the sequence is:\n\n\n\n$$\\alpha^m + \\frac{1-\\alpha^m}{2^n} = \\frac{2^n-1}{2^n}\\alpha^m + \\frac{1}{2^n} = A_0\\alpha^m + B_0$$\n\n\n\nIt follows that the probability of success, aka fidelity, decays exponentially with the sequence length, with exponent $\\alpha$.\n\nThe last statement is not necessarily true when the channel is other than the depolarizing channel. However, it turns out that if the gates are uniformly-randomized Clifford gates, then the noise of each gate behaves on average as if it was the depolarizing channel, with some parameter that can be computed from the channel, and we obtain the exponential decay of the fidelity.\n\nFormally, taking an average over a finite group $G$ (like the Clifford group) of a quantum channel $\\bar \\Lambda$ is also called a *twirl*:\n\n\n$$ W_G(\\bar \\Lambda) \\frac{1}{|G|} \\sum_{u \\in G} U^{\\dagger} \\circ \\bar \\Lambda \\circ U$$\n\n\nTwirling over the entire unitary group yields exactly the same result as the Clifford group. The Clifford group is a *2-design* of the unitary group.",
"_____no_output_____"
],
[
"## Simultaneous Randomized Benchmarking\n\n\nRB is designed to address fidelities in multiqubit systems in two ways. For one, RB over the full $n$-qubit space\ncan be performed by constructing sequences from the $n$-qubit Clifford group. Additionally, the $n$-qubit space\ncan be subdivided into sets of qubits $\\{n_i\\}$ and $n_i$-qubit RB performed in each subset simultaneously [4]. \nBoth methods give metrics of fidelity in the $n$-qubit space. \n\nFor example, it is common to perform 2Q RB on the subset of two-qubits defining a CNOT gate while the other qubits are quiescent. As explained in [4], this RB data will not necessarily decay exponentially because the other qubit subspaces are not twirled. Subsets are more rigorously characterized by simultaneous RB, which also measures some level of crosstalk error since all qubits are active.\n\nAn example of simultaneous RB (1Q RB and 2Q RB) can be found in: \nhttps://github.com/Qiskit/qiskit-tutorials/blob/master/qiskit/ignis/randomized_benchmarking.ipynb",
"_____no_output_____"
],
[
"## Predicted Gate Fidelity\n\nIf we know the errors on the underlying gates (the gateset) we can predict the fidelity. First we need to count the number of these gates per Clifford. \n\nThen, the two qubit Clifford gate error function gives the error per 2Q Clifford. It assumes that the error in the underlying gates is depolarizing. This function is derived in the supplement to [5]. ",
"_____no_output_____"
]
],
[
[
"#Count the number of single and 2Q gates in the 2Q Cliffords\ngates_per_cliff = rb.rb_utils.gates_per_clifford(qobj_list, xdata[0],basis_gates, rb_opts['rb_pattern'][0])\nfor i in range(len(basis_gates)):\n print(\"Number of %s gates per Clifford: %f\"%(basis_gates[i],\n np.mean([gates_per_cliff[0][i],gates_per_cliff[1][i]])))",
"Number of u1 gates per Clifford: 0.255895\nNumber of u2 gates per Clifford: 1.037882\nNumber of u3 gates per Clifford: 0.412664\nNumber of cx gates per Clifford: 1.487118\n"
],
[
"# Prepare lists of the number of qubits and the errors\nngates = np.zeros(7)\nngates[0:3] = gates_per_cliff[0][0:3]\nngates[3:6] = gates_per_cliff[1][0:3]\nngates[6] = gates_per_cliff[0][3]\ngate_qubits = np.array([0, 0, 0, 1, 1, 1, -1], dtype=int)\ngate_errs = np.zeros(len(gate_qubits))\ngate_errs[[1, 4]] = dp/2 #convert from depolarizing error to epg (1Q)\ngate_errs[[2, 5]] = 2*dp/2 #convert from depolarizing error to epg (1Q)\ngate_errs[6] = dp*3/4 #convert from depolarizing error to epg (2Q)\n\n#Calculate the predicted epc\npred_epc = rb.rb_utils.twoQ_clifford_error(ngates,gate_qubits,gate_errs)\nprint(\"Predicted 2Q Error per Clifford: %e\"%pred_epc)",
"Predicted 2Q Error per Clifford: 1.661579e-02\n"
],
[
"import qiskit\nqiskit.__qiskit_version__",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code",
"code",
"code"
]
] |
e7bb4ac41d3d4571d16a1fe839abe5c22ea2408f | 2,999 | ipynb | Jupyter Notebook | homework/homework-for-week-4-BLANKS.ipynb | jchapamalacara/fall21-students-practical-python | 3a1f4613b46933d3ab1d0741177f0d4ec6b6b600 | [
"MIT"
] | null | null | null | homework/homework-for-week-4-BLANKS.ipynb | jchapamalacara/fall21-students-practical-python | 3a1f4613b46933d3ab1d0741177f0d4ec6b6b600 | [
"MIT"
] | null | null | null | homework/homework-for-week-4-BLANKS.ipynb | jchapamalacara/fall21-students-practical-python | 3a1f4613b46933d3ab1d0741177f0d4ec6b6b600 | [
"MIT"
] | 1 | 2021-11-01T01:41:39.000Z | 2021-11-01T01:41:39.000Z | 19.730263 | 205 | 0.505168 | [
[
[
"# Homework for week 4",
"_____no_output_____"
],
[
"## Functions",
"_____no_output_____"
],
[
"#### 1. Convert String Number to Integer\nCreate a function that can take something like ```\"$5,356\"``` or ```\"$250,000\"``` or even ```\"$10.75\"``` and covert into integers like return ```5356```, ```250000``` and ```11``` respectively.",
"_____no_output_____"
]
],
[
[
"## build function here\n",
"_____no_output_____"
],
[
"## call the function on \"$5,356\"\n## Did you get 5356?\n",
"_____no_output_____"
],
[
"## call the function on \"$10.75\"\n## Did you get 11?\n\n",
"_____no_output_____"
],
[
"## Use map() to run your function on the following list\n## save result in a list called updated_list\nmy_amounts = [\"$12.24\", \"$4,576.33\", \"$23,629.01\"]",
"_____no_output_____"
]
],
[
[
"### 3. What if we encounter a list that has a mix of numbers as strings and integers and floats?",
"_____no_output_____"
]
],
[
[
"## Run this cell\nmore_amounts = [960, \"$12.24\", \"$4,576.33\", \"$23,629.01\", 23.656]",
"_____no_output_____"
],
[
"## tweaked the function here to handle all situations\n\n",
"_____no_output_____"
],
[
"## run it on more_amounts using map()\n",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
]
] |
e7bb59f4a6a1f0c9b087ca4f8f062ca82bcffc56 | 915,581 | ipynb | Jupyter Notebook | notebooks/figure/figF1.ipynb | mattkwiecien/jianbing | 0fbf82c973c6761d892115281b52ad9964c731db | [
"MIT"
] | 2 | 2021-08-14T17:33:07.000Z | 2021-08-18T20:53:40.000Z | notebooks/figure/figF1.ipynb | mattkwiecien/jianbing | 0fbf82c973c6761d892115281b52ad9964c731db | [
"MIT"
] | null | null | null | notebooks/figure/figF1.ipynb | mattkwiecien/jianbing | 0fbf82c973c6761d892115281b52ad9964c731db | [
"MIT"
] | null | null | null | 872.813155 | 171,912 | 0.950986 | [
[
[
"%matplotlib inline\n%load_ext autoreload\n%autoreload 2\n\nimport os\nimport sys\nimport copy \nimport pickle\n\nimport numpy as np \nimport matplotlib as mpl\nimport matplotlib.pyplot as plt\n\nfrom scipy import interpolate\nfrom astropy.table import Table, Column, vstack, join\n\nimport palettable\n\ncmap_1 = palettable.colorbrewer.sequential.Blues_7_r\ncmap_2 = palettable.colorbrewer.sequential.OrRd_7_r\ncmap_3 = palettable.colorbrewer.sequential.YlGn_7_r\ncmap_4 = palettable.colorbrewer.sequential.Purples_7_r\n\ncmap_list = [cmap_1, cmap_2, cmap_3, cmap_4]\n\ncolor_bins = [\"#377eb8\", \"#e41a1c\", \"#1b9e77\", \"#984ea3\"]",
"_____no_output_____"
],
[
"import jianbing\n\nfrom jianbing import hsc\nfrom jianbing import utils\nfrom jianbing import visual\nfrom jianbing import scatter\nfrom jianbing import catalog\nfrom jianbing import wlensing",
"_____no_output_____"
],
[
"data_dir = jianbing.DATA_DIR\n\nfig_dir = jianbing.FIG_DIR\n\nsim_dir = jianbing.SIM_DIR\nbin_dir = jianbing.BIN_DIR\nres_dir = jianbing.RES_DIR",
"_____no_output_____"
]
],
[
[
"### Compare the outer envelope stellar mass and SDSS redMaPPer clusters\n#### DSigma profiles of HSC massive galaxies",
"_____no_output_____"
]
],
[
[
"# DeltaSigma profiles of HSC massive galaxies\ntopn_massive = pickle.load(open(os.path.join(res_dir, 'topn_galaxies_sum.pkl'), 'rb'))\n\n# DeltaSigma profiles of redMaPPer and CAMIRA clusters\ntopn_cluster = pickle.load(open(os.path.join(res_dir, 'topn_clusters_cen_sum.pkl'), 'rb'))\n\n# For clusters, but using both central and satellite galaxies\ntopn_cluster_all = pickle.load(open(os.path.join(res_dir, 'topn_clusters_sum.pkl'), 'rb'))",
"_____no_output_____"
]
],
[
[
"#### DSigma profiles of mock galaxies",
"_____no_output_____"
]
],
[
[
"sim_dsig = Table.read(os.path.join(sim_dir, 'sim_merge_all_dsig.fits'))",
"_____no_output_____"
]
],
[
[
"#### Halo mass distributions",
"_____no_output_____"
]
],
[
[
"sim_mhalo = Table.read(os.path.join(sim_dir, 'sim_merge_mhalo_hist.fits'))",
"_____no_output_____"
]
],
[
[
"#### Pre-compute lensing results for HSC galaxies",
"_____no_output_____"
]
],
[
[
"# Pre-compute\ns16a_precompute = os.path.join(data_dir, 'topn_public_s16a_medium_precompute.hdf5')\n\nhsc_pre = Table.read(s16a_precompute, path='hsc_extra')\n\nred_sdss = Table.read(s16a_precompute, path='redm_sdss')\n\nred_hsc = Table.read(s16a_precompute, path='redm_hsc')",
"_____no_output_____"
]
],
[
[
"#### Pre-compute lensing results for randoms",
"_____no_output_____"
]
],
[
[
"# Lensing data using medium photo-z quality cut\ns16a_lensing = os.path.join(data_dir, 's16a_weak_lensing_medium.hdf5')\n\n# Random\ns16a_rand = Table.read(s16a_lensing, path='random')",
"_____no_output_____"
]
],
[
[
"#### Pre-defined number density bins",
"_____no_output_____"
]
],
[
[
"topn_bins = Table.read(os.path.join(bin_dir, 'topn_bins.fits'))",
"_____no_output_____"
]
],
[
[
"### Compute the DSigma profiles of SDSS redMaPPer clusters\n\n- 0.2 < z < 0.38 clusters",
"_____no_output_____"
]
],
[
[
"plt.scatter(red_sdss['z_best'], red_sdss['lambda_cluster_redm'], s=5, alpha=0.3)",
"_____no_output_____"
],
[
"mask_sdss_1 = (red_sdss['z_best'] >= 0.19) & (red_sdss['z_best'] <= 0.35)\nprint(mask_sdss_1.sum())\n\nsdss_bin_1 = Table(copy.deepcopy(topn_bins[0]))\n\nsdss_bin_1['n_obj'] = mask_sdss_1.sum()\nsdss_bin_1['rank_low'] = 1\nsdss_bin_1['rank_upp'] = mask_sdss_1.sum()\nsdss_bin_1['index_low'] = 0\nsdss_bin_1['index_upp'] = mask_sdss_1.sum() - 1",
"191\n"
],
[
"mask_sdss_2 = (red_sdss['z_best'] >= 0.19) & (red_sdss['z_best'] <= 0.50) & (red_sdss['lambda_cluster_redm'] >= 50)\nprint(mask_sdss_2.sum())\n\nsdss_bin_2 = Table(copy.deepcopy(topn_bins[0]))\n\nsdss_bin_2['n_obj'] = mask_sdss_2.sum()\nsdss_bin_2['rank_low'] = 1\nsdss_bin_2['rank_upp'] = mask_sdss_2.sum()\nsdss_bin_2['index_low'] = 0\nsdss_bin_2['index_upp'] = mask_sdss_2.sum() - 1",
"55\n"
],
[
"redm_sdss_dsig_1 = wlensing.gather_topn_dsigma_profiles(\n red_sdss, s16a_rand, sdss_bin_1, 'lambda_cluster_redm', \n mask=mask_sdss_1, n_rand=100000, n_boot=200, verbose=True)\n\nredm_sdss_sum_1 = scatter.compare_model_dsigma(\n redm_sdss_dsig_1, sim_dsig, model_err=False, poly=True, verbose=True)\n\nfig = visual.sum_plot_topn(\n redm_sdss_sum_1, r'$\\lambda_{\\rm SDSS}$', note=None, cov_type='jk', ref_tab=None)",
"\n# Using column: lambda_cluster_redm\n# Bin 1: 0 - 190\n# Dealing with Bin: 1\n"
],
[
"redm_sdss_dsig_2 = wlensing.gather_topn_dsigma_profiles(\n red_sdss, s16a_rand, sdss_bin_2, 'lambda_cluster_redm', \n mask=mask_sdss_2, n_rand=100000, n_boot=200, verbose=True)\n\nredm_sdss_sum_2 = scatter.compare_model_dsigma(\n redm_sdss_dsig_2, sim_dsig, model_err=False, poly=True, verbose=True)\n\nfig = visual.sum_plot_topn(\n redm_sdss_sum_2, r'$\\lambda_{\\rm SDSS}$', note=None, cov_type='jk', ref_tab=None)",
"\n# Using column: lambda_cluster_redm\n# Bin 1: 0 - 54\n# Dealing with Bin: 1\n"
]
],
[
[
"### Compute the DSigma profiles of HSC redMaPPer clusters",
"_____no_output_____"
]
],
[
[
"plt.scatter(red_hsc['z_best'], red_hsc['lambda'], s=5, alpha=0.3)",
"_____no_output_____"
],
[
"mask_hsc_1 = (red_hsc['z_best'] >= 0.19) & (red_hsc['z_best'] <= 0.35)\n\nredm_hsc_dsig_1 = wlensing.gather_topn_dsigma_profiles(\n red_hsc, s16a_rand, sdss_bin_1, 'lambda', \n mask=mask_hsc_1, n_rand=100000, n_boot=200, verbose=True)\n\nprint(np.min(redm_hsc_dsig['samples']))\n\nredm_hsc_sum_1 = scatter.compare_model_dsigma(\n redm_hsc_dsig_1, sim_dsig, model_err=False, poly=True, verbose=True)\n\nfig = visual.sum_plot_topn(\n redm_hsc_sum_1, r'$\\lambda_{\\rm HSC}$', note=None, cov_type='jk', ref_tab=None)\n",
"\n# Using column: lambda\n# Bin 1: 0 - 190\n14.115065\n# Dealing with Bin: 1\n"
],
[
"mask_hsc_2 = (red_hsc['z_best'] >= 0.19) & (red_hsc['z_best'] <= 0.5)\n\nredm_hsc_dsig_2 = wlensing.gather_topn_dsigma_profiles(\n red_hsc, s16a_rand, sdss_bin_2, 'lambda', \n mask=mask_hsc_2, n_rand=100000, n_boot=200, verbose=True)\n\nprint(np.min(redm_hsc_dsig['samples']))\n\nredm_hsc_sum_2 = scatter.compare_model_dsigma(\n redm_hsc_dsig_2, sim_dsig, model_err=False, poly=True, verbose=True)\n\nfig = visual.sum_plot_topn(\n redm_hsc_sum_2, r'$\\lambda_{\\rm HSC}$', note=None, cov_type='jk', ref_tab=None)",
"\n# Using column: lambda\n# Bin 1: 0 - 54\n14.115065\n# Dealing with Bin: 1\n"
]
],
[
[
"### Compute the DSigma profiles of HSC massive galaxies",
"_____no_output_____"
]
],
[
[
"# S18A bright star mask\nbsm_s18a = hsc_pre['flag'] > 0\n\n# General mask for HSC galaxies\nmask_mout_1 = (\n (hsc_pre['c82_100'] <= 18.) & (hsc_pre['logm_100'] - hsc_pre['logm_50'] <= 0.2) & (hsc_pre['logm_50_100'] > 0) &\n bsm_s18a & (hsc_pre['z'] >= 0.19) & (hsc_pre['z'] <= 0.35)\n)\n\nmask_mout_2 = (\n (hsc_pre['c82_100'] <= 18.) & (hsc_pre['logm_100'] - hsc_pre['logm_50'] <= 0.2) & (hsc_pre['logm_50_100'] > 0) &\n bsm_s18a & (hsc_pre['z'] >= 0.19) & (hsc_pre['z'] <= 0.50)\n)\n\n# Mask to select \"central\" galaxies\ncen_mask_1 = hsc_pre['cen_mask_1'] > 0\ncen_mask_2 = hsc_pre['cen_mask_2'] > 0\ncen_mask_3 = hsc_pre['cen_mask_3'] > 0",
"_____no_output_____"
],
[
"hsc_mout_dsig_1 = wlensing.gather_topn_dsigma_profiles(\n hsc_pre, s16a_rand, sdss_bin_1, 'logm_50_100', mask=mask_mout_1,\n n_rand=100000, n_boot=200, verbose=True)\n\nhsc_mout_sum_1 = scatter.compare_model_dsigma(\n hsc_mout_dsig_1, sim_dsig, model_err=False, poly=True, verbose=True)\n\nfig = visual.sum_plot_topn(\n hsc_mout_sum_1, r'$M_{\\star, [50,100]}$', note=None, cov_type='jk', ref_tab=None)",
"\n# Using column: logm_50_100\n# Bin 1: 0 - 190\n# Dealing with Bin: 1\n"
],
[
"hsc_mout_dsig_2 = wlensing.gather_topn_dsigma_profiles(\n hsc_pre, s16a_rand, sdss_bin_2, 'logm_50_100', mask=mask_mout_2,\n n_rand=100000, n_boot=200, verbose=True)\n\nhsc_mout_sum_2 = scatter.compare_model_dsigma(\n hsc_mout_dsig_2, sim_dsig, model_err=False, poly=True, verbose=True)\n\nfig = visual.sum_plot_topn(\n hsc_mout_sum_2, r'$M_{\\star, [50,100]}$', note=None, cov_type='jk', ref_tab=None)",
"\n# Using column: logm_50_100\n# Bin 1: 0 - 54\n# Dealing with Bin: 1\n"
]
],
[
[
"### Making a figure for the paper",
"_____no_output_____"
]
],
[
[
"def compare_sdss_redm_profiles(\n dsig_ref, dsig_cmp, sim_dsig, sig_type='bt', compare_to_model=True,\n label_ref=r'$\\rm Ref$', label_cmp=r'$\\rm Test$',\n sub_ref=r'{\\rm Ref}', sub_cmp=r'{\\rm Test}',\n cmap_list=None, color_bins=None, \n marker_ref='o', msize_ref=180,\n marker_cmp='P', msize_cmp=180, \n show_best_cmp=False, middle_title=None, mvir_min=None,\n dsig_cmp_2=None, label_cmp_2=r'$=rm Test 2$'):\n \"\"\"Compare the Dsigma profiles.\"\"\"\n\n def get_dsig_ratio(obs, ref, mod=None):\n \"\"\"\"\"\"\n obs_rand = np.random.normal(\n loc=obs['dsigma'][0], scale=obs['dsig_err_{:s}'.format(sig_type)][0])\n\n if mod is not None:\n ref_rand = np.random.normal(\n loc=mod['dsig'], scale=(mod['dsig_err'] * err_factor))\n\n ref_inter = 10.0 ** (\n interpolate.interp1d(\n mod['r_mpc'], np.log10(ref_rand), fill_value='extrapolate')(r_mpc_obs)\n )\n return obs_rand / ref_inter\n else:\n ref_rand = np.random.normal(\n loc=ref['dsigma'][0], scale=obs['dsig_err_{:s}'.format(sig_type)][0])\n return obs_rand / ref_rand\n\n\n # Color maps and bins\n if cmap_list is None:\n cmap_list = [\n palettable.colorbrewer.sequential.OrRd_7_r,\n palettable.colorbrewer.sequential.Blues_7_r,\n palettable.colorbrewer.sequential.YlGn_7_r,\n palettable.colorbrewer.sequential.Purples_7_r]\n\n if color_bins is None:\n color_bins = [\"#e41a1c\", \"#377eb8\", \"#1b9e77\", \"#984ea3\"]\n\n # Radius bin of the observed DSigma profiles\n r_mpc_obs = dsig_ref[0].meta['r_mpc']\n\n # ---- Start the figure ---- #\n\n # Setup the figure\n n_col, n_bins = 2, len(dsig_ref)\n fig_y = int(4.2 * n_bins + 2)\n left, right = 0.11, 0.995\n if n_bins == 4:\n bottom, top = 0.055, 0.96\n elif n_bins == 3:\n bottom, top = 0.08, 0.96\n elif n_bins == 2:\n bottom, top = 0.10, 0.93\n elif n_bins == 1:\n bottom, top = 0.16, 0.92\n \n x_space = 0.12\n x_size = (right - left - x_space * 1.05) / n_col\n y_size = (top - bottom) / n_bins\n \n fig = plt.figure(figsize=(12, fig_y))\n\n for idx, dsig in enumerate(dsig_ref):\n # Setup the three columns\n ax1 = fig.add_axes([left, top - y_size * (idx + 1), x_size, y_size])\n ax2 = fig.add_axes([left + x_space + x_size, top - y_size * (idx + 1), x_size, y_size])\n\n # Subplot title\n if idx == 0:\n ax1.set_title(r'$R \\times \\Delta\\Sigma\\ \\rm Profile$', fontsize=35, pad=10)\n if middle_title is None:\n ax2.set_title(r'${\\rm Richness\\ v.s.}\\ M_{\\star,\\ \\rm Outer}$', fontsize=35, pad=10)\n else:\n ax2.set_title(middle_title, fontsize=35, pad=10)\n\n # Color map\n cmap, color = cmap_list[idx], color_bins[idx]\n\n # MDPL halo mass information for this bin\n sim_dsig_bin = sim_dsig[sim_dsig['bin'] == 0]\n\n # DSigma result for this bin\n dsig_ref_bin = dsig_ref[idx]\n dsig_cmp_bin = dsig_cmp[idx]\n\n # Best fit DSigma profiles\n dsig_ref_best = sim_dsig_bin[\n np.argmin(\n np.abs(sim_dsig_bin['scatter'] - dsig_ref_bin['sig_med_{:s}'.format(sig_type)]))]\n dsig_cmp_best = sim_dsig_bin[\n np.argmin(\n np.abs(sim_dsig_bin['scatter'] - dsig_cmp_bin['sig_med_{:s}'.format(sig_type)]))]\n\n if dsig_ref_bin['sig_med_{:s}'.format(sig_type)] < 0.6:\n err_factor = 4.\n else:\n err_factor = 3.\n\n # Interpolated the reference model profile\n ref_model_inter = 10.0 ** (\n interpolate.interp1d(\n dsig_ref_best['r_mpc'], np.log10(dsig_ref_best['dsig']),\n fill_value='extrapolate')(r_mpc_obs)\n )\n\n if compare_to_model:\n ratio_sample = [\n get_dsig_ratio(\n dsig_cmp_bin, dsig_ref_bin, mod=dsig_ref_best) for i in np.arange(2000)]\n ratio_cmp = dsig_cmp_bin['dsigma'][0] / ref_model_inter\n else:\n ratio_sample = [\n get_dsig_ratio(dsig_cmp_bin, dsig_ref_bin, mod=None) for i in np.arange(2000)]\n ratio_cmp = dsig_cmp_bin['dsigma'][0] / dsig_ref_bin['dsigma'][0]\n\n ratio_cmp_err_low = ratio_cmp - np.nanpercentile(ratio_sample, 16, axis=0)\n ratio_cmp_err_upp = np.nanpercentile(ratio_sample, 84, axis=0) - ratio_cmp\n \n if dsig_cmp_2 is not None:\n try:\n dsig_cmp_2_bin = dsig_cmp_2[idx]\n dsig_cmp_2_best = sim_dsig_bin[\n np.argmin(\n np.abs(sim_dsig_bin['scatter'] - dsig_cmp_2_bin['sig_med_{:s}'.format(sig_type)]))]\n\n if compare_to_model:\n ratio_sample = [\n get_dsig_ratio(\n dsig_cmp_2_bin, dsig_ref_bin, mod=dsig_ref_best) for i in np.arange(2000)]\n ratio_cmp_2 = dsig_cmp_2_bin['dsigma'][0] / ref_model_inter\n else:\n ratio_sample = [\n get_dsig_ratio(dsig_cmp_2_bin, dsig_ref_bin, mod=None) for i in np.arange(2000)]\n ratio_cmp_2 = dsig_cmp_2_bin['dsigma'][0] / dsig_ref_bin['dsigma'][0]\n\n ratio_cmp_2_err_low = ratio_cmp_2 - np.nanpercentile(ratio_sample, 16, axis=0)\n ratio_cmp_2_err_upp = np.nanpercentile(ratio_sample, 84, axis=0) - ratio_cmp_2\n show_cmp_2 = True\n except Exception:\n show_cmp_2 = False\n else:\n show_cmp_2 = False\n\n # ----- Plot 1: R x DSigma plot ----- #\n ax1.set_xscale(\"log\", nonpositive='clip')\n\n # MDPL: Best-fit\n ax1.fill_between(\n dsig_ref_best['r_mpc'],\n dsig_ref_best['r_mpc'] * (\n dsig_ref_best['dsig'] - dsig_ref_best['dsig_err'] * err_factor),\n dsig_ref_best['r_mpc'] * (\n dsig_ref_best['dsig'] + dsig_ref_best['dsig_err'] * err_factor),\n alpha=0.2, edgecolor='grey', linewidth=2.0,\n label=r'__no_label__', facecolor='grey', linestyle='-', rasterized=True)\n\n if show_best_cmp:\n ax1.fill_between(\n dsig_cmp_best['r_mpc'],\n dsig_cmp_best['r_mpc'] * (\n dsig_cmp_best['dsig'] - dsig_cmp_best['dsig_err'] * err_factor),\n dsig_cmp_best['r_mpc'] * (\n dsig_cmp_best['dsig'] + dsig_cmp_best['dsig_err'] * err_factor),\n alpha=0.15, edgecolor='grey', linewidth=2.0,\n label=r'__no_label__', facecolor='grey', linestyle='--', rasterized=True)\n\n # Reference DSigma profile\n ax1.errorbar(\n r_mpc_obs,\n r_mpc_obs * dsig_ref_bin['dsigma'][0],\n yerr=(r_mpc_obs * dsig_ref_bin['dsig_err_{:s}'.format(sig_type)][0]),\n ecolor=cmap.mpl_colormap(0.6), color=cmap.mpl_colormap(0.6), alpha=0.9, \n capsize=4, capthick=2.5, elinewidth=2.5,\n label='__no_label__', fmt='o', zorder=0)\n ax1.scatter(\n r_mpc_obs,\n r_mpc_obs * dsig_ref_bin['dsigma'][0],\n s=msize_ref, alpha=0.9, facecolor=cmap.mpl_colormap(0.6), edgecolor='w', marker=marker_ref, \n linewidth=2.5, label=label_ref)\n\n # DSigma profiles to compare with\n ax1.errorbar(\n r_mpc_obs * 1.01,\n r_mpc_obs * dsig_cmp_bin['dsigma'][0],\n yerr=(r_mpc_obs * dsig_cmp_bin['dsig_err_{:s}'.format(sig_type)][0]),\n ecolor=color, color='w', alpha=0.9, capsize=4, capthick=2.5,\n elinewidth=2.5, label='__no_label__', fmt='o', zorder=0)\n ax1.scatter(\n r_mpc_obs * 1.01,\n r_mpc_obs * dsig_cmp_bin['dsigma'][0],\n s=msize_cmp, alpha=0.95, facecolor='w', edgecolor=color, \n marker=marker_cmp, linewidth=3.0, label=label_cmp)\n\n y_max = np.max(\n [np.max(dsig_ref_best['r_mpc'] * dsig_ref_best['dsig']), \n np.max(dsig_cmp_best['r_mpc'] * dsig_cmp_best['dsig'])]) * 1.47\n ax1.set_ylim(3.1, y_max)\n\n # Sample Info\n if idx == 1:\n _ = ax1.text(0.2, 0.08, r'$0.19 < z < 0.35$', fontsize=28, transform=ax1.transAxes)\n _ = ax2.text(0.16, 0.08, r'$\\lambda_{\\rm SDSS} \\geq 20;\\ N=191$', fontsize=28, transform=ax2.transAxes)\n \n elif idx == 0:\n _ = ax1.text(0.2, 0.08, r'$0.19 < z < 0.50$', fontsize=28, transform=ax1.transAxes)\n _ = ax2.text(0.16, 0.08, r'$\\lambda_{\\rm SDSS} \\geq 50;\\ N=55$', fontsize=28, transform=ax2.transAxes)\n \n if idx == 1:\n ax1.legend(loc='upper left', fontsize=22, handletextpad=0.04, ncol=2, mode=\"expand\")\n\n if idx == len(dsig_ref) - 1:\n _ = ax1.set_xlabel(r'$R\\ [\\mathrm{Mpc}]$', fontsize=30)\n else:\n ax1.set_xticklabels([])\n _ = ax1.set_ylabel(r'$R \\times \\Delta\\Sigma\\ [10^{6}\\ M_{\\odot}/\\mathrm{pc}]$', fontsize=32)\n\n\n # ----- Plot 2: Ratio of DSigma plot ----- #\n ax2.set_xscale(\"log\", nonpositive='clip')\n\n ax2.axhline(\n 1.0, linewidth=3.0, alpha=0.5, color='k', linestyle='--', label='__no_label__', )\n\n # Uncertainty of the model\n ax2.fill_between(\n dsig_ref_best['r_mpc'],\n 1.0 - (dsig_ref_best['dsig_err'] * err_factor / dsig_ref_best['dsig']),\n 1.0 + (dsig_ref_best['dsig_err'] * err_factor / dsig_ref_best['dsig']),\n alpha=0.2, edgecolor='none', linewidth=1.0, label='__no_label__',\n facecolor='grey', rasterized=True)\n\n if show_cmp_2:\n ax2.errorbar(\n r_mpc_obs * 1.2, ratio_cmp_2, yerr=[ratio_cmp_2_err_low, ratio_cmp_2_err_upp],\n ecolor=cmap.mpl_colormap(0.3), color='w', alpha=0.5, capsize=4, capthick=2.5,\n elinewidth=3.0, label='__no_label__', fmt='o', zorder=0)\n ax2.scatter(\n r_mpc_obs * 1.2, ratio_cmp_2,\n s=260, alpha=0.7, facecolor=cmap.mpl_colormap(0.3), edgecolor='w',\n marker='H', linewidth=3.0, label=label_cmp_2)\n\n ax2.errorbar(\n r_mpc_obs, ratio_cmp, yerr=[ratio_cmp_err_low, ratio_cmp_err_upp],\n ecolor=color, color='w', alpha=0.8, capsize=4, capthick=2.5,\n elinewidth=3.0, label='__no_label__', fmt='o', zorder=0)\n ax2.scatter(\n r_mpc_obs, ratio_cmp,\n s=msize_cmp, alpha=0.9, facecolor='w', edgecolor=color,\n marker=marker_cmp, linewidth=3.0, label=label_cmp)\n \n ax2.set_ylim(0.20, 2.49)\n\n if np.max(ratio_cmp) < 1.2:\n y_pos = 0.85\n else:\n y_pos = 0.15\n\n if idx == 1:\n ax2.legend(loc='upper left', fontsize=22, handletextpad=0.05)\n\n if idx == len(dsig_ref) - 1:\n _ = ax2.set_xlabel(r'$R\\ [\\mathrm{Mpc}]$', fontsize=30)\n else:\n ax2.set_xticklabels([])\n \n _ = ax2.set_ylabel(r'$\\Delta\\Sigma_{\\rm redM}/\\Delta\\Sigma_{[50, 100]}$', fontsize=35)\n\n for tick in ax1.xaxis.get_major_ticks():\n tick.label.set_fontsize(30)\n for tick in ax1.yaxis.get_major_ticks():\n tick.label.set_fontsize(30)\n\n for tick in ax2.xaxis.get_major_ticks():\n tick.label.set_fontsize(30)\n for tick in ax2.yaxis.get_major_ticks():\n tick.label.set_fontsize(30)\n\n return fig",
"_____no_output_____"
],
[
"dsig_cmp_2 = [redm_hsc_sum_2, redm_hsc_sum_1]\nlabel_cmp_2 = r'${\\rm redM\\ HSC}$'\n\ndsig_cmp = [redm_sdss_sum_2, redm_sdss_sum_1]\nlabel_cmp = r'${\\rm redM\\ SDSS}$'\nsub_cmp = r'{\\rm redM\\ SDSS}z'\n\ndsig_ref = [hsc_mout_sum_2, hsc_mout_sum_1]\nlabel_ref = r'$M_{\\star, [50, 100]}$'\nsub_ref = r'{[50, 100]}'\n\nfig = compare_sdss_redm_profiles(\n dsig_ref, dsig_cmp, sim_dsig, sig_type='bt', compare_to_model=True,\n label_ref=label_ref, label_cmp=label_cmp, sub_ref=sub_ref, sub_cmp=sub_cmp,\n marker_ref='o', marker_cmp='D', msize_ref=220, msize_cmp=160,\n dsig_cmp_2=dsig_cmp_2, label_cmp_2=label_cmp_2, mvir_min=12.8,\n middle_title=r'$\\rm HSC\\ v.s.\\ SDSS$')",
"<ipython-input-215-21f208b084b4>:23: RuntimeWarning: invalid value encountered in log10\n mod['r_mpc'], np.log10(ref_rand), fill_value='extrapolate')(r_mpc_obs)\n"
],
[
"fig.savefig(os.path.join(fig_dir, 'fig_F1.png'), dpi=120)\nfig.savefig(os.path.join(fig_dir, 'fig_F1.pdf'), dpi=120)",
"_____no_output_____"
],
[
"redm_hsc_dsig_1['samples'].min()",
"_____no_output_____"
],
[
"redm_hsc_dsig_2['samples'].min()",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
]
] |
e7bb650cbd1ad0562a1da4eb281b7e13ea189006 | 27,832 | ipynb | Jupyter Notebook | clase4/4-1_preprocessing.ipynb | jinchuika/umg-ai | c32c410e2dc2c4c1bde04f9f0d8100022d0e57f9 | [
"MIT"
] | 3 | 2020-05-04T19:09:52.000Z | 2021-10-05T23:13:26.000Z | clase4/4-1_preprocessing.ipynb | jinchuika/umg-ai | c32c410e2dc2c4c1bde04f9f0d8100022d0e57f9 | [
"MIT"
] | null | null | null | clase4/4-1_preprocessing.ipynb | jinchuika/umg-ai | c32c410e2dc2c4c1bde04f9f0d8100022d0e57f9 | [
"MIT"
] | null | null | null | 31.699317 | 345 | 0.390594 | [
[
[
"# Pre-procesamiento de datos\n\nTodos los datos operados por un algoritmo de inteligencia artificial deben ser numéricos en una forma específica. Debido a que la mayoría de datos se encuentran en un formato diferente, que no puede ser utilizado en un algoritmo, estos deben ser convertidos a un formato adecuado. Esta tarea se conoce como `preprocessing`.",
"_____no_output_____"
]
],
[
[
"import pandas as pd\nfrom sklearn.preprocessing import LabelEncoder",
"_____no_output_____"
],
[
"# Leer los datos del archivo CSV original\ndf = pd.read_csv('telco.csv')\ndf.info()",
"<class 'pandas.core.frame.DataFrame'>\nRangeIndex: 7043 entries, 0 to 7042\nData columns (total 21 columns):\ncustomerID 7043 non-null object\ngender 7043 non-null object\nSeniorCitizen 7043 non-null int64\nPartner 7043 non-null object\nDependents 7043 non-null object\ntenure 7043 non-null int64\nPhoneService 7043 non-null object\nMultipleLines 7043 non-null object\nInternetService 7043 non-null object\nOnlineSecurity 7043 non-null object\nOnlineBackup 7043 non-null object\nDeviceProtection 7043 non-null object\nTechSupport 7043 non-null object\nStreamingTV 7043 non-null object\nStreamingMovies 7043 non-null object\nContract 7043 non-null object\nPaperlessBilling 7043 non-null object\nPaymentMethod 7043 non-null object\nMonthlyCharges 7043 non-null float64\nTotalCharges 7043 non-null object\nChurn 7043 non-null object\ndtypes: float64(1), int64(2), object(18)\nmemory usage: 1.1+ MB\n"
],
[
"# Revisar la composición de datos en el DataFrame\ndf.head()",
"_____no_output_____"
]
],
[
[
"## Objetivo\n\nAplicaremos pre-procesamiento de datos para realizar una predicción en la columna `MonthlyCharges`, intentando predecir los pagos mensuales de un cliente. Para ello, seleccionamos las columnas que puedan servir como características (`X`) y nuestra variable objetivo (`y`).",
"_____no_output_____"
]
],
[
[
"# Elegir las columnas a utilizar\ncolumnas_importantes = [\n 'gender',\n 'Partner',\n 'Dependents',\n 'SeniorCitizen',\n 'PhoneService',\n 'MultipleLines',\n 'InternetService',\n 'MonthlyCharges'\n]\n\n# Nuevo DataFrame con las columnas seleccionadas\ndf_limpio = df[columnas_importantes]",
"_____no_output_____"
],
[
"# Revisamos una muestra de los datos de nuestro nuevo DataFrame\ndf_limpio.head()",
"_____no_output_____"
]
],
[
[
"Como podemos observar, la mayoría de columnas con datos categóricos se encuentran en formato de texto. Si deseamos utilizar esos datos en un algoritmo de inteligencia artificial, necesitamos convertirlos en representaciones numéricas. Para ello, realizaremos una tarea de pre-procesamiento utilizando un codificador de tipo `LabelEncoder`.",
"_____no_output_____"
]
],
[
[
"# Crear el encoder para la columna gender\ngender_encoder = LabelEncoder()\n\n# \"Aprender\" los datos para el encoder.\n# Este proceso reconoce las diferentes categorías en los datos.\n# Para cada categoría, asignará un número iniciando desde 0\ngender_encoder.fit(df_limpio['gender'])\n\n# Podemos transformar los datos originales en sus representaciones numéricas\ngender_encoder.fit_transform(df_limpio['gender'])",
"_____no_output_____"
],
[
"# Si quisiéramos transofrmar los datos de forma inversa, es decir,\n# obtener los datos originales a partir de sus representaciones,\n# podemos utilizar el método invevrse_transform\ngender_encoder.inverse_transform([0, 1, 1, 1, 0])",
"/home/chuik/venv/ml/lib/python3.6/site-packages/sklearn/preprocessing/label.py:151: DeprecationWarning: The truth value of an empty array is ambiguous. Returning False, but in future this will result in an error. Use `array.size > 0` to check that an array is not empty.\n if diff:\n"
],
[
"# Podemos visualizar las clases encontradas por el encoder\ngender_encoder.classes_",
"_____no_output_____"
]
],
[
[
"## Una forma más eficiente\n\nYa que necesitamos un codificador para cada columna, crearemos una estructura de datos que permita almacenar un codificador distinto para cada columna.",
"_____no_output_____"
]
],
[
[
"# Empezamos revisando la de columnas del DataFrame\nfor columna in df_limpio.columns:\n print(columna)",
"gender\nPartner\nDependents\nSeniorCitizen\nPhoneService\nMultipleLines\nInternetService\nMonthlyCharges\n"
]
],
[
[
"Ya que no todas las columnas necesitan ser codificadas (`SeniorCitizen` ya se encuentra codificada y `MonthlyCharges` no es un dato de tipo categórico), incluiremos únicamente las columnas con datos categóricos que necesitan ser codificados.",
"_____no_output_____"
]
],
[
[
"# Creamos un nuevo DataFrame que tendrá los datos convertidos\ndf_final = pd.DataFrame()\n\n# En un diccionario, almacenamos un codificador por cada columna\nencoders = {\n 'gender': LabelEncoder(),\n 'Partner': LabelEncoder(),\n 'Dependents': LabelEncoder(),\n 'PhoneService': LabelEncoder(),\n 'MultipleLines': LabelEncoder(),\n 'InternetService': LabelEncoder(),\n}\n\n# Codificar cada columna y agregarla al nuevo DataFrame\nfor columna, encoder in encoders.items():\n encoder.fit(df_limpio[columna])\n df_final[columna] = encoder.fit_transform(df_limpio[columna])\n\ndf_final.head()",
"_____no_output_____"
],
[
"# Revisión de todas las clases obtenidas por los codificadores\nfor column, encoder in encoders.items():\n print(column, encoder.classes_)\n print('=======')",
"gender ['Female' 'Male']\n=======\nPartner ['No' 'Yes']\n=======\nDependents ['No' 'Yes']\n=======\nPhoneService ['No' 'Yes']\n=======\nMultipleLines ['No' 'No phone service' 'Yes']\n=======\nInternetService ['DSL' 'Fiber optic' 'No']\n=======\n"
],
[
"# Agregamos las columnas que no transformamos al DataFrame final\ndf_final['SeniorCitizen'] = df_limpio['SeniorCitizen']\ndf_final['MonthlyCharges'] = df_limpio['MonthlyCharges']\ndf_final.head()",
"_____no_output_____"
],
[
"# Exportar los datos codificados a un nuevo archivo CSV\ndf_final.to_csv('datos_limpios.csv', index=None)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
]
] |
e7bb67ce193016c6366e5438195c0b2d36296a80 | 213,006 | ipynb | Jupyter Notebook | Ec_candidates_tweets_sentiment_an.ipynb | sayalaruano/Sentiment-analysis-of-Ecuadorian-political-tweets-from-2021election-with-Natural-Language-Processing | b0a9910e47cf5a9e9241a21d641172fd56a33fe8 | [
"MIT"
] | 1 | 2021-01-24T11:29:24.000Z | 2021-01-24T11:29:24.000Z | Ec_candidates_tweets_sentiment_an.ipynb | sayalaruano/Sentiment-analysis-of-Ecuadorian-political-tweets-from-2021election-with-Natural-Language-Processing | b0a9910e47cf5a9e9241a21d641172fd56a33fe8 | [
"MIT"
] | null | null | null | Ec_candidates_tweets_sentiment_an.ipynb | sayalaruano/Sentiment-analysis-of-Ecuadorian-political-tweets-from-2021election-with-Natural-Language-Processing | b0a9910e47cf5a9e9241a21d641172fd56a33fe8 | [
"MIT"
] | 1 | 2021-03-31T18:44:20.000Z | 2021-03-31T18:44:20.000Z | 239.063973 | 78,635 | 0.76741 | [
[
[
"# imports\r\nfrom google.colab import drive\r\nimport pandas as pd\r\nimport nltk\r\nfrom keras.preprocessing.text import Tokenizer\r\nfrom nltk.corpus import stopwords, words\r\nfrom matplotlib import pyplot as plt\r\nfrom keras.utils import to_categorical\r\nimport numpy as np\r\nfrom sklearn.model_selection import train_test_split\r\nfrom keras import models, layers\r\nfrom collections import Counter\r\n\r\n# configurations\r\npath_drive = '/content/drive/'\r\ndrive.mount(path_drive)",
"Mounted at /content/drive/\n"
],
[
"# Load joined TASS dataset\r\ntassDf = pd.read_csv(\"drive/MyDrive/AI_final_project_REAL/Project_dataset/ALL_TassDF.csv\", encoding='utf8').reset_index(drop=True)[['Text', 'Tag']]\r\n\r\n# Select tweets with tag of positive, neative or neutral\r\ntassDf = tassDf.loc[(tassDf.Tag == 'P') | (tassDf.Tag == 'N') | (tassDf.Tag == 'NEU')]\r\n\r\n# Verify the final dataset - 57454 tweets\r\nprint(tassDf, '\\n\\n', tassDf.shape)\r\nprint(tassDf.columns.values)\r\nprint(tassDf.values)",
" Text Tag\n0 @marizamora94 Ambas son mindblowing, lástima W... P\n1 Yo sé que cada quién hace lo que le da la puta... N\n2 @Phoenix2124 uno siente susto, asco, cólera et... N\n3 Hola @TutzyFF , muchas bendiciones, ves... si ... P\n4 Siempre la paz es lo mejor. De lo sucedido en ... N\n... ... ..\n81865 Reconozco que me encanta cuando Twitter corre.... P\n81866 @Juandecolmenero @angelrubioti @barbara_ruizp ... P\n81868 ¿A qué espera el alcalde promarihuana independ... N\n81869 Esto es lo que hay... Preocupante la flagrante... N\n81870 Rajoy, con el agua al cuello: España se asoma ... N\n\n[57454 rows x 2 columns] \n\n (57454, 2)\n['Text' 'Tag']\n[['@marizamora94 Ambas son mindblowing, lástima White Collar terminara así de repente, y esperando a la segunda par de la 5ta de Suits'\n 'P']\n ['Yo sé que cada quién hace lo que le da la puta gana pero hay mujeres que no deberían dejarlas pintarse las cejas.'\n 'N']\n ['@Phoenix2124 uno siente susto, asco, cólera etc.. y luego vienen los \"Porqué no le dijo\" \"Porqué no ..\" -.- Sean necios! Es una ruleta'\n 'N']\n ...\n ['¿A qué espera el alcalde promarihuana independentista de Rasquera para dimitir? Prometió que lo haría si fracasaba. http://t.co/4BcM4fQj'\n 'N']\n ['Esto es lo que hay... Preocupante la flagrante falta de liderazgo de Rajoy... Y no me vale que ZP fuera un desastre... http://t.co/c2uY3ukb'\n 'N']\n ['Rajoy, con el agua al cuello: España se asoma a la zona de rescate. http://t.co/w6qFgfJp#portadaEPC'\n 'N']]\n"
],
[
"# Load replies to tweets from two Ecuadorian presidential candidates\ncandidDf = pd.read_csv( 'drive/MyDrive/AI_final_project_REAL/Project_dataset/ALL_candidates.csv', encoding='utf8')\n\n# Merge TASS and canidiate datsets to create the corpus\njoinedDfTexts = candidDf['text'].append(tassDf['Text'], ignore_index=True) # continuous idxs\nprint(joinedDfTexts, '\\n\\n', joinedDfTexts.shape)\nprint(joinedDfTexts.values)",
"0 @ecuarauz es un payaso !😂😂 https://t.co/PgrRTh...\n1 @ecuarauz Todas y todos con INTERNET...un paso...\n2 @ecuarauz Cómo que nos oponemos? Cuidado con b...\n3 @ecuarauz ¿Alguna propuesta que no se haya ofr...\n4 @ecuarauz Vas a repatriar todo lo que los deli...\n ... \n59450 Reconozco que me encanta cuando Twitter corre....\n59451 @Juandecolmenero @angelrubioti @barbara_ruizp ...\n59452 ¿A qué espera el alcalde promarihuana independ...\n59453 Esto es lo que hay... Preocupante la flagrante...\n59454 Rajoy, con el agua al cuello: España se asoma ...\nLength: 59455, dtype: object \n\n (59455,)\n['@ecuarauz es un payaso !😂😂 https://t.co/PgrRThiPB5'\n '@ecuarauz Todas y todos con INTERNET...un paso más al desarrollo y progreso de nuestro Pais ☝️'\n '@ecuarauz Cómo que nos oponemos? Cuidado con bajarse de la camioneta de quien lo puso ahí.'\n ...\n '¿A qué espera el alcalde promarihuana independentista de Rasquera para dimitir? Prometió que lo haría si fracasaba. http://t.co/4BcM4fQj'\n 'Esto es lo que hay... Preocupante la flagrante falta de liderazgo de Rajoy... Y no me vale que ZP fuera un desastre... http://t.co/c2uY3ukb'\n 'Rajoy, con el agua al cuello: España se asoma a la zona de rescate. http://t.co/w6qFgfJp#portadaEPC']\n"
],
[
"# Build the one hot matrix considering TASS and candidate datasets\r\n# corpora downloads for nltk\r\nnltk.download('stopwords')\r\n\r\n# dictionary size\r\ndictionarySize = 7000\r\n\r\n# Function for build the one hot matrix\r\ndef build_corpus(tweets):\r\n # tokenize and get frequency\r\n topUniqueWordsFiltered = []\r\n tok = Tokenizer(filters='!\"#$%&()*+,-./:;<=>?[\\\\]^_`{|}~\\t\\n')\r\n tok.fit_on_texts(tweets)\r\n # print(tok.__dict__.items())\r\n topUniqueWords = sorted(tok.word_counts.items(), key=lambda x: x[1], reverse=True)\r\n # print(topUniqueWords)\r\n for word,_ in topUniqueWords:\r\n if len(word)> 3 and ('@') not in word and 'http' not in word and word not in stopwords.words('spanish') and not word in topUniqueWordsFiltered:\r\n topUniqueWordsFiltered.append(word) \r\n return topUniqueWordsFiltered[:dictionarySize]",
"[nltk_data] Downloading package stopwords to /root/nltk_data...\n[nltk_data] Unzipping corpora/stopwords.zip.\n7000 ['gracias', 'rajoy', 'gobierno', 'mejor', 'ahora', 'mañana', 'gran', 'días', 'psoe', 'bien', 'dice', 'españa', 'nuevo', 'feliz', 'años', 'hace', 'madrid', 'hacer', 'bueno', 'buenos', 'solo', 'siempre', 'menos', 'buen', 'rubalcaba', 'reforma', 'congreso', 'quiero', 'puede', 'buena', 'gente', 'buenas', 'andalucía', 'laboral', 'aquí', 'déficit', 'vida', 'trabajo', 'vamos', 'millones', 'parece', 'verdad', 'noche', 'presidente', 'abrazo', 'cada', 'cosas', 'empleo', 'mundo', 'casa', 'semana', 'enhorabuena', 'espero', 'sido', 'pues', 'crisis', 'ayer', 'muchas', 'partido', 'noches', 'país', 'sólo', 'nueva', 'creo', 'nunca', 'tiempo', 'grande', 'tener', 'amigos', 'mismo', 'después', 'gusta', 'foto', 'tras', 'huelga', 'cont', 'personas', '2012', 'hecho', 'cambio', 'igual', 'toda', 'primera', 'twitter', 'decir', 'elcambioandaluz', 'política', 'paro', 'quiere', 'tarde', 'falta', 'recortes', 'primer', 'amigo', 'claro', 'felicidades', 'apoyo', 'navidad', 'nadie', 'ministro', 'dinero', 'peor', 'caso', 'familia', 'puedo', 'poder', 'público', 'junta', 'impuestos', 'aunque', 'garzón', 'todas', 'chacón', 'acuerdo', 'luego', 'alguien', 'ramirez', 'cómo', 'equipo', 'hora', 'final', 'debe', 'jajaja', 'euros', 'suerte', 'debate', 'sigue', 'pais', 'horas', 'parte', 'cuenta', 'momento', 'social', 'grandes', 'busta', 'casi', 'pide', 'montoro', 'educación', 'valencia', 'amaiur', 'siento', 'hablar', 'queda', 'según', 'orbyt', 'investidura', 'seguro', 'malaga', 'justicia', 'pasado', 'veces', 'favor', 'interesante', 'dicen', 'general', '2011', 'mejores', 'viernes', 'salir', 'cierto', 'futuro', 'plan', 'mucha', 'disponible', 'triste', 'sino', 'seguir', 'prensa', 'visto', 'discurso', 'fiscal', 'amor', 'fuerte', 'españoles', 'europa', 'acabo', 'asturias', 'pasa', 'dicho', 'sanidad', 'hola', 'mujeres', 'elecciones', 'programa', 'real', 'campaña', 'guindos', 'trabajar', 'economía', 'genial', 'deuda', 'juez', 'mientras', 'vaya', 'historia', 'único', 'encanta', 'mujer', 'sevilla', 'ganas', 'persona', 'sabe', 'tres', 'besos', 'libro', 'acaba', 'dormir', 'cara', 'miedo', 'mayor', 'haber', 'hacen', 'medidas', 'calle', 'problema', 'pronto', 'dejo', 'jaja', 'frente', 'grupo', 'sociales', 'viene', 'sindicatos', 'presupuestos', 'domingo', 'noticia', 'derecho', 'entrevista', 'lunes', 'deja', 'especial', 'dijo', 'bonito', 'zapatero', 'usted', 'nuevos', 'gana', 'libertad', 'dios', 'camino', 'dias', 'empresas', 'meses', 'hombre', '¿qué', 'paso', 'artículo', 'blog', 'cosa', 'importante', 'mala', 'llegar', 'víctimas', 'griñán', 'salud', 'directo', 'portada', 'anuncia', 'político', 'medio', 'reyes', 'periodistas', 'premio', 'pasar', 'saber', 'malo', 'haciendo', 'reformas', 'pregunta', 'viendo', 'gasto', 'habla', 'posible', 'beso', 'pena', 'derechos', 'consejo', 'fuerza', 'último', 'parados', 'pagar', 'ciudadanos', 'extraño', 'arenas', 'gallardón', 'ministros', 'trabajadores', 'democracia', 'cree', 'pueblo', 'corrupción', 'pueden', 'nacional', 'andalucia', 'violencia', 'serio', 'fotos', 'lindo', 'málaga', 'pone', 'primero', 'espera', 'ganar', 'urdangarin', 'proyecto', 'forma', 'izquierda', 'sale', 'andaluces', 'vale', 'deseo', 'leer', 'cumple', 'ajuste', 'quieren', 'palabras', 'digo', 'punto', 'lado', 'empieza', 'información', 'concierto', 'periodismo', 'saludos', 'políticos', 'regalo', 'ciudad', 'historias', 'recorte', 'medios', 'socialistas', 'corazón', 'mayoría', 'español', 'compañeros', 'española', 'cariño', 'realidad', 'pública', 'oposición', 'llama', 'situación', 'electoral', 'barcelona', 'unas', 'victoria', 'difícil', 'sevillahoy', 'camps', 'llega', 'duda', 'riesgo', 'gratis', 'lleva', 'hizo', 'policía', 'niños', 'subida', 'bruselas', 'dejar', 'vuelta', 'dentro', 'siendo', 'blanco', 'poner', 'interior', 'internet', 'datos', 'empezar', 'dado', 'video', 'toca', 'soraya', 'servicios', 'constitución', 'diputados', 'escuchar', 'banco', 'minutos', 'amiga', 'seguridad', 'madre', 'tampoco', 'nuevas', 'volver', 'razón', 'quién', 'aqui', 'media', 'tipo', 'marzo', 'algún', 'idea', 'moreno', 'objetivo', 'centro', 'igualdad', 'fraga', 'mano', 'ayuda', 'sueño', 'duro', 'respeto', 'pacto', 'propone', 'necesita', 'banca', 'problemas', 'votos', 'merkel', 'subir', 'recuerdo', 'vídeo', 'además', 'públicos', 'ojalá', 'padre', 'encima', 'defensa', 'vuelve', 'cospedal', 'queremos', 'gusto', 'enero', 'homenaje', 'muerte', 'debería', 'derecha', 'acto', 'frío', '17congresopp', 'imposible', 'rato', 'nota', 'ejemplo', 'podemos', 'enorme', 'demás', 'misma', 'felices', 'jóvenes', 'cambiar', 'entiendo', 'josé', 'mesa', 'crear', 'alguna', 'confianza', 'noticias', 'guapa', 'nombre', 'económica', 'puntos', 'placer', 'sábado', 'mercados', 'carlos', 'jajajaja', 'fraude', 'culpa', '¿por', 'facebook', 'viva', 'sola', 'puedes', 'modelo', 'hacia', 'espectacular', 'rbcb', 'voto', 'cumpleaños', 'guerra', 'haga', 'junto', 'tema', 'éxito', 'justo', 'pobre', 'juntos', 'puesto', 'lugar', 'supremo', 'socialista', 'hijo', 'atención', 'cine', 'lucha', 'segundo', 'sistema', 'internacional', 'trabajando', 'quedan', 'impresionante', 'despido', 'hablando', 'ideas', 'cualquier', 'libre', 'pago', 'última', 'fondo', 'perdido', 'pedir', 'tambien', 'lista', 'tierra', 'jueves', 'excelente', 'esperanza', 'única', 'mensaje', 'comer', 'javier', 'sabes', 'agua', 'cerca', 'candidato', 'manera', 'comisión', '¡noticias', 'descombacantes', 'ecuador', 'entonces', 'jefe', 'demasiado', 'empresa', 'compromiso', 'increíble', 'juicio', 'timeline', 'momentos', 'bancos', 'clase', 'periodista', 'euro', 'total', 'contigo', 'mierda', 'control', 'cuanto', 'necesito', 'ccaa', 'barça', 'ayuntamiento', 'diputado', 'propuesta', 'líder', 'hijos', 'diga', 'vemos', 'baja', 'merece', 'encuentro', 'abrazos', 'denuncia', 'portavoz', 'aldia', 'señor', 'número', 'costa', 'alonso', 'sonia', 'sentencia', 'esfuerzo', 'post', 'andaluz', 'odio', 'fácil', 'ayudas', 'copa', 'griñan', 'sociedad', 'transparencia', 'error', 'seguimos', 'juan', 'prima', 'luis', 'rivera', 'pdwfyyra', 'manos', 'saludo', 'pueda', 'políticas', 'teatro', 'reunión', 'muerto', 'director', 'bajo', 'familias', 'esperando', 'crecimiento', 'martes', 'carta', '38congresopsoe', 'adelante', 'mamá', 'vivir', 'cuentas', 'todavía', 'opinión', 'comienza', 'sigo', 'miércoles', 'grecia', 'conmigo', 'propuestas', 'últimos', 'hará', 'sube', 'gestión', 'canal', 'próximo', 'recuerda', 'vergüenza', 'conocer', 'rosa', 'francia', 'lasso', 'evitar', 'recomiendo', 'jeje', 'cabeza', 'primeros', 'tiempos', 'papá', 'sector', 'presupuesto', 'cuatro', 'cierre', 'pleno', 'podría', 'quería', 'mercado', 'recortar', 'followercetes', 'botella', 'contamos', 'disco', 'manuel', 'cataluña', 'sarkozy', 'ustedes', 'imagen', 'resto', 'resultado', 'santa', 'llevo', 'calidad', 'preguntas', 'servicio', 'verte', 'ello', 'dejado', 'siria', 'mira', 'quieres', 'ningún', 'allí', 'diario', 'rueda', 'bonita', 'austeridad', 'antonio', 'mundial', 'ganado', 'jugar', 'fútbol', 'recomendable', 'foro', 'decisión', 'siguen', 'bono', 'muertos', 'poquito', 'partir', 'cumplir', 'película', 'radio', 'perdón', 'hago', 'segunda', 'febrero', 'encantado', 'extremadura', 'música', 'comentarios', 'pase', 'ilusión', 'dolor', 'ministra', 'presenta', 'yeswespainisdifferent', 'tomar', 'diez', 'eeuu', 'padres', 'hacerlo', 'bastante', 'entrar', 'pensar', 'recesión', 'mando', 'juego', 'análisis', 'aznar', 'parlamento', 'perder', 'sabemos', 'digital', 'asegura', 'necesario', 'gustaría', 'felicidad', 'upyd', 'urdangarín', 'fiestas', 'publico', 'moncloa', 'cádiz', 'nivel', 'comida', 'oficial', 'comunidad', 'sueldo', 'memoria', 'pensiones', 'cargo', 'dije', 'responsabilidad', 'largo', 'abierto', 'puerta', 'bdías', 'arte', 'respuesta', 'públicas', 'llorar', 'saben', 'hombres', 'salida', 'llegado', 'partidos', 'garcía', 'intervención', 'terrorismo', 'países', 'personal', 'gustado', 'nadal', 'lelo', 'seria', 'popular', 'fiscales', 'hermoso', 'económico', 'funcionarios', 'acabar', 'cena', 'editorial', 'cascos', 'cmin', 'miles', 'presos', 'esperar', 'busca', 'ánimo', 'honor', 'animo', 'condena', 'venezuela', 'jornada', 'sacar', 'pierde', 'ninguna', 'cultura', 'alemania', 'rtve', 'pido', 'pedro', 'marcha', 'oportunidades', 'alto', 'seguidores', 'alegría', 'irpf', 'puta', 'existe', 'alma', 'vacaciones', 'pesar', 'listo', 'fiesta', 'obama', 'confirma', 'aguirre', 'dando', 'correa', 'cárcel', 'tanta', 'frase', 'solidaridad', 'felipe', 'empezamos', 'gonzález', 'votar', 'anterior', 'recibe', 'clases', 'defender', 'maravilla', 'valor', 'negro', 'lópez', 'anoche', 'toma', 'dura', 'oferta', 'dónde', 'propio', 'alcalde', 'tele', 'ipad', 'unidad', 'europea', 'votapp', 'besazo', 'secretario', 'lleno', 'comprar', 'guste', 'redes', 'vivo', 'hermano', 'visita', 'normal', 'miguel', '0l0hzkdb', 'civil', 'común', 'decía', 'pasando', 'fondos', 'cristina', 'chicos', 'compartir', 'andaluzas', 'defiende', 'supuesto', 'interés', 'disfrutar', 'mitad', 'diciembre', 'semanas', 'comunicación', 'viaje', 'veremos', 'bilbao', 'gómez', 'sera', 'haces', 'perfecto', 'argentina', 'escuchando', 'fernández', 'resultados', 'david', 'deben', 'doble', 'paga', 'cifra', 'libros', 'premios', 'abre', 'suena', 'mensajes', 'niño', 'muere', 'gustan', 'matas', 'estabilidad', 'oportunidad', 'palabra', 'tuits', 'presentación', 'ataque', 'audiencia', 'vasco', '2010', 'tribunal', 'senado', 'central', 'debemos', 'dejan', 'empresarios', 'hija', 'profesionales', 'grave', 'critica', 'santamaría', 'amnistía', 'otero', 'spanair', 'necesitamos', 'inversión', 'duele', 'anda', 'felicitaciones', 'humor', 'losdesayunosdetve', 'explica', 'dices', 'estrategia', 'capacidad', 'ayudar', 'alegro', 'precio', 'zona', 'querido', 'verlo', 'dudas', 'wert', 'puro', 'raro', 'impuesto', 'varios', 'investigación', 'aborto', 'hablamos', 'clásico', 'pobres', 'entrada', 'súper', 'leído', 'contrario', 'amenaza', 'maría', 'dulce', 'anuncio', 'ajustes', 'hacemos', 'causa', 'exige', 'hacienda', 'intereses', 'allá', 'ingresos', 'piensa', 'imágenes', 'pasan', 'pelo', 'carrera', 'atrás', 'canción', 'crack', 'marca', 'promete', 'explicar', 'apoya', 'financiera', 'candidatura', 'mentiras', 'llevar', 'responde', 'administración', 'tantas', 'pilar', 'café', 'club', 'sala', 'carmen', 'portadaepc', 'conseguir', 'ofrece', 'tercer', 'entiende', 'estan', 'mejorar', 'reducir', 'maravilloso', 'iniciativa', 'muestra', 'podido', 'clave', 'papel', 'cinco', 'serie', 'perfil', 'entradas', 'tardes', 'vecinos', 'salamanca', 'acusa', 'niega', 'aprueba', 'hablan', 'propia', 'ministerio', 'darle', 'creer', 'daño', 'capaz', 'pensando', 'gobernar', 'detalles', 'venir', 'profesional', 'informe', 'contento', 'edición', 'campo', 'cama', 'gira', 'estupendo', 'vicepresidenta', 'goya', 'canciones', 'arauz', 'recuperar', 'mentira', 'bienestar', 'diciendo', 'pienso', 'favorito', '2017', 'esperamos', 'abril', '¿cómo', \"'público'\", 'rico', 'cáncer', 'próxima', 'fracaso', 'china', 'niña', 'dirección', 'cámara', 'coche', 'europeo', 'publica', 'irán', 'delegada', 'apuesta', 'palma', 'judicial', 'mismos', 'cuento', 'apenas', 'edad', 'crédito', 'cero', 'hermosa', 'ambiente', 'verano', 'sorpresa', 'leyendo', 'lectura', 'manifestación', 'elprimernaufragio', 'legislatura', 'pedazo', 'llena', 'liga', 'prisión', 'copago', 'marta', 'hambre', 'decisiones', 'siga', 'malas', 'tuit', 'joven', 'sueldos', 'alfonso', 'participación', 'dato', 'trata', 'linda', 'descansar', 'entrega', 'brutal', 'jorge', 'absoluta', 'siguiendo', 'messi', 'sabía', 'realmente', 'compañero', 'desempleo', 'vino', 'rechaza', 'piden', 'llegan', 'prueba', 'curioso', 'temporada', 'estreno', 'encontrar', 'rubal', 'athletic', 'cuantos', 'tantos', 'lejos', 'venga', 'tranquilo', 'calles', 'obra', 'siguiente', 'gobiernos', 'talento', 'escándalo', 'peli', 'chaves', 'bajar', 'creen', 'experiencia', 'plaza', 'herencia', 'aire', 'declaración', 'politica', 'contrato', 'sentir', 'cita', 'sitio', 'guapo', 'lujo', 'guerrero', 'periódico', 'afganistán', 'candidatos', 'plata', 'super', 'quedar', 'tremendo', 'apoyar', 'pocos', 'universidad', 'méxico', 'diferencia', 'primeras', 'condenado', 'díaz', 'vuelvo', 'batalla', 'ejecutiva', 'valenciana', 'decreto', 'base', 'hagan', 'móvil', 'negocio', 'fijo', 'luna', 'mayores', 'arriba', 'quedo', 'recibir', 'preciosa', 'incluso', 'celebrar', 'tasa', 'generalitat', 'reformalaboral', 'mantener', 'presidencia', 'loco', 'risa', 'llegue', 'crea', 'silencio', 'hice', '¡qué', 'chicas', 'entra', 'novia', 'precioso', 'heridos', 'podéis', 'penal', 'jurado', 'cierra', 'barato', 'inteligente', 'opción', 'máximo', 'estudiantes', 'sonrisa', 'terminar', 'malos', 'manda', 'alta', 'hablado', 'inocente', 'campeón', 'ignacio', 'vuelto', 'fuerzas', 'mato', 'rafa', 'parlamentario', 'patrimonio', 'insiste', 'cuarto', 'salen', 'entender', 'vienen', 'quedado', 'recordar', 'pensé', 'mantiene', 'actual', 'través', 'llego', 'conozco', 'responsable', 'página', 'gracia', 'puse', 'venta', 'pongo', 'reflexión', 'posada', 'tribuna', 'reconoce', 'quizá', 'plena', 'orgullo', 'legal', 'presente', 'estudiar', 'buscar', 'recién', 'funciona', 'salga', 'escribir', 'pequeño', 'ganó', 'generales', 'útil', 'sesión', 'tono', 'ayto', 'báñez', 'sueños', 'verde', 'jamás', 'digan', 'especialmente', 'afirma', 'cuesta', 'moral', 'despues', 'quiera', 'fuentes', 'pequeña', 'ciudadanía', 'capacidadparacambiar', 'derrota', 'razones', 'cámaras', 'divertido', 'publicado', 'julio', 'golpe', 'bella', 'imprescindible', 'díez', 'constitucional', 'pendiente', 'participar', 'proveedores', 'regalos', 'iphone', 'llevan', 'pymes', 'rápido', 'podía', 'faltan', 'fans', 'mariano', 'europeos', 'brillante', 'admite', 'navarro', 'larga', 'ecuatorianos', 'perro', 'escuela', 'menor', 'salido', 'chica', 'cambia', 'elegir', 'vista', 'relación', 'conseguido', 'ojala', 'hotel', 'breve', 'militantes', 'oscar', 'buenasnoches', 'pepe', 'formación', 'rebaja', 'cgpj', 'cargos', 'empleos', 'siquiera', 'sede', 'broma', 'salario', 'grupos', 'armas', 'basura', 'bienvenida', 'contar', 'turismo', 'lamentable', 'finde', 'trama', 'patio', 'medida', 'investidurarajoy', 'firma', 'guardia', 'debo', 'iglesia', 'ceoe', 'patxi', 'criterio', 'pasó', 'pura', 'privada', 'tweet', 'diferente', 'barrio', 'recuperación', 'alcaldesa', 'italia', 'termina', 'compañía', 'versión', 'bienvenido', 'familiar', 'jueces', 'columna', 'telediario', 'navarra', 'cumbre', 'autonomías', 'prioridad', 'hicieron', 'presidenta', 'querer', 'margen', 'lluvia', 'cuba', 'energía', 'resulta', 'protesta', 'emprendedores', 'caída', 'patronal', 'orgulloso', 'varias', 'ojos', 'paseo', 'tertulia', 'magos', 'cenar', 'escrito', 'gala', 'diálogo', 'deseos', 'jesús', 'salgado', 'etarras', 'ayuntamientos', 'basta', 'publicidad', 'proceso', 'importa', 'delito', 'viejo', 'preocupa', 'tarea', 'supera', 'feriado', 'encuesta', 'acabó', 'maravillosa', 'salvo', 'onda', 'autónomos', 'subvenciones', 'monti', 'consejero', 'debateand', 'deberían', 'asunto', 'demagogia', 'millón', 'guillermo', 'contratos', 'cobrar', 'cuerpo', 'dará', 'gane', 'mandar', 'deje', 'estudio', 'gastos', 'importantes', 'creación', 'función', 'actividad', '¡gracias', 'conocido', 'detalle', 'señal', 'ando', 'santiago', 'hermana', 'jejeje', 'apple', 'encontrado', 'querida', 'spain', 'tomás', 'errores', 'estrena', 'aprobado', 'crítica', 'tesoro', 'considera', 'vota', 'pablo', 'gordo', 'objetivos', 'cuándo', 'simplemente', 'innovación', 'interesa', 'acceso', 'hospital', 'cambios', 'toledo', 'sirve', 'pude', 'alternativa', 'declaraciones', 'lástima', 'casos', 'horrible', 'rodríguez', 'minuto', 'sorteo', 'récord', 'risas', 'piel', 'seis', 'primavera', 'maestro', 'resumen', '13tv', 'ricos', 'banda', 'provincia', 'recursos', 'rumbo', 'fuertes', 'orden', 'pedido', 'puedan', 'bancario', 'pruebas', 'espacio', 'responsabilidades', 'abrir', 'playa', '2016', 'chico', 'recuerdos', 'tweets', 'acaban', 'aeropuerto', 'metro', 'detrás', 'pese', 'ultima', 'franquismo', 'desarrollo', 'cuidado', 'pregunto', 'dedicado', 'concurso', 'meter', 'ultimo', 'caja', 'verla', 'hablo', 'temas', 'privado', 'suficiente', 'bolsa', 'cifras', 'salarios', 'permite', 'llamar', 'preguntamos', 'leche', 'pinta', 'lara', 'menudo', 'mirar', 'alberto', 'león', 'crónica', 'articulo', 'humildad', 'deporte', 'vivienda', 'reconocimiento', 'escaño', 'posesión', 'policial', 'previsto', 'corruptos', 'responder', 'cuánto', 'dignidad', 'despilfarro', 'consumo', 'planes', 'quede', 'ponen', 'financiero', 'código', 'necesidad', 'vara', 'prefiero', 'color', 'plazo', 'sangre', 'amigas', 'reclama', 'mill', 'imputado', 'chacon', 'facturas', 'imagino', 'vuelva', 'boca', 'ciudadano', 'actitud', 'fernando', 'castro', 'pendientes', 'pocas', 'presentado', 'celular', 'mexico', 'google', 'techo', 'empezó', 'usar', 'preparando', 'fecha', 'muchísimo', 'besitos', 'escenario', 'manifestaciones', 'uruguay', 'fundación', 'delegados', 'baleares', 'pepa', 'puestos', 'principal', 'dejen', 'mínimo', 'intenso', 'elección', 'cuestión', 'formas', 'pagan', 'liderazgo', 'demuestra', 'posición', 'examen', 'morir', 'rica', 'inicio', 'supongo', 'aprender', 'muchísimas', 'permanente', 'línea', 'parque', 'colaboración', 'calendario', 'mallorca', 'rusia', 'galicia', 'llaman', 'financiación', 'elena', 'sanz', 'protestas', 'reportaje', 'exclusiva', 'castillo', 'humano', 'estilo', 'destrucción', 'poca', 'luchar', 'competencia', 'podrá', 'salvar', 'papa', 'significa', 'totalmente', 'garantizar', 'delante', 'cielo', 'conferencia', 'muero', 'estupenda', 'novela', 'azul', 'disfrutando', 'matrimonio', 'premiosgoya', 'unión', 'dirigentes', 'listas', '1000', 'éste', 'necesitan', 'valiente', 'condiciones', 'alerta', 'comunidades', 'empleados', 'logra', 'cajas', 'chile', 'caer', 'esperan', 'rescate', 'quita', 'abierta', 'tranquila', '¿alguien', 'fuego', 'cantar', 'dormido', 'calor', 'igualmente', 'espectáculo', 'link', 'presión', 'emocionante', 'santo', 'palacio', 'lotería', 'valladolid', 'alemán', 'castilla', 'fabra', 'bravo', 'eperiódico', 'hable', 'pendejo', 'falso', 'vieja', 'obras', 'reír', 'modo', '2013', 'olvidar', 'terrible', 'discapacidad', 'sabia', 'corto', 'líderes', 'delitos', 'recibido', 'ademas', 'labor', 'falsos', 'enfermedad', 'parecido', 'medias', 'debajo', 'inglés', 'temprano', 'musica', 'elegido', 'salgo', 'proyectos', 'faltaba', 'jose', 'manifiesto', 'altura', 'supone', 'importancia', 'margallo', 'ssanta12', 'romney', 'televisión', 'capital', 'pastor', 'éxitos', 'completo', 'parecer', 'vive', 'marido', 'dejó', 'mente', 'solución', 'presentar', 'pequeños', 'obliga', 'crecer', 'celebra', 'alguno', 'alegra', 'reconocer', 'plantea', 'preguntan', 'empiezo', 'recuento', 'descanso', 'accidente', 'secreto', 'love', 'agencia', 'cola', 'sánchez', 'comienzo', 'infantil', 'exteriores', 'aplausos', 'miembros', 'conflicto', 'invercaria', 'perdáis', 'prisa', 'detenidos', 'iglesias', 'consecuencias', 'envidia', 'jajajajaja', 'diría', 'exterior', 'buscando', 'saca', '¿quién', 'videos', 'escolar', 'intento', 'conversación', 'oído', 'texto', 'pasada', 'belleza', 'oficina', 'justifica', 'gracioso', 'abogados', 'cerrar', 'roto', 'decidido', 'responsables', 'bomba', 'petición', 'antigüedad', 'cortes', 'primarias', 'recurso', 'iberia', 'nuclear', 'catalán', 'bildu', 'interesantes', 'comparecencia', 'andaluza', 'aparte', 'mental', 'inteligencia', 'podrán', 'peligro', 'novio', 'acuerdos', 'pretende', 'pobreza', 'preparado', 'aquellos', 'suma', 'votado', 'soluciones', 'tarjeta', 'administraciones', 'comité', 'absolutamente', 'mayo', 'llamado', 'info', 'ocho', 'operación', 'lanza', 'ninguno', 'reina', 'planeta', 'intentar', 'tráfico', 'detenido', 'renuncia', 'coste', 'nombramiento', 'fomento', 'contador', 'directivos', 'enmienda', 'cooperación', 'concordia', 'fiscalía', 'despidos', 'encuestas', 'perdida', 'leyes', 'regalar', 'chiste', 'humanos', 'jajajajajaja', 'paguen', 'valores', 'urnas', 'presencia', 'convencido', 'dijeron', 'roja', 'motivo', 'respecto', 'superior', 'agradecer', 'haremos', 'colegio', 'entero', 'reto', 'contesta', 'películas', 'puente', 'llegando', 'horario', 'cansado', 'curiosidad', 'deseando', 'partidazo', 'hablaremos', 'mirando', 'exposición', 'instituto', 'retraso', 'carga', 'abogado', 'oficiales', 'alsina', 'gürtel', 'simancas', 'rentas', 'torres', 'iran', 'latre', 'eliminar', 'dólares', 'tonto', 'ambos', 'parecen', 'redacción', 'siglo', 'viejos', 'despedir', 'volvemos', 'materia', 'preguntando', 'urgente', '¿que', 'deficit', 'increible', 'revista', 'ponga', 'alcaldes', 'aplicación', 'necesaria', 'queridos', 'menores', 'gato', 'hacerme', 'tenia', 'anunciar', 'noviembre', 'acerca', 'enemigo', 'adoro', 'granada', 'fantástico', 'sorprende', 'ccoo', 'mingote', 'córdoba', 'autonómicas', 'polémica', 'banquero', 'histórica', 'escuchas', 'bonitas', 'asociación', 'conoce', 'créditos', 'ruido', 'jugadores', 'señora', 'youtube', 'industria', 'agradece', 'tocar', 'unidos', 'llamada', 'triunfo', 'tasas', 'ejercicio', 'dependencia', 'agenda', 'iguales', 'presupuestaria', 'amable', 'ropa', 'juega', 'auto', 'entrenamiento', 'mario', 'today', 'sensación', 'peso', 'ganador', 'presento', 'magnífico', 'militar', 'siete', 'municipal', 'subasta', 'impone', 'hormiguero', 'elorrio', 'tensión', 'infanta', 'puso', 'andar', 'devolver', 'dictadura', 'pensaba', 'caro', 'exacto', 'cumplen', 'investigar', 'economia', 'cualquiera', 'ganan', 'llegará', 'difíciles', 'comisiones', 'familiares', 'romero', 'tercera', 'laborales', 'vienes', 'culpable', 'sencillo', 'extrema', 'teléfono', 'abajo', 'visitar', 'expresión', 'compra', 'camiseta', 'esperemos', 'votación', 'justa', 'compañera', 'francisco', 'preguntar', 'tren', 'prometo', 'escucho', 'contenta', 'asuntos', 'posibilidad', 'coches', 'contestar', 'decide', 'superar', 'homs', 'desviación', 'monago', '25mrebélate', 'sáenz', 'enrique', 'atentado', 'hala', 'banquillo', 'actos', 'argumentos', 'claves', 'marruecos', 'renta', 'porqué', 'disfruta', 'negocios', 'banqueros', 'escribe', 'extraña', 'genio', 'lograr', 'vales', 'hahahaha', 'aquel', 'saldrá', 'avión', 'firme', 'acabe', 'depende', 'llevado', 'echo', 'comparto', 'joder', 'tranquilidad', 'atentos', 'blanca', 'puerto', 'norte', 'género', 'aviso', 'juro', 'caras', 'saliendo', 'embajador', 'titular', 'temo', 'acabamos', 'ratito', 'etapa', 'estrella', 'rojo', 'adelanto', 'renovación', 'rompe', 'tuiteros', 'miembro', 'aumenta', 'lectores', 'consigue', 'valenciano', 'aniversario', 'chatidieta', 'policías', 'dejaron', 'cerebro', 'pies', 'cuál', 'médicos', 'ruptura', 'libres', 'injusto', 'preparar', 'principio', 'altos', 'unico', 'centros', 'aumento', 'ahorro', 'deudas', 'acción', 'regreso', 'lecciones', 'salió', 'quisiera', 'cruz', 'favoritos', 'entorno', 'barca', 'efecto', 'emoción', 'duermo', 'comiendo', 'arranca', 'lengua', 'haré', 'caído', 'tope', 'followers', 'llegada', 'futbol', 'cuentan', 'charla', 'alumnos', 'cervantes', 'floriano', 'catalanes', 'credibilidad', 'soria', 'debatertva', 'hablas', 'gratuito', 'quito', 'trabajan', 'traer', 'voluntad', 'robo', 'cadena', 'podamos', 'dieron', 'principios', 'paula', 'puto', 'ocurre', 'usuarios', 'tipos', 'pierden', 'durán', 'crece', 'bello', 'carretera', 'espalda', 'instituciones', 'vacío', 'venido', 'curso', 'horror', 'baño', 'buenísimo', 'vecino', 'hierro', 'original', 'empezado', 'incendio', 'felicitar', 'entrenar', 'mejora', 'negociación', 'happy', 'adiós', 'disculpas', 'positiva', 'prepara', 'pilotos', 'gobierna', 'referencia', 'davis', 'isabel', 'salarial', 'enlace', 'pons', 'garzon', 'ejemplar', 'desastre', 'delincuentes', 'calla', 'terroristas', 'tremenda', 'poniendo', 'obvio', 'volverá', 'mismas', 'viajar', 'desigualdad', 'jajaj', 'verdadero', 'rechazo', 'informa', 'guille', 'aceptar', 'diferencias', 'online', 'tratar', 'diego', 'merecido', 'colombia', 'puesta', 'moda', 'nombres', 'llegó', 'cristiano', 'paco', 'generación', 'cabo', 'echa', 'catalunya', 'personaje', 'mirada', 'ganamos', 'completa', 'ánimos', 'nueve', 'time', 'apertura', 'york', 'twitteros', '2008', 'franceses', 'madrileños', 'portugal', 'amiguete', 'histórico', 'lachispadelavida', 'almería', 'socialismo', 'señores', 'octubre', 'crees', 'pudo', 'sigan', 'título', 'perros', 'puertas', 'ruina', 'chino', 'veras', 'piensan', 'emocionado', 'profesor', 'vende', 'local', 'precios', 'ridículo', 'vayan', 'propios', 'critican', 'criticar', 'tomando', 'empresario', 'cantidad', 'trae', 'activo', 'pérdida', 'cabe', 'preocupado', 'pidiendo', 'invitados', 'pelota', 'segura', 'mata', 'depresión', 'feria', 'avanza', 'irme', 'agradezco', 'esfuerzos', 'asesino', 'vídeos', 'injusticia', 'democrática', 'trimestre', 'locales', 'tranquilos', 'ventana', 'fallo', 'receta', 'diarios', 'resolver', 'parar', 'argumento', 'ejecutivo', 'censura', 'rodriguez', 'sumo', 'vergonzoso', 'paséis', 'autonómico', 'juvenil', 'municipios', 'abadillo', 'gaceta', 'estructural', 'enough', 'trajes', 'naufragio', 'avance', 'prevé', 'dación', 'aragón', 'algun', 'dale', 'universal', 'mami', 'llevamos', 'época', 'apoyando', 'insultos', 'casas', 'creemos', 'asamblea', 'trabaja', 'democrático', 'hecha', 'económicas', 'cayo', 'mentir', 'cobertura', 'aplicar', 'vender', 'quitar', 'gabinete', 'empezando', 'ganando', 'bendiciones', 'partes', 'acepta', 'reducción', 'profesión', 'pagado', 'irse', 'verdes', 'pases', 'segundos', 'rara', 'revisar', '¡feliz', 'dormida', 'nochebuena', 'corte', 'decidir', 'show', 'pelicula', 'grabar', 'echar', 'paciencia', 'rollo', 'teneis', 'concha', 'lima', 'expertos', 'goles', 'acertado', 'capitán', 'grados', 'tuiter', 'relaciones', 'cancion', 'efectos', 'nieve', 'creativo', 'desaparecido', 'actuación', 'últimas', 'fotito', 'alfredo', 'denuncian', 'mancha', 'explicaciones', 'previsiones', 'marivi', 'larazon', 'euskadi', 'abcdesevilla', 'museo', 'unido', 'montes', 'descanséis', 'régimen', 'agricultura', 'declara', 'escaños', 'bañez', 'sociedades', 'repago', 'descarta', 'grease', 'debates', 'gobiernodelcambio', 'podrían', 'idiota', 'ciudades', 'pilas', 'letra', 'comercio', 'permiso', 'cayó', 'peores', 'quieran', 'diferentes', 'definitivamente', 'terremoto', 'gastar', 'equipos', 'olvido', 'documento', 'tomado', 'salimos', 'incluye', 'tienda', 'terrorista', 'razonable', 'generar', 'comprometido', 'bandera', 'empiezan', 'hagamos', 'quedó', 'sepa', 'tristeza', 'positivo', 'corazon', '¿será', 'termine', 'conciertos', 'culo', 'finales', 'ruedas', 'griego', 'suelo', 'hicimos', 'antiguo', 'especiales', 'insulto', 'pelis', 'consejos', 'lógico', 'acierto', 'afición', 'despertar', 'nena', 'agentes', 'sorpresas', 'ritmo', 'retos', 'perú', 'relato', 'interna', 'bcna', 'consenso', 'times', 'eguiguren', 'guardiola', 'londres', 'desmiente', 'retirada', 'batasuna', 'subidas', 'intereconomía', 'megaupload', 'lamenta', 'sanitario', 'adelanta', 'demanda', 'movimiento', 'colegios', 'preso', 'población', 'robar', 'musical', 'condenados', 'huevo', 'económicos', 'enfermo', 'lugares', 'opciones', 'complicado', 'come', 'fuente', 'pensado', 'parada', 'lastima', 'anti', 'destino', 'cumplido', 'duros', 'convierte', 'desayuno', 'navideño', 'escribo', 'activa', 'alba', 'quedarse', 'autor', 'septiembre', 'sabéis', 'mola', 'móviles', 'artista', 'tocado', 'fatal', 'siesta', 'plural', 'gral', 'dispara', 'vicente', 'comercial', 'crean', 'causas', 'temporal', 'ataques', 'merkozy', 'erkoreka', 'previsión', 'informacion', 'juzgado', 'independencia', 'extorsión', 'gallardon', 'diputación', 'iñaki', 'liquidez', 'asambleamadrid', 'aprobará', 'fátima', 'leerlo', 'militares', 'eurovegas', 'populista', 'patria', 'andrés', 'maduro', 'pagó', 'avisa', 'paraísos', 'nervios', 'tesis', 'mentiroso', 'américa', 'pasen', 'seguirá', 'termino', 'aprobar', 'decirle', 'pérez', 'matar', 'plataforma', 'dejando', 'clara', 'sobra', 'soledad', 'límite', 'pagando', 'vean', 'comunicado', 'comprado', 'vivimos', 'incluido', 'intención', 'duran', 'gustó', 'partida', 'mérito', 'tirar', 'caliente', 'veía', 'bebé', 'víctima', 'periódicos', 'balance', 'tristes', 'asumir', 'dieta', 'acabado', 'subido', 'santander', 'promoción', 'esperaba', 'francesa', 'aplauso', 'cañete', 'falla', 'prado', 'peque', 'carnaval', 'advierte', 'autonómica', 'pactar', 'quitateeltop', 'cultural', 'logroño', 'iker', 'barreda', 'condenar', 'policiales', 'ramos', 'asturianos', 'excepción', 'israel', 'escucha', 'canta', 'electorales', 'promesas', 'dedo', 'cumplan', 'listos', 'sorry', 'quiebra', 'explicación', 'representa', 'renovables', 'tarifa', 'pierdan', 'revolución', 'estudios', 'hacía', 'engaño', 'buscan', 'formar', 'trampa', 'montón', 'lados', 'respaldo', 'panorama', 'anteriores', 'agradecido', 'descanse', 'dificil', 'preocupación', 'personajes', 'ahorita', 'celebramos', 'olvida', 'capítulo', 'dani', 'imaginar', 'orgullosa', 'drama', 'sección', 'navideña', 'festival', 'primo', 'correcto', 'japón', 'sobran', 'normalidad', 'ruiz', 'suben', 'encuentra', 'aparece', 'voces', 'autobús', 'abiertas', 'revés', 'sentimiento', 'francés', 'mail', 'jerez', 'champions', 'bernabéu', 'franco', 'independiente', 'costar', 'putin', 'investiga', 'contenido', 'presidentes', 'actor', 'agresión', 'despedida', 'asesinato', 'papeles', 'q9hzeylp', 'jaume', 'indulto', 'veracruz', 'morenés', 'organización', 'privilegios', 'reuters', 'artur', 'ofrecer', 'celulares', 'creado', 'persecución', 'visión', 'pagos', 'marco', 'enfermos', 'gratuita', 'repetir', 'digas', 'infraestructuras', 'ésta', 'maria', 'wifi', 'viven', 'cobra', 'apoyado', 'escuchado', 'tecnología', 'productos', 'cien', 'hacerse', 'consejeros', 'lidera', 'reduce', 'ministerios', 'agradecimiento', 'república', 'fundamental', 'murió', 'comenzar', 'harán', 'votantes', 'sanciones', 'afecta', 'exámenes', 'compro', 'recorta', 'artistas', 'reglamento', 'vengo', 'admiro', 'iban', 'conocerte', 'rioja', 'pareja', 'negra', 'jugando', 'saluda', 'queria', 'cameron', 'pasamos', 'pedimos', 'garcia', 'ramón', 'celebrando', 'podré', 'otegi', 'sabado', 'invierno', 'vuelven', 'frio', 'arma', 'seguimiento', 'continúa', 'denunciar', 'sergio', 'motivos', 'turno', 'logrado', 'descanses', 'debatir', 'numero', 'acusado', 'portavoces', 'integración', 'funciones', 'positivegeneration', 'entidades', 'nóos', 'rigor', 'risto', 'nombramientos', 'concentración', 'oculta', 'suscriptores', 'cocaína', 'ecuatoriano', 'invito', 'animal', 'maldito', 'robado', 'paraíso', 'loca', 'vidas', 'acaso', 'principales', 'permitir', 'darte', 'hermanos', 'jodido', 'sinvergüenza', 'hospitales', 'números', 'pasas', 'simple', 'valen', 'vendrá', 'cuantas', 'ideal', 'huelgas', 'cursos', 'coherencia', 'próximos', 'impacto', 'beneficios', 'prioridades', 'compromisos', 'intenta', 'agencias', 'exigir', 'llorando', 'estable', 'molesta', 'regla', 'geniales', 'coca', 'afectados', 'corta', 'cambian', 'extra', 'quedamos', 'mereces', 'triple', 'titulares', 'abuelo', 'willy', 'global', 'pérdidas', 'traído', 'colegas', 'encantan', 'barbaridad', 'coraje', 'pasillos', 'sonrisas', 'intensa', 'alejandro', 'letras', 'martin', 'convenio', 'dispuesto', 'sinde', 'nobel', 'trillo', 'competitividad', 'duque', 'barroso', 'antena', 'jaén', 'parlament', 'angel', 'parlamentaria', '”gracias', 'gestores', 'arenaspresidente', 'bueno90', 'congresos', 'queja', 'saneamiento', 'itunes', 'informativo', 'vascos', '1812', 'toulouse', 'secretaria', 'invitado', 'ladrones', 'caen', 'respetar', 'hogar', 'merecen', 'empiece', 'bonos', 'caballero', 'hagas', 'seres', 'defendiendo', 'pueblos', 'nación', 'tira', 'lider', 'asalto', 'mande', 'contundente', 'vera', 'ironía', 'viste', 'convertir', 'excusa', 'líneas', 'propuesto', 'defienden', 'ventaja', 'preparados', 'proteger', 'huevos', 'daba', 'cansada', 'lindos', 'actividades', 'apellido', 'lección', 'carro', 'belén', 'veré', 'lágrimas', 'pinche', 'auditorio', 'secret', 'avatar', 'agradable', 'define', 'camacho', 'obligación', 'email', 'nerviosa', 'linea', 'experto', 'conversaciones', 'espejo', 'murcia', 'reformar', 'recomendación', 'institucional', 'punta', 'mención', 'alicante', 'oreja', 'pasará', 'médico', 'cadiz', 'sufrir', 'ganará', 'cayendo', 'hechos', 'ahorrar', 'ricardo', 'brava', 'libertades', 'opina', 'declarar', 'embajada', 'rubalcaba38', 'acusación', 'absolución', 'castigo', 'aparato', 'regional', 'ciento', 'intereconomia', 'asume', 'presunto', 'elmundo', 'compromete', 'anunciado', 'pluralidad', 'vuelos', 'mínimos', 'india', 'dorribo', 'revisión', 'anima', 'piquetes', 'ladrón', 'comentario', 'combatir', 'asesor', 'promesa', 'aprende', 'construir', 'radical', 'pensamiento', 'olvide', 'entendido', 'dirigir', 'pagamos', 'asesores', 'nubes', 'perfecta', 'saco', 'rafael', 'existen', 'toque', 'reales', 'eléctrica', 'respuestas', 'sincero', 'dictador', 'prometió', 'absoluto', 'comerciales', 'exceso', 'facil', 'zonas', 'previo', 'cambiado', 'abusos', 'tratado', 'capaces', 'apoyos', 'haría', 'bancada', 'enviar', 'ocurrido', 'llame', 'pasos', 'cerrado', 'inversiones', 'ética', 'jubilación', 'echado', 'aunq', 'reglas', 'isla', 'decirlo', 'desprecio', 'orgullosos', 'utilizar', 'susto', 'tenes', 'page', 'personalmente', 'amanecer', 'bajas', 'abuela', 'humanidad', 'durmiendo', 'últimamente', 'extrañar', 'misión', 'restaurante', 'tenis', 'ufff', 'juegos', 'ruta', 'pollo', 'femenino', 'quedaba', 'suba', 'gorda', 'princesa', 'descubrir', 'natural', 'razon', 'luchando', 'gigante', 'terminado', 'coruña', 'estación', 'felicitación', 'alli', 'hablaba', 'gallego', 'páginas', 'amistad', 'sufren', '¿para', 'admiración', 'firmar', 'saludar', 'dolores', 'mama', 'oficio', 'reino', 'rubio', 'graves', 'contando', 'encantada', 'factura', 'tratamiento', 'reuniones', 'grito', 'republicanos', 'lenguaje', 'consejera', 'dejuan', 'casillas', 'arena', 'movilización', 'jugador', 'atacar', 'publicas', 'antequera', 'recetas', '2009', 'rosell', '7714', 'extraordinaria', 'viviendas', 'valientes', 'zarzuela', 'bastaya', 'defraudadores', 'imputados', 'castellón', 'transición', 'eleva', 'programas', 'documentos', 'flexibilidad', 'espinosa', 'cofradiasmlg', 'pedirá', 'progreso', 'seguramente', 'piensas', 'chinos', 'delincuente', 'voten', 'cuadra', 'organismos', 'piedra', 'publicar', 'mueve', 'proponer', 'directa', 'demagogo', 'impunidad', 'ciudadana', 'miseria', 'sobretodo', 'repito', 'tomo', 'recaudar', 'asco', 'conocimiento', 'gloria', 'enemigos', 'genera', 'estudia', 'joda', 'roban', 'repite', 'reúne', 'gabriel', 'duras', 'preocupante', 'prácticas', 'suficientes', 'finalmente', 'cientos', 'comido', 'dicha', 'tiro', 'competencias', 'reacción', 'impresión', 'semestre', 'justificar', 'peligroso', 'gritos', 'sufre', 'tradición', 'bonitos', 'profe', 'hacerle', 'ensayo', 'almuerzo', 'pista', 'pierda', 'hahaha', 'darme', 'romper', 'españolas', 'agosto', 'sexta', 'quizás', 'espana', 'grabado', 'injusta', 'toni', 'pantalla', 'perdon', 'insultar', 'débil', 'menuda', 'pedo', 'fiel', 'pierdo', 'decían', 'críticas', 'prudencia', 'ángel', '¡que', 'alza', 'cierran', 'esperado', 'peru', 'firmado', 'tuitera', 'tragedia', 'excepcional', 'transporte', 'ovación', 'protagonistas', 'inolvidable', 'federal', 'borde', 'parlamentarios', 'diariosur', 'expolio', 'mossos', 'suprimir', 'navidades', 'plantilla', 'monarquía', 'recupera', 'privatización', 'delegado', 'avales', 'hemiciclo', 'frenar', 'mitin', 'linchamiento', 'watching', 'etarra', 'bankia', 'destaca', 'catalana', 'once', 'fdez', 'teletipo', 'abertzale', 'rival', 'regresar', 'patrón', 'fomentar', 'moneda', 'necesidades', 'dudo', 'demostrado', 'corrupto', 'regalado', 'fuga', 'terreno', 'alcanza', 'populismo', 'derroche', 'arias', 'tome', 'digno', 'sierra', 'becas', 'claras', 'entro', 'analizar', 'metido', 'culpables', 'bachiller', 'socio', 'sacado', 'impresentable', 'candidata', 'obligado', 'seguido', 'dirá', 'sigamos', 'porfa', 'universidades', 'acciones', 'felicito', 'aquella', 'proximo', 'perfectamente', 'aprovechar', 'gasta', 'eficiencia', 'sigues', 'verás', 'autoridad', 'contentos', 'inocentes', 'teoría', 'sentí', 'aburrido', 'clima', 'definitiva', 'gripe', 'maldita', 'probable', 'gasolina', 'discutir', 'definitivo', 'equivocado', 'creciendo', 'sabina', 'hiciera', 'detiene', 'haberme', 'alcohol', 'misa', 'ponerme', 'rubia', 'encantaría', 'cantando', 'diseño', 'leyenda', 'sentado', 'arreglar', 'balón', 'indignados', 'zaragoza', 'legales', 'esteban', 'profunda', 'sana', 'fallecido', '¿cuál', 'neta', 'cumplo', 'inmenso', 'elige', 'flores', 'confirmado', 'mapa', 'parado', 'esencial', 'tuitear', 'trabajadora', 'soldado', 'pida', 'barco', 'impulso', 'mercedes', 'errekondo', 'gesto', 'dialogo', 'insomne', 'ilegales', 'presiones', 'mariví', 'ocultar', 'audiencias', 'civiles', 'sanitaria', 'detención', 'prestaciones', 'rural', 'veto', 'dificultades', 'enterado', 'irak', 'recibida', 'halamadrid', 'pasión', 'urgencia', 'ciudadjardin', 'autónomas', 'paris', 'quot', 'hernandez', 'coloca', 'tribunales', 'regeneración', 'barajas', 'protección', 'botín', 'méndez', 'guiñoles', 'unanimidad', 'suprime', 'señala', 'jordi', 'ortega', 'bicentenario', 'apela', 'presupuestario', 'apunta', 'imperativo', 'jugamos', 'jamas', 'recoger', 'pague', 'drogas', 'ajeno', 'velocidad', 'producción', 'quitan', 'venganza', 'ciencia', 'perdiendo', 'resistencia', 'deportistas', 'verga', 'cinismo', 'reciben', 'aquello', 'buscamos', 'decente', 'plazas', 'votaron', 'solidario', 'propaganda', 'naturaleza', 'falsas', 'impulsar', 'pones', 'privados', 'actuar', 'insisto', 'deberíamos', 'estudiante', 'límites', 'sensato', 'bases', 'círculo', 'debido', 'gestionar', 'martínez', 'propósito', 'from', 'madrugada', 'sirven', 'cole', 'impide', 'entran', 'decimos', 'abrazote', 'evidencia', 'cocina', 'correo', 'crítico', 'decirme', 'distintos', 'corona', 'series', 'llueve', 'concepto', '¿dónde', 'cercano', 'productivo', 'entrenador', 'please', 'rapido', '¡enhorabuena', 'oviedo', 'caracteres', 'cumpla', 'posibilidades', 'comenzamos', 'propongo', 'aburrida', 'alternativas', 'concejal', 'burgos', 'aventura', 'lamento', 'noooo', 'veis', 'ataca', 'pulso', 'roberto', 'actualización', 'caigo', 'ayudado', 'mourinho', 'dulces', 'toman', 'ronda', 'perdió', 'distinto', 'seguidos', 'madres', 'fijado', 'pedía', 'seguiremos', 'roma', 'daniel', 'mandan', 'live', 'palo', 'lemon', 'carme', 'ejército', 'dimisión', 'torre', 'ordenador', 'mirad', 'empresarial', 'teme', 'porquepúblicohacefalta', '¿quien', 'masiva', 'magnífica', 'zerolo', 'sindical', 'terratpack', 'gregorio', 'videocapítulo', 'reivindica', 'celebración', 'juzgados', 'telesucesos', 'eduardo', 'alucinante', 'canarias', 'calificación', 'cope', 'micrófono', 'declarado', 'directora', 'mixto', 'obispo', 'sexo', 'ponencia', 'iredes', 'hachazo', 'acreedores', 'nacionalistas', 'rata', 'obtener', 'altas', '2000', 'trabajador', 'patético', 'levantar', 'votan', 'apoyan', 'entrevistas', 'invento', 'sectores', 'debes', 'vives', 'llamo', 'miserable', 'títulos', 'compañeras', 'logro', 'manifestantes', 'utiliza', 'desempleados', 'básicos', 'privilegio', 'sumar', 'quinta', 'habra', 'inter', 'trabajos', 'novedades', 'contacto', 'figura', 'falsa', 'vendido', 'venden', 'full', 'limpieza', 'contratación', 'controles', 'modelos', 'colaborar', 'tratan', 'petróleo', 'solamente', 'miente', 'alvaro', 'cuello', 'estudiando', 'desgracia', 'estadio', 'fenómeno', 'correr', 'frases', 'ciclo', 'fiebre', 'crush', 'evento', 'sano', 'quedé', 'pasé', 'fechas', 'llevaba', 'similar', 'duelo', 'decís', 'sienta', 'llegamos', 'descansa', 'resolución', 'pidió', 'cargado', 'sentimientos', 'city', 'bici', 'back', 'ganadores', 'abiertos', 'pasta', 'colectivo', 'seguiré', 'usuario', 'prat', 'machista', 'atentados', 'consciente', 'salu2', 'discursos', 'manzano', 'levantado', 'choque', 'doce', 'optimismo', 'cristo', 'kilos', 'brasil', 'huele', 'pierdas', 'negar', 'políticamente', 'suscripción', 'opinar', 'martín', 'poeta', 'agresiva', '¿cuántos', 'sombra', 'interviene', 'actúa', 'restos', 'preguntado', 'insulta', 'yerno', 'mini', 'espíritu', 'plató', 'doctrina', 'costas', 'chiringuitos', 'sanción', 'sebastián', 'artist', 'acato', 'proponemos', 'previsible', 'liderar', 'estatuto', 'enmiendas', 'oeoeoeoeoeoeoe', 'solvencia', 'golazo', 'bipartidismo', 'consecuencia', 'activos', 'negativo', 'dona', 'presidir', 'esencia', 'fortunas', 'intelectual', 'trenes', 'jiménez', 'cohecho', 'magnifico', 'dopaje', 'basagoiti', 'llull', 'moción', 'valderas', 'asociaciones', 'indemnización', 'eurogrupo', 'vivido', 'sacan', 'usan', 'iremos', 'preparada', 'porq', 'tiendas', 'escuelas', 'izquierdas', 'exactamente', 'implica', 'droga', 'soñar', 'acuerda', 'mandato', 'verdadera', 'campañas', 'poderes', 'digitales', 'beber', 'escribiendo', 'humo', 'remedio', 'subiendo', 'despide', 'mandado', 'daré', 'presentamos', 'absurdo', 'pelea', 'financiar', 'desea', 'traiga', 'afiliados', 'refiero', 'carreteras', '¿hay', 'beneficio', 'carreras', 'corregir', 'habitual', 'testigos', 'ingreso', 'producto', 'regalito', 'traerá', 'gonzalo', 'profesores', 'volviendo', 'enseña', 'turistas', 'viajes', 'avanzar', 'medicina', 'eficacia', 'dejes', 'arco', 'conciencia', 'grado', 'sincera', 'costumbre', 'vestido', 'batería', 'deportivo', 'barrios', 'encontré', 'digamos', 'aeropuertos', 'horarios', 'sabor', 'habia', 'cartera', 'cristal', 'violentos', 'muerta', 'tranqui', 'documental', 'gafas', 'coger', 'adolescentes', 'guay', 'posibles', 'comedia', 'tormenta', 'mezcla', 'quieras', 'casita', 'castellano', 'sufrido', 'notas', 'infancia', 'multa', 'conexión', 'caixa', 'identidad', 'celebran', 'copas', 'visitas', 'villa', 'equilibrio', 'urge', 'favorita', 'legado', 'distancia', 'región', 'caballo', 'diré', 'levante', 'ausencia', 'manolo', '¿habrá', 'nervioso', 'mueren', 'regala', 'órganos', 'alcanzar', 'escritor', 'campeones', 'directamente', 'tercero', 'convoca', 'aplauden', 'multas', 'embargo', 'signos', 'lady', 'príncipe', 'podréis', 'pacientes', 'puentes', 'joan', 'telemadrid', 'hahahahaha', 'conocía', 'forojoly', 'contenidos', 'contaremos', 'oigo', 'vela', 'maternidad', 'italiano', 'herido', 'potro', 'euco', 'contado', 'teresa', 'liberal', 'oficialmente', 'salva', 'abandona', 'tropas', 'govern', 'coherente', 'hahahahahaha', 'califica', 'opiniones', 'crespo', 'pensión', 'permitirá', 'betis', 'mirandés', 'fernandez', 'solidaria', 'gurtel', 'reptiles', 'spiegel', 'movilizaciones', 'informativos', 'nacionalismoescrisis', 'bolsillo', 'barata', 'hablará', 'evolución', 'locos', 'propiedad', 'desgraciadamente', 'quedaron', 'invertir', 'teléfonos', 'pensamos', 'asesinos', 'robaron', 'aprobada', 'trabajado', 'famosa', 'mostrar', 'duerme', 'ilegal', 'territorio', 'jaime', 'práctica', 'riqueza', 'reparto', 'mafia', 'prosperidad', 'representantes', 'suicidio', 'dueño', 'cerveza', 'símbolo', 'inútil', 'pretenden', 'infierno', 'colega', 'piso', 'penas', 'únicos', 'sucia', 'aplica', 'pequeñas', 'podrías', 'caminar', 'perdona', 'cuidar', 'amplia', 'educativo', 'olvidado', 'sufrimiento', 'demostrar', 'felicita', 'aprovecha', 'pesadilla', 'acordado', 'intentando', 'material', 'avanzando', 'ambas', 'repente', 'empecé', 'sonreír', 'salí', 'lloro', 'asistir', 'suele', 'star', 'carne', 'paciente', 'profesora', 'árbol', 'unida', 'cafe', 'resignación', 'gustara', 'brazo', 'moto', 'parezca', 'extraordinario', 'berlín', 'manchester', 'terminó', 'convención', 'defensor', 'serlo', 'milagro', 'cenando', 'capítulos', 'vasca', 'cariñoso', 'edificio', 'alegre', 'entera', 'actores', 'queréis', 'lopez', 'rodrigo', 'convocatoria', 'paquete', 'consulta', 'máxima', 'suicida', 'parís', 'lucas', 'jornadas', 'toro', 'informes', 'álbum', 'prometido', 'confía', 'divertida', 'recibo', 'planta', 'técnicos', 'alex', 'deportes', 'césar', 'extranjeros', 'tortura', 'cuadro', 'herida', 'conocen', 'taxi', 'faltas', '¿nos', 'voluntarios', 'profundo', 'basket', 'penales', 'cuartos', 'fórmula', 'raúl', 'marketing', 'renunciar', 'descargas', 'represión', 'plenomlg', 'irregularidades', 'salariales', 'endesa', 'nacionalista', 'apasionante', 'aclara', 'zougam', 'génova', 'different', 'share', 'organizado', 'cumplirá', 'financieros', 'rojas', 'supresión', '000€', 'convivencia', 'recaudación', 'mediante', 'abstención', 'rosa10', 'disolución', 'despacho', 'feliz2012', 'mill€', 'populares', 'iniciativas', 'steve', 'jobs', 'dimitir', 'disciplina', 'bowie65', 'tecnologías', 'garantías', \"'caso\", 'áfrica', 'mássocialismo', 'turístico', 'atleti', 'baloncesto', 'genero', 'portero', 'acabará', 'curiosidaddelinsomne', 'quitado', 'llamazares', 'prevaricación', 'pais”', 'saldremos', 'toxo', 'aumentan', 'reestructuración', 'selección', 'expectativas', 'tuitea', '2004', 'agujero', 'correistas', 'sectarismo', 'bruto', 'vacía', 'chávez', 'paises', 'usando', 'volveremos', 'engañar', 'herramienta', 'causado', 'construcción', 'demas', 'osea', 'gustos', 'fundamentales', 'nariz', 'vivos', 'merecemos', 'economista', 'fila', 'sales', 'aparecen', 'respeta', 'produce', 'semanal', 'alcance', 'jardín', 'explique', 'gritar', 'tabaco', 'vargas', 'famoso', 'permitido', 'seguros', 'distrito', 'hiciste', 'cruel', 'vega', 'confiar', 'provincias', 'mayorías', 'votó', 'cambie', 'privatizar', 'disney', 'pudiera', 'ocasión', 'perjudica', 'perdieron', 'comidas', 'todavia', 'ponerse', 'acceder', 'equivoca', 'medicamentos', 'homosexuales', 'llevas', 'vamo', 'verá', 'calma', 'pata', 'ganadora', 'bailar', 'damos', 'aguas', 'bloqueo', 'locura', 'sentirse', 'caes', 'despierto', 'garganta', 'pesado', 'personales', 'inicia', 'quedarme', 'siii', 'entrando', 'asesinado', 'bona', 'huelva', 'bajado', 'magia', 'echan', 'acabaron', 'gonzalez', 'jesus', 'viejas', 'hacéis', 'acompañado', '2015', 'ficción', 'provocado', 'sensibilidad', 'dedico', 'chistes', 'realizar', 'creéis', 'origen', 'aprendido', 'luces', 'plis', 'with', 'acordar', 'chocolate', 'lindas', 'área', 'inauguración', 'levantarse', 'misterio', 'dedica', 'mágica', 'cantante', 'garantía', 'giro', 'levanta', '2005', 'próximas', 'joaquín', 'promo', 'distintas', 'sarko', 'vidal', 'amanece', 'brothers', 'disfrutéis', 'cabalgata', 'incremento', 'sacrificios', 'inaceptable', 'dietas', 'milagros', 'ferraz', 'ventas', 'nacionalismo', 'garantiza', 'celebrará', 'marbella', 'cura', 'integral', 'recoge', 'clemente', 'traspaso', 'italiana', 'suspende', 'madridistas', 'havel', 'expectación', 'subirá', 'ministrables', 'diputaciones', 'aportaciones', 'washington', 'riolobos', 'ensayos', 'inhabilitación', 'socialdemocracia', 'retira', 'judiciales', 'ejemplares', 'lehendakari', 'tijeretazo', 'autonomía', 'atenas', 'dixit', 'incidentes', 'excesos', 'fichaje', 'fundador', 'intolerable', 'sindicalistas', 'soberanía', 'loewe', 'chófer', 'tuitero', 'malestar', 'malagasanta', 'hollande', 'delegación', 'ofrecido', 'riesgos', 'pareces', 'tontos', 'prófugo', 'sentimos', 'enviado', 'entregar', 'permiten', 'sabían', 'mameluco', 'pegar', 'gays', 'extranjero', 'entrado', 'internacionales', 'acogida', 'carácter', 'vandalismo', 'llamas', 'educacion', 'idiotas', '2020', 'salvador', 'ofrecen', 'jefes', 'maestros', 'cuotas', 'entusiasmo', 'baje', 'comportamiento', 'viviendo', 'brecha', 'sigas', 'anticorrupción', 'creíble', 'criminal', 'prestado', 'partidario', 'rodeado', 'contaminación', 'dientes', 'acertada', 'volando', 'sinceramente', 'juventud', 'bancaria', 'chaolasso', 'anual', 'sostenible', 'ganaremos', 'aprobó', 'socios', 'imponer', 'conviene', 'mantienen', 'inmediata', 'debía', 'corriendo', 'actualidad', 'frontera', 'parejas', 'esposa', 'puedas', 'flaco', 'latina', 'emprendedor', 'pasaron', 'rutina', 'muestran', 'escena', 'ámbito', 'cerrada', 'aveces', 'viento', 'juntas', 'sopa', 'entró', 'nieto', 'traje', 'levantarme', 'emergencia', 'portadas', 'pésame', 'directos', 'encanto', 'costado', 'yendo', 'baby', 'podes', 'insta', 'emocionada', 'pillado', 'toco', 'sorprendente', 'combate', 'pokemon', 'precisamente', 'aportar', 'simpática', 'apunto', 'pesada', 'verdades', 'fotografía', 'bloqueado', 'quedada', 'largos', 'compras', 'explicado', 'talla', 'pavo', 'levanto', 'efectivo', 'triunfa', 'embajadas', 'superado', 'encantó', 'culturas', 'guapísima', 'bajada', 'recien', 'noel', 'consiste', 'tacos', 'controlar', 'tenerte', 'colores', 'bbva', 'ganaron', 'denuncias', 'aumentar', 'melchor', 'soberbia', 'sospecha', 'humana', 'influencia', 'exigen', 'inocencia', 'lealtad', 'sonido', 'tolerancia', 'pongan', 'provoca', 'ratos', 'disfruten', 'dirán', 'medalla', 'empate', 'federación', 'preferido', 'rebajar', 'urdanga', 'ejemplaridad', 'prevista', 'radicales', 'realizado', 'semper', 'malagueños', 'técnico', 'nombra', 'niegan', 'gomez', 'prestigio', 'ppsoe', 'sanchez', 'prescripción', 'asad', 'evasión', 'duplicidades', 'vais', 'convertido', 'retirar', 'bienvenidos', 'embudo', '¿cuánto', 'argentino', 'testigo', 'indignante', 'celebrarlo', 'confusión', 'directiva', 'querella', 'escandaloso', 'iesecampañas', 'exclusión', 'lema', 'cumplimiento', 'víctor', 'conjunto', 'iraq', 'badajoz', 'autocrítica', 'piqué', 'valencianos', 'privatizaciones', 'agrupación', 'liberales', \"moody's\", 'cartel', 'turística', 'aviones', 'obsesión', 'magnifica', 'intervenciones', 'juzga', 'fondodereptiles', 'aplaude', 'fallece', '38congesopsoe', 'alemana', 'compac', 'valentín', 'economist', 'coordinación', 'laponia', 'barbate', 'visitaelhierro', 'junio', 'creará', 'pujol', 'reunido', '29mhg', 'sevillanos', 'retrato', 'andreu', 'comarca', 'desaparecer', 'vueltas', 'andres', 'títere', 'papeleta', 'marcado', 'corrupcion', 'motiva', 'corazones', 'mediocre', 'votaremos', 'década', 'ignorante', 'nacido', 'dólar', 'ayude', 'ignorancia', 'esque', 'chavez', 'caos', 'mudo', 'circo', 'gobernantes', 'abuso', 'energías', 'requiere', 'dejas', 'prohibido', 'escuché', 'caminos', 'vayas', 'whatsapp', 'papelón', 'intenciones', 'ningun', 'fidel', 'ambición', 'dejará', 'piedad', 'padrino', 'trolls', 'sobrevivir', 'positivos', 'literal', 'llegué', 'propias', 'diablo', 'hacerte', 'reactivación', 'descubierto', 'revelación', 'limpia', 'notable', 'dedicar', 'nuevamente', 'hipócrita', 'inflación', 'quejan', 'valora', 'suman', 'comience', 'apoyará', 'completamente', 'niveles', 'desahucios', 'especulación', 'callar', 'dispuestos', 'convencer', 'cliente', 'sientes', 'disfrute', 'digna', 'probado', 'discapacitados', 'efectivamente', 'situaciones', 'demasiada', 'segun', 'bromas', 'perra', 'deliciosa', 'verme', 'news', 'laura', 'netflix', 'terminando', 'pizza', 'helado', 'perdí', 'arroz', 'favoritas', 'tela', 'desperté', 'decirte', '¡buenos', 'cerrando', 'intensidad', 'pasear', 'sepas', 'aprendiendo', 'satisfecho', 'rock', 'hablen', 'increíbles', 'habló', 'conclusión', 'responden', 'sabeis', 'solos', 'amores', 'preocupes', 'llamadas', 'bronca', 'funeral', 'impresionantes', 'dejamos', 'auténtica', 'curro', 'home', 'conozca', 'skype', 'nacho', 'aguantar', 'aportación', 'donar', 'máquina', 'albert', 'caza', 'juegan', 'árabe', 'método', 'encuentre', 'lorenzo', 'probablemente', 'casualidad', 'manual', 'refería', 'actuaciones', 'recuerdan', 'subió', 'firmas', 'inmensa', 'ibex', 'optimista', 'conoces', 'cierta', 'confunde', 'like', 'particular', 'hermosas', 'testimonio', 'relevante', 'this', 'plano', 'mejorando', 'amado', 'coño', 'extremadamente', 'sacrificio', '2006', 'arde', 'cantan', 'renovar', 'parcial', 'burocracia', 'encuentran', 'enseguida', 'abren', 'fake', 'acompañe', 'conducta', 'negativos', 'corre', 'entramos', 'valle', 'metáfora', 'fotógrafo', 'debilidad', 'bernabeu', 'premiosapp', 'shak', 'prejubilaciones', 'réplica', 'lehman', 'sirio', '¿pero', 'uriarte', 'merendolatuitera', 'tramo', 'cese', 'forotransfiere', 'lleida', 'demócratas', 'gestion', 'niñas', 'egipto', 'pactos', 'vamosrafa', 'negociar', 'potenciar', 'recibiendo', 'campos', 'flamenco', 'herrera', 'conciliación', 'victimas', 'reprocha', 'reducido', 'presentará', 'congela', 'congelación', '38rubalcaba', 'nochevieja', 'participo', 'periodico', 'alemanes', 'hemeroteca', 'municipales', 'facilitar', 'reformista', 'jack', 'aytos', 'ampliar', 'secretarios', 'abortar', 'impropio', 'logo', 'otan', 'pesca', 'revela', 'quecosas', 'legislación', 'taxista', 'novedad', 'sindicales', 'profundamente', 'griega', 'libroblancodeturismo', 'costes', '8demarzo', 'biografía', 'ocasiones', 'otegui', 'piquete', 'sida', 'colectiva', 'payaso', 'leon', 'costo', 'miserables', 'universitario', 'vago', 'copia', 'suspender', 'dime', 'seguirán', 'afuera', 'fumar', 'gano', '¡viva', 'comunista', 'cínico', 'destruir', 'todito', 'territorial', 'votando', 'busque', 'dedos', 'tonta', 'enseñar', 'inflado', 'ratas', 'olvides', 'escuche', 'grabación', 'billete', 'elimina', '2021', 'creando', 'patas', 'lean', 'tratando', 'faltado', 'proyección', 'humanitaria', 'deberia', 'comentar', 'adecuado', 'bendiga', 'organizaciones', 'lleguen', 'temor', 'sufriendo', 'chulquero', 'eligieron', 'abandonado', 'llevando', 'montaña', 'sepan', 'inmediato', 'mantenga', 'calcula', 'aliado', 'refiere', 'educativa', 'envía', 'gatos', 'mañanas', 'existir', 'canales', 'crímenes', 'cobran', 'vencer', 'esquina', 'gastado', 'excelencia', 'carajo', 'puntual', 'constitucion', 'camisa', 'antigua', 'episodio', 'sugerencia', 'terminamos', 'pack', 'gimnasio', 'serrano', 'probar', 'protagonista', 'seguirme', 'delicia', 'soñado', 'argentinos', 'huerta', 'virtual', 'mentales', 'cago', 'pondré', 'intentado', 'animales', 'avanzado', 'sensible', 'bill', 'anuncios', 'bolso', 'aguanta', 'abrazarte', 'guardar', 'recomienda', 'mate', 'irresponsable', 'homosexual', 'tamaño', 'alumno', 'acuerdan', 'casco', 'juguete', 'satisfacción', 'conocí', 'registrado', 'demostrando', 'árbitro', 'gustando', 'bomberos', 'maquillaje', 'maleta', 'opinion', '¡hola', 'lucia', 'largas', 'master', 'blas', 'cano', 'inmigrantes', 'vuelves', 'movil', 'quedará', 'truco', '¿cuándo', 'clasificación', 'dilema', 'necesitaba', 'tentación', 'deberes', 'sitios', 'pamplona', 'división', 'santos', 'interpretación', 'press', 'terror', 'domingos', 'acústica', 'necesitas', 'cotizaciones', 'oscuro', 'niebla', 'estare', 'compis', 'doctor', 'barba', 'cargas', 'boletos', 'podremos', 'great', 'resaca', 'cortesía', 'cadáver', 'desayunos', 'himno', 'descubriendo', 'nostalgia', 'lagrimas', 'negativa', 'reclaman', 'llamen', 'yeah', 'kennedy', 'elvira', 'alegria', 'circunstancias', 'good', 'músicos', 'prox', 'android', 'deber', 'muchisimas', 'buenísima', 'irene', 'valorar', 'vázquez', 'arranque', 'curiosa', 'eurobonos', 'federico', 'glez', 'baltasar', 'eurozona', 'convocan', 'cumplimos', 'analistas', 'sitúa', 'absuelto', 'emilio', 'recreo', 'eliminación', 'evita', 'fran', 'necesarios', 'wikileaks', 'desvela', 'indignado', 'cifuentes', 'prestación', 'ejercer', 'animar', 'oculto', 'secretos', 'pantoja', 'polonia', 'tsqv', 'hipoteca', 'afrontar', 'condenan', 'condición', 'comisario', 'opone', 'ficha', 'john', 'fronteras', 'veros', 'débiles', 'ibarra', 'unamuno', 'merecía', 'estructurales', 'contratar', 'gracias”', 'ouyeah', 'pepevergencia', 'suspensión', 'mafo', 'tensiones', 'criticas', 'mevoy', 'convocar', 'dedicada', 'pere', 'asturiano', 'ezhwi2qb', 'congresopsoe', 'indultos', 'arenasyaestaba', 'oportuno', 'whitney', 'camp', 'cúpula', 'retiro', 'tenerife', 'conojosdemujer', 'tarragona', 'presentes', 'literatura', 'asistencia', 'ópera', 'nombrado', 'asesinatos', 'escudero', 'totalidad', 'espectadores', 'bustamante', 'abaratar', 'comparece', 'ocultó', 'canon', 'moragas', 'camas', 'mediterráneo', 'lluvias', 'evidente', 'estúpido', 'fortalecer', 'unica', 'vuelvan', 'tirado', 'trabajamos', 'borregos', 'sentencias', 'imbécil', 'apoye', 'disparate', 'torno', 'acordarse', 'túnel', 'potencia', 'privadas', 'espacios', 'pandemia', 'aguante', 'prepotencia', 'volumen', 'quebrar', 'décadas', 'millonario', 'limpias', 'menciona', 'coma', 'estimado', 'meterse', 'topic', 'cerdo', 'incapaz', 'obtiene', 'millon', 'desean', 'proponen', 'traigan', 'informar', 'sacando', 'prometer', 'muestras', 'pensamientos', 'migrantes', 'pagas', 'atender', 'eterno', 'funcione', 'harta', 'estafa', 'llevará', 'caiga', 'correcta', 'tapar', 'salgan', 'cuarta', 'méritos', 'distinta', 'verguenza', 'demasiadas', '¿como', 'física', 'afecto', 'continuar', 'mínima', 'contactos', 'interesados', 'jajajja', 'silla', 'sabiendo', 'trámite', 'esperanzas', 'botas', 'promover', 'venda', 'informarse', 'taller', 'afortunadamente', 'ordena', 'cejas', 'infarto', 'gratitud', 'trailer', 'representante', 'suave', 'mega', 'have', 'queres', 'montero', 'lento', 'siiii', 'play', 'putas', 'servidor', 'trajo', 'vaso', 'recomendar', 'verlos', 'senador', 'rojos', 'peligrosa', 'montar', 'piscina', 'escribí', 'corriente', 'bodas', 'término', 'piano', 'esther', 'lobos', 'comprobar', 'lorca', 'nace', 'follow', 'single', 'albacete', 'reforzar', 'temporales', 'arbitro', 'rabia', 'extremo', 'partidas', 'muchísima', 'emocional', 'aceptado', 'acoso', 'recupere', 'carrillo', 'síndrome', 'equivoco', 'meto', 'pasaba', 'prontito', 'veáis', 'votada', 'fifa', 'emociones', 'matices', 'obligan', 'especie', 'toros', 'universo', 'miss', 'mexicana', 'flexible', 'trato', 'corresponde', 'corea', 'juzgar', 'mete', 'estrés', 'rosca', 'sorprendido', 'estrenar', 'besito', 'colectivos', 'asalariados', 'enseñanza', 'manifestarse', 'continua', 'lesión', 'tomas', 'abordar', 'recompensa', 'guadalajara', 'primas', 'gustazo', 'peña', 'baile', 'grita', 'autoridades', 'jefa', 'emociona', 'sherlock', 'cartas', 'únicas', 'gestos', 'acepto', 'invita', 'sonora', 'movida', 'cambió', 'cordoba', 'fallecidos', 'querían', 'enterar', 'previa', 'urgentes', 'jajjaja', 'extremeño', 'jurídico', 'folios', 'pascual', 'penitenciaria', 'masivo', 'vigilancia', 'ywsid', 'bipartito', 'primaveravalenciana', 'poli', 'leysinde', 'óscar', 'vamosamorirtodos', 'fape', 'presidenciales', 'cambiará', 'insuficiente', 'armada', 'protocolo', 'imputa', 'tecnócratas', 'cívica', 'rumores', 'indica', 'lisboa', 'shakira', 'honradez', 'fatima', 'instrucción', 'marlaska', 'mateos', 'cantabria', 'contará', 'deriva', 'borja', 'menéame', 'archivo', 'compartido', 'cartaalosreyesmagos', 'recargo', 'chispa', 'discriminación', 'insostenible', 'perseguir', 'fitur', 'massocialismo', 'fianza', 'superpromo', 'exministro', 'griñanmiente', 'referente', 'plenario', 'torrente', 'prevención', 'concordato', 'virgen', 'financieras', 'voluntariado', 'aviviranantapur', 'houston', 'franquista', 'marea', 'ilegalización', 'manipulación', 'avala', 'world', 'crearemos', 'consolidación', 'atocha', 'matanza', 'telefónica', 'elnumerouno', 'sequía', 'bate', 'pspv', 'críticos', 'orquesta', 'rechazan', 'montilla', 'vespertina', 'almunia', 'repaso', 'euskera', 'concluye', 'pedraza', 'aporta', 'mantendrá', 'corredor', 'dimensión', 'fallas', 'radiohead', 'nomas', 'lenin', 'raya', 'deberías', 'tarado', 'solito', 'guante', 'bélgica', 'tendencia', 'durísimo', 'bola', 'traducción', 'anular', 'aprobación', 'concreta', 'hipocresía', 'fines', 'abandonar', 'incapaces', 'claridad', 'venezolanos', 'crimen', 'ofertas', 'mire', 'contexto', 'andan', 'normas', 'hombro', 'entienden', 'empleado', 'definición', 'desesperado', 'amas', 'compré', 'mendoza', 'jodas', 'brindis', 'llevó', 'cuántas', 'cálculo', 'atraer', 'politicos', 'virus', 'pico', 'clarito', 'ordenar', 'asustado', 'infamia', 'montaje', 'electores', 'bajando', 'cortar', 'limpio', 'complejos', 'union', 'correos', 'exigencia', 'simpatizantes', 'sacó', 'afectan', 'nacer', 'hoja', 'sudor', 'camisetas', 'colapso', 'enamorada', 'viajo', 'tomé', 'masa', 'fresco', 'papás', 'regular', 'daría', 'amiguitos', 'brazos', 'cambiando', 'montado', 'consigo', 'infinito', 'embarazada', 'prestar', 'trump', 'urbano', 'decepción', 'podés', 'perrito', 'aparecer', 'evitarlo', 'ansiedad', 'papas', 'escrita', 'indirecta', 'divino', 'saquen', 'viejito', 'negros', 'santi', 'iniciar', 'odiosa', 'sábados', 'gris', 'darse', 'verse', 'fase', 'echarle', 'aprendo', 'susana', 'alicia', 'ocupa', 'camara', 'merezco', 'bowie', 'tocando', 'animado', 'pareció', 'aires', 'volveré', 'mutuo', 'descuento', 'recordado', 'mono', 'pantalones', 'enamorados', 'poema', 'contarlo', 'help', 'gozada', 'creas', 'cometer', 'machismo', 'jugado', 'mercadona', 'hilo', 'semifinal', 'party', 'fantasma', 'laico', 'lenta', 'heridas', 'cuántos', 'desagradable', 'sara', 'thanks', 'necesarias', 'informado', 'históricos', 'meta', 'parecía', 'explicando', 'comprando', 'vente', 'vistas', 'sentirme', 'jura', 'participan', 'vernos', 'estais', 'males', 'usado', 'sentada', 'gustaria', 'secundaria', 'ponemos', 'tradicional', 'raza', 'quise', 'maravillosas', 'edificios', 'tarda', 'stop', 'paulina', 'maratón', 'power', 'sabíamos', 'brujas', 'chamba', 'comenta', 'cancha', 'becerril', 'reir', 'balcón', 'terminan', 'simpático', 'hara', 'siglos', 'útiles', 'hacernos', 'inevitable', 'urbana', 'restaurantes', 'asesinados', 'demasiados', 'consultar', 'paul', 'complicada', 'unidas', 'pozo', 'moderado', 'indignación', 'compre', 'policia', 'fuengirola', 'aceptan', 'endiascomohoy', 'desmantelamiento', 'comparecer', 'hahahahahahahahah', 'rajuste', 'juicios', 'gravedad', 'bdias', 'adoctrinamiento', 'irresponsabilidad', 'riendo', 'goldman', 'sachs', 'hashtag', 'redessociales', 'ppvergencia', 'vazquez', 'dirigente', '2014', 'difundir', 'boyswillbeboys', 'retrasa', 'reflexiones', 'qatar', 'occidente', 'regionales', 'copadavis', 'accidentes', 'ynterviumarivi', 'herramientas', 'apetece', 'indulta', 'nóminas', 'uxue', 'ayudan', 'dirige', 'bosch', 'metroscopia', 'elpais', 'tuiteando', 'rechazar', 'reguladores', 'rebajas', 'twitcam', 'gene', 'justos', 'ético', 'conocemos', 'colell', 'competente', 'replica', 'vanguardia', 'hernández', 'congelar', 'felicitado', 'vegas', 'limita', 'faisán', 'sugerencias', 'julia', 'explosión', 'aupa', 'ideología', 'puig', 'eliminatoria', 'infantiles', 'debatimos', 'católicos', 'islandia', 'arzobispo', 'george', 'berlin', 'davos', 'epílogo', 'maquina', 'salvados', 'patadas', '2003', 'urkullu', 'temazo', 'limitar', 'especuladores', 'damasco', 'comprometidos', 'futura', 'católica', 'fortaleza', 'protestan', 'temperaturas', 'mecanismo', 'compensación', 'fija', 'ocde', 'hunde', 'adversario', 'raul', 'lapepa', 'soldados', 'incendios', 'telecinco', 'marina', 'procesión', 'santorum', 'diputada', 'ferrer', 'xavi', 'brotes', 'convenios', 'dimite', 'cobró', 'rectificación', 'vistazo', 'nucleares', 'cristóbal', 'sugiere', 'rebeldía', 'acusan', 'revisable', 'donamedula', 'gobernará', 'obviamente', 'ofreciendo', 'contó', 'inteligentes', 'esten', 'daños', 'típico', 'trago', 'alimentos', 'mantenido', 'vagos', 'pondrá', 'gobiernan', 'lleve', 'darán', 'roben', 'impedir', 'regalen', 'concretas', 'mintiendo', 'podrás', 'demuestran', 'queden', 'cuentos', 'actuales', 'acusaciones', 'motos', 'deseamos', 'mediocres', 'dieran', 'dejé', 'fama', 'suegro', 'entregado', 'tweeter', 'recomendado', 'comenzando', 'vengas', 'olvidamos', 'cobarde', 'comparte', 'nebot', 'amar', 'regresa', 'incapacidad', 'muchacho', 'cómplice', 'adultos', 'mendez', 'incluir', 'exista', 'órdenes', 'estaria', 'podria', 'universitaria', 'caravana', 'ríos', 'permita', 'volverán', 'derechas', 'alas', 'minas', 'respondió', 'podían', 'propuso', 'creador', 'supuesta', 'pertenece', 'valentía', 'hogares', 'honestidad', 'ahhh', 'lacra', 'abuelos', 'forman', 'haberlo', 'acompañan', 'caridad', 'despierta', 'sistemas', 'caña', 'necios', 'llamaba', 'vine', 'chiva', 'cañas', 'duró', 'uber', 'insoportable', 'pierna', 'comunes', 'volvió', 'hielo', 'encargado', 'mortal', 'emergencias', 'congelado', 'campeona', 'encontramos', 'personalidad', 'elementos', 'spotify', 'bolas', 'nublado', 'deprisa', 'maravillosos', 'tributo', 'arruinar', 'mundos', 'republicana', 'terminas', 'ambiguo', 'vientos', 'pibe', 'intercambio', 'camión', 'maja', 'amazon', 'extrañas', 'boda', 'constancia', 'antiguos', 'benidorm', 'colocar', 'welcome', 'expo', 'cojo', 'espanyol', 'mateo', 'pelos', 'olvidemos', 'partidarios', 'trafico', 'enserio', 'debí', 'madrugar', 'emisión', 'asegurar', 'acabando', 'llamó', 'piernas', 'instagram', 'pesimista', 'solar', 'presentarse', 'pared', 'gilipollas', 'imaginaba', 'trayectoria', 'jode', 'mortales', 'minoría', 'soporto', 'proporcional', 'obesidad', 'plus', 'chido', 'probando', 'gustar', 'agarro', 'cinturón', 'porfavor', 'diaz', 'apuestas', 'guía', 'denunciado', 'besote', 'portal', 'gustavo', 'vuelo', 'salio', 'consuelo', 'viaja', 'mayoria', 'fría', 'jurídica', 'sentarse', 'salidas', 'desfile', 'compara', 'nacimiento', 'confirmar', 'amarga', 'seriedad', 'programación', 'flor', 'motor', 'tajo', 'zapatos', 'ingenio', 'miro', 'trinidad', 'liceo', 'mundiales', 'miercoles', 'llover', 'dama', 'eficaz', 'clientes', 'tierno', 'movimientos', 'rayo', 'fotitos', 'religión', 'krugman', 'schmidt', 'finanzas', 'internacionalización', 'antoni', 'refuerza', 'resultar', 'estara', 'sondeo', 'crucero', 'excusas', '¿tiene', 'avisos', 'discreción', '000m€', 'sostiene', 'galardón', 'prostitución', 'irlanda', 'ranking', 'ertzaintza', 'cubano', 'ventajas', 'dedicatoria', 'carcaño', 'acercamiento', 'minijobs', 'terrat', 'compartiendo', 'precariedad', 'patronato', 'legalidad', 'martinez', 'narbona', 'cementerio', 'saenz', 'secretaría', 'santamaria', 'guión', 'consej', 'twitteroff', 'ramirez”', '000m', 'fotógrafos', 'realista', 'subvención', 'trolldeldía', 'trending', 'álvarez', 'imparable', 'gasolinera', 'autonómicos', 'telmo', 'dosis', 'danielmartin', 'inmovilismo', 'generará', 'artículos', 'disidente', 'ipad2', 'fuencarral', 'feijóo', 'clooney', 'comercios', 'presunta', 'reunirse', 'llosa', 'respetos', 'cracks', 'fukushima', 'esperpento', 'establece', 'profesionalidad', 'marcar', 'casablanca', 'fantástica', 'incumplimiento', 'griñanvetetu', 'atlético', 'suárez', 'inconstitucional', 'funcionario', 'modificación', 'avivir', 'favorece', 'flipo', 'jelo', 'concesión', 'exportaciones', 'vial', 'representación', 'congresopp', 'aplicaciones', 'pancarta', 'sangría', 'chavales', '¿cual', 'notario', 'encuentros', 'spot', 'afectar', 'barones', 'llorente', 'británico', 'eléctrico', 'huelgageneral', 'manjón', 'sirva', 'islas', 'plantear', 'defendemos', 'coalición', 'desgaste', 'valeriano', 'enfrenta', 'anuncian', 'eume', 'pajín', 'gastó', 'solvente', 'televisiones', 'llamativo', 'oyendo', 'carolina', 'inferior', 'participado', 'patriotismo', 'quero', 'auténtico', 'borrell', 'madera', 'breves', 'públicamente', 'zapaterismo', 'sindicato', 'celebrado', 'abrazo”', 'aclare', 'reserva', 'presuntos', 'cobo', 'rubianes', 'desfachatez', 'sanitarios', 'quemar', 'cómplices', 'rectifica', 'ajena', 'bajó', 'idioma', 'haciéndolo', 'devuelva', 'burro', 'correismo', 'llevarse', 'limbo', 'llenas', 'oiga', 'pillos', 'dile', 'leve', 'perpetua', 'vendrán', 'cool', 'modificar', 'billetes', 'street', 'vuela', 'musicales', 'torpe', 'traidor', 'divisas', 'mensual', 'organizada', 'creatividad', 'nube', 'wikipedia', 'absurda', 'saqueo', 'burla', 'claramente', 'vicepresidente', 'incondicional', 'esmeraldas', 'roba', 'pasajeros', 'recurre', 'opositores', 'municipio', 'lobo', 'monte', 'entienda', 'olviden', 'ultimos', 'lavar', 'cuenca', 'pelean', 'ratón', 'queso', 'ideológica', 'bote', 'ambicioso', 'pagará', 'atreve', 'escuchamos', 'institución', 'adicional', 'confundir', 'insultando', 'chiriboga', 'banderas', 'pasadas', 'bloquear', 'cancer', 'literalmente', 'celebro', 'observadores', 'darles', 'apoyó', 'fascista', 'sienten', 'donación', 'atencion', 'altamente', 'instalaciones', 'titanic', 'estructura', 'requisitos', 'quiénes', 'pasarlo', 'alianza', 'excelentes', 'lleven', 'mames', 'médica', 'hueco', '¿está', 'impiden', 'generaciones', 'confío', 'villancicos', 'rajado', 'tenés', 'disponibles', 'mataron', 'grabando', 'arregla', 'vibra', 'íbamos', 'alvarez', 'acostada', 'parezco', 'metida', 'azúcar', 'sofía', 'lloré', 'snap', 'terminé', 'traen', 'canto', 'odia', 'desayunar', 'hazme', 'haha', 'madruga', 'andrea', 'desayunando', 'tijera', 'crecido', 'grandísima', 'chilena', 'saludando', 'normales', 'aguanto', 'criterios', 'respondo', 'escobar', 'pegan', 'jajajajajajaja', 'venía', 'participando', 'james', 'metros', 'enormes', 'moderna', 'defienda', 'espada', 'enfadado', 'artes', 'textos', 'pudieras', 'currar', 'malagueño', 'sexy', 'sevillano', 'presume', 'aprecio', 'muriendo', 'carita', 'florida', 'estatal', '¡muchas', 'cobro', 'cojones', 'bonus', 'echando', 'sony', 'cuadros', 'consumidores', 'informativa', 'escapa', 'gobierne', 'mandas', 'veas', 'estaciones', 'emitir', 'maneras', 'actriz', 'prohibir', 'pedagogía', 'bajón', 'seguidor', 'intentaré', 'solas', 'torneo', 'jejejeje', 'alternativo', 'presentan', 'agotado', 'sofá', 'reconocido', 'cogido', 'olvidan', 'curar', 'cositas', 'promocionar', 'aciertos', 'conservadora', 'demonios', 'mandame', 'invitar', 'acompaña', 'primaria', 'kiko', 'coincido', 'sobrina', 'oido', 'noto', 'reus', 'cerradas', 'contribución', 'harto', 'vicio', 'presentando', 'creía', 'preferidas', 'abraham', 'inicial', 'nací', 'muñeco', 'ahre', 'vibras', 'revisa', 'condenada', 'piense', 'marcas', 'eterna', 'monumento', 'obligada', 'casino', 'adelantar', 'chat', 'quincena', '3000', 'sucio', 'chantaje', 'sesiones', 'dudes', 'entrevisto', 'verán', 'colgar', 'semifinales', 'quemado', 'coincidir', 'mari', 'uyyy', 'avances', 'hermanas', 'temperatura', 'recordando', 'convicción', '¿donde', 'aclarar', 'look', 'lola', 'suiza', 'fantásticos', 'pintor', 'ladrillo', 'cordial', 'soportar', 'colmo', 'mató', 'bebe', 'cabezas', 'dentista', 'agustín', 'consultas', 'gráficos', 'llora', 'incomprensible', 'seguirnos', 'ilusion', 'sólido', 'sostenibilidad', 'embajadores', 'ideologías', 'preside', 'quereis', 'equipazo', 'extraordinarias', 'incrementa', 'jardin', 'estrenando', 'forumeuropa', 'dolorosa', 'standard', 'iribarne', 'fusión', 'extremeños', 'augura', 'acudir', 'others', 'pluralismo', 'romano', 'oscars', 'consideran', 'reunion', 'sortu', 'casta', 'cofrade', 'escrutinio', 'austeros', 'fiscalidad', 'superación', 'recortando', 'moderno', 'moscú', 'explosivos', 'normativa', 'ordóñez', 'generoso', '¿debería', 'palco', 'cerrará', 'getafe', 'aemet', 'ahoraandalucia', 'términos', 'expresidente', 'operaciones', 'investigadores', 'absentismo', 'elegante', 'jurar', 'malaria', 'germán', 'récords', 'moderación', 'premia', 'bipartidista', 'dada', 'cometido', 'compatible', 'project', 'pakistán', 'oca0npkv', 'anteproyecto', 'recortan', 'reciente', 'campofrío', 'autores', 'discrepar', 'antón', 'noos', 'oeoeoe', 'rbcbenvalencia', 'malagueña', 'científicos', 'graciasfraga', 'ramírez', 'exigirá', 'sencillamente', 'magistral', 'acusados', 'anonymous', 'reitera', 'expedientes', 'intervencion', 'libia', 'creció', 'dramático', 'viñeta', 'tregua', 'antiterrorista', 'explicará', 'beneficia', 'comparar', 'facilita', 'videominuto', 'monólogo', 'conquistar', 'villar', 'estrenamos', 'ofensiva', 'copadelrey', 'sensatez', 'reproche', 'paraiso', 'pancartas', 'viera', 'angeles', 'arcos', 'recortado', 'vitoria', 'respalda', 'insultan', 'paran', 'mediática', 'merced', 'icsoria', 'talibán', 'alaya', 'elgrandebate', 'crackovia', 'gustará', 'serrat', 'pregón', 'publi', 'coacción', 'jubilados', 'saudí', 'bajos', \"'buzón\", \"voz'\", 'invitación', 'frena', 'confirman', 'sastre', 'porcentaje', 'cáceres', 'generosidad', 'victor', 'hungría', 'canaria', 'cibeles', 'equivocan', 'contaré', 'apostar', 'abrirá', 'compromis', 'guaje', 'descalificación', 'mérida', 'izda', 'desaparecidos', 'cuestiones', 'rita', 'americanos', 'somalia', 'injustos', 'alcalá', 'árbitros', 'merlos', 'palos', 'mamés', 'cumplió', 'mentirosos', 'indígenas', 'estupidez', 'inepto', 'préstamos', 'andate', 'gaby', 'tocó', 'vaca', 'dolarizacion', 'cuente', 'pierdes', 'correctamente', 'presi', 'miren', 'sabido', 'pillo', 'tenerlo', 'próspero', 'tierras', 'dueños', 'álvaro', 'lanzan', 'pana', 'robos', 'ingresa', 'dolares', 'meten', 'dominio', 'cubanos', 'malditos', 'llamando', 'capitales', 'delincuencia', 'intente', 'asambleístas', 'reflejo', 'venezolano', 'votará', 'gallo', 'educado', 'deseas', 'protestar', 'esconder', 'destrozar', 'reactivar', 'fracasado', 'medicinas', 'creyendo', 'maestra', 'ponerle', 'ganen', 'cobros', 'ciego', 'técnicas', 'angustia', 'recuperado', 'andas', 'uñas', 'pedirle', 'robó', 'movilidad', 'confirme', 'conocida', 'engañando', 'cuanta', 'eficiente', 'vomitar', 'primos', 'cumpliendo', 'seras', 'senescyt', 'playas', 'votemos', 'guayaquil', 'sostener', 'esconde', 'mejoras', 'laso', 'bloque', 'pagaron', 'respirar', 'aplaudir', 'apoyamos', 'existencia', 'metiendo', 'básica', 'aquellas', 'veinte', 'provinciales', 'verle', 'abandonados', 'calladito', 'contradice', 'favorecer', 'responda', 'haberse', 'cambiara', 'acompañar', 'tumba', 'reciba', 'apostamos', 'adquisitivo', 'votaciones', 'hectáreas', 'cuerda', 'cólera', 'adolescente', 'rostro', 'abuelita', 'black', 'huracán', 'basa', 'opino', 'horribles', 'rubí', 'cargador', 'dinámica', 'aparta', 'favorable', 'química', 'mota', 'icon', 'ocupan', 'mito', 'ocupo', 'amargo', 'busco', 'olor', 'solicitud', 'interesado', 'cerró', 'guarda', 'discusión', 'volví', 'leones', 'enamora', 'panza', '¡felicidades', 'aspecto', 'penúltimo', 'ceniza', 'mediodía', 'tsunami', 'preocupada', 'conservador', 'pereza', 'aparentemente', 'darlo', 'decepcionado', 'consiga', 'tour', 'sporting', 'usas', 'molesto', 'debut', 'pidan', 'complejo', 'saberlo', 'elemento', 'uvas', 'piezas', 'falleció', 'buscado', 'family', 'atrevido', 'cosita', 'predicciones', 'baratos', 'mago', 'volvería', 'rodillas', 'suelta', 'grotesco', 'dejarlo', 'asiento', 'cortan', 'comprendo', 'jugada', 'mero', 'salía', 'encuentras', 'silvia', 'tronos', 'faltó', 'clásicos', 'salón', 'publicará', 'stream', 'open', 'sarcasmo', 'blogs', 'cris', 'leal', 'hastag', 'fábrica', 'podríamos', 'impotencia', 'mazo', 'algeciras', 'tortilla', 'miras', 'soluciona', 'malita', 'suscrito', 'streaming', 'atardecer', 'made', 'dejéis', 'spam', 'vital', 'yolanda', 'salvas', 'subo', 'rusa', 'verso', 'chan', 'sentar', 'marcos', 'imaginas', 'dijiste', 'guitarra', 'expresa', 'nooo', 'tomamos', 'cerebral', 'cagada', 'suelen', 'empeño', 'republicano', 'pongas', 'vivas', 'gustas', 'ilusiones', 'alejandra', 'pasaje', 'fail', 'dibujo', 'luchador', 'hacían', 'serios', 'embarazo', 'celosa', 'luce', 'brújula', 'cuida', 'dirigido', 'alarma', 'fieles', 'discos', 'informando', 'atenta', 'gráfica', 'casero', 'cerrados', 'cuáles', 'duela', 'americana', 'sacaron', 'amarillo', 'quinto', 'mamita', 'pego', 'pudimos', 'quema', 'internas', 'stand', 'barranco', 'aprovechando', 'mandamos', 'navideñas', 'admito', 'presentó', 'casanova', 'espanto', 'conversar', 'shaky', 'patricio', 'volar', 'vieron', 'daremos', 'sabiduría', 'cañón', 'pistas', 'descripción', 'paradojas', 'respetan', 'critique', 'avisan', 'divina', 'matan', 'asia', 'america', 'forever', 'desp', 'buenisima', 'matando', 'ultimas', 'fede', 'break', 'escribió', 'opinan', 'uruguayo', 'señales', 'dchos', 'miami', 'cuenten', 'hinchas', '¡por', 'aspectos', 'inaugura', 'descubrimiento', 'morosidad', 'apuesto', 'espectaculares', 'corrección', 'doña', 'mienten', 'clubes', 'antena3', 'eperiodico', 'marsella', 'oponen', 'gobiernorajoy', '2002', 'girona', 'elude', 'rápida', \"poor's\", 'votad', '¿cuando', 'comunicar', 'albiol', 'retirado', 'mtro', 'tripartito', 'cheque', 'cobardes', 'picasso', 'desigualdades', 'recibidas', 'losada', 'asturianas', 'sumando', 'seguís', 'espías', 'daros', 'interventores', 'apoderados', 'recargos', 'harvard', 'conclusiones', 'recio', 'amazing', 'amiguetes', 'leido', 'sevillaciudadtalisman', 'feijoo', 'umbral', 'prefiere', 'traslado', 'zara', 'lector', 'competición', 'felicitats', 'sumario', 'corresponsal', 'acreditación', 'firman', 'cenas', 'villalobos', '2007', 'rompen', 'marcelino', 'concedido', 'criticando', 'ocultan', 'sanear', 'retroceso', 'sospechas', 'coincidencia', 'acertó', 'equivocó', 'prisas', 'photo', 'cómicos', 'caerá', 'mora', 'manifiestan', 'asusta', 'concede', 'fijar', 'alucino', 'anunciada', 'majos', 'mintió', 'consejería', 'honrado', 'demócrata', 'impresionado', 'piratas', 'ahmadineyad', 'despertado', 'colección', 'barna', 'burbuja', 'goku', 'vinculado', 'individual', 'separación', 'vertebrar', 'ajustar', 'participantes', 'transacciones', 'gemela', 'calefacción', 'defendido', 'mineros', 'draghi', 'comentaremos', 'confunden', 'admirada', 'manel', 'desear', 'vigente', 'defiendo', 'honra', 'amenazas', 'africa', 'entrevistamos', 'fiasco', 'imprescindibles', 'nacionales', 'incumple', 'amantes', 'ceja', 'corporativo', 'lucía', 'disposición', 'nevada', 'diccionario', 'resiste', 'lgtb', 'magistrados', 'institucionalizada', 'rehn', 'armario', 'vicepta', 'indefinido', 'liberados', 'decretazo', 'resume', 'clínico', 'reconozco', 'padel', 'profundidad', 'inmobiliario', 'momentodormir', 'brillan', 'jaen', 'cuartel', 'imputación', 'luisa', 'analiza', 'productividad', 'culturales', 'costará', 'prof', 'indultado', 'intervenir', 'devaluación', 'importe', 'desaparición', 'abidal', 'perdidos', 'flipando', 'descargar', 'sensata', 'rahola', 'socialdemócrata', 'legitimidad', 'dibujante', 'dificultad', 'juanma', 'pascua', 'arabia', 'barkos', 'acumula', 'cortés', 'conspiración', 'campeon', 'visitando', 'osasuna', 'cercanía', 'tratados', 'talavera', 'solucionar', 'reparte', 'planea', 'reflexionar', 'europeas', 'aprovecho', 'redondo', 'eléctricas', 'presunción', 'vocación', '¿estáis', 'tobin', 'recibidos', 'pino', 'fundacion', 'palau', 'negó', 'belen', 'dialogar', 'wyoming', 'descubre', '¿los', 'suplemento', 'inocentada', 'malversación', 'disturbios', 'triana', 'manifa', 'impulsará', 'asignatura', 'impagos', 'prefieren', 'directas', 'indicios', 'springsteen', 'gordillo', 'impulsaremos', 'peticiones', 'bebés', 'vaticano', '¿alguna', 'llevaron', 'acabas', 'hables', 'desesperación', 'confiamos', 'honestos', 'manta', 'robando', 'entiendan', 'vándalos', 'ahhhh', 'repugnante', 'harías', '1500', 'neoliberalismo', 'conseguimos', 'enseñaron', 'convence', 'dolarización', 'tocayo', 'wall', 'endeudamiento', 'belga', 'quedando', 'extranjera', 'emprendimiento', 'infraestructura', 'pusieron', 'preparación', 'quedas', 'bobo', 'preocupan', 'troll', 'pare', 'educativos', 'considero', 'estructuras', 'llenos', 'obligatorio', 'rencor', '¿sabes', 'mantenimiento', 'incluidos', 'postura', 'ocurren', 'estímulos', 'harás', 'confirmo', 'estudiantil', 'áreas', 'recordemos', 'escucharlo', 'constitucionales', 'porte', 'cansancio', 'ofreció', 'internos', 'agricultores', 'benefician', 'organizar', 'down', 'comenzó', 'engaños', 'mimos', 'clon', 'despierte', 'darnos', 'habitantes', 'jajajajaj', 'huevada', 'preocupados', 'terminará', 'honesto', 'servir', 'limitado', 'distinción', 'jovenes', 'click', 'inseguridad', 'funcionan', 'registro', 'pagaba', 'vengan', 'reconozca', 'potente', 'situacion', 'mediocridad', 'gobernando', 'pregunte', 'trabajas', 'pulmón', 'leyendas', 'quiso', 'manteniendo', 'tendra', 'adecuada', 'éstos', 'platos', 'metieron', 'resuelto', 'causó', 'unen', 'pagarán', 'mexicano', 'pesa', 'mejore', 'cobrando', 'incluya', 'oscuridad', 'convierta', 'cubrir', 'olvidada', 'desconoce', 'quite', 'fortuna', 'bachillerato', 'pensábamos', 'igualito', 'agarra', 'ganaría', 'jajaa', 'olvidé', 'regaló', 'saludito', 'bendito', 'tamal', 'vieras', 'moco', 'pastilla', 'audio', 'suene', 'pasaría', 'marihuana', 'emotivo', 'transmitir', 'calvo', 'diagnóstico', 'nintendo', 'halloween', 'jamón', 'durado', 'cometió']\n"
],
[
"# Build the one hot matrix considering TASS and candidate datasets\ncorpusDictionary = build_corpus(joinedDfTexts)\nprint (len(corpusDictionary), corpusDictionary)",
"_____no_output_____"
],
[
"# Observe Tass data set balance, to see if we apply any method for balancing\r\n# histogram to see dataset balance\r\nplt.hist(tassDf['Tag'])",
"_____no_output_____"
],
[
"# Transform target names (P, N, NEU) of TASS dataset into integer representation\r\ntassDf.loc[tassDf['Tag'] == 'N', 'Tag'] = 0\r\ntassDf.loc[tassDf['Tag'] == 'NEU', 'Tag'] = 1\r\ntassDf.loc[tassDf['Tag'] == 'P', 'Tag'] = 2\r\n\r\n# visualize transformation to numerical classes\r\nplt.hist(tassDf['Tag'])\r\n# transform numerical tags to binary representation\r\n# encoded_tags = to_categorical(tassDf['Tag'], num_classes=3)\r\n#tassDf['Tag'] = tassDf['Tag'].apply(to_categorical, num_classes=3)",
"_____no_output_____"
],
[
"# Function to represent tweets as numerical vectors considering the corpus of TASS and candidate datsets as reference\n# in this case we have only 1 column\n# each row is a tweet represented as vector, corresponding to corpus\ndef buildWordVectorMatrix(tweetsVect, corpusW):\n # empty numpy matrix of necesary size\n wordVectorMatrix = np.zeros((len(tweetsVect), len(corpusW)))\n # fill matrix, with binary representation of words\n for pos, tweetInPos in enumerate (tweetsVect):\n # split each tweet into a list of its words\n tweetWords = tweetInPos.lower().split()\n # assign value of 1 in matrix position corresponding to the current tweet\n # and the idx each of its contained words is located on the previously built dictionary\n for word in tweetWords:\n # only consider words that are part of the built dictionary\n if word in corpusDictionary:\n wordVectorMatrix[pos, corpusDictionary.index(word)] = 1\n return wordVectorMatrix",
"_____no_output_____"
],
[
"# Reprresent TASS dataset as vectors considering the corpus created before\r\nX_data = np.array(buildWordVectorMatrix(tassDf['Text'], corpusDictionary))\r\n# Select the y (target) of all data\r\ny_data = np.array(tassDf['Tag'])\r\n\r\nprint('X_data')\r\nprint(X_data)\r\nprint(X_data.shape)\r\nprint('y_data')\r\nprint(y_data)\r\nprint(y_data.shape)\r\nprint(tassDf['Text'])",
"X_data\n[[0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n ...\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]]\n(57454, 7000)\ny_data\n[2 0 0 ... 0 0 0]\n(57454,)\n0 @marizamora94 Ambas son mindblowing, lástima W...\n1 Yo sé que cada quién hace lo que le da la puta...\n2 @Phoenix2124 uno siente susto, asco, cólera et...\n3 Hola @TutzyFF , muchas bendiciones, ves... si ...\n4 Siempre la paz es lo mejor. De lo sucedido en ...\n ... \n81865 Reconozco que me encanta cuando Twitter corre....\n81866 @Juandecolmenero @angelrubioti @barbara_ruizp ...\n81868 ¿A qué espera el alcalde promarihuana independ...\n81869 Esto es lo que hay... Preocupante la flagrante...\n81870 Rajoy, con el agua al cuello: España se asoma ...\nName: Text, Length: 57454, dtype: object\n"
],
[
"# Divide TASS dataset into training (70%), validation (15%) and test (15%)\n# Training and validation-test\nX_train, X_other, y_train, y_other = train_test_split(X_data, y_data, test_size=0.50, random_state=1, stratify=y_data)\n\n# divide into validation and test datasets\nX_validation, X_test, y_validation, y_test = train_test_split(X_other, y_other, test_size=0.50, random_state=1, stratify=y_other)\n\nprint('X_train')\nprint(X_train)\nprint(X_train.shape)\nprint('y_train')\nprint(y_train)\nprint(y_train.shape)\nprint('X_validation')\nprint(X_validation)\nprint(X_validation.shape)\nprint('X_test')\nprint(X_test)\nprint(X_test.shape)\nprint('y_validation')\nprint(y_validation)\nprint(y_validation.shape)\nprint('y_test')\nprint(y_test)\nprint(y_test.shape)\n\n# visualize transformation to numerical classes\nplt.figure(1)\nplt.hist(y_train)\nplt.figure(2)\nplt.hist(y_validation)\nplt.figure(3)\nplt.hist(y_test)\n",
"X_train\n[[0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n ...\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]]\n(28727, 7000)\ny_train\n[0 2 2 ... 2 2 2]\n(28727,)\nX_validation\n[[0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n [0. 1. 0. ... 0. 0. 0.]\n ...\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]]\n(14363, 7000)\nX_test\n[[0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n ...\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]]\n(14364, 7000)\ny_validation\n[0 0 0 ... 0 2 0]\n(14363,)\ny_test\n[2 0 0 ... 2 1 2]\n(14364,)\n"
],
[
"# Convert tags to binnary representation using to categorical function from Keras\ny_train_bin = to_categorical(y_train, num_classes=3)\ny_test_bin = to_categorical(y_test, num_classes=3)\ny_validation_bin = to_categorical(y_validation, num_classes=3)",
"_____no_output_____"
],
[
"# Create the neuronal network model with keras Sequential class and add layer with given activation functions \nmodel = models.Sequential()\nmodel.add(layers.Dense(120, activation='relu', input_shape=(len(corpusDictionary),)))\nmodel.add(layers.Dense(30, activation='relu'))\nmodel.add(layers.Dense(5, activation='relu'))\nmodel.add(layers.Dense(3, activation='softmax'))\n\n# Associate the metrics to the model\nmodel.compile(optimizer='adam',\n loss='categorical_crossentropy',\n metrics=['acc', 'AUC'])\n\n# Run the model with processed data and selected metrics\nprint('xTest test', X_train.sum(axis=1))\nprint('X_test', X_train, X_train.shape)\nprint('y_train_bin', y_train_bin)\ntrain_log = model.fit(X_train, y_train_bin,\n epochs=10, batch_size=512,\n validation_data=(X_validation, y_validation_bin))",
"xTest test [9. 3. 2. ... 2. 3. 3.]\nX_test [[0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n ...\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]\n [0. 0. 0. ... 0. 0. 0.]] (28727, 7000)\ny_train_bin [[1. 0. 0.]\n [0. 0. 1.]\n [0. 0. 1.]\n ...\n [0. 0. 1.]\n [0. 0. 1.]\n [0. 0. 1.]]\nEpoch 1/10\n57/57 [==============================] - 5s 73ms/step - loss: 1.0219 - acc: 0.5636 - auc: 0.7355 - val_loss: 0.7137 - val_acc: 0.7397 - val_auc: 0.8703\nEpoch 2/10\n57/57 [==============================] - 3s 59ms/step - loss: 0.6317 - acc: 0.7735 - auc: 0.8965 - val_loss: 0.6360 - val_acc: 0.7523 - val_auc: 0.8927\nEpoch 3/10\n57/57 [==============================] - 3s 60ms/step - loss: 0.4970 - acc: 0.8131 - auc: 0.9368 - val_loss: 0.6395 - val_acc: 0.7515 - val_auc: 0.8946\nEpoch 4/10\n57/57 [==============================] - 4s 69ms/step - loss: 0.4155 - acc: 0.8368 - auc: 0.9560 - val_loss: 0.6702 - val_acc: 0.7544 - val_auc: 0.8924\nEpoch 5/10\n57/57 [==============================] - 3s 60ms/step - loss: 0.3289 - acc: 0.8815 - auc: 0.9730 - val_loss: 0.7290 - val_acc: 0.7510 - val_auc: 0.8875\nEpoch 6/10\n57/57 [==============================] - 3s 59ms/step - loss: 0.2477 - acc: 0.9215 - auc: 0.9852 - val_loss: 0.8171 - val_acc: 0.7475 - val_auc: 0.8828\nEpoch 7/10\n57/57 [==============================] - 3s 60ms/step - loss: 0.1765 - acc: 0.9468 - auc: 0.9925 - val_loss: 0.9264 - val_acc: 0.7503 - val_auc: 0.8801\nEpoch 8/10\n57/57 [==============================] - 3s 58ms/step - loss: 0.1350 - acc: 0.9593 - auc: 0.9955 - val_loss: 1.0252 - val_acc: 0.7476 - val_auc: 0.8769\nEpoch 9/10\n57/57 [==============================] - 3s 59ms/step - loss: 0.1000 - acc: 0.9698 - auc: 0.9975 - val_loss: 1.1162 - val_acc: 0.7430 - val_auc: 0.8724\nEpoch 10/10\n57/57 [==============================] - 3s 60ms/step - loss: 0.0806 - acc: 0.9758 - auc: 0.9984 - val_loss: 1.2082 - val_acc: 0.7432 - val_auc: 0.8703\n"
],
[
"# Model evaluation considering the accuracy of training and validations datasets\nacc = train_log.history['acc']\nval_acc = train_log.history['val_acc']\nloss = train_log.history['loss']\nval_loss = train_log.history['val_loss']\nepochs = range(1, len(acc) + 1)\nplt.plot(epochs, acc, 'r', label='Training acc')\nplt.plot(epochs, val_acc, 'b', label='Validation acc')\nplt.title('Training and validation accuracy')\nplt.legend()\nplt.figure()\nplt.plot(epochs, loss, 'r', label='Training loss')\nplt.plot(epochs, val_loss, 'b', label='Validation loss')\nplt.title('Training and validation loss')\nplt.legend()\nplt.show()",
"_____no_output_____"
],
[
"# Calculate the accuracy considering test dataset\ntest_accuracy = model.evaluate(X_test, y_test_bin)\nprint('Test Metrics')\nprint(test_accuracy)",
"449/449 [==============================] - 1s 3ms/step - loss: 1.2433 - acc: 0.7367 - auc: 0.8665\nTest Metrics\n[1.2433488368988037, 0.7367028594017029, 0.8665334582328796]\n"
],
[
"# Import data from replies to tweets of ecuadorian candidates\nlassoPath = 'drive/MyDrive/AI_final_project_REAL/Project_dataset/lasso_candidato.csv'\narauzPath = 'drive/MyDrive/AI_final_project_REAL/Project_dataset/arauz_candidato.csv'\nlassoDf = pd.read_csv(lassoPath, encoding='utf8').reset_index(drop=True)\narauzDf = pd.read_csv(arauzPath, encoding='utf8').reset_index(drop=True)\n\n# Transform candidate tweets into numercial values by hot encoding\n# guillermo lasso candidate\nX_lasso = np.array(buildWordVectorMatrix(lassoDf['text'], corpusDictionary))\n# predict classes function will be deprecated for multi class distribution use (model.predict(X_lasso) > 0.5).astype(\"int32\") in the future\n# or numpy argmax, could be good option\nclassResultsLasso = model.predict_classes(X_lasso)\nlassoPredDistribution = Counter(classResultsLasso).most_common()\nprint(lassoPredDistribution)\n# andres arauz candidate\nX_arauz = np.array(buildWordVectorMatrix(arauzDf['text'], corpusDictionary))\nclassResultsArauz = model.predict_classes(X_arauz) # predict classes function will be deprecated\narauzPredDistribution = Counter(classResultsArauz).most_common()\nprint(arauzPredDistribution)\n\n\n\n# Candidate results\n# (0 Negative, 1 neutral, 2 postive)\nprint('\\nEl Resultado para los tweets de Guillermo Lasso')\nprint(f'NEGATIVOS: {lassoPredDistribution[0][1]}')\nprint(f'POSITIVOS: {lassoPredDistribution[1][1]}')\nprint(f'NEUTROS: {lassoPredDistribution[2][1]}')\nprint('\\nEl Resultado para los tweets de Andres Arauz')\nprint(f'NEGATIVOS: {arauzPredDistribution[0][1]}')\nprint(f'POSITIVOS: {arauzPredDistribution[1][1]}')\nprint(f'NEUTROS: {arauzPredDistribution[2][1]}')\n\n# Pie charts\nlabels = dict()\nlabels[0] = 'Negativo'\nlabels[1] = 'Neutro'\nlabels[2] = 'Postivo'\n\n# Lasso\nkeysLasso = [ k for k,v in lassoPredDistribution ]\nvaluesLasso = [ v for k,v in lassoPredDistribution ]\n\nlabelsLasso = []\nlabelsLasso.append(labels[keysLasso[0]])\nlabelsLasso.append(labels[keysLasso[1]])\nlabelsLasso.append(labels[keysLasso[2]])\nprint(labelsLasso)\nplt.figure('Guillermo Lasso')\nfigLasso1, axLasso1 = plt.subplots()\naxLasso1.set_title('Guillermo Lasso')\naxLasso1.pie([valuesLasso], labels=labelsLasso, autopct='%1.1f%%', shadow=True, startangle=90)\nplt.show()\n\n# Arauz\nkeysArauz = [ k for k,v in arauzPredDistribution ]\nvaluesArauz = [ v for k,v in arauzPredDistribution ]\nlabelsArauz = []\nlabelsArauz.append(labels[keysArauz[0]])\nlabelsArauz.append(labels[keysArauz[1]])\nlabelsArauz.append(labels[keysArauz[2]])\nplt.figure('Andres Arauz')\nfigArauz1, axArauz1 = plt.subplots()\naxArauz1.set_title('Andres Arauz')\naxArauz1.pie(valuesArauz, labels=labelsArauz, autopct='%1.1f%%', shadow=True, startangle=90)\nplt.show()",
"/usr/local/lib/python3.6/dist-packages/tensorflow/python/keras/engine/sequential.py:450: UserWarning: `model.predict_classes()` is deprecated and will be removed after 2021-01-01. Please use instead:* `np.argmax(model.predict(x), axis=-1)`, if your model does multi-class classification (e.g. if it uses a `softmax` last-layer activation).* `(model.predict(x) > 0.5).astype(\"int32\")`, if your model does binary classification (e.g. if it uses a `sigmoid` last-layer activation).\n warnings.warn('`model.predict_classes()` is deprecated and '\n"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bb782b50bb4a70929978b60dac61d0330e65e0 | 102,179 | ipynb | Jupyter Notebook | TensorFlow_impl.ipynb | twhughes/Finite-Difference-Frequency-Domain | e97739a40860305259ee7f53a052174a2697df0d | [
"MIT"
] | 1 | 2019-11-10T05:17:39.000Z | 2019-11-10T05:17:39.000Z | TensorFlow_impl.ipynb | twhughes/Finite-Difference-Frequency-Domain | e97739a40860305259ee7f53a052174a2697df0d | [
"MIT"
] | null | null | null | TensorFlow_impl.ipynb | twhughes/Finite-Difference-Frequency-Domain | e97739a40860305259ee7f53a052174a2697df0d | [
"MIT"
] | null | null | null | 259.997455 | 40,928 | 0.884986 | [
[
[
"# Imports\nimport numpy as np\nimport matplotlib.pylab as plt\nimport tensorflow as tf\nfrom solveFDFD import solveFDFD\n%matplotlib inline",
"_____no_output_____"
],
[
"# Define E&M Parameters\npol = 'TM' # polarization (TM -> Ez,Hx,Hy) (TE -> Hz,Ex,Ey)\ntiming = True # whether to time FDFD steps\nL0 = 1e-6 # length scale (m)\nwvlen = 0.5 # wavelength (L0)\nxrange = [-2, 2] # x position boundaries (L0)\nyrange = [-2, 2] # y position boundaries (L0)\nNx = 120 # number of grid points in x\nNy = 120 # number of grid points in y\nL_box = 40 # x-size of device region in grid points (centered)\nH_box = 40 # y-size of device region in grid points (centered)\neps = 3. # maximum permittivity of region\nnpml = 10 # number of pml points on each side",
"_____no_output_____"
],
[
"# Define neural network parameters\nn_hidden = [5, 5] # list of hidden layer sizes\nbeta = 1 # sigmoid steepness",
"_____no_output_____"
],
[
"N_iters = 50\nlearning_rate = 100.",
"_____no_output_____"
],
[
"# Set Up Simulation Variables\nnx = int(Nx//2) # x halfway index\nny = int(Ny//2) # y halfway index\nk0 = 2*np.pi/wvlen # free-space wavevector\nsrc_pos_og = int((Nx/2.0-npml)//2) + npml # original source x-position\nsrc_pos_aj = Nx - int((Nx/2.0-npml)//2) + npml # adjoint source y-potision\neps_r = np.ones((Nx,Ny)) # relative permittivity grid\nSRC_og = np.zeros((Nx,Ny)) # original current source injection grid\nSRC_aj = np.zeros((Nx,Ny)) # adjoint current source injection grid\nSRC_og[src_pos_og,ny] = 1 # set original current source\nSRC_aj[src_pos_aj,ny] = 1 # set adjoint current source\nNpml = [npml,npml,npml,npml] # set pml boundaries\ndelta_device = np.zeros((Nx,Ny)) # set optimization/device region\ndelta_device[nx-int(L_box//2):nx+int(L_box//2), ny-int(H_box//2):ny+int(H_box//2)] = 1\n",
"_____no_output_____"
],
[
"# Setup neural network variables\nx = tf.placeholder(\"float\", [2,Nx*Ny]) # input matrix placeholder [(x,y),#points]\ny = tf.placeholder(\"float\", [1, 1]) # output matrix placeholder (single value)\nxpoints = np.linspace(xrange[0],xrange[1],Nx) # x probe positions (L0)\nypoints = np.linspace(yrange[0],yrange[1],Ny) # y probe positions (L0)\npositions = np.array([[xp,yp] for xp in xpoints for yp in ypoints]).T # array of (x,y) positions to feed to network\nN_hidden_layers = len(n_hidden) # get number of hidden layers\n",
"_____no_output_____"
],
[
"# Define and initialize weights and biases\nweights = {}\nbiases = {}\nn_prev = 2\nfor l in range(1,N_hidden_layers+2):\n weight_name = 'w' + str(l)\n bias_name = 'b' + str(l)\n if l == N_hidden_layers+1:\n n_curr = 1\n else:\n n_curr = n_hidden[l-1] \n weight_vals = tf.random_normal([n_curr,n_prev])\n bias_vals = tf.random_normal([n_curr,1])\n weights[weight_name] = tf.Variable(weight_vals)\n biases[bias_name] = tf.Variable(bias_vals)\n \n n_prev = n_curr ",
"_____no_output_____"
],
[
"# Define neural network (phi(x,y)) and gradients\ndef neural_net(x):\n layer_prev = tf.tanh(tf.add(tf.matmul(weights['w1'],x), biases['b1'])) \n for l in range(2,N_hidden_layers+1):\n layer_l = tf.tanh(tf.add(tf.matmul(weights['w'+str(l)],layer_prev), biases['b'+str(l)]))\n layer_prev = layer_l\n layer_end = tf.sigmoid(tf.multiply(tf.constant(beta*1.),tf.add(tf.matmul(weights['w'+str(N_hidden_layers+1)],layer_prev), biases['b'+str(N_hidden_layers+1)])))\n epsilon_unmasked = tf.add(tf.constant(1.), tf.multiply(tf.add(tf.constant(eps),tf.constant(-1.)),layer_end))\n return tf.add(tf.constant(np.reshape(1.*(delta_device==0),(1,Nx*Ny)),dtype='float32'), tf.multiply(tf.constant(np.reshape(delta_device,(1,Nx*Ny)),dtype='float32'),epsilon_unmasked))\n\ngrads = {}\nfor l in range(1,N_hidden_layers+2):\n weight_label = 'w' + str(l)\n bias_label = 'b' + str(l)\n grads[weight_label] = tf.gradients(neural_net(x), [weights[weight_label]])\n grads[bias_label] = tf.gradients(neural_net(x), [biases[bias_label]])\n\nsess = tf.Session()\nsess.run(tf.global_variables_initializer())",
"_____no_output_____"
],
[
"# Compute the phi level-set function\nphis = sess.run(neural_net(x), feed_dict={x:positions})\ngradients = {}\nfor l in range(1,N_hidden_layers+2):\n weight_label = 'w' + str(l)\n bias_label = 'b' + str(l)\n grads[weight_label] = tf.gradients(neural_net(x), [weights[weight_label]])\n grads[bias_label] = tf.gradients(neural_net(x), [biases[bias_label]])\n \n#dw1s = sess.run(grads['w1'], feed_dict={x:positions})\nphis = np.reshape(phis,(Nx,Ny))\nplt.imshow(phis)\nplt.colorbar()\nplt.show()",
"_____no_output_____"
],
[
"# Do original simulation and adjoint simulation\n(_, _, Ez_og, Hx_og, Hy_og, _, _) = solveFDFD(pol, L0, wvlen, xrange, yrange, eps_r, SRC_og, Npml, timing=timing)\n(_, _, Ez_aj, Hx_aj, Hy_aj, _, _) = solveFDFD(pol, L0, wvlen, xrange, yrange, eps_r, SRC_aj, Npml, timing=timing)",
"setup time : 0.000535011291504\nS-parameters : 0.00884985923767\nderivative matrices : 2.06166219711\nfinal system solving: 0.842641115189\nsetup time : 0.000136852264404\nS-parameters : 0.00420904159546\nderivative matrices : 1.91641712189\nfinal system solving: 0.658952951431\n"
],
[
"# Plot original fields and get field strength\nimgplot_og = plt.imshow(np.real(Ez_og), clim=(-1,1))\nimgplot_og.set_cmap('coolwarm')\nplt.show()\nmeas = np.sum(Ez_og.T*SRC_aj)",
"_____no_output_____"
],
[
"# Multiply the correct adjoint prefactor and plot adjoint fields\nEz_aj_norm = -2*np.multiply(np.conj(meas),Ez_aj)\nimgplot_aj = plt.imshow(np.real(Ez_aj_norm), clim=(-2,2))\nimgplot_aj.set_cmap('coolwarm')\nplt.show()",
"_____no_output_____"
],
[
"# Compute adjoint sensitivity and plot\ndJde = -k0**2*np.real(np.multiply(np.multiply(Ez_aj_norm,Ez_og),delta_device.T))\nimgplot_sens = plt.imshow(dJde,clim=(-2,2))\nimgplot_sens.set_cmap('RdBu')\nplt.show()",
"_____no_output_____"
],
[
"sess = tf.Session()\nsess.run(tf.global_variables_initializer())\ngradients = {}\nJs = []\nfor i in range(N_iters):\n # get epsilon distribution\n sess.run(tf.global_variables_initializer()) \n eps_r = np.reshape(sess.run(neural_net(x), feed_dict={x:positions}),(Nx,Ny))\n # Do original simulation and adjoint simulation\n (_, _, Ez_og, Hx_og, Hy_og, _, _) = solveFDFD(pol, L0, wvlen, xrange, yrange, eps_r, SRC_og, Npml, timing=False)\n meas = np.sum(Ez_og.T*SRC_aj) \n Js.append(np.abs(np.sum(np.sum(np.multiply(Ez_og.T,SRC_aj)))))\n print(Js[i])\n #plt.imshow(eps_r)\n #plt.show()\n (_, _, Ez_aj, Hx_aj, Hy_aj, _, _) = solveFDFD(pol, L0, wvlen, xrange, yrange, eps_r, SRC_aj, Npml, timing=False)\n Ez_aj_norm = -2.*np.multiply(np.conj(meas),Ez_aj) \n # compute weight gradients\n sensitivity_kernel = np.reshape(np.real(np.multiply(Ez_og,Ez_aj).T),(1,Nx*Ny))\n sensitivity_kernel = np.ones((1,Nx*Ny))\n for l in range(1,N_hidden_layers+2):\n weight_label = 'w' + str(l)\n bias_label = 'b' + str(l)\n wgrad = tf.gradients(tf.multiply(neural_net(x), sensitivity_kernel), [weights[weight_label]])\n gradients[weight_label] = sess.run(wgrad, feed_dict={x:positions})\n weights[weight_label] = tf.add(weights[weight_label],tf.multiply(learning_rate,gradients[weight_label][0] ))\n bgrad = tf.gradients(tf.multiply(neural_net(x), sensitivity_kernel), [biases[bias_label]])\n gradients[bias_label] = sess.run(bgrad, feed_dict={x:positions})\n biases[bias_label] = tf.add(biases[bias_label],tf.multiply(learning_rate,gradients[bias_label][0] ))\n \n ",
"0.267010359315\n0.267010359315\n"
],
[
"plt.plot(Js)\nplt.show()",
"_____no_output_____"
],
[
"plt.imshow(eps_r)",
"_____no_output_____"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bb9e0ff0016cb291d9904867d0382ddcc7749d | 16,699 | ipynb | Jupyter Notebook | module2-convolutional-neural-networks/LS_DS_432_Convolution_Neural_Networks_Assignment.ipynb | j-m-d-h/DS-Unit-4-Sprint-3-Deep-Learning | ad720b9593eb2e5f19ee112bec58ce55dde547ea | [
"MIT"
] | null | null | null | module2-convolutional-neural-networks/LS_DS_432_Convolution_Neural_Networks_Assignment.ipynb | j-m-d-h/DS-Unit-4-Sprint-3-Deep-Learning | ad720b9593eb2e5f19ee112bec58ce55dde547ea | [
"MIT"
] | null | null | null | module2-convolutional-neural-networks/LS_DS_432_Convolution_Neural_Networks_Assignment.ipynb | j-m-d-h/DS-Unit-4-Sprint-3-Deep-Learning | ad720b9593eb2e5f19ee112bec58ce55dde547ea | [
"MIT"
] | null | null | null | 36.302174 | 519 | 0.595305 | [
[
[
"<img align=\"left\" src=\"https://lever-client-logos.s3.amazonaws.com/864372b1-534c-480e-acd5-9711f850815c-1524247202159.png\" width=200>\n<br></br>\n<br></br>\n\n## *Data Science Unit 4 Sprint 3 Assignment 2*\n# Convolutional Neural Networks (CNNs)",
"_____no_output_____"
],
[
"# Assignment\n\n- <a href=\"#p1\">Part 1:</a> Pre-Trained Model\n- <a href=\"#p2\">Part 2:</a> Custom CNN Model\n- <a href=\"#p3\">Part 3:</a> CNN with Data Augmentation\n\n\nYou will apply three different CNN models to a binary image classification model using Keras. Classify images of Mountains (`./data/mountain/*`) and images of forests (`./data/forest/*`). Treat mountains as the postive class (1) and the forest images as the negative (zero). \n\n|Mountain (+)|Forest (-)|\n|---|---|\n|||\n\nThe problem is realively difficult given that the sample is tiny: there are about 350 observations per class. This sample size might be something that you can expect with prototyping an image classification problem/solution at work. Get accustomed to evaluating several differnet possible models.",
"_____no_output_____"
],
[
"# Pre - Trained Model\n<a id=\"p1\"></a>\n\nLoad a pretrained network from Keras, [ResNet50](https://tfhub.dev/google/imagenet/resnet_v1_50/classification/1) - a 50 layer deep network trained to recognize [1000 objects](https://storage.googleapis.com/download.tensorflow.org/data/ImageNetLabels.txt). Starting usage:\n\n```python\nimport numpy as np\n\nfrom tensorflow.keras.applications.resnet50 import ResNet50\nfrom tensorflow.keras.preprocessing import image\nfrom tensorflow.keras.applications.resnet50 import preprocess_input, decode_predictions\n\nfrom tensorflow.keras.layers import Dense, GlobalAveragePooling2D()\nfrom tensorflow.keras.models import Model # This is the functional API\n\nresnet = ResNet50(weights='imagenet', include_top=False)\n\n```\n\nThe `include_top` parameter in `ResNet50` will remove the full connected layers from the ResNet model. The next step is to turn off the training of the ResNet layers. We want to use the learned parameters without updating them in future training passes. \n\n```python\nfor layer in resnet.layers:\n layer.trainable = False\n```\n\nUsing the Keras functional API, we will need to additional additional full connected layers to our model. We we removed the top layers, we removed all preivous fully connected layers. In other words, we kept only the feature processing portions of our network. You can expert with additional layers beyond what's listed here. The `GlobalAveragePooling2D` layer functions as a really fancy flatten function by taking the average of each of the last convolutional layer outputs (which is two dimensional still). \n\n```python\nx = res.output\nx = GlobalAveragePooling2D()(x) # This layer is a really fancy flatten\nx = Dense(1024, activation='relu')(x)\npredictions = Dense(1, activation='sigmoid')(x)\nmodel = Model(res.input, predictions)\n```\n\nYour assignment is to apply the transfer learning above to classify images of Mountains (`./data/mountain/*`) and images of forests (`./data/forest/*`). Treat mountains as the postive class (1) and the forest images as the negative (zero). \n\nSteps to complete assignment: \n1. Load in Image Data into numpy arrays (`X`) \n2. Create a `y` for the labels\n3. Train your model with pretrained layers from resnet\n4. Report your model's accuracy",
"_____no_output_____"
],
[
"## Load in Data\n\n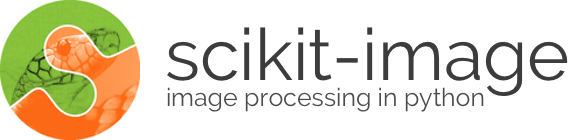\n\nCheck out out [`skimage`](https://scikit-image.org/) for useful functions related to processing the images. In particular checkout the documentation for `skimage.io.imread_collection` and `skimage.transform.resize`.",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport os\nfrom skimage import color, io\nfrom sklearn.model_selection import train_test_split\n\nfrom tensorflow.keras.applications.resnet50 import ResNet50\nfrom tensorflow.keras.preprocessing import image\nfrom tensorflow.keras.applications.resnet50 import preprocess_input, decode_predictions\n\nfrom tensorflow.keras.layers import Dense, GlobalAveragePooling2D\nfrom tensorflow.keras.models import Model\n\nresnet = ResNet50(weights='imagenet', include_top=False)",
"C:\\ProgramData\\Anaconda3\\envs\\U4-S2-NNF\\lib\\site-packages\\keras_applications\\resnet50.py:265: UserWarning: The output shape of `ResNet50(include_top=False)` has been changed since Keras 2.2.0.\n warnings.warn('The output shape of `ResNet50(include_top=False)` '\n"
],
[
"forests = io.imread_collection('./data/forest/*')\nmountains = io.imread_collection('./data/mountain/*')",
"_____no_output_____"
],
[
"len(forests), len(mountains)",
"_____no_output_____"
],
[
"forest_labels = np.zeros(len(forests))\nmountain_labels = np.ones(len(mountains))",
"_____no_output_____"
],
[
"labels = np.concatenate((forest_labels, mountain_labels), axis=0)\npics = np.concatenate((forests, mountains), axis=0)",
"_____no_output_____"
],
[
"labels_train = labels[:500]\nlabels_test = labels[500:]\n\npics_train = pics[:500]\npics_test = pics[500:]",
"_____no_output_____"
],
[
"len(labels_train), len(labels_test)",
"_____no_output_____"
]
],
[
[
"## Instatiate Model",
"_____no_output_____"
]
],
[
[
"for layer in resnet.layers:\n layer.trainable = False",
"_____no_output_____"
],
[
"x = resnet.output\nx = GlobalAveragePooling2D()(x)\nx = Dense(1024, activation='relu')(x)\npredictions = Dense(1, activation='sigmoid')(x)\nmodel = Model(resnet.input, predictions)\n\nmodel.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])",
"_____no_output_____"
]
],
[
[
"## Fit Model",
"_____no_output_____"
]
],
[
[
"model.fit(pics_train, labels_train, validation_data=(pics_test, labels_test), epochs=4)",
"Train on 500 samples, validate on 202 samples\nEpoch 1/4\n500/500 [==============================] - 115s 231ms/sample - loss: 0.0070 - acc: 0.9960 - val_loss: 0.1556 - val_acc: 0.9356\nEpoch 2/4\n500/500 [==============================] - 116s 233ms/sample - loss: 0.0191 - acc: 0.9900 - val_loss: 0.2591 - val_acc: 0.9010\nEpoch 3/4\n500/500 [==============================] - 113s 227ms/sample - loss: 0.0012 - acc: 1.0000 - val_loss: 0.4716 - val_acc: 0.8416\nEpoch 4/4\n500/500 [==============================] - 113s 225ms/sample - loss: 0.0148 - acc: 0.9960 - val_loss: 1.1197 - val_acc: 0.7475\n"
],
[
"model.predict(pics[206])",
"_____no_output_____"
]
],
[
[
"# Custom CNN Model\n\nIn this step, write and train your own convolutional neural network using Keras. You can use any architecture that suits you as long as it has at least one convolutional and one pooling layer at the beginning of the network - you can add more if you want. ",
"_____no_output_____"
]
],
[
[
"\n",
"_____no_output_____"
],
[
"\n",
"Train on 561 samples, validate on 141 samples\nEpoch 1/5\n561/561 [==============================] - 18s 32ms/sample - loss: 0.2667 - accuracy: 0.9073 - val_loss: 0.1186 - val_accuracy: 0.9858\nEpoch 2/5\n561/561 [==============================] - 18s 32ms/sample - loss: 0.2046 - accuracy: 0.9073 - val_loss: 0.3342 - val_accuracy: 0.8511\nEpoch 3/5\n561/561 [==============================] - 18s 32ms/sample - loss: 0.1778 - accuracy: 0.9287 - val_loss: 0.2746 - val_accuracy: 0.8723\nEpoch 4/5\n561/561 [==============================] - 18s 32ms/sample - loss: 0.1681 - accuracy: 0.9323 - val_loss: 0.8487 - val_accuracy: 0.5957\nEpoch 5/5\n561/561 [==============================] - 18s 32ms/sample - loss: 0.1606 - accuracy: 0.9394 - val_loss: 0.3903 - val_accuracy: 0.8582\n"
]
],
[
[
"# Custom CNN Model with Image Manipulations\n## *This a stretch goal, and it's relatively difficult*\n\nTo simulate an increase in a sample of image, you can apply image manipulation techniques: cropping, rotation, stretching, etc. Luckily Keras has some handy functions for us to apply these techniques to our mountain and forest example. Check out these resources to help you get started: \n\n1. [Keras `ImageGenerator` Class](https://keras.io/preprocessing/image/#imagedatagenerator-class)\n2. [Building a powerful image classifier with very little data](https://blog.keras.io/building-powerful-image-classification-models-using-very-little-data.html)\n ",
"_____no_output_____"
]
],
[
[
"# State Code for Image Manipulation Here",
"_____no_output_____"
]
],
[
[
"# Resources and Stretch Goals\n\nStretch goals\n- Enhance your code to use classes/functions and accept terms to search and classes to look for in recognizing the downloaded images (e.g. download images of parties, recognize all that contain balloons)\n- Check out [other available pretrained networks](https://tfhub.dev), try some and compare\n- Image recognition/classification is somewhat solved, but *relationships* between entities and describing an image is not - check out some of the extended resources (e.g. [Visual Genome](https://visualgenome.org/)) on the topic\n- Transfer learning - using images you source yourself, [retrain a classifier](https://www.tensorflow.org/hub/tutorials/image_retraining) with a new category\n- (Not CNN related) Use [piexif](https://pypi.org/project/piexif/) to check out the metadata of images passed in to your system - see if they're from a national park! (Note - many images lack GPS metadata, so this won't work in most cases, but still cool)\n\nResources\n- [Deep Residual Learning for Image Recognition](https://arxiv.org/abs/1512.03385) - influential paper (introduced ResNet)\n- [YOLO: Real-Time Object Detection](https://pjreddie.com/darknet/yolo/) - an influential convolution based object detection system, focused on inference speed (for applications to e.g. self driving vehicles)\n- [R-CNN, Fast R-CNN, Faster R-CNN, YOLO](https://towardsdatascience.com/r-cnn-fast-r-cnn-faster-r-cnn-yolo-object-detection-algorithms-36d53571365e) - comparison of object detection systems\n- [Common Objects in Context](http://cocodataset.org/) - a large-scale object detection, segmentation, and captioning dataset\n- [Visual Genome](https://visualgenome.org/) - a dataset, a knowledge base, an ongoing effort to connect structured image concepts to language",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
]
] |
e7bba7da66d2c125e7bfc9aa9931faabb1fccd3a | 40,544 | ipynb | Jupyter Notebook | va_circuit_court_eda.ipynb | mh4ey/va-case-expungement | 771810e20cfafd6cee691fb790601a8a691ed4e2 | [
"MIT"
] | null | null | null | va_circuit_court_eda.ipynb | mh4ey/va-case-expungement | 771810e20cfafd6cee691fb790601a8a691ed4e2 | [
"MIT"
] | null | null | null | va_circuit_court_eda.ipynb | mh4ey/va-case-expungement | 771810e20cfafd6cee691fb790601a8a691ed4e2 | [
"MIT"
] | null | null | null | 40.142574 | 1,585 | 0.51988 | [
[
[
"# Set Up",
"_____no_output_____"
]
],
[
[
"# imports and juppyter environment settings\n%run -i settings.py",
"Imports and display options set...\n"
],
[
"# setup environment variables\n%run -i setup.py",
"_____no_output_____"
],
[
"# To auto-reload modules in jupyter notebook (so that changes in files *.py doesn't require manual reloading):\n# for auto-reloading external modules\n# see http://stackoverflow.com/questions/1907993/autoreload-of-modules-in-ipython\n%reload_ext autoreload\n%autoreload 2\n\n# you can now enable 2x images by just adding the line:\n# see: https://gist.github.com/minrk/3301035\n%config InlineBackend.figure_format = 'png'",
"_____no_output_____"
],
[
"file = f'{BASE_DIR}/data/circuit_court_2009_2019.csv.gz'\ndf = pd.read_csv(file, parse_dates =['offense_date'])\ndf.head(5)",
"_____no_output_____"
]
],
[
[
"### Summary Statistics",
"_____no_output_____"
]
],
[
[
"# In this example, the data frame is described and [‘object’] is passed to include parameter\n# to see description of object series. [.20, .40, .60, .80] is passed to percentile parameter \n# to view the respective percentile of Numeric series.\n# see: https://www.geeksforgeeks.org/python-pandas-dataframe-describe-method/\nperc = [0.20, .40, .60, 0.80]\ninclude = ['object', 'float', 'int']\ndf.describe(percentiles= perc, include=include).T",
"_____no_output_____"
]
],
[
[
"## Data Cleaning",
"_____no_output_____"
]
],
[
[
"# number of na values in each column\ndf.isna().sum()",
"_____no_output_____"
]
],
[
[
"For our study, 'personId', 'offense_date', 'final_disposition', 'fips', 'race', 'gender', 'charge', 'fips_area' are mandatory columns.\nSo removing all records where these field values are missing will be removed",
"_____no_output_____"
]
],
[
[
"df.dropna(axis=0, subset=['final_disposition'], inplace=True)\ndf.dropna(axis=0, subset=['race'], inplace=True)",
"_____no_output_____"
],
[
"df.isna().sum()",
"_____no_output_____"
],
[
"# for the moment we consider case_class as important field, so we will impute missing value 'unknown'\ndf['ammended_charge'].fillna('unknown', inplace=True)",
"_____no_output_____"
],
[
"df.isna().sum()",
"_____no_output_____"
],
[
"duplicate_records = pd.DataFrame(df.duplicated(), columns=['isduplicate'])\nduplicate_records = duplicate_records.reset_index()\nduplicate_records.columns = [str(column) for column in duplicate_records.columns]\nduplicate_records.set_index('index')\nduplicate_records.groupby('isduplicate').count()",
"_____no_output_____"
]
],
[
[
"Our dataset has 974935 duplicate records, so we purge these records from our dataset",
"_____no_output_____"
]
],
[
[
"#drop duplicate records\ndf = df.drop_duplicates(['person_id', 'offense_date', 'final_disposition', 'fips', 'race', 'gender', 'charge', 'ammended_charge'])\nlen(df)",
"_____no_output_____"
]
],
[
[
"df",
"_____no_output_____"
],
[
"* We can see that hearing_date, fips, gender, charge_type and person_id do not have any missing data, however we need to address the missing data for other columns.\n\n",
"_____no_output_____"
]
],
[
[
"df.isna().sum()",
"_____no_output_____"
]
],
[
[
"Per our domain expert if ammended_charge is reduced to **'DWI'** then the case is a candidate for expungement.",
"_____no_output_____"
]
],
[
[
"dwi_ammended_charge_df = df [df.ammended_charge.str.contains('DWI') == True]\nlen(dwi_ammended_charge_df)",
"_____no_output_____"
]
],
[
[
"Our aim is to derive the cadidate cases for expungement based on hearing_results, let us examine the uniuqe values for hearing_result",
"_____no_output_____"
]
],
[
[
"# top 15 hearing results\nhearing_result_counts = df['final_disposition'].value_counts()\nsubset = hearing_result_counts[:15]\nsns.barplot(y=subset.index, x=subset.values)",
"_____no_output_____"
],
[
"# to 15 hearing results by gender\ndf_result_bygendger = df.groupby(['final_disposition', 'gender'])\\\n .size()\\\n .unstack()\\\n .fillna(0)\\\n .sort_values(['Female', 'Male'], ascending=False)\ndf_stacked = df_result_bygendger.head(15).stack()\ndf_stacked.name = 'total'\ndf_stacked= df_stacked.reset_index()\nsns.barplot(x='total', y='final_disposition', hue='gender', data= df_stacked)",
"_____no_output_____"
]
],
[
[
"## Preprocessing Data",
"_____no_output_____"
],
[
"### Adding fips_area name column",
"_____no_output_____"
]
],
[
[
"#load fips code table\nfips_file = 'reference-data/va-fips-codes.csv'\nfips_df = pd.read_csv(fips_file)\nfips_df = fips_df[['CountyFIPSCode', 'GUName']]\nfips_df",
"_____no_output_____"
],
[
"#add fips_GUName\ndf = pd.merge(df,fips_df,left_on='fips', right_on='CountyFIPSCode', how='left')\\\n .drop(columns=['CountyFIPSCode'], axis=1)\\\n .rename(columns={'GUName': 'fips_area'})\ndf",
"_____no_output_____"
]
],
[
[
"## Identifying the candidates for case expungement",
"_____no_output_____"
],
[
"Assumptions maded based on domain expert input:\n1. If the final_disposition is any the following values 'Dismissed','Noile Prosequi','Not Guilty', 'Withdrawn', 'Not Found', 'No Indictment Presented', 'No Longer Under Advisement', 'Not True Bill' then the case is candidate for expungement.\n2. **TODO:** If the charges are ammended to DWI then also it can be a candidate for expungement if the person does not have any prior felony charges \n",
"_____no_output_____"
]
],
[
[
"cand_list =['Dismissed','Noile Prosequi','Not Guilty', 'Withdrawn', 'Not Found', 'No Indictment Presented', 'No Longer Under Advisement', 'Not True Bill']",
"_____no_output_____"
],
[
"df['candidate'] = [1 if x in cand_list else 0 for x in df['final_disposition']]",
"_____no_output_____"
],
[
"df",
"_____no_output_____"
],
[
"df.groupby(['candidate']).count().head()",
"_____no_output_____"
],
[
"df.groupby(['candidate','race','gender']).count()",
"_____no_output_____"
],
[
"df.to_csv(\n PROCESSED_PATH + \"district_court_2009_2019_cleansed.csv.gz\",\n index=False,\n compression=\"gzip\",\n header=True,\n quotechar='\"',\n doublequote=True,\n line_terminator=\"\\n\",\n)",
"_____no_output_____"
],
[
"delete_file(PROCESSED_PATH + \"district_court_2009_2019.csv.gz\")",
"_____no_output_____"
],
[
"#!jupyter nbconvert va_circuit_court_eda.ipynb --to pdf",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bbc87896aeec8d070501d29b8871d932537791 | 75,812 | ipynb | Jupyter Notebook | ResNet.ipynb | harshithbelagur/ResNet | be4022e12e6b4702d19c711b24517601a6199591 | [
"MIT"
] | null | null | null | ResNet.ipynb | harshithbelagur/ResNet | be4022e12e6b4702d19c711b24517601a6199591 | [
"MIT"
] | null | null | null | ResNet.ipynb | harshithbelagur/ResNet | be4022e12e6b4702d19c711b24517601a6199591 | [
"MIT"
] | null | null | null | 53.164095 | 433 | 0.386311 | [
[
[
"import torch\nimport torch.nn as nn\n\nfrom functools import partial\nfrom dataclasses import dataclass\nfrom collections import OrderedDict",
"_____no_output_____"
]
],
[
[
"# Implementing ResNet in PyTorch\nToday we are going to implement the famous ResNet from Kaiming He et al. (Microsoft Research). It won the 1st place on the ILSVRC 2015 classification task.\n\nThe original paper can be read from [here ](https://arxiv.org/abs/1512.03385) and it is very easy to follow, additional material can be found in this [quora answer](https://www.quora.com/)\n\n\n*Deeper neural networks are more difficult to train.* Why? One big problem of deeper network is the vanishing gradient. Basically, the model is not able to learn anymore.\n\nTo solve this problem, the Authors proposed to use a reference to the previous layer to compute the output at a given layer. In ResNet, the output form the previous layer, called **residual**, is added to the output of the current layer. The following picture visualizes this operation\n\n\n\nWe are going to make our implementation **as scalable as possible** using one think think unknown to mostly of the data scientiest: **object orienting programming**",
"_____no_output_____"
],
[
"## Basic Block\n\nOkay, the first thing is to think about what we need. Well, first of all we need a convolution layer and since PyTorch does not have the 'auto' padding in Conv2d, so we have to code ourself!",
"_____no_output_____"
]
],
[
[
"class Conv2dAuto(nn.Conv2d):\n def __init__(self, *args, **kwargs):\n super().__init__(*args, **kwargs)\n self.padding = (self.kernel_size[0] // 2, self.kernel_size[1] // 2) # dynamic add padding based on the kernel_size\n \nconv3x3 = partial(Conv2dAuto, kernel_size=3, bias=False) \n ",
"_____no_output_____"
],
[
"conv = conv3x3(in_channels=32, out_channels=64)\nprint(conv)\ndel conv",
"Conv2dAuto(32, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)\n"
]
],
[
[
"## Residual Block\nTo make clean code is mandatory to think about the main building block of each application, or of the network in our case. The residual block takes an input with `in_channels`, applies some blocks of convolutional layers to reduce it to `out_channels` and sum it up to the original input. If their sizes mismatch, then the input goes into an `identity`. We can abstract this process and create a interface that can be extedend.",
"_____no_output_____"
]
],
[
[
"class ResidualBlock(nn.Module):\n def __init__(self, in_channels, out_channels):\n super().__init__()\n self.in_channels, self.out_channels = in_channels, out_channels\n self.blocks = nn.Identity()\n self.shortcut = nn.Identity() \n \n def forward(self, x):\n residual = x\n if self.should_apply_shortcut: residual = self.shortcut(x)\n x = self.blocks(x)\n x += residual\n return x\n \n @property\n def should_apply_shortcut(self):\n return self.in_channels != self.out_channels",
"_____no_output_____"
],
[
"ResidualBlock(32, 64)",
"_____no_output_____"
]
],
[
[
"Let's test it with a dummy vector with one one, we should get a vector with two",
"_____no_output_____"
]
],
[
[
"dummy = torch.ones((1, 1, 1, 1))\n\nblock = ResidualBlock(1, 64)\nblock(dummy)",
"_____no_output_____"
]
],
[
[
"In ResNet each block has a expansion parameter in order to increase the `out_channels`. Also, the identity is defined as a Convolution followed by an Activation layer, this is referred as `shortcut`. Then, we can just extend `ResidualBlock` and defined the `shortcut` function.",
"_____no_output_____"
]
],
[
[
"from collections import OrderedDict\n\nclass ResNetResidualBlock(ResidualBlock):\n def __init__(self, in_channels, out_channels, expansion=1, downsampling=1, conv=conv3x3, *args, **kwargs):\n super().__init__(in_channels, out_channels)\n self.expansion, self.downsampling, self.conv = expansion, downsampling, conv\n self.shortcut = nn.Sequential(OrderedDict(\n {\n 'conv' : nn.Conv2d(self.in_channels, self.expanded_channels, kernel_size=1,\n stride=self.downsampling, bias=False),\n 'bn' : nn.BatchNorm2d(self.expanded_channels)\n \n })) if self.should_apply_shortcut else None\n \n \n @property\n def expanded_channels(self):\n return self.out_channels * self.expansion\n \n @property\n def should_apply_shortcut(self):\n return self.in_channels != self.expanded_channels",
"_____no_output_____"
],
[
"ResNetResidualBlock(32, 64)",
"_____no_output_____"
]
],
[
[
"### Basic Block\nA basic ResNet block is composed by two layers of `3x3` convs/batchnorm/relu. In the picture, the lines represnet the residual operation. The dotted line means that the shortcut was applied to match the input and the output dimension.\n",
"_____no_output_____"
],
[
"Let's first create an handy function to stack one conv and batchnorm layer. Using `OrderedDict` to properly name each sublayer.",
"_____no_output_____"
]
],
[
[
"from collections import OrderedDict\ndef conv_bn(in_channels, out_channels, conv, *args, **kwargs):\n return nn.Sequential(OrderedDict({'conv': conv(in_channels, out_channels, *args, **kwargs), \n 'bn': nn.BatchNorm2d(out_channels) }))",
"_____no_output_____"
],
[
"conv_bn(3, 3, nn.Conv2d, kernel_size=3)",
"_____no_output_____"
],
[
"class ResNetBasicBlock(ResNetResidualBlock):\n expansion = 1\n def __init__(self, in_channels, out_channels, activation=nn.ReLU, *args, **kwargs):\n super().__init__(in_channels, out_channels, *args, **kwargs)\n self.blocks = nn.Sequential(\n conv_bn(self.in_channels, self.out_channels, conv=self.conv, bias=False, stride=self.downsampling),\n activation(),\n conv_bn(self.out_channels, self.expanded_channels, conv=self.conv, bias=False),\n )\n ",
"_____no_output_____"
],
[
"dummy = torch.ones((1, 32, 224, 224))\n\nblock = ResNetBasicBlock(32, 64)\nblock(dummy).shape\nprint(block)\n\n'''The shortcut is the residual (the solution for vanishing gradient, skipping the 2 layers\nand directly contributing to the output)'''",
"ResNetBasicBlock(\n (blocks): Sequential(\n (0): Sequential(\n (conv): Conv2dAuto(32, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)\n (bn): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)\n )\n (1): ReLU()\n (2): Sequential(\n (conv): Conv2dAuto(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)\n (bn): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)\n )\n )\n (shortcut): Sequential(\n (conv): Conv2d(32, 64, kernel_size=(1, 1), stride=(1, 1), bias=False)\n (bn): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)\n )\n)\n"
]
],
[
[
"### BottleNeck\nTo increase the network deepths but to decrese the number of parameters, the Authors defined a BottleNeck block that \n\"The three layers are 1x1, 3x3, and 1x1 convolutions, where the 1×1 layers are responsible for reducing and then increasing (restoring) dimensions, leaving the 3×3 layer a bottleneck with smaller input/output dimensions.\" We can extend the `ResNetResidualBlock` and create these blocks.",
"_____no_output_____"
]
],
[
[
"class ResNetBottleNeckBlock(ResNetResidualBlock):\n expansion = 4\n def __init__(self, in_channels, out_channels, activation=nn.ReLU, *args, **kwargs):\n super().__init__(in_channels, out_channels, expansion=4, *args, **kwargs)\n self.blocks = nn.Sequential(\n conv_bn(self.in_channels, self.out_channels, self.conv, kernel_size=1),\n activation(),\n conv_bn(self.out_channels, self.out_channels, self.conv, kernel_size=3, stride=self.downsampling),\n activation(),\n conv_bn(self.out_channels, self.expanded_channels, self.conv, kernel_size=1),\n )\n ",
"_____no_output_____"
],
[
"dummy = torch.ones((1, 32, 10, 10))\n\nblock = ResNetBottleNeckBlock(32, 64)\nblock(dummy).shape\nprint(block)",
"ResNetBottleNeckBlock(\n (blocks): Sequential(\n (0): Sequential(\n (conv): Conv2dAuto(32, 64, kernel_size=(1, 1), stride=(1, 1), bias=False)\n (bn): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)\n )\n (1): ReLU()\n (2): Sequential(\n (conv): Conv2dAuto(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)\n (bn): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)\n )\n (3): ReLU()\n (4): Sequential(\n (conv): Conv2dAuto(64, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)\n (bn): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)\n )\n )\n (shortcut): Sequential(\n (conv): Conv2d(32, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)\n (bn): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)\n )\n)\n"
]
],
[
[
"### Layer\nA ResNet's layer is composed by blocks stacked one after the other. \n\n\n\nWe can easily defined it by just stuck `n` blocks one after the other, just remember that the first convolution block has a stide of two since \"We perform downsampling directly by convolutional layers that have a stride of 2\".",
"_____no_output_____"
]
],
[
[
"class ResNetLayer(nn.Module):\n def __init__(self, in_channels, out_channels, block=ResNetBasicBlock, n=1, *args, **kwargs):\n super().__init__()\n # 'We perform downsampling directly by convolutional layers that have a stride of 2.'\n downsampling = 2 if in_channels != out_channels else 1\n \n self.blocks = nn.Sequential(\n block(in_channels , out_channels, *args, **kwargs, downsampling=downsampling),\n *[block(out_channels * block.expansion, \n out_channels, downsampling=1, *args, **kwargs) for _ in range(n - 1)]\n )\n\n def forward(self, x):\n x = self.blocks(x)\n return x",
"_____no_output_____"
],
[
"dummy = torch.ones((1, 32, 48, 48))\n\nlayer = ResNetLayer(64, 128, block=ResNetBasicBlock, n=3)\n# layer(dummy).shape\nlayer",
"_____no_output_____"
]
],
[
[
"### Encoder\nSimilarly, the encoder is composed by multiple layer at increasing features size.\n\n\n\n",
"_____no_output_____"
]
],
[
[
"class ResNetEncoder(nn.Module):\n \"\"\"\n ResNet encoder composed by increasing different layers with increasing features.\n \"\"\"\n def __init__(self, in_channels=3, blocks_sizes=[64, 128, 256, 512], deepths=[2,2,2,2], \n activation=nn.ReLU, block=ResNetBasicBlock, *args,**kwargs):\n super().__init__()\n \n self.blocks_sizes = blocks_sizes\n \n self.gate = nn.Sequential(\n nn.Conv2d(in_channels, self.blocks_sizes[0], kernel_size=7, stride=2, padding=3, bias=False),\n nn.BatchNorm2d(self.blocks_sizes[0]),\n activation(),\n nn.MaxPool2d(kernel_size=3, stride=2, padding=1)\n )\n \n self.in_out_block_sizes = list(zip(blocks_sizes, blocks_sizes[1:]))\n self.blocks = nn.ModuleList([ \n ResNetLayer(blocks_sizes[0], blocks_sizes[0], n=deepths[0], activation=activation, \n block=block, *args, **kwargs),\n *[ResNetLayer(in_channels * block.expansion, \n out_channels, n=n, activation=activation, \n block=block, *args, **kwargs) \n for (in_channels, out_channels), n in zip(self.in_out_block_sizes, deepths[1:])] \n ])\n \n \n def forward(self, x):\n x = self.gate(x)\n for block in self.blocks:\n x = block(x)\n return x",
"_____no_output_____"
]
],
[
[
"## Decoder\nThe decoder is the last piece we need to create the full network. It is a fully connected layer that maps the features learned by the network to their respective classes. Easily, we can defined it as:",
"_____no_output_____"
]
],
[
[
"class ResnetDecoder(nn.Module):\n \"\"\"\n This class represents the tail of ResNet. It performs a global pooling and maps the output to the\n correct class by using a fully connected layer.\n \"\"\"\n def __init__(self, in_features, n_classes):\n super().__init__()\n self.avg = nn.AdaptiveAvgPool2d((1, 1))\n self.decoder = nn.Linear(in_features, n_classes)\n\n def forward(self, x):\n x = self.avg(x)\n x = x.view(x.size(0), -1)\n x = self.decoder(x)\n return x\n",
"_____no_output_____"
]
],
[
[
"## ResNet\n\nFinal, we can put all the pieces together and create the final model.\n\n",
"_____no_output_____"
]
],
[
[
"class ResNet(nn.Module):\n \n def __init__(self, in_channels, n_classes, *args, **kwargs):\n super().__init__()\n self.encoder = ResNetEncoder(in_channels, *args, **kwargs)\n self.decoder = ResnetDecoder(self.encoder.blocks[-1].blocks[-1].expanded_channels, n_classes)\n \n def forward(self, x):\n x = self.encoder(x)\n x = self.decoder(x)\n return x",
"_____no_output_____"
]
],
[
[
"We can now defined the five models proposed by the Authors, `resnet18,34,50,101,152`",
"_____no_output_____"
]
],
[
[
"def resnet18(in_channels, n_classes):\n return ResNet(in_channels, n_classes, block=ResNetBasicBlock, deepths=[2, 2, 2, 2])\n\ndef resnet34(in_channels, n_classes):\n return ResNet(in_channels, n_classes, block=ResNetBasicBlock, deepths=[3, 4, 6, 3])\n\ndef resnet50(in_channels, n_classes):\n return ResNet(in_channels, n_classes, block=ResNetBottleNeckBlock, deepths=[3, 4, 6, 3])\n\ndef resnet101(in_channels, n_classes):\n return ResNet(in_channels, n_classes, block=ResNetBottleNeckBlock, deepths=[3, 4, 23, 3])\n\ndef resnet152(in_channels, n_classes):\n return ResNet(in_channels, n_classes, block=ResNetBottleNeckBlock, deepths=[3, 8, 36, 3])",
"_____no_output_____"
],
[
"from torchsummary import summary\n\nmodel = resnet101(3, 1000)\nsummary(model.cuda(), (3, 224, 224))",
"----------------------------------------------------------------\n Layer (type) Output Shape Param #\n================================================================\n Conv2d-1 [-1, 64, 112, 112] 9,408\n BatchNorm2d-2 [-1, 64, 112, 112] 128\n ReLU-3 [-1, 64, 112, 112] 0\n MaxPool2d-4 [-1, 64, 56, 56] 0\n Conv2d-5 [-1, 256, 56, 56] 16,384\n BatchNorm2d-6 [-1, 256, 56, 56] 512\n Conv2dAuto-7 [-1, 64, 56, 56] 4,096\n BatchNorm2d-8 [-1, 64, 56, 56] 128\n ReLU-9 [-1, 64, 56, 56] 0\n Conv2dAuto-10 [-1, 64, 56, 56] 36,864\n BatchNorm2d-11 [-1, 64, 56, 56] 128\n ReLU-12 [-1, 64, 56, 56] 0\n Conv2dAuto-13 [-1, 256, 56, 56] 16,384\n BatchNorm2d-14 [-1, 256, 56, 56] 512\nResNetBottleNeckBlock-15 [-1, 256, 56, 56] 0\n Conv2dAuto-16 [-1, 64, 56, 56] 16,384\n BatchNorm2d-17 [-1, 64, 56, 56] 128\n ReLU-18 [-1, 64, 56, 56] 0\n Conv2dAuto-19 [-1, 64, 56, 56] 36,864\n BatchNorm2d-20 [-1, 64, 56, 56] 128\n ReLU-21 [-1, 64, 56, 56] 0\n Conv2dAuto-22 [-1, 256, 56, 56] 16,384\n BatchNorm2d-23 [-1, 256, 56, 56] 512\nResNetBottleNeckBlock-24 [-1, 256, 56, 56] 0\n Conv2dAuto-25 [-1, 64, 56, 56] 16,384\n BatchNorm2d-26 [-1, 64, 56, 56] 128\n ReLU-27 [-1, 64, 56, 56] 0\n Conv2dAuto-28 [-1, 64, 56, 56] 36,864\n BatchNorm2d-29 [-1, 64, 56, 56] 128\n ReLU-30 [-1, 64, 56, 56] 0\n Conv2dAuto-31 [-1, 256, 56, 56] 16,384\n BatchNorm2d-32 [-1, 256, 56, 56] 512\nResNetBottleNeckBlock-33 [-1, 256, 56, 56] 0\n ResNetLayer-34 [-1, 256, 56, 56] 0\n Conv2d-35 [-1, 512, 28, 28] 131,072\n BatchNorm2d-36 [-1, 512, 28, 28] 1,024\n Conv2dAuto-37 [-1, 128, 56, 56] 32,768\n BatchNorm2d-38 [-1, 128, 56, 56] 256\n ReLU-39 [-1, 128, 56, 56] 0\n Conv2dAuto-40 [-1, 128, 28, 28] 147,456\n BatchNorm2d-41 [-1, 128, 28, 28] 256\n ReLU-42 [-1, 128, 28, 28] 0\n Conv2dAuto-43 [-1, 512, 28, 28] 65,536\n BatchNorm2d-44 [-1, 512, 28, 28] 1,024\nResNetBottleNeckBlock-45 [-1, 512, 28, 28] 0\n Conv2dAuto-46 [-1, 128, 28, 28] 65,536\n BatchNorm2d-47 [-1, 128, 28, 28] 256\n ReLU-48 [-1, 128, 28, 28] 0\n Conv2dAuto-49 [-1, 128, 28, 28] 147,456\n BatchNorm2d-50 [-1, 128, 28, 28] 256\n ReLU-51 [-1, 128, 28, 28] 0\n Conv2dAuto-52 [-1, 512, 28, 28] 65,536\n BatchNorm2d-53 [-1, 512, 28, 28] 1,024\nResNetBottleNeckBlock-54 [-1, 512, 28, 28] 0\n Conv2dAuto-55 [-1, 128, 28, 28] 65,536\n BatchNorm2d-56 [-1, 128, 28, 28] 256\n ReLU-57 [-1, 128, 28, 28] 0\n Conv2dAuto-58 [-1, 128, 28, 28] 147,456\n BatchNorm2d-59 [-1, 128, 28, 28] 256\n ReLU-60 [-1, 128, 28, 28] 0\n Conv2dAuto-61 [-1, 512, 28, 28] 65,536\n BatchNorm2d-62 [-1, 512, 28, 28] 1,024\nResNetBottleNeckBlock-63 [-1, 512, 28, 28] 0\n Conv2dAuto-64 [-1, 128, 28, 28] 65,536\n BatchNorm2d-65 [-1, 128, 28, 28] 256\n ReLU-66 [-1, 128, 28, 28] 0\n Conv2dAuto-67 [-1, 128, 28, 28] 147,456\n BatchNorm2d-68 [-1, 128, 28, 28] 256\n ReLU-69 [-1, 128, 28, 28] 0\n Conv2dAuto-70 [-1, 512, 28, 28] 65,536\n BatchNorm2d-71 [-1, 512, 28, 28] 1,024\nResNetBottleNeckBlock-72 [-1, 512, 28, 28] 0\n ResNetLayer-73 [-1, 512, 28, 28] 0\n Conv2d-74 [-1, 1024, 14, 14] 524,288\n BatchNorm2d-75 [-1, 1024, 14, 14] 2,048\n Conv2dAuto-76 [-1, 256, 28, 28] 131,072\n BatchNorm2d-77 [-1, 256, 28, 28] 512\n ReLU-78 [-1, 256, 28, 28] 0\n Conv2dAuto-79 [-1, 256, 14, 14] 589,824\n BatchNorm2d-80 [-1, 256, 14, 14] 512\n ReLU-81 [-1, 256, 14, 14] 0\n Conv2dAuto-82 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-83 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-84 [-1, 1024, 14, 14] 0\n Conv2dAuto-85 [-1, 256, 14, 14] 262,144\n BatchNorm2d-86 [-1, 256, 14, 14] 512\n ReLU-87 [-1, 256, 14, 14] 0\n Conv2dAuto-88 [-1, 256, 14, 14] 589,824\n BatchNorm2d-89 [-1, 256, 14, 14] 512\n ReLU-90 [-1, 256, 14, 14] 0\n Conv2dAuto-91 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-92 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-93 [-1, 1024, 14, 14] 0\n Conv2dAuto-94 [-1, 256, 14, 14] 262,144\n BatchNorm2d-95 [-1, 256, 14, 14] 512\n ReLU-96 [-1, 256, 14, 14] 0\n Conv2dAuto-97 [-1, 256, 14, 14] 589,824\n BatchNorm2d-98 [-1, 256, 14, 14] 512\n ReLU-99 [-1, 256, 14, 14] 0\n Conv2dAuto-100 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-101 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-102 [-1, 1024, 14, 14] 0\n Conv2dAuto-103 [-1, 256, 14, 14] 262,144\n BatchNorm2d-104 [-1, 256, 14, 14] 512\n ReLU-105 [-1, 256, 14, 14] 0\n Conv2dAuto-106 [-1, 256, 14, 14] 589,824\n BatchNorm2d-107 [-1, 256, 14, 14] 512\n ReLU-108 [-1, 256, 14, 14] 0\n Conv2dAuto-109 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-110 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-111 [-1, 1024, 14, 14] 0\n Conv2dAuto-112 [-1, 256, 14, 14] 262,144\n BatchNorm2d-113 [-1, 256, 14, 14] 512\n ReLU-114 [-1, 256, 14, 14] 0\n Conv2dAuto-115 [-1, 256, 14, 14] 589,824\n BatchNorm2d-116 [-1, 256, 14, 14] 512\n ReLU-117 [-1, 256, 14, 14] 0\n Conv2dAuto-118 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-119 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-120 [-1, 1024, 14, 14] 0\n Conv2dAuto-121 [-1, 256, 14, 14] 262,144\n BatchNorm2d-122 [-1, 256, 14, 14] 512\n ReLU-123 [-1, 256, 14, 14] 0\n Conv2dAuto-124 [-1, 256, 14, 14] 589,824\n BatchNorm2d-125 [-1, 256, 14, 14] 512\n ReLU-126 [-1, 256, 14, 14] 0\n Conv2dAuto-127 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-128 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-129 [-1, 1024, 14, 14] 0\n Conv2dAuto-130 [-1, 256, 14, 14] 262,144\n BatchNorm2d-131 [-1, 256, 14, 14] 512\n ReLU-132 [-1, 256, 14, 14] 0\n Conv2dAuto-133 [-1, 256, 14, 14] 589,824\n BatchNorm2d-134 [-1, 256, 14, 14] 512\n ReLU-135 [-1, 256, 14, 14] 0\n Conv2dAuto-136 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-137 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-138 [-1, 1024, 14, 14] 0\n Conv2dAuto-139 [-1, 256, 14, 14] 262,144\n BatchNorm2d-140 [-1, 256, 14, 14] 512\n ReLU-141 [-1, 256, 14, 14] 0\n Conv2dAuto-142 [-1, 256, 14, 14] 589,824\n BatchNorm2d-143 [-1, 256, 14, 14] 512\n ReLU-144 [-1, 256, 14, 14] 0\n Conv2dAuto-145 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-146 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-147 [-1, 1024, 14, 14] 0\n Conv2dAuto-148 [-1, 256, 14, 14] 262,144\n BatchNorm2d-149 [-1, 256, 14, 14] 512\n ReLU-150 [-1, 256, 14, 14] 0\n Conv2dAuto-151 [-1, 256, 14, 14] 589,824\n BatchNorm2d-152 [-1, 256, 14, 14] 512\n ReLU-153 [-1, 256, 14, 14] 0\n Conv2dAuto-154 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-155 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-156 [-1, 1024, 14, 14] 0\n Conv2dAuto-157 [-1, 256, 14, 14] 262,144\n BatchNorm2d-158 [-1, 256, 14, 14] 512\n ReLU-159 [-1, 256, 14, 14] 0\n Conv2dAuto-160 [-1, 256, 14, 14] 589,824\n BatchNorm2d-161 [-1, 256, 14, 14] 512\n ReLU-162 [-1, 256, 14, 14] 0\n Conv2dAuto-163 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-164 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-165 [-1, 1024, 14, 14] 0\n Conv2dAuto-166 [-1, 256, 14, 14] 262,144\n BatchNorm2d-167 [-1, 256, 14, 14] 512\n ReLU-168 [-1, 256, 14, 14] 0\n Conv2dAuto-169 [-1, 256, 14, 14] 589,824\n BatchNorm2d-170 [-1, 256, 14, 14] 512\n ReLU-171 [-1, 256, 14, 14] 0\n Conv2dAuto-172 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-173 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-174 [-1, 1024, 14, 14] 0\n Conv2dAuto-175 [-1, 256, 14, 14] 262,144\n BatchNorm2d-176 [-1, 256, 14, 14] 512\n ReLU-177 [-1, 256, 14, 14] 0\n Conv2dAuto-178 [-1, 256, 14, 14] 589,824\n BatchNorm2d-179 [-1, 256, 14, 14] 512\n ReLU-180 [-1, 256, 14, 14] 0\n Conv2dAuto-181 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-182 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-183 [-1, 1024, 14, 14] 0\n Conv2dAuto-184 [-1, 256, 14, 14] 262,144\n BatchNorm2d-185 [-1, 256, 14, 14] 512\n ReLU-186 [-1, 256, 14, 14] 0\n Conv2dAuto-187 [-1, 256, 14, 14] 589,824\n BatchNorm2d-188 [-1, 256, 14, 14] 512\n ReLU-189 [-1, 256, 14, 14] 0\n Conv2dAuto-190 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-191 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-192 [-1, 1024, 14, 14] 0\n Conv2dAuto-193 [-1, 256, 14, 14] 262,144\n BatchNorm2d-194 [-1, 256, 14, 14] 512\n ReLU-195 [-1, 256, 14, 14] 0\n Conv2dAuto-196 [-1, 256, 14, 14] 589,824\n BatchNorm2d-197 [-1, 256, 14, 14] 512\n ReLU-198 [-1, 256, 14, 14] 0\n Conv2dAuto-199 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-200 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-201 [-1, 1024, 14, 14] 0\n Conv2dAuto-202 [-1, 256, 14, 14] 262,144\n BatchNorm2d-203 [-1, 256, 14, 14] 512\n ReLU-204 [-1, 256, 14, 14] 0\n Conv2dAuto-205 [-1, 256, 14, 14] 589,824\n BatchNorm2d-206 [-1, 256, 14, 14] 512\n ReLU-207 [-1, 256, 14, 14] 0\n Conv2dAuto-208 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-209 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-210 [-1, 1024, 14, 14] 0\n Conv2dAuto-211 [-1, 256, 14, 14] 262,144\n BatchNorm2d-212 [-1, 256, 14, 14] 512\n ReLU-213 [-1, 256, 14, 14] 0\n Conv2dAuto-214 [-1, 256, 14, 14] 589,824\n BatchNorm2d-215 [-1, 256, 14, 14] 512\n ReLU-216 [-1, 256, 14, 14] 0\n Conv2dAuto-217 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-218 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-219 [-1, 1024, 14, 14] 0\n Conv2dAuto-220 [-1, 256, 14, 14] 262,144\n BatchNorm2d-221 [-1, 256, 14, 14] 512\n ReLU-222 [-1, 256, 14, 14] 0\n Conv2dAuto-223 [-1, 256, 14, 14] 589,824\n BatchNorm2d-224 [-1, 256, 14, 14] 512\n ReLU-225 [-1, 256, 14, 14] 0\n Conv2dAuto-226 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-227 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-228 [-1, 1024, 14, 14] 0\n Conv2dAuto-229 [-1, 256, 14, 14] 262,144\n BatchNorm2d-230 [-1, 256, 14, 14] 512\n ReLU-231 [-1, 256, 14, 14] 0\n Conv2dAuto-232 [-1, 256, 14, 14] 589,824\n BatchNorm2d-233 [-1, 256, 14, 14] 512\n ReLU-234 [-1, 256, 14, 14] 0\n Conv2dAuto-235 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-236 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-237 [-1, 1024, 14, 14] 0\n Conv2dAuto-238 [-1, 256, 14, 14] 262,144\n BatchNorm2d-239 [-1, 256, 14, 14] 512\n ReLU-240 [-1, 256, 14, 14] 0\n Conv2dAuto-241 [-1, 256, 14, 14] 589,824\n BatchNorm2d-242 [-1, 256, 14, 14] 512\n ReLU-243 [-1, 256, 14, 14] 0\n Conv2dAuto-244 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-245 [-1, 1024, 14, 14] 2,048\nResNetBottleNeckBlock-246 [-1, 1024, 14, 14] 0\n Conv2dAuto-247 [-1, 256, 14, 14] 262,144\n BatchNorm2d-248 [-1, 256, 14, 14] 512\n ReLU-249 [-1, 256, 14, 14] 0\n Conv2dAuto-250 [-1, 256, 14, 14] 589,824\n"
],
[
"import torchvision.models as models\n\n# resnet101(False)\n\nsummary(models.resnet101(False).cuda(), (3, 224, 224))",
"----------------------------------------------------------------\n Layer (type) Output Shape Param #\n================================================================\n Conv2d-1 [-1, 64, 112, 112] 9,408\n BatchNorm2d-2 [-1, 64, 112, 112] 128\n ReLU-3 [-1, 64, 112, 112] 0\n MaxPool2d-4 [-1, 64, 56, 56] 0\n Conv2d-5 [-1, 64, 56, 56] 4,096\n BatchNorm2d-6 [-1, 64, 56, 56] 128\n ReLU-7 [-1, 64, 56, 56] 0\n Conv2d-8 [-1, 64, 56, 56] 36,864\n BatchNorm2d-9 [-1, 64, 56, 56] 128\n ReLU-10 [-1, 64, 56, 56] 0\n Conv2d-11 [-1, 256, 56, 56] 16,384\n BatchNorm2d-12 [-1, 256, 56, 56] 512\n Conv2d-13 [-1, 256, 56, 56] 16,384\n BatchNorm2d-14 [-1, 256, 56, 56] 512\n ReLU-15 [-1, 256, 56, 56] 0\n Bottleneck-16 [-1, 256, 56, 56] 0\n Conv2d-17 [-1, 64, 56, 56] 16,384\n BatchNorm2d-18 [-1, 64, 56, 56] 128\n ReLU-19 [-1, 64, 56, 56] 0\n Conv2d-20 [-1, 64, 56, 56] 36,864\n BatchNorm2d-21 [-1, 64, 56, 56] 128\n ReLU-22 [-1, 64, 56, 56] 0\n Conv2d-23 [-1, 256, 56, 56] 16,384\n BatchNorm2d-24 [-1, 256, 56, 56] 512\n ReLU-25 [-1, 256, 56, 56] 0\n Bottleneck-26 [-1, 256, 56, 56] 0\n Conv2d-27 [-1, 64, 56, 56] 16,384\n BatchNorm2d-28 [-1, 64, 56, 56] 128\n ReLU-29 [-1, 64, 56, 56] 0\n Conv2d-30 [-1, 64, 56, 56] 36,864\n BatchNorm2d-31 [-1, 64, 56, 56] 128\n ReLU-32 [-1, 64, 56, 56] 0\n Conv2d-33 [-1, 256, 56, 56] 16,384\n BatchNorm2d-34 [-1, 256, 56, 56] 512\n ReLU-35 [-1, 256, 56, 56] 0\n Bottleneck-36 [-1, 256, 56, 56] 0\n Conv2d-37 [-1, 128, 56, 56] 32,768\n BatchNorm2d-38 [-1, 128, 56, 56] 256\n ReLU-39 [-1, 128, 56, 56] 0\n Conv2d-40 [-1, 128, 28, 28] 147,456\n BatchNorm2d-41 [-1, 128, 28, 28] 256\n ReLU-42 [-1, 128, 28, 28] 0\n Conv2d-43 [-1, 512, 28, 28] 65,536\n BatchNorm2d-44 [-1, 512, 28, 28] 1,024\n Conv2d-45 [-1, 512, 28, 28] 131,072\n BatchNorm2d-46 [-1, 512, 28, 28] 1,024\n ReLU-47 [-1, 512, 28, 28] 0\n Bottleneck-48 [-1, 512, 28, 28] 0\n Conv2d-49 [-1, 128, 28, 28] 65,536\n BatchNorm2d-50 [-1, 128, 28, 28] 256\n ReLU-51 [-1, 128, 28, 28] 0\n Conv2d-52 [-1, 128, 28, 28] 147,456\n BatchNorm2d-53 [-1, 128, 28, 28] 256\n ReLU-54 [-1, 128, 28, 28] 0\n Conv2d-55 [-1, 512, 28, 28] 65,536\n BatchNorm2d-56 [-1, 512, 28, 28] 1,024\n ReLU-57 [-1, 512, 28, 28] 0\n Bottleneck-58 [-1, 512, 28, 28] 0\n Conv2d-59 [-1, 128, 28, 28] 65,536\n BatchNorm2d-60 [-1, 128, 28, 28] 256\n ReLU-61 [-1, 128, 28, 28] 0\n Conv2d-62 [-1, 128, 28, 28] 147,456\n BatchNorm2d-63 [-1, 128, 28, 28] 256\n ReLU-64 [-1, 128, 28, 28] 0\n Conv2d-65 [-1, 512, 28, 28] 65,536\n BatchNorm2d-66 [-1, 512, 28, 28] 1,024\n ReLU-67 [-1, 512, 28, 28] 0\n Bottleneck-68 [-1, 512, 28, 28] 0\n Conv2d-69 [-1, 128, 28, 28] 65,536\n BatchNorm2d-70 [-1, 128, 28, 28] 256\n ReLU-71 [-1, 128, 28, 28] 0\n Conv2d-72 [-1, 128, 28, 28] 147,456\n BatchNorm2d-73 [-1, 128, 28, 28] 256\n ReLU-74 [-1, 128, 28, 28] 0\n Conv2d-75 [-1, 512, 28, 28] 65,536\n BatchNorm2d-76 [-1, 512, 28, 28] 1,024\n ReLU-77 [-1, 512, 28, 28] 0\n Bottleneck-78 [-1, 512, 28, 28] 0\n Conv2d-79 [-1, 256, 28, 28] 131,072\n BatchNorm2d-80 [-1, 256, 28, 28] 512\n ReLU-81 [-1, 256, 28, 28] 0\n Conv2d-82 [-1, 256, 14, 14] 589,824\n BatchNorm2d-83 [-1, 256, 14, 14] 512\n ReLU-84 [-1, 256, 14, 14] 0\n Conv2d-85 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-86 [-1, 1024, 14, 14] 2,048\n Conv2d-87 [-1, 1024, 14, 14] 524,288\n BatchNorm2d-88 [-1, 1024, 14, 14] 2,048\n ReLU-89 [-1, 1024, 14, 14] 0\n Bottleneck-90 [-1, 1024, 14, 14] 0\n Conv2d-91 [-1, 256, 14, 14] 262,144\n BatchNorm2d-92 [-1, 256, 14, 14] 512\n ReLU-93 [-1, 256, 14, 14] 0\n Conv2d-94 [-1, 256, 14, 14] 589,824\n BatchNorm2d-95 [-1, 256, 14, 14] 512\n ReLU-96 [-1, 256, 14, 14] 0\n Conv2d-97 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-98 [-1, 1024, 14, 14] 2,048\n ReLU-99 [-1, 1024, 14, 14] 0\n Bottleneck-100 [-1, 1024, 14, 14] 0\n Conv2d-101 [-1, 256, 14, 14] 262,144\n BatchNorm2d-102 [-1, 256, 14, 14] 512\n ReLU-103 [-1, 256, 14, 14] 0\n Conv2d-104 [-1, 256, 14, 14] 589,824\n BatchNorm2d-105 [-1, 256, 14, 14] 512\n ReLU-106 [-1, 256, 14, 14] 0\n Conv2d-107 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-108 [-1, 1024, 14, 14] 2,048\n ReLU-109 [-1, 1024, 14, 14] 0\n Bottleneck-110 [-1, 1024, 14, 14] 0\n Conv2d-111 [-1, 256, 14, 14] 262,144\n BatchNorm2d-112 [-1, 256, 14, 14] 512\n ReLU-113 [-1, 256, 14, 14] 0\n Conv2d-114 [-1, 256, 14, 14] 589,824\n BatchNorm2d-115 [-1, 256, 14, 14] 512\n ReLU-116 [-1, 256, 14, 14] 0\n Conv2d-117 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-118 [-1, 1024, 14, 14] 2,048\n ReLU-119 [-1, 1024, 14, 14] 0\n Bottleneck-120 [-1, 1024, 14, 14] 0\n Conv2d-121 [-1, 256, 14, 14] 262,144\n BatchNorm2d-122 [-1, 256, 14, 14] 512\n ReLU-123 [-1, 256, 14, 14] 0\n Conv2d-124 [-1, 256, 14, 14] 589,824\n BatchNorm2d-125 [-1, 256, 14, 14] 512\n ReLU-126 [-1, 256, 14, 14] 0\n Conv2d-127 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-128 [-1, 1024, 14, 14] 2,048\n ReLU-129 [-1, 1024, 14, 14] 0\n Bottleneck-130 [-1, 1024, 14, 14] 0\n Conv2d-131 [-1, 256, 14, 14] 262,144\n BatchNorm2d-132 [-1, 256, 14, 14] 512\n ReLU-133 [-1, 256, 14, 14] 0\n Conv2d-134 [-1, 256, 14, 14] 589,824\n BatchNorm2d-135 [-1, 256, 14, 14] 512\n ReLU-136 [-1, 256, 14, 14] 0\n Conv2d-137 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-138 [-1, 1024, 14, 14] 2,048\n ReLU-139 [-1, 1024, 14, 14] 0\n Bottleneck-140 [-1, 1024, 14, 14] 0\n Conv2d-141 [-1, 256, 14, 14] 262,144\n BatchNorm2d-142 [-1, 256, 14, 14] 512\n ReLU-143 [-1, 256, 14, 14] 0\n Conv2d-144 [-1, 256, 14, 14] 589,824\n BatchNorm2d-145 [-1, 256, 14, 14] 512\n ReLU-146 [-1, 256, 14, 14] 0\n Conv2d-147 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-148 [-1, 1024, 14, 14] 2,048\n ReLU-149 [-1, 1024, 14, 14] 0\n Bottleneck-150 [-1, 1024, 14, 14] 0\n Conv2d-151 [-1, 256, 14, 14] 262,144\n BatchNorm2d-152 [-1, 256, 14, 14] 512\n ReLU-153 [-1, 256, 14, 14] 0\n Conv2d-154 [-1, 256, 14, 14] 589,824\n BatchNorm2d-155 [-1, 256, 14, 14] 512\n ReLU-156 [-1, 256, 14, 14] 0\n Conv2d-157 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-158 [-1, 1024, 14, 14] 2,048\n ReLU-159 [-1, 1024, 14, 14] 0\n Bottleneck-160 [-1, 1024, 14, 14] 0\n Conv2d-161 [-1, 256, 14, 14] 262,144\n BatchNorm2d-162 [-1, 256, 14, 14] 512\n ReLU-163 [-1, 256, 14, 14] 0\n Conv2d-164 [-1, 256, 14, 14] 589,824\n BatchNorm2d-165 [-1, 256, 14, 14] 512\n ReLU-166 [-1, 256, 14, 14] 0\n Conv2d-167 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-168 [-1, 1024, 14, 14] 2,048\n ReLU-169 [-1, 1024, 14, 14] 0\n Bottleneck-170 [-1, 1024, 14, 14] 0\n Conv2d-171 [-1, 256, 14, 14] 262,144\n BatchNorm2d-172 [-1, 256, 14, 14] 512\n ReLU-173 [-1, 256, 14, 14] 0\n Conv2d-174 [-1, 256, 14, 14] 589,824\n BatchNorm2d-175 [-1, 256, 14, 14] 512\n ReLU-176 [-1, 256, 14, 14] 0\n Conv2d-177 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-178 [-1, 1024, 14, 14] 2,048\n ReLU-179 [-1, 1024, 14, 14] 0\n Bottleneck-180 [-1, 1024, 14, 14] 0\n Conv2d-181 [-1, 256, 14, 14] 262,144\n BatchNorm2d-182 [-1, 256, 14, 14] 512\n ReLU-183 [-1, 256, 14, 14] 0\n Conv2d-184 [-1, 256, 14, 14] 589,824\n BatchNorm2d-185 [-1, 256, 14, 14] 512\n ReLU-186 [-1, 256, 14, 14] 0\n Conv2d-187 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-188 [-1, 1024, 14, 14] 2,048\n ReLU-189 [-1, 1024, 14, 14] 0\n Bottleneck-190 [-1, 1024, 14, 14] 0\n Conv2d-191 [-1, 256, 14, 14] 262,144\n BatchNorm2d-192 [-1, 256, 14, 14] 512\n ReLU-193 [-1, 256, 14, 14] 0\n Conv2d-194 [-1, 256, 14, 14] 589,824\n BatchNorm2d-195 [-1, 256, 14, 14] 512\n ReLU-196 [-1, 256, 14, 14] 0\n Conv2d-197 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-198 [-1, 1024, 14, 14] 2,048\n ReLU-199 [-1, 1024, 14, 14] 0\n Bottleneck-200 [-1, 1024, 14, 14] 0\n Conv2d-201 [-1, 256, 14, 14] 262,144\n BatchNorm2d-202 [-1, 256, 14, 14] 512\n ReLU-203 [-1, 256, 14, 14] 0\n Conv2d-204 [-1, 256, 14, 14] 589,824\n BatchNorm2d-205 [-1, 256, 14, 14] 512\n ReLU-206 [-1, 256, 14, 14] 0\n Conv2d-207 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-208 [-1, 1024, 14, 14] 2,048\n ReLU-209 [-1, 1024, 14, 14] 0\n Bottleneck-210 [-1, 1024, 14, 14] 0\n Conv2d-211 [-1, 256, 14, 14] 262,144\n BatchNorm2d-212 [-1, 256, 14, 14] 512\n ReLU-213 [-1, 256, 14, 14] 0\n Conv2d-214 [-1, 256, 14, 14] 589,824\n BatchNorm2d-215 [-1, 256, 14, 14] 512\n ReLU-216 [-1, 256, 14, 14] 0\n Conv2d-217 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-218 [-1, 1024, 14, 14] 2,048\n ReLU-219 [-1, 1024, 14, 14] 0\n Bottleneck-220 [-1, 1024, 14, 14] 0\n Conv2d-221 [-1, 256, 14, 14] 262,144\n BatchNorm2d-222 [-1, 256, 14, 14] 512\n ReLU-223 [-1, 256, 14, 14] 0\n Conv2d-224 [-1, 256, 14, 14] 589,824\n BatchNorm2d-225 [-1, 256, 14, 14] 512\n ReLU-226 [-1, 256, 14, 14] 0\n Conv2d-227 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-228 [-1, 1024, 14, 14] 2,048\n ReLU-229 [-1, 1024, 14, 14] 0\n Bottleneck-230 [-1, 1024, 14, 14] 0\n Conv2d-231 [-1, 256, 14, 14] 262,144\n BatchNorm2d-232 [-1, 256, 14, 14] 512\n ReLU-233 [-1, 256, 14, 14] 0\n Conv2d-234 [-1, 256, 14, 14] 589,824\n BatchNorm2d-235 [-1, 256, 14, 14] 512\n ReLU-236 [-1, 256, 14, 14] 0\n Conv2d-237 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-238 [-1, 1024, 14, 14] 2,048\n ReLU-239 [-1, 1024, 14, 14] 0\n Bottleneck-240 [-1, 1024, 14, 14] 0\n Conv2d-241 [-1, 256, 14, 14] 262,144\n BatchNorm2d-242 [-1, 256, 14, 14] 512\n ReLU-243 [-1, 256, 14, 14] 0\n Conv2d-244 [-1, 256, 14, 14] 589,824\n BatchNorm2d-245 [-1, 256, 14, 14] 512\n ReLU-246 [-1, 256, 14, 14] 0\n Conv2d-247 [-1, 1024, 14, 14] 262,144\n BatchNorm2d-248 [-1, 1024, 14, 14] 2,048\n ReLU-249 [-1, 1024, 14, 14] 0\n Bottleneck-250 [-1, 1024, 14, 14] 0\n Conv2d-251 [-1, 256, 14, 14] 262,144\n BatchNorm2d-252 [-1, 256, 14, 14] 512\n"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
]
] |
e7bbcb7d9820657c4bad57f2982a3a548ff09391 | 62,616 | ipynb | Jupyter Notebook | 3-eq-ILP-lab-mini-suf-submatrices.ipynb | anpc/perfect-reductions | 7b0de1185d6b3ebf4c16650daea7c13d398fc3bb | [
"MIT"
] | null | null | null | 3-eq-ILP-lab-mini-suf-submatrices.ipynb | anpc/perfect-reductions | 7b0de1185d6b3ebf4c16650daea7c13d398fc3bb | [
"MIT"
] | null | null | null | 3-eq-ILP-lab-mini-suf-submatrices.ipynb | anpc/perfect-reductions | 7b0de1185d6b3ebf4c16650daea7c13d398fc3bb | [
"MIT"
] | null | null | null | 79.361217 | 140 | 0.45236 | [
[
[
"# Our modeling approach\n\n- We fully characterize the existence of a 1-round protocol by a Mixed Integer Linear Program.\n",
"_____no_output_____"
]
],
[
[
"from sage.all import *\nimport itertools\nimport random\nfrom sage.sat.solvers.satsolver import SAT\nfrom sage.sat.solvers.cryptominisat import CryptoMiniSat\nfrom sage.misc.temporary_file import atomic_write\nimport copy\nimport time",
"_____no_output_____"
],
[
"solver = SAT(solver=\"LP\")\nsolver.add_clause((-1,2))\nsolver.add_clause((1,3))\nsolution = solver()\nprint ' solution =',solution\nlst = ['play', 'ground']\nprint 'ground' in lst\n\nm = matrix(QQ, [[1,2],[4,2],[11,3]])\nprint 'm = ',m\nx = []\nfor v in m:\n x.append(v)\n \nx = matrix(QQ, x)\nprint 'x = ',x\n \npr = Permutations(20).random_element()\nprint pr, pr[4], pr[7]\n\n\nv = vector(QQ, [2,3,4])\nprint 'len = ',len(v)\n\nmin(5, 44)\n\nl = [1,2,3,4,5]\nprint l [2:4]",
" solution = [None, False, False, True]\nTrue\nm = [ 1 2]\n[ 4 2]\n[11 3]\nx = [ 1 2]\n[ 4 2]\n[11 3]\n[6, 7, 12, 16, 10, 9, 20, 11, 1, 15, 13, 8, 17, 19, 2, 18, 14, 3, 4, 5] 10 11\nlen = 3\n[3, 4]\n"
],
[
"# TODO: check correctness etc\ndef get_at(tup, a, l):\n \"\"\"Given 2*l elements flattened into a tuple, pick 1 out of each pair according to array of bits.\"\"\"\n assert len(tup) == 2*l\n assert len(a) == l\n for bit in a:\n assert bit in (0, 1)\n\n # tup structure is: $(t_{0,0}, t_{0,1}, t_{1,0}, t_{1,1}, t_{2,0}, t_{2,1})$\n return tuple(tup[2*i + a[i]] for i in range(l))\n\nassert get_at((3, 13, 85, 95), (0, 1), 2) == (3, 95)",
"_____no_output_____"
],
[
" \ndef tuples_fixed_proj(l, proj_ind, proj_val):\n# h = randint(1,4000)\n# if (h == 5):\n# print '==================== DEBUGGING ========== a,g = ', proj_ind, proj_val\n tuples = list()\n x = [0 for i in range(2*l)]\n for i in range(l):\n x[i*2 + proj_ind[i]] = proj_val[i]\n for g in itertools.product(range(2), repeat = l):\n for i in range(l):\n x[i*2 + 1 - proj_ind[i]] = g[i]\n# if (h == 5):\n# print 'modified x to ', x\n tuples.append(tuple(x[:]))\n return tuples \n\n# Hack from correctness and server security (!) \n# strength = 0 is consistent with standard LP. strength = 1 attempts to better sort things out \n\ndef add_no_id_a(solver, p0, q1, strength = 0):\n \n if strength == -1:\n return\n \n for i in range(3): \n for a in itertools.product(range(2), repeat = l):\n for j in range(3):\n if (j > i):\n # this is relaxed\n if strength == 0:\n solver.add_constraint(sum(q1[(i, a, zeros, b)] for b in range(2)) + sum(q1[(j, a, zeros, b)] \n for b in range(2)) <= 1) \n elif strength == 1:\n for b1 in range(2):\n for b2 in range(2):\n solver.add_constraint(p0[(i, a, zeros, b1)] + p0[(i, a, zeros, b2)] <= 1)\n \n# TODO: change the name to something more informative.\n# Hack #2 from correctness: For every i,a,b with p^i_a > 0, there exists g so that (i,a,g,b) > 0.\n# This requires some trial and error. Otherwise, one xi at least remains without TO-input options\n# This will eventually not be part of the client constraints. Currently used to `direct' the soutions.\n \n\n# Another hack from correctness. For all b, there exists at least one value of t, so \n# that every a reading this g will output b. Thus, the resulting sum of probabilities for\n# that g is 1. There may be additional g's (the g results from some V assigned to server for each output\n# value). \n\n\ndef get_rand_tup(len):\n return tuple([sage.misc.prandom.choice(range(2)) for i in range(len)])\n \n\n# i = -1 , search for an index\n# i = -2 : auxiliary for relaxed mode\n# [1,0,0]\n# [0,1,0]\n# [0,0,1]\n# [0,0,0]\n\ndef safe_insert(val_l, ind_l, (i,ind), v):\n to_search = []\n if i >= 0:\n to_search = [i]\n elif i == -1:\n to_search = [j for j in range(3)]\n elif i == -2:\n val_l[ind_l.index(ind)] = v\n return\n \n for j in to_search:\n if (j,ind) in ind_l:\n val_l[ind_l.index((j,ind))] = v\n\n\ndef find_set_server_vars(l, p0):\n try:\n print 'How much is server variable space limited by this solution & correctness?'\n print 'List = ',p0\n p1 = []\n for i in range(3): \n for t in itertools.product(range(2), repeat = 2*l):\n p1.append((i,t))\n\n for (y,(a,g,b)) in p0:\n for j in range(3):\n if ((b == 0) and (i == j)) or ((b == 1) and (i != j)):\n all = tuples_fixed_proj(l, a, g)\n for t in all:\n try:\n p1.remove((j, t))\n except Exception as e:\n pass\n\n print 'number of remaining server values =',len(p1)\n if (len(p1) > 0):\n print 'server variables are ',p1\n except: 'Uncaught exception!' \n\n\ndef permute_rows(m, b):\n \n nrows = m.nrows()\n perm = Permutations(nrows).random_element()\n pm = [row for row in m]\n pb = [v for v in b]\n \n new_m = matrix(QQ, [pm[perm[i] - 1] for i in range(nrows)])\n \n new_b = vector(QQ, [pb[perm[i] - 1] for i in range(nrows)])\n \n return (new_m, new_b)\n \n \n \ndef get_full_rank_sub(m, b):\n \n nrows = m.nrows()\n new_mat = []\n new_b = []\n \n # print 'into get_full_rank_sub',m.nrows(), m.ncols()\n \n for (i,row) in enumerate(m):\n so_far = (matrix(QQ, new_mat)).transpose()\n try:\n so_far\\row\n except Exception as e:\n# print 'at full rank sub Exception = ',e,i\n new_mat.append(row)\n new_b.append(b[i])\n \n new_mat = matrix(QQ, new_mat)\n new_b = vector(new_b)\n \n return (new_mat, new_b)\n\n\ndef print_matrix(m):\n\n if m.ncols < 37:\n print m\n return\n \n per_row = 30\n for row in m:\n n = ceil(QQ(m.nrows())/per_row)\n for i in range(n):\n ran = min(per_row, m.nrows() - i * per_row)\n print [row[i * per_row + j] for j in range(ran)]\n print '\\n' \n \n\ndef non_zeros(m): \n return sum(sum(1 for j in row if abs(j) != 0) for row in m) \n \n \ndef get_inv_sub_matrix(m, b):\n \n (new_mat, new_b) = get_full_rank_sub(m, b)\n \n ncols = new_mat.ncols()\n print 'new_mat rank / ',new_mat.rank(),'(',new_mat.nrows(),',',new_mat.ncols(),')'\n \n new_mat_tr = new_mat.transpose()\n b_dum = vector(QQ, [0 for i in range(ncols)]) \n \n \n (full_sub, b_dum) = permute_rows(new_mat_tr, b_dum)\n (full_sub, b_dum) = get_full_rank_sub(full_sub, b_dum)\n \n inv_sub = full_sub.inverse()\n \n print 'x = A^{-1}*b',\n # print full_sub\n \n det_A = full_sub.determinant()\n print '|A| = ', det_A\n # Finding independent columns\n print 'Sparsity parameters',non_zeros(full_sub),' => ',non_zeros(inv_sub)\n \n x = inv_sub * new_b\n print 'x = ',x\n print 'len(x) = ',len(x)\n \n if (full_sub.nrows() < 50 and abs(det_A) > 1) or (full_sub.ncols() < 40):\n print print_matrix(inv_sub)\n \n return x\n\ndef test_linear_solutions_sub(l, t = 3, p = 5):\n \n b = []\n constraints = []\n print 'generating constraints ...'\n \n freq = [0,0,0]\n ag_set = []\n a_set = []\n \n \n for a in itertools.product(range(2), repeat = l):\n i = sage.misc.prandom.choice(range(p))\n if (i <= t):\n a_set.append(a)\n for g in itertools.product(range(2), repeat = l):\n ag_set.append((a,g))\n \n ag_set_len = len(ag_set)\n \n print 'a_set = ',a_set\n \n for t in itertools.product(range(2), repeat = 2*l):\n cur_row = [0 for i in range(ag_set_len)]\n b.append(1)\n for a in a_set:\n cur_row[ag_set.index((a, get_at(t,a,l)))] = 1\n constraints.append(cur_row)\n \n distr = [[0 for j in range(ag_set_len)] for i in range(3)] \n present = [0,0,0]\n \n for a in a_set:\n y = sage.misc.prandom.choice(range(3))\n present[y] = 1\n for g in itertools.product(range(2), repeat = l):\n distr[y][ag_set.index((a,zeros))] = 1\n \n \n if sum(present[y] for y in range(3)) < 3:\n print 'Unluckly choice. Aborting execution...'\n \n for y in range(3):\n constraints.append(distr[y])\n b.append(1)\n \n m_constr = matrix(QQ,constraints)\n print_matrix(m_constr)\n right_side = vector(QQ, b)\n \n try:\n v = m_constr\\right_side \n print 'solution = ',v\n print 'len(solution) = ',len(v)\n nz = sum(1 for x in v if abs(x) > 0)\n print 'non-zeros ', nz\n except Exception as e:\n print 'Caught exception ',e\n \n \n\nmy_eps = QQ(1/10000)",
"_____no_output_____"
],
[
"import time \n \n\nfor l in range(3, 4):\n for step in range(5):\n t0 = time.time()\n print 'finding a solution for l=',l\n zeros = tuple((0 for i in range(l)))\n\n try:\n q = test_linear_solutions_sub(l, 4, 5)\n\n finally:\n print 'time =', time.time() - t0,'\\n'\n\n",
"finding a solution for l= 3\ngenerating constraints ...\na_set = [(0, 0, 0), (0, 0, 1), (0, 1, 0), (0, 1, 1), (1, 0, 0), (1, 0, 1), (1, 1, 0), (1, 1, 1)]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1]\n[0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]\nCaught exception matrix equation has no solutions\ntime = 0.0235588550568 \n\nfinding a solution for l= 3\ngenerating constraints ...\na_set = [(0, 0, 0), (0, 0, 1), (0, 1, 0), (0, 1, 1), (1, 0, 0), (1, 0, 1), (1, 1, 0), (1, 1, 1)]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]\n[1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1]\nCaught exception matrix equation has no solutions\ntime = 0.0284080505371 \n\nfinding a solution for l= 3\ngenerating constraints ...\na_set = [(0, 0, 0), (0, 0, 1), (0, 1, 0), (0, 1, 1), (1, 0, 0), (1, 0, 1), (1, 1, 0), (1, 1, 1)]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1]\n[0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]\nCaught exception matrix equation has no solutions\ntime = 0.0441679954529 \n\nfinding a solution for l= 3\ngenerating constraints ...\na_set = [(0, 0, 0), (0, 0, 1), (0, 1, 0), (0, 1, 1), (1, 0, 0), (1, 0, 1), (1, 1, 0), (1, 1, 1)]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1]\n[0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0]\nCaught exception matrix equation has no solutions\ntime = 0.0235769748688 \n\nfinding a solution for l= 3\ngenerating constraints ...\na_set = [(0, 0, 0), (0, 0, 1), (0, 1, 0), (0, 1, 1), (1, 0, 0), (1, 0, 1), (1, 1, 0), (1, 1, 1)]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 1 0]\n[0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 1]\n[1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0]\n[0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1]\n[0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]\nCaught exception matrix equation has no solutions\ntime = 0.0199320316315 \n\n"
]
]
] | [
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
]
] |
e7bbd78fa5ce8a0011fadbf77813146556443653 | 17,949 | ipynb | Jupyter Notebook | testing/sahel_cropmask/3_Train_fit_evaluate_classifier.ipynb | digitalearthafrica/crop-mask | 18ae773c4d5eb71c0add765260a1032c46c68a0e | [
"Apache-2.0"
] | 11 | 2020-12-15T04:09:41.000Z | 2022-01-19T11:07:21.000Z | testing/sahel_cropmask/3_Train_fit_evaluate_classifier.ipynb | digitalearthafrica/crop-mask | 18ae773c4d5eb71c0add765260a1032c46c68a0e | [
"Apache-2.0"
] | 15 | 2021-03-15T02:17:32.000Z | 2022-02-24T02:50:01.000Z | testing/sahel_cropmask/3_Train_fit_evaluate_classifier.ipynb | digitalearthafrica/crop-mask | 18ae773c4d5eb71c0add765260a1032c46c68a0e | [
"Apache-2.0"
] | 4 | 2020-12-16T04:48:36.000Z | 2021-03-30T16:51:37.000Z | 34.451056 | 581 | 0.603488 | [
[
[
"# Evaluate, optimize, and fit a classifier\n",
"_____no_output_____"
],
[
"## Background\n\nBefore we can begin making crop/non-crop predictions, we first need to evaluate, optimize, and fit a classifier. Because we are working with spatial data, and because the size of our training dataset is relatively small (by machine learning standards), we need to implement some lesser known methods for evaluating and optimizing our model. These include implementing spatially explicit k-fold cross-validation techniques, running nested k-fold cross validation, and fitting a model on our entire dataset.",
"_____no_output_____"
],
[
"## Description\n\nIn this notebook, we will use the training data collected in the first notebook (`1_Extract_training_data.ipynb`) to fit and evaluate a classifier. \n\nThe steps undertaken are:\n1. Spatially cluster our training data to visualize spatial groupings\n2. Calculate an unbiased performance estimate via **nested, k-fold cross-validation**. \n3. Optimize the hyperparameters of the model using `GridSearchCV`\n4. Fit a model on _all_ the data using the parameters identified in step 3\n5. Save the model to disk ",
"_____no_output_____"
],
[
"***\n## Getting started\n\nTo run this analysis, run all the cells in the notebook, starting with the \"Load packages\" cell. ",
"_____no_output_____"
],
[
"## Load packages",
"_____no_output_____"
]
],
[
[
"# from sklearn.svm import SVC\nfrom sklearn.ensemble import RandomForestClassifier\n\nimport os\nimport joblib\nimport numpy as np\nimport pandas as pd\nfrom joblib import dump\nfrom pprint import pprint\nimport matplotlib.pyplot as plt\nfrom odc.io.cgroups import get_cpu_quota\nfrom sklearn.model_selection import GridSearchCV, ShuffleSplit, KFold\nfrom sklearn.metrics import roc_curve, auc, f1_score, balanced_accuracy_score\n",
"_____no_output_____"
]
],
[
[
"## Analysis Parameters\n\n* `training_data`: Name and location of the training data `.txt` file output from runnning `1_Extract_training_data.ipynb`\n* `coordinate_data`: Name and location of the coordinate data `.txt` file output from runnning `1_Extract_training_data.ipynb`\n* `Classifier`: This parameter refers to the scikit-learn classification model to use, first uncomment the classifier of interest in the `Load Packages` section and then enter the function name into this parameter `e.g. Classifier = RandomForestClassifier` \n* `metric` : A single string that denotes the scorer used to optimize the model. See the scoring parameter page [here](https://scikit-learn.org/stable/modules/model_evaluation.html#scoring-parameter) for a pre-defined list of options. For binary classifications, 'F1' or 'balanced_accuracy' are good metrics.\n* `output_suffix`: A suffix to add to the exported model corresponding to the model iteration.\n",
"_____no_output_____"
]
],
[
[
"training_data = \"results/training_data/sahel_training_data_20211110.txt\"\n\nClassifier = RandomForestClassifier\n\nmetric = 'balanced_accuracy' \n\noutput_suffix = '20211110'",
"_____no_output_____"
]
],
[
[
"## K-Fold Cross Validation Parameters\n\n* `inner_cv_splits` : Number of cross-validation splits to do on the inner loop, e.g. `5`\n* `outer_cv_splits` : Number of cross-validation splits to do on the outer loop, e.g. `5`\n* `test_size` : This will determine what fraction of the dataset will be set aside as the testing dataset. There is a trade-off here between having a larger test set that will help us better determine the quality of our classifier, and leaving enough data to train the classifier. A good deafult is to set 10-20 % of your dataset aside for testing purposes.",
"_____no_output_____"
]
],
[
[
"inner_cv_splits = 5\n\nouter_cv_splits = 5\n\ntest_size = 0.20\n",
"_____no_output_____"
]
],
[
[
"### Automatically find the number of cpus",
"_____no_output_____"
]
],
[
[
"ncpus=round(get_cpu_quota())\nprint('ncpus = '+str(ncpus))",
"ncpus = 15\n"
]
],
[
[
"## Import training and coordinate data",
"_____no_output_____"
]
],
[
[
"# load the data\nmodel_input = np.loadtxt(training_data)\n# coordinates = np.loadtxt(coordinate_data)\n\n# load the column_names\nwith open(training_data, 'r') as file:\n header = file.readline()\n \ncolumn_names = header.split()[1:]\n\n# Extract relevant indices from training data\nmodel_col_indices = [column_names.index(var_name) for var_name in column_names[1:]]\n\n#convert variable names into sci-kit learn nomenclature\nX = model_input[:, model_col_indices]\ny = model_input[:, 0]",
"_____no_output_____"
]
],
[
[
"## Evaluating the classifier\n\nNow that we're happy with the spatial clustering, we can evaluate the classifier via _nested_, K-fold cross-validation.\n\nThe k-fold cross-validation procedure is used to estimate the performance of machine learning models when making predictions on data not used during training. However, when the same cross-validation procedure and dataset are used to both tune the hyperparameters and select a model, it is likely to lead to an optimistically biased evaluation of the model performance.\n\nOne approach to overcoming this bias is to nest the hyperparameter optimization procedure under the model selection procedure. This is called nested cross-validation and is the preferred way to evaluate and compare tuned machine learning models.\n \n_Figure 1: Nested K-Fold Cross Validation_ \n<img align=\"center\" src=\"../../figs/nested_CV.png\" width=\"400\">\n\n***\n\nBefore evaluating the model, we need to set up some hyperparameters to test during optimization. The `param_grid` object below is set up to test various important hyperparameters for a Random Forest model. \n\n> **Note**: the parameters in the `param_grid` object depend on the classifier being used. This notebook is set up for a random forest classifier, to adjust the paramaters to suit a different classifier, look up the important parameters under the relevant [sklearn documentation](https://scikit-learn.org/stable/supervised_learning.html). ",
"_____no_output_____"
]
],
[
[
"# Create the parameter grid\nparam_grid = {\n 'max_features': ['auto', 'log2', None],\n 'n_estimators': [150,200,250,300,350,400],\n 'criterion':['gini', 'entropy']\n}",
"_____no_output_____"
]
],
[
[
"Now we will conduct the nested CV using the SKCV function. This will take a while to run depending on the number of inner and outer cv splits",
"_____no_output_____"
]
],
[
[
"outer_cv = KFold(n_splits=outer_cv_splits, shuffle=True,\n random_state=0)\n\n# lists to store results of CV testing\nacc = []\nf1 = []\nroc_auc = []\ni = 1\nfor train_index, test_index in outer_cv.split(X, y):\n print(f\"Working on {i}/{outer_cv_splits} outer cv split\", end='\\r')\n model = Classifier(random_state=1)\n\n # index training, testing, and coordinate data\n X_tr, X_tt = X[train_index, :], X[test_index, :]\n y_tr, y_tt = y[train_index], y[test_index]\n \n # inner split on data within outer split\n inner_cv = KFold(n_splits=inner_cv_splits,\n shuffle=True,\n random_state=0)\n \n clf = GridSearchCV(\n estimator=model,\n param_grid=param_grid,\n scoring=metric,\n n_jobs=ncpus,\n refit=True,\n cv=inner_cv.split(X_tr, y_tr),\n )\n\n clf.fit(X_tr, y_tr)\n # predict using the best model\n best_model = clf.best_estimator_\n pred = best_model.predict(X_tt)\n\n # evaluate model w/ multiple metrics\n # ROC AUC\n probs = best_model.predict_proba(X_tt)\n probs = probs[:, 1]\n fpr, tpr, thresholds = roc_curve(y_tt, probs)\n auc_ = auc(fpr, tpr)\n roc_auc.append(auc_)\n # Overall accuracy\n ac = balanced_accuracy_score(y_tt, pred)\n acc.append(ac)\n # F1 scores\n f1_ = f1_score(y_tt, pred)\n f1.append(f1_)\n i += 1",
"Working on 5/5 outer cv split\r"
]
],
[
[
"#### The results of our model evaluation",
"_____no_output_____"
]
],
[
[
"print(\"=== Nested K-Fold Cross-Validation Scores ===\")\nprint(\"Mean balanced accuracy: \"+ str(round(np.mean(acc), 2)))\nprint(\"Std balanced accuracy: \"+ str(round(np.std(acc), 2)))\nprint('\\n')\nprint(\"Mean F1: \"+ str(round(np.mean(f1), 2)))\nprint(\"Std F1: \"+ str(round(np.std(f1), 2)))\nprint('\\n')\nprint(\"Mean roc_auc: \"+ str(round(np.mean(roc_auc), 3)))\nprint(\"Std roc_auc: \"+ str(round(np.std(roc_auc), 2)))",
"=== Nested K-Fold Cross-Validation Scores ===\nMean balanced accuracy: 0.82\nStd balanced accuracy: 0.01\n\n\nMean F1: 0.77\nStd F1: 0.01\n\n\nMean roc_auc: 0.916\nStd roc_auc: 0.01\n"
]
],
[
[
"These scores represent a robust estimate of the accuracy of our classifier. However, because we are using only a subset of data to fit and optimize the models, it is reasonable to expect these scores are an under-estimate of the final model's accuracy. Also, the _map_ accuracy will differ from the accuracies reported here since the training data is not a perfect representation of the data in the real world (e.g. if we have purposively over-sampled from hard-to-classify regions, or if the proportions of crop to non-crop do not match the proportions in the real world). ",
"_____no_output_____"
],
[
"## Optimize hyperparameters\n\nHyperparameter searches are a required process in machine learning. Machine learning models require certain “hyperparameters”; model parameters that can be learned from the data. Finding good values for these parameters is a “hyperparameter search” or an “hyperparameter optimization.”\n\nTo optimize the parameters in our model, we use [GridSearchCV](https://scikit-learn.org/stable/modules/generated/sklearn.model_selection.GridSearchCV.html) to exhaustively search through a set of parameters and determine the combination that will result in the highest accuracy based upon the accuracy metric defined.\n",
"_____no_output_____"
]
],
[
[
"#generate n_splits of train-test_split\nrs = ShuffleSplit(n_splits=outer_cv_splits, test_size=test_size, random_state=0)",
"_____no_output_____"
],
[
"# #instatiate a gridsearchCV\nclf = GridSearchCV(Classifier(),\n param_grid,\n scoring=metric,\n verbose=1,\n cv=rs.split(X, y),\n n_jobs=ncpus)\n\nclf.fit(X, y)\n\nprint(\"The most accurate combination of tested parameters is: \")\npprint(clf.best_params_)\nprint('\\n')\nprint(\"The \"+metric+\" score using these parameters is: \")\nprint(round(clf.best_score_, 2))",
"Fitting 5 folds for each of 36 candidates, totalling 180 fits\nThe most accurate combination of tested parameters is: \n{'criterion': 'entropy', 'max_features': None, 'n_estimators': 250}\n\n\nThe balanced_accuracy score using these parameters is: \n0.82\n"
]
],
[
[
"## Fit a model\n\nUsing the best parameters from our hyperparmeter optmization search, we now fit our model on all the data to give the best possible model.",
"_____no_output_____"
]
],
[
[
"#create a new model\n# new_model = Classifier(**clf.best_params_, random_state=1)\nnew_model = Classifier(**{'criterion': 'entropy', 'max_features': None, 'n_estimators': 200}, random_state=1)\nnew_model.fit(X, y)",
"_____no_output_____"
]
],
[
[
"## Save the model\n\nRunning this cell will export the classifier as a binary`.joblib` file. This will allow for importing the model in the subsequent script, `4_Predict.ipynb` \n",
"_____no_output_____"
]
],
[
[
"model_filename = 'results/sahel_ml_model_'+output_suffix+'.joblib'",
"_____no_output_____"
],
[
"dump(new_model, model_filename)",
"_____no_output_____"
]
],
[
[
"## Next steps\n\nTo continue working through the notebooks in this `Southern Africa Cropland Mask` workflow, go to the next notebook `4_Predict.ipynb`.\n\n1. [Extract_training_data](1_Extract_training_data.ipynb) \n2. [Inspect_training_data](2_Inspect_training_data.ipynb)\n3. **Train_fit_evaluate_classifier (this notebook)**\n4. [Predict](4_Predict.ipynb)\n",
"_____no_output_____"
],
[
"***\n\n## Additional information\n\n**License:** The code in this notebook is licensed under the [Apache License, Version 2.0](https://www.apache.org/licenses/LICENSE-2.0). \nDigital Earth Africa data is licensed under the [Creative Commons by Attribution 4.0](https://creativecommons.org/licenses/by/4.0/) license.\n\n**Contact:** If you need assistance, please post a question on the [Open Data Cube Slack channel](http://slack.opendatacube.org/) or on the [GIS Stack Exchange](https://gis.stackexchange.com/questions/ask?tags=open-data-cube) using the `open-data-cube` tag (you can view previously asked questions [here](https://gis.stackexchange.com/questions/tagged/open-data-cube)).\nIf you would like to report an issue with this notebook, you can file one on [Github](https://github.com/digitalearthafrica/deafrica-sandbox-notebooks).\n\n**Last modified:** Dec 2020\n",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
]
] |
e7bbd80dbc3e342c09ce3c8d0cd2277ac5a8655d | 54,049 | ipynb | Jupyter Notebook | SoftCosine_Results_Analysis.ipynb | CubasMike/wordnet_paraphrase | 199d3e1a43edb28827b771bfeded04fae82b58ef | [
"MIT"
] | 1 | 2018-06-12T01:09:39.000Z | 2018-06-12T01:09:39.000Z | SoftCosine_Results_Analysis.ipynb | CubasMike/wordnet_paraphrase | 199d3e1a43edb28827b771bfeded04fae82b58ef | [
"MIT"
] | null | null | null | SoftCosine_Results_Analysis.ipynb | CubasMike/wordnet_paraphrase | 199d3e1a43edb28827b771bfeded04fae82b58ef | [
"MIT"
] | null | null | null | 46.553833 | 187 | 0.433088 | [
[
[
"import pickle\nimport numpy as np\nimport re",
"_____no_output_____"
]
],
[
[
"### Loading results\nResults have the format:\n\n <dict> {conf:table_of_results},\n\nwhere **conf** represents the experimental setting and is the tuple \n \n (0-remove_stopwords,\n 1-remove_punctuation,\n 2-termsim_metric,\n 3-normalization,\n 4-termsim_th,\n 5-synsets_taken,\n 6-wscheme,\n 7-sim_metric)\n \nThere is another configuration for last experiments with did:\nConfiguration format\n0. Removing stopwords flag : [True, False]\n\n1. Removing punctuation flag : [True, False]\n\n2. Wordnet similarity metrics : [\"path\", \"lch\", \"wup\", \"res\", \"jcn\", \"lin\"]\n\n3. Features extracted to compute similarity : [\"token\", \"lemma\", \"stem\", \"lemmapos\"]\n\n4. Features used to extract synsets : [\"token\", \"lemma\", \"lemmapos\"]\n\n5. Information Content used in some WordNet metrics : [\"bnc_ic_2007\", \"bnc_ic_2000\", \"semcor_ic\", \"brown_ic\"]\n\n6. Normalization flag : [True, False]\n\n7. Term-Term similarity minimum threslhold : [0.0, 0.25, 0.5, 0.75] \n\n8. Synsets selection strategy (all-vs-all, first) : [\"all\", \"first\"]\n\n9. Features weighting scheme : [\"tf\", \"binary\"]\n\n10. Text similarity method : [\"mihalcea\", \"softcosine\", \"stevenson\"]\n \n**table_of_results** is a numpy 2d array with scores for each **similarity treshold** in the range **range(0, 101, 5)** sorted by the best accuracy. The **scores** (columns) are:\n\n scores = (0-threshold,\n 1-accuracy,\n 2-f_measure,\n 3-precision,\n 4-recall)",
"_____no_output_____"
]
],
[
[
"#experiments = pickle.load(open(\"./Results/results_20170620_complex_normalization.pickle\", 'rb'))\nexperiments = pickle.load(open(\"./Results/results_20170830_normalization_4termsimTh_Rada_Stevenson_Softcosine.pickle\", 'rb'))\nlen(experiments)",
"_____no_output_____"
],
[
"# res = (threshold, accuracy, f_measure, precision, recall)\nmax([(conf, res[0][0], res[0][1], res[0][2])\n for conf, res in experiments.items()\n #if conf[2] == \"path\"\n ]\n , key=lambda x:x[2])\n#[(conf, res[0][0], res[0][1]) for conf, res in all_scores.items()]",
"_____no_output_____"
],
[
"def extract_best_results(experiments, ktop=3, metric=\"accuracy\", wordnet_metric=None, text_metric=None):\n \"\"\"Extract best results\"\"\"\n # Maps score names to column index in the numpy 2d array\n # containing the results for a particular configuration\n scores_map = {\"accuracy\":1, \"f_measure\":2, \"precision\":3, \"recall\":4}\n \n best_results = np.zeros((ktop*2, 5))\n corresponding_confs = np.array([(None, None, None, None, None,\n None, None, None, None, None, None)]*(ktop*2), dtype=np.dtype('O'))\n #print(\"best_results:\", best_results.shape)\n #print(\"corresponding_confs:\", corresponding_confs.shape)\n #print(\"Starts\\n-------------------\")\n for conf, results in experiments.items():\n # Skip text sim metrics distinct to <text_metric>\n if text_metric and conf[10] != text_metric:\n continue\n # Skip wordnet sim metrics distinct to <wordnet_metric>\n if wordnet_metric and conf[2] != wordnet_metric:\n continue\n \n # Sort by desired score in descending order and select best ktop\n sorted_results = results[results[:,scores_map[metric]].argsort()[::-1]][:ktop, :]\n #print(\"sorted results\", sorted_results.shape)\n \n # Configuration of results\n results_conf = np.array([conf]*ktop, dtype=np.dtype('O'))\n #print(\"results conf\", sorted_results.shape)\n corresponding_confs[ktop:,:] = results_conf\n #print(\"corresponding_confs\", corresponding_confs.shape)\n \n #print(corresponding_confs)\n \n # Vertically stacking best and new results\n best_results[ktop:,:] = sorted_results[:ktop,:]\n #print(best_results)\n \n # Updating the best results if needed\n idx = best_results[:,scores_map[metric]].argsort(axis=0)[::-1]\n \n best_results = best_results[idx,:]\n corresponding_confs = corresponding_confs[idx,:]\n \n \n #print(\"New best results\")\n #print(best_results)\n \n #print(\"New corresponding configurations\")\n #print(corresponding_confs)\n \n # *** Declare best_results with twice the size of ktop and do everthing in-place ***\n # Then compare running times\n \n # Choose the new corresponding configurations to the new best results\n \n \n #break\n #print(\"\\n\")\n \n return best_results[:ktop,:], corresponding_confs[:ktop,:]",
"_____no_output_____"
],
[
"bres, bconf = extract_best_results(experiments, ktop=3, metric=\"accuracy\", wordnet_metric=None, text_metric=None)\nprint(bres)\nprint()\nprint(bconf)",
"[[0.7 0.74092247 0.81886792 0.77575561 0.86705412]\n [0.7 0.74092247 0.81886792 0.77575561 0.86705412]\n [0.7 0.74018646 0.81875749 0.77411003 0.86887032]]\n\n[[False True 'lin' 'lemma' 'lemma' 'bnc_ic_2007' False 0.5 'all' 'binary'\n 'mihalcea']\n [False True 'lin' 'lemma' 'lemma' 'bnc_ic_2007' False 0.5 'all' 'tf'\n 'mihalcea']\n [False True 'lin' 'stem' 'token' 'bnc_ic_2007' False 0.5 'all' 'tf'\n 'mihalcea']]\n"
]
],
[
[
"# Getting some results\nIn this cell we get the best results for each text similarity metric and 3-top best wordnet metrics",
"_____no_output_____"
]
],
[
[
"my_results = []\nfor text_metric in [\"mihalcea\", \"softcosine\", \"stevenson\"]:\n for wordnet_metric in [\"path\", \"lch\", \"wup\", \"res\", \"jcn\", \"lin\"]:\n print(\"------------------------------------------------------\")\n print(text_metric, wordnet_metric)\n res, conf = extract_best_results(experiments,\n ktop=1,\n metric=\"accuracy\",\n wordnet_metric=wordnet_metric,\n text_metric=text_metric)\n print(conf)\n print(res)\n print(\"\\n\")\n my_results.append(np.concatenate((conf[0,:], res[0,0:3]), axis=-1))",
"------------------------------------------------------\nmihalcea path\n[[False True 'path' 'stem' 'token' None False 0.5 'all' 'tf' 'mihalcea']]\n[[0.6 0.7392051 0.82095334 0.76538945 0.88521613]]\n\n\n------------------------------------------------------\nmihalcea lch\n[[False True 'lch' 'token' 'lemma' None True 0.0 'all' 'tf' 'mihalcea']]\n[[0.7 0.73454367 0.82145215 0.7526459 0.90410461]]\n\n\n------------------------------------------------------\nmihalcea wup\n[[False True 'wup' 'stem' 'token' None False 0.75 'all' 'tf' 'mihalcea']]\n[[0.65 0.73307164 0.82099375 0.75037594 0.90628405]]\n\n\n------------------------------------------------------\nmihalcea res\n[[False True 'res' 'lemma' 'lemma' 'brown_ic' True 0.0 'all' 'tf'\n 'mihalcea']]\n[[0.65 0.73675172 0.8173617 0.7690583 0.87213948]]\n\n\n------------------------------------------------------\nmihalcea jcn\n[[False True 'jcn' 'stem' 'token' 'brown_ic' True 0.5 'all' 'tf'\n 'mihalcea']]\n[[0.55 0.73478901 0.82448449 0.74544921 0.92226662]]\n\n\n------------------------------------------------------\nmihalcea lin\n[[False True 'lin' 'lemma' 'lemma' 'bnc_ic_2007' False 0.5 'all' 'tf'\n 'mihalcea']]\n[[0.7 0.74092247 0.81886792 0.77575561 0.86705412]]\n\n\n------------------------------------------------------\nsoftcosine path\n[[False True 'path' 'stem' 'token' None False 0.5 'all' 'binary'\n 'softcosine']]\n[[0.6 0.73356232 0.81717172 0.76153122 0.88158373]]\n\n\n------------------------------------------------------\nsoftcosine lch\n[[False True 'lch' 'lemma' 'lemmapos' None True 0.75 'all' 'binary'\n 'softcosine']]\n[[0.55 0.73184495 0.82031892 0.74924925 0.90628405]]\n\n\n------------------------------------------------------\nsoftcosine wup\n[[False True 'wup' 'stem' 'lemmapos' None False 0.75 'first' 'binary'\n 'softcosine']]\n[[0.55 0.72988224 0.81852645 0.74924562 0.90192517]]\n\n\n------------------------------------------------------\nsoftcosine res\n[[False True 'res' 'stem' 'token' 'bnc_ic_2007' True 0.25 'first'\n 'binary' 'softcosine']]\n[[0.55 0.7328263 0.82324298 0.74413146 0.9211769 ]]\n\n\n------------------------------------------------------\nsoftcosine jcn\n[[False True 'jcn' 'token' 'token' 'brown_ic' True 0.0 'first' 'binary'\n 'softcosine']]\n[[0.55 0.73233562 0.81874065 0.75443968 0.89502361]]\n\n\n------------------------------------------------------\nsoftcosine lin\n[[False True 'lin' 'stem' 'token' 'bnc_ic_2000' False 0.75 'all' 'binary'\n 'softcosine']]\n[[0.65 0.73552502 0.813301 0.77722608 0.85288776]]\n\n\n------------------------------------------------------\nstevenson path\n[[False True 'path' 'stem' 'token' None False 0.5 'first' 'binary'\n 'stevenson']]\n[[0.55 0.7328263 0.82079974 0.75030084 0.90592081]]\n\n\n------------------------------------------------------\nstevenson lch\n[[False True 'lch' 'stem' 'token' None True 0.75 'first' 'binary'\n 'stevenson']]\n[[0.55 0.73159961 0.81911376 0.75174507 0.89974573]]\n\n\n------------------------------------------------------\nstevenson wup\n[[False True 'wup' 'stem' 'lemmapos' None False 0.75 'first' 'binary'\n 'stevenson']]\n[[0.55 0.72669284 0.81809275 0.74310294 0.90991645]]\n\n\n------------------------------------------------------\nstevenson res\n[[False True 'res' 'lemma' 'lemma' 'brown_ic' True 0.75 'all' 'binary'\n 'stevenson']]\n[[0.55 0.72988224 0.8160401 0.75556931 0.88703233]]\n\n\n------------------------------------------------------\nstevenson jcn\n[[False True 'jcn' 'stem' 'token' 'semcor_ic' True 0.0 'all' 'binary'\n 'stevenson']]\n[[0.55 0.73405299 0.82561133 0.74097603 0.9320741 ]]\n\n\n------------------------------------------------------\nstevenson lin\n[[False True 'lin' 'token' 'token' 'semcor_ic' False 0.75 'first'\n 'binary' 'stevenson']]\n[[0.55 0.73331698 0.82447925 0.74215116 0.92735198]]\n\n\n"
],
[
"res = np.array(sorted(my_results, key=lambda x:x[-2], reverse=True))",
"_____no_output_____"
]
],
[
[
"# Saving results to latex table format",
"_____no_output_____"
],
[
"0. Removing stopwords flag : [True, False]\n\n1. Removing punctuation flag : [True, False]\n\n2. Wordnet similarity metrics : [\"path\", \"lch\", \"wup\", \"res\", \"jcn\", \"lin\"]\n\n3. Features extracted to compute similarity : [\"token\", \"lemma\", \"stem\", \"lemmapos\"]\n\n4. Features used to extract synsets : [\"token\", \"lemma\", \"stem\", \"lemmapos\"]\n\n5. Information Content used in some WordNet metrics : [\"bnc_ic_2007\", \"bnc_ic_2000\", \"semcor_ic\", \"brown_ic\"]\n\n6. Normalization flag : [True, False]\n\n7. Term-Term similarity minimum threslhold : [0.0, 0.25, 0.5, 0.75] \n\n8. Synsets selection strategy (all-vs-all, first) : [\"all\", \"first\"]\n\n9. Features weighting scheme : [\"tf\", \"binary\"]\n\n10. Text similarity method : [\"mihalcea\", \"softcosine\", \"stevenson\"]\n\n11. Text similarity threshold : Real\n\n12. Accuracy : Real\n\n13. F1-score : Real",
"_____no_output_____"
]
],
[
[
"ic_map = {None: \"\", \"bnc_ic_2007\": \"bnc07\", \"bnc_ic_2000\": \"bnc00\", \"semcor_ic\":\"semcor\", \"brown_ic\":\"brown\"}\nss_map = {\"first\":\"1\", \"all\":\"n\"}\nws_map = {\"tf\":\"tf\", \"binary\":\"bin\"}\nwith open(\"latex_out.txt\", \"w\") as fid:\n for row in res:\n line = \"{0} & {1} & {3} & {9} & {4} & {8} & {2} & {5} & {6} & {7:.2f} & {10} & {11:.2f} & {12:.3f} & {13:.3f}\".format(\n r\"\\checkmark\" if row[0] else \"\", \n r\"\\checkmark\" if row[1] else \"\",\n row[2],\n row[3],\n row[4],\n ic_map[row[5]],\n r\"\\checkmark\" if row[6] else \"\",\n row[7],\n ss_map[row[8]],\n ws_map[row[9]],\n row[10],\n row[11],\n row[12],\n row[13]\n )+\"\\\\\\\\\\n\"\n #line = \" & \".join([str(x) for x in row]) +\"\\\\\\\\\\n\"\n fid.write(line)",
"_____no_output_____"
],
[
"test_configurations = []\nfor row in res:\n test_configurations.append(tuple(row[:-3]))",
"_____no_output_____"
],
[
"with open(\"test_configurations.pickle\", \"wb\") as fid:\n pickle.dump(test_configurations, fid)",
"_____no_output_____"
]
],
[
[
"# Analyzing test scores",
"_____no_output_____"
]
],
[
[
"test_scores = pickle.load(open(\"./Results/test_results_20180504.pickle\",\"rb\"))",
"_____no_output_____"
],
[
"test_scores",
"_____no_output_____"
],
[
"ic_map = {None: \"\", \"bnc_ic_2007\": \"bnc07\", \"bnc_ic_2000\": \"bnc00\", \"semcor_ic\":\"semcor\", \"brown_ic\":\"brown\"}\nss_map = {\"first\":\"1\", \"all\":\"n\"}\nws_map = {\"tf\":\"tf\", \"binary\":\"bin\"}\nnew_scores = []\nfor old_row in res:\n conf = tuple(old_row[:-3])\n scores_mat = test_scores[conf]\n scores = [x for x in scores_mat if x[0] == old_row[-3]][0]\n new_scores.append((conf[-1], conf[2], scores[1], scores[2]))\nnew_scores.sort(key=lambda x:x[2], reverse=True)\nwith open(\"latex_test_out.txt\", \"w\") as fid:\n for row in new_scores:\n line = \"{0} & {1} & {2:.3f} & {3:.3f} \\\\\\\\\\n\".format(*row)\n fid.write(line)",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
]
] |
e7bbe58dbb8e8716045a16a7732c8b566ae88638 | 16,434 | ipynb | Jupyter Notebook | ds_test/SOAP/SOAP_pca/soap_taskd.ipynb | Suth-ICQMS/EPW-descriptor | a599a8c5666d4604b7db04c5f61dc031c65a5933 | [
"MIT"
] | 2 | 2022-01-05T12:52:46.000Z | 2022-02-28T07:40:30.000Z | ds_test/SOAP/SOAP_pca/soap_taskd.ipynb | Suth-ICQMS/EPW-descriptor | a599a8c5666d4604b7db04c5f61dc031c65a5933 | [
"MIT"
] | null | null | null | ds_test/SOAP/SOAP_pca/soap_taskd.ipynb | Suth-ICQMS/EPW-descriptor | a599a8c5666d4604b7db04c5f61dc031c65a5933 | [
"MIT"
] | null | null | null | 35.040512 | 90 | 0.363393 | [
[
[
"import pandas as pd\nimport numpy as np\nfrom sklearn.model_selection import StratifiedKFold\nfrom sklearn.model_selection import train_test_split\nfrom sklearn.ensemble import ExtraTreesClassifier as ET\nfrom sklearn.ensemble import RandomForestClassifier as RF\nfrom sklearn.metrics import roc_auc_score\nfrom sklearn.model_selection import cross_val_score\nfrom bayes_opt import BayesianOptimization",
"_____no_output_____"
],
[
"df1 = pd.read_csv(\"./SOAP_pca.csv\")\ndf2 = pd.read_csv(\"./id_prop.csv\",header=None)\ndf2.columns = [\"mpid\",\"target\"]\ndf3 = df2.merge(df1)\ndf3",
"_____no_output_____"
],
[
"X = df3.iloc[:,2:]\ny = df3.iloc[:,1]",
"_____no_output_____"
],
[
"from sklearn.model_selection import train_test_split\nX_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.25)\n\nrf = ET(n_estimators=300,criterion=\"entropy\",n_jobs = -1)\nprint(np.mean(cross_val_score(rf, X, y, cv=10, scoring='roc_auc')))",
"0.5827593604882619\n"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code"
]
] |
e7bbe7a5aca7ab1bf6ac954c2252ae317f5dd971 | 502,657 | ipynb | Jupyter Notebook | examples/liver_localization_training_with_chest.ipynb | mjirik/bodynavigation | 99db34cd4b8d9508784746df27356dda31468fe4 | [
"MIT"
] | 5 | 2017-03-26T20:24:59.000Z | 2021-04-10T04:13:05.000Z | examples/liver_localization_training_with_chest.ipynb | mjirik/bodynavigation | 99db34cd4b8d9508784746df27356dda31468fe4 | [
"MIT"
] | null | null | null | examples/liver_localization_training_with_chest.ipynb | mjirik/bodynavigation | 99db34cd4b8d9508784746df27356dda31468fe4 | [
"MIT"
] | 2 | 2017-03-27T17:24:52.000Z | 2022-01-28T01:55:20.000Z | 1,648.055738 | 494,276 | 0.944455 | [
[
[
"%pylab inline\n\nimport os.path as op\nimport sys\nimport matplotlib.pyplot as plt\nimport glob\n\nimport io3d\nimport sed3\n\n# sys.path.append(op.expanduser(\"~/projects/bodynavigation\"))\n# pt = op.expanduser(\"~/projects/imtools\")\n# sys.path.insert(0, pt)\n\nimport bodynavigation\nimport imtools\n# reload(imtools)\n# reload(bodynavigation)\n\nimport imtools.trainer3d\nimport imtools.datasets",
"Populating the interactive namespace from numpy and matplotlib\n"
],
[
"sliver_reference_dir = op.expanduser(\"~/data/medical/orig/sliver07/training/\")",
"_____no_output_____"
]
],
[
[
"# Other example with chest information",
"_____no_output_____"
],
[
"## Training",
"_____no_output_____"
],
[
"Prepare function to calculate feature vector from 3D to [1D x number_of_features]",
"_____no_output_____"
]
],
[
[
"def localization_fv2(data3d, voxelsize_mm): # scale\n fv = []\n # f0 = scipy.ndimage.filters.gaussian_filter(data3d, sigma=3).reshape(-1, 1)\n #f1 = scipy.ndimage.filters.gaussian_filter(data3dr, sigma=1).reshape(-1, 1) - f0\n #f2 = scipy.ndimage.filters.gaussian_filter(data3dr, sigma=5).reshape(-1, 1) - f0\n #f3 = scipy.ndimage.filters.gaussian_filter(data3dr, sigma=10).reshape(-1, 1) - f0\n #f4 = scipy.ndimage.filters.gaussian_filter(data3dr, sigma=20).reshape(-1, 1) - f0\n # position asdfas\n import bodynavigation as bn\n ss = bn.BodyNavigation(data3d, voxelsize_mm)\n fd1 = ss.dist_to_lungs().reshape(-1, 1)\n fd2 = ss.dist_to_spine().reshape(-1, 1)\n fd3 = ss.dist_sagittal().reshape(-1, 1)\n fd4 = ss.dist_coronal().reshape(-1, 1)\n fd5 = ss.dist_axial().reshape(-1, 1)\n fd6 = ss.dist_to_surface().reshape(-1, 1)\n fd7 = ss.dist_diaphragm().reshape(-1, 1)\n fd8 = ss.dist_to_chest().reshape(-1, 1)\n\n # f6 = scipy.ndimage.filters.gaussian_filter(data3d, sigma=[20, 1, 1]).reshape(-1, 1) - f0\n # f7 = scipy.ndimage.filters.gaussian_filter(data3d, sigma=[1, 20, 1]).reshape(-1, 1) - f0\n # f8 = scipy.ndimage.filters.gaussian_filter(data3d, sigma=[1, 1, 20]).reshape(-1, 1) - f0\n\n # print \"fv shapes \", f0.shape, fd2.shape, fd3.shape\n fv = np.concatenate([\n # f0,\n# f1, f2, f3, f4,\n fd1, fd2, fd3, fd4, fd5, fd6, fd7,\n #f6, f7, f8\n ], 1)\n\n\n return fv",
"_____no_output_____"
],
[
"import imtools.trainer3d\nimport imtools.datasets\nol = imtools.trainer3d.Trainer3D()\nol.feature_function = localization_fv2\n\nfor one in imtools.datasets.sliver_reader(\n \"*[0-2].mhd\", read_seg=True,\n sliver_reference_dir=sliver_reference_dir):\n \n numeric_label, vs_mm, oname, orig_data, rname, ref_data = one\n ol.add_train_data(orig_data, ref_data, voxelsize_mm=vs_mm)\n \nol.fit()",
"/Users/mjirik/miniconda/lib/python2.7/site-packages/numpy/core/numeric.py:190: VisibleDeprecationWarning: using a non-integer number instead of an integer will result in an error in the future\n a = empty(shape, dtype, order)\n/Users/mjirik/miniconda/lib/python2.7/site-packages/skimage/morphology/misc.py:122: UserWarning: Only one label was provided to `remove_small_objects`. Did you mean to use a boolean array?\n warn(\"Only one label was provided to `remove_small_objects`. \"\n/Users/mjirik/miniconda/lib/python2.7/site-packages/scipy/ndimage/interpolation.py:568: UserWarning: From scipy 0.13.0, the output shape of zoom() is calculated with round() instead of int() - for these inputs the size of the returned array has changed.\n \"the returned array has changed.\", UserWarning)\n"
]
],
[
[
"## Testing",
"_____no_output_____"
]
],
[
[
"one = list(imtools.datasets.sliver_reader(\"*019.mhd\", read_seg=True))[0]\nnumeric_label, vs_mm, oname, orig_data, rname, ref_data = one\nfit = ol.predict(orig_data, voxelsize_mm=vs_mm)",
"(156, 209, 209)\n(6814236,)\n"
],
[
"plt.figure(figsize=(15,10))\nsed3.show_slices(orig_data, fit, slice_step=20, axis=1, flipV=True)",
"_____no_output_____"
],
[
"import lisa.volumetry_evaluation\nlisa.volumetry_evaluation.compare_volumes_sliver(ref_data, fit, vs_mm)",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code",
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
]
] |
e7bc1b14d74ed9922a856874adae01ee725fcecc | 240,478 | ipynb | Jupyter Notebook | notebooks/SIR_modeling/.ipynb_checkpoints/0_SIR_modeling_intro-checkpoint.ipynb | ebinzacharias/ads_COVID-19 | bd1d5c73ef04012d2a2f83520de1c2261bb37b99 | [
"MIT"
] | null | null | null | notebooks/SIR_modeling/.ipynb_checkpoints/0_SIR_modeling_intro-checkpoint.ipynb | ebinzacharias/ads_COVID-19 | bd1d5c73ef04012d2a2f83520de1c2261bb37b99 | [
"MIT"
] | null | null | null | notebooks/SIR_modeling/.ipynb_checkpoints/0_SIR_modeling_intro-checkpoint.ipynb | ebinzacharias/ads_COVID-19 | bd1d5c73ef04012d2a2f83520de1c2261bb37b99 | [
"MIT"
] | null | null | null | 353.644118 | 91,424 | 0.923407 | [
[
[
"%matplotlib inline\n\nimport pandas as pd\nimport numpy as np\nfrom datetime import datetime\n\nimport matplotlib as mpl\nimport matplotlib.pyplot as plt\n\nimport seaborn as sns\n\nimport plotly.graph_objs as go\nimport plotly\nplotly.__version__",
"_____no_output_____"
],
[
"sns.set(style='darkgrid')\nmpl.rcParams['figure.figsize'] = (16,19)",
"_____no_output_____"
],
[
"dataPath_Raw = (\"data/PROF_small_flat_table.csv\")\n#dataPath_Processed = (\"../data/processed/\")\n\npd.set_option(\"display.max_rows\", 500)",
"_____no_output_____"
],
[
"df_analyse = pd.read_csv(dataPath_Raw, sep=\";\")\ndf_analyse.sort_values('date', ascending = True).head()",
"_____no_output_____"
],
[
"df_analyse.Germany[35]",
"_____no_output_____"
],
[
"N0 = 1000000\nbeta = 0.4\ngamma = 0.1\n\n# Reproduction number beta/gamma\n\n# S + I + R = N\n\nI0 = 27\nS0 = N0 - I0\nR0 = 0\n\nprint(S0)",
"999973\n"
],
[
"def SIR_model(SIR, beta, gamma):\n \n S,I,R = SIR\n dS = -beta * S * I/N0\n dI = beta * S * I/N0 - gamma * I\n dR = gamma * I\n \n return([dS, dI, dR])\n ",
"_____no_output_____"
],
[
"SIR = np.array([S0,I0,R0])\n\npropagation_rates = pd.DataFrame(columns={'Susceptible':S0,\n 'Infected':I0,\n 'Recovered':R0 \n })\n \nfor each in np.arange(100):\n new_delta_vector = SIR_model(SIR, beta, gamma)\n SIR = SIR + new_delta_vector\n \n propagation_rates = propagation_rates.append({'Susceptible':SIR[0],\n 'Infected':SIR[1],\n 'Recovered':SIR[2], \n },ignore_index=True\n )\n \n\n",
"_____no_output_____"
],
[
"fig, ax1 = plt.subplots(1,1)\n\nax1.plot(propagation_rates.index, propagation_rates.Infected, label = 'Infected', color= 'k')\nax1.plot(propagation_rates.index, propagation_rates.Recovered, label = 'Recovered')\nax1.plot(propagation_rates.index, propagation_rates.Susceptible, label = 'Susceptible')\n\nax1.set_ylim(10,1000000)\nax1.set_xlim(0,100)\nax1.set_yscale('linear')\nax1.set_title('SIR Simulation', size= 16)\nax1.set_xlabel('Number of days', size=16)\nax1.legend(loc='best',\n prop={'size':16})",
"_____no_output_____"
]
],
[
[
"## Fitting the parameters",
"_____no_output_____"
]
],
[
[
"from scipy import optimize\nfrom scipy import integrate",
"_____no_output_____"
],
[
"ydata = np.array(df_analyse.Germany[35:]) #90\ntime = np.arange(len(ydata))",
"_____no_output_____"
],
[
"I0 = ydata[0]\nS0 = 1000000\nR0 = 0\nbeta",
"_____no_output_____"
],
[
"print(I0)",
"27\n"
],
[
"def SIR_model_fit(SIR, time, beta, gamma):\n \n S,I,R = SIR\n dS = -beta * S * I/N0\n dI = beta * S * I/N0 - gamma * I\n dR = gamma * I\n \n return([dS, dI, dR])\n ",
"_____no_output_____"
],
[
"def fit_odeint(x,beta,gamma):\n return integrate.odeint(SIR_model_fit, (S0,I0,R0), time, args=(beta, gamma))[:,1]\n\n# [,:1] infected rate",
"_____no_output_____"
],
[
"# Integrate\n\npopt = [0.4, 0.1] #beta, gamma\n\nfit_odeint(time, *popt)",
"_____no_output_____"
],
[
"popt, pcov = optimize.curve_fit(fit_odeint, time, ydata)\nperr = np.sqrt(np.diag(pcov))\n",
"/usr/local/lib/python3.6/site-packages/scipy/integrate/odepack.py:248: ODEintWarning:\n\nExcess work done on this call (perhaps wrong Dfun type). Run with full_output = 1 to get quantitative information.\n\n"
],
[
"print('Standard deviation errors : ', str(perr), 'Infection Start : ', ydata[0])\n",
"Standard deviation errors : [0.00682282 0.00613163] Infection Start : 27\n"
],
[
"fitted = fit_odeint(time, *popt)",
"_____no_output_____"
],
[
"plt.semilogy(time, ydata, 'o')\nplt.semilogy(time, fitted)\nplt.title('SIR model for Germany')\nplt.ylabel('Number of infected people')\nplt.xlabel('Days')\nplt.show()\n\nprint('Optimal Parameters : beta = ', popt[0], 'gamma = ', popt[1])\nprint('Reproduction number, R0 : ', popt[0]/popt[1])",
"_____no_output_____"
]
],
[
[
"## Dynamic Beta ",
"_____no_output_____"
]
],
[
[
"t_initial = 25\nt_intro_measures = 14\nt_hold = 21\nt_relax = 21\n\nbeta_max = 0.4\nbeta_min = 0.11\ngamma = 0.1\n\n\npd_beta = np.concatenate((np.array(t_initial*[beta_max]),\n np.linspace(beta_max, beta_min, t_intro_measures),\n np.array(t_hold * [beta_min]),\n np.linspace(beta_min, beta_max, t_relax)\n ))\n\npd_beta",
"_____no_output_____"
],
[
"SIR = np.array([S0,I0,R0])\n\npropagation_rates = pd.DataFrame(columns={'Susceptible':S0,\n 'Infected':I0,\n 'Recovered':R0 \n })\n \nfor each_beta in pd_beta:\n new_delta_vector = SIR_model(SIR, each_beta, gamma)\n SIR = SIR + new_delta_vector\n \n propagation_rates = propagation_rates.append({'Susceptible':SIR[0],\n 'Infected':SIR[1],\n 'Recovered':SIR[2], \n },ignore_index=True\n )\n \n\n",
"_____no_output_____"
],
[
"fig, ax1 = plt.subplots(1,1)\n\nax1.plot(propagation_rates.index, propagation_rates.Infected, label = 'Infected', linewidth = 3)\n#ax1.plot(propagation_rates.index, propagation_rates.Recovered, label = 'Recovered')\n#ax1.plot(propagation_rates.index, propagation_rates.Susceptible, label = 'Susceptible')\n\nax1.bar(np.arange(len(ydata)), ydata, width=2, label = 'Actual cases in Germany', color = 'r')\nt_phases = np.array([t_initial, t_intro_measures, t_hold, t_relax]).cumsum()\n\nax1.axvspan(0, t_phases[0], facecolor='b', alpha=0.2, label=\"No Measures\")\nax1.axvspan(t_phases[0], t_phases[1], facecolor='b', alpha=0.3, label=\"Hard Measures\")\nax1.axvspan(t_phases[1], t_phases[2], facecolor='b', alpha=0.4, label=\"Holding Measures\")\nax1.axvspan(t_phases[2], t_phases[3], facecolor='b', alpha=0.5, label=\"Relaxed Measures\")\nax1.axvspan(t_phases[3], len(propagation_rates.Infected),facecolor='b', alpha=0.6, label=\"Hard Measures Again\")\n\nax1.set_ylim(10,1.5*max(propagation_rates.Infected))\n#ax1.set_xlim(0,100)\nax1.set_yscale('log')\nax1.set_title('SIR Simulation', size= 16)\nax1.set_xlabel('Number of days', size=16)\nax1.legend(loc='best',\n prop={'size':16})",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
]
] |
e7bc20d4b0eed91cc6034b876b9c5b759022d373 | 155,982 | ipynb | Jupyter Notebook | A1_part_1/Non Pipelined Tester.ipynb | ankurshaswat/COL772 | 503425e89290a8ac710cf053dae3c23aadf49ca7 | [
"MIT"
] | null | null | null | A1_part_1/Non Pipelined Tester.ipynb | ankurshaswat/COL772 | 503425e89290a8ac710cf053dae3c23aadf49ca7 | [
"MIT"
] | null | null | null | A1_part_1/Non Pipelined Tester.ipynb | ankurshaswat/COL772 | 503425e89290a8ac710cf053dae3c23aadf49ca7 | [
"MIT"
] | null | null | null | 51.564298 | 7,972 | 0.74013 | [
[
[
"# Imports",
"_____no_output_____"
]
],
[
[
"import json\nimport re\nimport string\nimport scipy\n\nimport matplotlib.pyplot as plt\nimport numpy as np\n\nfrom tqdm import tqdm_notebook as tqdm\nfrom nltk.sentiment.util import mark_negation\nfrom nltk import wordpunct_tokenize\nfrom nltk.tokenize import word_tokenize\nfrom nltk.corpus import stopwords\nfrom nltk.stem.snowball import SnowballStemmer\n\nfrom sklearn.linear_model import LinearRegression,SGDClassifier,ElasticNet,LogisticRegression\nfrom sklearn.ensemble import GradientBoostingClassifier,VotingClassifier\nfrom sklearn.naive_bayes import MultinomialNB\nfrom sklearn.metrics import accuracy_score,f1_score,mean_squared_error,confusion_matrix\nfrom sklearn.feature_extraction.text import TfidfVectorizer,CountVectorizer",
"_____no_output_____"
]
],
[
[
"# Constants",
"_____no_output_____"
]
],
[
[
"train_path = 'data/train.json'\ndev_path = 'data/dev.json'\n\ntranslator = str.maketrans(\"\",\"\", string.punctuation)\n# stemmer = SnowballStemmer(\"english\", ignore_stopwords=True)",
"_____no_output_____"
]
],
[
[
"# Function Defs",
"_____no_output_____"
]
],
[
[
"def read_file(path):\n data_X = []\n data_Y = []\n with open(path, 'r') as data_file:\n line = data_file.readline()\n while line:\n data = json.loads(line)\n data_X.append(data['review'])\n data_Y.append(data['ratings'])\n line = data_file.readline()\n return data_X,data_Y\n\ndef get_metrics_from_pred(y_pred,y_true):\n mse = mean_squared_error(y_pred,y_true)\n \n try:\n f1_scor = f1_score(y_true, y_pred, average='weighted')\n acc = accuracy_score(y_true, y_pred)\n conf_matrix = confusion_matrix(y_true,y_pred)\n \n except:\n y_pred = np.round(y_pred)\n \n f1_scor = f1_score(y_true, y_pred, average='weighted')\n acc = accuracy_score(y_true, y_pred)\n conf_matrix = confusion_matrix(y_true,y_pred)\n \n print(\"MSE = \",mse,\" F1 = \",f1_scor,\" Accuracy = \",acc)\n plt.matshow(conf_matrix)\n plt.colorbar()\n \ndef get_metrics(model,X,y_true):\n y_pred = model.predict(X)\n get_metrics_from_pred(y_pred,y_true)\n \ndef get_metrics_using_probs(model,X,y_true):\n y_pred = model.predict_proba(X)\n y_pred = np.average(y_pred,axis=1, weights=[1,2,3,4,5])*15\n get_metrics_from_pred(y_pred,y_true)\n \ndef remove_repeats(sentence):\n pattern = re.compile(r\"(.)\\1{2,}\")\n return pattern.sub(r\"\\1\\1\", sentence)\n\ndef tokenizer1(sentence):\n sentence = sentence.translate(translator) # Remove punctuations\n sentence = sentence.lower() # Convert to lowercase\n sentence = re.sub(r'\\d+', '', sentence) # Remove Numbers\n sentence = remove_repeats(sentence) # Remove repeated characters\n# sentence = sentence.strip() # Remove Whitespaces\n tokens = wordpunct_tokenize(sentence) # Tokenize\n# tokens = word_tokenize(sentence) # Tokenize\n \n# for i in range(len(tokens)): # Stem word\n# tokens[i] = stemmer.stem(tokens[i])\n return tokens",
"_____no_output_____"
],
[
"# emoticon_string = r\"\"\"\n# (?:\n# [<>]?\n# [:;=8] # eyes\n# [\\-o\\*\\']? # optional nose\n# [\\)\\]\\(\\[dDpP/\\:\\}\\{@\\|\\\\] # mouth \n# |\n# [\\)\\]\\(\\[dDpP/\\:\\}\\{@\\|\\\\] # mouth\n# [\\-o\\*\\']? # optional nose\n# [:;=8] # eyes\n# [<>]?\n# )\"\"\"\n\n# # The components of the tokenizer:\n# regex_strings = (\n# # Phone numbers:\n# r\"\"\"\n# (?:\n# (?: # (international)\n# \\+?[01]\n# [\\-\\s.]*\n# )? \n# (?: # (area code)\n# [\\(]?\n# \\d{3}\n# [\\-\\s.\\)]*\n# )? \n# \\d{3} # exchange\n# [\\-\\s.]* \n# \\d{4} # base\n# )\"\"\"\n# ,\n# # Emoticons:\n# emoticon_string\n# , \n# # HTML tags:\n# r\"\"\"<[^>]+>\"\"\"\n# ,\n# # Twitter username:\n# r\"\"\"(?:@[\\w_]+)\"\"\"\n# ,\n# # Twitter hashtags:\n# r\"\"\"(?:\\#+[\\w_]+[\\w\\'_\\-]*[\\w_]+)\"\"\"\n# ,\n# # Remaining word types:\n# r\"\"\"\n# (?:[a-z][a-z'\\-_]+[a-z]) # Words with apostrophes or dashes.\n# |\n# (?:[+\\-]?\\d+[,/.:-]\\d+[+\\-]?) # Numbers, including fractions, decimals.\n# |\n# (?:[\\w_]+) # Words without apostrophes or dashes.\n# |\n# (?:\\.(?:\\s*\\.){1,}) # Ellipsis dots. \n# |\n# (?:\\S) # Everything else that isn't whitespace.\n# \"\"\"\n# )\n\n# ######################################################################\n# # This is the core tokenizing regex:\n \n# word_re = re.compile(r\"\"\"(%s)\"\"\" % \"|\".join(regex_strings), re.VERBOSE | re.I | re.UNICODE)\n\n# # The emoticon string gets its own regex so that we can preserve case for them as needed:\n# emoticon_re = re.compile(regex_strings[1], re.VERBOSE | re.I | re.UNICODE)\n\n# # These are for regularizing HTML entities to Unicode:\n# html_entity_digit_re = re.compile(r\"&#\\d+;\")\n# html_entity_alpha_re = re.compile(r\"&\\w+;\")\n# amp = \"&\"\n\n# negation_re = re.compile(r\"\"\"\n# never|no|nothing|nowhere|noone|none|not|\n# havent|hasnt|hadnt|cant|couldnt|shouldnt|\n# wont|wouldnt|dont|doesnt|didnt|isnt|arent|aint|\n# n't|\n# haven't|hasn't|hadn't|can't|couldn't|shouldn't|\n# won't|wouldn't|don't|doesn't|didn't|isn't|aren't|ain't\n# \"\"\",re.VERBOSE )\n\n# clause_level_re = re.compile(r\"\"\"^[.:;!?]$\"\"\",re.VERBOSE )\n# ######################################################################\n\n# class Tokenizer:\n# def __init__(self, preserve_case=False):\n# self.preserve_case = preserve_case\n\n# def tokenize(self, s):\n# \"\"\"\n# Argument: s -- any string or unicode object\n# Value: a tokenize list of strings; conatenating this list returns the original string if preserve_case=False\n# \"\"\" \n# # Try to ensure unicode:\n# # try:\n# # s = unicode(s)\n# # except UnicodeDecodeError:\n# # s = str(s).encode('string_escape')\n# # s = unicode(s)\n# # Fix HTML character entitites:\n# # Tokenize:\n# words = word_re.findall(s)\n\n# # Possible alter the case, but avoid changing emoticons like :D into :d:\n# if not self.preserve_case: \n# words = list(map((lambda x : x if emoticon_re.search(x) else x.lower()), words))\n# # negator = False\n \n# # for i in range(len(words)):\n# # word = words[i]\n# # if(negation_re.match(word)):\n# # negator = !negator\n# # elif(clause_level_re.match(word)):\n# # negator = False\n# # elif(negator):\n# # words[i] = word+\"_NEG\"\n# return words\n \n \n# tok = Tokenizer().tokenize",
"_____no_output_____"
],
[
"tokenize = tokenizer1\n# tokenize = tok",
"_____no_output_____"
],
[
"# for i in tqdm(range(len(X_train))):\n# tokenize(X_train[i])\n\n# for i in range(200,600):\n# print(tokenize(X_train[i]))",
"_____no_output_____"
],
[
"X_train,Y_train = read_file(train_path)\nX_dev,Y_dev = read_file(dev_path)",
"_____no_output_____"
],
[
"# processed_stopwords = []\n\n# for word in stopwords.words('english'):\n# processed_stopwords += tokenize(word)\n \n# # print(processed_stopwords)",
"_____no_output_____"
],
[
"# vectorizer = TfidfVectorizer(strip_accents='ascii',\n# lowercase=True,\n# tokenizer=tokenize,\n# stop_words=processed_stopwords,\n# ngram_range=(1,1),\n# binary=True,\n# norm='l2',\n# analyzer='word')\n\n# vectorizer = TfidfVectorizer(binary=True,tokenizer=tokenize)\n\n# vectorizer = TfidfVectorizer(tokenizer=tokenize)\n\nvectorizer = TfidfVectorizer(tokenizer=tokenize,ngram_range=(1,2))\n\n# vectorizer = CountVectorizer(tokenizer=tokenize,ngram_range=(1,2))\n\nX_train_counts = vectorizer.fit_transform(X_train)\nX_dev_counts = vectorizer.transform(X_dev)",
"_____no_output_____"
],
[
"# print(X_train_counts)",
"_____no_output_____"
],
[
"# from sklearn import preprocessing\n\n# scaler = preprocessing.StandardScaler(with_mean=False).fit(X_train_counts)\n# X_train_counts = scaler.transform(X_train_counts)\n# X_dev_counts = scaler.transform(X_dev_counts)",
"_____no_output_____"
],
[
"# print(X_train_counts)",
"_____no_output_____"
]
],
[
[
"* Try Removeding whole numbers\n* Try seperating number and text\n* Try replacing 000ps by ooops\n* Try removing repeated characters like sssslllleeeepppp.",
"_____no_output_____"
],
[
"# Baseline",
"_____no_output_____"
]
],
[
[
"# all_5 = list(5*np.ones([len(Y_dev),]))\n# get_metrics_from_pred(all_5,Y_dev)",
"_____no_output_____"
]
],
[
[
"# Trying Multinomial Naive Bayes",
"_____no_output_____"
]
],
[
[
"# model = MultinomialNB()\n# model.fit(X_train_counts,Y_train)",
"_____no_output_____"
],
[
"# get_metrics(model,X_dev_counts,Y_dev)\n# get_metrics_using_probs(model,X_dev_counts,Y_dev)",
"_____no_output_____"
]
],
[
[
"# Trying Logistic Regression",
"_____no_output_____"
]
],
[
[
"model = LogisticRegression(verbose=1,n_jobs=7,solver='sag',multi_class='ovr')\nmodel.fit(X_train_counts,Y_train)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"get_metrics(model,X_dev_counts,Y_dev)\nget_metrics_using_probs(model,X_dev_counts,Y_dev)",
"MSE = 0.53488 F1 = 0.6910581415118381 Accuracy = 0.707255\nMSE = 0.39078704909456885 F1 = 0.6600416234970532 Accuracy = 0.647235\n"
],
[
"# model = LogisticRegression(verbose=1,n_jobs=7,class_weight='balanced',multi_class='ovr',solver='liblinear')\n# model.fit(X_train_counts,Y_train)",
"_____no_output_____"
],
[
"# get_metrics(model,X_dev_counts,Y_dev)\n# get_metrics_using_probs(model,X_dev_counts,Y_dev)",
"_____no_output_____"
],
[
"# model = LogisticRegression(verbose=1,n_jobs=7,class_weight='balanced',multi_class='multinomial',solver='lbfgs')\n# model.fit(X_train_counts,Y_train)",
"_____no_output_____"
],
[
"# get_metrics(model,X_dev_counts,Y_dev)\n# get_metrics_using_probs(model,X_dev_counts,Y_dev)",
"_____no_output_____"
],
[
"# model = LogisticRegression(verbose=1,n_jobs=7,class_weight='balanced',multi_class='ovr',solver='liblinear',penalty='l1')\n# model.fit(X_train_counts,Y_train)",
"_____no_output_____"
],
[
"# get_metrics(model,X_dev_counts,Y_dev)\n# get_metrics_using_probs(model,X_dev_counts,Y_dev)",
"_____no_output_____"
],
[
"# model = LogisticRegression(verbose=1,n_jobs=7,class_weight='balanced',multi_class='multinomial',solver='saga',penalty='l1')\n# model.fit(X_train_counts,Y_train)",
"_____no_output_____"
],
[
"# get_metrics(model,X_dev_counts,Y_dev)\n# get_metrics_using_probs(model,X_dev_counts,Y_dev)",
"_____no_output_____"
]
],
[
[
"# Linear Regression",
"_____no_output_____"
]
],
[
[
"# model = LinearRegression(n_jobs=7)\n# model.fit(X_train_counts,Y_train)",
"_____no_output_____"
],
[
"# get_metrics(model,X_dev_counts,Y_dev)",
"_____no_output_____"
]
],
[
[
"# SGD Classifier",
"_____no_output_____"
]
],
[
[
"# model = SGDClassifier(n_jobs=7,verbose=True)\n# model.fit(X_train_counts,Y_train)",
"_____no_output_____"
],
[
"# get_metrics(model,X_dev_counts,Y_dev)",
"_____no_output_____"
]
],
[
[
"# ElasticNet",
"_____no_output_____"
]
],
[
[
"# model = ElasticNet()\n# model.fit(X_train_counts,Y_train)",
"_____no_output_____"
],
[
"# get_metrics(model,X_dev_counts,Y_dev)",
"_____no_output_____"
]
],
[
[
"# GradientBoostingClassifier",
"_____no_output_____"
]
],
[
[
"# model = GradientBoostingClassifier(verbose=True)\n# model.fit(X_train_counts,Y_train)",
"_____no_output_____"
],
[
"# get_metrics(model,X_dev_counts,Y_dev)",
"_____no_output_____"
]
],
[
[
"# Complicated Model ( Tree with two branches 1-3 and 4-5)",
"_____no_output_____"
]
],
[
[
"indices = np.where(list(map(lambda x:x>3,Y_train)))[0]\nX_train_counts_4_5 = X_train_counts[indices]\nY_train_4_5 = [Y_train[j] for j in indices]\n\nindices = np.where(list(map(lambda x:x<=3,Y_train)))[0]\nX_train_counts_1_3 = X_train_counts[indices]\nY_train_1_3 = [Y_train[j] for j in indices]",
"_____no_output_____"
],
[
"Y_modified = list(map(lambda x:int(x>3),Y_train))",
"_____no_output_____"
],
[
"model1 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel1.fit(X_train_counts,Y_modified)",
"_____no_output_____"
],
[
"model2 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel2.fit(X_train_counts_4_5,Y_train_4_5)",
"_____no_output_____"
],
[
"model3 = LogisticRegression(verbose=1,n_jobs=7,solver='sag',multi_class='ovr')\nmodel3.fit(X_train_counts_1_3,Y_train_1_3)",
"_____no_output_____"
],
[
"pred1 = model1.predict(X_dev_counts)\npred2 = model2.predict_proba(X_dev_counts)\npred3 = model3.predict_proba(X_dev_counts)\n\npred = []\n\nfor i in tqdm(range(len(pred1))):\n if(pred1[i] == 1):\n pred.append(pred2[i][0]*4.0 + pred2[i][1]*5.0)\n else:\n pred.append(pred3[i][0]*1.0 + pred3[i][1]*2.0 + pred3[i][2]*3.0)\n\nget_metrics_from_pred(pred,Y_dev)",
"_____no_output_____"
]
],
[
[
"# Another Try (Tree with negative ,neutral and positive review)",
"_____no_output_____"
]
],
[
[
"indices = np.where(list(map(lambda x:x>3,Y_train)))[0]\nX_train_counts_4_5 = X_train_counts[indices]\nY_train_4_5 = [Y_train[j] for j in indices]\n\nindices = np.where(list(map(lambda x:x<3,Y_train)))[0]\nX_train_counts_1_2 = X_train_counts[indices]\nY_train_1_2 = [Y_train[j] for j in indices]\n\nindices = np.where(list(map(lambda x:x==3,Y_train)))[0]\nX_train_counts_3 = X_train_counts[indices]\nY_train_3 = [Y_train[j] for j in indices]",
"_____no_output_____"
],
[
"def modif(x):\n if (x==3):\n return 1\n elif(x>3):\n return 2\n else:\n return 0\n\nY_modified = list(map(lambda x: modif(x),Y_train))",
"_____no_output_____"
],
[
"model1 = LogisticRegression(verbose=1,n_jobs=7,solver='sag',multi_class='ovr')\nmodel1.fit(X_train_counts,Y_modified)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model2 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel2.fit(X_train_counts_4_5,Y_train_4_5)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model3 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel3.fit(X_train_counts_1_2,Y_train_1_2)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"pred1 = model1.predict(X_dev_counts)\npred1_p = model1.predict_proba(X_dev_counts)\npred2 = model2.predict_proba(X_dev_counts)\npred3 = model3.predict_proba(X_dev_counts)\n\npred = []\n\nfor i in tqdm(range(len(pred1))):\n if(pred1[i] == 0):\n pred.append(pred3[i][0]*1.0 + pred3[i][1]*2.0)\n elif(pred1[i] == 1):\n pred.append(pred1_p[i][0]*1.5 + pred1_p[i][1]*3 + pred1_p[i][2]*4.5)\n elif(pred1[i] == 2):\n pred.append(pred2[i][0]*4.0 + pred2[i][1]*5.0)\n\nget_metrics_from_pred(pred,Y_dev)",
"_____no_output_____"
],
[
"pred_n_3_p = model1.predict_proba(X_dev_counts)\npred_4_5 = model2.predict_proba(X_dev_counts)\npred_1_2 = model3.predict_proba(X_dev_counts)\n\npred = []\n\nfor i in tqdm(range(len(pred1))):\n pred.append(pred_n_3_p[i][0]*pred_1_2[i][0]*1.0 + pred_n_3_p[i][0]*pred_1_2[i][1]*2.0 + pred_n_3_p[i][1]*3.0 + pred_n_3_p[i][2]*pred_4_5[i][0]*4.0 + pred_n_3_p[i][2]*pred_4_5[i][1]*5.0)\n\nget_metrics_from_pred(pred,Y_dev)",
"_____no_output_____"
]
],
[
[
"# Voting Classifier (With simple ovr logistive regression and multinomial naive bayes)",
"_____no_output_____"
]
],
[
[
"# m1 = LogisticRegression(verbose=1,n_jobs=7,solver='sag',multi_class='ovr')\n# m2 = MultinomialNB()\n\n# model = VotingClassifier(estimators=[('lr', m1),('gnb', m2)],voting='soft')\n# model.fit(X_train_counts,Y_train) ",
"_____no_output_____"
],
[
"# get_metrics(model,X_dev_counts,Y_dev)\n# get_metrics_using_probs(model,X_dev_counts,Y_dev)",
"_____no_output_____"
]
],
[
[
"# Binary Logistics Everywhere (Tree with base classified as neutral or 1-3&4-5)",
"_____no_output_____"
]
],
[
[
"indices = np.where(list(map(lambda x: x!=3,Y_train)))[0]\nX_train_counts_p_n = X_train_counts[indices]\nY_train_p_n = [1 if Y_train[j]>3 else 0 for j in indices]\n\nindices = np.where(list(map(lambda x:x>3,Y_train)))[0]\nX_train_counts_4_5 = X_train_counts[indices]\nY_train_4_5 = [Y_train[j] for j in indices]\n\nindices = np.where(list(map(lambda x:x<3,Y_train)))[0]\nX_train_counts_1_2 = X_train_counts[indices]\nY_train_1_2 = [Y_train[j] for j in indices]",
"_____no_output_____"
],
[
"def modif(x):\n if (x==3):\n return 1\n else:\n return 0\n\nY_modified = list(map(lambda x: modif(x),Y_train))",
"_____no_output_____"
],
[
"model_neutral = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_neutral.fit(X_train_counts,Y_modified)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_n_p = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_n_p.fit(X_train_counts_p_n,Y_train_p_n)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_4_5 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_4_5.fit(X_train_counts_4_5,Y_train_4_5)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_1_2 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_1_2.fit(X_train_counts_1_2,Y_train_1_2)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"pred_neutral = model_neutral.predict_proba(X_dev_counts)\npred_n_p = model_n_p.predict_proba(X_dev_counts)\npred_1_2 = model_1_2.predict_proba(X_dev_counts)\npred_4_5 = model_4_5.predict_proba(X_dev_counts)\n\npred = []\n\nfor i in tqdm(range(len(pred_neutral))):\n pred.append(pred_neutral[i][1]*3.0 +\n pred_neutral[i][0]*pred_n_p[i][0]*pred_1_2[i][0]*1.0 +\n pred_neutral[i][0]*pred_n_p[i][0]*pred_1_2[i][1]*2.0+\n pred_neutral[i][0]*pred_n_p[i][1]*pred_4_5[i][0]*4.0+\n pred_neutral[i][0]*pred_n_p[i][1]*pred_4_5[i][1]*5.0)\nget_metrics_from_pred(pred,Y_dev)",
"_____no_output_____"
],
[
"pred_neutral_c = model_neutral.predict(X_dev_counts)\npred_neutral = model_neutral.predict_proba(X_dev_counts)\npred_n_p_c = model_n_p.predict(X_dev_counts)\npred_n_p = model_n_p.predict_proba(X_dev_counts)\npred_1_2_c = model_1_2.predict(X_dev_counts)\npred_1_2 = model_1_2.predict_proba(X_dev_counts)\npred_4_5_c = model_4_5.predict(X_dev_counts)\npred_4_5 = model_4_5.predict_proba(X_dev_counts)\n\npred = []\n\nfor i in tqdm(range(len(pred_neutral))):\n if(pred_neutral_c[i] == 1):\n pred.append(3)\n else:\n if(pred_n_p_c[i] == 0):\n pred.append(pred_1_2_c[i])\n else:\n pred.append(pred_4_5_c[i])\nget_metrics_from_pred(pred,Y_dev)",
"_____no_output_____"
]
],
[
[
"# Another Try (Full tree leaning towards 1)",
"_____no_output_____"
]
],
[
[
"indices = np.where(list(map(lambda x: x<=3,Y_train)))[0]\nX_train_counts_12_3 = X_train_counts[indices]\nY_train_12_3 = [1 if Y_train[j]==3 else 0 for j in indices]\n\nindices = np.where(list(map(lambda x:x>3,Y_train)))[0]\nX_train_counts_4_5 = X_train_counts[indices]\nY_train_4_5 = [Y_train[j] for j in indices]\n\nindices = np.where(list(map(lambda x:x<3,Y_train)))[0]\nX_train_counts_1_2 = X_train_counts[indices]\nY_train_1_2 = [Y_train[j] for j in indices]",
"_____no_output_____"
],
[
"def modif(x):\n if (x>3):\n return 1\n else:\n return 0\n\nY_modified = list(map(lambda x: modif(x),Y_train))",
"_____no_output_____"
],
[
"model_123_45 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_123_45.fit(X_train_counts,Y_modified)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_4_5 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_4_5.fit(X_train_counts_4_5,Y_train_4_5)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_12_3 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_12_3.fit(X_train_counts_12_3,Y_train_12_3)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_1_2 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_1_2.fit(X_train_counts_1_2,Y_train_1_2)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"pred_123_45 = model_123_45.predict_proba(X_dev_counts)\npred_12_3 = model_12_3.predict_proba(X_dev_counts)\npred_1_2 = model_1_2.predict_proba(X_dev_counts)\npred_4_5 = model_4_5.predict_proba(X_dev_counts)\n\npred = []\n\nfor i in tqdm(range(len(pred_neutral))):\n pred.append(pred_123_45[i][0]*pred_12_3[i][0]*pred_1_2[i][0]*1.0+\n pred_123_45[i][0]*pred_12_3[i][0]*pred_1_2[i][1]*2.0+\n pred_123_45[i][0]*pred_12_3[i][1]*3.0+\n pred_123_45[i][1]*pred_4_5[i][0]*4.0+\n pred_123_45[i][1]*pred_4_5[i][1]*5.0)\nget_metrics_from_pred(pred,Y_dev)",
"_____no_output_____"
]
],
[
[
"# Another Try (Full tree leaning towards 5)",
"_____no_output_____"
]
],
[
[
"indices = np.where(list(map(lambda x: x>=3,Y_train)))[0]\nX_train_counts_3_45 = X_train_counts[indices]\nY_train_3_45 = [0 if Y_train[j]==3 else 1 for j in indices]\n\nindices = np.where(list(map(lambda x:x>3,Y_train)))[0]\nX_train_counts_4_5 = X_train_counts[indices]\nY_train_4_5 = [Y_train[j] for j in indices]\n\nindices = np.where(list(map(lambda x:x<3,Y_train)))[0]\nX_train_counts_1_2 = X_train_counts[indices]\nY_train_1_2 = [Y_train[j] for j in indices]",
"_____no_output_____"
],
[
"# print(X_train_counts[0])\n# print('hey')\n# print(X_train_counts_1_2[0])",
"_____no_output_____"
],
[
"def modif(x):\n if (x>=3):\n return 1\n else:\n return 0\n\nY_modified = list(map(lambda x: modif(x),Y_train))",
"_____no_output_____"
],
[
"model_12_345 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_12_345.fit(X_train_counts,Y_modified)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_4_5 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_4_5.fit(X_train_counts_4_5,Y_train_4_5)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_3_45 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_3_45.fit(X_train_counts_3_45,Y_train_3_45)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_1_2 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_1_2.fit(X_train_counts_1_2,Y_train_1_2)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"pred_12_345 = model_12_345.predict_proba(X_dev_counts)\npred_3_45 = model_3_45.predict_proba(X_dev_counts)\npred_1_2 = model_1_2.predict_proba(X_dev_counts)\npred_4_5 = model_4_5.predict_proba(X_dev_counts)\n\npred = []\n\nfor i in tqdm(range(len(pred_12_345))):\n pred.append(pred_12_345[i][1]*pred_3_45[i][1]*pred_4_5[i][1]*5.0+\n pred_12_345[i][1]*pred_3_45[i][1]*pred_4_5[i][0]*4.0+\n pred_12_345[i][1]*pred_3_45[i][0]*3.0+\n pred_12_345[i][0]*pred_1_2[i][1]*2.0+\n pred_12_345[i][0]*pred_1_2[i][0]*1.0)\nget_metrics_from_pred(pred,Y_dev)",
"_____no_output_____"
]
],
[
[
"# Another Try",
"_____no_output_____"
]
],
[
[
"indices = np.where(list(map(lambda x: x>=3,Y_train)))[0]\nX_train_counts_3_45 = X_train_counts[indices]\nY_train_3_45 = [0 if Y_train[j]==3 else 1 for j in indices]\n\nindices = np.where(list(map(lambda x:x>3,Y_train)))[0]\nX_train_counts_4_5 = X_train_counts[indices]\nY_train_4_5 = [Y_train[j] for j in indices]\n\nindices = np.where(list(map(lambda x:x>1,Y_train)))[0]\nX_train_counts_2_345 = X_train_counts[indices]\nY_train_2_345 = [ 0 if Y_train[j]== 2 else 1 for j in indices]",
"_____no_output_____"
],
[
"def modif(x):\n if (x>1):\n return 1\n else:\n return 0\n\nY_modified = list(map(lambda x: modif(x),Y_train))",
"_____no_output_____"
],
[
"model_1_2345 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_1_2345.fit(X_train_counts,Y_modified)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_2_345 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_2_345.fit(X_train_counts_2_345,Y_train_2_345)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_3_45 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_3_45.fit(X_train_counts_3_45,Y_train_3_45)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_4_5 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_4_5.fit(X_train_counts_4_5,Y_train_4_5)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"pred_1_2345 = model_1_2345.predict_proba(X_dev_counts)\npred_2_345 = model_2_345.predict_proba(X_dev_counts)\npred_3_45 = model_3_45.predict_proba(X_dev_counts)\npred_4_5 = model_4_5.predict_proba(X_dev_counts)\n\npred = []\n\nfor i in tqdm(range(len(pred_1_2345))):\n pred.append(pred_1_2345[i][0]*1.0+\n pred_1_2345[i][1]*pred_2_345[i][0]*2.0+\n pred_1_2345[i][1]*pred_2_345[i][1]*pred_3_45[i][0]*3.0+\n pred_1_2345[i][1]*pred_2_345[i][1]*pred_3_45[i][1]*pred_4_5[i][0]*4.0+\n pred_1_2345[i][1]*pred_2_345[i][1]*pred_3_45[i][1]*pred_4_5[i][1]*5.0)\nget_metrics_from_pred(pred,Y_dev)",
"_____no_output_____"
]
],
[
[
"# Another Try",
"_____no_output_____"
]
],
[
[
"indices = np.where(list(map(lambda x: x<=3,Y_train)))[0]\nX_train_counts_12_3 = X_train_counts[indices]\nY_train_12_3 = [1 if Y_train[j]==3 else 0 for j in indices]\n\nindices = np.where(list(map(lambda x:x<3,Y_train)))[0]\nX_train_counts_1_2 = X_train_counts[indices]\nY_train_1_2 = [Y_train[j] for j in indices]\n\nindices = np.where(list(map(lambda x:x>1,Y_train)))[0]\nX_train_counts_123_4 = X_train_counts[indices]\nY_train_123_4 = [ 1 if Y_train[j]== 4 else 0 for j in indices]",
"_____no_output_____"
],
[
"def modif(x):\n if (x>4):\n return 1\n else:\n return 0\n\nY_modified = list(map(lambda x: modif(x),Y_train))",
"_____no_output_____"
],
[
"model_1234_5 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_1234_5.fit(X_train_counts,Y_modified)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_123_4 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_123_4.fit(X_train_counts_123_4,Y_train_123_4)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_12_3 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_12_3.fit(X_train_counts_12_3,Y_train_12_3)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"model_1_2 = LogisticRegression(verbose=1,n_jobs=7,solver='sag')\nmodel_1_2.fit(X_train_counts_1_2,Y_train_1_2)",
"[Parallel(n_jobs=7)]: Using backend ThreadingBackend with 7 concurrent workers.\n"
],
[
"pred_1234_5 = model_1234_5.predict_proba(X_dev_counts)\npred_123_4 = model_123_4.predict_proba(X_dev_counts)\npred_12_3 = model_12_3.predict_proba(X_dev_counts)\npred_1_2 = model_1_2.predict_proba(X_dev_counts)\n\npred = []\n\nfor i in tqdm(range(len(pred_1234_5))):\n pred.append(pred_1234_5[i][1]*5.0+\n pred_1234_5[i][0]*pred_123_4[i][1]*4.0+\n pred_1234_5[i][0]*pred_123_4[i][0]*pred_12_3[i][1]*3.0+\n pred_1234_5[i][0]*pred_123_4[i][0]*pred_12_3[i][0]*pred_1_2[i][1]*2.0+\n pred_1234_5[i][0]*pred_123_4[i][0]*pred_12_3[i][0]*pred_1_2[i][0]*1.0)\nget_metrics_from_pred(pred,Y_dev)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bc348f1e1ebedbb63539db0d337ccf660e64ad | 589,479 | ipynb | Jupyter Notebook | src/Practica2_LimpiezaYAnalisisDatos_Titanic.ipynb | luispicouoc/LimpiezayAnalisisDatos | 1c664b111b9f80492576a0b7bc4ef6a5015b7702 | [
"MIT"
] | null | null | null | src/Practica2_LimpiezaYAnalisisDatos_Titanic.ipynb | luispicouoc/LimpiezayAnalisisDatos | 1c664b111b9f80492576a0b7bc4ef6a5015b7702 | [
"MIT"
] | null | null | null | src/Practica2_LimpiezaYAnalisisDatos_Titanic.ipynb | luispicouoc/LimpiezayAnalisisDatos | 1c664b111b9f80492576a0b7bc4ef6a5015b7702 | [
"MIT"
] | null | null | null | 207.125439 | 164,564 | 0.881204 | [
[
[
"<div style=\"width: 100%; clear: both;\">\n<div style=\"float: left; width: 50%;\">\n<img src=\"http://www.uoc.edu/portal/_resources/common/imatges/marca_UOC/UOC_Masterbrand.jpg\", align=\"left\">\n</div>\n<div style=\"float: right; width: 50%;\">\n<p style=\"margin: 0; padding-top: 22px; text-align:right;\">Tipología de datos · Práctica 2</p>\n<p style=\"margin: 0; text-align:right;\">2021-6 · Máster universitario en Ciencia de datos (Data Science)</p>\n<p style=\"margin: 0; text-align:right; padding-button: 100px;\">Estudios de Informática, Multimedia y Telecomunicación</p>\n</div>\n</div>\n<div style=\"width:100%;\"> </div>\n\n\n# Práctica 2: Limpieza y Análisis de Datos\n\nEn esta práctica se elabora un caso práctico orientado a aprender a identificar los datos relevantes para un proyecto analítico y usar las herramientas de integración, limpieza, validación y análisis de las mismas.\n\n## Objetivos\n \n Los objetivos concretos de esta práctica son:\n \n - Aprender a aplicar los conocimientos adquiridos y su capacidad de resolución de problemas en entornos nuevos o poco conocidos dentro de contextos más amplios o multidisciplinares.\n - Saber identificar los datos relevantes y los tratamientos necesarios (integración, limpieza y validación) para llevar a cabo un proyecto analítico.\n - Aprender a analizar los datos adecuadamente para abordar la información contenida en los datos.\n - Identificar la mejor representación de los resultados para aportar conclusiones sobre el problema planteado en el proceso analítico.\n - Actuar con los principios éticos y legales relacionados con la manipulación de datos en el ámbito de aplicación.\n - Desarrollar las habilidades de aprendizaje que les permitan continuar estudiando de un modo que tendrá que ser en gran medida autodirigido o autónomo.\n - Desarrollar la capacidad de búsqueda, gestión y uso de información y recursos en el ámbito de la ciencia de datos.\n\n## Descripción de la Práctica a realizar\n \nEl objetivo de esta actividad será el tratamiento de un dataset, que puede ser el creado en la práctica 1 o bien cualquier dataset libre disponible en Kaggle (https://www.kaggle.com). Algunos ejemplos de dataset con los que podéis trabajar son:\n \n - Red Wine Quality (https://www.kaggle.com/uciml/red-wine-quality-cortez-et-al-2009)\n - Titanic: Machine Learning from Disaster (https://www.kaggle.com/c/titanic)\n \nEl último ejemplo corresponde a una competición activa de Kaggle de manera que, opcionalmente, podéis aprovechar el trabajo realizado durante la práctica para entrar en esta competición.\n \nSiguiendo las principales etapas de un proyecto analítico, las diferentes tareas a realizar (y justificar) son las siguientes:\n \n**1.** Descripción del dataset. ¿Por qué es importante y qué pregunta/problema pretende responder?\n \n**2.** Integración y selección de los datos de interés a analizar.\n \n**3.** Limpieza de los datos.\n - 3.1.¿Los datos contienen ceros o elementos vacíos? ¿Cómo gestionarías cada uno de estos casos?\n - 3.2.Identificación y tratamiento de valores extremos.\n \n**4.** Análisis de los datos.\n - 4.1.Selección de los grupos de datos que se quieren analizar/comparar (planificación de los análisis a aplicar).\n - 4.2.Comprobación de la normalidad y homogeneidad de la varianza.\n - 4.3.Aplicación de pruebas estadísticas para comparar los grupos de datos. En función de los datos y el objetivo del estudio, aplicar pruebas de contraste de hipótesis, correlaciones, regresiones, etc. Aplicar al menos tres métodos de análisis diferentes.\n \n**5.** Representación de los resultados a partir de tablas y gráficas.\n \n**6.** Resolución del problema. A partir de los resultados obtenidos, ¿cuáles son las conclusiones? ¿Los resultados permiten responder al problema?\n**7.** Código: Hay que adjuntar el código, preferiblemente en R, con el que se ha realizado la limpieza, análisis y representación de los datos. Si lo preferís, también podéis trabajar en Python. ",
"_____no_output_____"
],
[
"<div style=\"background-color: #FFFFCC; border-color: #7C9DBF; border-left: 5px solid #7C9DBF; padding: 0.5em;\">\n<font color=\"purple\"><strong>LUIS ALBERTO PICO</strong></font><br>\n</div>",
"_____no_output_____"
],
[
"### Configuración Inicial y Librerías",
"_____no_output_____"
]
],
[
[
"import pandas as pd\nimport numpy as np\nimport seaborn as sns\nimport matplotlib.pyplot as plt\nimport missingno as msno\nfrom statsmodels.graphics.gofplots import qqplot #Normalidad\nfrom scipy import stats #Pruebas Estadísticas\nfrom sklearn.preprocessing import OneHotEncoder\nfrom sklearn import preprocessing\nfrom sklearn.feature_selection import RFE\nfrom sklearn.linear_model import LogisticRegression\nimport statsmodels.api as sm\nfrom sklearn.linear_model import LogisticRegression\nfrom sklearn import metrics\nfrom sklearn.model_selection import train_test_split\nfrom sklearn.metrics import accuracy_score, confusion_matrix\n\ncustom_palette = [\"blue\", \"orange\", \"green\", \"purple\",\"yellow\",\"red\"]\nsns.set_palette(custom_palette)\nsns.set_context(\"notebook\")\nhue_colors = {0: \"red\",1: \"cyan\",2: \"yellow\"}",
"_____no_output_____"
]
],
[
[
"### 1. Descripción del Dataset\n\n#### Dataset\nTitanic \n\n#### Autor\nKaggle. Titanic - Machine Learning from Disaster. https://www.kaggle.com/c/titanic/overview\n\n#### Descripción\n\nEste dataset contiene dos conjuntos de datos similares que incluyen información del pasajero como nombre, edad, género, clase, etc. Un conjunto de datos se titula `train.csv` y el otro se titula `test.csv` .\n\n**train.csv** contiene los atributos de un subconjunto de pasajeros a bordo (891) y la variable objetivo que indica si sobrevivieron o no.\n\nEl conjunto de datos **test.csv** contiene información de los atributos de los pasajeros, más no si sobreviviron o no y este conjunto de datos nos servirá para determinar la precisión del modelo de predicción.\n\n#### Dimensiones\n**train.csv**. El Dataset esta compuesto de 891 registros (pasajeros) y 10 atributos (9 variables de entrada y 1 variable de salida) \n**test.csv** . El Dataset esta compuesto de 418 registros (pasajeros) y 9 atributos (9 variables de entrada)\n\n#### Atributos\n\nAtributo de salida: \n survival. 1 si pasajero sobrevivió y 0 de lo contrario \n\nAtributos de Entrada (pasajeros): \n * PassengerId. Identificador único de pasajero\n * Pclass. Clase asociada al boleto (1 = 1st, 2 = 2nd, 3 = 3rd) \n * Sex. Sexo del pasajero \n * Age. Edad en años \n * SibSp. Número de hermanos(as) /cónyuges a bordo.\n * Parch. Número de padres/hijos a bordo. \n * Ticket. Número de ticket \n * Fare. tarifa \n * Cabin. número de cabina \n * Embarked. Puerto de embarque (C = Cherbourg, Q = Queenstown, S = Southampton) \n \n#### Importancia\n\nEl hundimiento del Titanic es uno de los naufragios más infames de la historia.\n\nEl 15 de abril de 1912, durante su viaje inaugural, el RMS Titanic, ampliamente considerado \"insumergible\", se hundió después de chocar con un iceberg. Desafortunadamente, no había suficientes botes salvavidas para todos a bordo, lo que resultó en la muerte de 1,502 de los 2,224 pasajeros y la tripulación.\n\nSi bien hubo algún elemento de suerte involucrado en sobrevivir, parece que algunos grupos de personas tenían más probabilidades de sobrevivir que otros, esta hipótesis será corroborrada creando un modelo predictivo que responda a la pregunta: \"¿Qué tipo de personas tenían más probabilidades de sobrevivir?\" utilizando los datos de los pasajeros.\n\n\n### 2. Integración y selección de Datos\n\n#### 2.1 Carga del Conjunto de Datos\n\nProcedemos a realizar la lectura de los ficheros en formato CSV `train.csv` y `test.csv` previamente descargado desde Kaggle, los almacenaremos en los dataframes **Titanic_train** y **Titanic_test**, finalmente visualizamos una muestra de los datos que contienen.\n",
"_____no_output_____"
]
],
[
[
"titanic_train_original=pd.read_csv('train.csv')\ntitanic_test_original=pd.read_csv('test.csv')\ntitanic_train=titanic_train_original.copy()\ntitanic_test=titanic_test_original.copy()\ntitanic_train.head()",
"_____no_output_____"
],
[
"titanic_test.head()",
"_____no_output_____"
]
],
[
[
"Procedemos a revisar la estructura y tipos de datos que contiene de los conjuntos de datos, además de los valores únicos de cada atributo.",
"_____no_output_____"
]
],
[
[
"print('train \\n')\ntitanic_train.info()\nprint('\\n')\nprint('test \\n')\ntitanic_test.info()",
"train \n\n<class 'pandas.core.frame.DataFrame'>\nRangeIndex: 891 entries, 0 to 890\nData columns (total 12 columns):\n # Column Non-Null Count Dtype \n--- ------ -------------- ----- \n 0 PassengerId 891 non-null int64 \n 1 Survived 891 non-null int64 \n 2 Pclass 891 non-null int64 \n 3 Name 891 non-null object \n 4 Sex 891 non-null object \n 5 Age 714 non-null float64\n 6 SibSp 891 non-null int64 \n 7 Parch 891 non-null int64 \n 8 Ticket 891 non-null object \n 9 Fare 891 non-null float64\n 10 Cabin 204 non-null object \n 11 Embarked 889 non-null object \ndtypes: float64(2), int64(5), object(5)\nmemory usage: 83.7+ KB\n\n\ntest \n\n<class 'pandas.core.frame.DataFrame'>\nRangeIndex: 418 entries, 0 to 417\nData columns (total 11 columns):\n # Column Non-Null Count Dtype \n--- ------ -------------- ----- \n 0 PassengerId 418 non-null int64 \n 1 Pclass 418 non-null int64 \n 2 Name 418 non-null object \n 3 Sex 418 non-null object \n 4 Age 332 non-null float64\n 5 SibSp 418 non-null int64 \n 6 Parch 418 non-null int64 \n 7 Ticket 418 non-null object \n 8 Fare 417 non-null float64\n 9 Cabin 91 non-null object \n 10 Embarked 418 non-null object \ndtypes: float64(2), int64(4), object(5)\nmemory usage: 36.0+ KB\n"
],
[
"pd.DataFrame(titanic_train.nunique(),columns=['Valores Únicos'])",
"_____no_output_____"
],
[
"pd.DataFrame(titanic_test.nunique(),columns=['Valores Únicos'])",
"_____no_output_____"
]
],
[
[
"Los datos del pasajero estan consituidos por 5 atributos de texto y 6 atributos numéricos, se considera como atributos categóricos a `Pclass` y `Survived`, los nombres de los atributos los guardaremos en dos listas que indiquen cuales son cualitativos y cuales son cuantitativos, adicional el conjunto de train contiene la variable objetivo que toma un valor numérico. \nNo se consideran para el análisis los atributos `Name`,`PassengerId` y `Ticket` por cuanto son identificadores únicos de los pasajeros y sus boletos que no aportarán nada al análisis.",
"_____no_output_____"
]
],
[
[
"titanic_train['Pclass']=titanic_train['Pclass'].astype('category')\ntitanic_test['Pclass'] =titanic_test['Pclass'].astype('category')\ntitanic_train['Survived']=titanic_train['Survived'].astype('category')\n\n\natributos_cualitativos=['Sex','Cabin','Embarked','Pclass','Survived']\natributos_cuantitativos=['Age','SibSp','Parch','Fare']\n\ntitanic_train=titanic_train.drop(columns=['Name','PassengerId','Ticket']).copy()\ntitanic_test =titanic_test.drop(columns=['Name','PassengerId','Ticket']).copy()\ntitanic_train.info()",
"<class 'pandas.core.frame.DataFrame'>\nRangeIndex: 891 entries, 0 to 890\nData columns (total 9 columns):\n # Column Non-Null Count Dtype \n--- ------ -------------- ----- \n 0 Survived 891 non-null category\n 1 Pclass 891 non-null category\n 2 Sex 891 non-null object \n 3 Age 714 non-null float64 \n 4 SibSp 891 non-null int64 \n 5 Parch 891 non-null int64 \n 6 Fare 891 non-null float64 \n 7 Cabin 204 non-null object \n 8 Embarked 889 non-null object \ndtypes: category(2), float64(2), int64(2), object(3)\nmemory usage: 50.8+ KB\n"
]
],
[
[
"#### 2.2 Análisis estadístico básico\nProcedemos a visualizar estadístos básicos para los atributos cuantitativos `(media, mediana, desvianción estándar, mínimo, máximo, cuartiles)` a través de la función `describe` del dataframe.",
"_____no_output_____"
]
],
[
[
"titanic_train.describe()",
"_____no_output_____"
],
[
"titanic_test.describe()",
"_____no_output_____"
]
],
[
[
"Procedemos a visualizar estadístos básicos para los atributos cuanlitativos `unique(cantidad de valores únicos),top y frecuencia` a través de la función `describe` del dataframe.",
"_____no_output_____"
]
],
[
[
"titanic_train[atributos_cualitativos].describe()",
"_____no_output_____"
],
[
"titanic_test[atributos_cualitativos[:-1]].describe()",
"_____no_output_____"
]
],
[
[
"#### 2.3 Selección de Datos\nDado que el objetivo del análisis será generar una modelo predictivo que permita determinar si un pasajero sobrevivió o no en función de sus atributos sociodemográficos y del viaje, se utilizará como la variable objetivo o dependiente la variable `Survived`\n\n* **Sobrevivió (1)**. \n* **No Sobrevivió (0)**.\n\n\nLas variables independientes se definen por los siguientes atributos del conjunto de datos: `Sex`, `Cabin`, `Embarked`, `Pclass`, `Survived`, `Age`, `SibSp` ,`Parch` y `Fare`.",
"_____no_output_____"
],
[
"### 3. Limpieza de Datos\n\nProcederemos en este apartado a determinar si los datos contienen ceros o elementos vacíos y gestionarlos en caso de existir alguno, luego identificaremos y trataremos en la medida de los posible los valores extremos.\n\n#### 3.1 Ceros o Elementos Vacíos\n\n",
"_____no_output_____"
]
],
[
[
"pd.DataFrame(np.sum(titanic_train[atributos_cuantitativos]==0),columns=['Ceros'])",
"_____no_output_____"
],
[
"pd.DataFrame(np.sum(titanic_test[atributos_cuantitativos]==0),columns=['Ceros'])",
"_____no_output_____"
]
],
[
[
"Las variables `SibSp` (Número de hermanos(as) /cónyuges a bordo) ,`Parch` (Parch. Número de padres/hijos a bordo) y `Fare` (Tarifa) contienen valores cero, en función de sus definiciones el valor de cero es válido tanto para SibsSp y Parch, pero por la baja cantidad de valores cero se puede considerar que la tarifa fue cero para algunos invitados especiales, por lo que no se realizará ningún tipo de tratamiento para estos valores.\n\nPara deteminar si los datos contienen los elementos vacíos utilizaremos la función `matrix` y `bar` de la librería **missingno** y la función `isna` del dataframe asociado a nuestros conjuntos de datos.",
"_____no_output_____"
]
],
[
[
"fig = plt.figure(figsize=(4,2))\nfig.subplots_adjust(hspace=0.4, wspace=0.4)\nax = fig.add_subplot(1, 2, 1)\nmsno.matrix(titanic_train,ax=ax,sparkline=False)\nax = fig.add_subplot(1, 2, 2)\nmsno.bar(titanic_train)\nplt.show()",
"_____no_output_____"
],
[
"fig = plt.figure(figsize=(4,2))\nfig.subplots_adjust(hspace=0.4, wspace=0.4)\nax = fig.add_subplot(1, 2, 1)\nmsno.matrix(titanic_test,ax=ax,sparkline=False)\nax = fig.add_subplot(1, 2, 2)\nmsno.bar(titanic_test)\nplt.show()",
"_____no_output_____"
],
[
"pd.DataFrame(titanic_train.isna().sum(),columns=['Nulos'])",
"_____no_output_____"
],
[
"pd.DataFrame(titanic_test.isna().sum(),columns=['Nulos'])",
"_____no_output_____"
]
],
[
[
"Las variables `Age` (Edad), `Cabin` (Número de cabina), `Fare` (Tarifa) y `Embarked` contienen valores nulos, para los atributos `Age`, `Fare` y `Embarked` realizaremos un proceso de imputación, en cambio la variable `Cabin` será excluida del análisis por cuanto el **77.81%** (687/891) de los pasajeros no tienen un valor, el **16.49%** (147/891) corresponde a valores únicos y por tanto dado que es una variable cualitativa su aporte no será significativo para el modelo.",
"_____no_output_____"
]
],
[
[
"titanic_train=titanic_train.drop(columns=['Cabin']).copy()\ntitanic_test=titanic_test.drop(columns=['Cabin']).copy()",
"_____no_output_____"
]
],
[
[
"**Imputación Atributo Age**\n\nEl atributo `Age` lo imputaremos con el valor de la media de los pasajeros del conjunto de datos de train.",
"_____no_output_____"
]
],
[
[
"missing_age = titanic_train[titanic_train['Age'].isna()]\ncomplete_age = titanic_train[~titanic_train['Age'].isna()]\nprint('Missing')\nprint(missing_age.describe())\nprint('Complete')\nprint(complete_age.describe())\nmedia_age=titanic_train['Age'].mean()\ntitanic_train = titanic_train.fillna({'Age': media_age})\ntitanic_test = titanic_test.fillna({'Age': media_age})",
"Missing\n Age SibSp Parch Fare\ncount 0.0 177.000000 177.000000 177.000000\nmean NaN 0.564972 0.180791 22.158567\nstd NaN 1.626316 0.534145 31.874608\nmin NaN 0.000000 0.000000 0.000000\n25% NaN 0.000000 0.000000 7.750000\n50% NaN 0.000000 0.000000 8.050000\n75% NaN 0.000000 0.000000 24.150000\nmax NaN 8.000000 2.000000 227.525000\nComplete\n Age SibSp Parch Fare\ncount 714.000000 714.000000 714.000000 714.000000\nmean 29.699118 0.512605 0.431373 34.694514\nstd 14.526497 0.929783 0.853289 52.918930\nmin 0.420000 0.000000 0.000000 0.000000\n25% 20.125000 0.000000 0.000000 8.050000\n50% 28.000000 0.000000 0.000000 15.741700\n75% 38.000000 1.000000 1.000000 33.375000\nmax 80.000000 5.000000 6.000000 512.329200\n"
]
],
[
[
"**Imputación Atributo Fare**\n\nEl atributo `Fare` lo imputaremos con el valor de la media de los pasajeros del conjunto de datos de train.",
"_____no_output_____"
]
],
[
[
"missing_fare = titanic_test[titanic_test['Fare'].isna()]\ncomplete_fare = titanic_test[~titanic_test['Fare'].isna()]\nprint('Missing')\nprint(missing_fare.describe())\nprint('Complete')\nprint(complete_fare.describe())\nmedia_fare=titanic_train['Fare'].mean()\ntitanic_test = titanic_test.fillna({'Fare': media_fare})",
"Missing\n Age SibSp Parch Fare\ncount 1.0 1.0 1.0 0.0\nmean 60.5 0.0 0.0 NaN\nstd NaN NaN NaN NaN\nmin 60.5 0.0 0.0 NaN\n25% 60.5 0.0 0.0 NaN\n50% 60.5 0.0 0.0 NaN\n75% 60.5 0.0 0.0 NaN\nmax 60.5 0.0 0.0 NaN\nComplete\n Age SibSp Parch Fare\ncount 417.000000 417.000000 417.000000 417.000000\nmean 30.081832 0.448441 0.393285 35.627188\nstd 12.563849 0.897568 0.982419 55.907576\nmin 0.170000 0.000000 0.000000 0.000000\n25% 23.000000 0.000000 0.000000 7.895800\n50% 29.699118 0.000000 0.000000 14.454200\n75% 35.000000 1.000000 0.000000 31.500000\nmax 76.000000 8.000000 9.000000 512.329200\n"
]
],
[
[
"**Imputación Atributo Embarked**\n\nEl atributo `Embarked` lo imputaremos con el valor de la moda de los pasajeros del conjunto de datos de train.",
"_____no_output_____"
]
],
[
[
"missing_embarked = titanic_train[titanic_train['Embarked'].isna()]\ncomplete_embarked = titanic_train[~titanic_train['Embarked'].isna()]\nprint('Missing')\nprint(missing_embarked.describe())\nprint('Complete')\nprint(complete_embarked.describe())\nmoda_embarked='S'\ntitanic_train = titanic_train.fillna({'Embarked': moda_embarked})",
"Missing\n Age SibSp Parch Fare\ncount 2.000000 2.0 2.0 2.0\nmean 50.000000 0.0 0.0 80.0\nstd 16.970563 0.0 0.0 0.0\nmin 38.000000 0.0 0.0 80.0\n25% 44.000000 0.0 0.0 80.0\n50% 50.000000 0.0 0.0 80.0\n75% 56.000000 0.0 0.0 80.0\nmax 62.000000 0.0 0.0 80.0\nComplete\n Age SibSp Parch Fare\ncount 889.000000 889.000000 889.000000 889.000000\nmean 29.653446 0.524184 0.382452 32.096681\nstd 12.968366 1.103705 0.806761 49.697504\nmin 0.420000 0.000000 0.000000 0.000000\n25% 22.000000 0.000000 0.000000 7.895800\n50% 29.699118 0.000000 0.000000 14.454200\n75% 35.000000 1.000000 0.000000 31.000000\nmax 80.000000 8.000000 6.000000 512.329200\n"
],
[
"fig = plt.figure(figsize=(4,2))\nfig.subplots_adjust(hspace=0.4, wspace=0.4)\nax = fig.add_subplot(1, 2, 1)\nax.set_title('Train')\nmsno.bar(titanic_train)\nax = fig.add_subplot(1, 2, 2)\nax.set_title('Test')\nmsno.bar(titanic_test)\nplt.show()",
"_____no_output_____"
]
],
[
[
"#### 3.2 Valores Extremos\n\nProcedemos a identificar y dar tratamiento en la medidad de lo posible a los valores extremos que se identifiquen en el conjunto de datos, para esto se utilizará el diagrama de cajas para cada una de los atributos del dataset.",
"_____no_output_____"
]
],
[
[
"fig = plt.figure(figsize=(15,5))\nfig.subplots_adjust(hspace=0.4, wspace=0.4)\nfor i,atributo in enumerate(titanic_train[atributos_cuantitativos]):\n ax = fig.add_subplot(1, 4, i+1)\n sns.boxplot(data=titanic_train,y=atributo,ax=ax)\nplt.show()",
"_____no_output_____"
]
],
[
[
"En función de lo observado se identifica que los datos de todas las variables se encuentran en una escala de valores adecuado, por lo que no se sugiere realizar un tratamiento de valores extremos.",
"_____no_output_____"
]
],
[
[
"titanic_train.to_csv('titanic_train_clean.csv')\ntitanic_test.to_csv('titanic_test_clean.csv')",
"_____no_output_____"
]
],
[
[
"### 4. Análisis de los Datos\n\nDado que nuestro objetivo de análisis será generar un modelo que permita clasificar las personas que sobrevieron o no en función de sus atributos y con esto determinar si existían grupos de personas que tenían más probabilidades de sobrevivir que otros, procederemos a seleccionar los grupos de datos que se quieren para realizar el análisis y aplicaremos algunas pruebas estadísticas para comparar estos grupos.\n\n#### 4.1 Selección de los grupos de Datos\n\nSeleccionamos los grupos definidos inicialmente:\n* **Survived (1)**. Pasajero sobrevivió `sobrevivio_si`.\n* **Survived (0)**. Pasajero no sobrevivió `sobrevivio_no`.\n\nAdemás se discretizará el atributo edad en un nuevo atributo `age_range`",
"_____no_output_____"
]
],
[
[
"rangos = [0,16,40,60,np.inf]\ncategorias = ['0-16', '16-40', '40-60', '+60']\ntitanic_train['Age_range'] = pd.cut(titanic_train['Age'], bins=ranges,labels=group_names)\ntitanic_test['Age_range'] = pd.cut(titanic_test['Age'], bins=ranges,labels=group_names)\n\nsobrevivio_si=titanic_train[titanic_train['Survived']==1].copy()\nsobrevivio_no=titanic_train[titanic_train['Survived']==0].copy()",
"_____no_output_____"
]
],
[
[
"**Análisis de Atributos Cualitativos**",
"_____no_output_____"
]
],
[
[
"atributos_cualitativos=['Age_range','Sex','Embarked','Pclass']\n\nfig = plt.figure(figsize=(15,8))\nfig.subplots_adjust(hspace=0.4, wspace=0.4)\ni=1\nfor atributo in atributos_cualitativos:\n ax = fig.add_subplot(2,4,i)\n sns.countplot(data=titanic_train,x=atributo, ax=ax)\n ax = fig.add_subplot(2,4,i+1)\n sns.countplot(data=titanic_train,x=atributo,hue='Survived',ax=ax,palette=hue_colors)\n i=i+2\nplt.show()\nsns.set()",
"_____no_output_____"
]
],
[
[
"* **Rango de Edad** `range_Age` \nEn función del rango de edad podemos determinar que más del 50% de los pasajeros menores a 16 años sobrevivieron, en contraste con el resto de segmentos de edad, siendo las personas de más de 60 años las que en mayor proporción murieron. \n\n* **Sexo** `Sex` \nEn función del sexo podemos determinar que en proporción las mujeres sobrevivieron mucho más que en los hombres. \n\n* **Puerto de Embarqued** `Embarked` \nEn función del puerto de embarque podemos determinar que en proporción los pasajeros que embarcaron en el puerto **Cherbourg** sobrevivieron en mayor proporción que los embarcados en el resto de puertos. \n\n* **Calse** `Pclass` \nEn función de la clase en el que viajaron los pasajeros podemos determinar que los pasajeros de primera clase sobrevivieron en mayor proporción, en contraste con el resto de clases, siendo los pasajeros de tercera clase los que en mayor proporción murieron. ",
"_____no_output_____"
],
[
"**Análisis de Atributos Cuantitativos**",
"_____no_output_____"
]
],
[
[
"sns.pairplot(titanic_train,hue='Survived',palette=hue_colors)\nplt.show()",
"_____no_output_____"
]
],
[
[
"* **Edad** `Age` \nEn función de la edad del pasajero se ratifica que a menor edad existieron mayor probabilidad de sobrevivir. \n\n* **Número de hermanos/cónyuge a bordo** `Sibsip` \nEn función del número de hermanos/cónyuge a bordo podemos determinar que aquellos que tenían 1 tuvieron más probabilidad de sobrevivir. \n\n* **Número de padres/hijos a bordo** `Parch` \nEn función del número de padres/hijos a bordo podemos determinar que aquellos que tenían 1 tuvieron más probabilidad de sobrevivir.\n\n* **Tarifa** `Fare` \nEn función de la tarifa que pago el pasajero podemos determinar que los pasajeros cuyo boleto de mayor valor tuvieron mayor probabilidad de sobrevivir. ",
"_____no_output_____"
],
[
"### 4.2 Normalidad y Homogeneidad de la Varianza de los Datos\n\n#### 4.2.1 Normalidad\nDeterminamos la normalidad de las variables dependientes para esto se utilizará el método visual q-q plot y el método estadístico Shapiro-Wilk test.",
"_____no_output_____"
]
],
[
[
"fig = plt.figure(figsize=(15,4))\nfig.subplots_adjust(hspace=0.4, wspace=0.4)\nfor i,variable in enumerate(atributos_cuantitativos):\n ax = fig.add_subplot(1, 4, i+1)\n ax.set_title(variable)\n qqplot(titanic_train[variable], line='s',ax=ax) # q-q plot\n stat, p = stats.shapiro(titanic_train[variable]) \n ax.set_xlabel('Statistics=%.2f, p=%.2E' % (stat, p))\nplt.show()",
"_____no_output_____"
]
],
[
[
"Dado que para todas las variable en el test de `Shapiro-Wilk` se obtiene un *p-valor* **inferior** al nivel de\nsignificancia **α = 0.05**, entonces se determina que ninguna variable analizada sigue una distribución normal.\n\n##### 4.2.2 Homogeneidad de la Varianza de los Datos\n\nSe realizará el test de homogeneidad de la varianza para los atributos `Age` y `Fare` con realción a si sobrevivió o no el pasajero, para esto se utilizará la mediana como métrica dado que sus distribuciones no son normales.",
"_____no_output_____"
]
],
[
[
"statistic,pvalue = stats.levene(titanic_train.loc[sobrevivio_si.index,'Age'],titanic_train.loc[sobrevivio_no.index,'Age'], center='median')\nprint('Age : Statistics=%.2f, p-value=%.2f' % (statistic,pvalue))\n\nstatistic,pvalue = stats.levene(titanic_train.loc[sobrevivio_si.index,'Fare'],titanic_train.loc[sobrevivio_no.index,'Fare'], center='median')\nprint('Fare : Statistics=%.2f, p-value=%.2f' % (statistic,pvalue))",
"Age : Statistics=5.48, p-value=0.02\nFare : Statistics=45.10, p-value=0.00\n"
]
],
[
[
"En función de los test realizados determinamos que los atributos `Age` y `Fare` no tienen homogeneidad de la varianza en sus datos con relación a si el pasajero sobrevivió o no, por cuanto su estadístico *p-valor* es **inferior** al nivel de significancia **α <= 0.05**.\n\n\n### 4.3 Pruebas Estadisticas\n\nEn función del objetivo del estudio procederemos a realizar pruebas estadísticas para comparar los grupos de datos definidos.\n\n#### 4.3.1 Test de Igualdad de Medianas\n\nDado que los atributos `Age` y `Fare` no siguen una distribución normal se utliza la prueba H de Kruskal-Wallis que prueba la hipótesis nula de que la mediana de la población de todos los grupos es igual, utilizamos el parámetro `equal_var=False` dado que las varianzas no son iguales en estos atributos para los grupos analizados.",
"_____no_output_____"
]
],
[
[
"statistic,pvalue = stats.kruskal(titanic_train.loc[sobrevivio_si.index,'Age'],titanic_train.loc[sobrevivio_no.index,'Age'], equal_var =False)\nprint('Age : Statistics=%.2f, p-value=%.2f' % (statistic,pvalue))\n\nstatistic,pvalue = stats.kruskal(titanic_train.loc[sobrevivio_si.index,'Fare'],titanic_train.loc[sobrevivio_no.index,'Fare'], equal_var =False)\nprint('Fare : Statistics=%.2f, p-value=%.2f' % (statistic,pvalue))",
"Age : Statistics=1.36, p-value=0.24\nFare : Statistics=93.28, p-value=0.00\n"
]
],
[
[
"En función del test realizado determinamos que el atributo `Age` tiene medianas iguales entre los dos grupos de análisis (sobrevivio_si, sobrevivio_no) por cuanto su estadístico *p-valor* es **superior** al nivel de significancia **α >= 0.05**, no así el atributo `Fare`.\n\n#### 4.3.2 Correlación de Variables",
"_____no_output_____"
]
],
[
[
"fig = plt.figure(figsize=(8,5))\nsns.heatmap(titanic_train.corr(),cmap='Blues',annot=True,cbar=False)\nplt.title('Matriz de Correlación')\nplt.show()",
"_____no_output_____"
]
],
[
[
"Como se puede visualizar no existe alguna correlación fuerte entre las variables analizadas.",
"_____no_output_____"
],
[
"#### 4.4 Regresión Logística\n\nProcederemos a realizar el modelo predictivo a través de una regresión logística, para esto realizaremos el proceso para codificar los atributos cuanlitativos a datos numéricos a través de `OneHotEncoder` y luego normalizaremos todas las variables a través de `StandardScaler`.",
"_____no_output_____"
]
],
[
[
"encoder = OneHotEncoder(drop='first')\ncodificacion=encoder.fit_transform(titanic_train[['Pclass','Sex','Embarked']]).toarray()\ntitanic_train_encoding = pd.DataFrame(codificacion,columns=np.hstack(['2','3','male','Q','S']))# encoder.categories_\ntitanic_train=titanic_train.join(titanic_train_encoding)\ntitanic_train.drop(['Pclass','Sex','Embarked','Age_range'],axis=1,inplace=True)\n\ncodificacion=encoder.fit_transform(titanic_test[['Pclass','Sex','Embarked']]).toarray()\ntitanic_test_encoding = pd.DataFrame(codificacion,columns=np.hstack(['2','3','male','Q','S'])) #encoder.categories_\ntitanic_test=titanic_test.join(titanic_test_encoding)\ntitanic_test.drop(['Pclass','Sex','Embarked','Age_range'],axis=1,inplace=True)\n\nsurvived=titanic_train['Survived']\ntitanic_train.drop(['Survived'],axis=1,inplace=True)\n\ntitanic_train = pd.DataFrame(preprocessing.StandardScaler().fit_transform(titanic_train),columns=titanic_train.columns)\ntitanic_test = pd.DataFrame(preprocessing.StandardScaler().fit_transform(titanic_test),columns=titanic_test.columns)",
"_____no_output_____"
]
],
[
[
"Para determinar seleccionar los atributos que mayor aportación generen al modelo utilizaremos el proceso de eliminación de atributos recursivo `RFE (feature_selection)`.",
"_____no_output_____"
]
],
[
[
"logisticRegression = LogisticRegression()\nrecursiveFeatureElimination = RFE(logisticRegression)\nrecursiveFeatureElimination = recursiveFeatureElimination.fit(titanic_train, survived.values.ravel())\nprint(recursiveFeatureElimination.support_)\nprint(recursiveFeatureElimination.ranking_)",
"[ True False False False True True True False False]\n[1 2 5 4 1 1 1 6 3]\n"
],
[
"titanic_train=titanic_train.loc[:,recursiveFeatureElimination.support_].copy()\ntitanic_test=titanic_test.loc[:,recursiveFeatureElimination.support_].copy()\ntitanic_train.head()",
"_____no_output_____"
]
],
[
[
"Como resultado de la selección de atributos, se seleccionaron: `Age` , `Pclass` y `Sex`.\n\n**Coeficientes y odds** \n\nUtilizaremos el modelo Logit para determinar los coeficientes del modelo.",
"_____no_output_____"
]
],
[
[
"logit_model=sm.Logit(survived,titanic_train)\nresultado=logit_model.fit()\nprint(resultado.summary2())\nprint('Odds Ratios')\nprint(np.exp(resultado.params))",
"Optimization terminated successfully.\n Current function value: 0.483180\n Iterations 6\n Results: Logit\n=================================================================\nModel: Logit Pseudo R-squared: 0.274 \nDependent Variable: Survived AIC: 869.0267 \nDate: 2021-06-05 18:38 BIC: 888.1961 \nNo. Observations: 891 Log-Likelihood: -430.51 \nDf Model: 3 LL-Null: -593.33 \nDf Residuals: 887 LLR p-value: 2.8205e-70\nConverged: 1.0000 Scale: 1.0000 \nNo. Iterations: 6.0000 \n-------------------------------------------------------------------\n Coef. Std.Err. z P>|z| [0.025 0.975]\n-------------------------------------------------------------------\nAge -0.4131 0.0929 -4.4477 0.0000 -0.5951 -0.2311\n2 -0.4623 0.1050 -4.4035 0.0000 -0.6681 -0.2565\n3 -1.1064 0.1175 -9.4131 0.0000 -1.3368 -0.8760\nmale -1.2518 0.0908 -13.7811 0.0000 -1.4298 -1.0738\n=================================================================\n\nOdds Ratios\nAge 0.661592\n2 0.629827\n3 0.330750\nmale 0.285995\ndtype: float64\n"
]
],
[
[
"**Interpretación de Odds Ratio**\n\nEn función de los odds ratio del modelo de regresión logística, se puede concluir que:\n\n* **Age**. Por cada año de incremento en la edad, la probabilidad de sobrevivir es 0.66 veces menor.\n\n* **Pclass (2)**. La probabilidad de sobrevivir es 0.62 veces menor para la pasajeros de segunda clase, en relación con los pasajeros de las otras clases.\n\n* **Pclass (3)**. La probabilidad de sobrevivir es 0.33 veces menor para la pasajeros de tercera clase, en relación con los pasajeros de las otras clases.\n\n* **Sex (male)**. La probabilidad de sobrevivir es 0.28 veces menor para la pasajeros de sexo masculino, en relación con los pasajeros de sexo femenino.",
"_____no_output_____"
]
],
[
[
"X_train, X_test, y_train, y_test = train_test_split(titanic_train,survived,test_size=0.3,stratify=survived,random_state=24)\n\nlogisticRegression = LogisticRegression()\nlogisticRegression.fit(X_train, y_train)\nprint('Accuracy of logistic regression classifier on train set: {:.2f} %'.format(logisticRegression.score(X_train, y_train)*100))",
"Accuracy of logistic regression classifier on train set: 79.61 %\n"
]
],
[
[
"Como resultado del entrenamiento del modelo se obtuvo un accuracy en el conjunto de entrenamiento de **79.61%**\n\n### 5. Resultados\n\nCon el modelo entrenado determinamos:\n* El accuracy en el conjunto de test.\n* La matriz de confusión de los resultados del modelo.",
"_____no_output_____"
]
],
[
[
"print('Accuracy of logistic regression classifier on test set: {:.2f}%'.format(logisticRegression.score(X_test, y_test)*100))\nmatriz_confusion = confusion_matrix(y_test, logisticRegression.predict(X_test))\nprint('Matriz de Confusión:')\nsns.heatmap(matriz_confusion,annot=True,fmt=\"d\",cbar=False,cmap=\"Blues\")\nplt.xlabel('Observado')\nplt.ylabel('Estimado')\nplt.show()",
"Accuracy of logistic regression classifier on test set: 76.12%\nMatriz de Confusión:\n"
]
],
[
[
"Como el conjunto de test se obtuvo un accuracy de **76.12%**, finalmente procedemos a calcular si sobrevivieron o no los pasajeros del conjunto `titanic_test`.",
"_____no_output_____"
]
],
[
[
"titanic_test_original['Survived_Prediction']=logisticRegression.predict(titanic_test)\ntitanic_test_original",
"_____no_output_____"
]
],
[
[
"### 6. Conclusiones\n\nEn función de los resultados, podemos concluir que se obtiene un modelo relativamente bueno para clasificar si un pasajero sobrevivió o no, la precisión global del modelo es del **76.12%**. Esta precisión podría mejorarse utilizando modelos más avanzados bsados en árboles o redes neuronales.\n\nAdemás hemos determinado que los atributos más importantes a la hora de clasificar a los pasajeros si sobrevivieron o no son: `Age`, `Pclass` y `Fare`.\n\nFinalmente en función de los odds ratio del modelo de regresión logística, se puede concluir que:\n\n* **Age**. Por cada año de incremento en la edad, la probabilidad de sobrevivir es 0.66 veces menor.\n\n* **Pclass (2)**. La probabilidad de sobrevivir es 0.62 veces menor para la pasajeros de segunda clase, en relación con los pasajeros de las otras clases.\n\n* **Pclass (3)**. La probabilidad de sobrevivir es 0.33 veces menor para la pasajeros de tercera clase, en relación con los pasajeros de las otras clases.\n\n* **Sex (male)**. La probabilidad de sobrevivir es 0.28 veces menor para la pasajeros de sexo masculino, en relación con los pasajeros de sexo femenino.",
"_____no_output_____"
]
],
[
[
"pd.DataFrame({'CONTRIBUCIONES':['Investigación Previa','Redacción de las Respuestas','Desarrollo código'],\n 'FIRMA':['LP','LP','LP']})",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7bc3e5bcd394a85bff353ffc506b16974919853 | 3,361 | ipynb | Jupyter Notebook | Introduction to Airflow in Python/Intro to Airflow/practice/Untitled.ipynb | BakrFrag/DataCamp-Big-Data-Track- | 3f48078ed1a3bfd3f8e82d1ef7c1193d4338c612 | [
"MIT"
] | null | null | null | Introduction to Airflow in Python/Intro to Airflow/practice/Untitled.ipynb | BakrFrag/DataCamp-Big-Data-Track- | 3f48078ed1a3bfd3f8e82d1ef7c1193d4338c612 | [
"MIT"
] | null | null | null | Introduction to Airflow in Python/Intro to Airflow/practice/Untitled.ipynb | BakrFrag/DataCamp-Big-Data-Track- | 3f48078ed1a3bfd3f8e82d1ef7c1193d4338c612 | [
"MIT"
] | null | null | null | 26.888 | 150 | 0.507289 | [
[
[
"# Define Simple Dag With Python Code",
"_____no_output_____"
],
[
"from airflow.models import DAG\nfrom datetime import datetime",
"_____no_output_____"
],
[
"default_args={\n \"owner\":\"BK\",\n \"email\":\"bakr.frag\",\n \"start_date\":datetime(2021,2,10)\n}",
"_____no_output_____"
],
[
"etl_dag=DAG(\"simple_dag\",default_args=default_args)",
"_____no_output_____"
],
[
"!airflow run etl_dag",
"usage: airflow [-h] GROUP_OR_COMMAND ...\r\n\r\npositional arguments:\r\n GROUP_OR_COMMAND\r\n\r\n Groups:\r\n celery Celery components\r\n config View configuration\r\n connections Manage connections\r\n dags Manage DAGs\r\n db Database operations\r\n kubernetes Tools to help run the KubernetesExecutor\r\n pools Manage pools\r\n providers Display providers\r\n roles Manage roles\r\n tasks Manage tasks\r\n users Manage users\r\n variables Manage variables\r\n\r\n Commands:\r\n cheat-sheet Display cheat sheet\r\n info Show information about current Airflow and environment\r\n kerberos Start a kerberos ticket renewer\r\n plugins Dump information about loaded plugins\r\n rotate-fernet-key\r\n Rotate encrypted connection credentials and variables\r\n scheduler Start a scheduler instance\r\n sync-perm Update permissions for existing roles and DAGs\r\n version Show the version\r\n webserver Start a Airflow webserver instance\r\n\r\noptional arguments:\r\n -h, --help show this help message and exit\r\n\r\nairflow command error: argument GROUP_OR_COMMAND: `airflow run` command, has been removed, please use `airflow tasks run`, see help above.\r\n"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code"
]
] |
e7bc3eab96f5e1a3f81ba42e35e1a14b7b8c7f28 | 3,350 | ipynb | Jupyter Notebook | demos/mappers/FlatMapChainsDemo.ipynb | sbliven/mmtf-pyspark | 3d444178bdc0d5128aafdb1326fec12b5d7634b5 | [
"Apache-2.0"
] | 59 | 2018-01-28T06:50:56.000Z | 2022-02-10T06:07:12.000Z | demos/mappers/FlatMapChainsDemo.ipynb | sbliven/mmtf-pyspark | 3d444178bdc0d5128aafdb1326fec12b5d7634b5 | [
"Apache-2.0"
] | 101 | 2018-02-01T20:51:10.000Z | 2022-01-24T00:50:29.000Z | demos/mappers/FlatMapChainsDemo.ipynb | sbliven/mmtf-pyspark | 3d444178bdc0d5128aafdb1326fec12b5d7634b5 | [
"Apache-2.0"
] | 29 | 2018-01-29T10:09:51.000Z | 2022-01-23T18:53:28.000Z | 24.275362 | 227 | 0.521791 | [
[
[
"# Flat Map Chains Demo\n\nExample demonstrateing how to extract protein chains from PDB entries. This example uses a flatMap function to transform a structure to its polymer chains.\n\n\n## Imports",
"_____no_output_____"
]
],
[
[
"from pyspark.sql import SparkSession\nfrom mmtfPyspark.filters import PolymerComposition\nfrom mmtfPyspark.io import mmtfReader\nfrom mmtfPyspark.mappers import StructureToPolymerChains",
"_____no_output_____"
]
],
[
[
"#### Configure Spark ",
"_____no_output_____"
]
],
[
[
"spark = SparkSession.builder.appName(\"FlatMapChainsDemo\").getOrCreate()",
"_____no_output_____"
]
],
[
[
"## Read in MMTF files",
"_____no_output_____"
]
],
[
[
"path = \"../../resources/mmtf_reduced_sample/\"\n\npdb = mmtfReader.read_sequence_file(path)",
"_____no_output_____"
]
],
[
[
"## flat map structure to polymer chains, filter by polymer composition and count\n\n### Supported polymer composition type:\n\n** polymerComposition.AMINO_ACIDS_20 **= [\"ALA\",\"ARG\",\"ASN\",\"ASP\",\"CYS\",\"GLN\",\"GLU\",\"GLY\",\"HIS\",\"ILE\",\"LEU\",\"LYS\",\"MET\",\"PHE\",\"PRO\",\"SER\",\"THR\",\"TRP\",\"TYR\",\"VAL\"]\n\n** polymerComposition.AMINO_ACIDS_22 **= [\"ALA\",\"ARG\",\"ASN\",\"ASP\",\"CYS\",\"GLN\",\"GLU\",\"GLY\",\"HIS\",\"ILE\",\"LEU\",\"LYS\",\"MET\",\"PHE\",\"PRO\",\"SER\",\"THR\",\"TRP\",\"TYR\",\"VAL\",\"SEC\",\"PYL\"]\n\n** polymerComposition.DNA_STD_NUCLEOTIDES **= [\"DA\",\"DC\",\"DG\",\"DT\"]\n\n** polymerComposition.RNA_STD_NUCLEOTIDES **= [\"A\",\"C\",\"G\",\"U\"]\n",
"_____no_output_____"
]
],
[
[
"count = pdb.flatMap(StructureToPolymerChains(False, True)) \\\n .filter(PolymerComposition(PolymerComposition.AMINO_ACIDS_20)) \\\n .count()\n \nprint(f\"Chains with standard amino acids: {count}\")",
"Chains with standard amino acids: 8346\n"
]
],
[
[
"## Terminate Spark",
"_____no_output_____"
]
],
[
[
"spark.stop()",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7bc49241837f57b9f3d0f86a976fc1db337c35d | 4,871 | ipynb | Jupyter Notebook | courses/machine_learning/deepdive/06_structured/7_pipelines.ipynb | ranshiloni/training-data-analyst | 9d63e653dd9ddbe3f0f97796997ceaff6ae90c05 | [
"Apache-2.0"
] | 1 | 2020-02-06T19:38:49.000Z | 2020-02-06T19:38:49.000Z | courses/machine_learning/deepdive/06_structured/7_pipelines.ipynb | shethsameer/training-data-analyst | 62da9c2c3d5e2fd3f1fc998660773f2d151b4be5 | [
"Apache-2.0"
] | null | null | null | courses/machine_learning/deepdive/06_structured/7_pipelines.ipynb | shethsameer/training-data-analyst | 62da9c2c3d5e2fd3f1fc998660773f2d151b4be5 | [
"Apache-2.0"
] | null | null | null | 25.772487 | 177 | 0.584274 | [
[
[
"## Kubeflow pipelines\n\nThis notebook goes through the steps of using Kubeflow pipelines using the Python3 interpreter (command-line) to preprocess, train, tune and deploy the babyweight model.\n",
"_____no_output_____"
],
[
"### 1. Start Hosted Pipelines and Notebook\n\nTo try out this notebook, first launch Kubeflow Hosted Pipelines and an AI Platform Notebooks instance.\nFollow the instructions in this [README.md](pipelines/README.md) file.",
"_____no_output_____"
],
[
"### 2. Install necessary packages",
"_____no_output_____"
]
],
[
[
"%pip install --quiet kfp python-dateutil --upgrade",
"_____no_output_____"
]
],
[
[
"Make sure to *restart the kernel* to pick up new packages (look for button in the ribbon of icons above this notebook)",
"_____no_output_____"
],
[
"### 3. Connect to the Hosted Pipelines\n\nVisit https://console.cloud.google.com/ai-platform/pipelines/clusters\nand get the hostname for your cluster. You can get it by clicking on the Settings icon.\nAlternately, click on the Open Pipelines Dashboard link and look at the URL.\nChange the settings in the following cell",
"_____no_output_____"
]
],
[
[
"# CHANGE THESE\nPIPELINES_HOST='447cdd24f70c9541-dot-us-central1.notebooks.googleusercontent.com'\nPROJECT='ai-analytics-solutions'\nBUCKET='ai-analytics-solutions-kfpdemo'",
"_____no_output_____"
],
[
"import kfp\nclient = kfp.Client(host=PIPELINES_HOST)\n#client.list_pipelines()",
"_____no_output_____"
]
],
[
[
"## 4. [Optional] Build Docker containers\n\nI have made my containers public, so you can simply use those.",
"_____no_output_____"
]
],
[
[
"%%bash\ncd pipelines/containers\n#bash build_all.sh",
"_____no_output_____"
]
],
[
[
"Check that the Docker images work properly ...",
"_____no_output_____"
]
],
[
[
"#!docker pull gcr.io/cloud-training-demos/babyweight-pipeline-bqtocsv:latest\n#!docker run -t gcr.io/cloud-training-demos/babyweight-pipeline-bqtocsv:latest --project $PROJECT --bucket $BUCKET --local",
"_____no_output_____"
]
],
[
[
"### 5. Upload and execute pipeline\n\nUpload to the Kubeflow pipeline cluster",
"_____no_output_____"
]
],
[
[
"from pipelines import mlp_babyweight\n\npipeline = client.create_run_from_pipeline_func(mlp_babyweight.train_and_deploy, \n arguments={'project': PROJECT, 'bucket': BUCKET})",
"_____no_output_____"
],
[
"# Copyright 2020 Google LLC\n#\n# Licensed under the Apache License, Version 2.0 (the \"License\");\n# you may not use this file except in compliance with the License.\n# You may obtain a copy of the License at\n#\n# http://www.apache.org/licenses/LICENSE-2.0\n#\n# Unless required by applicable law or agreed to in writing, software\n# distributed under the License is distributed on an \"AS IS\" BASIS,\n# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.\n# See the License for the specific language governing permissions and\n# limitations under the License.",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
]
] |
e7bc51226d2e10f7541446ac95de49fd40dda5c6 | 1,033,297 | ipynb | Jupyter Notebook | BOX_OFFICE_ANALYSIS_WITH_PLOTLY_AND_PY.ipynb | itsdharmil/box-office-analysis-with-plotly-and-python | ff02c0a070d6123bf33fab2cd6e0203f09eaa528 | [
"MIT"
] | 1 | 2020-07-04T18:40:10.000Z | 2020-07-04T18:40:10.000Z | BOX_OFFICE_ANALYSIS_WITH_PLOTLY_AND_PY.ipynb | itsdharmil/box-office-analysis-with-plotly-and-python | ff02c0a070d6123bf33fab2cd6e0203f09eaa528 | [
"MIT"
] | null | null | null | BOX_OFFICE_ANALYSIS_WITH_PLOTLY_AND_PY.ipynb | itsdharmil/box-office-analysis-with-plotly-and-python | ff02c0a070d6123bf33fab2cd6e0203f09eaa528 | [
"MIT"
] | null | null | null | 155.593585 | 259,822 | 0.772107 | [
[
[
"<h2 align=center>Analyze Worldwide Box Office Revenue with Plotly and Python</h2>\n",
"_____no_output_____"
],
[
"###Libraries",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport pandas as pd\npd.set_option('max_columns', None)\nimport matplotlib.pyplot as plt\nimport seaborn as sns\n%matplotlib inline\nplt.style.use('ggplot')\nimport datetime\nimport lightgbm as lgb\nfrom scipy import stats\nfrom scipy.sparse import hstack, csr_matrix\nfrom sklearn.model_selection import train_test_split, KFold\nfrom wordcloud import WordCloud\nfrom collections import Counter\nfrom nltk.corpus import stopwords\nfrom nltk.util import ngrams\nfrom sklearn.feature_extraction.text import TfidfVectorizer, CountVectorizer\nfrom sklearn.preprocessing import StandardScaler\nimport nltk\nnltk.download('stopwords')\nstop = set(stopwords.words('english'))\nimport os\nimport plotly.offline as py\npy.init_notebook_mode(connected=True)\nimport plotly.graph_objs as go\nimport plotly.tools as tls\nimport xgboost as xgb\nimport lightgbm as lgb\nfrom sklearn import model_selection\nfrom sklearn.metrics import accuracy_score\nimport json\nimport ast\nfrom urllib.request import urlopen\nfrom PIL import Image\nfrom sklearn.preprocessing import LabelEncoder\nimport time\nfrom sklearn.metrics import mean_squared_error\nfrom sklearn.linear_model import LinearRegression\nfrom sklearn import linear_model",
"/usr/local/lib/python3.6/dist-packages/statsmodels/tools/_testing.py:19: FutureWarning: pandas.util.testing is deprecated. Use the functions in the public API at pandas.testing instead.\n import pandas.util.testing as tm\n"
]
],
[
[
"### Data Loading and Exploration",
"_____no_output_____"
]
],
[
[
"train = pd.read_csv('train.csv')\ntest = pd.read_csv('test.csv')",
"_____no_output_____"
],
[
"train.head()",
"_____no_output_____"
]
],
[
[
"###Visualizing the Target Distribution",
"_____no_output_____"
]
],
[
[
"fig, ax = plt.subplots(figsize = (16, 6))\nplt.subplot(1, 2, 1)\nplt.hist(train['revenue']);\nplt.title('Distribution of revenue');\nplt.subplot(1, 2, 2)\nplt.hist(np.log1p(train['revenue']));\nplt.title('Distribution of log of revenue');",
"_____no_output_____"
],
[
"train['log_revenue'] = np.log1p(train['revenue'])",
"_____no_output_____"
]
],
[
[
"###Relationship between Film Revenue and Budget",
"_____no_output_____"
]
],
[
[
"fig, ax = plt.subplots(figsize = (16, 6))\nplt.subplot(1, 2, 1)\nplt.hist(train['budget']);\nplt.title('Distribution of budget');\nplt.subplot(1, 2, 2)\nplt.hist(np.log1p(train['budget']));\nplt.title('Distribution of log of budget');",
"_____no_output_____"
],
[
"plt.figure(figsize=(16, 8))\nplt.subplot(1, 2, 1)\nplt.scatter(train['budget'], train['revenue'])\nplt.title('Revenue vs budget');\nplt.subplot(1, 2, 2)\nplt.scatter(np.log1p(train['budget']), train['log_revenue'])\nplt.title('Log Revenue vs log budget');",
"_____no_output_____"
],
[
"train['log_budget'] = np.log1p(train['budget'])\ntest['log_budget'] = np.log1p(test['budget'])",
"_____no_output_____"
]
],
[
[
"###Does having an Official Homepage Affect Revenue?",
"_____no_output_____"
]
],
[
[
"train['homepage'].value_counts().head(10)",
"_____no_output_____"
],
[
"train['has_homepage'] = 0\ntrain.loc[train['homepage'].isnull() == False, 'has_homepage'] = 1\ntest['has_homepage'] = 0\ntest.loc[test['homepage'].isnull() == False, 'has_homepage'] = 1",
"_____no_output_____"
],
[
"sns.catplot(x='has_homepage', y='revenue', data=train);\nplt.title('Revenue for film with and without homepage');",
"_____no_output_____"
]
],
[
[
"###Distribution of Languages in Film",
"_____no_output_____"
]
],
[
[
"plt.figure(figsize=(16, 8))\nplt.subplot(1, 2, 1)\nsns.boxplot(x='original_language', y='revenue', data=train.loc[train['original_language'].isin(train['original_language'].value_counts().head(10).index)]);\nplt.title('Mean revenue per language');\nplt.subplot(1, 2, 2)\nsns.boxplot(x='original_language', y='log_revenue', data=train.loc[train['original_language'].isin(train['original_language'].value_counts().head(10).index)]);\nplt.title('Mean log revenue per language');",
"_____no_output_____"
]
],
[
[
"### Frequent Words in Film Titles and Discriptions",
"_____no_output_____"
]
],
[
[
"plt.figure(figsize = (12, 12))\ntext = ' '.join(train['original_title'].values)\nwordcloud = WordCloud(max_font_size=None, background_color='white', width=1200, height=1000).generate(text)\nplt.imshow(wordcloud)\nplt.title('Top words in titles')\nplt.axis(\"off\")\nplt.show()",
"_____no_output_____"
],
[
"plt.figure(figsize = (12, 12))\ntext = ' '.join(train['overview'].fillna('').values)\nwordcloud = WordCloud(max_font_size=None, background_color='white', width=1200, height=1000).generate(text)\nplt.imshow(wordcloud)\nplt.title('Top words in overview')\nplt.axis(\"off\")\nplt.show()",
"_____no_output_____"
]
],
[
[
"###Do Film Descriptions Impact Revenue?",
"_____no_output_____"
]
],
[
[
"import eli5\n\nvectorizer = TfidfVectorizer(\n sublinear_tf=True,\n analyzer='word',\n token_pattern=r'\\w{1,}',\n ngram_range=(1, 2),\n min_df=5)\n\noverview_text = vectorizer.fit_transform(train['overview'].fillna(''))\nlinreg = LinearRegression()\nlinreg.fit(overview_text, train['log_revenue'])\neli5.show_weights(linreg, vec=vectorizer, top=20, feature_filter=lambda x: x != '<BIAS>')",
"_____no_output_____"
],
[
"print('Target value:', train['log_revenue'][1000])\neli5.show_prediction(linreg, doc=train['overview'].values[1000], vec=vectorizer)",
"Target value: 16.44583954907521\n"
]
],
[
[
"###Analyzing Movie Release Dates\n",
"_____no_output_____"
]
],
[
[
"test.loc[test['release_date'].isnull()==False,'release_date'].head()",
"_____no_output_____"
]
],
[
[
"###Preprocessing Features",
"_____no_output_____"
]
],
[
[
"def fix_date(x):\n \n year = x.split('/')[2]\n if int(year)<=19:\n return x[:-2] + '20' + year\n else:\n return x[:-2] + '19' + year",
"_____no_output_____"
],
[
"test.loc[test['release_date'].isnull() == True].head()",
"_____no_output_____"
],
[
"test.loc[test['release_date'].isnull() == True, 'release_date'] = '05/01/00'",
"_____no_output_____"
],
[
"train['release_date'] = train['release_date'].apply(lambda x: fix_date(x))",
"_____no_output_____"
],
[
"test['release_date'] = test['release_date'].apply(lambda x: fix_date(x))",
"_____no_output_____"
]
],
[
[
"###Creating Features Based on Release Date",
"_____no_output_____"
]
],
[
[
"train['release_date'] = pd.to_datetime(train['release_date'])\ntest['release_date'] = pd.to_datetime(test['release_date'])",
"_____no_output_____"
],
[
"def process_date(df):\n date_parts = ['year','weekday','month','weekofyear','day','quarter']\n for part in date_parts:\n part_col = 'release_date' + '_' + part\n df[part_col] = getattr(df['release_date'].dt,part).astype(int)\n return df\ntrain = process_date(train)\ntest= process_date(test)",
"_____no_output_____"
]
],
[
[
"###Using Plotly to Visualize the Number of Films Per Year",
"_____no_output_____"
]
],
[
[
"d1=train['release_date_year'].value_counts().sort_index()\nd2=test['release_date_year'].value_counts().sort_index()",
"_____no_output_____"
],
[
"import plotly.offline as py\npy.init_notebook_mode(connected=True)\nimport plotly.graph_objs as go\ndata = [go.Scatter(x=d1.index, y =d1.values, name='train'),\n go.Scatter(x=d2.index,y=d2.values,name='test')]\nlayout = go.Layout(dict(title= 'Number of flims per year',\n xaxis = dict(title = 'Year'),\n yaxis=dict(title='Count'),\n ),legend= dict(orientation='v'))\npy.iplot(dict(data=data,layout=layout))",
"_____no_output_____"
]
],
[
[
"###Number of Films and Revenue Per Year",
"_____no_output_____"
]
],
[
[
"#d1 = train['release_date_year'].value.counts().sort_index()\nd1=train['release_date_year'].value_counts().sort_index()\nd2 = train.groupby(['release_date_year'])['revenue'].sum()\n\ndata = [go.Scatter (x=d1.index,y=d1.values,name='film count'),\n go.Scatter(x=d2.index, y=d2.values,name='total revenue',yaxis='y2')]\n\nlayout = go.Layout(dict(title = 'Number of flims and revenue per year',\n xaxis = dict(title = 'Year'),\n yaxis = dict(title = 'count'),\n yaxis2=dict(title='total revenue',overlaying='y', side='right')),\n legend=dict(orientation='v'))\npy.iplot(dict(data=data, layout=layout))",
"_____no_output_____"
],
[
"#d1 = train['release_date_year'].value.counts().sort_index()\nd1=train['release_date_year'].value_counts().sort_index()\nd2 = train.groupby(['release_date_year'])['revenue'].mean()\n\ndata = [go.Scatter (x=d1.index,y=d1.values,name='film count'),\n go.Scatter(x=d2.index, y=d2.values,name='average revenue',yaxis='y2')]\n\nlayout = go.Layout(dict(title = 'Number of flims and revenue per year',\n xaxis = dict(title = 'Year'),\n yaxis = dict(title = 'count'),\n yaxis2=dict(title='average revenue',overlaying='y', side='right')),\n legend=dict(orientation='v'))\npy.iplot(dict(data=data, layout=layout))",
"_____no_output_____"
]
],
[
[
"###Do Release Days Impact Revenue?",
"_____no_output_____"
]
],
[
[
"sns.catplot(x='release_date_weekday', y='revenue',data=train);\nplt.title('revenue of dif days of the week')",
"_____no_output_____"
]
],
[
[
"### Relationship between Runtime and Revenue",
"_____no_output_____"
]
],
[
[
"sns.distplot(train['runtime'].fillna(0)/60,bins=(40),kde=False);\nplt.title('distribution of flims in hrs')",
"_____no_output_____"
],
[
"sns.scatterplot(train['runtime'].fillna(0)/60, train['revenue'])\nplt.title('runtime vs revenue')",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
]
] |
e7bc53aeb7088e167d435fe52753f7a3fe4f2912 | 69,422 | ipynb | Jupyter Notebook | _development/tutorials/automate-excel/4-large-file.ipynb | dsfm-org/code-bank | 01a6a542c2801856b52f222a52b1d6e9215dc00d | [
"MIT"
] | 10 | 2020-07-03T06:21:39.000Z | 2021-12-12T11:33:13.000Z | _development/tutorials/automate-excel/4-large-file.ipynb | dsfm-org/code-bank | 01a6a542c2801856b52f222a52b1d6e9215dc00d | [
"MIT"
] | 5 | 2020-08-17T09:37:44.000Z | 2021-08-25T16:10:04.000Z | _development/tutorials/automate-excel/4-large-file.ipynb | dsfm-org/code-bank | 01a6a542c2801856b52f222a52b1d6e9215dc00d | [
"MIT"
] | 4 | 2020-08-06T10:30:13.000Z | 2021-09-10T13:35:00.000Z | 31.186882 | 293 | 0.439328 | [
[
[
"# Large File Input",
"_____no_output_____"
],
[
"Source: [https://github.com/d-insight/code-bank.git](https://github.com/d-insight/code-bank.git) \nLicense: [MIT License](https://opensource.org/licenses/MIT). See open source [license](LICENSE) in the Code Bank repository. ",
"_____no_output_____"
],
[
"---",
"_____no_output_____"
],
[
"### Import libraries",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport pandas as pd\nfrom pathlib import Path\nimport csv\nfrom pprint import pprint \nimport sqlite3\nfrom sqlalchemy import create_engine",
"_____no_output_____"
]
],
[
[
"### Set data directory",
"_____no_output_____"
]
],
[
[
"DIR = 'data'\nFILE = '/yellow_tripdata_2016-03.csv'\n\ncsv_file = '{}{}'.format(DIR, FILE)\n\nprint('File directory: {}'.format(csv_file))",
"File directory: data/yellow_tripdata_2016-03.csv\n"
]
],
[
[
"#### How large is the dataset on disk?",
"_____no_output_____"
]
],
[
[
"size = Path(csv_file).stat().st_size",
"_____no_output_____"
],
[
"f'{size:,} bytes'",
"_____no_output_____"
]
],
[
[
"#### How many rows of data?",
"_____no_output_____"
]
],
[
[
"with open(csv_file) as f:\n row_count = sum(1 for row in f) # generator expression",
"_____no_output_____"
],
[
"f'{row_count:,} rows'",
"_____no_output_____"
]
],
[
[
"#### Inspect Raw Data",
"_____no_output_____"
]
],
[
[
"with open(csv_file) as f:\n for i, row in enumerate(f):\n pprint(row)\n if i == 3:\n break",
"'VendorID,tpep_pickup_datetime,tpep_dropoff_datetime,passenger_count,trip_distance,pickup_longitude,pickup_latitude,RatecodeID,store_and_fwd_flag,dropoff_longitude,dropoff_latitude,payment_type,fare_amount,extra,mta_tax,tip_amount,tolls_amount,improvement_surcharge,total_amount\\n'\n('1,2016-03-01 00:00:00,2016-03-01 '\n '00:07:55,1,2.50,-73.97674560546875,40.765151977539062,1,N,-74.004264831542969,40.746128082275391,1,9,0.5,0.5,2.05,0,0.3,12.35\\n')\n('1,2016-03-01 00:00:00,2016-03-01 '\n '00:11:06,1,2.90,-73.983482360839844,40.767925262451172,1,N,-74.005943298339844,40.733165740966797,1,11,0.5,0.5,3.05,0,0.3,15.35\\n')\n('2,2016-03-01 00:00:00,2016-03-01 '\n '00:31:06,2,19.98,-73.782020568847656,40.644809722900391,1,N,-73.974540710449219,40.675769805908203,1,54.5,0.5,0.5,8,0,0.3,63.8\\n')\n"
]
],
[
[
"Cleaner view",
"_____no_output_____"
]
],
[
[
"with open(csv_file) as f:\n csv_dict = csv.DictReader(f)\n for i, row in enumerate(csv_dict):\n pprint(row)\n if i ==3:\n break",
"{'RatecodeID': '1',\n 'VendorID': '1',\n 'dropoff_latitude': '40.746128082275391',\n 'dropoff_longitude': '-74.004264831542969',\n 'extra': '0.5',\n 'fare_amount': '9',\n 'improvement_surcharge': '0.3',\n 'mta_tax': '0.5',\n 'passenger_count': '1',\n 'payment_type': '1',\n 'pickup_latitude': '40.765151977539062',\n 'pickup_longitude': '-73.97674560546875',\n 'store_and_fwd_flag': 'N',\n 'tip_amount': '2.05',\n 'tolls_amount': '0',\n 'total_amount': '12.35',\n 'tpep_dropoff_datetime': '2016-03-01 00:07:55',\n 'tpep_pickup_datetime': '2016-03-01 00:00:00',\n 'trip_distance': '2.50'}\n{'RatecodeID': '1',\n 'VendorID': '1',\n 'dropoff_latitude': '40.733165740966797',\n 'dropoff_longitude': '-74.005943298339844',\n 'extra': '0.5',\n 'fare_amount': '11',\n 'improvement_surcharge': '0.3',\n 'mta_tax': '0.5',\n 'passenger_count': '1',\n 'payment_type': '1',\n 'pickup_latitude': '40.767925262451172',\n 'pickup_longitude': '-73.983482360839844',\n 'store_and_fwd_flag': 'N',\n 'tip_amount': '3.05',\n 'tolls_amount': '0',\n 'total_amount': '15.35',\n 'tpep_dropoff_datetime': '2016-03-01 00:11:06',\n 'tpep_pickup_datetime': '2016-03-01 00:00:00',\n 'trip_distance': '2.90'}\n{'RatecodeID': '1',\n 'VendorID': '2',\n 'dropoff_latitude': '40.675769805908203',\n 'dropoff_longitude': '-73.974540710449219',\n 'extra': '0.5',\n 'fare_amount': '54.5',\n 'improvement_surcharge': '0.3',\n 'mta_tax': '0.5',\n 'passenger_count': '2',\n 'payment_type': '1',\n 'pickup_latitude': '40.644809722900391',\n 'pickup_longitude': '-73.782020568847656',\n 'store_and_fwd_flag': 'N',\n 'tip_amount': '8',\n 'tolls_amount': '0',\n 'total_amount': '63.8',\n 'tpep_dropoff_datetime': '2016-03-01 00:31:06',\n 'tpep_pickup_datetime': '2016-03-01 00:00:00',\n 'trip_distance': '19.98'}\n{'RatecodeID': '1',\n 'VendorID': '2',\n 'dropoff_latitude': '40.757766723632812',\n 'dropoff_longitude': '-73.969650268554688',\n 'extra': '0',\n 'fare_amount': '31.5',\n 'improvement_surcharge': '0.3',\n 'mta_tax': '0.5',\n 'passenger_count': '3',\n 'payment_type': '1',\n 'pickup_latitude': '40.769813537597656',\n 'pickup_longitude': '-73.863418579101562',\n 'store_and_fwd_flag': 'N',\n 'tip_amount': '3.78',\n 'tolls_amount': '5.54',\n 'total_amount': '41.62',\n 'tpep_dropoff_datetime': '2016-03-01 00:00:00',\n 'tpep_pickup_datetime': '2016-03-01 00:00:00',\n 'trip_distance': '10.78'}\n"
]
],
[
[
"#### Import few rows using pandas",
"_____no_output_____"
]
],
[
[
"print(pd.read_csv(csv_file, nrows=2))",
" VendorID tpep_pickup_datetime tpep_dropoff_datetime passenger_count \\\n0 1 2016-03-01 00:00:00 2016-03-01 00:07:55 1 \n1 1 2016-03-01 00:00:00 2016-03-01 00:11:06 1 \n\n trip_distance pickup_longitude pickup_latitude RatecodeID \\\n0 2.5 -73.976746 40.765152 1 \n1 2.9 -73.983482 40.767925 1 \n\n store_and_fwd_flag dropoff_longitude dropoff_latitude payment_type \\\n0 N -74.004265 40.746128 1 \n1 N -74.005943 40.733166 1 \n\n fare_amount extra mta_tax tip_amount tolls_amount \\\n0 9 0.5 0.5 2.05 0 \n1 11 0.5 0.5 3.05 0 \n\n improvement_surcharge total_amount \n0 0.3 12.35 \n1 0.3 15.35 \n"
]
],
[
[
"Loaded succesfully!",
"_____no_output_____"
],
[
"### Calculating Size",
"_____no_output_____"
],
[
"Let’s load the first 10,000 lines into pandas and measure the amount of memory being used. Then we can calculate the total amount of memory needed to load the complete file",
"_____no_output_____"
]
],
[
[
"nrows = 10_000\nMB = 2**20 # 1 MB = 2**20 bytes = 1,048,576 bytes\ndf = pd.read_csv(csv_file, nrows=nrows)\ndf_mb = df.memory_usage(deep=True).sum() / MB \ndf_mb # how much memory the DataFrame is consuming in MB",
"_____no_output_____"
],
[
"df_mb * (row_count / nrows) # total memory consumption for the whole data file. ~4GB",
"_____no_output_____"
]
],
[
[
"### Import",
"_____no_output_____"
],
[
"#### Standard way -> Memory Error!",
"_____no_output_____"
]
],
[
[
"#df = pd.read_csv(file)",
"_____no_output_____"
],
[
"df.shape",
"_____no_output_____"
]
],
[
[
"<img src=\"img/memoryError.png\">",
"_____no_output_____"
]
],
[
[
"#df.info()",
"_____no_output_____"
]
],
[
[
"#### Solution A: Chunking!",
"_____no_output_____"
]
],
[
[
"chunk_size=50000\nbatch_no=1\nfor chunk in pd.read_csv(csv_file,chunksize=chunk_size):\n chunk.to_csv(DIR+'chunk'+str(batch_no)+'.csv',index=False)\n batch_no+=1",
"_____no_output_____"
]
],
[
[
"Importing a single chunk file into pandas dataframe:",
"_____no_output_____"
]
],
[
[
"DIR",
"_____no_output_____"
],
[
"df1 = pd.read_csv(DIR+'chunk2.csv')\ndf1.head()",
"_____no_output_____"
]
],
[
[
"#### Solution B: SQL!\n\n1. Create a Connector to a Database\n2. Build the Database (load CSV File) by Chunking\n3. Construct Pandas DF from Database using SQL query",
"_____no_output_____"
]
],
[
[
"# Create a Connector to a Database (creates it in Memory - not an SQL db)\ncsv_database = create_engine('sqlite:///csv_database.db')",
"_____no_output_____"
],
[
"# Build the Database (load CSV File) by Chunking\nchunk_size=10000\ni=0\nj=0\n\nfor df in pd.read_csv(csv_file, chunksize=chunk_size, iterator=True):\n df = df.rename(columns= {c: c.replace(' ','') for c in df.columns})\n # remove spaces in col names is important to make SQL run well\n df.index += j\n \n# Put all data in data_sql Table (temp table), using engine to fire it in background. Temp db sitting in Memory\n df.to_sql('data_sql', csv_database, if_exists = 'append') \n j = df.index[-1]+1\n \n print('| index: {}'.format(j))",
"dex: 223660000\n| index: 225780000\n| index: 227910000\n| index: 230050000\n| index: 232200000\n| index: 234360000\n| index: 236530000\n| index: 238710000\n| index: 240900000\n| index: 243100000\n| index: 245310000\n| index: 247530000\n| index: 249760000\n| index: 252000000\n| index: 254250000\n| index: 256510000\n| index: 258780000\n| index: 261060000\n| index: 263350000\n| index: 265650000\n| index: 267960000\n| index: 270280000\n| index: 272610000\n| index: 274950000\n| index: 277300000\n| index: 279660000\n| index: 282030000\n| index: 284410000\n| index: 286800000\n| index: 289200000\n| index: 291610000\n| index: 294030000\n| index: 296460000\n| index: 298900000\n| index: 301350000\n| index: 303810000\n| index: 306280000\n| index: 308760000\n| index: 311250000\n| index: 313750000\n| index: 316260000\n| index: 318780000\n| index: 321310000\n| index: 323850000\n| index: 326400000\n| index: 328960000\n| index: 331530000\n| index: 334110000\n| index: 336700000\n| index: 339300000\n| index: 341910000\n| index: 344530000\n| index: 347160000\n| index: 349800000\n| index: 352450000\n| index: 355110000\n| index: 357780000\n| index: 360460000\n| index: 363150000\n| index: 365850000\n| index: 368560000\n| index: 371280000\n| index: 374010000\n| index: 376750000\n| index: 379500000\n| index: 382260000\n| index: 385030000\n| index: 387810000\n| index: 390600000\n| index: 393400000\n| index: 396210000\n| index: 399030000\n| index: 401860000\n| index: 404700000\n| index: 407550000\n| index: 410410000\n| index: 413280000\n| index: 416160000\n| index: 419050000\n| index: 421950000\n| index: 424860000\n| index: 427780000\n| index: 430710000\n| index: 433650000\n| index: 436600000\n| index: 439560000\n| index: 442530000\n| index: 445510000\n| index: 448500000\n| index: 451500000\n| index: 454510000\n| index: 457530000\n| index: 460560000\n| index: 463600000\n| index: 466650000\n| index: 469710000\n| index: 472780000\n| index: 475860000\n| index: 478950000\n| index: 482050000\n| index: 485160000\n| index: 488280000\n| index: 491410000\n| index: 494550000\n| index: 497700000\n| index: 500860000\n| index: 504030000\n| index: 507210000\n| index: 510400000\n| index: 513600000\n| index: 516810000\n| index: 520030000\n| index: 523260000\n| index: 526500000\n| index: 529750000\n| index: 533010000\n| index: 536280000\n| index: 539560000\n| index: 542850000\n| index: 546150000\n| index: 549460000\n| index: 552780000\n| index: 556110000\n| index: 559450000\n| index: 562800000\n| index: 566160000\n| index: 569530000\n| index: 572910000\n| index: 576300000\n| index: 579700000\n| index: 583110000\n| index: 586530000\n| index: 589960000\n| index: 593400000\n| index: 596850000\n| index: 600310000\n| index: 603780000\n| index: 607260000\n| index: 610750000\n| index: 614250000\n| index: 617760000\n| index: 621280000\n| index: 624810000\n| index: 628350000\n| index: 631900000\n| index: 635460000\n| index: 639030000\n| index: 642610000\n| index: 646200000\n| index: 649800000\n| index: 653410000\n| index: 657030000\n| index: 660660000\n| index: 664300000\n| index: 667950000\n| index: 671610000\n| index: 675280000\n| index: 678960000\n| index: 682650000\n| index: 686350000\n| index: 690060000\n| index: 693780000\n| index: 697510000\n| index: 701250000\n| index: 705000000\n| index: 708760000\n| index: 712530000\n| index: 716310000\n| index: 720100000\n| index: 723900000\n| index: 727710000\n| index: 731530000\n| index: 735360000\n| index: 739200000\n| index: 743050000\n| index: 746910000\n| index: 750780000\n| index: 754660000\n| index: 758550000\n| index: 762450000\n| index: 766360000\n| index: 770280000\n| index: 774210000\n| index: 778150000\n| index: 782100000\n| index: 786060000\n| index: 790030000\n| index: 794010000\n| index: 798000000\n| index: 802000000\n| index: 806010000\n| index: 810030000\n| index: 814060000\n| index: 818100000\n| index: 822150000\n| index: 826210000\n| index: 830280000\n| index: 834360000\n| index: 838450000\n| index: 842550000\n| index: 846660000\n| index: 850780000\n| index: 854910000\n| index: 859050000\n| index: 863200000\n| index: 867360000\n| index: 871530000\n| index: 875710000\n| index: 879900000\n| index: 884100000\n| index: 888310000\n| index: 892530000\n| index: 896760000\n| index: 901000000\n| index: 905250000\n| index: 909510000\n| index: 913780000\n| index: 918060000\n| index: 922350000\n| index: 926650000\n| index: 930960000\n| index: 935280000\n| index: 939610000\n| index: 943950000\n| index: 948300000\n| index: 952660000\n| index: 957030000\n| index: 961410000\n| index: 965800000\n| index: 970200000\n| index: 974610000\n| index: 979030000\n| index: 983460000\n| index: 987900000\n| index: 992350000\n| index: 996810000\n| index: 1001280000\n| index: 1005760000\n| index: 1010250000\n| index: 1014750000\n| index: 1019260000\n| index: 1023780000\n| index: 1028310000\n| index: 1032850000\n| index: 1037400000\n| index: 1041960000\n| index: 1046530000\n| index: 1051110000\n| index: 1055700000\n| index: 1060300000\n| index: 1064910000\n| index: 1069530000\n| index: 1074160000\n| index: 1078800000\n| index: 1083450000\n| index: 1088110000\n| index: 1092780000\n| index: 1097460000\n| index: 1102150000\n| index: 1106850000\n| index: 1111560000\n| index: 1116280000\n| index: 1121010000\n| index: 1125750000\n| index: 1130500000\n| index: 1135260000\n| index: 1140030000\n| index: 1144810000\n| index: 1149600000\n| index: 1154400000\n| index: 1159210000\n| index: 1164030000\n| index: 1168860000\n| index: 1173700000\n| index: 1178550000\n| index: 1183410000\n| index: 1188280000\n| index: 1193160000\n| index: 1198050000\n| index: 1202950000\n| index: 1207860000\n| index: 1212780000\n| index: 1217710000\n| index: 1222650000\n| index: 1227600000\n| index: 1232560000\n| index: 1237530000\n| index: 1242510000\n| index: 1247500000\n| index: 1252500000\n| index: 1257510000\n| index: 1262530000\n| index: 1267560000\n| index: 1272600000\n| index: 1277650000\n| index: 1282710000\n| index: 1287780000\n| index: 1292860000\n| index: 1297950000\n| index: 1303050000\n| index: 1308160000\n| index: 1313280000\n| index: 1318410000\n| index: 1323550000\n| index: 1328700000\n| index: 1333860000\n| index: 1339030000\n| index: 1344210000\n| index: 1349400000\n| index: 1354600000\n| index: 1359810000\n| index: 1365030000\n| index: 1370260000\n| index: 1375500000\n| index: 1380750000\n| index: 1386010000\n| index: 1391280000\n| index: 1396560000\n| index: 1401850000\n| index: 1407150000\n| index: 1412460000\n| index: 1417780000\n| index: 1423110000\n| index: 1428450000\n| index: 1433800000\n| index: 1439160000\n| index: 1444530000\n| index: 1449910000\n| index: 1455300000\n| index: 1460700000\n| index: 1466110000\n| index: 1471530000\n| index: 1476960000\n| index: 1482400000\n| index: 1487850000\n| index: 1493310000\n| index: 1498780000\n| index: 1504260000\n| index: 1509750000\n| index: 1515250000\n| index: 1520760000\n| index: 1526280000\n| index: 1531810000\n| index: 1537350000\n| index: 1542900000\n| index: 1548460000\n| index: 1554030000\n| index: 1559610000\n| index: 1565200000\n| index: 1570800000\n| index: 1576410000\n| index: 1582030000\n| index: 1587660000\n| index: 1593300000\n| index: 1598950000\n| index: 1604610000\n| index: 1610280000\n| index: 1615960000\n| index: 1621650000\n| index: 1627350000\n| index: 1633060000\n| index: 1638780000\n| index: 1644510000\n| index: 1650250000\n| index: 1656000000\n| index: 1661760000\n| index: 1667530000\n| index: 1673310000\n| index: 1679100000\n| index: 1684900000\n| index: 1690710000\n| index: 1696530000\n| index: 1702360000\n| index: 1708200000\n| index: 1714050000\n| index: 1719910000\n| index: 1725780000\n| index: 1731660000\n| index: 1737550000\n| index: 1743450000\n| index: 1749360000\n| index: 1755280000\n| index: 1761210000\n| index: 1767150000\n| index: 1773100000\n| index: 1779060000\n| index: 1785030000\n| index: 1791010000\n| index: 1797000000\n| index: 1803000000\n| index: 1809010000\n| index: 1815030000\n| index: 1821060000\n| index: 1827100000\n| index: 1833150000\n| index: 1839210000\n| index: 1845280000\n| index: 1851360000\n| index: 1857450000\n| index: 1863550000\n| index: 1869660000\n| index: 1875780000\n| index: 1881910000\n| index: 1888050000\n| index: 1894200000\n| index: 1900360000\n| index: 1906530000\n| index: 1912710000\n| index: 1918900000\n| index: 1925100000\n| index: 1931310000\n| index: 1937530000\n| index: 1943760000\n| index: 1950000000\n| index: 1956250000\n| index: 1962510000\n| index: 1968780000\n| index: 1975060000\n| index: 1981350000\n| index: 1987650000\n| index: 1993960000\n| index: 2000280000\n| index: 2006610000\n| index: 2012950000\n| index: 2019300000\n| index: 2025660000\n| index: 2032030000\n| index: 2038410000\n| index: 2044800000\n| index: 2051200000\n| index: 2057610000\n| index: 2064030000\n| index: 2070460000\n| index: 2076900000\n| index: 2083350000\n| index: 2089810000\n| index: 2096280000\n| index: 2102760000\n| index: 2109250000\n| index: 2115750000\n| index: 2122260000\n| index: 2128780000\n| index: 2135310000\n| index: 2141850000\n| index: 2148400000\n| index: 2154960000\n| index: 2161530000\n| index: 2168110000\n| index: 2174700000\n| index: 2181300000\n| index: 2187910000\n| index: 2194530000\n| index: 2201160000\n| index: 2207800000\n| index: 2214450000\n| index: 2221110000\n| index: 2227780000\n| index: 2234460000\n| index: 2241150000\n| index: 2247850000\n| index: 2254560000\n| index: 2261280000\n| index: 2268010000\n| index: 2274750000\n| index: 2281500000\n| index: 2288260000\n| index: 2295030000\n| index: 2301810000\n| index: 2308600000\n| index: 2315400000\n| index: 2322210000\n| index: 2329030000\n| index: 2335860000\n| index: 2342700000\n| index: 2349550000\n| index: 2356410000\n| index: 2363280000\n| index: 2370160000\n| index: 2377050000\n| index: 2383950000\n| index: 2390860000\n| index: 2397780000\n| index: 2404710000\n| index: 2411650000\n| index: 2418600000\n| index: 2425560000\n| index: 2432530000\n| index: 2439510000\n| index: 2446500000\n| index: 2453500000\n| index: 2460510000\n| index: 2467530000\n| index: 2474560000\n| index: 2481600000\n| index: 2488650000\n| index: 2495710000\n| index: 2502780000\n| index: 2509860000\n| index: 2516950000\n| index: 2524050000\n| index: 2531160000\n| index: 2538280000\n| index: 2545410000\n| index: 2552550000\n| index: 2559700000\n| index: 2566860000\n| index: 2574030000\n| index: 2581210000\n| index: 2588400000\n| index: 2595600000\n| index: 2602810000\n| index: 2610030000\n| index: 2617260000\n| index: 2624500000\n| index: 2631750000\n| index: 2639010000\n| index: 2646280000\n| index: 2653560000\n| index: 2660850000\n| index: 2668150000\n| index: 2675460000\n| index: 2682780000\n| index: 2690110000\n| index: 2697450000\n| index: 2704800000\n| index: 2712160000\n| index: 2719530000\n| index: 2726910000\n| index: 2734300000\n| index: 2741700000\n| index: 2749110000\n| index: 2756530000\n| index: 2763960000\n| index: 2771400000\n| index: 2778850000\n| index: 2786310000\n| index: 2793780000\n| index: 2801260000\n| index: 2808750000\n| index: 2816250000\n| index: 2823760000\n| index: 2831280000\n| index: 2838810000\n| index: 2846350000\n| index: 2853900000\n| index: 2861460000\n| index: 2869030000\n| index: 2876610000\n| index: 2884200000\n| index: 2891800000\n| index: 2899410000\n| index: 2907030000\n| index: 2914660000\n| index: 2922300000\n| index: 2929950000\n| index: 2937610000\n| index: 2945280000\n| index: 2952960000\n| index: 2960650000\n| index: 2968350000\n| index: 2976060000\n| index: 2983780000\n| index: 2991510000\n| index: 2999250000\n| index: 3007000000\n| index: 3014760000\n| index: 3022530000\n| index: 3030310000\n| index: 3038100000\n| index: 3045900000\n| index: 3053710000\n| index: 3061530000\n| index: 3069360000\n| index: 3077200000\n| index: 3085050000\n| index: 3092910000\n| index: 3100780000\n| index: 3108660000\n| index: 3116550000\n| index: 3124450000\n| index: 3132360000\n| index: 3140280000\n| index: 3148210000\n| index: 3156150000\n| index: 3164100000\n| index: 3172060000\n| index: 3180030000\n| index: 3188010000\n| index: 3196000000\n| index: 3204000000\n| index: 3212010000\n| index: 3220030000\n| index: 3228060000\n| index: 3236100000\n| index: 3244150000\n| index: 3252210000\n| index: 3260280000\n| index: 3268360000\n| index: 3276450000\n| index: 3284550000\n| index: 3292660000\n| index: 3300780000\n| index: 3308910000\n| index: 3317050000\n| index: 3325200000\n| index: 3333360000\n| index: 3341530000\n| index: 3349710000\n| index: 3357900000\n| index: 3366100000\n| index: 3374310000\n| index: 3382530000\n| index: 3390760000\n| index: 3399000000\n| index: 3407250000\n| index: 3415510000\n| index: 3423780000\n| index: 3432060000\n| index: 3440350000\n| index: 3448650000\n| index: 3456960000\n| index: 3465280000\n| index: 3473610000\n| index: 3481950000\n| index: 3490300000\n| index: 3498660000\n| index: 3507030000\n| index: 3515410000\n| index: 3523800000\n| index: 3532200000\n| index: 3540610000\n| index: 3549030000\n| index: 3557460000\n| index: 3565900000\n| index: 3574350000\n| index: 3582810000\n| index: 3591280000\n| index: 3599760000\n| index: 3608250000\n| index: 3616750000\n| index: 3625260000\n| index: 3633780000\n| index: 3642310000\n| index: 3650850000\n| index: 3659400000\n| index: 3667960000\n| index: 3676530000\n| index: 3685110000\n| index: 3693700000\n| index: 3702300000\n| index: 3710910000\n| index: 3719530000\n| index: 3728160000\n| index: 3736800000\n| index: 3745450000\n| index: 3754110000\n| index: 3762780000\n| index: 3771460000\n| index: 3780150000\n| index: 3788850000\n| index: 3797560000\n| index: 3806280000\n| index: 3815010000\n| index: 3823750000\n| index: 3832500000\n| index: 3841260000\n| index: 3850030000\n| index: 3858810000\n| index: 3867600000\n| index: 3876400000\n| index: 3885210000\n| index: 3894030000\n| index: 3902860000\n| index: 3911700000\n| index: 3920550000\n| index: 3929410000\n| index: 3938280000\n| index: 3947160000\n| index: 3956050000\n| index: 3964950000\n| index: 3973860000\n| index: 3982780000\n| index: 3991710000\n| index: 4000650000\n| index: 4009600000\n| index: 4018560000\n| index: 4027530000\n| index: 4036510000\n| index: 4045500000\n| index: 4054500000\n| index: 4063510000\n| index: 4072530000\n| index: 4081560000\n| index: 4090600000\n| index: 4099650000\n| index: 4108710000\n| index: 4117780000\n| index: 4126860000\n| index: 4135950000\n| index: 4145050000\n| index: 4154160000\n| index: 4163280000\n| index: 4172410000\n| index: 4181550000\n| index: 4190700000\n| index: 4199860000\n| index: 4209030000\n| index: 4218210000\n| index: 4227400000\n| index: 4236600000\n| index: 4245810000\n| index: 4255030000\n| index: 4264260000\n| index: 4273500000\n| index: 4282750000\n| index: 4292010000\n| index: 4301280000\n| index: 4310560000\n| index: 4319850000\n| index: 4329150000\n| index: 4338460000\n| index: 4347780000\n| index: 4357110000\n| index: 4366450000\n| index: 4375800000\n| index: 4385160000\n| index: 4394530000\n| index: 4403910000\n| index: 4413300000\n| index: 4422700000\n| index: 4432110000\n| index: 4441530000\n| index: 4450960000\n| index: 4460400000\n| index: 4469850000\n| index: 4479310000\n| index: 4488780000\n| index: 4498260000\n| index: 4507750000\n| index: 4517250000\n| index: 4526760000\n| index: 4536280000\n| index: 4545810000\n| index: 4555350000\n| index: 4564900000\n| index: 4574460000\n| index: 4584030000\n| index: 4593610000\n| index: 4603200000\n| index: 4612800000\n| index: 4622410000\n| index: 4632030000\n| index: 4641660000\n| index: 4651300000\n| index: 4660950000\n| index: 4670610000\n| index: 4680280000\n| index: 4689960000\n| index: 4699650000\n| index: 4709350000\n| index: 4719060000\n| index: 4728780000\n| index: 4738510000\n| index: 4748250000\n| index: 4758000000\n| index: 4767760000\n| index: 4777530000\n| index: 4787310000\n| index: 4797100000\n| index: 4806900000\n| index: 4816710000\n| index: 4826530000\n| index: 4836360000\n| index: 4846200000\n| index: 4856050000\n| index: 4865910000\n| index: 4875780000\n| index: 4885660000\n| index: 4895550000\n| index: 4905450000\n| index: 4915360000\n| index: 4925280000\n| index: 4935210000\n| index: 4945150000\n| index: 4955100000\n| index: 4965060000\n| index: 4975030000\n| index: 4985010000\n| index: 4995000000\n| index: 5005000000\n| index: 5015010000\n| index: 5025030000\n| index: 5035060000\n| index: 5045100000\n| index: 5055150000\n| index: 5065210000\n| index: 5075280000\n| index: 5085360000\n| index: 5095450000\n| index: 5105550000\n| index: 5115660000\n| index: 5125780000\n| index: 5135910000\n| index: 5146050000\n| index: 5156200000\n| index: 5166360000\n| index: 5176530000\n| index: 5186710000\n| index: 5196900000\n| index: 5207100000\n| index: 5217310000\n| index: 5227530000\n| index: 5237760000\n| index: 5248000000\n| index: 5258250000\n| index: 5268510000\n| index: 5278780000\n| index: 5289060000\n| index: 5299350000\n| index: 5309650000\n| index: 5319960000\n| index: 5330280000\n| index: 5340610000\n| index: 5350950000\n| index: 5361300000\n| index: 5371660000\n| index: 5382030000\n| index: 5392410000\n| index: 5402800000\n| index: 5413200000\n| index: 5423610000\n| index: 5434030000\n| index: 5444460000\n| index: 5454900000\n| index: 5465350000\n| index: 5475810000\n| index: 5486280000\n| index: 5496760000\n| index: 5507250000\n| index: 5517750000\n| index: 5528260000\n| index: 5538780000\n| index: 5549310000\n| index: 5559850000\n| index: 5570400000\n| index: 5580960000\n| index: 5591530000\n| index: 5602110000\n| index: 5612700000\n| index: 5623300000\n| index: 5633910000\n| index: 5644530000\n| index: 5655160000\n| index: 5665800000\n| index: 5676450000\n| index: 5687110000\n| index: 5697780000\n| index: 5708460000\n| index: 5719150000\n| index: 5729850000\n| index: 5740560000\n| index: 5751280000\n| index: 5762010000\n| index: 5772750000\n| index: 5783500000\n| index: 5794260000\n| index: 5805030000\n| index: 5815810000\n| index: 5826600000\n| index: 5837400000\n| index: 5848210000\n| index: 5859030000\n| index: 5869860000\n| index: 5880700000\n| index: 5891550000\n| index: 5902410000\n| index: 5913280000\n| index: 5924160000\n| index: 5935050000\n| index: 5945950000\n| index: 5956860000\n| index: 5967780000\n| index: 5978710000\n| index: 5989650000\n| index: 6000600000\n| index: 6011560000\n| index: 6022530000\n| index: 6033510000\n| index: 6044500000\n| index: 6055500000\n| index: 6066510000\n| index: 6077530000\n| index: 6088560000\n| index: 6099600000\n| index: 6110650000\n| index: 6121710000\n| index: 6132780000\n| index: 6143860000\n| index: 6154950000\n| index: 6166050000\n| index: 6177160000\n| index: 6188280000\n| index: 6199410000\n| index: 6210550000\n| index: 6221700000\n| index: 6232860000\n| index: 6244030000\n| index: 6255210000\n| index: 6266400000\n| index: 6277600000\n| index: 6288810000\n| index: 6300030000\n| index: 6311260000\n| index: 6322500000\n| index: 6333750000\n| index: 6345010000\n| index: 6356280000\n| index: 6367560000\n| index: 6378850000\n| index: 6390150000\n| index: 6401460000\n| index: 6412780000\n| index: 6424110000\n| index: 6435450000\n| index: 6446800000\n| index: 6458160000\n| index: 6469530000\n| index: 6480910000\n| index: 6492300000\n| index: 6503700000\n| index: 6515110000\n| index: 6526530000\n| index: 6537960000\n| index: 6549400000\n| index: 6560850000\n| index: 6572310000\n| index: 6583780000\n| index: 6595260000\n| index: 6606750000\n| index: 6618250000\n| index: 6629760000\n| index: 6641280000\n| index: 6652810000\n| index: 6664350000\n| index: 6675900000\n| index: 6687460000\n| index: 6699030000\n| index: 6710610000\n| index: 6722200000\n| index: 6733800000\n| index: 6745410000\n| index: 6757030000\n| index: 6768660000\n| index: 6780300000\n| index: 6791950000\n| index: 6803610000\n| index: 6815280000\n| index: 6826960000\n| index: 6838650000\n| index: 6850350000\n| index: 6862060000\n| index: 6873780000\n| index: 6885510000\n| index: 6897250000\n| index: 6909000000\n| index: 6920760000\n| index: 6932530000\n| index: 6944310000\n| index: 6956100000\n| index: 6967900000\n| index: 6979710000\n| index: 6991530000\n| index: 7003360000\n| index: 7015200000\n| index: 7027050000\n| index: 7038910000\n| index: 7050780000\n| index: 7062660000\n| index: 7074550000\n| index: 7086450000\n| index: 7098360000\n| index: 7110280000\n| index: 7122210000\n| index: 7134150000\n| index: 7146100000\n| index: 7158060000\n| index: 7170030000\n| index: 7182010000\n| index: 7194000000\n| index: 7206000000\n| index: 7218010000\n| index: 7230030000\n| index: 7242060000\n| index: 7254100000\n| index: 7266150000\n| index: 7278210000\n| index: 7290280000\n| index: 7302360000\n| index: 7314450000\n| index: 7326550000\n| index: 7338660000\n| index: 7350780000\n| index: 7362910000\n| index: 7375050000\n| index: 7387200000\n| index: 7399360000\n| index: 7411530000\n| index: 7423710000\n| index: 7435900000\n| index: 7448100000\n| index: 7460310000\n| index: 7472520952\n"
],
[
"df2 = pd.read_sql_query('SELECT * FROM data_sql WHERE VendorID=1 AND trip_distance=2.90', csv_database)",
"_____no_output_____"
],
[
"df2",
"_____no_output_____"
],
[
"df2.columns",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
]
] |
e7bc569e8fc186b1101c1a8a2fe31e8e04de2ced | 4,111 | ipynb | Jupyter Notebook | 0.16/_downloads/plot_decoding_time_generalization_conditions.ipynb | drammock/mne-tools.github.io | 5d3a104d174255644d8d5335f58036e32695e85d | [
"BSD-3-Clause"
] | null | null | null | 0.16/_downloads/plot_decoding_time_generalization_conditions.ipynb | drammock/mne-tools.github.io | 5d3a104d174255644d8d5335f58036e32695e85d | [
"BSD-3-Clause"
] | null | null | null | 0.16/_downloads/plot_decoding_time_generalization_conditions.ipynb | drammock/mne-tools.github.io | 5d3a104d174255644d8d5335f58036e32695e85d | [
"BSD-3-Clause"
] | null | null | null | 76.12963 | 2,507 | 0.657991 | [
[
[
"%matplotlib inline",
"_____no_output_____"
]
],
[
[
"\n# Decoding sensor space data with generalization across time and conditions\n\n\nThis example runs the analysis described in [1]_. It illustrates how one can\nfit a linear classifier to identify a discriminatory topography at a given time\ninstant and subsequently assess whether this linear model can accurately\npredict all of the time samples of a second set of conditions.\n\nReferences\n----------\n\n.. [1] King & Dehaene (2014) 'Characterizing the dynamics of mental\n representations: the Temporal Generalization method', Trends In\n Cognitive Sciences, 18(4), 203-210. doi: 10.1016/j.tics.2014.01.002.\n\n",
"_____no_output_____"
]
],
[
[
"# Authors: Jean-Remi King <[email protected]>\n# Alexandre Gramfort <[email protected]>\n# Denis Engemann <[email protected]>\n#\n# License: BSD (3-clause)\n\nimport matplotlib.pyplot as plt\n\nfrom sklearn.pipeline import make_pipeline\nfrom sklearn.preprocessing import StandardScaler\nfrom sklearn.linear_model import LogisticRegression\n\nimport mne\nfrom mne.datasets import sample\nfrom mne.decoding import GeneralizingEstimator\n\nprint(__doc__)\n\n# Preprocess data\ndata_path = sample.data_path()\n# Load and filter data, set up epochs\nraw_fname = data_path + '/MEG/sample/sample_audvis_filt-0-40_raw.fif'\nevents_fname = data_path + '/MEG/sample/sample_audvis_filt-0-40_raw-eve.fif'\nraw = mne.io.read_raw_fif(raw_fname, preload=True)\npicks = mne.pick_types(raw.info, meg=True, exclude='bads') # Pick MEG channels\nraw.filter(1., 30., fir_design='firwin') # Band pass filtering signals\nevents = mne.read_events(events_fname)\nevent_id = {'Auditory/Left': 1, 'Auditory/Right': 2,\n 'Visual/Left': 3, 'Visual/Right': 4}\ntmin = -0.050\ntmax = 0.400\ndecim = 2 # decimate to make the example faster to run\nepochs = mne.Epochs(raw, events, event_id=event_id, tmin=tmin, tmax=tmax,\n proj=True, picks=picks, baseline=None, preload=True,\n reject=dict(mag=5e-12), decim=decim)\n\n# We will train the classifier on all left visual vs auditory trials\n# and test on all right visual vs auditory trials.\nclf = make_pipeline(StandardScaler(), LogisticRegression())\ntime_gen = GeneralizingEstimator(clf, scoring='roc_auc', n_jobs=1)\n\n# Fit classifiers on the epochs where the stimulus was presented to the left.\n# Note that the experimental condition y indicates auditory or visual\ntime_gen.fit(X=epochs['Left'].get_data(),\n y=epochs['Left'].events[:, 2] > 2)\n\n# Score on the epochs where the stimulus was presented to the right.\nscores = time_gen.score(X=epochs['Right'].get_data(),\n y=epochs['Right'].events[:, 2] > 2)\n\n# Plot\nfig, ax = plt.subplots(1)\nim = ax.matshow(scores, vmin=0, vmax=1., cmap='RdBu_r', origin='lower',\n extent=epochs.times[[0, -1, 0, -1]])\nax.axhline(0., color='k')\nax.axvline(0., color='k')\nax.xaxis.set_ticks_position('bottom')\nax.set_xlabel('Testing Time (s)')\nax.set_ylabel('Training Time (s)')\nax.set_title('Generalization across time and condition')\nplt.colorbar(im, ax=ax)\nplt.show()",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code"
] | [
[
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7bc5ecf7e83bc4329949c7808ccfd3dd63e9ffd | 1,734 | ipynb | Jupyter Notebook | docs/notebooks/05_Abstracting.ipynb | TheV1rtuoso/debuggingbook | dd4a1605cff793f614e67fbaef74de7d20e0519c | [
"MIT"
] | 1 | 2021-06-20T05:07:35.000Z | 2021-06-20T05:07:35.000Z | docs/notebooks/05_Abstracting.ipynb | TheV1rtuoso/debuggingbook | dd4a1605cff793f614e67fbaef74de7d20e0519c | [
"MIT"
] | null | null | null | docs/notebooks/05_Abstracting.ipynb | TheV1rtuoso/debuggingbook | dd4a1605cff793f614e67fbaef74de7d20e0519c | [
"MIT"
] | null | null | null | 25.5 | 202 | 0.61707 | [
[
[
"# Part V: Abstracting Failures\n\nIn this part, we show how to determine abstract failure conditions.\n\n* [Statistical Debugging](StatisticalDebugger.ipynb) is a technique to determine events during execution that _correlate with failure_ – for instance, the coverage of individual code locations.\n\n* [Mining Function Specifications](DynamicInvariants.ipynb) shows how to learn preconditions and postconditions from given executions.\n\n* In [Generalizing Failure Circumstances](DDSetDebugger.ipynb), we discuss how to automatically determine the (input) conditions under which the failure occurs.",
"_____no_output_____"
]
]
] | [
"markdown"
] | [
[
"markdown"
]
] |
e7bc65bdc8823289d6cc8f5aeb935df71a28f36e | 44,549 | ipynb | Jupyter Notebook | site/el/guide/keras/overview.ipynb | KarimaTouati/docs-l10n | 583c00a412cefd168a19975bf2f4cef2e2fc9dca | [
"Apache-2.0"
] | null | null | null | site/el/guide/keras/overview.ipynb | KarimaTouati/docs-l10n | 583c00a412cefd168a19975bf2f4cef2e2fc9dca | [
"Apache-2.0"
] | null | null | null | site/el/guide/keras/overview.ipynb | KarimaTouati/docs-l10n | 583c00a412cefd168a19975bf2f4cef2e2fc9dca | [
"Apache-2.0"
] | null | null | null | 37.65765 | 499 | 0.565041 | [
[
[
"##### Copyright 2019 The TensorFlow Authors.",
"_____no_output_____"
]
],
[
[
"#@title Licensed under the Apache License, Version 2.0 (the \"License\");\n# you may not use this file except in compliance with the License.\n# You may obtain a copy of the License at\n#\n# https://www.apache.org/licenses/LICENSE-2.0\n#\n# Unless required by applicable law or agreed to in writing, software\n# distributed under the License is distributed on an \"AS IS\" BASIS,\n# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.\n# See the License for the specific language governing permissions and\n# limitations under the License.",
"_____no_output_____"
]
],
[
[
"# Σύνοψη Keras\n",
"_____no_output_____"
],
[
"<table class=\"tfo-notebook-buttons\" align=\"left\">\n <td>\n <a target=\"_blank\" href=\"https://www.tensorflow.org/guide/keras/overview\"><img src=\"https://www.tensorflow.org/images/tf_logo_32px.png\" /> Άνοιγμα στο TensorFlow.org</a>\n </td>\n <td>\n <a target=\"_blank\" href=\"https://colab.research.google.com/github/tensorflow/docs/blob/master/site/e1/guide/keras/overview.ipynb\"><img src=\"https://www.tensorflow.org/images/colab_logo_32px.png\" /> Εκτέλεση στο Google Colab</a>\n </td>\n <td>\n <a target=\"_blank\" href=\"https://github.com/tensorflow/docs/blob/master/site/e1/guide/keras/overview.ipynb\"><img src=\"https://www.tensorflow.org/images/GitHub-Mark-32px.png\" /> Προβολή πηγαίου στο GitHub</a>\n </td>\n <td>\n <a href=\"https://storage.googleapis.com/tensorflow_docs/docs/site/e1/guide/keras/overview.ipynb\"><img src=\"https://www.tensorflow.org/images/download_logo_32px.png\" /> Λήψη \"σημειωματάριου\"</a>\n </td>\n</table>",
"_____no_output_____"
],
[
"Note: Η κοινότητα του TensorFlow έχει μεταφράσει αυτά τα έγγραφα. Καθότι οι μεταφράσεις αυτές αποτελούν την καλύτερη δυνατή προσπάθεια , δεν υπάρχει εγγύηση ότι θα παραμείνουν ενημερωμένες σε σχέση με τα [επίσημα Αγγλικά έγγραφα](https://www.tensorflow.org/?hl=en).\nΑν έχετε υποδείξεις για βελτίωση των αρχείων αυτών , δημιουργήστε ένα pull request στο [tensorflow/docs](https://github.com/tensorflow/docs) GitHub repository . Για να συμμετέχετε στη σύνταξη ή στην αναθεώρηση των μεταφράσεων της κοινότητας , επικοινωνήστε με το [[email protected] list](https://groups.google.com/a/tensorflow.org/forum/#!forum/docs).",
"_____no_output_____"
],
[
"Ο οδήγός αυτός προσφέρει τα βασικά ώστε να ξεκινήσετε να χρησιμοποιείτε το Keras. Η διάρκεια ανάγνωσης του είναι περίπου 10 λεπτά.",
"_____no_output_____"
],
[
"# Εισαγωγή tf.keras\n\n`tf.keras` . Πρόκειται για την υλοποίησης Διασύνδεσης Προγραμματισμού Εφαρμογών (API) του Keras. Είναι ένα API υψηλού επιπέδου που μπορεί να δημιουργήσει και να εκπαιδεύσει μοντέλα τα οποία θα υποστηρίζουν άμεσα τις υπόλοιπες λειτουργίες του TensorFlow , όπως η [\"ενθουσιώδης\" εκτέλεση](https://www.tensorflow.org/guide/eager) , `tf.data` αγωγοί , και [Εκτιμητές](https://www.tensorflow.org/guide/estimator)\n\nΤο `tf.keras` καθιστά το TensorFlow ευκολότερο στη χρήση , χωρίς να θυσιάζει σε προσαρμοστικότητα και απόδοση\n\nΓια να ξεκινήσετε , εισάγετε το `tf.keras` ως μέρος του προγράμματος TensorFlow\nσας : ",
"_____no_output_____"
]
],
[
[
"from __future__ import absolute_import, division, print_function, unicode_literals\n\ntry:\n # %tensorflow_version only exists in Colab.\n %tensorflow_version 2.x\nexcept Exception:\n pass\nimport tensorflow as tf\n\nfrom tensorflow import keras",
"_____no_output_____"
]
],
[
[
"Το tf.keras μπορέι να τρέξει οποιοδήποτε κομμάτι συμβατού κώδικα Keras , ωστόσο να έχετε υπόψιν σας τα εξής : \n\n* Η έκδοση `tf.keras` της τελευταίας έκδοσης του TensorFlow μπορεί να μην ταυτίζεται με την τελευταία έκδοση `keras` από το PyPI. Ελέγξτε το `tf.keras.__version__.`\n* Όταν [αποθηκεύετε τα βάρη ενός μοντέλου](https://colab.research.google.com/github/tensorflow/docs/blob/master/site/en/guide/keras/save_and_serialize.ipynb) το `tf.keras` θα προεπιλέγει την [μορφοποίηση σημείων ελέγχου](https://colab.research.google.com/github/tensorflow/docs/blob/master/site/en/guide/checkpoint.ipynb). Θέτοντας `save_format='h5'` χρησιμοποιείται η μορφοποίηση HDF5 (εναλλακτικά, ορίστε την επέκταση του αρχείου σε `.h5`)",
"_____no_output_____"
],
[
"# Δημιουργία απλού μοντέλου \n\n## Σειριακό μοντέλο\n\nΣτο Keras , συναθροίζοντας στρώματα(layers) δημιουργούνται τα *μοντελα (models)*. Ένα μοντέλο είναι (συνήθως) ένα γράφημα στρωμάτων. Ο πιο συνηθισμένος τύπος μοντέλου είναι η στοίβα (stack) στρωμάτων : το `tf.keras.Sequential` μοντέλο\n\nΓια την κατασκευή ενός απλού, πλήρως συνδεδεμένου (νευρωνικού) δικτύου (για παράδειγμα : πολυεπίπεδο perceptron): \n",
"_____no_output_____"
]
],
[
[
"from tensorflow.keras import layers\n\nmodel = tf.keras.Sequential()\n# Adds a densely-connected layer with 64 units to the model:\nmodel.add(layers.Dense(64, activation='relu'))\n# Add another:\nmodel.add(layers.Dense(64, activation='relu'))\n# Add a softmax layer with 10 output units:\nmodel.add(layers.Dense(10, activation='softmax'))",
"_____no_output_____"
]
],
[
[
"Μπορείτε να βρείτε ένα ολοκληρωμένο και σύντομο παράδειγμα για το πώς χρησιμοποιούνται τα σειριακά μοντέλα [εδώ](https://www.tensorflow.org/tutorials/quickstart/beginner)\n\nΓια να μάθετε να δημιουργείτε πιο προχωρημένα μοντέλα από τα σειριακά , δέιτε τα εξής :\n* [Οδηγός για το Keras functional API link text](https://colab.research.google.com/github/tensorflow/docs/blob/master/site/en/guide/keras/functional.ipynb)\n*[Οδηγός για τη σύνθεση επιπέδων και μοντέλων από το μηδέν μέσω της ενθυλάκωσης](https://colab.research.google.com/github/tensorflow/docs/blob/master/site/en/guide/keras/custom_layers_and_models.ipynb)",
"_____no_output_____"
],
[
"# Διαμόρφωση των επιπέδων\n\nΥπάρχουν πολλά επίπεδα `tf.keras.layers` διαθέσιμα. Τα περισσότερα από αυτά έχουν συνήθη ορίσματα στον κατασκευαστή : \n\n* `activation` : Θέτει τη συνάρτηση ενεργοποίησης το επίπεδο. Η παράμετρος αυτή διασαφηνίζεται από το όνομα μίας προεγκατεστημένης συνάρτησης , διαφορετικά ως ένα προς κλήση αντικείμενο. Ως προεπιλογή , δεν εφαρμόζεται activation\n* `kernel_initializer` και `bias_initializer` : Τα σχέδια αρχικοποίησης που δημιουργούν τα βάρη του επιπέδου (kernel και bias). Η παράμετρος αυτή είναι είτε όνομα είτε αντικείμενο που μπορεί να κληθεί . Αρχικοποιείται , ως προεπιλογή , στον `\"Glorot uniform\"` αρχικοποιητή.\n* `kernel_regularizer` και `bias_regularizer` : Τα σχέδια ρύθμισης που εφαρμόζουν τα βάρη του επιπέδου (kernel και bias), όπως για παράδειγμα οι L1 or\nL2 regularization (L1 ή L2 ρυθμίσεις). Ως προεπιλογή , δεν εφαρμόζεται regularization\n\nΟ ακόλουθος κώδικας δίνει υπόσταση στα `tf.keras.layers.Dense` επίπεδα με τη χρήση ορισμάτων σε κατασκευαστές :",
"_____no_output_____"
]
],
[
[
"# Create a sigmoid layer:\nlayers.Dense(64, activation='sigmoid')\n# Or:\nlayers.Dense(64, activation=tf.keras.activations.sigmoid)\n\n# A linear layer with L1 regularization of factor 0.01 applied to the kernel matrix:\nlayers.Dense(64, kernel_regularizer=tf.keras.regularizers.l1(0.01))\n\n# A linear layer with L2 regularization of factor 0.01 applied to the bias vector:\nlayers.Dense(64, bias_regularizer=tf.keras.regularizers.l2(0.01))\n\n# A linear layer with a kernel initialized to a random orthogonal matrix:\nlayers.Dense(64, kernel_initializer='orthogonal')\n\n# A linear layer with a bias vector initialized to 2.0s:\nlayers.Dense(64, bias_initializer=tf.keras.initializers.Constant(2.0))",
"_____no_output_____"
]
],
[
[
"# Μάθηση και αξιολόγηση \n\n## Στήνοντας την μάθηση\n\nΑφότου έχει κατασκευαστεί το μοντέλο, ορίστε την διαδικασία μάθησης καλώντας την μέθοδο `compile`:\n",
"_____no_output_____"
]
],
[
[
"model = tf.keras.Sequential([\n# Adds a densely-connected layer with 64 units to the model:\nlayers.Dense(64, activation='relu', input_shape=(32,)),\n# Add another:\nlayers.Dense(64, activation='relu'),\n# Add a softmax layer with 10 output units:\nlayers.Dense(10, activation='softmax')])\n\nmodel.compile(optimizer=tf.keras.optimizers.Adam(0.01),\n loss='categorical_crossentropy',\n metrics=['accuracy'])",
"_____no_output_____"
]
],
[
[
"Η μέθοδος `tf.keras.Model.compile` δέχεται 3 σημαντικά ορίσματα : \n\n* `optimizer` : Το αντικείμενο αυτό προσδιορίζει την διαδικασία μάθησης. Περάστε στιγμιότυπα βελτιστοποίησης (optimizer instances) από το άρθρωμα (module) `tf.keras.optimizers` , όπως `tf.keras.optimizers.Adam` ή `tf.keras.optimizers.SGD`. Αν θέλετε να χρησιμοποιήσετε τις προεπιλεγμένες παραμέτρους , μπορείτε να προσδιορίσετε βελτιστοποιητές μέσω συμβολοσειρών(strings) , όπως `adam` ή `sgd`.\n* `loss` : Η συνάρτηση που πρέπει να ελαχιστοποιείται κατά τη βελτιστοποίηση. Συνήθεις επιλογές είναι η μέση τετραγωνική απόκλιση(mse) ,`categorical_crossentropy`, και `binary_crossentropy`. Oι loss functions προσδιορίζονται μέσω του ονόματος ή παιρνώντας ένα αντικείμενο από το άρθρωμα `tf.keras.losses`.\n* `metrics`: Χρησιμοποιείται για την παρακολούθηση της διαδικασίας. Πρόκειται για συμβολοσειρές ή για προς κλήση αντικείμενα από το άρθρωμα `tf.keras.metrics`\n* Επιπρόσθετα , για να βεβαιωθείτε ότι το μοντέλο μαθαίνει και αξιολογεί eagerly(\"ενθουσιωδώς\"), μπορείτε να θέσετε `run_eagerly=True` ως παράμετρο κατά την μεταγλώττιση\n\nΟ ακόλουθος κώδικας υλοποιεί μερικά παραδείγματα παραμετροποίησης μοντέλου για μάθηση : ",
"_____no_output_____"
]
],
[
[
"# Configure a model for mean-squared error regression.\nmodel.compile(optimizer=tf.keras.optimizers.Adam(0.01),\n loss='mse', # mean squared error\n metrics=['mae']) # mean absolute error\n\n# Configure a model for categorical classification.\nmodel.compile(optimizer=tf.keras.optimizers.RMSprop(0.01),\n loss=tf.keras.losses.CategoricalCrossentropy(),\n metrics=[tf.keras.metrics.CategoricalAccuracy()])",
"_____no_output_____"
]
],
[
[
"# Μάθηση από NumPy δεδομένα\n\nΣε μικρό όγκο δεδομένων,συνιστάται η χρήση των in-memory πινάκων [NumPy](https://numpy.org/) για την μάθηση και την αξιολόγηση ενός μοντέλου. Το μοντέλο \"ταιριάζει\" στα δεδομένα εκμάθησης μέσω της μεθόδου `fit` :",
"_____no_output_____"
]
],
[
[
"import numpy as np\n\ndata = np.random.random((1000, 32))\nlabels = np.random.random((1000, 10))\n\nmodel.fit(data, labels, epochs=10, batch_size=32)",
"_____no_output_____"
]
],
[
[
"Η μέθοδος `tf.keras.Model.fit` δέχεται 3 ορίσματα : \n\n* `epochs` : Η μάθηση οργανώνεται σε *εποχές* (epochs). *Εποχή* είναι μία επανάληψη σε όλο τον όγκο των δεδομένων εισόδου(αυτό γίνεται πρώτα σε μικρότερες \"δεσμίδες\" δεδομένων)\n* `batch_size` : Όταν περνάνε τα δεδομένα NumPy , το μοντέλο τα τεμαχίζει σε (μικρότερες) \"δεσμίδες\" και εκτελεί επαναλήψεις πάνω σε αυτές κατά την εκμάθηση.\n* `validation_data` : Όταν δημιουργείτε ένα πρωτότυπο ενός μοντέλους , θα ήταν επιθυμητό να παρακολουθείτε με ευκολία την απόδοσή του σε μερικά δεδομένα επαλήθευσης (validation data). Περνώντας αυτό το όρισμα -μία πλειάδα εισόδων και \"ετικετών\"- επιτρέπετε στο μοντέλο να δείχνει την απώλεια και τις μετρήσεις σε \"συμπερασματική\" λειτουργία (inference mode) για τα εισαγώμενα δεδομένα, στο τέλος κάθε *εποχής*\n\nΑκολουθεί παράδειγμα με την χρήση της `validation_data` :\n\n\n\n",
"_____no_output_____"
]
],
[
[
"import numpy as np\n\ndata = np.random.random((1000, 32))\nlabels = np.random.random((1000, 10))\n\nval_data = np.random.random((100, 32))\nval_labels = np.random.random((100, 10))\n\nmodel.fit(data, labels, epochs=10, batch_size=32,\n validation_data=(val_data, val_labels))",
"_____no_output_____"
]
],
[
[
"# Μάθηση από σύνολα δεδομένων(datasets) tf.data\n\nΧρησιμοποιείστε τo [Datasets API](https://colab.research.google.com/github/tensorflow/docs/blob/master/site/en/guide/data.ipynb) για να κλιμακώσετε μεγάλα σύνολα δεδομένων ή μάθηση σε πολλές συσκευές. Περάστε ένα `tf.data.Dataset` στιγμιότυπο στην μέθοδο `fit` : \n",
"_____no_output_____"
]
],
[
[
"# Instantiates a toy dataset instance:\ndataset = tf.data.Dataset.from_tensor_slices((data, labels))\ndataset = dataset.batch(32)\n\nmodel.fit(dataset, epochs=10)",
"_____no_output_____"
]
],
[
[
"Από τη στιγμή που το `Dataset` παράγει \"δεσμίδες\" δεδομένων , αυτό το απόσπασμα δεν απαίτει το `batch_size`. \n\nΤα σύνολα δεδομένων μπορούν να χρησιμοποιηθούν και για επιβεβαίωση :",
"_____no_output_____"
]
],
[
[
"dataset = tf.data.Dataset.from_tensor_slices((data, labels))\ndataset = dataset.batch(32)\n\nval_dataset = tf.data.Dataset.from_tensor_slices((val_data, val_labels))\nval_dataset = val_dataset.batch(32)\n\nmodel.fit(dataset, epochs=10,\n validation_data=val_dataset)",
"_____no_output_____"
]
],
[
[
"#Αξιολόγηση και πρόβλεψη\n\nΟι `tf.keras.Model.evaluate` και `tf.keras.Model.predict` μέθοδοι μπορούν να χρησιμοποιήσουν δεδομένα NumPy και ένα `tf.data.Dataset`.\n\nΑκολουθεί ο τρόπος αξιολόγησης \"συμπερασματικής\" λειτουργίας των απωλειών και των μετρήσεων για τα παρεχόμενα δεδομένα : ",
"_____no_output_____"
]
],
[
[
"# With Numpy arrays\ndata = np.random.random((1000, 32))\nlabels = np.random.random((1000, 10))\n\nmodel.evaluate(data, labels, batch_size=32)\n\n# With a Dataset\ndataset = tf.data.Dataset.from_tensor_slices((data, labels))\ndataset = dataset.batch(32)\n\nmodel.evaluate(dataset)",
"_____no_output_____"
]
],
[
[
"Με αυτό το απόσπασμα μπορείτε να προβλέψετε την έξοδο του τελευταίου επιπέδου σε inference για τα παρεχόμενα δεδομένα , ως έναν πίνακα NumPy :",
"_____no_output_____"
]
],
[
[
"result = model.predict(data, batch_size=32)\nprint(result.shape)",
"_____no_output_____"
]
],
[
[
"Για έναν ολοκληρωμένο οδηγό στην εκμάθηση και την αξιολόγηση , ο οποίος περιλαμβάνει και οδηγίες για συγγραφή προσαρμοσμένων loops μαθήσεως από το μηδέν , δείτε τον οδηγό [αυτό](https://colab.research.google.com/github/tensorflow/docs/blob/master/site/en/guide/keras/train_and_evaluate.ipynb).",
"_____no_output_____"
],
[
"# Δημιουργία σύνθετων μοντέλων \n\n## To Functional API\n\nΤο μοντέλο `tf.keras.Sequential` είναι μία απλή στοίβα επιπέδων η οποία δεν μπορεί να εκπροσωπήσει αυθαίρετα μοντέλα .\nΜε τη χρήση του [Keras functional API](https://colab.research.google.com/github/tensorflow/docs/blob/master/site/en/guide/keras/functional.ipynb#scrollTo=vCMYwDIE9dTT) μπορείτε να φτιάξετε σύνθετες τοπολογίες μοντέλων όπως :\n* Μοντέλα πολλαπλών εισόδων,\n* Μοντέλα πολλαπλών εξόδων,\n* Μοντέλα με κοινόχρηστα επίπεδα (το ίδιο επίπεδο να καλείται πολλαπλές φορές),\n* Μοντέλα με μη σειριακή ροή δεδομένων (π.χ residual connections)\n\nH δημιουργία μοντέλων με το functional API ακολουθεί το εξής : \n1. Ένα στιγμιότυπο ενός επιπέδου καλείται και επιστρέφει έναν τανυστή(tensor).\n2. Τανυστές εισόδου και εξόδου χρησιμοποιούνται για να ορίσουν μία κατάσταση της `tf.keras.Model`.\n3. Το μοντέλο μαθαίνει κατά τον ίδιο τρόπο με το `Σειριακό` μοντέλο.\n\nΤο ακόλουθο παράδειγμα χρησιμοποιεί το functional API για να φτιάξει ένα απλό, πλήρως συνδεδεμένο (νευρωνικό) δίκτυο : \n\n\n\n",
"_____no_output_____"
]
],
[
[
"inputs = tf.keras.Input(shape=(32,)) # Returns an input placeholder\n\n# A layer instance is callable on a tensor, and returns a tensor.\nx = layers.Dense(64, activation='relu')(inputs)\nx = layers.Dense(64, activation='relu')(x)\npredictions = layers.Dense(10, activation='softmax')(x)",
"_____no_output_____"
]
],
[
[
"Δώστε υπόσταση στο μοντέλο με είσοδο και έξοδο:\n\n",
"_____no_output_____"
]
],
[
[
"model = tf.keras.Model(inputs=inputs, outputs=predictions)\n\n# The compile step specifies the training configuration.\nmodel.compile(optimizer=tf.keras.optimizers.RMSprop(0.001),\n loss='categorical_crossentropy',\n metrics=['accuracy'])\n\n# Trains for 5 epochs\nmodel.fit(data, labels, batch_size=32, epochs=5)",
"_____no_output_____"
]
],
[
[
"# Υποκατηγοριοποίηση στα μοντέλα\n\nΦτιάξτε ένα πλήρως παραμετροποιήσιμο μοντέλο υποκατηγοριοποιώντας την `tf.keras.Model` και ορίζοντας το δικό σας \"προς τα εμπρος πέρασμα\"(forward pass,κοινώς διαδικασία υπολογισμού η οποία ξεκινάει από το 1ο προς το τελευταίο επίπεδο). Κατασκευάστε επίπεδα στην μέθοδο `__init__` και θέστε τα ως ιδιότητες της κλάσης . Ορίστε έπειτα το \"προς τα εμπρος πέρασμα\"(forward pass)στην μέθοδο `call`.\n\nΗ υποκατηγοριοποίηση στα μοντέλα είναι ιδιαίτερα χρήσιμη όταν η [\"ενθουσιώδης\" εκτέλεση](https://colab.research.google.com/github/tensorflow/docs/blob/master/site/en/guide/eager.ipynb) είναι ενεργοποιημένη ,καθώς επιτρέπει το \"προς τα εμπρος πέρασμα\"(forward pass) να εγγράφεται αναγκαστικά.\n\nΣημείωση: αν το μοντέλο σας χρειάζεται να τρέχει *πάντα* αναγκαστικά, μπορείτε να θέσετε `dynamic=True` όταν καλείται ο `super` κατασκευαστής.\n\n\n> Σημείο κλειδί : Χρησιμοποιήστε το σωστό , ανάλογα με την δουλειά , API . Παρόλο που η χρήση της υποκατηγοριοποίηση προσφέρει ευελιξία , κοστίζει σε πολυπλοκότητα και σε μεγαλύτερα περιθώρια για σφάλματα χρήστη. Αν είναι εφικ΄το , προτιμήστε το functional API. \n\nΤο ακόλουθο παράδειγμα δείχνει μια υποκλάση `tf.keras.Model` που χρησιμοποιεί \n\"προς τα εμπρός πέρασμα\" το οποίο δεν χρειάζεται να εκτελείται αναγκαστικά: \n\n\n\n",
"_____no_output_____"
]
],
[
[
"class MyModel(tf.keras.Model):\n\n def __init__(self, num_classes=10):\n super(MyModel, self).__init__(name='my_model')\n self.num_classes = num_classes\n # Define your layers here.\n self.dense_1 = layers.Dense(32, activation='relu')\n self.dense_2 = layers.Dense(num_classes, activation='sigmoid')\n\n def call(self, inputs):\n # Define your forward pass here,\n # using layers you previously defined (in `__init__`).\n x = self.dense_1(inputs)\n return self.dense_2(x)",
"_____no_output_____"
]
],
[
[
"Η νέα κλάση model λαμβάνει υπόσταση : ",
"_____no_output_____"
]
],
[
[
"model = MyModel(num_classes=10)\n\n# The compile step specifies the training configuration.\nmodel.compile(optimizer=tf.keras.optimizers.RMSprop(0.001),\n loss='categorical_crossentropy',\n metrics=['accuracy'])\n\n# Trains for 5 epochs.\nmodel.fit(data, labels, batch_size=32, epochs=5)",
"_____no_output_____"
]
],
[
[
"# Παραμετροποιήσιμα επίπεδα\n\nΔημιουργήστε ένα παραμετροποιήσιμο επίπεδο υποκατηγοριοποιώντας την `tf.keras.layers.Layer` και υλοποιώντας τις παρακάτω μεθόδους :\n\n* `__init__` : (Προαιρετικά) ορίστε τα υποεπίπεδα που θα χρησιμοποιηθούν από το επίπεδο\n* `build` : Δημιουργεί τα βάρη του επιπέδου. Προσθέστε βάρη με την μέθοδο `add_weight`.\n* `call` : Ορίστε το \"προς τα εμπρός πέρασμα\"\n* Προαιρετικά, ένα επίπεδο μπορεί να σειριοποιηθεί με την υλοποίηση της μεθόδου `get_config` και της μεθόδου κλάσης `from_config`.\n\nΑκολουθεί παράδειγμα ενός παραμετροποιήσιμου επιπέδου το οποίο υλοποιεί ένα `matmul` με ορίσματα μία είσοδο και έναν kernel (πυρήνα)",
"_____no_output_____"
]
],
[
[
"class MyLayer(layers.Layer):\n\n def __init__(self, output_dim, **kwargs):\n self.output_dim = output_dim\n super(MyLayer, self).__init__(**kwargs)\n\n def build(self, input_shape):\n # Create a trainable weight variable for this layer.\n self.kernel = self.add_weight(name='kernel',\n shape=(input_shape[1], self.output_dim),\n initializer='uniform',\n trainable=True)\n\n def call(self, inputs):\n return tf.matmul(inputs, self.kernel)\n\n def get_config(self):\n base_config = super(MyLayer, self).get_config()\n base_config['output_dim'] = self.output_dim\n return base_config\n\n @classmethod\n def from_config(cls, config):\n return cls(**config)",
"_____no_output_____"
]
],
[
[
"Δημιουργήστε ένα μοντέλο χρησιμοποιώντας το δικό σας επίπεδο :",
"_____no_output_____"
]
],
[
[
"model = tf.keras.Sequential([\n MyLayer(10),\n layers.Activation('softmax')])\n\n# The compile step specifies the training configuration\nmodel.compile(optimizer=tf.keras.optimizers.RMSprop(0.001),\n loss='categorical_crossentropy',\n metrics=['accuracy'])\n\n# Trains for 5 epochs.\nmodel.fit(data, labels, batch_size=32, epochs=5)",
"_____no_output_____"
]
],
[
[
"#Επανακλήσεις\n\nΜία επανάκληση(callback) είναι ένα αντικείμενο το οποίο περνάει σε ένα μοντέλο για να τροποποιήσει και να επεκτείνει τη συμπεριφορά του κατά την διάρκεια της μάθησης. Μπορείτε να γράψετε τις δικές σας επανακλήσεις ή να χρησιμοποιήσετε την `tf.keras.callbacks` η οποία περιλαμβάνει :\n\n* `tf.keras.callbacks.ModelCheckpoint`: Αποθηκεύστε το μοντέλο σας ανά τακτά διαστήματα.\n* `tf.keras.callbacks.LearningRateScheduler`: Δυναμικά, αλλάξτε τον ρυθμό μάθησης.\n* `tf.keras.callbacks.EarlyStopping`: Διακόψτε την εκμάθηση όταν η απόδοση επαλήθευσης (validation performance) έχει σταματήσει να βελτιώνεται.\n* `tf.keras.callbacks.TensorBoard`: Παρακολουθήστε την συμπεριφορά του μοντέλου με τη χρήση του [TensorBoard](https://tensorflow.org/tensorboard).\n\nΓια να χρησιμοποιήσετε την `tf.keras.callbacks.Callback` , περάστε την στην μέθοδο `fit` του μοντέλου : \n",
"_____no_output_____"
]
],
[
[
"callbacks = [\n # Interrupt training if `val_loss` stops improving for over 2 epochs\n tf.keras.callbacks.EarlyStopping(patience=2, monitor='val_loss'),\n # Write TensorBoard logs to `./logs` directory\n tf.keras.callbacks.TensorBoard(log_dir='./logs')\n]\nmodel.fit(data, labels, batch_size=32, epochs=5, callbacks=callbacks,\n validation_data=(val_data, val_labels))",
"_____no_output_____"
]
],
[
[
"# Αποθήκευση και επαναφορά\n",
"_____no_output_____"
],
[
"## Αποθήκευση μόνο των τιμών των βαρών\n\nΑποθηκεύστε και φορτώστε τα βάρη ενός μοντέλου με τη χρήση της `tf.keras.Model.save_weights`:\n",
"_____no_output_____"
]
],
[
[
"model = tf.keras.Sequential([\nlayers.Dense(64, activation='relu', input_shape=(32,)),\nlayers.Dense(10, activation='softmax')])\n\nmodel.compile(optimizer=tf.keras.optimizers.Adam(0.001),\n loss='categorical_crossentropy',\n metrics=['accuracy'])",
"_____no_output_____"
],
[
"# Save weights to a TensorFlow Checkpoint file\nmodel.save_weights('./weights/my_model')\n\n# Restore the model's state,\n# this requires a model with the same architecture.\nmodel.load_weights('./weights/my_model')",
"_____no_output_____"
]
],
[
[
"Ως προεπιλογή , το παραπάνω αποθηκεύει τα βάρη του μοντέλου σε μορφοποίηση [TensorFlow checkpoint](../checkpoint.ipynb). Εναλλακτικά , μπορούν να αποθηκευτούν χρησιμοποιώντας την μορφοποιήση Keras HDF5(η προεπιλογή για την υλοποίηση του συστήματος υποστήριξης του Keras):",
"_____no_output_____"
]
],
[
[
"# Save weights to a HDF5 file\nmodel.save_weights('my_model.h5', save_format='h5')\n\n# Restore the model's state\nmodel.load_weights('my_model.h5')",
"_____no_output_____"
]
],
[
[
"## Αποθήκευση μόνο των ρυθμίσεων του μοντέλου\n\nΟι ρυθμίσεις ενός μοντέλου μπορούν να αποθηκευτούν-αυτό σειριοποιεί την αρχιτεκτονική του μοντέλου χωρίς βάρη. Οι αποθηκευμένες ρυθμίσεις μπορόυν να αναπαράγουν και να αρχικοποιήσουν το ίδιο μοντέλο, ακόμη και χωρίς τον κώδικα που όρισε το πρότυπο μοντέλο. To Keras υποστηρίζει τα JSON και YAML ως μορφές σειριοποίησης:",
"_____no_output_____"
]
],
[
[
"# Serialize a model to JSON format\njson_string = model.to_json()\njson_string",
"_____no_output_____"
],
[
"import json\nimport pprint\npprint.pprint(json.loads(json_string))",
"_____no_output_____"
]
],
[
[
"Αναπαραγωγή του μοντέλου(νεότερα αρχικοποιήθεντος) από το JSON :",
"_____no_output_____"
]
],
[
[
"fresh_model = tf.keras.models.model_from_json(json_string)",
"_____no_output_____"
]
],
[
[
"Η σειριοποίηση μοντέλου σε μορφή YAML απαιτεί την εγκατάσταση της `pyyaml` πριν γίνει είσοδος της TensorFlow: ",
"_____no_output_____"
]
],
[
[
"yaml_string = model.to_yaml()\nprint(yaml_string)",
"_____no_output_____"
]
],
[
[
"Αναπαραγωγή του μοντέλου από το YAML : \n",
"_____no_output_____"
]
],
[
[
"fresh_model = tf.keras.models.model_from_yaml(yaml_string)",
"_____no_output_____"
]
],
[
[
"Προσοχή : Μοντέλα ως υποκλάσεις δεν σειριοποιούνται διότι η αρχιτεκτονική τους ορίζεται από τον κώδικα Python στο σώμα τις μεθόδου `call`.",
"_____no_output_____"
],
[
"## Αποθήκευση ολόκληρου του μοντέλου σε ένα αρχείο\n\nΟλόκληρο το μοντέλο μπορεί να αποθηκευτεί σε ένα μόνο αρχείο το οποίο περιέχει τα βάρη , τις ρυθμίσεις του μοντέλου , ακόμη και τις ρυθμίσεις του βελτιστοποιητή. Έτσι , μπορείτε να θέσετε ένα σημείο ελέγχου σε ένα μοντέλο και να συνεχίσετε την εκμάθηση αργότερα - από την ακριβώς ίδια κατάσταση - χωρίς πρόσβαση στον πρωταρχικό κώδικα :",
"_____no_output_____"
]
],
[
[
"# Create a simple model\nmodel = tf.keras.Sequential([\n layers.Dense(10, activation='softmax', input_shape=(32,)),\n layers.Dense(10, activation='softmax')\n])\nmodel.compile(optimizer='rmsprop',\n loss='categorical_crossentropy',\n metrics=['accuracy'])\nmodel.fit(data, labels, batch_size=32, epochs=5)\n\n\n# Save entire model to a HDF5 file\nmodel.save('my_model.h5')\n\n# Recreate the exact same model, including weights and optimizer.\nmodel = tf.keras.models.load_model('my_model.h5')",
"_____no_output_____"
]
],
[
[
"Για να μάθετε περισσότερα για την αποθήκευση και την σειριοποίηση , πατήστε [εδώ](./save_and_serialize.ipynb).",
"_____no_output_____"
],
[
"# \"Ενθουσιώδης\" εκτέλεση(Eager execution)\n\nH [\"Ενθουσιώδης\" εκτέλεση](https://github.com/tensorflow/docs/blob/master/site/en/guide/eager.ipynb) είναι ένα (επιτακτικό) περιβάλλον προγραμματισμού το οποίο αξιολογεί λειτουργίες αμέσως. Αυτό δεν απαιτείται από το Keras , ωστόσο η `tf.keras` παρέχει υποστήριξη και χρησιμεύει για την επιθεώρηση και την εκσφαλμάτωση του προγράμματος.\n\nΌλα τα API της `tf.keras` για την κατασκευή μοντέλων είναι συμβατά με την \"ενθουσιώδη εκτέλεση\". Και καθώς τα `Σειριακά` και τα λειτουργικά (functional) API μπορούν να χρησιμοποιηθούν, η \"ενθουσιώδης\" εκτέλεση επωφελεί ιδιαίτερα την υποκατηγοριοποίηση μοντέλων και την κατασκευή παραμετροποιήσιμων επιπέδων-τα ΑPI's που σας προτρέπουν να γράψετε το \"προς τα εμπρος πέρασμα\" ως κώδικα (σε αντίθεση με τα API's που δημιουργούν μοντέλα μέσω της σύνθεσης των ήδη υπάρχοντων επιπέδων) \n\nΔείτε τον οδηγό [αυτό](https://github.com/tensorflow/docs/blob/master/site/en/guide/eager.ipynb) για παραδείγματα χρήσης μοντέλων Keras με custom training looops και την `tf.GradientTape`. Ακόμη , μπορείτε να δείτε ένα ολοκληρωμένο και σύντομο παράδειγμα [εδώ](https://www.tensorflow.org/tutorials/quickstart/advanced).\n",
"_____no_output_____"
],
[
"# Διανομή \n\nΤα μοντέλα της `tf.keras` μπορούν να εκτελεστούν σε πολλαπλές GPUs ,με την χρήση της `tf.distribute.Strategy`. Το API αυτό κατανείμει την μάθηση σε πολλαπλές κάρτες γραφικών με σχεδόν καθόλου αλλαγές στον υπάρχον κώδικα.\n\nΕπί του παρόντος, η `tf.distribute.MirroredStrategy` αποτελεί την μόνη στρατηγική κατανομής που υποστηρίζεται. Η `MirroredStrategy` πραγματοποιεί in-graph replication μαζί με συγχρονισμένη μάθηση, χρησιμοποιώντας all-reduce σε ένα μηχάνημα. Για να χρησιμοποιήσετε τη `distribute.Strategy`, εμφωλεύστε την αρχικοποίηση του βελτιστοποιητή, τον κατασκευαστή του μοντέλου, και το `compile` σε ένα μπλοκ κώδικα `Strategy.scope()`. Έπειτα , προχωρήστε στην εκμάθηση του μοντέλου.\n\nΤο ακόλουθο παράδειγμα κατανείμει ένα `tf.keras.Model` σε πολλαπλές κάρτες γραφικών σε ένα μηχάνημα.\nΠρώτα , ορίζεται ένα μοντέλο εντός της `distribute.Strategy`:\n",
"_____no_output_____"
]
],
[
[
"strategy = tf.distribute.MirroredStrategy()\n\nwith strategy.scope():\n model = tf.keras.Sequential()\n model.add(layers.Dense(16, activation='relu', input_shape=(10,)))\n model.add(layers.Dense(1, activation='sigmoid'))\n\n optimizer = tf.keras.optimizers.SGD(0.2)\n\n model.compile(loss='binary_crossentropy', optimizer=optimizer)\n\nmodel.summary()",
"_____no_output_____"
]
],
[
[
"Στη συνέχεια , εξασκείστε το μοντέλο πάνω σε δεδομένα κατά τα γνωστά :",
"_____no_output_____"
]
],
[
[
"x = np.random.random((1024, 10))\ny = np.random.randint(2, size=(1024, 1))\nx = tf.cast(x, tf.float32)\ndataset = tf.data.Dataset.from_tensor_slices((x, y))\ndataset = dataset.shuffle(buffer_size=1024).batch(32)\n\nmodel.fit(dataset, epochs=1)",
"_____no_output_____"
]
],
[
[
"Για περισσότερες πληροφορίες , δείτε τον [πλήρη οδηγό για την Κατανεμημένη Μάθηση με TensorFlow](../distributed_training.ipynb).",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown",
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
]
] |
e7bc6b060b0c4af76c7588f47e233bfe4565b012 | 53,724 | ipynb | Jupyter Notebook | src/For testing purposes.ipynb | fica-ps/fica-case-studies | 91fbd494a49d6dbd06abf3d06e7539cc32f019cc | [
"MIT"
] | null | null | null | src/For testing purposes.ipynb | fica-ps/fica-case-studies | 91fbd494a49d6dbd06abf3d06e7539cc32f019cc | [
"MIT"
] | null | null | null | src/For testing purposes.ipynb | fica-ps/fica-case-studies | 91fbd494a49d6dbd06abf3d06e7539cc32f019cc | [
"MIT"
] | null | null | null | 58.714754 | 229 | 0.568089 | [
[
[
"empty"
]
]
] | [
"empty"
] | [
[
"empty"
]
] |
e7bc7311df67cc9b96748f5e9c78306d32d91a2a | 9,418 | ipynb | Jupyter Notebook | TOI/Doc2Vec.ipynb | aashish-jain/Social-unrest-prediction | fa7898060528f77e27271ed9072145c95ded2e78 | [
"MIT"
] | 8 | 2019-05-16T04:22:23.000Z | 2021-02-10T05:14:21.000Z | TOI/Doc2Vec.ipynb | aashish-jain/Social-unrest-prediction | fa7898060528f77e27271ed9072145c95ded2e78 | [
"MIT"
] | null | null | null | TOI/Doc2Vec.ipynb | aashish-jain/Social-unrest-prediction | fa7898060528f77e27271ed9072145c95ded2e78 | [
"MIT"
] | 1 | 2019-05-17T18:38:49.000Z | 2019-05-17T18:38:49.000Z | 25.317204 | 185 | 0.54725 | [
[
[
"### Imports",
"_____no_output_____"
]
],
[
[
"!pip install gensim\n!pip nltk",
"_____no_output_____"
],
[
"# Import the libraries\nimport gensim\nfrom glob import glob\nimport pandas as pd\nfrom tqdm import tqdm, tqdm_notebook\nfrom gensim.test.utils import common_texts\nfrom gensim.models.doc2vec import Doc2Vec, TaggedDocument\nimport nltk\nfrom nltk.tokenize import word_tokenize\nfrom nltk.corpus import stopwords\nimport string\n\nnp = pd.np",
"_____no_output_____"
],
[
"# Load data for text processing\n\n#For removing punctuation\ntable = str.maketrans('', '', string.punctuation)\nnltk.download('stopwords')\nstop_words = set(stopwords.words('english'))\n\n#For Displaying progress\ntqdm_notebook(disable = True).pandas()",
"_____no_output_____"
],
[
"'''\n Reads articles from the given path.\n path : path format from which files have to be read. e.g. ./*.cvs will read all csvs\n Params:\n show_progress : Shows the progress using tqdm if True else nothing displayed\n'''\ndef read_articles(path, show_progress = True):\n df_list = []\n for file_name in tqdm_notebook(glob(path), disable = not show_progress):\n temp_df = pd.read_csv(file_name, index_col=0)\n temp_df[\"date\"] = file_name.split(\"/\")[-1].split('.')[0]\n df_list.append(temp_df)\n df = pd.concat(df_list, ignore_index=True)\n df[\"date\"] = pd.to_datetime(df[\"date\"])\n return df\n'''\n For the given text, returns a list of words representing the text\n with all words in lower case and punctuation along with stopwords \n removed\n Params:\n text : Text for which vocubulary has to be generated\n'''\ndef generate_document_vocabulary(text):\n vocabulary = []\n for word in word_tokenize(text):\n w = word.translate(table).lower()\n if w.isalpha() and w not in stop_words:\n vocabulary.append(w)\n return vocabulary",
"_____no_output_____"
]
],
[
[
"### All the CSVs contain the following data\ndate, title(headline), location, text(full article)",
"_____no_output_____"
],
[
"### Data pre-processing for doc2vec",
"_____no_output_____"
]
],
[
[
"# Read articles\ndf = read_articles(\"../data/TOI/*.csv\")\n# Take training data (until 1-Jan-2019)\ndf = df[df[\"date\"] < pd.to_datetime(\"1-Jan-2019\")]",
"_____no_output_____"
],
[
"# Get the vocabulary from the given text\ndf['vocabulary'] = df['text'].progress_apply(generate_document_vocabulary)",
"_____no_output_____"
],
[
"#Convert the vocabulary into Tagged document for doc2vec model\ndocuments = []\nfor i, row in df.iterrows():\n document = TaggedDocument(row['vocabulary'], [i])\n documents.append(document)",
"_____no_output_____"
]
],
[
[
"### Training the model",
"_____no_output_____"
]
],
[
[
"max_epochs = 100\nvec_size = 50\nalpha = 0.025\n\n# Distributed memory model\nmodel = Doc2Vec(vector_size=vec_size,\n alpha=alpha,\n min_alpha=0.00025,\n min_count=1,\n dm=1,\n workers=8)",
"_____no_output_____"
],
[
"# Initialize the model\nmodel.build_vocab(documents)",
"_____no_output_____"
],
[
"# Train the model\nfor _ in tqdm_notebook(range(max_epochs)):\n model.train(documents,\n total_examples=model.corpus_count,\n epochs=model.epochs,)\n # Deacying learning rate\n model.alpha -= 0.0002\n # fix the learning rate, no decay\n model.min_alpha = model.alpha",
"_____no_output_____"
],
[
"# Save the model\nmodel.save(\"article.d2v\")",
"_____no_output_____"
]
],
[
[
"### Generate the document dictionary that can be used to access a document by tag",
"_____no_output_____"
]
],
[
[
"# Generate the document dictionary that can be used to access a document by tag\ndocument_dic = {}\nfor doc,tag in documents:\n document_dic[tag[0]] = doc",
"_____no_output_____"
]
],
[
[
"### Test the model for Random Data from ACLED after Jan-1-2019",
"_____no_output_____"
]
],
[
[
"article = \"On July 15, a long protest march by farmers, from Mandsaur in Madhya Pradesh to New Delhi, demanding loan waiver and fair price for their produce, reached Jaipur.\"\narticle = ' '.join(generate_document_vocabulary(article))",
"_____no_output_____"
]
],
[
[
"### Find the closest Document\n",
"_____no_output_____"
]
],
[
[
"# Reference : https://github.com/RaRe-Technologies/gensim/blob/develop/docs/notebooks/doc2vec-lee.ipynb\n\n# find the vector\nvec = model.infer_vector(sabri)\n\n#find 10 closest documents\nsims = model.docvecs.most_similar([vec])",
"_____no_output_____"
],
[
"# Print the document\nfor doc_tag,score in sims:\n print(\"Document has score : \"score, \"\\nContent : \" document_dic[doc_tag] + \"\\n\\n\")",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
]
] |
e7bc82d2359b522b9005aed368b219c42bfeaa59 | 62,156 | ipynb | Jupyter Notebook | Notebooks/Binomial_MGWR_approaches_tried.ipynb | mehak-sachdeva/MGWR_book | d702a04c7e5f9101e0f04b6d84295bce86579059 | [
"MIT-0"
] | 1 | 2021-01-21T08:29:53.000Z | 2021-01-21T08:29:53.000Z | Notebooks/Binomial_MGWR_approaches_tried.ipynb | TaylorOshan/MGWR_book | c59db902b34d625af4d0e1b90fbc95018a3de579 | [
"MIT-0"
] | null | null | null | Notebooks/Binomial_MGWR_approaches_tried.ipynb | TaylorOshan/MGWR_book | c59db902b34d625af4d0e1b90fbc95018a3de579 | [
"MIT-0"
] | 1 | 2021-06-07T20:57:56.000Z | 2021-06-07T20:57:56.000Z | 81.891963 | 13,812 | 0.792538 | [
[
[
"https://github.com/pysal/mgwr/pull/56",
"_____no_output_____"
]
],
[
[
"import sys\nsys.path.append(\"C:/Users/msachde1/Downloads/Research/Development/mgwr\")",
"_____no_output_____"
],
[
"import warnings\nwarnings.filterwarnings(\"ignore\")\nimport pandas as pd\nimport numpy as np\n\nfrom mgwr.gwr import GWR\nfrom spglm.family import Gaussian, Binomial, Poisson\nfrom mgwr.gwr import MGWR\nfrom mgwr.sel_bw import Sel_BW\nimport multiprocessing as mp\npool = mp.Pool()\nfrom scipy import linalg\nimport numpy.linalg as la\nfrom scipy import sparse as sp\nfrom scipy.sparse import linalg as spla\nfrom spreg.utils import spdot, spmultiply\nfrom scipy import special\nimport libpysal as ps\nimport seaborn as sns\nimport matplotlib.pyplot as plt\nfrom copy import deepcopy\nimport copy\nfrom collections import namedtuple\nimport spglm",
"_____no_output_____"
]
],
[
[
"#### Fundamental equation",
"_____no_output_____"
],
[
"By simple algebraic manipulation, the probability that Y=1 is:",
"_____no_output_____"
],
[
"\\begin{align}\np = 1 / (1 + exp (-{\\beta} & _k x _{k,i}) ) \\\\\n\\end{align}",
"_____no_output_____"
],
[
"### Approaches tried:\n\n1. Changing XB to : `1 / (1 + np.exp (-1*np.sum(np.multiply(X,params),axis=1)))` - these are the predicted probabilities ~(0,1)\n\n\n2. Changing XB as above and writing a function to create temp_y as a binary variable using condition `1 if BXi > 0 else 0.`\n\n\n3. Derived manipulations to temp_y as in iwls for Logistic regression as below:\n\n `v = np.sum(np.multiply(X,params),axis=1)`\n \n `mu = 1/(1+(np.exp(-v)))`\n \n `z = v + (1/(mu * (1-mu)) * (y-mu))` -- this becomes the temp_y\n \n Then a simple linear regression can be run as z as the temp dependent variable\n \n \n4. Taken from GAM logistic model literature:\n \n `y=exp(b0+b1*x1+...+bm*xm)/{1+exp(b0+b1*x1+...+bm*xm)}`\n\n Applying the logistic link function to the probability p (ranging between 0 and 1):\n\n `p' = log {p/(1-p)}`\n\n By applying the logistic link function, we can now rewrite the model as:\n\n `p' = b0 + b1*X1 + ... + bm*Xm`\n\n Finally, we substitute the simple single-parameter additive terms to derive the generalized additive logistic model:\n\n `p' = b0 + f1(X1) + ... + fm(Xm)`\n \n (http://www.statsoft.com/textbook/generalized-additive-models#gam)\n \n This is the current approach in the latest commit:\n \n `XB = 1 / (1 + np.exp (-1*(np.multiply(X,params))))`\n \n XB is now the probability and is normally distributed\n \n Run MGWR (Gaussian) on this as the dependent variable for the partial models.\n ",
"_____no_output_____"
],
[
"### Data",
"_____no_output_____"
],
[
"#### Clearwater data - downloaded from link: https://sgsup.asu.edu/sparc/multiscale-gwr",
"_____no_output_____"
]
],
[
[
"data_p = pd.read_csv(\"C:/Users/msachde1/Downloads/logistic_mgwr_data/landslides.csv\") ",
"_____no_output_____"
],
[
"data_p.head()",
"_____no_output_____"
]
],
[
[
"#### Helper functions - hardcoded here for simplicity in the notebook workflow\nPlease note: A separate bw_func_b will not be required when changes will be made in the repository",
"_____no_output_____"
]
],
[
[
"kernel='bisquare'\nfixed=False\nspherical=False\nsearch_method='golden_section'\ncriterion='AICc'\ninterval=None\ntol=1e-06\nmax_iter=500\nX_glob=[]",
"_____no_output_____"
],
[
"def gwr_func(y, X, bw,family=Gaussian(),offset=None):\n return GWR(coords, y, X, bw, family,offset,kernel=kernel,\n fixed=fixed, constant=False,\n spherical=spherical, hat_matrix=False).fit(\n lite=True, pool=pool)\n\ndef gwr_func_g(y, X, bw):\n return GWR(coords, y, X, bw, family=Gaussian(),offset=None,kernel=kernel,\n fixed=fixed, constant=False,\n spherical=spherical, hat_matrix=False).fit(\n lite=True, pool=pool)\n\n\ndef bw_func_b(coords,y, X):\n selector = Sel_BW(coords,y, X,family=Binomial(),offset=None, X_glob=[],\n kernel=kernel, fixed=fixed,\n constant=False, spherical=spherical)\n return selector\n\ndef bw_func_p(coords,y, X):\n selector = Sel_BW(coords,y, X,family=Poisson(),offset=off, X_glob=[],\n kernel=kernel, fixed=fixed,\n constant=False, spherical=spherical)\n return selector\n\ndef bw_func(coords,y,X):\n selector = Sel_BW(coords,y,X,X_glob=[],\n kernel=kernel, fixed=fixed,\n constant=False, spherical=spherical)\n return selector\n\ndef sel_func(bw_func, bw_min=None, bw_max=None):\n return bw_func.search(\n search_method=search_method, criterion=criterion,\n bw_min=bw_min, bw_max=bw_max, interval=interval, tol=tol,\n max_iter=max_iter, pool=pool, verbose=False)",
"_____no_output_____"
]
],
[
[
"### GWR Binomial model with independent variable, x = slope",
"_____no_output_____"
]
],
[
[
"coords = list(zip(data_p['X'],data_p['Y']))\ny = np.array(data_p['Landslid']).reshape((-1,1)) \nelev = np.array(data_p['Elev']).reshape((-1,1))\nslope = np.array(data_p['Slope']).reshape((-1,1))\nSinAspct = np.array(data_p['SinAspct']).reshape(-1,1)\nCosAspct = np.array(data_p['CosAspct']).reshape(-1,1)\nX = np.hstack([elev,slope,SinAspct,CosAspct])\nx = SinAspct\n\nX_std = (X-X.mean(axis=0))/X.std(axis=0)\nx_std = (x-x.mean(axis=0))/x.std(axis=0)\ny_std = (y-y.mean(axis=0))/y.std(axis=0)",
"_____no_output_____"
],
[
"bw_gwbr=Sel_BW(coords,y,x_std,family=Binomial(),constant=False).search()",
"_____no_output_____"
],
[
"gwbr_model=GWR(coords,y,x_std,bw=bw_gwbr,family=Binomial(),constant=False).fit()",
"_____no_output_____"
],
[
"bw_gwbr",
"_____no_output_____"
],
[
"predy = 1/(1+np.exp(-1*np.sum(gwbr_model.X * gwbr_model.params, axis=1).reshape(-1, 1)))",
"_____no_output_____"
],
[
"sns.distplot(predy)",
"_____no_output_____"
],
[
"(predy==gwbr_model.predy).all()",
"_____no_output_____"
],
[
"sns.distplot(gwbr_model.y)",
"_____no_output_____"
]
],
[
[
"#### Multi_bw changes",
"_____no_output_____"
]
],
[
[
"def multi_bw(init,coords,y, X, n, k, family=Gaussian(),offset=None, tol=1e-06, max_iter=20, multi_bw_min=[None], multi_bw_max=[None],rss_score=True,bws_same_times=3,\n verbose=True):\n\n\n if multi_bw_min==[None]:\n multi_bw_min = multi_bw_min*X.shape[1]\n \n if multi_bw_max==[None]:\n multi_bw_max = multi_bw_max*X.shape[1]\n \n if isinstance(family,spglm.family.Poisson):\n bw = sel_func(bw_func_p(coords,y,X))\n optim_model=gwr_func(y,X,bw,family=Poisson(),offset=offset)\n err = optim_model.resid_response.reshape((-1, 1))\n param = optim_model.params\n #This change for the Poisson model follows from equation (1) above\n XB = offset*np.exp(np.multiply(param, X))\n \n \n elif isinstance(family,spglm.family.Binomial):\n bw = sel_func(bw_func_b(coords,y,X))\n optim_model=gwr_func(y,X,bw,family=Binomial())\n err = optim_model.resid_response.reshape((-1, 1))\n param = optim_model.params\n XB = 1/(1+np.exp(-1*np.multiply(optim_model.params,X)))\n print(\"first family: \"+str(optim_model.family))\n \n else:\n bw=sel_func(bw_func(coords,y,X))\n optim_model=gwr_func(y,X,bw)\n err = optim_model.resid_response.reshape((-1, 1))\n param = optim_model.params\n XB = np.multiply(param, X)\n \n bw_gwr = bw\n XB=XB\n \n if rss_score:\n rss = np.sum((err)**2)\n iters = 0\n scores = []\n delta = 1e6\n BWs = []\n bw_stable_counter = np.ones(k)\n bws = np.empty(k)\n\n try:\n from tqdm.auto import tqdm #if they have it, let users have a progress bar\n except ImportError:\n\n def tqdm(x, desc=''): #otherwise, just passthrough the range\n return x\n\n for iters in tqdm(range(1, max_iter + 1), desc='Backfitting'):\n new_XB = np.zeros_like(X)\n neww_XB = np.zeros_like(X)\n\n params = np.zeros_like(X)\n\n for j in range(k):\n temp_y = XB[:, j].reshape((-1, 1))\n temp_y = temp_y + err\n \n temp_X = X[:, j].reshape((-1, 1))\n \n #The step below will not be necessary once the bw_func is changed in the repo to accept family and offset as attributes\n if isinstance(family,spglm.family.Poisson):\n\n bw_class = bw_func_p(coords,temp_y, temp_X)\n \n else:\n bw_class = bw_func(coords,temp_y, temp_X)\n print(bw_class.family)\n\n if np.all(bw_stable_counter == bws_same_times):\n #If in backfitting, all bws not changing in bws_same_times (default 3) iterations\n bw = bws[j]\n else:\n bw = sel_func(bw_class, multi_bw_min[j], multi_bw_max[j])\n if bw == bws[j]:\n bw_stable_counter[j] += 1\n else:\n bw_stable_counter = np.ones(k)\n\n optim_model = gwr_func_g(temp_y, temp_X, bw)\n print(optim_model.family)\n \n err = optim_model.resid_response.reshape((-1, 1))\n param = optim_model.params.reshape((-1, ))\n\n new_XB[:,j]=optim_model.predy.reshape(-1)\n params[:, j] = param\n bws[j] = bw\n\n num = np.sum((new_XB - XB)**2) / n\n print(\"num = \"+str(num))\n den = np.sum(np.sum(new_XB, axis=1)**2)\n score = (num / den)**0.5\n print(score)\n XB = new_XB\n\n if rss_score:\n print(\"here\")\n predy = 1/(1+np.exp(-1*np.sum(X * params, axis=1).reshape(-1, 1)))\n new_rss = np.sum((y - predy)**2)\n score = np.abs((new_rss - rss) / new_rss)\n rss = new_rss\n scores.append(deepcopy(score))\n delta = score\n print(delta)\n BWs.append(deepcopy(bws))\n\n if verbose:\n print(\"Current iteration:\", iters, \",SOC:\", np.round(score, 7))\n print(\"Bandwidths:\", ', '.join([str(bw) for bw in bws]))\n\n if delta < tol:\n break\n \n print(\"iters = \"+str(iters))\n opt_bws = BWs[-1]\n print(\"opt_bws = \"+str(opt_bws))\n print(bw_gwr)\n return (opt_bws, np.array(BWs), np.array(scores), params, err, bw_gwr)",
"_____no_output_____"
],
[
"mgwbr = multi_bw(init=None,coords=coords,y=y, X=x_std, n=239, k=x.shape[1], family=Binomial())",
"first family: <spglm.family.Binomial object at 0x0000017B8F302588>\n<spglm.family.Gaussian object at 0x0000017B8DA45940>\n<spglm.family.Gaussian object at 0x0000017B8F341828>\nnum = 0.2261860620412264\n0.1493438159109546\nhere\n0.019261197296944955\nCurrent iteration: 1 ,SOC: 0.0192612\nBandwidths: 82.0\n<spglm.family.Gaussian object at 0x0000017B8DA45940>\n<spglm.family.Gaussian object at 0x0000017B8F2BD4A8>\nnum = 0.0\n0.0\nhere\n0.0\nCurrent iteration: 2 ,SOC: 0.0\nBandwidths: 82.0\niters = 2\nopt_bws = [82.]\n100.0\n"
],
[
"param = mgwbr[3]",
"_____no_output_____"
],
[
"predy = 1/(1+np.exp(-1*np.sum(x_std * param, axis=1).reshape(-1, 1)))",
"_____no_output_____"
],
[
"sns.distplot(predy)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
]
] |
e7bc9343fdcd4a988b4026672b9463a88b70c069 | 12,488 | ipynb | Jupyter Notebook | deep_learning/new-intro-to-pytorch/Part 8 - Transfer Learning (Solution).ipynb | willcanniford/python-learning | 1c2a33ab976e589fc6f801de2b6bd740d3aca2d7 | [
"MIT"
] | null | null | null | deep_learning/new-intro-to-pytorch/Part 8 - Transfer Learning (Solution).ipynb | willcanniford/python-learning | 1c2a33ab976e589fc6f801de2b6bd740d3aca2d7 | [
"MIT"
] | null | null | null | deep_learning/new-intro-to-pytorch/Part 8 - Transfer Learning (Solution).ipynb | willcanniford/python-learning | 1c2a33ab976e589fc6f801de2b6bd740d3aca2d7 | [
"MIT"
] | null | null | null | 42.332203 | 662 | 0.564302 | [
[
[
"# Transfer Learning\n\nIn this notebook, you'll learn how to use pre-trained networks to solved challenging problems in computer vision. Specifically, you'll use networks trained on [ImageNet](http://www.image-net.org/) [available from torchvision](http://pytorch.org/docs/0.3.0/torchvision/models.html). \n\nImageNet is a massive dataset with over 1 million labeled images in 1000 categories. It's used to train deep neural networks using an architecture called convolutional layers. I'm not going to get into the details of convolutional networks here, but if you want to learn more about them, please [watch this](https://www.youtube.com/watch?v=2-Ol7ZB0MmU).\n\nOnce trained, these models work astonishingly well as feature detectors for images they weren't trained on. Using a pre-trained network on images not in the training set is called transfer learning. Here we'll use transfer learning to train a network that can classify our cat and dog photos with near perfect accuracy.\n\nWith `torchvision.models` you can download these pre-trained networks and use them in your applications. We'll include `models` in our imports now.",
"_____no_output_____"
]
],
[
[
"%matplotlib inline\n%config InlineBackend.figure_format = 'retina'\n\nimport matplotlib.pyplot as plt\n\nimport torch\nfrom torch import nn\nfrom torch import optim\nimport torch.nn.functional as F\nfrom torchvision import datasets, transforms, models",
"_____no_output_____"
]
],
[
[
"Most of the pretrained models require the input to be 224x224 images. Also, we'll need to match the normalization used when the models were trained. Each color channel was normalized separately, the means are `[0.485, 0.456, 0.406]` and the standard deviations are `[0.229, 0.224, 0.225]`.",
"_____no_output_____"
]
],
[
[
"data_dir = 'Cat_Dog_data'\n\n# TODO: Define transforms for the training data and testing data\ntrain_transforms = transforms.Compose([transforms.RandomRotation(30),\n transforms.RandomResizedCrop(224),\n transforms.RandomHorizontalFlip(),\n transforms.ToTensor(),\n transforms.Normalize([0.485, 0.456, 0.406],\n [0.229, 0.224, 0.225])])\n\ntest_transforms = transforms.Compose([transforms.Resize(255),\n transforms.CenterCrop(224),\n transforms.ToTensor(),\n transforms.Normalize([0.485, 0.456, 0.406],\n [0.229, 0.224, 0.225])])\n\n# Pass transforms in here, then run the next cell to see how the transforms look\ntrain_data = datasets.ImageFolder(data_dir + '/train', transform=train_transforms)\ntest_data = datasets.ImageFolder(data_dir + '/test', transform=test_transforms)\n\ntrainloader = torch.utils.data.DataLoader(train_data, batch_size=64, shuffle=True)\ntestloader = torch.utils.data.DataLoader(test_data, batch_size=64)",
"_____no_output_____"
]
],
[
[
"We can load in a model such as [DenseNet](http://pytorch.org/docs/0.3.0/torchvision/models.html#id5). Let's print out the model architecture so we can see what's going on.",
"_____no_output_____"
]
],
[
[
"model = models.densenet121(pretrained=True)\nmodel",
"_____no_output_____"
]
],
[
[
"This model is built out of two main parts, the features and the classifier. The features part is a stack of convolutional layers and overall works as a feature detector that can be fed into a classifier. The classifier part is a single fully-connected layer `(classifier): Linear(in_features=1024, out_features=1000)`. This layer was trained on the ImageNet dataset, so it won't work for our specific problem. That means we need to replace the classifier, but the features will work perfectly on their own. In general, I think about pre-trained networks as amazingly good feature detectors that can be used as the input for simple feed-forward classifiers.",
"_____no_output_____"
]
],
[
[
"# Freeze parameters so we don't backprop through them\nfor param in model.parameters():\n param.requires_grad = False\n\nfrom collections import OrderedDict\nclassifier = nn.Sequential(OrderedDict([\n ('fc1', nn.Linear(1024, 500)),\n ('relu', nn.ReLU()),\n ('fc2', nn.Linear(500, 2)),\n ('output', nn.LogSoftmax(dim=1))\n ]))\n \nmodel.classifier = classifier",
"_____no_output_____"
]
],
[
[
"With our model built, we need to train the classifier. However, now we're using a **really deep** neural network. If you try to train this on a CPU like normal, it will take a long, long time. Instead, we're going to use the GPU to do the calculations. The linear algebra computations are done in parallel on the GPU leading to 100x increased training speeds. It's also possible to train on multiple GPUs, further decreasing training time.\n\nPyTorch, along with pretty much every other deep learning framework, uses [CUDA](https://developer.nvidia.com/cuda-zone) to efficiently compute the forward and backwards passes on the GPU. In PyTorch, you move your model parameters and other tensors to the GPU memory using `model.to('cuda')`. You can move them back from the GPU with `model.to('cpu')` which you'll commonly do when you need to operate on the network output outside of PyTorch. As a demonstration of the increased speed, I'll compare how long it takes to perform a forward and backward pass with and without a GPU.",
"_____no_output_____"
]
],
[
[
"import time",
"_____no_output_____"
],
[
"for device in ['cpu', 'cuda']:\n\n criterion = nn.NLLLoss()\n # Only train the classifier parameters, feature parameters are frozen\n optimizer = optim.Adam(model.classifier.parameters(), lr=0.001)\n\n model.to(device)\n\n for ii, (inputs, labels) in enumerate(trainloader):\n\n # Move input and label tensors to the GPU\n inputs, labels = inputs.to(device), labels.to(device)\n\n start = time.time()\n\n outputs = model.forward(inputs)\n loss = criterion(outputs, labels)\n loss.backward()\n optimizer.step()\n\n if ii==3:\n break\n \n print(f\"Device = {device}; Time per batch: {(time.time() - start)/3:.3f} seconds\")",
"_____no_output_____"
]
],
[
[
"You can write device agnostic code which will automatically use CUDA if it's enabled like so:\n```python\n# at beginning of the script\ndevice = torch.device(\"cuda:0\" if torch.cuda.is_available() else \"cpu\")\n\n...\n\n# then whenever you get a new Tensor or Module\n# this won't copy if they are already on the desired device\ninput = data.to(device)\nmodel = MyModule(...).to(device)\n```\n\nFrom here, I'll let you finish training the model. The process is the same as before except now your model is much more powerful. You should get better than 95% accuracy easily.\n\n>**Exercise:** Train a pretrained models to classify the cat and dog images. Continue with the DenseNet model, or try ResNet, it's also a good model to try out first. Make sure you are only training the classifier and the parameters for the features part are frozen.",
"_____no_output_____"
]
],
[
[
"# Use GPU if it's available\ndevice = torch.device(\"cuda\" if torch.cuda.is_available() else \"cpu\")\n\nmodel = models.densenet121(pretrained=True)\n\n# Freeze parameters so we don't backprop through them\nfor param in model.parameters():\n param.requires_grad = False\n \nmodel.classifier = nn.Sequential(nn.Linear(1024, 256),\n nn.ReLU(),\n nn.Dropout(0.2),\n nn.Linear(256, 2),\n nn.LogSoftmax(dim=1))\n\ncriterion = nn.NLLLoss()\n\n# Only train the classifier parameters, feature parameters are frozen\noptimizer = optim.Adam(model.classifier.parameters(), lr=0.003)\n\nmodel.to(device);",
"_____no_output_____"
],
[
"epochs = 1\nsteps = 0\nrunning_loss = 0\nprint_every = 5\nfor epoch in range(epochs):\n for inputs, labels in trainloader:\n steps += 1\n # Move input and label tensors to the default device\n inputs, labels = inputs.to(device), labels.to(device)\n \n optimizer.zero_grad()\n \n logps = model.forward(inputs)\n loss = criterion(logps, labels)\n loss.backward()\n optimizer.step()\n\n running_loss += loss.item()\n \n if steps % print_every == 0:\n test_loss = 0\n accuracy = 0\n model.eval()\n with torch.no_grad():\n for inputs, labels in testloader:\n inputs, labels = inputs.to(device), labels.to(device)\n logps = model.forward(inputs)\n batch_loss = criterion(logps, labels)\n \n test_loss += batch_loss.item()\n \n # Calculate accuracy\n ps = torch.exp(logps)\n top_p, top_class = ps.topk(1, dim=1)\n equals = top_class == labels.view(*top_class.shape)\n accuracy += torch.mean(equals.type(torch.FloatTensor)).item()\n \n print(f\"Epoch {epoch+1}/{epochs}.. \"\n f\"Train loss: {running_loss/print_every:.3f}.. \"\n f\"Test loss: {test_loss/len(testloader):.3f}.. \"\n f\"Test accuracy: {accuracy/len(testloader):.3f}\")\n running_loss = 0\n model.train()",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
]
] |
e7bc99969d9b32920adb2f76bf978948485a97d3 | 368,785 | ipynb | Jupyter Notebook | analysis/distribution-analysis.ipynb | HuckleyLab/phyto-mhw | 8e067c73310fb4a4520d5a72f68717030ce90e14 | [
"MIT"
] | 2 | 2020-10-13T02:37:26.000Z | 2021-04-27T04:41:09.000Z | analysis/distribution-analysis.ipynb | HuckleyLab/phyto-mhw | 8e067c73310fb4a4520d5a72f68717030ce90e14 | [
"MIT"
] | 8 | 2020-07-19T10:54:37.000Z | 2021-10-17T19:53:09.000Z | analysis/distribution-analysis.ipynb | HuckleyLab/phyto-mhw | 8e067c73310fb4a4520d5a72f68717030ce90e14 | [
"MIT"
] | null | null | null | 145.305359 | 66,728 | 0.864932 | [
[
[
"! pip install pygbif\n",
"Collecting pygbif\n Using cached https://files.pythonhosted.org/packages/7a/25/222531f22cf162409c38f55c48b13ab0c4128cf13ac05290de8878d639b1/pygbif-0.4.0-py2.py3-none-any.whl\nCollecting geomet\n Using cached https://files.pythonhosted.org/packages/c9/81/156ca48f950f833ddc392f8e3677ca50a18cb9d5db38ccb4ecea55a9303f/geomet-0.2.1.post1-py3-none-any.whl\nCollecting geojson-rewind\n Using cached https://files.pythonhosted.org/packages/f7/5b/55fb64433477ebc983546e2d84ce04473b8750525dab67b2b18c6959a1ae/geojson_rewind-0.2.0-py3-none-any.whl\nCollecting requests-cache\n Using cached https://files.pythonhosted.org/packages/7f/55/9b1c40eb83c16d8fc79c5f6c2ffade04208b080670fbfc35e0a5effb5a92/requests_cache-0.5.2-py2.py3-none-any.whl\nRequirement already satisfied: matplotlib in /srv/conda/envs/notebook/lib/python3.7/site-packages (from pygbif) (3.1.2)\nRequirement already satisfied: appdirs>=1.4.3 in /srv/conda/envs/notebook/lib/python3.7/site-packages (from pygbif) (1.4.3)\nRequirement already satisfied: requests>2.7 in /srv/conda/envs/notebook/lib/python3.7/site-packages (from pygbif) (2.22.0)\nRequirement already satisfied: six in /srv/conda/envs/notebook/lib/python3.7/site-packages (from geomet->pygbif) (1.14.0)\nRequirement already satisfied: click in /srv/conda/envs/notebook/lib/python3.7/site-packages (from geomet->pygbif) (7.0)\nRequirement already satisfied: kiwisolver>=1.0.1 in /srv/conda/envs/notebook/lib/python3.7/site-packages (from matplotlib->pygbif) (1.1.0)\nRequirement already satisfied: pyparsing!=2.0.4,!=2.1.2,!=2.1.6,>=2.0.1 in /srv/conda/envs/notebook/lib/python3.7/site-packages (from matplotlib->pygbif) (2.4.6)\nRequirement already satisfied: python-dateutil>=2.1 in /srv/conda/envs/notebook/lib/python3.7/site-packages (from matplotlib->pygbif) (2.7.5)\nRequirement already satisfied: numpy>=1.11 in /srv/conda/envs/notebook/lib/python3.7/site-packages (from matplotlib->pygbif) (1.17.3)\nRequirement already satisfied: cycler>=0.10 in /srv/conda/envs/notebook/lib/python3.7/site-packages (from matplotlib->pygbif) (0.10.0)\nRequirement already satisfied: chardet<3.1.0,>=3.0.2 in /srv/conda/envs/notebook/lib/python3.7/site-packages (from requests>2.7->pygbif) (3.0.4)\nRequirement already satisfied: urllib3!=1.25.0,!=1.25.1,<1.26,>=1.21.1 in /srv/conda/envs/notebook/lib/python3.7/site-packages (from requests>2.7->pygbif) (1.25.7)\nRequirement already satisfied: idna<2.9,>=2.5 in /srv/conda/envs/notebook/lib/python3.7/site-packages (from requests>2.7->pygbif) (2.8)\nRequirement already satisfied: certifi>=2017.4.17 in /srv/conda/envs/notebook/lib/python3.7/site-packages (from requests>2.7->pygbif) (2019.11.28)\nRequirement already satisfied: setuptools in /srv/conda/envs/notebook/lib/python3.7/site-packages (from kiwisolver>=1.0.1->matplotlib->pygbif) (45.1.0.post20200119)\nInstalling collected packages: geomet, geojson-rewind, requests-cache, pygbif\nSuccessfully installed geojson-rewind-0.2.0 geomet-0.2.1.post1 pygbif-0.4.0 requests-cache-0.5.2\n"
],
[
"import os\n\nimport pandas as pd\nimport seaborn as sns\n\nimport matplotlib.pyplot as plt\nfrom matplotlib import colors\nfrom matplotlib.animation import FuncAnimation\nsns.set_style('ticks', {'font_scale': 1.1})\n\nimport random\nimport numpy as np\n\nimport gcsfs\n\nfrom datetime import timedelta, datetime\n\nfrom functools import partial \n\nimport cartopy.crs as ccrs\nimport cartopy.feature as cf\n\nfrom tqdm import tqdm\n\nimport xarray as xr\nfrom dask.distributed import Client\n\n%matplotlib inline\n\nPNW_LAT = slice(1.52, 63.4)\nPNW_LON =slice(-170, -103)\n\nimport pygbif",
"_____no_output_____"
],
[
"GCP_PROJECT_ID = '170771369993'\nOISST_GCP = 'oisst/oisst.zarr'",
"_____no_output_____"
]
],
[
[
"# PNW Focal Species Sanity Check\nWe'll use GBIF to select a species with lots of occurrences in the PNW and assess the impact of MHWs on that species. ",
"_____no_output_____"
]
],
[
[
"plankton = pd.read_csv(\"../data/Phytoplankton_temperature_growth_rate_dataset_2016_01_29/traits_derived_2016_01_29.csv\", engine='python')",
"_____no_output_____"
],
[
"plankton = plankton[(plankton.minqual == \"good\") &\n (plankton.maxqual == \"good\") &\n (plankton.curvequal == \"good\")]",
"_____no_output_____"
],
[
"plankton = plankton[plankton.habitat == 'marine']",
"_____no_output_____"
],
[
"print(len(plankton))\nprint(len(set(list(zip(plankton.genus, plankton.species)))))",
"96\n62\n"
],
[
"plankton.genus.unique()",
"_____no_output_____"
],
[
"plankton['mu.c.opt.list'].plot(kind='hist')",
"_____no_output_____"
],
[
"plankton[plankton['mu.c.opt.list'].between(13, 16)]",
"_____no_output_____"
],
[
"sample_loc = 178",
"_____no_output_____"
],
[
"plankton.columns",
"_____no_output_____"
]
],
[
[
"## GBIF Occurrences\n*For locating PNW species...*",
"_____no_output_____"
]
],
[
[
"genera = plankton.genus.dropna().unique()\ngenera",
"_____no_output_____"
],
[
"genera = [pygbif.species.name_lookup(q=g, rank='genus') for g in genera]",
"_____no_output_____"
],
[
"genera = [g for g in genera if len(g['results']) > 0]",
"_____no_output_____"
],
[
"genera = [max(g['results'], key=lambda x: x['numDescendants']) for g in genera]",
"_____no_output_____"
],
[
"[g['key'] for g in genera]",
"_____no_output_____"
],
[
"genera_counts = [pygbif.occurrences.count(taxonKey=g['key']) for g in genera]",
"_____no_output_____"
],
[
"genera_counts",
"_____no_output_____"
],
[
"occs = []\nfor genus, genus_count in zip(genera, genera_counts):\n print(genus['genus'], genus_count)\n if genus_count > 5:\n occs.append(pygbif.occurrences.search(\n taxonKey=genus['key'],\n decimalLatitude=f\"{PNW_LAT.start},{PNW_LAT.stop}\",\n decimalLongitude=f\"{PNW_LON.start},{PNW_LON.stop}\"\n ))\n else: \n occs.append([])",
"Koliella 0\nKlebsormidium 1260\nPhaeocystis 15529\nNitzschia 0\nEmiliania 16685\nFibrocapsa 0\nGephyrocapsa 33714\nCalcidiscus 31506\nIsochrysis 1281\nTrichodesmium 0\nPernambugia 18\nSkeletonema 63424\nOdontella 44286\nChaetoceros 246661\nRhizosolenia 199674\nThalassionema 101496\nLeonella 0\nGymnodinium 44541\nCrocosphaera 0\nHelicotheca 2519\nKarenia 5\nThalassiosira 198039\nSynechococcus 18960\nAmphiprora 935\nLeptocylindrus 62691\nProchlorococcus 15007\nChattonella 1520\nAkashiwo 0\nCochlodinium 12674\nBiceratium 0\nNeoceratium 0\nPeridinium 19577\nProrocentrum 73640\nConticribra 105\nCylindrotheca 31771\nAlexandrium 23326\nMicromonas 0\nDiacronema 464\nIpsiura 641\nProboscia 23761\nNannochloris 1958\n"
],
[
"occs = [o for o in occs if type(o) == dict]",
"_____no_output_____"
],
[
"max_genus = max(occs, key=lambda x: x['count'])['results'][0]",
"_____no_output_____"
],
[
"max_genus['genus']",
"_____no_output_____"
],
[
"max_genus_name = \"Skeletonema\"",
"_____no_output_____"
]
],
[
[
"## TPC From Genus",
"_____no_output_____"
]
],
[
[
"def tpc(T, a, b, z, w):\n '''\n https://science.sciencemag.org/content/sci/suppl/2012/10/25/science.1224836.DC1/Thomas.SM.pdf\n '''\n return a * np.exp(b*T) * (1 - ((T - z)/(w / 2))**2)\ndef plot_tpc(sample, ax=None):\n T = np.arange(sample['mu.c.opt.list'] - (sample['mu.wlist'] / 2), sample['mu.c.opt.list'] + (sample['mu.wlist'] / 2), 0.1)\n perf = tpc(T, sample['mu.alist'], sample['mu.blist'], sample['mu.c.opt.list'], sample['mu.wlist'])\n try:\n plotTitle = \"{} {} [{}]\".format(sample.genus, sample.species, sample['isolate.code'])\n except AttributeError as e: \n plotTitle = \"\"\n if ax:\n ax.plot(T, perf)\n ax.set_title(plotTitle)\n ax.set_xlabel(\"$T$\")\n sns.despine(ax=ax)\n else:\n plt.plot(T, perf)\n plt.title(plotTitle)\n plt.xlabel(\"$T$ [${^\\circ}C$]\")\n sns.despine()",
"_____no_output_____"
],
[
"this_genus = plankton[plankton.genus == max_genus_name]",
"_____no_output_____"
],
[
"this_genus",
"_____no_output_____"
]
],
[
[
"## **NOTE** Overrode above analysis and chose a single species",
"_____no_output_____"
]
],
[
[
"sample_species = this_genus.sample(1).iloc[0]",
"_____no_output_____"
],
[
"plot_tpc(sample_species)",
"_____no_output_____"
]
],
[
[
"## Enter MHW and SST",
"_____no_output_____"
]
],
[
[
"mhw_time = slice('2014-01-01', '2015-05-31')",
"_____no_output_____"
],
[
"mhws = xr.open_dataset(\"../mhw_pipeline/pnw_mhw_intensity.nc\").rename({\n '__xarray_dataarray_variable__': 'mhw_intensity'\n})\n",
"_____no_output_____"
],
[
"mhws",
"_____no_output_____"
],
[
"mhw_recent = mhws.sel(time=mhw_time)",
"_____no_output_____"
],
[
"\n\nmhw_count = mhw_recent.median(dim='time').mhw_intensity",
"_____no_output_____"
],
[
"plt.figure(figsize=(10,10))\nax = plt.axes(projection=ccrs.Mercator().GOOGLE)\nplot = mhw_count.plot(norm=colors.LogNorm(vmin=1, vmax=mhw_count.max()), cmap='PuBu_r', ax=ax)",
"/srv/conda/envs/notebook/lib/python3.7/site-packages/matplotlib/colors.py:1110: RuntimeWarning: invalid value encountered in less_equal\n mask |= resdat <= 0\n"
],
[
"mhw_count.lon.max()",
"_____no_output_____"
],
[
"plt.figure(figsize=(10,10))\nax = plt.axes(projection=ccrs.Mercator().GOOGLE)\nmhw_count.plot.contourf(cmap='Spectral', ax=ax, transform=ccrs.PlateCarree())\nax.gridlines(alpha=0.7)\nax.add_feature(cf.COASTLINE, facecolor='black')",
"_____no_output_____"
]
],
[
[
"### SST",
"_____no_output_____"
]
],
[
[
"fs = gcsfs.GCSFileSystem(project=GCP_PROJECT_ID, token=\"/home/jovyan/gc-pangeo.json\")\noisst = xr.open_zarr(fs.get_mapper(OISST_GCP))\noisst = oisst.assign_coords(lon=(((oisst.lon + 180) % 360) - 180)).sortby('lon')",
"_____no_output_____"
],
[
"PNW_LAT = slice(mhw_count.lat.min(), mhw_count.lat.max())\nPNW_LON = slice(mhw_count.lon.min(), mhw_count.lon.max())",
"_____no_output_____"
],
[
"oisst_pnw = oisst.sel(lat = PNW_LAT, lon = PNW_LON).persist()\n",
"_____no_output_____"
],
[
"recent_sst = oisst_pnw.sel(time=mhw_time)",
"_____no_output_____"
],
[
"plt.figure(figsize=(10,10))\nax = plt.axes(projection=ccrs.Mercator().GOOGLE)\nrecent_sst.sst.max(dim='time',).plot( ax=ax, transform=ccrs.PlateCarree())\nax.add_feature(cf.COASTLINE)",
"/srv/conda/envs/notebook/lib/python3.7/site-packages/dask/utils.py:29: RuntimeWarning: All-NaN slice encountered\n return func(*args, **kwargs)\n/srv/conda/envs/notebook/lib/python3.7/site-packages/toolz/functoolz.py:488: RuntimeWarning: All-NaN slice encountered\n ret = f(ret)\n"
],
[
"\nplt.figure(figsize=(10,10))\nax = plt.axes(projection=ccrs.Mercator().GOOGLE)\nrecent_sst.sst.sel(time=mhw_time.stop).plot.contourf( ax=ax, transform=ccrs.PlateCarree(), levels=10)\nax.add_feature(cf.COASTLINE)",
"_____no_output_____"
],
[
"dates_for_plot = [pd.to_datetime(mhw_time.start) + timedelta(n) for n in range(len(recent_sst.time))]",
"_____no_output_____"
],
[
"mhws.sel(time=mhw_time.start).mhw.plot.contourf(cmap='binary',vmax=1, levels=3)\n",
"_____no_output_____"
],
[
"fig = plt.figure()\n\ndef anim_func(i):\n fig.clf()\n print(i)\n ax = plt.axes(projection=ccrs.Mercator().GOOGLE)\n mhws.sel(time=i).mhw.plot.contourf(cmap='binary', ax=ax, transform=ccrs.PlateCarree(), vmax=1, levels=3)\n\n \n \n \nanim = FuncAnimation(fig, anim_func, frames=dates_for_plot, interval=100)\nanim.save(\"test.gif\")",
"MovieWriter ffmpeg unavailable; trying to use <class 'matplotlib.animation.PillowWriter'> instead.\n"
]
],
[
[
"## Compute Performance Detriment\n<!-- We're calling it like this: $$P_{det} = P(T_{opt}) - P(T)$$ where $P(T)$ is the value of the thermal performance curve at temperature $T$. -->",
"_____no_output_____"
]
],
[
[
"def perf_det(T, T_opt, tpc, axis=1):\n \n return tpc(T_opt) - tpc(T)\n\ndef tsm(T, T_opt, axis):\n return T - T_opt\n\ndef plot_det(s, ax):\n this_tpc = partial(tpc, a=s['mu.alist'], b=s['mu.blist'], z=s['mu.c.opt.list'], w=s['mu.wlist'])\n T = np.arange(s['mu.g.opt.list'] - (s['mu.wlist'] / 2), s['mu.c.opt.list'] + (s['mu.wlist'] / 2), 0.1)\n \n perf = this_tpc(T)\n \n max_perf = this_tpc(s['mu.g.opt.list'])\n \n randomT = np.random.choice(T, size=1)\n perf_T = this_tpc(randomT)\n det_T = perf_det(randomT, s['mu.g.opt.list'], this_tpc)\n\n plt.plot(T, perf)\n plt.vlines(randomT,max_perf, max_perf - det_T)\n plt.axhline(max_perf)",
"_____no_output_____"
],
[
"mean_species=sample_species",
"_____no_output_____"
],
[
"this_det = partial(\n perf_det,\n T_opt = mean_species['mu.g.opt.list'],\n tpc = partial(tpc,\n a=mean_species['mu.alist'],\n b=mean_species['mu.blist'],\n z=mean_species['mu.c.opt.list'],\n w=mean_species['mu.wlist'])\n)",
"_____no_output_____"
],
[
"this_tsm = partial(\n tsm,\n T_opt = mean_species['mu.g.opt.list']\n)",
"_____no_output_____"
],
[
"ans = recent_sst.sst.reduce(this_det, dim='time').compute()",
"_____no_output_____"
],
[
"tsm_ans = recent_sst.sst.reduce(this_tsm, dim='time').compute()",
"_____no_output_____"
],
[
"plt.figure(figsize=(10,10))\nax = plt.axes(projection=ccrs.Mercator().GOOGLE)\nans.sel(time=slice('2014-01-01', '2014-05-28')).sum(dim='time').plot.contourf(ax=ax, cmap='viridis', vmin=-10, vmax=10)\nax.gridlines(alpha=0.7)\nax.add_feature(cf.COASTLINE, facecolor='black', zorder=10)\nplt.show()",
"_____no_output_____"
],
[
"plt.figure(figsize=(10,10))\nax = plt.axes(projection=ccrs.Mercator().GOOGLE)\ntsm_ans.mean(dim='time').plot.contourf(ax=ax, cmap='viridis', vmin=-1, vmax=1)\nax.gridlines(alpha=0.7)\nax.add_feature(cf.COASTLINE, facecolor='black')\n",
"_____no_output_____"
],
[
"plt.figure(figsize=(10,10))\nax = plt.axes(projection=ccrs.Mercator().GOOGLE)\nans.sel(time=slice('2014-10-25', '2014-12-31')).std(dim='time').plot.contourf(ax=ax)\nax.gridlines(alpha=0.7)\nax.add_feature(cf.COASTLINE, facecolor='black')\n",
"_____no_output_____"
],
[
"example_mhw = slice('2014-01-01', '2014-5-31')\nans_cumsum = ans.sel(time=example_mhw).cumsum(dim='time')\nmhw_cumsum = mhws.sel(time=example_mhw).mhw.cumsum(dim='time')",
"_____no_output_____"
],
[
"def daterange(start_date, end_date):\n for n in range(int ((pd.to_datetime(end_date) - pd.to_datetime(start_date)).days)):\n yield pd.to_datetime(start_date) + timedelta(n)",
"_____no_output_____"
],
[
"fig = plt.figure(figsize=(10,10))\n\ndef anim_func(i):\n print(i)\n fig.clf()\n ax = fig.subplots(nrows =1, ncols = 2, subplot_kw={\"projection\" : ccrs.PlateCarree()})\n mhws.mhw.sel(time=i).plot(cmap='Spectral', ax=ax[0], transform=ccrs.PlateCarree(), vmax=1)\n ans_norm = colors.DivergingNorm(vmin=-1, vcenter=0, vmax=1)\n ans.sel(time=i).plot(cmap='bwr', ax=ax[1], transform=ccrs.PlateCarree(), norm=ans_norm)\n ax[0].add_feature(cf.COASTLINE, facecolor='black')\n ax[1].add_feature(cf.COASTLINE, facecolor='black')\n\n\nframes = list(daterange(example_mhw.start, example_mhw.stop))\n\nanim = FuncAnimation(fig, anim_func, frames=frames, interval=100, save_count=len(frames))\nanim.save(\"test.gif\")",
"MovieWriter ffmpeg unavailable; trying to use <class 'matplotlib.animation.PillowWriter'> instead.\n"
],
[
"\n",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bcb0af428ab76c4c4bccfe76da9f4ebf2c702a | 391,057 | ipynb | Jupyter Notebook | analysis/.ipynb_checkpoints/Task_5-checkpoint_LOCAL_1236.ipynb | data301-2020-winter2/course-project-group_1001 | c0e548c9f5132f738ce4ccb8e822ac58b83f84b9 | [
"MIT"
] | null | null | null | analysis/.ipynb_checkpoints/Task_5-checkpoint_LOCAL_1236.ipynb | data301-2020-winter2/course-project-group_1001 | c0e548c9f5132f738ce4ccb8e822ac58b83f84b9 | [
"MIT"
] | 1 | 2021-03-23T23:41:04.000Z | 2021-03-29T02:28:19.000Z | analysis/.ipynb_checkpoints/Task_5-checkpoint_LOCAL_1236.ipynb | data301-2020-winter2/course-project-group_1001 | c0e548c9f5132f738ce4ccb8e822ac58b83f84b9 | [
"MIT"
] | 1 | 2021-02-07T23:23:55.000Z | 2021-02-07T23:23:55.000Z | 629.721417 | 98,568 | 0.941947 | [
[
[
"# Data Analysis\n\n## Research Question: Examine the top 3 countries who have competed at the olympics, and examine their preformance over the past 120 years.\n#### For Each top 3 country, these aspects should be examined in order to answer the question:\n- Which years did this country win the most medals? \n - Most gold medals?\n- Which years did this country win the least amount of medals?\n- When did this country first appear in the olympics?\n- How many olympics has this country competed in?\n- How many athletes of that country have competed? \n - How many each year? \n - How many male or female athletes?\n- How many athletes of that country have won medals?\n - How many won each year? \n - How many male or female athletes have won medals?\n - How many gold, silver, bronze medals have been won by this country?\n- How many athletes of this country have competed during summer olympics compared to winter olympics? \n - How does this compare yearly?\n- How many medals were won by athletes of this country during the summer olympics compared to the winter olympics? \n - How does this compare yearly?\n- What are the ages of the oldest and youngest athletes of this country to compete, and what is the average age of competing athletes of this country?\n- What are the ages of the oldest and youngest athletes of this country who have won medals, and what is the average age of athletes who have won medals for this country?\n- What is the average age of completing athletes per year of this country?\n- What is the average age of athletes who have won medals for this country per year?\n- What are the top 5 competed sports for this country?\n- What are the top 5 most successful sports for this country?\n",
"_____no_output_____"
],
[
"Starting with some basic imports, as well as the dataset import and a simple query, we can determine what are the 3 most sucessful countries during the olympics.",
"_____no_output_____"
]
],
[
[
"import pandas as pd\nimport numpy as np\nfrom matplotlib import pyplot\nimport matplotlib.pyplot as plt\nimport seaborn as sns\ndata = pd.read_csv('../data/raw/olympic_dataset.csv', low_memory=False, encoding = 'utf-8')\ntop3Countries=(data.dropna(subset=['Medal'])).value_counts(subset=['NOC'])\ntop3Countries.head(3)",
"_____no_output_____"
]
],
[
[
"Based on this, we can see that the United States leads the dataset with the most amount of medals, with the Soviet Union coming in second, and Germany in third. Therefore, our data analysis will focus on these three countries.\n\nAlthough, with the Soviet Union and Germany, there have been many historical changes to those two countries over the past 120 years, which could affect medal counts. So, in order to remain competitive with the United States, as well as accurate, previous and modern versions of these two countries olympic teams will be considered as well. For the Soviet Union, this means including modern Russian teams, teams that belonged to the Empire of Russia, as well as the Unified Soviet Team. For Germany, this means including the Empire of Germany, East Germany, West Germany, and the Saar Protectorate, alongside its modern form. Now that we are considering all modern and historical versions of the countries mentioned, we can start with the analysis.",
"_____no_output_____"
],
[
"## United States(Funmi)",
"_____no_output_____"
]
],
[
[
"us =(data[\"NOC\"] == \"USA\")\nathletes=data[(us)]\nprint(\"Number of events particpated in by American athletes: \"+str(athletes.shape[0]))\nathletesUnique=data[us].drop_duplicates(subset=['Name'])\nprint(\"Number of American athletes who have competed at the olympics: \"+str(athletesUnique.shape[0]))\nmaleAthletesUnique=data[us&(data['Sex'] == 'M')].drop_duplicates(subset=['Name'])\nprint(\"Number of male American athletes who have competed in the olympics: \"+str(maleAthletesUnique.shape[0]))\nfemaleAthletesUnique=data[us&(data['Sex'] == 'F')].drop_duplicates(subset=['Name'])\nprint(\"Number of female American athletes who have competed in the olympics: \"+str(femaleAthletesUnique.shape[0])+\"\\n\")\nmedals=athletes.dropna(subset=[\"Medal\"])\nprint(\"Number medals won by American athletes: \"+str(medals.shape[0]))\nmaleAthletesMedals=data[us&(data['Sex'] == 'M')].dropna(subset=[\"Medal\"])\nprint(\"Number of medals won by male American athletes: \"+str(maleAthletesMedals.shape[0]))\nfemaleAthletesMedals=data[us&(data['Sex'] == 'F')].dropna(subset=[\"Medal\"])\nprint(\"Number of medals won by female American athletes: \"+str(femaleAthletesMedals.shape[0])+\"\\n\")\nAthletesMedalsUnique=medals.drop_duplicates(subset=['Name'])\nprint(\"Number of American athletes who won medals: \"+str(AthletesMedalsUnique.shape[0]))\nmaleAthletesMedalsUnique=maleAthletesMedals.drop_duplicates(subset=['Name'])\nprint(\"Number of male American athletes who won medals: \"+str(maleAthletesMedalsUnique.shape[0]))\nfemaleAthletesMedalsUnique=femaleAthletesMedals.drop_duplicates(subset=['Name'])\nprint(\"Number of female American athletes who won medals: \"+str(femaleAthletesMedalsUnique.shape[0])+\"\\n\")\nmtype=medals.value_counts(subset=['Medal'])\nprint(\"Types of medals won by American athletes\")\nprint(mtype)",
"Number of events particpated in by American athletes: 18853\nNumber of American athletes who have competed at the olympics: 9652\nNumber of male American athletes who have competed in the olympics: 7039\nNumber of female American athletes who have competed in the olympics: 2613\n\nNumber medals won by American athletes: 5637\nNumber of medals won by male American athletes: 3832\nNumber of medals won by female American athletes: 1805\n\nNumber of American athletes who won medals: 3836\nNumber of male American athletes who won medals: 2742\nNumber of female American athletes who won medals: 1094\n\nTypes of medals won by American athletes\nMedal \nGold 2638\nSilver 1641\nBronze 1358\ndtype: int64\n"
],
[
"top5c=athletes.value_counts(['Sport'])\nprint(\"top 5 most competed sports by American athletes:\")\nprint(top5c.iloc[:5])\nprint(\"\\ntop top 5 most successful sports for American athletes:\")\ntop5m=medals.value_counts(['Sport'])\nprint(top5m.iloc[:5])\ncstats=athletesUnique.groupby('Sex').agg({'Age': ['mean', 'min', 'max']})\nprint(\"\\nAverage, minimum, and maximum ages of American athletes who have competed at the olympics:\")\nprint(cstats)\nmstats=medals.groupby('Sex').agg({'Age': ['mean', 'min', 'max']})\nprint(\"\\nAverage, minimum, and maximum ages of American athletes who have won medals at the olympics:\")\nprint(mstats)",
"top 5 most competed sports by American athletes:\nSport \nAthletics 3211\nGymnastics 2013\nSwimming 1638\nRowing 828\nFencing 690\ndtype: int64\n\ntop top 5 most successful sports for American athletes:\nSport \nAthletics 1080\nSwimming 1078\nRowing 375\nBasketball 341\nIce Hockey 276\ndtype: int64\n\nAverage, minimum, and maximum ages of American athletes who have competed at the olympics:\n Age \n mean min max\nSex \nF 23.588778 12.0 65.0\nM 25.413283 13.0 97.0\n\nAverage, minimum, and maximum ages of American athletes who have won medals at the olympics:\n Age \n mean min max\nSex \nF 23.722838 12.0 63.0\nM 25.455649 14.0 68.0\n"
],
[
"sns.lineplot(data=athletesUnique, x=\"Year\", y='Age')\nplt.title('Average Age of American Olympic Athletes per Year')\nsns.set(rc={'figure.figsize':(15,10)})",
"_____no_output_____"
],
[
"sns.lineplot(data=medals, x=\"Year\", y='Age')\nplt.title(\"Average age of American medal winning athletes per year\")",
"_____no_output_____"
],
[
"sns.countplot(data=medals, x=\"Year\", hue=\"Medal\", palette='rocket')\nplt.title(\"Medals won by American olympic athletes per year\")",
"_____no_output_____"
],
[
"sns.countplot(data=athletesUnique, x=\"Year\")\nplt.title(\"American olympic athletes per year\")",
"_____no_output_____"
],
[
"sns.countplot(data=AthletesMedalsUnique, x=\"Year\")\nplt.title(\"Americans that won that medals per year\")",
"_____no_output_____"
],
[
"sns.countplot(data=athletesUnique, x=\"Year\", hue=\"Season\", palette='dark')\nplt.title(\"American olympic athletes per year during summer vs winter olympics\")",
"_____no_output_____"
],
[
"sns.countplot(data=medals, x=\"Year\", hue=\"Season\", palette='dark')\nplt.title(\"American medal winning athletes per year during winter vs summer olympics\")",
"_____no_output_____"
]
],
[
[
"# Other Graphs to Note",
"_____no_output_____"
]
],
[
[
"sns.countplot(data=medals, x=\"Medal\", hue=\"Sex\", palette='pastel')\nplt.title(\"Types of medals won by male and female American athletes\")",
"_____no_output_____"
],
[
"sns.countplot(data=medals, x=\"Year\", hue=\"Sex\", palette='pastel')\nplt.title(\"Medals won by American olympic athletes per year compared to sex\")",
"_____no_output_____"
]
],
[
[
"## With all this information we can now answer those questions",
"_____no_output_____"
],
[
"Which years did this country win the most medals?\n- According to the graph in 1984 the US won the most gold medals. In 1904 they won the most silver and bronze medals.\n\nMost gold medals?\n- They won the most gold in at the 1984 olympics.\n\nWhich years did this country win the least amount of medals?\n- They won the least amount of medals in 1906 and 1994.\n\nWhen did this country first appear in the olympics?\n- America first appear at the olympics in 1896.\n\nHow many olympics has this country competed in?\n- They have competed in 35 olympics.\n\nHow many athletes of that country have competed?\n- 9652 American athletes have competed at the olympics\n\nHow many each year?\n- According to the graph \"American olympic athletes per year\" (4th graph) it varies from year to year. There have years where there were as little as around 20 people to as high as almost 600 people. These numbers also affected how many medals were won that year in the USA.\n\nHow many male or female athletes?\n- There have been about 7039 athletes\n- There have been about 2613 female athletes\n\nHow many athletes of that country have won medals?\n- There have been 3836 American Athletes that have won medals\n\nHow many won each year?\n- It can go as low as almost 10 to as high as almost 300 medals won\n\nHow many male or female athletes have won medals?\n- 2742 males and 1094 females have won medals in the olympics\n\nHow many gold, silver, bronze medals have been won by this country?\n- 2638 gold medals, 1641 silver medals and 1358 bronze medals\n\nHow many athletes of this country have competed during summer olympics compared to winter olympics?\n- There have been 100s more athletes that compete in the summer olympics compared winters olympics\n\nHow does this compare yearly?\n- This varies but as time progressed more athletes competed in the winter, but overall more athletes compete in the summer olympics.\n\nHow many medals were won by athletes of this country during the summer olympics compared to the winter olympics?\n- There have been 100s more medals one in summer compared to winter\n\nHow does this compare yearly?\n- This varies from year to year but it is based on the athletes that compete each year\n\nWhat are the ages of the oldest and youngest athletes of this country to compete, and what is the average age of competing athletes of this country?\n- For Females the youngest was 12y/o and the oldest was 65y/o\n- For Males the youngest was 13y/o and the oldest was 97y/o\n\nWhat are the ages of the oldest and youngest athletes of this country who have won medals, and what is the average age of athletes who have won medals for this country?\n- For Females the youngest was 12y/o and the oldest was 63y/o\n- For Males the youngest was 14y/o and the oldest was 68y/o\n\nWhat is the average age of completing athletes per year of this country?\n- For Females is around 24 years old and for Men it is around 25 years old\n\nWhat is the average age of athletes who have won medals for this country per year?\n- For Females is around 24 years old and for Men it is around 25 years old\n\nWhat are the top 5 competed sports for this country?\n- These sports are Athletics, Gymnastics, Swimming, Rowing, Fencing\n\nWhat are the top 5 most successful sports for this country?\n- The top 5 most successful sports for Americans would be Athletics, Swimming, Rowing, Basketball, Ice Hockey",
"_____no_output_____"
],
[
"## Soviet Union/Russia(name here)",
"_____no_output_____"
],
[
"## Germany(Christian)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown",
"markdown",
"markdown"
]
] |
e7bcb91408abbab09696e2a6158f3368c7ea6b90 | 18,758 | ipynb | Jupyter Notebook | examples/XNSFE_Solver/HeatedWall_VariableDensity/HeatedWall90DegVariableDensity.ipynb | FDYdarmstadt/BoSSS | 974f3eee826424a213e68d8d456d380aeb7cd7e9 | [
"Apache-2.0"
] | 22 | 2017-06-08T05:53:17.000Z | 2021-05-25T13:12:17.000Z | examples/XNSFE_Solver/HeatedWall_VariableDensity/HeatedWall90DegVariableDensity.ipynb | FDYdarmstadt/BoSSS | 974f3eee826424a213e68d8d456d380aeb7cd7e9 | [
"Apache-2.0"
] | 1 | 2020-07-20T15:32:56.000Z | 2020-07-20T15:34:22.000Z | examples/XNSFE_Solver/HeatedWall_VariableDensity/HeatedWall90DegVariableDensity.ipynb | FDYdarmstadt/BoSSS | 974f3eee826424a213e68d8d456d380aeb7cd7e9 | [
"Apache-2.0"
] | 12 | 2018-01-05T19:52:35.000Z | 2021-05-07T07:49:27.000Z | 40.081197 | 2,524 | 0.494829 | [
[
[
"# K26 - Heated Wall\r\n\r\nInterface at 90°. \r\nVariable fluid densities => but very small variations \r\nAlso no Heat capacity => infinitely fast heat conduction \r\nHeight of the domain is reduced \r\nImplicit RK timestepping, to allow for an adaption of the maximum step size \r\nAlso only the \"start\" of the process is simulated, i.e. tE = 1",
"_____no_output_____"
]
],
[
[
"#r \"..\\..\\..\\src\\L4-application\\BoSSSpad\\bin\\Release\\net5.0\\BoSSSpad.dll\"\r\nusing System;\r\nusing System.Collections.Generic;\r\nusing System.Linq;\r\nusing ilPSP;\r\nusing ilPSP.Utils;\r\nusing BoSSS.Platform;\r\nusing BoSSS.Foundation;\r\nusing BoSSS.Foundation.XDG;\r\nusing BoSSS.Foundation.Grid;\r\nusing BoSSS.Foundation.Grid.Classic;\r\nusing BoSSS.Foundation.IO;\r\nusing BoSSS.Solution;\r\nusing BoSSS.Solution.Control;\r\nusing BoSSS.Solution.GridImport;\r\nusing BoSSS.Solution.Statistic;\r\nusing BoSSS.Solution.Utils;\r\nusing BoSSS.Solution.AdvancedSolvers;\r\nusing BoSSS.Solution.Gnuplot;\r\nusing BoSSS.Application.BoSSSpad;\r\nusing BoSSS.Application.XNSE_Solver;\r\nusing static BoSSS.Application.BoSSSpad.BoSSSshell;\r\nInit();",
"_____no_output_____"
]
],
[
[
"## Setup Workflowmanagement, Batchprocessor and Database",
"_____no_output_____"
]
],
[
[
"ExecutionQueues",
"_____no_output_____"
],
[
"static var myBatch = BoSSSshell.GetDefaultQueue();",
"_____no_output_____"
],
[
"static var myDb = myBatch.CreateOrOpenCompatibleDatabase(\"XNSFE_HeatedWall\");",
"_____no_output_____"
],
[
"myDb.Path",
"Opening existing database '\\\\hpccluster\\hpccluster-scratch\\rieckmann\\XNSFE_HeatedWall'.\r\n"
],
[
"BoSSSshell.WorkflowMgm.Init($\"HeatedWall_VariableDensity\");",
"Project name is set to 'HeatedWall_VariableDensity'.\r\n"
]
],
[
[
"## Setup Simulationcontrols",
"_____no_output_____"
]
],
[
[
"using BoSSS.Application.XNSFE_Solver;",
"_____no_output_____"
],
[
"int[] hRes = {16, 24, 32, 48};\r\nint[] pDeg = {2};\r\ndouble[] Q = {0.2};\r\ndouble[] dRho_B = {0.0, -1e-3, -5e-3, -1e-2, -5e-2, -1e-1};",
"_____no_output_____"
],
[
"List<XNSFE_Control> Controls = new List<XNSFE_Control>();\r\nforeach(int h in hRes){\r\n foreach(int p in pDeg){\r\n foreach(double q in Q){\r\n foreach(double dR in dRho_B){\r\n\r\n var ctrl = new XNSFE_Control();\r\n ctrl.Paramstudy_CaseIdentification.Add(new Tuple<string, object>(\"HeatFlux\", q));\r\n\r\n ctrl.DbPath = null;\r\n ctrl.SessionName = $\"HeatedWall_VariableDensity_res:{h}_p:{p}_dR:{dR}\";\r\n ctrl.ProjectName = $\"HeatedWall_VariableDensity\";\r\n ctrl.SetDatabase(myDb);\r\n ctrl.savetodb = true; \r\n\r\n ctrl.FieldOptions.Add(\"VelocityX\", new FieldOpts() {\r\n Degree = p,\r\n SaveToDB = FieldOpts.SaveToDBOpt.TRUE\r\n });\r\n ctrl.FieldOptions.Add(\"VelocityY\", new FieldOpts() {\r\n Degree = p,\r\n SaveToDB = FieldOpts.SaveToDBOpt.TRUE\r\n });\r\n ctrl.FieldOptions.Add(\"GravityX#A\", new FieldOpts() {\r\n SaveToDB = FieldOpts.SaveToDBOpt.TRUE\r\n });\r\n ctrl.FieldOptions.Add(\"GravityY#A\", new FieldOpts() {\r\n SaveToDB = FieldOpts.SaveToDBOpt.TRUE\r\n });\r\n ctrl.FieldOptions.Add(\"GravityX#B\", new FieldOpts() {\r\n SaveToDB = FieldOpts.SaveToDBOpt.TRUE\r\n });\r\n ctrl.FieldOptions.Add(\"GravityY#B\", new FieldOpts() {\r\n SaveToDB = FieldOpts.SaveToDBOpt.TRUE\r\n });\r\n ctrl.FieldOptions.Add(\"Pressure\", new FieldOpts() {\r\n Degree = p - 1,\r\n SaveToDB = FieldOpts.SaveToDBOpt.TRUE\r\n });\r\n ctrl.FieldOptions.Add(\"PhiDG\", new FieldOpts() {\r\n SaveToDB = FieldOpts.SaveToDBOpt.TRUE\r\n });\r\n ctrl.FieldOptions.Add(\"Phi\", new FieldOpts() {\r\n Degree = Math.Max(p, 2),\r\n SaveToDB = FieldOpts.SaveToDBOpt.TRUE\r\n });\r\n ctrl.FieldOptions.Add(\"Temperature\", new FieldOpts() {\r\n Degree = p,\r\n SaveToDB = FieldOpts.SaveToDBOpt.TRUE\r\n });\r\n\r\n #region grid\r\n double L = 5.0;\r\n int kelemR = h;\r\n string[] Bndy = new string[] { \"Inner\",\r\n \"NavierSlip_linear_ConstantHeatFlux_right\",\r\n \"pressure_outlet_ZeroGradient_top\",\r\n \"freeslip_ZeroGradient_left\",\r\n \"pressure_outlet_ZeroGradient_bottom\"};\r\n\r\n ctrl.GridFunc = delegate () {\r\n double[] Xnodes = GenericBlas.Linspace(-L, 0, kelemR + 1);\r\n double[] Ynodes = GenericBlas.Linspace(0, L, kelemR + 1);\r\n var grd = Grid2D.Cartesian2DGrid(Xnodes, Ynodes);\r\n\r\n for(byte i= 1; i < Bndy.Count(); i++) {\r\n grd.EdgeTagNames.Add(i, Bndy[i]);\r\n }\r\n\r\n grd.DefineEdgeTags(delegate (double[] X) {\r\n byte et = 0;\r\n if(Math.Abs(X[0] - Xnodes.Last()) < 1e-8)\r\n return 1;\r\n if(Math.Abs(X[0] - Xnodes.First()) < 1e-8)\r\n return 3;\r\n if(Math.Abs(X[1] - Ynodes.Last()) < 1e-8)\r\n return 2;\r\n if(Math.Abs(X[1] - Ynodes.First()) < 1e-8)\r\n return 4;\r\n return et;\r\n });\r\n\r\n return grd;\r\n };\r\n #endregion\r\n\r\n #region material\r\n ctrl.PhysicalParameters = new BoSSS.Solution.XNSECommon.PhysicalParameters() {\r\n rho_A = 1.0, // 958.0\r\n rho_B = 1.0 + dR, // 0.59,\r\n\r\n mu_A = 1, //2.82 * 1e-4,\r\n mu_B = 0.001, //1.23 * 1e-6,\r\n\r\n Sigma = 1.0,\r\n betaS_A = 1000, // sliplength is mu/beta\r\n betaS_B = 1000,\r\n };\r\n\r\n ctrl.ThermalParameters = new BoSSS.Solution.XheatCommon.ThermalParameters() {\r\n rho_A = 1.0, // 958.0\r\n rho_B = 1.0 + dR, //0.59,\r\n\r\n k_A = 1.0, // 0.6\r\n k_B = 1.0, // 0.026,\r\n\r\n c_A = 0.0,\r\n c_B = 0.0,\r\n\r\n hVap = 1,//2.257 * 1e6,\r\n T_sat = 0.0 // 373.0\r\n };\r\n\r\n ctrl.PhysicalParameters.IncludeConvection = true;\r\n ctrl.ThermalParameters.IncludeConvection = true;\r\n ctrl.PhysicalParameters.Material = false;\r\n #endregion\r\n\r\n #region Initial Condition - Exact Solution\r\n\r\n // solution for massflux and velocity at level set\r\n double y0 = 0.2 * L;\r\n\r\n // inital values\r\n double g = 4;\r\n ctrl.AddInitialValue(\"Phi\", $\"(X, t) => -{y0} + X[1]\", true);\r\n ctrl.AddInitialValue(\"Temperature#A\", $\"(X, t) => {ctrl.ThermalParameters.T_sat}\", true);\r\n ctrl.AddInitialValue(\"Temperature#B\", $\"(X, t) => {ctrl.ThermalParameters.T_sat}\", true);\r\n ctrl.AddInitialValue(\"GravityY#A\", $\"(X, t) => -{g}\", true);\r\n\r\n #endregion\r\n\r\n #region Boundary Conditions\r\n\r\n double v = 1.0;\r\n ctrl.AddBoundaryValue(Bndy[1], \"HeatFluxX#A\", $\"(X, t) => {q}\", true);\r\n ctrl.AddBoundaryValue(Bndy[1], \"VelocityY#A\", $\"(X, t) => {v}\", true);\r\n ctrl.AddBoundaryValue(Bndy[1], \"VelocityY#B\", $\"(X, t) => {v}\", true);\r\n\r\n\r\n ctrl.AddBoundaryValue(Bndy[3]);\r\n ctrl.AddBoundaryValue(Bndy[2]);\r\n ctrl.AddBoundaryValue(Bndy[4], \"Pressure#A\", $\"(X, t) => {y0} * {ctrl.PhysicalParameters.rho_A} * {g}\", true);\r\n\r\n #endregion\r\n\r\n #region AMR\r\n\r\n // No AMR\r\n int level = 0;\r\n ctrl.AdaptiveMeshRefinement = level > 0;\r\n ctrl.activeAMRlevelIndicators.Add(new BoSSS.Solution.LevelSetTools.SolverWithLevelSetUpdater.AMRonNarrowband() { maxRefinementLevel = level });\r\n ctrl.AMR_startUpSweeps = level;\r\n\r\n #endregion\r\n\r\n #region Timestepping\r\n\r\n ctrl.AdvancedDiscretizationOptions.SST_isotropicMode = BoSSS.Solution.XNSECommon.SurfaceStressTensor_IsotropicMode.LaplaceBeltrami_ContactLine;\r\n ctrl.Option_LevelSetEvolution = BoSSS.Solution.LevelSetTools.LevelSetEvolution.FastMarching;\r\n ctrl.Timestepper_LevelSetHandling = BoSSS.Solution.XdgTimestepping.LevelSetHandling.LieSplitting;\r\n\r\n ctrl.NonLinearSolver.SolverCode = NonLinearSolverCode.Newton;\r\n ctrl.NonLinearSolver.Globalization = BoSSS.Solution.AdvancedSolvers.Newton.GlobalizationOption.Dogleg;\r\n ctrl.NonLinearSolver.ConvergenceCriterion = 1e-8;\r\n ctrl.NonLinearSolver.MaxSolverIterations = 10;\r\n\r\n ctrl.SkipSolveAndEvaluateResidual = false;\r\n\r\n ctrl.TimeSteppingScheme = BoSSS.Solution.XdgTimestepping.TimeSteppingScheme.RK_ImplicitEuler;\r\n ctrl.TimesteppingMode = BoSSS.Solution.Control.AppControl._TimesteppingMode.Transient;\r\n ctrl.dtFixed = 0.01;\r\n ctrl.Endtime = 1.0;\r\n ctrl.NoOfTimesteps = int.MaxValue; // timesteps can be adapted, simulate until endtime is reached\r\n\r\n #endregion\r\n ctrl.PostprocessingModules.Add(new BoSSS.Application.XNSFE_Solver.PhysicalBasedTestcases.MassfluxLogging() { LogPeriod = 1 });\r\n ctrl.PostprocessingModules.Add(new BoSSS.Application.XNSFE_Solver.PhysicalBasedTestcases.MovingContactLineLogging() { LogPeriod = 1 });\r\n\r\n Controls.Add(ctrl);\r\n }\r\n }\r\n }\r\n}",
"_____no_output_____"
],
[
"Controls.Count",
"_____no_output_____"
]
],
[
[
"## Start simulations on Batch processor",
"_____no_output_____"
]
],
[
[
"foreach(var C in Controls) {\r\n Type solver = typeof(BoSSS.Application.XNSFE_Solver.XNSFE<XNSFE_Control>);\r\n\r\n string jobName = C.SessionName;\r\n var oneJob = new Job(jobName, solver);\r\n oneJob.NumberOfMPIProcs = 1; \r\n oneJob.SetControlObject(C);\r\n oneJob.Activate(myBatch, true);\r\n}",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
]
] |
e7bcbb99946721e7d786de0aacbccb9a1caecfbd | 11,132 | ipynb | Jupyter Notebook | .ipynb_checkpoints/Hyperexponential Case-checkpoint.ipynb | Roshanmahes/Appointment-Scheduling | 2b8d7c8584bfbbf0a9496f6907622e240d2278b8 | [
"MIT"
] | 1 | 2021-06-17T06:37:54.000Z | 2021-06-17T06:37:54.000Z | .ipynb_checkpoints/Hyperexponential Case-checkpoint.ipynb | Roshanmahes/Appointment-Scheduling | 2b8d7c8584bfbbf0a9496f6907622e240d2278b8 | [
"MIT"
] | null | null | null | .ipynb_checkpoints/Hyperexponential Case-checkpoint.ipynb | Roshanmahes/Appointment-Scheduling | 2b8d7c8584bfbbf0a9496f6907622e240d2278b8 | [
"MIT"
] | null | null | null | 26.759615 | 158 | 0.452749 | [
[
[
"## Hyperexponential Case\n\nThroughout this document, the following packages are required:",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport scipy\nimport math\nfrom scipy.stats import binom, erlang, poisson\nfrom scipy.optimize import minimize\nfrom functools import lru_cache",
"_____no_output_____"
]
],
[
[
"### Plot Phase-Type Fit",
"_____no_output_____"
]
],
[
[
"from ipywidgets import interact, interactive, fixed, interact_manual\nimport ipywidgets as widgets\nimport matplotlib.pyplot as plt",
"_____no_output_____"
],
[
"def SCV_to_params(SCV):\n \n # weighted Erlang case\n if SCV <= 1:\n K = math.floor(1/SCV)\n p = ((K + 1) * SCV - math.sqrt((K + 1) * (1 - K * SCV))) / (SCV + 1)\n mu = K + (1 - p) * (K + 1)\n \n return K, p, mu\n \n # hyperexponential case\n else:\n p = 0.5 * (1 + np.sqrt((SCV - 1) / (SCV + 1)))\n mu = 1 # 1 / mean\n mu1 = 2 * p * mu\n mu2 = 2 * (1 - p) * mu\n \n return p, mu1, mu2",
"_____no_output_____"
],
[
"# for i in range(81):\n# SCV = 1 + 0.1 * i\n# print(round(SCV,2),SCV_to_params(SCV))",
"_____no_output_____"
],
[
"def density_WE(x, K, p, mu):\n return p * erlang.pdf(x, K, scale=1/mu) + (1 - p) * erlang.pdf(x, K+1, scale=1/mu)\n\ndef density_HE(x, p, mu1, mu2):\n return p * mu1 * np.exp(-mu1 * x) + (1 - p) * mu2 * np.exp(-mu2 * x)",
"_____no_output_____"
],
[
"x = np.linspace(0,4,1001)\n\ndef plot_f(SCV=1):\n \n if SCV <= 1:\n K, p, mu = SCV_to_params(SCV)\n f_x = density_WE(x, K, p, mu)\n title = f'SCV = {SCV}\\n p = {p:.2f}, $K$ = {K}, $\\mu$ = {mu:.2f}'\n else:\n p, mu1, mu2 = SCV_to_params(SCV)\n f_x = density_HE(x, p, mu1, mu2)\n title = f'SCV = {SCV}\\n p = {p:.2f}, $\\mu_1$ = {mu1:.2f}, $\\mu_2$ = {mu2:.2f}'\n \n plt.plot(x,f_x)\n plt.title(title)\n plt.xlabel('$x$')\n plt.ylabel('density')\n plt.ylim(0,2)\n",
"_____no_output_____"
],
[
"interact(plot_f, SCV=(0.01,2,0.01));",
"_____no_output_____"
]
],
[
[
"The recursion of the dynamic program is given as follows. For $i=1,\\dots,n-1$, $k=1,\\dots,i$, and $m\\in\\mathbb{N}_0$,\n\n\\begin{align*}\n\\xi_i(k,m) &= \\inf_{t\\in \\mathbb{N}_0}\n\\Big(\n\\omega \\bar{f}^{\\circ}_{k,m\\Delta}(t\\Delta) + (1-\\omega)\\bar{h}^{\\circ}_{k,m\\Delta} +\n\\sum_{\\ell=2}^{k}\\sum_{j=0}^{t}\\bar{q}_{k\\ell,mj}(t)\\xi_{i+1}(\\ell,j) +\nP^{\\downarrow}_{k,m\\Delta}(t\\Delta)\\xi_{i+1}(1,0) +\nP^{\\uparrow}_{k,m\\Delta}(t\\Delta)\\xi_{i+1}(k+1,m+t)\n\\Big),\n\\end{align*}\n\nwhereas, for $k=1,\\dots,n$ and $m\\in \\mathbb{N}_0$,\n\n\\begin{align*}\n\\xi_n(k,m) = (1-\\omega)\\bar{h}^{\\circ}_{k,m\\Delta}.\n\\end{align*}",
"_____no_output_____"
],
[
"We will implement this dynamic program step by step. First, we implement all functions in the equation above.\n\nOur formulas rely heavily on the survival function $\\mathbb{P}(B>t)$ and $\\gamma_z(t) = \\mathbb{P}(Z_t = z\\mid B>t)$:",
"_____no_output_____"
]
],
[
[
"@lru_cache(maxsize=128)\ndef B_sf(t):\n \"\"\"The survival function P(B > t).\"\"\"\n return p * np.exp(-mu1 * t) + (1 - p) * np.exp(-mu2 * t)\n\n@lru_cache(maxsize=128)\ndef gamma(z, t):\n \"\"\"Computes P(Z_t = z | B > t).\"\"\"\n \n gamma_circ = B_sf(t)\n \n if z == 1:\n return p * np.exp(-mu1 * t) / gamma_circ\n elif z == 2:\n return (1 - p) * np.exp(-mu2 * t) / gamma_circ",
"_____no_output_____"
]
],
[
[
"Next, we implement $\\bar{f}^{\\circ}_{k,u}(t)$, which depends on $\\bar{f}_{k,z}(t)$:",
"_____no_output_____"
]
],
[
[
"@lru_cache(maxsize=128)\ndef f_bar(k,z,t):\n \n if z == 1:\n return sum([binom.pmf(m, k-1, p) * sigma(t, m+1, k-1-m) for m in range(k)])\n elif z == 2:\n return sum([binom.pmf(m, k-1, p) * sigma(t, m, k-m) for m in range(k)])\n\n@lru_cache(maxsize=128)\ndef f_circ(k, u, t):\n return gamma(1, u) * f_bar(k, 1, t) + gamma(2, u) * f_bar(k, 2, t)",
"_____no_output_____"
]
],
[
[
"In here, we need to evaluate the object $\\sigma_{t}[m,k]$, which depends on $\\rho_{t}[m,k]$:",
"_____no_output_____"
]
],
[
[
"@lru_cache(maxsize=512)\ndef sigma(t,m,k):\n \n return (t - k / mu2) * erlang.cdf(t, m, scale=1/mu1) - (m / mu1) * erlang.cdf(t, m+1, mu1) + \\\n (mu1 / mu2) * sum([(k-i) * rho_t(t, m-1, i) for i in range(k)])\n\n@lru_cache(maxsize=512)\ndef rho_t(t,m,k):\n \n if not k:\n return np.exp(-mu2 * t) * (mu1 ** m) / ((mu1 - mu2) ** (m + 1)) * erlang.cdf(t, m+1, scale=1/(mu1 - mu2))\n elif not m:\n return np.exp(-mu1 * t) * (mu2 ** k) / ((mu1 - mu2) ** (k + 1)) * erlang.cdf(t, k+1, scale=1/(mu1 - mu2))\n else:\n return (mu1 * rho(t,a,m-1,k) - mu2 * rho(t,a,m,k-1)) / (mu1 - mu2)\n \n\n@lru_cache(maxsize=512)\ndef rho(t,a,m,k):\n \n if not k:\n return np.exp(-mu2 * t) * (mu1 ** m) / ((mu1 - mu2) ** (m + 1)) * erlang.cdf(a, m+1, scale=1/(mu1 - mu2))\n elif not m:\n return np.exp(-mu1 * t) * (mu2 ** k) / ((mu1 - mu2) ** (k + 1)) * \\\n (erlang.cdf(t, k+1, scale=1/(mu1 - mu2)) - erlang.cdf(t-a, k+1, scale=1/(mu1 - mu2)))\n else:\n return (mu1 * rho(t,a,m-1,k) - mu2 * rho(t,a,m,k-1) - r(t,a,m,k)) / (mu1 - mu2)\n\n\n@lru_cache(maxsize=512)\ndef r(t,s,m,k):\n return poisson.pmf(m,mu1*s) * poisson.pmf(k,t-s)",
"_____no_output_____"
]
],
[
[
"We do the same for $\\bar{h}^{\\circ}_{k,u}(t)$, which only depends on $\\bar{h}_{k,z}$:",
"_____no_output_____"
]
],
[
[
"@lru_cache(maxsize=128)\ndef h_bar(k, z):\n\n if k == 1:\n return 0\n elif z <= K:\n return ((k - 1) * (K + 1 - p) + 1 - z) / mu\n elif z == K + 1:\n return ((k - 2) * (K + 1 - p) + 1) / mu\n\n@lru_cache(maxsize=128)\ndef h_circ(k, u):\n return gamma(1, u) * h_bar() sum([gamma(z, u) * h_bar(k, z) for z in range(1, K+2)])",
"_____no_output_____"
]
],
[
[
"The next objective is to implement $\\bar{q}_{k\\ell,mj}(t)$. This function depends on $q_{k\\ell,z,v}(t)$, which depends on $\\psi_{vt}[k,\\ell]$: TODO",
"_____no_output_____"
]
],
[
[
"# TODO",
"_____no_output_____"
],
[
"poisson.pmf(3,0)",
"_____no_output_____"
]
],
[
[
"Finally, we implement the remaining transition probabilities $P^{\\uparrow}_{k,u}(t)$ and $P^{\\downarrow}_{k,u}(t)$:",
"_____no_output_____"
]
],
[
[
"# @lru_cache(maxsize=128)\ndef P_up(k, u, t):\n \"\"\"Computes P(N_t- = k | N_0 = k, B_0 = u).\"\"\"\n return B_sf(u + t) / B_sf(u)\n\n@lru_cache(maxsize=128)\ndef P_down(k, u, t):\n \"\"\"Computes P(N_t- = 0 | N_0 = k, B_0 = u).\"\"\"\n return sum([binom.pmf(m, k, p) * Psi(t, m, k-m) for m in range(k+1)])\n\n@lru_cache(maxsize=128)\ndef Psi(t, m, k):\n return erlang.cdf(t, m, scale=1/mu1) - mu1 * sum([rho_t(t, m-1, i) for i in range(k)])",
"_____no_output_____"
],
[
"erlang.cdf(0,1,1)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
]
] |
e7bcbc99e725c4dfc27086bb9de1484df989ea7a | 27,419 | ipynb | Jupyter Notebook | publication/scripts/convert_genes.ipynb | gerlichlab/HiCognition | dff022025b7c83732b9510ff5ca8232d30aa5304 | [
"MIT"
] | null | null | null | publication/scripts/convert_genes.ipynb | gerlichlab/HiCognition | dff022025b7c83732b9510ff5ca8232d30aa5304 | [
"MIT"
] | 5 | 2022-03-31T11:54:12.000Z | 2022-03-31T12:04:29.000Z | publication/scripts/convert_genes.ipynb | gerlichlab/HiCognition | dff022025b7c83732b9510ff5ca8232d30aa5304 | [
"MIT"
] | null | null | null | 34.359649 | 168 | 0.363142 | [
[
[
"import pandas as pd\nimport numpy as np\nimport matplotlib.pyplot as plt\nimport seaborn as sbn\nimport re",
"_____no_output_____"
],
[
"result = pd.read_csv(\"../../data/hg19_genes/gencode.v38lift37.basic.annotation.gtf.gz\", comment=\"#\", sep=\"\\t\", header=None)",
"_____no_output_____"
],
[
"renamed = result.rename(columns={0: \"chrom\", 1: \"source\", 2: \"type\",3:\"start\",4:\"end\", 6:\"strand\"})\nrenamed",
"_____no_output_____"
],
[
"subset = renamed.query(\"type == 'gene'\").copy()\nsubset",
"_____no_output_____"
],
[
"subset.loc[:, \"gene_type\"] = subset.apply(lambda x: re.findall(r\"gene_type ([^\\;]*)\", x[8])[0].strip(\"\\\"\"), axis=1)",
"_____no_output_____"
],
[
"subset_protein = subset.query(\"gene_type == 'protein_coding'\").copy()",
"_____no_output_____"
],
[
"subset_protein.loc[:, \"size\"] = subset_protein[\"end\"] - subset_protein[\"start\"]\nsubset_protein",
"_____no_output_____"
],
[
"all_genes_forward = subset_protein.query(\"strand == '+'\")\nall_genes_reverse = subset_protein.query(\"strand == '-'\")",
"_____no_output_____"
],
[
"all_genes_forward[[\"chrom\", \"start\", \"end\"]].to_csv(\"../../data/hg19_genes/all_genes_forward.bed\", sep=\"\\t\", header=None, index=False)",
"_____no_output_____"
],
[
"all_genes_reverse[[\"chrom\", \"start\", \"end\"]].to_csv(\"../../data/hg19_genes/all_genes_reverse.bed\", sep=\"\\t\", header=None, index=False)",
"_____no_output_____"
],
[
"all_genes_forward[[\"chrom\", \"start\", \"start\"]].to_csv(\"../../data/hg19_genes/protein_coding_genes_forward_tss.bed\", sep=\"\\t\", header=None, index=False)",
"_____no_output_____"
],
[
"all_genes_reverse[[\"chrom\", \"end\", \"end\"]].to_csv(\"../../data/hg19_genes/protein_coding_genes_reverse_tss.bed\", sep=\"\\t\", header=None, index=False)",
"_____no_output_____"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bcdcee6c1cafbe355a09b668ac6f5ecfa86469 | 13,283 | ipynb | Jupyter Notebook | Lesson 6 - File access in Python3.ipynb | mjmtnez/Accelerated_Intro_to_CompBio_Part_2 | 72ffa7bf30b4ac769d0196036682f246d227c73b | [
"CC-BY-4.0"
] | 3 | 2020-01-23T08:52:56.000Z | 2021-10-01T09:13:07.000Z | Lesson 6 - File access in Python3.ipynb | mjmtnez/Accelerated_Intro_to_CompBio_Part_2 | 72ffa7bf30b4ac769d0196036682f246d227c73b | [
"CC-BY-4.0"
] | null | null | null | Lesson 6 - File access in Python3.ipynb | mjmtnez/Accelerated_Intro_to_CompBio_Part_2 | 72ffa7bf30b4ac769d0196036682f246d227c73b | [
"CC-BY-4.0"
] | 71 | 2018-09-05T15:37:10.000Z | 2021-09-08T10:50:49.000Z | 33.627848 | 438 | 0.567342 | [
[
[
"# Reading and Writing files\n\nSo far, we have typed all of our \"data\" into the code of our software (e.g. the names of the students, and their ages.\n\nMost of the time, this kind of data is stored in files. We need to read (and write) files so that we can create and use permanent copies of these data (and exchange these data with other people or software)\n\nThe function we will use to open a file is called (surprise!) \"open\"\n\nopen takes two arguments\":\n1. the path to the file\n2. \"how\" to open the file (for read? for write? for \"append\"? for read and write?)\n\nit looks like this:\n\n myfile = open(\"/path/to/file.csv\", \"r\") # opens file.csv for \"read\"\n \nI have already created a file called students.csv that we can open now:",
"_____no_output_____"
]
],
[
[
"studentfile = open(\"students.csv\", \"r\")\nprint(studentfile)",
"_____no_output_____"
]
],
[
[
"\nDoes the output of that print statement surprise you? What it tells you is that 'studentfile' is a Python \"object\" (again, we will discuss objects in more detail later, but you will start to see how they work now...)\n\nstudentfile is an object of type \"TextIOWrapper\" (from the \"io\" Python library, which is automatically installed in all Python distributions). It knows what its filename is, it knows that it is open for \"read\", and it also has guessed the \"encoding\" of the file (UTF-8 is a kind of text encoding that allows extended text characters like the German umlaut's, and greek alpha, beta, etc. This is a good default for us!)\n\n# Reading information from a file\n\nsurprise! The most basic method used to read information is.... 'read'! This reads **the entire file**\n\n print(studentfile.read())\n \n",
"_____no_output_____"
]
],
[
[
"print(studentfile.read())",
"_____no_output_____"
]
],
[
[
"\nNow we need to talk about a feature of file input/output, called a \"pointer\". The pointer is the position where the code \"is\" in the file - is it at the beginning? is it at the end\"? Is it at line 5?\n\nWhere is the pointer now? Let's try the same command again!",
"_____no_output_____"
]
],
[
[
"\nprint(studentfile.read())",
"_____no_output_____"
]
],
[
[
"\nNada de nada! That's because the pointer is at the end of the file - when we say file.read it tries to read starting from the end of the file...and of course, there is nothing there. \n\nTo reset back to the beginning, we will use the \"seek\" function, and set it to position '0':\n\n",
"_____no_output_____"
]
],
[
[
"studentfile.seek(0)\nprint(studentfile.read())\n\n",
"_____no_output_____"
]
],
[
[
"\n## More refined file access - line-by-line\n\nMost of the time, you do not want to read the enire file into memory (tell me why this can be very very bad!.... please)\n\nMOST of the time, a file will have one \"record\" per line. e.g. our CSV file has the \"name,age\" for one student per line. We want to read those lines one-at-a-time and do something useful with each record.\n\nThe method we want to use is called \"readlines()\"\n\n print(studentfile.readlines())",
"_____no_output_____"
]
],
[
[
"studentfile.seek(0) # set it back to the beginning again for this lesson...\n\nprint(studentfile.readlines())",
"_____no_output_____"
]
],
[
[
"\nYou will see that this returns a list, which means we can use it in a FOR loop...\n",
"_____no_output_____"
]
],
[
[
"studentfile.seek(0) # set it back to the beginning again for this lesson...\n\nfor line in studentfile.readlines():\n print(\"the current record is\", line)\n",
"_____no_output_____"
]
],
[
[
"\nWe're getting closer to what we want! We have each record as a string in the format \"Mark,50\". What we want is to separate the \"Mark\" and the \"50\" so that we could put them into separate variables (e.g. *name* and *age*)\n\nThere is a ***correct*** way to this, but you already know one way to solve this problem! \n\nIn the box below, use regular expressions to capture the name and the age into the variables *name* and *age*\n\n<p style=\"visibility: hidden;\">\n#!/usr/bin/python3\nimport re # this brings the python regular expression object into your program\n\nstudentfile.seek(0) # set it back to the beginning again for this lesson...\n\nfor line in studentfile.readlines():\n #print(\"the current record is\", line)\n matchObj = re.search( r'(\\w+),(\\d+)', line) # match the index letter, then CAPTURE the rest of the sentence\n if matchObj:\n name = matchObj.group(1)\n age = matchObj.group(2)\n print(\"Name: \", name, \" Age: \", age)\n else:\n print (\"No match!!\")\n</p>",
"_____no_output_____"
]
],
[
[
"# put your amazing solution here!\n",
"_____no_output_____"
]
],
[
[
"\nOK, so now you have solved the problem using regular expressions, however... the solution isn't very \"abstract\". In another case, you might have a more complex record:\n\n Mark,50,190cm,95kg,163483,113mmhg,29mg/ml\n\nYour regular expression would start to get ugly! What is the one thing that is constant in this CSV file? (in fact,the name of the file-type tells you!)\n\nIn cases like this, there is a method called \"split\", which will take a string and split it based on whatever separator you give it. In this case, the comma.\n\n \n for line in studentfile.readlines():\n print(\"the current record is\", line)\n name, age = line.split(',')\n ",
"_____no_output_____"
]
],
[
[
"studentfile.seek(0) # set it back to the beginning again for this lesson...\n\nfor line in studentfile.readlines():\n print(\"the current record is\", line)\n name, age = line.split(',')\n print(\"Name:\", name, \" Age:\", age)\n",
"_____no_output_____"
]
],
[
[
"\nMuch better! But... *Still not quite right!!* What are all of those blank lines? We didn't ask for blank lines...\n\nRemember just a few minutes ago we looked at the output from readlines():\n\n studentfile.seek(0) # set it back to the beginning again for this lesson...\n print(studentfile.readlines())\n \n ==> ['Mark,50\\n', 'Alejandro,25\\n', 'Julia,26\\n', 'Denise,23\\n', 'Josef,21\\n']\n \nThose blank lines are because of the \\n (newline) character at the end of every line. What is happening is that the print statements above ACTUALLY look like this:\n\n the current record is Alejandro,25\\n\n Name: Alejandro Age: 25\\n <----- the value of the age variable after the spit is '25\\n'\n \nCan we discard this newline? Sure! The method *rstrip()* will strip all blank space (including newlines) from the end (right-hand end --> **r**strip() ) of the line:\n\n",
"_____no_output_____"
]
],
[
[
"studentfile.seek(0) # set it back to the beginning again for this lesson...\n\nfor line in studentfile.readlines():\n line = line.rstrip()\n print(\"the current record is\", line)\n name, age = line.split(',')\n print(\"Name:\", name, \" Age:\", age)\n",
"_____no_output_____"
]
],
[
[
"\n<pre>\n\n\n</pre>\nWhen you have finished with an open file, it is a very good idea to close it!\n\n studentfile.close() # it's a good idea to close a file once you are finished with it! We are...\n ",
"_____no_output_____"
]
],
[
[
"studentfile.close() # it's a good idea to close a file once you are finished with it! We are...",
"_____no_output_____"
]
],
[
[
"\n<pre>\n\n\n</pre>\n\n## Writing to a file\n\nWriting to a file is very straightforward. Use the same \"open\" command that you have already learned, but using the \"w\" flag (\"open for **w**rite), then write information to that open file using the *write()* method.\n\nPython will help you by creating the file if it doesn't exist. For example, the box below will create a file named \"OLDERstudents.csv\" if that file doesn't exist. ***IF IT DOES EXIST, IT WILL BE DESTROYED!!!!! YOU CANNOT GET THE CONTENT BACK!!!! BE CAREFUL!!!***\n\nThe file pointer is set to the beginning of the file.\n\nHere is how easy it is:",
"_____no_output_____"
]
],
[
[
"olderstudents = open(\"OLDERstudents.csv\", \"w\")\nolderstudents.write(\"hello, I am writing stuff to a file!\\nThis is very cool!\") # the write function, using \\n (newline)\nolderstudents.close()\n\ncheckcontent = open(\"OLDERstudents.csv\", \"r\")\nprint(checkcontent.read()) # print the content of the file\ncheckcontent.close()",
"_____no_output_____"
]
],
[
[
"<pre>\n\n\n</pre>\n## Now you!\n\n* create the file OLDERstudents.csv\n* using the data from the original students.csv, make everyone 5 years older\n* write the new older student data to the OLDERstudents.csv file, in an identical format (Mark,55....)\n* do this again, but this time, create a \"header line\" (Student Name, Student Age)\n Student Name, Student Age\n Mark,55\n Alejandro,35\n ...\n ...\n \n* do this again, but instead of creating a CSV (comma-separated value) file, create a TSV (tab-separated value)\n * call it ***OLDERstudents.tsv***\n * You need to know: the symbol for TAB is \\t\n * these are the two most common structured text-file formats\n * both of these can be imported into software like MS Excel",
"_____no_output_____"
]
],
[
[
"# put your amazing code here!\n",
"_____no_output_____"
]
],
[
[
"## Append\n\nThe final mode for writing to a file is \"append\", which means, \"open the file, and put the pointer at the END of the file\". This allows you to open a file and add new information to it, without destroying the existing information.\n\nThe append flag is 'a'\n\n olderstudents = open(\"OLDERstudents.csv\", \"a\") # open for append\n \nWe wont go through an example here, but... you might have a question about this on your exam, so try it yourself! :-)",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
]
] |
e7bcdd9fe9b83dc2e4ef324d84277fe3ea561a48 | 212,553 | ipynb | Jupyter Notebook | files/FinancialStatementAnalysis.ipynb | travischoy/travischoy.github.io | be04b6b30e12e8b968efcc30ef1682c275e623c8 | [
"CC-BY-3.0"
] | null | null | null | files/FinancialStatementAnalysis.ipynb | travischoy/travischoy.github.io | be04b6b30e12e8b968efcc30ef1682c275e623c8 | [
"CC-BY-3.0"
] | null | null | null | files/FinancialStatementAnalysis.ipynb | travischoy/travischoy.github.io | be04b6b30e12e8b968efcc30ef1682c275e623c8 | [
"CC-BY-3.0"
] | null | null | null | 67.498571 | 41,199 | 0.67865 | [
[
[
"pip install yfinance",
"_____no_output_____"
],
[
"pip install plotly",
"_____no_output_____"
],
[
"import yfinance as yf\nimport matplotlib.pyplot as plt\nimport plotly.express as px\nimport plotly.graph_objects as go\nimport numpy as np\n\nticker = yf.Ticker('MSFT')",
"_____no_output_____"
],
[
"# show balance sheet\nbalance = ticker.balance_sheet\nbalance = balance.T\n\nbalance['Current Ratio'] = np.divide(balance['Total Current Assets'], balance['Total Current Liabilities'])\nbalance['Working Capital'] = np.subtract(balance['Total Current Assets'], balance['Total Current Liabilities'])\nbalance['Debt to Equity'] = np.divide(balance['Total Liab'], balance['Total Stockholder Equity'])\n\nbalance.head(len(balance))\n",
"_____no_output_____"
],
[
"# show financial statement\nfinancials = ticker.financials\nfinancials = financials.T\n\nfinancials['Operating Margin'] = np.divide(financials['Operating Income'], financials['Total Revenue'])\n\nfinancials.head(len(financials))\n",
"_____no_output_____"
],
[
"plt.plot(balance['Current Ratio'])\nplt.title('Current Ratio for the Past 4 Years')\nplt.xticks(rotation = 45)\nplt.xlabel('Date')\nplt.ylabel('Current Ratio')",
"_____no_output_____"
],
[
"plt.plot(balance['Working Capital'])\nplt.title('Working Capital for the Past 4 Years')\nplt.xticks(rotation = 45)\nplt.xlabel('Date')\nplt.ylabel('Working Capital')",
"_____no_output_____"
],
[
"plt.plot(balance['Debt to Equity'])\nplt.title('Debt to Equity Ratio for the Past 4 Years')\nplt.xticks(rotation = 45)\nplt.xlabel('Date')\nplt.ylabel('Debt to Equity Ratio')",
"_____no_output_____"
],
[
"plt.plot(financials['Operating Margin'])\nplt.title('Operating Margin for the Past 4 Years')\nplt.xticks(rotation = 45)\nplt.xlabel('Date')\nplt.ylabel('Operating Margin')",
"_____no_output_____"
],
[
"# share price\nold = ticker.history(start='2018-09-29', end='2021-09-25')\n#old.head()\nold = old.reset_index()\nfor i in ['Open', 'High', 'Close', 'Low']: \n old[i] = old[i].astype('float64')\n\nfig = px.line(old, x='Date', y='Open', title='Microsoft Stock Prices')\nfig.show()\n",
"_____no_output_____"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bce31317466b02f49f03142c3434b88f7fc4e2 | 64,892 | ipynb | Jupyter Notebook | notebooks/python-data-science/sympy/sympy.ipynb | sparkboom/my_jupyter_notes | 9255e4236b27f0419cdd2c8a2159738d8fc383be | [
"MIT"
] | null | null | null | notebooks/python-data-science/sympy/sympy.ipynb | sparkboom/my_jupyter_notes | 9255e4236b27f0419cdd2c8a2159738d8fc383be | [
"MIT"
] | null | null | null | notebooks/python-data-science/sympy/sympy.ipynb | sparkboom/my_jupyter_notes | 9255e4236b27f0419cdd2c8a2159738d8fc383be | [
"MIT"
] | null | null | null | 60.420857 | 6,284 | 0.768816 | [
[
[
"from sympy import *\n# from sympy.abc import *\nfrom IPython.display import display\ninit_printing()",
"_____no_output_____"
]
],
[
[
"# SymPy\n\n## Symbolic Computation\nFree, Open Source, Python\n- solve equations - simplify expressions\n- compute derivatives, integrals, limits\n- work with matrices, - plotting & printing\n- code gen - physics - statitics - combinatorics\n- number theory - geometry - logic\n\n----\n\n## Modules\n[SymPy Core](http://docs.sympy.org/latest/modules/core.html) - [Combinatorics](http://docs.sympy.org/latest/modules/combinatorics/index.html) - [Number Theory](http://docs.sympy.org/latest/modules/ntheory.html) - [Basic Cryptography](http://docs.sympy.org/latest/modules/crypto.html) - [Concrete Maths](http://docs.sympy.org/latest/modules/concrete.html) - [Numerical Evaluation](http://docs.sympy.org/latest/modules/evalf.html) - [Code Gen](http://docs.sympy.org/latest/modules/codegen.html) - [Numeric Computation](http://docs.sympy.org/latest/modules/numeric-computation.html) - [Functions](http://docs.sympy.org/latest/modules/functions/index.html) - [Geometry](http://docs.sympy.org/latest/modules/geometry/index.html) - [Holonomic Functions](http://docs.sympy.org/latest/modules/holonomic/index.html) - [Symbolic Integrals](http://docs.sympy.org/latest/modules/integrals/integrals.html) - [Numeric Integrals](http://docs.sympy.org/latest/modules/integrals/integrals.html#numeric-integrals) - [Lie Algebra](http://docs.sympy.org/latest/modules/liealgebras/index.html) - [Logic](http://docs.sympy.org/latest/modules/logic.html) - [Matricies](http://docs.sympy.org/latest/modules/matrices/index.html) - [Polynomials](http://docs.sympy.org/latest/modules/polys/index.html) - [Printing](http://docs.sympy.org/latest/modules/printing.html) - [Plotting](http://docs.sympy.org/latest/modules/plotting.html) - [Pyglet Plotting](http://docs.sympy.org/latest/modules/plotting.html#module-sympy.plotting.pygletplot) - [Assumptions](http://docs.sympy.org/latest/modules/assumptions/index.html) - [Term Rewriting](http://docs.sympy.org/latest/modules/rewriting.html) - [Series Module](http://docs.sympy.org/latest/modules/series/index.html) - [Sets](http://docs.sympy.org/latest/modules/sets.html) - [Symplify](http://docs.sympy.org/latest/modules/simplify/simplify.html) - [Hypergeometrtic](http://docs.sympy.org/latest/modules/simplify/hyperexpand.html) - [Stats](http://docs.sympy.org/latest/modules/stats.html) - [ODE](http://docs.sympy.org/latest/modules/solvers/ode.html) - [PDE](http://docs.sympy.org/latest/modules/solvers/pde.html) - [Solvers](http://docs.sympy.org/latest/modules/solvers/solvers.html) - [Diophantine](http://docs.sympy.org/latest/modules/solvers/diophantine.html) - [Inequality Solvers](http://docs.sympy.org/latest/modules/solvers/inequalities.html) - [Solveset](http://docs.sympy.org/latest/modules/solvers/solveset.html) - [Tensor](http://docs.sympy.org/latest/modules/tensor/index.html) - [Utilities](http://docs.sympy.org/latest/modules/utilities/index.html) - [Parsing Input](http://docs.sympy.org/latest/modules/parsing.html) - [Calculus](http://docs.sympy.org/latest/modules/calculus/index.html) - [Physics](http://docs.sympy.org/latest/modules/physics/index.html) - [Categrory Theory](http://docs.sympy.org/latest/modules/categories.html) - [Differential Geometry](http://docs.sympy.org/latest/modules/diffgeom.html) - [Vector](http://docs.sympy.org/latest/modules/vector/index.html)",
"_____no_output_____"
],
[
"----\n## Simple Expressions",
"_____no_output_____"
]
],
[
[
"# declare variable first\nx, y = symbols('x y')\n\n# Declare expression\nexpr = x + 3*y\n\n# Print expressions\nprint(\"expr =\", expr)\nprint(\"expr + 1 =\", expr + 1)\nprint(\"expr - x =\", expr - x) # auto-simplify\nprint(\"x * expr =\", x * expr)",
"expr = x + 3*y\nexpr + 1 = x + 3*y + 1\nexpr - x = 3*y\nx * expr = x*(x + 3*y)\n"
]
],
[
[
"\n----\n## Substitution",
"_____no_output_____"
]
],
[
[
"x = symbols('x')\n\nexpr = x + 1\nprint(expr)\ndisplay(expr)",
"x + 1\n"
],
[
"# Evaluate expression at a point\nprint(\"expr(2)=\", expr.subs(x, 2))",
"expr(2)= 3\n"
],
[
"# Replace sub expression with another sub expression\n# 1. For expressions with symmetry\nx, y = symbols('x y')\nexpr2 = x ** y\nexpr2 = expr2.subs(y, x**y)\nexpr2 = expr2.subs(y, x**x)\ndisplay(expr2)",
"_____no_output_____"
],
[
"# 2. Controlled simplifcation\nexpr3 = sin(2*x) + cos(2*x)\nprint(\"expr3\")\ndisplay(expr3)\nprint(\" \")\n\nprint(\"expand_trig(expr3)\")\ndisplay(expand_trig(expr3))\nprint(\" \")\n\nprint(\"use this to only expand sin(2*x) if desired\")\nprint(\"expr3.subs(sin(2*x), 2*sin(x)*cos(x))\")\ndisplay(expr3.subs(sin(2*x), 2*sin(x)*cos(x)))",
"expr3\n"
],
[
"# multi-substitute\nexpr4 = x**3 + 4*x*y - z\nargs = [(x,2), (y,4), (z,0)]\nexpr5 = expr4.subs(args)\ndisplay(expr4)\nprint(\"args = \", args)\ndisplay(expr5)",
"_____no_output_____"
],
[
"expr6 = x**4 - 4*x**3 + 4 * x ** 2 - 2 * x + 3\nargs = [(x**i, y**i) for i in range(5) if i%2 == 0]\ndisplay(expr6)\nprint(args)\ndisplay(expr6.subs(args))",
"_____no_output_____"
]
],
[
[
"----\n## Equality & Equivalence",
"_____no_output_____"
]
],
[
[
"# do not use == between symbols and variables, will return false\n\nx = symbols('x')\nx+1==4",
"_____no_output_____"
],
[
"# Create a symbolic equality expression\nexpr2 = Eq(x+1, 4)\nprint(expr2)\ndisplay(expr2)\n\nprint(\"if x=3, then\", expr2.subs(x,3))",
"Eq(x + 1, 4)\n"
],
[
"# two equivalent formulas\nexpr3 = (x + 1)**2 # we use pythons ** exponentiation (instead of ^)\nexpr4 = x**2 + 2*x + 1\neq34 = Eq(expr3, expr4)\n\nprint(\"expr3\")\ndisplay(expr3)\n\nprint(\" ≡ expr4\")\ndisplay(expr4)\nprint(\"\")\n\nprint(\"(expr3 == expr4) => \", expr3 == expr4)\nprint(\"(these are equivalent, but not the same symbolically)\")\nprint(\"\")\n\nprint(\"Equal by negating, simplifying and comparing to 0\")\nprint(\"expr3 - expr4 => \", expr3 - expr4)\nprint(\"simplify(expr3-expr4)==0=> \", simplify(expr3 - expr4)==0 )\nprint(\"\")\n\nprint(\"Equals (test by evaluating 2 random points)\")\nprint(\"expr3.equals(expr4) => \", expr3.equals(expr4))\n",
"expr3\n"
]
],
[
[
"----\n## SymPy Types & Casting",
"_____no_output_____"
]
],
[
[
"print( \"1 =\", type(1) )\nprint( \"1.0 =\", type(1.0) )\nprint( \"Integer(1) =\", type(Integer(1)) )\nprint( \"Integer(1)/Integer(3) =\", type(Integer(1)/Integer(3)) )\nprint( \"Rational(0.5) =\", type(Rational(0.5)) )\nprint( \"Rational(1/3) =\", type(Rational(1,3)) )",
"1 = <class 'int'>\n1.0 = <class 'float'>\nInteger(1) = <class 'sympy.core.numbers.One'>\nInteger(1)/Integer(3) = <class 'sympy.core.numbers.Rational'>\nRational(0.5) = <class 'sympy.core.numbers.Half'>\nRational(1/3) = <class 'sympy.core.numbers.Rational'>\n"
],
[
"# string to SymPy\nsympify(\"x**2 + 3*x - 1/2\")",
"_____no_output_____"
]
],
[
[
"----\n\n## Evaluating Expressions",
"_____no_output_____"
]
],
[
[
"# evaluate as float using .evalf(), and N\ndisplay( sqrt(8) )\ndisplay( sqrt(8).evalf() )\ndisplay( sympy.N(sqrt(8)) )",
"_____no_output_____"
],
[
"# evaluate as float to nearest n decimals\ndisplay(sympy.pi)\ndisplay(sympy.pi.evalf(100))",
"_____no_output_____"
]
],
[
[
"----\n\n## SymPy Types\n\n#### Number Class\n[Number](http://docs.sympy.org/latest/modules/core.html#number) - [Float](http://docs.sympy.org/latest/modules/core.html#float) - [Rational](http://docs.sympy.org/latest/modules/core.html#rational) - [Integer](http://docs.sympy.org/latest/modules/core.html#integer) - [RealNumber](http://docs.sympy.org/latest/modules/core.html#realnumber)\n\n#### Numbers\n[Zero](http://docs.sympy.org/latest/modules/core.html#zero) - [One](http://docs.sympy.org/latest/modules/core.html#one) - [Negative One](http://docs.sympy.org/latest/modules/core.html#negativeone) - [Half](http://docs.sympy.org/latest/modules/core.html#half) - [NaN](http://docs.sympy.org/latest/modules/core.html#nan) - [Infinity](http://docs.sympy.org/latest/modules/core.html#infinity) - [Negative Infinity](http://docs.sympy.org/latest/modules/core.html#negativeinfinity) - [Complex Infinity](http://docs.sympy.org/latest/modules/core.html#complexinfinity) \n\n#### Constants\n[E (Transcedental Constant)](http://docs.sympy.org/latest/modules/core.html#exp1) - [I (Imaginary Unit)](http://docs.sympy.org/latest/modules/core.html#imaginaryunit) - [Pi](http://docs.sympy.org/latest/modules/core.html#pi) - [EulerGamma (Euler-Mascheroni constant)](http://docs.sympy.org/latest/modules/core.html#eulergamma) - [Catalan (Catalan's Constant)](http://docs.sympy.org/latest/modules/core.html#catalan) - [Golden Ratio](http://docs.sympy.org/latest/modules/core.html#goldenratio)\n",
"_____no_output_____"
],
[
"### Rational Numbers",
"_____no_output_____"
]
],
[
[
"# Rational Numbers\nexpr_rational = Rational(1)/3\nprint(\"expr_rational\")\ndisplay( type(expr_rational) )\ndisplay( expr_rational )\n\neval_rational = expr_rational.evalf()\nprint(\"eval_rational\")\ndisplay( type(eval_rational) )\ndisplay( eval_rational )\n\nneval_rational = N(expr_rational)\nprint(\"neval_rational\")\ndisplay( type(neval_rational) )\ndisplay( neval_rational )",
"expr_rational\n"
]
],
[
[
"### Complex Numbers",
"_____no_output_____"
]
],
[
[
"# Complex Numbers supported.\nexpr_cplx = 2.0 + 2*sympy.I\n\nprint(\"expr_cplx\")\ndisplay( type(expr_cplx) )\ndisplay( expr_cplx )\n\nprint(\"expr_cplx.evalf()\")\ndisplay( type(expr_cplx.evalf()) )\ndisplay( expr_cplx.evalf() )\n\nprint(\"float() - errors\")\nprint(\" \")\n# this errors complex cannot be converted to float\n#display( float(sym_cplx) )\n\nprint(\"complex() - evaluated to complex number\")\ndisplay( complex(expr_cplx) )\ndisplay( type(complex(expr_cplx)) )",
"expr_cplx\n"
],
[
"# Partial Evaluation if cannot be evaluated as float\ndisplay( (sympy.pi*x**2 + x/3).evalf(2) )",
"_____no_output_____"
],
[
"# use substitution in evalf\nexpr = cos(2*x)\nexpr.evalf(subs={x:2.4})",
"_____no_output_____"
],
[
"# sometimes there are round-offs smaller than the desired precision\none = cos(1)**2 + sin(1)**2\ndisplay( (one-1).evalf() )\n# chop=True can remove these errors\ndisplay( (one-1).evalf(chop=True) )",
"_____no_output_____"
],
[
"import sys\n'gmpy2' in sys.modules.keys()",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
]
] |
e7bce530fcb6548a6afe84e1510963337ade48bf | 83,499 | ipynb | Jupyter Notebook | StatRegistration_multiNLP.ipynb | thagusta/LEEM-analysis-1 | 907a80a95753bb9a965d37725bdd0f4117b44cff | [
"MIT"
] | null | null | null | StatRegistration_multiNLP.ipynb | thagusta/LEEM-analysis-1 | 907a80a95753bb9a965d37725bdd0f4117b44cff | [
"MIT"
] | null | null | null | StatRegistration_multiNLP.ipynb | thagusta/LEEM-analysis-1 | 907a80a95753bb9a965d37725bdd0f4117b44cff | [
"MIT"
] | null | null | null | 97.09186 | 48,064 | 0.743925 | [
[
[
"This is statregistration.py but then for multiple .nlp's. Edited from Tobias' script:\n\n Calculates shifts from CPcorrected and create a shift corrected stack. The calculations implements the drift\n correction algorithm as described in section 4 of the paper.\n https://doi.org/10.1016/j.ultramic.2019.112913\n https://github.com/TAdeJong/LEEM-analysis/blob/master/2%20-%20Driftcorrection.ipynb\n\n It uses cross correlation of all pairs of\n images after applying digital smoothing and edge detection filters to align\n Low Energy Electron Microscopy images with each other. When applied correctly,\n this allows for sub-pixel accurate image registration.\n\n Config parameters:\n SAVEFIG, boolean whether to save the figures\n stride: A stride larger than 1 takes 1 every stride images of the total dataset, This decreases computation time\n by a factor of stride**2, but decreases accuracy\n blocksize: dE is the blocksize used by dask, the number of images computed for at once.\n fftsize: The size of the image for which the drift correction is calculated\n startI: Starting frame for which the drift correction is calculated\n endI: Ending frame for which the drift correction is calculated\n sigma: the gaussian width over which the images are smoothened\n\nAdded napari to choose a rectangular patch on which to perform drift correction.",
"_____no_output_____"
]
],
[
[
"import numpy as np\nimport matplotlib.pyplot as plt\nimport dask.array as da\nfrom dask.distributed import Client, LocalCluster\nimport scipy.ndimage as ndi\n\nimport os\nimport time\n\nimport napari \n%gui qt\n\nfrom pyL5.lib.analysis.container import Container\nfrom pyL5.analysis.CorrectChannelPlate.CorrectChannelPlate import CorrectChannelPlate\nimport pyL5.lib.analysis.Registration as Reg\n\ncluster = LocalCluster(n_workers=1, threads_per_worker=6)\nclient = Client(cluster)\nclient",
"C:\\Users\\wgstam\\Anaconda3\\envs\\pyL5\\lib\\site-packages\\distributed\\node.py:160: UserWarning: Port 8787 is already in use.\nPerhaps you already have a cluster running?\nHosting the HTTP server on port 62459 instead\n warnings.warn(\n"
],
[
"def plot_masking(DX_DY, W_n, coords, dx, dy, shifts, min_normed_weight, sigma):\n \"\"\"Plot W, DX and DY to pick a value for W_{min} (Step 7 of algorithm)\"\"\"\n extent = [startI, endI, endI, startI]\n fig, axs = plt.subplots(1, 4, figsize=(12, 3), constrained_layout=True)\n im = {}\n im[0] = axs[0].imshow(DX_DY[0], cmap='seismic', extent=extent, interpolation='none')\n im[1] = axs[1].imshow(DX_DY[1], cmap='seismic', extent=extent, interpolation='none')\n im[2] = axs[2].imshow(W_n - np.diag(np.diag(W_n)), cmap='inferno',\n extent=extent, clim=(0.0, None), interpolation='none')\n axs[3].plot(coords, dx, 'x', label='dx')\n axs[3].plot(coords, dy, 'x', label='dy')\n axs[3].plot(shifts[:, 0], color='C0')\n axs[3].plot(shifts[:, 1], color='C1')\n axs[3].set_xlabel('frames')\n axs[3].set_ylabel('shift (pixels)')\n axs[3].set_box_aspect(1)\n axs[3].legend()\n \n axs[0].set_ylabel('$j$')\n fig.colorbar(im[0], ax=axs[:2], shrink=0.82, fraction=0.1)\n axs[0].contourf(W_n, [0, min_normed_weight], colors='black', alpha=0.6, extent=extent, origin='upper')\n axs[1].contourf(W_n, [0, min_normed_weight], colors='black', alpha=0.6, extent=extent, origin='upper')\n CF = axs[2].contourf(W_n, [0, min_normed_weight], colors='white', alpha=0.2, extent=extent, origin='upper')\n cbar = fig.colorbar(im[2], ax=axs[2], shrink=0.82, fraction=0.1)\n cbar.ax.fill_between([0, 1], 0, min_normed_weight, color='white', alpha=0.2)\n axs[0].set_title('$DX_{ij}$')\n axs[1].set_title('$DY_{ij}$')\n axs[2].set_title('$W_{ij}$')\n plt.show()\n return min_normed_weight",
"_____no_output_____"
],
[
"folder = 'D:\\\\20220210-36-CuGrKalbac-old\\\\growth'\n#folder = 'D:\\\\20211130-27-Si111SbPLD\\\\PLD2_100mJ_400C\\\\growthIVs'\nnames = [f.name for f in os.scandir(folder) if f.is_file() and f.name[-4:] == \".nlp\"]",
"_____no_output_____"
],
[
"for name in names:\n script = CorrectChannelPlate(os.path.join(folder, name))\n script.start()",
"_____no_output_____"
],
[
"conts = [Container(os.path.join(folder,f)) for f in names]\n#original = da.stack([cont.getStack().getDaskArray() for cont in conts])\n#original = da.stack([cont.getStack('CPcorrected').getDaskArray() for cont in conts])\noriginal = da.image.imread(os.path.join(folder+'\\driftcorrected01\\*'))\nm = 1\nsubfolder = 'driftcorrected%02d' %m + 'it2'\n#original = original[:,m]\noriginal",
"_____no_output_____"
],
[
"# config\nSAVEFIG = True\nstride = 1\ndE = 20\nfftsize = 256\nstartI, endI = 0, -1\nEslice = slice(startI,endI,stride)\nsigma = 10\nmin_norm = 0.4 #minimum",
"_____no_output_____"
]
],
[
[
"## Step 0: choose area\nChoose the (rectangular) area on which to perform drift correction.",
"_____no_output_____"
]
],
[
[
"center = [dim//2 for dim in original.shape[1:]]\nextent = (center[0]-fftsize, center[0]+fftsize, center[1]-fftsize, center[1]+fftsize)\nextent",
"_____no_output_____"
],
[
"viewer = napari.view_image(np.swapaxes(original, -1, -2), name='original')\n\n# create the square in napari\ncenter = np.array(original.shape[1:]) // 2\nsquare = np.array([[center[1]+fftsize, center[0]+fftsize],\n [center[1]-fftsize, center[0]+fftsize],\n [center[1]-fftsize, center[0]-fftsize],\n [center[1]+fftsize, center[0]-fftsize] \n ])\nshapes_layer = viewer.add_shapes(square, shape_type='polygon', edge_width=2,\n edge_color='white')\nshapes_layer._fixed_aspect = True # Keep it square",
"_____no_output_____"
],
[
"# load the outer coordinates of napari\ncoords = np.flip(np.array(shapes_layer.data).astype(int)[0])\nextent = np.min(coords[:,0]), np.max(coords[:,0]), np.min(coords[:,1]), np.max(coords[:,1]) #xmin, xmax, ymin, ymax\nfftsize = max(extent[1]-extent[0], extent[3]-extent[2]) //2 #This is basically for \nprint('The extent in x,y is:', extent, 'pixels, which makes the largest side/2', fftsize, 'pixels.')\nviewer.close()",
"The extent in x,y is: (214, 726, 646, 1158) pixels, which makes the largest side/2 256 pixels.\n"
]
],
[
[
"## Now starting the steps of the algorithm",
"_____no_output_____"
]
],
[
[
"def crop_and_filter_extent(images, extent, sigma=11, mode='nearest'):\n \"\"\"Crop images to extent chosen and apply the filters. Cropping is initially with a margin of sigma,\n to prevent edge effects of the filters. extent = minx,maxx,miny,maxy of ROI\"\"\"\n result = images[:, extent[0]-sigma:extent[1]+sigma,\n extent[2]-sigma:extent[3]+sigma]\n result = result.map_blocks(filter_block, dtype=np.float64,\n sigma=sigma, mode=mode)\n if sigma > 0:\n result = result[:, sigma:-sigma, sigma:-sigma]\n return result",
"_____no_output_____"
],
[
"# Step 1 to 3 of the algorithm as described in section 4 of the paper.\nsobel = crop_and_filter_extent(original[Eslice, ...].rechunk({0: dE}), extent, sigma=sigma)\nsobel = (sobel - sobel.mean(axis=(1, 2), keepdims=True)) # .persist()\n\n# Step 4 of the algorithm as described in paper.\nCorr = Reg.dask_cross_corr(sobel)\n\n# Step 5 of the algorithm\nweights, argmax = Reg.max_and_argmax(Corr)",
"_____no_output_____"
],
[
"# Do actual computations\nt = time.monotonic()\nW, DX_DY = Reg.calculate_halfmatrices(weights, argmax, fftsize=fftsize)\nprint(time.monotonic() - t, ' seconds')",
"memory backpressure assumed\n39.07800000003772 seconds\n"
],
[
"# Step 6 of the algorithm\nw_diag = np.atleast_2d(np.diag(W))\nW_n = W / np.sqrt(w_diag.T*w_diag)\n\n# Step 7 of the algorithm\nnr = np.arange(W.shape[0])*stride + startI\ncoords2, weightmatrix, DX, DY, row_mask = Reg.threshold_and_mask(min_norm, W, DX_DY, nr)\n\n# Step 8 of the algorithm: reduce the shift matrix to two vectors of absolute shifts\ndx, dy = Reg.calc_shift_vectors(DX, DY, weightmatrix)\n\n# Interpolate the shifts for all values not in coords\nshifts = np.stack(Reg.interp_shifts(coords2, [dx, dy], n=original.shape[0]), axis=1)\nneededMargins = np.ceil(shifts.max(axis=0)).astype(int)\n\nplot_masking(DX_DY, W_n, coords2, dx, dy, shifts, min_norm, sigma)\n\nprint(\"shiftshape\", shifts.shape)\nshifts = da.from_array(shifts, chunks=(dE, -1))",
"_____no_output_____"
],
[
"# Step 9, the actual shifting of the original images\n\n# Inferring output dtype is not supported in dask yet, so we need original.dtype here.\[email protected]_gufunc(signature=\"(i,j),(2)->(i,j)\", output_dtypes=original.dtype, vectorize=True)\ndef shift_images(image, shift):\n \"\"\"Shift `image` by `shift` pixels.\"\"\"\n return ndi.shift(image, shift=shift, order=1)\n\npadded = da.pad(original.rechunk({0: dE}),\n ((0, 0),\n (0, neededMargins[0]),\n (0, neededMargins[1])\n ),\n mode='constant'\n )\ncorrected = shift_images(padded.rechunk({1: -1, 2: -1}), shifts)\ncorrected",
"_____no_output_____"
],
[
"# Optional crop of images TODO",
"_____no_output_____"
],
[
"# Save as png with dask\nfrom pyL5.lib.analysis.stack import da_imsave\nos.makedirs(os.path.join(folder, subfolder), exist_ok=True)\nda_imsave(os.path.join(folder, subfolder, 'image{:04d}.png'),corrected, compute=True)",
"_____no_output_____"
],
[
"from pyL5.solidsnakephysics.helperFunctions import save_movie\nsave_movie(corrected,os.path.join(folder, subfolder))",
"Saving stack as .png\nNow saving movie stack.mp4\n"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bce5b65a7170c34b402afdd87e173868299d49 | 10,128 | ipynb | Jupyter Notebook | Ananthakrishnan_Python_Class&Objects.ipynb | VarunBhaaskar/Open-contributions | 95cad386b931fd128f65b6506394c6457a03d7f2 | [
"MIT"
] | 61 | 2020-09-10T05:16:19.000Z | 2021-11-07T00:22:46.000Z | Ananthakrishnan_Python_Class&Objects.ipynb | VarunBhaaskar/Open-contributions | 95cad386b931fd128f65b6506394c6457a03d7f2 | [
"MIT"
] | 72 | 2020-09-12T09:34:19.000Z | 2021-08-01T17:48:46.000Z | Ananthakrishnan_Python_Class&Objects.ipynb | VarunBhaaskar/Open-contributions | 95cad386b931fd128f65b6506394c6457a03d7f2 | [
"MIT"
] | 571 | 2020-09-10T01:52:56.000Z | 2022-03-26T17:26:23.000Z | 25.131514 | 565 | 0.475711 | [
[
[
"## Create a Class\n### To create a class, use the keyword class:",
"_____no_output_____"
]
],
[
[
"class car:\n x = 5\n\nprint(car)",
"<class '__main__.car'>\n"
]
],
[
[
"## Object Creation",
"_____no_output_____"
]
],
[
[
"class car:\n x = 5\n company=\"Maruti\"\n\np1 = car()\nprint(p1.x)\nprint(p1.company)",
"5\nMaruti\n"
]
],
[
[
"## Object Creation & The self Parameter",
"_____no_output_____"
]
],
[
[
"class Jeep: \n \n# A simple class \n# attribute \n\n company = \"Maruti\"\n location = \"India\"\n \n # A sample method \n def test(self): \n print(\"I'm from\", self.company) \n print(\"I'm made in \", self.location) \n \n\n # Object instantiation \njimny = Jeep() \n \n # Accessing class attributes \n # and method through objects \n \nprint(jimny.company) \njimny.test() ",
"Maruti\nI'm from Maruti\nI'm made in India\n"
]
],
[
[
"## The self Parameter",
"_____no_output_____"
],
[
"#### It does not have to be named *self* , you can call it whatever you like, but it has to be the first parameter of any function in the class:",
"_____no_output_____"
]
],
[
[
"class Car:\n def __init__(sampleobject, name, price):\n sampleobject.name = name\n sampleobject.price = price\n\n def myfunc(abc):\n print(\"Hello , my car is \" + abc.name)\n\np1 = Car(\"BMW\", 1)\np1.myfunc()\n",
"Hello , my car is BMW\n"
]
],
[
[
"\n ## The __init__() Function\n ##### Note: The __init__() function is called automatically every time the class is being used to create a new object.",
"_____no_output_____"
]
],
[
[
" class Car:\n def __init__(self, company):\n self.company = company \n \n\np1 = Car(\"Maruti\" )\nprint(p1.company)",
"Maruti\n"
]
],
[
[
"\n## Modify Object Properties",
"_____no_output_____"
]
],
[
[
"class Car:\n def __init__(self, model, rate):\n self.model = model\n self.rate = rate\n\n def myfunc(self):\n print(\"Hello my name is \" + self.model)\n\np1 = Car(\"Swift\", 500000)\np1.myfunc()\nprint(p1.model)\nprint(\"Previous rate of my model was \" , p1.rate) \np1.rate = 400000\nprint(\"New rate is\" , p1.rate)\n",
"Hello my name is Swift\nSwift\nPrevious rate of my model was 500000\nNew rate is 400000\n"
]
],
[
[
"## Delete Object Properties\n#### You can delete properties on objects by using the del keyword:",
"_____no_output_____"
]
],
[
[
"class Car:\n def __init__(self, model, rate):\n self.model = model\n self.rate = rate\n\n def myfunc(self):\n print(\"Hello my name is \" + self.model)\n\np1 = Car(\"Swift\",500000 )\n\n\ndel p1.rate\nprint(p1.rate)",
"_____no_output_____"
]
],
[
[
"## Delete Object\n#### You can delete objects by using the del keyword:",
"_____no_output_____"
]
],
[
[
"class Car:\n def __init__(self, model, rate):\n self.model = model\n self.rate = rate\n\n def myfunc(self):\n print(\"Hello my name is \" + self.model)\n\np1 = Car(\"Swift\",500000 )\n\n\ndel p1\nprint(p1.rate)",
"_____no_output_____"
]
],
[
[
"## The pass Statement",
"_____no_output_____"
]
],
[
[
"class Person:\n pass",
"_____no_output_____"
],
[
"class Car: \n \n # Class Variable \n fav_car = 'Verna' \n \n # The init method or constructor \n def __init__(self, model, color): \n \n # Instance Variable \n self.model = model \n self.color = color \n \n# Objects of Dog class \nModerna = Car(\"swift\", \"brown\") \nBuziga = Car(\"wagonR\", \"black\") \n \nprint('Moderna details:') \nprint('Moderna is a', Moderna.fav_car) \nprint('Model: ', Moderna.model) \nprint('Color: ', Moderna.color) \n \nprint('\\nBuziga details:') \nprint('Buziga is a', Buziga.fav_car) \nprint('Model: ', Buziga.model) \nprint('Color: ', Buziga.color) \n \n# Class variables can be accessed using class \n# name also \nprint(\"\\nAccessing class variable using class name\") \nprint(Car.fav_car)",
"Moderna details:\nModerna is a Verna\nModel: swift\nColor: brown\n\nBuziga details:\nBuziga is a Verna\nModel: wagonR\nColor: black\n\nAccessing class variable using class name\nVerna\n"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code"
] | [
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
]
] |
e7bce6b4c44fedba9e90a7a1a47eb8b2891e55ad | 989,629 | ipynb | Jupyter Notebook | module2-survival-analysis/LS_DS_232_Survival_Analysis.ipynb | macscheffer/DS-Unit-2-Sprint-3-Advanced-Regression | 9524f7824dafe5c8102f3596d344752c1ffd12c2 | [
"MIT"
] | null | null | null | module2-survival-analysis/LS_DS_232_Survival_Analysis.ipynb | macscheffer/DS-Unit-2-Sprint-3-Advanced-Regression | 9524f7824dafe5c8102f3596d344752c1ffd12c2 | [
"MIT"
] | null | null | null | module2-survival-analysis/LS_DS_232_Survival_Analysis.ipynb | macscheffer/DS-Unit-2-Sprint-3-Advanced-Regression | 9524f7824dafe5c8102f3596d344752c1ffd12c2 | [
"MIT"
] | null | null | null | 208.474616 | 110,420 | 0.845569 | [
[
[
"<a href=\"https://colab.research.google.com/github/macscheffer/DS-Unit-2-Sprint-3-Advanced-Regression/blob/master/module2-survival-analysis/LS_DS_232_Survival_Analysis.ipynb\" target=\"_parent\"><img src=\"https://colab.research.google.com/assets/colab-badge.svg\" alt=\"Open In Colab\"/></a>",
"_____no_output_____"
],
[
"# Lambda School Data Science - Survival Analysis\n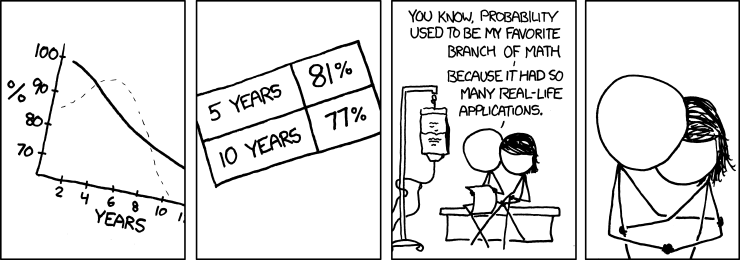\n\nhttps://xkcd.com/881/\n\nThe aim of survival analysis is to analyze the effect of different risk factors and use them to predict the duration of time between one event (\"birth\") and another (\"death\").",
"_____no_output_____"
],
[
"# Lecture\n\nSurvival analysis was first developed by actuaries and medical professionals to predict (as its name implies) how long individuals would survive. However, it has expanded into include many different applications.\n* it is referred to as **reliability analysis** in engineering\n* it can be referred to more generally as **time-to-event analysis**\n\nIn the general sense, it can be thought of as a way to model anything with a finite duration - retention, churn, completion, etc. The culmination of this duration may have a \"good\" or \"bad\" (or \"neutral\") connotation, depending on the situation. However old habits die hard, so most often it is called survival analysis and the following definitions are still commonly used:\n\n* birth: the event that marks the beginning of the time period for observation\n* death: the event of interest, which then marks the end of the observation period for an individual\n\n### Examples\n* Customer churn\n * birth event: customer subscribes to a service\n * death event: customer leaves the service\n* Employee retention\n * birth event: employee is hired\n * death event: employee quits\n* Engineering, part reliability\n * birth event: part is put in use\n * death event: part fails\n* Program completion\n * birth event: student begins PhD program\n * death event: student earns PhD\n* Response time\n * birth event: 911 call is made\n * death event: police arrive\n* Lambda School\n * birth event: student graduates LambdaSchool\n * death event: student gets a job!\n \nTake a moment and try to come up with your own specific example or two.\n\n#### So... if all we're predicting here is a length of time between two events, why can't we just use regular old Linear Regression?\nWell... if you have all the data, go for it. In some situations it may be reasonably effective.\n\n#### But, data for survival times are often highly skewed and, more importantly, we don't always get a chance to observe the \"death\" event. The current time or other factors interfere with our ability to observe the time of the event of interest. These observations are said to be _censored_.\n\nAdditionally, the occurrence or non-occurrence of an event is binary - so, while the time is continuous, the event itself is in some ways similar to a binary event in logistic regression.\n\n## Censorship in Data\n\nSuppose a new cancer treatment is developed. Researchers select 50 individuals for the study to undergo treatment and participate in post-treatment obsesrvation.\n\n##### Birth Event = Participant begins trial\n##### Death Event = Participant dies due to cancer or complications of cancer\nDuring the study:\n1. Some participants die during the course of the study--triggering their death event \n2. Some participants drop out or the researchers otherwise lose contact with them. The researchers have their data up intil the time they dropped out, but they don't have a death event to record\n3. Some participants are still be alive at the end of the observation period. So again, researchers have their data up until some point, but there is no death event to record\n\nWe only know the interval between the \"birth\" event and the \"death\" event for participants in category 1. All others we only know that they survived _up to_ a certain point.\n\n### Dealing with Censored Data\n\nWithout survival analysis, we could deal with censored data in two ways:\n* We could just treat the end of the observation period as the time of the death event\n* (Even worse) We could drop the censored data using the rationale that we have \"incomplete data\" for those observations\n\nBut... both of these will underestimate survival rates for the purpose of the study. We **know** that all those individuals \"survived\" the \"death event\" past a certain point.\n\nLuckily, in the 1980s a pair of smarty pants named David (main author Cox and coauthor Oakes) did the hard math work to make it possible to incorporate additional features as predictive measures to survival time probabilities. (Fun fact, the one named Cox also came up with logistic regression with non-David coauthor, Joyce Snell.)\n\n## lifelines\nIt wasn't until 2014 that some other smart people made an implementation of survival analysis in Python called lifelines. \nIt is built over Pandas and follows the same conventions for usage as scikit-learn.\n\n_Additional note: scikit pushed out a survival analysis implementation last year (2018) named scikit-survival that is imported by the name `sksurv`. It's super new so it may/may not have a bunch of bugs... but if you're interested you can check it out in the future. (For comparison, scikit originally came out in 2007 and Pandas came out in 2008)._",
"_____no_output_____"
]
],
[
[
"!pip install lifelines",
"Requirement already satisfied: lifelines in /usr/local/lib/python3.6/dist-packages (0.17.0)\nRequirement already satisfied: pandas>=0.18 in /usr/local/lib/python3.6/dist-packages (from lifelines) (0.22.0)\nCollecting matplotlib<3.0,>=2.0 (from lifelines)\n Using cached https://files.pythonhosted.org/packages/9e/59/f235ab21bbe7b7c6570c4abf17ffb893071f4fa3b9cf557b09b60359ad9a/matplotlib-2.2.3-cp36-cp36m-manylinux1_x86_64.whl\nRequirement already satisfied: scipy>=1.0 in /usr/local/lib/python3.6/dist-packages (from lifelines) (1.1.0)\nRequirement already satisfied: numpy in /usr/local/lib/python3.6/dist-packages (from lifelines) (1.14.6)\nRequirement already satisfied: python-dateutil>=2 in /usr/local/lib/python3.6/dist-packages (from pandas>=0.18->lifelines) (2.5.3)\nRequirement already satisfied: pytz>=2011k in /usr/local/lib/python3.6/dist-packages (from pandas>=0.18->lifelines) (2018.9)\nRequirement already satisfied: kiwisolver>=1.0.1 in /usr/local/lib/python3.6/dist-packages (from matplotlib<3.0,>=2.0->lifelines) (1.0.1)\nRequirement already satisfied: six>=1.10 in /usr/local/lib/python3.6/dist-packages (from matplotlib<3.0,>=2.0->lifelines) (1.11.0)\nRequirement already satisfied: cycler>=0.10 in /usr/local/lib/python3.6/dist-packages (from matplotlib<3.0,>=2.0->lifelines) (0.10.0)\nRequirement already satisfied: pyparsing!=2.0.4,!=2.1.2,!=2.1.6,>=2.0.1 in /usr/local/lib/python3.6/dist-packages (from matplotlib<3.0,>=2.0->lifelines) (2.3.1)\nRequirement already satisfied: setuptools in /usr/local/lib/python3.6/dist-packages (from kiwisolver>=1.0.1->matplotlib<3.0,>=2.0->lifelines) (40.6.3)\nInstalling collected packages: matplotlib\n Found existing installation: matplotlib 3.0.2\n Uninstalling matplotlib-3.0.2:\n Successfully uninstalled matplotlib-3.0.2\nSuccessfully installed matplotlib-2.2.3\n\u001b[0;31;1mWARNING: The following packages were previously imported in this runtime:\n [matplotlib, mpl_toolkits]\nYou must restart the runtime in order to use newly installed versions.\u001b[0m\n"
],
[
"import numpy as np\nimport pandas as pd\nimport matplotlib.pyplot as plt\nimport lifelines",
"_____no_output_____"
],
[
"# lifelines comes with some datasets to get you started playing around with it.\n# Most of the datasets are cleaned-up versions of real datasets. Here we will\n# use their Leukemia dataset comparing 2 different treatments taken from\n# http://web1.sph.emory.edu/dkleinb/allDatasets/surv2datasets/anderson.dat\n\nfrom lifelines.datasets import load_leukemia\nleukemia = load_leukemia()\n\nleukemia.head()",
"_____no_output_____"
]
],
[
[
"### You can you any Pandas DataFrame with lifelines. \n### The only requirement is that the DataFrame includes features that describe:\n* a duration of time for the observation\n* a binary column regarding censorship (`1` if the death event was observed, `0` if the death event was not observed)\n\nSometimes, you will have to engineer these features. How might you go about that? What information would you need?",
"_____no_output_____"
]
],
[
[
"leukemia.info()",
"<class 'pandas.core.frame.DataFrame'>\nRangeIndex: 42 entries, 0 to 41\nData columns (total 5 columns):\nt 42 non-null int64\nstatus 42 non-null int64\nsex 42 non-null int64\nlogWBC 42 non-null float64\nRx 42 non-null int64\ndtypes: float64(1), int64(4)\nmemory usage: 1.7 KB\n"
],
[
"leukemia.describe()",
"_____no_output_____"
],
[
"time = leukemia.t.values\nevent = leukemia.status.values\n\nax = lifelines.plotting.plot_lifetimes(time, event_observed=event)\nax.set_xlim(0, 40)\nax.grid(axis='x')\nax.set_xlabel(\"Time in Months\")\nax.set_title(\"Lifelines for Survival of Leukemia Patients\");\nplt.plot();",
"_____no_output_____"
]
],
[
[
"## Kaplan-Meier survival estimate\n \nThe Kaplan-Meier method estimates survival probability from observed survival times. It results in a step function that changes value only at the time of each event, and confidence intervals can be computer for the survival probabilities. \n\nThe KM survival curve,a plot of KM survival probability against time, provides a useful summary of the data.\nIt can be used to estimate measures such as median survival time.\n\nIt CANNOT account for risk factors and is NOT regression. It is *non-parametric* (does not involve parameters).\n\nHowever it is a good way to visualize a survival dataset, and can be useful to compare the effects of a single categorical variable.",
"_____no_output_____"
]
],
[
[
"kmf = lifelines.KaplanMeierFitter()\n\nkmf.fit(time, event_observed=event)",
"_____no_output_____"
],
[
"!pip install -U matplotlib # Colab has matplotlib 2.2.3, we need >3",
"Collecting matplotlib\n Using cached https://files.pythonhosted.org/packages/71/07/16d781df15be30df4acfd536c479268f1208b2dfbc91e9ca5d92c9caf673/matplotlib-3.0.2-cp36-cp36m-manylinux1_x86_64.whl\nRequirement already satisfied, skipping upgrade: kiwisolver>=1.0.1 in /usr/local/lib/python3.6/dist-packages (from matplotlib) (1.0.1)\nRequirement already satisfied, skipping upgrade: numpy>=1.10.0 in /usr/local/lib/python3.6/dist-packages (from matplotlib) (1.14.6)\nRequirement already satisfied, skipping upgrade: python-dateutil>=2.1 in /usr/local/lib/python3.6/dist-packages (from matplotlib) (2.5.3)\nRequirement already satisfied, skipping upgrade: pyparsing!=2.0.4,!=2.1.2,!=2.1.6,>=2.0.1 in /usr/local/lib/python3.6/dist-packages (from matplotlib) (2.3.1)\nRequirement already satisfied, skipping upgrade: cycler>=0.10 in /usr/local/lib/python3.6/dist-packages (from matplotlib) (0.10.0)\nRequirement already satisfied, skipping upgrade: setuptools in /usr/local/lib/python3.6/dist-packages (from kiwisolver>=1.0.1->matplotlib) (40.6.3)\nRequirement already satisfied, skipping upgrade: six>=1.5 in /usr/local/lib/python3.6/dist-packages (from python-dateutil>=2.1->matplotlib) (1.11.0)\n\u001b[31myellowbrick 0.9 has requirement matplotlib<3.0,>=1.5.1, but you'll have matplotlib 3.0.2 which is incompatible.\u001b[0m\n\u001b[31mlifelines 0.17.0 has requirement matplotlib<3.0,>=2.0, but you'll have matplotlib 3.0.2 which is incompatible.\u001b[0m\nInstalling collected packages: matplotlib\n Found existing installation: matplotlib 2.2.3\n Uninstalling matplotlib-2.2.3:\n Successfully uninstalled matplotlib-2.2.3\nSuccessfully installed matplotlib-3.0.2\n\u001b[0;31;1mWARNING: The following packages were previously imported in this runtime:\n [matplotlib, mpl_toolkits]\nYou must restart the runtime in order to use newly installed versions.\u001b[0m\n"
],
[
"kmf.survival_function_.plot()\nplt.title('Survival Function Leukemia Patients');\nprint(f'Median Survival: {kmf.median_} months after treatment')",
"Median Survival: 12.0 months after treatment\n"
],
[
"kmf.survival_function_.plot.line()",
"_____no_output_____"
],
[
"ax = plt.subplot(111)\n\ntreatment = (leukemia[\"Rx\"] == 1)\nkmf.fit(time[treatment], event_observed=event[treatment], label=\"Treatment 1\")\nkmf.plot(ax=ax)\nprint(f'Median survival time with Treatment 1: {kmf.median_} months')\n\nkmf.fit(time[~treatment], event_observed=event[~treatment], label=\"Treatment 0\")\nkmf.plot(ax=ax)\nprint(f'Median survival time with Treatment 0: {kmf.median_} months')\n\nplt.ylim(0, 1);\nplt.title(\"Survival Times for Leukemia Treatments\");",
"Median survival time with Treatment 1: 8.0 months\nMedian survival time with Treatment 0: 23.0 months\n"
]
],
[
[
"## Cox Proportional Hazards Model -- Survival Regression\nIt assumes the ratio of death event risks (hazard) of two groups remains about the same over time.\nThis ratio is called the hazards ratio or the relative risk.\n\nAll Cox regression requires is an assumption that ratio of hazards is constant over time across groups.\n\n*The good news* — we don’t need to know anything about overall shape of risk/hazard over time\n\n*The bad news* — the proportionality assumption can be restrictive",
"_____no_output_____"
]
],
[
[
"# Using Cox Proportional Hazards model\ncph = lifelines.CoxPHFitter()\ncph.fit(leukemia, 't', event_col='status')\ncph.print_summary()",
"<lifelines.CoxPHFitter: fitted with 42 observations, 12 censored>\n duration col = 't'\n event col = 'status'\nnumber of subjects = 42\n number of events = 30\n log-likelihood = -69.59\n time fit was run = 2019-01-20 19:09:48 UTC\n\n---\n coef exp(coef) se(coef) z p log(p) lower 0.95 upper 0.95 \nsex 0.31 1.37 0.45 0.69 0.49 -0.72 -0.58 1.21 \nlogWBC 1.68 5.38 0.34 5.00 <0.005 -14.36 1.02 2.34 ***\nRx 1.50 4.50 0.46 3.26 <0.005 -6.79 0.60 2.41 *\n---\nSignif. codes: 0 '***' 0.0001 '**' 0.001 '*' 0.01 '.' 0.05 ' ' 1\n\nConcordance = 0.85\nLikelihood ratio test = 47.19 on 3 df, log(p)=-21.87\n"
]
],
[
[
"## Interpreting the Results\n`coef`: usually denoted with $b$, the coefficient\n\n`exp(coef)`: $e^{b}$, equals the estimate of the hazard ratio. Here, we can say that participants who received treatment 1 had ~4.5 times the hazard risk (risk of death) compared to those who received treatment 2. And for every unit the `logWBC` increased, the hazard risk increased >5 times.\n\n`se(coef)`: standard error of the coefficient (used for calculating z-score and therefore p-value)\n\n`z`: z-score $\\frac{b}{se(b)}$\n\n`p`: p-value. derived from z-score. describes statistical significance. more specifically, it is the likelihood that the variable has no effect on the outcome\n\n`log(p)`: natural logarithm of p-value... used to more easily see differences in significance\n\n`lower/upper 0.95`: confidence levels for the coefficients. in this case, we can confidently say that the coefficient for `logWBC` is somewhere _between_ 1.02 and 2.34.\n\n`Signif. codes`: easily, visually identify significant variables! The more stars, the more solid (simply based on p-value). Here `logWBC` is highly significant, `Rx` is significant, and `sex` has no statistical significance\n\n`Concordance`: a measure of predictive power for classification problems (here looking at the `status` column. a value from 0 to 1 where values above 0.6 are considered good fits (the higher the better)\n\n`Likelihood ratio (LR) test`: this is a measure of how likely it is that the coefficients are not zero, and can compare the goodness of fit of a model versus an alternative null model. Is often actually calculated as a logarithm, resulting in the log-likelihood ratio statistic and allowing the distribution of the test statistic to be approximated with [Wilks' theorem](https://en.wikipedia.org/wiki/Wilks%27_theorem).",
"_____no_output_____"
]
],
[
[
"cph.plot_covariate_groups(covariate='logWBC', groups=np.arange(1.5,5,.5))",
"_____no_output_____"
],
[
"cph.plot_covariate_groups(covariate='sex', groups=[0,1])",
"_____no_output_____"
]
],
[
[
"### Remember how the Cox model assumes the ratio of death events between groups remains constant over time?\nWell we can check for that.",
"_____no_output_____"
]
],
[
[
"cph.check_assumptions(leukemia)",
"\n<lifelines.StatisticalResult>\n test_name = proportional_hazard_test\n null_distribution = chi squared\ndegrees_of_freedom = 1\n\n---\n test_statistic p log(p) \nRx identity 0.11 0.74 -0.30 \n km 0.23 0.63 -0.46 \n log 0.59 0.44 -0.81 \n rank 0.44 0.51 -0.68 \nlogWBC identity 0.21 0.64 -0.44 \n km 0.10 0.76 -0.28 \n log 0.30 0.59 -0.53 \n rank 0.16 0.68 -0.38 \nsex identity 2.56 0.11 -2.21 \n km 3.78 0.05 -2.96 \n log 3.94 0.05 -3.05 .\n rank 4.91 0.03 -3.62 .\n---\nSignif. codes: 0 '***' 0.0001 '**' 0.001 '*' 0.01 '.' 0.05 ' ' 1\n\n1. Variable 'sex' failed the non-proportional test, p=0.0267.\n Advice: with so few unique values (only 2), you can try `strata_col=['sex']` in the call in `.fit`. See documentation here: https://lifelines.readthedocs.io/en/latest/jupyter_notebooks/Proportional%20hazard%20assumption.html\n"
],
[
"# We can see that the sex variable is not very useful by plotting the coefficients\ncph.plot()",
"_____no_output_____"
],
[
"# Let's do what the check_assumptions function suggested\ncph = lifelines.CoxPHFitter()\ncph.fit(leukemia, 't', event_col='status', strata=['sex'])\ncph.print_summary()\ncph.baseline_cumulative_hazard_.shape",
"<lifelines.CoxPHFitter: fitted with 42 observations, 12 censored>\n duration col = 't'\n event col = 'status'\n strata = ['sex']\nnumber of subjects = 42\n number of events = 30\n log-likelihood = -55.73\n time fit was run = 2019-01-20 19:09:51 UTC\n\n---\n coef exp(coef) se(coef) z p log(p) lower 0.95 upper 0.95 \nlogWBC 1.45 4.28 0.34 4.23 <0.005 -10.64 0.78 2.13 ***\nRx 1.00 2.71 0.47 2.11 0.04 -3.35 0.07 1.93 .\n---\nSignif. codes: 0 '***' 0.0001 '**' 0.001 '*' 0.01 '.' 0.05 ' ' 1\n\nConcordance = 0.85\nLikelihood ratio test = 74.90 on 2 df, log(p)=-37.45\n"
]
],
[
[
"Notice that this regression has `Likelihood ratio test = 74.90 on 2 df, log(p)=-37.45`, while the one that included `sex` had `Likelihood ratio test = 47.19 on 3 df, log(p)=-21.87`. The LRT is higher and log(p) is lower, meaning this is likely a better fitting model.",
"_____no_output_____"
]
],
[
[
"cph.plot()",
"_____no_output_____"
],
[
"cph.compute_residuals(leukemia, kind='score')",
"_____no_output_____"
],
[
"cph.predict_cumulative_hazard(leukemia[:5])",
"_____no_output_____"
],
[
"surv_func = cph.predict_survival_function(leukemia[:5])\n\nexp_lifetime = cph.predict_expectation(leukemia[:5])\n\nplt.plot(surv_func)\n\nexp_lifetime",
"_____no_output_____"
],
[
"# lifelines comes with some datasets to get you started playing around with it\n# The Rossi dataset originally comes from Rossi et al. (1980), \n# and is used as an example in Allison (1995). \n\n# The data pertain to 432 convicts who were released from Maryland state prisons \n# in the 1970s and who were followed up for one year after release. Half the \n# released convicts were assigned at random to an experimental treatment in \n# which they were given financial aid; half did not receive aid.\n\nfrom lifelines.datasets import load_rossi\nrecidivism = load_rossi()\n\nrecidivism.head()\n\n# Looking at the Rossi dataset, how long do you think the study lasted?\n\n# All features are coded with numerical values, but which features do you think \n# are actually categorical?",
"_____no_output_____"
],
[
"recidivism.info()",
"<class 'pandas.core.frame.DataFrame'>\nRangeIndex: 432 entries, 0 to 431\nData columns (total 9 columns):\nweek 432 non-null int64\narrest 432 non-null int64\nfin 432 non-null int64\nage 432 non-null int64\nrace 432 non-null int64\nwexp 432 non-null int64\nmar 432 non-null int64\nparo 432 non-null int64\nprio 432 non-null int64\ndtypes: int64(9)\nmemory usage: 30.5 KB\n"
],
[
"recidivism.describe()",
"_____no_output_____"
]
],
[
[
"### These are the \"lifelines\" of the study participants as they attempt to avoid recidivism",
"_____no_output_____"
]
],
[
[
"recidivism_sample = recidivism.sample(n=25)\n\nduration = recidivism_sample.week.values\narrested = recidivism_sample.arrest.values\n\nax = lifelines.plotting.plot_lifetimes(duration, event_observed=arrested)\nax.set_xlim(0, 78)\nax.grid(axis='x')\nax.vlines(52, 0, 25, lw=2, linestyles='--')\nax.set_xlabel(\"Time in Weeks\")\nax.set_title(\"Recidivism Rates\");\nplt.plot();",
"_____no_output_____"
],
[
"kmf = lifelines.KaplanMeierFitter()\n\nduration = recidivism.week\narrested = recidivism.arrest\n\nkmf.fit(duration, arrested)",
"_____no_output_____"
],
[
"kmf.survival_function_.plot()\nplt.title('Survival Curve:\\nRecidivism of Recently Released Prisoners');",
"_____no_output_____"
],
[
"kmf.plot()\nplt.title('Survival Function of Recidivism Data');",
"_____no_output_____"
],
[
"print(f'Median time before recidivism: {kmf.median_} weeks')",
"Median time before recidivism: inf weeks\n"
],
[
"kmf_w_aid = lifelines.KaplanMeierFitter()\nkmf_no_aid = lifelines.KaplanMeierFitter()\n\nax = plt.subplot(111)\n\nw_aid = (recidivism['fin']==1)\n\nt = np.linspace(0, 70, 71)\nkmf_w_aid.fit(duration[w_aid], event_observed=arrested[w_aid], timeline=t, label=\"Received Financial Aid\")\nax = kmf_w_aid.plot(ax=ax)\n#print(\"Median survival time of democratic:\", kmf.median_)\n\nkmf_no_aid.fit(duration[~w_aid], event_observed=arrested[~w_aid], timeline=t, label=\"No Financial Aid\")\nax = kmf_no_aid.plot(ax=ax)\n#print(\"Median survival time of non-democratic:\", kmf.median_)\n\nplt.ylim(.5,1)\nplt.title(\"Recidivism for Participants Who Received Financial Aid \\vs. Those Who Did Not\");",
"_____no_output_____"
],
[
"naf = lifelines.NelsonAalenFitter()\nnaf.fit(duration, arrested)\n\nprint(naf.cumulative_hazard_.head())\nnaf.plot()",
" NA_estimate\ntimeline \n0.0 0.000000\n1.0 0.002315\n2.0 0.004635\n3.0 0.006961\n4.0 0.009292\n"
],
[
"naf_w_aid = lifelines.NelsonAalenFitter()\nnaf_no_aid = lifelines.NelsonAalenFitter()\n\nnaf_w_aid.fit(duration[w_aid], event_observed=arrested[w_aid], timeline=t, label=\"Received Financial Aid\")\nax = naf_w_aid.plot(loc=slice(0, 50))\nnaf_no_aid.fit(duration[~w_aid], event_observed=arrested[~w_aid], timeline=t, label=\"No Financial Aid\")\nax = naf_no_aid.plot(ax=ax, loc=slice(0, 50))\nplt.title(\"Recidivism Cumulative Hazard\\nfor Participants Who Received Financial Aid \\nvs. Those Who Did Not\");\nplt.show()",
"_____no_output_____"
],
[
"cph = lifelines.CoxPHFitter()\ncph.fit(recidivism, duration_col='week', event_col='arrest', show_progress=True)\n\ncph.print_summary()",
"Iteration 1: norm_delta = 0.48337, step_size = 0.95000, ll = -675.38063, newton_decrement = 16.76434, seconds_since_start = 0.0\nIteration 2: norm_delta = 0.13160, step_size = 0.95000, ll = -659.79004, newton_decrement = 0.99157, seconds_since_start = 0.0\nIteration 3: norm_delta = 0.01712, step_size = 0.95000, ll = -658.76197, newton_decrement = 0.01422, seconds_since_start = 0.0\nIteration 4: norm_delta = 0.00103, step_size = 0.95000, ll = -658.74771, newton_decrement = 0.00005, seconds_since_start = 0.0\nIteration 5: norm_delta = 0.00005, step_size = 0.95000, ll = -658.74766, newton_decrement = 0.00000, seconds_since_start = 0.0\nConvergence completed after 5 iterations.\n<lifelines.CoxPHFitter: fitted with 432 observations, 318 censored>\n duration col = 'week'\n event col = 'arrest'\nnumber of subjects = 432\n number of events = 114\n log-likelihood = -658.75\n time fit was run = 2019-01-20 19:09:54 UTC\n\n---\n coef exp(coef) se(coef) z p log(p) lower 0.95 upper 0.95 \nfin -0.38 0.68 0.19 -1.98 0.05 -3.05 -0.75 -0.00 .\nage -0.06 0.94 0.02 -2.61 0.01 -4.71 -0.10 -0.01 *\nrace 0.31 1.37 0.31 1.02 0.31 -1.18 -0.29 0.92 \nwexp -0.15 0.86 0.21 -0.71 0.48 -0.73 -0.57 0.27 \nmar -0.43 0.65 0.38 -1.14 0.26 -1.36 -1.18 0.31 \nparo -0.08 0.92 0.20 -0.43 0.66 -0.41 -0.47 0.30 \nprio 0.09 1.10 0.03 3.19 <0.005 -6.57 0.04 0.15 *\n---\nSignif. codes: 0 '***' 0.0001 '**' 0.001 '*' 0.01 '.' 0.05 ' ' 1\n\nConcordance = 0.64\nLikelihood ratio test = 33.27 on 7 df, log(p)=-10.65\n"
],
[
"cph.plot()",
"_____no_output_____"
],
[
"cph.plot_covariate_groups('fin', [0, 1])",
"_____no_output_____"
],
[
"cph.plot_covariate_groups('prio', [0, 5, 10, 15])",
"_____no_output_____"
],
[
"r = cph.compute_residuals(recidivism, 'martingale')\nr.head()",
"_____no_output_____"
],
[
"cph = lifelines.CoxPHFitter()\ncph.fit(recidivism, duration_col='week', event_col='arrest', show_progress=True)\n\ncph.print_summary()",
"Iteration 1: norm_delta = 0.48337, step_size = 0.95000, ll = -675.38063, newton_decrement = 16.76434, seconds_since_start = 0.0\nIteration 2: norm_delta = 0.13160, step_size = 0.95000, ll = -659.79004, newton_decrement = 0.99157, seconds_since_start = 0.0\nIteration 3: norm_delta = 0.01712, step_size = 0.95000, ll = -658.76197, newton_decrement = 0.01422, seconds_since_start = 0.0\nIteration 4: norm_delta = 0.00103, step_size = 0.95000, ll = -658.74771, newton_decrement = 0.00005, seconds_since_start = 0.0\nIteration 5: norm_delta = 0.00005, step_size = 0.95000, ll = -658.74766, newton_decrement = 0.00000, seconds_since_start = 0.0\nConvergence completed after 5 iterations.\n<lifelines.CoxPHFitter: fitted with 432 observations, 318 censored>\n duration col = 'week'\n event col = 'arrest'\nnumber of subjects = 432\n number of events = 114\n log-likelihood = -658.75\n time fit was run = 2019-01-20 19:11:37 UTC\n\n---\n coef exp(coef) se(coef) z p log(p) lower 0.95 upper 0.95 \nfin -0.38 0.68 0.19 -1.98 0.05 -3.05 -0.75 -0.00 .\nage -0.06 0.94 0.02 -2.61 0.01 -4.71 -0.10 -0.01 *\nrace 0.31 1.37 0.31 1.02 0.31 -1.18 -0.29 0.92 \nwexp -0.15 0.86 0.21 -0.71 0.48 -0.73 -0.57 0.27 \nmar -0.43 0.65 0.38 -1.14 0.26 -1.36 -1.18 0.31 \nparo -0.08 0.92 0.20 -0.43 0.66 -0.41 -0.47 0.30 \nprio 0.09 1.10 0.03 3.19 <0.005 -6.57 0.04 0.15 *\n---\nSignif. codes: 0 '***' 0.0001 '**' 0.001 '*' 0.01 '.' 0.05 ' ' 1\n\nConcordance = 0.64\nLikelihood ratio test = 33.27 on 7 df, log(p)=-10.65\n"
],
[
"cph.plot();",
"_____no_output_____"
],
[
"cph.plot_covariate_groups('prio', [0, 5, 10, 15]);",
"_____no_output_____"
],
[
"cph.check_assumptions(recidivism)",
"\n<lifelines.StatisticalResult>\n test_name = proportional_hazard_test\n null_distribution = chi squared\ndegrees_of_freedom = 1\n\n---\n test_statistic p log(p) \nage identity 12.06 <0.005 -7.57 **\n km 11.03 <0.005 -7.02 **\n log 13.07 <0.005 -8.11 **\n rank 11.09 <0.005 -7.05 **\nfin identity 0.06 0.81 -0.21 \n km 0.02 0.89 -0.12 \n log 0.49 0.48 -0.73 \n rank 0.02 0.90 -0.11 \nmar identity 0.75 0.39 -0.95 \n km 0.60 0.44 -0.82 \n log 1.03 0.31 -1.17 \n rank 0.67 0.41 -0.88 \nparo identity 0.12 0.73 -0.32 \n km 0.12 0.73 -0.31 \n log 0.01 0.92 -0.09 \n rank 0.14 0.71 -0.34 \nprio identity 0.01 0.92 -0.09 \n km 0.02 0.88 -0.13 \n log 0.38 0.54 -0.62 \n rank 0.02 0.88 -0.13 \nrace identity 1.49 0.22 -1.50 \n km 1.44 0.23 -1.47 \n log 1.03 0.31 -1.17 \n rank 1.46 0.23 -1.48 \nwexp identity 6.93 0.01 -4.77 *\n km 7.48 0.01 -5.07 *\n log 5.54 0.02 -3.99 .\n rank 7.18 0.01 -4.91 *\n---\nSignif. codes: 0 '***' 0.0001 '**' 0.001 '*' 0.01 '.' 0.05 ' ' 1\n\n1. Variable 'age' failed the non-proportional test, p=0.0003.\n Advice: try binning the variable 'age' using pd.cut, and then specify it in `strata_col=['age']` in the call in `.fit`. See more documentation here: https://lifelines.readthedocs.io/en/latest/jupyter_notebooks/Proportional%20hazard%20assumption.html\n Advice: try adding an interaction term with your time variable. See more documentation here: https://lifelines.readthedocs.io/en/latest/jupyter_notebooks/Proportional%20hazard%20assumption.html\n2. Variable 'wexp' failed the non-proportional test, p=0.0063.\n Advice: with so few unique values (only 2), you can try `strata_col=['wexp']` in the call in `.fit`. See documentation here: https://lifelines.readthedocs.io/en/latest/jupyter_notebooks/Proportional%20hazard%20assumption.html\n"
]
],
[
[
"## The Intuition - Hazard and Survival Functions\n\n### Hazard Function - the dangerous bathtub\n\nThe hazard function represents the *instantaneous* likelihood of failure. It can be treated as a PDF (probability density function), and with real-world data comes in three typical shapes.\n\n\n\nIncreasing and decreasing failure rate are fairly intuitive - the \"bathtub\" shaped is perhaps the most surprising, but actually models many real-world situations. In fact, life expectancy in general, and most threats to it, assume this shape.\n\nWhat the \"bathtub\" means is that - threats are highest at youth (e.g. infant mortality), but then decrease and stabilize at maturity, only to eventually re-emerge in old age. Many diseases primarily threaten children and elderly, and middle aged people are also more robust to physical trauma.\n\nThe \"bathtub\" is also suitable for many non-human situations - often with reliability analysis, mechanical parts either fail early (due to manufacturing defects), or they survive and have a relatively long lifetime to eventually fail out of age and use.\n\n### Survival Function (aka reliability function) - it's just a (backwards) CDF\n\nSince the hazard function can be treated as a probability density function, it makes sense to think about the corresponding cumulative distribution function (CDF). But because we're modeling time to failure, it's actually more interesting to look at the CDF backwards - this is called the complementary cumulative distribution function.\n\nIn survival analysis there's a special name for it - the survival function - and it gives the probability that the object being studied will survive beyond a given time.\n\n\n\nAs you can see they all start at 1 for time 0 - at the beginning, all things are alive. Then they all move down over time to eventually approach and converge to 0. The different shapes reflect the average/expected retention of a population subject to this function over time, and as such this is a particularly useful visualization when modeling overall retention/churn situations.\n\n### Ways to estimate/model survival analysis - terms to be aware of\nKey Components Necessary for these models - duration, and whether observation is censored.\n\n- Kaplan Meier Estimator\n- Nelson-Aalen Estimator\n- Proportional Hazards (Cox Model, integrates covariates)\n- Additive Hazards Model (Aalen's Additive Model, when covariates are time-dependent)\n\nAs with most statistics, these are all refinements of the general principles, with the math to back them up. Software packages will tend to select reasonable defaults, and allow you to use parameters to tune or select things. The math for these gets varied and deep - but feel free to [dive in](https://en.wikipedia.org/wiki/Survival_analysis) if you're curious!",
"_____no_output_____"
],
[
"## Live! Let's try modeling heart attack survival\n\nhttps://archive.ics.uci.edu/ml/datasets/echocardiogram",
"_____no_output_____"
]
],
[
[
"# TODO - Live! (As time permits)",
"_____no_output_____"
]
],
[
[
"# Assignment - Customer Churn\n\nTreselle Systems, a data consulting service, [analyzed customer churn data using logistic regression](http://www.treselle.com/blog/customer-churn-logistic-regression-with-r/). For simply modeling whether or not a customer left this can work, but if we want to model the actual tenure of a customer, survival analysis is more appropriate.\n\nThe \"tenure\" feature represents the duration that a given customer has been with them, and \"churn\" represents whether or not that customer left (i.e. the \"event\", from a survival analysis perspective). So, any situation where churn is \"no\" means that a customer is still active, and so from a survival analysis perspective the observation is censored (we have their tenure up to now, but we don't know their *true* duration until event).\n\nYour assignment is to [use their data](https://github.com/treselle-systems/customer_churn_analysis) to fit a survival model, and answer the following questions:\n\n- What features best model customer churn?\n- What would you characterize as the \"warning signs\" that a customer may discontinue service?\n- What actions would you recommend to this business to try to improve their customer retention?\n\nPlease create at least *3* plots or visualizations to support your findings, and in general write your summary/results targeting an \"interested layperson\" (e.g. your hypothetical business manager) as your audience.\n\nThis means that, as is often the case in data science, there isn't a single objective right answer - your goal is to *support* your answer, whatever it is, with data and reasoning.\n\nGood luck!",
"_____no_output_____"
]
],
[
[
"import pandas as pd",
"_____no_output_____"
],
[
"# Loading the data to get you started\ndf = pd.read_csv(\n 'https://raw.githubusercontent.com/treselle-systems/'\n 'customer_churn_analysis/master/WA_Fn-UseC_-Telco-Customer-Churn.csv')\ndf.head()",
"_____no_output_____"
],
[
"df.info() # A lot of these are \"object\" - some may need to be fixed...",
"<class 'pandas.core.frame.DataFrame'>\nRangeIndex: 7043 entries, 0 to 7042\nData columns (total 21 columns):\ncustomerID 7043 non-null object\ngender 7043 non-null object\nSeniorCitizen 7043 non-null int64\nPartner 7043 non-null object\nDependents 7043 non-null object\ntenure 7043 non-null int64\nPhoneService 7043 non-null object\nMultipleLines 7043 non-null object\nInternetService 7043 non-null object\nOnlineSecurity 7043 non-null object\nOnlineBackup 7043 non-null object\nDeviceProtection 7043 non-null object\nTechSupport 7043 non-null object\nStreamingTV 7043 non-null object\nStreamingMovies 7043 non-null object\nContract 7043 non-null object\nPaperlessBilling 7043 non-null object\nPaymentMethod 7043 non-null object\nMonthlyCharges 7043 non-null float64\nTotalCharges 7043 non-null object\nChurn 7043 non-null object\ndtypes: float64(1), int64(2), object(18)\nmemory usage: 1.1+ MB\n"
],
[
"pd.set_option('display.max_rows', 500)\npd.set_option('display.max_columns', 500)",
"_____no_output_____"
],
[
"df.head()",
"_____no_output_____"
],
[
"df.Churn = df.Churn.map({'No': 0, 'Yes':1})",
"_____no_output_____"
],
[
"df.Churn.mean()",
"_____no_output_____"
],
[
"df.pivot_table(index='PaperlessBilling', values='Churn')",
"_____no_output_____"
],
[
"df.pivot_table(index='Contract', values='Churn')",
"_____no_output_____"
],
[
"df.pivot_table(index='gender', values='Churn')",
"_____no_output_____"
],
[
"df.head()",
"_____no_output_____"
],
[
"df.columns",
"_____no_output_____"
],
[
"categorical = ['gender', 'SeniorCitizen', 'Partner', 'Dependents', \n 'PhoneService', 'MultipleLines', 'InternetService',\n 'OnlineSecurity', 'OnlineBackup', 'DeviceProtection', 'TechSupport',\n 'StreamingTV', 'StreamingMovies', 'Contract', 'PaperlessBilling',\n 'PaymentMethod']\n\nfor col in categorical:\n \n df = pd.get_dummies(df, columns=[col])",
"_____no_output_____"
],
[
"df.shape",
"_____no_output_____"
],
[
"df.head()",
"_____no_output_____"
],
[
"from sklearn.linear_model.logistic import LogisticRegression\nfrom sklearn.model_selection import train_test_split\n\nX = df.drop(['Churn', 'customerID','TotalCharges'],axis=1)\ny = df.Churn\n\nX_train, X_test, y_train, y_test = train_test_split(X, y, test_size=.2, random_state=42)\n\nlr = LogisticRegression()\nlr.fit(X_train, y_train)\n\nlr.score(X_test, y_test)",
"/usr/local/lib/python3.6/dist-packages/sklearn/linear_model/logistic.py:433: FutureWarning: Default solver will be changed to 'lbfgs' in 0.22. Specify a solver to silence this warning.\n FutureWarning)\n"
]
],
[
[
"# Resources and stretch goals\n\nResources:\n- [Wikipedia on Survival analysis](https://en.wikipedia.org/wiki/Survival_analysis)\n- [Wikipedia on Survival functions](https://en.wikipedia.org/wiki/Survival_function)\n- [Summary of survival analysis by a biostatistician](http://sphweb.bumc.bu.edu/otlt/MPH-Modules/BS/BS704_Survival/BS704_Survival_print.html)\n- [Another medical statistics article on survival analysis](https://www.sciencedirect.com/science/article/pii/S1756231716300639)\n- [Survival analysis using R lecture slides](http://www.stat.columbia.edu/~madigan/W2025/notes/survival.pdf)\n\nStretch goals:\n- Make ~5 slides that summarize and deliver your findings, as if you were to present them in a business meeting\n- Revisit any of the data from the lecture material, and explore/dig deeper\n- Write your own Python functions to calculate a simple hazard or survival function, and try to generate and plot data with them",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown",
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
]
] |
e7bcea317768916ed34b32920cd9989043f916ab | 33,262 | ipynb | Jupyter Notebook | Twitter Sentiment Analysis/Untitled2.ipynb | mmaleki/Deep-Learning-with-Tensorfow | 3e2ece806d43432959c6b3585cfea6aceaf49b52 | [
"MIT"
] | null | null | null | Twitter Sentiment Analysis/Untitled2.ipynb | mmaleki/Deep-Learning-with-Tensorfow | 3e2ece806d43432959c6b3585cfea6aceaf49b52 | [
"MIT"
] | null | null | null | Twitter Sentiment Analysis/Untitled2.ipynb | mmaleki/Deep-Learning-with-Tensorfow | 3e2ece806d43432959c6b3585cfea6aceaf49b52 | [
"MIT"
] | null | null | null | 48.771261 | 18,020 | 0.725843 | [
[
[
"import numpy as np\nimport pandas as pd\nimport matplotlib.pyplot as plt\nfrom nltk import word_tokenize\nfrom nltk import sent_tokenize\nfrom nltk import FreqDist\nfrom nltk.tokenize import RegexpTokenizer",
"_____no_output_____"
],
[
"twitter=pd.read_csv('twitter.csv',header=None,encoding='latin-1')",
"_____no_output_____"
],
[
"twitter=twitter.sample(frac=1)",
"_____no_output_____"
],
[
"twitter.to_csv('twitter5.csv',header=None)",
"_____no_output_____"
],
[
"data=pd.read_csv('twitter5.csv',header=None,encoding='latin-1')",
"_____no_output_____"
],
[
"twitter=data[:500000]",
"_____no_output_____"
],
[
"twitter.head(7)",
"_____no_output_____"
],
[
"tag=twitter[1]\nsen=twitter[6]",
"_____no_output_____"
],
[
"sent=[sen[i].lower() for i in range(len(twitter))]",
"_____no_output_____"
],
[
"def p(v):\n temp=len(tag[tag==v])\n prob=temp/len(twitter)\n return prob",
"_____no_output_____"
],
[
"p(0)",
"_____no_output_____"
],
[
"p(4)",
"_____no_output_____"
],
[
"def concatData(size):\n ls={}\n for i in range(size):\n ls['l{}'.format(i)]=''\n for s in sent[i*100000:(i+1)*100000]:\n ls['l{}'.format(i)]=s+ls['l{}'.format(i)]\n l=''\n for i in range(size):\n l=ls['l{}'.format(i)]+l\n return l",
"_____no_output_____"
],
[
"l=concatData(4)",
"_____no_output_____"
],
[
"allsent=l",
"_____no_output_____"
],
[
"vocabulary=word_tokenize(allsent)",
"_____no_output_____"
],
[
"#vocabulary",
"_____no_output_____"
],
[
"import re\ns='sent with @punct and? @someone with #teeth ate people in 1979! and 3.14 is pi number'\ns2=re.sub('\\w*[0-9]\\w*|@\\w*|[!,?,#,~,&,%,£]|\\.','',s)",
"_____no_output_____"
],
[
"s2",
"_____no_output_____"
],
[
"filter_all=re.sub('\\w*[0-9]\\w*|@\\w*|[!,?,#,~,&,%,£]|\\.','',allsent)",
"_____no_output_____"
],
[
"#filter_all",
"_____no_output_____"
],
[
"new_all=word_tokenize(filter_all)",
"_____no_output_____"
],
[
"frdis=FreqDist(new_all)",
"_____no_output_____"
],
[
"frdis.plot(10)",
"_____no_output_____"
],
[
"dictionary=set(filter_all)",
"_____no_output_____"
],
[
"whole_sent=np.concatenate([sent])",
"_____no_output_____"
],
[
"dc={}\nfor i in range(len(whole_sent)):\n dc['s{}'.format(i)]=word_tokenize(whole_sent[i])\n",
"_____no_output_____"
],
[
"sent_0=[]\nsent_4=[]\nfor i in range(len(whole_sent)):\n if tag[i]==0:\n sent_0.append(dc['s{}'.format(i)])\n elif tag[i]==4:\n sent_4.append(dc['s{}'.format(i)])\n \n ",
"_____no_output_____"
],
[
"text_0=set(np.concatenate(sent_0))\ntext_4=set(np.concatenate(sent_4))",
"_____no_output_____"
],
[
"voc1=set(new_all)",
"_____no_output_____"
],
[
"#word_0",
"_____no_output_____"
]
],
[
[
"$$p(word|C_k)$$",
"_____no_output_____"
]
],
[
[
"def nk(word,given_tag,data):\n if word not in voc1:\n return 0\n else:\n word_in_sen=[(word in dc['s{}'.format(i)]) and (tag[i]==given_tag) for i in range(len(data))]\n return sum(word_in_sen)",
"_____no_output_____"
],
[
"nk('find',4,twitter)",
"_____no_output_____"
],
[
"def n(given_tag):\n if given_tag==0:\n return len(text_0)\n elif given_tag==4:\n return len(text_4)\n else:\n return 0",
"_____no_output_____"
],
[
"def pr(w,given_tag,data):\n n_k=nk(w,given_tag,data)\n n_n=n(given_tag)\n prob=(n_k+1)/(n_n+len(voc1))\n return prob",
"_____no_output_____"
],
[
"pr('@',0,twitter)",
"_____no_output_____"
],
[
"def sentiment(new,data):\n tkn=word_tokenize(new)\n p1=p(0)\n q1=p(4)\n for w in tkn:\n p1=p1*pr(w,0,data)\n q1=q1*pr(w,4,data)\n \n if p1>q1:\n return \"Sentence is Negative\"\n else:\n return \"Sentence is Positive\"\n \n \n ",
"_____no_output_____"
],
[
"sentiment('I love nature',twitter)",
"_____no_output_____"
],
[
"sentiment('trust',twitter)",
"_____no_output_____"
]
]
] | [
"code",
"markdown",
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
],
[
"markdown"
],
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bcec79a93e9de1bb60a2d48d0da11e8bf2a8d1 | 179,477 | ipynb | Jupyter Notebook | m04_machine_learning/m04_c06_ml_workflow/m04_c06_lab.ipynb | Crhlb/mat281_repositorio | d5b7e93977dccc12b747c2e537c8153c0ddf1746 | [
"MIT"
] | null | null | null | m04_machine_learning/m04_c06_ml_workflow/m04_c06_lab.ipynb | Crhlb/mat281_repositorio | d5b7e93977dccc12b747c2e537c8153c0ddf1746 | [
"MIT"
] | null | null | null | m04_machine_learning/m04_c06_ml_workflow/m04_c06_lab.ipynb | Crhlb/mat281_repositorio | d5b7e93977dccc12b747c2e537c8153c0ddf1746 | [
"MIT"
] | null | null | null | 302.659359 | 72,172 | 0.927985 | [
[
[
"<img src=\"https://upload.wikimedia.org/wikipedia/commons/4/47/Logo_UTFSM.png\" width=\"200\" alt=\"utfsm-logo\" align=\"left\"/>\n\n# MAT281\n### Aplicaciones de la Matemática en la Ingeniería",
"_____no_output_____"
],
[
"## Módulo 04\n## Laboratorio Clase 06: Proyectos de Machine Learning",
"_____no_output_____"
],
[
"### Instrucciones\n\n\n* Completa tus datos personales (nombre y rol USM) en siguiente celda.\n* La escala es de 0 a 4 considerando solo valores enteros.\n* Debes _pushear_ tus cambios a tu repositorio personal del curso.\n* Como respaldo, debes enviar un archivo .zip con el siguiente formato `mXX_cYY_lab_apellido_nombre.zip` a [email protected], debe contener todo lo necesario para que se ejecute correctamente cada celda, ya sea datos, imágenes, scripts, etc.\n* Se evaluará:\n - Soluciones\n - Código\n - Que Binder esté bien configurado.\n - Al presionar `Kernel -> Restart Kernel and Run All Cells` deben ejecutarse todas las celdas sin error.\n* __La entrega es al final de esta clase.__",
"_____no_output_____"
],
[
"__Nombre__: Cristóbal Loyola\n\n__Rol__: 201510008-K",
"_____no_output_____"
],
[
"## GapMinder",
"_____no_output_____"
]
],
[
[
"import pandas as pd\nimport altair as alt\nimport numpy as np\n\nfrom vega_datasets import data\n\nalt.themes.enable('opaque')\n\n%matplotlib inline",
"_____no_output_____"
],
[
"gapminder = data.gapminder_health_income()\ngapminder.head()",
"_____no_output_____"
]
],
[
[
"### 1. Análisis exploratorio (1 pto)\n\nComo mínimo, realizar un `describe` del dataframe y una visualización adecuada, una _scatter matrix_ con los valores numéricos.",
"_____no_output_____"
]
],
[
[
"desc=gapminder.describe()\nprint(desc)",
" income health population\ncount 187.000000 187.000000 1.870000e+02\nmean 17232.240642 71.673262 3.908887e+07\nstd 19310.632882 7.748959 1.432898e+08\nmin 599.000000 48.500000 5.299300e+04\n25% 3585.500000 65.500000 2.248920e+06\n50% 10996.000000 73.130000 8.544586e+06\n75% 23834.000000 77.700000 2.769388e+07\nmax 132877.000000 84.100000 1.376049e+09\n"
],
[
"pd.plotting.scatter_matrix(gapminder, alpha=0.3, figsize=(9,9), diagonal='kde')",
"_____no_output_____"
]
],
[
[
"### 2. Preprocesamiento (1 pto)",
"_____no_output_____"
],
[
"Aplicar un escalamiento a los datos antes de aplicar nuestro algoritmo de clustering. Para ello, definir la variable `X_raw` que corresponde a un `numpy.array` con los valores del dataframe `gapminder` en las columnas _income_, _health_ y _population_. Luego, definir la variable `X` que deben ser los datos escalados de `X_raw`.",
"_____no_output_____"
]
],
[
[
"from sklearn.preprocessing import StandardScaler",
"_____no_output_____"
],
[
"X_raw = gapminder.drop('country', axis=1).values\nX = StandardScaler().fit_transform(X_raw)",
"_____no_output_____"
]
],
[
[
"### 3. Clustering (1 pto)",
"_____no_output_____"
]
],
[
[
"from sklearn.cluster import KMeans",
"_____no_output_____"
]
],
[
[
"Definir un _estimator_ `KMeans` con `k=3` y `random_state=42`, luego ajustar con `X` y finalmente, agregar los _labels_ obtenidos a una nueva columna del dataframe `gapminder` llamada `cluster`. Finalmente, realizar el mismo gráfico del principio pero coloreado por los clusters obtenidos.\n\n",
"_____no_output_____"
]
],
[
[
"k = 3\nkmeans = KMeans(k, random_state=42)\nkmeans.fit(X)\nclusters = kmeans.labels_\ngapminder['cluster']=clusters\n##gapminder.head()",
"_____no_output_____"
],
[
"colors_palette = {0: \"orange\", 1: \"green\", 2: \"violet\"}\ncolors = [colors_palette[c] for c in list(gapminder['cluster'])]\npd.plotting.scatter_matrix(gapminder, alpha=0.3, figsize=(9,9), c=colors, diagonal='kde')",
"_____no_output_____"
]
],
[
[
"### 4. Regla del codo (1 pto)",
"_____no_output_____"
],
[
"__¿Cómo escoger la mejor cantidad de _clusters_?__\n\nEn este ejercicio hemos utilizado que el número de clusters es igual a 3. El ajuste del modelo siempre será mejor al aumentar el número de clusters, pero ello no significa que el número de clusters sea el apropiado. De hecho, si tenemos que ajustar $n$ puntos, claramente tomar $n$ clusters generaría un ajuste perfecto, pero no permitiría representar si existen realmente agrupaciones de datos.\n\nCuando no se conoce el número de clusters a priori, se utiliza la [regla del codo](https://jarroba.com/seleccion-del-numero-optimo-clusters/), que indica que el número más apropiado es aquel donde \"cambia la pendiente\" de decrecimiento de la la suma de las distancias a los clusters para cada punto, en función del número de clusters.\n\nA continuación se provee el código para el caso de clustering sobre los datos estandarizados, leídos directamente de un archivo preparado especialmente.",
"_____no_output_____"
]
],
[
[
"elbow = pd.Series(name=\"inertia\").rename_axis(index=\"k\")\nfor k in range(1, 10):\n kmeans = KMeans(n_clusters=k, random_state=42).fit(X)\n elbow.loc[k] = kmeans.inertia_ # Inertia: Sum of distances of samples to their closest cluster center\nelbow = elbow.reset_index()",
"_____no_output_____"
],
[
"alt.Chart(elbow).mark_line(point=True).encode(\n x=\"k:O\",\n y=\"inertia:Q\"\n).properties(\n height=600,\n width=800\n)",
"_____no_output_____"
]
],
[
[
"__Pregunta__\n\nConsiderando los datos (países) y el gráfico anterior, ¿Cuántos clusters escogerías?",
"_____no_output_____"
],
[
"Escogería 4 clusters, pues a partir de k=4 no se ve un cambio de pendiente significativo respecto a lo que se puede ver en $[0, 3]$.",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown",
"markdown",
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
],
[
"code",
"code"
],
[
"markdown",
"markdown"
]
] |
e7bcf1ef7cfcda06875070e3631164114d5ad33b | 10,044 | ipynb | Jupyter Notebook | Experiments/Transformer_Class.ipynb | venelink/augment-acl21 | 43fcafb73ab8f5bbe46e721a29c611ede224b897 | [
"CC-BY-4.0"
] | null | null | null | Experiments/Transformer_Class.ipynb | venelink/augment-acl21 | 43fcafb73ab8f5bbe46e721a29c611ede224b897 | [
"CC-BY-4.0"
] | null | null | null | Experiments/Transformer_Class.ipynb | venelink/augment-acl21 | 43fcafb73ab8f5bbe46e721a29c611ede224b897 | [
"CC-BY-4.0"
] | null | null | null | 33.148515 | 164 | 0.576065 | [
[
[
"import pandas as pd\nimport numpy as np\nimport scipy\nimport nltk\nimport spacy\nimport gensim\nimport glob\nimport csv\nfrom spellchecker import SpellChecker\nimport matplotlib\nimport matplotlib.pyplot as plt\nimport sklearn\nfrom sklearn.model_selection import cross_val_score\nimport sklearn.model_selection\nimport sklearn.pipeline\nimport re\nfrom sklearn import svm\nfrom sklearn import *\nfrom sklearn.feature_selection import SelectKBest, VarianceThreshold\nfrom sklearn.feature_selection import chi2\nfrom sklearn.base import BaseEstimator, TransformerMixin\nimport gensim.models.wrappers.fasttext\nfrom scipy import sparse\nimport tensorflow_datasets as tfds\nimport tensorflow as tf\nimport collections\nfrom tensorflow.keras.preprocessing.text import Tokenizer\nfrom tensorflow.keras.preprocessing.sequence import pad_sequences\nfrom sklearn.model_selection import LeaveOneOut,KFold,train_test_split\nimport ktrain\nfrom ktrain import text\nfrom sklearn.metrics import accuracy_score\nimport simplejson\nimport pprint\n\n\n# Custom imports\nfrom mr_generic_scripts import *\nfrom mr_cls_Transformer import *",
"_____no_output_____"
],
[
"# Path to xlsx files folder\n\n# Original MIND-CA corpus\npath_to_raw_files = f_path + 'Data/raw_relabeled/'\n# MIND-CA + human augment\npath_to_plus_files = f_path + 'Data/raw_plus/'\n# UK-MIND-20\npath_to_wp1 = f_path + 'Data/wp1/'\n\n# Augmented data\n# augmentations, 125 examples per QA pair\npath_to_aug = f_path + 'Data/aug_data/all/'\n# augmentations, 500 examples per QA pair\npath_to_aug_hq_os = f_path + 'Data/aug_data_os/all/'\n# augmentation, no sampling - 1500 total examples per question\npath_to_aug_joint = f_path + 'Data/aug_data_joint/all/'\n\n# Merged xlsx files with multiple augmentations\npath_to_set_files = f_path + 'Data/aug_data/sets/'",
"_____no_output_____"
],
[
"# List of augmentations by category\nhq_data = ['reord','phrase','dict']\nlq_data = ['wordnet','ppdb','glove','fasttext']\nset_data = ['ab_lq','ab_hq','all_lq','all_hq','all_aug']",
"_____no_output_____"
],
[
"# General config of the training run (!)\n\n# List of data to use for training\n\n# All possible train sets\n# train_sets = ['orig','plus','reord','phrase','dict','wordnet','ppdb','glove','fasttext','ab_lq','ab_hq','all_lq','all_hq']\n\n# Selective train set\n#train_sets = ['reord','phrase','dict']\ntrain_sets = ['wordnet','ppdb','glove','fasttext']\n#train_sets = ['wp1']\n\n# Alias path to aug data (either 125 or 500 examples or the 1500 joint)\naug_path = path_to_aug_joint\n\n# Training parameters\n# Number of folds for k-fold cross validation\nn_k_fold = 10\n\n# Only answers (False) or questions + answers (True)\nmind_qa = True",
"_____no_output_____"
],
[
"# Get the datasets in dataframes\ndatasets = {}\n\n# Check if we load only answers or questions plus answers\nif mind_qa:\n # Always load MIND-CA + human aug, this is the base set\n datasets['plus'] = mr_get_qa_data(path_to_plus_files)\n\n # Always load UK-MIND-20, we need it for testing\n datasets['wp1'] = mr_get_qa_data(path_to_wp1)\n\n # If comparison is needed, load MIND-CA without any aug\n if 'orig' in train_sets:\n datasets['orig'] = mr_get_qa_data(path_to_raw_files)\n\n # Load augmented data\n for at_set in train_sets:\n if at_set in ['orig','plus','wp1']:\n continue\n path_to_aug = aug_path + at_set + \"/\"\n datasets[at_set] = mr_get_qa_data(path_to_aug)\n\n \n# Only the answer\nelse:\n # Always load MIND-CA + human aug, this is the base set\n datasets['plus'] = mr_get_data(path_to_plus_files)\n\n # Always load UK-MIND-20, we need it for testing\n datasets['wp1'] = mr_get_data(path_to_wp1)\n\n # If comparison is needed, load MIND-CA without any aug\n if 'orig' in train_sets:\n datasets['orig'] = mr_get_data(path_to_raw_files)\n\n # Load augmented data\n aug_dataset = {}\n for at_set in train_sets:\n if at_set in ['orig','plus','wp1']:\n continue\n path_to_aug = aug_path + at_set + \"/\"\n datasets[at_set] = mr_get_data(path_to_aug)",
"_____no_output_____"
],
[
"# Sanity check\nfor d_id in train_sets:\n print(len(datasets[d_id][-1][1]))\n if at_set in ['orig','plus','wp1']:\n continue\n # Augmented datasets have additional column that needs to be dropped\n datasets[d_id][-1][1].drop([\"Aug_ID\"],axis=1,inplace=True)",
"_____no_output_____"
],
[
"def mr_proc_results(raw_results):\n # Process the results from the 10 runs\n # result format: [acc, acc per q, acc per age], [f1, f1 per q, f1 per age], [acc, acc per q, acc per age] (for wp1), [f1, f1 per q, f1 per age] (for wp1)\n # Ignore ages as they seem to be mostly consistent with global average\n # Ignore accs per question and age as averaging them seems to be consistent with global average\n # Report global acc, global macro f1, average of macro f1 per question; same for wp1\n pr_results = [[[acc_score, f1_score,round(sum(qf_s)/11,2)],[acc_score_wp1, f1_score_wp1,round(sum(qf_s_wp1)/11,2)]] \n for ([acc_score, qa_s, aa_s], [f1_score, qf_s, af_s],\n [acc_score_wp1, qa_s_wp1, aa_s_wp1], [f1_score_wp1, qf_s_wp1, af_s_wp1]) in raw_results]\n\n # Throw the list in an np array\n pr_arr = np.array(pr_results)\n\n # Print the results\n pp = pprint.PrettyPrinter(indent=4)\n\n pp.pprint(pr_results)\n pp.pprint(np.mean(pr_arr,axis=0))",
"_____no_output_____"
],
[
"# Initialize the classifier\nif mind_qa:\n # ages 8 to 13, removing outliers; allowed classes 0,1,2; max len 35\n tr_cls = MR_transformer(text_cols,[8,9,10,11,12,13],[0,1,2],35)\nelse:\n # ages 8 to 13, removing outliers; allowed classes 0,1,2; max len 20\n tr_cls = MR_transformer(text_cols,[8,9,10,11,12,13],[0,1,2],20)\n\n# Configure eval parameters - eval by age and questions, do not return examples with errors (not fully implemented in current version) \ntr_cls.mr_set_eval_vars(True,True,False)",
"_____no_output_____"
],
[
"# Initialize the results variable \nresults = {}",
"_____no_output_____"
],
[
"# Run all train-test combos\nfor at_set in train_sets:\n print(\"Current train: \" + str(at_set) + \"\\n\")\n\n if at_set in ['orig','plus','wp1']:\n # For orig and plus we directly train and test using kfold validation\n results[at_set] = tr_cls.mr_kfold_pre_split(datasets[at_set][-1][1],datasets['wp1'][-1][1],0.25,n_k_fold)\n else:\n # For augmented data we need to also provide the \"plus\" set for evaluation and organizing the split\n results[at_set] = tr_cls.mr_kfold_aug_pre_split(datasets['plus'][-1][1],datasets[at_set][-1][1],datasets['wp1'][-1][1],0.25,n_k_fold)\n\n # Save the results in a file\n rs_path = 'Results/split_eval_joint/tr_qa_os_'\n s_path = rs_path + at_set + '.txt'\n with open(s_path,'w') as op:\n simplejson.dump(results[at_set],op)",
"_____no_output_____"
],
[
"# Visualize the results\nfor at_set in train_sets:\n print(at_set)\n mr_proc_results(results[at_set])",
"_____no_output_____"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
e7bd0529a8f9cf8c2990f5119e5777f33aff5fc8 | 18,688 | ipynb | Jupyter Notebook | 03_scikit-learn-intelex_Image_Cluster/03_ImageClustering.ipynb | IntelSoftware/scikit-learn_essentials | 93bbedb8e44cef27061440b49449bc49fc99f0f0 | [
"MIT"
] | 1 | 2021-12-09T21:31:38.000Z | 2021-12-09T21:31:38.000Z | 03_scikit-learn-intelex_Image_Cluster/03_ImageClustering.ipynb | IntelSoftware/scikit-learn_essentials | 93bbedb8e44cef27061440b49449bc49fc99f0f0 | [
"MIT"
] | null | null | null | 03_scikit-learn-intelex_Image_Cluster/03_ImageClustering.ipynb | IntelSoftware/scikit-learn_essentials | 93bbedb8e44cef27061440b49449bc49fc99f0f0 | [
"MIT"
] | null | null | null | 30.486134 | 330 | 0.602526 | [
[
[
"# 03 ImageClustering using PCA, Kmeans, DBSCAN\n\n\n",
"_____no_output_____"
],
[
"\n## Learning Objectives\n\n* Explore and interpret the image dataset\n\n* Apply Intel® Extension for Scikit-learn* patches to Principal Components Analysis (PCA), Kmeans,and DBSCAN algorithms and target GPU\n\n## Library Dependencies:\n\n - **pip install pillow**\n - **pip install seaborn**\n - also requires these libraries if they are not already installed: **matplotlib, numpy, pandas, sklearn**\n\n<a id='Back_to_Sections'></a>\n\n## Sections\n\n- _Code:_ [Read Images](#Define-image-manipulation-and-Reading-functions)\n- _Code:_ [Submit batch_clustering_Streamlined.py as a batch job](#Submit-batch_clustering_Streamlined.py-as-a-batch-job)\n- _Code:_ [Read the results of the dictionary after GPU computation](#Read-the-results-of-the-dictionary-after-GPU-computation)\n- _Code:_ [Plot Kmeans using GPU results](#Plot-Kmeans)\n- _Code:_ [Plot DBSCAN using GPU results](#Plot-DBSCAN)\n",
"_____no_output_____"
]
],
[
[
"from __future__ import print_function\ndata_path = ['data']\n\n# Notebook time start\nfrom datetime import datetime\n\nstart_time = datetime.now()\n\ncurrent_time = start_time.strftime(\"%H:%M:%S\")\nprint(\"Current Time =\", current_time)",
"_____no_output_____"
]
],
[
[
"# Define image manipulation and Reading functions\n",
"_____no_output_____"
]
],
[
[
"from lab.Read_Transform_Images import *\n",
"_____no_output_____"
]
],
[
[
"<a id='Actually-read-the-images'></a>\n# Actually read the images\n\n- [Back to Sections](#Back_to_Sections)",
"_____no_output_____"
]
],
[
[
"resultsDict = {}\n#resultsDict = Read_Transform_Images(resultsDict,imagesFilenameList = imagesFilenameList)\nresultsDict = Read_Transform_Images(resultsDict)\n#resultsDict.keys()",
"_____no_output_____"
]
],
[
[
"# Display ImageGrid Random Sampling\n\nThis should give an idea of how closely or differently the various images appear. Notice that some of the collard lizard images have much differnet white balance and this will affect the clustering. For this dataset the images are clustered based on the similarity in RGB colorspace only.",
"_____no_output_____"
]
],
[
[
"img_arr = []\nncols = 8\nimageGrid=(ncols,3)\nfor pil in random.sample(resultsDict['list_PIL_Images'], imageGrid[0]*imageGrid[1]) :\n img_arr.append(np.array(pil))\n#displayImageGrid(img_arr, imageGrid=imageGrid)\ndisplayImageGrid2(img_arr, ncols=ncols)",
"_____no_output_____"
]
],
[
[
"<a id='Review_python_batch_file_prior_to_submission'></a>\n\n# Review Python file prior to submission\n### Set SYCL Device Context\n\n\n- [Back to Sections](#Back-to-Sections)\n\nPaste this code in cell below and run it (twice) once to load, once to execute the cell:\n\n%load batch_clustering_Streamlined.py",
"_____no_output_____"
]
],
[
[
"%load batch_clustering_Streamlined.py",
"_____no_output_____"
]
],
[
[
"<a id='Submit-batch_clustering_Streamlined.py-as-a-batch-job'></a>\n# Submit batch_clustering_Streamlined.py\n\n- [Back to Sections](#Back_to_Sections)\n\nbatch_clustering_Streamlined.py executed with a Python* command inside a shell script - run_clustering_streamlined.sh.\n\nrun_clustering_streamlined.sh is submitted as a batch job to a node which has GPUs",
"_____no_output_____"
]
],
[
[
"#!python batch_kmeans.py # works on Windows\n! chmod 755 q; chmod 755 run_clustering_streamlined.sh; if [ -x \"$(command -v qsub)\" ]; then ./q run_clustering_streamlined.sh; else ./run_clustering_streamlined.sh; fi",
"_____no_output_____"
]
],
[
[
"<a id='Read-the-results-of-the-dictionary-after-GPU-computation'></a>\n# Read Results of the Dictionary After GPU Computation\n\n- [Back to Sections](#Back_to_Sections)\n",
"_____no_output_____"
]
],
[
[
"# read results from json file in results folder\nresultsDict = read_results_json()\n# get list_PIL_Images from Read_Tansform_Images\nresultsDict = Read_Transform_Images(resultsDict)",
"_____no_output_____"
]
],
[
[
"<a id='Plot-Kmeans'></a>\n# Plot Kmeans Clusters \n\nPlot a histogram of the using GPU results\n- [Back to Sections](#Back_to_Sections)",
"_____no_output_____"
]
],
[
[
"resultsDict.keys()",
"_____no_output_____"
],
[
"#resultsDict = Compute_kmeans_db_histogram_labels(resultsDict, knee = 6, gpu_available = gpu_available) #knee = 5\ncounts = np.asarray(resultsDict['counts'])\nbins = np.asarray(resultsDict['bins'])\nplt.xlabel(\"Weight\")\nplt.ylabel(\"Probability\")\nplt.title(\"Histogram with Probability Plot}\")\nslice = min(counts.shape[0], bins.shape[0])\nplt.bar(bins[:slice],counts[:slice])\nplt.grid()\nplt.show()",
"_____no_output_____"
]
],
[
[
"# Print Kmeans Related Data as a Sanity Check",
"_____no_output_____"
]
],
[
[
"print(resultsDict['bins'])\nprint(resultsDict['counts'])\nresultsDict.keys()",
"_____no_output_____"
]
],
[
[
"# Display Similar Images\n\nVisually compare image which have been clustered by the allgorithm",
"_____no_output_____"
]
],
[
[
"clusterRank = 2\nd = {i:cts for i, cts in enumerate(resultsDict['counts'])}\nsorted_d = sorted(d.items(), key=operator.itemgetter(1), reverse=True)\n\nid = sorted_d[clusterRank][0]\nindexCluster = np.where(np.asarray(resultsDict['km_labels']) == id )[0].tolist()\nimg_arr = []\nfor idx in indexCluster:\n img_arr.append(np.array((resultsDict['list_PIL_Images'][idx])))\nimg_arr = np.asarray(img_arr)\ndisplayImageGrid(img_arr)",
"_____no_output_____"
]
],
[
[
"# PlotPlot Seaborn Kmeans Clusters\n\nIndicates numbers of images that are close in color space",
"_____no_output_____"
]
],
[
[
"%matplotlib inline\n\nn_components = 4\n\ncolumns = ['PC{:0d}'.format(c) for c in range(n_components)]\ndata = pd.DataFrame(np.asarray(resultsDict['PCA_fit_transform'])[:,:n_components], columns = columns)\n#k_means = resultsDict['model']\ndata['cluster'] = resultsDict['km_labels']\ndata.head()\n# similarlyNamedImages = [9,6,6,8,6,4,8,3]\n# print('number of similarly named images: ', similarlyNamedImages)\n\ncolumns.append('cluster')\nplt.figure(figsize=(8,8))\n\nsns.set_context('notebook');\ng = sns.pairplot(data[columns], hue=\"cluster\", palette=\"Paired\", diag_kws=dict(hue=None));\ng.fig.suptitle(\"KMEANS pairplot\");",
"_____no_output_____"
]
],
[
[
"# Find DBSCAN EPS parameter\n\nDensity-Based Spatial Clustering of Applications with Noise (DBSCAN) finds core samples of high density and expands clusters from them. Good for data which contains clusters of similar density.\n\nEPS: \"epsilon\" value in sklearn is the maximum distance between two samples for one to be considered as in the neighborhood of the other.\n\nAt least a first value to start. We are using kNN to find distances commonly occuring in the dataset. Values of EPS below this threshold distance will be considered as lyig within a given cluster. This means we should look for long flat plateaus and read the y coordinate off the kNN plot to get a starting value for EPS.\n\nDifferent datasets can have wildly different sweet spots for EPS. Some datasets require EPS values of .001 other datasets may work best with values of EPS of several thousand. We use this trick to get in the right or approximate neighborhood of the EPS.\n",
"_____no_output_____"
]
],
[
[
"from sklearn.neighbors import NearestNeighbors\n\nPCA_images = resultsDict['PCA_fit_transform']\n\nneighbors = NearestNeighbors(n_neighbors=2)\n#X = StandardScaler().fit_transform(PCA_images)\nneighbors_fit = neighbors.fit(PCA_images)\ndistances, indices = neighbors_fit.kneighbors(PCA_images)\n\ndistances = np.sort(distances, axis=0)\nplt.xlabel('number of images')\nplt.ylabel('distances')\nplt.title('KNN Distances Plot')\nplt.plot(distances[:,1])\nplt.grid()",
"_____no_output_____"
]
],
[
[
"# Use DBSCAN to find clusters\n\nwe will use initial estiamtes from KNN above (find elbow) to given initial trial for DBSCAN EPS values \n\nIn the plot above, there is a plateau in the y values somewherre near 350 indicating that a cluster distance (aka EPS) might work well somewhere near this value. We used this value in the batch_clustering_Streamlined.py file when computing DBSCAN.\n\n**EPS:** Two points are neighbors if the distance between the two points is below a threshold.\n**n:** The minimum number of neighbors a given point should have in order to be classified as a core point. \nThe point itself is included in the minimum number of samples.\n\n# Below: Sort DBSCAN Results by Cluster Size",
"_____no_output_____"
]
],
[
[
"#%%write_and_run lab/compute_DBSCANClusterRank.py \ndef compute_DBSCANClusterRank(n, EPS):\n d = {index-1:int(cnt) for index, cnt in enumerate(counts )}\n sorted_d = sorted(d.items(), key=operator.itemgetter(1), reverse=True)\n for i in range(0, len(d)):\n idx = sorted_d[i][0]\n print('cluster = ', idx, ' occurs', int(sorted_d[i][1]), ' times')\n return db, counts, bins, sorted_d\n\nn_components = 4\n\ncolumns = ['PC{:0d}'.format(c) for c in range(n_components)]\ndata = pd.DataFrame(np.asarray(resultsDict['PCA_fit_transform'])[:,:n_components], columns = columns)\n\ncolumns.append('cluster')\ndata['cluster'] = resultsDict['db_labels'] \ndata.head()",
"_____no_output_____"
]
],
[
[
"<a id='Plot-DBSCAN'></a>\n# DBSCAN Cluster Plot\n\nPlot a histogram of the using GPU results\n- [Back to Sections](#Back_to_Sections)\n\n \n\nTo indicate numbers of images in each cluster. color each point by its membership in a cluster",
"_____no_output_____"
]
],
[
[
"%matplotlib inline\n\nsns.set_context('notebook');\ng = sns.pairplot(data[columns], hue=\"cluster\", palette=\"Paired\", diag_kws=dict(hue=None));\ng.fig.suptitle(\"DBSCAN pairplot\");",
"_____no_output_____"
]
],
[
[
"# Print Filenames of Outliers",
"_____no_output_____"
]
],
[
[
"print('Outlier/problematic images are: \\n', \n [resultsDict['imagesFilenameList'][f] for f in list(data[data['cluster'] == -1].index)]\n )",
"_____no_output_____"
]
],
[
[
"# Final Thoughts and next steps...\n\nYou may have noticed how difficult it is to get decent clustering on even 30 or 40 images using only RGB or HSV as the feature set\n.\nIf all the images are well separated in either RGB, or HSV color space, then these features are useful for clustering.\n\nHowever, a suggested next step—or next training—would be to encode the data differently. Perhaps using image classification with VGG16, but removing the last layer as a preprocess prior to k-means or DBSCAN.\n\n\n.",
"_____no_output_____"
],
[
"# Notices & Disclaimers \n\nIntel technologies may require enabled hardware, software or service activation.\nNo product or component can be absolutely secure.\n\nYour costs and results may vary.\n\n© Intel Corporation. Intel, the Intel logo, and other Intel marks are trademarks of Intel Corporation or its subsidiaries. \n*Other names and brands may be claimed as the property of others.\n\n",
"_____no_output_____"
]
]
] | [
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown",
"code",
"markdown"
] | [
[
"markdown",
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code",
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown"
],
[
"code"
],
[
"markdown",
"markdown"
]
] |
e7bd06c55ec1ccc27b8f14047b0212390903f2fc | 14,962 | ipynb | Jupyter Notebook | US_KLINE.ipynb | Ahrli/other_API | ec35e3641f3ec28e96472312aef1d7080bb8645b | [
"Apache-2.0"
] | 1 | 2021-10-04T15:53:13.000Z | 2021-10-04T15:53:13.000Z | US_KLINE.ipynb | Ahrli/other_API | ec35e3641f3ec28e96472312aef1d7080bb8645b | [
"Apache-2.0"
] | null | null | null | US_KLINE.ipynb | Ahrli/other_API | ec35e3641f3ec28e96472312aef1d7080bb8645b | [
"Apache-2.0"
] | 2 | 2021-10-04T15:53:15.000Z | 2022-03-27T10:41:00.000Z | 41.104396 | 1,672 | 0.532616 | [
[
[
"import tiingo",
"_____no_output_____"
],
[
"get_ipython().system('pip show tiingo')",
"Name: tiingo\r\nVersion: 0.8.0\r\nSummary: REST Client for Tiingo Data Platform API\r\nHome-page: https://github.com/hydrosquall/tiingo-python\r\nAuthor: Cameron Yick\r\nAuthor-email: [email protected]\r\nLicense: MIT license\r\nLocation: /home/ahrli/anaconda3/lib/python3.7/site-packages\r\nRequires: requests\r\nRequired-by: \r\n"
],
[
"from tiingo import TiingoClient\nconfig = {}\n",
"_____no_output_____"
],
[
"# To reuse the same HTTP Session across API calls (and have better performance), include a session key.\nconfig['session'] = True\n\n# If you don't have your API key as an environment variable,\n# pass it in via a configuration dictionary.\nconfig['api_key'] = \"5ad45e5247e9f3ddc3a85005ea92c69d1bc4a552\"\n",
"_____no_output_____"
],
[
"# Initialize\nclient = TiingoClient(config)",
"_____no_output_____"
],
[
"\n\ndata = client.get_dataframe('GOOGL',\n #\"000001.sh\",\n # tickers='000001',\n #frequency='1Min',\n #frequency='day',\n startDate='2019-01-15',\n endDate='2019-01-18')",
"_____no_output_____"
],
[
"print(data)",
" adjClose adjHigh adjLow adjOpen adjVolume close divCash \\\ndate \n2019-01-15 1086.51 1088.27 1054.44 1058.01 1875852 1086.51 0.0 \n2019-01-16 1089.51 1102.36 1088.01 1090.00 1914041 1089.51 0.0 \n2019-01-17 1099.12 1100.70 1083.26 1087.99 1244801 1099.12 0.0 \n2019-01-18 1107.30 1118.00 1099.28 1108.59 2244569 1107.30 0.0 \n\n high low open splitFactor volume \ndate \n2019-01-15 1088.27 1054.44 1058.01 1.0 1875852 \n2019-01-16 1102.36 1088.01 1090.00 1.0 1914041 \n2019-01-17 1100.70 1083.26 1087.99 1.0 1244801 \n2019-01-18 1118.00 1099.28 1108.59 1.0 2244569 \n"
],
[
"print(type(data))\ndata1=data.loc[:,['high','open','low','close','volume']]\nprint(data1)",
"<class 'pandas.core.frame.DataFrame'>\n high open low close volume\ndate \n2019-01-15 1088.27 1058.01 1054.44 1086.51 1875852\n2019-01-16 1102.36 1090.00 1088.01 1089.51 1914041\n2019-01-17 1100.70 1087.99 1083.26 1099.12 1244801\n2019-01-18 1118.00 1108.59 1099.28 1107.30 2244569\n"
],
[
"import arrow\ndata1['code'] = 'US.GOOGL'\ndata1['create_time'] = data1.index\ndata1['create_time'] = data1['create_time'].apply(lambda create_time: arrow.get(create_time).format('YYYY-MM-DD HH:mm:ss'))\nprint(data1)\nimport pendulum",
" high open low close volume code \\\ndate \n2019-01-15 1088.27 1058.01 1054.44 1086.51 1875852 US.GOOGL \n2019-01-16 1102.36 1090.00 1088.01 1089.51 1914041 US.GOOGL \n2019-01-17 1100.70 1087.99 1083.26 1099.12 1244801 US.GOOGL \n2019-01-18 1118.00 1108.59 1099.28 1107.30 2244569 US.GOOGL \n\n create_time \ndate \n2019-01-15 2019-01-15 00:00:00 \n2019-01-16 2019-01-16 00:00:00 \n2019-01-17 2019-01-17 00:00:00 \n2019-01-18 2019-01-18 00:00:00 \n"
],
[
"\ndata1['time_key'] = data1['create_time'].apply(lambda create_time: int(pendulum.from_format(create_time, '%Y-%m-%d %H:%M:%S', tz='America/New_York').timestamp()))\n\nprint(data1)",
" high open low close volume code \\\ndate \n2019-01-15 1088.27 1058.01 1054.44 1086.51 1875852 US.GOOGL \n2019-01-16 1102.36 1090.00 1088.01 1089.51 1914041 US.GOOGL \n2019-01-17 1100.70 1087.99 1083.26 1099.12 1244801 US.GOOGL \n2019-01-18 1118.00 1108.59 1099.28 1107.30 2244569 US.GOOGL \n\n create_time time_key \ndate \n2019-01-15 2019-01-15 00:00:00 1547528400 \n2019-01-16 2019-01-16 00:00:00 1547614800 \n2019-01-17 2019-01-17 00:00:00 1547701200 \n2019-01-18 2019-01-18 00:00:00 1547787600 \n"
],
[
"data1.to_dict('records') ",
"_____no_output_____"
],
[
"startDate = arrow.now().format('YYYY-MM-DD')\nendDate = arrow.now().shift(hours=-72).format('YYYY-MM-DD')\nprint(startDate)\nprint(endDate)",
"2019-01-25\n2019-01-22\n"
],
[
"\nfrom tiingo import TiingoClient\nimport pandas as pd\nconfig = {}\n\n# To reuse the same HTTP Session across API calls (and have better performance), include a session key.\nconfig['session'] = True\n\n# If you don't have your API key as an environment variable,\n# pass it in via a configuration dictionary.\nconfig['api_key'] = \"5ad45e5247e9f3ddc3a85005ea92c69d1bc4a552\"\n\n# Initialize\nclient = TiingoClient(config)\na = None\nfor i in ['BABA','BIDU']:\n data = client.get_dataframe(i,\n # \"000001.sh\",\n # tickers='000001',\n # frequency='1Min',\n frequency='day',\n startDate='2019-01-15',\n endDate='2019-01-18')\n try:\n a= a.append(data,ignore_index=True)\n\n except Exception as e:\n a=data\n\nprint(a)",
"_____no_output_____"
]
]
] | [
"code"
] | [
[
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code",
"code"
]
] |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.